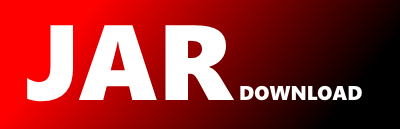
static.smart-ui.expand.core-expand.js Maven / Gradle / Ivy
(function(){
"use strict";
/**时间拓展----开始*/
var dateUtils = {
formatDate:function(date,format){
if(!date || !(date instanceof Date)){
return "";
}
if(!format){
format = "yyyy-MM-dd HH:mm:ss";
}
var fields = ["yyyy","yy","y","MM","M","dd","d","HH","H","hh","h","mm","m","ss","s","fff","ff","f"];
var yyyy = date.getFullYear();
var data = {
y:yyyy - parseInt(yyyy/100)*100,
M:date.getMonth()+1,
d:date.getDate(),
H:date.getHours(),
h:date.getHours()>12?date.getHours()-12:date.getHours(),
m:date.getMinutes(),
s:date.getSeconds(),
f:date.getMilliseconds()
};
for(var key in data){
data[key+key] = data[key]<10?"0"+data[key]:""+data[key];
}
data.yyyy = yyyy;
data.fff = data.f<100?(data.f<10?"00"+data.f:"0"+data.f):data.f;
for(var i=0;i=this.addMonths(cha).getTime()){
return cha;
}else{
return cha-1;
}
}
Date.prototype.betweenYears=function(x){
var cha = x.getFullYear()-this.getFullYear();
if(x.getTime()>=this.addYears(cha).getTime()){
return cha;
}else{
return cha-1;
}
}
Date.prototype.format = function(format){
return dateUtils.formatDate(this,format);
};
Date.parseDate = function(str,ignoreTimeZone){
if(Date.parse){
var number = Date.parse(str);
if(!isNaN(number)){
return new Date(number);
}
}
return dateUtils.parseDate(str,ignoreTimeZone);
};
/**时间拓展----结束*/
/*改变json格式化--开始*/
Date.prototype.toJSON = function () {
return this.formatDate("yyyy-MM-dd HH:mm:ss.fff");
};
if(!window.JSON){
/*增加json*/
window.JSON = {
stringify:function(obj){
return jsonStrByData(obj);
function jsonStrByData(data){
var type = Object.prototype.toString.call(data);
if(type =="[object Array]"){
var result = "[";
for(var i=0;i0){
result += ",";
}
result += jsonStrByData(data[i]);
}
result += "]";
return result;
}else if(type == "[object Date]"){
return '"'+data.toJSON()+'"';
}else if(type == "[object Object]"){
if(!data){
var res = "undefined";
if(data === null){
res = "null";
}
return res;
}
var result = "{";
var isFirst=true;
for(var key in data){
var str = jsonStrByData(data[key]);
if(str == "undefined"){
continue;
}
if(!isFirst){
result += ",";
}
result += "\""+key+"\":"+str;
isFirst = false;
}
result+="}";
return result;
}else if(type == "[object String]"){
return '"'+data+'"';
}else if(type == "[object Number]" || type == "[object Boolean]" || type == "[object Null]"){
return String(data);
}else{
return "undefined";
}
}
},
parse:function(str){
if(typeof str == "object"){
return str;
}
var map = {data:eval("("+str+")")};
parseDateDg(map);
function parseDateDg(data){
if(!data){
return;
}
var type = Object.prototype.toString.call(data);
if(type =="[object Array]"){
for(var i=0;i0) {
nums -= 1;
str += "0"
}
return str;
}
function fillZeroPosition(value, fixedNums, isRightFill) {
value = value + "";
fixedNums = typeof fixedNums == "number" ? fixedNums : 2;
var fillNums = fixedNums - value.length;
if (fillNums > 0) {
var str = joinZeroStr(fillNums);
return isRightFill? value + str : str + value;
}
return value;
}
function getFormatValue(value, format, formatMap) {
var regExp = /[^0#]/g;
value = Math.abs(value);
var hasDh = format.indexOf(",") != -1;
var formatSplit = format.split(".");
var integralFormat = (formatSplit[0] || "").replace(regExp, "");
var fractionalFormat = (formatSplit[1] || "").replace(regExp, "");
var formatValue = "";
var decimalsSeparator = formatMap.decimalsSeparator;
var groupSeparator = formatMap.groupSeparator;
var hasZero = integralFormat.indexOf("0");
integralFormat = hasZero == -1 ? "0" : (integralFormat.substr(hasZero) || "0");
var fractionalLength = fractionalFormat.length;
var fillZreoLenth = fractionalFormat.substr(0, fractionalFormat.lastIndexOf("0") + 1).length;
function numRound(number, fractionDigits) {
return Math.round(number * Math.pow(10, fractionDigits))/Math.pow(10, fractionDigits);
}
value = numRound(value, fractionalLength);
var valueSz = String(value).split(".");
var integralValue = valueSz[0];
var fractionalValue = valueSz[1] || "";
if (integralValue) {
integralValue = fillZeroPosition(integralValue, integralFormat.length);
if (hasDh) {
for(var i = 0; i < Math.floor((integralValue.length - (1 + i)) / 3); i++){
integralValue = integralValue.substring(0, integralValue.length - (4 * i + 3)) + groupSeparator + integralValue.substring(integralValue.length - (4 * i + 3));
}
}
formatValue += integralValue
}
if (fractionalLength > 0) {
fractionalValue = fillZeroPosition(fractionalValue.substr(0, fractionalLength), fillZreoLenth, true);
if (fractionalValue !== "") {
formatValue += decimalsSeparator;
formatValue += fractionalValue;
}
}
return formatValue;
}
function getFormatIndex(format) {
if (!format) {
return null;
}
function getZeroIndexs(format) {
var zeroIndex = format.indexOf("0");
var hashIndex = format.indexOf("#");
if (zeroIndex == -1 || (hashIndex != -1 && hashIndex < zeroIndex)) {
zeroIndex = hashIndex;
}
var lastZeroIndex = format.lastIndexOf("0");
var lastHashIndex = format.lastIndexOf("#");
if (lastZeroIndex == -1 || (lastHashIndex != -1 && lastHashIndex > lastZeroIndex)) {
lastZeroIndex = lastHashIndex;
}
return [zeroIndex, lastZeroIndex];
}
var zeroIndexs = getZeroIndexs(format);
var zeroIndex = zeroIndexs[0];
var lastZeroIndex = zeroIndexs[1];
return zeroIndex > -1 ? {begin: zeroIndex, end: lastZeroIndex, format: format.substring(zeroIndex, lastZeroIndex + 1)} : null
}
function hasZeroOrHash(value) {
return value.indexOf("0") != -1 || value.indexOf("#") != -1;
}
function getCommonFormat(format, number, formatMap, formatSz) {
var ncpReg = /^(n|c|p)(\d*)$/i;
var formatAttrs = formatMap.number;
var ncpRes = ncpReg.exec(format);
if (ncpRes != null) {
var ncpValue = ncpRes[1];
var ncpCount = ncpRes[2];
if (ncpValue == "p") {
formatAttrs = formatMap.percent;
} else if (ncpValue == "c") {
formatAttrs = formatMap.currency;
}
var pointCount = ncpCount ? parseInt(ncpCount) : formatAttrs.decimals;
var plusMinusFormat = formatAttrs.pattern[number < 0 ? 1 : 0];
plusMinusFormat = plusMinusFormat.replace("n", "#,#" + (pointCount > 0 ? "." + joinZeroStr(pointCount) : ""));
format = format.replace(ncpValue + ncpCount, plusMinusFormat).replace("$", formatMap.currency.symbol).replace("%", formatMap.percent.symbol);
} else if (hasZeroOrHash(format)){
if (number < 0 && !formatSz[1]) {
format = "-" + format;
}
}
return format;
}
var indexNumberReg = /^(e)(\d*)$/i;
if (typeof number != "number") {
return "";
}
if (!format) {
return String(number);
}
var formatSz = format.split(";");
format = formatSz[0];
if (number < 0 && formatSz.length >= 2) {
format = formatSz[1];
}
if (number == 0 && formatSz.length >= 3) {
format = formatSz[2];
}
var formatMap = {"number":{"pattern":["n","-n"],"decimals":2,"decimalsSeparator":".","groupSeparator":",","groupSize":[3]},"percent":{"pattern":["n%","-n%"],"decimals":2,"decimalsSeparator":".","groupSeparator":",","groupSize":[3],"symbol":"%"},"currency":{"pattern":["$n","$-n"],"decimals":2,"decimalsSeparator":".","groupSeparator":",","groupSize":[3],"symbol":"¥"}};
var numberFormat = formatMap.number;
var percentFormat = formatMap.percent;
var currencyFormat = formatMap.currency;
var commonFormat = getCommonFormat(format, number, formatMap, formatSz);
var hasCurrencySymbol = commonFormat.indexOf(currencyFormat.symbol) != -1;
var hasPercentSymbol = commonFormat.indexOf(percentFormat.symbol) != -1;
var hasZeroHash = hasZeroOrHash(commonFormat);
var realFormat = hasCurrencySymbol ? currencyFormat : (hasPercentSymbol ? currencyFormat : numberFormat);
var formatNumber = hasPercentSymbol ? number * 100 : number;
var indexNumberRes = indexNumberReg.exec(commonFormat);
if (indexNumberRes) {
var indexCount = parseInt(indexNumberRes[2]);
return isNaN(indexCount) ? formatNumber.toExponential() : formatNumber.toExponential(indexCount);
}
if (!hasZeroHash) {
return commonFormat;
}
var formatValue = "";
var formatIndex = getFormatIndex(commonFormat);
if (formatIndex != null) {
formatValue = getFormatValue(formatNumber, formatIndex.format, realFormat);
formatValue = commonFormat.substr(0, formatIndex.begin) + formatValue + commonFormat.substr(formatIndex.end + 1);
} else {
formatValue = commonFormat;
}
return formatValue;
}
};
Number.prototype.format = function(format){
return numberUtils.formatNumber(this,format);
};
var numberMethods = ["floor","round","ceil"];
for(var i=0;i15){
maxJd = 15;
}
type = type || "round";
if(type != "floor" && type != "round" && type != "ceil"){
console.warn(type+" is not a number,will be reset to 'round'");
type = "round";
}
function getAssist(v){
var str = String(v);
var index = str.indexOf(".");
var res = index != -1?str.length - index -1:0;
if(res>maxJd){
res = maxJd;
}
var pow = Math.pow(10,res);
var num = v[type](res);
return {pow:pow,num:num};
}
var a = getAssist(this);
var b = getAssist(x);
var max = a.pow>b.pow?a.pow:b.pow;
var jg = NaN;
if(f=="add"){
jg = (a.num*max+b.num*max)/max;
}else if(f=="subtract"){
jg = (a.num*max-b.num*max)/max;
}else if(f=="multiply"){
jg = (a.num*a.pow)*(b.num*b.pow)/(a.pow*b.pow);
}else if(f=="divide"){
jg = (a.num*max)/(b.num*max);
}
return jg;
}
})(field);
}
/**精准计算拓展---结束*/
})();
© 2015 - 2025 Weber Informatics LLC | Privacy Policy