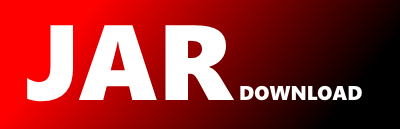
javascript.jasperreport.report.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
function openModal(elementId) {
console.log(elementId);
const modal = document.getElementById('myModal');
modal.style.display = 'block';
const image = document.getElementById(elementId);
const modalImage = document.getElementById('modal_image');
modalImage.src = image.src;
document.getElementById('imgCont').style.display = 'block';
}
function openModalVid(elementId) {
console.log(elementId);
const modalVid = document.getElementById('myModalVid');
modalVid.style.display = 'block';
const vid = document.getElementById(elementId);
const modalVideo = document.getElementById('modal_video');
modalVideo.setAttribute('controls', 'controls');
modalVideo.src = vid.src;
document.getElementById('vidCont').style.display = 'block';
}
function closeModal(modalId) {
const modal = document.getElementById(modalId);
if (modal && modal.style.display === 'block') {
modal.style.display = 'none';
}
}
function adjustCommentSectionHeights() {
let reportLevel = document.getElementById('report-level').textContent;
if (reportLevel === '1') {
let elementNodeListOf = document.querySelectorAll("[id='component-info']");
Array.from(elementNodeListOf).filter(el => el.innerText !== 'ActionComment').forEach(el => {
let firstParentDiv = el.parentElement.parentElement.parentElement.parentElement.parentElement.parentElement;
firstParentDiv.parentElement.parentElement.parentElement.style.height = '70px';
firstParentDiv.style.height = '70px';
Array.from(document.getElementsByTagName("tr")).filter(el => el.clientHeight > elementNodeListOf.length * 70)
.forEach(el => el.style.height = (el.clientHeight - elementNodeListOf.length * 70) + 'px');
let parentElement = el.parentElement.parentElement.parentElement.parentElement.parentElement.parentElement.parentElement.parentElement.parentElement;
parentElement.getElementsByTagName('svg')[0].setAttribute('height', 70);
parentElement.getElementsByTagName('svg')[0].getElementsByTagName('rect')[0].setAttribute('height', 70);
});
}
Array.from(document.querySelectorAll("[id='action-comment-section']")).forEach(el => {
const actualHeight = el.clientHeight;
if (actualHeight > 70) {
adjustCommentSection(el, actualHeight);
}
let table = el.parentElement.parentElement.parentElement.parentElement;
const tableHeight = table.clientHeight;
console.log('table height ' + tableHeight);
if (tableHeight > 170) {
let rows = table.rows;
let rowsToBeDeleted = 2;
if (reportLevel === '3') {
rowsToBeDeleted = 3;
}
for (let i = 0; i <= rowsToBeDeleted; i++) {
table.deleteRow(rows.length - 1);
console.log("row deleted");
}
let currentHeight = parseInt(rows[rows.length - 1].style.height);
let newHeight = currentHeight + 25;
rows[rows.length - 1].style.height = newHeight + 'px';
}
});
}
function adjustCommentSection(element, height) {
element.parentElement.style.height = '';
element.parentElement.parentElement.parentElement.style.height = height;
const parentLayout = element.parentElement.parentElement.parentElement.parentElement.parentElement.parentElement.parentElement.parentElement.parentElement;
const targetSvg = parentLayout.getElementsByTagName('svg')[0];
targetSvg.setAttribute('height', height + 100);
targetSvg.getElementsByTagName('rect')[0].setAttribute('height', height + 100);
Array.from(parentLayout.getElementsByTagName('span')).filter(el => el.innerText === 'No Image').forEach(el => {
el.parentElement.style.paddingTop = (height - el.clientHeight) / 2 + 10;
});
const icon = parentLayout.getElementsByClassName('api-icon')[0];
if (icon) {
icon.style.paddingBottom = height + 55;
icon.parentElement.style.height = height + 55;
}
}
function adjustVideoAndImageStyles() {
adjustVideoStyles();
adjustImageStyles();
}
function adjustVideoStyles() {
const videos = Array.from(document.getElementsByTagName('video')).filter(video => video.id !== 'modal_video');
videos.forEach(video => {
const parent = video.parentElement.parentElement;
parent.style.display = 'flex';
parent.style.alignItems = 'center';
parent.style.backgroundColor = '';
parent.parentElement.style.backgroundColor = '';
parent.parentElement.parentElement.style.backgroundColor = '';
});
}
function adjustImageStyles() {
let images = Array.from(document.getElementsByTagName('img'));
images = images.filter(image => image.title.match(/screen_/));
if (images.length > 0) {
images.forEach(image => {
adjustImage(image);
});
}
}
function adjustImage(imageElement) {
imageElement.setAttribute('id', imageElement.title);
imageElement.setAttribute('class', 'cursor image-style image');
imageElement.parentElement.setAttribute('style', 'pointer-events: auto;');
const topWrapper = createTopWrapper(imageElement);
const wrapper = createImageWrapper(imageElement);
const divOverLay = createOverlay(imageElement);
wrapper.appendChild(divOverLay);
imageElement.parentNode.replaceChild(wrapper, imageElement);
topWrapper.appendChild(wrapper.cloneNode(true));
wrapper.parentNode.replaceChild(topWrapper, wrapper);
const topParent = topWrapper.parentNode.parentNode.parentNode.parentNode.parentNode;
if (topParent.clientHeight > 172) {
topWrapper.style.paddingTop = (topParent.clientHeight - 150) / 2 - 10;
}
}
function createTopWrapper(imageElement) {
const topWrapper = document.createElement('div');
topWrapper.setAttribute('style', 'width:200px;height:150px;display: flex;align-items: center; overflow:hidden;');
return topWrapper;
}
function createImageWrapper(imageElement) {
const wrapper = document.createElement('div');
wrapper.setAttribute('class', 'overlay-image');
wrapper.appendChild(imageElement.cloneNode(true));
return wrapper;
}
function createOverlay(imageElement) {
const divOverLay = document.createElement('div');
divOverLay.setAttribute('class', 'hover cursor');
divOverLay.setAttribute('onClick', `openModal('${imageElement.title}')`);
return divOverLay;
}
function addLinkIconToHrefs() {
let maxLength = 800;
if (document.getElementById('report-level').textContent === "3") {
maxLength = 600;
}
let aElements = Array.from(document.getElementsByTagName('a'));
aElements.forEach(el => {
el.firstChild.className = 'ats-icon-hl-12-grey';
});
aElements.filter(el => el.offsetWidth >= maxLength).forEach(el => {
let childSpan = el.firstElementChild;
el.title = childSpan.textContent;
let divElement = document.createElement("div");
divElement.textContent = childSpan.textContent;
childSpan.parentNode.replaceChild(divElement, childSpan);
divElement.style.fontSize = '12px';
divElement.style.fontFamily = childSpan.style.fontFamily;
divElement.style.fontWeight = 'Bold';
divElement.style.color = childSpan.style.color;
divElement.style.lineHeight = childSpan.style.lineHeight;
divElement.className = 'ats-icon-hl-12-grey header-truncate action-link-truncate';
if (maxLength === 600) {
divElement.className = 'ats-icon-hl-12-grey header-truncate action-link-truncate action-link-truncate-short';
}
});
}
function addTooltipToHeader() {
let headerSpan = document.getElementsByTagName('span')[2];
let elementWidth = headerSpan.offsetWidth;
let headerText = headerSpan.textContent;
if (elementWidth > 980) {
headerSpan.style.fontSize = 18;
headerSpan.className = 'header-truncate';
let tooltipSpan = document.createElement("span");
tooltipSpan.textContent = headerText;
tooltipSpan.className = 'tooltiptext';
let headerParent = headerSpan.parentElement;
headerParent.className = 'tooltip';
headerParent.appendChild(tooltipSpan);
}
}
function applyIconsToStatusBar() {
let statusBars = Array.from(document.querySelectorAll("[id='header-icon-div']"));
statusBars.filter(el => !el.textContent.startsWith("com.ats.script.actions.ActionComment"))
.forEach(el => {
let props = el.textContent.split(' ');
if (props[1] === 'false' && props[2] === 'true') {
el.parentElement.className = 'false-icon';
} else if (props[1] === "false" && props[2] === 'false') {
el.parentElement.className = 'no-stop-icon';
} else if (props[1] === 'true') {
el.parentElement.className = 'true-icon';
}
})
}
document.addEventListener('DOMContentLoaded', function () {
adjustCommentSectionHeights();
adjustVideoAndImageStyles();
addLinkIconToHrefs();
addTooltipToHeader();
applyIconsToStatusBar();
document.getElementById('loader').style.display = 'none';
});
© 2015 - 2024 Weber Informatics LLC | Privacy Policy