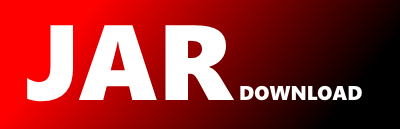
META-INF.resources.ajaxjs-ui-output.all.js Maven / Gradle / Ivy
The newest version!
// build date:Tue Jan 22 18:41:21 GMT+08:00 2019
ajaxjs = aj = function(cssSelector, fn) {
return Element.prototype.$.apply(document, arguments);
}
Element.prototype.$ = function(cssSelector, fn) {
if (typeof fn == 'function') {
var children = this.querySelectorAll(cssSelector);
if (children && fn)
Array.prototype.forEach.call(children, fn);
return children;
} else {
return this.querySelector.apply(this, arguments);
}
}
Element.prototype.die = function() {
this.parentNode.removeChild(this);
}
Element.prototype.up = function(tagName, className) {
if (tagName && className)
throw '只能任选一种参数,不能同时传';
var el = this.parentNode;
tagName = tagName && tagName.toUpperCase();
while (el) {
if (tagName && el.tagName == tagName)
return el;
if (className && el.className && ~el.className.indexOf(className))
return el;
el = el.parentNode;
}
return null;
}
Element.prototype.insertAfter = function(newElement) {
var targetElement = this, parent = targetElement.parentNode;
if (parent.lastChild == targetElement)
parent.insertBefore(newElement);
else
parent.insertBefore(newElement, targetElement.nextSibling);
}
ajaxjs.apply = function(a, b) {
for ( var i in b)
a[i] = b[i];
return a;
}
Function.prototype.delegate = function() {
var self = this, scope = this.scope, args = arguments, aLength = arguments.length, fnToken = 'function';
return function() {
var bLength = arguments.length, Length = (aLength > bLength) ? aLength : bLength;
for (var i = 0; i < Length; i++)
if (arguments[i])
args[i] = arguments[i];
args.length = Length;
for (var i = 0, j = args.length; i < j; i++) {
var _arg = args[i];
if (_arg && typeof _arg == fnToken && _arg.late == true)
args[i] = _arg.apply(scope || this, args);
}
return self.apply(scope || this, args);
};
}
Function.prototype.after = function(composeFn, isForceCall, scope) {
var self = this;
return function() {
var result = self.apply(scope || this, arguments);
if (isForceCall) {
return composeFn.call(this, result);
}
return result && (typeof result.pop != 'undefined')&& (typeof result.pop != 'unknown') ? composeFn.apply(this, result) : composeFn.call(this, result);
};
}
ajaxjs.params = {
json2url : function(json, appendUrl) {
var params = [];
for ( var i in json)
params.push(i + '=' + json[i]);
params = params.join('&');
if (appendUrl)
params = ~appendUrl.indexOf('?') ? appendUrl + '&' + params : appendUrl + '?' + params;
return params;
},
get : function(search, hash) {
search = search || window.location.search;
hash = hash || window.location.hash;
var fn = function(str, reg) {
if (str) {
var data = {};
str.replace(reg, function($0, $1, $2, $3) {
data[$1] = $3;
});
return data;
}
};
return {
search : fn(search, new RegExp("([^?=&]+)(=([^&]*))?", "g")) || {},
hash : fn(hash, new RegExp("([^#=&]+)(=([^&]*))?", "g")) || {}
};
}
};
if (!window.URLSearchParams) {
URLSearchParams = function() {
}
}
ajaxjs.xhr = {
json2url : ajaxjs.params.json2url,
request : function(url, cb, args, cfg, method) {
var params = this.json2url(args), xhr = new XMLHttpRequest();
method = method.toUpperCase();
if (method == 'POST' || method == 'PUT') {
xhr.open(method, url);
} else
xhr.open(method, url + (params ? '?' + params : ''));
cb.url = url;
xhr.onreadystatechange = this.callback.delegate(null, cb, cfg && cfg.parseContentType);
if (method == 'POST' || method == 'PUT') {
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.send(params);
} else {
xhr.send(null);
}
},
callback : function(event, cb, parseContentType) {
if (this.readyState === 4 && this.status === 200) {
var responseText = this.responseText.trim();
try {
if (!responseText)
throw '服务端返回空的字符串!';
var data = null;
switch (parseContentType) {
case 'text':
data = this.responseText;
break;
case 'xml':
data = this.responseXML;
break;
case 'json':
default:
try{
data = JSON.parse(responseText);
} catch(e) {
try{
data = eval("TEMP_VAR = " + responseText);
} catch(e) {
throw e;
}
}
}
} catch (e) {
alert('XHR 错误:\n' + e + '\nUrl is:' + cb.url);
}
if (!cb)
throw '你未提供回调函数';
cb(data, this);
}
if (this.readyState === 4 && this.status == 500)
alert('服务端 500 错误!');
},
jsonp : function(url, params, cb, cfg) {
var globalMethod_Token = 'globalMethod_' + parseInt(Math.random() * (200000 - 10000 + 1) + 10000);
if (!window.$$_jsonp)
window.$$_jsonp = {};
window.$$_jsonp[globalMethod_Token] = cb;
params = params || {};
params[cfg && cfg.callBackField || 'callBack'] = '$$_jsonp.' + globalMethod_Token;
var scriptTag = document.createElement('script');
scriptTag.src = this.json2url(params, url);
document.getElementsByTagName('head')[0].appendChild(scriptTag);
},
form : function(form, cb, cfg) {
cb = cb || ajaxjs.xhr.defaultCallBack;
cfg = cfg || {};
if(!form) return;
if (typeof form == 'string')
form = aj(form);
if (!form.action)
throw 'Please fill the url in ACTION attribute.';
var method;
if(form.getAttribute('method'))
method = form.getAttribute('method').toLowerCase();
cfg.method = method || cfg.method || 'post';
form.addEventListener('submit', function(e, cb, cfg) {
e.preventDefault();
var form = e.target;
var json = ajaxjs.xhr.serializeForm(form, cfg);
if (cfg && cfg.beforeSubmit && cfg.beforeSubmit(form, json) === false)
return;
if (cfg && cfg.method == 'put')
ajaxjs.xhr.put(form.action, cb, json);
else
ajaxjs.xhr.post(form.action, cb, json);
}.delegate(null, cb, cfg));
}
};
ajaxjs.xhr.get = ajaxjs.xhr.request.delegate(null, null, null, null, 'GET');
ajaxjs.xhr.post = ajaxjs.xhr.request.delegate(null, null, null, null, 'POST');
ajaxjs.xhr.put = ajaxjs.xhr.request.delegate(null, null, null, null, 'PUT');
ajaxjs.xhr.dele = ajaxjs.xhr.request.delegate(null, null, null, null, 'DELETE');
ajaxjs.xhr.serializeForm = function(form, cfg) {
var json = {};
if (window.FormData && FormData.prototype.forEach) {
var formData = new FormData(form);
formData.forEach(function(value, name) {
if (cfg && cfg.ignoreField != name)
json[name] = encodeURIComponent(value);
});
} else {
for (var i = 0, len = form.elements.length; i < len; i++) {
var formElement = form.elements[i], name = formElement.name, value = formElement.value;
if (formElement.name === '' || formElement.disabled || (cfg && cfg.ignoreField == name))
continue;
switch (formElement.nodeName.toLowerCase()) {
case 'input':
switch (formElement.type) {
case 'number':
case 'text':
case 'hidden':
case 'password':
json[name] = encodeURIComponent(value);
break;
case 'checkbox':
case 'radio':
if (formElement.checked)
json[name] = encodeURIComponent(value);
break;
}
break;
case 'textarea':
case 'select':
json[name] = encodeURIComponent(value);
break;
}
}
}
return json;
}
ajaxjs.xhr.defaultCallBack = function(json) {
if (json) {
if (json.isOk) {
ajaxjs.alert.show(json.msg || '操作成功!');
} else {
ajaxjs.alert.show(json.msg || '执行失败!原因未知!');
}
} else {
ajaxjs.alert.show('ServerSide Error!');
}
}
ajaxjs.throttle = {
event : {},
handler : [],
init : function(fn, eventType) {
eventType = eventType || 'resize';
this.handler.push(fn);
if (!this.event[eventType])
this.event[eventType] = true;
(function() {
var me = arguments.callee;
window.addEventListener(eventType, function() {
window.removeEventListener(eventType, arguments.callee);
var args = arguments;
window.setTimeout(function() {
for (var i = 0, j = ajaxjs.throttle.handler.length; i < j; i++) {
var obj = ajaxjs.throttle.handler[i];
if (typeof obj == 'function') {
obj.apply(this, args);
} else if (typeof obj.fn == 'function' && !obj.executeOnce) {
obj.fn.call(obj);
}
}
me();
}, 300);
});
})();
var obj = fn;
if (typeof obj == 'function') {
obj();
} else if (typeof obj.fn == 'function' && !obj.done) {
obj.fn.call(obj);
}
}
};
ajaxjs.throttle.onEl_in_viewport = function(el, fn) {
setTimeout(function() {
UserEvent2.onWinResizeFree({
executeOnce : false,
fn : function() {
var scrollTop = document.body.scrollTop, docHeight = window.innerHeight;
var elTop = el.getBoundingClientRect().top, isFirstPage = docHeight > elTop, isInPage = scrollTop > elTop;
if (isFirstPage || isInPage) {
this.executeOnce = true;
fn();
}
}
}, 'scroll');
}, 1500);
}
ajaxjs.throttle.onEl_in_viewport.actions = [];
tppl = function(tpl, data){
var fn = function(d) {
var i, k = [], v = [];
for (i in d) {
k.push(i);
v.push(d[i]);
};
return (new Function(k, fn.$)).apply(d, v);
};
if(!fn.$){
var tpls = tpl.split('[:');
fn.$ = "var $=''";
for(var t = 0;t < tpls.length;t++){
var p = tpls[t].split(':]');
if(t!=0){
fn.$ += '='==p[0].charAt(0)
? "+("+p[0].substr(1)+")"
: ";"+p[0].replace(/\r\n/g, '')+"$=$"
}
fn.$ += "+'"+p[p.length-1].replace(/\'/g,"\\'").replace(/\r\n/g, '\\n').replace(/\n/g, '\\n').replace(/\r/g, '\\n')+"'";
}
fn.$ += ";return $;";
}
return data ? fn(data) : fn;
}
ajaxjs.tab = function(cfg) {
ajaxjs.apply(this, cfg);
var mover = this.el.querySelector(this.moverTagName || 'div');
var children = mover.children, len = children.length;
var stepWidth = this.stepWidth || mover.parentNode.clientWidth || window.innerWidth;
if(this.isMagic)
mover.style.width = this.isUsePx ? (stepWidth * 2) +'px' : '200%';
else
mover.style.width = this.isUsePx ? (stepWidth * len) +'px' : len + '00%';
var tabWidth = this.isUsePx ? stepWidth + 'px' : (1 / len * 100).toFixed(5) + '%';
for(var i = 0; i < len; i++)
children[i].style.width = this.isMagic ? '50%' : tabWidth;
this.isUsePx && ajaxjs.throttle.init(onResize.bind(this));
this.tabHeader = this.el.$('header');
if (this.tabHeader && !this.disableTabHeaderJump)
this.tabHeader.onclick = onTabHeaderPress.bind(this);
var isWebkit = navigator.userAgent.toLowerCase().indexOf('webkit') != -1;
this.go = function(i) {
if(this.isGetCurrentHeight) {
for(var p = 0; p < len; p++) {
if(i == p) {
this.children[p].style.height = 'initial';
}else{
this.children[p].style.height = '1px';
}
}
}
if(this.isMagic) {
for(var p = 0; p < len; p++) {
if(this.currentIndex == p) {
continue;
}else if(i == p) {
children[p].classList.remove('hide');
}else {
children[p].classList.add('hide');
}
}
var cssText = i > this.currentIndex
? 'translate3d({0}, 0px, 0px)'.replace('{0}', '-50%')
: 'translate3d({0}, 0px, 0px)'.replace('{0}', '0%');
mover.style.webkitTransition = '-webkit-transform 400ms linear';
mover.style.webkitTransform = cssText;
}else{
var leftValue = this.isUsePx ? ('-' + (i * stepWidth) + 'px') : ('-' + (1 / len * 100 * i).toFixed(2) + '%');
mover.style[isWebkit ? 'webkitTransform' : 'transform'] = 'translate3d({0}, 0px, 0px)'.replace('{0}', leftValue);
}
this.currentIndex = i;
this.onItemSwitch && this.onItemSwitch.call(this, i, children[i]);
}
this.goPrevious = function() {
this.currentIndex--;
if (this.currentIndex < 0)this.currentIndex = len - 1;
this.go(this.currentIndex);
}
this.goNext = function() {
this.currentIndex++;
if (this.currentIndex == len)this.currentIndex = 0;
this.go(this.currentIndex);
}
this.loop = function() {
if(this.isEnableLoop)
this.loopTimer = window.setInterval(this.goNext.bind(this), this.autoLoop);
}
this.goTab = function(index) {
onTabHeaderPress.call(this, {
target : this.el.querySelectorAll('header ul li')[index]
});
}
function onResize() {
var stepWidth = mover.parentNode.clientWidth;
mover.style.width = this.isUsePx ? (stepWidth * len) +'px' : len + '00%';
for(var i = 0; i < len; i++)
children[i].style.width = stepWidth + 'px';
}
function onTabHeaderPress(e) {
var target = e.target,
li = target.tagName == 'LI' ? target : target.up('li');
if(!li)return;
var arr = this.tabHeader.$('ul').children, index;
for(var i = 0, j = arr.length; i < j; i++) {
if(li == arr[i]) {
arr[i].classList.add('active');
index = i;
} else
arr[i].classList.remove('active');
}
this.go(index);
this.currentIndex = index;
var nextItem = children[index];
this.onTabHeaderSwitch && this.onTabHeaderSwitch.call(this, li, nextItem, target, index);
autoHeight.call(this, nextItem);
}
function autoHeight(nextItem) {
if(this.autoHeight) {
var tabHeaderHeight = 0;
if(this.tabHeader)
tabHeaderHeight = this.tabHeader.scrollHeight;
this.el.style.height = (nextItem.scrollHeight + tabHeaderHeight + 50) + 'px';
}
}
}
ajaxjs.tab.prototype = {
isMagic : false,
isUsePx : false,
autoHeight : false,
disableTabHeaderJump : false,
isGetCurrentHeigh : true,
currentIndex : 0,
autoLoop : 4000,
initFirstTab : function() {
this.goTab(0);
}
};
ajaxjs.banner = function(cfg) {
cfg.isUsePx = true;
cfg.isEnableLoop = true;
cfg.isGetCurrentHeight = false;
ajaxjs.tab.call(this, cfg);
this.initIndicator = function() {
var ol = this.el.$('ol');
this.onItemSwitch = function(index) {
if (ol) ol.$('li', function(li, i) {
if(index == i)
li.classList.add('active');
else
li.classList.remove('active');
});
}
var self = this;
ol.onclick = function(e) {
var el = e.target;
if(el.tagName != 'LI')return;
if (ol) ol.$('li', function(li, i) {
if(el == li) {
self.go(i);
return;
}
});
}
}
this.loop();
this.initIndicator();
}
ajaxjs.banner.prototype = ajaxjs.tab.prototype;
ajaxjs.list = function(cfg) {
ajaxjs.apply(this, cfg);
var args = {};
args.start = 0;
args.limit = 10;
this.baseParam && ajaxjs.apply(args, this.baseParam);
if (!this.jsonInit)
this.jsonInit = function(json) {
if (json) {
if (!json.isOk)
ajaxjs.alert(json.msg || '执行失败!原因未知!');
} else {
ajaxjs.alert('ServerSide Error!');
}
return {
data : json.result,
total : json.total
};
}
this.load = function() {
ajaxjs.xhr.get(this.url, function(json) {
var result = this.jsonInit(json);
var data = result.data;
renderer.call(this, data);
args.start += args.limit;
this.afterDataLoad && typeof this.afterDataLoad == 'function' && this.afterDataLoad.call(this, result);
}.bind(this), args);
}
function renderer(data) {
if (!data || data.length <= 0)
return;
var lis = [];
for (var i = 0; i < data.length; i++) {
var _data = this.renderer ? this.renderer(data[i]) : data[i];
if (_data === false)
continue;
var li = tppl(this.tpl, _data);
if (this.isAppend) {
var loadingIndicator = this.el.$('.loadingIndicator');
if (loadingIndicator)
this.el.removeChild(loadingIndicator);
var temp = document.createElement('div');
temp.innerHTML = li;
this.el.appendChild(temp.$('li'));
temp = null;
} else {
lis.push(li);
}
}
if (!this.isAppend)
this.el.innerHTML = lis.join('');
}
function getCellRequestWidth() {
window.devicePixelRatio = window.devicePixelRatio || 1;
var screenWidth = window.innerWidth;
var columns = 3;
var cellWidth = screenWidth * ( 1 / columns );
var cellHight = cellWidth / (4 / 3);
var reqeustWidth = cellWidth * window.devicePixelRatio;
reqeustWidth = Math.floor(reqeustWidth);
var MaxWidth = 500;
return reqeustWidth;
}
function autoHeight() {
var firstHeight = arguments.callee.firstHeight;
for(var i = 0 , j = imgs.length; i < j; i++) {
var imgObj = imgs[i];
console.log(imgObj.el.complete);
if(i == 0 && !firstHeight)
firstHeight = arguments.callee.firstHeight = imgObj.el.height;
else
imgObj.el.height = firstHeight;
}
}
function fixImgHeigtBy() {
window.innerWidth * 0.3 / 1.333
}
}
ajaxjs.list.thumbFadeIn = function() {
var imgs = [];
this.el.$('img[data-src^="https://"]', function(img, index) {
img.onload = function(){ this.classList.add('tran') };
imgs.push({
index : index,
el : img,
src : img.getAttribute('data-src')
});
});
Step(function() {
for(var i = 0 , j = imgs.length; i < j; i++) {
if(imgs[i].src) {
var img = new Image();
img.onload = this.parallel();
img.src = imgs[i].src;
}
}
}, function() {
var i = 0;
var nextStep = this;
var id = setInterval(function() {
var imgObj = imgs[i++];
imgObj.el.src = imgObj.src;
if(i == imgs.length) {
clearInterval(id);
nextStep();
}
}, 300);
}, function() {
});
}
Vue.component('ajaxjs-admin-header', {
props : {
isCreate : Boolean,
uiName : String,
infoId : {
type: Number,
required: false
}
},
template :
'\
\
\
新窗口打开\
\
\
'
})
Vue.component('ajaxjs-admin-info-btns', {
props : {
isCreate : Boolean
},
template :
'\
\
\
\
',
methods : {
del : function () {
if (confirm('确定删除?'))
ajaxjs.xhr.dele('.', function(json) {
if (json && json.isOk) {
alert(json.msg);
location.assign('../list/');
}
});
}
}
});
Vue.component('aj-admin-filter-panel', {
props : {
label : {
type : String,
required : false
},
catelogId :{
type: Number,
required: false
},
selectedCatelogId :{
type: Number,
required: false
},
noCatelog :{
type : Boolean,
default : false
}
},
template:
'\
\
{{label||\'分类\'}}: \
'
});
aj.admin = {
del : function(id, title) {
if (confirm('请确定删除记录:\n' + title + ' ?')) {
ajaxjs.xhr.dele('../' + id + '/', function(json) {
if (json.isOk) {
alert('删除成功!');
location.reload();
}
});
}
},
setStatus : function(id, status) {
ajaxjs.xhr.post('../setStatus/' + id + '/', function(json) {
if (json.isOk) {
}
}, {
status : status
});
}
};
aj._carousel = {
props : {
isMagic : {
type : Boolean,
default : false
},
isUsePx : {
type : Boolean,
default : false
},
autoHeight : {
type : Boolean,
default : false
},
disableTabHeaderJump : {
type : Boolean,
default : false
},
isGetCurrentHeight : {
type : Boolean,
default : true
},
initItems : Array
},
data : function() {
return {
selected : 0
};
},
mounted: function() {
this.mover = this.$el.$('div.content');
var mover = this.mover, children = mover.children, len = children.length;
setTimeout(function() {
var stepWidth = this.setItemWidth();
if(this.isMagic)
mover.style.width = this.isUsePx ? (stepWidth * 2) +'px' : '200%';
else
mover.style.width = this.isUsePx ? (stepWidth * len) +'px' : len + '00%';
var tabWidth = this.isUsePx ? stepWidth + 'px' : (1 / len * 100).toFixed(5) + '%';
this.tabWidth = tabWidth;
for(var i = 0; i < len; i++)
children[i].style.width = this.isMagic ? '50%' : tabWidth;
var headerUl = this.$el.$('header ul');
if(headerUl)
for(var i = 0; i < len; i++)
headerUl.children[i].style.width = tabWidth;
this.doHeight(this.selected);
}.bind(this), 400);
},
watch:{
'selected' : function(index, oldIndex) {
if(this.$isStop)
return;
var children = this.$el.$('header ul').children;
var contentChild = this.$el.$('.content').children;
if(children && contentChild && children[oldIndex] && contentChild[oldIndex]) {
children[oldIndex].classList.remove('active');
contentChild[oldIndex].classList.remove('active');
children[index].classList.add('active');
contentChild[index].classList.add('active');
this.go(index);
}
}
},
methods : {
setItemWidth : function() {
this.stepWidth = this.stepWidth || this.mover.parentNode.clientWidth || window.innerWidth;
return this.stepWidth;
},
changeTab : function(index) {
this.selected = index;
this.go(index);
},
go : function(i) {
this.$emit('before-carousel-item-switch', this, i);
if(this.$isStop)
return;
var mover = this.mover, children = mover.children, len = children.length;
this.doHeight(i);
if(this.isMagic) {
for(var p = 0; p < len; p++) {
if(this.selected == p) {
continue;
}else if(i == p) {
children[p].classList.remove('hide');
}else {
children[p].classList.add('hide');
}
}
var cssText = i > this.selected
? 'translate3d({0}, 0px, 0px)'.replace('{0}', '-50%')
: 'translate3d({0}, 0px, 0px)'.replace('{0}', '0%');
mover.style.webkitTransition = '-webkit-transform 400ms linear';
mover.style.webkitTransform = cssText;
}else{
var isWebkit = navigator.userAgent.toLowerCase().indexOf('webkit') != -1;
var leftValue = this.isUsePx ? ('-' + (i * this.stepWidth) + 'px') : ('-' + (1 / len * 100 * i).toFixed(2) + '%');
mover.style[this.isWebkit ? 'webkitTransform' : 'transform'] = 'translate3d({0}, 0px, 0px)'.replace('{0}', leftValue);
}
this.$emit('carousel-item-switch', this, i, children[i]);
},
goPrevious : function() {
if(this.$isStop)
return;
var len = this.mover.children.length;
this.selected--;
if (this.selected < 0)
this.selected = len - 1;
this.go(this.selected);
},
goNext : function() {
if(this.$isStop)
return;
var len = this.mover.children.length;
this.selected++;
if (this.selected == len)
this.selected = 0;
this.go(this.selected);
},
onResize : function () {
var stepWidth = this.mover.parentNode.clientWidth;
this.mover.style.width = this.isUsePx ? (stepWidth * this.len) +'px' : this.len + '00%';
for(var i = 0; i < this.len; i++)
this.children[i].style.width = stepWidth + 'px';
},
doHeight: function(i) {
if(this.isGetCurrentHeight) {
var mover = this.mover, children = mover.children, len = children.length;
for(var p = 0; p < len; p++) {
if(i == p) {
children[p].style.height = 'initial';
}else{
children[p].style.height = '1px';
}
}
}
},
doAutoHeight : function (nextItem) {
if(this.autoHeight) {
var tabHeaderHeight = 0;
if(this.tabHeader)
tabHeaderHeight = this.tabHeader.scrollHeight;
this.el.style.height = (nextItem.scrollHeight + tabHeaderHeight + 50) + 'px';
}
},
autoChangeTab: function(e) {
if(this.$isStop)
return;
var el = e.currentTarget;
var children = el.parentNode.children;
for(var i = 0, j = children.length; i < j; i++) {
if(el == children[i]) {
break;
}
}
this.selected = i;
}
}
};
Vue.component('aj-carousel', {
mixins: [aj._carousel],
template :
'\
\
- {{item.name}}
\
\
\
\
\
',
data : function() {
return {
items : this.initItems || [
{name : '杜甫:望岳', content : '岱宗夫如何,齊魯青未了。
\
造化鐘神秀,陰陽割昏曉。
\
蕩胸生層云,決眥入歸鳥,
\
會當凌絕頂,一覽眾山小。'
},
{name : '资质证照', content : '之所以如此,端在于中国传统中存在着发达的契约。予谓不信,可看看早年由福建师范大学内部印行的两册本《明清福建经济契约文书选集》,中国社会科学出版社出版的《自贡盐业契约档案选集》,花山文艺出版社出版的共二十册的《徽州千年契约文书》;再看看近些年由安徽师范大学出版社出版的十卷本的《千年徽州契约文书集萃》,广西师范大学出版社出版的四辑共四十册《徽州文书》、三辑共三十册的《清水江文书》,民族出版社出版的多卷本《贵州清水江流域明清契约文书》,凤凰出版社出版的《敦煌契约文书辑校》,天津古籍出版社出版的《清代宁波契约文书辑校》,浙江大学出版社出版的《清代浙东契约文书辑选》……不难发现,中国传统契约文书的整理出版,完全可称为如雨后春笋般在迅速成长!'},
{name : '资质证照', content : '笔者出于个人兴趣,在关注这些已经整理出版的、卷帙浩繁的契约文献的同时,也游走各地,或亲自搜集各类契约文书,或到一些地方档案馆查看其业已编辑成册、内部印行的传统契约文书,如在台湾宜兰、高雄,山东青岛、威海,贵州锦屏、从江等地档案机构或民间都见到过相关契约文献。记忆尤深的一次,是我和几位学生共游山东浮来山。在一处值班室里,居然发现有人以清代契约文本粘糊墙壁!足见只要我们稍加留意,在这个文明发展历经数千年的国度,不时可以发现一些令人称心的古代契约文献。'}
]
};
}
});
Vue.component('aj-banner', {
mixins: [aj._carousel],
props : {
isUsePx : {
default: true
},
isGetCurrentHeight : {
default: false
},
autoLoop : {
type : Number,
default : 4000
},
showTitle : {
type: Boolean,
default : false
},
showDot : {
type : Boolean,
default : true
}
},
template :
' ',
data : function() {
return {
items : this.initItems || [
{name : '杜甫:望岳', content : '
', href : 'http://qq.com'},
{name : '资质证照', content : '
', href : 'javascript:alert(9);'},
{name : '资质证照', content : '
', href: '#'}
]
};
},
mounted: function() {
this.loop();
},
methods:{
loop : function() {
this.loopTimer = window.setInterval(this.goNext.bind(this), this.autoLoop);
},
getContent : function(content, href) {
if(!href)
return content;
else
return '' + content + '';
}
}
});
Vue.component('aj-page-captcha', {
props : {
imgSrc : {
type: String,
required: false,
},
fieldName : {
type: String,
required: false,
default : 'captcha'
}
},
template :
'\
\
\
\
\
',
methods : {
onClk : function(e) {
var img = e.target;
img.src = img.src.replace(/\?\d+$/, '') + '?' + new Date().valueOf();
}
}
});
Vue.component('aj-form-calendar', {
data: function() {
return {
year : 2017,
month : 2,
day : 1
};
},
props : {
},
template :
'',
mounted : function () {
aj.apply(this, this.getDate('now'));
this.render();
},
methods : {
render : function () {
var el = this.$el;
var arr = this.getDateArr();
this.days = [];
var frag = document.createDocumentFragment();
while (arr.length) {
var row = document.createElement("tr");
for (var i = 1; i <= 7; i++) {
var cell = document.createElement("td");
if (arr.length) {
var d = arr.shift();
if (d) {
cell.innerHTML = d;
cell.className = 'day day_' + this.year + '-' + this.month + '-' + d;
cell.title = this.year + '-' + this.month + '-' + d;
this.days[d] = cell;
var on = new Date(this.year, this.month - 1, d);
if (this.isSameDay(on, this.date)) {
cell.classList.add('onToday');
this.onToday && this.onToday(cell);
}
this.selectDay && this.onSelectDay && this.isSameDay(on, this.selectDay) && this.onSelectDay(cell);
}
}
row.appendChild(cell);
}
frag.appendChild(row);
}
var tbody = el.$("table tbody");
tbody.innerHTML = '';
tbody.appendChild(frag);
this.onFinish && this.onFinish();
},
setYear : function(type) {
this.year = this.getDate(type).year;
},
nextYear : function() {
},
getDate: function (dateType) {
var now = new Date(), date, nowYear = now.getFullYear(), nowMonth = now.getMonth() + 1;
switch (dateType) {
case 'now':
date = now;
break;
case 'preMonth':
date = new Date(nowYear, nowMonth - 2, 1);
break;
case 'nextMonth':
date = new Date(nowYear, nowMonth, 1);
break;
case 'preYear':
date = new Date(nowYear - 1, nowMonth - 1, 1);
break;
case 'nextYear':
date = new Date(nowYear + 1, nowMonth - 1, 1);
break;
}
return {
date : date,
year : date.getFullYear(),
month : date.getMonth() + 1,
};
},
getDateArr: function () {
var arr = [];
for (var i = 1, firstDay = new Date(this.year, this.month - 1, 1).getDay(); i <= firstDay; i++)
arr.push(0);
for (var i = 1, monthDay = new Date(this.year, this.month, 0).getDate(); i <= monthDay; i++)
arr.push(i);
return arr;
},
pickDay : function(e) {
var el = e.target, date = el.title;
this.$emit('pick-date', date);
return date;
},
isSameDay: function (d1, d2) {
return (d1.getFullYear() == d2.getFullYear() && d1.getMonth() == d2.getMonth() && d1.getDate() == d2.getDate());
}
}
});
Vue.component('aj-form-calendar-input', {
data : function(){
return {
date : this.fieldValue
}
},
props : {
fieldName : {
type: String,
required: true
},
fieldValue : {
type: String,
required: false
},
positionFixed : Boolean
},
template :
'\
\
\
\
',
mounted : function() {
if(this.positionFixed) {
this.$el.$('.aj-form-calendar').classList.add('positionFixed');
}
},
methods : {
recEvent: function(date) {
this.date = date;
},
onMouseOver : function(e) {
if(this.positionFixed) {
var el = e.currentTarget;
var b = el.getBoundingClientRect();
var c = this.$el.$('.aj-form-calendar');
c.style.top = (b.top + el.clientHeight - 0) + 'px';
c.style.left = ((b.left - 0) + 0) + 'px';
}
}
}
});
Vue.component('aj-form-html-editor', {
template : '',
props : {
fieldName : {
type: String,
required: true
},
content : {
type: String,
required: false
},
basePath : {
type: String,
required: false,
default : ''
}
},
beforeCreate : function() {
var xhr = new XMLHttpRequest();
xhr.open("GET", this.ajResources.libraryUse + '/htmleditor-tag.htm', false);
xhr.send(null);
this.$options.template = xhr.responseText;
},
mounted : function() {
var el = this.$el;
this.iframeEl = el.$('iframe');
this.sourceEditor = el.$('textarea');
this.iframeWin = this.iframeEl.contentWindow;
this.mode = 'iframe';
this.toolbarEl = el.$('.toolbar');
this.iframeWin.onload = function() {
this.iframeDoc = this.iframeWin.document;
this.iframeDoc.designMode = 'on';
this.iframeBody = this.iframeDoc.body;
this.sourceEditor.value && this.setValue(this.sourceEditor.value);
}.bind(this);
},
methods: {
onToolBarClk : function(e) {
var el = e.target, clsName = el.className;
clsName = clsName.split(' ').shift();
switch(clsName) {
case 'createLink':
var result = prompt("请输入 URL 地址");
if(result)
this.format("createLink", result);
break;
case 'insertImage':
if(window.isCreate)
aj.alert.show('请保存记录后再上传图片。');
else {
var self = this;
App.$refs.uploadLayer.show(function(imgUrl) {
if(imgUrl)
self.format("insertImage", imgUrl);
});
}
break;
case 'switchMode':
this.setMode();
break;
default:
this.format(clsName);
}
},
format : function (type, para) {
this.iframeWin.focus();
if (!para) {
if (document.all)
this.iframeDoc.execCommand(type);
else
this.iframeDoc.execCommand(type, false, false);
} else
this.iframeDoc.execCommand(type, false, para);
this.iframeWin.focus();
},
insertEl : function (html) {
this.iframeDoc.body.innerHTML = html;
},
setValue : function(v) {
var self = this;
setTimeout(function() {
self.iframeWin.document.body.innerHTML = v;
}, 500);
},
getValue : function(cfg) {
var result = this.iframeBody.innerHTML;
if(cfg && cfg.cleanWord)
result = this.cleanPaste(result);
if(cfg && cfg.encode)
result = encodeURIComponent(result);
return result;
},
cleanPaste : function(html) {
html = html.replace(/<(\/)*(\\?xml:|meta|link|span|font|del|ins|st1:|[ovwxp]:)((.|\s)*?)>/gi, '');
html = html.replace(/(class|style|type|start)=("(.*?)"|(\w*))/gi, '');
html = html.replace(/";
printHTML += aj('article').innerHTML;
printHTML += "