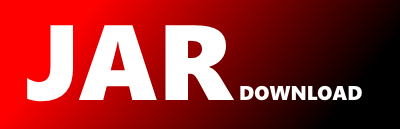
LExpress4.4.0.0-beta.1.source-code.QLParserVisitor Maven / Gradle / Ivy
// Generated from QLParser.g4 by ANTLR 4.9.3
package com.alibaba.qlexpress4.aparser;
import static com.alibaba.qlexpress4.aparser.ParserOperatorManager.OpType.*;
import static com.alibaba.qlexpress4.QLPrecedences.*;
import static com.alibaba.qlexpress4.aparser.InterpolationMode.*;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link QLParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface QLParserVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link QLParser#program}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitProgram(QLParser.ProgramContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#blockStatements}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBlockStatements(QLParser.BlockStatementsContext ctx);
/**
* Visit a parse tree produced by the {@code localVariableDeclarationStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLocalVariableDeclarationStatement(QLParser.LocalVariableDeclarationStatementContext ctx);
/**
* Visit a parse tree produced by the {@code throwStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitThrowStatement(QLParser.ThrowStatementContext ctx);
/**
* Visit a parse tree produced by the {@code whileStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhileStatement(QLParser.WhileStatementContext ctx);
/**
* Visit a parse tree produced by the {@code traditionalForStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTraditionalForStatement(QLParser.TraditionalForStatementContext ctx);
/**
* Visit a parse tree produced by the {@code forEachStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitForEachStatement(QLParser.ForEachStatementContext ctx);
/**
* Visit a parse tree produced by the {@code functionStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionStatement(QLParser.FunctionStatementContext ctx);
/**
* Visit a parse tree produced by the {@code macroStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMacroStatement(QLParser.MacroStatementContext ctx);
/**
* Visit a parse tree produced by the {@code breakContinueStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBreakContinueStatement(QLParser.BreakContinueStatementContext ctx);
/**
* Visit a parse tree produced by the {@code returnStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReturnStatement(QLParser.ReturnStatementContext ctx);
/**
* Visit a parse tree produced by the {@code emptyStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEmptyStatement(QLParser.EmptyStatementContext ctx);
/**
* Visit a parse tree produced by the {@code expressionStatement}
* labeled alternative in {@link QLParser#blockStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpressionStatement(QLParser.ExpressionStatementContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#localVariableDeclaration}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLocalVariableDeclaration(QLParser.LocalVariableDeclarationContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#forInit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitForInit(QLParser.ForInitContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#variableDeclaratorList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVariableDeclaratorList(QLParser.VariableDeclaratorListContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#variableDeclarator}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVariableDeclarator(QLParser.VariableDeclaratorContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#variableDeclaratorId}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVariableDeclaratorId(QLParser.VariableDeclaratorIdContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#variableInitializer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVariableInitializer(QLParser.VariableInitializerContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#arrayInitializer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArrayInitializer(QLParser.ArrayInitializerContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#variableInitializerList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVariableInitializerList(QLParser.VariableInitializerListContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#declType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDeclType(QLParser.DeclTypeContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#declTypeNoArr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDeclTypeNoArr(QLParser.DeclTypeNoArrContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#primitiveType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrimitiveType(QLParser.PrimitiveTypeContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#referenceType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReferenceType(QLParser.ReferenceTypeContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#dims}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDims(QLParser.DimsContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#clsTypeNoTypeArguments}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitClsTypeNoTypeArguments(QLParser.ClsTypeNoTypeArgumentsContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#clsType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitClsType(QLParser.ClsTypeContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#typeArguments}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeArguments(QLParser.TypeArgumentsContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#typeArgumentList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeArgumentList(QLParser.TypeArgumentListContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#typeArgument}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeArgument(QLParser.TypeArgumentContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#wildcard}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWildcard(QLParser.WildcardContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#wildcardBounds}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWildcardBounds(QLParser.WildcardBoundsContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpression(QLParser.ExpressionContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#leftHandSide}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLeftHandSide(QLParser.LeftHandSideContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#ternaryExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTernaryExpr(QLParser.TernaryExprContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#baseExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBaseExpr(QLParser.BaseExprContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#leftAsso}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLeftAsso(QLParser.LeftAssoContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#binaryop}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBinaryop(QLParser.BinaryopContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#primary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrimary(QLParser.PrimaryContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#prefixExpress}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPrefixExpress(QLParser.PrefixExpressContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#suffixExpress}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSuffixExpress(QLParser.SuffixExpressContext ctx);
/**
* Visit a parse tree produced by the {@code constExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConstExpr(QLParser.ConstExprContext ctx);
/**
* Visit a parse tree produced by the {@code castExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCastExpr(QLParser.CastExprContext ctx);
/**
* Visit a parse tree produced by the {@code groupExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGroupExpr(QLParser.GroupExprContext ctx);
/**
* Visit a parse tree produced by the {@code newObjExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNewObjExpr(QLParser.NewObjExprContext ctx);
/**
* Visit a parse tree produced by the {@code newEmptyArrExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNewEmptyArrExpr(QLParser.NewEmptyArrExprContext ctx);
/**
* Visit a parse tree produced by the {@code newInitArrExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNewInitArrExpr(QLParser.NewInitArrExprContext ctx);
/**
* Visit a parse tree produced by the {@code lambdaExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLambdaExpr(QLParser.LambdaExprContext ctx);
/**
* Visit a parse tree produced by the {@code varIdExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVarIdExpr(QLParser.VarIdExprContext ctx);
/**
* Visit a parse tree produced by the {@code typeExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeExpr(QLParser.TypeExprContext ctx);
/**
* Visit a parse tree produced by the {@code listExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitListExpr(QLParser.ListExprContext ctx);
/**
* Visit a parse tree produced by the {@code mapExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMapExpr(QLParser.MapExprContext ctx);
/**
* Visit a parse tree produced by the {@code blockExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBlockExpr(QLParser.BlockExprContext ctx);
/**
* Visit a parse tree produced by the {@code ifExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIfExpr(QLParser.IfExprContext ctx);
/**
* Visit a parse tree produced by the {@code tryCatchExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTryCatchExpr(QLParser.TryCatchExprContext ctx);
/**
* Visit a parse tree produced by the {@code contextSelectExpr}
* labeled alternative in {@link QLParser#primaryNoFix}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitContextSelectExpr(QLParser.ContextSelectExprContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#ifBody}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIfBody(QLParser.IfBodyContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#listItems}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitListItems(QLParser.ListItemsContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#dimExprs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDimExprs(QLParser.DimExprsContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#tryCatches}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTryCatches(QLParser.TryCatchesContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#tryCatch}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTryCatch(QLParser.TryCatchContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#catchParams}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCatchParams(QLParser.CatchParamsContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#tryFinally}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTryFinally(QLParser.TryFinallyContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#mapEntries}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMapEntries(QLParser.MapEntriesContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#mapEntry}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMapEntry(QLParser.MapEntryContext ctx);
/**
* Visit a parse tree produced by the {@code clsValue}
* labeled alternative in {@link QLParser#mapValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitClsValue(QLParser.ClsValueContext ctx);
/**
* Visit a parse tree produced by the {@code eValue}
* labeled alternative in {@link QLParser#mapValue}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEValue(QLParser.EValueContext ctx);
/**
* Visit a parse tree produced by the {@code idKey}
* labeled alternative in {@link QLParser#mapKey}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIdKey(QLParser.IdKeyContext ctx);
/**
* Visit a parse tree produced by the {@code stringKey}
* labeled alternative in {@link QLParser#mapKey}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringKey(QLParser.StringKeyContext ctx);
/**
* Visit a parse tree produced by the {@code quoteStringKey}
* labeled alternative in {@link QLParser#mapKey}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQuoteStringKey(QLParser.QuoteStringKeyContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#idMapKey}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIdMapKey(QLParser.IdMapKeyContext ctx);
/**
* Visit a parse tree produced by the {@code methodInvoke}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMethodInvoke(QLParser.MethodInvokeContext ctx);
/**
* Visit a parse tree produced by the {@code optionalMethodInvoke}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOptionalMethodInvoke(QLParser.OptionalMethodInvokeContext ctx);
/**
* Visit a parse tree produced by the {@code spreadMethodInvoke}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSpreadMethodInvoke(QLParser.SpreadMethodInvokeContext ctx);
/**
* Visit a parse tree produced by the {@code fieldAccess}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFieldAccess(QLParser.FieldAccessContext ctx);
/**
* Visit a parse tree produced by the {@code optionalFieldAccess}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOptionalFieldAccess(QLParser.OptionalFieldAccessContext ctx);
/**
* Visit a parse tree produced by the {@code spreadFieldAccess}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSpreadFieldAccess(QLParser.SpreadFieldAccessContext ctx);
/**
* Visit a parse tree produced by the {@code methodAccess}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMethodAccess(QLParser.MethodAccessContext ctx);
/**
* Visit a parse tree produced by the {@code callExpr}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCallExpr(QLParser.CallExprContext ctx);
/**
* Visit a parse tree produced by the {@code indexExpr}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIndexExpr(QLParser.IndexExprContext ctx);
/**
* Visit a parse tree produced by the {@code customPath}
* labeled alternative in {@link QLParser#pathPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCustomPath(QLParser.CustomPathContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#fieldId}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFieldId(QLParser.FieldIdContext ctx);
/**
* Visit a parse tree produced by the {@code singleIndex}
* labeled alternative in {@link QLParser#indexValueExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSingleIndex(QLParser.SingleIndexContext ctx);
/**
* Visit a parse tree produced by the {@code sliceIndex}
* labeled alternative in {@link QLParser#indexValueExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSliceIndex(QLParser.SliceIndexContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#argumentList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArgumentList(QLParser.ArgumentListContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteral(QLParser.LiteralContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#doubleQuoteStringLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDoubleQuoteStringLiteral(QLParser.DoubleQuoteStringLiteralContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#stringExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringExpression(QLParser.StringExpressionContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#boolenLiteral}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBoolenLiteral(QLParser.BoolenLiteralContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#lambdaParameters}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLambdaParameters(QLParser.LambdaParametersContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#formalOrInferredParameterList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFormalOrInferredParameterList(QLParser.FormalOrInferredParameterListContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#formalOrInferredParameter}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFormalOrInferredParameter(QLParser.FormalOrInferredParameterContext ctx);
/**
* Visit a parse tree produced by the {@code importCls}
* labeled alternative in {@link QLParser#importDeclaration}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImportCls(QLParser.ImportClsContext ctx);
/**
* Visit a parse tree produced by the {@code importPack}
* labeled alternative in {@link QLParser#importDeclaration}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImportPack(QLParser.ImportPackContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#assignOperator}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAssignOperator(QLParser.AssignOperatorContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#opId}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOpId(QLParser.OpIdContext ctx);
/**
* Visit a parse tree produced by {@link QLParser#varId}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVarId(QLParser.VarIdContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy