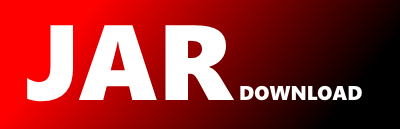
.experiment-jvm-server.1.6.1.source-code.RemoteEvaluationClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of experiment-jvm-server Show documentation
Show all versions of experiment-jvm-server Show documentation
Amplitude Experiment server-side SDK for JVM (Java, Kotlin)
The newest version!
package com.amplitude.experiment
import com.amplitude.experiment.evaluation.EvaluationVariant
import com.amplitude.experiment.util.BackoffConfig
import com.amplitude.experiment.util.FetchException
import com.amplitude.experiment.util.Logger
import com.amplitude.experiment.util.backoff
import com.amplitude.experiment.util.json
import com.amplitude.experiment.util.toJson
import com.amplitude.experiment.util.toVariant
import kotlinx.serialization.decodeFromString
import okhttp3.Call
import okhttp3.Callback
import okhttp3.HttpUrl
import okhttp3.HttpUrl.Companion.toHttpUrl
import okhttp3.OkHttpClient
import okhttp3.Request
import okhttp3.Response
import okio.IOException
import java.util.Base64
import java.util.concurrent.CompletableFuture
import java.util.concurrent.TimeUnit
class RemoteEvaluationClient internal constructor(
private val apiKey: String,
private val config: RemoteEvaluationConfig = RemoteEvaluationConfig(),
) {
private val httpClient = OkHttpClient.Builder().proxy(config.httpProxy).build()
private val retry: Boolean = config.fetchRetries > 0
private val serverUrl: HttpUrl = getServerUrl(config)
private val backoffConfig = BackoffConfig(
attempts = config.fetchRetries,
min = config.fetchRetryBackoffMinMillis,
max = config.fetchRetryBackoffMaxMillis,
scalar = config.fetchRetryBackoffScalar,
)
fun fetch(user: ExperimentUser): CompletableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy