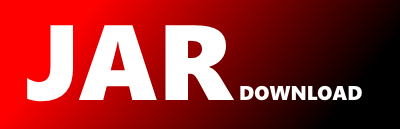
META-INF.resources.aoindustries.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ao-messaging-http-client-js
Show all versions of ao-messaging-http-client-js
JavaScript client for asynchronous bidirectional messaging over HTTP.
/*
* ao-messaging-http-client-js - JavaScript client for asynchronous bidirectional messaging over HTTP.
* Copyright (C) 2014, 2016 AO Industries, Inc.
* [email protected]
* 7262 Bull Pen Cir
* Mobile, AL 36695
*
* This file is part of ao-messaging-http-client-js.
*
* ao-messaging-http-client-js is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* ao-messaging-http-client-js is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with ao-messaging-http-client-js. If not, see .
*/
if(typeof aoindustries === 'undefined') aoindustries = {};
aoindustries.lang = new function() {
//
/**
* @constructor
*/
var Exception = this.Exception = function(message) {
this.message = message;
};
Exception.prototype.type = 'aoindustries.lang.Exception';
Exception.prototype.toString = function() {
return (this.message !== undefined)
? (this.type + ": " + this.message)
: this.type;
};
//
//
/**
* @constructor
*/
var AssertionError = this.AssertionError = function(message) {
Exception.call(this, message);
};
AssertionError.prototype = new Exception();
AssertionError.prototype.constructor = AssertionError;
AssertionError.prototype.type = 'aoindustries.lang.AssertionError';
//
//
/**
* @constructor
*/
var AbstractMethodError = this.AbstractMethodError = function(methodName) {
Exception.call(
this,
(methodName !== undefined)
? ("Abstract method not implemented: " + methodName)
: "Abstract method not implemented"
);
};
AbstractMethodError.prototype = new Exception();
AbstractMethodError.prototype.constructor = AbstractMethodError;
AbstractMethodError.prototype.type = 'aoindustries.lang.AbstractMethodError';
//
//
/**
* @constructor
*/
var IllegalArgumentException = this.IllegalArgumentException = function(message) {
Exception.call(this, message);
};
IllegalArgumentException.prototype = new Exception();
IllegalArgumentException.prototype.constructor = IllegalArgumentException;
IllegalArgumentException.prototype.type = 'aoindustries.lang.IllegalArgumentException';
//
};
aoindustries.io = new function() {
//
/**
* @constructor
*/
var IOException = this.IOException = function(message) {
aoindustries.lang.Exception.call(this, message);
};
IOException.prototype = new aoindustries.lang.Exception();
IOException.prototype.constructor = IOException;
IOException.prototype.type = 'aoindustries.io.IOException';
//
};
aoindustries.messaging = new function() {
//
/**
* @constructor
*/
var SocketException = this.SocketException = function(message) {
aoindustries.lang.Exception.call(this, message);
};
SocketException.prototype = new aoindustries.lang.Exception();
SocketException.prototype.constructor = SocketException;
SocketException.prototype.type = 'aoindustries.messaging.SocketException';
//
};
aoindustries.messaging.http = new function() {
//
/**
* @constructor
*/
var HttpSocket = this.HttpSocket = function(id, connectTime, endpoint) {
/** Server should normally respond within 60 seconds even if no data coming back. */
var READ_TIMEOUT = 2 * 60 * 1000;
var thisSocket = this;
this.id = id;
this.connectTime = connectTime;
this.endpoint = endpoint;
var inQueue = {};
var inSeq = 1;
var outQueue = new Array();
var outSeq = 1;
var closeTime = null;
/**
* The set of all active requests.
*/
var requests = new Array();
var close = this.close = function() {
if(closeTime === null) {
closeTime = (new Date()).getTime();
// Cancel all current requests
for(var i=0; i 0) outQueue.pop();
// Contact the server
var currentRequest = $.ajax({
cache : false,
timeout : READ_TIMEOUT,
type : "POST",
url : endpoint,
data : data,
dataType : "xml",
success : function(data, textStatus, jqXHR) {
if(!isClosed()) {
// Parse the response
// Add all messages to the inQueue by sequence to handle out-of-order messages
$(data).find('messages').find('message').each(function() {
var messageElem = $(this);
// Get the sequence
var seq = messageElem.attr("seq");
// Get the type
var type = messageElem.attr("type");
// Get the message string
var message;
if(type==="s") {
message = messageElem.text();
} else {
throw new aoindustries.lang.AssertionError("Unsupported message type: " + type);
}
if(inQueue[seq] !== undefined) {
throw new aoindustries.io.IOException("Duplicate incoming sequence: " + seq);
}
inQueue[seq] = message;
});
// Gather as many messages that have been delivered in-order
var messages=new Array();
while(true) {
var message = inQueue[inSeq.toString()];
if(message !== undefined) {
delete inQueue[inSeq.toString()];
messages.push(message);
inSeq++;
} else {
// Break in the sequence
break;
}
}
if(messages.length !== 0) {
for(var i=0; i
//
/**
* Client component for bi-directional messaging over HTTP.
*/
this.HttpSocketClient = new function() {
/**
* Asynchronously connects.
*/
this.connect = function(endpoint, timeout, onConnect, onError) {
var connectTime = (new Date()).getTime();
$.ajax({
cache : false,
timeout : timeout,
type : "POST",
url : endpoint,
data : {
action : "connect"
},
dataType : "xml",
success : function(data, textStatus, jqXHR) {
// Parse the response
var id = $(data).find('connection').attr('id');
if(onConnect) onConnect(new HttpSocket(id, connectTime, endpoint));
},
error : function(jqXHR, textStatus, errorThrown) {
if(onError) onError(new aoindustries.io.IOException(jqXHR.status + " " + errorThrown));
}
});
};
};
//
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy