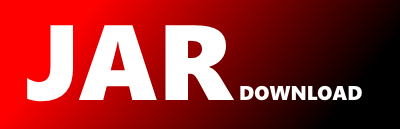
view.lib.cginput.js Maven / Gradle / Ivy
Show all versions of runner Show documentation
!function(n,t){"object"==typeof exports&&"object"==typeof module?module.exports=t():"function"==typeof define&&define.amd?define([],t):"object"==typeof exports?exports.cginput=t():n.cginput=t()}(window,(function(){return function(n){var t={};function e(i){if(t[i])return t[i].exports;var r=t[i]={i:i,l:!1,exports:{}};return n[i].call(r.exports,r,r.exports,e),r.l=!0,r.exports}return e.m=n,e.c=t,e.d=function(n,t,i){e.o(n,t)||Object.defineProperty(n,t,{enumerable:!0,get:i})},e.r=function(n){"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(n,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(n,"__esModule",{value:!0})},e.t=function(n,t){if(1&t&&(n=e(n)),8&t)return n;if(4&t&&"object"==typeof n&&n&&n.__esModule)return n;var i=Object.create(null);if(e.r(i),Object.defineProperty(i,"default",{enumerable:!0,value:n}),2&t&&"string"!=typeof n)for(var r in n)e.d(i,r,function(t){return n[t]}.bind(null,r));return i},e.n=function(n){var t=n&&n.__esModule?function(){return n.default}:function(){return n};return e.d(t,"a",t),t},e.o=function(n,t){return Object.prototype.hasOwnProperty.call(n,t)},e.p="",e(e.s=10)}([function(n,t,e){n.exports=function(){"use strict";
/*!
* mustache.js - Logic-less {{mustache}} templates with JavaScript
* http://github.com/janl/mustache.js
*/var n=Object.prototype.toString,t=Array.isArray||function(t){return"[object Array]"===n.call(t)};function e(n){return"function"==typeof n}function i(n){return n.replace(/[\-\[\]{}()*+?.,\\\^$|#\s]/g,"\\$&")}function r(n,t){return null!=n&&"object"==typeof n&&t in n}var p=RegExp.prototype.test,a=/\S/;function o(n){return!function(n,t){return p.call(n,t)}(a,n)}var s={"&":"&","<":"<",">":">",'"':""","'":"'","/":"/","`":"`","=":"="},l=/\s*/,h=/\s+/,d=/\s*=/,u=/\s*\}/,c=/#|\^|\/|>|\{|&|=|!/;function f(n){this.string=n,this.tail=n,this.pos=0}function m(n,t){this.view=n,this.cache={".":this.view},this.parent=t}function y(){this.cache={}}f.prototype.eos=function(){return""===this.tail},f.prototype.scan=function(n){var t=this.tail.match(n);if(!t||0!==t.index)return"";var e=t[0];return this.tail=this.tail.substring(e.length),this.pos+=e.length,e},f.prototype.scanUntil=function(n){var t,e=this.tail.search(n);switch(e){case-1:t=this.tail,this.tail="";break;case 0:t="";break;default:t=this.tail.substring(0,e),this.tail=this.tail.substring(e)}return this.pos+=t.length,t},m.prototype.push=function(n){return new m(n,this)},m.prototype.lookup=function(n){var t,i,p,a=this.cache;if(a.hasOwnProperty(n))t=a[n];else{for(var o,s,l,h=this,d=!1;h;){if(n.indexOf(".")>0)for(o=h.view,s=n.split("."),l=0;null!=o&&l"==T?[T,I,A,D.pos,O,R,s]:[T,I,A,D.pos],R++,y.push(_),"#"===T||"^"===T)m.push(_);else if("/"===T){if(!(w=m.pop()))throw new Error('Unopened section "'+I+'" at '+A);if(w[1]!==I)throw new Error('Unclosed section "'+w[1]+'" at '+A)}else"name"===T||"{"===T||"&"===T?L=!0:"="===T&&N(I)}if(b(),w=m.pop())throw new Error('Unclosed section "'+w[1]+'" at '+D.pos);return function(n){for(var t,e=[],i=e,r=[],p=0,a=n.length;p0?r[r.length-1][4]:e;break;default:i.push(t)}return e}(function(n){for(var t,e,i=[],r=0,p=n.length;r"===a?o=this.renderPartial(p,t,e,r):"&"===a?o=this.unescapedValue(p,t):"name"===a?o=this.escapedValue(p,t):"text"===a&&(o=this.rawValue(p)),void 0!==o&&(s+=o);return s},y.prototype.renderSection=function(n,i,r,p){var a=this,o="",s=i.lookup(n[1]);if(s){if(t(s))for(var l=0,h=s.length;l0||!e)&&(r[p]=i+r[p]);return r.join("\n")},y.prototype.renderPartial=function(n,t,i,r){if(i){var p=e(i)?i(n[1]):i[n[1]];if(null!=p){var a=n[6],o=n[5],s=n[4],l=p;return 0==o&&s&&(l=this.indentPartial(p,s,a)),this.renderTokens(this.parse(l,r),t,i,l)}}},y.prototype.unescapedValue=function(n,t){var e=t.lookup(n[1]);if(null!=e)return e},y.prototype.escapedValue=function(n,t){var e=t.lookup(n[1]);if(null!=e)return g.escape(e)},y.prototype.rawValue=function(n){return n[1]};var g={name:"mustache.js",version:"3.2.1",tags:["{{","}}"],clearCache:void 0,escape:void 0,parse:void 0,render:void 0,to_html:void 0,Scanner:void 0,Context:void 0,Writer:void 0},v=new y;return g.clearCache=function(){return v.clearCache()},g.parse=function(n,t){return v.parse(n,t)},g.render=function(n,e,i,r){if("string"!=typeof n)throw new TypeError('Invalid template! Template should be a "string" but "'+(t(p=n)?"array":typeof p)+'" was given as the first argument for mustache#render(template, view, partials)');var p;return v.render(n,e,i,r)},g.to_html=function(n,t,i,r){var p=g.render(n,t,i);if(!e(r))return p;r(p)},g.escape=function(n){return String(n).replace(/[&<>"'`=\/]/g,(function(n){return s[n]}))},g.Scanner=f,g.Context=m,g.Writer=y,g}()},function(n,t){function e(t,i){return n.exports=e=Object.setPrototypeOf||function(n,t){return n.__proto__=t,n},e(t,i)}n.exports=e},function(n,t){function e(t){return"function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?n.exports=e=function(n){return typeof n}:n.exports=e=function(n){return n&&"function"==typeof Symbol&&n.constructor===Symbol&&n!==Symbol.prototype?"symbol":typeof n},e(t)}n.exports=e},function(n,t){function e(t){return n.exports=e=Object.setPrototypeOf?Object.getPrototypeOf:function(n){return n.__proto__||Object.getPrototypeOf(n)},e(t)}n.exports=e},function(n,t,e){var i=e(1);n.exports=function(n,t){if("function"!=typeof t&&null!==t)throw new TypeError("Super expression must either be null or a function");n.prototype=Object.create(t&&t.prototype,{constructor:{value:n,writable:!0,configurable:!0}}),t&&i(n,t)}},function(n,t,e){var i=e(3),r=e(1),p=e(8),a=e(9);function o(t){var e="function"==typeof Map?new Map:void 0;return n.exports=o=function(n){if(null===n||!p(n))return n;if("function"!=typeof n)throw new TypeError("Super expression must either be null or a function");if(void 0!==e){if(e.has(n))return e.get(n);e.set(n,t)}function t(){return a(n,arguments,i(this).constructor)}return t.prototype=Object.create(n.prototype,{constructor:{value:t,enumerable:!1,writable:!0,configurable:!0}}),r(t,n)},o(t)}n.exports=o},function(n,t,e){var i=e(2),r=e(7);n.exports=function(n,t){return!t||"object"!==i(t)&&"function"!=typeof t?r(n):t}},function(n,t){n.exports=function(n){if(void 0===n)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return n}},function(n,t){n.exports=function(n){return-1!==Function.toString.call(n).indexOf("[native code]")}},function(n,t,e){var i=e(1);function r(t,e,p){return!function(){if("undefined"==typeof Reflect||!Reflect.construct)return!1;if(Reflect.construct.sham)return!1;if("function"==typeof Proxy)return!0;try{return Date.prototype.toString.call(Reflect.construct(Date,[],(function(){}))),!0}catch(n){return!1}}()?n.exports=r=function(n,t,e){var r=[null];r.push.apply(r,t);var p=new(Function.bind.apply(n,r));return e&&i(p,e.prototype),p}:n.exports=r=Reflect.construct,r.apply(null,arguments)}n.exports=r},function(n,t,e){"use strict";e.r(t);var i,r=e(0),p=e(2),a=e.n(p),o=e(4),s=e.n(o),l=(e(6),e(3),e(5)),h=e.n(l),d=function(){for(var n=0,t=0,e=arguments.length;t0},n.prototype.isAReadString=function(){return!(!this.hasChildren()||"read"!==this.name)&&(this.children[0].name===i.string||this.children[0].name===i.array&&this.children[0].param.type===i.string)},n.prototype.containsAReadString=function(){return this.hasChildren()&&(this.isAReadString()||this.children[0].containsAReadString())},n.prototype.isLast=function(){return null!=this.parent&&this.parent.children[this.parent.children.length-1]===this},n.prototype.containsARead=function(){return[i.read,i.readline].includes(this.name)||this.children.some((function(n){return n.containsARead()}))},n.prototype.print=function(){this._printTree()},n.prototype._printTree=function(n){var t=this;void 0===n&&(n=[]);var e=n.slice(1).reduce((function(n,t){return n+(t.isLast()?" ":"│")+" "}),""),i=0===n.length?"":this.isLast()?"└── ":"├── ",r=this.name+(null!=this.param?" "+JSON.stringify(this.param):"");console.log(""+e+i+r),this.children.forEach((function(e){e._printTree(d(n,[t]))}))},n}(),c=function(){function n(){this.needSolveFunction=!1,this.root=new u,this.vars={},this.args={},this.loops=[],this.maxNestedLoop=0,this.statement="Auto-generated code below aims at helping you parse\nthe standard input according to the problem statement.",this.help="Write an {{#turnBased}}action{{/turnBased}}{{^turnBased}}answer{{/turnBased}} using {{{printStdout}}}\nTo debug: {{{printStderr}}}",this.containsVarString=!1}return n.prototype.getVarType=function(n){return this.vars[n].parent.name},n.prototype.getVar=function(n){return this.vars[n].parent},n.prototype.getVarComment=function(n){return this.vars[n].comment},n.prototype.addVar=function(n,t){this.vars[n]=t,t.original=t.name},n.prototype.addArg=function(n,t){this.args[n]=t,t.original=t.name},n.prototype.addLoop=function(n){this.loops.push(n),n.original=n.param},n.prototype.setMaxNestedLoop=function(n){this.maxNestedLoop0},n.prototype.hasString=function(){return this.containsVarString},n}();function f(n,t){f=function(n,t){return new p(n,void 0,t)};var e=h()(RegExp),i=RegExp.prototype,r=new WeakMap;function p(n,t,i){var p=e.call(this,n,t);return r.set(p,i||r.get(n)),p}function o(n,t){var e=r.get(t);return Object.keys(e).reduce((function(t,i){return t[i]=n[e[i]],t}),Object.create(null))}return s()(p,e),p.prototype.exec=function(n){var t=i.exec.call(this,n);return t&&(t.groups=o(t,this)),t},p.prototype[Symbol.replace]=function(n,t){if("string"==typeof t){var e=r.get(this);return i[Symbol.replace].call(this,n,t.replace(/\$<([^>]+)>/g,(function(n,t){return"$"+e[t]})))}if("function"==typeof t){var p=this;return i[Symbol.replace].call(this,n,(function(){var n=[];return n.push.apply(n,arguments),"object"!==a()(n[n.length-1])&&n.push(o(n,p)),t.apply(this,n)}))}return i[Symbol.replace].call(this,n,t)},f.apply(this,arguments)}function m(n,t){this.message=n+": "+JSON.stringify(t),this.name=n,this.params=t}var y=function(){function n(){this.cursor=0,this.allowType=["int","long","float","string","word","bool","array"]}return n.prototype.getCodeName=function(n,t){for(var e=0;n[t+"@>"+e];)e++;return t+"@>"+e},n.prototype.getFirstCodeName=function(n){return n+"@>0"},n.prototype.parse=function(n,t){try{this.write=[],this.vars={},this.code=n;var e=new c;for(e.turnBased=t;this.hasNext();)this.parseTree(e.root,e,0);return e}finally{this.write=null,this.code=null,this.cursor=0}},n.prototype.read=function(n,t){for(var e=0,r=this.nextLine().split(" ");e1&&t.children.some((function(n){return"string"===n.name})))throw new Error("InvalidStringRead")},n.prototype.call=function(n,t){var e=this.nextLine(),i=f(/(?:([\0-\x08\x0E-\x1F!-'\*-\x9F\xA1-\u167F\u1681-\u1FFF\u200B-\u2027\u202A-\u202E\u2030-\u205E\u2060-\u2FFF\u3001-\uFEFE\uFF00-\uFFFF]+):([\0-\x08\x0E-\x1F!-\x9F\xA1-\u167F\u1681-\u1FFF\u200B-\u2027\u202A-\u202E\u2030-\u205E\u2060-\u2FFF\u3001-\uFEFE\uFF00-\uFFFF]+) )?([\0-\x08\x0E-\x1F!-'\*-\x9F\xA1-\u167F\u1681-\u1FFF\u200B-\u2027\u202A-\u202E\u2030-\u205E\u2060-\u2FFF\u3001-\uFEFE\uFF00-\uFFFF]+)(\(([\0-'\*-\uFFFF]*)\))/,{res:1,restype:2,name:3,dim:5}).exec(e);if(null==i)throw new m("InvalidCallArguments",{arguments:e,line:this.getLineNumber()});var r=i.groups.res,p=i.groups.restype,a=i.groups.name,o=i.groups.dim.trim().split(/\s*,\s*/);if(!/^[$a-zA-Z_][0-9a-zA-Z_$]*$/.test(a))throw new m("InvalidFunction",{function:a,line:this.getLineNumber()});var s=new u(a,t);if(s.param={ret:r},s=new u(p,s),/^array\[[a-z]+(\(([a-zA-Z0-9](,\s*)?)*\))?\]\(([a-zA-Z0-9]*(,\s*)?)*\)$/.test(p))this.createArrayNode(n,s,a,p);else if(p&&!this.allowType.includes(p))throw new m("InvalidReturnType",{function:a,type:p,line:this.getLineNumber()});for(var l=0,h=o;l0&&this.write.forEach((function(n){n.comment||(n.comment=N.join("\n"))}));break;case"STATEMENT":g=[];for(this.hasNext()&&this.nextLine();this.hasNext();){if(0===(A=this.nextLine()).trim().length)break;g.push(A)}t.statement=g.join("\n");break;case"HELP":g=[];for(this.hasNext()&&this.nextLine();this.hasNext();){var A;if(0===(A=this.nextLine()).trim().length)break;g.push(A)}t.help=g.join("\n");break;default:throw new m("InvalidKeyword",{param:p,line:this.getLineNumber()})}}},n.prototype.next=function(){for(;this.hasNext()&&(" "===this.code.charAt(this.cursor)||"\n"===this.code.charAt(this.cursor));)this.cursor++;for(var n=this.cursor;this.hasNext()&&" "!==this.code.charAt(this.cursor)&&"\n"!==this.code.charAt(this.cursor);)this.cursor++;return this.code.substring(n,this.cursor).trim()},n.prototype.nextLine=function(){for(var n=this.cursor;this.hasNext()&&"\n"!==this.code.charAt(this.cursor);)this.cursor++;return this.code.substring(n,this.cursor++).trim()},n.prototype.hasNext=function(){return this.cursor0&&e>="0"&&e<="9"?"_"+e+i:""+e.toUpperCase()+i}function b(n,t){return void 0===t&&(t={}),function(n,t){void 0===t&&(t={});for(var e=t.splitRegexp,i=void 0===e?S:e,r=t.stripRegexp,p=void 0===r?L:r,a=t.transform,o=void 0===a?v:a,s=t.delimiter,l=void 0===s?" ":s,h=O(O(n,i,"$1\0$2"),p,"\0"),d=0,u=h.length;"\0"===h.charAt(d);)d++;for(;"\0"===h.charAt(u-1);)u--;return h.slice(d,u).split("\0").map(o).join(l)}(n,g({delimiter:"",transform:R},t))}function N(n,t){return 0===t?n.toLowerCase():R(n,t)}var A,T=function(){function n(){var t=this;this.READ="read",this.READLINE="readline",this.LOOP="loop",this.LOOPLINE="loopline",this.WRITE="write",this.GAME_LOOP="gameloop",this.INT="int",this.LONG="long",this.FLOAT="float",this.STRING="string",this.WORD="word",this.BOOL="bool",this.ARRAY="array",this.CALL="call",this.DUMP="dump",this.methodCaseConversion=n.CASE_CONVERSIONS.AS_IS,this.caseConversion=n.CASE_CONVERSIONS.AS_IS,this.reservedWords=[],this.escapeReservedWords=function(n){return this.reservedWords.includes(n)?"_"+n:n},this.indentSize=4,this.sletters="ijklmnopqrstuvwxyzabcdefgh",this.typeMapping={},this.ignoreCodeComment="Ignore and do not change the code below",this.mapNodeToVariable=function(n){var e={name:n.children[0].name,comment:n.children[0].comment,type:{name:n.name}};return e.type.name===t.ARRAY?(e.type.dimensions=n.param.dim,e.type.innerType={name:n.param.type,dimensions:n.param.typeDim}):e.type.dimensions=n.param,e}}return n.prototype.begin=function(n){},n.prototype.end=function(){},n.prototype.write=function(n){switch(n.name){case this.DUMP:if(n.param.node){var t=n.param.node.children[0],e=n.param.node,i={name:t.name,type:{name:e.name}};i.type.name===this.ARRAY&&(i.type.dimensions=e.param.dim,i.type.innerType={name:e.param.type,dimensions:e.param.typeDim}),this.handleDump(i)}else this.handleDump(n.param.value);break;case this.CALL:var r=this.parseFunctionData(n);this.handleCall(r.functionName,r.argumentVariables,r.returnVariable);break;case this.READ:this.handleRead(n.children.map(this.mapNodeToVariable),n);break;case this.READLINE:this.handleReadLine(n.children.map(this.mapNodeToVariable),n);break;case this.GAME_LOOP:this.handleGameLoop(n.children);break;case this.LOOP:this.handleLoop(n);break;case this.LOOPLINE:this.handleLoopLine(n);break;case this.WRITE:this.handleWrite(n.param,n.paramSeparator,n.comment)}},n.prototype.handleRead=function(n,t){},n.prototype.handleReadLine=function(n,t){this.handleRead(n,t)},n.prototype.handleLoop=function(n){},n.prototype.handleLoopLine=function(n){this.handleLoop(n)},n.prototype.handleGameLoop=function(n){},n.prototype.handleWrite=function(n,t,e){},n.prototype.handleDump=function(n){},n.prototype.handleCall=function(n,t,e){},n.prototype.append=function(n){return this.code.push(n),this},n.prototype.setIndent=function(n){this.level=n},n.prototype.incIndent=function(){this.level++},n.prototype.decIndent=function(){this.level--},n.prototype.indent=function(n){n&&(this.level+=n);var t=this.level*this.indentSize;return t>0&&this.code.push(" ".repeat(t)),this},n.prototype.indentString=function(n,t){var e=" ".repeat(t*this.indentSize);return n.split("\n").map((function(n){return/^{{?[a-zA-Z0-9&{}]*}}$/.test(n)?n:e+n})).join("\n")},n.prototype.nl=function(){return this.code.push("\n"),this},n.prototype.formatVarNames=function(){var n=this,t=function(t){return n.escapeReservedWords(n.caseConversion(t))},e=this.input.vars||{};for(var i in e)e[i].name=t(e[i].original);for(var r=0,p=this.input.loops||[];r0&&i.split("\n").forEach((function(n){e.indent().append(e.commentLine(n)).nl()}))},n.CASE_CONVERSIONS={AS_IS:function(n){return n},CAMEL:function(n){return n===n.toUpperCase()?n:(void 0===t&&(t={}),b(n,g({transform:N},t)));var t},SNAKE:function(n){return n.replace(/([a-z])([A-Z0-9])/g,"$1_$2").toLowerCase()},SNAKE_KEEP_CONSTANTS:function(t){return t===t.toUpperCase()?t:n.CASE_CONVERSIONS.SNAKE(t)},LOWER:function(n){return n.toLowerCase()},PASCAL:b},n}(),I=(A=function(n,t){return(A=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(n,t)},function(n,t){function e(){this.constructor=n}A(n,t),n.prototype=null===t?Object.create(t):(e.prototype=t.prototype,new e)}),C=function(n){function t(){var t=null!==n&&n.apply(this,arguments)||this;return t.loop="i".charCodeAt(0),t}return I(t,n),t.prototype.begin=function(){if(this.input.hasStatement())for(var n=0,t=this.input.statement.split("\n");n1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===r?"":"+"+r)+"))",a=n[r].name;this.nl().indent().append(a+"=${myArray["+p+"]}")}},t.prototype.handleLoopLine=function(n){this.nl().indent().append("read -r -a myArray"),this.handleLoop(n)},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop),e=isNaN(n.param)?"$"+n.param:n.param;this.nl().indent().append("for (( "+t+"=0; "+t+"<"+e+"; "+t+"++ )); do"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("done")},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("# game loop").nl(),this.indent().append("while true; do"),this.incIndent();for(var t=0,e=n;t&2'),this.nl(),this.doMarkup&&this.append("").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?'"'+t+'"':"").replace(/""/g,"")),null==e||i||this.append(" # ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('echo "').append(o[0]).append('"'),null==e||i||this.append(" # ").append(e);for(var s=1;s")},t.prototype.commentLine=function(n){return"# "+n},t}(T),_=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),w=function(n){function t(){var t=null!==n&&n.apply(this,arguments)||this;return t.loop="i".charCodeAt(0),t.indentSize=2,t.toClose=0,t.inRead=!1,t}return _(t,n),t.prototype.begin=function(){if(this.append("(ns ").append(this.input.turnBased?"Player":"Solution"),this.incIndent(),this.nl().append("(:gen-class))"),this.decIndent(),this.nl(),this.input.hasStatement()){var n=this.input.statement.replace(/\n/g,"\n; ");this.nl().append("; ").append(n),this.nl()}this.nl().append("(defn output [msg] (println msg) (flush))"),this.nl().append("(defn debug [msg] (binding [*out* *err*] (println msg) (flush)))"),this.nl(),this.nl().append("(defn -main [& args]"),this.incIndent(),this.toClose++},t.prototype.nl=function(){return this.code.push("\n"),this.indent(),this},t.prototype.end=function(){this.append(")".repeat(this.toClose))},t.prototype.getNext=function(n){var t=n.parent.children,e=t.indexOf(n);return t[e+1]},t.prototype.handleRead=function(n,t){var e=this.getNext(t);this.inRead||(this.inRead=!0,this.comments=[],this.nl().append("(let ["),this.last=null,this.newLineNeeded=!1);for(var i=t.children.length,r=0;r "+t+" 0)"),this.incIndent(),this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().append("(recur (dec "+t+"))))"),"read"!==n.children[0].name&&"readline"!==n.children[0].name||(this.append(")"),this.toClose--),this.decIndent(),this.decIndent()},t.prototype.handleGameLoop=function(n){this.nl().append("(while true"),this.incIndent();for(var t=0,e=n;t"),null!=e)for(var r=0,p=e.split("\n");r0&&i.nl().append('(print "'+t+'")'),i.nl().append("(print "),n.type){case"VAR":i.append(n.node.children[0].name);break;case"CONST":i.append('"'+n.value+'"')}i.append(")")})),this.nl().append('(output "")');else{var o=n.split("\n");if(o.length>1||o[0].length>0)for(var s=0,l=o;s").nl()},t.prototype.commentLine=function(n){return"; "+n},t}(T),D=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),E=function(n){function t(){return null!==n&&n.apply(this,arguments)||this}return D(t,n),t.prototype.begin=function(){this.append('.name "zork"\n.comment "just a basic living prog"\n.extend\n\nl2: sti r1,%:live,%0x1\n\tand r1,%0,r1\nlive: live %1\n\tzjmp %:live\n\n.code 42 DE AD C0 DE 12 34 61 34 61 23 61\n')},t}(T),M=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),V=function(){return(V=Object.assign||function(n){for(var t,e=1,i=arguments.length;e{{/dim}}",t),e.methodCaseConversion=T.CASE_CONVERSIONS.SNAKE,e.reservedWords=["and","and_eq","asm","bitand","bitor","bool","break","case","catch","char","compl","const","const_cast","continue","do","double","dynamic_cast","else","enum","explicit","false","float","for","friend","goto","if","int","long","namespace","new","not","not_eq","operator","or","or_eq","private","protected","public","reinterpret_cast","return","short","signed","static","static_cast","switch","template","this","throw","true","try","typedef","typeid","typename","union","unsigned","virtual","void","volatile","wchar_t","while","xor","xor_eq"],e.loopTpl="for (int {{{counter}}} = {{{start}}}; {{{counter}}} < {{{end}}}; {{{counter}}}++) {\n{{{body}}}\n}",e.voidReturnType="void",e}return M(t,n),t.prototype.begin=function(){if(this.append("#include ").nl(),this.append("#include ").nl(),this.append("#include ").nl(),this.append("#include ").nl(),this.input.needSolveFunction&&this.append("#include ").nl(),this.nl(),this.append("using namespace std;").nl().nl(),this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' << "'+t+'" << ':" << ").replace(/" << "/g,"")),this.append(" << endl;"),null==e||i||this.append(" // ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('cout << "').append(o[0]).append('" << endl;'),null==e||i||this.append(" // ").append(e);for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.rawOutput(n));else if(n.type.name===this.ARRAY){for(var t=n.name,e=this.loopTpl,i=n.type.dimensions.length,p=0;p> "+n.name+";"},t.prototype.variableOutput=function(n){return"cout << "+this.formattedVarName(n.name)+" << endl;"},t.prototype.rawOutput=function(n){return"cout << "+n+" << endl;"},t.prototype.appendReadStatement=function(n){this.nl().indent().append("cin"),this.append(" >> ").append(n.join(" >> ")).append("; cin.ignore();")},t.prototype.functionReturnType=function(n){return n.type.name?n.type.name===this.ARRAY?r.render(this.typeMapping[n.type.name],{dim:n.type.dimensions,type:this.typeMapping[n.type.innerType.name]}):r.render(this.typeMapping[n.type.name],{}):this.voidReturnType},t.prototype.functionSignatureSimple=function(n,t){return n+"("+t+")"},t.prototype.functionSignatureFull=function(n,t,e){return t+" "+this.functionSignatureSimple(n,e)},t.prototype.functionDeclaration=function(n,t,e,i){return"\n"+this.functionSignatureFull(n,t,e)+'\n{\n // Write your code here\n // To debug: cerr << "Debug messages..." << endl;\n '+i+"\n}\n"},t.prototype.defaultReturnStatement=function(n){var t,e=((t={})[this.INT]="0",t[this.LONG]="0",t[this.FLOAT]="0.0",t[this.WORD]='""',t[this.STRING]='""',t[this.ARRAY]=this.typeDeclaration(n)+"()",t);return n.name?"\n return "+e[n.name]+";":""},t.prototype.functionCall=function(n,t,e,i){return r.render("int std_out_fd = dup(1);\ndup2(2, 1);\n{{#type}}{{{type}}} {{{res}}} = {{/type}}{{{name}}}({{{params}}});\ndup2(std_out_fd, 1);",{name:n,type:t,res:e,params:i})},t.prototype.buildReadLoop=function(n,t,e,i){var p=n.type.dimensions.length;if(0===i)return t;var a=p-i,o="";if(1===i){var s={name:n.name+"_tmp",type:{name:n.type.innerType.name,dimensions:n.type.innerType.dimensions}};o=this.variableDefinitionStatement(s)+"\n",o+=this.variableRead(s)+"\n",o+=e+".push_back("+s.name+");"}else{var l=V(V({},n.type),{dimensions:n.type.dimensions.slice(0,-(a+1))});o=e+(".push_back("+this.typeDeclaration(l)+"());\n")+this.loopTpl}var h=r.render(t,{counter:this.sletters[a],start:0,end:n.type.dimensions[a],body:this.indentString(o,a+1)});return this.buildReadLoop(n,h,e+"["+this.sletters[a]+"]",i-1)},t.prototype.buildStatementData=function(n,t,e){var i=this,r=t.map((function(n){return i.variableDefinition(n)})).join(", "),p=t.map((function(n){return n.name})).join(", "),a=this.functionReturnType(e);return{functionNomenclature:"function",arrayNomenclature:"vector",null:"NULL",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.typeDeclaration(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,p),functionFull:this.functionSignatureFull(n,a,r),returnType:a}},t}(T),x=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),j=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.typeMapping=((t={})[i.FLOAT]="float",t[i.INT]="int",t[i.LONG]="long",t[i.STRING]="string",t[i.WORD]="string",t[i.BOOL]="bool",t[i.ARRAY]="{{&type}}[{{&dim}}]",t),i.readMapping=((e={})[i.INT]="int.Parse(%s)",e[i.LONG]="long.Parse(%s)",e[i.WORD]="%s",e[i.STRING]="%s",e[i.FLOAT]="float.Parse(%s)",e[i.BOOL]='%s != "0"',e[i.ARRAY]="new {{&type}}[{{&dim}}]",e),i.MAGIC_TOKEN="@@ C# is evil @@",i.EVIL_TOKEN="@@ C# is magic @@",i.evilIndentation=0,i.needWizard=0,i.loopLine=!1,i.caseConversion=T.CASE_CONVERSIONS.CAMEL,i.methodCaseConversion=T.CASE_CONVERSIONS.PASCAL,i.reservedWords=["abstract","bool","continue","decimal","default","event","explicit","extern","char","checked","class","const","break","as","base","delegate","is","lock","long","num","byte","case","catch","false","finally","fixed","float","for","as","foreach","goto","if","implicit","in","int","interface","internal","do","double","else","namespace","new","null","object","operator","out","override","params","private","protected","public","readonly","sealed","short","sizeof","ref","return","sbyte","stackalloc","static","string","struct","void","volatile","while","true","try","switch","this","throw","unchecked","unsafe","ushort","using","virtual","typeof","uint","ulong","out"],i.loopTpl="for (int {{&counter}} = {{&start}}; {{&counter}} < {{&end}}; {{&counter}}++)\n{\n{{&body}}\n}",i.voidReturnType="void",i}return x(t,n),t.prototype.begin=function(){if(this.append("using System;").nl(),this.append("using System.Linq;").nl(),this.append("using System.IO;").nl(),this.append("using System.Text;").nl(),this.append("using System.Collections;").nl(),this.append("using System.Collections.Generic;").nl(),this.nl(),this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n1?"\n string[] inputs;":"")).replace(this.EVIL_TOKEN,1===this.needWizard?"string[] ":""),this.code=[n]},t.prototype.handleRead=function(n){if(this.nl().indent(),n.length>1){0===this.needWizard?(this.evilIndentation=this.level,this.append(this.EVIL_TOKEN).append("inputs = Console.ReadLine().Split(' ');")):this.append("inputs = Console.ReadLine().Split(' ');"),this.needWizard++;for(var t=0,e=0,i=n;e1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===t?"":"+"+t),r=this.format(this.readMapping[e.type.name],["inputs["+i+"]"]);this.nl().indent().append(this.typeDeclaration(e.type)+" ").append(e.name).append(" = ").append(r).append(";"),null!=e.comment&&this.append("// ").append(e.comment)}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop);this.nl().indent().append("for (int "+t+" = 0; "+t+" < "+n.param+"; "+t+"++)").nl().indent().append("{"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("}")},t.prototype.handleLoopLine=function(n){0===this.needWizard?(this.evilIndentation=this.level,this.nl().indent().append(this.EVIL_TOKEN).append("inputs = Console.ReadLine().Split(' ');")):this.nl().indent().append("inputs = Console.ReadLine().Split(' ');"),this.needWizard++,this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop").nl(),this.indent().append("while (true)").nl().indent().append("{"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' + "'+t+'" + ':" + ").replace(/" \+ "/g,"")),this.append(");"),null==e||i||this.append(" // ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('Console.WriteLine("').append(o[0]).append('");'),null==e||i||this.append(" // ").append(e).nl();for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildDumpLoop(n,n.name,t);this.nl().append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().indent().append(this.variableOutput(i))}},t.prototype.handleCall=function(n,t,e){var i=this,r=t.map((function(n){return i.typeDeclaration(n.type)+" "+i.formattedVarName(n.name)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[a]=this.functionDeclaration(n,r,this.typeDeclaration(e.type),this.defaultReturnStatement(e.type));var o=this.functionCall(n,e.type.name!==this.voidReturnType?this.typeDeclaration(e.type):"",e.name,p);this.nl().append(this.indentString(o,this.level))},t.prototype.buildReadLoop=function(n,t,e,i){var p=n.type.dimensions.length,a="";if(a=0===this.needWizard?this.EVIL_TOKEN+"inputs = Console.ReadLine().Split(' ');\n":"inputs = Console.ReadLine().Split(' ');\n",this.needWizard++,0===i)return t;var o=p-i,s="",l=e;l+=i===p?"["+this.sletters[o]:","+this.sletters[o],1===i?s=n.type.innerType.name===this.STRING?l+"] = Console.ReadLine();":l+"] = "+this.format(this.readMapping[n.type.innerType.name],["inputs["+this.sletters[o]+"]"])+";":(2===i&&n.type.innerType.name!==this.STRING&&(s=a),s+=this.loopTpl);var h="";return 1===p&&n.type.innerType.name!==this.STRING&&(h=a),h+=r.render(t,{counter:this.sletters[o],start:0,end:this.formattedVarName(n.type.dimensions[o]),body:this.indentString(s,o+1)}),this.buildReadLoop(n,h,l,i-1)},t.prototype.buildDumpLoop=function(n,t,e){var i=n.type.dimensions.length,p=i-e,a="",o=t;return e===i&&(o+="["),1===e?(o+=this.sletters[p]+"]",a=this.variableOutput(o)):(o+=this.sletters[p]+",",a=this.buildDumpLoop(n,o,e-1)),r.render(this.loopTpl,{counter:this.sletters[p],start:0,end:n.type.dimensions[p]?this.formattedVarName(n.type.dimensions[p]):this.formattedVarName(n.name)+".GetLength("+p+")",body:this.indentString(a,1)})},t.prototype.variableOutput=function(n){return"Console.WriteLine("+n+");"},t.prototype.functionSignatureSimple=function(n,t){return n+"("+t+")"},t.prototype.functionSignatureFull=function(n,t,e){return e+" "+this.functionSignatureSimple(n,t)},t.prototype.functionDeclaration=function(n,t,e,i){return"\n public static "+this.functionSignatureFull(n,t,e)+'\n {\n // Write your code here\n // To debug: Console.Error.WriteLine("Debug messages...");\n '+i+"\n }\n\n"},t.prototype.defaultReturnStatement=function(n){return n.name?"\n return default("+this.typeDeclaration(n)+");":""},t.prototype.functionCall=function(n,t,e,i){return r.render("var stdtoutWriter = Console.Out;\nConsole.SetOut(Console.Error);\n{{#type}}{{&type}} {{&res}} = {{/type}}{{&name}}({{¶ms}});\nConsole.SetOut(stdtoutWriter);",{name:n,type:t,res:e,params:i})},t.prototype.typeDeclaration=function(n){if(n.name){var t=this.typeMapping[n.name];return n.name===this.ARRAY?r.render(t,{dim:",".repeat(n.dimensions.length-1),type:this.typeMapping[n.innerType.name]}):r.render(t,{})}return this.voidReturnType},t.prototype.variableDefinition=function(n){var t=this.typeDeclaration(n.type)+" "+n.name;return n.type.name===this.ARRAY?t+" = "+r.render(this.readMapping[this.ARRAY],{dim:n.type.dimensions.join(","),type:this.typeMapping[n.type.innerType.name]}):t},t.prototype.variableDefinitionStatement=function(n){return this.variableDefinition(n)+";"},t.prototype.buildStatementData=function(n,t,e){var i=this,r=t.map((function(n){return i.typeDeclaration(n.type)+" "+i.formattedVarName(n.name)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=this.typeDeclaration(e.type);return{functionNomenclature:"method",arrayNomenclature:"array",null:"null",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.typeDeclaration(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,p),functionFull:this.functionSignatureFull(n,r,a),returnType:a}},t}(T),G=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),W=function(){return(W=Object.assign||function(n){for(var t,e=1,i=arguments.length;e","#include ","#include ","#include "],i}return G(t,n),t.prototype.begin=function(){var n=this;if((this.input.needSolveFunction?k(this.headers,["#include "]):this.headers).forEach((function(t){n.append(t).nl()})),this.nl(),this.input.hasStatement()){this.append("/**").nl();for(var t=0,e=this.input.statement.split("\n");t0&&(e.appendReadStatement(i,p,a),a=[],i=[],p=[])},s=0,l=n;s").nl();var r=null!=e&&(e.length>50||e.split("\n").length>1);if(r){for(var p=0,a=e.split("\n");p1||s[0].length>0){this.indent().append('printf("').append(s[0]).append('\\n");'),null==e||r||this.append(" // ").append(e);for(var l=1;l")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.rawOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildDumpLoop(n,n.name,t);this.nl().append(this.indentString(e,this.level))}else this.nl().indent().append(this.variableOutput(n))},t.prototype.handleCall=function(n,t,e){this.returnVariableName=e.name;var i=this.createFunctionArguments(t,e),r=i.args,p=i.params;this.declareReturnVariableDimensionVariables(e);var a=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[a]=this.functionDeclaration(n,r.join(", "),e.type.name?this.returnTypeDefinition(e):this.voidReturnType,this.defaultReturnStatement(e.type.name));var o=this.functionCall(n,e.name?this.returnTypeDefinition(e):"",e.name,p.join(", "));this.nl().append(this.indentString(o,this.level))},t.prototype.computeDimension=function(n){var t=parseInt(n,10);return Number.isNaN(t)?n+" + 1":t+1},t.prototype.declareReturnVariableDimensionVariables=function(n){if(n.name&&n.type.name===this.ARRAY){var t=n.type.dimensions.length;if(1===t){var e=n.name+"_count";this.nl().indent().append("int "+e+";")}else for(var i=0;i1?p:"")+"_count":this.formattedVarName(n.type.dimensions[p]);return r.render(this.loopTpl,{counter:this.sletters[p],start:0,end:s,body:this.indentString(a,1)})},t.prototype.variableOutput=function(n){return'printf("'+this.typeSpecifiers[n.type.name!==this.ARRAY?n.type.name:n.type.innerType.name]+'\\n", '+this.formattedVarName(n.name)+");"},t.prototype.rawOutput=function(n){return"printf("+n+");"},t.prototype.functionSignatureSimple=function(n,t){return n+"("+t+")"},t.prototype.functionSignatureFull=function(n,t,e){return e+" "+this.functionSignatureSimple(n,t)},t.prototype.functionDeclaration=function(n,t,e,i){return"\n"+this.functionSignatureFull(n,t,e)+'\n{\n // Write your code here\n // To debug: fprintf(stderr, "Debug messages...\\n");\'\n '+i+"\n}\n"},t.prototype.defaultReturnStatement=function(n){var t,e=((t={})[this.INT]="0",t[this.LONG]="0",t[this.FLOAT]="0.0",t[this.WORD]='""',t[this.STRING]='""',t[this.ARRAY]="NULL",t);return n?"\n return "+e[n]+";":""},t.prototype.functionCall=function(n,t,e,i){return r.render("int std_out_fd = dup(1);\ndup2(2, 1);\n{{#type}}{{&type}} {{&res}} = {{/type}}{{&name}}({{¶ms}});\ndup2(std_out_fd, 1);",{name:n,type:t,res:e,params:i})},t.prototype.variableDefinition=function(n,t){var e=this;if([this.ARRAY,this.STRING,this.WORD].includes(n.type.name)){var i=n.type.innerType&&n.type.innerType.dimensions?[this.computeDimension(n.type.innerType.dimensions)]:[],p=n.type.name===this.ARRAY?n.type.dimensions:[this.computeDimension(n.type.dimensions)],a=k(p,i).map((function(n){return e.formattedVarName(String(n))})),o=n.type.name===this.ARRAY?n.type.innerType.name:n.type.name;return r.render(this.typeMapping[this.ARRAY],{name:t?this.formattedVarName(n.name):"",dim:a,type:this.typeMapping[o]})}return this.typeMapping[n.type.name]+(t?" "+this.formattedVarName(n.name):"")},t.prototype.returnTypeDefinition=function(n){if([this.STRING,this.WORD,this.ARRAY].includes(n.type.name)){var t=this.arrayPointersTemplate,e=n.type.name===this.ARRAY?n.type.innerType.name:n.type.name,i=n.type.innerType&&n.type.innerType.dimensions?[this.computeDimension(n.type.innerType.dimensions)]:[],p=n.type.name===this.ARRAY?n.type.dimensions:[this.computeDimension(n.type.dimensions)],a=k(p,i);return r.render(t,{type:this.typeMapping[e],dim:a})}return this.variableDefinition(n,!1)},t.prototype.buildStatementData=function(n,t,e){var i=this,r=this.createFunctionArguments(t,e),p=r.args,a=r.params,o=e.type.name?this.returnTypeDefinition(e):this.voidReturnType;return{functionNomenclature:"function",arrayNomenclature:"array",null:"NULL",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.variableDefinition(n,!1)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,a.join(", ")),functionFull:this.functionSignatureFull(n,p.join(", "),o),returnType:o}},t}(T),$=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),Y=function(n){function t(){var t,e=null!==n&&n.apply(this,arguments)||this;return e.loop="i".charCodeAt(0),e.typeMapping=((t={})[e.INT]="int",t[e.LONG]="long",t[e.STRING]="string",t[e.WORD]="string",t[e.FLOAT]="float",t[e.BOOL]="int",t),e.typeSpecifiers={int:"%d",long:"%d",float:"%f",string:"%s",word:"%s",bool:"%d"},e.methodCaseConversion=T.CASE_CONVERSIONS.CAMEL,e.caseConversion=T.CASE_CONVERSIONS.CAMEL,e.inputsCounter=0,e}return $(t,n),t.prototype.begin=function(){if(this.append("import std;").nl(),this.nl(),this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n1&&(this.inputsCounter++,t="inputs"+(this.inputsCounter>1?this.inputsCounter:""),this.nl().indent().append("auto "+t+" = readln.split;"));for(var e=0;e1?t+"["+e+"]":"readln.chomp";this.nl().indent().append(this.variableDefinition(i,!0)+" = "+this.cast(r,i.type.name)+";"),null!=i.comment&&this.append(" // "+i.comment)}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop);this.nl().indent().append("for (int "+t+" = 0; "+t+" < "+n.param+"; "+t+"++) {"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("}")},t.prototype.handleLoopLine=function(n){this.inputsCounter++;var t="inputs"+(this.inputsCounter>1?this.inputsCounter:"");this.nl().indent().append("auto "+t+" = readln.split;"),this.handleLoop(n)},t.prototype.handleReadLine=function(n){for(var t=0;t1?this.inputsCounter:"")+"["+((n.length>1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===t?"":"+"+t))+"]";this.nl().indent().append(this.variableDefinition(e,!0)+" = "+this.cast(i,e.type.name)+";"),null!=e.comment&&this.append(" // "+e.comment)}},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop").nl(),this.indent().append("while (1) {"),this.incIndent();for(var t=0,e=n;t").nl();var r=null!=e&&(e.length>50||e.split("\n").length>1);if(r){for(var p=0,a=e.split("\n");p1||h[0].length>0){this.indent().append('writeln("'+h[0]+'");'),null==e||r||this.append(" // ").append(e);for(var d=1;d")},t.prototype.handleDump=function(n){"string"==typeof n?this.nl().indent().append(this.rawOutput(n)):this.nl().indent().append(this.variableOutput(n))},t.prototype.variableOutput=function(n){return"writeln("+this.formattedVarName(n.name)+");"},t.prototype.rawOutput=function(n){return"writeln("+n+");"},t.prototype.variableDefinition=function(n,t){return this.typeMapping[n.type.name]+(t?" "+this.formattedVarName(n.name):"")},t.prototype.cast=function(n,t){return[this.INT,this.LONG,this.FLOAT,this.BOOL].includes(t)?n+".to!"+this.typeMapping[t]:n},t}(T),B=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),U=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.typeMapping=((t={})[i.FLOAT]="double",t[i.INT]="int",t[i.LONG]="int",t[i.STRING]="String",t[i.WORD]="String",t[i.BOOL]="int",t),i.readMapping=((e={})[i.INT]="int.parse(%s)",e[i.LONG]="int.parse(%s)",e[i.WORD]="%s",e[i.STRING]="%s",e[i.FLOAT]="double.parse(%s)",e[i.BOOL]="int.parse(%s)",e),i.PLACEHOLDER_INPUTS="--- PLACEHOLDER INPUTS ---",i.needInputsDeclaration=!1,i}return B(t,n),t.prototype.begin=function(){if(this.append("import 'dart:io';").nl(),this.append("import 'dart:math';").nl(),this.nl(),this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n1){this.needInputsDeclaration=!0,this.append("inputs = stdin.readLineSync().split(' ');");for(var t=0,e=0,i=n;e1?t.children.length+"*":"")+String.fromCharCode(this.loop-1)+(0===e?"":"+"+e),a=this.format(this.readMapping[i.name],["inputs["+p+"]"]);this.nl().indent().append(this.typeMapping[i.name]+" "+r.name+" = "+a+";"),null!=r.comment&&this.append(" // "+r.comment)}},t.prototype.handleLoopLine=function(n){this.nl().indent(),this.append("inputs = stdin.readLineSync().split(' ');"),this.handleLoop(n)},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop);this.nl().indent().append("for (int "+t+" = 0; "+t+" < "+n.param+"; "+t+"++) {"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("}")},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop").nl(),this.indent().append("while (true) {"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r1||o[0].length>0){this.indent().append("print('").append(o[0]).append("');"),null==e||i||this.append(" // ").append(e);for(var s=1;s")},t}(T),z=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),H=function(n){function t(){var t=null!==n&&n.apply(this,arguments)||this;return t.loop="i".charCodeAt(0),t.looplineUsed=!1,t.tempVariable="token",t.tempVariableCount=0,t}return z(t,n),t.prototype.begin=function(){if(this.input.hasStatement())for(var n=0,t=this.input.statement.split("\n");n1){for(var e=0,i=t.children;e1?n.length+" * ":"")+String.fromCharCode(this.loop-1)+(0===i?"":" + "+i);"word"===p.name?this.nl().indent().append("let "+r.name+" = words.["+a+"]"):this.nl().indent().append("let "+r.name+" = "+p.name+"(words.["+a+"])")}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop);this.nl().indent().append("for "+t+" in 0 .. "+n.param+" - 1 do"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.nl().indent().append("()"),this.decIndent(),this.nl()},t.prototype.handleLoopLine=function(n){var t=this.looplineUsed?"":"let ";this.looplineUsed=!0,this.nl().indent().append(t+"words = (Console.In.ReadLine()).Split [|' '|]"),this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("(* game loop *)").nl(),this.indent().append("while true do"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r1||o[0].length>0){this.indent().append('printfn "').append(o[0]).append('"'),null==e||i||this.append(" (* ").append(e).append(" *)");for(var s=1;s")},t.prototype.commentLine=function(n){return"(* "+n+" *)"},t}(T),K=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),Z=function(n){function t(){var t,e=null!==n&&n.apply(this,arguments)||this;return e.loop="i".charCodeAt(0),e.typeMapping=((t={})[e.INT]="int",t[e.FLOAT]="float64",t[e.LONG]="int",t[e.WORD]="string",t[e.STRING]="string",t[e.BOOL]="bool",t),e.needScanner=!1,e.useSplit=!1,e.useConv=!1,e.useStrings=!1,e.needFmt=!1,e.looplineUsed=!1,e.isInLoop=!1,e.DECLARE_TOKEN="{{scanner := bufio.NewScanner(os.Stdin)}}",e.caseConversion=T.CASE_CONVERSIONS.CAMEL,e.methodCaseConversion=T.CASE_CONVERSIONS.PASCAL,e.loopTpl="for {{&counter}} := {{&start}}; {{&counter}} < {{&end}}; {{&counter}}++ {\n{{&body}}\n}",e.voidReturnType="void",e}return K(t,n),t.prototype.begin=function(){if(this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===e?"":"+"+e)+"]"),e++,o.type.name===this.INT||o.type.name===this.LONG?(this.append(",10,32)"),this.nl().append("_ = "+o.name)):o.type.name===this.FLOAT&&(this.append(",10,64)"),this.nl().append("_ = "+o.name))}},t.prototype.handleLoop=function(n){this.isInLoop=!0;var t=String.fromCharCode(this.loop);this.nl().append("for "+t+" := 0; "+t+" < "+n.param+"; "+t+"++ {"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().append("}")},t.prototype.handleLoopLine=function(n){this.needScanner=!0,this.useSplit=!0;var t=this.looplineUsed?"inputs = ":"inputs := ";this.nl().append("scanner.Scan()"),this.useStrings=!0,this.nl().append(t+'strings.Split(scanner.Text()," ")'),this.looplineUsed=!0,this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl().append("for {"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r1||o[0].length>0){this.append('fmt.Println("'+o[0]+'")'),i||(null!=e?this.append(" // ").append(e):this.append(this.input.turnBased?"// Write action to stdout":"// Write answer to stdout"));for(var s=1;s")},t.prototype.handleDump=function(n){if(this.needFmt=!0,"string"==typeof n)this.nl().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildDumpLoop(n,n.name,t);this.code.push("\n"),this.append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().append(this.variableOutput(i))}},t.prototype.handleCall=function(n,t,e){var i=this,r=t.map((function(n){return i.formattedVarName(n.name)+" "+i.functionArgumentType(n.type)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=this.functionDeclaration(n,r,this.functionArgumentType(e.type),this.defaultReturnStatement(e.type)),o=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[o]=a;var s=this.functionCall(n,e.type.name!==this.voidReturnType?e.type.name:"",e.name,p);this.append("\n").append(this.indentString(s,this.level))},t.prototype.buildReadStringLoop=function(n,t,e,i){var p=n.type.dimensions.length;if(0===i)return t;var a=p-i,o="",s=e+"["+this.sletters[a]+"]";o=1===i?"scanner.Scan()\n"+s+" = scanner.Text()":this.buildArrayMakingStatement(s,"=",this.typeMapping[n.type.innerType.name],i-1,n.type.dimensions[a])+this.loopTpl;var l=0===a?this.buildArrayMakingStatement(e,":=",this.typeMapping[n.type.innerType.name],i,n.type.dimensions[a])+t:t,h=r.render(l,{counter:this.sletters[a],start:0,end:this.formattedVarName(n.type.dimensions[a]),body:this.indentString(o,a+1)});return this.buildReadStringLoop(n,h,s,i-1)},t.prototype.buildReadNonStringLoop=function(n,t,e){var i=n.type.dimensions.length,p=i-e;this.useStrings=!0;var a="",o=t+"["+this.sletters[p]+"]";if(0===e)return n.type.innerType.name!==this.WORD?(this.useConv=!0,n.type.innerType.name===this.INT?t+",_ = strconv.Atoi(inputs["+this.sletters[p-1]+"])":t+",_ = strconv.ParseFloat(inputs["+this.sletters[p-1]+"],64)"):t+" = inputs["+this.sletters[p-1]+"]";a=this.buildReadNonStringLoop(n,o,e-1);var s=e===i?":=":"=",l=this.buildArrayMakingStatement(t,s,this.typeMapping[n.type.innerType.name],e,n.type.dimensions[p])+this.loopTpl;return 1===n.type.dimensions.length&&(this.useStrings=!0,l='scanner.Scan()\ninputs := strings.Split(scanner.Text(), " ")\n'+l),r.render(l,{counter:this.sletters[p],start:0,end:this.formattedVarName(n.type.dimensions[p]),body:this.indentString((2===e?'scanner.Scan()\ninputs := strings.Split(scanner.Text(), " ")\n':"")+a,1)})},t.prototype.buildDumpLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="";return p=1===e?this.variableOutput(t+"["+this.sletters[i]+"]"):this.buildDumpLoop(n,t+"["+this.sletters[i]+"]",e-1),r.render(this.loopTpl,{counter:this.sletters[i],start:0,end:n.type.dimensions[i]?this.formattedVarName(n.type.dimensions[i]):"len("+t+")",body:this.indentString(p,1)})},t.prototype.variableOutput=function(n){return"fmt.Println("+n+")"},t.prototype.functionSignatureSimple=function(n,t){return n+"("+t+")"},t.prototype.functionSignatureFull=function(n,t,e){return this.functionSignatureSimple(n,t)+(e?" "+e:"")},t.prototype.functionDeclaration=function(n,t,e,i){return"\nfunc "+this.functionSignatureFull(n,t,e)+' {\n // Write your code here\n // fmt.Fprintln(os.Stderr, "Debug messages...")\n '+i+"\n}\n"},t.prototype.defaultReturnStatement=function(n){var t,e=this,i=((t={})[this.BOOL]=function(){return"false"},t[this.INT]=function(){return"0"},t[this.LONG]=function(){return"0"},t[this.FLOAT]=function(){return"0.0"},t[this.WORD]=function(){return'""'},t[this.STRING]=function(){return'""'},t[this.ARRAY]=function(n){return"[]".repeat(n.dimensions.length)+e.typeMapping[n.innerType.name]+"{}"},t);return n.name?"\n return "+i[n.name](n):""},t.prototype.functionCall=function(n,t,e,i){return r.render("stdoutWriter := os.Stdout\nos.Stdout = os.Stderr\n{{#type}}{{&res}} := {{/type}}{{&name}}({{¶ms}})\nos.Stdout = stdoutWriter",{name:n,type:t,res:e,params:i})},t.prototype.functionArgumentType=function(n){return n.name===this.voidReturnType?"":n.name===this.ARRAY?"[]".repeat(n.dimensions.length)+this.typeMapping[n.innerType.name]:this.typeMapping[n.name]},t.prototype.typeDeclaration=function(n){return n.name===this.voidReturnType?"":n.name===this.ARRAY?"["+n.dimensions.join("][")+"]"+this.typeMapping[n.innerType.name]:this.typeMapping[n.name]},t.prototype.buildArrayMakingStatement=function(n,t,e,i,r){return n+" "+t+" make("+"[]".repeat(i)+e+", "+r+")\n"},t.prototype.buildStatementData=function(n,t,e){var i=this,r=t.map((function(n){return i.formattedVarName(n.name)+" "+i.functionArgumentType(n.type)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=this.functionArgumentType(e.type);return{functionNomenclature:"function",arrayNomenclature:"array",null:"nil",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.functionArgumentType(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,p),functionFull:this.functionSignatureFull(n,r,a),returnType:a}},t.prototype.groupVariablesByType=function(n){return n.reduce((function(n,t){return n.length>0&&n[n.length-1][0].type.name===t.type.name?n[n.length-1].push(t):n.push([t]),n}),[])},t}(T),q=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),J=function(n){function t(){var t,e=null!==n&&n.apply(this,arguments)||this;return e.loop="i".charCodeAt(0),e.readMapping=((t={})[e.INT]="input.nextInt()",t[e.LONG]="input.nextLong()",t[e.FLOAT]="input.nextFloat()",t[e.STRING]="input.nextLine()",t[e.WORD]="input.next()",t[e.BOOL]="input.nextInt()",t),e}return q(t,n),t.prototype.begin=function(n){if(n.containsRead&&(this.append("input = new Scanner(System.in);").nl(),this.nl()),this.input.hasStatement()){this.append("/**").nl();for(var t=0,e=this.input.statement.split("\n");t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' + "'+t+'" + ':" + ").replace(/" \+ "/g,"")),null==e||i||this.append(" // ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('println "').append(o[0]).append('"'),null==e||i||this.append(" // ").append(e);for(var s=1;s")},t}(T),Q=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),X=function(n){function t(){var t,e=null!==n&&n.apply(this,arguments)||this;return e.typeMapping=((t={})[e.INT]="Int",t[e.LONG]="Int",t[e.FLOAT]="Float",t[e.STRING]="String",t[e.WORD]="String",t[e.BOOL]="Int",t),e.loop="i".charCodeAt(0),e.inRead=!1,e.gameLoopParams=[],e.varsOutOfLoop=[],e.inGameLoop=!1,e.caseConversion=T.CASE_CONVERSIONS.LOWER,e}return Q(t,n),t.prototype.begin=function(){if(this.append("import System.IO").nl(),this.append("import Control.Monad").nl(),this.nl(),this.append("main :: IO ()").nl(),this.append("main = do"),this.incIndent(),this.nl().append("hSetBuffering stdout NoBuffering -- DO NOT REMOVE"),this.nl(),this.input.hasStatement()){for(var n=0,t=this.input.statement.split("\n");n1){this.nl().append("let input = words input_line");for(var t=0;t1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===t?"":"+"+t)])):this.nl().append(this.format("let %s = read (input!!(%s)) :: %s",[r,(n.length>1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===t?"":"+"+t),this.typeMapping[i.name]])),e.comment&&this.append(" -- ").append(e.comment),this.inGameLoop||this.varsOutOfLoop.push(r)}},t.prototype.handleLoop=function(n){var t=n.param.toLowerCase();this.inGameLoop&&-1===this.gameLoopParams.indexOf(t)&&-1!==this.varsOutOfLoop.indexOf(t)&&parseInt(t,10).toString()!==t&&this.gameLoopParams.push(t),this.nl().nl().append("replicateM "+t+" $ do"),this.incIndent(),this.write(n.children[0]),this.nl().append("return ()"),this.decIndent()},t.prototype.handleLoopLine=function(n){var t=String.fromCharCode(this.loop);this.loop++,this.nl().append("input_line <- getLine"),this.nl().append("let input = words input_line");var e=n.param.toLowerCase();this.inGameLoop&&-1===this.gameLoopParams.indexOf(e)&&-1!==this.varsOutOfLoop.indexOf(e)&&parseInt(e,10).toString()!==e&&this.gameLoopParams.push(e),this.nl().nl().append("forM [0..("+e+"-1)] $ \\"+t+" -> do"),this.incIndent(),this.write(n.children[0]),this.nl().append("return ()"),this.loop--,this.decIndent()},t.prototype.handleGameLoop=function(n){this.inGameLoop=!0,this.nl(),this.nl().append("-- game loop"),this.nl().append("forever $ do"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' ++ "'+t+'" ++ ':" ++ ").replace(/" \++ "/g,"")),this.append(")");else{var o=n.split("\n");if(o.length>1||o[0].length>0){i||(null!=e?this.nl().append("-- ").append(e):this.nl().append(this.input.turnBased?"-- Write action to stdout":"-- Write answer to stdout"));for(var s=0,l=o;s")},t}(T),nn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),tn=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.typeMapping=((t={})[i.BOOL]="boolean",t[i.INT]="number",t[i.FLOAT]="number",t[i.LONG]="number",t[i.WORD]="string",t[i.STRING]="string",t[i.ARRAY]="{{&type}}{{#dim}}[]{{/dim}}",t),i.readMapping=((e={})[i.INT]="parseInt(%s)",e[i.LONG]="parseInt(%s)",e[i.FLOAT]="parseFloat(%s)",e[i.BOOL]="%s !== '0'",e[i.WORD]="%s",e[i.STRING]="%s",e),i.methodCaseConversion=T.CASE_CONVERSIONS.CAMEL,i.caseConversion=T.CASE_CONVERSIONS.CAMEL,i.reservedWords=["abstract","arguments","await","boolean","break","byte","case","catch","char","class","const","continue","debugger","default","delete","do","double","else","enum","eval","export","extends","false","final","finally","float","for","function","goto","if","implements","import","in","instanceof","int","interface","let","long","native","new","null","package","private","protected","public","return","short","static","super","switch","synchronized","this","throw","throws","transient","true","try","typeof","var","void","volatile","while","with","yield","eval","uneval","isFinite","isNaN","parseFloat","parseInt","decodeURI","decodeURIComponent","encodeURI","encodeURIComponent","escape","unescape"],i.loopTpl="for (let {{&counter}} = {{&start}}; {{&counter}} < {{&end}}; {{&counter}}++) {\n{{&body}}\n}",i.voidReturnType="",i}return nn(t,n),t.prototype.begin=function(){if(this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n1){this.append("var inputs = readline().split(' ');");for(var t=0,e=0,i=n;e1||o.type.innerType.name===this.STRING?(this.append("const "+o.name+" = "+"[".repeat(s)+"]".repeat(s)+";"),this.nl().append(this.indentString(l,this.level))):this.append("const "+l)}}},t.prototype.handleReadLine=function(n){for(var t=0;t1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===t?"":"+"+t)+"]"]);this.nl().indent().append("const ").append(e.name).append(" = ").append(i).append(";"),null!=e.comment&&this.append("// ").append(e.comment)}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop);this.nl().indent().append("for (let "+t+" = 0; "+t+" < "+n.param+"; "+t+"++) {"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("}")},t.prototype.handleLoopLine=function(n){this.nl().indent(),this.append("var inputs = readline().split(' ');"),this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop").nl(),this.indent().append("while (true) {"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?" + '"+t+"' + ":" + ").replace(/' \+ '/g,"")),this.append(");"),null==e||i||this.append(" // ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append("console.log('").append(o[0]).append("');"),null==e||i||this.indent().append(" // ").append(e).nl();for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildDumpLoop(n,n.name,t);this.nl().append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().indent().append(this.variableOutput(i))}},t.prototype.handleCall=function(n,t,e){var i=this,r=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[a]=this.functionDeclaration(n,r,this.defaultReturnStatement(e.type));var o=this.functionCall(n,e.type.name!==this.voidReturnType?e.type.name:"",e.name,p);this.nl().append(this.indentString(o,this.level))},t.prototype.buildReadStringLoop=function(n,t,e,i){var p=n.type.dimensions.length;if(0===i)return t;var a=p-i,o="",s=e+"["+this.sletters[a]+"]";o=1===i?s+" = readline();":this.loopTpl;var l=r.render(t,{counter:this.sletters[a],start:0,end:this.formattedVarName(n.type.dimensions[a]),body:this.indentString(o,a+1)});return this.buildReadStringLoop(n,l,s,i-1)},t.prototype.buildReadNonStringLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="";if(1===e){var a=n.type.innerType.name!==this.WORD?".map("+this.sletters[i+1]+" => "+this.format(this.readMapping[n.type.innerType.name],[this.sletters[i+1]])+")":"",o=parseInt(n.type.dimensions[i],10);return t+" = "+("readline().split(' ')"+a+(isNaN(o)?".slice(0, "+n.type.dimensions[i]+")":"")+";")}var s=t+"["+this.sletters[i]+"]";return p=this.buildReadNonStringLoop(n,s,e-1),r.render(this.loopTpl,{counter:this.sletters[i],start:0,end:this.formattedVarName(n.type.dimensions[i]),body:this.indentString(p,1)})},t.prototype.buildDumpLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="";return p=1===e?this.variableOutput(t+"["+this.sletters[i]+"]"):this.buildDumpLoop(n,t+"["+this.sletters[i]+"]",e-1),r.render(this.loopTpl,{counter:this.sletters[i],start:0,end:n.type.dimensions[i]?this.formattedVarName(n.type.dimensions[i]):t+".length",body:this.indentString(p,1)})},t.prototype.variableOutput=function(n){return"console.log("+n+");"},t.prototype.functionSignatureSimple=function(n,t){return n+"("+t+")"},t.prototype.functionDeclaration=function(n,t,e){return"\nfunction "+this.functionSignatureSimple(n,t)+" {\n"+this.indentString("// Write your code here\n// To debug: console.error('Debug messages...');\n"+e,1)+"\n}\n"},t.prototype.defaultReturnStatement=function(n){var t,e=((t={})[this.BOOL]=function(){return"false"},t[this.INT]=function(){return"0"},t[this.LONG]=function(){return"0"},t[this.FLOAT]=function(){return"0.0"},t[this.STRING]=function(){return"''"},t[this.WORD]=function(){return"''"},t[this.ARRAY]=function(n){return"[".repeat(n.dimensions.length)+"]".repeat(n.dimensions.length)},t);return n.name?"\nreturn "+e[n.name](n)+";":""},t.prototype.functionCall=function(n,t,e,i){return r.render("const oldWrite = process.stdout.write;\nprocess.stdout.write = chunk => console.error(chunk);\n{{#type}}var {{&res}} = {{/type}}{{&name}}({{¶ms}});\nprocess.stdout.write = oldWrite;",{name:n,type:t,res:e,params:i})},t.prototype.buildStatementData=function(n,t,e){var i=this,r=t.map((function(n){return i.formattedVarName(n.name)})).join(", ");return{functionNomenclature:"function",arrayNomenclature:"array",null:"null",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.formatType(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,r),functionFull:this.functionSignatureSimple(n,r),returnType:this.formatType(e.type)}},t}(T),en=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),rn=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.typeMapping=((t={})[i.INT]="int",t[i.LONG]="long",t[i.STRING]="String",t[i.WORD]="String",t[i.FLOAT]="float",t[i.BOOL]="boolean",t[i.ARRAY]="{{&type}}{{#dim}}[]{{/dim}}",t),i.readMapping=((e={})[i.FLOAT]="in.nextFloat();",e[i.INT]="in.nextInt();",e[i.LONG]="in.nextLong();",e[i.STRING]="in.nextLine();",e[i.WORD]="in.next();",e[i.BOOL]="in.nextInt() != 0;",e[i.ARRAY]="new {{&type}}{{#dim}}[{{.}}]{{/dim}};",e),i.caseConversion=T.CASE_CONVERSIONS.CAMEL,i.methodCaseConversion=T.CASE_CONVERSIONS.CAMEL,i.reservedWords=["abstract","assert","boolean","break","byte","case","catch","char","class","const","default","do","double","else","enum","extends","false","final","finally","float","for","goto","if","implements","import","instanceof","int","interface","long","native","new","null","package","private","protected","public","return","short","static","strictfp","super","switch","synchronized","this","throw","throws","transient","true","try","void","volatile","while","continue"],i.loopTpl="for (int {{&counter}} = {{&start}}; {{&counter}} < {{&end}}; {{&counter}}++) {\n{{&body}}\n}",i.voidReturnType="void",i}return en(t,n),t.prototype.begin=function(n){var t=n.containsRead;if(this.append("import java.util.*;").nl(),this.append("import java.io.*;").nl(),this.append("import java.math.*;").nl(),this.nl(),this.input.hasStatement()){this.append("/**").nl();for(var e=0,i=this.input.statement.split("\n");e").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' + "'+t+'" + ':" + ").replace(/" \+ "/g,"")),this.append(");"),null==e||i||this.append(" // ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('System.out.println("').append(o[0]).append('");'),null==e||i||this.append(" // ").append(e);for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildDumpLoop(n,n.name,t);this.nl().append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().indent().append(this.variableOutput(i))}},t.prototype.handleCall=function(n,t,e){var i=this,r=t.map((function(n){return i.typeDeclaration(n.type)+" "+i.formattedVarName(n.name)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[a]=this.functionDeclaration(n,r,e.type.name?this.typeDeclaration(e.type):this.voidReturnType,this.defaultReturnStatement(e.type));var o=this.functionCall(n,e.name?this.typeDeclaration(e.type):"",e.name,p);this.nl().append(this.indentString(o,this.level))},t.prototype.buildReadLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="";return p=1===e?t+"["+this.sletters[i]+"] = "+this.readMapping[n.type.innerType.name]:this.buildReadLoop(n,t+"["+this.sletters[i]+"]",e-1),r.render(this.loopTpl,{counter:this.sletters[i],start:0,end:this.formattedVarName(n.type.dimensions[i]),body:this.indentString(p,1)})},t.prototype.buildDumpLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="";return p=1===e?this.variableOutput(t+"["+this.sletters[i]+"]"):this.buildDumpLoop(n,t+"["+this.sletters[i]+"]",e-1),r.render(this.loopTpl,{counter:this.sletters[i],start:0,end:n.type.dimensions[i]?this.formattedVarName(n.type.dimensions[i]):t+".length",body:this.indentString(p,1)})},t.prototype.variableOutput=function(n){return"System.out.println("+n+");"},t.prototype.functionSignatureSimple=function(n,t){return n+"("+t+")"},t.prototype.functionSignatureFull=function(n,t,e){return e+" "+this.functionSignatureSimple(n,t)},t.prototype.functionDeclaration=function(n,t,e,i){return"\n public static "+this.functionSignatureFull(n,t,e)+' {\n // Write your code here\n // To debug: System.err.println("Debug messages...");\n '+i+"\n }\n"},t.prototype.defaultReturnStatement=function(n){var t,e=this,i=((t={})[this.BOOL]=function(){return"false"},t[this.INT]=function(){return"0"},t[this.LONG]=function(){return"0"},t[this.FLOAT]=function(){return"0.0"},t[this.WORD]=function(){return'""'},t[this.STRING]=function(){return'""'},t[this.ARRAY]=function(n){return r.render(e.readMapping[e.ARRAY],{dim:n.dimensions,type:e.typeMapping[n.innerType.name]}).slice(0,-1)},t);return n.name?"\n return "+i[n.name](n)+";":""},t.prototype.functionCall=function(n,t,e,i){return r.render("PrintStream outStream = System.out;\nSystem.setOut(System.err);\n{{#type}}{{&type}} {{&res}} = {{/type}}{{&name}}({{¶ms}});\nSystem.setOut(outStream);",{name:n,type:t,res:e,params:i})},t.prototype.typeDeclaration=function(n){var t=this.typeMapping[n.name];return n.name===this.ARRAY?r.render(t,{dim:n.dimensions,type:this.typeMapping[n.innerType.name]}):r.render(t,{})},t.prototype.variableDefinition=function(n){var t=this.typeDeclaration(n.type)+" "+n.name;return n.type.name===this.ARRAY?t+" = "+r.render(this.readMapping[this.ARRAY],{dim:n.type.dimensions,type:this.typeMapping[n.type.innerType.name]}):t+" = "+this.readMapping[n.type.name]},t.prototype.buildStatementData=function(n,t,e){var i=this,r=t.map((function(n){return i.typeDeclaration(n.type)+" "+i.formattedVarName(n.name)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=e.type.name?this.typeDeclaration(e.type):this.voidReturnType;return{functionNomenclature:"method",arrayNomenclature:"array",null:"null",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.typeDeclaration(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,p),functionFull:this.functionSignatureFull(n,r,a),returnType:a}},t}(T),pn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),an=function(n){function t(){var t,e,i,r=null!==n&&n.apply(this,arguments)||this;return r.loop="i".charCodeAt(0),r.typeMapping=((t={})[r.BOOL]="Boolean",t[r.INT]="Int",t[r.LONG]="Long",t[r.STRING]="String",t[r.WORD]="String",t[r.FLOAT]="Float",t[r.ARRAY]="{{#dim}}Array<{{/dim}}{{{type}}}{{#dim}}>{{/dim}}",t),r.readMapping=((e={})[r.FLOAT]="input.nextFloat()",e[r.INT]="input.nextInt()",e[r.LONG]="input.nextLong()",e[r.STRING]="input.nextLine()",e[r.WORD]="input.next()",e[r.BOOL]="input.nextInt() != 0",e),r.defaultReturnMapping=((i={})[r.BOOL]=function(){return"false"},i[r.INT]=function(){return"0"},i[r.LONG]=function(){return"0"},i[r.FLOAT]=function(){return"0.0"},i[r.STRING]=function(){return'""'},i[r.WORD]=function(){return'""'},i[r.ARRAY]=function(n){return"arrayOf<"+r.formatType(n.innerType)+">()"},i),r.methodCaseConversion=T.CASE_CONVERSIONS.CAMEL,r.caseConversion=T.CASE_CONVERSIONS.CAMEL,r}return pn(t,n),t.prototype.begin=function(n){var t=n.containsRead;if(this.append("import java.util.*").nl(),this.append("import java.io.*").nl(),this.append("import java.math.*").nl(),this.nl(),this.input.hasStatement()){this.append("/**").nl();for(var e=0,i=this.input.statement.split("\n");e) {").nl(),this.incIndent(),t&&this.indent().append("val input = Scanner(System.`in`)")},t.prototype.end=function(){this.decIndent(),this.nl().indent().append("}")},t.prototype.handleRead=function(n,t){for(var e=null,i=0,r=n;i").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?t:"")),this.append('")'),null==e||i||this.append(" // ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('println("').append(o[0]).append('")'),null==e||i||this.append(" // ").append(e);for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildArrayDumpLoop(n,t);this.nl().append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().indent().append(this.variableOutput(i))}},t.prototype.variableOutput=function(n){return"println("+n+")"},t.prototype.buildArrayDumpLoop=function(n,t){return 0===t?this.variableOutput("it"):(n.type.dimensions.length===t?n.name:"it")+".forEach { "+this.buildArrayDumpLoop(n,t-1)+" }"},t.prototype.handleCall=function(n,t,e){var i=this.functionDeclaration(n,t,e.type),r=this.functionCall(n,t,e),p=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[p]=i,this.nl().append(this.indentString(r,this.level))},t.prototype.functionCall=function(n,t,e){var i=this,r=t.map((function(n){return i.formattedVarName(n.name)})).join(", ");return"val outStream = System.out\nSystem.setOut(System.err)\nval "+this.variableDefinition(e)+" = "+n+"("+r+")\nSystem.setOut(outStream)"},t.prototype.functionDeclaration=function(n,t,e){return"fun "+this.functionSignatureFull(n,t,e)+' {\n // Write your code here\n // To debug: System.err.println("Debug messages...");\n\n return '+this.defaultReturnMapping[e.name](e)+"\n}\n"},t.prototype.functionSignatureSimple=function(n,t,e){var i=this;return n+" ("+t.map((function(n){return i.formattedVarName(n.name)})).join(", ")+")"},t.prototype.functionSignatureFull=function(n,t,e){var i=this;return n+" ("+t.map((function(n){return i.variableDefinition(n)})).join(", ")+"): "+this.formatType(e)},t.prototype.variableDefinition=function(n){return this.formattedVarName(n.name)+": "+this.formatType(n.type)},t.prototype.buildStatementData=function(n,t,e){var i=this;return{functionNomenclature:"function",arrayNomenclature:"array",null:"null",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.formatType(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,t,e.type),functionFull:this.functionSignatureFull(n,t,e.type),returnType:this.formatType(e.type)}},t}(T),on=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),sn=function(n){function t(){var t=null!==n&&n.apply(this,arguments)||this;return t.loop="i".charCodeAt(0),t}return on(t,n),t.prototype.begin=function(){if(this.input.hasStatement())for(var n=0,t=this.input.statement.split("\n");n1){for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' .. "'+t+'" .. ':" .. ").replace(/" \.. "/g,"")),this.append(");"),null==e||i||this.append(" -- ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('print("').append(o[0]).append('")'),null==e||i||this.append(" -- ").append(e);for(var s=1;s")},t.prototype.commentLine=function(n){return"-- "+n},t}(T),ln=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),hn=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.typeMapping=((t={})[i.INT]="int %s;",t[i.LONG]="long long %s;",t[i.STRING]="char %s[%s];",t[i.WORD]="char %s[%s];",t[i.FLOAT]="float %s;",t[i.BOOL]="int %s;",t),i.readMapping=((e={})[i.INT]="%d",e[i.FLOAT]="%f",e[i.LONG]="%lld",e[i.WORD]="%s",e[i.BOOL]="%d",e),i}return ln(t,n),t.prototype.begin=function(){if(this.append("#include ").nl().nl(),this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r1||o[0].length>0){this.indent().append('printf([@"').append(o[0]).append('\\n" UTF8String]);'),null==e||i||this.append(" // ").append(e);for(var s=1;s")},t}(T),dn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),un={int:"%d",long:"%ld",float:"%f",word:"%s",string:"%s",bool:"%d"},cn=function(n){function t(){var t=null!==n&&n.apply(this,arguments)||this;return t.loop="i".charCodeAt(0),t.caseConversion=T.CASE_CONVERSIONS.LOWER,t}return dn(t,n),t.prototype.begin=function(){var n=this;this.input.hasStatement()&&this.input.statement.split("\n").forEach((function(t){n.append("(* "+t+" *)").nl()}))},t.prototype.handleRead=function(n){var t=this;if(n.length>1){n.forEach((function(n){null!=n.comment&&t.nl().indent().append("(* "+n.name.toLowerCase()+": "+n.comment+" *)")}));var e=n.map((function(n){return n.name.toLowerCase()})),i=n.map((function(n){return un[n.type.name]}));this.nl(),this.nl().indent().append("let line = input_line stdin in"),this.nl().indent().append("let "+e.join(", ")+' = Scanf.sscanf line "'+i.join(" ")+'" (fun '+e.join(" ")+" -> ("+e.join(", ")+")) in")}else{var r=n[0];switch(this.nl().indent().append("let "+r.name.toLowerCase()+" = "),r.type.name){case this.INT:case this.BOOL:case this.LONG:this.append("int_of_string (input_line stdin) in");break;case this.FLOAT:this.append("float_of_string (input_line stdin) in");break;default:this.append("input_line stdin in")}r.comment&&this.append(" (* "+r.comment+" *)")}},t.prototype.handleReadLine=function(n){var t=this;n.forEach((function(n){null!=n.comment&&t.nl().indent().append("(* "+n.name.toLowerCase()+": "+n.comment+" *)")}));var e=n.map((function(n){return n.name.toLowerCase()})),i=n.map((function(n){return un[n.type.name]}));this.nl().indent().append("let "+e.join(", ")+' = Scanf.sscanf line "'+i.join(" ")+'" (fun '+e.join(" ")+" -> ("+e.join(", ")+")) in")},t.prototype.handleLoop=function(n){var t=this,e=String.fromCharCode(this.loop),i=parseInt(n.param,10);if(isNaN(i)?i=n.param.toLowerCase()+" - 1":i--,this.nl().indent().append("for "+e.toLowerCase()+" = 0 to "+i+" do"),this.incIndent(),this.loop++,n.name!==this.LOOPLINE)this.write(n.children[0]);else{var r=n.children[0],p=r.children.map((function(n){return n.children[0]}));p.forEach((function(n){null!=n.comment&&t.nl().indent().append("(* "+n.name.toLowerCase()+": "+n.comment+" *)")}));var a=p.map((function(n){return n.name.toLowerCase()})),o=r.children.map((function(n){return un[n.name]}));this.nl().indent().append("let "+a.join(", ")+' = Scanf.scanf " '+o.join(" ")+'" (fun '+a.join(" ")+" -> ("+a.join(", ")+")) in")}this.loop--,this.nl().indent().append("();"),this.decIndent(),this.nl().indent().append("done;"),this.nl()},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("(* game loop *)").nl(),this.indent().append("while true do"),this.incIndent();for(var t=0,e=n;t").nl();var r=null!=e&&(e.length>50||e.split("\n").length>1);r&&(e.split("\n").forEach((function(n){i.nl().indent().append("(* "+n+" *)")})),this.nl());if(Array.isArray(n))this.indent().append('Printf.printf "'),this.append(n.map((function(n){return"VAR"===n.type?un[n.node.name]:n.value})).join(t)),this.append('" '),this.append(n.filter((function(n){return"VAR"===n.type})).map((function(n){return n.node.children[0].name})).join(" ")),this.append(";"),null==e||r||this.append(" (* "+e+" *)"),this.nl().indent().append('print_endline "";');else{var p=n.split("\n");if(p.length>1||p[0].length>0){this.indent().append('print_endline "'+p[0]+'";'),null==e||r||this.append(" (* "+e+" *)");for(var a=1;a")},t.prototype.commentLine=function(n){return"(* "+n+" *)"},t}(T),fn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),mn=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.typeMapping=((t={})[i.INT]="Int32",t[i.LONG]="Int64",t[i.STRING]="String",t[i.WORD]="String",t[i.FLOAT]="Extended",t[i.BOOL]="Int32",t),i.readMapping=((e={})[i.FLOAT]="StrToFloat(Inputs[%s]);",e[i.INT]="StrToInt(Inputs[%s]);",e[i.LONG]="StrToInt64(Inputs[%s]);",e[i.STRING]="readln();",e[i.WORD]="Inputs[%s];",e[i.BOOL]="StrToInt(Inputs[%s]);",e),i}return fn(t,n),t.prototype.begin=function(n){var t=n.containsRead,e=this.input;if(this.input.hasStatement())for(var i=0,r=this.input.statement.split("\n");i1?t.children.length+"*":"")+String.fromCharCode(this.loop-1)+(0===e?"":"+"+e)]);this.nl().indent().append(r.name).append(" := ").append(p)}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop),e=parseInt(n.param,10);isNaN(e)?e=n.param+"-1":e--,this.nl().indent().append("for "+t+" := 0 to "+e+" do"),this.nl().indent().append("begin"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("end;")},t.prototype.handleLoopLine=function(n){this.nl().indent().append("ParseIn(Inputs);"),this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop").nl(),this.indent().append("while true do"),this.nl().indent().append("begin"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r1||o[0].length>0){this.indent().append("writeln('").append(o[0]).append("');"),null==e||i||this.append(" // ").append(e);for(var s=1;s"),this.nl().indent().append("flush(StdErr); flush(output); // DO NOT REMOVE")},t}(T),yn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),gn=function(n){function t(){var t=null!==n&&n.apply(this,arguments)||this;return t.loop="i".charCodeAt(0),t.caseConversion=T.CASE_CONVERSIONS.SNAKE,t.tokensUsed=!1,t}return yn(t,n),t.prototype.begin=function(){if(this.append("use strict;\nuse warnings;\n#use diagnostics;\nuse 5.20.1;\n\nselect(STDOUT); $| = 1; # DO NOT REMOVE").nl(),this.nl(),this.input.hasStatement())for(var n=0,t=this.input.statement.split("\n");n1){for(var t=0,e=n;t);"),this.tokensUsed=!0;var i=n.map((function(n){return"$"+n.name})).join(", ");this.nl().indent().append("my ("+i+") = split(/ /,$tokens);")}else{var r=n[0];this.nl().indent().append("chomp(my $"+r.name+" = );"),r.comment&&this.append(" # "+r.comment)}},t.prototype.handleReadLine=function(n){for(var t=0,e=n;t1?n.length+"*":"")+"$"+p+(0===i?"":"+"+i);this.nl().indent().append("my $"+r.name+" = $inputs["+a+"];")}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop),e=isNaN(n.param)?"$"+n.param+"-1":n.param-1;this.nl().indent().append("for my $"+t+" (0.."+e+") {"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("}")},t.prototype.handleLoopLine=function(n){this.tokensUsed?this.nl().indent().append("chomp($tokens=);"):(this.nl().indent().append("chomp($tokens=);"),this.tokensUsed=!0),this.nl().indent().append("my @inputs = split(/ /,$tokens);");var t=n.children[0].children;if(1===t.length)return this.nl().indent().append("for my $"+t[0].children[0].name+" (@inputs) {"),this.incIndent(),this.loop++,this.nl().indent(),this.loop--,this.decIndent(),void this.nl().indent().append("}");this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("# game loop").nl(),this.indent().append("while (1) {"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?".'"+t+"'.":".").replace(/'\.'/g,"")),this.append(";"),null==e||i||this.append(" # ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.nl().indent().append('print "').append(o[0]).append('\\n";'),null==e||i||this.append(" # ").append(e);for(var s=1;s")},t.prototype.commentLine=function(n){return"# "+n},t}(T),vn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),Sn=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.typeMapping=((t={})[i.BOOL]="boolean",t[i.INT]="integer",t[i.FLOAT]="float",t[i.LONG]="integer",t[i.WORD]="string",t[i.STRING]="string",t[i.ARRAY]="{{#dim}}array({{/dim}}{{&type}}{{#dim}}){{/dim}}",t),i.readMapping=((e={})[i.BOOL]="%d",e[i.INT]="%d",e[i.LONG]="%d",e[i.FLOAT]="%f",e[i.WORD]="%s",e),i.caseConversion=T.CASE_CONVERSIONS.CAMEL,i.methodCaseConversion=T.CASE_CONVERSIONS.CAMEL,i.loopTpl="{{&beforeLoop}}for ({{&counter}} = {{&start}}; {{&counter}} < {{&end}}; {{&counter}}++) {\n{{&body}}\n}",i.voidReturnType="void",i}return vn(t,n),t.prototype.cast=function(n,t){return this.INT===n||this.LONG===n?"intval("+t+")":this.FLOAT===n?"floatval("+t+")":t},t.prototype.begin=function(){if(this.append("")},t.prototype.handleRead=function(n){var t=this;if(this.STRING!==n[0].type.name&&this.ARRAY!==n[0].type.name){for(var e=0,i=n;e=1&&this.nl().indent().append("$"+s.name+" = array();");var o=this.buildReadLoop(s,this.loopTpl,s.name,a);this.nl().append(this.indentString(o,this.level))}else{var s=n[0],l=Number.isNaN(parseInt(s.type.dimensions,10))?"$":"";this.nl().indent().append("$"+s.name+" = stream_get_line(STDIN, "+l+s.type.dimensions+' + 1, "\\n");'),null!=s.comment&&this.append("// "+s.comment)}},t.prototype.handleReadLine=function(n){for(var t=0;t1?n.length+"*":"")+"$"+String.fromCharCode(this.loop-1)+(0===t?"":"+"+t)]);this.nl().indent().append("$").append(n[t].name).append(" = "),this.INT===n[t].type.name||this.LONG===n[t].type.name?this.append("intval"):this.FLOAT===n[t].type.name&&this.append("floatval"),this.append(e),null!=n[t].comment&&this.append(" // ").append(n[t].comment)}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop),e=isNaN(n.param)?"$"+n.param:n.param;this.nl().indent().append("for ($"+t+" = 0; $"+t+" < "+e+"; $"+t+"++)"),this.nl().indent().append("{"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("}")},t.prototype.handleLoopLine=function(n){this.nl().indent().append('$inputs = explode(" ", fgets(STDIN));'),this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop"),this.nl().indent().append("while (TRUE)"),this.nl().indent().append("{"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' . "'+t+'" . ':" . ")+' . "\\n"').replace(/" \. "/g,"")),this.append(");"),null==e||i||this.append(" // ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('echo("').append(o[0]).append('\\n");'),null==e||i||this.append(" // ").append(e);for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.rawOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildDumpLoop(n,n.name,t);this.nl().append(this.indentString(e,this.level))}else this.nl().indent().append(this.variableOutput(n))},t.prototype.handleCall=function(n,t,e){var i=this,r=t.map((function(n){return(n.type.name===i.ARRAY?"array $":"$")+i.formattedVarName(n.name)})).join(", "),p=t.map((function(n){return"$"+i.formattedVarName(n.name)})).join(", "),a=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[a]=this.functionDeclaration(n,r,this.defaultReturnStatement(e.type));var o=this.functionCall(n,e.type.name!==this.voidReturnType?e.type.name:"","$"+e.name,p);this.nl().append(this.indentString(o,this.level))},t.prototype.buildReadLoop=function(n,t,e,i){var p=n.type.dimensions.length;if(0===i)return t;var a=p-i,o=null,s=null,l=e+"[$"+this.sletters[a]+"]";if(1===i)if(this.STRING===n.type.innerType.name)s="$"+l+" = fgets(STDIN);";else{var h="$inputs[$"+this.sletters[a]+"]";o='$inputs = explode(" ", fgets(STDIN));',s="$"+l+" = "+this.cast(n.type.innerType.name,h)+";"}else s=this.loopTpl;var d=r.render(t,{beforeLoop:null!=o?o+"\n"+this.indentString("",a):"",counter:"$"+this.sletters[a],start:0,end:(isNaN(n.type.dimensions[a])?"$":"")+this.formattedVarName(n.type.dimensions[a]),body:this.indentString(s,a+1)});return this.buildReadLoop(n,d,l,i-1)},t.prototype.buildDumpLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="",a=t+"[$"+this.sletters[i]+"]";return p=1===e?this.rawOutput("$"+a):this.buildDumpLoop(n,a,e-1),r.render(this.loopTpl,{counter:"$"+this.sletters[i],start:0,end:n.type.dimensions[i]?(isNaN(n.type.dimensions[i])?"$":"")+this.formattedVarName(n.type.dimensions[i]):"count($"+t+")",body:this.indentString(p,1)})},t.prototype.variableOutput=function(n){return'echo "$'+this.formattedVarName(n.name)+'\n";'},t.prototype.rawOutput=function(n){return"echo "+n+' . "\\n";'},t.prototype.functionSignatureSimple=function(n,t){return n+"("+t+")"},t.prototype.functionDeclaration=function(n,t,e){return"\nfunction "+this.functionSignatureSimple(n,t)+" {\n // Write your code here\n // To debug (equivalent to var_dump): error_log(var_export($var, true));\n "+e+"\n}\n"},t.prototype.defaultReturnStatement=function(n){var t,e=((t={})[this.INT]=function(){return"0"},t[this.LONG]=function(){return"0"},t[this.FLOAT]=function(){return"0.0"},t[this.WORD]=function(){return'""'},t[this.STRING]=function(){return'""'},t[this.ARRAY]=function(n){return"[".repeat(n.dimensions.length)+"]".repeat(n.dimensions.length)},t);return n.name?"\n return "+e[n.name](n)+";":""},t.prototype.functionCall=function(n,t,e,i){return r.render("$oldStdout = fopen('php://stdout', 'wb');\neio_dup2(STDERR, STDOUT);\neio_event_loop();\n{{#type}}{{&res}} = {{/type}}{{&name}}({{¶ms}});\neio_dup2($oldStdout, STDOUT);\neio_event_loop();",{name:n,type:t,res:e,params:i})},t.prototype.buildStatementData=function(n,t,e){var i=this,r=t.map((function(n){return(n.type.name===i.ARRAY?"array $":"$")+i.formattedVarName(n.name)})).join(", "),p=t.map((function(n){return"$"+i.formattedVarName(n.name)})).join(", "),a=e.type.name?this.formatType(e.type):this.voidReturnType;return{functionNomenclature:"function",arrayNomenclature:"array",null:"NULL",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.formatType(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,p),functionFull:this.functionSignatureSimple(n,r),returnType:a}},t}(T),Ln=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),On=function(n){function t(){var t=null!==n&&n.apply(this,arguments)||this;return t.loop="i".charCodeAt(0),t.keyWords={en:{read:"read {var}",for:"for {var}={begin} to {end}",endfor:"end for",while:"while {var}",endwhile:"end while",true:"true",false:"false"},fr:{read:"lire {var}",for:"pour {var} de {begin} jusqu'à {end}",endfor:"end pour",while:"tant que {var}",endwhile:"fin tant que",true:"vrai",false:"faux"}},t}return Ln(t,n),t.prototype.handleRead=function(n){for(var t=0,e=n;t1){for(var e=0,i=n;e1||l.type.innerType.name===this.STRING&&c>=1)&&this.nl().indent().append(l.name+" = []"),l.comment&&this.append(" # ").append(l.comment);var f=void 0;f=l.type.innerType.name===this.STRING?this.buildReadStringLoop(l,this.loopTpl,l.name,c):this.buildReadNonStringLoop(l,l.name,c),this.nl().append(this.indentString(f,this.level))}}},t.prototype.handleReadLine=function(n){for(var t=0,e=n;t1?n.length+"*":"")+p+(0===i?"":"+"+i))+"]";this.nl().indent().append(r.name+" = "+this.format(this.readMapping[r.type.name],[a]))}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop);this.nl().indent().append("for "+t+" in range("+n.param+"):"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent()},t.prototype.handleLoopLine=function(n){if(1===n.children[0].children.length){var t=String.fromCharCode(this.loop),e=n.children[0].children[0],i=e.children[0];e.name===this.WORD?this.nl().indent().append("for "+i.name+" in input().split():"):this.nl().indent().append("for "+t+" in input().split():"),this.incIndent(),null!=i.comment&&this.nl().indent().append("# "+i.name+": "+i.comment),e.name===this.WORD?this.nl().indent().append("pass"):this.nl().indent().append(i.name+" = "+this.format(this.readMapping[e.name],[t])),this.decIndent()}else this.nl().indent().append("inputs = input().split()"),this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("# game loop").nl(),this.indent().append("while True:"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' + "'+t+'" + ':" + ").replace(/" \+ "/g,"")),this.append(")");else{var o=n.split("\n");if(o.length>1||o[0].length>0){null==e||i||this.indent().append("# ").append(e).nl(),this.indent().append('print("').append(o[0]).append('")');for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildDumpLoop(n,n.name,t);this.nl().append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().indent().append(this.variableOutput(i))}},t.prototype.handleCall=function(n,t,e){var i=this,r=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[a]=this.functionDeclaration(n,r,this.defaultReturnStatement(e.type));var o=this.functionCall(n,e.type.name!==this.voidReturnType?e.name:"",e.name,p);this.nl().append(this.indentString(o,this.level))},t.prototype.buildReadStringLoop=function(n,t,e,i){var p=n.type.dimensions.length;if(0===i)return t;var a=p-i,o="";1===i?o=e+".append(input())":o=e+".append([])\n"+this.loopTpl;var s=r.render(t,{counter:this.sletters[a],start:0,end:this.formattedVarName(n.type.dimensions[a]),body:this.indentString(o,a+1)});return this.buildReadStringLoop(n,s,e+"["+this.sletters[a]+"]",i-1)},t.prototype.buildReadNonStringLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="["+this.format(this.readMapping[n.type.innerType.name],[this.sletters[i]])+" for "+this.sletters[i]+" in input().split()]",a="";return 1===e?1===n.type.dimensions.length?t+" = "+p:t+".append("+p+")":(a=e>2?t+".append([])\n"+this.buildReadNonStringLoop(n,t+"["+this.sletters[i]+"]",e-1):this.buildReadNonStringLoop(n,t,e-1),r.render(this.loopTpl,{counter:this.sletters[i],start:0,end:this.formattedVarName(n.type.dimensions[i]),body:this.indentString(a,1)}))},t.prototype.buildDumpLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="";return p=1===e?this.variableOutput(t+"["+this.sletters[i]+"]"):this.buildDumpLoop(n,t+"["+this.sletters[i]+"]",e-1),r.render(this.loopTpl,{counter:this.sletters[i],start:0,end:n.type.dimensions[i]?this.formattedVarName(n.type.dimensions[i]):"len("+t+")",body:this.indentString(p,1)})},t.prototype.variableOutput=function(n){return"print("+n+")"},t.prototype.functionSignatureSimple=function(n,t){return n+"("+t+")"},t.prototype.functionDeclaration=function(n,t,e){return"\ndef "+this.functionSignatureSimple(n,t)+':\n # Write your code here\n # To debug: print("Debug messages...", file=sys.stderr)\n '+e+"\n"},t.prototype.defaultReturnStatement=function(n){var t,e=((t={})[this.INT]=function(){return"0"},t[this.LONG]=function(){return"0"},t[this.FLOAT]=function(){return"0.0"},t[this.STRING]=function(){return"''"},t[this.WORD]=function(){return"''"},t[this.BOOL]=function(){return"False"},t[this.ARRAY]=function(n){return"[".repeat(n.dimensions.length)+"]".repeat(n.dimensions.length)},t);return n.name?"return "+e[n.name](n):"pass"},t.prototype.functionCall=function(n,t,e,i){return r.render("with redirect_stdout(sys.stderr):\n {{#type}}{{&res}} = {{/type}}{{&name}}({{¶ms}})",{name:n,type:t,res:e,params:i})},t.prototype.buildStatementData=function(n,t,e){var i=this,r=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=e.type.name?this.formatType(e.type):this.voidReturnType;return{functionNomenclature:"function",arrayNomenclature:"list",null:"None",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.formatType(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,p),functionFull:this.functionSignatureSimple(n,r),returnType:a}},t.prototype.commentLine=function(n){return"# "+n},t}(T),Nn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),An=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.caseConversion=T.CASE_CONVERSIONS.SNAKE,i.typeMapping=((t={})[i.BOOL]="Boolean",t[i.INT]="Integer",t[i.FLOAT]="Float",t[i.LONG]="Integer",t[i.WORD]="String",t[i.STRING]="String",t[i.ARRAY]="Array",t),i.defaultReturnMapping=((e={})[i.BOOL]="false",e[i.INT]="0",e[i.LONG]="0",e[i.FLOAT]="0.0",e[i.STRING]='""',e[i.WORD]='""',e[i.ARRAY]="[]",e),i}return Nn(t,n),t.prototype.begin=function(){if(this.input.turnBased&&(this.append("STDOUT.sync = true # DO NOT REMOVE"),this.nl()),this.input.hasStatement())for(var n=0,t=this.input.statement.split("\n");n1){for(var e=0,i=n;e1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===r?"":"+"+r)+"]"),p.type.name===this.FLOAT?this.append(".to_f"):p.type.name===this.BOOL?this.append(".to_i == 1"):p.type.name!==this.INT&&p.type.name!==this.LONG||this.append(".to_i")}},t.prototype.handleLoop=function(n){var t=n.param;this.nl().indent().append(t+".times do"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("end")},t.prototype.handleLoopLine=function(n){this.nl().indent().append('inputs = gets.split(" ")');var t=String.fromCharCode(this.loop),e=n.param;this.nl().indent().append("for "+t+" in 0..("+e+"-1)"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("end")},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("# game loop").nl(),this.indent().append("loop do"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r1||o[0].length>0){this.indent().append('puts "').append(o[0]).append('"'),null==e||i||this.append(" # ").append(e);for(var s=1;s")},t.prototype.handleCall=function(n,t,e){var i=this.functionDeclaration(n,t,e.type),r=this.functionCall(n,t,e),p=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[p]=i,this.nl().append(this.indentString(r,this.level))},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildArrayDumpLoop(n,t);this.nl().append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().indent().append(this.variableOutput(i))}},t.prototype.variableOutput=function(n){return"puts "+n},t.prototype.buildArrayDumpLoop=function(n,t){return 0===t?this.variableOutput("arr"):(n.type.dimensions.length===t?n.name:"arr")+".each { |arr| "+this.buildArrayDumpLoop(n,t-1)+" }"},t.prototype.functionCall=function(n,t,e){var i=this.functionSignatureFull(n,t);return"out_stream = $stdout\n$stdout = $stderr\n"+e.name+" = "+i+"\n$stdout = out_stream"},t.prototype.functionDeclaration=function(n,t,e){return"def "+this.functionSignatureFull(n,t)+'\n # Write your code here\n # To debug: $stderr.puts "Debug messages...";\n\n '+this.defaultReturnMapping[e.name]+"\nend\n"},t.prototype.functionSignatureFull=function(n,t){var e=this;return this.caseConversion(n)+"("+t.map((function(n){return e.formattedVarName(n.name)})).join(", ")+")"},t.prototype.buildStatementData=function(n,t,e){var i=this;return{functionNomenclature:"method",arrayNomenclature:"array",null:"nil",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.formatType(n.type)}})),functionName:this.caseConversion(n),functionSimple:this.functionSignatureFull(n,t),functionFull:this.functionSignatureFull(n,t),returnType:this.formatType(e.type)}},t.prototype.commentLine=function(n){return"# "+n},t}(T),Tn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),In=function(n){function t(){var t,e=null!==n&&n.apply(this,arguments)||this;return e.loop="i".charCodeAt(0),e.readMapping=((t={})[e.INT]="parse_input!(%s, i32)",t[e.LONG]="parse_input!(%s, i64)",t[e.FLOAT]="parse_input!(%s, f64)",t[e.WORD]="%s.trim().to_string()",t[e.STRING]="%s.trim_end().to_string()",t[e.BOOL]="parse_input!(%s, i32)",t),e.caseConversion=T.CASE_CONVERSIONS.SNAKE,e}return Tn(t,n),t.prototype.begin=function(n){var t=n.containsRead;if(this.append("use std::io;").nl().nl(),t&&(this.append("macro_rules! parse_input {").nl().incIndent(),this.indent().append("($x:expr, $t:ident) => ($x.trim().parse::<$t>().unwrap())").nl().decIndent(),this.indent().append("}").nl().nl()),this.input.hasStatement()){this.append("/**").nl();for(var e=0,i=this.input.statement.split("\n");e1){this.append("let mut input_line = String::new();").nl().indent(),this.append("io::stdin().read_line(&mut input_line).unwrap();").nl().indent(),this.append('let inputs = input_line.split(" ").collect::>();');for(var t=0,e=0,i=n;e1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===t?"":"+"+t)+"]";this.nl().indent().append("let ").append(e.name+" = "+this.format(this.readMapping[e.type.name],[i])+";"),null!=e.comment&&this.append(" // ").append(e.comment)}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop);this.nl().indent().append("for "+t+" in 0.."+n.param+" as usize {"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("}")},t.prototype.handleLoopLine=function(n){if(this.nl().indent(),1===n.children[0].children.length){this.append("let mut inputs = String::new();"),this.nl().indent(),this.append("io::stdin().read_line(&mut inputs).unwrap();");var t=String.fromCharCode(this.loop),e=n.children[0].children[0],i=e.children[0];return e.name===this.WORD?this.nl().indent().append("for ").append(i.name).append(" in inputs.split_whitespace() {"):this.nl().indent().append("for ").append(t).append(" in inputs.split_whitespace() {"),this.incIndent(),e.name!==this.WORD&&this.nl().indent().append("let "+i.name+" = "+this.format(this.readMapping[e.name],[t])+";"),this.decIndent(),void this.nl().indent().append("}")}this.append("let mut input_line = String::new();").nl().indent(),this.append("io::stdin().read_line(&mut input_line).unwrap();").nl().indent(),this.append("let inputs = input_line.split_whitespace().collect::>();"),this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop").nl(),this.indent().append("loop {"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r1||o[0].length>0){this.indent().append('println!("').append(o[0]).append('");'),null==e||i||this.append(" // ").append(e);for(var s=1;s")},t}(T),Cn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),_n=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.typeMapping=((t={})[i.BOOL]="Boolean",t[i.INT]="Int",t[i.LONG]="Long",t[i.STRING]="String",t[i.WORD]="String",t[i.FLOAT]="Float",t[i.ARRAY]="{{#dim}}Array[{{/dim}}{{{type}}}{{#dim}}]{{/dim}}",t),i.defaultReturnMapping=((e={})[i.BOOL]="false",e[i.INT]="0",e[i.LONG]="0",e[i.FLOAT]="0.0",e[i.STRING]='""',e[i.WORD]='""',e[i.ARRAY]="Array()",e),i.looplineUsed=!1,i.caseConversion=T.CASE_CONVERSIONS.CAMEL,i}return Cn(t,n),t.prototype.begin=function(){if(this.append("import math._").nl(),this.append("import scala.util._").nl(),this.append("import scala.io.StdIn._").nl(),this.nl(),this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n1){for(var e=0,i=n;e "+this.buildArrayReadLoop(n,t-1)+" }"},t.prototype.getParseFunction=function(n){return this.INT===n.name?"toInt":this.LONG===n.name?"toLong":this.FLOAT===n.name?"toFloat":this.BOOL===n.name?"toInt != 0":null},t.prototype.handleReadLine=function(n,t){for(var e=0,i=n;e1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===r?"":"+"+r)+")");var s=this.getParseFunction(n[0].type);null!=s&&this.append("."+s)}}this.nl().indent()},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop);this.nl().indent().append("for("+t+" <- 0 until "+this.formattedVarName(n.param)+") {"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("}")},t.prototype.handleLoopLine=function(n){var t=this.looplineUsed?"":"var ";this.looplineUsed=!0,this.nl().indent().append(t+'inputs = readLine split " "'),this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop").nl(),this.indent().append("while(true) {"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' + "'+t+'" + ':" + ").replace(/" \+ "/g,"")),this.append(")"),null==e||i||this.append(" // ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('println("').append(o[0]).append('")'),null==e||i||this.append(" // ").append(e);for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildArrayDumpLoop(n,t);this.nl().append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().indent().append(this.variableOutput(i))}},t.prototype.buildArrayDumpLoop=function(n,t){return 0===t?"println":(n.type.dimensions.length===t?n.name:"_")+".foreach("+this.buildArrayDumpLoop(n,t-1)+")"},t.prototype.variableOutput=function(n){return"println("+n+")"},t.prototype.handleCall=function(n,t,e){var i=this.functionDeclaration(n,t,e.type),r=this.functionCall(n,t,e),p=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[p]=i,this.nl().append(this.indentString(r,this.level))},t.prototype.functionCall=function(n,t,e){var i=this.functionSignatureSimple(n,t);return"val outStream = System.out\nSystem.setOut(System.err)\nval "+this.variableDefinition(e)+" = "+i+"\nSystem.setOut(outStream)"},t.prototype.functionDeclaration=function(n,t,e){return"\n def "+this.functionSignatureFull(n,t,e)+' = {\n // Write your code here\n // To debug: System.err.println("Debug messages...");\n\n return '+this.defaultReturnMapping[e.name]+"\n }\n"},t.prototype.functionSignatureSimple=function(n,t){var e=this;return n+"("+t.map((function(n){return e.formattedVarName(n.name)})).join(", ")+")"},t.prototype.functionSignatureFull=function(n,t,e){var i=this;return n+"("+t.map((function(n){return i.variableDefinition(n)})).join(", ")+"): "+this.formatType(e)},t.prototype.variableDefinition=function(n){return this.formattedVarName(n.name)+": "+this.formatType(n.type)},t.prototype.buildStatementData=function(n,t,e){var i=this;return{functionNomenclature:"method",arrayNomenclature:"array",null:"null",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.formatType(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,t),functionFull:this.functionSignatureFull(n,t,e.type),returnType:this.formatType(e.type)}},t}(T),wn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),Dn=function(n){function t(){var t,e,i=null!==n&&n.apply(this,arguments)||this;return i.loop="i".charCodeAt(0),i.typeMapping=((t={})[i.INT]="Int",t[i.FLOAT]="Float",t[i.LONG]="Long",t[i.WORD]="String",t[i.BOOL]="Bool",t[i.STRING]="String",t),i.readMapping=((e={})[i.INT]="Int(%s)!",e[i.LONG]="Int(%s)!",e[i.FLOAT]="Float(%s)!",e[i.BOOL]='%s != "0"',e[i.WORD]="%s",e[i.STRING]="%s",e),i.caseConversion=T.CASE_CONVERSIONS.CAMEL,i.methodCaseConversion=T.CASE_CONVERSIONS.CAMEL,i.loopTpl="for {{&counter}} in {{&start}}..<{{&end}} {\n{{&body}}\n}",i.inputsCounter=0,i.voidReturnType="Void",i}return wn(t,n),t.prototype.begin=function(){if(this.append("import Glibc").nl(),this.append("import Foundation").nl().nl(),this.append("public struct StderrOutputStream: TextOutputStream {").nl().incIndent(),this.indent().append("public mutating func write(_ string: String) { fputs(string, stderr) }").nl().decIndent(),this.append("}").nl(),this.append("public var errStream = StderrOutputStream()").nl().nl(),this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n1){var t="inputs"+(++this.inputsCounter>1?this.inputsCounter:"");this.append("let "+t+' = (readLine()!).split(separator: " ").map(String.init)');for(var e=0,i=0,r=n;i=1&&this.append(this.variableDefinition(s));var h="";h=s.type.innerType.name===this.STRING?this.buildReadStringLoop(s,this.loopTpl,s.name,l):this.buildReadNonStringLoop(s,s.name,l),this.nl().append(this.indentString(h,this.level))}}},t.prototype.handleReadLine=function(n){for(var t=0;t1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===t?"":"+"+t)+"]"]);this.nl().indent().append("let ").append(e.name).append(" = ").append(i),null!=e.comment&&this.append(" // ").append(e.comment)}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop),e=!1,i=parseInt(n.param,10);isNaN(i)?(i="("+n.param+"-1)",this.nl().indent().append("if "+n.param+" > 0 {"),this.incIndent(),e=!0):i--,this.nl().indent().append("for "+t+" in 0..."+i+" {"),this.incIndent(),this.loop++;var r=this.inputsCounter;this.inputsCounter=0,this.write(n.children[0]),this.inputsCounter=r,this.loop--,this.decIndent(),this.nl().indent().append("}"),e&&(this.decIndent(),this.nl().indent().append("}"))},t.prototype.handleLoopLine=function(n){if(this.nl().indent(),1===n.children[0].children.length){var t=String.fromCharCode(this.loop),e=n.children[0].children[0],i=e.children[0];e.name===this.WORD?(this.append("for "+i.name+' in ((readLine()!).split(separator: " ").map(String.init)) {'),this.nl().indent().append("}")):(this.append("for "+t+' in ((readLine()!).split(separator: " ").map(String.init)) {').incIndent(),this.nl().indent().append("let "+i.name+" = "+this.format(this.readMapping[e.name],[t])).decIndent(),this.nl().indent().append("}"))}else this.append('let inputs = (readLine()!).split(separator: " ").map(String.init)'),this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop").nl(),this.indent().append("while true {"),this.incIndent();var t=this.inputsCounter;this.inputsCounter=0;for(var e=0,i=n;e").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r1||o[0].length>0){this.indent().append('print("').append(o[0]).append('");'),null==e||i||this.indent().append(" // ").append(e).nl();for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildDumpLoop(n,n.name,t);this.nl().append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().indent().append(this.variableOutput(i))}},t.prototype.handleCall=function(n,t,e){var i=this,r=t.map((function(n){return"_ "+i.formattedVarName(n.name)+": "+i.typeDeclaration(n.type)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[a]=this.functionDeclaration(n,r,e.name?this.typeDeclaration(e.type):this.voidReturnType);var o=this.functionCall(n,e.type.name!==this.voidReturnType?e.type.name:"",e.name,p);this.nl().append(this.indentString(o,this.level))},t.prototype.buildReadStringLoop=function(n,t,e,i){var p=n.type.dimensions.length;if(0===i)return t;var a=p-i,o="",s=e+"["+this.sletters[a]+"]";o=1===i?s+" = readLine()!":this.loopTpl;var l=r.render(t,{counter:this.sletters[a],start:0,end:this.formattedVarName(n.type.dimensions[a]),body:this.indentString(o,a+1)});return this.buildReadStringLoop(n,l,s,i-1)},t.prototype.buildReadNonStringLoop=function(n,t,e){var i=n.type.dimensions.length-e,p='(readLine()!).split(separator: " ").map(String.init)';n.type.innerType.name!==this.WORD&&(p='(readLine()!).split(separator: " ").map{'+this.typeMapping[n.type.innerType.name]+"(String($0))!}");var a="",o=t+"["+this.sletters[i]+"]";return 1===e?(n.type.dimensions.length,t+" = "+p):(a=this.buildReadNonStringLoop(n,o,e-1),r.render(this.loopTpl,{counter:this.sletters[i],start:0,end:this.formattedVarName(n.type.dimensions[i]),body:this.indentString(a,1)}))},t.prototype.buildDumpLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="";return p=1===e?this.variableOutput(t+"["+this.sletters[i]+"]"):this.buildDumpLoop(n,t+"["+this.sletters[i]+"]",e-1),r.render(this.loopTpl,{counter:this.sletters[i],start:0,end:n.type.dimensions[i]?this.formattedVarName(n.type.dimensions[i]):t+".count",body:this.indentString(p,1)})},t.prototype.variableOutput=function(n){return"print("+n+")"},t.prototype.functionSignatureSimple=function(n,t){return n+"("+t+")"},t.prototype.functionSignatureFull=function(n,t,e){return this.functionSignatureSimple(n,t)+" -> "+e},t.prototype.functionDeclaration=function(n,t,e){return"\nfunc "+this.functionSignatureFull(n,t,e)+' {\n // Write your code here\n // To debug: print("Debug messages...", to: &errStream)\n\n return '+e+"()\n}\n"},t.prototype.functionCall=function(n,t,e,i){return r.render("var stdoutRescue = stdout\nstdout = stderr\n{{#type}}let {{&res}} = {{/type}}{{&name}}({{¶ms}})\nstdout = stdoutRescue",{name:n,type:t,res:e,params:i})},t.prototype.typeDeclaration=function(n){return n.name===this.ARRAY?"[".repeat(n.dimensions.length)+this.typeMapping[n.innerType.name]+"]".repeat(n.dimensions.length):this.typeMapping[n.name]},t.prototype.variableDefinition=function(n){return(n.type.name===this.ARRAY?"var ":"let ")+n.name+" = "+this.typeDeclaration(n.type)+"()"},t.prototype.buildStatementData=function(n,t,e){var i=this,r=t.map((function(n){return i.formattedVarName(n.name)+": "+i.typeDeclaration(n.type)})).join(", "),p=t.map((function(n){return i.formattedVarName(n.name)})).join(", "),a=e.name?this.typeDeclaration(e.type):this.voidReturnType;return{functionNomenclature:"function",arrayNomenclature:"array",null:"nil",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.typeDeclaration(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,p),functionFull:this.functionSignatureFull(n,r,a),returnType:a}},t}(T),En=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),Mn=function(n){function t(){var t,e,i,r=null!==n&&n.apply(this,arguments)||this;return r.loop="i".charCodeAt(0),r.typeMapping=((t={})[r.BOOL]="boolean",t[r.INT]="number",t[r.FLOAT]="number",t[r.LONG]="number",t[r.WORD]="string",t[r.STRING]="string",t[r.ARRAY]="{{&type}}{{#dim}}[]{{/dim}}",t),r.readMapping=((e={})[r.INT]="parseInt(%s)",e[r.LONG]="parseInt(%s)",e[r.FLOAT]="parseFloat(%s)",e[r.BOOL]="%s !== '0'",e[r.WORD]="%s",e[r.STRING]="%s",e),r.defaultReturnMapping=((i={})[r.BOOL]=function(){return"false"},i[r.INT]=function(){return"0"},i[r.LONG]=function(){return"0"},i[r.FLOAT]=function(){return"0.0"},i[r.STRING]=function(){return"''"},i[r.WORD]=function(){return"''"},i[r.ARRAY]=function(n){return"[".repeat(n.dimensions.length)+"]".repeat(n.dimensions.length)},i),r.methodCaseConversion=T.CASE_CONVERSIONS.CAMEL,r.caseConversion=T.CASE_CONVERSIONS.CAMEL,r.reservedWords=["abstract","arguments","await","boolean","break","byte","case","catch","char","class","const","continue","debugger","default","delete","do","double","else","enum","eval","export","extends","false","final","finally","float","for","function","goto","if","implements","import","in","instanceof","int","interface","let","long","native","new","null","package","private","protected","public","return","short","static","super","switch","synchronized","this","throw","throws","transient","true","try","typeof","var","void","volatile","while","with","yield","eval","uneval","isFinite","isNaN","parseFloat","parseInt","decodeURI","decodeURIComponent","encodeURI","encodeURIComponent","escape","unescape"],r.loopTpl="for (let {{&counter}} = {{&start}}; {{&counter}} < {{&end}}; {{&counter}}++) {\n{{&body}}\n}",r.voidReturnType="",r}return En(t,n),t.prototype.begin=function(){if(this.input.hasStatement()){this.append("/**").nl();for(var n=0,t=this.input.statement.split("\n");n1){this.append("var inputs: string[] = readline().split(' ');");for(var t=0,e=0,i=n;e1||o.type.innerType.name===this.STRING?(this.append("const "+this.variableDefinition(o)+" = "+"[".repeat(s)+"]".repeat(s)+";"),this.nl().append(this.indentString(l,this.level))):this.append("const "+l)}}},t.prototype.handleReadLine=function(n){for(var t=0;t1?n.length+"*":"")+i+(0===t?"":"+"+t),p=this.format(this.readMapping[e.type.name],["inputs["+r+"]"]),a=this.variableDefinition(e);this.nl().indent().append("const "+a+" = "+p+";"),null!=e.comment&&this.append("// ").append(e.comment)}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop);this.nl().indent().append("for (let "+t+" = 0; "+t+" < "+n.param+"; "+t+"++) {"),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("}")},t.prototype.handleLoopLine=function(n){this.nl().indent(),this.append("var inputs: string[] = readline().split(' ');"),this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl(),this.nl().indent().append("// game loop").nl(),this.indent().append("while (true) {"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?" + '"+t+"' + ":" + ").replace(/' \+ '/g,"")),this.append(");"),null==e||i||this.append(" // ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append("console.log('").append(o[0]).append("');"),null==e||i||this.indent().append(" // ").append(e).nl();for(var s=1;s")},t.prototype.handleDump=function(n){if("string"==typeof n)this.nl().indent().append(this.variableOutput(n));else if(n.type.name===this.ARRAY){var t=n.type.dimensions.length,e=this.buildDumpLoop(n,n.name,t);this.nl().append(this.indentString(e,this.level))}else{var i=this.formattedVarName(n.name);this.nl().indent().append(this.variableOutput(i))}},t.prototype.handleCall=function(n,t,e){var i=this.functionDeclaration(n,t,e.type),r=this.functionCall(n,t,e),p=this.code.findIndex((function(n){return"{{{solve}}}"===n}));this.code[p]=i,this.nl().append(this.indentString(r,this.level))},t.prototype.buildReadStringLoop=function(n,t,e,i){var p=n.type.dimensions.length;if(0===i)return t;var a=p-i,o="",s=e+"["+this.sletters[a]+"]";o=1===i?s+" = readline();":this.loopTpl;var l=r.render(t,{counter:this.sletters[a],start:0,end:this.formattedVarName(n.type.dimensions[a]),body:this.indentString(o,a+1)});return this.buildReadStringLoop(n,l,s,i-1)},t.prototype.buildReadNonStringLoop=function(n,t,e){var i=n.type.dimensions.length-e,p="";if(1===e){var a=n.type.innerType.name!==this.WORD?".map("+this.sletters[i+1]+" => "+this.format(this.readMapping[n.type.innerType.name],[this.sletters[i+1]])+")":"",o=parseInt(n.type.dimensions[i],10),s="readline().split(' ')"+a+(isNaN(o)?".slice(0, "+n.type.dimensions[i]+")":"")+";";if(e===t)return this.variableDefinition(n)+" = "+s;for(var l="",h=0;h console.error(chunk);\nconst "+this.variableDefinition(e)+" = "+n+"("+r+");\nprocess.stdout.write = oldWrite;"},t.prototype.variableDefinition=function(n){return this.formattedVarName(n.name)+": "+this.formatType(n.type)},t.prototype.buildStatementData=function(n,t,e){var i=this;return{functionNomenclature:"function",arrayNomenclature:"array",null:"null",parameters:t.map((function(n){return{name:n.name,label:i.formattedVarName(n.name),type:i.formatType(n.type)}})),functionName:n,functionSimple:this.functionSignatureSimple(n,t,e.type),functionFull:this.functionSignatureSimple(n,t,e.type),returnType:this.formatType(e.type)}},t}(T),Vn=function(){var n=function(t,e){return(n=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(n,t){n.__proto__=t}||function(n,t){for(var e in t)t.hasOwnProperty(e)&&(n[e]=t[e])})(t,e)};return function(t,e){function i(){this.constructor=t}n(t,e),t.prototype=null===e?Object.create(e):(i.prototype=e.prototype,new i)}}(),Fn=function(n){function t(){var t,e=null!==n&&n.apply(this,arguments)||this;return e.loop="i".charCodeAt(0),e.typeMapping=((t={})[e.INT]="Integer",t[e.LONG]="Long",t[e.STRING]="String",t[e.WORD]="String",t[e.FLOAT]="Double",t),e.SPLITLINE_TOKEN="{{Dim inputs as String()}}",e.EVIL_TOKEN="@@ VB.NET is magic @@",e.evilIndentation=0,e.needSplitline=0,e}return Vn(t,n),t.prototype.begin=function(){if(this.append("Module ").append(this.input.turnBased?"Player":"Solution").nl(),this.input.hasStatement())for(var n=0,t=this.input.statement.split("\n");n1?"Dim inputs as String()":"");var t=new Array(this.evilIndentation*this.indentSize+1).join(" ");n=n.replace(this.EVIL_TOKEN,1===this.needSplitline?t+"Dim inputs as String()":""),this.code=[n]},t.prototype.handleRead=function(n){if(n.length>1){0===this.needSplitline&&(this.evilIndentation=this.level,this.nl().append(this.EVIL_TOKEN)),this.needSplitline++;for(var t=0,e=n;t1?n.length+"*":"")+String.fromCharCode(this.loop-1)+(0===r?"":"+"+r)).append(")")}},t.prototype.handleLoop=function(n){var t=String.fromCharCode(this.loop),e=parseInt(n.param,10);isNaN(e)?e=n.param+"-1":e--,this.nl().indent().append("For "+t+" as Integer = 0 To "+e),this.incIndent(),this.loop++,this.write(n.children[0]),this.loop--,this.decIndent(),this.nl().indent().append("Next"),this.nl()},t.prototype.handleLoopLine=function(n){0===this.needSplitline&&(this.evilIndentation=this.level,this.nl().append(this.EVIL_TOKEN)),this.nl().indent().append('inputs = Console.ReadLine().Split(" ")'),this.needSplitline++,this.handleLoop(n)},t.prototype.handleGameLoop=function(n){this.nl().indent().append("' game loop").nl(),this.indent().append("While True"),this.incIndent();for(var t=0,e=n;t").nl();var i=null!=e&&(e.length>50||e.split("\n").length>1);if(i){for(var r=0,p=e.split("\n");r0?' & "'+t+'" & ':" & ").replace(/" & "/g,"")),this.append(")"),null==e||i||this.append(" ' ").append(e);else{var o=n.split("\n");if(o.length>1||o[0].length>0){this.indent().append('Console.WriteLine("').append(o[0]).append('")'),null==e||i||this.append(" ' ").append(e);for(var s=1;s")},t.prototype.commentLine=function(n){return"' "+n},t}(T);e.d(t,"generateStub",(function(){return Wn})),e.d(t,"generateStatementData",(function(){return kn})),e.d(t,"generateStubAndStatementData",(function(){return $n})),e.d(t,"parseStubInput",(function(){return Yn})),e.d(t,"generateStatement",(function(){return Un}));var xn=function(){return(xn=Object.assign||function(n){for(var t,e=1,i=arguments.length;e