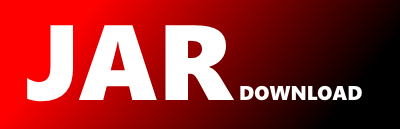
astra.astra-db-java.1.5.0.source-code.overview.html Maven / Gradle / Ivy
Java Client Library for DataAPI
Overview
This client library provides a simplified way to interact with Data API with DataStax AstraDB or local deployments ( Stargate + Cassandra).
More resources can be found:
- In Datastax Astra Client documentation page
- In the github repository with an {@code examples} section.
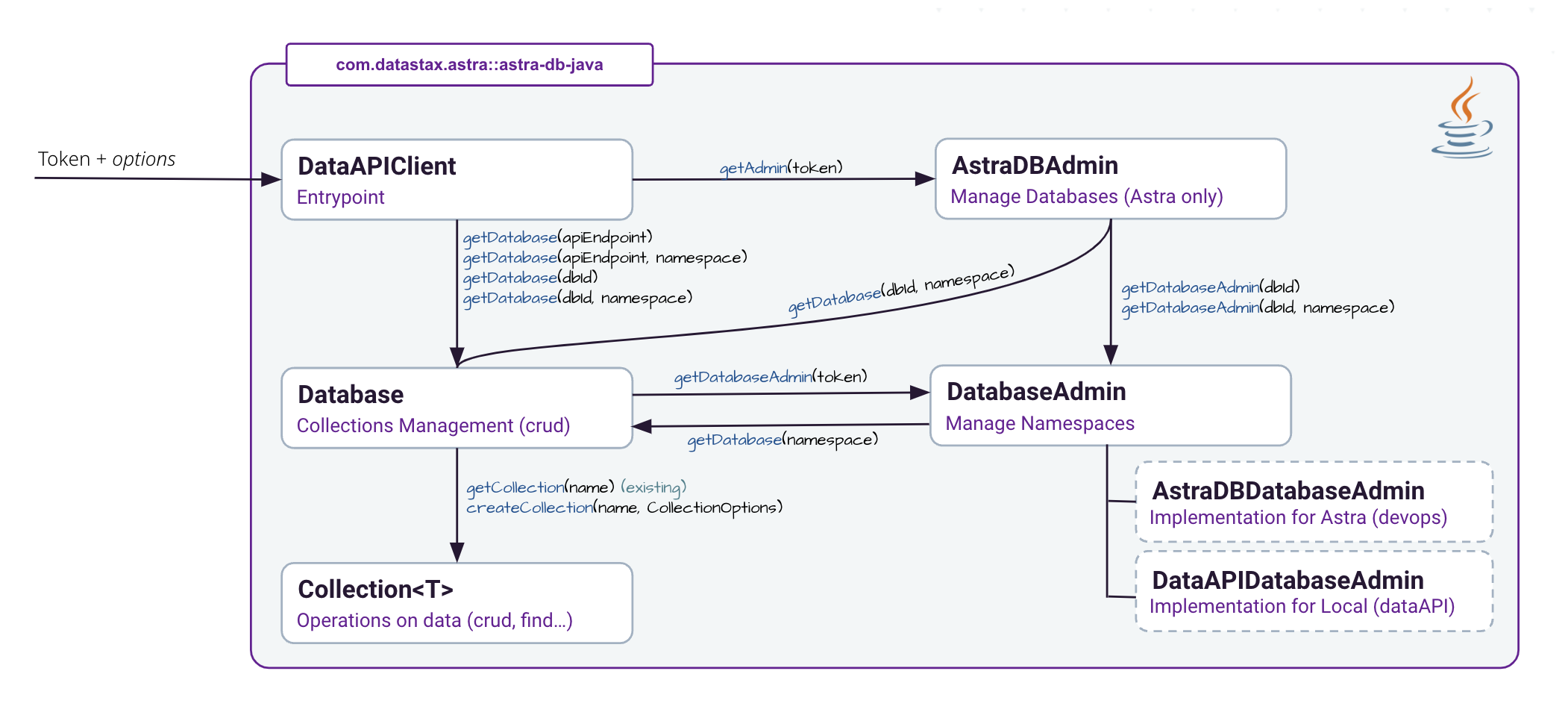
Installation
For Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>com.datastax.astra</groupId>
<artifactId>astra-db-java</artifactId>
<version>1.0.0-SNAPSHOT</version>
</dependency>
QuickStart
import com.datastax.astra.client.DataAPIClient;
import com.datastax.astra.client.Collection;
import com.datastax.astra.client.Database;
import com.datastax.astra.client.model.Document;
import com.datastax.astra.client.model.FindIterable;
import java.util.List;
import static com.datastax.astra.client.model.Filters.eq;
import static com.datastax.astra.client.model.SimilarityMetric.cosine;
public class GettingStarted {
public static void main(String[] args) {
// Initializing client with a token
DataAPIClient client = new DataAPIClient("my_token");
// Accessing the Database through the HTTP endpoint
Database db = client.getDatabase("http://db-region.apps.astra.datastax.com");
// Create collection with vector support
Collection<Document> col = db.createCollection("demo", 2, cosine);
// Insert documents with embeddings
col.insertMany(List.of(
new Document("doc1").vector(new float[]{.1f, 0.2f}).append("key", "value1"),
new Document().id("doc2").vector(new float[]{.2f, 0.4f}).append("hello", "world"),
new Document("doc3").vector(new float[]{.5f, 0.6f}).append("key", "value1"))
);
// Semantic Search with metadata filtering
FindIterable<Document> docs = col.find(
eq("key", "value1"), // metadata filter
new float[] {.5f, .5f}, //vector
10); // maxRecord
// Iterate over and print your results
for (Document doc : docs) System.out.println(doc);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy