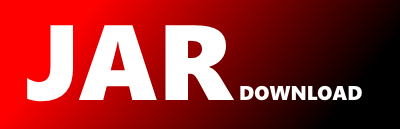
xsuite.xmaven.bash-maven-plugin.1.0.4.source-code.test-framework.sh Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bash-maven-plugin Show documentation
Show all versions of bash-maven-plugin Show documentation
Bash Maven Plugin is used to generate documentation as well as to run unit test for bash scripts.
#!/bin/bash
###
# #%L
# Bash Unit Test Plugin
# %%
# Copyright (C) 2016 - 2017 Exadatum Software Services Pvt. Ltd.
# %%
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# #L%
###
###############################################################################
# Documentation #
###############################################################################
# #
# Description #
# : #
# #
###############################################################################
# Function Definitions #
###############################################################################
###
# Integer representation of boolean true
# @Type : Integer
# @Final : true
BOOLEAN_TRUE=1
###
# Integer representation of boolean false
# @Type : Integer
# @Final : true
BOOLEAN_FALSE=0
###
# Constant to represent flag to fail in case of
# some error situation.
# @Type : Integer
# @Final : true
FAIL_ON_ERROR=1
###
# Constant to represent exit code returned by
# successful execution of command/function
# @Type : Integer
# @Final : true
EXIT_CODE_SUCCESS=0
###
# Constant to represent exit code returned by
# un-successful execution of command/function
# @Type : Integer
# @Final : true
EXIT_CODE_FAIL=1
###
# Log any message
#
# Arguments:
# message*
# - string message
#
function fn_test_log(){
#Message to be logged should be passed as first argument to
#this function
message=$1
#Print message along with the current timestamp
echo "[`date`]" ${message}
}
###
# Log information message
#
# Arguments:
# message*
# - string message
#
function fn_test_log_info(){
#Message to be logged should be passed as first argument to
#this function
message=$1
#Log message with INFO label
fn_test_log "INFO ${message}"
}
###
# Log warning message
#
# Arguments:
# message*
# - string message
#
function fn_test_log_warn(){
#Message to be logged should be passed as first argument to
#this function
message=$1
#Log message with WARN label
fn_test_log "WARN ${message}"
}
###
# Log error message
#
# Arguments:
# message*
# - string message
#
function fn_test_log_error(){
#Message to be logged should be passed as first argument to
#this function
message=$1
#Log message with ERROR label
fn_test_log "ERROR ${message}"
}
TEST_FAILED_EXIT_CODE=255
TEST_CASE_NAME=
TEST_CASE_MESSAGE=
TEST_CASE_RESULT=
TEST_CASE_TIME_TAKEN=
function fn_test_exit(){
exit_code=$1
if [ "${exit_code}" == "${TEST_FAILED_EXIT_CODE}" ]
then
TEST_CASE_RESULT="FAILED"
else
TEST_CASE_RESULT "UNEXPECTED_EXIT"
fi
}
function run_test(){
# USER _####_ as concatenation string to split the message
#reset test variables for every test case
TEST_CASE_NAME="$1"
TEST_CASE_RESULT="SUCCESSFUL"
TEST_CASE_TIME_TAKEN=""
TEST_CASE_MESSAGE=""
function_name=$1
start_time=`date +%s`
"${function_name}" "${@:2}"
end_time=`date +%s`
TEST_CASE_TIME_TAKEN="$((end_time - start_time))"
echo "TEST_RESULT=${TEST_CASE_RESULT}_####_${TEST_CASE_MESSAGE}_####_${TEST_CASE_NAME}_####_${TEST_CASE_TIME_TAKEN}"
}
function test_assert_variable_is_set(){
variable_name=$1
variable_value=$2
if [ "${variable_value}" == "" ]
then
TEST_CASE_MESSAGE="${variable_name} variable is not set"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_equals(){
message="$1"
obj1="$2"
obj2="$3"
if [ "${obj1}" != "${obj2}" ]
then
TEST_CASE_MESSAGE="${message}"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_local_file_exists(){
file_path="$1"
test_assert_variable_is_set "file_path" "${file_path}"
if [ ! -f "${file_path}" ];
then
TEST_CASE_MESSAGE="File ${file_path} does not exists"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_local_directory_exists(){
dir_path="$1"
test_assert_variable_is_set "dir_path" "${dir_path}"
if [ ! -d "${dir_path}" ];
then
TEST_CASE_MESSAGE="Directory ${dir_path} does not exists"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_local_file_not_empty(){
file_to_be_checked="${1}"
test_assert_variable_is_set "file_to_be_checked" "${file_to_be_checked}"
test_assert_local_file_exists "${file_to_be_checked}" "${BOOLEAN_TRUE}"
if [ ! -s "${file_to_be_checked}" ];
then
TEST_CASE_MESSAGE="File ${file_to_be_checked} is empty"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_local_executable_exists() {
executable=$1
test_assert_variable_is_set "executable" "${executable}"
if ! type "${executable}" > /dev/null;
then
TEST_CASE_MESSAGE="Executable ${executable} does not exists"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_hadoop_directory_exists(){
test_assert_local_executable_exists "hdfs"
dir_path="$1"
test_assert_variable_is_set "dir_path" "${dir_path}"
hdfs dfs -test -e "${dir_path}"
exit_code=$?
if [ "${exit_code}" != "$EXIT_CODE_SUCCESS" ]
then
TEST_CASE_MESSAGE="Directory ${dir_path} does not exists"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_hadoop_directory_does_not_exist(){
test_assert_local_executable_exists "hdfs"
dir_path="$1"
test_assert_variable_is_set "dir_path" "${dir_path}"
hdfs dfs -test -e "${dir_path}"
exit_code=$?
if [ "${exit_code}" == "$EXIT_CODE_SUCCESS" ]
then
TEST_CASE_MESSAGE="Directory ${dir_path} exists"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_hadoop_file_exists(){
test_assert_local_executable_exists "hdfs"
file_path="$1"
test_assert_variable_is_set "file_path" "${file_path}"
hdfs dfs -test -e "${file_path}"
exit_code=$?
if [ "${exit_code}" != "$EXIT_CODE_SUCCESS" ]
then
TEST_CASE_MESSAGE="File ${file_path} does not exists"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_hadoop_file_does_not_exist(){
test_assert_local_executable_exists "hdfs"
file_path="$1"
test_assert_variable_is_set "file_path" "${file_path}"
hdfs dfs -test -e "${file_path}"
exit_code=$?
if [ "${exit_code}" == "$EXIT_CODE_SUCCESS" ]
then
TEST_CASE_MESSAGE="File ${file_path} exists"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
fi
}
function test_assert_hadoop_file_not_empty(){
file_to_be_checked="${1}"
test_assert_variable_is_set "file_to_be_checked" "${file_to_be_checked}"
test_assert_hadoop_file_exists "${file_to_be_checked}"
TEST_CASE_MESSAGE="Yet to be implemented"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
}
function test_assert_hadoop_directory_not_empty(){
directory_to_be_checked="${1}"
test_assert_variable_is_set "directory_to_be_checked" "${directory_to_be_checked}"
test_assert_hadoop_file_exists "${directory_to_be_checked}"
TEST_CASE_MESSAGE="Yet to be implemented"
fn_test_exit ${TEST_FAILED_EXIT_CODE}
}
###############################################################################
# End #
###############################################################################
© 2015 - 2025 Weber Informatics LLC | Privacy Policy