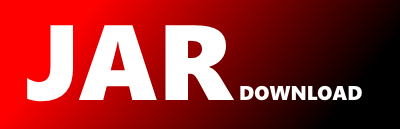
META-INF.resources.demo.client.js.FaceApi.js Maven / Gradle / Ivy
The newest version!
//
// Autogenerated by Thrift Compiler (0.13.0)
//
// DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
//
if (typeof Int64 === 'undefined' && typeof require === 'function') {
var Int64 = require('node-int64');
}
//HELPER FUNCTIONS AND STRUCTURES
FaceApi_compare2Face_args = function(args) {
this.imgData1 = null;
this.facePos1 = null;
this.imgData2 = null;
this.facePos2 = null;
if (args) {
if (args.imgData1 !== undefined && args.imgData1 !== null) {
this.imgData1 = args.imgData1;
}
if (args.facePos1 !== undefined && args.facePos1 !== null) {
this.facePos1 = new CodeInfo(args.facePos1);
}
if (args.imgData2 !== undefined && args.imgData2 !== null) {
this.imgData2 = args.imgData2;
}
if (args.facePos2 !== undefined && args.facePos2 !== null) {
this.facePos2 = new CodeInfo(args.facePos2);
}
}
};
FaceApi_compare2Face_args.prototype = {};
FaceApi_compare2Face_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData1 = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.facePos1 = new CodeInfo();
this.facePos1.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRING) {
this.imgData2 = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.STRUCT) {
this.facePos2 = new CodeInfo();
this.facePos2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compare2Face_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compare2Face_args');
if (this.imgData1 !== null && this.imgData1 !== undefined) {
output.writeFieldBegin('imgData1', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData1);
output.writeFieldEnd();
}
if (this.facePos1 !== null && this.facePos1 !== undefined) {
output.writeFieldBegin('facePos1', Thrift.Type.STRUCT, 2);
this.facePos1.write(output);
output.writeFieldEnd();
}
if (this.imgData2 !== null && this.imgData2 !== undefined) {
output.writeFieldBegin('imgData2', Thrift.Type.STRING, 3);
output.writeBinary(this.imgData2);
output.writeFieldEnd();
}
if (this.facePos2 !== null && this.facePos2 !== undefined) {
output.writeFieldBegin('facePos2', Thrift.Type.STRUCT, 4);
this.facePos2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_compare2Face_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
this.ex3 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof NotFaceDetectedException) {
this.ex2 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex3 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
if (args.ex3 !== undefined && args.ex3 !== null) {
this.ex3 = args.ex3;
}
}
};
FaceApi_compare2Face_result.prototype = {};
FaceApi_compare2Face_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.DOUBLE) {
this.success = input.readDouble().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new NotFaceDetectedException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRUCT) {
this.ex3 = new ServiceRuntimeException();
this.ex3.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compare2Face_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compare2Face_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.DOUBLE, 0);
output.writeDouble(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
if (this.ex3 !== null && this.ex3 !== undefined) {
output.writeFieldBegin('ex3', Thrift.Type.STRUCT, 3);
this.ex3.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_compareCode_args = function(args) {
this.code1 = null;
this.code2 = null;
if (args) {
if (args.code1 !== undefined && args.code1 !== null) {
this.code1 = args.code1;
}
if (args.code2 !== undefined && args.code2 !== null) {
this.code2 = args.code2;
}
}
};
FaceApi_compareCode_args.prototype = {};
FaceApi_compareCode_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.code1 = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.code2 = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compareCode_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compareCode_args');
if (this.code1 !== null && this.code1 !== undefined) {
output.writeFieldBegin('code1', Thrift.Type.STRING, 1);
output.writeBinary(this.code1);
output.writeFieldEnd();
}
if (this.code2 !== null && this.code2 !== undefined) {
output.writeFieldBegin('code2', Thrift.Type.STRING, 2);
output.writeBinary(this.code2);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_compareCode_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_compareCode_result.prototype = {};
FaceApi_compareCode_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.DOUBLE) {
this.success = input.readDouble().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compareCode_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compareCode_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.DOUBLE, 0);
output.writeDouble(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_compareCodes_args = function(args) {
this.code1 = null;
this.codes = null;
if (args) {
if (args.code1 !== undefined && args.code1 !== null) {
this.code1 = args.code1;
}
if (args.codes !== undefined && args.codes !== null) {
this.codes = Thrift.copyList(args.codes, [CodeInfo]);
}
}
};
FaceApi_compareCodes_args.prototype = {};
FaceApi_compareCodes_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.code1 = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.LIST) {
this.codes = [];
var _rtmp311 = input.readListBegin();
var _size10 = _rtmp311.size || 0;
for (var _i12 = 0; _i12 < _size10; ++_i12) {
var elem13 = null;
elem13 = new CodeInfo();
elem13.read(input);
this.codes.push(elem13);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compareCodes_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compareCodes_args');
if (this.code1 !== null && this.code1 !== undefined) {
output.writeFieldBegin('code1', Thrift.Type.STRING, 1);
output.writeBinary(this.code1);
output.writeFieldEnd();
}
if (this.codes !== null && this.codes !== undefined) {
output.writeFieldBegin('codes', Thrift.Type.LIST, 2);
output.writeListBegin(Thrift.Type.STRUCT, this.codes.length);
for (var iter14 in this.codes) {
if (this.codes.hasOwnProperty(iter14)) {
iter14 = this.codes[iter14];
iter14.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_compareCodes_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [null]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_compareCodes_result.prototype = {};
FaceApi_compareCodes_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp316 = input.readListBegin();
var _size15 = _rtmp316.size || 0;
for (var _i17 = 0; _i17 < _size15; ++_i17) {
var elem18 = null;
elem18 = input.readDouble().value;
this.success.push(elem18);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compareCodes_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compareCodes_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.DOUBLE, this.success.length);
for (var iter19 in this.success) {
if (this.success.hasOwnProperty(iter19)) {
iter19 = this.success[iter19];
output.writeDouble(iter19);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_compareFaces_args = function(args) {
this.code = null;
this.imgData = null;
this.faceNum = null;
if (args) {
if (args.code !== undefined && args.code !== null) {
this.code = args.code;
}
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
if (args.faceNum !== undefined && args.faceNum !== null) {
this.faceNum = args.faceNum;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field faceNum is unset!');
}
}
};
FaceApi_compareFaces_args.prototype = {};
FaceApi_compareFaces_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.code = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.faceNum = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compareFaces_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compareFaces_args');
if (this.code !== null && this.code !== undefined) {
output.writeFieldBegin('code', Thrift.Type.STRING, 1);
output.writeBinary(this.code);
output.writeFieldEnd();
}
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 2);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
if (this.faceNum !== null && this.faceNum !== undefined) {
output.writeFieldBegin('faceNum', Thrift.Type.I32, 3);
output.writeI32(this.faceNum);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_compareFaces_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
this.ex3 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof NotFaceDetectedException) {
this.ex2 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex3 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CompareResult(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
if (args.ex3 !== undefined && args.ex3 !== null) {
this.ex3 = args.ex3;
}
}
};
FaceApi_compareFaces_result.prototype = {};
FaceApi_compareFaces_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CompareResult();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new NotFaceDetectedException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRUCT) {
this.ex3 = new ServiceRuntimeException();
this.ex3.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compareFaces_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compareFaces_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
if (this.ex3 !== null && this.ex3 !== undefined) {
output.writeFieldBegin('ex3', Thrift.Type.STRUCT, 3);
this.ex3.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_compareFeatures_args = function(args) {
this.code1 = null;
this.codes = null;
if (args) {
if (args.code1 !== undefined && args.code1 !== null) {
this.code1 = args.code1;
}
if (args.codes !== undefined && args.codes !== null) {
this.codes = Thrift.copyList(args.codes, [null]);
}
}
};
FaceApi_compareFeatures_args.prototype = {};
FaceApi_compareFeatures_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.code1 = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.LIST) {
this.codes = [];
var _rtmp321 = input.readListBegin();
var _size20 = _rtmp321.size || 0;
for (var _i22 = 0; _i22 < _size20; ++_i22) {
var elem23 = null;
elem23 = input.readBinary().value;
this.codes.push(elem23);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compareFeatures_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compareFeatures_args');
if (this.code1 !== null && this.code1 !== undefined) {
output.writeFieldBegin('code1', Thrift.Type.STRING, 1);
output.writeBinary(this.code1);
output.writeFieldEnd();
}
if (this.codes !== null && this.codes !== undefined) {
output.writeFieldBegin('codes', Thrift.Type.LIST, 2);
output.writeListBegin(Thrift.Type.STRING, this.codes.length);
for (var iter24 in this.codes) {
if (this.codes.hasOwnProperty(iter24)) {
iter24 = this.codes[iter24];
output.writeBinary(iter24);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_compareFeatures_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [null]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_compareFeatures_result.prototype = {};
FaceApi_compareFeatures_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp326 = input.readListBegin();
var _size25 = _rtmp326.size || 0;
for (var _i27 = 0; _i27 < _size25; ++_i27) {
var elem28 = null;
elem28 = input.readDouble().value;
this.success.push(elem28);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_compareFeatures_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_compareFeatures_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.DOUBLE, this.success.length);
for (var iter29 in this.success) {
if (this.success.hasOwnProperty(iter29)) {
iter29 = this.success[iter29];
output.writeDouble(iter29);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectAndCompare2Face_args = function(args) {
this.imgData1 = null;
this.detectRect1 = null;
this.imgData2 = null;
this.detectRect2 = null;
if (args) {
if (args.imgData1 !== undefined && args.imgData1 !== null) {
this.imgData1 = args.imgData1;
}
if (args.detectRect1 !== undefined && args.detectRect1 !== null) {
this.detectRect1 = new FRect(args.detectRect1);
}
if (args.imgData2 !== undefined && args.imgData2 !== null) {
this.imgData2 = args.imgData2;
}
if (args.detectRect2 !== undefined && args.detectRect2 !== null) {
this.detectRect2 = new FRect(args.detectRect2);
}
}
};
FaceApi_detectAndCompare2Face_args.prototype = {};
FaceApi_detectAndCompare2Face_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData1 = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.detectRect1 = new FRect();
this.detectRect1.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRING) {
this.imgData2 = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.STRUCT) {
this.detectRect2 = new FRect();
this.detectRect2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectAndCompare2Face_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectAndCompare2Face_args');
if (this.imgData1 !== null && this.imgData1 !== undefined) {
output.writeFieldBegin('imgData1', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData1);
output.writeFieldEnd();
}
if (this.detectRect1 !== null && this.detectRect1 !== undefined) {
output.writeFieldBegin('detectRect1', Thrift.Type.STRUCT, 2);
this.detectRect1.write(output);
output.writeFieldEnd();
}
if (this.imgData2 !== null && this.imgData2 !== undefined) {
output.writeFieldBegin('imgData2', Thrift.Type.STRING, 3);
output.writeBinary(this.imgData2);
output.writeFieldEnd();
}
if (this.detectRect2 !== null && this.detectRect2 !== undefined) {
output.writeFieldBegin('detectRect2', Thrift.Type.STRUCT, 4);
this.detectRect2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectAndCompare2Face_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
this.ex3 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof NotFaceDetectedException) {
this.ex2 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex3 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
if (args.ex3 !== undefined && args.ex3 !== null) {
this.ex3 = args.ex3;
}
}
};
FaceApi_detectAndCompare2Face_result.prototype = {};
FaceApi_detectAndCompare2Face_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.DOUBLE) {
this.success = input.readDouble().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new NotFaceDetectedException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRUCT) {
this.ex3 = new ServiceRuntimeException();
this.ex3.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectAndCompare2Face_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectAndCompare2Face_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.DOUBLE, 0);
output.writeDouble(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
if (this.ex3 !== null && this.ex3 !== undefined) {
output.writeFieldBegin('ex3', Thrift.Type.STRUCT, 3);
this.ex3.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectAndGetCodeInfo_args = function(args) {
this.imgData = null;
this.faceNum = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
if (args.faceNum !== undefined && args.faceNum !== null) {
this.faceNum = args.faceNum;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field faceNum is unset!');
}
}
};
FaceApi_detectAndGetCodeInfo_args.prototype = {};
FaceApi_detectAndGetCodeInfo_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.I32) {
this.faceNum = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectAndGetCodeInfo_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectAndGetCodeInfo_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
if (this.faceNum !== null && this.faceNum !== undefined) {
output.writeFieldBegin('faceNum', Thrift.Type.I32, 2);
output.writeI32(this.faceNum);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectAndGetCodeInfo_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
this.ex3 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof NotFaceDetectedException) {
this.ex2 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex3 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeInfo]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
if (args.ex3 !== undefined && args.ex3 !== null) {
this.ex3 = args.ex3;
}
}
};
FaceApi_detectAndGetCodeInfo_result.prototype = {};
FaceApi_detectAndGetCodeInfo_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp331 = input.readListBegin();
var _size30 = _rtmp331.size || 0;
for (var _i32 = 0; _i32 < _size30; ++_i32) {
var elem33 = null;
elem33 = new CodeInfo();
elem33.read(input);
this.success.push(elem33);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new NotFaceDetectedException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRUCT) {
this.ex3 = new ServiceRuntimeException();
this.ex3.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectAndGetCodeInfo_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectAndGetCodeInfo_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter34 in this.success) {
if (this.success.hasOwnProperty(iter34)) {
iter34 = this.success[iter34];
iter34.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
if (this.ex3 !== null && this.ex3 !== undefined) {
output.writeFieldBegin('ex3', Thrift.Type.STRUCT, 3);
this.ex3.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectCenterFace_args = function(args) {
this.imgData = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
}
};
FaceApi_detectCenterFace_args.prototype = {};
FaceApi_detectCenterFace_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectCenterFace_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectCenterFace_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectCenterFace_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
this.ex3 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof NotFaceDetectedException) {
this.ex2 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex3 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CodeInfo(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
if (args.ex3 !== undefined && args.ex3 !== null) {
this.ex3 = args.ex3;
}
}
};
FaceApi_detectCenterFace_result.prototype = {};
FaceApi_detectCenterFace_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CodeInfo();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new NotFaceDetectedException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRUCT) {
this.ex3 = new ServiceRuntimeException();
this.ex3.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectCenterFace_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectCenterFace_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
if (this.ex3 !== null && this.ex3 !== undefined) {
output.writeFieldBegin('ex3', Thrift.Type.STRUCT, 3);
this.ex3.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectFace_args = function(args) {
this.imgData = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
}
};
FaceApi_detectFace_args.prototype = {};
FaceApi_detectFace_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectFace_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectFace_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectFace_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeInfo]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_detectFace_result.prototype = {};
FaceApi_detectFace_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp336 = input.readListBegin();
var _size35 = _rtmp336.size || 0;
for (var _i37 = 0; _i37 < _size35; ++_i37) {
var elem38 = null;
elem38 = new CodeInfo();
elem38.read(input);
this.success.push(elem38);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectFace_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectFace_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter39 in this.success) {
if (this.success.hasOwnProperty(iter39)) {
iter39 = this.success[iter39];
iter39.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectFaceWithOptions_args = function(args) {
this.imgData = null;
this.jsonOptions = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
if (args.jsonOptions !== undefined && args.jsonOptions !== null) {
this.jsonOptions = args.jsonOptions;
}
}
};
FaceApi_detectFaceWithOptions_args.prototype = {};
FaceApi_detectFaceWithOptions_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.jsonOptions = input.readString().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectFaceWithOptions_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectFaceWithOptions_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
if (this.jsonOptions !== null && this.jsonOptions !== undefined) {
output.writeFieldBegin('jsonOptions', Thrift.Type.STRING, 2);
output.writeString(this.jsonOptions);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectFaceWithOptions_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeInfo]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_detectFaceWithOptions_result.prototype = {};
FaceApi_detectFaceWithOptions_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp341 = input.readListBegin();
var _size40 = _rtmp341.size || 0;
for (var _i42 = 0; _i42 < _size40; ++_i42) {
var elem43 = null;
elem43 = new CodeInfo();
elem43.read(input);
this.success.push(elem43);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectFaceWithOptions_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectFaceWithOptions_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter44 in this.success) {
if (this.success.hasOwnProperty(iter44)) {
iter44 = this.success[iter44];
iter44.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectMaxFace_args = function(args) {
this.imgData = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
}
};
FaceApi_detectMaxFace_args.prototype = {};
FaceApi_detectMaxFace_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectMaxFace_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectMaxFace_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectMaxFace_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
this.ex3 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof NotFaceDetectedException) {
this.ex2 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex3 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CodeInfo(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
if (args.ex3 !== undefined && args.ex3 !== null) {
this.ex3 = args.ex3;
}
}
};
FaceApi_detectMaxFace_result.prototype = {};
FaceApi_detectMaxFace_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CodeInfo();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new NotFaceDetectedException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRUCT) {
this.ex3 = new ServiceRuntimeException();
this.ex3.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectMaxFace_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectMaxFace_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
if (this.ex3 !== null && this.ex3 !== undefined) {
output.writeFieldBegin('ex3', Thrift.Type.STRUCT, 3);
this.ex3.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectMaxFaceAndGetCodeInfo_args = function(args) {
this.imgData = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
}
};
FaceApi_detectMaxFaceAndGetCodeInfo_args.prototype = {};
FaceApi_detectMaxFaceAndGetCodeInfo_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectMaxFaceAndGetCodeInfo_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectMaxFaceAndGetCodeInfo_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_detectMaxFaceAndGetCodeInfo_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
this.ex3 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof NotFaceDetectedException) {
this.ex2 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex3 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CodeInfo(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
if (args.ex3 !== undefined && args.ex3 !== null) {
this.ex3 = args.ex3;
}
}
};
FaceApi_detectMaxFaceAndGetCodeInfo_result.prototype = {};
FaceApi_detectMaxFaceAndGetCodeInfo_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CodeInfo();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new NotFaceDetectedException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRUCT) {
this.ex3 = new ServiceRuntimeException();
this.ex3.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_detectMaxFaceAndGetCodeInfo_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_detectMaxFaceAndGetCodeInfo_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
if (this.ex3 !== null && this.ex3 !== undefined) {
output.writeFieldBegin('ex3', Thrift.Type.STRUCT, 3);
this.ex3.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_getCodeInfo_args = function(args) {
this.imgData = null;
this.faceNum = null;
this.facePos = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
if (args.faceNum !== undefined && args.faceNum !== null) {
this.faceNum = args.faceNum;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field faceNum is unset!');
}
if (args.facePos !== undefined && args.facePos !== null) {
this.facePos = Thrift.copyList(args.facePos, [CodeInfo]);
}
}
};
FaceApi_getCodeInfo_args.prototype = {};
FaceApi_getCodeInfo_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.I32) {
this.faceNum = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.LIST) {
this.facePos = [];
var _rtmp346 = input.readListBegin();
var _size45 = _rtmp346.size || 0;
for (var _i47 = 0; _i47 < _size45; ++_i47) {
var elem48 = null;
elem48 = new CodeInfo();
elem48.read(input);
this.facePos.push(elem48);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_getCodeInfo_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_getCodeInfo_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
if (this.faceNum !== null && this.faceNum !== undefined) {
output.writeFieldBegin('faceNum', Thrift.Type.I32, 2);
output.writeI32(this.faceNum);
output.writeFieldEnd();
}
if (this.facePos !== null && this.facePos !== undefined) {
output.writeFieldBegin('facePos', Thrift.Type.LIST, 3);
output.writeListBegin(Thrift.Type.STRUCT, this.facePos.length);
for (var iter49 in this.facePos) {
if (this.facePos.hasOwnProperty(iter49)) {
iter49 = this.facePos[iter49];
iter49.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_getCodeInfo_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof NotFaceDetectedException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeInfo]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_getCodeInfo_result.prototype = {};
FaceApi_getCodeInfo_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp351 = input.readListBegin();
var _size50 = _rtmp351.size || 0;
for (var _i52 = 0; _i52 < _size50; ++_i52) {
var elem53 = null;
elem53 = new CodeInfo();
elem53.read(input);
this.success.push(elem53);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new NotFaceDetectedException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_getCodeInfo_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_getCodeInfo_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter54 in this.success) {
if (this.success.hasOwnProperty(iter54)) {
iter54 = this.success[iter54];
iter54.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_getCodeInfoSingle_args = function(args) {
this.imgData = null;
this.facePos = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
if (args.facePos !== undefined && args.facePos !== null) {
this.facePos = new CodeInfo(args.facePos);
}
}
};
FaceApi_getCodeInfoSingle_args.prototype = {};
FaceApi_getCodeInfoSingle_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.facePos = new CodeInfo();
this.facePos.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_getCodeInfoSingle_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_getCodeInfoSingle_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
if (this.facePos !== null && this.facePos !== undefined) {
output.writeFieldBegin('facePos', Thrift.Type.STRUCT, 2);
this.facePos.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_getCodeInfoSingle_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CodeInfo(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_getCodeInfoSingle_result.prototype = {};
FaceApi_getCodeInfoSingle_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CodeInfo();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_getCodeInfoSingle_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_getCodeInfoSingle_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_getFeature_args = function(args) {
this.faces = null;
if (args) {
if (args.faces !== undefined && args.faces !== null) {
this.faces = Thrift.copyMap(args.faces, [CodeInfo]);
}
}
};
FaceApi_getFeature_args.prototype = {};
FaceApi_getFeature_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.MAP) {
this.faces = {};
var _rtmp356 = input.readMapBegin();
var _size55 = _rtmp356.size || 0;
for (var _i57 = 0; _i57 < _size55; ++_i57) {
if (_i57 > 0 ) {
if (input.rstack.length > input.rpos[input.rpos.length -1] + 1) {
input.rstack.pop();
}
}
var key58 = null;
var val59 = null;
key58 = input.readBinary().value;
val59 = new CodeInfo();
val59.read(input);
this.faces[key58] = val59;
}
input.readMapEnd();
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_getFeature_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_getFeature_args');
if (this.faces !== null && this.faces !== undefined) {
output.writeFieldBegin('faces', Thrift.Type.MAP, 1);
output.writeMapBegin(Thrift.Type.STRING, Thrift.Type.STRUCT, Thrift.objectLength(this.faces));
for (var kiter60 in this.faces) {
if (this.faces.hasOwnProperty(kiter60)) {
var viter61 = this.faces[kiter60];
output.writeBinary(kiter60);
viter61.write(output);
}
}
output.writeMapEnd();
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_getFeature_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof NotFaceDetectedException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_getFeature_result.prototype = {};
FaceApi_getFeature_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRING) {
this.success = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new NotFaceDetectedException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_getFeature_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_getFeature_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRING, 0);
output.writeBinary(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_hasFace_args = function(args) {
this.imgData = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
}
};
FaceApi_hasFace_args.prototype = {};
FaceApi_hasFace_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_hasFace_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_hasFace_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_hasFace_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_hasFace_result.prototype = {};
FaceApi_hasFace_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_hasFace_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_hasFace_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_isLocal_args = function(args) {
};
FaceApi_isLocal_args.prototype = {};
FaceApi_isLocal_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
if (ftype == Thrift.Type.STOP) {
break;
}
input.skip(ftype);
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_isLocal_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_isLocal_args');
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_isLocal_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_isLocal_result.prototype = {};
FaceApi_isLocal_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_isLocal_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_isLocal_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectAndGetCodeInfo_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
this.faceNum = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
if (args.faceNum !== undefined && args.faceNum !== null) {
this.faceNum = args.faceNum;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field faceNum is unset!');
}
}
};
FaceApi_matDetectAndGetCodeInfo_args.prototype = {};
FaceApi_matDetectAndGetCodeInfo_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 5:
if (ftype == Thrift.Type.I32) {
this.faceNum = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectAndGetCodeInfo_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectAndGetCodeInfo_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
if (this.faceNum !== null && this.faceNum !== undefined) {
output.writeFieldBegin('faceNum', Thrift.Type.I32, 5);
output.writeI32(this.faceNum);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectAndGetCodeInfo_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof NotFaceDetectedException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeInfo]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_matDetectAndGetCodeInfo_result.prototype = {};
FaceApi_matDetectAndGetCodeInfo_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp363 = input.readListBegin();
var _size62 = _rtmp363.size || 0;
for (var _i64 = 0; _i64 < _size62; ++_i64) {
var elem65 = null;
elem65 = new CodeInfo();
elem65.read(input);
this.success.push(elem65);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new NotFaceDetectedException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectAndGetCodeInfo_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectAndGetCodeInfo_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter66 in this.success) {
if (this.success.hasOwnProperty(iter66)) {
iter66 = this.success[iter66];
iter66.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectAndGetCodeInfoUnchecked_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
}
};
FaceApi_matDetectAndGetCodeInfoUnchecked_args.prototype = {};
FaceApi_matDetectAndGetCodeInfoUnchecked_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectAndGetCodeInfoUnchecked_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectAndGetCodeInfoUnchecked_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectAndGetCodeInfoUnchecked_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeInfo]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_matDetectAndGetCodeInfoUnchecked_result.prototype = {};
FaceApi_matDetectAndGetCodeInfoUnchecked_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp368 = input.readListBegin();
var _size67 = _rtmp368.size || 0;
for (var _i69 = 0; _i69 < _size67; ++_i69) {
var elem70 = null;
elem70 = new CodeInfo();
elem70.read(input);
this.success.push(elem70);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectAndGetCodeInfoUnchecked_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectAndGetCodeInfoUnchecked_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter71 in this.success) {
if (this.success.hasOwnProperty(iter71)) {
iter71 = this.success[iter71];
iter71.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectFace_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
}
};
FaceApi_matDetectFace_args.prototype = {};
FaceApi_matDetectFace_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectFace_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectFace_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectFace_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeInfo]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_matDetectFace_result.prototype = {};
FaceApi_matDetectFace_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp373 = input.readListBegin();
var _size72 = _rtmp373.size || 0;
for (var _i74 = 0; _i74 < _size72; ++_i74) {
var elem75 = null;
elem75 = new CodeInfo();
elem75.read(input);
this.success.push(elem75);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectFace_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectFace_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter76 in this.success) {
if (this.success.hasOwnProperty(iter76)) {
iter76 = this.success[iter76];
iter76.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectFaceFacenum_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
this.facenum = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
if (args.facenum !== undefined && args.facenum !== null) {
this.facenum = args.facenum;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field facenum is unset!');
}
}
};
FaceApi_matDetectFaceFacenum_args.prototype = {};
FaceApi_matDetectFaceFacenum_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 5:
if (ftype == Thrift.Type.I32) {
this.facenum = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectFaceFacenum_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectFaceFacenum_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
if (this.facenum !== null && this.facenum !== undefined) {
output.writeFieldBegin('facenum', Thrift.Type.I32, 5);
output.writeI32(this.facenum);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectFaceFacenum_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof NotFaceDetectedException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeInfo]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_matDetectFaceFacenum_result.prototype = {};
FaceApi_matDetectFaceFacenum_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp378 = input.readListBegin();
var _size77 = _rtmp378.size || 0;
for (var _i79 = 0; _i79 < _size77; ++_i79) {
var elem80 = null;
elem80 = new CodeInfo();
elem80.read(input);
this.success.push(elem80);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new NotFaceDetectedException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectFaceFacenum_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectFaceFacenum_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter81 in this.success) {
if (this.success.hasOwnProperty(iter81)) {
iter81 = this.success[iter81];
iter81.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectMaxFace_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
}
};
FaceApi_matDetectMaxFace_args.prototype = {};
FaceApi_matDetectMaxFace_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectMaxFace_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectMaxFace_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectMaxFace_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof NotFaceDetectedException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CodeInfo(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_matDetectMaxFace_result.prototype = {};
FaceApi_matDetectMaxFace_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CodeInfo();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new NotFaceDetectedException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectMaxFace_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectMaxFace_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectMaxFaceAndGetCodeInfo_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
}
};
FaceApi_matDetectMaxFaceAndGetCodeInfo_args.prototype = {};
FaceApi_matDetectMaxFaceAndGetCodeInfo_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectMaxFaceAndGetCodeInfo_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectMaxFaceAndGetCodeInfo_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matDetectMaxFaceAndGetCodeInfo_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof NotFaceDetectedException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CodeInfo(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_matDetectMaxFaceAndGetCodeInfo_result.prototype = {};
FaceApi_matDetectMaxFaceAndGetCodeInfo_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CodeInfo();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new NotFaceDetectedException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matDetectMaxFaceAndGetCodeInfo_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matDetectMaxFaceAndGetCodeInfo_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matGetCodeInfo_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
this.facenum = null;
this.facePos = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
if (args.facenum !== undefined && args.facenum !== null) {
this.facenum = args.facenum;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field facenum is unset!');
}
if (args.facePos !== undefined && args.facePos !== null) {
this.facePos = Thrift.copyList(args.facePos, [CodeInfo]);
}
}
};
FaceApi_matGetCodeInfo_args.prototype = {};
FaceApi_matGetCodeInfo_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 5:
if (ftype == Thrift.Type.I32) {
this.facenum = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 6:
if (ftype == Thrift.Type.LIST) {
this.facePos = [];
var _rtmp383 = input.readListBegin();
var _size82 = _rtmp383.size || 0;
for (var _i84 = 0; _i84 < _size82; ++_i84) {
var elem85 = null;
elem85 = new CodeInfo();
elem85.read(input);
this.facePos.push(elem85);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matGetCodeInfo_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matGetCodeInfo_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
if (this.facenum !== null && this.facenum !== undefined) {
output.writeFieldBegin('facenum', Thrift.Type.I32, 5);
output.writeI32(this.facenum);
output.writeFieldEnd();
}
if (this.facePos !== null && this.facePos !== undefined) {
output.writeFieldBegin('facePos', Thrift.Type.LIST, 6);
output.writeListBegin(Thrift.Type.STRUCT, this.facePos.length);
for (var iter86 in this.facePos) {
if (this.facePos.hasOwnProperty(iter86)) {
iter86 = this.facePos[iter86];
iter86.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matGetCodeInfo_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof NotFaceDetectedException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeInfo]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_matGetCodeInfo_result.prototype = {};
FaceApi_matGetCodeInfo_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp388 = input.readListBegin();
var _size87 = _rtmp388.size || 0;
for (var _i89 = 0; _i89 < _size87; ++_i89) {
var elem90 = null;
elem90 = new CodeInfo();
elem90.read(input);
this.success.push(elem90);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new NotFaceDetectedException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matGetCodeInfo_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matGetCodeInfo_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter91 in this.success) {
if (this.success.hasOwnProperty(iter91)) {
iter91 = this.success[iter91];
iter91.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matGetCodeInfoSingle_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
this.facePos = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
if (args.facePos !== undefined && args.facePos !== null) {
this.facePos = new CodeInfo(args.facePos);
}
}
};
FaceApi_matGetCodeInfoSingle_args.prototype = {};
FaceApi_matGetCodeInfoSingle_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 5:
if (ftype == Thrift.Type.STRUCT) {
this.facePos = new CodeInfo();
this.facePos.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matGetCodeInfoSingle_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matGetCodeInfoSingle_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
if (this.facePos !== null && this.facePos !== undefined) {
output.writeFieldBegin('facePos', Thrift.Type.STRUCT, 5);
this.facePos.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matGetCodeInfoSingle_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CodeInfo(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_matGetCodeInfoSingle_result.prototype = {};
FaceApi_matGetCodeInfoSingle_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CodeInfo();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matGetCodeInfoSingle_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matGetCodeInfoSingle_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matHasFace_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
}
};
FaceApi_matHasFace_args.prototype = {};
FaceApi_matHasFace_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matHasFace_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matHasFace_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matHasFace_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_matHasFace_result.prototype = {};
FaceApi_matHasFace_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matHasFace_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matHasFace_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matSearchFaces_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
this.facePos = null;
this.similarty = null;
this.rows = null;
this.imgMD5Set = null;
this.group = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
if (args.facePos !== undefined && args.facePos !== null) {
this.facePos = new CodeInfo(args.facePos);
}
if (args.similarty !== undefined && args.similarty !== null) {
this.similarty = args.similarty;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field similarty is unset!');
}
if (args.rows !== undefined && args.rows !== null) {
this.rows = args.rows;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field rows is unset!');
}
if (args.imgMD5Set !== undefined && args.imgMD5Set !== null) {
this.imgMD5Set = Thrift.copyList(args.imgMD5Set, [null]);
}
if (args.group !== undefined && args.group !== null) {
this.group = args.group;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field group is unset!');
}
}
};
FaceApi_matSearchFaces_args.prototype = {};
FaceApi_matSearchFaces_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 5:
if (ftype == Thrift.Type.STRUCT) {
this.facePos = new CodeInfo();
this.facePos.read(input);
} else {
input.skip(ftype);
}
break;
case 6:
if (ftype == Thrift.Type.DOUBLE) {
this.similarty = input.readDouble().value;
} else {
input.skip(ftype);
}
break;
case 7:
if (ftype == Thrift.Type.I32) {
this.rows = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 8:
if (ftype == Thrift.Type.LIST) {
this.imgMD5Set = [];
var _rtmp393 = input.readListBegin();
var _size92 = _rtmp393.size || 0;
for (var _i94 = 0; _i94 < _size92; ++_i94) {
var elem95 = null;
elem95 = input.readString().value;
this.imgMD5Set.push(elem95);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 9:
if (ftype == Thrift.Type.I32) {
this.group = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matSearchFaces_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matSearchFaces_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
if (this.facePos !== null && this.facePos !== undefined) {
output.writeFieldBegin('facePos', Thrift.Type.STRUCT, 5);
this.facePos.write(output);
output.writeFieldEnd();
}
if (this.similarty !== null && this.similarty !== undefined) {
output.writeFieldBegin('similarty', Thrift.Type.DOUBLE, 6);
output.writeDouble(this.similarty);
output.writeFieldEnd();
}
if (this.rows !== null && this.rows !== undefined) {
output.writeFieldBegin('rows', Thrift.Type.I32, 7);
output.writeI32(this.rows);
output.writeFieldEnd();
}
if (this.imgMD5Set !== null && this.imgMD5Set !== undefined) {
output.writeFieldBegin('imgMD5Set', Thrift.Type.LIST, 8);
output.writeListBegin(Thrift.Type.STRING, this.imgMD5Set.length);
for (var iter96 in this.imgMD5Set) {
if (this.imgMD5Set.hasOwnProperty(iter96)) {
iter96 = this.imgMD5Set[iter96];
output.writeString(iter96);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.group !== null && this.group !== undefined) {
output.writeFieldBegin('group', Thrift.Type.I32, 9);
output.writeI32(this.group);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matSearchFaces_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
this.ex3 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof NotFaceDetectedException) {
this.ex2 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex3 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [FseResult]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
if (args.ex3 !== undefined && args.ex3 !== null) {
this.ex3 = args.ex3;
}
}
};
FaceApi_matSearchFaces_result.prototype = {};
FaceApi_matSearchFaces_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp398 = input.readListBegin();
var _size97 = _rtmp398.size || 0;
for (var _i99 = 0; _i99 < _size97; ++_i99) {
var elem100 = null;
elem100 = new FseResult();
elem100.read(input);
this.success.push(elem100);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new NotFaceDetectedException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRUCT) {
this.ex3 = new ServiceRuntimeException();
this.ex3.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matSearchFaces_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matSearchFaces_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter101 in this.success) {
if (this.success.hasOwnProperty(iter101)) {
iter101 = this.success[iter101];
iter101.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
if (this.ex3 !== null && this.ex3 !== undefined) {
output.writeFieldBegin('ex3', Thrift.Type.STRUCT, 3);
this.ex3.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matWearMask_args = function(args) {
this.matType = null;
this.matData = null;
this.width = null;
this.height = null;
this.faceInfo = null;
if (args) {
if (args.matType !== undefined && args.matType !== null) {
this.matType = args.matType;
}
if (args.matData !== undefined && args.matData !== null) {
this.matData = args.matData;
}
if (args.width !== undefined && args.width !== null) {
this.width = args.width;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field width is unset!');
}
if (args.height !== undefined && args.height !== null) {
this.height = args.height;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field height is unset!');
}
if (args.faceInfo !== undefined && args.faceInfo !== null) {
this.faceInfo = new CodeInfo(args.faceInfo);
}
}
};
FaceApi_matWearMask_args.prototype = {};
FaceApi_matWearMask_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.I32) {
this.matType = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.matData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.width = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.height = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 5:
if (ftype == Thrift.Type.STRUCT) {
this.faceInfo = new CodeInfo();
this.faceInfo.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matWearMask_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matWearMask_args');
if (this.matType !== null && this.matType !== undefined) {
output.writeFieldBegin('matType', Thrift.Type.I32, 1);
output.writeI32(this.matType);
output.writeFieldEnd();
}
if (this.matData !== null && this.matData !== undefined) {
output.writeFieldBegin('matData', Thrift.Type.STRING, 2);
output.writeBinary(this.matData);
output.writeFieldEnd();
}
if (this.width !== null && this.width !== undefined) {
output.writeFieldBegin('width', Thrift.Type.I32, 3);
output.writeI32(this.width);
output.writeFieldEnd();
}
if (this.height !== null && this.height !== undefined) {
output.writeFieldBegin('height', Thrift.Type.I32, 4);
output.writeI32(this.height);
output.writeFieldEnd();
}
if (this.faceInfo !== null && this.faceInfo !== undefined) {
output.writeFieldBegin('faceInfo', Thrift.Type.STRUCT, 5);
this.faceInfo.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_matWearMask_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_matWearMask_result.prototype = {};
FaceApi_matWearMask_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_matWearMask_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_matWearMask_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_sdkCapacity_args = function(args) {
};
FaceApi_sdkCapacity_args.prototype = {};
FaceApi_sdkCapacity_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
if (ftype == Thrift.Type.STOP) {
break;
}
input.skip(ftype);
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_sdkCapacity_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_sdkCapacity_args');
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_sdkCapacity_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyMap(args.success, [null]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_sdkCapacity_result.prototype = {};
FaceApi_sdkCapacity_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.MAP) {
this.success = {};
var _rtmp3103 = input.readMapBegin();
var _size102 = _rtmp3103.size || 0;
for (var _i104 = 0; _i104 < _size102; ++_i104) {
if (_i104 > 0 ) {
if (input.rstack.length > input.rpos[input.rpos.length -1] + 1) {
input.rstack.pop();
}
}
var key105 = null;
var val106 = null;
key105 = input.readString().value;
val106 = input.readString().value;
this.success[key105] = val106;
}
input.readMapEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_sdkCapacity_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_sdkCapacity_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.MAP, 0);
output.writeMapBegin(Thrift.Type.STRING, Thrift.Type.STRING, Thrift.objectLength(this.success));
for (var kiter107 in this.success) {
if (this.success.hasOwnProperty(kiter107)) {
var viter108 = this.success[kiter107];
output.writeString(kiter107);
output.writeString(viter108);
}
}
output.writeMapEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_searchFaces_args = function(args) {
this.imgData = null;
this.facePos = null;
this.similarty = null;
this.rows = null;
this.imgMD5Set = null;
this.group = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
if (args.facePos !== undefined && args.facePos !== null) {
this.facePos = new CodeInfo(args.facePos);
}
if (args.similarty !== undefined && args.similarty !== null) {
this.similarty = args.similarty;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field similarty is unset!');
}
if (args.rows !== undefined && args.rows !== null) {
this.rows = args.rows;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field rows is unset!');
}
if (args.imgMD5Set !== undefined && args.imgMD5Set !== null) {
this.imgMD5Set = Thrift.copyList(args.imgMD5Set, [null]);
}
if (args.group !== undefined && args.group !== null) {
this.group = args.group;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field group is unset!');
}
}
};
FaceApi_searchFaces_args.prototype = {};
FaceApi_searchFaces_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.facePos = new CodeInfo();
this.facePos.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.DOUBLE) {
this.similarty = input.readDouble().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.rows = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 5:
if (ftype == Thrift.Type.LIST) {
this.imgMD5Set = [];
var _rtmp3110 = input.readListBegin();
var _size109 = _rtmp3110.size || 0;
for (var _i111 = 0; _i111 < _size109; ++_i111) {
var elem112 = null;
elem112 = input.readString().value;
this.imgMD5Set.push(elem112);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 6:
if (ftype == Thrift.Type.I32) {
this.group = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_searchFaces_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_searchFaces_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
if (this.facePos !== null && this.facePos !== undefined) {
output.writeFieldBegin('facePos', Thrift.Type.STRUCT, 2);
this.facePos.write(output);
output.writeFieldEnd();
}
if (this.similarty !== null && this.similarty !== undefined) {
output.writeFieldBegin('similarty', Thrift.Type.DOUBLE, 3);
output.writeDouble(this.similarty);
output.writeFieldEnd();
}
if (this.rows !== null && this.rows !== undefined) {
output.writeFieldBegin('rows', Thrift.Type.I32, 4);
output.writeI32(this.rows);
output.writeFieldEnd();
}
if (this.imgMD5Set !== null && this.imgMD5Set !== undefined) {
output.writeFieldBegin('imgMD5Set', Thrift.Type.LIST, 5);
output.writeListBegin(Thrift.Type.STRING, this.imgMD5Set.length);
for (var iter113 in this.imgMD5Set) {
if (this.imgMD5Set.hasOwnProperty(iter113)) {
iter113 = this.imgMD5Set[iter113];
output.writeString(iter113);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.group !== null && this.group !== undefined) {
output.writeFieldBegin('group', Thrift.Type.I32, 6);
output.writeI32(this.group);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_searchFaces_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
this.ex3 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof NotFaceDetectedException) {
this.ex2 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex3 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [FseResult]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
if (args.ex3 !== undefined && args.ex3 !== null) {
this.ex3 = args.ex3;
}
}
};
FaceApi_searchFaces_result.prototype = {};
FaceApi_searchFaces_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp3115 = input.readListBegin();
var _size114 = _rtmp3115.size || 0;
for (var _i116 = 0; _i116 < _size114; ++_i116) {
var elem117 = null;
elem117 = new FseResult();
elem117.read(input);
this.success.push(elem117);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new NotFaceDetectedException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRUCT) {
this.ex3 = new ServiceRuntimeException();
this.ex3.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_searchFaces_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_searchFaces_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter118 in this.success) {
if (this.success.hasOwnProperty(iter118)) {
iter118 = this.success[iter118];
iter118.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
if (this.ex3 !== null && this.ex3 !== undefined) {
output.writeFieldBegin('ex3', Thrift.Type.STRUCT, 3);
this.ex3.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_searchFeatures_args = function(args) {
this.feature = null;
this.similarity = null;
this.rows = null;
this.imgMD5Set = null;
this.group = null;
if (args) {
if (args.feature !== undefined && args.feature !== null) {
this.feature = args.feature;
}
if (args.similarity !== undefined && args.similarity !== null) {
this.similarity = args.similarity;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field similarity is unset!');
}
if (args.rows !== undefined && args.rows !== null) {
this.rows = args.rows;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field rows is unset!');
}
if (args.imgMD5Set !== undefined && args.imgMD5Set !== null) {
this.imgMD5Set = Thrift.copyList(args.imgMD5Set, [null]);
}
if (args.group !== undefined && args.group !== null) {
this.group = args.group;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field group is unset!');
}
}
};
FaceApi_searchFeatures_args.prototype = {};
FaceApi_searchFeatures_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.feature = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.DOUBLE) {
this.similarity = input.readDouble().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.rows = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.LIST) {
this.imgMD5Set = [];
var _rtmp3120 = input.readListBegin();
var _size119 = _rtmp3120.size || 0;
for (var _i121 = 0; _i121 < _size119; ++_i121) {
var elem122 = null;
elem122 = input.readString().value;
this.imgMD5Set.push(elem122);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 5:
if (ftype == Thrift.Type.I32) {
this.group = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_searchFeatures_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_searchFeatures_args');
if (this.feature !== null && this.feature !== undefined) {
output.writeFieldBegin('feature', Thrift.Type.STRING, 1);
output.writeBinary(this.feature);
output.writeFieldEnd();
}
if (this.similarity !== null && this.similarity !== undefined) {
output.writeFieldBegin('similarity', Thrift.Type.DOUBLE, 2);
output.writeDouble(this.similarity);
output.writeFieldEnd();
}
if (this.rows !== null && this.rows !== undefined) {
output.writeFieldBegin('rows', Thrift.Type.I32, 3);
output.writeI32(this.rows);
output.writeFieldEnd();
}
if (this.imgMD5Set !== null && this.imgMD5Set !== undefined) {
output.writeFieldBegin('imgMD5Set', Thrift.Type.LIST, 4);
output.writeListBegin(Thrift.Type.STRING, this.imgMD5Set.length);
for (var iter123 in this.imgMD5Set) {
if (this.imgMD5Set.hasOwnProperty(iter123)) {
iter123 = this.imgMD5Set[iter123];
output.writeString(iter123);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.group !== null && this.group !== undefined) {
output.writeFieldBegin('group', Thrift.Type.I32, 5);
output.writeI32(this.group);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_searchFeatures_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [FseResult]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FaceApi_searchFeatures_result.prototype = {};
FaceApi_searchFeatures_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp3125 = input.readListBegin();
var _size124 = _rtmp3125.size || 0;
for (var _i126 = 0; _i126 < _size124; ++_i126) {
var elem127 = null;
elem127 = new FseResult();
elem127.read(input);
this.success.push(elem127);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_searchFeatures_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_searchFeatures_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter128 in this.success) {
if (this.success.hasOwnProperty(iter128)) {
iter128 = this.success[iter128];
iter128.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_wearMask_args = function(args) {
this.imgData = null;
this.faceInfo = null;
if (args) {
if (args.imgData !== undefined && args.imgData !== null) {
this.imgData = args.imgData;
}
if (args.faceInfo !== undefined && args.faceInfo !== null) {
this.faceInfo = new CodeInfo(args.faceInfo);
}
}
};
FaceApi_wearMask_args.prototype = {};
FaceApi_wearMask_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.imgData = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.faceInfo = new CodeInfo();
this.faceInfo.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_wearMask_args.prototype.write = function(output) {
output.writeStructBegin('FaceApi_wearMask_args');
if (this.imgData !== null && this.imgData !== undefined) {
output.writeFieldBegin('imgData', Thrift.Type.STRING, 1);
output.writeBinary(this.imgData);
output.writeFieldEnd();
}
if (this.faceInfo !== null && this.faceInfo !== undefined) {
output.writeFieldBegin('faceInfo', Thrift.Type.STRUCT, 2);
this.faceInfo.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApi_wearMask_result = function(args) {
this.success = null;
this.ex1 = null;
this.ex2 = null;
if (args instanceof ImageErrorException) {
this.ex1 = args;
return;
}
if (args instanceof ServiceRuntimeException) {
this.ex2 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
if (args.ex2 !== undefined && args.ex2 !== null) {
this.ex2 = args.ex2;
}
}
};
FaceApi_wearMask_result.prototype = {};
FaceApi_wearMask_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ImageErrorException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRUCT) {
this.ex2 = new ServiceRuntimeException();
this.ex2.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FaceApi_wearMask_result.prototype.write = function(output) {
output.writeStructBegin('FaceApi_wearMask_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
if (this.ex2 !== null && this.ex2 !== undefined) {
output.writeFieldBegin('ex2', Thrift.Type.STRUCT, 2);
this.ex2.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FaceApiClient = function(input, output) {
this.input = input;
this.output = (!output) ? input : output;
this.seqid = 0;
};
FaceApiClient.prototype = {};
FaceApiClient.prototype.compare2Face = function(imgData1, facePos1, imgData2, facePos2, callback) {
if (callback === undefined) {
this.send_compare2Face(imgData1, facePos1, imgData2, facePos2);
return this.recv_compare2Face();
} else {
var postData = this.send_compare2Face(imgData1, facePos1, imgData2, facePos2, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_compare2Face);
}
};
FaceApiClient.prototype.send_compare2Face = function(imgData1, facePos1, imgData2, facePos2, callback) {
var params = {
imgData1: imgData1,
facePos1: facePos1,
imgData2: imgData2,
facePos2: facePos2
};
var args = new FaceApi_compare2Face_args(params);
try {
this.output.writeMessageBegin('compare2Face', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_compare2Face = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_compare2Face_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.ex3) {
throw result.ex3;
}
if (null !== result.success) {
return result.success;
}
throw 'compare2Face failed: unknown result';
};
FaceApiClient.prototype.compareCode = function(code1, code2, callback) {
if (callback === undefined) {
this.send_compareCode(code1, code2);
return this.recv_compareCode();
} else {
var postData = this.send_compareCode(code1, code2, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_compareCode);
}
};
FaceApiClient.prototype.send_compareCode = function(code1, code2, callback) {
var params = {
code1: code1,
code2: code2
};
var args = new FaceApi_compareCode_args(params);
try {
this.output.writeMessageBegin('compareCode', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_compareCode = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_compareCode_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'compareCode failed: unknown result';
};
FaceApiClient.prototype.compareCodes = function(code1, codes, callback) {
if (callback === undefined) {
this.send_compareCodes(code1, codes);
return this.recv_compareCodes();
} else {
var postData = this.send_compareCodes(code1, codes, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_compareCodes);
}
};
FaceApiClient.prototype.send_compareCodes = function(code1, codes, callback) {
var params = {
code1: code1,
codes: codes
};
var args = new FaceApi_compareCodes_args(params);
try {
this.output.writeMessageBegin('compareCodes', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_compareCodes = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_compareCodes_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'compareCodes failed: unknown result';
};
FaceApiClient.prototype.compareFaces = function(code, imgData, faceNum, callback) {
if (callback === undefined) {
this.send_compareFaces(code, imgData, faceNum);
return this.recv_compareFaces();
} else {
var postData = this.send_compareFaces(code, imgData, faceNum, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_compareFaces);
}
};
FaceApiClient.prototype.send_compareFaces = function(code, imgData, faceNum, callback) {
var params = {
code: code,
imgData: imgData,
faceNum: faceNum
};
var args = new FaceApi_compareFaces_args(params);
try {
this.output.writeMessageBegin('compareFaces', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_compareFaces = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_compareFaces_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.ex3) {
throw result.ex3;
}
if (null !== result.success) {
return result.success;
}
throw 'compareFaces failed: unknown result';
};
FaceApiClient.prototype.compareFeatures = function(code1, codes, callback) {
if (callback === undefined) {
this.send_compareFeatures(code1, codes);
return this.recv_compareFeatures();
} else {
var postData = this.send_compareFeatures(code1, codes, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_compareFeatures);
}
};
FaceApiClient.prototype.send_compareFeatures = function(code1, codes, callback) {
var params = {
code1: code1,
codes: codes
};
var args = new FaceApi_compareFeatures_args(params);
try {
this.output.writeMessageBegin('compareFeatures', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_compareFeatures = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_compareFeatures_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'compareFeatures failed: unknown result';
};
FaceApiClient.prototype.detectAndCompare2Face = function(imgData1, detectRect1, imgData2, detectRect2, callback) {
if (callback === undefined) {
this.send_detectAndCompare2Face(imgData1, detectRect1, imgData2, detectRect2);
return this.recv_detectAndCompare2Face();
} else {
var postData = this.send_detectAndCompare2Face(imgData1, detectRect1, imgData2, detectRect2, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_detectAndCompare2Face);
}
};
FaceApiClient.prototype.send_detectAndCompare2Face = function(imgData1, detectRect1, imgData2, detectRect2, callback) {
var params = {
imgData1: imgData1,
detectRect1: detectRect1,
imgData2: imgData2,
detectRect2: detectRect2
};
var args = new FaceApi_detectAndCompare2Face_args(params);
try {
this.output.writeMessageBegin('detectAndCompare2Face', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_detectAndCompare2Face = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_detectAndCompare2Face_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.ex3) {
throw result.ex3;
}
if (null !== result.success) {
return result.success;
}
throw 'detectAndCompare2Face failed: unknown result';
};
FaceApiClient.prototype.detectAndGetCodeInfo = function(imgData, faceNum, callback) {
if (callback === undefined) {
this.send_detectAndGetCodeInfo(imgData, faceNum);
return this.recv_detectAndGetCodeInfo();
} else {
var postData = this.send_detectAndGetCodeInfo(imgData, faceNum, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_detectAndGetCodeInfo);
}
};
FaceApiClient.prototype.send_detectAndGetCodeInfo = function(imgData, faceNum, callback) {
var params = {
imgData: imgData,
faceNum: faceNum
};
var args = new FaceApi_detectAndGetCodeInfo_args(params);
try {
this.output.writeMessageBegin('detectAndGetCodeInfo', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_detectAndGetCodeInfo = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_detectAndGetCodeInfo_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.ex3) {
throw result.ex3;
}
if (null !== result.success) {
return result.success;
}
throw 'detectAndGetCodeInfo failed: unknown result';
};
FaceApiClient.prototype.detectCenterFace = function(imgData, callback) {
if (callback === undefined) {
this.send_detectCenterFace(imgData);
return this.recv_detectCenterFace();
} else {
var postData = this.send_detectCenterFace(imgData, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_detectCenterFace);
}
};
FaceApiClient.prototype.send_detectCenterFace = function(imgData, callback) {
var params = {
imgData: imgData
};
var args = new FaceApi_detectCenterFace_args(params);
try {
this.output.writeMessageBegin('detectCenterFace', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_detectCenterFace = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_detectCenterFace_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.ex3) {
throw result.ex3;
}
if (null !== result.success) {
return result.success;
}
throw 'detectCenterFace failed: unknown result';
};
FaceApiClient.prototype.detectFace = function(imgData, callback) {
if (callback === undefined) {
this.send_detectFace(imgData);
return this.recv_detectFace();
} else {
var postData = this.send_detectFace(imgData, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_detectFace);
}
};
FaceApiClient.prototype.send_detectFace = function(imgData, callback) {
var params = {
imgData: imgData
};
var args = new FaceApi_detectFace_args(params);
try {
this.output.writeMessageBegin('detectFace', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_detectFace = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_detectFace_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'detectFace failed: unknown result';
};
FaceApiClient.prototype.detectFaceWithOptions = function(imgData, jsonOptions, callback) {
if (callback === undefined) {
this.send_detectFaceWithOptions(imgData, jsonOptions);
return this.recv_detectFaceWithOptions();
} else {
var postData = this.send_detectFaceWithOptions(imgData, jsonOptions, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_detectFaceWithOptions);
}
};
FaceApiClient.prototype.send_detectFaceWithOptions = function(imgData, jsonOptions, callback) {
var params = {
imgData: imgData,
jsonOptions: jsonOptions
};
var args = new FaceApi_detectFaceWithOptions_args(params);
try {
this.output.writeMessageBegin('detectFaceWithOptions', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_detectFaceWithOptions = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_detectFaceWithOptions_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'detectFaceWithOptions failed: unknown result';
};
FaceApiClient.prototype.detectMaxFace = function(imgData, callback) {
if (callback === undefined) {
this.send_detectMaxFace(imgData);
return this.recv_detectMaxFace();
} else {
var postData = this.send_detectMaxFace(imgData, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_detectMaxFace);
}
};
FaceApiClient.prototype.send_detectMaxFace = function(imgData, callback) {
var params = {
imgData: imgData
};
var args = new FaceApi_detectMaxFace_args(params);
try {
this.output.writeMessageBegin('detectMaxFace', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_detectMaxFace = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_detectMaxFace_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.ex3) {
throw result.ex3;
}
if (null !== result.success) {
return result.success;
}
throw 'detectMaxFace failed: unknown result';
};
FaceApiClient.prototype.detectMaxFaceAndGetCodeInfo = function(imgData, callback) {
if (callback === undefined) {
this.send_detectMaxFaceAndGetCodeInfo(imgData);
return this.recv_detectMaxFaceAndGetCodeInfo();
} else {
var postData = this.send_detectMaxFaceAndGetCodeInfo(imgData, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_detectMaxFaceAndGetCodeInfo);
}
};
FaceApiClient.prototype.send_detectMaxFaceAndGetCodeInfo = function(imgData, callback) {
var params = {
imgData: imgData
};
var args = new FaceApi_detectMaxFaceAndGetCodeInfo_args(params);
try {
this.output.writeMessageBegin('detectMaxFaceAndGetCodeInfo', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_detectMaxFaceAndGetCodeInfo = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_detectMaxFaceAndGetCodeInfo_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.ex3) {
throw result.ex3;
}
if (null !== result.success) {
return result.success;
}
throw 'detectMaxFaceAndGetCodeInfo failed: unknown result';
};
FaceApiClient.prototype.getCodeInfo = function(imgData, faceNum, facePos, callback) {
if (callback === undefined) {
this.send_getCodeInfo(imgData, faceNum, facePos);
return this.recv_getCodeInfo();
} else {
var postData = this.send_getCodeInfo(imgData, faceNum, facePos, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_getCodeInfo);
}
};
FaceApiClient.prototype.send_getCodeInfo = function(imgData, faceNum, facePos, callback) {
var params = {
imgData: imgData,
faceNum: faceNum,
facePos: facePos
};
var args = new FaceApi_getCodeInfo_args(params);
try {
this.output.writeMessageBegin('getCodeInfo', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_getCodeInfo = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_getCodeInfo_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'getCodeInfo failed: unknown result';
};
FaceApiClient.prototype.getCodeInfoSingle = function(imgData, facePos, callback) {
if (callback === undefined) {
this.send_getCodeInfoSingle(imgData, facePos);
return this.recv_getCodeInfoSingle();
} else {
var postData = this.send_getCodeInfoSingle(imgData, facePos, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_getCodeInfoSingle);
}
};
FaceApiClient.prototype.send_getCodeInfoSingle = function(imgData, facePos, callback) {
var params = {
imgData: imgData,
facePos: facePos
};
var args = new FaceApi_getCodeInfoSingle_args(params);
try {
this.output.writeMessageBegin('getCodeInfoSingle', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_getCodeInfoSingle = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_getCodeInfoSingle_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'getCodeInfoSingle failed: unknown result';
};
FaceApiClient.prototype.getFeature = function(faces, callback) {
if (callback === undefined) {
this.send_getFeature(faces);
return this.recv_getFeature();
} else {
var postData = this.send_getFeature(faces, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_getFeature);
}
};
FaceApiClient.prototype.send_getFeature = function(faces, callback) {
var params = {
faces: faces
};
var args = new FaceApi_getFeature_args(params);
try {
this.output.writeMessageBegin('getFeature', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_getFeature = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_getFeature_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'getFeature failed: unknown result';
};
FaceApiClient.prototype.hasFace = function(imgData, callback) {
if (callback === undefined) {
this.send_hasFace(imgData);
return this.recv_hasFace();
} else {
var postData = this.send_hasFace(imgData, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_hasFace);
}
};
FaceApiClient.prototype.send_hasFace = function(imgData, callback) {
var params = {
imgData: imgData
};
var args = new FaceApi_hasFace_args(params);
try {
this.output.writeMessageBegin('hasFace', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_hasFace = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_hasFace_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'hasFace failed: unknown result';
};
FaceApiClient.prototype.isLocal = function(callback) {
if (callback === undefined) {
this.send_isLocal();
return this.recv_isLocal();
} else {
var postData = this.send_isLocal(true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_isLocal);
}
};
FaceApiClient.prototype.send_isLocal = function(callback) {
var args = new FaceApi_isLocal_args();
try {
this.output.writeMessageBegin('isLocal', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_isLocal = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_isLocal_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'isLocal failed: unknown result';
};
FaceApiClient.prototype.matDetectAndGetCodeInfo = function(matType, matData, width, height, faceNum, callback) {
if (callback === undefined) {
this.send_matDetectAndGetCodeInfo(matType, matData, width, height, faceNum);
return this.recv_matDetectAndGetCodeInfo();
} else {
var postData = this.send_matDetectAndGetCodeInfo(matType, matData, width, height, faceNum, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matDetectAndGetCodeInfo);
}
};
FaceApiClient.prototype.send_matDetectAndGetCodeInfo = function(matType, matData, width, height, faceNum, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height,
faceNum: faceNum
};
var args = new FaceApi_matDetectAndGetCodeInfo_args(params);
try {
this.output.writeMessageBegin('matDetectAndGetCodeInfo', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matDetectAndGetCodeInfo = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matDetectAndGetCodeInfo_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'matDetectAndGetCodeInfo failed: unknown result';
};
FaceApiClient.prototype.matDetectAndGetCodeInfoUnchecked = function(matType, matData, width, height, callback) {
if (callback === undefined) {
this.send_matDetectAndGetCodeInfoUnchecked(matType, matData, width, height);
return this.recv_matDetectAndGetCodeInfoUnchecked();
} else {
var postData = this.send_matDetectAndGetCodeInfoUnchecked(matType, matData, width, height, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matDetectAndGetCodeInfoUnchecked);
}
};
FaceApiClient.prototype.send_matDetectAndGetCodeInfoUnchecked = function(matType, matData, width, height, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height
};
var args = new FaceApi_matDetectAndGetCodeInfoUnchecked_args(params);
try {
this.output.writeMessageBegin('matDetectAndGetCodeInfoUnchecked', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matDetectAndGetCodeInfoUnchecked = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matDetectAndGetCodeInfoUnchecked_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'matDetectAndGetCodeInfoUnchecked failed: unknown result';
};
FaceApiClient.prototype.matDetectFace = function(matType, matData, width, height, callback) {
if (callback === undefined) {
this.send_matDetectFace(matType, matData, width, height);
return this.recv_matDetectFace();
} else {
var postData = this.send_matDetectFace(matType, matData, width, height, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matDetectFace);
}
};
FaceApiClient.prototype.send_matDetectFace = function(matType, matData, width, height, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height
};
var args = new FaceApi_matDetectFace_args(params);
try {
this.output.writeMessageBegin('matDetectFace', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matDetectFace = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matDetectFace_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'matDetectFace failed: unknown result';
};
FaceApiClient.prototype.matDetectFaceFacenum = function(matType, matData, width, height, facenum, callback) {
if (callback === undefined) {
this.send_matDetectFaceFacenum(matType, matData, width, height, facenum);
return this.recv_matDetectFaceFacenum();
} else {
var postData = this.send_matDetectFaceFacenum(matType, matData, width, height, facenum, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matDetectFaceFacenum);
}
};
FaceApiClient.prototype.send_matDetectFaceFacenum = function(matType, matData, width, height, facenum, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height,
facenum: facenum
};
var args = new FaceApi_matDetectFaceFacenum_args(params);
try {
this.output.writeMessageBegin('matDetectFaceFacenum', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matDetectFaceFacenum = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matDetectFaceFacenum_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'matDetectFaceFacenum failed: unknown result';
};
FaceApiClient.prototype.matDetectMaxFace = function(matType, matData, width, height, callback) {
if (callback === undefined) {
this.send_matDetectMaxFace(matType, matData, width, height);
return this.recv_matDetectMaxFace();
} else {
var postData = this.send_matDetectMaxFace(matType, matData, width, height, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matDetectMaxFace);
}
};
FaceApiClient.prototype.send_matDetectMaxFace = function(matType, matData, width, height, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height
};
var args = new FaceApi_matDetectMaxFace_args(params);
try {
this.output.writeMessageBegin('matDetectMaxFace', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matDetectMaxFace = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matDetectMaxFace_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'matDetectMaxFace failed: unknown result';
};
FaceApiClient.prototype.matDetectMaxFaceAndGetCodeInfo = function(matType, matData, width, height, callback) {
if (callback === undefined) {
this.send_matDetectMaxFaceAndGetCodeInfo(matType, matData, width, height);
return this.recv_matDetectMaxFaceAndGetCodeInfo();
} else {
var postData = this.send_matDetectMaxFaceAndGetCodeInfo(matType, matData, width, height, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matDetectMaxFaceAndGetCodeInfo);
}
};
FaceApiClient.prototype.send_matDetectMaxFaceAndGetCodeInfo = function(matType, matData, width, height, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height
};
var args = new FaceApi_matDetectMaxFaceAndGetCodeInfo_args(params);
try {
this.output.writeMessageBegin('matDetectMaxFaceAndGetCodeInfo', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matDetectMaxFaceAndGetCodeInfo = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matDetectMaxFaceAndGetCodeInfo_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'matDetectMaxFaceAndGetCodeInfo failed: unknown result';
};
FaceApiClient.prototype.matGetCodeInfo = function(matType, matData, width, height, facenum, facePos, callback) {
if (callback === undefined) {
this.send_matGetCodeInfo(matType, matData, width, height, facenum, facePos);
return this.recv_matGetCodeInfo();
} else {
var postData = this.send_matGetCodeInfo(matType, matData, width, height, facenum, facePos, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matGetCodeInfo);
}
};
FaceApiClient.prototype.send_matGetCodeInfo = function(matType, matData, width, height, facenum, facePos, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height,
facenum: facenum,
facePos: facePos
};
var args = new FaceApi_matGetCodeInfo_args(params);
try {
this.output.writeMessageBegin('matGetCodeInfo', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matGetCodeInfo = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matGetCodeInfo_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'matGetCodeInfo failed: unknown result';
};
FaceApiClient.prototype.matGetCodeInfoSingle = function(matType, matData, width, height, facePos, callback) {
if (callback === undefined) {
this.send_matGetCodeInfoSingle(matType, matData, width, height, facePos);
return this.recv_matGetCodeInfoSingle();
} else {
var postData = this.send_matGetCodeInfoSingle(matType, matData, width, height, facePos, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matGetCodeInfoSingle);
}
};
FaceApiClient.prototype.send_matGetCodeInfoSingle = function(matType, matData, width, height, facePos, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height,
facePos: facePos
};
var args = new FaceApi_matGetCodeInfoSingle_args(params);
try {
this.output.writeMessageBegin('matGetCodeInfoSingle', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matGetCodeInfoSingle = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matGetCodeInfoSingle_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'matGetCodeInfoSingle failed: unknown result';
};
FaceApiClient.prototype.matHasFace = function(matType, matData, width, height, callback) {
if (callback === undefined) {
this.send_matHasFace(matType, matData, width, height);
return this.recv_matHasFace();
} else {
var postData = this.send_matHasFace(matType, matData, width, height, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matHasFace);
}
};
FaceApiClient.prototype.send_matHasFace = function(matType, matData, width, height, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height
};
var args = new FaceApi_matHasFace_args(params);
try {
this.output.writeMessageBegin('matHasFace', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matHasFace = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matHasFace_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'matHasFace failed: unknown result';
};
FaceApiClient.prototype.matSearchFaces = function(matType, matData, width, height, facePos, similarty, rows, imgMD5Set, group, callback) {
if (callback === undefined) {
this.send_matSearchFaces(matType, matData, width, height, facePos, similarty, rows, imgMD5Set, group);
return this.recv_matSearchFaces();
} else {
var postData = this.send_matSearchFaces(matType, matData, width, height, facePos, similarty, rows, imgMD5Set, group, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matSearchFaces);
}
};
FaceApiClient.prototype.send_matSearchFaces = function(matType, matData, width, height, facePos, similarty, rows, imgMD5Set, group, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height,
facePos: facePos,
similarty: similarty,
rows: rows,
imgMD5Set: imgMD5Set,
group: group
};
var args = new FaceApi_matSearchFaces_args(params);
try {
this.output.writeMessageBegin('matSearchFaces', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matSearchFaces = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matSearchFaces_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.ex3) {
throw result.ex3;
}
if (null !== result.success) {
return result.success;
}
throw 'matSearchFaces failed: unknown result';
};
FaceApiClient.prototype.matWearMask = function(matType, matData, width, height, faceInfo, callback) {
if (callback === undefined) {
this.send_matWearMask(matType, matData, width, height, faceInfo);
return this.recv_matWearMask();
} else {
var postData = this.send_matWearMask(matType, matData, width, height, faceInfo, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_matWearMask);
}
};
FaceApiClient.prototype.send_matWearMask = function(matType, matData, width, height, faceInfo, callback) {
var params = {
matType: matType,
matData: matData,
width: width,
height: height,
faceInfo: faceInfo
};
var args = new FaceApi_matWearMask_args(params);
try {
this.output.writeMessageBegin('matWearMask', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_matWearMask = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_matWearMask_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'matWearMask failed: unknown result';
};
FaceApiClient.prototype.sdkCapacity = function(callback) {
if (callback === undefined) {
this.send_sdkCapacity();
return this.recv_sdkCapacity();
} else {
var postData = this.send_sdkCapacity(true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_sdkCapacity);
}
};
FaceApiClient.prototype.send_sdkCapacity = function(callback) {
var args = new FaceApi_sdkCapacity_args();
try {
this.output.writeMessageBegin('sdkCapacity', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_sdkCapacity = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_sdkCapacity_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'sdkCapacity failed: unknown result';
};
FaceApiClient.prototype.searchFaces = function(imgData, facePos, similarty, rows, imgMD5Set, group, callback) {
if (callback === undefined) {
this.send_searchFaces(imgData, facePos, similarty, rows, imgMD5Set, group);
return this.recv_searchFaces();
} else {
var postData = this.send_searchFaces(imgData, facePos, similarty, rows, imgMD5Set, group, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_searchFaces);
}
};
FaceApiClient.prototype.send_searchFaces = function(imgData, facePos, similarty, rows, imgMD5Set, group, callback) {
var params = {
imgData: imgData,
facePos: facePos,
similarty: similarty,
rows: rows,
imgMD5Set: imgMD5Set,
group: group
};
var args = new FaceApi_searchFaces_args(params);
try {
this.output.writeMessageBegin('searchFaces', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_searchFaces = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_searchFaces_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.ex3) {
throw result.ex3;
}
if (null !== result.success) {
return result.success;
}
throw 'searchFaces failed: unknown result';
};
FaceApiClient.prototype.searchFeatures = function(feature, similarity, rows, imgMD5Set, group, callback) {
if (callback === undefined) {
this.send_searchFeatures(feature, similarity, rows, imgMD5Set, group);
return this.recv_searchFeatures();
} else {
var postData = this.send_searchFeatures(feature, similarity, rows, imgMD5Set, group, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_searchFeatures);
}
};
FaceApiClient.prototype.send_searchFeatures = function(feature, similarity, rows, imgMD5Set, group, callback) {
var params = {
feature: feature,
similarity: similarity,
rows: rows,
imgMD5Set: imgMD5Set,
group: group
};
var args = new FaceApi_searchFeatures_args(params);
try {
this.output.writeMessageBegin('searchFeatures', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_searchFeatures = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_searchFeatures_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'searchFeatures failed: unknown result';
};
FaceApiClient.prototype.wearMask = function(imgData, faceInfo, callback) {
if (callback === undefined) {
this.send_wearMask(imgData, faceInfo);
return this.recv_wearMask();
} else {
var postData = this.send_wearMask(imgData, faceInfo, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_wearMask);
}
};
FaceApiClient.prototype.send_wearMask = function(imgData, faceInfo, callback) {
var params = {
imgData: imgData,
faceInfo: faceInfo
};
var args = new FaceApi_wearMask_args(params);
try {
this.output.writeMessageBegin('wearMask', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FaceApiClient.prototype.recv_wearMask = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FaceApi_wearMask_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.ex2) {
throw result.ex2;
}
if (null !== result.success) {
return result.success;
}
throw 'wearMask failed: unknown result';
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy