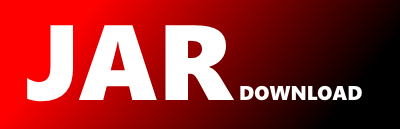
META-INF.resources.demo.client.js.FeatureSe.js Maven / Gradle / Ivy
The newest version!
//
// Autogenerated by Thrift Compiler (0.13.0)
//
// DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
//
if (typeof Int64 === 'undefined' && typeof require === 'function') {
var Int64 = require('node-int64');
}
//HELPER FUNCTIONS AND STRUCTURES
FeatureSe_addFeatureToFse_args = function(args) {
this.featureId = null;
this.feature = null;
this.imgMD5 = null;
this.group = null;
if (args) {
if (args.featureId !== undefined && args.featureId !== null) {
this.featureId = args.featureId;
}
if (args.feature !== undefined && args.feature !== null) {
this.feature = args.feature;
}
if (args.imgMD5 !== undefined && args.imgMD5 !== null) {
this.imgMD5 = args.imgMD5;
}
if (args.group !== undefined && args.group !== null) {
this.group = args.group;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field group is unset!');
}
}
};
FeatureSe_addFeatureToFse_args.prototype = {};
FeatureSe_addFeatureToFse_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.featureId = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.feature = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.STRING) {
this.imgMD5 = input.readString().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.group = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_addFeatureToFse_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_addFeatureToFse_args');
if (this.featureId !== null && this.featureId !== undefined) {
output.writeFieldBegin('featureId', Thrift.Type.STRING, 1);
output.writeBinary(this.featureId);
output.writeFieldEnd();
}
if (this.feature !== null && this.feature !== undefined) {
output.writeFieldBegin('feature', Thrift.Type.STRING, 2);
output.writeBinary(this.feature);
output.writeFieldEnd();
}
if (this.imgMD5 !== null && this.imgMD5 !== undefined) {
output.writeFieldBegin('imgMD5', Thrift.Type.STRING, 3);
output.writeString(this.imgMD5);
output.writeFieldEnd();
}
if (this.group !== null && this.group !== undefined) {
output.writeFieldBegin('group', Thrift.Type.I32, 4);
output.writeI32(this.group);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_addFeatureToFse_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_addFeatureToFse_result.prototype = {};
FeatureSe_addFeatureToFse_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_addFeatureToFse_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_addFeatureToFse_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_addFeatureToFseWithAppId_args = function(args) {
this.featureId = null;
this.feature = null;
this.appid = null;
this.group = null;
if (args) {
if (args.featureId !== undefined && args.featureId !== null) {
this.featureId = args.featureId;
}
if (args.feature !== undefined && args.feature !== null) {
this.feature = args.feature;
}
if (args.appid !== undefined && args.appid !== null) {
this.appid = args.appid;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field appid is unset!');
}
if (args.group !== undefined && args.group !== null) {
this.group = args.group;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field group is unset!');
}
}
};
FeatureSe_addFeatureToFseWithAppId_args.prototype = {};
FeatureSe_addFeatureToFseWithAppId_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.featureId = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.STRING) {
this.feature = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I64) {
this.appid = input.readI64().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.I32) {
this.group = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_addFeatureToFseWithAppId_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_addFeatureToFseWithAppId_args');
if (this.featureId !== null && this.featureId !== undefined) {
output.writeFieldBegin('featureId', Thrift.Type.STRING, 1);
output.writeBinary(this.featureId);
output.writeFieldEnd();
}
if (this.feature !== null && this.feature !== undefined) {
output.writeFieldBegin('feature', Thrift.Type.STRING, 2);
output.writeBinary(this.feature);
output.writeFieldEnd();
}
if (this.appid !== null && this.appid !== undefined) {
output.writeFieldBegin('appid', Thrift.Type.I64, 3);
output.writeI64(this.appid);
output.writeFieldEnd();
}
if (this.group !== null && this.group !== undefined) {
output.writeFieldBegin('group', Thrift.Type.I32, 4);
output.writeI32(this.group);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_addFeatureToFseWithAppId_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_addFeatureToFseWithAppId_result.prototype = {};
FeatureSe_addFeatureToFseWithAppId_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_addFeatureToFseWithAppId_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_addFeatureToFseWithAppId_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_clearAllOfFse_args = function(args) {
};
FeatureSe_clearAllOfFse_args.prototype = {};
FeatureSe_clearAllOfFse_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
if (ftype == Thrift.Type.STOP) {
break;
}
input.skip(ftype);
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_clearAllOfFse_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_clearAllOfFse_args');
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_clearAllOfFse_result = function(args) {
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_clearAllOfFse_result.prototype = {};
FeatureSe_clearAllOfFse_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_clearAllOfFse_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_clearAllOfFse_result');
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_getFeatureByHexFromFse_args = function(args) {
this.featureId = null;
if (args) {
if (args.featureId !== undefined && args.featureId !== null) {
this.featureId = args.featureId;
}
}
};
FeatureSe_getFeatureByHexFromFse_args.prototype = {};
FeatureSe_getFeatureByHexFromFse_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.featureId = input.readString().value;
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_getFeatureByHexFromFse_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_getFeatureByHexFromFse_args');
if (this.featureId !== null && this.featureId !== undefined) {
output.writeFieldBegin('featureId', Thrift.Type.STRING, 1);
output.writeString(this.featureId);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_getFeatureByHexFromFse_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CodeBean(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_getFeatureByHexFromFse_result.prototype = {};
FeatureSe_getFeatureByHexFromFse_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CodeBean();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_getFeatureByHexFromFse_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_getFeatureByHexFromFse_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_getFeatureFromFse_args = function(args) {
this.featureId = null;
if (args) {
if (args.featureId !== undefined && args.featureId !== null) {
this.featureId = args.featureId;
}
}
};
FeatureSe_getFeatureFromFse_args.prototype = {};
FeatureSe_getFeatureFromFse_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.featureId = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_getFeatureFromFse_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_getFeatureFromFse_args');
if (this.featureId !== null && this.featureId !== undefined) {
output.writeFieldBegin('featureId', Thrift.Type.STRING, 1);
output.writeBinary(this.featureId);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_getFeatureFromFse_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = new CodeBean(args.success);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_getFeatureFromFse_result.prototype = {};
FeatureSe_getFeatureFromFse_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.STRUCT) {
this.success = new CodeBean();
this.success.read(input);
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_getFeatureFromFse_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_getFeatureFromFse_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.STRUCT, 0);
this.success.write(output);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_removeFeatureByHexFromFse_args = function(args) {
this.featureId = null;
if (args) {
if (args.featureId !== undefined && args.featureId !== null) {
this.featureId = args.featureId;
}
}
};
FeatureSe_removeFeatureByHexFromFse_args.prototype = {};
FeatureSe_removeFeatureByHexFromFse_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.featureId = input.readString().value;
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_removeFeatureByHexFromFse_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_removeFeatureByHexFromFse_args');
if (this.featureId !== null && this.featureId !== undefined) {
output.writeFieldBegin('featureId', Thrift.Type.STRING, 1);
output.writeString(this.featureId);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_removeFeatureByHexFromFse_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_removeFeatureByHexFromFse_result.prototype = {};
FeatureSe_removeFeatureByHexFromFse_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_removeFeatureByHexFromFse_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_removeFeatureByHexFromFse_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_removeFeatureFromFse_args = function(args) {
this.featureId = null;
if (args) {
if (args.featureId !== undefined && args.featureId !== null) {
this.featureId = args.featureId;
}
}
};
FeatureSe_removeFeatureFromFse_args.prototype = {};
FeatureSe_removeFeatureFromFse_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.featureId = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 0:
input.skip(ftype);
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_removeFeatureFromFse_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_removeFeatureFromFse_args');
if (this.featureId !== null && this.featureId !== undefined) {
output.writeFieldBegin('featureId', Thrift.Type.STRING, 1);
output.writeBinary(this.featureId);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_removeFeatureFromFse_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_removeFeatureFromFse_result.prototype = {};
FeatureSe_removeFeatureFromFse_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_removeFeatureFromFse_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_removeFeatureFromFse_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_searchCodeFromFse_args = function(args) {
this.code = null;
this.sim = null;
this.rows = null;
this.imgMD5Set = null;
this.group = null;
if (args) {
if (args.code !== undefined && args.code !== null) {
this.code = args.code;
}
if (args.sim !== undefined && args.sim !== null) {
this.sim = args.sim;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field sim is unset!');
}
if (args.rows !== undefined && args.rows !== null) {
this.rows = args.rows;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field rows is unset!');
}
if (args.imgMD5Set !== undefined && args.imgMD5Set !== null) {
this.imgMD5Set = Thrift.copyList(args.imgMD5Set, [null]);
}
if (args.group !== undefined && args.group !== null) {
this.group = args.group;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field group is unset!');
}
}
};
FeatureSe_searchCodeFromFse_args.prototype = {};
FeatureSe_searchCodeFromFse_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.code = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.DOUBLE) {
this.sim = input.readDouble().value;
} else {
input.skip(ftype);
}
break;
case 3:
if (ftype == Thrift.Type.I32) {
this.rows = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 4:
if (ftype == Thrift.Type.LIST) {
this.imgMD5Set = [];
var _rtmp31 = input.readListBegin();
var _size0 = _rtmp31.size || 0;
for (var _i2 = 0; _i2 < _size0; ++_i2) {
var elem3 = null;
elem3 = input.readString().value;
this.imgMD5Set.push(elem3);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 5:
if (ftype == Thrift.Type.I32) {
this.group = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_searchCodeFromFse_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_searchCodeFromFse_args');
if (this.code !== null && this.code !== undefined) {
output.writeFieldBegin('code', Thrift.Type.STRING, 1);
output.writeBinary(this.code);
output.writeFieldEnd();
}
if (this.sim !== null && this.sim !== undefined) {
output.writeFieldBegin('sim', Thrift.Type.DOUBLE, 2);
output.writeDouble(this.sim);
output.writeFieldEnd();
}
if (this.rows !== null && this.rows !== undefined) {
output.writeFieldBegin('rows', Thrift.Type.I32, 3);
output.writeI32(this.rows);
output.writeFieldEnd();
}
if (this.imgMD5Set !== null && this.imgMD5Set !== undefined) {
output.writeFieldBegin('imgMD5Set', Thrift.Type.LIST, 4);
output.writeListBegin(Thrift.Type.STRING, this.imgMD5Set.length);
for (var iter4 in this.imgMD5Set) {
if (this.imgMD5Set.hasOwnProperty(iter4)) {
iter4 = this.imgMD5Set[iter4];
output.writeString(iter4);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.group !== null && this.group !== undefined) {
output.writeFieldBegin('group', Thrift.Type.I32, 5);
output.writeI32(this.group);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_searchCodeFromFse_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = Thrift.copyList(args.success, [CodeBean]);
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_searchCodeFromFse_result.prototype = {};
FeatureSe_searchCodeFromFse_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.LIST) {
this.success = [];
var _rtmp36 = input.readListBegin();
var _size5 = _rtmp36.size || 0;
for (var _i7 = 0; _i7 < _size5; ++_i7) {
var elem8 = null;
elem8 = new CodeBean();
elem8.read(input);
this.success.push(elem8);
}
input.readListEnd();
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_searchCodeFromFse_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_searchCodeFromFse_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.LIST, 0);
output.writeListBegin(Thrift.Type.STRUCT, this.success.length);
for (var iter9 in this.success) {
if (this.success.hasOwnProperty(iter9)) {
iter9 = this.success[iter9];
iter9.write(output);
}
}
output.writeListEnd();
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_sizeOfFse_args = function(args) {
};
FeatureSe_sizeOfFse_args.prototype = {};
FeatureSe_sizeOfFse_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
if (ftype == Thrift.Type.STOP) {
break;
}
input.skip(ftype);
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_sizeOfFse_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_sizeOfFse_args');
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_sizeOfFse_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_sizeOfFse_result.prototype = {};
FeatureSe_sizeOfFse_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.I32) {
this.success = input.readI32().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_sizeOfFse_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_sizeOfFse_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.I32, 0);
output.writeI32(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_updateGroup_args = function(args) {
this.featureId = null;
this.group = null;
if (args) {
if (args.featureId !== undefined && args.featureId !== null) {
this.featureId = args.featureId;
}
if (args.group !== undefined && args.group !== null) {
this.group = args.group;
} else {
throw new Thrift.TProtocolException(Thrift.TProtocolExceptionType.UNKNOWN, 'Required field group is unset!');
}
}
};
FeatureSe_updateGroup_args.prototype = {};
FeatureSe_updateGroup_args.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 1:
if (ftype == Thrift.Type.STRING) {
this.featureId = input.readBinary().value;
} else {
input.skip(ftype);
}
break;
case 2:
if (ftype == Thrift.Type.I32) {
this.group = input.readI32().value;
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_updateGroup_args.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_updateGroup_args');
if (this.featureId !== null && this.featureId !== undefined) {
output.writeFieldBegin('featureId', Thrift.Type.STRING, 1);
output.writeBinary(this.featureId);
output.writeFieldEnd();
}
if (this.group !== null && this.group !== undefined) {
output.writeFieldBegin('group', Thrift.Type.I32, 2);
output.writeI32(this.group);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSe_updateGroup_result = function(args) {
this.success = null;
this.ex1 = null;
if (args instanceof ServiceRuntimeException) {
this.ex1 = args;
return;
}
if (args) {
if (args.success !== undefined && args.success !== null) {
this.success = args.success;
}
if (args.ex1 !== undefined && args.ex1 !== null) {
this.ex1 = args.ex1;
}
}
};
FeatureSe_updateGroup_result.prototype = {};
FeatureSe_updateGroup_result.prototype.read = function(input) {
input.readStructBegin();
while (true) {
var ret = input.readFieldBegin();
var ftype = ret.ftype;
var fid = ret.fid;
if (ftype == Thrift.Type.STOP) {
break;
}
switch (fid) {
case 0:
if (ftype == Thrift.Type.BOOL) {
this.success = input.readBool().value;
} else {
input.skip(ftype);
}
break;
case 1:
if (ftype == Thrift.Type.STRUCT) {
this.ex1 = new ServiceRuntimeException();
this.ex1.read(input);
} else {
input.skip(ftype);
}
break;
default:
input.skip(ftype);
}
input.readFieldEnd();
}
input.readStructEnd();
return;
};
FeatureSe_updateGroup_result.prototype.write = function(output) {
output.writeStructBegin('FeatureSe_updateGroup_result');
if (this.success !== null && this.success !== undefined) {
output.writeFieldBegin('success', Thrift.Type.BOOL, 0);
output.writeBool(this.success);
output.writeFieldEnd();
}
if (this.ex1 !== null && this.ex1 !== undefined) {
output.writeFieldBegin('ex1', Thrift.Type.STRUCT, 1);
this.ex1.write(output);
output.writeFieldEnd();
}
output.writeFieldStop();
output.writeStructEnd();
return;
};
FeatureSeClient = function(input, output) {
this.input = input;
this.output = (!output) ? input : output;
this.seqid = 0;
};
FeatureSeClient.prototype = {};
FeatureSeClient.prototype.addFeatureToFse = function(featureId, feature, imgMD5, group, callback) {
if (callback === undefined) {
this.send_addFeatureToFse(featureId, feature, imgMD5, group);
return this.recv_addFeatureToFse();
} else {
var postData = this.send_addFeatureToFse(featureId, feature, imgMD5, group, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_addFeatureToFse);
}
};
FeatureSeClient.prototype.send_addFeatureToFse = function(featureId, feature, imgMD5, group, callback) {
var params = {
featureId: featureId,
feature: feature,
imgMD5: imgMD5,
group: group
};
var args = new FeatureSe_addFeatureToFse_args(params);
try {
this.output.writeMessageBegin('addFeatureToFse', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_addFeatureToFse = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_addFeatureToFse_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'addFeatureToFse failed: unknown result';
};
FeatureSeClient.prototype.addFeatureToFseWithAppId = function(featureId, feature, appid, group, callback) {
if (callback === undefined) {
this.send_addFeatureToFseWithAppId(featureId, feature, appid, group);
return this.recv_addFeatureToFseWithAppId();
} else {
var postData = this.send_addFeatureToFseWithAppId(featureId, feature, appid, group, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_addFeatureToFseWithAppId);
}
};
FeatureSeClient.prototype.send_addFeatureToFseWithAppId = function(featureId, feature, appid, group, callback) {
var params = {
featureId: featureId,
feature: feature,
appid: appid,
group: group
};
var args = new FeatureSe_addFeatureToFseWithAppId_args(params);
try {
this.output.writeMessageBegin('addFeatureToFseWithAppId', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_addFeatureToFseWithAppId = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_addFeatureToFseWithAppId_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'addFeatureToFseWithAppId failed: unknown result';
};
FeatureSeClient.prototype.clearAllOfFse = function(callback) {
if (callback === undefined) {
this.send_clearAllOfFse();
this.recv_clearAllOfFse();
} else {
var postData = this.send_clearAllOfFse(true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_clearAllOfFse);
}
};
FeatureSeClient.prototype.send_clearAllOfFse = function(callback) {
var args = new FeatureSe_clearAllOfFse_args();
try {
this.output.writeMessageBegin('clearAllOfFse', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_clearAllOfFse = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_clearAllOfFse_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
return;
};
FeatureSeClient.prototype.getFeatureByHexFromFse = function(featureId, callback) {
if (callback === undefined) {
this.send_getFeatureByHexFromFse(featureId);
return this.recv_getFeatureByHexFromFse();
} else {
var postData = this.send_getFeatureByHexFromFse(featureId, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_getFeatureByHexFromFse);
}
};
FeatureSeClient.prototype.send_getFeatureByHexFromFse = function(featureId, callback) {
var params = {
featureId: featureId
};
var args = new FeatureSe_getFeatureByHexFromFse_args(params);
try {
this.output.writeMessageBegin('getFeatureByHexFromFse', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_getFeatureByHexFromFse = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_getFeatureByHexFromFse_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'getFeatureByHexFromFse failed: unknown result';
};
FeatureSeClient.prototype.getFeatureFromFse = function(featureId, callback) {
if (callback === undefined) {
this.send_getFeatureFromFse(featureId);
return this.recv_getFeatureFromFse();
} else {
var postData = this.send_getFeatureFromFse(featureId, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_getFeatureFromFse);
}
};
FeatureSeClient.prototype.send_getFeatureFromFse = function(featureId, callback) {
var params = {
featureId: featureId
};
var args = new FeatureSe_getFeatureFromFse_args(params);
try {
this.output.writeMessageBegin('getFeatureFromFse', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_getFeatureFromFse = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_getFeatureFromFse_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'getFeatureFromFse failed: unknown result';
};
FeatureSeClient.prototype.removeFeatureByHexFromFse = function(featureId, callback) {
if (callback === undefined) {
this.send_removeFeatureByHexFromFse(featureId);
return this.recv_removeFeatureByHexFromFse();
} else {
var postData = this.send_removeFeatureByHexFromFse(featureId, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_removeFeatureByHexFromFse);
}
};
FeatureSeClient.prototype.send_removeFeatureByHexFromFse = function(featureId, callback) {
var params = {
featureId: featureId
};
var args = new FeatureSe_removeFeatureByHexFromFse_args(params);
try {
this.output.writeMessageBegin('removeFeatureByHexFromFse', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_removeFeatureByHexFromFse = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_removeFeatureByHexFromFse_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'removeFeatureByHexFromFse failed: unknown result';
};
FeatureSeClient.prototype.removeFeatureFromFse = function(featureId, callback) {
if (callback === undefined) {
this.send_removeFeatureFromFse(featureId);
return this.recv_removeFeatureFromFse();
} else {
var postData = this.send_removeFeatureFromFse(featureId, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_removeFeatureFromFse);
}
};
FeatureSeClient.prototype.send_removeFeatureFromFse = function(featureId, callback) {
var params = {
featureId: featureId
};
var args = new FeatureSe_removeFeatureFromFse_args(params);
try {
this.output.writeMessageBegin('removeFeatureFromFse', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_removeFeatureFromFse = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_removeFeatureFromFse_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'removeFeatureFromFse failed: unknown result';
};
FeatureSeClient.prototype.searchCodeFromFse = function(code, sim, rows, imgMD5Set, group, callback) {
if (callback === undefined) {
this.send_searchCodeFromFse(code, sim, rows, imgMD5Set, group);
return this.recv_searchCodeFromFse();
} else {
var postData = this.send_searchCodeFromFse(code, sim, rows, imgMD5Set, group, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_searchCodeFromFse);
}
};
FeatureSeClient.prototype.send_searchCodeFromFse = function(code, sim, rows, imgMD5Set, group, callback) {
var params = {
code: code,
sim: sim,
rows: rows,
imgMD5Set: imgMD5Set,
group: group
};
var args = new FeatureSe_searchCodeFromFse_args(params);
try {
this.output.writeMessageBegin('searchCodeFromFse', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_searchCodeFromFse = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_searchCodeFromFse_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'searchCodeFromFse failed: unknown result';
};
FeatureSeClient.prototype.sizeOfFse = function(callback) {
if (callback === undefined) {
this.send_sizeOfFse();
return this.recv_sizeOfFse();
} else {
var postData = this.send_sizeOfFse(true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_sizeOfFse);
}
};
FeatureSeClient.prototype.send_sizeOfFse = function(callback) {
var args = new FeatureSe_sizeOfFse_args();
try {
this.output.writeMessageBegin('sizeOfFse', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_sizeOfFse = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_sizeOfFse_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'sizeOfFse failed: unknown result';
};
FeatureSeClient.prototype.updateGroup = function(featureId, group, callback) {
if (callback === undefined) {
this.send_updateGroup(featureId, group);
return this.recv_updateGroup();
} else {
var postData = this.send_updateGroup(featureId, group, true);
return this.output.getTransport()
.jqRequest(this, postData, arguments, this.recv_updateGroup);
}
};
FeatureSeClient.prototype.send_updateGroup = function(featureId, group, callback) {
var params = {
featureId: featureId,
group: group
};
var args = new FeatureSe_updateGroup_args(params);
try {
this.output.writeMessageBegin('updateGroup', Thrift.MessageType.CALL, this.seqid);
args.write(this.output);
this.output.writeMessageEnd();
return this.output.getTransport().flush(callback);
}
catch (e) {
if (typeof this.output.getTransport().reset === 'function') {
this.output.getTransport().reset();
}
throw e;
}
};
FeatureSeClient.prototype.recv_updateGroup = function() {
var ret = this.input.readMessageBegin();
var mtype = ret.mtype;
if (mtype == Thrift.MessageType.EXCEPTION) {
var x = new Thrift.TApplicationException();
x.read(this.input);
this.input.readMessageEnd();
throw x;
}
var result = new FeatureSe_updateGroup_result();
result.read(this.input);
this.input.readMessageEnd();
if (null !== result.ex1) {
throw result.ex1;
}
if (null !== result.success) {
return result.success;
}
throw 'updateGroup failed: unknown result';
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy