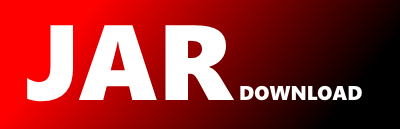
rortega.cf4j-recsys.1.1.0.source-code.Item Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cf4j-recsys Show documentation
Show all versions of cf4j-recsys Show documentation
A Java's Collaborative Filtering library to carry out experiments in research of Collaborative Filtering based Recommender Systems. The library has been designed from researchers to researchers.
The newest version!
package cf4j;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import cf4j.utils.Methods;
/**
* Defines an item. An item is composed by:
*
* - Item code
* - Item index in the items array
* - A map where we can save any type of information
* - Array of users who have rated the item
* - Array of ratings that the item have received
*
* @author Fernando Ortega
*/
public class Item implements Serializable {
private static final long serialVersionUID = 20171018L;
/**
* Item code
*/
protected int itemCode;
/**
* Item index
*/
protected int itemIndex;
/**
* Map of the item
*/
protected Map map;
/**
* Users that have rated this item
*/
protected int [] users;
/**
* Ratings of the users
*/
protected double [] ratings;
/**
* Rating average of the item
*/
protected double ratingAverage;
/**
* Standard deviation of this item
*/
protected double ratingStandardDeviation;
/**
* Creates a new instance of an item. This constructor should not be users by developers.
* @param itemCode Item code
* @param itemIndex Item index
* @param users Users that have rated this item
* @param ratings Ratings of the users
*/
public Item (int itemCode, int itemIndex, int [] users, double [] ratings) {
this.itemCode = itemCode;
this.itemIndex = itemIndex;
this.map = new HashMap();
this.users = users;
this.ratings = ratings;
this.ratingAverage = Methods.arrayAverage(ratings);
this.ratingStandardDeviation = Methods.arrayStandardDeviation(ratings);
}
/**
* Write a data in the item map.
* @param key Key associated to the value
* @param value Value to be written in the map
* @return Previously value of the key if exists or null
*/
public synchronized Object put (String key, Object value) {
return map.put(key, value);
}
/**
* Retrieves a value from a key.
* @param key Key of the saved object
* @return The value associated to the key if exists or null
*/
public synchronized Object get(String key) {
return map.get(key);
}
/**
* Average of the ratings received by the item.
* @return Rating average
*/
public double getRatingAverage() {
return this.ratingAverage;
}
/**
* Standard deviation of the ratings received by the item.
* @return Rating standard deviation
*/
public double getRatingStandardDeviation() {
return this.ratingStandardDeviation;
}
/**
* Return the item code.
* @return Item code
*/
public int getItemCode() {
return this.itemCode;
}
/**
* Return the item index.
* @return Item index
*/
public int getItemIndex() {
return this.itemIndex;
}
/**
* Get the map of the item. It is recommended using put(...) and get(...) instead of
* this method.
* @return Map of the item
*/
public Map getMap() {
return map;
}
/**
* Get the users that have rated the item.
* @return Test users codes sorted from low to high.
*/
public int [] getUsers() {
return this.users;
}
/**
* Get the ratings of the users to the item. The indexes of the array overlaps
* with indexes of the getUsers() array.
* @return Training users ratings
*/
public double [] getRatings() {
return this.ratings;
}
/**
* Get the index of an user code at the user's item array.
* @param user_code User code
* @return User index in the user's item array if the user has rated the item or -1 if not
*/
public int getUserIndex (int user_code) {
return Methods.getIndex(this.users, user_code);
}
/**
* Get the number of ratings that the item have received.
* @return Number of ratings received
*/
public int getNumberOfRatings () {
return this.ratings.length;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy