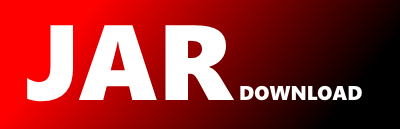
carosellini.rJava.REngine.0.9-7.source-code.REXPEnvironment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of REngine Show documentation
Show all versions of REngine Show documentation
Rserve is a TCP/IP server which allows other programs to use facilities of R (see www.r-project.org) from various languages without the need to initialize R or link against R library. Every connection has a separate workspace and working directory. Client-side implementations are available for popular languages such as C/C++, PHP and Java. Rserve supports remote connection, authentication and file transfer. Typical use is to integrate R backend for computation of statstical models, plots etc. in other applications.
The newest version!
package org.rosuda.REngine;
// environments are like REXPReferences except that they cannot be resolved
/** REXPEnvironment represents an environment in R. Very much like {@link org.rosuda.REngine.REXPReference} this is a proxy object to the actual object on the R side. It provides methods for accessing the content of the environment. The actual implementation may vary by the back-end used and not all engines support environments. Check {@link org.rosuda.REngine.REngine.supportsEnvironments()} for the given engine. Environments are specific for a given engine, they cannot be passed across engines.
*/
public class REXPEnvironment extends REXP {
/** engine associated with this environment */
REngine eng;
/** transparent handle that can be used by the engine to indentify the environment. It is not used by REngine API itself. */
Object handle;
/** create a new environemnt reference - this constructor should never be used directly, use {@link REngine.newEnvironment()} instead.
* @param eng engine responsible for this environment
* @param handle handle used by the engine to identify this environment
*/
public REXPEnvironment(REngine eng, Object handle) {
super();
this.eng = eng;
this.handle = handle;
}
public boolean isEnvironment() { return true; }
/** returns the handle used to identify this environemnt in the engine - for internal use by engine implementations only
* @return handle of this environment */
public Object getHandle() { return handle; }
/** get a value from this environment
* @param name name of the value
* @param resolve if false
returns a reference to the object, if false
the reference is resolved
* @return value corresponding to the symbol name or possibly null
if the value is unbound (the latter is currently engine-specific) */
public REXP get(String name, boolean resolve) throws REngineException {
try {
return eng.get(name, this, resolve);
} catch (REXPMismatchException e) { // this should never happen because this is always guaranteed to be REXPEnv
throw(new REngineException(eng, "REXPMismatchException:"+e+" in get()"));
}
}
/** get a value from this environment - equavalent to get(name, true)
.
* @param name name of the value
* @return value (see {@link #get(String,boolean)}) */
public REXP get(String name) throws REngineException {
return get(name, true);
}
/** assigns a value to a given symbol name
* @param name symbol name
* @param value value */
public void assign(String name, REXP value) throws REngineException, REXPMismatchException {
eng.assign(name, value, this);
}
/** returns the parent environment or a reference to it
* @param resolve if true
returns the environemnt, otherwise a reference.
* @return parent environemnt (or a reference to it) */
public REXP parent(boolean resolve) throws REngineException {
try {
return eng.getParentEnvironment(this, resolve);
} catch (REXPMismatchException e) { // this should never happen because this is always guaranteed to be REXPEnv
throw(new REngineException(eng, "REXPMismatchException:"+e+" in parent()"));
}
}
/** returns the parent environment. This is equivalent to parent(true)
.
* @return parent environemnt */
public REXPEnvironment parent() throws REngineException {
return (REXPEnvironment) parent(true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy