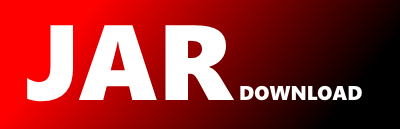
rahbuisson.kotlin-parser.1.5.source-code.KotlinParserBaseListener Maven / Gradle / Ivy
// Generated from KotlinParser.g4 by ANTLR 4.7
package com.github.sarahbuisson.kotlinparser;
import org.antlr.v4.runtime.ParserRuleContext;
import org.antlr.v4.runtime.tree.ErrorNode;
import org.antlr.v4.runtime.tree.TerminalNode;
/**
* This class provides an empty implementation of {@link KotlinParserListener},
* which can be extended to create a listener which only needs to handle a subset
* of the available methods.
*/
public class KotlinParserBaseListener implements KotlinParserListener {
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterKotlinFile(KotlinParser.KotlinFileContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitKotlinFile(KotlinParser.KotlinFileContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterScript(KotlinParser.ScriptContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitScript(KotlinParser.ScriptContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFileAnnotation(KotlinParser.FileAnnotationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFileAnnotation(KotlinParser.FileAnnotationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPackageHeader(KotlinParser.PackageHeaderContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPackageHeader(KotlinParser.PackageHeaderContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterImportList(KotlinParser.ImportListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitImportList(KotlinParser.ImportListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterImportHeader(KotlinParser.ImportHeaderContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitImportHeader(KotlinParser.ImportHeaderContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterImportAlias(KotlinParser.ImportAliasContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitImportAlias(KotlinParser.ImportAliasContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTopLevelObject(KotlinParser.TopLevelObjectContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTopLevelObject(KotlinParser.TopLevelObjectContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterClassDeclaration(KotlinParser.ClassDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitClassDeclaration(KotlinParser.ClassDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPrimaryConstructor(KotlinParser.PrimaryConstructorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPrimaryConstructor(KotlinParser.PrimaryConstructorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterClassParameters(KotlinParser.ClassParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitClassParameters(KotlinParser.ClassParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterClassParameter(KotlinParser.ClassParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitClassParameter(KotlinParser.ClassParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterDelegationSpecifiers(KotlinParser.DelegationSpecifiersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitDelegationSpecifiers(KotlinParser.DelegationSpecifiersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterDelegationSpecifier(KotlinParser.DelegationSpecifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitDelegationSpecifier(KotlinParser.DelegationSpecifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterConstructorInvocation(KotlinParser.ConstructorInvocationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitConstructorInvocation(KotlinParser.ConstructorInvocationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterExplicitDelegation(KotlinParser.ExplicitDelegationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitExplicitDelegation(KotlinParser.ExplicitDelegationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterClassBody(KotlinParser.ClassBodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitClassBody(KotlinParser.ClassBodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterClassMemberDeclaration(KotlinParser.ClassMemberDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitClassMemberDeclaration(KotlinParser.ClassMemberDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAnonymousInitializer(KotlinParser.AnonymousInitializerContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAnonymousInitializer(KotlinParser.AnonymousInitializerContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSecondaryConstructor(KotlinParser.SecondaryConstructorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSecondaryConstructor(KotlinParser.SecondaryConstructorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterConstructorDelegationCall(KotlinParser.ConstructorDelegationCallContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitConstructorDelegationCall(KotlinParser.ConstructorDelegationCallContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterEnumClassBody(KotlinParser.EnumClassBodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitEnumClassBody(KotlinParser.EnumClassBodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterEnumEntries(KotlinParser.EnumEntriesContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitEnumEntries(KotlinParser.EnumEntriesContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterEnumEntry(KotlinParser.EnumEntryContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitEnumEntry(KotlinParser.EnumEntryContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFunctionDeclaration(KotlinParser.FunctionDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFunctionDeclaration(KotlinParser.FunctionDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFunctionValueParameters(KotlinParser.FunctionValueParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFunctionValueParameters(KotlinParser.FunctionValueParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFunctionValueParameter(KotlinParser.FunctionValueParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFunctionValueParameter(KotlinParser.FunctionValueParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterParameter(KotlinParser.ParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitParameter(KotlinParser.ParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFunctionBody(KotlinParser.FunctionBodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFunctionBody(KotlinParser.FunctionBodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterObjectDeclaration(KotlinParser.ObjectDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitObjectDeclaration(KotlinParser.ObjectDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterCompanionObject(KotlinParser.CompanionObjectContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitCompanionObject(KotlinParser.CompanionObjectContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPropertyDeclaration(KotlinParser.PropertyDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPropertyDeclaration(KotlinParser.PropertyDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterMultiVariableDeclaration(KotlinParser.MultiVariableDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitMultiVariableDeclaration(KotlinParser.MultiVariableDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterVariableDeclaration(KotlinParser.VariableDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitVariableDeclaration(KotlinParser.VariableDeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterGetter(KotlinParser.GetterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitGetter(KotlinParser.GetterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSetter(KotlinParser.SetterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSetter(KotlinParser.SetterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeAlias(KotlinParser.TypeAliasContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeAlias(KotlinParser.TypeAliasContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeParameters(KotlinParser.TypeParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeParameters(KotlinParser.TypeParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeParameter(KotlinParser.TypeParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeParameter(KotlinParser.TypeParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterType(KotlinParser.TypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitType(KotlinParser.TypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeModifierList(KotlinParser.TypeModifierListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeModifierList(KotlinParser.TypeModifierListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterParenthesizedType(KotlinParser.ParenthesizedTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitParenthesizedType(KotlinParser.ParenthesizedTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterNullableType(KotlinParser.NullableTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitNullableType(KotlinParser.NullableTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeReference(KotlinParser.TypeReferenceContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeReference(KotlinParser.TypeReferenceContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFunctionType(KotlinParser.FunctionTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFunctionType(KotlinParser.FunctionTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFunctionTypeReceiver(KotlinParser.FunctionTypeReceiverContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFunctionTypeReceiver(KotlinParser.FunctionTypeReceiverContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterUserType(KotlinParser.UserTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitUserType(KotlinParser.UserTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSimpleUserType(KotlinParser.SimpleUserTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSimpleUserType(KotlinParser.SimpleUserTypeContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFunctionTypeParameters(KotlinParser.FunctionTypeParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFunctionTypeParameters(KotlinParser.FunctionTypeParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeConstraints(KotlinParser.TypeConstraintsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeConstraints(KotlinParser.TypeConstraintsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeConstraint(KotlinParser.TypeConstraintContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeConstraint(KotlinParser.TypeConstraintContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterBlock(KotlinParser.BlockContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitBlock(KotlinParser.BlockContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterStatements(KotlinParser.StatementsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitStatements(KotlinParser.StatementsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterStatement(KotlinParser.StatementContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitStatement(KotlinParser.StatementContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterDeclaration(KotlinParser.DeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitDeclaration(KotlinParser.DeclarationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAssignment(KotlinParser.AssignmentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAssignment(KotlinParser.AssignmentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterExpression(KotlinParser.ExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitExpression(KotlinParser.ExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterDisjunction(KotlinParser.DisjunctionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitDisjunction(KotlinParser.DisjunctionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterConjunction(KotlinParser.ConjunctionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitConjunction(KotlinParser.ConjunctionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterEquality(KotlinParser.EqualityContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitEquality(KotlinParser.EqualityContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterComparison(KotlinParser.ComparisonContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitComparison(KotlinParser.ComparisonContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterInfixOperation(KotlinParser.InfixOperationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitInfixOperation(KotlinParser.InfixOperationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterElvisExpression(KotlinParser.ElvisExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitElvisExpression(KotlinParser.ElvisExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterInfixFunctionCall(KotlinParser.InfixFunctionCallContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitInfixFunctionCall(KotlinParser.InfixFunctionCallContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterRangeExpression(KotlinParser.RangeExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitRangeExpression(KotlinParser.RangeExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAdditiveExpression(KotlinParser.AdditiveExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAdditiveExpression(KotlinParser.AdditiveExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterMultiplicativeExpression(KotlinParser.MultiplicativeExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitMultiplicativeExpression(KotlinParser.MultiplicativeExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAsExpression(KotlinParser.AsExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAsExpression(KotlinParser.AsExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAsExpressionTail(KotlinParser.AsExpressionTailContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAsExpressionTail(KotlinParser.AsExpressionTailContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPrefixUnaryExpression(KotlinParser.PrefixUnaryExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPrefixUnaryExpression(KotlinParser.PrefixUnaryExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPostfixUnaryExpression(KotlinParser.PostfixUnaryExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPostfixUnaryExpression(KotlinParser.PostfixUnaryExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterCallExpression(KotlinParser.CallExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitCallExpression(KotlinParser.CallExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLabeledExpression(KotlinParser.LabeledExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLabeledExpression(KotlinParser.LabeledExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterDotQualifiedExpression(KotlinParser.DotQualifiedExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitDotQualifiedExpression(KotlinParser.DotQualifiedExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAssignableExpression(KotlinParser.AssignableExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAssignableExpression(KotlinParser.AssignableExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterIndexingExpression(KotlinParser.IndexingExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitIndexingExpression(KotlinParser.IndexingExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterCallSuffix(KotlinParser.CallSuffixContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitCallSuffix(KotlinParser.CallSuffixContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAnnotatedLambda(KotlinParser.AnnotatedLambdaContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAnnotatedLambda(KotlinParser.AnnotatedLambdaContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterArrayAccess(KotlinParser.ArrayAccessContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitArrayAccess(KotlinParser.ArrayAccessContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterValueArguments(KotlinParser.ValueArgumentsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitValueArguments(KotlinParser.ValueArgumentsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeArguments(KotlinParser.TypeArgumentsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeArguments(KotlinParser.TypeArgumentsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeProjection(KotlinParser.TypeProjectionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeProjection(KotlinParser.TypeProjectionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeProjectionModifierList(KotlinParser.TypeProjectionModifierListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeProjectionModifierList(KotlinParser.TypeProjectionModifierListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterValueArgument(KotlinParser.ValueArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitValueArgument(KotlinParser.ValueArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPrimaryExpression(KotlinParser.PrimaryExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPrimaryExpression(KotlinParser.PrimaryExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterParenthesizedExpression(KotlinParser.ParenthesizedExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitParenthesizedExpression(KotlinParser.ParenthesizedExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLiteralConstant(KotlinParser.LiteralConstantContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLiteralConstant(KotlinParser.LiteralConstantContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterStringLiteral(KotlinParser.StringLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitStringLiteral(KotlinParser.StringLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLineStringLiteral(KotlinParser.LineStringLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLineStringLiteral(KotlinParser.LineStringLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterMultiLineStringLiteral(KotlinParser.MultiLineStringLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitMultiLineStringLiteral(KotlinParser.MultiLineStringLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLineStringContent(KotlinParser.LineStringContentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLineStringContent(KotlinParser.LineStringContentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLineStringExpression(KotlinParser.LineStringExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLineStringExpression(KotlinParser.LineStringExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterMultiLineStringContent(KotlinParser.MultiLineStringContentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitMultiLineStringContent(KotlinParser.MultiLineStringContentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterMultiLineStringExpression(KotlinParser.MultiLineStringExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitMultiLineStringExpression(KotlinParser.MultiLineStringExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFunctionLiteral(KotlinParser.FunctionLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFunctionLiteral(KotlinParser.FunctionLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLambdaParameters(KotlinParser.LambdaParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLambdaParameters(KotlinParser.LambdaParametersContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLambdaParameter(KotlinParser.LambdaParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLambdaParameter(KotlinParser.LambdaParameterContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterObjectLiteral(KotlinParser.ObjectLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitObjectLiteral(KotlinParser.ObjectLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterCollectionLiteral(KotlinParser.CollectionLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitCollectionLiteral(KotlinParser.CollectionLiteralContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterThisExpression(KotlinParser.ThisExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitThisExpression(KotlinParser.ThisExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSuperExpression(KotlinParser.SuperExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSuperExpression(KotlinParser.SuperExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterConditionalExpression(KotlinParser.ConditionalExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitConditionalExpression(KotlinParser.ConditionalExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterIfExpression(KotlinParser.IfExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitIfExpression(KotlinParser.IfExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterControlStructureBody(KotlinParser.ControlStructureBodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitControlStructureBody(KotlinParser.ControlStructureBodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterWhenExpression(KotlinParser.WhenExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitWhenExpression(KotlinParser.WhenExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterWhenEntry(KotlinParser.WhenEntryContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitWhenEntry(KotlinParser.WhenEntryContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterWhenCondition(KotlinParser.WhenConditionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitWhenCondition(KotlinParser.WhenConditionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterRangeTest(KotlinParser.RangeTestContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitRangeTest(KotlinParser.RangeTestContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeTest(KotlinParser.TypeTestContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeTest(KotlinParser.TypeTestContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTryExpression(KotlinParser.TryExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTryExpression(KotlinParser.TryExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterCatchBlock(KotlinParser.CatchBlockContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitCatchBlock(KotlinParser.CatchBlockContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFinallyBlock(KotlinParser.FinallyBlockContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFinallyBlock(KotlinParser.FinallyBlockContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLoopExpression(KotlinParser.LoopExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLoopExpression(KotlinParser.LoopExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterForExpression(KotlinParser.ForExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitForExpression(KotlinParser.ForExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterWhileExpression(KotlinParser.WhileExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitWhileExpression(KotlinParser.WhileExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterDoWhileExpression(KotlinParser.DoWhileExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitDoWhileExpression(KotlinParser.DoWhileExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterJumpExpression(KotlinParser.JumpExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitJumpExpression(KotlinParser.JumpExpressionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterCallableReference(KotlinParser.CallableReferenceContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitCallableReference(KotlinParser.CallableReferenceContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAssignmentOperator(KotlinParser.AssignmentOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAssignmentOperator(KotlinParser.AssignmentOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterEqualityOperator(KotlinParser.EqualityOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitEqualityOperator(KotlinParser.EqualityOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterComparisonOperator(KotlinParser.ComparisonOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitComparisonOperator(KotlinParser.ComparisonOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterInOperator(KotlinParser.InOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitInOperator(KotlinParser.InOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterIsOperator(KotlinParser.IsOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitIsOperator(KotlinParser.IsOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAdditiveOperator(KotlinParser.AdditiveOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAdditiveOperator(KotlinParser.AdditiveOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterMultiplicativeOperator(KotlinParser.MultiplicativeOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitMultiplicativeOperator(KotlinParser.MultiplicativeOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAsOperator(KotlinParser.AsOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAsOperator(KotlinParser.AsOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPrefixUnaryOperator(KotlinParser.PrefixUnaryOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPrefixUnaryOperator(KotlinParser.PrefixUnaryOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPostfixUnaryOperator(KotlinParser.PostfixUnaryOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPostfixUnaryOperator(KotlinParser.PostfixUnaryOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterMemberAccessOperator(KotlinParser.MemberAccessOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitMemberAccessOperator(KotlinParser.MemberAccessOperatorContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterModifierList(KotlinParser.ModifierListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitModifierList(KotlinParser.ModifierListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterModifier(KotlinParser.ModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitModifier(KotlinParser.ModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterClassModifier(KotlinParser.ClassModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitClassModifier(KotlinParser.ClassModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterMemberModifier(KotlinParser.MemberModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitMemberModifier(KotlinParser.MemberModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterVisibilityModifier(KotlinParser.VisibilityModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitVisibilityModifier(KotlinParser.VisibilityModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterVarianceAnnotation(KotlinParser.VarianceAnnotationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitVarianceAnnotation(KotlinParser.VarianceAnnotationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFunctionModifier(KotlinParser.FunctionModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFunctionModifier(KotlinParser.FunctionModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPropertyModifier(KotlinParser.PropertyModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPropertyModifier(KotlinParser.PropertyModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterInheritanceModifier(KotlinParser.InheritanceModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitInheritanceModifier(KotlinParser.InheritanceModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterParameterModifier(KotlinParser.ParameterModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitParameterModifier(KotlinParser.ParameterModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterTypeParameterModifier(KotlinParser.TypeParameterModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitTypeParameterModifier(KotlinParser.TypeParameterModifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLabelDefinition(KotlinParser.LabelDefinitionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLabelDefinition(KotlinParser.LabelDefinitionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAnnotations(KotlinParser.AnnotationsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAnnotations(KotlinParser.AnnotationsContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAnnotation(KotlinParser.AnnotationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAnnotation(KotlinParser.AnnotationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAnnotationList(KotlinParser.AnnotationListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAnnotationList(KotlinParser.AnnotationListContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAnnotationUseSiteTarget(KotlinParser.AnnotationUseSiteTargetContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAnnotationUseSiteTarget(KotlinParser.AnnotationUseSiteTargetContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterUnescapedAnnotation(KotlinParser.UnescapedAnnotationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitUnescapedAnnotation(KotlinParser.UnescapedAnnotationContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterIdentifier(KotlinParser.IdentifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitIdentifier(KotlinParser.IdentifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSimpleIdentifier(KotlinParser.SimpleIdentifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSimpleIdentifier(KotlinParser.SimpleIdentifierContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSemi(KotlinParser.SemiContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSemi(KotlinParser.SemiContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterEveryRule(ParserRuleContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitEveryRule(ParserRuleContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void visitTerminal(TerminalNode node) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void visitErrorNode(ErrorNode node) { }
}