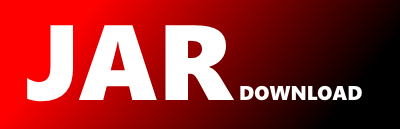
node_modules.apollo-codegen.lib.generate.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of apollo-client-maven-plugin Show documentation
Show all versions of apollo-client-maven-plugin Show documentation
Maven plugin for generating graphql clients
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
const fs = require("fs");
const path = require("path");
const rimraf = require("rimraf");
const loading_1 = require("apollo-codegen-core/lib/loading");
const validation_1 = require("./validation");
const compiler_1 = require("apollo-codegen-core/lib/compiler");
const legacyIR_1 = require("apollo-codegen-core/lib/compiler/legacyIR");
const serializeToJSON_1 = require("apollo-codegen-core/lib/serializeToJSON");
const apollo_codegen_swift_1 = require("apollo-codegen-swift");
const apollo_codegen_typescript_legacy_1 = require("apollo-codegen-typescript-legacy");
const apollo_codegen_flow_legacy_1 = require("apollo-codegen-flow-legacy");
const apollo_codegen_flow_1 = require("apollo-codegen-flow");
const apollo_codegen_typescript_1 = require("apollo-codegen-typescript");
const apollo_codegen_scala_1 = require("apollo-codegen-scala");
function generate(inputPaths, schemaPath, outputPath, only, target, tagName, projectName, options) {
const schema = schemaPath == null
? loading_1.loadSchemaFromConfig(projectName)
: loading_1.loadSchema(schemaPath);
const document = loading_1.loadAndMergeQueryDocuments(inputPaths, tagName);
validation_1.validateQueryDocument(schema, document);
if (target === 'swift') {
options.addTypename = true;
const context = compiler_1.compileToIR(schema, document, options);
const outputIndividualFiles = fs.existsSync(outputPath) && fs.statSync(outputPath).isDirectory();
const generator = apollo_codegen_swift_1.generateSource(context, outputIndividualFiles, only);
if (outputIndividualFiles) {
writeGeneratedFiles(generator.generatedFiles, outputPath);
}
else {
fs.writeFileSync(outputPath, generator.output);
}
if (options.generateOperationIds) {
writeOperationIdsMap(context);
}
}
else if (target === 'flow' || target === 'typescript' || target === 'ts') {
const context = compiler_1.compileToIR(schema, document, options);
const { generatedFiles, common } = target === 'flow'
? apollo_codegen_flow_1.generateSource(context)
: apollo_codegen_typescript_1.generateSource(context);
const outFiles = {};
const outputIndividualFiles = fs.existsSync(outputPath) && fs.statSync(outputPath).isDirectory();
if (outputIndividualFiles) {
Object.keys(generatedFiles)
.forEach((filePath) => {
outFiles[path.basename(filePath)] = {
output: generatedFiles[filePath].fileContents + common
};
});
writeGeneratedFiles(outFiles, outputPath);
}
else {
fs.writeFileSync(outputPath, Object.values(generatedFiles).map(v => v.fileContents).join("\n") + common);
}
}
else {
let output;
const context = legacyIR_1.compileToLegacyIR(schema, document, options);
switch (target) {
case 'json':
output = serializeToJSON_1.default(context);
break;
case 'ts-legacy':
case 'typescript-legacy':
output = apollo_codegen_typescript_legacy_1.generateSource(context);
break;
case 'flow-legacy':
output = apollo_codegen_flow_legacy_1.generateSource(context);
break;
case 'scala':
output = apollo_codegen_scala_1.generateSource(context);
}
if (outputPath) {
fs.writeFileSync(outputPath, output);
}
else {
console.log(output);
}
}
}
exports.default = generate;
function writeGeneratedFiles(generatedFiles, outputDirectory) {
rimraf.sync(outputDirectory);
fs.mkdirSync(outputDirectory);
for (const [fileName, generatedFile] of Object.entries(generatedFiles)) {
fs.writeFileSync(path.join(outputDirectory, fileName), generatedFile.output);
}
}
function writeOperationIdsMap(context) {
let operationIdsMap = {};
Object.keys(context.operations).map(k => context.operations[k]).forEach(operation => {
operationIdsMap[operation.operationId] = {
name: operation.operationName,
source: operation.sourceWithFragments
};
});
fs.writeFileSync(context.options.operationIdsPath, JSON.stringify(operationIdsMap, null, 2));
}
//# sourceMappingURL=generate.js.map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy