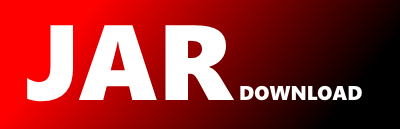
public.assets.vs.language.html.htmlWorker.js Maven / Gradle / Ivy
The newest version!
"use strict";/*!-----------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Version: 0.34.1(547870b6881302c5b4ff32173c16d06009e3588f)
* Released under the MIT license
* https://github.com/microsoft/monaco-editor/blob/main/LICENSE.txt
*-----------------------------------------------------------------------------*/
define("vs/language/html/htmlWorker", ["require","require"],(require)=>{
var moduleExports=(()=>{var Xe=Object.defineProperty;var xn=Object.getOwnPropertyDescriptor;var Dn=Object.getOwnPropertyNames;var En=Object.prototype.hasOwnProperty;var Cn=(t,i)=>{for(var o in i)Xe(t,o,{get:i[o],enumerable:!0})},Ln=(t,i,o,n)=>{if(i&&typeof i=="object"||typeof i=="function")for(let e of Dn(i))!En.call(t,e)&&e!==o&&Xe(t,e,{get:()=>i[e],enumerable:!(n=xn(i,e))||n.enumerable});return t};var Mn=t=>Ln(Xe({},"__esModule",{value:!0}),t);var Ri={};Cn(Ri,{HTMLWorker:()=>Je,create:()=>Mi});function Rn(t,i){let o;return i.length===0?o=t:o=t.replace(/\{(\d+)\}/g,(n,e)=>{let a=e[0];return typeof i[a]<"u"?i[a]:n}),o}function zn(t,i,...o){return Rn(i,o)}function be(t){return zn}var yt;(function(t){t.MIN_VALUE=-2147483648,t.MAX_VALUE=2147483647})(yt||(yt={}));var Ue;(function(t){t.MIN_VALUE=0,t.MAX_VALUE=2147483647})(Ue||(Ue={}));var X;(function(t){function i(n,e){return n===Number.MAX_VALUE&&(n=Ue.MAX_VALUE),e===Number.MAX_VALUE&&(e=Ue.MAX_VALUE),{line:n,character:e}}t.create=i;function o(n){var e=n;return _.objectLiteral(e)&&_.uinteger(e.line)&&_.uinteger(e.character)}t.is=o})(X||(X={}));var P;(function(t){function i(n,e,a,c){if(_.uinteger(n)&&_.uinteger(e)&&_.uinteger(a)&&_.uinteger(c))return{start:X.create(n,e),end:X.create(a,c)};if(X.is(n)&&X.is(e))return{start:n,end:e};throw new Error("Range#create called with invalid arguments["+n+", "+e+", "+a+", "+c+"]")}t.create=i;function o(n){var e=n;return _.objectLiteral(e)&&X.is(e.start)&&X.is(e.end)}t.is=o})(P||(P={}));var we;(function(t){function i(n,e){return{uri:n,range:e}}t.create=i;function o(n){var e=n;return _.defined(e)&&P.is(e.range)&&(_.string(e.uri)||_.undefined(e.uri))}t.is=o})(we||(we={}));var Tt;(function(t){function i(n,e,a,c){return{targetUri:n,targetRange:e,targetSelectionRange:a,originSelectionRange:c}}t.create=i;function o(n){var e=n;return _.defined(e)&&P.is(e.targetRange)&&_.string(e.targetUri)&&(P.is(e.targetSelectionRange)||_.undefined(e.targetSelectionRange))&&(P.is(e.originSelectionRange)||_.undefined(e.originSelectionRange))}t.is=o})(Tt||(Tt={}));var We;(function(t){function i(n,e,a,c){return{red:n,green:e,blue:a,alpha:c}}t.create=i;function o(n){var e=n;return _.numberRange(e.red,0,1)&&_.numberRange(e.green,0,1)&&_.numberRange(e.blue,0,1)&&_.numberRange(e.alpha,0,1)}t.is=o})(We||(We={}));var $e;(function(t){function i(n,e){return{range:n,color:e}}t.create=i;function o(n){var e=n;return P.is(e.range)&&We.is(e.color)}t.is=o})($e||($e={}));var Qe;(function(t){function i(n,e,a){return{label:n,textEdit:e,additionalTextEdits:a}}t.create=i;function o(n){var e=n;return _.string(e.label)&&(_.undefined(e.textEdit)||Y.is(e))&&(_.undefined(e.additionalTextEdits)||_.typedArray(e.additionalTextEdits,Y.is))}t.is=o})(Qe||(Qe={}));var _e;(function(t){t.Comment="comment",t.Imports="imports",t.Region="region"})(_e||(_e={}));var Ze;(function(t){function i(n,e,a,c,l){var r={startLine:n,endLine:e};return _.defined(a)&&(r.startCharacter=a),_.defined(c)&&(r.endCharacter=c),_.defined(l)&&(r.kind=l),r}t.create=i;function o(n){var e=n;return _.uinteger(e.startLine)&&_.uinteger(e.startLine)&&(_.undefined(e.startCharacter)||_.uinteger(e.startCharacter))&&(_.undefined(e.endCharacter)||_.uinteger(e.endCharacter))&&(_.undefined(e.kind)||_.string(e.kind))}t.is=o})(Ze||(Ze={}));var Ke;(function(t){function i(n,e){return{location:n,message:e}}t.create=i;function o(n){var e=n;return _.defined(e)&&we.is(e.location)&&_.string(e.message)}t.is=o})(Ke||(Ke={}));var kt;(function(t){t.Error=1,t.Warning=2,t.Information=3,t.Hint=4})(kt||(kt={}));var St;(function(t){t.Unnecessary=1,t.Deprecated=2})(St||(St={}));var At;(function(t){function i(o){var n=o;return n!=null&&_.string(n.href)}t.is=i})(At||(At={}));var xe;(function(t){function i(n,e,a,c,l,r){var s={range:n,message:e};return _.defined(a)&&(s.severity=a),_.defined(c)&&(s.code=c),_.defined(l)&&(s.source=l),_.defined(r)&&(s.relatedInformation=r),s}t.create=i;function o(n){var e,a=n;return _.defined(a)&&P.is(a.range)&&_.string(a.message)&&(_.number(a.severity)||_.undefined(a.severity))&&(_.integer(a.code)||_.string(a.code)||_.undefined(a.code))&&(_.undefined(a.codeDescription)||_.string((e=a.codeDescription)===null||e===void 0?void 0:e.href))&&(_.string(a.source)||_.undefined(a.source))&&(_.undefined(a.relatedInformation)||_.typedArray(a.relatedInformation,Ke.is))}t.is=o})(xe||(xe={}));var ye;(function(t){function i(n,e){for(var a=[],c=2;c0&&(l.arguments=a),l}t.create=i;function o(n){var e=n;return _.defined(e)&&_.string(e.title)&&_.string(e.command)}t.is=o})(ye||(ye={}));var Y;(function(t){function i(a,c){return{range:a,newText:c}}t.replace=i;function o(a,c){return{range:{start:a,end:a},newText:c}}t.insert=o;function n(a){return{range:a,newText:""}}t.del=n;function e(a){var c=a;return _.objectLiteral(c)&&_.string(c.newText)&&P.is(c.range)}t.is=e})(Y||(Y={}));var ve;(function(t){function i(n,e,a){var c={label:n};return e!==void 0&&(c.needsConfirmation=e),a!==void 0&&(c.description=a),c}t.create=i;function o(n){var e=n;return e!==void 0&&_.objectLiteral(e)&&_.string(e.label)&&(_.boolean(e.needsConfirmation)||e.needsConfirmation===void 0)&&(_.string(e.description)||e.description===void 0)}t.is=o})(ve||(ve={}));var Z;(function(t){function i(o){var n=o;return typeof n=="string"}t.is=i})(Z||(Z={}));var ce;(function(t){function i(a,c,l){return{range:a,newText:c,annotationId:l}}t.replace=i;function o(a,c,l){return{range:{start:a,end:a},newText:c,annotationId:l}}t.insert=o;function n(a,c){return{range:a,newText:"",annotationId:c}}t.del=n;function e(a){var c=a;return Y.is(c)&&(ve.is(c.annotationId)||Z.is(c.annotationId))}t.is=e})(ce||(ce={}));var Be;(function(t){function i(n,e){return{textDocument:n,edits:e}}t.create=i;function o(n){var e=n;return _.defined(e)&&Pe.is(e.textDocument)&&Array.isArray(e.edits)}t.is=o})(Be||(Be={}));var De;(function(t){function i(n,e,a){var c={kind:"create",uri:n};return e!==void 0&&(e.overwrite!==void 0||e.ignoreIfExists!==void 0)&&(c.options=e),a!==void 0&&(c.annotationId=a),c}t.create=i;function o(n){var e=n;return e&&e.kind==="create"&&_.string(e.uri)&&(e.options===void 0||(e.options.overwrite===void 0||_.boolean(e.options.overwrite))&&(e.options.ignoreIfExists===void 0||_.boolean(e.options.ignoreIfExists)))&&(e.annotationId===void 0||Z.is(e.annotationId))}t.is=o})(De||(De={}));var Ee;(function(t){function i(n,e,a,c){var l={kind:"rename",oldUri:n,newUri:e};return a!==void 0&&(a.overwrite!==void 0||a.ignoreIfExists!==void 0)&&(l.options=a),c!==void 0&&(l.annotationId=c),l}t.create=i;function o(n){var e=n;return e&&e.kind==="rename"&&_.string(e.oldUri)&&_.string(e.newUri)&&(e.options===void 0||(e.options.overwrite===void 0||_.boolean(e.options.overwrite))&&(e.options.ignoreIfExists===void 0||_.boolean(e.options.ignoreIfExists)))&&(e.annotationId===void 0||Z.is(e.annotationId))}t.is=o})(Ee||(Ee={}));var Ce;(function(t){function i(n,e,a){var c={kind:"delete",uri:n};return e!==void 0&&(e.recursive!==void 0||e.ignoreIfNotExists!==void 0)&&(c.options=e),a!==void 0&&(c.annotationId=a),c}t.create=i;function o(n){var e=n;return e&&e.kind==="delete"&&_.string(e.uri)&&(e.options===void 0||(e.options.recursive===void 0||_.boolean(e.options.recursive))&&(e.options.ignoreIfNotExists===void 0||_.boolean(e.options.ignoreIfNotExists)))&&(e.annotationId===void 0||Z.is(e.annotationId))}t.is=o})(Ce||(Ce={}));var Fe;(function(t){function i(o){var n=o;return n&&(n.changes!==void 0||n.documentChanges!==void 0)&&(n.documentChanges===void 0||n.documentChanges.every(function(e){return _.string(e.kind)?De.is(e)||Ee.is(e)||Ce.is(e):Be.is(e)}))}t.is=i})(Fe||(Fe={}));var Ie=function(){function t(i,o){this.edits=i,this.changeAnnotations=o}return t.prototype.insert=function(i,o,n){var e,a;if(n===void 0?e=Y.insert(i,o):Z.is(n)?(a=n,e=ce.insert(i,o,n)):(this.assertChangeAnnotations(this.changeAnnotations),a=this.changeAnnotations.manage(n),e=ce.insert(i,o,a)),this.edits.push(e),a!==void 0)return a},t.prototype.replace=function(i,o,n){var e,a;if(n===void 0?e=Y.replace(i,o):Z.is(n)?(a=n,e=ce.replace(i,o,n)):(this.assertChangeAnnotations(this.changeAnnotations),a=this.changeAnnotations.manage(n),e=ce.replace(i,o,a)),this.edits.push(e),a!==void 0)return a},t.prototype.delete=function(i,o){var n,e;if(o===void 0?n=Y.del(i):Z.is(o)?(e=o,n=ce.del(i,o)):(this.assertChangeAnnotations(this.changeAnnotations),e=this.changeAnnotations.manage(o),n=ce.del(i,e)),this.edits.push(n),e!==void 0)return e},t.prototype.add=function(i){this.edits.push(i)},t.prototype.all=function(){return this.edits},t.prototype.clear=function(){this.edits.splice(0,this.edits.length)},t.prototype.assertChangeAnnotations=function(i){if(i===void 0)throw new Error("Text edit change is not configured to manage change annotations.")},t}(),xt=function(){function t(i){this._annotations=i===void 0?Object.create(null):i,this._counter=0,this._size=0}return t.prototype.all=function(){return this._annotations},Object.defineProperty(t.prototype,"size",{get:function(){return this._size},enumerable:!1,configurable:!0}),t.prototype.manage=function(i,o){var n;if(Z.is(i)?n=i:(n=this.nextId(),o=i),this._annotations[n]!==void 0)throw new Error("Id "+n+" is already in use.");if(o===void 0)throw new Error("No annotation provided for id "+n);return this._annotations[n]=o,this._size++,n},t.prototype.nextId=function(){return this._counter++,this._counter.toString()},t}(),Hi=function(){function t(i){var o=this;this._textEditChanges=Object.create(null),i!==void 0?(this._workspaceEdit=i,i.documentChanges?(this._changeAnnotations=new xt(i.changeAnnotations),i.changeAnnotations=this._changeAnnotations.all(),i.documentChanges.forEach(function(n){if(Be.is(n)){var e=new Ie(n.edits,o._changeAnnotations);o._textEditChanges[n.textDocument.uri]=e}})):i.changes&&Object.keys(i.changes).forEach(function(n){var e=new Ie(i.changes[n]);o._textEditChanges[n]=e})):this._workspaceEdit={}}return Object.defineProperty(t.prototype,"edit",{get:function(){return this.initDocumentChanges(),this._changeAnnotations!==void 0&&(this._changeAnnotations.size===0?this._workspaceEdit.changeAnnotations=void 0:this._workspaceEdit.changeAnnotations=this._changeAnnotations.all()),this._workspaceEdit},enumerable:!1,configurable:!0}),t.prototype.getTextEditChange=function(i){if(Pe.is(i)){if(this.initDocumentChanges(),this._workspaceEdit.documentChanges===void 0)throw new Error("Workspace edit is not configured for document changes.");var o={uri:i.uri,version:i.version},n=this._textEditChanges[o.uri];if(!n){var e=[],a={textDocument:o,edits:e};this._workspaceEdit.documentChanges.push(a),n=new Ie(e,this._changeAnnotations),this._textEditChanges[o.uri]=n}return n}else{if(this.initChanges(),this._workspaceEdit.changes===void 0)throw new Error("Workspace edit is not configured for normal text edit changes.");var n=this._textEditChanges[i];if(!n){var e=[];this._workspaceEdit.changes[i]=e,n=new Ie(e),this._textEditChanges[i]=n}return n}},t.prototype.initDocumentChanges=function(){this._workspaceEdit.documentChanges===void 0&&this._workspaceEdit.changes===void 0&&(this._changeAnnotations=new xt,this._workspaceEdit.documentChanges=[],this._workspaceEdit.changeAnnotations=this._changeAnnotations.all())},t.prototype.initChanges=function(){this._workspaceEdit.documentChanges===void 0&&this._workspaceEdit.changes===void 0&&(this._workspaceEdit.changes=Object.create(null))},t.prototype.createFile=function(i,o,n){if(this.initDocumentChanges(),this._workspaceEdit.documentChanges===void 0)throw new Error("Workspace edit is not configured for document changes.");var e;ve.is(o)||Z.is(o)?e=o:n=o;var a,c;if(e===void 0?a=De.create(i,n):(c=Z.is(e)?e:this._changeAnnotations.manage(e),a=De.create(i,n,c)),this._workspaceEdit.documentChanges.push(a),c!==void 0)return c},t.prototype.renameFile=function(i,o,n,e){if(this.initDocumentChanges(),this._workspaceEdit.documentChanges===void 0)throw new Error("Workspace edit is not configured for document changes.");var a;ve.is(n)||Z.is(n)?a=n:e=n;var c,l;if(a===void 0?c=Ee.create(i,o,e):(l=Z.is(a)?a:this._changeAnnotations.manage(a),c=Ee.create(i,o,e,l)),this._workspaceEdit.documentChanges.push(c),l!==void 0)return l},t.prototype.deleteFile=function(i,o,n){if(this.initDocumentChanges(),this._workspaceEdit.documentChanges===void 0)throw new Error("Workspace edit is not configured for document changes.");var e;ve.is(o)||Z.is(o)?e=o:n=o;var a,c;if(e===void 0?a=Ce.create(i,n):(c=Z.is(e)?e:this._changeAnnotations.manage(e),a=Ce.create(i,n,c)),this._workspaceEdit.documentChanges.push(a),c!==void 0)return c},t}();var Dt;(function(t){function i(n){return{uri:n}}t.create=i;function o(n){var e=n;return _.defined(e)&&_.string(e.uri)}t.is=o})(Dt||(Dt={}));var Et;(function(t){function i(n,e){return{uri:n,version:e}}t.create=i;function o(n){var e=n;return _.defined(e)&&_.string(e.uri)&&_.integer(e.version)}t.is=o})(Et||(Et={}));var Pe;(function(t){function i(n,e){return{uri:n,version:e}}t.create=i;function o(n){var e=n;return _.defined(e)&&_.string(e.uri)&&(e.version===null||_.integer(e.version))}t.is=o})(Pe||(Pe={}));var Ct;(function(t){function i(n,e,a,c){return{uri:n,languageId:e,version:a,text:c}}t.create=i;function o(n){var e=n;return _.defined(e)&&_.string(e.uri)&&_.string(e.languageId)&&_.integer(e.version)&&_.string(e.text)}t.is=o})(Ct||(Ct={}));var ee;(function(t){t.PlainText="plaintext",t.Markdown="markdown"})(ee||(ee={}));(function(t){function i(o){var n=o;return n===t.PlainText||n===t.Markdown}t.is=i})(ee||(ee={}));var Ne;(function(t){function i(o){var n=o;return _.objectLiteral(o)&&ee.is(n.kind)&&_.string(n.value)}t.is=i})(Ne||(Ne={}));var Q;(function(t){t.Text=1,t.Method=2,t.Function=3,t.Constructor=4,t.Field=5,t.Variable=6,t.Class=7,t.Interface=8,t.Module=9,t.Property=10,t.Unit=11,t.Value=12,t.Enum=13,t.Keyword=14,t.Snippet=15,t.Color=16,t.File=17,t.Reference=18,t.Folder=19,t.EnumMember=20,t.Constant=21,t.Struct=22,t.Event=23,t.Operator=24,t.TypeParameter=25})(Q||(Q={}));var ne;(function(t){t.PlainText=1,t.Snippet=2})(ne||(ne={}));var et;(function(t){t.Deprecated=1})(et||(et={}));var tt;(function(t){function i(n,e,a){return{newText:n,insert:e,replace:a}}t.create=i;function o(n){var e=n;return e&&_.string(e.newText)&&P.is(e.insert)&&P.is(e.replace)}t.is=o})(tt||(tt={}));var nt;(function(t){t.asIs=1,t.adjustIndentation=2})(nt||(nt={}));var it;(function(t){function i(o){return{label:o}}t.create=i})(it||(it={}));var rt;(function(t){function i(o,n){return{items:o||[],isIncomplete:!!n}}t.create=i})(rt||(rt={}));var Le;(function(t){function i(n){return n.replace(/[\\`*_{}[\]()#+\-.!]/g,"\\$&")}t.fromPlainText=i;function o(n){var e=n;return _.string(e)||_.objectLiteral(e)&&_.string(e.language)&&_.string(e.value)}t.is=o})(Le||(Le={}));var at;(function(t){function i(o){var n=o;return!!n&&_.objectLiteral(n)&&(Ne.is(n.contents)||Le.is(n.contents)||_.typedArray(n.contents,Le.is))&&(o.range===void 0||P.is(o.range))}t.is=i})(at||(at={}));var Lt;(function(t){function i(o,n){return n?{label:o,documentation:n}:{label:o}}t.create=i})(Lt||(Lt={}));var Mt;(function(t){function i(o,n){for(var e=[],a=2;a=0;u--){var h=r[u],d=a.offsetAt(h.range.start),g=a.offsetAt(h.range.end);if(g<=s)l=l.substring(0,d)+h.newText+l.substring(g,l.length);else throw new Error("Overlapping edit");s=d}return l}t.applyEdits=n;function e(a,c){if(a.length<=1)return a;var l=a.length/2|0,r=a.slice(0,l),s=a.slice(l);e(r,c),e(s,c);for(var u=0,h=0,d=0;u0&&i.push(o.length),this._lineOffsets=i}return this._lineOffsets},t.prototype.positionAt=function(i){i=Math.max(Math.min(i,this._content.length),0);var o=this.getLineOffsets(),n=0,e=o.length;if(e===0)return X.create(0,i);for(;ni?e=a:n=a+1}var c=n-1;return X.create(c,i-o[c])},t.prototype.offsetAt=function(i){var o=this.getLineOffsets();if(i.line>=o.length)return this._content.length;if(i.line<0)return 0;var n=o[i.line],e=i.line+1"u"}t.undefined=n;function e(g){return g===!0||g===!1}t.boolean=e;function a(g){return i.call(g)==="[object String]"}t.string=a;function c(g){return i.call(g)==="[object Number]"}t.number=c;function l(g,y,m){return i.call(g)==="[object Number]"&&y<=g&&g<=m}t.numberRange=l;function r(g){return i.call(g)==="[object Number]"&&-2147483648<=g&&g<=2147483647}t.integer=r;function s(g){return i.call(g)==="[object Number]"&&0<=g&&g<=2147483647}t.uinteger=s;function u(g){return i.call(g)==="[object Function]"}t.func=u;function h(g){return g!==null&&typeof g=="object"}t.objectLiteral=h;function d(g,y){return Array.isArray(g)&&g.every(y)}t.typedArray=d})(_||(_={}));var pe=class{constructor(i,o,n,e){this._uri=i,this._languageId=o,this._version=n,this._content=e,this._lineOffsets=void 0}get uri(){return this._uri}get languageId(){return this._languageId}get version(){return this._version}getText(i){if(i){let o=this.offsetAt(i.start),n=this.offsetAt(i.end);return this._content.substring(o,n)}return this._content}update(i,o){for(let n of i)if(pe.isIncremental(n)){let e=Pt(n.range),a=this.offsetAt(e.start),c=this.offsetAt(e.end);this._content=this._content.substring(0,a)+n.text+this._content.substring(c,this._content.length);let l=Math.max(e.start.line,0),r=Math.max(e.end.line,0),s=this._lineOffsets,u=Ft(n.text,!1,a);if(r-l===u.length)for(let d=0,g=u.length;di?e=c:n=c+1}let a=n-1;return{line:a,character:i-o[a]}}offsetAt(i){let o=this.getLineOffsets();if(i.line>=o.length)return this._content.length;if(i.line<0)return 0;let n=o[i.line],e=i.line+1{let d=u.range.start.line-h.range.start.line;return d===0?u.range.start.character-h.range.start.character:d}),r=0,s=[];for(let u of l){let h=e.offsetAt(u.range.start);if(hr&&s.push(c.substring(r,h)),u.newText.length&&s.push(u.newText),r=e.offsetAt(u.range.end)}return s.push(c.substr(r)),s.join("")}t.applyEdits=n})(Re||(Re={}));function ct(t,i){if(t.length<=1)return t;let o=t.length/2|0,n=t.slice(0,o),e=t.slice(o);ct(n,i),ct(e,i);let a=0,c=0,l=0;for(;ao.line||i.line===o.line&&i.character>o.character?{start:o,end:i}:t}function In(t){let i=Pt(t.range);return i!==t.range?{newText:t.newText,range:i}:t}var S;(function(t){t[t.StartCommentTag=0]="StartCommentTag",t[t.Comment=1]="Comment",t[t.EndCommentTag=2]="EndCommentTag",t[t.StartTagOpen=3]="StartTagOpen",t[t.StartTagClose=4]="StartTagClose",t[t.StartTagSelfClose=5]="StartTagSelfClose",t[t.StartTag=6]="StartTag",t[t.EndTagOpen=7]="EndTagOpen",t[t.EndTagClose=8]="EndTagClose",t[t.EndTag=9]="EndTag",t[t.DelimiterAssign=10]="DelimiterAssign",t[t.AttributeName=11]="AttributeName",t[t.AttributeValue=12]="AttributeValue",t[t.StartDoctypeTag=13]="StartDoctypeTag",t[t.Doctype=14]="Doctype",t[t.EndDoctypeTag=15]="EndDoctypeTag",t[t.Content=16]="Content",t[t.Whitespace=17]="Whitespace",t[t.Unknown=18]="Unknown",t[t.Script=19]="Script",t[t.Styles=20]="Styles",t[t.EOS=21]="EOS"})(S||(S={}));var W;(function(t){t[t.WithinContent=0]="WithinContent",t[t.AfterOpeningStartTag=1]="AfterOpeningStartTag",t[t.AfterOpeningEndTag=2]="AfterOpeningEndTag",t[t.WithinDoctype=3]="WithinDoctype",t[t.WithinTag=4]="WithinTag",t[t.WithinEndTag=5]="WithinEndTag",t[t.WithinComment=6]="WithinComment",t[t.WithinScriptContent=7]="WithinScriptContent",t[t.WithinStyleContent=8]="WithinStyleContent",t[t.AfterAttributeName=9]="AfterAttributeName",t[t.BeforeAttributeValue=10]="BeforeAttributeValue"})(W||(W={}));var Nt;(function(t){t.LATEST={textDocument:{completion:{completionItem:{documentationFormat:[ee.Markdown,ee.PlainText]}},hover:{contentFormat:[ee.Markdown,ee.PlainText]}}}})(Nt||(Nt={}));var Oe;(function(t){t[t.Unknown=0]="Unknown",t[t.File=1]="File",t[t.Directory=2]="Directory",t[t.SymbolicLink=64]="SymbolicLink"})(Oe||(Oe={}));var he=be(),Un=function(){function t(i,o){this.source=i,this.len=i.length,this.position=o}return t.prototype.eos=function(){return this.len<=this.position},t.prototype.getSource=function(){return this.source},t.prototype.pos=function(){return this.position},t.prototype.goBackTo=function(i){this.position=i},t.prototype.goBack=function(i){this.position-=i},t.prototype.advance=function(i){this.position+=i},t.prototype.goToEnd=function(){this.position=this.source.length},t.prototype.nextChar=function(){return this.source.charCodeAt(this.position++)||0},t.prototype.peekChar=function(i){return i===void 0&&(i=0),this.source.charCodeAt(this.position+i)||0},t.prototype.advanceIfChar=function(i){return i===this.source.charCodeAt(this.position)?(this.position++,!0):!1},t.prototype.advanceIfChars=function(i){var o;if(this.position+i.length>this.source.length)return!1;for(o=0;o0},t.prototype.advanceWhileChar=function(i){for(var o=this.position;this.position".charCodeAt(0),ht="/".charCodeAt(0),Wn="=".charCodeAt(0),Bn='"'.charCodeAt(0),Fn="'".charCodeAt(0),Pn=`
`.charCodeAt(0),Nn="\r".charCodeAt(0),On="\f".charCodeAt(0),qn=" ".charCodeAt(0),jn=" ".charCodeAt(0),Gn={"text/x-handlebars-template":!0,"text/html":!0};function $(t,i,o,n){i===void 0&&(i=0),o===void 0&&(o=W.WithinContent),n===void 0&&(n=!1);var e=new Un(t,i),a=o,c=0,l=S.Unknown,r,s,u,h,d;function g(){return e.advanceIfRegExp(/^[_:\w][_:\w-.\d]*/).toLowerCase()}function y(){return e.advanceIfRegExp(/^[^\s"'>=\x00-\x0F\x7F\x80-\x9F]*/).toLowerCase()}function m(w,M,B){return l=M,c=w,r=B,M}function A(){var w=e.pos(),M=a,B=E();return B!==S.EOS&&w===e.pos()&&!(n&&(B===S.StartTagClose||B===S.EndTagClose))?(console.log("Scanner.scan has not advanced at offset "+w+", state before: "+M+" after: "+a),e.advance(1),m(w,S.Unknown)):B}function E(){var w=e.pos();if(e.eos())return m(w,S.EOS);var M;switch(a){case W.WithinComment:return e.advanceIfChars([Se,Se,se])?(a=W.WithinContent,m(w,S.EndCommentTag)):(e.advanceUntilChars([Se,Se,se]),m(w,S.Comment));case W.WithinDoctype:return e.advanceIfChar(se)?(a=W.WithinContent,m(w,S.EndDoctypeTag)):(e.advanceUntilChar(se),m(w,S.Doctype));case W.WithinContent:if(e.advanceIfChar(qe)){if(!e.eos()&&e.peekChar()===Ot){if(e.advanceIfChars([Ot,Se,Se]))return a=W.WithinComment,m(w,S.StartCommentTag);if(e.advanceIfRegExp(/^!doctype/i))return a=W.WithinDoctype,m(w,S.StartDoctypeTag)}return e.advanceIfChar(ht)?(a=W.AfterOpeningEndTag,m(w,S.EndTagOpen)):(a=W.AfterOpeningStartTag,m(w,S.StartTagOpen))}return e.advanceUntilChar(qe),m(w,S.Content);case W.AfterOpeningEndTag:var B=g();return B.length>0?(a=W.WithinEndTag,m(w,S.EndTag)):e.skipWhitespace()?m(w,S.Whitespace,he("error.unexpectedWhitespace","Tag name must directly follow the open bracket.")):(a=W.WithinEndTag,e.advanceUntilChar(se),w0?(s=!1,a=W.WithinTag,m(w,S.StartTag)):e.skipWhitespace()?m(w,S.Whitespace,he("error.unexpectedWhitespace","Tag name must directly follow the open bracket.")):(a=W.WithinTag,e.advanceUntilChar(se),w0)?(a=W.AfterAttributeName,s=!1,m(w,S.AttributeName)):e.advanceIfChars([ht,se])?(a=W.WithinContent,m(w,S.StartTagSelfClose)):e.advanceIfChar(se)?(u==="script"?d&&Gn[d]?a=W.WithinContent:a=W.WithinScriptContent:u==="style"?a=W.WithinStyleContent:a=W.WithinContent,m(w,S.StartTagClose)):n&&e.peekChar()===qe?(a=W.WithinContent,m(w,S.StartTagClose,he("error.closingBracketMissing","Closing bracket missing."))):(e.advance(1),m(w,S.Unknown,he("error.unexpectedCharacterInTag","Unexpected character in tag.")));case W.AfterAttributeName:return e.skipWhitespace()?(s=!0,m(w,S.Whitespace)):e.advanceIfChar(Wn)?(a=W.BeforeAttributeValue,m(w,S.DelimiterAssign)):(a=W.WithinTag,E());case W.BeforeAttributeValue:if(e.skipWhitespace())return m(w,S.Whitespace);var G=e.advanceIfRegExp(/^[^\s"'`=<>]+/);if(G.length>0)return e.peekChar()===se&&e.peekChar(-1)===ht&&(e.goBack(1),G=G.substr(0,G.length-1)),h==="type"&&(d=G),a=W.WithinTag,s=!1,m(w,S.AttributeValue);var J=e.peekChar();return J===Fn||J===Bn?(e.advance(1),e.advanceUntilChar(J)&&e.advance(1),h==="type"&&(d=e.getSource().substring(w+1,e.pos()-1)),a=W.WithinTag,s=!1,m(w,S.AttributeValue)):(a=W.WithinTag,s=!1,E());case W.WithinScriptContent:for(var f=1;!e.eos();){var p=e.advanceIfRegExp(/|<\/?script\s*\/?>?/i);if(p.length===0)return e.goToEnd(),m(w,S.Script);if(p==="")f=1;else if(p[1]!=="/")f===2&&(f=3);else if(f===3)f=2;else{e.goBack(p.length);break}}return a=W.WithinContent,w0)e=a-1;else return a}return-(n+1)}var Jn=["area","base","br","col","embed","hr","img","input","keygen","link","menuitem","meta","param","source","track","wbr"];function me(t){return!!t&&qt(Jn,t.toLowerCase(),function(i,o){return i.localeCompare(o)})>=0}var jt=function(){function t(i,o,n,e){this.start=i,this.end=o,this.children=n,this.parent=e,this.closed=!1}return Object.defineProperty(t.prototype,"attributeNames",{get:function(){return this.attributes?Object.keys(this.attributes):[]},enumerable:!1,configurable:!0}),t.prototype.isSameTag=function(i){return this.tag===void 0?i===void 0:i!==void 0&&this.tag.length===i.length&&this.tag.toLowerCase()===i},Object.defineProperty(t.prototype,"firstChild",{get:function(){return this.children[0]},enumerable:!1,configurable:!0}),Object.defineProperty(t.prototype,"lastChild",{get:function(){return this.children.length?this.children[this.children.length-1]:void 0},enumerable:!1,configurable:!0}),t.prototype.findNodeBefore=function(i){var o=dt(this.children,function(a){return i<=a.start})-1;if(o>=0){var n=this.children[o];if(i>n.start){if(i=0){var n=this.children[o];if(i>n.start&&i<=n.end)return n.findNodeAt(i)}return this},t}();function je(t){for(var i=$(t,void 0,void 0,!0),o=new jt(0,t.length,[],void 0),n=o,e=-1,a=void 0,c=null,l=i.scan();l!==S.EOS;){switch(l){case S.StartTagOpen:var r=new jt(i.getTokenOffset(),t.length,[],n);n.children.push(r),n=r;break;case S.StartTag:n.tag=i.getTokenText();break;case S.StartTagClose:n.parent&&(n.end=i.getTokenEnd(),i.getTokenLength()?(n.startTagEnd=i.getTokenEnd(),n.tag&&me(n.tag)&&(n.closed=!0,n=n.parent)):n=n.parent);break;case S.StartTagSelfClose:n.parent&&(n.closed=!0,n.end=i.getTokenEnd(),n.startTagEnd=i.getTokenEnd(),n=n.parent);break;case S.EndTagOpen:e=i.getTokenOffset(),a=void 0;break;case S.EndTag:a=i.getTokenText().toLowerCase();break;case S.EndTagClose:for(var s=n;!s.isSameTag(a)&&s.parent;)s=s.parent;if(s.parent){for(;n!==s;)n.end=e,n.closed=!1,n=n.parent;n.closed=!0,n.endTagStart=e,n.end=i.getTokenEnd(),n=n.parent}break;case S.AttributeName:{c=i.getTokenText();var u=n.attributes;u||(n.attributes=u={}),u[c]=null;break}case S.AttributeValue:{var h=i.getTokenText(),u=n.attributes;u&&c&&(u[c]=h,c=null);break}}l=i.scan()}for(;n.parent;)n.end=t.length,n.closed=!1,n=n.parent;return{roots:o.children,findNodeBefore:o.findNodeBefore.bind(o),findNodeAt:o.findNodeAt.bind(o)}}var fe={"Aacute;":"\xC1",Aacute:"\xC1","aacute;":"\xE1",aacute:"\xE1","Abreve;":"\u0102","abreve;":"\u0103","ac;":"\u223E","acd;":"\u223F","acE;":"\u223E\u0333","Acirc;":"\xC2",Acirc:"\xC2","acirc;":"\xE2",acirc:"\xE2","acute;":"\xB4",acute:"\xB4","Acy;":"\u0410","acy;":"\u0430","AElig;":"\xC6",AElig:"\xC6","aelig;":"\xE6",aelig:"\xE6","af;":"\u2061","Afr;":"\u{1D504}","afr;":"\u{1D51E}","Agrave;":"\xC0",Agrave:"\xC0","agrave;":"\xE0",agrave:"\xE0","alefsym;":"\u2135","aleph;":"\u2135","Alpha;":"\u0391","alpha;":"\u03B1","Amacr;":"\u0100","amacr;":"\u0101","amalg;":"\u2A3F","AMP;":"&",AMP:"&","amp;":"&",amp:"&","And;":"\u2A53","and;":"\u2227","andand;":"\u2A55","andd;":"\u2A5C","andslope;":"\u2A58","andv;":"\u2A5A","ang;":"\u2220","ange;":"\u29A4","angle;":"\u2220","angmsd;":"\u2221","angmsdaa;":"\u29A8","angmsdab;":"\u29A9","angmsdac;":"\u29AA","angmsdad;":"\u29AB","angmsdae;":"\u29AC","angmsdaf;":"\u29AD","angmsdag;":"\u29AE","angmsdah;":"\u29AF","angrt;":"\u221F","angrtvb;":"\u22BE","angrtvbd;":"\u299D","angsph;":"\u2222","angst;":"\xC5","angzarr;":"\u237C","Aogon;":"\u0104","aogon;":"\u0105","Aopf;":"\u{1D538}","aopf;":"\u{1D552}","ap;":"\u2248","apacir;":"\u2A6F","apE;":"\u2A70","ape;":"\u224A","apid;":"\u224B","apos;":"'","ApplyFunction;":"\u2061","approx;":"\u2248","approxeq;":"\u224A","Aring;":"\xC5",Aring:"\xC5","aring;":"\xE5",aring:"\xE5","Ascr;":"\u{1D49C}","ascr;":"\u{1D4B6}","Assign;":"\u2254","ast;":"*","asymp;":"\u2248","asympeq;":"\u224D","Atilde;":"\xC3",Atilde:"\xC3","atilde;":"\xE3",atilde:"\xE3","Auml;":"\xC4",Auml:"\xC4","auml;":"\xE4",auml:"\xE4","awconint;":"\u2233","awint;":"\u2A11","backcong;":"\u224C","backepsilon;":"\u03F6","backprime;":"\u2035","backsim;":"\u223D","backsimeq;":"\u22CD","Backslash;":"\u2216","Barv;":"\u2AE7","barvee;":"\u22BD","Barwed;":"\u2306","barwed;":"\u2305","barwedge;":"\u2305","bbrk;":"\u23B5","bbrktbrk;":"\u23B6","bcong;":"\u224C","Bcy;":"\u0411","bcy;":"\u0431","bdquo;":"\u201E","becaus;":"\u2235","Because;":"\u2235","because;":"\u2235","bemptyv;":"\u29B0","bepsi;":"\u03F6","bernou;":"\u212C","Bernoullis;":"\u212C","Beta;":"\u0392","beta;":"\u03B2","beth;":"\u2136","between;":"\u226C","Bfr;":"\u{1D505}","bfr;":"\u{1D51F}","bigcap;":"\u22C2","bigcirc;":"\u25EF","bigcup;":"\u22C3","bigodot;":"\u2A00","bigoplus;":"\u2A01","bigotimes;":"\u2A02","bigsqcup;":"\u2A06","bigstar;":"\u2605","bigtriangledown;":"\u25BD","bigtriangleup;":"\u25B3","biguplus;":"\u2A04","bigvee;":"\u22C1","bigwedge;":"\u22C0","bkarow;":"\u290D","blacklozenge;":"\u29EB","blacksquare;":"\u25AA","blacktriangle;":"\u25B4","blacktriangledown;":"\u25BE","blacktriangleleft;":"\u25C2","blacktriangleright;":"\u25B8","blank;":"\u2423","blk12;":"\u2592","blk14;":"\u2591","blk34;":"\u2593","block;":"\u2588","bne;":"=\u20E5","bnequiv;":"\u2261\u20E5","bNot;":"\u2AED","bnot;":"\u2310","Bopf;":"\u{1D539}","bopf;":"\u{1D553}","bot;":"\u22A5","bottom;":"\u22A5","bowtie;":"\u22C8","boxbox;":"\u29C9","boxDL;":"\u2557","boxDl;":"\u2556","boxdL;":"\u2555","boxdl;":"\u2510","boxDR;":"\u2554","boxDr;":"\u2553","boxdR;":"\u2552","boxdr;":"\u250C","boxH;":"\u2550","boxh;":"\u2500","boxHD;":"\u2566","boxHd;":"\u2564","boxhD;":"\u2565","boxhd;":"\u252C","boxHU;":"\u2569","boxHu;":"\u2567","boxhU;":"\u2568","boxhu;":"\u2534","boxminus;":"\u229F","boxplus;":"\u229E","boxtimes;":"\u22A0","boxUL;":"\u255D","boxUl;":"\u255C","boxuL;":"\u255B","boxul;":"\u2518","boxUR;":"\u255A","boxUr;":"\u2559","boxuR;":"\u2558","boxur;":"\u2514","boxV;":"\u2551","boxv;":"\u2502","boxVH;":"\u256C","boxVh;":"\u256B","boxvH;":"\u256A","boxvh;":"\u253C","boxVL;":"\u2563","boxVl;":"\u2562","boxvL;":"\u2561","boxvl;":"\u2524","boxVR;":"\u2560","boxVr;":"\u255F","boxvR;":"\u255E","boxvr;":"\u251C","bprime;":"\u2035","Breve;":"\u02D8","breve;":"\u02D8","brvbar;":"\xA6",brvbar:"\xA6","Bscr;":"\u212C","bscr;":"\u{1D4B7}","bsemi;":"\u204F","bsim;":"\u223D","bsime;":"\u22CD","bsol;":"\\","bsolb;":"\u29C5","bsolhsub;":"\u27C8","bull;":"\u2022","bullet;":"\u2022","bump;":"\u224E","bumpE;":"\u2AAE","bumpe;":"\u224F","Bumpeq;":"\u224E","bumpeq;":"\u224F","Cacute;":"\u0106","cacute;":"\u0107","Cap;":"\u22D2","cap;":"\u2229","capand;":"\u2A44","capbrcup;":"\u2A49","capcap;":"\u2A4B","capcup;":"\u2A47","capdot;":"\u2A40","CapitalDifferentialD;":"\u2145","caps;":"\u2229\uFE00","caret;":"\u2041","caron;":"\u02C7","Cayleys;":"\u212D","ccaps;":"\u2A4D","Ccaron;":"\u010C","ccaron;":"\u010D","Ccedil;":"\xC7",Ccedil:"\xC7","ccedil;":"\xE7",ccedil:"\xE7","Ccirc;":"\u0108","ccirc;":"\u0109","Cconint;":"\u2230","ccups;":"\u2A4C","ccupssm;":"\u2A50","Cdot;":"\u010A","cdot;":"\u010B","cedil;":"\xB8",cedil:"\xB8","Cedilla;":"\xB8","cemptyv;":"\u29B2","cent;":"\xA2",cent:"\xA2","CenterDot;":"\xB7","centerdot;":"\xB7","Cfr;":"\u212D","cfr;":"\u{1D520}","CHcy;":"\u0427","chcy;":"\u0447","check;":"\u2713","checkmark;":"\u2713","Chi;":"\u03A7","chi;":"\u03C7","cir;":"\u25CB","circ;":"\u02C6","circeq;":"\u2257","circlearrowleft;":"\u21BA","circlearrowright;":"\u21BB","circledast;":"\u229B","circledcirc;":"\u229A","circleddash;":"\u229D","CircleDot;":"\u2299","circledR;":"\xAE","circledS;":"\u24C8","CircleMinus;":"\u2296","CirclePlus;":"\u2295","CircleTimes;":"\u2297","cirE;":"\u29C3","cire;":"\u2257","cirfnint;":"\u2A10","cirmid;":"\u2AEF","cirscir;":"\u29C2","ClockwiseContourIntegral;":"\u2232","CloseCurlyDoubleQuote;":"\u201D","CloseCurlyQuote;":"\u2019","clubs;":"\u2663","clubsuit;":"\u2663","Colon;":"\u2237","colon;":":","Colone;":"\u2A74","colone;":"\u2254","coloneq;":"\u2254","comma;":",","commat;":"@","comp;":"\u2201","compfn;":"\u2218","complement;":"\u2201","complexes;":"\u2102","cong;":"\u2245","congdot;":"\u2A6D","Congruent;":"\u2261","Conint;":"\u222F","conint;":"\u222E","ContourIntegral;":"\u222E","Copf;":"\u2102","copf;":"\u{1D554}","coprod;":"\u2210","Coproduct;":"\u2210","COPY;":"\xA9",COPY:"\xA9","copy;":"\xA9",copy:"\xA9","copysr;":"\u2117","CounterClockwiseContourIntegral;":"\u2233","crarr;":"\u21B5","Cross;":"\u2A2F","cross;":"\u2717","Cscr;":"\u{1D49E}","cscr;":"\u{1D4B8}","csub;":"\u2ACF","csube;":"\u2AD1","csup;":"\u2AD0","csupe;":"\u2AD2","ctdot;":"\u22EF","cudarrl;":"\u2938","cudarrr;":"\u2935","cuepr;":"\u22DE","cuesc;":"\u22DF","cularr;":"\u21B6","cularrp;":"\u293D","Cup;":"\u22D3","cup;":"\u222A","cupbrcap;":"\u2A48","CupCap;":"\u224D","cupcap;":"\u2A46","cupcup;":"\u2A4A","cupdot;":"\u228D","cupor;":"\u2A45","cups;":"\u222A\uFE00","curarr;":"\u21B7","curarrm;":"\u293C","curlyeqprec;":"\u22DE","curlyeqsucc;":"\u22DF","curlyvee;":"\u22CE","curlywedge;":"\u22CF","curren;":"\xA4",curren:"\xA4","curvearrowleft;":"\u21B6","curvearrowright;":"\u21B7","cuvee;":"\u22CE","cuwed;":"\u22CF","cwconint;":"\u2232","cwint;":"\u2231","cylcty;":"\u232D","Dagger;":"\u2021","dagger;":"\u2020","daleth;":"\u2138","Darr;":"\u21A1","dArr;":"\u21D3","darr;":"\u2193","dash;":"\u2010","Dashv;":"\u2AE4","dashv;":"\u22A3","dbkarow;":"\u290F","dblac;":"\u02DD","Dcaron;":"\u010E","dcaron;":"\u010F","Dcy;":"\u0414","dcy;":"\u0434","DD;":"\u2145","dd;":"\u2146","ddagger;":"\u2021","ddarr;":"\u21CA","DDotrahd;":"\u2911","ddotseq;":"\u2A77","deg;":"\xB0",deg:"\xB0","Del;":"\u2207","Delta;":"\u0394","delta;":"\u03B4","demptyv;":"\u29B1","dfisht;":"\u297F","Dfr;":"\u{1D507}","dfr;":"\u{1D521}","dHar;":"\u2965","dharl;":"\u21C3","dharr;":"\u21C2","DiacriticalAcute;":"\xB4","DiacriticalDot;":"\u02D9","DiacriticalDoubleAcute;":"\u02DD","DiacriticalGrave;":"`","DiacriticalTilde;":"\u02DC","diam;":"\u22C4","Diamond;":"\u22C4","diamond;":"\u22C4","diamondsuit;":"\u2666","diams;":"\u2666","die;":"\xA8","DifferentialD;":"\u2146","digamma;":"\u03DD","disin;":"\u22F2","div;":"\xF7","divide;":"\xF7",divide:"\xF7","divideontimes;":"\u22C7","divonx;":"\u22C7","DJcy;":"\u0402","djcy;":"\u0452","dlcorn;":"\u231E","dlcrop;":"\u230D","dollar;":"$","Dopf;":"\u{1D53B}","dopf;":"\u{1D555}","Dot;":"\xA8","dot;":"\u02D9","DotDot;":"\u20DC","doteq;":"\u2250","doteqdot;":"\u2251","DotEqual;":"\u2250","dotminus;":"\u2238","dotplus;":"\u2214","dotsquare;":"\u22A1","doublebarwedge;":"\u2306","DoubleContourIntegral;":"\u222F","DoubleDot;":"\xA8","DoubleDownArrow;":"\u21D3","DoubleLeftArrow;":"\u21D0","DoubleLeftRightArrow;":"\u21D4","DoubleLeftTee;":"\u2AE4","DoubleLongLeftArrow;":"\u27F8","DoubleLongLeftRightArrow;":"\u27FA","DoubleLongRightArrow;":"\u27F9","DoubleRightArrow;":"\u21D2","DoubleRightTee;":"\u22A8","DoubleUpArrow;":"\u21D1","DoubleUpDownArrow;":"\u21D5","DoubleVerticalBar;":"\u2225","DownArrow;":"\u2193","Downarrow;":"\u21D3","downarrow;":"\u2193","DownArrowBar;":"\u2913","DownArrowUpArrow;":"\u21F5","DownBreve;":"\u0311","downdownarrows;":"\u21CA","downharpoonleft;":"\u21C3","downharpoonright;":"\u21C2","DownLeftRightVector;":"\u2950","DownLeftTeeVector;":"\u295E","DownLeftVector;":"\u21BD","DownLeftVectorBar;":"\u2956","DownRightTeeVector;":"\u295F","DownRightVector;":"\u21C1","DownRightVectorBar;":"\u2957","DownTee;":"\u22A4","DownTeeArrow;":"\u21A7","drbkarow;":"\u2910","drcorn;":"\u231F","drcrop;":"\u230C","Dscr;":"\u{1D49F}","dscr;":"\u{1D4B9}","DScy;":"\u0405","dscy;":"\u0455","dsol;":"\u29F6","Dstrok;":"\u0110","dstrok;":"\u0111","dtdot;":"\u22F1","dtri;":"\u25BF","dtrif;":"\u25BE","duarr;":"\u21F5","duhar;":"\u296F","dwangle;":"\u29A6","DZcy;":"\u040F","dzcy;":"\u045F","dzigrarr;":"\u27FF","Eacute;":"\xC9",Eacute:"\xC9","eacute;":"\xE9",eacute:"\xE9","easter;":"\u2A6E","Ecaron;":"\u011A","ecaron;":"\u011B","ecir;":"\u2256","Ecirc;":"\xCA",Ecirc:"\xCA","ecirc;":"\xEA",ecirc:"\xEA","ecolon;":"\u2255","Ecy;":"\u042D","ecy;":"\u044D","eDDot;":"\u2A77","Edot;":"\u0116","eDot;":"\u2251","edot;":"\u0117","ee;":"\u2147","efDot;":"\u2252","Efr;":"\u{1D508}","efr;":"\u{1D522}","eg;":"\u2A9A","Egrave;":"\xC8",Egrave:"\xC8","egrave;":"\xE8",egrave:"\xE8","egs;":"\u2A96","egsdot;":"\u2A98","el;":"\u2A99","Element;":"\u2208","elinters;":"\u23E7","ell;":"\u2113","els;":"\u2A95","elsdot;":"\u2A97","Emacr;":"\u0112","emacr;":"\u0113","empty;":"\u2205","emptyset;":"\u2205","EmptySmallSquare;":"\u25FB","emptyv;":"\u2205","EmptyVerySmallSquare;":"\u25AB","emsp;":"\u2003","emsp13;":"\u2004","emsp14;":"\u2005","ENG;":"\u014A","eng;":"\u014B","ensp;":"\u2002","Eogon;":"\u0118","eogon;":"\u0119","Eopf;":"\u{1D53C}","eopf;":"\u{1D556}","epar;":"\u22D5","eparsl;":"\u29E3","eplus;":"\u2A71","epsi;":"\u03B5","Epsilon;":"\u0395","epsilon;":"\u03B5","epsiv;":"\u03F5","eqcirc;":"\u2256","eqcolon;":"\u2255","eqsim;":"\u2242","eqslantgtr;":"\u2A96","eqslantless;":"\u2A95","Equal;":"\u2A75","equals;":"=","EqualTilde;":"\u2242","equest;":"\u225F","Equilibrium;":"\u21CC","equiv;":"\u2261","equivDD;":"\u2A78","eqvparsl;":"\u29E5","erarr;":"\u2971","erDot;":"\u2253","Escr;":"\u2130","escr;":"\u212F","esdot;":"\u2250","Esim;":"\u2A73","esim;":"\u2242","Eta;":"\u0397","eta;":"\u03B7","ETH;":"\xD0",ETH:"\xD0","eth;":"\xF0",eth:"\xF0","Euml;":"\xCB",Euml:"\xCB","euml;":"\xEB",euml:"\xEB","euro;":"\u20AC","excl;":"!","exist;":"\u2203","Exists;":"\u2203","expectation;":"\u2130","ExponentialE;":"\u2147","exponentiale;":"\u2147","fallingdotseq;":"\u2252","Fcy;":"\u0424","fcy;":"\u0444","female;":"\u2640","ffilig;":"\uFB03","fflig;":"\uFB00","ffllig;":"\uFB04","Ffr;":"\u{1D509}","ffr;":"\u{1D523}","filig;":"\uFB01","FilledSmallSquare;":"\u25FC","FilledVerySmallSquare;":"\u25AA","fjlig;":"fj","flat;":"\u266D","fllig;":"\uFB02","fltns;":"\u25B1","fnof;":"\u0192","Fopf;":"\u{1D53D}","fopf;":"\u{1D557}","ForAll;":"\u2200","forall;":"\u2200","fork;":"\u22D4","forkv;":"\u2AD9","Fouriertrf;":"\u2131","fpartint;":"\u2A0D","frac12;":"\xBD",frac12:"\xBD","frac13;":"\u2153","frac14;":"\xBC",frac14:"\xBC","frac15;":"\u2155","frac16;":"\u2159","frac18;":"\u215B","frac23;":"\u2154","frac25;":"\u2156","frac34;":"\xBE",frac34:"\xBE","frac35;":"\u2157","frac38;":"\u215C","frac45;":"\u2158","frac56;":"\u215A","frac58;":"\u215D","frac78;":"\u215E","frasl;":"\u2044","frown;":"\u2322","Fscr;":"\u2131","fscr;":"\u{1D4BB}","gacute;":"\u01F5","Gamma;":"\u0393","gamma;":"\u03B3","Gammad;":"\u03DC","gammad;":"\u03DD","gap;":"\u2A86","Gbreve;":"\u011E","gbreve;":"\u011F","Gcedil;":"\u0122","Gcirc;":"\u011C","gcirc;":"\u011D","Gcy;":"\u0413","gcy;":"\u0433","Gdot;":"\u0120","gdot;":"\u0121","gE;":"\u2267","ge;":"\u2265","gEl;":"\u2A8C","gel;":"\u22DB","geq;":"\u2265","geqq;":"\u2267","geqslant;":"\u2A7E","ges;":"\u2A7E","gescc;":"\u2AA9","gesdot;":"\u2A80","gesdoto;":"\u2A82","gesdotol;":"\u2A84","gesl;":"\u22DB\uFE00","gesles;":"\u2A94","Gfr;":"\u{1D50A}","gfr;":"\u{1D524}","Gg;":"\u22D9","gg;":"\u226B","ggg;":"\u22D9","gimel;":"\u2137","GJcy;":"\u0403","gjcy;":"\u0453","gl;":"\u2277","gla;":"\u2AA5","glE;":"\u2A92","glj;":"\u2AA4","gnap;":"\u2A8A","gnapprox;":"\u2A8A","gnE;":"\u2269","gne;":"\u2A88","gneq;":"\u2A88","gneqq;":"\u2269","gnsim;":"\u22E7","Gopf;":"\u{1D53E}","gopf;":"\u{1D558}","grave;":"`","GreaterEqual;":"\u2265","GreaterEqualLess;":"\u22DB","GreaterFullEqual;":"\u2267","GreaterGreater;":"\u2AA2","GreaterLess;":"\u2277","GreaterSlantEqual;":"\u2A7E","GreaterTilde;":"\u2273","Gscr;":"\u{1D4A2}","gscr;":"\u210A","gsim;":"\u2273","gsime;":"\u2A8E","gsiml;":"\u2A90","GT;":">",GT:">","Gt;":"\u226B","gt;":">",gt:">","gtcc;":"\u2AA7","gtcir;":"\u2A7A","gtdot;":"\u22D7","gtlPar;":"\u2995","gtquest;":"\u2A7C","gtrapprox;":"\u2A86","gtrarr;":"\u2978","gtrdot;":"\u22D7","gtreqless;":"\u22DB","gtreqqless;":"\u2A8C","gtrless;":"\u2277","gtrsim;":"\u2273","gvertneqq;":"\u2269\uFE00","gvnE;":"\u2269\uFE00","Hacek;":"\u02C7","hairsp;":"\u200A","half;":"\xBD","hamilt;":"\u210B","HARDcy;":"\u042A","hardcy;":"\u044A","hArr;":"\u21D4","harr;":"\u2194","harrcir;":"\u2948","harrw;":"\u21AD","Hat;":"^","hbar;":"\u210F","Hcirc;":"\u0124","hcirc;":"\u0125","hearts;":"\u2665","heartsuit;":"\u2665","hellip;":"\u2026","hercon;":"\u22B9","Hfr;":"\u210C","hfr;":"\u{1D525}","HilbertSpace;":"\u210B","hksearow;":"\u2925","hkswarow;":"\u2926","hoarr;":"\u21FF","homtht;":"\u223B","hookleftarrow;":"\u21A9","hookrightarrow;":"\u21AA","Hopf;":"\u210D","hopf;":"\u{1D559}","horbar;":"\u2015","HorizontalLine;":"\u2500","Hscr;":"\u210B","hscr;":"\u{1D4BD}","hslash;":"\u210F","Hstrok;":"\u0126","hstrok;":"\u0127","HumpDownHump;":"\u224E","HumpEqual;":"\u224F","hybull;":"\u2043","hyphen;":"\u2010","Iacute;":"\xCD",Iacute:"\xCD","iacute;":"\xED",iacute:"\xED","ic;":"\u2063","Icirc;":"\xCE",Icirc:"\xCE","icirc;":"\xEE",icirc:"\xEE","Icy;":"\u0418","icy;":"\u0438","Idot;":"\u0130","IEcy;":"\u0415","iecy;":"\u0435","iexcl;":"\xA1",iexcl:"\xA1","iff;":"\u21D4","Ifr;":"\u2111","ifr;":"\u{1D526}","Igrave;":"\xCC",Igrave:"\xCC","igrave;":"\xEC",igrave:"\xEC","ii;":"\u2148","iiiint;":"\u2A0C","iiint;":"\u222D","iinfin;":"\u29DC","iiota;":"\u2129","IJlig;":"\u0132","ijlig;":"\u0133","Im;":"\u2111","Imacr;":"\u012A","imacr;":"\u012B","image;":"\u2111","ImaginaryI;":"\u2148","imagline;":"\u2110","imagpart;":"\u2111","imath;":"\u0131","imof;":"\u22B7","imped;":"\u01B5","Implies;":"\u21D2","in;":"\u2208","incare;":"\u2105","infin;":"\u221E","infintie;":"\u29DD","inodot;":"\u0131","Int;":"\u222C","int;":"\u222B","intcal;":"\u22BA","integers;":"\u2124","Integral;":"\u222B","intercal;":"\u22BA","Intersection;":"\u22C2","intlarhk;":"\u2A17","intprod;":"\u2A3C","InvisibleComma;":"\u2063","InvisibleTimes;":"\u2062","IOcy;":"\u0401","iocy;":"\u0451","Iogon;":"\u012E","iogon;":"\u012F","Iopf;":"\u{1D540}","iopf;":"\u{1D55A}","Iota;":"\u0399","iota;":"\u03B9","iprod;":"\u2A3C","iquest;":"\xBF",iquest:"\xBF","Iscr;":"\u2110","iscr;":"\u{1D4BE}","isin;":"\u2208","isindot;":"\u22F5","isinE;":"\u22F9","isins;":"\u22F4","isinsv;":"\u22F3","isinv;":"\u2208","it;":"\u2062","Itilde;":"\u0128","itilde;":"\u0129","Iukcy;":"\u0406","iukcy;":"\u0456","Iuml;":"\xCF",Iuml:"\xCF","iuml;":"\xEF",iuml:"\xEF","Jcirc;":"\u0134","jcirc;":"\u0135","Jcy;":"\u0419","jcy;":"\u0439","Jfr;":"\u{1D50D}","jfr;":"\u{1D527}","jmath;":"\u0237","Jopf;":"\u{1D541}","jopf;":"\u{1D55B}","Jscr;":"\u{1D4A5}","jscr;":"\u{1D4BF}","Jsercy;":"\u0408","jsercy;":"\u0458","Jukcy;":"\u0404","jukcy;":"\u0454","Kappa;":"\u039A","kappa;":"\u03BA","kappav;":"\u03F0","Kcedil;":"\u0136","kcedil;":"\u0137","Kcy;":"\u041A","kcy;":"\u043A","Kfr;":"\u{1D50E}","kfr;":"\u{1D528}","kgreen;":"\u0138","KHcy;":"\u0425","khcy;":"\u0445","KJcy;":"\u040C","kjcy;":"\u045C","Kopf;":"\u{1D542}","kopf;":"\u{1D55C}","Kscr;":"\u{1D4A6}","kscr;":"\u{1D4C0}","lAarr;":"\u21DA","Lacute;":"\u0139","lacute;":"\u013A","laemptyv;":"\u29B4","lagran;":"\u2112","Lambda;":"\u039B","lambda;":"\u03BB","Lang;":"\u27EA","lang;":"\u27E8","langd;":"\u2991","langle;":"\u27E8","lap;":"\u2A85","Laplacetrf;":"\u2112","laquo;":"\xAB",laquo:"\xAB","Larr;":"\u219E","lArr;":"\u21D0","larr;":"\u2190","larrb;":"\u21E4","larrbfs;":"\u291F","larrfs;":"\u291D","larrhk;":"\u21A9","larrlp;":"\u21AB","larrpl;":"\u2939","larrsim;":"\u2973","larrtl;":"\u21A2","lat;":"\u2AAB","lAtail;":"\u291B","latail;":"\u2919","late;":"\u2AAD","lates;":"\u2AAD\uFE00","lBarr;":"\u290E","lbarr;":"\u290C","lbbrk;":"\u2772","lbrace;":"{","lbrack;":"[","lbrke;":"\u298B","lbrksld;":"\u298F","lbrkslu;":"\u298D","Lcaron;":"\u013D","lcaron;":"\u013E","Lcedil;":"\u013B","lcedil;":"\u013C","lceil;":"\u2308","lcub;":"{","Lcy;":"\u041B","lcy;":"\u043B","ldca;":"\u2936","ldquo;":"\u201C","ldquor;":"\u201E","ldrdhar;":"\u2967","ldrushar;":"\u294B","ldsh;":"\u21B2","lE;":"\u2266","le;":"\u2264","LeftAngleBracket;":"\u27E8","LeftArrow;":"\u2190","Leftarrow;":"\u21D0","leftarrow;":"\u2190","LeftArrowBar;":"\u21E4","LeftArrowRightArrow;":"\u21C6","leftarrowtail;":"\u21A2","LeftCeiling;":"\u2308","LeftDoubleBracket;":"\u27E6","LeftDownTeeVector;":"\u2961","LeftDownVector;":"\u21C3","LeftDownVectorBar;":"\u2959","LeftFloor;":"\u230A","leftharpoondown;":"\u21BD","leftharpoonup;":"\u21BC","leftleftarrows;":"\u21C7","LeftRightArrow;":"\u2194","Leftrightarrow;":"\u21D4","leftrightarrow;":"\u2194","leftrightarrows;":"\u21C6","leftrightharpoons;":"\u21CB","leftrightsquigarrow;":"\u21AD","LeftRightVector;":"\u294E","LeftTee;":"\u22A3","LeftTeeArrow;":"\u21A4","LeftTeeVector;":"\u295A","leftthreetimes;":"\u22CB","LeftTriangle;":"\u22B2","LeftTriangleBar;":"\u29CF","LeftTriangleEqual;":"\u22B4","LeftUpDownVector;":"\u2951","LeftUpTeeVector;":"\u2960","LeftUpVector;":"\u21BF","LeftUpVectorBar;":"\u2958","LeftVector;":"\u21BC","LeftVectorBar;":"\u2952","lEg;":"\u2A8B","leg;":"\u22DA","leq;":"\u2264","leqq;":"\u2266","leqslant;":"\u2A7D","les;":"\u2A7D","lescc;":"\u2AA8","lesdot;":"\u2A7F","lesdoto;":"\u2A81","lesdotor;":"\u2A83","lesg;":"\u22DA\uFE00","lesges;":"\u2A93","lessapprox;":"\u2A85","lessdot;":"\u22D6","lesseqgtr;":"\u22DA","lesseqqgtr;":"\u2A8B","LessEqualGreater;":"\u22DA","LessFullEqual;":"\u2266","LessGreater;":"\u2276","lessgtr;":"\u2276","LessLess;":"\u2AA1","lesssim;":"\u2272","LessSlantEqual;":"\u2A7D","LessTilde;":"\u2272","lfisht;":"\u297C","lfloor;":"\u230A","Lfr;":"\u{1D50F}","lfr;":"\u{1D529}","lg;":"\u2276","lgE;":"\u2A91","lHar;":"\u2962","lhard;":"\u21BD","lharu;":"\u21BC","lharul;":"\u296A","lhblk;":"\u2584","LJcy;":"\u0409","ljcy;":"\u0459","Ll;":"\u22D8","ll;":"\u226A","llarr;":"\u21C7","llcorner;":"\u231E","Lleftarrow;":"\u21DA","llhard;":"\u296B","lltri;":"\u25FA","Lmidot;":"\u013F","lmidot;":"\u0140","lmoust;":"\u23B0","lmoustache;":"\u23B0","lnap;":"\u2A89","lnapprox;":"\u2A89","lnE;":"\u2268","lne;":"\u2A87","lneq;":"\u2A87","lneqq;":"\u2268","lnsim;":"\u22E6","loang;":"\u27EC","loarr;":"\u21FD","lobrk;":"\u27E6","LongLeftArrow;":"\u27F5","Longleftarrow;":"\u27F8","longleftarrow;":"\u27F5","LongLeftRightArrow;":"\u27F7","Longleftrightarrow;":"\u27FA","longleftrightarrow;":"\u27F7","longmapsto;":"\u27FC","LongRightArrow;":"\u27F6","Longrightarrow;":"\u27F9","longrightarrow;":"\u27F6","looparrowleft;":"\u21AB","looparrowright;":"\u21AC","lopar;":"\u2985","Lopf;":"\u{1D543}","lopf;":"\u{1D55D}","loplus;":"\u2A2D","lotimes;":"\u2A34","lowast;":"\u2217","lowbar;":"_","LowerLeftArrow;":"\u2199","LowerRightArrow;":"\u2198","loz;":"\u25CA","lozenge;":"\u25CA","lozf;":"\u29EB","lpar;":"(","lparlt;":"\u2993","lrarr;":"\u21C6","lrcorner;":"\u231F","lrhar;":"\u21CB","lrhard;":"\u296D","lrm;":"\u200E","lrtri;":"\u22BF","lsaquo;":"\u2039","Lscr;":"\u2112","lscr;":"\u{1D4C1}","Lsh;":"\u21B0","lsh;":"\u21B0","lsim;":"\u2272","lsime;":"\u2A8D","lsimg;":"\u2A8F","lsqb;":"[","lsquo;":"\u2018","lsquor;":"\u201A","Lstrok;":"\u0141","lstrok;":"\u0142","LT;":"<",LT:"<","Lt;":"\u226A","lt;":"<",lt:"<","ltcc;":"\u2AA6","ltcir;":"\u2A79","ltdot;":"\u22D6","lthree;":"\u22CB","ltimes;":"\u22C9","ltlarr;":"\u2976","ltquest;":"\u2A7B","ltri;":"\u25C3","ltrie;":"\u22B4","ltrif;":"\u25C2","ltrPar;":"\u2996","lurdshar;":"\u294A","luruhar;":"\u2966","lvertneqq;":"\u2268\uFE00","lvnE;":"\u2268\uFE00","macr;":"\xAF",macr:"\xAF","male;":"\u2642","malt;":"\u2720","maltese;":"\u2720","Map;":"\u2905","map;":"\u21A6","mapsto;":"\u21A6","mapstodown;":"\u21A7","mapstoleft;":"\u21A4","mapstoup;":"\u21A5","marker;":"\u25AE","mcomma;":"\u2A29","Mcy;":"\u041C","mcy;":"\u043C","mdash;":"\u2014","mDDot;":"\u223A","measuredangle;":"\u2221","MediumSpace;":"\u205F","Mellintrf;":"\u2133","Mfr;":"\u{1D510}","mfr;":"\u{1D52A}","mho;":"\u2127","micro;":"\xB5",micro:"\xB5","mid;":"\u2223","midast;":"*","midcir;":"\u2AF0","middot;":"\xB7",middot:"\xB7","minus;":"\u2212","minusb;":"\u229F","minusd;":"\u2238","minusdu;":"\u2A2A","MinusPlus;":"\u2213","mlcp;":"\u2ADB","mldr;":"\u2026","mnplus;":"\u2213","models;":"\u22A7","Mopf;":"\u{1D544}","mopf;":"\u{1D55E}","mp;":"\u2213","Mscr;":"\u2133","mscr;":"\u{1D4C2}","mstpos;":"\u223E","Mu;":"\u039C","mu;":"\u03BC","multimap;":"\u22B8","mumap;":"\u22B8","nabla;":"\u2207","Nacute;":"\u0143","nacute;":"\u0144","nang;":"\u2220\u20D2","nap;":"\u2249","napE;":"\u2A70\u0338","napid;":"\u224B\u0338","napos;":"\u0149","napprox;":"\u2249","natur;":"\u266E","natural;":"\u266E","naturals;":"\u2115","nbsp;":"\xA0",nbsp:"\xA0","nbump;":"\u224E\u0338","nbumpe;":"\u224F\u0338","ncap;":"\u2A43","Ncaron;":"\u0147","ncaron;":"\u0148","Ncedil;":"\u0145","ncedil;":"\u0146","ncong;":"\u2247","ncongdot;":"\u2A6D\u0338","ncup;":"\u2A42","Ncy;":"\u041D","ncy;":"\u043D","ndash;":"\u2013","ne;":"\u2260","nearhk;":"\u2924","neArr;":"\u21D7","nearr;":"\u2197","nearrow;":"\u2197","nedot;":"\u2250\u0338","NegativeMediumSpace;":"\u200B","NegativeThickSpace;":"\u200B","NegativeThinSpace;":"\u200B","NegativeVeryThinSpace;":"\u200B","nequiv;":"\u2262","nesear;":"\u2928","nesim;":"\u2242\u0338","NestedGreaterGreater;":"\u226B","NestedLessLess;":"\u226A","NewLine;":`
`,"nexist;":"\u2204","nexists;":"\u2204","Nfr;":"\u{1D511}","nfr;":"\u{1D52B}","ngE;":"\u2267\u0338","nge;":"\u2271","ngeq;":"\u2271","ngeqq;":"\u2267\u0338","ngeqslant;":"\u2A7E\u0338","nges;":"\u2A7E\u0338","nGg;":"\u22D9\u0338","ngsim;":"\u2275","nGt;":"\u226B\u20D2","ngt;":"\u226F","ngtr;":"\u226F","nGtv;":"\u226B\u0338","nhArr;":"\u21CE","nharr;":"\u21AE","nhpar;":"\u2AF2","ni;":"\u220B","nis;":"\u22FC","nisd;":"\u22FA","niv;":"\u220B","NJcy;":"\u040A","njcy;":"\u045A","nlArr;":"\u21CD","nlarr;":"\u219A","nldr;":"\u2025","nlE;":"\u2266\u0338","nle;":"\u2270","nLeftarrow;":"\u21CD","nleftarrow;":"\u219A","nLeftrightarrow;":"\u21CE","nleftrightarrow;":"\u21AE","nleq;":"\u2270","nleqq;":"\u2266\u0338","nleqslant;":"\u2A7D\u0338","nles;":"\u2A7D\u0338","nless;":"\u226E","nLl;":"\u22D8\u0338","nlsim;":"\u2274","nLt;":"\u226A\u20D2","nlt;":"\u226E","nltri;":"\u22EA","nltrie;":"\u22EC","nLtv;":"\u226A\u0338","nmid;":"\u2224","NoBreak;":"\u2060","NonBreakingSpace;":"\xA0","Nopf;":"\u2115","nopf;":"\u{1D55F}","Not;":"\u2AEC","not;":"\xAC",not:"\xAC","NotCongruent;":"\u2262","NotCupCap;":"\u226D","NotDoubleVerticalBar;":"\u2226","NotElement;":"\u2209","NotEqual;":"\u2260","NotEqualTilde;":"\u2242\u0338","NotExists;":"\u2204","NotGreater;":"\u226F","NotGreaterEqual;":"\u2271","NotGreaterFullEqual;":"\u2267\u0338","NotGreaterGreater;":"\u226B\u0338","NotGreaterLess;":"\u2279","NotGreaterSlantEqual;":"\u2A7E\u0338","NotGreaterTilde;":"\u2275","NotHumpDownHump;":"\u224E\u0338","NotHumpEqual;":"\u224F\u0338","notin;":"\u2209","notindot;":"\u22F5\u0338","notinE;":"\u22F9\u0338","notinva;":"\u2209","notinvb;":"\u22F7","notinvc;":"\u22F6","NotLeftTriangle;":"\u22EA","NotLeftTriangleBar;":"\u29CF\u0338","NotLeftTriangleEqual;":"\u22EC","NotLess;":"\u226E","NotLessEqual;":"\u2270","NotLessGreater;":"\u2278","NotLessLess;":"\u226A\u0338","NotLessSlantEqual;":"\u2A7D\u0338","NotLessTilde;":"\u2274","NotNestedGreaterGreater;":"\u2AA2\u0338","NotNestedLessLess;":"\u2AA1\u0338","notni;":"\u220C","notniva;":"\u220C","notnivb;":"\u22FE","notnivc;":"\u22FD","NotPrecedes;":"\u2280","NotPrecedesEqual;":"\u2AAF\u0338","NotPrecedesSlantEqual;":"\u22E0","NotReverseElement;":"\u220C","NotRightTriangle;":"\u22EB","NotRightTriangleBar;":"\u29D0\u0338","NotRightTriangleEqual;":"\u22ED","NotSquareSubset;":"\u228F\u0338","NotSquareSubsetEqual;":"\u22E2","NotSquareSuperset;":"\u2290\u0338","NotSquareSupersetEqual;":"\u22E3","NotSubset;":"\u2282\u20D2","NotSubsetEqual;":"\u2288","NotSucceeds;":"\u2281","NotSucceedsEqual;":"\u2AB0\u0338","NotSucceedsSlantEqual;":"\u22E1","NotSucceedsTilde;":"\u227F\u0338","NotSuperset;":"\u2283\u20D2","NotSupersetEqual;":"\u2289","NotTilde;":"\u2241","NotTildeEqual;":"\u2244","NotTildeFullEqual;":"\u2247","NotTildeTilde;":"\u2249","NotVerticalBar;":"\u2224","npar;":"\u2226","nparallel;":"\u2226","nparsl;":"\u2AFD\u20E5","npart;":"\u2202\u0338","npolint;":"\u2A14","npr;":"\u2280","nprcue;":"\u22E0","npre;":"\u2AAF\u0338","nprec;":"\u2280","npreceq;":"\u2AAF\u0338","nrArr;":"\u21CF","nrarr;":"\u219B","nrarrc;":"\u2933\u0338","nrarrw;":"\u219D\u0338","nRightarrow;":"\u21CF","nrightarrow;":"\u219B","nrtri;":"\u22EB","nrtrie;":"\u22ED","nsc;":"\u2281","nsccue;":"\u22E1","nsce;":"\u2AB0\u0338","Nscr;":"\u{1D4A9}","nscr;":"\u{1D4C3}","nshortmid;":"\u2224","nshortparallel;":"\u2226","nsim;":"\u2241","nsime;":"\u2244","nsimeq;":"\u2244","nsmid;":"\u2224","nspar;":"\u2226","nsqsube;":"\u22E2","nsqsupe;":"\u22E3","nsub;":"\u2284","nsubE;":"\u2AC5\u0338","nsube;":"\u2288","nsubset;":"\u2282\u20D2","nsubseteq;":"\u2288","nsubseteqq;":"\u2AC5\u0338","nsucc;":"\u2281","nsucceq;":"\u2AB0\u0338","nsup;":"\u2285","nsupE;":"\u2AC6\u0338","nsupe;":"\u2289","nsupset;":"\u2283\u20D2","nsupseteq;":"\u2289","nsupseteqq;":"\u2AC6\u0338","ntgl;":"\u2279","Ntilde;":"\xD1",Ntilde:"\xD1","ntilde;":"\xF1",ntilde:"\xF1","ntlg;":"\u2278","ntriangleleft;":"\u22EA","ntrianglelefteq;":"\u22EC","ntriangleright;":"\u22EB","ntrianglerighteq;":"\u22ED","Nu;":"\u039D","nu;":"\u03BD","num;":"#","numero;":"\u2116","numsp;":"\u2007","nvap;":"\u224D\u20D2","nVDash;":"\u22AF","nVdash;":"\u22AE","nvDash;":"\u22AD","nvdash;":"\u22AC","nvge;":"\u2265\u20D2","nvgt;":">\u20D2","nvHarr;":"\u2904","nvinfin;":"\u29DE","nvlArr;":"\u2902","nvle;":"\u2264\u20D2","nvlt;":"<\u20D2","nvltrie;":"\u22B4\u20D2","nvrArr;":"\u2903","nvrtrie;":"\u22B5\u20D2","nvsim;":"\u223C\u20D2","nwarhk;":"\u2923","nwArr;":"\u21D6","nwarr;":"\u2196","nwarrow;":"\u2196","nwnear;":"\u2927","Oacute;":"\xD3",Oacute:"\xD3","oacute;":"\xF3",oacute:"\xF3","oast;":"\u229B","ocir;":"\u229A","Ocirc;":"\xD4",Ocirc:"\xD4","ocirc;":"\xF4",ocirc:"\xF4","Ocy;":"\u041E","ocy;":"\u043E","odash;":"\u229D","Odblac;":"\u0150","odblac;":"\u0151","odiv;":"\u2A38","odot;":"\u2299","odsold;":"\u29BC","OElig;":"\u0152","oelig;":"\u0153","ofcir;":"\u29BF","Ofr;":"\u{1D512}","ofr;":"\u{1D52C}","ogon;":"\u02DB","Ograve;":"\xD2",Ograve:"\xD2","ograve;":"\xF2",ograve:"\xF2","ogt;":"\u29C1","ohbar;":"\u29B5","ohm;":"\u03A9","oint;":"\u222E","olarr;":"\u21BA","olcir;":"\u29BE","olcross;":"\u29BB","oline;":"\u203E","olt;":"\u29C0","Omacr;":"\u014C","omacr;":"\u014D","Omega;":"\u03A9","omega;":"\u03C9","Omicron;":"\u039F","omicron;":"\u03BF","omid;":"\u29B6","ominus;":"\u2296","Oopf;":"\u{1D546}","oopf;":"\u{1D560}","opar;":"\u29B7","OpenCurlyDoubleQuote;":"\u201C","OpenCurlyQuote;":"\u2018","operp;":"\u29B9","oplus;":"\u2295","Or;":"\u2A54","or;":"\u2228","orarr;":"\u21BB","ord;":"\u2A5D","order;":"\u2134","orderof;":"\u2134","ordf;":"\xAA",ordf:"\xAA","ordm;":"\xBA",ordm:"\xBA","origof;":"\u22B6","oror;":"\u2A56","orslope;":"\u2A57","orv;":"\u2A5B","oS;":"\u24C8","Oscr;":"\u{1D4AA}","oscr;":"\u2134","Oslash;":"\xD8",Oslash:"\xD8","oslash;":"\xF8",oslash:"\xF8","osol;":"\u2298","Otilde;":"\xD5",Otilde:"\xD5","otilde;":"\xF5",otilde:"\xF5","Otimes;":"\u2A37","otimes;":"\u2297","otimesas;":"\u2A36","Ouml;":"\xD6",Ouml:"\xD6","ouml;":"\xF6",ouml:"\xF6","ovbar;":"\u233D","OverBar;":"\u203E","OverBrace;":"\u23DE","OverBracket;":"\u23B4","OverParenthesis;":"\u23DC","par;":"\u2225","para;":"\xB6",para:"\xB6","parallel;":"\u2225","parsim;":"\u2AF3","parsl;":"\u2AFD","part;":"\u2202","PartialD;":"\u2202","Pcy;":"\u041F","pcy;":"\u043F","percnt;":"%","period;":".","permil;":"\u2030","perp;":"\u22A5","pertenk;":"\u2031","Pfr;":"\u{1D513}","pfr;":"\u{1D52D}","Phi;":"\u03A6","phi;":"\u03C6","phiv;":"\u03D5","phmmat;":"\u2133","phone;":"\u260E","Pi;":"\u03A0","pi;":"\u03C0","pitchfork;":"\u22D4","piv;":"\u03D6","planck;":"\u210F","planckh;":"\u210E","plankv;":"\u210F","plus;":"+","plusacir;":"\u2A23","plusb;":"\u229E","pluscir;":"\u2A22","plusdo;":"\u2214","plusdu;":"\u2A25","pluse;":"\u2A72","PlusMinus;":"\xB1","plusmn;":"\xB1",plusmn:"\xB1","plussim;":"\u2A26","plustwo;":"\u2A27","pm;":"\xB1","Poincareplane;":"\u210C","pointint;":"\u2A15","Popf;":"\u2119","popf;":"\u{1D561}","pound;":"\xA3",pound:"\xA3","Pr;":"\u2ABB","pr;":"\u227A","prap;":"\u2AB7","prcue;":"\u227C","prE;":"\u2AB3","pre;":"\u2AAF","prec;":"\u227A","precapprox;":"\u2AB7","preccurlyeq;":"\u227C","Precedes;":"\u227A","PrecedesEqual;":"\u2AAF","PrecedesSlantEqual;":"\u227C","PrecedesTilde;":"\u227E","preceq;":"\u2AAF","precnapprox;":"\u2AB9","precneqq;":"\u2AB5","precnsim;":"\u22E8","precsim;":"\u227E","Prime;":"\u2033","prime;":"\u2032","primes;":"\u2119","prnap;":"\u2AB9","prnE;":"\u2AB5","prnsim;":"\u22E8","prod;":"\u220F","Product;":"\u220F","profalar;":"\u232E","profline;":"\u2312","profsurf;":"\u2313","prop;":"\u221D","Proportion;":"\u2237","Proportional;":"\u221D","propto;":"\u221D","prsim;":"\u227E","prurel;":"\u22B0","Pscr;":"\u{1D4AB}","pscr;":"\u{1D4C5}","Psi;":"\u03A8","psi;":"\u03C8","puncsp;":"\u2008","Qfr;":"\u{1D514}","qfr;":"\u{1D52E}","qint;":"\u2A0C","Qopf;":"\u211A","qopf;":"\u{1D562}","qprime;":"\u2057","Qscr;":"\u{1D4AC}","qscr;":"\u{1D4C6}","quaternions;":"\u210D","quatint;":"\u2A16","quest;":"?","questeq;":"\u225F","QUOT;":'"',QUOT:'"',"quot;":'"',quot:'"',"rAarr;":"\u21DB","race;":"\u223D\u0331","Racute;":"\u0154","racute;":"\u0155","radic;":"\u221A","raemptyv;":"\u29B3","Rang;":"\u27EB","rang;":"\u27E9","rangd;":"\u2992","range;":"\u29A5","rangle;":"\u27E9","raquo;":"\xBB",raquo:"\xBB","Rarr;":"\u21A0","rArr;":"\u21D2","rarr;":"\u2192","rarrap;":"\u2975","rarrb;":"\u21E5","rarrbfs;":"\u2920","rarrc;":"\u2933","rarrfs;":"\u291E","rarrhk;":"\u21AA","rarrlp;":"\u21AC","rarrpl;":"\u2945","rarrsim;":"\u2974","Rarrtl;":"\u2916","rarrtl;":"\u21A3","rarrw;":"\u219D","rAtail;":"\u291C","ratail;":"\u291A","ratio;":"\u2236","rationals;":"\u211A","RBarr;":"\u2910","rBarr;":"\u290F","rbarr;":"\u290D","rbbrk;":"\u2773","rbrace;":"}","rbrack;":"]","rbrke;":"\u298C","rbrksld;":"\u298E","rbrkslu;":"\u2990","Rcaron;":"\u0158","rcaron;":"\u0159","Rcedil;":"\u0156","rcedil;":"\u0157","rceil;":"\u2309","rcub;":"}","Rcy;":"\u0420","rcy;":"\u0440","rdca;":"\u2937","rdldhar;":"\u2969","rdquo;":"\u201D","rdquor;":"\u201D","rdsh;":"\u21B3","Re;":"\u211C","real;":"\u211C","realine;":"\u211B","realpart;":"\u211C","reals;":"\u211D","rect;":"\u25AD","REG;":"\xAE",REG:"\xAE","reg;":"\xAE",reg:"\xAE","ReverseElement;":"\u220B","ReverseEquilibrium;":"\u21CB","ReverseUpEquilibrium;":"\u296F","rfisht;":"\u297D","rfloor;":"\u230B","Rfr;":"\u211C","rfr;":"\u{1D52F}","rHar;":"\u2964","rhard;":"\u21C1","rharu;":"\u21C0","rharul;":"\u296C","Rho;":"\u03A1","rho;":"\u03C1","rhov;":"\u03F1","RightAngleBracket;":"\u27E9","RightArrow;":"\u2192","Rightarrow;":"\u21D2","rightarrow;":"\u2192","RightArrowBar;":"\u21E5","RightArrowLeftArrow;":"\u21C4","rightarrowtail;":"\u21A3","RightCeiling;":"\u2309","RightDoubleBracket;":"\u27E7","RightDownTeeVector;":"\u295D","RightDownVector;":"\u21C2","RightDownVectorBar;":"\u2955","RightFloor;":"\u230B","rightharpoondown;":"\u21C1","rightharpoonup;":"\u21C0","rightleftarrows;":"\u21C4","rightleftharpoons;":"\u21CC","rightrightarrows;":"\u21C9","rightsquigarrow;":"\u219D","RightTee;":"\u22A2","RightTeeArrow;":"\u21A6","RightTeeVector;":"\u295B","rightthreetimes;":"\u22CC","RightTriangle;":"\u22B3","RightTriangleBar;":"\u29D0","RightTriangleEqual;":"\u22B5","RightUpDownVector;":"\u294F","RightUpTeeVector;":"\u295C","RightUpVector;":"\u21BE","RightUpVectorBar;":"\u2954","RightVector;":"\u21C0","RightVectorBar;":"\u2953","ring;":"\u02DA","risingdotseq;":"\u2253","rlarr;":"\u21C4","rlhar;":"\u21CC","rlm;":"\u200F","rmoust;":"\u23B1","rmoustache;":"\u23B1","rnmid;":"\u2AEE","roang;":"\u27ED","roarr;":"\u21FE","robrk;":"\u27E7","ropar;":"\u2986","Ropf;":"\u211D","ropf;":"\u{1D563}","roplus;":"\u2A2E","rotimes;":"\u2A35","RoundImplies;":"\u2970","rpar;":")","rpargt;":"\u2994","rppolint;":"\u2A12","rrarr;":"\u21C9","Rrightarrow;":"\u21DB","rsaquo;":"\u203A","Rscr;":"\u211B","rscr;":"\u{1D4C7}","Rsh;":"\u21B1","rsh;":"\u21B1","rsqb;":"]","rsquo;":"\u2019","rsquor;":"\u2019","rthree;":"\u22CC","rtimes;":"\u22CA","rtri;":"\u25B9","rtrie;":"\u22B5","rtrif;":"\u25B8","rtriltri;":"\u29CE","RuleDelayed;":"\u29F4","ruluhar;":"\u2968","rx;":"\u211E","Sacute;":"\u015A","sacute;":"\u015B","sbquo;":"\u201A","Sc;":"\u2ABC","sc;":"\u227B","scap;":"\u2AB8","Scaron;":"\u0160","scaron;":"\u0161","sccue;":"\u227D","scE;":"\u2AB4","sce;":"\u2AB0","Scedil;":"\u015E","scedil;":"\u015F","Scirc;":"\u015C","scirc;":"\u015D","scnap;":"\u2ABA","scnE;":"\u2AB6","scnsim;":"\u22E9","scpolint;":"\u2A13","scsim;":"\u227F","Scy;":"\u0421","scy;":"\u0441","sdot;":"\u22C5","sdotb;":"\u22A1","sdote;":"\u2A66","searhk;":"\u2925","seArr;":"\u21D8","searr;":"\u2198","searrow;":"\u2198","sect;":"\xA7",sect:"\xA7","semi;":";","seswar;":"\u2929","setminus;":"\u2216","setmn;":"\u2216","sext;":"\u2736","Sfr;":"\u{1D516}","sfr;":"\u{1D530}","sfrown;":"\u2322","sharp;":"\u266F","SHCHcy;":"\u0429","shchcy;":"\u0449","SHcy;":"\u0428","shcy;":"\u0448","ShortDownArrow;":"\u2193","ShortLeftArrow;":"\u2190","shortmid;":"\u2223","shortparallel;":"\u2225","ShortRightArrow;":"\u2192","ShortUpArrow;":"\u2191","shy;":"\xAD",shy:"\xAD","Sigma;":"\u03A3","sigma;":"\u03C3","sigmaf;":"\u03C2","sigmav;":"\u03C2","sim;":"\u223C","simdot;":"\u2A6A","sime;":"\u2243","simeq;":"\u2243","simg;":"\u2A9E","simgE;":"\u2AA0","siml;":"\u2A9D","simlE;":"\u2A9F","simne;":"\u2246","simplus;":"\u2A24","simrarr;":"\u2972","slarr;":"\u2190","SmallCircle;":"\u2218","smallsetminus;":"\u2216","smashp;":"\u2A33","smeparsl;":"\u29E4","smid;":"\u2223","smile;":"\u2323","smt;":"\u2AAA","smte;":"\u2AAC","smtes;":"\u2AAC\uFE00","SOFTcy;":"\u042C","softcy;":"\u044C","sol;":"/","solb;":"\u29C4","solbar;":"\u233F","Sopf;":"\u{1D54A}","sopf;":"\u{1D564}","spades;":"\u2660","spadesuit;":"\u2660","spar;":"\u2225","sqcap;":"\u2293","sqcaps;":"\u2293\uFE00","sqcup;":"\u2294","sqcups;":"\u2294\uFE00","Sqrt;":"\u221A","sqsub;":"\u228F","sqsube;":"\u2291","sqsubset;":"\u228F","sqsubseteq;":"\u2291","sqsup;":"\u2290","sqsupe;":"\u2292","sqsupset;":"\u2290","sqsupseteq;":"\u2292","squ;":"\u25A1","Square;":"\u25A1","square;":"\u25A1","SquareIntersection;":"\u2293","SquareSubset;":"\u228F","SquareSubsetEqual;":"\u2291","SquareSuperset;":"\u2290","SquareSupersetEqual;":"\u2292","SquareUnion;":"\u2294","squarf;":"\u25AA","squf;":"\u25AA","srarr;":"\u2192","Sscr;":"\u{1D4AE}","sscr;":"\u{1D4C8}","ssetmn;":"\u2216","ssmile;":"\u2323","sstarf;":"\u22C6","Star;":"\u22C6","star;":"\u2606","starf;":"\u2605","straightepsilon;":"\u03F5","straightphi;":"\u03D5","strns;":"\xAF","Sub;":"\u22D0","sub;":"\u2282","subdot;":"\u2ABD","subE;":"\u2AC5","sube;":"\u2286","subedot;":"\u2AC3","submult;":"\u2AC1","subnE;":"\u2ACB","subne;":"\u228A","subplus;":"\u2ABF","subrarr;":"\u2979","Subset;":"\u22D0","subset;":"\u2282","subseteq;":"\u2286","subseteqq;":"\u2AC5","SubsetEqual;":"\u2286","subsetneq;":"\u228A","subsetneqq;":"\u2ACB","subsim;":"\u2AC7","subsub;":"\u2AD5","subsup;":"\u2AD3","succ;":"\u227B","succapprox;":"\u2AB8","succcurlyeq;":"\u227D","Succeeds;":"\u227B","SucceedsEqual;":"\u2AB0","SucceedsSlantEqual;":"\u227D","SucceedsTilde;":"\u227F","succeq;":"\u2AB0","succnapprox;":"\u2ABA","succneqq;":"\u2AB6","succnsim;":"\u22E9","succsim;":"\u227F","SuchThat;":"\u220B","Sum;":"\u2211","sum;":"\u2211","sung;":"\u266A","Sup;":"\u22D1","sup;":"\u2283","sup1;":"\xB9",sup1:"\xB9","sup2;":"\xB2",sup2:"\xB2","sup3;":"\xB3",sup3:"\xB3","supdot;":"\u2ABE","supdsub;":"\u2AD8","supE;":"\u2AC6","supe;":"\u2287","supedot;":"\u2AC4","Superset;":"\u2283","SupersetEqual;":"\u2287","suphsol;":"\u27C9","suphsub;":"\u2AD7","suplarr;":"\u297B","supmult;":"\u2AC2","supnE;":"\u2ACC","supne;":"\u228B","supplus;":"\u2AC0","Supset;":"\u22D1","supset;":"\u2283","supseteq;":"\u2287","supseteqq;":"\u2AC6","supsetneq;":"\u228B","supsetneqq;":"\u2ACC","supsim;":"\u2AC8","supsub;":"\u2AD4","supsup;":"\u2AD6","swarhk;":"\u2926","swArr;":"\u21D9","swarr;":"\u2199","swarrow;":"\u2199","swnwar;":"\u292A","szlig;":"\xDF",szlig:"\xDF","Tab;":" ","target;":"\u2316","Tau;":"\u03A4","tau;":"\u03C4","tbrk;":"\u23B4","Tcaron;":"\u0164","tcaron;":"\u0165","Tcedil;":"\u0162","tcedil;":"\u0163","Tcy;":"\u0422","tcy;":"\u0442","tdot;":"\u20DB","telrec;":"\u2315","Tfr;":"\u{1D517}","tfr;":"\u{1D531}","there4;":"\u2234","Therefore;":"\u2234","therefore;":"\u2234","Theta;":"\u0398","theta;":"\u03B8","thetasym;":"\u03D1","thetav;":"\u03D1","thickapprox;":"\u2248","thicksim;":"\u223C","ThickSpace;":"\u205F\u200A","thinsp;":"\u2009","ThinSpace;":"\u2009","thkap;":"\u2248","thksim;":"\u223C","THORN;":"\xDE",THORN:"\xDE","thorn;":"\xFE",thorn:"\xFE","Tilde;":"\u223C","tilde;":"\u02DC","TildeEqual;":"\u2243","TildeFullEqual;":"\u2245","TildeTilde;":"\u2248","times;":"\xD7",times:"\xD7","timesb;":"\u22A0","timesbar;":"\u2A31","timesd;":"\u2A30","tint;":"\u222D","toea;":"\u2928","top;":"\u22A4","topbot;":"\u2336","topcir;":"\u2AF1","Topf;":"\u{1D54B}","topf;":"\u{1D565}","topfork;":"\u2ADA","tosa;":"\u2929","tprime;":"\u2034","TRADE;":"\u2122","trade;":"\u2122","triangle;":"\u25B5","triangledown;":"\u25BF","triangleleft;":"\u25C3","trianglelefteq;":"\u22B4","triangleq;":"\u225C","triangleright;":"\u25B9","trianglerighteq;":"\u22B5","tridot;":"\u25EC","trie;":"\u225C","triminus;":"\u2A3A","TripleDot;":"\u20DB","triplus;":"\u2A39","trisb;":"\u29CD","tritime;":"\u2A3B","trpezium;":"\u23E2","Tscr;":"\u{1D4AF}","tscr;":"\u{1D4C9}","TScy;":"\u0426","tscy;":"\u0446","TSHcy;":"\u040B","tshcy;":"\u045B","Tstrok;":"\u0166","tstrok;":"\u0167","twixt;":"\u226C","twoheadleftarrow;":"\u219E","twoheadrightarrow;":"\u21A0","Uacute;":"\xDA",Uacute:"\xDA","uacute;":"\xFA",uacute:"\xFA","Uarr;":"\u219F","uArr;":"\u21D1","uarr;":"\u2191","Uarrocir;":"\u2949","Ubrcy;":"\u040E","ubrcy;":"\u045E","Ubreve;":"\u016C","ubreve;":"\u016D","Ucirc;":"\xDB",Ucirc:"\xDB","ucirc;":"\xFB",ucirc:"\xFB","Ucy;":"\u0423","ucy;":"\u0443","udarr;":"\u21C5","Udblac;":"\u0170","udblac;":"\u0171","udhar;":"\u296E","ufisht;":"\u297E","Ufr;":"\u{1D518}","ufr;":"\u{1D532}","Ugrave;":"\xD9",Ugrave:"\xD9","ugrave;":"\xF9",ugrave:"\xF9","uHar;":"\u2963","uharl;":"\u21BF","uharr;":"\u21BE","uhblk;":"\u2580","ulcorn;":"\u231C","ulcorner;":"\u231C","ulcrop;":"\u230F","ultri;":"\u25F8","Umacr;":"\u016A","umacr;":"\u016B","uml;":"\xA8",uml:"\xA8","UnderBar;":"_","UnderBrace;":"\u23DF","UnderBracket;":"\u23B5","UnderParenthesis;":"\u23DD","Union;":"\u22C3","UnionPlus;":"\u228E","Uogon;":"\u0172","uogon;":"\u0173","Uopf;":"\u{1D54C}","uopf;":"\u{1D566}","UpArrow;":"\u2191","Uparrow;":"\u21D1","uparrow;":"\u2191","UpArrowBar;":"\u2912","UpArrowDownArrow;":"\u21C5","UpDownArrow;":"\u2195","Updownarrow;":"\u21D5","updownarrow;":"\u2195","UpEquilibrium;":"\u296E","upharpoonleft;":"\u21BF","upharpoonright;":"\u21BE","uplus;":"\u228E","UpperLeftArrow;":"\u2196","UpperRightArrow;":"\u2197","Upsi;":"\u03D2","upsi;":"\u03C5","upsih;":"\u03D2","Upsilon;":"\u03A5","upsilon;":"\u03C5","UpTee;":"\u22A5","UpTeeArrow;":"\u21A5","upuparrows;":"\u21C8","urcorn;":"\u231D","urcorner;":"\u231D","urcrop;":"\u230E","Uring;":"\u016E","uring;":"\u016F","urtri;":"\u25F9","Uscr;":"\u{1D4B0}","uscr;":"\u{1D4CA}","utdot;":"\u22F0","Utilde;":"\u0168","utilde;":"\u0169","utri;":"\u25B5","utrif;":"\u25B4","uuarr;":"\u21C8","Uuml;":"\xDC",Uuml:"\xDC","uuml;":"\xFC",uuml:"\xFC","uwangle;":"\u29A7","vangrt;":"\u299C","varepsilon;":"\u03F5","varkappa;":"\u03F0","varnothing;":"\u2205","varphi;":"\u03D5","varpi;":"\u03D6","varpropto;":"\u221D","vArr;":"\u21D5","varr;":"\u2195","varrho;":"\u03F1","varsigma;":"\u03C2","varsubsetneq;":"\u228A\uFE00","varsubsetneqq;":"\u2ACB\uFE00","varsupsetneq;":"\u228B\uFE00","varsupsetneqq;":"\u2ACC\uFE00","vartheta;":"\u03D1","vartriangleleft;":"\u22B2","vartriangleright;":"\u22B3","Vbar;":"\u2AEB","vBar;":"\u2AE8","vBarv;":"\u2AE9","Vcy;":"\u0412","vcy;":"\u0432","VDash;":"\u22AB","Vdash;":"\u22A9","vDash;":"\u22A8","vdash;":"\u22A2","Vdashl;":"\u2AE6","Vee;":"\u22C1","vee;":"\u2228","veebar;":"\u22BB","veeeq;":"\u225A","vellip;":"\u22EE","Verbar;":"\u2016","verbar;":"|","Vert;":"\u2016","vert;":"|","VerticalBar;":"\u2223","VerticalLine;":"|","VerticalSeparator;":"\u2758","VerticalTilde;":"\u2240","VeryThinSpace;":"\u200A","Vfr;":"\u{1D519}","vfr;":"\u{1D533}","vltri;":"\u22B2","vnsub;":"\u2282\u20D2","vnsup;":"\u2283\u20D2","Vopf;":"\u{1D54D}","vopf;":"\u{1D567}","vprop;":"\u221D","vrtri;":"\u22B3","Vscr;":"\u{1D4B1}","vscr;":"\u{1D4CB}","vsubnE;":"\u2ACB\uFE00","vsubne;":"\u228A\uFE00","vsupnE;":"\u2ACC\uFE00","vsupne;":"\u228B\uFE00","Vvdash;":"\u22AA","vzigzag;":"\u299A","Wcirc;":"\u0174","wcirc;":"\u0175","wedbar;":"\u2A5F","Wedge;":"\u22C0","wedge;":"\u2227","wedgeq;":"\u2259","weierp;":"\u2118","Wfr;":"\u{1D51A}","wfr;":"\u{1D534}","Wopf;":"\u{1D54E}","wopf;":"\u{1D568}","wp;":"\u2118","wr;":"\u2240","wreath;":"\u2240","Wscr;":"\u{1D4B2}","wscr;":"\u{1D4CC}","xcap;":"\u22C2","xcirc;":"\u25EF","xcup;":"\u22C3","xdtri;":"\u25BD","Xfr;":"\u{1D51B}","xfr;":"\u{1D535}","xhArr;":"\u27FA","xharr;":"\u27F7","Xi;":"\u039E","xi;":"\u03BE","xlArr;":"\u27F8","xlarr;":"\u27F5","xmap;":"\u27FC","xnis;":"\u22FB","xodot;":"\u2A00","Xopf;":"\u{1D54F}","xopf;":"\u{1D569}","xoplus;":"\u2A01","xotime;":"\u2A02","xrArr;":"\u27F9","xrarr;":"\u27F6","Xscr;":"\u{1D4B3}","xscr;":"\u{1D4CD}","xsqcup;":"\u2A06","xuplus;":"\u2A04","xutri;":"\u25B3","xvee;":"\u22C1","xwedge;":"\u22C0","Yacute;":"\xDD",Yacute:"\xDD","yacute;":"\xFD",yacute:"\xFD","YAcy;":"\u042F","yacy;":"\u044F","Ycirc;":"\u0176","ycirc;":"\u0177","Ycy;":"\u042B","ycy;":"\u044B","yen;":"\xA5",yen:"\xA5","Yfr;":"\u{1D51C}","yfr;":"\u{1D536}","YIcy;":"\u0407","yicy;":"\u0457","Yopf;":"\u{1D550}","yopf;":"\u{1D56A}","Yscr;":"\u{1D4B4}","yscr;":"\u{1D4CE}","YUcy;":"\u042E","yucy;":"\u044E","Yuml;":"\u0178","yuml;":"\xFF",yuml:"\xFF","Zacute;":"\u0179","zacute;":"\u017A","Zcaron;":"\u017D","zcaron;":"\u017E","Zcy;":"\u0417","zcy;":"\u0437","Zdot;":"\u017B","zdot;":"\u017C","zeetrf;":"\u2128","ZeroWidthSpace;":"\u200B","Zeta;":"\u0396","zeta;":"\u03B6","Zfr;":"\u2128","zfr;":"\u{1D537}","ZHcy;":"\u0416","zhcy;":"\u0436","zigrarr;":"\u21DD","Zopf;":"\u2124","zopf;":"\u{1D56B}","Zscr;":"\u{1D4B5}","zscr;":"\u{1D4CF}","zwj;":"\u200D","zwnj;":"\u200C"};function ae(t,i){if(t.length0?t.lastIndexOf(i)===o:o===0?t===i:!1}function pt(t,i){for(var o="";i>0;)(i&1)===1&&(o+=t),t+=t,i=i>>>1;return o}var Xn="a".charCodeAt(0),Yn="z".charCodeAt(0),$n="A".charCodeAt(0),Qn="Z".charCodeAt(0),Zn="0".charCodeAt(0),Kn="9".charCodeAt(0);function ge(t,i){var o=t.charCodeAt(i);return Xn<=o&&o<=Yn||$n<=o&&o<=Qn||Zn<=o&&o<=Kn}function Ae(t){return typeof t<"u"}function Vt(t){if(!!t)return typeof t=="string"?{kind:"markdown",value:t}:{kind:"markdown",value:t.value}}var Ge=function(){function t(i,o){var n=this;this.id=i,this._tags=[],this._tagMap={},this._valueSetMap={},this._tags=o.tags||[],this._globalAttributes=o.globalAttributes||[],this._tags.forEach(function(e){n._tagMap[e.name.toLowerCase()]=e}),o.valueSets&&o.valueSets.forEach(function(e){n._valueSetMap[e.name]=e.values})}return t.prototype.isApplicable=function(){return!0},t.prototype.getId=function(){return this.id},t.prototype.provideTags=function(){return this._tags},t.prototype.provideAttributes=function(i){var o=[],n=function(a){o.push(a)},e=this._tagMap[i.toLowerCase()];return e&&e.attributes.forEach(n),this._globalAttributes.forEach(n),o},t.prototype.provideValues=function(i,o){var n=this,e=[];o=o.toLowerCase();var a=function(l){l.forEach(function(r){r.name.toLowerCase()===o&&(r.values&&r.values.forEach(function(s){e.push(s)}),r.valueSet&&n._valueSetMap[r.valueSet]&&n._valueSetMap[r.valueSet].forEach(function(s){e.push(s)}))})},c=this._tagMap[i.toLowerCase()];return c&&a(c.attributes),a(this._globalAttributes),e},t}();function le(t,i,o){i===void 0&&(i={});var n={kind:o?"markdown":"plaintext",value:""};if(t.description&&i.documentation!==!1){var e=Vt(t.description);e&&(n.value+=e.value)}if(t.references&&t.references.length>0&&i.references!==!1&&(n.value.length&&(n.value+=`
`),o?n.value+=t.references.map(function(a){return"[".concat(a.name,"](").concat(a.url,")")}).join(" | "):n.value+=t.references.map(function(a){return"".concat(a.name,": ").concat(a.url)}).join(`
`)),n.value!=="")return n}var Jt=function(t,i,o,n){function e(a){return a instanceof o?a:new o(function(c){c(a)})}return new(o||(o=Promise))(function(a,c){function l(u){try{s(n.next(u))}catch(h){c(h)}}function r(u){try{s(n.throw(u))}catch(h){c(h)}}function s(u){u.done?a(u.value):e(u.value).then(l,r)}s((n=n.apply(t,i||[])).next())})},Xt=function(t,i){var o={label:0,sent:function(){if(a[0]&1)throw a[1];return a[1]},trys:[],ops:[]},n,e,a,c;return c={next:l(0),throw:l(1),return:l(2)},typeof Symbol=="function"&&(c[Symbol.iterator]=function(){return this}),c;function l(s){return function(u){return r([s,u])}}function r(s){if(n)throw new TypeError("Generator is already executing.");for(;o;)try{if(n=1,e&&(a=s[0]&2?e.return:s[0]?e.throw||((a=e.return)&&a.call(e),0):e.next)&&!(a=a.call(e,s[1])).done)return a;switch(e=0,a&&(s=[s[0]&2,a.value]),s[0]){case 0:case 1:a=s;break;case 4:return o.label++,{value:s[1],done:!1};case 5:o.label++,e=s[1],s=[0];continue;case 7:s=o.ops.pop(),o.trys.pop();continue;default:if(a=o.trys,!(a=a.length>0&&a[a.length-1])&&(s[0]===6||s[0]===2)){o=0;continue}if(s[0]===3&&(!a||s[1]>a[0]&&s[1]0&&a[a.length-1])&&(s[0]===6||s[0]===2)){o=0;continue}if(s[0]===3&&(!a||s[1]>a[0]&&s[1]u&&(x=u),{start:i.positionAt(x),end:i.positionAt(D)}}function A(x,D){var L=m(x,D);return l.forEach(function(q){q.provideTags().forEach(function(j){a.items.push({label:j.name,kind:Q.Property,documentation:le(j,void 0,r),textEdit:Y.replace(L,j.name),insertTextFormat:ne.PlainText})})}),a}function E(x){for(var D=x;D>0;){var L=s.charAt(D-1);if(`
\r`.indexOf(L)>=0)return s.substring(D,x);if(!Ve(L))return null;D--}return s.substring(0,x)}function w(x,D,L){L===void 0&&(L=u);var q=m(x,L),j=$t(s,L,W.WithinEndTag,S.EndTagClose)?"":">",O=h;for(D&&(O=O.parent);O;){var V=O.tag;if(V&&(!O.closed||O.endTagStart&&O.endTagStart>u)){var K={label:"/"+V,kind:Q.Property,filterText:"/"+V,textEdit:Y.replace(q,"/"+V+j),insertTextFormat:ne.PlainText},oe=E(O.start),ue=E(x-1);if(oe!==null&&ue!==null&&oe!==ue){var te=oe+""+V+j;K.textEdit=Y.replace(m(x-1-ue.length),te),K.filterText=ue+""+V}return a.items.push(K),a}O=O.parent}return D||l.forEach(function(de){de.provideTags().forEach(function(re){a.items.push({label:"/"+re.name,kind:Q.Property,documentation:le(re,void 0,r),filterText:"/"+re.name+j,textEdit:Y.replace(q,"/"+re.name+j),insertTextFormat:ne.PlainText})})}),a}function M(x,D){if(e&&e.hideAutoCompleteProposals)return a;if(!me(D)){var L=i.positionAt(x);a.items.push({label:""+D+">",kind:Q.Property,filterText:""+D+">",textEdit:Y.insert(L,"$0"+D+">"),insertTextFormat:ne.Snippet})}return a}function B(x,D){return A(x,D),w(x,!0,D),a}function G(){var x=Object.create(null);return h.attributeNames.forEach(function(D){x[D]=!0}),x}function J(x,D){var L;D===void 0&&(D=u);for(var q=u;qx&&u<=D&&di(s[x])){var O=x+1,V=D;D>x&&s[D-1]===s[x]&&V--;var K=pi(s,u,O),oe=mi(s,u,V);L=m(K,oe),j=u>=O&&u<=V?s.substring(O,u):"",q=!1}else L=m(x,D),j=s.substring(x,u),q=!0;if(c.length>0)for(var ue=g.toLowerCase(),te=y.toLowerCase(),de=m(x,D),re=0,vt=c;re=0&&ge(s,x);)x--,D--;if(x>=0&&s[x]==="&"){var L=P.create(X.create(o.line,D-1),o);for(var q in fe)if(Gt(q,";")){var j="&"+q;a.items.push({label:j,kind:Q.Keyword,documentation:hi("entity.propose","Character entity representing '".concat(fe[q],"'")),textEdit:Y.replace(L,j),insertTextFormat:ne.PlainText})}}return a}function U(x,D){var L=m(x,D);a.items.push({label:"!DOCTYPE",kind:Q.Property,documentation:"A preamble for an HTML document.",textEdit:Y.replace(L,"!DOCTYPE html>"),insertTextFormat:ne.PlainText})}for(var H=d.scan();H!==S.EOS&&d.getTokenOffset()<=u;){switch(H){case S.StartTagOpen:if(d.getTokenEnd()===u){var z=b(S.StartTag);return o.line===0&&U(u,z),B(u,z)}break;case S.StartTag:if(d.getTokenOffset()<=u&&u<=d.getTokenEnd())return A(d.getTokenOffset(),d.getTokenEnd());g=d.getTokenText();break;case S.AttributeName:if(d.getTokenOffset()<=u&&u<=d.getTokenEnd())return J(d.getTokenOffset(),d.getTokenEnd());y=d.getTokenText();break;case S.DelimiterAssign:if(d.getTokenEnd()===u){var z=b(S.AttributeValue);return p(u,z)}break;case S.AttributeValue:if(d.getTokenOffset()<=u&&u<=d.getTokenEnd())return p(d.getTokenOffset(),d.getTokenEnd());break;case S.Whitespace:if(u<=d.getTokenEnd())switch(d.getScannerState()){case W.AfterOpeningStartTag:var I=d.getTokenOffset(),F=b(S.StartTag);return B(I,F);case W.WithinTag:case W.AfterAttributeName:return J(d.getTokenEnd());case W.BeforeAttributeValue:return p(d.getTokenEnd());case W.AfterOpeningEndTag:return w(d.getTokenOffset()-1,!1);case W.WithinContent:return N()}break;case S.EndTagOpen:if(u<=d.getTokenEnd()){var T=d.getTokenOffset()+1,v=b(S.EndTag);return w(T,!1,v)}break;case S.EndTag:if(u<=d.getTokenEnd())for(var k=d.getTokenOffset()-1;k>=0;){var C=s.charAt(k);if(C==="/")return w(k,!1,d.getTokenEnd());if(!Ve(C))break;k--}break;case S.StartTagClose:if(u<=d.getTokenEnd()&&g)return M(d.getTokenEnd(),g);break;case S.Content:if(u<=d.getTokenEnd())return N();break;default:if(u<=d.getTokenEnd())return a;break}H=d.scan()}return a},t.prototype.doQuoteComplete=function(i,o,n,e){var a,c=i.offsetAt(o);if(c<=0)return null;var l=(a=e?.attributeDefaultValue)!==null&&a!==void 0?a:"doublequotes";if(l==="empty")return null;var r=i.getText().charAt(c-1);if(r!=="=")return null;var s=l==="doublequotes"?'"$1"':"'$1'",u=n.findNodeBefore(c);if(u&&u.attributes&&u.startc))for(var h=$(i.getText(),u.start),d=h.scan();d!==S.EOS&&h.getTokenEnd()<=c;){if(d===S.AttributeName&&h.getTokenEnd()===c-1)return d=h.scan(),d!==S.DelimiterAssign||(d=h.scan(),d===S.Unknown||d===S.AttributeValue)?null:s;d=h.scan()}return null},t.prototype.doTagComplete=function(i,o,n){var e=i.offsetAt(o);if(e<=0)return null;var a=i.getText().charAt(e-1);if(a===">"){var c=n.findNodeBefore(e);if(c&&c.tag&&!me(c.tag)&&c.starte))for(var l=$(i.getText(),c.start),r=l.scan();r!==S.EOS&&l.getTokenEnd()<=e;){if(r===S.StartTagClose&&l.getTokenEnd()===e)return"$0".concat(c.tag,">");r=l.scan()}}else if(a==="/"){for(var c=n.findNodeBefore(e);c&&c.closed&&!(c.endTagStart&&c.endTagStart>e);)c=c.parent;if(c&&c.tag)for(var l=$(i.getText(),c.start),r=l.scan();r!==S.EOS&&l.getTokenEnd()<=e;){if(r===S.EndTagOpen&&l.getTokenEnd()===e)return"".concat(c.tag,">");r=l.scan()}}return null},t.prototype.convertCompletionList=function(i){return this.doesSupportMarkdown()||i.items.forEach(function(o){o.documentation&&typeof o.documentation!="string"&&(o.documentation={kind:"plaintext",value:o.documentation.value})}),i},t.prototype.doesSupportMarkdown=function(){var i,o,n;if(!Ae(this.supportsMarkdown)){if(!Ae(this.lsOptions.clientCapabilities))return this.supportsMarkdown=!0,this.supportsMarkdown;var e=(n=(o=(i=this.lsOptions.clientCapabilities.textDocument)===null||i===void 0?void 0:i.completion)===null||o===void 0?void 0:o.completionItem)===null||n===void 0?void 0:n.documentationFormat;this.supportsMarkdown=Array.isArray(e)&&e.indexOf(ee.Markdown)!==-1}return this.supportsMarkdown},t}();function di(t){return/^["']*$/.test(t)}function Ve(t){return/^\s*$/.test(t)}function $t(t,i,o,n){for(var e=$(t,i,o),a=e.scan();a===S.Whitespace;)a=e.scan();return a===n}function pi(t,i,o){for(;i>o&&!Ve(t[i-1]);)i--;return i}function mi(t,i,o){for(;i=0&&ge(s,U);)U--,H--;for(var z=U+1,I=H;ge(s,z);)z++,I++;if(U>=0&&s[U]==="&"){var F=null;return s[z]===";"?F=P.create(X.create(o.line,H),X.create(o.line,I+1)):F=P.create(X.create(o.line,H),X.create(o.line,I)),F}return null}function E(U){for(var H=l-1,z="&";H>=0&&ge(U,H);)H--;for(H=H+1;ge(U,H);)z+=U[H],H+=1;return z+=";",z}if(r.endTagStart&&l>=r.endTagStart){var w=m(S.EndTag,r.endTagStart);return w?h(r.tag,w,!1):null}var M=m(S.StartTag,r.start);if(M)return h(r.tag,M,!0);var B=m(S.AttributeName,r.start);if(B){var G=r.tag,J=i.getText(B);return d(G,J,B)}var f=A();if(f)return y(s,f);function p(U,H){for(var z=$(i.getText(),U),I=z.scan(),F=void 0;I!==S.EOS&&z.getTokenEnd()<=H;)I=z.scan(),I===S.AttributeName&&(F=z.getTokenText());return F}var b=m(S.AttributeValue,r.start);if(b){var G=r.tag,N=gi(i.getText(b)),R=p(r.start,i.offsetAt(b.start));if(R)return g(G,R,N,b)}return null},t.prototype.convertContents=function(i){if(!this.doesSupportMarkdown()){if(typeof i=="string")return i;if("kind"in i)return{kind:"plaintext",value:i.value};if(Array.isArray(i))i.map(function(o){return typeof o=="string"?o:o.value});else return i.value}return i},t.prototype.doesSupportMarkdown=function(){var i,o,n;if(!Ae(this.supportsMarkdown)){if(!Ae(this.lsOptions.clientCapabilities))return this.supportsMarkdown=!0,this.supportsMarkdown;var e=(n=(o=(i=this.lsOptions.clientCapabilities)===null||i===void 0?void 0:i.textDocument)===null||o===void 0?void 0:o.hover)===null||n===void 0?void 0:n.contentFormat;this.supportsMarkdown=Array.isArray(e)&&e.indexOf(ee.Markdown)!==-1}return this.supportsMarkdown},t}();function gi(t){return t.length<=1?t.replace(/['"]/,""):((t[0]==="'"||t[0]==='"')&&(t=t.slice(1)),(t[t.length-1]==="'"||t[t.length-1]==='"')&&(t=t.slice(0,-1)),t)}function Kt(t,i){return t}var en;(function(){"use strict";var t=[,,function(e){function a(r){this.__parent=r,this.__character_count=0,this.__indent_count=-1,this.__alignment_count=0,this.__wrap_point_index=0,this.__wrap_point_character_count=0,this.__wrap_point_indent_count=-1,this.__wrap_point_alignment_count=0,this.__items=[]}a.prototype.clone_empty=function(){var r=new a(this.__parent);return r.set_indent(this.__indent_count,this.__alignment_count),r},a.prototype.item=function(r){return r<0?this.__items[this.__items.length+r]:this.__items[r]},a.prototype.has_match=function(r){for(var s=this.__items.length-1;s>=0;s--)if(this.__items[s].match(r))return!0;return!1},a.prototype.set_indent=function(r,s){this.is_empty()&&(this.__indent_count=r||0,this.__alignment_count=s||0,this.__character_count=this.__parent.get_indent_size(this.__indent_count,this.__alignment_count))},a.prototype._set_wrap_point=function(){this.__parent.wrap_line_length&&(this.__wrap_point_index=this.__items.length,this.__wrap_point_character_count=this.__character_count,this.__wrap_point_indent_count=this.__parent.next_line.__indent_count,this.__wrap_point_alignment_count=this.__parent.next_line.__alignment_count)},a.prototype._should_wrap=function(){return this.__wrap_point_index&&this.__character_count>this.__parent.wrap_line_length&&this.__wrap_point_character_count>this.__parent.next_line.__character_count},a.prototype._allow_wrap=function(){if(this._should_wrap()){this.__parent.add_new_line();var r=this.__parent.current_line;return r.set_indent(this.__wrap_point_indent_count,this.__wrap_point_alignment_count),r.__items=this.__items.slice(this.__wrap_point_index),this.__items=this.__items.slice(0,this.__wrap_point_index),r.__character_count+=this.__character_count-this.__wrap_point_character_count,this.__character_count=this.__wrap_point_character_count,r.__items[0]===" "&&(r.__items.splice(0,1),r.__character_count-=1),!0}return!1},a.prototype.is_empty=function(){return this.__items.length===0},a.prototype.last=function(){return this.is_empty()?null:this.__items[this.__items.length-1]},a.prototype.push=function(r){this.__items.push(r);var s=r.lastIndexOf(`
`);s!==-1?this.__character_count=r.length-s:this.__character_count+=r.length},a.prototype.pop=function(){var r=null;return this.is_empty()||(r=this.__items.pop(),this.__character_count-=r.length),r},a.prototype._remove_indent=function(){this.__indent_count>0&&(this.__indent_count-=1,this.__character_count-=this.__parent.indent_size)},a.prototype._remove_wrap_indent=function(){this.__wrap_point_indent_count>0&&(this.__wrap_point_indent_count-=1)},a.prototype.trim=function(){for(;this.last()===" ";)this.__items.pop(),this.__character_count-=1},a.prototype.toString=function(){var r="";return this.is_empty()?this.__parent.indent_empty_lines&&(r=this.__parent.get_indent_string(this.__indent_count)):(r=this.__parent.get_indent_string(this.__indent_count,this.__alignment_count),r+=this.__items.join("")),r};function c(r,s){this.__cache=[""],this.__indent_size=r.indent_size,this.__indent_string=r.indent_char,r.indent_with_tabs||(this.__indent_string=new Array(r.indent_size+1).join(r.indent_char)),s=s||"",r.indent_level>0&&(s=new Array(r.indent_level+1).join(this.__indent_string)),this.__base_string=s,this.__base_string_length=s.length}c.prototype.get_indent_size=function(r,s){var u=this.__base_string_length;return s=s||0,r<0&&(u=0),u+=r*this.__indent_size,u+=s,u},c.prototype.get_indent_string=function(r,s){var u=this.__base_string;return s=s||0,r<0&&(r=0,u=""),s+=r*this.__indent_size,this.__ensure_cache(s),u+=this.__cache[s],u},c.prototype.__ensure_cache=function(r){for(;r>=this.__cache.length;)this.__add_column()},c.prototype.__add_column=function(){var r=this.__cache.length,s=0,u="";this.__indent_size&&r>=this.__indent_size&&(s=Math.floor(r/this.__indent_size),r-=s*this.__indent_size,u=new Array(s+1).join(this.__indent_string)),r&&(u+=new Array(r+1).join(" ")),this.__cache.push(u)};function l(r,s){this.__indent_cache=new c(r,s),this.raw=!1,this._end_with_newline=r.end_with_newline,this.indent_size=r.indent_size,this.wrap_line_length=r.wrap_line_length,this.indent_empty_lines=r.indent_empty_lines,this.__lines=[],this.previous_line=null,this.current_line=null,this.next_line=new a(this),this.space_before_token=!1,this.non_breaking_space=!1,this.previous_token_wrapped=!1,this.__add_outputline()}l.prototype.__add_outputline=function(){this.previous_line=this.current_line,this.current_line=this.next_line.clone_empty(),this.__lines.push(this.current_line)},l.prototype.get_line_number=function(){return this.__lines.length},l.prototype.get_indent_string=function(r,s){return this.__indent_cache.get_indent_string(r,s)},l.prototype.get_indent_size=function(r,s){return this.__indent_cache.get_indent_size(r,s)},l.prototype.is_empty=function(){return!this.previous_line&&this.current_line.is_empty()},l.prototype.add_new_line=function(r){return this.is_empty()||!r&&this.just_added_newline()?!1:(this.raw||this.__add_outputline(),!0)},l.prototype.get_code=function(r){this.trim(!0);var s=this.current_line.pop();s&&(s[s.length-1]===`
`&&(s=s.replace(/\n+$/g,"")),this.current_line.push(s)),this._end_with_newline&&this.__add_outputline();var u=this.__lines.join(`
`);return r!==`
`&&(u=u.replace(/[\n]/g,r)),u},l.prototype.set_wrap_point=function(){this.current_line._set_wrap_point()},l.prototype.set_indent=function(r,s){return r=r||0,s=s||0,this.next_line.set_indent(r,s),this.__lines.length>1?(this.current_line.set_indent(r,s),!0):(this.current_line.set_indent(),!1)},l.prototype.add_raw_token=function(r){for(var s=0;s1&&this.current_line.is_empty();)this.__lines.pop(),this.current_line=this.__lines[this.__lines.length-1],this.current_line.trim();this.previous_line=this.__lines.length>1?this.__lines[this.__lines.length-2]:null},l.prototype.just_added_newline=function(){return this.current_line.is_empty()},l.prototype.just_added_blankline=function(){return this.is_empty()||this.current_line.is_empty()&&this.previous_line.is_empty()},l.prototype.ensure_empty_line_above=function(r,s){for(var u=this.__lines.length-2;u>=0;){var h=this.__lines[u];if(h.is_empty())break;if(h.item(0).indexOf(r)!==0&&h.item(-1)!==s){this.__lines.splice(u+1,0,new a(this)),this.previous_line=this.__lines[this.__lines.length-2];break}u--}},e.exports.Output=l},,,,function(e){function a(r,s){this.raw_options=c(r,s),this.disabled=this._get_boolean("disabled"),this.eol=this._get_characters("eol","auto"),this.end_with_newline=this._get_boolean("end_with_newline"),this.indent_size=this._get_number("indent_size",4),this.indent_char=this._get_characters("indent_char"," "),this.indent_level=this._get_number("indent_level"),this.preserve_newlines=this._get_boolean("preserve_newlines",!0),this.max_preserve_newlines=this._get_number("max_preserve_newlines",32786),this.preserve_newlines||(this.max_preserve_newlines=0),this.indent_with_tabs=this._get_boolean("indent_with_tabs",this.indent_char===" "),this.indent_with_tabs&&(this.indent_char=" ",this.indent_size===1&&(this.indent_size=4)),this.wrap_line_length=this._get_number("wrap_line_length",this._get_number("max_char")),this.indent_empty_lines=this._get_boolean("indent_empty_lines"),this.templating=this._get_selection_list("templating",["auto","none","django","erb","handlebars","php","smarty"],["auto"])}a.prototype._get_array=function(r,s){var u=this.raw_options[r],h=s||[];return typeof u=="object"?u!==null&&typeof u.concat=="function"&&(h=u.concat()):typeof u=="string"&&(h=u.split(/[^a-zA-Z0-9_\/\-]+/)),h},a.prototype._get_boolean=function(r,s){var u=this.raw_options[r],h=u===void 0?!!s:!!u;return h},a.prototype._get_characters=function(r,s){var u=this.raw_options[r],h=s||"";return typeof u=="string"&&(h=u.replace(/\\r/,"\r").replace(/\\n/,`
`).replace(/\\t/," ")),h},a.prototype._get_number=function(r,s){var u=this.raw_options[r];s=parseInt(s,10),isNaN(s)&&(s=0);var h=parseInt(u,10);return isNaN(h)&&(h=s),h},a.prototype._get_selection=function(r,s,u){var h=this._get_selection_list(r,s,u);if(h.length!==1)throw new Error("Invalid Option Value: The option '"+r+`' can only be one of the following values:
`+s+`
You passed in: '`+this.raw_options[r]+"'");return h[0]},a.prototype._get_selection_list=function(r,s,u){if(!s||s.length===0)throw new Error("Selection list cannot be empty.");if(u=u||[s[0]],!this._is_valid_selection(u,s))throw new Error("Invalid Default Value!");var h=this._get_array(r,u);if(!this._is_valid_selection(h,s))throw new Error("Invalid Option Value: The option '"+r+`' can contain only the following values:
`+s+`
You passed in: '`+this.raw_options[r]+"'");return h},a.prototype._is_valid_selection=function(r,s){return r.length&&s.length&&!r.some(function(u){return s.indexOf(u)===-1})};function c(r,s){var u={};r=l(r);var h;for(h in r)h!==s&&(u[h]=r[h]);if(s&&r[s])for(h in r[s])u[h]=r[s][h];return u}function l(r){var s={},u;for(u in r){var h=u.replace(/-/g,"_");s[h]=r[u]}return s}e.exports.Options=a,e.exports.normalizeOpts=l,e.exports.mergeOpts=c},,function(e){var a=RegExp.prototype.hasOwnProperty("sticky");function c(l){this.__input=l||"",this.__input_length=this.__input.length,this.__position=0}c.prototype.restart=function(){this.__position=0},c.prototype.back=function(){this.__position>0&&(this.__position-=1)},c.prototype.hasNext=function(){return this.__position=0&&l=0&&r=l.length&&this.__input.substring(r-l.length,r).toLowerCase()===l},e.exports.InputScanner=c},,,,,function(e){function a(c,l){c=typeof c=="string"?c:c.source,l=typeof l=="string"?l:l.source,this.__directives_block_pattern=new RegExp(c+/ beautify( \w+[:]\w+)+ /.source+l,"g"),this.__directive_pattern=/ (\w+)[:](\w+)/g,this.__directives_end_ignore_pattern=new RegExp(c+/\sbeautify\signore:end\s/.source+l,"g")}a.prototype.get_directives=function(c){if(!c.match(this.__directives_block_pattern))return null;var l={};this.__directive_pattern.lastIndex=0;for(var r=this.__directive_pattern.exec(c);r;)l[r[1]]=r[2],r=this.__directive_pattern.exec(c);return l},a.prototype.readIgnored=function(c){return c.readUntilAfter(this.__directives_end_ignore_pattern)},e.exports.Directives=a},,function(e,a,c){var l=c(16).Beautifier,r=c(17).Options;function s(u,h){var d=new l(u,h);return d.beautify()}e.exports=s,e.exports.defaultOptions=function(){return new r}},function(e,a,c){var l=c(17).Options,r=c(2).Output,s=c(8).InputScanner,u=c(13).Directives,h=new u(/\/\*/,/\*\//),d=/\r\n|[\r\n]/,g=/\r\n|[\r\n]/g,y=/\s/,m=/(?:\s|\n)+/g,A=/\/\*(?:[\s\S]*?)((?:\*\/)|$)/g,E=/\/\/(?:[^\n\r\u2028\u2029]*)/g;function w(M,B){this._source_text=M||"",this._options=new l(B),this._ch=null,this._input=null,this.NESTED_AT_RULE={"@page":!0,"@font-face":!0,"@keyframes":!0,"@media":!0,"@supports":!0,"@document":!0},this.CONDITIONAL_GROUP_RULE={"@media":!0,"@supports":!0,"@document":!0}}w.prototype.eatString=function(M){var B="";for(this._ch=this._input.next();this._ch;){if(B+=this._ch,this._ch==="\\")B+=this._input.next();else if(M.indexOf(this._ch)!==-1||this._ch===`
`)break;this._ch=this._input.next()}return B},w.prototype.eatWhitespace=function(M){for(var B=y.test(this._input.peek()),G=0;y.test(this._input.peek());)this._ch=this._input.next(),M&&this._ch===`
`&&(G===0||G0&&this._indentLevel--},w.prototype.beautify=function(){if(this._options.disabled)return this._source_text;var M=this._source_text,B=this._options.eol;B==="auto"&&(B=`
`,M&&d.test(M||"")&&(B=M.match(d)[0])),M=M.replace(g,`
`);var G=M.match(/^[\t ]*/)[0];this._output=new r(this._options,G),this._input=new s(M),this._indentLevel=0,this._nestedLevel=0,this._ch=null;for(var J=0,f=!1,p=!1,b=!1,N=!1,R=!1,U=this._ch,H,z,I;H=this._input.read(m),z=H!=="",I=U,this._ch=this._input.next(),this._ch==="\\"&&this._input.hasNext()&&(this._ch+=this._input.next()),U=this._ch,this._ch;)if(this._ch==="/"&&this._input.peek()==="*"){this._output.add_new_line(),this._input.back();var F=this._input.read(A),T=h.get_directives(F);T&&T.ignore==="start"&&(F+=h.readIgnored(this._input)),this.print_string(F),this.eatWhitespace(!0),this._output.add_new_line()}else if(this._ch==="/"&&this._input.peek()==="/")this._output.space_before_token=!0,this._input.back(),this.print_string(this._input.read(E)),this.eatWhitespace(!0);else if(this._ch==="@")if(this.preserveSingleSpace(z),this._input.peek()==="{")this.print_string(this._ch+this.eatString("}"));else{this.print_string(this._ch);var v=this._input.peekUntilAfter(/[: ,;{}()[\]\/='"]/g);v.match(/[ :]$/)&&(v=this.eatString(": ").replace(/\s$/,""),this.print_string(v),this._output.space_before_token=!0),v=v.replace(/\s$/,""),v==="extend"?N=!0:v==="import"&&(R=!0),v in this.NESTED_AT_RULE?(this._nestedLevel+=1,v in this.CONDITIONAL_GROUP_RULE&&(b=!0)):!f&&J===0&&v.indexOf(":")!==-1&&(p=!0,this.indent())}else this._ch==="#"&&this._input.peek()==="{"?(this.preserveSingleSpace(z),this.print_string(this._ch+this.eatString("}"))):this._ch==="{"?(p&&(p=!1,this.outdent()),b?(b=!1,f=this._indentLevel>=this._nestedLevel):f=this._indentLevel>=this._nestedLevel-1,this._options.newline_between_rules&&f&&this._output.previous_line&&this._output.previous_line.item(-1)!=="{"&&this._output.ensure_empty_line_above("/",","),this._output.space_before_token=!0,this._options.brace_style==="expand"?(this._output.add_new_line(),this.print_string(this._ch),this.indent(),this._output.set_indent(this._indentLevel)):(this.indent(),this.print_string(this._ch)),this.eatWhitespace(!0),this._output.add_new_line()):this._ch==="}"?(this.outdent(),this._output.add_new_line(),I==="{"&&this._output.trim(!0),R=!1,N=!1,p&&(this.outdent(),p=!1),this.print_string(this._ch),f=!1,this._nestedLevel&&this._nestedLevel--,this.eatWhitespace(!0),this._output.add_new_line(),this._options.newline_between_rules&&!this._output.just_added_blankline()&&this._input.peek()!=="}"&&this._output.add_new_line(!0)):this._ch===":"?(f||b)&&!(this._input.lookBack("&")||this.foundNestedPseudoClass())&&!this._input.lookBack("(")&&!N&&J===0?(this.print_string(":"),p||(p=!0,this._output.space_before_token=!0,this.eatWhitespace(!0),this.indent())):(this._input.lookBack(" ")&&(this._output.space_before_token=!0),this._input.peek()===":"?(this._ch=this._input.next(),this.print_string("::")):this.print_string(":")):this._ch==='"'||this._ch==="'"?(this.preserveSingleSpace(z),this.print_string(this._ch+this.eatString(this._ch)),this.eatWhitespace(!0)):this._ch===";"?J===0?(p&&(this.outdent(),p=!1),N=!1,R=!1,this.print_string(this._ch),this.eatWhitespace(!0),this._input.peek()!=="/"&&this._output.add_new_line()):(this.print_string(this._ch),this.eatWhitespace(!0),this._output.space_before_token=!0):this._ch==="("?this._input.lookBack("url")?(this.print_string(this._ch),this.eatWhitespace(),J++,this.indent(),this._ch=this._input.next(),this._ch===")"||this._ch==='"'||this._ch==="'"?this._input.back():this._ch&&(this.print_string(this._ch+this.eatString(")")),J&&(J--,this.outdent()))):(this.preserveSingleSpace(z),this.print_string(this._ch),this.eatWhitespace(),J++,this.indent()):this._ch===")"?(J&&(J--,this.outdent()),this.print_string(this._ch)):this._ch===","?(this.print_string(this._ch),this.eatWhitespace(!0),this._options.selector_separator_newline&&!p&&J===0&&!R&&!N?this._output.add_new_line():this._output.space_before_token=!0):(this._ch===">"||this._ch==="+"||this._ch==="~")&&!p&&J===0?this._options.space_around_combinator?(this._output.space_before_token=!0,this.print_string(this._ch),this._output.space_before_token=!0):(this.print_string(this._ch),this.eatWhitespace(),this._ch&&y.test(this._ch)&&(this._ch="")):this._ch==="]"?this.print_string(this._ch):this._ch==="["?(this.preserveSingleSpace(z),this.print_string(this._ch)):this._ch==="="?(this.eatWhitespace(),this.print_string("="),y.test(this._ch)&&(this._ch="")):this._ch==="!"&&!this._input.lookBack("\\")?(this.print_string(" "),this.print_string(this._ch)):(this.preserveSingleSpace(z),this.print_string(this._ch));var k=this._output.get_code(B);return k},e.exports.Beautifier=w},function(e,a,c){var l=c(6).Options;function r(s){l.call(this,s,"css"),this.selector_separator_newline=this._get_boolean("selector_separator_newline",!0),this.newline_between_rules=this._get_boolean("newline_between_rules",!0);var u=this._get_boolean("space_around_selector_separator");this.space_around_combinator=this._get_boolean("space_around_combinator")||u;var h=this._get_selection_list("brace_style",["collapse","expand","end-expand","none","preserve-inline"]);this.brace_style="collapse";for(var d=0;d=0;s--)if(this.__items[s].match(r))return!0;return!1},a.prototype.set_indent=function(r,s){this.is_empty()&&(this.__indent_count=r||0,this.__alignment_count=s||0,this.__character_count=this.__parent.get_indent_size(this.__indent_count,this.__alignment_count))},a.prototype._set_wrap_point=function(){this.__parent.wrap_line_length&&(this.__wrap_point_index=this.__items.length,this.__wrap_point_character_count=this.__character_count,this.__wrap_point_indent_count=this.__parent.next_line.__indent_count,this.__wrap_point_alignment_count=this.__parent.next_line.__alignment_count)},a.prototype._should_wrap=function(){return this.__wrap_point_index&&this.__character_count>this.__parent.wrap_line_length&&this.__wrap_point_character_count>this.__parent.next_line.__character_count},a.prototype._allow_wrap=function(){if(this._should_wrap()){this.__parent.add_new_line();var r=this.__parent.current_line;return r.set_indent(this.__wrap_point_indent_count,this.__wrap_point_alignment_count),r.__items=this.__items.slice(this.__wrap_point_index),this.__items=this.__items.slice(0,this.__wrap_point_index),r.__character_count+=this.__character_count-this.__wrap_point_character_count,this.__character_count=this.__wrap_point_character_count,r.__items[0]===" "&&(r.__items.splice(0,1),r.__character_count-=1),!0}return!1},a.prototype.is_empty=function(){return this.__items.length===0},a.prototype.last=function(){return this.is_empty()?null:this.__items[this.__items.length-1]},a.prototype.push=function(r){this.__items.push(r);var s=r.lastIndexOf(`
`);s!==-1?this.__character_count=r.length-s:this.__character_count+=r.length},a.prototype.pop=function(){var r=null;return this.is_empty()||(r=this.__items.pop(),this.__character_count-=r.length),r},a.prototype._remove_indent=function(){this.__indent_count>0&&(this.__indent_count-=1,this.__character_count-=this.__parent.indent_size)},a.prototype._remove_wrap_indent=function(){this.__wrap_point_indent_count>0&&(this.__wrap_point_indent_count-=1)},a.prototype.trim=function(){for(;this.last()===" ";)this.__items.pop(),this.__character_count-=1},a.prototype.toString=function(){var r="";return this.is_empty()?this.__parent.indent_empty_lines&&(r=this.__parent.get_indent_string(this.__indent_count)):(r=this.__parent.get_indent_string(this.__indent_count,this.__alignment_count),r+=this.__items.join("")),r};function c(r,s){this.__cache=[""],this.__indent_size=r.indent_size,this.__indent_string=r.indent_char,r.indent_with_tabs||(this.__indent_string=new Array(r.indent_size+1).join(r.indent_char)),s=s||"",r.indent_level>0&&(s=new Array(r.indent_level+1).join(this.__indent_string)),this.__base_string=s,this.__base_string_length=s.length}c.prototype.get_indent_size=function(r,s){var u=this.__base_string_length;return s=s||0,r<0&&(u=0),u+=r*this.__indent_size,u+=s,u},c.prototype.get_indent_string=function(r,s){var u=this.__base_string;return s=s||0,r<0&&(r=0,u=""),s+=r*this.__indent_size,this.__ensure_cache(s),u+=this.__cache[s],u},c.prototype.__ensure_cache=function(r){for(;r>=this.__cache.length;)this.__add_column()},c.prototype.__add_column=function(){var r=this.__cache.length,s=0,u="";this.__indent_size&&r>=this.__indent_size&&(s=Math.floor(r/this.__indent_size),r-=s*this.__indent_size,u=new Array(s+1).join(this.__indent_string)),r&&(u+=new Array(r+1).join(" ")),this.__cache.push(u)};function l(r,s){this.__indent_cache=new c(r,s),this.raw=!1,this._end_with_newline=r.end_with_newline,this.indent_size=r.indent_size,this.wrap_line_length=r.wrap_line_length,this.indent_empty_lines=r.indent_empty_lines,this.__lines=[],this.previous_line=null,this.current_line=null,this.next_line=new a(this),this.space_before_token=!1,this.non_breaking_space=!1,this.previous_token_wrapped=!1,this.__add_outputline()}l.prototype.__add_outputline=function(){this.previous_line=this.current_line,this.current_line=this.next_line.clone_empty(),this.__lines.push(this.current_line)},l.prototype.get_line_number=function(){return this.__lines.length},l.prototype.get_indent_string=function(r,s){return this.__indent_cache.get_indent_string(r,s)},l.prototype.get_indent_size=function(r,s){return this.__indent_cache.get_indent_size(r,s)},l.prototype.is_empty=function(){return!this.previous_line&&this.current_line.is_empty()},l.prototype.add_new_line=function(r){return this.is_empty()||!r&&this.just_added_newline()?!1:(this.raw||this.__add_outputline(),!0)},l.prototype.get_code=function(r){this.trim(!0);var s=this.current_line.pop();s&&(s[s.length-1]===`
`&&(s=s.replace(/\n+$/g,"")),this.current_line.push(s)),this._end_with_newline&&this.__add_outputline();var u=this.__lines.join(`
`);return r!==`
`&&(u=u.replace(/[\n]/g,r)),u},l.prototype.set_wrap_point=function(){this.current_line._set_wrap_point()},l.prototype.set_indent=function(r,s){return r=r||0,s=s||0,this.next_line.set_indent(r,s),this.__lines.length>1?(this.current_line.set_indent(r,s),!0):(this.current_line.set_indent(),!1)},l.prototype.add_raw_token=function(r){for(var s=0;s1&&this.current_line.is_empty();)this.__lines.pop(),this.current_line=this.__lines[this.__lines.length-1],this.current_line.trim();this.previous_line=this.__lines.length>1?this.__lines[this.__lines.length-2]:null},l.prototype.just_added_newline=function(){return this.current_line.is_empty()},l.prototype.just_added_blankline=function(){return this.is_empty()||this.current_line.is_empty()&&this.previous_line.is_empty()},l.prototype.ensure_empty_line_above=function(r,s){for(var u=this.__lines.length-2;u>=0;){var h=this.__lines[u];if(h.is_empty())break;if(h.item(0).indexOf(r)!==0&&h.item(-1)!==s){this.__lines.splice(u+1,0,new a(this)),this.previous_line=this.__lines[this.__lines.length-2];break}u--}},e.exports.Output=l},function(e){function a(c,l,r,s){this.type=c,this.text=l,this.comments_before=null,this.newlines=r||0,this.whitespace_before=s||"",this.parent=null,this.next=null,this.previous=null,this.opened=null,this.closed=null,this.directives=null}e.exports.Token=a},,,function(e){function a(r,s){this.raw_options=c(r,s),this.disabled=this._get_boolean("disabled"),this.eol=this._get_characters("eol","auto"),this.end_with_newline=this._get_boolean("end_with_newline"),this.indent_size=this._get_number("indent_size",4),this.indent_char=this._get_characters("indent_char"," "),this.indent_level=this._get_number("indent_level"),this.preserve_newlines=this._get_boolean("preserve_newlines",!0),this.max_preserve_newlines=this._get_number("max_preserve_newlines",32786),this.preserve_newlines||(this.max_preserve_newlines=0),this.indent_with_tabs=this._get_boolean("indent_with_tabs",this.indent_char===" "),this.indent_with_tabs&&(this.indent_char=" ",this.indent_size===1&&(this.indent_size=4)),this.wrap_line_length=this._get_number("wrap_line_length",this._get_number("max_char")),this.indent_empty_lines=this._get_boolean("indent_empty_lines"),this.templating=this._get_selection_list("templating",["auto","none","django","erb","handlebars","php","smarty"],["auto"])}a.prototype._get_array=function(r,s){var u=this.raw_options[r],h=s||[];return typeof u=="object"?u!==null&&typeof u.concat=="function"&&(h=u.concat()):typeof u=="string"&&(h=u.split(/[^a-zA-Z0-9_\/\-]+/)),h},a.prototype._get_boolean=function(r,s){var u=this.raw_options[r],h=u===void 0?!!s:!!u;return h},a.prototype._get_characters=function(r,s){var u=this.raw_options[r],h=s||"";return typeof u=="string"&&(h=u.replace(/\\r/,"\r").replace(/\\n/,`
`).replace(/\\t/," ")),h},a.prototype._get_number=function(r,s){var u=this.raw_options[r];s=parseInt(s,10),isNaN(s)&&(s=0);var h=parseInt(u,10);return isNaN(h)&&(h=s),h},a.prototype._get_selection=function(r,s,u){var h=this._get_selection_list(r,s,u);if(h.length!==1)throw new Error("Invalid Option Value: The option '"+r+`' can only be one of the following values:
`+s+`
You passed in: '`+this.raw_options[r]+"'");return h[0]},a.prototype._get_selection_list=function(r,s,u){if(!s||s.length===0)throw new Error("Selection list cannot be empty.");if(u=u||[s[0]],!this._is_valid_selection(u,s))throw new Error("Invalid Default Value!");var h=this._get_array(r,u);if(!this._is_valid_selection(h,s))throw new Error("Invalid Option Value: The option '"+r+`' can contain only the following values:
`+s+`
You passed in: '`+this.raw_options[r]+"'");return h},a.prototype._is_valid_selection=function(r,s){return r.length&&s.length&&!r.some(function(u){return s.indexOf(u)===-1})};function c(r,s){var u={};r=l(r);var h;for(h in r)h!==s&&(u[h]=r[h]);if(s&&r[s])for(h in r[s])u[h]=r[s][h];return u}function l(r){var s={},u;for(u in r){var h=u.replace(/-/g,"_");s[h]=r[u]}return s}e.exports.Options=a,e.exports.normalizeOpts=l,e.exports.mergeOpts=c},,function(e){var a=RegExp.prototype.hasOwnProperty("sticky");function c(l){this.__input=l||"",this.__input_length=this.__input.length,this.__position=0}c.prototype.restart=function(){this.__position=0},c.prototype.back=function(){this.__position>0&&(this.__position-=1)},c.prototype.hasNext=function(){return this.__position=0&&l=0&&r=l.length&&this.__input.substring(r-l.length,r).toLowerCase()===l},e.exports.InputScanner=c},function(e,a,c){var l=c(8).InputScanner,r=c(3).Token,s=c(10).TokenStream,u=c(11).WhitespacePattern,h={START:"TK_START",RAW:"TK_RAW",EOF:"TK_EOF"},d=function(g,y){this._input=new l(g),this._options=y||{},this.__tokens=null,this._patterns={},this._patterns.whitespace=new u(this._input)};d.prototype.tokenize=function(){this._input.restart(),this.__tokens=new s,this._reset();for(var g,y=new r(h.START,""),m=null,A=[],E=new s;y.type!==h.EOF;){for(g=this._get_next_token(y,m);this._is_comment(g);)E.add(g),g=this._get_next_token(y,m);E.isEmpty()||(g.comments_before=E,E=new s),g.parent=m,this._is_opening(g)?(A.push(m),m=g):m&&this._is_closing(g,m)&&(g.opened=m,m.closed=g,m=A.pop(),g.parent=m),g.previous=y,y.next=g,this.__tokens.add(g),y=g}return this.__tokens},d.prototype._is_first_token=function(){return this.__tokens.isEmpty()},d.prototype._reset=function(){},d.prototype._get_next_token=function(g,y){this._readWhitespace();var m=this._input.read(/.+/g);return m?this._create_token(h.RAW,m):this._create_token(h.EOF,"")},d.prototype._is_comment=function(g){return!1},d.prototype._is_opening=function(g){return!1},d.prototype._is_closing=function(g,y){return!1},d.prototype._create_token=function(g,y){var m=new r(g,y,this._patterns.whitespace.newline_count,this._patterns.whitespace.whitespace_before_token);return m},d.prototype._readWhitespace=function(){return this._patterns.whitespace.read()},e.exports.Tokenizer=d,e.exports.TOKEN=h},function(e){function a(c){this.__tokens=[],this.__tokens_length=this.__tokens.length,this.__position=0,this.__parent_token=c}a.prototype.restart=function(){this.__position=0},a.prototype.isEmpty=function(){return this.__tokens_length===0},a.prototype.hasNext=function(){return this.__position=0&&c/),erb:d.starting_with(/<%[^%]/).until_after(/[^%]%>/),django:d.starting_with(/{%/).until_after(/%}/),django_value:d.starting_with(/{{/).until_after(/}}/),django_comment:d.starting_with(/{#/).until_after(/#}/),smarty:d.starting_with(/{(?=[^}{\s\n])/).until_after(/[^\s\n]}/),smarty_comment:d.starting_with(/{\*/).until_after(/\*}/),smarty_literal:d.starting_with(/{literal}/).until_after(/{\/literal}/)}}s.prototype=new l,s.prototype._create=function(){return new s(this._input,this)},s.prototype._update=function(){this.__set_templated_pattern()},s.prototype.disable=function(u){var h=this._create();return h._disabled[u]=!0,h._update(),h},s.prototype.read_options=function(u){var h=this._create();for(var d in r)h._disabled[d]=u.templating.indexOf(d)===-1;return h._update(),h},s.prototype.exclude=function(u){var h=this._create();return h._excluded[u]=!0,h._update(),h},s.prototype.read=function(){var u="";this._match_pattern?u=this._input.read(this._starting_pattern):u=this._input.read(this._starting_pattern,this.__template_pattern);for(var h=this._read_template();h;)this._match_pattern?h+=this._input.read(this._match_pattern):h+=this._input.readUntil(this.__template_pattern),u+=h,h=this._read_template();return this._until_after&&(u+=this._input.readUntilAfter(this._until_pattern)),u},s.prototype.__set_templated_pattern=function(){var u=[];this._disabled.php||u.push(this.__patterns.php._starting_pattern.source),this._disabled.handlebars||u.push(this.__patterns.handlebars._starting_pattern.source),this._disabled.erb||u.push(this.__patterns.erb._starting_pattern.source),this._disabled.django||(u.push(this.__patterns.django._starting_pattern.source),u.push(this.__patterns.django_value._starting_pattern.source),u.push(this.__patterns.django_comment._starting_pattern.source)),this._disabled.smarty||u.push(this.__patterns.smarty._starting_pattern.source),this._until_pattern&&u.push(this._until_pattern.source),this.__template_pattern=this._input.get_regexp("(?:"+u.join("|")+")")},s.prototype._read_template=function(){var u="",h=this._input.peek();if(h==="<"){var d=this._input.peek(1);!this._disabled.php&&!this._excluded.php&&d==="?"&&(u=u||this.__patterns.php.read()),!this._disabled.erb&&!this._excluded.erb&&d==="%"&&(u=u||this.__patterns.erb.read())}else h==="{"&&(!this._disabled.handlebars&&!this._excluded.handlebars&&(u=u||this.__patterns.handlebars_comment.read(),u=u||this.__patterns.handlebars_unescaped.read(),u=u||this.__patterns.handlebars.read()),this._disabled.django||(!this._excluded.django&&!this._excluded.handlebars&&(u=u||this.__patterns.django_value.read()),this._excluded.django||(u=u||this.__patterns.django_comment.read(),u=u||this.__patterns.django.read())),this._disabled.smarty||this._disabled.django&&this._disabled.handlebars&&(u=u||this.__patterns.smarty_comment.read(),u=u||this.__patterns.smarty_literal.read(),u=u||this.__patterns.smarty.read()));return u},e.exports.TemplatablePattern=s},,,,function(e,a,c){var l=c(19).Beautifier,r=c(20).Options;function s(u,h,d,g){var y=new l(u,h,d,g);return y.beautify()}e.exports=s,e.exports.defaultOptions=function(){return new r}},function(e,a,c){var l=c(20).Options,r=c(2).Output,s=c(21).Tokenizer,u=c(21).TOKEN,h=/\r\n|[\r\n]/,d=/\r\n|[\r\n]/g,g=function(f,p){this.indent_level=0,this.alignment_size=0,this.max_preserve_newlines=f.max_preserve_newlines,this.preserve_newlines=f.preserve_newlines,this._output=new r(f,p)};g.prototype.current_line_has_match=function(f){return this._output.current_line.has_match(f)},g.prototype.set_space_before_token=function(f,p){this._output.space_before_token=f,this._output.non_breaking_space=p},g.prototype.set_wrap_point=function(){this._output.set_indent(this.indent_level,this.alignment_size),this._output.set_wrap_point()},g.prototype.add_raw_token=function(f){this._output.add_raw_token(f)},g.prototype.print_preserved_newlines=function(f){var p=0;f.type!==u.TEXT&&f.previous.type!==u.TEXT&&(p=f.newlines?1:0),this.preserve_newlines&&(p=f.newlines0);return p!==0},g.prototype.traverse_whitespace=function(f){return f.whitespace_before||f.newlines?(this.print_preserved_newlines(f)||(this._output.space_before_token=!0),!0):!1},g.prototype.previous_token_wrapped=function(){return this._output.previous_token_wrapped},g.prototype.print_newline=function(f){this._output.add_new_line(f)},g.prototype.print_token=function(f){f.text&&(this._output.set_indent(this.indent_level,this.alignment_size),this._output.add_token(f.text))},g.prototype.indent=function(){this.indent_level++},g.prototype.get_full_indent=function(f){return f=this.indent_level+(f||0),f<1?"":this._output.get_indent_string(f)};var y=function(f){for(var p=null,b=f.next;b.type!==u.EOF&&f.closed!==b;){if(b.type===u.ATTRIBUTE&&b.text==="type"){b.next&&b.next.type===u.EQUALS&&b.next.next&&b.next.next.type===u.VALUE&&(p=b.next.next.text);break}b=b.next}return p},m=function(f,p){var b=null,N=null;return p.closed?(f==="script"?b="text/javascript":f==="style"&&(b="text/css"),b=y(p)||b,b.search("text/css")>-1?N="css":b.search(/module|((text|application|dojo)\/(x-)?(javascript|ecmascript|jscript|livescript|(ld\+)?json|method|aspect))/)>-1?N="javascript":b.search(/(text|application|dojo)\/(x-)?(html)/)>-1?N="html":b.search(/test\/null/)>-1&&(N="null"),N):null};function A(f,p){return p.indexOf(f)!==-1}function E(f,p,b){this.parent=f||null,this.tag=p?p.tag_name:"",this.indent_level=b||0,this.parser_token=p||null}function w(f){this._printer=f,this._current_frame=null}w.prototype.get_parser_token=function(){return this._current_frame?this._current_frame.parser_token:null},w.prototype.record_tag=function(f){var p=new E(this._current_frame,f,this._printer.indent_level);this._current_frame=p},w.prototype._try_pop_frame=function(f){var p=null;return f&&(p=f.parser_token,this._printer.indent_level=f.indent_level,this._current_frame=f.parent),p},w.prototype._get_frame=function(f,p){for(var b=this._current_frame;b&&f.indexOf(b.tag)===-1;){if(p&&p.indexOf(b.tag)!==-1){b=null;break}b=b.parent}return b},w.prototype.try_pop=function(f,p){var b=this._get_frame([f],p);return this._try_pop_frame(b)},w.prototype.indent_to_tag=function(f){var p=this._get_frame(f);p&&(this._printer.indent_level=p.indent_level)};function M(f,p,b,N){this._source_text=f||"",p=p||{},this._js_beautify=b,this._css_beautify=N,this._tag_stack=null;var R=new l(p,"html");this._options=R,this._is_wrap_attributes_force=this._options.wrap_attributes.substr(0,5)==="force",this._is_wrap_attributes_force_expand_multiline=this._options.wrap_attributes==="force-expand-multiline",this._is_wrap_attributes_force_aligned=this._options.wrap_attributes==="force-aligned",this._is_wrap_attributes_aligned_multiple=this._options.wrap_attributes==="aligned-multiple",this._is_wrap_attributes_preserve=this._options.wrap_attributes.substr(0,8)==="preserve",this._is_wrap_attributes_preserve_aligned=this._options.wrap_attributes==="preserve-aligned"}M.prototype.beautify=function(){if(this._options.disabled)return this._source_text;var f=this._source_text,p=this._options.eol;this._options.eol==="auto"&&(p=`
`,f&&h.test(f)&&(p=f.match(h)[0])),f=f.replace(d,`
`);var b=f.match(/^[\t ]*/)[0],N={text:"",type:""},R=new B,U=new g(this._options,b),H=new s(f,this._options).tokenize();this._tag_stack=new w(U);for(var z=null,I=H.next();I.type!==u.EOF;)I.type===u.TAG_OPEN||I.type===u.COMMENT?(z=this._handle_tag_open(U,I,R,N),R=z):I.type===u.ATTRIBUTE||I.type===u.EQUALS||I.type===u.VALUE||I.type===u.TEXT&&!R.tag_complete?z=this._handle_inside_tag(U,I,R,H):I.type===u.TAG_CLOSE?z=this._handle_tag_close(U,I,R):I.type===u.TEXT?z=this._handle_text(U,I,R):U.add_raw_token(I),N=z,I=H.next();var F=U._output.get_code(p);return F},M.prototype._handle_tag_close=function(f,p,b){var N={text:p.text,type:p.type};return f.alignment_size=0,b.tag_complete=!0,f.set_space_before_token(p.newlines||p.whitespace_before!=="",!0),b.is_unformatted?f.add_raw_token(p):(b.tag_start_char==="<"&&(f.set_space_before_token(p.text[0]==="/",!0),this._is_wrap_attributes_force_expand_multiline&&b.has_wrapped_attrs&&f.print_newline(!1)),f.print_token(p)),b.indent_content&&!(b.is_unformatted||b.is_content_unformatted)&&(f.indent(),b.indent_content=!1),!b.is_inline_element&&!(b.is_unformatted||b.is_content_unformatted)&&f.set_wrap_point(),N},M.prototype._handle_inside_tag=function(f,p,b,N){var R=b.has_wrapped_attrs,U={text:p.text,type:p.type};if(f.set_space_before_token(p.newlines||p.whitespace_before!=="",!0),b.is_unformatted)f.add_raw_token(p);else if(b.tag_start_char==="{"&&p.type===u.TEXT)f.print_preserved_newlines(p)?(p.newlines=0,f.add_raw_token(p)):f.print_token(p);else{if(p.type===u.ATTRIBUTE?(f.set_space_before_token(!0),b.attr_count+=1):(p.type===u.EQUALS||p.type===u.VALUE&&p.previous.type===u.EQUALS)&&f.set_space_before_token(!1),p.type===u.ATTRIBUTE&&b.tag_start_char==="<"&&((this._is_wrap_attributes_preserve||this._is_wrap_attributes_preserve_aligned)&&(f.traverse_whitespace(p),R=R||p.newlines!==0),this._is_wrap_attributes_force)){var H=b.attr_count>1;if(this._is_wrap_attributes_force_expand_multiline&&b.attr_count===1){var z=!0,I=0,F;do{if(F=N.peek(I),F.type===u.ATTRIBUTE){z=!1;break}I+=1}while(I<4&&F.type!==u.EOF&&F.type!==u.TAG_CLOSE);H=!z}H&&(f.print_newline(!1),R=!0)}f.print_token(p),R=R||f.previous_token_wrapped(),b.has_wrapped_attrs=R}return U},M.prototype._handle_text=function(f,p,b){var N={text:p.text,type:"TK_CONTENT"};return b.custom_beautifier_name?this._print_custom_beatifier_text(f,p,b):b.is_unformatted||b.is_content_unformatted?f.add_raw_token(p):(f.traverse_whitespace(p),f.print_token(p)),N},M.prototype._print_custom_beatifier_text=function(f,p,b){var N=this;if(p.text!==""){var R=p.text,U,H=1,z="",I="";b.custom_beautifier_name==="javascript"&&typeof this._js_beautify=="function"?U=this._js_beautify:b.custom_beautifier_name==="css"&&typeof this._css_beautify=="function"?U=this._css_beautify:b.custom_beautifier_name==="html"&&(U=function(x,D){var L=new M(x,D,N._js_beautify,N._css_beautify);return L.beautify()}),this._options.indent_scripts==="keep"?H=0:this._options.indent_scripts==="separate"&&(H=-f.indent_level);var F=f.get_full_indent(H);if(R=R.replace(/\n[ \t]*$/,""),b.custom_beautifier_name!=="html"&&R[0]==="<"&&R.match(/^(|]]>)$/.exec(R);if(!T){f.add_raw_token(p);return}z=F+T[1]+`
`,R=T[4],T[5]&&(I=F+T[5]),R=R.replace(/\n[ \t]*$/,""),(T[2]||T[3].indexOf(`
`)!==-1)&&(T=T[3].match(/[ \t]+$/),T&&(p.whitespace_before=T[0]))}if(R)if(U){var v=function(){this.eol=`
`};v.prototype=this._options.raw_options;var k=new v;R=U(F+R,k)}else{var C=p.whitespace_before;C&&(R=R.replace(new RegExp(`
(`+C+")?","g"),`
`)),R=F+R.replace(/\n/g,`
`+F)}z&&(R?R=z+R+`
`+I:R=z+I),f.print_newline(!1),R&&(p.text=R,p.whitespace_before="",p.newlines=0,f.add_raw_token(p),f.print_newline(!0))}},M.prototype._handle_tag_open=function(f,p,b,N){var R=this._get_tag_open_token(p);return(b.is_unformatted||b.is_content_unformatted)&&!b.is_empty_element&&p.type===u.TAG_OPEN&&p.text.indexOf("")===0?(f.add_raw_token(p),R.start_tag_token=this._tag_stack.try_pop(R.tag_name)):(f.traverse_whitespace(p),this._set_tag_position(f,p,R,b,N),R.is_inline_element||f.set_wrap_point(),f.print_token(p)),(this._is_wrap_attributes_force_aligned||this._is_wrap_attributes_aligned_multiple||this._is_wrap_attributes_preserve_aligned)&&(R.alignment_size=p.text.length+1),!R.tag_complete&&!R.is_unformatted&&(f.alignment_size=R.alignment_size),R};var B=function(f,p){if(this.parent=f||null,this.text="",this.type="TK_TAG_OPEN",this.tag_name="",this.is_inline_element=!1,this.is_unformatted=!1,this.is_content_unformatted=!1,this.is_empty_element=!1,this.is_start_tag=!1,this.is_end_tag=!1,this.indent_content=!1,this.multiline_content=!1,this.custom_beautifier_name=null,this.start_tag_token=null,this.attr_count=0,this.has_wrapped_attrs=!1,this.alignment_size=0,this.tag_complete=!1,this.tag_start_char="",this.tag_check="",!p)this.tag_complete=!0;else{var b;this.tag_start_char=p.text[0],this.text=p.text,this.tag_start_char==="<"?(b=p.text.match(/^<([^\s>]*)/),this.tag_check=b?b[1]:""):(b=p.text.match(/^{{(?:[\^]|#\*?)?([^\s}]+)/),this.tag_check=b?b[1]:"",p.text==="{{#>"&&this.tag_check===">"&&p.next!==null&&(this.tag_check=p.next.text)),this.tag_check=this.tag_check.toLowerCase(),p.type===u.COMMENT&&(this.tag_complete=!0),this.is_start_tag=this.tag_check.charAt(0)!=="/",this.tag_name=this.is_start_tag?this.tag_check:this.tag_check.substr(1),this.is_end_tag=!this.is_start_tag||p.closed&&p.closed.text==="/>",this.is_end_tag=this.is_end_tag||this.tag_start_char==="{"&&(this.text.length<3||/[^#\^]/.test(this.text.charAt(2)))}};M.prototype._get_tag_open_token=function(f){var p=new B(this._tag_stack.get_parser_token(),f);return p.alignment_size=this._options.wrap_attributes_indent_size,p.is_end_tag=p.is_end_tag||A(p.tag_check,this._options.void_elements),p.is_empty_element=p.tag_complete||p.is_start_tag&&p.is_end_tag,p.is_unformatted=!p.tag_complete&&A(p.tag_check,this._options.unformatted),p.is_content_unformatted=!p.is_empty_element&&A(p.tag_check,this._options.content_unformatted),p.is_inline_element=A(p.tag_name,this._options.inline)||p.tag_start_char==="{",p},M.prototype._set_tag_position=function(f,p,b,N,R){if(b.is_empty_element||(b.is_end_tag?b.start_tag_token=this._tag_stack.try_pop(b.tag_name):(this._do_optional_end_element(b)&&(b.is_inline_element||f.print_newline(!1)),this._tag_stack.record_tag(b),(b.tag_name==="script"||b.tag_name==="style")&&!(b.is_unformatted||b.is_content_unformatted)&&(b.custom_beautifier_name=m(b.tag_check,p)))),A(b.tag_check,this._options.extra_liners)&&(f.print_newline(!1),f._output.just_added_blankline()||f.print_newline(!0)),b.is_empty_element){if(b.tag_start_char==="{"&&b.tag_check==="else"){this._tag_stack.indent_to_tag(["if","unless","each"]),b.indent_content=!0;var U=f.current_line_has_match(/{{#if/);U||f.print_newline(!1)}b.tag_name==="!--"&&R.type===u.TAG_CLOSE&&N.is_end_tag&&b.text.indexOf(`
`)===-1||(b.is_inline_element||b.is_unformatted||f.print_newline(!1),this._calcluate_parent_multiline(f,b))}else if(b.is_end_tag){var H=!1;H=b.start_tag_token&&b.start_tag_token.multiline_content,H=H||!b.is_inline_element&&!(N.is_inline_element||N.is_unformatted)&&!(R.type===u.TAG_CLOSE&&b.start_tag_token===N)&&R.type!=="TK_CONTENT",(b.is_content_unformatted||b.is_unformatted)&&(H=!1),H&&f.print_newline(!1)}else b.indent_content=!b.custom_beautifier_name,b.tag_start_char==="<"&&(b.tag_name==="html"?b.indent_content=this._options.indent_inner_html:b.tag_name==="head"?b.indent_content=this._options.indent_head_inner_html:b.tag_name==="body"&&(b.indent_content=this._options.indent_body_inner_html)),!(b.is_inline_element||b.is_unformatted)&&(R.type!=="TK_CONTENT"||b.is_content_unformatted)&&f.print_newline(!1),this._calcluate_parent_multiline(f,b)},M.prototype._calcluate_parent_multiline=function(f,p){p.parent&&f._output.just_added_newline()&&!((p.is_inline_element||p.is_unformatted)&&p.parent.is_inline_element)&&(p.parent.multiline_content=!0)};var G=["address","article","aside","blockquote","details","div","dl","fieldset","figcaption","figure","footer","form","h1","h2","h3","h4","h5","h6","header","hr","main","nav","ol","p","pre","section","table","ul"],J=["a","audio","del","ins","map","noscript","video"];M.prototype._do_optional_end_element=function(f){var p=null;if(!(f.is_empty_element||!f.is_start_tag||!f.parent)){if(f.tag_name==="body")p=p||this._tag_stack.try_pop("head");else if(f.tag_name==="li")p=p||this._tag_stack.try_pop("li",["ol","ul"]);else if(f.tag_name==="dd"||f.tag_name==="dt")p=p||this._tag_stack.try_pop("dt",["dl"]),p=p||this._tag_stack.try_pop("dd",["dl"]);else if(f.parent.tag_name==="p"&&G.indexOf(f.tag_name)!==-1){var b=f.parent.parent;(!b||J.indexOf(b.tag_name)===-1)&&(p=p||this._tag_stack.try_pop("p"))}else f.tag_name==="rp"||f.tag_name==="rt"?(p=p||this._tag_stack.try_pop("rt",["ruby","rtc"]),p=p||this._tag_stack.try_pop("rp",["ruby","rtc"])):f.tag_name==="optgroup"?p=p||this._tag_stack.try_pop("optgroup",["select"]):f.tag_name==="option"?p=p||this._tag_stack.try_pop("option",["select","datalist","optgroup"]):f.tag_name==="colgroup"?p=p||this._tag_stack.try_pop("caption",["table"]):f.tag_name==="thead"?(p=p||this._tag_stack.try_pop("caption",["table"]),p=p||this._tag_stack.try_pop("colgroup",["table"])):f.tag_name==="tbody"||f.tag_name==="tfoot"?(p=p||this._tag_stack.try_pop("caption",["table"]),p=p||this._tag_stack.try_pop("colgroup",["table"]),p=p||this._tag_stack.try_pop("thead",["table"]),p=p||this._tag_stack.try_pop("tbody",["table"])):f.tag_name==="tr"?(p=p||this._tag_stack.try_pop("caption",["table"]),p=p||this._tag_stack.try_pop("colgroup",["table"]),p=p||this._tag_stack.try_pop("tr",["table","thead","tbody","tfoot"])):(f.tag_name==="th"||f.tag_name==="td")&&(p=p||this._tag_stack.try_pop("td",["table","thead","tbody","tfoot","tr"]),p=p||this._tag_stack.try_pop("th",["table","thead","tbody","tfoot","tr"]));return f.parent=this._tag_stack.get_parser_token(),p}},e.exports.Beautifier=M},function(e,a,c){var l=c(6).Options;function r(s){l.call(this,s,"html"),this.templating.length===1&&this.templating[0]==="auto"&&(this.templating=["django","erb","handlebars","php"]),this.indent_inner_html=this._get_boolean("indent_inner_html"),this.indent_body_inner_html=this._get_boolean("indent_body_inner_html",!0),this.indent_head_inner_html=this._get_boolean("indent_head_inner_html",!0),this.indent_handlebars=this._get_boolean("indent_handlebars",!0),this.wrap_attributes=this._get_selection("wrap_attributes",["auto","force","force-aligned","force-expand-multiline","aligned-multiple","preserve","preserve-aligned"]),this.wrap_attributes_indent_size=this._get_number("wrap_attributes_indent_size",this.indent_size),this.extra_liners=this._get_array("extra_liners",["head","body","/html"]),this.inline=this._get_array("inline",["a","abbr","area","audio","b","bdi","bdo","br","button","canvas","cite","code","data","datalist","del","dfn","em","embed","i","iframe","img","input","ins","kbd","keygen","label","map","mark","math","meter","noscript","object","output","progress","q","ruby","s","samp","select","small","span","strong","sub","sup","svg","template","textarea","time","u","var","video","wbr","text","acronym","big","strike","tt"]),this.void_elements=this._get_array("void_elements",["area","base","br","col","embed","hr","img","input","keygen","link","menuitem","meta","param","source","track","wbr","!doctype","?xml","basefont","isindex"]),this.unformatted=this._get_array("unformatted",[]),this.content_unformatted=this._get_array("content_unformatted",["pre","textarea"]),this.unformatted_content_delimiter=this._get_characters("unformatted_content_delimiter"),this.indent_scripts=this._get_selection("indent_scripts",["normal","keep","separate"])}r.prototype=new l,e.exports.Options=r},function(e,a,c){var l=c(9).Tokenizer,r=c(9).TOKEN,s=c(13).Directives,u=c(14).TemplatablePattern,h=c(12).Pattern,d={TAG_OPEN:"TK_TAG_OPEN",TAG_CLOSE:"TK_TAG_CLOSE",ATTRIBUTE:"TK_ATTRIBUTE",EQUALS:"TK_EQUALS",VALUE:"TK_VALUE",COMMENT:"TK_COMMENT",TEXT:"TK_TEXT",UNKNOWN:"TK_UNKNOWN",START:r.START,RAW:r.RAW,EOF:r.EOF},g=new s(/<\!--/,/-->/),y=function(m,A){l.call(this,m,A),this._current_tag_name="";var E=new u(this._input).read_options(this._options),w=new h(this._input);if(this.__patterns={word:E.until(/[\n\r\t <]/),single_quote:E.until_after(/'/),double_quote:E.until_after(/"/),attribute:E.until(/[\n\r\t =>]|\/>/),element_name:E.until(/[\n\r\t >\/]/),handlebars_comment:w.starting_with(/{{!--/).until_after(/--}}/),handlebars:w.starting_with(/{{/).until_after(/}}/),handlebars_open:w.until(/[\n\r\t }]/),handlebars_raw_close:w.until(/}}/),comment:w.starting_with(//),cdata:w.starting_with(//),conditional_comment:w.starting_with(//),processing:w.starting_with(/<\?/).until_after(/\?>/)},this._options.indent_handlebars&&(this.__patterns.word=this.__patterns.word.exclude("handlebars")),this._unformatted_content_delimiter=null,this._options.unformatted_content_delimiter){var M=this._input.get_literal_regexp(this._options.unformatted_content_delimiter);this.__patterns.unformatted_content_delimiter=w.matching(M).until_after(M)}};y.prototype=new l,y.prototype._is_comment=function(m){return!1},y.prototype._is_opening=function(m){return m.type===d.TAG_OPEN},y.prototype._is_closing=function(m,A){return m.type===d.TAG_CLOSE&&A&&((m.text===">"||m.text==="/>")&&A.text[0]==="<"||m.text==="}}"&&A.text[0]==="{"&&A.text[1]==="{")},y.prototype._reset=function(){this._current_tag_name=""},y.prototype._get_next_token=function(m,A){var E=null;this._readWhitespace();var w=this._input.peek();return w===null?this._create_token(d.EOF,""):(E=E||this._read_open_handlebars(w,A),E=E||this._read_attribute(w,m,A),E=E||this._read_close(w,A),E=E||this._read_raw_content(w,m,A),E=E||this._read_content_word(w),E=E||this._read_comment_or_cdata(w),E=E||this._read_processing(w),E=E||this._read_open(w,A),E=E||this._create_token(d.UNKNOWN,this._input.next()),E)},y.prototype._read_comment_or_cdata=function(m){var A=null,E=null,w=null;if(m==="<"){var M=this._input.peek(1);M==="!"&&(E=this.__patterns.comment.read(),E?(w=g.get_directives(E),w&&w.ignore==="start"&&(E+=g.readIgnored(this._input))):E=this.__patterns.cdata.read()),E&&(A=this._create_token(d.COMMENT,E),A.directives=w)}return A},y.prototype._read_processing=function(m){var A=null,E=null,w=null;if(m==="<"){var M=this._input.peek(1);(M==="!"||M==="?")&&(E=this.__patterns.conditional_comment.read(),E=E||this.__patterns.processing.read()),E&&(A=this._create_token(d.COMMENT,E),A.directives=w)}return A},y.prototype._read_open=function(m,A){var E=null,w=null;return A||m==="<"&&(E=this._input.next(),this._input.peek()==="/"&&(E+=this._input.next()),E+=this.__patterns.element_name.read(),w=this._create_token(d.TAG_OPEN,E)),w},y.prototype._read_open_handlebars=function(m,A){var E=null,w=null;return A||this._options.indent_handlebars&&m==="{"&&this._input.peek(1)==="{"&&(this._input.peek(2)==="!"?(E=this.__patterns.handlebars_comment.read(),E=E||this.__patterns.handlebars.read(),w=this._create_token(d.COMMENT,E)):(E=this.__patterns.handlebars_open.read(),w=this._create_token(d.TAG_OPEN,E))),w},y.prototype._read_close=function(m,A){var E=null,w=null;return A&&(A.text[0]==="<"&&(m===">"||m==="/"&&this._input.peek(1)===">")?(E=this._input.next(),m==="/"&&(E+=this._input.next()),w=this._create_token(d.TAG_CLOSE,E)):A.text[0]==="{"&&m==="}"&&this._input.peek(1)==="}"&&(this._input.next(),this._input.next(),w=this._create_token(d.TAG_CLOSE,"}}"))),w},y.prototype._read_attribute=function(m,A,E){var w=null,M="";if(E&&E.text[0]==="<")if(m==="=")w=this._create_token(d.EQUALS,this._input.next());else if(m==='"'||m==="'"){var B=this._input.next();m==='"'?B+=this.__patterns.double_quote.read():B+=this.__patterns.single_quote.read(),w=this._create_token(d.VALUE,B)}else M=this.__patterns.attribute.read(),M&&(A.type===d.EQUALS?w=this._create_token(d.VALUE,M):w=this._create_token(d.ATTRIBUTE,M));return w},y.prototype._is_content_unformatted=function(m){return this._options.void_elements.indexOf(m)===-1&&(this._options.content_unformatted.indexOf(m)!==-1||this._options.unformatted.indexOf(m)!==-1)},y.prototype._read_raw_content=function(m,A,E){var w="";if(E&&E.text[0]==="{")w=this.__patterns.handlebars_raw_close.read();else if(A.type===d.TAG_CLOSE&&A.opened.text[0]==="<"&&A.text[0]!=="/"){var M=A.opened.text.substr(1).toLowerCase();if(M==="script"||M==="style"){var B=this._read_comment_or_cdata(m);if(B)return B.type=d.TEXT,B;w=this._input.readUntil(new RegExp(""+M+"[\\n\\r\\t ]*?>","ig"))}else this._is_content_unformatted(M)&&(w=this._input.readUntil(new RegExp(""+M+"[\\n\\r\\t ]*?>","ig")))}return w?this._create_token(d.TEXT,w):null},y.prototype._read_content_word=function(m){var A="";if(this._options.unformatted_content_delimiter&&m===this._options.unformatted_content_delimiter[0]&&(A=this.__patterns.unformatted_content_delimiter.read()),A||(A=this.__patterns.word.read()),A)return this._create_token(d.TEXT,A)},e.exports.Tokenizer=y,e.exports.TOKEN=d}],i={};function o(e){var a=i[e];if(a!==void 0)return a.exports;var c=i[e]={exports:{}};return t[e](c,c.exports,o),c.exports}var n=o(18);nn=n})();function rn(t,i){return nn(t,i,Kt,tn)}function sn(t,i,o){var n=t.getText(),e=!0,a=0,c=o.tabSize||4;if(i){for(var l=t.offsetAt(i.start),r=l;r>0&&on(n,r-1);)r--;r===0||an(n,r-1)?l=r:r]*$/).test(h))return n=n.substring(l,s),[{range:i,newText:n}];if(e=s===n.length,n=n.substring(l,s),l!==0){var d=t.offsetAt(X.create(i.start.line,0));a=wi(t.getText(),d,o)}}else i=P.create(X.create(0,0),t.positionAt(n.length));var g={indent_size:c,indent_char:o.insertSpaces?" ":" ",indent_empty_lines:ie(o,"indentEmptyLines",!1),wrap_line_length:ie(o,"wrapLineLength",120),unformatted:mt(o,"unformatted",void 0),content_unformatted:mt(o,"contentUnformatted",void 0),indent_inner_html:ie(o,"indentInnerHtml",!1),preserve_newlines:ie(o,"preserveNewLines",!0),max_preserve_newlines:ie(o,"maxPreserveNewLines",32786),indent_handlebars:ie(o,"indentHandlebars",!1),end_with_newline:e&&ie(o,"endWithNewline",!1),extra_liners:mt(o,"extraLiners",void 0),wrap_attributes:ie(o,"wrapAttributes","auto"),wrap_attributes_indent_size:ie(o,"wrapAttributesIndentSize",void 0),eol:`
`,indent_scripts:ie(o,"indentScripts","normal"),templating:vi(o,"all"),unformatted_content_delimiter:ie(o,"unformattedContentDelimiter","")},y=rn(bi(n),g);if(a>0){var m=o.insertSpaces?pt(" ",c*a):pt(" ",a);y=y.split(`
`).join(`
`+m),i.start.character===0&&(y=m+y)}return[{range:i,newText:y}]}function bi(t){return t.replace(/^\s+/,"")}function ie(t,i,o){if(t&&t.hasOwnProperty(i)){var n=t[i];if(n!==null)return n}return o}function mt(t,i,o){var n=ie(t,i,null);return typeof n=="string"?n.length>0?n.split(",").map(function(e){return e.trim().toLowerCase()}):[]:o}function vi(t,i){var o=ie(t,"templating",i);return o===!0?["auto"]:["none"]}function wi(t,i,o){for(var n=i,e=0,a=o.tabSize||4;n{"use strict";var t={470:n=>{function e(l){if(typeof l!="string")throw new TypeError("Path must be a string. Received "+JSON.stringify(l))}function a(l,r){for(var s,u="",h=0,d=-1,g=0,y=0;y<=l.length;++y){if(y2){var m=u.lastIndexOf("/");if(m!==u.length-1){m===-1?(u="",h=0):h=(u=u.slice(0,m)).length-1-u.lastIndexOf("/"),d=y,g=0;continue}}else if(u.length===2||u.length===1){u="",h=0,d=y,g=0;continue}}r&&(u.length>0?u+="/..":u="..",h=2)}else u.length>0?u+="/"+l.slice(d+1,y):u=l.slice(d+1,y),h=y-d-1;d=y,g=0}else s===46&&g!==-1?++g:g=-1}return u}var c={resolve:function(){for(var l,r="",s=!1,u=arguments.length-1;u>=-1&&!s;u--){var h;u>=0?h=arguments[u]:(l===void 0&&(l=process.cwd()),h=l),e(h),h.length!==0&&(r=h+"/"+r,s=h.charCodeAt(0)===47)}return r=a(r,!s),s?r.length>0?"/"+r:"/":r.length>0?r:"."},normalize:function(l){if(e(l),l.length===0)return".";var r=l.charCodeAt(0)===47,s=l.charCodeAt(l.length-1)===47;return(l=a(l,!r)).length!==0||r||(l="."),l.length>0&&s&&(l+="/"),r?"/"+l:l},isAbsolute:function(l){return e(l),l.length>0&&l.charCodeAt(0)===47},join:function(){if(arguments.length===0)return".";for(var l,r=0;r0&&(l===void 0?l=s:l+="/"+s)}return l===void 0?".":c.normalize(l)},relative:function(l,r){if(e(l),e(r),l===r||(l=c.resolve(l))===(r=c.resolve(r)))return"";for(var s=1;sy){if(r.charCodeAt(d+A)===47)return r.slice(d+A+1);if(A===0)return r.slice(d+A)}else h>y&&(l.charCodeAt(s+A)===47?m=A:A===0&&(m=0));break}var E=l.charCodeAt(s+A);if(E!==r.charCodeAt(d+A))break;E===47&&(m=A)}var w="";for(A=s+m+1;A<=u;++A)A!==u&&l.charCodeAt(A)!==47||(w.length===0?w+="..":w+="/..");return w.length>0?w+r.slice(d+m):(d+=m,r.charCodeAt(d)===47&&++d,r.slice(d))},_makeLong:function(l){return l},dirname:function(l){if(e(l),l.length===0)return".";for(var r=l.charCodeAt(0),s=r===47,u=-1,h=!0,d=l.length-1;d>=1;--d)if((r=l.charCodeAt(d))===47){if(!h){u=d;break}}else h=!1;return u===-1?s?"/":".":s&&u===1?"//":l.slice(0,u)},basename:function(l,r){if(r!==void 0&&typeof r!="string")throw new TypeError('"ext" argument must be a string');e(l);var s,u=0,h=-1,d=!0;if(r!==void 0&&r.length>0&&r.length<=l.length){if(r.length===l.length&&r===l)return"";var g=r.length-1,y=-1;for(s=l.length-1;s>=0;--s){var m=l.charCodeAt(s);if(m===47){if(!d){u=s+1;break}}else y===-1&&(d=!1,y=s+1),g>=0&&(m===r.charCodeAt(g)?--g==-1&&(h=s):(g=-1,h=y))}return u===h?h=y:h===-1&&(h=l.length),l.slice(u,h)}for(s=l.length-1;s>=0;--s)if(l.charCodeAt(s)===47){if(!d){u=s+1;break}}else h===-1&&(d=!1,h=s+1);return h===-1?"":l.slice(u,h)},extname:function(l){e(l);for(var r=-1,s=0,u=-1,h=!0,d=0,g=l.length-1;g>=0;--g){var y=l.charCodeAt(g);if(y!==47)u===-1&&(h=!1,u=g+1),y===46?r===-1?r=g:d!==1&&(d=1):r!==-1&&(d=-1);else if(!h){s=g+1;break}}return r===-1||u===-1||d===0||d===1&&r===u-1&&r===s+1?"":l.slice(r,u)},format:function(l){if(l===null||typeof l!="object")throw new TypeError('The "pathObject" argument must be of type Object. Received type '+typeof l);return function(r,s){var u=s.dir||s.root,h=s.base||(s.name||"")+(s.ext||"");return u?u===s.root?u+h:u+"/"+h:h}(0,l)},parse:function(l){e(l);var r={root:"",dir:"",base:"",ext:"",name:""};if(l.length===0)return r;var s,u=l.charCodeAt(0),h=u===47;h?(r.root="/",s=1):s=0;for(var d=-1,g=0,y=-1,m=!0,A=l.length-1,E=0;A>=s;--A)if((u=l.charCodeAt(A))!==47)y===-1&&(m=!1,y=A+1),u===46?d===-1?d=A:E!==1&&(E=1):d!==-1&&(E=-1);else if(!m){g=A+1;break}return d===-1||y===-1||E===0||E===1&&d===y-1&&d===g+1?y!==-1&&(r.base=r.name=g===0&&h?l.slice(1,y):l.slice(g,y)):(g===0&&h?(r.name=l.slice(1,d),r.base=l.slice(1,y)):(r.name=l.slice(g,d),r.base=l.slice(g,y)),r.ext=l.slice(d,y)),g>0?r.dir=l.slice(0,g-1):h&&(r.dir="/"),r},sep:"/",delimiter:":",win32:null,posix:null};c.posix=c,n.exports=c},447:(n,e,a)=>{var c;if(a.r(e),a.d(e,{URI:()=>w,Utils:()=>H}),typeof process=="object")c=process.platform==="win32";else if(typeof navigator=="object"){var l=navigator.userAgent;c=l.indexOf("Windows")>=0}var r,s,u=(r=function(T,v){return(r=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(k,C){k.__proto__=C}||function(k,C){for(var x in C)Object.prototype.hasOwnProperty.call(C,x)&&(k[x]=C[x])})(T,v)},function(T,v){if(typeof v!="function"&&v!==null)throw new TypeError("Class extends value "+String(v)+" is not a constructor or null");function k(){this.constructor=T}r(T,v),T.prototype=v===null?Object.create(v):(k.prototype=v.prototype,new k)}),h=/^\w[\w\d+.-]*$/,d=/^\//,g=/^\/\//;function y(T,v){if(!T.scheme&&v)throw new Error('[UriError]: Scheme is missing: {scheme: "", authority: "'.concat(T.authority,'", path: "').concat(T.path,'", query: "').concat(T.query,'", fragment: "').concat(T.fragment,'"}'));if(T.scheme&&!h.test(T.scheme))throw new Error("[UriError]: Scheme contains illegal characters.");if(T.path){if(T.authority){if(!d.test(T.path))throw new Error('[UriError]: If a URI contains an authority component, then the path component must either be empty or begin with a slash ("/") character')}else if(g.test(T.path))throw new Error('[UriError]: If a URI does not contain an authority component, then the path cannot begin with two slash characters ("//")')}}var m="",A="/",E=/^(([^:/?#]+?):)?(\/\/([^/?#]*))?([^?#]*)(\?([^#]*))?(#(.*))?/,w=function(){function T(v,k,C,x,D,L){L===void 0&&(L=!1),typeof v=="object"?(this.scheme=v.scheme||m,this.authority=v.authority||m,this.path=v.path||m,this.query=v.query||m,this.fragment=v.fragment||m):(this.scheme=function(q,j){return q||j?q:"file"}(v,L),this.authority=k||m,this.path=function(q,j){switch(q){case"https":case"http":case"file":j?j[0]!==A&&(j=A+j):j=A}return j}(this.scheme,C||m),this.query=x||m,this.fragment=D||m,y(this,L))}return T.isUri=function(v){return v instanceof T||!!v&&typeof v.authority=="string"&&typeof v.fragment=="string"&&typeof v.path=="string"&&typeof v.query=="string"&&typeof v.scheme=="string"&&typeof v.fsPath=="string"&&typeof v.with=="function"&&typeof v.toString=="function"},Object.defineProperty(T.prototype,"fsPath",{get:function(){return p(this,!1)},enumerable:!1,configurable:!0}),T.prototype.with=function(v){if(!v)return this;var k=v.scheme,C=v.authority,x=v.path,D=v.query,L=v.fragment;return k===void 0?k=this.scheme:k===null&&(k=m),C===void 0?C=this.authority:C===null&&(C=m),x===void 0?x=this.path:x===null&&(x=m),D===void 0?D=this.query:D===null&&(D=m),L===void 0?L=this.fragment:L===null&&(L=m),k===this.scheme&&C===this.authority&&x===this.path&&D===this.query&&L===this.fragment?this:new B(k,C,x,D,L)},T.parse=function(v,k){k===void 0&&(k=!1);var C=E.exec(v);return C?new B(C[2]||m,U(C[4]||m),U(C[5]||m),U(C[7]||m),U(C[9]||m),k):new B(m,m,m,m,m)},T.file=function(v){var k=m;if(c&&(v=v.replace(/\\/g,A)),v[0]===A&&v[1]===A){var C=v.indexOf(A,2);C===-1?(k=v.substring(2),v=A):(k=v.substring(2,C),v=v.substring(C)||A)}return new B("file",k,v,m,m)},T.from=function(v){var k=new B(v.scheme,v.authority,v.path,v.query,v.fragment);return y(k,!0),k},T.prototype.toString=function(v){return v===void 0&&(v=!1),b(this,v)},T.prototype.toJSON=function(){return this},T.revive=function(v){if(v){if(v instanceof T)return v;var k=new B(v);return k._formatted=v.external,k._fsPath=v._sep===M?v.fsPath:null,k}return v},T}(),M=c?1:void 0,B=function(T){function v(){var k=T!==null&&T.apply(this,arguments)||this;return k._formatted=null,k._fsPath=null,k}return u(v,T),Object.defineProperty(v.prototype,"fsPath",{get:function(){return this._fsPath||(this._fsPath=p(this,!1)),this._fsPath},enumerable:!1,configurable:!0}),v.prototype.toString=function(k){return k===void 0&&(k=!1),k?b(this,!0):(this._formatted||(this._formatted=b(this,!1)),this._formatted)},v.prototype.toJSON=function(){var k={$mid:1};return this._fsPath&&(k.fsPath=this._fsPath,k._sep=M),this._formatted&&(k.external=this._formatted),this.path&&(k.path=this.path),this.scheme&&(k.scheme=this.scheme),this.authority&&(k.authority=this.authority),this.query&&(k.query=this.query),this.fragment&&(k.fragment=this.fragment),k},v}(w),G=((s={})[58]="%3A",s[47]="%2F",s[63]="%3F",s[35]="%23",s[91]="%5B",s[93]="%5D",s[64]="%40",s[33]="%21",s[36]="%24",s[38]="%26",s[39]="%27",s[40]="%28",s[41]="%29",s[42]="%2A",s[43]="%2B",s[44]="%2C",s[59]="%3B",s[61]="%3D",s[32]="%20",s);function J(T,v){for(var k=void 0,C=-1,x=0;x=97&&D<=122||D>=65&&D<=90||D>=48&&D<=57||D===45||D===46||D===95||D===126||v&&D===47)C!==-1&&(k+=encodeURIComponent(T.substring(C,x)),C=-1),k!==void 0&&(k+=T.charAt(x));else{k===void 0&&(k=T.substr(0,x));var L=G[D];L!==void 0?(C!==-1&&(k+=encodeURIComponent(T.substring(C,x)),C=-1),k+=L):C===-1&&(C=x)}}return C!==-1&&(k+=encodeURIComponent(T.substring(C))),k!==void 0?k:T}function f(T){for(var v=void 0,k=0;k1&&T.scheme==="file"?"//".concat(T.authority).concat(T.path):T.path.charCodeAt(0)===47&&(T.path.charCodeAt(1)>=65&&T.path.charCodeAt(1)<=90||T.path.charCodeAt(1)>=97&&T.path.charCodeAt(1)<=122)&&T.path.charCodeAt(2)===58?v?T.path.substr(1):T.path[1].toLowerCase()+T.path.substr(2):T.path,c&&(k=k.replace(/\//g,"\\")),k}function b(T,v){var k=v?f:J,C="",x=T.scheme,D=T.authority,L=T.path,q=T.query,j=T.fragment;if(x&&(C+=x,C+=":"),(D||x==="file")&&(C+=A,C+=A),D){var O=D.indexOf("@");if(O!==-1){var V=D.substr(0,O);D=D.substr(O+1),(O=V.indexOf(":"))===-1?C+=k(V,!1):(C+=k(V.substr(0,O),!1),C+=":",C+=k(V.substr(O+1),!1)),C+="@"}(O=(D=D.toLowerCase()).indexOf(":"))===-1?C+=k(D,!1):(C+=k(D.substr(0,O),!1),C+=D.substr(O))}if(L){if(L.length>=3&&L.charCodeAt(0)===47&&L.charCodeAt(2)===58)(K=L.charCodeAt(1))>=65&&K<=90&&(L="/".concat(String.fromCharCode(K+32),":").concat(L.substr(3)));else if(L.length>=2&&L.charCodeAt(1)===58){var K;(K=L.charCodeAt(0))>=65&&K<=90&&(L="".concat(String.fromCharCode(K+32),":").concat(L.substr(2)))}C+=k(L,!0)}return q&&(C+="?",C+=k(q,!1)),j&&(C+="#",C+=v?j:J(j,!1)),C}function N(T){try{return decodeURIComponent(T)}catch{return T.length>3?T.substr(0,3)+N(T.substr(3)):T}}var R=/(%[0-9A-Za-z][0-9A-Za-z])+/g;function U(T){return T.match(R)?T.replace(R,function(v){return N(v)}):T}var H,z=a(470),I=function(T,v,k){if(k||arguments.length===2)for(var C,x=0,D=v.length;x{for(var a in e)o.o(e,a)&&!o.o(n,a)&&Object.defineProperty(n,a,{enumerable:!0,get:e[a]})},o.o=(n,e)=>Object.prototype.hasOwnProperty.call(n,e),o.r=n=>{typeof Symbol<"u"&&Symbol.toStringTag&&Object.defineProperty(n,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(n,"__esModule",{value:!0})},o(447)})();var{URI:un,Utils:Dr}=ln;function ft(t){var i=t[0],o=t[t.length-1];return i===o&&(i==="'"||i==='"')&&(t=t.substr(1,t.length-2)),t}function _i(t,i){return!t.length||i==="handlebars"&&/{{|}}/.test(t)?!1:/\b(w[\w\d+.-]*:\/\/)?[^\s()<>]+(?:\([\w\d]+\)|([^[:punct:]\s]|\/?))/.test(t)}function yi(t,i,o,n){if(!(/^\s*javascript\:/i.test(i)||/[\n\r]/.test(i))){if(i=i.replace(/^\s*/g,""),/^https?:\/\//i.test(i)||/^file:\/\//i.test(i))return i;if(/^\#/i.test(i))return t+i;if(/^\/\//i.test(i)){var e=ae(t,"https://")?"https":"http";return e+":"+i.replace(/^\s*/g,"")}return o?o.resolveReference(i,n||t):i}}function Ti(t,i,o,n,e,a){var c=ft(o);if(!!_i(c,t.languageId)){c.length"u"&&(l=ft(u),l&&i&&(l=i.resolveReference(l,t.uri))),c=!1,a=void 0}else if(a==="id"){var d=ft(n.getTokenText());r[d]=n.getTokenOffset()}break}e=n.scan()}for(var g=0,y=o;go.startLine){if(r.endLine<=o.endLine)n.push(o),o=r,c(l,n.length);else if(r.startLine>o.endLine){do o=n.pop();while(o&&r.startLine>o.endLine);o&&n.push(o),o=r,c(l,n.length)}}}for(var s=0,u=0,l=0;li){u=l;break}s+=h}}for(var d=[],l=0;l=0&&a[h].tagName!==c;)h--;if(h>=0){var d=a[h];a.length=h;var g=t.positionAt(o.getTokenOffset()).line,u=d.startLine,y=g-1;y>u&&l!==u&&r({startLine:u,endLine:y})}break}case S.Comment:{var u=t.positionAt(o.getTokenOffset()).line,m=o.getTokenText(),A=m.match(/^\s*#(region\b)|(endregion\b)/);if(A)if(A[1])a.push({startLine:u,tagName:""});else{for(var h=a.length-1;h>=0&&a[h].tagName.length;)h--;if(h>=0){var d=a[h];a.length=h;var y=u;u=d.startLine,y>u&&l!==u&&r({startLine:u,endLine:y,kind:_e.Region})}}else{var y=t.positionAt(o.getTokenOffset()+o.getTokenLength()).line;uE?xi(e,E):e}function yn(t,i){function o(n){for(var e=Di(t,n),a=void 0,c=void 0,l=e.length-1;l>=0;l--){var r=e[l];(!a||r[0]!==a[0]||r[1]!==a[1])&&(c=ke.create(P.create(t.positionAt(e[l][0]),t.positionAt(e[l][1])),c)),a=r}return c||(c=ke.create(P.create(n,n))),c}return i.map(o)}function Di(t,i){var o=je(t.getText()),n=t.offsetAt(i),e=o.findNodeAt(n),a=Ei(e);if(e.startTagEnd&&!e.endTagStart){if(e.startTagEnd!==e.end)return[[e.start,e.end]];var c=P.create(t.positionAt(e.startTagEnd-2),t.positionAt(e.startTagEnd)),l=t.getText(c);l==="/>"?a.unshift([e.start+1,e.startTagEnd-2]):a.unshift([e.start+1,e.startTagEnd-1]);var r=_n(t,e,n);return a=r.concat(a),a}if(!e.startTagEnd||!e.endTagStart)return a;if(a.unshift([e.start,e.end]),e.start=e.endTagStart+2&&a.unshift([e.endTagStart+2,e.end-1]),a)}function Ei(t){for(var i=t,o=function(e){return e.startTagEnd&&e.endTagStart&&e.startTagEnd=c.getTokenOffset()&&a<=c.getTokenEnd()&&(s.unshift([c.getTokenOffset(),c.getTokenEnd()]),(d[0]==='"'&&d[d.length-1]==='"'||d[0]==="'"&&d[d.length-1]==="'")&&a>=c.getTokenOffset()+1&&a<=c.getTokenEnd()-1&&s.unshift([c.getTokenOffset()+1,c.getTokenEnd()-1]),s.push([h,c.getTokenEnd()]));break}}l=c.scan()}return s.map(function(g){return[g[0]+r,g[1]+r]})}var bt={version:1.1,tags:[{name:"html",description:{kind:"markdown",value:"The html element represents the root of an HTML document."},attributes:[{name:"manifest",description:{kind:"markdown",value:"Specifies the URI of a resource manifest indicating resources that should be cached locally. See [Using the application cache](https://developer.mozilla.org/en-US/docs/Web/HTML/Using_the_application_cache) for details."}},{name:"version",description:'Specifies the version of the HTML [Document Type Definition](https://developer.mozilla.org/en-US/docs/Glossary/DTD "Document Type Definition: In HTML, the doctype is the required "" preamble found at the top of all documents. Its sole purpose is to prevent a browser from switching into so-called \u201Cquirks mode\u201D when rendering a document; that is, the "" doctype ensures that the browser makes a best-effort attempt at following the relevant specifications, rather than using a different rendering mode that is incompatible with some specifications.") that governs the current document. This attribute is not needed, because it is redundant with the version information in the document type declaration.'},{name:"xmlns",description:'Specifies the XML Namespace of the document. Default value is `"http://www.w3.org/1999/xhtml"`. This is required in documents parsed with XML parsers, and optional in text/html documents.'}],references:[{name:"MDN Reference",url:"https://developer.mozilla.org/docs/Web/HTML/Element/html"}]},{name:"head",description:{kind:"markdown",value:"The head element represents a collection of metadata for the Document."},attributes:[{name:"profile",description:"The URIs of one or more metadata profiles, separated by white space."}],references:[{name:"MDN Reference",url:"https://developer.mozilla.org/docs/Web/HTML/Element/head"}]},{name:"title",description:{kind:"markdown",value:"The title element represents the document's title or name. Authors should use titles that identify their documents even when they are used out of context, for example in a user's history or bookmarks, or in search results. The document's title is often different from its first heading, since the first heading does not have to stand alone when taken out of context."},attributes:[],references:[{name:"MDN Reference",url:"https://developer.mozilla.org/docs/Web/HTML/Element/title"}]},{name:"base",description:{kind:"markdown",value:"The base element allows authors to specify the document base URL for the purposes of resolving relative URLs, and the name of the default browsing context for the purposes of following hyperlinks. The element does not represent any content beyond this information."},attributes:[{name:"href",description:{kind:"markdown",value:"The base URL to be used throughout the document for relative URL addresses. If this attribute is specified, this element must come before any other elements with attributes whose values are URLs. Absolute and relative URLs are allowed."}},{name:"target",description:{kind:"markdown",value:"A name or keyword indicating the default location to display the result when hyperlinks or forms cause navigation, for elements that do not have an explicit target reference. It is a name of, or keyword for, a _browsing context_ (for example: tab, window, or inline frame). The following keywords have special meanings:\n\n* `_self`: Load the result into the same browsing context as the current one. This value is the default if the attribute is not specified.\n* `_blank`: Load the result into a new unnamed browsing context.\n* `_parent`: Load the result into the parent browsing context of the current one. If there is no parent, this option behaves the same way as `_self`.\n* `_top`: Load the result into the top-level browsing context (that is, the browsing context that is an ancestor of the current one, and has no parent). If there is no parent, this option behaves the same way as `_self`.\n\nIf this attribute is specified, this element must come before any other elements with attributes whose values are URLs."}}],references:[{name:"MDN Reference",url:"https://developer.mozilla.org/docs/Web/HTML/Element/base"}]},{name:"link",description:{kind:"markdown",value:"The link element allows authors to link their document to other resources."},attributes:[{name:"href",description:{kind:"markdown",value:'This attribute specifies the [URL](https://developer.mozilla.org/en-US/docs/Glossary/URL "URL: Uniform Resource Locator (URL) is a text string specifying where a resource can be found on the Internet.") of the linked resource. A URL can be absolute or relative.'}},{name:"crossorigin",valueSet:"xo",description:{kind:"markdown",value:'This enumerated attribute indicates whether [CORS](https://developer.mozilla.org/en-US/docs/Glossary/CORS "CORS: CORS (Cross-Origin Resource Sharing) is a system, consisting of transmitting HTTP headers, that determines whether browsers block frontend JavaScript code from accessing responses for cross-origin requests.") must be used when fetching the resource. [CORS-enabled images](https://developer.mozilla.org/en-US/docs/Web/HTML/CORS_Enabled_Image) can be reused in the [`