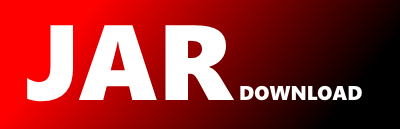
rvinh.java-plugins.1.2.source-code.PluginLoader Maven / Gradle / Ivy
import com.github.javaparser.JavaParser;
import com.github.javaparser.ParseResult;
import com.github.javaparser.ast.CompilationUnit;
import com.github.javaparser.ast.Modifier;
import com.github.javaparser.ast.body.TypeDeclaration;
import org.apache.commons.io.FileUtils;
import javax.tools.JavaCompiler;
import javax.tools.ToolProvider;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLClassLoader;
import java.nio.file.Files;
import java.util.Arrays;
import java.util.Set;
import java.util.stream.Collectors;
/**
* This class loads plugins and instantiates them
*/
public class PluginLoader {
public static class LoadingException extends Exception{
public LoadingException(String message) {
super(message);
}
public LoadingException(Throwable cause) {
super(cause);
}
}
/**
* Compiles a plugin given as a java class in source code form and loads it into the Java Runtime.
* It only loads classes that extend the given java class.
* After successfully loading it, an instance of each found class that extends the given class
* will be returned to the caller.
* If a class already exists, it will be updated if its code has changed. If the code has
* not changed, the loading is skipped and a new instance of the class is returned.
* @param plugin The string containing the source code of the plugin class
* @param pluginInterface The interface/class the plugin class has to implement/extend
* @return OBJECT The new instance of the class
* @throws LoadingException When something goes wrong while compiling or loading the class
*/
public
© 2015 - 2025 Weber Informatics LLC | Privacy Policy