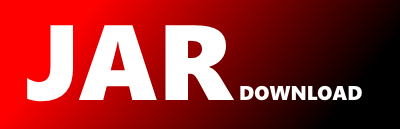
chs.jdmn.jdmn-core.2.5.1.source-code.SFEELParser Maven / Gradle / Ivy
// Generated from SFEELParser.g4 by ANTLR 4.7.1
package com.gs.dmn.feel.analysis.syntax.antlrv4;
import com.gs.dmn.feel.analysis.syntax.ast.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.arithmetic.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.comparison.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.function.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.literal.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.logic.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.textual.*;
import com.gs.dmn.feel.analysis.syntax.ast.test.*;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class SFEELParser extends Parser {
static { RuntimeMetaData.checkVersion("4.7.1", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
BLOCK_COMMENT=1, LINE_COMMENT=2, WS=3, STRING=4, NUMBER=5, EQ=6, NE=7,
LT=8, GT=9, LE=10, GE=11, PLUS=12, MINUS=13, STAR=14, FORWARD_SLASH=15,
STAR_STAR=16, DOT_DOT=17, DOT=18, COMMA=19, PAREN_OPEN=20, PAREN_CLOSE=21,
BRACKET_OPEN=22, BRACKET_CLOSE=23, NOT=24, TRUE=25, FALSE=26, NAME=27;
public static final int
RULE_simpleUnaryTestsRoot = 0, RULE_expressionRoot = 1, RULE_simpleExpressionsRoot = 2,
RULE_simpleUnaryTests = 3, RULE_simplePositiveUnaryTests = 4, RULE_simplePositiveUnaryTest = 5,
RULE_interval = 6, RULE_intervalStartPar = 7, RULE_intervalEndPar = 8,
RULE_endpoint = 9, RULE_expression = 10, RULE_simpleExpressions = 11,
RULE_simpleExpression = 12, RULE_comparison = 13, RULE_arithmeticExpression = 14,
RULE_addition = 15, RULE_multiplication = 16, RULE_exponentiation = 17,
RULE_arithmeticNegation = 18, RULE_primaryExpression = 19, RULE_simpleValue = 20,
RULE_qualifiedName = 21, RULE_simpleLiteral = 22, RULE_stringLiteral = 23,
RULE_booleanLiteral = 24, RULE_numericLiteral = 25, RULE_dateTimeLiteral = 26,
RULE_identifier = 27;
public static final String[] ruleNames = {
"simpleUnaryTestsRoot", "expressionRoot", "simpleExpressionsRoot", "simpleUnaryTests",
"simplePositiveUnaryTests", "simplePositiveUnaryTest", "interval", "intervalStartPar",
"intervalEndPar", "endpoint", "expression", "simpleExpressions", "simpleExpression",
"comparison", "arithmeticExpression", "addition", "multiplication", "exponentiation",
"arithmeticNegation", "primaryExpression", "simpleValue", "qualifiedName",
"simpleLiteral", "stringLiteral", "booleanLiteral", "numericLiteral",
"dateTimeLiteral", "identifier"
};
private static final String[] _LITERAL_NAMES = {
null, null, null, null, null, null, null, "'!='", "'<'", "'>'", "'<='",
"'>='", "'+'", "'-'", "'*'", "'/'", "'**'", "'..'", "'.'", "','", "'('",
"')'", "'['", "']'", "'not'", "'true'", "'false'"
};
private static final String[] _SYMBOLIC_NAMES = {
null, "BLOCK_COMMENT", "LINE_COMMENT", "WS", "STRING", "NUMBER", "EQ",
"NE", "LT", "GT", "LE", "GE", "PLUS", "MINUS", "STAR", "FORWARD_SLASH",
"STAR_STAR", "DOT_DOT", "DOT", "COMMA", "PAREN_OPEN", "PAREN_CLOSE", "BRACKET_OPEN",
"BRACKET_CLOSE", "NOT", "TRUE", "FALSE", "NAME"
};
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "SFEELParser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
private ASTFactory astFactory;
public SFEELParser(TokenStream input, ASTFactory astFactory) {
this(input);
this.astFactory = astFactory;
}
public ASTFactory getASTFactory() {
return astFactory;
}
public SFEELParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class SimpleUnaryTestsRootContext extends ParserRuleContext {
public SimpleUnaryTests ast;
public SimpleUnaryTestsContext simpleUnaryTests;
public SimpleUnaryTestsContext simpleUnaryTests() {
return getRuleContext(SimpleUnaryTestsContext.class,0);
}
public TerminalNode EOF() { return getToken(SFEELParser.EOF, 0); }
public SimpleUnaryTestsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleUnaryTestsRoot; }
}
public final SimpleUnaryTestsRootContext simpleUnaryTestsRoot() throws RecognitionException {
SimpleUnaryTestsRootContext _localctx = new SimpleUnaryTestsRootContext(_ctx, getState());
enterRule(_localctx, 0, RULE_simpleUnaryTestsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(56);
((SimpleUnaryTestsRootContext)_localctx).simpleUnaryTests = simpleUnaryTests();
((SimpleUnaryTestsRootContext)_localctx).ast = ((SimpleUnaryTestsRootContext)_localctx).simpleUnaryTests.ast;
setState(58);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExpressionRootContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext expression;
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode EOF() { return getToken(SFEELParser.EOF, 0); }
public ExpressionRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expressionRoot; }
}
public final ExpressionRootContext expressionRoot() throws RecognitionException {
ExpressionRootContext _localctx = new ExpressionRootContext(_ctx, getState());
enterRule(_localctx, 2, RULE_expressionRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(60);
((ExpressionRootContext)_localctx).expression = expression();
((ExpressionRootContext)_localctx).ast = ((ExpressionRootContext)_localctx).expression.ast;
setState(62);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleExpressionsRootContext extends ParserRuleContext {
public Expression ast;
public SimpleExpressionsContext simpleExpressions;
public SimpleExpressionsContext simpleExpressions() {
return getRuleContext(SimpleExpressionsContext.class,0);
}
public TerminalNode EOF() { return getToken(SFEELParser.EOF, 0); }
public SimpleExpressionsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleExpressionsRoot; }
}
public final SimpleExpressionsRootContext simpleExpressionsRoot() throws RecognitionException {
SimpleExpressionsRootContext _localctx = new SimpleExpressionsRootContext(_ctx, getState());
enterRule(_localctx, 4, RULE_simpleExpressionsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(64);
((SimpleExpressionsRootContext)_localctx).simpleExpressions = simpleExpressions();
((SimpleExpressionsRootContext)_localctx).ast = ((SimpleExpressionsRootContext)_localctx).simpleExpressions.ast;
setState(66);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleUnaryTestsContext extends ParserRuleContext {
public SimpleUnaryTests ast;
public SimplePositiveUnaryTestsContext tests;
public TerminalNode NOT() { return getToken(SFEELParser.NOT, 0); }
public TerminalNode PAREN_OPEN() { return getToken(SFEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(SFEELParser.PAREN_CLOSE, 0); }
public SimplePositiveUnaryTestsContext simplePositiveUnaryTests() {
return getRuleContext(SimplePositiveUnaryTestsContext.class,0);
}
public TerminalNode MINUS() { return getToken(SFEELParser.MINUS, 0); }
public SimpleUnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleUnaryTests; }
}
public final SimpleUnaryTestsContext simpleUnaryTests() throws RecognitionException {
SimpleUnaryTestsContext _localctx = new SimpleUnaryTestsContext(_ctx, getState());
enterRule(_localctx, 6, RULE_simpleUnaryTests);
try {
setState(79);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,0,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(68);
match(NOT);
setState(69);
match(PAREN_OPEN);
setState(70);
((SimpleUnaryTestsContext)_localctx).tests = simplePositiveUnaryTests();
setState(71);
match(PAREN_CLOSE);
((SimpleUnaryTestsContext)_localctx).ast = astFactory.toNegatedSimpleUnaryTests(((SimpleUnaryTestsContext)_localctx).tests.ast);
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(74);
((SimpleUnaryTestsContext)_localctx).tests = simplePositiveUnaryTests();
((SimpleUnaryTestsContext)_localctx).ast = ((SimpleUnaryTestsContext)_localctx).tests.ast;
}
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
{
setState(77);
match(MINUS);
((SimpleUnaryTestsContext)_localctx).ast = astFactory.toAny();
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimplePositiveUnaryTestsContext extends ParserRuleContext {
public SimplePositiveUnaryTests ast;
public SimplePositiveUnaryTestContext test;
public List simplePositiveUnaryTest() {
return getRuleContexts(SimplePositiveUnaryTestContext.class);
}
public SimplePositiveUnaryTestContext simplePositiveUnaryTest(int i) {
return getRuleContext(SimplePositiveUnaryTestContext.class,i);
}
public List COMMA() { return getTokens(SFEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(SFEELParser.COMMA, i);
}
public SimplePositiveUnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simplePositiveUnaryTests; }
}
public final SimplePositiveUnaryTestsContext simplePositiveUnaryTests() throws RecognitionException {
SimplePositiveUnaryTestsContext _localctx = new SimplePositiveUnaryTestsContext(_ctx, getState());
enterRule(_localctx, 8, RULE_simplePositiveUnaryTests);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List tests = new ArrayList<>();
setState(82);
((SimplePositiveUnaryTestsContext)_localctx).test = simplePositiveUnaryTest();
tests.add(((SimplePositiveUnaryTestsContext)_localctx).test.ast);
setState(90);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(84);
match(COMMA);
setState(85);
((SimplePositiveUnaryTestsContext)_localctx).test = simplePositiveUnaryTest();
tests.add(((SimplePositiveUnaryTestsContext)_localctx).test.ast);
}
}
setState(92);
_errHandler.sync(this);
_la = _input.LA(1);
}
((SimplePositiveUnaryTestsContext)_localctx).ast = astFactory.toSimplePositiveUnaryTests(tests);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimplePositiveUnaryTestContext extends ParserRuleContext {
public SimplePositiveUnaryTest ast;
public Token op;
public EndpointContext opd;
public IntervalContext opd2;
public EndpointContext endpoint() {
return getRuleContext(EndpointContext.class,0);
}
public TerminalNode LT() { return getToken(SFEELParser.LT, 0); }
public TerminalNode LE() { return getToken(SFEELParser.LE, 0); }
public TerminalNode GT() { return getToken(SFEELParser.GT, 0); }
public TerminalNode GE() { return getToken(SFEELParser.GE, 0); }
public IntervalContext interval() {
return getRuleContext(IntervalContext.class,0);
}
public SimplePositiveUnaryTestContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simplePositiveUnaryTest; }
}
public final SimplePositiveUnaryTestContext simplePositiveUnaryTest() throws RecognitionException {
SimplePositiveUnaryTestContext _localctx = new SimplePositiveUnaryTestContext(_ctx, getState());
enterRule(_localctx, 10, RULE_simplePositiveUnaryTest);
try {
setState(107);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING:
case NUMBER:
case LT:
case GT:
case LE:
case GE:
case MINUS:
case TRUE:
case FALSE:
case NAME:
enterOuterAlt(_localctx, 1);
{
{
setState(99);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LT:
{
setState(95);
((SimplePositiveUnaryTestContext)_localctx).op = match(LT);
}
break;
case LE:
{
setState(96);
((SimplePositiveUnaryTestContext)_localctx).op = match(LE);
}
break;
case GT:
{
setState(97);
((SimplePositiveUnaryTestContext)_localctx).op = match(GT);
}
break;
case GE:
{
setState(98);
((SimplePositiveUnaryTestContext)_localctx).op = match(GE);
}
break;
case STRING:
case NUMBER:
case MINUS:
case TRUE:
case FALSE:
case NAME:
break;
default:
break;
}
setState(101);
((SimplePositiveUnaryTestContext)_localctx).opd = endpoint();
((SimplePositiveUnaryTestContext)_localctx).ast = ((SimplePositiveUnaryTestContext)_localctx).op == null ? astFactory.toOperatorTest(null, ((SimplePositiveUnaryTestContext)_localctx).opd.ast) : astFactory.toOperatorTest((((SimplePositiveUnaryTestContext)_localctx).op!=null?((SimplePositiveUnaryTestContext)_localctx).op.getText():null), ((SimplePositiveUnaryTestContext)_localctx).opd.ast);
}
}
break;
case PAREN_OPEN:
case BRACKET_OPEN:
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 2);
{
{
setState(104);
((SimplePositiveUnaryTestContext)_localctx).opd2 = interval();
((SimplePositiveUnaryTestContext)_localctx).ast = ((SimplePositiveUnaryTestContext)_localctx).opd2.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntervalContext extends ParserRuleContext {
public RangeTest ast;
public IntervalStartParContext leftPar;
public EndpointContext ep1;
public EndpointContext ep2;
public IntervalEndParContext rightPar;
public TerminalNode DOT_DOT() { return getToken(SFEELParser.DOT_DOT, 0); }
public IntervalStartParContext intervalStartPar() {
return getRuleContext(IntervalStartParContext.class,0);
}
public List endpoint() {
return getRuleContexts(EndpointContext.class);
}
public EndpointContext endpoint(int i) {
return getRuleContext(EndpointContext.class,i);
}
public IntervalEndParContext intervalEndPar() {
return getRuleContext(IntervalEndParContext.class,0);
}
public IntervalContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_interval; }
}
public final IntervalContext interval() throws RecognitionException {
IntervalContext _localctx = new IntervalContext(_ctx, getState());
enterRule(_localctx, 12, RULE_interval);
try {
enterOuterAlt(_localctx, 1);
{
setState(109);
((IntervalContext)_localctx).leftPar = intervalStartPar();
setState(110);
((IntervalContext)_localctx).ep1 = endpoint();
setState(111);
match(DOT_DOT);
setState(112);
((IntervalContext)_localctx).ep2 = endpoint();
setState(113);
((IntervalContext)_localctx).rightPar = intervalEndPar();
((IntervalContext)_localctx).ast = astFactory.toIntervalTest(((IntervalContext)_localctx).leftPar.ast, ((IntervalContext)_localctx).ep1.ast, ((IntervalContext)_localctx).rightPar.ast, ((IntervalContext)_localctx).ep2.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntervalStartParContext extends ParserRuleContext {
public String ast;
public Token token;
public TerminalNode PAREN_OPEN() { return getToken(SFEELParser.PAREN_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(SFEELParser.BRACKET_CLOSE, 0); }
public TerminalNode BRACKET_OPEN() { return getToken(SFEELParser.BRACKET_OPEN, 0); }
public IntervalStartParContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intervalStartPar; }
}
public final IntervalStartParContext intervalStartPar() throws RecognitionException {
IntervalStartParContext _localctx = new IntervalStartParContext(_ctx, getState());
enterRule(_localctx, 14, RULE_intervalStartPar);
try {
setState(122);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PAREN_OPEN:
enterOuterAlt(_localctx, 1);
{
{
setState(116);
((IntervalStartParContext)_localctx).token = match(PAREN_OPEN);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 2);
{
{
setState(118);
((IntervalStartParContext)_localctx).token = match(BRACKET_CLOSE);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_OPEN:
enterOuterAlt(_localctx, 3);
{
{
setState(120);
((IntervalStartParContext)_localctx).token = match(BRACKET_OPEN);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntervalEndParContext extends ParserRuleContext {
public String ast;
public Token token;
public TerminalNode PAREN_CLOSE() { return getToken(SFEELParser.PAREN_CLOSE, 0); }
public TerminalNode BRACKET_OPEN() { return getToken(SFEELParser.BRACKET_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(SFEELParser.BRACKET_CLOSE, 0); }
public IntervalEndParContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intervalEndPar; }
}
public final IntervalEndParContext intervalEndPar() throws RecognitionException {
IntervalEndParContext _localctx = new IntervalEndParContext(_ctx, getState());
enterRule(_localctx, 16, RULE_intervalEndPar);
try {
setState(130);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PAREN_CLOSE:
enterOuterAlt(_localctx, 1);
{
{
setState(124);
((IntervalEndParContext)_localctx).token = match(PAREN_CLOSE);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_OPEN:
enterOuterAlt(_localctx, 2);
{
{
setState(126);
((IntervalEndParContext)_localctx).token = match(BRACKET_OPEN);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 3);
{
{
setState(128);
((IntervalEndParContext)_localctx).token = match(BRACKET_CLOSE);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EndpointContext extends ParserRuleContext {
public Expression ast;
public Token op;
public SimpleValueContext opd;
public SimpleValueContext simpleValue() {
return getRuleContext(SimpleValueContext.class,0);
}
public TerminalNode MINUS() { return getToken(SFEELParser.MINUS, 0); }
public EndpointContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_endpoint; }
}
public final EndpointContext endpoint() throws RecognitionException {
EndpointContext _localctx = new EndpointContext(_ctx, getState());
enterRule(_localctx, 18, RULE_endpoint);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(133);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==MINUS) {
{
setState(132);
((EndpointContext)_localctx).op = match(MINUS);
}
}
setState(135);
((EndpointContext)_localctx).opd = simpleValue();
((EndpointContext)_localctx).ast = (((EndpointContext)_localctx).op == null) ? ((EndpointContext)_localctx).opd.ast : astFactory.toNegation((((EndpointContext)_localctx).op!=null?((EndpointContext)_localctx).op.getText():null), ((EndpointContext)_localctx).opd.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExpressionContext extends ParserRuleContext {
public Expression ast;
public SimpleExpressionContext left;
public SimpleExpressionContext simpleExpression() {
return getRuleContext(SimpleExpressionContext.class,0);
}
public ExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression; }
}
public final ExpressionContext expression() throws RecognitionException {
ExpressionContext _localctx = new ExpressionContext(_ctx, getState());
enterRule(_localctx, 20, RULE_expression);
try {
enterOuterAlt(_localctx, 1);
{
setState(138);
((ExpressionContext)_localctx).left = simpleExpression();
((ExpressionContext)_localctx).ast = ((ExpressionContext)_localctx).left.ast;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleExpressionsContext extends ParserRuleContext {
public Expression ast;
public SimpleExpressionContext exp;
public List simpleExpression() {
return getRuleContexts(SimpleExpressionContext.class);
}
public SimpleExpressionContext simpleExpression(int i) {
return getRuleContext(SimpleExpressionContext.class,i);
}
public List COMMA() { return getTokens(SFEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(SFEELParser.COMMA, i);
}
public SimpleExpressionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleExpressions; }
}
public final SimpleExpressionsContext simpleExpressions() throws RecognitionException {
SimpleExpressionsContext _localctx = new SimpleExpressionsContext(_ctx, getState());
enterRule(_localctx, 22, RULE_simpleExpressions);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List expressionList = new ArrayList<>();
setState(142);
((SimpleExpressionsContext)_localctx).exp = simpleExpression();
expressionList.add(((SimpleExpressionsContext)_localctx).exp.ast);
setState(150);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(144);
match(COMMA);
setState(145);
((SimpleExpressionsContext)_localctx).exp = simpleExpression();
expressionList.add(((SimpleExpressionsContext)_localctx).exp.ast);
}
}
setState(152);
_errHandler.sync(this);
_la = _input.LA(1);
}
((SimpleExpressionsContext)_localctx).ast = astFactory.toExpressionList(expressionList);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleExpressionContext extends ParserRuleContext {
public Expression ast;
public ComparisonContext left;
public ComparisonContext comparison() {
return getRuleContext(ComparisonContext.class,0);
}
public SimpleExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleExpression; }
}
public final SimpleExpressionContext simpleExpression() throws RecognitionException {
SimpleExpressionContext _localctx = new SimpleExpressionContext(_ctx, getState());
enterRule(_localctx, 24, RULE_simpleExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(155);
((SimpleExpressionContext)_localctx).left = comparison();
((SimpleExpressionContext)_localctx).ast = ((SimpleExpressionContext)_localctx).left.ast;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ComparisonContext extends ParserRuleContext {
public Expression ast;
public ArithmeticExpressionContext ae1;
public Token op;
public ArithmeticExpressionContext ae2;
public List arithmeticExpression() {
return getRuleContexts(ArithmeticExpressionContext.class);
}
public ArithmeticExpressionContext arithmeticExpression(int i) {
return getRuleContext(ArithmeticExpressionContext.class,i);
}
public TerminalNode EQ() { return getToken(SFEELParser.EQ, 0); }
public TerminalNode NE() { return getToken(SFEELParser.NE, 0); }
public TerminalNode LT() { return getToken(SFEELParser.LT, 0); }
public TerminalNode GT() { return getToken(SFEELParser.GT, 0); }
public TerminalNode LE() { return getToken(SFEELParser.LE, 0); }
public TerminalNode GE() { return getToken(SFEELParser.GE, 0); }
public ComparisonContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_comparison; }
}
public final ComparisonContext comparison() throws RecognitionException {
ComparisonContext _localctx = new ComparisonContext(_ctx, getState());
enterRule(_localctx, 26, RULE_comparison);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(158);
((ComparisonContext)_localctx).ae1 = arithmeticExpression();
((ComparisonContext)_localctx).ast = ((ComparisonContext)_localctx).ae1.ast;
setState(171);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << EQ) | (1L << NE) | (1L << LT) | (1L << GT) | (1L << LE) | (1L << GE))) != 0)) {
{
setState(166);
_errHandler.sync(this);
switch (_input.LA(1)) {
case EQ:
{
setState(160);
((ComparisonContext)_localctx).op = match(EQ);
}
break;
case NE:
{
setState(161);
((ComparisonContext)_localctx).op = match(NE);
}
break;
case LT:
{
setState(162);
((ComparisonContext)_localctx).op = match(LT);
}
break;
case GT:
{
setState(163);
((ComparisonContext)_localctx).op = match(GT);
}
break;
case LE:
{
setState(164);
((ComparisonContext)_localctx).op = match(LE);
}
break;
case GE:
{
setState(165);
((ComparisonContext)_localctx).op = match(GE);
}
break;
default:
throw new NoViableAltException(this);
}
setState(168);
((ComparisonContext)_localctx).ae2 = arithmeticExpression();
((ComparisonContext)_localctx).ast = astFactory.toComparison((((ComparisonContext)_localctx).op!=null?((ComparisonContext)_localctx).op.getText():null), ((ComparisonContext)_localctx).ae1.ast, ((ComparisonContext)_localctx).ae2.ast);
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArithmeticExpressionContext extends ParserRuleContext {
public Expression ast;
public AdditionContext addition;
public AdditionContext addition() {
return getRuleContext(AdditionContext.class,0);
}
public ArithmeticExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arithmeticExpression; }
}
public final ArithmeticExpressionContext arithmeticExpression() throws RecognitionException {
ArithmeticExpressionContext _localctx = new ArithmeticExpressionContext(_ctx, getState());
enterRule(_localctx, 28, RULE_arithmeticExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(173);
((ArithmeticExpressionContext)_localctx).addition = addition();
((ArithmeticExpressionContext)_localctx).ast = ((ArithmeticExpressionContext)_localctx).addition.ast;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AdditionContext extends ParserRuleContext {
public Expression ast;
public MultiplicationContext left;
public Token op;
public MultiplicationContext right;
public List multiplication() {
return getRuleContexts(MultiplicationContext.class);
}
public MultiplicationContext multiplication(int i) {
return getRuleContext(MultiplicationContext.class,i);
}
public List PLUS() { return getTokens(SFEELParser.PLUS); }
public TerminalNode PLUS(int i) {
return getToken(SFEELParser.PLUS, i);
}
public List MINUS() { return getTokens(SFEELParser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(SFEELParser.MINUS, i);
}
public AdditionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_addition; }
}
public final AdditionContext addition() throws RecognitionException {
AdditionContext _localctx = new AdditionContext(_ctx, getState());
enterRule(_localctx, 30, RULE_addition);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(176);
((AdditionContext)_localctx).left = multiplication();
((AdditionContext)_localctx).ast = ((AdditionContext)_localctx).left.ast;
setState(187);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==PLUS || _la==MINUS) {
{
{
setState(180);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PLUS:
{
setState(178);
((AdditionContext)_localctx).op = match(PLUS);
}
break;
case MINUS:
{
setState(179);
((AdditionContext)_localctx).op = match(MINUS);
}
break;
default:
throw new NoViableAltException(this);
}
setState(182);
((AdditionContext)_localctx).right = multiplication();
((AdditionContext)_localctx).ast = astFactory.toAddition((((AdditionContext)_localctx).op!=null?((AdditionContext)_localctx).op.getText():null), _localctx.ast, ((AdditionContext)_localctx).right.ast);
}
}
setState(189);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class MultiplicationContext extends ParserRuleContext {
public Expression ast;
public ExponentiationContext left;
public Token op;
public ExponentiationContext right;
public List exponentiation() {
return getRuleContexts(ExponentiationContext.class);
}
public ExponentiationContext exponentiation(int i) {
return getRuleContext(ExponentiationContext.class,i);
}
public List STAR() { return getTokens(SFEELParser.STAR); }
public TerminalNode STAR(int i) {
return getToken(SFEELParser.STAR, i);
}
public List FORWARD_SLASH() { return getTokens(SFEELParser.FORWARD_SLASH); }
public TerminalNode FORWARD_SLASH(int i) {
return getToken(SFEELParser.FORWARD_SLASH, i);
}
public MultiplicationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_multiplication; }
}
public final MultiplicationContext multiplication() throws RecognitionException {
MultiplicationContext _localctx = new MultiplicationContext(_ctx, getState());
enterRule(_localctx, 32, RULE_multiplication);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(190);
((MultiplicationContext)_localctx).left = exponentiation();
((MultiplicationContext)_localctx).ast = ((MultiplicationContext)_localctx).left.ast;
setState(201);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==STAR || _la==FORWARD_SLASH) {
{
{
setState(194);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STAR:
{
setState(192);
((MultiplicationContext)_localctx).op = match(STAR);
}
break;
case FORWARD_SLASH:
{
setState(193);
((MultiplicationContext)_localctx).op = match(FORWARD_SLASH);
}
break;
default:
throw new NoViableAltException(this);
}
setState(196);
((MultiplicationContext)_localctx).right = exponentiation();
((MultiplicationContext)_localctx).ast = astFactory.toMultiplication((((MultiplicationContext)_localctx).op!=null?((MultiplicationContext)_localctx).op.getText():null), _localctx.ast, ((MultiplicationContext)_localctx).right.ast);
}
}
setState(203);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExponentiationContext extends ParserRuleContext {
public Expression ast;
public ArithmeticNegationContext left;
public ArithmeticNegationContext right;
public List arithmeticNegation() {
return getRuleContexts(ArithmeticNegationContext.class);
}
public ArithmeticNegationContext arithmeticNegation(int i) {
return getRuleContext(ArithmeticNegationContext.class,i);
}
public List STAR_STAR() { return getTokens(SFEELParser.STAR_STAR); }
public TerminalNode STAR_STAR(int i) {
return getToken(SFEELParser.STAR_STAR, i);
}
public ExponentiationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_exponentiation; }
}
public final ExponentiationContext exponentiation() throws RecognitionException {
ExponentiationContext _localctx = new ExponentiationContext(_ctx, getState());
enterRule(_localctx, 34, RULE_exponentiation);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(204);
((ExponentiationContext)_localctx).left = arithmeticNegation();
((ExponentiationContext)_localctx).ast = ((ExponentiationContext)_localctx).left.ast;
setState(212);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==STAR_STAR) {
{
{
setState(206);
match(STAR_STAR);
setState(207);
((ExponentiationContext)_localctx).right = arithmeticNegation();
((ExponentiationContext)_localctx).ast = astFactory.toExponentiation(_localctx.ast, ((ExponentiationContext)_localctx).right.ast);
}
}
setState(214);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArithmeticNegationContext extends ParserRuleContext {
public Expression ast;
public PrimaryExpressionContext opd;
public PrimaryExpressionContext primaryExpression() {
return getRuleContext(PrimaryExpressionContext.class,0);
}
public List MINUS() { return getTokens(SFEELParser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(SFEELParser.MINUS, i);
}
public ArithmeticNegationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arithmeticNegation; }
}
public final ArithmeticNegationContext arithmeticNegation() throws RecognitionException {
ArithmeticNegationContext _localctx = new ArithmeticNegationContext(_ctx, getState());
enterRule(_localctx, 36, RULE_arithmeticNegation);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List prefixOperators = new ArrayList<>();
setState(220);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==MINUS) {
{
{
{
setState(216);
match(MINUS);
prefixOperators.add("-");
}
}
}
setState(222);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(223);
((ArithmeticNegationContext)_localctx).opd = primaryExpression();
((ArithmeticNegationContext)_localctx).ast = astFactory.toNegation(prefixOperators, ((ArithmeticNegationContext)_localctx).opd.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PrimaryExpressionContext extends ParserRuleContext {
public Expression ast;
public SimpleValueContext simpleValue;
public ExpressionContext expression;
public SimpleValueContext simpleValue() {
return getRuleContext(SimpleValueContext.class,0);
}
public TerminalNode PAREN_OPEN() { return getToken(SFEELParser.PAREN_OPEN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode PAREN_CLOSE() { return getToken(SFEELParser.PAREN_CLOSE, 0); }
public PrimaryExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_primaryExpression; }
}
public final PrimaryExpressionContext primaryExpression() throws RecognitionException {
PrimaryExpressionContext _localctx = new PrimaryExpressionContext(_ctx, getState());
enterRule(_localctx, 38, RULE_primaryExpression);
try {
setState(234);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING:
case NUMBER:
case TRUE:
case FALSE:
case NAME:
enterOuterAlt(_localctx, 1);
{
{
setState(226);
((PrimaryExpressionContext)_localctx).simpleValue = simpleValue();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).simpleValue.ast;
}
}
break;
case PAREN_OPEN:
enterOuterAlt(_localctx, 2);
{
{
setState(229);
match(PAREN_OPEN);
setState(230);
((PrimaryExpressionContext)_localctx).expression = expression();
setState(231);
match(PAREN_CLOSE);
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).expression.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleValueContext extends ParserRuleContext {
public Expression ast;
public SimpleLiteralContext simpleLiteral;
public QualifiedNameContext qualifiedName;
public SimpleLiteralContext simpleLiteral() {
return getRuleContext(SimpleLiteralContext.class,0);
}
public QualifiedNameContext qualifiedName() {
return getRuleContext(QualifiedNameContext.class,0);
}
public SimpleValueContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleValue; }
}
public final SimpleValueContext simpleValue() throws RecognitionException {
SimpleValueContext _localctx = new SimpleValueContext(_ctx, getState());
enterRule(_localctx, 40, RULE_simpleValue);
try {
setState(242);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,17,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(236);
((SimpleValueContext)_localctx).simpleLiteral = simpleLiteral();
((SimpleValueContext)_localctx).ast = ((SimpleValueContext)_localctx).simpleLiteral.ast;
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(239);
((SimpleValueContext)_localctx).qualifiedName = qualifiedName();
((SimpleValueContext)_localctx).ast = ((SimpleValueContext)_localctx).qualifiedName.ast;
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class QualifiedNameContext extends ParserRuleContext {
public Expression ast;
public IdentifierContext name;
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List DOT() { return getTokens(SFEELParser.DOT); }
public TerminalNode DOT(int i) {
return getToken(SFEELParser.DOT, i);
}
public QualifiedNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_qualifiedName; }
}
public final QualifiedNameContext qualifiedName() throws RecognitionException {
QualifiedNameContext _localctx = new QualifiedNameContext(_ctx, getState());
enterRule(_localctx, 42, RULE_qualifiedName);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List names = new ArrayList<>();
setState(245);
((QualifiedNameContext)_localctx).name = identifier();
names.add((((QualifiedNameContext)_localctx).name!=null?_input.getText(((QualifiedNameContext)_localctx).name.start,((QualifiedNameContext)_localctx).name.stop):null));
setState(253);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==DOT) {
{
{
setState(247);
match(DOT);
setState(248);
((QualifiedNameContext)_localctx).name = identifier();
names.add((((QualifiedNameContext)_localctx).name!=null?_input.getText(((QualifiedNameContext)_localctx).name.start,((QualifiedNameContext)_localctx).name.stop):null));
}
}
setState(255);
_errHandler.sync(this);
_la = _input.LA(1);
}
((QualifiedNameContext)_localctx).ast = astFactory.toQualifiedName(names);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleLiteralContext extends ParserRuleContext {
public Expression ast;
public NumericLiteralContext numericLiteral;
public StringLiteralContext stringLiteral;
public BooleanLiteralContext booleanLiteral;
public DateTimeLiteralContext dateTimeLiteral;
public NumericLiteralContext numericLiteral() {
return getRuleContext(NumericLiteralContext.class,0);
}
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public BooleanLiteralContext booleanLiteral() {
return getRuleContext(BooleanLiteralContext.class,0);
}
public DateTimeLiteralContext dateTimeLiteral() {
return getRuleContext(DateTimeLiteralContext.class,0);
}
public SimpleLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleLiteral; }
}
public final SimpleLiteralContext simpleLiteral() throws RecognitionException {
SimpleLiteralContext _localctx = new SimpleLiteralContext(_ctx, getState());
enterRule(_localctx, 44, RULE_simpleLiteral);
try {
setState(270);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NUMBER:
enterOuterAlt(_localctx, 1);
{
{
setState(258);
((SimpleLiteralContext)_localctx).numericLiteral = numericLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).numericLiteral.ast;
}
}
break;
case STRING:
enterOuterAlt(_localctx, 2);
{
{
setState(261);
((SimpleLiteralContext)_localctx).stringLiteral = stringLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).stringLiteral.ast;
}
}
break;
case TRUE:
case FALSE:
enterOuterAlt(_localctx, 3);
{
{
setState(264);
((SimpleLiteralContext)_localctx).booleanLiteral = booleanLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).booleanLiteral.ast;
}
}
break;
case NAME:
enterOuterAlt(_localctx, 4);
{
{
setState(267);
((SimpleLiteralContext)_localctx).dateTimeLiteral = dateTimeLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).dateTimeLiteral.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StringLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode STRING() { return getToken(SFEELParser.STRING, 0); }
public StringLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringLiteral; }
}
public final StringLiteralContext stringLiteral() throws RecognitionException {
StringLiteralContext _localctx = new StringLiteralContext(_ctx, getState());
enterRule(_localctx, 46, RULE_stringLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(272);
((StringLiteralContext)_localctx).lit = match(STRING);
((StringLiteralContext)_localctx).ast = astFactory.toStringLiteral((((StringLiteralContext)_localctx).lit!=null?((StringLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BooleanLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode TRUE() { return getToken(SFEELParser.TRUE, 0); }
public TerminalNode FALSE() { return getToken(SFEELParser.FALSE, 0); }
public BooleanLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_booleanLiteral; }
}
public final BooleanLiteralContext booleanLiteral() throws RecognitionException {
BooleanLiteralContext _localctx = new BooleanLiteralContext(_ctx, getState());
enterRule(_localctx, 48, RULE_booleanLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(277);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TRUE:
{
setState(275);
((BooleanLiteralContext)_localctx).lit = match(TRUE);
}
break;
case FALSE:
{
setState(276);
((BooleanLiteralContext)_localctx).lit = match(FALSE);
}
break;
default:
throw new NoViableAltException(this);
}
((BooleanLiteralContext)_localctx).ast = astFactory.toBooleanLiteral((((BooleanLiteralContext)_localctx).lit!=null?((BooleanLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NumericLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode NUMBER() { return getToken(SFEELParser.NUMBER, 0); }
public NumericLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_numericLiteral; }
}
public final NumericLiteralContext numericLiteral() throws RecognitionException {
NumericLiteralContext _localctx = new NumericLiteralContext(_ctx, getState());
enterRule(_localctx, 50, RULE_numericLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(281);
((NumericLiteralContext)_localctx).lit = match(NUMBER);
((NumericLiteralContext)_localctx).ast = astFactory.toNumericLiteral((((NumericLiteralContext)_localctx).lit!=null?((NumericLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DateTimeLiteralContext extends ParserRuleContext {
public Expression ast;
public IdentifierContext kind;
public StringLiteralContext stringLiteral;
public TerminalNode PAREN_OPEN() { return getToken(SFEELParser.PAREN_OPEN, 0); }
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public TerminalNode PAREN_CLOSE() { return getToken(SFEELParser.PAREN_CLOSE, 0); }
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public DateTimeLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dateTimeLiteral; }
}
public final DateTimeLiteralContext dateTimeLiteral() throws RecognitionException {
DateTimeLiteralContext _localctx = new DateTimeLiteralContext(_ctx, getState());
enterRule(_localctx, 52, RULE_dateTimeLiteral);
try {
enterOuterAlt(_localctx, 1);
{
{
setState(284);
((DateTimeLiteralContext)_localctx).kind = identifier();
}
setState(285);
match(PAREN_OPEN);
setState(286);
((DateTimeLiteralContext)_localctx).stringLiteral = stringLiteral();
setState(287);
match(PAREN_CLOSE);
((DateTimeLiteralContext)_localctx).ast = astFactory.toDateTimeLiteral((((DateTimeLiteralContext)_localctx).kind!=null?_input.getText(((DateTimeLiteralContext)_localctx).kind.start,((DateTimeLiteralContext)_localctx).kind.stop):null), ((DateTimeLiteralContext)_localctx).stringLiteral.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IdentifierContext extends ParserRuleContext {
public Token ast;
public Token token;
public TerminalNode NAME() { return getToken(SFEELParser.NAME, 0); }
public IdentifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_identifier; }
}
public final IdentifierContext identifier() throws RecognitionException {
IdentifierContext _localctx = new IdentifierContext(_ctx, getState());
enterRule(_localctx, 54, RULE_identifier);
try {
enterOuterAlt(_localctx, 1);
{
setState(290);
((IdentifierContext)_localctx).token = match(NAME);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3\35\u0128\4\2\t\2"+
"\4\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13"+
"\t\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\4\32\t\32\4\33\t\33\4\34\t\34\4\35\t\35\3\2\3\2\3\2\3\2\3\3\3\3\3\3\3"+
"\3\3\4\3\4\3\4\3\4\3\5\3\5\3\5\3\5\3\5\3\5\3\5\3\5\3\5\3\5\3\5\5\5R\n"+
"\5\3\6\3\6\3\6\3\6\3\6\3\6\3\6\7\6[\n\6\f\6\16\6^\13\6\3\6\3\6\3\7\3\7"+
"\3\7\3\7\5\7f\n\7\3\7\3\7\3\7\3\7\3\7\3\7\5\7n\n\7\3\b\3\b\3\b\3\b\3\b"+
"\3\b\3\b\3\t\3\t\3\t\3\t\3\t\3\t\5\t}\n\t\3\n\3\n\3\n\3\n\3\n\3\n\5\n"+
"\u0085\n\n\3\13\5\13\u0088\n\13\3\13\3\13\3\13\3\f\3\f\3\f\3\r\3\r\3\r"+
"\3\r\3\r\3\r\3\r\7\r\u0097\n\r\f\r\16\r\u009a\13\r\3\r\3\r\3\16\3\16\3"+
"\16\3\17\3\17\3\17\3\17\3\17\3\17\3\17\3\17\5\17\u00a9\n\17\3\17\3\17"+
"\3\17\5\17\u00ae\n\17\3\20\3\20\3\20\3\21\3\21\3\21\3\21\5\21\u00b7\n"+
"\21\3\21\3\21\3\21\7\21\u00bc\n\21\f\21\16\21\u00bf\13\21\3\22\3\22\3"+
"\22\3\22\5\22\u00c5\n\22\3\22\3\22\3\22\7\22\u00ca\n\22\f\22\16\22\u00cd"+
"\13\22\3\23\3\23\3\23\3\23\3\23\3\23\7\23\u00d5\n\23\f\23\16\23\u00d8"+
"\13\23\3\24\3\24\3\24\7\24\u00dd\n\24\f\24\16\24\u00e0\13\24\3\24\3\24"+
"\3\24\3\25\3\25\3\25\3\25\3\25\3\25\3\25\3\25\5\25\u00ed\n\25\3\26\3\26"+
"\3\26\3\26\3\26\3\26\5\26\u00f5\n\26\3\27\3\27\3\27\3\27\3\27\3\27\3\27"+
"\7\27\u00fe\n\27\f\27\16\27\u0101\13\27\3\27\3\27\3\30\3\30\3\30\3\30"+
"\3\30\3\30\3\30\3\30\3\30\3\30\3\30\3\30\5\30\u0111\n\30\3\31\3\31\3\31"+
"\3\32\3\32\5\32\u0118\n\32\3\32\3\32\3\33\3\33\3\33\3\34\3\34\3\34\3\34"+
"\3\34\3\34\3\35\3\35\3\35\3\35\2\2\36\2\4\6\b\n\f\16\20\22\24\26\30\32"+
"\34\36 \"$&(*,.\60\62\64\668\2\2\2\u012c\2:\3\2\2\2\4>\3\2\2\2\6B\3\2"+
"\2\2\bQ\3\2\2\2\nS\3\2\2\2\fm\3\2\2\2\16o\3\2\2\2\20|\3\2\2\2\22\u0084"+
"\3\2\2\2\24\u0087\3\2\2\2\26\u008c\3\2\2\2\30\u008f\3\2\2\2\32\u009d\3"+
"\2\2\2\34\u00a0\3\2\2\2\36\u00af\3\2\2\2 \u00b2\3\2\2\2\"\u00c0\3\2\2"+
"\2$\u00ce\3\2\2\2&\u00d9\3\2\2\2(\u00ec\3\2\2\2*\u00f4\3\2\2\2,\u00f6"+
"\3\2\2\2.\u0110\3\2\2\2\60\u0112\3\2\2\2\62\u0117\3\2\2\2\64\u011b\3\2"+
"\2\2\66\u011e\3\2\2\28\u0124\3\2\2\2:;\5\b\5\2;<\b\2\1\2<=\7\2\2\3=\3"+
"\3\2\2\2>?\5\26\f\2?@\b\3\1\2@A\7\2\2\3A\5\3\2\2\2BC\5\30\r\2CD\b\4\1"+
"\2DE\7\2\2\3E\7\3\2\2\2FG\7\32\2\2GH\7\26\2\2HI\5\n\6\2IJ\7\27\2\2JK\b"+
"\5\1\2KR\3\2\2\2LM\5\n\6\2MN\b\5\1\2NR\3\2\2\2OP\7\17\2\2PR\b\5\1\2QF"+
"\3\2\2\2QL\3\2\2\2QO\3\2\2\2R\t\3\2\2\2ST\b\6\1\2TU\5\f\7\2U\\\b\6\1\2"+
"VW\7\25\2\2WX\5\f\7\2XY\b\6\1\2Y[\3\2\2\2ZV\3\2\2\2[^\3\2\2\2\\Z\3\2\2"+
"\2\\]\3\2\2\2]_\3\2\2\2^\\\3\2\2\2_`\b\6\1\2`\13\3\2\2\2af\7\n\2\2bf\7"+
"\f\2\2cf\7\13\2\2df\7\r\2\2ea\3\2\2\2eb\3\2\2\2ec\3\2\2\2ed\3\2\2\2ef"+
"\3\2\2\2fg\3\2\2\2gh\5\24\13\2hi\b\7\1\2in\3\2\2\2jk\5\16\b\2kl\b\7\1"+
"\2ln\3\2\2\2me\3\2\2\2mj\3\2\2\2n\r\3\2\2\2op\5\20\t\2pq\5\24\13\2qr\7"+
"\23\2\2rs\5\24\13\2st\5\22\n\2tu\b\b\1\2u\17\3\2\2\2vw\7\26\2\2w}\b\t"+
"\1\2xy\7\31\2\2y}\b\t\1\2z{\7\30\2\2{}\b\t\1\2|v\3\2\2\2|x\3\2\2\2|z\3"+
"\2\2\2}\21\3\2\2\2~\177\7\27\2\2\177\u0085\b\n\1\2\u0080\u0081\7\30\2"+
"\2\u0081\u0085\b\n\1\2\u0082\u0083\7\31\2\2\u0083\u0085\b\n\1\2\u0084"+
"~\3\2\2\2\u0084\u0080\3\2\2\2\u0084\u0082\3\2\2\2\u0085\23\3\2\2\2\u0086"+
"\u0088\7\17\2\2\u0087\u0086\3\2\2\2\u0087\u0088\3\2\2\2\u0088\u0089\3"+
"\2\2\2\u0089\u008a\5*\26\2\u008a\u008b\b\13\1\2\u008b\25\3\2\2\2\u008c"+
"\u008d\5\32\16\2\u008d\u008e\b\f\1\2\u008e\27\3\2\2\2\u008f\u0090\b\r"+
"\1\2\u0090\u0091\5\32\16\2\u0091\u0098\b\r\1\2\u0092\u0093\7\25\2\2\u0093"+
"\u0094\5\32\16\2\u0094\u0095\b\r\1\2\u0095\u0097\3\2\2\2\u0096\u0092\3"+
"\2\2\2\u0097\u009a\3\2\2\2\u0098\u0096\3\2\2\2\u0098\u0099\3\2\2\2\u0099"+
"\u009b\3\2\2\2\u009a\u0098\3\2\2\2\u009b\u009c\b\r\1\2\u009c\31\3\2\2"+
"\2\u009d\u009e\5\34\17\2\u009e\u009f\b\16\1\2\u009f\33\3\2\2\2\u00a0\u00a1"+
"\5\36\20\2\u00a1\u00ad\b\17\1\2\u00a2\u00a9\7\b\2\2\u00a3\u00a9\7\t\2"+
"\2\u00a4\u00a9\7\n\2\2\u00a5\u00a9\7\13\2\2\u00a6\u00a9\7\f\2\2\u00a7"+
"\u00a9\7\r\2\2\u00a8\u00a2\3\2\2\2\u00a8\u00a3\3\2\2\2\u00a8\u00a4\3\2"+
"\2\2\u00a8\u00a5\3\2\2\2\u00a8\u00a6\3\2\2\2\u00a8\u00a7\3\2\2\2\u00a9"+
"\u00aa\3\2\2\2\u00aa\u00ab\5\36\20\2\u00ab\u00ac\b\17\1\2\u00ac\u00ae"+
"\3\2\2\2\u00ad\u00a8\3\2\2\2\u00ad\u00ae\3\2\2\2\u00ae\35\3\2\2\2\u00af"+
"\u00b0\5 \21\2\u00b0\u00b1\b\20\1\2\u00b1\37\3\2\2\2\u00b2\u00b3\5\"\22"+
"\2\u00b3\u00bd\b\21\1\2\u00b4\u00b7\7\16\2\2\u00b5\u00b7\7\17\2\2\u00b6"+
"\u00b4\3\2\2\2\u00b6\u00b5\3\2\2\2\u00b7\u00b8\3\2\2\2\u00b8\u00b9\5\""+
"\22\2\u00b9\u00ba\b\21\1\2\u00ba\u00bc\3\2\2\2\u00bb\u00b6\3\2\2\2\u00bc"+
"\u00bf\3\2\2\2\u00bd\u00bb\3\2\2\2\u00bd\u00be\3\2\2\2\u00be!\3\2\2\2"+
"\u00bf\u00bd\3\2\2\2\u00c0\u00c1\5$\23\2\u00c1\u00cb\b\22\1\2\u00c2\u00c5"+
"\7\20\2\2\u00c3\u00c5\7\21\2\2\u00c4\u00c2\3\2\2\2\u00c4\u00c3\3\2\2\2"+
"\u00c5\u00c6\3\2\2\2\u00c6\u00c7\5$\23\2\u00c7\u00c8\b\22\1\2\u00c8\u00ca"+
"\3\2\2\2\u00c9\u00c4\3\2\2\2\u00ca\u00cd\3\2\2\2\u00cb\u00c9\3\2\2\2\u00cb"+
"\u00cc\3\2\2\2\u00cc#\3\2\2\2\u00cd\u00cb\3\2\2\2\u00ce\u00cf\5&\24\2"+
"\u00cf\u00d6\b\23\1\2\u00d0\u00d1\7\22\2\2\u00d1\u00d2\5&\24\2\u00d2\u00d3"+
"\b\23\1\2\u00d3\u00d5\3\2\2\2\u00d4\u00d0\3\2\2\2\u00d5\u00d8\3\2\2\2"+
"\u00d6\u00d4\3\2\2\2\u00d6\u00d7\3\2\2\2\u00d7%\3\2\2\2\u00d8\u00d6\3"+
"\2\2\2\u00d9\u00de\b\24\1\2\u00da\u00db\7\17\2\2\u00db\u00dd\b\24\1\2"+
"\u00dc\u00da\3\2\2\2\u00dd\u00e0\3\2\2\2\u00de\u00dc\3\2\2\2\u00de\u00df"+
"\3\2\2\2\u00df\u00e1\3\2\2\2\u00e0\u00de\3\2\2\2\u00e1\u00e2\5(\25\2\u00e2"+
"\u00e3\b\24\1\2\u00e3\'\3\2\2\2\u00e4\u00e5\5*\26\2\u00e5\u00e6\b\25\1"+
"\2\u00e6\u00ed\3\2\2\2\u00e7\u00e8\7\26\2\2\u00e8\u00e9\5\26\f\2\u00e9"+
"\u00ea\7\27\2\2\u00ea\u00eb\b\25\1\2\u00eb\u00ed\3\2\2\2\u00ec\u00e4\3"+
"\2\2\2\u00ec\u00e7\3\2\2\2\u00ed)\3\2\2\2\u00ee\u00ef\5.\30\2\u00ef\u00f0"+
"\b\26\1\2\u00f0\u00f5\3\2\2\2\u00f1\u00f2\5,\27\2\u00f2\u00f3\b\26\1\2"+
"\u00f3\u00f5\3\2\2\2\u00f4\u00ee\3\2\2\2\u00f4\u00f1\3\2\2\2\u00f5+\3"+
"\2\2\2\u00f6\u00f7\b\27\1\2\u00f7\u00f8\58\35\2\u00f8\u00ff\b\27\1\2\u00f9"+
"\u00fa\7\24\2\2\u00fa\u00fb\58\35\2\u00fb\u00fc\b\27\1\2\u00fc\u00fe\3"+
"\2\2\2\u00fd\u00f9\3\2\2\2\u00fe\u0101\3\2\2\2\u00ff\u00fd\3\2\2\2\u00ff"+
"\u0100\3\2\2\2\u0100\u0102\3\2\2\2\u0101\u00ff\3\2\2\2\u0102\u0103\b\27"+
"\1\2\u0103-\3\2\2\2\u0104\u0105\5\64\33\2\u0105\u0106\b\30\1\2\u0106\u0111"+
"\3\2\2\2\u0107\u0108\5\60\31\2\u0108\u0109\b\30\1\2\u0109\u0111\3\2\2"+
"\2\u010a\u010b\5\62\32\2\u010b\u010c\b\30\1\2\u010c\u0111\3\2\2\2\u010d"+
"\u010e\5\66\34\2\u010e\u010f\b\30\1\2\u010f\u0111\3\2\2\2\u0110\u0104"+
"\3\2\2\2\u0110\u0107\3\2\2\2\u0110\u010a\3\2\2\2\u0110\u010d\3\2\2\2\u0111"+
"/\3\2\2\2\u0112\u0113\7\6\2\2\u0113\u0114\b\31\1\2\u0114\61\3\2\2\2\u0115"+
"\u0118\7\33\2\2\u0116\u0118\7\34\2\2\u0117\u0115\3\2\2\2\u0117\u0116\3"+
"\2\2\2\u0118\u0119\3\2\2\2\u0119\u011a\b\32\1\2\u011a\63\3\2\2\2\u011b"+
"\u011c\7\7\2\2\u011c\u011d\b\33\1\2\u011d\65\3\2\2\2\u011e\u011f\58\35"+
"\2\u011f\u0120\7\26\2\2\u0120\u0121\5\60\31\2\u0121\u0122\7\27\2\2\u0122"+
"\u0123\b\34\1\2\u0123\67\3\2\2\2\u0124\u0125\7\35\2\2\u0125\u0126\b\35"+
"\1\2\u01269\3\2\2\2\27Q\\em|\u0084\u0087\u0098\u00a8\u00ad\u00b6\u00bd"+
"\u00c4\u00cb\u00d6\u00de\u00ec\u00f4\u00ff\u0110\u0117";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy