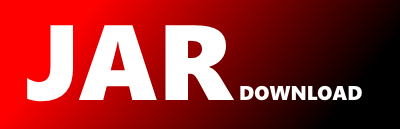
chs.jdmn.jdmn-core.3.3.1.source-code.FEELParser Maven / Gradle / Ivy
// Generated from FEELParser.g4 by ANTLR 4.7.2
package com.gs.dmn.feel.analysis.syntax.antlrv4;
import java.util.*;
import com.gs.dmn.feel.analysis.syntax.ast.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.arithmetic.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.comparison.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.function.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.literal.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.logic.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.textual.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.type.*;
import com.gs.dmn.feel.analysis.syntax.ast.test.*;
import com.gs.dmn.feel.analysis.semantics.type.*;
import com.gs.dmn.runtime.Pair;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class FEELParser extends Parser {
static { RuntimeMetaData.checkVersion("4.7.2", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
BLOCK_COMMENT=1, LINE_COMMENT=2, WS=3, STRING=4, NUMBER=5, EQ=6, NE=7,
LT=8, GT=9, LE=10, GE=11, PLUS=12, MINUS=13, STAR=14, FORWARD_SLASH=15,
STAR_STAR=16, DOT_DOT=17, DOT=18, COMMA=19, PAREN_OPEN=20, PAREN_CLOSE=21,
BRACKET_OPEN=22, BRACKET_CLOSE=23, BRACE_OPEN=24, BRACE_CLOSE=25, COLON=26,
ARROW=27, NOT=28, TRUE=29, FALSE=30, NULL=31, FUNCTION=32, EXTERNAL=33,
FOR=34, IN=35, RETURN=36, IF=37, THEN=38, ELSE=39, SOME=40, EVERY=41,
SATISFIES=42, AND=43, OR=44, BETWEEN=45, INSTANCE_OF=46, NAME=47;
public static final int
RULE_unaryTestsRoot = 0, RULE_simpleUnaryTestsRoot = 1, RULE_expressionRoot = 2,
RULE_simpleExpressionsRoot = 3, RULE_textualExpressionsRoot = 4, RULE_boxedExpressionRoot = 5,
RULE_unaryTests = 6, RULE_positiveUnaryTests = 7, RULE_positiveUnaryTest = 8,
RULE_simpleUnaryTests = 9, RULE_simplePositiveUnaryTests = 10, RULE_simplePositiveUnaryTest = 11,
RULE_interval = 12, RULE_intervalStartPar = 13, RULE_intervalEndPar = 14,
RULE_endpoint = 15, RULE_simpleExpressions = 16, RULE_simpleExpression = 17,
RULE_expression = 18, RULE_textualExpressions = 19, RULE_textualExpression = 20,
RULE_functionDefinition = 21, RULE_formalParameter = 22, RULE_forExpression = 23,
RULE_iterationDomain = 24, RULE_ifExpression = 25, RULE_quantifiedExpression = 26,
RULE_disjunction = 27, RULE_conjunction = 28, RULE_comparison = 29, RULE_arithmeticExpression = 30,
RULE_addition = 31, RULE_multiplication = 32, RULE_exponentiation = 33,
RULE_arithmeticNegation = 34, RULE_instanceOf = 35, RULE_type = 36, RULE_postfixExpression = 37,
RULE_parameters = 38, RULE_namedParameters = 39, RULE_parameterName = 40,
RULE_positionalParameters = 41, RULE_primaryExpression = 42, RULE_simpleValue = 43,
RULE_qualifiedName = 44, RULE_literal = 45, RULE_simpleLiteral = 46, RULE_stringLiteral = 47,
RULE_booleanLiteral = 48, RULE_numericLiteral = 49, RULE_boxedExpression = 50,
RULE_list = 51, RULE_context = 52, RULE_contextEntry = 53, RULE_key = 54,
RULE_dateTimeLiteral = 55, RULE_identifier = 56;
private static String[] makeRuleNames() {
return new String[] {
"unaryTestsRoot", "simpleUnaryTestsRoot", "expressionRoot", "simpleExpressionsRoot",
"textualExpressionsRoot", "boxedExpressionRoot", "unaryTests", "positiveUnaryTests",
"positiveUnaryTest", "simpleUnaryTests", "simplePositiveUnaryTests",
"simplePositiveUnaryTest", "interval", "intervalStartPar", "intervalEndPar",
"endpoint", "simpleExpressions", "simpleExpression", "expression", "textualExpressions",
"textualExpression", "functionDefinition", "formalParameter", "forExpression",
"iterationDomain", "ifExpression", "quantifiedExpression", "disjunction",
"conjunction", "comparison", "arithmeticExpression", "addition", "multiplication",
"exponentiation", "arithmeticNegation", "instanceOf", "type", "postfixExpression",
"parameters", "namedParameters", "parameterName", "positionalParameters",
"primaryExpression", "simpleValue", "qualifiedName", "literal", "simpleLiteral",
"stringLiteral", "booleanLiteral", "numericLiteral", "boxedExpression",
"list", "context", "contextEntry", "key", "dateTimeLiteral", "identifier"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, null, null, null, null, null, null, "'!='", "'<'", "'>'", "'<='",
"'>='", "'+'", "'-'", "'*'", "'/'", "'**'", "'..'", "'.'", "','", "'('",
"')'", "'['", "']'", "'{'", "'}'", "':'", "'->'", "'not'", "'true'",
"'false'", "'null'", "'function'", "'external'", "'for'", "'in'", "'return'",
"'if'", "'then'", "'else'", "'some'", "'every'", "'satisfies'", "'and'",
"'or'", "'between'", "'instance of'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "BLOCK_COMMENT", "LINE_COMMENT", "WS", "STRING", "NUMBER", "EQ",
"NE", "LT", "GT", "LE", "GE", "PLUS", "MINUS", "STAR", "FORWARD_SLASH",
"STAR_STAR", "DOT_DOT", "DOT", "COMMA", "PAREN_OPEN", "PAREN_CLOSE",
"BRACKET_OPEN", "BRACKET_CLOSE", "BRACE_OPEN", "BRACE_CLOSE", "COLON",
"ARROW", "NOT", "TRUE", "FALSE", "NULL", "FUNCTION", "EXTERNAL", "FOR",
"IN", "RETURN", "IF", "THEN", "ELSE", "SOME", "EVERY", "SATISFIES", "AND",
"OR", "BETWEEN", "INSTANCE_OF", "NAME"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "FEELParser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
private ASTFactory astFactory;
public FEELParser(TokenStream input, ASTFactory astFactory) {
this(input);
this.astFactory = astFactory;
}
public ASTFactory getASTFactory() {
return astFactory;
}
public FEELParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class UnaryTestsRootContext extends ParserRuleContext {
public UnaryTests ast;
public UnaryTestsContext unaryTests;
public UnaryTestsContext unaryTests() {
return getRuleContext(UnaryTestsContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public UnaryTestsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unaryTestsRoot; }
}
public final UnaryTestsRootContext unaryTestsRoot() throws RecognitionException {
UnaryTestsRootContext _localctx = new UnaryTestsRootContext(_ctx, getState());
enterRule(_localctx, 0, RULE_unaryTestsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(114);
((UnaryTestsRootContext)_localctx).unaryTests = unaryTests();
((UnaryTestsRootContext)_localctx).ast = ((UnaryTestsRootContext)_localctx).unaryTests.ast;
setState(116);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleUnaryTestsRootContext extends ParserRuleContext {
public SimpleUnaryTests ast;
public SimpleUnaryTestsContext simpleUnaryTests;
public SimpleUnaryTestsContext simpleUnaryTests() {
return getRuleContext(SimpleUnaryTestsContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public SimpleUnaryTestsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleUnaryTestsRoot; }
}
public final SimpleUnaryTestsRootContext simpleUnaryTestsRoot() throws RecognitionException {
SimpleUnaryTestsRootContext _localctx = new SimpleUnaryTestsRootContext(_ctx, getState());
enterRule(_localctx, 2, RULE_simpleUnaryTestsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(118);
((SimpleUnaryTestsRootContext)_localctx).simpleUnaryTests = simpleUnaryTests();
((SimpleUnaryTestsRootContext)_localctx).ast = ((SimpleUnaryTestsRootContext)_localctx).simpleUnaryTests.ast;
setState(120);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExpressionRootContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext expression;
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public ExpressionRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expressionRoot; }
}
public final ExpressionRootContext expressionRoot() throws RecognitionException {
ExpressionRootContext _localctx = new ExpressionRootContext(_ctx, getState());
enterRule(_localctx, 4, RULE_expressionRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(122);
((ExpressionRootContext)_localctx).expression = expression();
((ExpressionRootContext)_localctx).ast = ((ExpressionRootContext)_localctx).expression.ast;
setState(124);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleExpressionsRootContext extends ParserRuleContext {
public Expression ast;
public SimpleExpressionsContext simpleExpressions;
public SimpleExpressionsContext simpleExpressions() {
return getRuleContext(SimpleExpressionsContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public SimpleExpressionsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleExpressionsRoot; }
}
public final SimpleExpressionsRootContext simpleExpressionsRoot() throws RecognitionException {
SimpleExpressionsRootContext _localctx = new SimpleExpressionsRootContext(_ctx, getState());
enterRule(_localctx, 6, RULE_simpleExpressionsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(126);
((SimpleExpressionsRootContext)_localctx).simpleExpressions = simpleExpressions();
((SimpleExpressionsRootContext)_localctx).ast = ((SimpleExpressionsRootContext)_localctx).simpleExpressions.ast;
setState(128);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TextualExpressionsRootContext extends ParserRuleContext {
public Expression ast;
public TextualExpressionsContext textualExpressions;
public TextualExpressionsContext textualExpressions() {
return getRuleContext(TextualExpressionsContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public TextualExpressionsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_textualExpressionsRoot; }
}
public final TextualExpressionsRootContext textualExpressionsRoot() throws RecognitionException {
TextualExpressionsRootContext _localctx = new TextualExpressionsRootContext(_ctx, getState());
enterRule(_localctx, 8, RULE_textualExpressionsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(130);
((TextualExpressionsRootContext)_localctx).textualExpressions = textualExpressions();
((TextualExpressionsRootContext)_localctx).ast = ((TextualExpressionsRootContext)_localctx).textualExpressions.ast;
setState(132);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BoxedExpressionRootContext extends ParserRuleContext {
public Expression ast;
public BoxedExpressionContext boxedExpression;
public BoxedExpressionContext boxedExpression() {
return getRuleContext(BoxedExpressionContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public BoxedExpressionRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_boxedExpressionRoot; }
}
public final BoxedExpressionRootContext boxedExpressionRoot() throws RecognitionException {
BoxedExpressionRootContext _localctx = new BoxedExpressionRootContext(_ctx, getState());
enterRule(_localctx, 10, RULE_boxedExpressionRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(134);
((BoxedExpressionRootContext)_localctx).boxedExpression = boxedExpression();
((BoxedExpressionRootContext)_localctx).ast = ((BoxedExpressionRootContext)_localctx).boxedExpression.ast;
setState(136);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class UnaryTestsContext extends ParserRuleContext {
public UnaryTests ast;
public PositiveUnaryTestsContext tests;
public TerminalNode NOT() { return getToken(FEELParser.NOT, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public PositiveUnaryTestsContext positiveUnaryTests() {
return getRuleContext(PositiveUnaryTestsContext.class,0);
}
public TerminalNode MINUS() { return getToken(FEELParser.MINUS, 0); }
public UnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unaryTests; }
}
public final UnaryTestsContext unaryTests() throws RecognitionException {
UnaryTestsContext _localctx = new UnaryTestsContext(_ctx, getState());
enterRule(_localctx, 12, RULE_unaryTests);
try {
setState(149);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,0,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(138);
match(NOT);
setState(139);
match(PAREN_OPEN);
setState(140);
((UnaryTestsContext)_localctx).tests = positiveUnaryTests();
setState(141);
match(PAREN_CLOSE);
((UnaryTestsContext)_localctx).ast = astFactory.toNegatedUnaryTests(((UnaryTestsContext)_localctx).tests.ast);
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(144);
((UnaryTestsContext)_localctx).tests = positiveUnaryTests();
((UnaryTestsContext)_localctx).ast = ((UnaryTestsContext)_localctx).tests.ast;
}
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
{
setState(147);
match(MINUS);
((UnaryTestsContext)_localctx).ast = astFactory.toAny();
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PositiveUnaryTestsContext extends ParserRuleContext {
public PositiveUnaryTests ast;
public PositiveUnaryTestContext test;
public List positiveUnaryTest() {
return getRuleContexts(PositiveUnaryTestContext.class);
}
public PositiveUnaryTestContext positiveUnaryTest(int i) {
return getRuleContext(PositiveUnaryTestContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public PositiveUnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positiveUnaryTests; }
}
public final PositiveUnaryTestsContext positiveUnaryTests() throws RecognitionException {
PositiveUnaryTestsContext _localctx = new PositiveUnaryTestsContext(_ctx, getState());
enterRule(_localctx, 14, RULE_positiveUnaryTests);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List tests = new ArrayList<>();
setState(152);
((PositiveUnaryTestsContext)_localctx).test = positiveUnaryTest();
tests.add(((PositiveUnaryTestsContext)_localctx).test.ast);
setState(160);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(154);
match(COMMA);
setState(155);
((PositiveUnaryTestsContext)_localctx).test = positiveUnaryTest();
tests.add(((PositiveUnaryTestsContext)_localctx).test.ast);
}
}
setState(162);
_errHandler.sync(this);
_la = _input.LA(1);
}
((PositiveUnaryTestsContext)_localctx).ast = astFactory.toPositiveUnaryTests(tests);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PositiveUnaryTestContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext expression;
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public PositiveUnaryTestContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positiveUnaryTest; }
}
public final PositiveUnaryTestContext positiveUnaryTest() throws RecognitionException {
PositiveUnaryTestContext _localctx = new PositiveUnaryTestContext(_ctx, getState());
enterRule(_localctx, 16, RULE_positiveUnaryTest);
try {
enterOuterAlt(_localctx, 1);
{
{
setState(165);
((PositiveUnaryTestContext)_localctx).expression = expression();
((PositiveUnaryTestContext)_localctx).ast = astFactory.toPositiveUnaryTest(((PositiveUnaryTestContext)_localctx).expression.ast);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleUnaryTestsContext extends ParserRuleContext {
public SimpleUnaryTests ast;
public SimplePositiveUnaryTestsContext tests;
public TerminalNode NOT() { return getToken(FEELParser.NOT, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public SimplePositiveUnaryTestsContext simplePositiveUnaryTests() {
return getRuleContext(SimplePositiveUnaryTestsContext.class,0);
}
public TerminalNode MINUS() { return getToken(FEELParser.MINUS, 0); }
public SimpleUnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleUnaryTests; }
}
public final SimpleUnaryTestsContext simpleUnaryTests() throws RecognitionException {
SimpleUnaryTestsContext _localctx = new SimpleUnaryTestsContext(_ctx, getState());
enterRule(_localctx, 18, RULE_simpleUnaryTests);
try {
setState(179);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,2,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(168);
match(NOT);
setState(169);
match(PAREN_OPEN);
setState(170);
((SimpleUnaryTestsContext)_localctx).tests = simplePositiveUnaryTests();
setState(171);
match(PAREN_CLOSE);
((SimpleUnaryTestsContext)_localctx).ast = astFactory.toNegatedSimpleUnaryTests(((SimpleUnaryTestsContext)_localctx).tests.ast);
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(174);
((SimpleUnaryTestsContext)_localctx).tests = simplePositiveUnaryTests();
((SimpleUnaryTestsContext)_localctx).ast = ((SimpleUnaryTestsContext)_localctx).tests.ast;
}
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
{
setState(177);
match(MINUS);
((SimpleUnaryTestsContext)_localctx).ast = astFactory.toAny();
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimplePositiveUnaryTestsContext extends ParserRuleContext {
public SimplePositiveUnaryTests ast;
public SimplePositiveUnaryTestContext test;
public List simplePositiveUnaryTest() {
return getRuleContexts(SimplePositiveUnaryTestContext.class);
}
public SimplePositiveUnaryTestContext simplePositiveUnaryTest(int i) {
return getRuleContext(SimplePositiveUnaryTestContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public SimplePositiveUnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simplePositiveUnaryTests; }
}
public final SimplePositiveUnaryTestsContext simplePositiveUnaryTests() throws RecognitionException {
SimplePositiveUnaryTestsContext _localctx = new SimplePositiveUnaryTestsContext(_ctx, getState());
enterRule(_localctx, 20, RULE_simplePositiveUnaryTests);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List tests = new ArrayList<>();
setState(182);
((SimplePositiveUnaryTestsContext)_localctx).test = simplePositiveUnaryTest();
tests.add(((SimplePositiveUnaryTestsContext)_localctx).test.ast);
setState(190);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(184);
match(COMMA);
setState(185);
((SimplePositiveUnaryTestsContext)_localctx).test = simplePositiveUnaryTest();
tests.add(((SimplePositiveUnaryTestsContext)_localctx).test.ast);
}
}
setState(192);
_errHandler.sync(this);
_la = _input.LA(1);
}
((SimplePositiveUnaryTestsContext)_localctx).ast = astFactory.toSimplePositiveUnaryTests(tests);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimplePositiveUnaryTestContext extends ParserRuleContext {
public Expression ast;
public Token op;
public EndpointContext opd;
public IntervalContext opd2;
public EndpointContext endpoint() {
return getRuleContext(EndpointContext.class,0);
}
public TerminalNode LT() { return getToken(FEELParser.LT, 0); }
public TerminalNode LE() { return getToken(FEELParser.LE, 0); }
public TerminalNode GT() { return getToken(FEELParser.GT, 0); }
public TerminalNode GE() { return getToken(FEELParser.GE, 0); }
public IntervalContext interval() {
return getRuleContext(IntervalContext.class,0);
}
public SimplePositiveUnaryTestContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simplePositiveUnaryTest; }
}
public final SimplePositiveUnaryTestContext simplePositiveUnaryTest() throws RecognitionException {
SimplePositiveUnaryTestContext _localctx = new SimplePositiveUnaryTestContext(_ctx, getState());
enterRule(_localctx, 22, RULE_simplePositiveUnaryTest);
try {
setState(207);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING:
case NUMBER:
case LT:
case GT:
case LE:
case GE:
case MINUS:
case TRUE:
case FALSE:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 1);
{
{
setState(199);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LT:
{
setState(195);
((SimplePositiveUnaryTestContext)_localctx).op = match(LT);
}
break;
case LE:
{
setState(196);
((SimplePositiveUnaryTestContext)_localctx).op = match(LE);
}
break;
case GT:
{
setState(197);
((SimplePositiveUnaryTestContext)_localctx).op = match(GT);
}
break;
case GE:
{
setState(198);
((SimplePositiveUnaryTestContext)_localctx).op = match(GE);
}
break;
case STRING:
case NUMBER:
case MINUS:
case TRUE:
case FALSE:
case FUNCTION:
case AND:
case OR:
case NAME:
break;
default:
break;
}
setState(201);
((SimplePositiveUnaryTestContext)_localctx).opd = endpoint();
((SimplePositiveUnaryTestContext)_localctx).ast = ((SimplePositiveUnaryTestContext)_localctx).op == null ? astFactory.toOperatorTest(null, ((SimplePositiveUnaryTestContext)_localctx).opd.ast) : astFactory.toOperatorTest((((SimplePositiveUnaryTestContext)_localctx).op!=null?((SimplePositiveUnaryTestContext)_localctx).op.getText():null), ((SimplePositiveUnaryTestContext)_localctx).opd.ast);
}
}
break;
case PAREN_OPEN:
case BRACKET_OPEN:
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 2);
{
{
setState(204);
((SimplePositiveUnaryTestContext)_localctx).opd2 = interval();
((SimplePositiveUnaryTestContext)_localctx).ast = ((SimplePositiveUnaryTestContext)_localctx).opd2.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntervalContext extends ParserRuleContext {
public RangeTest ast;
public IntervalStartParContext leftPar;
public EndpointContext ep1;
public EndpointContext ep2;
public IntervalEndParContext rightPar;
public TerminalNode DOT_DOT() { return getToken(FEELParser.DOT_DOT, 0); }
public IntervalStartParContext intervalStartPar() {
return getRuleContext(IntervalStartParContext.class,0);
}
public List endpoint() {
return getRuleContexts(EndpointContext.class);
}
public EndpointContext endpoint(int i) {
return getRuleContext(EndpointContext.class,i);
}
public IntervalEndParContext intervalEndPar() {
return getRuleContext(IntervalEndParContext.class,0);
}
public IntervalContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_interval; }
}
public final IntervalContext interval() throws RecognitionException {
IntervalContext _localctx = new IntervalContext(_ctx, getState());
enterRule(_localctx, 24, RULE_interval);
try {
enterOuterAlt(_localctx, 1);
{
setState(209);
((IntervalContext)_localctx).leftPar = intervalStartPar();
setState(210);
((IntervalContext)_localctx).ep1 = endpoint();
setState(211);
match(DOT_DOT);
setState(212);
((IntervalContext)_localctx).ep2 = endpoint();
setState(213);
((IntervalContext)_localctx).rightPar = intervalEndPar();
((IntervalContext)_localctx).ast = astFactory.toIntervalTest(((IntervalContext)_localctx).leftPar.ast, ((IntervalContext)_localctx).ep1.ast, ((IntervalContext)_localctx).rightPar.ast, ((IntervalContext)_localctx).ep2.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntervalStartParContext extends ParserRuleContext {
public String ast;
public Token token;
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(FEELParser.BRACKET_CLOSE, 0); }
public TerminalNode BRACKET_OPEN() { return getToken(FEELParser.BRACKET_OPEN, 0); }
public IntervalStartParContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intervalStartPar; }
}
public final IntervalStartParContext intervalStartPar() throws RecognitionException {
IntervalStartParContext _localctx = new IntervalStartParContext(_ctx, getState());
enterRule(_localctx, 26, RULE_intervalStartPar);
try {
setState(222);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PAREN_OPEN:
enterOuterAlt(_localctx, 1);
{
{
setState(216);
((IntervalStartParContext)_localctx).token = match(PAREN_OPEN);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 2);
{
{
setState(218);
((IntervalStartParContext)_localctx).token = match(BRACKET_CLOSE);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_OPEN:
enterOuterAlt(_localctx, 3);
{
{
setState(220);
((IntervalStartParContext)_localctx).token = match(BRACKET_OPEN);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntervalEndParContext extends ParserRuleContext {
public String ast;
public Token token;
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public TerminalNode BRACKET_OPEN() { return getToken(FEELParser.BRACKET_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(FEELParser.BRACKET_CLOSE, 0); }
public IntervalEndParContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intervalEndPar; }
}
public final IntervalEndParContext intervalEndPar() throws RecognitionException {
IntervalEndParContext _localctx = new IntervalEndParContext(_ctx, getState());
enterRule(_localctx, 28, RULE_intervalEndPar);
try {
setState(230);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PAREN_CLOSE:
enterOuterAlt(_localctx, 1);
{
{
setState(224);
((IntervalEndParContext)_localctx).token = match(PAREN_CLOSE);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_OPEN:
enterOuterAlt(_localctx, 2);
{
{
setState(226);
((IntervalEndParContext)_localctx).token = match(BRACKET_OPEN);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 3);
{
{
setState(228);
((IntervalEndParContext)_localctx).token = match(BRACKET_CLOSE);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EndpointContext extends ParserRuleContext {
public Expression ast;
public Token op;
public SimpleValueContext opd;
public SimpleValueContext simpleValue() {
return getRuleContext(SimpleValueContext.class,0);
}
public TerminalNode MINUS() { return getToken(FEELParser.MINUS, 0); }
public EndpointContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_endpoint; }
}
public final EndpointContext endpoint() throws RecognitionException {
EndpointContext _localctx = new EndpointContext(_ctx, getState());
enterRule(_localctx, 30, RULE_endpoint);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
{
setState(233);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==MINUS) {
{
setState(232);
((EndpointContext)_localctx).op = match(MINUS);
}
}
setState(235);
((EndpointContext)_localctx).opd = simpleValue();
((EndpointContext)_localctx).ast = (((EndpointContext)_localctx).op == null) ? ((EndpointContext)_localctx).opd.ast : astFactory.toNegation((((EndpointContext)_localctx).op!=null?((EndpointContext)_localctx).op.getText():null), ((EndpointContext)_localctx).opd.ast);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleExpressionsContext extends ParserRuleContext {
public Expression ast;
public SimpleExpressionContext exp;
public List simpleExpression() {
return getRuleContexts(SimpleExpressionContext.class);
}
public SimpleExpressionContext simpleExpression(int i) {
return getRuleContext(SimpleExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public SimpleExpressionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleExpressions; }
}
public final SimpleExpressionsContext simpleExpressions() throws RecognitionException {
SimpleExpressionsContext _localctx = new SimpleExpressionsContext(_ctx, getState());
enterRule(_localctx, 32, RULE_simpleExpressions);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List expressionList = new ArrayList<>();
setState(239);
((SimpleExpressionsContext)_localctx).exp = simpleExpression();
expressionList.add(((SimpleExpressionsContext)_localctx).exp.ast);
setState(247);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(241);
match(COMMA);
setState(242);
((SimpleExpressionsContext)_localctx).exp = simpleExpression();
expressionList.add(((SimpleExpressionsContext)_localctx).exp.ast);
}
}
setState(249);
_errHandler.sync(this);
_la = _input.LA(1);
}
((SimpleExpressionsContext)_localctx).ast = astFactory.toExpressionList(expressionList);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleExpressionContext extends ParserRuleContext {
public Expression ast;
public ArithmeticExpressionContext arithmeticExpression;
public SimpleValueContext simpleValue;
public ArithmeticExpressionContext arithmeticExpression() {
return getRuleContext(ArithmeticExpressionContext.class,0);
}
public SimpleValueContext simpleValue() {
return getRuleContext(SimpleValueContext.class,0);
}
public SimpleExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleExpression; }
}
public final SimpleExpressionContext simpleExpression() throws RecognitionException {
SimpleExpressionContext _localctx = new SimpleExpressionContext(_ctx, getState());
enterRule(_localctx, 34, RULE_simpleExpression);
try {
setState(258);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,10,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(252);
((SimpleExpressionContext)_localctx).arithmeticExpression = arithmeticExpression();
((SimpleExpressionContext)_localctx).ast = ((SimpleExpressionContext)_localctx).arithmeticExpression.ast;
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(255);
((SimpleExpressionContext)_localctx).simpleValue = simpleValue();
((SimpleExpressionContext)_localctx).ast = ((SimpleExpressionContext)_localctx).simpleValue.ast;
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExpressionContext extends ParserRuleContext {
public Expression ast;
public TextualExpressionContext textualExpression;
public TextualExpressionContext textualExpression() {
return getRuleContext(TextualExpressionContext.class,0);
}
public ExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression; }
}
public final ExpressionContext expression() throws RecognitionException {
ExpressionContext _localctx = new ExpressionContext(_ctx, getState());
enterRule(_localctx, 36, RULE_expression);
try {
enterOuterAlt(_localctx, 1);
{
{
setState(260);
((ExpressionContext)_localctx).textualExpression = textualExpression();
((ExpressionContext)_localctx).ast = ((ExpressionContext)_localctx).textualExpression.ast;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TextualExpressionsContext extends ParserRuleContext {
public Expression ast;
public TextualExpressionContext exp;
public List textualExpression() {
return getRuleContexts(TextualExpressionContext.class);
}
public TextualExpressionContext textualExpression(int i) {
return getRuleContext(TextualExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public TextualExpressionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_textualExpressions; }
}
public final TextualExpressionsContext textualExpressions() throws RecognitionException {
TextualExpressionsContext _localctx = new TextualExpressionsContext(_ctx, getState());
enterRule(_localctx, 38, RULE_textualExpressions);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List expressionList = new ArrayList<>();
setState(264);
((TextualExpressionsContext)_localctx).exp = textualExpression();
expressionList.add(((TextualExpressionsContext)_localctx).exp.ast);
setState(272);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(266);
match(COMMA);
setState(267);
((TextualExpressionsContext)_localctx).exp = textualExpression();
expressionList.add(((TextualExpressionsContext)_localctx).exp.ast);
}
}
setState(274);
_errHandler.sync(this);
_la = _input.LA(1);
}
((TextualExpressionsContext)_localctx).ast = astFactory.toExpressionList(expressionList);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TextualExpressionContext extends ParserRuleContext {
public Expression ast;
public ForExpressionContext forExpression;
public IfExpressionContext ifExpression;
public QuantifiedExpressionContext quantifiedExpression;
public DisjunctionContext disjunction;
public ForExpressionContext forExpression() {
return getRuleContext(ForExpressionContext.class,0);
}
public IfExpressionContext ifExpression() {
return getRuleContext(IfExpressionContext.class,0);
}
public QuantifiedExpressionContext quantifiedExpression() {
return getRuleContext(QuantifiedExpressionContext.class,0);
}
public DisjunctionContext disjunction() {
return getRuleContext(DisjunctionContext.class,0);
}
public TextualExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_textualExpression; }
}
public final TextualExpressionContext textualExpression() throws RecognitionException {
TextualExpressionContext _localctx = new TextualExpressionContext(_ctx, getState());
enterRule(_localctx, 40, RULE_textualExpression);
try {
setState(289);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FOR:
enterOuterAlt(_localctx, 1);
{
{
setState(277);
((TextualExpressionContext)_localctx).forExpression = forExpression();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).forExpression.ast;
}
}
break;
case IF:
enterOuterAlt(_localctx, 2);
{
{
setState(280);
((TextualExpressionContext)_localctx).ifExpression = ifExpression();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).ifExpression.ast;
}
}
break;
case SOME:
case EVERY:
enterOuterAlt(_localctx, 3);
{
{
setState(283);
((TextualExpressionContext)_localctx).quantifiedExpression = quantifiedExpression();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).quantifiedExpression.ast;
}
}
break;
case STRING:
case NUMBER:
case LT:
case GT:
case LE:
case GE:
case MINUS:
case PAREN_OPEN:
case BRACKET_OPEN:
case BRACKET_CLOSE:
case BRACE_OPEN:
case NOT:
case TRUE:
case FALSE:
case NULL:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 4);
{
{
setState(286);
((TextualExpressionContext)_localctx).disjunction = disjunction();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).disjunction.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FunctionDefinitionContext extends ParserRuleContext {
public Expression ast;
public FormalParameterContext param;
public ExpressionContext body;
public TerminalNode FUNCTION() { return getToken(FEELParser.FUNCTION, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode EXTERNAL() { return getToken(FEELParser.EXTERNAL, 0); }
public List formalParameter() {
return getRuleContexts(FormalParameterContext.class);
}
public FormalParameterContext formalParameter(int i) {
return getRuleContext(FormalParameterContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public FunctionDefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionDefinition; }
}
public final FunctionDefinitionContext functionDefinition() throws RecognitionException {
FunctionDefinitionContext _localctx = new FunctionDefinitionContext(_ctx, getState());
enterRule(_localctx, 42, RULE_functionDefinition);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List parameters = new ArrayList<>();
boolean external = false;
setState(293);
match(FUNCTION);
setState(294);
match(PAREN_OPEN);
setState(306);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << FUNCTION) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
setState(295);
((FunctionDefinitionContext)_localctx).param = formalParameter();
parameters.add(((FunctionDefinitionContext)_localctx).param.ast);
setState(303);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(297);
match(COMMA);
setState(298);
((FunctionDefinitionContext)_localctx).param = formalParameter();
parameters.add(((FunctionDefinitionContext)_localctx).param.ast);
}
}
setState(305);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(308);
match(PAREN_CLOSE);
setState(311);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==EXTERNAL) {
{
setState(309);
match(EXTERNAL);
external = true;
}
}
setState(313);
((FunctionDefinitionContext)_localctx).body = expression();
((FunctionDefinitionContext)_localctx).ast = astFactory.toFunctionDefinition(parameters, ((FunctionDefinitionContext)_localctx).body.ast, external);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FormalParameterContext extends ParserRuleContext {
public FormalParameter ast;
public ParameterNameContext name;
public TypeContext type;
public ParameterNameContext parameterName() {
return getRuleContext(ParameterNameContext.class,0);
}
public TerminalNode COLON() { return getToken(FEELParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public FormalParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_formalParameter; }
}
public final FormalParameterContext formalParameter() throws RecognitionException {
FormalParameterContext _localctx = new FormalParameterContext(_ctx, getState());
enterRule(_localctx, 44, RULE_formalParameter);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
TypeExpression typeExp = null;
setState(317);
((FormalParameterContext)_localctx).name = parameterName();
setState(322);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON) {
{
setState(318);
match(COLON);
setState(319);
((FormalParameterContext)_localctx).type = type();
typeExp = ((FormalParameterContext)_localctx).type.ast;
}
}
((FormalParameterContext)_localctx).ast = astFactory.toFormalParameter(((FormalParameterContext)_localctx).name.ast, typeExp);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ForExpressionContext extends ParserRuleContext {
public Expression ast;
public IdentifierContext var;
public IterationDomainContext domain;
public ExpressionContext body;
public TerminalNode FOR() { return getToken(FEELParser.FOR, 0); }
public List IN() { return getTokens(FEELParser.IN); }
public TerminalNode IN(int i) {
return getToken(FEELParser.IN, i);
}
public TerminalNode RETURN() { return getToken(FEELParser.RETURN, 0); }
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List iterationDomain() {
return getRuleContexts(IterationDomainContext.class);
}
public IterationDomainContext iterationDomain(int i) {
return getRuleContext(IterationDomainContext.class,i);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public ForExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_forExpression; }
}
public final ForExpressionContext forExpression() throws RecognitionException {
ForExpressionContext _localctx = new ForExpressionContext(_ctx, getState());
enterRule(_localctx, 46, RULE_forExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List iterators = new ArrayList<>();
setState(327);
match(FOR);
setState(328);
((ForExpressionContext)_localctx).var = identifier();
setState(329);
match(IN);
setState(330);
((ForExpressionContext)_localctx).domain = iterationDomain();
iterators.add(astFactory.toIterator((((ForExpressionContext)_localctx).var!=null?_input.getText(((ForExpressionContext)_localctx).var.start,((ForExpressionContext)_localctx).var.stop):null), ((ForExpressionContext)_localctx).domain.ast));
setState(340);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(332);
match(COMMA);
setState(333);
((ForExpressionContext)_localctx).var = identifier();
setState(334);
match(IN);
setState(335);
((ForExpressionContext)_localctx).domain = iterationDomain();
iterators.add(astFactory.toIterator((((ForExpressionContext)_localctx).var!=null?_input.getText(((ForExpressionContext)_localctx).var.start,((ForExpressionContext)_localctx).var.stop):null), ((ForExpressionContext)_localctx).domain.ast));
}
}
setState(342);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(343);
match(RETURN);
setState(344);
((ForExpressionContext)_localctx).body = expression();
((ForExpressionContext)_localctx).ast = astFactory.toForExpression(iterators, ((ForExpressionContext)_localctx).body.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IterationDomainContext extends ParserRuleContext {
public IteratorDomain ast;
public ExpressionContext exp1;
public ExpressionContext exp2;
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode DOT_DOT() { return getToken(FEELParser.DOT_DOT, 0); }
public IterationDomainContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_iterationDomain; }
}
public final IterationDomainContext iterationDomain() throws RecognitionException {
IterationDomainContext _localctx = new IterationDomainContext(_ctx, getState());
enterRule(_localctx, 48, RULE_iterationDomain);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
Expression end = null;
setState(348);
((IterationDomainContext)_localctx).exp1 = expression();
setState(353);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DOT_DOT) {
{
setState(349);
match(DOT_DOT);
setState(350);
((IterationDomainContext)_localctx).exp2 = expression();
end = ((IterationDomainContext)_localctx).exp2.ast;
}
}
((IterationDomainContext)_localctx).ast = astFactory.toIteratorDomain(((IterationDomainContext)_localctx).exp1.ast, end);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IfExpressionContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext cond;
public ExpressionContext exp1;
public ExpressionContext exp2;
public TerminalNode IF() { return getToken(FEELParser.IF, 0); }
public TerminalNode THEN() { return getToken(FEELParser.THEN, 0); }
public TerminalNode ELSE() { return getToken(FEELParser.ELSE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public IfExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ifExpression; }
}
public final IfExpressionContext ifExpression() throws RecognitionException {
IfExpressionContext _localctx = new IfExpressionContext(_ctx, getState());
enterRule(_localctx, 50, RULE_ifExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(357);
match(IF);
setState(358);
((IfExpressionContext)_localctx).cond = expression();
setState(359);
match(THEN);
setState(360);
((IfExpressionContext)_localctx).exp1 = expression();
setState(361);
match(ELSE);
setState(362);
((IfExpressionContext)_localctx).exp2 = expression();
((IfExpressionContext)_localctx).ast = astFactory.toIfExpression(((IfExpressionContext)_localctx).cond.ast, ((IfExpressionContext)_localctx).exp1.ast, ((IfExpressionContext)_localctx).exp2.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class QuantifiedExpressionContext extends ParserRuleContext {
public Expression ast;
public Token op;
public IdentifierContext var;
public ExpressionContext domain;
public ExpressionContext body;
public List IN() { return getTokens(FEELParser.IN); }
public TerminalNode IN(int i) {
return getToken(FEELParser.IN, i);
}
public TerminalNode SATISFIES() { return getToken(FEELParser.SATISFIES, 0); }
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode SOME() { return getToken(FEELParser.SOME, 0); }
public TerminalNode EVERY() { return getToken(FEELParser.EVERY, 0); }
public QuantifiedExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_quantifiedExpression; }
}
public final QuantifiedExpressionContext quantifiedExpression() throws RecognitionException {
QuantifiedExpressionContext _localctx = new QuantifiedExpressionContext(_ctx, getState());
enterRule(_localctx, 52, RULE_quantifiedExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List iterators = new ArrayList<>();
setState(368);
_errHandler.sync(this);
switch (_input.LA(1)) {
case SOME:
{
setState(366);
((QuantifiedExpressionContext)_localctx).op = match(SOME);
}
break;
case EVERY:
{
setState(367);
((QuantifiedExpressionContext)_localctx).op = match(EVERY);
}
break;
default:
throw new NoViableAltException(this);
}
setState(370);
((QuantifiedExpressionContext)_localctx).var = identifier();
setState(371);
match(IN);
setState(372);
((QuantifiedExpressionContext)_localctx).domain = expression();
iterators.add(astFactory.toIterator((((QuantifiedExpressionContext)_localctx).var!=null?_input.getText(((QuantifiedExpressionContext)_localctx).var.start,((QuantifiedExpressionContext)_localctx).var.stop):null), ((QuantifiedExpressionContext)_localctx).domain.ast));
setState(381);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << FUNCTION) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
{
setState(374);
((QuantifiedExpressionContext)_localctx).var = identifier();
setState(375);
match(IN);
setState(376);
((QuantifiedExpressionContext)_localctx).domain = expression();
iterators.add(astFactory.toIterator((((QuantifiedExpressionContext)_localctx).var!=null?_input.getText(((QuantifiedExpressionContext)_localctx).var.start,((QuantifiedExpressionContext)_localctx).var.stop):null), ((QuantifiedExpressionContext)_localctx).domain.ast));
}
}
setState(383);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(384);
match(SATISFIES);
setState(385);
((QuantifiedExpressionContext)_localctx).body = expression();
((QuantifiedExpressionContext)_localctx).ast = astFactory.toQuantifiedExpression((((QuantifiedExpressionContext)_localctx).op!=null?((QuantifiedExpressionContext)_localctx).op.getText():null), iterators, ((QuantifiedExpressionContext)_localctx).body.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DisjunctionContext extends ParserRuleContext {
public Expression ast;
public ConjunctionContext left;
public ConjunctionContext right;
public List conjunction() {
return getRuleContexts(ConjunctionContext.class);
}
public ConjunctionContext conjunction(int i) {
return getRuleContext(ConjunctionContext.class,i);
}
public List OR() { return getTokens(FEELParser.OR); }
public TerminalNode OR(int i) {
return getToken(FEELParser.OR, i);
}
public DisjunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_disjunction; }
}
public final DisjunctionContext disjunction() throws RecognitionException {
DisjunctionContext _localctx = new DisjunctionContext(_ctx, getState());
enterRule(_localctx, 54, RULE_disjunction);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(388);
((DisjunctionContext)_localctx).left = conjunction();
((DisjunctionContext)_localctx).ast = ((DisjunctionContext)_localctx).left.ast;
setState(396);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,21,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(390);
match(OR);
setState(391);
((DisjunctionContext)_localctx).right = conjunction();
((DisjunctionContext)_localctx).ast = astFactory.toDisjunction(_localctx.ast, ((DisjunctionContext)_localctx).right.ast);
}
}
}
setState(398);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,21,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ConjunctionContext extends ParserRuleContext {
public Expression ast;
public ComparisonContext left;
public ComparisonContext right;
public List comparison() {
return getRuleContexts(ComparisonContext.class);
}
public ComparisonContext comparison(int i) {
return getRuleContext(ComparisonContext.class,i);
}
public List AND() { return getTokens(FEELParser.AND); }
public TerminalNode AND(int i) {
return getToken(FEELParser.AND, i);
}
public ConjunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_conjunction; }
}
public final ConjunctionContext conjunction() throws RecognitionException {
ConjunctionContext _localctx = new ConjunctionContext(_ctx, getState());
enterRule(_localctx, 56, RULE_conjunction);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(399);
((ConjunctionContext)_localctx).left = comparison();
((ConjunctionContext)_localctx).ast = ((ConjunctionContext)_localctx).left.ast;
setState(407);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,22,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(401);
match(AND);
setState(402);
((ConjunctionContext)_localctx).right = comparison();
((ConjunctionContext)_localctx).ast = astFactory.toConjunction(_localctx.ast, ((ConjunctionContext)_localctx).right.ast);
}
}
}
setState(409);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,22,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ComparisonContext extends ParserRuleContext {
public Expression ast;
public ArithmeticExpressionContext ae1;
public Token op;
public ArithmeticExpressionContext ae2;
public ExpressionContext leftEndpoint;
public ExpressionContext rightEndpoint;
public PositiveUnaryTestContext test;
public PositiveUnaryTestsContext tests;
public List arithmeticExpression() {
return getRuleContexts(ArithmeticExpressionContext.class);
}
public ArithmeticExpressionContext arithmeticExpression(int i) {
return getRuleContext(ArithmeticExpressionContext.class,i);
}
public TerminalNode BETWEEN() { return getToken(FEELParser.BETWEEN, 0); }
public TerminalNode AND() { return getToken(FEELParser.AND, 0); }
public TerminalNode IN() { return getToken(FEELParser.IN, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public PositiveUnaryTestContext positiveUnaryTest() {
return getRuleContext(PositiveUnaryTestContext.class,0);
}
public PositiveUnaryTestsContext positiveUnaryTests() {
return getRuleContext(PositiveUnaryTestsContext.class,0);
}
public TerminalNode EQ() { return getToken(FEELParser.EQ, 0); }
public TerminalNode NE() { return getToken(FEELParser.NE, 0); }
public TerminalNode LT() { return getToken(FEELParser.LT, 0); }
public TerminalNode GT() { return getToken(FEELParser.GT, 0); }
public TerminalNode LE() { return getToken(FEELParser.LE, 0); }
public TerminalNode GE() { return getToken(FEELParser.GE, 0); }
public ComparisonContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_comparison; }
}
public final ComparisonContext comparison() throws RecognitionException {
ComparisonContext _localctx = new ComparisonContext(_ctx, getState());
enterRule(_localctx, 58, RULE_comparison);
try {
enterOuterAlt(_localctx, 1);
{
setState(410);
((ComparisonContext)_localctx).ae1 = arithmeticExpression();
((ComparisonContext)_localctx).ast = ((ComparisonContext)_localctx).ae1.ast;
setState(439);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,24,_ctx) ) {
case 1:
{
{
setState(418);
_errHandler.sync(this);
switch (_input.LA(1)) {
case EQ:
{
setState(412);
((ComparisonContext)_localctx).op = match(EQ);
}
break;
case NE:
{
setState(413);
((ComparisonContext)_localctx).op = match(NE);
}
break;
case LT:
{
setState(414);
((ComparisonContext)_localctx).op = match(LT);
}
break;
case GT:
{
setState(415);
((ComparisonContext)_localctx).op = match(GT);
}
break;
case LE:
{
setState(416);
((ComparisonContext)_localctx).op = match(LE);
}
break;
case GE:
{
setState(417);
((ComparisonContext)_localctx).op = match(GE);
}
break;
default:
throw new NoViableAltException(this);
}
setState(420);
((ComparisonContext)_localctx).ae2 = arithmeticExpression();
((ComparisonContext)_localctx).ast = astFactory.toComparison((((ComparisonContext)_localctx).op!=null?((ComparisonContext)_localctx).op.getText():null), ((ComparisonContext)_localctx).ae1.ast, ((ComparisonContext)_localctx).ae2.ast);
}
}
break;
case 2:
{
{
setState(423);
match(BETWEEN);
setState(424);
((ComparisonContext)_localctx).leftEndpoint = expression();
setState(425);
match(AND);
setState(426);
((ComparisonContext)_localctx).rightEndpoint = expression();
((ComparisonContext)_localctx).ast = astFactory.toBetweenExpression(_localctx.ast, ((ComparisonContext)_localctx).leftEndpoint.ast, ((ComparisonContext)_localctx).rightEndpoint.ast);
}
}
break;
case 3:
{
{
setState(429);
match(IN);
setState(430);
((ComparisonContext)_localctx).test = positiveUnaryTest();
((ComparisonContext)_localctx).ast = astFactory.toInExpression(_localctx.ast, ((ComparisonContext)_localctx).test.ast);
}
}
break;
case 4:
{
{
setState(433);
match(IN);
setState(434);
match(PAREN_OPEN);
setState(435);
((ComparisonContext)_localctx).tests = positiveUnaryTests();
setState(436);
match(PAREN_CLOSE);
((ComparisonContext)_localctx).ast = astFactory.toInExpression(_localctx.ast, ((ComparisonContext)_localctx).tests.ast);
}
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArithmeticExpressionContext extends ParserRuleContext {
public Expression ast;
public AdditionContext addition;
public AdditionContext addition() {
return getRuleContext(AdditionContext.class,0);
}
public ArithmeticExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arithmeticExpression; }
}
public final ArithmeticExpressionContext arithmeticExpression() throws RecognitionException {
ArithmeticExpressionContext _localctx = new ArithmeticExpressionContext(_ctx, getState());
enterRule(_localctx, 60, RULE_arithmeticExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(441);
((ArithmeticExpressionContext)_localctx).addition = addition();
((ArithmeticExpressionContext)_localctx).ast = ((ArithmeticExpressionContext)_localctx).addition.ast;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AdditionContext extends ParserRuleContext {
public Expression ast;
public MultiplicationContext left;
public Token op;
public MultiplicationContext right;
public List multiplication() {
return getRuleContexts(MultiplicationContext.class);
}
public MultiplicationContext multiplication(int i) {
return getRuleContext(MultiplicationContext.class,i);
}
public List PLUS() { return getTokens(FEELParser.PLUS); }
public TerminalNode PLUS(int i) {
return getToken(FEELParser.PLUS, i);
}
public List MINUS() { return getTokens(FEELParser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(FEELParser.MINUS, i);
}
public AdditionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_addition; }
}
public final AdditionContext addition() throws RecognitionException {
AdditionContext _localctx = new AdditionContext(_ctx, getState());
enterRule(_localctx, 62, RULE_addition);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(444);
((AdditionContext)_localctx).left = multiplication();
((AdditionContext)_localctx).ast = ((AdditionContext)_localctx).left.ast;
setState(455);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,26,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(448);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PLUS:
{
setState(446);
((AdditionContext)_localctx).op = match(PLUS);
}
break;
case MINUS:
{
setState(447);
((AdditionContext)_localctx).op = match(MINUS);
}
break;
default:
throw new NoViableAltException(this);
}
setState(450);
((AdditionContext)_localctx).right = multiplication();
((AdditionContext)_localctx).ast = astFactory.toAddition((((AdditionContext)_localctx).op!=null?((AdditionContext)_localctx).op.getText():null), _localctx.ast, ((AdditionContext)_localctx).right.ast);
}
}
}
setState(457);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,26,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class MultiplicationContext extends ParserRuleContext {
public Expression ast;
public ExponentiationContext left;
public Token op;
public ExponentiationContext right;
public List exponentiation() {
return getRuleContexts(ExponentiationContext.class);
}
public ExponentiationContext exponentiation(int i) {
return getRuleContext(ExponentiationContext.class,i);
}
public List STAR() { return getTokens(FEELParser.STAR); }
public TerminalNode STAR(int i) {
return getToken(FEELParser.STAR, i);
}
public List FORWARD_SLASH() { return getTokens(FEELParser.FORWARD_SLASH); }
public TerminalNode FORWARD_SLASH(int i) {
return getToken(FEELParser.FORWARD_SLASH, i);
}
public MultiplicationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_multiplication; }
}
public final MultiplicationContext multiplication() throws RecognitionException {
MultiplicationContext _localctx = new MultiplicationContext(_ctx, getState());
enterRule(_localctx, 64, RULE_multiplication);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(458);
((MultiplicationContext)_localctx).left = exponentiation();
((MultiplicationContext)_localctx).ast = ((MultiplicationContext)_localctx).left.ast;
setState(469);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,28,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(462);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STAR:
{
setState(460);
((MultiplicationContext)_localctx).op = match(STAR);
}
break;
case FORWARD_SLASH:
{
setState(461);
((MultiplicationContext)_localctx).op = match(FORWARD_SLASH);
}
break;
default:
throw new NoViableAltException(this);
}
setState(464);
((MultiplicationContext)_localctx).right = exponentiation();
((MultiplicationContext)_localctx).ast = astFactory.toMultiplication((((MultiplicationContext)_localctx).op!=null?((MultiplicationContext)_localctx).op.getText():null), _localctx.ast, ((MultiplicationContext)_localctx).right.ast);
}
}
}
setState(471);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,28,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExponentiationContext extends ParserRuleContext {
public Expression ast;
public ArithmeticNegationContext left;
public ArithmeticNegationContext right;
public List arithmeticNegation() {
return getRuleContexts(ArithmeticNegationContext.class);
}
public ArithmeticNegationContext arithmeticNegation(int i) {
return getRuleContext(ArithmeticNegationContext.class,i);
}
public List STAR_STAR() { return getTokens(FEELParser.STAR_STAR); }
public TerminalNode STAR_STAR(int i) {
return getToken(FEELParser.STAR_STAR, i);
}
public ExponentiationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_exponentiation; }
}
public final ExponentiationContext exponentiation() throws RecognitionException {
ExponentiationContext _localctx = new ExponentiationContext(_ctx, getState());
enterRule(_localctx, 66, RULE_exponentiation);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(472);
((ExponentiationContext)_localctx).left = arithmeticNegation();
((ExponentiationContext)_localctx).ast = ((ExponentiationContext)_localctx).left.ast;
setState(480);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,29,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(474);
match(STAR_STAR);
setState(475);
((ExponentiationContext)_localctx).right = arithmeticNegation();
((ExponentiationContext)_localctx).ast = astFactory.toExponentiation(_localctx.ast, ((ExponentiationContext)_localctx).right.ast);
}
}
}
setState(482);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,29,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArithmeticNegationContext extends ParserRuleContext {
public Expression ast;
public InstanceOfContext opd;
public InstanceOfContext instanceOf() {
return getRuleContext(InstanceOfContext.class,0);
}
public List MINUS() { return getTokens(FEELParser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(FEELParser.MINUS, i);
}
public List NOT() { return getTokens(FEELParser.NOT); }
public TerminalNode NOT(int i) {
return getToken(FEELParser.NOT, i);
}
public ArithmeticNegationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arithmeticNegation; }
}
public final ArithmeticNegationContext arithmeticNegation() throws RecognitionException {
ArithmeticNegationContext _localctx = new ArithmeticNegationContext(_ctx, getState());
enterRule(_localctx, 68, RULE_arithmeticNegation);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
List prefixOperators = new ArrayList<>();
setState(490);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,31,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
setState(488);
_errHandler.sync(this);
switch (_input.LA(1)) {
case MINUS:
{
{
setState(484);
match(MINUS);
prefixOperators.add("-");
}
}
break;
case NOT:
{
{
setState(486);
match(NOT);
prefixOperators.add("not");
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(492);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,31,_ctx);
}
setState(493);
((ArithmeticNegationContext)_localctx).opd = instanceOf();
((ArithmeticNegationContext)_localctx).ast = astFactory.toNegation(prefixOperators, ((ArithmeticNegationContext)_localctx).opd.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class InstanceOfContext extends ParserRuleContext {
public Expression ast;
public PostfixExpressionContext exp;
public TypeContext type;
public PostfixExpressionContext postfixExpression() {
return getRuleContext(PostfixExpressionContext.class,0);
}
public TerminalNode INSTANCE_OF() { return getToken(FEELParser.INSTANCE_OF, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public InstanceOfContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_instanceOf; }
}
public final InstanceOfContext instanceOf() throws RecognitionException {
InstanceOfContext _localctx = new InstanceOfContext(_ctx, getState());
enterRule(_localctx, 70, RULE_instanceOf);
try {
enterOuterAlt(_localctx, 1);
{
setState(496);
((InstanceOfContext)_localctx).exp = postfixExpression();
((InstanceOfContext)_localctx).ast = ((InstanceOfContext)_localctx).exp.ast;
setState(502);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,32,_ctx) ) {
case 1:
{
setState(498);
match(INSTANCE_OF);
{
setState(499);
((InstanceOfContext)_localctx).type = type();
((InstanceOfContext)_localctx).ast = astFactory.toInstanceOf(_localctx.ast, ((InstanceOfContext)_localctx).type.ast);
}
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TypeContext extends ParserRuleContext {
public TypeExpression ast;
public QualifiedNameContext qName;
public TypeContext type;
public IdentifierContext id1;
public TypeContext t1;
public IdentifierContext id2;
public TypeContext t2;
public TypeContext returnType;
public QualifiedNameContext qualifiedName() {
return getRuleContext(QualifiedNameContext.class,0);
}
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public TerminalNode LT() { return getToken(FEELParser.LT, 0); }
public List type() {
return getRuleContexts(TypeContext.class);
}
public TypeContext type(int i) {
return getRuleContext(TypeContext.class,i);
}
public TerminalNode GT() { return getToken(FEELParser.GT, 0); }
public List COLON() { return getTokens(FEELParser.COLON); }
public TerminalNode COLON(int i) {
return getToken(FEELParser.COLON, i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public TerminalNode ARROW() { return getToken(FEELParser.ARROW, 0); }
public TypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_type; }
}
public final TypeContext type() throws RecognitionException {
TypeContext _localctx = new TypeContext(_ctx, getState());
enterRule(_localctx, 72, RULE_type);
int _la;
try {
setState(555);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,36,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(504);
((TypeContext)_localctx).qName = qualifiedName();
((TypeContext)_localctx).ast = astFactory.toNamedTypeExpression(((TypeContext)_localctx).qName.ast);
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(507);
identifier();
setState(508);
match(LT);
setState(509);
((TypeContext)_localctx).type = type();
setState(510);
match(GT);
((TypeContext)_localctx).ast = astFactory.toListTypeExpression(((TypeContext)_localctx).type.ast);
}
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
{
List> members = new ArrayList<>();
setState(514);
identifier();
setState(515);
match(LT);
setState(516);
((TypeContext)_localctx).id1 = identifier();
setState(517);
match(COLON);
setState(518);
((TypeContext)_localctx).t1 = type();
members.add(new Pair(((TypeContext)_localctx).id1.ast.getText(), ((TypeContext)_localctx).t1.ast));
setState(528);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(520);
match(COMMA);
setState(521);
((TypeContext)_localctx).id2 = identifier();
setState(522);
match(COLON);
setState(523);
((TypeContext)_localctx).t2 = type();
members.add(new Pair(((TypeContext)_localctx).id2.ast.getText(), ((TypeContext)_localctx).t2.ast));
}
}
setState(530);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(531);
match(GT);
((TypeContext)_localctx).ast = astFactory.toContextTypeExpression(members);
}
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
{
List parameters = new ArrayList<>();
setState(535);
identifier();
setState(536);
match(LT);
setState(548);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << FUNCTION) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
setState(537);
((TypeContext)_localctx).t1 = type();
parameters.add(((TypeContext)_localctx).t1.ast);
setState(543);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(539);
match(COMMA);
setState(540);
((TypeContext)_localctx).t2 = type();
}
}
setState(545);
_errHandler.sync(this);
_la = _input.LA(1);
}
parameters.add(((TypeContext)_localctx).t2.ast);
}
}
setState(550);
match(GT);
setState(551);
match(ARROW);
setState(552);
((TypeContext)_localctx).returnType = type();
((TypeContext)_localctx).ast = astFactory.toFunctionTypeExpression(parameters, ((TypeContext)_localctx).returnType.ast);
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PostfixExpressionContext extends ParserRuleContext {
public Expression ast;
public PrimaryExpressionContext primaryExpression;
public ExpressionContext filter;
public ParametersContext parameters;
public IdentifierContext name;
public PrimaryExpressionContext primaryExpression() {
return getRuleContext(PrimaryExpressionContext.class,0);
}
public List BRACKET_OPEN() { return getTokens(FEELParser.BRACKET_OPEN); }
public TerminalNode BRACKET_OPEN(int i) {
return getToken(FEELParser.BRACKET_OPEN, i);
}
public List BRACKET_CLOSE() { return getTokens(FEELParser.BRACKET_CLOSE); }
public TerminalNode BRACKET_CLOSE(int i) {
return getToken(FEELParser.BRACKET_CLOSE, i);
}
public List parameters() {
return getRuleContexts(ParametersContext.class);
}
public ParametersContext parameters(int i) {
return getRuleContext(ParametersContext.class,i);
}
public List DOT() { return getTokens(FEELParser.DOT); }
public TerminalNode DOT(int i) {
return getToken(FEELParser.DOT, i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public PostfixExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_postfixExpression; }
}
public final PostfixExpressionContext postfixExpression() throws RecognitionException {
PostfixExpressionContext _localctx = new PostfixExpressionContext(_ctx, getState());
enterRule(_localctx, 74, RULE_postfixExpression);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
{
{
setState(557);
((PostfixExpressionContext)_localctx).primaryExpression = primaryExpression();
((PostfixExpressionContext)_localctx).ast = ((PostfixExpressionContext)_localctx).primaryExpression.ast;
}
}
setState(574);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,38,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
setState(572);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BRACKET_OPEN:
{
{
setState(560);
match(BRACKET_OPEN);
setState(561);
((PostfixExpressionContext)_localctx).filter = expression();
setState(562);
match(BRACKET_CLOSE);
((PostfixExpressionContext)_localctx).ast = astFactory.toFilterExpression(_localctx.ast, ((PostfixExpressionContext)_localctx).filter.ast);
}
}
break;
case PAREN_OPEN:
{
{
setState(565);
((PostfixExpressionContext)_localctx).parameters = parameters();
((PostfixExpressionContext)_localctx).ast = astFactory.toFunctionInvocation(_localctx.ast, ((PostfixExpressionContext)_localctx).parameters.ast);
}
}
break;
case DOT:
{
{
setState(568);
match(DOT);
setState(569);
((PostfixExpressionContext)_localctx).name = identifier();
((PostfixExpressionContext)_localctx).ast = astFactory.toPathExpression(_localctx.ast, (((PostfixExpressionContext)_localctx).name!=null?_input.getText(((PostfixExpressionContext)_localctx).name.start,((PostfixExpressionContext)_localctx).name.stop):null));
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(576);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,38,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ParametersContext extends ParserRuleContext {
public Parameters ast;
public NamedParametersContext namedParameters;
public PositionalParametersContext positionalParameters;
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public NamedParametersContext namedParameters() {
return getRuleContext(NamedParametersContext.class,0);
}
public PositionalParametersContext positionalParameters() {
return getRuleContext(PositionalParametersContext.class,0);
}
public ParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parameters; }
}
public final ParametersContext parameters() throws RecognitionException {
ParametersContext _localctx = new ParametersContext(_ctx, getState());
enterRule(_localctx, 76, RULE_parameters);
try {
enterOuterAlt(_localctx, 1);
{
setState(577);
match(PAREN_OPEN);
setState(584);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,39,_ctx) ) {
case 1:
{
{
setState(578);
((ParametersContext)_localctx).namedParameters = namedParameters();
((ParametersContext)_localctx).ast = ((ParametersContext)_localctx).namedParameters.ast;
}
}
break;
case 2:
{
{
setState(581);
((ParametersContext)_localctx).positionalParameters = positionalParameters();
((ParametersContext)_localctx).ast = ((ParametersContext)_localctx).positionalParameters.ast;
}
}
break;
}
setState(586);
match(PAREN_CLOSE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NamedParametersContext extends ParserRuleContext {
public NamedParameters ast;
public ParameterNameContext name;
public ExpressionContext value;
public List COLON() { return getTokens(FEELParser.COLON); }
public TerminalNode COLON(int i) {
return getToken(FEELParser.COLON, i);
}
public List parameterName() {
return getRuleContexts(ParameterNameContext.class);
}
public ParameterNameContext parameterName(int i) {
return getRuleContext(ParameterNameContext.class,i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public NamedParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_namedParameters; }
}
public final NamedParametersContext namedParameters() throws RecognitionException {
NamedParametersContext _localctx = new NamedParametersContext(_ctx, getState());
enterRule(_localctx, 78, RULE_namedParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
Map parameters = new LinkedHashMap<>();
setState(589);
((NamedParametersContext)_localctx).name = parameterName();
setState(590);
match(COLON);
setState(591);
((NamedParametersContext)_localctx).value = expression();
parameters.put((((NamedParametersContext)_localctx).name!=null?_input.getText(((NamedParametersContext)_localctx).name.start,((NamedParametersContext)_localctx).name.stop):null), ((NamedParametersContext)_localctx).value.ast);
setState(601);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(593);
match(COMMA);
setState(594);
((NamedParametersContext)_localctx).name = parameterName();
setState(595);
match(COLON);
setState(596);
((NamedParametersContext)_localctx).value = expression();
parameters.put((((NamedParametersContext)_localctx).name!=null?_input.getText(((NamedParametersContext)_localctx).name.start,((NamedParametersContext)_localctx).name.stop):null), ((NamedParametersContext)_localctx).value.ast);
}
}
setState(603);
_errHandler.sync(this);
_la = _input.LA(1);
}
((NamedParametersContext)_localctx).ast = astFactory.toNamedParameters(parameters);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ParameterNameContext extends ParserRuleContext {
public String ast;
public IdentifierContext name;
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public ParameterNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parameterName; }
}
public final ParameterNameContext parameterName() throws RecognitionException {
ParameterNameContext _localctx = new ParameterNameContext(_ctx, getState());
enterRule(_localctx, 80, RULE_parameterName);
try {
enterOuterAlt(_localctx, 1);
{
setState(606);
((ParameterNameContext)_localctx).name = identifier();
((ParameterNameContext)_localctx).ast = (((ParameterNameContext)_localctx).name!=null?_input.getText(((ParameterNameContext)_localctx).name.start,((ParameterNameContext)_localctx).name.stop):null);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PositionalParametersContext extends ParserRuleContext {
public PositionalParameters ast;
public ExpressionContext param;
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public PositionalParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positionalParameters; }
}
public final PositionalParametersContext positionalParameters() throws RecognitionException {
PositionalParametersContext _localctx = new PositionalParametersContext(_ctx, getState());
enterRule(_localctx, 82, RULE_positionalParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List parameters = new ArrayList<>();
setState(621);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << STRING) | (1L << NUMBER) | (1L << LT) | (1L << GT) | (1L << LE) | (1L << GE) | (1L << MINUS) | (1L << PAREN_OPEN) | (1L << BRACKET_OPEN) | (1L << BRACKET_CLOSE) | (1L << BRACE_OPEN) | (1L << NOT) | (1L << TRUE) | (1L << FALSE) | (1L << NULL) | (1L << FUNCTION) | (1L << FOR) | (1L << IF) | (1L << SOME) | (1L << EVERY) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
setState(610);
((PositionalParametersContext)_localctx).param = expression();
parameters.add(((PositionalParametersContext)_localctx).param.ast);
setState(618);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(612);
match(COMMA);
setState(613);
((PositionalParametersContext)_localctx).param = expression();
parameters.add(((PositionalParametersContext)_localctx).param.ast);
}
}
setState(620);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
((PositionalParametersContext)_localctx).ast = astFactory.toPositionalParameters(parameters);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PrimaryExpressionContext extends ParserRuleContext {
public Expression ast;
public LiteralContext literal;
public IdentifierContext name;
public ExpressionContext exp;
public BoxedExpressionContext boxedExpression;
public SimplePositiveUnaryTestContext simplePositiveUnaryTest;
public LiteralContext literal() {
return getRuleContext(LiteralContext.class,0);
}
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public BoxedExpressionContext boxedExpression() {
return getRuleContext(BoxedExpressionContext.class,0);
}
public SimplePositiveUnaryTestContext simplePositiveUnaryTest() {
return getRuleContext(SimplePositiveUnaryTestContext.class,0);
}
public PrimaryExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_primaryExpression; }
}
public final PrimaryExpressionContext primaryExpression() throws RecognitionException {
PrimaryExpressionContext _localctx = new PrimaryExpressionContext(_ctx, getState());
enterRule(_localctx, 84, RULE_primaryExpression);
try {
setState(642);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,43,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(625);
((PrimaryExpressionContext)_localctx).literal = literal();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).literal.ast;
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(628);
((PrimaryExpressionContext)_localctx).name = identifier();
((PrimaryExpressionContext)_localctx).ast = astFactory.toName((((PrimaryExpressionContext)_localctx).name!=null?_input.getText(((PrimaryExpressionContext)_localctx).name.start,((PrimaryExpressionContext)_localctx).name.stop):null));
}
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
{
setState(631);
match(PAREN_OPEN);
setState(632);
((PrimaryExpressionContext)_localctx).exp = expression();
setState(633);
match(PAREN_CLOSE);
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).exp.ast;
}
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
{
setState(636);
((PrimaryExpressionContext)_localctx).boxedExpression = boxedExpression();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).boxedExpression.ast;
}
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
{
setState(639);
((PrimaryExpressionContext)_localctx).simplePositiveUnaryTest = simplePositiveUnaryTest();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).simplePositiveUnaryTest.ast;
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleValueContext extends ParserRuleContext {
public Expression ast;
public SimpleLiteralContext simpleLiteral;
public QualifiedNameContext qualifiedName;
public SimpleLiteralContext simpleLiteral() {
return getRuleContext(SimpleLiteralContext.class,0);
}
public QualifiedNameContext qualifiedName() {
return getRuleContext(QualifiedNameContext.class,0);
}
public SimpleValueContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleValue; }
}
public final SimpleValueContext simpleValue() throws RecognitionException {
SimpleValueContext _localctx = new SimpleValueContext(_ctx, getState());
enterRule(_localctx, 86, RULE_simpleValue);
try {
setState(650);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,44,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(644);
((SimpleValueContext)_localctx).simpleLiteral = simpleLiteral();
((SimpleValueContext)_localctx).ast = ((SimpleValueContext)_localctx).simpleLiteral.ast;
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(647);
((SimpleValueContext)_localctx).qualifiedName = qualifiedName();
((SimpleValueContext)_localctx).ast = ((SimpleValueContext)_localctx).qualifiedName.ast;
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class QualifiedNameContext extends ParserRuleContext {
public Expression ast;
public IdentifierContext name;
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List DOT() { return getTokens(FEELParser.DOT); }
public TerminalNode DOT(int i) {
return getToken(FEELParser.DOT, i);
}
public QualifiedNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_qualifiedName; }
}
public final QualifiedNameContext qualifiedName() throws RecognitionException {
QualifiedNameContext _localctx = new QualifiedNameContext(_ctx, getState());
enterRule(_localctx, 88, RULE_qualifiedName);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
List names = new ArrayList<>();
setState(653);
((QualifiedNameContext)_localctx).name = identifier();
names.add((((QualifiedNameContext)_localctx).name!=null?_input.getText(((QualifiedNameContext)_localctx).name.start,((QualifiedNameContext)_localctx).name.stop):null));
setState(661);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,45,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(655);
match(DOT);
setState(656);
((QualifiedNameContext)_localctx).name = identifier();
names.add((((QualifiedNameContext)_localctx).name!=null?_input.getText(((QualifiedNameContext)_localctx).name.start,((QualifiedNameContext)_localctx).name.stop):null));
}
}
}
setState(663);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,45,_ctx);
}
((QualifiedNameContext)_localctx).ast = astFactory.toQualifiedName(names);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class LiteralContext extends ParserRuleContext {
public Expression ast;
public SimpleLiteralContext simpleLiteral;
public SimpleLiteralContext simpleLiteral() {
return getRuleContext(SimpleLiteralContext.class,0);
}
public TerminalNode NULL() { return getToken(FEELParser.NULL, 0); }
public LiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_literal; }
}
public final LiteralContext literal() throws RecognitionException {
LiteralContext _localctx = new LiteralContext(_ctx, getState());
enterRule(_localctx, 90, RULE_literal);
try {
enterOuterAlt(_localctx, 1);
{
setState(671);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING:
case NUMBER:
case TRUE:
case FALSE:
case FUNCTION:
case AND:
case OR:
case NAME:
{
{
setState(666);
((LiteralContext)_localctx).simpleLiteral = simpleLiteral();
((LiteralContext)_localctx).ast = ((LiteralContext)_localctx).simpleLiteral.ast;
}
}
break;
case NULL:
{
{
setState(669);
match(NULL);
((LiteralContext)_localctx).ast = astFactory.toNullLiteral();
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleLiteralContext extends ParserRuleContext {
public Expression ast;
public NumericLiteralContext numericLiteral;
public StringLiteralContext stringLiteral;
public BooleanLiteralContext booleanLiteral;
public DateTimeLiteralContext dateTimeLiteral;
public NumericLiteralContext numericLiteral() {
return getRuleContext(NumericLiteralContext.class,0);
}
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public BooleanLiteralContext booleanLiteral() {
return getRuleContext(BooleanLiteralContext.class,0);
}
public DateTimeLiteralContext dateTimeLiteral() {
return getRuleContext(DateTimeLiteralContext.class,0);
}
public SimpleLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleLiteral; }
}
public final SimpleLiteralContext simpleLiteral() throws RecognitionException {
SimpleLiteralContext _localctx = new SimpleLiteralContext(_ctx, getState());
enterRule(_localctx, 92, RULE_simpleLiteral);
try {
setState(685);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NUMBER:
enterOuterAlt(_localctx, 1);
{
{
setState(673);
((SimpleLiteralContext)_localctx).numericLiteral = numericLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).numericLiteral.ast;
}
}
break;
case STRING:
enterOuterAlt(_localctx, 2);
{
{
setState(676);
((SimpleLiteralContext)_localctx).stringLiteral = stringLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).stringLiteral.ast;
}
}
break;
case TRUE:
case FALSE:
enterOuterAlt(_localctx, 3);
{
{
setState(679);
((SimpleLiteralContext)_localctx).booleanLiteral = booleanLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).booleanLiteral.ast;
}
}
break;
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 4);
{
{
setState(682);
((SimpleLiteralContext)_localctx).dateTimeLiteral = dateTimeLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).dateTimeLiteral.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StringLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode STRING() { return getToken(FEELParser.STRING, 0); }
public StringLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringLiteral; }
}
public final StringLiteralContext stringLiteral() throws RecognitionException {
StringLiteralContext _localctx = new StringLiteralContext(_ctx, getState());
enterRule(_localctx, 94, RULE_stringLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(687);
((StringLiteralContext)_localctx).lit = match(STRING);
((StringLiteralContext)_localctx).ast = astFactory.toStringLiteral((((StringLiteralContext)_localctx).lit!=null?((StringLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BooleanLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode TRUE() { return getToken(FEELParser.TRUE, 0); }
public TerminalNode FALSE() { return getToken(FEELParser.FALSE, 0); }
public BooleanLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_booleanLiteral; }
}
public final BooleanLiteralContext booleanLiteral() throws RecognitionException {
BooleanLiteralContext _localctx = new BooleanLiteralContext(_ctx, getState());
enterRule(_localctx, 96, RULE_booleanLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(692);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TRUE:
{
setState(690);
((BooleanLiteralContext)_localctx).lit = match(TRUE);
}
break;
case FALSE:
{
setState(691);
((BooleanLiteralContext)_localctx).lit = match(FALSE);
}
break;
default:
throw new NoViableAltException(this);
}
((BooleanLiteralContext)_localctx).ast = astFactory.toBooleanLiteral((((BooleanLiteralContext)_localctx).lit!=null?((BooleanLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NumericLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode NUMBER() { return getToken(FEELParser.NUMBER, 0); }
public NumericLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_numericLiteral; }
}
public final NumericLiteralContext numericLiteral() throws RecognitionException {
NumericLiteralContext _localctx = new NumericLiteralContext(_ctx, getState());
enterRule(_localctx, 98, RULE_numericLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(696);
((NumericLiteralContext)_localctx).lit = match(NUMBER);
((NumericLiteralContext)_localctx).ast = astFactory.toNumericLiteral((((NumericLiteralContext)_localctx).lit!=null?((NumericLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BoxedExpressionContext extends ParserRuleContext {
public Expression ast;
public ListContext list;
public FunctionDefinitionContext functionDefinition;
public ContextContext context;
public ListContext list() {
return getRuleContext(ListContext.class,0);
}
public FunctionDefinitionContext functionDefinition() {
return getRuleContext(FunctionDefinitionContext.class,0);
}
public ContextContext context() {
return getRuleContext(ContextContext.class,0);
}
public BoxedExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_boxedExpression; }
}
public final BoxedExpressionContext boxedExpression() throws RecognitionException {
BoxedExpressionContext _localctx = new BoxedExpressionContext(_ctx, getState());
enterRule(_localctx, 100, RULE_boxedExpression);
try {
setState(708);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BRACKET_OPEN:
enterOuterAlt(_localctx, 1);
{
{
setState(699);
((BoxedExpressionContext)_localctx).list = list();
((BoxedExpressionContext)_localctx).ast = ((BoxedExpressionContext)_localctx).list.ast;
}
}
break;
case FUNCTION:
enterOuterAlt(_localctx, 2);
{
{
setState(702);
((BoxedExpressionContext)_localctx).functionDefinition = functionDefinition();
((BoxedExpressionContext)_localctx).ast = ((BoxedExpressionContext)_localctx).functionDefinition.ast;
}
}
break;
case BRACE_OPEN:
enterOuterAlt(_localctx, 3);
{
{
setState(705);
((BoxedExpressionContext)_localctx).context = context();
((BoxedExpressionContext)_localctx).ast = ((BoxedExpressionContext)_localctx).context.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ListContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext exp;
public TerminalNode BRACKET_OPEN() { return getToken(FEELParser.BRACKET_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(FEELParser.BRACKET_CLOSE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public ListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_list; }
}
public final ListContext list() throws RecognitionException {
ListContext _localctx = new ListContext(_ctx, getState());
enterRule(_localctx, 102, RULE_list);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List expressions = new ArrayList<>();
setState(711);
match(BRACKET_OPEN);
setState(723);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,51,_ctx) ) {
case 1:
{
setState(712);
((ListContext)_localctx).exp = expression();
expressions.add(((ListContext)_localctx).exp.ast);
setState(720);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(714);
match(COMMA);
setState(715);
((ListContext)_localctx).exp = expression();
expressions.add(((ListContext)_localctx).exp.ast);
}
}
setState(722);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
}
setState(725);
match(BRACKET_CLOSE);
((ListContext)_localctx).ast = astFactory.toListLiteral(expressions);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ContextContext extends ParserRuleContext {
public Expression ast;
public ContextEntryContext entry;
public TerminalNode BRACE_OPEN() { return getToken(FEELParser.BRACE_OPEN, 0); }
public TerminalNode BRACE_CLOSE() { return getToken(FEELParser.BRACE_CLOSE, 0); }
public List contextEntry() {
return getRuleContexts(ContextEntryContext.class);
}
public ContextEntryContext contextEntry(int i) {
return getRuleContext(ContextEntryContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public ContextContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_context; }
}
public final ContextContext context() throws RecognitionException {
ContextContext _localctx = new ContextContext(_ctx, getState());
enterRule(_localctx, 104, RULE_context);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List entries = new ArrayList<>();
setState(729);
match(BRACE_OPEN);
setState(741);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << STRING) | (1L << FUNCTION) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
setState(730);
((ContextContext)_localctx).entry = contextEntry();
entries.add(((ContextContext)_localctx).entry.ast);
setState(738);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(732);
match(COMMA);
setState(733);
((ContextContext)_localctx).entry = contextEntry();
entries.add(((ContextContext)_localctx).entry.ast);
}
}
setState(740);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(743);
match(BRACE_CLOSE);
((ContextContext)_localctx).ast = astFactory.toContext(entries);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ContextEntryContext extends ParserRuleContext {
public ContextEntry ast;
public KeyContext key;
public ExpressionContext expression;
public KeyContext key() {
return getRuleContext(KeyContext.class,0);
}
public TerminalNode COLON() { return getToken(FEELParser.COLON, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public ContextEntryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_contextEntry; }
}
public final ContextEntryContext contextEntry() throws RecognitionException {
ContextEntryContext _localctx = new ContextEntryContext(_ctx, getState());
enterRule(_localctx, 106, RULE_contextEntry);
try {
enterOuterAlt(_localctx, 1);
{
setState(746);
((ContextEntryContext)_localctx).key = key();
setState(747);
match(COLON);
setState(748);
((ContextEntryContext)_localctx).expression = expression();
((ContextEntryContext)_localctx).ast = astFactory.toContextEntry(((ContextEntryContext)_localctx).key.ast, ((ContextEntryContext)_localctx).expression.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class KeyContext extends ParserRuleContext {
public ContextEntryKey ast;
public IdentifierContext name;
public StringLiteralContext stringLiteral;
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public KeyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_key; }
}
public final KeyContext key() throws RecognitionException {
KeyContext _localctx = new KeyContext(_ctx, getState());
enterRule(_localctx, 108, RULE_key);
try {
setState(757);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 1);
{
{
setState(751);
((KeyContext)_localctx).name = identifier();
((KeyContext)_localctx).ast = astFactory.toContextEntryKey((((KeyContext)_localctx).name!=null?_input.getText(((KeyContext)_localctx).name.start,((KeyContext)_localctx).name.stop):null));
}
}
break;
case STRING:
enterOuterAlt(_localctx, 2);
{
{
setState(754);
((KeyContext)_localctx).stringLiteral = stringLiteral();
((KeyContext)_localctx).ast = astFactory.toContextEntryKey((((KeyContext)_localctx).stringLiteral!=null?_input.getText(((KeyContext)_localctx).stringLiteral.start,((KeyContext)_localctx).stringLiteral.stop):null));
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DateTimeLiteralContext extends ParserRuleContext {
public Expression ast;
public IdentifierContext kind;
public StringLiteralContext stringLiteral;
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public DateTimeLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dateTimeLiteral; }
}
public final DateTimeLiteralContext dateTimeLiteral() throws RecognitionException {
DateTimeLiteralContext _localctx = new DateTimeLiteralContext(_ctx, getState());
enterRule(_localctx, 110, RULE_dateTimeLiteral);
try {
enterOuterAlt(_localctx, 1);
{
{
setState(759);
((DateTimeLiteralContext)_localctx).kind = identifier();
}
setState(760);
match(PAREN_OPEN);
setState(761);
((DateTimeLiteralContext)_localctx).stringLiteral = stringLiteral();
setState(762);
match(PAREN_CLOSE);
((DateTimeLiteralContext)_localctx).ast = astFactory.toDateTimeLiteral((((DateTimeLiteralContext)_localctx).kind!=null?_input.getText(((DateTimeLiteralContext)_localctx).kind.start,((DateTimeLiteralContext)_localctx).kind.stop):null), ((DateTimeLiteralContext)_localctx).stringLiteral.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IdentifierContext extends ParserRuleContext {
public Token ast;
public Token token;
public TerminalNode NAME() { return getToken(FEELParser.NAME, 0); }
public TerminalNode OR() { return getToken(FEELParser.OR, 0); }
public TerminalNode AND() { return getToken(FEELParser.AND, 0); }
public TerminalNode FUNCTION() { return getToken(FEELParser.FUNCTION, 0); }
public IdentifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_identifier; }
}
public final IdentifierContext identifier() throws RecognitionException {
IdentifierContext _localctx = new IdentifierContext(_ctx, getState());
enterRule(_localctx, 112, RULE_identifier);
try {
setState(773);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NAME:
enterOuterAlt(_localctx, 1);
{
{
setState(765);
((IdentifierContext)_localctx).token = match(NAME);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
}
break;
case OR:
enterOuterAlt(_localctx, 2);
{
{
setState(767);
((IdentifierContext)_localctx).token = match(OR);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
}
break;
case AND:
enterOuterAlt(_localctx, 3);
{
{
setState(769);
((IdentifierContext)_localctx).token = match(AND);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
}
break;
case FUNCTION:
enterOuterAlt(_localctx, 4);
{
{
setState(771);
((IdentifierContext)_localctx).token = match(FUNCTION);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3\61\u030a\4\2\t\2"+
"\4\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13"+
"\t\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\4\32\t\32\4\33\t\33\4\34\t\34\4\35\t\35\4\36\t\36\4\37\t\37\4 \t \4!"+
"\t!\4\"\t\"\4#\t#\4$\t$\4%\t%\4&\t&\4\'\t\'\4(\t(\4)\t)\4*\t*\4+\t+\4"+
",\t,\4-\t-\4.\t.\4/\t/\4\60\t\60\4\61\t\61\4\62\t\62\4\63\t\63\4\64\t"+
"\64\4\65\t\65\4\66\t\66\4\67\t\67\48\t8\49\t9\4:\t:\3\2\3\2\3\2\3\2\3"+
"\3\3\3\3\3\3\3\3\4\3\4\3\4\3\4\3\5\3\5\3\5\3\5\3\6\3\6\3\6\3\6\3\7\3\7"+
"\3\7\3\7\3\b\3\b\3\b\3\b\3\b\3\b\3\b\3\b\3\b\3\b\3\b\5\b\u0098\n\b\3\t"+
"\3\t\3\t\3\t\3\t\3\t\3\t\7\t\u00a1\n\t\f\t\16\t\u00a4\13\t\3\t\3\t\3\n"+
"\3\n\3\n\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\3\13\5\13\u00b6"+
"\n\13\3\f\3\f\3\f\3\f\3\f\3\f\3\f\7\f\u00bf\n\f\f\f\16\f\u00c2\13\f\3"+
"\f\3\f\3\r\3\r\3\r\3\r\5\r\u00ca\n\r\3\r\3\r\3\r\3\r\3\r\3\r\5\r\u00d2"+
"\n\r\3\16\3\16\3\16\3\16\3\16\3\16\3\16\3\17\3\17\3\17\3\17\3\17\3\17"+
"\5\17\u00e1\n\17\3\20\3\20\3\20\3\20\3\20\3\20\5\20\u00e9\n\20\3\21\5"+
"\21\u00ec\n\21\3\21\3\21\3\21\3\22\3\22\3\22\3\22\3\22\3\22\3\22\7\22"+
"\u00f8\n\22\f\22\16\22\u00fb\13\22\3\22\3\22\3\23\3\23\3\23\3\23\3\23"+
"\3\23\5\23\u0105\n\23\3\24\3\24\3\24\3\25\3\25\3\25\3\25\3\25\3\25\3\25"+
"\7\25\u0111\n\25\f\25\16\25\u0114\13\25\3\25\3\25\3\26\3\26\3\26\3\26"+
"\3\26\3\26\3\26\3\26\3\26\3\26\3\26\3\26\5\26\u0124\n\26\3\27\3\27\3\27"+
"\3\27\3\27\3\27\3\27\3\27\3\27\3\27\7\27\u0130\n\27\f\27\16\27\u0133\13"+
"\27\5\27\u0135\n\27\3\27\3\27\3\27\5\27\u013a\n\27\3\27\3\27\3\27\3\30"+
"\3\30\3\30\3\30\3\30\3\30\5\30\u0145\n\30\3\30\3\30\3\31\3\31\3\31\3\31"+
"\3\31\3\31\3\31\3\31\3\31\3\31\3\31\3\31\7\31\u0155\n\31\f\31\16\31\u0158"+
"\13\31\3\31\3\31\3\31\3\31\3\32\3\32\3\32\3\32\3\32\3\32\5\32\u0164\n"+
"\32\3\32\3\32\3\33\3\33\3\33\3\33\3\33\3\33\3\33\3\33\3\34\3\34\3\34\5"+
"\34\u0173\n\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34\3\34\7\34\u017e"+
"\n\34\f\34\16\34\u0181\13\34\3\34\3\34\3\34\3\34\3\35\3\35\3\35\3\35\3"+
"\35\3\35\7\35\u018d\n\35\f\35\16\35\u0190\13\35\3\36\3\36\3\36\3\36\3"+
"\36\3\36\7\36\u0198\n\36\f\36\16\36\u019b\13\36\3\37\3\37\3\37\3\37\3"+
"\37\3\37\3\37\3\37\5\37\u01a5\n\37\3\37\3\37\3\37\3\37\3\37\3\37\3\37"+
"\3\37\3\37\3\37\3\37\3\37\3\37\3\37\3\37\3\37\3\37\3\37\3\37\5\37\u01ba"+
"\n\37\3 \3 \3 \3!\3!\3!\3!\5!\u01c3\n!\3!\3!\3!\7!\u01c8\n!\f!\16!\u01cb"+
"\13!\3\"\3\"\3\"\3\"\5\"\u01d1\n\"\3\"\3\"\3\"\7\"\u01d6\n\"\f\"\16\""+
"\u01d9\13\"\3#\3#\3#\3#\3#\3#\7#\u01e1\n#\f#\16#\u01e4\13#\3$\3$\3$\3"+
"$\3$\7$\u01eb\n$\f$\16$\u01ee\13$\3$\3$\3$\3%\3%\3%\3%\3%\3%\5%\u01f9"+
"\n%\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&"+
"\7&\u0211\n&\f&\16&\u0214\13&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\7&\u0220\n"+
"&\f&\16&\u0223\13&\3&\3&\5&\u0227\n&\3&\3&\3&\3&\3&\5&\u022e\n&\3\'\3"+
"\'\3\'\3\'\3\'\3\'\3\'\3\'\3\'\3\'\3\'\3\'\3\'\3\'\3\'\7\'\u023f\n\'\f"+
"\'\16\'\u0242\13\'\3(\3(\3(\3(\3(\3(\3(\5(\u024b\n(\3(\3(\3)\3)\3)\3)"+
"\3)\3)\3)\3)\3)\3)\3)\7)\u025a\n)\f)\16)\u025d\13)\3)\3)\3*\3*\3*\3+\3"+
"+\3+\3+\3+\3+\3+\7+\u026b\n+\f+\16+\u026e\13+\5+\u0270\n+\3+\3+\3,\3,"+
"\3,\3,\3,\3,\3,\3,\3,\3,\3,\3,\3,\3,\3,\3,\3,\5,\u0285\n,\3-\3-\3-\3-"+
"\3-\3-\5-\u028d\n-\3.\3.\3.\3.\3.\3.\3.\7.\u0296\n.\f.\16.\u0299\13.\3"+
".\3.\3/\3/\3/\3/\3/\5/\u02a2\n/\3\60\3\60\3\60\3\60\3\60\3\60\3\60\3\60"+
"\3\60\3\60\3\60\3\60\5\60\u02b0\n\60\3\61\3\61\3\61\3\62\3\62\5\62\u02b7"+
"\n\62\3\62\3\62\3\63\3\63\3\63\3\64\3\64\3\64\3\64\3\64\3\64\3\64\3\64"+
"\3\64\5\64\u02c7\n\64\3\65\3\65\3\65\3\65\3\65\3\65\3\65\3\65\7\65\u02d1"+
"\n\65\f\65\16\65\u02d4\13\65\5\65\u02d6\n\65\3\65\3\65\3\65\3\66\3\66"+
"\3\66\3\66\3\66\3\66\3\66\3\66\7\66\u02e3\n\66\f\66\16\66\u02e6\13\66"+
"\5\66\u02e8\n\66\3\66\3\66\3\66\3\67\3\67\3\67\3\67\3\67\38\38\38\38\3"+
"8\38\58\u02f8\n8\39\39\39\39\39\39\3:\3:\3:\3:\3:\3:\3:\3:\5:\u0308\n"+
":\3:\2\2;\2\4\6\b\n\f\16\20\22\24\26\30\32\34\36 \"$&(*,.\60\62\64\66"+
"8:<>@BDFHJLNPRTVXZ\\^`bdfhjlnpr\2\2\2\u0323\2t\3\2\2\2\4x\3\2\2\2\6|\3"+
"\2\2\2\b\u0080\3\2\2\2\n\u0084\3\2\2\2\f\u0088\3\2\2\2\16\u0097\3\2\2"+
"\2\20\u0099\3\2\2\2\22\u00a7\3\2\2\2\24\u00b5\3\2\2\2\26\u00b7\3\2\2\2"+
"\30\u00d1\3\2\2\2\32\u00d3\3\2\2\2\34\u00e0\3\2\2\2\36\u00e8\3\2\2\2 "+
"\u00eb\3\2\2\2\"\u00f0\3\2\2\2$\u0104\3\2\2\2&\u0106\3\2\2\2(\u0109\3"+
"\2\2\2*\u0123\3\2\2\2,\u0125\3\2\2\2.\u013e\3\2\2\2\60\u0148\3\2\2\2\62"+
"\u015d\3\2\2\2\64\u0167\3\2\2\2\66\u016f\3\2\2\28\u0186\3\2\2\2:\u0191"+
"\3\2\2\2<\u019c\3\2\2\2>\u01bb\3\2\2\2@\u01be\3\2\2\2B\u01cc\3\2\2\2D"+
"\u01da\3\2\2\2F\u01e5\3\2\2\2H\u01f2\3\2\2\2J\u022d\3\2\2\2L\u022f\3\2"+
"\2\2N\u0243\3\2\2\2P\u024e\3\2\2\2R\u0260\3\2\2\2T\u0263\3\2\2\2V\u0284"+
"\3\2\2\2X\u028c\3\2\2\2Z\u028e\3\2\2\2\\\u02a1\3\2\2\2^\u02af\3\2\2\2"+
"`\u02b1\3\2\2\2b\u02b6\3\2\2\2d\u02ba\3\2\2\2f\u02c6\3\2\2\2h\u02c8\3"+
"\2\2\2j\u02da\3\2\2\2l\u02ec\3\2\2\2n\u02f7\3\2\2\2p\u02f9\3\2\2\2r\u0307"+
"\3\2\2\2tu\5\16\b\2uv\b\2\1\2vw\7\2\2\3w\3\3\2\2\2xy\5\24\13\2yz\b\3\1"+
"\2z{\7\2\2\3{\5\3\2\2\2|}\5&\24\2}~\b\4\1\2~\177\7\2\2\3\177\7\3\2\2\2"+
"\u0080\u0081\5\"\22\2\u0081\u0082\b\5\1\2\u0082\u0083\7\2\2\3\u0083\t"+
"\3\2\2\2\u0084\u0085\5(\25\2\u0085\u0086\b\6\1\2\u0086\u0087\7\2\2\3\u0087"+
"\13\3\2\2\2\u0088\u0089\5f\64\2\u0089\u008a\b\7\1\2\u008a\u008b\7\2\2"+
"\3\u008b\r\3\2\2\2\u008c\u008d\7\36\2\2\u008d\u008e\7\26\2\2\u008e\u008f"+
"\5\20\t\2\u008f\u0090\7\27\2\2\u0090\u0091\b\b\1\2\u0091\u0098\3\2\2\2"+
"\u0092\u0093\5\20\t\2\u0093\u0094\b\b\1\2\u0094\u0098\3\2\2\2\u0095\u0096"+
"\7\17\2\2\u0096\u0098\b\b\1\2\u0097\u008c\3\2\2\2\u0097\u0092\3\2\2\2"+
"\u0097\u0095\3\2\2\2\u0098\17\3\2\2\2\u0099\u009a\b\t\1\2\u009a\u009b"+
"\5\22\n\2\u009b\u00a2\b\t\1\2\u009c\u009d\7\25\2\2\u009d\u009e\5\22\n"+
"\2\u009e\u009f\b\t\1\2\u009f\u00a1\3\2\2\2\u00a0\u009c\3\2\2\2\u00a1\u00a4"+
"\3\2\2\2\u00a2\u00a0\3\2\2\2\u00a2\u00a3\3\2\2\2\u00a3\u00a5\3\2\2\2\u00a4"+
"\u00a2\3\2\2\2\u00a5\u00a6\b\t\1\2\u00a6\21\3\2\2\2\u00a7\u00a8\5&\24"+
"\2\u00a8\u00a9\b\n\1\2\u00a9\23\3\2\2\2\u00aa\u00ab\7\36\2\2\u00ab\u00ac"+
"\7\26\2\2\u00ac\u00ad\5\26\f\2\u00ad\u00ae\7\27\2\2\u00ae\u00af\b\13\1"+
"\2\u00af\u00b6\3\2\2\2\u00b0\u00b1\5\26\f\2\u00b1\u00b2\b\13\1\2\u00b2"+
"\u00b6\3\2\2\2\u00b3\u00b4\7\17\2\2\u00b4\u00b6\b\13\1\2\u00b5\u00aa\3"+
"\2\2\2\u00b5\u00b0\3\2\2\2\u00b5\u00b3\3\2\2\2\u00b6\25\3\2\2\2\u00b7"+
"\u00b8\b\f\1\2\u00b8\u00b9\5\30\r\2\u00b9\u00c0\b\f\1\2\u00ba\u00bb\7"+
"\25\2\2\u00bb\u00bc\5\30\r\2\u00bc\u00bd\b\f\1\2\u00bd\u00bf\3\2\2\2\u00be"+
"\u00ba\3\2\2\2\u00bf\u00c2\3\2\2\2\u00c0\u00be\3\2\2\2\u00c0\u00c1\3\2"+
"\2\2\u00c1\u00c3\3\2\2\2\u00c2\u00c0\3\2\2\2\u00c3\u00c4\b\f\1\2\u00c4"+
"\27\3\2\2\2\u00c5\u00ca\7\n\2\2\u00c6\u00ca\7\f\2\2\u00c7\u00ca\7\13\2"+
"\2\u00c8\u00ca\7\r\2\2\u00c9\u00c5\3\2\2\2\u00c9\u00c6\3\2\2\2\u00c9\u00c7"+
"\3\2\2\2\u00c9\u00c8\3\2\2\2\u00c9\u00ca\3\2\2\2\u00ca\u00cb\3\2\2\2\u00cb"+
"\u00cc\5 \21\2\u00cc\u00cd\b\r\1\2\u00cd\u00d2\3\2\2\2\u00ce\u00cf\5\32"+
"\16\2\u00cf\u00d0\b\r\1\2\u00d0\u00d2\3\2\2\2\u00d1\u00c9\3\2\2\2\u00d1"+
"\u00ce\3\2\2\2\u00d2\31\3\2\2\2\u00d3\u00d4\5\34\17\2\u00d4\u00d5\5 \21"+
"\2\u00d5\u00d6\7\23\2\2\u00d6\u00d7\5 \21\2\u00d7\u00d8\5\36\20\2\u00d8"+
"\u00d9\b\16\1\2\u00d9\33\3\2\2\2\u00da\u00db\7\26\2\2\u00db\u00e1\b\17"+
"\1\2\u00dc\u00dd\7\31\2\2\u00dd\u00e1\b\17\1\2\u00de\u00df\7\30\2\2\u00df"+
"\u00e1\b\17\1\2\u00e0\u00da\3\2\2\2\u00e0\u00dc\3\2\2\2\u00e0\u00de\3"+
"\2\2\2\u00e1\35\3\2\2\2\u00e2\u00e3\7\27\2\2\u00e3\u00e9\b\20\1\2\u00e4"+
"\u00e5\7\30\2\2\u00e5\u00e9\b\20\1\2\u00e6\u00e7\7\31\2\2\u00e7\u00e9"+
"\b\20\1\2\u00e8\u00e2\3\2\2\2\u00e8\u00e4\3\2\2\2\u00e8\u00e6\3\2\2\2"+
"\u00e9\37\3\2\2\2\u00ea\u00ec\7\17\2\2\u00eb\u00ea\3\2\2\2\u00eb\u00ec"+
"\3\2\2\2\u00ec\u00ed\3\2\2\2\u00ed\u00ee\5X-\2\u00ee\u00ef\b\21\1\2\u00ef"+
"!\3\2\2\2\u00f0\u00f1\b\22\1\2\u00f1\u00f2\5$\23\2\u00f2\u00f9\b\22\1"+
"\2\u00f3\u00f4\7\25\2\2\u00f4\u00f5\5$\23\2\u00f5\u00f6\b\22\1\2\u00f6"+
"\u00f8\3\2\2\2\u00f7\u00f3\3\2\2\2\u00f8\u00fb\3\2\2\2\u00f9\u00f7\3\2"+
"\2\2\u00f9\u00fa\3\2\2\2\u00fa\u00fc\3\2\2\2\u00fb\u00f9\3\2\2\2\u00fc"+
"\u00fd\b\22\1\2\u00fd#\3\2\2\2\u00fe\u00ff\5> \2\u00ff\u0100\b\23\1\2"+
"\u0100\u0105\3\2\2\2\u0101\u0102\5X-\2\u0102\u0103\b\23\1\2\u0103\u0105"+
"\3\2\2\2\u0104\u00fe\3\2\2\2\u0104\u0101\3\2\2\2\u0105%\3\2\2\2\u0106"+
"\u0107\5*\26\2\u0107\u0108\b\24\1\2\u0108\'\3\2\2\2\u0109\u010a\b\25\1"+
"\2\u010a\u010b\5*\26\2\u010b\u0112\b\25\1\2\u010c\u010d\7\25\2\2\u010d"+
"\u010e\5*\26\2\u010e\u010f\b\25\1\2\u010f\u0111\3\2\2\2\u0110\u010c\3"+
"\2\2\2\u0111\u0114\3\2\2\2\u0112\u0110\3\2\2\2\u0112\u0113\3\2\2\2\u0113"+
"\u0115\3\2\2\2\u0114\u0112\3\2\2\2\u0115\u0116\b\25\1\2\u0116)\3\2\2\2"+
"\u0117\u0118\5\60\31\2\u0118\u0119\b\26\1\2\u0119\u0124\3\2\2\2\u011a"+
"\u011b\5\64\33\2\u011b\u011c\b\26\1\2\u011c\u0124\3\2\2\2\u011d\u011e"+
"\5\66\34\2\u011e\u011f\b\26\1\2\u011f\u0124\3\2\2\2\u0120\u0121\58\35"+
"\2\u0121\u0122\b\26\1\2\u0122\u0124\3\2\2\2\u0123\u0117\3\2\2\2\u0123"+
"\u011a\3\2\2\2\u0123\u011d\3\2\2\2\u0123\u0120\3\2\2\2\u0124+\3\2\2\2"+
"\u0125\u0126\b\27\1\2\u0126\u0127\b\27\1\2\u0127\u0128\7\"\2\2\u0128\u0134"+
"\7\26\2\2\u0129\u012a\5.\30\2\u012a\u0131\b\27\1\2\u012b\u012c\7\25\2"+
"\2\u012c\u012d\5.\30\2\u012d\u012e\b\27\1\2\u012e\u0130\3\2\2\2\u012f"+
"\u012b\3\2\2\2\u0130\u0133\3\2\2\2\u0131\u012f\3\2\2\2\u0131\u0132\3\2"+
"\2\2\u0132\u0135\3\2\2\2\u0133\u0131\3\2\2\2\u0134\u0129\3\2\2\2\u0134"+
"\u0135\3\2\2\2\u0135\u0136\3\2\2\2\u0136\u0139\7\27\2\2\u0137\u0138\7"+
"#\2\2\u0138\u013a\b\27\1\2\u0139\u0137\3\2\2\2\u0139\u013a\3\2\2\2\u013a"+
"\u013b\3\2\2\2\u013b\u013c\5&\24\2\u013c\u013d\b\27\1\2\u013d-\3\2\2\2"+
"\u013e\u013f\b\30\1\2\u013f\u0144\5R*\2\u0140\u0141\7\34\2\2\u0141\u0142"+
"\5J&\2\u0142\u0143\b\30\1\2\u0143\u0145\3\2\2\2\u0144\u0140\3\2\2\2\u0144"+
"\u0145\3\2\2\2\u0145\u0146\3\2\2\2\u0146\u0147\b\30\1\2\u0147/\3\2\2\2"+
"\u0148\u0149\b\31\1\2\u0149\u014a\7$\2\2\u014a\u014b\5r:\2\u014b\u014c"+
"\7%\2\2\u014c\u014d\5\62\32\2\u014d\u0156\b\31\1\2\u014e\u014f\7\25\2"+
"\2\u014f\u0150\5r:\2\u0150\u0151\7%\2\2\u0151\u0152\5\62\32\2\u0152\u0153"+
"\b\31\1\2\u0153\u0155\3\2\2\2\u0154\u014e\3\2\2\2\u0155\u0158\3\2\2\2"+
"\u0156\u0154\3\2\2\2\u0156\u0157\3\2\2\2\u0157\u0159\3\2\2\2\u0158\u0156"+
"\3\2\2\2\u0159\u015a\7&\2\2\u015a\u015b\5&\24\2\u015b\u015c\b\31\1\2\u015c"+
"\61\3\2\2\2\u015d\u015e\b\32\1\2\u015e\u0163\5&\24\2\u015f\u0160\7\23"+
"\2\2\u0160\u0161\5&\24\2\u0161\u0162\b\32\1\2\u0162\u0164\3\2\2\2\u0163"+
"\u015f\3\2\2\2\u0163\u0164\3\2\2\2\u0164\u0165\3\2\2\2\u0165\u0166\b\32"+
"\1\2\u0166\63\3\2\2\2\u0167\u0168\7\'\2\2\u0168\u0169\5&\24\2\u0169\u016a"+
"\7(\2\2\u016a\u016b\5&\24\2\u016b\u016c\7)\2\2\u016c\u016d\5&\24\2\u016d"+
"\u016e\b\33\1\2\u016e\65\3\2\2\2\u016f\u0172\b\34\1\2\u0170\u0173\7*\2"+
"\2\u0171\u0173\7+\2\2\u0172\u0170\3\2\2\2\u0172\u0171\3\2\2\2\u0173\u0174"+
"\3\2\2\2\u0174\u0175\5r:\2\u0175\u0176\7%\2\2\u0176\u0177\5&\24\2\u0177"+
"\u017f\b\34\1\2\u0178\u0179\5r:\2\u0179\u017a\7%\2\2\u017a\u017b\5&\24"+
"\2\u017b\u017c\b\34\1\2\u017c\u017e\3\2\2\2\u017d\u0178\3\2\2\2\u017e"+
"\u0181\3\2\2\2\u017f\u017d\3\2\2\2\u017f\u0180\3\2\2\2\u0180\u0182\3\2"+
"\2\2\u0181\u017f\3\2\2\2\u0182\u0183\7,\2\2\u0183\u0184\5&\24\2\u0184"+
"\u0185\b\34\1\2\u0185\67\3\2\2\2\u0186\u0187\5:\36\2\u0187\u018e\b\35"+
"\1\2\u0188\u0189\7.\2\2\u0189\u018a\5:\36\2\u018a\u018b\b\35\1\2\u018b"+
"\u018d\3\2\2\2\u018c\u0188\3\2\2\2\u018d\u0190\3\2\2\2\u018e\u018c\3\2"+
"\2\2\u018e\u018f\3\2\2\2\u018f9\3\2\2\2\u0190\u018e\3\2\2\2\u0191\u0192"+
"\5<\37\2\u0192\u0199\b\36\1\2\u0193\u0194\7-\2\2\u0194\u0195\5<\37\2\u0195"+
"\u0196\b\36\1\2\u0196\u0198\3\2\2\2\u0197\u0193\3\2\2\2\u0198\u019b\3"+
"\2\2\2\u0199\u0197\3\2\2\2\u0199\u019a\3\2\2\2\u019a;\3\2\2\2\u019b\u0199"+
"\3\2\2\2\u019c\u019d\5> \2\u019d\u01b9\b\37\1\2\u019e\u01a5\7\b\2\2\u019f"+
"\u01a5\7\t\2\2\u01a0\u01a5\7\n\2\2\u01a1\u01a5\7\13\2\2\u01a2\u01a5\7"+
"\f\2\2\u01a3\u01a5\7\r\2\2\u01a4\u019e\3\2\2\2\u01a4\u019f\3\2\2\2\u01a4"+
"\u01a0\3\2\2\2\u01a4\u01a1\3\2\2\2\u01a4\u01a2\3\2\2\2\u01a4\u01a3\3\2"+
"\2\2\u01a5\u01a6\3\2\2\2\u01a6\u01a7\5> \2\u01a7\u01a8\b\37\1\2\u01a8"+
"\u01ba\3\2\2\2\u01a9\u01aa\7/\2\2\u01aa\u01ab\5&\24\2\u01ab\u01ac\7-\2"+
"\2\u01ac\u01ad\5&\24\2\u01ad\u01ae\b\37\1\2\u01ae\u01ba\3\2\2\2\u01af"+
"\u01b0\7%\2\2\u01b0\u01b1\5\22\n\2\u01b1\u01b2\b\37\1\2\u01b2\u01ba\3"+
"\2\2\2\u01b3\u01b4\7%\2\2\u01b4\u01b5\7\26\2\2\u01b5\u01b6\5\20\t\2\u01b6"+
"\u01b7\7\27\2\2\u01b7\u01b8\b\37\1\2\u01b8\u01ba\3\2\2\2\u01b9\u01a4\3"+
"\2\2\2\u01b9\u01a9\3\2\2\2\u01b9\u01af\3\2\2\2\u01b9\u01b3\3\2\2\2\u01b9"+
"\u01ba\3\2\2\2\u01ba=\3\2\2\2\u01bb\u01bc\5@!\2\u01bc\u01bd\b \1\2\u01bd"+
"?\3\2\2\2\u01be\u01bf\5B\"\2\u01bf\u01c9\b!\1\2\u01c0\u01c3\7\16\2\2\u01c1"+
"\u01c3\7\17\2\2\u01c2\u01c0\3\2\2\2\u01c2\u01c1\3\2\2\2\u01c3\u01c4\3"+
"\2\2\2\u01c4\u01c5\5B\"\2\u01c5\u01c6\b!\1\2\u01c6\u01c8\3\2\2\2\u01c7"+
"\u01c2\3\2\2\2\u01c8\u01cb\3\2\2\2\u01c9\u01c7\3\2\2\2\u01c9\u01ca\3\2"+
"\2\2\u01caA\3\2\2\2\u01cb\u01c9\3\2\2\2\u01cc\u01cd\5D#\2\u01cd\u01d7"+
"\b\"\1\2\u01ce\u01d1\7\20\2\2\u01cf\u01d1\7\21\2\2\u01d0\u01ce\3\2\2\2"+
"\u01d0\u01cf\3\2\2\2\u01d1\u01d2\3\2\2\2\u01d2\u01d3\5D#\2\u01d3\u01d4"+
"\b\"\1\2\u01d4\u01d6\3\2\2\2\u01d5\u01d0\3\2\2\2\u01d6\u01d9\3\2\2\2\u01d7"+
"\u01d5\3\2\2\2\u01d7\u01d8\3\2\2\2\u01d8C\3\2\2\2\u01d9\u01d7\3\2\2\2"+
"\u01da\u01db\5F$\2\u01db\u01e2\b#\1\2\u01dc\u01dd\7\22\2\2\u01dd\u01de"+
"\5F$\2\u01de\u01df\b#\1\2\u01df\u01e1\3\2\2\2\u01e0\u01dc\3\2\2\2\u01e1"+
"\u01e4\3\2\2\2\u01e2\u01e0\3\2\2\2\u01e2\u01e3\3\2\2\2\u01e3E\3\2\2\2"+
"\u01e4\u01e2\3\2\2\2\u01e5\u01ec\b$\1\2\u01e6\u01e7\7\17\2\2\u01e7\u01eb"+
"\b$\1\2\u01e8\u01e9\7\36\2\2\u01e9\u01eb\b$\1\2\u01ea\u01e6\3\2\2\2\u01ea"+
"\u01e8\3\2\2\2\u01eb\u01ee\3\2\2\2\u01ec\u01ea\3\2\2\2\u01ec\u01ed\3\2"+
"\2\2\u01ed\u01ef\3\2\2\2\u01ee\u01ec\3\2\2\2\u01ef\u01f0\5H%\2\u01f0\u01f1"+
"\b$\1\2\u01f1G\3\2\2\2\u01f2\u01f3\5L\'\2\u01f3\u01f8\b%\1\2\u01f4\u01f5"+
"\7\60\2\2\u01f5\u01f6\5J&\2\u01f6\u01f7\b%\1\2\u01f7\u01f9\3\2\2\2\u01f8"+
"\u01f4\3\2\2\2\u01f8\u01f9\3\2\2\2\u01f9I\3\2\2\2\u01fa\u01fb\5Z.\2\u01fb"+
"\u01fc\b&\1\2\u01fc\u022e\3\2\2\2\u01fd\u01fe\5r:\2\u01fe\u01ff\7\n\2"+
"\2\u01ff\u0200\5J&\2\u0200\u0201\7\13\2\2\u0201\u0202\b&\1\2\u0202\u022e"+
"\3\2\2\2\u0203\u0204\b&\1\2\u0204\u0205\5r:\2\u0205\u0206\7\n\2\2\u0206"+
"\u0207\5r:\2\u0207\u0208\7\34\2\2\u0208\u0209\5J&\2\u0209\u0212\b&\1\2"+
"\u020a\u020b\7\25\2\2\u020b\u020c\5r:\2\u020c\u020d\7\34\2\2\u020d\u020e"+
"\5J&\2\u020e\u020f\b&\1\2\u020f\u0211\3\2\2\2\u0210\u020a\3\2\2\2\u0211"+
"\u0214\3\2\2\2\u0212\u0210\3\2\2\2\u0212\u0213\3\2\2\2\u0213\u0215\3\2"+
"\2\2\u0214\u0212\3\2\2\2\u0215\u0216\7\13\2\2\u0216\u0217\b&\1\2\u0217"+
"\u022e\3\2\2\2\u0218\u0219\b&\1\2\u0219\u021a\5r:\2\u021a\u0226\7\n\2"+
"\2\u021b\u021c\5J&\2\u021c\u0221\b&\1\2\u021d\u021e\7\25\2\2\u021e\u0220"+
"\5J&\2\u021f\u021d\3\2\2\2\u0220\u0223\3\2\2\2\u0221\u021f\3\2\2\2\u0221"+
"\u0222\3\2\2\2\u0222\u0224\3\2\2\2\u0223\u0221\3\2\2\2\u0224\u0225\b&"+
"\1\2\u0225\u0227\3\2\2\2\u0226\u021b\3\2\2\2\u0226\u0227\3\2\2\2\u0227"+
"\u0228\3\2\2\2\u0228\u0229\7\13\2\2\u0229\u022a\7\35\2\2\u022a\u022b\5"+
"J&\2\u022b\u022c\b&\1\2\u022c\u022e\3\2\2\2\u022d\u01fa\3\2\2\2\u022d"+
"\u01fd\3\2\2\2\u022d\u0203\3\2\2\2\u022d\u0218\3\2\2\2\u022eK\3\2\2\2"+
"\u022f\u0230\5V,\2\u0230\u0231\b\'\1\2\u0231\u0240\3\2\2\2\u0232\u0233"+
"\7\30\2\2\u0233\u0234\5&\24\2\u0234\u0235\7\31\2\2\u0235\u0236\b\'\1\2"+
"\u0236\u023f\3\2\2\2\u0237\u0238\5N(\2\u0238\u0239\b\'\1\2\u0239\u023f"+
"\3\2\2\2\u023a\u023b\7\24\2\2\u023b\u023c\5r:\2\u023c\u023d\b\'\1\2\u023d"+
"\u023f\3\2\2\2\u023e\u0232\3\2\2\2\u023e\u0237\3\2\2\2\u023e\u023a\3\2"+
"\2\2\u023f\u0242\3\2\2\2\u0240\u023e\3\2\2\2\u0240\u0241\3\2\2\2\u0241"+
"M\3\2\2\2\u0242\u0240\3\2\2\2\u0243\u024a\7\26\2\2\u0244\u0245\5P)\2\u0245"+
"\u0246\b(\1\2\u0246\u024b\3\2\2\2\u0247\u0248\5T+\2\u0248\u0249\b(\1\2"+
"\u0249\u024b\3\2\2\2\u024a\u0244\3\2\2\2\u024a\u0247\3\2\2\2\u024b\u024c"+
"\3\2\2\2\u024c\u024d\7\27\2\2\u024dO\3\2\2\2\u024e\u024f\b)\1\2\u024f"+
"\u0250\5R*\2\u0250\u0251\7\34\2\2\u0251\u0252\5&\24\2\u0252\u025b\b)\1"+
"\2\u0253\u0254\7\25\2\2\u0254\u0255\5R*\2\u0255\u0256\7\34\2\2\u0256\u0257"+
"\5&\24\2\u0257\u0258\b)\1\2\u0258\u025a\3\2\2\2\u0259\u0253\3\2\2\2\u025a"+
"\u025d\3\2\2\2\u025b\u0259\3\2\2\2\u025b\u025c\3\2\2\2\u025c\u025e\3\2"+
"\2\2\u025d\u025b\3\2\2\2\u025e\u025f\b)\1\2\u025fQ\3\2\2\2\u0260\u0261"+
"\5r:\2\u0261\u0262\b*\1\2\u0262S\3\2\2\2\u0263\u026f\b+\1\2\u0264\u0265"+
"\5&\24\2\u0265\u026c\b+\1\2\u0266\u0267\7\25\2\2\u0267\u0268\5&\24\2\u0268"+
"\u0269\b+\1\2\u0269\u026b\3\2\2\2\u026a\u0266\3\2\2\2\u026b\u026e\3\2"+
"\2\2\u026c\u026a\3\2\2\2\u026c\u026d\3\2\2\2\u026d\u0270\3\2\2\2\u026e"+
"\u026c\3\2\2\2\u026f\u0264\3\2\2\2\u026f\u0270\3\2\2\2\u0270\u0271\3\2"+
"\2\2\u0271\u0272\b+\1\2\u0272U\3\2\2\2\u0273\u0274\5\\/\2\u0274\u0275"+
"\b,\1\2\u0275\u0285\3\2\2\2\u0276\u0277\5r:\2\u0277\u0278\b,\1\2\u0278"+
"\u0285\3\2\2\2\u0279\u027a\7\26\2\2\u027a\u027b\5&\24\2\u027b\u027c\7"+
"\27\2\2\u027c\u027d\b,\1\2\u027d\u0285\3\2\2\2\u027e\u027f\5f\64\2\u027f"+
"\u0280\b,\1\2\u0280\u0285\3\2\2\2\u0281\u0282\5\30\r\2\u0282\u0283\b,"+
"\1\2\u0283\u0285\3\2\2\2\u0284\u0273\3\2\2\2\u0284\u0276\3\2\2\2\u0284"+
"\u0279\3\2\2\2\u0284\u027e\3\2\2\2\u0284\u0281\3\2\2\2\u0285W\3\2\2\2"+
"\u0286\u0287\5^\60\2\u0287\u0288\b-\1\2\u0288\u028d\3\2\2\2\u0289\u028a"+
"\5Z.\2\u028a\u028b\b-\1\2\u028b\u028d\3\2\2\2\u028c\u0286\3\2\2\2\u028c"+
"\u0289\3\2\2\2\u028dY\3\2\2\2\u028e\u028f\b.\1\2\u028f\u0290\5r:\2\u0290"+
"\u0297\b.\1\2\u0291\u0292\7\24\2\2\u0292\u0293\5r:\2\u0293\u0294\b.\1"+
"\2\u0294\u0296\3\2\2\2\u0295\u0291\3\2\2\2\u0296\u0299\3\2\2\2\u0297\u0295"+
"\3\2\2\2\u0297\u0298\3\2\2\2\u0298\u029a\3\2\2\2\u0299\u0297\3\2\2\2\u029a"+
"\u029b\b.\1\2\u029b[\3\2\2\2\u029c\u029d\5^\60\2\u029d\u029e\b/\1\2\u029e"+
"\u02a2\3\2\2\2\u029f\u02a0\7!\2\2\u02a0\u02a2\b/\1\2\u02a1\u029c\3\2\2"+
"\2\u02a1\u029f\3\2\2\2\u02a2]\3\2\2\2\u02a3\u02a4\5d\63\2\u02a4\u02a5"+
"\b\60\1\2\u02a5\u02b0\3\2\2\2\u02a6\u02a7\5`\61\2\u02a7\u02a8\b\60\1\2"+
"\u02a8\u02b0\3\2\2\2\u02a9\u02aa\5b\62\2\u02aa\u02ab\b\60\1\2\u02ab\u02b0"+
"\3\2\2\2\u02ac\u02ad\5p9\2\u02ad\u02ae\b\60\1\2\u02ae\u02b0\3\2\2\2\u02af"+
"\u02a3\3\2\2\2\u02af\u02a6\3\2\2\2\u02af\u02a9\3\2\2\2\u02af\u02ac\3\2"+
"\2\2\u02b0_\3\2\2\2\u02b1\u02b2\7\6\2\2\u02b2\u02b3\b\61\1\2\u02b3a\3"+
"\2\2\2\u02b4\u02b7\7\37\2\2\u02b5\u02b7\7 \2\2\u02b6\u02b4\3\2\2\2\u02b6"+
"\u02b5\3\2\2\2\u02b7\u02b8\3\2\2\2\u02b8\u02b9\b\62\1\2\u02b9c\3\2\2\2"+
"\u02ba\u02bb\7\7\2\2\u02bb\u02bc\b\63\1\2\u02bce\3\2\2\2\u02bd\u02be\5"+
"h\65\2\u02be\u02bf\b\64\1\2\u02bf\u02c7\3\2\2\2\u02c0\u02c1\5,\27\2\u02c1"+
"\u02c2\b\64\1\2\u02c2\u02c7\3\2\2\2\u02c3\u02c4\5j\66\2\u02c4\u02c5\b"+
"\64\1\2\u02c5\u02c7\3\2\2\2\u02c6\u02bd\3\2\2\2\u02c6\u02c0\3\2\2\2\u02c6"+
"\u02c3\3\2\2\2\u02c7g\3\2\2\2\u02c8\u02c9\b\65\1\2\u02c9\u02d5\7\30\2"+
"\2\u02ca\u02cb\5&\24\2\u02cb\u02d2\b\65\1\2\u02cc\u02cd\7\25\2\2\u02cd"+
"\u02ce\5&\24\2\u02ce\u02cf\b\65\1\2\u02cf\u02d1\3\2\2\2\u02d0\u02cc\3"+
"\2\2\2\u02d1\u02d4\3\2\2\2\u02d2\u02d0\3\2\2\2\u02d2\u02d3\3\2\2\2\u02d3"+
"\u02d6\3\2\2\2\u02d4\u02d2\3\2\2\2\u02d5\u02ca\3\2\2\2\u02d5\u02d6\3\2"+
"\2\2\u02d6\u02d7\3\2\2\2\u02d7\u02d8\7\31\2\2\u02d8\u02d9\b\65\1\2\u02d9"+
"i\3\2\2\2\u02da\u02db\b\66\1\2\u02db\u02e7\7\32\2\2\u02dc\u02dd\5l\67"+
"\2\u02dd\u02e4\b\66\1\2\u02de\u02df\7\25\2\2\u02df\u02e0\5l\67\2\u02e0"+
"\u02e1\b\66\1\2\u02e1\u02e3\3\2\2\2\u02e2\u02de\3\2\2\2\u02e3\u02e6\3"+
"\2\2\2\u02e4\u02e2\3\2\2\2\u02e4\u02e5\3\2\2\2\u02e5\u02e8\3\2\2\2\u02e6"+
"\u02e4\3\2\2\2\u02e7\u02dc\3\2\2\2\u02e7\u02e8\3\2\2\2\u02e8\u02e9\3\2"+
"\2\2\u02e9\u02ea\7\33\2\2\u02ea\u02eb\b\66\1\2\u02ebk\3\2\2\2\u02ec\u02ed"+
"\5n8\2\u02ed\u02ee\7\34\2\2\u02ee\u02ef\5&\24\2\u02ef\u02f0\b\67\1\2\u02f0"+
"m\3\2\2\2\u02f1\u02f2\5r:\2\u02f2\u02f3\b8\1\2\u02f3\u02f8\3\2\2\2\u02f4"+
"\u02f5\5`\61\2\u02f5\u02f6\b8\1\2\u02f6\u02f8\3\2\2\2\u02f7\u02f1\3\2"+
"\2\2\u02f7\u02f4\3\2\2\2\u02f8o\3\2\2\2\u02f9\u02fa\5r:\2\u02fa\u02fb"+
"\7\26\2\2\u02fb\u02fc\5`\61\2\u02fc\u02fd\7\27\2\2\u02fd\u02fe\b9\1\2"+
"\u02feq\3\2\2\2\u02ff\u0300\7\61\2\2\u0300\u0308\b:\1\2\u0301\u0302\7"+
".\2\2\u0302\u0308\b:\1\2\u0303\u0304\7-\2\2\u0304\u0308\b:\1\2\u0305\u0306"+
"\7\"\2\2\u0306\u0308\b:\1\2\u0307\u02ff\3\2\2\2\u0307\u0301\3\2\2\2\u0307"+
"\u0303\3\2\2\2\u0307\u0305\3\2\2\2\u0308s\3\2\2\2:\u0097\u00a2\u00b5\u00c0"+
"\u00c9\u00d1\u00e0\u00e8\u00eb\u00f9\u0104\u0112\u0123\u0131\u0134\u0139"+
"\u0144\u0156\u0163\u0172\u017f\u018e\u0199\u01a4\u01b9\u01c2\u01c9\u01d0"+
"\u01d7\u01e2\u01ea\u01ec\u01f8\u0212\u0221\u0226\u022d\u023e\u0240\u024a"+
"\u025b\u026c\u026f\u0284\u028c\u0297\u02a1\u02af\u02b6\u02c6\u02d2\u02d5"+
"\u02e4\u02e7\u02f7\u0307";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy