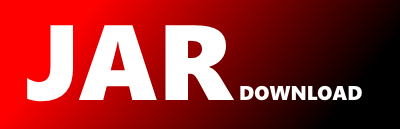
chs.jdmn.jdmn-core.6.0.0.source-code.FEELParser Maven / Gradle / Ivy
// Generated from FEELParser.g4 by ANTLR 4.9.3
package com.gs.dmn.feel.analysis.syntax.antlrv4;
import java.util.*;
import com.gs.dmn.feel.analysis.syntax.ast.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.arithmetic.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.comparison.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.context.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.function.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.literal.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.logic.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.textual.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.type.*;
import com.gs.dmn.feel.analysis.syntax.ast.test.*;
import com.gs.dmn.runtime.Pair;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class FEELParser extends Parser {
static { RuntimeMetaData.checkVersion("4.9.3", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
BLOCK_COMMENT=1, LINE_COMMENT=2, WS=3, STRING=4, NUMBER=5, TEMPORAL=6,
EQ=7, NE=8, LT=9, GT=10, LE=11, GE=12, PLUS=13, MINUS=14, STAR=15, FORWARD_SLASH=16,
STAR_STAR=17, DOT_DOT=18, DOT=19, COMMA=20, PAREN_OPEN=21, PAREN_CLOSE=22,
BRACKET_OPEN=23, BRACKET_CLOSE=24, BRACE_OPEN=25, BRACE_CLOSE=26, COLON=27,
ARROW=28, NOT=29, TRUE=30, FALSE=31, NULL=32, FUNCTION=33, EXTERNAL=34,
FOR=35, IN=36, RETURN=37, IF=38, THEN=39, ELSE=40, SOME=41, EVERY=42,
SATISFIES=43, AND=44, OR=45, BETWEEN=46, INSTANCE_OF=47, NAME=48;
public static final int
RULE_unaryTestsRoot = 0, RULE_expressionRoot = 1, RULE_textualExpressionsRoot = 2,
RULE_boxedExpressionRoot = 3, RULE_unaryTests = 4, RULE_positiveUnaryTests = 5,
RULE_positiveUnaryTest = 6, RULE_simplePositiveUnaryTest = 7, RULE_interval = 8,
RULE_intervalStartPar = 9, RULE_intervalEndPar = 10, RULE_endpoint = 11,
RULE_expression = 12, RULE_textualExpressions = 13, RULE_textualExpression = 14,
RULE_functionDefinition = 15, RULE_formalParameter = 16, RULE_forExpression = 17,
RULE_iterationDomain = 18, RULE_ifExpression = 19, RULE_quantifiedExpression = 20,
RULE_disjunction = 21, RULE_conjunction = 22, RULE_comparison = 23, RULE_arithmeticExpression = 24,
RULE_addition = 25, RULE_multiplication = 26, RULE_exponentiation = 27,
RULE_arithmeticNegation = 28, RULE_instanceOf = 29, RULE_type = 30, RULE_postfixExpression = 31,
RULE_parameters = 32, RULE_namedParameters = 33, RULE_parameterName = 34,
RULE_positionalParameters = 35, RULE_primaryExpression = 36, RULE_simpleValue = 37,
RULE_qualifiedName = 38, RULE_literal = 39, RULE_simpleLiteral = 40, RULE_stringLiteral = 41,
RULE_booleanLiteral = 42, RULE_numericLiteral = 43, RULE_boxedExpression = 44,
RULE_list = 45, RULE_context = 46, RULE_contextEntry = 47, RULE_key = 48,
RULE_dateTimeLiteral = 49, RULE_identifier = 50;
private static String[] makeRuleNames() {
return new String[] {
"unaryTestsRoot", "expressionRoot", "textualExpressionsRoot", "boxedExpressionRoot",
"unaryTests", "positiveUnaryTests", "positiveUnaryTest", "simplePositiveUnaryTest",
"interval", "intervalStartPar", "intervalEndPar", "endpoint", "expression",
"textualExpressions", "textualExpression", "functionDefinition", "formalParameter",
"forExpression", "iterationDomain", "ifExpression", "quantifiedExpression",
"disjunction", "conjunction", "comparison", "arithmeticExpression", "addition",
"multiplication", "exponentiation", "arithmeticNegation", "instanceOf",
"type", "postfixExpression", "parameters", "namedParameters", "parameterName",
"positionalParameters", "primaryExpression", "simpleValue", "qualifiedName",
"literal", "simpleLiteral", "stringLiteral", "booleanLiteral", "numericLiteral",
"boxedExpression", "list", "context", "contextEntry", "key", "dateTimeLiteral",
"identifier"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, null, null, null, null, null, null, null, "'!='", "'<'", "'>'",
"'<='", "'>='", "'+'", "'-'", "'*'", "'/'", "'**'", "'..'", "'.'", "','",
"'('", "')'", "'['", "']'", "'{'", "'}'", "':'", "'->'", "'not'", "'true'",
"'false'", "'null'", "'function'", "'external'", "'for'", "'in'", "'return'",
"'if'", "'then'", "'else'", "'some'", "'every'", "'satisfies'", "'and'",
"'or'", "'between'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "BLOCK_COMMENT", "LINE_COMMENT", "WS", "STRING", "NUMBER", "TEMPORAL",
"EQ", "NE", "LT", "GT", "LE", "GE", "PLUS", "MINUS", "STAR", "FORWARD_SLASH",
"STAR_STAR", "DOT_DOT", "DOT", "COMMA", "PAREN_OPEN", "PAREN_CLOSE",
"BRACKET_OPEN", "BRACKET_CLOSE", "BRACE_OPEN", "BRACE_CLOSE", "COLON",
"ARROW", "NOT", "TRUE", "FALSE", "NULL", "FUNCTION", "EXTERNAL", "FOR",
"IN", "RETURN", "IF", "THEN", "ELSE", "SOME", "EVERY", "SATISFIES", "AND",
"OR", "BETWEEN", "INSTANCE_OF", "NAME"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "FEELParser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
private ASTFactory astFactory;
public FEELParser(TokenStream input, ASTFactory astFactory) {
this(input);
this.astFactory = astFactory;
}
public ASTFactory getASTFactory() {
return astFactory;
}
public FEELParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class UnaryTestsRootContext extends ParserRuleContext {
public UnaryTests ast;
public UnaryTestsContext unaryTests;
public UnaryTestsContext unaryTests() {
return getRuleContext(UnaryTestsContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public UnaryTestsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unaryTestsRoot; }
}
public final UnaryTestsRootContext unaryTestsRoot() throws RecognitionException {
UnaryTestsRootContext _localctx = new UnaryTestsRootContext(_ctx, getState());
enterRule(_localctx, 0, RULE_unaryTestsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(102);
((UnaryTestsRootContext)_localctx).unaryTests = unaryTests();
((UnaryTestsRootContext)_localctx).ast = ((UnaryTestsRootContext)_localctx).unaryTests.ast;
setState(104);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExpressionRootContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext expression;
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public ExpressionRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expressionRoot; }
}
public final ExpressionRootContext expressionRoot() throws RecognitionException {
ExpressionRootContext _localctx = new ExpressionRootContext(_ctx, getState());
enterRule(_localctx, 2, RULE_expressionRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(106);
((ExpressionRootContext)_localctx).expression = expression();
((ExpressionRootContext)_localctx).ast = ((ExpressionRootContext)_localctx).expression.ast;
setState(108);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TextualExpressionsRootContext extends ParserRuleContext {
public Expression ast;
public TextualExpressionsContext textualExpressions;
public TextualExpressionsContext textualExpressions() {
return getRuleContext(TextualExpressionsContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public TextualExpressionsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_textualExpressionsRoot; }
}
public final TextualExpressionsRootContext textualExpressionsRoot() throws RecognitionException {
TextualExpressionsRootContext _localctx = new TextualExpressionsRootContext(_ctx, getState());
enterRule(_localctx, 4, RULE_textualExpressionsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(110);
((TextualExpressionsRootContext)_localctx).textualExpressions = textualExpressions();
((TextualExpressionsRootContext)_localctx).ast = ((TextualExpressionsRootContext)_localctx).textualExpressions.ast;
setState(112);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BoxedExpressionRootContext extends ParserRuleContext {
public Expression ast;
public BoxedExpressionContext boxedExpression;
public BoxedExpressionContext boxedExpression() {
return getRuleContext(BoxedExpressionContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public BoxedExpressionRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_boxedExpressionRoot; }
}
public final BoxedExpressionRootContext boxedExpressionRoot() throws RecognitionException {
BoxedExpressionRootContext _localctx = new BoxedExpressionRootContext(_ctx, getState());
enterRule(_localctx, 6, RULE_boxedExpressionRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(114);
((BoxedExpressionRootContext)_localctx).boxedExpression = boxedExpression();
((BoxedExpressionRootContext)_localctx).ast = ((BoxedExpressionRootContext)_localctx).boxedExpression.ast;
setState(116);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class UnaryTestsContext extends ParserRuleContext {
public UnaryTests ast;
public PositiveUnaryTestsContext tests;
public TerminalNode NOT() { return getToken(FEELParser.NOT, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public PositiveUnaryTestsContext positiveUnaryTests() {
return getRuleContext(PositiveUnaryTestsContext.class,0);
}
public TerminalNode MINUS() { return getToken(FEELParser.MINUS, 0); }
public UnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unaryTests; }
}
public final UnaryTestsContext unaryTests() throws RecognitionException {
UnaryTestsContext _localctx = new UnaryTestsContext(_ctx, getState());
enterRule(_localctx, 8, RULE_unaryTests);
try {
setState(129);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,0,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(118);
match(NOT);
setState(119);
match(PAREN_OPEN);
setState(120);
((UnaryTestsContext)_localctx).tests = positiveUnaryTests();
setState(121);
match(PAREN_CLOSE);
((UnaryTestsContext)_localctx).ast = astFactory.toNegatedUnaryTests(((UnaryTestsContext)_localctx).tests.ast);
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(124);
((UnaryTestsContext)_localctx).tests = positiveUnaryTests();
((UnaryTestsContext)_localctx).ast = ((UnaryTestsContext)_localctx).tests.ast;
}
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
{
setState(127);
match(MINUS);
((UnaryTestsContext)_localctx).ast = astFactory.toAny();
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PositiveUnaryTestsContext extends ParserRuleContext {
public PositiveUnaryTests ast;
public PositiveUnaryTestContext test;
public List positiveUnaryTest() {
return getRuleContexts(PositiveUnaryTestContext.class);
}
public PositiveUnaryTestContext positiveUnaryTest(int i) {
return getRuleContext(PositiveUnaryTestContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public PositiveUnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positiveUnaryTests; }
}
public final PositiveUnaryTestsContext positiveUnaryTests() throws RecognitionException {
PositiveUnaryTestsContext _localctx = new PositiveUnaryTestsContext(_ctx, getState());
enterRule(_localctx, 10, RULE_positiveUnaryTests);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List tests = new ArrayList<>();
setState(132);
((PositiveUnaryTestsContext)_localctx).test = positiveUnaryTest();
tests.add(((PositiveUnaryTestsContext)_localctx).test.ast);
setState(140);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(134);
match(COMMA);
setState(135);
((PositiveUnaryTestsContext)_localctx).test = positiveUnaryTest();
tests.add(((PositiveUnaryTestsContext)_localctx).test.ast);
}
}
setState(142);
_errHandler.sync(this);
_la = _input.LA(1);
}
((PositiveUnaryTestsContext)_localctx).ast = astFactory.toPositiveUnaryTests(tests);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PositiveUnaryTestContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext expression;
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public PositiveUnaryTestContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positiveUnaryTest; }
}
public final PositiveUnaryTestContext positiveUnaryTest() throws RecognitionException {
PositiveUnaryTestContext _localctx = new PositiveUnaryTestContext(_ctx, getState());
enterRule(_localctx, 12, RULE_positiveUnaryTest);
try {
enterOuterAlt(_localctx, 1);
{
{
setState(145);
((PositiveUnaryTestContext)_localctx).expression = expression();
((PositiveUnaryTestContext)_localctx).ast = astFactory.toPositiveUnaryTest(((PositiveUnaryTestContext)_localctx).expression.ast);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimplePositiveUnaryTestContext extends ParserRuleContext {
public Expression ast;
public Token op;
public EndpointContext opd;
public IntervalContext opd2;
public EndpointContext endpoint() {
return getRuleContext(EndpointContext.class,0);
}
public TerminalNode LT() { return getToken(FEELParser.LT, 0); }
public TerminalNode LE() { return getToken(FEELParser.LE, 0); }
public TerminalNode GT() { return getToken(FEELParser.GT, 0); }
public TerminalNode GE() { return getToken(FEELParser.GE, 0); }
public IntervalContext interval() {
return getRuleContext(IntervalContext.class,0);
}
public SimplePositiveUnaryTestContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simplePositiveUnaryTest; }
}
public final SimplePositiveUnaryTestContext simplePositiveUnaryTest() throws RecognitionException {
SimplePositiveUnaryTestContext _localctx = new SimplePositiveUnaryTestContext(_ctx, getState());
enterRule(_localctx, 14, RULE_simplePositiveUnaryTest);
try {
setState(160);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING:
case NUMBER:
case TEMPORAL:
case LT:
case GT:
case LE:
case GE:
case MINUS:
case TRUE:
case FALSE:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 1);
{
{
setState(152);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LT:
{
setState(148);
((SimplePositiveUnaryTestContext)_localctx).op = match(LT);
}
break;
case LE:
{
setState(149);
((SimplePositiveUnaryTestContext)_localctx).op = match(LE);
}
break;
case GT:
{
setState(150);
((SimplePositiveUnaryTestContext)_localctx).op = match(GT);
}
break;
case GE:
{
setState(151);
((SimplePositiveUnaryTestContext)_localctx).op = match(GE);
}
break;
case STRING:
case NUMBER:
case TEMPORAL:
case MINUS:
case TRUE:
case FALSE:
case FUNCTION:
case AND:
case OR:
case NAME:
break;
default:
break;
}
setState(154);
((SimplePositiveUnaryTestContext)_localctx).opd = endpoint();
((SimplePositiveUnaryTestContext)_localctx).ast = ((SimplePositiveUnaryTestContext)_localctx).op == null ? astFactory.toOperatorRange(null, ((SimplePositiveUnaryTestContext)_localctx).opd.ast) : astFactory.toOperatorRange((((SimplePositiveUnaryTestContext)_localctx).op!=null?((SimplePositiveUnaryTestContext)_localctx).op.getText():null), ((SimplePositiveUnaryTestContext)_localctx).opd.ast);
}
}
break;
case PAREN_OPEN:
case BRACKET_OPEN:
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 2);
{
{
setState(157);
((SimplePositiveUnaryTestContext)_localctx).opd2 = interval();
((SimplePositiveUnaryTestContext)_localctx).ast = ((SimplePositiveUnaryTestContext)_localctx).opd2.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntervalContext extends ParserRuleContext {
public EndpointsRange ast;
public IntervalStartParContext leftPar;
public EndpointContext ep1;
public EndpointContext ep2;
public IntervalEndParContext rightPar;
public TerminalNode DOT_DOT() { return getToken(FEELParser.DOT_DOT, 0); }
public IntervalStartParContext intervalStartPar() {
return getRuleContext(IntervalStartParContext.class,0);
}
public List endpoint() {
return getRuleContexts(EndpointContext.class);
}
public EndpointContext endpoint(int i) {
return getRuleContext(EndpointContext.class,i);
}
public IntervalEndParContext intervalEndPar() {
return getRuleContext(IntervalEndParContext.class,0);
}
public IntervalContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_interval; }
}
public final IntervalContext interval() throws RecognitionException {
IntervalContext _localctx = new IntervalContext(_ctx, getState());
enterRule(_localctx, 16, RULE_interval);
try {
enterOuterAlt(_localctx, 1);
{
setState(162);
((IntervalContext)_localctx).leftPar = intervalStartPar();
setState(163);
((IntervalContext)_localctx).ep1 = endpoint();
setState(164);
match(DOT_DOT);
setState(165);
((IntervalContext)_localctx).ep2 = endpoint();
setState(166);
((IntervalContext)_localctx).rightPar = intervalEndPar();
((IntervalContext)_localctx).ast = astFactory.toEndpointsRange(((IntervalContext)_localctx).leftPar.ast, ((IntervalContext)_localctx).ep1.ast, ((IntervalContext)_localctx).rightPar.ast, ((IntervalContext)_localctx).ep2.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntervalStartParContext extends ParserRuleContext {
public String ast;
public Token token;
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(FEELParser.BRACKET_CLOSE, 0); }
public TerminalNode BRACKET_OPEN() { return getToken(FEELParser.BRACKET_OPEN, 0); }
public IntervalStartParContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intervalStartPar; }
}
public final IntervalStartParContext intervalStartPar() throws RecognitionException {
IntervalStartParContext _localctx = new IntervalStartParContext(_ctx, getState());
enterRule(_localctx, 18, RULE_intervalStartPar);
try {
setState(175);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PAREN_OPEN:
enterOuterAlt(_localctx, 1);
{
{
setState(169);
((IntervalStartParContext)_localctx).token = match(PAREN_OPEN);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 2);
{
{
setState(171);
((IntervalStartParContext)_localctx).token = match(BRACKET_CLOSE);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_OPEN:
enterOuterAlt(_localctx, 3);
{
{
setState(173);
((IntervalStartParContext)_localctx).token = match(BRACKET_OPEN);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntervalEndParContext extends ParserRuleContext {
public String ast;
public Token token;
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public TerminalNode BRACKET_OPEN() { return getToken(FEELParser.BRACKET_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(FEELParser.BRACKET_CLOSE, 0); }
public IntervalEndParContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intervalEndPar; }
}
public final IntervalEndParContext intervalEndPar() throws RecognitionException {
IntervalEndParContext _localctx = new IntervalEndParContext(_ctx, getState());
enterRule(_localctx, 20, RULE_intervalEndPar);
try {
setState(183);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PAREN_CLOSE:
enterOuterAlt(_localctx, 1);
{
{
setState(177);
((IntervalEndParContext)_localctx).token = match(PAREN_CLOSE);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_OPEN:
enterOuterAlt(_localctx, 2);
{
{
setState(179);
((IntervalEndParContext)_localctx).token = match(BRACKET_OPEN);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
}
break;
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 3);
{
{
setState(181);
((IntervalEndParContext)_localctx).token = match(BRACKET_CLOSE);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EndpointContext extends ParserRuleContext {
public Expression ast;
public Token op;
public SimpleValueContext opd;
public SimpleValueContext simpleValue() {
return getRuleContext(SimpleValueContext.class,0);
}
public TerminalNode MINUS() { return getToken(FEELParser.MINUS, 0); }
public EndpointContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_endpoint; }
}
public final EndpointContext endpoint() throws RecognitionException {
EndpointContext _localctx = new EndpointContext(_ctx, getState());
enterRule(_localctx, 22, RULE_endpoint);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
{
setState(186);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==MINUS) {
{
setState(185);
((EndpointContext)_localctx).op = match(MINUS);
}
}
setState(188);
((EndpointContext)_localctx).opd = simpleValue();
((EndpointContext)_localctx).ast = (((EndpointContext)_localctx).op == null) ? ((EndpointContext)_localctx).opd.ast : astFactory.toNegation((((EndpointContext)_localctx).op!=null?((EndpointContext)_localctx).op.getText():null), ((EndpointContext)_localctx).opd.ast);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExpressionContext extends ParserRuleContext {
public Expression ast;
public TextualExpressionContext textualExpression;
public TextualExpressionContext textualExpression() {
return getRuleContext(TextualExpressionContext.class,0);
}
public ExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression; }
}
public final ExpressionContext expression() throws RecognitionException {
ExpressionContext _localctx = new ExpressionContext(_ctx, getState());
enterRule(_localctx, 24, RULE_expression);
try {
enterOuterAlt(_localctx, 1);
{
{
setState(191);
((ExpressionContext)_localctx).textualExpression = textualExpression();
((ExpressionContext)_localctx).ast = ((ExpressionContext)_localctx).textualExpression.ast;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TextualExpressionsContext extends ParserRuleContext {
public Expression ast;
public TextualExpressionContext exp;
public List textualExpression() {
return getRuleContexts(TextualExpressionContext.class);
}
public TextualExpressionContext textualExpression(int i) {
return getRuleContext(TextualExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public TextualExpressionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_textualExpressions; }
}
public final TextualExpressionsContext textualExpressions() throws RecognitionException {
TextualExpressionsContext _localctx = new TextualExpressionsContext(_ctx, getState());
enterRule(_localctx, 26, RULE_textualExpressions);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List expressionList = new ArrayList<>();
setState(195);
((TextualExpressionsContext)_localctx).exp = textualExpression();
expressionList.add(((TextualExpressionsContext)_localctx).exp.ast);
setState(203);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(197);
match(COMMA);
setState(198);
((TextualExpressionsContext)_localctx).exp = textualExpression();
expressionList.add(((TextualExpressionsContext)_localctx).exp.ast);
}
}
setState(205);
_errHandler.sync(this);
_la = _input.LA(1);
}
((TextualExpressionsContext)_localctx).ast = astFactory.toExpressionList(expressionList);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TextualExpressionContext extends ParserRuleContext {
public Expression ast;
public ForExpressionContext forExpression;
public IfExpressionContext ifExpression;
public QuantifiedExpressionContext quantifiedExpression;
public DisjunctionContext disjunction;
public ForExpressionContext forExpression() {
return getRuleContext(ForExpressionContext.class,0);
}
public IfExpressionContext ifExpression() {
return getRuleContext(IfExpressionContext.class,0);
}
public QuantifiedExpressionContext quantifiedExpression() {
return getRuleContext(QuantifiedExpressionContext.class,0);
}
public DisjunctionContext disjunction() {
return getRuleContext(DisjunctionContext.class,0);
}
public TextualExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_textualExpression; }
}
public final TextualExpressionContext textualExpression() throws RecognitionException {
TextualExpressionContext _localctx = new TextualExpressionContext(_ctx, getState());
enterRule(_localctx, 28, RULE_textualExpression);
try {
setState(220);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FOR:
enterOuterAlt(_localctx, 1);
{
{
setState(208);
((TextualExpressionContext)_localctx).forExpression = forExpression();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).forExpression.ast;
}
}
break;
case IF:
enterOuterAlt(_localctx, 2);
{
{
setState(211);
((TextualExpressionContext)_localctx).ifExpression = ifExpression();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).ifExpression.ast;
}
}
break;
case SOME:
case EVERY:
enterOuterAlt(_localctx, 3);
{
{
setState(214);
((TextualExpressionContext)_localctx).quantifiedExpression = quantifiedExpression();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).quantifiedExpression.ast;
}
}
break;
case STRING:
case NUMBER:
case TEMPORAL:
case LT:
case GT:
case LE:
case GE:
case MINUS:
case PAREN_OPEN:
case BRACKET_OPEN:
case BRACKET_CLOSE:
case BRACE_OPEN:
case NOT:
case TRUE:
case FALSE:
case NULL:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 4);
{
{
setState(217);
((TextualExpressionContext)_localctx).disjunction = disjunction();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).disjunction.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FunctionDefinitionContext extends ParserRuleContext {
public Expression ast;
public FormalParameterContext param;
public TypeContext type;
public ExpressionContext body;
public TerminalNode FUNCTION() { return getToken(FEELParser.FUNCTION, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode COLON() { return getToken(FEELParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TerminalNode EXTERNAL() { return getToken(FEELParser.EXTERNAL, 0); }
public List formalParameter() {
return getRuleContexts(FormalParameterContext.class);
}
public FormalParameterContext formalParameter(int i) {
return getRuleContext(FormalParameterContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public FunctionDefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionDefinition; }
}
public final FunctionDefinitionContext functionDefinition() throws RecognitionException {
FunctionDefinitionContext _localctx = new FunctionDefinitionContext(_ctx, getState());
enterRule(_localctx, 30, RULE_functionDefinition);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List parameters = new ArrayList<>();
boolean external = false;
TypeExpression returnTypeExp = null;
setState(225);
match(FUNCTION);
setState(226);
match(PAREN_OPEN);
setState(238);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << FUNCTION) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
setState(227);
((FunctionDefinitionContext)_localctx).param = formalParameter();
parameters.add(((FunctionDefinitionContext)_localctx).param.ast);
setState(235);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(229);
match(COMMA);
setState(230);
((FunctionDefinitionContext)_localctx).param = formalParameter();
parameters.add(((FunctionDefinitionContext)_localctx).param.ast);
}
}
setState(237);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(240);
match(PAREN_CLOSE);
setState(245);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON) {
{
setState(241);
match(COLON);
setState(242);
((FunctionDefinitionContext)_localctx).type = type();
returnTypeExp = ((FunctionDefinitionContext)_localctx).type.ast;
}
}
setState(249);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==EXTERNAL) {
{
setState(247);
match(EXTERNAL);
external = true;
}
}
setState(251);
((FunctionDefinitionContext)_localctx).body = expression();
((FunctionDefinitionContext)_localctx).ast = astFactory.toFunctionDefinition(parameters, returnTypeExp, ((FunctionDefinitionContext)_localctx).body.ast, external);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FormalParameterContext extends ParserRuleContext {
public FormalParameter ast;
public ParameterNameContext name;
public TypeContext type;
public ParameterNameContext parameterName() {
return getRuleContext(ParameterNameContext.class,0);
}
public TerminalNode COLON() { return getToken(FEELParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public FormalParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_formalParameter; }
}
public final FormalParameterContext formalParameter() throws RecognitionException {
FormalParameterContext _localctx = new FormalParameterContext(_ctx, getState());
enterRule(_localctx, 32, RULE_formalParameter);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
TypeExpression typeExp = null;
setState(255);
((FormalParameterContext)_localctx).name = parameterName();
setState(260);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON) {
{
setState(256);
match(COLON);
setState(257);
((FormalParameterContext)_localctx).type = type();
typeExp = ((FormalParameterContext)_localctx).type.ast;
}
}
((FormalParameterContext)_localctx).ast = astFactory.toFormalParameter(((FormalParameterContext)_localctx).name.ast, typeExp);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ForExpressionContext extends ParserRuleContext {
public Expression ast;
public IdentifierContext var;
public IterationDomainContext domain;
public ExpressionContext body;
public TerminalNode FOR() { return getToken(FEELParser.FOR, 0); }
public List IN() { return getTokens(FEELParser.IN); }
public TerminalNode IN(int i) {
return getToken(FEELParser.IN, i);
}
public TerminalNode RETURN() { return getToken(FEELParser.RETURN, 0); }
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List iterationDomain() {
return getRuleContexts(IterationDomainContext.class);
}
public IterationDomainContext iterationDomain(int i) {
return getRuleContext(IterationDomainContext.class,i);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public ForExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_forExpression; }
}
public final ForExpressionContext forExpression() throws RecognitionException {
ForExpressionContext _localctx = new ForExpressionContext(_ctx, getState());
enterRule(_localctx, 34, RULE_forExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List iterators = new ArrayList<>();
setState(265);
match(FOR);
setState(266);
((ForExpressionContext)_localctx).var = identifier();
setState(267);
match(IN);
setState(268);
((ForExpressionContext)_localctx).domain = iterationDomain();
iterators.add(astFactory.toIterator((((ForExpressionContext)_localctx).var!=null?_input.getText(((ForExpressionContext)_localctx).var.start,((ForExpressionContext)_localctx).var.stop):null), ((ForExpressionContext)_localctx).domain.ast));
setState(278);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(270);
match(COMMA);
setState(271);
((ForExpressionContext)_localctx).var = identifier();
setState(272);
match(IN);
setState(273);
((ForExpressionContext)_localctx).domain = iterationDomain();
iterators.add(astFactory.toIterator((((ForExpressionContext)_localctx).var!=null?_input.getText(((ForExpressionContext)_localctx).var.start,((ForExpressionContext)_localctx).var.stop):null), ((ForExpressionContext)_localctx).domain.ast));
}
}
setState(280);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(281);
match(RETURN);
setState(282);
((ForExpressionContext)_localctx).body = expression();
((ForExpressionContext)_localctx).ast = astFactory.toForExpression(iterators, ((ForExpressionContext)_localctx).body.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IterationDomainContext extends ParserRuleContext {
public IteratorDomain ast;
public ExpressionContext exp1;
public ExpressionContext exp2;
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode DOT_DOT() { return getToken(FEELParser.DOT_DOT, 0); }
public IterationDomainContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_iterationDomain; }
}
public final IterationDomainContext iterationDomain() throws RecognitionException {
IterationDomainContext _localctx = new IterationDomainContext(_ctx, getState());
enterRule(_localctx, 36, RULE_iterationDomain);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
Expression end = null;
setState(286);
((IterationDomainContext)_localctx).exp1 = expression();
setState(291);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DOT_DOT) {
{
setState(287);
match(DOT_DOT);
setState(288);
((IterationDomainContext)_localctx).exp2 = expression();
end = ((IterationDomainContext)_localctx).exp2.ast;
}
}
((IterationDomainContext)_localctx).ast = astFactory.toIteratorDomain(((IterationDomainContext)_localctx).exp1.ast, end);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IfExpressionContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext cond;
public ExpressionContext exp1;
public ExpressionContext exp2;
public TerminalNode IF() { return getToken(FEELParser.IF, 0); }
public TerminalNode THEN() { return getToken(FEELParser.THEN, 0); }
public TerminalNode ELSE() { return getToken(FEELParser.ELSE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public IfExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ifExpression; }
}
public final IfExpressionContext ifExpression() throws RecognitionException {
IfExpressionContext _localctx = new IfExpressionContext(_ctx, getState());
enterRule(_localctx, 38, RULE_ifExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(295);
match(IF);
setState(296);
((IfExpressionContext)_localctx).cond = expression();
setState(297);
match(THEN);
setState(298);
((IfExpressionContext)_localctx).exp1 = expression();
setState(299);
match(ELSE);
setState(300);
((IfExpressionContext)_localctx).exp2 = expression();
((IfExpressionContext)_localctx).ast = astFactory.toIfExpression(((IfExpressionContext)_localctx).cond.ast, ((IfExpressionContext)_localctx).exp1.ast, ((IfExpressionContext)_localctx).exp2.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class QuantifiedExpressionContext extends ParserRuleContext {
public Expression ast;
public Token op;
public IdentifierContext var;
public ExpressionContext domain;
public ExpressionContext body;
public List IN() { return getTokens(FEELParser.IN); }
public TerminalNode IN(int i) {
return getToken(FEELParser.IN, i);
}
public TerminalNode SATISFIES() { return getToken(FEELParser.SATISFIES, 0); }
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode SOME() { return getToken(FEELParser.SOME, 0); }
public TerminalNode EVERY() { return getToken(FEELParser.EVERY, 0); }
public QuantifiedExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_quantifiedExpression; }
}
public final QuantifiedExpressionContext quantifiedExpression() throws RecognitionException {
QuantifiedExpressionContext _localctx = new QuantifiedExpressionContext(_ctx, getState());
enterRule(_localctx, 40, RULE_quantifiedExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List iterators = new ArrayList<>();
setState(306);
_errHandler.sync(this);
switch (_input.LA(1)) {
case SOME:
{
setState(304);
((QuantifiedExpressionContext)_localctx).op = match(SOME);
}
break;
case EVERY:
{
setState(305);
((QuantifiedExpressionContext)_localctx).op = match(EVERY);
}
break;
default:
throw new NoViableAltException(this);
}
setState(308);
((QuantifiedExpressionContext)_localctx).var = identifier();
setState(309);
match(IN);
setState(310);
((QuantifiedExpressionContext)_localctx).domain = expression();
iterators.add(astFactory.toIterator((((QuantifiedExpressionContext)_localctx).var!=null?_input.getText(((QuantifiedExpressionContext)_localctx).var.start,((QuantifiedExpressionContext)_localctx).var.stop):null), ((QuantifiedExpressionContext)_localctx).domain.ast));
setState(319);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << FUNCTION) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
{
setState(312);
((QuantifiedExpressionContext)_localctx).var = identifier();
setState(313);
match(IN);
setState(314);
((QuantifiedExpressionContext)_localctx).domain = expression();
iterators.add(astFactory.toIterator((((QuantifiedExpressionContext)_localctx).var!=null?_input.getText(((QuantifiedExpressionContext)_localctx).var.start,((QuantifiedExpressionContext)_localctx).var.stop):null), ((QuantifiedExpressionContext)_localctx).domain.ast));
}
}
setState(321);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(322);
match(SATISFIES);
setState(323);
((QuantifiedExpressionContext)_localctx).body = expression();
((QuantifiedExpressionContext)_localctx).ast = astFactory.toQuantifiedExpression((((QuantifiedExpressionContext)_localctx).op!=null?((QuantifiedExpressionContext)_localctx).op.getText():null), iterators, ((QuantifiedExpressionContext)_localctx).body.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DisjunctionContext extends ParserRuleContext {
public Expression ast;
public ConjunctionContext left;
public ConjunctionContext right;
public List conjunction() {
return getRuleContexts(ConjunctionContext.class);
}
public ConjunctionContext conjunction(int i) {
return getRuleContext(ConjunctionContext.class,i);
}
public List OR() { return getTokens(FEELParser.OR); }
public TerminalNode OR(int i) {
return getToken(FEELParser.OR, i);
}
public DisjunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_disjunction; }
}
public final DisjunctionContext disjunction() throws RecognitionException {
DisjunctionContext _localctx = new DisjunctionContext(_ctx, getState());
enterRule(_localctx, 42, RULE_disjunction);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(326);
((DisjunctionContext)_localctx).left = conjunction();
((DisjunctionContext)_localctx).ast = ((DisjunctionContext)_localctx).left.ast;
setState(334);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,18,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(328);
match(OR);
setState(329);
((DisjunctionContext)_localctx).right = conjunction();
((DisjunctionContext)_localctx).ast = astFactory.toDisjunction(_localctx.ast, ((DisjunctionContext)_localctx).right.ast);
}
}
}
setState(336);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,18,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ConjunctionContext extends ParserRuleContext {
public Expression ast;
public ComparisonContext left;
public ComparisonContext right;
public List comparison() {
return getRuleContexts(ComparisonContext.class);
}
public ComparisonContext comparison(int i) {
return getRuleContext(ComparisonContext.class,i);
}
public List AND() { return getTokens(FEELParser.AND); }
public TerminalNode AND(int i) {
return getToken(FEELParser.AND, i);
}
public ConjunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_conjunction; }
}
public final ConjunctionContext conjunction() throws RecognitionException {
ConjunctionContext _localctx = new ConjunctionContext(_ctx, getState());
enterRule(_localctx, 44, RULE_conjunction);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(337);
((ConjunctionContext)_localctx).left = comparison();
((ConjunctionContext)_localctx).ast = ((ConjunctionContext)_localctx).left.ast;
setState(345);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,19,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(339);
match(AND);
setState(340);
((ConjunctionContext)_localctx).right = comparison();
((ConjunctionContext)_localctx).ast = astFactory.toConjunction(_localctx.ast, ((ConjunctionContext)_localctx).right.ast);
}
}
}
setState(347);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,19,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ComparisonContext extends ParserRuleContext {
public Expression ast;
public ArithmeticExpressionContext ae1;
public Token op;
public ArithmeticExpressionContext ae2;
public ExpressionContext leftEndpoint;
public ExpressionContext rightEndpoint;
public PositiveUnaryTestContext test;
public PositiveUnaryTestsContext tests;
public List arithmeticExpression() {
return getRuleContexts(ArithmeticExpressionContext.class);
}
public ArithmeticExpressionContext arithmeticExpression(int i) {
return getRuleContext(ArithmeticExpressionContext.class,i);
}
public TerminalNode BETWEEN() { return getToken(FEELParser.BETWEEN, 0); }
public TerminalNode AND() { return getToken(FEELParser.AND, 0); }
public TerminalNode IN() { return getToken(FEELParser.IN, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public PositiveUnaryTestContext positiveUnaryTest() {
return getRuleContext(PositiveUnaryTestContext.class,0);
}
public PositiveUnaryTestsContext positiveUnaryTests() {
return getRuleContext(PositiveUnaryTestsContext.class,0);
}
public TerminalNode EQ() { return getToken(FEELParser.EQ, 0); }
public TerminalNode NE() { return getToken(FEELParser.NE, 0); }
public TerminalNode LT() { return getToken(FEELParser.LT, 0); }
public TerminalNode GT() { return getToken(FEELParser.GT, 0); }
public TerminalNode LE() { return getToken(FEELParser.LE, 0); }
public TerminalNode GE() { return getToken(FEELParser.GE, 0); }
public ComparisonContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_comparison; }
}
public final ComparisonContext comparison() throws RecognitionException {
ComparisonContext _localctx = new ComparisonContext(_ctx, getState());
enterRule(_localctx, 46, RULE_comparison);
try {
enterOuterAlt(_localctx, 1);
{
setState(348);
((ComparisonContext)_localctx).ae1 = arithmeticExpression();
((ComparisonContext)_localctx).ast = ((ComparisonContext)_localctx).ae1.ast;
setState(377);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,21,_ctx) ) {
case 1:
{
{
setState(356);
_errHandler.sync(this);
switch (_input.LA(1)) {
case EQ:
{
setState(350);
((ComparisonContext)_localctx).op = match(EQ);
}
break;
case NE:
{
setState(351);
((ComparisonContext)_localctx).op = match(NE);
}
break;
case LT:
{
setState(352);
((ComparisonContext)_localctx).op = match(LT);
}
break;
case GT:
{
setState(353);
((ComparisonContext)_localctx).op = match(GT);
}
break;
case LE:
{
setState(354);
((ComparisonContext)_localctx).op = match(LE);
}
break;
case GE:
{
setState(355);
((ComparisonContext)_localctx).op = match(GE);
}
break;
default:
throw new NoViableAltException(this);
}
setState(358);
((ComparisonContext)_localctx).ae2 = arithmeticExpression();
((ComparisonContext)_localctx).ast = astFactory.toComparison((((ComparisonContext)_localctx).op!=null?((ComparisonContext)_localctx).op.getText():null), ((ComparisonContext)_localctx).ae1.ast, ((ComparisonContext)_localctx).ae2.ast);
}
}
break;
case 2:
{
{
setState(361);
match(BETWEEN);
setState(362);
((ComparisonContext)_localctx).leftEndpoint = expression();
setState(363);
match(AND);
setState(364);
((ComparisonContext)_localctx).rightEndpoint = expression();
((ComparisonContext)_localctx).ast = astFactory.toBetweenExpression(_localctx.ast, ((ComparisonContext)_localctx).leftEndpoint.ast, ((ComparisonContext)_localctx).rightEndpoint.ast);
}
}
break;
case 3:
{
{
setState(367);
match(IN);
setState(368);
((ComparisonContext)_localctx).test = positiveUnaryTest();
((ComparisonContext)_localctx).ast = astFactory.toInExpression(_localctx.ast, ((ComparisonContext)_localctx).test.ast);
}
}
break;
case 4:
{
{
setState(371);
match(IN);
setState(372);
match(PAREN_OPEN);
setState(373);
((ComparisonContext)_localctx).tests = positiveUnaryTests();
setState(374);
match(PAREN_CLOSE);
((ComparisonContext)_localctx).ast = astFactory.toInExpression(_localctx.ast, ((ComparisonContext)_localctx).tests.ast);
}
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArithmeticExpressionContext extends ParserRuleContext {
public Expression ast;
public AdditionContext addition;
public AdditionContext addition() {
return getRuleContext(AdditionContext.class,0);
}
public ArithmeticExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arithmeticExpression; }
}
public final ArithmeticExpressionContext arithmeticExpression() throws RecognitionException {
ArithmeticExpressionContext _localctx = new ArithmeticExpressionContext(_ctx, getState());
enterRule(_localctx, 48, RULE_arithmeticExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(379);
((ArithmeticExpressionContext)_localctx).addition = addition();
((ArithmeticExpressionContext)_localctx).ast = ((ArithmeticExpressionContext)_localctx).addition.ast;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AdditionContext extends ParserRuleContext {
public Expression ast;
public MultiplicationContext left;
public Token op;
public MultiplicationContext right;
public List multiplication() {
return getRuleContexts(MultiplicationContext.class);
}
public MultiplicationContext multiplication(int i) {
return getRuleContext(MultiplicationContext.class,i);
}
public List PLUS() { return getTokens(FEELParser.PLUS); }
public TerminalNode PLUS(int i) {
return getToken(FEELParser.PLUS, i);
}
public List MINUS() { return getTokens(FEELParser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(FEELParser.MINUS, i);
}
public AdditionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_addition; }
}
public final AdditionContext addition() throws RecognitionException {
AdditionContext _localctx = new AdditionContext(_ctx, getState());
enterRule(_localctx, 50, RULE_addition);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(382);
((AdditionContext)_localctx).left = multiplication();
((AdditionContext)_localctx).ast = ((AdditionContext)_localctx).left.ast;
setState(393);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,23,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(386);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PLUS:
{
setState(384);
((AdditionContext)_localctx).op = match(PLUS);
}
break;
case MINUS:
{
setState(385);
((AdditionContext)_localctx).op = match(MINUS);
}
break;
default:
throw new NoViableAltException(this);
}
setState(388);
((AdditionContext)_localctx).right = multiplication();
((AdditionContext)_localctx).ast = astFactory.toAddition((((AdditionContext)_localctx).op!=null?((AdditionContext)_localctx).op.getText():null), _localctx.ast, ((AdditionContext)_localctx).right.ast);
}
}
}
setState(395);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,23,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class MultiplicationContext extends ParserRuleContext {
public Expression ast;
public ExponentiationContext left;
public Token op;
public ExponentiationContext right;
public List exponentiation() {
return getRuleContexts(ExponentiationContext.class);
}
public ExponentiationContext exponentiation(int i) {
return getRuleContext(ExponentiationContext.class,i);
}
public List STAR() { return getTokens(FEELParser.STAR); }
public TerminalNode STAR(int i) {
return getToken(FEELParser.STAR, i);
}
public List FORWARD_SLASH() { return getTokens(FEELParser.FORWARD_SLASH); }
public TerminalNode FORWARD_SLASH(int i) {
return getToken(FEELParser.FORWARD_SLASH, i);
}
public MultiplicationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_multiplication; }
}
public final MultiplicationContext multiplication() throws RecognitionException {
MultiplicationContext _localctx = new MultiplicationContext(_ctx, getState());
enterRule(_localctx, 52, RULE_multiplication);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(396);
((MultiplicationContext)_localctx).left = exponentiation();
((MultiplicationContext)_localctx).ast = ((MultiplicationContext)_localctx).left.ast;
setState(407);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,25,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(400);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STAR:
{
setState(398);
((MultiplicationContext)_localctx).op = match(STAR);
}
break;
case FORWARD_SLASH:
{
setState(399);
((MultiplicationContext)_localctx).op = match(FORWARD_SLASH);
}
break;
default:
throw new NoViableAltException(this);
}
setState(402);
((MultiplicationContext)_localctx).right = exponentiation();
((MultiplicationContext)_localctx).ast = astFactory.toMultiplication((((MultiplicationContext)_localctx).op!=null?((MultiplicationContext)_localctx).op.getText():null), _localctx.ast, ((MultiplicationContext)_localctx).right.ast);
}
}
}
setState(409);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,25,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExponentiationContext extends ParserRuleContext {
public Expression ast;
public ArithmeticNegationContext left;
public ArithmeticNegationContext right;
public List arithmeticNegation() {
return getRuleContexts(ArithmeticNegationContext.class);
}
public ArithmeticNegationContext arithmeticNegation(int i) {
return getRuleContext(ArithmeticNegationContext.class,i);
}
public List STAR_STAR() { return getTokens(FEELParser.STAR_STAR); }
public TerminalNode STAR_STAR(int i) {
return getToken(FEELParser.STAR_STAR, i);
}
public ExponentiationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_exponentiation; }
}
public final ExponentiationContext exponentiation() throws RecognitionException {
ExponentiationContext _localctx = new ExponentiationContext(_ctx, getState());
enterRule(_localctx, 54, RULE_exponentiation);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(410);
((ExponentiationContext)_localctx).left = arithmeticNegation();
((ExponentiationContext)_localctx).ast = ((ExponentiationContext)_localctx).left.ast;
setState(418);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,26,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(412);
match(STAR_STAR);
setState(413);
((ExponentiationContext)_localctx).right = arithmeticNegation();
((ExponentiationContext)_localctx).ast = astFactory.toExponentiation(_localctx.ast, ((ExponentiationContext)_localctx).right.ast);
}
}
}
setState(420);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,26,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ArithmeticNegationContext extends ParserRuleContext {
public Expression ast;
public InstanceOfContext opd;
public InstanceOfContext instanceOf() {
return getRuleContext(InstanceOfContext.class,0);
}
public List MINUS() { return getTokens(FEELParser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(FEELParser.MINUS, i);
}
public List NOT() { return getTokens(FEELParser.NOT); }
public TerminalNode NOT(int i) {
return getToken(FEELParser.NOT, i);
}
public ArithmeticNegationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arithmeticNegation; }
}
public final ArithmeticNegationContext arithmeticNegation() throws RecognitionException {
ArithmeticNegationContext _localctx = new ArithmeticNegationContext(_ctx, getState());
enterRule(_localctx, 56, RULE_arithmeticNegation);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
List prefixOperators = new ArrayList<>();
setState(428);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,28,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
setState(426);
_errHandler.sync(this);
switch (_input.LA(1)) {
case MINUS:
{
{
setState(422);
match(MINUS);
prefixOperators.add("-");
}
}
break;
case NOT:
{
{
setState(424);
match(NOT);
prefixOperators.add("not");
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(430);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,28,_ctx);
}
setState(431);
((ArithmeticNegationContext)_localctx).opd = instanceOf();
((ArithmeticNegationContext)_localctx).ast = astFactory.toNegation(prefixOperators, ((ArithmeticNegationContext)_localctx).opd.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class InstanceOfContext extends ParserRuleContext {
public Expression ast;
public PostfixExpressionContext exp;
public TypeContext type;
public PostfixExpressionContext postfixExpression() {
return getRuleContext(PostfixExpressionContext.class,0);
}
public TerminalNode INSTANCE_OF() { return getToken(FEELParser.INSTANCE_OF, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public InstanceOfContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_instanceOf; }
}
public final InstanceOfContext instanceOf() throws RecognitionException {
InstanceOfContext _localctx = new InstanceOfContext(_ctx, getState());
enterRule(_localctx, 58, RULE_instanceOf);
try {
enterOuterAlt(_localctx, 1);
{
setState(434);
((InstanceOfContext)_localctx).exp = postfixExpression();
((InstanceOfContext)_localctx).ast = ((InstanceOfContext)_localctx).exp.ast;
setState(440);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,29,_ctx) ) {
case 1:
{
setState(436);
match(INSTANCE_OF);
{
setState(437);
((InstanceOfContext)_localctx).type = type();
((InstanceOfContext)_localctx).ast = astFactory.toInstanceOf(_localctx.ast, ((InstanceOfContext)_localctx).type.ast);
}
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TypeContext extends ParserRuleContext {
public TypeExpression ast;
public QualifiedNameContext qName;
public IdentifierContext typeName;
public TypeContext type;
public IdentifierContext id1;
public TypeContext t1;
public IdentifierContext id2;
public TypeContext t2;
public TypeContext returnType;
public QualifiedNameContext qualifiedName() {
return getRuleContext(QualifiedNameContext.class,0);
}
public TerminalNode LT() { return getToken(FEELParser.LT, 0); }
public List type() {
return getRuleContexts(TypeContext.class);
}
public TypeContext type(int i) {
return getRuleContext(TypeContext.class,i);
}
public TerminalNode GT() { return getToken(FEELParser.GT, 0); }
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List COLON() { return getTokens(FEELParser.COLON); }
public TerminalNode COLON(int i) {
return getToken(FEELParser.COLON, i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public TerminalNode ARROW() { return getToken(FEELParser.ARROW, 0); }
public TypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_type; }
}
public final TypeContext type() throws RecognitionException {
TypeContext _localctx = new TypeContext(_ctx, getState());
enterRule(_localctx, 60, RULE_type);
int _la;
try {
setState(496);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,33,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(442);
((TypeContext)_localctx).qName = qualifiedName();
((TypeContext)_localctx).ast = astFactory.toNamedTypeExpression(((TypeContext)_localctx).qName.ast);
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(445);
((TypeContext)_localctx).typeName = identifier();
setState(446);
if (!("list".equals(((TypeContext)_localctx).typeName.ast.getText()))) throw new FailedPredicateException(this, "\"list\".equals($typeName.ast.getText())");
setState(447);
match(LT);
setState(448);
((TypeContext)_localctx).type = type();
setState(449);
match(GT);
((TypeContext)_localctx).ast = astFactory.toListTypeExpression(((TypeContext)_localctx).type.ast);
}
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
{
List> members = new ArrayList<>();
setState(453);
((TypeContext)_localctx).typeName = identifier();
setState(454);
if (!("context".equals(((TypeContext)_localctx).typeName.ast.getText()))) throw new FailedPredicateException(this, "\"context\".equals($typeName.ast.getText())");
setState(455);
match(LT);
setState(456);
((TypeContext)_localctx).id1 = identifier();
setState(457);
match(COLON);
setState(458);
((TypeContext)_localctx).t1 = type();
members.add(new Pair(((TypeContext)_localctx).id1.ast.getText(), ((TypeContext)_localctx).t1.ast));
setState(468);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(460);
match(COMMA);
setState(461);
((TypeContext)_localctx).id2 = identifier();
setState(462);
match(COLON);
setState(463);
((TypeContext)_localctx).t2 = type();
members.add(new Pair(((TypeContext)_localctx).id2.ast.getText(), ((TypeContext)_localctx).t2.ast));
}
}
setState(470);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(471);
match(GT);
((TypeContext)_localctx).ast = astFactory.toContextTypeExpression(members);
}
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
{
List parameters = new ArrayList<>();
setState(475);
((TypeContext)_localctx).typeName = identifier();
setState(476);
if (!("function".equals(((TypeContext)_localctx).typeName.ast.getText()))) throw new FailedPredicateException(this, "\"function\".equals($typeName.ast.getText())");
setState(477);
match(LT);
setState(489);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << FUNCTION) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
setState(478);
((TypeContext)_localctx).t1 = type();
parameters.add(((TypeContext)_localctx).t1.ast);
setState(484);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(480);
match(COMMA);
setState(481);
((TypeContext)_localctx).t2 = type();
}
}
setState(486);
_errHandler.sync(this);
_la = _input.LA(1);
}
parameters.add(((TypeContext)_localctx).t2.ast);
}
}
setState(491);
match(GT);
setState(492);
match(ARROW);
setState(493);
((TypeContext)_localctx).returnType = type();
((TypeContext)_localctx).ast = astFactory.toFunctionTypeExpression(parameters, ((TypeContext)_localctx).returnType.ast);
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PostfixExpressionContext extends ParserRuleContext {
public Expression ast;
public PrimaryExpressionContext primaryExpression;
public ExpressionContext filter;
public ParametersContext parameters;
public IdentifierContext name;
public PrimaryExpressionContext primaryExpression() {
return getRuleContext(PrimaryExpressionContext.class,0);
}
public List BRACKET_OPEN() { return getTokens(FEELParser.BRACKET_OPEN); }
public TerminalNode BRACKET_OPEN(int i) {
return getToken(FEELParser.BRACKET_OPEN, i);
}
public List BRACKET_CLOSE() { return getTokens(FEELParser.BRACKET_CLOSE); }
public TerminalNode BRACKET_CLOSE(int i) {
return getToken(FEELParser.BRACKET_CLOSE, i);
}
public List parameters() {
return getRuleContexts(ParametersContext.class);
}
public ParametersContext parameters(int i) {
return getRuleContext(ParametersContext.class,i);
}
public List DOT() { return getTokens(FEELParser.DOT); }
public TerminalNode DOT(int i) {
return getToken(FEELParser.DOT, i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public PostfixExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_postfixExpression; }
}
public final PostfixExpressionContext postfixExpression() throws RecognitionException {
PostfixExpressionContext _localctx = new PostfixExpressionContext(_ctx, getState());
enterRule(_localctx, 62, RULE_postfixExpression);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
{
{
setState(498);
((PostfixExpressionContext)_localctx).primaryExpression = primaryExpression();
((PostfixExpressionContext)_localctx).ast = ((PostfixExpressionContext)_localctx).primaryExpression.ast;
}
}
setState(515);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,35,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
setState(513);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BRACKET_OPEN:
{
{
setState(501);
match(BRACKET_OPEN);
setState(502);
((PostfixExpressionContext)_localctx).filter = expression();
setState(503);
match(BRACKET_CLOSE);
((PostfixExpressionContext)_localctx).ast = astFactory.toFilterExpression(_localctx.ast, ((PostfixExpressionContext)_localctx).filter.ast);
}
}
break;
case PAREN_OPEN:
{
{
setState(506);
((PostfixExpressionContext)_localctx).parameters = parameters();
((PostfixExpressionContext)_localctx).ast = astFactory.toFunctionInvocation(_localctx.ast, ((PostfixExpressionContext)_localctx).parameters.ast);
}
}
break;
case DOT:
{
{
setState(509);
match(DOT);
setState(510);
((PostfixExpressionContext)_localctx).name = identifier();
((PostfixExpressionContext)_localctx).ast = astFactory.toPathExpression(_localctx.ast, (((PostfixExpressionContext)_localctx).name!=null?_input.getText(((PostfixExpressionContext)_localctx).name.start,((PostfixExpressionContext)_localctx).name.stop):null));
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(517);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,35,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ParametersContext extends ParserRuleContext {
public Parameters ast;
public NamedParametersContext namedParameters;
public PositionalParametersContext positionalParameters;
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public NamedParametersContext namedParameters() {
return getRuleContext(NamedParametersContext.class,0);
}
public PositionalParametersContext positionalParameters() {
return getRuleContext(PositionalParametersContext.class,0);
}
public ParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parameters; }
}
public final ParametersContext parameters() throws RecognitionException {
ParametersContext _localctx = new ParametersContext(_ctx, getState());
enterRule(_localctx, 64, RULE_parameters);
try {
enterOuterAlt(_localctx, 1);
{
setState(518);
match(PAREN_OPEN);
setState(525);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,36,_ctx) ) {
case 1:
{
{
setState(519);
((ParametersContext)_localctx).namedParameters = namedParameters();
((ParametersContext)_localctx).ast = ((ParametersContext)_localctx).namedParameters.ast;
}
}
break;
case 2:
{
{
setState(522);
((ParametersContext)_localctx).positionalParameters = positionalParameters();
((ParametersContext)_localctx).ast = ((ParametersContext)_localctx).positionalParameters.ast;
}
}
break;
}
setState(527);
match(PAREN_CLOSE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NamedParametersContext extends ParserRuleContext {
public NamedParameters ast;
public ParameterNameContext name;
public ExpressionContext value;
public List COLON() { return getTokens(FEELParser.COLON); }
public TerminalNode COLON(int i) {
return getToken(FEELParser.COLON, i);
}
public List parameterName() {
return getRuleContexts(ParameterNameContext.class);
}
public ParameterNameContext parameterName(int i) {
return getRuleContext(ParameterNameContext.class,i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public NamedParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_namedParameters; }
}
public final NamedParametersContext namedParameters() throws RecognitionException {
NamedParametersContext _localctx = new NamedParametersContext(_ctx, getState());
enterRule(_localctx, 66, RULE_namedParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
Map parameters = new LinkedHashMap<>();
setState(530);
((NamedParametersContext)_localctx).name = parameterName();
setState(531);
match(COLON);
setState(532);
((NamedParametersContext)_localctx).value = expression();
parameters.put((((NamedParametersContext)_localctx).name!=null?_input.getText(((NamedParametersContext)_localctx).name.start,((NamedParametersContext)_localctx).name.stop):null), ((NamedParametersContext)_localctx).value.ast);
setState(542);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(534);
match(COMMA);
setState(535);
((NamedParametersContext)_localctx).name = parameterName();
setState(536);
match(COLON);
setState(537);
((NamedParametersContext)_localctx).value = expression();
parameters.put((((NamedParametersContext)_localctx).name!=null?_input.getText(((NamedParametersContext)_localctx).name.start,((NamedParametersContext)_localctx).name.stop):null), ((NamedParametersContext)_localctx).value.ast);
}
}
setState(544);
_errHandler.sync(this);
_la = _input.LA(1);
}
((NamedParametersContext)_localctx).ast = astFactory.toNamedParameters(parameters);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ParameterNameContext extends ParserRuleContext {
public String ast;
public IdentifierContext name;
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public ParameterNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parameterName; }
}
public final ParameterNameContext parameterName() throws RecognitionException {
ParameterNameContext _localctx = new ParameterNameContext(_ctx, getState());
enterRule(_localctx, 68, RULE_parameterName);
try {
enterOuterAlt(_localctx, 1);
{
setState(547);
((ParameterNameContext)_localctx).name = identifier();
((ParameterNameContext)_localctx).ast = (((ParameterNameContext)_localctx).name!=null?_input.getText(((ParameterNameContext)_localctx).name.start,((ParameterNameContext)_localctx).name.stop):null);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PositionalParametersContext extends ParserRuleContext {
public PositionalParameters ast;
public ExpressionContext param;
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public PositionalParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positionalParameters; }
}
public final PositionalParametersContext positionalParameters() throws RecognitionException {
PositionalParametersContext _localctx = new PositionalParametersContext(_ctx, getState());
enterRule(_localctx, 70, RULE_positionalParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List parameters = new ArrayList<>();
setState(562);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << STRING) | (1L << NUMBER) | (1L << TEMPORAL) | (1L << LT) | (1L << GT) | (1L << LE) | (1L << GE) | (1L << MINUS) | (1L << PAREN_OPEN) | (1L << BRACKET_OPEN) | (1L << BRACKET_CLOSE) | (1L << BRACE_OPEN) | (1L << NOT) | (1L << TRUE) | (1L << FALSE) | (1L << NULL) | (1L << FUNCTION) | (1L << FOR) | (1L << IF) | (1L << SOME) | (1L << EVERY) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
setState(551);
((PositionalParametersContext)_localctx).param = expression();
parameters.add(((PositionalParametersContext)_localctx).param.ast);
setState(559);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(553);
match(COMMA);
setState(554);
((PositionalParametersContext)_localctx).param = expression();
parameters.add(((PositionalParametersContext)_localctx).param.ast);
}
}
setState(561);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
((PositionalParametersContext)_localctx).ast = astFactory.toPositionalParameters(parameters);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PrimaryExpressionContext extends ParserRuleContext {
public Expression ast;
public LiteralContext literal;
public IdentifierContext name;
public ExpressionContext exp;
public BoxedExpressionContext boxedExpression;
public SimplePositiveUnaryTestContext simplePositiveUnaryTest;
public LiteralContext literal() {
return getRuleContext(LiteralContext.class,0);
}
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public BoxedExpressionContext boxedExpression() {
return getRuleContext(BoxedExpressionContext.class,0);
}
public SimplePositiveUnaryTestContext simplePositiveUnaryTest() {
return getRuleContext(SimplePositiveUnaryTestContext.class,0);
}
public PrimaryExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_primaryExpression; }
}
public final PrimaryExpressionContext primaryExpression() throws RecognitionException {
PrimaryExpressionContext _localctx = new PrimaryExpressionContext(_ctx, getState());
enterRule(_localctx, 72, RULE_primaryExpression);
try {
setState(583);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,40,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(566);
((PrimaryExpressionContext)_localctx).literal = literal();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).literal.ast;
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(569);
((PrimaryExpressionContext)_localctx).name = identifier();
((PrimaryExpressionContext)_localctx).ast = astFactory.toName((((PrimaryExpressionContext)_localctx).name!=null?_input.getText(((PrimaryExpressionContext)_localctx).name.start,((PrimaryExpressionContext)_localctx).name.stop):null));
}
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
{
setState(572);
match(PAREN_OPEN);
setState(573);
((PrimaryExpressionContext)_localctx).exp = expression();
setState(574);
match(PAREN_CLOSE);
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).exp.ast;
}
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
{
setState(577);
((PrimaryExpressionContext)_localctx).boxedExpression = boxedExpression();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).boxedExpression.ast;
}
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
{
setState(580);
((PrimaryExpressionContext)_localctx).simplePositiveUnaryTest = simplePositiveUnaryTest();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).simplePositiveUnaryTest.ast;
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleValueContext extends ParserRuleContext {
public Expression ast;
public SimpleLiteralContext simpleLiteral;
public QualifiedNameContext qualifiedName;
public SimpleLiteralContext simpleLiteral() {
return getRuleContext(SimpleLiteralContext.class,0);
}
public QualifiedNameContext qualifiedName() {
return getRuleContext(QualifiedNameContext.class,0);
}
public SimpleValueContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleValue; }
}
public final SimpleValueContext simpleValue() throws RecognitionException {
SimpleValueContext _localctx = new SimpleValueContext(_ctx, getState());
enterRule(_localctx, 74, RULE_simpleValue);
try {
setState(591);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,41,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
{
setState(585);
((SimpleValueContext)_localctx).simpleLiteral = simpleLiteral();
((SimpleValueContext)_localctx).ast = ((SimpleValueContext)_localctx).simpleLiteral.ast;
}
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
{
setState(588);
((SimpleValueContext)_localctx).qualifiedName = qualifiedName();
((SimpleValueContext)_localctx).ast = ((SimpleValueContext)_localctx).qualifiedName.ast;
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class QualifiedNameContext extends ParserRuleContext {
public Expression ast;
public IdentifierContext name;
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List DOT() { return getTokens(FEELParser.DOT); }
public TerminalNode DOT(int i) {
return getToken(FEELParser.DOT, i);
}
public QualifiedNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_qualifiedName; }
}
public final QualifiedNameContext qualifiedName() throws RecognitionException {
QualifiedNameContext _localctx = new QualifiedNameContext(_ctx, getState());
enterRule(_localctx, 76, RULE_qualifiedName);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
List names = new ArrayList<>();
setState(594);
((QualifiedNameContext)_localctx).name = identifier();
names.add((((QualifiedNameContext)_localctx).name!=null?_input.getText(((QualifiedNameContext)_localctx).name.start,((QualifiedNameContext)_localctx).name.stop):null));
setState(602);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,42,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(596);
match(DOT);
setState(597);
((QualifiedNameContext)_localctx).name = identifier();
names.add((((QualifiedNameContext)_localctx).name!=null?_input.getText(((QualifiedNameContext)_localctx).name.start,((QualifiedNameContext)_localctx).name.stop):null));
}
}
}
setState(604);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,42,_ctx);
}
((QualifiedNameContext)_localctx).ast = astFactory.toQualifiedName(names);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class LiteralContext extends ParserRuleContext {
public Expression ast;
public SimpleLiteralContext simpleLiteral;
public SimpleLiteralContext simpleLiteral() {
return getRuleContext(SimpleLiteralContext.class,0);
}
public TerminalNode NULL() { return getToken(FEELParser.NULL, 0); }
public LiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_literal; }
}
public final LiteralContext literal() throws RecognitionException {
LiteralContext _localctx = new LiteralContext(_ctx, getState());
enterRule(_localctx, 78, RULE_literal);
try {
enterOuterAlt(_localctx, 1);
{
setState(612);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING:
case NUMBER:
case TEMPORAL:
case TRUE:
case FALSE:
case FUNCTION:
case AND:
case OR:
case NAME:
{
{
setState(607);
((LiteralContext)_localctx).simpleLiteral = simpleLiteral();
((LiteralContext)_localctx).ast = ((LiteralContext)_localctx).simpleLiteral.ast;
}
}
break;
case NULL:
{
{
setState(610);
match(NULL);
((LiteralContext)_localctx).ast = astFactory.toNullLiteral();
}
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SimpleLiteralContext extends ParserRuleContext {
public Expression ast;
public NumericLiteralContext numericLiteral;
public StringLiteralContext stringLiteral;
public BooleanLiteralContext booleanLiteral;
public DateTimeLiteralContext dateTimeLiteral;
public NumericLiteralContext numericLiteral() {
return getRuleContext(NumericLiteralContext.class,0);
}
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public BooleanLiteralContext booleanLiteral() {
return getRuleContext(BooleanLiteralContext.class,0);
}
public DateTimeLiteralContext dateTimeLiteral() {
return getRuleContext(DateTimeLiteralContext.class,0);
}
public SimpleLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleLiteral; }
}
public final SimpleLiteralContext simpleLiteral() throws RecognitionException {
SimpleLiteralContext _localctx = new SimpleLiteralContext(_ctx, getState());
enterRule(_localctx, 80, RULE_simpleLiteral);
try {
setState(626);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NUMBER:
enterOuterAlt(_localctx, 1);
{
{
setState(614);
((SimpleLiteralContext)_localctx).numericLiteral = numericLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).numericLiteral.ast;
}
}
break;
case STRING:
enterOuterAlt(_localctx, 2);
{
{
setState(617);
((SimpleLiteralContext)_localctx).stringLiteral = stringLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).stringLiteral.ast;
}
}
break;
case TRUE:
case FALSE:
enterOuterAlt(_localctx, 3);
{
{
setState(620);
((SimpleLiteralContext)_localctx).booleanLiteral = booleanLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).booleanLiteral.ast;
}
}
break;
case TEMPORAL:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 4);
{
{
setState(623);
((SimpleLiteralContext)_localctx).dateTimeLiteral = dateTimeLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).dateTimeLiteral.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StringLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode STRING() { return getToken(FEELParser.STRING, 0); }
public StringLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringLiteral; }
}
public final StringLiteralContext stringLiteral() throws RecognitionException {
StringLiteralContext _localctx = new StringLiteralContext(_ctx, getState());
enterRule(_localctx, 82, RULE_stringLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(628);
((StringLiteralContext)_localctx).lit = match(STRING);
((StringLiteralContext)_localctx).ast = astFactory.toStringLiteral((((StringLiteralContext)_localctx).lit!=null?((StringLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BooleanLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode TRUE() { return getToken(FEELParser.TRUE, 0); }
public TerminalNode FALSE() { return getToken(FEELParser.FALSE, 0); }
public BooleanLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_booleanLiteral; }
}
public final BooleanLiteralContext booleanLiteral() throws RecognitionException {
BooleanLiteralContext _localctx = new BooleanLiteralContext(_ctx, getState());
enterRule(_localctx, 84, RULE_booleanLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(633);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TRUE:
{
setState(631);
((BooleanLiteralContext)_localctx).lit = match(TRUE);
}
break;
case FALSE:
{
setState(632);
((BooleanLiteralContext)_localctx).lit = match(FALSE);
}
break;
default:
throw new NoViableAltException(this);
}
((BooleanLiteralContext)_localctx).ast = astFactory.toBooleanLiteral((((BooleanLiteralContext)_localctx).lit!=null?((BooleanLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NumericLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode NUMBER() { return getToken(FEELParser.NUMBER, 0); }
public NumericLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_numericLiteral; }
}
public final NumericLiteralContext numericLiteral() throws RecognitionException {
NumericLiteralContext _localctx = new NumericLiteralContext(_ctx, getState());
enterRule(_localctx, 86, RULE_numericLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(637);
((NumericLiteralContext)_localctx).lit = match(NUMBER);
((NumericLiteralContext)_localctx).ast = astFactory.toNumericLiteral((((NumericLiteralContext)_localctx).lit!=null?((NumericLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BoxedExpressionContext extends ParserRuleContext {
public Expression ast;
public ListContext list;
public FunctionDefinitionContext functionDefinition;
public ContextContext context;
public ListContext list() {
return getRuleContext(ListContext.class,0);
}
public FunctionDefinitionContext functionDefinition() {
return getRuleContext(FunctionDefinitionContext.class,0);
}
public ContextContext context() {
return getRuleContext(ContextContext.class,0);
}
public BoxedExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_boxedExpression; }
}
public final BoxedExpressionContext boxedExpression() throws RecognitionException {
BoxedExpressionContext _localctx = new BoxedExpressionContext(_ctx, getState());
enterRule(_localctx, 88, RULE_boxedExpression);
try {
setState(649);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BRACKET_OPEN:
enterOuterAlt(_localctx, 1);
{
{
setState(640);
((BoxedExpressionContext)_localctx).list = list();
((BoxedExpressionContext)_localctx).ast = ((BoxedExpressionContext)_localctx).list.ast;
}
}
break;
case FUNCTION:
enterOuterAlt(_localctx, 2);
{
{
setState(643);
((BoxedExpressionContext)_localctx).functionDefinition = functionDefinition();
((BoxedExpressionContext)_localctx).ast = ((BoxedExpressionContext)_localctx).functionDefinition.ast;
}
}
break;
case BRACE_OPEN:
enterOuterAlt(_localctx, 3);
{
{
setState(646);
((BoxedExpressionContext)_localctx).context = context();
((BoxedExpressionContext)_localctx).ast = ((BoxedExpressionContext)_localctx).context.ast;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ListContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext exp;
public TerminalNode BRACKET_OPEN() { return getToken(FEELParser.BRACKET_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(FEELParser.BRACKET_CLOSE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public ListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_list; }
}
public final ListContext list() throws RecognitionException {
ListContext _localctx = new ListContext(_ctx, getState());
enterRule(_localctx, 90, RULE_list);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List expressions = new ArrayList<>();
setState(652);
match(BRACKET_OPEN);
setState(664);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,48,_ctx) ) {
case 1:
{
setState(653);
((ListContext)_localctx).exp = expression();
expressions.add(((ListContext)_localctx).exp.ast);
setState(661);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(655);
match(COMMA);
setState(656);
((ListContext)_localctx).exp = expression();
expressions.add(((ListContext)_localctx).exp.ast);
}
}
setState(663);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
}
setState(666);
match(BRACKET_CLOSE);
((ListContext)_localctx).ast = astFactory.toListLiteral(expressions);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ContextContext extends ParserRuleContext {
public Expression ast;
public ContextEntryContext entry;
public TerminalNode BRACE_OPEN() { return getToken(FEELParser.BRACE_OPEN, 0); }
public TerminalNode BRACE_CLOSE() { return getToken(FEELParser.BRACE_CLOSE, 0); }
public List contextEntry() {
return getRuleContexts(ContextEntryContext.class);
}
public ContextEntryContext contextEntry(int i) {
return getRuleContext(ContextEntryContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public ContextContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_context; }
}
public final ContextContext context() throws RecognitionException {
ContextContext _localctx = new ContextContext(_ctx, getState());
enterRule(_localctx, 92, RULE_context);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List entries = new ArrayList<>();
setState(670);
match(BRACE_OPEN);
setState(682);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << STRING) | (1L << FUNCTION) | (1L << AND) | (1L << OR) | (1L << NAME))) != 0)) {
{
setState(671);
((ContextContext)_localctx).entry = contextEntry();
entries.add(((ContextContext)_localctx).entry.ast);
setState(679);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(673);
match(COMMA);
setState(674);
((ContextContext)_localctx).entry = contextEntry();
entries.add(((ContextContext)_localctx).entry.ast);
}
}
setState(681);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(684);
match(BRACE_CLOSE);
((ContextContext)_localctx).ast = astFactory.toContext(entries);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ContextEntryContext extends ParserRuleContext {
public ContextEntry ast;
public KeyContext key;
public ExpressionContext expression;
public KeyContext key() {
return getRuleContext(KeyContext.class,0);
}
public TerminalNode COLON() { return getToken(FEELParser.COLON, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public ContextEntryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_contextEntry; }
}
public final ContextEntryContext contextEntry() throws RecognitionException {
ContextEntryContext _localctx = new ContextEntryContext(_ctx, getState());
enterRule(_localctx, 94, RULE_contextEntry);
try {
enterOuterAlt(_localctx, 1);
{
setState(687);
((ContextEntryContext)_localctx).key = key();
setState(688);
match(COLON);
setState(689);
((ContextEntryContext)_localctx).expression = expression();
((ContextEntryContext)_localctx).ast = astFactory.toContextEntry(((ContextEntryContext)_localctx).key.ast, ((ContextEntryContext)_localctx).expression.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class KeyContext extends ParserRuleContext {
public ContextEntryKey ast;
public IdentifierContext name;
public StringLiteralContext stringLiteral;
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public KeyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_key; }
}
public final KeyContext key() throws RecognitionException {
KeyContext _localctx = new KeyContext(_ctx, getState());
enterRule(_localctx, 96, RULE_key);
try {
setState(698);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 1);
{
{
setState(692);
((KeyContext)_localctx).name = identifier();
((KeyContext)_localctx).ast = astFactory.toContextEntryKey((((KeyContext)_localctx).name!=null?_input.getText(((KeyContext)_localctx).name.start,((KeyContext)_localctx).name.stop):null));
}
}
break;
case STRING:
enterOuterAlt(_localctx, 2);
{
{
setState(695);
((KeyContext)_localctx).stringLiteral = stringLiteral();
((KeyContext)_localctx).ast = astFactory.toContextEntryKey((((KeyContext)_localctx).stringLiteral!=null?_input.getText(((KeyContext)_localctx).stringLiteral.start,((KeyContext)_localctx).stringLiteral.stop):null));
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DateTimeLiteralContext extends ParserRuleContext {
public Expression ast;
public Token token;
public IdentifierContext kind;
public ExpressionContext expression;
public TerminalNode TEMPORAL() { return getToken(FEELParser.TEMPORAL, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public DateTimeLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dateTimeLiteral; }
}
public final DateTimeLiteralContext dateTimeLiteral() throws RecognitionException {
DateTimeLiteralContext _localctx = new DateTimeLiteralContext(_ctx, getState());
enterRule(_localctx, 98, RULE_dateTimeLiteral);
try {
setState(708);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TEMPORAL:
enterOuterAlt(_localctx, 1);
{
setState(700);
((DateTimeLiteralContext)_localctx).token = match(TEMPORAL);
{
((DateTimeLiteralContext)_localctx).ast = astFactory.toDateTimeLiteral((((DateTimeLiteralContext)_localctx).token!=null?((DateTimeLiteralContext)_localctx).token.getText():null));
}
}
break;
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 2);
{
{
{
setState(702);
((DateTimeLiteralContext)_localctx).kind = identifier();
}
setState(703);
match(PAREN_OPEN);
setState(704);
((DateTimeLiteralContext)_localctx).expression = expression();
setState(705);
match(PAREN_CLOSE);
((DateTimeLiteralContext)_localctx).ast = astFactory.toDateTimeLiteral((((DateTimeLiteralContext)_localctx).kind!=null?_input.getText(((DateTimeLiteralContext)_localctx).kind.start,((DateTimeLiteralContext)_localctx).kind.stop):null), ((DateTimeLiteralContext)_localctx).expression.ast);
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IdentifierContext extends ParserRuleContext {
public Token ast;
public Token token;
public TerminalNode NAME() { return getToken(FEELParser.NAME, 0); }
public TerminalNode OR() { return getToken(FEELParser.OR, 0); }
public TerminalNode AND() { return getToken(FEELParser.AND, 0); }
public TerminalNode FUNCTION() { return getToken(FEELParser.FUNCTION, 0); }
public IdentifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_identifier; }
}
public final IdentifierContext identifier() throws RecognitionException {
IdentifierContext _localctx = new IdentifierContext(_ctx, getState());
enterRule(_localctx, 100, RULE_identifier);
try {
setState(718);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NAME:
enterOuterAlt(_localctx, 1);
{
{
setState(710);
((IdentifierContext)_localctx).token = match(NAME);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
}
break;
case OR:
enterOuterAlt(_localctx, 2);
{
{
setState(712);
((IdentifierContext)_localctx).token = match(OR);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
}
break;
case AND:
enterOuterAlt(_localctx, 3);
{
{
setState(714);
((IdentifierContext)_localctx).token = match(AND);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
}
break;
case FUNCTION:
enterOuterAlt(_localctx, 4);
{
{
setState(716);
((IdentifierContext)_localctx).token = match(FUNCTION);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public boolean sempred(RuleContext _localctx, int ruleIndex, int predIndex) {
switch (ruleIndex) {
case 30:
return type_sempred((TypeContext)_localctx, predIndex);
}
return true;
}
private boolean type_sempred(TypeContext _localctx, int predIndex) {
switch (predIndex) {
case 0:
return "list".equals(((TypeContext)_localctx).typeName.ast.getText());
case 1:
return "context".equals(((TypeContext)_localctx).typeName.ast.getText());
case 2:
return "function".equals(((TypeContext)_localctx).typeName.ast.getText());
}
return true;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3\62\u02d3\4\2\t\2"+
"\4\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13"+
"\t\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\4\32\t\32\4\33\t\33\4\34\t\34\4\35\t\35\4\36\t\36\4\37\t\37\4 \t \4!"+
"\t!\4\"\t\"\4#\t#\4$\t$\4%\t%\4&\t&\4\'\t\'\4(\t(\4)\t)\4*\t*\4+\t+\4"+
",\t,\4-\t-\4.\t.\4/\t/\4\60\t\60\4\61\t\61\4\62\t\62\4\63\t\63\4\64\t"+
"\64\3\2\3\2\3\2\3\2\3\3\3\3\3\3\3\3\3\4\3\4\3\4\3\4\3\5\3\5\3\5\3\5\3"+
"\6\3\6\3\6\3\6\3\6\3\6\3\6\3\6\3\6\3\6\3\6\5\6\u0084\n\6\3\7\3\7\3\7\3"+
"\7\3\7\3\7\3\7\7\7\u008d\n\7\f\7\16\7\u0090\13\7\3\7\3\7\3\b\3\b\3\b\3"+
"\t\3\t\3\t\3\t\5\t\u009b\n\t\3\t\3\t\3\t\3\t\3\t\3\t\5\t\u00a3\n\t\3\n"+
"\3\n\3\n\3\n\3\n\3\n\3\n\3\13\3\13\3\13\3\13\3\13\3\13\5\13\u00b2\n\13"+
"\3\f\3\f\3\f\3\f\3\f\3\f\5\f\u00ba\n\f\3\r\5\r\u00bd\n\r\3\r\3\r\3\r\3"+
"\16\3\16\3\16\3\17\3\17\3\17\3\17\3\17\3\17\3\17\7\17\u00cc\n\17\f\17"+
"\16\17\u00cf\13\17\3\17\3\17\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3\20\3"+
"\20\3\20\3\20\3\20\5\20\u00df\n\20\3\21\3\21\3\21\3\21\3\21\3\21\3\21"+
"\3\21\3\21\3\21\3\21\7\21\u00ec\n\21\f\21\16\21\u00ef\13\21\5\21\u00f1"+
"\n\21\3\21\3\21\3\21\3\21\3\21\5\21\u00f8\n\21\3\21\3\21\5\21\u00fc\n"+
"\21\3\21\3\21\3\21\3\22\3\22\3\22\3\22\3\22\3\22\5\22\u0107\n\22\3\22"+
"\3\22\3\23\3\23\3\23\3\23\3\23\3\23\3\23\3\23\3\23\3\23\3\23\3\23\7\23"+
"\u0117\n\23\f\23\16\23\u011a\13\23\3\23\3\23\3\23\3\23\3\24\3\24\3\24"+
"\3\24\3\24\3\24\5\24\u0126\n\24\3\24\3\24\3\25\3\25\3\25\3\25\3\25\3\25"+
"\3\25\3\25\3\26\3\26\3\26\5\26\u0135\n\26\3\26\3\26\3\26\3\26\3\26\3\26"+
"\3\26\3\26\3\26\7\26\u0140\n\26\f\26\16\26\u0143\13\26\3\26\3\26\3\26"+
"\3\26\3\27\3\27\3\27\3\27\3\27\3\27\7\27\u014f\n\27\f\27\16\27\u0152\13"+
"\27\3\30\3\30\3\30\3\30\3\30\3\30\7\30\u015a\n\30\f\30\16\30\u015d\13"+
"\30\3\31\3\31\3\31\3\31\3\31\3\31\3\31\3\31\5\31\u0167\n\31\3\31\3\31"+
"\3\31\3\31\3\31\3\31\3\31\3\31\3\31\3\31\3\31\3\31\3\31\3\31\3\31\3\31"+
"\3\31\3\31\3\31\5\31\u017c\n\31\3\32\3\32\3\32\3\33\3\33\3\33\3\33\5\33"+
"\u0185\n\33\3\33\3\33\3\33\7\33\u018a\n\33\f\33\16\33\u018d\13\33\3\34"+
"\3\34\3\34\3\34\5\34\u0193\n\34\3\34\3\34\3\34\7\34\u0198\n\34\f\34\16"+
"\34\u019b\13\34\3\35\3\35\3\35\3\35\3\35\3\35\7\35\u01a3\n\35\f\35\16"+
"\35\u01a6\13\35\3\36\3\36\3\36\3\36\3\36\7\36\u01ad\n\36\f\36\16\36\u01b0"+
"\13\36\3\36\3\36\3\36\3\37\3\37\3\37\3\37\3\37\3\37\5\37\u01bb\n\37\3"+
" \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3"+
" \7 \u01d5\n \f \16 \u01d8\13 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \3 \7 \u01e5"+
"\n \f \16 \u01e8\13 \3 \3 \5 \u01ec\n \3 \3 \3 \3 \3 \5 \u01f3\n \3!\3"+
"!\3!\3!\3!\3!\3!\3!\3!\3!\3!\3!\3!\3!\3!\7!\u0204\n!\f!\16!\u0207\13!"+
"\3\"\3\"\3\"\3\"\3\"\3\"\3\"\5\"\u0210\n\"\3\"\3\"\3#\3#\3#\3#\3#\3#\3"+
"#\3#\3#\3#\3#\7#\u021f\n#\f#\16#\u0222\13#\3#\3#\3$\3$\3$\3%\3%\3%\3%"+
"\3%\3%\3%\7%\u0230\n%\f%\16%\u0233\13%\5%\u0235\n%\3%\3%\3&\3&\3&\3&\3"+
"&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\3&\5&\u024a\n&\3\'\3\'\3\'\3\'\3\'"+
"\3\'\5\'\u0252\n\'\3(\3(\3(\3(\3(\3(\3(\7(\u025b\n(\f(\16(\u025e\13(\3"+
"(\3(\3)\3)\3)\3)\3)\5)\u0267\n)\3*\3*\3*\3*\3*\3*\3*\3*\3*\3*\3*\3*\5"+
"*\u0275\n*\3+\3+\3+\3,\3,\5,\u027c\n,\3,\3,\3-\3-\3-\3.\3.\3.\3.\3.\3"+
".\3.\3.\3.\5.\u028c\n.\3/\3/\3/\3/\3/\3/\3/\3/\7/\u0296\n/\f/\16/\u0299"+
"\13/\5/\u029b\n/\3/\3/\3/\3\60\3\60\3\60\3\60\3\60\3\60\3\60\3\60\7\60"+
"\u02a8\n\60\f\60\16\60\u02ab\13\60\5\60\u02ad\n\60\3\60\3\60\3\60\3\61"+
"\3\61\3\61\3\61\3\61\3\62\3\62\3\62\3\62\3\62\3\62\5\62\u02bd\n\62\3\63"+
"\3\63\3\63\3\63\3\63\3\63\3\63\3\63\5\63\u02c7\n\63\3\64\3\64\3\64\3\64"+
"\3\64\3\64\3\64\3\64\5\64\u02d1\n\64\3\64\2\2\65\2\4\6\b\n\f\16\20\22"+
"\24\26\30\32\34\36 \"$&(*,.\60\62\64\668:<>@BDFHJLNPRTVXZ\\^`bdf\2\2\2"+
"\u02ef\2h\3\2\2\2\4l\3\2\2\2\6p\3\2\2\2\bt\3\2\2\2\n\u0083\3\2\2\2\f\u0085"+
"\3\2\2\2\16\u0093\3\2\2\2\20\u00a2\3\2\2\2\22\u00a4\3\2\2\2\24\u00b1\3"+
"\2\2\2\26\u00b9\3\2\2\2\30\u00bc\3\2\2\2\32\u00c1\3\2\2\2\34\u00c4\3\2"+
"\2\2\36\u00de\3\2\2\2 \u00e0\3\2\2\2\"\u0100\3\2\2\2$\u010a\3\2\2\2&\u011f"+
"\3\2\2\2(\u0129\3\2\2\2*\u0131\3\2\2\2,\u0148\3\2\2\2.\u0153\3\2\2\2\60"+
"\u015e\3\2\2\2\62\u017d\3\2\2\2\64\u0180\3\2\2\2\66\u018e\3\2\2\28\u019c"+
"\3\2\2\2:\u01a7\3\2\2\2<\u01b4\3\2\2\2>\u01f2\3\2\2\2@\u01f4\3\2\2\2B"+
"\u0208\3\2\2\2D\u0213\3\2\2\2F\u0225\3\2\2\2H\u0228\3\2\2\2J\u0249\3\2"+
"\2\2L\u0251\3\2\2\2N\u0253\3\2\2\2P\u0266\3\2\2\2R\u0274\3\2\2\2T\u0276"+
"\3\2\2\2V\u027b\3\2\2\2X\u027f\3\2\2\2Z\u028b\3\2\2\2\\\u028d\3\2\2\2"+
"^\u029f\3\2\2\2`\u02b1\3\2\2\2b\u02bc\3\2\2\2d\u02c6\3\2\2\2f\u02d0\3"+
"\2\2\2hi\5\n\6\2ij\b\2\1\2jk\7\2\2\3k\3\3\2\2\2lm\5\32\16\2mn\b\3\1\2"+
"no\7\2\2\3o\5\3\2\2\2pq\5\34\17\2qr\b\4\1\2rs\7\2\2\3s\7\3\2\2\2tu\5Z"+
".\2uv\b\5\1\2vw\7\2\2\3w\t\3\2\2\2xy\7\37\2\2yz\7\27\2\2z{\5\f\7\2{|\7"+
"\30\2\2|}\b\6\1\2}\u0084\3\2\2\2~\177\5\f\7\2\177\u0080\b\6\1\2\u0080"+
"\u0084\3\2\2\2\u0081\u0082\7\20\2\2\u0082\u0084\b\6\1\2\u0083x\3\2\2\2"+
"\u0083~\3\2\2\2\u0083\u0081\3\2\2\2\u0084\13\3\2\2\2\u0085\u0086\b\7\1"+
"\2\u0086\u0087\5\16\b\2\u0087\u008e\b\7\1\2\u0088\u0089\7\26\2\2\u0089"+
"\u008a\5\16\b\2\u008a\u008b\b\7\1\2\u008b\u008d\3\2\2\2\u008c\u0088\3"+
"\2\2\2\u008d\u0090\3\2\2\2\u008e\u008c\3\2\2\2\u008e\u008f\3\2\2\2\u008f"+
"\u0091\3\2\2\2\u0090\u008e\3\2\2\2\u0091\u0092\b\7\1\2\u0092\r\3\2\2\2"+
"\u0093\u0094\5\32\16\2\u0094\u0095\b\b\1\2\u0095\17\3\2\2\2\u0096\u009b"+
"\7\13\2\2\u0097\u009b\7\r\2\2\u0098\u009b\7\f\2\2\u0099\u009b\7\16\2\2"+
"\u009a\u0096\3\2\2\2\u009a\u0097\3\2\2\2\u009a\u0098\3\2\2\2\u009a\u0099"+
"\3\2\2\2\u009a\u009b\3\2\2\2\u009b\u009c\3\2\2\2\u009c\u009d\5\30\r\2"+
"\u009d\u009e\b\t\1\2\u009e\u00a3\3\2\2\2\u009f\u00a0\5\22\n\2\u00a0\u00a1"+
"\b\t\1\2\u00a1\u00a3\3\2\2\2\u00a2\u009a\3\2\2\2\u00a2\u009f\3\2\2\2\u00a3"+
"\21\3\2\2\2\u00a4\u00a5\5\24\13\2\u00a5\u00a6\5\30\r\2\u00a6\u00a7\7\24"+
"\2\2\u00a7\u00a8\5\30\r\2\u00a8\u00a9\5\26\f\2\u00a9\u00aa\b\n\1\2\u00aa"+
"\23\3\2\2\2\u00ab\u00ac\7\27\2\2\u00ac\u00b2\b\13\1\2\u00ad\u00ae\7\32"+
"\2\2\u00ae\u00b2\b\13\1\2\u00af\u00b0\7\31\2\2\u00b0\u00b2\b\13\1\2\u00b1"+
"\u00ab\3\2\2\2\u00b1\u00ad\3\2\2\2\u00b1\u00af\3\2\2\2\u00b2\25\3\2\2"+
"\2\u00b3\u00b4\7\30\2\2\u00b4\u00ba\b\f\1\2\u00b5\u00b6\7\31\2\2\u00b6"+
"\u00ba\b\f\1\2\u00b7\u00b8\7\32\2\2\u00b8\u00ba\b\f\1\2\u00b9\u00b3\3"+
"\2\2\2\u00b9\u00b5\3\2\2\2\u00b9\u00b7\3\2\2\2\u00ba\27\3\2\2\2\u00bb"+
"\u00bd\7\20\2\2\u00bc\u00bb\3\2\2\2\u00bc\u00bd\3\2\2\2\u00bd\u00be\3"+
"\2\2\2\u00be\u00bf\5L\'\2\u00bf\u00c0\b\r\1\2\u00c0\31\3\2\2\2\u00c1\u00c2"+
"\5\36\20\2\u00c2\u00c3\b\16\1\2\u00c3\33\3\2\2\2\u00c4\u00c5\b\17\1\2"+
"\u00c5\u00c6\5\36\20\2\u00c6\u00cd\b\17\1\2\u00c7\u00c8\7\26\2\2\u00c8"+
"\u00c9\5\36\20\2\u00c9\u00ca\b\17\1\2\u00ca\u00cc\3\2\2\2\u00cb\u00c7"+
"\3\2\2\2\u00cc\u00cf\3\2\2\2\u00cd\u00cb\3\2\2\2\u00cd\u00ce\3\2\2\2\u00ce"+
"\u00d0\3\2\2\2\u00cf\u00cd\3\2\2\2\u00d0\u00d1\b\17\1\2\u00d1\35\3\2\2"+
"\2\u00d2\u00d3\5$\23\2\u00d3\u00d4\b\20\1\2\u00d4\u00df\3\2\2\2\u00d5"+
"\u00d6\5(\25\2\u00d6\u00d7\b\20\1\2\u00d7\u00df\3\2\2\2\u00d8\u00d9\5"+
"*\26\2\u00d9\u00da\b\20\1\2\u00da\u00df\3\2\2\2\u00db\u00dc\5,\27\2\u00dc"+
"\u00dd\b\20\1\2\u00dd\u00df\3\2\2\2\u00de\u00d2\3\2\2\2\u00de\u00d5\3"+
"\2\2\2\u00de\u00d8\3\2\2\2\u00de\u00db\3\2\2\2\u00df\37\3\2\2\2\u00e0"+
"\u00e1\b\21\1\2\u00e1\u00e2\b\21\1\2\u00e2\u00e3\b\21\1\2\u00e3\u00e4"+
"\7#\2\2\u00e4\u00f0\7\27\2\2\u00e5\u00e6\5\"\22\2\u00e6\u00ed\b\21\1\2"+
"\u00e7\u00e8\7\26\2\2\u00e8\u00e9\5\"\22\2\u00e9\u00ea\b\21\1\2\u00ea"+
"\u00ec\3\2\2\2\u00eb\u00e7\3\2\2\2\u00ec\u00ef\3\2\2\2\u00ed\u00eb\3\2"+
"\2\2\u00ed\u00ee\3\2\2\2\u00ee\u00f1\3\2\2\2\u00ef\u00ed\3\2\2\2\u00f0"+
"\u00e5\3\2\2\2\u00f0\u00f1\3\2\2\2\u00f1\u00f2\3\2\2\2\u00f2\u00f7\7\30"+
"\2\2\u00f3\u00f4\7\35\2\2\u00f4\u00f5\5> \2\u00f5\u00f6\b\21\1\2\u00f6"+
"\u00f8\3\2\2\2\u00f7\u00f3\3\2\2\2\u00f7\u00f8\3\2\2\2\u00f8\u00fb\3\2"+
"\2\2\u00f9\u00fa\7$\2\2\u00fa\u00fc\b\21\1\2\u00fb\u00f9\3\2\2\2\u00fb"+
"\u00fc\3\2\2\2\u00fc\u00fd\3\2\2\2\u00fd\u00fe\5\32\16\2\u00fe\u00ff\b"+
"\21\1\2\u00ff!\3\2\2\2\u0100\u0101\b\22\1\2\u0101\u0106\5F$\2\u0102\u0103"+
"\7\35\2\2\u0103\u0104\5> \2\u0104\u0105\b\22\1\2\u0105\u0107\3\2\2\2\u0106"+
"\u0102\3\2\2\2\u0106\u0107\3\2\2\2\u0107\u0108\3\2\2\2\u0108\u0109\b\22"+
"\1\2\u0109#\3\2\2\2\u010a\u010b\b\23\1\2\u010b\u010c\7%\2\2\u010c\u010d"+
"\5f\64\2\u010d\u010e\7&\2\2\u010e\u010f\5&\24\2\u010f\u0118\b\23\1\2\u0110"+
"\u0111\7\26\2\2\u0111\u0112\5f\64\2\u0112\u0113\7&\2\2\u0113\u0114\5&"+
"\24\2\u0114\u0115\b\23\1\2\u0115\u0117\3\2\2\2\u0116\u0110\3\2\2\2\u0117"+
"\u011a\3\2\2\2\u0118\u0116\3\2\2\2\u0118\u0119\3\2\2\2\u0119\u011b\3\2"+
"\2\2\u011a\u0118\3\2\2\2\u011b\u011c\7\'\2\2\u011c\u011d\5\32\16\2\u011d"+
"\u011e\b\23\1\2\u011e%\3\2\2\2\u011f\u0120\b\24\1\2\u0120\u0125\5\32\16"+
"\2\u0121\u0122\7\24\2\2\u0122\u0123\5\32\16\2\u0123\u0124\b\24\1\2\u0124"+
"\u0126\3\2\2\2\u0125\u0121\3\2\2\2\u0125\u0126\3\2\2\2\u0126\u0127\3\2"+
"\2\2\u0127\u0128\b\24\1\2\u0128\'\3\2\2\2\u0129\u012a\7(\2\2\u012a\u012b"+
"\5\32\16\2\u012b\u012c\7)\2\2\u012c\u012d\5\32\16\2\u012d\u012e\7*\2\2"+
"\u012e\u012f\5\32\16\2\u012f\u0130\b\25\1\2\u0130)\3\2\2\2\u0131\u0134"+
"\b\26\1\2\u0132\u0135\7+\2\2\u0133\u0135\7,\2\2\u0134\u0132\3\2\2\2\u0134"+
"\u0133\3\2\2\2\u0135\u0136\3\2\2\2\u0136\u0137\5f\64\2\u0137\u0138\7&"+
"\2\2\u0138\u0139\5\32\16\2\u0139\u0141\b\26\1\2\u013a\u013b\5f\64\2\u013b"+
"\u013c\7&\2\2\u013c\u013d\5\32\16\2\u013d\u013e\b\26\1\2\u013e\u0140\3"+
"\2\2\2\u013f\u013a\3\2\2\2\u0140\u0143\3\2\2\2\u0141\u013f\3\2\2\2\u0141"+
"\u0142\3\2\2\2\u0142\u0144\3\2\2\2\u0143\u0141\3\2\2\2\u0144\u0145\7-"+
"\2\2\u0145\u0146\5\32\16\2\u0146\u0147\b\26\1\2\u0147+\3\2\2\2\u0148\u0149"+
"\5.\30\2\u0149\u0150\b\27\1\2\u014a\u014b\7/\2\2\u014b\u014c\5.\30\2\u014c"+
"\u014d\b\27\1\2\u014d\u014f\3\2\2\2\u014e\u014a\3\2\2\2\u014f\u0152\3"+
"\2\2\2\u0150\u014e\3\2\2\2\u0150\u0151\3\2\2\2\u0151-\3\2\2\2\u0152\u0150"+
"\3\2\2\2\u0153\u0154\5\60\31\2\u0154\u015b\b\30\1\2\u0155\u0156\7.\2\2"+
"\u0156\u0157\5\60\31\2\u0157\u0158\b\30\1\2\u0158\u015a\3\2\2\2\u0159"+
"\u0155\3\2\2\2\u015a\u015d\3\2\2\2\u015b\u0159\3\2\2\2\u015b\u015c\3\2"+
"\2\2\u015c/\3\2\2\2\u015d\u015b\3\2\2\2\u015e\u015f\5\62\32\2\u015f\u017b"+
"\b\31\1\2\u0160\u0167\7\t\2\2\u0161\u0167\7\n\2\2\u0162\u0167\7\13\2\2"+
"\u0163\u0167\7\f\2\2\u0164\u0167\7\r\2\2\u0165\u0167\7\16\2\2\u0166\u0160"+
"\3\2\2\2\u0166\u0161\3\2\2\2\u0166\u0162\3\2\2\2\u0166\u0163\3\2\2\2\u0166"+
"\u0164\3\2\2\2\u0166\u0165\3\2\2\2\u0167\u0168\3\2\2\2\u0168\u0169\5\62"+
"\32\2\u0169\u016a\b\31\1\2\u016a\u017c\3\2\2\2\u016b\u016c\7\60\2\2\u016c"+
"\u016d\5\32\16\2\u016d\u016e\7.\2\2\u016e\u016f\5\32\16\2\u016f\u0170"+
"\b\31\1\2\u0170\u017c\3\2\2\2\u0171\u0172\7&\2\2\u0172\u0173\5\16\b\2"+
"\u0173\u0174\b\31\1\2\u0174\u017c\3\2\2\2\u0175\u0176\7&\2\2\u0176\u0177"+
"\7\27\2\2\u0177\u0178\5\f\7\2\u0178\u0179\7\30\2\2\u0179\u017a\b\31\1"+
"\2\u017a\u017c\3\2\2\2\u017b\u0166\3\2\2\2\u017b\u016b\3\2\2\2\u017b\u0171"+
"\3\2\2\2\u017b\u0175\3\2\2\2\u017b\u017c\3\2\2\2\u017c\61\3\2\2\2\u017d"+
"\u017e\5\64\33\2\u017e\u017f\b\32\1\2\u017f\63\3\2\2\2\u0180\u0181\5\66"+
"\34\2\u0181\u018b\b\33\1\2\u0182\u0185\7\17\2\2\u0183\u0185\7\20\2\2\u0184"+
"\u0182\3\2\2\2\u0184\u0183\3\2\2\2\u0185\u0186\3\2\2\2\u0186\u0187\5\66"+
"\34\2\u0187\u0188\b\33\1\2\u0188\u018a\3\2\2\2\u0189\u0184\3\2\2\2\u018a"+
"\u018d\3\2\2\2\u018b\u0189\3\2\2\2\u018b\u018c\3\2\2\2\u018c\65\3\2\2"+
"\2\u018d\u018b\3\2\2\2\u018e\u018f\58\35\2\u018f\u0199\b\34\1\2\u0190"+
"\u0193\7\21\2\2\u0191\u0193\7\22\2\2\u0192\u0190\3\2\2\2\u0192\u0191\3"+
"\2\2\2\u0193\u0194\3\2\2\2\u0194\u0195\58\35\2\u0195\u0196\b\34\1\2\u0196"+
"\u0198\3\2\2\2\u0197\u0192\3\2\2\2\u0198\u019b\3\2\2\2\u0199\u0197\3\2"+
"\2\2\u0199\u019a\3\2\2\2\u019a\67\3\2\2\2\u019b\u0199\3\2\2\2\u019c\u019d"+
"\5:\36\2\u019d\u01a4\b\35\1\2\u019e\u019f\7\23\2\2\u019f\u01a0\5:\36\2"+
"\u01a0\u01a1\b\35\1\2\u01a1\u01a3\3\2\2\2\u01a2\u019e\3\2\2\2\u01a3\u01a6"+
"\3\2\2\2\u01a4\u01a2\3\2\2\2\u01a4\u01a5\3\2\2\2\u01a59\3\2\2\2\u01a6"+
"\u01a4\3\2\2\2\u01a7\u01ae\b\36\1\2\u01a8\u01a9\7\20\2\2\u01a9\u01ad\b"+
"\36\1\2\u01aa\u01ab\7\37\2\2\u01ab\u01ad\b\36\1\2\u01ac\u01a8\3\2\2\2"+
"\u01ac\u01aa\3\2\2\2\u01ad\u01b0\3\2\2\2\u01ae\u01ac\3\2\2\2\u01ae\u01af"+
"\3\2\2\2\u01af\u01b1\3\2\2\2\u01b0\u01ae\3\2\2\2\u01b1\u01b2\5<\37\2\u01b2"+
"\u01b3\b\36\1\2\u01b3;\3\2\2\2\u01b4\u01b5\5@!\2\u01b5\u01ba\b\37\1\2"+
"\u01b6\u01b7\7\61\2\2\u01b7\u01b8\5> \2\u01b8\u01b9\b\37\1\2\u01b9\u01bb"+
"\3\2\2\2\u01ba\u01b6\3\2\2\2\u01ba\u01bb\3\2\2\2\u01bb=\3\2\2\2\u01bc"+
"\u01bd\5N(\2\u01bd\u01be\b \1\2\u01be\u01f3\3\2\2\2\u01bf\u01c0\5f\64"+
"\2\u01c0\u01c1\6 \2\3\u01c1\u01c2\7\13\2\2\u01c2\u01c3\5> \2\u01c3\u01c4"+
"\7\f\2\2\u01c4\u01c5\b \1\2\u01c5\u01f3\3\2\2\2\u01c6\u01c7\b \1\2\u01c7"+
"\u01c8\5f\64\2\u01c8\u01c9\6 \3\3\u01c9\u01ca\7\13\2\2\u01ca\u01cb\5f"+
"\64\2\u01cb\u01cc\7\35\2\2\u01cc\u01cd\5> \2\u01cd\u01d6\b \1\2\u01ce"+
"\u01cf\7\26\2\2\u01cf\u01d0\5f\64\2\u01d0\u01d1\7\35\2\2\u01d1\u01d2\5"+
"> \2\u01d2\u01d3\b \1\2\u01d3\u01d5\3\2\2\2\u01d4\u01ce\3\2\2\2\u01d5"+
"\u01d8\3\2\2\2\u01d6\u01d4\3\2\2\2\u01d6\u01d7\3\2\2\2\u01d7\u01d9\3\2"+
"\2\2\u01d8\u01d6\3\2\2\2\u01d9\u01da\7\f\2\2\u01da\u01db\b \1\2\u01db"+
"\u01f3\3\2\2\2\u01dc\u01dd\b \1\2\u01dd\u01de\5f\64\2\u01de\u01df\6 \4"+
"\3\u01df\u01eb\7\13\2\2\u01e0\u01e1\5> \2\u01e1\u01e6\b \1\2\u01e2\u01e3"+
"\7\26\2\2\u01e3\u01e5\5> \2\u01e4\u01e2\3\2\2\2\u01e5\u01e8\3\2\2\2\u01e6"+
"\u01e4\3\2\2\2\u01e6\u01e7\3\2\2\2\u01e7\u01e9\3\2\2\2\u01e8\u01e6\3\2"+
"\2\2\u01e9\u01ea\b \1\2\u01ea\u01ec\3\2\2\2\u01eb\u01e0\3\2\2\2\u01eb"+
"\u01ec\3\2\2\2\u01ec\u01ed\3\2\2\2\u01ed\u01ee\7\f\2\2\u01ee\u01ef\7\36"+
"\2\2\u01ef\u01f0\5> \2\u01f0\u01f1\b \1\2\u01f1\u01f3\3\2\2\2\u01f2\u01bc"+
"\3\2\2\2\u01f2\u01bf\3\2\2\2\u01f2\u01c6\3\2\2\2\u01f2\u01dc\3\2\2\2\u01f3"+
"?\3\2\2\2\u01f4\u01f5\5J&\2\u01f5\u01f6\b!\1\2\u01f6\u0205\3\2\2\2\u01f7"+
"\u01f8\7\31\2\2\u01f8\u01f9\5\32\16\2\u01f9\u01fa\7\32\2\2\u01fa\u01fb"+
"\b!\1\2\u01fb\u0204\3\2\2\2\u01fc\u01fd\5B\"\2\u01fd\u01fe\b!\1\2\u01fe"+
"\u0204\3\2\2\2\u01ff\u0200\7\25\2\2\u0200\u0201\5f\64\2\u0201\u0202\b"+
"!\1\2\u0202\u0204\3\2\2\2\u0203\u01f7\3\2\2\2\u0203\u01fc\3\2\2\2\u0203"+
"\u01ff\3\2\2\2\u0204\u0207\3\2\2\2\u0205\u0203\3\2\2\2\u0205\u0206\3\2"+
"\2\2\u0206A\3\2\2\2\u0207\u0205\3\2\2\2\u0208\u020f\7\27\2\2\u0209\u020a"+
"\5D#\2\u020a\u020b\b\"\1\2\u020b\u0210\3\2\2\2\u020c\u020d\5H%\2\u020d"+
"\u020e\b\"\1\2\u020e\u0210\3\2\2\2\u020f\u0209\3\2\2\2\u020f\u020c\3\2"+
"\2\2\u0210\u0211\3\2\2\2\u0211\u0212\7\30\2\2\u0212C\3\2\2\2\u0213\u0214"+
"\b#\1\2\u0214\u0215\5F$\2\u0215\u0216\7\35\2\2\u0216\u0217\5\32\16\2\u0217"+
"\u0220\b#\1\2\u0218\u0219\7\26\2\2\u0219\u021a\5F$\2\u021a\u021b\7\35"+
"\2\2\u021b\u021c\5\32\16\2\u021c\u021d\b#\1\2\u021d\u021f\3\2\2\2\u021e"+
"\u0218\3\2\2\2\u021f\u0222\3\2\2\2\u0220\u021e\3\2\2\2\u0220\u0221\3\2"+
"\2\2\u0221\u0223\3\2\2\2\u0222\u0220\3\2\2\2\u0223\u0224\b#\1\2\u0224"+
"E\3\2\2\2\u0225\u0226\5f\64\2\u0226\u0227\b$\1\2\u0227G\3\2\2\2\u0228"+
"\u0234\b%\1\2\u0229\u022a\5\32\16\2\u022a\u0231\b%\1\2\u022b\u022c\7\26"+
"\2\2\u022c\u022d\5\32\16\2\u022d\u022e\b%\1\2\u022e\u0230\3\2\2\2\u022f"+
"\u022b\3\2\2\2\u0230\u0233\3\2\2\2\u0231\u022f\3\2\2\2\u0231\u0232\3\2"+
"\2\2\u0232\u0235\3\2\2\2\u0233\u0231\3\2\2\2\u0234\u0229\3\2\2\2\u0234"+
"\u0235\3\2\2\2\u0235\u0236\3\2\2\2\u0236\u0237\b%\1\2\u0237I\3\2\2\2\u0238"+
"\u0239\5P)\2\u0239\u023a\b&\1\2\u023a\u024a\3\2\2\2\u023b\u023c\5f\64"+
"\2\u023c\u023d\b&\1\2\u023d\u024a\3\2\2\2\u023e\u023f\7\27\2\2\u023f\u0240"+
"\5\32\16\2\u0240\u0241\7\30\2\2\u0241\u0242\b&\1\2\u0242\u024a\3\2\2\2"+
"\u0243\u0244\5Z.\2\u0244\u0245\b&\1\2\u0245\u024a\3\2\2\2\u0246\u0247"+
"\5\20\t\2\u0247\u0248\b&\1\2\u0248\u024a\3\2\2\2\u0249\u0238\3\2\2\2\u0249"+
"\u023b\3\2\2\2\u0249\u023e\3\2\2\2\u0249\u0243\3\2\2\2\u0249\u0246\3\2"+
"\2\2\u024aK\3\2\2\2\u024b\u024c\5R*\2\u024c\u024d\b\'\1\2\u024d\u0252"+
"\3\2\2\2\u024e\u024f\5N(\2\u024f\u0250\b\'\1\2\u0250\u0252\3\2\2\2\u0251"+
"\u024b\3\2\2\2\u0251\u024e\3\2\2\2\u0252M\3\2\2\2\u0253\u0254\b(\1\2\u0254"+
"\u0255\5f\64\2\u0255\u025c\b(\1\2\u0256\u0257\7\25\2\2\u0257\u0258\5f"+
"\64\2\u0258\u0259\b(\1\2\u0259\u025b\3\2\2\2\u025a\u0256\3\2\2\2\u025b"+
"\u025e\3\2\2\2\u025c\u025a\3\2\2\2\u025c\u025d\3\2\2\2\u025d\u025f\3\2"+
"\2\2\u025e\u025c\3\2\2\2\u025f\u0260\b(\1\2\u0260O\3\2\2\2\u0261\u0262"+
"\5R*\2\u0262\u0263\b)\1\2\u0263\u0267\3\2\2\2\u0264\u0265\7\"\2\2\u0265"+
"\u0267\b)\1\2\u0266\u0261\3\2\2\2\u0266\u0264\3\2\2\2\u0267Q\3\2\2\2\u0268"+
"\u0269\5X-\2\u0269\u026a\b*\1\2\u026a\u0275\3\2\2\2\u026b\u026c\5T+\2"+
"\u026c\u026d\b*\1\2\u026d\u0275\3\2\2\2\u026e\u026f\5V,\2\u026f\u0270"+
"\b*\1\2\u0270\u0275\3\2\2\2\u0271\u0272\5d\63\2\u0272\u0273\b*\1\2\u0273"+
"\u0275\3\2\2\2\u0274\u0268\3\2\2\2\u0274\u026b\3\2\2\2\u0274\u026e\3\2"+
"\2\2\u0274\u0271\3\2\2\2\u0275S\3\2\2\2\u0276\u0277\7\6\2\2\u0277\u0278"+
"\b+\1\2\u0278U\3\2\2\2\u0279\u027c\7 \2\2\u027a\u027c\7!\2\2\u027b\u0279"+
"\3\2\2\2\u027b\u027a\3\2\2\2\u027c\u027d\3\2\2\2\u027d\u027e\b,\1\2\u027e"+
"W\3\2\2\2\u027f\u0280\7\7\2\2\u0280\u0281\b-\1\2\u0281Y\3\2\2\2\u0282"+
"\u0283\5\\/\2\u0283\u0284\b.\1\2\u0284\u028c\3\2\2\2\u0285\u0286\5 \21"+
"\2\u0286\u0287\b.\1\2\u0287\u028c\3\2\2\2\u0288\u0289\5^\60\2\u0289\u028a"+
"\b.\1\2\u028a\u028c\3\2\2\2\u028b\u0282\3\2\2\2\u028b\u0285\3\2\2\2\u028b"+
"\u0288\3\2\2\2\u028c[\3\2\2\2\u028d\u028e\b/\1\2\u028e\u029a\7\31\2\2"+
"\u028f\u0290\5\32\16\2\u0290\u0297\b/\1\2\u0291\u0292\7\26\2\2\u0292\u0293"+
"\5\32\16\2\u0293\u0294\b/\1\2\u0294\u0296\3\2\2\2\u0295\u0291\3\2\2\2"+
"\u0296\u0299\3\2\2\2\u0297\u0295\3\2\2\2\u0297\u0298\3\2\2\2\u0298\u029b"+
"\3\2\2\2\u0299\u0297\3\2\2\2\u029a\u028f\3\2\2\2\u029a\u029b\3\2\2\2\u029b"+
"\u029c\3\2\2\2\u029c\u029d\7\32\2\2\u029d\u029e\b/\1\2\u029e]\3\2\2\2"+
"\u029f\u02a0\b\60\1\2\u02a0\u02ac\7\33\2\2\u02a1\u02a2\5`\61\2\u02a2\u02a9"+
"\b\60\1\2\u02a3\u02a4\7\26\2\2\u02a4\u02a5\5`\61\2\u02a5\u02a6\b\60\1"+
"\2\u02a6\u02a8\3\2\2\2\u02a7\u02a3\3\2\2\2\u02a8\u02ab\3\2\2\2\u02a9\u02a7"+
"\3\2\2\2\u02a9\u02aa\3\2\2\2\u02aa\u02ad\3\2\2\2\u02ab\u02a9\3\2\2\2\u02ac"+
"\u02a1\3\2\2\2\u02ac\u02ad\3\2\2\2\u02ad\u02ae\3\2\2\2\u02ae\u02af\7\34"+
"\2\2\u02af\u02b0\b\60\1\2\u02b0_\3\2\2\2\u02b1\u02b2\5b\62\2\u02b2\u02b3"+
"\7\35\2\2\u02b3\u02b4\5\32\16\2\u02b4\u02b5\b\61\1\2\u02b5a\3\2\2\2\u02b6"+
"\u02b7\5f\64\2\u02b7\u02b8\b\62\1\2\u02b8\u02bd\3\2\2\2\u02b9\u02ba\5"+
"T+\2\u02ba\u02bb\b\62\1\2\u02bb\u02bd\3\2\2\2\u02bc\u02b6\3\2\2\2\u02bc"+
"\u02b9\3\2\2\2\u02bdc\3\2\2\2\u02be\u02bf\7\b\2\2\u02bf\u02c7\b\63\1\2"+
"\u02c0\u02c1\5f\64\2\u02c1\u02c2\7\27\2\2\u02c2\u02c3\5\32\16\2\u02c3"+
"\u02c4\7\30\2\2\u02c4\u02c5\b\63\1\2\u02c5\u02c7\3\2\2\2\u02c6\u02be\3"+
"\2\2\2\u02c6\u02c0\3\2\2\2\u02c7e\3\2\2\2\u02c8\u02c9\7\62\2\2\u02c9\u02d1"+
"\b\64\1\2\u02ca\u02cb\7/\2\2\u02cb\u02d1\b\64\1\2\u02cc\u02cd\7.\2\2\u02cd"+
"\u02d1\b\64\1\2\u02ce\u02cf\7#\2\2\u02cf\u02d1\b\64\1\2\u02d0\u02c8\3"+
"\2\2\2\u02d0\u02ca\3\2\2\2\u02d0\u02cc\3\2\2\2\u02d0\u02ce\3\2\2\2\u02d1"+
"g\3\2\2\28\u0083\u008e\u009a\u00a2\u00b1\u00b9\u00bc\u00cd\u00de\u00ed"+
"\u00f0\u00f7\u00fb\u0106\u0118\u0125\u0134\u0141\u0150\u015b\u0166\u017b"+
"\u0184\u018b\u0192\u0199\u01a4\u01ac\u01ae\u01ba\u01d6\u01e6\u01eb\u01f2"+
"\u0203\u0205\u020f\u0220\u0231\u0234\u0249\u0251\u025c\u0266\u0274\u027b"+
"\u028b\u0297\u029a\u02a9\u02ac\u02bc\u02c6\u02d0";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy