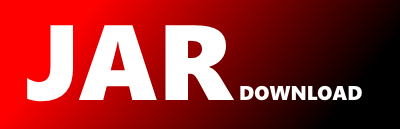
chs.jdmn.jdmn-core.8.7.3.source-code.FEELParser Maven / Gradle / Ivy
The newest version!
// Generated from FEELParser.g4 by ANTLR 4.13.1
package com.gs.dmn.feel.analysis.syntax.antlrv4;
import java.util.*;
import com.gs.dmn.feel.analysis.syntax.ast.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.arithmetic.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.comparison.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.context.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.function.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.literal.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.logic.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.textual.*;
import com.gs.dmn.feel.analysis.syntax.ast.expression.type.*;
import com.gs.dmn.feel.analysis.syntax.ast.test.*;
import com.gs.dmn.runtime.Pair;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast", "CheckReturnValue"})
public class FEELParser extends Parser {
static { RuntimeMetaData.checkVersion("4.13.1", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
BLOCK_COMMENT=1, LINE_COMMENT=2, WS=3, STRING=4, NUMBER=5, TEMPORAL=6,
EQ=7, NE=8, LT=9, GT=10, LE=11, GE=12, PLUS=13, MINUS=14, STAR=15, FORWARD_SLASH=16,
STAR_STAR=17, DOT_DOT=18, DOT=19, COMMA=20, PAREN_OPEN=21, PAREN_CLOSE=22,
BRACKET_OPEN=23, BRACKET_CLOSE=24, BRACE_OPEN=25, BRACE_CLOSE=26, COLON=27,
ARROW=28, NOT=29, TRUE=30, FALSE=31, NULL=32, FUNCTION=33, EXTERNAL=34,
FOR=35, IN=36, RETURN=37, IF=38, THEN=39, ELSE=40, SOME=41, EVERY=42,
SATISFIES=43, AND=44, OR=45, BETWEEN=46, INSTANCE_OF=47, NAME=48;
public static final int
RULE_unaryTestsRoot = 0, RULE_expressionRoot = 1, RULE_textualExpressionsRoot = 2,
RULE_boxedExpressionRoot = 3, RULE_unaryTests = 4, RULE_positiveUnaryTests = 5,
RULE_positiveUnaryTest = 6, RULE_simplePositiveUnaryTest = 7, RULE_interval = 8,
RULE_intervalStartPar = 9, RULE_intervalEndPar = 10, RULE_endpoint = 11,
RULE_expression = 12, RULE_textualExpressions = 13, RULE_textualExpression = 14,
RULE_functionDefinition = 15, RULE_formalParameter = 16, RULE_forExpression = 17,
RULE_iterationDomain = 18, RULE_ifExpression = 19, RULE_quantifiedExpression = 20,
RULE_disjunction = 21, RULE_conjunction = 22, RULE_comparison = 23, RULE_arithmeticExpression = 24,
RULE_addition = 25, RULE_multiplication = 26, RULE_exponentiation = 27,
RULE_arithmeticNegation = 28, RULE_instanceOf = 29, RULE_type = 30, RULE_postfixExpression = 31,
RULE_parameters = 32, RULE_namedParameters = 33, RULE_parameterName = 34,
RULE_positionalParameters = 35, RULE_primaryExpression = 36, RULE_simpleValue = 37,
RULE_qualifiedName = 38, RULE_literal = 39, RULE_simpleLiteral = 40, RULE_stringLiteral = 41,
RULE_booleanLiteral = 42, RULE_numericLiteral = 43, RULE_boxedExpression = 44,
RULE_list = 45, RULE_context = 46, RULE_contextEntry = 47, RULE_key = 48,
RULE_dateTimeLiteral = 49, RULE_identifier = 50;
private static String[] makeRuleNames() {
return new String[] {
"unaryTestsRoot", "expressionRoot", "textualExpressionsRoot", "boxedExpressionRoot",
"unaryTests", "positiveUnaryTests", "positiveUnaryTest", "simplePositiveUnaryTest",
"interval", "intervalStartPar", "intervalEndPar", "endpoint", "expression",
"textualExpressions", "textualExpression", "functionDefinition", "formalParameter",
"forExpression", "iterationDomain", "ifExpression", "quantifiedExpression",
"disjunction", "conjunction", "comparison", "arithmeticExpression", "addition",
"multiplication", "exponentiation", "arithmeticNegation", "instanceOf",
"type", "postfixExpression", "parameters", "namedParameters", "parameterName",
"positionalParameters", "primaryExpression", "simpleValue", "qualifiedName",
"literal", "simpleLiteral", "stringLiteral", "booleanLiteral", "numericLiteral",
"boxedExpression", "list", "context", "contextEntry", "key", "dateTimeLiteral",
"identifier"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, null, null, null, null, null, null, null, "'!='", "'<'", "'>'",
"'<='", "'>='", "'+'", "'-'", "'*'", "'/'", "'**'", "'..'", "'.'", "','",
"'('", "')'", "'['", "']'", "'{'", "'}'", "':'", "'->'", "'not'", "'true'",
"'false'", "'null'", "'function'", "'external'", "'for'", "'in'", "'return'",
"'if'", "'then'", "'else'", "'some'", "'every'", "'satisfies'", "'and'",
"'or'", "'between'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "BLOCK_COMMENT", "LINE_COMMENT", "WS", "STRING", "NUMBER", "TEMPORAL",
"EQ", "NE", "LT", "GT", "LE", "GE", "PLUS", "MINUS", "STAR", "FORWARD_SLASH",
"STAR_STAR", "DOT_DOT", "DOT", "COMMA", "PAREN_OPEN", "PAREN_CLOSE",
"BRACKET_OPEN", "BRACKET_CLOSE", "BRACE_OPEN", "BRACE_CLOSE", "COLON",
"ARROW", "NOT", "TRUE", "FALSE", "NULL", "FUNCTION", "EXTERNAL", "FOR",
"IN", "RETURN", "IF", "THEN", "ELSE", "SOME", "EVERY", "SATISFIES", "AND",
"OR", "BETWEEN", "INSTANCE_OF", "NAME"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "FEELParser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
private ASTFactory astFactory;
public FEELParser(TokenStream input, ASTFactory astFactory) {
this(input);
this.astFactory = astFactory;
}
public FEELParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
@SuppressWarnings("CheckReturnValue")
public static class UnaryTestsRootContext extends ParserRuleContext {
public UnaryTests ast;
public UnaryTestsContext unaryTests;
public UnaryTestsContext unaryTests() {
return getRuleContext(UnaryTestsContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public UnaryTestsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unaryTestsRoot; }
}
public final UnaryTestsRootContext unaryTestsRoot() throws RecognitionException {
UnaryTestsRootContext _localctx = new UnaryTestsRootContext(_ctx, getState());
enterRule(_localctx, 0, RULE_unaryTestsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(102);
((UnaryTestsRootContext)_localctx).unaryTests = unaryTests();
((UnaryTestsRootContext)_localctx).ast = ((UnaryTestsRootContext)_localctx).unaryTests.ast;
setState(104);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExpressionRootContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext expression;
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public ExpressionRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expressionRoot; }
}
public final ExpressionRootContext expressionRoot() throws RecognitionException {
ExpressionRootContext _localctx = new ExpressionRootContext(_ctx, getState());
enterRule(_localctx, 2, RULE_expressionRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(106);
((ExpressionRootContext)_localctx).expression = expression();
((ExpressionRootContext)_localctx).ast = ((ExpressionRootContext)_localctx).expression.ast;
setState(108);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TextualExpressionsRootContext extends ParserRuleContext {
public Expression ast;
public TextualExpressionsContext textualExpressions;
public TextualExpressionsContext textualExpressions() {
return getRuleContext(TextualExpressionsContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public TextualExpressionsRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_textualExpressionsRoot; }
}
public final TextualExpressionsRootContext textualExpressionsRoot() throws RecognitionException {
TextualExpressionsRootContext _localctx = new TextualExpressionsRootContext(_ctx, getState());
enterRule(_localctx, 4, RULE_textualExpressionsRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(110);
((TextualExpressionsRootContext)_localctx).textualExpressions = textualExpressions();
((TextualExpressionsRootContext)_localctx).ast = ((TextualExpressionsRootContext)_localctx).textualExpressions.ast;
setState(112);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class BoxedExpressionRootContext extends ParserRuleContext {
public Expression ast;
public BoxedExpressionContext boxedExpression;
public BoxedExpressionContext boxedExpression() {
return getRuleContext(BoxedExpressionContext.class,0);
}
public TerminalNode EOF() { return getToken(FEELParser.EOF, 0); }
public BoxedExpressionRootContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_boxedExpressionRoot; }
}
public final BoxedExpressionRootContext boxedExpressionRoot() throws RecognitionException {
BoxedExpressionRootContext _localctx = new BoxedExpressionRootContext(_ctx, getState());
enterRule(_localctx, 6, RULE_boxedExpressionRoot);
try {
enterOuterAlt(_localctx, 1);
{
setState(114);
((BoxedExpressionRootContext)_localctx).boxedExpression = boxedExpression();
((BoxedExpressionRootContext)_localctx).ast = ((BoxedExpressionRootContext)_localctx).boxedExpression.ast;
setState(116);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class UnaryTestsContext extends ParserRuleContext {
public UnaryTests ast;
public PositiveUnaryTestsContext tests;
public TerminalNode NOT() { return getToken(FEELParser.NOT, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public PositiveUnaryTestsContext positiveUnaryTests() {
return getRuleContext(PositiveUnaryTestsContext.class,0);
}
public TerminalNode MINUS() { return getToken(FEELParser.MINUS, 0); }
public UnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unaryTests; }
}
public final UnaryTestsContext unaryTests() throws RecognitionException {
UnaryTestsContext _localctx = new UnaryTestsContext(_ctx, getState());
enterRule(_localctx, 8, RULE_unaryTests);
try {
setState(129);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,0,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(118);
match(NOT);
setState(119);
match(PAREN_OPEN);
setState(120);
((UnaryTestsContext)_localctx).tests = positiveUnaryTests();
setState(121);
match(PAREN_CLOSE);
((UnaryTestsContext)_localctx).ast = astFactory.toNegatedUnaryTests(((UnaryTestsContext)_localctx).tests.ast);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(124);
((UnaryTestsContext)_localctx).tests = positiveUnaryTests();
((UnaryTestsContext)_localctx).ast = ((UnaryTestsContext)_localctx).tests.ast;
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(127);
match(MINUS);
((UnaryTestsContext)_localctx).ast = astFactory.toAny();
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PositiveUnaryTestsContext extends ParserRuleContext {
public PositiveUnaryTests ast;
public PositiveUnaryTestContext test;
public List positiveUnaryTest() {
return getRuleContexts(PositiveUnaryTestContext.class);
}
public PositiveUnaryTestContext positiveUnaryTest(int i) {
return getRuleContext(PositiveUnaryTestContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public PositiveUnaryTestsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positiveUnaryTests; }
}
public final PositiveUnaryTestsContext positiveUnaryTests() throws RecognitionException {
PositiveUnaryTestsContext _localctx = new PositiveUnaryTestsContext(_ctx, getState());
enterRule(_localctx, 10, RULE_positiveUnaryTests);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List tests = new ArrayList<>();
setState(132);
((PositiveUnaryTestsContext)_localctx).test = positiveUnaryTest();
tests.add(((PositiveUnaryTestsContext)_localctx).test.ast);
setState(140);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(134);
match(COMMA);
setState(135);
((PositiveUnaryTestsContext)_localctx).test = positiveUnaryTest();
tests.add(((PositiveUnaryTestsContext)_localctx).test.ast);
}
}
setState(142);
_errHandler.sync(this);
_la = _input.LA(1);
}
((PositiveUnaryTestsContext)_localctx).ast = astFactory.toPositiveUnaryTests(tests);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PositiveUnaryTestContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext expression;
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public PositiveUnaryTestContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positiveUnaryTest; }
}
public final PositiveUnaryTestContext positiveUnaryTest() throws RecognitionException {
PositiveUnaryTestContext _localctx = new PositiveUnaryTestContext(_ctx, getState());
enterRule(_localctx, 12, RULE_positiveUnaryTest);
try {
enterOuterAlt(_localctx, 1);
{
setState(145);
((PositiveUnaryTestContext)_localctx).expression = expression();
((PositiveUnaryTestContext)_localctx).ast = astFactory.toPositiveUnaryTest(((PositiveUnaryTestContext)_localctx).expression.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SimplePositiveUnaryTestContext extends ParserRuleContext {
public Expression ast;
public Token op;
public EndpointContext opd;
public IntervalContext opd2;
public EndpointContext endpoint() {
return getRuleContext(EndpointContext.class,0);
}
public TerminalNode LT() { return getToken(FEELParser.LT, 0); }
public TerminalNode LE() { return getToken(FEELParser.LE, 0); }
public TerminalNode GT() { return getToken(FEELParser.GT, 0); }
public TerminalNode GE() { return getToken(FEELParser.GE, 0); }
public IntervalContext interval() {
return getRuleContext(IntervalContext.class,0);
}
public SimplePositiveUnaryTestContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simplePositiveUnaryTest; }
}
public final SimplePositiveUnaryTestContext simplePositiveUnaryTest() throws RecognitionException {
SimplePositiveUnaryTestContext _localctx = new SimplePositiveUnaryTestContext(_ctx, getState());
enterRule(_localctx, 14, RULE_simplePositiveUnaryTest);
try {
setState(160);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LT:
case GT:
case LE:
case GE:
enterOuterAlt(_localctx, 1);
{
setState(152);
_errHandler.sync(this);
switch (_input.LA(1)) {
case LT:
{
setState(148);
((SimplePositiveUnaryTestContext)_localctx).op = match(LT);
}
break;
case LE:
{
setState(149);
((SimplePositiveUnaryTestContext)_localctx).op = match(LE);
}
break;
case GT:
{
setState(150);
((SimplePositiveUnaryTestContext)_localctx).op = match(GT);
}
break;
case GE:
{
setState(151);
((SimplePositiveUnaryTestContext)_localctx).op = match(GE);
}
break;
default:
throw new NoViableAltException(this);
}
setState(154);
((SimplePositiveUnaryTestContext)_localctx).opd = endpoint();
((SimplePositiveUnaryTestContext)_localctx).ast = ((SimplePositiveUnaryTestContext)_localctx).op == null ? astFactory.toOperatorRange(null, ((SimplePositiveUnaryTestContext)_localctx).opd.ast) : astFactory.toOperatorRange((((SimplePositiveUnaryTestContext)_localctx).op!=null?((SimplePositiveUnaryTestContext)_localctx).op.getText():null), ((SimplePositiveUnaryTestContext)_localctx).opd.ast);
}
break;
case PAREN_OPEN:
case BRACKET_OPEN:
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 2);
{
setState(157);
((SimplePositiveUnaryTestContext)_localctx).opd2 = interval();
((SimplePositiveUnaryTestContext)_localctx).ast = ((SimplePositiveUnaryTestContext)_localctx).opd2.ast;
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class IntervalContext extends ParserRuleContext {
public EndpointsRange ast;
public IntervalStartParContext leftPar;
public EndpointContext ep1;
public EndpointContext ep2;
public IntervalEndParContext rightPar;
public TerminalNode DOT_DOT() { return getToken(FEELParser.DOT_DOT, 0); }
public IntervalStartParContext intervalStartPar() {
return getRuleContext(IntervalStartParContext.class,0);
}
public List endpoint() {
return getRuleContexts(EndpointContext.class);
}
public EndpointContext endpoint(int i) {
return getRuleContext(EndpointContext.class,i);
}
public IntervalEndParContext intervalEndPar() {
return getRuleContext(IntervalEndParContext.class,0);
}
public IntervalContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_interval; }
}
public final IntervalContext interval() throws RecognitionException {
IntervalContext _localctx = new IntervalContext(_ctx, getState());
enterRule(_localctx, 16, RULE_interval);
try {
enterOuterAlt(_localctx, 1);
{
setState(162);
((IntervalContext)_localctx).leftPar = intervalStartPar();
setState(163);
((IntervalContext)_localctx).ep1 = endpoint();
setState(164);
match(DOT_DOT);
setState(165);
((IntervalContext)_localctx).ep2 = endpoint();
setState(166);
((IntervalContext)_localctx).rightPar = intervalEndPar();
((IntervalContext)_localctx).ast = astFactory.toEndpointsRange(((IntervalContext)_localctx).leftPar.ast, ((IntervalContext)_localctx).ep1.ast, ((IntervalContext)_localctx).rightPar.ast, ((IntervalContext)_localctx).ep2.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class IntervalStartParContext extends ParserRuleContext {
public String ast;
public Token token;
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(FEELParser.BRACKET_CLOSE, 0); }
public TerminalNode BRACKET_OPEN() { return getToken(FEELParser.BRACKET_OPEN, 0); }
public IntervalStartParContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intervalStartPar; }
}
public final IntervalStartParContext intervalStartPar() throws RecognitionException {
IntervalStartParContext _localctx = new IntervalStartParContext(_ctx, getState());
enterRule(_localctx, 18, RULE_intervalStartPar);
try {
setState(175);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PAREN_OPEN:
enterOuterAlt(_localctx, 1);
{
setState(169);
((IntervalStartParContext)_localctx).token = match(PAREN_OPEN);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
break;
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 2);
{
setState(171);
((IntervalStartParContext)_localctx).token = match(BRACKET_CLOSE);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
break;
case BRACKET_OPEN:
enterOuterAlt(_localctx, 3);
{
setState(173);
((IntervalStartParContext)_localctx).token = match(BRACKET_OPEN);
((IntervalStartParContext)_localctx).ast = (((IntervalStartParContext)_localctx).token!=null?((IntervalStartParContext)_localctx).token.getText():null);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class IntervalEndParContext extends ParserRuleContext {
public String ast;
public Token token;
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public TerminalNode BRACKET_OPEN() { return getToken(FEELParser.BRACKET_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(FEELParser.BRACKET_CLOSE, 0); }
public IntervalEndParContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intervalEndPar; }
}
public final IntervalEndParContext intervalEndPar() throws RecognitionException {
IntervalEndParContext _localctx = new IntervalEndParContext(_ctx, getState());
enterRule(_localctx, 20, RULE_intervalEndPar);
try {
setState(183);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PAREN_CLOSE:
enterOuterAlt(_localctx, 1);
{
setState(177);
((IntervalEndParContext)_localctx).token = match(PAREN_CLOSE);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
break;
case BRACKET_OPEN:
enterOuterAlt(_localctx, 2);
{
setState(179);
((IntervalEndParContext)_localctx).token = match(BRACKET_OPEN);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
break;
case BRACKET_CLOSE:
enterOuterAlt(_localctx, 3);
{
setState(181);
((IntervalEndParContext)_localctx).token = match(BRACKET_CLOSE);
((IntervalEndParContext)_localctx).ast = (((IntervalEndParContext)_localctx).token!=null?((IntervalEndParContext)_localctx).token.getText():null);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class EndpointContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext expression;
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public EndpointContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_endpoint; }
}
public final EndpointContext endpoint() throws RecognitionException {
EndpointContext _localctx = new EndpointContext(_ctx, getState());
enterRule(_localctx, 22, RULE_endpoint);
try {
enterOuterAlt(_localctx, 1);
{
setState(185);
((EndpointContext)_localctx).expression = expression();
((EndpointContext)_localctx).ast = ((EndpointContext)_localctx).expression.ast;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExpressionContext extends ParserRuleContext {
public Expression ast;
public TextualExpressionContext textualExpression;
public TextualExpressionContext textualExpression() {
return getRuleContext(TextualExpressionContext.class,0);
}
public ExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression; }
}
public final ExpressionContext expression() throws RecognitionException {
ExpressionContext _localctx = new ExpressionContext(_ctx, getState());
enterRule(_localctx, 24, RULE_expression);
try {
enterOuterAlt(_localctx, 1);
{
setState(188);
((ExpressionContext)_localctx).textualExpression = textualExpression();
((ExpressionContext)_localctx).ast = ((ExpressionContext)_localctx).textualExpression.ast;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TextualExpressionsContext extends ParserRuleContext {
public Expression ast;
public TextualExpressionContext exp;
public List textualExpression() {
return getRuleContexts(TextualExpressionContext.class);
}
public TextualExpressionContext textualExpression(int i) {
return getRuleContext(TextualExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public TextualExpressionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_textualExpressions; }
}
public final TextualExpressionsContext textualExpressions() throws RecognitionException {
TextualExpressionsContext _localctx = new TextualExpressionsContext(_ctx, getState());
enterRule(_localctx, 26, RULE_textualExpressions);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List expressionList = new ArrayList<>();
setState(192);
((TextualExpressionsContext)_localctx).exp = textualExpression();
expressionList.add(((TextualExpressionsContext)_localctx).exp.ast);
setState(200);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(194);
match(COMMA);
setState(195);
((TextualExpressionsContext)_localctx).exp = textualExpression();
expressionList.add(((TextualExpressionsContext)_localctx).exp.ast);
}
}
setState(202);
_errHandler.sync(this);
_la = _input.LA(1);
}
((TextualExpressionsContext)_localctx).ast = astFactory.toExpressionList(expressionList);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TextualExpressionContext extends ParserRuleContext {
public Expression ast;
public ForExpressionContext forExpression;
public IfExpressionContext ifExpression;
public QuantifiedExpressionContext quantifiedExpression;
public DisjunctionContext disjunction;
public ForExpressionContext forExpression() {
return getRuleContext(ForExpressionContext.class,0);
}
public IfExpressionContext ifExpression() {
return getRuleContext(IfExpressionContext.class,0);
}
public QuantifiedExpressionContext quantifiedExpression() {
return getRuleContext(QuantifiedExpressionContext.class,0);
}
public DisjunctionContext disjunction() {
return getRuleContext(DisjunctionContext.class,0);
}
public TextualExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_textualExpression; }
}
public final TextualExpressionContext textualExpression() throws RecognitionException {
TextualExpressionContext _localctx = new TextualExpressionContext(_ctx, getState());
enterRule(_localctx, 28, RULE_textualExpression);
try {
setState(217);
_errHandler.sync(this);
switch (_input.LA(1)) {
case FOR:
enterOuterAlt(_localctx, 1);
{
setState(205);
((TextualExpressionContext)_localctx).forExpression = forExpression();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).forExpression.ast;
}
break;
case IF:
enterOuterAlt(_localctx, 2);
{
setState(208);
((TextualExpressionContext)_localctx).ifExpression = ifExpression();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).ifExpression.ast;
}
break;
case SOME:
case EVERY:
enterOuterAlt(_localctx, 3);
{
setState(211);
((TextualExpressionContext)_localctx).quantifiedExpression = quantifiedExpression();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).quantifiedExpression.ast;
}
break;
case STRING:
case NUMBER:
case TEMPORAL:
case LT:
case GT:
case LE:
case GE:
case MINUS:
case PAREN_OPEN:
case BRACKET_OPEN:
case BRACKET_CLOSE:
case BRACE_OPEN:
case NOT:
case TRUE:
case FALSE:
case NULL:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 4);
{
setState(214);
((TextualExpressionContext)_localctx).disjunction = disjunction();
((TextualExpressionContext)_localctx).ast = ((TextualExpressionContext)_localctx).disjunction.ast;
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FunctionDefinitionContext extends ParserRuleContext {
public Expression ast;
public FormalParameterContext param;
public TypeContext type;
public ExpressionContext body;
public TerminalNode FUNCTION() { return getToken(FEELParser.FUNCTION, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode COLON() { return getToken(FEELParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public TerminalNode EXTERNAL() { return getToken(FEELParser.EXTERNAL, 0); }
public List formalParameter() {
return getRuleContexts(FormalParameterContext.class);
}
public FormalParameterContext formalParameter(int i) {
return getRuleContext(FormalParameterContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public FunctionDefinitionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_functionDefinition; }
}
public final FunctionDefinitionContext functionDefinition() throws RecognitionException {
FunctionDefinitionContext _localctx = new FunctionDefinitionContext(_ctx, getState());
enterRule(_localctx, 30, RULE_functionDefinition);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List parameters = new ArrayList<>();
boolean external = false;
TypeExpression returnTypeExp = null;
setState(222);
match(FUNCTION);
setState(223);
match(PAREN_OPEN);
setState(235);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & 334260661649408L) != 0)) {
{
setState(224);
((FunctionDefinitionContext)_localctx).param = formalParameter();
parameters.add(((FunctionDefinitionContext)_localctx).param.ast);
setState(232);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(226);
match(COMMA);
setState(227);
((FunctionDefinitionContext)_localctx).param = formalParameter();
parameters.add(((FunctionDefinitionContext)_localctx).param.ast);
}
}
setState(234);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(237);
match(PAREN_CLOSE);
setState(242);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON) {
{
setState(238);
match(COLON);
setState(239);
((FunctionDefinitionContext)_localctx).type = type();
returnTypeExp = ((FunctionDefinitionContext)_localctx).type.ast;
}
}
setState(246);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==EXTERNAL) {
{
setState(244);
match(EXTERNAL);
external = true;
}
}
setState(248);
((FunctionDefinitionContext)_localctx).body = expression();
((FunctionDefinitionContext)_localctx).ast = astFactory.toFunctionDefinition(parameters, returnTypeExp, ((FunctionDefinitionContext)_localctx).body.ast, external);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FormalParameterContext extends ParserRuleContext {
public FormalParameter ast;
public ParameterNameContext name;
public TypeContext type;
public ParameterNameContext parameterName() {
return getRuleContext(ParameterNameContext.class,0);
}
public TerminalNode COLON() { return getToken(FEELParser.COLON, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public FormalParameterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_formalParameter; }
}
public final FormalParameterContext formalParameter() throws RecognitionException {
FormalParameterContext _localctx = new FormalParameterContext(_ctx, getState());
enterRule(_localctx, 32, RULE_formalParameter);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
TypeExpression typeExp = null;
setState(252);
((FormalParameterContext)_localctx).name = parameterName();
setState(257);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==COLON) {
{
setState(253);
match(COLON);
setState(254);
((FormalParameterContext)_localctx).type = type();
typeExp = ((FormalParameterContext)_localctx).type.ast;
}
}
((FormalParameterContext)_localctx).ast = astFactory.toFormalParameter(((FormalParameterContext)_localctx).name.ast, typeExp);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ForExpressionContext extends ParserRuleContext {
public Expression ast;
public IdentifierContext var;
public IterationDomainContext domain;
public ExpressionContext body;
public TerminalNode FOR() { return getToken(FEELParser.FOR, 0); }
public List IN() { return getTokens(FEELParser.IN); }
public TerminalNode IN(int i) {
return getToken(FEELParser.IN, i);
}
public TerminalNode RETURN() { return getToken(FEELParser.RETURN, 0); }
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List iterationDomain() {
return getRuleContexts(IterationDomainContext.class);
}
public IterationDomainContext iterationDomain(int i) {
return getRuleContext(IterationDomainContext.class,i);
}
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public ForExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_forExpression; }
}
public final ForExpressionContext forExpression() throws RecognitionException {
ForExpressionContext _localctx = new ForExpressionContext(_ctx, getState());
enterRule(_localctx, 34, RULE_forExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List iterators = new ArrayList<>();
setState(262);
match(FOR);
setState(263);
((ForExpressionContext)_localctx).var = identifier();
setState(264);
match(IN);
setState(265);
((ForExpressionContext)_localctx).domain = iterationDomain();
iterators.add(astFactory.toIterator((((ForExpressionContext)_localctx).var!=null?_input.getText(((ForExpressionContext)_localctx).var.start,((ForExpressionContext)_localctx).var.stop):null), ((ForExpressionContext)_localctx).domain.ast));
setState(275);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(267);
match(COMMA);
setState(268);
((ForExpressionContext)_localctx).var = identifier();
setState(269);
match(IN);
setState(270);
((ForExpressionContext)_localctx).domain = iterationDomain();
iterators.add(astFactory.toIterator((((ForExpressionContext)_localctx).var!=null?_input.getText(((ForExpressionContext)_localctx).var.start,((ForExpressionContext)_localctx).var.stop):null), ((ForExpressionContext)_localctx).domain.ast));
}
}
setState(277);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(278);
match(RETURN);
setState(279);
((ForExpressionContext)_localctx).body = expression();
((ForExpressionContext)_localctx).ast = astFactory.toForExpression(iterators, ((ForExpressionContext)_localctx).body.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class IterationDomainContext extends ParserRuleContext {
public IteratorDomain ast;
public ExpressionContext exp1;
public ExpressionContext exp2;
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode DOT_DOT() { return getToken(FEELParser.DOT_DOT, 0); }
public IterationDomainContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_iterationDomain; }
}
public final IterationDomainContext iterationDomain() throws RecognitionException {
IterationDomainContext _localctx = new IterationDomainContext(_ctx, getState());
enterRule(_localctx, 36, RULE_iterationDomain);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
Expression end = null;
setState(283);
((IterationDomainContext)_localctx).exp1 = expression();
setState(288);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DOT_DOT) {
{
setState(284);
match(DOT_DOT);
setState(285);
((IterationDomainContext)_localctx).exp2 = expression();
end = ((IterationDomainContext)_localctx).exp2.ast;
}
}
((IterationDomainContext)_localctx).ast = astFactory.toIteratorDomain(((IterationDomainContext)_localctx).exp1.ast, end);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class IfExpressionContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext cond;
public ExpressionContext exp1;
public ExpressionContext exp2;
public TerminalNode IF() { return getToken(FEELParser.IF, 0); }
public TerminalNode THEN() { return getToken(FEELParser.THEN, 0); }
public TerminalNode ELSE() { return getToken(FEELParser.ELSE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public IfExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ifExpression; }
}
public final IfExpressionContext ifExpression() throws RecognitionException {
IfExpressionContext _localctx = new IfExpressionContext(_ctx, getState());
enterRule(_localctx, 38, RULE_ifExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(292);
match(IF);
setState(293);
((IfExpressionContext)_localctx).cond = expression();
setState(294);
match(THEN);
setState(295);
((IfExpressionContext)_localctx).exp1 = expression();
setState(296);
match(ELSE);
setState(297);
((IfExpressionContext)_localctx).exp2 = expression();
((IfExpressionContext)_localctx).ast = astFactory.toIfExpression(((IfExpressionContext)_localctx).cond.ast, ((IfExpressionContext)_localctx).exp1.ast, ((IfExpressionContext)_localctx).exp2.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class QuantifiedExpressionContext extends ParserRuleContext {
public Expression ast;
public Token op;
public IdentifierContext var;
public ExpressionContext domain;
public ExpressionContext body;
public List IN() { return getTokens(FEELParser.IN); }
public TerminalNode IN(int i) {
return getToken(FEELParser.IN, i);
}
public TerminalNode SATISFIES() { return getToken(FEELParser.SATISFIES, 0); }
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public TerminalNode SOME() { return getToken(FEELParser.SOME, 0); }
public TerminalNode EVERY() { return getToken(FEELParser.EVERY, 0); }
public QuantifiedExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_quantifiedExpression; }
}
public final QuantifiedExpressionContext quantifiedExpression() throws RecognitionException {
QuantifiedExpressionContext _localctx = new QuantifiedExpressionContext(_ctx, getState());
enterRule(_localctx, 40, RULE_quantifiedExpression);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List iterators = new ArrayList<>();
setState(303);
_errHandler.sync(this);
switch (_input.LA(1)) {
case SOME:
{
setState(301);
((QuantifiedExpressionContext)_localctx).op = match(SOME);
}
break;
case EVERY:
{
setState(302);
((QuantifiedExpressionContext)_localctx).op = match(EVERY);
}
break;
default:
throw new NoViableAltException(this);
}
setState(305);
((QuantifiedExpressionContext)_localctx).var = identifier();
setState(306);
match(IN);
setState(307);
((QuantifiedExpressionContext)_localctx).domain = expression();
iterators.add(astFactory.toIterator((((QuantifiedExpressionContext)_localctx).var!=null?_input.getText(((QuantifiedExpressionContext)_localctx).var.start,((QuantifiedExpressionContext)_localctx).var.stop):null), ((QuantifiedExpressionContext)_localctx).domain.ast));
setState(316);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & 334260661649408L) != 0)) {
{
{
setState(309);
((QuantifiedExpressionContext)_localctx).var = identifier();
setState(310);
match(IN);
setState(311);
((QuantifiedExpressionContext)_localctx).domain = expression();
iterators.add(astFactory.toIterator((((QuantifiedExpressionContext)_localctx).var!=null?_input.getText(((QuantifiedExpressionContext)_localctx).var.start,((QuantifiedExpressionContext)_localctx).var.stop):null), ((QuantifiedExpressionContext)_localctx).domain.ast));
}
}
setState(318);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(319);
match(SATISFIES);
setState(320);
((QuantifiedExpressionContext)_localctx).body = expression();
((QuantifiedExpressionContext)_localctx).ast = astFactory.toQuantifiedExpression((((QuantifiedExpressionContext)_localctx).op!=null?((QuantifiedExpressionContext)_localctx).op.getText():null), iterators, ((QuantifiedExpressionContext)_localctx).body.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DisjunctionContext extends ParserRuleContext {
public Expression ast;
public ConjunctionContext left;
public ConjunctionContext right;
public List conjunction() {
return getRuleContexts(ConjunctionContext.class);
}
public ConjunctionContext conjunction(int i) {
return getRuleContext(ConjunctionContext.class,i);
}
public List OR() { return getTokens(FEELParser.OR); }
public TerminalNode OR(int i) {
return getToken(FEELParser.OR, i);
}
public DisjunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_disjunction; }
}
public final DisjunctionContext disjunction() throws RecognitionException {
DisjunctionContext _localctx = new DisjunctionContext(_ctx, getState());
enterRule(_localctx, 42, RULE_disjunction);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(323);
((DisjunctionContext)_localctx).left = conjunction();
((DisjunctionContext)_localctx).ast = ((DisjunctionContext)_localctx).left.ast;
setState(331);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,17,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(325);
match(OR);
setState(326);
((DisjunctionContext)_localctx).right = conjunction();
((DisjunctionContext)_localctx).ast = astFactory.toDisjunction(_localctx.ast, ((DisjunctionContext)_localctx).right.ast);
}
}
}
setState(333);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,17,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ConjunctionContext extends ParserRuleContext {
public Expression ast;
public ComparisonContext left;
public ComparisonContext right;
public List comparison() {
return getRuleContexts(ComparisonContext.class);
}
public ComparisonContext comparison(int i) {
return getRuleContext(ComparisonContext.class,i);
}
public List AND() { return getTokens(FEELParser.AND); }
public TerminalNode AND(int i) {
return getToken(FEELParser.AND, i);
}
public ConjunctionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_conjunction; }
}
public final ConjunctionContext conjunction() throws RecognitionException {
ConjunctionContext _localctx = new ConjunctionContext(_ctx, getState());
enterRule(_localctx, 44, RULE_conjunction);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(334);
((ConjunctionContext)_localctx).left = comparison();
((ConjunctionContext)_localctx).ast = ((ConjunctionContext)_localctx).left.ast;
setState(342);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,18,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(336);
match(AND);
setState(337);
((ConjunctionContext)_localctx).right = comparison();
((ConjunctionContext)_localctx).ast = astFactory.toConjunction(_localctx.ast, ((ConjunctionContext)_localctx).right.ast);
}
}
}
setState(344);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,18,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ComparisonContext extends ParserRuleContext {
public Expression ast;
public ArithmeticExpressionContext ae1;
public Token op;
public ArithmeticExpressionContext ae2;
public ExpressionContext leftEndpoint;
public ExpressionContext rightEndpoint;
public PositiveUnaryTestContext test;
public PositiveUnaryTestsContext tests;
public List arithmeticExpression() {
return getRuleContexts(ArithmeticExpressionContext.class);
}
public ArithmeticExpressionContext arithmeticExpression(int i) {
return getRuleContext(ArithmeticExpressionContext.class,i);
}
public TerminalNode BETWEEN() { return getToken(FEELParser.BETWEEN, 0); }
public TerminalNode AND() { return getToken(FEELParser.AND, 0); }
public TerminalNode IN() { return getToken(FEELParser.IN, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public PositiveUnaryTestContext positiveUnaryTest() {
return getRuleContext(PositiveUnaryTestContext.class,0);
}
public PositiveUnaryTestsContext positiveUnaryTests() {
return getRuleContext(PositiveUnaryTestsContext.class,0);
}
public TerminalNode EQ() { return getToken(FEELParser.EQ, 0); }
public TerminalNode NE() { return getToken(FEELParser.NE, 0); }
public TerminalNode LT() { return getToken(FEELParser.LT, 0); }
public TerminalNode GT() { return getToken(FEELParser.GT, 0); }
public TerminalNode LE() { return getToken(FEELParser.LE, 0); }
public TerminalNode GE() { return getToken(FEELParser.GE, 0); }
public ComparisonContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_comparison; }
}
public final ComparisonContext comparison() throws RecognitionException {
ComparisonContext _localctx = new ComparisonContext(_ctx, getState());
enterRule(_localctx, 46, RULE_comparison);
try {
enterOuterAlt(_localctx, 1);
{
setState(345);
((ComparisonContext)_localctx).ae1 = arithmeticExpression();
((ComparisonContext)_localctx).ast = ((ComparisonContext)_localctx).ae1.ast;
setState(374);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,20,_ctx) ) {
case 1:
{
setState(353);
_errHandler.sync(this);
switch (_input.LA(1)) {
case EQ:
{
setState(347);
((ComparisonContext)_localctx).op = match(EQ);
}
break;
case NE:
{
setState(348);
((ComparisonContext)_localctx).op = match(NE);
}
break;
case LT:
{
setState(349);
((ComparisonContext)_localctx).op = match(LT);
}
break;
case GT:
{
setState(350);
((ComparisonContext)_localctx).op = match(GT);
}
break;
case LE:
{
setState(351);
((ComparisonContext)_localctx).op = match(LE);
}
break;
case GE:
{
setState(352);
((ComparisonContext)_localctx).op = match(GE);
}
break;
default:
throw new NoViableAltException(this);
}
setState(355);
((ComparisonContext)_localctx).ae2 = arithmeticExpression();
((ComparisonContext)_localctx).ast = astFactory.toComparison((((ComparisonContext)_localctx).op!=null?((ComparisonContext)_localctx).op.getText():null), ((ComparisonContext)_localctx).ae1.ast, ((ComparisonContext)_localctx).ae2.ast);
}
break;
case 2:
{
setState(358);
match(BETWEEN);
setState(359);
((ComparisonContext)_localctx).leftEndpoint = expression();
setState(360);
match(AND);
setState(361);
((ComparisonContext)_localctx).rightEndpoint = expression();
((ComparisonContext)_localctx).ast = astFactory.toBetweenExpression(_localctx.ast, ((ComparisonContext)_localctx).leftEndpoint.ast, ((ComparisonContext)_localctx).rightEndpoint.ast);
}
break;
case 3:
{
setState(364);
match(IN);
setState(365);
((ComparisonContext)_localctx).test = positiveUnaryTest();
((ComparisonContext)_localctx).ast = astFactory.toInExpression(_localctx.ast, ((ComparisonContext)_localctx).test.ast);
}
break;
case 4:
{
setState(368);
match(IN);
setState(369);
match(PAREN_OPEN);
setState(370);
((ComparisonContext)_localctx).tests = positiveUnaryTests();
setState(371);
match(PAREN_CLOSE);
((ComparisonContext)_localctx).ast = astFactory.toInExpression(_localctx.ast, ((ComparisonContext)_localctx).tests.ast);
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ArithmeticExpressionContext extends ParserRuleContext {
public Expression ast;
public AdditionContext addition;
public AdditionContext addition() {
return getRuleContext(AdditionContext.class,0);
}
public ArithmeticExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arithmeticExpression; }
}
public final ArithmeticExpressionContext arithmeticExpression() throws RecognitionException {
ArithmeticExpressionContext _localctx = new ArithmeticExpressionContext(_ctx, getState());
enterRule(_localctx, 48, RULE_arithmeticExpression);
try {
enterOuterAlt(_localctx, 1);
{
setState(376);
((ArithmeticExpressionContext)_localctx).addition = addition();
((ArithmeticExpressionContext)_localctx).ast = ((ArithmeticExpressionContext)_localctx).addition.ast;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class AdditionContext extends ParserRuleContext {
public Expression ast;
public MultiplicationContext left;
public Token op;
public MultiplicationContext right;
public List multiplication() {
return getRuleContexts(MultiplicationContext.class);
}
public MultiplicationContext multiplication(int i) {
return getRuleContext(MultiplicationContext.class,i);
}
public List PLUS() { return getTokens(FEELParser.PLUS); }
public TerminalNode PLUS(int i) {
return getToken(FEELParser.PLUS, i);
}
public List MINUS() { return getTokens(FEELParser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(FEELParser.MINUS, i);
}
public AdditionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_addition; }
}
public final AdditionContext addition() throws RecognitionException {
AdditionContext _localctx = new AdditionContext(_ctx, getState());
enterRule(_localctx, 50, RULE_addition);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(379);
((AdditionContext)_localctx).left = multiplication();
((AdditionContext)_localctx).ast = ((AdditionContext)_localctx).left.ast;
setState(390);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,22,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(383);
_errHandler.sync(this);
switch (_input.LA(1)) {
case PLUS:
{
setState(381);
((AdditionContext)_localctx).op = match(PLUS);
}
break;
case MINUS:
{
setState(382);
((AdditionContext)_localctx).op = match(MINUS);
}
break;
default:
throw new NoViableAltException(this);
}
setState(385);
((AdditionContext)_localctx).right = multiplication();
((AdditionContext)_localctx).ast = astFactory.toAddition((((AdditionContext)_localctx).op!=null?((AdditionContext)_localctx).op.getText():null), _localctx.ast, ((AdditionContext)_localctx).right.ast);
}
}
}
setState(392);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,22,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class MultiplicationContext extends ParserRuleContext {
public Expression ast;
public ExponentiationContext left;
public Token op;
public ExponentiationContext right;
public List exponentiation() {
return getRuleContexts(ExponentiationContext.class);
}
public ExponentiationContext exponentiation(int i) {
return getRuleContext(ExponentiationContext.class,i);
}
public List STAR() { return getTokens(FEELParser.STAR); }
public TerminalNode STAR(int i) {
return getToken(FEELParser.STAR, i);
}
public List FORWARD_SLASH() { return getTokens(FEELParser.FORWARD_SLASH); }
public TerminalNode FORWARD_SLASH(int i) {
return getToken(FEELParser.FORWARD_SLASH, i);
}
public MultiplicationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_multiplication; }
}
public final MultiplicationContext multiplication() throws RecognitionException {
MultiplicationContext _localctx = new MultiplicationContext(_ctx, getState());
enterRule(_localctx, 52, RULE_multiplication);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(393);
((MultiplicationContext)_localctx).left = exponentiation();
((MultiplicationContext)_localctx).ast = ((MultiplicationContext)_localctx).left.ast;
setState(404);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,24,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(397);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STAR:
{
setState(395);
((MultiplicationContext)_localctx).op = match(STAR);
}
break;
case FORWARD_SLASH:
{
setState(396);
((MultiplicationContext)_localctx).op = match(FORWARD_SLASH);
}
break;
default:
throw new NoViableAltException(this);
}
setState(399);
((MultiplicationContext)_localctx).right = exponentiation();
((MultiplicationContext)_localctx).ast = astFactory.toMultiplication((((MultiplicationContext)_localctx).op!=null?((MultiplicationContext)_localctx).op.getText():null), _localctx.ast, ((MultiplicationContext)_localctx).right.ast);
}
}
}
setState(406);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,24,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ExponentiationContext extends ParserRuleContext {
public Expression ast;
public ArithmeticNegationContext left;
public ArithmeticNegationContext right;
public List arithmeticNegation() {
return getRuleContexts(ArithmeticNegationContext.class);
}
public ArithmeticNegationContext arithmeticNegation(int i) {
return getRuleContext(ArithmeticNegationContext.class,i);
}
public List STAR_STAR() { return getTokens(FEELParser.STAR_STAR); }
public TerminalNode STAR_STAR(int i) {
return getToken(FEELParser.STAR_STAR, i);
}
public ExponentiationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_exponentiation; }
}
public final ExponentiationContext exponentiation() throws RecognitionException {
ExponentiationContext _localctx = new ExponentiationContext(_ctx, getState());
enterRule(_localctx, 54, RULE_exponentiation);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(407);
((ExponentiationContext)_localctx).left = arithmeticNegation();
((ExponentiationContext)_localctx).ast = ((ExponentiationContext)_localctx).left.ast;
setState(415);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,25,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(409);
match(STAR_STAR);
setState(410);
((ExponentiationContext)_localctx).right = arithmeticNegation();
((ExponentiationContext)_localctx).ast = astFactory.toExponentiation(_localctx.ast, ((ExponentiationContext)_localctx).right.ast);
}
}
}
setState(417);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,25,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ArithmeticNegationContext extends ParserRuleContext {
public Expression ast;
public InstanceOfContext opd;
public InstanceOfContext instanceOf() {
return getRuleContext(InstanceOfContext.class,0);
}
public List MINUS() { return getTokens(FEELParser.MINUS); }
public TerminalNode MINUS(int i) {
return getToken(FEELParser.MINUS, i);
}
public List NOT() { return getTokens(FEELParser.NOT); }
public TerminalNode NOT(int i) {
return getToken(FEELParser.NOT, i);
}
public ArithmeticNegationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_arithmeticNegation; }
}
public final ArithmeticNegationContext arithmeticNegation() throws RecognitionException {
ArithmeticNegationContext _localctx = new ArithmeticNegationContext(_ctx, getState());
enterRule(_localctx, 56, RULE_arithmeticNegation);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
List prefixOperators = new ArrayList<>();
setState(425);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,27,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
setState(423);
_errHandler.sync(this);
switch (_input.LA(1)) {
case MINUS:
{
setState(419);
match(MINUS);
prefixOperators.add("-");
}
break;
case NOT:
{
setState(421);
match(NOT);
prefixOperators.add("not");
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(427);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,27,_ctx);
}
setState(428);
((ArithmeticNegationContext)_localctx).opd = instanceOf();
((ArithmeticNegationContext)_localctx).ast = astFactory.toNegation(prefixOperators, ((ArithmeticNegationContext)_localctx).opd.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class InstanceOfContext extends ParserRuleContext {
public Expression ast;
public PostfixExpressionContext exp;
public TypeContext type;
public PostfixExpressionContext postfixExpression() {
return getRuleContext(PostfixExpressionContext.class,0);
}
public TerminalNode INSTANCE_OF() { return getToken(FEELParser.INSTANCE_OF, 0); }
public TypeContext type() {
return getRuleContext(TypeContext.class,0);
}
public InstanceOfContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_instanceOf; }
}
public final InstanceOfContext instanceOf() throws RecognitionException {
InstanceOfContext _localctx = new InstanceOfContext(_ctx, getState());
enterRule(_localctx, 58, RULE_instanceOf);
try {
enterOuterAlt(_localctx, 1);
{
setState(431);
((InstanceOfContext)_localctx).exp = postfixExpression();
((InstanceOfContext)_localctx).ast = ((InstanceOfContext)_localctx).exp.ast;
setState(437);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,28,_ctx) ) {
case 1:
{
setState(433);
match(INSTANCE_OF);
setState(434);
((InstanceOfContext)_localctx).type = type();
((InstanceOfContext)_localctx).ast = astFactory.toInstanceOf(_localctx.ast, ((InstanceOfContext)_localctx).type.ast);
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class TypeContext extends ParserRuleContext {
public TypeExpression ast;
public QualifiedNameContext qName;
public IdentifierContext typeName;
public TypeContext type;
public IdentifierContext id1;
public TypeContext t1;
public IdentifierContext id2;
public TypeContext t2;
public TypeContext returnType;
public QualifiedNameContext qualifiedName() {
return getRuleContext(QualifiedNameContext.class,0);
}
public TerminalNode LT() { return getToken(FEELParser.LT, 0); }
public List type() {
return getRuleContexts(TypeContext.class);
}
public TypeContext type(int i) {
return getRuleContext(TypeContext.class,i);
}
public TerminalNode GT() { return getToken(FEELParser.GT, 0); }
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List COLON() { return getTokens(FEELParser.COLON); }
public TerminalNode COLON(int i) {
return getToken(FEELParser.COLON, i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public TerminalNode ARROW() { return getToken(FEELParser.ARROW, 0); }
public TypeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_type; }
}
public final TypeContext type() throws RecognitionException {
TypeContext _localctx = new TypeContext(_ctx, getState());
enterRule(_localctx, 60, RULE_type);
int _la;
try {
setState(493);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,32,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(439);
((TypeContext)_localctx).qName = qualifiedName();
((TypeContext)_localctx).ast = astFactory.toNamedTypeExpression(((TypeContext)_localctx).qName.ast);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(442);
((TypeContext)_localctx).typeName = identifier();
setState(443);
if (!("range".equals(((TypeContext)_localctx).typeName.ast.getText()) || "list".equals(((TypeContext)_localctx).typeName.ast.getText()))) throw new FailedPredicateException(this, "\"range\".equals($typeName.ast.getText()) || \"list\".equals($typeName.ast.getText())");
setState(444);
match(LT);
setState(445);
((TypeContext)_localctx).type = type();
setState(446);
match(GT);
((TypeContext)_localctx).ast = astFactory.toTypeExpression(((TypeContext)_localctx).typeName.ast.getText(), ((TypeContext)_localctx).type.ast);
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
List> members = new ArrayList<>();
setState(450);
((TypeContext)_localctx).typeName = identifier();
setState(451);
if (!("context".equals(((TypeContext)_localctx).typeName.ast.getText()))) throw new FailedPredicateException(this, "\"context\".equals($typeName.ast.getText())");
setState(452);
match(LT);
setState(453);
((TypeContext)_localctx).id1 = identifier();
setState(454);
match(COLON);
setState(455);
((TypeContext)_localctx).t1 = type();
members.add(new Pair(((TypeContext)_localctx).id1.ast.getText(), ((TypeContext)_localctx).t1.ast));
setState(465);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(457);
match(COMMA);
setState(458);
((TypeContext)_localctx).id2 = identifier();
setState(459);
match(COLON);
setState(460);
((TypeContext)_localctx).t2 = type();
members.add(new Pair(((TypeContext)_localctx).id2.ast.getText(), ((TypeContext)_localctx).t2.ast));
}
}
setState(467);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(468);
match(GT);
((TypeContext)_localctx).ast = astFactory.toContextTypeExpression(members);
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
List parameters = new ArrayList<>();
setState(472);
((TypeContext)_localctx).typeName = identifier();
setState(473);
if (!("function".equals(((TypeContext)_localctx).typeName.ast.getText()))) throw new FailedPredicateException(this, "\"function\".equals($typeName.ast.getText())");
setState(474);
match(LT);
setState(486);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & 334260661649408L) != 0)) {
{
setState(475);
((TypeContext)_localctx).t1 = type();
parameters.add(((TypeContext)_localctx).t1.ast);
setState(481);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(477);
match(COMMA);
setState(478);
((TypeContext)_localctx).t2 = type();
}
}
setState(483);
_errHandler.sync(this);
_la = _input.LA(1);
}
parameters.add(((TypeContext)_localctx).t2.ast);
}
}
setState(488);
match(GT);
setState(489);
match(ARROW);
setState(490);
((TypeContext)_localctx).returnType = type();
((TypeContext)_localctx).ast = astFactory.toFunctionTypeExpression(parameters, ((TypeContext)_localctx).returnType.ast);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PostfixExpressionContext extends ParserRuleContext {
public Expression ast;
public PrimaryExpressionContext primaryExpression;
public ExpressionContext filter;
public ParametersContext parameters;
public IdentifierContext name;
public PrimaryExpressionContext primaryExpression() {
return getRuleContext(PrimaryExpressionContext.class,0);
}
public List BRACKET_OPEN() { return getTokens(FEELParser.BRACKET_OPEN); }
public TerminalNode BRACKET_OPEN(int i) {
return getToken(FEELParser.BRACKET_OPEN, i);
}
public List BRACKET_CLOSE() { return getTokens(FEELParser.BRACKET_CLOSE); }
public TerminalNode BRACKET_CLOSE(int i) {
return getToken(FEELParser.BRACKET_CLOSE, i);
}
public List parameters() {
return getRuleContexts(ParametersContext.class);
}
public ParametersContext parameters(int i) {
return getRuleContext(ParametersContext.class,i);
}
public List DOT() { return getTokens(FEELParser.DOT); }
public TerminalNode DOT(int i) {
return getToken(FEELParser.DOT, i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public PostfixExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_postfixExpression; }
}
public final PostfixExpressionContext postfixExpression() throws RecognitionException {
PostfixExpressionContext _localctx = new PostfixExpressionContext(_ctx, getState());
enterRule(_localctx, 62, RULE_postfixExpression);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(495);
((PostfixExpressionContext)_localctx).primaryExpression = primaryExpression();
((PostfixExpressionContext)_localctx).ast = ((PostfixExpressionContext)_localctx).primaryExpression.ast;
setState(511);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,34,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
setState(509);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BRACKET_OPEN:
{
setState(497);
match(BRACKET_OPEN);
setState(498);
((PostfixExpressionContext)_localctx).filter = expression();
setState(499);
match(BRACKET_CLOSE);
((PostfixExpressionContext)_localctx).ast = astFactory.toFilterExpression(_localctx.ast, ((PostfixExpressionContext)_localctx).filter.ast);
}
break;
case PAREN_OPEN:
{
setState(502);
((PostfixExpressionContext)_localctx).parameters = parameters();
((PostfixExpressionContext)_localctx).ast = astFactory.toFunctionInvocation(_localctx.ast, ((PostfixExpressionContext)_localctx).parameters.ast);
}
break;
case DOT:
{
setState(505);
match(DOT);
setState(506);
((PostfixExpressionContext)_localctx).name = identifier();
((PostfixExpressionContext)_localctx).ast = astFactory.toPathExpression(_localctx.ast, (((PostfixExpressionContext)_localctx).name!=null?_input.getText(((PostfixExpressionContext)_localctx).name.start,((PostfixExpressionContext)_localctx).name.stop):null));
}
break;
default:
throw new NoViableAltException(this);
}
}
}
setState(513);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,34,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParametersContext extends ParserRuleContext {
public Parameters ast;
public NamedParametersContext namedParameters;
public PositionalParametersContext positionalParameters;
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public NamedParametersContext namedParameters() {
return getRuleContext(NamedParametersContext.class,0);
}
public PositionalParametersContext positionalParameters() {
return getRuleContext(PositionalParametersContext.class,0);
}
public ParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parameters; }
}
public final ParametersContext parameters() throws RecognitionException {
ParametersContext _localctx = new ParametersContext(_ctx, getState());
enterRule(_localctx, 64, RULE_parameters);
try {
enterOuterAlt(_localctx, 1);
{
setState(514);
match(PAREN_OPEN);
setState(521);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,35,_ctx) ) {
case 1:
{
setState(515);
((ParametersContext)_localctx).namedParameters = namedParameters();
((ParametersContext)_localctx).ast = ((ParametersContext)_localctx).namedParameters.ast;
}
break;
case 2:
{
setState(518);
((ParametersContext)_localctx).positionalParameters = positionalParameters();
((ParametersContext)_localctx).ast = ((ParametersContext)_localctx).positionalParameters.ast;
}
break;
}
setState(523);
match(PAREN_CLOSE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NamedParametersContext extends ParserRuleContext {
public NamedParameters ast;
public ParameterNameContext name;
public ExpressionContext value;
public List COLON() { return getTokens(FEELParser.COLON); }
public TerminalNode COLON(int i) {
return getToken(FEELParser.COLON, i);
}
public List parameterName() {
return getRuleContexts(ParameterNameContext.class);
}
public ParameterNameContext parameterName(int i) {
return getRuleContext(ParameterNameContext.class,i);
}
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public NamedParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_namedParameters; }
}
public final NamedParametersContext namedParameters() throws RecognitionException {
NamedParametersContext _localctx = new NamedParametersContext(_ctx, getState());
enterRule(_localctx, 66, RULE_namedParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
Map parameters = new LinkedHashMap<>();
setState(526);
((NamedParametersContext)_localctx).name = parameterName();
setState(527);
match(COLON);
setState(528);
((NamedParametersContext)_localctx).value = expression();
parameters.put((((NamedParametersContext)_localctx).name!=null?_input.getText(((NamedParametersContext)_localctx).name.start,((NamedParametersContext)_localctx).name.stop):null), ((NamedParametersContext)_localctx).value.ast);
setState(538);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(530);
match(COMMA);
setState(531);
((NamedParametersContext)_localctx).name = parameterName();
setState(532);
match(COLON);
setState(533);
((NamedParametersContext)_localctx).value = expression();
parameters.put((((NamedParametersContext)_localctx).name!=null?_input.getText(((NamedParametersContext)_localctx).name.start,((NamedParametersContext)_localctx).name.stop):null), ((NamedParametersContext)_localctx).value.ast);
}
}
setState(540);
_errHandler.sync(this);
_la = _input.LA(1);
}
((NamedParametersContext)_localctx).ast = astFactory.toNamedParameters(parameters);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ParameterNameContext extends ParserRuleContext {
public String ast;
public IdentifierContext name;
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public ParameterNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parameterName; }
}
public final ParameterNameContext parameterName() throws RecognitionException {
ParameterNameContext _localctx = new ParameterNameContext(_ctx, getState());
enterRule(_localctx, 68, RULE_parameterName);
try {
enterOuterAlt(_localctx, 1);
{
setState(543);
((ParameterNameContext)_localctx).name = identifier();
((ParameterNameContext)_localctx).ast = (((ParameterNameContext)_localctx).name!=null?_input.getText(((ParameterNameContext)_localctx).name.start,((ParameterNameContext)_localctx).name.stop):null);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PositionalParametersContext extends ParserRuleContext {
public PositionalParameters ast;
public ExpressionContext param;
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public PositionalParametersContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positionalParameters; }
}
public final PositionalParametersContext positionalParameters() throws RecognitionException {
PositionalParametersContext _localctx = new PositionalParametersContext(_ctx, getState());
enterRule(_localctx, 70, RULE_positionalParameters);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List parameters = new ArrayList<>();
setState(558);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & 341174546095728L) != 0)) {
{
setState(547);
((PositionalParametersContext)_localctx).param = expression();
parameters.add(((PositionalParametersContext)_localctx).param.ast);
setState(555);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(549);
match(COMMA);
setState(550);
((PositionalParametersContext)_localctx).param = expression();
parameters.add(((PositionalParametersContext)_localctx).param.ast);
}
}
setState(557);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
((PositionalParametersContext)_localctx).ast = astFactory.toPositionalParameters(parameters);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class PrimaryExpressionContext extends ParserRuleContext {
public Expression ast;
public LiteralContext literal;
public IdentifierContext name;
public ExpressionContext exp;
public BoxedExpressionContext boxedExpression;
public SimplePositiveUnaryTestContext simplePositiveUnaryTest;
public LiteralContext literal() {
return getRuleContext(LiteralContext.class,0);
}
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public BoxedExpressionContext boxedExpression() {
return getRuleContext(BoxedExpressionContext.class,0);
}
public SimplePositiveUnaryTestContext simplePositiveUnaryTest() {
return getRuleContext(SimplePositiveUnaryTestContext.class,0);
}
public PrimaryExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_primaryExpression; }
}
public final PrimaryExpressionContext primaryExpression() throws RecognitionException {
PrimaryExpressionContext _localctx = new PrimaryExpressionContext(_ctx, getState());
enterRule(_localctx, 72, RULE_primaryExpression);
try {
setState(579);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,39,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(562);
((PrimaryExpressionContext)_localctx).literal = literal();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).literal.ast;
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(565);
((PrimaryExpressionContext)_localctx).name = identifier();
((PrimaryExpressionContext)_localctx).ast = astFactory.toName((((PrimaryExpressionContext)_localctx).name!=null?_input.getText(((PrimaryExpressionContext)_localctx).name.start,((PrimaryExpressionContext)_localctx).name.stop):null));
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(568);
match(PAREN_OPEN);
setState(569);
((PrimaryExpressionContext)_localctx).exp = expression();
setState(570);
match(PAREN_CLOSE);
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).exp.ast;
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(573);
((PrimaryExpressionContext)_localctx).boxedExpression = boxedExpression();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).boxedExpression.ast;
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(576);
((PrimaryExpressionContext)_localctx).simplePositiveUnaryTest = simplePositiveUnaryTest();
((PrimaryExpressionContext)_localctx).ast = ((PrimaryExpressionContext)_localctx).simplePositiveUnaryTest.ast;
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SimpleValueContext extends ParserRuleContext {
public Expression ast;
public SimpleLiteralContext simpleLiteral;
public QualifiedNameContext qualifiedName;
public SimpleLiteralContext simpleLiteral() {
return getRuleContext(SimpleLiteralContext.class,0);
}
public QualifiedNameContext qualifiedName() {
return getRuleContext(QualifiedNameContext.class,0);
}
public SimpleValueContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleValue; }
}
public final SimpleValueContext simpleValue() throws RecognitionException {
SimpleValueContext _localctx = new SimpleValueContext(_ctx, getState());
enterRule(_localctx, 74, RULE_simpleValue);
try {
setState(587);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,40,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(581);
((SimpleValueContext)_localctx).simpleLiteral = simpleLiteral();
((SimpleValueContext)_localctx).ast = ((SimpleValueContext)_localctx).simpleLiteral.ast;
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(584);
((SimpleValueContext)_localctx).qualifiedName = qualifiedName();
((SimpleValueContext)_localctx).ast = ((SimpleValueContext)_localctx).qualifiedName.ast;
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class QualifiedNameContext extends ParserRuleContext {
public Expression ast;
public IdentifierContext name;
public List identifier() {
return getRuleContexts(IdentifierContext.class);
}
public IdentifierContext identifier(int i) {
return getRuleContext(IdentifierContext.class,i);
}
public List DOT() { return getTokens(FEELParser.DOT); }
public TerminalNode DOT(int i) {
return getToken(FEELParser.DOT, i);
}
public QualifiedNameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_qualifiedName; }
}
public final QualifiedNameContext qualifiedName() throws RecognitionException {
QualifiedNameContext _localctx = new QualifiedNameContext(_ctx, getState());
enterRule(_localctx, 76, RULE_qualifiedName);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
List names = new ArrayList<>();
setState(590);
((QualifiedNameContext)_localctx).name = identifier();
names.add((((QualifiedNameContext)_localctx).name!=null?_input.getText(((QualifiedNameContext)_localctx).name.start,((QualifiedNameContext)_localctx).name.stop):null));
setState(598);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,41,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(592);
match(DOT);
setState(593);
((QualifiedNameContext)_localctx).name = identifier();
names.add((((QualifiedNameContext)_localctx).name!=null?_input.getText(((QualifiedNameContext)_localctx).name.start,((QualifiedNameContext)_localctx).name.stop):null));
}
}
}
setState(600);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,41,_ctx);
}
((QualifiedNameContext)_localctx).ast = astFactory.toQualifiedName(names);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class LiteralContext extends ParserRuleContext {
public Expression ast;
public SimpleLiteralContext simpleLiteral;
public SimpleLiteralContext simpleLiteral() {
return getRuleContext(SimpleLiteralContext.class,0);
}
public TerminalNode NULL() { return getToken(FEELParser.NULL, 0); }
public LiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_literal; }
}
public final LiteralContext literal() throws RecognitionException {
LiteralContext _localctx = new LiteralContext(_ctx, getState());
enterRule(_localctx, 78, RULE_literal);
try {
setState(608);
_errHandler.sync(this);
switch (_input.LA(1)) {
case STRING:
case NUMBER:
case TEMPORAL:
case NOT:
case TRUE:
case FALSE:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 1);
{
setState(603);
((LiteralContext)_localctx).simpleLiteral = simpleLiteral();
((LiteralContext)_localctx).ast = ((LiteralContext)_localctx).simpleLiteral.ast;
}
break;
case NULL:
enterOuterAlt(_localctx, 2);
{
setState(606);
match(NULL);
((LiteralContext)_localctx).ast = astFactory.toNullLiteral();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class SimpleLiteralContext extends ParserRuleContext {
public Expression ast;
public NumericLiteralContext numericLiteral;
public StringLiteralContext stringLiteral;
public BooleanLiteralContext booleanLiteral;
public DateTimeLiteralContext dateTimeLiteral;
public NumericLiteralContext numericLiteral() {
return getRuleContext(NumericLiteralContext.class,0);
}
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public BooleanLiteralContext booleanLiteral() {
return getRuleContext(BooleanLiteralContext.class,0);
}
public DateTimeLiteralContext dateTimeLiteral() {
return getRuleContext(DateTimeLiteralContext.class,0);
}
public SimpleLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_simpleLiteral; }
}
public final SimpleLiteralContext simpleLiteral() throws RecognitionException {
SimpleLiteralContext _localctx = new SimpleLiteralContext(_ctx, getState());
enterRule(_localctx, 80, RULE_simpleLiteral);
try {
setState(622);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NUMBER:
enterOuterAlt(_localctx, 1);
{
setState(610);
((SimpleLiteralContext)_localctx).numericLiteral = numericLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).numericLiteral.ast;
}
break;
case STRING:
enterOuterAlt(_localctx, 2);
{
setState(613);
((SimpleLiteralContext)_localctx).stringLiteral = stringLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).stringLiteral.ast;
}
break;
case TRUE:
case FALSE:
enterOuterAlt(_localctx, 3);
{
setState(616);
((SimpleLiteralContext)_localctx).booleanLiteral = booleanLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).booleanLiteral.ast;
}
break;
case TEMPORAL:
case NOT:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 4);
{
setState(619);
((SimpleLiteralContext)_localctx).dateTimeLiteral = dateTimeLiteral();
((SimpleLiteralContext)_localctx).ast = ((SimpleLiteralContext)_localctx).dateTimeLiteral.ast;
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class StringLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode STRING() { return getToken(FEELParser.STRING, 0); }
public StringLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringLiteral; }
}
public final StringLiteralContext stringLiteral() throws RecognitionException {
StringLiteralContext _localctx = new StringLiteralContext(_ctx, getState());
enterRule(_localctx, 82, RULE_stringLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(624);
((StringLiteralContext)_localctx).lit = match(STRING);
((StringLiteralContext)_localctx).ast = astFactory.toStringLiteral((((StringLiteralContext)_localctx).lit!=null?((StringLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class BooleanLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode TRUE() { return getToken(FEELParser.TRUE, 0); }
public TerminalNode FALSE() { return getToken(FEELParser.FALSE, 0); }
public BooleanLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_booleanLiteral; }
}
public final BooleanLiteralContext booleanLiteral() throws RecognitionException {
BooleanLiteralContext _localctx = new BooleanLiteralContext(_ctx, getState());
enterRule(_localctx, 84, RULE_booleanLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(629);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TRUE:
{
setState(627);
((BooleanLiteralContext)_localctx).lit = match(TRUE);
}
break;
case FALSE:
{
setState(628);
((BooleanLiteralContext)_localctx).lit = match(FALSE);
}
break;
default:
throw new NoViableAltException(this);
}
((BooleanLiteralContext)_localctx).ast = astFactory.toBooleanLiteral((((BooleanLiteralContext)_localctx).lit!=null?((BooleanLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NumericLiteralContext extends ParserRuleContext {
public Expression ast;
public Token lit;
public TerminalNode NUMBER() { return getToken(FEELParser.NUMBER, 0); }
public NumericLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_numericLiteral; }
}
public final NumericLiteralContext numericLiteral() throws RecognitionException {
NumericLiteralContext _localctx = new NumericLiteralContext(_ctx, getState());
enterRule(_localctx, 86, RULE_numericLiteral);
try {
enterOuterAlt(_localctx, 1);
{
setState(633);
((NumericLiteralContext)_localctx).lit = match(NUMBER);
((NumericLiteralContext)_localctx).ast = astFactory.toNumericLiteral((((NumericLiteralContext)_localctx).lit!=null?((NumericLiteralContext)_localctx).lit.getText():null));
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class BoxedExpressionContext extends ParserRuleContext {
public Expression ast;
public ListContext list;
public FunctionDefinitionContext functionDefinition;
public ContextContext context;
public ListContext list() {
return getRuleContext(ListContext.class,0);
}
public FunctionDefinitionContext functionDefinition() {
return getRuleContext(FunctionDefinitionContext.class,0);
}
public ContextContext context() {
return getRuleContext(ContextContext.class,0);
}
public BoxedExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_boxedExpression; }
}
public final BoxedExpressionContext boxedExpression() throws RecognitionException {
BoxedExpressionContext _localctx = new BoxedExpressionContext(_ctx, getState());
enterRule(_localctx, 88, RULE_boxedExpression);
try {
setState(645);
_errHandler.sync(this);
switch (_input.LA(1)) {
case BRACKET_OPEN:
enterOuterAlt(_localctx, 1);
{
setState(636);
((BoxedExpressionContext)_localctx).list = list();
((BoxedExpressionContext)_localctx).ast = ((BoxedExpressionContext)_localctx).list.ast;
}
break;
case FUNCTION:
enterOuterAlt(_localctx, 2);
{
setState(639);
((BoxedExpressionContext)_localctx).functionDefinition = functionDefinition();
((BoxedExpressionContext)_localctx).ast = ((BoxedExpressionContext)_localctx).functionDefinition.ast;
}
break;
case BRACE_OPEN:
enterOuterAlt(_localctx, 3);
{
setState(642);
((BoxedExpressionContext)_localctx).context = context();
((BoxedExpressionContext)_localctx).ast = ((BoxedExpressionContext)_localctx).context.ast;
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ListContext extends ParserRuleContext {
public Expression ast;
public ExpressionContext exp;
public TerminalNode BRACKET_OPEN() { return getToken(FEELParser.BRACKET_OPEN, 0); }
public TerminalNode BRACKET_CLOSE() { return getToken(FEELParser.BRACKET_CLOSE, 0); }
public List expression() {
return getRuleContexts(ExpressionContext.class);
}
public ExpressionContext expression(int i) {
return getRuleContext(ExpressionContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public ListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_list; }
}
public final ListContext list() throws RecognitionException {
ListContext _localctx = new ListContext(_ctx, getState());
enterRule(_localctx, 90, RULE_list);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List expressions = new ArrayList<>();
setState(648);
match(BRACKET_OPEN);
setState(660);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,47,_ctx) ) {
case 1:
{
setState(649);
((ListContext)_localctx).exp = expression();
expressions.add(((ListContext)_localctx).exp.ast);
setState(657);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(651);
match(COMMA);
setState(652);
((ListContext)_localctx).exp = expression();
expressions.add(((ListContext)_localctx).exp.ast);
}
}
setState(659);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
break;
}
setState(662);
match(BRACKET_CLOSE);
((ListContext)_localctx).ast = astFactory.toListLiteral(expressions);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ContextContext extends ParserRuleContext {
public Expression ast;
public ContextEntryContext entry;
public TerminalNode BRACE_OPEN() { return getToken(FEELParser.BRACE_OPEN, 0); }
public TerminalNode BRACE_CLOSE() { return getToken(FEELParser.BRACE_CLOSE, 0); }
public List contextEntry() {
return getRuleContexts(ContextEntryContext.class);
}
public ContextEntryContext contextEntry(int i) {
return getRuleContext(ContextEntryContext.class,i);
}
public List COMMA() { return getTokens(FEELParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(FEELParser.COMMA, i);
}
public ContextContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_context; }
}
public final ContextContext context() throws RecognitionException {
ContextContext _localctx = new ContextContext(_ctx, getState());
enterRule(_localctx, 92, RULE_context);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
List entries = new ArrayList<>();
setState(666);
match(BRACE_OPEN);
setState(678);
_errHandler.sync(this);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & 334260661649424L) != 0)) {
{
setState(667);
((ContextContext)_localctx).entry = contextEntry();
entries.add(((ContextContext)_localctx).entry.ast);
setState(675);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==COMMA) {
{
{
setState(669);
match(COMMA);
setState(670);
((ContextContext)_localctx).entry = contextEntry();
entries.add(((ContextContext)_localctx).entry.ast);
}
}
setState(677);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
setState(680);
match(BRACE_CLOSE);
((ContextContext)_localctx).ast = astFactory.toContext(entries);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ContextEntryContext extends ParserRuleContext {
public ContextEntry ast;
public KeyContext key;
public ExpressionContext expression;
public KeyContext key() {
return getRuleContext(KeyContext.class,0);
}
public TerminalNode COLON() { return getToken(FEELParser.COLON, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public ContextEntryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_contextEntry; }
}
public final ContextEntryContext contextEntry() throws RecognitionException {
ContextEntryContext _localctx = new ContextEntryContext(_ctx, getState());
enterRule(_localctx, 94, RULE_contextEntry);
try {
enterOuterAlt(_localctx, 1);
{
setState(683);
((ContextEntryContext)_localctx).key = key();
setState(684);
match(COLON);
setState(685);
((ContextEntryContext)_localctx).expression = expression();
((ContextEntryContext)_localctx).ast = astFactory.toContextEntry(((ContextEntryContext)_localctx).key.ast, ((ContextEntryContext)_localctx).expression.ast);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class KeyContext extends ParserRuleContext {
public ContextEntryKey ast;
public IdentifierContext name;
public StringLiteralContext stringLiteral;
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public StringLiteralContext stringLiteral() {
return getRuleContext(StringLiteralContext.class,0);
}
public KeyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_key; }
}
public final KeyContext key() throws RecognitionException {
KeyContext _localctx = new KeyContext(_ctx, getState());
enterRule(_localctx, 96, RULE_key);
try {
setState(694);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NOT:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 1);
{
setState(688);
((KeyContext)_localctx).name = identifier();
((KeyContext)_localctx).ast = astFactory.toContextEntryKey((((KeyContext)_localctx).name!=null?_input.getText(((KeyContext)_localctx).name.start,((KeyContext)_localctx).name.stop):null));
}
break;
case STRING:
enterOuterAlt(_localctx, 2);
{
setState(691);
((KeyContext)_localctx).stringLiteral = stringLiteral();
((KeyContext)_localctx).ast = astFactory.toContextEntryKey((((KeyContext)_localctx).stringLiteral!=null?_input.getText(((KeyContext)_localctx).stringLiteral.start,((KeyContext)_localctx).stringLiteral.stop):null));
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DateTimeLiteralContext extends ParserRuleContext {
public Expression ast;
public Token token;
public IdentifierContext kind;
public ExpressionContext expression;
public TerminalNode TEMPORAL() { return getToken(FEELParser.TEMPORAL, 0); }
public TerminalNode PAREN_OPEN() { return getToken(FEELParser.PAREN_OPEN, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode PAREN_CLOSE() { return getToken(FEELParser.PAREN_CLOSE, 0); }
public IdentifierContext identifier() {
return getRuleContext(IdentifierContext.class,0);
}
public DateTimeLiteralContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dateTimeLiteral; }
}
public final DateTimeLiteralContext dateTimeLiteral() throws RecognitionException {
DateTimeLiteralContext _localctx = new DateTimeLiteralContext(_ctx, getState());
enterRule(_localctx, 98, RULE_dateTimeLiteral);
try {
setState(704);
_errHandler.sync(this);
switch (_input.LA(1)) {
case TEMPORAL:
enterOuterAlt(_localctx, 1);
{
setState(696);
((DateTimeLiteralContext)_localctx).token = match(TEMPORAL);
((DateTimeLiteralContext)_localctx).ast = astFactory.toDateTimeLiteral((((DateTimeLiteralContext)_localctx).token!=null?((DateTimeLiteralContext)_localctx).token.getText():null));
}
break;
case NOT:
case FUNCTION:
case AND:
case OR:
case NAME:
enterOuterAlt(_localctx, 2);
{
setState(698);
((DateTimeLiteralContext)_localctx).kind = identifier();
setState(699);
match(PAREN_OPEN);
setState(700);
((DateTimeLiteralContext)_localctx).expression = expression();
setState(701);
match(PAREN_CLOSE);
((DateTimeLiteralContext)_localctx).ast = astFactory.toDateTimeLiteral((((DateTimeLiteralContext)_localctx).kind!=null?_input.getText(((DateTimeLiteralContext)_localctx).kind.start,((DateTimeLiteralContext)_localctx).kind.stop):null), ((DateTimeLiteralContext)_localctx).expression.ast);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class IdentifierContext extends ParserRuleContext {
public Token ast;
public Token token;
public TerminalNode NAME() { return getToken(FEELParser.NAME, 0); }
public TerminalNode NOT() { return getToken(FEELParser.NOT, 0); }
public TerminalNode OR() { return getToken(FEELParser.OR, 0); }
public TerminalNode AND() { return getToken(FEELParser.AND, 0); }
public TerminalNode FUNCTION() { return getToken(FEELParser.FUNCTION, 0); }
public IdentifierContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_identifier; }
}
public final IdentifierContext identifier() throws RecognitionException {
IdentifierContext _localctx = new IdentifierContext(_ctx, getState());
enterRule(_localctx, 100, RULE_identifier);
try {
setState(716);
_errHandler.sync(this);
switch (_input.LA(1)) {
case NAME:
enterOuterAlt(_localctx, 1);
{
setState(706);
((IdentifierContext)_localctx).token = match(NAME);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
break;
case NOT:
enterOuterAlt(_localctx, 2);
{
setState(708);
((IdentifierContext)_localctx).token = match(NOT);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
break;
case OR:
enterOuterAlt(_localctx, 3);
{
setState(710);
((IdentifierContext)_localctx).token = match(OR);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
break;
case AND:
enterOuterAlt(_localctx, 4);
{
setState(712);
((IdentifierContext)_localctx).token = match(AND);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
break;
case FUNCTION:
enterOuterAlt(_localctx, 5);
{
setState(714);
((IdentifierContext)_localctx).token = match(FUNCTION);
((IdentifierContext)_localctx).ast = ((IdentifierContext)_localctx).token;
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public boolean sempred(RuleContext _localctx, int ruleIndex, int predIndex) {
switch (ruleIndex) {
case 30:
return type_sempred((TypeContext)_localctx, predIndex);
}
return true;
}
private boolean type_sempred(TypeContext _localctx, int predIndex) {
switch (predIndex) {
case 0:
return "range".equals(((TypeContext)_localctx).typeName.ast.getText()) || "list".equals(((TypeContext)_localctx).typeName.ast.getText());
case 1:
return "context".equals(((TypeContext)_localctx).typeName.ast.getText());
case 2:
return "function".equals(((TypeContext)_localctx).typeName.ast.getText());
}
return true;
}
public static final String _serializedATN =
"\u0004\u00010\u02cf\u0002\u0000\u0007\u0000\u0002\u0001\u0007\u0001\u0002"+
"\u0002\u0007\u0002\u0002\u0003\u0007\u0003\u0002\u0004\u0007\u0004\u0002"+
"\u0005\u0007\u0005\u0002\u0006\u0007\u0006\u0002\u0007\u0007\u0007\u0002"+
"\b\u0007\b\u0002\t\u0007\t\u0002\n\u0007\n\u0002\u000b\u0007\u000b\u0002"+
"\f\u0007\f\u0002\r\u0007\r\u0002\u000e\u0007\u000e\u0002\u000f\u0007\u000f"+
"\u0002\u0010\u0007\u0010\u0002\u0011\u0007\u0011\u0002\u0012\u0007\u0012"+
"\u0002\u0013\u0007\u0013\u0002\u0014\u0007\u0014\u0002\u0015\u0007\u0015"+
"\u0002\u0016\u0007\u0016\u0002\u0017\u0007\u0017\u0002\u0018\u0007\u0018"+
"\u0002\u0019\u0007\u0019\u0002\u001a\u0007\u001a\u0002\u001b\u0007\u001b"+
"\u0002\u001c\u0007\u001c\u0002\u001d\u0007\u001d\u0002\u001e\u0007\u001e"+
"\u0002\u001f\u0007\u001f\u0002 \u0007 \u0002!\u0007!\u0002\"\u0007\"\u0002"+
"#\u0007#\u0002$\u0007$\u0002%\u0007%\u0002&\u0007&\u0002\'\u0007\'\u0002"+
"(\u0007(\u0002)\u0007)\u0002*\u0007*\u0002+\u0007+\u0002,\u0007,\u0002"+
"-\u0007-\u0002.\u0007.\u0002/\u0007/\u00020\u00070\u00021\u00071\u0002"+
"2\u00072\u0001\u0000\u0001\u0000\u0001\u0000\u0001\u0000\u0001\u0001\u0001"+
"\u0001\u0001\u0001\u0001\u0001\u0001\u0002\u0001\u0002\u0001\u0002\u0001"+
"\u0002\u0001\u0003\u0001\u0003\u0001\u0003\u0001\u0003\u0001\u0004\u0001"+
"\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001"+
"\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0003\u0004\u0082\b\u0004\u0001"+
"\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001"+
"\u0005\u0005\u0005\u008b\b\u0005\n\u0005\f\u0005\u008e\t\u0005\u0001\u0005"+
"\u0001\u0005\u0001\u0006\u0001\u0006\u0001\u0006\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0003\u0007\u0099\b\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0003\u0007\u00a1\b\u0007"+
"\u0001\b\u0001\b\u0001\b\u0001\b\u0001\b\u0001\b\u0001\b\u0001\t\u0001"+
"\t\u0001\t\u0001\t\u0001\t\u0001\t\u0003\t\u00b0\b\t\u0001\n\u0001\n\u0001"+
"\n\u0001\n\u0001\n\u0001\n\u0003\n\u00b8\b\n\u0001\u000b\u0001\u000b\u0001"+
"\u000b\u0001\f\u0001\f\u0001\f\u0001\r\u0001\r\u0001\r\u0001\r\u0001\r"+
"\u0001\r\u0001\r\u0005\r\u00c7\b\r\n\r\f\r\u00ca\t\r\u0001\r\u0001\r\u0001"+
"\u000e\u0001\u000e\u0001\u000e\u0001\u000e\u0001\u000e\u0001\u000e\u0001"+
"\u000e\u0001\u000e\u0001\u000e\u0001\u000e\u0001\u000e\u0001\u000e\u0003"+
"\u000e\u00da\b\u000e\u0001\u000f\u0001\u000f\u0001\u000f\u0001\u000f\u0001"+
"\u000f\u0001\u000f\u0001\u000f\u0001\u000f\u0001\u000f\u0001\u000f\u0001"+
"\u000f\u0005\u000f\u00e7\b\u000f\n\u000f\f\u000f\u00ea\t\u000f\u0003\u000f"+
"\u00ec\b\u000f\u0001\u000f\u0001\u000f\u0001\u000f\u0001\u000f\u0001\u000f"+
"\u0003\u000f\u00f3\b\u000f\u0001\u000f\u0001\u000f\u0003\u000f\u00f7\b"+
"\u000f\u0001\u000f\u0001\u000f\u0001\u000f\u0001\u0010\u0001\u0010\u0001"+
"\u0010\u0001\u0010\u0001\u0010\u0001\u0010\u0003\u0010\u0102\b\u0010\u0001"+
"\u0010\u0001\u0010\u0001\u0011\u0001\u0011\u0001\u0011\u0001\u0011\u0001"+
"\u0011\u0001\u0011\u0001\u0011\u0001\u0011\u0001\u0011\u0001\u0011\u0001"+
"\u0011\u0001\u0011\u0005\u0011\u0112\b\u0011\n\u0011\f\u0011\u0115\t\u0011"+
"\u0001\u0011\u0001\u0011\u0001\u0011\u0001\u0011\u0001\u0012\u0001\u0012"+
"\u0001\u0012\u0001\u0012\u0001\u0012\u0001\u0012\u0003\u0012\u0121\b\u0012"+
"\u0001\u0012\u0001\u0012\u0001\u0013\u0001\u0013\u0001\u0013\u0001\u0013"+
"\u0001\u0013\u0001\u0013\u0001\u0013\u0001\u0013\u0001\u0014\u0001\u0014"+
"\u0001\u0014\u0003\u0014\u0130\b\u0014\u0001\u0014\u0001\u0014\u0001\u0014"+
"\u0001\u0014\u0001\u0014\u0001\u0014\u0001\u0014\u0001\u0014\u0001\u0014"+
"\u0005\u0014\u013b\b\u0014\n\u0014\f\u0014\u013e\t\u0014\u0001\u0014\u0001"+
"\u0014\u0001\u0014\u0001\u0014\u0001\u0015\u0001\u0015\u0001\u0015\u0001"+
"\u0015\u0001\u0015\u0001\u0015\u0005\u0015\u014a\b\u0015\n\u0015\f\u0015"+
"\u014d\t\u0015\u0001\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0001\u0016"+
"\u0001\u0016\u0005\u0016\u0155\b\u0016\n\u0016\f\u0016\u0158\t\u0016\u0001"+
"\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001"+
"\u0017\u0001\u0017\u0003\u0017\u0162\b\u0017\u0001\u0017\u0001\u0017\u0001"+
"\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001"+
"\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001"+
"\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0003\u0017\u0177"+
"\b\u0017\u0001\u0018\u0001\u0018\u0001\u0018\u0001\u0019\u0001\u0019\u0001"+
"\u0019\u0001\u0019\u0003\u0019\u0180\b\u0019\u0001\u0019\u0001\u0019\u0001"+
"\u0019\u0005\u0019\u0185\b\u0019\n\u0019\f\u0019\u0188\t\u0019\u0001\u001a"+
"\u0001\u001a\u0001\u001a\u0001\u001a\u0003\u001a\u018e\b\u001a\u0001\u001a"+
"\u0001\u001a\u0001\u001a\u0005\u001a\u0193\b\u001a\n\u001a\f\u001a\u0196"+
"\t\u001a\u0001\u001b\u0001\u001b\u0001\u001b\u0001\u001b\u0001\u001b\u0001"+
"\u001b\u0005\u001b\u019e\b\u001b\n\u001b\f\u001b\u01a1\t\u001b\u0001\u001c"+
"\u0001\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0005\u001c\u01a8\b\u001c"+
"\n\u001c\f\u001c\u01ab\t\u001c\u0001\u001c\u0001\u001c\u0001\u001c\u0001"+
"\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0001\u001d\u0003"+
"\u001d\u01b6\b\u001d\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001"+
"\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001"+
"\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001"+
"\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001"+
"\u001e\u0001\u001e\u0005\u001e\u01d0\b\u001e\n\u001e\f\u001e\u01d3\t\u001e"+
"\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e"+
"\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0005\u001e"+
"\u01e0\b\u001e\n\u001e\f\u001e\u01e3\t\u001e\u0001\u001e\u0001\u001e\u0003"+
"\u001e\u01e7\b\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001\u001e\u0001"+
"\u001e\u0003\u001e\u01ee\b\u001e\u0001\u001f\u0001\u001f\u0001\u001f\u0001"+
"\u001f\u0001\u001f\u0001\u001f\u0001\u001f\u0001\u001f\u0001\u001f\u0001"+
"\u001f\u0001\u001f\u0001\u001f\u0001\u001f\u0001\u001f\u0005\u001f\u01fe"+
"\b\u001f\n\u001f\f\u001f\u0201\t\u001f\u0001 \u0001 \u0001 \u0001 \u0001"+
" \u0001 \u0001 \u0003 \u020a\b \u0001 \u0001 \u0001!\u0001!\u0001!\u0001"+
"!\u0001!\u0001!\u0001!\u0001!\u0001!\u0001!\u0001!\u0005!\u0219\b!\n!"+
"\f!\u021c\t!\u0001!\u0001!\u0001\"\u0001\"\u0001\"\u0001#\u0001#\u0001"+
"#\u0001#\u0001#\u0001#\u0001#\u0005#\u022a\b#\n#\f#\u022d\t#\u0003#\u022f"+
"\b#\u0001#\u0001#\u0001$\u0001$\u0001$\u0001$\u0001$\u0001$\u0001$\u0001"+
"$\u0001$\u0001$\u0001$\u0001$\u0001$\u0001$\u0001$\u0001$\u0001$\u0003"+
"$\u0244\b$\u0001%\u0001%\u0001%\u0001%\u0001%\u0001%\u0003%\u024c\b%\u0001"+
"&\u0001&\u0001&\u0001&\u0001&\u0001&\u0001&\u0005&\u0255\b&\n&\f&\u0258"+
"\t&\u0001&\u0001&\u0001\'\u0001\'\u0001\'\u0001\'\u0001\'\u0003\'\u0261"+
"\b\'\u0001(\u0001(\u0001(\u0001(\u0001(\u0001(\u0001(\u0001(\u0001(\u0001"+
"(\u0001(\u0001(\u0003(\u026f\b(\u0001)\u0001)\u0001)\u0001*\u0001*\u0003"+
"*\u0276\b*\u0001*\u0001*\u0001+\u0001+\u0001+\u0001,\u0001,\u0001,\u0001"+
",\u0001,\u0001,\u0001,\u0001,\u0001,\u0003,\u0286\b,\u0001-\u0001-\u0001"+
"-\u0001-\u0001-\u0001-\u0001-\u0001-\u0005-\u0290\b-\n-\f-\u0293\t-\u0003"+
"-\u0295\b-\u0001-\u0001-\u0001-\u0001.\u0001.\u0001.\u0001.\u0001.\u0001"+
".\u0001.\u0001.\u0005.\u02a2\b.\n.\f.\u02a5\t.\u0003.\u02a7\b.\u0001."+
"\u0001.\u0001.\u0001/\u0001/\u0001/\u0001/\u0001/\u00010\u00010\u0001"+
"0\u00010\u00010\u00010\u00030\u02b7\b0\u00011\u00011\u00011\u00011\u0001"+
"1\u00011\u00011\u00011\u00031\u02c1\b1\u00012\u00012\u00012\u00012\u0001"+
"2\u00012\u00012\u00012\u00012\u00012\u00032\u02cd\b2\u00012\u0000\u0000"+
"3\u0000\u0002\u0004\u0006\b\n\f\u000e\u0010\u0012\u0014\u0016\u0018\u001a"+
"\u001c\u001e \"$&(*,.02468:<>@BDFHJLNPRTVXZ\\^`bd\u0000\u0000\u02ea\u0000"+
"f\u0001\u0000\u0000\u0000\u0002j\u0001\u0000\u0000\u0000\u0004n\u0001"+
"\u0000\u0000\u0000\u0006r\u0001\u0000\u0000\u0000\b\u0081\u0001\u0000"+
"\u0000\u0000\n\u0083\u0001\u0000\u0000\u0000\f\u0091\u0001\u0000\u0000"+
"\u0000\u000e\u00a0\u0001\u0000\u0000\u0000\u0010\u00a2\u0001\u0000\u0000"+
"\u0000\u0012\u00af\u0001\u0000\u0000\u0000\u0014\u00b7\u0001\u0000\u0000"+
"\u0000\u0016\u00b9\u0001\u0000\u0000\u0000\u0018\u00bc\u0001\u0000\u0000"+
"\u0000\u001a\u00bf\u0001\u0000\u0000\u0000\u001c\u00d9\u0001\u0000\u0000"+
"\u0000\u001e\u00db\u0001\u0000\u0000\u0000 \u00fb\u0001\u0000\u0000\u0000"+
"\"\u0105\u0001\u0000\u0000\u0000$\u011a\u0001\u0000\u0000\u0000&\u0124"+
"\u0001\u0000\u0000\u0000(\u012c\u0001\u0000\u0000\u0000*\u0143\u0001\u0000"+
"\u0000\u0000,\u014e\u0001\u0000\u0000\u0000.\u0159\u0001\u0000\u0000\u0000"+
"0\u0178\u0001\u0000\u0000\u00002\u017b\u0001\u0000\u0000\u00004\u0189"+
"\u0001\u0000\u0000\u00006\u0197\u0001\u0000\u0000\u00008\u01a2\u0001\u0000"+
"\u0000\u0000:\u01af\u0001\u0000\u0000\u0000<\u01ed\u0001\u0000\u0000\u0000"+
">\u01ef\u0001\u0000\u0000\u0000@\u0202\u0001\u0000\u0000\u0000B\u020d"+
"\u0001\u0000\u0000\u0000D\u021f\u0001\u0000\u0000\u0000F\u0222\u0001\u0000"+
"\u0000\u0000H\u0243\u0001\u0000\u0000\u0000J\u024b\u0001\u0000\u0000\u0000"+
"L\u024d\u0001\u0000\u0000\u0000N\u0260\u0001\u0000\u0000\u0000P\u026e"+
"\u0001\u0000\u0000\u0000R\u0270\u0001\u0000\u0000\u0000T\u0275\u0001\u0000"+
"\u0000\u0000V\u0279\u0001\u0000\u0000\u0000X\u0285\u0001\u0000\u0000\u0000"+
"Z\u0287\u0001\u0000\u0000\u0000\\\u0299\u0001\u0000\u0000\u0000^\u02ab"+
"\u0001\u0000\u0000\u0000`\u02b6\u0001\u0000\u0000\u0000b\u02c0\u0001\u0000"+
"\u0000\u0000d\u02cc\u0001\u0000\u0000\u0000fg\u0003\b\u0004\u0000gh\u0006"+
"\u0000\uffff\uffff\u0000hi\u0005\u0000\u0000\u0001i\u0001\u0001\u0000"+
"\u0000\u0000jk\u0003\u0018\f\u0000kl\u0006\u0001\uffff\uffff\u0000lm\u0005"+
"\u0000\u0000\u0001m\u0003\u0001\u0000\u0000\u0000no\u0003\u001a\r\u0000"+
"op\u0006\u0002\uffff\uffff\u0000pq\u0005\u0000\u0000\u0001q\u0005\u0001"+
"\u0000\u0000\u0000rs\u0003X,\u0000st\u0006\u0003\uffff\uffff\u0000tu\u0005"+
"\u0000\u0000\u0001u\u0007\u0001\u0000\u0000\u0000vw\u0005\u001d\u0000"+
"\u0000wx\u0005\u0015\u0000\u0000xy\u0003\n\u0005\u0000yz\u0005\u0016\u0000"+
"\u0000z{\u0006\u0004\uffff\uffff\u0000{\u0082\u0001\u0000\u0000\u0000"+
"|}\u0003\n\u0005\u0000}~\u0006\u0004\uffff\uffff\u0000~\u0082\u0001\u0000"+
"\u0000\u0000\u007f\u0080\u0005\u000e\u0000\u0000\u0080\u0082\u0006\u0004"+
"\uffff\uffff\u0000\u0081v\u0001\u0000\u0000\u0000\u0081|\u0001\u0000\u0000"+
"\u0000\u0081\u007f\u0001\u0000\u0000\u0000\u0082\t\u0001\u0000\u0000\u0000"+
"\u0083\u0084\u0006\u0005\uffff\uffff\u0000\u0084\u0085\u0003\f\u0006\u0000"+
"\u0085\u008c\u0006\u0005\uffff\uffff\u0000\u0086\u0087\u0005\u0014\u0000"+
"\u0000\u0087\u0088\u0003\f\u0006\u0000\u0088\u0089\u0006\u0005\uffff\uffff"+
"\u0000\u0089\u008b\u0001\u0000\u0000\u0000\u008a\u0086\u0001\u0000\u0000"+
"\u0000\u008b\u008e\u0001\u0000\u0000\u0000\u008c\u008a\u0001\u0000\u0000"+
"\u0000\u008c\u008d\u0001\u0000\u0000\u0000\u008d\u008f\u0001\u0000\u0000"+
"\u0000\u008e\u008c\u0001\u0000\u0000\u0000\u008f\u0090\u0006\u0005\uffff"+
"\uffff\u0000\u0090\u000b\u0001\u0000\u0000\u0000\u0091\u0092\u0003\u0018"+
"\f\u0000\u0092\u0093\u0006\u0006\uffff\uffff\u0000\u0093\r\u0001\u0000"+
"\u0000\u0000\u0094\u0099\u0005\t\u0000\u0000\u0095\u0099\u0005\u000b\u0000"+
"\u0000\u0096\u0099\u0005\n\u0000\u0000\u0097\u0099\u0005\f\u0000\u0000"+
"\u0098\u0094\u0001\u0000\u0000\u0000\u0098\u0095\u0001\u0000\u0000\u0000"+
"\u0098\u0096\u0001\u0000\u0000\u0000\u0098\u0097\u0001\u0000\u0000\u0000"+
"\u0099\u009a\u0001\u0000\u0000\u0000\u009a\u009b\u0003\u0016\u000b\u0000"+
"\u009b\u009c\u0006\u0007\uffff\uffff\u0000\u009c\u00a1\u0001\u0000\u0000"+
"\u0000\u009d\u009e\u0003\u0010\b\u0000\u009e\u009f\u0006\u0007\uffff\uffff"+
"\u0000\u009f\u00a1\u0001\u0000\u0000\u0000\u00a0\u0098\u0001\u0000\u0000"+
"\u0000\u00a0\u009d\u0001\u0000\u0000\u0000\u00a1\u000f\u0001\u0000\u0000"+
"\u0000\u00a2\u00a3\u0003\u0012\t\u0000\u00a3\u00a4\u0003\u0016\u000b\u0000"+
"\u00a4\u00a5\u0005\u0012\u0000\u0000\u00a5\u00a6\u0003\u0016\u000b\u0000"+
"\u00a6\u00a7\u0003\u0014\n\u0000\u00a7\u00a8\u0006\b\uffff\uffff\u0000"+
"\u00a8\u0011\u0001\u0000\u0000\u0000\u00a9\u00aa\u0005\u0015\u0000\u0000"+
"\u00aa\u00b0\u0006\t\uffff\uffff\u0000\u00ab\u00ac\u0005\u0018\u0000\u0000"+
"\u00ac\u00b0\u0006\t\uffff\uffff\u0000\u00ad\u00ae\u0005\u0017\u0000\u0000"+
"\u00ae\u00b0\u0006\t\uffff\uffff\u0000\u00af\u00a9\u0001\u0000\u0000\u0000"+
"\u00af\u00ab\u0001\u0000\u0000\u0000\u00af\u00ad\u0001\u0000\u0000\u0000"+
"\u00b0\u0013\u0001\u0000\u0000\u0000\u00b1\u00b2\u0005\u0016\u0000\u0000"+
"\u00b2\u00b8\u0006\n\uffff\uffff\u0000\u00b3\u00b4\u0005\u0017\u0000\u0000"+
"\u00b4\u00b8\u0006\n\uffff\uffff\u0000\u00b5\u00b6\u0005\u0018\u0000\u0000"+
"\u00b6\u00b8\u0006\n\uffff\uffff\u0000\u00b7\u00b1\u0001\u0000\u0000\u0000"+
"\u00b7\u00b3\u0001\u0000\u0000\u0000\u00b7\u00b5\u0001\u0000\u0000\u0000"+
"\u00b8\u0015\u0001\u0000\u0000\u0000\u00b9\u00ba\u0003\u0018\f\u0000\u00ba"+
"\u00bb\u0006\u000b\uffff\uffff\u0000\u00bb\u0017\u0001\u0000\u0000\u0000"+
"\u00bc\u00bd\u0003\u001c\u000e\u0000\u00bd\u00be\u0006\f\uffff\uffff\u0000"+
"\u00be\u0019\u0001\u0000\u0000\u0000\u00bf\u00c0\u0006\r\uffff\uffff\u0000"+
"\u00c0\u00c1\u0003\u001c\u000e\u0000\u00c1\u00c8\u0006\r\uffff\uffff\u0000"+
"\u00c2\u00c3\u0005\u0014\u0000\u0000\u00c3\u00c4\u0003\u001c\u000e\u0000"+
"\u00c4\u00c5\u0006\r\uffff\uffff\u0000\u00c5\u00c7\u0001\u0000\u0000\u0000"+
"\u00c6\u00c2\u0001\u0000\u0000\u0000\u00c7\u00ca\u0001\u0000\u0000\u0000"+
"\u00c8\u00c6\u0001\u0000\u0000\u0000\u00c8\u00c9\u0001\u0000\u0000\u0000"+
"\u00c9\u00cb\u0001\u0000\u0000\u0000\u00ca\u00c8\u0001\u0000\u0000\u0000"+
"\u00cb\u00cc\u0006\r\uffff\uffff\u0000\u00cc\u001b\u0001\u0000\u0000\u0000"+
"\u00cd\u00ce\u0003\"\u0011\u0000\u00ce\u00cf\u0006\u000e\uffff\uffff\u0000"+
"\u00cf\u00da\u0001\u0000\u0000\u0000\u00d0\u00d1\u0003&\u0013\u0000\u00d1"+
"\u00d2\u0006\u000e\uffff\uffff\u0000\u00d2\u00da\u0001\u0000\u0000\u0000"+
"\u00d3\u00d4\u0003(\u0014\u0000\u00d4\u00d5\u0006\u000e\uffff\uffff\u0000"+
"\u00d5\u00da\u0001\u0000\u0000\u0000\u00d6\u00d7\u0003*\u0015\u0000\u00d7"+
"\u00d8\u0006\u000e\uffff\uffff\u0000\u00d8\u00da\u0001\u0000\u0000\u0000"+
"\u00d9\u00cd\u0001\u0000\u0000\u0000\u00d9\u00d0\u0001\u0000\u0000\u0000"+
"\u00d9\u00d3\u0001\u0000\u0000\u0000\u00d9\u00d6\u0001\u0000\u0000\u0000"+
"\u00da\u001d\u0001\u0000\u0000\u0000\u00db\u00dc\u0006\u000f\uffff\uffff"+
"\u0000\u00dc\u00dd\u0006\u000f\uffff\uffff\u0000\u00dd\u00de\u0006\u000f"+
"\uffff\uffff\u0000\u00de\u00df\u0005!\u0000\u0000\u00df\u00eb\u0005\u0015"+
"\u0000\u0000\u00e0\u00e1\u0003 \u0010\u0000\u00e1\u00e8\u0006\u000f\uffff"+
"\uffff\u0000\u00e2\u00e3\u0005\u0014\u0000\u0000\u00e3\u00e4\u0003 \u0010"+
"\u0000\u00e4\u00e5\u0006\u000f\uffff\uffff\u0000\u00e5\u00e7\u0001\u0000"+
"\u0000\u0000\u00e6\u00e2\u0001\u0000\u0000\u0000\u00e7\u00ea\u0001\u0000"+
"\u0000\u0000\u00e8\u00e6\u0001\u0000\u0000\u0000\u00e8\u00e9\u0001\u0000"+
"\u0000\u0000\u00e9\u00ec\u0001\u0000\u0000\u0000\u00ea\u00e8\u0001\u0000"+
"\u0000\u0000\u00eb\u00e0\u0001\u0000\u0000\u0000\u00eb\u00ec\u0001\u0000"+
"\u0000\u0000\u00ec\u00ed\u0001\u0000\u0000\u0000\u00ed\u00f2\u0005\u0016"+
"\u0000\u0000\u00ee\u00ef\u0005\u001b\u0000\u0000\u00ef\u00f0\u0003<\u001e"+
"\u0000\u00f0\u00f1\u0006\u000f\uffff\uffff\u0000\u00f1\u00f3\u0001\u0000"+
"\u0000\u0000\u00f2\u00ee\u0001\u0000\u0000\u0000\u00f2\u00f3\u0001\u0000"+
"\u0000\u0000\u00f3\u00f6\u0001\u0000\u0000\u0000\u00f4\u00f5\u0005\"\u0000"+
"\u0000\u00f5\u00f7\u0006\u000f\uffff\uffff\u0000\u00f6\u00f4\u0001\u0000"+
"\u0000\u0000\u00f6\u00f7\u0001\u0000\u0000\u0000\u00f7\u00f8\u0001\u0000"+
"\u0000\u0000\u00f8\u00f9\u0003\u0018\f\u0000\u00f9\u00fa\u0006\u000f\uffff"+
"\uffff\u0000\u00fa\u001f\u0001\u0000\u0000\u0000\u00fb\u00fc\u0006\u0010"+
"\uffff\uffff\u0000\u00fc\u0101\u0003D\"\u0000\u00fd\u00fe\u0005\u001b"+
"\u0000\u0000\u00fe\u00ff\u0003<\u001e\u0000\u00ff\u0100\u0006\u0010\uffff"+
"\uffff\u0000\u0100\u0102\u0001\u0000\u0000\u0000\u0101\u00fd\u0001\u0000"+
"\u0000\u0000\u0101\u0102\u0001\u0000\u0000\u0000\u0102\u0103\u0001\u0000"+
"\u0000\u0000\u0103\u0104\u0006\u0010\uffff\uffff\u0000\u0104!\u0001\u0000"+
"\u0000\u0000\u0105\u0106\u0006\u0011\uffff\uffff\u0000\u0106\u0107\u0005"+
"#\u0000\u0000\u0107\u0108\u0003d2\u0000\u0108\u0109\u0005$\u0000\u0000"+
"\u0109\u010a\u0003$\u0012\u0000\u010a\u0113\u0006\u0011\uffff\uffff\u0000"+
"\u010b\u010c\u0005\u0014\u0000\u0000\u010c\u010d\u0003d2\u0000\u010d\u010e"+
"\u0005$\u0000\u0000\u010e\u010f\u0003$\u0012\u0000\u010f\u0110\u0006\u0011"+
"\uffff\uffff\u0000\u0110\u0112\u0001\u0000\u0000\u0000\u0111\u010b\u0001"+
"\u0000\u0000\u0000\u0112\u0115\u0001\u0000\u0000\u0000\u0113\u0111\u0001"+
"\u0000\u0000\u0000\u0113\u0114\u0001\u0000\u0000\u0000\u0114\u0116\u0001"+
"\u0000\u0000\u0000\u0115\u0113\u0001\u0000\u0000\u0000\u0116\u0117\u0005"+
"%\u0000\u0000\u0117\u0118\u0003\u0018\f\u0000\u0118\u0119\u0006\u0011"+
"\uffff\uffff\u0000\u0119#\u0001\u0000\u0000\u0000\u011a\u011b\u0006\u0012"+
"\uffff\uffff\u0000\u011b\u0120\u0003\u0018\f\u0000\u011c\u011d\u0005\u0012"+
"\u0000\u0000\u011d\u011e\u0003\u0018\f\u0000\u011e\u011f\u0006\u0012\uffff"+
"\uffff\u0000\u011f\u0121\u0001\u0000\u0000\u0000\u0120\u011c\u0001\u0000"+
"\u0000\u0000\u0120\u0121\u0001\u0000\u0000\u0000\u0121\u0122\u0001\u0000"+
"\u0000\u0000\u0122\u0123\u0006\u0012\uffff\uffff\u0000\u0123%\u0001\u0000"+
"\u0000\u0000\u0124\u0125\u0005&\u0000\u0000\u0125\u0126\u0003\u0018\f"+
"\u0000\u0126\u0127\u0005\'\u0000\u0000\u0127\u0128\u0003\u0018\f\u0000"+
"\u0128\u0129\u0005(\u0000\u0000\u0129\u012a\u0003\u0018\f\u0000\u012a"+
"\u012b\u0006\u0013\uffff\uffff\u0000\u012b\'\u0001\u0000\u0000\u0000\u012c"+
"\u012f\u0006\u0014\uffff\uffff\u0000\u012d\u0130\u0005)\u0000\u0000\u012e"+
"\u0130\u0005*\u0000\u0000\u012f\u012d\u0001\u0000\u0000\u0000\u012f\u012e"+
"\u0001\u0000\u0000\u0000\u0130\u0131\u0001\u0000\u0000\u0000\u0131\u0132"+
"\u0003d2\u0000\u0132\u0133\u0005$\u0000\u0000\u0133\u0134\u0003\u0018"+
"\f\u0000\u0134\u013c\u0006\u0014\uffff\uffff\u0000\u0135\u0136\u0003d"+
"2\u0000\u0136\u0137\u0005$\u0000\u0000\u0137\u0138\u0003\u0018\f\u0000"+
"\u0138\u0139\u0006\u0014\uffff\uffff\u0000\u0139\u013b\u0001\u0000\u0000"+
"\u0000\u013a\u0135\u0001\u0000\u0000\u0000\u013b\u013e\u0001\u0000\u0000"+
"\u0000\u013c\u013a\u0001\u0000\u0000\u0000\u013c\u013d\u0001\u0000\u0000"+
"\u0000\u013d\u013f\u0001\u0000\u0000\u0000\u013e\u013c\u0001\u0000\u0000"+
"\u0000\u013f\u0140\u0005+\u0000\u0000\u0140\u0141\u0003\u0018\f\u0000"+
"\u0141\u0142\u0006\u0014\uffff\uffff\u0000\u0142)\u0001\u0000\u0000\u0000"+
"\u0143\u0144\u0003,\u0016\u0000\u0144\u014b\u0006\u0015\uffff\uffff\u0000"+
"\u0145\u0146\u0005-\u0000\u0000\u0146\u0147\u0003,\u0016\u0000\u0147\u0148"+
"\u0006\u0015\uffff\uffff\u0000\u0148\u014a\u0001\u0000\u0000\u0000\u0149"+
"\u0145\u0001\u0000\u0000\u0000\u014a\u014d\u0001\u0000\u0000\u0000\u014b"+
"\u0149\u0001\u0000\u0000\u0000\u014b\u014c\u0001\u0000\u0000\u0000\u014c"+
"+\u0001\u0000\u0000\u0000\u014d\u014b\u0001\u0000\u0000\u0000\u014e\u014f"+
"\u0003.\u0017\u0000\u014f\u0156\u0006\u0016\uffff\uffff\u0000\u0150\u0151"+
"\u0005,\u0000\u0000\u0151\u0152\u0003.\u0017\u0000\u0152\u0153\u0006\u0016"+
"\uffff\uffff\u0000\u0153\u0155\u0001\u0000\u0000\u0000\u0154\u0150\u0001"+
"\u0000\u0000\u0000\u0155\u0158\u0001\u0000\u0000\u0000\u0156\u0154\u0001"+
"\u0000\u0000\u0000\u0156\u0157\u0001\u0000\u0000\u0000\u0157-\u0001\u0000"+
"\u0000\u0000\u0158\u0156\u0001\u0000\u0000\u0000\u0159\u015a\u00030\u0018"+
"\u0000\u015a\u0176\u0006\u0017\uffff\uffff\u0000\u015b\u0162\u0005\u0007"+
"\u0000\u0000\u015c\u0162\u0005\b\u0000\u0000\u015d\u0162\u0005\t\u0000"+
"\u0000\u015e\u0162\u0005\n\u0000\u0000\u015f\u0162\u0005\u000b\u0000\u0000"+
"\u0160\u0162\u0005\f\u0000\u0000\u0161\u015b\u0001\u0000\u0000\u0000\u0161"+
"\u015c\u0001\u0000\u0000\u0000\u0161\u015d\u0001\u0000\u0000\u0000\u0161"+
"\u015e\u0001\u0000\u0000\u0000\u0161\u015f\u0001\u0000\u0000\u0000\u0161"+
"\u0160\u0001\u0000\u0000\u0000\u0162\u0163\u0001\u0000\u0000\u0000\u0163"+
"\u0164\u00030\u0018\u0000\u0164\u0165\u0006\u0017\uffff\uffff\u0000\u0165"+
"\u0177\u0001\u0000\u0000\u0000\u0166\u0167\u0005.\u0000\u0000\u0167\u0168"+
"\u0003\u0018\f\u0000\u0168\u0169\u0005,\u0000\u0000\u0169\u016a\u0003"+
"\u0018\f\u0000\u016a\u016b\u0006\u0017\uffff\uffff\u0000\u016b\u0177\u0001"+
"\u0000\u0000\u0000\u016c\u016d\u0005$\u0000\u0000\u016d\u016e\u0003\f"+
"\u0006\u0000\u016e\u016f\u0006\u0017\uffff\uffff\u0000\u016f\u0177\u0001"+
"\u0000\u0000\u0000\u0170\u0171\u0005$\u0000\u0000\u0171\u0172\u0005\u0015"+
"\u0000\u0000\u0172\u0173\u0003\n\u0005\u0000\u0173\u0174\u0005\u0016\u0000"+
"\u0000\u0174\u0175\u0006\u0017\uffff\uffff\u0000\u0175\u0177\u0001\u0000"+
"\u0000\u0000\u0176\u0161\u0001\u0000\u0000\u0000\u0176\u0166\u0001\u0000"+
"\u0000\u0000\u0176\u016c\u0001\u0000\u0000\u0000\u0176\u0170\u0001\u0000"+
"\u0000\u0000\u0176\u0177\u0001\u0000\u0000\u0000\u0177/\u0001\u0000\u0000"+
"\u0000\u0178\u0179\u00032\u0019\u0000\u0179\u017a\u0006\u0018\uffff\uffff"+
"\u0000\u017a1\u0001\u0000\u0000\u0000\u017b\u017c\u00034\u001a\u0000\u017c"+
"\u0186\u0006\u0019\uffff\uffff\u0000\u017d\u0180\u0005\r\u0000\u0000\u017e"+
"\u0180\u0005\u000e\u0000\u0000\u017f\u017d\u0001\u0000\u0000\u0000\u017f"+
"\u017e\u0001\u0000\u0000\u0000\u0180\u0181\u0001\u0000\u0000\u0000\u0181"+
"\u0182\u00034\u001a\u0000\u0182\u0183\u0006\u0019\uffff\uffff\u0000\u0183"+
"\u0185\u0001\u0000\u0000\u0000\u0184\u017f\u0001\u0000\u0000\u0000\u0185"+
"\u0188\u0001\u0000\u0000\u0000\u0186\u0184\u0001\u0000\u0000\u0000\u0186"+
"\u0187\u0001\u0000\u0000\u0000\u01873\u0001\u0000\u0000\u0000\u0188\u0186"+
"\u0001\u0000\u0000\u0000\u0189\u018a\u00036\u001b\u0000\u018a\u0194\u0006"+
"\u001a\uffff\uffff\u0000\u018b\u018e\u0005\u000f\u0000\u0000\u018c\u018e"+
"\u0005\u0010\u0000\u0000\u018d\u018b\u0001\u0000\u0000\u0000\u018d\u018c"+
"\u0001\u0000\u0000\u0000\u018e\u018f\u0001\u0000\u0000\u0000\u018f\u0190"+
"\u00036\u001b\u0000\u0190\u0191\u0006\u001a\uffff\uffff\u0000\u0191\u0193"+
"\u0001\u0000\u0000\u0000\u0192\u018d\u0001\u0000\u0000\u0000\u0193\u0196"+
"\u0001\u0000\u0000\u0000\u0194\u0192\u0001\u0000\u0000\u0000\u0194\u0195"+
"\u0001\u0000\u0000\u0000\u01955\u0001\u0000\u0000\u0000\u0196\u0194\u0001"+
"\u0000\u0000\u0000\u0197\u0198\u00038\u001c\u0000\u0198\u019f\u0006\u001b"+
"\uffff\uffff\u0000\u0199\u019a\u0005\u0011\u0000\u0000\u019a\u019b\u0003"+
"8\u001c\u0000\u019b\u019c\u0006\u001b\uffff\uffff\u0000\u019c\u019e\u0001"+
"\u0000\u0000\u0000\u019d\u0199\u0001\u0000\u0000\u0000\u019e\u01a1\u0001"+
"\u0000\u0000\u0000\u019f\u019d\u0001\u0000\u0000\u0000\u019f\u01a0\u0001"+
"\u0000\u0000\u0000\u01a07\u0001\u0000\u0000\u0000\u01a1\u019f\u0001\u0000"+
"\u0000\u0000\u01a2\u01a9\u0006\u001c\uffff\uffff\u0000\u01a3\u01a4\u0005"+
"\u000e\u0000\u0000\u01a4\u01a8\u0006\u001c\uffff\uffff\u0000\u01a5\u01a6"+
"\u0005\u001d\u0000\u0000\u01a6\u01a8\u0006\u001c\uffff\uffff\u0000\u01a7"+
"\u01a3\u0001\u0000\u0000\u0000\u01a7\u01a5\u0001\u0000\u0000\u0000\u01a8"+
"\u01ab\u0001\u0000\u0000\u0000\u01a9\u01a7\u0001\u0000\u0000\u0000\u01a9"+
"\u01aa\u0001\u0000\u0000\u0000\u01aa\u01ac\u0001\u0000\u0000\u0000\u01ab"+
"\u01a9\u0001\u0000\u0000\u0000\u01ac\u01ad\u0003:\u001d\u0000\u01ad\u01ae"+
"\u0006\u001c\uffff\uffff\u0000\u01ae9\u0001\u0000\u0000\u0000\u01af\u01b0"+
"\u0003>\u001f\u0000\u01b0\u01b5\u0006\u001d\uffff\uffff\u0000\u01b1\u01b2"+
"\u0005/\u0000\u0000\u01b2\u01b3\u0003<\u001e\u0000\u01b3\u01b4\u0006\u001d"+
"\uffff\uffff\u0000\u01b4\u01b6\u0001\u0000\u0000\u0000\u01b5\u01b1\u0001"+
"\u0000\u0000\u0000\u01b5\u01b6\u0001\u0000\u0000\u0000\u01b6;\u0001\u0000"+
"\u0000\u0000\u01b7\u01b8\u0003L&\u0000\u01b8\u01b9\u0006\u001e\uffff\uffff"+
"\u0000\u01b9\u01ee\u0001\u0000\u0000\u0000\u01ba\u01bb\u0003d2\u0000\u01bb"+
"\u01bc\u0004\u001e\u0000\u0001\u01bc\u01bd\u0005\t\u0000\u0000\u01bd\u01be"+
"\u0003<\u001e\u0000\u01be\u01bf\u0005\n\u0000\u0000\u01bf\u01c0\u0006"+
"\u001e\uffff\uffff\u0000\u01c0\u01ee\u0001\u0000\u0000\u0000\u01c1\u01c2"+
"\u0006\u001e\uffff\uffff\u0000\u01c2\u01c3\u0003d2\u0000\u01c3\u01c4\u0004"+
"\u001e\u0001\u0001\u01c4\u01c5\u0005\t\u0000\u0000\u01c5\u01c6\u0003d"+
"2\u0000\u01c6\u01c7\u0005\u001b\u0000\u0000\u01c7\u01c8\u0003<\u001e\u0000"+
"\u01c8\u01d1\u0006\u001e\uffff\uffff\u0000\u01c9\u01ca\u0005\u0014\u0000"+
"\u0000\u01ca\u01cb\u0003d2\u0000\u01cb\u01cc\u0005\u001b\u0000\u0000\u01cc"+
"\u01cd\u0003<\u001e\u0000\u01cd\u01ce\u0006\u001e\uffff\uffff\u0000\u01ce"+
"\u01d0\u0001\u0000\u0000\u0000\u01cf\u01c9\u0001\u0000\u0000\u0000\u01d0"+
"\u01d3\u0001\u0000\u0000\u0000\u01d1\u01cf\u0001\u0000\u0000\u0000\u01d1"+
"\u01d2\u0001\u0000\u0000\u0000\u01d2\u01d4\u0001\u0000\u0000\u0000\u01d3"+
"\u01d1\u0001\u0000\u0000\u0000\u01d4\u01d5\u0005\n\u0000\u0000\u01d5\u01d6"+
"\u0006\u001e\uffff\uffff\u0000\u01d6\u01ee\u0001\u0000\u0000\u0000\u01d7"+
"\u01d8\u0006\u001e\uffff\uffff\u0000\u01d8\u01d9\u0003d2\u0000\u01d9\u01da"+
"\u0004\u001e\u0002\u0001\u01da\u01e6\u0005\t\u0000\u0000\u01db\u01dc\u0003"+
"<\u001e\u0000\u01dc\u01e1\u0006\u001e\uffff\uffff\u0000\u01dd\u01de\u0005"+
"\u0014\u0000\u0000\u01de\u01e0\u0003<\u001e\u0000\u01df\u01dd\u0001\u0000"+
"\u0000\u0000\u01e0\u01e3\u0001\u0000\u0000\u0000\u01e1\u01df\u0001\u0000"+
"\u0000\u0000\u01e1\u01e2\u0001\u0000\u0000\u0000\u01e2\u01e4\u0001\u0000"+
"\u0000\u0000\u01e3\u01e1\u0001\u0000\u0000\u0000\u01e4\u01e5\u0006\u001e"+
"\uffff\uffff\u0000\u01e5\u01e7\u0001\u0000\u0000\u0000\u01e6\u01db\u0001"+
"\u0000\u0000\u0000\u01e6\u01e7\u0001\u0000\u0000\u0000\u01e7\u01e8\u0001"+
"\u0000\u0000\u0000\u01e8\u01e9\u0005\n\u0000\u0000\u01e9\u01ea\u0005\u001c"+
"\u0000\u0000\u01ea\u01eb\u0003<\u001e\u0000\u01eb\u01ec\u0006\u001e\uffff"+
"\uffff\u0000\u01ec\u01ee\u0001\u0000\u0000\u0000\u01ed\u01b7\u0001\u0000"+
"\u0000\u0000\u01ed\u01ba\u0001\u0000\u0000\u0000\u01ed\u01c1\u0001\u0000"+
"\u0000\u0000\u01ed\u01d7\u0001\u0000\u0000\u0000\u01ee=\u0001\u0000\u0000"+
"\u0000\u01ef\u01f0\u0003H$\u0000\u01f0\u01ff\u0006\u001f\uffff\uffff\u0000"+
"\u01f1\u01f2\u0005\u0017\u0000\u0000\u01f2\u01f3\u0003\u0018\f\u0000\u01f3"+
"\u01f4\u0005\u0018\u0000\u0000\u01f4\u01f5\u0006\u001f\uffff\uffff\u0000"+
"\u01f5\u01fe\u0001\u0000\u0000\u0000\u01f6\u01f7\u0003@ \u0000\u01f7\u01f8"+
"\u0006\u001f\uffff\uffff\u0000\u01f8\u01fe\u0001\u0000\u0000\u0000\u01f9"+
"\u01fa\u0005\u0013\u0000\u0000\u01fa\u01fb\u0003d2\u0000\u01fb\u01fc\u0006"+
"\u001f\uffff\uffff\u0000\u01fc\u01fe\u0001\u0000\u0000\u0000\u01fd\u01f1"+
"\u0001\u0000\u0000\u0000\u01fd\u01f6\u0001\u0000\u0000\u0000\u01fd\u01f9"+
"\u0001\u0000\u0000\u0000\u01fe\u0201\u0001\u0000\u0000\u0000\u01ff\u01fd"+
"\u0001\u0000\u0000\u0000\u01ff\u0200\u0001\u0000\u0000\u0000\u0200?\u0001"+
"\u0000\u0000\u0000\u0201\u01ff\u0001\u0000\u0000\u0000\u0202\u0209\u0005"+
"\u0015\u0000\u0000\u0203\u0204\u0003B!\u0000\u0204\u0205\u0006 \uffff"+
"\uffff\u0000\u0205\u020a\u0001\u0000\u0000\u0000\u0206\u0207\u0003F#\u0000"+
"\u0207\u0208\u0006 \uffff\uffff\u0000\u0208\u020a\u0001\u0000\u0000\u0000"+
"\u0209\u0203\u0001\u0000\u0000\u0000\u0209\u0206\u0001\u0000\u0000\u0000"+
"\u020a\u020b\u0001\u0000\u0000\u0000\u020b\u020c\u0005\u0016\u0000\u0000"+
"\u020cA\u0001\u0000\u0000\u0000\u020d\u020e\u0006!\uffff\uffff\u0000\u020e"+
"\u020f\u0003D\"\u0000\u020f\u0210\u0005\u001b\u0000\u0000\u0210\u0211"+
"\u0003\u0018\f\u0000\u0211\u021a\u0006!\uffff\uffff\u0000\u0212\u0213"+
"\u0005\u0014\u0000\u0000\u0213\u0214\u0003D\"\u0000\u0214\u0215\u0005"+
"\u001b\u0000\u0000\u0215\u0216\u0003\u0018\f\u0000\u0216\u0217\u0006!"+
"\uffff\uffff\u0000\u0217\u0219\u0001\u0000\u0000\u0000\u0218\u0212\u0001"+
"\u0000\u0000\u0000\u0219\u021c\u0001\u0000\u0000\u0000\u021a\u0218\u0001"+
"\u0000\u0000\u0000\u021a\u021b\u0001\u0000\u0000\u0000\u021b\u021d\u0001"+
"\u0000\u0000\u0000\u021c\u021a\u0001\u0000\u0000\u0000\u021d\u021e\u0006"+
"!\uffff\uffff\u0000\u021eC\u0001\u0000\u0000\u0000\u021f\u0220\u0003d"+
"2\u0000\u0220\u0221\u0006\"\uffff\uffff\u0000\u0221E\u0001\u0000\u0000"+
"\u0000\u0222\u022e\u0006#\uffff\uffff\u0000\u0223\u0224\u0003\u0018\f"+
"\u0000\u0224\u022b\u0006#\uffff\uffff\u0000\u0225\u0226\u0005\u0014\u0000"+
"\u0000\u0226\u0227\u0003\u0018\f\u0000\u0227\u0228\u0006#\uffff\uffff"+
"\u0000\u0228\u022a\u0001\u0000\u0000\u0000\u0229\u0225\u0001\u0000\u0000"+
"\u0000\u022a\u022d\u0001\u0000\u0000\u0000\u022b\u0229\u0001\u0000\u0000"+
"\u0000\u022b\u022c\u0001\u0000\u0000\u0000\u022c\u022f\u0001\u0000\u0000"+
"\u0000\u022d\u022b\u0001\u0000\u0000\u0000\u022e\u0223\u0001\u0000\u0000"+
"\u0000\u022e\u022f\u0001\u0000\u0000\u0000\u022f\u0230\u0001\u0000\u0000"+
"\u0000\u0230\u0231\u0006#\uffff\uffff\u0000\u0231G\u0001\u0000\u0000\u0000"+
"\u0232\u0233\u0003N\'\u0000\u0233\u0234\u0006$\uffff\uffff\u0000\u0234"+
"\u0244\u0001\u0000\u0000\u0000\u0235\u0236\u0003d2\u0000\u0236\u0237\u0006"+
"$\uffff\uffff\u0000\u0237\u0244\u0001\u0000\u0000\u0000\u0238\u0239\u0005"+
"\u0015\u0000\u0000\u0239\u023a\u0003\u0018\f\u0000\u023a\u023b\u0005\u0016"+
"\u0000\u0000\u023b\u023c\u0006$\uffff\uffff\u0000\u023c\u0244\u0001\u0000"+
"\u0000\u0000\u023d\u023e\u0003X,\u0000\u023e\u023f\u0006$\uffff\uffff"+
"\u0000\u023f\u0244\u0001\u0000\u0000\u0000\u0240\u0241\u0003\u000e\u0007"+
"\u0000\u0241\u0242\u0006$\uffff\uffff\u0000\u0242\u0244\u0001\u0000\u0000"+
"\u0000\u0243\u0232\u0001\u0000\u0000\u0000\u0243\u0235\u0001\u0000\u0000"+
"\u0000\u0243\u0238\u0001\u0000\u0000\u0000\u0243\u023d\u0001\u0000\u0000"+
"\u0000\u0243\u0240\u0001\u0000\u0000\u0000\u0244I\u0001\u0000\u0000\u0000"+
"\u0245\u0246\u0003P(\u0000\u0246\u0247\u0006%\uffff\uffff\u0000\u0247"+
"\u024c\u0001\u0000\u0000\u0000\u0248\u0249\u0003L&\u0000\u0249\u024a\u0006"+
"%\uffff\uffff\u0000\u024a\u024c\u0001\u0000\u0000\u0000\u024b\u0245\u0001"+
"\u0000\u0000\u0000\u024b\u0248\u0001\u0000\u0000\u0000\u024cK\u0001\u0000"+
"\u0000\u0000\u024d\u024e\u0006&\uffff\uffff\u0000\u024e\u024f\u0003d2"+
"\u0000\u024f\u0256\u0006&\uffff\uffff\u0000\u0250\u0251\u0005\u0013\u0000"+
"\u0000\u0251\u0252\u0003d2\u0000\u0252\u0253\u0006&\uffff\uffff\u0000"+
"\u0253\u0255\u0001\u0000\u0000\u0000\u0254\u0250\u0001\u0000\u0000\u0000"+
"\u0255\u0258\u0001\u0000\u0000\u0000\u0256\u0254\u0001\u0000\u0000\u0000"+
"\u0256\u0257\u0001\u0000\u0000\u0000\u0257\u0259\u0001\u0000\u0000\u0000"+
"\u0258\u0256\u0001\u0000\u0000\u0000\u0259\u025a\u0006&\uffff\uffff\u0000"+
"\u025aM\u0001\u0000\u0000\u0000\u025b\u025c\u0003P(\u0000\u025c\u025d"+
"\u0006\'\uffff\uffff\u0000\u025d\u0261\u0001\u0000\u0000\u0000\u025e\u025f"+
"\u0005 \u0000\u0000\u025f\u0261\u0006\'\uffff\uffff\u0000\u0260\u025b"+
"\u0001\u0000\u0000\u0000\u0260\u025e\u0001\u0000\u0000\u0000\u0261O\u0001"+
"\u0000\u0000\u0000\u0262\u0263\u0003V+\u0000\u0263\u0264\u0006(\uffff"+
"\uffff\u0000\u0264\u026f\u0001\u0000\u0000\u0000\u0265\u0266\u0003R)\u0000"+
"\u0266\u0267\u0006(\uffff\uffff\u0000\u0267\u026f\u0001\u0000\u0000\u0000"+
"\u0268\u0269\u0003T*\u0000\u0269\u026a\u0006(\uffff\uffff\u0000\u026a"+
"\u026f\u0001\u0000\u0000\u0000\u026b\u026c\u0003b1\u0000\u026c\u026d\u0006"+
"(\uffff\uffff\u0000\u026d\u026f\u0001\u0000\u0000\u0000\u026e\u0262\u0001"+
"\u0000\u0000\u0000\u026e\u0265\u0001\u0000\u0000\u0000\u026e\u0268\u0001"+
"\u0000\u0000\u0000\u026e\u026b\u0001\u0000\u0000\u0000\u026fQ\u0001\u0000"+
"\u0000\u0000\u0270\u0271\u0005\u0004\u0000\u0000\u0271\u0272\u0006)\uffff"+
"\uffff\u0000\u0272S\u0001\u0000\u0000\u0000\u0273\u0276\u0005\u001e\u0000"+
"\u0000\u0274\u0276\u0005\u001f\u0000\u0000\u0275\u0273\u0001\u0000\u0000"+
"\u0000\u0275\u0274\u0001\u0000\u0000\u0000\u0276\u0277\u0001\u0000\u0000"+
"\u0000\u0277\u0278\u0006*\uffff\uffff\u0000\u0278U\u0001\u0000\u0000\u0000"+
"\u0279\u027a\u0005\u0005\u0000\u0000\u027a\u027b\u0006+\uffff\uffff\u0000"+
"\u027bW\u0001\u0000\u0000\u0000\u027c\u027d\u0003Z-\u0000\u027d\u027e"+
"\u0006,\uffff\uffff\u0000\u027e\u0286\u0001\u0000\u0000\u0000\u027f\u0280"+
"\u0003\u001e\u000f\u0000\u0280\u0281\u0006,\uffff\uffff\u0000\u0281\u0286"+
"\u0001\u0000\u0000\u0000\u0282\u0283\u0003\\.\u0000\u0283\u0284\u0006"+
",\uffff\uffff\u0000\u0284\u0286\u0001\u0000\u0000\u0000\u0285\u027c\u0001"+
"\u0000\u0000\u0000\u0285\u027f\u0001\u0000\u0000\u0000\u0285\u0282\u0001"+
"\u0000\u0000\u0000\u0286Y\u0001\u0000\u0000\u0000\u0287\u0288\u0006-\uffff"+
"\uffff\u0000\u0288\u0294\u0005\u0017\u0000\u0000\u0289\u028a\u0003\u0018"+
"\f\u0000\u028a\u0291\u0006-\uffff\uffff\u0000\u028b\u028c\u0005\u0014"+
"\u0000\u0000\u028c\u028d\u0003\u0018\f\u0000\u028d\u028e\u0006-\uffff"+
"\uffff\u0000\u028e\u0290\u0001\u0000\u0000\u0000\u028f\u028b\u0001\u0000"+
"\u0000\u0000\u0290\u0293\u0001\u0000\u0000\u0000\u0291\u028f\u0001\u0000"+
"\u0000\u0000\u0291\u0292\u0001\u0000\u0000\u0000\u0292\u0295\u0001\u0000"+
"\u0000\u0000\u0293\u0291\u0001\u0000\u0000\u0000\u0294\u0289\u0001\u0000"+
"\u0000\u0000\u0294\u0295\u0001\u0000\u0000\u0000\u0295\u0296\u0001\u0000"+
"\u0000\u0000\u0296\u0297\u0005\u0018\u0000\u0000\u0297\u0298\u0006-\uffff"+
"\uffff\u0000\u0298[\u0001\u0000\u0000\u0000\u0299\u029a\u0006.\uffff\uffff"+
"\u0000\u029a\u02a6\u0005\u0019\u0000\u0000\u029b\u029c\u0003^/\u0000\u029c"+
"\u02a3\u0006.\uffff\uffff\u0000\u029d\u029e\u0005\u0014\u0000\u0000\u029e"+
"\u029f\u0003^/\u0000\u029f\u02a0\u0006.\uffff\uffff\u0000\u02a0\u02a2"+
"\u0001\u0000\u0000\u0000\u02a1\u029d\u0001\u0000\u0000\u0000\u02a2\u02a5"+
"\u0001\u0000\u0000\u0000\u02a3\u02a1\u0001\u0000\u0000\u0000\u02a3\u02a4"+
"\u0001\u0000\u0000\u0000\u02a4\u02a7\u0001\u0000\u0000\u0000\u02a5\u02a3"+
"\u0001\u0000\u0000\u0000\u02a6\u029b\u0001\u0000\u0000\u0000\u02a6\u02a7"+
"\u0001\u0000\u0000\u0000\u02a7\u02a8\u0001\u0000\u0000\u0000\u02a8\u02a9"+
"\u0005\u001a\u0000\u0000\u02a9\u02aa\u0006.\uffff\uffff\u0000\u02aa]\u0001"+
"\u0000\u0000\u0000\u02ab\u02ac\u0003`0\u0000\u02ac\u02ad\u0005\u001b\u0000"+
"\u0000\u02ad\u02ae\u0003\u0018\f\u0000\u02ae\u02af\u0006/\uffff\uffff"+
"\u0000\u02af_\u0001\u0000\u0000\u0000\u02b0\u02b1\u0003d2\u0000\u02b1"+
"\u02b2\u00060\uffff\uffff\u0000\u02b2\u02b7\u0001\u0000\u0000\u0000\u02b3"+
"\u02b4\u0003R)\u0000\u02b4\u02b5\u00060\uffff\uffff\u0000\u02b5\u02b7"+
"\u0001\u0000\u0000\u0000\u02b6\u02b0\u0001\u0000\u0000\u0000\u02b6\u02b3"+
"\u0001\u0000\u0000\u0000\u02b7a\u0001\u0000\u0000\u0000\u02b8\u02b9\u0005"+
"\u0006\u0000\u0000\u02b9\u02c1\u00061\uffff\uffff\u0000\u02ba\u02bb\u0003"+
"d2\u0000\u02bb\u02bc\u0005\u0015\u0000\u0000\u02bc\u02bd\u0003\u0018\f"+
"\u0000\u02bd\u02be\u0005\u0016\u0000\u0000\u02be\u02bf\u00061\uffff\uffff"+
"\u0000\u02bf\u02c1\u0001\u0000\u0000\u0000\u02c0\u02b8\u0001\u0000\u0000"+
"\u0000\u02c0\u02ba\u0001\u0000\u0000\u0000\u02c1c\u0001\u0000\u0000\u0000"+
"\u02c2\u02c3\u00050\u0000\u0000\u02c3\u02cd\u00062\uffff\uffff\u0000\u02c4"+
"\u02c5\u0005\u001d\u0000\u0000\u02c5\u02cd\u00062\uffff\uffff\u0000\u02c6"+
"\u02c7\u0005-\u0000\u0000\u02c7\u02cd\u00062\uffff\uffff\u0000\u02c8\u02c9"+
"\u0005,\u0000\u0000\u02c9\u02cd\u00062\uffff\uffff\u0000\u02ca\u02cb\u0005"+
"!\u0000\u0000\u02cb\u02cd\u00062\uffff\uffff\u0000\u02cc\u02c2\u0001\u0000"+
"\u0000\u0000\u02cc\u02c4\u0001\u0000\u0000\u0000\u02cc\u02c6\u0001\u0000"+
"\u0000\u0000\u02cc\u02c8\u0001\u0000\u0000\u0000\u02cc\u02ca\u0001\u0000"+
"\u0000\u0000\u02cde\u0001\u0000\u0000\u00005\u0081\u008c\u0098\u00a0\u00af"+
"\u00b7\u00c8\u00d9\u00e8\u00eb\u00f2\u00f6\u0101\u0113\u0120\u012f\u013c"+
"\u014b\u0156\u0161\u0176\u017f\u0186\u018d\u0194\u019f\u01a7\u01a9\u01b5"+
"\u01d1\u01e1\u01e6\u01ed\u01fd\u01ff\u0209\u021a\u022b\u022e\u0243\u024b"+
"\u0256\u0260\u026e\u0275\u0285\u0291\u0294\u02a3\u02a6\u02b6\u02c0\u02cc";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy