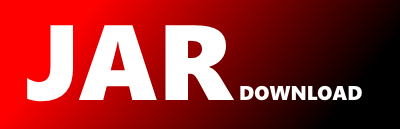
META-INF.resources.js.components.criteria_builder.CriteriaGroup.js Maven / Gradle / Ivy
The newest version!
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
import ClayIcon from '@clayui/icon';
import classNames from 'classnames';
import {PropTypes} from 'prop-types';
import React, {Fragment} from 'react';
import useDragSource from '../../hooks/useDragSource';
import {CONJUNCTIONS} from '../../utils/constants';
import {DragTypes} from '../../utils/dragTypes';
import {
generateGroupId,
getChildGroupIds,
getSupportedOperatorsFromType,
insertAtIndex,
replaceAtIndex,
} from '../../utils/utils';
import Conjunction from './Conjunction.es';
import CriteriaRow from './CriteriaRow';
import DropZone from './DropZone';
import EmptyDropZone from './EmptyDropZone';
export default function CriteriaGroup({
criteria,
draggingItem,
editing,
emptyContributors,
entityName,
groupId,
index,
modelLabel,
onChange,
onMove,
parentGroupId,
propertyKey,
renderEmptyValuesErrors = false,
root = false,
supportedProperties,
}) {
const _handleConjunctionSelect = (conjunctionName) => {
onChange({
...criteria,
conjunctionName,
});
};
const {handlerRef, isDragging} = useDragSource({
item: {
childGroupIds: getChildGroupIds(criteria),
criterion: criteria,
groupId: parentGroupId,
index,
type: DragTypes.CRITERIA_GROUP,
},
});
/**
* Adds a new criterion in a group at the specified index. If the criteria
* was previously empty and is being added to the root group, a new group
* will be added as well.
* @param {number} index The position the criterion will be inserted in.
* @param {object} criterion The criterion that will be added.
* @memberof CriteriaGroup
*/
const _handleCriterionAdd = (index, criterion) => {
const {
defaultValue = '',
operatorName,
propertyName,
type,
value,
} = criterion;
const criterionValue = value || defaultValue;
const operators = getSupportedOperatorsFromType(type);
const newCriterion = {
operatorName: operatorName ? operatorName : operators[0].name,
propertyName,
type,
value: criterionValue,
};
if (root && !criteria) {
onChange({
conjunctionName: CONJUNCTIONS.AND,
groupId: generateGroupId(),
items: [newCriterion],
});
}
else {
onChange({
...criteria,
items: insertAtIndex(newCriterion, criteria.items, index),
});
}
};
const _handleCriterionChange = (index) => (newCriterion) => {
onChange({
...criteria,
items: replaceAtIndex(newCriterion, criteria.items, index),
});
};
const _handleCriterionDelete = (index) => {
onChange({
...criteria,
items: criteria.items.filter((fItem, fIndex) => fIndex !== index),
});
};
const _isCriteriaEmpty = () => {
return criteria ? !criteria.items.length : true;
};
const _renderConjunction = (index) => {
return (
<>
>
);
};
const _renderCriterion = (criterion, index) => {
return (
{criterion.items ? (
) : (
)}
);
};
const singleRow = criteria && criteria.items && criteria.items.length === 1;
const disabled =
isDragging ||
(draggingItem && draggingItem.propertyKey !== propertyKey);
return (
{_isCriteriaEmpty() ? (
) : (
<>
{editing && singleRow && !root && (
)}
{criteria.items &&
criteria.items.map((criterion, index) => {
return (
{index !== 0 && _renderConjunction(index)}
{_renderCriterion(criterion, index)}
);
})}
>
)}
);
}
CriteriaGroup.propTypes = {
connectDragPreview: PropTypes.func,
criteria: PropTypes.object,
dragging: PropTypes.bool,
editing: PropTypes.bool,
emptyContributors: PropTypes.bool,
entityName: PropTypes.string,
groupId: PropTypes.string,
index: PropTypes.number,
modelLabel: PropTypes.string,
onChange: PropTypes.func,
onMove: PropTypes.func,
parentGroupId: PropTypes.string,
propertyKey: PropTypes.string.isRequired,
renderEmptyValuesErrors: PropTypes.bool,
root: PropTypes.bool,
supportedProperties: PropTypes.array,
};
© 2015 - 2024 Weber Informatics LLC | Privacy Policy