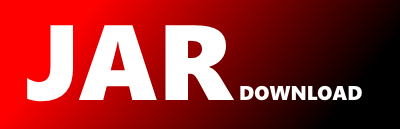
META-INF.resources.js.components.criteria_builder.CriteriaRowEditable.js Maven / Gradle / Ivy
The newest version!
/**
* SPDX-FileCopyrightText: (c) 2000 Liferay, Inc. https://liferay.com
* SPDX-License-Identifier: LGPL-2.1-or-later OR LicenseRef-Liferay-DXP-EULA-2.0.0-2023-06
*/
import ClayButton, {ClayButtonWithIcon} from '@clayui/button';
import {ClaySelectWithOption} from '@clayui/form';
import ClayIcon from '@clayui/icon';
import classNames from 'classnames';
import {PropTypes} from 'prop-types';
import React from 'react';
import {
useDisableKeyboardMovement,
useMovementSource,
useSetMovementSource,
} from '../../contexts/KeyboardMovementContext';
import useDragSource from '../../hooks/useDragSource';
import {PROPERTY_TYPES} from '../../utils/constants';
import {DragTypes} from '../../utils/dragTypes';
import {TYPE_ICONS} from '../../utils/typeIcons';
import {createNewGroup, getSupportedOperatorsFromType} from '../../utils/utils';
import BooleanInput from '../inputs/BooleanInput';
import CollectionInput from '../inputs/CollectionInput';
import DateTimeInput from '../inputs/DateTimeInput';
import DecimalInput from '../inputs/DecimalInput';
import IntegerInput from '../inputs/IntegerInput';
import SelectEntityInput from '../inputs/SelectEntityInput';
import StringInput from '../inputs/StringInput';
export default function CriteriaRowEditable({
criterion = {},
error,
groupId,
index,
item,
onAdd,
onChange,
onDelete,
propertyKey,
renderEmptyValuesErrors,
selectedOperator,
selectedProperty,
}) {
const itemIcon = item.icon || TYPE_ICONS[item.type] || 'text';
const {handlerRef, isDragging} = useDragSource({
item: {
criterion,
groupId,
icon: itemIcon,
index,
name: item.label,
propertyKey,
type: DragTypes.CRITERIA_ROW,
},
});
const _handleDelete = (event) => {
event.preventDefault();
onDelete(index);
};
const _handleDuplicate = (event) => {
event.preventDefault();
onAdd(index + 1, criterion);
};
const _handleInputChange = (propertyName) => (event) => {
onChange({
...criterion,
[propertyName]: event.target.value,
});
};
/**
* Updates the criteria with a criterion value change. The param 'value'
* will only be an array when selecting multiple entities (see
* {@link SelectEntityInput.es.js}). And in the case of an array, a new
* group with multiple criterion rows will be created.
* @param {Array|object} value The properties or list of objects with
* properties to update.
*/
const _handleTypedInputChange = (value) => {
if (Array.isArray(value)) {
const items = value.map((item) => ({
...criterion,
...item,
}));
onChange(createNewGroup(items));
}
else {
onChange({
...criterion,
...value,
});
}
};
const _renderEditableProperty = ({
error,
propertyLabel,
selectedOperator,
selectedProperty,
value,
}) => {
const disabledInput = !!error;
const propertyType = selectedProperty ? selectedProperty.type : '';
const filteredSupportedOperators =
getSupportedOperatorsFromType(propertyType);
return (
<>
{propertyLabel}
({
label,
value: name,
})
)}
value={selectedOperator && selectedOperator.name}
/>
{_renderValueInput(
disabledInput,
propertyLabel,
renderEmptyValuesErrors,
selectedProperty,
value
)}
>
);
};
const _renderValueInput = (
disabled,
propertyLabel,
renderEmptyValuesErrors,
selectedProperty,
value
) => {
const inputComponentsMap = {
[PROPERTY_TYPES.BOOLEAN]: BooleanInput,
[PROPERTY_TYPES.COLLECTION]: CollectionInput,
[PROPERTY_TYPES.DATE]: DateTimeInput,
[PROPERTY_TYPES.DATE_TIME]: DateTimeInput,
[PROPERTY_TYPES.DOUBLE]: DecimalInput,
[PROPERTY_TYPES.ID]: SelectEntityInput,
[PROPERTY_TYPES.INTEGER]: IntegerInput,
[PROPERTY_TYPES.STRING]: StringInput,
};
const InputComponent =
inputComponentsMap[selectedProperty.type] ||
inputComponentsMap[PROPERTY_TYPES.STRING];
return (
);
};
const value = criterion.value;
const propertyLabel = selectedProperty ? selectedProperty.label : '';
const setMovementSource = useSetMovementSource();
const movementSource = useMovementSource();
const disableMovement = useDisableKeyboardMovement();
const isKeyboardSource =
movementSource?.groupId === groupId && movementSource?.index === index;
return (
setMovementSource({
groupId,
icon: itemIcon,
index,
label: item.label,
propertyKey,
})
}
ref={handlerRef}
size="sm"
symbol="drag"
/>
{_renderEditableProperty({
error,
propertyLabel,
selectedOperator,
selectedProperty,
value,
})}
{error ? (
{Liferay.Language.get('delete-segment-property')}
) : (
<>
>
)}
);
}
CriteriaRowEditable.propTypes = {
criterion: PropTypes.object.isRequired,
error: PropTypes.bool,
index: PropTypes.number.isRequired,
onAdd: PropTypes.func.isRequired,
onChange: PropTypes.func.isRequired,
onDelete: PropTypes.func.isRequired,
renderEmptyValuesErrors: PropTypes.bool,
selectedOperator: PropTypes.object,
selectedProperty: PropTypes.object.isRequired,
};
© 2015 - 2024 Weber Informatics LLC | Privacy Policy