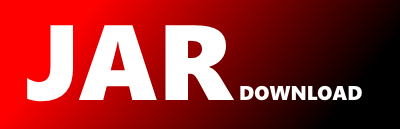
features.opensocial-reference.activity.js Maven / Gradle / Ivy
Show all versions of shindig-features Show documentation
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/*global opensocial */
/**
* @class
* Representation of an activity.
*
* Activities are rendered with a title and an optional activity body.
*
* You may set the title and body directly as strings when calling
* opensocial.createActivity.
*
* However, it is usually beneficial to create activities using
* Activity Templates for the title and body. Activity Templates support:
*
* - Internationalization
* - Replacement variables in the message
* - Activity Summaries, which are message variations used to summarize
* repeated activities that share something in common.
*
*
* Activity Templates are defined as messages in the gadget specification.
* To define messages, you create and reference message bundle XML files for
* each locale you support.
*
* Example module spec in gadget XML:
*
* <ModulePrefs title="ListenToThis">
* <Locale messages="http://www.listentostuff.com/messages.xml"/>
* <Locale lang="de" messages="http://www.listentostuff.com/messages-DE.xml"/>
* </ModulePrefs>
*
*
*
* Example message bundle:
*
* <messagebundle>
* <msg name="LISTEN_TO_THIS_SONG">
* ${Subject.DisplayName} told ${Owner.DisplayName} to
* listen to a song!
* </msg>
* </messagebundle>
*
*
*
* You can set custom key/value string pairs when posting an activity.
* These values will be used for variable substitution in the templates.
* Example JS call:
*
* var owner = ...;
* var viewer = ...;
* var activity = opensocial.newActivity('LISTEN_TO_THIS_SONG',
* {Song: 'Do That There - (Young Einstein hoo-hoo mix)',
* Artist: 'Lyrics Born', Subject: viewer, Owner: owner})
*
*
*
* Associated message:
*
* <msg name="LISTEN_TO_THIS_SONG">
* ${Subject.DisplayName} told ${Owner.DisplayName} to listen
* to ${Song} by ${Artist}
* </msg>
*
*
*
* People can also be set as values in key/value pairs when posting
* an activity. You can then reference the following fields on a person:
*
* - ${Person.DisplayName} The person's name
* - ${Person.Id} The user ID of the person
* - ${Person.ProfileUrl} The profile URL of the person
* - ${Person} This will show the display name, but containers may optionally
* provide special formatting, such as showing the name as a link
*
*
* Users will have many activities in their activity streams, and containers
* will not show every activity that is visible to a user. To help display
* large numbers of activities, containers will summarize a list of activities
* from a given source to a single entry.
*
* You can provide Activity Summaries to customize the text shown when
* multiple activities are summarized. If no customization is provided, a
* container may ignore your activities altogether or provide default text
* such as "Bob changed his status message + 20 other events like this."
*
* - Activity Summaries will always summarize around a specific key in a
* key/value pair. This is so that the summary can say something concrete
* (this is clearer in the example below).
* - Other variables will have synthetic "Count" variables created with
* the total number of items summarized.
* - Message ID of the summary is the message ID of the main template + ":" +
* the data key
*
*
* Example summaries:
*
* <messagebundle>
* <msg name="LISTEN_TO_THIS_SONG:Artist">
* ${Subject.Count} of your friends have suggested listening to songs
* by ${Artist}!
* </msg>
* <msg name="LISTEN_TO_THIS_SONG:Song">
* ${Subject.Count} of your friends have suggested listening to ${Song}
* !</msg>
* <msg name="LISTEN_TO_THIS_SONG:Subject">
* ${Subject.DisplayName} has recommended ${Song.Count} songs to you.
* </msg>
* </messagebundle>
*
*
* Activity Templates may only have the following HTML tags: <b>,
* <i>, <a>, <span>. The container also has the option
* to strip out these tags when rendering the activity.
*
*
* See also:
* opensocial.newActivity(),
*
* opensocial.requestCreateActivity()
*
* @name opensocial.Activity
*/
/**
* Base interface for all activity objects.
*
* Private, see opensocial.createActivity() for usage.
*
* @param {Object.} params Parameters defining the activity.
* @private
* @constructor
*/
opensocial.Activity = function(params) {
this.fields_ = params;
};
/**
* @static
* @class
* All of the fields that activities can have.
*
* It is only required to set one of TITLE_ID or TITLE. In addition, if you
* are using any variables in your title or title template,
* you must set TEMPLATE_PARAMS.
*
* Other possible fields to set are: URL, MEDIA_ITEMS, BODY_ID, BODY,
* EXTERNAL_ID, PRIORITY, STREAM_TITLE, STREAM_URL, STREAM_SOURCE_URL,
* and STREAM_FAVICON_URL.
*
* Containers are only required to use TITLE_ID or TITLE, they may ignore
* additional parameters.
*
*
* See also:
* opensocial.Activity.getField()
*
*
* @name opensocial.Activity.Field
* @enum {string}
*/
opensocial.Activity.Field = {
/**
* A string specifying the title template message ID in the gadget
* spec.
*
* The title is the primary text of an activity.
*
* Titles may only have the following HTML tags: <b> <i>,
* <a>, <span>.
* The container may ignore this formatting when rendering the activity.
*
* @member opensocial.Activity.Field
*/
TITLE_ID : 'titleId',
/**
* A string specifying the primary text of an activity.
*
* Titles may only have the following HTML tags: <b> <i>,
* <a>, <span>.
* The container may ignore this formatting when rendering the activity.
*
* @member opensocial.Activity.Field
*/
TITLE : 'title',
/**
* A map of custom keys to values associated with this activity.
* These will be used for evaluation in templates.
*
* The data has type Map<String, Object>
. The
* object may be either a String or an opensocial.Person.
*
* When passing in a person with key PersonKey, can use the following
* replacement variables in the template:
*
* - PersonKey.DisplayName - Display name for the person
* - PersonKey.ProfileUrl. URL of the person's profile
* - PersonKey.Id - The ID of the person
* - PersonKey - Container may replace with DisplayName, but may also
* optionally link to the user.
*
*
* @member opensocial.Activity.Field
*/
TEMPLATE_PARAMS : 'templateParams',
/**
* A string specifying the
* URL that represents this activity.
* @member opensocial.Activity.Field
*/
URL : 'url',
/**
* Any photos, videos, or images that should be associated
* with the activity. Higher priority ones are higher in the list.
* The data has type Array<
* MediaItem>
.
* @member opensocial.Activity.Field
*/
MEDIA_ITEMS : 'mediaItems',
/**
* A string specifying the body template message ID in the gadget spec.
*
* The body is an optional expanded version of an activity.
*
* Bodies may only have the following HTML tags: <b> <i>,
* <a>, <span>.
* The container may ignore this formatting when rendering the activity.
*
* @member opensocial.Activity.Field
*/
BODY_ID : 'bodyId',
/**
* A string specifying an optional expanded version of an activity.
*
* Bodies may only have the following HTML tags: <b> <i>,
* <a>, <span>.
* The container may ignore this formatting when rendering the activity.
*
* @member opensocial.Activity.Field
*/
BODY : 'body',
/**
* An optional string ID generated by the posting application.
* @member opensocial.Activity.Field
*/
EXTERNAL_ID : 'externalId',
/**
* A string specifing the title of the stream.
* @member opensocial.Activity.Field
*/
STREAM_TITLE : 'streamTitle',
/**
* A string specifying the stream's URL.
* @member opensocial.Activity.Field
*/
STREAM_URL : 'streamUrl',
/**
* A string specifying the stream's source URL.
* @member opensocial.Activity.Field
*/
STREAM_SOURCE_URL : 'streamSourceUrl',
/**
* A string specifying the URL for the stream's favicon.
* @member opensocial.Activity.Field
*/
STREAM_FAVICON_URL : 'streamFaviconUrl',
/**
* A number between 0 and 1 representing the relative priority of
* this activity in relation to other activities from the same source
* @member opensocial.Activity.Field
*/
PRIORITY : 'priority',
/**
* A string ID that is permanently associated with this activity.
* This value can not be set.
* @member opensocial.Activity.Field
*/
ID : 'id',
/**
* The string ID of the user who this activity is for.
* This value can not be set.
* @member opensocial.Activity.Field
*/
USER_ID : 'userId',
/**
* A string specifying the application that this activity is associated with.
* This value can not be set.
* @member opensocial.Activity.Field
*/
APP_ID : 'appId',
/**
* A string specifying the time at which this activity took place
* in milliseconds since the epoch.
* This value can not be set.
* @member opensocial.Activity.Field
*/
POSTED_TIME : 'postedTime'
};
/**
* Gets an ID that can be permanently associated with this activity.
*
* @return {string} The ID
* @member opensocial.Activity
*/
opensocial.Activity.prototype.getId = function() {
return this.getField(opensocial.Activity.Field.ID);
};
/**
* Gets the activity data that's associated with the specified key.
*
* @param {string} key The key to get data for;
* see the Field class
* for possible values
* @param {Object.=}
* opt_params Additional
* params
* to pass to the request.
* @return {string} The data
* @member opensocial.Activity
*/
opensocial.Activity.prototype.getField = function(key, opt_params) {
return opensocial.Container.getField(this.fields_, key, opt_params);
};
/**
* Sets data for this activity associated with the given key.
*
* @param {string} key The key to set data for
* @param {string} data The data to set
*/
opensocial.Activity.prototype.setField = function(key, data) {
return (this.fields_[key] = data);
};