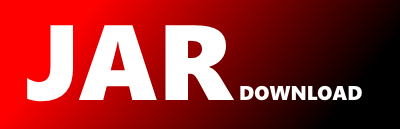
features.opensocial-reference.responseitem.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shindig-features Show documentation
Show all versions of shindig-features Show documentation
Packages all the features that shindig provides into a single jar file to allow
loading from the classpath
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
/*global opensocial */
/**
* @fileoverview ResponseItem containing information about a specific response
* from the server.
*/
/**
* @class
* Represents a response that was generated
* by processing a data request item on the server.
*
* @name opensocial.ResponseItem
*/
/**
* Represents a response that was generated by processing a data request item
* on the server.
*
* @private
* @constructor
*/
opensocial.ResponseItem = function(originalDataRequest, data,
opt_errorCode, opt_errorMessage) {
this.originalDataRequest_ = originalDataRequest;
this.data_ = data;
this.errorCode_ = opt_errorCode;
this.errorMessage_ = opt_errorMessage;
};
/**
* Returns true if there was an error in fetching this data from the server.
*
* @return {boolean} True if there was an error; otherwise, false
*/
opensocial.ResponseItem.prototype.hadError = function() {
return !!this.errorCode_;
};
/**
* @static
* @class
*
* Error codes that a response item can return.
*
* @name opensocial.ResponseItem.Error
*/
opensocial.ResponseItem.Error = {
/**
* This container does not support the request that was made.
*
* @member opensocial.ResponseItem.Error
*/
NOT_IMPLEMENTED : 'notImplemented',
/**
* The gadget does not have access to the requested data.
* To get access, use
*
* opensocial.requestPermission().
*
* @member opensocial.ResponseItem.Error
*/
UNAUTHORIZED : 'unauthorized',
/**
* The gadget can never have access to the requested data.
*
* @member opensocial.ResponseItem.Error
*/
FORBIDDEN : 'forbidden',
/**
* The request was invalid. Example: 'max' was -1.
*
* @member opensocial.ResponseItem.Error
*/
BAD_REQUEST : 'badRequest',
/**
* The request encountered an unexpected condition that
* prevented it from fulfilling the request.
*
* @member opensocial.ResponseItem.Error
*/
INTERNAL_ERROR : 'internalError',
/**
* The gadget exceeded a quota on the request. Example quotas include a
* max number of calls per day, calls per user per day, calls within a
* certain time period and so forth.
*
* @member opensocial.ResponseItem.Error
*/
LIMIT_EXCEEDED : 'limitExceeded'
};
/**
* If the request had an error, returns the error code.
* The error code can be container-specific
* or one of the values defined by
* Error
.
*
* @return {string} The error code, or null if no error occurred
*/
opensocial.ResponseItem.prototype.getErrorCode = function() {
return this.errorCode_;
};
/**
* If the request had an error, returns the error message.
*
* @return {string} A human-readable description of the error that occurred;
* can be null, even if an error occurred
*/
opensocial.ResponseItem.prototype.getErrorMessage = function() {
return this.errorMessage_;
};
/**
* Returns the original data request.
*
* @return {opensocial.DataRequest} The data request used to fetch this data
* response
*/
opensocial.ResponseItem.prototype.getOriginalDataRequest = function() {
return this.originalDataRequest_;
};
/**
* Gets the response data.
*
* @return {Object} The requested value calculated by the server; the type of
* this value is defined by the type of request that was made
*/
opensocial.ResponseItem.prototype.getData = function() {
return this.data_;
};
© 2015 - 2024 Weber Informatics LLC | Privacy Policy