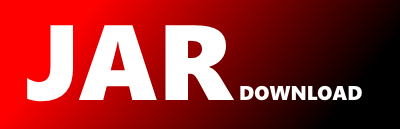
dashboard.dashboard-vad-onfilter.js Maven / Gradle / Ivy
The newest version!
let isFilterActive = filteredElementCount !== sheetsMap.size;
let initialCvssSeverityPerAllVulnerabilityCounts = new Array(cvssRanges.length).fill(0);
let contextCvssSeverityPerAllVulnerabilityCounts = new Array(cvssRanges.length).fill(0);
let contextCvssSeverityPerReviewedVulnerabilityCounts = new Array(cvssRanges.length).fill(0);
let contextCvssSeverityPerInReviewVulnerabilityCounts = new Array(cvssRanges.length).fill(0);
let currentCveStatusCounts = new Array(statuses.length).fill(0);
/* method for finding a cvss range for a specific score */
function checkForRange(score, ranges) {
for (let i = 0; i < ranges.length; i++) {
if (ranges[i].floor <= score && ranges[i].ceil >= score) {
return ranges[i];
}
}
return ranges[0];
}
let navigationListElements = document.getElementsByClassName('navigation-entry');
let initialCvssOverallColumnIndex = findNavigationTableColumnIndex('Initial Overall');
let contextCvssOverallColumnIndex = findNavigationTableColumnIndex('Context Overall');
let statusHeaderIndex = findNavigationTableColumnIndex('Status');
for (let i = 0, max = navigationListElements.length; i < max; i++) {
try {
if (navigationListElements[i].classList.contains('hidden')) {
continue;
}
let isReviewed = false;
let isInReview = false;
let isVoid = false;
let isNotApplicable = false;
if (statusHeaderIndex !== -1) {
let baseStatus = navigationListElements[i].childNodes[statusHeaderIndex].innerText;
let mappedStatus = reviewStateMappingFunction(baseStatus);
let index = statuses.indexOf(mappedStatus);
currentCveStatusCounts[index]++;
isReviewed = mappedStatus === 'applicable';
isInReview = mappedStatus === 'in review';
isVoid = baseStatus === 'void';
isNotApplicable = baseStatus === 'not applicable';
} else {
console.error('Could not find the column for the status in navigation table.');
}
if (initialCvssOverallColumnIndex !== -1) {
let score = parseFloat(navigationListElements[i].childNodes[initialCvssOverallColumnIndex].innerText);
let index = cvssRanges.indexOf(checkForRange(score, cvssRanges));
initialCvssSeverityPerAllVulnerabilityCounts[index]++;
} else {
console.error('Could not find the column for the unmodified scores in navigation table.');
}
if (contextCvssOverallColumnIndex !== -1) {
let score = parseFloat(navigationListElements[i].childNodes[contextCvssOverallColumnIndex].innerText);
if (isVoid || isNotApplicable) {
score = 0;
}
let index = cvssRanges.indexOf(checkForRange(score, cvssRanges));
contextCvssSeverityPerAllVulnerabilityCounts[index]++;
if (isReviewed) {
contextCvssSeverityPerReviewedVulnerabilityCounts[index]++;
} else if (isInReview) {
contextCvssSeverityPerInReviewVulnerabilityCounts[index]++;
}
} else {
console.error('Could not find the column for the modified scores in navigation table.');
}
} catch (e) {
console.error('Error while processing navigationListElements[', i, ']:', navigationListElements[i], e);
}
}
/* apply the new values to the chart */
vulnerabilityOverviewChartCvssSeverityInitial.data.datasets[0].data = initialCvssSeverityPerAllVulnerabilityCounts;
vulnerabilityOverviewChartCvssSeverityInitial.data.labels = cvssRanges.map(e => e.name);
vulnerabilityOverviewChartCvssSeverityContext.data.datasets[0].data = contextCvssSeverityPerAllVulnerabilityCounts;
vulnerabilityOverviewChartCvssSeverityContext.data.labels = cvssRanges.map(e => e.name);
vulnerabilityOverviewChartCvssSeverityContextByStatusApplicable.data.datasets[0].data = contextCvssSeverityPerReviewedVulnerabilityCounts;
vulnerabilityOverviewChartCvssSeverityContextByStatusApplicable.data.labels = cvssRanges.map(e => e.name);
vulnerabilityOverviewChartCvssSeverityContextByStatusInReview.data.datasets[0].data = contextCvssSeverityPerInReviewVulnerabilityCounts;
vulnerabilityOverviewChartCvssSeverityContextByStatusInReview.data.labels = cvssRanges.map(e => e.name);
vulnerabilityOverviewChartVulnerabilityStatus.data.datasets[0].data = currentCveStatusCounts;
if (isFilterActive) {
let createDiagonalPattern = function (color = 'black') {
let shape = document.createElement('canvas');
shape.width = 10;
shape.height = 10;
let c = shape.getContext('2d');
c.fillStyle = color;
c.fillRect(0, 0, shape.width, shape.height);
c.strokeStyle = 'rgba(255,255,255,0.82)';
c.beginPath();
c.moveTo(2, 0);
c.lineTo(10, 8);
c.stroke();
c.beginPath();
c.moveTo(0, 8);
c.lineTo(2, 10);
c.stroke();
return c.createPattern(shape, 'repeat');
};
vulnerabilityOverviewChartCvssSeverityInitial.data.datasets[0].backgroundColor = cvssRanges.map(e => createDiagonalPattern(e.color));
vulnerabilityOverviewChartCvssSeverityContext.data.datasets[0].backgroundColor = cvssRanges.map(e => createDiagonalPattern(e.color));
vulnerabilityOverviewChartCvssSeverityContextByStatusApplicable.data.datasets[0].backgroundColor = cvssRanges.map(e => createDiagonalPattern(e.color));
vulnerabilityOverviewChartCvssSeverityContextByStatusInReview.data.datasets[0].backgroundColor = cvssRanges.map(e => createDiagonalPattern(e.color));
if (!vulnerabilityOverviewChartVulnerabilityStatus.data.datasets[0].originalBackgroundColors) {
vulnerabilityOverviewChartVulnerabilityStatus.data.datasets[0].originalBackgroundColors = [...vulnerabilityOverviewChartVulnerabilityStatus.data.datasets[0].backgroundColor];
}
vulnerabilityOverviewChartVulnerabilityStatus.data.datasets[0].backgroundColor = vulnerabilityOverviewChartVulnerabilityStatus.data.datasets[0].originalBackgroundColors.map(e => createDiagonalPattern(e));
} else {
vulnerabilityOverviewChartCvssSeverityInitial.data.datasets[0].backgroundColor = cvssRanges.map(e => e.color);
vulnerabilityOverviewChartCvssSeverityContext.data.datasets[0].backgroundColor = cvssRanges.map(e => e.color);
vulnerabilityOverviewChartCvssSeverityContextByStatusApplicable.data.datasets[0].backgroundColor = cvssRanges.map(e => e.color);
vulnerabilityOverviewChartCvssSeverityContextByStatusInReview.data.datasets[0].backgroundColor = cvssRanges.map(e => e.color);
if (vulnerabilityOverviewChartVulnerabilityStatus.data.datasets[0].originalBackgroundColors) {
vulnerabilityOverviewChartVulnerabilityStatus.data.datasets[0].backgroundColor = vulnerabilityOverviewChartVulnerabilityStatus.data.datasets[0].originalBackgroundColors;
}
}
/* apply the updated values to the charts */
vulnerabilityOverviewChartCvssSeverityInitial.update();
vulnerabilityOverviewChartCvssSeverityContext.update();
vulnerabilityOverviewChartCvssSeverityContextByStatusApplicable.update();
vulnerabilityOverviewChartCvssSeverityContextByStatusInReview.update();
vulnerabilityOverviewChartVulnerabilityStatus.update();
hideOverviewChartsWithoutData(); /* for hiding/showing charts that are empty */
if (isFilterActive) { /* update the hint on the overview charts */
document.getElementById('overview-charts-are-filtered-hint').classList.remove('hidden');
} else {
document.getElementById('overview-charts-are-filtered-hint').classList.add('hidden');
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy