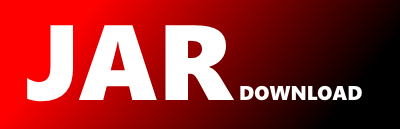
report.inventory-overview-html-report.additional-report-scripts.js Maven / Gradle / Ivy
function setupReport() {
const decompressionStart = performance.now();
const compressedLength = stringByteLength(compressedData);
DecompressBlob(base64toBlob(compressedData))
.then(e => {
e.text().then(data => {
const decompressionEnd = performance.now();
const decompressedLength = stringByteLength(data);
logDecompressionStatistics('Report data', decompressionStart, decompressionEnd, compressedLength, decompressedLength);
document.getElementById('report.data-model').remove();
try {
data = JSON.parse(data);
} catch (e) {
console.error('Failed to parse report data:', e);
reportLoadingCompleted(false, 'report-parse', e);
return;
}
const initialisationStart = performance.now();
try {
new InventoryOverviewReport.InventoryOverviewReport(data);
const initialisationEnd = performance.now();
console.log('Report initialisation statistics:');
console.log(' Time:', initialisationEnd - initialisationStart, 'ms');
console.log(' Page size:', formatBytes(document.body.innerHTML.length));
reportLoadingCompleted(true);
} catch (e) {
console.error('Failed to initialize report:', e);
reportLoadingCompleted(false, 'report-init', e);
}
})
.catch(e => {
console.error('Failed to access and decompress report data:', e);
reportLoadingCompleted(false, 'report-access', e);
});
})
.catch(e => {
console.error('Failed to access and decompress report data: ', e);
reportLoadingCompleted(false, 'report-access', e);
});
}
function setupScripts(completedCallback) {
const decompressionStart = performance.now();
const compressedLength = stringByteLength(compressedJsBundle);
DecompressBlob(base64toBlob(compressedJsBundle))
.then(e => {
e.text().then(data => {
const decompressionEnd = performance.now();
const decompressedLength = stringByteLength(data);
logDecompressionStatistics('Scripts', decompressionStart, decompressionEnd, compressedLength, decompressedLength);
document.getElementById('resource.ae-npm-inventory-overview-html-report').remove();
try {
eval(data);
if (completedCallback) {
completedCallback();
}
} catch (e) {
console.error('Failed to evaluate scripts:', e);
reportLoadingCompleted(false, 'eval-scripts', e);
}
})
.catch(e => {
console.error('Failed to access and decompress scripts:', e);
reportLoadingCompleted(false, 'access-scripts', e);
});
})
.catch(e => {
console.error('Failed to access and decompress scripts: ', e);
reportLoadingCompleted(false, 'access-scripts', e);
});
}
function logDecompressionStatistics(compressedDataName, decompressionStart, decompressionEnd, compressedLength, decompressedLength) {
const compressionRatio = compressedLength / decompressedLength;
console.log(compressedDataName, 'decompression statistics:');
console.log(' Time:', decompressionEnd - decompressionStart, 'ms');
console.log(' Compressed:', formatBytes(compressedLength));
console.log(' Decompressed:', formatBytes(decompressedLength));
console.log(' Compression ratio:', (compressionRatio * 100).toFixed(3), '% (lower is better)');
console.log(' Decompression rate:', ((decompressedLength / 1024) / (decompressionEnd - decompressionStart)).toFixed(3), 'KB/ms');
}
function reportLoadingCompleted(success, errorType = undefined, error = undefined) {
const loadingSpinner = document.getElementById('full-report-loading-spinner');
if (loadingSpinner) {
loadingSpinner.remove();
}
Array.from(document.getElementsByClassName('show-on-load')).forEach(e => e.classList.remove('hidden'));
Array.from(document.getElementsByClassName('hide-on-load')).forEach(e => e.classList.add('hidden'));
if (!success) {
const overlay = document.createElement('div');
overlay.id = 'report-loading-overlay';
const errorContainer = document.createElement('div');
errorContainer.id = 'report-loading-error';
const errorHeader = document.createElement('h1');
errorHeader.innerText = 'Oops! Something went wrong.';
const errorDetails = document.createElement('p');
if (!success) {
switch (errorType) {
case 'report-parse':
errorDetails.innerText = 'An issue occurred while parsing the report data. This might be due to a corrupted or invalid report.';
break;
case 'report-init':
errorDetails.innerText = 'The report data has successfully been decompressed, but an error occurred while initializing the report.';
break;
case 'report-access':
errorDetails.innerText = 'The report data could not be accessed or decompressed. Please try again or contact support for assistance.';
break;
case 'access-scripts':
errorDetails.innerText = 'The report scripts could not be accessed or decompressed. Please try again or contact support for assistance.';
break;
case 'eval-scripts':
errorDetails.innerText = 'An error occurred while evaluating the report scripts. This might be due to a corrupted or invalid report.';
break;
default:
errorDetails.innerText = 'An unexpected error occurred.';
break;
}
}
const closeButton = document.createElement('button');
closeButton.innerText = 'There\'s nothing that can be done about it here.';
closeButton.onclick = function () {
overlay.remove();
};
errorContainer.appendChild(errorHeader);
errorContainer.appendChild(errorDetails);
if (error) {
const stackTraceContainer = document.createElement('div');
stackTraceContainer.style.marginTop = '15px';
const stackTraceLabel = document.createElement('p');
stackTraceLabel.innerText = 'Error: ' + error.message;
stackTraceLabel.style.fontWeight = 'bold';
const technicalDetails = document.createElement('pre');
technicalDetails.style.overflow = 'auto';
technicalDetails.style.maxHeight = '150px';
technicalDetails.style.margin = '0';
technicalDetails.style.padding = '10px';
technicalDetails.style.backgroundColor = '#f8f9fa';
technicalDetails.style.border = '1px solid #dee2e6';
technicalDetails.style.borderRadius = '5px';
technicalDetails.style.fontFamily = 'monospace';
let stackTrace = error.stack || error.stackTrace || error.stacktrace;
if (stackTrace) {
technicalDetails.textContent = stackTrace;
}
stackTraceContainer.appendChild(stackTraceLabel);
stackTraceContainer.appendChild(technicalDetails);
errorContainer.appendChild(stackTraceContainer);
}
errorContainer.appendChild(closeButton);
overlay.appendChild(errorContainer);
document.body.appendChild(overlay);
}
}
document.addEventListener('DOMContentLoaded', function () {
setTimeout(() => {
setupScripts(setupReport);
}, 50);
});
function stringByteLength(str) {
try {
return (new TextEncoder().encode(str)).length;
} catch (e) {
return str.length;
}
}
function formatBytes(bytes, decimals = 2) {
if (bytes === 0) return '0 Bytes';
const k = 1024;
const dm = decimals < 0 ? 0 : decimals;
const sizes = ['Bytes', 'KB', 'MB', 'GB', 'TB', 'PB', 'EB', 'ZB', 'YB'];
const i = Math.floor(Math.log(bytes) / Math.log(k));
return parseFloat((bytes / Math.pow(k, i)).toFixed(dm)) + ' ' + sizes[i];
}
async function DecompressBlob(blob) {
const ds = new DecompressionStream("gzip");
const decompressedStream = blob.stream().pipeThrough(ds);
return await new Response(decompressedStream).blob();
}
/**
* https://stackoverflow.com/questions/16245767/creating-a-blob-from-a-base64-string-in-javascript
* Convert a base64 string to a Blob object.
* @param base64Data {string}
* @param contentType {string}
* @returns {Blob}
*/
function base64toBlob(base64Data, contentType) {
contentType = contentType || '';
const sliceSize = 1024;
const byteCharacters = atob(base64Data);
const bytesLength = byteCharacters.length;
const slicesCount = Math.ceil(bytesLength / sliceSize);
const byteArrays = new Array(slicesCount);
for (let sliceIndex = 0; sliceIndex < slicesCount; ++sliceIndex) {
const begin = sliceIndex * sliceSize;
const end = Math.min(begin + sliceSize, bytesLength);
const bytes = new Array(end - begin);
for (let offset = begin, i = 0; offset < end; ++i, ++offset) {
bytes[i] = byteCharacters[offset].charCodeAt(0);
}
byteArrays[sliceIndex] = new Uint8Array(bytes);
}
return new Blob(byteArrays, {type: contentType});
}
function setPageLanguage(lang) {
sessionStorage.setItem('translationService.language', lang);
location.reload();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy