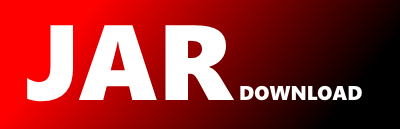
tajin.tajin.core.js Maven / Gradle / Ivy
/*
* Copyright (C) 2011 Ovea
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*jslint white: true, browser: true, devel: false, indent: 4, plusplus: true */
/*global jQuery, window, console*/
(function (w, $) {
"use strict";
w.Tajin = function () {
var self = this, status = 'new', listeners = [], modules = [];
this.options = {};
$.extend(self, {
status: function () {
return status;
},
uninstall: function (name) {
if (name && typeof name !== 'string') {
name = name.name;
}
if (!name) {
throw new Error('Module name is missing');
}
var i;
for (i = 0; i < modules.length; i++) {
if (modules[i].name === name) {
modules.splice(i, 1);
delete this[name];
break;
}
}
},
install: function (module) {
if (status === 'initializing') {
throw new Error('Initializing...');
}
if (!module.name) {
throw new Error('Module name is missing');
}
self.uninstall(module.name);
if (module.requires) {
var missing = $.isArray(module.requires) ? module.requires.slice(0) : module.requires.split(','), p, i;
for (i = 0; i < modules.length; i++) {
p = $.inArray(modules[i].name, missing);
if (p >= 0) {
missing.splice(p, 1);
}
}
if (missing.length) {
throw new Error("Error loading module '" + module.name + "': missing modules: " + missing);
}
}
if (!module.exports) {
module.exports = {};
}
self.options[module.name] = self.options[module.name] || {};
self[module.name] = module.exports;
if ($.isFunction(module.oninstall)) {
module.oninstall(self);
}
if (status === 'ready') {
if($.isFunction(module.onconfigure)) {
module.onconfigure($.noop, self.options[module.name], self);
} else {
module.tajin_init = 'success';
}
}
modules.push(module);
},
configure: function (opts) {
if (status === 'new' || status === 'initializing') {
if (status === 'new') {
self.options = $.extend(true, {
debug: false,
onready: $.noop
}, self.options || {}, w.tajin_init || {}, opts || {});
}
status = 'initializing';
var n, i = -1,
inits = $.grep(modules, function (m) {
return m.tajin_init !== 'success' && $.isFunction(m.onconfigure);
}),
next = function () {
i++;
if (i > 0 && i <= inits.length) {
// exports previously suceed module
self[n] = inits[i - 1].exports;
inits[i - 1].tajin_init = 'success';
}
if (i < inits.length) {
n = inits[i].name;
self.options[n] = self.options[n] || {};
if (self.options.debug) {
console.log('[tajin.core] init', n, self.options[n]);
}
try {
inits[i].onconfigure(next, self.options[n], self);
} catch (e) {
inits[i].tajin_init = e;
if ($.isFunction(self.options.onerror)) {
self.options.onerror(self, e);
}
throw e;
}
} else if (i === inits.length) {
if (self.options.debug) {
console.log('[tajin.core] onready - init completed with options', self.options);
}
status = 'ready';
if ($.isFunction(self.options.onready)) {
self.options.onready(self);
}
while (listeners.length) {
listeners.shift()(self);
}
}
};
next();
}
},
toString: function () {
return "Tajin Framework, version ${project.version}, modules: " + self.modules();
},
modules: function () {
return $.map(modules, function (e) {
return e.name;
});
},
ready: function (fn) {
if ($.isFunction(fn)) {
if (status === 'ready') {
fn(this);
} else {
listeners.push(fn);
}
}
}
});
};
w.tajin = new w.Tajin();
}(window, jQuery));
© 2015 - 2025 Weber Informatics LLC | Privacy Policy