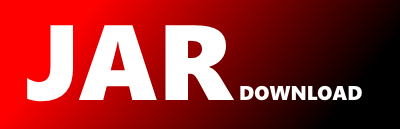
com.smartclient.debug.public.sc.client.widgets.Element.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartgwt Show documentation
Show all versions of smartgwt Show documentation
SmartGWT - GWT API's for SmartClient
The newest version!
/*
* Isomorphic SmartClient
* Version SC_SNAPSHOT-2011-08-08 (2011-08-08)
* Copyright(c) 1998 and beyond Isomorphic Software, Inc. All rights reserved.
* "SmartClient" is a trademark of Isomorphic Software, Inc.
*
* [email protected]
*
* http://smartclient.com/license
*/
//> @class Element
//
// Helper class containing methods for direct DOM interaction. Note that even if
// +link{isc, isc_useSimpleNames} is true, this class is not available in the global scope
// as window.Element
- to access it developers must always use
// isc.Element
//
// @treeLocation Client Reference/Foundation
// @visibility internal
//<
// Currently has no exposed methods - Developers typically will only need to interact with
// higher level canvas methods
isc.ClassFactory.defineClass("Element", null, null, true);
isc.Element.addClassMethods({
// --------------------------------------------------------------------------------------------
// DOM Access / Manipulation
//> @classMethod Element.get()
// Like the DOM method document.getElementById(), but works in all supported browsers.
//<
get : function (id, doc) {
doc = doc || this.getDocument();
if (isc.Browser.isDOM) return doc.getElementById(id);
},
// _getElementFromSelection()
// Determine which DOM element contains the current selection.
// 'doc' param allows caller to pass in a pointer to the document element - (may be document
// element from some frame/iframe - if not specified the main page document is used).
_getElementFromSelection : function (doc) {
if (!doc) doc = document;
if (isc.Browser.isIE ) {
var selection = doc.selection,
type = selection.type.toLowerCase(),
isText = (type == "text" || type == "none");
if (!selection) return null;
// If it's a text range use the 'parentElement()' method to determine what element
// contains the text.
// NOTE: an empty selection will be reported as type "None", but can be used to create
// a zero char text range, so we treat it like a "Text" selection.
if (isText) {
var range;
try {
range = selection.createRange();
} catch (e) {
}
return range ? range.parentElement() : null;
// If it's a control range, we can get at the elements in the control range
// by index. Iterate through the elements and find the common ancester.
} else {
// If this is a control range
// We're interested in the first common ancestor of the elements
var range = selection.createRange(),
commonParent;
for (var i = 0; i < range.length; i++) {
if (!commonParent) {
commonParent = range(i).parentElement;
} else {
// To determine whether the element is contained by the common parent,
// we're creating a textRange from both elements and using the inRange
// method.
while (!commonParent.contains(range(i))) {
commonParent = commonParent.parentElement;
}
}
}
return commonParent;
}
}
},
// Given an element attribute, iterate recursively through child nodes till you find a match.
// May be slow for deep hierarchies
findAttribute : function (element, attribute, value) {
if (!element) return null;
if (element[attribute] == value ||
(element.getAttribute && element.getAttribute(attribute) == value)) {
return element;
}
var children = element.childNodes;
for (var i = 0; i < children.length; i++) {
var subElement = this.findAttribute(children[i], attribute, value);
if (subElement) return subElement;
}
return null;
},
// helpers for createAbsoluteElement
_insertAfterBodyStart : window.isc_insertAfterBodyStart,
_globalInsertionMarker : "isc_global_insertion_marker",
getInsertionMarkerHTML : function () {
return "";
},
getInsertionMarker : function () {
return document.getElementById(this._globalInsertionMarker);
},
// create a new, absolutely positioned element, after page load
_$afterBegin : "afterBegin",
_$afterEnd : "afterEnd",
_$beforeBegin: "beforeBegin",
_$beforeEnd : "beforeEnd",
createAbsoluteElement : function (html, targetWindow) {
var wd = targetWindow || this.getWindow(),
body = this.getDocumentBody(true);
//>DEBUG
// if there's no body tag, we bail
if (body == null && !isc.Element.noBodyTagMessageShown) {
isc.Element.noBodyTagMessageShown = true;
var msg ="Error: Attempt to write content into a page outside the BODY tag. Isomorphic " +
"SmartClient requires this tag be present and all widgets be written out inside " +
"it.\r" +
"Please ensure your file has a BODY tag and any code to draw SmartClient widgets " +
"is enclosed in this tag."
;
//alert(msg);
this.logError(msg);
return;
}
// element with no enclosing