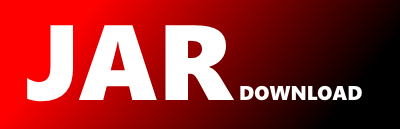
oc.runtime-components.0.9.0.source-code.IntLongOverflowChecks.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime-components Show documentation
Show all versions of runtime-components Show documentation
Adds some utilities to code, developed originally for Advent of Code solving
The newest version!
@file:Suppress("NOTHING_TO_INLINE")
package com.sschr15.aoc.annotations
inline fun plus(a: Int, b: Int): Int = Math.addExact(a, b)
inline fun minus(a: Int, b: Int): Int = Math.subtractExact(a, b)
inline fun times(a: Int, b: Int): Int = Math.multiplyExact(a, b)
inline fun inc(a: Int): Int = Math.incrementExact(a)
inline fun dec(a: Int): Int = Math.decrementExact(a)
inline fun unaryMinus(a: Int): Int = Math.negateExact(a)
inline fun abs(a: Int): Int = Math.absExact(a)
inline fun rem(a: Int, b: Int): Int {
if (a < 0 && b != a && System.getProperty("aoc.warnOnNegativeRemainder") != "false") {
System.err.println("Warning: Remainder of $a (a negative number)")
System.setProperty("aoc.warnOnNegativeRemainder", "false")
}
return a % b
}
inline fun toFloat(a: Int): Float =
if (a > 16777216 || a < -16777216) throw ArithmeticException("Not enough precision") else a.toFloat()
inline fun plus(a: Long, b: Long): Long = Math.addExact(a, b)
inline fun minus(a: Long, b: Long): Long = Math.subtractExact(a, b)
inline fun times(a: Long, b: Long): Long = Math.multiplyExact(a, b)
inline fun inc(a: Long): Long = Math.incrementExact(a)
inline fun dec(a: Long): Long = Math.decrementExact(a)
inline fun unaryMinus(a: Long): Long = Math.negateExact(a)
inline fun abs(a: Long): Long = Math.absExact(a)
inline fun rem(a: Long, b: Long): Long {
if (a < 0 && b != a && System.getProperty("aoc.warnOnNegativeRemainder") != "false") {
System.err.println("Warning: Remainder of $a (a negative number)")
System.setProperty("aoc.warnOnNegativeRemainder", "false")
}
return a % b
}
inline fun toInt(a: Long): Int = Math.toIntExact(a)
inline fun toFloat(a: Long): Float =
if (a > 16777216 || a < -16777216) throw ArithmeticException("Not enough precision") else a.toFloat()
inline fun toDouble(a: Long): Double =
if (a > 9007199254740992 || a < -9007199254740992) throw ArithmeticException("Not enough precision") else a.toDouble()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy