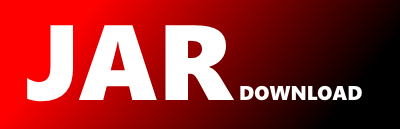
com.vaadin.polymer.public.bower_components.pouchdb.dist.pouchdb.fruitdown.js Maven / Gradle / Ivy
The newest version!
// PouchDB fruitdown plugin 6.3.4
// Based on FruitDOWN: https://github.com/nolanlawson/fruitdown
//
// (c) 2012-2017 Dale Harvey and the PouchDB team
// PouchDB may be freely distributed under the Apache license, version 2.0.
// For all details and documentation:
// http://pouchdb.com
(function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o 0) {
throw new Error('Invalid string. Length must be a multiple of 4')
}
// the number of equal signs (place holders)
// if there are two placeholders, than the two characters before it
// represent one byte
// if there is only one, then the three characters before it represent 2 bytes
// this is just a cheap hack to not do indexOf twice
return b64[len - 2] === '=' ? 2 : b64[len - 1] === '=' ? 1 : 0
}
function byteLength (b64) {
// base64 is 4/3 + up to two characters of the original data
return (b64.length * 3 / 4) - placeHoldersCount(b64)
}
function toByteArray (b64) {
var i, l, tmp, placeHolders, arr
var len = b64.length
placeHolders = placeHoldersCount(b64)
arr = new Arr((len * 3 / 4) - placeHolders)
// if there are placeholders, only get up to the last complete 4 chars
l = placeHolders > 0 ? len - 4 : len
var L = 0
for (i = 0; i < l; i += 4) {
tmp = (revLookup[b64.charCodeAt(i)] << 18) | (revLookup[b64.charCodeAt(i + 1)] << 12) | (revLookup[b64.charCodeAt(i + 2)] << 6) | revLookup[b64.charCodeAt(i + 3)]
arr[L++] = (tmp >> 16) & 0xFF
arr[L++] = (tmp >> 8) & 0xFF
arr[L++] = tmp & 0xFF
}
if (placeHolders === 2) {
tmp = (revLookup[b64.charCodeAt(i)] << 2) | (revLookup[b64.charCodeAt(i + 1)] >> 4)
arr[L++] = tmp & 0xFF
} else if (placeHolders === 1) {
tmp = (revLookup[b64.charCodeAt(i)] << 10) | (revLookup[b64.charCodeAt(i + 1)] << 4) | (revLookup[b64.charCodeAt(i + 2)] >> 2)
arr[L++] = (tmp >> 8) & 0xFF
arr[L++] = tmp & 0xFF
}
return arr
}
function tripletToBase64 (num) {
return lookup[num >> 18 & 0x3F] + lookup[num >> 12 & 0x3F] + lookup[num >> 6 & 0x3F] + lookup[num & 0x3F]
}
function encodeChunk (uint8, start, end) {
var tmp
var output = []
for (var i = start; i < end; i += 3) {
tmp = (uint8[i] << 16) + (uint8[i + 1] << 8) + (uint8[i + 2])
output.push(tripletToBase64(tmp))
}
return output.join('')
}
function fromByteArray (uint8) {
var tmp
var len = uint8.length
var extraBytes = len % 3 // if we have 1 byte left, pad 2 bytes
var output = ''
var parts = []
var maxChunkLength = 16383 // must be multiple of 3
// go through the array every three bytes, we'll deal with trailing stuff later
for (var i = 0, len2 = len - extraBytes; i < len2; i += maxChunkLength) {
parts.push(encodeChunk(uint8, i, (i + maxChunkLength) > len2 ? len2 : (i + maxChunkLength)))
}
// pad the end with zeros, but make sure to not forget the extra bytes
if (extraBytes === 1) {
tmp = uint8[len - 1]
output += lookup[tmp >> 2]
output += lookup[(tmp << 4) & 0x3F]
output += '=='
} else if (extraBytes === 2) {
tmp = (uint8[len - 2] << 8) + (uint8[len - 1])
output += lookup[tmp >> 10]
output += lookup[(tmp >> 4) & 0x3F]
output += lookup[(tmp << 2) & 0x3F]
output += '='
}
parts.push(output)
return parts.join('')
}
},{}],6:[function(_dereq_,module,exports){
},{}],7:[function(_dereq_,module,exports){
(function (Buffer){
var isArrayBuffer = _dereq_(34)
var isModern = (
typeof Buffer.alloc === 'function' &&
typeof Buffer.allocUnsafe === 'function' &&
typeof Buffer.from === 'function'
)
function fromArrayBuffer (obj, byteOffset, length) {
byteOffset >>>= 0
var maxLength = obj.byteLength - byteOffset
if (maxLength < 0) {
throw new RangeError("'offset' is out of bounds")
}
if (length === undefined) {
length = maxLength
} else {
length >>>= 0
if (length > maxLength) {
throw new RangeError("'length' is out of bounds")
}
}
return isModern
? Buffer.from(obj.slice(byteOffset, byteOffset + length))
: new Buffer(new Uint8Array(obj.slice(byteOffset, byteOffset + length)))
}
function fromString (string, encoding) {
if (typeof encoding !== 'string' || encoding === '') {
encoding = 'utf8'
}
if (!Buffer.isEncoding(encoding)) {
throw new TypeError('"encoding" must be a valid string encoding')
}
return isModern
? Buffer.from(string, encoding)
: new Buffer(string, encoding)
}
function bufferFrom (value, encodingOrOffset, length) {
if (typeof value === 'number') {
throw new TypeError('"value" argument must not be a number')
}
if (isArrayBuffer(value)) {
return fromArrayBuffer(value, encodingOrOffset, length)
}
if (typeof value === 'string') {
return fromString(value, encodingOrOffset)
}
return isModern
? Buffer.from(value)
: new Buffer(value)
}
module.exports = bufferFrom
}).call(this,_dereq_(8).Buffer)
},{"34":34,"8":8}],8:[function(_dereq_,module,exports){
/*!
* The buffer module from node.js, for the browser.
*
* @author Feross Aboukhadijeh
* @license MIT
*/
/* eslint-disable no-proto */
'use strict'
var base64 = _dereq_(5)
var ieee754 = _dereq_(31)
exports.Buffer = Buffer
exports.SlowBuffer = SlowBuffer
exports.INSPECT_MAX_BYTES = 50
var K_MAX_LENGTH = 0x7fffffff
exports.kMaxLength = K_MAX_LENGTH
/**
* If `Buffer.TYPED_ARRAY_SUPPORT`:
* === true Use Uint8Array implementation (fastest)
* === false Print warning and recommend using `buffer` v4.x which has an Object
* implementation (most compatible, even IE6)
*
* Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,
* Opera 11.6+, iOS 4.2+.
*
* We report that the browser does not support typed arrays if the are not subclassable
* using __proto__. Firefox 4-29 lacks support for adding new properties to `Uint8Array`
* (See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438). IE 10 lacks support
* for __proto__ and has a buggy typed array implementation.
*/
Buffer.TYPED_ARRAY_SUPPORT = typedArraySupport()
if (!Buffer.TYPED_ARRAY_SUPPORT && typeof console !== 'undefined' &&
typeof console.error === 'function') {
console.error(
'This browser lacks typed array (Uint8Array) support which is required by ' +
'`buffer` v5.x. Use `buffer` v4.x if you require old browser support.'
)
}
function typedArraySupport () {
// Can typed array instances can be augmented?
try {
var arr = new Uint8Array(1)
arr.__proto__ = {__proto__: Uint8Array.prototype, foo: function () { return 42 }}
return arr.foo() === 42
} catch (e) {
return false
}
}
function createBuffer (length) {
if (length > K_MAX_LENGTH) {
throw new RangeError('Invalid typed array length')
}
// Return an augmented `Uint8Array` instance
var buf = new Uint8Array(length)
buf.__proto__ = Buffer.prototype
return buf
}
/**
* The Buffer constructor returns instances of `Uint8Array` that have their
* prototype changed to `Buffer.prototype`. Furthermore, `Buffer` is a subclass of
* `Uint8Array`, so the returned instances will have all the node `Buffer` methods
* and the `Uint8Array` methods. Square bracket notation works as expected -- it
* returns a single octet.
*
* The `Uint8Array` prototype remains unmodified.
*/
function Buffer (arg, encodingOrOffset, length) {
// Common case.
if (typeof arg === 'number') {
if (typeof encodingOrOffset === 'string') {
throw new Error(
'If encoding is specified then the first argument must be a string'
)
}
return allocUnsafe(arg)
}
return from(arg, encodingOrOffset, length)
}
// Fix subarray() in ES2016. See: https://github.com/feross/buffer/pull/97
if (typeof Symbol !== 'undefined' && Symbol.species &&
Buffer[Symbol.species] === Buffer) {
Object.defineProperty(Buffer, Symbol.species, {
value: null,
configurable: true,
enumerable: false,
writable: false
})
}
Buffer.poolSize = 8192 // not used by this implementation
function from (value, encodingOrOffset, length) {
if (typeof value === 'number') {
throw new TypeError('"value" argument must not be a number')
}
if (value instanceof ArrayBuffer) {
return fromArrayBuffer(value, encodingOrOffset, length)
}
if (typeof value === 'string') {
return fromString(value, encodingOrOffset)
}
return fromObject(value)
}
/**
* Functionally equivalent to Buffer(arg, encoding) but throws a TypeError
* if value is a number.
* Buffer.from(str[, encoding])
* Buffer.from(array)
* Buffer.from(buffer)
* Buffer.from(arrayBuffer[, byteOffset[, length]])
**/
Buffer.from = function (value, encodingOrOffset, length) {
return from(value, encodingOrOffset, length)
}
// Note: Change prototype *after* Buffer.from is defined to workaround Chrome bug:
// https://github.com/feross/buffer/pull/148
Buffer.prototype.__proto__ = Uint8Array.prototype
Buffer.__proto__ = Uint8Array
function assertSize (size) {
if (typeof size !== 'number') {
throw new TypeError('"size" argument must be a number')
} else if (size < 0) {
throw new RangeError('"size" argument must not be negative')
}
}
function alloc (size, fill, encoding) {
assertSize(size)
if (size <= 0) {
return createBuffer(size)
}
if (fill !== undefined) {
// Only pay attention to encoding if it's a string. This
// prevents accidentally sending in a number that would
// be interpretted as a start offset.
return typeof encoding === 'string'
? createBuffer(size).fill(fill, encoding)
: createBuffer(size).fill(fill)
}
return createBuffer(size)
}
/**
* Creates a new filled Buffer instance.
* alloc(size[, fill[, encoding]])
**/
Buffer.alloc = function (size, fill, encoding) {
return alloc(size, fill, encoding)
}
function allocUnsafe (size) {
assertSize(size)
return createBuffer(size < 0 ? 0 : checked(size) | 0)
}
/**
* Equivalent to Buffer(num), by default creates a non-zero-filled Buffer instance.
* */
Buffer.allocUnsafe = function (size) {
return allocUnsafe(size)
}
/**
* Equivalent to SlowBuffer(num), by default creates a non-zero-filled Buffer instance.
*/
Buffer.allocUnsafeSlow = function (size) {
return allocUnsafe(size)
}
function fromString (string, encoding) {
if (typeof encoding !== 'string' || encoding === '') {
encoding = 'utf8'
}
if (!Buffer.isEncoding(encoding)) {
throw new TypeError('"encoding" must be a valid string encoding')
}
var length = byteLength(string, encoding) | 0
var buf = createBuffer(length)
var actual = buf.write(string, encoding)
if (actual !== length) {
// Writing a hex string, for example, that contains invalid characters will
// cause everything after the first invalid character to be ignored. (e.g.
// 'abxxcd' will be treated as 'ab')
buf = buf.slice(0, actual)
}
return buf
}
function fromArrayLike (array) {
var length = array.length < 0 ? 0 : checked(array.length) | 0
var buf = createBuffer(length)
for (var i = 0; i < length; i += 1) {
buf[i] = array[i] & 255
}
return buf
}
function fromArrayBuffer (array, byteOffset, length) {
if (byteOffset < 0 || array.byteLength < byteOffset) {
throw new RangeError('\'offset\' is out of bounds')
}
if (array.byteLength < byteOffset + (length || 0)) {
throw new RangeError('\'length\' is out of bounds')
}
var buf
if (byteOffset === undefined && length === undefined) {
buf = new Uint8Array(array)
} else if (length === undefined) {
buf = new Uint8Array(array, byteOffset)
} else {
buf = new Uint8Array(array, byteOffset, length)
}
// Return an augmented `Uint8Array` instance
buf.__proto__ = Buffer.prototype
return buf
}
function fromObject (obj) {
if (Buffer.isBuffer(obj)) {
var len = checked(obj.length) | 0
var buf = createBuffer(len)
if (buf.length === 0) {
return buf
}
obj.copy(buf, 0, 0, len)
return buf
}
if (obj) {
if (isArrayBufferView(obj) || 'length' in obj) {
if (typeof obj.length !== 'number' || numberIsNaN(obj.length)) {
return createBuffer(0)
}
return fromArrayLike(obj)
}
if (obj.type === 'Buffer' && Array.isArray(obj.data)) {
return fromArrayLike(obj.data)
}
}
throw new TypeError('First argument must be a string, Buffer, ArrayBuffer, Array, or array-like object.')
}
function checked (length) {
// Note: cannot use `length < K_MAX_LENGTH` here because that fails when
// length is NaN (which is otherwise coerced to zero.)
if (length >= K_MAX_LENGTH) {
throw new RangeError('Attempt to allocate Buffer larger than maximum ' +
'size: 0x' + K_MAX_LENGTH.toString(16) + ' bytes')
}
return length | 0
}
function SlowBuffer (length) {
if (+length != length) { // eslint-disable-line eqeqeq
length = 0
}
return Buffer.alloc(+length)
}
Buffer.isBuffer = function isBuffer (b) {
return b != null && b._isBuffer === true
}
Buffer.compare = function compare (a, b) {
if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {
throw new TypeError('Arguments must be Buffers')
}
if (a === b) return 0
var x = a.length
var y = b.length
for (var i = 0, len = Math.min(x, y); i < len; ++i) {
if (a[i] !== b[i]) {
x = a[i]
y = b[i]
break
}
}
if (x < y) return -1
if (y < x) return 1
return 0
}
Buffer.isEncoding = function isEncoding (encoding) {
switch (String(encoding).toLowerCase()) {
case 'hex':
case 'utf8':
case 'utf-8':
case 'ascii':
case 'latin1':
case 'binary':
case 'base64':
case 'ucs2':
case 'ucs-2':
case 'utf16le':
case 'utf-16le':
return true
default:
return false
}
}
Buffer.concat = function concat (list, length) {
if (!Array.isArray(list)) {
throw new TypeError('"list" argument must be an Array of Buffers')
}
if (list.length === 0) {
return Buffer.alloc(0)
}
var i
if (length === undefined) {
length = 0
for (i = 0; i < list.length; ++i) {
length += list[i].length
}
}
var buffer = Buffer.allocUnsafe(length)
var pos = 0
for (i = 0; i < list.length; ++i) {
var buf = list[i]
if (!Buffer.isBuffer(buf)) {
throw new TypeError('"list" argument must be an Array of Buffers')
}
buf.copy(buffer, pos)
pos += buf.length
}
return buffer
}
function byteLength (string, encoding) {
if (Buffer.isBuffer(string)) {
return string.length
}
if (isArrayBufferView(string) || string instanceof ArrayBuffer) {
return string.byteLength
}
if (typeof string !== 'string') {
string = '' + string
}
var len = string.length
if (len === 0) return 0
// Use a for loop to avoid recursion
var loweredCase = false
for (;;) {
switch (encoding) {
case 'ascii':
case 'latin1':
case 'binary':
return len
case 'utf8':
case 'utf-8':
case undefined:
return utf8ToBytes(string).length
case 'ucs2':
case 'ucs-2':
case 'utf16le':
case 'utf-16le':
return len * 2
case 'hex':
return len >>> 1
case 'base64':
return base64ToBytes(string).length
default:
if (loweredCase) return utf8ToBytes(string).length // assume utf8
encoding = ('' + encoding).toLowerCase()
loweredCase = true
}
}
}
Buffer.byteLength = byteLength
function slowToString (encoding, start, end) {
var loweredCase = false
// No need to verify that "this.length <= MAX_UINT32" since it's a read-only
// property of a typed array.
// This behaves neither like String nor Uint8Array in that we set start/end
// to their upper/lower bounds if the value passed is out of range.
// undefined is handled specially as per ECMA-262 6th Edition,
// Section 13.3.3.7 Runtime Semantics: KeyedBindingInitialization.
if (start === undefined || start < 0) {
start = 0
}
// Return early if start > this.length. Done here to prevent potential uint32
// coercion fail below.
if (start > this.length) {
return ''
}
if (end === undefined || end > this.length) {
end = this.length
}
if (end <= 0) {
return ''
}
// Force coersion to uint32. This will also coerce falsey/NaN values to 0.
end >>>= 0
start >>>= 0
if (end <= start) {
return ''
}
if (!encoding) encoding = 'utf8'
while (true) {
switch (encoding) {
case 'hex':
return hexSlice(this, start, end)
case 'utf8':
case 'utf-8':
return utf8Slice(this, start, end)
case 'ascii':
return asciiSlice(this, start, end)
case 'latin1':
case 'binary':
return latin1Slice(this, start, end)
case 'base64':
return base64Slice(this, start, end)
case 'ucs2':
case 'ucs-2':
case 'utf16le':
case 'utf-16le':
return utf16leSlice(this, start, end)
default:
if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
encoding = (encoding + '').toLowerCase()
loweredCase = true
}
}
}
// This property is used by `Buffer.isBuffer` (and the `is-buffer` npm package)
// to detect a Buffer instance. It's not possible to use `instanceof Buffer`
// reliably in a browserify context because there could be multiple different
// copies of the 'buffer' package in use. This method works even for Buffer
// instances that were created from another copy of the `buffer` package.
// See: https://github.com/feross/buffer/issues/154
Buffer.prototype._isBuffer = true
function swap (b, n, m) {
var i = b[n]
b[n] = b[m]
b[m] = i
}
Buffer.prototype.swap16 = function swap16 () {
var len = this.length
if (len % 2 !== 0) {
throw new RangeError('Buffer size must be a multiple of 16-bits')
}
for (var i = 0; i < len; i += 2) {
swap(this, i, i + 1)
}
return this
}
Buffer.prototype.swap32 = function swap32 () {
var len = this.length
if (len % 4 !== 0) {
throw new RangeError('Buffer size must be a multiple of 32-bits')
}
for (var i = 0; i < len; i += 4) {
swap(this, i, i + 3)
swap(this, i + 1, i + 2)
}
return this
}
Buffer.prototype.swap64 = function swap64 () {
var len = this.length
if (len % 8 !== 0) {
throw new RangeError('Buffer size must be a multiple of 64-bits')
}
for (var i = 0; i < len; i += 8) {
swap(this, i, i + 7)
swap(this, i + 1, i + 6)
swap(this, i + 2, i + 5)
swap(this, i + 3, i + 4)
}
return this
}
Buffer.prototype.toString = function toString () {
var length = this.length
if (length === 0) return ''
if (arguments.length === 0) return utf8Slice(this, 0, length)
return slowToString.apply(this, arguments)
}
Buffer.prototype.equals = function equals (b) {
if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')
if (this === b) return true
return Buffer.compare(this, b) === 0
}
Buffer.prototype.inspect = function inspect () {
var str = ''
var max = exports.INSPECT_MAX_BYTES
if (this.length > 0) {
str = this.toString('hex', 0, max).match(/.{2}/g).join(' ')
if (this.length > max) str += ' ... '
}
return ''
}
Buffer.prototype.compare = function compare (target, start, end, thisStart, thisEnd) {
if (!Buffer.isBuffer(target)) {
throw new TypeError('Argument must be a Buffer')
}
if (start === undefined) {
start = 0
}
if (end === undefined) {
end = target ? target.length : 0
}
if (thisStart === undefined) {
thisStart = 0
}
if (thisEnd === undefined) {
thisEnd = this.length
}
if (start < 0 || end > target.length || thisStart < 0 || thisEnd > this.length) {
throw new RangeError('out of range index')
}
if (thisStart >= thisEnd && start >= end) {
return 0
}
if (thisStart >= thisEnd) {
return -1
}
if (start >= end) {
return 1
}
start >>>= 0
end >>>= 0
thisStart >>>= 0
thisEnd >>>= 0
if (this === target) return 0
var x = thisEnd - thisStart
var y = end - start
var len = Math.min(x, y)
var thisCopy = this.slice(thisStart, thisEnd)
var targetCopy = target.slice(start, end)
for (var i = 0; i < len; ++i) {
if (thisCopy[i] !== targetCopy[i]) {
x = thisCopy[i]
y = targetCopy[i]
break
}
}
if (x < y) return -1
if (y < x) return 1
return 0
}
// Finds either the first index of `val` in `buffer` at offset >= `byteOffset`,
// OR the last index of `val` in `buffer` at offset <= `byteOffset`.
//
// Arguments:
// - buffer - a Buffer to search
// - val - a string, Buffer, or number
// - byteOffset - an index into `buffer`; will be clamped to an int32
// - encoding - an optional encoding, relevant is val is a string
// - dir - true for indexOf, false for lastIndexOf
function bidirectionalIndexOf (buffer, val, byteOffset, encoding, dir) {
// Empty buffer means no match
if (buffer.length === 0) return -1
// Normalize byteOffset
if (typeof byteOffset === 'string') {
encoding = byteOffset
byteOffset = 0
} else if (byteOffset > 0x7fffffff) {
byteOffset = 0x7fffffff
} else if (byteOffset < -0x80000000) {
byteOffset = -0x80000000
}
byteOffset = +byteOffset // Coerce to Number.
if (numberIsNaN(byteOffset)) {
// byteOffset: it it's undefined, null, NaN, "foo", etc, search whole buffer
byteOffset = dir ? 0 : (buffer.length - 1)
}
// Normalize byteOffset: negative offsets start from the end of the buffer
if (byteOffset < 0) byteOffset = buffer.length + byteOffset
if (byteOffset >= buffer.length) {
if (dir) return -1
else byteOffset = buffer.length - 1
} else if (byteOffset < 0) {
if (dir) byteOffset = 0
else return -1
}
// Normalize val
if (typeof val === 'string') {
val = Buffer.from(val, encoding)
}
// Finally, search either indexOf (if dir is true) or lastIndexOf
if (Buffer.isBuffer(val)) {
// Special case: looking for empty string/buffer always fails
if (val.length === 0) {
return -1
}
return arrayIndexOf(buffer, val, byteOffset, encoding, dir)
} else if (typeof val === 'number') {
val = val & 0xFF // Search for a byte value [0-255]
if (typeof Uint8Array.prototype.indexOf === 'function') {
if (dir) {
return Uint8Array.prototype.indexOf.call(buffer, val, byteOffset)
} else {
return Uint8Array.prototype.lastIndexOf.call(buffer, val, byteOffset)
}
}
return arrayIndexOf(buffer, [ val ], byteOffset, encoding, dir)
}
throw new TypeError('val must be string, number or Buffer')
}
function arrayIndexOf (arr, val, byteOffset, encoding, dir) {
var indexSize = 1
var arrLength = arr.length
var valLength = val.length
if (encoding !== undefined) {
encoding = String(encoding).toLowerCase()
if (encoding === 'ucs2' || encoding === 'ucs-2' ||
encoding === 'utf16le' || encoding === 'utf-16le') {
if (arr.length < 2 || val.length < 2) {
return -1
}
indexSize = 2
arrLength /= 2
valLength /= 2
byteOffset /= 2
}
}
function read (buf, i) {
if (indexSize === 1) {
return buf[i]
} else {
return buf.readUInt16BE(i * indexSize)
}
}
var i
if (dir) {
var foundIndex = -1
for (i = byteOffset; i < arrLength; i++) {
if (read(arr, i) === read(val, foundIndex === -1 ? 0 : i - foundIndex)) {
if (foundIndex === -1) foundIndex = i
if (i - foundIndex + 1 === valLength) return foundIndex * indexSize
} else {
if (foundIndex !== -1) i -= i - foundIndex
foundIndex = -1
}
}
} else {
if (byteOffset + valLength > arrLength) byteOffset = arrLength - valLength
for (i = byteOffset; i >= 0; i--) {
var found = true
for (var j = 0; j < valLength; j++) {
if (read(arr, i + j) !== read(val, j)) {
found = false
break
}
}
if (found) return i
}
}
return -1
}
Buffer.prototype.includes = function includes (val, byteOffset, encoding) {
return this.indexOf(val, byteOffset, encoding) !== -1
}
Buffer.prototype.indexOf = function indexOf (val, byteOffset, encoding) {
return bidirectionalIndexOf(this, val, byteOffset, encoding, true)
}
Buffer.prototype.lastIndexOf = function lastIndexOf (val, byteOffset, encoding) {
return bidirectionalIndexOf(this, val, byteOffset, encoding, false)
}
function hexWrite (buf, string, offset, length) {
offset = Number(offset) || 0
var remaining = buf.length - offset
if (!length) {
length = remaining
} else {
length = Number(length)
if (length > remaining) {
length = remaining
}
}
// must be an even number of digits
var strLen = string.length
if (strLen % 2 !== 0) throw new TypeError('Invalid hex string')
if (length > strLen / 2) {
length = strLen / 2
}
for (var i = 0; i < length; ++i) {
var parsed = parseInt(string.substr(i * 2, 2), 16)
if (numberIsNaN(parsed)) return i
buf[offset + i] = parsed
}
return i
}
function utf8Write (buf, string, offset, length) {
return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length)
}
function asciiWrite (buf, string, offset, length) {
return blitBuffer(asciiToBytes(string), buf, offset, length)
}
function latin1Write (buf, string, offset, length) {
return asciiWrite(buf, string, offset, length)
}
function base64Write (buf, string, offset, length) {
return blitBuffer(base64ToBytes(string), buf, offset, length)
}
function ucs2Write (buf, string, offset, length) {
return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length)
}
Buffer.prototype.write = function write (string, offset, length, encoding) {
// Buffer#write(string)
if (offset === undefined) {
encoding = 'utf8'
length = this.length
offset = 0
// Buffer#write(string, encoding)
} else if (length === undefined && typeof offset === 'string') {
encoding = offset
length = this.length
offset = 0
// Buffer#write(string, offset[, length][, encoding])
} else if (isFinite(offset)) {
offset = offset >>> 0
if (isFinite(length)) {
length = length >>> 0
if (encoding === undefined) encoding = 'utf8'
} else {
encoding = length
length = undefined
}
} else {
throw new Error(
'Buffer.write(string, encoding, offset[, length]) is no longer supported'
)
}
var remaining = this.length - offset
if (length === undefined || length > remaining) length = remaining
if ((string.length > 0 && (length < 0 || offset < 0)) || offset > this.length) {
throw new RangeError('Attempt to write outside buffer bounds')
}
if (!encoding) encoding = 'utf8'
var loweredCase = false
for (;;) {
switch (encoding) {
case 'hex':
return hexWrite(this, string, offset, length)
case 'utf8':
case 'utf-8':
return utf8Write(this, string, offset, length)
case 'ascii':
return asciiWrite(this, string, offset, length)
case 'latin1':
case 'binary':
return latin1Write(this, string, offset, length)
case 'base64':
// Warning: maxLength not taken into account in base64Write
return base64Write(this, string, offset, length)
case 'ucs2':
case 'ucs-2':
case 'utf16le':
case 'utf-16le':
return ucs2Write(this, string, offset, length)
default:
if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
encoding = ('' + encoding).toLowerCase()
loweredCase = true
}
}
}
Buffer.prototype.toJSON = function toJSON () {
return {
type: 'Buffer',
data: Array.prototype.slice.call(this._arr || this, 0)
}
}
function base64Slice (buf, start, end) {
if (start === 0 && end === buf.length) {
return base64.fromByteArray(buf)
} else {
return base64.fromByteArray(buf.slice(start, end))
}
}
function utf8Slice (buf, start, end) {
end = Math.min(buf.length, end)
var res = []
var i = start
while (i < end) {
var firstByte = buf[i]
var codePoint = null
var bytesPerSequence = (firstByte > 0xEF) ? 4
: (firstByte > 0xDF) ? 3
: (firstByte > 0xBF) ? 2
: 1
if (i + bytesPerSequence <= end) {
var secondByte, thirdByte, fourthByte, tempCodePoint
switch (bytesPerSequence) {
case 1:
if (firstByte < 0x80) {
codePoint = firstByte
}
break
case 2:
secondByte = buf[i + 1]
if ((secondByte & 0xC0) === 0x80) {
tempCodePoint = (firstByte & 0x1F) << 0x6 | (secondByte & 0x3F)
if (tempCodePoint > 0x7F) {
codePoint = tempCodePoint
}
}
break
case 3:
secondByte = buf[i + 1]
thirdByte = buf[i + 2]
if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {
tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | (thirdByte & 0x3F)
if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {
codePoint = tempCodePoint
}
}
break
case 4:
secondByte = buf[i + 1]
thirdByte = buf[i + 2]
fourthByte = buf[i + 3]
if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {
tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | (fourthByte & 0x3F)
if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {
codePoint = tempCodePoint
}
}
}
}
if (codePoint === null) {
// we did not generate a valid codePoint so insert a
// replacement char (U+FFFD) and advance only 1 byte
codePoint = 0xFFFD
bytesPerSequence = 1
} else if (codePoint > 0xFFFF) {
// encode to utf16 (surrogate pair dance)
codePoint -= 0x10000
res.push(codePoint >>> 10 & 0x3FF | 0xD800)
codePoint = 0xDC00 | codePoint & 0x3FF
}
res.push(codePoint)
i += bytesPerSequence
}
return decodeCodePointsArray(res)
}
// Based on http://stackoverflow.com/a/22747272/680742, the browser with
// the lowest limit is Chrome, with 0x10000 args.
// We go 1 magnitude less, for safety
var MAX_ARGUMENTS_LENGTH = 0x1000
function decodeCodePointsArray (codePoints) {
var len = codePoints.length
if (len <= MAX_ARGUMENTS_LENGTH) {
return String.fromCharCode.apply(String, codePoints) // avoid extra slice()
}
// Decode in chunks to avoid "call stack size exceeded".
var res = ''
var i = 0
while (i < len) {
res += String.fromCharCode.apply(
String,
codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH)
)
}
return res
}
function asciiSlice (buf, start, end) {
var ret = ''
end = Math.min(buf.length, end)
for (var i = start; i < end; ++i) {
ret += String.fromCharCode(buf[i] & 0x7F)
}
return ret
}
function latin1Slice (buf, start, end) {
var ret = ''
end = Math.min(buf.length, end)
for (var i = start; i < end; ++i) {
ret += String.fromCharCode(buf[i])
}
return ret
}
function hexSlice (buf, start, end) {
var len = buf.length
if (!start || start < 0) start = 0
if (!end || end < 0 || end > len) end = len
var out = ''
for (var i = start; i < end; ++i) {
out += toHex(buf[i])
}
return out
}
function utf16leSlice (buf, start, end) {
var bytes = buf.slice(start, end)
var res = ''
for (var i = 0; i < bytes.length; i += 2) {
res += String.fromCharCode(bytes[i] + (bytes[i + 1] * 256))
}
return res
}
Buffer.prototype.slice = function slice (start, end) {
var len = this.length
start = ~~start
end = end === undefined ? len : ~~end
if (start < 0) {
start += len
if (start < 0) start = 0
} else if (start > len) {
start = len
}
if (end < 0) {
end += len
if (end < 0) end = 0
} else if (end > len) {
end = len
}
if (end < start) end = start
var newBuf = this.subarray(start, end)
// Return an augmented `Uint8Array` instance
newBuf.__proto__ = Buffer.prototype
return newBuf
}
/*
* Need to make sure that buffer isn't trying to write out of bounds.
*/
function checkOffset (offset, ext, length) {
if ((offset % 1) !== 0 || offset < 0) throw new RangeError('offset is not uint')
if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length')
}
Buffer.prototype.readUIntLE = function readUIntLE (offset, byteLength, noAssert) {
offset = offset >>> 0
byteLength = byteLength >>> 0
if (!noAssert) checkOffset(offset, byteLength, this.length)
var val = this[offset]
var mul = 1
var i = 0
while (++i < byteLength && (mul *= 0x100)) {
val += this[offset + i] * mul
}
return val
}
Buffer.prototype.readUIntBE = function readUIntBE (offset, byteLength, noAssert) {
offset = offset >>> 0
byteLength = byteLength >>> 0
if (!noAssert) {
checkOffset(offset, byteLength, this.length)
}
var val = this[offset + --byteLength]
var mul = 1
while (byteLength > 0 && (mul *= 0x100)) {
val += this[offset + --byteLength] * mul
}
return val
}
Buffer.prototype.readUInt8 = function readUInt8 (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 1, this.length)
return this[offset]
}
Buffer.prototype.readUInt16LE = function readUInt16LE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 2, this.length)
return this[offset] | (this[offset + 1] << 8)
}
Buffer.prototype.readUInt16BE = function readUInt16BE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 2, this.length)
return (this[offset] << 8) | this[offset + 1]
}
Buffer.prototype.readUInt32LE = function readUInt32LE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 4, this.length)
return ((this[offset]) |
(this[offset + 1] << 8) |
(this[offset + 2] << 16)) +
(this[offset + 3] * 0x1000000)
}
Buffer.prototype.readUInt32BE = function readUInt32BE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 4, this.length)
return (this[offset] * 0x1000000) +
((this[offset + 1] << 16) |
(this[offset + 2] << 8) |
this[offset + 3])
}
Buffer.prototype.readIntLE = function readIntLE (offset, byteLength, noAssert) {
offset = offset >>> 0
byteLength = byteLength >>> 0
if (!noAssert) checkOffset(offset, byteLength, this.length)
var val = this[offset]
var mul = 1
var i = 0
while (++i < byteLength && (mul *= 0x100)) {
val += this[offset + i] * mul
}
mul *= 0x80
if (val >= mul) val -= Math.pow(2, 8 * byteLength)
return val
}
Buffer.prototype.readIntBE = function readIntBE (offset, byteLength, noAssert) {
offset = offset >>> 0
byteLength = byteLength >>> 0
if (!noAssert) checkOffset(offset, byteLength, this.length)
var i = byteLength
var mul = 1
var val = this[offset + --i]
while (i > 0 && (mul *= 0x100)) {
val += this[offset + --i] * mul
}
mul *= 0x80
if (val >= mul) val -= Math.pow(2, 8 * byteLength)
return val
}
Buffer.prototype.readInt8 = function readInt8 (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 1, this.length)
if (!(this[offset] & 0x80)) return (this[offset])
return ((0xff - this[offset] + 1) * -1)
}
Buffer.prototype.readInt16LE = function readInt16LE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 2, this.length)
var val = this[offset] | (this[offset + 1] << 8)
return (val & 0x8000) ? val | 0xFFFF0000 : val
}
Buffer.prototype.readInt16BE = function readInt16BE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 2, this.length)
var val = this[offset + 1] | (this[offset] << 8)
return (val & 0x8000) ? val | 0xFFFF0000 : val
}
Buffer.prototype.readInt32LE = function readInt32LE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 4, this.length)
return (this[offset]) |
(this[offset + 1] << 8) |
(this[offset + 2] << 16) |
(this[offset + 3] << 24)
}
Buffer.prototype.readInt32BE = function readInt32BE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 4, this.length)
return (this[offset] << 24) |
(this[offset + 1] << 16) |
(this[offset + 2] << 8) |
(this[offset + 3])
}
Buffer.prototype.readFloatLE = function readFloatLE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 4, this.length)
return ieee754.read(this, offset, true, 23, 4)
}
Buffer.prototype.readFloatBE = function readFloatBE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 4, this.length)
return ieee754.read(this, offset, false, 23, 4)
}
Buffer.prototype.readDoubleLE = function readDoubleLE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 8, this.length)
return ieee754.read(this, offset, true, 52, 8)
}
Buffer.prototype.readDoubleBE = function readDoubleBE (offset, noAssert) {
offset = offset >>> 0
if (!noAssert) checkOffset(offset, 8, this.length)
return ieee754.read(this, offset, false, 52, 8)
}
function checkInt (buf, value, offset, ext, max, min) {
if (!Buffer.isBuffer(buf)) throw new TypeError('"buffer" argument must be a Buffer instance')
if (value > max || value < min) throw new RangeError('"value" argument is out of bounds')
if (offset + ext > buf.length) throw new RangeError('Index out of range')
}
Buffer.prototype.writeUIntLE = function writeUIntLE (value, offset, byteLength, noAssert) {
value = +value
offset = offset >>> 0
byteLength = byteLength >>> 0
if (!noAssert) {
var maxBytes = Math.pow(2, 8 * byteLength) - 1
checkInt(this, value, offset, byteLength, maxBytes, 0)
}
var mul = 1
var i = 0
this[offset] = value & 0xFF
while (++i < byteLength && (mul *= 0x100)) {
this[offset + i] = (value / mul) & 0xFF
}
return offset + byteLength
}
Buffer.prototype.writeUIntBE = function writeUIntBE (value, offset, byteLength, noAssert) {
value = +value
offset = offset >>> 0
byteLength = byteLength >>> 0
if (!noAssert) {
var maxBytes = Math.pow(2, 8 * byteLength) - 1
checkInt(this, value, offset, byteLength, maxBytes, 0)
}
var i = byteLength - 1
var mul = 1
this[offset + i] = value & 0xFF
while (--i >= 0 && (mul *= 0x100)) {
this[offset + i] = (value / mul) & 0xFF
}
return offset + byteLength
}
Buffer.prototype.writeUInt8 = function writeUInt8 (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0)
this[offset] = (value & 0xff)
return offset + 1
}
Buffer.prototype.writeUInt16LE = function writeUInt16LE (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
this[offset] = (value & 0xff)
this[offset + 1] = (value >>> 8)
return offset + 2
}
Buffer.prototype.writeUInt16BE = function writeUInt16BE (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
this[offset] = (value >>> 8)
this[offset + 1] = (value & 0xff)
return offset + 2
}
Buffer.prototype.writeUInt32LE = function writeUInt32LE (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
this[offset + 3] = (value >>> 24)
this[offset + 2] = (value >>> 16)
this[offset + 1] = (value >>> 8)
this[offset] = (value & 0xff)
return offset + 4
}
Buffer.prototype.writeUInt32BE = function writeUInt32BE (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
this[offset] = (value >>> 24)
this[offset + 1] = (value >>> 16)
this[offset + 2] = (value >>> 8)
this[offset + 3] = (value & 0xff)
return offset + 4
}
Buffer.prototype.writeIntLE = function writeIntLE (value, offset, byteLength, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) {
var limit = Math.pow(2, (8 * byteLength) - 1)
checkInt(this, value, offset, byteLength, limit - 1, -limit)
}
var i = 0
var mul = 1
var sub = 0
this[offset] = value & 0xFF
while (++i < byteLength && (mul *= 0x100)) {
if (value < 0 && sub === 0 && this[offset + i - 1] !== 0) {
sub = 1
}
this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
}
return offset + byteLength
}
Buffer.prototype.writeIntBE = function writeIntBE (value, offset, byteLength, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) {
var limit = Math.pow(2, (8 * byteLength) - 1)
checkInt(this, value, offset, byteLength, limit - 1, -limit)
}
var i = byteLength - 1
var mul = 1
var sub = 0
this[offset + i] = value & 0xFF
while (--i >= 0 && (mul *= 0x100)) {
if (value < 0 && sub === 0 && this[offset + i + 1] !== 0) {
sub = 1
}
this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
}
return offset + byteLength
}
Buffer.prototype.writeInt8 = function writeInt8 (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80)
if (value < 0) value = 0xff + value + 1
this[offset] = (value & 0xff)
return offset + 1
}
Buffer.prototype.writeInt16LE = function writeInt16LE (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
this[offset] = (value & 0xff)
this[offset + 1] = (value >>> 8)
return offset + 2
}
Buffer.prototype.writeInt16BE = function writeInt16BE (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
this[offset] = (value >>> 8)
this[offset + 1] = (value & 0xff)
return offset + 2
}
Buffer.prototype.writeInt32LE = function writeInt32LE (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
this[offset] = (value & 0xff)
this[offset + 1] = (value >>> 8)
this[offset + 2] = (value >>> 16)
this[offset + 3] = (value >>> 24)
return offset + 4
}
Buffer.prototype.writeInt32BE = function writeInt32BE (value, offset, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
if (value < 0) value = 0xffffffff + value + 1
this[offset] = (value >>> 24)
this[offset + 1] = (value >>> 16)
this[offset + 2] = (value >>> 8)
this[offset + 3] = (value & 0xff)
return offset + 4
}
function checkIEEE754 (buf, value, offset, ext, max, min) {
if (offset + ext > buf.length) throw new RangeError('Index out of range')
if (offset < 0) throw new RangeError('Index out of range')
}
function writeFloat (buf, value, offset, littleEndian, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) {
checkIEEE754(buf, value, offset, 4, 3.4028234663852886e+38, -3.4028234663852886e+38)
}
ieee754.write(buf, value, offset, littleEndian, 23, 4)
return offset + 4
}
Buffer.prototype.writeFloatLE = function writeFloatLE (value, offset, noAssert) {
return writeFloat(this, value, offset, true, noAssert)
}
Buffer.prototype.writeFloatBE = function writeFloatBE (value, offset, noAssert) {
return writeFloat(this, value, offset, false, noAssert)
}
function writeDouble (buf, value, offset, littleEndian, noAssert) {
value = +value
offset = offset >>> 0
if (!noAssert) {
checkIEEE754(buf, value, offset, 8, 1.7976931348623157E+308, -1.7976931348623157E+308)
}
ieee754.write(buf, value, offset, littleEndian, 52, 8)
return offset + 8
}
Buffer.prototype.writeDoubleLE = function writeDoubleLE (value, offset, noAssert) {
return writeDouble(this, value, offset, true, noAssert)
}
Buffer.prototype.writeDoubleBE = function writeDoubleBE (value, offset, noAssert) {
return writeDouble(this, value, offset, false, noAssert)
}
// copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)
Buffer.prototype.copy = function copy (target, targetStart, start, end) {
if (!start) start = 0
if (!end && end !== 0) end = this.length
if (targetStart >= target.length) targetStart = target.length
if (!targetStart) targetStart = 0
if (end > 0 && end < start) end = start
// Copy 0 bytes; we're done
if (end === start) return 0
if (target.length === 0 || this.length === 0) return 0
// Fatal error conditions
if (targetStart < 0) {
throw new RangeError('targetStart out of bounds')
}
if (start < 0 || start >= this.length) throw new RangeError('sourceStart out of bounds')
if (end < 0) throw new RangeError('sourceEnd out of bounds')
// Are we oob?
if (end > this.length) end = this.length
if (target.length - targetStart < end - start) {
end = target.length - targetStart + start
}
var len = end - start
var i
if (this === target && start < targetStart && targetStart < end) {
// descending copy from end
for (i = len - 1; i >= 0; --i) {
target[i + targetStart] = this[i + start]
}
} else if (len < 1000) {
// ascending copy from start
for (i = 0; i < len; ++i) {
target[i + targetStart] = this[i + start]
}
} else {
Uint8Array.prototype.set.call(
target,
this.subarray(start, start + len),
targetStart
)
}
return len
}
// Usage:
// buffer.fill(number[, offset[, end]])
// buffer.fill(buffer[, offset[, end]])
// buffer.fill(string[, offset[, end]][, encoding])
Buffer.prototype.fill = function fill (val, start, end, encoding) {
// Handle string cases:
if (typeof val === 'string') {
if (typeof start === 'string') {
encoding = start
start = 0
end = this.length
} else if (typeof end === 'string') {
encoding = end
end = this.length
}
if (val.length === 1) {
var code = val.charCodeAt(0)
if (code < 256) {
val = code
}
}
if (encoding !== undefined && typeof encoding !== 'string') {
throw new TypeError('encoding must be a string')
}
if (typeof encoding === 'string' && !Buffer.isEncoding(encoding)) {
throw new TypeError('Unknown encoding: ' + encoding)
}
} else if (typeof val === 'number') {
val = val & 255
}
// Invalid ranges are not set to a default, so can range check early.
if (start < 0 || this.length < start || this.length < end) {
throw new RangeError('Out of range index')
}
if (end <= start) {
return this
}
start = start >>> 0
end = end === undefined ? this.length : end >>> 0
if (!val) val = 0
var i
if (typeof val === 'number') {
for (i = start; i < end; ++i) {
this[i] = val
}
} else {
var bytes = Buffer.isBuffer(val)
? val
: new Buffer(val, encoding)
var len = bytes.length
for (i = 0; i < end - start; ++i) {
this[i + start] = bytes[i % len]
}
}
return this
}
// HELPER FUNCTIONS
// ================
var INVALID_BASE64_RE = /[^+/0-9A-Za-z-_]/g
function base64clean (str) {
// Node strips out invalid characters like \n and \t from the string, base64-js does not
str = str.trim().replace(INVALID_BASE64_RE, '')
// Node converts strings with length < 2 to ''
if (str.length < 2) return ''
// Node allows for non-padded base64 strings (missing trailing ===), base64-js does not
while (str.length % 4 !== 0) {
str = str + '='
}
return str
}
function toHex (n) {
if (n < 16) return '0' + n.toString(16)
return n.toString(16)
}
function utf8ToBytes (string, units) {
units = units || Infinity
var codePoint
var length = string.length
var leadSurrogate = null
var bytes = []
for (var i = 0; i < length; ++i) {
codePoint = string.charCodeAt(i)
// is surrogate component
if (codePoint > 0xD7FF && codePoint < 0xE000) {
// last char was a lead
if (!leadSurrogate) {
// no lead yet
if (codePoint > 0xDBFF) {
// unexpected trail
if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
continue
} else if (i + 1 === length) {
// unpaired lead
if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
continue
}
// valid lead
leadSurrogate = codePoint
continue
}
// 2 leads in a row
if (codePoint < 0xDC00) {
if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
leadSurrogate = codePoint
continue
}
// valid surrogate pair
codePoint = (leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00) + 0x10000
} else if (leadSurrogate) {
// valid bmp char, but last char was a lead
if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
}
leadSurrogate = null
// encode utf8
if (codePoint < 0x80) {
if ((units -= 1) < 0) break
bytes.push(codePoint)
} else if (codePoint < 0x800) {
if ((units -= 2) < 0) break
bytes.push(
codePoint >> 0x6 | 0xC0,
codePoint & 0x3F | 0x80
)
} else if (codePoint < 0x10000) {
if ((units -= 3) < 0) break
bytes.push(
codePoint >> 0xC | 0xE0,
codePoint >> 0x6 & 0x3F | 0x80,
codePoint & 0x3F | 0x80
)
} else if (codePoint < 0x110000) {
if ((units -= 4) < 0) break
bytes.push(
codePoint >> 0x12 | 0xF0,
codePoint >> 0xC & 0x3F | 0x80,
codePoint >> 0x6 & 0x3F | 0x80,
codePoint & 0x3F | 0x80
)
} else {
throw new Error('Invalid code point')
}
}
return bytes
}
function asciiToBytes (str) {
var byteArray = []
for (var i = 0; i < str.length; ++i) {
// Node's code seems to be doing this and not & 0x7F..
byteArray.push(str.charCodeAt(i) & 0xFF)
}
return byteArray
}
function utf16leToBytes (str, units) {
var c, hi, lo
var byteArray = []
for (var i = 0; i < str.length; ++i) {
if ((units -= 2) < 0) break
c = str.charCodeAt(i)
hi = c >> 8
lo = c % 256
byteArray.push(lo)
byteArray.push(hi)
}
return byteArray
}
function base64ToBytes (str) {
return base64.toByteArray(base64clean(str))
}
function blitBuffer (src, dst, offset, length) {
for (var i = 0; i < length; ++i) {
if ((i + offset >= dst.length) || (i >= src.length)) break
dst[i + offset] = src[i]
}
return i
}
// Node 0.10 supports `ArrayBuffer` but lacks `ArrayBuffer.isView`
function isArrayBufferView (obj) {
return (typeof ArrayBuffer.isView === 'function') && ArrayBuffer.isView(obj)
}
function numberIsNaN (obj) {
return obj !== obj // eslint-disable-line no-self-compare
}
},{"31":31,"5":5}],9:[function(_dereq_,module,exports){
(function (Buffer){
// Copyright Joyent, Inc. and other Node contributors.
//
// Permission is hereby granted, free of charge, to any person obtaining a
// copy of this software and associated documentation files (the
// "Software"), to deal in the Software without restriction, including
// without limitation the rights to use, copy, modify, merge, publish,
// distribute, sublicense, and/or sell copies of the Software, and to permit
// persons to whom the Software is furnished to do so, subject to the
// following conditions:
//
// The above copyright notice and this permission notice shall be included
// in all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN
// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
// USE OR OTHER DEALINGS IN THE SOFTWARE.
// NOTE: These type checking functions intentionally don't use `instanceof`
// because it is fragile and can be easily faked with `Object.create()`.
function isArray(arg) {
if (Array.isArray) {
return Array.isArray(arg);
}
return objectToString(arg) === '[object Array]';
}
exports.isArray = isArray;
function isBoolean(arg) {
return typeof arg === 'boolean';
}
exports.isBoolean = isBoolean;
function isNull(arg) {
return arg === null;
}
exports.isNull = isNull;
function isNullOrUndefined(arg) {
return arg == null;
}
exports.isNullOrUndefined = isNullOrUndefined;
function isNumber(arg) {
return typeof arg === 'number';
}
exports.isNumber = isNumber;
function isString(arg) {
return typeof arg === 'string';
}
exports.isString = isString;
function isSymbol(arg) {
return typeof arg === 'symbol';
}
exports.isSymbol = isSymbol;
function isUndefined(arg) {
return arg === void 0;
}
exports.isUndefined = isUndefined;
function isRegExp(re) {
return objectToString(re) === '[object RegExp]';
}
exports.isRegExp = isRegExp;
function isObject(arg) {
return typeof arg === 'object' && arg !== null;
}
exports.isObject = isObject;
function isDate(d) {
return objectToString(d) === '[object Date]';
}
exports.isDate = isDate;
function isError(e) {
return (objectToString(e) === '[object Error]' || e instanceof Error);
}
exports.isError = isError;
function isFunction(arg) {
return typeof arg === 'function';
}
exports.isFunction = isFunction;
function isPrimitive(arg) {
return arg === null ||
typeof arg === 'boolean' ||
typeof arg === 'number' ||
typeof arg === 'string' ||
typeof arg === 'symbol' || // ES6 symbol
typeof arg === 'undefined';
}
exports.isPrimitive = isPrimitive;
exports.isBuffer = Buffer.isBuffer;
function objectToString(o) {
return Object.prototype.toString.call(o);
}
}).call(this,{"isBuffer":_dereq_(35)})
},{"35":35}],10:[function(_dereq_,module,exports){
var Buffer = _dereq_(8).Buffer
var CHARS = '.PYFGCRLAOEUIDHTNSQJKXBMWVZ_pyfgcrlaoeuidhtnsqjkxbmwvz1234567890'
.split('').sort().join('')
module.exports = function (chars, exports) {
chars = chars || CHARS
exports = exports || {}
if(chars.length !== 64) throw new Error('a base 64 encoding requires 64 chars')
var codeToIndex = new Buffer(128)
codeToIndex.fill()
for(var i = 0; i < 64; i++) {
var code = chars.charCodeAt(i)
codeToIndex[code] = i
}
exports.encode = function (data) {
var s = '', l = data.length, hang = 0
for(var i = 0; i < l; i++) {
var v = data[i]
switch (i % 3) {
case 0:
s += chars[v >> 2]
hang = (v & 3) << 4
break;
case 1:
s += chars[hang | v >> 4]
hang = (v & 0xf) << 2
break;
case 2:
s += chars[hang | v >> 6]
s += chars[v & 0x3f]
hang = 0
break;
}
}
if(l%3) s += chars[hang]
return s
}
exports.decode = function (str) {
var l = str.length, j = 0
var b = new Buffer(~~((l/4)*3)), hang = 0
for(var i = 0; i < l; i++) {
var v = codeToIndex[str.charCodeAt(i)]
switch (i % 4) {
case 0:
hang = v << 2;
break;
case 1:
b[j++] = hang | v >> 4
hang = (v << 4) & 0xff
break;
case 2:
b[j++] = hang | v >> 2
hang = (v << 6) & 0xff
break;
case 3:
b[j++] = hang | v
break;
}
}
return b
}
return exports
}
module.exports(CHARS, module.exports)
},{"8":8}],11:[function(_dereq_,module,exports){
var util = _dereq_(100)
, AbstractIterator = _dereq_(16).AbstractIterator
function DeferredIterator (options) {
AbstractIterator.call(this, options)
this._options = options
this._iterator = null
this._operations = []
}
util.inherits(DeferredIterator, AbstractIterator)
DeferredIterator.prototype.setDb = function (db) {
var it = this._iterator = db.iterator(this._options)
this._operations.forEach(function (op) {
it[op.method].apply(it, op.args)
})
}
DeferredIterator.prototype._operation = function (method, args) {
if (this._iterator)
return this._iterator[method].apply(this._iterator, args)
this._operations.push({ method: method, args: args })
}
'next end'.split(' ').forEach(function (m) {
DeferredIterator.prototype['_' + m] = function () {
this._operation(m, arguments)
}
})
module.exports = DeferredIterator;
},{"100":100,"16":16}],12:[function(_dereq_,module,exports){
(function (Buffer,process){
var util = _dereq_(100)
, AbstractLevelDOWN = _dereq_(16).AbstractLevelDOWN
, DeferredIterator = _dereq_(11)
function DeferredLevelDOWN (location) {
AbstractLevelDOWN.call(this, typeof location == 'string' ? location : '') // optional location, who cares?
this._db = undefined
this._operations = []
this._iterators = []
}
util.inherits(DeferredLevelDOWN, AbstractLevelDOWN)
// called by LevelUP when we have a real DB to take its place
DeferredLevelDOWN.prototype.setDb = function (db) {
this._db = db
this._operations.forEach(function (op) {
db[op.method].apply(db, op.args)
})
this._iterators.forEach(function (it) {
it.setDb(db)
})
}
DeferredLevelDOWN.prototype._open = function (options, callback) {
return process.nextTick(callback)
}
// queue a new deferred operation
DeferredLevelDOWN.prototype._operation = function (method, args) {
if (this._db)
return this._db[method].apply(this._db, args)
this._operations.push({ method: method, args: args })
}
// deferrables
'put get del batch approximateSize'.split(' ').forEach(function (m) {
DeferredLevelDOWN.prototype['_' + m] = function () {
this._operation(m, arguments)
}
})
DeferredLevelDOWN.prototype._isBuffer = function (obj) {
return Buffer.isBuffer(obj)
}
DeferredLevelDOWN.prototype._iterator = function (options) {
if (this._db)
return this._db.iterator.apply(this._db, arguments)
var it = new DeferredIterator(options)
this._iterators.push(it)
return it
}
module.exports = DeferredLevelDOWN
module.exports.DeferredIterator = DeferredIterator
}).call(this,{"isBuffer":_dereq_(35)},_dereq_(55))
},{"100":100,"11":11,"16":16,"35":35,"55":55}],13:[function(_dereq_,module,exports){
(function (process){
/* Copyright (c) 2013 Rod Vagg, MIT License */
function AbstractChainedBatch (db) {
this._db = db
this._operations = []
this._written = false
}
AbstractChainedBatch.prototype._checkWritten = function () {
if (this._written)
throw new Error('write() already called on this batch')
}
AbstractChainedBatch.prototype.put = function (key, value) {
this._checkWritten()
var err = this._db._checkKey(key, 'key', this._db._isBuffer)
if (err)
throw err
if (!this._db._isBuffer(key)) key = String(key)
if (!this._db._isBuffer(value)) value = String(value)
if (typeof this._put == 'function' )
this._put(key, value)
else
this._operations.push({ type: 'put', key: key, value: value })
return this
}
AbstractChainedBatch.prototype.del = function (key) {
this._checkWritten()
var err = this._db._checkKey(key, 'key', this._db._isBuffer)
if (err) throw err
if (!this._db._isBuffer(key)) key = String(key)
if (typeof this._del == 'function' )
this._del(key)
else
this._operations.push({ type: 'del', key: key })
return this
}
AbstractChainedBatch.prototype.clear = function () {
this._checkWritten()
this._operations = []
if (typeof this._clear == 'function' )
this._clear()
return this
}
AbstractChainedBatch.prototype.write = function (options, callback) {
this._checkWritten()
if (typeof options == 'function')
callback = options
if (typeof callback != 'function')
throw new Error('write() requires a callback argument')
if (typeof options != 'object')
options = {}
this._written = true
if (typeof this._write == 'function' )
return this._write(callback)
if (typeof this._db._batch == 'function')
return this._db._batch(this._operations, options, callback)
process.nextTick(callback)
}
module.exports = AbstractChainedBatch
}).call(this,_dereq_(55))
},{"55":55}],14:[function(_dereq_,module,exports){
arguments[4][2][0].apply(exports,arguments)
},{"2":2,"55":55}],15:[function(_dereq_,module,exports){
(function (Buffer,process){
/* Copyright (c) 2013 Rod Vagg, MIT License */
var xtend = _dereq_(18)
, AbstractIterator = _dereq_(14)
, AbstractChainedBatch = _dereq_(13)
function AbstractLevelDOWN (location) {
if (!arguments.length || location === undefined)
throw new Error('constructor requires at least a location argument')
if (typeof location != 'string')
throw new Error('constructor requires a location string argument')
this.location = location
this.status = 'new'
}
AbstractLevelDOWN.prototype.open = function (options, callback) {
var self = this
, oldStatus = this.status
if (typeof options == 'function')
callback = options
if (typeof callback != 'function')
throw new Error('open() requires a callback argument')
if (typeof options != 'object')
options = {}
options.createIfMissing = options.createIfMissing != false
options.errorIfExists = !!options.errorIfExists
if (typeof this._open == 'function') {
this.status = 'opening'
this._open(options, function (err) {
if (err) {
self.status = oldStatus
return callback(err)
}
self.status = 'open'
callback()
})
} else {
this.status = 'open'
process.nextTick(callback)
}
}
AbstractLevelDOWN.prototype.close = function (callback) {
var self = this
, oldStatus = this.status
if (typeof callback != 'function')
throw new Error('close() requires a callback argument')
if (typeof this._close == 'function') {
this.status = 'closing'
this._close(function (err) {
if (err) {
self.status = oldStatus
return callback(err)
}
self.status = 'closed'
callback()
})
} else {
this.status = 'closed'
process.nextTick(callback)
}
}
AbstractLevelDOWN.prototype.get = function (key, options, callback) {
var err
if (typeof options == 'function')
callback = options
if (typeof callback != 'function')
throw new Error('get() requires a callback argument')
if (err = this._checkKey(key, 'key', this._isBuffer))
return callback(err)
if (!this._isBuffer(key))
key = String(key)
if (typeof options != 'object')
options = {}
options.asBuffer = options.asBuffer != false
if (typeof this._get == 'function')
return this._get(key, options, callback)
process.nextTick(function () { callback(new Error('NotFound')) })
}
AbstractLevelDOWN.prototype.put = function (key, value, options, callback) {
var err
if (typeof options == 'function')
callback = options
if (typeof callback != 'function')
throw new Error('put() requires a callback argument')
if (err = this._checkKey(key, 'key', this._isBuffer))
return callback(err)
if (!this._isBuffer(key))
key = String(key)
// coerce value to string in node, don't touch it in browser
// (indexeddb can store any JS type)
if (value != null && !this._isBuffer(value) && !process.browser)
value = String(value)
if (typeof options != 'object')
options = {}
if (typeof this._put == 'function')
return this._put(key, value, options, callback)
process.nextTick(callback)
}
AbstractLevelDOWN.prototype.del = function (key, options, callback) {
var err
if (typeof options == 'function')
callback = options
if (typeof callback != 'function')
throw new Error('del() requires a callback argument')
if (err = this._checkKey(key, 'key', this._isBuffer))
return callback(err)
if (!this._isBuffer(key))
key = String(key)
if (typeof options != 'object')
options = {}
if (typeof this._del == 'function')
return this._del(key, options, callback)
process.nextTick(callback)
}
AbstractLevelDOWN.prototype.batch = function (array, options, callback) {
if (!arguments.length)
return this._chainedBatch()
if (typeof options == 'function')
callback = options
if (typeof array == 'function')
callback = array
if (typeof callback != 'function')
throw new Error('batch(array) requires a callback argument')
if (!Array.isArray(array))
return callback(new Error('batch(array) requires an array argument'))
if (!options || typeof options != 'object')
options = {}
var i = 0
, l = array.length
, e
, err
for (; i < l; i++) {
e = array[i]
if (typeof e != 'object')
continue
if (err = this._checkKey(e.type, 'type', this._isBuffer))
return callback(err)
if (err = this._checkKey(e.key, 'key', this._isBuffer))
return callback(err)
}
if (typeof this._batch == 'function')
return this._batch(array, options, callback)
process.nextTick(callback)
}
//TODO: remove from here, not a necessary primitive
AbstractLevelDOWN.prototype.approximateSize = function (start, end, callback) {
if ( start == null
|| end == null
|| typeof start == 'function'
|| typeof end == 'function') {
throw new Error('approximateSize() requires valid `start`, `end` and `callback` arguments')
}
if (typeof callback != 'function')
throw new Error('approximateSize() requires a callback argument')
if (!this._isBuffer(start))
start = String(start)
if (!this._isBuffer(end))
end = String(end)
if (typeof this._approximateSize == 'function')
return this._approximateSize(start, end, callback)
process.nextTick(function () {
callback(null, 0)
})
}
AbstractLevelDOWN.prototype._setupIteratorOptions = function (options) {
var self = this
options = xtend(options)
;[ 'start', 'end', 'gt', 'gte', 'lt', 'lte' ].forEach(function (o) {
if (options[o] && self._isBuffer(options[o]) && options[o].length === 0)
delete options[o]
})
options.reverse = !!options.reverse
options.keys = options.keys != false
options.values = options.values != false
options.limit = 'limit' in options ? options.limit : -1
options.keyAsBuffer = options.keyAsBuffer != false
options.valueAsBuffer = options.valueAsBuffer != false
return options
}
AbstractLevelDOWN.prototype.iterator = function (options) {
if (typeof options != 'object')
options = {}
options = this._setupIteratorOptions(options)
if (typeof this._iterator == 'function')
return this._iterator(options)
return new AbstractIterator(this)
}
AbstractLevelDOWN.prototype._chainedBatch = function () {
return new AbstractChainedBatch(this)
}
AbstractLevelDOWN.prototype._isBuffer = function (obj) {
return Buffer.isBuffer(obj)
}
AbstractLevelDOWN.prototype._checkKey = function (obj, type) {
if (obj === null || obj === undefined)
return new Error(type + ' cannot be `null` or `undefined`')
if (this._isBuffer(obj)) {
if (obj.length === 0)
return new Error(type + ' cannot be an empty Buffer')
} else if (String(obj) === '')
return new Error(type + ' cannot be an empty String')
}
module.exports = AbstractLevelDOWN
}).call(this,{"isBuffer":_dereq_(35)},_dereq_(55))
},{"13":13,"14":14,"18":18,"35":35,"55":55}],16:[function(_dereq_,module,exports){
exports.AbstractLevelDOWN = _dereq_(15)
exports.AbstractIterator = _dereq_(14)
exports.AbstractChainedBatch = _dereq_(13)
exports.isLevelDOWN = _dereq_(17)
},{"13":13,"14":14,"15":15,"17":17}],17:[function(_dereq_,module,exports){
var AbstractLevelDOWN = _dereq_(15)
function isLevelDOWN (db) {
if (!db || typeof db !== 'object')
return false
return Object.keys(AbstractLevelDOWN.prototype).filter(function (name) {
// TODO remove approximateSize check when method is gone
return name[0] != '_' && name != 'approximateSize'
}).every(function (name) {
return typeof db[name] == 'function'
})
}
module.exports = isLevelDOWN
},{"15":15}],18:[function(_dereq_,module,exports){
module.exports = extend
var hasOwnProperty = Object.prototype.hasOwnProperty;
function extend() {
var target = {}
for (var i = 0; i < arguments.length; i++) {
var source = arguments[i]
for (var key in source) {
if (hasOwnProperty.call(source, key)) {
target[key] = source[key]
}
}
}
return target
}
},{}],19:[function(_dereq_,module,exports){
/**
* Copyright (c) 2013 Petka Antonov
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
"use strict";
function Deque(capacity) {
this._capacity = getCapacity(capacity);
this._length = 0;
this._front = 0;
if (isArray(capacity)) {
var len = capacity.length;
for (var i = 0; i < len; ++i) {
this[i] = capacity[i];
}
this._length = len;
}
}
Deque.prototype.toArray = function Deque$toArray() {
var len = this._length;
var ret = new Array(len);
var front = this._front;
var capacity = this._capacity;
for (var j = 0; j < len; ++j) {
ret[j] = this[(front + j) & (capacity - 1)];
}
return ret;
};
Deque.prototype.push = function Deque$push(item) {
var argsLength = arguments.length;
var length = this._length;
if (argsLength > 1) {
var capacity = this._capacity;
if (length + argsLength > capacity) {
for (var i = 0; i < argsLength; ++i) {
this._checkCapacity(length + 1);
var j = (this._front + length) & (this._capacity - 1);
this[j] = arguments[i];
length++;
this._length = length;
}
return length;
}
else {
var j = this._front;
for (var i = 0; i < argsLength; ++i) {
this[(j + length) & (capacity - 1)] = arguments[i];
j++;
}
this._length = length + argsLength;
return length + argsLength;
}
}
if (argsLength === 0) return length;
this._checkCapacity(length + 1);
var i = (this._front + length) & (this._capacity - 1);
this[i] = item;
this._length = length + 1;
return length + 1;
};
Deque.prototype.pop = function Deque$pop() {
var length = this._length;
if (length === 0) {
return void 0;
}
var i = (this._front + length - 1) & (this._capacity - 1);
var ret = this[i];
this[i] = void 0;
this._length = length - 1;
return ret;
};
Deque.prototype.shift = function Deque$shift() {
var length = this._length;
if (length === 0) {
return void 0;
}
var front = this._front;
var ret = this[front];
this[front] = void 0;
this._front = (front + 1) & (this._capacity - 1);
this._length = length - 1;
return ret;
};
Deque.prototype.unshift = function Deque$unshift(item) {
var length = this._length;
var argsLength = arguments.length;
if (argsLength > 1) {
var capacity = this._capacity;
if (length + argsLength > capacity) {
for (var i = argsLength - 1; i >= 0; i--) {
this._checkCapacity(length + 1);
var capacity = this._capacity;
var j = (((( this._front - 1 ) &
( capacity - 1) ) ^ capacity ) - capacity );
this[j] = arguments[i];
length++;
this._length = length;
this._front = j;
}
return length;
}
else {
var front = this._front;
for (var i = argsLength - 1; i >= 0; i--) {
var j = (((( front - 1 ) &
( capacity - 1) ) ^ capacity ) - capacity );
this[j] = arguments[i];
front = j;
}
this._front = front;
this._length = length + argsLength;
return length + argsLength;
}
}
if (argsLength === 0) return length;
this._checkCapacity(length + 1);
var capacity = this._capacity;
var i = (((( this._front - 1 ) &
( capacity - 1) ) ^ capacity ) - capacity );
this[i] = item;
this._length = length + 1;
this._front = i;
return length + 1;
};
Deque.prototype.peekBack = function Deque$peekBack() {
var length = this._length;
if (length === 0) {
return void 0;
}
var index = (this._front + length - 1) & (this._capacity - 1);
return this[index];
};
Deque.prototype.peekFront = function Deque$peekFront() {
if (this._length === 0) {
return void 0;
}
return this[this._front];
};
Deque.prototype.get = function Deque$get(index) {
var i = index;
if ((i !== (i | 0))) {
return void 0;
}
var len = this._length;
if (i < 0) {
i = i + len;
}
if (i < 0 || i >= len) {
return void 0;
}
return this[(this._front + i) & (this._capacity - 1)];
};
Deque.prototype.isEmpty = function Deque$isEmpty() {
return this._length === 0;
};
Deque.prototype.clear = function Deque$clear() {
var len = this._length;
var front = this._front;
var capacity = this._capacity;
for (var j = 0; j < len; ++j) {
this[(front + j) & (capacity - 1)] = void 0;
}
this._length = 0;
this._front = 0;
};
Deque.prototype.toString = function Deque$toString() {
return this.toArray().toString();
};
Deque.prototype.valueOf = Deque.prototype.toString;
Deque.prototype.removeFront = Deque.prototype.shift;
Deque.prototype.removeBack = Deque.prototype.pop;
Deque.prototype.insertFront = Deque.prototype.unshift;
Deque.prototype.insertBack = Deque.prototype.push;
Deque.prototype.enqueue = Deque.prototype.push;
Deque.prototype.dequeue = Deque.prototype.shift;
Deque.prototype.toJSON = Deque.prototype.toArray;
Object.defineProperty(Deque.prototype, "length", {
get: function() {
return this._length;
},
set: function() {
throw new RangeError("");
}
});
Deque.prototype._checkCapacity = function Deque$_checkCapacity(size) {
if (this._capacity < size) {
this._resizeTo(getCapacity(this._capacity * 1.5 + 16));
}
};
Deque.prototype._resizeTo = function Deque$_resizeTo(capacity) {
var oldCapacity = this._capacity;
this._capacity = capacity;
var front = this._front;
var length = this._length;
if (front + length > oldCapacity) {
var moveItemsCount = (front + length) & (oldCapacity - 1);
arrayMove(this, 0, this, oldCapacity, moveItemsCount);
}
};
var isArray = Array.isArray;
function arrayMove(src, srcIndex, dst, dstIndex, len) {
for (var j = 0; j < len; ++j) {
dst[j + dstIndex] = src[j + srcIndex];
src[j + srcIndex] = void 0;
}
}
function pow2AtLeast(n) {
n = n >>> 0;
n = n - 1;
n = n | (n >> 1);
n = n | (n >> 2);
n = n | (n >> 4);
n = n | (n >> 8);
n = n | (n >> 16);
return n + 1;
}
function getCapacity(capacity) {
if (typeof capacity !== "number") {
if (isArray(capacity)) {
capacity = capacity.length;
}
else {
return 16;
}
}
return pow2AtLeast(
Math.min(
Math.max(16, capacity), 1073741824)
);
}
module.exports = Deque;
},{}],20:[function(_dereq_,module,exports){
var prr = _dereq_(22)
function init (type, message, cause) {
prr(this, {
type : type
, name : type
// can be passed just a 'cause'
, cause : typeof message != 'string' ? message : cause
, message : !!message && typeof message != 'string' ? message.message : message
}, 'ewr')
}
// generic prototype, not intended to be actually used - helpful for `instanceof`
function CustomError (message, cause) {
Error.call(this)
if (Error.captureStackTrace)
Error.captureStackTrace(this, arguments.callee)
init.call(this, 'CustomError', message, cause)
}
CustomError.prototype = new Error()
function createError (errno, type, proto) {
var err = function (message, cause) {
init.call(this, type, message, cause)
//TODO: the specificity here is stupid, errno should be available everywhere
if (type == 'FilesystemError') {
this.code = this.cause.code
this.path = this.cause.path
this.errno = this.cause.errno
this.message =
(errno.errno[this.cause.errno]
? errno.errno[this.cause.errno].description
: this.cause.message)
+ (this.cause.path ? ' [' + this.cause.path + ']' : '')
}
Error.call(this)
if (Error.captureStackTrace)
Error.captureStackTrace(this, arguments.callee)
}
err.prototype = !!proto ? new proto() : new CustomError()
return err
}
module.exports = function (errno) {
var ce = function (type, proto) {
return createError(errno, type, proto)
}
return {
CustomError : CustomError
, FilesystemError : ce('FilesystemError')
, createError : ce
}
}
},{"22":22}],21:[function(_dereq_,module,exports){
var all = module.exports.all = [
{
errno: -2,
code: 'ENOENT',
description: 'no such file or directory'
},
{
errno: -1,
code: 'UNKNOWN',
description: 'unknown error'
},
{
errno: 0,
code: 'OK',
description: 'success'
},
{
errno: 1,
code: 'EOF',
description: 'end of file'
},
{
errno: 2,
code: 'EADDRINFO',
description: 'getaddrinfo error'
},
{
errno: 3,
code: 'EACCES',
description: 'permission denied'
},
{
errno: 4,
code: 'EAGAIN',
description: 'resource temporarily unavailable'
},
{
errno: 5,
code: 'EADDRINUSE',
description: 'address already in use'
},
{
errno: 6,
code: 'EADDRNOTAVAIL',
description: 'address not available'
},
{
errno: 7,
code: 'EAFNOSUPPORT',
description: 'address family not supported'
},
{
errno: 8,
code: 'EALREADY',
description: 'connection already in progress'
},
{
errno: 9,
code: 'EBADF',
description: 'bad file descriptor'
},
{
errno: 10,
code: 'EBUSY',
description: 'resource busy or locked'
},
{
errno: 11,
code: 'ECONNABORTED',
description: 'software caused connection abort'
},
{
errno: 12,
code: 'ECONNREFUSED',
description: 'connection refused'
},
{
errno: 13,
code: 'ECONNRESET',
description: 'connection reset by peer'
},
{
errno: 14,
code: 'EDESTADDRREQ',
description: 'destination address required'
},
{
errno: 15,
code: 'EFAULT',
description: 'bad address in system call argument'
},
{
errno: 16,
code: 'EHOSTUNREACH',
description: 'host is unreachable'
},
{
errno: 17,
code: 'EINTR',
description: 'interrupted system call'
},
{
errno: 18,
code: 'EINVAL',
description: 'invalid argument'
},
{
errno: 19,
code: 'EISCONN',
description: 'socket is already connected'
},
{
errno: 20,
code: 'EMFILE',
description: 'too many open files'
},
{
errno: 21,
code: 'EMSGSIZE',
description: 'message too long'
},
{
errno: 22,
code: 'ENETDOWN',
description: 'network is down'
},
{
errno: 23,
code: 'ENETUNREACH',
description: 'network is unreachable'
},
{
errno: 24,
code: 'ENFILE',
description: 'file table overflow'
},
{
errno: 25,
code: 'ENOBUFS',
description: 'no buffer space available'
},
{
errno: 26,
code: 'ENOMEM',
description: 'not enough memory'
},
{
errno: 27,
code: 'ENOTDIR',
description: 'not a directory'
},
{
errno: 28,
code: 'EISDIR',
description: 'illegal operation on a directory'
},
{
errno: 29,
code: 'ENONET',
description: 'machine is not on the network'
},
{
errno: 31,
code: 'ENOTCONN',
description: 'socket is not connected'
},
{
errno: 32,
code: 'ENOTSOCK',
description: 'socket operation on non-socket'
},
{
errno: 33,
code: 'ENOTSUP',
description: 'operation not supported on socket'
},
{
errno: 34,
code: 'ENOENT',
description: 'no such file or directory'
},
{
errno: 35,
code: 'ENOSYS',
description: 'function not implemented'
},
{
errno: 36,
code: 'EPIPE',
description: 'broken pipe'
},
{
errno: 37,
code: 'EPROTO',
description: 'protocol error'
},
{
errno: 38,
code: 'EPROTONOSUPPORT',
description: 'protocol not supported'
},
{
errno: 39,
code: 'EPROTOTYPE',
description: 'protocol wrong type for socket'
},
{
errno: 40,
code: 'ETIMEDOUT',
description: 'connection timed out'
},
{
errno: 41,
code: 'ECHARSET',
description: 'invalid Unicode character'
},
{
errno: 42,
code: 'EAIFAMNOSUPPORT',
description: 'address family for hostname not supported'
},
{
errno: 44,
code: 'EAISERVICE',
description: 'servname not supported for ai_socktype'
},
{
errno: 45,
code: 'EAISOCKTYPE',
description: 'ai_socktype not supported'
},
{
errno: 46,
code: 'ESHUTDOWN',
description: 'cannot send after transport endpoint shutdown'
},
{
errno: 47,
code: 'EEXIST',
description: 'file already exists'
},
{
errno: 48,
code: 'ESRCH',
description: 'no such process'
},
{
errno: 49,
code: 'ENAMETOOLONG',
description: 'name too long'
},
{
errno: 50,
code: 'EPERM',
description: 'operation not permitted'
},
{
errno: 51,
code: 'ELOOP',
description: 'too many symbolic links encountered'
},
{
errno: 52,
code: 'EXDEV',
description: 'cross-device link not permitted'
},
{
errno: 53,
code: 'ENOTEMPTY',
description: 'directory not empty'
},
{
errno: 54,
code: 'ENOSPC',
description: 'no space left on device'
},
{
errno: 55,
code: 'EIO',
description: 'i/o error'
},
{
errno: 56,
code: 'EROFS',
description: 'read-only file system'
},
{
errno: 57,
code: 'ENODEV',
description: 'no such device'
},
{
errno: 58,
code: 'ESPIPE',
description: 'invalid seek'
},
{
errno: 59,
code: 'ECANCELED',
description: 'operation canceled'
}
]
module.exports.errno = {}
module.exports.code = {}
all.forEach(function (error) {
module.exports.errno[error.errno] = error
module.exports.code[error.code] = error
})
module.exports.custom = _dereq_(20)(module.exports)
module.exports.create = module.exports.custom.createError
},{"20":20}],22:[function(_dereq_,module,exports){
/*!
* prr
* (c) 2013 Rod Vagg
* https://github.com/rvagg/prr
* License: MIT
*/
(function (name, context, definition) {
if (typeof module != 'undefined' && module.exports)
module.exports = definition()
else
context[name] = definition()
})('prr', this, function() {
var setProperty = typeof Object.defineProperty == 'function'
? function (obj, key, options) {
Object.defineProperty(obj, key, options)
return obj
}
: function (obj, key, options) { // < es5
obj[key] = options.value
return obj
}
, makeOptions = function (value, options) {
var oo = typeof options == 'object'
, os = !oo && typeof options == 'string'
, op = function (p) {
return oo
? !!options[p]
: os
? options.indexOf(p[0]) > -1
: false
}
return {
enumerable : op('enumerable')
, configurable : op('configurable')
, writable : op('writable')
, value : value
}
}
, prr = function (obj, key, value, options) {
var k
options = makeOptions(value, options)
if (typeof key == 'object') {
for (k in key) {
if (Object.hasOwnProperty.call(key, k)) {
options.value = key[k]
setProperty(obj, k, options)
}
}
return obj
}
return setProperty(obj, key, options)
}
return prr
})
},{}],23:[function(_dereq_,module,exports){
// Copyright Joyent, Inc. and other Node contributors.
//
// Permission is hereby granted, free of charge, to any person obtaining a
// copy of this software and associated documentation files (the
// "Software"), to deal in the Software without restriction, including
// without limitation the rights to use, copy, modify, merge, publish,
// distribute, sublicense, and/or sell copies of the Software, and to permit
// persons to whom the Software is furnished to do so, subject to the
// following conditions:
//
// The above copyright notice and this permission notice shall be included
// in all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN
// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
// USE OR OTHER DEALINGS IN THE SOFTWARE.
function EventEmitter() {
this._events = this._events || {};
this._maxListeners = this._maxListeners || undefined;
}
module.exports = EventEmitter;
// Backwards-compat with node 0.10.x
EventEmitter.EventEmitter = EventEmitter;
EventEmitter.prototype._events = undefined;
EventEmitter.prototype._maxListeners = undefined;
// By default EventEmitters will print a warning if more than 10 listeners are
// added to it. This is a useful default which helps finding memory leaks.
EventEmitter.defaultMaxListeners = 10;
// Obviously not all Emitters should be limited to 10. This function allows
// that to be increased. Set to zero for unlimited.
EventEmitter.prototype.setMaxListeners = function(n) {
if (!isNumber(n) || n < 0 || isNaN(n))
throw TypeError('n must be a positive number');
this._maxListeners = n;
return this;
};
EventEmitter.prototype.emit = function(type) {
var er, handler, len, args, i, listeners;
if (!this._events)
this._events = {};
// If there is no 'error' event listener then throw.
if (type === 'error') {
if (!this._events.error ||
(isObject(this._events.error) && !this._events.error.length)) {
er = arguments[1];
if (er instanceof Error) {
throw er; // Unhandled 'error' event
} else {
// At least give some kind of context to the user
var err = new Error('Uncaught, unspecified "error" event. (' + er + ')');
err.context = er;
throw err;
}
}
}
handler = this._events[type];
if (isUndefined(handler))
return false;
if (isFunction(handler)) {
switch (arguments.length) {
// fast cases
case 1:
handler.call(this);
break;
case 2:
handler.call(this, arguments[1]);
break;
case 3:
handler.call(this, arguments[1], arguments[2]);
break;
// slower
default:
args = Array.prototype.slice.call(arguments, 1);
handler.apply(this, args);
}
} else if (isObject(handler)) {
args = Array.prototype.slice.call(arguments, 1);
listeners = handler.slice();
len = listeners.length;
for (i = 0; i < len; i++)
listeners[i].apply(this, args);
}
return true;
};
EventEmitter.prototype.addListener = function(type, listener) {
var m;
if (!isFunction(listener))
throw TypeError('listener must be a function');
if (!this._events)
this._events = {};
// To avoid recursion in the case that type === "newListener"! Before
// adding it to the listeners, first emit "newListener".
if (this._events.newListener)
this.emit('newListener', type,
isFunction(listener.listener) ?
listener.listener : listener);
if (!this._events[type])
// Optimize the case of one listener. Don't need the extra array object.
this._events[type] = listener;
else if (isObject(this._events[type]))
// If we've already got an array, just append.
this._events[type].push(listener);
else
// Adding the second element, need to change to array.
this._events[type] = [this._events[type], listener];
// Check for listener leak
if (isObject(this._events[type]) && !this._events[type].warned) {
if (!isUndefined(this._maxListeners)) {
m = this._maxListeners;
} else {
m = EventEmitter.defaultMaxListeners;
}
if (m && m > 0 && this._events[type].length > m) {
this._events[type].warned = true;
console.error('(node) warning: possible EventEmitter memory ' +
'leak detected. %d listeners added. ' +
'Use emitter.setMaxListeners() to increase limit.',
this._events[type].length);
if (typeof console.trace === 'function') {
// not supported in IE 10
console.trace();
}
}
}
return this;
};
EventEmitter.prototype.on = EventEmitter.prototype.addListener;
EventEmitter.prototype.once = function(type, listener) {
if (!isFunction(listener))
throw TypeError('listener must be a function');
var fired = false;
function g() {
this.removeListener(type, g);
if (!fired) {
fired = true;
listener.apply(this, arguments);
}
}
g.listener = listener;
this.on(type, g);
return this;
};
// emits a 'removeListener' event iff the listener was removed
EventEmitter.prototype.removeListener = function(type, listener) {
var list, position, length, i;
if (!isFunction(listener))
throw TypeError('listener must be a function');
if (!this._events || !this._events[type])
return this;
list = this._events[type];
length = list.length;
position = -1;
if (list === listener ||
(isFunction(list.listener) && list.listener === listener)) {
delete this._events[type];
if (this._events.removeListener)
this.emit('removeListener', type, listener);
} else if (isObject(list)) {
for (i = length; i-- > 0;) {
if (list[i] === listener ||
(list[i].listener && list[i].listener === listener)) {
position = i;
break;
}
}
if (position < 0)
return this;
if (list.length === 1) {
list.length = 0;
delete this._events[type];
} else {
list.splice(position, 1);
}
if (this._events.removeListener)
this.emit('removeListener', type, listener);
}
return this;
};
EventEmitter.prototype.removeAllListeners = function(type) {
var key, listeners;
if (!this._events)
return this;
// not listening for removeListener, no need to emit
if (!this._events.removeListener) {
if (arguments.length === 0)
this._events = {};
else if (this._events[type])
delete this._events[type];
return this;
}
// emit removeListener for all listeners on all events
if (arguments.length === 0) {
for (key in this._events) {
if (key === 'removeListener') continue;
this.removeAllListeners(key);
}
this.removeAllListeners('removeListener');
this._events = {};
return this;
}
listeners = this._events[type];
if (isFunction(listeners)) {
this.removeListener(type, listeners);
} else if (listeners) {
// LIFO order
while (listeners.length)
this.removeListener(type, listeners[listeners.length - 1]);
}
delete this._events[type];
return this;
};
EventEmitter.prototype.listeners = function(type) {
var ret;
if (!this._events || !this._events[type])
ret = [];
else if (isFunction(this._events[type]))
ret = [this._events[type]];
else
ret = this._events[type].slice();
return ret;
};
EventEmitter.prototype.listenerCount = function(type) {
if (this._events) {
var evlistener = this._events[type];
if (isFunction(evlistener))
return 1;
else if (evlistener)
return evlistener.length;
}
return 0;
};
EventEmitter.listenerCount = function(emitter, type) {
return emitter.listenerCount(type);
};
function isFunction(arg) {
return typeof arg === 'function';
}
function isNumber(arg) {
return typeof arg === 'number';
}
function isObject(arg) {
return typeof arg === 'object' && arg !== null;
}
function isUndefined(arg) {
return arg === void 0;
}
},{}],24:[function(_dereq_,module,exports){
(function (process,global){
'use strict';
//
// Class that should contain everything necessary to interact
// with IndexedDB as a generic key-value store (based on LocalStorage).
//
/* global indexedDB */
var STORE = 'fruitdown';
// see http://stackoverflow.com/a/15349865/680742
var nextTick = global.setImmediate || process.nextTick;
// IE has race conditions, which is what these two caches work around
// https://gist.github.com/nolanlawson/a841ee23436410f37168
var cachedDBs = {};
var openReqList = {};
function StorageCore(dbName) {
this._dbName = dbName;
}
function getDatabase(dbName, callback) {
if (cachedDBs[dbName]) {
return nextTick(function () {
callback(null, cachedDBs[dbName]);
});
}
var req = indexedDB.open(dbName, 1);
openReqList[dbName] = req;
req.onupgradeneeded = function (e) {
var db = e.target.result;
// Apple migration bug (https://bugs.webkit.org/show_bug.cgi?id=136888)
// This will be null the first time rather than 0, so check for 1
if (e.oldVersion === 1) {
return;
}
// Create a superfluous secondary index purely so we can do
// openKeyCursor(). (Limitation of the IndexedDB API, fixed in v2.0.)
// We would also use this for detecting ConstraintErrors, but that
// doesn't work in Safari: https://bugs.webkit.org/show_bug.cgi?id=149107
db.createObjectStore(STORE).createIndex('fakeKey', 'fakeKey');
};
req.onsuccess = function (e) {
var db = cachedDBs[dbName] = e.target.result;
callback(null, db);
};
req.onerror = function(e) {
var msg = 'Failed to open indexedDB, are you in private browsing mode?';
console.error(msg);
callback(e);
};
}
function openTransactionSafely(db, mode) {
try {
return {
txn: db.transaction(STORE, mode)
};
} catch (err) {
return {
error: err
};
}
}
StorageCore.prototype.getKeys = function (callback) {
getDatabase(this._dbName, function (err, db) {
if (err) {
return callback(err);
}
var txnRes = openTransactionSafely(db, 'readonly');
if (txnRes.error) {
return callback(txnRes.error);
}
var txn = txnRes.txn;
var store = txn.objectStore(STORE);
txn.onerror = callback;
var keys = [];
txn.oncomplete = function () {
// Safari has a bug where these keys aren't returned in sorted
// order, so we have to sort them explicitly
// https://bugs.webkit.org/show_bug.cgi?id=149205
callback(null, keys.sort());
};
// using openKeyCursor avoids reading in the whole value,
// which may be large
var req = store.index('fakeKey').openKeyCursor();
req.onsuccess = function (e) {
var cursor = e.target.result;
if (!cursor) {
return;
}
keys.push(cursor.primaryKey);
cursor.continue();
};
});
};
StorageCore.prototype.put = function (key, value, callback) {
getDatabase(this._dbName, function (err, db) {
if (err) {
return callback(err);
}
var txnRes = openTransactionSafely(db, 'readwrite');
if (txnRes.error) {
return callback(txnRes.error);
}
var txn = txnRes.txn;
var store = txn.objectStore(STORE);
var valueToStore = typeof value === 'string' ? value : value.toString();
txn.onerror = callback;
txn.oncomplete = function () {
callback();
};
store.put({value: valueToStore, fakeKey: 0}, key);
});
};
StorageCore.prototype.get = function (key, callback) {
getDatabase(this._dbName, function (err, db) {
if (err) {
return callback(err);
}
var txnRes = openTransactionSafely(db, 'readonly');
if (txnRes.error) {
return callback(txnRes.error);
}
var txn = txnRes.txn;
var store = txn.objectStore(STORE);
var gotten;
var req = store.get(key);
req.onsuccess = function (e) {
if (e.target.result) {
gotten = e.target.result.value;
}
};
txn.onerror = callback;
txn.oncomplete = function () {
callback(null, gotten);
};
});
};
StorageCore.prototype.remove = function (key, callback) {
getDatabase(this._dbName, function (err, db) {
if (err) {
return callback(err);
}
var txnRes = openTransactionSafely(db, 'readwrite');
if (txnRes.error) {
return callback(txnRes.error);
}
var txn = txnRes.txn;
var store = txn.objectStore(STORE);
store.delete(key);
txn.onerror = callback;
txn.oncomplete = function () {
callback();
};
});
};
StorageCore.destroy = function (dbName, callback) {
nextTick(function () {
//Close open request for "dbName" database to fix ie delay.
if (openReqList[dbName] && openReqList[dbName].result) {
openReqList[dbName].result.close();
delete cachedDBs[dbName];
}
var req = indexedDB.deleteDatabase(dbName);
req.onsuccess = function () {
//Remove open request from the list.
if (openReqList[dbName]) {
openReqList[dbName] = null;
}
callback(null);
};
req.onerror = callback;
});
};
module.exports = StorageCore;
}).call(this,_dereq_(55),typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {})
},{"55":55}],25:[function(_dereq_,module,exports){
(function (Buffer){
'use strict';
// ArrayBuffer/Uint8Array are old formats that date back to before we
// had a proper browserified buffer type. they may be removed later
var arrayBuffPrefix = 'ArrayBuffer:';
var arrayBuffRegex = new RegExp('^' + arrayBuffPrefix);
var uintPrefix = 'Uint8Array:';
var uintRegex = new RegExp('^' + uintPrefix);
// this is the new encoding format used going forward
var bufferPrefix = 'Buff:';
var bufferRegex = new RegExp('^' + bufferPrefix);
var utils = _dereq_(28);
var DatabaseCore = _dereq_(24);
var TaskQueue = _dereq_(27);
var d64 = _dereq_(10);
function Database(dbname) {
this._store = new DatabaseCore(dbname);
this._queue = new TaskQueue();
}
Database.prototype.sequentialize = function (callback, fun) {
this._queue.add(fun, callback);
};
Database.prototype.init = function (callback) {
var self = this;
self.sequentialize(callback, function (callback) {
self._store.getKeys(function (err, keys) {
if (err) {
return callback(err);
}
self._keys = keys;
return callback();
});
});
};
Database.prototype.keys = function (callback) {
var self = this;
self.sequentialize(callback, function (callback) {
callback(null, self._keys.slice());
});
};
//setItem: Saves and item at the key provided.
Database.prototype.setItem = function (key, value, callback) {
var self = this;
self.sequentialize(callback, function (callback) {
if (Buffer.isBuffer(value)) {
value = bufferPrefix + d64.encode(value);
}
var idx = utils.sortedIndexOf(self._keys, key);
if (self._keys[idx] !== key) {
self._keys.splice(idx, 0, key);
}
self._store.put(key, value, callback);
});
};
//getItem: Returns the item identified by it's key.
Database.prototype.getItem = function (key, callback) {
var self = this;
self.sequentialize(callback, function (callback) {
self._store.get(key, function (err, retval) {
if (err) {
return callback(err);
}
if (typeof retval === 'undefined' || retval === null) {
// 'NotFound' error, consistent with LevelDOWN API
return callback(new Error('NotFound'));
}
if (typeof retval !== 'undefined') {
if (bufferRegex.test(retval)) {
retval = d64.decode(retval.substring(bufferPrefix.length));
} else if (arrayBuffRegex.test(retval)) {
// this type is kept for backwards
// compatibility with older databases, but may be removed
// after a major version bump
retval = retval.substring(arrayBuffPrefix.length);
retval = new ArrayBuffer(atob(retval).split('').map(function (c) {
return c.charCodeAt(0);
}));
} else if (uintRegex.test(retval)) {
// ditto
retval = retval.substring(uintPrefix.length);
retval = new Uint8Array(atob(retval).split('').map(function (c) {
return c.charCodeAt(0);
}));
}
}
callback(null, retval);
});
});
};
//removeItem: Removes the item identified by it's key.
Database.prototype.removeItem = function (key, callback) {
var self = this;
self.sequentialize(callback, function (callback) {
var idx = utils.sortedIndexOf(self._keys, key);
if (self._keys[idx] === key) {
self._keys.splice(idx, 1);
self._store.remove(key, function (err) {
if (err) {
return callback(err);
}
callback();
});
} else {
callback();
}
});
};
Database.prototype.length = function (callback) {
var self = this;
self.sequentialize(callback, function (callback) {
callback(null, self._keys.length);
});
};
module.exports = Database;
}).call(this,{"isBuffer":_dereq_(35)})
},{"10":10,"24":24,"27":27,"28":28,"35":35}],26:[function(_dereq_,module,exports){
(function (process,global,Buffer){
'use strict';
var inherits = _dereq_(33);
var AbstractLevelDOWN = _dereq_(3).AbstractLevelDOWN;
var AbstractIterator = _dereq_(3).AbstractIterator;
var Database = _dereq_(25);
var DatabaseCore = _dereq_(24);
var utils = _dereq_(28);
// see http://stackoverflow.com/a/15349865/680742
var nextTick = global.setImmediate || process.nextTick;
function DatabaseIterator(db, options) {
AbstractIterator.call(this, db);
this._reverse = !!options.reverse;
this._endkey = options.end;
this._startkey = options.start;
this._gt = options.gt;
this._gte = options.gte;
this._lt = options.lt;
this._lte = options.lte;
this._exclusiveStart = options.exclusiveStart;
this._limit = options.limit;
this._count = 0;
this.onInitCompleteListeners = [];
}
inherits(DatabaseIterator, AbstractIterator);
DatabaseIterator.prototype._init = function (callback) {
nextTick(function () {
callback();
});
};
DatabaseIterator.prototype._next = function (callback) {
var self = this;
function onInitComplete() {
if (self._pos === self._keys.length || self._pos < 0) { // done reading
return callback();
}
var key = self._keys[self._pos];
if (!!self._endkey && (self._reverse ? key < self._endkey : key > self._endkey)) {
return callback();
}
if (!!self._limit && self._limit > 0 && self._count++ >= self._limit) {
return callback();
}
if ((self._lt && key >= self._lt) ||
(self._lte && key > self._lte) ||
(self._gt && key <= self._gt) ||
(self._gte && key < self._gte)) {
return callback();
}
self._pos += self._reverse ? -1 : 1;
self.db.container.getItem(key, function (err, value) {
if (err) {
if (err.message === 'NotFound') {
return nextTick(function () {
self._next(callback);
});
}
return callback(err);
}
callback(null, key, value);
});
}
if (!self.initStarted) {
self.initStarted = true;
self._init(function (err) {
if (err) {
return callback(err);
}
self.db.container.keys(function (err, keys) {
if (err) {
return callback(err);
}
self._keys = keys;
if (self._startkey) {
var index = utils.sortedIndexOf(self._keys, self._startkey);
var startkey = (index >= self._keys.length || index < 0) ?
undefined : self._keys[index];
self._pos = index;
if (self._reverse) {
if (self._exclusiveStart || startkey !== self._startkey) {
self._pos--;
}
} else if (self._exclusiveStart && startkey === self._startkey) {
self._pos++;
}
} else {
self._pos = self._reverse ? self._keys.length - 1 : 0;
}
onInitComplete();
self.initCompleted = true;
var i = -1;
while (++i < self.onInitCompleteListeners) {
nextTick(self.onInitCompleteListeners[i]);
}
});
});
} else if (!self.initCompleted) {
self.onInitCompleteListeners.push(onInitComplete);
} else {
onInitComplete();
}
};
function FruitDown(location) {
if (!(this instanceof FruitDown)) {
return new FruitDown(location);
}
AbstractLevelDOWN.call(this, location);
this.container = new Database(location);
}
inherits(FruitDown, AbstractLevelDOWN);
FruitDown.prototype._open = function (options, callback) {
this.container.init(callback);
};
FruitDown.prototype._put = function (key, value, options, callback) {
var err = checkKeyValue(key, 'key');
if (err) {
return nextTick(function () {
callback(err);
});
}
err = checkKeyValue(value, 'value');
if (err) {
return nextTick(function () {
callback(err);
});
}
if (typeof value === 'object' && !Buffer.isBuffer(value) && value.buffer === undefined) {
var obj = {};
obj.storetype = "json";
obj.data = value;
value = JSON.stringify(obj);
}
this.container.setItem(key, value, callback);
};
FruitDown.prototype._get = function (key, options, callback) {
var err = checkKeyValue(key, 'key');
if (err) {
return nextTick(function () {
callback(err);
});
}
if (!Buffer.isBuffer(key)) {
key = String(key);
}
this.container.getItem(key, function (err, value) {
if (err) {
return callback(err);
}
if (options.asBuffer !== false && !Buffer.isBuffer(value)) {
value = new Buffer(value);
}
if (options.asBuffer === false) {
if (value.indexOf("{\"storetype\":\"json\",\"data\"") > -1) {
var res = JSON.parse(value);
value = res.data;
}
}
callback(null, value);
});
};
FruitDown.prototype._del = function (key, options, callback) {
var err = checkKeyValue(key, 'key');
if (err) {
return nextTick(function () {
callback(err);
});
}
if (!Buffer.isBuffer(key)) {
key = String(key);
}
this.container.removeItem(key, callback);
};
FruitDown.prototype._batch = function (array, options, callback) {
var self = this;
nextTick(function () {
var err;
var key;
var value;
var numDone = 0;
var overallErr;
function checkDone() {
if (++numDone === array.length) {
callback(overallErr);
}
}
if (Array.isArray(array) && array.length) {
for (var i = 0; i < array.length; i++) {
var task = array[i];
if (task) {
key = Buffer.isBuffer(task.key) ? task.key : String(task.key);
err = checkKeyValue(key, 'key');
if (err) {
overallErr = err;
checkDone();
} else if (task.type === 'del') {
self._del(task.key, options, checkDone);
} else if (task.type === 'put') {
value = Buffer.isBuffer(task.value) ? task.value : String(task.value);
err = checkKeyValue(value, 'value');
if (err) {
overallErr = err;
checkDone();
} else {
self._put(key, value, options, checkDone);
}
}
} else {
checkDone();
}
}
} else {
callback();
}
});
};
FruitDown.prototype._iterator = function (options) {
return new DatabaseIterator(this, options);
};
FruitDown.destroy = function (name, callback) {
DatabaseCore.destroy(name, callback);
};
function checkKeyValue(obj, type) {
if (obj === null || obj === undefined) {
return new Error(type + ' cannot be `null` or `undefined`');
}
if (obj === null || obj === undefined) {
return new Error(type + ' cannot be `null` or `undefined`');
}
if (type === 'key') {
if (obj instanceof Boolean) {
return new Error(type + ' cannot be `null` or `undefined`');
}
if (obj === '') {
return new Error(type + ' cannot be empty');
}
}
if (obj.toString().indexOf("[object ArrayBuffer]") === 0) {
if (obj.byteLength === 0 || obj.byteLength === undefined) {
return new Error(type + ' cannot be an empty Buffer');
}
}
if (Buffer.isBuffer(obj)) {
if (obj.length === 0) {
return new Error(type + ' cannot be an empty Buffer');
}
} else if (String(obj) === '') {
return new Error(type + ' cannot be an empty String');
}
}
module.exports = FruitDown;
}).call(this,_dereq_(55),typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {},_dereq_(8).Buffer)
},{"24":24,"25":25,"28":28,"3":3,"33":33,"55":55,"8":8}],27:[function(_dereq_,module,exports){
(function (process,global){
'use strict';
var argsarray = _dereq_(4);
var Queue = _dereq_(95);
// see http://stackoverflow.com/a/15349865/680742
var nextTick = global.setImmediate || process.nextTick;
function TaskQueue() {
this.queue = new Queue();
this.running = false;
}
TaskQueue.prototype.add = function (fun, callback) {
this.queue.push({fun: fun, callback: callback});
this.processNext();
};
TaskQueue.prototype.processNext = function () {
var self = this;
if (self.running || !self.queue.length) {
return;
}
self.running = true;
var task = self.queue.shift();
nextTick(function () {
task.fun(argsarray(function (args) {
task.callback.apply(null, args);
self.running = false;
self.processNext();
}));
});
};
module.exports = TaskQueue;
}).call(this,_dereq_(55),typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {})
},{"4":4,"55":55,"95":95}],28:[function(_dereq_,module,exports){
'use strict';
// taken from rvagg/memdown commit 2078b40
exports.sortedIndexOf = function(arr, item) {
var low = 0;
var high = arr.length;
var mid;
while (low < high) {
mid = (low + high) >>> 1;
if (arr[mid] < item) {
low = mid + 1;
} else {
high = mid;
}
}
return low;
};
},{}],29:[function(_dereq_,module,exports){
/**
* @file Tests if ES6 Symbol is supported.
* @version 1.4.0
* @author Xotic750
* @copyright Xotic750
* @license {@link MIT}
* @module has-symbol-support-x
*/
'use strict';
/**
* Indicates if `Symbol`exists and creates the correct type.
* `true`, if it exists and creates the correct type, otherwise `false`.
*
* @type boolean
*/
module.exports = typeof Symbol === 'function' && typeof Symbol('') === 'symbol';
},{}],30:[function(_dereq_,module,exports){
/**
* @file Tests if ES6 @@toStringTag is supported.
* @see {@link http://www.ecma-international.org/ecma-262/6.0/#sec-@@tostringtag|26.3.1 @@toStringTag}
* @version 1.4.0
* @author Xotic750
* @copyright Xotic750
* @license {@link MIT}
* @module has-to-string-tag-x
*/
'use strict';
/**
* Indicates if `Symbol.toStringTag`exists and is the correct type.
* `true`, if it exists and is the correct type, otherwise `false`.
*
* @type boolean
*/
module.exports = _dereq_(29) && typeof Symbol.toStringTag === 'symbol';
},{"29":29}],31:[function(_dereq_,module,exports){
exports.read = function (buffer, offset, isLE, mLen, nBytes) {
var e, m
var eLen = nBytes * 8 - mLen - 1
var eMax = (1 << eLen) - 1
var eBias = eMax >> 1
var nBits = -7
var i = isLE ? (nBytes - 1) : 0
var d = isLE ? -1 : 1
var s = buffer[offset + i]
i += d
e = s & ((1 << (-nBits)) - 1)
s >>= (-nBits)
nBits += eLen
for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8) {}
m = e & ((1 << (-nBits)) - 1)
e >>= (-nBits)
nBits += mLen
for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8) {}
if (e === 0) {
e = 1 - eBias
} else if (e === eMax) {
return m ? NaN : ((s ? -1 : 1) * Infinity)
} else {
m = m + Math.pow(2, mLen)
e = e - eBias
}
return (s ? -1 : 1) * m * Math.pow(2, e - mLen)
}
exports.write = function (buffer, value, offset, isLE, mLen, nBytes) {
var e, m, c
var eLen = nBytes * 8 - mLen - 1
var eMax = (1 << eLen) - 1
var eBias = eMax >> 1
var rt = (mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0)
var i = isLE ? 0 : (nBytes - 1)
var d = isLE ? 1 : -1
var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0
value = Math.abs(value)
if (isNaN(value) || value === Infinity) {
m = isNaN(value) ? 1 : 0
e = eMax
} else {
e = Math.floor(Math.log(value) / Math.LN2)
if (value * (c = Math.pow(2, -e)) < 1) {
e--
c *= 2
}
if (e + eBias >= 1) {
value += rt / c
} else {
value += rt * Math.pow(2, 1 - eBias)
}
if (value * c >= 2) {
e++
c /= 2
}
if (e + eBias >= eMax) {
m = 0
e = eMax
} else if (e + eBias >= 1) {
m = (value * c - 1) * Math.pow(2, mLen)
e = e + eBias
} else {
m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen)
e = 0
}
}
for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}
e = (e << mLen) | m
eLen += mLen
for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}
buffer[offset + i - d] |= s * 128
}
},{}],32:[function(_dereq_,module,exports){
(function (global){
'use strict';
var Mutation = global.MutationObserver || global.WebKitMutationObserver;
var scheduleDrain;
{
if (Mutation) {
var called = 0;
var observer = new Mutation(nextTick);
var element = global.document.createTextNode('');
observer.observe(element, {
characterData: true
});
scheduleDrain = function () {
element.data = (called = ++called % 2);
};
} else if (!global.setImmediate && typeof global.MessageChannel !== 'undefined') {
var channel = new global.MessageChannel();
channel.port1.onmessage = nextTick;
scheduleDrain = function () {
channel.port2.postMessage(0);
};
} else if ('document' in global && 'onreadystatechange' in global.document.createElement('script')) {
scheduleDrain = function () {
// Create a