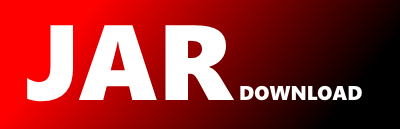
META-INF.frontend.generated.convert.js Maven / Gradle / Ivy
#!/usr/bin/env node
var __create = Object.create;
var __defProp = Object.defineProperty;
var __getOwnPropDesc = Object.getOwnPropertyDescriptor;
var __getOwnPropNames = Object.getOwnPropertyNames;
var __getProtoOf = Object.getPrototypeOf;
var __hasOwnProp = Object.prototype.hasOwnProperty;
var __commonJS = (cb, mod) => function __require() {
return mod || (0, cb[__getOwnPropNames(cb)[0]])((mod = { exports: {} }).exports, mod), mod.exports;
};
var __copyProps = (to, from, except, desc) => {
if (from && typeof from === "object" || typeof from === "function") {
for (let key of __getOwnPropNames(from))
if (!__hasOwnProp.call(to, key) && key !== except)
__defProp(to, key, { get: () => from[key], enumerable: !(desc = __getOwnPropDesc(from, key)) || desc.enumerable });
}
return to;
};
var __toESM = (mod, isNodeMode, target) => (target = mod != null ? __create(__getProtoOf(mod)) : {}, __copyProps(
isNodeMode || !mod || !mod.__esModule ? __defProp(target, "default", { value: mod, enumerable: true }) : target,
mod
));
// node_modules/acorn/dist/acorn.js
var require_acorn = __commonJS({
"node_modules/acorn/dist/acorn.js"(exports, module2) {
(function(global2, factory) {
typeof exports === "object" && typeof module2 !== "undefined" ? factory(exports) : typeof define === "function" && define.amd ? define(["exports"], factory) : (global2 = global2 || self, factory(global2.acorn = {}));
})(exports, function(exports2) {
"use strict";
var reservedWords = {
3: "abstract boolean byte char class double enum export extends final float goto implements import int interface long native package private protected public short static super synchronized throws transient volatile",
5: "class enum extends super const export import",
6: "enum",
strict: "implements interface let package private protected public static yield",
strictBind: "eval arguments"
};
var ecma5AndLessKeywords = "break case catch continue debugger default do else finally for function if return switch throw try var while with null true false instanceof typeof void delete new in this";
var keywords = {
5: ecma5AndLessKeywords,
"5module": ecma5AndLessKeywords + " export import",
6: ecma5AndLessKeywords + " const class extends export import super"
};
var keywordRelationalOperator = /^in(stanceof)?$/;
var nonASCIIidentifierStartChars = "\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0370-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u048A-\u052F\u0531-\u0556\u0559\u0560-\u0588\u05D0-\u05EA\u05EF-\u05F2\u0620-\u064A\u066E\u066F\u0671-\u06D3\u06D5\u06E5\u06E6\u06EE\u06EF\u06FA-\u06FC\u06FF\u0710\u0712-\u072F\u074D-\u07A5\u07B1\u07CA-\u07EA\u07F4\u07F5\u07FA\u0800-\u0815\u081A\u0824\u0828\u0840-\u0858\u0860-\u086A\u08A0-\u08B4\u08B6-\u08C7\u0904-\u0939\u093D\u0950\u0958-\u0961\u0971-\u0980\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD\u09CE\u09DC\u09DD\u09DF-\u09E1\u09F0\u09F1\u09FC\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A59-\u0A5C\u0A5E\u0A72-\u0A74\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABD\u0AD0\u0AE0\u0AE1\u0AF9\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3D\u0B5C\u0B5D\u0B5F-\u0B61\u0B71\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BD0\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C58-\u0C5A\u0C60\u0C61\u0C80\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CDE\u0CE0\u0CE1\u0CF1\u0CF2\u0D04-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D\u0D4E\u0D54-\u0D56\u0D5F-\u0D61\u0D7A-\u0D7F\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0E01-\u0E30\u0E32\u0E33\u0E40-\u0E46\u0E81\u0E82\u0E84\u0E86-\u0E8A\u0E8C-\u0EA3\u0EA5\u0EA7-\u0EB0\u0EB2\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6\u0EDC-\u0EDF\u0F00\u0F40-\u0F47\u0F49-\u0F6C\u0F88-\u0F8C\u1000-\u102A\u103F\u1050-\u1055\u105A-\u105D\u1061\u1065\u1066\u106E-\u1070\u1075-\u1081\u108E\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u170C\u170E-\u1711\u1720-\u1731\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17D7\u17DC\u1820-\u1878\u1880-\u18A8\u18AA\u18B0-\u18F5\u1900-\u191E\u1950-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u1A00-\u1A16\u1A20-\u1A54\u1AA7\u1B05-\u1B33\u1B45-\u1B4B\u1B83-\u1BA0\u1BAE\u1BAF\u1BBA-\u1BE5\u1C00-\u1C23\u1C4D-\u1C4F\u1C5A-\u1C7D\u1C80-\u1C88\u1C90-\u1CBA\u1CBD-\u1CBF\u1CE9-\u1CEC\u1CEE-\u1CF3\u1CF5\u1CF6\u1CFA\u1D00-\u1DBF\u1E00-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2118-\u211D\u2124\u2126\u2128\u212A-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2C2E\u2C30-\u2C5E\u2C60-\u2CE4\u2CEB-\u2CEE\u2CF2\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u3005-\u3007\u3021-\u3029\u3031-\u3035\u3038-\u303C\u3041-\u3096\u309B-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312F\u3131-\u318E\u31A0-\u31BF\u31F0-\u31FF\u3400-\u4DBF\u4E00-\u9FFC\uA000-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA61F\uA62A\uA62B\uA640-\uA66E\uA67F-\uA69D\uA6A0-\uA6EF\uA717-\uA71F\uA722-\uA788\uA78B-\uA7BF\uA7C2-\uA7CA\uA7F5-\uA801\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA840-\uA873\uA882-\uA8B3\uA8F2-\uA8F7\uA8FB\uA8FD\uA8FE\uA90A-\uA925\uA930-\uA946\uA960-\uA97C\uA984-\uA9B2\uA9CF\uA9E0-\uA9E4\uA9E6-\uA9EF\uA9FA-\uA9FE\uAA00-\uAA28\uAA40-\uAA42\uAA44-\uAA4B\uAA60-\uAA76\uAA7A\uAA7E-\uAAAF\uAAB1\uAAB5\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADD\uAAE0-\uAAEA\uAAF2-\uAAF4\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB69\uAB70-\uABE2\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE70-\uFE74\uFE76-\uFEFC\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC";
var nonASCIIidentifierChars = "\u200C\u200D\xB7\u0300-\u036F\u0387\u0483-\u0487\u0591-\u05BD\u05BF\u05C1\u05C2\u05C4\u05C5\u05C7\u0610-\u061A\u064B-\u0669\u0670\u06D6-\u06DC\u06DF-\u06E4\u06E7\u06E8\u06EA-\u06ED\u06F0-\u06F9\u0711\u0730-\u074A\u07A6-\u07B0\u07C0-\u07C9\u07EB-\u07F3\u07FD\u0816-\u0819\u081B-\u0823\u0825-\u0827\u0829-\u082D\u0859-\u085B\u08D3-\u08E1\u08E3-\u0903\u093A-\u093C\u093E-\u094F\u0951-\u0957\u0962\u0963\u0966-\u096F\u0981-\u0983\u09BC\u09BE-\u09C4\u09C7\u09C8\u09CB-\u09CD\u09D7\u09E2\u09E3\u09E6-\u09EF\u09FE\u0A01-\u0A03\u0A3C\u0A3E-\u0A42\u0A47\u0A48\u0A4B-\u0A4D\u0A51\u0A66-\u0A71\u0A75\u0A81-\u0A83\u0ABC\u0ABE-\u0AC5\u0AC7-\u0AC9\u0ACB-\u0ACD\u0AE2\u0AE3\u0AE6-\u0AEF\u0AFA-\u0AFF\u0B01-\u0B03\u0B3C\u0B3E-\u0B44\u0B47\u0B48\u0B4B-\u0B4D\u0B55-\u0B57\u0B62\u0B63\u0B66-\u0B6F\u0B82\u0BBE-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCD\u0BD7\u0BE6-\u0BEF\u0C00-\u0C04\u0C3E-\u0C44\u0C46-\u0C48\u0C4A-\u0C4D\u0C55\u0C56\u0C62\u0C63\u0C66-\u0C6F\u0C81-\u0C83\u0CBC\u0CBE-\u0CC4\u0CC6-\u0CC8\u0CCA-\u0CCD\u0CD5\u0CD6\u0CE2\u0CE3\u0CE6-\u0CEF\u0D00-\u0D03\u0D3B\u0D3C\u0D3E-\u0D44\u0D46-\u0D48\u0D4A-\u0D4D\u0D57\u0D62\u0D63\u0D66-\u0D6F\u0D81-\u0D83\u0DCA\u0DCF-\u0DD4\u0DD6\u0DD8-\u0DDF\u0DE6-\u0DEF\u0DF2\u0DF3\u0E31\u0E34-\u0E3A\u0E47-\u0E4E\u0E50-\u0E59\u0EB1\u0EB4-\u0EBC\u0EC8-\u0ECD\u0ED0-\u0ED9\u0F18\u0F19\u0F20-\u0F29\u0F35\u0F37\u0F39\u0F3E\u0F3F\u0F71-\u0F84\u0F86\u0F87\u0F8D-\u0F97\u0F99-\u0FBC\u0FC6\u102B-\u103E\u1040-\u1049\u1056-\u1059\u105E-\u1060\u1062-\u1064\u1067-\u106D\u1071-\u1074\u1082-\u108D\u108F-\u109D\u135D-\u135F\u1369-\u1371\u1712-\u1714\u1732-\u1734\u1752\u1753\u1772\u1773\u17B4-\u17D3\u17DD\u17E0-\u17E9\u180B-\u180D\u1810-\u1819\u18A9\u1920-\u192B\u1930-\u193B\u1946-\u194F\u19D0-\u19DA\u1A17-\u1A1B\u1A55-\u1A5E\u1A60-\u1A7C\u1A7F-\u1A89\u1A90-\u1A99\u1AB0-\u1ABD\u1ABF\u1AC0\u1B00-\u1B04\u1B34-\u1B44\u1B50-\u1B59\u1B6B-\u1B73\u1B80-\u1B82\u1BA1-\u1BAD\u1BB0-\u1BB9\u1BE6-\u1BF3\u1C24-\u1C37\u1C40-\u1C49\u1C50-\u1C59\u1CD0-\u1CD2\u1CD4-\u1CE8\u1CED\u1CF4\u1CF7-\u1CF9\u1DC0-\u1DF9\u1DFB-\u1DFF\u203F\u2040\u2054\u20D0-\u20DC\u20E1\u20E5-\u20F0\u2CEF-\u2CF1\u2D7F\u2DE0-\u2DFF\u302A-\u302F\u3099\u309A\uA620-\uA629\uA66F\uA674-\uA67D\uA69E\uA69F\uA6F0\uA6F1\uA802\uA806\uA80B\uA823-\uA827\uA82C\uA880\uA881\uA8B4-\uA8C5\uA8D0-\uA8D9\uA8E0-\uA8F1\uA8FF-\uA909\uA926-\uA92D\uA947-\uA953\uA980-\uA983\uA9B3-\uA9C0\uA9D0-\uA9D9\uA9E5\uA9F0-\uA9F9\uAA29-\uAA36\uAA43\uAA4C\uAA4D\uAA50-\uAA59\uAA7B-\uAA7D\uAAB0\uAAB2-\uAAB4\uAAB7\uAAB8\uAABE\uAABF\uAAC1\uAAEB-\uAAEF\uAAF5\uAAF6\uABE3-\uABEA\uABEC\uABED\uABF0-\uABF9\uFB1E\uFE00-\uFE0F\uFE20-\uFE2F\uFE33\uFE34\uFE4D-\uFE4F\uFF10-\uFF19\uFF3F";
var nonASCIIidentifierStart = new RegExp("[" + nonASCIIidentifierStartChars + "]");
var nonASCIIidentifier = new RegExp("[" + nonASCIIidentifierStartChars + nonASCIIidentifierChars + "]");
nonASCIIidentifierStartChars = nonASCIIidentifierChars = null;
var astralIdentifierStartCodes = [0, 11, 2, 25, 2, 18, 2, 1, 2, 14, 3, 13, 35, 122, 70, 52, 268, 28, 4, 48, 48, 31, 14, 29, 6, 37, 11, 29, 3, 35, 5, 7, 2, 4, 43, 157, 19, 35, 5, 35, 5, 39, 9, 51, 157, 310, 10, 21, 11, 7, 153, 5, 3, 0, 2, 43, 2, 1, 4, 0, 3, 22, 11, 22, 10, 30, 66, 18, 2, 1, 11, 21, 11, 25, 71, 55, 7, 1, 65, 0, 16, 3, 2, 2, 2, 28, 43, 28, 4, 28, 36, 7, 2, 27, 28, 53, 11, 21, 11, 18, 14, 17, 111, 72, 56, 50, 14, 50, 14, 35, 349, 41, 7, 1, 79, 28, 11, 0, 9, 21, 107, 20, 28, 22, 13, 52, 76, 44, 33, 24, 27, 35, 30, 0, 3, 0, 9, 34, 4, 0, 13, 47, 15, 3, 22, 0, 2, 0, 36, 17, 2, 24, 85, 6, 2, 0, 2, 3, 2, 14, 2, 9, 8, 46, 39, 7, 3, 1, 3, 21, 2, 6, 2, 1, 2, 4, 4, 0, 19, 0, 13, 4, 159, 52, 19, 3, 21, 2, 31, 47, 21, 1, 2, 0, 185, 46, 42, 3, 37, 47, 21, 0, 60, 42, 14, 0, 72, 26, 230, 43, 117, 63, 32, 7, 3, 0, 3, 7, 2, 1, 2, 23, 16, 0, 2, 0, 95, 7, 3, 38, 17, 0, 2, 0, 29, 0, 11, 39, 8, 0, 22, 0, 12, 45, 20, 0, 35, 56, 264, 8, 2, 36, 18, 0, 50, 29, 113, 6, 2, 1, 2, 37, 22, 0, 26, 5, 2, 1, 2, 31, 15, 0, 328, 18, 190, 0, 80, 921, 103, 110, 18, 195, 2749, 1070, 4050, 582, 8634, 568, 8, 30, 114, 29, 19, 47, 17, 3, 32, 20, 6, 18, 689, 63, 129, 74, 6, 0, 67, 12, 65, 1, 2, 0, 29, 6135, 9, 1237, 43, 8, 8952, 286, 50, 2, 18, 3, 9, 395, 2309, 106, 6, 12, 4, 8, 8, 9, 5991, 84, 2, 70, 2, 1, 3, 0, 3, 1, 3, 3, 2, 11, 2, 0, 2, 6, 2, 64, 2, 3, 3, 7, 2, 6, 2, 27, 2, 3, 2, 4, 2, 0, 4, 6, 2, 339, 3, 24, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 7, 2357, 44, 11, 6, 17, 0, 370, 43, 1301, 196, 60, 67, 8, 0, 1205, 3, 2, 26, 2, 1, 2, 0, 3, 0, 2, 9, 2, 3, 2, 0, 2, 0, 7, 0, 5, 0, 2, 0, 2, 0, 2, 2, 2, 1, 2, 0, 3, 0, 2, 0, 2, 0, 2, 0, 2, 0, 2, 1, 2, 0, 3, 3, 2, 6, 2, 3, 2, 3, 2, 0, 2, 9, 2, 16, 6, 2, 2, 4, 2, 16, 4421, 42717, 35, 4148, 12, 221, 3, 5761, 15, 7472, 3104, 541, 1507, 4938];
var astralIdentifierCodes = [509, 0, 227, 0, 150, 4, 294, 9, 1368, 2, 2, 1, 6, 3, 41, 2, 5, 0, 166, 1, 574, 3, 9, 9, 370, 1, 154, 10, 176, 2, 54, 14, 32, 9, 16, 3, 46, 10, 54, 9, 7, 2, 37, 13, 2, 9, 6, 1, 45, 0, 13, 2, 49, 13, 9, 3, 2, 11, 83, 11, 7, 0, 161, 11, 6, 9, 7, 3, 56, 1, 2, 6, 3, 1, 3, 2, 10, 0, 11, 1, 3, 6, 4, 4, 193, 17, 10, 9, 5, 0, 82, 19, 13, 9, 214, 6, 3, 8, 28, 1, 83, 16, 16, 9, 82, 12, 9, 9, 84, 14, 5, 9, 243, 14, 166, 9, 71, 5, 2, 1, 3, 3, 2, 0, 2, 1, 13, 9, 120, 6, 3, 6, 4, 0, 29, 9, 41, 6, 2, 3, 9, 0, 10, 10, 47, 15, 406, 7, 2, 7, 17, 9, 57, 21, 2, 13, 123, 5, 4, 0, 2, 1, 2, 6, 2, 0, 9, 9, 49, 4, 2, 1, 2, 4, 9, 9, 330, 3, 19306, 9, 135, 4, 60, 6, 26, 9, 1014, 0, 2, 54, 8, 3, 82, 0, 12, 1, 19628, 1, 5319, 4, 4, 5, 9, 7, 3, 6, 31, 3, 149, 2, 1418, 49, 513, 54, 5, 49, 9, 0, 15, 0, 23, 4, 2, 14, 1361, 6, 2, 16, 3, 6, 2, 1, 2, 4, 262, 6, 10, 9, 419, 13, 1495, 6, 110, 6, 6, 9, 4759, 9, 787719, 239];
function isInAstralSet(code, set) {
var pos = 65536;
for (var i = 0; i < set.length; i += 2) {
pos += set[i];
if (pos > code) {
return false;
}
pos += set[i + 1];
if (pos >= code) {
return true;
}
}
}
function isIdentifierStart(code, astral) {
if (code < 65) {
return code === 36;
}
if (code < 91) {
return true;
}
if (code < 97) {
return code === 95;
}
if (code < 123) {
return true;
}
if (code <= 65535) {
return code >= 170 && nonASCIIidentifierStart.test(String.fromCharCode(code));
}
if (astral === false) {
return false;
}
return isInAstralSet(code, astralIdentifierStartCodes);
}
function isIdentifierChar(code, astral) {
if (code < 48) {
return code === 36;
}
if (code < 58) {
return true;
}
if (code < 65) {
return false;
}
if (code < 91) {
return true;
}
if (code < 97) {
return code === 95;
}
if (code < 123) {
return true;
}
if (code <= 65535) {
return code >= 170 && nonASCIIidentifier.test(String.fromCharCode(code));
}
if (astral === false) {
return false;
}
return isInAstralSet(code, astralIdentifierStartCodes) || isInAstralSet(code, astralIdentifierCodes);
}
var TokenType = function TokenType2(label, conf) {
if (conf === void 0)
conf = {};
this.label = label;
this.keyword = conf.keyword;
this.beforeExpr = !!conf.beforeExpr;
this.startsExpr = !!conf.startsExpr;
this.isLoop = !!conf.isLoop;
this.isAssign = !!conf.isAssign;
this.prefix = !!conf.prefix;
this.postfix = !!conf.postfix;
this.binop = conf.binop || null;
this.updateContext = null;
};
function binop(name, prec) {
return new TokenType(name, { beforeExpr: true, binop: prec });
}
var beforeExpr = { beforeExpr: true }, startsExpr = { startsExpr: true };
var keywords$1 = {};
function kw(name, options) {
if (options === void 0)
options = {};
options.keyword = name;
return keywords$1[name] = new TokenType(name, options);
}
var types = {
num: new TokenType("num", startsExpr),
regexp: new TokenType("regexp", startsExpr),
string: new TokenType("string", startsExpr),
name: new TokenType("name", startsExpr),
eof: new TokenType("eof"),
bracketL: new TokenType("[", { beforeExpr: true, startsExpr: true }),
bracketR: new TokenType("]"),
braceL: new TokenType("{", { beforeExpr: true, startsExpr: true }),
braceR: new TokenType("}"),
parenL: new TokenType("(", { beforeExpr: true, startsExpr: true }),
parenR: new TokenType(")"),
comma: new TokenType(",", beforeExpr),
semi: new TokenType(";", beforeExpr),
colon: new TokenType(":", beforeExpr),
dot: new TokenType("."),
question: new TokenType("?", beforeExpr),
questionDot: new TokenType("?."),
arrow: new TokenType("=>", beforeExpr),
template: new TokenType("template"),
invalidTemplate: new TokenType("invalidTemplate"),
ellipsis: new TokenType("...", beforeExpr),
backQuote: new TokenType("`", startsExpr),
dollarBraceL: new TokenType("${", { beforeExpr: true, startsExpr: true }),
eq: new TokenType("=", { beforeExpr: true, isAssign: true }),
assign: new TokenType("_=", { beforeExpr: true, isAssign: true }),
incDec: new TokenType("++/--", { prefix: true, postfix: true, startsExpr: true }),
prefix: new TokenType("!/~", { beforeExpr: true, prefix: true, startsExpr: true }),
logicalOR: binop("||", 1),
logicalAND: binop("&&", 2),
bitwiseOR: binop("|", 3),
bitwiseXOR: binop("^", 4),
bitwiseAND: binop("&", 5),
equality: binop("==/!=/===/!==", 6),
relational: binop(">/<=/>=", 7),
bitShift: binop("<>>/>>>", 8),
plusMin: new TokenType("+/-", { beforeExpr: true, binop: 9, prefix: true, startsExpr: true }),
modulo: binop("%", 10),
star: binop("*", 10),
slash: binop("/", 10),
starstar: new TokenType("**", { beforeExpr: true }),
coalesce: binop("??", 1),
_break: kw("break"),
_case: kw("case", beforeExpr),
_catch: kw("catch"),
_continue: kw("continue"),
_debugger: kw("debugger"),
_default: kw("default", beforeExpr),
_do: kw("do", { isLoop: true, beforeExpr: true }),
_else: kw("else", beforeExpr),
_finally: kw("finally"),
_for: kw("for", { isLoop: true }),
_function: kw("function", startsExpr),
_if: kw("if"),
_return: kw("return", beforeExpr),
_switch: kw("switch"),
_throw: kw("throw", beforeExpr),
_try: kw("try"),
_var: kw("var"),
_const: kw("const"),
_while: kw("while", { isLoop: true }),
_with: kw("with"),
_new: kw("new", { beforeExpr: true, startsExpr: true }),
_this: kw("this", startsExpr),
_super: kw("super", startsExpr),
_class: kw("class", startsExpr),
_extends: kw("extends", beforeExpr),
_export: kw("export"),
_import: kw("import", startsExpr),
_null: kw("null", startsExpr),
_true: kw("true", startsExpr),
_false: kw("false", startsExpr),
_in: kw("in", { beforeExpr: true, binop: 7 }),
_instanceof: kw("instanceof", { beforeExpr: true, binop: 7 }),
_typeof: kw("typeof", { beforeExpr: true, prefix: true, startsExpr: true }),
_void: kw("void", { beforeExpr: true, prefix: true, startsExpr: true }),
_delete: kw("delete", { beforeExpr: true, prefix: true, startsExpr: true })
};
var lineBreak = /\r\n?|\n|\u2028|\u2029/;
var lineBreakG = new RegExp(lineBreak.source, "g");
function isNewLine(code, ecma2019String) {
return code === 10 || code === 13 || !ecma2019String && (code === 8232 || code === 8233);
}
var nonASCIIwhitespace = /[\u1680\u2000-\u200a\u202f\u205f\u3000\ufeff]/;
var skipWhiteSpace = /(?:\s|\/\/.*|\/\*[^]*?\*\/)*/g;
var ref = Object.prototype;
var hasOwnProperty = ref.hasOwnProperty;
var toString2 = ref.toString;
function has(obj, propName) {
return hasOwnProperty.call(obj, propName);
}
var isArray = Array.isArray || function(obj) {
return toString2.call(obj) === "[object Array]";
};
function wordsRegexp(words) {
return new RegExp("^(?:" + words.replace(/ /g, "|") + ")$");
}
var Position = function Position2(line, col) {
this.line = line;
this.column = col;
};
Position.prototype.offset = function offset(n2) {
return new Position(this.line, this.column + n2);
};
var SourceLocation = function SourceLocation2(p, start, end) {
this.start = start;
this.end = end;
if (p.sourceFile !== null) {
this.source = p.sourceFile;
}
};
function getLineInfo2(input, offset) {
for (var line = 1, cur = 0; ; ) {
lineBreakG.lastIndex = cur;
var match = lineBreakG.exec(input);
if (match && match.index < offset) {
++line;
cur = match.index + match[0].length;
} else {
return new Position(line, offset - cur);
}
}
}
var defaultOptions = {
ecmaVersion: 10,
sourceType: "script",
onInsertedSemicolon: null,
onTrailingComma: null,
allowReserved: null,
allowReturnOutsideFunction: false,
allowImportExportEverywhere: false,
allowAwaitOutsideFunction: false,
allowHashBang: false,
locations: false,
onToken: null,
onComment: null,
ranges: false,
program: null,
sourceFile: null,
directSourceFile: null,
preserveParens: false
};
function getOptions(opts) {
var options = {};
for (var opt in defaultOptions) {
options[opt] = opts && has(opts, opt) ? opts[opt] : defaultOptions[opt];
}
if (options.ecmaVersion >= 2015) {
options.ecmaVersion -= 2009;
}
if (options.allowReserved == null) {
options.allowReserved = options.ecmaVersion < 5;
}
if (isArray(options.onToken)) {
var tokens = options.onToken;
options.onToken = function(token) {
return tokens.push(token);
};
}
if (isArray(options.onComment)) {
options.onComment = pushComment(options, options.onComment);
}
return options;
}
function pushComment(options, array) {
return function(block, text, start, end, startLoc, endLoc) {
var comment = {
type: block ? "Block" : "Line",
value: text,
start,
end
};
if (options.locations) {
comment.loc = new SourceLocation(this, startLoc, endLoc);
}
if (options.ranges) {
comment.range = [start, end];
}
array.push(comment);
};
}
var SCOPE_TOP = 1, SCOPE_FUNCTION = 2, SCOPE_VAR = SCOPE_TOP | SCOPE_FUNCTION, SCOPE_ASYNC = 4, SCOPE_GENERATOR = 8, SCOPE_ARROW = 16, SCOPE_SIMPLE_CATCH = 32, SCOPE_SUPER = 64, SCOPE_DIRECT_SUPER = 128;
function functionFlags(async, generator) {
return SCOPE_FUNCTION | (async ? SCOPE_ASYNC : 0) | (generator ? SCOPE_GENERATOR : 0);
}
var BIND_NONE = 0, BIND_VAR = 1, BIND_LEXICAL = 2, BIND_FUNCTION = 3, BIND_SIMPLE_CATCH = 4, BIND_OUTSIDE = 5;
var Parser = function Parser2(options, input, startPos) {
this.options = options = getOptions(options);
this.sourceFile = options.sourceFile;
this.keywords = wordsRegexp(keywords[options.ecmaVersion >= 6 ? 6 : options.sourceType === "module" ? "5module" : 5]);
var reserved = "";
if (options.allowReserved !== true) {
for (var v = options.ecmaVersion; ; v--) {
if (reserved = reservedWords[v]) {
break;
}
}
if (options.sourceType === "module") {
reserved += " await";
}
}
this.reservedWords = wordsRegexp(reserved);
var reservedStrict = (reserved ? reserved + " " : "") + reservedWords.strict;
this.reservedWordsStrict = wordsRegexp(reservedStrict);
this.reservedWordsStrictBind = wordsRegexp(reservedStrict + " " + reservedWords.strictBind);
this.input = String(input);
this.containsEsc = false;
if (startPos) {
this.pos = startPos;
this.lineStart = this.input.lastIndexOf("\n", startPos - 1) + 1;
this.curLine = this.input.slice(0, this.lineStart).split(lineBreak).length;
} else {
this.pos = this.lineStart = 0;
this.curLine = 1;
}
this.type = types.eof;
this.value = null;
this.start = this.end = this.pos;
this.startLoc = this.endLoc = this.curPosition();
this.lastTokEndLoc = this.lastTokStartLoc = null;
this.lastTokStart = this.lastTokEnd = this.pos;
this.context = this.initialContext();
this.exprAllowed = true;
this.inModule = options.sourceType === "module";
this.strict = this.inModule || this.strictDirective(this.pos);
this.potentialArrowAt = -1;
this.yieldPos = this.awaitPos = this.awaitIdentPos = 0;
this.labels = [];
this.undefinedExports = {};
if (this.pos === 0 && options.allowHashBang && this.input.slice(0, 2) === "#!") {
this.skipLineComment(2);
}
this.scopeStack = [];
this.enterScope(SCOPE_TOP);
this.regexpState = null;
};
var prototypeAccessors = { inFunction: { configurable: true }, inGenerator: { configurable: true }, inAsync: { configurable: true }, allowSuper: { configurable: true }, allowDirectSuper: { configurable: true }, treatFunctionsAsVar: { configurable: true } };
Parser.prototype.parse = function parse3() {
var node = this.options.program || this.startNode();
this.nextToken();
return this.parseTopLevel(node);
};
prototypeAccessors.inFunction.get = function() {
return (this.currentVarScope().flags & SCOPE_FUNCTION) > 0;
};
prototypeAccessors.inGenerator.get = function() {
return (this.currentVarScope().flags & SCOPE_GENERATOR) > 0;
};
prototypeAccessors.inAsync.get = function() {
return (this.currentVarScope().flags & SCOPE_ASYNC) > 0;
};
prototypeAccessors.allowSuper.get = function() {
return (this.currentThisScope().flags & SCOPE_SUPER) > 0;
};
prototypeAccessors.allowDirectSuper.get = function() {
return (this.currentThisScope().flags & SCOPE_DIRECT_SUPER) > 0;
};
prototypeAccessors.treatFunctionsAsVar.get = function() {
return this.treatFunctionsAsVarInScope(this.currentScope());
};
Parser.prototype.inNonArrowFunction = function inNonArrowFunction() {
return (this.currentThisScope().flags & SCOPE_FUNCTION) > 0;
};
Parser.extend = function extend() {
var plugins = [], len = arguments.length;
while (len--)
plugins[len] = arguments[len];
var cls = this;
for (var i = 0; i < plugins.length; i++) {
cls = plugins[i](cls);
}
return cls;
};
Parser.parse = function parse3(input, options) {
return new this(options, input).parse();
};
Parser.parseExpressionAt = function parseExpressionAt2(input, pos, options) {
var parser = new this(options, input, pos);
parser.nextToken();
return parser.parseExpression();
};
Parser.tokenizer = function tokenizer2(input, options) {
return new this(options, input);
};
Object.defineProperties(Parser.prototype, prototypeAccessors);
var pp = Parser.prototype;
var literal = /^(?:'((?:\\.|[^'\\])*?)'|"((?:\\.|[^"\\])*?)")/;
pp.strictDirective = function(start) {
for (; ; ) {
skipWhiteSpace.lastIndex = start;
start += skipWhiteSpace.exec(this.input)[0].length;
var match = literal.exec(this.input.slice(start));
if (!match) {
return false;
}
if ((match[1] || match[2]) === "use strict") {
skipWhiteSpace.lastIndex = start + match[0].length;
var spaceAfter = skipWhiteSpace.exec(this.input), end = spaceAfter.index + spaceAfter[0].length;
var next = this.input.charAt(end);
return next === ";" || next === "}" || lineBreak.test(spaceAfter[0]) && !(/[(`.[+\-/*%<>=,?^&]/.test(next) || next === "!" && this.input.charAt(end + 1) === "=");
}
start += match[0].length;
skipWhiteSpace.lastIndex = start;
start += skipWhiteSpace.exec(this.input)[0].length;
if (this.input[start] === ";") {
start++;
}
}
};
pp.eat = function(type) {
if (this.type === type) {
this.next();
return true;
} else {
return false;
}
};
pp.isContextual = function(name) {
return this.type === types.name && this.value === name && !this.containsEsc;
};
pp.eatContextual = function(name) {
if (!this.isContextual(name)) {
return false;
}
this.next();
return true;
};
pp.expectContextual = function(name) {
if (!this.eatContextual(name)) {
this.unexpected();
}
};
pp.canInsertSemicolon = function() {
return this.type === types.eof || this.type === types.braceR || lineBreak.test(this.input.slice(this.lastTokEnd, this.start));
};
pp.insertSemicolon = function() {
if (this.canInsertSemicolon()) {
if (this.options.onInsertedSemicolon) {
this.options.onInsertedSemicolon(this.lastTokEnd, this.lastTokEndLoc);
}
return true;
}
};
pp.semicolon = function() {
if (!this.eat(types.semi) && !this.insertSemicolon()) {
this.unexpected();
}
};
pp.afterTrailingComma = function(tokType, notNext) {
if (this.type === tokType) {
if (this.options.onTrailingComma) {
this.options.onTrailingComma(this.lastTokStart, this.lastTokStartLoc);
}
if (!notNext) {
this.next();
}
return true;
}
};
pp.expect = function(type) {
this.eat(type) || this.unexpected();
};
pp.unexpected = function(pos) {
this.raise(pos != null ? pos : this.start, "Unexpected token");
};
function DestructuringErrors() {
this.shorthandAssign = this.trailingComma = this.parenthesizedAssign = this.parenthesizedBind = this.doubleProto = -1;
}
pp.checkPatternErrors = function(refDestructuringErrors, isAssign) {
if (!refDestructuringErrors) {
return;
}
if (refDestructuringErrors.trailingComma > -1) {
this.raiseRecoverable(refDestructuringErrors.trailingComma, "Comma is not permitted after the rest element");
}
var parens = isAssign ? refDestructuringErrors.parenthesizedAssign : refDestructuringErrors.parenthesizedBind;
if (parens > -1) {
this.raiseRecoverable(parens, "Parenthesized pattern");
}
};
pp.checkExpressionErrors = function(refDestructuringErrors, andThrow) {
if (!refDestructuringErrors) {
return false;
}
var shorthandAssign = refDestructuringErrors.shorthandAssign;
var doubleProto = refDestructuringErrors.doubleProto;
if (!andThrow) {
return shorthandAssign >= 0 || doubleProto >= 0;
}
if (shorthandAssign >= 0) {
this.raise(shorthandAssign, "Shorthand property assignments are valid only in destructuring patterns");
}
if (doubleProto >= 0) {
this.raiseRecoverable(doubleProto, "Redefinition of __proto__ property");
}
};
pp.checkYieldAwaitInDefaultParams = function() {
if (this.yieldPos && (!this.awaitPos || this.yieldPos < this.awaitPos)) {
this.raise(this.yieldPos, "Yield expression cannot be a default value");
}
if (this.awaitPos) {
this.raise(this.awaitPos, "Await expression cannot be a default value");
}
};
pp.isSimpleAssignTarget = function(expr) {
if (expr.type === "ParenthesizedExpression") {
return this.isSimpleAssignTarget(expr.expression);
}
return expr.type === "Identifier" || expr.type === "MemberExpression";
};
var pp$1 = Parser.prototype;
pp$1.parseTopLevel = function(node) {
var exports3 = {};
if (!node.body) {
node.body = [];
}
while (this.type !== types.eof) {
var stmt = this.parseStatement(null, true, exports3);
node.body.push(stmt);
}
if (this.inModule) {
for (var i = 0, list = Object.keys(this.undefinedExports); i < list.length; i += 1) {
var name = list[i];
this.raiseRecoverable(this.undefinedExports[name].start, "Export '" + name + "' is not defined");
}
}
this.adaptDirectivePrologue(node.body);
this.next();
node.sourceType = this.options.sourceType;
return this.finishNode(node, "Program");
};
var loopLabel = { kind: "loop" }, switchLabel = { kind: "switch" };
pp$1.isLet = function(context) {
if (this.options.ecmaVersion < 6 || !this.isContextual("let")) {
return false;
}
skipWhiteSpace.lastIndex = this.pos;
var skip = skipWhiteSpace.exec(this.input);
var next = this.pos + skip[0].length, nextCh = this.input.charCodeAt(next);
if (nextCh === 91) {
return true;
}
if (context) {
return false;
}
if (nextCh === 123) {
return true;
}
if (isIdentifierStart(nextCh, true)) {
var pos = next + 1;
while (isIdentifierChar(this.input.charCodeAt(pos), true)) {
++pos;
}
var ident = this.input.slice(next, pos);
if (!keywordRelationalOperator.test(ident)) {
return true;
}
}
return false;
};
pp$1.isAsyncFunction = function() {
if (this.options.ecmaVersion < 8 || !this.isContextual("async")) {
return false;
}
skipWhiteSpace.lastIndex = this.pos;
var skip = skipWhiteSpace.exec(this.input);
var next = this.pos + skip[0].length;
return !lineBreak.test(this.input.slice(this.pos, next)) && this.input.slice(next, next + 8) === "function" && (next + 8 === this.input.length || !isIdentifierChar(this.input.charAt(next + 8)));
};
pp$1.parseStatement = function(context, topLevel, exports3) {
var starttype = this.type, node = this.startNode(), kind;
if (this.isLet(context)) {
starttype = types._var;
kind = "let";
}
switch (starttype) {
case types._break:
case types._continue:
return this.parseBreakContinueStatement(node, starttype.keyword);
case types._debugger:
return this.parseDebuggerStatement(node);
case types._do:
return this.parseDoStatement(node);
case types._for:
return this.parseForStatement(node);
case types._function:
if (context && (this.strict || context !== "if" && context !== "label") && this.options.ecmaVersion >= 6) {
this.unexpected();
}
return this.parseFunctionStatement(node, false, !context);
case types._class:
if (context) {
this.unexpected();
}
return this.parseClass(node, true);
case types._if:
return this.parseIfStatement(node);
case types._return:
return this.parseReturnStatement(node);
case types._switch:
return this.parseSwitchStatement(node);
case types._throw:
return this.parseThrowStatement(node);
case types._try:
return this.parseTryStatement(node);
case types._const:
case types._var:
kind = kind || this.value;
if (context && kind !== "var") {
this.unexpected();
}
return this.parseVarStatement(node, kind);
case types._while:
return this.parseWhileStatement(node);
case types._with:
return this.parseWithStatement(node);
case types.braceL:
return this.parseBlock(true, node);
case types.semi:
return this.parseEmptyStatement(node);
case types._export:
case types._import:
if (this.options.ecmaVersion > 10 && starttype === types._import) {
skipWhiteSpace.lastIndex = this.pos;
var skip = skipWhiteSpace.exec(this.input);
var next = this.pos + skip[0].length, nextCh = this.input.charCodeAt(next);
if (nextCh === 40 || nextCh === 46) {
return this.parseExpressionStatement(node, this.parseExpression());
}
}
if (!this.options.allowImportExportEverywhere) {
if (!topLevel) {
this.raise(this.start, "'import' and 'export' may only appear at the top level");
}
if (!this.inModule) {
this.raise(this.start, "'import' and 'export' may appear only with 'sourceType: module'");
}
}
return starttype === types._import ? this.parseImport(node) : this.parseExport(node, exports3);
default:
if (this.isAsyncFunction()) {
if (context) {
this.unexpected();
}
this.next();
return this.parseFunctionStatement(node, true, !context);
}
var maybeName = this.value, expr = this.parseExpression();
if (starttype === types.name && expr.type === "Identifier" && this.eat(types.colon)) {
return this.parseLabeledStatement(node, maybeName, expr, context);
} else {
return this.parseExpressionStatement(node, expr);
}
}
};
pp$1.parseBreakContinueStatement = function(node, keyword) {
var isBreak = keyword === "break";
this.next();
if (this.eat(types.semi) || this.insertSemicolon()) {
node.label = null;
} else if (this.type !== types.name) {
this.unexpected();
} else {
node.label = this.parseIdent();
this.semicolon();
}
var i = 0;
for (; i < this.labels.length; ++i) {
var lab = this.labels[i];
if (node.label == null || lab.name === node.label.name) {
if (lab.kind != null && (isBreak || lab.kind === "loop")) {
break;
}
if (node.label && isBreak) {
break;
}
}
}
if (i === this.labels.length) {
this.raise(node.start, "Unsyntactic " + keyword);
}
return this.finishNode(node, isBreak ? "BreakStatement" : "ContinueStatement");
};
pp$1.parseDebuggerStatement = function(node) {
this.next();
this.semicolon();
return this.finishNode(node, "DebuggerStatement");
};
pp$1.parseDoStatement = function(node) {
this.next();
this.labels.push(loopLabel);
node.body = this.parseStatement("do");
this.labels.pop();
this.expect(types._while);
node.test = this.parseParenExpression();
if (this.options.ecmaVersion >= 6) {
this.eat(types.semi);
} else {
this.semicolon();
}
return this.finishNode(node, "DoWhileStatement");
};
pp$1.parseForStatement = function(node) {
this.next();
var awaitAt = this.options.ecmaVersion >= 9 && (this.inAsync || !this.inFunction && this.options.allowAwaitOutsideFunction) && this.eatContextual("await") ? this.lastTokStart : -1;
this.labels.push(loopLabel);
this.enterScope(0);
this.expect(types.parenL);
if (this.type === types.semi) {
if (awaitAt > -1) {
this.unexpected(awaitAt);
}
return this.parseFor(node, null);
}
var isLet = this.isLet();
if (this.type === types._var || this.type === types._const || isLet) {
var init$1 = this.startNode(), kind = isLet ? "let" : this.value;
this.next();
this.parseVar(init$1, true, kind);
this.finishNode(init$1, "VariableDeclaration");
if ((this.type === types._in || this.options.ecmaVersion >= 6 && this.isContextual("of")) && init$1.declarations.length === 1) {
if (this.options.ecmaVersion >= 9) {
if (this.type === types._in) {
if (awaitAt > -1) {
this.unexpected(awaitAt);
}
} else {
node.await = awaitAt > -1;
}
}
return this.parseForIn(node, init$1);
}
if (awaitAt > -1) {
this.unexpected(awaitAt);
}
return this.parseFor(node, init$1);
}
var refDestructuringErrors = new DestructuringErrors();
var init = this.parseExpression(true, refDestructuringErrors);
if (this.type === types._in || this.options.ecmaVersion >= 6 && this.isContextual("of")) {
if (this.options.ecmaVersion >= 9) {
if (this.type === types._in) {
if (awaitAt > -1) {
this.unexpected(awaitAt);
}
} else {
node.await = awaitAt > -1;
}
}
this.toAssignable(init, false, refDestructuringErrors);
this.checkLVal(init);
return this.parseForIn(node, init);
} else {
this.checkExpressionErrors(refDestructuringErrors, true);
}
if (awaitAt > -1) {
this.unexpected(awaitAt);
}
return this.parseFor(node, init);
};
pp$1.parseFunctionStatement = function(node, isAsync, declarationPosition) {
this.next();
return this.parseFunction(node, FUNC_STATEMENT | (declarationPosition ? 0 : FUNC_HANGING_STATEMENT), false, isAsync);
};
pp$1.parseIfStatement = function(node) {
this.next();
node.test = this.parseParenExpression();
node.consequent = this.parseStatement("if");
node.alternate = this.eat(types._else) ? this.parseStatement("if") : null;
return this.finishNode(node, "IfStatement");
};
pp$1.parseReturnStatement = function(node) {
if (!this.inFunction && !this.options.allowReturnOutsideFunction) {
this.raise(this.start, "'return' outside of function");
}
this.next();
if (this.eat(types.semi) || this.insertSemicolon()) {
node.argument = null;
} else {
node.argument = this.parseExpression();
this.semicolon();
}
return this.finishNode(node, "ReturnStatement");
};
pp$1.parseSwitchStatement = function(node) {
this.next();
node.discriminant = this.parseParenExpression();
node.cases = [];
this.expect(types.braceL);
this.labels.push(switchLabel);
this.enterScope(0);
var cur;
for (var sawDefault = false; this.type !== types.braceR; ) {
if (this.type === types._case || this.type === types._default) {
var isCase = this.type === types._case;
if (cur) {
this.finishNode(cur, "SwitchCase");
}
node.cases.push(cur = this.startNode());
cur.consequent = [];
this.next();
if (isCase) {
cur.test = this.parseExpression();
} else {
if (sawDefault) {
this.raiseRecoverable(this.lastTokStart, "Multiple default clauses");
}
sawDefault = true;
cur.test = null;
}
this.expect(types.colon);
} else {
if (!cur) {
this.unexpected();
}
cur.consequent.push(this.parseStatement(null));
}
}
this.exitScope();
if (cur) {
this.finishNode(cur, "SwitchCase");
}
this.next();
this.labels.pop();
return this.finishNode(node, "SwitchStatement");
};
pp$1.parseThrowStatement = function(node) {
this.next();
if (lineBreak.test(this.input.slice(this.lastTokEnd, this.start))) {
this.raise(this.lastTokEnd, "Illegal newline after throw");
}
node.argument = this.parseExpression();
this.semicolon();
return this.finishNode(node, "ThrowStatement");
};
var empty = [];
pp$1.parseTryStatement = function(node) {
this.next();
node.block = this.parseBlock();
node.handler = null;
if (this.type === types._catch) {
var clause = this.startNode();
this.next();
if (this.eat(types.parenL)) {
clause.param = this.parseBindingAtom();
var simple = clause.param.type === "Identifier";
this.enterScope(simple ? SCOPE_SIMPLE_CATCH : 0);
this.checkLVal(clause.param, simple ? BIND_SIMPLE_CATCH : BIND_LEXICAL);
this.expect(types.parenR);
} else {
if (this.options.ecmaVersion < 10) {
this.unexpected();
}
clause.param = null;
this.enterScope(0);
}
clause.body = this.parseBlock(false);
this.exitScope();
node.handler = this.finishNode(clause, "CatchClause");
}
node.finalizer = this.eat(types._finally) ? this.parseBlock() : null;
if (!node.handler && !node.finalizer) {
this.raise(node.start, "Missing catch or finally clause");
}
return this.finishNode(node, "TryStatement");
};
pp$1.parseVarStatement = function(node, kind) {
this.next();
this.parseVar(node, false, kind);
this.semicolon();
return this.finishNode(node, "VariableDeclaration");
};
pp$1.parseWhileStatement = function(node) {
this.next();
node.test = this.parseParenExpression();
this.labels.push(loopLabel);
node.body = this.parseStatement("while");
this.labels.pop();
return this.finishNode(node, "WhileStatement");
};
pp$1.parseWithStatement = function(node) {
if (this.strict) {
this.raise(this.start, "'with' in strict mode");
}
this.next();
node.object = this.parseParenExpression();
node.body = this.parseStatement("with");
return this.finishNode(node, "WithStatement");
};
pp$1.parseEmptyStatement = function(node) {
this.next();
return this.finishNode(node, "EmptyStatement");
};
pp$1.parseLabeledStatement = function(node, maybeName, expr, context) {
for (var i$1 = 0, list = this.labels; i$1 < list.length; i$1 += 1) {
var label = list[i$1];
if (label.name === maybeName) {
this.raise(expr.start, "Label '" + maybeName + "' is already declared");
}
}
var kind = this.type.isLoop ? "loop" : this.type === types._switch ? "switch" : null;
for (var i = this.labels.length - 1; i >= 0; i--) {
var label$1 = this.labels[i];
if (label$1.statementStart === node.start) {
label$1.statementStart = this.start;
label$1.kind = kind;
} else {
break;
}
}
this.labels.push({ name: maybeName, kind, statementStart: this.start });
node.body = this.parseStatement(context ? context.indexOf("label") === -1 ? context + "label" : context : "label");
this.labels.pop();
node.label = expr;
return this.finishNode(node, "LabeledStatement");
};
pp$1.parseExpressionStatement = function(node, expr) {
node.expression = expr;
this.semicolon();
return this.finishNode(node, "ExpressionStatement");
};
pp$1.parseBlock = function(createNewLexicalScope, node, exitStrict) {
if (createNewLexicalScope === void 0)
createNewLexicalScope = true;
if (node === void 0)
node = this.startNode();
node.body = [];
this.expect(types.braceL);
if (createNewLexicalScope) {
this.enterScope(0);
}
while (this.type !== types.braceR) {
var stmt = this.parseStatement(null);
node.body.push(stmt);
}
if (exitStrict) {
this.strict = false;
}
this.next();
if (createNewLexicalScope) {
this.exitScope();
}
return this.finishNode(node, "BlockStatement");
};
pp$1.parseFor = function(node, init) {
node.init = init;
this.expect(types.semi);
node.test = this.type === types.semi ? null : this.parseExpression();
this.expect(types.semi);
node.update = this.type === types.parenR ? null : this.parseExpression();
this.expect(types.parenR);
node.body = this.parseStatement("for");
this.exitScope();
this.labels.pop();
return this.finishNode(node, "ForStatement");
};
pp$1.parseForIn = function(node, init) {
var isForIn = this.type === types._in;
this.next();
if (init.type === "VariableDeclaration" && init.declarations[0].init != null && (!isForIn || this.options.ecmaVersion < 8 || this.strict || init.kind !== "var" || init.declarations[0].id.type !== "Identifier")) {
this.raise(
init.start,
(isForIn ? "for-in" : "for-of") + " loop variable declaration may not have an initializer"
);
} else if (init.type === "AssignmentPattern") {
this.raise(init.start, "Invalid left-hand side in for-loop");
}
node.left = init;
node.right = isForIn ? this.parseExpression() : this.parseMaybeAssign();
this.expect(types.parenR);
node.body = this.parseStatement("for");
this.exitScope();
this.labels.pop();
return this.finishNode(node, isForIn ? "ForInStatement" : "ForOfStatement");
};
pp$1.parseVar = function(node, isFor, kind) {
node.declarations = [];
node.kind = kind;
for (; ; ) {
var decl = this.startNode();
this.parseVarId(decl, kind);
if (this.eat(types.eq)) {
decl.init = this.parseMaybeAssign(isFor);
} else if (kind === "const" && !(this.type === types._in || this.options.ecmaVersion >= 6 && this.isContextual("of"))) {
this.unexpected();
} else if (decl.id.type !== "Identifier" && !(isFor && (this.type === types._in || this.isContextual("of")))) {
this.raise(this.lastTokEnd, "Complex binding patterns require an initialization value");
} else {
decl.init = null;
}
node.declarations.push(this.finishNode(decl, "VariableDeclarator"));
if (!this.eat(types.comma)) {
break;
}
}
return node;
};
pp$1.parseVarId = function(decl, kind) {
decl.id = this.parseBindingAtom();
this.checkLVal(decl.id, kind === "var" ? BIND_VAR : BIND_LEXICAL, false);
};
var FUNC_STATEMENT = 1, FUNC_HANGING_STATEMENT = 2, FUNC_NULLABLE_ID = 4;
pp$1.parseFunction = function(node, statement, allowExpressionBody, isAsync) {
this.initFunction(node);
if (this.options.ecmaVersion >= 9 || this.options.ecmaVersion >= 6 && !isAsync) {
if (this.type === types.star && statement & FUNC_HANGING_STATEMENT) {
this.unexpected();
}
node.generator = this.eat(types.star);
}
if (this.options.ecmaVersion >= 8) {
node.async = !!isAsync;
}
if (statement & FUNC_STATEMENT) {
node.id = statement & FUNC_NULLABLE_ID && this.type !== types.name ? null : this.parseIdent();
if (node.id && !(statement & FUNC_HANGING_STATEMENT)) {
this.checkLVal(node.id, this.strict || node.generator || node.async ? this.treatFunctionsAsVar ? BIND_VAR : BIND_LEXICAL : BIND_FUNCTION);
}
}
var oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, oldAwaitIdentPos = this.awaitIdentPos;
this.yieldPos = 0;
this.awaitPos = 0;
this.awaitIdentPos = 0;
this.enterScope(functionFlags(node.async, node.generator));
if (!(statement & FUNC_STATEMENT)) {
node.id = this.type === types.name ? this.parseIdent() : null;
}
this.parseFunctionParams(node);
this.parseFunctionBody(node, allowExpressionBody, false);
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
this.awaitIdentPos = oldAwaitIdentPos;
return this.finishNode(node, statement & FUNC_STATEMENT ? "FunctionDeclaration" : "FunctionExpression");
};
pp$1.parseFunctionParams = function(node) {
this.expect(types.parenL);
node.params = this.parseBindingList(types.parenR, false, this.options.ecmaVersion >= 8);
this.checkYieldAwaitInDefaultParams();
};
pp$1.parseClass = function(node, isStatement) {
this.next();
var oldStrict = this.strict;
this.strict = true;
this.parseClassId(node, isStatement);
this.parseClassSuper(node);
var classBody = this.startNode();
var hadConstructor = false;
classBody.body = [];
this.expect(types.braceL);
while (this.type !== types.braceR) {
var element = this.parseClassElement(node.superClass !== null);
if (element) {
classBody.body.push(element);
if (element.type === "MethodDefinition" && element.kind === "constructor") {
if (hadConstructor) {
this.raise(element.start, "Duplicate constructor in the same class");
}
hadConstructor = true;
}
}
}
this.strict = oldStrict;
this.next();
node.body = this.finishNode(classBody, "ClassBody");
return this.finishNode(node, isStatement ? "ClassDeclaration" : "ClassExpression");
};
pp$1.parseClassElement = function(constructorAllowsSuper) {
var this$1 = this;
if (this.eat(types.semi)) {
return null;
}
var method = this.startNode();
var tryContextual = function(k, noLineBreak) {
if (noLineBreak === void 0)
noLineBreak = false;
var start = this$1.start, startLoc = this$1.startLoc;
if (!this$1.eatContextual(k)) {
return false;
}
if (this$1.type !== types.parenL && (!noLineBreak || !this$1.canInsertSemicolon())) {
return true;
}
if (method.key) {
this$1.unexpected();
}
method.computed = false;
method.key = this$1.startNodeAt(start, startLoc);
method.key.name = k;
this$1.finishNode(method.key, "Identifier");
return false;
};
method.kind = "method";
method.static = tryContextual("static");
var isGenerator = this.eat(types.star);
var isAsync = false;
if (!isGenerator) {
if (this.options.ecmaVersion >= 8 && tryContextual("async", true)) {
isAsync = true;
isGenerator = this.options.ecmaVersion >= 9 && this.eat(types.star);
} else if (tryContextual("get")) {
method.kind = "get";
} else if (tryContextual("set")) {
method.kind = "set";
}
}
if (!method.key) {
this.parsePropertyName(method);
}
var key = method.key;
var allowsDirectSuper = false;
if (!method.computed && !method.static && (key.type === "Identifier" && key.name === "constructor" || key.type === "Literal" && key.value === "constructor")) {
if (method.kind !== "method") {
this.raise(key.start, "Constructor can't have get/set modifier");
}
if (isGenerator) {
this.raise(key.start, "Constructor can't be a generator");
}
if (isAsync) {
this.raise(key.start, "Constructor can't be an async method");
}
method.kind = "constructor";
allowsDirectSuper = constructorAllowsSuper;
} else if (method.static && key.type === "Identifier" && key.name === "prototype") {
this.raise(key.start, "Classes may not have a static property named prototype");
}
this.parseClassMethod(method, isGenerator, isAsync, allowsDirectSuper);
if (method.kind === "get" && method.value.params.length !== 0) {
this.raiseRecoverable(method.value.start, "getter should have no params");
}
if (method.kind === "set" && method.value.params.length !== 1) {
this.raiseRecoverable(method.value.start, "setter should have exactly one param");
}
if (method.kind === "set" && method.value.params[0].type === "RestElement") {
this.raiseRecoverable(method.value.params[0].start, "Setter cannot use rest params");
}
return method;
};
pp$1.parseClassMethod = function(method, isGenerator, isAsync, allowsDirectSuper) {
method.value = this.parseMethod(isGenerator, isAsync, allowsDirectSuper);
return this.finishNode(method, "MethodDefinition");
};
pp$1.parseClassId = function(node, isStatement) {
if (this.type === types.name) {
node.id = this.parseIdent();
if (isStatement) {
this.checkLVal(node.id, BIND_LEXICAL, false);
}
} else {
if (isStatement === true) {
this.unexpected();
}
node.id = null;
}
};
pp$1.parseClassSuper = function(node) {
node.superClass = this.eat(types._extends) ? this.parseExprSubscripts() : null;
};
pp$1.parseExport = function(node, exports3) {
this.next();
if (this.eat(types.star)) {
if (this.options.ecmaVersion >= 11) {
if (this.eatContextual("as")) {
node.exported = this.parseIdent(true);
this.checkExport(exports3, node.exported.name, this.lastTokStart);
} else {
node.exported = null;
}
}
this.expectContextual("from");
if (this.type !== types.string) {
this.unexpected();
}
node.source = this.parseExprAtom();
this.semicolon();
return this.finishNode(node, "ExportAllDeclaration");
}
if (this.eat(types._default)) {
this.checkExport(exports3, "default", this.lastTokStart);
var isAsync;
if (this.type === types._function || (isAsync = this.isAsyncFunction())) {
var fNode = this.startNode();
this.next();
if (isAsync) {
this.next();
}
node.declaration = this.parseFunction(fNode, FUNC_STATEMENT | FUNC_NULLABLE_ID, false, isAsync);
} else if (this.type === types._class) {
var cNode = this.startNode();
node.declaration = this.parseClass(cNode, "nullableID");
} else {
node.declaration = this.parseMaybeAssign();
this.semicolon();
}
return this.finishNode(node, "ExportDefaultDeclaration");
}
if (this.shouldParseExportStatement()) {
node.declaration = this.parseStatement(null);
if (node.declaration.type === "VariableDeclaration") {
this.checkVariableExport(exports3, node.declaration.declarations);
} else {
this.checkExport(exports3, node.declaration.id.name, node.declaration.id.start);
}
node.specifiers = [];
node.source = null;
} else {
node.declaration = null;
node.specifiers = this.parseExportSpecifiers(exports3);
if (this.eatContextual("from")) {
if (this.type !== types.string) {
this.unexpected();
}
node.source = this.parseExprAtom();
} else {
for (var i = 0, list = node.specifiers; i < list.length; i += 1) {
var spec = list[i];
this.checkUnreserved(spec.local);
this.checkLocalExport(spec.local);
}
node.source = null;
}
this.semicolon();
}
return this.finishNode(node, "ExportNamedDeclaration");
};
pp$1.checkExport = function(exports3, name, pos) {
if (!exports3) {
return;
}
if (has(exports3, name)) {
this.raiseRecoverable(pos, "Duplicate export '" + name + "'");
}
exports3[name] = true;
};
pp$1.checkPatternExport = function(exports3, pat) {
var type = pat.type;
if (type === "Identifier") {
this.checkExport(exports3, pat.name, pat.start);
} else if (type === "ObjectPattern") {
for (var i = 0, list = pat.properties; i < list.length; i += 1) {
var prop = list[i];
this.checkPatternExport(exports3, prop);
}
} else if (type === "ArrayPattern") {
for (var i$1 = 0, list$1 = pat.elements; i$1 < list$1.length; i$1 += 1) {
var elt = list$1[i$1];
if (elt) {
this.checkPatternExport(exports3, elt);
}
}
} else if (type === "Property") {
this.checkPatternExport(exports3, pat.value);
} else if (type === "AssignmentPattern") {
this.checkPatternExport(exports3, pat.left);
} else if (type === "RestElement") {
this.checkPatternExport(exports3, pat.argument);
} else if (type === "ParenthesizedExpression") {
this.checkPatternExport(exports3, pat.expression);
}
};
pp$1.checkVariableExport = function(exports3, decls) {
if (!exports3) {
return;
}
for (var i = 0, list = decls; i < list.length; i += 1) {
var decl = list[i];
this.checkPatternExport(exports3, decl.id);
}
};
pp$1.shouldParseExportStatement = function() {
return this.type.keyword === "var" || this.type.keyword === "const" || this.type.keyword === "class" || this.type.keyword === "function" || this.isLet() || this.isAsyncFunction();
};
pp$1.parseExportSpecifiers = function(exports3) {
var nodes = [], first = true;
this.expect(types.braceL);
while (!this.eat(types.braceR)) {
if (!first) {
this.expect(types.comma);
if (this.afterTrailingComma(types.braceR)) {
break;
}
} else {
first = false;
}
var node = this.startNode();
node.local = this.parseIdent(true);
node.exported = this.eatContextual("as") ? this.parseIdent(true) : node.local;
this.checkExport(exports3, node.exported.name, node.exported.start);
nodes.push(this.finishNode(node, "ExportSpecifier"));
}
return nodes;
};
pp$1.parseImport = function(node) {
this.next();
if (this.type === types.string) {
node.specifiers = empty;
node.source = this.parseExprAtom();
} else {
node.specifiers = this.parseImportSpecifiers();
this.expectContextual("from");
node.source = this.type === types.string ? this.parseExprAtom() : this.unexpected();
}
this.semicolon();
return this.finishNode(node, "ImportDeclaration");
};
pp$1.parseImportSpecifiers = function() {
var nodes = [], first = true;
if (this.type === types.name) {
var node = this.startNode();
node.local = this.parseIdent();
this.checkLVal(node.local, BIND_LEXICAL);
nodes.push(this.finishNode(node, "ImportDefaultSpecifier"));
if (!this.eat(types.comma)) {
return nodes;
}
}
if (this.type === types.star) {
var node$1 = this.startNode();
this.next();
this.expectContextual("as");
node$1.local = this.parseIdent();
this.checkLVal(node$1.local, BIND_LEXICAL);
nodes.push(this.finishNode(node$1, "ImportNamespaceSpecifier"));
return nodes;
}
this.expect(types.braceL);
while (!this.eat(types.braceR)) {
if (!first) {
this.expect(types.comma);
if (this.afterTrailingComma(types.braceR)) {
break;
}
} else {
first = false;
}
var node$2 = this.startNode();
node$2.imported = this.parseIdent(true);
if (this.eatContextual("as")) {
node$2.local = this.parseIdent();
} else {
this.checkUnreserved(node$2.imported);
node$2.local = node$2.imported;
}
this.checkLVal(node$2.local, BIND_LEXICAL);
nodes.push(this.finishNode(node$2, "ImportSpecifier"));
}
return nodes;
};
pp$1.adaptDirectivePrologue = function(statements) {
for (var i = 0; i < statements.length && this.isDirectiveCandidate(statements[i]); ++i) {
statements[i].directive = statements[i].expression.raw.slice(1, -1);
}
};
pp$1.isDirectiveCandidate = function(statement) {
return statement.type === "ExpressionStatement" && statement.expression.type === "Literal" && typeof statement.expression.value === "string" && (this.input[statement.start] === '"' || this.input[statement.start] === "'");
};
var pp$2 = Parser.prototype;
pp$2.toAssignable = function(node, isBinding, refDestructuringErrors) {
if (this.options.ecmaVersion >= 6 && node) {
switch (node.type) {
case "Identifier":
if (this.inAsync && node.name === "await") {
this.raise(node.start, "Cannot use 'await' as identifier inside an async function");
}
break;
case "ObjectPattern":
case "ArrayPattern":
case "RestElement":
break;
case "ObjectExpression":
node.type = "ObjectPattern";
if (refDestructuringErrors) {
this.checkPatternErrors(refDestructuringErrors, true);
}
for (var i = 0, list = node.properties; i < list.length; i += 1) {
var prop = list[i];
this.toAssignable(prop, isBinding);
if (prop.type === "RestElement" && (prop.argument.type === "ArrayPattern" || prop.argument.type === "ObjectPattern")) {
this.raise(prop.argument.start, "Unexpected token");
}
}
break;
case "Property":
if (node.kind !== "init") {
this.raise(node.key.start, "Object pattern can't contain getter or setter");
}
this.toAssignable(node.value, isBinding);
break;
case "ArrayExpression":
node.type = "ArrayPattern";
if (refDestructuringErrors) {
this.checkPatternErrors(refDestructuringErrors, true);
}
this.toAssignableList(node.elements, isBinding);
break;
case "SpreadElement":
node.type = "RestElement";
this.toAssignable(node.argument, isBinding);
if (node.argument.type === "AssignmentPattern") {
this.raise(node.argument.start, "Rest elements cannot have a default value");
}
break;
case "AssignmentExpression":
if (node.operator !== "=") {
this.raise(node.left.end, "Only '=' operator can be used for specifying default value.");
}
node.type = "AssignmentPattern";
delete node.operator;
this.toAssignable(node.left, isBinding);
case "AssignmentPattern":
break;
case "ParenthesizedExpression":
this.toAssignable(node.expression, isBinding, refDestructuringErrors);
break;
case "ChainExpression":
this.raiseRecoverable(node.start, "Optional chaining cannot appear in left-hand side");
break;
case "MemberExpression":
if (!isBinding) {
break;
}
default:
this.raise(node.start, "Assigning to rvalue");
}
} else if (refDestructuringErrors) {
this.checkPatternErrors(refDestructuringErrors, true);
}
return node;
};
pp$2.toAssignableList = function(exprList, isBinding) {
var end = exprList.length;
for (var i = 0; i < end; i++) {
var elt = exprList[i];
if (elt) {
this.toAssignable(elt, isBinding);
}
}
if (end) {
var last = exprList[end - 1];
if (this.options.ecmaVersion === 6 && isBinding && last && last.type === "RestElement" && last.argument.type !== "Identifier") {
this.unexpected(last.argument.start);
}
}
return exprList;
};
pp$2.parseSpread = function(refDestructuringErrors) {
var node = this.startNode();
this.next();
node.argument = this.parseMaybeAssign(false, refDestructuringErrors);
return this.finishNode(node, "SpreadElement");
};
pp$2.parseRestBinding = function() {
var node = this.startNode();
this.next();
if (this.options.ecmaVersion === 6 && this.type !== types.name) {
this.unexpected();
}
node.argument = this.parseBindingAtom();
return this.finishNode(node, "RestElement");
};
pp$2.parseBindingAtom = function() {
if (this.options.ecmaVersion >= 6) {
switch (this.type) {
case types.bracketL:
var node = this.startNode();
this.next();
node.elements = this.parseBindingList(types.bracketR, true, true);
return this.finishNode(node, "ArrayPattern");
case types.braceL:
return this.parseObj(true);
}
}
return this.parseIdent();
};
pp$2.parseBindingList = function(close, allowEmpty, allowTrailingComma) {
var elts = [], first = true;
while (!this.eat(close)) {
if (first) {
first = false;
} else {
this.expect(types.comma);
}
if (allowEmpty && this.type === types.comma) {
elts.push(null);
} else if (allowTrailingComma && this.afterTrailingComma(close)) {
break;
} else if (this.type === types.ellipsis) {
var rest = this.parseRestBinding();
this.parseBindingListItem(rest);
elts.push(rest);
if (this.type === types.comma) {
this.raise(this.start, "Comma is not permitted after the rest element");
}
this.expect(close);
break;
} else {
var elem = this.parseMaybeDefault(this.start, this.startLoc);
this.parseBindingListItem(elem);
elts.push(elem);
}
}
return elts;
};
pp$2.parseBindingListItem = function(param) {
return param;
};
pp$2.parseMaybeDefault = function(startPos, startLoc, left) {
left = left || this.parseBindingAtom();
if (this.options.ecmaVersion < 6 || !this.eat(types.eq)) {
return left;
}
var node = this.startNodeAt(startPos, startLoc);
node.left = left;
node.right = this.parseMaybeAssign();
return this.finishNode(node, "AssignmentPattern");
};
pp$2.checkLVal = function(expr, bindingType, checkClashes) {
if (bindingType === void 0)
bindingType = BIND_NONE;
switch (expr.type) {
case "Identifier":
if (bindingType === BIND_LEXICAL && expr.name === "let") {
this.raiseRecoverable(expr.start, "let is disallowed as a lexically bound name");
}
if (this.strict && this.reservedWordsStrictBind.test(expr.name)) {
this.raiseRecoverable(expr.start, (bindingType ? "Binding " : "Assigning to ") + expr.name + " in strict mode");
}
if (checkClashes) {
if (has(checkClashes, expr.name)) {
this.raiseRecoverable(expr.start, "Argument name clash");
}
checkClashes[expr.name] = true;
}
if (bindingType !== BIND_NONE && bindingType !== BIND_OUTSIDE) {
this.declareName(expr.name, bindingType, expr.start);
}
break;
case "ChainExpression":
this.raiseRecoverable(expr.start, "Optional chaining cannot appear in left-hand side");
break;
case "MemberExpression":
if (bindingType) {
this.raiseRecoverable(expr.start, "Binding member expression");
}
break;
case "ObjectPattern":
for (var i = 0, list = expr.properties; i < list.length; i += 1) {
var prop = list[i];
this.checkLVal(prop, bindingType, checkClashes);
}
break;
case "Property":
this.checkLVal(expr.value, bindingType, checkClashes);
break;
case "ArrayPattern":
for (var i$1 = 0, list$1 = expr.elements; i$1 < list$1.length; i$1 += 1) {
var elem = list$1[i$1];
if (elem) {
this.checkLVal(elem, bindingType, checkClashes);
}
}
break;
case "AssignmentPattern":
this.checkLVal(expr.left, bindingType, checkClashes);
break;
case "RestElement":
this.checkLVal(expr.argument, bindingType, checkClashes);
break;
case "ParenthesizedExpression":
this.checkLVal(expr.expression, bindingType, checkClashes);
break;
default:
this.raise(expr.start, (bindingType ? "Binding" : "Assigning to") + " rvalue");
}
};
var pp$3 = Parser.prototype;
pp$3.checkPropClash = function(prop, propHash, refDestructuringErrors) {
if (this.options.ecmaVersion >= 9 && prop.type === "SpreadElement") {
return;
}
if (this.options.ecmaVersion >= 6 && (prop.computed || prop.method || prop.shorthand)) {
return;
}
var key = prop.key;
var name;
switch (key.type) {
case "Identifier":
name = key.name;
break;
case "Literal":
name = String(key.value);
break;
default:
return;
}
var kind = prop.kind;
if (this.options.ecmaVersion >= 6) {
if (name === "__proto__" && kind === "init") {
if (propHash.proto) {
if (refDestructuringErrors) {
if (refDestructuringErrors.doubleProto < 0) {
refDestructuringErrors.doubleProto = key.start;
}
} else {
this.raiseRecoverable(key.start, "Redefinition of __proto__ property");
}
}
propHash.proto = true;
}
return;
}
name = "$" + name;
var other = propHash[name];
if (other) {
var redefinition;
if (kind === "init") {
redefinition = this.strict && other.init || other.get || other.set;
} else {
redefinition = other.init || other[kind];
}
if (redefinition) {
this.raiseRecoverable(key.start, "Redefinition of property");
}
} else {
other = propHash[name] = {
init: false,
get: false,
set: false
};
}
other[kind] = true;
};
pp$3.parseExpression = function(noIn, refDestructuringErrors) {
var startPos = this.start, startLoc = this.startLoc;
var expr = this.parseMaybeAssign(noIn, refDestructuringErrors);
if (this.type === types.comma) {
var node = this.startNodeAt(startPos, startLoc);
node.expressions = [expr];
while (this.eat(types.comma)) {
node.expressions.push(this.parseMaybeAssign(noIn, refDestructuringErrors));
}
return this.finishNode(node, "SequenceExpression");
}
return expr;
};
pp$3.parseMaybeAssign = function(noIn, refDestructuringErrors, afterLeftParse) {
if (this.isContextual("yield")) {
if (this.inGenerator) {
return this.parseYield(noIn);
} else {
this.exprAllowed = false;
}
}
var ownDestructuringErrors = false, oldParenAssign = -1, oldTrailingComma = -1;
if (refDestructuringErrors) {
oldParenAssign = refDestructuringErrors.parenthesizedAssign;
oldTrailingComma = refDestructuringErrors.trailingComma;
refDestructuringErrors.parenthesizedAssign = refDestructuringErrors.trailingComma = -1;
} else {
refDestructuringErrors = new DestructuringErrors();
ownDestructuringErrors = true;
}
var startPos = this.start, startLoc = this.startLoc;
if (this.type === types.parenL || this.type === types.name) {
this.potentialArrowAt = this.start;
}
var left = this.parseMaybeConditional(noIn, refDestructuringErrors);
if (afterLeftParse) {
left = afterLeftParse.call(this, left, startPos, startLoc);
}
if (this.type.isAssign) {
var node = this.startNodeAt(startPos, startLoc);
node.operator = this.value;
node.left = this.type === types.eq ? this.toAssignable(left, false, refDestructuringErrors) : left;
if (!ownDestructuringErrors) {
refDestructuringErrors.parenthesizedAssign = refDestructuringErrors.trailingComma = refDestructuringErrors.doubleProto = -1;
}
if (refDestructuringErrors.shorthandAssign >= node.left.start) {
refDestructuringErrors.shorthandAssign = -1;
}
this.checkLVal(left);
this.next();
node.right = this.parseMaybeAssign(noIn);
return this.finishNode(node, "AssignmentExpression");
} else {
if (ownDestructuringErrors) {
this.checkExpressionErrors(refDestructuringErrors, true);
}
}
if (oldParenAssign > -1) {
refDestructuringErrors.parenthesizedAssign = oldParenAssign;
}
if (oldTrailingComma > -1) {
refDestructuringErrors.trailingComma = oldTrailingComma;
}
return left;
};
pp$3.parseMaybeConditional = function(noIn, refDestructuringErrors) {
var startPos = this.start, startLoc = this.startLoc;
var expr = this.parseExprOps(noIn, refDestructuringErrors);
if (this.checkExpressionErrors(refDestructuringErrors)) {
return expr;
}
if (this.eat(types.question)) {
var node = this.startNodeAt(startPos, startLoc);
node.test = expr;
node.consequent = this.parseMaybeAssign();
this.expect(types.colon);
node.alternate = this.parseMaybeAssign(noIn);
return this.finishNode(node, "ConditionalExpression");
}
return expr;
};
pp$3.parseExprOps = function(noIn, refDestructuringErrors) {
var startPos = this.start, startLoc = this.startLoc;
var expr = this.parseMaybeUnary(refDestructuringErrors, false);
if (this.checkExpressionErrors(refDestructuringErrors)) {
return expr;
}
return expr.start === startPos && expr.type === "ArrowFunctionExpression" ? expr : this.parseExprOp(expr, startPos, startLoc, -1, noIn);
};
pp$3.parseExprOp = function(left, leftStartPos, leftStartLoc, minPrec, noIn) {
var prec = this.type.binop;
if (prec != null && (!noIn || this.type !== types._in)) {
if (prec > minPrec) {
var logical = this.type === types.logicalOR || this.type === types.logicalAND;
var coalesce = this.type === types.coalesce;
if (coalesce) {
prec = types.logicalAND.binop;
}
var op = this.value;
this.next();
var startPos = this.start, startLoc = this.startLoc;
var right = this.parseExprOp(this.parseMaybeUnary(null, false), startPos, startLoc, prec, noIn);
var node = this.buildBinary(leftStartPos, leftStartLoc, left, right, op, logical || coalesce);
if (logical && this.type === types.coalesce || coalesce && (this.type === types.logicalOR || this.type === types.logicalAND)) {
this.raiseRecoverable(this.start, "Logical expressions and coalesce expressions cannot be mixed. Wrap either by parentheses");
}
return this.parseExprOp(node, leftStartPos, leftStartLoc, minPrec, noIn);
}
}
return left;
};
pp$3.buildBinary = function(startPos, startLoc, left, right, op, logical) {
var node = this.startNodeAt(startPos, startLoc);
node.left = left;
node.operator = op;
node.right = right;
return this.finishNode(node, logical ? "LogicalExpression" : "BinaryExpression");
};
pp$3.parseMaybeUnary = function(refDestructuringErrors, sawUnary) {
var startPos = this.start, startLoc = this.startLoc, expr;
if (this.isContextual("await") && (this.inAsync || !this.inFunction && this.options.allowAwaitOutsideFunction)) {
expr = this.parseAwait();
sawUnary = true;
} else if (this.type.prefix) {
var node = this.startNode(), update = this.type === types.incDec;
node.operator = this.value;
node.prefix = true;
this.next();
node.argument = this.parseMaybeUnary(null, true);
this.checkExpressionErrors(refDestructuringErrors, true);
if (update) {
this.checkLVal(node.argument);
} else if (this.strict && node.operator === "delete" && node.argument.type === "Identifier") {
this.raiseRecoverable(node.start, "Deleting local variable in strict mode");
} else {
sawUnary = true;
}
expr = this.finishNode(node, update ? "UpdateExpression" : "UnaryExpression");
} else {
expr = this.parseExprSubscripts(refDestructuringErrors);
if (this.checkExpressionErrors(refDestructuringErrors)) {
return expr;
}
while (this.type.postfix && !this.canInsertSemicolon()) {
var node$1 = this.startNodeAt(startPos, startLoc);
node$1.operator = this.value;
node$1.prefix = false;
node$1.argument = expr;
this.checkLVal(expr);
this.next();
expr = this.finishNode(node$1, "UpdateExpression");
}
}
if (!sawUnary && this.eat(types.starstar)) {
return this.buildBinary(startPos, startLoc, expr, this.parseMaybeUnary(null, false), "**", false);
} else {
return expr;
}
};
pp$3.parseExprSubscripts = function(refDestructuringErrors) {
var startPos = this.start, startLoc = this.startLoc;
var expr = this.parseExprAtom(refDestructuringErrors);
if (expr.type === "ArrowFunctionExpression" && this.input.slice(this.lastTokStart, this.lastTokEnd) !== ")") {
return expr;
}
var result = this.parseSubscripts(expr, startPos, startLoc);
if (refDestructuringErrors && result.type === "MemberExpression") {
if (refDestructuringErrors.parenthesizedAssign >= result.start) {
refDestructuringErrors.parenthesizedAssign = -1;
}
if (refDestructuringErrors.parenthesizedBind >= result.start) {
refDestructuringErrors.parenthesizedBind = -1;
}
}
return result;
};
pp$3.parseSubscripts = function(base, startPos, startLoc, noCalls) {
var maybeAsyncArrow = this.options.ecmaVersion >= 8 && base.type === "Identifier" && base.name === "async" && this.lastTokEnd === base.end && !this.canInsertSemicolon() && base.end - base.start === 5 && this.potentialArrowAt === base.start;
var optionalChained = false;
while (true) {
var element = this.parseSubscript(base, startPos, startLoc, noCalls, maybeAsyncArrow, optionalChained);
if (element.optional) {
optionalChained = true;
}
if (element === base || element.type === "ArrowFunctionExpression") {
if (optionalChained) {
var chainNode = this.startNodeAt(startPos, startLoc);
chainNode.expression = element;
element = this.finishNode(chainNode, "ChainExpression");
}
return element;
}
base = element;
}
};
pp$3.parseSubscript = function(base, startPos, startLoc, noCalls, maybeAsyncArrow, optionalChained) {
var optionalSupported = this.options.ecmaVersion >= 11;
var optional = optionalSupported && this.eat(types.questionDot);
if (noCalls && optional) {
this.raise(this.lastTokStart, "Optional chaining cannot appear in the callee of new expressions");
}
var computed = this.eat(types.bracketL);
if (computed || optional && this.type !== types.parenL && this.type !== types.backQuote || this.eat(types.dot)) {
var node = this.startNodeAt(startPos, startLoc);
node.object = base;
node.property = computed ? this.parseExpression() : this.parseIdent(this.options.allowReserved !== "never");
node.computed = !!computed;
if (computed) {
this.expect(types.bracketR);
}
if (optionalSupported) {
node.optional = optional;
}
base = this.finishNode(node, "MemberExpression");
} else if (!noCalls && this.eat(types.parenL)) {
var refDestructuringErrors = new DestructuringErrors(), oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, oldAwaitIdentPos = this.awaitIdentPos;
this.yieldPos = 0;
this.awaitPos = 0;
this.awaitIdentPos = 0;
var exprList = this.parseExprList(types.parenR, this.options.ecmaVersion >= 8, false, refDestructuringErrors);
if (maybeAsyncArrow && !optional && !this.canInsertSemicolon() && this.eat(types.arrow)) {
this.checkPatternErrors(refDestructuringErrors, false);
this.checkYieldAwaitInDefaultParams();
if (this.awaitIdentPos > 0) {
this.raise(this.awaitIdentPos, "Cannot use 'await' as identifier inside an async function");
}
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
this.awaitIdentPos = oldAwaitIdentPos;
return this.parseArrowExpression(this.startNodeAt(startPos, startLoc), exprList, true);
}
this.checkExpressionErrors(refDestructuringErrors, true);
this.yieldPos = oldYieldPos || this.yieldPos;
this.awaitPos = oldAwaitPos || this.awaitPos;
this.awaitIdentPos = oldAwaitIdentPos || this.awaitIdentPos;
var node$1 = this.startNodeAt(startPos, startLoc);
node$1.callee = base;
node$1.arguments = exprList;
if (optionalSupported) {
node$1.optional = optional;
}
base = this.finishNode(node$1, "CallExpression");
} else if (this.type === types.backQuote) {
if (optional || optionalChained) {
this.raise(this.start, "Optional chaining cannot appear in the tag of tagged template expressions");
}
var node$2 = this.startNodeAt(startPos, startLoc);
node$2.tag = base;
node$2.quasi = this.parseTemplate({ isTagged: true });
base = this.finishNode(node$2, "TaggedTemplateExpression");
}
return base;
};
pp$3.parseExprAtom = function(refDestructuringErrors) {
if (this.type === types.slash) {
this.readRegexp();
}
var node, canBeArrow = this.potentialArrowAt === this.start;
switch (this.type) {
case types._super:
if (!this.allowSuper) {
this.raise(this.start, "'super' keyword outside a method");
}
node = this.startNode();
this.next();
if (this.type === types.parenL && !this.allowDirectSuper) {
this.raise(node.start, "super() call outside constructor of a subclass");
}
if (this.type !== types.dot && this.type !== types.bracketL && this.type !== types.parenL) {
this.unexpected();
}
return this.finishNode(node, "Super");
case types._this:
node = this.startNode();
this.next();
return this.finishNode(node, "ThisExpression");
case types.name:
var startPos = this.start, startLoc = this.startLoc, containsEsc = this.containsEsc;
var id = this.parseIdent(false);
if (this.options.ecmaVersion >= 8 && !containsEsc && id.name === "async" && !this.canInsertSemicolon() && this.eat(types._function)) {
return this.parseFunction(this.startNodeAt(startPos, startLoc), 0, false, true);
}
if (canBeArrow && !this.canInsertSemicolon()) {
if (this.eat(types.arrow)) {
return this.parseArrowExpression(this.startNodeAt(startPos, startLoc), [id], false);
}
if (this.options.ecmaVersion >= 8 && id.name === "async" && this.type === types.name && !containsEsc) {
id = this.parseIdent(false);
if (this.canInsertSemicolon() || !this.eat(types.arrow)) {
this.unexpected();
}
return this.parseArrowExpression(this.startNodeAt(startPos, startLoc), [id], true);
}
}
return id;
case types.regexp:
var value = this.value;
node = this.parseLiteral(value.value);
node.regex = { pattern: value.pattern, flags: value.flags };
return node;
case types.num:
case types.string:
return this.parseLiteral(this.value);
case types._null:
case types._true:
case types._false:
node = this.startNode();
node.value = this.type === types._null ? null : this.type === types._true;
node.raw = this.type.keyword;
this.next();
return this.finishNode(node, "Literal");
case types.parenL:
var start = this.start, expr = this.parseParenAndDistinguishExpression(canBeArrow);
if (refDestructuringErrors) {
if (refDestructuringErrors.parenthesizedAssign < 0 && !this.isSimpleAssignTarget(expr)) {
refDestructuringErrors.parenthesizedAssign = start;
}
if (refDestructuringErrors.parenthesizedBind < 0) {
refDestructuringErrors.parenthesizedBind = start;
}
}
return expr;
case types.bracketL:
node = this.startNode();
this.next();
node.elements = this.parseExprList(types.bracketR, true, true, refDestructuringErrors);
return this.finishNode(node, "ArrayExpression");
case types.braceL:
return this.parseObj(false, refDestructuringErrors);
case types._function:
node = this.startNode();
this.next();
return this.parseFunction(node, 0);
case types._class:
return this.parseClass(this.startNode(), false);
case types._new:
return this.parseNew();
case types.backQuote:
return this.parseTemplate();
case types._import:
if (this.options.ecmaVersion >= 11) {
return this.parseExprImport();
} else {
return this.unexpected();
}
default:
this.unexpected();
}
};
pp$3.parseExprImport = function() {
var node = this.startNode();
if (this.containsEsc) {
this.raiseRecoverable(this.start, "Escape sequence in keyword import");
}
var meta = this.parseIdent(true);
switch (this.type) {
case types.parenL:
return this.parseDynamicImport(node);
case types.dot:
node.meta = meta;
return this.parseImportMeta(node);
default:
this.unexpected();
}
};
pp$3.parseDynamicImport = function(node) {
this.next();
node.source = this.parseMaybeAssign();
if (!this.eat(types.parenR)) {
var errorPos = this.start;
if (this.eat(types.comma) && this.eat(types.parenR)) {
this.raiseRecoverable(errorPos, "Trailing comma is not allowed in import()");
} else {
this.unexpected(errorPos);
}
}
return this.finishNode(node, "ImportExpression");
};
pp$3.parseImportMeta = function(node) {
this.next();
var containsEsc = this.containsEsc;
node.property = this.parseIdent(true);
if (node.property.name !== "meta") {
this.raiseRecoverable(node.property.start, "The only valid meta property for import is 'import.meta'");
}
if (containsEsc) {
this.raiseRecoverable(node.start, "'import.meta' must not contain escaped characters");
}
if (this.options.sourceType !== "module") {
this.raiseRecoverable(node.start, "Cannot use 'import.meta' outside a module");
}
return this.finishNode(node, "MetaProperty");
};
pp$3.parseLiteral = function(value) {
var node = this.startNode();
node.value = value;
node.raw = this.input.slice(this.start, this.end);
if (node.raw.charCodeAt(node.raw.length - 1) === 110) {
node.bigint = node.raw.slice(0, -1).replace(/_/g, "");
}
this.next();
return this.finishNode(node, "Literal");
};
pp$3.parseParenExpression = function() {
this.expect(types.parenL);
var val = this.parseExpression();
this.expect(types.parenR);
return val;
};
pp$3.parseParenAndDistinguishExpression = function(canBeArrow) {
var startPos = this.start, startLoc = this.startLoc, val, allowTrailingComma = this.options.ecmaVersion >= 8;
if (this.options.ecmaVersion >= 6) {
this.next();
var innerStartPos = this.start, innerStartLoc = this.startLoc;
var exprList = [], first = true, lastIsComma = false;
var refDestructuringErrors = new DestructuringErrors(), oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, spreadStart;
this.yieldPos = 0;
this.awaitPos = 0;
while (this.type !== types.parenR) {
first ? first = false : this.expect(types.comma);
if (allowTrailingComma && this.afterTrailingComma(types.parenR, true)) {
lastIsComma = true;
break;
} else if (this.type === types.ellipsis) {
spreadStart = this.start;
exprList.push(this.parseParenItem(this.parseRestBinding()));
if (this.type === types.comma) {
this.raise(this.start, "Comma is not permitted after the rest element");
}
break;
} else {
exprList.push(this.parseMaybeAssign(false, refDestructuringErrors, this.parseParenItem));
}
}
var innerEndPos = this.start, innerEndLoc = this.startLoc;
this.expect(types.parenR);
if (canBeArrow && !this.canInsertSemicolon() && this.eat(types.arrow)) {
this.checkPatternErrors(refDestructuringErrors, false);
this.checkYieldAwaitInDefaultParams();
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
return this.parseParenArrowList(startPos, startLoc, exprList);
}
if (!exprList.length || lastIsComma) {
this.unexpected(this.lastTokStart);
}
if (spreadStart) {
this.unexpected(spreadStart);
}
this.checkExpressionErrors(refDestructuringErrors, true);
this.yieldPos = oldYieldPos || this.yieldPos;
this.awaitPos = oldAwaitPos || this.awaitPos;
if (exprList.length > 1) {
val = this.startNodeAt(innerStartPos, innerStartLoc);
val.expressions = exprList;
this.finishNodeAt(val, "SequenceExpression", innerEndPos, innerEndLoc);
} else {
val = exprList[0];
}
} else {
val = this.parseParenExpression();
}
if (this.options.preserveParens) {
var par = this.startNodeAt(startPos, startLoc);
par.expression = val;
return this.finishNode(par, "ParenthesizedExpression");
} else {
return val;
}
};
pp$3.parseParenItem = function(item) {
return item;
};
pp$3.parseParenArrowList = function(startPos, startLoc, exprList) {
return this.parseArrowExpression(this.startNodeAt(startPos, startLoc), exprList);
};
var empty$1 = [];
pp$3.parseNew = function() {
if (this.containsEsc) {
this.raiseRecoverable(this.start, "Escape sequence in keyword new");
}
var node = this.startNode();
var meta = this.parseIdent(true);
if (this.options.ecmaVersion >= 6 && this.eat(types.dot)) {
node.meta = meta;
var containsEsc = this.containsEsc;
node.property = this.parseIdent(true);
if (node.property.name !== "target") {
this.raiseRecoverable(node.property.start, "The only valid meta property for new is 'new.target'");
}
if (containsEsc) {
this.raiseRecoverable(node.start, "'new.target' must not contain escaped characters");
}
if (!this.inNonArrowFunction()) {
this.raiseRecoverable(node.start, "'new.target' can only be used in functions");
}
return this.finishNode(node, "MetaProperty");
}
var startPos = this.start, startLoc = this.startLoc, isImport = this.type === types._import;
node.callee = this.parseSubscripts(this.parseExprAtom(), startPos, startLoc, true);
if (isImport && node.callee.type === "ImportExpression") {
this.raise(startPos, "Cannot use new with import()");
}
if (this.eat(types.parenL)) {
node.arguments = this.parseExprList(types.parenR, this.options.ecmaVersion >= 8, false);
} else {
node.arguments = empty$1;
}
return this.finishNode(node, "NewExpression");
};
pp$3.parseTemplateElement = function(ref2) {
var isTagged = ref2.isTagged;
var elem = this.startNode();
if (this.type === types.invalidTemplate) {
if (!isTagged) {
this.raiseRecoverable(this.start, "Bad escape sequence in untagged template literal");
}
elem.value = {
raw: this.value,
cooked: null
};
} else {
elem.value = {
raw: this.input.slice(this.start, this.end).replace(/\r\n?/g, "\n"),
cooked: this.value
};
}
this.next();
elem.tail = this.type === types.backQuote;
return this.finishNode(elem, "TemplateElement");
};
pp$3.parseTemplate = function(ref2) {
if (ref2 === void 0)
ref2 = {};
var isTagged = ref2.isTagged;
if (isTagged === void 0)
isTagged = false;
var node = this.startNode();
this.next();
node.expressions = [];
var curElt = this.parseTemplateElement({ isTagged });
node.quasis = [curElt];
while (!curElt.tail) {
if (this.type === types.eof) {
this.raise(this.pos, "Unterminated template literal");
}
this.expect(types.dollarBraceL);
node.expressions.push(this.parseExpression());
this.expect(types.braceR);
node.quasis.push(curElt = this.parseTemplateElement({ isTagged }));
}
this.next();
return this.finishNode(node, "TemplateLiteral");
};
pp$3.isAsyncProp = function(prop) {
return !prop.computed && prop.key.type === "Identifier" && prop.key.name === "async" && (this.type === types.name || this.type === types.num || this.type === types.string || this.type === types.bracketL || this.type.keyword || this.options.ecmaVersion >= 9 && this.type === types.star) && !lineBreak.test(this.input.slice(this.lastTokEnd, this.start));
};
pp$3.parseObj = function(isPattern, refDestructuringErrors) {
var node = this.startNode(), first = true, propHash = {};
node.properties = [];
this.next();
while (!this.eat(types.braceR)) {
if (!first) {
this.expect(types.comma);
if (this.options.ecmaVersion >= 5 && this.afterTrailingComma(types.braceR)) {
break;
}
} else {
first = false;
}
var prop = this.parseProperty(isPattern, refDestructuringErrors);
if (!isPattern) {
this.checkPropClash(prop, propHash, refDestructuringErrors);
}
node.properties.push(prop);
}
return this.finishNode(node, isPattern ? "ObjectPattern" : "ObjectExpression");
};
pp$3.parseProperty = function(isPattern, refDestructuringErrors) {
var prop = this.startNode(), isGenerator, isAsync, startPos, startLoc;
if (this.options.ecmaVersion >= 9 && this.eat(types.ellipsis)) {
if (isPattern) {
prop.argument = this.parseIdent(false);
if (this.type === types.comma) {
this.raise(this.start, "Comma is not permitted after the rest element");
}
return this.finishNode(prop, "RestElement");
}
if (this.type === types.parenL && refDestructuringErrors) {
if (refDestructuringErrors.parenthesizedAssign < 0) {
refDestructuringErrors.parenthesizedAssign = this.start;
}
if (refDestructuringErrors.parenthesizedBind < 0) {
refDestructuringErrors.parenthesizedBind = this.start;
}
}
prop.argument = this.parseMaybeAssign(false, refDestructuringErrors);
if (this.type === types.comma && refDestructuringErrors && refDestructuringErrors.trailingComma < 0) {
refDestructuringErrors.trailingComma = this.start;
}
return this.finishNode(prop, "SpreadElement");
}
if (this.options.ecmaVersion >= 6) {
prop.method = false;
prop.shorthand = false;
if (isPattern || refDestructuringErrors) {
startPos = this.start;
startLoc = this.startLoc;
}
if (!isPattern) {
isGenerator = this.eat(types.star);
}
}
var containsEsc = this.containsEsc;
this.parsePropertyName(prop);
if (!isPattern && !containsEsc && this.options.ecmaVersion >= 8 && !isGenerator && this.isAsyncProp(prop)) {
isAsync = true;
isGenerator = this.options.ecmaVersion >= 9 && this.eat(types.star);
this.parsePropertyName(prop, refDestructuringErrors);
} else {
isAsync = false;
}
this.parsePropertyValue(prop, isPattern, isGenerator, isAsync, startPos, startLoc, refDestructuringErrors, containsEsc);
return this.finishNode(prop, "Property");
};
pp$3.parsePropertyValue = function(prop, isPattern, isGenerator, isAsync, startPos, startLoc, refDestructuringErrors, containsEsc) {
if ((isGenerator || isAsync) && this.type === types.colon) {
this.unexpected();
}
if (this.eat(types.colon)) {
prop.value = isPattern ? this.parseMaybeDefault(this.start, this.startLoc) : this.parseMaybeAssign(false, refDestructuringErrors);
prop.kind = "init";
} else if (this.options.ecmaVersion >= 6 && this.type === types.parenL) {
if (isPattern) {
this.unexpected();
}
prop.kind = "init";
prop.method = true;
prop.value = this.parseMethod(isGenerator, isAsync);
} else if (!isPattern && !containsEsc && this.options.ecmaVersion >= 5 && !prop.computed && prop.key.type === "Identifier" && (prop.key.name === "get" || prop.key.name === "set") && (this.type !== types.comma && this.type !== types.braceR && this.type !== types.eq)) {
if (isGenerator || isAsync) {
this.unexpected();
}
prop.kind = prop.key.name;
this.parsePropertyName(prop);
prop.value = this.parseMethod(false);
var paramCount = prop.kind === "get" ? 0 : 1;
if (prop.value.params.length !== paramCount) {
var start = prop.value.start;
if (prop.kind === "get") {
this.raiseRecoverable(start, "getter should have no params");
} else {
this.raiseRecoverable(start, "setter should have exactly one param");
}
} else {
if (prop.kind === "set" && prop.value.params[0].type === "RestElement") {
this.raiseRecoverable(prop.value.params[0].start, "Setter cannot use rest params");
}
}
} else if (this.options.ecmaVersion >= 6 && !prop.computed && prop.key.type === "Identifier") {
if (isGenerator || isAsync) {
this.unexpected();
}
this.checkUnreserved(prop.key);
if (prop.key.name === "await" && !this.awaitIdentPos) {
this.awaitIdentPos = startPos;
}
prop.kind = "init";
if (isPattern) {
prop.value = this.parseMaybeDefault(startPos, startLoc, prop.key);
} else if (this.type === types.eq && refDestructuringErrors) {
if (refDestructuringErrors.shorthandAssign < 0) {
refDestructuringErrors.shorthandAssign = this.start;
}
prop.value = this.parseMaybeDefault(startPos, startLoc, prop.key);
} else {
prop.value = prop.key;
}
prop.shorthand = true;
} else {
this.unexpected();
}
};
pp$3.parsePropertyName = function(prop) {
if (this.options.ecmaVersion >= 6) {
if (this.eat(types.bracketL)) {
prop.computed = true;
prop.key = this.parseMaybeAssign();
this.expect(types.bracketR);
return prop.key;
} else {
prop.computed = false;
}
}
return prop.key = this.type === types.num || this.type === types.string ? this.parseExprAtom() : this.parseIdent(this.options.allowReserved !== "never");
};
pp$3.initFunction = function(node) {
node.id = null;
if (this.options.ecmaVersion >= 6) {
node.generator = node.expression = false;
}
if (this.options.ecmaVersion >= 8) {
node.async = false;
}
};
pp$3.parseMethod = function(isGenerator, isAsync, allowDirectSuper) {
var node = this.startNode(), oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, oldAwaitIdentPos = this.awaitIdentPos;
this.initFunction(node);
if (this.options.ecmaVersion >= 6) {
node.generator = isGenerator;
}
if (this.options.ecmaVersion >= 8) {
node.async = !!isAsync;
}
this.yieldPos = 0;
this.awaitPos = 0;
this.awaitIdentPos = 0;
this.enterScope(functionFlags(isAsync, node.generator) | SCOPE_SUPER | (allowDirectSuper ? SCOPE_DIRECT_SUPER : 0));
this.expect(types.parenL);
node.params = this.parseBindingList(types.parenR, false, this.options.ecmaVersion >= 8);
this.checkYieldAwaitInDefaultParams();
this.parseFunctionBody(node, false, true);
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
this.awaitIdentPos = oldAwaitIdentPos;
return this.finishNode(node, "FunctionExpression");
};
pp$3.parseArrowExpression = function(node, params, isAsync) {
var oldYieldPos = this.yieldPos, oldAwaitPos = this.awaitPos, oldAwaitIdentPos = this.awaitIdentPos;
this.enterScope(functionFlags(isAsync, false) | SCOPE_ARROW);
this.initFunction(node);
if (this.options.ecmaVersion >= 8) {
node.async = !!isAsync;
}
this.yieldPos = 0;
this.awaitPos = 0;
this.awaitIdentPos = 0;
node.params = this.toAssignableList(params, true);
this.parseFunctionBody(node, true, false);
this.yieldPos = oldYieldPos;
this.awaitPos = oldAwaitPos;
this.awaitIdentPos = oldAwaitIdentPos;
return this.finishNode(node, "ArrowFunctionExpression");
};
pp$3.parseFunctionBody = function(node, isArrowFunction, isMethod) {
var isExpression = isArrowFunction && this.type !== types.braceL;
var oldStrict = this.strict, useStrict = false;
if (isExpression) {
node.body = this.parseMaybeAssign();
node.expression = true;
this.checkParams(node, false);
} else {
var nonSimple = this.options.ecmaVersion >= 7 && !this.isSimpleParamList(node.params);
if (!oldStrict || nonSimple) {
useStrict = this.strictDirective(this.end);
if (useStrict && nonSimple) {
this.raiseRecoverable(node.start, "Illegal 'use strict' directive in function with non-simple parameter list");
}
}
var oldLabels = this.labels;
this.labels = [];
if (useStrict) {
this.strict = true;
}
this.checkParams(node, !oldStrict && !useStrict && !isArrowFunction && !isMethod && this.isSimpleParamList(node.params));
if (this.strict && node.id) {
this.checkLVal(node.id, BIND_OUTSIDE);
}
node.body = this.parseBlock(false, void 0, useStrict && !oldStrict);
node.expression = false;
this.adaptDirectivePrologue(node.body.body);
this.labels = oldLabels;
}
this.exitScope();
};
pp$3.isSimpleParamList = function(params) {
for (var i = 0, list = params; i < list.length; i += 1) {
var param = list[i];
if (param.type !== "Identifier") {
return false;
}
}
return true;
};
pp$3.checkParams = function(node, allowDuplicates) {
var nameHash = {};
for (var i = 0, list = node.params; i < list.length; i += 1) {
var param = list[i];
this.checkLVal(param, BIND_VAR, allowDuplicates ? null : nameHash);
}
};
pp$3.parseExprList = function(close, allowTrailingComma, allowEmpty, refDestructuringErrors) {
var elts = [], first = true;
while (!this.eat(close)) {
if (!first) {
this.expect(types.comma);
if (allowTrailingComma && this.afterTrailingComma(close)) {
break;
}
} else {
first = false;
}
var elt = void 0;
if (allowEmpty && this.type === types.comma) {
elt = null;
} else if (this.type === types.ellipsis) {
elt = this.parseSpread(refDestructuringErrors);
if (refDestructuringErrors && this.type === types.comma && refDestructuringErrors.trailingComma < 0) {
refDestructuringErrors.trailingComma = this.start;
}
} else {
elt = this.parseMaybeAssign(false, refDestructuringErrors);
}
elts.push(elt);
}
return elts;
};
pp$3.checkUnreserved = function(ref2) {
var start = ref2.start;
var end = ref2.end;
var name = ref2.name;
if (this.inGenerator && name === "yield") {
this.raiseRecoverable(start, "Cannot use 'yield' as identifier inside a generator");
}
if (this.inAsync && name === "await") {
this.raiseRecoverable(start, "Cannot use 'await' as identifier inside an async function");
}
if (this.keywords.test(name)) {
this.raise(start, "Unexpected keyword '" + name + "'");
}
if (this.options.ecmaVersion < 6 && this.input.slice(start, end).indexOf("\\") !== -1) {
return;
}
var re = this.strict ? this.reservedWordsStrict : this.reservedWords;
if (re.test(name)) {
if (!this.inAsync && name === "await") {
this.raiseRecoverable(start, "Cannot use keyword 'await' outside an async function");
}
this.raiseRecoverable(start, "The keyword '" + name + "' is reserved");
}
};
pp$3.parseIdent = function(liberal, isBinding) {
var node = this.startNode();
if (this.type === types.name) {
node.name = this.value;
} else if (this.type.keyword) {
node.name = this.type.keyword;
if ((node.name === "class" || node.name === "function") && (this.lastTokEnd !== this.lastTokStart + 1 || this.input.charCodeAt(this.lastTokStart) !== 46)) {
this.context.pop();
}
} else {
this.unexpected();
}
this.next(!!liberal);
this.finishNode(node, "Identifier");
if (!liberal) {
this.checkUnreserved(node);
if (node.name === "await" && !this.awaitIdentPos) {
this.awaitIdentPos = node.start;
}
}
return node;
};
pp$3.parseYield = function(noIn) {
if (!this.yieldPos) {
this.yieldPos = this.start;
}
var node = this.startNode();
this.next();
if (this.type === types.semi || this.canInsertSemicolon() || this.type !== types.star && !this.type.startsExpr) {
node.delegate = false;
node.argument = null;
} else {
node.delegate = this.eat(types.star);
node.argument = this.parseMaybeAssign(noIn);
}
return this.finishNode(node, "YieldExpression");
};
pp$3.parseAwait = function() {
if (!this.awaitPos) {
this.awaitPos = this.start;
}
var node = this.startNode();
this.next();
node.argument = this.parseMaybeUnary(null, false);
return this.finishNode(node, "AwaitExpression");
};
var pp$4 = Parser.prototype;
pp$4.raise = function(pos, message) {
var loc = getLineInfo2(this.input, pos);
message += " (" + loc.line + ":" + loc.column + ")";
var err = new SyntaxError(message);
err.pos = pos;
err.loc = loc;
err.raisedAt = this.pos;
throw err;
};
pp$4.raiseRecoverable = pp$4.raise;
pp$4.curPosition = function() {
if (this.options.locations) {
return new Position(this.curLine, this.pos - this.lineStart);
}
};
var pp$5 = Parser.prototype;
var Scope = function Scope2(flags) {
this.flags = flags;
this.var = [];
this.lexical = [];
this.functions = [];
};
pp$5.enterScope = function(flags) {
this.scopeStack.push(new Scope(flags));
};
pp$5.exitScope = function() {
this.scopeStack.pop();
};
pp$5.treatFunctionsAsVarInScope = function(scope) {
return scope.flags & SCOPE_FUNCTION || !this.inModule && scope.flags & SCOPE_TOP;
};
pp$5.declareName = function(name, bindingType, pos) {
var redeclared = false;
if (bindingType === BIND_LEXICAL) {
var scope = this.currentScope();
redeclared = scope.lexical.indexOf(name) > -1 || scope.functions.indexOf(name) > -1 || scope.var.indexOf(name) > -1;
scope.lexical.push(name);
if (this.inModule && scope.flags & SCOPE_TOP) {
delete this.undefinedExports[name];
}
} else if (bindingType === BIND_SIMPLE_CATCH) {
var scope$1 = this.currentScope();
scope$1.lexical.push(name);
} else if (bindingType === BIND_FUNCTION) {
var scope$2 = this.currentScope();
if (this.treatFunctionsAsVar) {
redeclared = scope$2.lexical.indexOf(name) > -1;
} else {
redeclared = scope$2.lexical.indexOf(name) > -1 || scope$2.var.indexOf(name) > -1;
}
scope$2.functions.push(name);
} else {
for (var i = this.scopeStack.length - 1; i >= 0; --i) {
var scope$3 = this.scopeStack[i];
if (scope$3.lexical.indexOf(name) > -1 && !(scope$3.flags & SCOPE_SIMPLE_CATCH && scope$3.lexical[0] === name) || !this.treatFunctionsAsVarInScope(scope$3) && scope$3.functions.indexOf(name) > -1) {
redeclared = true;
break;
}
scope$3.var.push(name);
if (this.inModule && scope$3.flags & SCOPE_TOP) {
delete this.undefinedExports[name];
}
if (scope$3.flags & SCOPE_VAR) {
break;
}
}
}
if (redeclared) {
this.raiseRecoverable(pos, "Identifier '" + name + "' has already been declared");
}
};
pp$5.checkLocalExport = function(id) {
if (this.scopeStack[0].lexical.indexOf(id.name) === -1 && this.scopeStack[0].var.indexOf(id.name) === -1) {
this.undefinedExports[id.name] = id;
}
};
pp$5.currentScope = function() {
return this.scopeStack[this.scopeStack.length - 1];
};
pp$5.currentVarScope = function() {
for (var i = this.scopeStack.length - 1; ; i--) {
var scope = this.scopeStack[i];
if (scope.flags & SCOPE_VAR) {
return scope;
}
}
};
pp$5.currentThisScope = function() {
for (var i = this.scopeStack.length - 1; ; i--) {
var scope = this.scopeStack[i];
if (scope.flags & SCOPE_VAR && !(scope.flags & SCOPE_ARROW)) {
return scope;
}
}
};
var Node = function Node2(parser, pos, loc) {
this.type = "";
this.start = pos;
this.end = 0;
if (parser.options.locations) {
this.loc = new SourceLocation(parser, loc);
}
if (parser.options.directSourceFile) {
this.sourceFile = parser.options.directSourceFile;
}
if (parser.options.ranges) {
this.range = [pos, 0];
}
};
var pp$6 = Parser.prototype;
pp$6.startNode = function() {
return new Node(this, this.start, this.startLoc);
};
pp$6.startNodeAt = function(pos, loc) {
return new Node(this, pos, loc);
};
function finishNodeAt(node, type, pos, loc) {
node.type = type;
node.end = pos;
if (this.options.locations) {
node.loc.end = loc;
}
if (this.options.ranges) {
node.range[1] = pos;
}
return node;
}
pp$6.finishNode = function(node, type) {
return finishNodeAt.call(this, node, type, this.lastTokEnd, this.lastTokEndLoc);
};
pp$6.finishNodeAt = function(node, type, pos, loc) {
return finishNodeAt.call(this, node, type, pos, loc);
};
var TokContext = function TokContext2(token, isExpr, preserveSpace, override, generator) {
this.token = token;
this.isExpr = !!isExpr;
this.preserveSpace = !!preserveSpace;
this.override = override;
this.generator = !!generator;
};
var types$1 = {
b_stat: new TokContext("{", false),
b_expr: new TokContext("{", true),
b_tmpl: new TokContext("${", false),
p_stat: new TokContext("(", false),
p_expr: new TokContext("(", true),
q_tmpl: new TokContext("`", true, true, function(p) {
return p.tryReadTemplateToken();
}),
f_stat: new TokContext("function", false),
f_expr: new TokContext("function", true),
f_expr_gen: new TokContext("function", true, false, null, true),
f_gen: new TokContext("function", false, false, null, true)
};
var pp$7 = Parser.prototype;
pp$7.initialContext = function() {
return [types$1.b_stat];
};
pp$7.braceIsBlock = function(prevType) {
var parent = this.curContext();
if (parent === types$1.f_expr || parent === types$1.f_stat) {
return true;
}
if (prevType === types.colon && (parent === types$1.b_stat || parent === types$1.b_expr)) {
return !parent.isExpr;
}
if (prevType === types._return || prevType === types.name && this.exprAllowed) {
return lineBreak.test(this.input.slice(this.lastTokEnd, this.start));
}
if (prevType === types._else || prevType === types.semi || prevType === types.eof || prevType === types.parenR || prevType === types.arrow) {
return true;
}
if (prevType === types.braceL) {
return parent === types$1.b_stat;
}
if (prevType === types._var || prevType === types._const || prevType === types.name) {
return false;
}
return !this.exprAllowed;
};
pp$7.inGeneratorContext = function() {
for (var i = this.context.length - 1; i >= 1; i--) {
var context = this.context[i];
if (context.token === "function") {
return context.generator;
}
}
return false;
};
pp$7.updateContext = function(prevType) {
var update, type = this.type;
if (type.keyword && prevType === types.dot) {
this.exprAllowed = false;
} else if (update = type.updateContext) {
update.call(this, prevType);
} else {
this.exprAllowed = type.beforeExpr;
}
};
types.parenR.updateContext = types.braceR.updateContext = function() {
if (this.context.length === 1) {
this.exprAllowed = true;
return;
}
var out = this.context.pop();
if (out === types$1.b_stat && this.curContext().token === "function") {
out = this.context.pop();
}
this.exprAllowed = !out.isExpr;
};
types.braceL.updateContext = function(prevType) {
this.context.push(this.braceIsBlock(prevType) ? types$1.b_stat : types$1.b_expr);
this.exprAllowed = true;
};
types.dollarBraceL.updateContext = function() {
this.context.push(types$1.b_tmpl);
this.exprAllowed = true;
};
types.parenL.updateContext = function(prevType) {
var statementParens = prevType === types._if || prevType === types._for || prevType === types._with || prevType === types._while;
this.context.push(statementParens ? types$1.p_stat : types$1.p_expr);
this.exprAllowed = true;
};
types.incDec.updateContext = function() {
};
types._function.updateContext = types._class.updateContext = function(prevType) {
if (prevType.beforeExpr && prevType !== types.semi && prevType !== types._else && !(prevType === types._return && lineBreak.test(this.input.slice(this.lastTokEnd, this.start))) && !((prevType === types.colon || prevType === types.braceL) && this.curContext() === types$1.b_stat)) {
this.context.push(types$1.f_expr);
} else {
this.context.push(types$1.f_stat);
}
this.exprAllowed = false;
};
types.backQuote.updateContext = function() {
if (this.curContext() === types$1.q_tmpl) {
this.context.pop();
} else {
this.context.push(types$1.q_tmpl);
}
this.exprAllowed = false;
};
types.star.updateContext = function(prevType) {
if (prevType === types._function) {
var index = this.context.length - 1;
if (this.context[index] === types$1.f_expr) {
this.context[index] = types$1.f_expr_gen;
} else {
this.context[index] = types$1.f_gen;
}
}
this.exprAllowed = true;
};
types.name.updateContext = function(prevType) {
var allowed = false;
if (this.options.ecmaVersion >= 6 && prevType !== types.dot) {
if (this.value === "of" && !this.exprAllowed || this.value === "yield" && this.inGeneratorContext()) {
allowed = true;
}
}
this.exprAllowed = allowed;
};
var ecma9BinaryProperties = "ASCII ASCII_Hex_Digit AHex Alphabetic Alpha Any Assigned Bidi_Control Bidi_C Bidi_Mirrored Bidi_M Case_Ignorable CI Cased Changes_When_Casefolded CWCF Changes_When_Casemapped CWCM Changes_When_Lowercased CWL Changes_When_NFKC_Casefolded CWKCF Changes_When_Titlecased CWT Changes_When_Uppercased CWU Dash Default_Ignorable_Code_Point DI Deprecated Dep Diacritic Dia Emoji Emoji_Component Emoji_Modifier Emoji_Modifier_Base Emoji_Presentation Extender Ext Grapheme_Base Gr_Base Grapheme_Extend Gr_Ext Hex_Digit Hex IDS_Binary_Operator IDSB IDS_Trinary_Operator IDST ID_Continue IDC ID_Start IDS Ideographic Ideo Join_Control Join_C Logical_Order_Exception LOE Lowercase Lower Math Noncharacter_Code_Point NChar Pattern_Syntax Pat_Syn Pattern_White_Space Pat_WS Quotation_Mark QMark Radical Regional_Indicator RI Sentence_Terminal STerm Soft_Dotted SD Terminal_Punctuation Term Unified_Ideograph UIdeo Uppercase Upper Variation_Selector VS White_Space space XID_Continue XIDC XID_Start XIDS";
var ecma10BinaryProperties = ecma9BinaryProperties + " Extended_Pictographic";
var ecma11BinaryProperties = ecma10BinaryProperties;
var unicodeBinaryProperties = {
9: ecma9BinaryProperties,
10: ecma10BinaryProperties,
11: ecma11BinaryProperties
};
var unicodeGeneralCategoryValues = "Cased_Letter LC Close_Punctuation Pe Connector_Punctuation Pc Control Cc cntrl Currency_Symbol Sc Dash_Punctuation Pd Decimal_Number Nd digit Enclosing_Mark Me Final_Punctuation Pf Format Cf Initial_Punctuation Pi Letter L Letter_Number Nl Line_Separator Zl Lowercase_Letter Ll Mark M Combining_Mark Math_Symbol Sm Modifier_Letter Lm Modifier_Symbol Sk Nonspacing_Mark Mn Number N Open_Punctuation Ps Other C Other_Letter Lo Other_Number No Other_Punctuation Po Other_Symbol So Paragraph_Separator Zp Private_Use Co Punctuation P punct Separator Z Space_Separator Zs Spacing_Mark Mc Surrogate Cs Symbol S Titlecase_Letter Lt Unassigned Cn Uppercase_Letter Lu";
var ecma9ScriptValues = "Adlam Adlm Ahom Ahom Anatolian_Hieroglyphs Hluw Arabic Arab Armenian Armn Avestan Avst Balinese Bali Bamum Bamu Bassa_Vah Bass Batak Batk Bengali Beng Bhaiksuki Bhks Bopomofo Bopo Brahmi Brah Braille Brai Buginese Bugi Buhid Buhd Canadian_Aboriginal Cans Carian Cari Caucasian_Albanian Aghb Chakma Cakm Cham Cham Cherokee Cher Common Zyyy Coptic Copt Qaac Cuneiform Xsux Cypriot Cprt Cyrillic Cyrl Deseret Dsrt Devanagari Deva Duployan Dupl Egyptian_Hieroglyphs Egyp Elbasan Elba Ethiopic Ethi Georgian Geor Glagolitic Glag Gothic Goth Grantha Gran Greek Grek Gujarati Gujr Gurmukhi Guru Han Hani Hangul Hang Hanunoo Hano Hatran Hatr Hebrew Hebr Hiragana Hira Imperial_Aramaic Armi Inherited Zinh Qaai Inscriptional_Pahlavi Phli Inscriptional_Parthian Prti Javanese Java Kaithi Kthi Kannada Knda Katakana Kana Kayah_Li Kali Kharoshthi Khar Khmer Khmr Khojki Khoj Khudawadi Sind Lao Laoo Latin Latn Lepcha Lepc Limbu Limb Linear_A Lina Linear_B Linb Lisu Lisu Lycian Lyci Lydian Lydi Mahajani Mahj Malayalam Mlym Mandaic Mand Manichaean Mani Marchen Marc Masaram_Gondi Gonm Meetei_Mayek Mtei Mende_Kikakui Mend Meroitic_Cursive Merc Meroitic_Hieroglyphs Mero Miao Plrd Modi Modi Mongolian Mong Mro Mroo Multani Mult Myanmar Mymr Nabataean Nbat New_Tai_Lue Talu Newa Newa Nko Nkoo Nushu Nshu Ogham Ogam Ol_Chiki Olck Old_Hungarian Hung Old_Italic Ital Old_North_Arabian Narb Old_Permic Perm Old_Persian Xpeo Old_South_Arabian Sarb Old_Turkic Orkh Oriya Orya Osage Osge Osmanya Osma Pahawh_Hmong Hmng Palmyrene Palm Pau_Cin_Hau Pauc Phags_Pa Phag Phoenician Phnx Psalter_Pahlavi Phlp Rejang Rjng Runic Runr Samaritan Samr Saurashtra Saur Sharada Shrd Shavian Shaw Siddham Sidd SignWriting Sgnw Sinhala Sinh Sora_Sompeng Sora Soyombo Soyo Sundanese Sund Syloti_Nagri Sylo Syriac Syrc Tagalog Tglg Tagbanwa Tagb Tai_Le Tale Tai_Tham Lana Tai_Viet Tavt Takri Takr Tamil Taml Tangut Tang Telugu Telu Thaana Thaa Thai Thai Tibetan Tibt Tifinagh Tfng Tirhuta Tirh Ugaritic Ugar Vai Vaii Warang_Citi Wara Yi Yiii Zanabazar_Square Zanb";
var ecma10ScriptValues = ecma9ScriptValues + " Dogra Dogr Gunjala_Gondi Gong Hanifi_Rohingya Rohg Makasar Maka Medefaidrin Medf Old_Sogdian Sogo Sogdian Sogd";
var ecma11ScriptValues = ecma10ScriptValues + " Elymaic Elym Nandinagari Nand Nyiakeng_Puachue_Hmong Hmnp Wancho Wcho";
var unicodeScriptValues = {
9: ecma9ScriptValues,
10: ecma10ScriptValues,
11: ecma11ScriptValues
};
var data = {};
function buildUnicodeData(ecmaVersion) {
var d = data[ecmaVersion] = {
binary: wordsRegexp(unicodeBinaryProperties[ecmaVersion] + " " + unicodeGeneralCategoryValues),
nonBinary: {
General_Category: wordsRegexp(unicodeGeneralCategoryValues),
Script: wordsRegexp(unicodeScriptValues[ecmaVersion])
}
};
d.nonBinary.Script_Extensions = d.nonBinary.Script;
d.nonBinary.gc = d.nonBinary.General_Category;
d.nonBinary.sc = d.nonBinary.Script;
d.nonBinary.scx = d.nonBinary.Script_Extensions;
}
buildUnicodeData(9);
buildUnicodeData(10);
buildUnicodeData(11);
var pp$8 = Parser.prototype;
var RegExpValidationState = function RegExpValidationState2(parser) {
this.parser = parser;
this.validFlags = "gim" + (parser.options.ecmaVersion >= 6 ? "uy" : "") + (parser.options.ecmaVersion >= 9 ? "s" : "");
this.unicodeProperties = data[parser.options.ecmaVersion >= 11 ? 11 : parser.options.ecmaVersion];
this.source = "";
this.flags = "";
this.start = 0;
this.switchU = false;
this.switchN = false;
this.pos = 0;
this.lastIntValue = 0;
this.lastStringValue = "";
this.lastAssertionIsQuantifiable = false;
this.numCapturingParens = 0;
this.maxBackReference = 0;
this.groupNames = [];
this.backReferenceNames = [];
};
RegExpValidationState.prototype.reset = function reset(start, pattern, flags) {
var unicode = flags.indexOf("u") !== -1;
this.start = start | 0;
this.source = pattern + "";
this.flags = flags;
this.switchU = unicode && this.parser.options.ecmaVersion >= 6;
this.switchN = unicode && this.parser.options.ecmaVersion >= 9;
};
RegExpValidationState.prototype.raise = function raise(message) {
this.parser.raiseRecoverable(this.start, "Invalid regular expression: /" + this.source + "/: " + message);
};
RegExpValidationState.prototype.at = function at(i, forceU) {
if (forceU === void 0)
forceU = false;
var s = this.source;
var l = s.length;
if (i >= l) {
return -1;
}
var c = s.charCodeAt(i);
if (!(forceU || this.switchU) || c <= 55295 || c >= 57344 || i + 1 >= l) {
return c;
}
var next = s.charCodeAt(i + 1);
return next >= 56320 && next <= 57343 ? (c << 10) + next - 56613888 : c;
};
RegExpValidationState.prototype.nextIndex = function nextIndex(i, forceU) {
if (forceU === void 0)
forceU = false;
var s = this.source;
var l = s.length;
if (i >= l) {
return l;
}
var c = s.charCodeAt(i), next;
if (!(forceU || this.switchU) || c <= 55295 || c >= 57344 || i + 1 >= l || (next = s.charCodeAt(i + 1)) < 56320 || next > 57343) {
return i + 1;
}
return i + 2;
};
RegExpValidationState.prototype.current = function current(forceU) {
if (forceU === void 0)
forceU = false;
return this.at(this.pos, forceU);
};
RegExpValidationState.prototype.lookahead = function lookahead(forceU) {
if (forceU === void 0)
forceU = false;
return this.at(this.nextIndex(this.pos, forceU), forceU);
};
RegExpValidationState.prototype.advance = function advance(forceU) {
if (forceU === void 0)
forceU = false;
this.pos = this.nextIndex(this.pos, forceU);
};
RegExpValidationState.prototype.eat = function eat(ch, forceU) {
if (forceU === void 0)
forceU = false;
if (this.current(forceU) === ch) {
this.advance(forceU);
return true;
}
return false;
};
function codePointToString(ch) {
if (ch <= 65535) {
return String.fromCharCode(ch);
}
ch -= 65536;
return String.fromCharCode((ch >> 10) + 55296, (ch & 1023) + 56320);
}
pp$8.validateRegExpFlags = function(state) {
var validFlags = state.validFlags;
var flags = state.flags;
for (var i = 0; i < flags.length; i++) {
var flag = flags.charAt(i);
if (validFlags.indexOf(flag) === -1) {
this.raise(state.start, "Invalid regular expression flag");
}
if (flags.indexOf(flag, i + 1) > -1) {
this.raise(state.start, "Duplicate regular expression flag");
}
}
};
pp$8.validateRegExpPattern = function(state) {
this.regexp_pattern(state);
if (!state.switchN && this.options.ecmaVersion >= 9 && state.groupNames.length > 0) {
state.switchN = true;
this.regexp_pattern(state);
}
};
pp$8.regexp_pattern = function(state) {
state.pos = 0;
state.lastIntValue = 0;
state.lastStringValue = "";
state.lastAssertionIsQuantifiable = false;
state.numCapturingParens = 0;
state.maxBackReference = 0;
state.groupNames.length = 0;
state.backReferenceNames.length = 0;
this.regexp_disjunction(state);
if (state.pos !== state.source.length) {
if (state.eat(41)) {
state.raise("Unmatched ')'");
}
if (state.eat(93) || state.eat(125)) {
state.raise("Lone quantifier brackets");
}
}
if (state.maxBackReference > state.numCapturingParens) {
state.raise("Invalid escape");
}
for (var i = 0, list = state.backReferenceNames; i < list.length; i += 1) {
var name = list[i];
if (state.groupNames.indexOf(name) === -1) {
state.raise("Invalid named capture referenced");
}
}
};
pp$8.regexp_disjunction = function(state) {
this.regexp_alternative(state);
while (state.eat(124)) {
this.regexp_alternative(state);
}
if (this.regexp_eatQuantifier(state, true)) {
state.raise("Nothing to repeat");
}
if (state.eat(123)) {
state.raise("Lone quantifier brackets");
}
};
pp$8.regexp_alternative = function(state) {
while (state.pos < state.source.length && this.regexp_eatTerm(state)) {
}
};
pp$8.regexp_eatTerm = function(state) {
if (this.regexp_eatAssertion(state)) {
if (state.lastAssertionIsQuantifiable && this.regexp_eatQuantifier(state)) {
if (state.switchU) {
state.raise("Invalid quantifier");
}
}
return true;
}
if (state.switchU ? this.regexp_eatAtom(state) : this.regexp_eatExtendedAtom(state)) {
this.regexp_eatQuantifier(state);
return true;
}
return false;
};
pp$8.regexp_eatAssertion = function(state) {
var start = state.pos;
state.lastAssertionIsQuantifiable = false;
if (state.eat(94) || state.eat(36)) {
return true;
}
if (state.eat(92)) {
if (state.eat(66) || state.eat(98)) {
return true;
}
state.pos = start;
}
if (state.eat(40) && state.eat(63)) {
var lookbehind = false;
if (this.options.ecmaVersion >= 9) {
lookbehind = state.eat(60);
}
if (state.eat(61) || state.eat(33)) {
this.regexp_disjunction(state);
if (!state.eat(41)) {
state.raise("Unterminated group");
}
state.lastAssertionIsQuantifiable = !lookbehind;
return true;
}
}
state.pos = start;
return false;
};
pp$8.regexp_eatQuantifier = function(state, noError) {
if (noError === void 0)
noError = false;
if (this.regexp_eatQuantifierPrefix(state, noError)) {
state.eat(63);
return true;
}
return false;
};
pp$8.regexp_eatQuantifierPrefix = function(state, noError) {
return state.eat(42) || state.eat(43) || state.eat(63) || this.regexp_eatBracedQuantifier(state, noError);
};
pp$8.regexp_eatBracedQuantifier = function(state, noError) {
var start = state.pos;
if (state.eat(123)) {
var min = 0, max = -1;
if (this.regexp_eatDecimalDigits(state)) {
min = state.lastIntValue;
if (state.eat(44) && this.regexp_eatDecimalDigits(state)) {
max = state.lastIntValue;
}
if (state.eat(125)) {
if (max !== -1 && max < min && !noError) {
state.raise("numbers out of order in {} quantifier");
}
return true;
}
}
if (state.switchU && !noError) {
state.raise("Incomplete quantifier");
}
state.pos = start;
}
return false;
};
pp$8.regexp_eatAtom = function(state) {
return this.regexp_eatPatternCharacters(state) || state.eat(46) || this.regexp_eatReverseSolidusAtomEscape(state) || this.regexp_eatCharacterClass(state) || this.regexp_eatUncapturingGroup(state) || this.regexp_eatCapturingGroup(state);
};
pp$8.regexp_eatReverseSolidusAtomEscape = function(state) {
var start = state.pos;
if (state.eat(92)) {
if (this.regexp_eatAtomEscape(state)) {
return true;
}
state.pos = start;
}
return false;
};
pp$8.regexp_eatUncapturingGroup = function(state) {
var start = state.pos;
if (state.eat(40)) {
if (state.eat(63) && state.eat(58)) {
this.regexp_disjunction(state);
if (state.eat(41)) {
return true;
}
state.raise("Unterminated group");
}
state.pos = start;
}
return false;
};
pp$8.regexp_eatCapturingGroup = function(state) {
if (state.eat(40)) {
if (this.options.ecmaVersion >= 9) {
this.regexp_groupSpecifier(state);
} else if (state.current() === 63) {
state.raise("Invalid group");
}
this.regexp_disjunction(state);
if (state.eat(41)) {
state.numCapturingParens += 1;
return true;
}
state.raise("Unterminated group");
}
return false;
};
pp$8.regexp_eatExtendedAtom = function(state) {
return state.eat(46) || this.regexp_eatReverseSolidusAtomEscape(state) || this.regexp_eatCharacterClass(state) || this.regexp_eatUncapturingGroup(state) || this.regexp_eatCapturingGroup(state) || this.regexp_eatInvalidBracedQuantifier(state) || this.regexp_eatExtendedPatternCharacter(state);
};
pp$8.regexp_eatInvalidBracedQuantifier = function(state) {
if (this.regexp_eatBracedQuantifier(state, true)) {
state.raise("Nothing to repeat");
}
return false;
};
pp$8.regexp_eatSyntaxCharacter = function(state) {
var ch = state.current();
if (isSyntaxCharacter(ch)) {
state.lastIntValue = ch;
state.advance();
return true;
}
return false;
};
function isSyntaxCharacter(ch) {
return ch === 36 || ch >= 40 && ch <= 43 || ch === 46 || ch === 63 || ch >= 91 && ch <= 94 || ch >= 123 && ch <= 125;
}
pp$8.regexp_eatPatternCharacters = function(state) {
var start = state.pos;
var ch = 0;
while ((ch = state.current()) !== -1 && !isSyntaxCharacter(ch)) {
state.advance();
}
return state.pos !== start;
};
pp$8.regexp_eatExtendedPatternCharacter = function(state) {
var ch = state.current();
if (ch !== -1 && ch !== 36 && !(ch >= 40 && ch <= 43) && ch !== 46 && ch !== 63 && ch !== 91 && ch !== 94 && ch !== 124) {
state.advance();
return true;
}
return false;
};
pp$8.regexp_groupSpecifier = function(state) {
if (state.eat(63)) {
if (this.regexp_eatGroupName(state)) {
if (state.groupNames.indexOf(state.lastStringValue) !== -1) {
state.raise("Duplicate capture group name");
}
state.groupNames.push(state.lastStringValue);
return;
}
state.raise("Invalid group");
}
};
pp$8.regexp_eatGroupName = function(state) {
state.lastStringValue = "";
if (state.eat(60)) {
if (this.regexp_eatRegExpIdentifierName(state) && state.eat(62)) {
return true;
}
state.raise("Invalid capture group name");
}
return false;
};
pp$8.regexp_eatRegExpIdentifierName = function(state) {
state.lastStringValue = "";
if (this.regexp_eatRegExpIdentifierStart(state)) {
state.lastStringValue += codePointToString(state.lastIntValue);
while (this.regexp_eatRegExpIdentifierPart(state)) {
state.lastStringValue += codePointToString(state.lastIntValue);
}
return true;
}
return false;
};
pp$8.regexp_eatRegExpIdentifierStart = function(state) {
var start = state.pos;
var forceU = this.options.ecmaVersion >= 11;
var ch = state.current(forceU);
state.advance(forceU);
if (ch === 92 && this.regexp_eatRegExpUnicodeEscapeSequence(state, forceU)) {
ch = state.lastIntValue;
}
if (isRegExpIdentifierStart(ch)) {
state.lastIntValue = ch;
return true;
}
state.pos = start;
return false;
};
function isRegExpIdentifierStart(ch) {
return isIdentifierStart(ch, true) || ch === 36 || ch === 95;
}
pp$8.regexp_eatRegExpIdentifierPart = function(state) {
var start = state.pos;
var forceU = this.options.ecmaVersion >= 11;
var ch = state.current(forceU);
state.advance(forceU);
if (ch === 92 && this.regexp_eatRegExpUnicodeEscapeSequence(state, forceU)) {
ch = state.lastIntValue;
}
if (isRegExpIdentifierPart(ch)) {
state.lastIntValue = ch;
return true;
}
state.pos = start;
return false;
};
function isRegExpIdentifierPart(ch) {
return isIdentifierChar(ch, true) || ch === 36 || ch === 95 || ch === 8204 || ch === 8205;
}
pp$8.regexp_eatAtomEscape = function(state) {
if (this.regexp_eatBackReference(state) || this.regexp_eatCharacterClassEscape(state) || this.regexp_eatCharacterEscape(state) || state.switchN && this.regexp_eatKGroupName(state)) {
return true;
}
if (state.switchU) {
if (state.current() === 99) {
state.raise("Invalid unicode escape");
}
state.raise("Invalid escape");
}
return false;
};
pp$8.regexp_eatBackReference = function(state) {
var start = state.pos;
if (this.regexp_eatDecimalEscape(state)) {
var n2 = state.lastIntValue;
if (state.switchU) {
if (n2 > state.maxBackReference) {
state.maxBackReference = n2;
}
return true;
}
if (n2 <= state.numCapturingParens) {
return true;
}
state.pos = start;
}
return false;
};
pp$8.regexp_eatKGroupName = function(state) {
if (state.eat(107)) {
if (this.regexp_eatGroupName(state)) {
state.backReferenceNames.push(state.lastStringValue);
return true;
}
state.raise("Invalid named reference");
}
return false;
};
pp$8.regexp_eatCharacterEscape = function(state) {
return this.regexp_eatControlEscape(state) || this.regexp_eatCControlLetter(state) || this.regexp_eatZero(state) || this.regexp_eatHexEscapeSequence(state) || this.regexp_eatRegExpUnicodeEscapeSequence(state, false) || !state.switchU && this.regexp_eatLegacyOctalEscapeSequence(state) || this.regexp_eatIdentityEscape(state);
};
pp$8.regexp_eatCControlLetter = function(state) {
var start = state.pos;
if (state.eat(99)) {
if (this.regexp_eatControlLetter(state)) {
return true;
}
state.pos = start;
}
return false;
};
pp$8.regexp_eatZero = function(state) {
if (state.current() === 48 && !isDecimalDigit(state.lookahead())) {
state.lastIntValue = 0;
state.advance();
return true;
}
return false;
};
pp$8.regexp_eatControlEscape = function(state) {
var ch = state.current();
if (ch === 116) {
state.lastIntValue = 9;
state.advance();
return true;
}
if (ch === 110) {
state.lastIntValue = 10;
state.advance();
return true;
}
if (ch === 118) {
state.lastIntValue = 11;
state.advance();
return true;
}
if (ch === 102) {
state.lastIntValue = 12;
state.advance();
return true;
}
if (ch === 114) {
state.lastIntValue = 13;
state.advance();
return true;
}
return false;
};
pp$8.regexp_eatControlLetter = function(state) {
var ch = state.current();
if (isControlLetter(ch)) {
state.lastIntValue = ch % 32;
state.advance();
return true;
}
return false;
};
function isControlLetter(ch) {
return ch >= 65 && ch <= 90 || ch >= 97 && ch <= 122;
}
pp$8.regexp_eatRegExpUnicodeEscapeSequence = function(state, forceU) {
if (forceU === void 0)
forceU = false;
var start = state.pos;
var switchU = forceU || state.switchU;
if (state.eat(117)) {
if (this.regexp_eatFixedHexDigits(state, 4)) {
var lead = state.lastIntValue;
if (switchU && lead >= 55296 && lead <= 56319) {
var leadSurrogateEnd = state.pos;
if (state.eat(92) && state.eat(117) && this.regexp_eatFixedHexDigits(state, 4)) {
var trail = state.lastIntValue;
if (trail >= 56320 && trail <= 57343) {
state.lastIntValue = (lead - 55296) * 1024 + (trail - 56320) + 65536;
return true;
}
}
state.pos = leadSurrogateEnd;
state.lastIntValue = lead;
}
return true;
}
if (switchU && state.eat(123) && this.regexp_eatHexDigits(state) && state.eat(125) && isValidUnicode(state.lastIntValue)) {
return true;
}
if (switchU) {
state.raise("Invalid unicode escape");
}
state.pos = start;
}
return false;
};
function isValidUnicode(ch) {
return ch >= 0 && ch <= 1114111;
}
pp$8.regexp_eatIdentityEscape = function(state) {
if (state.switchU) {
if (this.regexp_eatSyntaxCharacter(state)) {
return true;
}
if (state.eat(47)) {
state.lastIntValue = 47;
return true;
}
return false;
}
var ch = state.current();
if (ch !== 99 && (!state.switchN || ch !== 107)) {
state.lastIntValue = ch;
state.advance();
return true;
}
return false;
};
pp$8.regexp_eatDecimalEscape = function(state) {
state.lastIntValue = 0;
var ch = state.current();
if (ch >= 49 && ch <= 57) {
do {
state.lastIntValue = 10 * state.lastIntValue + (ch - 48);
state.advance();
} while ((ch = state.current()) >= 48 && ch <= 57);
return true;
}
return false;
};
pp$8.regexp_eatCharacterClassEscape = function(state) {
var ch = state.current();
if (isCharacterClassEscape(ch)) {
state.lastIntValue = -1;
state.advance();
return true;
}
if (state.switchU && this.options.ecmaVersion >= 9 && (ch === 80 || ch === 112)) {
state.lastIntValue = -1;
state.advance();
if (state.eat(123) && this.regexp_eatUnicodePropertyValueExpression(state) && state.eat(125)) {
return true;
}
state.raise("Invalid property name");
}
return false;
};
function isCharacterClassEscape(ch) {
return ch === 100 || ch === 68 || ch === 115 || ch === 83 || ch === 119 || ch === 87;
}
pp$8.regexp_eatUnicodePropertyValueExpression = function(state) {
var start = state.pos;
if (this.regexp_eatUnicodePropertyName(state) && state.eat(61)) {
var name = state.lastStringValue;
if (this.regexp_eatUnicodePropertyValue(state)) {
var value = state.lastStringValue;
this.regexp_validateUnicodePropertyNameAndValue(state, name, value);
return true;
}
}
state.pos = start;
if (this.regexp_eatLoneUnicodePropertyNameOrValue(state)) {
var nameOrValue = state.lastStringValue;
this.regexp_validateUnicodePropertyNameOrValue(state, nameOrValue);
return true;
}
return false;
};
pp$8.regexp_validateUnicodePropertyNameAndValue = function(state, name, value) {
if (!has(state.unicodeProperties.nonBinary, name)) {
state.raise("Invalid property name");
}
if (!state.unicodeProperties.nonBinary[name].test(value)) {
state.raise("Invalid property value");
}
};
pp$8.regexp_validateUnicodePropertyNameOrValue = function(state, nameOrValue) {
if (!state.unicodeProperties.binary.test(nameOrValue)) {
state.raise("Invalid property name");
}
};
pp$8.regexp_eatUnicodePropertyName = function(state) {
var ch = 0;
state.lastStringValue = "";
while (isUnicodePropertyNameCharacter(ch = state.current())) {
state.lastStringValue += codePointToString(ch);
state.advance();
}
return state.lastStringValue !== "";
};
function isUnicodePropertyNameCharacter(ch) {
return isControlLetter(ch) || ch === 95;
}
pp$8.regexp_eatUnicodePropertyValue = function(state) {
var ch = 0;
state.lastStringValue = "";
while (isUnicodePropertyValueCharacter(ch = state.current())) {
state.lastStringValue += codePointToString(ch);
state.advance();
}
return state.lastStringValue !== "";
};
function isUnicodePropertyValueCharacter(ch) {
return isUnicodePropertyNameCharacter(ch) || isDecimalDigit(ch);
}
pp$8.regexp_eatLoneUnicodePropertyNameOrValue = function(state) {
return this.regexp_eatUnicodePropertyValue(state);
};
pp$8.regexp_eatCharacterClass = function(state) {
if (state.eat(91)) {
state.eat(94);
this.regexp_classRanges(state);
if (state.eat(93)) {
return true;
}
state.raise("Unterminated character class");
}
return false;
};
pp$8.regexp_classRanges = function(state) {
while (this.regexp_eatClassAtom(state)) {
var left = state.lastIntValue;
if (state.eat(45) && this.regexp_eatClassAtom(state)) {
var right = state.lastIntValue;
if (state.switchU && (left === -1 || right === -1)) {
state.raise("Invalid character class");
}
if (left !== -1 && right !== -1 && left > right) {
state.raise("Range out of order in character class");
}
}
}
};
pp$8.regexp_eatClassAtom = function(state) {
var start = state.pos;
if (state.eat(92)) {
if (this.regexp_eatClassEscape(state)) {
return true;
}
if (state.switchU) {
var ch$1 = state.current();
if (ch$1 === 99 || isOctalDigit(ch$1)) {
state.raise("Invalid class escape");
}
state.raise("Invalid escape");
}
state.pos = start;
}
var ch = state.current();
if (ch !== 93) {
state.lastIntValue = ch;
state.advance();
return true;
}
return false;
};
pp$8.regexp_eatClassEscape = function(state) {
var start = state.pos;
if (state.eat(98)) {
state.lastIntValue = 8;
return true;
}
if (state.switchU && state.eat(45)) {
state.lastIntValue = 45;
return true;
}
if (!state.switchU && state.eat(99)) {
if (this.regexp_eatClassControlLetter(state)) {
return true;
}
state.pos = start;
}
return this.regexp_eatCharacterClassEscape(state) || this.regexp_eatCharacterEscape(state);
};
pp$8.regexp_eatClassControlLetter = function(state) {
var ch = state.current();
if (isDecimalDigit(ch) || ch === 95) {
state.lastIntValue = ch % 32;
state.advance();
return true;
}
return false;
};
pp$8.regexp_eatHexEscapeSequence = function(state) {
var start = state.pos;
if (state.eat(120)) {
if (this.regexp_eatFixedHexDigits(state, 2)) {
return true;
}
if (state.switchU) {
state.raise("Invalid escape");
}
state.pos = start;
}
return false;
};
pp$8.regexp_eatDecimalDigits = function(state) {
var start = state.pos;
var ch = 0;
state.lastIntValue = 0;
while (isDecimalDigit(ch = state.current())) {
state.lastIntValue = 10 * state.lastIntValue + (ch - 48);
state.advance();
}
return state.pos !== start;
};
function isDecimalDigit(ch) {
return ch >= 48 && ch <= 57;
}
pp$8.regexp_eatHexDigits = function(state) {
var start = state.pos;
var ch = 0;
state.lastIntValue = 0;
while (isHexDigit(ch = state.current())) {
state.lastIntValue = 16 * state.lastIntValue + hexToInt(ch);
state.advance();
}
return state.pos !== start;
};
function isHexDigit(ch) {
return ch >= 48 && ch <= 57 || ch >= 65 && ch <= 70 || ch >= 97 && ch <= 102;
}
function hexToInt(ch) {
if (ch >= 65 && ch <= 70) {
return 10 + (ch - 65);
}
if (ch >= 97 && ch <= 102) {
return 10 + (ch - 97);
}
return ch - 48;
}
pp$8.regexp_eatLegacyOctalEscapeSequence = function(state) {
if (this.regexp_eatOctalDigit(state)) {
var n1 = state.lastIntValue;
if (this.regexp_eatOctalDigit(state)) {
var n2 = state.lastIntValue;
if (n1 <= 3 && this.regexp_eatOctalDigit(state)) {
state.lastIntValue = n1 * 64 + n2 * 8 + state.lastIntValue;
} else {
state.lastIntValue = n1 * 8 + n2;
}
} else {
state.lastIntValue = n1;
}
return true;
}
return false;
};
pp$8.regexp_eatOctalDigit = function(state) {
var ch = state.current();
if (isOctalDigit(ch)) {
state.lastIntValue = ch - 48;
state.advance();
return true;
}
state.lastIntValue = 0;
return false;
};
function isOctalDigit(ch) {
return ch >= 48 && ch <= 55;
}
pp$8.regexp_eatFixedHexDigits = function(state, length) {
var start = state.pos;
state.lastIntValue = 0;
for (var i = 0; i < length; ++i) {
var ch = state.current();
if (!isHexDigit(ch)) {
state.pos = start;
return false;
}
state.lastIntValue = 16 * state.lastIntValue + hexToInt(ch);
state.advance();
}
return true;
};
var Token = function Token2(p) {
this.type = p.type;
this.value = p.value;
this.start = p.start;
this.end = p.end;
if (p.options.locations) {
this.loc = new SourceLocation(p, p.startLoc, p.endLoc);
}
if (p.options.ranges) {
this.range = [p.start, p.end];
}
};
var pp$9 = Parser.prototype;
pp$9.next = function(ignoreEscapeSequenceInKeyword) {
if (!ignoreEscapeSequenceInKeyword && this.type.keyword && this.containsEsc) {
this.raiseRecoverable(this.start, "Escape sequence in keyword " + this.type.keyword);
}
if (this.options.onToken) {
this.options.onToken(new Token(this));
}
this.lastTokEnd = this.end;
this.lastTokStart = this.start;
this.lastTokEndLoc = this.endLoc;
this.lastTokStartLoc = this.startLoc;
this.nextToken();
};
pp$9.getToken = function() {
this.next();
return new Token(this);
};
if (typeof Symbol !== "undefined") {
pp$9[Symbol.iterator] = function() {
var this$1 = this;
return {
next: function() {
var token = this$1.getToken();
return {
done: token.type === types.eof,
value: token
};
}
};
};
}
pp$9.curContext = function() {
return this.context[this.context.length - 1];
};
pp$9.nextToken = function() {
var curContext = this.curContext();
if (!curContext || !curContext.preserveSpace) {
this.skipSpace();
}
this.start = this.pos;
if (this.options.locations) {
this.startLoc = this.curPosition();
}
if (this.pos >= this.input.length) {
return this.finishToken(types.eof);
}
if (curContext.override) {
return curContext.override(this);
} else {
this.readToken(this.fullCharCodeAtPos());
}
};
pp$9.readToken = function(code) {
if (isIdentifierStart(code, this.options.ecmaVersion >= 6) || code === 92) {
return this.readWord();
}
return this.getTokenFromCode(code);
};
pp$9.fullCharCodeAtPos = function() {
var code = this.input.charCodeAt(this.pos);
if (code <= 55295 || code >= 57344) {
return code;
}
var next = this.input.charCodeAt(this.pos + 1);
return (code << 10) + next - 56613888;
};
pp$9.skipBlockComment = function() {
var startLoc = this.options.onComment && this.curPosition();
var start = this.pos, end = this.input.indexOf("*/", this.pos += 2);
if (end === -1) {
this.raise(this.pos - 2, "Unterminated comment");
}
this.pos = end + 2;
if (this.options.locations) {
lineBreakG.lastIndex = start;
var match;
while ((match = lineBreakG.exec(this.input)) && match.index < this.pos) {
++this.curLine;
this.lineStart = match.index + match[0].length;
}
}
if (this.options.onComment) {
this.options.onComment(
true,
this.input.slice(start + 2, end),
start,
this.pos,
startLoc,
this.curPosition()
);
}
};
pp$9.skipLineComment = function(startSkip) {
var start = this.pos;
var startLoc = this.options.onComment && this.curPosition();
var ch = this.input.charCodeAt(this.pos += startSkip);
while (this.pos < this.input.length && !isNewLine(ch)) {
ch = this.input.charCodeAt(++this.pos);
}
if (this.options.onComment) {
this.options.onComment(
false,
this.input.slice(start + startSkip, this.pos),
start,
this.pos,
startLoc,
this.curPosition()
);
}
};
pp$9.skipSpace = function() {
loop:
while (this.pos < this.input.length) {
var ch = this.input.charCodeAt(this.pos);
switch (ch) {
case 32:
case 160:
++this.pos;
break;
case 13:
if (this.input.charCodeAt(this.pos + 1) === 10) {
++this.pos;
}
case 10:
case 8232:
case 8233:
++this.pos;
if (this.options.locations) {
++this.curLine;
this.lineStart = this.pos;
}
break;
case 47:
switch (this.input.charCodeAt(this.pos + 1)) {
case 42:
this.skipBlockComment();
break;
case 47:
this.skipLineComment(2);
break;
default:
break loop;
}
break;
default:
if (ch > 8 && ch < 14 || ch >= 5760 && nonASCIIwhitespace.test(String.fromCharCode(ch))) {
++this.pos;
} else {
break loop;
}
}
}
};
pp$9.finishToken = function(type, val) {
this.end = this.pos;
if (this.options.locations) {
this.endLoc = this.curPosition();
}
var prevType = this.type;
this.type = type;
this.value = val;
this.updateContext(prevType);
};
pp$9.readToken_dot = function() {
var next = this.input.charCodeAt(this.pos + 1);
if (next >= 48 && next <= 57) {
return this.readNumber(true);
}
var next2 = this.input.charCodeAt(this.pos + 2);
if (this.options.ecmaVersion >= 6 && next === 46 && next2 === 46) {
this.pos += 3;
return this.finishToken(types.ellipsis);
} else {
++this.pos;
return this.finishToken(types.dot);
}
};
pp$9.readToken_slash = function() {
var next = this.input.charCodeAt(this.pos + 1);
if (this.exprAllowed) {
++this.pos;
return this.readRegexp();
}
if (next === 61) {
return this.finishOp(types.assign, 2);
}
return this.finishOp(types.slash, 1);
};
pp$9.readToken_mult_modulo_exp = function(code) {
var next = this.input.charCodeAt(this.pos + 1);
var size = 1;
var tokentype = code === 42 ? types.star : types.modulo;
if (this.options.ecmaVersion >= 7 && code === 42 && next === 42) {
++size;
tokentype = types.starstar;
next = this.input.charCodeAt(this.pos + 2);
}
if (next === 61) {
return this.finishOp(types.assign, size + 1);
}
return this.finishOp(tokentype, size);
};
pp$9.readToken_pipe_amp = function(code) {
var next = this.input.charCodeAt(this.pos + 1);
if (next === code) {
if (this.options.ecmaVersion >= 12) {
var next2 = this.input.charCodeAt(this.pos + 2);
if (next2 === 61) {
return this.finishOp(types.assign, 3);
}
}
return this.finishOp(code === 124 ? types.logicalOR : types.logicalAND, 2);
}
if (next === 61) {
return this.finishOp(types.assign, 2);
}
return this.finishOp(code === 124 ? types.bitwiseOR : types.bitwiseAND, 1);
};
pp$9.readToken_caret = function() {
var next = this.input.charCodeAt(this.pos + 1);
if (next === 61) {
return this.finishOp(types.assign, 2);
}
return this.finishOp(types.bitwiseXOR, 1);
};
pp$9.readToken_plus_min = function(code) {
var next = this.input.charCodeAt(this.pos + 1);
if (next === code) {
if (next === 45 && !this.inModule && this.input.charCodeAt(this.pos + 2) === 62 && (this.lastTokEnd === 0 || lineBreak.test(this.input.slice(this.lastTokEnd, this.pos)))) {
this.skipLineComment(3);
this.skipSpace();
return this.nextToken();
}
return this.finishOp(types.incDec, 2);
}
if (next === 61) {
return this.finishOp(types.assign, 2);
}
return this.finishOp(types.plusMin, 1);
};
pp$9.readToken_lt_gt = function(code) {
var next = this.input.charCodeAt(this.pos + 1);
var size = 1;
if (next === code) {
size = code === 62 && this.input.charCodeAt(this.pos + 2) === 62 ? 3 : 2;
if (this.input.charCodeAt(this.pos + size) === 61) {
return this.finishOp(types.assign, size + 1);
}
return this.finishOp(types.bitShift, size);
}
if (next === 33 && code === 60 && !this.inModule && this.input.charCodeAt(this.pos + 2) === 45 && this.input.charCodeAt(this.pos + 3) === 45) {
this.skipLineComment(4);
this.skipSpace();
return this.nextToken();
}
if (next === 61) {
size = 2;
}
return this.finishOp(types.relational, size);
};
pp$9.readToken_eq_excl = function(code) {
var next = this.input.charCodeAt(this.pos + 1);
if (next === 61) {
return this.finishOp(types.equality, this.input.charCodeAt(this.pos + 2) === 61 ? 3 : 2);
}
if (code === 61 && next === 62 && this.options.ecmaVersion >= 6) {
this.pos += 2;
return this.finishToken(types.arrow);
}
return this.finishOp(code === 61 ? types.eq : types.prefix, 1);
};
pp$9.readToken_question = function() {
var ecmaVersion = this.options.ecmaVersion;
if (ecmaVersion >= 11) {
var next = this.input.charCodeAt(this.pos + 1);
if (next === 46) {
var next2 = this.input.charCodeAt(this.pos + 2);
if (next2 < 48 || next2 > 57) {
return this.finishOp(types.questionDot, 2);
}
}
if (next === 63) {
if (ecmaVersion >= 12) {
var next2$1 = this.input.charCodeAt(this.pos + 2);
if (next2$1 === 61) {
return this.finishOp(types.assign, 3);
}
}
return this.finishOp(types.coalesce, 2);
}
}
return this.finishOp(types.question, 1);
};
pp$9.getTokenFromCode = function(code) {
switch (code) {
case 46:
return this.readToken_dot();
case 40:
++this.pos;
return this.finishToken(types.parenL);
case 41:
++this.pos;
return this.finishToken(types.parenR);
case 59:
++this.pos;
return this.finishToken(types.semi);
case 44:
++this.pos;
return this.finishToken(types.comma);
case 91:
++this.pos;
return this.finishToken(types.bracketL);
case 93:
++this.pos;
return this.finishToken(types.bracketR);
case 123:
++this.pos;
return this.finishToken(types.braceL);
case 125:
++this.pos;
return this.finishToken(types.braceR);
case 58:
++this.pos;
return this.finishToken(types.colon);
case 96:
if (this.options.ecmaVersion < 6) {
break;
}
++this.pos;
return this.finishToken(types.backQuote);
case 48:
var next = this.input.charCodeAt(this.pos + 1);
if (next === 120 || next === 88) {
return this.readRadixNumber(16);
}
if (this.options.ecmaVersion >= 6) {
if (next === 111 || next === 79) {
return this.readRadixNumber(8);
}
if (next === 98 || next === 66) {
return this.readRadixNumber(2);
}
}
case 49:
case 50:
case 51:
case 52:
case 53:
case 54:
case 55:
case 56:
case 57:
return this.readNumber(false);
case 34:
case 39:
return this.readString(code);
case 47:
return this.readToken_slash();
case 37:
case 42:
return this.readToken_mult_modulo_exp(code);
case 124:
case 38:
return this.readToken_pipe_amp(code);
case 94:
return this.readToken_caret();
case 43:
case 45:
return this.readToken_plus_min(code);
case 60:
case 62:
return this.readToken_lt_gt(code);
case 61:
case 33:
return this.readToken_eq_excl(code);
case 63:
return this.readToken_question();
case 126:
return this.finishOp(types.prefix, 1);
}
this.raise(this.pos, "Unexpected character '" + codePointToString$1(code) + "'");
};
pp$9.finishOp = function(type, size) {
var str = this.input.slice(this.pos, this.pos + size);
this.pos += size;
return this.finishToken(type, str);
};
pp$9.readRegexp = function() {
var escaped, inClass, start = this.pos;
for (; ; ) {
if (this.pos >= this.input.length) {
this.raise(start, "Unterminated regular expression");
}
var ch = this.input.charAt(this.pos);
if (lineBreak.test(ch)) {
this.raise(start, "Unterminated regular expression");
}
if (!escaped) {
if (ch === "[") {
inClass = true;
} else if (ch === "]" && inClass) {
inClass = false;
} else if (ch === "/" && !inClass) {
break;
}
escaped = ch === "\\";
} else {
escaped = false;
}
++this.pos;
}
var pattern = this.input.slice(start, this.pos);
++this.pos;
var flagsStart = this.pos;
var flags = this.readWord1();
if (this.containsEsc) {
this.unexpected(flagsStart);
}
var state = this.regexpState || (this.regexpState = new RegExpValidationState(this));
state.reset(start, pattern, flags);
this.validateRegExpFlags(state);
this.validateRegExpPattern(state);
var value = null;
try {
value = new RegExp(pattern, flags);
} catch (e) {
}
return this.finishToken(types.regexp, { pattern, flags, value });
};
pp$9.readInt = function(radix, len, maybeLegacyOctalNumericLiteral) {
var allowSeparators = this.options.ecmaVersion >= 12 && len === void 0;
var isLegacyOctalNumericLiteral = maybeLegacyOctalNumericLiteral && this.input.charCodeAt(this.pos) === 48;
var start = this.pos, total = 0, lastCode = 0;
for (var i = 0, e = len == null ? Infinity : len; i < e; ++i, ++this.pos) {
var code = this.input.charCodeAt(this.pos), val = void 0;
if (allowSeparators && code === 95) {
if (isLegacyOctalNumericLiteral) {
this.raiseRecoverable(this.pos, "Numeric separator is not allowed in legacy octal numeric literals");
}
if (lastCode === 95) {
this.raiseRecoverable(this.pos, "Numeric separator must be exactly one underscore");
}
if (i === 0) {
this.raiseRecoverable(this.pos, "Numeric separator is not allowed at the first of digits");
}
lastCode = code;
continue;
}
if (code >= 97) {
val = code - 97 + 10;
} else if (code >= 65) {
val = code - 65 + 10;
} else if (code >= 48 && code <= 57) {
val = code - 48;
} else {
val = Infinity;
}
if (val >= radix) {
break;
}
lastCode = code;
total = total * radix + val;
}
if (allowSeparators && lastCode === 95) {
this.raiseRecoverable(this.pos - 1, "Numeric separator is not allowed at the last of digits");
}
if (this.pos === start || len != null && this.pos - start !== len) {
return null;
}
return total;
};
function stringToNumber(str, isLegacyOctalNumericLiteral) {
if (isLegacyOctalNumericLiteral) {
return parseInt(str, 8);
}
return parseFloat(str.replace(/_/g, ""));
}
function stringToBigInt(str) {
if (typeof BigInt !== "function") {
return null;
}
return BigInt(str.replace(/_/g, ""));
}
pp$9.readRadixNumber = function(radix) {
var start = this.pos;
this.pos += 2;
var val = this.readInt(radix);
if (val == null) {
this.raise(this.start + 2, "Expected number in radix " + radix);
}
if (this.options.ecmaVersion >= 11 && this.input.charCodeAt(this.pos) === 110) {
val = stringToBigInt(this.input.slice(start, this.pos));
++this.pos;
} else if (isIdentifierStart(this.fullCharCodeAtPos())) {
this.raise(this.pos, "Identifier directly after number");
}
return this.finishToken(types.num, val);
};
pp$9.readNumber = function(startsWithDot) {
var start = this.pos;
if (!startsWithDot && this.readInt(10, void 0, true) === null) {
this.raise(start, "Invalid number");
}
var octal = this.pos - start >= 2 && this.input.charCodeAt(start) === 48;
if (octal && this.strict) {
this.raise(start, "Invalid number");
}
var next = this.input.charCodeAt(this.pos);
if (!octal && !startsWithDot && this.options.ecmaVersion >= 11 && next === 110) {
var val$1 = stringToBigInt(this.input.slice(start, this.pos));
++this.pos;
if (isIdentifierStart(this.fullCharCodeAtPos())) {
this.raise(this.pos, "Identifier directly after number");
}
return this.finishToken(types.num, val$1);
}
if (octal && /[89]/.test(this.input.slice(start, this.pos))) {
octal = false;
}
if (next === 46 && !octal) {
++this.pos;
this.readInt(10);
next = this.input.charCodeAt(this.pos);
}
if ((next === 69 || next === 101) && !octal) {
next = this.input.charCodeAt(++this.pos);
if (next === 43 || next === 45) {
++this.pos;
}
if (this.readInt(10) === null) {
this.raise(start, "Invalid number");
}
}
if (isIdentifierStart(this.fullCharCodeAtPos())) {
this.raise(this.pos, "Identifier directly after number");
}
var val = stringToNumber(this.input.slice(start, this.pos), octal);
return this.finishToken(types.num, val);
};
pp$9.readCodePoint = function() {
var ch = this.input.charCodeAt(this.pos), code;
if (ch === 123) {
if (this.options.ecmaVersion < 6) {
this.unexpected();
}
var codePos = ++this.pos;
code = this.readHexChar(this.input.indexOf("}", this.pos) - this.pos);
++this.pos;
if (code > 1114111) {
this.invalidStringToken(codePos, "Code point out of bounds");
}
} else {
code = this.readHexChar(4);
}
return code;
};
function codePointToString$1(code) {
if (code <= 65535) {
return String.fromCharCode(code);
}
code -= 65536;
return String.fromCharCode((code >> 10) + 55296, (code & 1023) + 56320);
}
pp$9.readString = function(quote2) {
var out = "", chunkStart = ++this.pos;
for (; ; ) {
if (this.pos >= this.input.length) {
this.raise(this.start, "Unterminated string constant");
}
var ch = this.input.charCodeAt(this.pos);
if (ch === quote2) {
break;
}
if (ch === 92) {
out += this.input.slice(chunkStart, this.pos);
out += this.readEscapedChar(false);
chunkStart = this.pos;
} else {
if (isNewLine(ch, this.options.ecmaVersion >= 10)) {
this.raise(this.start, "Unterminated string constant");
}
++this.pos;
}
}
out += this.input.slice(chunkStart, this.pos++);
return this.finishToken(types.string, out);
};
var INVALID_TEMPLATE_ESCAPE_ERROR = {};
pp$9.tryReadTemplateToken = function() {
this.inTemplateElement = true;
try {
this.readTmplToken();
} catch (err) {
if (err === INVALID_TEMPLATE_ESCAPE_ERROR) {
this.readInvalidTemplateToken();
} else {
throw err;
}
}
this.inTemplateElement = false;
};
pp$9.invalidStringToken = function(position, message) {
if (this.inTemplateElement && this.options.ecmaVersion >= 9) {
throw INVALID_TEMPLATE_ESCAPE_ERROR;
} else {
this.raise(position, message);
}
};
pp$9.readTmplToken = function() {
var out = "", chunkStart = this.pos;
for (; ; ) {
if (this.pos >= this.input.length) {
this.raise(this.start, "Unterminated template");
}
var ch = this.input.charCodeAt(this.pos);
if (ch === 96 || ch === 36 && this.input.charCodeAt(this.pos + 1) === 123) {
if (this.pos === this.start && (this.type === types.template || this.type === types.invalidTemplate)) {
if (ch === 36) {
this.pos += 2;
return this.finishToken(types.dollarBraceL);
} else {
++this.pos;
return this.finishToken(types.backQuote);
}
}
out += this.input.slice(chunkStart, this.pos);
return this.finishToken(types.template, out);
}
if (ch === 92) {
out += this.input.slice(chunkStart, this.pos);
out += this.readEscapedChar(true);
chunkStart = this.pos;
} else if (isNewLine(ch)) {
out += this.input.slice(chunkStart, this.pos);
++this.pos;
switch (ch) {
case 13:
if (this.input.charCodeAt(this.pos) === 10) {
++this.pos;
}
case 10:
out += "\n";
break;
default:
out += String.fromCharCode(ch);
break;
}
if (this.options.locations) {
++this.curLine;
this.lineStart = this.pos;
}
chunkStart = this.pos;
} else {
++this.pos;
}
}
};
pp$9.readInvalidTemplateToken = function() {
for (; this.pos < this.input.length; this.pos++) {
switch (this.input[this.pos]) {
case "\\":
++this.pos;
break;
case "$":
if (this.input[this.pos + 1] !== "{") {
break;
}
case "`":
return this.finishToken(types.invalidTemplate, this.input.slice(this.start, this.pos));
}
}
this.raise(this.start, "Unterminated template");
};
pp$9.readEscapedChar = function(inTemplate) {
var ch = this.input.charCodeAt(++this.pos);
++this.pos;
switch (ch) {
case 110:
return "\n";
case 114:
return "\r";
case 120:
return String.fromCharCode(this.readHexChar(2));
case 117:
return codePointToString$1(this.readCodePoint());
case 116:
return " ";
case 98:
return "\b";
case 118:
return "\v";
case 102:
return "\f";
case 13:
if (this.input.charCodeAt(this.pos) === 10) {
++this.pos;
}
case 10:
if (this.options.locations) {
this.lineStart = this.pos;
++this.curLine;
}
return "";
case 56:
case 57:
if (inTemplate) {
var codePos = this.pos - 1;
this.invalidStringToken(
codePos,
"Invalid escape sequence in template string"
);
return null;
}
default:
if (ch >= 48 && ch <= 55) {
var octalStr = this.input.substr(this.pos - 1, 3).match(/^[0-7]+/)[0];
var octal = parseInt(octalStr, 8);
if (octal > 255) {
octalStr = octalStr.slice(0, -1);
octal = parseInt(octalStr, 8);
}
this.pos += octalStr.length - 1;
ch = this.input.charCodeAt(this.pos);
if ((octalStr !== "0" || ch === 56 || ch === 57) && (this.strict || inTemplate)) {
this.invalidStringToken(
this.pos - 1 - octalStr.length,
inTemplate ? "Octal literal in template string" : "Octal literal in strict mode"
);
}
return String.fromCharCode(octal);
}
if (isNewLine(ch)) {
return "";
}
return String.fromCharCode(ch);
}
};
pp$9.readHexChar = function(len) {
var codePos = this.pos;
var n2 = this.readInt(16, len);
if (n2 === null) {
this.invalidStringToken(codePos, "Bad character escape sequence");
}
return n2;
};
pp$9.readWord1 = function() {
this.containsEsc = false;
var word = "", first = true, chunkStart = this.pos;
var astral = this.options.ecmaVersion >= 6;
while (this.pos < this.input.length) {
var ch = this.fullCharCodeAtPos();
if (isIdentifierChar(ch, astral)) {
this.pos += ch <= 65535 ? 1 : 2;
} else if (ch === 92) {
this.containsEsc = true;
word += this.input.slice(chunkStart, this.pos);
var escStart = this.pos;
if (this.input.charCodeAt(++this.pos) !== 117) {
this.invalidStringToken(this.pos, "Expecting Unicode escape sequence \\uXXXX");
}
++this.pos;
var esc = this.readCodePoint();
if (!(first ? isIdentifierStart : isIdentifierChar)(esc, astral)) {
this.invalidStringToken(escStart, "Invalid Unicode escape");
}
word += codePointToString$1(esc);
chunkStart = this.pos;
} else {
break;
}
first = false;
}
return word + this.input.slice(chunkStart, this.pos);
};
pp$9.readWord = function() {
var word = this.readWord1();
var type = types.name;
if (this.keywords.test(word)) {
type = keywords$1[word];
}
return this.finishToken(type, word);
};
var version = "7.4.1";
Parser.acorn = {
Parser,
version,
defaultOptions,
Position,
SourceLocation,
getLineInfo: getLineInfo2,
Node,
TokenType,
tokTypes: types,
keywordTypes: keywords$1,
TokContext,
tokContexts: types$1,
isIdentifierChar,
isIdentifierStart,
Token,
isNewLine,
lineBreak,
lineBreakG,
nonASCIIwhitespace
};
function parse2(input, options) {
return Parser.parse(input, options);
}
function parseExpressionAt(input, pos, options) {
return Parser.parseExpressionAt(input, pos, options);
}
function tokenizer(input, options) {
return Parser.tokenizer(input, options);
}
exports2.Node = Node;
exports2.Parser = Parser;
exports2.Position = Position;
exports2.SourceLocation = SourceLocation;
exports2.TokContext = TokContext;
exports2.Token = Token;
exports2.TokenType = TokenType;
exports2.defaultOptions = defaultOptions;
exports2.getLineInfo = getLineInfo2;
exports2.isIdentifierChar = isIdentifierChar;
exports2.isIdentifierStart = isIdentifierStart;
exports2.isNewLine = isNewLine;
exports2.keywordTypes = keywords$1;
exports2.lineBreak = lineBreak;
exports2.lineBreakG = lineBreakG;
exports2.nonASCIIwhitespace = nonASCIIwhitespace;
exports2.parse = parse2;
exports2.parseExpressionAt = parseExpressionAt;
exports2.tokContexts = types$1;
exports2.tokTypes = types;
exports2.tokenizer = tokenizer;
exports2.version = version;
Object.defineProperty(exports2, "__esModule", { value: true });
});
}
});
// node_modules/sourcemap-codec/dist/sourcemap-codec.umd.js
var require_sourcemap_codec_umd = __commonJS({
"node_modules/sourcemap-codec/dist/sourcemap-codec.umd.js"(exports, module2) {
(function(global2, factory) {
typeof exports === "object" && typeof module2 !== "undefined" ? factory(exports) : typeof define === "function" && define.amd ? define(["exports"], factory) : (global2 = global2 || self, factory(global2.sourcemapCodec = {}));
})(exports, function(exports2) {
"use strict";
var charToInteger = {};
var chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=";
for (var i = 0; i < chars.length; i++) {
charToInteger[chars.charCodeAt(i)] = i;
}
function decode(mappings) {
var decoded = [];
var line = [];
var segment = [
0,
0,
0,
0,
0
];
var j = 0;
for (var i2 = 0, shift = 0, value = 0; i2 < mappings.length; i2++) {
var c = mappings.charCodeAt(i2);
if (c === 44) {
segmentify(line, segment, j);
j = 0;
} else if (c === 59) {
segmentify(line, segment, j);
j = 0;
decoded.push(line);
line = [];
segment[0] = 0;
} else {
var integer = charToInteger[c];
if (integer === void 0) {
throw new Error("Invalid character (" + String.fromCharCode(c) + ")");
}
var hasContinuationBit = integer & 32;
integer &= 31;
value += integer << shift;
if (hasContinuationBit) {
shift += 5;
} else {
var shouldNegate = value & 1;
value >>>= 1;
if (shouldNegate) {
value = value === 0 ? -2147483648 : -value;
}
segment[j] += value;
j++;
value = shift = 0;
}
}
}
segmentify(line, segment, j);
decoded.push(line);
return decoded;
}
function segmentify(line, segment, j) {
if (j === 4)
line.push([segment[0], segment[1], segment[2], segment[3]]);
else if (j === 5)
line.push([segment[0], segment[1], segment[2], segment[3], segment[4]]);
else if (j === 1)
line.push([segment[0]]);
}
function encode2(decoded) {
var sourceFileIndex = 0;
var sourceCodeLine = 0;
var sourceCodeColumn = 0;
var nameIndex = 0;
var mappings = "";
for (var i2 = 0; i2 < decoded.length; i2++) {
var line = decoded[i2];
if (i2 > 0)
mappings += ";";
if (line.length === 0)
continue;
var generatedCodeColumn = 0;
var lineMappings = [];
for (var _i = 0, line_1 = line; _i < line_1.length; _i++) {
var segment = line_1[_i];
var segmentMappings = encodeInteger(segment[0] - generatedCodeColumn);
generatedCodeColumn = segment[0];
if (segment.length > 1) {
segmentMappings += encodeInteger(segment[1] - sourceFileIndex) + encodeInteger(segment[2] - sourceCodeLine) + encodeInteger(segment[3] - sourceCodeColumn);
sourceFileIndex = segment[1];
sourceCodeLine = segment[2];
sourceCodeColumn = segment[3];
}
if (segment.length === 5) {
segmentMappings += encodeInteger(segment[4] - nameIndex);
nameIndex = segment[4];
}
lineMappings.push(segmentMappings);
}
mappings += lineMappings.join(",");
}
return mappings;
}
function encodeInteger(num) {
var result = "";
num = num < 0 ? -num << 1 | 1 : num << 1;
do {
var clamped = num & 31;
num >>>= 5;
if (num > 0) {
clamped |= 32;
}
result += chars[clamped];
} while (num > 0);
return result;
}
exports2.decode = decode;
exports2.encode = encode2;
Object.defineProperty(exports2, "__esModule", { value: true });
});
}
});
// node_modules/prettier/package.json
var require_package = __commonJS({
"node_modules/prettier/package.json"(exports, module2) {
module2.exports = {
name: "prettier",
version: "2.8.8",
description: "Prettier is an opinionated code formatter",
bin: "./bin-prettier.js",
repository: "prettier/prettier",
funding: "https://github.com/prettier/prettier?sponsor=1",
homepage: "https://prettier.io",
author: "James Long",
license: "MIT",
main: "./index.js",
browser: "./standalone.js",
unpkg: "./standalone.js",
engines: {
node: ">=10.13.0"
},
files: [
"*.js",
"esm/*.mjs"
]
};
}
});
// node_modules/prettier/doc.js
var require_doc = __commonJS({
"node_modules/prettier/doc.js"(exports, module2) {
(function(factory) {
if (typeof exports === "object" && typeof module2 === "object") {
module2.exports = factory();
} else if (typeof define === "function" && define.amd) {
define(factory);
} else {
var root = typeof globalThis !== "undefined" ? globalThis : typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : this || {};
root.doc = factory();
}
})(function() {
"use strict";
var __getOwnPropNames2 = Object.getOwnPropertyNames;
var __commonJS2 = (cb, mod) => function __require() {
return mod || (0, cb[__getOwnPropNames2(cb)[0]])((mod = { exports: {} }).exports, mod), mod.exports;
};
var require_doc_js_umd = __commonJS2({
"dist/_doc.js.umd.js"(exports2, module3) {
var __create2 = Object.create;
var __defProp2 = Object.defineProperty;
var __getOwnPropDesc2 = Object.getOwnPropertyDescriptor;
var __getOwnPropNames22 = Object.getOwnPropertyNames;
var __getProtoOf2 = Object.getPrototypeOf;
var __hasOwnProp2 = Object.prototype.hasOwnProperty;
var __esm = (fn, res) => function __init() {
return fn && (res = (0, fn[__getOwnPropNames22(fn)[0]])(fn = 0)), res;
};
var __commonJS22 = (cb, mod) => function __require() {
return mod || (0, cb[__getOwnPropNames22(cb)[0]])((mod = {
exports: {}
}).exports, mod), mod.exports;
};
var __export = (target, all) => {
for (var name in all)
__defProp2(target, name, {
get: all[name],
enumerable: true
});
};
var __copyProps2 = (to, from, except, desc) => {
if (from && typeof from === "object" || typeof from === "function") {
for (let key of __getOwnPropNames22(from))
if (!__hasOwnProp2.call(to, key) && key !== except)
__defProp2(to, key, {
get: () => from[key],
enumerable: !(desc = __getOwnPropDesc2(from, key)) || desc.enumerable
});
}
return to;
};
var __toESM2 = (mod, isNodeMode, target) => (target = mod != null ? __create2(__getProtoOf2(mod)) : {}, __copyProps2(isNodeMode || !mod || !mod.__esModule ? __defProp2(target, "default", {
value: mod,
enumerable: true
}) : target, mod));
var __toCommonJS = (mod) => __copyProps2(__defProp2({}, "__esModule", {
value: true
}), mod);
var init_define_process = __esm({
""() {
}
});
var require_doc_builders = __commonJS22({
"src/document/doc-builders.js"(exports22, module22) {
"use strict";
init_define_process();
function concat(parts) {
if (false) {
for (const part of parts) {
assertDoc(part);
}
}
return {
type: "concat",
parts
};
}
function indent(contents) {
if (false) {
assertDoc(contents);
}
return {
type: "indent",
contents
};
}
function align(widthOrString, contents) {
if (false) {
assertDoc(contents);
}
return {
type: "align",
contents,
n: widthOrString
};
}
function group(contents) {
let opts = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
if (false) {
assertDoc(contents);
}
return {
type: "group",
id: opts.id,
contents,
break: Boolean(opts.shouldBreak),
expandedStates: opts.expandedStates
};
}
function dedentToRoot(contents) {
return align(Number.NEGATIVE_INFINITY, contents);
}
function markAsRoot(contents) {
return align({
type: "root"
}, contents);
}
function dedent(contents) {
return align(-1, contents);
}
function conditionalGroup(states, opts) {
return group(states[0], Object.assign(Object.assign({}, opts), {}, {
expandedStates: states
}));
}
function fill(parts) {
if (false) {
for (const part of parts) {
assertDoc(part);
}
}
return {
type: "fill",
parts
};
}
function ifBreak(breakContents, flatContents) {
let opts = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {};
if (false) {
if (breakContents) {
assertDoc(breakContents);
}
if (flatContents) {
assertDoc(flatContents);
}
}
return {
type: "if-break",
breakContents,
flatContents,
groupId: opts.groupId
};
}
function indentIfBreak(contents, opts) {
return {
type: "indent-if-break",
contents,
groupId: opts.groupId,
negate: opts.negate
};
}
function lineSuffix(contents) {
if (false) {
assertDoc(contents);
}
return {
type: "line-suffix",
contents
};
}
var lineSuffixBoundary = {
type: "line-suffix-boundary"
};
var breakParent = {
type: "break-parent"
};
var trim = {
type: "trim"
};
var hardlineWithoutBreakParent = {
type: "line",
hard: true
};
var literallineWithoutBreakParent = {
type: "line",
hard: true,
literal: true
};
var line = {
type: "line"
};
var softline = {
type: "line",
soft: true
};
var hardline = concat([hardlineWithoutBreakParent, breakParent]);
var literalline = concat([literallineWithoutBreakParent, breakParent]);
var cursor = {
type: "cursor",
placeholder: Symbol("cursor")
};
function join(sep, arr) {
const res = [];
for (let i = 0; i < arr.length; i++) {
if (i !== 0) {
res.push(sep);
}
res.push(arr[i]);
}
return concat(res);
}
function addAlignmentToDoc(doc, size, tabWidth) {
let aligned = doc;
if (size > 0) {
for (let i = 0; i < Math.floor(size / tabWidth); ++i) {
aligned = indent(aligned);
}
aligned = align(size % tabWidth, aligned);
aligned = align(Number.NEGATIVE_INFINITY, aligned);
}
return aligned;
}
function label(label2, contents) {
return {
type: "label",
label: label2,
contents
};
}
module22.exports = {
concat,
join,
line,
softline,
hardline,
literalline,
group,
conditionalGroup,
fill,
lineSuffix,
lineSuffixBoundary,
cursor,
breakParent,
ifBreak,
trim,
indent,
indentIfBreak,
align,
addAlignmentToDoc,
markAsRoot,
dedentToRoot,
dedent,
hardlineWithoutBreakParent,
literallineWithoutBreakParent,
label
};
}
});
var require_end_of_line = __commonJS22({
"src/common/end-of-line.js"(exports22, module22) {
"use strict";
init_define_process();
function guessEndOfLine(text) {
const index = text.indexOf("\r");
if (index >= 0) {
return text.charAt(index + 1) === "\n" ? "crlf" : "cr";
}
return "lf";
}
function convertEndOfLineToChars(value) {
switch (value) {
case "cr":
return "\r";
case "crlf":
return "\r\n";
default:
return "\n";
}
}
function countEndOfLineChars(text, eol) {
let regex;
switch (eol) {
case "\n":
regex = /\n/g;
break;
case "\r":
regex = /\r/g;
break;
case "\r\n":
regex = /\r\n/g;
break;
default:
throw new Error(`Unexpected "eol" ${JSON.stringify(eol)}.`);
}
const endOfLines = text.match(regex);
return endOfLines ? endOfLines.length : 0;
}
function normalizeEndOfLine(text) {
return text.replace(/\r\n?/g, "\n");
}
module22.exports = {
guessEndOfLine,
convertEndOfLineToChars,
countEndOfLineChars,
normalizeEndOfLine
};
}
});
var require_get_last = __commonJS22({
"src/utils/get-last.js"(exports22, module22) {
"use strict";
init_define_process();
var getLast = (arr) => arr[arr.length - 1];
module22.exports = getLast;
}
});
function ansiRegex() {
let {
onlyFirst = false
} = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {};
const pattern = ["[\\u001B\\u009B][[\\]()#;?]*(?:(?:(?:(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]+)*|[a-zA-Z\\d]+(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]*)*)?\\u0007)", "(?:(?:\\d{1,4}(?:;\\d{0,4})*)?[\\dA-PR-TZcf-ntqry=><~]))"].join("|");
return new RegExp(pattern, onlyFirst ? void 0 : "g");
}
var init_ansi_regex = __esm({
"node_modules/strip-ansi/node_modules/ansi-regex/index.js"() {
init_define_process();
}
});
function stripAnsi(string) {
if (typeof string !== "string") {
throw new TypeError(`Expected a \`string\`, got \`${typeof string}\``);
}
return string.replace(ansiRegex(), "");
}
var init_strip_ansi = __esm({
"node_modules/strip-ansi/index.js"() {
init_define_process();
init_ansi_regex();
}
});
function isFullwidthCodePoint(codePoint) {
if (!Number.isInteger(codePoint)) {
return false;
}
return codePoint >= 4352 && (codePoint <= 4447 || codePoint === 9001 || codePoint === 9002 || 11904 <= codePoint && codePoint <= 12871 && codePoint !== 12351 || 12880 <= codePoint && codePoint <= 19903 || 19968 <= codePoint && codePoint <= 42182 || 43360 <= codePoint && codePoint <= 43388 || 44032 <= codePoint && codePoint <= 55203 || 63744 <= codePoint && codePoint <= 64255 || 65040 <= codePoint && codePoint <= 65049 || 65072 <= codePoint && codePoint <= 65131 || 65281 <= codePoint && codePoint <= 65376 || 65504 <= codePoint && codePoint <= 65510 || 110592 <= codePoint && codePoint <= 110593 || 127488 <= codePoint && codePoint <= 127569 || 131072 <= codePoint && codePoint <= 262141);
}
var init_is_fullwidth_code_point = __esm({
"node_modules/is-fullwidth-code-point/index.js"() {
init_define_process();
}
});
var require_emoji_regex = __commonJS22({
"node_modules/emoji-regex/index.js"(exports22, module22) {
"use strict";
init_define_process();
module22.exports = function() {
return /\uD83C\uDFF4\uDB40\uDC67\uDB40\uDC62(?:\uDB40\uDC77\uDB40\uDC6C\uDB40\uDC73|\uDB40\uDC73\uDB40\uDC63\uDB40\uDC74|\uDB40\uDC65\uDB40\uDC6E\uDB40\uDC67)\uDB40\uDC7F|(?:\uD83E\uDDD1\uD83C\uDFFF\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFF\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB-\uDFFE])|(?:\uD83E\uDDD1\uD83C\uDFFE\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFE\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB-\uDFFD\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFD\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFD\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB\uDFFC\uDFFE\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFC\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFC\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB\uDFFD-\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFB\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFB\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFC-\uDFFF])|\uD83D\uDC68(?:\uD83C\uDFFB(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF]))|\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFC-\uDFFF])|[\u2695\u2696\u2708]\uFE0F|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))?|(?:\uD83C[\uDFFC-\uDFFF])\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF]))|\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83D\uDC68|(?:\uD83D[\uDC68\uDC69])\u200D(?:\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67]))|\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFE])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFD\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB\uDFFC\uDFFE\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB\uDFFD-\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])\uFE0F|\u200D(?:(?:\uD83D[\uDC68\uDC69])\u200D(?:\uD83D[\uDC66\uDC67])|\uD83D[\uDC66\uDC67])|\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC)?|(?:\uD83D\uDC69(?:\uD83C\uDFFB\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69])|(?:\uD83C[\uDFFC-\uDFFF])\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69]))|\uD83E\uDDD1(?:\uD83C[\uDFFB-\uDFFF])\u200D\uD83E\uDD1D\u200D\uD83E\uDDD1)(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC69\u200D\uD83D\uDC69\u200D(?:\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67]))|\uD83D\uDC69(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFB\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))|\uD83E\uDDD1(?:\u200D(?:\uD83E\uDD1D\u200D\uD83E\uDDD1|\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFB\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))|\uD83D\uDC69\u200D\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC69\u200D\uD83D\uDC69\u200D(?:\uD83D[\uDC66\uDC67])|\uD83D\uDC69\u200D\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|(?:\uD83D\uDC41\uFE0F\u200D\uD83D\uDDE8|\uD83E\uDDD1(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\uD83C\uDFFB\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])|\uD83D\uDC69(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\uD83C\uDFFB\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])|\uD83D\uDE36\u200D\uD83C\uDF2B|\uD83C\uDFF3\uFE0F\u200D\u26A7|\uD83D\uDC3B\u200D\u2744|(?:(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC6F|\uD83E[\uDD3C\uDDDE\uDDDF])\u200D[\u2640\u2642]|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])\u200D[\u2640\u2642]|\uD83C\uDFF4\u200D\u2620|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])\u200D[\u2640\u2642]|[\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u2328\u23CF\u23ED-\u23EF\u23F1\u23F2\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB\u25FC\u2600-\u2604\u260E\u2611\u2618\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u265F\u2660\u2663\u2665\u2666\u2668\u267B\u267E\u2692\u2694-\u2697\u2699\u269B\u269C\u26A0\u26A7\u26B0\u26B1\u26C8\u26CF\u26D1\u26D3\u26E9\u26F0\u26F1\u26F4\u26F7\u26F8\u2702\u2708\u2709\u270F\u2712\u2714\u2716\u271D\u2721\u2733\u2734\u2744\u2747\u2763\u27A1\u2934\u2935\u2B05-\u2B07\u3030\u303D\u3297\u3299]|\uD83C[\uDD70\uDD71\uDD7E\uDD7F\uDE02\uDE37\uDF21\uDF24-\uDF2C\uDF36\uDF7D\uDF96\uDF97\uDF99-\uDF9B\uDF9E\uDF9F\uDFCD\uDFCE\uDFD4-\uDFDF\uDFF5\uDFF7]|\uD83D[\uDC3F\uDCFD\uDD49\uDD4A\uDD6F\uDD70\uDD73\uDD76-\uDD79\uDD87\uDD8A-\uDD8D\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA\uDECB\uDECD-\uDECF\uDEE0-\uDEE5\uDEE9\uDEF0\uDEF3])\uFE0F|\uD83C\uDFF3\uFE0F\u200D\uD83C\uDF08|\uD83D\uDC69\u200D\uD83D\uDC67|\uD83D\uDC69\u200D\uD83D\uDC66|\uD83D\uDE35\u200D\uD83D\uDCAB|\uD83D\uDE2E\u200D\uD83D\uDCA8|\uD83D\uDC15\u200D\uD83E\uDDBA|\uD83E\uDDD1(?:\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC|\uD83C\uDFFB)?|\uD83D\uDC69(?:\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC|\uD83C\uDFFB)?|\uD83C\uDDFD\uD83C\uDDF0|\uD83C\uDDF6\uD83C\uDDE6|\uD83C\uDDF4\uD83C\uDDF2|\uD83D\uDC08\u200D\u2B1B|\u2764\uFE0F\u200D(?:\uD83D\uDD25|\uD83E\uDE79)|\uD83D\uDC41\uFE0F|\uD83C\uDFF3\uFE0F|\uD83C\uDDFF(?:\uD83C[\uDDE6\uDDF2\uDDFC])|\uD83C\uDDFE(?:\uD83C[\uDDEA\uDDF9])|\uD83C\uDDFC(?:\uD83C[\uDDEB\uDDF8])|\uD83C\uDDFB(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDEE\uDDF3\uDDFA])|\uD83C\uDDFA(?:\uD83C[\uDDE6\uDDEC\uDDF2\uDDF3\uDDF8\uDDFE\uDDFF])|\uD83C\uDDF9(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDED\uDDEF-\uDDF4\uDDF7\uDDF9\uDDFB\uDDFC\uDDFF])|\uD83C\uDDF8(?:\uD83C[\uDDE6-\uDDEA\uDDEC-\uDDF4\uDDF7-\uDDF9\uDDFB\uDDFD-\uDDFF])|\uD83C\uDDF7(?:\uD83C[\uDDEA\uDDF4\uDDF8\uDDFA\uDDFC])|\uD83C\uDDF5(?:\uD83C[\uDDE6\uDDEA-\uDDED\uDDF0-\uDDF3\uDDF7-\uDDF9\uDDFC\uDDFE])|\uD83C\uDDF3(?:\uD83C[\uDDE6\uDDE8\uDDEA-\uDDEC\uDDEE\uDDF1\uDDF4\uDDF5\uDDF7\uDDFA\uDDFF])|\uD83C\uDDF2(?:\uD83C[\uDDE6\uDDE8-\uDDED\uDDF0-\uDDFF])|\uD83C\uDDF1(?:\uD83C[\uDDE6-\uDDE8\uDDEE\uDDF0\uDDF7-\uDDFB\uDDFE])|\uD83C\uDDF0(?:\uD83C[\uDDEA\uDDEC-\uDDEE\uDDF2\uDDF3\uDDF5\uDDF7\uDDFC\uDDFE\uDDFF])|\uD83C\uDDEF(?:\uD83C[\uDDEA\uDDF2\uDDF4\uDDF5])|\uD83C\uDDEE(?:\uD83C[\uDDE8-\uDDEA\uDDF1-\uDDF4\uDDF6-\uDDF9])|\uD83C\uDDED(?:\uD83C[\uDDF0\uDDF2\uDDF3\uDDF7\uDDF9\uDDFA])|\uD83C\uDDEC(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEE\uDDF1-\uDDF3\uDDF5-\uDDFA\uDDFC\uDDFE])|\uD83C\uDDEB(?:\uD83C[\uDDEE-\uDDF0\uDDF2\uDDF4\uDDF7])|\uD83C\uDDEA(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDED\uDDF7-\uDDFA])|\uD83C\uDDE9(?:\uD83C[\uDDEA\uDDEC\uDDEF\uDDF0\uDDF2\uDDF4\uDDFF])|\uD83C\uDDE8(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDEE\uDDF0-\uDDF5\uDDF7\uDDFA-\uDDFF])|\uD83C\uDDE7(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEF\uDDF1-\uDDF4\uDDF6-\uDDF9\uDDFB\uDDFC\uDDFE\uDDFF])|\uD83C\uDDE6(?:\uD83C[\uDDE8-\uDDEC\uDDEE\uDDF1\uDDF2\uDDF4\uDDF6-\uDDFA\uDDFC\uDDFD\uDDFF])|[#\*0-9]\uFE0F\u20E3|\u2764\uFE0F|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])|\uD83C\uDFF4|(?:[\u270A\u270B]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC6B-\uDC6D\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDC8F\uDC91\uDCAA\uDD7A\uDD95\uDD96\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD34\uDD36\uDD77\uDDB5\uDDB6\uDDBB\uDDD2\uDDD3\uDDD5])(?:\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u270C\u270D]|\uD83D[\uDD74\uDD90])(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])|[\u270A\u270B]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC08\uDC15\uDC3B\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC6B-\uDC6D\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDC8F\uDC91\uDCAA\uDD7A\uDD95\uDD96\uDE2E\uDE35\uDE36\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD34\uDD36\uDD77\uDDB5\uDDB6\uDDBB\uDDD2\uDDD3\uDDD5]|\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD]|\uD83D\uDC6F|\uD83E[\uDD3C\uDDDE\uDDDF]|[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF84\uDF86-\uDF93\uDFA0-\uDFC1\uDFC5\uDFC6\uDFC8\uDFC9\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC07\uDC09-\uDC14\uDC16-\uDC3A\uDC3C-\uDC3E\uDC40\uDC44\uDC45\uDC51-\uDC65\uDC6A\uDC79-\uDC7B\uDC7D-\uDC80\uDC84\uDC88-\uDC8E\uDC90\uDC92-\uDCA9\uDCAB-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDDA4\uDDFB-\uDE2D\uDE2F-\uDE34\uDE37-\uDE44\uDE48-\uDE4A\uDE80-\uDEA2\uDEA4-\uDEB3\uDEB7-\uDEBF\uDEC1-\uDEC5\uDED0-\uDED2\uDED5-\uDED7\uDEEB\uDEEC\uDEF4-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0D\uDD0E\uDD10-\uDD17\uDD1D\uDD20-\uDD25\uDD27-\uDD2F\uDD3A\uDD3F-\uDD45\uDD47-\uDD76\uDD78\uDD7A-\uDDB4\uDDB7\uDDBA\uDDBC-\uDDCB\uDDD0\uDDE0-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6]|(?:[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u270A\u270B\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF93\uDFA0-\uDFCA\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF4\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC3E\uDC40\uDC42-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDD7A\uDD95\uDD96\uDDA4\uDDFB-\uDE4F\uDE80-\uDEC5\uDECC\uDED0-\uDED2\uDED5-\uDED7\uDEEB\uDEEC\uDEF4-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0C-\uDD3A\uDD3C-\uDD45\uDD47-\uDD78\uDD7A-\uDDCB\uDDCD-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6])|(?:[#\*0-9\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u231A\u231B\u2328\u23CF\u23E9-\u23F3\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB-\u25FE\u2600-\u2604\u260E\u2611\u2614\u2615\u2618\u261D\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u2648-\u2653\u265F\u2660\u2663\u2665\u2666\u2668\u267B\u267E\u267F\u2692-\u2697\u2699\u269B\u269C\u26A0\u26A1\u26A7\u26AA\u26AB\u26B0\u26B1\u26BD\u26BE\u26C4\u26C5\u26C8\u26CE\u26CF\u26D1\u26D3\u26D4\u26E9\u26EA\u26F0-\u26F5\u26F7-\u26FA\u26FD\u2702\u2705\u2708-\u270D\u270F\u2712\u2714\u2716\u271D\u2721\u2728\u2733\u2734\u2744\u2747\u274C\u274E\u2753-\u2755\u2757\u2763\u2764\u2795-\u2797\u27A1\u27B0\u27BF\u2934\u2935\u2B05-\u2B07\u2B1B\u2B1C\u2B50\u2B55\u3030\u303D\u3297\u3299]|\uD83C[\uDC04\uDCCF\uDD70\uDD71\uDD7E\uDD7F\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE02\uDE1A\uDE2F\uDE32-\uDE3A\uDE50\uDE51\uDF00-\uDF21\uDF24-\uDF93\uDF96\uDF97\uDF99-\uDF9B\uDF9E-\uDFF0\uDFF3-\uDFF5\uDFF7-\uDFFF]|\uD83D[\uDC00-\uDCFD\uDCFF-\uDD3D\uDD49-\uDD4E\uDD50-\uDD67\uDD6F\uDD70\uDD73-\uDD7A\uDD87\uDD8A-\uDD8D\uDD90\uDD95\uDD96\uDDA4\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA-\uDE4F\uDE80-\uDEC5\uDECB-\uDED2\uDED5-\uDED7\uDEE0-\uDEE5\uDEE9\uDEEB\uDEEC\uDEF0\uDEF3-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0C-\uDD3A\uDD3C-\uDD45\uDD47-\uDD78\uDD7A-\uDDCB\uDDCD-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6])\uFE0F|(?:[\u261D\u26F9\u270A-\u270D]|\uD83C[\uDF85\uDFC2-\uDFC4\uDFC7\uDFCA-\uDFCC]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66-\uDC78\uDC7C\uDC81-\uDC83\uDC85-\uDC87\uDC8F\uDC91\uDCAA\uDD74\uDD75\uDD7A\uDD90\uDD95\uDD96\uDE45-\uDE47\uDE4B-\uDE4F\uDEA3\uDEB4-\uDEB6\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1F\uDD26\uDD30-\uDD39\uDD3C-\uDD3E\uDD77\uDDB5\uDDB6\uDDB8\uDDB9\uDDBB\uDDCD-\uDDCF\uDDD1-\uDDDD])/g;
};
}
});
var string_width_exports = {};
__export(string_width_exports, {
default: () => stringWidth
});
function stringWidth(string) {
if (typeof string !== "string" || string.length === 0) {
return 0;
}
string = stripAnsi(string);
if (string.length === 0) {
return 0;
}
string = string.replace((0, import_emoji_regex.default)(), " ");
let width = 0;
for (let index = 0; index < string.length; index++) {
const codePoint = string.codePointAt(index);
if (codePoint <= 31 || codePoint >= 127 && codePoint <= 159) {
continue;
}
if (codePoint >= 768 && codePoint <= 879) {
continue;
}
if (codePoint > 65535) {
index++;
}
width += isFullwidthCodePoint(codePoint) ? 2 : 1;
}
return width;
}
var import_emoji_regex;
var init_string_width = __esm({
"node_modules/string-width/index.js"() {
init_define_process();
init_strip_ansi();
init_is_fullwidth_code_point();
import_emoji_regex = __toESM2(require_emoji_regex());
}
});
var require_get_string_width = __commonJS22({
"src/utils/get-string-width.js"(exports22, module22) {
"use strict";
init_define_process();
var stringWidth2 = (init_string_width(), __toCommonJS(string_width_exports)).default;
var notAsciiRegex = /[^\x20-\x7F]/;
function getStringWidth(text) {
if (!text) {
return 0;
}
if (!notAsciiRegex.test(text)) {
return text.length;
}
return stringWidth2(text);
}
module22.exports = getStringWidth;
}
});
var require_doc_utils = __commonJS22({
"src/document/doc-utils.js"(exports22, module22) {
"use strict";
init_define_process();
var getLast = require_get_last();
var {
literalline,
join
} = require_doc_builders();
var isConcat = (doc) => Array.isArray(doc) || doc && doc.type === "concat";
var getDocParts = (doc) => {
if (Array.isArray(doc)) {
return doc;
}
if (doc.type !== "concat" && doc.type !== "fill") {
throw new Error("Expect doc type to be `concat` or `fill`.");
}
return doc.parts;
};
var traverseDocOnExitStackMarker = {};
function traverseDoc(doc, onEnter, onExit, shouldTraverseConditionalGroups) {
const docsStack = [doc];
while (docsStack.length > 0) {
const doc2 = docsStack.pop();
if (doc2 === traverseDocOnExitStackMarker) {
onExit(docsStack.pop());
continue;
}
if (onExit) {
docsStack.push(doc2, traverseDocOnExitStackMarker);
}
if (!onEnter || onEnter(doc2) !== false) {
if (isConcat(doc2) || doc2.type === "fill") {
const parts = getDocParts(doc2);
for (let ic = parts.length, i = ic - 1; i >= 0; --i) {
docsStack.push(parts[i]);
}
} else if (doc2.type === "if-break") {
if (doc2.flatContents) {
docsStack.push(doc2.flatContents);
}
if (doc2.breakContents) {
docsStack.push(doc2.breakContents);
}
} else if (doc2.type === "group" && doc2.expandedStates) {
if (shouldTraverseConditionalGroups) {
for (let ic = doc2.expandedStates.length, i = ic - 1; i >= 0; --i) {
docsStack.push(doc2.expandedStates[i]);
}
} else {
docsStack.push(doc2.contents);
}
} else if (doc2.contents) {
docsStack.push(doc2.contents);
}
}
}
}
function mapDoc(doc, cb) {
const mapped = /* @__PURE__ */ new Map();
return rec(doc);
function rec(doc2) {
if (mapped.has(doc2)) {
return mapped.get(doc2);
}
const result = process2(doc2);
mapped.set(doc2, result);
return result;
}
function process2(doc2) {
if (Array.isArray(doc2)) {
return cb(doc2.map(rec));
}
if (doc2.type === "concat" || doc2.type === "fill") {
const parts = doc2.parts.map(rec);
return cb(Object.assign(Object.assign({}, doc2), {}, {
parts
}));
}
if (doc2.type === "if-break") {
const breakContents = doc2.breakContents && rec(doc2.breakContents);
const flatContents = doc2.flatContents && rec(doc2.flatContents);
return cb(Object.assign(Object.assign({}, doc2), {}, {
breakContents,
flatContents
}));
}
if (doc2.type === "group" && doc2.expandedStates) {
const expandedStates = doc2.expandedStates.map(rec);
const contents = expandedStates[0];
return cb(Object.assign(Object.assign({}, doc2), {}, {
contents,
expandedStates
}));
}
if (doc2.contents) {
const contents = rec(doc2.contents);
return cb(Object.assign(Object.assign({}, doc2), {}, {
contents
}));
}
return cb(doc2);
}
}
function findInDoc(doc, fn, defaultValue) {
let result = defaultValue;
let hasStopped = false;
function findInDocOnEnterFn(doc2) {
const maybeResult = fn(doc2);
if (maybeResult !== void 0) {
hasStopped = true;
result = maybeResult;
}
if (hasStopped) {
return false;
}
}
traverseDoc(doc, findInDocOnEnterFn);
return result;
}
function willBreakFn(doc) {
if (doc.type === "group" && doc.break) {
return true;
}
if (doc.type === "line" && doc.hard) {
return true;
}
if (doc.type === "break-parent") {
return true;
}
}
function willBreak(doc) {
return findInDoc(doc, willBreakFn, false);
}
function breakParentGroup(groupStack) {
if (groupStack.length > 0) {
const parentGroup = getLast(groupStack);
if (!parentGroup.expandedStates && !parentGroup.break) {
parentGroup.break = "propagated";
}
}
return null;
}
function propagateBreaks(doc) {
const alreadyVisitedSet = /* @__PURE__ */ new Set();
const groupStack = [];
function propagateBreaksOnEnterFn(doc2) {
if (doc2.type === "break-parent") {
breakParentGroup(groupStack);
}
if (doc2.type === "group") {
groupStack.push(doc2);
if (alreadyVisitedSet.has(doc2)) {
return false;
}
alreadyVisitedSet.add(doc2);
}
}
function propagateBreaksOnExitFn(doc2) {
if (doc2.type === "group") {
const group = groupStack.pop();
if (group.break) {
breakParentGroup(groupStack);
}
}
}
traverseDoc(doc, propagateBreaksOnEnterFn, propagateBreaksOnExitFn, true);
}
function removeLinesFn(doc) {
if (doc.type === "line" && !doc.hard) {
return doc.soft ? "" : " ";
}
if (doc.type === "if-break") {
return doc.flatContents || "";
}
return doc;
}
function removeLines(doc) {
return mapDoc(doc, removeLinesFn);
}
var isHardline = (doc, nextDoc) => doc && doc.type === "line" && doc.hard && nextDoc && nextDoc.type === "break-parent";
function stripDocTrailingHardlineFromDoc(doc) {
if (!doc) {
return doc;
}
if (isConcat(doc) || doc.type === "fill") {
const parts = getDocParts(doc);
while (parts.length > 1 && isHardline(...parts.slice(-2))) {
parts.length -= 2;
}
if (parts.length > 0) {
const lastPart = stripDocTrailingHardlineFromDoc(getLast(parts));
parts[parts.length - 1] = lastPart;
}
return Array.isArray(doc) ? parts : Object.assign(Object.assign({}, doc), {}, {
parts
});
}
switch (doc.type) {
case "align":
case "indent":
case "indent-if-break":
case "group":
case "line-suffix":
case "label": {
const contents = stripDocTrailingHardlineFromDoc(doc.contents);
return Object.assign(Object.assign({}, doc), {}, {
contents
});
}
case "if-break": {
const breakContents = stripDocTrailingHardlineFromDoc(doc.breakContents);
const flatContents = stripDocTrailingHardlineFromDoc(doc.flatContents);
return Object.assign(Object.assign({}, doc), {}, {
breakContents,
flatContents
});
}
}
return doc;
}
function stripTrailingHardline(doc) {
return stripDocTrailingHardlineFromDoc(cleanDoc(doc));
}
function cleanDocFn(doc) {
switch (doc.type) {
case "fill":
if (doc.parts.every((part) => part === "")) {
return "";
}
break;
case "group":
if (!doc.contents && !doc.id && !doc.break && !doc.expandedStates) {
return "";
}
if (doc.contents.type === "group" && doc.contents.id === doc.id && doc.contents.break === doc.break && doc.contents.expandedStates === doc.expandedStates) {
return doc.contents;
}
break;
case "align":
case "indent":
case "indent-if-break":
case "line-suffix":
if (!doc.contents) {
return "";
}
break;
case "if-break":
if (!doc.flatContents && !doc.breakContents) {
return "";
}
break;
}
if (!isConcat(doc)) {
return doc;
}
const parts = [];
for (const part of getDocParts(doc)) {
if (!part) {
continue;
}
const [currentPart, ...restParts] = isConcat(part) ? getDocParts(part) : [part];
if (typeof currentPart === "string" && typeof getLast(parts) === "string") {
parts[parts.length - 1] += currentPart;
} else {
parts.push(currentPart);
}
parts.push(...restParts);
}
if (parts.length === 0) {
return "";
}
if (parts.length === 1) {
return parts[0];
}
return Array.isArray(doc) ? parts : Object.assign(Object.assign({}, doc), {}, {
parts
});
}
function cleanDoc(doc) {
return mapDoc(doc, (currentDoc) => cleanDocFn(currentDoc));
}
function normalizeParts(parts) {
const newParts = [];
const restParts = parts.filter(Boolean);
while (restParts.length > 0) {
const part = restParts.shift();
if (!part) {
continue;
}
if (isConcat(part)) {
restParts.unshift(...getDocParts(part));
continue;
}
if (newParts.length > 0 && typeof getLast(newParts) === "string" && typeof part === "string") {
newParts[newParts.length - 1] += part;
continue;
}
newParts.push(part);
}
return newParts;
}
function normalizeDoc(doc) {
return mapDoc(doc, (currentDoc) => {
if (Array.isArray(currentDoc)) {
return normalizeParts(currentDoc);
}
if (!currentDoc.parts) {
return currentDoc;
}
return Object.assign(Object.assign({}, currentDoc), {}, {
parts: normalizeParts(currentDoc.parts)
});
});
}
function replaceEndOfLine(doc) {
return mapDoc(doc, (currentDoc) => typeof currentDoc === "string" && currentDoc.includes("\n") ? replaceTextEndOfLine(currentDoc) : currentDoc);
}
function replaceTextEndOfLine(text) {
let replacement = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : literalline;
return join(replacement, text.split("\n")).parts;
}
function canBreakFn(doc) {
if (doc.type === "line") {
return true;
}
}
function canBreak(doc) {
return findInDoc(doc, canBreakFn, false);
}
module22.exports = {
isConcat,
getDocParts,
willBreak,
traverseDoc,
findInDoc,
mapDoc,
propagateBreaks,
removeLines,
stripTrailingHardline,
normalizeParts,
normalizeDoc,
cleanDoc,
replaceTextEndOfLine,
replaceEndOfLine,
canBreak
};
}
});
var require_doc_printer = __commonJS22({
"src/document/doc-printer.js"(exports22, module22) {
"use strict";
init_define_process();
var {
convertEndOfLineToChars
} = require_end_of_line();
var getLast = require_get_last();
var getStringWidth = require_get_string_width();
var {
fill,
cursor,
indent
} = require_doc_builders();
var {
isConcat,
getDocParts
} = require_doc_utils();
var groupModeMap;
var MODE_BREAK = 1;
var MODE_FLAT = 2;
function rootIndent() {
return {
value: "",
length: 0,
queue: []
};
}
function makeIndent(ind, options) {
return generateInd(ind, {
type: "indent"
}, options);
}
function makeAlign(indent2, widthOrDoc, options) {
if (widthOrDoc === Number.NEGATIVE_INFINITY) {
return indent2.root || rootIndent();
}
if (widthOrDoc < 0) {
return generateInd(indent2, {
type: "dedent"
}, options);
}
if (!widthOrDoc) {
return indent2;
}
if (widthOrDoc.type === "root") {
return Object.assign(Object.assign({}, indent2), {}, {
root: indent2
});
}
const alignType = typeof widthOrDoc === "string" ? "stringAlign" : "numberAlign";
return generateInd(indent2, {
type: alignType,
n: widthOrDoc
}, options);
}
function generateInd(ind, newPart, options) {
const queue = newPart.type === "dedent" ? ind.queue.slice(0, -1) : [...ind.queue, newPart];
let value = "";
let length = 0;
let lastTabs = 0;
let lastSpaces = 0;
for (const part of queue) {
switch (part.type) {
case "indent":
flush();
if (options.useTabs) {
addTabs(1);
} else {
addSpaces(options.tabWidth);
}
break;
case "stringAlign":
flush();
value += part.n;
length += part.n.length;
break;
case "numberAlign":
lastTabs += 1;
lastSpaces += part.n;
break;
default:
throw new Error(`Unexpected type '${part.type}'`);
}
}
flushSpaces();
return Object.assign(Object.assign({}, ind), {}, {
value,
length,
queue
});
function addTabs(count) {
value += " ".repeat(count);
length += options.tabWidth * count;
}
function addSpaces(count) {
value += " ".repeat(count);
length += count;
}
function flush() {
if (options.useTabs) {
flushTabs();
} else {
flushSpaces();
}
}
function flushTabs() {
if (lastTabs > 0) {
addTabs(lastTabs);
}
resetLast();
}
function flushSpaces() {
if (lastSpaces > 0) {
addSpaces(lastSpaces);
}
resetLast();
}
function resetLast() {
lastTabs = 0;
lastSpaces = 0;
}
}
function trim(out) {
if (out.length === 0) {
return 0;
}
let trimCount = 0;
while (out.length > 0 && typeof getLast(out) === "string" && /^[\t ]*$/.test(getLast(out))) {
trimCount += out.pop().length;
}
if (out.length > 0 && typeof getLast(out) === "string") {
const trimmed = getLast(out).replace(/[\t ]*$/, "");
trimCount += getLast(out).length - trimmed.length;
out[out.length - 1] = trimmed;
}
return trimCount;
}
function fits(next, restCommands, width, hasLineSuffix, mustBeFlat) {
let restIdx = restCommands.length;
const cmds = [next];
const out = [];
while (width >= 0) {
if (cmds.length === 0) {
if (restIdx === 0) {
return true;
}
cmds.push(restCommands[--restIdx]);
continue;
}
const {
mode,
doc
} = cmds.pop();
if (typeof doc === "string") {
out.push(doc);
width -= getStringWidth(doc);
} else if (isConcat(doc) || doc.type === "fill") {
const parts = getDocParts(doc);
for (let i = parts.length - 1; i >= 0; i--) {
cmds.push({
mode,
doc: parts[i]
});
}
} else {
switch (doc.type) {
case "indent":
case "align":
case "indent-if-break":
case "label":
cmds.push({
mode,
doc: doc.contents
});
break;
case "trim":
width += trim(out);
break;
case "group": {
if (mustBeFlat && doc.break) {
return false;
}
const groupMode = doc.break ? MODE_BREAK : mode;
const contents = doc.expandedStates && groupMode === MODE_BREAK ? getLast(doc.expandedStates) : doc.contents;
cmds.push({
mode: groupMode,
doc: contents
});
break;
}
case "if-break": {
const groupMode = doc.groupId ? groupModeMap[doc.groupId] || MODE_FLAT : mode;
const contents = groupMode === MODE_BREAK ? doc.breakContents : doc.flatContents;
if (contents) {
cmds.push({
mode,
doc: contents
});
}
break;
}
case "line":
if (mode === MODE_BREAK || doc.hard) {
return true;
}
if (!doc.soft) {
out.push(" ");
width--;
}
break;
case "line-suffix":
hasLineSuffix = true;
break;
case "line-suffix-boundary":
if (hasLineSuffix) {
return false;
}
break;
}
}
}
return false;
}
function printDocToString(doc, options) {
groupModeMap = {};
const width = options.printWidth;
const newLine = convertEndOfLineToChars(options.endOfLine);
let pos = 0;
const cmds = [{
ind: rootIndent(),
mode: MODE_BREAK,
doc
}];
const out = [];
let shouldRemeasure = false;
const lineSuffix = [];
while (cmds.length > 0) {
const {
ind,
mode,
doc: doc2
} = cmds.pop();
if (typeof doc2 === "string") {
const formatted = newLine !== "\n" ? doc2.replace(/\n/g, newLine) : doc2;
out.push(formatted);
pos += getStringWidth(formatted);
} else if (isConcat(doc2)) {
const parts = getDocParts(doc2);
for (let i = parts.length - 1; i >= 0; i--) {
cmds.push({
ind,
mode,
doc: parts[i]
});
}
} else {
switch (doc2.type) {
case "cursor":
out.push(cursor.placeholder);
break;
case "indent":
cmds.push({
ind: makeIndent(ind, options),
mode,
doc: doc2.contents
});
break;
case "align":
cmds.push({
ind: makeAlign(ind, doc2.n, options),
mode,
doc: doc2.contents
});
break;
case "trim":
pos -= trim(out);
break;
case "group":
switch (mode) {
case MODE_FLAT:
if (!shouldRemeasure) {
cmds.push({
ind,
mode: doc2.break ? MODE_BREAK : MODE_FLAT,
doc: doc2.contents
});
break;
}
case MODE_BREAK: {
shouldRemeasure = false;
const next = {
ind,
mode: MODE_FLAT,
doc: doc2.contents
};
const rem = width - pos;
const hasLineSuffix = lineSuffix.length > 0;
if (!doc2.break && fits(next, cmds, rem, hasLineSuffix)) {
cmds.push(next);
} else {
if (doc2.expandedStates) {
const mostExpanded = getLast(doc2.expandedStates);
if (doc2.break) {
cmds.push({
ind,
mode: MODE_BREAK,
doc: mostExpanded
});
break;
} else {
for (let i = 1; i < doc2.expandedStates.length + 1; i++) {
if (i >= doc2.expandedStates.length) {
cmds.push({
ind,
mode: MODE_BREAK,
doc: mostExpanded
});
break;
} else {
const state = doc2.expandedStates[i];
const cmd = {
ind,
mode: MODE_FLAT,
doc: state
};
if (fits(cmd, cmds, rem, hasLineSuffix)) {
cmds.push(cmd);
break;
}
}
}
}
} else {
cmds.push({
ind,
mode: MODE_BREAK,
doc: doc2.contents
});
}
}
break;
}
}
if (doc2.id) {
groupModeMap[doc2.id] = getLast(cmds).mode;
}
break;
case "fill": {
const rem = width - pos;
const {
parts
} = doc2;
if (parts.length === 0) {
break;
}
const [content, whitespace] = parts;
const contentFlatCmd = {
ind,
mode: MODE_FLAT,
doc: content
};
const contentBreakCmd = {
ind,
mode: MODE_BREAK,
doc: content
};
const contentFits = fits(contentFlatCmd, [], rem, lineSuffix.length > 0, true);
if (parts.length === 1) {
if (contentFits) {
cmds.push(contentFlatCmd);
} else {
cmds.push(contentBreakCmd);
}
break;
}
const whitespaceFlatCmd = {
ind,
mode: MODE_FLAT,
doc: whitespace
};
const whitespaceBreakCmd = {
ind,
mode: MODE_BREAK,
doc: whitespace
};
if (parts.length === 2) {
if (contentFits) {
cmds.push(whitespaceFlatCmd, contentFlatCmd);
} else {
cmds.push(whitespaceBreakCmd, contentBreakCmd);
}
break;
}
parts.splice(0, 2);
const remainingCmd = {
ind,
mode,
doc: fill(parts)
};
const secondContent = parts[0];
const firstAndSecondContentFlatCmd = {
ind,
mode: MODE_FLAT,
doc: [content, whitespace, secondContent]
};
const firstAndSecondContentFits = fits(firstAndSecondContentFlatCmd, [], rem, lineSuffix.length > 0, true);
if (firstAndSecondContentFits) {
cmds.push(remainingCmd, whitespaceFlatCmd, contentFlatCmd);
} else if (contentFits) {
cmds.push(remainingCmd, whitespaceBreakCmd, contentFlatCmd);
} else {
cmds.push(remainingCmd, whitespaceBreakCmd, contentBreakCmd);
}
break;
}
case "if-break":
case "indent-if-break": {
const groupMode = doc2.groupId ? groupModeMap[doc2.groupId] : mode;
if (groupMode === MODE_BREAK) {
const breakContents = doc2.type === "if-break" ? doc2.breakContents : doc2.negate ? doc2.contents : indent(doc2.contents);
if (breakContents) {
cmds.push({
ind,
mode,
doc: breakContents
});
}
}
if (groupMode === MODE_FLAT) {
const flatContents = doc2.type === "if-break" ? doc2.flatContents : doc2.negate ? indent(doc2.contents) : doc2.contents;
if (flatContents) {
cmds.push({
ind,
mode,
doc: flatContents
});
}
}
break;
}
case "line-suffix":
lineSuffix.push({
ind,
mode,
doc: doc2.contents
});
break;
case "line-suffix-boundary":
if (lineSuffix.length > 0) {
cmds.push({
ind,
mode,
doc: {
type: "line",
hard: true
}
});
}
break;
case "line":
switch (mode) {
case MODE_FLAT:
if (!doc2.hard) {
if (!doc2.soft) {
out.push(" ");
pos += 1;
}
break;
} else {
shouldRemeasure = true;
}
case MODE_BREAK:
if (lineSuffix.length > 0) {
cmds.push({
ind,
mode,
doc: doc2
}, ...lineSuffix.reverse());
lineSuffix.length = 0;
break;
}
if (doc2.literal) {
if (ind.root) {
out.push(newLine, ind.root.value);
pos = ind.root.length;
} else {
out.push(newLine);
pos = 0;
}
} else {
pos -= trim(out);
out.push(newLine + ind.value);
pos = ind.length;
}
break;
}
break;
case "label":
cmds.push({
ind,
mode,
doc: doc2.contents
});
break;
default:
}
}
if (cmds.length === 0 && lineSuffix.length > 0) {
cmds.push(...lineSuffix.reverse());
lineSuffix.length = 0;
}
}
const cursorPlaceholderIndex = out.indexOf(cursor.placeholder);
if (cursorPlaceholderIndex !== -1) {
const otherCursorPlaceholderIndex = out.indexOf(cursor.placeholder, cursorPlaceholderIndex + 1);
const beforeCursor = out.slice(0, cursorPlaceholderIndex).join("");
const aroundCursor = out.slice(cursorPlaceholderIndex + 1, otherCursorPlaceholderIndex).join("");
const afterCursor = out.slice(otherCursorPlaceholderIndex + 1).join("");
return {
formatted: beforeCursor + aroundCursor + afterCursor,
cursorNodeStart: beforeCursor.length,
cursorNodeText: aroundCursor
};
}
return {
formatted: out.join("")
};
}
module22.exports = {
printDocToString
};
}
});
var require_doc_debug = __commonJS22({
"src/document/doc-debug.js"(exports22, module22) {
"use strict";
init_define_process();
var {
isConcat,
getDocParts
} = require_doc_utils();
function flattenDoc(doc) {
if (!doc) {
return "";
}
if (isConcat(doc)) {
const res = [];
for (const part of getDocParts(doc)) {
if (isConcat(part)) {
res.push(...flattenDoc(part).parts);
} else {
const flattened = flattenDoc(part);
if (flattened !== "") {
res.push(flattened);
}
}
}
return {
type: "concat",
parts: res
};
}
if (doc.type === "if-break") {
return Object.assign(Object.assign({}, doc), {}, {
breakContents: flattenDoc(doc.breakContents),
flatContents: flattenDoc(doc.flatContents)
});
}
if (doc.type === "group") {
return Object.assign(Object.assign({}, doc), {}, {
contents: flattenDoc(doc.contents),
expandedStates: doc.expandedStates && doc.expandedStates.map(flattenDoc)
});
}
if (doc.type === "fill") {
return {
type: "fill",
parts: doc.parts.map(flattenDoc)
};
}
if (doc.contents) {
return Object.assign(Object.assign({}, doc), {}, {
contents: flattenDoc(doc.contents)
});
}
return doc;
}
function printDocToDebug(doc) {
const printedSymbols = /* @__PURE__ */ Object.create(null);
const usedKeysForSymbols = /* @__PURE__ */ new Set();
return printDoc(flattenDoc(doc));
function printDoc(doc2, index, parentParts) {
if (typeof doc2 === "string") {
return JSON.stringify(doc2);
}
if (isConcat(doc2)) {
const printed = getDocParts(doc2).map(printDoc).filter(Boolean);
return printed.length === 1 ? printed[0] : `[${printed.join(", ")}]`;
}
if (doc2.type === "line") {
const withBreakParent = Array.isArray(parentParts) && parentParts[index + 1] && parentParts[index + 1].type === "break-parent";
if (doc2.literal) {
return withBreakParent ? "literalline" : "literallineWithoutBreakParent";
}
if (doc2.hard) {
return withBreakParent ? "hardline" : "hardlineWithoutBreakParent";
}
if (doc2.soft) {
return "softline";
}
return "line";
}
if (doc2.type === "break-parent") {
const afterHardline = Array.isArray(parentParts) && parentParts[index - 1] && parentParts[index - 1].type === "line" && parentParts[index - 1].hard;
return afterHardline ? void 0 : "breakParent";
}
if (doc2.type === "trim") {
return "trim";
}
if (doc2.type === "indent") {
return "indent(" + printDoc(doc2.contents) + ")";
}
if (doc2.type === "align") {
return doc2.n === Number.NEGATIVE_INFINITY ? "dedentToRoot(" + printDoc(doc2.contents) + ")" : doc2.n < 0 ? "dedent(" + printDoc(doc2.contents) + ")" : doc2.n.type === "root" ? "markAsRoot(" + printDoc(doc2.contents) + ")" : "align(" + JSON.stringify(doc2.n) + ", " + printDoc(doc2.contents) + ")";
}
if (doc2.type === "if-break") {
return "ifBreak(" + printDoc(doc2.breakContents) + (doc2.flatContents ? ", " + printDoc(doc2.flatContents) : "") + (doc2.groupId ? (!doc2.flatContents ? ', ""' : "") + `, { groupId: ${printGroupId(doc2.groupId)} }` : "") + ")";
}
if (doc2.type === "indent-if-break") {
const optionsParts = [];
if (doc2.negate) {
optionsParts.push("negate: true");
}
if (doc2.groupId) {
optionsParts.push(`groupId: ${printGroupId(doc2.groupId)}`);
}
const options = optionsParts.length > 0 ? `, { ${optionsParts.join(", ")} }` : "";
return `indentIfBreak(${printDoc(doc2.contents)}${options})`;
}
if (doc2.type === "group") {
const optionsParts = [];
if (doc2.break && doc2.break !== "propagated") {
optionsParts.push("shouldBreak: true");
}
if (doc2.id) {
optionsParts.push(`id: ${printGroupId(doc2.id)}`);
}
const options = optionsParts.length > 0 ? `, { ${optionsParts.join(", ")} }` : "";
if (doc2.expandedStates) {
return `conditionalGroup([${doc2.expandedStates.map((part) => printDoc(part)).join(",")}]${options})`;
}
return `group(${printDoc(doc2.contents)}${options})`;
}
if (doc2.type === "fill") {
return `fill([${doc2.parts.map((part) => printDoc(part)).join(", ")}])`;
}
if (doc2.type === "line-suffix") {
return "lineSuffix(" + printDoc(doc2.contents) + ")";
}
if (doc2.type === "line-suffix-boundary") {
return "lineSuffixBoundary";
}
if (doc2.type === "label") {
return `label(${JSON.stringify(doc2.label)}, ${printDoc(doc2.contents)})`;
}
throw new Error("Unknown doc type " + doc2.type);
}
function printGroupId(id) {
if (typeof id !== "symbol") {
return JSON.stringify(String(id));
}
if (id in printedSymbols) {
return printedSymbols[id];
}
const prefix = String(id).slice(7, -1) || "symbol";
for (let counter = 0; ; counter++) {
const key = prefix + (counter > 0 ? ` #${counter}` : "");
if (!usedKeysForSymbols.has(key)) {
usedKeysForSymbols.add(key);
return printedSymbols[id] = `Symbol.for(${JSON.stringify(key)})`;
}
}
}
}
module22.exports = {
printDocToDebug
};
}
});
init_define_process();
module3.exports = {
builders: require_doc_builders(),
printer: require_doc_printer(),
utils: require_doc_utils(),
debug: require_doc_debug()
};
}
});
return require_doc_js_umd();
});
}
});
// node_modules/prettier/third-party.js
var require_third_party = __commonJS({
"node_modules/prettier/third-party.js"(exports, module2) {
"use strict";
var __getOwnPropNames2 = Object.getOwnPropertyNames;
var __commonJS2 = (cb, mod) => function __require() {
return mod || (0, cb[__getOwnPropNames2(cb)[0]])((mod = {
exports: {}
}).exports, mod), mod.exports;
};
var require_resolve_from = __commonJS2({
"node_modules/import-fresh/node_modules/resolve-from/index.js"(exports2, module22) {
"use strict";
var path = require("path");
var Module = require("module");
var fs2 = require("fs");
var resolveFrom = (fromDir, moduleId, silent) => {
if (typeof fromDir !== "string") {
throw new TypeError(`Expected \`fromDir\` to be of type \`string\`, got \`${typeof fromDir}\``);
}
if (typeof moduleId !== "string") {
throw new TypeError(`Expected \`moduleId\` to be of type \`string\`, got \`${typeof moduleId}\``);
}
try {
fromDir = fs2.realpathSync(fromDir);
} catch (err) {
if (err.code === "ENOENT") {
fromDir = path.resolve(fromDir);
} else if (silent) {
return null;
} else {
throw err;
}
}
const fromFile = path.join(fromDir, "noop.js");
const resolveFileName = () => Module._resolveFilename(moduleId, {
id: fromFile,
filename: fromFile,
paths: Module._nodeModulePaths(fromDir)
});
if (silent) {
try {
return resolveFileName();
} catch (err) {
return null;
}
}
return resolveFileName();
};
module22.exports = (fromDir, moduleId) => resolveFrom(fromDir, moduleId);
module22.exports.silent = (fromDir, moduleId) => resolveFrom(fromDir, moduleId, true);
}
});
var require_parent_module = __commonJS2({
"scripts/build/shims/parent-module.cjs"(exports2, module22) {
"use strict";
module22.exports = (file) => file;
}
});
var require_import_fresh = __commonJS2({
"node_modules/import-fresh/index.js"(exports2, module22) {
"use strict";
var path = require("path");
var resolveFrom = require_resolve_from();
var parentModule = require_parent_module();
module22.exports = (moduleId) => {
if (typeof moduleId !== "string") {
throw new TypeError("Expected a string");
}
const parentPath = parentModule(__filename);
const cwd = parentPath ? path.dirname(parentPath) : __dirname;
const filePath2 = resolveFrom(cwd, moduleId);
const oldModule = require.cache[filePath2];
if (oldModule && oldModule.parent) {
let i = oldModule.parent.children.length;
while (i--) {
if (oldModule.parent.children[i].id === filePath2) {
oldModule.parent.children.splice(i, 1);
}
}
}
delete require.cache[filePath2];
const parent = require.cache[parentPath];
return parent === void 0 ? require(filePath2) : parent.require(filePath2);
};
}
});
var require_is_arrayish = __commonJS2({
"node_modules/is-arrayish/index.js"(exports2, module22) {
"use strict";
module22.exports = function isArrayish(obj) {
if (!obj) {
return false;
}
return obj instanceof Array || Array.isArray(obj) || obj.length >= 0 && obj.splice instanceof Function;
};
}
});
var require_error_ex = __commonJS2({
"node_modules/error-ex/index.js"(exports2, module22) {
"use strict";
var util = require("util");
var isArrayish = require_is_arrayish();
var errorEx = function errorEx2(name, properties) {
if (!name || name.constructor !== String) {
properties = name || {};
name = Error.name;
}
var errorExError = function ErrorEXError(message) {
if (!this) {
return new ErrorEXError(message);
}
message = message instanceof Error ? message.message : message || this.message;
Error.call(this, message);
Error.captureStackTrace(this, errorExError);
this.name = name;
Object.defineProperty(this, "message", {
configurable: true,
enumerable: false,
get: function() {
var newMessage = message.split(/\r?\n/g);
for (var key in properties) {
if (!properties.hasOwnProperty(key)) {
continue;
}
var modifier = properties[key];
if ("message" in modifier) {
newMessage = modifier.message(this[key], newMessage) || newMessage;
if (!isArrayish(newMessage)) {
newMessage = [newMessage];
}
}
}
return newMessage.join("\n");
},
set: function(v) {
message = v;
}
});
var overwrittenStack = null;
var stackDescriptor = Object.getOwnPropertyDescriptor(this, "stack");
var stackGetter = stackDescriptor.get;
var stackValue = stackDescriptor.value;
delete stackDescriptor.value;
delete stackDescriptor.writable;
stackDescriptor.set = function(newstack) {
overwrittenStack = newstack;
};
stackDescriptor.get = function() {
var stack = (overwrittenStack || (stackGetter ? stackGetter.call(this) : stackValue)).split(/\r?\n+/g);
if (!overwrittenStack) {
stack[0] = this.name + ": " + this.message;
}
var lineCount = 1;
for (var key in properties) {
if (!properties.hasOwnProperty(key)) {
continue;
}
var modifier = properties[key];
if ("line" in modifier) {
var line = modifier.line(this[key]);
if (line) {
stack.splice(lineCount++, 0, " " + line);
}
}
if ("stack" in modifier) {
modifier.stack(this[key], stack);
}
}
return stack.join("\n");
};
Object.defineProperty(this, "stack", stackDescriptor);
};
if (Object.setPrototypeOf) {
Object.setPrototypeOf(errorExError.prototype, Error.prototype);
Object.setPrototypeOf(errorExError, Error);
} else {
util.inherits(errorExError, Error);
}
return errorExError;
};
errorEx.append = function(str, def) {
return {
message: function(v, message) {
v = v || def;
if (v) {
message[0] += " " + str.replace("%s", v.toString());
}
return message;
}
};
};
errorEx.line = function(str, def) {
return {
line: function(v) {
v = v || def;
if (v) {
return str.replace("%s", v.toString());
}
return null;
}
};
};
module22.exports = errorEx;
}
});
var require_json_parse_even_better_errors = __commonJS2({
"node_modules/json-parse-even-better-errors/index.js"(exports2, module22) {
"use strict";
var hexify = (char2) => {
const h = char2.charCodeAt(0).toString(16).toUpperCase();
return "0x" + (h.length % 2 ? "0" : "") + h;
};
var parseError = (e, txt, context) => {
if (!txt) {
return {
message: e.message + " while parsing empty string",
position: 0
};
}
const badToken = e.message.match(/^Unexpected token (.) .*position\s+(\d+)/i);
const errIdx = badToken ? +badToken[2] : e.message.match(/^Unexpected end of JSON.*/i) ? txt.length - 1 : null;
const msg = badToken ? e.message.replace(/^Unexpected token ./, `Unexpected token ${JSON.stringify(badToken[1])} (${hexify(badToken[1])})`) : e.message;
if (errIdx !== null && errIdx !== void 0) {
const start = errIdx <= context ? 0 : errIdx - context;
const end = errIdx + context >= txt.length ? txt.length : errIdx + context;
const slice = (start === 0 ? "" : "...") + txt.slice(start, end) + (end === txt.length ? "" : "...");
const near = txt === slice ? "" : "near ";
return {
message: msg + ` while parsing ${near}${JSON.stringify(slice)}`,
position: errIdx
};
} else {
return {
message: msg + ` while parsing '${txt.slice(0, context * 2)}'`,
position: 0
};
}
};
var JSONParseError = class extends SyntaxError {
constructor(er, txt, context, caller) {
context = context || 20;
const metadata = parseError(er, txt, context);
super(metadata.message);
Object.assign(this, metadata);
this.code = "EJSONPARSE";
this.systemError = er;
Error.captureStackTrace(this, caller || this.constructor);
}
get name() {
return this.constructor.name;
}
set name(n2) {
}
get [Symbol.toStringTag]() {
return this.constructor.name;
}
};
var kIndent = Symbol.for("indent");
var kNewline = Symbol.for("newline");
var formatRE = /^\s*[{\[]((?:\r?\n)+)([\s\t]*)/;
var emptyRE = /^(?:\{\}|\[\])((?:\r?\n)+)?$/;
var parseJson = (txt, reviver, context) => {
const parseText = stripBOM(txt);
context = context || 20;
try {
const [, newline = "\n", indent = " "] = parseText.match(emptyRE) || parseText.match(formatRE) || [, "", ""];
const result = JSON.parse(parseText, reviver);
if (result && typeof result === "object") {
result[kNewline] = newline;
result[kIndent] = indent;
}
return result;
} catch (e) {
if (typeof txt !== "string" && !Buffer.isBuffer(txt)) {
const isEmptyArray = Array.isArray(txt) && txt.length === 0;
throw Object.assign(new TypeError(`Cannot parse ${isEmptyArray ? "an empty array" : String(txt)}`), {
code: "EJSONPARSE",
systemError: e
});
}
throw new JSONParseError(e, parseText, context, parseJson);
}
};
var stripBOM = (txt) => String(txt).replace(/^\uFEFF/, "");
module22.exports = parseJson;
parseJson.JSONParseError = JSONParseError;
parseJson.noExceptions = (txt, reviver) => {
try {
return JSON.parse(stripBOM(txt), reviver);
} catch (e) {
}
};
}
});
var require_build = __commonJS2({
"node_modules/parse-json/node_modules/lines-and-columns/build/index.js"(exports2) {
"use strict";
exports2.__esModule = true;
exports2.LinesAndColumns = void 0;
var LF = "\n";
var CR = "\r";
var LinesAndColumns = function() {
function LinesAndColumns2(string) {
this.string = string;
var offsets = [0];
for (var offset = 0; offset < string.length; ) {
switch (string[offset]) {
case LF:
offset += LF.length;
offsets.push(offset);
break;
case CR:
offset += CR.length;
if (string[offset] === LF) {
offset += LF.length;
}
offsets.push(offset);
break;
default:
offset++;
break;
}
}
this.offsets = offsets;
}
LinesAndColumns2.prototype.locationForIndex = function(index) {
if (index < 0 || index > this.string.length) {
return null;
}
var line = 0;
var offsets = this.offsets;
while (offsets[line + 1] <= index) {
line++;
}
var column = index - offsets[line];
return {
line,
column
};
};
LinesAndColumns2.prototype.indexForLocation = function(location) {
var line = location.line, column = location.column;
if (line < 0 || line >= this.offsets.length) {
return null;
}
if (column < 0 || column > this.lengthOfLine(line)) {
return null;
}
return this.offsets[line] + column;
};
LinesAndColumns2.prototype.lengthOfLine = function(line) {
var offset = this.offsets[line];
var nextOffset = line === this.offsets.length - 1 ? this.string.length : this.offsets[line + 1];
return nextOffset - offset;
};
return LinesAndColumns2;
}();
exports2.LinesAndColumns = LinesAndColumns;
exports2["default"] = LinesAndColumns;
}
});
var require_js_tokens = __commonJS2({
"node_modules/js-tokens/index.js"(exports2) {
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.default = /((['"])(?:(?!\2|\\).|\\(?:\r\n|[\s\S]))*(\2)?|`(?:[^`\\$]|\\[\s\S]|\$(?!\{)|\$\{(?:[^{}]|\{[^}]*\}?)*\}?)*(`)?)|(\/\/.*)|(\/\*(?:[^*]|\*(?!\/))*(\*\/)?)|(\/(?!\*)(?:\[(?:(?![\]\\]).|\\.)*\]|(?![\/\]\\]).|\\.)+\/(?:(?!\s*(?:\b|[\u0080-\uFFFF$\\'"~({]|[+\-!](?!=)|\.?\d))|[gmiyus]{1,6}\b(?![\u0080-\uFFFF$\\]|\s*(?:[+\-*%&|^<>!=?({]|\/(?![\/*])))))|(0[xX][\da-fA-F]+|0[oO][0-7]+|0[bB][01]+|(?:\d*\.\d+|\d+\.?)(?:[eE][+-]?\d+)?)|((?!\d)(?:(?!\s)[$\w\u0080-\uFFFF]|\\u[\da-fA-F]{4}|\\u\{[\da-fA-F]+\})+)|(--|\+\+|&&|\|\||=>|\.{3}|(?:[+\-\/%&|^]|\*{1,2}|<{1,2}|>{1,3}|!=?|={1,2})=?|[?~.,:;[\](){}])|(\s+)|(^$|[\s\S])/g;
exports2.matchToToken = function(match) {
var token = {
type: "invalid",
value: match[0],
closed: void 0
};
if (match[1])
token.type = "string", token.closed = !!(match[3] || match[4]);
else if (match[5])
token.type = "comment";
else if (match[6])
token.type = "comment", token.closed = !!match[7];
else if (match[8])
token.type = "regex";
else if (match[9])
token.type = "number";
else if (match[10])
token.type = "name";
else if (match[11])
token.type = "punctuator";
else if (match[12])
token.type = "whitespace";
return token;
};
}
});
var require_identifier = __commonJS2({
"node_modules/@babel/helper-validator-identifier/lib/identifier.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.isIdentifierChar = isIdentifierChar;
exports2.isIdentifierName = isIdentifierName;
exports2.isIdentifierStart = isIdentifierStart;
var nonASCIIidentifierStartChars = "\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0370-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u048A-\u052F\u0531-\u0556\u0559\u0560-\u0588\u05D0-\u05EA\u05EF-\u05F2\u0620-\u064A\u066E\u066F\u0671-\u06D3\u06D5\u06E5\u06E6\u06EE\u06EF\u06FA-\u06FC\u06FF\u0710\u0712-\u072F\u074D-\u07A5\u07B1\u07CA-\u07EA\u07F4\u07F5\u07FA\u0800-\u0815\u081A\u0824\u0828\u0840-\u0858\u0860-\u086A\u0870-\u0887\u0889-\u088E\u08A0-\u08C9\u0904-\u0939\u093D\u0950\u0958-\u0961\u0971-\u0980\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD\u09CE\u09DC\u09DD\u09DF-\u09E1\u09F0\u09F1\u09FC\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A59-\u0A5C\u0A5E\u0A72-\u0A74\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABD\u0AD0\u0AE0\u0AE1\u0AF9\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3D\u0B5C\u0B5D\u0B5F-\u0B61\u0B71\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BD0\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C58-\u0C5A\u0C5D\u0C60\u0C61\u0C80\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CDD\u0CDE\u0CE0\u0CE1\u0CF1\u0CF2\u0D04-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D\u0D4E\u0D54-\u0D56\u0D5F-\u0D61\u0D7A-\u0D7F\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0E01-\u0E30\u0E32\u0E33\u0E40-\u0E46\u0E81\u0E82\u0E84\u0E86-\u0E8A\u0E8C-\u0EA3\u0EA5\u0EA7-\u0EB0\u0EB2\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6\u0EDC-\u0EDF\u0F00\u0F40-\u0F47\u0F49-\u0F6C\u0F88-\u0F8C\u1000-\u102A\u103F\u1050-\u1055\u105A-\u105D\u1061\u1065\u1066\u106E-\u1070\u1075-\u1081\u108E\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u1711\u171F-\u1731\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17D7\u17DC\u1820-\u1878\u1880-\u18A8\u18AA\u18B0-\u18F5\u1900-\u191E\u1950-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u1A00-\u1A16\u1A20-\u1A54\u1AA7\u1B05-\u1B33\u1B45-\u1B4C\u1B83-\u1BA0\u1BAE\u1BAF\u1BBA-\u1BE5\u1C00-\u1C23\u1C4D-\u1C4F\u1C5A-\u1C7D\u1C80-\u1C88\u1C90-\u1CBA\u1CBD-\u1CBF\u1CE9-\u1CEC\u1CEE-\u1CF3\u1CF5\u1CF6\u1CFA\u1D00-\u1DBF\u1E00-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2118-\u211D\u2124\u2126\u2128\u212A-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2CE4\u2CEB-\u2CEE\u2CF2\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u3005-\u3007\u3021-\u3029\u3031-\u3035\u3038-\u303C\u3041-\u3096\u309B-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312F\u3131-\u318E\u31A0-\u31BF\u31F0-\u31FF\u3400-\u4DBF\u4E00-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA61F\uA62A\uA62B\uA640-\uA66E\uA67F-\uA69D\uA6A0-\uA6EF\uA717-\uA71F\uA722-\uA788\uA78B-\uA7CA\uA7D0\uA7D1\uA7D3\uA7D5-\uA7D9\uA7F2-\uA801\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA840-\uA873\uA882-\uA8B3\uA8F2-\uA8F7\uA8FB\uA8FD\uA8FE\uA90A-\uA925\uA930-\uA946\uA960-\uA97C\uA984-\uA9B2\uA9CF\uA9E0-\uA9E4\uA9E6-\uA9EF\uA9FA-\uA9FE\uAA00-\uAA28\uAA40-\uAA42\uAA44-\uAA4B\uAA60-\uAA76\uAA7A\uAA7E-\uAAAF\uAAB1\uAAB5\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADD\uAAE0-\uAAEA\uAAF2-\uAAF4\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB69\uAB70-\uABE2\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE70-\uFE74\uFE76-\uFEFC\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC";
var nonASCIIidentifierChars = "\u200C\u200D\xB7\u0300-\u036F\u0387\u0483-\u0487\u0591-\u05BD\u05BF\u05C1\u05C2\u05C4\u05C5\u05C7\u0610-\u061A\u064B-\u0669\u0670\u06D6-\u06DC\u06DF-\u06E4\u06E7\u06E8\u06EA-\u06ED\u06F0-\u06F9\u0711\u0730-\u074A\u07A6-\u07B0\u07C0-\u07C9\u07EB-\u07F3\u07FD\u0816-\u0819\u081B-\u0823\u0825-\u0827\u0829-\u082D\u0859-\u085B\u0898-\u089F\u08CA-\u08E1\u08E3-\u0903\u093A-\u093C\u093E-\u094F\u0951-\u0957\u0962\u0963\u0966-\u096F\u0981-\u0983\u09BC\u09BE-\u09C4\u09C7\u09C8\u09CB-\u09CD\u09D7\u09E2\u09E3\u09E6-\u09EF\u09FE\u0A01-\u0A03\u0A3C\u0A3E-\u0A42\u0A47\u0A48\u0A4B-\u0A4D\u0A51\u0A66-\u0A71\u0A75\u0A81-\u0A83\u0ABC\u0ABE-\u0AC5\u0AC7-\u0AC9\u0ACB-\u0ACD\u0AE2\u0AE3\u0AE6-\u0AEF\u0AFA-\u0AFF\u0B01-\u0B03\u0B3C\u0B3E-\u0B44\u0B47\u0B48\u0B4B-\u0B4D\u0B55-\u0B57\u0B62\u0B63\u0B66-\u0B6F\u0B82\u0BBE-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCD\u0BD7\u0BE6-\u0BEF\u0C00-\u0C04\u0C3C\u0C3E-\u0C44\u0C46-\u0C48\u0C4A-\u0C4D\u0C55\u0C56\u0C62\u0C63\u0C66-\u0C6F\u0C81-\u0C83\u0CBC\u0CBE-\u0CC4\u0CC6-\u0CC8\u0CCA-\u0CCD\u0CD5\u0CD6\u0CE2\u0CE3\u0CE6-\u0CEF\u0CF3\u0D00-\u0D03\u0D3B\u0D3C\u0D3E-\u0D44\u0D46-\u0D48\u0D4A-\u0D4D\u0D57\u0D62\u0D63\u0D66-\u0D6F\u0D81-\u0D83\u0DCA\u0DCF-\u0DD4\u0DD6\u0DD8-\u0DDF\u0DE6-\u0DEF\u0DF2\u0DF3\u0E31\u0E34-\u0E3A\u0E47-\u0E4E\u0E50-\u0E59\u0EB1\u0EB4-\u0EBC\u0EC8-\u0ECE\u0ED0-\u0ED9\u0F18\u0F19\u0F20-\u0F29\u0F35\u0F37\u0F39\u0F3E\u0F3F\u0F71-\u0F84\u0F86\u0F87\u0F8D-\u0F97\u0F99-\u0FBC\u0FC6\u102B-\u103E\u1040-\u1049\u1056-\u1059\u105E-\u1060\u1062-\u1064\u1067-\u106D\u1071-\u1074\u1082-\u108D\u108F-\u109D\u135D-\u135F\u1369-\u1371\u1712-\u1715\u1732-\u1734\u1752\u1753\u1772\u1773\u17B4-\u17D3\u17DD\u17E0-\u17E9\u180B-\u180D\u180F-\u1819\u18A9\u1920-\u192B\u1930-\u193B\u1946-\u194F\u19D0-\u19DA\u1A17-\u1A1B\u1A55-\u1A5E\u1A60-\u1A7C\u1A7F-\u1A89\u1A90-\u1A99\u1AB0-\u1ABD\u1ABF-\u1ACE\u1B00-\u1B04\u1B34-\u1B44\u1B50-\u1B59\u1B6B-\u1B73\u1B80-\u1B82\u1BA1-\u1BAD\u1BB0-\u1BB9\u1BE6-\u1BF3\u1C24-\u1C37\u1C40-\u1C49\u1C50-\u1C59\u1CD0-\u1CD2\u1CD4-\u1CE8\u1CED\u1CF4\u1CF7-\u1CF9\u1DC0-\u1DFF\u203F\u2040\u2054\u20D0-\u20DC\u20E1\u20E5-\u20F0\u2CEF-\u2CF1\u2D7F\u2DE0-\u2DFF\u302A-\u302F\u3099\u309A\uA620-\uA629\uA66F\uA674-\uA67D\uA69E\uA69F\uA6F0\uA6F1\uA802\uA806\uA80B\uA823-\uA827\uA82C\uA880\uA881\uA8B4-\uA8C5\uA8D0-\uA8D9\uA8E0-\uA8F1\uA8FF-\uA909\uA926-\uA92D\uA947-\uA953\uA980-\uA983\uA9B3-\uA9C0\uA9D0-\uA9D9\uA9E5\uA9F0-\uA9F9\uAA29-\uAA36\uAA43\uAA4C\uAA4D\uAA50-\uAA59\uAA7B-\uAA7D\uAAB0\uAAB2-\uAAB4\uAAB7\uAAB8\uAABE\uAABF\uAAC1\uAAEB-\uAAEF\uAAF5\uAAF6\uABE3-\uABEA\uABEC\uABED\uABF0-\uABF9\uFB1E\uFE00-\uFE0F\uFE20-\uFE2F\uFE33\uFE34\uFE4D-\uFE4F\uFF10-\uFF19\uFF3F";
var nonASCIIidentifierStart = new RegExp("[" + nonASCIIidentifierStartChars + "]");
var nonASCIIidentifier = new RegExp("[" + nonASCIIidentifierStartChars + nonASCIIidentifierChars + "]");
nonASCIIidentifierStartChars = nonASCIIidentifierChars = null;
var astralIdentifierStartCodes = [0, 11, 2, 25, 2, 18, 2, 1, 2, 14, 3, 13, 35, 122, 70, 52, 268, 28, 4, 48, 48, 31, 14, 29, 6, 37, 11, 29, 3, 35, 5, 7, 2, 4, 43, 157, 19, 35, 5, 35, 5, 39, 9, 51, 13, 10, 2, 14, 2, 6, 2, 1, 2, 10, 2, 14, 2, 6, 2, 1, 68, 310, 10, 21, 11, 7, 25, 5, 2, 41, 2, 8, 70, 5, 3, 0, 2, 43, 2, 1, 4, 0, 3, 22, 11, 22, 10, 30, 66, 18, 2, 1, 11, 21, 11, 25, 71, 55, 7, 1, 65, 0, 16, 3, 2, 2, 2, 28, 43, 28, 4, 28, 36, 7, 2, 27, 28, 53, 11, 21, 11, 18, 14, 17, 111, 72, 56, 50, 14, 50, 14, 35, 349, 41, 7, 1, 79, 28, 11, 0, 9, 21, 43, 17, 47, 20, 28, 22, 13, 52, 58, 1, 3, 0, 14, 44, 33, 24, 27, 35, 30, 0, 3, 0, 9, 34, 4, 0, 13, 47, 15, 3, 22, 0, 2, 0, 36, 17, 2, 24, 20, 1, 64, 6, 2, 0, 2, 3, 2, 14, 2, 9, 8, 46, 39, 7, 3, 1, 3, 21, 2, 6, 2, 1, 2, 4, 4, 0, 19, 0, 13, 4, 159, 52, 19, 3, 21, 2, 31, 47, 21, 1, 2, 0, 185, 46, 42, 3, 37, 47, 21, 0, 60, 42, 14, 0, 72, 26, 38, 6, 186, 43, 117, 63, 32, 7, 3, 0, 3, 7, 2, 1, 2, 23, 16, 0, 2, 0, 95, 7, 3, 38, 17, 0, 2, 0, 29, 0, 11, 39, 8, 0, 22, 0, 12, 45, 20, 0, 19, 72, 264, 8, 2, 36, 18, 0, 50, 29, 113, 6, 2, 1, 2, 37, 22, 0, 26, 5, 2, 1, 2, 31, 15, 0, 328, 18, 16, 0, 2, 12, 2, 33, 125, 0, 80, 921, 103, 110, 18, 195, 2637, 96, 16, 1071, 18, 5, 4026, 582, 8634, 568, 8, 30, 18, 78, 18, 29, 19, 47, 17, 3, 32, 20, 6, 18, 689, 63, 129, 74, 6, 0, 67, 12, 65, 1, 2, 0, 29, 6135, 9, 1237, 43, 8, 8936, 3, 2, 6, 2, 1, 2, 290, 16, 0, 30, 2, 3, 0, 15, 3, 9, 395, 2309, 106, 6, 12, 4, 8, 8, 9, 5991, 84, 2, 70, 2, 1, 3, 0, 3, 1, 3, 3, 2, 11, 2, 0, 2, 6, 2, 64, 2, 3, 3, 7, 2, 6, 2, 27, 2, 3, 2, 4, 2, 0, 4, 6, 2, 339, 3, 24, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 7, 1845, 30, 7, 5, 262, 61, 147, 44, 11, 6, 17, 0, 322, 29, 19, 43, 485, 27, 757, 6, 2, 3, 2, 1, 2, 14, 2, 196, 60, 67, 8, 0, 1205, 3, 2, 26, 2, 1, 2, 0, 3, 0, 2, 9, 2, 3, 2, 0, 2, 0, 7, 0, 5, 0, 2, 0, 2, 0, 2, 2, 2, 1, 2, 0, 3, 0, 2, 0, 2, 0, 2, 0, 2, 0, 2, 1, 2, 0, 3, 3, 2, 6, 2, 3, 2, 3, 2, 0, 2, 9, 2, 16, 6, 2, 2, 4, 2, 16, 4421, 42719, 33, 4153, 7, 221, 3, 5761, 15, 7472, 3104, 541, 1507, 4938, 6, 4191];
var astralIdentifierCodes = [509, 0, 227, 0, 150, 4, 294, 9, 1368, 2, 2, 1, 6, 3, 41, 2, 5, 0, 166, 1, 574, 3, 9, 9, 370, 1, 81, 2, 71, 10, 50, 3, 123, 2, 54, 14, 32, 10, 3, 1, 11, 3, 46, 10, 8, 0, 46, 9, 7, 2, 37, 13, 2, 9, 6, 1, 45, 0, 13, 2, 49, 13, 9, 3, 2, 11, 83, 11, 7, 0, 3, 0, 158, 11, 6, 9, 7, 3, 56, 1, 2, 6, 3, 1, 3, 2, 10, 0, 11, 1, 3, 6, 4, 4, 193, 17, 10, 9, 5, 0, 82, 19, 13, 9, 214, 6, 3, 8, 28, 1, 83, 16, 16, 9, 82, 12, 9, 9, 84, 14, 5, 9, 243, 14, 166, 9, 71, 5, 2, 1, 3, 3, 2, 0, 2, 1, 13, 9, 120, 6, 3, 6, 4, 0, 29, 9, 41, 6, 2, 3, 9, 0, 10, 10, 47, 15, 406, 7, 2, 7, 17, 9, 57, 21, 2, 13, 123, 5, 4, 0, 2, 1, 2, 6, 2, 0, 9, 9, 49, 4, 2, 1, 2, 4, 9, 9, 330, 3, 10, 1, 2, 0, 49, 6, 4, 4, 14, 9, 5351, 0, 7, 14, 13835, 9, 87, 9, 39, 4, 60, 6, 26, 9, 1014, 0, 2, 54, 8, 3, 82, 0, 12, 1, 19628, 1, 4706, 45, 3, 22, 543, 4, 4, 5, 9, 7, 3, 6, 31, 3, 149, 2, 1418, 49, 513, 54, 5, 49, 9, 0, 15, 0, 23, 4, 2, 14, 1361, 6, 2, 16, 3, 6, 2, 1, 2, 4, 101, 0, 161, 6, 10, 9, 357, 0, 62, 13, 499, 13, 983, 6, 110, 6, 6, 9, 4759, 9, 787719, 239];
function isInAstralSet(code, set) {
let pos = 65536;
for (let i = 0, length = set.length; i < length; i += 2) {
pos += set[i];
if (pos > code)
return false;
pos += set[i + 1];
if (pos >= code)
return true;
}
return false;
}
function isIdentifierStart(code) {
if (code < 65)
return code === 36;
if (code <= 90)
return true;
if (code < 97)
return code === 95;
if (code <= 122)
return true;
if (code <= 65535) {
return code >= 170 && nonASCIIidentifierStart.test(String.fromCharCode(code));
}
return isInAstralSet(code, astralIdentifierStartCodes);
}
function isIdentifierChar(code) {
if (code < 48)
return code === 36;
if (code < 58)
return true;
if (code < 65)
return false;
if (code <= 90)
return true;
if (code < 97)
return code === 95;
if (code <= 122)
return true;
if (code <= 65535) {
return code >= 170 && nonASCIIidentifier.test(String.fromCharCode(code));
}
return isInAstralSet(code, astralIdentifierStartCodes) || isInAstralSet(code, astralIdentifierCodes);
}
function isIdentifierName(name) {
let isFirst = true;
for (let i = 0; i < name.length; i++) {
let cp = name.charCodeAt(i);
if ((cp & 64512) === 55296 && i + 1 < name.length) {
const trail = name.charCodeAt(++i);
if ((trail & 64512) === 56320) {
cp = 65536 + ((cp & 1023) << 10) + (trail & 1023);
}
}
if (isFirst) {
isFirst = false;
if (!isIdentifierStart(cp)) {
return false;
}
} else if (!isIdentifierChar(cp)) {
return false;
}
}
return !isFirst;
}
}
});
var require_keyword = __commonJS2({
"node_modules/@babel/helper-validator-identifier/lib/keyword.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.isKeyword = isKeyword;
exports2.isReservedWord = isReservedWord;
exports2.isStrictBindOnlyReservedWord = isStrictBindOnlyReservedWord;
exports2.isStrictBindReservedWord = isStrictBindReservedWord;
exports2.isStrictReservedWord = isStrictReservedWord;
var reservedWords = {
keyword: ["break", "case", "catch", "continue", "debugger", "default", "do", "else", "finally", "for", "function", "if", "return", "switch", "throw", "try", "var", "const", "while", "with", "new", "this", "super", "class", "extends", "export", "import", "null", "true", "false", "in", "instanceof", "typeof", "void", "delete"],
strict: ["implements", "interface", "let", "package", "private", "protected", "public", "static", "yield"],
strictBind: ["eval", "arguments"]
};
var keywords = new Set(reservedWords.keyword);
var reservedWordsStrictSet = new Set(reservedWords.strict);
var reservedWordsStrictBindSet = new Set(reservedWords.strictBind);
function isReservedWord(word, inModule) {
return inModule && word === "await" || word === "enum";
}
function isStrictReservedWord(word, inModule) {
return isReservedWord(word, inModule) || reservedWordsStrictSet.has(word);
}
function isStrictBindOnlyReservedWord(word) {
return reservedWordsStrictBindSet.has(word);
}
function isStrictBindReservedWord(word, inModule) {
return isStrictReservedWord(word, inModule) || isStrictBindOnlyReservedWord(word);
}
function isKeyword(word) {
return keywords.has(word);
}
}
});
var require_lib8 = __commonJS2({
"node_modules/@babel/helper-validator-identifier/lib/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
Object.defineProperty(exports2, "isIdentifierChar", {
enumerable: true,
get: function() {
return _identifier.isIdentifierChar;
}
});
Object.defineProperty(exports2, "isIdentifierName", {
enumerable: true,
get: function() {
return _identifier.isIdentifierName;
}
});
Object.defineProperty(exports2, "isIdentifierStart", {
enumerable: true,
get: function() {
return _identifier.isIdentifierStart;
}
});
Object.defineProperty(exports2, "isKeyword", {
enumerable: true,
get: function() {
return _keyword.isKeyword;
}
});
Object.defineProperty(exports2, "isReservedWord", {
enumerable: true,
get: function() {
return _keyword.isReservedWord;
}
});
Object.defineProperty(exports2, "isStrictBindOnlyReservedWord", {
enumerable: true,
get: function() {
return _keyword.isStrictBindOnlyReservedWord;
}
});
Object.defineProperty(exports2, "isStrictBindReservedWord", {
enumerable: true,
get: function() {
return _keyword.isStrictBindReservedWord;
}
});
Object.defineProperty(exports2, "isStrictReservedWord", {
enumerable: true,
get: function() {
return _keyword.isStrictReservedWord;
}
});
var _identifier = require_identifier();
var _keyword = require_keyword();
}
});
var require_escape_string_regexp = __commonJS2({
"node_modules/@babel/highlight/node_modules/escape-string-regexp/index.js"(exports2, module22) {
"use strict";
var matchOperatorsRe = /[|\\{}()[\]^$+*?.]/g;
module22.exports = function(str) {
if (typeof str !== "string") {
throw new TypeError("Expected a string");
}
return str.replace(matchOperatorsRe, "\\$&");
};
}
});
var require_color_name = __commonJS2({
"node_modules/color-name/index.js"(exports2, module22) {
"use strict";
module22.exports = {
"aliceblue": [240, 248, 255],
"antiquewhite": [250, 235, 215],
"aqua": [0, 255, 255],
"aquamarine": [127, 255, 212],
"azure": [240, 255, 255],
"beige": [245, 245, 220],
"bisque": [255, 228, 196],
"black": [0, 0, 0],
"blanchedalmond": [255, 235, 205],
"blue": [0, 0, 255],
"blueviolet": [138, 43, 226],
"brown": [165, 42, 42],
"burlywood": [222, 184, 135],
"cadetblue": [95, 158, 160],
"chartreuse": [127, 255, 0],
"chocolate": [210, 105, 30],
"coral": [255, 127, 80],
"cornflowerblue": [100, 149, 237],
"cornsilk": [255, 248, 220],
"crimson": [220, 20, 60],
"cyan": [0, 255, 255],
"darkblue": [0, 0, 139],
"darkcyan": [0, 139, 139],
"darkgoldenrod": [184, 134, 11],
"darkgray": [169, 169, 169],
"darkgreen": [0, 100, 0],
"darkgrey": [169, 169, 169],
"darkkhaki": [189, 183, 107],
"darkmagenta": [139, 0, 139],
"darkolivegreen": [85, 107, 47],
"darkorange": [255, 140, 0],
"darkorchid": [153, 50, 204],
"darkred": [139, 0, 0],
"darksalmon": [233, 150, 122],
"darkseagreen": [143, 188, 143],
"darkslateblue": [72, 61, 139],
"darkslategray": [47, 79, 79],
"darkslategrey": [47, 79, 79],
"darkturquoise": [0, 206, 209],
"darkviolet": [148, 0, 211],
"deeppink": [255, 20, 147],
"deepskyblue": [0, 191, 255],
"dimgray": [105, 105, 105],
"dimgrey": [105, 105, 105],
"dodgerblue": [30, 144, 255],
"firebrick": [178, 34, 34],
"floralwhite": [255, 250, 240],
"forestgreen": [34, 139, 34],
"fuchsia": [255, 0, 255],
"gainsboro": [220, 220, 220],
"ghostwhite": [248, 248, 255],
"gold": [255, 215, 0],
"goldenrod": [218, 165, 32],
"gray": [128, 128, 128],
"green": [0, 128, 0],
"greenyellow": [173, 255, 47],
"grey": [128, 128, 128],
"honeydew": [240, 255, 240],
"hotpink": [255, 105, 180],
"indianred": [205, 92, 92],
"indigo": [75, 0, 130],
"ivory": [255, 255, 240],
"khaki": [240, 230, 140],
"lavender": [230, 230, 250],
"lavenderblush": [255, 240, 245],
"lawngreen": [124, 252, 0],
"lemonchiffon": [255, 250, 205],
"lightblue": [173, 216, 230],
"lightcoral": [240, 128, 128],
"lightcyan": [224, 255, 255],
"lightgoldenrodyellow": [250, 250, 210],
"lightgray": [211, 211, 211],
"lightgreen": [144, 238, 144],
"lightgrey": [211, 211, 211],
"lightpink": [255, 182, 193],
"lightsalmon": [255, 160, 122],
"lightseagreen": [32, 178, 170],
"lightskyblue": [135, 206, 250],
"lightslategray": [119, 136, 153],
"lightslategrey": [119, 136, 153],
"lightsteelblue": [176, 196, 222],
"lightyellow": [255, 255, 224],
"lime": [0, 255, 0],
"limegreen": [50, 205, 50],
"linen": [250, 240, 230],
"magenta": [255, 0, 255],
"maroon": [128, 0, 0],
"mediumaquamarine": [102, 205, 170],
"mediumblue": [0, 0, 205],
"mediumorchid": [186, 85, 211],
"mediumpurple": [147, 112, 219],
"mediumseagreen": [60, 179, 113],
"mediumslateblue": [123, 104, 238],
"mediumspringgreen": [0, 250, 154],
"mediumturquoise": [72, 209, 204],
"mediumvioletred": [199, 21, 133],
"midnightblue": [25, 25, 112],
"mintcream": [245, 255, 250],
"mistyrose": [255, 228, 225],
"moccasin": [255, 228, 181],
"navajowhite": [255, 222, 173],
"navy": [0, 0, 128],
"oldlace": [253, 245, 230],
"olive": [128, 128, 0],
"olivedrab": [107, 142, 35],
"orange": [255, 165, 0],
"orangered": [255, 69, 0],
"orchid": [218, 112, 214],
"palegoldenrod": [238, 232, 170],
"palegreen": [152, 251, 152],
"paleturquoise": [175, 238, 238],
"palevioletred": [219, 112, 147],
"papayawhip": [255, 239, 213],
"peachpuff": [255, 218, 185],
"peru": [205, 133, 63],
"pink": [255, 192, 203],
"plum": [221, 160, 221],
"powderblue": [176, 224, 230],
"purple": [128, 0, 128],
"rebeccapurple": [102, 51, 153],
"red": [255, 0, 0],
"rosybrown": [188, 143, 143],
"royalblue": [65, 105, 225],
"saddlebrown": [139, 69, 19],
"salmon": [250, 128, 114],
"sandybrown": [244, 164, 96],
"seagreen": [46, 139, 87],
"seashell": [255, 245, 238],
"sienna": [160, 82, 45],
"silver": [192, 192, 192],
"skyblue": [135, 206, 235],
"slateblue": [106, 90, 205],
"slategray": [112, 128, 144],
"slategrey": [112, 128, 144],
"snow": [255, 250, 250],
"springgreen": [0, 255, 127],
"steelblue": [70, 130, 180],
"tan": [210, 180, 140],
"teal": [0, 128, 128],
"thistle": [216, 191, 216],
"tomato": [255, 99, 71],
"turquoise": [64, 224, 208],
"violet": [238, 130, 238],
"wheat": [245, 222, 179],
"white": [255, 255, 255],
"whitesmoke": [245, 245, 245],
"yellow": [255, 255, 0],
"yellowgreen": [154, 205, 50]
};
}
});
var require_conversions = __commonJS2({
"node_modules/color-convert/conversions.js"(exports2, module22) {
var cssKeywords = require_color_name();
var reverseKeywords = {};
for (key in cssKeywords) {
if (cssKeywords.hasOwnProperty(key)) {
reverseKeywords[cssKeywords[key]] = key;
}
}
var key;
var convert = module22.exports = {
rgb: {
channels: 3,
labels: "rgb"
},
hsl: {
channels: 3,
labels: "hsl"
},
hsv: {
channels: 3,
labels: "hsv"
},
hwb: {
channels: 3,
labels: "hwb"
},
cmyk: {
channels: 4,
labels: "cmyk"
},
xyz: {
channels: 3,
labels: "xyz"
},
lab: {
channels: 3,
labels: "lab"
},
lch: {
channels: 3,
labels: "lch"
},
hex: {
channels: 1,
labels: ["hex"]
},
keyword: {
channels: 1,
labels: ["keyword"]
},
ansi16: {
channels: 1,
labels: ["ansi16"]
},
ansi256: {
channels: 1,
labels: ["ansi256"]
},
hcg: {
channels: 3,
labels: ["h", "c", "g"]
},
apple: {
channels: 3,
labels: ["r16", "g16", "b16"]
},
gray: {
channels: 1,
labels: ["gray"]
}
};
for (model in convert) {
if (convert.hasOwnProperty(model)) {
if (!("channels" in convert[model])) {
throw new Error("missing channels property: " + model);
}
if (!("labels" in convert[model])) {
throw new Error("missing channel labels property: " + model);
}
if (convert[model].labels.length !== convert[model].channels) {
throw new Error("channel and label counts mismatch: " + model);
}
channels = convert[model].channels;
labels = convert[model].labels;
delete convert[model].channels;
delete convert[model].labels;
Object.defineProperty(convert[model], "channels", {
value: channels
});
Object.defineProperty(convert[model], "labels", {
value: labels
});
}
}
var channels;
var labels;
var model;
convert.rgb.hsl = function(rgb) {
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
var min = Math.min(r, g, b);
var max = Math.max(r, g, b);
var delta = max - min;
var h;
var s;
var l;
if (max === min) {
h = 0;
} else if (r === max) {
h = (g - b) / delta;
} else if (g === max) {
h = 2 + (b - r) / delta;
} else if (b === max) {
h = 4 + (r - g) / delta;
}
h = Math.min(h * 60, 360);
if (h < 0) {
h += 360;
}
l = (min + max) / 2;
if (max === min) {
s = 0;
} else if (l <= 0.5) {
s = delta / (max + min);
} else {
s = delta / (2 - max - min);
}
return [h, s * 100, l * 100];
};
convert.rgb.hsv = function(rgb) {
var rdif;
var gdif;
var bdif;
var h;
var s;
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
var v = Math.max(r, g, b);
var diff = v - Math.min(r, g, b);
var diffc = function(c) {
return (v - c) / 6 / diff + 1 / 2;
};
if (diff === 0) {
h = s = 0;
} else {
s = diff / v;
rdif = diffc(r);
gdif = diffc(g);
bdif = diffc(b);
if (r === v) {
h = bdif - gdif;
} else if (g === v) {
h = 1 / 3 + rdif - bdif;
} else if (b === v) {
h = 2 / 3 + gdif - rdif;
}
if (h < 0) {
h += 1;
} else if (h > 1) {
h -= 1;
}
}
return [h * 360, s * 100, v * 100];
};
convert.rgb.hwb = function(rgb) {
var r = rgb[0];
var g = rgb[1];
var b = rgb[2];
var h = convert.rgb.hsl(rgb)[0];
var w = 1 / 255 * Math.min(r, Math.min(g, b));
b = 1 - 1 / 255 * Math.max(r, Math.max(g, b));
return [h, w * 100, b * 100];
};
convert.rgb.cmyk = function(rgb) {
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
var c;
var m;
var y;
var k;
k = Math.min(1 - r, 1 - g, 1 - b);
c = (1 - r - k) / (1 - k) || 0;
m = (1 - g - k) / (1 - k) || 0;
y = (1 - b - k) / (1 - k) || 0;
return [c * 100, m * 100, y * 100, k * 100];
};
function comparativeDistance(x, y) {
return Math.pow(x[0] - y[0], 2) + Math.pow(x[1] - y[1], 2) + Math.pow(x[2] - y[2], 2);
}
convert.rgb.keyword = function(rgb) {
var reversed = reverseKeywords[rgb];
if (reversed) {
return reversed;
}
var currentClosestDistance = Infinity;
var currentClosestKeyword;
for (var keyword in cssKeywords) {
if (cssKeywords.hasOwnProperty(keyword)) {
var value = cssKeywords[keyword];
var distance = comparativeDistance(rgb, value);
if (distance < currentClosestDistance) {
currentClosestDistance = distance;
currentClosestKeyword = keyword;
}
}
}
return currentClosestKeyword;
};
convert.keyword.rgb = function(keyword) {
return cssKeywords[keyword];
};
convert.rgb.xyz = function(rgb) {
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
r = r > 0.04045 ? Math.pow((r + 0.055) / 1.055, 2.4) : r / 12.92;
g = g > 0.04045 ? Math.pow((g + 0.055) / 1.055, 2.4) : g / 12.92;
b = b > 0.04045 ? Math.pow((b + 0.055) / 1.055, 2.4) : b / 12.92;
var x = r * 0.4124 + g * 0.3576 + b * 0.1805;
var y = r * 0.2126 + g * 0.7152 + b * 0.0722;
var z = r * 0.0193 + g * 0.1192 + b * 0.9505;
return [x * 100, y * 100, z * 100];
};
convert.rgb.lab = function(rgb) {
var xyz = convert.rgb.xyz(rgb);
var x = xyz[0];
var y = xyz[1];
var z = xyz[2];
var l;
var a;
var b;
x /= 95.047;
y /= 100;
z /= 108.883;
x = x > 8856e-6 ? Math.pow(x, 1 / 3) : 7.787 * x + 16 / 116;
y = y > 8856e-6 ? Math.pow(y, 1 / 3) : 7.787 * y + 16 / 116;
z = z > 8856e-6 ? Math.pow(z, 1 / 3) : 7.787 * z + 16 / 116;
l = 116 * y - 16;
a = 500 * (x - y);
b = 200 * (y - z);
return [l, a, b];
};
convert.hsl.rgb = function(hsl) {
var h = hsl[0] / 360;
var s = hsl[1] / 100;
var l = hsl[2] / 100;
var t1;
var t2;
var t3;
var rgb;
var val;
if (s === 0) {
val = l * 255;
return [val, val, val];
}
if (l < 0.5) {
t2 = l * (1 + s);
} else {
t2 = l + s - l * s;
}
t1 = 2 * l - t2;
rgb = [0, 0, 0];
for (var i = 0; i < 3; i++) {
t3 = h + 1 / 3 * -(i - 1);
if (t3 < 0) {
t3++;
}
if (t3 > 1) {
t3--;
}
if (6 * t3 < 1) {
val = t1 + (t2 - t1) * 6 * t3;
} else if (2 * t3 < 1) {
val = t2;
} else if (3 * t3 < 2) {
val = t1 + (t2 - t1) * (2 / 3 - t3) * 6;
} else {
val = t1;
}
rgb[i] = val * 255;
}
return rgb;
};
convert.hsl.hsv = function(hsl) {
var h = hsl[0];
var s = hsl[1] / 100;
var l = hsl[2] / 100;
var smin = s;
var lmin = Math.max(l, 0.01);
var sv;
var v;
l *= 2;
s *= l <= 1 ? l : 2 - l;
smin *= lmin <= 1 ? lmin : 2 - lmin;
v = (l + s) / 2;
sv = l === 0 ? 2 * smin / (lmin + smin) : 2 * s / (l + s);
return [h, sv * 100, v * 100];
};
convert.hsv.rgb = function(hsv) {
var h = hsv[0] / 60;
var s = hsv[1] / 100;
var v = hsv[2] / 100;
var hi = Math.floor(h) % 6;
var f = h - Math.floor(h);
var p = 255 * v * (1 - s);
var q = 255 * v * (1 - s * f);
var t = 255 * v * (1 - s * (1 - f));
v *= 255;
switch (hi) {
case 0:
return [v, t, p];
case 1:
return [q, v, p];
case 2:
return [p, v, t];
case 3:
return [p, q, v];
case 4:
return [t, p, v];
case 5:
return [v, p, q];
}
};
convert.hsv.hsl = function(hsv) {
var h = hsv[0];
var s = hsv[1] / 100;
var v = hsv[2] / 100;
var vmin = Math.max(v, 0.01);
var lmin;
var sl;
var l;
l = (2 - s) * v;
lmin = (2 - s) * vmin;
sl = s * vmin;
sl /= lmin <= 1 ? lmin : 2 - lmin;
sl = sl || 0;
l /= 2;
return [h, sl * 100, l * 100];
};
convert.hwb.rgb = function(hwb) {
var h = hwb[0] / 360;
var wh = hwb[1] / 100;
var bl = hwb[2] / 100;
var ratio = wh + bl;
var i;
var v;
var f;
var n2;
if (ratio > 1) {
wh /= ratio;
bl /= ratio;
}
i = Math.floor(6 * h);
v = 1 - bl;
f = 6 * h - i;
if ((i & 1) !== 0) {
f = 1 - f;
}
n2 = wh + f * (v - wh);
var r;
var g;
var b;
switch (i) {
default:
case 6:
case 0:
r = v;
g = n2;
b = wh;
break;
case 1:
r = n2;
g = v;
b = wh;
break;
case 2:
r = wh;
g = v;
b = n2;
break;
case 3:
r = wh;
g = n2;
b = v;
break;
case 4:
r = n2;
g = wh;
b = v;
break;
case 5:
r = v;
g = wh;
b = n2;
break;
}
return [r * 255, g * 255, b * 255];
};
convert.cmyk.rgb = function(cmyk) {
var c = cmyk[0] / 100;
var m = cmyk[1] / 100;
var y = cmyk[2] / 100;
var k = cmyk[3] / 100;
var r;
var g;
var b;
r = 1 - Math.min(1, c * (1 - k) + k);
g = 1 - Math.min(1, m * (1 - k) + k);
b = 1 - Math.min(1, y * (1 - k) + k);
return [r * 255, g * 255, b * 255];
};
convert.xyz.rgb = function(xyz) {
var x = xyz[0] / 100;
var y = xyz[1] / 100;
var z = xyz[2] / 100;
var r;
var g;
var b;
r = x * 3.2406 + y * -1.5372 + z * -0.4986;
g = x * -0.9689 + y * 1.8758 + z * 0.0415;
b = x * 0.0557 + y * -0.204 + z * 1.057;
r = r > 31308e-7 ? 1.055 * Math.pow(r, 1 / 2.4) - 0.055 : r * 12.92;
g = g > 31308e-7 ? 1.055 * Math.pow(g, 1 / 2.4) - 0.055 : g * 12.92;
b = b > 31308e-7 ? 1.055 * Math.pow(b, 1 / 2.4) - 0.055 : b * 12.92;
r = Math.min(Math.max(0, r), 1);
g = Math.min(Math.max(0, g), 1);
b = Math.min(Math.max(0, b), 1);
return [r * 255, g * 255, b * 255];
};
convert.xyz.lab = function(xyz) {
var x = xyz[0];
var y = xyz[1];
var z = xyz[2];
var l;
var a;
var b;
x /= 95.047;
y /= 100;
z /= 108.883;
x = x > 8856e-6 ? Math.pow(x, 1 / 3) : 7.787 * x + 16 / 116;
y = y > 8856e-6 ? Math.pow(y, 1 / 3) : 7.787 * y + 16 / 116;
z = z > 8856e-6 ? Math.pow(z, 1 / 3) : 7.787 * z + 16 / 116;
l = 116 * y - 16;
a = 500 * (x - y);
b = 200 * (y - z);
return [l, a, b];
};
convert.lab.xyz = function(lab) {
var l = lab[0];
var a = lab[1];
var b = lab[2];
var x;
var y;
var z;
y = (l + 16) / 116;
x = a / 500 + y;
z = y - b / 200;
var y2 = Math.pow(y, 3);
var x2 = Math.pow(x, 3);
var z2 = Math.pow(z, 3);
y = y2 > 8856e-6 ? y2 : (y - 16 / 116) / 7.787;
x = x2 > 8856e-6 ? x2 : (x - 16 / 116) / 7.787;
z = z2 > 8856e-6 ? z2 : (z - 16 / 116) / 7.787;
x *= 95.047;
y *= 100;
z *= 108.883;
return [x, y, z];
};
convert.lab.lch = function(lab) {
var l = lab[0];
var a = lab[1];
var b = lab[2];
var hr;
var h;
var c;
hr = Math.atan2(b, a);
h = hr * 360 / 2 / Math.PI;
if (h < 0) {
h += 360;
}
c = Math.sqrt(a * a + b * b);
return [l, c, h];
};
convert.lch.lab = function(lch) {
var l = lch[0];
var c = lch[1];
var h = lch[2];
var a;
var b;
var hr;
hr = h / 360 * 2 * Math.PI;
a = c * Math.cos(hr);
b = c * Math.sin(hr);
return [l, a, b];
};
convert.rgb.ansi16 = function(args) {
var r = args[0];
var g = args[1];
var b = args[2];
var value = 1 in arguments ? arguments[1] : convert.rgb.hsv(args)[2];
value = Math.round(value / 50);
if (value === 0) {
return 30;
}
var ansi = 30 + (Math.round(b / 255) << 2 | Math.round(g / 255) << 1 | Math.round(r / 255));
if (value === 2) {
ansi += 60;
}
return ansi;
};
convert.hsv.ansi16 = function(args) {
return convert.rgb.ansi16(convert.hsv.rgb(args), args[2]);
};
convert.rgb.ansi256 = function(args) {
var r = args[0];
var g = args[1];
var b = args[2];
if (r === g && g === b) {
if (r < 8) {
return 16;
}
if (r > 248) {
return 231;
}
return Math.round((r - 8) / 247 * 24) + 232;
}
var ansi = 16 + 36 * Math.round(r / 255 * 5) + 6 * Math.round(g / 255 * 5) + Math.round(b / 255 * 5);
return ansi;
};
convert.ansi16.rgb = function(args) {
var color = args % 10;
if (color === 0 || color === 7) {
if (args > 50) {
color += 3.5;
}
color = color / 10.5 * 255;
return [color, color, color];
}
var mult = (~~(args > 50) + 1) * 0.5;
var r = (color & 1) * mult * 255;
var g = (color >> 1 & 1) * mult * 255;
var b = (color >> 2 & 1) * mult * 255;
return [r, g, b];
};
convert.ansi256.rgb = function(args) {
if (args >= 232) {
var c = (args - 232) * 10 + 8;
return [c, c, c];
}
args -= 16;
var rem;
var r = Math.floor(args / 36) / 5 * 255;
var g = Math.floor((rem = args % 36) / 6) / 5 * 255;
var b = rem % 6 / 5 * 255;
return [r, g, b];
};
convert.rgb.hex = function(args) {
var integer = ((Math.round(args[0]) & 255) << 16) + ((Math.round(args[1]) & 255) << 8) + (Math.round(args[2]) & 255);
var string = integer.toString(16).toUpperCase();
return "000000".substring(string.length) + string;
};
convert.hex.rgb = function(args) {
var match = args.toString(16).match(/[a-f0-9]{6}|[a-f0-9]{3}/i);
if (!match) {
return [0, 0, 0];
}
var colorString = match[0];
if (match[0].length === 3) {
colorString = colorString.split("").map(function(char2) {
return char2 + char2;
}).join("");
}
var integer = parseInt(colorString, 16);
var r = integer >> 16 & 255;
var g = integer >> 8 & 255;
var b = integer & 255;
return [r, g, b];
};
convert.rgb.hcg = function(rgb) {
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
var max = Math.max(Math.max(r, g), b);
var min = Math.min(Math.min(r, g), b);
var chroma = max - min;
var grayscale;
var hue;
if (chroma < 1) {
grayscale = min / (1 - chroma);
} else {
grayscale = 0;
}
if (chroma <= 0) {
hue = 0;
} else if (max === r) {
hue = (g - b) / chroma % 6;
} else if (max === g) {
hue = 2 + (b - r) / chroma;
} else {
hue = 4 + (r - g) / chroma + 4;
}
hue /= 6;
hue %= 1;
return [hue * 360, chroma * 100, grayscale * 100];
};
convert.hsl.hcg = function(hsl) {
var s = hsl[1] / 100;
var l = hsl[2] / 100;
var c = 1;
var f = 0;
if (l < 0.5) {
c = 2 * s * l;
} else {
c = 2 * s * (1 - l);
}
if (c < 1) {
f = (l - 0.5 * c) / (1 - c);
}
return [hsl[0], c * 100, f * 100];
};
convert.hsv.hcg = function(hsv) {
var s = hsv[1] / 100;
var v = hsv[2] / 100;
var c = s * v;
var f = 0;
if (c < 1) {
f = (v - c) / (1 - c);
}
return [hsv[0], c * 100, f * 100];
};
convert.hcg.rgb = function(hcg) {
var h = hcg[0] / 360;
var c = hcg[1] / 100;
var g = hcg[2] / 100;
if (c === 0) {
return [g * 255, g * 255, g * 255];
}
var pure = [0, 0, 0];
var hi = h % 1 * 6;
var v = hi % 1;
var w = 1 - v;
var mg = 0;
switch (Math.floor(hi)) {
case 0:
pure[0] = 1;
pure[1] = v;
pure[2] = 0;
break;
case 1:
pure[0] = w;
pure[1] = 1;
pure[2] = 0;
break;
case 2:
pure[0] = 0;
pure[1] = 1;
pure[2] = v;
break;
case 3:
pure[0] = 0;
pure[1] = w;
pure[2] = 1;
break;
case 4:
pure[0] = v;
pure[1] = 0;
pure[2] = 1;
break;
default:
pure[0] = 1;
pure[1] = 0;
pure[2] = w;
}
mg = (1 - c) * g;
return [(c * pure[0] + mg) * 255, (c * pure[1] + mg) * 255, (c * pure[2] + mg) * 255];
};
convert.hcg.hsv = function(hcg) {
var c = hcg[1] / 100;
var g = hcg[2] / 100;
var v = c + g * (1 - c);
var f = 0;
if (v > 0) {
f = c / v;
}
return [hcg[0], f * 100, v * 100];
};
convert.hcg.hsl = function(hcg) {
var c = hcg[1] / 100;
var g = hcg[2] / 100;
var l = g * (1 - c) + 0.5 * c;
var s = 0;
if (l > 0 && l < 0.5) {
s = c / (2 * l);
} else if (l >= 0.5 && l < 1) {
s = c / (2 * (1 - l));
}
return [hcg[0], s * 100, l * 100];
};
convert.hcg.hwb = function(hcg) {
var c = hcg[1] / 100;
var g = hcg[2] / 100;
var v = c + g * (1 - c);
return [hcg[0], (v - c) * 100, (1 - v) * 100];
};
convert.hwb.hcg = function(hwb) {
var w = hwb[1] / 100;
var b = hwb[2] / 100;
var v = 1 - b;
var c = v - w;
var g = 0;
if (c < 1) {
g = (v - c) / (1 - c);
}
return [hwb[0], c * 100, g * 100];
};
convert.apple.rgb = function(apple) {
return [apple[0] / 65535 * 255, apple[1] / 65535 * 255, apple[2] / 65535 * 255];
};
convert.rgb.apple = function(rgb) {
return [rgb[0] / 255 * 65535, rgb[1] / 255 * 65535, rgb[2] / 255 * 65535];
};
convert.gray.rgb = function(args) {
return [args[0] / 100 * 255, args[0] / 100 * 255, args[0] / 100 * 255];
};
convert.gray.hsl = convert.gray.hsv = function(args) {
return [0, 0, args[0]];
};
convert.gray.hwb = function(gray) {
return [0, 100, gray[0]];
};
convert.gray.cmyk = function(gray) {
return [0, 0, 0, gray[0]];
};
convert.gray.lab = function(gray) {
return [gray[0], 0, 0];
};
convert.gray.hex = function(gray) {
var val = Math.round(gray[0] / 100 * 255) & 255;
var integer = (val << 16) + (val << 8) + val;
var string = integer.toString(16).toUpperCase();
return "000000".substring(string.length) + string;
};
convert.rgb.gray = function(rgb) {
var val = (rgb[0] + rgb[1] + rgb[2]) / 3;
return [val / 255 * 100];
};
}
});
var require_route = __commonJS2({
"node_modules/color-convert/route.js"(exports2, module22) {
var conversions = require_conversions();
function buildGraph() {
var graph = {};
var models = Object.keys(conversions);
for (var len = models.length, i = 0; i < len; i++) {
graph[models[i]] = {
distance: -1,
parent: null
};
}
return graph;
}
function deriveBFS(fromModel) {
var graph = buildGraph();
var queue = [fromModel];
graph[fromModel].distance = 0;
while (queue.length) {
var current = queue.pop();
var adjacents = Object.keys(conversions[current]);
for (var len = adjacents.length, i = 0; i < len; i++) {
var adjacent = adjacents[i];
var node = graph[adjacent];
if (node.distance === -1) {
node.distance = graph[current].distance + 1;
node.parent = current;
queue.unshift(adjacent);
}
}
}
return graph;
}
function link(from, to) {
return function(args) {
return to(from(args));
};
}
function wrapConversion(toModel, graph) {
var path = [graph[toModel].parent, toModel];
var fn = conversions[graph[toModel].parent][toModel];
var cur = graph[toModel].parent;
while (graph[cur].parent) {
path.unshift(graph[cur].parent);
fn = link(conversions[graph[cur].parent][cur], fn);
cur = graph[cur].parent;
}
fn.conversion = path;
return fn;
}
module22.exports = function(fromModel) {
var graph = deriveBFS(fromModel);
var conversion = {};
var models = Object.keys(graph);
for (var len = models.length, i = 0; i < len; i++) {
var toModel = models[i];
var node = graph[toModel];
if (node.parent === null) {
continue;
}
conversion[toModel] = wrapConversion(toModel, graph);
}
return conversion;
};
}
});
var require_color_convert = __commonJS2({
"node_modules/color-convert/index.js"(exports2, module22) {
var conversions = require_conversions();
var route = require_route();
var convert = {};
var models = Object.keys(conversions);
function wrapRaw(fn) {
var wrappedFn = function(args) {
if (args === void 0 || args === null) {
return args;
}
if (arguments.length > 1) {
args = Array.prototype.slice.call(arguments);
}
return fn(args);
};
if ("conversion" in fn) {
wrappedFn.conversion = fn.conversion;
}
return wrappedFn;
}
function wrapRounded(fn) {
var wrappedFn = function(args) {
if (args === void 0 || args === null) {
return args;
}
if (arguments.length > 1) {
args = Array.prototype.slice.call(arguments);
}
var result = fn(args);
if (typeof result === "object") {
for (var len = result.length, i = 0; i < len; i++) {
result[i] = Math.round(result[i]);
}
}
return result;
};
if ("conversion" in fn) {
wrappedFn.conversion = fn.conversion;
}
return wrappedFn;
}
models.forEach(function(fromModel) {
convert[fromModel] = {};
Object.defineProperty(convert[fromModel], "channels", {
value: conversions[fromModel].channels
});
Object.defineProperty(convert[fromModel], "labels", {
value: conversions[fromModel].labels
});
var routes = route(fromModel);
var routeModels = Object.keys(routes);
routeModels.forEach(function(toModel) {
var fn = routes[toModel];
convert[fromModel][toModel] = wrapRounded(fn);
convert[fromModel][toModel].raw = wrapRaw(fn);
});
});
module22.exports = convert;
}
});
var require_ansi_styles = __commonJS2({
"node_modules/ansi-styles/index.js"(exports2, module22) {
"use strict";
var colorConvert = require_color_convert();
var wrapAnsi16 = (fn, offset) => function() {
const code = fn.apply(colorConvert, arguments);
return `\x1B[${code + offset}m`;
};
var wrapAnsi256 = (fn, offset) => function() {
const code = fn.apply(colorConvert, arguments);
return `\x1B[${38 + offset};5;${code}m`;
};
var wrapAnsi16m = (fn, offset) => function() {
const rgb = fn.apply(colorConvert, arguments);
return `\x1B[${38 + offset};2;${rgb[0]};${rgb[1]};${rgb[2]}m`;
};
function assembleStyles() {
const codes = /* @__PURE__ */ new Map();
const styles = {
modifier: {
reset: [0, 0],
bold: [1, 22],
dim: [2, 22],
italic: [3, 23],
underline: [4, 24],
inverse: [7, 27],
hidden: [8, 28],
strikethrough: [9, 29]
},
color: {
black: [30, 39],
red: [31, 39],
green: [32, 39],
yellow: [33, 39],
blue: [34, 39],
magenta: [35, 39],
cyan: [36, 39],
white: [37, 39],
gray: [90, 39],
redBright: [91, 39],
greenBright: [92, 39],
yellowBright: [93, 39],
blueBright: [94, 39],
magentaBright: [95, 39],
cyanBright: [96, 39],
whiteBright: [97, 39]
},
bgColor: {
bgBlack: [40, 49],
bgRed: [41, 49],
bgGreen: [42, 49],
bgYellow: [43, 49],
bgBlue: [44, 49],
bgMagenta: [45, 49],
bgCyan: [46, 49],
bgWhite: [47, 49],
bgBlackBright: [100, 49],
bgRedBright: [101, 49],
bgGreenBright: [102, 49],
bgYellowBright: [103, 49],
bgBlueBright: [104, 49],
bgMagentaBright: [105, 49],
bgCyanBright: [106, 49],
bgWhiteBright: [107, 49]
}
};
styles.color.grey = styles.color.gray;
for (const groupName of Object.keys(styles)) {
const group = styles[groupName];
for (const styleName of Object.keys(group)) {
const style = group[styleName];
styles[styleName] = {
open: `\x1B[${style[0]}m`,
close: `\x1B[${style[1]}m`
};
group[styleName] = styles[styleName];
codes.set(style[0], style[1]);
}
Object.defineProperty(styles, groupName, {
value: group,
enumerable: false
});
Object.defineProperty(styles, "codes", {
value: codes,
enumerable: false
});
}
const ansi2ansi = (n2) => n2;
const rgb2rgb = (r, g, b) => [r, g, b];
styles.color.close = "\x1B[39m";
styles.bgColor.close = "\x1B[49m";
styles.color.ansi = {
ansi: wrapAnsi16(ansi2ansi, 0)
};
styles.color.ansi256 = {
ansi256: wrapAnsi256(ansi2ansi, 0)
};
styles.color.ansi16m = {
rgb: wrapAnsi16m(rgb2rgb, 0)
};
styles.bgColor.ansi = {
ansi: wrapAnsi16(ansi2ansi, 10)
};
styles.bgColor.ansi256 = {
ansi256: wrapAnsi256(ansi2ansi, 10)
};
styles.bgColor.ansi16m = {
rgb: wrapAnsi16m(rgb2rgb, 10)
};
for (let key of Object.keys(colorConvert)) {
if (typeof colorConvert[key] !== "object") {
continue;
}
const suite = colorConvert[key];
if (key === "ansi16") {
key = "ansi";
}
if ("ansi16" in suite) {
styles.color.ansi[key] = wrapAnsi16(suite.ansi16, 0);
styles.bgColor.ansi[key] = wrapAnsi16(suite.ansi16, 10);
}
if ("ansi256" in suite) {
styles.color.ansi256[key] = wrapAnsi256(suite.ansi256, 0);
styles.bgColor.ansi256[key] = wrapAnsi256(suite.ansi256, 10);
}
if ("rgb" in suite) {
styles.color.ansi16m[key] = wrapAnsi16m(suite.rgb, 0);
styles.bgColor.ansi16m[key] = wrapAnsi16m(suite.rgb, 10);
}
}
return styles;
}
Object.defineProperty(module22, "exports", {
enumerable: true,
get: assembleStyles
});
}
});
var require_has_flag = __commonJS2({
"node_modules/@babel/highlight/node_modules/has-flag/index.js"(exports2, module22) {
"use strict";
module22.exports = (flag, argv) => {
argv = argv || process.argv;
const prefix = flag.startsWith("-") ? "" : flag.length === 1 ? "-" : "--";
const pos = argv.indexOf(prefix + flag);
const terminatorPos = argv.indexOf("--");
return pos !== -1 && (terminatorPos === -1 ? true : pos < terminatorPos);
};
}
});
var require_supports_color = __commonJS2({
"node_modules/@babel/highlight/node_modules/supports-color/index.js"(exports2, module22) {
"use strict";
var os = require("os");
var hasFlag = require_has_flag();
var env = process.env;
var forceColor;
if (hasFlag("no-color") || hasFlag("no-colors") || hasFlag("color=false")) {
forceColor = false;
} else if (hasFlag("color") || hasFlag("colors") || hasFlag("color=true") || hasFlag("color=always")) {
forceColor = true;
}
if ("FORCE_COLOR" in env) {
forceColor = env.FORCE_COLOR.length === 0 || parseInt(env.FORCE_COLOR, 10) !== 0;
}
function translateLevel(level) {
if (level === 0) {
return false;
}
return {
level,
hasBasic: true,
has256: level >= 2,
has16m: level >= 3
};
}
function supportsColor(stream) {
if (forceColor === false) {
return 0;
}
if (hasFlag("color=16m") || hasFlag("color=full") || hasFlag("color=truecolor")) {
return 3;
}
if (hasFlag("color=256")) {
return 2;
}
if (stream && !stream.isTTY && forceColor !== true) {
return 0;
}
const min = forceColor ? 1 : 0;
if (process.platform === "win32") {
const osRelease = os.release().split(".");
if (Number(process.versions.node.split(".")[0]) >= 8 && Number(osRelease[0]) >= 10 && Number(osRelease[2]) >= 10586) {
return Number(osRelease[2]) >= 14931 ? 3 : 2;
}
return 1;
}
if ("CI" in env) {
if (["TRAVIS", "CIRCLECI", "APPVEYOR", "GITLAB_CI"].some((sign) => sign in env) || env.CI_NAME === "codeship") {
return 1;
}
return min;
}
if ("TEAMCITY_VERSION" in env) {
return /^(9\.(0*[1-9]\d*)\.|\d{2,}\.)/.test(env.TEAMCITY_VERSION) ? 1 : 0;
}
if (env.COLORTERM === "truecolor") {
return 3;
}
if ("TERM_PROGRAM" in env) {
const version = parseInt((env.TERM_PROGRAM_VERSION || "").split(".")[0], 10);
switch (env.TERM_PROGRAM) {
case "iTerm.app":
return version >= 3 ? 3 : 2;
case "Apple_Terminal":
return 2;
}
}
if (/-256(color)?$/i.test(env.TERM)) {
return 2;
}
if (/^screen|^xterm|^vt100|^vt220|^rxvt|color|ansi|cygwin|linux/i.test(env.TERM)) {
return 1;
}
if ("COLORTERM" in env) {
return 1;
}
if (env.TERM === "dumb") {
return min;
}
return min;
}
function getSupportLevel(stream) {
const level = supportsColor(stream);
return translateLevel(level);
}
module22.exports = {
supportsColor: getSupportLevel,
stdout: getSupportLevel(process.stdout),
stderr: getSupportLevel(process.stderr)
};
}
});
var require_templates = __commonJS2({
"node_modules/@babel/highlight/node_modules/chalk/templates.js"(exports2, module22) {
"use strict";
var TEMPLATE_REGEX = /(?:\\(u[a-f\d]{4}|x[a-f\d]{2}|.))|(?:\{(~)?(\w+(?:\([^)]*\))?(?:\.\w+(?:\([^)]*\))?)*)(?:[ \t]|(?=\r?\n)))|(\})|((?:.|[\r\n\f])+?)/gi;
var STYLE_REGEX = /(?:^|\.)(\w+)(?:\(([^)]*)\))?/g;
var STRING_REGEX = /^(['"])((?:\\.|(?!\1)[^\\])*)\1$/;
var ESCAPE_REGEX = /\\(u[a-f\d]{4}|x[a-f\d]{2}|.)|([^\\])/gi;
var ESCAPES = /* @__PURE__ */ new Map([["n", "\n"], ["r", "\r"], ["t", " "], ["b", "\b"], ["f", "\f"], ["v", "\v"], ["0", "\0"], ["\\", "\\"], ["e", "\x1B"], ["a", "\x07"]]);
function unescape2(c) {
if (c[0] === "u" && c.length === 5 || c[0] === "x" && c.length === 3) {
return String.fromCharCode(parseInt(c.slice(1), 16));
}
return ESCAPES.get(c) || c;
}
function parseArguments(name, args) {
const results = [];
const chunks = args.trim().split(/\s*,\s*/g);
let matches;
for (const chunk of chunks) {
if (!isNaN(chunk)) {
results.push(Number(chunk));
} else if (matches = chunk.match(STRING_REGEX)) {
results.push(matches[2].replace(ESCAPE_REGEX, (m, escape, chr) => escape ? unescape2(escape) : chr));
} else {
throw new Error(`Invalid Chalk template style argument: ${chunk} (in style '${name}')`);
}
}
return results;
}
function parseStyle(style) {
STYLE_REGEX.lastIndex = 0;
const results = [];
let matches;
while ((matches = STYLE_REGEX.exec(style)) !== null) {
const name = matches[1];
if (matches[2]) {
const args = parseArguments(name, matches[2]);
results.push([name].concat(args));
} else {
results.push([name]);
}
}
return results;
}
function buildStyle(chalk, styles) {
const enabled = {};
for (const layer of styles) {
for (const style of layer.styles) {
enabled[style[0]] = layer.inverse ? null : style.slice(1);
}
}
let current = chalk;
for (const styleName of Object.keys(enabled)) {
if (Array.isArray(enabled[styleName])) {
if (!(styleName in current)) {
throw new Error(`Unknown Chalk style: ${styleName}`);
}
if (enabled[styleName].length > 0) {
current = current[styleName].apply(current, enabled[styleName]);
} else {
current = current[styleName];
}
}
}
return current;
}
module22.exports = (chalk, tmp) => {
const styles = [];
const chunks = [];
let chunk = [];
tmp.replace(TEMPLATE_REGEX, (m, escapeChar, inverse, style, close, chr) => {
if (escapeChar) {
chunk.push(unescape2(escapeChar));
} else if (style) {
const str = chunk.join("");
chunk = [];
chunks.push(styles.length === 0 ? str : buildStyle(chalk, styles)(str));
styles.push({
inverse,
styles: parseStyle(style)
});
} else if (close) {
if (styles.length === 0) {
throw new Error("Found extraneous } in Chalk template literal");
}
chunks.push(buildStyle(chalk, styles)(chunk.join("")));
chunk = [];
styles.pop();
} else {
chunk.push(chr);
}
});
chunks.push(chunk.join(""));
if (styles.length > 0) {
const errMsg = `Chalk template literal is missing ${styles.length} closing bracket${styles.length === 1 ? "" : "s"} (\`}\`)`;
throw new Error(errMsg);
}
return chunks.join("");
};
}
});
var require_chalk = __commonJS2({
"node_modules/@babel/highlight/node_modules/chalk/index.js"(exports2, module22) {
"use strict";
var escapeStringRegexp = require_escape_string_regexp();
var ansiStyles = require_ansi_styles();
var stdoutColor = require_supports_color().stdout;
var template = require_templates();
var isSimpleWindowsTerm = process.platform === "win32" && !(process.env.TERM || "").toLowerCase().startsWith("xterm");
var levelMapping = ["ansi", "ansi", "ansi256", "ansi16m"];
var skipModels = /* @__PURE__ */ new Set(["gray"]);
var styles = /* @__PURE__ */ Object.create(null);
function applyOptions(obj, options) {
options = options || {};
const scLevel = stdoutColor ? stdoutColor.level : 0;
obj.level = options.level === void 0 ? scLevel : options.level;
obj.enabled = "enabled" in options ? options.enabled : obj.level > 0;
}
function Chalk(options) {
if (!this || !(this instanceof Chalk) || this.template) {
const chalk = {};
applyOptions(chalk, options);
chalk.template = function() {
const args = [].slice.call(arguments);
return chalkTag.apply(null, [chalk.template].concat(args));
};
Object.setPrototypeOf(chalk, Chalk.prototype);
Object.setPrototypeOf(chalk.template, chalk);
chalk.template.constructor = Chalk;
return chalk.template;
}
applyOptions(this, options);
}
if (isSimpleWindowsTerm) {
ansiStyles.blue.open = "\x1B[94m";
}
for (const key of Object.keys(ansiStyles)) {
ansiStyles[key].closeRe = new RegExp(escapeStringRegexp(ansiStyles[key].close), "g");
styles[key] = {
get() {
const codes = ansiStyles[key];
return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, key);
}
};
}
styles.visible = {
get() {
return build.call(this, this._styles || [], true, "visible");
}
};
ansiStyles.color.closeRe = new RegExp(escapeStringRegexp(ansiStyles.color.close), "g");
for (const model of Object.keys(ansiStyles.color.ansi)) {
if (skipModels.has(model)) {
continue;
}
styles[model] = {
get() {
const level = this.level;
return function() {
const open = ansiStyles.color[levelMapping[level]][model].apply(null, arguments);
const codes = {
open,
close: ansiStyles.color.close,
closeRe: ansiStyles.color.closeRe
};
return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, model);
};
}
};
}
ansiStyles.bgColor.closeRe = new RegExp(escapeStringRegexp(ansiStyles.bgColor.close), "g");
for (const model of Object.keys(ansiStyles.bgColor.ansi)) {
if (skipModels.has(model)) {
continue;
}
const bgModel = "bg" + model[0].toUpperCase() + model.slice(1);
styles[bgModel] = {
get() {
const level = this.level;
return function() {
const open = ansiStyles.bgColor[levelMapping[level]][model].apply(null, arguments);
const codes = {
open,
close: ansiStyles.bgColor.close,
closeRe: ansiStyles.bgColor.closeRe
};
return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, model);
};
}
};
}
var proto = Object.defineProperties(() => {
}, styles);
function build(_styles, _empty, key) {
const builder = function() {
return applyStyle.apply(builder, arguments);
};
builder._styles = _styles;
builder._empty = _empty;
const self2 = this;
Object.defineProperty(builder, "level", {
enumerable: true,
get() {
return self2.level;
},
set(level) {
self2.level = level;
}
});
Object.defineProperty(builder, "enabled", {
enumerable: true,
get() {
return self2.enabled;
},
set(enabled) {
self2.enabled = enabled;
}
});
builder.hasGrey = this.hasGrey || key === "gray" || key === "grey";
builder.__proto__ = proto;
return builder;
}
function applyStyle() {
const args = arguments;
const argsLen = args.length;
let str = String(arguments[0]);
if (argsLen === 0) {
return "";
}
if (argsLen > 1) {
for (let a = 1; a < argsLen; a++) {
str += " " + args[a];
}
}
if (!this.enabled || this.level <= 0 || !str) {
return this._empty ? "" : str;
}
const originalDim = ansiStyles.dim.open;
if (isSimpleWindowsTerm && this.hasGrey) {
ansiStyles.dim.open = "";
}
for (const code of this._styles.slice().reverse()) {
str = code.open + str.replace(code.closeRe, code.open) + code.close;
str = str.replace(/\r?\n/g, `${code.close}$&${code.open}`);
}
ansiStyles.dim.open = originalDim;
return str;
}
function chalkTag(chalk, strings) {
if (!Array.isArray(strings)) {
return [].slice.call(arguments, 1).join(" ");
}
const args = [].slice.call(arguments, 2);
const parts = [strings.raw[0]];
for (let i = 1; i < strings.length; i++) {
parts.push(String(args[i - 1]).replace(/[{}\\]/g, "\\$&"));
parts.push(String(strings.raw[i]));
}
return template(chalk, parts.join(""));
}
Object.defineProperties(Chalk.prototype, styles);
module22.exports = Chalk();
module22.exports.supportsColor = stdoutColor;
module22.exports.default = module22.exports;
}
});
var require_lib22 = __commonJS2({
"node_modules/@babel/highlight/lib/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.default = highlight;
exports2.getChalk = getChalk;
exports2.shouldHighlight = shouldHighlight;
var _jsTokens = require_js_tokens();
var _helperValidatorIdentifier = require_lib8();
var _chalk = require_chalk();
var sometimesKeywords = /* @__PURE__ */ new Set(["as", "async", "from", "get", "of", "set"]);
function getDefs(chalk) {
return {
keyword: chalk.cyan,
capitalized: chalk.yellow,
jsxIdentifier: chalk.yellow,
punctuator: chalk.yellow,
number: chalk.magenta,
string: chalk.green,
regex: chalk.magenta,
comment: chalk.grey,
invalid: chalk.white.bgRed.bold
};
}
var NEWLINE = /\r\n|[\n\r\u2028\u2029]/;
var BRACKET = /^[()[\]{}]$/;
var tokenize;
{
const JSX_TAG = /^[a-z][\w-]*$/i;
const getTokenType = function(token, offset, text) {
if (token.type === "name") {
if ((0, _helperValidatorIdentifier.isKeyword)(token.value) || (0, _helperValidatorIdentifier.isStrictReservedWord)(token.value, true) || sometimesKeywords.has(token.value)) {
return "keyword";
}
if (JSX_TAG.test(token.value) && (text[offset - 1] === "<" || text.slice(offset - 2, offset) == "")) {
return "jsxIdentifier";
}
if (token.value[0] !== token.value[0].toLowerCase()) {
return "capitalized";
}
}
if (token.type === "punctuator" && BRACKET.test(token.value)) {
return "bracket";
}
if (token.type === "invalid" && (token.value === "@" || token.value === "#")) {
return "punctuator";
}
return token.type;
};
tokenize = function* (text) {
let match;
while (match = _jsTokens.default.exec(text)) {
const token = _jsTokens.matchToToken(match);
yield {
type: getTokenType(token, match.index, text),
value: token.value
};
}
};
}
function highlightTokens(defs, text) {
let highlighted = "";
for (const {
type,
value
} of tokenize(text)) {
const colorize = defs[type];
if (colorize) {
highlighted += value.split(NEWLINE).map((str) => colorize(str)).join("\n");
} else {
highlighted += value;
}
}
return highlighted;
}
function shouldHighlight(options) {
return !!_chalk.supportsColor || options.forceColor;
}
function getChalk(options) {
return options.forceColor ? new _chalk.constructor({
enabled: true,
level: 1
}) : _chalk;
}
function highlight(code, options = {}) {
if (code !== "" && shouldHighlight(options)) {
const chalk = getChalk(options);
const defs = getDefs(chalk);
return highlightTokens(defs, code);
} else {
return code;
}
}
}
});
var require_lib32 = __commonJS2({
"node_modules/@babel/code-frame/lib/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.codeFrameColumns = codeFrameColumns;
exports2.default = _default;
var _highlight = require_lib22();
var deprecationWarningShown = false;
function getDefs(chalk) {
return {
gutter: chalk.grey,
marker: chalk.red.bold,
message: chalk.red.bold
};
}
var NEWLINE = /\r\n|[\n\r\u2028\u2029]/;
function getMarkerLines(loc, source, opts) {
const startLoc = Object.assign({
column: 0,
line: -1
}, loc.start);
const endLoc = Object.assign({}, startLoc, loc.end);
const {
linesAbove = 2,
linesBelow = 3
} = opts || {};
const startLine = startLoc.line;
const startColumn = startLoc.column;
const endLine = endLoc.line;
const endColumn = endLoc.column;
let start = Math.max(startLine - (linesAbove + 1), 0);
let end = Math.min(source.length, endLine + linesBelow);
if (startLine === -1) {
start = 0;
}
if (endLine === -1) {
end = source.length;
}
const lineDiff = endLine - startLine;
const markerLines = {};
if (lineDiff) {
for (let i = 0; i <= lineDiff; i++) {
const lineNumber = i + startLine;
if (!startColumn) {
markerLines[lineNumber] = true;
} else if (i === 0) {
const sourceLength = source[lineNumber - 1].length;
markerLines[lineNumber] = [startColumn, sourceLength - startColumn + 1];
} else if (i === lineDiff) {
markerLines[lineNumber] = [0, endColumn];
} else {
const sourceLength = source[lineNumber - i].length;
markerLines[lineNumber] = [0, sourceLength];
}
}
} else {
if (startColumn === endColumn) {
if (startColumn) {
markerLines[startLine] = [startColumn, 0];
} else {
markerLines[startLine] = true;
}
} else {
markerLines[startLine] = [startColumn, endColumn - startColumn];
}
}
return {
start,
end,
markerLines
};
}
function codeFrameColumns(rawLines, loc, opts = {}) {
const highlighted = (opts.highlightCode || opts.forceColor) && (0, _highlight.shouldHighlight)(opts);
const chalk = (0, _highlight.getChalk)(opts);
const defs = getDefs(chalk);
const maybeHighlight = (chalkFn, string) => {
return highlighted ? chalkFn(string) : string;
};
const lines = rawLines.split(NEWLINE);
const {
start,
end,
markerLines
} = getMarkerLines(loc, lines, opts);
const hasColumns = loc.start && typeof loc.start.column === "number";
const numberMaxWidth = String(end).length;
const highlightedLines = highlighted ? (0, _highlight.default)(rawLines, opts) : rawLines;
let frame = highlightedLines.split(NEWLINE, end).slice(start, end).map((line, index) => {
const number = start + 1 + index;
const paddedNumber = ` ${number}`.slice(-numberMaxWidth);
const gutter = ` ${paddedNumber} |`;
const hasMarker = markerLines[number];
const lastMarkerLine = !markerLines[number + 1];
if (hasMarker) {
let markerLine = "";
if (Array.isArray(hasMarker)) {
const markerSpacing = line.slice(0, Math.max(hasMarker[0] - 1, 0)).replace(/[^\t]/g, " ");
const numberOfMarkers = hasMarker[1] || 1;
markerLine = ["\n ", maybeHighlight(defs.gutter, gutter.replace(/\d/g, " ")), " ", markerSpacing, maybeHighlight(defs.marker, "^").repeat(numberOfMarkers)].join("");
if (lastMarkerLine && opts.message) {
markerLine += " " + maybeHighlight(defs.message, opts.message);
}
}
return [maybeHighlight(defs.marker, ">"), maybeHighlight(defs.gutter, gutter), line.length > 0 ? ` ${line}` : "", markerLine].join("");
} else {
return ` ${maybeHighlight(defs.gutter, gutter)}${line.length > 0 ? ` ${line}` : ""}`;
}
}).join("\n");
if (opts.message && !hasColumns) {
frame = `${" ".repeat(numberMaxWidth + 1)}${opts.message}
${frame}`;
}
if (highlighted) {
return chalk.reset(frame);
} else {
return frame;
}
}
function _default(rawLines, lineNumber, colNumber, opts = {}) {
if (!deprecationWarningShown) {
deprecationWarningShown = true;
const message = "Passing lineNumber and colNumber is deprecated to @babel/code-frame. Please use `codeFrameColumns`.";
if (process.emitWarning) {
process.emitWarning(message, "DeprecationWarning");
} else {
const deprecationError = new Error(message);
deprecationError.name = "DeprecationWarning";
console.warn(new Error(message));
}
}
colNumber = Math.max(colNumber, 0);
const location = {
start: {
column: colNumber,
line: lineNumber
}
};
return codeFrameColumns(rawLines, location, opts);
}
}
});
var require_parse_json = __commonJS2({
"node_modules/parse-json/index.js"(exports2, module22) {
"use strict";
var errorEx = require_error_ex();
var fallback = require_json_parse_even_better_errors();
var {
default: LinesAndColumns
} = require_build();
var {
codeFrameColumns
} = require_lib32();
var JSONError = errorEx("JSONError", {
fileName: errorEx.append("in %s"),
codeFrame: errorEx.append("\n\n%s\n")
});
var parseJson = (string, reviver, filename) => {
if (typeof reviver === "string") {
filename = reviver;
reviver = null;
}
try {
try {
return JSON.parse(string, reviver);
} catch (error) {
fallback(string, reviver);
throw error;
}
} catch (error) {
error.message = error.message.replace(/\n/g, "");
const indexMatch = error.message.match(/in JSON at position (\d+) while parsing/);
const jsonError = new JSONError(error);
if (filename) {
jsonError.fileName = filename;
}
if (indexMatch && indexMatch.length > 0) {
const lines = new LinesAndColumns(string);
const index = Number(indexMatch[1]);
const location = lines.locationForIndex(index);
const codeFrame = codeFrameColumns(string, {
start: {
line: location.line + 1,
column: location.column + 1
}
}, {
highlightCode: true
});
jsonError.codeFrame = codeFrame;
}
throw jsonError;
}
};
parseJson.JSONError = JSONError;
module22.exports = parseJson;
}
});
var require_PlainValue_ec8e588e = __commonJS2({
"node_modules/yaml/dist/PlainValue-ec8e588e.js"(exports2) {
"use strict";
var Char = {
ANCHOR: "&",
COMMENT: "#",
TAG: "!",
DIRECTIVES_END: "-",
DOCUMENT_END: "."
};
var Type = {
ALIAS: "ALIAS",
BLANK_LINE: "BLANK_LINE",
BLOCK_FOLDED: "BLOCK_FOLDED",
BLOCK_LITERAL: "BLOCK_LITERAL",
COMMENT: "COMMENT",
DIRECTIVE: "DIRECTIVE",
DOCUMENT: "DOCUMENT",
FLOW_MAP: "FLOW_MAP",
FLOW_SEQ: "FLOW_SEQ",
MAP: "MAP",
MAP_KEY: "MAP_KEY",
MAP_VALUE: "MAP_VALUE",
PLAIN: "PLAIN",
QUOTE_DOUBLE: "QUOTE_DOUBLE",
QUOTE_SINGLE: "QUOTE_SINGLE",
SEQ: "SEQ",
SEQ_ITEM: "SEQ_ITEM"
};
var defaultTagPrefix = "tag:yaml.org,2002:";
var defaultTags = {
MAP: "tag:yaml.org,2002:map",
SEQ: "tag:yaml.org,2002:seq",
STR: "tag:yaml.org,2002:str"
};
function findLineStarts(src) {
const ls = [0];
let offset = src.indexOf("\n");
while (offset !== -1) {
offset += 1;
ls.push(offset);
offset = src.indexOf("\n", offset);
}
return ls;
}
function getSrcInfo(cst) {
let lineStarts, src;
if (typeof cst === "string") {
lineStarts = findLineStarts(cst);
src = cst;
} else {
if (Array.isArray(cst))
cst = cst[0];
if (cst && cst.context) {
if (!cst.lineStarts)
cst.lineStarts = findLineStarts(cst.context.src);
lineStarts = cst.lineStarts;
src = cst.context.src;
}
}
return {
lineStarts,
src
};
}
function getLinePos(offset, cst) {
if (typeof offset !== "number" || offset < 0)
return null;
const {
lineStarts,
src
} = getSrcInfo(cst);
if (!lineStarts || !src || offset > src.length)
return null;
for (let i = 0; i < lineStarts.length; ++i) {
const start = lineStarts[i];
if (offset < start) {
return {
line: i,
col: offset - lineStarts[i - 1] + 1
};
}
if (offset === start)
return {
line: i + 1,
col: 1
};
}
const line = lineStarts.length;
return {
line,
col: offset - lineStarts[line - 1] + 1
};
}
function getLine(line, cst) {
const {
lineStarts,
src
} = getSrcInfo(cst);
if (!lineStarts || !(line >= 1) || line > lineStarts.length)
return null;
const start = lineStarts[line - 1];
let end = lineStarts[line];
while (end && end > start && src[end - 1] === "\n")
--end;
return src.slice(start, end);
}
function getPrettyContext({
start,
end
}, cst, maxWidth = 80) {
let src = getLine(start.line, cst);
if (!src)
return null;
let {
col
} = start;
if (src.length > maxWidth) {
if (col <= maxWidth - 10) {
src = src.substr(0, maxWidth - 1) + "\u2026";
} else {
const halfWidth = Math.round(maxWidth / 2);
if (src.length > col + halfWidth)
src = src.substr(0, col + halfWidth - 1) + "\u2026";
col -= src.length - maxWidth;
src = "\u2026" + src.substr(1 - maxWidth);
}
}
let errLen = 1;
let errEnd = "";
if (end) {
if (end.line === start.line && col + (end.col - start.col) <= maxWidth + 1) {
errLen = end.col - start.col;
} else {
errLen = Math.min(src.length + 1, maxWidth) - col;
errEnd = "\u2026";
}
}
const offset = col > 1 ? " ".repeat(col - 1) : "";
const err = "^".repeat(errLen);
return `${src}
${offset}${err}${errEnd}`;
}
var Range = class {
static copy(orig) {
return new Range(orig.start, orig.end);
}
constructor(start, end) {
this.start = start;
this.end = end || start;
}
isEmpty() {
return typeof this.start !== "number" || !this.end || this.end <= this.start;
}
setOrigRange(cr, offset) {
const {
start,
end
} = this;
if (cr.length === 0 || end <= cr[0]) {
this.origStart = start;
this.origEnd = end;
return offset;
}
let i = offset;
while (i < cr.length) {
if (cr[i] > start)
break;
else
++i;
}
this.origStart = start + i;
const nextOffset = i;
while (i < cr.length) {
if (cr[i] >= end)
break;
else
++i;
}
this.origEnd = end + i;
return nextOffset;
}
};
var Node = class {
static addStringTerminator(src, offset, str) {
if (str[str.length - 1] === "\n")
return str;
const next = Node.endOfWhiteSpace(src, offset);
return next >= src.length || src[next] === "\n" ? str + "\n" : str;
}
static atDocumentBoundary(src, offset, sep) {
const ch0 = src[offset];
if (!ch0)
return true;
const prev = src[offset - 1];
if (prev && prev !== "\n")
return false;
if (sep) {
if (ch0 !== sep)
return false;
} else {
if (ch0 !== Char.DIRECTIVES_END && ch0 !== Char.DOCUMENT_END)
return false;
}
const ch1 = src[offset + 1];
const ch2 = src[offset + 2];
if (ch1 !== ch0 || ch2 !== ch0)
return false;
const ch3 = src[offset + 3];
return !ch3 || ch3 === "\n" || ch3 === " " || ch3 === " ";
}
static endOfIdentifier(src, offset) {
let ch = src[offset];
const isVerbatim = ch === "<";
const notOk = isVerbatim ? ["\n", " ", " ", ">"] : ["\n", " ", " ", "[", "]", "{", "}", ","];
while (ch && notOk.indexOf(ch) === -1)
ch = src[offset += 1];
if (isVerbatim && ch === ">")
offset += 1;
return offset;
}
static endOfIndent(src, offset) {
let ch = src[offset];
while (ch === " ")
ch = src[offset += 1];
return offset;
}
static endOfLine(src, offset) {
let ch = src[offset];
while (ch && ch !== "\n")
ch = src[offset += 1];
return offset;
}
static endOfWhiteSpace(src, offset) {
let ch = src[offset];
while (ch === " " || ch === " ")
ch = src[offset += 1];
return offset;
}
static startOfLine(src, offset) {
let ch = src[offset - 1];
if (ch === "\n")
return offset;
while (ch && ch !== "\n")
ch = src[offset -= 1];
return offset + 1;
}
static endOfBlockIndent(src, indent, lineStart) {
const inEnd = Node.endOfIndent(src, lineStart);
if (inEnd > lineStart + indent) {
return inEnd;
} else {
const wsEnd = Node.endOfWhiteSpace(src, inEnd);
const ch = src[wsEnd];
if (!ch || ch === "\n")
return wsEnd;
}
return null;
}
static atBlank(src, offset, endAsBlank) {
const ch = src[offset];
return ch === "\n" || ch === " " || ch === " " || endAsBlank && !ch;
}
static nextNodeIsIndented(ch, indentDiff, indicatorAsIndent) {
if (!ch || indentDiff < 0)
return false;
if (indentDiff > 0)
return true;
return indicatorAsIndent && ch === "-";
}
static normalizeOffset(src, offset) {
const ch = src[offset];
return !ch ? offset : ch !== "\n" && src[offset - 1] === "\n" ? offset - 1 : Node.endOfWhiteSpace(src, offset);
}
static foldNewline(src, offset, indent) {
let inCount = 0;
let error = false;
let fold = "";
let ch = src[offset + 1];
while (ch === " " || ch === " " || ch === "\n") {
switch (ch) {
case "\n":
inCount = 0;
offset += 1;
fold += "\n";
break;
case " ":
if (inCount <= indent)
error = true;
offset = Node.endOfWhiteSpace(src, offset + 2) - 1;
break;
case " ":
inCount += 1;
offset += 1;
break;
}
ch = src[offset + 1];
}
if (!fold)
fold = " ";
if (ch && inCount <= indent)
error = true;
return {
fold,
offset,
error
};
}
constructor(type, props, context) {
Object.defineProperty(this, "context", {
value: context || null,
writable: true
});
this.error = null;
this.range = null;
this.valueRange = null;
this.props = props || [];
this.type = type;
this.value = null;
}
getPropValue(idx, key, skipKey) {
if (!this.context)
return null;
const {
src
} = this.context;
const prop = this.props[idx];
return prop && src[prop.start] === key ? src.slice(prop.start + (skipKey ? 1 : 0), prop.end) : null;
}
get anchor() {
for (let i = 0; i < this.props.length; ++i) {
const anchor = this.getPropValue(i, Char.ANCHOR, true);
if (anchor != null)
return anchor;
}
return null;
}
get comment() {
const comments = [];
for (let i = 0; i < this.props.length; ++i) {
const comment = this.getPropValue(i, Char.COMMENT, true);
if (comment != null)
comments.push(comment);
}
return comments.length > 0 ? comments.join("\n") : null;
}
commentHasRequiredWhitespace(start) {
const {
src
} = this.context;
if (this.header && start === this.header.end)
return false;
if (!this.valueRange)
return false;
const {
end
} = this.valueRange;
return start !== end || Node.atBlank(src, end - 1);
}
get hasComment() {
if (this.context) {
const {
src
} = this.context;
for (let i = 0; i < this.props.length; ++i) {
if (src[this.props[i].start] === Char.COMMENT)
return true;
}
}
return false;
}
get hasProps() {
if (this.context) {
const {
src
} = this.context;
for (let i = 0; i < this.props.length; ++i) {
if (src[this.props[i].start] !== Char.COMMENT)
return true;
}
}
return false;
}
get includesTrailingLines() {
return false;
}
get jsonLike() {
const jsonLikeTypes = [Type.FLOW_MAP, Type.FLOW_SEQ, Type.QUOTE_DOUBLE, Type.QUOTE_SINGLE];
return jsonLikeTypes.indexOf(this.type) !== -1;
}
get rangeAsLinePos() {
if (!this.range || !this.context)
return void 0;
const start = getLinePos(this.range.start, this.context.root);
if (!start)
return void 0;
const end = getLinePos(this.range.end, this.context.root);
return {
start,
end
};
}
get rawValue() {
if (!this.valueRange || !this.context)
return null;
const {
start,
end
} = this.valueRange;
return this.context.src.slice(start, end);
}
get tag() {
for (let i = 0; i < this.props.length; ++i) {
const tag = this.getPropValue(i, Char.TAG, false);
if (tag != null) {
if (tag[1] === "<") {
return {
verbatim: tag.slice(2, -1)
};
} else {
const [_, handle, suffix] = tag.match(/^(.*!)([^!]*)$/);
return {
handle,
suffix
};
}
}
}
return null;
}
get valueRangeContainsNewline() {
if (!this.valueRange || !this.context)
return false;
const {
start,
end
} = this.valueRange;
const {
src
} = this.context;
for (let i = start; i < end; ++i) {
if (src[i] === "\n")
return true;
}
return false;
}
parseComment(start) {
const {
src
} = this.context;
if (src[start] === Char.COMMENT) {
const end = Node.endOfLine(src, start + 1);
const commentRange = new Range(start, end);
this.props.push(commentRange);
return end;
}
return start;
}
setOrigRanges(cr, offset) {
if (this.range)
offset = this.range.setOrigRange(cr, offset);
if (this.valueRange)
this.valueRange.setOrigRange(cr, offset);
this.props.forEach((prop) => prop.setOrigRange(cr, offset));
return offset;
}
toString() {
const {
context: {
src
},
range,
value
} = this;
if (value != null)
return value;
const str = src.slice(range.start, range.end);
return Node.addStringTerminator(src, range.end, str);
}
};
var YAMLError = class extends Error {
constructor(name, source, message) {
if (!message || !(source instanceof Node))
throw new Error(`Invalid arguments for new ${name}`);
super();
this.name = name;
this.message = message;
this.source = source;
}
makePretty() {
if (!this.source)
return;
this.nodeType = this.source.type;
const cst = this.source.context && this.source.context.root;
if (typeof this.offset === "number") {
this.range = new Range(this.offset, this.offset + 1);
const start = cst && getLinePos(this.offset, cst);
if (start) {
const end = {
line: start.line,
col: start.col + 1
};
this.linePos = {
start,
end
};
}
delete this.offset;
} else {
this.range = this.source.range;
this.linePos = this.source.rangeAsLinePos;
}
if (this.linePos) {
const {
line,
col
} = this.linePos.start;
this.message += ` at line ${line}, column ${col}`;
const ctx = cst && getPrettyContext(this.linePos, cst);
if (ctx)
this.message += `:
${ctx}
`;
}
delete this.source;
}
};
var YAMLReferenceError = class extends YAMLError {
constructor(source, message) {
super("YAMLReferenceError", source, message);
}
};
var YAMLSemanticError = class extends YAMLError {
constructor(source, message) {
super("YAMLSemanticError", source, message);
}
};
var YAMLSyntaxError = class extends YAMLError {
constructor(source, message) {
super("YAMLSyntaxError", source, message);
}
};
var YAMLWarning = class extends YAMLError {
constructor(source, message) {
super("YAMLWarning", source, message);
}
};
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, {
value,
enumerable: true,
configurable: true,
writable: true
});
} else {
obj[key] = value;
}
return obj;
}
var PlainValue = class extends Node {
static endOfLine(src, start, inFlow) {
let ch = src[start];
let offset = start;
while (ch && ch !== "\n") {
if (inFlow && (ch === "[" || ch === "]" || ch === "{" || ch === "}" || ch === ","))
break;
const next = src[offset + 1];
if (ch === ":" && (!next || next === "\n" || next === " " || next === " " || inFlow && next === ","))
break;
if ((ch === " " || ch === " ") && next === "#")
break;
offset += 1;
ch = next;
}
return offset;
}
get strValue() {
if (!this.valueRange || !this.context)
return null;
let {
start,
end
} = this.valueRange;
const {
src
} = this.context;
let ch = src[end - 1];
while (start < end && (ch === "\n" || ch === " " || ch === " "))
ch = src[--end - 1];
let str = "";
for (let i = start; i < end; ++i) {
const ch2 = src[i];
if (ch2 === "\n") {
const {
fold,
offset
} = Node.foldNewline(src, i, -1);
str += fold;
i = offset;
} else if (ch2 === " " || ch2 === " ") {
const wsStart = i;
let next = src[i + 1];
while (i < end && (next === " " || next === " ")) {
i += 1;
next = src[i + 1];
}
if (next !== "\n")
str += i > wsStart ? src.slice(wsStart, i + 1) : ch2;
} else {
str += ch2;
}
}
const ch0 = src[start];
switch (ch0) {
case " ": {
const msg = "Plain value cannot start with a tab character";
const errors = [new YAMLSemanticError(this, msg)];
return {
errors,
str
};
}
case "@":
case "`": {
const msg = `Plain value cannot start with reserved character ${ch0}`;
const errors = [new YAMLSemanticError(this, msg)];
return {
errors,
str
};
}
default:
return str;
}
}
parseBlockValue(start) {
const {
indent,
inFlow,
src
} = this.context;
let offset = start;
let valueEnd = start;
for (let ch = src[offset]; ch === "\n"; ch = src[offset]) {
if (Node.atDocumentBoundary(src, offset + 1))
break;
const end = Node.endOfBlockIndent(src, indent, offset + 1);
if (end === null || src[end] === "#")
break;
if (src[end] === "\n") {
offset = end;
} else {
valueEnd = PlainValue.endOfLine(src, end, inFlow);
offset = valueEnd;
}
}
if (this.valueRange.isEmpty())
this.valueRange.start = start;
this.valueRange.end = valueEnd;
return valueEnd;
}
parse(context, start) {
this.context = context;
const {
inFlow,
src
} = context;
let offset = start;
const ch = src[offset];
if (ch && ch !== "#" && ch !== "\n") {
offset = PlainValue.endOfLine(src, start, inFlow);
}
this.valueRange = new Range(start, offset);
offset = Node.endOfWhiteSpace(src, offset);
offset = this.parseComment(offset);
if (!this.hasComment || this.valueRange.isEmpty()) {
offset = this.parseBlockValue(offset);
}
return offset;
}
};
exports2.Char = Char;
exports2.Node = Node;
exports2.PlainValue = PlainValue;
exports2.Range = Range;
exports2.Type = Type;
exports2.YAMLError = YAMLError;
exports2.YAMLReferenceError = YAMLReferenceError;
exports2.YAMLSemanticError = YAMLSemanticError;
exports2.YAMLSyntaxError = YAMLSyntaxError;
exports2.YAMLWarning = YAMLWarning;
exports2._defineProperty = _defineProperty;
exports2.defaultTagPrefix = defaultTagPrefix;
exports2.defaultTags = defaultTags;
}
});
var require_parse_cst = __commonJS2({
"node_modules/yaml/dist/parse-cst.js"(exports2) {
"use strict";
var PlainValue = require_PlainValue_ec8e588e();
var BlankLine = class extends PlainValue.Node {
constructor() {
super(PlainValue.Type.BLANK_LINE);
}
get includesTrailingLines() {
return true;
}
parse(context, start) {
this.context = context;
this.range = new PlainValue.Range(start, start + 1);
return start + 1;
}
};
var CollectionItem = class extends PlainValue.Node {
constructor(type, props) {
super(type, props);
this.node = null;
}
get includesTrailingLines() {
return !!this.node && this.node.includesTrailingLines;
}
parse(context, start) {
this.context = context;
const {
parseNode,
src
} = context;
let {
atLineStart,
lineStart
} = context;
if (!atLineStart && this.type === PlainValue.Type.SEQ_ITEM)
this.error = new PlainValue.YAMLSemanticError(this, "Sequence items must not have preceding content on the same line");
const indent = atLineStart ? start - lineStart : context.indent;
let offset = PlainValue.Node.endOfWhiteSpace(src, start + 1);
let ch = src[offset];
const inlineComment = ch === "#";
const comments = [];
let blankLine = null;
while (ch === "\n" || ch === "#") {
if (ch === "#") {
const end2 = PlainValue.Node.endOfLine(src, offset + 1);
comments.push(new PlainValue.Range(offset, end2));
offset = end2;
} else {
atLineStart = true;
lineStart = offset + 1;
const wsEnd = PlainValue.Node.endOfWhiteSpace(src, lineStart);
if (src[wsEnd] === "\n" && comments.length === 0) {
blankLine = new BlankLine();
lineStart = blankLine.parse({
src
}, lineStart);
}
offset = PlainValue.Node.endOfIndent(src, lineStart);
}
ch = src[offset];
}
if (PlainValue.Node.nextNodeIsIndented(ch, offset - (lineStart + indent), this.type !== PlainValue.Type.SEQ_ITEM)) {
this.node = parseNode({
atLineStart,
inCollection: false,
indent,
lineStart,
parent: this
}, offset);
} else if (ch && lineStart > start + 1) {
offset = lineStart - 1;
}
if (this.node) {
if (blankLine) {
const items = context.parent.items || context.parent.contents;
if (items)
items.push(blankLine);
}
if (comments.length)
Array.prototype.push.apply(this.props, comments);
offset = this.node.range.end;
} else {
if (inlineComment) {
const c = comments[0];
this.props.push(c);
offset = c.end;
} else {
offset = PlainValue.Node.endOfLine(src, start + 1);
}
}
const end = this.node ? this.node.valueRange.end : offset;
this.valueRange = new PlainValue.Range(start, end);
return offset;
}
setOrigRanges(cr, offset) {
offset = super.setOrigRanges(cr, offset);
return this.node ? this.node.setOrigRanges(cr, offset) : offset;
}
toString() {
const {
context: {
src
},
node,
range,
value
} = this;
if (value != null)
return value;
const str = node ? src.slice(range.start, node.range.start) + String(node) : src.slice(range.start, range.end);
return PlainValue.Node.addStringTerminator(src, range.end, str);
}
};
var Comment = class extends PlainValue.Node {
constructor() {
super(PlainValue.Type.COMMENT);
}
parse(context, start) {
this.context = context;
const offset = this.parseComment(start);
this.range = new PlainValue.Range(start, offset);
return offset;
}
};
function grabCollectionEndComments(node) {
let cnode = node;
while (cnode instanceof CollectionItem)
cnode = cnode.node;
if (!(cnode instanceof Collection))
return null;
const len = cnode.items.length;
let ci = -1;
for (let i = len - 1; i >= 0; --i) {
const n2 = cnode.items[i];
if (n2.type === PlainValue.Type.COMMENT) {
const {
indent,
lineStart
} = n2.context;
if (indent > 0 && n2.range.start >= lineStart + indent)
break;
ci = i;
} else if (n2.type === PlainValue.Type.BLANK_LINE)
ci = i;
else
break;
}
if (ci === -1)
return null;
const ca = cnode.items.splice(ci, len - ci);
const prevEnd = ca[0].range.start;
while (true) {
cnode.range.end = prevEnd;
if (cnode.valueRange && cnode.valueRange.end > prevEnd)
cnode.valueRange.end = prevEnd;
if (cnode === node)
break;
cnode = cnode.context.parent;
}
return ca;
}
var Collection = class extends PlainValue.Node {
static nextContentHasIndent(src, offset, indent) {
const lineStart = PlainValue.Node.endOfLine(src, offset) + 1;
offset = PlainValue.Node.endOfWhiteSpace(src, lineStart);
const ch = src[offset];
if (!ch)
return false;
if (offset >= lineStart + indent)
return true;
if (ch !== "#" && ch !== "\n")
return false;
return Collection.nextContentHasIndent(src, offset, indent);
}
constructor(firstItem) {
super(firstItem.type === PlainValue.Type.SEQ_ITEM ? PlainValue.Type.SEQ : PlainValue.Type.MAP);
for (let i = firstItem.props.length - 1; i >= 0; --i) {
if (firstItem.props[i].start < firstItem.context.lineStart) {
this.props = firstItem.props.slice(0, i + 1);
firstItem.props = firstItem.props.slice(i + 1);
const itemRange = firstItem.props[0] || firstItem.valueRange;
firstItem.range.start = itemRange.start;
break;
}
}
this.items = [firstItem];
const ec = grabCollectionEndComments(firstItem);
if (ec)
Array.prototype.push.apply(this.items, ec);
}
get includesTrailingLines() {
return this.items.length > 0;
}
parse(context, start) {
this.context = context;
const {
parseNode,
src
} = context;
let lineStart = PlainValue.Node.startOfLine(src, start);
const firstItem = this.items[0];
firstItem.context.parent = this;
this.valueRange = PlainValue.Range.copy(firstItem.valueRange);
const indent = firstItem.range.start - firstItem.context.lineStart;
let offset = start;
offset = PlainValue.Node.normalizeOffset(src, offset);
let ch = src[offset];
let atLineStart = PlainValue.Node.endOfWhiteSpace(src, lineStart) === offset;
let prevIncludesTrailingLines = false;
while (ch) {
while (ch === "\n" || ch === "#") {
if (atLineStart && ch === "\n" && !prevIncludesTrailingLines) {
const blankLine = new BlankLine();
offset = blankLine.parse({
src
}, offset);
this.valueRange.end = offset;
if (offset >= src.length) {
ch = null;
break;
}
this.items.push(blankLine);
offset -= 1;
} else if (ch === "#") {
if (offset < lineStart + indent && !Collection.nextContentHasIndent(src, offset, indent)) {
return offset;
}
const comment = new Comment();
offset = comment.parse({
indent,
lineStart,
src
}, offset);
this.items.push(comment);
this.valueRange.end = offset;
if (offset >= src.length) {
ch = null;
break;
}
}
lineStart = offset + 1;
offset = PlainValue.Node.endOfIndent(src, lineStart);
if (PlainValue.Node.atBlank(src, offset)) {
const wsEnd = PlainValue.Node.endOfWhiteSpace(src, offset);
const next = src[wsEnd];
if (!next || next === "\n" || next === "#") {
offset = wsEnd;
}
}
ch = src[offset];
atLineStart = true;
}
if (!ch) {
break;
}
if (offset !== lineStart + indent && (atLineStart || ch !== ":")) {
if (offset < lineStart + indent) {
if (lineStart > start)
offset = lineStart;
break;
} else if (!this.error) {
const msg = "All collection items must start at the same column";
this.error = new PlainValue.YAMLSyntaxError(this, msg);
}
}
if (firstItem.type === PlainValue.Type.SEQ_ITEM) {
if (ch !== "-") {
if (lineStart > start)
offset = lineStart;
break;
}
} else if (ch === "-" && !this.error) {
const next = src[offset + 1];
if (!next || next === "\n" || next === " " || next === " ") {
const msg = "A collection cannot be both a mapping and a sequence";
this.error = new PlainValue.YAMLSyntaxError(this, msg);
}
}
const node = parseNode({
atLineStart,
inCollection: true,
indent,
lineStart,
parent: this
}, offset);
if (!node)
return offset;
this.items.push(node);
this.valueRange.end = node.valueRange.end;
offset = PlainValue.Node.normalizeOffset(src, node.range.end);
ch = src[offset];
atLineStart = false;
prevIncludesTrailingLines = node.includesTrailingLines;
if (ch) {
let ls = offset - 1;
let prev = src[ls];
while (prev === " " || prev === " ")
prev = src[--ls];
if (prev === "\n") {
lineStart = ls + 1;
atLineStart = true;
}
}
const ec = grabCollectionEndComments(node);
if (ec)
Array.prototype.push.apply(this.items, ec);
}
return offset;
}
setOrigRanges(cr, offset) {
offset = super.setOrigRanges(cr, offset);
this.items.forEach((node) => {
offset = node.setOrigRanges(cr, offset);
});
return offset;
}
toString() {
const {
context: {
src
},
items,
range,
value
} = this;
if (value != null)
return value;
let str = src.slice(range.start, items[0].range.start) + String(items[0]);
for (let i = 1; i < items.length; ++i) {
const item = items[i];
const {
atLineStart,
indent
} = item.context;
if (atLineStart)
for (let i2 = 0; i2 < indent; ++i2)
str += " ";
str += String(item);
}
return PlainValue.Node.addStringTerminator(src, range.end, str);
}
};
var Directive = class extends PlainValue.Node {
constructor() {
super(PlainValue.Type.DIRECTIVE);
this.name = null;
}
get parameters() {
const raw = this.rawValue;
return raw ? raw.trim().split(/[ \t]+/) : [];
}
parseName(start) {
const {
src
} = this.context;
let offset = start;
let ch = src[offset];
while (ch && ch !== "\n" && ch !== " " && ch !== " ")
ch = src[offset += 1];
this.name = src.slice(start, offset);
return offset;
}
parseParameters(start) {
const {
src
} = this.context;
let offset = start;
let ch = src[offset];
while (ch && ch !== "\n" && ch !== "#")
ch = src[offset += 1];
this.valueRange = new PlainValue.Range(start, offset);
return offset;
}
parse(context, start) {
this.context = context;
let offset = this.parseName(start + 1);
offset = this.parseParameters(offset);
offset = this.parseComment(offset);
this.range = new PlainValue.Range(start, offset);
return offset;
}
};
var Document = class extends PlainValue.Node {
static startCommentOrEndBlankLine(src, start) {
const offset = PlainValue.Node.endOfWhiteSpace(src, start);
const ch = src[offset];
return ch === "#" || ch === "\n" ? offset : start;
}
constructor() {
super(PlainValue.Type.DOCUMENT);
this.directives = null;
this.contents = null;
this.directivesEndMarker = null;
this.documentEndMarker = null;
}
parseDirectives(start) {
const {
src
} = this.context;
this.directives = [];
let atLineStart = true;
let hasDirectives = false;
let offset = start;
while (!PlainValue.Node.atDocumentBoundary(src, offset, PlainValue.Char.DIRECTIVES_END)) {
offset = Document.startCommentOrEndBlankLine(src, offset);
switch (src[offset]) {
case "\n":
if (atLineStart) {
const blankLine = new BlankLine();
offset = blankLine.parse({
src
}, offset);
if (offset < src.length) {
this.directives.push(blankLine);
}
} else {
offset += 1;
atLineStart = true;
}
break;
case "#":
{
const comment = new Comment();
offset = comment.parse({
src
}, offset);
this.directives.push(comment);
atLineStart = false;
}
break;
case "%":
{
const directive = new Directive();
offset = directive.parse({
parent: this,
src
}, offset);
this.directives.push(directive);
hasDirectives = true;
atLineStart = false;
}
break;
default:
if (hasDirectives) {
this.error = new PlainValue.YAMLSemanticError(this, "Missing directives-end indicator line");
} else if (this.directives.length > 0) {
this.contents = this.directives;
this.directives = [];
}
return offset;
}
}
if (src[offset]) {
this.directivesEndMarker = new PlainValue.Range(offset, offset + 3);
return offset + 3;
}
if (hasDirectives) {
this.error = new PlainValue.YAMLSemanticError(this, "Missing directives-end indicator line");
} else if (this.directives.length > 0) {
this.contents = this.directives;
this.directives = [];
}
return offset;
}
parseContents(start) {
const {
parseNode,
src
} = this.context;
if (!this.contents)
this.contents = [];
let lineStart = start;
while (src[lineStart - 1] === "-")
lineStart -= 1;
let offset = PlainValue.Node.endOfWhiteSpace(src, start);
let atLineStart = lineStart === start;
this.valueRange = new PlainValue.Range(offset);
while (!PlainValue.Node.atDocumentBoundary(src, offset, PlainValue.Char.DOCUMENT_END)) {
switch (src[offset]) {
case "\n":
if (atLineStart) {
const blankLine = new BlankLine();
offset = blankLine.parse({
src
}, offset);
if (offset < src.length) {
this.contents.push(blankLine);
}
} else {
offset += 1;
atLineStart = true;
}
lineStart = offset;
break;
case "#":
{
const comment = new Comment();
offset = comment.parse({
src
}, offset);
this.contents.push(comment);
atLineStart = false;
}
break;
default: {
const iEnd = PlainValue.Node.endOfIndent(src, offset);
const context = {
atLineStart,
indent: -1,
inFlow: false,
inCollection: false,
lineStart,
parent: this
};
const node = parseNode(context, iEnd);
if (!node)
return this.valueRange.end = iEnd;
this.contents.push(node);
offset = node.range.end;
atLineStart = false;
const ec = grabCollectionEndComments(node);
if (ec)
Array.prototype.push.apply(this.contents, ec);
}
}
offset = Document.startCommentOrEndBlankLine(src, offset);
}
this.valueRange.end = offset;
if (src[offset]) {
this.documentEndMarker = new PlainValue.Range(offset, offset + 3);
offset += 3;
if (src[offset]) {
offset = PlainValue.Node.endOfWhiteSpace(src, offset);
if (src[offset] === "#") {
const comment = new Comment();
offset = comment.parse({
src
}, offset);
this.contents.push(comment);
}
switch (src[offset]) {
case "\n":
offset += 1;
break;
case void 0:
break;
default:
this.error = new PlainValue.YAMLSyntaxError(this, "Document end marker line cannot have a non-comment suffix");
}
}
}
return offset;
}
parse(context, start) {
context.root = this;
this.context = context;
const {
src
} = context;
let offset = src.charCodeAt(start) === 65279 ? start + 1 : start;
offset = this.parseDirectives(offset);
offset = this.parseContents(offset);
return offset;
}
setOrigRanges(cr, offset) {
offset = super.setOrigRanges(cr, offset);
this.directives.forEach((node) => {
offset = node.setOrigRanges(cr, offset);
});
if (this.directivesEndMarker)
offset = this.directivesEndMarker.setOrigRange(cr, offset);
this.contents.forEach((node) => {
offset = node.setOrigRanges(cr, offset);
});
if (this.documentEndMarker)
offset = this.documentEndMarker.setOrigRange(cr, offset);
return offset;
}
toString() {
const {
contents,
directives,
value
} = this;
if (value != null)
return value;
let str = directives.join("");
if (contents.length > 0) {
if (directives.length > 0 || contents[0].type === PlainValue.Type.COMMENT)
str += "---\n";
str += contents.join("");
}
if (str[str.length - 1] !== "\n")
str += "\n";
return str;
}
};
var Alias = class extends PlainValue.Node {
parse(context, start) {
this.context = context;
const {
src
} = context;
let offset = PlainValue.Node.endOfIdentifier(src, start + 1);
this.valueRange = new PlainValue.Range(start + 1, offset);
offset = PlainValue.Node.endOfWhiteSpace(src, offset);
offset = this.parseComment(offset);
return offset;
}
};
var Chomp = {
CLIP: "CLIP",
KEEP: "KEEP",
STRIP: "STRIP"
};
var BlockValue = class extends PlainValue.Node {
constructor(type, props) {
super(type, props);
this.blockIndent = null;
this.chomping = Chomp.CLIP;
this.header = null;
}
get includesTrailingLines() {
return this.chomping === Chomp.KEEP;
}
get strValue() {
if (!this.valueRange || !this.context)
return null;
let {
start,
end
} = this.valueRange;
const {
indent,
src
} = this.context;
if (this.valueRange.isEmpty())
return "";
let lastNewLine = null;
let ch = src[end - 1];
while (ch === "\n" || ch === " " || ch === " ") {
end -= 1;
if (end <= start) {
if (this.chomping === Chomp.KEEP)
break;
else
return "";
}
if (ch === "\n")
lastNewLine = end;
ch = src[end - 1];
}
let keepStart = end + 1;
if (lastNewLine) {
if (this.chomping === Chomp.KEEP) {
keepStart = lastNewLine;
end = this.valueRange.end;
} else {
end = lastNewLine;
}
}
const bi = indent + this.blockIndent;
const folded = this.type === PlainValue.Type.BLOCK_FOLDED;
let atStart = true;
let str = "";
let sep = "";
let prevMoreIndented = false;
for (let i = start; i < end; ++i) {
for (let j = 0; j < bi; ++j) {
if (src[i] !== " ")
break;
i += 1;
}
const ch2 = src[i];
if (ch2 === "\n") {
if (sep === "\n")
str += "\n";
else
sep = "\n";
} else {
const lineEnd = PlainValue.Node.endOfLine(src, i);
const line = src.slice(i, lineEnd);
i = lineEnd;
if (folded && (ch2 === " " || ch2 === " ") && i < keepStart) {
if (sep === " ")
sep = "\n";
else if (!prevMoreIndented && !atStart && sep === "\n")
sep = "\n\n";
str += sep + line;
sep = lineEnd < end && src[lineEnd] || "";
prevMoreIndented = true;
} else {
str += sep + line;
sep = folded && i < keepStart ? " " : "\n";
prevMoreIndented = false;
}
if (atStart && line !== "")
atStart = false;
}
}
return this.chomping === Chomp.STRIP ? str : str + "\n";
}
parseBlockHeader(start) {
const {
src
} = this.context;
let offset = start + 1;
let bi = "";
while (true) {
const ch = src[offset];
switch (ch) {
case "-":
this.chomping = Chomp.STRIP;
break;
case "+":
this.chomping = Chomp.KEEP;
break;
case "0":
case "1":
case "2":
case "3":
case "4":
case "5":
case "6":
case "7":
case "8":
case "9":
bi += ch;
break;
default:
this.blockIndent = Number(bi) || null;
this.header = new PlainValue.Range(start, offset);
return offset;
}
offset += 1;
}
}
parseBlockValue(start) {
const {
indent,
src
} = this.context;
const explicit = !!this.blockIndent;
let offset = start;
let valueEnd = start;
let minBlockIndent = 1;
for (let ch = src[offset]; ch === "\n"; ch = src[offset]) {
offset += 1;
if (PlainValue.Node.atDocumentBoundary(src, offset))
break;
const end = PlainValue.Node.endOfBlockIndent(src, indent, offset);
if (end === null)
break;
const ch2 = src[end];
const lineIndent = end - (offset + indent);
if (!this.blockIndent) {
if (src[end] !== "\n") {
if (lineIndent < minBlockIndent) {
const msg = "Block scalars with more-indented leading empty lines must use an explicit indentation indicator";
this.error = new PlainValue.YAMLSemanticError(this, msg);
}
this.blockIndent = lineIndent;
} else if (lineIndent > minBlockIndent) {
minBlockIndent = lineIndent;
}
} else if (ch2 && ch2 !== "\n" && lineIndent < this.blockIndent) {
if (src[end] === "#")
break;
if (!this.error) {
const src2 = explicit ? "explicit indentation indicator" : "first line";
const msg = `Block scalars must not be less indented than their ${src2}`;
this.error = new PlainValue.YAMLSemanticError(this, msg);
}
}
if (src[end] === "\n") {
offset = end;
} else {
offset = valueEnd = PlainValue.Node.endOfLine(src, end);
}
}
if (this.chomping !== Chomp.KEEP) {
offset = src[valueEnd] ? valueEnd + 1 : valueEnd;
}
this.valueRange = new PlainValue.Range(start + 1, offset);
return offset;
}
parse(context, start) {
this.context = context;
const {
src
} = context;
let offset = this.parseBlockHeader(start);
offset = PlainValue.Node.endOfWhiteSpace(src, offset);
offset = this.parseComment(offset);
offset = this.parseBlockValue(offset);
return offset;
}
setOrigRanges(cr, offset) {
offset = super.setOrigRanges(cr, offset);
return this.header ? this.header.setOrigRange(cr, offset) : offset;
}
};
var FlowCollection = class extends PlainValue.Node {
constructor(type, props) {
super(type, props);
this.items = null;
}
prevNodeIsJsonLike(idx = this.items.length) {
const node = this.items[idx - 1];
return !!node && (node.jsonLike || node.type === PlainValue.Type.COMMENT && this.prevNodeIsJsonLike(idx - 1));
}
parse(context, start) {
this.context = context;
const {
parseNode,
src
} = context;
let {
indent,
lineStart
} = context;
let char2 = src[start];
this.items = [{
char: char2,
offset: start
}];
let offset = PlainValue.Node.endOfWhiteSpace(src, start + 1);
char2 = src[offset];
while (char2 && char2 !== "]" && char2 !== "}") {
switch (char2) {
case "\n":
{
lineStart = offset + 1;
const wsEnd = PlainValue.Node.endOfWhiteSpace(src, lineStart);
if (src[wsEnd] === "\n") {
const blankLine = new BlankLine();
lineStart = blankLine.parse({
src
}, lineStart);
this.items.push(blankLine);
}
offset = PlainValue.Node.endOfIndent(src, lineStart);
if (offset <= lineStart + indent) {
char2 = src[offset];
if (offset < lineStart + indent || char2 !== "]" && char2 !== "}") {
const msg = "Insufficient indentation in flow collection";
this.error = new PlainValue.YAMLSemanticError(this, msg);
}
}
}
break;
case ",":
{
this.items.push({
char: char2,
offset
});
offset += 1;
}
break;
case "#":
{
const comment = new Comment();
offset = comment.parse({
src
}, offset);
this.items.push(comment);
}
break;
case "?":
case ":": {
const next = src[offset + 1];
if (next === "\n" || next === " " || next === " " || next === "," || char2 === ":" && this.prevNodeIsJsonLike()) {
this.items.push({
char: char2,
offset
});
offset += 1;
break;
}
}
default: {
const node = parseNode({
atLineStart: false,
inCollection: false,
inFlow: true,
indent: -1,
lineStart,
parent: this
}, offset);
if (!node) {
this.valueRange = new PlainValue.Range(start, offset);
return offset;
}
this.items.push(node);
offset = PlainValue.Node.normalizeOffset(src, node.range.end);
}
}
offset = PlainValue.Node.endOfWhiteSpace(src, offset);
char2 = src[offset];
}
this.valueRange = new PlainValue.Range(start, offset + 1);
if (char2) {
this.items.push({
char: char2,
offset
});
offset = PlainValue.Node.endOfWhiteSpace(src, offset + 1);
offset = this.parseComment(offset);
}
return offset;
}
setOrigRanges(cr, offset) {
offset = super.setOrigRanges(cr, offset);
this.items.forEach((node) => {
if (node instanceof PlainValue.Node) {
offset = node.setOrigRanges(cr, offset);
} else if (cr.length === 0) {
node.origOffset = node.offset;
} else {
let i = offset;
while (i < cr.length) {
if (cr[i] > node.offset)
break;
else
++i;
}
node.origOffset = node.offset + i;
offset = i;
}
});
return offset;
}
toString() {
const {
context: {
src
},
items,
range,
value
} = this;
if (value != null)
return value;
const nodes = items.filter((item) => item instanceof PlainValue.Node);
let str = "";
let prevEnd = range.start;
nodes.forEach((node) => {
const prefix = src.slice(prevEnd, node.range.start);
prevEnd = node.range.end;
str += prefix + String(node);
if (str[str.length - 1] === "\n" && src[prevEnd - 1] !== "\n" && src[prevEnd] === "\n") {
prevEnd += 1;
}
});
str += src.slice(prevEnd, range.end);
return PlainValue.Node.addStringTerminator(src, range.end, str);
}
};
var QuoteDouble = class extends PlainValue.Node {
static endOfQuote(src, offset) {
let ch = src[offset];
while (ch && ch !== '"') {
offset += ch === "\\" ? 2 : 1;
ch = src[offset];
}
return offset + 1;
}
get strValue() {
if (!this.valueRange || !this.context)
return null;
const errors = [];
const {
start,
end
} = this.valueRange;
const {
indent,
src
} = this.context;
if (src[end - 1] !== '"')
errors.push(new PlainValue.YAMLSyntaxError(this, 'Missing closing "quote'));
let str = "";
for (let i = start + 1; i < end - 1; ++i) {
const ch = src[i];
if (ch === "\n") {
if (PlainValue.Node.atDocumentBoundary(src, i + 1))
errors.push(new PlainValue.YAMLSemanticError(this, "Document boundary indicators are not allowed within string values"));
const {
fold,
offset,
error
} = PlainValue.Node.foldNewline(src, i, indent);
str += fold;
i = offset;
if (error)
errors.push(new PlainValue.YAMLSemanticError(this, "Multi-line double-quoted string needs to be sufficiently indented"));
} else if (ch === "\\") {
i += 1;
switch (src[i]) {
case "0":
str += "\0";
break;
case "a":
str += "\x07";
break;
case "b":
str += "\b";
break;
case "e":
str += "\x1B";
break;
case "f":
str += "\f";
break;
case "n":
str += "\n";
break;
case "r":
str += "\r";
break;
case "t":
str += " ";
break;
case "v":
str += "\v";
break;
case "N":
str += "\x85";
break;
case "_":
str += "\xA0";
break;
case "L":
str += "\u2028";
break;
case "P":
str += "\u2029";
break;
case " ":
str += " ";
break;
case '"':
str += '"';
break;
case "/":
str += "/";
break;
case "\\":
str += "\\";
break;
case " ":
str += " ";
break;
case "x":
str += this.parseCharCode(i + 1, 2, errors);
i += 2;
break;
case "u":
str += this.parseCharCode(i + 1, 4, errors);
i += 4;
break;
case "U":
str += this.parseCharCode(i + 1, 8, errors);
i += 8;
break;
case "\n":
while (src[i + 1] === " " || src[i + 1] === " ")
i += 1;
break;
default:
errors.push(new PlainValue.YAMLSyntaxError(this, `Invalid escape sequence ${src.substr(i - 1, 2)}`));
str += "\\" + src[i];
}
} else if (ch === " " || ch === " ") {
const wsStart = i;
let next = src[i + 1];
while (next === " " || next === " ") {
i += 1;
next = src[i + 1];
}
if (next !== "\n")
str += i > wsStart ? src.slice(wsStart, i + 1) : ch;
} else {
str += ch;
}
}
return errors.length > 0 ? {
errors,
str
} : str;
}
parseCharCode(offset, length, errors) {
const {
src
} = this.context;
const cc = src.substr(offset, length);
const ok = cc.length === length && /^[0-9a-fA-F]+$/.test(cc);
const code = ok ? parseInt(cc, 16) : NaN;
if (isNaN(code)) {
errors.push(new PlainValue.YAMLSyntaxError(this, `Invalid escape sequence ${src.substr(offset - 2, length + 2)}`));
return src.substr(offset - 2, length + 2);
}
return String.fromCodePoint(code);
}
parse(context, start) {
this.context = context;
const {
src
} = context;
let offset = QuoteDouble.endOfQuote(src, start + 1);
this.valueRange = new PlainValue.Range(start, offset);
offset = PlainValue.Node.endOfWhiteSpace(src, offset);
offset = this.parseComment(offset);
return offset;
}
};
var QuoteSingle = class extends PlainValue.Node {
static endOfQuote(src, offset) {
let ch = src[offset];
while (ch) {
if (ch === "'") {
if (src[offset + 1] !== "'")
break;
ch = src[offset += 2];
} else {
ch = src[offset += 1];
}
}
return offset + 1;
}
get strValue() {
if (!this.valueRange || !this.context)
return null;
const errors = [];
const {
start,
end
} = this.valueRange;
const {
indent,
src
} = this.context;
if (src[end - 1] !== "'")
errors.push(new PlainValue.YAMLSyntaxError(this, "Missing closing 'quote"));
let str = "";
for (let i = start + 1; i < end - 1; ++i) {
const ch = src[i];
if (ch === "\n") {
if (PlainValue.Node.atDocumentBoundary(src, i + 1))
errors.push(new PlainValue.YAMLSemanticError(this, "Document boundary indicators are not allowed within string values"));
const {
fold,
offset,
error
} = PlainValue.Node.foldNewline(src, i, indent);
str += fold;
i = offset;
if (error)
errors.push(new PlainValue.YAMLSemanticError(this, "Multi-line single-quoted string needs to be sufficiently indented"));
} else if (ch === "'") {
str += ch;
i += 1;
if (src[i] !== "'")
errors.push(new PlainValue.YAMLSyntaxError(this, "Unescaped single quote? This should not happen."));
} else if (ch === " " || ch === " ") {
const wsStart = i;
let next = src[i + 1];
while (next === " " || next === " ") {
i += 1;
next = src[i + 1];
}
if (next !== "\n")
str += i > wsStart ? src.slice(wsStart, i + 1) : ch;
} else {
str += ch;
}
}
return errors.length > 0 ? {
errors,
str
} : str;
}
parse(context, start) {
this.context = context;
const {
src
} = context;
let offset = QuoteSingle.endOfQuote(src, start + 1);
this.valueRange = new PlainValue.Range(start, offset);
offset = PlainValue.Node.endOfWhiteSpace(src, offset);
offset = this.parseComment(offset);
return offset;
}
};
function createNewNode(type, props) {
switch (type) {
case PlainValue.Type.ALIAS:
return new Alias(type, props);
case PlainValue.Type.BLOCK_FOLDED:
case PlainValue.Type.BLOCK_LITERAL:
return new BlockValue(type, props);
case PlainValue.Type.FLOW_MAP:
case PlainValue.Type.FLOW_SEQ:
return new FlowCollection(type, props);
case PlainValue.Type.MAP_KEY:
case PlainValue.Type.MAP_VALUE:
case PlainValue.Type.SEQ_ITEM:
return new CollectionItem(type, props);
case PlainValue.Type.COMMENT:
case PlainValue.Type.PLAIN:
return new PlainValue.PlainValue(type, props);
case PlainValue.Type.QUOTE_DOUBLE:
return new QuoteDouble(type, props);
case PlainValue.Type.QUOTE_SINGLE:
return new QuoteSingle(type, props);
default:
return null;
}
}
var ParseContext = class {
static parseType(src, offset, inFlow) {
switch (src[offset]) {
case "*":
return PlainValue.Type.ALIAS;
case ">":
return PlainValue.Type.BLOCK_FOLDED;
case "|":
return PlainValue.Type.BLOCK_LITERAL;
case "{":
return PlainValue.Type.FLOW_MAP;
case "[":
return PlainValue.Type.FLOW_SEQ;
case "?":
return !inFlow && PlainValue.Node.atBlank(src, offset + 1, true) ? PlainValue.Type.MAP_KEY : PlainValue.Type.PLAIN;
case ":":
return !inFlow && PlainValue.Node.atBlank(src, offset + 1, true) ? PlainValue.Type.MAP_VALUE : PlainValue.Type.PLAIN;
case "-":
return !inFlow && PlainValue.Node.atBlank(src, offset + 1, true) ? PlainValue.Type.SEQ_ITEM : PlainValue.Type.PLAIN;
case '"':
return PlainValue.Type.QUOTE_DOUBLE;
case "'":
return PlainValue.Type.QUOTE_SINGLE;
default:
return PlainValue.Type.PLAIN;
}
}
constructor(orig = {}, {
atLineStart,
inCollection,
inFlow,
indent,
lineStart,
parent
} = {}) {
PlainValue._defineProperty(this, "parseNode", (overlay, start) => {
if (PlainValue.Node.atDocumentBoundary(this.src, start))
return null;
const context = new ParseContext(this, overlay);
const {
props,
type,
valueStart
} = context.parseProps(start);
const node = createNewNode(type, props);
let offset = node.parse(context, valueStart);
node.range = new PlainValue.Range(start, offset);
if (offset <= start) {
node.error = new Error(`Node#parse consumed no characters`);
node.error.parseEnd = offset;
node.error.source = node;
node.range.end = start + 1;
}
if (context.nodeStartsCollection(node)) {
if (!node.error && !context.atLineStart && context.parent.type === PlainValue.Type.DOCUMENT) {
node.error = new PlainValue.YAMLSyntaxError(node, "Block collection must not have preceding content here (e.g. directives-end indicator)");
}
const collection = new Collection(node);
offset = collection.parse(new ParseContext(context), offset);
collection.range = new PlainValue.Range(start, offset);
return collection;
}
return node;
});
this.atLineStart = atLineStart != null ? atLineStart : orig.atLineStart || false;
this.inCollection = inCollection != null ? inCollection : orig.inCollection || false;
this.inFlow = inFlow != null ? inFlow : orig.inFlow || false;
this.indent = indent != null ? indent : orig.indent;
this.lineStart = lineStart != null ? lineStart : orig.lineStart;
this.parent = parent != null ? parent : orig.parent || {};
this.root = orig.root;
this.src = orig.src;
}
nodeStartsCollection(node) {
const {
inCollection,
inFlow,
src
} = this;
if (inCollection || inFlow)
return false;
if (node instanceof CollectionItem)
return true;
let offset = node.range.end;
if (src[offset] === "\n" || src[offset - 1] === "\n")
return false;
offset = PlainValue.Node.endOfWhiteSpace(src, offset);
return src[offset] === ":";
}
parseProps(offset) {
const {
inFlow,
parent,
src
} = this;
const props = [];
let lineHasProps = false;
offset = this.atLineStart ? PlainValue.Node.endOfIndent(src, offset) : PlainValue.Node.endOfWhiteSpace(src, offset);
let ch = src[offset];
while (ch === PlainValue.Char.ANCHOR || ch === PlainValue.Char.COMMENT || ch === PlainValue.Char.TAG || ch === "\n") {
if (ch === "\n") {
let inEnd = offset;
let lineStart;
do {
lineStart = inEnd + 1;
inEnd = PlainValue.Node.endOfIndent(src, lineStart);
} while (src[inEnd] === "\n");
const indentDiff = inEnd - (lineStart + this.indent);
const noIndicatorAsIndent = parent.type === PlainValue.Type.SEQ_ITEM && parent.context.atLineStart;
if (src[inEnd] !== "#" && !PlainValue.Node.nextNodeIsIndented(src[inEnd], indentDiff, !noIndicatorAsIndent))
break;
this.atLineStart = true;
this.lineStart = lineStart;
lineHasProps = false;
offset = inEnd;
} else if (ch === PlainValue.Char.COMMENT) {
const end = PlainValue.Node.endOfLine(src, offset + 1);
props.push(new PlainValue.Range(offset, end));
offset = end;
} else {
let end = PlainValue.Node.endOfIdentifier(src, offset + 1);
if (ch === PlainValue.Char.TAG && src[end] === "," && /^[a-zA-Z0-9-]+\.[a-zA-Z0-9-]+,\d\d\d\d(-\d\d){0,2}\/\S/.test(src.slice(offset + 1, end + 13))) {
end = PlainValue.Node.endOfIdentifier(src, end + 5);
}
props.push(new PlainValue.Range(offset, end));
lineHasProps = true;
offset = PlainValue.Node.endOfWhiteSpace(src, end);
}
ch = src[offset];
}
if (lineHasProps && ch === ":" && PlainValue.Node.atBlank(src, offset + 1, true))
offset -= 1;
const type = ParseContext.parseType(src, offset, inFlow);
return {
props,
type,
valueStart: offset
};
}
};
function parse2(src) {
const cr = [];
if (src.indexOf("\r") !== -1) {
src = src.replace(/\r\n?/g, (match, offset2) => {
if (match.length > 1)
cr.push(offset2);
return "\n";
});
}
const documents = [];
let offset = 0;
do {
const doc = new Document();
const context = new ParseContext({
src
});
offset = doc.parse(context, offset);
documents.push(doc);
} while (offset < src.length);
documents.setOrigRanges = () => {
if (cr.length === 0)
return false;
for (let i = 1; i < cr.length; ++i)
cr[i] -= i;
let crOffset = 0;
for (let i = 0; i < documents.length; ++i) {
crOffset = documents[i].setOrigRanges(cr, crOffset);
}
cr.splice(0, cr.length);
return true;
};
documents.toString = () => documents.join("...\n");
return documents;
}
exports2.parse = parse2;
}
});
var require_resolveSeq_d03cb037 = __commonJS2({
"node_modules/yaml/dist/resolveSeq-d03cb037.js"(exports2) {
"use strict";
var PlainValue = require_PlainValue_ec8e588e();
function addCommentBefore(str, indent, comment) {
if (!comment)
return str;
const cc = comment.replace(/[\s\S]^/gm, `$&${indent}#`);
return `#${cc}
${indent}${str}`;
}
function addComment(str, indent, comment) {
return !comment ? str : comment.indexOf("\n") === -1 ? `${str} #${comment}` : `${str}
` + comment.replace(/^/gm, `${indent || ""}#`);
}
var Node = class {
};
function toJSON(value, arg, ctx) {
if (Array.isArray(value))
return value.map((v, i) => toJSON(v, String(i), ctx));
if (value && typeof value.toJSON === "function") {
const anchor = ctx && ctx.anchors && ctx.anchors.get(value);
if (anchor)
ctx.onCreate = (res2) => {
anchor.res = res2;
delete ctx.onCreate;
};
const res = value.toJSON(arg, ctx);
if (anchor && ctx.onCreate)
ctx.onCreate(res);
return res;
}
if ((!ctx || !ctx.keep) && typeof value === "bigint")
return Number(value);
return value;
}
var Scalar = class extends Node {
constructor(value) {
super();
this.value = value;
}
toJSON(arg, ctx) {
return ctx && ctx.keep ? this.value : toJSON(this.value, arg, ctx);
}
toString() {
return String(this.value);
}
};
function collectionFromPath(schema, path, value) {
let v = value;
for (let i = path.length - 1; i >= 0; --i) {
const k = path[i];
if (Number.isInteger(k) && k >= 0) {
const a = [];
a[k] = v;
v = a;
} else {
const o = {};
Object.defineProperty(o, k, {
value: v,
writable: true,
enumerable: true,
configurable: true
});
v = o;
}
}
return schema.createNode(v, false);
}
var isEmptyPath = (path) => path == null || typeof path === "object" && path[Symbol.iterator]().next().done;
var Collection = class extends Node {
constructor(schema) {
super();
PlainValue._defineProperty(this, "items", []);
this.schema = schema;
}
addIn(path, value) {
if (isEmptyPath(path))
this.add(value);
else {
const [key, ...rest] = path;
const node = this.get(key, true);
if (node instanceof Collection)
node.addIn(rest, value);
else if (node === void 0 && this.schema)
this.set(key, collectionFromPath(this.schema, rest, value));
else
throw new Error(`Expected YAML collection at ${key}. Remaining path: ${rest}`);
}
}
deleteIn([key, ...rest]) {
if (rest.length === 0)
return this.delete(key);
const node = this.get(key, true);
if (node instanceof Collection)
return node.deleteIn(rest);
else
throw new Error(`Expected YAML collection at ${key}. Remaining path: ${rest}`);
}
getIn([key, ...rest], keepScalar) {
const node = this.get(key, true);
if (rest.length === 0)
return !keepScalar && node instanceof Scalar ? node.value : node;
else
return node instanceof Collection ? node.getIn(rest, keepScalar) : void 0;
}
hasAllNullValues() {
return this.items.every((node) => {
if (!node || node.type !== "PAIR")
return false;
const n2 = node.value;
return n2 == null || n2 instanceof Scalar && n2.value == null && !n2.commentBefore && !n2.comment && !n2.tag;
});
}
hasIn([key, ...rest]) {
if (rest.length === 0)
return this.has(key);
const node = this.get(key, true);
return node instanceof Collection ? node.hasIn(rest) : false;
}
setIn([key, ...rest], value) {
if (rest.length === 0) {
this.set(key, value);
} else {
const node = this.get(key, true);
if (node instanceof Collection)
node.setIn(rest, value);
else if (node === void 0 && this.schema)
this.set(key, collectionFromPath(this.schema, rest, value));
else
throw new Error(`Expected YAML collection at ${key}. Remaining path: ${rest}`);
}
}
toJSON() {
return null;
}
toString(ctx, {
blockItem,
flowChars,
isMap,
itemIndent
}, onComment, onChompKeep) {
const {
indent,
indentStep,
stringify
} = ctx;
const inFlow = this.type === PlainValue.Type.FLOW_MAP || this.type === PlainValue.Type.FLOW_SEQ || ctx.inFlow;
if (inFlow)
itemIndent += indentStep;
const allNullValues = isMap && this.hasAllNullValues();
ctx = Object.assign({}, ctx, {
allNullValues,
indent: itemIndent,
inFlow,
type: null
});
let chompKeep = false;
let hasItemWithNewLine = false;
const nodes = this.items.reduce((nodes2, item, i) => {
let comment;
if (item) {
if (!chompKeep && item.spaceBefore)
nodes2.push({
type: "comment",
str: ""
});
if (item.commentBefore)
item.commentBefore.match(/^.*$/gm).forEach((line) => {
nodes2.push({
type: "comment",
str: `#${line}`
});
});
if (item.comment)
comment = item.comment;
if (inFlow && (!chompKeep && item.spaceBefore || item.commentBefore || item.comment || item.key && (item.key.commentBefore || item.key.comment) || item.value && (item.value.commentBefore || item.value.comment)))
hasItemWithNewLine = true;
}
chompKeep = false;
let str2 = stringify(item, ctx, () => comment = null, () => chompKeep = true);
if (inFlow && !hasItemWithNewLine && str2.includes("\n"))
hasItemWithNewLine = true;
if (inFlow && i < this.items.length - 1)
str2 += ",";
str2 = addComment(str2, itemIndent, comment);
if (chompKeep && (comment || inFlow))
chompKeep = false;
nodes2.push({
type: "item",
str: str2
});
return nodes2;
}, []);
let str;
if (nodes.length === 0) {
str = flowChars.start + flowChars.end;
} else if (inFlow) {
const {
start,
end
} = flowChars;
const strings = nodes.map((n2) => n2.str);
if (hasItemWithNewLine || strings.reduce((sum, str2) => sum + str2.length + 2, 2) > Collection.maxFlowStringSingleLineLength) {
str = start;
for (const s of strings) {
str += s ? `
${indentStep}${indent}${s}` : "\n";
}
str += `
${indent}${end}`;
} else {
str = `${start} ${strings.join(" ")} ${end}`;
}
} else {
const strings = nodes.map(blockItem);
str = strings.shift();
for (const s of strings)
str += s ? `
${indent}${s}` : "\n";
}
if (this.comment) {
str += "\n" + this.comment.replace(/^/gm, `${indent}#`);
if (onComment)
onComment();
} else if (chompKeep && onChompKeep)
onChompKeep();
return str;
}
};
PlainValue._defineProperty(Collection, "maxFlowStringSingleLineLength", 60);
function asItemIndex(key) {
let idx = key instanceof Scalar ? key.value : key;
if (idx && typeof idx === "string")
idx = Number(idx);
return Number.isInteger(idx) && idx >= 0 ? idx : null;
}
var YAMLSeq = class extends Collection {
add(value) {
this.items.push(value);
}
delete(key) {
const idx = asItemIndex(key);
if (typeof idx !== "number")
return false;
const del = this.items.splice(idx, 1);
return del.length > 0;
}
get(key, keepScalar) {
const idx = asItemIndex(key);
if (typeof idx !== "number")
return void 0;
const it = this.items[idx];
return !keepScalar && it instanceof Scalar ? it.value : it;
}
has(key) {
const idx = asItemIndex(key);
return typeof idx === "number" && idx < this.items.length;
}
set(key, value) {
const idx = asItemIndex(key);
if (typeof idx !== "number")
throw new Error(`Expected a valid index, not ${key}.`);
this.items[idx] = value;
}
toJSON(_, ctx) {
const seq = [];
if (ctx && ctx.onCreate)
ctx.onCreate(seq);
let i = 0;
for (const item of this.items)
seq.push(toJSON(item, String(i++), ctx));
return seq;
}
toString(ctx, onComment, onChompKeep) {
if (!ctx)
return JSON.stringify(this);
return super.toString(ctx, {
blockItem: (n2) => n2.type === "comment" ? n2.str : `- ${n2.str}`,
flowChars: {
start: "[",
end: "]"
},
isMap: false,
itemIndent: (ctx.indent || "") + " "
}, onComment, onChompKeep);
}
};
var stringifyKey = (key, jsKey, ctx) => {
if (jsKey === null)
return "";
if (typeof jsKey !== "object")
return String(jsKey);
if (key instanceof Node && ctx && ctx.doc)
return key.toString({
anchors: /* @__PURE__ */ Object.create(null),
doc: ctx.doc,
indent: "",
indentStep: ctx.indentStep,
inFlow: true,
inStringifyKey: true,
stringify: ctx.stringify
});
return JSON.stringify(jsKey);
};
var Pair = class extends Node {
constructor(key, value = null) {
super();
this.key = key;
this.value = value;
this.type = Pair.Type.PAIR;
}
get commentBefore() {
return this.key instanceof Node ? this.key.commentBefore : void 0;
}
set commentBefore(cb) {
if (this.key == null)
this.key = new Scalar(null);
if (this.key instanceof Node)
this.key.commentBefore = cb;
else {
const msg = "Pair.commentBefore is an alias for Pair.key.commentBefore. To set it, the key must be a Node.";
throw new Error(msg);
}
}
addToJSMap(ctx, map) {
const key = toJSON(this.key, "", ctx);
if (map instanceof Map) {
const value = toJSON(this.value, key, ctx);
map.set(key, value);
} else if (map instanceof Set) {
map.add(key);
} else {
const stringKey = stringifyKey(this.key, key, ctx);
const value = toJSON(this.value, stringKey, ctx);
if (stringKey in map)
Object.defineProperty(map, stringKey, {
value,
writable: true,
enumerable: true,
configurable: true
});
else
map[stringKey] = value;
}
return map;
}
toJSON(_, ctx) {
const pair = ctx && ctx.mapAsMap ? /* @__PURE__ */ new Map() : {};
return this.addToJSMap(ctx, pair);
}
toString(ctx, onComment, onChompKeep) {
if (!ctx || !ctx.doc)
return JSON.stringify(this);
const {
indent: indentSize,
indentSeq,
simpleKeys
} = ctx.doc.options;
let {
key,
value
} = this;
let keyComment = key instanceof Node && key.comment;
if (simpleKeys) {
if (keyComment) {
throw new Error("With simple keys, key nodes cannot have comments");
}
if (key instanceof Collection) {
const msg = "With simple keys, collection cannot be used as a key value";
throw new Error(msg);
}
}
let explicitKey = !simpleKeys && (!key || keyComment || (key instanceof Node ? key instanceof Collection || key.type === PlainValue.Type.BLOCK_FOLDED || key.type === PlainValue.Type.BLOCK_LITERAL : typeof key === "object"));
const {
doc,
indent,
indentStep,
stringify
} = ctx;
ctx = Object.assign({}, ctx, {
implicitKey: !explicitKey,
indent: indent + indentStep
});
let chompKeep = false;
let str = stringify(key, ctx, () => keyComment = null, () => chompKeep = true);
str = addComment(str, ctx.indent, keyComment);
if (!explicitKey && str.length > 1024) {
if (simpleKeys)
throw new Error("With simple keys, single line scalar must not span more than 1024 characters");
explicitKey = true;
}
if (ctx.allNullValues && !simpleKeys) {
if (this.comment) {
str = addComment(str, ctx.indent, this.comment);
if (onComment)
onComment();
} else if (chompKeep && !keyComment && onChompKeep)
onChompKeep();
return ctx.inFlow && !explicitKey ? str : `? ${str}`;
}
str = explicitKey ? `? ${str}
${indent}:` : `${str}:`;
if (this.comment) {
str = addComment(str, ctx.indent, this.comment);
if (onComment)
onComment();
}
let vcb = "";
let valueComment = null;
if (value instanceof Node) {
if (value.spaceBefore)
vcb = "\n";
if (value.commentBefore) {
const cs = value.commentBefore.replace(/^/gm, `${ctx.indent}#`);
vcb += `
${cs}`;
}
valueComment = value.comment;
} else if (value && typeof value === "object") {
value = doc.schema.createNode(value, true);
}
ctx.implicitKey = false;
if (!explicitKey && !this.comment && value instanceof Scalar)
ctx.indentAtStart = str.length + 1;
chompKeep = false;
if (!indentSeq && indentSize >= 2 && !ctx.inFlow && !explicitKey && value instanceof YAMLSeq && value.type !== PlainValue.Type.FLOW_SEQ && !value.tag && !doc.anchors.getName(value)) {
ctx.indent = ctx.indent.substr(2);
}
const valueStr = stringify(value, ctx, () => valueComment = null, () => chompKeep = true);
let ws = " ";
if (vcb || this.comment) {
ws = `${vcb}
${ctx.indent}`;
} else if (!explicitKey && value instanceof Collection) {
const flow = valueStr[0] === "[" || valueStr[0] === "{";
if (!flow || valueStr.includes("\n"))
ws = `
${ctx.indent}`;
} else if (valueStr[0] === "\n")
ws = "";
if (chompKeep && !valueComment && onChompKeep)
onChompKeep();
return addComment(str + ws + valueStr, ctx.indent, valueComment);
}
};
PlainValue._defineProperty(Pair, "Type", {
PAIR: "PAIR",
MERGE_PAIR: "MERGE_PAIR"
});
var getAliasCount = (node, anchors) => {
if (node instanceof Alias) {
const anchor = anchors.get(node.source);
return anchor.count * anchor.aliasCount;
} else if (node instanceof Collection) {
let count = 0;
for (const item of node.items) {
const c = getAliasCount(item, anchors);
if (c > count)
count = c;
}
return count;
} else if (node instanceof Pair) {
const kc = getAliasCount(node.key, anchors);
const vc = getAliasCount(node.value, anchors);
return Math.max(kc, vc);
}
return 1;
};
var Alias = class extends Node {
static stringify({
range,
source
}, {
anchors,
doc,
implicitKey,
inStringifyKey
}) {
let anchor = Object.keys(anchors).find((a) => anchors[a] === source);
if (!anchor && inStringifyKey)
anchor = doc.anchors.getName(source) || doc.anchors.newName();
if (anchor)
return `*${anchor}${implicitKey ? " " : ""}`;
const msg = doc.anchors.getName(source) ? "Alias node must be after source node" : "Source node not found for alias node";
throw new Error(`${msg} [${range}]`);
}
constructor(source) {
super();
this.source = source;
this.type = PlainValue.Type.ALIAS;
}
set tag(t) {
throw new Error("Alias nodes cannot have tags");
}
toJSON(arg, ctx) {
if (!ctx)
return toJSON(this.source, arg, ctx);
const {
anchors,
maxAliasCount
} = ctx;
const anchor = anchors.get(this.source);
if (!anchor || anchor.res === void 0) {
const msg = "This should not happen: Alias anchor was not resolved?";
if (this.cstNode)
throw new PlainValue.YAMLReferenceError(this.cstNode, msg);
else
throw new ReferenceError(msg);
}
if (maxAliasCount >= 0) {
anchor.count += 1;
if (anchor.aliasCount === 0)
anchor.aliasCount = getAliasCount(this.source, anchors);
if (anchor.count * anchor.aliasCount > maxAliasCount) {
const msg = "Excessive alias count indicates a resource exhaustion attack";
if (this.cstNode)
throw new PlainValue.YAMLReferenceError(this.cstNode, msg);
else
throw new ReferenceError(msg);
}
}
return anchor.res;
}
toString(ctx) {
return Alias.stringify(this, ctx);
}
};
PlainValue._defineProperty(Alias, "default", true);
function findPair(items, key) {
const k = key instanceof Scalar ? key.value : key;
for (const it of items) {
if (it instanceof Pair) {
if (it.key === key || it.key === k)
return it;
if (it.key && it.key.value === k)
return it;
}
}
return void 0;
}
var YAMLMap = class extends Collection {
add(pair, overwrite) {
if (!pair)
pair = new Pair(pair);
else if (!(pair instanceof Pair))
pair = new Pair(pair.key || pair, pair.value);
const prev = findPair(this.items, pair.key);
const sortEntries = this.schema && this.schema.sortMapEntries;
if (prev) {
if (overwrite)
prev.value = pair.value;
else
throw new Error(`Key ${pair.key} already set`);
} else if (sortEntries) {
const i = this.items.findIndex((item) => sortEntries(pair, item) < 0);
if (i === -1)
this.items.push(pair);
else
this.items.splice(i, 0, pair);
} else {
this.items.push(pair);
}
}
delete(key) {
const it = findPair(this.items, key);
if (!it)
return false;
const del = this.items.splice(this.items.indexOf(it), 1);
return del.length > 0;
}
get(key, keepScalar) {
const it = findPair(this.items, key);
const node = it && it.value;
return !keepScalar && node instanceof Scalar ? node.value : node;
}
has(key) {
return !!findPair(this.items, key);
}
set(key, value) {
this.add(new Pair(key, value), true);
}
toJSON(_, ctx, Type) {
const map = Type ? new Type() : ctx && ctx.mapAsMap ? /* @__PURE__ */ new Map() : {};
if (ctx && ctx.onCreate)
ctx.onCreate(map);
for (const item of this.items)
item.addToJSMap(ctx, map);
return map;
}
toString(ctx, onComment, onChompKeep) {
if (!ctx)
return JSON.stringify(this);
for (const item of this.items) {
if (!(item instanceof Pair))
throw new Error(`Map items must all be pairs; found ${JSON.stringify(item)} instead`);
}
return super.toString(ctx, {
blockItem: (n2) => n2.str,
flowChars: {
start: "{",
end: "}"
},
isMap: true,
itemIndent: ctx.indent || ""
}, onComment, onChompKeep);
}
};
var MERGE_KEY = "<<";
var Merge = class extends Pair {
constructor(pair) {
if (pair instanceof Pair) {
let seq = pair.value;
if (!(seq instanceof YAMLSeq)) {
seq = new YAMLSeq();
seq.items.push(pair.value);
seq.range = pair.value.range;
}
super(pair.key, seq);
this.range = pair.range;
} else {
super(new Scalar(MERGE_KEY), new YAMLSeq());
}
this.type = Pair.Type.MERGE_PAIR;
}
addToJSMap(ctx, map) {
for (const {
source
} of this.value.items) {
if (!(source instanceof YAMLMap))
throw new Error("Merge sources must be maps");
const srcMap = source.toJSON(null, ctx, Map);
for (const [key, value] of srcMap) {
if (map instanceof Map) {
if (!map.has(key))
map.set(key, value);
} else if (map instanceof Set) {
map.add(key);
} else if (!Object.prototype.hasOwnProperty.call(map, key)) {
Object.defineProperty(map, key, {
value,
writable: true,
enumerable: true,
configurable: true
});
}
}
}
return map;
}
toString(ctx, onComment) {
const seq = this.value;
if (seq.items.length > 1)
return super.toString(ctx, onComment);
this.value = seq.items[0];
const str = super.toString(ctx, onComment);
this.value = seq;
return str;
}
};
var binaryOptions = {
defaultType: PlainValue.Type.BLOCK_LITERAL,
lineWidth: 76
};
var boolOptions = {
trueStr: "true",
falseStr: "false"
};
var intOptions = {
asBigInt: false
};
var nullOptions = {
nullStr: "null"
};
var strOptions = {
defaultType: PlainValue.Type.PLAIN,
doubleQuoted: {
jsonEncoding: false,
minMultiLineLength: 40
},
fold: {
lineWidth: 80,
minContentWidth: 20
}
};
function resolveScalar(str, tags, scalarFallback) {
for (const {
format: format2,
test,
resolve
} of tags) {
if (test) {
const match = str.match(test);
if (match) {
let res = resolve.apply(null, match);
if (!(res instanceof Scalar))
res = new Scalar(res);
if (format2)
res.format = format2;
return res;
}
}
}
if (scalarFallback)
str = scalarFallback(str);
return new Scalar(str);
}
var FOLD_FLOW = "flow";
var FOLD_BLOCK = "block";
var FOLD_QUOTED = "quoted";
var consumeMoreIndentedLines = (text, i) => {
let ch = text[i + 1];
while (ch === " " || ch === " ") {
do {
ch = text[i += 1];
} while (ch && ch !== "\n");
ch = text[i + 1];
}
return i;
};
function foldFlowLines(text, indent, mode, {
indentAtStart,
lineWidth = 80,
minContentWidth = 20,
onFold,
onOverflow
}) {
if (!lineWidth || lineWidth < 0)
return text;
const endStep = Math.max(1 + minContentWidth, 1 + lineWidth - indent.length);
if (text.length <= endStep)
return text;
const folds = [];
const escapedFolds = {};
let end = lineWidth - indent.length;
if (typeof indentAtStart === "number") {
if (indentAtStart > lineWidth - Math.max(2, minContentWidth))
folds.push(0);
else
end = lineWidth - indentAtStart;
}
let split = void 0;
let prev = void 0;
let overflow = false;
let i = -1;
let escStart = -1;
let escEnd = -1;
if (mode === FOLD_BLOCK) {
i = consumeMoreIndentedLines(text, i);
if (i !== -1)
end = i + endStep;
}
for (let ch; ch = text[i += 1]; ) {
if (mode === FOLD_QUOTED && ch === "\\") {
escStart = i;
switch (text[i + 1]) {
case "x":
i += 3;
break;
case "u":
i += 5;
break;
case "U":
i += 9;
break;
default:
i += 1;
}
escEnd = i;
}
if (ch === "\n") {
if (mode === FOLD_BLOCK)
i = consumeMoreIndentedLines(text, i);
end = i + endStep;
split = void 0;
} else {
if (ch === " " && prev && prev !== " " && prev !== "\n" && prev !== " ") {
const next = text[i + 1];
if (next && next !== " " && next !== "\n" && next !== " ")
split = i;
}
if (i >= end) {
if (split) {
folds.push(split);
end = split + endStep;
split = void 0;
} else if (mode === FOLD_QUOTED) {
while (prev === " " || prev === " ") {
prev = ch;
ch = text[i += 1];
overflow = true;
}
const j = i > escEnd + 1 ? i - 2 : escStart - 1;
if (escapedFolds[j])
return text;
folds.push(j);
escapedFolds[j] = true;
end = j + endStep;
split = void 0;
} else {
overflow = true;
}
}
}
prev = ch;
}
if (overflow && onOverflow)
onOverflow();
if (folds.length === 0)
return text;
if (onFold)
onFold();
let res = text.slice(0, folds[0]);
for (let i2 = 0; i2 < folds.length; ++i2) {
const fold = folds[i2];
const end2 = folds[i2 + 1] || text.length;
if (fold === 0)
res = `
${indent}${text.slice(0, end2)}`;
else {
if (mode === FOLD_QUOTED && escapedFolds[fold])
res += `${text[fold]}\\`;
res += `
${indent}${text.slice(fold + 1, end2)}`;
}
}
return res;
}
var getFoldOptions = ({
indentAtStart
}) => indentAtStart ? Object.assign({
indentAtStart
}, strOptions.fold) : strOptions.fold;
var containsDocumentMarker = (str) => /^(%|---|\.\.\.)/m.test(str);
function lineLengthOverLimit(str, lineWidth, indentLength) {
if (!lineWidth || lineWidth < 0)
return false;
const limit = lineWidth - indentLength;
const strLen = str.length;
if (strLen <= limit)
return false;
for (let i = 0, start = 0; i < strLen; ++i) {
if (str[i] === "\n") {
if (i - start > limit)
return true;
start = i + 1;
if (strLen - start <= limit)
return false;
}
}
return true;
}
function doubleQuotedString(value, ctx) {
const {
implicitKey
} = ctx;
const {
jsonEncoding,
minMultiLineLength
} = strOptions.doubleQuoted;
const json = JSON.stringify(value);
if (jsonEncoding)
return json;
const indent = ctx.indent || (containsDocumentMarker(value) ? " " : "");
let str = "";
let start = 0;
for (let i = 0, ch = json[i]; ch; ch = json[++i]) {
if (ch === " " && json[i + 1] === "\\" && json[i + 2] === "n") {
str += json.slice(start, i) + "\\ ";
i += 1;
start = i;
ch = "\\";
}
if (ch === "\\")
switch (json[i + 1]) {
case "u":
{
str += json.slice(start, i);
const code = json.substr(i + 2, 4);
switch (code) {
case "0000":
str += "\\0";
break;
case "0007":
str += "\\a";
break;
case "000b":
str += "\\v";
break;
case "001b":
str += "\\e";
break;
case "0085":
str += "\\N";
break;
case "00a0":
str += "\\_";
break;
case "2028":
str += "\\L";
break;
case "2029":
str += "\\P";
break;
default:
if (code.substr(0, 2) === "00")
str += "\\x" + code.substr(2);
else
str += json.substr(i, 6);
}
i += 5;
start = i + 1;
}
break;
case "n":
if (implicitKey || json[i + 2] === '"' || json.length < minMultiLineLength) {
i += 1;
} else {
str += json.slice(start, i) + "\n\n";
while (json[i + 2] === "\\" && json[i + 3] === "n" && json[i + 4] !== '"') {
str += "\n";
i += 2;
}
str += indent;
if (json[i + 2] === " ")
str += "\\";
i += 1;
start = i + 1;
}
break;
default:
i += 1;
}
}
str = start ? str + json.slice(start) : json;
return implicitKey ? str : foldFlowLines(str, indent, FOLD_QUOTED, getFoldOptions(ctx));
}
function singleQuotedString(value, ctx) {
if (ctx.implicitKey) {
if (/\n/.test(value))
return doubleQuotedString(value, ctx);
} else {
if (/[ \t]\n|\n[ \t]/.test(value))
return doubleQuotedString(value, ctx);
}
const indent = ctx.indent || (containsDocumentMarker(value) ? " " : "");
const res = "'" + value.replace(/'/g, "''").replace(/\n+/g, `$&
${indent}`) + "'";
return ctx.implicitKey ? res : foldFlowLines(res, indent, FOLD_FLOW, getFoldOptions(ctx));
}
function blockString({
comment,
type,
value
}, ctx, onComment, onChompKeep) {
if (/\n[\t ]+$/.test(value) || /^\s*$/.test(value)) {
return doubleQuotedString(value, ctx);
}
const indent = ctx.indent || (ctx.forceBlockIndent || containsDocumentMarker(value) ? " " : "");
const indentSize = indent ? "2" : "1";
const literal = type === PlainValue.Type.BLOCK_FOLDED ? false : type === PlainValue.Type.BLOCK_LITERAL ? true : !lineLengthOverLimit(value, strOptions.fold.lineWidth, indent.length);
let header = literal ? "|" : ">";
if (!value)
return header + "\n";
let wsStart = "";
let wsEnd = "";
value = value.replace(/[\n\t ]*$/, (ws) => {
const n2 = ws.indexOf("\n");
if (n2 === -1) {
header += "-";
} else if (value === ws || n2 !== ws.length - 1) {
header += "+";
if (onChompKeep)
onChompKeep();
}
wsEnd = ws.replace(/\n$/, "");
return "";
}).replace(/^[\n ]*/, (ws) => {
if (ws.indexOf(" ") !== -1)
header += indentSize;
const m = ws.match(/ +$/);
if (m) {
wsStart = ws.slice(0, -m[0].length);
return m[0];
} else {
wsStart = ws;
return "";
}
});
if (wsEnd)
wsEnd = wsEnd.replace(/\n+(?!\n|$)/g, `$&${indent}`);
if (wsStart)
wsStart = wsStart.replace(/\n+/g, `$&${indent}`);
if (comment) {
header += " #" + comment.replace(/ ?[\r\n]+/g, " ");
if (onComment)
onComment();
}
if (!value)
return `${header}${indentSize}
${indent}${wsEnd}`;
if (literal) {
value = value.replace(/\n+/g, `$&${indent}`);
return `${header}
${indent}${wsStart}${value}${wsEnd}`;
}
value = value.replace(/\n+/g, "\n$&").replace(/(?:^|\n)([\t ].*)(?:([\n\t ]*)\n(?![\n\t ]))?/g, "$1$2").replace(/\n+/g, `$&${indent}`);
const body = foldFlowLines(`${wsStart}${value}${wsEnd}`, indent, FOLD_BLOCK, strOptions.fold);
return `${header}
${indent}${body}`;
}
function plainString(item, ctx, onComment, onChompKeep) {
const {
comment,
type,
value
} = item;
const {
actualString,
implicitKey,
indent,
inFlow
} = ctx;
if (implicitKey && /[\n[\]{},]/.test(value) || inFlow && /[[\]{},]/.test(value)) {
return doubleQuotedString(value, ctx);
}
if (!value || /^[\n\t ,[\]{}#&*!|>'"%@`]|^[?-]$|^[?-][ \t]|[\n:][ \t]|[ \t]\n|[\n\t ]#|[\n\t :]$/.test(value)) {
return implicitKey || inFlow || value.indexOf("\n") === -1 ? value.indexOf('"') !== -1 && value.indexOf("'") === -1 ? singleQuotedString(value, ctx) : doubleQuotedString(value, ctx) : blockString(item, ctx, onComment, onChompKeep);
}
if (!implicitKey && !inFlow && type !== PlainValue.Type.PLAIN && value.indexOf("\n") !== -1) {
return blockString(item, ctx, onComment, onChompKeep);
}
if (indent === "" && containsDocumentMarker(value)) {
ctx.forceBlockIndent = true;
return blockString(item, ctx, onComment, onChompKeep);
}
const str = value.replace(/\n+/g, `$&
${indent}`);
if (actualString) {
const {
tags
} = ctx.doc.schema;
const resolved = resolveScalar(str, tags, tags.scalarFallback).value;
if (typeof resolved !== "string")
return doubleQuotedString(value, ctx);
}
const body = implicitKey ? str : foldFlowLines(str, indent, FOLD_FLOW, getFoldOptions(ctx));
if (comment && !inFlow && (body.indexOf("\n") !== -1 || comment.indexOf("\n") !== -1)) {
if (onComment)
onComment();
return addCommentBefore(body, indent, comment);
}
return body;
}
function stringifyString(item, ctx, onComment, onChompKeep) {
const {
defaultType
} = strOptions;
const {
implicitKey,
inFlow
} = ctx;
let {
type,
value
} = item;
if (typeof value !== "string") {
value = String(value);
item = Object.assign({}, item, {
value
});
}
const _stringify = (_type) => {
switch (_type) {
case PlainValue.Type.BLOCK_FOLDED:
case PlainValue.Type.BLOCK_LITERAL:
return blockString(item, ctx, onComment, onChompKeep);
case PlainValue.Type.QUOTE_DOUBLE:
return doubleQuotedString(value, ctx);
case PlainValue.Type.QUOTE_SINGLE:
return singleQuotedString(value, ctx);
case PlainValue.Type.PLAIN:
return plainString(item, ctx, onComment, onChompKeep);
default:
return null;
}
};
if (type !== PlainValue.Type.QUOTE_DOUBLE && /[\x00-\x08\x0b-\x1f\x7f-\x9f]/.test(value)) {
type = PlainValue.Type.QUOTE_DOUBLE;
} else if ((implicitKey || inFlow) && (type === PlainValue.Type.BLOCK_FOLDED || type === PlainValue.Type.BLOCK_LITERAL)) {
type = PlainValue.Type.QUOTE_DOUBLE;
}
let res = _stringify(type);
if (res === null) {
res = _stringify(defaultType);
if (res === null)
throw new Error(`Unsupported default string type ${defaultType}`);
}
return res;
}
function stringifyNumber({
format: format2,
minFractionDigits,
tag,
value
}) {
if (typeof value === "bigint")
return String(value);
if (!isFinite(value))
return isNaN(value) ? ".nan" : value < 0 ? "-.inf" : ".inf";
let n2 = JSON.stringify(value);
if (!format2 && minFractionDigits && (!tag || tag === "tag:yaml.org,2002:float") && /^\d/.test(n2)) {
let i = n2.indexOf(".");
if (i < 0) {
i = n2.length;
n2 += ".";
}
let d = minFractionDigits - (n2.length - i - 1);
while (d-- > 0)
n2 += "0";
}
return n2;
}
function checkFlowCollectionEnd(errors, cst) {
let char2, name;
switch (cst.type) {
case PlainValue.Type.FLOW_MAP:
char2 = "}";
name = "flow map";
break;
case PlainValue.Type.FLOW_SEQ:
char2 = "]";
name = "flow sequence";
break;
default:
errors.push(new PlainValue.YAMLSemanticError(cst, "Not a flow collection!?"));
return;
}
let lastItem;
for (let i = cst.items.length - 1; i >= 0; --i) {
const item = cst.items[i];
if (!item || item.type !== PlainValue.Type.COMMENT) {
lastItem = item;
break;
}
}
if (lastItem && lastItem.char !== char2) {
const msg = `Expected ${name} to end with ${char2}`;
let err;
if (typeof lastItem.offset === "number") {
err = new PlainValue.YAMLSemanticError(cst, msg);
err.offset = lastItem.offset + 1;
} else {
err = new PlainValue.YAMLSemanticError(lastItem, msg);
if (lastItem.range && lastItem.range.end)
err.offset = lastItem.range.end - lastItem.range.start;
}
errors.push(err);
}
}
function checkFlowCommentSpace(errors, comment) {
const prev = comment.context.src[comment.range.start - 1];
if (prev !== "\n" && prev !== " " && prev !== " ") {
const msg = "Comments must be separated from other tokens by white space characters";
errors.push(new PlainValue.YAMLSemanticError(comment, msg));
}
}
function getLongKeyError(source, key) {
const sk = String(key);
const k = sk.substr(0, 8) + "..." + sk.substr(-8);
return new PlainValue.YAMLSemanticError(source, `The "${k}" key is too long`);
}
function resolveComments(collection, comments) {
for (const {
afterKey,
before,
comment
} of comments) {
let item = collection.items[before];
if (!item) {
if (comment !== void 0) {
if (collection.comment)
collection.comment += "\n" + comment;
else
collection.comment = comment;
}
} else {
if (afterKey && item.value)
item = item.value;
if (comment === void 0) {
if (afterKey || !item.commentBefore)
item.spaceBefore = true;
} else {
if (item.commentBefore)
item.commentBefore += "\n" + comment;
else
item.commentBefore = comment;
}
}
}
}
function resolveString(doc, node) {
const res = node.strValue;
if (!res)
return "";
if (typeof res === "string")
return res;
res.errors.forEach((error) => {
if (!error.source)
error.source = node;
doc.errors.push(error);
});
return res.str;
}
function resolveTagHandle(doc, node) {
const {
handle,
suffix
} = node.tag;
let prefix = doc.tagPrefixes.find((p) => p.handle === handle);
if (!prefix) {
const dtp = doc.getDefaults().tagPrefixes;
if (dtp)
prefix = dtp.find((p) => p.handle === handle);
if (!prefix)
throw new PlainValue.YAMLSemanticError(node, `The ${handle} tag handle is non-default and was not declared.`);
}
if (!suffix)
throw new PlainValue.YAMLSemanticError(node, `The ${handle} tag has no suffix.`);
if (handle === "!" && (doc.version || doc.options.version) === "1.0") {
if (suffix[0] === "^") {
doc.warnings.push(new PlainValue.YAMLWarning(node, "YAML 1.0 ^ tag expansion is not supported"));
return suffix;
}
if (/[:/]/.test(suffix)) {
const vocab = suffix.match(/^([a-z0-9-]+)\/(.*)/i);
return vocab ? `tag:${vocab[1]}.yaml.org,2002:${vocab[2]}` : `tag:${suffix}`;
}
}
return prefix.prefix + decodeURIComponent(suffix);
}
function resolveTagName(doc, node) {
const {
tag,
type
} = node;
let nonSpecific = false;
if (tag) {
const {
handle,
suffix,
verbatim
} = tag;
if (verbatim) {
if (verbatim !== "!" && verbatim !== "!!")
return verbatim;
const msg = `Verbatim tags aren't resolved, so ${verbatim} is invalid.`;
doc.errors.push(new PlainValue.YAMLSemanticError(node, msg));
} else if (handle === "!" && !suffix) {
nonSpecific = true;
} else {
try {
return resolveTagHandle(doc, node);
} catch (error) {
doc.errors.push(error);
}
}
}
switch (type) {
case PlainValue.Type.BLOCK_FOLDED:
case PlainValue.Type.BLOCK_LITERAL:
case PlainValue.Type.QUOTE_DOUBLE:
case PlainValue.Type.QUOTE_SINGLE:
return PlainValue.defaultTags.STR;
case PlainValue.Type.FLOW_MAP:
case PlainValue.Type.MAP:
return PlainValue.defaultTags.MAP;
case PlainValue.Type.FLOW_SEQ:
case PlainValue.Type.SEQ:
return PlainValue.defaultTags.SEQ;
case PlainValue.Type.PLAIN:
return nonSpecific ? PlainValue.defaultTags.STR : null;
default:
return null;
}
}
function resolveByTagName(doc, node, tagName) {
const {
tags
} = doc.schema;
const matchWithTest = [];
for (const tag of tags) {
if (tag.tag === tagName) {
if (tag.test)
matchWithTest.push(tag);
else {
const res = tag.resolve(doc, node);
return res instanceof Collection ? res : new Scalar(res);
}
}
}
const str = resolveString(doc, node);
if (typeof str === "string" && matchWithTest.length > 0)
return resolveScalar(str, matchWithTest, tags.scalarFallback);
return null;
}
function getFallbackTagName({
type
}) {
switch (type) {
case PlainValue.Type.FLOW_MAP:
case PlainValue.Type.MAP:
return PlainValue.defaultTags.MAP;
case PlainValue.Type.FLOW_SEQ:
case PlainValue.Type.SEQ:
return PlainValue.defaultTags.SEQ;
default:
return PlainValue.defaultTags.STR;
}
}
function resolveTag(doc, node, tagName) {
try {
const res = resolveByTagName(doc, node, tagName);
if (res) {
if (tagName && node.tag)
res.tag = tagName;
return res;
}
} catch (error) {
if (!error.source)
error.source = node;
doc.errors.push(error);
return null;
}
try {
const fallback = getFallbackTagName(node);
if (!fallback)
throw new Error(`The tag ${tagName} is unavailable`);
const msg = `The tag ${tagName} is unavailable, falling back to ${fallback}`;
doc.warnings.push(new PlainValue.YAMLWarning(node, msg));
const res = resolveByTagName(doc, node, fallback);
res.tag = tagName;
return res;
} catch (error) {
const refError = new PlainValue.YAMLReferenceError(node, error.message);
refError.stack = error.stack;
doc.errors.push(refError);
return null;
}
}
var isCollectionItem = (node) => {
if (!node)
return false;
const {
type
} = node;
return type === PlainValue.Type.MAP_KEY || type === PlainValue.Type.MAP_VALUE || type === PlainValue.Type.SEQ_ITEM;
};
function resolveNodeProps(errors, node) {
const comments = {
before: [],
after: []
};
let hasAnchor = false;
let hasTag = false;
const props = isCollectionItem(node.context.parent) ? node.context.parent.props.concat(node.props) : node.props;
for (const {
start,
end
} of props) {
switch (node.context.src[start]) {
case PlainValue.Char.COMMENT: {
if (!node.commentHasRequiredWhitespace(start)) {
const msg = "Comments must be separated from other tokens by white space characters";
errors.push(new PlainValue.YAMLSemanticError(node, msg));
}
const {
header,
valueRange
} = node;
const cc = valueRange && (start > valueRange.start || header && start > header.start) ? comments.after : comments.before;
cc.push(node.context.src.slice(start + 1, end));
break;
}
case PlainValue.Char.ANCHOR:
if (hasAnchor) {
const msg = "A node can have at most one anchor";
errors.push(new PlainValue.YAMLSemanticError(node, msg));
}
hasAnchor = true;
break;
case PlainValue.Char.TAG:
if (hasTag) {
const msg = "A node can have at most one tag";
errors.push(new PlainValue.YAMLSemanticError(node, msg));
}
hasTag = true;
break;
}
}
return {
comments,
hasAnchor,
hasTag
};
}
function resolveNodeValue(doc, node) {
const {
anchors,
errors,
schema
} = doc;
if (node.type === PlainValue.Type.ALIAS) {
const name = node.rawValue;
const src = anchors.getNode(name);
if (!src) {
const msg = `Aliased anchor not found: ${name}`;
errors.push(new PlainValue.YAMLReferenceError(node, msg));
return null;
}
const res = new Alias(src);
anchors._cstAliases.push(res);
return res;
}
const tagName = resolveTagName(doc, node);
if (tagName)
return resolveTag(doc, node, tagName);
if (node.type !== PlainValue.Type.PLAIN) {
const msg = `Failed to resolve ${node.type} node here`;
errors.push(new PlainValue.YAMLSyntaxError(node, msg));
return null;
}
try {
const str = resolveString(doc, node);
return resolveScalar(str, schema.tags, schema.tags.scalarFallback);
} catch (error) {
if (!error.source)
error.source = node;
errors.push(error);
return null;
}
}
function resolveNode(doc, node) {
if (!node)
return null;
if (node.error)
doc.errors.push(node.error);
const {
comments,
hasAnchor,
hasTag
} = resolveNodeProps(doc.errors, node);
if (hasAnchor) {
const {
anchors
} = doc;
const name = node.anchor;
const prev = anchors.getNode(name);
if (prev)
anchors.map[anchors.newName(name)] = prev;
anchors.map[name] = node;
}
if (node.type === PlainValue.Type.ALIAS && (hasAnchor || hasTag)) {
const msg = "An alias node must not specify any properties";
doc.errors.push(new PlainValue.YAMLSemanticError(node, msg));
}
const res = resolveNodeValue(doc, node);
if (res) {
res.range = [node.range.start, node.range.end];
if (doc.options.keepCstNodes)
res.cstNode = node;
if (doc.options.keepNodeTypes)
res.type = node.type;
const cb = comments.before.join("\n");
if (cb) {
res.commentBefore = res.commentBefore ? `${res.commentBefore}
${cb}` : cb;
}
const ca = comments.after.join("\n");
if (ca)
res.comment = res.comment ? `${res.comment}
${ca}` : ca;
}
return node.resolved = res;
}
function resolveMap(doc, cst) {
if (cst.type !== PlainValue.Type.MAP && cst.type !== PlainValue.Type.FLOW_MAP) {
const msg = `A ${cst.type} node cannot be resolved as a mapping`;
doc.errors.push(new PlainValue.YAMLSyntaxError(cst, msg));
return null;
}
const {
comments,
items
} = cst.type === PlainValue.Type.FLOW_MAP ? resolveFlowMapItems(doc, cst) : resolveBlockMapItems(doc, cst);
const map = new YAMLMap();
map.items = items;
resolveComments(map, comments);
let hasCollectionKey = false;
for (let i = 0; i < items.length; ++i) {
const {
key: iKey
} = items[i];
if (iKey instanceof Collection)
hasCollectionKey = true;
if (doc.schema.merge && iKey && iKey.value === MERGE_KEY) {
items[i] = new Merge(items[i]);
const sources = items[i].value.items;
let error = null;
sources.some((node) => {
if (node instanceof Alias) {
const {
type
} = node.source;
if (type === PlainValue.Type.MAP || type === PlainValue.Type.FLOW_MAP)
return false;
return error = "Merge nodes aliases can only point to maps";
}
return error = "Merge nodes can only have Alias nodes as values";
});
if (error)
doc.errors.push(new PlainValue.YAMLSemanticError(cst, error));
} else {
for (let j = i + 1; j < items.length; ++j) {
const {
key: jKey
} = items[j];
if (iKey === jKey || iKey && jKey && Object.prototype.hasOwnProperty.call(iKey, "value") && iKey.value === jKey.value) {
const msg = `Map keys must be unique; "${iKey}" is repeated`;
doc.errors.push(new PlainValue.YAMLSemanticError(cst, msg));
break;
}
}
}
}
if (hasCollectionKey && !doc.options.mapAsMap) {
const warn = "Keys with collection values will be stringified as YAML due to JS Object restrictions. Use mapAsMap: true to avoid this.";
doc.warnings.push(new PlainValue.YAMLWarning(cst, warn));
}
cst.resolved = map;
return map;
}
var valueHasPairComment = ({
context: {
lineStart,
node,
src
},
props
}) => {
if (props.length === 0)
return false;
const {
start
} = props[0];
if (node && start > node.valueRange.start)
return false;
if (src[start] !== PlainValue.Char.COMMENT)
return false;
for (let i = lineStart; i < start; ++i)
if (src[i] === "\n")
return false;
return true;
};
function resolvePairComment(item, pair) {
if (!valueHasPairComment(item))
return;
const comment = item.getPropValue(0, PlainValue.Char.COMMENT, true);
let found = false;
const cb = pair.value.commentBefore;
if (cb && cb.startsWith(comment)) {
pair.value.commentBefore = cb.substr(comment.length + 1);
found = true;
} else {
const cc = pair.value.comment;
if (!item.node && cc && cc.startsWith(comment)) {
pair.value.comment = cc.substr(comment.length + 1);
found = true;
}
}
if (found)
pair.comment = comment;
}
function resolveBlockMapItems(doc, cst) {
const comments = [];
const items = [];
let key = void 0;
let keyStart = null;
for (let i = 0; i < cst.items.length; ++i) {
const item = cst.items[i];
switch (item.type) {
case PlainValue.Type.BLANK_LINE:
comments.push({
afterKey: !!key,
before: items.length
});
break;
case PlainValue.Type.COMMENT:
comments.push({
afterKey: !!key,
before: items.length,
comment: item.comment
});
break;
case PlainValue.Type.MAP_KEY:
if (key !== void 0)
items.push(new Pair(key));
if (item.error)
doc.errors.push(item.error);
key = resolveNode(doc, item.node);
keyStart = null;
break;
case PlainValue.Type.MAP_VALUE:
{
if (key === void 0)
key = null;
if (item.error)
doc.errors.push(item.error);
if (!item.context.atLineStart && item.node && item.node.type === PlainValue.Type.MAP && !item.node.context.atLineStart) {
const msg = "Nested mappings are not allowed in compact mappings";
doc.errors.push(new PlainValue.YAMLSemanticError(item.node, msg));
}
let valueNode = item.node;
if (!valueNode && item.props.length > 0) {
valueNode = new PlainValue.PlainValue(PlainValue.Type.PLAIN, []);
valueNode.context = {
parent: item,
src: item.context.src
};
const pos = item.range.start + 1;
valueNode.range = {
start: pos,
end: pos
};
valueNode.valueRange = {
start: pos,
end: pos
};
if (typeof item.range.origStart === "number") {
const origPos = item.range.origStart + 1;
valueNode.range.origStart = valueNode.range.origEnd = origPos;
valueNode.valueRange.origStart = valueNode.valueRange.origEnd = origPos;
}
}
const pair = new Pair(key, resolveNode(doc, valueNode));
resolvePairComment(item, pair);
items.push(pair);
if (key && typeof keyStart === "number") {
if (item.range.start > keyStart + 1024)
doc.errors.push(getLongKeyError(cst, key));
}
key = void 0;
keyStart = null;
}
break;
default:
if (key !== void 0)
items.push(new Pair(key));
key = resolveNode(doc, item);
keyStart = item.range.start;
if (item.error)
doc.errors.push(item.error);
next:
for (let j = i + 1; ; ++j) {
const nextItem = cst.items[j];
switch (nextItem && nextItem.type) {
case PlainValue.Type.BLANK_LINE:
case PlainValue.Type.COMMENT:
continue next;
case PlainValue.Type.MAP_VALUE:
break next;
default: {
const msg = "Implicit map keys need to be followed by map values";
doc.errors.push(new PlainValue.YAMLSemanticError(item, msg));
break next;
}
}
}
if (item.valueRangeContainsNewline) {
const msg = "Implicit map keys need to be on a single line";
doc.errors.push(new PlainValue.YAMLSemanticError(item, msg));
}
}
}
if (key !== void 0)
items.push(new Pair(key));
return {
comments,
items
};
}
function resolveFlowMapItems(doc, cst) {
const comments = [];
const items = [];
let key = void 0;
let explicitKey = false;
let next = "{";
for (let i = 0; i < cst.items.length; ++i) {
const item = cst.items[i];
if (typeof item.char === "string") {
const {
char: char2,
offset
} = item;
if (char2 === "?" && key === void 0 && !explicitKey) {
explicitKey = true;
next = ":";
continue;
}
if (char2 === ":") {
if (key === void 0)
key = null;
if (next === ":") {
next = ",";
continue;
}
} else {
if (explicitKey) {
if (key === void 0 && char2 !== ",")
key = null;
explicitKey = false;
}
if (key !== void 0) {
items.push(new Pair(key));
key = void 0;
if (char2 === ",") {
next = ":";
continue;
}
}
}
if (char2 === "}") {
if (i === cst.items.length - 1)
continue;
} else if (char2 === next) {
next = ":";
continue;
}
const msg = `Flow map contains an unexpected ${char2}`;
const err = new PlainValue.YAMLSyntaxError(cst, msg);
err.offset = offset;
doc.errors.push(err);
} else if (item.type === PlainValue.Type.BLANK_LINE) {
comments.push({
afterKey: !!key,
before: items.length
});
} else if (item.type === PlainValue.Type.COMMENT) {
checkFlowCommentSpace(doc.errors, item);
comments.push({
afterKey: !!key,
before: items.length,
comment: item.comment
});
} else if (key === void 0) {
if (next === ",")
doc.errors.push(new PlainValue.YAMLSemanticError(item, "Separator , missing in flow map"));
key = resolveNode(doc, item);
} else {
if (next !== ",")
doc.errors.push(new PlainValue.YAMLSemanticError(item, "Indicator : missing in flow map entry"));
items.push(new Pair(key, resolveNode(doc, item)));
key = void 0;
explicitKey = false;
}
}
checkFlowCollectionEnd(doc.errors, cst);
if (key !== void 0)
items.push(new Pair(key));
return {
comments,
items
};
}
function resolveSeq(doc, cst) {
if (cst.type !== PlainValue.Type.SEQ && cst.type !== PlainValue.Type.FLOW_SEQ) {
const msg = `A ${cst.type} node cannot be resolved as a sequence`;
doc.errors.push(new PlainValue.YAMLSyntaxError(cst, msg));
return null;
}
const {
comments,
items
} = cst.type === PlainValue.Type.FLOW_SEQ ? resolveFlowSeqItems(doc, cst) : resolveBlockSeqItems(doc, cst);
const seq = new YAMLSeq();
seq.items = items;
resolveComments(seq, comments);
if (!doc.options.mapAsMap && items.some((it) => it instanceof Pair && it.key instanceof Collection)) {
const warn = "Keys with collection values will be stringified as YAML due to JS Object restrictions. Use mapAsMap: true to avoid this.";
doc.warnings.push(new PlainValue.YAMLWarning(cst, warn));
}
cst.resolved = seq;
return seq;
}
function resolveBlockSeqItems(doc, cst) {
const comments = [];
const items = [];
for (let i = 0; i < cst.items.length; ++i) {
const item = cst.items[i];
switch (item.type) {
case PlainValue.Type.BLANK_LINE:
comments.push({
before: items.length
});
break;
case PlainValue.Type.COMMENT:
comments.push({
comment: item.comment,
before: items.length
});
break;
case PlainValue.Type.SEQ_ITEM:
if (item.error)
doc.errors.push(item.error);
items.push(resolveNode(doc, item.node));
if (item.hasProps) {
const msg = "Sequence items cannot have tags or anchors before the - indicator";
doc.errors.push(new PlainValue.YAMLSemanticError(item, msg));
}
break;
default:
if (item.error)
doc.errors.push(item.error);
doc.errors.push(new PlainValue.YAMLSyntaxError(item, `Unexpected ${item.type} node in sequence`));
}
}
return {
comments,
items
};
}
function resolveFlowSeqItems(doc, cst) {
const comments = [];
const items = [];
let explicitKey = false;
let key = void 0;
let keyStart = null;
let next = "[";
let prevItem = null;
for (let i = 0; i < cst.items.length; ++i) {
const item = cst.items[i];
if (typeof item.char === "string") {
const {
char: char2,
offset
} = item;
if (char2 !== ":" && (explicitKey || key !== void 0)) {
if (explicitKey && key === void 0)
key = next ? items.pop() : null;
items.push(new Pair(key));
explicitKey = false;
key = void 0;
keyStart = null;
}
if (char2 === next) {
next = null;
} else if (!next && char2 === "?") {
explicitKey = true;
} else if (next !== "[" && char2 === ":" && key === void 0) {
if (next === ",") {
key = items.pop();
if (key instanceof Pair) {
const msg = "Chaining flow sequence pairs is invalid";
const err = new PlainValue.YAMLSemanticError(cst, msg);
err.offset = offset;
doc.errors.push(err);
}
if (!explicitKey && typeof keyStart === "number") {
const keyEnd = item.range ? item.range.start : item.offset;
if (keyEnd > keyStart + 1024)
doc.errors.push(getLongKeyError(cst, key));
const {
src
} = prevItem.context;
for (let i2 = keyStart; i2 < keyEnd; ++i2)
if (src[i2] === "\n") {
const msg = "Implicit keys of flow sequence pairs need to be on a single line";
doc.errors.push(new PlainValue.YAMLSemanticError(prevItem, msg));
break;
}
}
} else {
key = null;
}
keyStart = null;
explicitKey = false;
next = null;
} else if (next === "[" || char2 !== "]" || i < cst.items.length - 1) {
const msg = `Flow sequence contains an unexpected ${char2}`;
const err = new PlainValue.YAMLSyntaxError(cst, msg);
err.offset = offset;
doc.errors.push(err);
}
} else if (item.type === PlainValue.Type.BLANK_LINE) {
comments.push({
before: items.length
});
} else if (item.type === PlainValue.Type.COMMENT) {
checkFlowCommentSpace(doc.errors, item);
comments.push({
comment: item.comment,
before: items.length
});
} else {
if (next) {
const msg = `Expected a ${next} in flow sequence`;
doc.errors.push(new PlainValue.YAMLSemanticError(item, msg));
}
const value = resolveNode(doc, item);
if (key === void 0) {
items.push(value);
prevItem = item;
} else {
items.push(new Pair(key, value));
key = void 0;
}
keyStart = item.range.start;
next = ",";
}
}
checkFlowCollectionEnd(doc.errors, cst);
if (key !== void 0)
items.push(new Pair(key));
return {
comments,
items
};
}
exports2.Alias = Alias;
exports2.Collection = Collection;
exports2.Merge = Merge;
exports2.Node = Node;
exports2.Pair = Pair;
exports2.Scalar = Scalar;
exports2.YAMLMap = YAMLMap;
exports2.YAMLSeq = YAMLSeq;
exports2.addComment = addComment;
exports2.binaryOptions = binaryOptions;
exports2.boolOptions = boolOptions;
exports2.findPair = findPair;
exports2.intOptions = intOptions;
exports2.isEmptyPath = isEmptyPath;
exports2.nullOptions = nullOptions;
exports2.resolveMap = resolveMap;
exports2.resolveNode = resolveNode;
exports2.resolveSeq = resolveSeq;
exports2.resolveString = resolveString;
exports2.strOptions = strOptions;
exports2.stringifyNumber = stringifyNumber;
exports2.stringifyString = stringifyString;
exports2.toJSON = toJSON;
}
});
var require_warnings_1000a372 = __commonJS2({
"node_modules/yaml/dist/warnings-1000a372.js"(exports2) {
"use strict";
var PlainValue = require_PlainValue_ec8e588e();
var resolveSeq = require_resolveSeq_d03cb037();
var binary = {
identify: (value) => value instanceof Uint8Array,
default: false,
tag: "tag:yaml.org,2002:binary",
resolve: (doc, node) => {
const src = resolveSeq.resolveString(doc, node);
if (typeof Buffer === "function") {
return Buffer.from(src, "base64");
} else if (typeof atob === "function") {
const str = atob(src.replace(/[\n\r]/g, ""));
const buffer = new Uint8Array(str.length);
for (let i = 0; i < str.length; ++i)
buffer[i] = str.charCodeAt(i);
return buffer;
} else {
const msg = "This environment does not support reading binary tags; either Buffer or atob is required";
doc.errors.push(new PlainValue.YAMLReferenceError(node, msg));
return null;
}
},
options: resolveSeq.binaryOptions,
stringify: ({
comment,
type,
value
}, ctx, onComment, onChompKeep) => {
let src;
if (typeof Buffer === "function") {
src = value instanceof Buffer ? value.toString("base64") : Buffer.from(value.buffer).toString("base64");
} else if (typeof btoa === "function") {
let s = "";
for (let i = 0; i < value.length; ++i)
s += String.fromCharCode(value[i]);
src = btoa(s);
} else {
throw new Error("This environment does not support writing binary tags; either Buffer or btoa is required");
}
if (!type)
type = resolveSeq.binaryOptions.defaultType;
if (type === PlainValue.Type.QUOTE_DOUBLE) {
value = src;
} else {
const {
lineWidth
} = resolveSeq.binaryOptions;
const n2 = Math.ceil(src.length / lineWidth);
const lines = new Array(n2);
for (let i = 0, o = 0; i < n2; ++i, o += lineWidth) {
lines[i] = src.substr(o, lineWidth);
}
value = lines.join(type === PlainValue.Type.BLOCK_LITERAL ? "\n" : " ");
}
return resolveSeq.stringifyString({
comment,
type,
value
}, ctx, onComment, onChompKeep);
}
};
function parsePairs(doc, cst) {
const seq = resolveSeq.resolveSeq(doc, cst);
for (let i = 0; i < seq.items.length; ++i) {
let item = seq.items[i];
if (item instanceof resolveSeq.Pair)
continue;
else if (item instanceof resolveSeq.YAMLMap) {
if (item.items.length > 1) {
const msg = "Each pair must have its own sequence indicator";
throw new PlainValue.YAMLSemanticError(cst, msg);
}
const pair = item.items[0] || new resolveSeq.Pair();
if (item.commentBefore)
pair.commentBefore = pair.commentBefore ? `${item.commentBefore}
${pair.commentBefore}` : item.commentBefore;
if (item.comment)
pair.comment = pair.comment ? `${item.comment}
${pair.comment}` : item.comment;
item = pair;
}
seq.items[i] = item instanceof resolveSeq.Pair ? item : new resolveSeq.Pair(item);
}
return seq;
}
function createPairs(schema, iterable, ctx) {
const pairs2 = new resolveSeq.YAMLSeq(schema);
pairs2.tag = "tag:yaml.org,2002:pairs";
for (const it of iterable) {
let key, value;
if (Array.isArray(it)) {
if (it.length === 2) {
key = it[0];
value = it[1];
} else
throw new TypeError(`Expected [key, value] tuple: ${it}`);
} else if (it && it instanceof Object) {
const keys = Object.keys(it);
if (keys.length === 1) {
key = keys[0];
value = it[key];
} else
throw new TypeError(`Expected { key: value } tuple: ${it}`);
} else {
key = it;
}
const pair = schema.createPair(key, value, ctx);
pairs2.items.push(pair);
}
return pairs2;
}
var pairs = {
default: false,
tag: "tag:yaml.org,2002:pairs",
resolve: parsePairs,
createNode: createPairs
};
var YAMLOMap = class extends resolveSeq.YAMLSeq {
constructor() {
super();
PlainValue._defineProperty(this, "add", resolveSeq.YAMLMap.prototype.add.bind(this));
PlainValue._defineProperty(this, "delete", resolveSeq.YAMLMap.prototype.delete.bind(this));
PlainValue._defineProperty(this, "get", resolveSeq.YAMLMap.prototype.get.bind(this));
PlainValue._defineProperty(this, "has", resolveSeq.YAMLMap.prototype.has.bind(this));
PlainValue._defineProperty(this, "set", resolveSeq.YAMLMap.prototype.set.bind(this));
this.tag = YAMLOMap.tag;
}
toJSON(_, ctx) {
const map = /* @__PURE__ */ new Map();
if (ctx && ctx.onCreate)
ctx.onCreate(map);
for (const pair of this.items) {
let key, value;
if (pair instanceof resolveSeq.Pair) {
key = resolveSeq.toJSON(pair.key, "", ctx);
value = resolveSeq.toJSON(pair.value, key, ctx);
} else {
key = resolveSeq.toJSON(pair, "", ctx);
}
if (map.has(key))
throw new Error("Ordered maps must not include duplicate keys");
map.set(key, value);
}
return map;
}
};
PlainValue._defineProperty(YAMLOMap, "tag", "tag:yaml.org,2002:omap");
function parseOMap(doc, cst) {
const pairs2 = parsePairs(doc, cst);
const seenKeys = [];
for (const {
key
} of pairs2.items) {
if (key instanceof resolveSeq.Scalar) {
if (seenKeys.includes(key.value)) {
const msg = "Ordered maps must not include duplicate keys";
throw new PlainValue.YAMLSemanticError(cst, msg);
} else {
seenKeys.push(key.value);
}
}
}
return Object.assign(new YAMLOMap(), pairs2);
}
function createOMap(schema, iterable, ctx) {
const pairs2 = createPairs(schema, iterable, ctx);
const omap2 = new YAMLOMap();
omap2.items = pairs2.items;
return omap2;
}
var omap = {
identify: (value) => value instanceof Map,
nodeClass: YAMLOMap,
default: false,
tag: "tag:yaml.org,2002:omap",
resolve: parseOMap,
createNode: createOMap
};
var YAMLSet = class extends resolveSeq.YAMLMap {
constructor() {
super();
this.tag = YAMLSet.tag;
}
add(key) {
const pair = key instanceof resolveSeq.Pair ? key : new resolveSeq.Pair(key);
const prev = resolveSeq.findPair(this.items, pair.key);
if (!prev)
this.items.push(pair);
}
get(key, keepPair) {
const pair = resolveSeq.findPair(this.items, key);
return !keepPair && pair instanceof resolveSeq.Pair ? pair.key instanceof resolveSeq.Scalar ? pair.key.value : pair.key : pair;
}
set(key, value) {
if (typeof value !== "boolean")
throw new Error(`Expected boolean value for set(key, value) in a YAML set, not ${typeof value}`);
const prev = resolveSeq.findPair(this.items, key);
if (prev && !value) {
this.items.splice(this.items.indexOf(prev), 1);
} else if (!prev && value) {
this.items.push(new resolveSeq.Pair(key));
}
}
toJSON(_, ctx) {
return super.toJSON(_, ctx, Set);
}
toString(ctx, onComment, onChompKeep) {
if (!ctx)
return JSON.stringify(this);
if (this.hasAllNullValues())
return super.toString(ctx, onComment, onChompKeep);
else
throw new Error("Set items must all have null values");
}
};
PlainValue._defineProperty(YAMLSet, "tag", "tag:yaml.org,2002:set");
function parseSet(doc, cst) {
const map = resolveSeq.resolveMap(doc, cst);
if (!map.hasAllNullValues())
throw new PlainValue.YAMLSemanticError(cst, "Set items must all have null values");
return Object.assign(new YAMLSet(), map);
}
function createSet(schema, iterable, ctx) {
const set2 = new YAMLSet();
for (const value of iterable)
set2.items.push(schema.createPair(value, null, ctx));
return set2;
}
var set = {
identify: (value) => value instanceof Set,
nodeClass: YAMLSet,
default: false,
tag: "tag:yaml.org,2002:set",
resolve: parseSet,
createNode: createSet
};
var parseSexagesimal = (sign, parts) => {
const n2 = parts.split(":").reduce((n22, p) => n22 * 60 + Number(p), 0);
return sign === "-" ? -n2 : n2;
};
var stringifySexagesimal = ({
value
}) => {
if (isNaN(value) || !isFinite(value))
return resolveSeq.stringifyNumber(value);
let sign = "";
if (value < 0) {
sign = "-";
value = Math.abs(value);
}
const parts = [value % 60];
if (value < 60) {
parts.unshift(0);
} else {
value = Math.round((value - parts[0]) / 60);
parts.unshift(value % 60);
if (value >= 60) {
value = Math.round((value - parts[0]) / 60);
parts.unshift(value);
}
}
return sign + parts.map((n2) => n2 < 10 ? "0" + String(n2) : String(n2)).join(":").replace(/000000\d*$/, "");
};
var intTime = {
identify: (value) => typeof value === "number",
default: true,
tag: "tag:yaml.org,2002:int",
format: "TIME",
test: /^([-+]?)([0-9][0-9_]*(?::[0-5]?[0-9])+)$/,
resolve: (str, sign, parts) => parseSexagesimal(sign, parts.replace(/_/g, "")),
stringify: stringifySexagesimal
};
var floatTime = {
identify: (value) => typeof value === "number",
default: true,
tag: "tag:yaml.org,2002:float",
format: "TIME",
test: /^([-+]?)([0-9][0-9_]*(?::[0-5]?[0-9])+\.[0-9_]*)$/,
resolve: (str, sign, parts) => parseSexagesimal(sign, parts.replace(/_/g, "")),
stringify: stringifySexagesimal
};
var timestamp = {
identify: (value) => value instanceof Date,
default: true,
tag: "tag:yaml.org,2002:timestamp",
test: RegExp("^(?:([0-9]{4})-([0-9]{1,2})-([0-9]{1,2})(?:(?:t|T|[ \\t]+)([0-9]{1,2}):([0-9]{1,2}):([0-9]{1,2}(\\.[0-9]+)?)(?:[ \\t]*(Z|[-+][012]?[0-9](?::[0-9]{2})?))?)?)$"),
resolve: (str, year, month, day, hour, minute, second, millisec, tz) => {
if (millisec)
millisec = (millisec + "00").substr(1, 3);
let date = Date.UTC(year, month - 1, day, hour || 0, minute || 0, second || 0, millisec || 0);
if (tz && tz !== "Z") {
let d = parseSexagesimal(tz[0], tz.slice(1));
if (Math.abs(d) < 30)
d *= 60;
date -= 6e4 * d;
}
return new Date(date);
},
stringify: ({
value
}) => value.toISOString().replace(/((T00:00)?:00)?\.000Z$/, "")
};
function shouldWarn(deprecation) {
const env = typeof process !== "undefined" && process.env || {};
if (deprecation) {
if (typeof YAML_SILENCE_DEPRECATION_WARNINGS !== "undefined")
return !YAML_SILENCE_DEPRECATION_WARNINGS;
return !env.YAML_SILENCE_DEPRECATION_WARNINGS;
}
if (typeof YAML_SILENCE_WARNINGS !== "undefined")
return !YAML_SILENCE_WARNINGS;
return !env.YAML_SILENCE_WARNINGS;
}
function warn(warning, type) {
if (shouldWarn(false)) {
const emit = typeof process !== "undefined" && process.emitWarning;
if (emit)
emit(warning, type);
else {
console.warn(type ? `${type}: ${warning}` : warning);
}
}
}
function warnFileDeprecation(filename) {
if (shouldWarn(true)) {
const path = filename.replace(/.*yaml[/\\]/i, "").replace(/\.js$/, "").replace(/\\/g, "/");
warn(`The endpoint 'yaml/${path}' will be removed in a future release.`, "DeprecationWarning");
}
}
var warned2 = {};
function warnOptionDeprecation(name, alternative) {
if (!warned2[name] && shouldWarn(true)) {
warned2[name] = true;
let msg = `The option '${name}' will be removed in a future release`;
msg += alternative ? `, use '${alternative}' instead.` : ".";
warn(msg, "DeprecationWarning");
}
}
exports2.binary = binary;
exports2.floatTime = floatTime;
exports2.intTime = intTime;
exports2.omap = omap;
exports2.pairs = pairs;
exports2.set = set;
exports2.timestamp = timestamp;
exports2.warn = warn;
exports2.warnFileDeprecation = warnFileDeprecation;
exports2.warnOptionDeprecation = warnOptionDeprecation;
}
});
var require_Schema_88e323a7 = __commonJS2({
"node_modules/yaml/dist/Schema-88e323a7.js"(exports2) {
"use strict";
var PlainValue = require_PlainValue_ec8e588e();
var resolveSeq = require_resolveSeq_d03cb037();
var warnings = require_warnings_1000a372();
function createMap(schema, obj, ctx) {
const map2 = new resolveSeq.YAMLMap(schema);
if (obj instanceof Map) {
for (const [key, value] of obj)
map2.items.push(schema.createPair(key, value, ctx));
} else if (obj && typeof obj === "object") {
for (const key of Object.keys(obj))
map2.items.push(schema.createPair(key, obj[key], ctx));
}
if (typeof schema.sortMapEntries === "function") {
map2.items.sort(schema.sortMapEntries);
}
return map2;
}
var map = {
createNode: createMap,
default: true,
nodeClass: resolveSeq.YAMLMap,
tag: "tag:yaml.org,2002:map",
resolve: resolveSeq.resolveMap
};
function createSeq(schema, obj, ctx) {
const seq2 = new resolveSeq.YAMLSeq(schema);
if (obj && obj[Symbol.iterator]) {
for (const it of obj) {
const v = schema.createNode(it, ctx.wrapScalars, null, ctx);
seq2.items.push(v);
}
}
return seq2;
}
var seq = {
createNode: createSeq,
default: true,
nodeClass: resolveSeq.YAMLSeq,
tag: "tag:yaml.org,2002:seq",
resolve: resolveSeq.resolveSeq
};
var string = {
identify: (value) => typeof value === "string",
default: true,
tag: "tag:yaml.org,2002:str",
resolve: resolveSeq.resolveString,
stringify(item, ctx, onComment, onChompKeep) {
ctx = Object.assign({
actualString: true
}, ctx);
return resolveSeq.stringifyString(item, ctx, onComment, onChompKeep);
},
options: resolveSeq.strOptions
};
var failsafe = [map, seq, string];
var intIdentify$2 = (value) => typeof value === "bigint" || Number.isInteger(value);
var intResolve$1 = (src, part, radix) => resolveSeq.intOptions.asBigInt ? BigInt(src) : parseInt(part, radix);
function intStringify$1(node, radix, prefix) {
const {
value
} = node;
if (intIdentify$2(value) && value >= 0)
return prefix + value.toString(radix);
return resolveSeq.stringifyNumber(node);
}
var nullObj = {
identify: (value) => value == null,
createNode: (schema, value, ctx) => ctx.wrapScalars ? new resolveSeq.Scalar(null) : null,
default: true,
tag: "tag:yaml.org,2002:null",
test: /^(?:~|[Nn]ull|NULL)?$/,
resolve: () => null,
options: resolveSeq.nullOptions,
stringify: () => resolveSeq.nullOptions.nullStr
};
var boolObj = {
identify: (value) => typeof value === "boolean",
default: true,
tag: "tag:yaml.org,2002:bool",
test: /^(?:[Tt]rue|TRUE|[Ff]alse|FALSE)$/,
resolve: (str) => str[0] === "t" || str[0] === "T",
options: resolveSeq.boolOptions,
stringify: ({
value
}) => value ? resolveSeq.boolOptions.trueStr : resolveSeq.boolOptions.falseStr
};
var octObj = {
identify: (value) => intIdentify$2(value) && value >= 0,
default: true,
tag: "tag:yaml.org,2002:int",
format: "OCT",
test: /^0o([0-7]+)$/,
resolve: (str, oct) => intResolve$1(str, oct, 8),
options: resolveSeq.intOptions,
stringify: (node) => intStringify$1(node, 8, "0o")
};
var intObj = {
identify: intIdentify$2,
default: true,
tag: "tag:yaml.org,2002:int",
test: /^[-+]?[0-9]+$/,
resolve: (str) => intResolve$1(str, str, 10),
options: resolveSeq.intOptions,
stringify: resolveSeq.stringifyNumber
};
var hexObj = {
identify: (value) => intIdentify$2(value) && value >= 0,
default: true,
tag: "tag:yaml.org,2002:int",
format: "HEX",
test: /^0x([0-9a-fA-F]+)$/,
resolve: (str, hex) => intResolve$1(str, hex, 16),
options: resolveSeq.intOptions,
stringify: (node) => intStringify$1(node, 16, "0x")
};
var nanObj = {
identify: (value) => typeof value === "number",
default: true,
tag: "tag:yaml.org,2002:float",
test: /^(?:[-+]?\.inf|(\.nan))$/i,
resolve: (str, nan) => nan ? NaN : str[0] === "-" ? Number.NEGATIVE_INFINITY : Number.POSITIVE_INFINITY,
stringify: resolveSeq.stringifyNumber
};
var expObj = {
identify: (value) => typeof value === "number",
default: true,
tag: "tag:yaml.org,2002:float",
format: "EXP",
test: /^[-+]?(?:\.[0-9]+|[0-9]+(?:\.[0-9]*)?)[eE][-+]?[0-9]+$/,
resolve: (str) => parseFloat(str),
stringify: ({
value
}) => Number(value).toExponential()
};
var floatObj = {
identify: (value) => typeof value === "number",
default: true,
tag: "tag:yaml.org,2002:float",
test: /^[-+]?(?:\.([0-9]+)|[0-9]+\.([0-9]*))$/,
resolve(str, frac1, frac2) {
const frac = frac1 || frac2;
const node = new resolveSeq.Scalar(parseFloat(str));
if (frac && frac[frac.length - 1] === "0")
node.minFractionDigits = frac.length;
return node;
},
stringify: resolveSeq.stringifyNumber
};
var core = failsafe.concat([nullObj, boolObj, octObj, intObj, hexObj, nanObj, expObj, floatObj]);
var intIdentify$1 = (value) => typeof value === "bigint" || Number.isInteger(value);
var stringifyJSON = ({
value
}) => JSON.stringify(value);
var json = [map, seq, {
identify: (value) => typeof value === "string",
default: true,
tag: "tag:yaml.org,2002:str",
resolve: resolveSeq.resolveString,
stringify: stringifyJSON
}, {
identify: (value) => value == null,
createNode: (schema, value, ctx) => ctx.wrapScalars ? new resolveSeq.Scalar(null) : null,
default: true,
tag: "tag:yaml.org,2002:null",
test: /^null$/,
resolve: () => null,
stringify: stringifyJSON
}, {
identify: (value) => typeof value === "boolean",
default: true,
tag: "tag:yaml.org,2002:bool",
test: /^true|false$/,
resolve: (str) => str === "true",
stringify: stringifyJSON
}, {
identify: intIdentify$1,
default: true,
tag: "tag:yaml.org,2002:int",
test: /^-?(?:0|[1-9][0-9]*)$/,
resolve: (str) => resolveSeq.intOptions.asBigInt ? BigInt(str) : parseInt(str, 10),
stringify: ({
value
}) => intIdentify$1(value) ? value.toString() : JSON.stringify(value)
}, {
identify: (value) => typeof value === "number",
default: true,
tag: "tag:yaml.org,2002:float",
test: /^-?(?:0|[1-9][0-9]*)(?:\.[0-9]*)?(?:[eE][-+]?[0-9]+)?$/,
resolve: (str) => parseFloat(str),
stringify: stringifyJSON
}];
json.scalarFallback = (str) => {
throw new SyntaxError(`Unresolved plain scalar ${JSON.stringify(str)}`);
};
var boolStringify = ({
value
}) => value ? resolveSeq.boolOptions.trueStr : resolveSeq.boolOptions.falseStr;
var intIdentify = (value) => typeof value === "bigint" || Number.isInteger(value);
function intResolve(sign, src, radix) {
let str = src.replace(/_/g, "");
if (resolveSeq.intOptions.asBigInt) {
switch (radix) {
case 2:
str = `0b${str}`;
break;
case 8:
str = `0o${str}`;
break;
case 16:
str = `0x${str}`;
break;
}
const n22 = BigInt(str);
return sign === "-" ? BigInt(-1) * n22 : n22;
}
const n2 = parseInt(str, radix);
return sign === "-" ? -1 * n2 : n2;
}
function intStringify(node, radix, prefix) {
const {
value
} = node;
if (intIdentify(value)) {
const str = value.toString(radix);
return value < 0 ? "-" + prefix + str.substr(1) : prefix + str;
}
return resolveSeq.stringifyNumber(node);
}
var yaml11 = failsafe.concat([{
identify: (value) => value == null,
createNode: (schema, value, ctx) => ctx.wrapScalars ? new resolveSeq.Scalar(null) : null,
default: true,
tag: "tag:yaml.org,2002:null",
test: /^(?:~|[Nn]ull|NULL)?$/,
resolve: () => null,
options: resolveSeq.nullOptions,
stringify: () => resolveSeq.nullOptions.nullStr
}, {
identify: (value) => typeof value === "boolean",
default: true,
tag: "tag:yaml.org,2002:bool",
test: /^(?:Y|y|[Yy]es|YES|[Tt]rue|TRUE|[Oo]n|ON)$/,
resolve: () => true,
options: resolveSeq.boolOptions,
stringify: boolStringify
}, {
identify: (value) => typeof value === "boolean",
default: true,
tag: "tag:yaml.org,2002:bool",
test: /^(?:N|n|[Nn]o|NO|[Ff]alse|FALSE|[Oo]ff|OFF)$/i,
resolve: () => false,
options: resolveSeq.boolOptions,
stringify: boolStringify
}, {
identify: intIdentify,
default: true,
tag: "tag:yaml.org,2002:int",
format: "BIN",
test: /^([-+]?)0b([0-1_]+)$/,
resolve: (str, sign, bin) => intResolve(sign, bin, 2),
stringify: (node) => intStringify(node, 2, "0b")
}, {
identify: intIdentify,
default: true,
tag: "tag:yaml.org,2002:int",
format: "OCT",
test: /^([-+]?)0([0-7_]+)$/,
resolve: (str, sign, oct) => intResolve(sign, oct, 8),
stringify: (node) => intStringify(node, 8, "0")
}, {
identify: intIdentify,
default: true,
tag: "tag:yaml.org,2002:int",
test: /^([-+]?)([0-9][0-9_]*)$/,
resolve: (str, sign, abs) => intResolve(sign, abs, 10),
stringify: resolveSeq.stringifyNumber
}, {
identify: intIdentify,
default: true,
tag: "tag:yaml.org,2002:int",
format: "HEX",
test: /^([-+]?)0x([0-9a-fA-F_]+)$/,
resolve: (str, sign, hex) => intResolve(sign, hex, 16),
stringify: (node) => intStringify(node, 16, "0x")
}, {
identify: (value) => typeof value === "number",
default: true,
tag: "tag:yaml.org,2002:float",
test: /^(?:[-+]?\.inf|(\.nan))$/i,
resolve: (str, nan) => nan ? NaN : str[0] === "-" ? Number.NEGATIVE_INFINITY : Number.POSITIVE_INFINITY,
stringify: resolveSeq.stringifyNumber
}, {
identify: (value) => typeof value === "number",
default: true,
tag: "tag:yaml.org,2002:float",
format: "EXP",
test: /^[-+]?([0-9][0-9_]*)?(\.[0-9_]*)?[eE][-+]?[0-9]+$/,
resolve: (str) => parseFloat(str.replace(/_/g, "")),
stringify: ({
value
}) => Number(value).toExponential()
}, {
identify: (value) => typeof value === "number",
default: true,
tag: "tag:yaml.org,2002:float",
test: /^[-+]?(?:[0-9][0-9_]*)?\.([0-9_]*)$/,
resolve(str, frac) {
const node = new resolveSeq.Scalar(parseFloat(str.replace(/_/g, "")));
if (frac) {
const f = frac.replace(/_/g, "");
if (f[f.length - 1] === "0")
node.minFractionDigits = f.length;
}
return node;
},
stringify: resolveSeq.stringifyNumber
}], warnings.binary, warnings.omap, warnings.pairs, warnings.set, warnings.intTime, warnings.floatTime, warnings.timestamp);
var schemas = {
core,
failsafe,
json,
yaml11
};
var tags = {
binary: warnings.binary,
bool: boolObj,
float: floatObj,
floatExp: expObj,
floatNaN: nanObj,
floatTime: warnings.floatTime,
int: intObj,
intHex: hexObj,
intOct: octObj,
intTime: warnings.intTime,
map,
null: nullObj,
omap: warnings.omap,
pairs: warnings.pairs,
seq,
set: warnings.set,
timestamp: warnings.timestamp
};
function findTagObject(value, tagName, tags2) {
if (tagName) {
const match = tags2.filter((t) => t.tag === tagName);
const tagObj = match.find((t) => !t.format) || match[0];
if (!tagObj)
throw new Error(`Tag ${tagName} not found`);
return tagObj;
}
return tags2.find((t) => (t.identify && t.identify(value) || t.class && value instanceof t.class) && !t.format);
}
function createNode(value, tagName, ctx) {
if (value instanceof resolveSeq.Node)
return value;
const {
defaultPrefix,
onTagObj,
prevObjects,
schema,
wrapScalars
} = ctx;
if (tagName && tagName.startsWith("!!"))
tagName = defaultPrefix + tagName.slice(2);
let tagObj = findTagObject(value, tagName, schema.tags);
if (!tagObj) {
if (typeof value.toJSON === "function")
value = value.toJSON();
if (!value || typeof value !== "object")
return wrapScalars ? new resolveSeq.Scalar(value) : value;
tagObj = value instanceof Map ? map : value[Symbol.iterator] ? seq : map;
}
if (onTagObj) {
onTagObj(tagObj);
delete ctx.onTagObj;
}
const obj = {
value: void 0,
node: void 0
};
if (value && typeof value === "object" && prevObjects) {
const prev = prevObjects.get(value);
if (prev) {
const alias = new resolveSeq.Alias(prev);
ctx.aliasNodes.push(alias);
return alias;
}
obj.value = value;
prevObjects.set(value, obj);
}
obj.node = tagObj.createNode ? tagObj.createNode(ctx.schema, value, ctx) : wrapScalars ? new resolveSeq.Scalar(value) : value;
if (tagName && obj.node instanceof resolveSeq.Node)
obj.node.tag = tagName;
return obj.node;
}
function getSchemaTags(schemas2, knownTags, customTags, schemaId) {
let tags2 = schemas2[schemaId.replace(/\W/g, "")];
if (!tags2) {
const keys = Object.keys(schemas2).map((key) => JSON.stringify(key)).join(", ");
throw new Error(`Unknown schema "${schemaId}"; use one of ${keys}`);
}
if (Array.isArray(customTags)) {
for (const tag of customTags)
tags2 = tags2.concat(tag);
} else if (typeof customTags === "function") {
tags2 = customTags(tags2.slice());
}
for (let i = 0; i < tags2.length; ++i) {
const tag = tags2[i];
if (typeof tag === "string") {
const tagObj = knownTags[tag];
if (!tagObj) {
const keys = Object.keys(knownTags).map((key) => JSON.stringify(key)).join(", ");
throw new Error(`Unknown custom tag "${tag}"; use one of ${keys}`);
}
tags2[i] = tagObj;
}
}
return tags2;
}
var sortMapEntriesByKey = (a, b) => a.key < b.key ? -1 : a.key > b.key ? 1 : 0;
var Schema = class {
constructor({
customTags,
merge,
schema,
sortMapEntries,
tags: deprecatedCustomTags
}) {
this.merge = !!merge;
this.name = schema;
this.sortMapEntries = sortMapEntries === true ? sortMapEntriesByKey : sortMapEntries || null;
if (!customTags && deprecatedCustomTags)
warnings.warnOptionDeprecation("tags", "customTags");
this.tags = getSchemaTags(schemas, tags, customTags || deprecatedCustomTags, schema);
}
createNode(value, wrapScalars, tagName, ctx) {
const baseCtx = {
defaultPrefix: Schema.defaultPrefix,
schema: this,
wrapScalars
};
const createCtx = ctx ? Object.assign(ctx, baseCtx) : baseCtx;
return createNode(value, tagName, createCtx);
}
createPair(key, value, ctx) {
if (!ctx)
ctx = {
wrapScalars: true
};
const k = this.createNode(key, ctx.wrapScalars, null, ctx);
const v = this.createNode(value, ctx.wrapScalars, null, ctx);
return new resolveSeq.Pair(k, v);
}
};
PlainValue._defineProperty(Schema, "defaultPrefix", PlainValue.defaultTagPrefix);
PlainValue._defineProperty(Schema, "defaultTags", PlainValue.defaultTags);
exports2.Schema = Schema;
}
});
var require_Document_9b4560a1 = __commonJS2({
"node_modules/yaml/dist/Document-9b4560a1.js"(exports2) {
"use strict";
var PlainValue = require_PlainValue_ec8e588e();
var resolveSeq = require_resolveSeq_d03cb037();
var Schema = require_Schema_88e323a7();
var defaultOptions = {
anchorPrefix: "a",
customTags: null,
indent: 2,
indentSeq: true,
keepCstNodes: false,
keepNodeTypes: true,
keepBlobsInJSON: true,
mapAsMap: false,
maxAliasCount: 100,
prettyErrors: false,
simpleKeys: false,
version: "1.2"
};
var scalarOptions = {
get binary() {
return resolveSeq.binaryOptions;
},
set binary(opt) {
Object.assign(resolveSeq.binaryOptions, opt);
},
get bool() {
return resolveSeq.boolOptions;
},
set bool(opt) {
Object.assign(resolveSeq.boolOptions, opt);
},
get int() {
return resolveSeq.intOptions;
},
set int(opt) {
Object.assign(resolveSeq.intOptions, opt);
},
get null() {
return resolveSeq.nullOptions;
},
set null(opt) {
Object.assign(resolveSeq.nullOptions, opt);
},
get str() {
return resolveSeq.strOptions;
},
set str(opt) {
Object.assign(resolveSeq.strOptions, opt);
}
};
var documentOptions = {
"1.0": {
schema: "yaml-1.1",
merge: true,
tagPrefixes: [{
handle: "!",
prefix: PlainValue.defaultTagPrefix
}, {
handle: "!!",
prefix: "tag:private.yaml.org,2002:"
}]
},
1.1: {
schema: "yaml-1.1",
merge: true,
tagPrefixes: [{
handle: "!",
prefix: "!"
}, {
handle: "!!",
prefix: PlainValue.defaultTagPrefix
}]
},
1.2: {
schema: "core",
merge: false,
tagPrefixes: [{
handle: "!",
prefix: "!"
}, {
handle: "!!",
prefix: PlainValue.defaultTagPrefix
}]
}
};
function stringifyTag(doc, tag) {
if ((doc.version || doc.options.version) === "1.0") {
const priv = tag.match(/^tag:private\.yaml\.org,2002:([^:/]+)$/);
if (priv)
return "!" + priv[1];
const vocab = tag.match(/^tag:([a-zA-Z0-9-]+)\.yaml\.org,2002:(.*)/);
return vocab ? `!${vocab[1]}/${vocab[2]}` : `!${tag.replace(/^tag:/, "")}`;
}
let p = doc.tagPrefixes.find((p2) => tag.indexOf(p2.prefix) === 0);
if (!p) {
const dtp = doc.getDefaults().tagPrefixes;
p = dtp && dtp.find((p2) => tag.indexOf(p2.prefix) === 0);
}
if (!p)
return tag[0] === "!" ? tag : `!<${tag}>`;
const suffix = tag.substr(p.prefix.length).replace(/[!,[\]{}]/g, (ch) => ({
"!": "%21",
",": "%2C",
"[": "%5B",
"]": "%5D",
"{": "%7B",
"}": "%7D"
})[ch]);
return p.handle + suffix;
}
function getTagObject(tags, item) {
if (item instanceof resolveSeq.Alias)
return resolveSeq.Alias;
if (item.tag) {
const match = tags.filter((t) => t.tag === item.tag);
if (match.length > 0)
return match.find((t) => t.format === item.format) || match[0];
}
let tagObj, obj;
if (item instanceof resolveSeq.Scalar) {
obj = item.value;
const match = tags.filter((t) => t.identify && t.identify(obj) || t.class && obj instanceof t.class);
tagObj = match.find((t) => t.format === item.format) || match.find((t) => !t.format);
} else {
obj = item;
tagObj = tags.find((t) => t.nodeClass && obj instanceof t.nodeClass);
}
if (!tagObj) {
const name = obj && obj.constructor ? obj.constructor.name : typeof obj;
throw new Error(`Tag not resolved for ${name} value`);
}
return tagObj;
}
function stringifyProps(node, tagObj, {
anchors,
doc
}) {
const props = [];
const anchor = doc.anchors.getName(node);
if (anchor) {
anchors[anchor] = node;
props.push(`&${anchor}`);
}
if (node.tag) {
props.push(stringifyTag(doc, node.tag));
} else if (!tagObj.default) {
props.push(stringifyTag(doc, tagObj.tag));
}
return props.join(" ");
}
function stringify(item, ctx, onComment, onChompKeep) {
const {
anchors,
schema
} = ctx.doc;
let tagObj;
if (!(item instanceof resolveSeq.Node)) {
const createCtx = {
aliasNodes: [],
onTagObj: (o) => tagObj = o,
prevObjects: /* @__PURE__ */ new Map()
};
item = schema.createNode(item, true, null, createCtx);
for (const alias of createCtx.aliasNodes) {
alias.source = alias.source.node;
let name = anchors.getName(alias.source);
if (!name) {
name = anchors.newName();
anchors.map[name] = alias.source;
}
}
}
if (item instanceof resolveSeq.Pair)
return item.toString(ctx, onComment, onChompKeep);
if (!tagObj)
tagObj = getTagObject(schema.tags, item);
const props = stringifyProps(item, tagObj, ctx);
if (props.length > 0)
ctx.indentAtStart = (ctx.indentAtStart || 0) + props.length + 1;
const str = typeof tagObj.stringify === "function" ? tagObj.stringify(item, ctx, onComment, onChompKeep) : item instanceof resolveSeq.Scalar ? resolveSeq.stringifyString(item, ctx, onComment, onChompKeep) : item.toString(ctx, onComment, onChompKeep);
if (!props)
return str;
return item instanceof resolveSeq.Scalar || str[0] === "{" || str[0] === "[" ? `${props} ${str}` : `${props}
${ctx.indent}${str}`;
}
var Anchors = class {
static validAnchorNode(node) {
return node instanceof resolveSeq.Scalar || node instanceof resolveSeq.YAMLSeq || node instanceof resolveSeq.YAMLMap;
}
constructor(prefix) {
PlainValue._defineProperty(this, "map", /* @__PURE__ */ Object.create(null));
this.prefix = prefix;
}
createAlias(node, name) {
this.setAnchor(node, name);
return new resolveSeq.Alias(node);
}
createMergePair(...sources) {
const merge = new resolveSeq.Merge();
merge.value.items = sources.map((s) => {
if (s instanceof resolveSeq.Alias) {
if (s.source instanceof resolveSeq.YAMLMap)
return s;
} else if (s instanceof resolveSeq.YAMLMap) {
return this.createAlias(s);
}
throw new Error("Merge sources must be Map nodes or their Aliases");
});
return merge;
}
getName(node) {
const {
map
} = this;
return Object.keys(map).find((a) => map[a] === node);
}
getNames() {
return Object.keys(this.map);
}
getNode(name) {
return this.map[name];
}
newName(prefix) {
if (!prefix)
prefix = this.prefix;
const names = Object.keys(this.map);
for (let i = 1; true; ++i) {
const name = `${prefix}${i}`;
if (!names.includes(name))
return name;
}
}
resolveNodes() {
const {
map,
_cstAliases
} = this;
Object.keys(map).forEach((a) => {
map[a] = map[a].resolved;
});
_cstAliases.forEach((a) => {
a.source = a.source.resolved;
});
delete this._cstAliases;
}
setAnchor(node, name) {
if (node != null && !Anchors.validAnchorNode(node)) {
throw new Error("Anchors may only be set for Scalar, Seq and Map nodes");
}
if (name && /[\x00-\x19\s,[\]{}]/.test(name)) {
throw new Error("Anchor names must not contain whitespace or control characters");
}
const {
map
} = this;
const prev = node && Object.keys(map).find((a) => map[a] === node);
if (prev) {
if (!name) {
return prev;
} else if (prev !== name) {
delete map[prev];
map[name] = node;
}
} else {
if (!name) {
if (!node)
return null;
name = this.newName();
}
map[name] = node;
}
return name;
}
};
var visit = (node, tags) => {
if (node && typeof node === "object") {
const {
tag
} = node;
if (node instanceof resolveSeq.Collection) {
if (tag)
tags[tag] = true;
node.items.forEach((n2) => visit(n2, tags));
} else if (node instanceof resolveSeq.Pair) {
visit(node.key, tags);
visit(node.value, tags);
} else if (node instanceof resolveSeq.Scalar) {
if (tag)
tags[tag] = true;
}
}
return tags;
};
var listTagNames = (node) => Object.keys(visit(node, {}));
function parseContents(doc, contents) {
const comments = {
before: [],
after: []
};
let body = void 0;
let spaceBefore = false;
for (const node of contents) {
if (node.valueRange) {
if (body !== void 0) {
const msg = "Document contains trailing content not separated by a ... or --- line";
doc.errors.push(new PlainValue.YAMLSyntaxError(node, msg));
break;
}
const res = resolveSeq.resolveNode(doc, node);
if (spaceBefore) {
res.spaceBefore = true;
spaceBefore = false;
}
body = res;
} else if (node.comment !== null) {
const cc = body === void 0 ? comments.before : comments.after;
cc.push(node.comment);
} else if (node.type === PlainValue.Type.BLANK_LINE) {
spaceBefore = true;
if (body === void 0 && comments.before.length > 0 && !doc.commentBefore) {
doc.commentBefore = comments.before.join("\n");
comments.before = [];
}
}
}
doc.contents = body || null;
if (!body) {
doc.comment = comments.before.concat(comments.after).join("\n") || null;
} else {
const cb = comments.before.join("\n");
if (cb) {
const cbNode = body instanceof resolveSeq.Collection && body.items[0] ? body.items[0] : body;
cbNode.commentBefore = cbNode.commentBefore ? `${cb}
${cbNode.commentBefore}` : cb;
}
doc.comment = comments.after.join("\n") || null;
}
}
function resolveTagDirective({
tagPrefixes
}, directive) {
const [handle, prefix] = directive.parameters;
if (!handle || !prefix) {
const msg = "Insufficient parameters given for %TAG directive";
throw new PlainValue.YAMLSemanticError(directive, msg);
}
if (tagPrefixes.some((p) => p.handle === handle)) {
const msg = "The %TAG directive must only be given at most once per handle in the same document.";
throw new PlainValue.YAMLSemanticError(directive, msg);
}
return {
handle,
prefix
};
}
function resolveYamlDirective(doc, directive) {
let [version] = directive.parameters;
if (directive.name === "YAML:1.0")
version = "1.0";
if (!version) {
const msg = "Insufficient parameters given for %YAML directive";
throw new PlainValue.YAMLSemanticError(directive, msg);
}
if (!documentOptions[version]) {
const v0 = doc.version || doc.options.version;
const msg = `Document will be parsed as YAML ${v0} rather than YAML ${version}`;
doc.warnings.push(new PlainValue.YAMLWarning(directive, msg));
}
return version;
}
function parseDirectives(doc, directives, prevDoc) {
const directiveComments = [];
let hasDirectives = false;
for (const directive of directives) {
const {
comment,
name
} = directive;
switch (name) {
case "TAG":
try {
doc.tagPrefixes.push(resolveTagDirective(doc, directive));
} catch (error) {
doc.errors.push(error);
}
hasDirectives = true;
break;
case "YAML":
case "YAML:1.0":
if (doc.version) {
const msg = "The %YAML directive must only be given at most once per document.";
doc.errors.push(new PlainValue.YAMLSemanticError(directive, msg));
}
try {
doc.version = resolveYamlDirective(doc, directive);
} catch (error) {
doc.errors.push(error);
}
hasDirectives = true;
break;
default:
if (name) {
const msg = `YAML only supports %TAG and %YAML directives, and not %${name}`;
doc.warnings.push(new PlainValue.YAMLWarning(directive, msg));
}
}
if (comment)
directiveComments.push(comment);
}
if (prevDoc && !hasDirectives && "1.1" === (doc.version || prevDoc.version || doc.options.version)) {
const copyTagPrefix = ({
handle,
prefix
}) => ({
handle,
prefix
});
doc.tagPrefixes = prevDoc.tagPrefixes.map(copyTagPrefix);
doc.version = prevDoc.version;
}
doc.commentBefore = directiveComments.join("\n") || null;
}
function assertCollection(contents) {
if (contents instanceof resolveSeq.Collection)
return true;
throw new Error("Expected a YAML collection as document contents");
}
var Document = class {
constructor(options) {
this.anchors = new Anchors(options.anchorPrefix);
this.commentBefore = null;
this.comment = null;
this.contents = null;
this.directivesEndMarker = null;
this.errors = [];
this.options = options;
this.schema = null;
this.tagPrefixes = [];
this.version = null;
this.warnings = [];
}
add(value) {
assertCollection(this.contents);
return this.contents.add(value);
}
addIn(path, value) {
assertCollection(this.contents);
this.contents.addIn(path, value);
}
delete(key) {
assertCollection(this.contents);
return this.contents.delete(key);
}
deleteIn(path) {
if (resolveSeq.isEmptyPath(path)) {
if (this.contents == null)
return false;
this.contents = null;
return true;
}
assertCollection(this.contents);
return this.contents.deleteIn(path);
}
getDefaults() {
return Document.defaults[this.version] || Document.defaults[this.options.version] || {};
}
get(key, keepScalar) {
return this.contents instanceof resolveSeq.Collection ? this.contents.get(key, keepScalar) : void 0;
}
getIn(path, keepScalar) {
if (resolveSeq.isEmptyPath(path))
return !keepScalar && this.contents instanceof resolveSeq.Scalar ? this.contents.value : this.contents;
return this.contents instanceof resolveSeq.Collection ? this.contents.getIn(path, keepScalar) : void 0;
}
has(key) {
return this.contents instanceof resolveSeq.Collection ? this.contents.has(key) : false;
}
hasIn(path) {
if (resolveSeq.isEmptyPath(path))
return this.contents !== void 0;
return this.contents instanceof resolveSeq.Collection ? this.contents.hasIn(path) : false;
}
set(key, value) {
assertCollection(this.contents);
this.contents.set(key, value);
}
setIn(path, value) {
if (resolveSeq.isEmptyPath(path))
this.contents = value;
else {
assertCollection(this.contents);
this.contents.setIn(path, value);
}
}
setSchema(id, customTags) {
if (!id && !customTags && this.schema)
return;
if (typeof id === "number")
id = id.toFixed(1);
if (id === "1.0" || id === "1.1" || id === "1.2") {
if (this.version)
this.version = id;
else
this.options.version = id;
delete this.options.schema;
} else if (id && typeof id === "string") {
this.options.schema = id;
}
if (Array.isArray(customTags))
this.options.customTags = customTags;
const opt = Object.assign({}, this.getDefaults(), this.options);
this.schema = new Schema.Schema(opt);
}
parse(node, prevDoc) {
if (this.options.keepCstNodes)
this.cstNode = node;
if (this.options.keepNodeTypes)
this.type = "DOCUMENT";
const {
directives = [],
contents = [],
directivesEndMarker,
error,
valueRange
} = node;
if (error) {
if (!error.source)
error.source = this;
this.errors.push(error);
}
parseDirectives(this, directives, prevDoc);
if (directivesEndMarker)
this.directivesEndMarker = true;
this.range = valueRange ? [valueRange.start, valueRange.end] : null;
this.setSchema();
this.anchors._cstAliases = [];
parseContents(this, contents);
this.anchors.resolveNodes();
if (this.options.prettyErrors) {
for (const error2 of this.errors)
if (error2 instanceof PlainValue.YAMLError)
error2.makePretty();
for (const warn of this.warnings)
if (warn instanceof PlainValue.YAMLError)
warn.makePretty();
}
return this;
}
listNonDefaultTags() {
return listTagNames(this.contents).filter((t) => t.indexOf(Schema.Schema.defaultPrefix) !== 0);
}
setTagPrefix(handle, prefix) {
if (handle[0] !== "!" || handle[handle.length - 1] !== "!")
throw new Error("Handle must start and end with !");
if (prefix) {
const prev = this.tagPrefixes.find((p) => p.handle === handle);
if (prev)
prev.prefix = prefix;
else
this.tagPrefixes.push({
handle,
prefix
});
} else {
this.tagPrefixes = this.tagPrefixes.filter((p) => p.handle !== handle);
}
}
toJSON(arg, onAnchor) {
const {
keepBlobsInJSON,
mapAsMap,
maxAliasCount
} = this.options;
const keep = keepBlobsInJSON && (typeof arg !== "string" || !(this.contents instanceof resolveSeq.Scalar));
const ctx = {
doc: this,
indentStep: " ",
keep,
mapAsMap: keep && !!mapAsMap,
maxAliasCount,
stringify
};
const anchorNames = Object.keys(this.anchors.map);
if (anchorNames.length > 0)
ctx.anchors = new Map(anchorNames.map((name) => [this.anchors.map[name], {
alias: [],
aliasCount: 0,
count: 1
}]));
const res = resolveSeq.toJSON(this.contents, arg, ctx);
if (typeof onAnchor === "function" && ctx.anchors)
for (const {
count,
res: res2
} of ctx.anchors.values())
onAnchor(res2, count);
return res;
}
toString() {
if (this.errors.length > 0)
throw new Error("Document with errors cannot be stringified");
const indentSize = this.options.indent;
if (!Number.isInteger(indentSize) || indentSize <= 0) {
const s = JSON.stringify(indentSize);
throw new Error(`"indent" option must be a positive integer, not ${s}`);
}
this.setSchema();
const lines = [];
let hasDirectives = false;
if (this.version) {
let vd = "%YAML 1.2";
if (this.schema.name === "yaml-1.1") {
if (this.version === "1.0")
vd = "%YAML:1.0";
else if (this.version === "1.1")
vd = "%YAML 1.1";
}
lines.push(vd);
hasDirectives = true;
}
const tagNames = this.listNonDefaultTags();
this.tagPrefixes.forEach(({
handle,
prefix
}) => {
if (tagNames.some((t) => t.indexOf(prefix) === 0)) {
lines.push(`%TAG ${handle} ${prefix}`);
hasDirectives = true;
}
});
if (hasDirectives || this.directivesEndMarker)
lines.push("---");
if (this.commentBefore) {
if (hasDirectives || !this.directivesEndMarker)
lines.unshift("");
lines.unshift(this.commentBefore.replace(/^/gm, "#"));
}
const ctx = {
anchors: /* @__PURE__ */ Object.create(null),
doc: this,
indent: "",
indentStep: " ".repeat(indentSize),
stringify
};
let chompKeep = false;
let contentComment = null;
if (this.contents) {
if (this.contents instanceof resolveSeq.Node) {
if (this.contents.spaceBefore && (hasDirectives || this.directivesEndMarker))
lines.push("");
if (this.contents.commentBefore)
lines.push(this.contents.commentBefore.replace(/^/gm, "#"));
ctx.forceBlockIndent = !!this.comment;
contentComment = this.contents.comment;
}
const onChompKeep = contentComment ? null : () => chompKeep = true;
const body = stringify(this.contents, ctx, () => contentComment = null, onChompKeep);
lines.push(resolveSeq.addComment(body, "", contentComment));
} else if (this.contents !== void 0) {
lines.push(stringify(this.contents, ctx));
}
if (this.comment) {
if ((!chompKeep || contentComment) && lines[lines.length - 1] !== "")
lines.push("");
lines.push(this.comment.replace(/^/gm, "#"));
}
return lines.join("\n") + "\n";
}
};
PlainValue._defineProperty(Document, "defaults", documentOptions);
exports2.Document = Document;
exports2.defaultOptions = defaultOptions;
exports2.scalarOptions = scalarOptions;
}
});
var require_dist2 = __commonJS2({
"node_modules/yaml/dist/index.js"(exports2) {
"use strict";
var parseCst = require_parse_cst();
var Document$1 = require_Document_9b4560a1();
var Schema = require_Schema_88e323a7();
var PlainValue = require_PlainValue_ec8e588e();
var warnings = require_warnings_1000a372();
require_resolveSeq_d03cb037();
function createNode(value, wrapScalars = true, tag) {
if (tag === void 0 && typeof wrapScalars === "string") {
tag = wrapScalars;
wrapScalars = true;
}
const options = Object.assign({}, Document$1.Document.defaults[Document$1.defaultOptions.version], Document$1.defaultOptions);
const schema = new Schema.Schema(options);
return schema.createNode(value, wrapScalars, tag);
}
var Document = class extends Document$1.Document {
constructor(options) {
super(Object.assign({}, Document$1.defaultOptions, options));
}
};
function parseAllDocuments(src, options) {
const stream = [];
let prev;
for (const cstDoc of parseCst.parse(src)) {
const doc = new Document(options);
doc.parse(cstDoc, prev);
stream.push(doc);
prev = doc;
}
return stream;
}
function parseDocument(src, options) {
const cst = parseCst.parse(src);
const doc = new Document(options).parse(cst[0]);
if (cst.length > 1) {
const errMsg = "Source contains multiple documents; please use YAML.parseAllDocuments()";
doc.errors.unshift(new PlainValue.YAMLSemanticError(cst[1], errMsg));
}
return doc;
}
function parse2(src, options) {
const doc = parseDocument(src, options);
doc.warnings.forEach((warning) => warnings.warn(warning));
if (doc.errors.length > 0)
throw doc.errors[0];
return doc.toJSON();
}
function stringify(value, options) {
const doc = new Document(options);
doc.contents = value;
return String(doc);
}
var YAML = {
createNode,
defaultOptions: Document$1.defaultOptions,
Document,
parse: parse2,
parseAllDocuments,
parseCST: parseCst.parse,
parseDocument,
scalarOptions: Document$1.scalarOptions,
stringify
};
exports2.YAML = YAML;
}
});
var require_yaml = __commonJS2({
"node_modules/yaml/index.js"(exports2, module22) {
module22.exports = require_dist2().YAML;
}
});
var require_loaders = __commonJS2({
"node_modules/cosmiconfig/dist/loaders.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.loaders = void 0;
var importFresh;
var loadJs = function loadJs2(filepath) {
if (importFresh === void 0) {
importFresh = require_import_fresh();
}
const result = importFresh(filepath);
return result;
};
var parseJson;
var loadJson = function loadJson2(filepath, content) {
if (parseJson === void 0) {
parseJson = require_parse_json();
}
try {
const result = parseJson(content);
return result;
} catch (error) {
error.message = `JSON Error in ${filepath}:
${error.message}`;
throw error;
}
};
var yaml;
var loadYaml = function loadYaml2(filepath, content) {
if (yaml === void 0) {
yaml = require_yaml();
}
try {
const result = yaml.parse(content, {
prettyErrors: true
});
return result;
} catch (error) {
error.message = `YAML Error in ${filepath}:
${error.message}`;
throw error;
}
};
var loaders = {
loadJs,
loadJson,
loadYaml
};
exports2.loaders = loaders;
}
});
var require_getPropertyByPath = __commonJS2({
"node_modules/cosmiconfig/dist/getPropertyByPath.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.getPropertyByPath = getPropertyByPath;
function getPropertyByPath(source, path) {
if (typeof path === "string" && Object.prototype.hasOwnProperty.call(source, path)) {
return source[path];
}
const parsedPath = typeof path === "string" ? path.split(".") : path;
return parsedPath.reduce((previous, key) => {
if (previous === void 0) {
return previous;
}
return previous[key];
}, source);
}
}
});
var require_ExplorerBase = __commonJS2({
"node_modules/cosmiconfig/dist/ExplorerBase.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.getExtensionDescription = getExtensionDescription;
exports2.ExplorerBase = void 0;
var _path = _interopRequireDefault(require("path"));
var _loaders = require_loaders();
var _getPropertyByPath = require_getPropertyByPath();
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : {
default: obj
};
}
var ExplorerBase = class {
constructor(options) {
if (options.cache === true) {
this.loadCache = /* @__PURE__ */ new Map();
this.searchCache = /* @__PURE__ */ new Map();
}
this.config = options;
this.validateConfig();
}
clearLoadCache() {
if (this.loadCache) {
this.loadCache.clear();
}
}
clearSearchCache() {
if (this.searchCache) {
this.searchCache.clear();
}
}
clearCaches() {
this.clearLoadCache();
this.clearSearchCache();
}
validateConfig() {
const config = this.config;
config.searchPlaces.forEach((place) => {
const loaderKey = _path.default.extname(place) || "noExt";
const loader = config.loaders[loaderKey];
if (!loader) {
throw new Error(`No loader specified for ${getExtensionDescription(place)}, so searchPlaces item "${place}" is invalid`);
}
if (typeof loader !== "function") {
throw new Error(`loader for ${getExtensionDescription(place)} is not a function (type provided: "${typeof loader}"), so searchPlaces item "${place}" is invalid`);
}
});
}
shouldSearchStopWithResult(result) {
if (result === null)
return false;
if (result.isEmpty && this.config.ignoreEmptySearchPlaces)
return false;
return true;
}
nextDirectoryToSearch(currentDir, currentResult) {
if (this.shouldSearchStopWithResult(currentResult)) {
return null;
}
const nextDir = nextDirUp(currentDir);
if (nextDir === currentDir || currentDir === this.config.stopDir) {
return null;
}
return nextDir;
}
loadPackageProp(filepath, content) {
const parsedContent = _loaders.loaders.loadJson(filepath, content);
const packagePropValue = (0, _getPropertyByPath.getPropertyByPath)(parsedContent, this.config.packageProp);
return packagePropValue || null;
}
getLoaderEntryForFile(filepath) {
if (_path.default.basename(filepath) === "package.json") {
const loader2 = this.loadPackageProp.bind(this);
return loader2;
}
const loaderKey = _path.default.extname(filepath) || "noExt";
const loader = this.config.loaders[loaderKey];
if (!loader) {
throw new Error(`No loader specified for ${getExtensionDescription(filepath)}`);
}
return loader;
}
loadedContentToCosmiconfigResult(filepath, loadedContent) {
if (loadedContent === null) {
return null;
}
if (loadedContent === void 0) {
return {
filepath,
config: void 0,
isEmpty: true
};
}
return {
config: loadedContent,
filepath
};
}
validateFilePath(filepath) {
if (!filepath) {
throw new Error("load must pass a non-empty string");
}
}
};
exports2.ExplorerBase = ExplorerBase;
function nextDirUp(dir) {
return _path.default.dirname(dir);
}
function getExtensionDescription(filepath) {
const ext = _path.default.extname(filepath);
return ext ? `extension "${ext}"` : "files without extensions";
}
}
});
var require_readFile = __commonJS2({
"node_modules/cosmiconfig/dist/readFile.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.readFile = readFile;
exports2.readFileSync = readFileSync2;
var _fs = _interopRequireDefault(require("fs"));
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : {
default: obj
};
}
async function fsReadFileAsync(pathname, encoding) {
return new Promise((resolve, reject) => {
_fs.default.readFile(pathname, encoding, (error, contents) => {
if (error) {
reject(error);
return;
}
resolve(contents);
});
});
}
async function readFile(filepath, options = {}) {
const throwNotFound = options.throwNotFound === true;
try {
const content = await fsReadFileAsync(filepath, "utf8");
return content;
} catch (error) {
if (throwNotFound === false && (error.code === "ENOENT" || error.code === "EISDIR")) {
return null;
}
throw error;
}
}
function readFileSync2(filepath, options = {}) {
const throwNotFound = options.throwNotFound === true;
try {
const content = _fs.default.readFileSync(filepath, "utf8");
return content;
} catch (error) {
if (throwNotFound === false && (error.code === "ENOENT" || error.code === "EISDIR")) {
return null;
}
throw error;
}
}
}
});
var require_cacheWrapper = __commonJS2({
"node_modules/cosmiconfig/dist/cacheWrapper.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.cacheWrapper = cacheWrapper;
exports2.cacheWrapperSync = cacheWrapperSync;
async function cacheWrapper(cache, key, fn) {
const cached = cache.get(key);
if (cached !== void 0) {
return cached;
}
const result = await fn();
cache.set(key, result);
return result;
}
function cacheWrapperSync(cache, key, fn) {
const cached = cache.get(key);
if (cached !== void 0) {
return cached;
}
const result = fn();
cache.set(key, result);
return result;
}
}
});
var require_path_type = __commonJS2({
"node_modules/path-type/index.js"(exports2) {
"use strict";
var {
promisify
} = require("util");
var fs2 = require("fs");
async function isType(fsStatType, statsMethodName, filePath2) {
if (typeof filePath2 !== "string") {
throw new TypeError(`Expected a string, got ${typeof filePath2}`);
}
try {
const stats = await promisify(fs2[fsStatType])(filePath2);
return stats[statsMethodName]();
} catch (error) {
if (error.code === "ENOENT") {
return false;
}
throw error;
}
}
function isTypeSync(fsStatType, statsMethodName, filePath2) {
if (typeof filePath2 !== "string") {
throw new TypeError(`Expected a string, got ${typeof filePath2}`);
}
try {
return fs2[fsStatType](filePath2)[statsMethodName]();
} catch (error) {
if (error.code === "ENOENT") {
return false;
}
throw error;
}
}
exports2.isFile = isType.bind(null, "stat", "isFile");
exports2.isDirectory = isType.bind(null, "stat", "isDirectory");
exports2.isSymlink = isType.bind(null, "lstat", "isSymbolicLink");
exports2.isFileSync = isTypeSync.bind(null, "statSync", "isFile");
exports2.isDirectorySync = isTypeSync.bind(null, "statSync", "isDirectory");
exports2.isSymlinkSync = isTypeSync.bind(null, "lstatSync", "isSymbolicLink");
}
});
var require_getDirectory = __commonJS2({
"node_modules/cosmiconfig/dist/getDirectory.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.getDirectory = getDirectory;
exports2.getDirectorySync = getDirectorySync;
var _path = _interopRequireDefault(require("path"));
var _pathType = require_path_type();
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : {
default: obj
};
}
async function getDirectory(filepath) {
const filePathIsDirectory = await (0, _pathType.isDirectory)(filepath);
if (filePathIsDirectory === true) {
return filepath;
}
const directory = _path.default.dirname(filepath);
return directory;
}
function getDirectorySync(filepath) {
const filePathIsDirectory = (0, _pathType.isDirectorySync)(filepath);
if (filePathIsDirectory === true) {
return filepath;
}
const directory = _path.default.dirname(filepath);
return directory;
}
}
});
var require_Explorer = __commonJS2({
"node_modules/cosmiconfig/dist/Explorer.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.Explorer = void 0;
var _path = _interopRequireDefault(require("path"));
var _ExplorerBase = require_ExplorerBase();
var _readFile = require_readFile();
var _cacheWrapper = require_cacheWrapper();
var _getDirectory = require_getDirectory();
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : {
default: obj
};
}
var Explorer = class extends _ExplorerBase.ExplorerBase {
constructor(options) {
super(options);
}
async search(searchFrom = process.cwd()) {
const startDirectory = await (0, _getDirectory.getDirectory)(searchFrom);
const result = await this.searchFromDirectory(startDirectory);
return result;
}
async searchFromDirectory(dir) {
const absoluteDir = _path.default.resolve(process.cwd(), dir);
const run = async () => {
const result = await this.searchDirectory(absoluteDir);
const nextDir = this.nextDirectoryToSearch(absoluteDir, result);
if (nextDir) {
return this.searchFromDirectory(nextDir);
}
const transformResult = await this.config.transform(result);
return transformResult;
};
if (this.searchCache) {
return (0, _cacheWrapper.cacheWrapper)(this.searchCache, absoluteDir, run);
}
return run();
}
async searchDirectory(dir) {
for await (const place of this.config.searchPlaces) {
const placeResult = await this.loadSearchPlace(dir, place);
if (this.shouldSearchStopWithResult(placeResult) === true) {
return placeResult;
}
}
return null;
}
async loadSearchPlace(dir, place) {
const filepath = _path.default.join(dir, place);
const fileContents = await (0, _readFile.readFile)(filepath);
const result = await this.createCosmiconfigResult(filepath, fileContents);
return result;
}
async loadFileContent(filepath, content) {
if (content === null) {
return null;
}
if (content.trim() === "") {
return void 0;
}
const loader = this.getLoaderEntryForFile(filepath);
const loaderResult = await loader(filepath, content);
return loaderResult;
}
async createCosmiconfigResult(filepath, content) {
const fileContent = await this.loadFileContent(filepath, content);
const result = this.loadedContentToCosmiconfigResult(filepath, fileContent);
return result;
}
async load(filepath) {
this.validateFilePath(filepath);
const absoluteFilePath = _path.default.resolve(process.cwd(), filepath);
const runLoad = async () => {
const fileContents = await (0, _readFile.readFile)(absoluteFilePath, {
throwNotFound: true
});
const result = await this.createCosmiconfigResult(absoluteFilePath, fileContents);
const transformResult = await this.config.transform(result);
return transformResult;
};
if (this.loadCache) {
return (0, _cacheWrapper.cacheWrapper)(this.loadCache, absoluteFilePath, runLoad);
}
return runLoad();
}
};
exports2.Explorer = Explorer;
}
});
var require_ExplorerSync = __commonJS2({
"node_modules/cosmiconfig/dist/ExplorerSync.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.ExplorerSync = void 0;
var _path = _interopRequireDefault(require("path"));
var _ExplorerBase = require_ExplorerBase();
var _readFile = require_readFile();
var _cacheWrapper = require_cacheWrapper();
var _getDirectory = require_getDirectory();
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : {
default: obj
};
}
var ExplorerSync = class extends _ExplorerBase.ExplorerBase {
constructor(options) {
super(options);
}
searchSync(searchFrom = process.cwd()) {
const startDirectory = (0, _getDirectory.getDirectorySync)(searchFrom);
const result = this.searchFromDirectorySync(startDirectory);
return result;
}
searchFromDirectorySync(dir) {
const absoluteDir = _path.default.resolve(process.cwd(), dir);
const run = () => {
const result = this.searchDirectorySync(absoluteDir);
const nextDir = this.nextDirectoryToSearch(absoluteDir, result);
if (nextDir) {
return this.searchFromDirectorySync(nextDir);
}
const transformResult = this.config.transform(result);
return transformResult;
};
if (this.searchCache) {
return (0, _cacheWrapper.cacheWrapperSync)(this.searchCache, absoluteDir, run);
}
return run();
}
searchDirectorySync(dir) {
for (const place of this.config.searchPlaces) {
const placeResult = this.loadSearchPlaceSync(dir, place);
if (this.shouldSearchStopWithResult(placeResult) === true) {
return placeResult;
}
}
return null;
}
loadSearchPlaceSync(dir, place) {
const filepath = _path.default.join(dir, place);
const content = (0, _readFile.readFileSync)(filepath);
const result = this.createCosmiconfigResultSync(filepath, content);
return result;
}
loadFileContentSync(filepath, content) {
if (content === null) {
return null;
}
if (content.trim() === "") {
return void 0;
}
const loader = this.getLoaderEntryForFile(filepath);
const loaderResult = loader(filepath, content);
return loaderResult;
}
createCosmiconfigResultSync(filepath, content) {
const fileContent = this.loadFileContentSync(filepath, content);
const result = this.loadedContentToCosmiconfigResult(filepath, fileContent);
return result;
}
loadSync(filepath) {
this.validateFilePath(filepath);
const absoluteFilePath = _path.default.resolve(process.cwd(), filepath);
const runLoadSync = () => {
const content = (0, _readFile.readFileSync)(absoluteFilePath, {
throwNotFound: true
});
const cosmiconfigResult = this.createCosmiconfigResultSync(absoluteFilePath, content);
const transformResult = this.config.transform(cosmiconfigResult);
return transformResult;
};
if (this.loadCache) {
return (0, _cacheWrapper.cacheWrapperSync)(this.loadCache, absoluteFilePath, runLoadSync);
}
return runLoadSync();
}
};
exports2.ExplorerSync = ExplorerSync;
}
});
var require_types2 = __commonJS2({
"node_modules/cosmiconfig/dist/types.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
}
});
var require_dist22 = __commonJS2({
"node_modules/cosmiconfig/dist/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.cosmiconfig = cosmiconfig;
exports2.cosmiconfigSync = cosmiconfigSync;
exports2.defaultLoaders = void 0;
var _os = _interopRequireDefault(require("os"));
var _Explorer = require_Explorer();
var _ExplorerSync = require_ExplorerSync();
var _loaders = require_loaders();
var _types = require_types2();
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : {
default: obj
};
}
function cosmiconfig(moduleName, options = {}) {
const normalizedOptions = normalizeOptions(moduleName, options);
const explorer = new _Explorer.Explorer(normalizedOptions);
return {
search: explorer.search.bind(explorer),
load: explorer.load.bind(explorer),
clearLoadCache: explorer.clearLoadCache.bind(explorer),
clearSearchCache: explorer.clearSearchCache.bind(explorer),
clearCaches: explorer.clearCaches.bind(explorer)
};
}
function cosmiconfigSync(moduleName, options = {}) {
const normalizedOptions = normalizeOptions(moduleName, options);
const explorerSync = new _ExplorerSync.ExplorerSync(normalizedOptions);
return {
search: explorerSync.searchSync.bind(explorerSync),
load: explorerSync.loadSync.bind(explorerSync),
clearLoadCache: explorerSync.clearLoadCache.bind(explorerSync),
clearSearchCache: explorerSync.clearSearchCache.bind(explorerSync),
clearCaches: explorerSync.clearCaches.bind(explorerSync)
};
}
var defaultLoaders = Object.freeze({
".cjs": _loaders.loaders.loadJs,
".js": _loaders.loaders.loadJs,
".json": _loaders.loaders.loadJson,
".yaml": _loaders.loaders.loadYaml,
".yml": _loaders.loaders.loadYaml,
noExt: _loaders.loaders.loadYaml
});
exports2.defaultLoaders = defaultLoaders;
var identity = function identity2(x) {
return x;
};
function normalizeOptions(moduleName, options) {
const defaults = {
packageProp: moduleName,
searchPlaces: ["package.json", `.${moduleName}rc`, `.${moduleName}rc.json`, `.${moduleName}rc.yaml`, `.${moduleName}rc.yml`, `.${moduleName}rc.js`, `.${moduleName}rc.cjs`, `${moduleName}.config.js`, `${moduleName}.config.cjs`],
ignoreEmptySearchPlaces: true,
stopDir: _os.default.homedir(),
cache: true,
transform: identity,
loaders: defaultLoaders
};
const normalizedOptions = Object.assign(Object.assign(Object.assign({}, defaults), options), {}, {
loaders: Object.assign(Object.assign({}, defaults.loaders), options.loaders)
});
return normalizedOptions;
}
}
});
var require_find_parent_dir = __commonJS2({
"node_modules/find-parent-dir/index.js"(exports2, module22) {
"use strict";
var path = require("path");
var fs2 = require("fs");
var exists = fs2.exists || path.exists;
var existsSync = fs2.existsSync || path.existsSync;
function splitPath(path2) {
var parts = path2.split(/(\/|\\)/);
if (!parts.length)
return parts;
return !parts[0].length ? parts.slice(1) : parts;
}
exports2 = module22.exports = function(currentFullPath, clue, cb) {
function testDir(parts) {
if (parts.length === 0)
return cb(null, null);
var p = parts.join("");
exists(path.join(p, clue), function(itdoes) {
if (itdoes)
return cb(null, p);
testDir(parts.slice(0, -1));
});
}
testDir(splitPath(currentFullPath));
};
exports2.sync = function(currentFullPath, clue) {
function testDir(parts) {
if (parts.length === 0)
return null;
var p = parts.join("");
var itdoes = existsSync(path.join(p, clue));
return itdoes ? p : testDir(parts.slice(0, -1));
}
return testDir(splitPath(currentFullPath));
};
}
});
var require_get_stdin = __commonJS2({
"node_modules/get-stdin/index.js"(exports2, module22) {
"use strict";
var {
stdin
} = process;
module22.exports = async () => {
let result = "";
if (stdin.isTTY) {
return result;
}
stdin.setEncoding("utf8");
for await (const chunk of stdin) {
result += chunk;
}
return result;
};
module22.exports.buffer = async () => {
const result = [];
let length = 0;
if (stdin.isTTY) {
return Buffer.concat([]);
}
for await (const chunk of stdin) {
result.push(chunk);
length += chunk.length;
}
return Buffer.concat(result, length);
};
}
});
var require_vendors = __commonJS2({
"node_modules/ci-info/vendors.json"(exports2, module22) {
module22.exports = [{
name: "AppVeyor",
constant: "APPVEYOR",
env: "APPVEYOR",
pr: "APPVEYOR_PULL_REQUEST_NUMBER"
}, {
name: "Azure Pipelines",
constant: "AZURE_PIPELINES",
env: "SYSTEM_TEAMFOUNDATIONCOLLECTIONURI",
pr: "SYSTEM_PULLREQUEST_PULLREQUESTID"
}, {
name: "Appcircle",
constant: "APPCIRCLE",
env: "AC_APPCIRCLE"
}, {
name: "Bamboo",
constant: "BAMBOO",
env: "bamboo_planKey"
}, {
name: "Bitbucket Pipelines",
constant: "BITBUCKET",
env: "BITBUCKET_COMMIT",
pr: "BITBUCKET_PR_ID"
}, {
name: "Bitrise",
constant: "BITRISE",
env: "BITRISE_IO",
pr: "BITRISE_PULL_REQUEST"
}, {
name: "Buddy",
constant: "BUDDY",
env: "BUDDY_WORKSPACE_ID",
pr: "BUDDY_EXECUTION_PULL_REQUEST_ID"
}, {
name: "Buildkite",
constant: "BUILDKITE",
env: "BUILDKITE",
pr: {
env: "BUILDKITE_PULL_REQUEST",
ne: "false"
}
}, {
name: "CircleCI",
constant: "CIRCLE",
env: "CIRCLECI",
pr: "CIRCLE_PULL_REQUEST"
}, {
name: "Cirrus CI",
constant: "CIRRUS",
env: "CIRRUS_CI",
pr: "CIRRUS_PR"
}, {
name: "AWS CodeBuild",
constant: "CODEBUILD",
env: "CODEBUILD_BUILD_ARN"
}, {
name: "Codefresh",
constant: "CODEFRESH",
env: "CF_BUILD_ID",
pr: {
any: ["CF_PULL_REQUEST_NUMBER", "CF_PULL_REQUEST_ID"]
}
}, {
name: "Codeship",
constant: "CODESHIP",
env: {
CI_NAME: "codeship"
}
}, {
name: "Drone",
constant: "DRONE",
env: "DRONE",
pr: {
DRONE_BUILD_EVENT: "pull_request"
}
}, {
name: "dsari",
constant: "DSARI",
env: "DSARI"
}, {
name: "Expo Application Services",
constant: "EAS",
env: "EAS_BUILD"
}, {
name: "GitHub Actions",
constant: "GITHUB_ACTIONS",
env: "GITHUB_ACTIONS",
pr: {
GITHUB_EVENT_NAME: "pull_request"
}
}, {
name: "GitLab CI",
constant: "GITLAB",
env: "GITLAB_CI",
pr: "CI_MERGE_REQUEST_ID"
}, {
name: "GoCD",
constant: "GOCD",
env: "GO_PIPELINE_LABEL"
}, {
name: "LayerCI",
constant: "LAYERCI",
env: "LAYERCI",
pr: "LAYERCI_PULL_REQUEST"
}, {
name: "Hudson",
constant: "HUDSON",
env: "HUDSON_URL"
}, {
name: "Jenkins",
constant: "JENKINS",
env: ["JENKINS_URL", "BUILD_ID"],
pr: {
any: ["ghprbPullId", "CHANGE_ID"]
}
}, {
name: "Magnum CI",
constant: "MAGNUM",
env: "MAGNUM"
}, {
name: "Netlify CI",
constant: "NETLIFY",
env: "NETLIFY",
pr: {
env: "PULL_REQUEST",
ne: "false"
}
}, {
name: "Nevercode",
constant: "NEVERCODE",
env: "NEVERCODE",
pr: {
env: "NEVERCODE_PULL_REQUEST",
ne: "false"
}
}, {
name: "Render",
constant: "RENDER",
env: "RENDER",
pr: {
IS_PULL_REQUEST: "true"
}
}, {
name: "Sail CI",
constant: "SAIL",
env: "SAILCI",
pr: "SAIL_PULL_REQUEST_NUMBER"
}, {
name: "Semaphore",
constant: "SEMAPHORE",
env: "SEMAPHORE",
pr: "PULL_REQUEST_NUMBER"
}, {
name: "Screwdriver",
constant: "SCREWDRIVER",
env: "SCREWDRIVER",
pr: {
env: "SD_PULL_REQUEST",
ne: "false"
}
}, {
name: "Shippable",
constant: "SHIPPABLE",
env: "SHIPPABLE",
pr: {
IS_PULL_REQUEST: "true"
}
}, {
name: "Solano CI",
constant: "SOLANO",
env: "TDDIUM",
pr: "TDDIUM_PR_ID"
}, {
name: "Strider CD",
constant: "STRIDER",
env: "STRIDER"
}, {
name: "TaskCluster",
constant: "TASKCLUSTER",
env: ["TASK_ID", "RUN_ID"]
}, {
name: "TeamCity",
constant: "TEAMCITY",
env: "TEAMCITY_VERSION"
}, {
name: "Travis CI",
constant: "TRAVIS",
env: "TRAVIS",
pr: {
env: "TRAVIS_PULL_REQUEST",
ne: "false"
}
}, {
name: "Vercel",
constant: "VERCEL",
env: "NOW_BUILDER"
}, {
name: "Visual Studio App Center",
constant: "APPCENTER",
env: "APPCENTER_BUILD_ID"
}];
}
});
var require_ci_info = __commonJS2({
"node_modules/ci-info/index.js"(exports2) {
"use strict";
var vendors = require_vendors();
var env = process.env;
Object.defineProperty(exports2, "_vendors", {
value: vendors.map(function(v) {
return v.constant;
})
});
exports2.name = null;
exports2.isPR = null;
vendors.forEach(function(vendor) {
const envs = Array.isArray(vendor.env) ? vendor.env : [vendor.env];
const isCI = envs.every(function(obj) {
return checkEnv(obj);
});
exports2[vendor.constant] = isCI;
if (isCI) {
exports2.name = vendor.name;
switch (typeof vendor.pr) {
case "string":
exports2.isPR = !!env[vendor.pr];
break;
case "object":
if ("env" in vendor.pr) {
exports2.isPR = vendor.pr.env in env && env[vendor.pr.env] !== vendor.pr.ne;
} else if ("any" in vendor.pr) {
exports2.isPR = vendor.pr.any.some(function(key) {
return !!env[key];
});
} else {
exports2.isPR = checkEnv(vendor.pr);
}
break;
default:
exports2.isPR = null;
}
}
});
exports2.isCI = !!(env.CI || env.CONTINUOUS_INTEGRATION || env.BUILD_NUMBER || env.RUN_ID || exports2.name || false);
function checkEnv(obj) {
if (typeof obj === "string")
return !!env[obj];
return Object.keys(obj).every(function(k) {
return env[k] === obj[k];
});
}
}
});
module2.exports = {
cosmiconfig: require_dist22().cosmiconfig,
cosmiconfigSync: require_dist22().cosmiconfigSync,
findParentDir: require_find_parent_dir().sync,
getStdin: require_get_stdin(),
isCI: () => require_ci_info().isCI
};
}
});
// node_modules/prettier/parser-babel.js
var require_parser_babel = __commonJS({
"node_modules/prettier/parser-babel.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.babel = e();
}
})(function() {
"use strict";
var E = (l, h) => () => (h || l((h = { exports: {} }).exports, h), h.exports);
var re = E((xd, Zr) => {
var Ct = function(l) {
return l && l.Math == Math && l;
};
Zr.exports = Ct(typeof globalThis == "object" && globalThis) || Ct(typeof window == "object" && window) || Ct(typeof self == "object" && self) || Ct(typeof global == "object" && global) || function() {
return this;
}() || Function("return this")();
});
var ie = E((gd, ei) => {
ei.exports = function(l) {
try {
return !!l();
} catch {
return true;
}
};
});
var ye = E((Pd, ti) => {
var kh = ie();
ti.exports = !kh(function() {
return Object.defineProperty({}, 1, { get: function() {
return 7;
} })[1] != 7;
});
});
var bt = E((Ad, si) => {
var Dh = ie();
si.exports = !Dh(function() {
var l = function() {
}.bind();
return typeof l != "function" || l.hasOwnProperty("prototype");
});
});
var wt = E((Td, ri) => {
var Fh = bt(), St = Function.prototype.call;
ri.exports = Fh ? St.bind(St) : function() {
return St.apply(St, arguments);
};
});
var oi = E((ni) => {
"use strict";
var ii = {}.propertyIsEnumerable, ai = Object.getOwnPropertyDescriptor, Lh = ai && !ii.call({ 1: 2 }, 1);
ni.f = Lh ? function(h) {
var p = ai(this, h);
return !!p && p.enumerable;
} : ii;
});
var fs2 = E((Ed, li) => {
li.exports = function(l, h) {
return { enumerable: !(l & 1), configurable: !(l & 2), writable: !(l & 4), value: h };
};
});
var ae = E((Cd, ci) => {
var hi = bt(), ui = Function.prototype, ds = ui.call, Oh = hi && ui.bind.bind(ds, ds);
ci.exports = hi ? Oh : function(l) {
return function() {
return ds.apply(l, arguments);
};
};
});
var Ye = E((bd, fi) => {
var pi = ae(), Bh = pi({}.toString), Mh = pi("".slice);
fi.exports = function(l) {
return Mh(Bh(l), 8, -1);
};
});
var mi = E((Sd, di) => {
var _h = ae(), Rh = ie(), jh = Ye(), ms = Object, qh = _h("".split);
di.exports = Rh(function() {
return !ms("z").propertyIsEnumerable(0);
}) ? function(l) {
return jh(l) == "String" ? qh(l, "") : ms(l);
} : ms;
});
var ys = E((wd, yi) => {
yi.exports = function(l) {
return l == null;
};
});
var xs = E((Id, xi) => {
var Uh = ys(), $h = TypeError;
xi.exports = function(l) {
if (Uh(l))
throw $h("Can't call method on " + l);
return l;
};
});
var It = E((Nd, gi) => {
var Hh = mi(), zh = xs();
gi.exports = function(l) {
return Hh(zh(l));
};
});
var Ps = E((kd, Pi) => {
var gs = typeof document == "object" && document.all, Vh = typeof gs > "u" && gs !== void 0;
Pi.exports = { all: gs, IS_HTMLDDA: Vh };
});
var ee = E((Dd, Ti) => {
var Ai = Ps(), Kh = Ai.all;
Ti.exports = Ai.IS_HTMLDDA ? function(l) {
return typeof l == "function" || l === Kh;
} : function(l) {
return typeof l == "function";
};
});
var Ie = E((Fd, Ci) => {
var vi = ee(), Ei = Ps(), Wh = Ei.all;
Ci.exports = Ei.IS_HTMLDDA ? function(l) {
return typeof l == "object" ? l !== null : vi(l) || l === Wh;
} : function(l) {
return typeof l == "object" ? l !== null : vi(l);
};
});
var Qe = E((Ld, bi) => {
var As = re(), Gh = ee(), Jh = function(l) {
return Gh(l) ? l : void 0;
};
bi.exports = function(l, h) {
return arguments.length < 2 ? Jh(As[l]) : As[l] && As[l][h];
};
});
var wi = E((Od, Si) => {
var Xh = ae();
Si.exports = Xh({}.isPrototypeOf);
});
var Ni = E((Bd, Ii) => {
var Yh = Qe();
Ii.exports = Yh("navigator", "userAgent") || "";
});
var Mi = E((Md, Bi) => {
var Oi = re(), Ts = Ni(), ki = Oi.process, Di = Oi.Deno, Fi = ki && ki.versions || Di && Di.version, Li = Fi && Fi.v8, ne, Nt;
Li && (ne = Li.split("."), Nt = ne[0] > 0 && ne[0] < 4 ? 1 : +(ne[0] + ne[1]));
!Nt && Ts && (ne = Ts.match(/Edge\/(\d+)/), (!ne || ne[1] >= 74) && (ne = Ts.match(/Chrome\/(\d+)/), ne && (Nt = +ne[1])));
Bi.exports = Nt;
});
var vs = E((_d, Ri) => {
var _i = Mi(), Qh = ie();
Ri.exports = !!Object.getOwnPropertySymbols && !Qh(function() {
var l = Symbol();
return !String(l) || !(Object(l) instanceof Symbol) || !Symbol.sham && _i && _i < 41;
});
});
var Es = E((Rd, ji) => {
var Zh = vs();
ji.exports = Zh && !Symbol.sham && typeof Symbol.iterator == "symbol";
});
var Cs = E((jd, qi) => {
var eu = Qe(), tu = ee(), su = wi(), ru = Es(), iu = Object;
qi.exports = ru ? function(l) {
return typeof l == "symbol";
} : function(l) {
var h = eu("Symbol");
return tu(h) && su(h.prototype, iu(l));
};
});
var $i = E((qd, Ui) => {
var au = String;
Ui.exports = function(l) {
try {
return au(l);
} catch {
return "Object";
}
};
});
var kt = E((Ud, Hi) => {
var nu = ee(), ou = $i(), lu = TypeError;
Hi.exports = function(l) {
if (nu(l))
return l;
throw lu(ou(l) + " is not a function");
};
});
var Vi = E(($d, zi) => {
var hu = kt(), uu = ys();
zi.exports = function(l, h) {
var p = l[h];
return uu(p) ? void 0 : hu(p);
};
});
var Wi = E((Hd, Ki) => {
var bs = wt(), Ss = ee(), ws = Ie(), cu = TypeError;
Ki.exports = function(l, h) {
var p, d;
if (h === "string" && Ss(p = l.toString) && !ws(d = bs(p, l)) || Ss(p = l.valueOf) && !ws(d = bs(p, l)) || h !== "string" && Ss(p = l.toString) && !ws(d = bs(p, l)))
return d;
throw cu("Can't convert object to primitive value");
};
});
var Ji = E((zd, Gi) => {
Gi.exports = false;
});
var Dt = E((Vd, Yi) => {
var Xi = re(), pu = Object.defineProperty;
Yi.exports = function(l, h) {
try {
pu(Xi, l, { value: h, configurable: true, writable: true });
} catch {
Xi[l] = h;
}
return h;
};
});
var Ft = E((Kd, Zi) => {
var fu = re(), du = Dt(), Qi = "__core-js_shared__", mu = fu[Qi] || du(Qi, {});
Zi.exports = mu;
});
var Is = E((Wd, ta) => {
var yu = Ji(), ea = Ft();
(ta.exports = function(l, h) {
return ea[l] || (ea[l] = h !== void 0 ? h : {});
})("versions", []).push({ version: "3.26.1", mode: yu ? "pure" : "global", copyright: "\xA9 2014-2022 Denis Pushkarev (zloirock.ru)", license: "https://github.com/zloirock/core-js/blob/v3.26.1/LICENSE", source: "https://github.com/zloirock/core-js" });
});
var Ns = E((Gd, sa) => {
var xu = xs(), gu = Object;
sa.exports = function(l) {
return gu(xu(l));
};
});
var ve = E((Jd, ra) => {
var Pu = ae(), Au = Ns(), Tu = Pu({}.hasOwnProperty);
ra.exports = Object.hasOwn || function(h, p) {
return Tu(Au(h), p);
};
});
var ks = E((Xd, ia) => {
var vu = ae(), Eu = 0, Cu = Math.random(), bu = vu(1 .toString);
ia.exports = function(l) {
return "Symbol(" + (l === void 0 ? "" : l) + ")_" + bu(++Eu + Cu, 36);
};
});
var Ze = E((Yd, ha) => {
var Su = re(), wu = Is(), aa = ve(), Iu = ks(), na = vs(), la = Es(), qe = wu("wks"), Ne = Su.Symbol, oa = Ne && Ne.for, Nu = la ? Ne : Ne && Ne.withoutSetter || Iu;
ha.exports = function(l) {
if (!aa(qe, l) || !(na || typeof qe[l] == "string")) {
var h = "Symbol." + l;
na && aa(Ne, l) ? qe[l] = Ne[l] : la && oa ? qe[l] = oa(h) : qe[l] = Nu(h);
}
return qe[l];
};
});
var fa = E((Qd, pa) => {
var ku = wt(), ua = Ie(), ca = Cs(), Du = Vi(), Fu = Wi(), Lu = Ze(), Ou = TypeError, Bu = Lu("toPrimitive");
pa.exports = function(l, h) {
if (!ua(l) || ca(l))
return l;
var p = Du(l, Bu), d;
if (p) {
if (h === void 0 && (h = "default"), d = ku(p, l, h), !ua(d) || ca(d))
return d;
throw Ou("Can't convert object to primitive value");
}
return h === void 0 && (h = "number"), Fu(l, h);
};
});
var Ds = E((Zd, da) => {
var Mu = fa(), _u = Cs();
da.exports = function(l) {
var h = Mu(l, "string");
return _u(h) ? h : h + "";
};
});
var xa = E((em, ya) => {
var Ru = re(), ma = Ie(), Fs = Ru.document, ju = ma(Fs) && ma(Fs.createElement);
ya.exports = function(l) {
return ju ? Fs.createElement(l) : {};
};
});
var Ls = E((tm, ga) => {
var qu = ye(), Uu = ie(), $u = xa();
ga.exports = !qu && !Uu(function() {
return Object.defineProperty($u("div"), "a", { get: function() {
return 7;
} }).a != 7;
});
});
var Os = E((Aa) => {
var Hu = ye(), zu = wt(), Vu = oi(), Ku = fs2(), Wu = It(), Gu = Ds(), Ju = ve(), Xu = Ls(), Pa = Object.getOwnPropertyDescriptor;
Aa.f = Hu ? Pa : function(h, p) {
if (h = Wu(h), p = Gu(p), Xu)
try {
return Pa(h, p);
} catch {
}
if (Ju(h, p))
return Ku(!zu(Vu.f, h, p), h[p]);
};
});
var va = E((rm, Ta) => {
var Yu = ye(), Qu = ie();
Ta.exports = Yu && Qu(function() {
return Object.defineProperty(function() {
}, "prototype", { value: 42, writable: false }).prototype != 42;
});
});
var Lt = E((im, Ea) => {
var Zu = Ie(), ec = String, tc = TypeError;
Ea.exports = function(l) {
if (Zu(l))
return l;
throw tc(ec(l) + " is not an object");
};
});
var et = E((ba) => {
var sc = ye(), rc = Ls(), ic = va(), Ot = Lt(), Ca = Ds(), ac = TypeError, Bs = Object.defineProperty, nc = Object.getOwnPropertyDescriptor, Ms = "enumerable", _s = "configurable", Rs = "writable";
ba.f = sc ? ic ? function(h, p, d) {
if (Ot(h), p = Ca(p), Ot(d), typeof h == "function" && p === "prototype" && "value" in d && Rs in d && !d[Rs]) {
var x = nc(h, p);
x && x[Rs] && (h[p] = d.value, d = { configurable: _s in d ? d[_s] : x[_s], enumerable: Ms in d ? d[Ms] : x[Ms], writable: false });
}
return Bs(h, p, d);
} : Bs : function(h, p, d) {
if (Ot(h), p = Ca(p), Ot(d), rc)
try {
return Bs(h, p, d);
} catch {
}
if ("get" in d || "set" in d)
throw ac("Accessors not supported");
return "value" in d && (h[p] = d.value), h;
};
});
var js = E((nm, Sa) => {
var oc = ye(), lc = et(), hc = fs2();
Sa.exports = oc ? function(l, h, p) {
return lc.f(l, h, hc(1, p));
} : function(l, h, p) {
return l[h] = p, l;
};
});
var Na = E((om, Ia) => {
var qs = ye(), uc = ve(), wa = Function.prototype, cc = qs && Object.getOwnPropertyDescriptor, Us = uc(wa, "name"), pc = Us && function() {
}.name === "something", fc = Us && (!qs || qs && cc(wa, "name").configurable);
Ia.exports = { EXISTS: Us, PROPER: pc, CONFIGURABLE: fc };
});
var Hs = E((lm, ka) => {
var dc = ae(), mc = ee(), $s = Ft(), yc = dc(Function.toString);
mc($s.inspectSource) || ($s.inspectSource = function(l) {
return yc(l);
});
ka.exports = $s.inspectSource;
});
var La = E((hm, Fa) => {
var xc = re(), gc = ee(), Da = xc.WeakMap;
Fa.exports = gc(Da) && /native code/.test(String(Da));
});
var Ma = E((um, Ba) => {
var Pc = Is(), Ac = ks(), Oa = Pc("keys");
Ba.exports = function(l) {
return Oa[l] || (Oa[l] = Ac(l));
};
});
var zs = E((cm, _a) => {
_a.exports = {};
});
var Ua = E((pm, qa) => {
var Tc = La(), ja = re(), vc = Ie(), Ec = js(), Vs = ve(), Ks = Ft(), Cc = Ma(), bc = zs(), Ra = "Object already initialized", Ws = ja.TypeError, Sc = ja.WeakMap, Bt, tt, Mt, wc = function(l) {
return Mt(l) ? tt(l) : Bt(l, {});
}, Ic = function(l) {
return function(h) {
var p;
if (!vc(h) || (p = tt(h)).type !== l)
throw Ws("Incompatible receiver, " + l + " required");
return p;
};
};
Tc || Ks.state ? (oe = Ks.state || (Ks.state = new Sc()), oe.get = oe.get, oe.has = oe.has, oe.set = oe.set, Bt = function(l, h) {
if (oe.has(l))
throw Ws(Ra);
return h.facade = l, oe.set(l, h), h;
}, tt = function(l) {
return oe.get(l) || {};
}, Mt = function(l) {
return oe.has(l);
}) : (ke = Cc("state"), bc[ke] = true, Bt = function(l, h) {
if (Vs(l, ke))
throw Ws(Ra);
return h.facade = l, Ec(l, ke, h), h;
}, tt = function(l) {
return Vs(l, ke) ? l[ke] : {};
}, Mt = function(l) {
return Vs(l, ke);
});
var oe, ke;
qa.exports = { set: Bt, get: tt, has: Mt, enforce: wc, getterFor: Ic };
});
var Js = E((fm, Ha) => {
var Nc = ie(), kc = ee(), _t = ve(), Gs = ye(), Dc = Na().CONFIGURABLE, Fc = Hs(), $a = Ua(), Lc = $a.enforce, Oc = $a.get, Rt = Object.defineProperty, Bc = Gs && !Nc(function() {
return Rt(function() {
}, "length", { value: 8 }).length !== 8;
}), Mc = String(String).split("String"), _c = Ha.exports = function(l, h, p) {
String(h).slice(0, 7) === "Symbol(" && (h = "[" + String(h).replace(/^Symbol\(([^)]*)\)/, "$1") + "]"), p && p.getter && (h = "get " + h), p && p.setter && (h = "set " + h), (!_t(l, "name") || Dc && l.name !== h) && (Gs ? Rt(l, "name", { value: h, configurable: true }) : l.name = h), Bc && p && _t(p, "arity") && l.length !== p.arity && Rt(l, "length", { value: p.arity });
try {
p && _t(p, "constructor") && p.constructor ? Gs && Rt(l, "prototype", { writable: false }) : l.prototype && (l.prototype = void 0);
} catch {
}
var d = Lc(l);
return _t(d, "source") || (d.source = Mc.join(typeof h == "string" ? h : "")), l;
};
Function.prototype.toString = _c(function() {
return kc(this) && Oc(this).source || Fc(this);
}, "toString");
});
var Va = E((dm, za) => {
var Rc = ee(), jc = et(), qc = Js(), Uc = Dt();
za.exports = function(l, h, p, d) {
d || (d = {});
var x = d.enumerable, P = d.name !== void 0 ? d.name : h;
if (Rc(p) && qc(p, P, d), d.global)
x ? l[h] = p : Uc(h, p);
else {
try {
d.unsafe ? l[h] && (x = true) : delete l[h];
} catch {
}
x ? l[h] = p : jc.f(l, h, { value: p, enumerable: false, configurable: !d.nonConfigurable, writable: !d.nonWritable });
}
return l;
};
});
var Wa = E((mm, Ka) => {
var $c = Math.ceil, Hc = Math.floor;
Ka.exports = Math.trunc || function(h) {
var p = +h;
return (p > 0 ? Hc : $c)(p);
};
});
var Xs = E((ym, Ga) => {
var zc = Wa();
Ga.exports = function(l) {
var h = +l;
return h !== h || h === 0 ? 0 : zc(h);
};
});
var Xa = E((xm, Ja) => {
var Vc = Xs(), Kc = Math.max, Wc = Math.min;
Ja.exports = function(l, h) {
var p = Vc(l);
return p < 0 ? Kc(p + h, 0) : Wc(p, h);
};
});
var Qa = E((gm, Ya) => {
var Gc = Xs(), Jc = Math.min;
Ya.exports = function(l) {
return l > 0 ? Jc(Gc(l), 9007199254740991) : 0;
};
});
var jt = E((Pm, Za) => {
var Xc = Qa();
Za.exports = function(l) {
return Xc(l.length);
};
});
var sn = E((Am, tn) => {
var Yc = It(), Qc = Xa(), Zc = jt(), en = function(l) {
return function(h, p, d) {
var x = Yc(h), P = Zc(x), m = Qc(d, P), v;
if (l && p != p) {
for (; P > m; )
if (v = x[m++], v != v)
return true;
} else
for (; P > m; m++)
if ((l || m in x) && x[m] === p)
return l || m || 0;
return !l && -1;
};
};
tn.exports = { includes: en(true), indexOf: en(false) };
});
var nn = E((Tm, an) => {
var ep = ae(), Ys = ve(), tp = It(), sp = sn().indexOf, rp = zs(), rn = ep([].push);
an.exports = function(l, h) {
var p = tp(l), d = 0, x = [], P;
for (P in p)
!Ys(rp, P) && Ys(p, P) && rn(x, P);
for (; h.length > d; )
Ys(p, P = h[d++]) && (~sp(x, P) || rn(x, P));
return x;
};
});
var ln = E((vm, on) => {
on.exports = ["constructor", "hasOwnProperty", "isPrototypeOf", "propertyIsEnumerable", "toLocaleString", "toString", "valueOf"];
});
var un = E((hn) => {
var ip = nn(), ap = ln(), np = ap.concat("length", "prototype");
hn.f = Object.getOwnPropertyNames || function(h) {
return ip(h, np);
};
});
var pn = E((cn) => {
cn.f = Object.getOwnPropertySymbols;
});
var dn = E((bm, fn) => {
var op = Qe(), lp = ae(), hp = un(), up = pn(), cp = Lt(), pp = lp([].concat);
fn.exports = op("Reflect", "ownKeys") || function(h) {
var p = hp.f(cp(h)), d = up.f;
return d ? pp(p, d(h)) : p;
};
});
var xn = E((Sm, yn) => {
var mn = ve(), fp = dn(), dp = Os(), mp = et();
yn.exports = function(l, h, p) {
for (var d = fp(h), x = mp.f, P = dp.f, m = 0; m < d.length; m++) {
var v = d[m];
!mn(l, v) && !(p && mn(p, v)) && x(l, v, P(h, v));
}
};
});
var Pn = E((wm, gn) => {
var yp = ie(), xp = ee(), gp = /#|\.prototype\./, st = function(l, h) {
var p = Ap[Pp(l)];
return p == vp ? true : p == Tp ? false : xp(h) ? yp(h) : !!h;
}, Pp = st.normalize = function(l) {
return String(l).replace(gp, ".").toLowerCase();
}, Ap = st.data = {}, Tp = st.NATIVE = "N", vp = st.POLYFILL = "P";
gn.exports = st;
});
var Zs = E((Im, An) => {
var Qs = re(), Ep = Os().f, Cp = js(), bp = Va(), Sp = Dt(), wp = xn(), Ip = Pn();
An.exports = function(l, h) {
var p = l.target, d = l.global, x = l.stat, P, m, v, S, k, F;
if (d ? m = Qs : x ? m = Qs[p] || Sp(p, {}) : m = (Qs[p] || {}).prototype, m)
for (v in h) {
if (k = h[v], l.dontCallGetSet ? (F = Ep(m, v), S = F && F.value) : S = m[v], P = Ip(d ? v : p + (x ? "." : "#") + v, l.forced), !P && S !== void 0) {
if (typeof k == typeof S)
continue;
wp(k, S);
}
(l.sham || S && S.sham) && Cp(k, "sham", true), bp(m, v, k, l);
}
};
});
var Tn = E(() => {
var Np = Zs(), er = re();
Np({ global: true, forced: er.globalThis !== er }, { globalThis: er });
});
var vn = E(() => {
Tn();
});
var bn = E((Lm, Cn) => {
var En = Js(), kp = et();
Cn.exports = function(l, h, p) {
return p.get && En(p.get, h, { getter: true }), p.set && En(p.set, h, { setter: true }), kp.f(l, h, p);
};
});
var wn = E((Om, Sn) => {
"use strict";
var Dp = Lt();
Sn.exports = function() {
var l = Dp(this), h = "";
return l.hasIndices && (h += "d"), l.global && (h += "g"), l.ignoreCase && (h += "i"), l.multiline && (h += "m"), l.dotAll && (h += "s"), l.unicode && (h += "u"), l.unicodeSets && (h += "v"), l.sticky && (h += "y"), h;
};
});
var kn = E(() => {
var Fp = re(), Lp = ye(), Op = bn(), Bp = wn(), Mp = ie(), In = Fp.RegExp, Nn = In.prototype, _p = Lp && Mp(function() {
var l = true;
try {
In(".", "d");
} catch {
l = false;
}
var h = {}, p = "", d = l ? "dgimsy" : "gimsy", x = function(S, k) {
Object.defineProperty(h, S, { get: function() {
return p += k, true;
} });
}, P = { dotAll: "s", global: "g", ignoreCase: "i", multiline: "m", sticky: "y" };
l && (P.hasIndices = "d");
for (var m in P)
x(m, P[m]);
var v = Object.getOwnPropertyDescriptor(Nn, "flags").get.call(h);
return v !== d || p !== d;
});
_p && Op(Nn, "flags", { configurable: true, get: Bp });
});
var tr = E((_m, Dn) => {
var Rp = Ye();
Dn.exports = Array.isArray || function(h) {
return Rp(h) == "Array";
};
});
var Ln = E((Rm, Fn) => {
var jp = TypeError, qp = 9007199254740991;
Fn.exports = function(l) {
if (l > qp)
throw jp("Maximum allowed index exceeded");
return l;
};
});
var Bn = E((jm, On) => {
var Up = Ye(), $p = ae();
On.exports = function(l) {
if (Up(l) === "Function")
return $p(l);
};
});
var Rn = E((qm, _n) => {
var Mn = Bn(), Hp = kt(), zp = bt(), Vp = Mn(Mn.bind);
_n.exports = function(l, h) {
return Hp(l), h === void 0 ? l : zp ? Vp(l, h) : function() {
return l.apply(h, arguments);
};
};
});
var Un = E((Um, qn) => {
"use strict";
var Kp = tr(), Wp = jt(), Gp = Ln(), Jp = Rn(), jn = function(l, h, p, d, x, P, m, v) {
for (var S = x, k = 0, F = m ? Jp(m, v) : false, w, L; k < d; )
k in p && (w = F ? F(p[k], k, h) : p[k], P > 0 && Kp(w) ? (L = Wp(w), S = jn(l, h, w, L, S, P - 1) - 1) : (Gp(S + 1), l[S] = w), S++), k++;
return S;
};
qn.exports = jn;
});
var zn = E(($m, Hn) => {
var Xp = Ze(), Yp = Xp("toStringTag"), $n = {};
$n[Yp] = "z";
Hn.exports = String($n) === "[object z]";
});
var Kn = E((Hm, Vn) => {
var Qp = zn(), Zp = ee(), qt = Ye(), ef = Ze(), tf = ef("toStringTag"), sf = Object, rf = qt(function() {
return arguments;
}()) == "Arguments", af = function(l, h) {
try {
return l[h];
} catch {
}
};
Vn.exports = Qp ? qt : function(l) {
var h, p, d;
return l === void 0 ? "Undefined" : l === null ? "Null" : typeof (p = af(h = sf(l), tf)) == "string" ? p : rf ? qt(h) : (d = qt(h)) == "Object" && Zp(h.callee) ? "Arguments" : d;
};
});
var Qn = E((zm, Yn) => {
var nf = ae(), of = ie(), Wn = ee(), lf = Kn(), hf = Qe(), uf = Hs(), Gn = function() {
}, cf = [], Jn = hf("Reflect", "construct"), sr = /^\s*(?:class|function)\b/, pf = nf(sr.exec), ff = !sr.exec(Gn), rt = function(h) {
if (!Wn(h))
return false;
try {
return Jn(Gn, cf, h), true;
} catch {
return false;
}
}, Xn = function(h) {
if (!Wn(h))
return false;
switch (lf(h)) {
case "AsyncFunction":
case "GeneratorFunction":
case "AsyncGeneratorFunction":
return false;
}
try {
return ff || !!pf(sr, uf(h));
} catch {
return true;
}
};
Xn.sham = true;
Yn.exports = !Jn || of(function() {
var l;
return rt(rt.call) || !rt(Object) || !rt(function() {
l = true;
}) || l;
}) ? Xn : rt;
});
var so = E((Vm, to) => {
var Zn = tr(), df = Qn(), mf = Ie(), yf = Ze(), xf = yf("species"), eo = Array;
to.exports = function(l) {
var h;
return Zn(l) && (h = l.constructor, df(h) && (h === eo || Zn(h.prototype)) ? h = void 0 : mf(h) && (h = h[xf], h === null && (h = void 0))), h === void 0 ? eo : h;
};
});
var io = E((Km, ro) => {
var gf = so();
ro.exports = function(l, h) {
return new (gf(l))(h === 0 ? 0 : h);
};
});
var ao = E(() => {
"use strict";
var Pf = Zs(), Af = Un(), Tf = kt(), vf = Ns(), Ef = jt(), Cf = io();
Pf({ target: "Array", proto: true }, { flatMap: function(h) {
var p = vf(this), d = Ef(p), x;
return Tf(h), x = Cf(p, 0), x.length = Af(x, p, p, d, 0, 1, h, arguments.length > 1 ? arguments[1] : void 0), x;
} });
});
var md = E((ty, Oo) => {
vn();
kn();
ao();
var nr = Object.defineProperty, bf = Object.getOwnPropertyDescriptor, or = Object.getOwnPropertyNames, Sf = Object.prototype.hasOwnProperty, co = (l, h) => function() {
return l && (h = (0, l[or(l)[0]])(l = 0)), h;
}, $ = (l, h) => function() {
return h || (0, l[or(l)[0]])((h = { exports: {} }).exports, h), h.exports;
}, wf = (l, h) => {
for (var p in h)
nr(l, p, { get: h[p], enumerable: true });
}, If = (l, h, p, d) => {
if (h && typeof h == "object" || typeof h == "function")
for (let x of or(h))
!Sf.call(l, x) && x !== p && nr(l, x, { get: () => h[x], enumerable: !(d = bf(h, x)) || d.enumerable });
return l;
}, Nf = (l) => If(nr({}, "__esModule", { value: true }), l), U = co({ ""() {
} }), kf = $({ "src/utils/try-combinations.js"(l, h) {
"use strict";
U();
function p() {
let d;
for (var x = arguments.length, P = new Array(x), m = 0; m < x; m++)
P[m] = arguments[m];
for (let [v, S] of P.entries())
try {
return { result: S() };
} catch (k) {
v === 0 && (d = k);
}
return { error: d };
}
h.exports = p;
} }), po = $({ "src/language-js/utils/get-shebang.js"(l, h) {
"use strict";
U();
function p(d) {
if (!d.startsWith("#!"))
return "";
let x = d.indexOf(`
`);
return x === -1 ? d : d.slice(0, x);
}
h.exports = p;
} }), Df = $({ "src/utils/text/skip-inline-comment.js"(l, h) {
"use strict";
U();
function p(d, x) {
if (x === false)
return false;
if (d.charAt(x) === "/" && d.charAt(x + 1) === "*") {
for (let P = x + 2; P < d.length; ++P)
if (d.charAt(P) === "*" && d.charAt(P + 1) === "/")
return P + 2;
}
return x;
}
h.exports = p;
} }), Ff = $({ "src/utils/text/skip-newline.js"(l, h) {
"use strict";
U();
function p(d, x, P) {
let m = P && P.backwards;
if (x === false)
return false;
let v = d.charAt(x);
if (m) {
if (d.charAt(x - 1) === "\r" && v === `
`)
return x - 2;
if (v === `
` || v === "\r" || v === "\u2028" || v === "\u2029")
return x - 1;
} else {
if (v === "\r" && d.charAt(x + 1) === `
`)
return x + 2;
if (v === `
` || v === "\r" || v === "\u2028" || v === "\u2029")
return x + 1;
}
return x;
}
h.exports = p;
} }), fo = $({ "src/utils/text/skip.js"(l, h) {
"use strict";
U();
function p(v) {
return (S, k, F) => {
let w = F && F.backwards;
if (k === false)
return false;
let { length: L } = S, A = k;
for (; A >= 0 && A < L; ) {
let _ = S.charAt(A);
if (v instanceof RegExp) {
if (!v.test(_))
return A;
} else if (!v.includes(_))
return A;
w ? A-- : A++;
}
return A === -1 || A === L ? A : false;
};
}
var d = p(/\s/), x = p(" "), P = p(",; "), m = p(/[^\n\r]/);
h.exports = { skipWhitespace: d, skipSpaces: x, skipToLineEnd: P, skipEverythingButNewLine: m };
} }), Lf = $({ "src/utils/text/skip-trailing-comment.js"(l, h) {
"use strict";
U();
var { skipEverythingButNewLine: p } = fo();
function d(x, P) {
return P === false ? false : x.charAt(P) === "/" && x.charAt(P + 1) === "/" ? p(x, P) : P;
}
h.exports = d;
} }), Of = $({ "src/utils/text/get-next-non-space-non-comment-character-index-with-start-index.js"(l, h) {
"use strict";
U();
var p = Df(), d = Ff(), x = Lf(), { skipSpaces: P } = fo();
function m(v, S) {
let k = null, F = S;
for (; F !== k; )
k = F, F = P(v, F), F = p(v, F), F = x(v, F), F = d(v, F);
return F;
}
h.exports = m;
} }), mo = {};
wf(mo, { EOL: () => ar, arch: () => Bf, cpus: () => vo, default: () => wo, endianness: () => yo, freemem: () => Ao, getNetworkInterfaces: () => So, hostname: () => xo, loadavg: () => go, networkInterfaces: () => bo, platform: () => Mf, release: () => Co, tmpDir: () => rr, tmpdir: () => ir, totalmem: () => To, type: () => Eo, uptime: () => Po });
function yo() {
if (typeof Ut > "u") {
var l = new ArrayBuffer(2), h = new Uint8Array(l), p = new Uint16Array(l);
if (h[0] = 1, h[1] = 2, p[0] === 258)
Ut = "BE";
else if (p[0] === 513)
Ut = "LE";
else
throw new Error("unable to figure out endianess");
}
return Ut;
}
function xo() {
return typeof globalThis.location < "u" ? globalThis.location.hostname : "";
}
function go() {
return [];
}
function Po() {
return 0;
}
function Ao() {
return Number.MAX_VALUE;
}
function To() {
return Number.MAX_VALUE;
}
function vo() {
return [];
}
function Eo() {
return "Browser";
}
function Co() {
return typeof globalThis.navigator < "u" ? globalThis.navigator.appVersion : "";
}
function bo() {
}
function So() {
}
function Bf() {
return "javascript";
}
function Mf() {
return "browser";
}
function rr() {
return "/tmp";
}
var Ut, ir, ar, wo, _f = co({ "node-modules-polyfills:os"() {
U(), ir = rr, ar = `
`, wo = { EOL: ar, tmpdir: ir, tmpDir: rr, networkInterfaces: bo, getNetworkInterfaces: So, release: Co, type: Eo, cpus: vo, totalmem: To, freemem: Ao, uptime: Po, loadavg: go, hostname: xo, endianness: yo };
} }), Rf = $({ "node-modules-polyfills-commonjs:os"(l, h) {
U();
var p = (_f(), Nf(mo));
if (p && p.default) {
h.exports = p.default;
for (let d in p)
h.exports[d] = p[d];
} else
p && (h.exports = p);
} }), jf = $({ "node_modules/detect-newline/index.js"(l, h) {
"use strict";
U();
var p = (d) => {
if (typeof d != "string")
throw new TypeError("Expected a string");
let x = d.match(/(?:\r?\n)/g) || [];
if (x.length === 0)
return;
let P = x.filter((v) => v === `\r
`).length, m = x.length - P;
return P > m ? `\r
` : `
`;
};
h.exports = p, h.exports.graceful = (d) => typeof d == "string" && p(d) || `
`;
} }), qf = $({ "node_modules/jest-docblock/build/index.js"(l) {
"use strict";
U(), Object.defineProperty(l, "__esModule", { value: true }), l.extract = A, l.parse = G, l.parseWithComments = N, l.print = O, l.strip = _;
function h() {
let R = Rf();
return h = function() {
return R;
}, R;
}
function p() {
let R = d(jf());
return p = function() {
return R;
}, R;
}
function d(R) {
return R && R.__esModule ? R : { default: R };
}
var x = /\*\/$/, P = /^\/\*\*?/, m = /^\s*(\/\*\*?(.|\r?\n)*?\*\/)/, v = /(^|\s+)\/\/([^\r\n]*)/g, S = /^(\r?\n)+/, k = /(?:^|\r?\n) *(@[^\r\n]*?) *\r?\n *(?![^@\r\n]*\/\/[^]*)([^@\r\n\s][^@\r\n]+?) *\r?\n/g, F = /(?:^|\r?\n) *@(\S+) *([^\r\n]*)/g, w = /(\r?\n|^) *\* ?/g, L = [];
function A(R) {
let z = R.match(m);
return z ? z[0].trimLeft() : "";
}
function _(R) {
let z = R.match(m);
return z && z[0] ? R.substring(z[0].length) : R;
}
function G(R) {
return N(R).pragmas;
}
function N(R) {
let z = (0, p().default)(R) || h().EOL;
R = R.replace(P, "").replace(x, "").replace(w, "$1");
let Q = "";
for (; Q !== R; )
Q = R, R = R.replace(k, `${z}$1 $2${z}`);
R = R.replace(S, "").trimRight();
let b = /* @__PURE__ */ Object.create(null), B = R.replace(F, "").replace(S, "").trimRight(), Z;
for (; Z = F.exec(R); ) {
let q = Z[2].replace(v, "");
typeof b[Z[1]] == "string" || Array.isArray(b[Z[1]]) ? b[Z[1]] = L.concat(b[Z[1]], q) : b[Z[1]] = q;
}
return { comments: B, pragmas: b };
}
function O(R) {
let { comments: z = "", pragmas: Q = {} } = R, b = (0, p().default)(z) || h().EOL, B = "/**", Z = " *", q = " */", ue = Object.keys(Q), te = ue.map((se) => H(se, Q[se])).reduce((se, He) => se.concat(He), []).map((se) => `${Z} ${se}${b}`).join("");
if (!z) {
if (ue.length === 0)
return "";
if (ue.length === 1 && !Array.isArray(Q[ue[0]])) {
let se = Q[ue[0]];
return `${B} ${H(ue[0], se)[0]}${q}`;
}
}
let it = z.split(b).map((se) => `${Z} ${se}`).join(b) + b;
return B + b + (z ? it : "") + (z && ue.length ? Z + b : "") + te + q;
}
function H(R, z) {
return L.concat(z).map((Q) => `@${R} ${Q}`.trim());
}
} }), Uf = $({ "src/common/end-of-line.js"(l, h) {
"use strict";
U();
function p(m) {
let v = m.indexOf("\r");
return v >= 0 ? m.charAt(v + 1) === `
` ? "crlf" : "cr" : "lf";
}
function d(m) {
switch (m) {
case "cr":
return "\r";
case "crlf":
return `\r
`;
default:
return `
`;
}
}
function x(m, v) {
let S;
switch (v) {
case `
`:
S = /\n/g;
break;
case "\r":
S = /\r/g;
break;
case `\r
`:
S = /\r\n/g;
break;
default:
throw new Error(`Unexpected "eol" ${JSON.stringify(v)}.`);
}
let k = m.match(S);
return k ? k.length : 0;
}
function P(m) {
return m.replace(/\r\n?/g, `
`);
}
h.exports = { guessEndOfLine: p, convertEndOfLineToChars: d, countEndOfLineChars: x, normalizeEndOfLine: P };
} }), $f = $({ "src/language-js/pragma.js"(l, h) {
"use strict";
U();
var { parseWithComments: p, strip: d, extract: x, print: P } = qf(), { normalizeEndOfLine: m } = Uf(), v = po();
function S(w) {
let L = v(w);
L && (w = w.slice(L.length + 1));
let A = x(w), { pragmas: _, comments: G } = p(A);
return { shebang: L, text: w, pragmas: _, comments: G };
}
function k(w) {
let L = Object.keys(S(w).pragmas);
return L.includes("prettier") || L.includes("format");
}
function F(w) {
let { shebang: L, text: A, pragmas: _, comments: G } = S(w), N = d(A), O = P({ pragmas: Object.assign({ format: "" }, _), comments: G.trimStart() });
return (L ? `${L}
` : "") + m(O) + (N.startsWith(`
`) ? `
` : `
`) + N;
}
h.exports = { hasPragma: k, insertPragma: F };
} }), Io = $({ "src/utils/is-non-empty-array.js"(l, h) {
"use strict";
U();
function p(d) {
return Array.isArray(d) && d.length > 0;
}
h.exports = p;
} }), No = $({ "src/language-js/loc.js"(l, h) {
"use strict";
U();
var p = Io();
function d(S) {
var k, F;
let w = S.range ? S.range[0] : S.start, L = (k = (F = S.declaration) === null || F === void 0 ? void 0 : F.decorators) !== null && k !== void 0 ? k : S.decorators;
return p(L) ? Math.min(d(L[0]), w) : w;
}
function x(S) {
return S.range ? S.range[1] : S.end;
}
function P(S, k) {
let F = d(S);
return Number.isInteger(F) && F === d(k);
}
function m(S, k) {
let F = x(S);
return Number.isInteger(F) && F === x(k);
}
function v(S, k) {
return P(S, k) && m(S, k);
}
h.exports = { locStart: d, locEnd: x, hasSameLocStart: P, hasSameLoc: v };
} }), ko = $({ "src/language-js/parse/utils/create-parser.js"(l, h) {
"use strict";
U();
var { hasPragma: p } = $f(), { locStart: d, locEnd: x } = No();
function P(m) {
return m = typeof m == "function" ? { parse: m } : m, Object.assign({ astFormat: "estree", hasPragma: p, locStart: d, locEnd: x }, m);
}
h.exports = P;
} }), lr = $({ "src/common/parser-create-error.js"(l, h) {
"use strict";
U();
function p(d, x) {
let P = new SyntaxError(d + " (" + x.start.line + ":" + x.start.column + ")");
return P.loc = x, P;
}
h.exports = p;
} }), Do = $({ "src/language-js/parse/utils/create-babel-parse-error.js"(l, h) {
"use strict";
U();
var p = lr();
function d(x) {
let { message: P, loc: m } = x;
return p(P.replace(/ \(.*\)/, ""), { start: { line: m ? m.line : 0, column: m ? m.column + 1 : 0 } });
}
h.exports = d;
} }), Hf = $({ "src/language-js/utils/is-ts-keyword-type.js"(l, h) {
"use strict";
U();
function p(d) {
let { type: x } = d;
return x.startsWith("TS") && x.endsWith("Keyword");
}
h.exports = p;
} }), zf = $({ "src/language-js/utils/is-block-comment.js"(l, h) {
"use strict";
U();
var p = /* @__PURE__ */ new Set(["Block", "CommentBlock", "MultiLine"]), d = (x) => p.has(x == null ? void 0 : x.type);
h.exports = d;
} }), Vf = $({ "src/language-js/utils/is-type-cast-comment.js"(l, h) {
"use strict";
U();
var p = zf();
function d(x) {
return p(x) && x.value[0] === "*" && /@(?:type|satisfies)\b/.test(x.value);
}
h.exports = d;
} }), Kf = $({ "src/utils/get-last.js"(l, h) {
"use strict";
U();
var p = (d) => d[d.length - 1];
h.exports = p;
} }), Wf = $({ "src/language-js/parse/postprocess/visit-node.js"(l, h) {
"use strict";
U();
function p(d, x) {
if (Array.isArray(d)) {
for (let P = 0; P < d.length; P++)
d[P] = p(d[P], x);
return d;
}
if (d && typeof d == "object" && typeof d.type == "string") {
let P = Object.keys(d);
for (let m = 0; m < P.length; m++)
d[P[m]] = p(d[P[m]], x);
return x(d) || d;
}
return d;
}
h.exports = p;
} }), Gf = $({ "src/language-js/parse/postprocess/throw-syntax-error.js"(l, h) {
"use strict";
U();
var p = lr();
function d(x, P) {
let { start: m, end: v } = x.loc;
throw p(P, { start: { line: m.line, column: m.column + 1 }, end: { line: v.line, column: v.column + 1 } });
}
h.exports = d;
} }), Jf = $({ "src/language-js/parse/postprocess/index.js"(l, h) {
"use strict";
U();
var { locStart: p, locEnd: d } = No(), x = Hf(), P = Vf(), m = Kf(), v = Wf(), S = Gf();
function k(A, _) {
if (_.parser !== "typescript" && _.parser !== "flow" && _.parser !== "acorn" && _.parser !== "espree" && _.parser !== "meriyah") {
let N = /* @__PURE__ */ new Set();
A = v(A, (O) => {
O.leadingComments && O.leadingComments.some(P) && N.add(p(O));
}), A = v(A, (O) => {
if (O.type === "ParenthesizedExpression") {
let { expression: H } = O;
if (H.type === "TypeCastExpression")
return H.range = O.range, H;
let R = p(O);
if (!N.has(R))
return H.extra = Object.assign(Object.assign({}, H.extra), {}, { parenthesized: true }), H;
}
});
}
return A = v(A, (N) => {
switch (N.type) {
case "ChainExpression":
return F(N.expression);
case "LogicalExpression": {
if (w(N))
return L(N);
break;
}
case "VariableDeclaration": {
let O = m(N.declarations);
O && O.init && G(N, O);
break;
}
case "TSParenthesizedType":
return x(N.typeAnnotation) || N.typeAnnotation.type === "TSThisType" || (N.typeAnnotation.range = [p(N), d(N)]), N.typeAnnotation;
case "TSTypeParameter":
if (typeof N.name == "string") {
let O = p(N);
N.name = { type: "Identifier", name: N.name, range: [O, O + N.name.length] };
}
break;
case "ObjectExpression":
if (_.parser === "typescript") {
let O = N.properties.find((H) => H.type === "Property" && H.value.type === "TSEmptyBodyFunctionExpression");
O && S(O.value, "Unexpected token.");
}
break;
case "SequenceExpression": {
let O = m(N.expressions);
N.range = [p(N), Math.min(d(O), d(N))];
break;
}
case "TopicReference":
_.__isUsingHackPipeline = true;
break;
case "ExportAllDeclaration": {
let { exported: O } = N;
if (_.parser === "meriyah" && O && O.type === "Identifier") {
let H = _.originalText.slice(p(O), d(O));
(H.startsWith('"') || H.startsWith("'")) && (N.exported = Object.assign(Object.assign({}, N.exported), {}, { type: "Literal", value: N.exported.name, raw: H }));
}
break;
}
case "PropertyDefinition":
if (_.parser === "meriyah" && N.static && !N.computed && !N.key) {
let O = "static", H = p(N);
Object.assign(N, { static: false, key: { type: "Identifier", name: O, range: [H, H + O.length] } });
}
break;
}
}), A;
function G(N, O) {
_.originalText[d(O)] !== ";" && (N.range = [p(N), d(O)]);
}
}
function F(A) {
switch (A.type) {
case "CallExpression":
A.type = "OptionalCallExpression", A.callee = F(A.callee);
break;
case "MemberExpression":
A.type = "OptionalMemberExpression", A.object = F(A.object);
break;
case "TSNonNullExpression":
A.expression = F(A.expression);
break;
}
return A;
}
function w(A) {
return A.type === "LogicalExpression" && A.right.type === "LogicalExpression" && A.operator === A.right.operator;
}
function L(A) {
return w(A) ? L({ type: "LogicalExpression", operator: A.operator, left: L({ type: "LogicalExpression", operator: A.operator, left: A.left, right: A.right.left, range: [p(A.left), d(A.right.left)] }), right: A.right.right, range: [p(A), d(A)] }) : A;
}
h.exports = k;
} }), Fo = $({ "node_modules/@babel/parser/lib/index.js"(l) {
"use strict";
U(), Object.defineProperty(l, "__esModule", { value: true });
var h = { sourceType: "script", sourceFilename: void 0, startColumn: 0, startLine: 1, allowAwaitOutsideFunction: false, allowReturnOutsideFunction: false, allowNewTargetOutsideFunction: false, allowImportExportEverywhere: false, allowSuperOutsideMethod: false, allowUndeclaredExports: false, plugins: [], strictMode: null, ranges: false, tokens: false, createParenthesizedExpressions: false, errorRecovery: false, attachComment: true, annexB: true };
function p(t) {
if (t && t.annexB != null && t.annexB !== false)
throw new Error("The `annexB` option can only be set to `false`.");
let r = {};
for (let e of Object.keys(h))
r[e] = t && t[e] != null ? t[e] : h[e];
return r;
}
var d = class {
constructor(t, r) {
this.token = void 0, this.preserveSpace = void 0, this.token = t, this.preserveSpace = !!r;
}
}, x = { brace: new d("{"), j_oTag: new d("... ", true) };
x.template = new d("`", true);
var P = true, m = true, v = true, S = true, k = true, F = true, w = class {
constructor(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
this.label = void 0, this.keyword = void 0, this.beforeExpr = void 0, this.startsExpr = void 0, this.rightAssociative = void 0, this.isLoop = void 0, this.isAssign = void 0, this.prefix = void 0, this.postfix = void 0, this.binop = void 0, this.label = t, this.keyword = r.keyword, this.beforeExpr = !!r.beforeExpr, this.startsExpr = !!r.startsExpr, this.rightAssociative = !!r.rightAssociative, this.isLoop = !!r.isLoop, this.isAssign = !!r.isAssign, this.prefix = !!r.prefix, this.postfix = !!r.postfix, this.binop = r.binop != null ? r.binop : null, this.updateContext = null;
}
}, L = /* @__PURE__ */ new Map();
function A(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
r.keyword = t;
let e = b(t, r);
return L.set(t, e), e;
}
function _(t, r) {
return b(t, { beforeExpr: P, binop: r });
}
var G = -1, N = [], O = [], H = [], R = [], z = [], Q = [];
function b(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
var e, s, i, a;
return ++G, O.push(t), H.push((e = r.binop) != null ? e : -1), R.push((s = r.beforeExpr) != null ? s : false), z.push((i = r.startsExpr) != null ? i : false), Q.push((a = r.prefix) != null ? a : false), N.push(new w(t, r)), G;
}
function B(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
var e, s, i, a;
return ++G, L.set(t, G), O.push(t), H.push((e = r.binop) != null ? e : -1), R.push((s = r.beforeExpr) != null ? s : false), z.push((i = r.startsExpr) != null ? i : false), Q.push((a = r.prefix) != null ? a : false), N.push(new w("name", r)), G;
}
var Z = { bracketL: b("[", { beforeExpr: P, startsExpr: m }), bracketHashL: b("#[", { beforeExpr: P, startsExpr: m }), bracketBarL: b("[|", { beforeExpr: P, startsExpr: m }), bracketR: b("]"), bracketBarR: b("|]"), braceL: b("{", { beforeExpr: P, startsExpr: m }), braceBarL: b("{|", { beforeExpr: P, startsExpr: m }), braceHashL: b("#{", { beforeExpr: P, startsExpr: m }), braceR: b("}"), braceBarR: b("|}"), parenL: b("(", { beforeExpr: P, startsExpr: m }), parenR: b(")"), comma: b(",", { beforeExpr: P }), semi: b(";", { beforeExpr: P }), colon: b(":", { beforeExpr: P }), doubleColon: b("::", { beforeExpr: P }), dot: b("."), question: b("?", { beforeExpr: P }), questionDot: b("?."), arrow: b("=>", { beforeExpr: P }), template: b("template"), ellipsis: b("...", { beforeExpr: P }), backQuote: b("`", { startsExpr: m }), dollarBraceL: b("${", { beforeExpr: P, startsExpr: m }), templateTail: b("...`", { startsExpr: m }), templateNonTail: b("...${", { beforeExpr: P, startsExpr: m }), at: b("@"), hash: b("#", { startsExpr: m }), interpreterDirective: b("#!..."), eq: b("=", { beforeExpr: P, isAssign: S }), assign: b("_=", { beforeExpr: P, isAssign: S }), slashAssign: b("_=", { beforeExpr: P, isAssign: S }), xorAssign: b("_=", { beforeExpr: P, isAssign: S }), moduloAssign: b("_=", { beforeExpr: P, isAssign: S }), incDec: b("++/--", { prefix: k, postfix: F, startsExpr: m }), bang: b("!", { beforeExpr: P, prefix: k, startsExpr: m }), tilde: b("~", { beforeExpr: P, prefix: k, startsExpr: m }), doubleCaret: b("^^", { startsExpr: m }), doubleAt: b("@@", { startsExpr: m }), pipeline: _("|>", 0), nullishCoalescing: _("??", 1), logicalOR: _("||", 1), logicalAND: _("&&", 2), bitwiseOR: _("|", 3), bitwiseXOR: _("^", 4), bitwiseAND: _("&", 5), equality: _("==/!=/===/!==", 6), lt: _(">/<=/>=", 7), gt: _(">/<=/>=", 7), relational: _(">/<=/>=", 7), bitShift: _("<>>/>>>", 8), bitShiftL: _("<>>/>>>", 8), bitShiftR: _("<>>/>>>", 8), plusMin: b("+/-", { beforeExpr: P, binop: 9, prefix: k, startsExpr: m }), modulo: b("%", { binop: 10, startsExpr: m }), star: b("*", { binop: 10 }), slash: _("/", 10), exponent: b("**", { beforeExpr: P, binop: 11, rightAssociative: true }), _in: A("in", { beforeExpr: P, binop: 7 }), _instanceof: A("instanceof", { beforeExpr: P, binop: 7 }), _break: A("break"), _case: A("case", { beforeExpr: P }), _catch: A("catch"), _continue: A("continue"), _debugger: A("debugger"), _default: A("default", { beforeExpr: P }), _else: A("else", { beforeExpr: P }), _finally: A("finally"), _function: A("function", { startsExpr: m }), _if: A("if"), _return: A("return", { beforeExpr: P }), _switch: A("switch"), _throw: A("throw", { beforeExpr: P, prefix: k, startsExpr: m }), _try: A("try"), _var: A("var"), _const: A("const"), _with: A("with"), _new: A("new", { beforeExpr: P, startsExpr: m }), _this: A("this", { startsExpr: m }), _super: A("super", { startsExpr: m }), _class: A("class", { startsExpr: m }), _extends: A("extends", { beforeExpr: P }), _export: A("export"), _import: A("import", { startsExpr: m }), _null: A("null", { startsExpr: m }), _true: A("true", { startsExpr: m }), _false: A("false", { startsExpr: m }), _typeof: A("typeof", { beforeExpr: P, prefix: k, startsExpr: m }), _void: A("void", { beforeExpr: P, prefix: k, startsExpr: m }), _delete: A("delete", { beforeExpr: P, prefix: k, startsExpr: m }), _do: A("do", { isLoop: v, beforeExpr: P }), _for: A("for", { isLoop: v }), _while: A("while", { isLoop: v }), _as: B("as", { startsExpr: m }), _assert: B("assert", { startsExpr: m }), _async: B("async", { startsExpr: m }), _await: B("await", { startsExpr: m }), _from: B("from", { startsExpr: m }), _get: B("get", { startsExpr: m }), _let: B("let", { startsExpr: m }), _meta: B("meta", { startsExpr: m }), _of: B("of", { startsExpr: m }), _sent: B("sent", { startsExpr: m }), _set: B("set", { startsExpr: m }), _static: B("static", { startsExpr: m }), _using: B("using", { startsExpr: m }), _yield: B("yield", { startsExpr: m }), _asserts: B("asserts", { startsExpr: m }), _checks: B("checks", { startsExpr: m }), _exports: B("exports", { startsExpr: m }), _global: B("global", { startsExpr: m }), _implements: B("implements", { startsExpr: m }), _intrinsic: B("intrinsic", { startsExpr: m }), _infer: B("infer", { startsExpr: m }), _is: B("is", { startsExpr: m }), _mixins: B("mixins", { startsExpr: m }), _proto: B("proto", { startsExpr: m }), _require: B("require", { startsExpr: m }), _satisfies: B("satisfies", { startsExpr: m }), _keyof: B("keyof", { startsExpr: m }), _readonly: B("readonly", { startsExpr: m }), _unique: B("unique", { startsExpr: m }), _abstract: B("abstract", { startsExpr: m }), _declare: B("declare", { startsExpr: m }), _enum: B("enum", { startsExpr: m }), _module: B("module", { startsExpr: m }), _namespace: B("namespace", { startsExpr: m }), _interface: B("interface", { startsExpr: m }), _type: B("type", { startsExpr: m }), _opaque: B("opaque", { startsExpr: m }), name: b("name", { startsExpr: m }), string: b("string", { startsExpr: m }), num: b("num", { startsExpr: m }), bigint: b("bigint", { startsExpr: m }), decimal: b("decimal", { startsExpr: m }), regexp: b("regexp", { startsExpr: m }), privateName: b("#name", { startsExpr: m }), eof: b("eof"), jsxName: b("jsxName"), jsxText: b("jsxText", { beforeExpr: true }), jsxTagStart: b("jsxTagStart", { startsExpr: true }), jsxTagEnd: b("jsxTagEnd"), placeholder: b("%%", { startsExpr: true }) };
function q(t) {
return t >= 93 && t <= 130;
}
function ue(t) {
return t <= 92;
}
function te(t) {
return t >= 58 && t <= 130;
}
function it(t) {
return t >= 58 && t <= 134;
}
function se(t) {
return R[t];
}
function He(t) {
return z[t];
}
function Bo(t) {
return t >= 29 && t <= 33;
}
function hr(t) {
return t >= 127 && t <= 129;
}
function Mo(t) {
return t >= 90 && t <= 92;
}
function $t(t) {
return t >= 58 && t <= 92;
}
function _o(t) {
return t >= 39 && t <= 59;
}
function Ro(t) {
return t === 34;
}
function jo(t) {
return Q[t];
}
function qo(t) {
return t >= 119 && t <= 121;
}
function Uo(t) {
return t >= 122 && t <= 128;
}
function xe(t) {
return O[t];
}
function at(t) {
return H[t];
}
function $o(t) {
return t === 57;
}
function nt(t) {
return t >= 24 && t <= 25;
}
function ce(t) {
return N[t];
}
N[8].updateContext = (t) => {
t.pop();
}, N[5].updateContext = N[7].updateContext = N[23].updateContext = (t) => {
t.push(x.brace);
}, N[22].updateContext = (t) => {
t[t.length - 1] === x.template ? t.pop() : t.push(x.template);
}, N[140].updateContext = (t) => {
t.push(x.j_expr, x.j_oTag);
};
function ot(t, r) {
if (t == null)
return {};
var e = {}, s = Object.keys(t), i, a;
for (a = 0; a < s.length; a++)
i = s[a], !(r.indexOf(i) >= 0) && (e[i] = t[i]);
return e;
}
var ge = class {
constructor(t, r, e) {
this.line = void 0, this.column = void 0, this.index = void 0, this.line = t, this.column = r, this.index = e;
}
}, lt = class {
constructor(t, r) {
this.start = void 0, this.end = void 0, this.filename = void 0, this.identifierName = void 0, this.start = t, this.end = r;
}
};
function Y(t, r) {
let { line: e, column: s, index: i } = t;
return new ge(e, s + r, i + r);
}
var Ht = { SyntaxError: "BABEL_PARSER_SYNTAX_ERROR", SourceTypeModuleError: "BABEL_PARSER_SOURCETYPE_MODULE_REQUIRED" }, Ho = function(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : t.length - 1;
return { get() {
return t.reduce((e, s) => e[s], this);
}, set(e) {
t.reduce((s, i, a) => a === r ? s[i] = e : s[i], this);
} };
}, zo = (t, r, e) => Object.keys(e).map((s) => [s, e[s]]).filter((s) => {
let [, i] = s;
return !!i;
}).map((s) => {
let [i, a] = s;
return [i, typeof a == "function" ? { value: a, enumerable: false } : typeof a.reflect == "string" ? Object.assign({}, a, Ho(a.reflect.split("."))) : a];
}).reduce((s, i) => {
let [a, n2] = i;
return Object.defineProperty(s, a, Object.assign({ configurable: true }, n2));
}, Object.assign(new t(), r)), Vo = { ImportMetaOutsideModule: { message: `import.meta may appear only with 'sourceType: "module"'`, code: Ht.SourceTypeModuleError }, ImportOutsideModule: { message: `'import' and 'export' may appear only with 'sourceType: "module"'`, code: Ht.SourceTypeModuleError } }, ur = { ArrayPattern: "array destructuring pattern", AssignmentExpression: "assignment expression", AssignmentPattern: "assignment expression", ArrowFunctionExpression: "arrow function expression", ConditionalExpression: "conditional expression", CatchClause: "catch clause", ForOfStatement: "for-of statement", ForInStatement: "for-in statement", ForStatement: "for-loop", FormalParameters: "function parameter list", Identifier: "identifier", ImportSpecifier: "import specifier", ImportDefaultSpecifier: "import default specifier", ImportNamespaceSpecifier: "import namespace specifier", ObjectPattern: "object destructuring pattern", ParenthesizedExpression: "parenthesized expression", RestElement: "rest element", UpdateExpression: { true: "prefix operation", false: "postfix operation" }, VariableDeclarator: "variable declaration", YieldExpression: "yield expression" }, zt = (t) => {
let { type: r, prefix: e } = t;
return r === "UpdateExpression" ? ur.UpdateExpression[String(e)] : ur[r];
}, Ko = { AccessorIsGenerator: (t) => {
let { kind: r } = t;
return `A ${r}ter cannot be a generator.`;
}, ArgumentsInClass: "'arguments' is only allowed in functions and class methods.", AsyncFunctionInSingleStatementContext: "Async functions can only be declared at the top level or inside a block.", AwaitBindingIdentifier: "Can not use 'await' as identifier inside an async function.", AwaitBindingIdentifierInStaticBlock: "Can not use 'await' as identifier inside a static block.", AwaitExpressionFormalParameter: "'await' is not allowed in async function parameters.", AwaitInUsingBinding: "'await' is not allowed to be used as a name in 'using' declarations.", AwaitNotInAsyncContext: "'await' is only allowed within async functions and at the top levels of modules.", AwaitNotInAsyncFunction: "'await' is only allowed within async functions.", BadGetterArity: "A 'get' accessor must not have any formal parameters.", BadSetterArity: "A 'set' accessor must have exactly one formal parameter.", BadSetterRestParameter: "A 'set' accessor function argument must not be a rest parameter.", ConstructorClassField: "Classes may not have a field named 'constructor'.", ConstructorClassPrivateField: "Classes may not have a private field named '#constructor'.", ConstructorIsAccessor: "Class constructor may not be an accessor.", ConstructorIsAsync: "Constructor can't be an async function.", ConstructorIsGenerator: "Constructor can't be a generator.", DeclarationMissingInitializer: (t) => {
let { kind: r } = t;
return `Missing initializer in ${r} declaration.`;
}, DecoratorArgumentsOutsideParentheses: "Decorator arguments must be moved inside parentheses: use '@(decorator(args))' instead of '@(decorator)(args)'.", DecoratorBeforeExport: "Decorators must be placed *before* the 'export' keyword. Remove the 'decoratorsBeforeExport: true' option to use the 'export @decorator class {}' syntax.", DecoratorsBeforeAfterExport: "Decorators can be placed *either* before or after the 'export' keyword, but not in both locations at the same time.", DecoratorConstructor: "Decorators can't be used with a constructor. Did you mean '@dec class { ... }'?", DecoratorExportClass: "Decorators must be placed *after* the 'export' keyword. Remove the 'decoratorsBeforeExport: false' option to use the '@decorator export class {}' syntax.", DecoratorSemicolon: "Decorators must not be followed by a semicolon.", DecoratorStaticBlock: "Decorators can't be used with a static block.", DeletePrivateField: "Deleting a private field is not allowed.", DestructureNamedImport: "ES2015 named imports do not destructure. Use another statement for destructuring after the import.", DuplicateConstructor: "Duplicate constructor in the same class.", DuplicateDefaultExport: "Only one default export allowed per module.", DuplicateExport: (t) => {
let { exportName: r } = t;
return `\`${r}\` has already been exported. Exported identifiers must be unique.`;
}, DuplicateProto: "Redefinition of __proto__ property.", DuplicateRegExpFlags: "Duplicate regular expression flag.", ElementAfterRest: "Rest element must be last element.", EscapedCharNotAnIdentifier: "Invalid Unicode escape.", ExportBindingIsString: (t) => {
let { localName: r, exportName: e } = t;
return `A string literal cannot be used as an exported binding without \`from\`.
- Did you mean \`export { '${r}' as '${e}' } from 'some-module'\`?`;
}, ExportDefaultFromAsIdentifier: "'from' is not allowed as an identifier after 'export default'.", ForInOfLoopInitializer: (t) => {
let { type: r } = t;
return `'${r === "ForInStatement" ? "for-in" : "for-of"}' loop variable declaration may not have an initializer.`;
}, ForInUsing: "For-in loop may not start with 'using' declaration.", ForOfAsync: "The left-hand side of a for-of loop may not be 'async'.", ForOfLet: "The left-hand side of a for-of loop may not start with 'let'.", GeneratorInSingleStatementContext: "Generators can only be declared at the top level or inside a block.", IllegalBreakContinue: (t) => {
let { type: r } = t;
return `Unsyntactic ${r === "BreakStatement" ? "break" : "continue"}.`;
}, IllegalLanguageModeDirective: "Illegal 'use strict' directive in function with non-simple parameter list.", IllegalReturn: "'return' outside of function.", ImportBindingIsString: (t) => {
let { importName: r } = t;
return `A string literal cannot be used as an imported binding.
- Did you mean \`import { "${r}" as foo }\`?`;
}, ImportCallArgumentTrailingComma: "Trailing comma is disallowed inside import(...) arguments.", ImportCallArity: (t) => {
let { maxArgumentCount: r } = t;
return `\`import()\` requires exactly ${r === 1 ? "one argument" : "one or two arguments"}.`;
}, ImportCallNotNewExpression: "Cannot use new with import(...).", ImportCallSpreadArgument: "`...` is not allowed in `import()`.", ImportJSONBindingNotDefault: "A JSON module can only be imported with `default`.", ImportReflectionHasAssertion: "`import module x` cannot have assertions.", ImportReflectionNotBinding: 'Only `import module x from "./module"` is valid.', IncompatibleRegExpUVFlags: "The 'u' and 'v' regular expression flags cannot be enabled at the same time.", InvalidBigIntLiteral: "Invalid BigIntLiteral.", InvalidCodePoint: "Code point out of bounds.", InvalidCoverInitializedName: "Invalid shorthand property initializer.", InvalidDecimal: "Invalid decimal.", InvalidDigit: (t) => {
let { radix: r } = t;
return `Expected number in radix ${r}.`;
}, InvalidEscapeSequence: "Bad character escape sequence.", InvalidEscapeSequenceTemplate: "Invalid escape sequence in template.", InvalidEscapedReservedWord: (t) => {
let { reservedWord: r } = t;
return `Escape sequence in keyword ${r}.`;
}, InvalidIdentifier: (t) => {
let { identifierName: r } = t;
return `Invalid identifier ${r}.`;
}, InvalidLhs: (t) => {
let { ancestor: r } = t;
return `Invalid left-hand side in ${zt(r)}.`;
}, InvalidLhsBinding: (t) => {
let { ancestor: r } = t;
return `Binding invalid left-hand side in ${zt(r)}.`;
}, InvalidNumber: "Invalid number.", InvalidOrMissingExponent: "Floating-point numbers require a valid exponent after the 'e'.", InvalidOrUnexpectedToken: (t) => {
let { unexpected: r } = t;
return `Unexpected character '${r}'.`;
}, InvalidParenthesizedAssignment: "Invalid parenthesized assignment pattern.", InvalidPrivateFieldResolution: (t) => {
let { identifierName: r } = t;
return `Private name #${r} is not defined.`;
}, InvalidPropertyBindingPattern: "Binding member expression.", InvalidRecordProperty: "Only properties and spread elements are allowed in record definitions.", InvalidRestAssignmentPattern: "Invalid rest operator's argument.", LabelRedeclaration: (t) => {
let { labelName: r } = t;
return `Label '${r}' is already declared.`;
}, LetInLexicalBinding: "'let' is not allowed to be used as a name in 'let' or 'const' declarations.", LineTerminatorBeforeArrow: "No line break is allowed before '=>'.", MalformedRegExpFlags: "Invalid regular expression flag.", MissingClassName: "A class name is required.", MissingEqInAssignment: "Only '=' operator can be used for specifying default value.", MissingSemicolon: "Missing semicolon.", MissingPlugin: (t) => {
let { missingPlugin: r } = t;
return `This experimental syntax requires enabling the parser plugin: ${r.map((e) => JSON.stringify(e)).join(", ")}.`;
}, MissingOneOfPlugins: (t) => {
let { missingPlugin: r } = t;
return `This experimental syntax requires enabling one of the following parser plugin(s): ${r.map((e) => JSON.stringify(e)).join(", ")}.`;
}, MissingUnicodeEscape: "Expecting Unicode escape sequence \\uXXXX.", MixingCoalesceWithLogical: "Nullish coalescing operator(??) requires parens when mixing with logical operators.", ModuleAttributeDifferentFromType: "The only accepted module attribute is `type`.", ModuleAttributeInvalidValue: "Only string literals are allowed as module attribute values.", ModuleAttributesWithDuplicateKeys: (t) => {
let { key: r } = t;
return `Duplicate key "${r}" is not allowed in module attributes.`;
}, ModuleExportNameHasLoneSurrogate: (t) => {
let { surrogateCharCode: r } = t;
return `An export name cannot include a lone surrogate, found '\\u${r.toString(16)}'.`;
}, ModuleExportUndefined: (t) => {
let { localName: r } = t;
return `Export '${r}' is not defined.`;
}, MultipleDefaultsInSwitch: "Multiple default clauses.", NewlineAfterThrow: "Illegal newline after throw.", NoCatchOrFinally: "Missing catch or finally clause.", NumberIdentifier: "Identifier directly after number.", NumericSeparatorInEscapeSequence: "Numeric separators are not allowed inside unicode escape sequences or hex escape sequences.", ObsoleteAwaitStar: "'await*' has been removed from the async functions proposal. Use Promise.all() instead.", OptionalChainingNoNew: "Constructors in/after an Optional Chain are not allowed.", OptionalChainingNoTemplate: "Tagged Template Literals are not allowed in optionalChain.", OverrideOnConstructor: "'override' modifier cannot appear on a constructor declaration.", ParamDupe: "Argument name clash.", PatternHasAccessor: "Object pattern can't contain getter or setter.", PatternHasMethod: "Object pattern can't contain methods.", PrivateInExpectedIn: (t) => {
let { identifierName: r } = t;
return `Private names are only allowed in property accesses (\`obj.#${r}\`) or in \`in\` expressions (\`#${r} in obj\`).`;
}, PrivateNameRedeclaration: (t) => {
let { identifierName: r } = t;
return `Duplicate private name #${r}.`;
}, RecordExpressionBarIncorrectEndSyntaxType: "Record expressions ending with '|}' are only allowed when the 'syntaxType' option of the 'recordAndTuple' plugin is set to 'bar'.", RecordExpressionBarIncorrectStartSyntaxType: "Record expressions starting with '{|' are only allowed when the 'syntaxType' option of the 'recordAndTuple' plugin is set to 'bar'.", RecordExpressionHashIncorrectStartSyntaxType: "Record expressions starting with '#{' are only allowed when the 'syntaxType' option of the 'recordAndTuple' plugin is set to 'hash'.", RecordNoProto: "'__proto__' is not allowed in Record expressions.", RestTrailingComma: "Unexpected trailing comma after rest element.", SloppyFunction: "In non-strict mode code, functions can only be declared at top level or inside a block.", SloppyFunctionAnnexB: "In non-strict mode code, functions can only be declared at top level, inside a block, or as the body of an if statement.", StaticPrototype: "Classes may not have static property named prototype.", SuperNotAllowed: "`super()` is only valid inside a class constructor of a subclass. Maybe a typo in the method name ('constructor') or not extending another class?", SuperPrivateField: "Private fields can't be accessed on super.", TrailingDecorator: "Decorators must be attached to a class element.", TupleExpressionBarIncorrectEndSyntaxType: "Tuple expressions ending with '|]' are only allowed when the 'syntaxType' option of the 'recordAndTuple' plugin is set to 'bar'.", TupleExpressionBarIncorrectStartSyntaxType: "Tuple expressions starting with '[|' are only allowed when the 'syntaxType' option of the 'recordAndTuple' plugin is set to 'bar'.", TupleExpressionHashIncorrectStartSyntaxType: "Tuple expressions starting with '#[' are only allowed when the 'syntaxType' option of the 'recordAndTuple' plugin is set to 'hash'.", UnexpectedArgumentPlaceholder: "Unexpected argument placeholder.", UnexpectedAwaitAfterPipelineBody: 'Unexpected "await" after pipeline body; await must have parentheses in minimal proposal.', UnexpectedDigitAfterHash: "Unexpected digit after hash token.", UnexpectedImportExport: "'import' and 'export' may only appear at the top level.", UnexpectedKeyword: (t) => {
let { keyword: r } = t;
return `Unexpected keyword '${r}'.`;
}, UnexpectedLeadingDecorator: "Leading decorators must be attached to a class declaration.", UnexpectedLexicalDeclaration: "Lexical declaration cannot appear in a single-statement context.", UnexpectedNewTarget: "`new.target` can only be used in functions or class properties.", UnexpectedNumericSeparator: "A numeric separator is only allowed between two digits.", UnexpectedPrivateField: "Unexpected private name.", UnexpectedReservedWord: (t) => {
let { reservedWord: r } = t;
return `Unexpected reserved word '${r}'.`;
}, UnexpectedSuper: "'super' is only allowed in object methods and classes.", UnexpectedToken: (t) => {
let { expected: r, unexpected: e } = t;
return `Unexpected token${e ? ` '${e}'.` : ""}${r ? `, expected "${r}"` : ""}`;
}, UnexpectedTokenUnaryExponentiation: "Illegal expression. Wrap left hand side or entire exponentiation in parentheses.", UnexpectedUsingDeclaration: "Using declaration cannot appear in the top level when source type is `script`.", UnsupportedBind: "Binding should be performed on object property.", UnsupportedDecoratorExport: "A decorated export must export a class declaration.", UnsupportedDefaultExport: "Only expressions, functions or classes are allowed as the `default` export.", UnsupportedImport: "`import` can only be used in `import()` or `import.meta`.", UnsupportedMetaProperty: (t) => {
let { target: r, onlyValidPropertyName: e } = t;
return `The only valid meta property for ${r} is ${r}.${e}.`;
}, UnsupportedParameterDecorator: "Decorators cannot be used to decorate parameters.", UnsupportedPropertyDecorator: "Decorators cannot be used to decorate object literal properties.", UnsupportedSuper: "'super' can only be used with function calls (i.e. super()) or in property accesses (i.e. super.prop or super[prop]).", UnterminatedComment: "Unterminated comment.", UnterminatedRegExp: "Unterminated regular expression.", UnterminatedString: "Unterminated string constant.", UnterminatedTemplate: "Unterminated template.", UsingDeclarationHasBindingPattern: "Using declaration cannot have destructuring patterns.", VarRedeclaration: (t) => {
let { identifierName: r } = t;
return `Identifier '${r}' has already been declared.`;
}, YieldBindingIdentifier: "Can not use 'yield' as identifier inside a generator.", YieldInParameter: "Yield expression is not allowed in formal parameters.", ZeroDigitNumericSeparator: "Numeric separator can not be used after leading 0." }, Wo = { StrictDelete: "Deleting local variable in strict mode.", StrictEvalArguments: (t) => {
let { referenceName: r } = t;
return `Assigning to '${r}' in strict mode.`;
}, StrictEvalArgumentsBinding: (t) => {
let { bindingName: r } = t;
return `Binding '${r}' in strict mode.`;
}, StrictFunction: "In strict mode code, functions can only be declared at top level or inside a block.", StrictNumericEscape: "The only valid numeric escape in strict mode is '\\0'.", StrictOctalLiteral: "Legacy octal literals are not allowed in strict mode.", StrictWith: "'with' in strict mode." }, Go = /* @__PURE__ */ new Set(["ArrowFunctionExpression", "AssignmentExpression", "ConditionalExpression", "YieldExpression"]), Jo = { PipeBodyIsTighter: "Unexpected yield after pipeline body; any yield expression acting as Hack-style pipe body must be parenthesized due to its loose operator precedence.", PipeTopicRequiresHackPipes: 'Topic reference is used, but the pipelineOperator plugin was not passed a "proposal": "hack" or "smart" option.', PipeTopicUnbound: "Topic reference is unbound; it must be inside a pipe body.", PipeTopicUnconfiguredToken: (t) => {
let { token: r } = t;
return `Invalid topic token ${r}. In order to use ${r} as a topic reference, the pipelineOperator plugin must be configured with { "proposal": "hack", "topicToken": "${r}" }.`;
}, PipeTopicUnused: "Hack-style pipe body does not contain a topic reference; Hack-style pipes must use topic at least once.", PipeUnparenthesizedBody: (t) => {
let { type: r } = t;
return `Hack-style pipe body cannot be an unparenthesized ${zt({ type: r })}; please wrap it in parentheses.`;
}, PipelineBodyNoArrow: 'Unexpected arrow "=>" after pipeline body; arrow function in pipeline body must be parenthesized.', PipelineBodySequenceExpression: "Pipeline body may not be a comma-separated sequence expression.", PipelineHeadSequenceExpression: "Pipeline head should not be a comma-separated sequence expression.", PipelineTopicUnused: "Pipeline is in topic style but does not use topic reference.", PrimaryTopicNotAllowed: "Topic reference was used in a lexical context without topic binding.", PrimaryTopicRequiresSmartPipeline: 'Topic reference is used, but the pipelineOperator plugin was not passed a "proposal": "hack" or "smart" option.' }, Xo = ["toMessage"], Yo = ["message"];
function Qo(t) {
let { toMessage: r } = t, e = ot(t, Xo);
return function s(i) {
let { loc: a, details: n2 } = i;
return zo(SyntaxError, Object.assign({}, e, { loc: a }), { clone() {
let o = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {}, u = o.loc || {};
return s({ loc: new ge("line" in u ? u.line : this.loc.line, "column" in u ? u.column : this.loc.column, "index" in u ? u.index : this.loc.index), details: Object.assign({}, this.details, o.details) });
}, details: { value: n2, enumerable: false }, message: { get() {
return `${r(this.details)} (${this.loc.line}:${this.loc.column})`;
}, set(o) {
Object.defineProperty(this, "message", { value: o });
} }, pos: { reflect: "loc.index", enumerable: true }, missingPlugin: "missingPlugin" in n2 && { reflect: "details.missingPlugin", enumerable: true } });
};
}
function pe(t, r) {
if (Array.isArray(t))
return (s) => pe(s, t[0]);
let e = {};
for (let s of Object.keys(t)) {
let i = t[s], a = typeof i == "string" ? { message: () => i } : typeof i == "function" ? { message: i } : i, { message: n2 } = a, o = ot(a, Yo), u = typeof n2 == "string" ? () => n2 : n2;
e[s] = Qo(Object.assign({ code: Ht.SyntaxError, reasonCode: s, toMessage: u }, r ? { syntaxPlugin: r } : {}, o));
}
return e;
}
var f = Object.assign({}, pe(Vo), pe(Ko), pe(Wo), pe`pipelineOperator`(Jo)), { defineProperty: Zo } = Object, cr = (t, r) => Zo(t, r, { enumerable: false, value: t[r] });
function ze(t) {
return t.loc.start && cr(t.loc.start, "index"), t.loc.end && cr(t.loc.end, "index"), t;
}
var el = (t) => class extends t {
parse() {
let e = ze(super.parse());
return this.options.tokens && (e.tokens = e.tokens.map(ze)), e;
}
parseRegExpLiteral(e) {
let { pattern: s, flags: i } = e, a = null;
try {
a = new RegExp(s, i);
} catch {
}
let n2 = this.estreeParseLiteral(a);
return n2.regex = { pattern: s, flags: i }, n2;
}
parseBigIntLiteral(e) {
let s;
try {
s = BigInt(e);
} catch {
s = null;
}
let i = this.estreeParseLiteral(s);
return i.bigint = String(i.value || e), i;
}
parseDecimalLiteral(e) {
let i = this.estreeParseLiteral(null);
return i.decimal = String(i.value || e), i;
}
estreeParseLiteral(e) {
return this.parseLiteral(e, "Literal");
}
parseStringLiteral(e) {
return this.estreeParseLiteral(e);
}
parseNumericLiteral(e) {
return this.estreeParseLiteral(e);
}
parseNullLiteral() {
return this.estreeParseLiteral(null);
}
parseBooleanLiteral(e) {
return this.estreeParseLiteral(e);
}
directiveToStmt(e) {
let s = e.value;
delete e.value, s.type = "Literal", s.raw = s.extra.raw, s.value = s.extra.expressionValue;
let i = e;
return i.type = "ExpressionStatement", i.expression = s, i.directive = s.extra.rawValue, delete s.extra, i;
}
initFunction(e, s) {
super.initFunction(e, s), e.expression = false;
}
checkDeclaration(e) {
e != null && this.isObjectProperty(e) ? this.checkDeclaration(e.value) : super.checkDeclaration(e);
}
getObjectOrClassMethodParams(e) {
return e.value.params;
}
isValidDirective(e) {
var s;
return e.type === "ExpressionStatement" && e.expression.type === "Literal" && typeof e.expression.value == "string" && !((s = e.expression.extra) != null && s.parenthesized);
}
parseBlockBody(e, s, i, a, n2) {
super.parseBlockBody(e, s, i, a, n2);
let o = e.directives.map((u) => this.directiveToStmt(u));
e.body = o.concat(e.body), delete e.directives;
}
pushClassMethod(e, s, i, a, n2, o) {
this.parseMethod(s, i, a, n2, o, "ClassMethod", true), s.typeParameters && (s.value.typeParameters = s.typeParameters, delete s.typeParameters), e.body.push(s);
}
parsePrivateName() {
let e = super.parsePrivateName();
return this.getPluginOption("estree", "classFeatures") ? this.convertPrivateNameToPrivateIdentifier(e) : e;
}
convertPrivateNameToPrivateIdentifier(e) {
let s = super.getPrivateNameSV(e);
return e = e, delete e.id, e.name = s, e.type = "PrivateIdentifier", e;
}
isPrivateName(e) {
return this.getPluginOption("estree", "classFeatures") ? e.type === "PrivateIdentifier" : super.isPrivateName(e);
}
getPrivateNameSV(e) {
return this.getPluginOption("estree", "classFeatures") ? e.name : super.getPrivateNameSV(e);
}
parseLiteral(e, s) {
let i = super.parseLiteral(e, s);
return i.raw = i.extra.raw, delete i.extra, i;
}
parseFunctionBody(e, s) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
super.parseFunctionBody(e, s, i), e.expression = e.body.type !== "BlockStatement";
}
parseMethod(e, s, i, a, n2, o) {
let u = arguments.length > 6 && arguments[6] !== void 0 ? arguments[6] : false, c = this.startNode();
return c.kind = e.kind, c = super.parseMethod(c, s, i, a, n2, o, u), c.type = "FunctionExpression", delete c.kind, e.value = c, o === "ClassPrivateMethod" && (e.computed = false), this.finishNode(e, "MethodDefinition");
}
parseClassProperty() {
let e = super.parseClassProperty(...arguments);
return this.getPluginOption("estree", "classFeatures") && (e.type = "PropertyDefinition"), e;
}
parseClassPrivateProperty() {
let e = super.parseClassPrivateProperty(...arguments);
return this.getPluginOption("estree", "classFeatures") && (e.type = "PropertyDefinition", e.computed = false), e;
}
parseObjectMethod(e, s, i, a, n2) {
let o = super.parseObjectMethod(e, s, i, a, n2);
return o && (o.type = "Property", o.kind === "method" && (o.kind = "init"), o.shorthand = false), o;
}
parseObjectProperty(e, s, i, a) {
let n2 = super.parseObjectProperty(e, s, i, a);
return n2 && (n2.kind = "init", n2.type = "Property"), n2;
}
isValidLVal(e, s, i) {
return e === "Property" ? "value" : super.isValidLVal(e, s, i);
}
isAssignable(e, s) {
return e != null && this.isObjectProperty(e) ? this.isAssignable(e.value, s) : super.isAssignable(e, s);
}
toAssignable(e) {
let s = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
if (e != null && this.isObjectProperty(e)) {
let { key: i, value: a } = e;
this.isPrivateName(i) && this.classScope.usePrivateName(this.getPrivateNameSV(i), i.loc.start), this.toAssignable(a, s);
} else
super.toAssignable(e, s);
}
toAssignableObjectExpressionProp(e, s, i) {
e.kind === "get" || e.kind === "set" ? this.raise(f.PatternHasAccessor, { at: e.key }) : e.method ? this.raise(f.PatternHasMethod, { at: e.key }) : super.toAssignableObjectExpressionProp(e, s, i);
}
finishCallExpression(e, s) {
let i = super.finishCallExpression(e, s);
if (i.callee.type === "Import") {
if (i.type = "ImportExpression", i.source = i.arguments[0], this.hasPlugin("importAssertions")) {
var a;
i.attributes = (a = i.arguments[1]) != null ? a : null;
}
delete i.arguments, delete i.callee;
}
return i;
}
toReferencedArguments(e) {
e.type !== "ImportExpression" && super.toReferencedArguments(e);
}
parseExport(e, s) {
let i = this.state.lastTokStartLoc, a = super.parseExport(e, s);
switch (a.type) {
case "ExportAllDeclaration":
a.exported = null;
break;
case "ExportNamedDeclaration":
a.specifiers.length === 1 && a.specifiers[0].type === "ExportNamespaceSpecifier" && (a.type = "ExportAllDeclaration", a.exported = a.specifiers[0].exported, delete a.specifiers);
case "ExportDefaultDeclaration":
{
var n2;
let { declaration: o } = a;
(o == null ? void 0 : o.type) === "ClassDeclaration" && ((n2 = o.decorators) == null ? void 0 : n2.length) > 0 && o.start === a.start && this.resetStartLocation(a, i);
}
break;
}
return a;
}
parseSubscript(e, s, i, a) {
let n2 = super.parseSubscript(e, s, i, a);
if (a.optionalChainMember) {
if ((n2.type === "OptionalMemberExpression" || n2.type === "OptionalCallExpression") && (n2.type = n2.type.substring(8)), a.stop) {
let o = this.startNodeAtNode(n2);
return o.expression = n2, this.finishNode(o, "ChainExpression");
}
} else
(n2.type === "MemberExpression" || n2.type === "CallExpression") && (n2.optional = false);
return n2;
}
hasPropertyAsPrivateName(e) {
return e.type === "ChainExpression" && (e = e.expression), super.hasPropertyAsPrivateName(e);
}
isObjectProperty(e) {
return e.type === "Property" && e.kind === "init" && !e.method;
}
isObjectMethod(e) {
return e.method || e.kind === "get" || e.kind === "set";
}
finishNodeAt(e, s, i) {
return ze(super.finishNodeAt(e, s, i));
}
resetStartLocation(e, s) {
super.resetStartLocation(e, s), ze(e);
}
resetEndLocation(e) {
let s = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : this.state.lastTokEndLoc;
super.resetEndLocation(e, s), ze(e);
}
}, Vt = "\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0370-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u048A-\u052F\u0531-\u0556\u0559\u0560-\u0588\u05D0-\u05EA\u05EF-\u05F2\u0620-\u064A\u066E\u066F\u0671-\u06D3\u06D5\u06E5\u06E6\u06EE\u06EF\u06FA-\u06FC\u06FF\u0710\u0712-\u072F\u074D-\u07A5\u07B1\u07CA-\u07EA\u07F4\u07F5\u07FA\u0800-\u0815\u081A\u0824\u0828\u0840-\u0858\u0860-\u086A\u0870-\u0887\u0889-\u088E\u08A0-\u08C9\u0904-\u0939\u093D\u0950\u0958-\u0961\u0971-\u0980\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD\u09CE\u09DC\u09DD\u09DF-\u09E1\u09F0\u09F1\u09FC\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A59-\u0A5C\u0A5E\u0A72-\u0A74\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABD\u0AD0\u0AE0\u0AE1\u0AF9\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3D\u0B5C\u0B5D\u0B5F-\u0B61\u0B71\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BD0\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C58-\u0C5A\u0C5D\u0C60\u0C61\u0C80\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CDD\u0CDE\u0CE0\u0CE1\u0CF1\u0CF2\u0D04-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D\u0D4E\u0D54-\u0D56\u0D5F-\u0D61\u0D7A-\u0D7F\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0E01-\u0E30\u0E32\u0E33\u0E40-\u0E46\u0E81\u0E82\u0E84\u0E86-\u0E8A\u0E8C-\u0EA3\u0EA5\u0EA7-\u0EB0\u0EB2\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6\u0EDC-\u0EDF\u0F00\u0F40-\u0F47\u0F49-\u0F6C\u0F88-\u0F8C\u1000-\u102A\u103F\u1050-\u1055\u105A-\u105D\u1061\u1065\u1066\u106E-\u1070\u1075-\u1081\u108E\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u1711\u171F-\u1731\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17D7\u17DC\u1820-\u1878\u1880-\u18A8\u18AA\u18B0-\u18F5\u1900-\u191E\u1950-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u1A00-\u1A16\u1A20-\u1A54\u1AA7\u1B05-\u1B33\u1B45-\u1B4C\u1B83-\u1BA0\u1BAE\u1BAF\u1BBA-\u1BE5\u1C00-\u1C23\u1C4D-\u1C4F\u1C5A-\u1C7D\u1C80-\u1C88\u1C90-\u1CBA\u1CBD-\u1CBF\u1CE9-\u1CEC\u1CEE-\u1CF3\u1CF5\u1CF6\u1CFA\u1D00-\u1DBF\u1E00-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2118-\u211D\u2124\u2126\u2128\u212A-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2CE4\u2CEB-\u2CEE\u2CF2\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u3005-\u3007\u3021-\u3029\u3031-\u3035\u3038-\u303C\u3041-\u3096\u309B-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312F\u3131-\u318E\u31A0-\u31BF\u31F0-\u31FF\u3400-\u4DBF\u4E00-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA61F\uA62A\uA62B\uA640-\uA66E\uA67F-\uA69D\uA6A0-\uA6EF\uA717-\uA71F\uA722-\uA788\uA78B-\uA7CA\uA7D0\uA7D1\uA7D3\uA7D5-\uA7D9\uA7F2-\uA801\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA840-\uA873\uA882-\uA8B3\uA8F2-\uA8F7\uA8FB\uA8FD\uA8FE\uA90A-\uA925\uA930-\uA946\uA960-\uA97C\uA984-\uA9B2\uA9CF\uA9E0-\uA9E4\uA9E6-\uA9EF\uA9FA-\uA9FE\uAA00-\uAA28\uAA40-\uAA42\uAA44-\uAA4B\uAA60-\uAA76\uAA7A\uAA7E-\uAAAF\uAAB1\uAAB5\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADD\uAAE0-\uAAEA\uAAF2-\uAAF4\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB69\uAB70-\uABE2\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE70-\uFE74\uFE76-\uFEFC\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC", pr = "\u200C\u200D\xB7\u0300-\u036F\u0387\u0483-\u0487\u0591-\u05BD\u05BF\u05C1\u05C2\u05C4\u05C5\u05C7\u0610-\u061A\u064B-\u0669\u0670\u06D6-\u06DC\u06DF-\u06E4\u06E7\u06E8\u06EA-\u06ED\u06F0-\u06F9\u0711\u0730-\u074A\u07A6-\u07B0\u07C0-\u07C9\u07EB-\u07F3\u07FD\u0816-\u0819\u081B-\u0823\u0825-\u0827\u0829-\u082D\u0859-\u085B\u0898-\u089F\u08CA-\u08E1\u08E3-\u0903\u093A-\u093C\u093E-\u094F\u0951-\u0957\u0962\u0963\u0966-\u096F\u0981-\u0983\u09BC\u09BE-\u09C4\u09C7\u09C8\u09CB-\u09CD\u09D7\u09E2\u09E3\u09E6-\u09EF\u09FE\u0A01-\u0A03\u0A3C\u0A3E-\u0A42\u0A47\u0A48\u0A4B-\u0A4D\u0A51\u0A66-\u0A71\u0A75\u0A81-\u0A83\u0ABC\u0ABE-\u0AC5\u0AC7-\u0AC9\u0ACB-\u0ACD\u0AE2\u0AE3\u0AE6-\u0AEF\u0AFA-\u0AFF\u0B01-\u0B03\u0B3C\u0B3E-\u0B44\u0B47\u0B48\u0B4B-\u0B4D\u0B55-\u0B57\u0B62\u0B63\u0B66-\u0B6F\u0B82\u0BBE-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCD\u0BD7\u0BE6-\u0BEF\u0C00-\u0C04\u0C3C\u0C3E-\u0C44\u0C46-\u0C48\u0C4A-\u0C4D\u0C55\u0C56\u0C62\u0C63\u0C66-\u0C6F\u0C81-\u0C83\u0CBC\u0CBE-\u0CC4\u0CC6-\u0CC8\u0CCA-\u0CCD\u0CD5\u0CD6\u0CE2\u0CE3\u0CE6-\u0CEF\u0CF3\u0D00-\u0D03\u0D3B\u0D3C\u0D3E-\u0D44\u0D46-\u0D48\u0D4A-\u0D4D\u0D57\u0D62\u0D63\u0D66-\u0D6F\u0D81-\u0D83\u0DCA\u0DCF-\u0DD4\u0DD6\u0DD8-\u0DDF\u0DE6-\u0DEF\u0DF2\u0DF3\u0E31\u0E34-\u0E3A\u0E47-\u0E4E\u0E50-\u0E59\u0EB1\u0EB4-\u0EBC\u0EC8-\u0ECE\u0ED0-\u0ED9\u0F18\u0F19\u0F20-\u0F29\u0F35\u0F37\u0F39\u0F3E\u0F3F\u0F71-\u0F84\u0F86\u0F87\u0F8D-\u0F97\u0F99-\u0FBC\u0FC6\u102B-\u103E\u1040-\u1049\u1056-\u1059\u105E-\u1060\u1062-\u1064\u1067-\u106D\u1071-\u1074\u1082-\u108D\u108F-\u109D\u135D-\u135F\u1369-\u1371\u1712-\u1715\u1732-\u1734\u1752\u1753\u1772\u1773\u17B4-\u17D3\u17DD\u17E0-\u17E9\u180B-\u180D\u180F-\u1819\u18A9\u1920-\u192B\u1930-\u193B\u1946-\u194F\u19D0-\u19DA\u1A17-\u1A1B\u1A55-\u1A5E\u1A60-\u1A7C\u1A7F-\u1A89\u1A90-\u1A99\u1AB0-\u1ABD\u1ABF-\u1ACE\u1B00-\u1B04\u1B34-\u1B44\u1B50-\u1B59\u1B6B-\u1B73\u1B80-\u1B82\u1BA1-\u1BAD\u1BB0-\u1BB9\u1BE6-\u1BF3\u1C24-\u1C37\u1C40-\u1C49\u1C50-\u1C59\u1CD0-\u1CD2\u1CD4-\u1CE8\u1CED\u1CF4\u1CF7-\u1CF9\u1DC0-\u1DFF\u203F\u2040\u2054\u20D0-\u20DC\u20E1\u20E5-\u20F0\u2CEF-\u2CF1\u2D7F\u2DE0-\u2DFF\u302A-\u302F\u3099\u309A\uA620-\uA629\uA66F\uA674-\uA67D\uA69E\uA69F\uA6F0\uA6F1\uA802\uA806\uA80B\uA823-\uA827\uA82C\uA880\uA881\uA8B4-\uA8C5\uA8D0-\uA8D9\uA8E0-\uA8F1\uA8FF-\uA909\uA926-\uA92D\uA947-\uA953\uA980-\uA983\uA9B3-\uA9C0\uA9D0-\uA9D9\uA9E5\uA9F0-\uA9F9\uAA29-\uAA36\uAA43\uAA4C\uAA4D\uAA50-\uAA59\uAA7B-\uAA7D\uAAB0\uAAB2-\uAAB4\uAAB7\uAAB8\uAABE\uAABF\uAAC1\uAAEB-\uAAEF\uAAF5\uAAF6\uABE3-\uABEA\uABEC\uABED\uABF0-\uABF9\uFB1E\uFE00-\uFE0F\uFE20-\uFE2F\uFE33\uFE34\uFE4D-\uFE4F\uFF10-\uFF19\uFF3F", tl = new RegExp("[" + Vt + "]"), sl = new RegExp("[" + Vt + pr + "]");
Vt = pr = null;
var fr = [0, 11, 2, 25, 2, 18, 2, 1, 2, 14, 3, 13, 35, 122, 70, 52, 268, 28, 4, 48, 48, 31, 14, 29, 6, 37, 11, 29, 3, 35, 5, 7, 2, 4, 43, 157, 19, 35, 5, 35, 5, 39, 9, 51, 13, 10, 2, 14, 2, 6, 2, 1, 2, 10, 2, 14, 2, 6, 2, 1, 68, 310, 10, 21, 11, 7, 25, 5, 2, 41, 2, 8, 70, 5, 3, 0, 2, 43, 2, 1, 4, 0, 3, 22, 11, 22, 10, 30, 66, 18, 2, 1, 11, 21, 11, 25, 71, 55, 7, 1, 65, 0, 16, 3, 2, 2, 2, 28, 43, 28, 4, 28, 36, 7, 2, 27, 28, 53, 11, 21, 11, 18, 14, 17, 111, 72, 56, 50, 14, 50, 14, 35, 349, 41, 7, 1, 79, 28, 11, 0, 9, 21, 43, 17, 47, 20, 28, 22, 13, 52, 58, 1, 3, 0, 14, 44, 33, 24, 27, 35, 30, 0, 3, 0, 9, 34, 4, 0, 13, 47, 15, 3, 22, 0, 2, 0, 36, 17, 2, 24, 20, 1, 64, 6, 2, 0, 2, 3, 2, 14, 2, 9, 8, 46, 39, 7, 3, 1, 3, 21, 2, 6, 2, 1, 2, 4, 4, 0, 19, 0, 13, 4, 159, 52, 19, 3, 21, 2, 31, 47, 21, 1, 2, 0, 185, 46, 42, 3, 37, 47, 21, 0, 60, 42, 14, 0, 72, 26, 38, 6, 186, 43, 117, 63, 32, 7, 3, 0, 3, 7, 2, 1, 2, 23, 16, 0, 2, 0, 95, 7, 3, 38, 17, 0, 2, 0, 29, 0, 11, 39, 8, 0, 22, 0, 12, 45, 20, 0, 19, 72, 264, 8, 2, 36, 18, 0, 50, 29, 113, 6, 2, 1, 2, 37, 22, 0, 26, 5, 2, 1, 2, 31, 15, 0, 328, 18, 16, 0, 2, 12, 2, 33, 125, 0, 80, 921, 103, 110, 18, 195, 2637, 96, 16, 1071, 18, 5, 4026, 582, 8634, 568, 8, 30, 18, 78, 18, 29, 19, 47, 17, 3, 32, 20, 6, 18, 689, 63, 129, 74, 6, 0, 67, 12, 65, 1, 2, 0, 29, 6135, 9, 1237, 43, 8, 8936, 3, 2, 6, 2, 1, 2, 290, 16, 0, 30, 2, 3, 0, 15, 3, 9, 395, 2309, 106, 6, 12, 4, 8, 8, 9, 5991, 84, 2, 70, 2, 1, 3, 0, 3, 1, 3, 3, 2, 11, 2, 0, 2, 6, 2, 64, 2, 3, 3, 7, 2, 6, 2, 27, 2, 3, 2, 4, 2, 0, 4, 6, 2, 339, 3, 24, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 7, 1845, 30, 7, 5, 262, 61, 147, 44, 11, 6, 17, 0, 322, 29, 19, 43, 485, 27, 757, 6, 2, 3, 2, 1, 2, 14, 2, 196, 60, 67, 8, 0, 1205, 3, 2, 26, 2, 1, 2, 0, 3, 0, 2, 9, 2, 3, 2, 0, 2, 0, 7, 0, 5, 0, 2, 0, 2, 0, 2, 2, 2, 1, 2, 0, 3, 0, 2, 0, 2, 0, 2, 0, 2, 0, 2, 1, 2, 0, 3, 3, 2, 6, 2, 3, 2, 3, 2, 0, 2, 9, 2, 16, 6, 2, 2, 4, 2, 16, 4421, 42719, 33, 4153, 7, 221, 3, 5761, 15, 7472, 3104, 541, 1507, 4938, 6, 4191], rl = [509, 0, 227, 0, 150, 4, 294, 9, 1368, 2, 2, 1, 6, 3, 41, 2, 5, 0, 166, 1, 574, 3, 9, 9, 370, 1, 81, 2, 71, 10, 50, 3, 123, 2, 54, 14, 32, 10, 3, 1, 11, 3, 46, 10, 8, 0, 46, 9, 7, 2, 37, 13, 2, 9, 6, 1, 45, 0, 13, 2, 49, 13, 9, 3, 2, 11, 83, 11, 7, 0, 3, 0, 158, 11, 6, 9, 7, 3, 56, 1, 2, 6, 3, 1, 3, 2, 10, 0, 11, 1, 3, 6, 4, 4, 193, 17, 10, 9, 5, 0, 82, 19, 13, 9, 214, 6, 3, 8, 28, 1, 83, 16, 16, 9, 82, 12, 9, 9, 84, 14, 5, 9, 243, 14, 166, 9, 71, 5, 2, 1, 3, 3, 2, 0, 2, 1, 13, 9, 120, 6, 3, 6, 4, 0, 29, 9, 41, 6, 2, 3, 9, 0, 10, 10, 47, 15, 406, 7, 2, 7, 17, 9, 57, 21, 2, 13, 123, 5, 4, 0, 2, 1, 2, 6, 2, 0, 9, 9, 49, 4, 2, 1, 2, 4, 9, 9, 330, 3, 10, 1, 2, 0, 49, 6, 4, 4, 14, 9, 5351, 0, 7, 14, 13835, 9, 87, 9, 39, 4, 60, 6, 26, 9, 1014, 0, 2, 54, 8, 3, 82, 0, 12, 1, 19628, 1, 4706, 45, 3, 22, 543, 4, 4, 5, 9, 7, 3, 6, 31, 3, 149, 2, 1418, 49, 513, 54, 5, 49, 9, 0, 15, 0, 23, 4, 2, 14, 1361, 6, 2, 16, 3, 6, 2, 1, 2, 4, 101, 0, 161, 6, 10, 9, 357, 0, 62, 13, 499, 13, 983, 6, 110, 6, 6, 9, 4759, 9, 787719, 239];
function Kt(t, r) {
let e = 65536;
for (let s = 0, i = r.length; s < i; s += 2) {
if (e += r[s], e > t)
return false;
if (e += r[s + 1], e >= t)
return true;
}
return false;
}
function fe(t) {
return t < 65 ? t === 36 : t <= 90 ? true : t < 97 ? t === 95 : t <= 122 ? true : t <= 65535 ? t >= 170 && tl.test(String.fromCharCode(t)) : Kt(t, fr);
}
function De(t) {
return t < 48 ? t === 36 : t < 58 ? true : t < 65 ? false : t <= 90 ? true : t < 97 ? t === 95 : t <= 122 ? true : t <= 65535 ? t >= 170 && sl.test(String.fromCharCode(t)) : Kt(t, fr) || Kt(t, rl);
}
var Wt = { keyword: ["break", "case", "catch", "continue", "debugger", "default", "do", "else", "finally", "for", "function", "if", "return", "switch", "throw", "try", "var", "const", "while", "with", "new", "this", "super", "class", "extends", "export", "import", "null", "true", "false", "in", "instanceof", "typeof", "void", "delete"], strict: ["implements", "interface", "let", "package", "private", "protected", "public", "static", "yield"], strictBind: ["eval", "arguments"] }, il = new Set(Wt.keyword), al = new Set(Wt.strict), nl = new Set(Wt.strictBind);
function dr(t, r) {
return r && t === "await" || t === "enum";
}
function mr(t, r) {
return dr(t, r) || al.has(t);
}
function yr(t) {
return nl.has(t);
}
function xr(t, r) {
return mr(t, r) || yr(t);
}
function ol(t) {
return il.has(t);
}
function ll(t, r, e) {
return t === 64 && r === 64 && fe(e);
}
var hl = /* @__PURE__ */ new Set(["break", "case", "catch", "continue", "debugger", "default", "do", "else", "finally", "for", "function", "if", "return", "switch", "throw", "try", "var", "const", "while", "with", "new", "this", "super", "class", "extends", "export", "import", "null", "true", "false", "in", "instanceof", "typeof", "void", "delete", "implements", "interface", "let", "package", "private", "protected", "public", "static", "yield", "eval", "arguments", "enum", "await"]);
function ul(t) {
return hl.has(t);
}
var Fe = 0, Le = 1, de = 2, Gt = 4, gr = 8, ht = 16, Pr = 32, Ee = 64, ut = 128, Oe = 256, ct = Le | de | ut | Oe, le = 1, Ce = 2, Ar = 4, be = 8, pt = 16, Tr = 64, ft = 128, Jt = 256, Xt = 512, Yt = 1024, Qt = 2048, Ve = 4096, dt = 8192, vr = le | Ce | be | ft | dt, Be = le | 0 | be | dt, cl = le | 0 | be | 0, mt = le | 0 | Ar | 0, Er = le | 0 | pt | 0, pl = 0 | Ce | 0 | ft, fl = 0 | Ce | 0 | 0, Cr = le | Ce | be | Jt | dt, br = 0 | Yt, Pe = 0 | Tr, dl = le | 0 | 0 | Tr, ml = Cr | Xt, yl = 0 | Yt, Sr = 0 | Ce | 0 | Ve, xl = Qt, yt = 4, Zt = 2, es = 1, ts = Zt | es, gl = Zt | yt, Pl = es | yt, Al = Zt, Tl = es, ss = 0, rs = class {
constructor(t) {
this.var = /* @__PURE__ */ new Set(), this.lexical = /* @__PURE__ */ new Set(), this.functions = /* @__PURE__ */ new Set(), this.flags = t;
}
}, is = class {
constructor(t, r) {
this.parser = void 0, this.scopeStack = [], this.inModule = void 0, this.undefinedExports = /* @__PURE__ */ new Map(), this.parser = t, this.inModule = r;
}
get inTopLevel() {
return (this.currentScope().flags & Le) > 0;
}
get inFunction() {
return (this.currentVarScopeFlags() & de) > 0;
}
get allowSuper() {
return (this.currentThisScopeFlags() & ht) > 0;
}
get allowDirectSuper() {
return (this.currentThisScopeFlags() & Pr) > 0;
}
get inClass() {
return (this.currentThisScopeFlags() & Ee) > 0;
}
get inClassAndNotInNonArrowFunction() {
let t = this.currentThisScopeFlags();
return (t & Ee) > 0 && (t & de) === 0;
}
get inStaticBlock() {
for (let t = this.scopeStack.length - 1; ; t--) {
let { flags: r } = this.scopeStack[t];
if (r & ut)
return true;
if (r & (ct | Ee))
return false;
}
}
get inNonArrowFunction() {
return (this.currentThisScopeFlags() & de) > 0;
}
get treatFunctionsAsVar() {
return this.treatFunctionsAsVarInScope(this.currentScope());
}
createScope(t) {
return new rs(t);
}
enter(t) {
this.scopeStack.push(this.createScope(t));
}
exit() {
return this.scopeStack.pop().flags;
}
treatFunctionsAsVarInScope(t) {
return !!(t.flags & (de | ut) || !this.parser.inModule && t.flags & Le);
}
declareName(t, r, e) {
let s = this.currentScope();
if (r & be || r & pt)
this.checkRedeclarationInScope(s, t, r, e), r & pt ? s.functions.add(t) : s.lexical.add(t), r & be && this.maybeExportDefined(s, t);
else if (r & Ar)
for (let i = this.scopeStack.length - 1; i >= 0 && (s = this.scopeStack[i], this.checkRedeclarationInScope(s, t, r, e), s.var.add(t), this.maybeExportDefined(s, t), !(s.flags & ct)); --i)
;
this.parser.inModule && s.flags & Le && this.undefinedExports.delete(t);
}
maybeExportDefined(t, r) {
this.parser.inModule && t.flags & Le && this.undefinedExports.delete(r);
}
checkRedeclarationInScope(t, r, e, s) {
this.isRedeclaredInScope(t, r, e) && this.parser.raise(f.VarRedeclaration, { at: s, identifierName: r });
}
isRedeclaredInScope(t, r, e) {
return e & le ? e & be ? t.lexical.has(r) || t.functions.has(r) || t.var.has(r) : e & pt ? t.lexical.has(r) || !this.treatFunctionsAsVarInScope(t) && t.var.has(r) : t.lexical.has(r) && !(t.flags & gr && t.lexical.values().next().value === r) || !this.treatFunctionsAsVarInScope(t) && t.functions.has(r) : false;
}
checkLocalExport(t) {
let { name: r } = t, e = this.scopeStack[0];
!e.lexical.has(r) && !e.var.has(r) && !e.functions.has(r) && this.undefinedExports.set(r, t.loc.start);
}
currentScope() {
return this.scopeStack[this.scopeStack.length - 1];
}
currentVarScopeFlags() {
for (let t = this.scopeStack.length - 1; ; t--) {
let { flags: r } = this.scopeStack[t];
if (r & ct)
return r;
}
}
currentThisScopeFlags() {
for (let t = this.scopeStack.length - 1; ; t--) {
let { flags: r } = this.scopeStack[t];
if (r & (ct | Ee) && !(r & Gt))
return r;
}
}
}, vl = class extends rs {
constructor() {
super(...arguments), this.declareFunctions = /* @__PURE__ */ new Set();
}
}, El = class extends is {
createScope(t) {
return new vl(t);
}
declareName(t, r, e) {
let s = this.currentScope();
if (r & Qt) {
this.checkRedeclarationInScope(s, t, r, e), this.maybeExportDefined(s, t), s.declareFunctions.add(t);
return;
}
super.declareName(t, r, e);
}
isRedeclaredInScope(t, r, e) {
return super.isRedeclaredInScope(t, r, e) ? true : e & Qt ? !t.declareFunctions.has(r) && (t.lexical.has(r) || t.functions.has(r)) : false;
}
checkLocalExport(t) {
this.scopeStack[0].declareFunctions.has(t.name) || super.checkLocalExport(t);
}
}, Cl = class {
constructor() {
this.sawUnambiguousESM = false, this.ambiguousScriptDifferentAst = false;
}
hasPlugin(t) {
if (typeof t == "string")
return this.plugins.has(t);
{
let [r, e] = t;
if (!this.hasPlugin(r))
return false;
let s = this.plugins.get(r);
for (let i of Object.keys(e))
if ((s == null ? void 0 : s[i]) !== e[i])
return false;
return true;
}
}
getPluginOption(t, r) {
var e;
return (e = this.plugins.get(t)) == null ? void 0 : e[r];
}
};
function wr(t, r) {
t.trailingComments === void 0 ? t.trailingComments = r : t.trailingComments.unshift(...r);
}
function bl(t, r) {
t.leadingComments === void 0 ? t.leadingComments = r : t.leadingComments.unshift(...r);
}
function Ke(t, r) {
t.innerComments === void 0 ? t.innerComments = r : t.innerComments.unshift(...r);
}
function We(t, r, e) {
let s = null, i = r.length;
for (; s === null && i > 0; )
s = r[--i];
s === null || s.start > e.start ? Ke(t, e.comments) : wr(s, e.comments);
}
var Sl = class extends Cl {
addComment(t) {
this.filename && (t.loc.filename = this.filename), this.state.comments.push(t);
}
processComment(t) {
let { commentStack: r } = this.state, e = r.length;
if (e === 0)
return;
let s = e - 1, i = r[s];
i.start === t.end && (i.leadingNode = t, s--);
let { start: a } = t;
for (; s >= 0; s--) {
let n2 = r[s], o = n2.end;
if (o > a)
n2.containingNode = t, this.finalizeComment(n2), r.splice(s, 1);
else {
o === a && (n2.trailingNode = t);
break;
}
}
}
finalizeComment(t) {
let { comments: r } = t;
if (t.leadingNode !== null || t.trailingNode !== null)
t.leadingNode !== null && wr(t.leadingNode, r), t.trailingNode !== null && bl(t.trailingNode, r);
else {
let { containingNode: e, start: s } = t;
if (this.input.charCodeAt(s - 1) === 44)
switch (e.type) {
case "ObjectExpression":
case "ObjectPattern":
case "RecordExpression":
We(e, e.properties, t);
break;
case "CallExpression":
case "OptionalCallExpression":
We(e, e.arguments, t);
break;
case "FunctionDeclaration":
case "FunctionExpression":
case "ArrowFunctionExpression":
case "ObjectMethod":
case "ClassMethod":
case "ClassPrivateMethod":
We(e, e.params, t);
break;
case "ArrayExpression":
case "ArrayPattern":
case "TupleExpression":
We(e, e.elements, t);
break;
case "ExportNamedDeclaration":
case "ImportDeclaration":
We(e, e.specifiers, t);
break;
default:
Ke(e, r);
}
else
Ke(e, r);
}
}
finalizeRemainingComments() {
let { commentStack: t } = this.state;
for (let r = t.length - 1; r >= 0; r--)
this.finalizeComment(t[r]);
this.state.commentStack = [];
}
resetPreviousNodeTrailingComments(t) {
let { commentStack: r } = this.state, { length: e } = r;
if (e === 0)
return;
let s = r[e - 1];
s.leadingNode === t && (s.leadingNode = null);
}
takeSurroundingComments(t, r, e) {
let { commentStack: s } = this.state, i = s.length;
if (i === 0)
return;
let a = i - 1;
for (; a >= 0; a--) {
let n2 = s[a], o = n2.end;
if (n2.start === e)
n2.leadingNode = t;
else if (o === r)
n2.trailingNode = t;
else if (o < r)
break;
}
}
}, as = /\r\n?|[\n\u2028\u2029]/, xt = new RegExp(as.source, "g");
function Ge(t) {
switch (t) {
case 10:
case 13:
case 8232:
case 8233:
return true;
default:
return false;
}
}
var ns = /(?:\s|\/\/.*|\/\*[^]*?\*\/)*/g, wl = /(?:[^\S\n\r\u2028\u2029]|\/\/.*|\/\*.*?\*\/)*/y, Ir = new RegExp("(?=(" + wl.source + "))\\1" + /(?=[\n\r\u2028\u2029]|\/\*(?!.*?\*\/)|$)/.source, "y");
function Il(t) {
switch (t) {
case 9:
case 11:
case 12:
case 32:
case 160:
case 5760:
case 8192:
case 8193:
case 8194:
case 8195:
case 8196:
case 8197:
case 8198:
case 8199:
case 8200:
case 8201:
case 8202:
case 8239:
case 8287:
case 12288:
case 65279:
return true;
default:
return false;
}
}
var Nr = class {
constructor() {
this.strict = void 0, this.curLine = void 0, this.lineStart = void 0, this.startLoc = void 0, this.endLoc = void 0, this.errors = [], this.potentialArrowAt = -1, this.noArrowAt = [], this.noArrowParamsConversionAt = [], this.maybeInArrowParameters = false, this.inType = false, this.noAnonFunctionType = false, this.hasFlowComment = false, this.isAmbientContext = false, this.inAbstractClass = false, this.inDisallowConditionalTypesContext = false, this.topicContext = { maxNumOfResolvableTopics: 0, maxTopicIndex: null }, this.soloAwait = false, this.inFSharpPipelineDirectBody = false, this.labels = [], this.comments = [], this.commentStack = [], this.pos = 0, this.type = 137, this.value = null, this.start = 0, this.end = 0, this.lastTokEndLoc = null, this.lastTokStartLoc = null, this.lastTokStart = 0, this.context = [x.brace], this.canStartJSXElement = true, this.containsEsc = false, this.firstInvalidTemplateEscapePos = null, this.strictErrors = /* @__PURE__ */ new Map(), this.tokensLength = 0;
}
init(t) {
let { strictMode: r, sourceType: e, startLine: s, startColumn: i } = t;
this.strict = r === false ? false : r === true ? true : e === "module", this.curLine = s, this.lineStart = -i, this.startLoc = this.endLoc = new ge(s, i, 0);
}
curPosition() {
return new ge(this.curLine, this.pos - this.lineStart, this.pos);
}
clone(t) {
let r = new Nr(), e = Object.keys(this);
for (let s = 0, i = e.length; s < i; s++) {
let a = e[s], n2 = this[a];
!t && Array.isArray(n2) && (n2 = n2.slice()), r[a] = n2;
}
return r;
}
}, Nl = function(r) {
return r >= 48 && r <= 57;
}, kr = { decBinOct: /* @__PURE__ */ new Set([46, 66, 69, 79, 95, 98, 101, 111]), hex: /* @__PURE__ */ new Set([46, 88, 95, 120]) }, gt = { bin: (t) => t === 48 || t === 49, oct: (t) => t >= 48 && t <= 55, dec: (t) => t >= 48 && t <= 57, hex: (t) => t >= 48 && t <= 57 || t >= 65 && t <= 70 || t >= 97 && t <= 102 };
function Dr(t, r, e, s, i, a) {
let n2 = e, o = s, u = i, c = "", y = null, g = e, { length: T } = r;
for (; ; ) {
if (e >= T) {
a.unterminated(n2, o, u), c += r.slice(g, e);
break;
}
let C = r.charCodeAt(e);
if (kl(t, C, r, e)) {
c += r.slice(g, e);
break;
}
if (C === 92) {
c += r.slice(g, e);
let M = Dl(r, e, s, i, t === "template", a);
M.ch === null && !y ? y = { pos: e, lineStart: s, curLine: i } : c += M.ch, { pos: e, lineStart: s, curLine: i } = M, g = e;
} else
C === 8232 || C === 8233 ? (++e, ++i, s = e) : C === 10 || C === 13 ? t === "template" ? (c += r.slice(g, e) + `
`, ++e, C === 13 && r.charCodeAt(e) === 10 && ++e, ++i, g = s = e) : a.unterminated(n2, o, u) : ++e;
}
return { pos: e, str: c, firstInvalidLoc: y, lineStart: s, curLine: i, containsInvalid: !!y };
}
function kl(t, r, e, s) {
return t === "template" ? r === 96 || r === 36 && e.charCodeAt(s + 1) === 123 : r === (t === "double" ? 34 : 39);
}
function Dl(t, r, e, s, i, a) {
let n2 = !i;
r++;
let o = (c) => ({ pos: r, ch: c, lineStart: e, curLine: s }), u = t.charCodeAt(r++);
switch (u) {
case 110:
return o(`
`);
case 114:
return o("\r");
case 120: {
let c;
return { code: c, pos: r } = os(t, r, e, s, 2, false, n2, a), o(c === null ? null : String.fromCharCode(c));
}
case 117: {
let c;
return { code: c, pos: r } = Lr(t, r, e, s, n2, a), o(c === null ? null : String.fromCodePoint(c));
}
case 116:
return o(" ");
case 98:
return o("\b");
case 118:
return o("\v");
case 102:
return o("\f");
case 13:
t.charCodeAt(r) === 10 && ++r;
case 10:
e = r, ++s;
case 8232:
case 8233:
return o("");
case 56:
case 57:
if (i)
return o(null);
a.strictNumericEscape(r - 1, e, s);
default:
if (u >= 48 && u <= 55) {
let c = r - 1, g = t.slice(c, r + 2).match(/^[0-7]+/)[0], T = parseInt(g, 8);
T > 255 && (g = g.slice(0, -1), T = parseInt(g, 8)), r += g.length - 1;
let C = t.charCodeAt(r);
if (g !== "0" || C === 56 || C === 57) {
if (i)
return o(null);
a.strictNumericEscape(c, e, s);
}
return o(String.fromCharCode(T));
}
return o(String.fromCharCode(u));
}
}
function os(t, r, e, s, i, a, n2, o) {
let u = r, c;
return { n: c, pos: r } = Fr(t, r, e, s, 16, i, a, false, o, !n2), c === null && (n2 ? o.invalidEscapeSequence(u, e, s) : r = u - 1), { code: c, pos: r };
}
function Fr(t, r, e, s, i, a, n2, o, u, c) {
let y = r, g = i === 16 ? kr.hex : kr.decBinOct, T = i === 16 ? gt.hex : i === 10 ? gt.dec : i === 8 ? gt.oct : gt.bin, C = false, M = 0;
for (let j = 0, K = a == null ? 1 / 0 : a; j < K; ++j) {
let W = t.charCodeAt(r), V;
if (W === 95 && o !== "bail") {
let X = t.charCodeAt(r - 1), je = t.charCodeAt(r + 1);
if (o) {
if (Number.isNaN(je) || !T(je) || g.has(X) || g.has(je)) {
if (c)
return { n: null, pos: r };
u.unexpectedNumericSeparator(r, e, s);
}
} else {
if (c)
return { n: null, pos: r };
u.numericSeparatorInEscapeSequence(r, e, s);
}
++r;
continue;
}
if (W >= 97 ? V = W - 97 + 10 : W >= 65 ? V = W - 65 + 10 : Nl(W) ? V = W - 48 : V = 1 / 0, V >= i) {
if (V <= 9 && c)
return { n: null, pos: r };
if (V <= 9 && u.invalidDigit(r, e, s, i))
V = 0;
else if (n2)
V = 0, C = true;
else
break;
}
++r, M = M * i + V;
}
return r === y || a != null && r - y !== a || C ? { n: null, pos: r } : { n: M, pos: r };
}
function Lr(t, r, e, s, i, a) {
let n2 = t.charCodeAt(r), o;
if (n2 === 123) {
if (++r, { code: o, pos: r } = os(t, r, e, s, t.indexOf("}", r) - r, true, i, a), ++r, o !== null && o > 1114111)
if (i)
a.invalidCodePoint(r, e, s);
else
return { code: null, pos: r };
} else
({ code: o, pos: r } = os(t, r, e, s, 4, false, i, a));
return { code: o, pos: r };
}
var Fl = ["at"], Ll = ["at"];
function Je(t, r, e) {
return new ge(e, t - r, t);
}
var Ol = /* @__PURE__ */ new Set([103, 109, 115, 105, 121, 117, 100, 118]), Ae = class {
constructor(t) {
this.type = t.type, this.value = t.value, this.start = t.start, this.end = t.end, this.loc = new lt(t.startLoc, t.endLoc);
}
}, Bl = class extends Sl {
constructor(t, r) {
super(), this.isLookahead = void 0, this.tokens = [], this.errorHandlers_readInt = { invalidDigit: (e, s, i, a) => this.options.errorRecovery ? (this.raise(f.InvalidDigit, { at: Je(e, s, i), radix: a }), true) : false, numericSeparatorInEscapeSequence: this.errorBuilder(f.NumericSeparatorInEscapeSequence), unexpectedNumericSeparator: this.errorBuilder(f.UnexpectedNumericSeparator) }, this.errorHandlers_readCodePoint = Object.assign({}, this.errorHandlers_readInt, { invalidEscapeSequence: this.errorBuilder(f.InvalidEscapeSequence), invalidCodePoint: this.errorBuilder(f.InvalidCodePoint) }), this.errorHandlers_readStringContents_string = Object.assign({}, this.errorHandlers_readCodePoint, { strictNumericEscape: (e, s, i) => {
this.recordStrictModeErrors(f.StrictNumericEscape, { at: Je(e, s, i) });
}, unterminated: (e, s, i) => {
throw this.raise(f.UnterminatedString, { at: Je(e - 1, s, i) });
} }), this.errorHandlers_readStringContents_template = Object.assign({}, this.errorHandlers_readCodePoint, { strictNumericEscape: this.errorBuilder(f.StrictNumericEscape), unterminated: (e, s, i) => {
throw this.raise(f.UnterminatedTemplate, { at: Je(e, s, i) });
} }), this.state = new Nr(), this.state.init(t), this.input = r, this.length = r.length, this.isLookahead = false;
}
pushToken(t) {
this.tokens.length = this.state.tokensLength, this.tokens.push(t), ++this.state.tokensLength;
}
next() {
this.checkKeywordEscapes(), this.options.tokens && this.pushToken(new Ae(this.state)), this.state.lastTokStart = this.state.start, this.state.lastTokEndLoc = this.state.endLoc, this.state.lastTokStartLoc = this.state.startLoc, this.nextToken();
}
eat(t) {
return this.match(t) ? (this.next(), true) : false;
}
match(t) {
return this.state.type === t;
}
createLookaheadState(t) {
return { pos: t.pos, value: null, type: t.type, start: t.start, end: t.end, context: [this.curContext()], inType: t.inType, startLoc: t.startLoc, lastTokEndLoc: t.lastTokEndLoc, curLine: t.curLine, lineStart: t.lineStart, curPosition: t.curPosition };
}
lookahead() {
let t = this.state;
this.state = this.createLookaheadState(t), this.isLookahead = true, this.nextToken(), this.isLookahead = false;
let r = this.state;
return this.state = t, r;
}
nextTokenStart() {
return this.nextTokenStartSince(this.state.pos);
}
nextTokenStartSince(t) {
return ns.lastIndex = t, ns.test(this.input) ? ns.lastIndex : t;
}
lookaheadCharCode() {
return this.input.charCodeAt(this.nextTokenStart());
}
codePointAtPos(t) {
let r = this.input.charCodeAt(t);
if ((r & 64512) === 55296 && ++t < this.input.length) {
let e = this.input.charCodeAt(t);
(e & 64512) === 56320 && (r = 65536 + ((r & 1023) << 10) + (e & 1023));
}
return r;
}
setStrict(t) {
this.state.strict = t, t && (this.state.strictErrors.forEach((r) => {
let [e, s] = r;
return this.raise(e, { at: s });
}), this.state.strictErrors.clear());
}
curContext() {
return this.state.context[this.state.context.length - 1];
}
nextToken() {
if (this.skipSpace(), this.state.start = this.state.pos, this.isLookahead || (this.state.startLoc = this.state.curPosition()), this.state.pos >= this.length) {
this.finishToken(137);
return;
}
this.getTokenFromCode(this.codePointAtPos(this.state.pos));
}
skipBlockComment(t) {
let r;
this.isLookahead || (r = this.state.curPosition());
let e = this.state.pos, s = this.input.indexOf(t, e + 2);
if (s === -1)
throw this.raise(f.UnterminatedComment, { at: this.state.curPosition() });
for (this.state.pos = s + t.length, xt.lastIndex = e + 2; xt.test(this.input) && xt.lastIndex <= s; )
++this.state.curLine, this.state.lineStart = xt.lastIndex;
if (this.isLookahead)
return;
let i = { type: "CommentBlock", value: this.input.slice(e + 2, s), start: e, end: s + t.length, loc: new lt(r, this.state.curPosition()) };
return this.options.tokens && this.pushToken(i), i;
}
skipLineComment(t) {
let r = this.state.pos, e;
this.isLookahead || (e = this.state.curPosition());
let s = this.input.charCodeAt(this.state.pos += t);
if (this.state.pos < this.length)
for (; !Ge(s) && ++this.state.pos < this.length; )
s = this.input.charCodeAt(this.state.pos);
if (this.isLookahead)
return;
let i = this.state.pos, n2 = { type: "CommentLine", value: this.input.slice(r + t, i), start: r, end: i, loc: new lt(e, this.state.curPosition()) };
return this.options.tokens && this.pushToken(n2), n2;
}
skipSpace() {
let t = this.state.pos, r = [];
e:
for (; this.state.pos < this.length; ) {
let e = this.input.charCodeAt(this.state.pos);
switch (e) {
case 32:
case 160:
case 9:
++this.state.pos;
break;
case 13:
this.input.charCodeAt(this.state.pos + 1) === 10 && ++this.state.pos;
case 10:
case 8232:
case 8233:
++this.state.pos, ++this.state.curLine, this.state.lineStart = this.state.pos;
break;
case 47:
switch (this.input.charCodeAt(this.state.pos + 1)) {
case 42: {
let s = this.skipBlockComment("*/");
s !== void 0 && (this.addComment(s), this.options.attachComment && r.push(s));
break;
}
case 47: {
let s = this.skipLineComment(2);
s !== void 0 && (this.addComment(s), this.options.attachComment && r.push(s));
break;
}
default:
break e;
}
break;
default:
if (Il(e))
++this.state.pos;
else if (e === 45 && !this.inModule && this.options.annexB) {
let s = this.state.pos;
if (this.input.charCodeAt(s + 1) === 45 && this.input.charCodeAt(s + 2) === 62 && (t === 0 || this.state.lineStart > t)) {
let i = this.skipLineComment(3);
i !== void 0 && (this.addComment(i), this.options.attachComment && r.push(i));
} else
break e;
} else if (e === 60 && !this.inModule && this.options.annexB) {
let s = this.state.pos;
if (this.input.charCodeAt(s + 1) === 33 && this.input.charCodeAt(s + 2) === 45 && this.input.charCodeAt(s + 3) === 45) {
let i = this.skipLineComment(4);
i !== void 0 && (this.addComment(i), this.options.attachComment && r.push(i));
} else
break e;
} else
break e;
}
}
if (r.length > 0) {
let e = this.state.pos, s = { start: t, end: e, comments: r, leadingNode: null, trailingNode: null, containingNode: null };
this.state.commentStack.push(s);
}
}
finishToken(t, r) {
this.state.end = this.state.pos, this.state.endLoc = this.state.curPosition();
let e = this.state.type;
this.state.type = t, this.state.value = r, this.isLookahead || this.updateContext(e);
}
replaceToken(t) {
this.state.type = t, this.updateContext();
}
readToken_numberSign() {
if (this.state.pos === 0 && this.readToken_interpreter())
return;
let t = this.state.pos + 1, r = this.codePointAtPos(t);
if (r >= 48 && r <= 57)
throw this.raise(f.UnexpectedDigitAfterHash, { at: this.state.curPosition() });
if (r === 123 || r === 91 && this.hasPlugin("recordAndTuple")) {
if (this.expectPlugin("recordAndTuple"), this.getPluginOption("recordAndTuple", "syntaxType") === "bar")
throw this.raise(r === 123 ? f.RecordExpressionHashIncorrectStartSyntaxType : f.TupleExpressionHashIncorrectStartSyntaxType, { at: this.state.curPosition() });
this.state.pos += 2, r === 123 ? this.finishToken(7) : this.finishToken(1);
} else
fe(r) ? (++this.state.pos, this.finishToken(136, this.readWord1(r))) : r === 92 ? (++this.state.pos, this.finishToken(136, this.readWord1())) : this.finishOp(27, 1);
}
readToken_dot() {
let t = this.input.charCodeAt(this.state.pos + 1);
if (t >= 48 && t <= 57) {
this.readNumber(true);
return;
}
t === 46 && this.input.charCodeAt(this.state.pos + 2) === 46 ? (this.state.pos += 3, this.finishToken(21)) : (++this.state.pos, this.finishToken(16));
}
readToken_slash() {
this.input.charCodeAt(this.state.pos + 1) === 61 ? this.finishOp(31, 2) : this.finishOp(56, 1);
}
readToken_interpreter() {
if (this.state.pos !== 0 || this.length < 2)
return false;
let t = this.input.charCodeAt(this.state.pos + 1);
if (t !== 33)
return false;
let r = this.state.pos;
for (this.state.pos += 1; !Ge(t) && ++this.state.pos < this.length; )
t = this.input.charCodeAt(this.state.pos);
let e = this.input.slice(r + 2, this.state.pos);
return this.finishToken(28, e), true;
}
readToken_mult_modulo(t) {
let r = t === 42 ? 55 : 54, e = 1, s = this.input.charCodeAt(this.state.pos + 1);
t === 42 && s === 42 && (e++, s = this.input.charCodeAt(this.state.pos + 2), r = 57), s === 61 && !this.state.inType && (e++, r = t === 37 ? 33 : 30), this.finishOp(r, e);
}
readToken_pipe_amp(t) {
let r = this.input.charCodeAt(this.state.pos + 1);
if (r === t) {
this.input.charCodeAt(this.state.pos + 2) === 61 ? this.finishOp(30, 3) : this.finishOp(t === 124 ? 41 : 42, 2);
return;
}
if (t === 124) {
if (r === 62) {
this.finishOp(39, 2);
return;
}
if (this.hasPlugin("recordAndTuple") && r === 125) {
if (this.getPluginOption("recordAndTuple", "syntaxType") !== "bar")
throw this.raise(f.RecordExpressionBarIncorrectEndSyntaxType, { at: this.state.curPosition() });
this.state.pos += 2, this.finishToken(9);
return;
}
if (this.hasPlugin("recordAndTuple") && r === 93) {
if (this.getPluginOption("recordAndTuple", "syntaxType") !== "bar")
throw this.raise(f.TupleExpressionBarIncorrectEndSyntaxType, { at: this.state.curPosition() });
this.state.pos += 2, this.finishToken(4);
return;
}
}
if (r === 61) {
this.finishOp(30, 2);
return;
}
this.finishOp(t === 124 ? 43 : 45, 1);
}
readToken_caret() {
let t = this.input.charCodeAt(this.state.pos + 1);
t === 61 && !this.state.inType ? this.finishOp(32, 2) : t === 94 && this.hasPlugin(["pipelineOperator", { proposal: "hack", topicToken: "^^" }]) ? (this.finishOp(37, 2), this.input.codePointAt(this.state.pos) === 94 && this.unexpected()) : this.finishOp(44, 1);
}
readToken_atSign() {
this.input.charCodeAt(this.state.pos + 1) === 64 && this.hasPlugin(["pipelineOperator", { proposal: "hack", topicToken: "@@" }]) ? this.finishOp(38, 2) : this.finishOp(26, 1);
}
readToken_plus_min(t) {
let r = this.input.charCodeAt(this.state.pos + 1);
if (r === t) {
this.finishOp(34, 2);
return;
}
r === 61 ? this.finishOp(30, 2) : this.finishOp(53, 1);
}
readToken_lt() {
let { pos: t } = this.state, r = this.input.charCodeAt(t + 1);
if (r === 60) {
if (this.input.charCodeAt(t + 2) === 61) {
this.finishOp(30, 3);
return;
}
this.finishOp(51, 2);
return;
}
if (r === 61) {
this.finishOp(49, 2);
return;
}
this.finishOp(47, 1);
}
readToken_gt() {
let { pos: t } = this.state, r = this.input.charCodeAt(t + 1);
if (r === 62) {
let e = this.input.charCodeAt(t + 2) === 62 ? 3 : 2;
if (this.input.charCodeAt(t + e) === 61) {
this.finishOp(30, e + 1);
return;
}
this.finishOp(52, e);
return;
}
if (r === 61) {
this.finishOp(49, 2);
return;
}
this.finishOp(48, 1);
}
readToken_eq_excl(t) {
let r = this.input.charCodeAt(this.state.pos + 1);
if (r === 61) {
this.finishOp(46, this.input.charCodeAt(this.state.pos + 2) === 61 ? 3 : 2);
return;
}
if (t === 61 && r === 62) {
this.state.pos += 2, this.finishToken(19);
return;
}
this.finishOp(t === 61 ? 29 : 35, 1);
}
readToken_question() {
let t = this.input.charCodeAt(this.state.pos + 1), r = this.input.charCodeAt(this.state.pos + 2);
t === 63 ? r === 61 ? this.finishOp(30, 3) : this.finishOp(40, 2) : t === 46 && !(r >= 48 && r <= 57) ? (this.state.pos += 2, this.finishToken(18)) : (++this.state.pos, this.finishToken(17));
}
getTokenFromCode(t) {
switch (t) {
case 46:
this.readToken_dot();
return;
case 40:
++this.state.pos, this.finishToken(10);
return;
case 41:
++this.state.pos, this.finishToken(11);
return;
case 59:
++this.state.pos, this.finishToken(13);
return;
case 44:
++this.state.pos, this.finishToken(12);
return;
case 91:
if (this.hasPlugin("recordAndTuple") && this.input.charCodeAt(this.state.pos + 1) === 124) {
if (this.getPluginOption("recordAndTuple", "syntaxType") !== "bar")
throw this.raise(f.TupleExpressionBarIncorrectStartSyntaxType, { at: this.state.curPosition() });
this.state.pos += 2, this.finishToken(2);
} else
++this.state.pos, this.finishToken(0);
return;
case 93:
++this.state.pos, this.finishToken(3);
return;
case 123:
if (this.hasPlugin("recordAndTuple") && this.input.charCodeAt(this.state.pos + 1) === 124) {
if (this.getPluginOption("recordAndTuple", "syntaxType") !== "bar")
throw this.raise(f.RecordExpressionBarIncorrectStartSyntaxType, { at: this.state.curPosition() });
this.state.pos += 2, this.finishToken(6);
} else
++this.state.pos, this.finishToken(5);
return;
case 125:
++this.state.pos, this.finishToken(8);
return;
case 58:
this.hasPlugin("functionBind") && this.input.charCodeAt(this.state.pos + 1) === 58 ? this.finishOp(15, 2) : (++this.state.pos, this.finishToken(14));
return;
case 63:
this.readToken_question();
return;
case 96:
this.readTemplateToken();
return;
case 48: {
let r = this.input.charCodeAt(this.state.pos + 1);
if (r === 120 || r === 88) {
this.readRadixNumber(16);
return;
}
if (r === 111 || r === 79) {
this.readRadixNumber(8);
return;
}
if (r === 98 || r === 66) {
this.readRadixNumber(2);
return;
}
}
case 49:
case 50:
case 51:
case 52:
case 53:
case 54:
case 55:
case 56:
case 57:
this.readNumber(false);
return;
case 34:
case 39:
this.readString(t);
return;
case 47:
this.readToken_slash();
return;
case 37:
case 42:
this.readToken_mult_modulo(t);
return;
case 124:
case 38:
this.readToken_pipe_amp(t);
return;
case 94:
this.readToken_caret();
return;
case 43:
case 45:
this.readToken_plus_min(t);
return;
case 60:
this.readToken_lt();
return;
case 62:
this.readToken_gt();
return;
case 61:
case 33:
this.readToken_eq_excl(t);
return;
case 126:
this.finishOp(36, 1);
return;
case 64:
this.readToken_atSign();
return;
case 35:
this.readToken_numberSign();
return;
case 92:
this.readWord();
return;
default:
if (fe(t)) {
this.readWord(t);
return;
}
}
throw this.raise(f.InvalidOrUnexpectedToken, { at: this.state.curPosition(), unexpected: String.fromCodePoint(t) });
}
finishOp(t, r) {
let e = this.input.slice(this.state.pos, this.state.pos + r);
this.state.pos += r, this.finishToken(t, e);
}
readRegexp() {
let t = this.state.startLoc, r = this.state.start + 1, e, s, { pos: i } = this.state;
for (; ; ++i) {
if (i >= this.length)
throw this.raise(f.UnterminatedRegExp, { at: Y(t, 1) });
let u = this.input.charCodeAt(i);
if (Ge(u))
throw this.raise(f.UnterminatedRegExp, { at: Y(t, 1) });
if (e)
e = false;
else {
if (u === 91)
s = true;
else if (u === 93 && s)
s = false;
else if (u === 47 && !s)
break;
e = u === 92;
}
}
let a = this.input.slice(r, i);
++i;
let n2 = "", o = () => Y(t, i + 2 - r);
for (; i < this.length; ) {
let u = this.codePointAtPos(i), c = String.fromCharCode(u);
if (Ol.has(u))
u === 118 ? (this.expectPlugin("regexpUnicodeSets", o()), n2.includes("u") && this.raise(f.IncompatibleRegExpUVFlags, { at: o() })) : u === 117 && n2.includes("v") && this.raise(f.IncompatibleRegExpUVFlags, { at: o() }), n2.includes(c) && this.raise(f.DuplicateRegExpFlags, { at: o() });
else if (De(u) || u === 92)
this.raise(f.MalformedRegExpFlags, { at: o() });
else
break;
++i, n2 += c;
}
this.state.pos = i, this.finishToken(135, { pattern: a, flags: n2 });
}
readInt(t, r) {
let e = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false, s = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : true, { n: i, pos: a } = Fr(this.input, this.state.pos, this.state.lineStart, this.state.curLine, t, r, e, s, this.errorHandlers_readInt, false);
return this.state.pos = a, i;
}
readRadixNumber(t) {
let r = this.state.curPosition(), e = false;
this.state.pos += 2;
let s = this.readInt(t);
s == null && this.raise(f.InvalidDigit, { at: Y(r, 2), radix: t });
let i = this.input.charCodeAt(this.state.pos);
if (i === 110)
++this.state.pos, e = true;
else if (i === 109)
throw this.raise(f.InvalidDecimal, { at: r });
if (fe(this.codePointAtPos(this.state.pos)))
throw this.raise(f.NumberIdentifier, { at: this.state.curPosition() });
if (e) {
let a = this.input.slice(r.index, this.state.pos).replace(/[_n]/g, "");
this.finishToken(133, a);
return;
}
this.finishToken(132, s);
}
readNumber(t) {
let r = this.state.pos, e = this.state.curPosition(), s = false, i = false, a = false, n2 = false, o = false;
!t && this.readInt(10) === null && this.raise(f.InvalidNumber, { at: this.state.curPosition() });
let u = this.state.pos - r >= 2 && this.input.charCodeAt(r) === 48;
if (u) {
let T = this.input.slice(r, this.state.pos);
if (this.recordStrictModeErrors(f.StrictOctalLiteral, { at: e }), !this.state.strict) {
let C = T.indexOf("_");
C > 0 && this.raise(f.ZeroDigitNumericSeparator, { at: Y(e, C) });
}
o = u && !/[89]/.test(T);
}
let c = this.input.charCodeAt(this.state.pos);
if (c === 46 && !o && (++this.state.pos, this.readInt(10), s = true, c = this.input.charCodeAt(this.state.pos)), (c === 69 || c === 101) && !o && (c = this.input.charCodeAt(++this.state.pos), (c === 43 || c === 45) && ++this.state.pos, this.readInt(10) === null && this.raise(f.InvalidOrMissingExponent, { at: e }), s = true, n2 = true, c = this.input.charCodeAt(this.state.pos)), c === 110 && ((s || u) && this.raise(f.InvalidBigIntLiteral, { at: e }), ++this.state.pos, i = true), c === 109 && (this.expectPlugin("decimal", this.state.curPosition()), (n2 || u) && this.raise(f.InvalidDecimal, { at: e }), ++this.state.pos, a = true), fe(this.codePointAtPos(this.state.pos)))
throw this.raise(f.NumberIdentifier, { at: this.state.curPosition() });
let y = this.input.slice(r, this.state.pos).replace(/[_mn]/g, "");
if (i) {
this.finishToken(133, y);
return;
}
if (a) {
this.finishToken(134, y);
return;
}
let g = o ? parseInt(y, 8) : parseFloat(y);
this.finishToken(132, g);
}
readCodePoint(t) {
let { code: r, pos: e } = Lr(this.input, this.state.pos, this.state.lineStart, this.state.curLine, t, this.errorHandlers_readCodePoint);
return this.state.pos = e, r;
}
readString(t) {
let { str: r, pos: e, curLine: s, lineStart: i } = Dr(t === 34 ? "double" : "single", this.input, this.state.pos + 1, this.state.lineStart, this.state.curLine, this.errorHandlers_readStringContents_string);
this.state.pos = e + 1, this.state.lineStart = i, this.state.curLine = s, this.finishToken(131, r);
}
readTemplateContinuation() {
this.match(8) || this.unexpected(null, 8), this.state.pos--, this.readTemplateToken();
}
readTemplateToken() {
let t = this.input[this.state.pos], { str: r, firstInvalidLoc: e, pos: s, curLine: i, lineStart: a } = Dr("template", this.input, this.state.pos + 1, this.state.lineStart, this.state.curLine, this.errorHandlers_readStringContents_template);
this.state.pos = s + 1, this.state.lineStart = a, this.state.curLine = i, e && (this.state.firstInvalidTemplateEscapePos = new ge(e.curLine, e.pos - e.lineStart, e.pos)), this.input.codePointAt(s) === 96 ? this.finishToken(24, e ? null : t + r + "`") : (this.state.pos++, this.finishToken(25, e ? null : t + r + "${"));
}
recordStrictModeErrors(t, r) {
let { at: e } = r, s = e.index;
this.state.strict && !this.state.strictErrors.has(s) ? this.raise(t, { at: e }) : this.state.strictErrors.set(s, [t, e]);
}
readWord1(t) {
this.state.containsEsc = false;
let r = "", e = this.state.pos, s = this.state.pos;
for (t !== void 0 && (this.state.pos += t <= 65535 ? 1 : 2); this.state.pos < this.length; ) {
let i = this.codePointAtPos(this.state.pos);
if (De(i))
this.state.pos += i <= 65535 ? 1 : 2;
else if (i === 92) {
this.state.containsEsc = true, r += this.input.slice(s, this.state.pos);
let a = this.state.curPosition(), n2 = this.state.pos === e ? fe : De;
if (this.input.charCodeAt(++this.state.pos) !== 117) {
this.raise(f.MissingUnicodeEscape, { at: this.state.curPosition() }), s = this.state.pos - 1;
continue;
}
++this.state.pos;
let o = this.readCodePoint(true);
o !== null && (n2(o) || this.raise(f.EscapedCharNotAnIdentifier, { at: a }), r += String.fromCodePoint(o)), s = this.state.pos;
} else
break;
}
return r + this.input.slice(s, this.state.pos);
}
readWord(t) {
let r = this.readWord1(t), e = L.get(r);
e !== void 0 ? this.finishToken(e, xe(e)) : this.finishToken(130, r);
}
checkKeywordEscapes() {
let { type: t } = this.state;
$t(t) && this.state.containsEsc && this.raise(f.InvalidEscapedReservedWord, { at: this.state.startLoc, reservedWord: xe(t) });
}
raise(t, r) {
let { at: e } = r, s = ot(r, Fl), i = e instanceof ge ? e : e.loc.start, a = t({ loc: i, details: s });
if (!this.options.errorRecovery)
throw a;
return this.isLookahead || this.state.errors.push(a), a;
}
raiseOverwrite(t, r) {
let { at: e } = r, s = ot(r, Ll), i = e instanceof ge ? e : e.loc.start, a = i.index, n2 = this.state.errors;
for (let o = n2.length - 1; o >= 0; o--) {
let u = n2[o];
if (u.loc.index === a)
return n2[o] = t({ loc: i, details: s });
if (u.loc.index < a)
break;
}
return this.raise(t, r);
}
updateContext(t) {
}
unexpected(t, r) {
throw this.raise(f.UnexpectedToken, { expected: r ? xe(r) : null, at: t != null ? t : this.state.startLoc });
}
expectPlugin(t, r) {
if (this.hasPlugin(t))
return true;
throw this.raise(f.MissingPlugin, { at: r != null ? r : this.state.startLoc, missingPlugin: [t] });
}
expectOnePlugin(t) {
if (!t.some((r) => this.hasPlugin(r)))
throw this.raise(f.MissingOneOfPlugins, { at: this.state.startLoc, missingPlugin: t });
}
errorBuilder(t) {
return (r, e, s) => {
this.raise(t, { at: Je(r, e, s) });
};
}
}, Ml = class {
constructor() {
this.privateNames = /* @__PURE__ */ new Set(), this.loneAccessors = /* @__PURE__ */ new Map(), this.undefinedPrivateNames = /* @__PURE__ */ new Map();
}
}, _l = class {
constructor(t) {
this.parser = void 0, this.stack = [], this.undefinedPrivateNames = /* @__PURE__ */ new Map(), this.parser = t;
}
current() {
return this.stack[this.stack.length - 1];
}
enter() {
this.stack.push(new Ml());
}
exit() {
let t = this.stack.pop(), r = this.current();
for (let [e, s] of Array.from(t.undefinedPrivateNames))
r ? r.undefinedPrivateNames.has(e) || r.undefinedPrivateNames.set(e, s) : this.parser.raise(f.InvalidPrivateFieldResolution, { at: s, identifierName: e });
}
declarePrivateName(t, r, e) {
let { privateNames: s, loneAccessors: i, undefinedPrivateNames: a } = this.current(), n2 = s.has(t);
if (r & ts) {
let o = n2 && i.get(t);
if (o) {
let u = o & yt, c = r & yt, y = o & ts, g = r & ts;
n2 = y === g || u !== c, n2 || i.delete(t);
} else
n2 || i.set(t, r);
}
n2 && this.parser.raise(f.PrivateNameRedeclaration, { at: e, identifierName: t }), s.add(t), a.delete(t);
}
usePrivateName(t, r) {
let e;
for (e of this.stack)
if (e.privateNames.has(t))
return;
e ? e.undefinedPrivateNames.set(t, r) : this.parser.raise(f.InvalidPrivateFieldResolution, { at: r, identifierName: t });
}
}, Rl = 0, Or = 1, ls = 2, Br = 3, Pt = class {
constructor() {
let t = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : Rl;
this.type = void 0, this.type = t;
}
canBeArrowParameterDeclaration() {
return this.type === ls || this.type === Or;
}
isCertainlyParameterDeclaration() {
return this.type === Br;
}
}, Mr = class extends Pt {
constructor(t) {
super(t), this.declarationErrors = /* @__PURE__ */ new Map();
}
recordDeclarationError(t, r) {
let { at: e } = r, s = e.index;
this.declarationErrors.set(s, [t, e]);
}
clearDeclarationError(t) {
this.declarationErrors.delete(t);
}
iterateErrors(t) {
this.declarationErrors.forEach(t);
}
}, jl = class {
constructor(t) {
this.parser = void 0, this.stack = [new Pt()], this.parser = t;
}
enter(t) {
this.stack.push(t);
}
exit() {
this.stack.pop();
}
recordParameterInitializerError(t, r) {
let { at: e } = r, s = { at: e.loc.start }, { stack: i } = this, a = i.length - 1, n2 = i[a];
for (; !n2.isCertainlyParameterDeclaration(); ) {
if (n2.canBeArrowParameterDeclaration())
n2.recordDeclarationError(t, s);
else
return;
n2 = i[--a];
}
this.parser.raise(t, s);
}
recordArrowParameterBindingError(t, r) {
let { at: e } = r, { stack: s } = this, i = s[s.length - 1], a = { at: e.loc.start };
if (i.isCertainlyParameterDeclaration())
this.parser.raise(t, a);
else if (i.canBeArrowParameterDeclaration())
i.recordDeclarationError(t, a);
else
return;
}
recordAsyncArrowParametersError(t) {
let { at: r } = t, { stack: e } = this, s = e.length - 1, i = e[s];
for (; i.canBeArrowParameterDeclaration(); )
i.type === ls && i.recordDeclarationError(f.AwaitBindingIdentifier, { at: r }), i = e[--s];
}
validateAsPattern() {
let { stack: t } = this, r = t[t.length - 1];
r.canBeArrowParameterDeclaration() && r.iterateErrors((e) => {
let [s, i] = e;
this.parser.raise(s, { at: i });
let a = t.length - 2, n2 = t[a];
for (; n2.canBeArrowParameterDeclaration(); )
n2.clearDeclarationError(i.index), n2 = t[--a];
});
}
};
function ql() {
return new Pt(Br);
}
function Ul() {
return new Mr(Or);
}
function $l() {
return new Mr(ls);
}
function _r() {
return new Pt();
}
var Me = 0, Rr = 1, At = 2, jr = 4, _e = 8, Hl = class {
constructor() {
this.stacks = [];
}
enter(t) {
this.stacks.push(t);
}
exit() {
this.stacks.pop();
}
currentFlags() {
return this.stacks[this.stacks.length - 1];
}
get hasAwait() {
return (this.currentFlags() & At) > 0;
}
get hasYield() {
return (this.currentFlags() & Rr) > 0;
}
get hasReturn() {
return (this.currentFlags() & jr) > 0;
}
get hasIn() {
return (this.currentFlags() & _e) > 0;
}
};
function Tt(t, r) {
return (t ? At : 0) | (r ? Rr : 0);
}
var zl = class extends Bl {
addExtra(t, r, e) {
let s = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : true;
if (!t)
return;
let i = t.extra = t.extra || {};
s ? i[r] = e : Object.defineProperty(i, r, { enumerable: s, value: e });
}
isContextual(t) {
return this.state.type === t && !this.state.containsEsc;
}
isUnparsedContextual(t, r) {
let e = t + r.length;
if (this.input.slice(t, e) === r) {
let s = this.input.charCodeAt(e);
return !(De(s) || (s & 64512) === 55296);
}
return false;
}
isLookaheadContextual(t) {
let r = this.nextTokenStart();
return this.isUnparsedContextual(r, t);
}
eatContextual(t) {
return this.isContextual(t) ? (this.next(), true) : false;
}
expectContextual(t, r) {
if (!this.eatContextual(t)) {
if (r != null)
throw this.raise(r, { at: this.state.startLoc });
this.unexpected(null, t);
}
}
canInsertSemicolon() {
return this.match(137) || this.match(8) || this.hasPrecedingLineBreak();
}
hasPrecedingLineBreak() {
return as.test(this.input.slice(this.state.lastTokEndLoc.index, this.state.start));
}
hasFollowingLineBreak() {
return Ir.lastIndex = this.state.end, Ir.test(this.input);
}
isLineTerminator() {
return this.eat(13) || this.canInsertSemicolon();
}
semicolon() {
((arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : true) ? this.isLineTerminator() : this.eat(13)) || this.raise(f.MissingSemicolon, { at: this.state.lastTokEndLoc });
}
expect(t, r) {
this.eat(t) || this.unexpected(r, t);
}
tryParse(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : this.state.clone(), e = { node: null };
try {
let s = t(function() {
let i = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : null;
throw e.node = i, e;
});
if (this.state.errors.length > r.errors.length) {
let i = this.state;
return this.state = r, this.state.tokensLength = i.tokensLength, { node: s, error: i.errors[r.errors.length], thrown: false, aborted: false, failState: i };
}
return { node: s, error: null, thrown: false, aborted: false, failState: null };
} catch (s) {
let i = this.state;
if (this.state = r, s instanceof SyntaxError)
return { node: null, error: s, thrown: true, aborted: false, failState: i };
if (s === e)
return { node: e.node, error: null, thrown: false, aborted: true, failState: i };
throw s;
}
}
checkExpressionErrors(t, r) {
if (!t)
return false;
let { shorthandAssignLoc: e, doubleProtoLoc: s, privateKeyLoc: i, optionalParametersLoc: a } = t, n2 = !!e || !!s || !!a || !!i;
if (!r)
return n2;
e != null && this.raise(f.InvalidCoverInitializedName, { at: e }), s != null && this.raise(f.DuplicateProto, { at: s }), i != null && this.raise(f.UnexpectedPrivateField, { at: i }), a != null && this.unexpected(a);
}
isLiteralPropertyName() {
return it(this.state.type);
}
isPrivateName(t) {
return t.type === "PrivateName";
}
getPrivateNameSV(t) {
return t.id.name;
}
hasPropertyAsPrivateName(t) {
return (t.type === "MemberExpression" || t.type === "OptionalMemberExpression") && this.isPrivateName(t.property);
}
isObjectProperty(t) {
return t.type === "ObjectProperty";
}
isObjectMethod(t) {
return t.type === "ObjectMethod";
}
initializeScopes() {
let t = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : this.options.sourceType === "module", r = this.state.labels;
this.state.labels = [];
let e = this.exportedIdentifiers;
this.exportedIdentifiers = /* @__PURE__ */ new Set();
let s = this.inModule;
this.inModule = t;
let i = this.scope, a = this.getScopeHandler();
this.scope = new a(this, t);
let n2 = this.prodParam;
this.prodParam = new Hl();
let o = this.classScope;
this.classScope = new _l(this);
let u = this.expressionScope;
return this.expressionScope = new jl(this), () => {
this.state.labels = r, this.exportedIdentifiers = e, this.inModule = s, this.scope = i, this.prodParam = n2, this.classScope = o, this.expressionScope = u;
};
}
enterInitialScopes() {
let t = Me;
this.inModule && (t |= At), this.scope.enter(Le), this.prodParam.enter(t);
}
checkDestructuringPrivate(t) {
let { privateKeyLoc: r } = t;
r !== null && this.expectPlugin("destructuringPrivate", r);
}
}, vt = class {
constructor() {
this.shorthandAssignLoc = null, this.doubleProtoLoc = null, this.privateKeyLoc = null, this.optionalParametersLoc = null;
}
}, Et = class {
constructor(t, r, e) {
this.type = "", this.start = r, this.end = 0, this.loc = new lt(e), t != null && t.options.ranges && (this.range = [r, 0]), t != null && t.filename && (this.loc.filename = t.filename);
}
}, hs = Et.prototype;
hs.__clone = function() {
let t = new Et(void 0, this.start, this.loc.start), r = Object.keys(this);
for (let e = 0, s = r.length; e < s; e++) {
let i = r[e];
i !== "leadingComments" && i !== "trailingComments" && i !== "innerComments" && (t[i] = this[i]);
}
return t;
};
function Vl(t) {
return me(t);
}
function me(t) {
let { type: r, start: e, end: s, loc: i, range: a, extra: n2, name: o } = t, u = Object.create(hs);
return u.type = r, u.start = e, u.end = s, u.loc = i, u.range = a, u.extra = n2, u.name = o, r === "Placeholder" && (u.expectedNode = t.expectedNode), u;
}
function Kl(t) {
let { type: r, start: e, end: s, loc: i, range: a, extra: n2 } = t;
if (r === "Placeholder")
return Vl(t);
let o = Object.create(hs);
return o.type = r, o.start = e, o.end = s, o.loc = i, o.range = a, t.raw !== void 0 ? o.raw = t.raw : o.extra = n2, o.value = t.value, o;
}
var Wl = class extends zl {
startNode() {
return new Et(this, this.state.start, this.state.startLoc);
}
startNodeAt(t) {
return new Et(this, t.index, t);
}
startNodeAtNode(t) {
return this.startNodeAt(t.loc.start);
}
finishNode(t, r) {
return this.finishNodeAt(t, r, this.state.lastTokEndLoc);
}
finishNodeAt(t, r, e) {
return t.type = r, t.end = e.index, t.loc.end = e, this.options.ranges && (t.range[1] = e.index), this.options.attachComment && this.processComment(t), t;
}
resetStartLocation(t, r) {
t.start = r.index, t.loc.start = r, this.options.ranges && (t.range[0] = r.index);
}
resetEndLocation(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : this.state.lastTokEndLoc;
t.end = r.index, t.loc.end = r, this.options.ranges && (t.range[1] = r.index);
}
resetStartLocationFromNode(t, r) {
this.resetStartLocation(t, r.loc.start);
}
}, Gl = /* @__PURE__ */ new Set(["_", "any", "bool", "boolean", "empty", "extends", "false", "interface", "mixed", "null", "number", "static", "string", "true", "typeof", "void"]), D = pe`flow`({ AmbiguousConditionalArrow: "Ambiguous expression: wrap the arrow functions in parentheses to disambiguate.", AmbiguousDeclareModuleKind: "Found both `declare module.exports` and `declare export` in the same module. Modules can only have 1 since they are either an ES module or they are a CommonJS module.", AssignReservedType: (t) => {
let { reservedType: r } = t;
return `Cannot overwrite reserved type ${r}.`;
}, DeclareClassElement: "The `declare` modifier can only appear on class fields.", DeclareClassFieldInitializer: "Initializers are not allowed in fields with the `declare` modifier.", DuplicateDeclareModuleExports: "Duplicate `declare module.exports` statement.", EnumBooleanMemberNotInitialized: (t) => {
let { memberName: r, enumName: e } = t;
return `Boolean enum members need to be initialized. Use either \`${r} = true,\` or \`${r} = false,\` in enum \`${e}\`.`;
}, EnumDuplicateMemberName: (t) => {
let { memberName: r, enumName: e } = t;
return `Enum member names need to be unique, but the name \`${r}\` has already been used before in enum \`${e}\`.`;
}, EnumInconsistentMemberValues: (t) => {
let { enumName: r } = t;
return `Enum \`${r}\` has inconsistent member initializers. Either use no initializers, or consistently use literals (either booleans, numbers, or strings) for all member initializers.`;
}, EnumInvalidExplicitType: (t) => {
let { invalidEnumType: r, enumName: e } = t;
return `Enum type \`${r}\` is not valid. Use one of \`boolean\`, \`number\`, \`string\`, or \`symbol\` in enum \`${e}\`.`;
}, EnumInvalidExplicitTypeUnknownSupplied: (t) => {
let { enumName: r } = t;
return `Supplied enum type is not valid. Use one of \`boolean\`, \`number\`, \`string\`, or \`symbol\` in enum \`${r}\`.`;
}, EnumInvalidMemberInitializerPrimaryType: (t) => {
let { enumName: r, memberName: e, explicitType: s } = t;
return `Enum \`${r}\` has type \`${s}\`, so the initializer of \`${e}\` needs to be a ${s} literal.`;
}, EnumInvalidMemberInitializerSymbolType: (t) => {
let { enumName: r, memberName: e } = t;
return `Symbol enum members cannot be initialized. Use \`${e},\` in enum \`${r}\`.`;
}, EnumInvalidMemberInitializerUnknownType: (t) => {
let { enumName: r, memberName: e } = t;
return `The enum member initializer for \`${e}\` needs to be a literal (either a boolean, number, or string) in enum \`${r}\`.`;
}, EnumInvalidMemberName: (t) => {
let { enumName: r, memberName: e, suggestion: s } = t;
return `Enum member names cannot start with lowercase 'a' through 'z'. Instead of using \`${e}\`, consider using \`${s}\`, in enum \`${r}\`.`;
}, EnumNumberMemberNotInitialized: (t) => {
let { enumName: r, memberName: e } = t;
return `Number enum members need to be initialized, e.g. \`${e} = 1\` in enum \`${r}\`.`;
}, EnumStringMemberInconsistentlyInitailized: (t) => {
let { enumName: r } = t;
return `String enum members need to consistently either all use initializers, or use no initializers, in enum \`${r}\`.`;
}, GetterMayNotHaveThisParam: "A getter cannot have a `this` parameter.", ImportReflectionHasImportType: "An `import module` declaration can not use `type` or `typeof` keyword.", ImportTypeShorthandOnlyInPureImport: "The `type` and `typeof` keywords on named imports can only be used on regular `import` statements. It cannot be used with `import type` or `import typeof` statements.", InexactInsideExact: "Explicit inexact syntax cannot appear inside an explicit exact object type.", InexactInsideNonObject: "Explicit inexact syntax cannot appear in class or interface definitions.", InexactVariance: "Explicit inexact syntax cannot have variance.", InvalidNonTypeImportInDeclareModule: "Imports within a `declare module` body must always be `import type` or `import typeof`.", MissingTypeParamDefault: "Type parameter declaration needs a default, since a preceding type parameter declaration has a default.", NestedDeclareModule: "`declare module` cannot be used inside another `declare module`.", NestedFlowComment: "Cannot have a flow comment inside another flow comment.", PatternIsOptional: Object.assign({ message: "A binding pattern parameter cannot be optional in an implementation signature." }, { reasonCode: "OptionalBindingPattern" }), SetterMayNotHaveThisParam: "A setter cannot have a `this` parameter.", SpreadVariance: "Spread properties cannot have variance.", ThisParamAnnotationRequired: "A type annotation is required for the `this` parameter.", ThisParamBannedInConstructor: "Constructors cannot have a `this` parameter; constructors don't bind `this` like other functions.", ThisParamMayNotBeOptional: "The `this` parameter cannot be optional.", ThisParamMustBeFirst: "The `this` parameter must be the first function parameter.", ThisParamNoDefault: "The `this` parameter may not have a default value.", TypeBeforeInitializer: "Type annotations must come before default assignments, e.g. instead of `age = 25: number` use `age: number = 25`.", TypeCastInPattern: "The type cast expression is expected to be wrapped with parenthesis.", UnexpectedExplicitInexactInObject: "Explicit inexact syntax must appear at the end of an inexact object.", UnexpectedReservedType: (t) => {
let { reservedType: r } = t;
return `Unexpected reserved type ${r}.`;
}, UnexpectedReservedUnderscore: "`_` is only allowed as a type argument to call or new.", UnexpectedSpaceBetweenModuloChecks: "Spaces between `%` and `checks` are not allowed here.", UnexpectedSpreadType: "Spread operator cannot appear in class or interface definitions.", UnexpectedSubtractionOperand: 'Unexpected token, expected "number" or "bigint".', UnexpectedTokenAfterTypeParameter: "Expected an arrow function after this type parameter declaration.", UnexpectedTypeParameterBeforeAsyncArrowFunction: "Type parameters must come after the async keyword, e.g. instead of ` async () => {}`, use `async () => {}`.", UnsupportedDeclareExportKind: (t) => {
let { unsupportedExportKind: r, suggestion: e } = t;
return `\`declare export ${r}\` is not supported. Use \`${e}\` instead.`;
}, UnsupportedStatementInDeclareModule: "Only declares and type imports are allowed inside declare module.", UnterminatedFlowComment: "Unterminated flow-comment." });
function Jl(t) {
return t.type === "DeclareExportAllDeclaration" || t.type === "DeclareExportDeclaration" && (!t.declaration || t.declaration.type !== "TypeAlias" && t.declaration.type !== "InterfaceDeclaration");
}
function us(t) {
return t.importKind === "type" || t.importKind === "typeof";
}
function qr(t) {
return te(t) && t !== 97;
}
var Xl = { const: "declare export var", let: "declare export var", type: "export type", interface: "export interface" };
function Yl(t, r) {
let e = [], s = [];
for (let i = 0; i < t.length; i++)
(r(t[i], i, t) ? e : s).push(t[i]);
return [e, s];
}
var Ql = /\*?\s*@((?:no)?flow)\b/, Zl = (t) => class extends t {
constructor() {
super(...arguments), this.flowPragma = void 0;
}
getScopeHandler() {
return El;
}
shouldParseTypes() {
return this.getPluginOption("flow", "all") || this.flowPragma === "flow";
}
shouldParseEnums() {
return !!this.getPluginOption("flow", "enums");
}
finishToken(e, s) {
e !== 131 && e !== 13 && e !== 28 && this.flowPragma === void 0 && (this.flowPragma = null), super.finishToken(e, s);
}
addComment(e) {
if (this.flowPragma === void 0) {
let s = Ql.exec(e.value);
if (s)
if (s[1] === "flow")
this.flowPragma = "flow";
else if (s[1] === "noflow")
this.flowPragma = "noflow";
else
throw new Error("Unexpected flow pragma");
}
super.addComment(e);
}
flowParseTypeInitialiser(e) {
let s = this.state.inType;
this.state.inType = true, this.expect(e || 14);
let i = this.flowParseType();
return this.state.inType = s, i;
}
flowParsePredicate() {
let e = this.startNode(), s = this.state.startLoc;
return this.next(), this.expectContextual(108), this.state.lastTokStart > s.index + 1 && this.raise(D.UnexpectedSpaceBetweenModuloChecks, { at: s }), this.eat(10) ? (e.value = super.parseExpression(), this.expect(11), this.finishNode(e, "DeclaredPredicate")) : this.finishNode(e, "InferredPredicate");
}
flowParseTypeAndPredicateInitialiser() {
let e = this.state.inType;
this.state.inType = true, this.expect(14);
let s = null, i = null;
return this.match(54) ? (this.state.inType = e, i = this.flowParsePredicate()) : (s = this.flowParseType(), this.state.inType = e, this.match(54) && (i = this.flowParsePredicate())), [s, i];
}
flowParseDeclareClass(e) {
return this.next(), this.flowParseInterfaceish(e, true), this.finishNode(e, "DeclareClass");
}
flowParseDeclareFunction(e) {
this.next();
let s = e.id = this.parseIdentifier(), i = this.startNode(), a = this.startNode();
this.match(47) ? i.typeParameters = this.flowParseTypeParameterDeclaration() : i.typeParameters = null, this.expect(10);
let n2 = this.flowParseFunctionTypeParams();
return i.params = n2.params, i.rest = n2.rest, i.this = n2._this, this.expect(11), [i.returnType, e.predicate] = this.flowParseTypeAndPredicateInitialiser(), a.typeAnnotation = this.finishNode(i, "FunctionTypeAnnotation"), s.typeAnnotation = this.finishNode(a, "TypeAnnotation"), this.resetEndLocation(s), this.semicolon(), this.scope.declareName(e.id.name, xl, e.id.loc.start), this.finishNode(e, "DeclareFunction");
}
flowParseDeclare(e, s) {
if (this.match(80))
return this.flowParseDeclareClass(e);
if (this.match(68))
return this.flowParseDeclareFunction(e);
if (this.match(74))
return this.flowParseDeclareVariable(e);
if (this.eatContextual(125))
return this.match(16) ? this.flowParseDeclareModuleExports(e) : (s && this.raise(D.NestedDeclareModule, { at: this.state.lastTokStartLoc }), this.flowParseDeclareModule(e));
if (this.isContextual(128))
return this.flowParseDeclareTypeAlias(e);
if (this.isContextual(129))
return this.flowParseDeclareOpaqueType(e);
if (this.isContextual(127))
return this.flowParseDeclareInterface(e);
if (this.match(82))
return this.flowParseDeclareExportDeclaration(e, s);
this.unexpected();
}
flowParseDeclareVariable(e) {
return this.next(), e.id = this.flowParseTypeAnnotatableIdentifier(true), this.scope.declareName(e.id.name, mt, e.id.loc.start), this.semicolon(), this.finishNode(e, "DeclareVariable");
}
flowParseDeclareModule(e) {
this.scope.enter(Fe), this.match(131) ? e.id = super.parseExprAtom() : e.id = this.parseIdentifier();
let s = e.body = this.startNode(), i = s.body = [];
for (this.expect(5); !this.match(8); ) {
let o = this.startNode();
this.match(83) ? (this.next(), !this.isContextual(128) && !this.match(87) && this.raise(D.InvalidNonTypeImportInDeclareModule, { at: this.state.lastTokStartLoc }), super.parseImport(o)) : (this.expectContextual(123, D.UnsupportedStatementInDeclareModule), o = this.flowParseDeclare(o, true)), i.push(o);
}
this.scope.exit(), this.expect(8), this.finishNode(s, "BlockStatement");
let a = null, n2 = false;
return i.forEach((o) => {
Jl(o) ? (a === "CommonJS" && this.raise(D.AmbiguousDeclareModuleKind, { at: o }), a = "ES") : o.type === "DeclareModuleExports" && (n2 && this.raise(D.DuplicateDeclareModuleExports, { at: o }), a === "ES" && this.raise(D.AmbiguousDeclareModuleKind, { at: o }), a = "CommonJS", n2 = true);
}), e.kind = a || "CommonJS", this.finishNode(e, "DeclareModule");
}
flowParseDeclareExportDeclaration(e, s) {
if (this.expect(82), this.eat(65))
return this.match(68) || this.match(80) ? e.declaration = this.flowParseDeclare(this.startNode()) : (e.declaration = this.flowParseType(), this.semicolon()), e.default = true, this.finishNode(e, "DeclareExportDeclaration");
if (this.match(75) || this.isLet() || (this.isContextual(128) || this.isContextual(127)) && !s) {
let i = this.state.value;
throw this.raise(D.UnsupportedDeclareExportKind, { at: this.state.startLoc, unsupportedExportKind: i, suggestion: Xl[i] });
}
if (this.match(74) || this.match(68) || this.match(80) || this.isContextual(129))
return e.declaration = this.flowParseDeclare(this.startNode()), e.default = false, this.finishNode(e, "DeclareExportDeclaration");
if (this.match(55) || this.match(5) || this.isContextual(127) || this.isContextual(128) || this.isContextual(129))
return e = this.parseExport(e, null), e.type === "ExportNamedDeclaration" && (e.type = "ExportDeclaration", e.default = false, delete e.exportKind), e.type = "Declare" + e.type, e;
this.unexpected();
}
flowParseDeclareModuleExports(e) {
return this.next(), this.expectContextual(109), e.typeAnnotation = this.flowParseTypeAnnotation(), this.semicolon(), this.finishNode(e, "DeclareModuleExports");
}
flowParseDeclareTypeAlias(e) {
this.next();
let s = this.flowParseTypeAlias(e);
return s.type = "DeclareTypeAlias", s;
}
flowParseDeclareOpaqueType(e) {
this.next();
let s = this.flowParseOpaqueType(e, true);
return s.type = "DeclareOpaqueType", s;
}
flowParseDeclareInterface(e) {
return this.next(), this.flowParseInterfaceish(e, false), this.finishNode(e, "DeclareInterface");
}
flowParseInterfaceish(e, s) {
if (e.id = this.flowParseRestrictedIdentifier(!s, true), this.scope.declareName(e.id.name, s ? Er : Be, e.id.loc.start), this.match(47) ? e.typeParameters = this.flowParseTypeParameterDeclaration() : e.typeParameters = null, e.extends = [], e.implements = [], e.mixins = [], this.eat(81))
do
e.extends.push(this.flowParseInterfaceExtends());
while (!s && this.eat(12));
if (s) {
if (this.eatContextual(115))
do
e.mixins.push(this.flowParseInterfaceExtends());
while (this.eat(12));
if (this.eatContextual(111))
do
e.implements.push(this.flowParseInterfaceExtends());
while (this.eat(12));
}
e.body = this.flowParseObjectType({ allowStatic: s, allowExact: false, allowSpread: false, allowProto: s, allowInexact: false });
}
flowParseInterfaceExtends() {
let e = this.startNode();
return e.id = this.flowParseQualifiedTypeIdentifier(), this.match(47) ? e.typeParameters = this.flowParseTypeParameterInstantiation() : e.typeParameters = null, this.finishNode(e, "InterfaceExtends");
}
flowParseInterface(e) {
return this.flowParseInterfaceish(e, false), this.finishNode(e, "InterfaceDeclaration");
}
checkNotUnderscore(e) {
e === "_" && this.raise(D.UnexpectedReservedUnderscore, { at: this.state.startLoc });
}
checkReservedType(e, s, i) {
Gl.has(e) && this.raise(i ? D.AssignReservedType : D.UnexpectedReservedType, { at: s, reservedType: e });
}
flowParseRestrictedIdentifier(e, s) {
return this.checkReservedType(this.state.value, this.state.startLoc, s), this.parseIdentifier(e);
}
flowParseTypeAlias(e) {
return e.id = this.flowParseRestrictedIdentifier(false, true), this.scope.declareName(e.id.name, Be, e.id.loc.start), this.match(47) ? e.typeParameters = this.flowParseTypeParameterDeclaration() : e.typeParameters = null, e.right = this.flowParseTypeInitialiser(29), this.semicolon(), this.finishNode(e, "TypeAlias");
}
flowParseOpaqueType(e, s) {
return this.expectContextual(128), e.id = this.flowParseRestrictedIdentifier(true, true), this.scope.declareName(e.id.name, Be, e.id.loc.start), this.match(47) ? e.typeParameters = this.flowParseTypeParameterDeclaration() : e.typeParameters = null, e.supertype = null, this.match(14) && (e.supertype = this.flowParseTypeInitialiser(14)), e.impltype = null, s || (e.impltype = this.flowParseTypeInitialiser(29)), this.semicolon(), this.finishNode(e, "OpaqueType");
}
flowParseTypeParameter() {
let e = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : false, s = this.state.startLoc, i = this.startNode(), a = this.flowParseVariance(), n2 = this.flowParseTypeAnnotatableIdentifier();
return i.name = n2.name, i.variance = a, i.bound = n2.typeAnnotation, this.match(29) ? (this.eat(29), i.default = this.flowParseType()) : e && this.raise(D.MissingTypeParamDefault, { at: s }), this.finishNode(i, "TypeParameter");
}
flowParseTypeParameterDeclaration() {
let e = this.state.inType, s = this.startNode();
s.params = [], this.state.inType = true, this.match(47) || this.match(140) ? this.next() : this.unexpected();
let i = false;
do {
let a = this.flowParseTypeParameter(i);
s.params.push(a), a.default && (i = true), this.match(48) || this.expect(12);
} while (!this.match(48));
return this.expect(48), this.state.inType = e, this.finishNode(s, "TypeParameterDeclaration");
}
flowParseTypeParameterInstantiation() {
let e = this.startNode(), s = this.state.inType;
e.params = [], this.state.inType = true, this.expect(47);
let i = this.state.noAnonFunctionType;
for (this.state.noAnonFunctionType = false; !this.match(48); )
e.params.push(this.flowParseType()), this.match(48) || this.expect(12);
return this.state.noAnonFunctionType = i, this.expect(48), this.state.inType = s, this.finishNode(e, "TypeParameterInstantiation");
}
flowParseTypeParameterInstantiationCallOrNew() {
let e = this.startNode(), s = this.state.inType;
for (e.params = [], this.state.inType = true, this.expect(47); !this.match(48); )
e.params.push(this.flowParseTypeOrImplicitInstantiation()), this.match(48) || this.expect(12);
return this.expect(48), this.state.inType = s, this.finishNode(e, "TypeParameterInstantiation");
}
flowParseInterfaceType() {
let e = this.startNode();
if (this.expectContextual(127), e.extends = [], this.eat(81))
do
e.extends.push(this.flowParseInterfaceExtends());
while (this.eat(12));
return e.body = this.flowParseObjectType({ allowStatic: false, allowExact: false, allowSpread: false, allowProto: false, allowInexact: false }), this.finishNode(e, "InterfaceTypeAnnotation");
}
flowParseObjectPropertyKey() {
return this.match(132) || this.match(131) ? super.parseExprAtom() : this.parseIdentifier(true);
}
flowParseObjectTypeIndexer(e, s, i) {
return e.static = s, this.lookahead().type === 14 ? (e.id = this.flowParseObjectPropertyKey(), e.key = this.flowParseTypeInitialiser()) : (e.id = null, e.key = this.flowParseType()), this.expect(3), e.value = this.flowParseTypeInitialiser(), e.variance = i, this.finishNode(e, "ObjectTypeIndexer");
}
flowParseObjectTypeInternalSlot(e, s) {
return e.static = s, e.id = this.flowParseObjectPropertyKey(), this.expect(3), this.expect(3), this.match(47) || this.match(10) ? (e.method = true, e.optional = false, e.value = this.flowParseObjectTypeMethodish(this.startNodeAt(e.loc.start))) : (e.method = false, this.eat(17) && (e.optional = true), e.value = this.flowParseTypeInitialiser()), this.finishNode(e, "ObjectTypeInternalSlot");
}
flowParseObjectTypeMethodish(e) {
for (e.params = [], e.rest = null, e.typeParameters = null, e.this = null, this.match(47) && (e.typeParameters = this.flowParseTypeParameterDeclaration()), this.expect(10), this.match(78) && (e.this = this.flowParseFunctionTypeParam(true), e.this.name = null, this.match(11) || this.expect(12)); !this.match(11) && !this.match(21); )
e.params.push(this.flowParseFunctionTypeParam(false)), this.match(11) || this.expect(12);
return this.eat(21) && (e.rest = this.flowParseFunctionTypeParam(false)), this.expect(11), e.returnType = this.flowParseTypeInitialiser(), this.finishNode(e, "FunctionTypeAnnotation");
}
flowParseObjectTypeCallProperty(e, s) {
let i = this.startNode();
return e.static = s, e.value = this.flowParseObjectTypeMethodish(i), this.finishNode(e, "ObjectTypeCallProperty");
}
flowParseObjectType(e) {
let { allowStatic: s, allowExact: i, allowSpread: a, allowProto: n2, allowInexact: o } = e, u = this.state.inType;
this.state.inType = true;
let c = this.startNode();
c.callProperties = [], c.properties = [], c.indexers = [], c.internalSlots = [];
let y, g, T = false;
for (i && this.match(6) ? (this.expect(6), y = 9, g = true) : (this.expect(5), y = 8, g = false), c.exact = g; !this.match(y); ) {
let M = false, j = null, K = null, W = this.startNode();
if (n2 && this.isContextual(116)) {
let X = this.lookahead();
X.type !== 14 && X.type !== 17 && (this.next(), j = this.state.startLoc, s = false);
}
if (s && this.isContextual(104)) {
let X = this.lookahead();
X.type !== 14 && X.type !== 17 && (this.next(), M = true);
}
let V = this.flowParseVariance();
if (this.eat(0))
j != null && this.unexpected(j), this.eat(0) ? (V && this.unexpected(V.loc.start), c.internalSlots.push(this.flowParseObjectTypeInternalSlot(W, M))) : c.indexers.push(this.flowParseObjectTypeIndexer(W, M, V));
else if (this.match(10) || this.match(47))
j != null && this.unexpected(j), V && this.unexpected(V.loc.start), c.callProperties.push(this.flowParseObjectTypeCallProperty(W, M));
else {
let X = "init";
if (this.isContextual(98) || this.isContextual(103)) {
let Nh = this.lookahead();
it(Nh.type) && (X = this.state.value, this.next());
}
let je = this.flowParseObjectTypeProperty(W, M, j, V, X, a, o != null ? o : !g);
je === null ? (T = true, K = this.state.lastTokStartLoc) : c.properties.push(je);
}
this.flowObjectTypeSemicolon(), K && !this.match(8) && !this.match(9) && this.raise(D.UnexpectedExplicitInexactInObject, { at: K });
}
this.expect(y), a && (c.inexact = T);
let C = this.finishNode(c, "ObjectTypeAnnotation");
return this.state.inType = u, C;
}
flowParseObjectTypeProperty(e, s, i, a, n2, o, u) {
if (this.eat(21))
return this.match(12) || this.match(13) || this.match(8) || this.match(9) ? (o ? u || this.raise(D.InexactInsideExact, { at: this.state.lastTokStartLoc }) : this.raise(D.InexactInsideNonObject, { at: this.state.lastTokStartLoc }), a && this.raise(D.InexactVariance, { at: a }), null) : (o || this.raise(D.UnexpectedSpreadType, { at: this.state.lastTokStartLoc }), i != null && this.unexpected(i), a && this.raise(D.SpreadVariance, { at: a }), e.argument = this.flowParseType(), this.finishNode(e, "ObjectTypeSpreadProperty"));
{
e.key = this.flowParseObjectPropertyKey(), e.static = s, e.proto = i != null, e.kind = n2;
let c = false;
return this.match(47) || this.match(10) ? (e.method = true, i != null && this.unexpected(i), a && this.unexpected(a.loc.start), e.value = this.flowParseObjectTypeMethodish(this.startNodeAt(e.loc.start)), (n2 === "get" || n2 === "set") && this.flowCheckGetterSetterParams(e), !o && e.key.name === "constructor" && e.value.this && this.raise(D.ThisParamBannedInConstructor, { at: e.value.this })) : (n2 !== "init" && this.unexpected(), e.method = false, this.eat(17) && (c = true), e.value = this.flowParseTypeInitialiser(), e.variance = a), e.optional = c, this.finishNode(e, "ObjectTypeProperty");
}
}
flowCheckGetterSetterParams(e) {
let s = e.kind === "get" ? 0 : 1, i = e.value.params.length + (e.value.rest ? 1 : 0);
e.value.this && this.raise(e.kind === "get" ? D.GetterMayNotHaveThisParam : D.SetterMayNotHaveThisParam, { at: e.value.this }), i !== s && this.raise(e.kind === "get" ? f.BadGetterArity : f.BadSetterArity, { at: e }), e.kind === "set" && e.value.rest && this.raise(f.BadSetterRestParameter, { at: e });
}
flowObjectTypeSemicolon() {
!this.eat(13) && !this.eat(12) && !this.match(8) && !this.match(9) && this.unexpected();
}
flowParseQualifiedTypeIdentifier(e, s) {
var i;
(i = e) != null || (e = this.state.startLoc);
let a = s || this.flowParseRestrictedIdentifier(true);
for (; this.eat(16); ) {
let n2 = this.startNodeAt(e);
n2.qualification = a, n2.id = this.flowParseRestrictedIdentifier(true), a = this.finishNode(n2, "QualifiedTypeIdentifier");
}
return a;
}
flowParseGenericType(e, s) {
let i = this.startNodeAt(e);
return i.typeParameters = null, i.id = this.flowParseQualifiedTypeIdentifier(e, s), this.match(47) && (i.typeParameters = this.flowParseTypeParameterInstantiation()), this.finishNode(i, "GenericTypeAnnotation");
}
flowParseTypeofType() {
let e = this.startNode();
return this.expect(87), e.argument = this.flowParsePrimaryType(), this.finishNode(e, "TypeofTypeAnnotation");
}
flowParseTupleType() {
let e = this.startNode();
for (e.types = [], this.expect(0); this.state.pos < this.length && !this.match(3) && (e.types.push(this.flowParseType()), !this.match(3)); )
this.expect(12);
return this.expect(3), this.finishNode(e, "TupleTypeAnnotation");
}
flowParseFunctionTypeParam(e) {
let s = null, i = false, a = null, n2 = this.startNode(), o = this.lookahead(), u = this.state.type === 78;
return o.type === 14 || o.type === 17 ? (u && !e && this.raise(D.ThisParamMustBeFirst, { at: n2 }), s = this.parseIdentifier(u), this.eat(17) && (i = true, u && this.raise(D.ThisParamMayNotBeOptional, { at: n2 })), a = this.flowParseTypeInitialiser()) : a = this.flowParseType(), n2.name = s, n2.optional = i, n2.typeAnnotation = a, this.finishNode(n2, "FunctionTypeParam");
}
reinterpretTypeAsFunctionTypeParam(e) {
let s = this.startNodeAt(e.loc.start);
return s.name = null, s.optional = false, s.typeAnnotation = e, this.finishNode(s, "FunctionTypeParam");
}
flowParseFunctionTypeParams() {
let e = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : [], s = null, i = null;
for (this.match(78) && (i = this.flowParseFunctionTypeParam(true), i.name = null, this.match(11) || this.expect(12)); !this.match(11) && !this.match(21); )
e.push(this.flowParseFunctionTypeParam(false)), this.match(11) || this.expect(12);
return this.eat(21) && (s = this.flowParseFunctionTypeParam(false)), { params: e, rest: s, _this: i };
}
flowIdentToTypeAnnotation(e, s, i) {
switch (i.name) {
case "any":
return this.finishNode(s, "AnyTypeAnnotation");
case "bool":
case "boolean":
return this.finishNode(s, "BooleanTypeAnnotation");
case "mixed":
return this.finishNode(s, "MixedTypeAnnotation");
case "empty":
return this.finishNode(s, "EmptyTypeAnnotation");
case "number":
return this.finishNode(s, "NumberTypeAnnotation");
case "string":
return this.finishNode(s, "StringTypeAnnotation");
case "symbol":
return this.finishNode(s, "SymbolTypeAnnotation");
default:
return this.checkNotUnderscore(i.name), this.flowParseGenericType(e, i);
}
}
flowParsePrimaryType() {
let e = this.state.startLoc, s = this.startNode(), i, a, n2 = false, o = this.state.noAnonFunctionType;
switch (this.state.type) {
case 5:
return this.flowParseObjectType({ allowStatic: false, allowExact: false, allowSpread: true, allowProto: false, allowInexact: true });
case 6:
return this.flowParseObjectType({ allowStatic: false, allowExact: true, allowSpread: true, allowProto: false, allowInexact: false });
case 0:
return this.state.noAnonFunctionType = false, a = this.flowParseTupleType(), this.state.noAnonFunctionType = o, a;
case 47:
return s.typeParameters = this.flowParseTypeParameterDeclaration(), this.expect(10), i = this.flowParseFunctionTypeParams(), s.params = i.params, s.rest = i.rest, s.this = i._this, this.expect(11), this.expect(19), s.returnType = this.flowParseType(), this.finishNode(s, "FunctionTypeAnnotation");
case 10:
if (this.next(), !this.match(11) && !this.match(21))
if (q(this.state.type) || this.match(78)) {
let u = this.lookahead().type;
n2 = u !== 17 && u !== 14;
} else
n2 = true;
if (n2) {
if (this.state.noAnonFunctionType = false, a = this.flowParseType(), this.state.noAnonFunctionType = o, this.state.noAnonFunctionType || !(this.match(12) || this.match(11) && this.lookahead().type === 19))
return this.expect(11), a;
this.eat(12);
}
return a ? i = this.flowParseFunctionTypeParams([this.reinterpretTypeAsFunctionTypeParam(a)]) : i = this.flowParseFunctionTypeParams(), s.params = i.params, s.rest = i.rest, s.this = i._this, this.expect(11), this.expect(19), s.returnType = this.flowParseType(), s.typeParameters = null, this.finishNode(s, "FunctionTypeAnnotation");
case 131:
return this.parseLiteral(this.state.value, "StringLiteralTypeAnnotation");
case 85:
case 86:
return s.value = this.match(85), this.next(), this.finishNode(s, "BooleanLiteralTypeAnnotation");
case 53:
if (this.state.value === "-") {
if (this.next(), this.match(132))
return this.parseLiteralAtNode(-this.state.value, "NumberLiteralTypeAnnotation", s);
if (this.match(133))
return this.parseLiteralAtNode(-this.state.value, "BigIntLiteralTypeAnnotation", s);
throw this.raise(D.UnexpectedSubtractionOperand, { at: this.state.startLoc });
}
this.unexpected();
return;
case 132:
return this.parseLiteral(this.state.value, "NumberLiteralTypeAnnotation");
case 133:
return this.parseLiteral(this.state.value, "BigIntLiteralTypeAnnotation");
case 88:
return this.next(), this.finishNode(s, "VoidTypeAnnotation");
case 84:
return this.next(), this.finishNode(s, "NullLiteralTypeAnnotation");
case 78:
return this.next(), this.finishNode(s, "ThisTypeAnnotation");
case 55:
return this.next(), this.finishNode(s, "ExistsTypeAnnotation");
case 87:
return this.flowParseTypeofType();
default:
if ($t(this.state.type)) {
let u = xe(this.state.type);
return this.next(), super.createIdentifier(s, u);
} else if (q(this.state.type))
return this.isContextual(127) ? this.flowParseInterfaceType() : this.flowIdentToTypeAnnotation(e, s, this.parseIdentifier());
}
this.unexpected();
}
flowParsePostfixType() {
let e = this.state.startLoc, s = this.flowParsePrimaryType(), i = false;
for (; (this.match(0) || this.match(18)) && !this.canInsertSemicolon(); ) {
let a = this.startNodeAt(e), n2 = this.eat(18);
i = i || n2, this.expect(0), !n2 && this.match(3) ? (a.elementType = s, this.next(), s = this.finishNode(a, "ArrayTypeAnnotation")) : (a.objectType = s, a.indexType = this.flowParseType(), this.expect(3), i ? (a.optional = n2, s = this.finishNode(a, "OptionalIndexedAccessType")) : s = this.finishNode(a, "IndexedAccessType"));
}
return s;
}
flowParsePrefixType() {
let e = this.startNode();
return this.eat(17) ? (e.typeAnnotation = this.flowParsePrefixType(), this.finishNode(e, "NullableTypeAnnotation")) : this.flowParsePostfixType();
}
flowParseAnonFunctionWithoutParens() {
let e = this.flowParsePrefixType();
if (!this.state.noAnonFunctionType && this.eat(19)) {
let s = this.startNodeAt(e.loc.start);
return s.params = [this.reinterpretTypeAsFunctionTypeParam(e)], s.rest = null, s.this = null, s.returnType = this.flowParseType(), s.typeParameters = null, this.finishNode(s, "FunctionTypeAnnotation");
}
return e;
}
flowParseIntersectionType() {
let e = this.startNode();
this.eat(45);
let s = this.flowParseAnonFunctionWithoutParens();
for (e.types = [s]; this.eat(45); )
e.types.push(this.flowParseAnonFunctionWithoutParens());
return e.types.length === 1 ? s : this.finishNode(e, "IntersectionTypeAnnotation");
}
flowParseUnionType() {
let e = this.startNode();
this.eat(43);
let s = this.flowParseIntersectionType();
for (e.types = [s]; this.eat(43); )
e.types.push(this.flowParseIntersectionType());
return e.types.length === 1 ? s : this.finishNode(e, "UnionTypeAnnotation");
}
flowParseType() {
let e = this.state.inType;
this.state.inType = true;
let s = this.flowParseUnionType();
return this.state.inType = e, s;
}
flowParseTypeOrImplicitInstantiation() {
if (this.state.type === 130 && this.state.value === "_") {
let e = this.state.startLoc, s = this.parseIdentifier();
return this.flowParseGenericType(e, s);
} else
return this.flowParseType();
}
flowParseTypeAnnotation() {
let e = this.startNode();
return e.typeAnnotation = this.flowParseTypeInitialiser(), this.finishNode(e, "TypeAnnotation");
}
flowParseTypeAnnotatableIdentifier(e) {
let s = e ? this.parseIdentifier() : this.flowParseRestrictedIdentifier();
return this.match(14) && (s.typeAnnotation = this.flowParseTypeAnnotation(), this.resetEndLocation(s)), s;
}
typeCastToParameter(e) {
return e.expression.typeAnnotation = e.typeAnnotation, this.resetEndLocation(e.expression, e.typeAnnotation.loc.end), e.expression;
}
flowParseVariance() {
let e = null;
return this.match(53) ? (e = this.startNode(), this.state.value === "+" ? e.kind = "plus" : e.kind = "minus", this.next(), this.finishNode(e, "Variance")) : e;
}
parseFunctionBody(e, s) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
if (s) {
this.forwardNoArrowParamsConversionAt(e, () => super.parseFunctionBody(e, true, i));
return;
}
super.parseFunctionBody(e, false, i);
}
parseFunctionBodyAndFinish(e, s) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
if (this.match(14)) {
let a = this.startNode();
[a.typeAnnotation, e.predicate] = this.flowParseTypeAndPredicateInitialiser(), e.returnType = a.typeAnnotation ? this.finishNode(a, "TypeAnnotation") : null;
}
return super.parseFunctionBodyAndFinish(e, s, i);
}
parseStatementLike(e) {
if (this.state.strict && this.isContextual(127)) {
let i = this.lookahead();
if (te(i.type)) {
let a = this.startNode();
return this.next(), this.flowParseInterface(a);
}
} else if (this.shouldParseEnums() && this.isContextual(124)) {
let i = this.startNode();
return this.next(), this.flowParseEnumDeclaration(i);
}
let s = super.parseStatementLike(e);
return this.flowPragma === void 0 && !this.isValidDirective(s) && (this.flowPragma = null), s;
}
parseExpressionStatement(e, s, i) {
if (s.type === "Identifier") {
if (s.name === "declare") {
if (this.match(80) || q(this.state.type) || this.match(68) || this.match(74) || this.match(82))
return this.flowParseDeclare(e);
} else if (q(this.state.type)) {
if (s.name === "interface")
return this.flowParseInterface(e);
if (s.name === "type")
return this.flowParseTypeAlias(e);
if (s.name === "opaque")
return this.flowParseOpaqueType(e, false);
}
}
return super.parseExpressionStatement(e, s, i);
}
shouldParseExportDeclaration() {
let { type: e } = this.state;
return hr(e) || this.shouldParseEnums() && e === 124 ? !this.state.containsEsc : super.shouldParseExportDeclaration();
}
isExportDefaultSpecifier() {
let { type: e } = this.state;
return hr(e) || this.shouldParseEnums() && e === 124 ? this.state.containsEsc : super.isExportDefaultSpecifier();
}
parseExportDefaultExpression() {
if (this.shouldParseEnums() && this.isContextual(124)) {
let e = this.startNode();
return this.next(), this.flowParseEnumDeclaration(e);
}
return super.parseExportDefaultExpression();
}
parseConditional(e, s, i) {
if (!this.match(17))
return e;
if (this.state.maybeInArrowParameters) {
let T = this.lookaheadCharCode();
if (T === 44 || T === 61 || T === 58 || T === 41)
return this.setOptionalParametersError(i), e;
}
this.expect(17);
let a = this.state.clone(), n2 = this.state.noArrowAt, o = this.startNodeAt(s), { consequent: u, failed: c } = this.tryParseConditionalConsequent(), [y, g] = this.getArrowLikeExpressions(u);
if (c || g.length > 0) {
let T = [...n2];
if (g.length > 0) {
this.state = a, this.state.noArrowAt = T;
for (let C = 0; C < g.length; C++)
T.push(g[C].start);
({ consequent: u, failed: c } = this.tryParseConditionalConsequent()), [y, g] = this.getArrowLikeExpressions(u);
}
c && y.length > 1 && this.raise(D.AmbiguousConditionalArrow, { at: a.startLoc }), c && y.length === 1 && (this.state = a, T.push(y[0].start), this.state.noArrowAt = T, { consequent: u, failed: c } = this.tryParseConditionalConsequent());
}
return this.getArrowLikeExpressions(u, true), this.state.noArrowAt = n2, this.expect(14), o.test = e, o.consequent = u, o.alternate = this.forwardNoArrowParamsConversionAt(o, () => this.parseMaybeAssign(void 0, void 0)), this.finishNode(o, "ConditionalExpression");
}
tryParseConditionalConsequent() {
this.state.noArrowParamsConversionAt.push(this.state.start);
let e = this.parseMaybeAssignAllowIn(), s = !this.match(14);
return this.state.noArrowParamsConversionAt.pop(), { consequent: e, failed: s };
}
getArrowLikeExpressions(e, s) {
let i = [e], a = [];
for (; i.length !== 0; ) {
let n2 = i.pop();
n2.type === "ArrowFunctionExpression" ? (n2.typeParameters || !n2.returnType ? this.finishArrowValidation(n2) : a.push(n2), i.push(n2.body)) : n2.type === "ConditionalExpression" && (i.push(n2.consequent), i.push(n2.alternate));
}
return s ? (a.forEach((n2) => this.finishArrowValidation(n2)), [a, []]) : Yl(a, (n2) => n2.params.every((o) => this.isAssignable(o, true)));
}
finishArrowValidation(e) {
var s;
this.toAssignableList(e.params, (s = e.extra) == null ? void 0 : s.trailingCommaLoc, false), this.scope.enter(de | Gt), super.checkParams(e, false, true), this.scope.exit();
}
forwardNoArrowParamsConversionAt(e, s) {
let i;
return this.state.noArrowParamsConversionAt.indexOf(e.start) !== -1 ? (this.state.noArrowParamsConversionAt.push(this.state.start), i = s(), this.state.noArrowParamsConversionAt.pop()) : i = s(), i;
}
parseParenItem(e, s) {
if (e = super.parseParenItem(e, s), this.eat(17) && (e.optional = true, this.resetEndLocation(e)), this.match(14)) {
let i = this.startNodeAt(s);
return i.expression = e, i.typeAnnotation = this.flowParseTypeAnnotation(), this.finishNode(i, "TypeCastExpression");
}
return e;
}
assertModuleNodeAllowed(e) {
e.type === "ImportDeclaration" && (e.importKind === "type" || e.importKind === "typeof") || e.type === "ExportNamedDeclaration" && e.exportKind === "type" || e.type === "ExportAllDeclaration" && e.exportKind === "type" || super.assertModuleNodeAllowed(e);
}
parseExport(e, s) {
let i = super.parseExport(e, s);
return (i.type === "ExportNamedDeclaration" || i.type === "ExportAllDeclaration") && (i.exportKind = i.exportKind || "value"), i;
}
parseExportDeclaration(e) {
if (this.isContextual(128)) {
e.exportKind = "type";
let s = this.startNode();
return this.next(), this.match(5) ? (e.specifiers = this.parseExportSpecifiers(true), super.parseExportFrom(e), null) : this.flowParseTypeAlias(s);
} else if (this.isContextual(129)) {
e.exportKind = "type";
let s = this.startNode();
return this.next(), this.flowParseOpaqueType(s, false);
} else if (this.isContextual(127)) {
e.exportKind = "type";
let s = this.startNode();
return this.next(), this.flowParseInterface(s);
} else if (this.shouldParseEnums() && this.isContextual(124)) {
e.exportKind = "value";
let s = this.startNode();
return this.next(), this.flowParseEnumDeclaration(s);
} else
return super.parseExportDeclaration(e);
}
eatExportStar(e) {
return super.eatExportStar(e) ? true : this.isContextual(128) && this.lookahead().type === 55 ? (e.exportKind = "type", this.next(), this.next(), true) : false;
}
maybeParseExportNamespaceSpecifier(e) {
let { startLoc: s } = this.state, i = super.maybeParseExportNamespaceSpecifier(e);
return i && e.exportKind === "type" && this.unexpected(s), i;
}
parseClassId(e, s, i) {
super.parseClassId(e, s, i), this.match(47) && (e.typeParameters = this.flowParseTypeParameterDeclaration());
}
parseClassMember(e, s, i) {
let { startLoc: a } = this.state;
if (this.isContextual(123)) {
if (super.parseClassMemberFromModifier(e, s))
return;
s.declare = true;
}
super.parseClassMember(e, s, i), s.declare && (s.type !== "ClassProperty" && s.type !== "ClassPrivateProperty" && s.type !== "PropertyDefinition" ? this.raise(D.DeclareClassElement, { at: a }) : s.value && this.raise(D.DeclareClassFieldInitializer, { at: s.value }));
}
isIterator(e) {
return e === "iterator" || e === "asyncIterator";
}
readIterator() {
let e = super.readWord1(), s = "@@" + e;
(!this.isIterator(e) || !this.state.inType) && this.raise(f.InvalidIdentifier, { at: this.state.curPosition(), identifierName: s }), this.finishToken(130, s);
}
getTokenFromCode(e) {
let s = this.input.charCodeAt(this.state.pos + 1);
e === 123 && s === 124 ? this.finishOp(6, 2) : this.state.inType && (e === 62 || e === 60) ? this.finishOp(e === 62 ? 48 : 47, 1) : this.state.inType && e === 63 ? s === 46 ? this.finishOp(18, 2) : this.finishOp(17, 1) : ll(e, s, this.input.charCodeAt(this.state.pos + 2)) ? (this.state.pos += 2, this.readIterator()) : super.getTokenFromCode(e);
}
isAssignable(e, s) {
return e.type === "TypeCastExpression" ? this.isAssignable(e.expression, s) : super.isAssignable(e, s);
}
toAssignable(e) {
let s = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
!s && e.type === "AssignmentExpression" && e.left.type === "TypeCastExpression" && (e.left = this.typeCastToParameter(e.left)), super.toAssignable(e, s);
}
toAssignableList(e, s, i) {
for (let a = 0; a < e.length; a++) {
let n2 = e[a];
(n2 == null ? void 0 : n2.type) === "TypeCastExpression" && (e[a] = this.typeCastToParameter(n2));
}
super.toAssignableList(e, s, i);
}
toReferencedList(e, s) {
for (let a = 0; a < e.length; a++) {
var i;
let n2 = e[a];
n2 && n2.type === "TypeCastExpression" && !((i = n2.extra) != null && i.parenthesized) && (e.length > 1 || !s) && this.raise(D.TypeCastInPattern, { at: n2.typeAnnotation });
}
return e;
}
parseArrayLike(e, s, i, a) {
let n2 = super.parseArrayLike(e, s, i, a);
return s && !this.state.maybeInArrowParameters && this.toReferencedList(n2.elements), n2;
}
isValidLVal(e, s, i) {
return e === "TypeCastExpression" || super.isValidLVal(e, s, i);
}
parseClassProperty(e) {
return this.match(14) && (e.typeAnnotation = this.flowParseTypeAnnotation()), super.parseClassProperty(e);
}
parseClassPrivateProperty(e) {
return this.match(14) && (e.typeAnnotation = this.flowParseTypeAnnotation()), super.parseClassPrivateProperty(e);
}
isClassMethod() {
return this.match(47) || super.isClassMethod();
}
isClassProperty() {
return this.match(14) || super.isClassProperty();
}
isNonstaticConstructor(e) {
return !this.match(14) && super.isNonstaticConstructor(e);
}
pushClassMethod(e, s, i, a, n2, o) {
if (s.variance && this.unexpected(s.variance.loc.start), delete s.variance, this.match(47) && (s.typeParameters = this.flowParseTypeParameterDeclaration()), super.pushClassMethod(e, s, i, a, n2, o), s.params && n2) {
let u = s.params;
u.length > 0 && this.isThisParam(u[0]) && this.raise(D.ThisParamBannedInConstructor, { at: s });
} else if (s.type === "MethodDefinition" && n2 && s.value.params) {
let u = s.value.params;
u.length > 0 && this.isThisParam(u[0]) && this.raise(D.ThisParamBannedInConstructor, { at: s });
}
}
pushClassPrivateMethod(e, s, i, a) {
s.variance && this.unexpected(s.variance.loc.start), delete s.variance, this.match(47) && (s.typeParameters = this.flowParseTypeParameterDeclaration()), super.pushClassPrivateMethod(e, s, i, a);
}
parseClassSuper(e) {
if (super.parseClassSuper(e), e.superClass && this.match(47) && (e.superTypeParameters = this.flowParseTypeParameterInstantiation()), this.isContextual(111)) {
this.next();
let s = e.implements = [];
do {
let i = this.startNode();
i.id = this.flowParseRestrictedIdentifier(true), this.match(47) ? i.typeParameters = this.flowParseTypeParameterInstantiation() : i.typeParameters = null, s.push(this.finishNode(i, "ClassImplements"));
} while (this.eat(12));
}
}
checkGetterSetterParams(e) {
super.checkGetterSetterParams(e);
let s = this.getObjectOrClassMethodParams(e);
if (s.length > 0) {
let i = s[0];
this.isThisParam(i) && e.kind === "get" ? this.raise(D.GetterMayNotHaveThisParam, { at: i }) : this.isThisParam(i) && this.raise(D.SetterMayNotHaveThisParam, { at: i });
}
}
parsePropertyNamePrefixOperator(e) {
e.variance = this.flowParseVariance();
}
parseObjPropValue(e, s, i, a, n2, o, u) {
e.variance && this.unexpected(e.variance.loc.start), delete e.variance;
let c;
this.match(47) && !o && (c = this.flowParseTypeParameterDeclaration(), this.match(10) || this.unexpected());
let y = super.parseObjPropValue(e, s, i, a, n2, o, u);
return c && ((y.value || y).typeParameters = c), y;
}
parseAssignableListItemTypes(e) {
return this.eat(17) && (e.type !== "Identifier" && this.raise(D.PatternIsOptional, { at: e }), this.isThisParam(e) && this.raise(D.ThisParamMayNotBeOptional, { at: e }), e.optional = true), this.match(14) ? e.typeAnnotation = this.flowParseTypeAnnotation() : this.isThisParam(e) && this.raise(D.ThisParamAnnotationRequired, { at: e }), this.match(29) && this.isThisParam(e) && this.raise(D.ThisParamNoDefault, { at: e }), this.resetEndLocation(e), e;
}
parseMaybeDefault(e, s) {
let i = super.parseMaybeDefault(e, s);
return i.type === "AssignmentPattern" && i.typeAnnotation && i.right.start < i.typeAnnotation.start && this.raise(D.TypeBeforeInitializer, { at: i.typeAnnotation }), i;
}
shouldParseDefaultImport(e) {
return us(e) ? qr(this.state.type) : super.shouldParseDefaultImport(e);
}
checkImportReflection(e) {
super.checkImportReflection(e), e.module && e.importKind !== "value" && this.raise(D.ImportReflectionHasImportType, { at: e.specifiers[0].loc.start });
}
parseImportSpecifierLocal(e, s, i) {
s.local = us(e) ? this.flowParseRestrictedIdentifier(true, true) : this.parseIdentifier(), e.specifiers.push(this.finishImportSpecifier(s, i));
}
maybeParseDefaultImportSpecifier(e) {
e.importKind = "value";
let s = null;
if (this.match(87) ? s = "typeof" : this.isContextual(128) && (s = "type"), s) {
let i = this.lookahead(), { type: a } = i;
s === "type" && a === 55 && this.unexpected(null, i.type), (qr(a) || a === 5 || a === 55) && (this.next(), e.importKind = s);
}
return super.maybeParseDefaultImportSpecifier(e);
}
parseImportSpecifier(e, s, i, a, n2) {
let o = e.imported, u = null;
o.type === "Identifier" && (o.name === "type" ? u = "type" : o.name === "typeof" && (u = "typeof"));
let c = false;
if (this.isContextual(93) && !this.isLookaheadContextual("as")) {
let g = this.parseIdentifier(true);
u !== null && !te(this.state.type) ? (e.imported = g, e.importKind = u, e.local = me(g)) : (e.imported = o, e.importKind = null, e.local = this.parseIdentifier());
} else {
if (u !== null && te(this.state.type))
e.imported = this.parseIdentifier(true), e.importKind = u;
else {
if (s)
throw this.raise(f.ImportBindingIsString, { at: e, importName: o.value });
e.imported = o, e.importKind = null;
}
this.eatContextual(93) ? e.local = this.parseIdentifier() : (c = true, e.local = me(e.imported));
}
let y = us(e);
return i && y && this.raise(D.ImportTypeShorthandOnlyInPureImport, { at: e }), (i || y) && this.checkReservedType(e.local.name, e.local.loc.start, true), c && !i && !y && this.checkReservedWord(e.local.name, e.loc.start, true, true), this.finishImportSpecifier(e, "ImportSpecifier");
}
parseBindingAtom() {
switch (this.state.type) {
case 78:
return this.parseIdentifier(true);
default:
return super.parseBindingAtom();
}
}
parseFunctionParams(e, s) {
let i = e.kind;
i !== "get" && i !== "set" && this.match(47) && (e.typeParameters = this.flowParseTypeParameterDeclaration()), super.parseFunctionParams(e, s);
}
parseVarId(e, s) {
super.parseVarId(e, s), this.match(14) && (e.id.typeAnnotation = this.flowParseTypeAnnotation(), this.resetEndLocation(e.id));
}
parseAsyncArrowFromCallExpression(e, s) {
if (this.match(14)) {
let i = this.state.noAnonFunctionType;
this.state.noAnonFunctionType = true, e.returnType = this.flowParseTypeAnnotation(), this.state.noAnonFunctionType = i;
}
return super.parseAsyncArrowFromCallExpression(e, s);
}
shouldParseAsyncArrow() {
return this.match(14) || super.shouldParseAsyncArrow();
}
parseMaybeAssign(e, s) {
var i;
let a = null, n2;
if (this.hasPlugin("jsx") && (this.match(140) || this.match(47))) {
if (a = this.state.clone(), n2 = this.tryParse(() => super.parseMaybeAssign(e, s), a), !n2.error)
return n2.node;
let { context: c } = this.state, y = c[c.length - 1];
(y === x.j_oTag || y === x.j_expr) && c.pop();
}
if ((i = n2) != null && i.error || this.match(47)) {
var o, u;
a = a || this.state.clone();
let c, y = this.tryParse((T) => {
var C;
c = this.flowParseTypeParameterDeclaration();
let M = this.forwardNoArrowParamsConversionAt(c, () => {
let K = super.parseMaybeAssign(e, s);
return this.resetStartLocationFromNode(K, c), K;
});
(C = M.extra) != null && C.parenthesized && T();
let j = this.maybeUnwrapTypeCastExpression(M);
return j.type !== "ArrowFunctionExpression" && T(), j.typeParameters = c, this.resetStartLocationFromNode(j, c), M;
}, a), g = null;
if (y.node && this.maybeUnwrapTypeCastExpression(y.node).type === "ArrowFunctionExpression") {
if (!y.error && !y.aborted)
return y.node.async && this.raise(D.UnexpectedTypeParameterBeforeAsyncArrowFunction, { at: c }), y.node;
g = y.node;
}
if ((o = n2) != null && o.node)
return this.state = n2.failState, n2.node;
if (g)
return this.state = y.failState, g;
throw (u = n2) != null && u.thrown ? n2.error : y.thrown ? y.error : this.raise(D.UnexpectedTokenAfterTypeParameter, { at: c });
}
return super.parseMaybeAssign(e, s);
}
parseArrow(e) {
if (this.match(14)) {
let s = this.tryParse(() => {
let i = this.state.noAnonFunctionType;
this.state.noAnonFunctionType = true;
let a = this.startNode();
return [a.typeAnnotation, e.predicate] = this.flowParseTypeAndPredicateInitialiser(), this.state.noAnonFunctionType = i, this.canInsertSemicolon() && this.unexpected(), this.match(19) || this.unexpected(), a;
});
if (s.thrown)
return null;
s.error && (this.state = s.failState), e.returnType = s.node.typeAnnotation ? this.finishNode(s.node, "TypeAnnotation") : null;
}
return super.parseArrow(e);
}
shouldParseArrow(e) {
return this.match(14) || super.shouldParseArrow(e);
}
setArrowFunctionParameters(e, s) {
this.state.noArrowParamsConversionAt.indexOf(e.start) !== -1 ? e.params = s : super.setArrowFunctionParameters(e, s);
}
checkParams(e, s, i) {
let a = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : true;
if (!(i && this.state.noArrowParamsConversionAt.indexOf(e.start) !== -1)) {
for (let n2 = 0; n2 < e.params.length; n2++)
this.isThisParam(e.params[n2]) && n2 > 0 && this.raise(D.ThisParamMustBeFirst, { at: e.params[n2] });
super.checkParams(e, s, i, a);
}
}
parseParenAndDistinguishExpression(e) {
return super.parseParenAndDistinguishExpression(e && this.state.noArrowAt.indexOf(this.state.start) === -1);
}
parseSubscripts(e, s, i) {
if (e.type === "Identifier" && e.name === "async" && this.state.noArrowAt.indexOf(s.index) !== -1) {
this.next();
let a = this.startNodeAt(s);
a.callee = e, a.arguments = super.parseCallExpressionArguments(11, false), e = this.finishNode(a, "CallExpression");
} else if (e.type === "Identifier" && e.name === "async" && this.match(47)) {
let a = this.state.clone(), n2 = this.tryParse((u) => this.parseAsyncArrowWithTypeParameters(s) || u(), a);
if (!n2.error && !n2.aborted)
return n2.node;
let o = this.tryParse(() => super.parseSubscripts(e, s, i), a);
if (o.node && !o.error)
return o.node;
if (n2.node)
return this.state = n2.failState, n2.node;
if (o.node)
return this.state = o.failState, o.node;
throw n2.error || o.error;
}
return super.parseSubscripts(e, s, i);
}
parseSubscript(e, s, i, a) {
if (this.match(18) && this.isLookaheadToken_lt()) {
if (a.optionalChainMember = true, i)
return a.stop = true, e;
this.next();
let n2 = this.startNodeAt(s);
return n2.callee = e, n2.typeArguments = this.flowParseTypeParameterInstantiation(), this.expect(10), n2.arguments = this.parseCallExpressionArguments(11, false), n2.optional = true, this.finishCallExpression(n2, true);
} else if (!i && this.shouldParseTypes() && this.match(47)) {
let n2 = this.startNodeAt(s);
n2.callee = e;
let o = this.tryParse(() => (n2.typeArguments = this.flowParseTypeParameterInstantiationCallOrNew(), this.expect(10), n2.arguments = super.parseCallExpressionArguments(11, false), a.optionalChainMember && (n2.optional = false), this.finishCallExpression(n2, a.optionalChainMember)));
if (o.node)
return o.error && (this.state = o.failState), o.node;
}
return super.parseSubscript(e, s, i, a);
}
parseNewCallee(e) {
super.parseNewCallee(e);
let s = null;
this.shouldParseTypes() && this.match(47) && (s = this.tryParse(() => this.flowParseTypeParameterInstantiationCallOrNew()).node), e.typeArguments = s;
}
parseAsyncArrowWithTypeParameters(e) {
let s = this.startNodeAt(e);
if (this.parseFunctionParams(s, false), !!this.parseArrow(s))
return super.parseArrowExpression(s, void 0, true);
}
readToken_mult_modulo(e) {
let s = this.input.charCodeAt(this.state.pos + 1);
if (e === 42 && s === 47 && this.state.hasFlowComment) {
this.state.hasFlowComment = false, this.state.pos += 2, this.nextToken();
return;
}
super.readToken_mult_modulo(e);
}
readToken_pipe_amp(e) {
let s = this.input.charCodeAt(this.state.pos + 1);
if (e === 124 && s === 125) {
this.finishOp(9, 2);
return;
}
super.readToken_pipe_amp(e);
}
parseTopLevel(e, s) {
let i = super.parseTopLevel(e, s);
return this.state.hasFlowComment && this.raise(D.UnterminatedFlowComment, { at: this.state.curPosition() }), i;
}
skipBlockComment() {
if (this.hasPlugin("flowComments") && this.skipFlowComment()) {
if (this.state.hasFlowComment)
throw this.raise(D.NestedFlowComment, { at: this.state.startLoc });
this.hasFlowCommentCompletion();
let e = this.skipFlowComment();
e && (this.state.pos += e, this.state.hasFlowComment = true);
return;
}
return super.skipBlockComment(this.state.hasFlowComment ? "*-/" : "*/");
}
skipFlowComment() {
let { pos: e } = this.state, s = 2;
for (; [32, 9].includes(this.input.charCodeAt(e + s)); )
s++;
let i = this.input.charCodeAt(s + e), a = this.input.charCodeAt(s + e + 1);
return i === 58 && a === 58 ? s + 2 : this.input.slice(s + e, s + e + 12) === "flow-include" ? s + 12 : i === 58 && a !== 58 ? s : false;
}
hasFlowCommentCompletion() {
if (this.input.indexOf("*/", this.state.pos) === -1)
throw this.raise(f.UnterminatedComment, { at: this.state.curPosition() });
}
flowEnumErrorBooleanMemberNotInitialized(e, s) {
let { enumName: i, memberName: a } = s;
this.raise(D.EnumBooleanMemberNotInitialized, { at: e, memberName: a, enumName: i });
}
flowEnumErrorInvalidMemberInitializer(e, s) {
return this.raise(s.explicitType ? s.explicitType === "symbol" ? D.EnumInvalidMemberInitializerSymbolType : D.EnumInvalidMemberInitializerPrimaryType : D.EnumInvalidMemberInitializerUnknownType, Object.assign({ at: e }, s));
}
flowEnumErrorNumberMemberNotInitialized(e, s) {
let { enumName: i, memberName: a } = s;
this.raise(D.EnumNumberMemberNotInitialized, { at: e, enumName: i, memberName: a });
}
flowEnumErrorStringMemberInconsistentlyInitailized(e, s) {
let { enumName: i } = s;
this.raise(D.EnumStringMemberInconsistentlyInitailized, { at: e, enumName: i });
}
flowEnumMemberInit() {
let e = this.state.startLoc, s = () => this.match(12) || this.match(8);
switch (this.state.type) {
case 132: {
let i = this.parseNumericLiteral(this.state.value);
return s() ? { type: "number", loc: i.loc.start, value: i } : { type: "invalid", loc: e };
}
case 131: {
let i = this.parseStringLiteral(this.state.value);
return s() ? { type: "string", loc: i.loc.start, value: i } : { type: "invalid", loc: e };
}
case 85:
case 86: {
let i = this.parseBooleanLiteral(this.match(85));
return s() ? { type: "boolean", loc: i.loc.start, value: i } : { type: "invalid", loc: e };
}
default:
return { type: "invalid", loc: e };
}
}
flowEnumMemberRaw() {
let e = this.state.startLoc, s = this.parseIdentifier(true), i = this.eat(29) ? this.flowEnumMemberInit() : { type: "none", loc: e };
return { id: s, init: i };
}
flowEnumCheckExplicitTypeMismatch(e, s, i) {
let { explicitType: a } = s;
a !== null && a !== i && this.flowEnumErrorInvalidMemberInitializer(e, s);
}
flowEnumMembers(e) {
let { enumName: s, explicitType: i } = e, a = /* @__PURE__ */ new Set(), n2 = { booleanMembers: [], numberMembers: [], stringMembers: [], defaultedMembers: [] }, o = false;
for (; !this.match(8); ) {
if (this.eat(21)) {
o = true;
break;
}
let u = this.startNode(), { id: c, init: y } = this.flowEnumMemberRaw(), g = c.name;
if (g === "")
continue;
/^[a-z]/.test(g) && this.raise(D.EnumInvalidMemberName, { at: c, memberName: g, suggestion: g[0].toUpperCase() + g.slice(1), enumName: s }), a.has(g) && this.raise(D.EnumDuplicateMemberName, { at: c, memberName: g, enumName: s }), a.add(g);
let T = { enumName: s, explicitType: i, memberName: g };
switch (u.id = c, y.type) {
case "boolean": {
this.flowEnumCheckExplicitTypeMismatch(y.loc, T, "boolean"), u.init = y.value, n2.booleanMembers.push(this.finishNode(u, "EnumBooleanMember"));
break;
}
case "number": {
this.flowEnumCheckExplicitTypeMismatch(y.loc, T, "number"), u.init = y.value, n2.numberMembers.push(this.finishNode(u, "EnumNumberMember"));
break;
}
case "string": {
this.flowEnumCheckExplicitTypeMismatch(y.loc, T, "string"), u.init = y.value, n2.stringMembers.push(this.finishNode(u, "EnumStringMember"));
break;
}
case "invalid":
throw this.flowEnumErrorInvalidMemberInitializer(y.loc, T);
case "none":
switch (i) {
case "boolean":
this.flowEnumErrorBooleanMemberNotInitialized(y.loc, T);
break;
case "number":
this.flowEnumErrorNumberMemberNotInitialized(y.loc, T);
break;
default:
n2.defaultedMembers.push(this.finishNode(u, "EnumDefaultedMember"));
}
}
this.match(8) || this.expect(12);
}
return { members: n2, hasUnknownMembers: o };
}
flowEnumStringMembers(e, s, i) {
let { enumName: a } = i;
if (e.length === 0)
return s;
if (s.length === 0)
return e;
if (s.length > e.length) {
for (let n2 of e)
this.flowEnumErrorStringMemberInconsistentlyInitailized(n2, { enumName: a });
return s;
} else {
for (let n2 of s)
this.flowEnumErrorStringMemberInconsistentlyInitailized(n2, { enumName: a });
return e;
}
}
flowEnumParseExplicitType(e) {
let { enumName: s } = e;
if (!this.eatContextual(101))
return null;
if (!q(this.state.type))
throw this.raise(D.EnumInvalidExplicitTypeUnknownSupplied, { at: this.state.startLoc, enumName: s });
let { value: i } = this.state;
return this.next(), i !== "boolean" && i !== "number" && i !== "string" && i !== "symbol" && this.raise(D.EnumInvalidExplicitType, { at: this.state.startLoc, enumName: s, invalidEnumType: i }), i;
}
flowEnumBody(e, s) {
let i = s.name, a = s.loc.start, n2 = this.flowEnumParseExplicitType({ enumName: i });
this.expect(5);
let { members: o, hasUnknownMembers: u } = this.flowEnumMembers({ enumName: i, explicitType: n2 });
switch (e.hasUnknownMembers = u, n2) {
case "boolean":
return e.explicitType = true, e.members = o.booleanMembers, this.expect(8), this.finishNode(e, "EnumBooleanBody");
case "number":
return e.explicitType = true, e.members = o.numberMembers, this.expect(8), this.finishNode(e, "EnumNumberBody");
case "string":
return e.explicitType = true, e.members = this.flowEnumStringMembers(o.stringMembers, o.defaultedMembers, { enumName: i }), this.expect(8), this.finishNode(e, "EnumStringBody");
case "symbol":
return e.members = o.defaultedMembers, this.expect(8), this.finishNode(e, "EnumSymbolBody");
default: {
let c = () => (e.members = [], this.expect(8), this.finishNode(e, "EnumStringBody"));
e.explicitType = false;
let y = o.booleanMembers.length, g = o.numberMembers.length, T = o.stringMembers.length, C = o.defaultedMembers.length;
if (!y && !g && !T && !C)
return c();
if (!y && !g)
return e.members = this.flowEnumStringMembers(o.stringMembers, o.defaultedMembers, { enumName: i }), this.expect(8), this.finishNode(e, "EnumStringBody");
if (!g && !T && y >= C) {
for (let M of o.defaultedMembers)
this.flowEnumErrorBooleanMemberNotInitialized(M.loc.start, { enumName: i, memberName: M.id.name });
return e.members = o.booleanMembers, this.expect(8), this.finishNode(e, "EnumBooleanBody");
} else if (!y && !T && g >= C) {
for (let M of o.defaultedMembers)
this.flowEnumErrorNumberMemberNotInitialized(M.loc.start, { enumName: i, memberName: M.id.name });
return e.members = o.numberMembers, this.expect(8), this.finishNode(e, "EnumNumberBody");
} else
return this.raise(D.EnumInconsistentMemberValues, { at: a, enumName: i }), c();
}
}
}
flowParseEnumDeclaration(e) {
let s = this.parseIdentifier();
return e.id = s, e.body = this.flowEnumBody(this.startNode(), s), this.finishNode(e, "EnumDeclaration");
}
isLookaheadToken_lt() {
let e = this.nextTokenStart();
if (this.input.charCodeAt(e) === 60) {
let s = this.input.charCodeAt(e + 1);
return s !== 60 && s !== 61;
}
return false;
}
maybeUnwrapTypeCastExpression(e) {
return e.type === "TypeCastExpression" ? e.expression : e;
}
}, eh = { __proto__: null, quot: '"', amp: "&", apos: "'", lt: "<", gt: ">", nbsp: "\xA0", iexcl: "\xA1", cent: "\xA2", pound: "\xA3", curren: "\xA4", yen: "\xA5", brvbar: "\xA6", sect: "\xA7", uml: "\xA8", copy: "\xA9", ordf: "\xAA", laquo: "\xAB", not: "\xAC", shy: "\xAD", reg: "\xAE", macr: "\xAF", deg: "\xB0", plusmn: "\xB1", sup2: "\xB2", sup3: "\xB3", acute: "\xB4", micro: "\xB5", para: "\xB6", middot: "\xB7", cedil: "\xB8", sup1: "\xB9", ordm: "\xBA", raquo: "\xBB", frac14: "\xBC", frac12: "\xBD", frac34: "\xBE", iquest: "\xBF", Agrave: "\xC0", Aacute: "\xC1", Acirc: "\xC2", Atilde: "\xC3", Auml: "\xC4", Aring: "\xC5", AElig: "\xC6", Ccedil: "\xC7", Egrave: "\xC8", Eacute: "\xC9", Ecirc: "\xCA", Euml: "\xCB", Igrave: "\xCC", Iacute: "\xCD", Icirc: "\xCE", Iuml: "\xCF", ETH: "\xD0", Ntilde: "\xD1", Ograve: "\xD2", Oacute: "\xD3", Ocirc: "\xD4", Otilde: "\xD5", Ouml: "\xD6", times: "\xD7", Oslash: "\xD8", Ugrave: "\xD9", Uacute: "\xDA", Ucirc: "\xDB", Uuml: "\xDC", Yacute: "\xDD", THORN: "\xDE", szlig: "\xDF", agrave: "\xE0", aacute: "\xE1", acirc: "\xE2", atilde: "\xE3", auml: "\xE4", aring: "\xE5", aelig: "\xE6", ccedil: "\xE7", egrave: "\xE8", eacute: "\xE9", ecirc: "\xEA", euml: "\xEB", igrave: "\xEC", iacute: "\xED", icirc: "\xEE", iuml: "\xEF", eth: "\xF0", ntilde: "\xF1", ograve: "\xF2", oacute: "\xF3", ocirc: "\xF4", otilde: "\xF5", ouml: "\xF6", divide: "\xF7", oslash: "\xF8", ugrave: "\xF9", uacute: "\xFA", ucirc: "\xFB", uuml: "\xFC", yacute: "\xFD", thorn: "\xFE", yuml: "\xFF", OElig: "\u0152", oelig: "\u0153", Scaron: "\u0160", scaron: "\u0161", Yuml: "\u0178", fnof: "\u0192", circ: "\u02C6", tilde: "\u02DC", Alpha: "\u0391", Beta: "\u0392", Gamma: "\u0393", Delta: "\u0394", Epsilon: "\u0395", Zeta: "\u0396", Eta: "\u0397", Theta: "\u0398", Iota: "\u0399", Kappa: "\u039A", Lambda: "\u039B", Mu: "\u039C", Nu: "\u039D", Xi: "\u039E", Omicron: "\u039F", Pi: "\u03A0", Rho: "\u03A1", Sigma: "\u03A3", Tau: "\u03A4", Upsilon: "\u03A5", Phi: "\u03A6", Chi: "\u03A7", Psi: "\u03A8", Omega: "\u03A9", alpha: "\u03B1", beta: "\u03B2", gamma: "\u03B3", delta: "\u03B4", epsilon: "\u03B5", zeta: "\u03B6", eta: "\u03B7", theta: "\u03B8", iota: "\u03B9", kappa: "\u03BA", lambda: "\u03BB", mu: "\u03BC", nu: "\u03BD", xi: "\u03BE", omicron: "\u03BF", pi: "\u03C0", rho: "\u03C1", sigmaf: "\u03C2", sigma: "\u03C3", tau: "\u03C4", upsilon: "\u03C5", phi: "\u03C6", chi: "\u03C7", psi: "\u03C8", omega: "\u03C9", thetasym: "\u03D1", upsih: "\u03D2", piv: "\u03D6", ensp: "\u2002", emsp: "\u2003", thinsp: "\u2009", zwnj: "\u200C", zwj: "\u200D", lrm: "\u200E", rlm: "\u200F", ndash: "\u2013", mdash: "\u2014", lsquo: "\u2018", rsquo: "\u2019", sbquo: "\u201A", ldquo: "\u201C", rdquo: "\u201D", bdquo: "\u201E", dagger: "\u2020", Dagger: "\u2021", bull: "\u2022", hellip: "\u2026", permil: "\u2030", prime: "\u2032", Prime: "\u2033", lsaquo: "\u2039", rsaquo: "\u203A", oline: "\u203E", frasl: "\u2044", euro: "\u20AC", image: "\u2111", weierp: "\u2118", real: "\u211C", trade: "\u2122", alefsym: "\u2135", larr: "\u2190", uarr: "\u2191", rarr: "\u2192", darr: "\u2193", harr: "\u2194", crarr: "\u21B5", lArr: "\u21D0", uArr: "\u21D1", rArr: "\u21D2", dArr: "\u21D3", hArr: "\u21D4", forall: "\u2200", part: "\u2202", exist: "\u2203", empty: "\u2205", nabla: "\u2207", isin: "\u2208", notin: "\u2209", ni: "\u220B", prod: "\u220F", sum: "\u2211", minus: "\u2212", lowast: "\u2217", radic: "\u221A", prop: "\u221D", infin: "\u221E", ang: "\u2220", and: "\u2227", or: "\u2228", cap: "\u2229", cup: "\u222A", int: "\u222B", there4: "\u2234", sim: "\u223C", cong: "\u2245", asymp: "\u2248", ne: "\u2260", equiv: "\u2261", le: "\u2264", ge: "\u2265", sub: "\u2282", sup: "\u2283", nsub: "\u2284", sube: "\u2286", supe: "\u2287", oplus: "\u2295", otimes: "\u2297", perp: "\u22A5", sdot: "\u22C5", lceil: "\u2308", rceil: "\u2309", lfloor: "\u230A", rfloor: "\u230B", lang: "\u2329", rang: "\u232A", loz: "\u25CA", spades: "\u2660", clubs: "\u2663", hearts: "\u2665", diams: "\u2666" }, Se = pe`jsx`({ AttributeIsEmpty: "JSX attributes must only be assigned a non-empty expression.", MissingClosingTagElement: (t) => {
let { openingTagName: r } = t;
return `Expected corresponding JSX closing tag for <${r}>.`;
}, MissingClosingTagFragment: "Expected corresponding JSX closing tag for <>.", UnexpectedSequenceExpression: "Sequence expressions cannot be directly nested inside JSX. Did you mean to wrap it in parentheses (...)?", UnexpectedToken: (t) => {
let { unexpected: r, HTMLEntity: e } = t;
return `Unexpected token \`${r}\`. Did you mean \`${e}\` or \`{'${r}'}\`?`;
}, UnsupportedJsxValue: "JSX value should be either an expression or a quoted JSX text.", UnterminatedJsxContent: "Unterminated JSX contents.", UnwrappedAdjacentJSXElements: "Adjacent JSX elements must be wrapped in an enclosing tag. Did you want a JSX fragment <>...>?" });
function Te(t) {
return t ? t.type === "JSXOpeningFragment" || t.type === "JSXClosingFragment" : false;
}
function Re(t) {
if (t.type === "JSXIdentifier")
return t.name;
if (t.type === "JSXNamespacedName")
return t.namespace.name + ":" + t.name.name;
if (t.type === "JSXMemberExpression")
return Re(t.object) + "." + Re(t.property);
throw new Error("Node had unexpected type: " + t.type);
}
var th = (t) => class extends t {
jsxReadToken() {
let e = "", s = this.state.pos;
for (; ; ) {
if (this.state.pos >= this.length)
throw this.raise(Se.UnterminatedJsxContent, { at: this.state.startLoc });
let i = this.input.charCodeAt(this.state.pos);
switch (i) {
case 60:
case 123:
if (this.state.pos === this.state.start) {
i === 60 && this.state.canStartJSXElement ? (++this.state.pos, this.finishToken(140)) : super.getTokenFromCode(i);
return;
}
e += this.input.slice(s, this.state.pos), this.finishToken(139, e);
return;
case 38:
e += this.input.slice(s, this.state.pos), e += this.jsxReadEntity(), s = this.state.pos;
break;
case 62:
case 125:
default:
Ge(i) ? (e += this.input.slice(s, this.state.pos), e += this.jsxReadNewLine(true), s = this.state.pos) : ++this.state.pos;
}
}
}
jsxReadNewLine(e) {
let s = this.input.charCodeAt(this.state.pos), i;
return ++this.state.pos, s === 13 && this.input.charCodeAt(this.state.pos) === 10 ? (++this.state.pos, i = e ? `
` : `\r
`) : i = String.fromCharCode(s), ++this.state.curLine, this.state.lineStart = this.state.pos, i;
}
jsxReadString(e) {
let s = "", i = ++this.state.pos;
for (; ; ) {
if (this.state.pos >= this.length)
throw this.raise(f.UnterminatedString, { at: this.state.startLoc });
let a = this.input.charCodeAt(this.state.pos);
if (a === e)
break;
a === 38 ? (s += this.input.slice(i, this.state.pos), s += this.jsxReadEntity(), i = this.state.pos) : Ge(a) ? (s += this.input.slice(i, this.state.pos), s += this.jsxReadNewLine(false), i = this.state.pos) : ++this.state.pos;
}
s += this.input.slice(i, this.state.pos++), this.finishToken(131, s);
}
jsxReadEntity() {
let e = ++this.state.pos;
if (this.codePointAtPos(this.state.pos) === 35) {
++this.state.pos;
let s = 10;
this.codePointAtPos(this.state.pos) === 120 && (s = 16, ++this.state.pos);
let i = this.readInt(s, void 0, false, "bail");
if (i !== null && this.codePointAtPos(this.state.pos) === 59)
return ++this.state.pos, String.fromCodePoint(i);
} else {
let s = 0, i = false;
for (; s++ < 10 && this.state.pos < this.length && !(i = this.codePointAtPos(this.state.pos) == 59); )
++this.state.pos;
if (i) {
let a = this.input.slice(e, this.state.pos), n2 = eh[a];
if (++this.state.pos, n2)
return n2;
}
}
return this.state.pos = e, "&";
}
jsxReadWord() {
let e, s = this.state.pos;
do
e = this.input.charCodeAt(++this.state.pos);
while (De(e) || e === 45);
this.finishToken(138, this.input.slice(s, this.state.pos));
}
jsxParseIdentifier() {
let e = this.startNode();
return this.match(138) ? e.name = this.state.value : $t(this.state.type) ? e.name = xe(this.state.type) : this.unexpected(), this.next(), this.finishNode(e, "JSXIdentifier");
}
jsxParseNamespacedName() {
let e = this.state.startLoc, s = this.jsxParseIdentifier();
if (!this.eat(14))
return s;
let i = this.startNodeAt(e);
return i.namespace = s, i.name = this.jsxParseIdentifier(), this.finishNode(i, "JSXNamespacedName");
}
jsxParseElementName() {
let e = this.state.startLoc, s = this.jsxParseNamespacedName();
if (s.type === "JSXNamespacedName")
return s;
for (; this.eat(16); ) {
let i = this.startNodeAt(e);
i.object = s, i.property = this.jsxParseIdentifier(), s = this.finishNode(i, "JSXMemberExpression");
}
return s;
}
jsxParseAttributeValue() {
let e;
switch (this.state.type) {
case 5:
return e = this.startNode(), this.setContext(x.brace), this.next(), e = this.jsxParseExpressionContainer(e, x.j_oTag), e.expression.type === "JSXEmptyExpression" && this.raise(Se.AttributeIsEmpty, { at: e }), e;
case 140:
case 131:
return this.parseExprAtom();
default:
throw this.raise(Se.UnsupportedJsxValue, { at: this.state.startLoc });
}
}
jsxParseEmptyExpression() {
let e = this.startNodeAt(this.state.lastTokEndLoc);
return this.finishNodeAt(e, "JSXEmptyExpression", this.state.startLoc);
}
jsxParseSpreadChild(e) {
return this.next(), e.expression = this.parseExpression(), this.setContext(x.j_expr), this.state.canStartJSXElement = true, this.expect(8), this.finishNode(e, "JSXSpreadChild");
}
jsxParseExpressionContainer(e, s) {
if (this.match(8))
e.expression = this.jsxParseEmptyExpression();
else {
let i = this.parseExpression();
e.expression = i;
}
return this.setContext(s), this.state.canStartJSXElement = true, this.expect(8), this.finishNode(e, "JSXExpressionContainer");
}
jsxParseAttribute() {
let e = this.startNode();
return this.match(5) ? (this.setContext(x.brace), this.next(), this.expect(21), e.argument = this.parseMaybeAssignAllowIn(), this.setContext(x.j_oTag), this.state.canStartJSXElement = true, this.expect(8), this.finishNode(e, "JSXSpreadAttribute")) : (e.name = this.jsxParseNamespacedName(), e.value = this.eat(29) ? this.jsxParseAttributeValue() : null, this.finishNode(e, "JSXAttribute"));
}
jsxParseOpeningElementAt(e) {
let s = this.startNodeAt(e);
return this.eat(141) ? this.finishNode(s, "JSXOpeningFragment") : (s.name = this.jsxParseElementName(), this.jsxParseOpeningElementAfterName(s));
}
jsxParseOpeningElementAfterName(e) {
let s = [];
for (; !this.match(56) && !this.match(141); )
s.push(this.jsxParseAttribute());
return e.attributes = s, e.selfClosing = this.eat(56), this.expect(141), this.finishNode(e, "JSXOpeningElement");
}
jsxParseClosingElementAt(e) {
let s = this.startNodeAt(e);
return this.eat(141) ? this.finishNode(s, "JSXClosingFragment") : (s.name = this.jsxParseElementName(), this.expect(141), this.finishNode(s, "JSXClosingElement"));
}
jsxParseElementAt(e) {
let s = this.startNodeAt(e), i = [], a = this.jsxParseOpeningElementAt(e), n2 = null;
if (!a.selfClosing) {
e:
for (; ; )
switch (this.state.type) {
case 140:
if (e = this.state.startLoc, this.next(), this.eat(56)) {
n2 = this.jsxParseClosingElementAt(e);
break e;
}
i.push(this.jsxParseElementAt(e));
break;
case 139:
i.push(this.parseExprAtom());
break;
case 5: {
let o = this.startNode();
this.setContext(x.brace), this.next(), this.match(21) ? i.push(this.jsxParseSpreadChild(o)) : i.push(this.jsxParseExpressionContainer(o, x.j_expr));
break;
}
default:
this.unexpected();
}
Te(a) && !Te(n2) && n2 !== null ? this.raise(Se.MissingClosingTagFragment, { at: n2 }) : !Te(a) && Te(n2) ? this.raise(Se.MissingClosingTagElement, { at: n2, openingTagName: Re(a.name) }) : !Te(a) && !Te(n2) && Re(n2.name) !== Re(a.name) && this.raise(Se.MissingClosingTagElement, { at: n2, openingTagName: Re(a.name) });
}
if (Te(a) ? (s.openingFragment = a, s.closingFragment = n2) : (s.openingElement = a, s.closingElement = n2), s.children = i, this.match(47))
throw this.raise(Se.UnwrappedAdjacentJSXElements, { at: this.state.startLoc });
return Te(a) ? this.finishNode(s, "JSXFragment") : this.finishNode(s, "JSXElement");
}
jsxParseElement() {
let e = this.state.startLoc;
return this.next(), this.jsxParseElementAt(e);
}
setContext(e) {
let { context: s } = this.state;
s[s.length - 1] = e;
}
parseExprAtom(e) {
return this.match(139) ? this.parseLiteral(this.state.value, "JSXText") : this.match(140) ? this.jsxParseElement() : this.match(47) && this.input.charCodeAt(this.state.pos) !== 33 ? (this.replaceToken(140), this.jsxParseElement()) : super.parseExprAtom(e);
}
skipSpace() {
this.curContext().preserveSpace || super.skipSpace();
}
getTokenFromCode(e) {
let s = this.curContext();
if (s === x.j_expr) {
this.jsxReadToken();
return;
}
if (s === x.j_oTag || s === x.j_cTag) {
if (fe(e)) {
this.jsxReadWord();
return;
}
if (e === 62) {
++this.state.pos, this.finishToken(141);
return;
}
if ((e === 34 || e === 39) && s === x.j_oTag) {
this.jsxReadString(e);
return;
}
}
if (e === 60 && this.state.canStartJSXElement && this.input.charCodeAt(this.state.pos + 1) !== 33) {
++this.state.pos, this.finishToken(140);
return;
}
super.getTokenFromCode(e);
}
updateContext(e) {
let { context: s, type: i } = this.state;
if (i === 56 && e === 140)
s.splice(-2, 2, x.j_cTag), this.state.canStartJSXElement = false;
else if (i === 140)
s.push(x.j_oTag);
else if (i === 141) {
let a = s[s.length - 1];
a === x.j_oTag && e === 56 || a === x.j_cTag ? (s.pop(), this.state.canStartJSXElement = s[s.length - 1] === x.j_expr) : (this.setContext(x.j_expr), this.state.canStartJSXElement = true);
} else
this.state.canStartJSXElement = se(i);
}
}, sh = class extends rs {
constructor() {
super(...arguments), this.types = /* @__PURE__ */ new Set(), this.enums = /* @__PURE__ */ new Set(), this.constEnums = /* @__PURE__ */ new Set(), this.classes = /* @__PURE__ */ new Set(), this.exportOnlyBindings = /* @__PURE__ */ new Set();
}
}, rh = class extends is {
constructor() {
super(...arguments), this.importsStack = [];
}
createScope(t) {
return this.importsStack.push(/* @__PURE__ */ new Set()), new sh(t);
}
enter(t) {
t == Oe && this.importsStack.push(/* @__PURE__ */ new Set()), super.enter(t);
}
exit() {
let t = super.exit();
return t == Oe && this.importsStack.pop(), t;
}
hasImport(t, r) {
let e = this.importsStack.length;
if (this.importsStack[e - 1].has(t))
return true;
if (!r && e > 1) {
for (let s = 0; s < e - 1; s++)
if (this.importsStack[s].has(t))
return true;
}
return false;
}
declareName(t, r, e) {
if (r & Ve) {
this.hasImport(t, true) && this.parser.raise(f.VarRedeclaration, { at: e, identifierName: t }), this.importsStack[this.importsStack.length - 1].add(t);
return;
}
let s = this.currentScope();
if (r & Yt) {
this.maybeExportDefined(s, t), s.exportOnlyBindings.add(t);
return;
}
super.declareName(t, r, e), r & Ce && (r & le || (this.checkRedeclarationInScope(s, t, r, e), this.maybeExportDefined(s, t)), s.types.add(t)), r & Jt && s.enums.add(t), r & Xt && s.constEnums.add(t), r & ft && s.classes.add(t);
}
isRedeclaredInScope(t, r, e) {
if (t.enums.has(r)) {
if (e & Jt) {
let s = !!(e & Xt), i = t.constEnums.has(r);
return s !== i;
}
return true;
}
return e & ft && t.classes.has(r) ? t.lexical.has(r) ? !!(e & le) : false : e & Ce && t.types.has(r) ? true : super.isRedeclaredInScope(t, r, e);
}
checkLocalExport(t) {
let { name: r } = t;
if (this.hasImport(r))
return;
let e = this.scopeStack.length;
for (let s = e - 1; s >= 0; s--) {
let i = this.scopeStack[s];
if (i.types.has(r) || i.exportOnlyBindings.has(r))
return;
}
super.checkLocalExport(t);
}
}, ih = (t, r) => Object.hasOwnProperty.call(t, r) && t[r], Ur = (t) => t.type === "ParenthesizedExpression" ? Ur(t.expression) : t, ah = class extends Wl {
toAssignable(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
var e, s;
let i;
switch ((t.type === "ParenthesizedExpression" || (e = t.extra) != null && e.parenthesized) && (i = Ur(t), r ? i.type === "Identifier" ? this.expressionScope.recordArrowParameterBindingError(f.InvalidParenthesizedAssignment, { at: t }) : i.type !== "MemberExpression" && this.raise(f.InvalidParenthesizedAssignment, { at: t }) : this.raise(f.InvalidParenthesizedAssignment, { at: t })), t.type) {
case "Identifier":
case "ObjectPattern":
case "ArrayPattern":
case "AssignmentPattern":
case "RestElement":
break;
case "ObjectExpression":
t.type = "ObjectPattern";
for (let n2 = 0, o = t.properties.length, u = o - 1; n2 < o; n2++) {
var a;
let c = t.properties[n2], y = n2 === u;
this.toAssignableObjectExpressionProp(c, y, r), y && c.type === "RestElement" && (a = t.extra) != null && a.trailingCommaLoc && this.raise(f.RestTrailingComma, { at: t.extra.trailingCommaLoc });
}
break;
case "ObjectProperty": {
let { key: n2, value: o } = t;
this.isPrivateName(n2) && this.classScope.usePrivateName(this.getPrivateNameSV(n2), n2.loc.start), this.toAssignable(o, r);
break;
}
case "SpreadElement":
throw new Error("Internal @babel/parser error (this is a bug, please report it). SpreadElement should be converted by .toAssignable's caller.");
case "ArrayExpression":
t.type = "ArrayPattern", this.toAssignableList(t.elements, (s = t.extra) == null ? void 0 : s.trailingCommaLoc, r);
break;
case "AssignmentExpression":
t.operator !== "=" && this.raise(f.MissingEqInAssignment, { at: t.left.loc.end }), t.type = "AssignmentPattern", delete t.operator, this.toAssignable(t.left, r);
break;
case "ParenthesizedExpression":
this.toAssignable(i, r);
break;
}
}
toAssignableObjectExpressionProp(t, r, e) {
if (t.type === "ObjectMethod")
this.raise(t.kind === "get" || t.kind === "set" ? f.PatternHasAccessor : f.PatternHasMethod, { at: t.key });
else if (t.type === "SpreadElement") {
t.type = "RestElement";
let s = t.argument;
this.checkToRestConversion(s, false), this.toAssignable(s, e), r || this.raise(f.RestTrailingComma, { at: t });
} else
this.toAssignable(t, e);
}
toAssignableList(t, r, e) {
let s = t.length - 1;
for (let i = 0; i <= s; i++) {
let a = t[i];
if (a) {
if (a.type === "SpreadElement") {
a.type = "RestElement";
let n2 = a.argument;
this.checkToRestConversion(n2, true), this.toAssignable(n2, e);
} else
this.toAssignable(a, e);
a.type === "RestElement" && (i < s ? this.raise(f.RestTrailingComma, { at: a }) : r && this.raise(f.RestTrailingComma, { at: r }));
}
}
}
isAssignable(t, r) {
switch (t.type) {
case "Identifier":
case "ObjectPattern":
case "ArrayPattern":
case "AssignmentPattern":
case "RestElement":
return true;
case "ObjectExpression": {
let e = t.properties.length - 1;
return t.properties.every((s, i) => s.type !== "ObjectMethod" && (i === e || s.type !== "SpreadElement") && this.isAssignable(s));
}
case "ObjectProperty":
return this.isAssignable(t.value);
case "SpreadElement":
return this.isAssignable(t.argument);
case "ArrayExpression":
return t.elements.every((e) => e === null || this.isAssignable(e));
case "AssignmentExpression":
return t.operator === "=";
case "ParenthesizedExpression":
return this.isAssignable(t.expression);
case "MemberExpression":
case "OptionalMemberExpression":
return !r;
default:
return false;
}
}
toReferencedList(t, r) {
return t;
}
toReferencedListDeep(t, r) {
this.toReferencedList(t, r);
for (let e of t)
(e == null ? void 0 : e.type) === "ArrayExpression" && this.toReferencedListDeep(e.elements);
}
parseSpread(t) {
let r = this.startNode();
return this.next(), r.argument = this.parseMaybeAssignAllowIn(t, void 0), this.finishNode(r, "SpreadElement");
}
parseRestBinding() {
let t = this.startNode();
return this.next(), t.argument = this.parseBindingAtom(), this.finishNode(t, "RestElement");
}
parseBindingAtom() {
switch (this.state.type) {
case 0: {
let t = this.startNode();
return this.next(), t.elements = this.parseBindingList(3, 93, 1), this.finishNode(t, "ArrayPattern");
}
case 5:
return this.parseObjectLike(8, true);
}
return this.parseIdentifier();
}
parseBindingList(t, r, e) {
let s = e & 1, i = [], a = true;
for (; !this.eat(t); )
if (a ? a = false : this.expect(12), s && this.match(12))
i.push(null);
else {
if (this.eat(t))
break;
if (this.match(21)) {
if (i.push(this.parseAssignableListItemTypes(this.parseRestBinding(), e)), !this.checkCommaAfterRest(r)) {
this.expect(t);
break;
}
} else {
let n2 = [];
for (this.match(26) && this.hasPlugin("decorators") && this.raise(f.UnsupportedParameterDecorator, { at: this.state.startLoc }); this.match(26); )
n2.push(this.parseDecorator());
i.push(this.parseAssignableListItem(e, n2));
}
}
return i;
}
parseBindingRestProperty(t) {
return this.next(), t.argument = this.parseIdentifier(), this.checkCommaAfterRest(125), this.finishNode(t, "RestElement");
}
parseBindingProperty() {
let t = this.startNode(), { type: r, startLoc: e } = this.state;
return r === 21 ? this.parseBindingRestProperty(t) : (r === 136 ? (this.expectPlugin("destructuringPrivate", e), this.classScope.usePrivateName(this.state.value, e), t.key = this.parsePrivateName()) : this.parsePropertyName(t), t.method = false, this.parseObjPropValue(t, e, false, false, true, false));
}
parseAssignableListItem(t, r) {
let e = this.parseMaybeDefault();
this.parseAssignableListItemTypes(e, t);
let s = this.parseMaybeDefault(e.loc.start, e);
return r.length && (e.decorators = r), s;
}
parseAssignableListItemTypes(t, r) {
return t;
}
parseMaybeDefault(t, r) {
var e, s;
if ((e = t) != null || (t = this.state.startLoc), r = (s = r) != null ? s : this.parseBindingAtom(), !this.eat(29))
return r;
let i = this.startNodeAt(t);
return i.left = r, i.right = this.parseMaybeAssignAllowIn(), this.finishNode(i, "AssignmentPattern");
}
isValidLVal(t, r, e) {
return ih({ AssignmentPattern: "left", RestElement: "argument", ObjectProperty: "value", ParenthesizedExpression: "expression", ArrayPattern: "elements", ObjectPattern: "properties" }, t);
}
checkLVal(t, r) {
let { in: e, binding: s = Pe, checkClashes: i = false, strictModeChanged: a = false, hasParenthesizedAncestor: n2 = false } = r;
var o;
let u = t.type;
if (this.isObjectMethod(t))
return;
if (u === "MemberExpression") {
s !== Pe && this.raise(f.InvalidPropertyBindingPattern, { at: t });
return;
}
if (u === "Identifier") {
this.checkIdentifier(t, s, a);
let { name: C } = t;
i && (i.has(C) ? this.raise(f.ParamDupe, { at: t }) : i.add(C));
return;
}
let c = this.isValidLVal(u, !(n2 || (o = t.extra) != null && o.parenthesized) && e.type === "AssignmentExpression", s);
if (c === true)
return;
if (c === false) {
let C = s === Pe ? f.InvalidLhs : f.InvalidLhsBinding;
this.raise(C, { at: t, ancestor: e });
return;
}
let [y, g] = Array.isArray(c) ? c : [c, u === "ParenthesizedExpression"], T = u === "ArrayPattern" || u === "ObjectPattern" || u === "ParenthesizedExpression" ? { type: u } : e;
for (let C of [].concat(t[y]))
C && this.checkLVal(C, { in: T, binding: s, checkClashes: i, strictModeChanged: a, hasParenthesizedAncestor: g });
}
checkIdentifier(t, r) {
let e = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
this.state.strict && (e ? xr(t.name, this.inModule) : yr(t.name)) && (r === Pe ? this.raise(f.StrictEvalArguments, { at: t, referenceName: t.name }) : this.raise(f.StrictEvalArgumentsBinding, { at: t, bindingName: t.name })), r & dt && t.name === "let" && this.raise(f.LetInLexicalBinding, { at: t }), r & Pe || this.declareNameFromIdentifier(t, r);
}
declareNameFromIdentifier(t, r) {
this.scope.declareName(t.name, r, t.loc.start);
}
checkToRestConversion(t, r) {
switch (t.type) {
case "ParenthesizedExpression":
this.checkToRestConversion(t.expression, r);
break;
case "Identifier":
case "MemberExpression":
break;
case "ArrayExpression":
case "ObjectExpression":
if (r)
break;
default:
this.raise(f.InvalidRestAssignmentPattern, { at: t });
}
}
checkCommaAfterRest(t) {
return this.match(12) ? (this.raise(this.lookaheadCharCode() === t ? f.RestTrailingComma : f.ElementAfterRest, { at: this.state.startLoc }), true) : false;
}
}, nh = (t, r) => Object.hasOwnProperty.call(t, r) && t[r];
function oh(t) {
if (t == null)
throw new Error(`Unexpected ${t} value.`);
return t;
}
function $r(t) {
if (!t)
throw new Error("Assert fail");
}
var I = pe`typescript`({ AbstractMethodHasImplementation: (t) => {
let { methodName: r } = t;
return `Method '${r}' cannot have an implementation because it is marked abstract.`;
}, AbstractPropertyHasInitializer: (t) => {
let { propertyName: r } = t;
return `Property '${r}' cannot have an initializer because it is marked abstract.`;
}, AccesorCannotDeclareThisParameter: "'get' and 'set' accessors cannot declare 'this' parameters.", AccesorCannotHaveTypeParameters: "An accessor cannot have type parameters.", AccessorCannotBeOptional: "An 'accessor' property cannot be declared optional.", ClassMethodHasDeclare: "Class methods cannot have the 'declare' modifier.", ClassMethodHasReadonly: "Class methods cannot have the 'readonly' modifier.", ConstInitiailizerMustBeStringOrNumericLiteralOrLiteralEnumReference: "A 'const' initializer in an ambient context must be a string or numeric literal or literal enum reference.", ConstructorHasTypeParameters: "Type parameters cannot appear on a constructor declaration.", DeclareAccessor: (t) => {
let { kind: r } = t;
return `'declare' is not allowed in ${r}ters.`;
}, DeclareClassFieldHasInitializer: "Initializers are not allowed in ambient contexts.", DeclareFunctionHasImplementation: "An implementation cannot be declared in ambient contexts.", DuplicateAccessibilityModifier: (t) => {
let { modifier: r } = t;
return "Accessibility modifier already seen.";
}, DuplicateModifier: (t) => {
let { modifier: r } = t;
return `Duplicate modifier: '${r}'.`;
}, EmptyHeritageClauseType: (t) => {
let { token: r } = t;
return `'${r}' list cannot be empty.`;
}, EmptyTypeArguments: "Type argument list cannot be empty.", EmptyTypeParameters: "Type parameter list cannot be empty.", ExpectedAmbientAfterExportDeclare: "'export declare' must be followed by an ambient declaration.", ImportAliasHasImportType: "An import alias can not use 'import type'.", ImportReflectionHasImportType: "An `import module` declaration can not use `type` modifier", IncompatibleModifiers: (t) => {
let { modifiers: r } = t;
return `'${r[0]}' modifier cannot be used with '${r[1]}' modifier.`;
}, IndexSignatureHasAbstract: "Index signatures cannot have the 'abstract' modifier.", IndexSignatureHasAccessibility: (t) => {
let { modifier: r } = t;
return `Index signatures cannot have an accessibility modifier ('${r}').`;
}, IndexSignatureHasDeclare: "Index signatures cannot have the 'declare' modifier.", IndexSignatureHasOverride: "'override' modifier cannot appear on an index signature.", IndexSignatureHasStatic: "Index signatures cannot have the 'static' modifier.", InitializerNotAllowedInAmbientContext: "Initializers are not allowed in ambient contexts.", InvalidModifierOnTypeMember: (t) => {
let { modifier: r } = t;
return `'${r}' modifier cannot appear on a type member.`;
}, InvalidModifierOnTypeParameter: (t) => {
let { modifier: r } = t;
return `'${r}' modifier cannot appear on a type parameter.`;
}, InvalidModifierOnTypeParameterPositions: (t) => {
let { modifier: r } = t;
return `'${r}' modifier can only appear on a type parameter of a class, interface or type alias.`;
}, InvalidModifiersOrder: (t) => {
let { orderedModifiers: r } = t;
return `'${r[0]}' modifier must precede '${r[1]}' modifier.`;
}, InvalidPropertyAccessAfterInstantiationExpression: "Invalid property access after an instantiation expression. You can either wrap the instantiation expression in parentheses, or delete the type arguments.", InvalidTupleMemberLabel: "Tuple members must be labeled with a simple identifier.", MissingInterfaceName: "'interface' declarations must be followed by an identifier.", MixedLabeledAndUnlabeledElements: "Tuple members must all have names or all not have names.", NonAbstractClassHasAbstractMethod: "Abstract methods can only appear within an abstract class.", NonClassMethodPropertyHasAbstractModifer: "'abstract' modifier can only appear on a class, method, or property declaration.", OptionalTypeBeforeRequired: "A required element cannot follow an optional element.", OverrideNotInSubClass: "This member cannot have an 'override' modifier because its containing class does not extend another class.", PatternIsOptional: "A binding pattern parameter cannot be optional in an implementation signature.", PrivateElementHasAbstract: "Private elements cannot have the 'abstract' modifier.", PrivateElementHasAccessibility: (t) => {
let { modifier: r } = t;
return `Private elements cannot have an accessibility modifier ('${r}').`;
}, ReadonlyForMethodSignature: "'readonly' modifier can only appear on a property declaration or index signature.", ReservedArrowTypeParam: "This syntax is reserved in files with the .mts or .cts extension. Add a trailing comma, as in `() => ...`.", ReservedTypeAssertion: "This syntax is reserved in files with the .mts or .cts extension. Use an `as` expression instead.", SetAccesorCannotHaveOptionalParameter: "A 'set' accessor cannot have an optional parameter.", SetAccesorCannotHaveRestParameter: "A 'set' accessor cannot have rest parameter.", SetAccesorCannotHaveReturnType: "A 'set' accessor cannot have a return type annotation.", SingleTypeParameterWithoutTrailingComma: (t) => {
let { typeParameterName: r } = t;
return `Single type parameter ${r} should have a trailing comma. Example usage: <${r},>.`;
}, StaticBlockCannotHaveModifier: "Static class blocks cannot have any modifier.", TupleOptionalAfterType: "A labeled tuple optional element must be declared using a question mark after the name and before the colon (`name?: type`), rather than after the type (`name: type?`).", TypeAnnotationAfterAssign: "Type annotations must come before default assignments, e.g. instead of `age = 25: number` use `age: number = 25`.", TypeImportCannotSpecifyDefaultAndNamed: "A type-only import can specify a default import or named bindings, but not both.", TypeModifierIsUsedInTypeExports: "The 'type' modifier cannot be used on a named export when 'export type' is used on its export statement.", TypeModifierIsUsedInTypeImports: "The 'type' modifier cannot be used on a named import when 'import type' is used on its import statement.", UnexpectedParameterModifier: "A parameter property is only allowed in a constructor implementation.", UnexpectedReadonly: "'readonly' type modifier is only permitted on array and tuple literal types.", UnexpectedTypeAnnotation: "Did not expect a type annotation here.", UnexpectedTypeCastInParameter: "Unexpected type cast in parameter position.", UnsupportedImportTypeArgument: "Argument in a type import must be a string literal.", UnsupportedParameterPropertyKind: "A parameter property may not be declared using a binding pattern.", UnsupportedSignatureParameterKind: (t) => {
let { type: r } = t;
return `Name in a signature must be an Identifier, ObjectPattern or ArrayPattern, instead got ${r}.`;
} });
function lh(t) {
switch (t) {
case "any":
return "TSAnyKeyword";
case "boolean":
return "TSBooleanKeyword";
case "bigint":
return "TSBigIntKeyword";
case "never":
return "TSNeverKeyword";
case "number":
return "TSNumberKeyword";
case "object":
return "TSObjectKeyword";
case "string":
return "TSStringKeyword";
case "symbol":
return "TSSymbolKeyword";
case "undefined":
return "TSUndefinedKeyword";
case "unknown":
return "TSUnknownKeyword";
default:
return;
}
}
function Hr(t) {
return t === "private" || t === "public" || t === "protected";
}
function hh(t) {
return t === "in" || t === "out";
}
var uh = (t) => class extends t {
constructor() {
super(...arguments), this.tsParseInOutModifiers = this.tsParseModifiers.bind(this, { allowedModifiers: ["in", "out"], disallowedModifiers: ["const", "public", "private", "protected", "readonly", "declare", "abstract", "override"], errorTemplate: I.InvalidModifierOnTypeParameter }), this.tsParseConstModifier = this.tsParseModifiers.bind(this, { allowedModifiers: ["const"], disallowedModifiers: ["in", "out"], errorTemplate: I.InvalidModifierOnTypeParameterPositions }), this.tsParseInOutConstModifiers = this.tsParseModifiers.bind(this, { allowedModifiers: ["in", "out", "const"], disallowedModifiers: ["public", "private", "protected", "readonly", "declare", "abstract", "override"], errorTemplate: I.InvalidModifierOnTypeParameter });
}
getScopeHandler() {
return rh;
}
tsIsIdentifier() {
return q(this.state.type);
}
tsTokenCanFollowModifier() {
return (this.match(0) || this.match(5) || this.match(55) || this.match(21) || this.match(136) || this.isLiteralPropertyName()) && !this.hasPrecedingLineBreak();
}
tsNextTokenCanFollowModifier() {
return this.next(), this.tsTokenCanFollowModifier();
}
tsParseModifier(e, s) {
if (!q(this.state.type) && this.state.type !== 58 && this.state.type !== 75)
return;
let i = this.state.value;
if (e.indexOf(i) !== -1) {
if (s && this.tsIsStartOfStaticBlocks())
return;
if (this.tsTryParse(this.tsNextTokenCanFollowModifier.bind(this)))
return i;
}
}
tsParseModifiers(e, s) {
let { allowedModifiers: i, disallowedModifiers: a, stopOnStartOfClassStaticBlock: n2, errorTemplate: o = I.InvalidModifierOnTypeMember } = e, u = (y, g, T, C) => {
g === T && s[C] && this.raise(I.InvalidModifiersOrder, { at: y, orderedModifiers: [T, C] });
}, c = (y, g, T, C) => {
(s[T] && g === C || s[C] && g === T) && this.raise(I.IncompatibleModifiers, { at: y, modifiers: [T, C] });
};
for (; ; ) {
let { startLoc: y } = this.state, g = this.tsParseModifier(i.concat(a != null ? a : []), n2);
if (!g)
break;
Hr(g) ? s.accessibility ? this.raise(I.DuplicateAccessibilityModifier, { at: y, modifier: g }) : (u(y, g, g, "override"), u(y, g, g, "static"), u(y, g, g, "readonly"), s.accessibility = g) : hh(g) ? (s[g] && this.raise(I.DuplicateModifier, { at: y, modifier: g }), s[g] = true, u(y, g, "in", "out")) : (Object.hasOwnProperty.call(s, g) ? this.raise(I.DuplicateModifier, { at: y, modifier: g }) : (u(y, g, "static", "readonly"), u(y, g, "static", "override"), u(y, g, "override", "readonly"), u(y, g, "abstract", "override"), c(y, g, "declare", "override"), c(y, g, "static", "abstract")), s[g] = true), a != null && a.includes(g) && this.raise(o, { at: y, modifier: g });
}
}
tsIsListTerminator(e) {
switch (e) {
case "EnumMembers":
case "TypeMembers":
return this.match(8);
case "HeritageClauseElement":
return this.match(5);
case "TupleElementTypes":
return this.match(3);
case "TypeParametersOrArguments":
return this.match(48);
}
}
tsParseList(e, s) {
let i = [];
for (; !this.tsIsListTerminator(e); )
i.push(s());
return i;
}
tsParseDelimitedList(e, s, i) {
return oh(this.tsParseDelimitedListWorker(e, s, true, i));
}
tsParseDelimitedListWorker(e, s, i, a) {
let n2 = [], o = -1;
for (; !this.tsIsListTerminator(e); ) {
o = -1;
let u = s();
if (u == null)
return;
if (n2.push(u), this.eat(12)) {
o = this.state.lastTokStart;
continue;
}
if (this.tsIsListTerminator(e))
break;
i && this.expect(12);
return;
}
return a && (a.value = o), n2;
}
tsParseBracketedList(e, s, i, a, n2) {
a || (i ? this.expect(0) : this.expect(47));
let o = this.tsParseDelimitedList(e, s, n2);
return i ? this.expect(3) : this.expect(48), o;
}
tsParseImportType() {
let e = this.startNode();
return this.expect(83), this.expect(10), this.match(131) || this.raise(I.UnsupportedImportTypeArgument, { at: this.state.startLoc }), e.argument = super.parseExprAtom(), this.expect(11), this.eat(16) && (e.qualifier = this.tsParseEntityName()), this.match(47) && (e.typeParameters = this.tsParseTypeArguments()), this.finishNode(e, "TSImportType");
}
tsParseEntityName() {
let e = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : true, s = this.parseIdentifier(e);
for (; this.eat(16); ) {
let i = this.startNodeAtNode(s);
i.left = s, i.right = this.parseIdentifier(e), s = this.finishNode(i, "TSQualifiedName");
}
return s;
}
tsParseTypeReference() {
let e = this.startNode();
return e.typeName = this.tsParseEntityName(), !this.hasPrecedingLineBreak() && this.match(47) && (e.typeParameters = this.tsParseTypeArguments()), this.finishNode(e, "TSTypeReference");
}
tsParseThisTypePredicate(e) {
this.next();
let s = this.startNodeAtNode(e);
return s.parameterName = e, s.typeAnnotation = this.tsParseTypeAnnotation(false), s.asserts = false, this.finishNode(s, "TSTypePredicate");
}
tsParseThisTypeNode() {
let e = this.startNode();
return this.next(), this.finishNode(e, "TSThisType");
}
tsParseTypeQuery() {
let e = this.startNode();
return this.expect(87), this.match(83) ? e.exprName = this.tsParseImportType() : e.exprName = this.tsParseEntityName(), !this.hasPrecedingLineBreak() && this.match(47) && (e.typeParameters = this.tsParseTypeArguments()), this.finishNode(e, "TSTypeQuery");
}
tsParseTypeParameter(e) {
let s = this.startNode();
return e(s), s.name = this.tsParseTypeParameterName(), s.constraint = this.tsEatThenParseType(81), s.default = this.tsEatThenParseType(29), this.finishNode(s, "TSTypeParameter");
}
tsTryParseTypeParameters(e) {
if (this.match(47))
return this.tsParseTypeParameters(e);
}
tsParseTypeParameters(e) {
let s = this.startNode();
this.match(47) || this.match(140) ? this.next() : this.unexpected();
let i = { value: -1 };
return s.params = this.tsParseBracketedList("TypeParametersOrArguments", this.tsParseTypeParameter.bind(this, e), false, true, i), s.params.length === 0 && this.raise(I.EmptyTypeParameters, { at: s }), i.value !== -1 && this.addExtra(s, "trailingComma", i.value), this.finishNode(s, "TSTypeParameterDeclaration");
}
tsFillSignature(e, s) {
let i = e === 19, a = "parameters", n2 = "typeAnnotation";
s.typeParameters = this.tsTryParseTypeParameters(this.tsParseConstModifier), this.expect(10), s[a] = this.tsParseBindingListForSignature(), i ? s[n2] = this.tsParseTypeOrTypePredicateAnnotation(e) : this.match(e) && (s[n2] = this.tsParseTypeOrTypePredicateAnnotation(e));
}
tsParseBindingListForSignature() {
return super.parseBindingList(11, 41, 2).map((e) => (e.type !== "Identifier" && e.type !== "RestElement" && e.type !== "ObjectPattern" && e.type !== "ArrayPattern" && this.raise(I.UnsupportedSignatureParameterKind, { at: e, type: e.type }), e));
}
tsParseTypeMemberSemicolon() {
!this.eat(12) && !this.isLineTerminator() && this.expect(13);
}
tsParseSignatureMember(e, s) {
return this.tsFillSignature(14, s), this.tsParseTypeMemberSemicolon(), this.finishNode(s, e);
}
tsIsUnambiguouslyIndexSignature() {
return this.next(), q(this.state.type) ? (this.next(), this.match(14)) : false;
}
tsTryParseIndexSignature(e) {
if (!(this.match(0) && this.tsLookAhead(this.tsIsUnambiguouslyIndexSignature.bind(this))))
return;
this.expect(0);
let s = this.parseIdentifier();
s.typeAnnotation = this.tsParseTypeAnnotation(), this.resetEndLocation(s), this.expect(3), e.parameters = [s];
let i = this.tsTryParseTypeAnnotation();
return i && (e.typeAnnotation = i), this.tsParseTypeMemberSemicolon(), this.finishNode(e, "TSIndexSignature");
}
tsParsePropertyOrMethodSignature(e, s) {
this.eat(17) && (e.optional = true);
let i = e;
if (this.match(10) || this.match(47)) {
s && this.raise(I.ReadonlyForMethodSignature, { at: e });
let a = i;
a.kind && this.match(47) && this.raise(I.AccesorCannotHaveTypeParameters, { at: this.state.curPosition() }), this.tsFillSignature(14, a), this.tsParseTypeMemberSemicolon();
let n2 = "parameters", o = "typeAnnotation";
if (a.kind === "get")
a[n2].length > 0 && (this.raise(f.BadGetterArity, { at: this.state.curPosition() }), this.isThisParam(a[n2][0]) && this.raise(I.AccesorCannotDeclareThisParameter, { at: this.state.curPosition() }));
else if (a.kind === "set") {
if (a[n2].length !== 1)
this.raise(f.BadSetterArity, { at: this.state.curPosition() });
else {
let u = a[n2][0];
this.isThisParam(u) && this.raise(I.AccesorCannotDeclareThisParameter, { at: this.state.curPosition() }), u.type === "Identifier" && u.optional && this.raise(I.SetAccesorCannotHaveOptionalParameter, { at: this.state.curPosition() }), u.type === "RestElement" && this.raise(I.SetAccesorCannotHaveRestParameter, { at: this.state.curPosition() });
}
a[o] && this.raise(I.SetAccesorCannotHaveReturnType, { at: a[o] });
} else
a.kind = "method";
return this.finishNode(a, "TSMethodSignature");
} else {
let a = i;
s && (a.readonly = true);
let n2 = this.tsTryParseTypeAnnotation();
return n2 && (a.typeAnnotation = n2), this.tsParseTypeMemberSemicolon(), this.finishNode(a, "TSPropertySignature");
}
}
tsParseTypeMember() {
let e = this.startNode();
if (this.match(10) || this.match(47))
return this.tsParseSignatureMember("TSCallSignatureDeclaration", e);
if (this.match(77)) {
let i = this.startNode();
return this.next(), this.match(10) || this.match(47) ? this.tsParseSignatureMember("TSConstructSignatureDeclaration", e) : (e.key = this.createIdentifier(i, "new"), this.tsParsePropertyOrMethodSignature(e, false));
}
this.tsParseModifiers({ allowedModifiers: ["readonly"], disallowedModifiers: ["declare", "abstract", "private", "protected", "public", "static", "override"] }, e);
let s = this.tsTryParseIndexSignature(e);
return s || (super.parsePropertyName(e), !e.computed && e.key.type === "Identifier" && (e.key.name === "get" || e.key.name === "set") && this.tsTokenCanFollowModifier() && (e.kind = e.key.name, super.parsePropertyName(e)), this.tsParsePropertyOrMethodSignature(e, !!e.readonly));
}
tsParseTypeLiteral() {
let e = this.startNode();
return e.members = this.tsParseObjectTypeMembers(), this.finishNode(e, "TSTypeLiteral");
}
tsParseObjectTypeMembers() {
this.expect(5);
let e = this.tsParseList("TypeMembers", this.tsParseTypeMember.bind(this));
return this.expect(8), e;
}
tsIsStartOfMappedType() {
return this.next(), this.eat(53) ? this.isContextual(120) : (this.isContextual(120) && this.next(), !this.match(0) || (this.next(), !this.tsIsIdentifier()) ? false : (this.next(), this.match(58)));
}
tsParseMappedTypeParameter() {
let e = this.startNode();
return e.name = this.tsParseTypeParameterName(), e.constraint = this.tsExpectThenParseType(58), this.finishNode(e, "TSTypeParameter");
}
tsParseMappedType() {
let e = this.startNode();
return this.expect(5), this.match(53) ? (e.readonly = this.state.value, this.next(), this.expectContextual(120)) : this.eatContextual(120) && (e.readonly = true), this.expect(0), e.typeParameter = this.tsParseMappedTypeParameter(), e.nameType = this.eatContextual(93) ? this.tsParseType() : null, this.expect(3), this.match(53) ? (e.optional = this.state.value, this.next(), this.expect(17)) : this.eat(17) && (e.optional = true), e.typeAnnotation = this.tsTryParseType(), this.semicolon(), this.expect(8), this.finishNode(e, "TSMappedType");
}
tsParseTupleType() {
let e = this.startNode();
e.elementTypes = this.tsParseBracketedList("TupleElementTypes", this.tsParseTupleElementType.bind(this), true, false);
let s = false, i = null;
return e.elementTypes.forEach((a) => {
var n2;
let { type: o } = a;
s && o !== "TSRestType" && o !== "TSOptionalType" && !(o === "TSNamedTupleMember" && a.optional) && this.raise(I.OptionalTypeBeforeRequired, { at: a }), s || (s = o === "TSNamedTupleMember" && a.optional || o === "TSOptionalType");
let u = o;
o === "TSRestType" && (a = a.typeAnnotation, u = a.type);
let c = u === "TSNamedTupleMember";
(n2 = i) != null || (i = c), i !== c && this.raise(I.MixedLabeledAndUnlabeledElements, { at: a });
}), this.finishNode(e, "TSTupleType");
}
tsParseTupleElementType() {
let { startLoc: e } = this.state, s = this.eat(21), i, a, n2, o, c = te(this.state.type) ? this.lookaheadCharCode() : null;
if (c === 58)
i = true, n2 = false, a = this.parseIdentifier(true), this.expect(14), o = this.tsParseType();
else if (c === 63) {
n2 = true;
let y = this.state.startLoc, g = this.state.value, T = this.tsParseNonArrayType();
this.lookaheadCharCode() === 58 ? (i = true, a = this.createIdentifier(this.startNodeAt(y), g), this.expect(17), this.expect(14), o = this.tsParseType()) : (i = false, o = T, this.expect(17));
} else
o = this.tsParseType(), n2 = this.eat(17), i = this.eat(14);
if (i) {
let y;
a ? (y = this.startNodeAtNode(a), y.optional = n2, y.label = a, y.elementType = o, this.eat(17) && (y.optional = true, this.raise(I.TupleOptionalAfterType, { at: this.state.lastTokStartLoc }))) : (y = this.startNodeAtNode(o), y.optional = n2, this.raise(I.InvalidTupleMemberLabel, { at: o }), y.label = o, y.elementType = this.tsParseType()), o = this.finishNode(y, "TSNamedTupleMember");
} else if (n2) {
let y = this.startNodeAtNode(o);
y.typeAnnotation = o, o = this.finishNode(y, "TSOptionalType");
}
if (s) {
let y = this.startNodeAt(e);
y.typeAnnotation = o, o = this.finishNode(y, "TSRestType");
}
return o;
}
tsParseParenthesizedType() {
let e = this.startNode();
return this.expect(10), e.typeAnnotation = this.tsParseType(), this.expect(11), this.finishNode(e, "TSParenthesizedType");
}
tsParseFunctionOrConstructorType(e, s) {
let i = this.startNode();
return e === "TSConstructorType" && (i.abstract = !!s, s && this.next(), this.next()), this.tsInAllowConditionalTypesContext(() => this.tsFillSignature(19, i)), this.finishNode(i, e);
}
tsParseLiteralTypeNode() {
let e = this.startNode();
return e.literal = (() => {
switch (this.state.type) {
case 132:
case 133:
case 131:
case 85:
case 86:
return super.parseExprAtom();
default:
this.unexpected();
}
})(), this.finishNode(e, "TSLiteralType");
}
tsParseTemplateLiteralType() {
let e = this.startNode();
return e.literal = super.parseTemplate(false), this.finishNode(e, "TSLiteralType");
}
parseTemplateSubstitution() {
return this.state.inType ? this.tsParseType() : super.parseTemplateSubstitution();
}
tsParseThisTypeOrThisTypePredicate() {
let e = this.tsParseThisTypeNode();
return this.isContextual(114) && !this.hasPrecedingLineBreak() ? this.tsParseThisTypePredicate(e) : e;
}
tsParseNonArrayType() {
switch (this.state.type) {
case 131:
case 132:
case 133:
case 85:
case 86:
return this.tsParseLiteralTypeNode();
case 53:
if (this.state.value === "-") {
let e = this.startNode(), s = this.lookahead();
return s.type !== 132 && s.type !== 133 && this.unexpected(), e.literal = this.parseMaybeUnary(), this.finishNode(e, "TSLiteralType");
}
break;
case 78:
return this.tsParseThisTypeOrThisTypePredicate();
case 87:
return this.tsParseTypeQuery();
case 83:
return this.tsParseImportType();
case 5:
return this.tsLookAhead(this.tsIsStartOfMappedType.bind(this)) ? this.tsParseMappedType() : this.tsParseTypeLiteral();
case 0:
return this.tsParseTupleType();
case 10:
return this.tsParseParenthesizedType();
case 25:
case 24:
return this.tsParseTemplateLiteralType();
default: {
let { type: e } = this.state;
if (q(e) || e === 88 || e === 84) {
let s = e === 88 ? "TSVoidKeyword" : e === 84 ? "TSNullKeyword" : lh(this.state.value);
if (s !== void 0 && this.lookaheadCharCode() !== 46) {
let i = this.startNode();
return this.next(), this.finishNode(i, s);
}
return this.tsParseTypeReference();
}
}
}
this.unexpected();
}
tsParseArrayTypeOrHigher() {
let e = this.tsParseNonArrayType();
for (; !this.hasPrecedingLineBreak() && this.eat(0); )
if (this.match(3)) {
let s = this.startNodeAtNode(e);
s.elementType = e, this.expect(3), e = this.finishNode(s, "TSArrayType");
} else {
let s = this.startNodeAtNode(e);
s.objectType = e, s.indexType = this.tsParseType(), this.expect(3), e = this.finishNode(s, "TSIndexedAccessType");
}
return e;
}
tsParseTypeOperator() {
let e = this.startNode(), s = this.state.value;
return this.next(), e.operator = s, e.typeAnnotation = this.tsParseTypeOperatorOrHigher(), s === "readonly" && this.tsCheckTypeAnnotationForReadOnly(e), this.finishNode(e, "TSTypeOperator");
}
tsCheckTypeAnnotationForReadOnly(e) {
switch (e.typeAnnotation.type) {
case "TSTupleType":
case "TSArrayType":
return;
default:
this.raise(I.UnexpectedReadonly, { at: e });
}
}
tsParseInferType() {
let e = this.startNode();
this.expectContextual(113);
let s = this.startNode();
return s.name = this.tsParseTypeParameterName(), s.constraint = this.tsTryParse(() => this.tsParseConstraintForInferType()), e.typeParameter = this.finishNode(s, "TSTypeParameter"), this.finishNode(e, "TSInferType");
}
tsParseConstraintForInferType() {
if (this.eat(81)) {
let e = this.tsInDisallowConditionalTypesContext(() => this.tsParseType());
if (this.state.inDisallowConditionalTypesContext || !this.match(17))
return e;
}
}
tsParseTypeOperatorOrHigher() {
return qo(this.state.type) && !this.state.containsEsc ? this.tsParseTypeOperator() : this.isContextual(113) ? this.tsParseInferType() : this.tsInAllowConditionalTypesContext(() => this.tsParseArrayTypeOrHigher());
}
tsParseUnionOrIntersectionType(e, s, i) {
let a = this.startNode(), n2 = this.eat(i), o = [];
do
o.push(s());
while (this.eat(i));
return o.length === 1 && !n2 ? o[0] : (a.types = o, this.finishNode(a, e));
}
tsParseIntersectionTypeOrHigher() {
return this.tsParseUnionOrIntersectionType("TSIntersectionType", this.tsParseTypeOperatorOrHigher.bind(this), 45);
}
tsParseUnionTypeOrHigher() {
return this.tsParseUnionOrIntersectionType("TSUnionType", this.tsParseIntersectionTypeOrHigher.bind(this), 43);
}
tsIsStartOfFunctionType() {
return this.match(47) ? true : this.match(10) && this.tsLookAhead(this.tsIsUnambiguouslyStartOfFunctionType.bind(this));
}
tsSkipParameterStart() {
if (q(this.state.type) || this.match(78))
return this.next(), true;
if (this.match(5)) {
let { errors: e } = this.state, s = e.length;
try {
return this.parseObjectLike(8, true), e.length === s;
} catch {
return false;
}
}
if (this.match(0)) {
this.next();
let { errors: e } = this.state, s = e.length;
try {
return super.parseBindingList(3, 93, 1), e.length === s;
} catch {
return false;
}
}
return false;
}
tsIsUnambiguouslyStartOfFunctionType() {
return this.next(), !!(this.match(11) || this.match(21) || this.tsSkipParameterStart() && (this.match(14) || this.match(12) || this.match(17) || this.match(29) || this.match(11) && (this.next(), this.match(19))));
}
tsParseTypeOrTypePredicateAnnotation(e) {
return this.tsInType(() => {
let s = this.startNode();
this.expect(e);
let i = this.startNode(), a = !!this.tsTryParse(this.tsParseTypePredicateAsserts.bind(this));
if (a && this.match(78)) {
let u = this.tsParseThisTypeOrThisTypePredicate();
return u.type === "TSThisType" ? (i.parameterName = u, i.asserts = true, i.typeAnnotation = null, u = this.finishNode(i, "TSTypePredicate")) : (this.resetStartLocationFromNode(u, i), u.asserts = true), s.typeAnnotation = u, this.finishNode(s, "TSTypeAnnotation");
}
let n2 = this.tsIsIdentifier() && this.tsTryParse(this.tsParseTypePredicatePrefix.bind(this));
if (!n2)
return a ? (i.parameterName = this.parseIdentifier(), i.asserts = a, i.typeAnnotation = null, s.typeAnnotation = this.finishNode(i, "TSTypePredicate"), this.finishNode(s, "TSTypeAnnotation")) : this.tsParseTypeAnnotation(false, s);
let o = this.tsParseTypeAnnotation(false);
return i.parameterName = n2, i.typeAnnotation = o, i.asserts = a, s.typeAnnotation = this.finishNode(i, "TSTypePredicate"), this.finishNode(s, "TSTypeAnnotation");
});
}
tsTryParseTypeOrTypePredicateAnnotation() {
return this.match(14) ? this.tsParseTypeOrTypePredicateAnnotation(14) : void 0;
}
tsTryParseTypeAnnotation() {
return this.match(14) ? this.tsParseTypeAnnotation() : void 0;
}
tsTryParseType() {
return this.tsEatThenParseType(14);
}
tsParseTypePredicatePrefix() {
let e = this.parseIdentifier();
if (this.isContextual(114) && !this.hasPrecedingLineBreak())
return this.next(), e;
}
tsParseTypePredicateAsserts() {
if (this.state.type !== 107)
return false;
let e = this.state.containsEsc;
return this.next(), !q(this.state.type) && !this.match(78) ? false : (e && this.raise(f.InvalidEscapedReservedWord, { at: this.state.lastTokStartLoc, reservedWord: "asserts" }), true);
}
tsParseTypeAnnotation() {
let e = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : true, s = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : this.startNode();
return this.tsInType(() => {
e && this.expect(14), s.typeAnnotation = this.tsParseType();
}), this.finishNode(s, "TSTypeAnnotation");
}
tsParseType() {
$r(this.state.inType);
let e = this.tsParseNonConditionalType();
if (this.state.inDisallowConditionalTypesContext || this.hasPrecedingLineBreak() || !this.eat(81))
return e;
let s = this.startNodeAtNode(e);
return s.checkType = e, s.extendsType = this.tsInDisallowConditionalTypesContext(() => this.tsParseNonConditionalType()), this.expect(17), s.trueType = this.tsInAllowConditionalTypesContext(() => this.tsParseType()), this.expect(14), s.falseType = this.tsInAllowConditionalTypesContext(() => this.tsParseType()), this.finishNode(s, "TSConditionalType");
}
isAbstractConstructorSignature() {
return this.isContextual(122) && this.lookahead().type === 77;
}
tsParseNonConditionalType() {
return this.tsIsStartOfFunctionType() ? this.tsParseFunctionOrConstructorType("TSFunctionType") : this.match(77) ? this.tsParseFunctionOrConstructorType("TSConstructorType") : this.isAbstractConstructorSignature() ? this.tsParseFunctionOrConstructorType("TSConstructorType", true) : this.tsParseUnionTypeOrHigher();
}
tsParseTypeAssertion() {
this.getPluginOption("typescript", "disallowAmbiguousJSXLike") && this.raise(I.ReservedTypeAssertion, { at: this.state.startLoc });
let e = this.startNode();
return e.typeAnnotation = this.tsInType(() => (this.next(), this.match(75) ? this.tsParseTypeReference() : this.tsParseType())), this.expect(48), e.expression = this.parseMaybeUnary(), this.finishNode(e, "TSTypeAssertion");
}
tsParseHeritageClause(e) {
let s = this.state.startLoc, i = this.tsParseDelimitedList("HeritageClauseElement", () => {
let a = this.startNode();
return a.expression = this.tsParseEntityName(), this.match(47) && (a.typeParameters = this.tsParseTypeArguments()), this.finishNode(a, "TSExpressionWithTypeArguments");
});
return i.length || this.raise(I.EmptyHeritageClauseType, { at: s, token: e }), i;
}
tsParseInterfaceDeclaration(e) {
let s = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
if (this.hasFollowingLineBreak())
return null;
this.expectContextual(127), s.declare && (e.declare = true), q(this.state.type) ? (e.id = this.parseIdentifier(), this.checkIdentifier(e.id, pl)) : (e.id = null, this.raise(I.MissingInterfaceName, { at: this.state.startLoc })), e.typeParameters = this.tsTryParseTypeParameters(this.tsParseInOutConstModifiers), this.eat(81) && (e.extends = this.tsParseHeritageClause("extends"));
let i = this.startNode();
return i.body = this.tsInType(this.tsParseObjectTypeMembers.bind(this)), e.body = this.finishNode(i, "TSInterfaceBody"), this.finishNode(e, "TSInterfaceDeclaration");
}
tsParseTypeAliasDeclaration(e) {
return e.id = this.parseIdentifier(), this.checkIdentifier(e.id, fl), e.typeAnnotation = this.tsInType(() => {
if (e.typeParameters = this.tsTryParseTypeParameters(this.tsParseInOutModifiers), this.expect(29), this.isContextual(112) && this.lookahead().type !== 16) {
let s = this.startNode();
return this.next(), this.finishNode(s, "TSIntrinsicKeyword");
}
return this.tsParseType();
}), this.semicolon(), this.finishNode(e, "TSTypeAliasDeclaration");
}
tsInNoContext(e) {
let s = this.state.context;
this.state.context = [s[0]];
try {
return e();
} finally {
this.state.context = s;
}
}
tsInType(e) {
let s = this.state.inType;
this.state.inType = true;
try {
return e();
} finally {
this.state.inType = s;
}
}
tsInDisallowConditionalTypesContext(e) {
let s = this.state.inDisallowConditionalTypesContext;
this.state.inDisallowConditionalTypesContext = true;
try {
return e();
} finally {
this.state.inDisallowConditionalTypesContext = s;
}
}
tsInAllowConditionalTypesContext(e) {
let s = this.state.inDisallowConditionalTypesContext;
this.state.inDisallowConditionalTypesContext = false;
try {
return e();
} finally {
this.state.inDisallowConditionalTypesContext = s;
}
}
tsEatThenParseType(e) {
return this.match(e) ? this.tsNextThenParseType() : void 0;
}
tsExpectThenParseType(e) {
return this.tsDoThenParseType(() => this.expect(e));
}
tsNextThenParseType() {
return this.tsDoThenParseType(() => this.next());
}
tsDoThenParseType(e) {
return this.tsInType(() => (e(), this.tsParseType()));
}
tsParseEnumMember() {
let e = this.startNode();
return e.id = this.match(131) ? super.parseStringLiteral(this.state.value) : this.parseIdentifier(true), this.eat(29) && (e.initializer = super.parseMaybeAssignAllowIn()), this.finishNode(e, "TSEnumMember");
}
tsParseEnumDeclaration(e) {
let s = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
return s.const && (e.const = true), s.declare && (e.declare = true), this.expectContextual(124), e.id = this.parseIdentifier(), this.checkIdentifier(e.id, e.const ? ml : Cr), this.expect(5), e.members = this.tsParseDelimitedList("EnumMembers", this.tsParseEnumMember.bind(this)), this.expect(8), this.finishNode(e, "TSEnumDeclaration");
}
tsParseModuleBlock() {
let e = this.startNode();
return this.scope.enter(Fe), this.expect(5), super.parseBlockOrModuleBlockBody(e.body = [], void 0, true, 8), this.scope.exit(), this.finishNode(e, "TSModuleBlock");
}
tsParseModuleOrNamespaceDeclaration(e) {
let s = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
if (e.id = this.parseIdentifier(), s || this.checkIdentifier(e.id, yl), this.eat(16)) {
let i = this.startNode();
this.tsParseModuleOrNamespaceDeclaration(i, true), e.body = i;
} else
this.scope.enter(Oe), this.prodParam.enter(Me), e.body = this.tsParseModuleBlock(), this.prodParam.exit(), this.scope.exit();
return this.finishNode(e, "TSModuleDeclaration");
}
tsParseAmbientExternalModuleDeclaration(e) {
return this.isContextual(110) ? (e.global = true, e.id = this.parseIdentifier()) : this.match(131) ? e.id = super.parseStringLiteral(this.state.value) : this.unexpected(), this.match(5) ? (this.scope.enter(Oe), this.prodParam.enter(Me), e.body = this.tsParseModuleBlock(), this.prodParam.exit(), this.scope.exit()) : this.semicolon(), this.finishNode(e, "TSModuleDeclaration");
}
tsParseImportEqualsDeclaration(e, s) {
e.isExport = s || false, e.id = this.parseIdentifier(), this.checkIdentifier(e.id, Ve), this.expect(29);
let i = this.tsParseModuleReference();
return e.importKind === "type" && i.type !== "TSExternalModuleReference" && this.raise(I.ImportAliasHasImportType, { at: i }), e.moduleReference = i, this.semicolon(), this.finishNode(e, "TSImportEqualsDeclaration");
}
tsIsExternalModuleReference() {
return this.isContextual(117) && this.lookaheadCharCode() === 40;
}
tsParseModuleReference() {
return this.tsIsExternalModuleReference() ? this.tsParseExternalModuleReference() : this.tsParseEntityName(false);
}
tsParseExternalModuleReference() {
let e = this.startNode();
return this.expectContextual(117), this.expect(10), this.match(131) || this.unexpected(), e.expression = super.parseExprAtom(), this.expect(11), this.finishNode(e, "TSExternalModuleReference");
}
tsLookAhead(e) {
let s = this.state.clone(), i = e();
return this.state = s, i;
}
tsTryParseAndCatch(e) {
let s = this.tryParse((i) => e() || i());
if (!(s.aborted || !s.node))
return s.error && (this.state = s.failState), s.node;
}
tsTryParse(e) {
let s = this.state.clone(), i = e();
if (i !== void 0 && i !== false)
return i;
this.state = s;
}
tsTryParseDeclare(e) {
if (this.isLineTerminator())
return;
let s = this.state.type, i;
return this.isContextual(99) && (s = 74, i = "let"), this.tsInAmbientContext(() => {
if (s === 68)
return e.declare = true, super.parseFunctionStatement(e, false, false);
if (s === 80)
return e.declare = true, this.parseClass(e, true, false);
if (s === 124)
return this.tsParseEnumDeclaration(e, { declare: true });
if (s === 110)
return this.tsParseAmbientExternalModuleDeclaration(e);
if (s === 75 || s === 74)
return !this.match(75) || !this.isLookaheadContextual("enum") ? (e.declare = true, this.parseVarStatement(e, i || this.state.value, true)) : (this.expect(75), this.tsParseEnumDeclaration(e, { const: true, declare: true }));
if (s === 127) {
let a = this.tsParseInterfaceDeclaration(e, { declare: true });
if (a)
return a;
}
if (q(s))
return this.tsParseDeclaration(e, this.state.value, true, null);
});
}
tsTryParseExportDeclaration() {
return this.tsParseDeclaration(this.startNode(), this.state.value, true, null);
}
tsParseExpressionStatement(e, s, i) {
switch (s.name) {
case "declare": {
let a = this.tsTryParseDeclare(e);
if (a)
return a.declare = true, a;
break;
}
case "global":
if (this.match(5)) {
this.scope.enter(Oe), this.prodParam.enter(Me);
let a = e;
return a.global = true, a.id = s, a.body = this.tsParseModuleBlock(), this.scope.exit(), this.prodParam.exit(), this.finishNode(a, "TSModuleDeclaration");
}
break;
default:
return this.tsParseDeclaration(e, s.name, false, i);
}
}
tsParseDeclaration(e, s, i, a) {
switch (s) {
case "abstract":
if (this.tsCheckLineTerminator(i) && (this.match(80) || q(this.state.type)))
return this.tsParseAbstractDeclaration(e, a);
break;
case "module":
if (this.tsCheckLineTerminator(i)) {
if (this.match(131))
return this.tsParseAmbientExternalModuleDeclaration(e);
if (q(this.state.type))
return this.tsParseModuleOrNamespaceDeclaration(e);
}
break;
case "namespace":
if (this.tsCheckLineTerminator(i) && q(this.state.type))
return this.tsParseModuleOrNamespaceDeclaration(e);
break;
case "type":
if (this.tsCheckLineTerminator(i) && q(this.state.type))
return this.tsParseTypeAliasDeclaration(e);
break;
}
}
tsCheckLineTerminator(e) {
return e ? this.hasFollowingLineBreak() ? false : (this.next(), true) : !this.isLineTerminator();
}
tsTryParseGenericAsyncArrowFunction(e) {
if (!this.match(47))
return;
let s = this.state.maybeInArrowParameters;
this.state.maybeInArrowParameters = true;
let i = this.tsTryParseAndCatch(() => {
let a = this.startNodeAt(e);
return a.typeParameters = this.tsParseTypeParameters(this.tsParseConstModifier), super.parseFunctionParams(a), a.returnType = this.tsTryParseTypeOrTypePredicateAnnotation(), this.expect(19), a;
});
if (this.state.maybeInArrowParameters = s, !!i)
return super.parseArrowExpression(i, null, true);
}
tsParseTypeArgumentsInExpression() {
if (this.reScan_lt() === 47)
return this.tsParseTypeArguments();
}
tsParseTypeArguments() {
let e = this.startNode();
return e.params = this.tsInType(() => this.tsInNoContext(() => (this.expect(47), this.tsParseDelimitedList("TypeParametersOrArguments", this.tsParseType.bind(this))))), e.params.length === 0 && this.raise(I.EmptyTypeArguments, { at: e }), this.expect(48), this.finishNode(e, "TSTypeParameterInstantiation");
}
tsIsDeclarationStart() {
return Uo(this.state.type);
}
isExportDefaultSpecifier() {
return this.tsIsDeclarationStart() ? false : super.isExportDefaultSpecifier();
}
parseAssignableListItem(e, s) {
let i = this.state.startLoc, a = {};
this.tsParseModifiers({ allowedModifiers: ["public", "private", "protected", "override", "readonly"] }, a);
let n2 = a.accessibility, o = a.override, u = a.readonly;
!(e & 4) && (n2 || u || o) && this.raise(I.UnexpectedParameterModifier, { at: i });
let c = this.parseMaybeDefault();
this.parseAssignableListItemTypes(c, e);
let y = this.parseMaybeDefault(c.loc.start, c);
if (n2 || u || o) {
let g = this.startNodeAt(i);
return s.length && (g.decorators = s), n2 && (g.accessibility = n2), u && (g.readonly = u), o && (g.override = o), y.type !== "Identifier" && y.type !== "AssignmentPattern" && this.raise(I.UnsupportedParameterPropertyKind, { at: g }), g.parameter = y, this.finishNode(g, "TSParameterProperty");
}
return s.length && (c.decorators = s), y;
}
isSimpleParameter(e) {
return e.type === "TSParameterProperty" && super.isSimpleParameter(e.parameter) || super.isSimpleParameter(e);
}
tsDisallowOptionalPattern(e) {
for (let s of e.params)
s.type !== "Identifier" && s.optional && !this.state.isAmbientContext && this.raise(I.PatternIsOptional, { at: s });
}
setArrowFunctionParameters(e, s, i) {
super.setArrowFunctionParameters(e, s, i), this.tsDisallowOptionalPattern(e);
}
parseFunctionBodyAndFinish(e, s) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
this.match(14) && (e.returnType = this.tsParseTypeOrTypePredicateAnnotation(14));
let a = s === "FunctionDeclaration" ? "TSDeclareFunction" : s === "ClassMethod" || s === "ClassPrivateMethod" ? "TSDeclareMethod" : void 0;
return a && !this.match(5) && this.isLineTerminator() ? this.finishNode(e, a) : a === "TSDeclareFunction" && this.state.isAmbientContext && (this.raise(I.DeclareFunctionHasImplementation, { at: e }), e.declare) ? super.parseFunctionBodyAndFinish(e, a, i) : (this.tsDisallowOptionalPattern(e), super.parseFunctionBodyAndFinish(e, s, i));
}
registerFunctionStatementId(e) {
!e.body && e.id ? this.checkIdentifier(e.id, br) : super.registerFunctionStatementId(e);
}
tsCheckForInvalidTypeCasts(e) {
e.forEach((s) => {
(s == null ? void 0 : s.type) === "TSTypeCastExpression" && this.raise(I.UnexpectedTypeAnnotation, { at: s.typeAnnotation });
});
}
toReferencedList(e, s) {
return this.tsCheckForInvalidTypeCasts(e), e;
}
parseArrayLike(e, s, i, a) {
let n2 = super.parseArrayLike(e, s, i, a);
return n2.type === "ArrayExpression" && this.tsCheckForInvalidTypeCasts(n2.elements), n2;
}
parseSubscript(e, s, i, a) {
if (!this.hasPrecedingLineBreak() && this.match(35)) {
this.state.canStartJSXElement = false, this.next();
let o = this.startNodeAt(s);
return o.expression = e, this.finishNode(o, "TSNonNullExpression");
}
let n2 = false;
if (this.match(18) && this.lookaheadCharCode() === 60) {
if (i)
return a.stop = true, e;
a.optionalChainMember = n2 = true, this.next();
}
if (this.match(47) || this.match(51)) {
let o, u = this.tsTryParseAndCatch(() => {
if (!i && this.atPossibleAsyncArrow(e)) {
let T = this.tsTryParseGenericAsyncArrowFunction(s);
if (T)
return T;
}
let c = this.tsParseTypeArgumentsInExpression();
if (!c)
return;
if (n2 && !this.match(10)) {
o = this.state.curPosition();
return;
}
if (nt(this.state.type)) {
let T = super.parseTaggedTemplateExpression(e, s, a);
return T.typeParameters = c, T;
}
if (!i && this.eat(10)) {
let T = this.startNodeAt(s);
return T.callee = e, T.arguments = this.parseCallExpressionArguments(11, false), this.tsCheckForInvalidTypeCasts(T.arguments), T.typeParameters = c, a.optionalChainMember && (T.optional = n2), this.finishCallExpression(T, a.optionalChainMember);
}
let y = this.state.type;
if (y === 48 || y === 52 || y !== 10 && He(y) && !this.hasPrecedingLineBreak())
return;
let g = this.startNodeAt(s);
return g.expression = e, g.typeParameters = c, this.finishNode(g, "TSInstantiationExpression");
});
if (o && this.unexpected(o, 10), u)
return u.type === "TSInstantiationExpression" && (this.match(16) || this.match(18) && this.lookaheadCharCode() !== 40) && this.raise(I.InvalidPropertyAccessAfterInstantiationExpression, { at: this.state.startLoc }), u;
}
return super.parseSubscript(e, s, i, a);
}
parseNewCallee(e) {
var s;
super.parseNewCallee(e);
let { callee: i } = e;
i.type === "TSInstantiationExpression" && !((s = i.extra) != null && s.parenthesized) && (e.typeParameters = i.typeParameters, e.callee = i.expression);
}
parseExprOp(e, s, i) {
let a;
if (at(58) > i && !this.hasPrecedingLineBreak() && (this.isContextual(93) || (a = this.isContextual(118)))) {
let n2 = this.startNodeAt(s);
return n2.expression = e, n2.typeAnnotation = this.tsInType(() => (this.next(), this.match(75) ? (a && this.raise(f.UnexpectedKeyword, { at: this.state.startLoc, keyword: "const" }), this.tsParseTypeReference()) : this.tsParseType())), this.finishNode(n2, a ? "TSSatisfiesExpression" : "TSAsExpression"), this.reScan_lt_gt(), this.parseExprOp(n2, s, i);
}
return super.parseExprOp(e, s, i);
}
checkReservedWord(e, s, i, a) {
this.state.isAmbientContext || super.checkReservedWord(e, s, i, a);
}
checkImportReflection(e) {
super.checkImportReflection(e), e.module && e.importKind !== "value" && this.raise(I.ImportReflectionHasImportType, { at: e.specifiers[0].loc.start });
}
checkDuplicateExports() {
}
parseImport(e) {
if (e.importKind = "value", q(this.state.type) || this.match(55) || this.match(5)) {
let i = this.lookahead();
if (this.isContextual(128) && i.type !== 12 && i.type !== 97 && i.type !== 29 && (e.importKind = "type", this.next(), i = this.lookahead()), q(this.state.type) && i.type === 29)
return this.tsParseImportEqualsDeclaration(e);
}
let s = super.parseImport(e);
return s.importKind === "type" && s.specifiers.length > 1 && s.specifiers[0].type === "ImportDefaultSpecifier" && this.raise(I.TypeImportCannotSpecifyDefaultAndNamed, { at: s }), s;
}
parseExport(e, s) {
if (this.match(83))
return this.next(), this.isContextual(128) && this.lookaheadCharCode() !== 61 ? (e.importKind = "type", this.next()) : e.importKind = "value", this.tsParseImportEqualsDeclaration(e, true);
if (this.eat(29)) {
let i = e;
return i.expression = super.parseExpression(), this.semicolon(), this.finishNode(i, "TSExportAssignment");
} else if (this.eatContextual(93)) {
let i = e;
return this.expectContextual(126), i.id = this.parseIdentifier(), this.semicolon(), this.finishNode(i, "TSNamespaceExportDeclaration");
} else {
if (e.exportKind = "value", this.isContextual(128)) {
let i = this.lookaheadCharCode();
(i === 123 || i === 42) && (this.next(), e.exportKind = "type");
}
return super.parseExport(e, s);
}
}
isAbstractClass() {
return this.isContextual(122) && this.lookahead().type === 80;
}
parseExportDefaultExpression() {
if (this.isAbstractClass()) {
let e = this.startNode();
return this.next(), e.abstract = true, this.parseClass(e, true, true);
}
if (this.match(127)) {
let e = this.tsParseInterfaceDeclaration(this.startNode());
if (e)
return e;
}
return super.parseExportDefaultExpression();
}
parseVarStatement(e, s) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false, { isAmbientContext: a } = this.state, n2 = super.parseVarStatement(e, s, i || a);
if (!a)
return n2;
for (let { id: o, init: u } of n2.declarations)
u && (s !== "const" || o.typeAnnotation ? this.raise(I.InitializerNotAllowedInAmbientContext, { at: u }) : ph(u, this.hasPlugin("estree")) || this.raise(I.ConstInitiailizerMustBeStringOrNumericLiteralOrLiteralEnumReference, { at: u }));
return n2;
}
parseStatementContent(e, s) {
if (this.match(75) && this.isLookaheadContextual("enum")) {
let i = this.startNode();
return this.expect(75), this.tsParseEnumDeclaration(i, { const: true });
}
if (this.isContextual(124))
return this.tsParseEnumDeclaration(this.startNode());
if (this.isContextual(127)) {
let i = this.tsParseInterfaceDeclaration(this.startNode());
if (i)
return i;
}
return super.parseStatementContent(e, s);
}
parseAccessModifier() {
return this.tsParseModifier(["public", "protected", "private"]);
}
tsHasSomeModifiers(e, s) {
return s.some((i) => Hr(i) ? e.accessibility === i : !!e[i]);
}
tsIsStartOfStaticBlocks() {
return this.isContextual(104) && this.lookaheadCharCode() === 123;
}
parseClassMember(e, s, i) {
let a = ["declare", "private", "public", "protected", "override", "abstract", "readonly", "static"];
this.tsParseModifiers({ allowedModifiers: a, disallowedModifiers: ["in", "out"], stopOnStartOfClassStaticBlock: true, errorTemplate: I.InvalidModifierOnTypeParameterPositions }, s);
let n2 = () => {
this.tsIsStartOfStaticBlocks() ? (this.next(), this.next(), this.tsHasSomeModifiers(s, a) && this.raise(I.StaticBlockCannotHaveModifier, { at: this.state.curPosition() }), super.parseClassStaticBlock(e, s)) : this.parseClassMemberWithIsStatic(e, s, i, !!s.static);
};
s.declare ? this.tsInAmbientContext(n2) : n2();
}
parseClassMemberWithIsStatic(e, s, i, a) {
let n2 = this.tsTryParseIndexSignature(s);
if (n2) {
e.body.push(n2), s.abstract && this.raise(I.IndexSignatureHasAbstract, { at: s }), s.accessibility && this.raise(I.IndexSignatureHasAccessibility, { at: s, modifier: s.accessibility }), s.declare && this.raise(I.IndexSignatureHasDeclare, { at: s }), s.override && this.raise(I.IndexSignatureHasOverride, { at: s });
return;
}
!this.state.inAbstractClass && s.abstract && this.raise(I.NonAbstractClassHasAbstractMethod, { at: s }), s.override && (i.hadSuperClass || this.raise(I.OverrideNotInSubClass, { at: s })), super.parseClassMemberWithIsStatic(e, s, i, a);
}
parsePostMemberNameModifiers(e) {
this.eat(17) && (e.optional = true), e.readonly && this.match(10) && this.raise(I.ClassMethodHasReadonly, { at: e }), e.declare && this.match(10) && this.raise(I.ClassMethodHasDeclare, { at: e });
}
parseExpressionStatement(e, s, i) {
return (s.type === "Identifier" ? this.tsParseExpressionStatement(e, s, i) : void 0) || super.parseExpressionStatement(e, s, i);
}
shouldParseExportDeclaration() {
return this.tsIsDeclarationStart() ? true : super.shouldParseExportDeclaration();
}
parseConditional(e, s, i) {
if (!this.state.maybeInArrowParameters || !this.match(17))
return super.parseConditional(e, s, i);
let a = this.tryParse(() => super.parseConditional(e, s));
return a.node ? (a.error && (this.state = a.failState), a.node) : (a.error && super.setOptionalParametersError(i, a.error), e);
}
parseParenItem(e, s) {
if (e = super.parseParenItem(e, s), this.eat(17) && (e.optional = true, this.resetEndLocation(e)), this.match(14)) {
let i = this.startNodeAt(s);
return i.expression = e, i.typeAnnotation = this.tsParseTypeAnnotation(), this.finishNode(i, "TSTypeCastExpression");
}
return e;
}
parseExportDeclaration(e) {
if (!this.state.isAmbientContext && this.isContextual(123))
return this.tsInAmbientContext(() => this.parseExportDeclaration(e));
let s = this.state.startLoc, i = this.eatContextual(123);
if (i && (this.isContextual(123) || !this.shouldParseExportDeclaration()))
throw this.raise(I.ExpectedAmbientAfterExportDeclare, { at: this.state.startLoc });
let n2 = q(this.state.type) && this.tsTryParseExportDeclaration() || super.parseExportDeclaration(e);
return n2 ? ((n2.type === "TSInterfaceDeclaration" || n2.type === "TSTypeAliasDeclaration" || i) && (e.exportKind = "type"), i && (this.resetStartLocation(n2, s), n2.declare = true), n2) : null;
}
parseClassId(e, s, i, a) {
if ((!s || i) && this.isContextual(111))
return;
super.parseClassId(e, s, i, e.declare ? br : vr);
let n2 = this.tsTryParseTypeParameters(this.tsParseInOutConstModifiers);
n2 && (e.typeParameters = n2);
}
parseClassPropertyAnnotation(e) {
e.optional || (this.eat(35) ? e.definite = true : this.eat(17) && (e.optional = true));
let s = this.tsTryParseTypeAnnotation();
s && (e.typeAnnotation = s);
}
parseClassProperty(e) {
if (this.parseClassPropertyAnnotation(e), this.state.isAmbientContext && !(e.readonly && !e.typeAnnotation) && this.match(29) && this.raise(I.DeclareClassFieldHasInitializer, { at: this.state.startLoc }), e.abstract && this.match(29)) {
let { key: s } = e;
this.raise(I.AbstractPropertyHasInitializer, { at: this.state.startLoc, propertyName: s.type === "Identifier" && !e.computed ? s.name : `[${this.input.slice(s.start, s.end)}]` });
}
return super.parseClassProperty(e);
}
parseClassPrivateProperty(e) {
return e.abstract && this.raise(I.PrivateElementHasAbstract, { at: e }), e.accessibility && this.raise(I.PrivateElementHasAccessibility, { at: e, modifier: e.accessibility }), this.parseClassPropertyAnnotation(e), super.parseClassPrivateProperty(e);
}
parseClassAccessorProperty(e) {
return this.parseClassPropertyAnnotation(e), e.optional && this.raise(I.AccessorCannotBeOptional, { at: e }), super.parseClassAccessorProperty(e);
}
pushClassMethod(e, s, i, a, n2, o) {
let u = this.tsTryParseTypeParameters(this.tsParseConstModifier);
u && n2 && this.raise(I.ConstructorHasTypeParameters, { at: u });
let { declare: c = false, kind: y } = s;
c && (y === "get" || y === "set") && this.raise(I.DeclareAccessor, { at: s, kind: y }), u && (s.typeParameters = u), super.pushClassMethod(e, s, i, a, n2, o);
}
pushClassPrivateMethod(e, s, i, a) {
let n2 = this.tsTryParseTypeParameters(this.tsParseConstModifier);
n2 && (s.typeParameters = n2), super.pushClassPrivateMethod(e, s, i, a);
}
declareClassPrivateMethodInScope(e, s) {
e.type !== "TSDeclareMethod" && (e.type === "MethodDefinition" && !e.value.body || super.declareClassPrivateMethodInScope(e, s));
}
parseClassSuper(e) {
super.parseClassSuper(e), e.superClass && (this.match(47) || this.match(51)) && (e.superTypeParameters = this.tsParseTypeArgumentsInExpression()), this.eatContextual(111) && (e.implements = this.tsParseHeritageClause("implements"));
}
parseObjPropValue(e, s, i, a, n2, o, u) {
let c = this.tsTryParseTypeParameters(this.tsParseConstModifier);
return c && (e.typeParameters = c), super.parseObjPropValue(e, s, i, a, n2, o, u);
}
parseFunctionParams(e, s) {
let i = this.tsTryParseTypeParameters(this.tsParseConstModifier);
i && (e.typeParameters = i), super.parseFunctionParams(e, s);
}
parseVarId(e, s) {
super.parseVarId(e, s), e.id.type === "Identifier" && !this.hasPrecedingLineBreak() && this.eat(35) && (e.definite = true);
let i = this.tsTryParseTypeAnnotation();
i && (e.id.typeAnnotation = i, this.resetEndLocation(e.id));
}
parseAsyncArrowFromCallExpression(e, s) {
return this.match(14) && (e.returnType = this.tsParseTypeAnnotation()), super.parseAsyncArrowFromCallExpression(e, s);
}
parseMaybeAssign(e, s) {
var i, a, n2, o, u, c, y;
let g, T, C;
if (this.hasPlugin("jsx") && (this.match(140) || this.match(47))) {
if (g = this.state.clone(), T = this.tryParse(() => super.parseMaybeAssign(e, s), g), !T.error)
return T.node;
let { context: K } = this.state, W = K[K.length - 1];
(W === x.j_oTag || W === x.j_expr) && K.pop();
}
if (!((i = T) != null && i.error) && !this.match(47))
return super.parseMaybeAssign(e, s);
(!g || g === this.state) && (g = this.state.clone());
let M, j = this.tryParse((K) => {
var W, V;
M = this.tsParseTypeParameters(this.tsParseConstModifier);
let X = super.parseMaybeAssign(e, s);
return (X.type !== "ArrowFunctionExpression" || (W = X.extra) != null && W.parenthesized) && K(), ((V = M) == null ? void 0 : V.params.length) !== 0 && this.resetStartLocationFromNode(X, M), X.typeParameters = M, X;
}, g);
if (!j.error && !j.aborted)
return M && this.reportReservedArrowTypeParam(M), j.node;
if (!T && ($r(!this.hasPlugin("jsx")), C = this.tryParse(() => super.parseMaybeAssign(e, s), g), !C.error))
return C.node;
if ((a = T) != null && a.node)
return this.state = T.failState, T.node;
if (j.node)
return this.state = j.failState, M && this.reportReservedArrowTypeParam(M), j.node;
if ((n2 = C) != null && n2.node)
return this.state = C.failState, C.node;
throw (o = T) != null && o.thrown ? T.error : j.thrown ? j.error : (u = C) != null && u.thrown ? C.error : ((c = T) == null ? void 0 : c.error) || j.error || ((y = C) == null ? void 0 : y.error);
}
reportReservedArrowTypeParam(e) {
var s;
e.params.length === 1 && !e.params[0].constraint && !((s = e.extra) != null && s.trailingComma) && this.getPluginOption("typescript", "disallowAmbiguousJSXLike") && this.raise(I.ReservedArrowTypeParam, { at: e });
}
parseMaybeUnary(e, s) {
return !this.hasPlugin("jsx") && this.match(47) ? this.tsParseTypeAssertion() : super.parseMaybeUnary(e, s);
}
parseArrow(e) {
if (this.match(14)) {
let s = this.tryParse((i) => {
let a = this.tsParseTypeOrTypePredicateAnnotation(14);
return (this.canInsertSemicolon() || !this.match(19)) && i(), a;
});
if (s.aborted)
return;
s.thrown || (s.error && (this.state = s.failState), e.returnType = s.node);
}
return super.parseArrow(e);
}
parseAssignableListItemTypes(e, s) {
if (!(s & 2))
return e;
this.eat(17) && (e.optional = true);
let i = this.tsTryParseTypeAnnotation();
return i && (e.typeAnnotation = i), this.resetEndLocation(e), e;
}
isAssignable(e, s) {
switch (e.type) {
case "TSTypeCastExpression":
return this.isAssignable(e.expression, s);
case "TSParameterProperty":
return true;
default:
return super.isAssignable(e, s);
}
}
toAssignable(e) {
let s = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
switch (e.type) {
case "ParenthesizedExpression":
this.toAssignableParenthesizedExpression(e, s);
break;
case "TSAsExpression":
case "TSSatisfiesExpression":
case "TSNonNullExpression":
case "TSTypeAssertion":
s ? this.expressionScope.recordArrowParameterBindingError(I.UnexpectedTypeCastInParameter, { at: e }) : this.raise(I.UnexpectedTypeCastInParameter, { at: e }), this.toAssignable(e.expression, s);
break;
case "AssignmentExpression":
!s && e.left.type === "TSTypeCastExpression" && (e.left = this.typeCastToParameter(e.left));
default:
super.toAssignable(e, s);
}
}
toAssignableParenthesizedExpression(e, s) {
switch (e.expression.type) {
case "TSAsExpression":
case "TSSatisfiesExpression":
case "TSNonNullExpression":
case "TSTypeAssertion":
case "ParenthesizedExpression":
this.toAssignable(e.expression, s);
break;
default:
super.toAssignable(e, s);
}
}
checkToRestConversion(e, s) {
switch (e.type) {
case "TSAsExpression":
case "TSSatisfiesExpression":
case "TSTypeAssertion":
case "TSNonNullExpression":
this.checkToRestConversion(e.expression, false);
break;
default:
super.checkToRestConversion(e, s);
}
}
isValidLVal(e, s, i) {
return nh({ TSTypeCastExpression: true, TSParameterProperty: "parameter", TSNonNullExpression: "expression", TSAsExpression: (i !== Pe || !s) && ["expression", true], TSSatisfiesExpression: (i !== Pe || !s) && ["expression", true], TSTypeAssertion: (i !== Pe || !s) && ["expression", true] }, e) || super.isValidLVal(e, s, i);
}
parseBindingAtom() {
switch (this.state.type) {
case 78:
return this.parseIdentifier(true);
default:
return super.parseBindingAtom();
}
}
parseMaybeDecoratorArguments(e) {
if (this.match(47) || this.match(51)) {
let s = this.tsParseTypeArgumentsInExpression();
if (this.match(10)) {
let i = super.parseMaybeDecoratorArguments(e);
return i.typeParameters = s, i;
}
this.unexpected(null, 10);
}
return super.parseMaybeDecoratorArguments(e);
}
checkCommaAfterRest(e) {
return this.state.isAmbientContext && this.match(12) && this.lookaheadCharCode() === e ? (this.next(), false) : super.checkCommaAfterRest(e);
}
isClassMethod() {
return this.match(47) || super.isClassMethod();
}
isClassProperty() {
return this.match(35) || this.match(14) || super.isClassProperty();
}
parseMaybeDefault(e, s) {
let i = super.parseMaybeDefault(e, s);
return i.type === "AssignmentPattern" && i.typeAnnotation && i.right.start < i.typeAnnotation.start && this.raise(I.TypeAnnotationAfterAssign, { at: i.typeAnnotation }), i;
}
getTokenFromCode(e) {
if (this.state.inType) {
if (e === 62) {
this.finishOp(48, 1);
return;
}
if (e === 60) {
this.finishOp(47, 1);
return;
}
}
super.getTokenFromCode(e);
}
reScan_lt_gt() {
let { type: e } = this.state;
e === 47 ? (this.state.pos -= 1, this.readToken_lt()) : e === 48 && (this.state.pos -= 1, this.readToken_gt());
}
reScan_lt() {
let { type: e } = this.state;
return e === 51 ? (this.state.pos -= 2, this.finishOp(47, 1), 47) : e;
}
toAssignableList(e, s, i) {
for (let a = 0; a < e.length; a++) {
let n2 = e[a];
(n2 == null ? void 0 : n2.type) === "TSTypeCastExpression" && (e[a] = this.typeCastToParameter(n2));
}
super.toAssignableList(e, s, i);
}
typeCastToParameter(e) {
return e.expression.typeAnnotation = e.typeAnnotation, this.resetEndLocation(e.expression, e.typeAnnotation.loc.end), e.expression;
}
shouldParseArrow(e) {
return this.match(14) ? e.every((s) => this.isAssignable(s, true)) : super.shouldParseArrow(e);
}
shouldParseAsyncArrow() {
return this.match(14) || super.shouldParseAsyncArrow();
}
canHaveLeadingDecorator() {
return super.canHaveLeadingDecorator() || this.isAbstractClass();
}
jsxParseOpeningElementAfterName(e) {
if (this.match(47) || this.match(51)) {
let s = this.tsTryParseAndCatch(() => this.tsParseTypeArgumentsInExpression());
s && (e.typeParameters = s);
}
return super.jsxParseOpeningElementAfterName(e);
}
getGetterSetterExpectedParamCount(e) {
let s = super.getGetterSetterExpectedParamCount(e), a = this.getObjectOrClassMethodParams(e)[0];
return a && this.isThisParam(a) ? s + 1 : s;
}
parseCatchClauseParam() {
let e = super.parseCatchClauseParam(), s = this.tsTryParseTypeAnnotation();
return s && (e.typeAnnotation = s, this.resetEndLocation(e)), e;
}
tsInAmbientContext(e) {
let s = this.state.isAmbientContext;
this.state.isAmbientContext = true;
try {
return e();
} finally {
this.state.isAmbientContext = s;
}
}
parseClass(e, s, i) {
let a = this.state.inAbstractClass;
this.state.inAbstractClass = !!e.abstract;
try {
return super.parseClass(e, s, i);
} finally {
this.state.inAbstractClass = a;
}
}
tsParseAbstractDeclaration(e, s) {
if (this.match(80))
return e.abstract = true, this.maybeTakeDecorators(s, this.parseClass(e, true, false));
if (this.isContextual(127)) {
if (!this.hasFollowingLineBreak())
return e.abstract = true, this.raise(I.NonClassMethodPropertyHasAbstractModifer, { at: e }), this.tsParseInterfaceDeclaration(e);
} else
this.unexpected(null, 80);
}
parseMethod(e, s, i, a, n2, o, u) {
let c = super.parseMethod(e, s, i, a, n2, o, u);
if (c.abstract && (this.hasPlugin("estree") ? !!c.value.body : !!c.body)) {
let { key: g } = c;
this.raise(I.AbstractMethodHasImplementation, { at: c, methodName: g.type === "Identifier" && !c.computed ? g.name : `[${this.input.slice(g.start, g.end)}]` });
}
return c;
}
tsParseTypeParameterName() {
return this.parseIdentifier().name;
}
shouldParseAsAmbientContext() {
return !!this.getPluginOption("typescript", "dts");
}
parse() {
return this.shouldParseAsAmbientContext() && (this.state.isAmbientContext = true), super.parse();
}
getExpression() {
return this.shouldParseAsAmbientContext() && (this.state.isAmbientContext = true), super.getExpression();
}
parseExportSpecifier(e, s, i, a) {
return !s && a ? (this.parseTypeOnlyImportExportSpecifier(e, false, i), this.finishNode(e, "ExportSpecifier")) : (e.exportKind = "value", super.parseExportSpecifier(e, s, i, a));
}
parseImportSpecifier(e, s, i, a, n2) {
return !s && a ? (this.parseTypeOnlyImportExportSpecifier(e, true, i), this.finishNode(e, "ImportSpecifier")) : (e.importKind = "value", super.parseImportSpecifier(e, s, i, a, i ? Sr : Ve));
}
parseTypeOnlyImportExportSpecifier(e, s, i) {
let a = s ? "imported" : "local", n2 = s ? "local" : "exported", o = e[a], u, c = false, y = true, g = o.loc.start;
if (this.isContextual(93)) {
let C = this.parseIdentifier();
if (this.isContextual(93)) {
let M = this.parseIdentifier();
te(this.state.type) ? (c = true, o = C, u = s ? this.parseIdentifier() : this.parseModuleExportName(), y = false) : (u = M, y = false);
} else
te(this.state.type) ? (y = false, u = s ? this.parseIdentifier() : this.parseModuleExportName()) : (c = true, o = C);
} else
te(this.state.type) && (c = true, s ? (o = this.parseIdentifier(true), this.isContextual(93) || this.checkReservedWord(o.name, o.loc.start, true, true)) : o = this.parseModuleExportName());
c && i && this.raise(s ? I.TypeModifierIsUsedInTypeImports : I.TypeModifierIsUsedInTypeExports, { at: g }), e[a] = o, e[n2] = u;
let T = s ? "importKind" : "exportKind";
e[T] = c ? "type" : "value", y && this.eatContextual(93) && (e[n2] = s ? this.parseIdentifier() : this.parseModuleExportName()), e[n2] || (e[n2] = me(e[a])), s && this.checkIdentifier(e[n2], c ? Sr : Ve);
}
};
function ch(t) {
if (t.type !== "MemberExpression")
return false;
let { computed: r, property: e } = t;
return r && e.type !== "StringLiteral" && (e.type !== "TemplateLiteral" || e.expressions.length > 0) ? false : Vr(t.object);
}
function ph(t, r) {
var e;
let { type: s } = t;
if ((e = t.extra) != null && e.parenthesized)
return false;
if (r) {
if (s === "Literal") {
let { value: i } = t;
if (typeof i == "string" || typeof i == "boolean")
return true;
}
} else if (s === "StringLiteral" || s === "BooleanLiteral")
return true;
return !!(zr(t, r) || fh(t, r) || s === "TemplateLiteral" && t.expressions.length === 0 || ch(t));
}
function zr(t, r) {
return r ? t.type === "Literal" && (typeof t.value == "number" || "bigint" in t) : t.type === "NumericLiteral" || t.type === "BigIntLiteral";
}
function fh(t, r) {
if (t.type === "UnaryExpression") {
let { operator: e, argument: s } = t;
if (e === "-" && zr(s, r))
return true;
}
return false;
}
function Vr(t) {
return t.type === "Identifier" ? true : t.type !== "MemberExpression" || t.computed ? false : Vr(t.object);
}
var Kr = pe`placeholders`({ ClassNameIsRequired: "A class name is required.", UnexpectedSpace: "Unexpected space in placeholder." }), dh = (t) => class extends t {
parsePlaceholder(e) {
if (this.match(142)) {
let s = this.startNode();
return this.next(), this.assertNoSpace(), s.name = super.parseIdentifier(true), this.assertNoSpace(), this.expect(142), this.finishPlaceholder(s, e);
}
}
finishPlaceholder(e, s) {
let i = !!(e.expectedNode && e.type === "Placeholder");
return e.expectedNode = s, i ? e : this.finishNode(e, "Placeholder");
}
getTokenFromCode(e) {
e === 37 && this.input.charCodeAt(this.state.pos + 1) === 37 ? this.finishOp(142, 2) : super.getTokenFromCode(e);
}
parseExprAtom(e) {
return this.parsePlaceholder("Expression") || super.parseExprAtom(e);
}
parseIdentifier(e) {
return this.parsePlaceholder("Identifier") || super.parseIdentifier(e);
}
checkReservedWord(e, s, i, a) {
e !== void 0 && super.checkReservedWord(e, s, i, a);
}
parseBindingAtom() {
return this.parsePlaceholder("Pattern") || super.parseBindingAtom();
}
isValidLVal(e, s, i) {
return e === "Placeholder" || super.isValidLVal(e, s, i);
}
toAssignable(e, s) {
e && e.type === "Placeholder" && e.expectedNode === "Expression" ? e.expectedNode = "Pattern" : super.toAssignable(e, s);
}
chStartsBindingIdentifier(e, s) {
return !!(super.chStartsBindingIdentifier(e, s) || this.lookahead().type === 142);
}
verifyBreakContinue(e, s) {
e.label && e.label.type === "Placeholder" || super.verifyBreakContinue(e, s);
}
parseExpressionStatement(e, s) {
if (s.type !== "Placeholder" || s.extra && s.extra.parenthesized)
return super.parseExpressionStatement(e, s);
if (this.match(14)) {
let i = e;
return i.label = this.finishPlaceholder(s, "Identifier"), this.next(), i.body = super.parseStatementOrSloppyAnnexBFunctionDeclaration(), this.finishNode(i, "LabeledStatement");
}
return this.semicolon(), e.name = s.name, this.finishPlaceholder(e, "Statement");
}
parseBlock(e, s, i) {
return this.parsePlaceholder("BlockStatement") || super.parseBlock(e, s, i);
}
parseFunctionId(e) {
return this.parsePlaceholder("Identifier") || super.parseFunctionId(e);
}
parseClass(e, s, i) {
let a = s ? "ClassDeclaration" : "ClassExpression";
this.next();
let n2 = this.state.strict, o = this.parsePlaceholder("Identifier");
if (o)
if (this.match(81) || this.match(142) || this.match(5))
e.id = o;
else {
if (i || !s)
return e.id = null, e.body = this.finishPlaceholder(o, "ClassBody"), this.finishNode(e, a);
throw this.raise(Kr.ClassNameIsRequired, { at: this.state.startLoc });
}
else
this.parseClassId(e, s, i);
return super.parseClassSuper(e), e.body = this.parsePlaceholder("ClassBody") || super.parseClassBody(!!e.superClass, n2), this.finishNode(e, a);
}
parseExport(e, s) {
let i = this.parsePlaceholder("Identifier");
if (!i)
return super.parseExport(e, s);
if (!this.isContextual(97) && !this.match(12))
return e.specifiers = [], e.source = null, e.declaration = this.finishPlaceholder(i, "Declaration"), this.finishNode(e, "ExportNamedDeclaration");
this.expectPlugin("exportDefaultFrom");
let a = this.startNode();
return a.exported = i, e.specifiers = [this.finishNode(a, "ExportDefaultSpecifier")], super.parseExport(e, s);
}
isExportDefaultSpecifier() {
if (this.match(65)) {
let e = this.nextTokenStart();
if (this.isUnparsedContextual(e, "from") && this.input.startsWith(xe(142), this.nextTokenStartSince(e + 4)))
return true;
}
return super.isExportDefaultSpecifier();
}
maybeParseExportDefaultSpecifier(e) {
return e.specifiers && e.specifiers.length > 0 ? true : super.maybeParseExportDefaultSpecifier(e);
}
checkExport(e) {
let { specifiers: s } = e;
s != null && s.length && (e.specifiers = s.filter((i) => i.exported.type === "Placeholder")), super.checkExport(e), e.specifiers = s;
}
parseImport(e) {
let s = this.parsePlaceholder("Identifier");
if (!s)
return super.parseImport(e);
if (e.specifiers = [], !this.isContextual(97) && !this.match(12))
return e.source = this.finishPlaceholder(s, "StringLiteral"), this.semicolon(), this.finishNode(e, "ImportDeclaration");
let i = this.startNodeAtNode(s);
return i.local = s, e.specifiers.push(this.finishNode(i, "ImportDefaultSpecifier")), this.eat(12) && (this.maybeParseStarImportSpecifier(e) || this.parseNamedImportSpecifiers(e)), this.expectContextual(97), e.source = this.parseImportSource(), this.semicolon(), this.finishNode(e, "ImportDeclaration");
}
parseImportSource() {
return this.parsePlaceholder("StringLiteral") || super.parseImportSource();
}
assertNoSpace() {
this.state.start > this.state.lastTokEndLoc.index && this.raise(Kr.UnexpectedSpace, { at: this.state.lastTokEndLoc });
}
}, mh = (t) => class extends t {
parseV8Intrinsic() {
if (this.match(54)) {
let e = this.state.startLoc, s = this.startNode();
if (this.next(), q(this.state.type)) {
let i = this.parseIdentifierName(), a = this.createIdentifier(s, i);
if (a.type = "V8IntrinsicIdentifier", this.match(10))
return a;
}
this.unexpected(e);
}
}
parseExprAtom(e) {
return this.parseV8Intrinsic() || super.parseExprAtom(e);
}
};
function J(t, r) {
let [e, s] = typeof r == "string" ? [r, {}] : r, i = Object.keys(s), a = i.length === 0;
return t.some((n2) => {
if (typeof n2 == "string")
return a && n2 === e;
{
let [o, u] = n2;
if (o !== e)
return false;
for (let c of i)
if (u[c] !== s[c])
return false;
return true;
}
});
}
function we(t, r, e) {
let s = t.find((i) => Array.isArray(i) ? i[0] === r : i === r);
return s && Array.isArray(s) && s.length > 1 ? s[1][e] : null;
}
var Wr = ["minimal", "fsharp", "hack", "smart"], Gr = ["^^", "@@", "^", "%", "#"], Jr = ["hash", "bar"];
function yh(t) {
if (J(t, "decorators")) {
if (J(t, "decorators-legacy"))
throw new Error("Cannot use the decorators and decorators-legacy plugin together");
let r = we(t, "decorators", "decoratorsBeforeExport");
if (r != null && typeof r != "boolean")
throw new Error("'decoratorsBeforeExport' must be a boolean, if specified.");
let e = we(t, "decorators", "allowCallParenthesized");
if (e != null && typeof e != "boolean")
throw new Error("'allowCallParenthesized' must be a boolean.");
}
if (J(t, "flow") && J(t, "typescript"))
throw new Error("Cannot combine flow and typescript plugins.");
if (J(t, "placeholders") && J(t, "v8intrinsic"))
throw new Error("Cannot combine placeholders and v8intrinsic plugins.");
if (J(t, "pipelineOperator")) {
let r = we(t, "pipelineOperator", "proposal");
if (!Wr.includes(r)) {
let s = Wr.map((i) => `"${i}"`).join(", ");
throw new Error(`"pipelineOperator" requires "proposal" option whose value must be one of: ${s}.`);
}
let e = J(t, ["recordAndTuple", { syntaxType: "hash" }]);
if (r === "hack") {
if (J(t, "placeholders"))
throw new Error("Cannot combine placeholders plugin and Hack-style pipes.");
if (J(t, "v8intrinsic"))
throw new Error("Cannot combine v8intrinsic plugin and Hack-style pipes.");
let s = we(t, "pipelineOperator", "topicToken");
if (!Gr.includes(s)) {
let i = Gr.map((a) => `"${a}"`).join(", ");
throw new Error(`"pipelineOperator" in "proposal": "hack" mode also requires a "topicToken" option whose value must be one of: ${i}.`);
}
if (s === "#" && e)
throw new Error('Plugin conflict between `["pipelineOperator", { proposal: "hack", topicToken: "#" }]` and `["recordAndtuple", { syntaxType: "hash"}]`.');
} else if (r === "smart" && e)
throw new Error('Plugin conflict between `["pipelineOperator", { proposal: "smart" }]` and `["recordAndtuple", { syntaxType: "hash"}]`.');
}
if (J(t, "moduleAttributes")) {
if (J(t, "importAssertions"))
throw new Error("Cannot combine importAssertions and moduleAttributes plugins.");
if (we(t, "moduleAttributes", "version") !== "may-2020")
throw new Error("The 'moduleAttributes' plugin requires a 'version' option, representing the last proposal update. Currently, the only supported value is 'may-2020'.");
}
if (J(t, "recordAndTuple") && we(t, "recordAndTuple", "syntaxType") != null && !Jr.includes(we(t, "recordAndTuple", "syntaxType")))
throw new Error("The 'syntaxType' option of the 'recordAndTuple' plugin must be one of: " + Jr.map((r) => `'${r}'`).join(", "));
if (J(t, "asyncDoExpressions") && !J(t, "doExpressions")) {
let r = new Error("'asyncDoExpressions' requires 'doExpressions', please add 'doExpressions' to parser plugins.");
throw r.missingPlugins = "doExpressions", r;
}
}
var Xr = { estree: el, jsx: th, flow: Zl, typescript: uh, v8intrinsic: mh, placeholders: dh }, xh = Object.keys(Xr), gh = class extends ah {
checkProto(t, r, e, s) {
if (t.type === "SpreadElement" || this.isObjectMethod(t) || t.computed || t.shorthand)
return;
let i = t.key;
if ((i.type === "Identifier" ? i.name : i.value) === "__proto__") {
if (r) {
this.raise(f.RecordNoProto, { at: i });
return;
}
e.used && (s ? s.doubleProtoLoc === null && (s.doubleProtoLoc = i.loc.start) : this.raise(f.DuplicateProto, { at: i })), e.used = true;
}
}
shouldExitDescending(t, r) {
return t.type === "ArrowFunctionExpression" && t.start === r;
}
getExpression() {
this.enterInitialScopes(), this.nextToken();
let t = this.parseExpression();
return this.match(137) || this.unexpected(), this.finalizeRemainingComments(), t.comments = this.state.comments, t.errors = this.state.errors, this.options.tokens && (t.tokens = this.tokens), t;
}
parseExpression(t, r) {
return t ? this.disallowInAnd(() => this.parseExpressionBase(r)) : this.allowInAnd(() => this.parseExpressionBase(r));
}
parseExpressionBase(t) {
let r = this.state.startLoc, e = this.parseMaybeAssign(t);
if (this.match(12)) {
let s = this.startNodeAt(r);
for (s.expressions = [e]; this.eat(12); )
s.expressions.push(this.parseMaybeAssign(t));
return this.toReferencedList(s.expressions), this.finishNode(s, "SequenceExpression");
}
return e;
}
parseMaybeAssignDisallowIn(t, r) {
return this.disallowInAnd(() => this.parseMaybeAssign(t, r));
}
parseMaybeAssignAllowIn(t, r) {
return this.allowInAnd(() => this.parseMaybeAssign(t, r));
}
setOptionalParametersError(t, r) {
var e;
t.optionalParametersLoc = (e = r == null ? void 0 : r.loc) != null ? e : this.state.startLoc;
}
parseMaybeAssign(t, r) {
let e = this.state.startLoc;
if (this.isContextual(106) && this.prodParam.hasYield) {
let n2 = this.parseYield();
return r && (n2 = r.call(this, n2, e)), n2;
}
let s;
t ? s = false : (t = new vt(), s = true);
let { type: i } = this.state;
(i === 10 || q(i)) && (this.state.potentialArrowAt = this.state.start);
let a = this.parseMaybeConditional(t);
if (r && (a = r.call(this, a, e)), Bo(this.state.type)) {
let n2 = this.startNodeAt(e), o = this.state.value;
if (n2.operator = o, this.match(29)) {
this.toAssignable(a, true), n2.left = a;
let u = e.index;
t.doubleProtoLoc != null && t.doubleProtoLoc.index >= u && (t.doubleProtoLoc = null), t.shorthandAssignLoc != null && t.shorthandAssignLoc.index >= u && (t.shorthandAssignLoc = null), t.privateKeyLoc != null && t.privateKeyLoc.index >= u && (this.checkDestructuringPrivate(t), t.privateKeyLoc = null);
} else
n2.left = a;
return this.next(), n2.right = this.parseMaybeAssign(), this.checkLVal(a, { in: this.finishNode(n2, "AssignmentExpression") }), n2;
} else
s && this.checkExpressionErrors(t, true);
return a;
}
parseMaybeConditional(t) {
let r = this.state.startLoc, e = this.state.potentialArrowAt, s = this.parseExprOps(t);
return this.shouldExitDescending(s, e) ? s : this.parseConditional(s, r, t);
}
parseConditional(t, r, e) {
if (this.eat(17)) {
let s = this.startNodeAt(r);
return s.test = t, s.consequent = this.parseMaybeAssignAllowIn(), this.expect(14), s.alternate = this.parseMaybeAssign(), this.finishNode(s, "ConditionalExpression");
}
return t;
}
parseMaybeUnaryOrPrivate(t) {
return this.match(136) ? this.parsePrivateName() : this.parseMaybeUnary(t);
}
parseExprOps(t) {
let r = this.state.startLoc, e = this.state.potentialArrowAt, s = this.parseMaybeUnaryOrPrivate(t);
return this.shouldExitDescending(s, e) ? s : this.parseExprOp(s, r, -1);
}
parseExprOp(t, r, e) {
if (this.isPrivateName(t)) {
let i = this.getPrivateNameSV(t);
(e >= at(58) || !this.prodParam.hasIn || !this.match(58)) && this.raise(f.PrivateInExpectedIn, { at: t, identifierName: i }), this.classScope.usePrivateName(i, t.loc.start);
}
let s = this.state.type;
if (_o(s) && (this.prodParam.hasIn || !this.match(58))) {
let i = at(s);
if (i > e) {
if (s === 39) {
if (this.expectPlugin("pipelineOperator"), this.state.inFSharpPipelineDirectBody)
return t;
this.checkPipelineAtInfixOperator(t, r);
}
let a = this.startNodeAt(r);
a.left = t, a.operator = this.state.value;
let n2 = s === 41 || s === 42, o = s === 40;
if (o && (i = at(42)), this.next(), s === 39 && this.hasPlugin(["pipelineOperator", { proposal: "minimal" }]) && this.state.type === 96 && this.prodParam.hasAwait)
throw this.raise(f.UnexpectedAwaitAfterPipelineBody, { at: this.state.startLoc });
a.right = this.parseExprOpRightExpr(s, i);
let u = this.finishNode(a, n2 || o ? "LogicalExpression" : "BinaryExpression"), c = this.state.type;
if (o && (c === 41 || c === 42) || n2 && c === 40)
throw this.raise(f.MixingCoalesceWithLogical, { at: this.state.startLoc });
return this.parseExprOp(u, r, e);
}
}
return t;
}
parseExprOpRightExpr(t, r) {
let e = this.state.startLoc;
switch (t) {
case 39:
switch (this.getPluginOption("pipelineOperator", "proposal")) {
case "hack":
return this.withTopicBindingContext(() => this.parseHackPipeBody());
case "smart":
return this.withTopicBindingContext(() => {
if (this.prodParam.hasYield && this.isContextual(106))
throw this.raise(f.PipeBodyIsTighter, { at: this.state.startLoc });
return this.parseSmartPipelineBodyInStyle(this.parseExprOpBaseRightExpr(t, r), e);
});
case "fsharp":
return this.withSoloAwaitPermittingContext(() => this.parseFSharpPipelineBody(r));
}
default:
return this.parseExprOpBaseRightExpr(t, r);
}
}
parseExprOpBaseRightExpr(t, r) {
let e = this.state.startLoc;
return this.parseExprOp(this.parseMaybeUnaryOrPrivate(), e, $o(t) ? r - 1 : r);
}
parseHackPipeBody() {
var t;
let { startLoc: r } = this.state, e = this.parseMaybeAssign();
return Go.has(e.type) && !((t = e.extra) != null && t.parenthesized) && this.raise(f.PipeUnparenthesizedBody, { at: r, type: e.type }), this.topicReferenceWasUsedInCurrentContext() || this.raise(f.PipeTopicUnused, { at: r }), e;
}
checkExponentialAfterUnary(t) {
this.match(57) && this.raise(f.UnexpectedTokenUnaryExponentiation, { at: t.argument });
}
parseMaybeUnary(t, r) {
let e = this.state.startLoc, s = this.isContextual(96);
if (s && this.isAwaitAllowed()) {
this.next();
let o = this.parseAwait(e);
return r || this.checkExponentialAfterUnary(o), o;
}
let i = this.match(34), a = this.startNode();
if (jo(this.state.type)) {
a.operator = this.state.value, a.prefix = true, this.match(72) && this.expectPlugin("throwExpressions");
let o = this.match(89);
if (this.next(), a.argument = this.parseMaybeUnary(null, true), this.checkExpressionErrors(t, true), this.state.strict && o) {
let u = a.argument;
u.type === "Identifier" ? this.raise(f.StrictDelete, { at: a }) : this.hasPropertyAsPrivateName(u) && this.raise(f.DeletePrivateField, { at: a });
}
if (!i)
return r || this.checkExponentialAfterUnary(a), this.finishNode(a, "UnaryExpression");
}
let n2 = this.parseUpdate(a, i, t);
if (s) {
let { type: o } = this.state;
if ((this.hasPlugin("v8intrinsic") ? He(o) : He(o) && !this.match(54)) && !this.isAmbiguousAwait())
return this.raiseOverwrite(f.AwaitNotInAsyncContext, { at: e }), this.parseAwait(e);
}
return n2;
}
parseUpdate(t, r, e) {
if (r) {
let a = t;
return this.checkLVal(a.argument, { in: this.finishNode(a, "UpdateExpression") }), t;
}
let s = this.state.startLoc, i = this.parseExprSubscripts(e);
if (this.checkExpressionErrors(e, false))
return i;
for (; Ro(this.state.type) && !this.canInsertSemicolon(); ) {
let a = this.startNodeAt(s);
a.operator = this.state.value, a.prefix = false, a.argument = i, this.next(), this.checkLVal(i, { in: i = this.finishNode(a, "UpdateExpression") });
}
return i;
}
parseExprSubscripts(t) {
let r = this.state.startLoc, e = this.state.potentialArrowAt, s = this.parseExprAtom(t);
return this.shouldExitDescending(s, e) ? s : this.parseSubscripts(s, r);
}
parseSubscripts(t, r, e) {
let s = { optionalChainMember: false, maybeAsyncArrow: this.atPossibleAsyncArrow(t), stop: false };
do
t = this.parseSubscript(t, r, e, s), s.maybeAsyncArrow = false;
while (!s.stop);
return t;
}
parseSubscript(t, r, e, s) {
let { type: i } = this.state;
if (!e && i === 15)
return this.parseBind(t, r, e, s);
if (nt(i))
return this.parseTaggedTemplateExpression(t, r, s);
let a = false;
if (i === 18) {
if (e && (this.raise(f.OptionalChainingNoNew, { at: this.state.startLoc }), this.lookaheadCharCode() === 40))
return s.stop = true, t;
s.optionalChainMember = a = true, this.next();
}
if (!e && this.match(10))
return this.parseCoverCallAndAsyncArrowHead(t, r, s, a);
{
let n2 = this.eat(0);
return n2 || a || this.eat(16) ? this.parseMember(t, r, s, n2, a) : (s.stop = true, t);
}
}
parseMember(t, r, e, s, i) {
let a = this.startNodeAt(r);
return a.object = t, a.computed = s, s ? (a.property = this.parseExpression(), this.expect(3)) : this.match(136) ? (t.type === "Super" && this.raise(f.SuperPrivateField, { at: r }), this.classScope.usePrivateName(this.state.value, this.state.startLoc), a.property = this.parsePrivateName()) : a.property = this.parseIdentifier(true), e.optionalChainMember ? (a.optional = i, this.finishNode(a, "OptionalMemberExpression")) : this.finishNode(a, "MemberExpression");
}
parseBind(t, r, e, s) {
let i = this.startNodeAt(r);
return i.object = t, this.next(), i.callee = this.parseNoCallExpr(), s.stop = true, this.parseSubscripts(this.finishNode(i, "BindExpression"), r, e);
}
parseCoverCallAndAsyncArrowHead(t, r, e, s) {
let i = this.state.maybeInArrowParameters, a = null;
this.state.maybeInArrowParameters = true, this.next();
let n2 = this.startNodeAt(r);
n2.callee = t;
let { maybeAsyncArrow: o, optionalChainMember: u } = e;
o && (this.expressionScope.enter($l()), a = new vt()), u && (n2.optional = s), s ? n2.arguments = this.parseCallExpressionArguments(11) : n2.arguments = this.parseCallExpressionArguments(11, t.type === "Import", t.type !== "Super", n2, a);
let c = this.finishCallExpression(n2, u);
return o && this.shouldParseAsyncArrow() && !s ? (e.stop = true, this.checkDestructuringPrivate(a), this.expressionScope.validateAsPattern(), this.expressionScope.exit(), c = this.parseAsyncArrowFromCallExpression(this.startNodeAt(r), c)) : (o && (this.checkExpressionErrors(a, true), this.expressionScope.exit()), this.toReferencedArguments(c)), this.state.maybeInArrowParameters = i, c;
}
toReferencedArguments(t, r) {
this.toReferencedListDeep(t.arguments, r);
}
parseTaggedTemplateExpression(t, r, e) {
let s = this.startNodeAt(r);
return s.tag = t, s.quasi = this.parseTemplate(true), e.optionalChainMember && this.raise(f.OptionalChainingNoTemplate, { at: r }), this.finishNode(s, "TaggedTemplateExpression");
}
atPossibleAsyncArrow(t) {
return t.type === "Identifier" && t.name === "async" && this.state.lastTokEndLoc.index === t.end && !this.canInsertSemicolon() && t.end - t.start === 5 && t.start === this.state.potentialArrowAt;
}
finishCallExpression(t, r) {
if (t.callee.type === "Import")
if (t.arguments.length === 2 && (this.hasPlugin("moduleAttributes") || this.expectPlugin("importAssertions")), t.arguments.length === 0 || t.arguments.length > 2)
this.raise(f.ImportCallArity, { at: t, maxArgumentCount: this.hasPlugin("importAssertions") || this.hasPlugin("moduleAttributes") ? 2 : 1 });
else
for (let e of t.arguments)
e.type === "SpreadElement" && this.raise(f.ImportCallSpreadArgument, { at: e });
return this.finishNode(t, r ? "OptionalCallExpression" : "CallExpression");
}
parseCallExpressionArguments(t, r, e, s, i) {
let a = [], n2 = true, o = this.state.inFSharpPipelineDirectBody;
for (this.state.inFSharpPipelineDirectBody = false; !this.eat(t); ) {
if (n2)
n2 = false;
else if (this.expect(12), this.match(t)) {
r && !this.hasPlugin("importAssertions") && !this.hasPlugin("moduleAttributes") && this.raise(f.ImportCallArgumentTrailingComma, { at: this.state.lastTokStartLoc }), s && this.addTrailingCommaExtraToNode(s), this.next();
break;
}
a.push(this.parseExprListItem(false, i, e));
}
return this.state.inFSharpPipelineDirectBody = o, a;
}
shouldParseAsyncArrow() {
return this.match(19) && !this.canInsertSemicolon();
}
parseAsyncArrowFromCallExpression(t, r) {
var e;
return this.resetPreviousNodeTrailingComments(r), this.expect(19), this.parseArrowExpression(t, r.arguments, true, (e = r.extra) == null ? void 0 : e.trailingCommaLoc), r.innerComments && Ke(t, r.innerComments), r.callee.trailingComments && Ke(t, r.callee.trailingComments), t;
}
parseNoCallExpr() {
let t = this.state.startLoc;
return this.parseSubscripts(this.parseExprAtom(), t, true);
}
parseExprAtom(t) {
let r, e = null, { type: s } = this.state;
switch (s) {
case 79:
return this.parseSuper();
case 83:
return r = this.startNode(), this.next(), this.match(16) ? this.parseImportMetaProperty(r) : (this.match(10) || this.raise(f.UnsupportedImport, { at: this.state.lastTokStartLoc }), this.finishNode(r, "Import"));
case 78:
return r = this.startNode(), this.next(), this.finishNode(r, "ThisExpression");
case 90:
return this.parseDo(this.startNode(), false);
case 56:
case 31:
return this.readRegexp(), this.parseRegExpLiteral(this.state.value);
case 132:
return this.parseNumericLiteral(this.state.value);
case 133:
return this.parseBigIntLiteral(this.state.value);
case 134:
return this.parseDecimalLiteral(this.state.value);
case 131:
return this.parseStringLiteral(this.state.value);
case 84:
return this.parseNullLiteral();
case 85:
return this.parseBooleanLiteral(true);
case 86:
return this.parseBooleanLiteral(false);
case 10: {
let i = this.state.potentialArrowAt === this.state.start;
return this.parseParenAndDistinguishExpression(i);
}
case 2:
case 1:
return this.parseArrayLike(this.state.type === 2 ? 4 : 3, false, true);
case 0:
return this.parseArrayLike(3, true, false, t);
case 6:
case 7:
return this.parseObjectLike(this.state.type === 6 ? 9 : 8, false, true);
case 5:
return this.parseObjectLike(8, false, false, t);
case 68:
return this.parseFunctionOrFunctionSent();
case 26:
e = this.parseDecorators();
case 80:
return this.parseClass(this.maybeTakeDecorators(e, this.startNode()), false);
case 77:
return this.parseNewOrNewTarget();
case 25:
case 24:
return this.parseTemplate(false);
case 15: {
r = this.startNode(), this.next(), r.object = null;
let i = r.callee = this.parseNoCallExpr();
if (i.type === "MemberExpression")
return this.finishNode(r, "BindExpression");
throw this.raise(f.UnsupportedBind, { at: i });
}
case 136:
return this.raise(f.PrivateInExpectedIn, { at: this.state.startLoc, identifierName: this.state.value }), this.parsePrivateName();
case 33:
return this.parseTopicReferenceThenEqualsSign(54, "%");
case 32:
return this.parseTopicReferenceThenEqualsSign(44, "^");
case 37:
case 38:
return this.parseTopicReference("hack");
case 44:
case 54:
case 27: {
let i = this.getPluginOption("pipelineOperator", "proposal");
if (i)
return this.parseTopicReference(i);
this.unexpected();
break;
}
case 47: {
let i = this.input.codePointAt(this.nextTokenStart());
fe(i) || i === 62 ? this.expectOnePlugin(["jsx", "flow", "typescript"]) : this.unexpected();
break;
}
default:
if (q(s)) {
if (this.isContextual(125) && this.lookaheadCharCode() === 123 && !this.hasFollowingLineBreak())
return this.parseModuleExpression();
let i = this.state.potentialArrowAt === this.state.start, a = this.state.containsEsc, n2 = this.parseIdentifier();
if (!a && n2.name === "async" && !this.canInsertSemicolon()) {
let { type: o } = this.state;
if (o === 68)
return this.resetPreviousNodeTrailingComments(n2), this.next(), this.parseAsyncFunctionExpression(this.startNodeAtNode(n2));
if (q(o))
return this.lookaheadCharCode() === 61 ? this.parseAsyncArrowUnaryFunction(this.startNodeAtNode(n2)) : n2;
if (o === 90)
return this.resetPreviousNodeTrailingComments(n2), this.parseDo(this.startNodeAtNode(n2), true);
}
return i && this.match(19) && !this.canInsertSemicolon() ? (this.next(), this.parseArrowExpression(this.startNodeAtNode(n2), [n2], false)) : n2;
} else
this.unexpected();
}
}
parseTopicReferenceThenEqualsSign(t, r) {
let e = this.getPluginOption("pipelineOperator", "proposal");
if (e)
return this.state.type = t, this.state.value = r, this.state.pos--, this.state.end--, this.state.endLoc = Y(this.state.endLoc, -1), this.parseTopicReference(e);
this.unexpected();
}
parseTopicReference(t) {
let r = this.startNode(), e = this.state.startLoc, s = this.state.type;
return this.next(), this.finishTopicReference(r, e, t, s);
}
finishTopicReference(t, r, e, s) {
if (this.testTopicReferenceConfiguration(e, r, s)) {
let i = e === "smart" ? "PipelinePrimaryTopicReference" : "TopicReference";
return this.topicReferenceIsAllowedInCurrentContext() || this.raise(e === "smart" ? f.PrimaryTopicNotAllowed : f.PipeTopicUnbound, { at: r }), this.registerTopicReference(), this.finishNode(t, i);
} else
throw this.raise(f.PipeTopicUnconfiguredToken, { at: r, token: xe(s) });
}
testTopicReferenceConfiguration(t, r, e) {
switch (t) {
case "hack":
return this.hasPlugin(["pipelineOperator", { topicToken: xe(e) }]);
case "smart":
return e === 27;
default:
throw this.raise(f.PipeTopicRequiresHackPipes, { at: r });
}
}
parseAsyncArrowUnaryFunction(t) {
this.prodParam.enter(Tt(true, this.prodParam.hasYield));
let r = [this.parseIdentifier()];
return this.prodParam.exit(), this.hasPrecedingLineBreak() && this.raise(f.LineTerminatorBeforeArrow, { at: this.state.curPosition() }), this.expect(19), this.parseArrowExpression(t, r, true);
}
parseDo(t, r) {
this.expectPlugin("doExpressions"), r && this.expectPlugin("asyncDoExpressions"), t.async = r, this.next();
let e = this.state.labels;
return this.state.labels = [], r ? (this.prodParam.enter(At), t.body = this.parseBlock(), this.prodParam.exit()) : t.body = this.parseBlock(), this.state.labels = e, this.finishNode(t, "DoExpression");
}
parseSuper() {
let t = this.startNode();
return this.next(), this.match(10) && !this.scope.allowDirectSuper && !this.options.allowSuperOutsideMethod ? this.raise(f.SuperNotAllowed, { at: t }) : !this.scope.allowSuper && !this.options.allowSuperOutsideMethod && this.raise(f.UnexpectedSuper, { at: t }), !this.match(10) && !this.match(0) && !this.match(16) && this.raise(f.UnsupportedSuper, { at: t }), this.finishNode(t, "Super");
}
parsePrivateName() {
let t = this.startNode(), r = this.startNodeAt(Y(this.state.startLoc, 1)), e = this.state.value;
return this.next(), t.id = this.createIdentifier(r, e), this.finishNode(t, "PrivateName");
}
parseFunctionOrFunctionSent() {
let t = this.startNode();
if (this.next(), this.prodParam.hasYield && this.match(16)) {
let r = this.createIdentifier(this.startNodeAtNode(t), "function");
return this.next(), this.match(102) ? this.expectPlugin("functionSent") : this.hasPlugin("functionSent") || this.unexpected(), this.parseMetaProperty(t, r, "sent");
}
return this.parseFunction(t);
}
parseMetaProperty(t, r, e) {
t.meta = r;
let s = this.state.containsEsc;
return t.property = this.parseIdentifier(true), (t.property.name !== e || s) && this.raise(f.UnsupportedMetaProperty, { at: t.property, target: r.name, onlyValidPropertyName: e }), this.finishNode(t, "MetaProperty");
}
parseImportMetaProperty(t) {
let r = this.createIdentifier(this.startNodeAtNode(t), "import");
return this.next(), this.isContextual(100) && (this.inModule || this.raise(f.ImportMetaOutsideModule, { at: r }), this.sawUnambiguousESM = true), this.parseMetaProperty(t, r, "meta");
}
parseLiteralAtNode(t, r, e) {
return this.addExtra(e, "rawValue", t), this.addExtra(e, "raw", this.input.slice(e.start, this.state.end)), e.value = t, this.next(), this.finishNode(e, r);
}
parseLiteral(t, r) {
let e = this.startNode();
return this.parseLiteralAtNode(t, r, e);
}
parseStringLiteral(t) {
return this.parseLiteral(t, "StringLiteral");
}
parseNumericLiteral(t) {
return this.parseLiteral(t, "NumericLiteral");
}
parseBigIntLiteral(t) {
return this.parseLiteral(t, "BigIntLiteral");
}
parseDecimalLiteral(t) {
return this.parseLiteral(t, "DecimalLiteral");
}
parseRegExpLiteral(t) {
let r = this.parseLiteral(t.value, "RegExpLiteral");
return r.pattern = t.pattern, r.flags = t.flags, r;
}
parseBooleanLiteral(t) {
let r = this.startNode();
return r.value = t, this.next(), this.finishNode(r, "BooleanLiteral");
}
parseNullLiteral() {
let t = this.startNode();
return this.next(), this.finishNode(t, "NullLiteral");
}
parseParenAndDistinguishExpression(t) {
let r = this.state.startLoc, e;
this.next(), this.expressionScope.enter(Ul());
let s = this.state.maybeInArrowParameters, i = this.state.inFSharpPipelineDirectBody;
this.state.maybeInArrowParameters = true, this.state.inFSharpPipelineDirectBody = false;
let a = this.state.startLoc, n2 = [], o = new vt(), u = true, c, y;
for (; !this.match(11); ) {
if (u)
u = false;
else if (this.expect(12, o.optionalParametersLoc === null ? null : o.optionalParametersLoc), this.match(11)) {
y = this.state.startLoc;
break;
}
if (this.match(21)) {
let C = this.state.startLoc;
if (c = this.state.startLoc, n2.push(this.parseParenItem(this.parseRestBinding(), C)), !this.checkCommaAfterRest(41))
break;
} else
n2.push(this.parseMaybeAssignAllowIn(o, this.parseParenItem));
}
let g = this.state.lastTokEndLoc;
this.expect(11), this.state.maybeInArrowParameters = s, this.state.inFSharpPipelineDirectBody = i;
let T = this.startNodeAt(r);
return t && this.shouldParseArrow(n2) && (T = this.parseArrow(T)) ? (this.checkDestructuringPrivate(o), this.expressionScope.validateAsPattern(), this.expressionScope.exit(), this.parseArrowExpression(T, n2, false), T) : (this.expressionScope.exit(), n2.length || this.unexpected(this.state.lastTokStartLoc), y && this.unexpected(y), c && this.unexpected(c), this.checkExpressionErrors(o, true), this.toReferencedListDeep(n2, true), n2.length > 1 ? (e = this.startNodeAt(a), e.expressions = n2, this.finishNode(e, "SequenceExpression"), this.resetEndLocation(e, g)) : e = n2[0], this.wrapParenthesis(r, e));
}
wrapParenthesis(t, r) {
if (!this.options.createParenthesizedExpressions)
return this.addExtra(r, "parenthesized", true), this.addExtra(r, "parenStart", t.index), this.takeSurroundingComments(r, t.index, this.state.lastTokEndLoc.index), r;
let e = this.startNodeAt(t);
return e.expression = r, this.finishNode(e, "ParenthesizedExpression");
}
shouldParseArrow(t) {
return !this.canInsertSemicolon();
}
parseArrow(t) {
if (this.eat(19))
return t;
}
parseParenItem(t, r) {
return t;
}
parseNewOrNewTarget() {
let t = this.startNode();
if (this.next(), this.match(16)) {
let r = this.createIdentifier(this.startNodeAtNode(t), "new");
this.next();
let e = this.parseMetaProperty(t, r, "target");
return !this.scope.inNonArrowFunction && !this.scope.inClass && !this.options.allowNewTargetOutsideFunction && this.raise(f.UnexpectedNewTarget, { at: e }), e;
}
return this.parseNew(t);
}
parseNew(t) {
if (this.parseNewCallee(t), this.eat(10)) {
let r = this.parseExprList(11);
this.toReferencedList(r), t.arguments = r;
} else
t.arguments = [];
return this.finishNode(t, "NewExpression");
}
parseNewCallee(t) {
t.callee = this.parseNoCallExpr(), t.callee.type === "Import" && this.raise(f.ImportCallNotNewExpression, { at: t.callee });
}
parseTemplateElement(t) {
let { start: r, startLoc: e, end: s, value: i } = this.state, a = r + 1, n2 = this.startNodeAt(Y(e, 1));
i === null && (t || this.raise(f.InvalidEscapeSequenceTemplate, { at: Y(this.state.firstInvalidTemplateEscapePos, 1) }));
let o = this.match(24), u = o ? -1 : -2, c = s + u;
n2.value = { raw: this.input.slice(a, c).replace(/\r\n?/g, `
`), cooked: i === null ? null : i.slice(1, u) }, n2.tail = o, this.next();
let y = this.finishNode(n2, "TemplateElement");
return this.resetEndLocation(y, Y(this.state.lastTokEndLoc, u)), y;
}
parseTemplate(t) {
let r = this.startNode();
r.expressions = [];
let e = this.parseTemplateElement(t);
for (r.quasis = [e]; !e.tail; )
r.expressions.push(this.parseTemplateSubstitution()), this.readTemplateContinuation(), r.quasis.push(e = this.parseTemplateElement(t));
return this.finishNode(r, "TemplateLiteral");
}
parseTemplateSubstitution() {
return this.parseExpression();
}
parseObjectLike(t, r, e, s) {
e && this.expectPlugin("recordAndTuple");
let i = this.state.inFSharpPipelineDirectBody;
this.state.inFSharpPipelineDirectBody = false;
let a = /* @__PURE__ */ Object.create(null), n2 = true, o = this.startNode();
for (o.properties = [], this.next(); !this.match(t); ) {
if (n2)
n2 = false;
else if (this.expect(12), this.match(t)) {
this.addTrailingCommaExtraToNode(o);
break;
}
let c;
r ? c = this.parseBindingProperty() : (c = this.parsePropertyDefinition(s), this.checkProto(c, e, a, s)), e && !this.isObjectProperty(c) && c.type !== "SpreadElement" && this.raise(f.InvalidRecordProperty, { at: c }), c.shorthand && this.addExtra(c, "shorthand", true), o.properties.push(c);
}
this.next(), this.state.inFSharpPipelineDirectBody = i;
let u = "ObjectExpression";
return r ? u = "ObjectPattern" : e && (u = "RecordExpression"), this.finishNode(o, u);
}
addTrailingCommaExtraToNode(t) {
this.addExtra(t, "trailingComma", this.state.lastTokStart), this.addExtra(t, "trailingCommaLoc", this.state.lastTokStartLoc, false);
}
maybeAsyncOrAccessorProp(t) {
return !t.computed && t.key.type === "Identifier" && (this.isLiteralPropertyName() || this.match(0) || this.match(55));
}
parsePropertyDefinition(t) {
let r = [];
if (this.match(26))
for (this.hasPlugin("decorators") && this.raise(f.UnsupportedPropertyDecorator, { at: this.state.startLoc }); this.match(26); )
r.push(this.parseDecorator());
let e = this.startNode(), s = false, i = false, a;
if (this.match(21))
return r.length && this.unexpected(), this.parseSpread();
r.length && (e.decorators = r, r = []), e.method = false, t && (a = this.state.startLoc);
let n2 = this.eat(55);
this.parsePropertyNamePrefixOperator(e);
let o = this.state.containsEsc, u = this.parsePropertyName(e, t);
if (!n2 && !o && this.maybeAsyncOrAccessorProp(e)) {
let c = u.name;
c === "async" && !this.hasPrecedingLineBreak() && (s = true, this.resetPreviousNodeTrailingComments(u), n2 = this.eat(55), this.parsePropertyName(e)), (c === "get" || c === "set") && (i = true, this.resetPreviousNodeTrailingComments(u), e.kind = c, this.match(55) && (n2 = true, this.raise(f.AccessorIsGenerator, { at: this.state.curPosition(), kind: c }), this.next()), this.parsePropertyName(e));
}
return this.parseObjPropValue(e, a, n2, s, false, i, t);
}
getGetterSetterExpectedParamCount(t) {
return t.kind === "get" ? 0 : 1;
}
getObjectOrClassMethodParams(t) {
return t.params;
}
checkGetterSetterParams(t) {
var r;
let e = this.getGetterSetterExpectedParamCount(t), s = this.getObjectOrClassMethodParams(t);
s.length !== e && this.raise(t.kind === "get" ? f.BadGetterArity : f.BadSetterArity, { at: t }), t.kind === "set" && ((r = s[s.length - 1]) == null ? void 0 : r.type) === "RestElement" && this.raise(f.BadSetterRestParameter, { at: t });
}
parseObjectMethod(t, r, e, s, i) {
if (i) {
let a = this.parseMethod(t, r, false, false, false, "ObjectMethod");
return this.checkGetterSetterParams(a), a;
}
if (e || r || this.match(10))
return s && this.unexpected(), t.kind = "method", t.method = true, this.parseMethod(t, r, e, false, false, "ObjectMethod");
}
parseObjectProperty(t, r, e, s) {
if (t.shorthand = false, this.eat(14))
return t.value = e ? this.parseMaybeDefault(this.state.startLoc) : this.parseMaybeAssignAllowIn(s), this.finishNode(t, "ObjectProperty");
if (!t.computed && t.key.type === "Identifier") {
if (this.checkReservedWord(t.key.name, t.key.loc.start, true, false), e)
t.value = this.parseMaybeDefault(r, me(t.key));
else if (this.match(29)) {
let i = this.state.startLoc;
s != null ? s.shorthandAssignLoc === null && (s.shorthandAssignLoc = i) : this.raise(f.InvalidCoverInitializedName, { at: i }), t.value = this.parseMaybeDefault(r, me(t.key));
} else
t.value = me(t.key);
return t.shorthand = true, this.finishNode(t, "ObjectProperty");
}
}
parseObjPropValue(t, r, e, s, i, a, n2) {
let o = this.parseObjectMethod(t, e, s, i, a) || this.parseObjectProperty(t, r, i, n2);
return o || this.unexpected(), o;
}
parsePropertyName(t, r) {
if (this.eat(0))
t.computed = true, t.key = this.parseMaybeAssignAllowIn(), this.expect(3);
else {
let { type: e, value: s } = this.state, i;
if (te(e))
i = this.parseIdentifier(true);
else
switch (e) {
case 132:
i = this.parseNumericLiteral(s);
break;
case 131:
i = this.parseStringLiteral(s);
break;
case 133:
i = this.parseBigIntLiteral(s);
break;
case 134:
i = this.parseDecimalLiteral(s);
break;
case 136: {
let a = this.state.startLoc;
r != null ? r.privateKeyLoc === null && (r.privateKeyLoc = a) : this.raise(f.UnexpectedPrivateField, { at: a }), i = this.parsePrivateName();
break;
}
default:
this.unexpected();
}
t.key = i, e !== 136 && (t.computed = false);
}
return t.key;
}
initFunction(t, r) {
t.id = null, t.generator = false, t.async = r;
}
parseMethod(t, r, e, s, i, a) {
let n2 = arguments.length > 6 && arguments[6] !== void 0 ? arguments[6] : false;
this.initFunction(t, e), t.generator = r, this.scope.enter(de | ht | (n2 ? Ee : 0) | (i ? Pr : 0)), this.prodParam.enter(Tt(e, t.generator)), this.parseFunctionParams(t, s);
let o = this.parseFunctionBodyAndFinish(t, a, true);
return this.prodParam.exit(), this.scope.exit(), o;
}
parseArrayLike(t, r, e, s) {
e && this.expectPlugin("recordAndTuple");
let i = this.state.inFSharpPipelineDirectBody;
this.state.inFSharpPipelineDirectBody = false;
let a = this.startNode();
return this.next(), a.elements = this.parseExprList(t, !e, s, a), this.state.inFSharpPipelineDirectBody = i, this.finishNode(a, e ? "TupleExpression" : "ArrayExpression");
}
parseArrowExpression(t, r, e, s) {
this.scope.enter(de | Gt);
let i = Tt(e, false);
!this.match(5) && this.prodParam.hasIn && (i |= _e), this.prodParam.enter(i), this.initFunction(t, e);
let a = this.state.maybeInArrowParameters;
return r && (this.state.maybeInArrowParameters = true, this.setArrowFunctionParameters(t, r, s)), this.state.maybeInArrowParameters = false, this.parseFunctionBody(t, true), this.prodParam.exit(), this.scope.exit(), this.state.maybeInArrowParameters = a, this.finishNode(t, "ArrowFunctionExpression");
}
setArrowFunctionParameters(t, r, e) {
this.toAssignableList(r, e, false), t.params = r;
}
parseFunctionBodyAndFinish(t, r) {
let e = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
return this.parseFunctionBody(t, false, e), this.finishNode(t, r);
}
parseFunctionBody(t, r) {
let e = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false, s = r && !this.match(5);
if (this.expressionScope.enter(_r()), s)
t.body = this.parseMaybeAssign(), this.checkParams(t, false, r, false);
else {
let i = this.state.strict, a = this.state.labels;
this.state.labels = [], this.prodParam.enter(this.prodParam.currentFlags() | jr), t.body = this.parseBlock(true, false, (n2) => {
let o = !this.isSimpleParamList(t.params);
n2 && o && this.raise(f.IllegalLanguageModeDirective, { at: (t.kind === "method" || t.kind === "constructor") && t.key ? t.key.loc.end : t });
let u = !i && this.state.strict;
this.checkParams(t, !this.state.strict && !r && !e && !o, r, u), this.state.strict && t.id && this.checkIdentifier(t.id, dl, u);
}), this.prodParam.exit(), this.state.labels = a;
}
this.expressionScope.exit();
}
isSimpleParameter(t) {
return t.type === "Identifier";
}
isSimpleParamList(t) {
for (let r = 0, e = t.length; r < e; r++)
if (!this.isSimpleParameter(t[r]))
return false;
return true;
}
checkParams(t, r, e) {
let s = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : true, i = !r && /* @__PURE__ */ new Set(), a = { type: "FormalParameters" };
for (let n2 of t.params)
this.checkLVal(n2, { in: a, binding: mt, checkClashes: i, strictModeChanged: s });
}
parseExprList(t, r, e, s) {
let i = [], a = true;
for (; !this.eat(t); ) {
if (a)
a = false;
else if (this.expect(12), this.match(t)) {
s && this.addTrailingCommaExtraToNode(s), this.next();
break;
}
i.push(this.parseExprListItem(r, e));
}
return i;
}
parseExprListItem(t, r, e) {
let s;
if (this.match(12))
t || this.raise(f.UnexpectedToken, { at: this.state.curPosition(), unexpected: "," }), s = null;
else if (this.match(21)) {
let i = this.state.startLoc;
s = this.parseParenItem(this.parseSpread(r), i);
} else if (this.match(17)) {
this.expectPlugin("partialApplication"), e || this.raise(f.UnexpectedArgumentPlaceholder, { at: this.state.startLoc });
let i = this.startNode();
this.next(), s = this.finishNode(i, "ArgumentPlaceholder");
} else
s = this.parseMaybeAssignAllowIn(r, this.parseParenItem);
return s;
}
parseIdentifier(t) {
let r = this.startNode(), e = this.parseIdentifierName(t);
return this.createIdentifier(r, e);
}
createIdentifier(t, r) {
return t.name = r, t.loc.identifierName = r, this.finishNode(t, "Identifier");
}
parseIdentifierName(t) {
let r, { startLoc: e, type: s } = this.state;
te(s) ? r = this.state.value : this.unexpected();
let i = ue(s);
return t ? i && this.replaceToken(130) : this.checkReservedWord(r, e, i, false), this.next(), r;
}
checkReservedWord(t, r, e, s) {
if (t.length > 10 || !ul(t))
return;
if (e && ol(t)) {
this.raise(f.UnexpectedKeyword, { at: r, keyword: t });
return;
}
if ((this.state.strict ? s ? xr : mr : dr)(t, this.inModule)) {
this.raise(f.UnexpectedReservedWord, { at: r, reservedWord: t });
return;
} else if (t === "yield") {
if (this.prodParam.hasYield) {
this.raise(f.YieldBindingIdentifier, { at: r });
return;
}
} else if (t === "await") {
if (this.prodParam.hasAwait) {
this.raise(f.AwaitBindingIdentifier, { at: r });
return;
}
if (this.scope.inStaticBlock) {
this.raise(f.AwaitBindingIdentifierInStaticBlock, { at: r });
return;
}
this.expressionScope.recordAsyncArrowParametersError({ at: r });
} else if (t === "arguments" && this.scope.inClassAndNotInNonArrowFunction) {
this.raise(f.ArgumentsInClass, { at: r });
return;
}
}
isAwaitAllowed() {
return !!(this.prodParam.hasAwait || this.options.allowAwaitOutsideFunction && !this.scope.inFunction);
}
parseAwait(t) {
let r = this.startNodeAt(t);
return this.expressionScope.recordParameterInitializerError(f.AwaitExpressionFormalParameter, { at: r }), this.eat(55) && this.raise(f.ObsoleteAwaitStar, { at: r }), !this.scope.inFunction && !this.options.allowAwaitOutsideFunction && (this.isAmbiguousAwait() ? this.ambiguousScriptDifferentAst = true : this.sawUnambiguousESM = true), this.state.soloAwait || (r.argument = this.parseMaybeUnary(null, true)), this.finishNode(r, "AwaitExpression");
}
isAmbiguousAwait() {
if (this.hasPrecedingLineBreak())
return true;
let { type: t } = this.state;
return t === 53 || t === 10 || t === 0 || nt(t) || t === 101 && !this.state.containsEsc || t === 135 || t === 56 || this.hasPlugin("v8intrinsic") && t === 54;
}
parseYield() {
let t = this.startNode();
this.expressionScope.recordParameterInitializerError(f.YieldInParameter, { at: t }), this.next();
let r = false, e = null;
if (!this.hasPrecedingLineBreak())
switch (r = this.eat(55), this.state.type) {
case 13:
case 137:
case 8:
case 11:
case 3:
case 9:
case 14:
case 12:
if (!r)
break;
default:
e = this.parseMaybeAssign();
}
return t.delegate = r, t.argument = e, this.finishNode(t, "YieldExpression");
}
checkPipelineAtInfixOperator(t, r) {
this.hasPlugin(["pipelineOperator", { proposal: "smart" }]) && t.type === "SequenceExpression" && this.raise(f.PipelineHeadSequenceExpression, { at: r });
}
parseSmartPipelineBodyInStyle(t, r) {
if (this.isSimpleReference(t)) {
let e = this.startNodeAt(r);
return e.callee = t, this.finishNode(e, "PipelineBareFunction");
} else {
let e = this.startNodeAt(r);
return this.checkSmartPipeTopicBodyEarlyErrors(r), e.expression = t, this.finishNode(e, "PipelineTopicExpression");
}
}
isSimpleReference(t) {
switch (t.type) {
case "MemberExpression":
return !t.computed && this.isSimpleReference(t.object);
case "Identifier":
return true;
default:
return false;
}
}
checkSmartPipeTopicBodyEarlyErrors(t) {
if (this.match(19))
throw this.raise(f.PipelineBodyNoArrow, { at: this.state.startLoc });
this.topicReferenceWasUsedInCurrentContext() || this.raise(f.PipelineTopicUnused, { at: t });
}
withTopicBindingContext(t) {
let r = this.state.topicContext;
this.state.topicContext = { maxNumOfResolvableTopics: 1, maxTopicIndex: null };
try {
return t();
} finally {
this.state.topicContext = r;
}
}
withSmartMixTopicForbiddingContext(t) {
if (this.hasPlugin(["pipelineOperator", { proposal: "smart" }])) {
let r = this.state.topicContext;
this.state.topicContext = { maxNumOfResolvableTopics: 0, maxTopicIndex: null };
try {
return t();
} finally {
this.state.topicContext = r;
}
} else
return t();
}
withSoloAwaitPermittingContext(t) {
let r = this.state.soloAwait;
this.state.soloAwait = true;
try {
return t();
} finally {
this.state.soloAwait = r;
}
}
allowInAnd(t) {
let r = this.prodParam.currentFlags();
if (_e & ~r) {
this.prodParam.enter(r | _e);
try {
return t();
} finally {
this.prodParam.exit();
}
}
return t();
}
disallowInAnd(t) {
let r = this.prodParam.currentFlags();
if (_e & r) {
this.prodParam.enter(r & ~_e);
try {
return t();
} finally {
this.prodParam.exit();
}
}
return t();
}
registerTopicReference() {
this.state.topicContext.maxTopicIndex = 0;
}
topicReferenceIsAllowedInCurrentContext() {
return this.state.topicContext.maxNumOfResolvableTopics >= 1;
}
topicReferenceWasUsedInCurrentContext() {
return this.state.topicContext.maxTopicIndex != null && this.state.topicContext.maxTopicIndex >= 0;
}
parseFSharpPipelineBody(t) {
let r = this.state.startLoc;
this.state.potentialArrowAt = this.state.start;
let e = this.state.inFSharpPipelineDirectBody;
this.state.inFSharpPipelineDirectBody = true;
let s = this.parseExprOp(this.parseMaybeUnaryOrPrivate(), r, t);
return this.state.inFSharpPipelineDirectBody = e, s;
}
parseModuleExpression() {
this.expectPlugin("moduleBlocks");
let t = this.startNode();
this.next(), this.match(5) || this.unexpected(null, 5);
let r = this.startNodeAt(this.state.endLoc);
this.next();
let e = this.initializeScopes(true);
this.enterInitialScopes();
try {
t.body = this.parseProgram(r, 8, "module");
} finally {
e();
}
return this.finishNode(t, "ModuleExpression");
}
parsePropertyNamePrefixOperator(t) {
}
}, cs = { kind: "loop" }, Ph = { kind: "switch" }, Ah = /[\uD800-\uDFFF]/u, ps = /in(?:stanceof)?/y;
function Th(t, r) {
for (let e = 0; e < t.length; e++) {
let s = t[e], { type: i } = s;
if (typeof i == "number") {
{
if (i === 136) {
let { loc: a, start: n2, value: o, end: u } = s, c = n2 + 1, y = Y(a.start, 1);
t.splice(e, 1, new Ae({ type: ce(27), value: "#", start: n2, end: c, startLoc: a.start, endLoc: y }), new Ae({ type: ce(130), value: o, start: c, end: u, startLoc: y, endLoc: a.end })), e++;
continue;
}
if (nt(i)) {
let { loc: a, start: n2, value: o, end: u } = s, c = n2 + 1, y = Y(a.start, 1), g;
r.charCodeAt(n2) === 96 ? g = new Ae({ type: ce(22), value: "`", start: n2, end: c, startLoc: a.start, endLoc: y }) : g = new Ae({ type: ce(8), value: "}", start: n2, end: c, startLoc: a.start, endLoc: y });
let T, C, M, j;
i === 24 ? (C = u - 1, M = Y(a.end, -1), T = o === null ? null : o.slice(1, -1), j = new Ae({ type: ce(22), value: "`", start: C, end: u, startLoc: M, endLoc: a.end })) : (C = u - 2, M = Y(a.end, -2), T = o === null ? null : o.slice(1, -2), j = new Ae({ type: ce(23), value: "${", start: C, end: u, startLoc: M, endLoc: a.end })), t.splice(e, 1, g, new Ae({ type: ce(20), value: T, start: c, end: C, startLoc: y, endLoc: M }), j), e += 2;
continue;
}
}
s.type = ce(i);
}
}
return t;
}
var vh = class extends gh {
parseTopLevel(t, r) {
return t.program = this.parseProgram(r), t.comments = this.state.comments, this.options.tokens && (t.tokens = Th(this.tokens, this.input)), this.finishNode(t, "File");
}
parseProgram(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 137, e = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : this.options.sourceType;
if (t.sourceType = e, t.interpreter = this.parseInterpreterDirective(), this.parseBlockBody(t, true, true, r), this.inModule && !this.options.allowUndeclaredExports && this.scope.undefinedExports.size > 0)
for (let [i, a] of Array.from(this.scope.undefinedExports))
this.raise(f.ModuleExportUndefined, { at: a, localName: i });
let s;
return r === 137 ? s = this.finishNode(t, "Program") : s = this.finishNodeAt(t, "Program", Y(this.state.startLoc, -1)), s;
}
stmtToDirective(t) {
let r = t;
r.type = "Directive", r.value = r.expression, delete r.expression;
let e = r.value, s = e.value, i = this.input.slice(e.start, e.end), a = e.value = i.slice(1, -1);
return this.addExtra(e, "raw", i), this.addExtra(e, "rawValue", a), this.addExtra(e, "expressionValue", s), e.type = "DirectiveLiteral", r;
}
parseInterpreterDirective() {
if (!this.match(28))
return null;
let t = this.startNode();
return t.value = this.state.value, this.next(), this.finishNode(t, "InterpreterDirective");
}
isLet() {
return this.isContextual(99) ? this.hasFollowingBindingAtom() : false;
}
chStartsBindingIdentifier(t, r) {
if (fe(t)) {
if (ps.lastIndex = r, ps.test(this.input)) {
let e = this.codePointAtPos(ps.lastIndex);
if (!De(e) && e !== 92)
return false;
}
return true;
} else
return t === 92;
}
chStartsBindingPattern(t) {
return t === 91 || t === 123;
}
hasFollowingBindingAtom() {
let t = this.nextTokenStart(), r = this.codePointAtPos(t);
return this.chStartsBindingPattern(r) || this.chStartsBindingIdentifier(r, t);
}
hasFollowingBindingIdentifier() {
let t = this.nextTokenStart(), r = this.codePointAtPos(t);
return this.chStartsBindingIdentifier(r, t);
}
startsUsingForOf() {
let t = this.lookahead();
return t.type === 101 && !t.containsEsc ? false : (this.expectPlugin("explicitResourceManagement"), true);
}
parseModuleItem() {
return this.parseStatementLike(15);
}
parseStatementListItem() {
return this.parseStatementLike(6 | (!this.options.annexB || this.state.strict ? 0 : 8));
}
parseStatementOrSloppyAnnexBFunctionDeclaration() {
let t = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : false, r = 0;
return this.options.annexB && !this.state.strict && (r |= 4, t && (r |= 8)), this.parseStatementLike(r);
}
parseStatement() {
return this.parseStatementLike(0);
}
parseStatementLike(t) {
let r = null;
return this.match(26) && (r = this.parseDecorators(true)), this.parseStatementContent(t, r);
}
parseStatementContent(t, r) {
let e = this.state.type, s = this.startNode(), i = !!(t & 2), a = !!(t & 4), n2 = t & 1;
switch (e) {
case 60:
return this.parseBreakContinueStatement(s, true);
case 63:
return this.parseBreakContinueStatement(s, false);
case 64:
return this.parseDebuggerStatement(s);
case 90:
return this.parseDoWhileStatement(s);
case 91:
return this.parseForStatement(s);
case 68:
if (this.lookaheadCharCode() === 46)
break;
return a || this.raise(this.state.strict ? f.StrictFunction : this.options.annexB ? f.SloppyFunctionAnnexB : f.SloppyFunction, { at: this.state.startLoc }), this.parseFunctionStatement(s, false, !i && a);
case 80:
return i || this.unexpected(), this.parseClass(this.maybeTakeDecorators(r, s), true);
case 69:
return this.parseIfStatement(s);
case 70:
return this.parseReturnStatement(s);
case 71:
return this.parseSwitchStatement(s);
case 72:
return this.parseThrowStatement(s);
case 73:
return this.parseTryStatement(s);
case 105:
if (this.hasFollowingLineBreak() || this.state.containsEsc || !this.hasFollowingBindingIdentifier())
break;
return this.expectPlugin("explicitResourceManagement"), !this.scope.inModule && this.scope.inTopLevel ? this.raise(f.UnexpectedUsingDeclaration, { at: this.state.startLoc }) : i || this.raise(f.UnexpectedLexicalDeclaration, { at: this.state.startLoc }), this.parseVarStatement(s, "using");
case 99: {
if (this.state.containsEsc)
break;
let c = this.nextTokenStart(), y = this.codePointAtPos(c);
if (y !== 91 && (!i && this.hasFollowingLineBreak() || !this.chStartsBindingIdentifier(y, c) && y !== 123))
break;
}
case 75:
i || this.raise(f.UnexpectedLexicalDeclaration, { at: this.state.startLoc });
case 74: {
let c = this.state.value;
return this.parseVarStatement(s, c);
}
case 92:
return this.parseWhileStatement(s);
case 76:
return this.parseWithStatement(s);
case 5:
return this.parseBlock();
case 13:
return this.parseEmptyStatement(s);
case 83: {
let c = this.lookaheadCharCode();
if (c === 40 || c === 46)
break;
}
case 82: {
!this.options.allowImportExportEverywhere && !n2 && this.raise(f.UnexpectedImportExport, { at: this.state.startLoc }), this.next();
let c;
return e === 83 ? (c = this.parseImport(s), c.type === "ImportDeclaration" && (!c.importKind || c.importKind === "value") && (this.sawUnambiguousESM = true)) : (c = this.parseExport(s, r), (c.type === "ExportNamedDeclaration" && (!c.exportKind || c.exportKind === "value") || c.type === "ExportAllDeclaration" && (!c.exportKind || c.exportKind === "value") || c.type === "ExportDefaultDeclaration") && (this.sawUnambiguousESM = true)), this.assertModuleNodeAllowed(c), c;
}
default:
if (this.isAsyncFunction())
return i || this.raise(f.AsyncFunctionInSingleStatementContext, { at: this.state.startLoc }), this.next(), this.parseFunctionStatement(s, true, !i && a);
}
let o = this.state.value, u = this.parseExpression();
return q(e) && u.type === "Identifier" && this.eat(14) ? this.parseLabeledStatement(s, o, u, t) : this.parseExpressionStatement(s, u, r);
}
assertModuleNodeAllowed(t) {
!this.options.allowImportExportEverywhere && !this.inModule && this.raise(f.ImportOutsideModule, { at: t });
}
decoratorsEnabledBeforeExport() {
return this.hasPlugin("decorators-legacy") ? true : this.hasPlugin("decorators") && this.getPluginOption("decorators", "decoratorsBeforeExport") !== false;
}
maybeTakeDecorators(t, r, e) {
return t && (r.decorators && r.decorators.length > 0 ? (typeof this.getPluginOption("decorators", "decoratorsBeforeExport") != "boolean" && this.raise(f.DecoratorsBeforeAfterExport, { at: r.decorators[0] }), r.decorators.unshift(...t)) : r.decorators = t, this.resetStartLocationFromNode(r, t[0]), e && this.resetStartLocationFromNode(e, r)), r;
}
canHaveLeadingDecorator() {
return this.match(80);
}
parseDecorators(t) {
let r = [];
do
r.push(this.parseDecorator());
while (this.match(26));
if (this.match(82))
t || this.unexpected(), this.decoratorsEnabledBeforeExport() || this.raise(f.DecoratorExportClass, { at: this.state.startLoc });
else if (!this.canHaveLeadingDecorator())
throw this.raise(f.UnexpectedLeadingDecorator, { at: this.state.startLoc });
return r;
}
parseDecorator() {
this.expectOnePlugin(["decorators", "decorators-legacy"]);
let t = this.startNode();
if (this.next(), this.hasPlugin("decorators")) {
let r = this.state.startLoc, e;
if (this.match(10)) {
let s = this.state.startLoc;
this.next(), e = this.parseExpression(), this.expect(11), e = this.wrapParenthesis(s, e);
let i = this.state.startLoc;
t.expression = this.parseMaybeDecoratorArguments(e), this.getPluginOption("decorators", "allowCallParenthesized") === false && t.expression !== e && this.raise(f.DecoratorArgumentsOutsideParentheses, { at: i });
} else {
for (e = this.parseIdentifier(false); this.eat(16); ) {
let s = this.startNodeAt(r);
s.object = e, this.match(136) ? (this.classScope.usePrivateName(this.state.value, this.state.startLoc), s.property = this.parsePrivateName()) : s.property = this.parseIdentifier(true), s.computed = false, e = this.finishNode(s, "MemberExpression");
}
t.expression = this.parseMaybeDecoratorArguments(e);
}
} else
t.expression = this.parseExprSubscripts();
return this.finishNode(t, "Decorator");
}
parseMaybeDecoratorArguments(t) {
if (this.eat(10)) {
let r = this.startNodeAtNode(t);
return r.callee = t, r.arguments = this.parseCallExpressionArguments(11, false), this.toReferencedList(r.arguments), this.finishNode(r, "CallExpression");
}
return t;
}
parseBreakContinueStatement(t, r) {
return this.next(), this.isLineTerminator() ? t.label = null : (t.label = this.parseIdentifier(), this.semicolon()), this.verifyBreakContinue(t, r), this.finishNode(t, r ? "BreakStatement" : "ContinueStatement");
}
verifyBreakContinue(t, r) {
let e;
for (e = 0; e < this.state.labels.length; ++e) {
let s = this.state.labels[e];
if ((t.label == null || s.name === t.label.name) && (s.kind != null && (r || s.kind === "loop") || t.label && r))
break;
}
if (e === this.state.labels.length) {
let s = r ? "BreakStatement" : "ContinueStatement";
this.raise(f.IllegalBreakContinue, { at: t, type: s });
}
}
parseDebuggerStatement(t) {
return this.next(), this.semicolon(), this.finishNode(t, "DebuggerStatement");
}
parseHeaderExpression() {
this.expect(10);
let t = this.parseExpression();
return this.expect(11), t;
}
parseDoWhileStatement(t) {
return this.next(), this.state.labels.push(cs), t.body = this.withSmartMixTopicForbiddingContext(() => this.parseStatement()), this.state.labels.pop(), this.expect(92), t.test = this.parseHeaderExpression(), this.eat(13), this.finishNode(t, "DoWhileStatement");
}
parseForStatement(t) {
this.next(), this.state.labels.push(cs);
let r = null;
if (this.isAwaitAllowed() && this.eatContextual(96) && (r = this.state.lastTokStartLoc), this.scope.enter(Fe), this.expect(10), this.match(13))
return r !== null && this.unexpected(r), this.parseFor(t, null);
let e = this.isContextual(99), s = this.isContextual(105) && !this.hasFollowingLineBreak(), i = e && this.hasFollowingBindingAtom() || s && this.hasFollowingBindingIdentifier() && this.startsUsingForOf();
if (this.match(74) || this.match(75) || i) {
let c = this.startNode(), y = this.state.value;
this.next(), this.parseVar(c, true, y);
let g = this.finishNode(c, "VariableDeclaration"), T = this.match(58);
return T && s && this.raise(f.ForInUsing, { at: g }), (T || this.isContextual(101)) && g.declarations.length === 1 ? this.parseForIn(t, g, r) : (r !== null && this.unexpected(r), this.parseFor(t, g));
}
let a = this.isContextual(95), n2 = new vt(), o = this.parseExpression(true, n2), u = this.isContextual(101);
if (u && (e && this.raise(f.ForOfLet, { at: o }), r === null && a && o.type === "Identifier" && this.raise(f.ForOfAsync, { at: o })), u || this.match(58)) {
this.checkDestructuringPrivate(n2), this.toAssignable(o, true);
let c = u ? "ForOfStatement" : "ForInStatement";
return this.checkLVal(o, { in: { type: c } }), this.parseForIn(t, o, r);
} else
this.checkExpressionErrors(n2, true);
return r !== null && this.unexpected(r), this.parseFor(t, o);
}
parseFunctionStatement(t, r, e) {
return this.next(), this.parseFunction(t, 1 | (e ? 2 : 0) | (r ? 8 : 0));
}
parseIfStatement(t) {
return this.next(), t.test = this.parseHeaderExpression(), t.consequent = this.parseStatementOrSloppyAnnexBFunctionDeclaration(), t.alternate = this.eat(66) ? this.parseStatementOrSloppyAnnexBFunctionDeclaration() : null, this.finishNode(t, "IfStatement");
}
parseReturnStatement(t) {
return !this.prodParam.hasReturn && !this.options.allowReturnOutsideFunction && this.raise(f.IllegalReturn, { at: this.state.startLoc }), this.next(), this.isLineTerminator() ? t.argument = null : (t.argument = this.parseExpression(), this.semicolon()), this.finishNode(t, "ReturnStatement");
}
parseSwitchStatement(t) {
this.next(), t.discriminant = this.parseHeaderExpression();
let r = t.cases = [];
this.expect(5), this.state.labels.push(Ph), this.scope.enter(Fe);
let e;
for (let s; !this.match(8); )
if (this.match(61) || this.match(65)) {
let i = this.match(61);
e && this.finishNode(e, "SwitchCase"), r.push(e = this.startNode()), e.consequent = [], this.next(), i ? e.test = this.parseExpression() : (s && this.raise(f.MultipleDefaultsInSwitch, { at: this.state.lastTokStartLoc }), s = true, e.test = null), this.expect(14);
} else
e ? e.consequent.push(this.parseStatementListItem()) : this.unexpected();
return this.scope.exit(), e && this.finishNode(e, "SwitchCase"), this.next(), this.state.labels.pop(), this.finishNode(t, "SwitchStatement");
}
parseThrowStatement(t) {
return this.next(), this.hasPrecedingLineBreak() && this.raise(f.NewlineAfterThrow, { at: this.state.lastTokEndLoc }), t.argument = this.parseExpression(), this.semicolon(), this.finishNode(t, "ThrowStatement");
}
parseCatchClauseParam() {
let t = this.parseBindingAtom();
return this.scope.enter(this.options.annexB && t.type === "Identifier" ? gr : 0), this.checkLVal(t, { in: { type: "CatchClause" }, binding: cl }), t;
}
parseTryStatement(t) {
if (this.next(), t.block = this.parseBlock(), t.handler = null, this.match(62)) {
let r = this.startNode();
this.next(), this.match(10) ? (this.expect(10), r.param = this.parseCatchClauseParam(), this.expect(11)) : (r.param = null, this.scope.enter(Fe)), r.body = this.withSmartMixTopicForbiddingContext(() => this.parseBlock(false, false)), this.scope.exit(), t.handler = this.finishNode(r, "CatchClause");
}
return t.finalizer = this.eat(67) ? this.parseBlock() : null, !t.handler && !t.finalizer && this.raise(f.NoCatchOrFinally, { at: t }), this.finishNode(t, "TryStatement");
}
parseVarStatement(t, r) {
let e = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
return this.next(), this.parseVar(t, false, r, e), this.semicolon(), this.finishNode(t, "VariableDeclaration");
}
parseWhileStatement(t) {
return this.next(), t.test = this.parseHeaderExpression(), this.state.labels.push(cs), t.body = this.withSmartMixTopicForbiddingContext(() => this.parseStatement()), this.state.labels.pop(), this.finishNode(t, "WhileStatement");
}
parseWithStatement(t) {
return this.state.strict && this.raise(f.StrictWith, { at: this.state.startLoc }), this.next(), t.object = this.parseHeaderExpression(), t.body = this.withSmartMixTopicForbiddingContext(() => this.parseStatement()), this.finishNode(t, "WithStatement");
}
parseEmptyStatement(t) {
return this.next(), this.finishNode(t, "EmptyStatement");
}
parseLabeledStatement(t, r, e, s) {
for (let a of this.state.labels)
a.name === r && this.raise(f.LabelRedeclaration, { at: e, labelName: r });
let i = Mo(this.state.type) ? "loop" : this.match(71) ? "switch" : null;
for (let a = this.state.labels.length - 1; a >= 0; a--) {
let n2 = this.state.labels[a];
if (n2.statementStart === t.start)
n2.statementStart = this.state.start, n2.kind = i;
else
break;
}
return this.state.labels.push({ name: r, kind: i, statementStart: this.state.start }), t.body = s & 8 ? this.parseStatementOrSloppyAnnexBFunctionDeclaration(true) : this.parseStatement(), this.state.labels.pop(), t.label = e, this.finishNode(t, "LabeledStatement");
}
parseExpressionStatement(t, r, e) {
return t.expression = r, this.semicolon(), this.finishNode(t, "ExpressionStatement");
}
parseBlock() {
let t = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : false, r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : true, e = arguments.length > 2 ? arguments[2] : void 0, s = this.startNode();
return t && this.state.strictErrors.clear(), this.expect(5), r && this.scope.enter(Fe), this.parseBlockBody(s, t, false, 8, e), r && this.scope.exit(), this.finishNode(s, "BlockStatement");
}
isValidDirective(t) {
return t.type === "ExpressionStatement" && t.expression.type === "StringLiteral" && !t.expression.extra.parenthesized;
}
parseBlockBody(t, r, e, s, i) {
let a = t.body = [], n2 = t.directives = [];
this.parseBlockOrModuleBlockBody(a, r ? n2 : void 0, e, s, i);
}
parseBlockOrModuleBlockBody(t, r, e, s, i) {
let a = this.state.strict, n2 = false, o = false;
for (; !this.match(s); ) {
let u = e ? this.parseModuleItem() : this.parseStatementListItem();
if (r && !o) {
if (this.isValidDirective(u)) {
let c = this.stmtToDirective(u);
r.push(c), !n2 && c.value.value === "use strict" && (n2 = true, this.setStrict(true));
continue;
}
o = true, this.state.strictErrors.clear();
}
t.push(u);
}
i && i.call(this, n2), a || this.setStrict(false), this.next();
}
parseFor(t, r) {
return t.init = r, this.semicolon(false), t.test = this.match(13) ? null : this.parseExpression(), this.semicolon(false), t.update = this.match(11) ? null : this.parseExpression(), this.expect(11), t.body = this.withSmartMixTopicForbiddingContext(() => this.parseStatement()), this.scope.exit(), this.state.labels.pop(), this.finishNode(t, "ForStatement");
}
parseForIn(t, r, e) {
let s = this.match(58);
return this.next(), s ? e !== null && this.unexpected(e) : t.await = e !== null, r.type === "VariableDeclaration" && r.declarations[0].init != null && (!s || !this.options.annexB || this.state.strict || r.kind !== "var" || r.declarations[0].id.type !== "Identifier") && this.raise(f.ForInOfLoopInitializer, { at: r, type: s ? "ForInStatement" : "ForOfStatement" }), r.type === "AssignmentPattern" && this.raise(f.InvalidLhs, { at: r, ancestor: { type: "ForStatement" } }), t.left = r, t.right = s ? this.parseExpression() : this.parseMaybeAssignAllowIn(), this.expect(11), t.body = this.withSmartMixTopicForbiddingContext(() => this.parseStatement()), this.scope.exit(), this.state.labels.pop(), this.finishNode(t, s ? "ForInStatement" : "ForOfStatement");
}
parseVar(t, r, e) {
let s = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : false, i = t.declarations = [];
for (t.kind = e; ; ) {
let a = this.startNode();
if (this.parseVarId(a, e), a.init = this.eat(29) ? r ? this.parseMaybeAssignDisallowIn() : this.parseMaybeAssignAllowIn() : null, a.init === null && !s && (a.id.type !== "Identifier" && !(r && (this.match(58) || this.isContextual(101))) ? this.raise(f.DeclarationMissingInitializer, { at: this.state.lastTokEndLoc, kind: "destructuring" }) : e === "const" && !(this.match(58) || this.isContextual(101)) && this.raise(f.DeclarationMissingInitializer, { at: this.state.lastTokEndLoc, kind: "const" })), i.push(this.finishNode(a, "VariableDeclarator")), !this.eat(12))
break;
}
return t;
}
parseVarId(t, r) {
r === "using" && !this.inModule && this.match(96) && this.raise(f.AwaitInUsingBinding, { at: this.state.startLoc });
let e = this.parseBindingAtom();
this.checkLVal(e, { in: { type: "VariableDeclarator" }, binding: r === "var" ? mt : Be }), t.id = e;
}
parseAsyncFunctionExpression(t) {
return this.parseFunction(t, 8);
}
parseFunction(t) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 0, e = r & 2, s = !!(r & 1), i = s && !(r & 4), a = !!(r & 8);
this.initFunction(t, a), this.match(55) && (e && this.raise(f.GeneratorInSingleStatementContext, { at: this.state.startLoc }), this.next(), t.generator = true), s && (t.id = this.parseFunctionId(i));
let n2 = this.state.maybeInArrowParameters;
return this.state.maybeInArrowParameters = false, this.scope.enter(de), this.prodParam.enter(Tt(a, t.generator)), s || (t.id = this.parseFunctionId()), this.parseFunctionParams(t, false), this.withSmartMixTopicForbiddingContext(() => {
this.parseFunctionBodyAndFinish(t, s ? "FunctionDeclaration" : "FunctionExpression");
}), this.prodParam.exit(), this.scope.exit(), s && !e && this.registerFunctionStatementId(t), this.state.maybeInArrowParameters = n2, t;
}
parseFunctionId(t) {
return t || q(this.state.type) ? this.parseIdentifier() : null;
}
parseFunctionParams(t, r) {
this.expect(10), this.expressionScope.enter(ql()), t.params = this.parseBindingList(11, 41, 2 | (r ? 4 : 0)), this.expressionScope.exit();
}
registerFunctionStatementId(t) {
t.id && this.scope.declareName(t.id.name, !this.options.annexB || this.state.strict || t.generator || t.async ? this.scope.treatFunctionsAsVar ? mt : Be : Er, t.id.loc.start);
}
parseClass(t, r, e) {
this.next();
let s = this.state.strict;
return this.state.strict = true, this.parseClassId(t, r, e), this.parseClassSuper(t), t.body = this.parseClassBody(!!t.superClass, s), this.finishNode(t, r ? "ClassDeclaration" : "ClassExpression");
}
isClassProperty() {
return this.match(29) || this.match(13) || this.match(8);
}
isClassMethod() {
return this.match(10);
}
isNonstaticConstructor(t) {
return !t.computed && !t.static && (t.key.name === "constructor" || t.key.value === "constructor");
}
parseClassBody(t, r) {
this.classScope.enter();
let e = { hadConstructor: false, hadSuperClass: t }, s = [], i = this.startNode();
if (i.body = [], this.expect(5), this.withSmartMixTopicForbiddingContext(() => {
for (; !this.match(8); ) {
if (this.eat(13)) {
if (s.length > 0)
throw this.raise(f.DecoratorSemicolon, { at: this.state.lastTokEndLoc });
continue;
}
if (this.match(26)) {
s.push(this.parseDecorator());
continue;
}
let a = this.startNode();
s.length && (a.decorators = s, this.resetStartLocationFromNode(a, s[0]), s = []), this.parseClassMember(i, a, e), a.kind === "constructor" && a.decorators && a.decorators.length > 0 && this.raise(f.DecoratorConstructor, { at: a });
}
}), this.state.strict = r, this.next(), s.length)
throw this.raise(f.TrailingDecorator, { at: this.state.startLoc });
return this.classScope.exit(), this.finishNode(i, "ClassBody");
}
parseClassMemberFromModifier(t, r) {
let e = this.parseIdentifier(true);
if (this.isClassMethod()) {
let s = r;
return s.kind = "method", s.computed = false, s.key = e, s.static = false, this.pushClassMethod(t, s, false, false, false, false), true;
} else if (this.isClassProperty()) {
let s = r;
return s.computed = false, s.key = e, s.static = false, t.body.push(this.parseClassProperty(s)), true;
}
return this.resetPreviousNodeTrailingComments(e), false;
}
parseClassMember(t, r, e) {
let s = this.isContextual(104);
if (s) {
if (this.parseClassMemberFromModifier(t, r))
return;
if (this.eat(5)) {
this.parseClassStaticBlock(t, r);
return;
}
}
this.parseClassMemberWithIsStatic(t, r, e, s);
}
parseClassMemberWithIsStatic(t, r, e, s) {
let i = r, a = r, n2 = r, o = r, u = r, c = i, y = i;
if (r.static = s, this.parsePropertyNamePrefixOperator(r), this.eat(55)) {
c.kind = "method";
let j = this.match(136);
if (this.parseClassElementName(c), j) {
this.pushClassPrivateMethod(t, a, true, false);
return;
}
this.isNonstaticConstructor(i) && this.raise(f.ConstructorIsGenerator, { at: i.key }), this.pushClassMethod(t, i, true, false, false, false);
return;
}
let g = q(this.state.type) && !this.state.containsEsc, T = this.match(136), C = this.parseClassElementName(r), M = this.state.startLoc;
if (this.parsePostMemberNameModifiers(y), this.isClassMethod()) {
if (c.kind = "method", T) {
this.pushClassPrivateMethod(t, a, false, false);
return;
}
let j = this.isNonstaticConstructor(i), K = false;
j && (i.kind = "constructor", e.hadConstructor && !this.hasPlugin("typescript") && this.raise(f.DuplicateConstructor, { at: C }), j && this.hasPlugin("typescript") && r.override && this.raise(f.OverrideOnConstructor, { at: C }), e.hadConstructor = true, K = e.hadSuperClass), this.pushClassMethod(t, i, false, false, j, K);
} else if (this.isClassProperty())
T ? this.pushClassPrivateProperty(t, o) : this.pushClassProperty(t, n2);
else if (g && C.name === "async" && !this.isLineTerminator()) {
this.resetPreviousNodeTrailingComments(C);
let j = this.eat(55);
y.optional && this.unexpected(M), c.kind = "method";
let K = this.match(136);
this.parseClassElementName(c), this.parsePostMemberNameModifiers(y), K ? this.pushClassPrivateMethod(t, a, j, true) : (this.isNonstaticConstructor(i) && this.raise(f.ConstructorIsAsync, { at: i.key }), this.pushClassMethod(t, i, j, true, false, false));
} else if (g && (C.name === "get" || C.name === "set") && !(this.match(55) && this.isLineTerminator())) {
this.resetPreviousNodeTrailingComments(C), c.kind = C.name;
let j = this.match(136);
this.parseClassElementName(i), j ? this.pushClassPrivateMethod(t, a, false, false) : (this.isNonstaticConstructor(i) && this.raise(f.ConstructorIsAccessor, { at: i.key }), this.pushClassMethod(t, i, false, false, false, false)), this.checkGetterSetterParams(i);
} else if (g && C.name === "accessor" && !this.isLineTerminator()) {
this.expectPlugin("decoratorAutoAccessors"), this.resetPreviousNodeTrailingComments(C);
let j = this.match(136);
this.parseClassElementName(n2), this.pushClassAccessorProperty(t, u, j);
} else
this.isLineTerminator() ? T ? this.pushClassPrivateProperty(t, o) : this.pushClassProperty(t, n2) : this.unexpected();
}
parseClassElementName(t) {
let { type: r, value: e } = this.state;
if ((r === 130 || r === 131) && t.static && e === "prototype" && this.raise(f.StaticPrototype, { at: this.state.startLoc }), r === 136) {
e === "constructor" && this.raise(f.ConstructorClassPrivateField, { at: this.state.startLoc });
let s = this.parsePrivateName();
return t.key = s, s;
}
return this.parsePropertyName(t);
}
parseClassStaticBlock(t, r) {
var e;
this.scope.enter(Ee | ut | ht);
let s = this.state.labels;
this.state.labels = [], this.prodParam.enter(Me);
let i = r.body = [];
this.parseBlockOrModuleBlockBody(i, void 0, false, 8), this.prodParam.exit(), this.scope.exit(), this.state.labels = s, t.body.push(this.finishNode(r, "StaticBlock")), (e = r.decorators) != null && e.length && this.raise(f.DecoratorStaticBlock, { at: r });
}
pushClassProperty(t, r) {
!r.computed && (r.key.name === "constructor" || r.key.value === "constructor") && this.raise(f.ConstructorClassField, { at: r.key }), t.body.push(this.parseClassProperty(r));
}
pushClassPrivateProperty(t, r) {
let e = this.parseClassPrivateProperty(r);
t.body.push(e), this.classScope.declarePrivateName(this.getPrivateNameSV(e.key), ss, e.key.loc.start);
}
pushClassAccessorProperty(t, r, e) {
if (!e && !r.computed) {
let i = r.key;
(i.name === "constructor" || i.value === "constructor") && this.raise(f.ConstructorClassField, { at: i });
}
let s = this.parseClassAccessorProperty(r);
t.body.push(s), e && this.classScope.declarePrivateName(this.getPrivateNameSV(s.key), ss, s.key.loc.start);
}
pushClassMethod(t, r, e, s, i, a) {
t.body.push(this.parseMethod(r, e, s, i, a, "ClassMethod", true));
}
pushClassPrivateMethod(t, r, e, s) {
let i = this.parseMethod(r, e, s, false, false, "ClassPrivateMethod", true);
t.body.push(i);
let a = i.kind === "get" ? i.static ? gl : Al : i.kind === "set" ? i.static ? Pl : Tl : ss;
this.declareClassPrivateMethodInScope(i, a);
}
declareClassPrivateMethodInScope(t, r) {
this.classScope.declarePrivateName(this.getPrivateNameSV(t.key), r, t.key.loc.start);
}
parsePostMemberNameModifiers(t) {
}
parseClassPrivateProperty(t) {
return this.parseInitializer(t), this.semicolon(), this.finishNode(t, "ClassPrivateProperty");
}
parseClassProperty(t) {
return this.parseInitializer(t), this.semicolon(), this.finishNode(t, "ClassProperty");
}
parseClassAccessorProperty(t) {
return this.parseInitializer(t), this.semicolon(), this.finishNode(t, "ClassAccessorProperty");
}
parseInitializer(t) {
this.scope.enter(Ee | ht), this.expressionScope.enter(_r()), this.prodParam.enter(Me), t.value = this.eat(29) ? this.parseMaybeAssignAllowIn() : null, this.expressionScope.exit(), this.prodParam.exit(), this.scope.exit();
}
parseClassId(t, r, e) {
let s = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : vr;
if (q(this.state.type))
t.id = this.parseIdentifier(), r && this.declareNameFromIdentifier(t.id, s);
else if (e || !r)
t.id = null;
else
throw this.raise(f.MissingClassName, { at: this.state.startLoc });
}
parseClassSuper(t) {
t.superClass = this.eat(81) ? this.parseExprSubscripts() : null;
}
parseExport(t, r) {
let e = this.maybeParseExportDefaultSpecifier(t), s = !e || this.eat(12), i = s && this.eatExportStar(t), a = i && this.maybeParseExportNamespaceSpecifier(t), n2 = s && (!a || this.eat(12)), o = e || i;
if (i && !a) {
if (e && this.unexpected(), r)
throw this.raise(f.UnsupportedDecoratorExport, { at: t });
return this.parseExportFrom(t, true), this.finishNode(t, "ExportAllDeclaration");
}
let u = this.maybeParseExportNamedSpecifiers(t);
e && s && !i && !u && this.unexpected(null, 5), a && n2 && this.unexpected(null, 97);
let c;
if (o || u) {
if (c = false, r)
throw this.raise(f.UnsupportedDecoratorExport, { at: t });
this.parseExportFrom(t, o);
} else
c = this.maybeParseExportDeclaration(t);
if (o || u || c) {
var y;
let g = t;
if (this.checkExport(g, true, false, !!g.source), ((y = g.declaration) == null ? void 0 : y.type) === "ClassDeclaration")
this.maybeTakeDecorators(r, g.declaration, g);
else if (r)
throw this.raise(f.UnsupportedDecoratorExport, { at: t });
return this.finishNode(g, "ExportNamedDeclaration");
}
if (this.eat(65)) {
let g = t, T = this.parseExportDefaultExpression();
if (g.declaration = T, T.type === "ClassDeclaration")
this.maybeTakeDecorators(r, T, g);
else if (r)
throw this.raise(f.UnsupportedDecoratorExport, { at: t });
return this.checkExport(g, true, true), this.finishNode(g, "ExportDefaultDeclaration");
}
this.unexpected(null, 5);
}
eatExportStar(t) {
return this.eat(55);
}
maybeParseExportDefaultSpecifier(t) {
if (this.isExportDefaultSpecifier()) {
this.expectPlugin("exportDefaultFrom");
let r = this.startNode();
return r.exported = this.parseIdentifier(true), t.specifiers = [this.finishNode(r, "ExportDefaultSpecifier")], true;
}
return false;
}
maybeParseExportNamespaceSpecifier(t) {
if (this.isContextual(93)) {
t.specifiers || (t.specifiers = []);
let r = this.startNodeAt(this.state.lastTokStartLoc);
return this.next(), r.exported = this.parseModuleExportName(), t.specifiers.push(this.finishNode(r, "ExportNamespaceSpecifier")), true;
}
return false;
}
maybeParseExportNamedSpecifiers(t) {
if (this.match(5)) {
t.specifiers || (t.specifiers = []);
let r = t.exportKind === "type";
return t.specifiers.push(...this.parseExportSpecifiers(r)), t.source = null, t.declaration = null, this.hasPlugin("importAssertions") && (t.assertions = []), true;
}
return false;
}
maybeParseExportDeclaration(t) {
return this.shouldParseExportDeclaration() ? (t.specifiers = [], t.source = null, this.hasPlugin("importAssertions") && (t.assertions = []), t.declaration = this.parseExportDeclaration(t), true) : false;
}
isAsyncFunction() {
if (!this.isContextual(95))
return false;
let t = this.nextTokenStart();
return !as.test(this.input.slice(this.state.pos, t)) && this.isUnparsedContextual(t, "function");
}
parseExportDefaultExpression() {
let t = this.startNode();
if (this.match(68))
return this.next(), this.parseFunction(t, 5);
if (this.isAsyncFunction())
return this.next(), this.next(), this.parseFunction(t, 13);
if (this.match(80))
return this.parseClass(t, true, true);
if (this.match(26))
return this.hasPlugin("decorators") && this.getPluginOption("decorators", "decoratorsBeforeExport") === true && this.raise(f.DecoratorBeforeExport, { at: this.state.startLoc }), this.parseClass(this.maybeTakeDecorators(this.parseDecorators(false), this.startNode()), true, true);
if (this.match(75) || this.match(74) || this.isLet())
throw this.raise(f.UnsupportedDefaultExport, { at: this.state.startLoc });
let r = this.parseMaybeAssignAllowIn();
return this.semicolon(), r;
}
parseExportDeclaration(t) {
return this.match(80) ? this.parseClass(this.startNode(), true, false) : this.parseStatementListItem();
}
isExportDefaultSpecifier() {
let { type: t } = this.state;
if (q(t)) {
if (t === 95 && !this.state.containsEsc || t === 99)
return false;
if ((t === 128 || t === 127) && !this.state.containsEsc) {
let { type: s } = this.lookahead();
if (q(s) && s !== 97 || s === 5)
return this.expectOnePlugin(["flow", "typescript"]), false;
}
} else if (!this.match(65))
return false;
let r = this.nextTokenStart(), e = this.isUnparsedContextual(r, "from");
if (this.input.charCodeAt(r) === 44 || q(this.state.type) && e)
return true;
if (this.match(65) && e) {
let s = this.input.charCodeAt(this.nextTokenStartSince(r + 4));
return s === 34 || s === 39;
}
return false;
}
parseExportFrom(t, r) {
if (this.eatContextual(97)) {
t.source = this.parseImportSource(), this.checkExport(t);
let e = this.maybeParseImportAssertions();
e && (t.assertions = e, this.checkJSONModuleImport(t));
} else
r && this.unexpected();
this.semicolon();
}
shouldParseExportDeclaration() {
let { type: t } = this.state;
return t === 26 && (this.expectOnePlugin(["decorators", "decorators-legacy"]), this.hasPlugin("decorators")) ? (this.getPluginOption("decorators", "decoratorsBeforeExport") === true && this.raise(f.DecoratorBeforeExport, { at: this.state.startLoc }), true) : t === 74 || t === 75 || t === 68 || t === 80 || this.isLet() || this.isAsyncFunction();
}
checkExport(t, r, e, s) {
if (r) {
if (e) {
if (this.checkDuplicateExports(t, "default"), this.hasPlugin("exportDefaultFrom")) {
var i;
let a = t.declaration;
a.type === "Identifier" && a.name === "from" && a.end - a.start === 4 && !((i = a.extra) != null && i.parenthesized) && this.raise(f.ExportDefaultFromAsIdentifier, { at: a });
}
} else if (t.specifiers && t.specifiers.length)
for (let a of t.specifiers) {
let { exported: n2 } = a, o = n2.type === "Identifier" ? n2.name : n2.value;
if (this.checkDuplicateExports(a, o), !s && a.local) {
let { local: u } = a;
u.type !== "Identifier" ? this.raise(f.ExportBindingIsString, { at: a, localName: u.value, exportName: o }) : (this.checkReservedWord(u.name, u.loc.start, true, false), this.scope.checkLocalExport(u));
}
}
else if (t.declaration) {
if (t.declaration.type === "FunctionDeclaration" || t.declaration.type === "ClassDeclaration") {
let a = t.declaration.id;
if (!a)
throw new Error("Assertion failure");
this.checkDuplicateExports(t, a.name);
} else if (t.declaration.type === "VariableDeclaration")
for (let a of t.declaration.declarations)
this.checkDeclaration(a.id);
}
}
}
checkDeclaration(t) {
if (t.type === "Identifier")
this.checkDuplicateExports(t, t.name);
else if (t.type === "ObjectPattern")
for (let r of t.properties)
this.checkDeclaration(r);
else if (t.type === "ArrayPattern")
for (let r of t.elements)
r && this.checkDeclaration(r);
else
t.type === "ObjectProperty" ? this.checkDeclaration(t.value) : t.type === "RestElement" ? this.checkDeclaration(t.argument) : t.type === "AssignmentPattern" && this.checkDeclaration(t.left);
}
checkDuplicateExports(t, r) {
this.exportedIdentifiers.has(r) && (r === "default" ? this.raise(f.DuplicateDefaultExport, { at: t }) : this.raise(f.DuplicateExport, { at: t, exportName: r })), this.exportedIdentifiers.add(r);
}
parseExportSpecifiers(t) {
let r = [], e = true;
for (this.expect(5); !this.eat(8); ) {
if (e)
e = false;
else if (this.expect(12), this.eat(8))
break;
let s = this.isContextual(128), i = this.match(131), a = this.startNode();
a.local = this.parseModuleExportName(), r.push(this.parseExportSpecifier(a, i, t, s));
}
return r;
}
parseExportSpecifier(t, r, e, s) {
return this.eatContextual(93) ? t.exported = this.parseModuleExportName() : r ? t.exported = Kl(t.local) : t.exported || (t.exported = me(t.local)), this.finishNode(t, "ExportSpecifier");
}
parseModuleExportName() {
if (this.match(131)) {
let t = this.parseStringLiteral(this.state.value), r = t.value.match(Ah);
return r && this.raise(f.ModuleExportNameHasLoneSurrogate, { at: t, surrogateCharCode: r[0].charCodeAt(0) }), t;
}
return this.parseIdentifier(true);
}
isJSONModuleImport(t) {
return t.assertions != null ? t.assertions.some((r) => {
let { key: e, value: s } = r;
return s.value === "json" && (e.type === "Identifier" ? e.name === "type" : e.value === "type");
}) : false;
}
checkImportReflection(t) {
if (t.module) {
var r;
(t.specifiers.length !== 1 || t.specifiers[0].type !== "ImportDefaultSpecifier") && this.raise(f.ImportReflectionNotBinding, { at: t.specifiers[0].loc.start }), ((r = t.assertions) == null ? void 0 : r.length) > 0 && this.raise(f.ImportReflectionHasAssertion, { at: t.specifiers[0].loc.start });
}
}
checkJSONModuleImport(t) {
if (this.isJSONModuleImport(t) && t.type !== "ExportAllDeclaration") {
let { specifiers: r } = t;
if (r != null) {
let e = r.find((s) => {
let i;
if (s.type === "ExportSpecifier" ? i = s.local : s.type === "ImportSpecifier" && (i = s.imported), i !== void 0)
return i.type === "Identifier" ? i.name !== "default" : i.value !== "default";
});
e !== void 0 && this.raise(f.ImportJSONBindingNotDefault, { at: e.loc.start });
}
}
}
parseMaybeImportReflection(t) {
let r = false;
if (this.isContextual(125)) {
let e = this.lookahead(), s = e.type;
q(s) ? (s !== 97 || this.input.charCodeAt(this.nextTokenStartSince(e.end)) === 102) && (r = true) : s !== 12 && (r = true);
}
r ? (this.expectPlugin("importReflection"), this.next(), t.module = true) : this.hasPlugin("importReflection") && (t.module = false);
}
parseImport(t) {
if (t.specifiers = [], !this.match(131)) {
this.parseMaybeImportReflection(t);
let s = !this.maybeParseDefaultImportSpecifier(t) || this.eat(12), i = s && this.maybeParseStarImportSpecifier(t);
s && !i && this.parseNamedImportSpecifiers(t), this.expectContextual(97);
}
t.source = this.parseImportSource();
let r = this.maybeParseImportAssertions();
if (r)
t.assertions = r;
else {
let e = this.maybeParseModuleAttributes();
e && (t.attributes = e);
}
return this.checkImportReflection(t), this.checkJSONModuleImport(t), this.semicolon(), this.finishNode(t, "ImportDeclaration");
}
parseImportSource() {
return this.match(131) || this.unexpected(), this.parseExprAtom();
}
shouldParseDefaultImport(t) {
return q(this.state.type);
}
parseImportSpecifierLocal(t, r, e) {
r.local = this.parseIdentifier(), t.specifiers.push(this.finishImportSpecifier(r, e));
}
finishImportSpecifier(t, r) {
let e = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : Be;
return this.checkLVal(t.local, { in: { type: r }, binding: e }), this.finishNode(t, r);
}
parseAssertEntries() {
let t = [], r = /* @__PURE__ */ new Set();
do {
if (this.match(8))
break;
let e = this.startNode(), s = this.state.value;
if (r.has(s) && this.raise(f.ModuleAttributesWithDuplicateKeys, { at: this.state.startLoc, key: s }), r.add(s), this.match(131) ? e.key = this.parseStringLiteral(s) : e.key = this.parseIdentifier(true), this.expect(14), !this.match(131))
throw this.raise(f.ModuleAttributeInvalidValue, { at: this.state.startLoc });
e.value = this.parseStringLiteral(this.state.value), t.push(this.finishNode(e, "ImportAttribute"));
} while (this.eat(12));
return t;
}
maybeParseModuleAttributes() {
if (this.match(76) && !this.hasPrecedingLineBreak())
this.expectPlugin("moduleAttributes"), this.next();
else
return this.hasPlugin("moduleAttributes") ? [] : null;
let t = [], r = /* @__PURE__ */ new Set();
do {
let e = this.startNode();
if (e.key = this.parseIdentifier(true), e.key.name !== "type" && this.raise(f.ModuleAttributeDifferentFromType, { at: e.key }), r.has(e.key.name) && this.raise(f.ModuleAttributesWithDuplicateKeys, { at: e.key, key: e.key.name }), r.add(e.key.name), this.expect(14), !this.match(131))
throw this.raise(f.ModuleAttributeInvalidValue, { at: this.state.startLoc });
e.value = this.parseStringLiteral(this.state.value), this.finishNode(e, "ImportAttribute"), t.push(e);
} while (this.eat(12));
return t;
}
maybeParseImportAssertions() {
if (this.isContextual(94) && !this.hasPrecedingLineBreak())
this.expectPlugin("importAssertions"), this.next();
else
return this.hasPlugin("importAssertions") ? [] : null;
this.eat(5);
let t = this.parseAssertEntries();
return this.eat(8), t;
}
maybeParseDefaultImportSpecifier(t) {
return this.shouldParseDefaultImport(t) ? (this.parseImportSpecifierLocal(t, this.startNode(), "ImportDefaultSpecifier"), true) : false;
}
maybeParseStarImportSpecifier(t) {
if (this.match(55)) {
let r = this.startNode();
return this.next(), this.expectContextual(93), this.parseImportSpecifierLocal(t, r, "ImportNamespaceSpecifier"), true;
}
return false;
}
parseNamedImportSpecifiers(t) {
let r = true;
for (this.expect(5); !this.eat(8); ) {
if (r)
r = false;
else {
if (this.eat(14))
throw this.raise(f.DestructureNamedImport, { at: this.state.startLoc });
if (this.expect(12), this.eat(8))
break;
}
let e = this.startNode(), s = this.match(131), i = this.isContextual(128);
e.imported = this.parseModuleExportName();
let a = this.parseImportSpecifier(e, s, t.importKind === "type" || t.importKind === "typeof", i, void 0);
t.specifiers.push(a);
}
}
parseImportSpecifier(t, r, e, s, i) {
if (this.eatContextual(93))
t.local = this.parseIdentifier();
else {
let { imported: a } = t;
if (r)
throw this.raise(f.ImportBindingIsString, { at: t, importName: a.value });
this.checkReservedWord(a.name, t.loc.start, true, true), t.local || (t.local = me(a));
}
return this.finishImportSpecifier(t, "ImportSpecifier", i);
}
isThisParam(t) {
return t.type === "Identifier" && t.name === "this";
}
}, Yr = class extends vh {
constructor(t, r) {
t = p(t), super(t, r), this.options = t, this.initializeScopes(), this.plugins = Eh(this.options.plugins), this.filename = t.sourceFilename;
}
getScopeHandler() {
return is;
}
parse() {
this.enterInitialScopes();
let t = this.startNode(), r = this.startNode();
return this.nextToken(), t.errors = null, this.parseTopLevel(t, r), t.errors = this.state.errors, t;
}
};
function Eh(t) {
let r = /* @__PURE__ */ new Map();
for (let e of t) {
let [s, i] = Array.isArray(e) ? e : [e, {}];
r.has(s) || r.set(s, i || {});
}
return r;
}
function Ch(t, r) {
var e;
if (((e = r) == null ? void 0 : e.sourceType) === "unambiguous") {
r = Object.assign({}, r);
try {
r.sourceType = "module";
let s = Xe(r, t), i = s.parse();
if (s.sawUnambiguousESM)
return i;
if (s.ambiguousScriptDifferentAst)
try {
return r.sourceType = "script", Xe(r, t).parse();
} catch {
}
else
i.program.sourceType = "script";
return i;
} catch (s) {
try {
return r.sourceType = "script", Xe(r, t).parse();
} catch {
}
throw s;
}
} else
return Xe(r, t).parse();
}
function bh(t, r) {
let e = Xe(r, t);
return e.options.strictMode && (e.state.strict = true), e.getExpression();
}
function Sh(t) {
let r = {};
for (let e of Object.keys(t))
r[e] = ce(t[e]);
return r;
}
var wh = Sh(Z);
function Xe(t, r) {
let e = Yr;
return t != null && t.plugins && (yh(t.plugins), e = Ih(t.plugins)), new e(t, r);
}
var Qr = {};
function Ih(t) {
let r = xh.filter((i) => J(t, i)), e = r.join("/"), s = Qr[e];
if (!s) {
s = Yr;
for (let i of r)
s = Xr[i](s);
Qr[e] = s;
}
return s;
}
l.parse = Ch, l.parseExpression = bh, l.tokTypes = wh;
} }), Xf = $({ "src/language-js/parse/json.js"(l, h) {
"use strict";
U();
var p = Io(), d = lr(), x = ko(), P = Do();
function m() {
let w = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {}, { allowComments: L = true } = w;
return function(_) {
let { parseExpression: G } = Fo(), N;
try {
N = G(_, { tokens: true, ranges: true });
} catch (O) {
throw P(O);
}
if (!L && p(N.comments))
throw v(N.comments[0], "Comment");
return S(N), N;
};
}
function v(w, L) {
let [A, _] = [w.loc.start, w.loc.end].map((G) => {
let { line: N, column: O } = G;
return { line: N, column: O + 1 };
});
return d(`${L} is not allowed in JSON.`, { start: A, end: _ });
}
function S(w) {
switch (w.type) {
case "ArrayExpression":
for (let L of w.elements)
L !== null && S(L);
return;
case "ObjectExpression":
for (let L of w.properties)
S(L);
return;
case "ObjectProperty":
if (w.computed)
throw v(w.key, "Computed key");
if (w.shorthand)
throw v(w.key, "Shorthand property");
w.key.type !== "Identifier" && S(w.key), S(w.value);
return;
case "UnaryExpression": {
let { operator: L, argument: A } = w;
if (L !== "+" && L !== "-")
throw v(w, `Operator '${w.operator}'`);
if (A.type === "NumericLiteral" || A.type === "Identifier" && (A.name === "Infinity" || A.name === "NaN"))
return;
throw v(A, `Operator '${L}' before '${A.type}'`);
}
case "Identifier":
if (w.name !== "Infinity" && w.name !== "NaN" && w.name !== "undefined")
throw v(w, `Identifier '${w.name}'`);
return;
case "TemplateLiteral":
if (p(w.expressions))
throw v(w.expressions[0], "'TemplateLiteral' with expression");
for (let L of w.quasis)
S(L);
return;
case "NullLiteral":
case "BooleanLiteral":
case "NumericLiteral":
case "StringLiteral":
case "TemplateElement":
return;
default:
throw v(w, `'${w.type}'`);
}
}
var k = m(), F = { json: x({ parse: k, hasPragma() {
return true;
} }), json5: x(k), "json-stringify": x({ parse: m({ allowComments: false }), astFormat: "estree-json" }) };
h.exports = F;
} });
U();
var Yf = kf(), Qf = po(), Zf = Of(), Ue = ko(), ed = Do(), td = Jf(), sd = Xf(), rd = { sourceType: "module", allowImportExportEverywhere: true, allowReturnOutsideFunction: true, allowSuperOutsideMethod: true, allowUndeclaredExports: true, errorRecovery: true, createParenthesizedExpressions: true, plugins: ["doExpressions", "exportDefaultFrom", "functionBind", "functionSent", "throwExpressions", "partialApplication", ["decorators", { decoratorsBeforeExport: false }], "importAssertions", "decimal", "moduleBlocks", "asyncDoExpressions", "regexpUnicodeSets", "destructuringPrivate", "decoratorAutoAccessors"], tokens: true, ranges: true }, id = ["recordAndTuple", { syntaxType: "hash" }], no = "v8intrinsic", oo = [["pipelineOperator", { proposal: "hack", topicToken: "%" }], ["pipelineOperator", { proposal: "minimal" }], ["pipelineOperator", { proposal: "fsharp" }]], he = function(l) {
let h = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : rd;
return Object.assign(Object.assign({}, h), {}, { plugins: [...h.plugins, ...l] });
}, ad = /@(?:no)?flow\b/;
function nd(l, h) {
if (h.filepath && h.filepath.endsWith(".js.flow"))
return true;
let p = Qf(l);
p && (l = l.slice(p.length));
let d = Zf(l, 0);
return d !== false && (l = l.slice(0, d)), ad.test(l);
}
function od(l, h, p) {
let d = Fo()[l], x = d(h, p), P = x.errors.find((m) => !fd.has(m.reasonCode));
if (P)
throw P;
return x;
}
function $e(l) {
for (var h = arguments.length, p = new Array(h > 1 ? h - 1 : 0), d = 1; d < h; d++)
p[d - 1] = arguments[d];
return function(x, P) {
let m = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {};
if ((m.parser === "babel" || m.parser === "__babel_estree") && nd(x, m))
return m.parser = "babel-flow", Lo(x, P, m);
let v = p;
m.__babelSourceType === "script" && (v = v.map((w) => Object.assign(Object.assign({}, w), {}, { sourceType: "script" }))), /#[[{]/.test(x) && (v = v.map((w) => he([id], w)));
let S = /%[A-Z]/.test(x);
x.includes("|>") ? v = (S ? [...oo, no] : oo).flatMap((L) => v.map((A) => he([L], A))) : S && (v = v.map((w) => he([no], w)));
let { result: k, error: F } = Yf(...v.map((w) => () => od(l, x, w)));
if (!k)
throw ed(F);
return m.originalText = x, td(k, m);
};
}
var ld = $e("parse", he(["jsx", "flow"])), Lo = $e("parse", he(["jsx", ["flow", { all: true, enums: true }]])), hd = $e("parse", he(["jsx", "typescript"]), he(["typescript"])), ud = $e("parse", he(["jsx", "flow", "estree"])), cd = $e("parseExpression", he(["jsx"])), pd = $e("parseExpression", he(["typescript"])), fd = /* @__PURE__ */ new Set(["StrictNumericEscape", "StrictWith", "StrictOctalLiteral", "StrictDelete", "StrictEvalArguments", "StrictEvalArgumentsBinding", "StrictFunction", "EmptyTypeArguments", "EmptyTypeParameters", "ConstructorHasTypeParameters", "UnsupportedParameterPropertyKind", "UnexpectedParameterModifier", "MixedLabeledAndUnlabeledElements", "InvalidTupleMemberLabel", "NonClassMethodPropertyHasAbstractModifer", "ReadonlyForMethodSignature", "ClassMethodHasDeclare", "ClassMethodHasReadonly", "InvalidModifierOnTypeMember", "DuplicateAccessibilityModifier", "IndexSignatureHasDeclare", "DecoratorExportClass", "ParamDupe", "InvalidDecimal", "RestTrailingComma", "UnsupportedParameterDecorator", "UnterminatedJsxContent", "UnexpectedReservedWord", "ModuleAttributesWithDuplicateKeys", "LineTerminatorBeforeArrow", "InvalidEscapeSequenceTemplate", "NonAbstractClassHasAbstractMethod", "UnsupportedPropertyDecorator", "OptionalTypeBeforeRequired", "PatternIsOptional", "OptionalBindingPattern", "DeclareClassFieldHasInitializer", "TypeImportCannotSpecifyDefaultAndNamed", "DeclareFunctionHasImplementation", "ConstructorClassField", "VarRedeclaration", "InvalidPrivateFieldResolution", "DuplicateExport"]), lo = Ue(ld), ho = Ue(hd), uo = Ue(cd), dd = Ue(pd);
Oo.exports = { parsers: Object.assign(Object.assign({ babel: lo, "babel-flow": Ue(Lo), "babel-ts": ho }, sd), {}, { __js_expression: uo, __vue_expression: uo, __vue_ts_expression: dd, __vue_event_binding: lo, __vue_ts_event_binding: ho, __babel_estree: Ue(ud) }) };
});
return md();
});
}
});
// node_modules/prettier/parser-flow.js
var require_parser_flow = __commonJS({
"node_modules/prettier/parser-flow.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.flow = e();
}
})(function() {
"use strict";
var Ne = (A0, j0) => () => (j0 || A0((j0 = { exports: {} }).exports, j0), j0.exports);
var Ii = Ne((Mae, in0) => {
var h_ = function(A0) {
return A0 && A0.Math == Math && A0;
};
in0.exports = h_(typeof globalThis == "object" && globalThis) || h_(typeof window == "object" && window) || h_(typeof self == "object" && self) || h_(typeof global == "object" && global) || function() {
return this;
}() || Function("return this")();
});
var Wc = Ne((Bae, fn0) => {
fn0.exports = function(A0) {
try {
return !!A0();
} catch {
return true;
}
};
});
var ws = Ne((qae, xn0) => {
var V7e = Wc();
xn0.exports = !V7e(function() {
return Object.defineProperty({}, 1, { get: function() {
return 7;
} })[1] != 7;
});
});
var BR = Ne((Uae, an0) => {
var z7e = Wc();
an0.exports = !z7e(function() {
var A0 = function() {
}.bind();
return typeof A0 != "function" || A0.hasOwnProperty("prototype");
});
});
var w_ = Ne((Hae, on0) => {
var K7e = BR(), k_ = Function.prototype.call;
on0.exports = K7e ? k_.bind(k_) : function() {
return k_.apply(k_, arguments);
};
});
var ln0 = Ne((vn0) => {
"use strict";
var cn0 = {}.propertyIsEnumerable, sn0 = Object.getOwnPropertyDescriptor, W7e = sn0 && !cn0.call({ 1: 2 }, 1);
vn0.f = W7e ? function(j0) {
var ur = sn0(this, j0);
return !!ur && ur.enumerable;
} : cn0;
});
var qR = Ne((Yae, bn0) => {
bn0.exports = function(A0, j0) {
return { enumerable: !(A0 & 1), configurable: !(A0 & 2), writable: !(A0 & 4), value: j0 };
};
});
var Es = Ne((Vae, _n0) => {
var pn0 = BR(), mn0 = Function.prototype, UR = mn0.call, J7e = pn0 && mn0.bind.bind(UR, UR);
_n0.exports = pn0 ? J7e : function(A0) {
return function() {
return UR.apply(A0, arguments);
};
};
});
var hn0 = Ne((zae, dn0) => {
var yn0 = Es(), $7e = yn0({}.toString), Z7e = yn0("".slice);
dn0.exports = function(A0) {
return Z7e($7e(A0), 8, -1);
};
});
var wn0 = Ne((Kae, kn0) => {
var Q7e = Es(), rie = Wc(), eie = hn0(), HR = Object, nie = Q7e("".split);
kn0.exports = rie(function() {
return !HR("z").propertyIsEnumerable(0);
}) ? function(A0) {
return eie(A0) == "String" ? nie(A0, "") : HR(A0);
} : HR;
});
var XR = Ne((Wae, En0) => {
En0.exports = function(A0) {
return A0 == null;
};
});
var YR = Ne((Jae, Sn0) => {
var tie = XR(), uie = TypeError;
Sn0.exports = function(A0) {
if (tie(A0))
throw uie("Can't call method on " + A0);
return A0;
};
});
var E_ = Ne(($ae, gn0) => {
var iie = wn0(), fie = YR();
gn0.exports = function(A0) {
return iie(fie(A0));
};
});
var zR = Ne((Zae, Fn0) => {
var VR = typeof document == "object" && document.all, xie = typeof VR > "u" && VR !== void 0;
Fn0.exports = { all: VR, IS_HTMLDDA: xie };
});
var $i = Ne((Qae, On0) => {
var Tn0 = zR(), aie = Tn0.all;
On0.exports = Tn0.IS_HTMLDDA ? function(A0) {
return typeof A0 == "function" || A0 === aie;
} : function(A0) {
return typeof A0 == "function";
};
});
var S2 = Ne((roe, Nn0) => {
var In0 = $i(), An0 = zR(), oie = An0.all;
Nn0.exports = An0.IS_HTMLDDA ? function(A0) {
return typeof A0 == "object" ? A0 !== null : In0(A0) || A0 === oie;
} : function(A0) {
return typeof A0 == "object" ? A0 !== null : In0(A0);
};
});
var S_ = Ne((eoe, Cn0) => {
var KR = Ii(), cie = $i(), sie = function(A0) {
return cie(A0) ? A0 : void 0;
};
Cn0.exports = function(A0, j0) {
return arguments.length < 2 ? sie(KR[A0]) : KR[A0] && KR[A0][j0];
};
});
var Dn0 = Ne((noe, Pn0) => {
var vie = Es();
Pn0.exports = vie({}.isPrototypeOf);
});
var Rn0 = Ne((toe, Ln0) => {
var lie = S_();
Ln0.exports = lie("navigator", "userAgent") || "";
});
var Hn0 = Ne((uoe, Un0) => {
var qn0 = Ii(), WR = Rn0(), jn0 = qn0.process, Gn0 = qn0.Deno, Mn0 = jn0 && jn0.versions || Gn0 && Gn0.version, Bn0 = Mn0 && Mn0.v8, Zi, g_;
Bn0 && (Zi = Bn0.split("."), g_ = Zi[0] > 0 && Zi[0] < 4 ? 1 : +(Zi[0] + Zi[1]));
!g_ && WR && (Zi = WR.match(/Edge\/(\d+)/), (!Zi || Zi[1] >= 74) && (Zi = WR.match(/Chrome\/(\d+)/), Zi && (g_ = +Zi[1])));
Un0.exports = g_;
});
var JR = Ne((ioe, Yn0) => {
var Xn0 = Hn0(), bie = Wc();
Yn0.exports = !!Object.getOwnPropertySymbols && !bie(function() {
var A0 = Symbol();
return !String(A0) || !(Object(A0) instanceof Symbol) || !Symbol.sham && Xn0 && Xn0 < 41;
});
});
var $R = Ne((foe, Vn0) => {
var pie = JR();
Vn0.exports = pie && !Symbol.sham && typeof Symbol.iterator == "symbol";
});
var ZR = Ne((xoe, zn0) => {
var mie = S_(), _ie = $i(), yie = Dn0(), die = $R(), hie = Object;
zn0.exports = die ? function(A0) {
return typeof A0 == "symbol";
} : function(A0) {
var j0 = mie("Symbol");
return _ie(j0) && yie(j0.prototype, hie(A0));
};
});
var Wn0 = Ne((aoe, Kn0) => {
var kie = String;
Kn0.exports = function(A0) {
try {
return kie(A0);
} catch {
return "Object";
}
};
});
var $n0 = Ne((ooe, Jn0) => {
var wie = $i(), Eie = Wn0(), Sie = TypeError;
Jn0.exports = function(A0) {
if (wie(A0))
return A0;
throw Sie(Eie(A0) + " is not a function");
};
});
var Qn0 = Ne((coe, Zn0) => {
var gie = $n0(), Fie = XR();
Zn0.exports = function(A0, j0) {
var ur = A0[j0];
return Fie(ur) ? void 0 : gie(ur);
};
});
var et0 = Ne((soe, rt0) => {
var QR = w_(), rj = $i(), ej = S2(), Tie = TypeError;
rt0.exports = function(A0, j0) {
var ur, hr;
if (j0 === "string" && rj(ur = A0.toString) && !ej(hr = QR(ur, A0)) || rj(ur = A0.valueOf) && !ej(hr = QR(ur, A0)) || j0 !== "string" && rj(ur = A0.toString) && !ej(hr = QR(ur, A0)))
return hr;
throw Tie("Can't convert object to primitive value");
};
});
var tt0 = Ne((voe, nt0) => {
nt0.exports = false;
});
var F_ = Ne((loe, it0) => {
var ut0 = Ii(), Oie = Object.defineProperty;
it0.exports = function(A0, j0) {
try {
Oie(ut0, A0, { value: j0, configurable: true, writable: true });
} catch {
ut0[A0] = j0;
}
return j0;
};
});
var T_ = Ne((boe, xt0) => {
var Iie = Ii(), Aie = F_(), ft0 = "__core-js_shared__", Nie = Iie[ft0] || Aie(ft0, {});
xt0.exports = Nie;
});
var nj = Ne((poe, ot0) => {
var Cie = tt0(), at0 = T_();
(ot0.exports = function(A0, j0) {
return at0[A0] || (at0[A0] = j0 !== void 0 ? j0 : {});
})("versions", []).push({ version: "3.26.1", mode: Cie ? "pure" : "global", copyright: "\xA9 2014-2022 Denis Pushkarev (zloirock.ru)", license: "https://github.com/zloirock/core-js/blob/v3.26.1/LICENSE", source: "https://github.com/zloirock/core-js" });
});
var st0 = Ne((moe, ct0) => {
var Pie = YR(), Die = Object;
ct0.exports = function(A0) {
return Die(Pie(A0));
};
});
var n1 = Ne((_oe, vt0) => {
var Lie = Es(), Rie = st0(), jie = Lie({}.hasOwnProperty);
vt0.exports = Object.hasOwn || function(j0, ur) {
return jie(Rie(j0), ur);
};
});
var tj = Ne((yoe, lt0) => {
var Gie = Es(), Mie = 0, Bie = Math.random(), qie = Gie(1 .toString);
lt0.exports = function(A0) {
return "Symbol(" + (A0 === void 0 ? "" : A0) + ")_" + qie(++Mie + Bie, 36);
};
});
var dt0 = Ne((doe, yt0) => {
var Uie = Ii(), Hie = nj(), bt0 = n1(), Xie = tj(), pt0 = JR(), _t0 = $R(), g2 = Hie("wks"), xv = Uie.Symbol, mt0 = xv && xv.for, Yie = _t0 ? xv : xv && xv.withoutSetter || Xie;
yt0.exports = function(A0) {
if (!bt0(g2, A0) || !(pt0 || typeof g2[A0] == "string")) {
var j0 = "Symbol." + A0;
pt0 && bt0(xv, A0) ? g2[A0] = xv[A0] : _t0 && mt0 ? g2[A0] = mt0(j0) : g2[A0] = Yie(j0);
}
return g2[A0];
};
});
var Et0 = Ne((hoe, wt0) => {
var Vie = w_(), ht0 = S2(), kt0 = ZR(), zie = Qn0(), Kie = et0(), Wie = dt0(), Jie = TypeError, $ie = Wie("toPrimitive");
wt0.exports = function(A0, j0) {
if (!ht0(A0) || kt0(A0))
return A0;
var ur = zie(A0, $ie), hr;
if (ur) {
if (j0 === void 0 && (j0 = "default"), hr = Vie(ur, A0, j0), !ht0(hr) || kt0(hr))
return hr;
throw Jie("Can't convert object to primitive value");
}
return j0 === void 0 && (j0 = "number"), Kie(A0, j0);
};
});
var uj = Ne((koe, St0) => {
var Zie = Et0(), Qie = ZR();
St0.exports = function(A0) {
var j0 = Zie(A0, "string");
return Qie(j0) ? j0 : j0 + "";
};
});
var Tt0 = Ne((woe, Ft0) => {
var rfe = Ii(), gt0 = S2(), ij = rfe.document, efe = gt0(ij) && gt0(ij.createElement);
Ft0.exports = function(A0) {
return efe ? ij.createElement(A0) : {};
};
});
var fj = Ne((Eoe, Ot0) => {
var nfe = ws(), tfe = Wc(), ufe = Tt0();
Ot0.exports = !nfe && !tfe(function() {
return Object.defineProperty(ufe("div"), "a", { get: function() {
return 7;
} }).a != 7;
});
});
var xj = Ne((At0) => {
var ife = ws(), ffe = w_(), xfe = ln0(), afe = qR(), ofe = E_(), cfe = uj(), sfe = n1(), vfe = fj(), It0 = Object.getOwnPropertyDescriptor;
At0.f = ife ? It0 : function(j0, ur) {
if (j0 = ofe(j0), ur = cfe(ur), vfe)
try {
return It0(j0, ur);
} catch {
}
if (sfe(j0, ur))
return afe(!ffe(xfe.f, j0, ur), j0[ur]);
};
});
var Ct0 = Ne((goe, Nt0) => {
var lfe = ws(), bfe = Wc();
Nt0.exports = lfe && bfe(function() {
return Object.defineProperty(function() {
}, "prototype", { value: 42, writable: false }).prototype != 42;
});
});
var O_ = Ne((Foe, Pt0) => {
var pfe = S2(), mfe = String, _fe = TypeError;
Pt0.exports = function(A0) {
if (pfe(A0))
return A0;
throw _fe(mfe(A0) + " is not an object");
};
});
var o4 = Ne((Lt0) => {
var yfe = ws(), dfe = fj(), hfe = Ct0(), I_ = O_(), Dt0 = uj(), kfe = TypeError, aj = Object.defineProperty, wfe = Object.getOwnPropertyDescriptor, oj = "enumerable", cj = "configurable", sj = "writable";
Lt0.f = yfe ? hfe ? function(j0, ur, hr) {
if (I_(j0), ur = Dt0(ur), I_(hr), typeof j0 == "function" && ur === "prototype" && "value" in hr && sj in hr && !hr[sj]) {
var le = wfe(j0, ur);
le && le[sj] && (j0[ur] = hr.value, hr = { configurable: cj in hr ? hr[cj] : le[cj], enumerable: oj in hr ? hr[oj] : le[oj], writable: false });
}
return aj(j0, ur, hr);
} : aj : function(j0, ur, hr) {
if (I_(j0), ur = Dt0(ur), I_(hr), dfe)
try {
return aj(j0, ur, hr);
} catch {
}
if ("get" in hr || "set" in hr)
throw kfe("Accessors not supported");
return "value" in hr && (j0[ur] = hr.value), j0;
};
});
var vj = Ne((Ooe, Rt0) => {
var Efe = ws(), Sfe = o4(), gfe = qR();
Rt0.exports = Efe ? function(A0, j0, ur) {
return Sfe.f(A0, j0, gfe(1, ur));
} : function(A0, j0, ur) {
return A0[j0] = ur, A0;
};
});
var Mt0 = Ne((Ioe, Gt0) => {
var lj = ws(), Ffe = n1(), jt0 = Function.prototype, Tfe = lj && Object.getOwnPropertyDescriptor, bj = Ffe(jt0, "name"), Ofe = bj && function() {
}.name === "something", Ife = bj && (!lj || lj && Tfe(jt0, "name").configurable);
Gt0.exports = { EXISTS: bj, PROPER: Ofe, CONFIGURABLE: Ife };
});
var qt0 = Ne((Aoe, Bt0) => {
var Afe = Es(), Nfe = $i(), pj = T_(), Cfe = Afe(Function.toString);
Nfe(pj.inspectSource) || (pj.inspectSource = function(A0) {
return Cfe(A0);
});
Bt0.exports = pj.inspectSource;
});
var Xt0 = Ne((Noe, Ht0) => {
var Pfe = Ii(), Dfe = $i(), Ut0 = Pfe.WeakMap;
Ht0.exports = Dfe(Ut0) && /native code/.test(String(Ut0));
});
var zt0 = Ne((Coe, Vt0) => {
var Lfe = nj(), Rfe = tj(), Yt0 = Lfe("keys");
Vt0.exports = function(A0) {
return Yt0[A0] || (Yt0[A0] = Rfe(A0));
};
});
var mj = Ne((Poe, Kt0) => {
Kt0.exports = {};
});
var Zt0 = Ne((Doe, $t0) => {
var jfe = Xt0(), Jt0 = Ii(), Gfe = S2(), Mfe = vj(), _j = n1(), yj = T_(), Bfe = zt0(), qfe = mj(), Wt0 = "Object already initialized", dj = Jt0.TypeError, Ufe = Jt0.WeakMap, A_, c4, N_, Hfe = function(A0) {
return N_(A0) ? c4(A0) : A_(A0, {});
}, Xfe = function(A0) {
return function(j0) {
var ur;
if (!Gfe(j0) || (ur = c4(j0)).type !== A0)
throw dj("Incompatible receiver, " + A0 + " required");
return ur;
};
};
jfe || yj.state ? (Qi = yj.state || (yj.state = new Ufe()), Qi.get = Qi.get, Qi.has = Qi.has, Qi.set = Qi.set, A_ = function(A0, j0) {
if (Qi.has(A0))
throw dj(Wt0);
return j0.facade = A0, Qi.set(A0, j0), j0;
}, c4 = function(A0) {
return Qi.get(A0) || {};
}, N_ = function(A0) {
return Qi.has(A0);
}) : (av = Bfe("state"), qfe[av] = true, A_ = function(A0, j0) {
if (_j(A0, av))
throw dj(Wt0);
return j0.facade = A0, Mfe(A0, av, j0), j0;
}, c4 = function(A0) {
return _j(A0, av) ? A0[av] : {};
}, N_ = function(A0) {
return _j(A0, av);
});
var Qi, av;
$t0.exports = { set: A_, get: c4, has: N_, enforce: Hfe, getterFor: Xfe };
});
var kj = Ne((Loe, ru0) => {
var Yfe = Wc(), Vfe = $i(), C_ = n1(), hj = ws(), zfe = Mt0().CONFIGURABLE, Kfe = qt0(), Qt0 = Zt0(), Wfe = Qt0.enforce, Jfe = Qt0.get, P_ = Object.defineProperty, $fe = hj && !Yfe(function() {
return P_(function() {
}, "length", { value: 8 }).length !== 8;
}), Zfe = String(String).split("String"), Qfe = ru0.exports = function(A0, j0, ur) {
String(j0).slice(0, 7) === "Symbol(" && (j0 = "[" + String(j0).replace(/^Symbol\(([^)]*)\)/, "$1") + "]"), ur && ur.getter && (j0 = "get " + j0), ur && ur.setter && (j0 = "set " + j0), (!C_(A0, "name") || zfe && A0.name !== j0) && (hj ? P_(A0, "name", { value: j0, configurable: true }) : A0.name = j0), $fe && ur && C_(ur, "arity") && A0.length !== ur.arity && P_(A0, "length", { value: ur.arity });
try {
ur && C_(ur, "constructor") && ur.constructor ? hj && P_(A0, "prototype", { writable: false }) : A0.prototype && (A0.prototype = void 0);
} catch {
}
var hr = Wfe(A0);
return C_(hr, "source") || (hr.source = Zfe.join(typeof j0 == "string" ? j0 : "")), A0;
};
Function.prototype.toString = Qfe(function() {
return Vfe(this) && Jfe(this).source || Kfe(this);
}, "toString");
});
var nu0 = Ne((Roe, eu0) => {
var rxe = $i(), exe = o4(), nxe = kj(), txe = F_();
eu0.exports = function(A0, j0, ur, hr) {
hr || (hr = {});
var le = hr.enumerable, Ve = hr.name !== void 0 ? hr.name : j0;
if (rxe(ur) && nxe(ur, Ve, hr), hr.global)
le ? A0[j0] = ur : txe(j0, ur);
else {
try {
hr.unsafe ? A0[j0] && (le = true) : delete A0[j0];
} catch {
}
le ? A0[j0] = ur : exe.f(A0, j0, { value: ur, enumerable: false, configurable: !hr.nonConfigurable, writable: !hr.nonWritable });
}
return A0;
};
});
var uu0 = Ne((joe, tu0) => {
var uxe = Math.ceil, ixe = Math.floor;
tu0.exports = Math.trunc || function(j0) {
var ur = +j0;
return (ur > 0 ? ixe : uxe)(ur);
};
});
var wj = Ne((Goe, iu0) => {
var fxe = uu0();
iu0.exports = function(A0) {
var j0 = +A0;
return j0 !== j0 || j0 === 0 ? 0 : fxe(j0);
};
});
var xu0 = Ne((Moe, fu0) => {
var xxe = wj(), axe = Math.max, oxe = Math.min;
fu0.exports = function(A0, j0) {
var ur = xxe(A0);
return ur < 0 ? axe(ur + j0, 0) : oxe(ur, j0);
};
});
var ou0 = Ne((Boe, au0) => {
var cxe = wj(), sxe = Math.min;
au0.exports = function(A0) {
return A0 > 0 ? sxe(cxe(A0), 9007199254740991) : 0;
};
});
var su0 = Ne((qoe, cu0) => {
var vxe = ou0();
cu0.exports = function(A0) {
return vxe(A0.length);
};
});
var bu0 = Ne((Uoe, lu0) => {
var lxe = E_(), bxe = xu0(), pxe = su0(), vu0 = function(A0) {
return function(j0, ur, hr) {
var le = lxe(j0), Ve = pxe(le), Le = bxe(hr, Ve), Fn;
if (A0 && ur != ur) {
for (; Ve > Le; )
if (Fn = le[Le++], Fn != Fn)
return true;
} else
for (; Ve > Le; Le++)
if ((A0 || Le in le) && le[Le] === ur)
return A0 || Le || 0;
return !A0 && -1;
};
};
lu0.exports = { includes: vu0(true), indexOf: vu0(false) };
});
var _u0 = Ne((Hoe, mu0) => {
var mxe = Es(), Ej = n1(), _xe = E_(), yxe = bu0().indexOf, dxe = mj(), pu0 = mxe([].push);
mu0.exports = function(A0, j0) {
var ur = _xe(A0), hr = 0, le = [], Ve;
for (Ve in ur)
!Ej(dxe, Ve) && Ej(ur, Ve) && pu0(le, Ve);
for (; j0.length > hr; )
Ej(ur, Ve = j0[hr++]) && (~yxe(le, Ve) || pu0(le, Ve));
return le;
};
});
var du0 = Ne((Xoe, yu0) => {
yu0.exports = ["constructor", "hasOwnProperty", "isPrototypeOf", "propertyIsEnumerable", "toLocaleString", "toString", "valueOf"];
});
var ku0 = Ne((hu0) => {
var hxe = _u0(), kxe = du0(), wxe = kxe.concat("length", "prototype");
hu0.f = Object.getOwnPropertyNames || function(j0) {
return hxe(j0, wxe);
};
});
var Eu0 = Ne((wu0) => {
wu0.f = Object.getOwnPropertySymbols;
});
var gu0 = Ne((zoe, Su0) => {
var Exe = S_(), Sxe = Es(), gxe = ku0(), Fxe = Eu0(), Txe = O_(), Oxe = Sxe([].concat);
Su0.exports = Exe("Reflect", "ownKeys") || function(j0) {
var ur = gxe.f(Txe(j0)), hr = Fxe.f;
return hr ? Oxe(ur, hr(j0)) : ur;
};
});
var Ou0 = Ne((Koe, Tu0) => {
var Fu0 = n1(), Ixe = gu0(), Axe = xj(), Nxe = o4();
Tu0.exports = function(A0, j0, ur) {
for (var hr = Ixe(j0), le = Nxe.f, Ve = Axe.f, Le = 0; Le < hr.length; Le++) {
var Fn = hr[Le];
!Fu0(A0, Fn) && !(ur && Fu0(ur, Fn)) && le(A0, Fn, Ve(j0, Fn));
}
};
});
var Au0 = Ne((Woe, Iu0) => {
var Cxe = Wc(), Pxe = $i(), Dxe = /#|\.prototype\./, s4 = function(A0, j0) {
var ur = Rxe[Lxe(A0)];
return ur == Gxe ? true : ur == jxe ? false : Pxe(j0) ? Cxe(j0) : !!j0;
}, Lxe = s4.normalize = function(A0) {
return String(A0).replace(Dxe, ".").toLowerCase();
}, Rxe = s4.data = {}, jxe = s4.NATIVE = "N", Gxe = s4.POLYFILL = "P";
Iu0.exports = s4;
});
var Cu0 = Ne((Joe, Nu0) => {
var Sj = Ii(), Mxe = xj().f, Bxe = vj(), qxe = nu0(), Uxe = F_(), Hxe = Ou0(), Xxe = Au0();
Nu0.exports = function(A0, j0) {
var ur = A0.target, hr = A0.global, le = A0.stat, Ve, Le, Fn, gn, et, at;
if (hr ? Le = Sj : le ? Le = Sj[ur] || Uxe(ur, {}) : Le = (Sj[ur] || {}).prototype, Le)
for (Fn in j0) {
if (et = j0[Fn], A0.dontCallGetSet ? (at = Mxe(Le, Fn), gn = at && at.value) : gn = Le[Fn], Ve = Xxe(hr ? Fn : ur + (le ? "." : "#") + Fn, A0.forced), !Ve && gn !== void 0) {
if (typeof et == typeof gn)
continue;
Hxe(et, gn);
}
(A0.sham || gn && gn.sham) && Bxe(et, "sham", true), qxe(Le, Fn, et, A0);
}
};
});
var Pu0 = Ne(() => {
var Yxe = Cu0(), gj = Ii();
Yxe({ global: true, forced: gj.globalThis !== gj }, { globalThis: gj });
});
var Du0 = Ne(() => {
Pu0();
});
var ju0 = Ne((ece, Ru0) => {
var Lu0 = kj(), Vxe = o4();
Ru0.exports = function(A0, j0, ur) {
return ur.get && Lu0(ur.get, j0, { getter: true }), ur.set && Lu0(ur.set, j0, { setter: true }), Vxe.f(A0, j0, ur);
};
});
var Mu0 = Ne((nce, Gu0) => {
"use strict";
var zxe = O_();
Gu0.exports = function() {
var A0 = zxe(this), j0 = "";
return A0.hasIndices && (j0 += "d"), A0.global && (j0 += "g"), A0.ignoreCase && (j0 += "i"), A0.multiline && (j0 += "m"), A0.dotAll && (j0 += "s"), A0.unicode && (j0 += "u"), A0.unicodeSets && (j0 += "v"), A0.sticky && (j0 += "y"), j0;
};
});
var Uu0 = Ne(() => {
var Kxe = Ii(), Wxe = ws(), Jxe = ju0(), $xe = Mu0(), Zxe = Wc(), Bu0 = Kxe.RegExp, qu0 = Bu0.prototype, Qxe = Wxe && Zxe(function() {
var A0 = true;
try {
Bu0(".", "d");
} catch {
A0 = false;
}
var j0 = {}, ur = "", hr = A0 ? "dgimsy" : "gimsy", le = function(gn, et) {
Object.defineProperty(j0, gn, { get: function() {
return ur += et, true;
} });
}, Ve = { dotAll: "s", global: "g", ignoreCase: "i", multiline: "m", sticky: "y" };
A0 && (Ve.hasIndices = "d");
for (var Le in Ve)
le(Le, Ve[Le]);
var Fn = Object.getOwnPropertyDescriptor(qu0, "flags").get.call(j0);
return Fn !== hr || ur !== hr;
});
Qxe && Jxe(qu0, "flags", { configurable: true, get: $xe });
});
var Pae = Ne((ice, a70) => {
Du0();
Uu0();
var tU = Object.defineProperty, rae = Object.getOwnPropertyDescriptor, uU = Object.getOwnPropertyNames, eae = Object.prototype.hasOwnProperty, L_ = (A0, j0) => function() {
return A0 && (j0 = (0, A0[uU(A0)[0]])(A0 = 0)), j0;
}, au = (A0, j0) => function() {
return j0 || (0, A0[uU(A0)[0]])((j0 = { exports: {} }).exports, j0), j0.exports;
}, iU = (A0, j0) => {
for (var ur in j0)
tU(A0, ur, { get: j0[ur], enumerable: true });
}, nae = (A0, j0, ur, hr) => {
if (j0 && typeof j0 == "object" || typeof j0 == "function")
for (let le of uU(j0))
!eae.call(A0, le) && le !== ur && tU(A0, le, { get: () => j0[le], enumerable: !(hr = rae(j0, le)) || hr.enumerable });
return A0;
}, fU = (A0) => nae(tU({}, "__esModule", { value: true }), A0), Lt = L_({ ""() {
} }), Hu0 = au({ "src/common/parser-create-error.js"(A0, j0) {
"use strict";
Lt();
function ur(hr, le) {
let Ve = new SyntaxError(hr + " (" + le.start.line + ":" + le.start.column + ")");
return Ve.loc = le, Ve;
}
j0.exports = ur;
} }), Xu0 = {};
iU(Xu0, { EOL: () => Ij, arch: () => tae, cpus: () => $u0, default: () => n70, endianness: () => Yu0, freemem: () => Wu0, getNetworkInterfaces: () => e70, hostname: () => Vu0, loadavg: () => zu0, networkInterfaces: () => r70, platform: () => uae, release: () => Qu0, tmpDir: () => Tj, tmpdir: () => Oj, totalmem: () => Ju0, type: () => Zu0, uptime: () => Ku0 });
function Yu0() {
if (typeof D_ > "u") {
var A0 = new ArrayBuffer(2), j0 = new Uint8Array(A0), ur = new Uint16Array(A0);
if (j0[0] = 1, j0[1] = 2, ur[0] === 258)
D_ = "BE";
else if (ur[0] === 513)
D_ = "LE";
else
throw new Error("unable to figure out endianess");
}
return D_;
}
function Vu0() {
return typeof globalThis.location < "u" ? globalThis.location.hostname : "";
}
function zu0() {
return [];
}
function Ku0() {
return 0;
}
function Wu0() {
return Number.MAX_VALUE;
}
function Ju0() {
return Number.MAX_VALUE;
}
function $u0() {
return [];
}
function Zu0() {
return "Browser";
}
function Qu0() {
return typeof globalThis.navigator < "u" ? globalThis.navigator.appVersion : "";
}
function r70() {
}
function e70() {
}
function tae() {
return "javascript";
}
function uae() {
return "browser";
}
function Tj() {
return "/tmp";
}
var D_, Oj, Ij, n70, iae = L_({ "node-modules-polyfills:os"() {
Lt(), Oj = Tj, Ij = `
`, n70 = { EOL: Ij, tmpdir: Oj, tmpDir: Tj, networkInterfaces: r70, getNetworkInterfaces: e70, release: Qu0, type: Zu0, cpus: $u0, totalmem: Ju0, freemem: Wu0, uptime: Ku0, loadavg: zu0, hostname: Vu0, endianness: Yu0 };
} }), fae = au({ "node-modules-polyfills-commonjs:os"(A0, j0) {
Lt();
var ur = (iae(), fU(Xu0));
if (ur && ur.default) {
j0.exports = ur.default;
for (let hr in ur)
j0.exports[hr] = ur[hr];
} else
ur && (j0.exports = ur);
} }), xae = au({ "node_modules/detect-newline/index.js"(A0, j0) {
"use strict";
Lt();
var ur = (hr) => {
if (typeof hr != "string")
throw new TypeError("Expected a string");
let le = hr.match(/(?:\r?\n)/g) || [];
if (le.length === 0)
return;
let Ve = le.filter((Fn) => Fn === `\r
`).length, Le = le.length - Ve;
return Ve > Le ? `\r
` : `
`;
};
j0.exports = ur, j0.exports.graceful = (hr) => typeof hr == "string" && ur(hr) || `
`;
} }), aae = au({ "node_modules/jest-docblock/build/index.js"(A0) {
"use strict";
Lt(), Object.defineProperty(A0, "__esModule", { value: true }), A0.extract = kn, A0.parse = rf, A0.parseWithComments = hn, A0.print = Mn, A0.strip = Qt;
function j0() {
let On = fae();
return j0 = function() {
return On;
}, On;
}
function ur() {
let On = hr(xae());
return ur = function() {
return On;
}, On;
}
function hr(On) {
return On && On.__esModule ? On : { default: On };
}
var le = /\*\/$/, Ve = /^\/\*\*?/, Le = /^\s*(\/\*\*?(.|\r?\n)*?\*\/)/, Fn = /(^|\s+)\/\/([^\r\n]*)/g, gn = /^(\r?\n)+/, et = /(?:^|\r?\n) *(@[^\r\n]*?) *\r?\n *(?![^@\r\n]*\/\/[^]*)([^@\r\n\s][^@\r\n]+?) *\r?\n/g, at = /(?:^|\r?\n) *@(\S+) *([^\r\n]*)/g, Zt = /(\r?\n|^) *\* ?/g, Ut = [];
function kn(On) {
let ru = On.match(Le);
return ru ? ru[0].trimLeft() : "";
}
function Qt(On) {
let ru = On.match(Le);
return ru && ru[0] ? On.substring(ru[0].length) : On;
}
function rf(On) {
return hn(On).pragmas;
}
function hn(On) {
let ru = (0, ur().default)(On) || j0().EOL;
On = On.replace(Ve, "").replace(le, "").replace(Zt, "$1");
let E7 = "";
for (; E7 !== On; )
E7 = On, On = On.replace(et, `${ru}$1 $2${ru}`);
On = On.replace(gn, "").trimRight();
let Ct = /* @__PURE__ */ Object.create(null), Ss = On.replace(at, "").replace(gn, "").trimRight(), In;
for (; In = at.exec(On); ) {
let Jc = In[2].replace(Fn, "");
typeof Ct[In[1]] == "string" || Array.isArray(Ct[In[1]]) ? Ct[In[1]] = Ut.concat(Ct[In[1]], Jc) : Ct[In[1]] = Jc;
}
return { comments: Ss, pragmas: Ct };
}
function Mn(On) {
let { comments: ru = "", pragmas: E7 = {} } = On, Ct = (0, ur().default)(ru) || j0().EOL, Ss = "/**", In = " *", Jc = " */", Ai = Object.keys(E7), vi = Ai.map((S7) => ut(S7, E7[S7])).reduce((S7, ov) => S7.concat(ov), []).map((S7) => `${In} ${S7}${Ct}`).join("");
if (!ru) {
if (Ai.length === 0)
return "";
if (Ai.length === 1 && !Array.isArray(E7[Ai[0]])) {
let S7 = E7[Ai[0]];
return `${Ss} ${ut(Ai[0], S7)[0]}${Jc}`;
}
}
let Rt = ru.split(Ct).map((S7) => `${In} ${S7}`).join(Ct) + Ct;
return Ss + Ct + (ru ? Rt : "") + (ru && Ai.length ? In + Ct : "") + vi + Jc;
}
function ut(On, ru) {
return Ut.concat(ru).map((E7) => `@${On} ${E7}`.trim());
}
} }), oae = au({ "src/common/end-of-line.js"(A0, j0) {
"use strict";
Lt();
function ur(Le) {
let Fn = Le.indexOf("\r");
return Fn >= 0 ? Le.charAt(Fn + 1) === `
` ? "crlf" : "cr" : "lf";
}
function hr(Le) {
switch (Le) {
case "cr":
return "\r";
case "crlf":
return `\r
`;
default:
return `
`;
}
}
function le(Le, Fn) {
let gn;
switch (Fn) {
case `
`:
gn = /\n/g;
break;
case "\r":
gn = /\r/g;
break;
case `\r
`:
gn = /\r\n/g;
break;
default:
throw new Error(`Unexpected "eol" ${JSON.stringify(Fn)}.`);
}
let et = Le.match(gn);
return et ? et.length : 0;
}
function Ve(Le) {
return Le.replace(/\r\n?/g, `
`);
}
j0.exports = { guessEndOfLine: ur, convertEndOfLineToChars: hr, countEndOfLineChars: le, normalizeEndOfLine: Ve };
} }), cae = au({ "src/language-js/utils/get-shebang.js"(A0, j0) {
"use strict";
Lt();
function ur(hr) {
if (!hr.startsWith("#!"))
return "";
let le = hr.indexOf(`
`);
return le === -1 ? hr : hr.slice(0, le);
}
j0.exports = ur;
} }), sae = au({ "src/language-js/pragma.js"(A0, j0) {
"use strict";
Lt();
var { parseWithComments: ur, strip: hr, extract: le, print: Ve } = aae(), { normalizeEndOfLine: Le } = oae(), Fn = cae();
function gn(Zt) {
let Ut = Fn(Zt);
Ut && (Zt = Zt.slice(Ut.length + 1));
let kn = le(Zt), { pragmas: Qt, comments: rf } = ur(kn);
return { shebang: Ut, text: Zt, pragmas: Qt, comments: rf };
}
function et(Zt) {
let Ut = Object.keys(gn(Zt).pragmas);
return Ut.includes("prettier") || Ut.includes("format");
}
function at(Zt) {
let { shebang: Ut, text: kn, pragmas: Qt, comments: rf } = gn(Zt), hn = hr(kn), Mn = Ve({ pragmas: Object.assign({ format: "" }, Qt), comments: rf.trimStart() });
return (Ut ? `${Ut}
` : "") + Le(Mn) + (hn.startsWith(`
`) ? `
` : `
`) + hn;
}
j0.exports = { hasPragma: et, insertPragma: at };
} }), vae = au({ "src/utils/is-non-empty-array.js"(A0, j0) {
"use strict";
Lt();
function ur(hr) {
return Array.isArray(hr) && hr.length > 0;
}
j0.exports = ur;
} }), t70 = au({ "src/language-js/loc.js"(A0, j0) {
"use strict";
Lt();
var ur = vae();
function hr(gn) {
var et, at;
let Zt = gn.range ? gn.range[0] : gn.start, Ut = (et = (at = gn.declaration) === null || at === void 0 ? void 0 : at.decorators) !== null && et !== void 0 ? et : gn.decorators;
return ur(Ut) ? Math.min(hr(Ut[0]), Zt) : Zt;
}
function le(gn) {
return gn.range ? gn.range[1] : gn.end;
}
function Ve(gn, et) {
let at = hr(gn);
return Number.isInteger(at) && at === hr(et);
}
function Le(gn, et) {
let at = le(gn);
return Number.isInteger(at) && at === le(et);
}
function Fn(gn, et) {
return Ve(gn, et) && Le(gn, et);
}
j0.exports = { locStart: hr, locEnd: le, hasSameLocStart: Ve, hasSameLoc: Fn };
} }), lae = au({ "src/language-js/parse/utils/create-parser.js"(A0, j0) {
"use strict";
Lt();
var { hasPragma: ur } = sae(), { locStart: hr, locEnd: le } = t70();
function Ve(Le) {
return Le = typeof Le == "function" ? { parse: Le } : Le, Object.assign({ astFormat: "estree", hasPragma: ur, locStart: hr, locEnd: le }, Le);
}
j0.exports = Ve;
} }), bae = au({ "src/language-js/parse/utils/replace-hashbang.js"(A0, j0) {
"use strict";
Lt();
function ur(hr) {
return hr.charAt(0) === "#" && hr.charAt(1) === "!" ? "//" + hr.slice(2) : hr;
}
j0.exports = ur;
} }), pae = au({ "src/language-js/utils/is-ts-keyword-type.js"(A0, j0) {
"use strict";
Lt();
function ur(hr) {
let { type: le } = hr;
return le.startsWith("TS") && le.endsWith("Keyword");
}
j0.exports = ur;
} }), mae = au({ "src/language-js/utils/is-block-comment.js"(A0, j0) {
"use strict";
Lt();
var ur = /* @__PURE__ */ new Set(["Block", "CommentBlock", "MultiLine"]), hr = (le) => ur.has(le == null ? void 0 : le.type);
j0.exports = hr;
} }), _ae = au({ "src/language-js/utils/is-type-cast-comment.js"(A0, j0) {
"use strict";
Lt();
var ur = mae();
function hr(le) {
return ur(le) && le.value[0] === "*" && /@(?:type|satisfies)\b/.test(le.value);
}
j0.exports = hr;
} }), yae = au({ "src/utils/get-last.js"(A0, j0) {
"use strict";
Lt();
var ur = (hr) => hr[hr.length - 1];
j0.exports = ur;
} }), dae = au({ "src/language-js/parse/postprocess/visit-node.js"(A0, j0) {
"use strict";
Lt();
function ur(hr, le) {
if (Array.isArray(hr)) {
for (let Ve = 0; Ve < hr.length; Ve++)
hr[Ve] = ur(hr[Ve], le);
return hr;
}
if (hr && typeof hr == "object" && typeof hr.type == "string") {
let Ve = Object.keys(hr);
for (let Le = 0; Le < Ve.length; Le++)
hr[Ve[Le]] = ur(hr[Ve[Le]], le);
return le(hr) || hr;
}
return hr;
}
j0.exports = ur;
} }), hae = au({ "src/language-js/parse/postprocess/throw-syntax-error.js"(A0, j0) {
"use strict";
Lt();
var ur = Hu0();
function hr(le, Ve) {
let { start: Le, end: Fn } = le.loc;
throw ur(Ve, { start: { line: Le.line, column: Le.column + 1 }, end: { line: Fn.line, column: Fn.column + 1 } });
}
j0.exports = hr;
} }), kae = au({ "src/language-js/parse/postprocess/index.js"(A0, j0) {
"use strict";
Lt();
var { locStart: ur, locEnd: hr } = t70(), le = pae(), Ve = _ae(), Le = yae(), Fn = dae(), gn = hae();
function et(kn, Qt) {
if (Qt.parser !== "typescript" && Qt.parser !== "flow" && Qt.parser !== "acorn" && Qt.parser !== "espree" && Qt.parser !== "meriyah") {
let hn = /* @__PURE__ */ new Set();
kn = Fn(kn, (Mn) => {
Mn.leadingComments && Mn.leadingComments.some(Ve) && hn.add(ur(Mn));
}), kn = Fn(kn, (Mn) => {
if (Mn.type === "ParenthesizedExpression") {
let { expression: ut } = Mn;
if (ut.type === "TypeCastExpression")
return ut.range = Mn.range, ut;
let On = ur(Mn);
if (!hn.has(On))
return ut.extra = Object.assign(Object.assign({}, ut.extra), {}, { parenthesized: true }), ut;
}
});
}
return kn = Fn(kn, (hn) => {
switch (hn.type) {
case "ChainExpression":
return at(hn.expression);
case "LogicalExpression": {
if (Zt(hn))
return Ut(hn);
break;
}
case "VariableDeclaration": {
let Mn = Le(hn.declarations);
Mn && Mn.init && rf(hn, Mn);
break;
}
case "TSParenthesizedType":
return le(hn.typeAnnotation) || hn.typeAnnotation.type === "TSThisType" || (hn.typeAnnotation.range = [ur(hn), hr(hn)]), hn.typeAnnotation;
case "TSTypeParameter":
if (typeof hn.name == "string") {
let Mn = ur(hn);
hn.name = { type: "Identifier", name: hn.name, range: [Mn, Mn + hn.name.length] };
}
break;
case "ObjectExpression":
if (Qt.parser === "typescript") {
let Mn = hn.properties.find((ut) => ut.type === "Property" && ut.value.type === "TSEmptyBodyFunctionExpression");
Mn && gn(Mn.value, "Unexpected token.");
}
break;
case "SequenceExpression": {
let Mn = Le(hn.expressions);
hn.range = [ur(hn), Math.min(hr(Mn), hr(hn))];
break;
}
case "TopicReference":
Qt.__isUsingHackPipeline = true;
break;
case "ExportAllDeclaration": {
let { exported: Mn } = hn;
if (Qt.parser === "meriyah" && Mn && Mn.type === "Identifier") {
let ut = Qt.originalText.slice(ur(Mn), hr(Mn));
(ut.startsWith('"') || ut.startsWith("'")) && (hn.exported = Object.assign(Object.assign({}, hn.exported), {}, { type: "Literal", value: hn.exported.name, raw: ut }));
}
break;
}
case "PropertyDefinition":
if (Qt.parser === "meriyah" && hn.static && !hn.computed && !hn.key) {
let Mn = "static", ut = ur(hn);
Object.assign(hn, { static: false, key: { type: "Identifier", name: Mn, range: [ut, ut + Mn.length] } });
}
break;
}
}), kn;
function rf(hn, Mn) {
Qt.originalText[hr(Mn)] !== ";" && (hn.range = [ur(hn), hr(Mn)]);
}
}
function at(kn) {
switch (kn.type) {
case "CallExpression":
kn.type = "OptionalCallExpression", kn.callee = at(kn.callee);
break;
case "MemberExpression":
kn.type = "OptionalMemberExpression", kn.object = at(kn.object);
break;
case "TSNonNullExpression":
kn.expression = at(kn.expression);
break;
}
return kn;
}
function Zt(kn) {
return kn.type === "LogicalExpression" && kn.right.type === "LogicalExpression" && kn.operator === kn.right.operator;
}
function Ut(kn) {
return Zt(kn) ? Ut({ type: "LogicalExpression", operator: kn.operator, left: Ut({ type: "LogicalExpression", operator: kn.operator, left: kn.left, right: kn.right.left, range: [ur(kn.left), hr(kn.right.left)] }), right: kn.right.right, range: [ur(kn), hr(kn)] }) : kn;
}
j0.exports = et;
} }), u70 = {};
iU(u70, { default: () => i70 });
var i70, wae = L_({ "node-modules-polyfills:fs"() {
Lt(), i70 = {};
} }), Fj = au({ "node-modules-polyfills-commonjs:fs"(A0, j0) {
Lt();
var ur = (wae(), fU(u70));
if (ur && ur.default) {
j0.exports = ur.default;
for (let hr in ur)
j0.exports[hr] = ur[hr];
} else
ur && (j0.exports = ur);
} }), f70 = {};
iU(f70, { ALPN_ENABLED: () => Gq, COPYFILE_EXCL: () => jB, COPYFILE_FICLONE: () => MB, COPYFILE_FICLONE_FORCE: () => qB, DH_CHECK_P_NOT_PRIME: () => Lq, DH_CHECK_P_NOT_SAFE_PRIME: () => Dq, DH_NOT_SUITABLE_GENERATOR: () => jq, DH_UNABLE_TO_CHECK_GENERATOR: () => Rq, E2BIG: () => Dj, EACCES: () => Lj, EADDRINUSE: () => Rj, EADDRNOTAVAIL: () => jj, EAFNOSUPPORT: () => Gj, EAGAIN: () => Mj, EALREADY: () => Bj, EBADF: () => qj, EBADMSG: () => Uj, EBUSY: () => Hj, ECANCELED: () => Xj, ECHILD: () => Yj, ECONNABORTED: () => Vj, ECONNREFUSED: () => zj, ECONNRESET: () => Kj, EDEADLK: () => Wj, EDESTADDRREQ: () => Jj, EDOM: () => $j, EDQUOT: () => Zj, EEXIST: () => Qj, EFAULT: () => rG, EFBIG: () => eG, EHOSTUNREACH: () => nG, EIDRM: () => tG, EILSEQ: () => uG, EINPROGRESS: () => iG, EINTR: () => fG, EINVAL: () => xG, EIO: () => aG, EISCONN: () => oG, EISDIR: () => cG, ELOOP: () => sG, EMFILE: () => vG, EMLINK: () => lG, EMSGSIZE: () => bG, EMULTIHOP: () => pG, ENAMETOOLONG: () => mG, ENETDOWN: () => _G, ENETRESET: () => yG, ENETUNREACH: () => dG, ENFILE: () => hG, ENGINE_METHOD_ALL: () => Cq, ENGINE_METHOD_CIPHERS: () => Oq, ENGINE_METHOD_DH: () => gq, ENGINE_METHOD_DIGESTS: () => Iq, ENGINE_METHOD_DSA: () => Sq, ENGINE_METHOD_EC: () => Tq, ENGINE_METHOD_NONE: () => Pq, ENGINE_METHOD_PKEY_ASN1_METHS: () => Nq, ENGINE_METHOD_PKEY_METHS: () => Aq, ENGINE_METHOD_RAND: () => Fq, ENGINE_METHOD_RSA: () => Eq, ENOBUFS: () => kG, ENODATA: () => wG, ENODEV: () => EG, ENOENT: () => SG, ENOEXEC: () => gG, ENOLCK: () => FG, ENOLINK: () => TG, ENOMEM: () => OG, ENOMSG: () => IG, ENOPROTOOPT: () => AG, ENOSPC: () => NG, ENOSR: () => CG, ENOSTR: () => PG, ENOSYS: () => DG, ENOTCONN: () => LG, ENOTDIR: () => RG, ENOTEMPTY: () => jG, ENOTSOCK: () => GG, ENOTSUP: () => MG, ENOTTY: () => BG, ENXIO: () => qG, EOPNOTSUPP: () => UG, EOVERFLOW: () => HG, EPERM: () => XG, EPIPE: () => YG, EPROTO: () => VG, EPROTONOSUPPORT: () => zG, EPROTOTYPE: () => KG, ERANGE: () => WG, EROFS: () => JG, ESPIPE: () => $G, ESRCH: () => ZG, ESTALE: () => QG, ETIME: () => rM, ETIMEDOUT: () => eM, ETXTBSY: () => nM, EWOULDBLOCK: () => tM, EXDEV: () => uM, F_OK: () => CB, OPENSSL_VERSION_NUMBER: () => UB, O_APPEND: () => lB, O_CREAT: () => oB, O_DIRECTORY: () => bB, O_DSYNC: () => _B, O_EXCL: () => cB, O_NOCTTY: () => sB, O_NOFOLLOW: () => pB, O_NONBLOCK: () => dB, O_RDONLY: () => XM, O_RDWR: () => VM, O_SYMLINK: () => yB, O_SYNC: () => mB, O_TRUNC: () => vB, O_WRONLY: () => YM, POINT_CONVERSION_COMPRESSED: () => Qq, POINT_CONVERSION_HYBRID: () => eU, POINT_CONVERSION_UNCOMPRESSED: () => rU, PRIORITY_ABOVE_NORMAL: () => aM, PRIORITY_BELOW_NORMAL: () => fM, PRIORITY_HIGH: () => oM, PRIORITY_HIGHEST: () => cM, PRIORITY_LOW: () => iM, PRIORITY_NORMAL: () => xM, RSA_NO_PADDING: () => qq, RSA_PKCS1_OAEP_PADDING: () => Uq, RSA_PKCS1_PADDING: () => Mq, RSA_PKCS1_PSS_PADDING: () => Xq, RSA_PSS_SALTLEN_AUTO: () => zq, RSA_PSS_SALTLEN_DIGEST: () => Yq, RSA_PSS_SALTLEN_MAX_SIGN: () => Vq, RSA_SSLV23_PADDING: () => Bq, RSA_X931_PADDING: () => Hq, RTLD_GLOBAL: () => Cj, RTLD_LAZY: () => Aj, RTLD_LOCAL: () => Pj, RTLD_NOW: () => Nj, R_OK: () => PB, SIGABRT: () => mM, SIGALRM: () => gM, SIGBUS: () => yM, SIGCHLD: () => TM, SIGCONT: () => OM, SIGFPE: () => dM, SIGHUP: () => sM, SIGILL: () => bM, SIGINFO: () => BM, SIGINT: () => vM, SIGIO: () => MM, SIGIOT: () => _M, SIGKILL: () => hM, SIGPIPE: () => SM, SIGPROF: () => jM, SIGQUIT: () => lM, SIGSEGV: () => wM, SIGSTOP: () => IM, SIGSYS: () => qM, SIGTERM: () => FM, SIGTRAP: () => pM, SIGTSTP: () => AM, SIGTTIN: () => NM, SIGTTOU: () => CM, SIGURG: () => PM, SIGUSR1: () => kM, SIGUSR2: () => EM, SIGVTALRM: () => RM, SIGWINCH: () => GM, SIGXCPU: () => DM, SIGXFSZ: () => LM, SSL_OP_ALL: () => HB, SSL_OP_ALLOW_UNSAFE_LEGACY_RENEGOTIATION: () => XB, SSL_OP_CIPHER_SERVER_PREFERENCE: () => YB, SSL_OP_CISCO_ANYCONNECT: () => VB, SSL_OP_COOKIE_EXCHANGE: () => zB, SSL_OP_CRYPTOPRO_TLSEXT_BUG: () => KB, SSL_OP_DONT_INSERT_EMPTY_FRAGMENTS: () => WB, SSL_OP_EPHEMERAL_RSA: () => JB, SSL_OP_LEGACY_SERVER_CONNECT: () => $B, SSL_OP_MICROSOFT_BIG_SSLV3_BUFFER: () => ZB, SSL_OP_MICROSOFT_SESS_ID_BUG: () => QB, SSL_OP_MSIE_SSLV2_RSA_PADDING: () => rq, SSL_OP_NETSCAPE_CA_DN_BUG: () => eq, SSL_OP_NETSCAPE_CHALLENGE_BUG: () => nq, SSL_OP_NETSCAPE_DEMO_CIPHER_CHANGE_BUG: () => tq, SSL_OP_NETSCAPE_REUSE_CIPHER_CHANGE_BUG: () => uq, SSL_OP_NO_COMPRESSION: () => iq, SSL_OP_NO_QUERY_MTU: () => fq, SSL_OP_NO_SESSION_RESUMPTION_ON_RENEGOTIATION: () => xq, SSL_OP_NO_SSLv2: () => aq, SSL_OP_NO_SSLv3: () => oq, SSL_OP_NO_TICKET: () => cq, SSL_OP_NO_TLSv1: () => sq, SSL_OP_NO_TLSv1_1: () => vq, SSL_OP_NO_TLSv1_2: () => lq, SSL_OP_PKCS1_CHECK_1: () => bq, SSL_OP_PKCS1_CHECK_2: () => pq, SSL_OP_SINGLE_DH_USE: () => mq, SSL_OP_SINGLE_ECDH_USE: () => _q, SSL_OP_SSLEAY_080_CLIENT_DH_BUG: () => yq, SSL_OP_SSLREF2_REUSE_CERT_TYPE_BUG: () => dq, SSL_OP_TLS_BLOCK_PADDING_BUG: () => hq, SSL_OP_TLS_D5_BUG: () => kq, SSL_OP_TLS_ROLLBACK_BUG: () => wq, S_IFBLK: () => iB, S_IFCHR: () => uB, S_IFDIR: () => tB, S_IFIFO: () => fB, S_IFLNK: () => xB, S_IFMT: () => eB, S_IFREG: () => nB, S_IFSOCK: () => aB, S_IRGRP: () => gB, S_IROTH: () => IB, S_IRUSR: () => kB, S_IRWXG: () => SB, S_IRWXO: () => OB, S_IRWXU: () => hB, S_IWGRP: () => FB, S_IWOTH: () => AB, S_IWUSR: () => wB, S_IXGRP: () => TB, S_IXOTH: () => NB, S_IXUSR: () => EB, TLS1_1_VERSION: () => Jq, TLS1_2_VERSION: () => $q, TLS1_3_VERSION: () => Zq, TLS1_VERSION: () => Wq, UV_DIRENT_BLOCK: () => rB, UV_DIRENT_CHAR: () => QM, UV_DIRENT_DIR: () => WM, UV_DIRENT_FIFO: () => $M, UV_DIRENT_FILE: () => KM, UV_DIRENT_LINK: () => JM, UV_DIRENT_SOCKET: () => ZM, UV_DIRENT_UNKNOWN: () => zM, UV_FS_COPYFILE_EXCL: () => RB, UV_FS_COPYFILE_FICLONE: () => GB, UV_FS_COPYFILE_FICLONE_FORCE: () => BB, UV_FS_SYMLINK_DIR: () => UM, UV_FS_SYMLINK_JUNCTION: () => HM, W_OK: () => DB, X_OK: () => LB, default: () => x70, defaultCipherList: () => nU, defaultCoreCipherList: () => Kq });
var Aj, Nj, Cj, Pj, Dj, Lj, Rj, jj, Gj, Mj, Bj, qj, Uj, Hj, Xj, Yj, Vj, zj, Kj, Wj, Jj, $j, Zj, Qj, rG, eG, nG, tG, uG, iG, fG, xG, aG, oG, cG, sG, vG, lG, bG, pG, mG, _G, yG, dG, hG, kG, wG, EG, SG, gG, FG, TG, OG, IG, AG, NG, CG, PG, DG, LG, RG, jG, GG, MG, BG, qG, UG, HG, XG, YG, VG, zG, KG, WG, JG, $G, ZG, QG, rM, eM, nM, tM, uM, iM, fM, xM, aM, oM, cM, sM, vM, lM, bM, pM, mM, _M, yM, dM, hM, kM, wM, EM, SM, gM, FM, TM, OM, IM, AM, NM, CM, PM, DM, LM, RM, jM, GM, MM, BM, qM, UM, HM, XM, YM, VM, zM, KM, WM, JM, $M, ZM, QM, rB, eB, nB, tB, uB, iB, fB, xB, aB, oB, cB, sB, vB, lB, bB, pB, mB, _B, yB, dB, hB, kB, wB, EB, SB, gB, FB, TB, OB, IB, AB, NB, CB, PB, DB, LB, RB, jB, GB, MB, BB, qB, UB, HB, XB, YB, VB, zB, KB, WB, JB, $B, ZB, QB, rq, eq, nq, tq, uq, iq, fq, xq, aq, oq, cq, sq, vq, lq, bq, pq, mq, _q, yq, dq, hq, kq, wq, Eq, Sq, gq, Fq, Tq, Oq, Iq, Aq, Nq, Cq, Pq, Dq, Lq, Rq, jq, Gq, Mq, Bq, qq, Uq, Hq, Xq, Yq, Vq, zq, Kq, Wq, Jq, $q, Zq, Qq, rU, eU, nU, x70, Eae = L_({ "node-modules-polyfills:constants"() {
Lt(), Aj = 1, Nj = 2, Cj = 8, Pj = 4, Dj = 7, Lj = 13, Rj = 48, jj = 49, Gj = 47, Mj = 35, Bj = 37, qj = 9, Uj = 94, Hj = 16, Xj = 89, Yj = 10, Vj = 53, zj = 61, Kj = 54, Wj = 11, Jj = 39, $j = 33, Zj = 69, Qj = 17, rG = 14, eG = 27, nG = 65, tG = 90, uG = 92, iG = 36, fG = 4, xG = 22, aG = 5, oG = 56, cG = 21, sG = 62, vG = 24, lG = 31, bG = 40, pG = 95, mG = 63, _G = 50, yG = 52, dG = 51, hG = 23, kG = 55, wG = 96, EG = 19, SG = 2, gG = 8, FG = 77, TG = 97, OG = 12, IG = 91, AG = 42, NG = 28, CG = 98, PG = 99, DG = 78, LG = 57, RG = 20, jG = 66, GG = 38, MG = 45, BG = 25, qG = 6, UG = 102, HG = 84, XG = 1, YG = 32, VG = 100, zG = 43, KG = 41, WG = 34, JG = 30, $G = 29, ZG = 3, QG = 70, rM = 101, eM = 60, nM = 26, tM = 35, uM = 18, iM = 19, fM = 10, xM = 0, aM = -7, oM = -14, cM = -20, sM = 1, vM = 2, lM = 3, bM = 4, pM = 5, mM = 6, _M = 6, yM = 10, dM = 8, hM = 9, kM = 30, wM = 11, EM = 31, SM = 13, gM = 14, FM = 15, TM = 20, OM = 19, IM = 17, AM = 18, NM = 21, CM = 22, PM = 16, DM = 24, LM = 25, RM = 26, jM = 27, GM = 28, MM = 23, BM = 29, qM = 12, UM = 1, HM = 2, XM = 0, YM = 1, VM = 2, zM = 0, KM = 1, WM = 2, JM = 3, $M = 4, ZM = 5, QM = 6, rB = 7, eB = 61440, nB = 32768, tB = 16384, uB = 8192, iB = 24576, fB = 4096, xB = 40960, aB = 49152, oB = 512, cB = 2048, sB = 131072, vB = 1024, lB = 8, bB = 1048576, pB = 256, mB = 128, _B = 4194304, yB = 2097152, dB = 4, hB = 448, kB = 256, wB = 128, EB = 64, SB = 56, gB = 32, FB = 16, TB = 8, OB = 7, IB = 4, AB = 2, NB = 1, CB = 0, PB = 4, DB = 2, LB = 1, RB = 1, jB = 1, GB = 2, MB = 2, BB = 4, qB = 4, UB = 269488175, HB = 2147485780, XB = 262144, YB = 4194304, VB = 32768, zB = 8192, KB = 2147483648, WB = 2048, JB = 0, $B = 4, ZB = 0, QB = 0, rq = 0, eq = 0, nq = 0, tq = 0, uq = 0, iq = 131072, fq = 4096, xq = 65536, aq = 0, oq = 33554432, cq = 16384, sq = 67108864, vq = 268435456, lq = 134217728, bq = 0, pq = 0, mq = 0, _q = 0, yq = 0, dq = 0, hq = 0, kq = 0, wq = 8388608, Eq = 1, Sq = 2, gq = 4, Fq = 8, Tq = 2048, Oq = 64, Iq = 128, Aq = 512, Nq = 1024, Cq = 65535, Pq = 0, Dq = 2, Lq = 1, Rq = 4, jq = 8, Gq = 1, Mq = 1, Bq = 2, qq = 3, Uq = 4, Hq = 5, Xq = 6, Yq = -1, Vq = -2, zq = -2, Kq = "TLS_AES_256_GCM_SHA384:TLS_CHACHA20_POLY1305_SHA256:TLS_AES_128_GCM_SHA256:ECDHE-RSA-AES128-GCM-SHA256:ECDHE-ECDSA-AES128-GCM-SHA256:ECDHE-RSA-AES256-GCM-SHA384:ECDHE-ECDSA-AES256-GCM-SHA384:DHE-RSA-AES128-GCM-SHA256:ECDHE-RSA-AES128-SHA256:DHE-RSA-AES128-SHA256:ECDHE-RSA-AES256-SHA384:DHE-RSA-AES256-SHA384:ECDHE-RSA-AES256-SHA256:DHE-RSA-AES256-SHA256:HIGH:!aNULL:!eNULL:!EXPORT:!DES:!RC4:!MD5:!PSK:!SRP:!CAMELLIA", Wq = 769, Jq = 770, $q = 771, Zq = 772, Qq = 2, rU = 4, eU = 6, nU = "TLS_AES_256_GCM_SHA384:TLS_CHACHA20_POLY1305_SHA256:TLS_AES_128_GCM_SHA256:ECDHE-RSA-AES128-GCM-SHA256:ECDHE-ECDSA-AES128-GCM-SHA256:ECDHE-RSA-AES256-GCM-SHA384:ECDHE-ECDSA-AES256-GCM-SHA384:DHE-RSA-AES128-GCM-SHA256:ECDHE-RSA-AES128-SHA256:DHE-RSA-AES128-SHA256:ECDHE-RSA-AES256-SHA384:DHE-RSA-AES256-SHA384:ECDHE-RSA-AES256-SHA256:DHE-RSA-AES256-SHA256:HIGH:!aNULL:!eNULL:!EXPORT:!DES:!RC4:!MD5:!PSK:!SRP:!CAMELLIA", x70 = { RTLD_LAZY: Aj, RTLD_NOW: Nj, RTLD_GLOBAL: Cj, RTLD_LOCAL: Pj, E2BIG: Dj, EACCES: Lj, EADDRINUSE: Rj, EADDRNOTAVAIL: jj, EAFNOSUPPORT: Gj, EAGAIN: Mj, EALREADY: Bj, EBADF: qj, EBADMSG: Uj, EBUSY: Hj, ECANCELED: Xj, ECHILD: Yj, ECONNABORTED: Vj, ECONNREFUSED: zj, ECONNRESET: Kj, EDEADLK: Wj, EDESTADDRREQ: Jj, EDOM: $j, EDQUOT: Zj, EEXIST: Qj, EFAULT: rG, EFBIG: eG, EHOSTUNREACH: nG, EIDRM: tG, EILSEQ: uG, EINPROGRESS: iG, EINTR: fG, EINVAL: xG, EIO: aG, EISCONN: oG, EISDIR: cG, ELOOP: sG, EMFILE: vG, EMLINK: lG, EMSGSIZE: bG, EMULTIHOP: pG, ENAMETOOLONG: mG, ENETDOWN: _G, ENETRESET: yG, ENETUNREACH: dG, ENFILE: hG, ENOBUFS: kG, ENODATA: wG, ENODEV: EG, ENOENT: SG, ENOEXEC: gG, ENOLCK: FG, ENOLINK: TG, ENOMEM: OG, ENOMSG: IG, ENOPROTOOPT: AG, ENOSPC: NG, ENOSR: CG, ENOSTR: PG, ENOSYS: DG, ENOTCONN: LG, ENOTDIR: RG, ENOTEMPTY: jG, ENOTSOCK: GG, ENOTSUP: MG, ENOTTY: BG, ENXIO: qG, EOPNOTSUPP: UG, EOVERFLOW: HG, EPERM: XG, EPIPE: YG, EPROTO: VG, EPROTONOSUPPORT: zG, EPROTOTYPE: KG, ERANGE: WG, EROFS: JG, ESPIPE: $G, ESRCH: ZG, ESTALE: QG, ETIME: rM, ETIMEDOUT: eM, ETXTBSY: nM, EWOULDBLOCK: tM, EXDEV: uM, PRIORITY_LOW: iM, PRIORITY_BELOW_NORMAL: fM, PRIORITY_NORMAL: xM, PRIORITY_ABOVE_NORMAL: aM, PRIORITY_HIGH: oM, PRIORITY_HIGHEST: cM, SIGHUP: sM, SIGINT: vM, SIGQUIT: lM, SIGILL: bM, SIGTRAP: pM, SIGABRT: mM, SIGIOT: _M, SIGBUS: yM, SIGFPE: dM, SIGKILL: hM, SIGUSR1: kM, SIGSEGV: wM, SIGUSR2: EM, SIGPIPE: SM, SIGALRM: gM, SIGTERM: FM, SIGCHLD: TM, SIGCONT: OM, SIGSTOP: IM, SIGTSTP: AM, SIGTTIN: NM, SIGTTOU: CM, SIGURG: PM, SIGXCPU: DM, SIGXFSZ: LM, SIGVTALRM: RM, SIGPROF: jM, SIGWINCH: GM, SIGIO: MM, SIGINFO: BM, SIGSYS: qM, UV_FS_SYMLINK_DIR: UM, UV_FS_SYMLINK_JUNCTION: HM, O_RDONLY: XM, O_WRONLY: YM, O_RDWR: VM, UV_DIRENT_UNKNOWN: zM, UV_DIRENT_FILE: KM, UV_DIRENT_DIR: WM, UV_DIRENT_LINK: JM, UV_DIRENT_FIFO: $M, UV_DIRENT_SOCKET: ZM, UV_DIRENT_CHAR: QM, UV_DIRENT_BLOCK: rB, S_IFMT: eB, S_IFREG: nB, S_IFDIR: tB, S_IFCHR: uB, S_IFBLK: iB, S_IFIFO: fB, S_IFLNK: xB, S_IFSOCK: aB, O_CREAT: oB, O_EXCL: cB, O_NOCTTY: sB, O_TRUNC: vB, O_APPEND: lB, O_DIRECTORY: bB, O_NOFOLLOW: pB, O_SYNC: mB, O_DSYNC: _B, O_SYMLINK: yB, O_NONBLOCK: dB, S_IRWXU: hB, S_IRUSR: kB, S_IWUSR: wB, S_IXUSR: EB, S_IRWXG: SB, S_IRGRP: gB, S_IWGRP: FB, S_IXGRP: TB, S_IRWXO: OB, S_IROTH: IB, S_IWOTH: AB, S_IXOTH: NB, F_OK: CB, R_OK: PB, W_OK: DB, X_OK: LB, UV_FS_COPYFILE_EXCL: RB, COPYFILE_EXCL: jB, UV_FS_COPYFILE_FICLONE: GB, COPYFILE_FICLONE: MB, UV_FS_COPYFILE_FICLONE_FORCE: BB, COPYFILE_FICLONE_FORCE: qB, OPENSSL_VERSION_NUMBER: UB, SSL_OP_ALL: HB, SSL_OP_ALLOW_UNSAFE_LEGACY_RENEGOTIATION: XB, SSL_OP_CIPHER_SERVER_PREFERENCE: YB, SSL_OP_CISCO_ANYCONNECT: VB, SSL_OP_COOKIE_EXCHANGE: zB, SSL_OP_CRYPTOPRO_TLSEXT_BUG: KB, SSL_OP_DONT_INSERT_EMPTY_FRAGMENTS: WB, SSL_OP_EPHEMERAL_RSA: JB, SSL_OP_LEGACY_SERVER_CONNECT: $B, SSL_OP_MICROSOFT_BIG_SSLV3_BUFFER: ZB, SSL_OP_MICROSOFT_SESS_ID_BUG: QB, SSL_OP_MSIE_SSLV2_RSA_PADDING: rq, SSL_OP_NETSCAPE_CA_DN_BUG: eq, SSL_OP_NETSCAPE_CHALLENGE_BUG: nq, SSL_OP_NETSCAPE_DEMO_CIPHER_CHANGE_BUG: tq, SSL_OP_NETSCAPE_REUSE_CIPHER_CHANGE_BUG: uq, SSL_OP_NO_COMPRESSION: iq, SSL_OP_NO_QUERY_MTU: fq, SSL_OP_NO_SESSION_RESUMPTION_ON_RENEGOTIATION: xq, SSL_OP_NO_SSLv2: aq, SSL_OP_NO_SSLv3: oq, SSL_OP_NO_TICKET: cq, SSL_OP_NO_TLSv1: sq, SSL_OP_NO_TLSv1_1: vq, SSL_OP_NO_TLSv1_2: lq, SSL_OP_PKCS1_CHECK_1: bq, SSL_OP_PKCS1_CHECK_2: pq, SSL_OP_SINGLE_DH_USE: mq, SSL_OP_SINGLE_ECDH_USE: _q, SSL_OP_SSLEAY_080_CLIENT_DH_BUG: yq, SSL_OP_SSLREF2_REUSE_CERT_TYPE_BUG: dq, SSL_OP_TLS_BLOCK_PADDING_BUG: hq, SSL_OP_TLS_D5_BUG: kq, SSL_OP_TLS_ROLLBACK_BUG: wq, ENGINE_METHOD_RSA: Eq, ENGINE_METHOD_DSA: Sq, ENGINE_METHOD_DH: gq, ENGINE_METHOD_RAND: Fq, ENGINE_METHOD_EC: Tq, ENGINE_METHOD_CIPHERS: Oq, ENGINE_METHOD_DIGESTS: Iq, ENGINE_METHOD_PKEY_METHS: Aq, ENGINE_METHOD_PKEY_ASN1_METHS: Nq, ENGINE_METHOD_ALL: Cq, ENGINE_METHOD_NONE: Pq, DH_CHECK_P_NOT_SAFE_PRIME: Dq, DH_CHECK_P_NOT_PRIME: Lq, DH_UNABLE_TO_CHECK_GENERATOR: Rq, DH_NOT_SUITABLE_GENERATOR: jq, ALPN_ENABLED: Gq, RSA_PKCS1_PADDING: Mq, RSA_SSLV23_PADDING: Bq, RSA_NO_PADDING: qq, RSA_PKCS1_OAEP_PADDING: Uq, RSA_X931_PADDING: Hq, RSA_PKCS1_PSS_PADDING: Xq, RSA_PSS_SALTLEN_DIGEST: Yq, RSA_PSS_SALTLEN_MAX_SIGN: Vq, RSA_PSS_SALTLEN_AUTO: zq, defaultCoreCipherList: Kq, TLS1_VERSION: Wq, TLS1_1_VERSION: Jq, TLS1_2_VERSION: $q, TLS1_3_VERSION: Zq, POINT_CONVERSION_COMPRESSED: Qq, POINT_CONVERSION_UNCOMPRESSED: rU, POINT_CONVERSION_HYBRID: eU, defaultCipherList: nU };
} }), Sae = au({ "node-modules-polyfills-commonjs:constants"(A0, j0) {
Lt();
var ur = (Eae(), fU(f70));
if (ur && ur.default) {
j0.exports = ur.default;
for (let hr in ur)
j0.exports[hr] = ur[hr];
} else
ur && (j0.exports = ur);
} }), gae = au({ "node_modules/flow-parser/flow_parser.js"(A0) {
Lt(), function(j0) {
"use strict";
var ur = "member_property_expression", hr = 8483, le = 12538, Ve = "children", Le = "predicate_expression", Fn = "??", gn = "Identifier", et = 64311, at = 192, Zt = 11710, Ut = 122654, kn = 110947, Qt = 67591, rf = "!", hn = "directive", Mn = 163, ut = "block", On = 126553, ru = 12735, E7 = 68096, Ct = "params", Ss = 93071, In = 122, Jc = 72767, Ai = 181, vi = "for_statement", Rt = 128, S7 = "start", ov = 43867, xU = "_method", R_ = 70414, cv = ">", ef = "catch_body", j_ = 120121, aU = "the end of an expression statement (`;`)", G_ = 124907, oU = 1027, v4 = 126558, nf = "jsx_fragment", M_ = 42527, B_ = "decorators", q_ = 82943, U_ = 71039, H_ = 110882, X_ = 67514, cU = 8472, sU = "update", Y_ = 12783, V_ = 12438, z_ = 12352, K_ = 8511, W_ = 42961, F2 = "method", l4 = 120713, tf = 8191, uf = "function_param", J_ = 67871, g7 = "throw", $_ = 11507, ff = "class_extends", Z_ = 43470, xf = "object_key_literal", Q_ = 71903, ry = 65437, af = "jsx_child", ey = 43311, b4 = 119995, ny = 67637, p4 = 68116, ty = 66204, uy = 65470, vU = "<<=", iy = "e", fy = 67391, m4 = 11631, _4 = 69956, sv = "tparams", xy = 66735, ay = 64217, oy = 43697, lU = "Invalid binary/octal ", cy = -43, sy = 43255, y4 = "do", vy = 43301, of = "binding_pattern", ly = 120487, cf = "jsx_attribute_value_literal", d4 = "package", sf = "interface_declaration", by = 72750, py = 119892, bU = "tail", pU = -53, vf = 111, mU = 180, my = 119807, _y = 71959, _U = 8206, yy = 65613, $c = "type", dy = 55215, hy = -42, lf = "export_default_declaration_decl", h4 = 72970, yU = "filtered_out", ky = 70416, dU = 229, bf = "function_this_param", hU = "module", k4 = "try", wy = 70143, Ey = 125183, Sy = 70412, h0 = "@])", pf = "binary", kU = "infinity", w4 = "private", gy = 65500, E4 = "has_unknown_members", mf = "pattern_array_rest_element", wU = "Property", gs = "implements", Fy = 12548, EU = 211, _f = "if_alternate_statement", Ty = 124903, Oy = 43395, vv = "src/parser/type_parser.ml", Iy = 66915, S4 = 126552, Ay = 120712, g4 = 126555, Ny = 120596, o7 = "raw", F7 = 112, yf = "class_declaration", df = "statement", Cy = 126624, Py = 71235, hf = "meta_property", Dy = 44002, Ly = 8467, kf = "class_property_value", Ry = 8318, wf = "optional_call", jy = 43761, Zc = "kind", Ef = "class_identifier", Gy = 69955, My = 66378, By = 120512, qy = 68220, Ht = 110, Uy = 123583, T2 = "declare", Sf = "typeof_member_identifier", gf = "catch_clause", Hy = 11742, Xy = 70831, F4 = 8468, Ff = "for_in_assignment_pattern", SU = -32, Tf = "object_", Yy = 43262, Vy = "mixins", Of = "type_param", gU = "visit_trailing_comment", zy = 71839, O2 = "boolean", If = "call", FU = "expected *", Ky = 43010, Wy = 241, Au = "expression", I2 = "column", Jy = 43595, $y = 43258, Zy = 191456, Af = "member_type_identifier", A2 = 117, Qy = 43754, T4 = 126544, TU = "Assert_failure", rd = 66517, ed = 42964, Nf = "enum_number_member", OU = "a string", nd = 65855, td = 119993, ud = "opaque", IU = 870530776, id = 67711, fd = 66994, Cf = "enum_symbol_body", AU = 185, NU = 219, O4 = "filter", xd = 43615, I4 = 126560, ad = 19903, t1 = "get", od = 64316, CU = `Fatal error: exception %s
`, A4 = "exported", PU = ">=", Wu = "return", N4 = "members", C4 = 256, cd = 66962, sd = 64279, vd = 67829, DU = "Enum `", LU = "&&=", Pf = "object_property", ld = 67589, Df = "pattern_object_property", Lf = "template_literal_element", bd = 69551, Ni = 127343600, P4 = 70452, Rf = "class_element", pd = "ENOENT", md = 71131, RU = 200, _d = 120137, yd = 94098, D4 = 72349, jU = 1328, jf = "function_identifier", dd = 126543, Gf = "jsx_attribute_name", hd = 43487, kr = "@[<2>{ ", GU = "ENOTEMPTY", kd = 65908, wd = 72191, L4 = 120513, Ed = 92909, MU = "bound", Sd = 162, BU = 172, R4 = 120070, Mf = "enum_number_body", Bf = "update_expression", qf = "spread_element", Uf = "for_in_left_declaration", j4 = 64319, N2 = "%d", gd = 12703, G4 = 11687, qU = "@,))@]", Fd = 42239, Hf = "type_cast", Td = 42508, Xf = "class_implements_interface", Od = 67640, Id = 605857695, UU = "Cygwin", HU = "buffer.ml", Ad = 124908, XU = "handler", Nd = 66207, Cd = 66963, M4 = 11558, YU = "-=", Pn = 113, Pd = 113775, VU = "collect_comments", B4 = 126540, lv = "set", Yf = "assignment_pattern", Nu = "right", Vf = "object_key_identifier", q4 = 120133, Dd = "Invalid number ", Ld = 42963, U4 = 12539, Rd = 68023, jd = 43798, ni = 100, zf = "pattern_literal", Kf = "generic_type", zU = "*", Gd = 42783, Md = 42890, Bd = 230, H4 = "else", qd = 70851, Ud = 69289, KU = "the start of a statement", X4 = "properties", Hd = 43696, Xd = 110959, Wf = "declare_function", Y4 = 120597, Jf = "object_indexer_property_type", Yd = 70492, Vd = 2048, C2 = "arguments", Xr = "comments", zd = 43042, Qc = 107, Kd = 110575, WU = 161, Wd = 67431, V4 = "line", P2 = "declaration", eu = "static", $f = "pattern_identifier", Jd = 69958, JU = "the", $d = "Unix.Unix_error", Zd = 43814, rs = "annot", Qd = 65786, rh = 66303, eh = 64967, nh = 64255, th = 8584, z4 = 120655, $U = "Stack_overflow", uh = 43700, Zf = "syntax_opt", ZU = "/static/", Qf = "comprehension", ih = 253, QU = "Not_found", rH = "+=", eH = 235, fh = 68680, xh = 66954, ah = 64324, oh = 72966, nH = 174, tH = -1053382366, ch = "rest", rx = "pattern_array_element", ex = "jsx_attribute_value_expression", K4 = 65595, nx = "pattern_array_e", uH = 243, sh = 43711, vh = "rmdir", W4 = "symbol", lh = 69926, J4 = "*dummy method*", bh = 43741, T7 = "typeParameters", D2 = "const", iH = 1026, fH = 149, ph = 12341, mh = 72847, _h = 66993, xH = 202, Ci = "false", Xt = 106, yh = 120076, dh = 186, Pi = 128, hh = 125124, kh = "Fatal error: exception ", $4 = 67593, wh = 69297, Eh = 44031, aH = 234, Sh = 92927, gh = 68095, Ju = 8231, tx = "object_key_computed", ux = "labeled_statement", ix = "function_param_pattern", Z4 = 126590, Fh = 65481, Th = 43442, oH = "collect_comments_opt", fx = "variable_declarator", bv = "_", Oh = "compare: functional value", Ih = 67967, pv = "computed", xx = "object_property_type", mt = "id", Ah = 126562, u1 = 114, cH = "comment_bounds", Nh = 70853, Ch = 69247, ax = "class_private_field", Ph = 42237, Dh = 72329, sH = "Invalid_argument", Lh = 113770, Q4 = 94031, Rh = 120092, ox = "declare_class", jh = 67839, Gh = 72250, vH = "%ni", Mh = 92879, lH = "prototype", Fs = "`.", cx = 8287, r8 = 65344, Bh = "&", O7 = "debugger", sx = "type_identifier_reference", bH = "Internal Error: Found private field in object props", vx = "sequence", lx = "call_type_args", pH = 238, qh = 12348, mH = "++", Uh = 68863, Hh = 72001, Xh = 70084, Yh = "label", mv = -45, bx = "jsx_opening_attribute", Vh = 43583, e8 = "%F", zh = 43784, Kh = 113791, px = "call_arguments", n8 = 126503, Wh = 43743, $u = "0", Jh = 119967, t8 = 126538, mx = "new_", _v = 449540197, $h = 64109, Zh = 68466, Qh = 177983, wt = 248, _x = "program", Xe = "@,]@]", rk = 68031, yx = "function_type", dx = "type_", u8 = 8484, ek = 67382, nk = 42537, tk = 226, uk = 66559, ik = 42993, fk = 64274, i8 = 71236, xk = 120069, ak = 72105, ok = 126570, ck = "object", sk = 42959, I7 = "break", hx = "for_of_statement", vk = 43695, f8 = 126551, lk = 66955, x8 = 126520, bk = 66499, L2 = 1024, pk = 67455, mk = 43018, _H = 198, a8 = 126522, kx = "function_declaration", _k = 73064, wx = "await", yk = 92728, dk = 70418, hk = 68119, Ex = "function_rest_param", kk = 42653, o8 = 11703, li = "left", c8 = 70449, wk = 184, Sx = "declare_type_alias", gx = 16777215, s8 = 70302, yH = "/=", dH = "|=", Ek = 55242, Sk = 126583, gk = 124927, Fk = 124895, Tk = 72959, Ok = 65497, hH = "Invalid legacy octal ", es = "typeof", Ik = "explicit_type", Fx = "statement_list", Ak = 65495, Tx = "class_method", v8 = 8526, l8 = 244, Nk = 67861, b8 = 119994, p8 = "enum", kH = 2147483647, Ck = 69762, wH = 208, R2 = "in", Pk = 11702, m8 = 67638, EH = ", characters ", Dk = 70753, yv = "super", Lk = 92783, Rk = 8304, _8 = 126504, Ox = "import_specifier", jk = 68324, Gk = 101589, Mk = 67646, Ix = "expression_or_spread", Bk = 74879, qk = 43792, y8 = 43260, Uk = 93052, SH = "{", Hk = 65574, Xk = 125258, dv = 224, Ax = "jsx_element_name_member_expression", j2 = "instanceof", Yk = 69599, Vk = 43560, Nx = "function_expression", d8 = 223, zk = 72242, Kk = 11498, Wk = 126467, Jk = 73112, gH = 140, h8 = 70107, $k = 13311, Cx = "jsx_children", k8 = 126548, Zk = 63743, w8 = 43471, Px = "jsx_expression", Qk = 69864, rw = 71998, ew = 72e3, E8 = 126591, S8 = 12592, Dx = "type_params", nw = 126578, g8 = 126537, wr = "{ ", tw = 123627, Lx = "jsx_spread_attribute", Pe = "@,", uw = 70161, iw = 187, F8 = 126500, Rx = "label_identifier", fw = 42606, jx = "number_literal_type", T8 = 42999, xw = 64310, FH = -594953737, aw = 122623, O8 = "hasUnknownMembers", Gx = "array", TH = "^=", Mx = "enum_string_member", ow = 65536, cw = 65615, ns = "void", sw = 65135, Z0 = ")", OH = 138, vw = 70002, G2 = "let", lw = 70271, bw = "nan", W = "@[%s =@ ", pw = 194559, mw = 110579, Bx = "binding_type_identifier", _w = 42735, IH = 57343, Zu = "/", qx = "for_in_statement_lhs", yw = 43503, dw = 8516, hw = 66938, kw = "ENOTDIR", AH = "TypeParameterInstantiation", ww = 69749, Ew = 65381, Sw = 83526, hv = "number", gw = 12447, NH = 154, I8 = 70286, Fw = 72160, Tw = 43493, CH = 206, Ux = "enum_member_identifier", A8 = 70280, M2 = "function", N8 = 70162, Ow = 255, Iw = 67702, Aw = 66771, Nw = 70312, PH = "|", Cw = 93759, DH = "End_of_file", Pw = 43709, i1 = "new", LH = "Failure", B2 = "local", Dw = 101631, C8 = 8489, P8 = "with", Hx = "enum_declaration", Lw = 218, Rw = 70457, D8 = 8488, Xx = "member", L8 = 64325, jw = 247, Gw = 70448, Mw = 69967, R8 = 126535, Bw = 71934, Yx = "import_named_specifier", qw = 65312, Uw = 126619, Vx = "type_annotation", RH = 56320, Hw = 131071, Xw = 120770, Yw = 67002, zx = "with_", Kx = "statement_fork_point", jH = "finalizer", Vw = 12320, GH = "elements", Wx = "literal", zw = 68607, Kw = 8507, j8 = "each", MH = "Sys_error", Ww = 123535, Jw = 130, Jx = "bigint_literal_type", $w = 64829, G8 = 11727, Zw = 120538, $x = "member_private_name", Zx = "type_alias", BH = "Printexc.handle_uncaught_exception", M8 = 126556, Qx = "tagged_template", ra = "pattern_object_property_literal_key", Qw = 43881, B8 = 72192, rE = 67826, eE = 124910, nE = 66511, ts = "int_of_string", tE = 43249, nr = "None", qH = "FunctionTypeParam", ti = "name", uE = 70285, c7 = 103, iE = 120744, ea = 12288, na = "intersection_type", fE = 11679, q8 = 11559, UH = "callee", xE = 71295, aE = 70018, oE = 11567, cE = 42954, HH = "*-/", Qu = "predicate", ta = "expression_statement", XH = "regexp", sE = 65479, YH = 132, vE = 11389, Bu = "optional", VH = -602162310, z = "@]", lE = 120003, bE = 72249, zH = "Unexpected ", pE = 73008, U8 = "finally", ua = "toplevel_statement_list", KH = "end", mE = 178207, WH = "&=", _E = 70301, JH = "%Li", yE = 72161, dE = 69746, hE = 70460, kE = 12799, H8 = 65535, wE = "loc", EE = 69375, SE = 43518, $H = 205, gE = 65487, ia = "while_", FE = 183983, fa = "typeof_expression", TE = -673950933, OE = 42559, ZH = "||", IE = 124926, AE = 55291, xa = "jsx_element_name_identifier", aa = 8239, X8 = "mixed", QH = 136, NE = -253313196, CE = 11734, Y8 = 67827, PE = 68287, DE = 119976, rX = "**", J = " =", V8 = 888960333, LE = 124902, oa = "tuple_type", eX = 227, RE = 70726, jE = 73111, z8 = 126602, GE = 126529, ca = "object_property_value_type", C0 = "%a", nX = ", ", tX = "<=", ME = 69423, uX = 199, K8 = 11695, BE = 12294, W8 = 11711, qE = 67583, iX = 710, J8 = 126584, UE = 68295, HE = 72703, XE = "prefix", fX = -80, $8 = 69415, YE = 11492, q2 = "class", Z8 = 65575, A7 = "continue", VE = 65663, xX = 2047, Q8 = 68120, zE = 71086, KE = 19967, Di = 782176664, WE = 120779, r3 = 8486, bi = " ", aX = "||=", oX = "Undefined_recursive_module", JE = 66863, cX = "RestElement", e3 = 126634, $E = 66377, ZE = 74751, sa = "jsx_element_name_namespaced", QE = 43334, rS = 66815, N7 = "typeAnnotation", eS = 120126, va = "array_element", n3 = 64285, sX = 189, vX = "**=", Yr = "()", nS = 8543, la = "declare_module", ba = "export_batch_specifier", lX = "%i", bX = ">>>=", tS = 68029, pX = "importKind", C7 = "extends", uS = 64296, t3 = 43259, iS = 71679, fS = 64913, xS = 119969, aS = 94175, oS = 72440, u3 = 65141, pa = "function_", cS = 43071, sS = 42888, vS = 69807, ou = "variance", us = 123, ma = "import_default_specifier", mX = ">>>", lS = 43764, pi = "pattern", bS = 71947, pS = 70655, kv = "consequent", _X = 4096, mS = 183, _S = 68447, yS = 65473, is = 255, dS = 73648, _a = "call_type_arg", ya = 8238, hS = 68899, kS = 93026, Ye = "@[<2>[", wS = 110588, da = "comment", yX = 191, ha = "switch_case", dX = 175, ES = 71942, ka = "do_while", wv = "constructor", SS = 43587, gS = 43586, wu = "yield", FS = 67462, hX = "fd ", TS = -61, OS = "target", i3 = 72272, U2 = "var", kX = "impltype", f3 = 70108, H2 = "0o", IS = 119972, AS = 92991, x3 = 70441, a3 = 8450, NS = 120074, CS = 66717, wa = "interface_type", o3 = 43880, An = "%B", PS = 111355, Ev = 5760, DS = 11630, c3 = 126499, LS = "of", wX = ">>", EX = "Popping lex mode from empty stack", s3 = 120629, fs2 = 108, RS = 43002, SX = "%=", v3 = 126539, jS = 126502, Ea = "template_literal", GS = "src/parser/statement_parser.ml", MS = ": Not a directory", gX = "b", BS = 67461, qS = 11519, FX = "src/parser/flow_lexer.ml", TX = "Out_of_memory", US = 120570, Sa = 12287, HS = 126534, XS = "index out of bounds", YS = 73029, l3 = "_bigarr02", b3 = 126571, OX = "))", ga = "for_statement_init", IX = "supertype", Fa = "class_property", p3 = "}", f1 = "this", Ta = "declare_module_exports", AX = "@", Oa = "union_type", Li = 65535, Ia = "variance_opt", VS = 94032, NX = 222, zS = 42124, Aa = "this_expression", Na = "jsx_element", CX = "typeArguments", KS = 65019, WS = 125251, JS = 64111, $S = 8471, Ca = "typeof_qualified_identifier", ZS = 70497, PX = "EnumDefaultedMember", Pa = 8202, QS = 66927, P7 = "switch", rg = 69634, Da = "unary_expression", eg = 71215, DX = 126, ng = 67679, tg = 65597, LX = 207, ug = 120686, m3 = 72163, ig = 67001, fg = 42962, xg = 64262, X2 = 124, La = 65279, ag = 126495, RX = 169, og = 71944, jX = -10, _3 = "alternate", cg = 92975, sg = 65489, Y2 = 252, vg = 67807, lg = 43187, bg = 68850, y3 = "export", pg = 66383, GX = "===", Ra = ".", ja = "type_args", MX = 147, mg = 92159, BX = 240, Ga = "jsx_element_name", _g = 72283, yg = 171, x1 = 116, dg = 110587, d3 = 70279, hg = 75075, kg = 65338, Ma = "function_params", wg = 126627, qX = 213, h3 = 73065, Eg = 71352, k3 = 119970, Sg = 70005, gg = 12295, w3 = 120771, Fg = 71494, Tg = 11557, Og = 42191, UX = "flags", Ig = 68437, Ag = 70730, Ba = "optional_indexed_access", qa = "pattern_object_p", Ng = 42785, Ua = "nullable_type", Bn = "value", Cg = 12343, Pg = 68415, Dg = 11694, HX = 221, Lg = 11726, Ha = "syntax", Rg = 119964, XX = "&&", jg = 68497, Gg = 73097, xs = "null", E3 = 126523, Mg = 120084, Bg = 126601, qg = 8454, Ug = "expressions", Hg = 72144, V2 = '"', Zr = "(@[", YX = 1022, VX = 231, Xg = 170, S3 = 12448, Yg = 68786, g3 = "<", zX = 931, KX = "(", WX = 196, JX = 2048, F3 = "an identifier", T3 = 69959, Vg = 68799, $X = "leadingComments", zg = 72969, Kg = 182, Wg = 100351, Xa = "enum_defaulted_member", Jg = 69839, $g = 94026, Zg = 209, ZX = ">>=", Qg = 131, O3 = 12336, s7 = "empty", QX = 331416730, rY = 204, rF = 70479, eF = 69487, nF = 101640, tF = 43123, eY = "([^/]+)", I3 = 8319, nY = 165, Ya = "object_type_property_setter", tY = 909, uF = 15, iF = 12591, br = 125, fF = 92735, uY = "cases", xF = 183969, a1 = "bigint", iY = "Division_by_zero", aF = 67071, oF = 12329, A3 = 120004, cF = 69414, N3 = "if", sF = 126519, vF = "immediately within another function.", lF = 55238, bF = 126498, fY = "qualification", pF = 66256, Er = "@ }@]", z2 = 118, C3 = 11565, P3 = 120122, Va = "pattern_object_rest_property", mF = 74862, D3 = "'", _F = -26065557, yF = 124911, Sv = 119, D7 = 104, za = "assignment", dF = 8457, K2 = "from", hF = 64321, kF = 113817, wF = 65629, EF = 42655, Ri = 102, SF = 43137, gF = 11502, o0 = ";@ ", L7 = 101, Ka = "pattern_array_element_pattern", Wn = "body", Wa = "jsx_member_expression", FF = 65547, Ja = "jsx_attribute_value", $a = "jsx_namespaced_name", L3 = 72967, TF = 126550, gv = 254, OF = 43807, IF = 43738, R3 = 126589, j3 = 8455, G3 = 126628, AF = 11670, xY = "*=", M3 = 120134, Za = "conditional", aY = " : flags Open_text and Open_binary are not compatible", B3 = 119965, NF = 69890, CF = 72817, PF = 164, DF = 43822, q3 = 69744, oY = "\\\\", LF = 43638, RF = 93047, jF = "AssignmentPattern", U3 = 64322, GF = 123190, cY = 188, Qa = "object_spread_property_type", MF = 70783, BF = 113663, sY = 160, H3 = 42622, X3 = 43823, ji = "init", Fv = 109, qF = 66503, Y3 = "proto", UF = 74649, ro = "optional_member", HF = 40981, XF = 120654, v = "@ ", eo = "enum_boolean_body", no = "export_named_specifier", to = "declare_interface", YF = 70451, uo = "pattern_object_property_computed_key", V3 = -97, z3 = 120539, K3 = 64317, VF = 12543, io = "export_named_declaration_specifier", zF = 43359, W3 = 126530, J3 = 72713, KF = 113800, vY = 195, WF = 72367, JF = 72103, $F = 70278, fo = "if_consequent_statement", W2 = -85, $3 = 126496, xo = "try_catch", ao = "computed_key", oo = "class_", ZF = 173823, co = "pattern_object_property_identifier_key", lY = "f", so = "arrow_function", Z3 = 8485, QF = 126546, vo = "enum_boolean_member", rT = 94177, J2 = "delete", eT = 232, bY = "blocks", lo = "pattern_array_rest_element_pattern", nT = 78894, Q3 = 66512, tT = 94111, Tv = "string", Ts = "test", uT = 69572, iT = 66463, fT = 66335, xT = 72348, aT = 73061, o1 = ":", bo = "enum_body", oT = 110590, po = "function_this_param_type", cT = 215, sT = 77823, pY = "minus", mY = 201, vT = 119980, mo = "private_name", _o = "object_key", yo = "function_param_type", _Y = "<<", lT = 11718, c1 = "as", yY = "delegate", Gi = "true", bT = 67413, r6 = 70854, pT = 73439, mT = 43776, _T = 71723, yT = 11505, dT = 214, hT = 120628, kT = 43513, ho = "jsx_attribute_name_namespaced", e6 = 120127, n6 = "Map.bal", t6 = "any", dY = "@[", hY = "camlinternalMod.ml", u6 = 126559, qu = "import", i6 = 70404, ko = "jsx_spread_child", wT = 233, ET = 67897, ST = 119974, Uu = 8233, gT = 68405, f6 = 239, kY = "attributes", wY = 173, wo = "object_internal_slot_property_type", FT = 71351, TT = 242, OT = 67643, x6 = "shorthand", Eo = "for_in_statement", IT = 126463, AT = 71338, NT = 69445, CT = 65370, PT = 73055, DT = 167, LT = 64911, So = "pattern_object_property_pattern", EY = 212, SY = 197, a6 = 126579, RT = 64286, jT = "explicitType", GT = 67669, MT = 43866, gY = "Sys_blocked_io", o6 = "catch", BT = 123197, qT = 64466, UT = 65140, HT = 73030, XT = 69404, c6 = "protected", FY = 8204, YT = 67504, VT = 193, $2 = 246, zT = 43713, s6 = 120571, go = "array_type", TY = "%u", Fo = "export_default_declaration", To = "class_expression", OY = "quasi", Yt = "%S", KT = 8525, v6 = 126515, WT = 120485, l6 = 43519, b6 = 120745, p6 = 94178, JT = 126588, zn = 127, $T = 66855, IY = "@{", AY = "visit_leading_comment", ZT = 67742, NY = " : flags Open_rdonly and Open_wronly are not compatible", QT = 120144, m6 = "returnType", s1 = -744106340, v1 = 240, Oo = "-", _6 = 8469, Os = "async", y6 = 126521, rO = 72095, d6 = 216, CY = " : file already exists", eO = 178205, nO = 8449, h6 = 94179, tO = 42774, k6 = "case", uO = 66965, iO = 66431, PY = 190, Io = "declare_export_declaration", Z2 = "targs", Ao = "type_identifier", fO = 64284, xO = 43013, w6 = 43815, No = "function_body_any", aO = 66966, E6 = 120687, oO = 66939, cO = 66978, DY = 168, S6 = "public", sO = 68115, vO = 43712, g6 = 65598, F6 = 126547, lO = 110591, Co = "indexed_access", LY = 12520, r7 = "interface", RY = `(Program not linked with -g, cannot print stack backtrace)
`, l1 = -46, Po = "string_literal_type", Do = "import_namespace_specifier", bO = 120132, T6 = 11735, pO = 67505, O6 = 119893, I6 = "bool", Q2 = 1e3, mi = "default", mO = 236, C = "", _O = "exportKind", jY = "trailingComments", A6 = "^", yO = 71983, dO = 8348, hO = 66977, kO = 65594, Lo = "logical", Ro = "jsx_member_expression_identifier", N6 = 210, GY = "cooked", jo = "for_of_left_declaration", Ov = 63, wO = 72202, v7 = "argument", EO = 12442, SO = 43645, C6 = 120085, gO = 42539, P6 = 126468, MY = 166, BY = "Match_failure", FO = 68191, Eu = "src/parser/flow_ast.ml", D6 = 11647, Go = "declare_variable", as = "+", TO = 71127, L6 = 120145, Mo = "declare_export_declaration_decl", R6 = 64318, qY = 179, Bo = "class_implements", UY = "!=", HY = "inexact", XY = "%li", YY = 237, rl = "a", j6 = 73062, OO = 178, qo = 65278, Uo = "function_rest_param_type", IO = 77711, AO = 70066, NO = 43714, VY = -696510241, G6 = 70480, CO = 69748, PO = 113788, DO = 94207, zY = `\r
`, Ho = "class_body", LO = 126651, RO = 68735, jO = 43273, M6 = 119996, B6 = 67644, KY = 224, Xo = "catch_clause_pattern", Yo = "boolean_literal_type", q6 = 126554, U6 = 126557, GO = 113807, H6 = 126536, WY = "%", Iv = "property", MO = 71956, JY = "#", BO = 123213, el = "meta", Vo = "for_of_assignment_pattern", zo = "if_statement", qO = 66421, UO = 8505, HO = 225, nl = 250, XO = 100343, X6 = "Literal", YO = 42887, Av = 115, $Y = ";", VO = 1255, zO = "=", KO = 126566, WO = 93823, Ko = "opaque_type", ZY = "!==", Wo = "jsx_attribute", Jo = "type_annotation_hint", Mi = 32768, JO = 73727, QY = "range", rV = 245, $O = "jsError", Y6 = 70006, ZO = 43492, V6 = "@]}", tr = "(Some ", QO = 8477, eV = 129, rI = 71487, z6 = 126564, nV = `
`, eI = 126514, nI = 70080, $o = "generic_identifier_type", tI = 66811, Zo = "typeof_identifier", tV = "~", uI = 65007, Qo = "pattern_object_rest_property_pattern", iI = 194, uV = 1039100673, fI = 66461, xI = 70319, K6 = 11719, aI = 72271, zt = -48, rc = "enum_string_body", oI = 70461, ec = "export_named_declaration", cI = 110930, sI = 92862, iV = "??=", vI = 70440, W6 = "while", cu = "camlinternalFormat.ml", lI = 43782, fV = 203, bI = 173791, pI = 11263, mI = 1114111, _I = 42969, J6 = 70750, nc = "jsx_identifier", yI = 70105, dI = 43014, hI = 11564, tc = "typeof_type", xV = "EEXIST", kI = 64847, wI = 71167, EI = 42511, SI = 72712, gI = 92995, FI = 43704, tl = 121, uc = "object_call_property_type", TI = 64433, ul = "operator", $6 = 68296, ic = "class_decorator", fc = 120, xc = "for_of_statement_lhs", OI = 11623, II = 67004, AI = 71999, NI = 70708, CI = 512, PI = 110927, DI = 71423, aV = 32752, LI = 93951, RI = 12292, ac = "object_type", Z6 = "types", jI = 110580, oV = 177, GI = 126633, MI = 12686, oc = 8286, cV = 144, BI = 73647, sV = 228, Q6 = 70855, b1 = "0x", qI = 70366, UI = `
`, cc = "variable_declaration", HI = 65276, rp = 119981, XI = 71945, YI = 43887, R7 = 105, VI = 8335, zI = 123565, KI = 69505, WI = 70187, sc = "jsx_attribute_name_identifier", vc = "source", lc = "pattern_object_property_key", ep = 65548, JI = 66175, $I = 92766, bc = "pattern_assignment_pattern", pc = "object_type_property_getter", np = 8305, j7 = "generator", tp = "for", vV = "PropertyDefinition", lV = "--", su = -36, ZI = "mkdir", QI = 68223, mc = "generic_qualified_identifier_type", rA = 11686, _c = "jsx_closing_element", eA = 43790, up = ": No such file or directory", nA = 69687, tA = 66348, ip = 72162, uA = 43388, iA = 72768, fA = 68351, d = "<2>", fp = 64297, xA = 125259, aA = 220, zr = ",@ ", bV = "win32", xp = 70281, yc = "member_property_identifier", oA = 68149, cA = 68111, sA = 71450, vA = 43009, dc = "member_property", lA = 73458, _i = "identifier", bA = 67423, pA = 66775, mA = 110951, pV = "Internal Error: Found object private prop", hc = "super_expression", kc = "jsx_opening_element", _A = 177976, wc = "variable_declarator_pattern", Ec = "pattern_expression", Sc = "jsx_member_expression_object", yA = 68252, dA = 77808, Nv = -835925911, gc = "import_declaration", hA = 55203, mV = "Pervasives.do_at_exit", _V = "utf8", ui = "key", kA = 43702, Fc = "spread_property", ap = 126563, wA = 863850040, EA = 70106, op = 67592, Tc = "function_expression_or_method", SA = 71958, Oc = "for_init_declaration", gA = 71955, cp = 123214, FA = 68479, yV = "==", TA = 43019, OA = 123180, sp = 217, Cv = "specifiers", Ic = "function_body", IA = 69622, vp = 8487, AA = 43641, dV = "Unexpected token `", hV = "v", NA = 123135, CA = 69295, lp = 120093, PA = 8521, bp = 43642, kV = 176;
function o70(t, n4, e, i, x) {
if (i <= n4)
for (var c = 1; c <= x; c++)
e[i + c] = t[n4 + c];
else
for (var c = x; c >= 1; c--)
e[i + c] = t[n4 + c];
return 0;
}
function c70(t) {
for (var n4 = [0]; t !== 0; ) {
for (var e = t[1], i = 1; i < e.length; i++)
n4.push(e[i]);
t = t[2];
}
return n4;
}
function s70(t, n4, e) {
var i = new Array(e + 1);
i[0] = 0;
for (var x = 1, c = n4 + 1; x <= e; x++, c++)
i[x] = t[c];
return i;
}
function DA(t, n4, e) {
var i = String.fromCharCode;
if (n4 == 0 && e <= _X && e == t.length)
return i.apply(null, t);
for (var x = C; 0 < e; n4 += L2, e -= L2)
x += i.apply(null, t.slice(n4, n4 + Math.min(e, L2)));
return x;
}
function pp(t) {
if (j0.Uint8Array)
var n4 = new j0.Uint8Array(t.l);
else
var n4 = new Array(t.l);
for (var e = t.c, i = e.length, x = 0; x < i; x++)
n4[x] = e.charCodeAt(x);
for (i = t.l; x < i; x++)
n4[x] = 0;
return t.c = n4, t.t = 4, n4;
}
function Is(t, n4, e, i, x) {
if (x == 0)
return 0;
if (i == 0 && (x >= e.l || e.t == 2 && x >= e.c.length))
e.c = t.t == 4 ? DA(t.c, n4, x) : n4 == 0 && t.c.length == x ? t.c : t.c.substr(n4, x), e.t = e.c.length == e.l ? 0 : 2;
else if (e.t == 2 && i == e.c.length)
e.c += t.t == 4 ? DA(t.c, n4, x) : n4 == 0 && t.c.length == x ? t.c : t.c.substr(n4, x), e.t = e.c.length == e.l ? 0 : 2;
else {
e.t != 4 && pp(e);
var c = t.c, s = e.c;
if (t.t == 4)
if (i <= n4)
for (var p = 0; p < x; p++)
s[i + p] = c[n4 + p];
else
for (var p = x - 1; p >= 0; p--)
s[i + p] = c[n4 + p];
else {
for (var y = Math.min(x, c.length - n4), p = 0; p < y; p++)
s[i + p] = c.charCodeAt(n4 + p);
for (; p < x; p++)
s[i + p] = 0;
}
}
return 0;
}
function Dae(t) {
return t;
}
function As(t, n4, e, i, x) {
return Is(t, n4, e, i, x), 0;
}
function v70(t, n4) {
throw [0, t, n4];
}
function Pv(t, n4) {
if (t == 0)
return C;
if (n4.repeat)
return n4.repeat(t);
for (var e = C, i = 0; ; ) {
if (t & 1 && (e += n4), t >>= 1, t == 0)
return e;
n4 += n4, i++, i == 9 && n4.slice(0, 1);
}
}
function Dv(t) {
t.t == 2 ? t.c += Pv(t.l - t.c.length, "\0") : t.c = DA(t.c, 0, t.c.length), t.t = 0;
}
function wV(t) {
if (t.length < 24) {
for (var n4 = 0; n4 < t.length; n4++)
if (t.charCodeAt(n4) > zn)
return false;
return true;
} else
return !/[^\x00-\x7f]/.test(t);
}
function LA(t) {
for (var n4 = C, e = C, i, x, c, s, p = 0, y = t.length; p < y; p++) {
if (x = t.charCodeAt(p), x < Pi) {
for (var T = p + 1; T < y && (x = t.charCodeAt(T)) < Pi; T++)
;
if (T - p > CI ? (e.substr(0, 1), n4 += e, e = C, n4 += t.slice(p, T)) : e += t.slice(p, T), T == y)
break;
p = T;
}
s = 1, ++p < y && ((c = t.charCodeAt(p)) & -64) == Rt && (i = c + (x << 6), x < KY ? (s = i - 12416, s < Pi && (s = 1)) : (s = 2, ++p < y && ((c = t.charCodeAt(p)) & -64) == Rt && (i = c + (i << 6), x < BX ? (s = i - 925824, (s < JX || s >= 55295 && s < 57344) && (s = 2)) : (s = 3, ++p < y && ((c = t.charCodeAt(p)) & -64) == Rt && x < 245 && (s = c - 63447168 + (i << 6), (s < 65536 || s > 1114111) && (s = 3)))))), s < 4 ? (p -= s, e += "\uFFFD") : s > Li ? e += String.fromCharCode(55232 + (s >> 10), RH + (s & 1023)) : e += String.fromCharCode(s), e.length > L2 && (e.substr(0, 1), n4 += e, e = C);
}
return n4 + e;
}
function Ac(t, n4, e) {
this.t = t, this.c = n4, this.l = e;
}
Ac.prototype.toString = function() {
switch (this.t) {
case 9:
return this.c;
default:
Dv(this);
case 0:
if (wV(this.c))
return this.t = 9, this.c;
this.t = 8;
case 8:
return this.c;
}
}, Ac.prototype.toUtf16 = function() {
var t = this.toString();
return this.t == 9 ? t : LA(t);
}, Ac.prototype.slice = function() {
var t = this.t == 4 ? this.c.slice() : this.c;
return new Ac(this.t, t, this.l);
};
function EV(t) {
return new Ac(0, t, t.length);
}
function r(t) {
return EV(t);
}
function RA(t, n4) {
v70(t, r(n4));
}
var Vt = [0];
function vu(t) {
RA(Vt.Invalid_argument, t);
}
function SV() {
vu(XS);
}
function Jn(t, n4, e) {
if (e &= is, t.t != 4) {
if (n4 == t.c.length)
return t.c += String.fromCharCode(e), n4 + 1 == t.l && (t.t = 0), 0;
pp(t);
}
return t.c[n4] = e, 0;
}
function p1(t, n4, e) {
return n4 >>> 0 >= t.l && SV(), Jn(t, n4, e);
}
function Hu(t, n4) {
switch (t.t & 6) {
default:
if (n4 >= t.c.length)
return 0;
case 0:
return t.c.charCodeAt(n4);
case 4:
return t.c[n4];
}
}
function os(t, n4) {
if (t.fun)
return os(t.fun, n4);
if (typeof t != "function")
return t;
var e = t.length | 0;
if (e === 0)
return t.apply(null, n4);
var i = n4.length | 0, x = e - i | 0;
return x == 0 ? t.apply(null, n4) : x < 0 ? os(t.apply(null, n4.slice(0, e)), n4.slice(e)) : function() {
for (var c = arguments.length == 0 ? 1 : arguments.length, s = new Array(n4.length + c), p = 0; p < n4.length; p++)
s[p] = n4[p];
for (var p = 0; p < arguments.length; p++)
s[n4.length + p] = arguments[p];
return os(t, s);
};
}
function il() {
vu(XS);
}
function nu(t, n4) {
return n4 >>> 0 >= t.length - 1 && il(), t;
}
function l70(t) {
return isFinite(t) ? Math.abs(t) >= 22250738585072014e-324 ? 0 : t != 0 ? 1 : 2 : isNaN(t) ? 4 : 3;
}
function Nc(t) {
return t.t & 6 && Dv(t), t.c;
}
var b70 = Math.log2 && Math.log2(11235582092889474e291) == 1020;
function p70(t) {
if (b70)
return Math.floor(Math.log2(t));
var n4 = 0;
if (t == 0)
return -1 / 0;
if (t >= 1)
for (; t >= 2; )
t /= 2, n4++;
else
for (; t < 1; )
t *= 2, n4--;
return n4;
}
function jA(t) {
var n4 = new j0.Float32Array(1);
n4[0] = t;
var e = new j0.Int32Array(n4.buffer);
return e[0] | 0;
}
var gV = Math.pow(2, -24);
function FV(t) {
throw t;
}
function TV() {
FV(Vt.Division_by_zero);
}
function an(t, n4, e) {
this.lo = t & gx, this.mi = n4 & gx, this.hi = e & Li;
}
an.prototype.caml_custom = "_j", an.prototype.copy = function() {
return new an(this.lo, this.mi, this.hi);
}, an.prototype.ucompare = function(t) {
return this.hi > t.hi ? 1 : this.hi < t.hi ? -1 : this.mi > t.mi ? 1 : this.mi < t.mi ? -1 : this.lo > t.lo ? 1 : this.lo < t.lo ? -1 : 0;
}, an.prototype.compare = function(t) {
var n4 = this.hi << 16, e = t.hi << 16;
return n4 > e ? 1 : n4 < e ? -1 : this.mi > t.mi ? 1 : this.mi < t.mi ? -1 : this.lo > t.lo ? 1 : this.lo < t.lo ? -1 : 0;
}, an.prototype.neg = function() {
var t = -this.lo, n4 = -this.mi + (t >> 24), e = -this.hi + (n4 >> 24);
return new an(t, n4, e);
}, an.prototype.add = function(t) {
var n4 = this.lo + t.lo, e = this.mi + t.mi + (n4 >> 24), i = this.hi + t.hi + (e >> 24);
return new an(n4, e, i);
}, an.prototype.sub = function(t) {
var n4 = this.lo - t.lo, e = this.mi - t.mi + (n4 >> 24), i = this.hi - t.hi + (e >> 24);
return new an(n4, e, i);
}, an.prototype.mul = function(t) {
var n4 = this.lo * t.lo, e = (n4 * gV | 0) + this.mi * t.lo + this.lo * t.mi, i = (e * gV | 0) + this.hi * t.lo + this.mi * t.mi + this.lo * t.hi;
return new an(n4, e, i);
}, an.prototype.isZero = function() {
return (this.lo | this.mi | this.hi) == 0;
}, an.prototype.isNeg = function() {
return this.hi << 16 < 0;
}, an.prototype.and = function(t) {
return new an(this.lo & t.lo, this.mi & t.mi, this.hi & t.hi);
}, an.prototype.or = function(t) {
return new an(this.lo | t.lo, this.mi | t.mi, this.hi | t.hi);
}, an.prototype.xor = function(t) {
return new an(this.lo ^ t.lo, this.mi ^ t.mi, this.hi ^ t.hi);
}, an.prototype.shift_left = function(t) {
return t = t & 63, t == 0 ? this : t < 24 ? new an(this.lo << t, this.mi << t | this.lo >> 24 - t, this.hi << t | this.mi >> 24 - t) : t < 48 ? new an(0, this.lo << t - 24, this.mi << t - 24 | this.lo >> 48 - t) : new an(0, 0, this.lo << t - 48);
}, an.prototype.shift_right_unsigned = function(t) {
return t = t & 63, t == 0 ? this : t < 24 ? new an(this.lo >> t | this.mi << 24 - t, this.mi >> t | this.hi << 24 - t, this.hi >> t) : t < 48 ? new an(this.mi >> t - 24 | this.hi << 48 - t, this.hi >> t - 24, 0) : new an(this.hi >> t - 48, 0, 0);
}, an.prototype.shift_right = function(t) {
if (t = t & 63, t == 0)
return this;
var n4 = this.hi << 16 >> 16;
if (t < 24)
return new an(this.lo >> t | this.mi << 24 - t, this.mi >> t | n4 << 24 - t, this.hi << 16 >> t >>> 16);
var e = this.hi << 16 >> 31;
return t < 48 ? new an(this.mi >> t - 24 | this.hi << 48 - t, this.hi << 16 >> t - 24 >> 16, e & Li) : new an(this.hi << 16 >> t - 32, e, e);
}, an.prototype.lsl1 = function() {
this.hi = this.hi << 1 | this.mi >> 23, this.mi = (this.mi << 1 | this.lo >> 23) & gx, this.lo = this.lo << 1 & gx;
}, an.prototype.lsr1 = function() {
this.lo = (this.lo >>> 1 | this.mi << 23) & gx, this.mi = (this.mi >>> 1 | this.hi << 23) & gx, this.hi = this.hi >>> 1;
}, an.prototype.udivmod = function(t) {
for (var n4 = 0, e = this.copy(), i = t.copy(), x = new an(0, 0, 0); e.ucompare(i) > 0; )
n4++, i.lsl1();
for (; n4 >= 0; )
n4--, x.lsl1(), e.ucompare(i) >= 0 && (x.lo++, e = e.sub(i)), i.lsr1();
return { quotient: x, modulus: e };
}, an.prototype.div = function(t) {
var n4 = this;
t.isZero() && TV();
var e = n4.hi ^ t.hi;
n4.hi & Mi && (n4 = n4.neg()), t.hi & Mi && (t = t.neg());
var i = n4.udivmod(t).quotient;
return e & Mi && (i = i.neg()), i;
}, an.prototype.mod = function(t) {
var n4 = this;
t.isZero() && TV();
var e = n4.hi;
n4.hi & Mi && (n4 = n4.neg()), t.hi & Mi && (t = t.neg());
var i = n4.udivmod(t).modulus;
return e & Mi && (i = i.neg()), i;
}, an.prototype.toInt = function() {
return this.lo | this.mi << 24;
}, an.prototype.toFloat = function() {
return (this.hi << 16) * Math.pow(2, 32) + this.mi * Math.pow(2, 24) + this.lo;
}, an.prototype.toArray = function() {
return [this.hi >> 8, this.hi & is, this.mi >> 16, this.mi >> 8 & is, this.mi & is, this.lo >> 16, this.lo >> 8 & is, this.lo & is];
}, an.prototype.lo32 = function() {
return this.lo | (this.mi & is) << 24;
}, an.prototype.hi32 = function() {
return this.mi >>> 8 & Li | this.hi << 16;
};
function mp(t, n4, e) {
return new an(t, n4, e);
}
function _p(t) {
if (!isFinite(t))
return isNaN(t) ? mp(1, 0, aV) : t > 0 ? mp(0, 0, aV) : mp(0, 0, 65520);
var n4 = t == 0 && 1 / t == -1 / 0 ? Mi : t >= 0 ? 0 : Mi;
n4 && (t = -t);
var e = p70(t) + 1023;
e <= 0 ? (e = 0, t /= Math.pow(2, -iH)) : (t /= Math.pow(2, e - oU), t < 16 && (t *= 2, e -= 1), e == 0 && (t /= 2));
var i = Math.pow(2, 24), x = t | 0;
t = (t - x) * i;
var c = t | 0;
t = (t - c) * i;
var s = t | 0;
return x = x & uF | n4 | e << 4, mp(s, c, x);
}
function fl(t) {
return t.toArray();
}
function OV(t, n4, e) {
if (t.write(32, n4.dims.length), t.write(32, n4.kind | n4.layout << 8), n4.caml_custom == l3)
for (var i = 0; i < n4.dims.length; i++)
n4.dims[i] < Li ? t.write(16, n4.dims[i]) : (t.write(16, Li), t.write(32, 0), t.write(32, n4.dims[i]));
else
for (var i = 0; i < n4.dims.length; i++)
t.write(32, n4.dims[i]);
switch (n4.kind) {
case 2:
case 3:
case 12:
for (var i = 0; i < n4.data.length; i++)
t.write(8, n4.data[i]);
break;
case 4:
case 5:
for (var i = 0; i < n4.data.length; i++)
t.write(16, n4.data[i]);
break;
case 6:
for (var i = 0; i < n4.data.length; i++)
t.write(32, n4.data[i]);
break;
case 8:
case 9:
t.write(8, 0);
for (var i = 0; i < n4.data.length; i++)
t.write(32, n4.data[i]);
break;
case 7:
for (var i = 0; i < n4.data.length / 2; i++)
for (var x = fl(n4.get(i)), c = 0; c < 8; c++)
t.write(8, x[c]);
break;
case 1:
for (var i = 0; i < n4.data.length; i++)
for (var x = fl(_p(n4.get(i))), c = 0; c < 8; c++)
t.write(8, x[c]);
break;
case 0:
for (var i = 0; i < n4.data.length; i++) {
var x = jA(n4.get(i));
t.write(32, x);
}
break;
case 10:
for (var i = 0; i < n4.data.length / 2; i++) {
var c = n4.get(i);
t.write(32, jA(c[1])), t.write(32, jA(c[2]));
}
break;
case 11:
for (var i = 0; i < n4.data.length / 2; i++) {
for (var s = n4.get(i), x = fl(_p(s[1])), c = 0; c < 8; c++)
t.write(8, x[c]);
for (var x = fl(_p(s[2])), c = 0; c < 8; c++)
t.write(8, x[c]);
}
break;
}
e[0] = (4 + n4.dims.length) * 4, e[1] = (4 + n4.dims.length) * 8;
}
function IV(t) {
switch (t) {
case 7:
case 10:
case 11:
return 2;
default:
return 1;
}
}
function m70(t, n4) {
var e = j0, i;
switch (t) {
case 0:
i = e.Float32Array;
break;
case 1:
i = e.Float64Array;
break;
case 2:
i = e.Int8Array;
break;
case 3:
i = e.Uint8Array;
break;
case 4:
i = e.Int16Array;
break;
case 5:
i = e.Uint16Array;
break;
case 6:
i = e.Int32Array;
break;
case 7:
i = e.Int32Array;
break;
case 8:
i = e.Int32Array;
break;
case 9:
i = e.Int32Array;
break;
case 10:
i = e.Float32Array;
break;
case 11:
i = e.Float64Array;
break;
case 12:
i = e.Uint8Array;
break;
}
i || vu("Bigarray.create: unsupported kind");
var x = new i(n4 * IV(t));
return x;
}
function GA(t) {
var n4 = new j0.Int32Array(1);
n4[0] = t;
var e = new j0.Float32Array(n4.buffer);
return e[0];
}
function xl(t) {
return new an(t[7] << 0 | t[6] << 8 | t[5] << 16, t[4] << 0 | t[3] << 8 | t[2] << 16, t[1] << 0 | t[0] << 8);
}
function MA(t) {
var n4 = t.lo, e = t.mi, i = t.hi, x = (i & 32767) >> 4;
if (x == xX)
return n4 | e | i & uF ? NaN : i & Mi ? -1 / 0 : 1 / 0;
var c = Math.pow(2, -24), s = (n4 * c + e) * c + (i & uF);
return x > 0 ? (s += 16, s *= Math.pow(2, x - oU)) : s *= Math.pow(2, -iH), i & Mi && (s = -s), s;
}
function BA(t) {
for (var n4 = t.length, e = 1, i = 0; i < n4; i++)
t[i] < 0 && vu("Bigarray.create: negative dimension"), e = e * t[i];
return e;
}
function _70(t, n4) {
return new an(t & gx, t >>> 24 & is | (n4 & Li) << 8, n4 >>> 16 & Li);
}
function qA(t) {
return t.hi32();
}
function UA(t) {
return t.lo32();
}
var y70 = l3;
function Ns(t, n4, e, i) {
this.kind = t, this.layout = n4, this.dims = e, this.data = i;
}
Ns.prototype.caml_custom = y70, Ns.prototype.offset = function(t) {
var n4 = 0;
if (typeof t == "number" && (t = [t]), t instanceof Array || vu("bigarray.js: invalid offset"), this.dims.length != t.length && vu("Bigarray.get/set: bad number of dimensions"), this.layout == 0)
for (var e = 0; e < this.dims.length; e++)
(t[e] < 0 || t[e] >= this.dims[e]) && il(), n4 = n4 * this.dims[e] + t[e];
else
for (var e = this.dims.length - 1; e >= 0; e--)
(t[e] < 1 || t[e] > this.dims[e]) && il(), n4 = n4 * this.dims[e] + (t[e] - 1);
return n4;
}, Ns.prototype.get = function(t) {
switch (this.kind) {
case 7:
var n4 = this.data[t * 2 + 0], e = this.data[t * 2 + 1];
return _70(n4, e);
case 10:
case 11:
var i = this.data[t * 2 + 0], x = this.data[t * 2 + 1];
return [gv, i, x];
default:
return this.data[t];
}
}, Ns.prototype.set = function(t, n4) {
switch (this.kind) {
case 7:
this.data[t * 2 + 0] = UA(n4), this.data[t * 2 + 1] = qA(n4);
break;
case 10:
case 11:
this.data[t * 2 + 0] = n4[1], this.data[t * 2 + 1] = n4[2];
break;
default:
this.data[t] = n4;
break;
}
return 0;
}, Ns.prototype.fill = function(t) {
switch (this.kind) {
case 7:
var n4 = UA(t), e = qA(t);
if (n4 == e)
this.data.fill(n4);
else
for (var i = 0; i < this.data.length; i++)
this.data[i] = i % 2 == 0 ? n4 : e;
break;
case 10:
case 11:
var x = t[1], c = t[2];
if (x == c)
this.data.fill(x);
else
for (var i = 0; i < this.data.length; i++)
this.data[i] = i % 2 == 0 ? x : c;
break;
default:
this.data.fill(t);
break;
}
}, Ns.prototype.compare = function(t, n4) {
if (this.layout != t.layout || this.kind != t.kind) {
var e = this.kind | this.layout << 8, i = t.kind | t.layout << 8;
return i - e;
}
if (this.dims.length != t.dims.length)
return t.dims.length - this.dims.length;
for (var x = 0; x < this.dims.length; x++)
if (this.dims[x] != t.dims[x])
return this.dims[x] < t.dims[x] ? -1 : 1;
switch (this.kind) {
case 0:
case 1:
case 10:
case 11:
for (var c, s, x = 0; x < this.data.length; x++) {
if (c = this.data[x], s = t.data[x], c < s)
return -1;
if (c > s)
return 1;
if (c != s) {
if (!n4)
return NaN;
if (c == c)
return 1;
if (s == s)
return -1;
}
}
break;
case 7:
for (var x = 0; x < this.data.length; x += 2) {
if (this.data[x + 1] < t.data[x + 1])
return -1;
if (this.data[x + 1] > t.data[x + 1])
return 1;
if (this.data[x] >>> 0 < t.data[x] >>> 0)
return -1;
if (this.data[x] >>> 0 > t.data[x] >>> 0)
return 1;
}
break;
case 2:
case 3:
case 4:
case 5:
case 6:
case 8:
case 9:
case 12:
for (var x = 0; x < this.data.length; x++) {
if (this.data[x] < t.data[x])
return -1;
if (this.data[x] > t.data[x])
return 1;
}
break;
}
return 0;
};
function Lv(t, n4, e, i) {
this.kind = t, this.layout = n4, this.dims = e, this.data = i;
}
Lv.prototype = new Ns(), Lv.prototype.offset = function(t) {
return typeof t != "number" && (t instanceof Array && t.length == 1 ? t = t[0] : vu("Ml_Bigarray_c_1_1.offset")), (t < 0 || t >= this.dims[0]) && il(), t;
}, Lv.prototype.get = function(t) {
return this.data[t];
}, Lv.prototype.set = function(t, n4) {
return this.data[t] = n4, 0;
}, Lv.prototype.fill = function(t) {
return this.data.fill(t), 0;
};
function AV(t, n4, e, i) {
var x = IV(t);
return BA(e) * x != i.length && vu("length doesn't match dims"), n4 == 0 && e.length == 1 && x == 1 ? new Lv(t, n4, e, i) : new Ns(t, n4, e, i);
}
function e7(t) {
RA(Vt.Failure, t);
}
function NV(t, n4, e) {
var i = t.read32s();
(i < 0 || i > 16) && e7("input_value: wrong number of bigarray dimensions");
var x = t.read32s(), c = x & is, s = x >> 8 & 1, p = [];
if (e == l3)
for (var y = 0; y < i; y++) {
var T = t.read16u();
if (T == Li) {
var E = t.read32u(), h = t.read32u();
E != 0 && e7("input_value: bigarray dimension overflow in 32bit"), T = h;
}
p.push(T);
}
else
for (var y = 0; y < i; y++)
p.push(t.read32u());
var w = BA(p), G = m70(c, w), A = AV(c, s, p, G);
switch (c) {
case 2:
for (var y = 0; y < w; y++)
G[y] = t.read8s();
break;
case 3:
case 12:
for (var y = 0; y < w; y++)
G[y] = t.read8u();
break;
case 4:
for (var y = 0; y < w; y++)
G[y] = t.read16s();
break;
case 5:
for (var y = 0; y < w; y++)
G[y] = t.read16u();
break;
case 6:
for (var y = 0; y < w; y++)
G[y] = t.read32s();
break;
case 8:
case 9:
var S = t.read8u();
S && e7("input_value: cannot read bigarray with 64-bit OCaml ints");
for (var y = 0; y < w; y++)
G[y] = t.read32s();
break;
case 7:
for (var k0 = new Array(8), y = 0; y < w; y++) {
for (var M = 0; M < 8; M++)
k0[M] = t.read8u();
var K = xl(k0);
A.set(y, K);
}
break;
case 1:
for (var k0 = new Array(8), y = 0; y < w; y++) {
for (var M = 0; M < 8; M++)
k0[M] = t.read8u();
var V = MA(xl(k0));
A.set(y, V);
}
break;
case 0:
for (var y = 0; y < w; y++) {
var V = GA(t.read32s());
A.set(y, V);
}
break;
case 10:
for (var y = 0; y < w; y++) {
var f0 = GA(t.read32s()), m0 = GA(t.read32s());
A.set(y, [gv, f0, m0]);
}
break;
case 11:
for (var k0 = new Array(8), y = 0; y < w; y++) {
for (var M = 0; M < 8; M++)
k0[M] = t.read8u();
for (var f0 = MA(xl(k0)), M = 0; M < 8; M++)
k0[M] = t.read8u();
var m0 = MA(xl(k0));
A.set(y, [gv, f0, m0]);
}
break;
}
return n4[0] = (4 + i) * 4, AV(c, s, p, G);
}
function CV(t, n4, e) {
return t.compare(n4, e);
}
function PV(t, n4) {
return Math.imul(t, n4);
}
function cs(t, n4) {
return n4 = PV(n4, -862048943), n4 = n4 << 15 | n4 >>> 32 - 15, n4 = PV(n4, 461845907), t ^= n4, t = t << 13 | t >>> 32 - 13, (t + (t << 2) | 0) + -430675100 | 0;
}
function d70(t, n4) {
return t = cs(t, UA(n4)), t = cs(t, qA(n4)), t;
}
function DV(t, n4) {
return d70(t, _p(n4));
}
function LV(t) {
var n4 = BA(t.dims), e = 0;
switch (t.kind) {
case 2:
case 3:
case 12:
n4 > C4 && (n4 = C4);
var i = 0, x = 0;
for (x = 0; x + 4 <= t.data.length; x += 4)
i = t.data[x + 0] | t.data[x + 1] << 8 | t.data[x + 2] << 16 | t.data[x + 3] << 24, e = cs(e, i);
switch (i = 0, n4 & 3) {
case 3:
i = t.data[x + 2] << 16;
case 2:
i |= t.data[x + 1] << 8;
case 1:
i |= t.data[x + 0], e = cs(e, i);
}
break;
case 4:
case 5:
n4 > Rt && (n4 = Rt);
var i = 0, x = 0;
for (x = 0; x + 2 <= t.data.length; x += 2)
i = t.data[x + 0] | t.data[x + 1] << 16, e = cs(e, i);
n4 & 1 && (e = cs(e, t.data[x]));
break;
case 6:
n4 > 64 && (n4 = 64);
for (var x = 0; x < n4; x++)
e = cs(e, t.data[x]);
break;
case 8:
case 9:
n4 > 64 && (n4 = 64);
for (var x = 0; x < n4; x++)
e = cs(e, t.data[x]);
break;
case 7:
n4 > 32 && (n4 = 32), n4 *= 2;
for (var x = 0; x < n4; x++)
e = cs(e, t.data[x]);
break;
case 10:
n4 *= 2;
case 0:
n4 > 64 && (n4 = 64);
for (var x = 0; x < n4; x++)
e = DV(e, t.data[x]);
break;
case 11:
n4 *= 2;
case 1:
n4 > 32 && (n4 = 32);
for (var x = 0; x < n4; x++)
e = DV(e, t.data[x]);
break;
}
return e;
}
function h70(t, n4) {
return n4[0] = 4, t.read32s();
}
function k70(t, n4) {
switch (t.read8u()) {
case 1:
return n4[0] = 4, t.read32s();
case 2:
e7("input_value: native integer value too large");
default:
e7("input_value: ill-formed native integer");
}
}
function w70(t, n4) {
for (var e = new Array(8), i = 0; i < 8; i++)
e[i] = t.read8u();
return n4[0] = 8, xl(e);
}
function E70(t, n4, e) {
for (var i = fl(n4), x = 0; x < 8; x++)
t.write(8, i[x]);
e[0] = 8, e[1] = 8;
}
function S70(t, n4, e) {
return t.compare(n4);
}
function g70(t) {
return t.lo32() ^ t.hi32();
}
var RV = { _j: { deserialize: w70, serialize: E70, fixed_length: 8, compare: S70, hash: g70 }, _i: { deserialize: h70, fixed_length: 4 }, _n: { deserialize: k70, fixed_length: 4 }, _bigarray: { deserialize: function(t, n4) {
return NV(t, n4, "_bigarray");
}, serialize: OV, compare: CV, hash: LV }, _bigarr02: { deserialize: function(t, n4) {
return NV(t, n4, l3);
}, serialize: OV, compare: CV, hash: LV } };
function HA(t) {
return RV[t.caml_custom] && RV[t.caml_custom].compare;
}
function jV(t, n4, e, i) {
var x = HA(n4);
if (x) {
var c = e > 0 ? x(n4, t, i) : x(t, n4, i);
if (i && c != c)
return e;
if (+c != +c)
return +c;
if (c | 0)
return c | 0;
}
return e;
}
function yp(t) {
return t instanceof Ac;
}
function XA(t) {
return yp(t);
}
function GV(t) {
if (typeof t == "number")
return Q2;
if (yp(t))
return Y2;
if (XA(t))
return 1252;
if (t instanceof Array && t[0] === t[0] >>> 0 && t[0] <= Ow) {
var n4 = t[0] | 0;
return n4 == gv ? 0 : n4;
} else {
if (t instanceof String)
return LY;
if (typeof t == "string")
return LY;
if (t instanceof Number)
return Q2;
if (t && t.caml_custom)
return VO;
if (t && t.compare)
return 1256;
if (typeof t == "function")
return 1247;
if (typeof t == "symbol")
return 1251;
}
return 1001;
}
function Cc(t, n4) {
return t < n4 ? -1 : t == n4 ? 0 : 1;
}
function MV(t, n4) {
return t.t & 6 && Dv(t), n4.t & 6 && Dv(n4), t.c < n4.c ? -1 : t.c > n4.c ? 1 : 0;
}
function Ee(t, n4) {
return MV(t, n4);
}
function dp(t, n4, e) {
for (var i = []; ; ) {
if (!(e && t === n4)) {
var x = GV(t);
if (x == nl) {
t = t[1];
continue;
}
var c = GV(n4);
if (c == nl) {
n4 = n4[1];
continue;
}
if (x !== c)
return x == Q2 ? c == VO ? jV(t, n4, -1, e) : -1 : c == Q2 ? x == VO ? jV(n4, t, 1, e) : 1 : x < c ? -1 : 1;
switch (x) {
case 247:
vu(Oh);
break;
case 248:
var p = Cc(t[2], n4[2]);
if (p != 0)
return p | 0;
break;
case 249:
vu(Oh);
break;
case 250:
vu("equal: got Forward_tag, should not happen");
break;
case 251:
vu("equal: abstract value");
break;
case 252:
if (t !== n4) {
var p = MV(t, n4);
if (p != 0)
return p | 0;
}
break;
case 253:
vu("equal: got Double_tag, should not happen");
break;
case 254:
vu("equal: got Double_array_tag, should not happen");
break;
case 255:
vu("equal: got Custom_tag, should not happen");
break;
case 1247:
vu(Oh);
break;
case 1255:
var s = HA(t);
if (s != HA(n4))
return t.caml_custom < n4.caml_custom ? -1 : 1;
s || vu("compare: abstract value");
var p = s(t, n4, e);
if (p != p)
return e ? -1 : p;
if (p !== (p | 0))
return -1;
if (p != 0)
return p | 0;
break;
case 1256:
var p = t.compare(n4, e);
if (p != p)
return e ? -1 : p;
if (p !== (p | 0))
return -1;
if (p != 0)
return p | 0;
break;
case 1e3:
if (t = +t, n4 = +n4, t < n4)
return -1;
if (t > n4)
return 1;
if (t != n4) {
if (!e)
return NaN;
if (t == t)
return 1;
if (n4 == n4)
return -1;
}
break;
case 1001:
if (t < n4)
return -1;
if (t > n4)
return 1;
if (t != n4) {
if (!e)
return NaN;
if (t == t)
return 1;
if (n4 == n4)
return -1;
}
break;
case 1251:
if (t !== n4)
return e ? 1 : NaN;
break;
case 1252:
var t = Nc(t), n4 = Nc(n4);
if (t !== n4) {
if (t < n4)
return -1;
if (t > n4)
return 1;
}
break;
case 12520:
var t = t.toString(), n4 = n4.toString();
if (t !== n4) {
if (t < n4)
return -1;
if (t > n4)
return 1;
}
break;
case 246:
case 254:
default:
if (t.length != n4.length)
return t.length < n4.length ? -1 : 1;
t.length > 1 && i.push(t, n4, 1);
break;
}
}
if (i.length == 0)
return 0;
var y = i.pop();
n4 = i.pop(), t = i.pop(), y + 1 < t.length && i.push(t, n4, y + 1), t = t[y], n4 = n4[y];
}
}
function BV(t, n4) {
return dp(t, n4, true);
}
function F70() {
return [0];
}
function Pt(t) {
return t < 0 && vu("Bytes.create"), new Ac(t ? 2 : 9, C, t);
}
function qV(t, n4) {
return +(dp(t, n4, false) == 0);
}
function T70(t, n4, e, i) {
if (e > 0)
if (n4 == 0 && (e >= t.l || t.t == 2 && e >= t.c.length))
i == 0 ? (t.c = C, t.t = 2) : (t.c = Pv(e, String.fromCharCode(i)), t.t = e == t.l ? 0 : 2);
else
for (t.t != 4 && pp(t), e += n4; n4 < e; n4++)
t.c[n4] = i;
return 0;
}
function al(t) {
var n4;
if (t = Nc(t), n4 = +t, t.length > 0 && n4 === n4 || (t = t.replace(/_/g, C), n4 = +t, t.length > 0 && n4 === n4 || /^[+-]?nan$/i.test(t)))
return n4;
var e = /^ *([+-]?)0x([0-9a-f]+)\.?([0-9a-f]*)p([+-]?[0-9]+)/i.exec(t);
if (e) {
var i = e[3].replace(/0+$/, C), x = parseInt(e[1] + e[2] + i, 16), c = (e[4] | 0) - 4 * i.length;
return n4 = x * Math.pow(2, c), n4;
}
if (/^\+?inf(inity)?$/i.test(t))
return 1 / 0;
if (/^-inf(inity)?$/i.test(t))
return -1 / 0;
e7("float_of_string");
}
function YA(t) {
t = Nc(t);
var n4 = t.length;
n4 > 31 && vu("format_int: format too long");
for (var e = { justify: as, signstyle: Oo, filler: bi, alternate: false, base: 0, signedconv: false, width: 0, uppercase: false, sign: 1, prec: -1, conv: lY }, i = 0; i < n4; i++) {
var x = t.charAt(i);
switch (x) {
case "-":
e.justify = Oo;
break;
case "+":
case " ":
e.signstyle = x;
break;
case "0":
e.filler = $u;
break;
case "#":
e.alternate = true;
break;
case "1":
case "2":
case "3":
case "4":
case "5":
case "6":
case "7":
case "8":
case "9":
for (e.width = 0; x = t.charCodeAt(i) - 48, x >= 0 && x <= 9; )
e.width = e.width * 10 + x, i++;
i--;
break;
case ".":
for (e.prec = 0, i++; x = t.charCodeAt(i) - 48, x >= 0 && x <= 9; )
e.prec = e.prec * 10 + x, i++;
i--;
case "d":
case "i":
e.signedconv = true;
case "u":
e.base = 10;
break;
case "x":
e.base = 16;
break;
case "X":
e.base = 16, e.uppercase = true;
break;
case "o":
e.base = 8;
break;
case "e":
case "f":
case "g":
e.signedconv = true, e.conv = x;
break;
case "E":
case "F":
case "G":
e.signedconv = true, e.uppercase = true, e.conv = x.toLowerCase();
break;
}
}
return e;
}
function VA(t, n4) {
t.uppercase && (n4 = n4.toUpperCase());
var e = n4.length;
t.signedconv && (t.sign < 0 || t.signstyle != Oo) && e++, t.alternate && (t.base == 8 && (e += 1), t.base == 16 && (e += 2));
var i = C;
if (t.justify == as && t.filler == bi)
for (var x = e; x < t.width; x++)
i += bi;
if (t.signedconv && (t.sign < 0 ? i += Oo : t.signstyle != Oo && (i += t.signstyle)), t.alternate && t.base == 8 && (i += $u), t.alternate && t.base == 16 && (i += b1), t.justify == as && t.filler == $u)
for (var x = e; x < t.width; x++)
i += $u;
if (i += n4, t.justify == Oo)
for (var x = e; x < t.width; x++)
i += bi;
return r(i);
}
function zA(t, n4) {
function e(E, h) {
if (Math.abs(E) < 1)
return E.toFixed(h);
var w = parseInt(E.toString().split(as)[1]);
return w > 20 ? (w -= 20, E /= Math.pow(10, w), E += new Array(w + 1).join($u), h > 0 && (E = E + Ra + new Array(h + 1).join($u)), E) : E.toFixed(h);
}
var i, x = YA(t), c = x.prec < 0 ? 6 : x.prec;
if ((n4 < 0 || n4 == 0 && 1 / n4 == -1 / 0) && (x.sign = -1, n4 = -n4), isNaN(n4))
i = bw, x.filler = bi;
else if (!isFinite(n4))
i = "inf", x.filler = bi;
else
switch (x.conv) {
case "e":
var i = n4.toExponential(c), s = i.length;
i.charAt(s - 3) == iy && (i = i.slice(0, s - 1) + $u + i.slice(s - 1));
break;
case "f":
i = e(n4, c);
break;
case "g":
c = c || 1, i = n4.toExponential(c - 1);
var p = i.indexOf(iy), y = +i.slice(p + 1);
if (y < -4 || n4 >= 1e21 || n4.toFixed(0).length > c) {
for (var s = p - 1; i.charAt(s) == $u; )
s--;
i.charAt(s) == Ra && s--, i = i.slice(0, s + 1) + i.slice(p), s = i.length, i.charAt(s - 3) == iy && (i = i.slice(0, s - 1) + $u + i.slice(s - 1));
break;
} else {
var T = c;
if (y < 0)
T -= y + 1, i = n4.toFixed(T);
else
for (; i = n4.toFixed(T), i.length > c + 1; )
T--;
if (T) {
for (var s = i.length - 1; i.charAt(s) == $u; )
s--;
i.charAt(s) == Ra && s--, i = i.slice(0, s + 1);
}
}
break;
}
return VA(x, i);
}
function hp(t, n4) {
if (Nc(t) == N2)
return r(C + n4);
var e = YA(t);
n4 < 0 && (e.signedconv ? (e.sign = -1, n4 = -n4) : n4 >>>= 0);
var i = n4.toString(e.base);
if (e.prec >= 0) {
e.filler = bi;
var x = e.prec - i.length;
x > 0 && (i = Pv(x, $u) + i);
}
return VA(e, i);
}
var UV = 0;
function G7() {
return UV++;
}
function O70() {
return 0;
}
function HV() {
return [0];
}
var kp = [];
function Ze(t, n4, e) {
var i = t[1], x = kp[e];
if (x === void 0)
for (var c = kp.length; c < e; c++)
kp[c] = 0;
else if (i[x] === n4)
return i[x - 1];
for (var s = 3, p = i[1] * 2 + 1, y; s < p; )
y = s + p >> 1 | 1, n4 < i[y + 1] ? p = y - 2 : s = y;
return kp[e] = s + 1, n4 == i[s + 1] ? i[s] : 0;
}
function I70(t) {
for (var n4 = C, e = n4, i, x, c = 0, s = t.length; c < s; c++) {
if (i = t.charCodeAt(c), i < Pi) {
for (var p = c + 1; p < s && (i = t.charCodeAt(p)) < Pi; p++)
;
if (p - c > CI ? (e.substr(0, 1), n4 += e, e = C, n4 += t.slice(c, p)) : e += t.slice(c, p), p == s)
break;
c = p;
}
i < JX ? (e += String.fromCharCode(192 | i >> 6), e += String.fromCharCode(Pi | i & Ov)) : i < 55296 || i >= IH ? e += String.fromCharCode(KY | i >> 12, Pi | i >> 6 & Ov, Pi | i & Ov) : i >= 56319 || c + 1 == s || (x = t.charCodeAt(c + 1)) < RH || x > IH ? e += "\xEF\xBF\xBD" : (c++, i = (i << 10) + x - 56613888, e += String.fromCharCode(BX | i >> 18, Pi | i >> 12 & Ov, Pi | i >> 6 & Ov, Pi | i & Ov)), e.length > L2 && (e.substr(0, 1), n4 += e, e = C);
}
return n4 + e;
}
function A70(t) {
var n4 = 9;
return wV(t) || (n4 = 8, t = I70(t)), new Ac(n4, t, t.length);
}
function M7(t) {
return A70(t);
}
function N70(t, n4, e) {
if (!isFinite(t))
return isNaN(t) ? M7(bw) : M7(t > 0 ? kU : "-infinity");
var i = t == 0 && 1 / t == -1 / 0 ? 1 : t >= 0 ? 0 : 1;
i && (t = -t);
var x = 0;
if (t != 0)
if (t < 1)
for (; t < 1 && x > -YX; )
t *= 2, x--;
else
for (; t >= 2; )
t /= 2, x++;
var c = x < 0 ? C : as, s = C;
if (i)
s = Oo;
else
switch (e) {
case 43:
s = as;
break;
case 32:
s = bi;
break;
default:
break;
}
if (n4 >= 0 && n4 < 13) {
var p = Math.pow(2, n4 * 4);
t = Math.round(t * p) / p;
}
var y = t.toString(16);
if (n4 >= 0) {
var T = y.indexOf(Ra);
if (T < 0)
y += Ra + Pv(n4, $u);
else {
var E = T + 1 + n4;
y.length < E ? y += Pv(E - y.length, $u) : y = y.substr(0, E);
}
}
return M7(s + b1 + y + "p" + c + x.toString(10));
}
function C70(t) {
return +t.isZero();
}
function wp(t) {
return new an(t & gx, t >> 24 & gx, t >> 31 & Li);
}
function P70(t) {
return t.toInt();
}
function D70(t) {
return +t.isNeg();
}
function XV(t) {
return t.neg();
}
function L70(t, n4) {
var e = YA(t);
e.signedconv && D70(n4) && (e.sign = -1, n4 = XV(n4));
var i = C, x = wp(e.base), c = "0123456789abcdef";
do {
var s = n4.udivmod(x);
n4 = s.quotient, i = c.charAt(P70(s.modulus)) + i;
} while (!C70(n4));
if (e.prec >= 0) {
e.filler = bi;
var p = e.prec - i.length;
p > 0 && (i = Pv(p, $u) + i);
}
return VA(e, i);
}
function l7(t) {
return t.l;
}
function nn(t) {
return l7(t);
}
function Vr(t, n4) {
return Hu(t, n4);
}
function R70(t, n4) {
return t.add(n4);
}
function j70(t, n4) {
return t.mul(n4);
}
function KA(t, n4) {
return t.ucompare(n4) < 0;
}
function YV(t) {
var n4 = 0, e = nn(t), i = 10, x = 1;
if (e > 0)
switch (Vr(t, n4)) {
case 45:
n4++, x = -1;
break;
case 43:
n4++, x = 1;
break;
}
if (n4 + 1 < e && Vr(t, n4) == 48)
switch (Vr(t, n4 + 1)) {
case 120:
case 88:
i = 16, n4 += 2;
break;
case 111:
case 79:
i = 8, n4 += 2;
break;
case 98:
case 66:
i = 2, n4 += 2;
break;
case 117:
case 85:
n4 += 2;
break;
}
return [n4, x, i];
}
function Ep(t) {
return t >= 48 && t <= 57 ? t - 48 : t >= 65 && t <= 90 ? t - 55 : t >= 97 && t <= In ? t - 87 : -1;
}
function Rv(t) {
var n4 = YV(t), e = n4[0], i = n4[1], x = n4[2], c = wp(x), s = new an(gx, 268435455, Li).udivmod(c).quotient, p = Vr(t, e), y = Ep(p);
(y < 0 || y >= x) && e7(ts);
for (var T = wp(y); ; )
if (e++, p = Vr(t, e), p != 95) {
if (y = Ep(p), y < 0 || y >= x)
break;
KA(s, T) && e7(ts), y = wp(y), T = R70(j70(c, T), y), KA(T, y) && e7(ts);
}
return e != nn(t) && e7(ts), x == 10 && KA(new an(0, 0, Mi), T) && e7(ts), i < 0 && (T = XV(T)), T;
}
function jv(t) {
return t.toFloat();
}
function Bi(t) {
var n4 = YV(t), e = n4[0], i = n4[1], x = n4[2], c = nn(t), s = -1 >>> 0, p = e < c ? Vr(t, e) : 0, y = Ep(p);
(y < 0 || y >= x) && e7(ts);
var T = y;
for (e++; e < c; e++)
if (p = Vr(t, e), p != 95) {
if (y = Ep(p), y < 0 || y >= x)
break;
T = x * T + y, T > s && e7(ts);
}
return e != c && e7(ts), T = i * T, x == 10 && (T | 0) != T && e7(ts), T | 0;
}
function G70(t) {
return t.slice(1);
}
function M70(t) {
return !!t;
}
function sn(t) {
return t.toUtf16();
}
function B70(t) {
for (var n4 = {}, e = 1; e < t.length; e++) {
var i = t[e];
n4[sn(i[1])] = i[2];
}
return n4;
}
function q70(t, n4) {
return +(dp(t, n4, false) < 0);
}
function Gv(e, n4) {
e < 0 && il();
var e = e + 1 | 0, i = new Array(e);
i[0] = 0;
for (var x = 1; x < e; x++)
i[x] = n4;
return i;
}
function U70() {
return 0;
}
function ot(t) {
RA(Vt.Sys_error, t);
}
var Pc = new Array();
function m1(t) {
var n4 = Pc[t];
if (n4.opened || ot("Cannot flush a closed channel"), !n4.buffer || n4.buffer == C)
return 0;
if (n4.fd && Vt.fds[n4.fd] && Vt.fds[n4.fd].output) {
var e = Vt.fds[n4.fd].output;
switch (e.length) {
case 2:
e(t, n4.buffer);
break;
default:
e(n4.buffer);
}
}
return n4.buffer = C, 0;
}
function VV() {
return typeof j0.process < "u" && typeof j0.process.versions < "u" && typeof j0.process.versions.node < "u" && j0.process.platform !== "browser";
}
function H70() {
function t(e) {
if (e.charAt(0) === Zu)
return [C, e.substring(1)];
}
function n4(e) {
var i = /^([a-zA-Z]:|[\\/]{2}[^\\/]+[\\/]+[^\\/]+)?([\\/])?([\s\S]*?)$/, x = i.exec(e), c = x[1] || C, s = Boolean(c && c.charAt(1) !== o1);
if (Boolean(x[2] || s)) {
var p = x[1] || C, y = x[2] || C;
return [p, e.substring(p.length + y.length)];
}
}
return j0.process && j0.process.platform && j0.process.platform === bV ? n4 : t;
}
var WA = H70();
function zV(t) {
return t.slice(-1) !== Zu ? t + Zu : t;
}
if (j0.process && j0.process.cwd)
var ol = j0.process.cwd().replace(/\\/g, Zu);
else
var ol = "/static";
ol = zV(ol);
function X70(t) {
t = sn(t), WA(t) || (t = ol + t);
for (var n4 = WA(t), e = n4[1].split(Zu), i = [], x = 0; x < e.length; x++)
switch (e[x]) {
case "..":
i.length > 1 && i.pop();
break;
case ".":
break;
default:
i.push(e[x]);
break;
}
return i.unshift(n4[0]), i.orig = t, i;
}
var Y70 = ["E2BIG", "EACCES", "EAGAIN", "EBADF", "EBUSY", "ECHILD", "EDEADLK", "EDOM", xV, "EFAULT", "EFBIG", "EINTR", "EINVAL", "EIO", "EISDIR", "EMFILE", "EMLINK", "ENAMETOOLONG", "ENFILE", "ENODEV", pd, "ENOEXEC", "ENOLCK", "ENOMEM", "ENOSPC", "ENOSYS", kw, GU, "ENOTTY", "ENXIO", "EPERM", "EPIPE", "ERANGE", "EROFS", "ESPIPE", "ESRCH", "EXDEV", "EWOULDBLOCK", "EINPROGRESS", "EALREADY", "ENOTSOCK", "EDESTADDRREQ", "EMSGSIZE", "EPROTOTYPE", "ENOPROTOOPT", "EPROTONOSUPPORT", "ESOCKTNOSUPPORT", "EOPNOTSUPP", "EPFNOSUPPORT", "EAFNOSUPPORT", "EADDRINUSE", "EADDRNOTAVAIL", "ENETDOWN", "ENETUNREACH", "ENETRESET", "ECONNABORTED", "ECONNRESET", "ENOBUFS", "EISCONN", "ENOTCONN", "ESHUTDOWN", "ETOOMANYREFS", "ETIMEDOUT", "ECONNREFUSED", "EHOSTDOWN", "EHOSTUNREACH", "ELOOP", "EOVERFLOW"];
function _1(t, n4, e, i) {
var x = Y70.indexOf(t);
x < 0 && (i == null && (i = -9999), x = [0, i]);
var c = [x, M7(n4 || C), M7(e || C)];
return c;
}
var KV = {};
function y1(t) {
return KV[t];
}
function d1(t, n4) {
throw [0, t].concat(n4);
}
function V70(t) {
return new Ac(4, t, t.length);
}
function z70(t) {
t = Nc(t), ot(t + up);
}
function K70(t, n4) {
return n4 >>> 0 >= t.l && SV(), Hu(t, n4);
}
function WV() {
}
function Su(t) {
this.data = t;
}
Su.prototype = new WV(), Su.prototype.truncate = function(t) {
var n4 = this.data;
this.data = Pt(t | 0), Is(n4, 0, this.data, 0, t);
}, Su.prototype.length = function() {
return l7(this.data);
}, Su.prototype.write = function(t, n4, e, i) {
var x = this.length();
if (t + i >= x) {
var c = Pt(t + i), s = this.data;
this.data = c, Is(s, 0, this.data, 0, x);
}
return As(n4, e, this.data, t, i), 0;
}, Su.prototype.read = function(t, n4, e, i) {
var x = this.length();
return Is(this.data, t, n4, e, i), 0;
}, Su.prototype.read_one = function(t) {
return K70(this.data, t);
}, Su.prototype.close = function() {
}, Su.prototype.constructor = Su;
function n7(t, n4) {
this.content = {}, this.root = t, this.lookupFun = n4;
}
n7.prototype.nm = function(t) {
return this.root + t;
}, n7.prototype.create_dir_if_needed = function(t) {
for (var n4 = t.split(Zu), e = C, i = 0; i < n4.length - 1; i++)
e += n4[i] + Zu, !this.content[e] && (this.content[e] = Symbol("directory"));
}, n7.prototype.slash = function(t) {
return /\/$/.test(t) ? t : t + Zu;
}, n7.prototype.lookup = function(t) {
if (!this.content[t] && this.lookupFun) {
var n4 = this.lookupFun(r(this.root), r(t));
n4 !== 0 && (this.create_dir_if_needed(t), this.content[t] = new Su(n4[1]));
}
}, n7.prototype.exists = function(t) {
if (t == C)
return 1;
var n4 = this.slash(t);
return this.content[n4] ? 1 : (this.lookup(t), this.content[t] ? 1 : 0);
}, n7.prototype.mkdir = function(t, n4, e) {
var i = e && y1($d);
this.exists(t) && (i ? d1(i, _1(xV, ZI, this.nm(t))) : ot(t + ": File exists"));
var x = /^(.*)\/[^/]+/.exec(t);
x = x && x[1] || C, this.exists(x) || (i ? d1(i, _1(pd, ZI, this.nm(x))) : ot(x + up)), this.is_dir(x) || (i ? d1(i, _1(kw, ZI, this.nm(x))) : ot(x + MS)), this.create_dir_if_needed(this.slash(t));
}, n7.prototype.rmdir = function(t, n4) {
var e = n4 && y1($d), i = t == C ? C : this.slash(t), x = new RegExp(A6 + i + eY);
this.exists(t) || (e ? d1(e, _1(pd, vh, this.nm(t))) : ot(t + up)), this.is_dir(t) || (e ? d1(e, _1(kw, vh, this.nm(t))) : ot(t + MS));
for (var c in this.content)
c.match(x) && (e ? d1(e, _1(GU, vh, this.nm(t))) : ot(this.nm(t) + ": Directory not empty"));
delete this.content[i];
}, n7.prototype.readdir = function(t) {
var n4 = t == C ? C : this.slash(t);
this.exists(t) || ot(t + up), this.is_dir(t) || ot(t + MS);
var e = new RegExp(A6 + n4 + eY), i = {}, x = [];
for (var c in this.content) {
var s = c.match(e);
s && !i[s[1]] && (i[s[1]] = true, x.push(s[1]));
}
return x;
}, n7.prototype.is_dir = function(t) {
if (t == C)
return true;
var n4 = this.slash(t);
return this.content[n4] ? 1 : 0;
}, n7.prototype.unlink = function(t) {
var n4 = !!this.content[t];
return delete this.content[t], n4;
}, n7.prototype.open = function(t, n4) {
if (n4.rdonly && n4.wronly && ot(this.nm(t) + NY), n4.text && n4.binary && ot(this.nm(t) + aY), this.lookup(t), this.content[t]) {
this.is_dir(t) && ot(this.nm(t) + " : is a directory"), n4.create && n4.excl && ot(this.nm(t) + CY);
var e = this.content[t];
return n4.truncate && e.truncate(), e;
} else {
if (n4.create)
return this.create_dir_if_needed(t), this.content[t] = new Su(Pt(0)), this.content[t];
z70(this.nm(t));
}
}, n7.prototype.register = function(t, n4) {
var e;
if (this.content[t] && ot(this.nm(t) + CY), yp(n4) && (e = new Su(n4)), XA(n4))
e = new Su(n4);
else if (n4 instanceof Array)
e = new Su(V70(n4));
else if (typeof n4 == "string")
e = new Su(EV(n4));
else if (n4.toString) {
var i = M7(n4.toString());
e = new Su(i);
}
e ? (this.create_dir_if_needed(t), this.content[t] = e) : ot(this.nm(t) + " : registering file with invalid content type");
}, n7.prototype.constructor = n7;
function W70(t) {
for (var n4 = nn(t), e = new Array(n4), i = 0; i < n4; i++)
e[i] = Vr(t, i);
return e;
}
function J70(t) {
return t.t != 4 && pp(t), t.c;
}
function Dc(t) {
this.fs = Fj(), this.fd = t;
}
Dc.prototype = new WV(), Dc.prototype.truncate = function(t) {
try {
this.fs.ftruncateSync(this.fd, t | 0);
} catch (n4) {
ot(n4.toString());
}
}, Dc.prototype.length = function() {
try {
return this.fs.fstatSync(this.fd).size;
} catch (t) {
ot(t.toString());
}
}, Dc.prototype.write = function(t, n4, e, i) {
var x = W70(n4);
x instanceof j0.Uint8Array || (x = new j0.Uint8Array(x));
var c = j0.Buffer.from(x);
try {
this.fs.writeSync(this.fd, c, e, i, t);
} catch (s) {
ot(s.toString());
}
return 0;
}, Dc.prototype.read = function(t, n4, e, i) {
var x = J70(n4);
x instanceof j0.Uint8Array || (x = new j0.Uint8Array(x));
var c = j0.Buffer.from(x);
try {
this.fs.readSync(this.fd, c, e, i, t);
} catch (p) {
ot(p.toString());
}
for (var s = 0; s < i; s++)
p1(n4, e + s, c[e + s]);
return 0;
}, Dc.prototype.read_one = function(t) {
var n4 = new j0.Uint8Array(1), e = j0.Buffer.from(n4);
try {
this.fs.readSync(this.fd, e, 0, 1, t);
} catch (i) {
ot(i.toString());
}
return e[0];
}, Dc.prototype.close = function() {
try {
this.fs.closeSync(this.fd);
} catch (t) {
ot(t.toString());
}
}, Dc.prototype.constructor = Dc;
function gu(t) {
this.fs = Fj(), this.root = t;
}
gu.prototype.nm = function(t) {
return this.root + t;
}, gu.prototype.exists = function(t) {
try {
return this.fs.existsSync(this.nm(t)) ? 1 : 0;
} catch {
return 0;
}
}, gu.prototype.mkdir = function(t, n4, e) {
try {
return this.fs.mkdirSync(this.nm(t), { mode: n4 }), 0;
} catch (i) {
this.raise_nodejs_error(i, e);
}
}, gu.prototype.rmdir = function(t, n4) {
try {
return this.fs.rmdirSync(this.nm(t)), 0;
} catch (e) {
this.raise_nodejs_error(e, n4);
}
}, gu.prototype.readdir = function(t, n4) {
try {
return this.fs.readdirSync(this.nm(t));
} catch (e) {
this.raise_nodejs_error(e, n4);
}
}, gu.prototype.is_dir = function(t) {
try {
return this.fs.statSync(this.nm(t)).isDirectory() ? 1 : 0;
} catch (n4) {
ot(n4.toString());
}
}, gu.prototype.unlink = function(t, n4) {
try {
var e = this.fs.existsSync(this.nm(t)) ? 1 : 0;
return this.fs.unlinkSync(this.nm(t)), e;
} catch (i) {
this.raise_nodejs_error(i, n4);
}
}, gu.prototype.open = function(t, n4, e) {
var i = Sae(), x = 0;
for (var c in n4)
switch (c) {
case "rdonly":
x |= i.O_RDONLY;
break;
case "wronly":
x |= i.O_WRONLY;
break;
case "append":
x |= i.O_WRONLY | i.O_APPEND;
break;
case "create":
x |= i.O_CREAT;
break;
case "truncate":
x |= i.O_TRUNC;
break;
case "excl":
x |= i.O_EXCL;
break;
case "binary":
x |= i.O_BINARY;
break;
case "text":
x |= i.O_TEXT;
break;
case "nonblock":
x |= i.O_NONBLOCK;
break;
}
try {
var s = this.fs.openSync(this.nm(t), x);
return new Dc(s);
} catch (p) {
this.raise_nodejs_error(p, e);
}
}, gu.prototype.rename = function(t, n4, e) {
try {
this.fs.renameSync(this.nm(t), this.nm(n4));
} catch (i) {
this.raise_nodejs_error(i, e);
}
}, gu.prototype.stat = function(t, n4) {
try {
var e = this.fs.statSync(this.nm(t));
return this.stats_from_js(e);
} catch (i) {
this.raise_nodejs_error(i, n4);
}
}, gu.prototype.lstat = function(t, n4) {
try {
var e = this.fs.lstatSync(this.nm(t));
return this.stats_from_js(e);
} catch (i) {
this.raise_nodejs_error(i, n4);
}
}, gu.prototype.symlink = function(t, n4, e, i) {
try {
return this.fs.symlinkSync(this.nm(n4), this.nm(e), t ? "dir" : "file"), 0;
} catch (x) {
this.raise_nodejs_error(x, i);
}
}, gu.prototype.readlink = function(t, n4) {
try {
var e = this.fs.readlinkSync(this.nm(t), _V);
return M7(e);
} catch (i) {
this.raise_nodejs_error(i, n4);
}
}, gu.prototype.raise_nodejs_error = function(t, n4) {
var e = y1($d);
if (n4 && e) {
var i = _1(t.code, t.syscall, t.path, t.errno);
d1(e, i);
} else
ot(t.toString());
}, gu.prototype.stats_from_js = function(t) {
var n4;
return t.isFile() ? n4 = 0 : t.isDirectory() ? n4 = 1 : t.isCharacterDevice() ? n4 = 2 : t.isBlockDevice() ? n4 = 3 : t.isSymbolicLink() ? n4 = 4 : t.isFIFO() ? n4 = 5 : t.isSocket() && (n4 = 6), [0, t.dev, t.ino, n4, t.mode, t.nlink, t.uid, t.gid, t.rdev, t.size, t.atimeMs, t.mtimeMs, t.ctimeMs];
}, gu.prototype.constructor = gu;
function JV(t) {
var n4 = WA(t);
if (n4)
return n4[0] + Zu;
}
var Sp = JV(ol) || e7("unable to compute caml_root"), Mv = [];
VV() ? Mv.push({ path: Sp, device: new gu(Sp) }) : Mv.push({ path: Sp, device: new n7(Sp) }), Mv.push({ path: ZU, device: new n7(ZU) });
function $70(e) {
for (var n4 = X70(e), e = n4.join(Zu), i = zV(e), x, c = 0; c < Mv.length; c++) {
var s = Mv[c];
i.search(s.path) == 0 && (!x || x.path.length < s.path.length) && (x = { path: s.path, device: s.device, rest: e.substring(s.path.length, e.length) });
}
if (!x) {
var p = JV(e);
if (p && p.match(/^[a-zA-Z]:\/$/)) {
var s = { path: p, device: new gu(p) };
Mv.push(s), x = { path: s.path, device: s.device, rest: e.substring(s.path.length, e.length) };
}
}
if (x)
return x;
ot("no device found for " + i);
}
function $V(t, n4) {
var e = Pc[t], i = r(n4), x = nn(i);
return e.file.write(e.offset, i, 0, x), e.offset += x, 0;
}
function Z70(n4) {
var n4 = LA(n4), e = j0;
if (e.process && e.process.stdout && e.process.stdout.write)
e.process.stderr.write(n4);
else {
n4.charCodeAt(n4.length - 1) == 10 && (n4 = n4.substr(0, n4.length - 1));
var i = e.console;
i && i.error && i.error(n4);
}
}
function Q70(n4) {
var n4 = LA(n4), e = j0;
if (e.process && e.process.stdout && e.process.stdout.write)
e.process.stdout.write(n4);
else {
n4.charCodeAt(n4.length - 1) == 10 && (n4 = n4.substr(0, n4.length - 1));
var i = e.console;
i && i.log && i.log(n4);
}
}
function gp(t, n4, e, i) {
Vt.fds === void 0 && (Vt.fds = new Array()), i = i || {};
var x = {};
return x.file = e, x.offset = i.append ? e.length() : 0, x.flags = i, x.output = n4, Vt.fds[t] = x, (!Vt.fd_last_idx || t > Vt.fd_last_idx) && (Vt.fd_last_idx = t), t;
}
function Lae(t, n4, e) {
for (var i = {}; n4; ) {
switch (n4[1]) {
case 0:
i.rdonly = 1;
break;
case 1:
i.wronly = 1;
break;
case 2:
i.append = 1;
break;
case 3:
i.create = 1;
break;
case 4:
i.truncate = 1;
break;
case 5:
i.excl = 1;
break;
case 6:
i.binary = 1;
break;
case 7:
i.text = 1;
break;
case 8:
i.nonblock = 1;
break;
}
n4 = n4[2];
}
i.rdonly && i.wronly && ot(Nc(t) + NY), i.text && i.binary && ot(Nc(t) + aY);
var x = $70(t), c = x.device.open(x.rest, i), s = Vt.fd_last_idx ? Vt.fd_last_idx : 0;
return gp(s + 1, $V, c, i);
}
gp(0, $V, new Su(Pt(0))), gp(1, Q70, new Su(Pt(0))), gp(2, Z70, new Su(Pt(0)));
function ri0(t) {
var n4 = Vt.fds[t];
n4.flags.wronly && ot(hX + t + " is writeonly");
var e = null;
if (t == 0 && VV()) {
var i = Fj();
e = function() {
return M7(i.readFileSync(0, _V));
};
}
var x = { file: n4.file, offset: n4.offset, fd: t, opened: true, out: false, refill: e };
return Pc[x.fd] = x, x.fd;
}
function ZV(t) {
var n4 = Vt.fds[t];
n4.flags.rdonly && ot(hX + t + " is readonly");
var e = { file: n4.file, offset: n4.offset, fd: t, opened: true, out: true, buffer: C };
return Pc[e.fd] = e, e.fd;
}
function ei0() {
for (var t = 0, n4 = 0; n4 < Pc.length; n4++)
Pc[n4] && Pc[n4].opened && Pc[n4].out && (t = [0, Pc[n4].fd, t]);
return t;
}
function Rae(t) {
return t;
}
function ni0(t, n4, e, i) {
var x = Pc[t];
x.opened || ot("Cannot output to a closed channel");
var c;
e == 0 && l7(n4) == i ? c = n4 : (c = Pt(i), Is(n4, e, c, 0, i));
var s = c, p = Nc(s), y = p.lastIndexOf(nV);
return y < 0 ? x.buffer += p : (x.buffer += p.substr(0, y + 1), m1(t), x.buffer += p.substr(y + 1)), 0;
}
function JA(t, n4, e, i) {
return ni0(t, n4, e, i);
}
function QV(t, n4) {
var e = r(String.fromCharCode(n4));
return JA(t, e, 0, 1), 0;
}
function cl(t, n4) {
return +(dp(t, n4, false) != 0);
}
function $A(t, n4) {
var e = new Array(n4 + 1);
e[0] = t;
for (var i = 1; i <= n4; i++)
e[i] = 0;
return e;
}
function ti0(t, n4) {
return t[0] = nl, t[1] = n4, 0;
}
function h1(t) {
return t instanceof Array && t[0] == t[0] >>> 0 ? t[0] : yp(t) || XA(t) ? Y2 : t instanceof Function || typeof t == "function" ? jw : t && t.caml_custom ? Ow : Q2;
}
function yi(t, n4, e) {
e && j0.toplevelReloc && (t = j0.toplevelReloc(e)), Vt[t + 1] = n4, e && (Vt[e] = n4);
}
function ZA(t, n4) {
return KV[Nc(t)] = n4, 0;
}
function ui0(t) {
return t[2] = UV++, t;
}
function ii0(t, n4) {
return t === n4 ? 1 : (t.t & 6 && Dv(t), n4.t & 6 && Dv(n4), t.c == n4.c ? 1 : 0);
}
function qn(t, n4) {
return ii0(t, n4);
}
function fi0() {
vu(XS);
}
function Ot(t, n4) {
return n4 >>> 0 >= nn(t) && fi0(), Vr(t, n4);
}
function n0(t, n4) {
return 1 - qn(t, n4);
}
function xi0() {
return [0, r("js_of_ocaml")];
}
function ai0() {
return 2147483647 / 4 | 0;
}
function oi0(t) {
return 0;
}
var ci0 = j0.process && j0.process.platform && j0.process.platform == bV ? UU : "Unix";
function si0() {
return [0, r(ci0), 32, 0];
}
function vi0() {
FV(Vt.Not_found);
}
function rz(t) {
var n4 = j0, e = sn(t);
if (n4.process && n4.process.env && n4.process.env[e] != null)
return M7(n4.process.env[e]);
if (j0.jsoo_static_env && j0.jsoo_static_env[e])
return M7(j0.jsoo_static_env[e]);
vi0();
}
function QA(t) {
for (var n4 = 1; t && t.joo_tramp; )
t = t.joo_tramp.apply(null, t.joo_args), n4++;
return t;
}
function Fu(t, n4) {
return { joo_tramp: t, joo_args: n4 };
}
function N(t, n4) {
if (typeof n4 == "function")
return t.fun = n4, 0;
if (n4.fun)
return t.fun = n4.fun, 0;
for (var e = n4.length; e--; )
t[e] = n4[e];
return 0;
}
function jae(t) {
return t;
}
function Et(t) {
return t instanceof Array ? t : j0.RangeError && t instanceof j0.RangeError && t.message && t.message.match(/maximum call stack/i) || j0.InternalError && t instanceof j0.InternalError && t.message && t.message.match(/too much recursion/i) ? Vt.Stack_overflow : t instanceof j0.Error && y1($O) ? [0, y1($O), t] : [0, Vt.Failure, M7(String(t))];
}
function li0(t) {
switch (t[2]) {
case -8:
case -11:
case -12:
return 1;
default:
return 0;
}
}
function bi0(t) {
var n4 = C;
if (t[0] == 0) {
if (n4 += t[1][1], t.length == 3 && t[2][0] == 0 && li0(t[1]))
var i = t[2], e = 1;
else
var e = 2, i = t;
n4 += KX;
for (var x = e; x < i.length; x++) {
x > e && (n4 += nX);
var c = i[x];
typeof c == "number" ? n4 += c.toString() : c instanceof Ac || typeof c == "string" ? n4 += V2 + c.toString() + V2 : n4 += bv;
}
n4 += Z0;
} else
t[0] == wt && (n4 += t[1]);
return n4;
}
function ez(t) {
if (t instanceof Array && (t[0] == 0 || t[0] == wt)) {
var n4 = y1(BH);
if (n4)
n4(t, false);
else {
var e = bi0(t), i = y1(mV);
i && i(0), j0.console.error(kh + e + nV);
}
} else
throw t;
}
function pi0() {
var t = j0;
t.process && t.process.on ? t.process.on("uncaughtException", function(n4, e) {
ez(n4), t.process.exit(2);
}) : t.addEventListener && t.addEventListener("error", function(n4) {
n4.error && ez(n4.error);
});
}
pi0();
function u(t, n4) {
return t.length == 1 ? t(n4) : os(t, [n4]);
}
function a(t, n4, e) {
return t.length == 2 ? t(n4, e) : os(t, [n4, e]);
}
function ir(t, n4, e, i) {
return t.length == 3 ? t(n4, e, i) : os(t, [n4, e, i]);
}
function R(t, n4, e, i, x) {
return t.length == 4 ? t(n4, e, i, x) : os(t, [n4, e, i, x]);
}
function b7(t, n4, e, i, x, c) {
return t.length == 5 ? t(n4, e, i, x, c) : os(t, [n4, e, i, x, c]);
}
function mi0(t, n4, e, i, x, c, s, p) {
return t.length == 7 ? t(n4, e, i, x, c, s, p) : os(t, [n4, e, i, x, c, s, p]);
}
var rN = [wt, r(TX), -1], nz = [wt, r(MH), -2], B7 = [wt, r(LH), -3], eN = [wt, r(sH), -4], Kt = [wt, r(QU), -7], tz = [wt, r(BY), -8], uz = [wt, r($U), -9], wn = [wt, r(TU), -11], sl = [wt, r(oX), -12], iz = [0, c7], _i0 = [4, 0, 0, 0, [12, 45, [4, 0, 0, 0, 0]]], nN = [0, [11, r('File "'), [2, 0, [11, r('", line '), [4, 0, 0, 0, [11, r(EH), [4, 0, 0, 0, [12, 45, [4, 0, 0, 0, [11, r(": "), [2, 0, 0]]]]]]]]]], r('File "%s", line %d, characters %d-%d: %s')], fz = [0, 0, [0, 0, 0], [0, 0, 0]], tN = r(""), uN = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), Bv = [0, 0, 0, 0, 1, 0], xz = [0, r(Gx), r(va), r(go), r(so), r(za), r(Yf), r(Jx), r(pf), r(of), r(Bx), r(ut), r(Yo), r(I7), r(If), r(px), r(_a), r(lx), r(ef), r(gf), r(Xo), r(oo), r(Ho), r(yf), r(ic), r(Rf), r(To), r(ff), r(Ef), r(Bo), r(Xf), r(Tx), r(ax), r(Fa), r(kf), r(da), r(Qf), r(ao), r(Za), r(A7), r(O7), r(ox), r(Io), r(Mo), r(Wf), r(to), r(la), r(Ta), r(Sx), r(Go), r(ka), r(s7), r(bo), r(eo), r(vo), r(Hx), r(Xa), r(Ux), r(Mf), r(Nf), r(rc), r(Mx), r(Cf), r(ba), r(Fo), r(lf), r(ec), r(io), r(no), r(Au), r(Ix), r(ta), r(Ff), r(Uf), r(Eo), r(qx), r(Oc), r(Vo), r(jo), r(hx), r(xc), r(vi), r(ga), r(pa), r(Ic), r(No), r(kx), r(Nx), r(Tc), r(jf), r(uf), r(ix), r(yo), r(Ma), r(Ex), r(Uo), r(bf), r(po), r(yx), r(j7), r($o), r(mc), r(Kf), r(_i), r(_f), r(fo), r(zo), r(qu), r(gc), r(ma), r(Yx), r(Do), r(Ox), r(Co), r(r7), r(sf), r(wa), r(na), r(Wo), r(Gf), r(sc), r(ho), r(Ja), r(ex), r(cf), r(af), r(Cx), r(_c), r(Na), r(Ga), r(xa), r(Ax), r(sa), r(Px), r(nf), r(nc), r(Wa), r(Ro), r(Sc), r($a), r(bx), r(kc), r(Lx), r(ko), r(Rx), r(ux), r(Wx), r(Lo), r(Xx), r($x), r(dc), r(ur), r(yc), r(Af), r(hf), r(mx), r(Ua), r(jx), r(Tf), r(uc), r(Jf), r(wo), r(_o), r(tx), r(Vf), r(xf), r(Pf), r(xx), r(ca), r(Qa), r(ac), r(pc), r(Ya), r(Ko), r(wf), r(Ba), r(ro), r(pi), r(nx), r(rx), r(Ka), r(mf), r(lo), r(bc), r(Ec), r($f), r(zf), r(qa), r(Df), r(uo), r(co), r(lc), r(ra), r(So), r(Va), r(Qo), r(Qu), r(Le), r(mo), r(_x), r(Wu), r(vx), r(qf), r(Fc), r(df), r(Kx), r(Fx), r(Po), r(hc), r(P7), r(ha), r(Ha), r(Zf), r(Qx), r(Ea), r(Lf), r(Aa), r(g7), r(ua), r(xo), r(oa), r(dx), r(Zx), r(Vx), r(Jo), r(ja), r(Hf), r(Ao), r(sx), r(Of), r(Dx), r(fa), r(Zo), r(Sf), r(Ca), r(tc), r(Da), r(Oa), r(Bf), r(cc), r(fx), r(wc), r(ou), r(Ia), r(ia), r(zx), r(wu)], az = [0, r("first_leading"), r("last_trailing")], oz = [0, 0];
yi(11, sl, oX), yi(10, wn, TU), yi(9, [wt, r(gY), jX], gY), yi(8, uz, $U), yi(7, tz, BY), yi(6, Kt, QU), yi(5, [wt, r(iY), -6], iY), yi(4, [wt, r(DH), -5], DH), yi(3, eN, sH), yi(2, B7, LH), yi(1, nz, MH), yi(0, rN, TX);
var yi0 = r("output_substring"), di0 = r("%.12g"), hi0 = r(Ra), ki0 = r(Gi), wi0 = r(Ci), Ei0 = r(oY), Si0 = r("\\'"), gi0 = r("\\b"), Fi0 = r("\\t"), Ti0 = r("\\n"), Oi0 = r("\\r"), Ii0 = r("List.iter2"), Ai0 = r("tl"), Ni0 = r("hd"), Ci0 = r("String.blit / Bytes.blit_string"), Pi0 = r("Bytes.blit"), Di0 = r("String.sub / Bytes.sub"), Li0 = r("Array.blit"), Ri0 = r("Array.sub"), ji0 = r("Map.remove_min_elt"), Gi0 = [0, 0, 0, 0], Mi0 = [0, r("map.ml"), 400, 10], Bi0 = [0, 0, 0], qi0 = r(n6), Ui0 = r(n6), Hi0 = r(n6), Xi0 = r(n6), Yi0 = r("Stdlib.Queue.Empty"), Vi0 = r("CamlinternalLazy.Undefined"), zi0 = r("Buffer.add_substring/add_subbytes"), Ki0 = r("Buffer.add: cannot grow buffer"), Wi0 = [0, r(HU), 93, 2], Ji0 = [0, r(HU), 94, 2], $i0 = r("Buffer.sub"), Zi0 = r("%c"), Qi0 = r("%s"), rf0 = r(lX), ef0 = r(XY), nf0 = r(vH), tf0 = r(JH), uf0 = r("%f"), if0 = r(An), ff0 = r("%{"), xf0 = r("%}"), af0 = r("%("), of0 = r("%)"), cf0 = r(C0), sf0 = r("%t"), vf0 = r("%?"), lf0 = r("%r"), bf0 = r("%_r"), pf0 = [0, r(cu), 850, 23], mf0 = [0, r(cu), 814, 21], _f0 = [0, r(cu), 815, 21], yf0 = [0, r(cu), 818, 21], df0 = [0, r(cu), 819, 21], hf0 = [0, r(cu), 822, 19], kf0 = [0, r(cu), 823, 19], wf0 = [0, r(cu), 826, 22], Ef0 = [0, r(cu), 827, 22], Sf0 = [0, r(cu), 831, 30], gf0 = [0, r(cu), 832, 30], Ff0 = [0, r(cu), 836, 26], Tf0 = [0, r(cu), 837, 26], Of0 = [0, r(cu), 846, 28], If0 = [0, r(cu), 847, 28], Af0 = [0, r(cu), 851, 23], Nf0 = r(TY), Cf0 = [0, r(cu), 1558, 4], Pf0 = r("Printf: bad conversion %["), Df0 = [0, r(cu), 1626, 39], Lf0 = [0, r(cu), 1649, 31], Rf0 = [0, r(cu), 1650, 31], jf0 = r("Printf: bad conversion %_"), Gf0 = r(IY), Mf0 = r(dY), Bf0 = r(IY), qf0 = r(dY), Uf0 = [0, [11, r("invalid box description "), [3, 0, 0]], r("invalid box description %S")], Hf0 = r(C), Xf0 = [0, 0, 4], Yf0 = r(C), Vf0 = r(gX), zf0 = r("h"), Kf0 = r("hov"), Wf0 = r("hv"), Jf0 = r(hV), $f0 = r(bw), Zf0 = r("neg_infinity"), Qf0 = r(kU), rx0 = r(Ra), ex0 = r("%+nd"), nx0 = r("% nd"), tx0 = r("%+ni"), ux0 = r("% ni"), ix0 = r("%nx"), fx0 = r("%#nx"), xx0 = r("%nX"), ax0 = r("%#nX"), ox0 = r("%no"), cx0 = r("%#no"), sx0 = r("%nd"), vx0 = r(vH), lx0 = r("%nu"), bx0 = r("%+ld"), px0 = r("% ld"), mx0 = r("%+li"), _x0 = r("% li"), yx0 = r("%lx"), dx0 = r("%#lx"), hx0 = r("%lX"), kx0 = r("%#lX"), wx0 = r("%lo"), Ex0 = r("%#lo"), Sx0 = r("%ld"), gx0 = r(XY), Fx0 = r("%lu"), Tx0 = r("%+Ld"), Ox0 = r("% Ld"), Ix0 = r("%+Li"), Ax0 = r("% Li"), Nx0 = r("%Lx"), Cx0 = r("%#Lx"), Px0 = r("%LX"), Dx0 = r("%#LX"), Lx0 = r("%Lo"), Rx0 = r("%#Lo"), jx0 = r("%Ld"), Gx0 = r(JH), Mx0 = r("%Lu"), Bx0 = r("%+d"), qx0 = r("% d"), Ux0 = r("%+i"), Hx0 = r("% i"), Xx0 = r("%x"), Yx0 = r("%#x"), Vx0 = r("%X"), zx0 = r("%#X"), Kx0 = r("%o"), Wx0 = r("%#o"), Jx0 = r(N2), $x0 = r(lX), Zx0 = r(TY), Qx0 = r(z), ra0 = r("@}"), ea0 = r("@?"), na0 = r(`@
`), ta0 = r("@."), ua0 = r("@@"), ia0 = r("@%"), fa0 = r(AX), xa0 = r("CamlinternalFormat.Type_mismatch"), aa0 = r(C), oa0 = [0, [11, r(nX), [2, 0, [2, 0, 0]]], r(", %s%s")], ca0 = [0, [11, r(kh), [2, 0, [12, 10, 0]]], r(CU)], sa0 = [0, [11, r("Fatal error in uncaught exception handler: exception "), [2, 0, [12, 10, 0]]], r(`Fatal error in uncaught exception handler: exception %s
`)], va0 = r("Fatal error: out of memory in uncaught exception handler"), la0 = [0, [11, r(kh), [2, 0, [12, 10, 0]]], r(CU)], ba0 = [0, [2, 0, [12, 10, 0]], r(`%s
`)], pa0 = [0, [11, r(RY), 0], r(RY)], ma0 = r("Raised at"), _a0 = r("Re-raised at"), ya0 = r("Raised by primitive operation at"), da0 = r("Called from"), ha0 = r(" (inlined)"), ka0 = r(C), wa0 = [0, [2, 0, [12, 32, [2, 0, [11, r(' in file "'), [2, 0, [12, 34, [2, 0, [11, r(", line "), [4, 0, 0, 0, [11, r(EH), _i0]]]]]]]]]], r('%s %s in file "%s"%s, line %d, characters %d-%d')], Ea0 = [0, [2, 0, [11, r(" unknown location"), 0]], r("%s unknown location")], Sa0 = r("Out of memory"), ga0 = r("Stack overflow"), Fa0 = r("Pattern matching failed"), Ta0 = r("Assertion failed"), Oa0 = r("Undefined recursive module"), Ia0 = [0, [12, 40, [2, 0, [2, 0, [12, 41, 0]]]], r("(%s%s)")], Aa0 = r(C), Na0 = r(C), Ca0 = [0, [12, 40, [2, 0, [12, 41, 0]]], r("(%s)")], Pa0 = [0, [4, 0, 0, 0, 0], r(N2)], Da0 = [0, [3, 0, 0], r(Yt)], La0 = r(bv), Ra0 = [0, r(C), r(`(Cannot print locations:
bytecode executable program file not found)`), r(`(Cannot print locations:
bytecode executable program file appears to be corrupt)`), r(`(Cannot print locations:
bytecode executable program file has wrong magic number)`), r(`(Cannot print locations:
bytecode executable program file cannot be opened;
-- too many open files. Try running with OCAMLRUNPARAM=b=2)`)], ja0 = [3, 0, 3], Ga0 = r(Ra), Ma0 = r(cv), Ba0 = r(""), qa0 = r(C), Ua0 = r(cv), Ha0 = r(g3), Xa0 = r(C), Ya0 = r(UI), Va0 = r(C), za0 = r(C), Ka0 = r(C), Wa0 = r(C), Ja0 = [0, r(C)], $a0 = r(C), Za0 = r(C), Qa0 = r(C), ro0 = r(C), eo0 = [0, r(C), 0, r(C)], no0 = r(C), to0 = r("Stdlib.Format.String_tag"), uo0 = [0, r("camlinternalOO.ml"), 281, 50], io0 = r(C), fo0 = [0, r(hY), 72, 5], xo0 = [0, r(hY), 81, 2], ao0 = r("CamlinternalMod.update_mod: not a module"), oo0 = r("CamlinternalMod.init_mod: not a module"), co0 = r("TMPDIR"), so0 = r("TEMP"), vo0 = r(UU), lo0 = r("Win32"), bo0 = [0, r("src/wtf8.ml"), 65, 9], po0 = r("Js_of_ocaml__Js.Error"), mo0 = r($O), _o0 = [0, [15, 0], r(C0)], yo0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], do0 = r(Yr), ho0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ko0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], wo0 = r("Flow_ast.Program.statements"), Eo0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], So0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], go0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Fo0 = [0, [17, 0, 0], r(z)], To0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Oo0 = r(Xr), Io0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ao0 = r(tr), No0 = r(Z0), Co0 = r(nr), Po0 = [0, [17, 0, 0], r(z)], Do0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Lo0 = r("all_comments"), Ro0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], jo0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Go0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Mo0 = [0, [17, 0, 0], r(z)], Bo0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], qo0 = [0, [15, 0], r(C0)], Uo0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Ho0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Xo0 = [0, [17, 0, [12, 41, 0]], r(h0)], Yo0 = [0, [15, 0], r(C0)], Vo0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Function.BodyBlock"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Function.BodyBlock@ ")], zo0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Ko0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Wo0 = [0, [17, 0, [12, 41, 0]], r(h0)], Jo0 = [0, [17, 0, [12, 41, 0]], r(h0)], $o0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Function.BodyExpression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Function.BodyExpression@ ")], Zo0 = [0, [17, 0, [12, 41, 0]], r(h0)], Qo0 = [0, [15, 0], r(C0)], rc0 = r(Yr), ec0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], nc0 = r("Flow_ast.Function.id"), tc0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], uc0 = r(tr), ic0 = r(Z0), fc0 = r(nr), xc0 = [0, [17, 0, 0], r(z)], ac0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], oc0 = r(Ct), cc0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], sc0 = [0, [17, 0, 0], r(z)], vc0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], lc0 = r(Wn), bc0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], pc0 = [0, [17, 0, 0], r(z)], mc0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], _c0 = r(Os), yc0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], dc0 = [0, [9, 0, 0], r(An)], hc0 = [0, [17, 0, 0], r(z)], kc0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], wc0 = r(j7), Ec0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Sc0 = [0, [9, 0, 0], r(An)], gc0 = [0, [17, 0, 0], r(z)], Fc0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Tc0 = r(Qu), Oc0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ic0 = r(tr), Ac0 = r(Z0), Nc0 = r(nr), Cc0 = [0, [17, 0, 0], r(z)], Pc0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Dc0 = r(Wu), Lc0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Rc0 = [0, [17, 0, 0], r(z)], jc0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Gc0 = r(sv), Mc0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Bc0 = r(tr), qc0 = r(Z0), Uc0 = r(nr), Hc0 = [0, [17, 0, 0], r(z)], Xc0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Yc0 = r(Xr), Vc0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], zc0 = r(tr), Kc0 = r(Z0), Wc0 = r(nr), Jc0 = [0, [17, 0, 0], r(z)], $c0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Zc0 = r("sig_loc"), Qc0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], rs0 = [0, [17, 0, 0], r(z)], es0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], ns0 = [0, [15, 0], r(C0)], ts0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], us0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], is0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], fs0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], xs0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], as0 = r("Flow_ast.Function.Params.this_"), os0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], cs0 = r(tr), ss0 = r(Z0), vs0 = r(nr), ls0 = [0, [17, 0, 0], r(z)], bs0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ps0 = r(Ct), ms0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _s0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], ys0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], ds0 = [0, [17, 0, 0], r(z)], hs0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ks0 = r(ch), ws0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Es0 = r(tr), Ss0 = r(Z0), gs0 = r(nr), Fs0 = [0, [17, 0, 0], r(z)], Ts0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Os0 = r(Xr), Is0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], As0 = r(tr), Ns0 = r(Z0), Cs0 = r(nr), Ps0 = [0, [17, 0, 0], r(z)], Ds0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Ls0 = [0, [15, 0], r(C0)], Rs0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], js0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Gs0 = [0, [17, 0, [12, 41, 0]], r(h0)], Ms0 = [0, [15, 0], r(C0)], Bs0 = r(Yr), qs0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Us0 = r("Flow_ast.Function.ThisParam.annot"), Hs0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Xs0 = [0, [17, 0, 0], r(z)], Ys0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Vs0 = r(Xr), zs0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ks0 = r(tr), Ws0 = r(Z0), Js0 = r(nr), $s0 = [0, [17, 0, 0], r(z)], Zs0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Qs0 = [0, [15, 0], r(C0)], r10 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], e10 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], n10 = [0, [17, 0, [12, 41, 0]], r(h0)], t10 = [0, [15, 0], r(C0)], u10 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], i10 = r("Flow_ast.Function.Param.argument"), f10 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], x10 = [0, [17, 0, 0], r(z)], a10 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], o10 = r(mi), c10 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], s10 = r(tr), v10 = r(Z0), l10 = r(nr), b10 = [0, [17, 0, 0], r(z)], p10 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], m10 = [0, [15, 0], r(C0)], _10 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], y10 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], d10 = [0, [17, 0, [12, 41, 0]], r(h0)], h10 = [0, [15, 0], r(C0)], k10 = r(Yr), w10 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], E10 = r("Flow_ast.Function.RestParam.argument"), S10 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], g10 = [0, [17, 0, 0], r(z)], F10 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], T10 = r(Xr), O10 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], I10 = r(tr), A10 = r(Z0), N10 = r(nr), C10 = [0, [17, 0, 0], r(z)], P10 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], D10 = [0, [15, 0], r(C0)], L10 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], R10 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], j10 = [0, [17, 0, [12, 41, 0]], r(h0)], G10 = [0, [15, 0], r(C0)], M10 = r(Yr), B10 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], q10 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], U10 = r("Flow_ast.Class.id"), H10 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], X10 = r(tr), Y10 = r(Z0), V10 = r(nr), z10 = [0, [17, 0, 0], r(z)], K10 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], W10 = r(Wn), J10 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $10 = [0, [17, 0, 0], r(z)], Z10 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Q10 = r(sv), rv0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ev0 = r(tr), nv0 = r(Z0), tv0 = r(nr), uv0 = [0, [17, 0, 0], r(z)], iv0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], fv0 = r(C7), xv0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], av0 = r(tr), ov0 = r(Z0), cv0 = r(nr), sv0 = [0, [17, 0, 0], r(z)], vv0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], lv0 = r(gs), bv0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], pv0 = r(tr), mv0 = r(Z0), _v0 = r(nr), yv0 = [0, [17, 0, 0], r(z)], dv0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hv0 = r("class_decorators"), kv0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], wv0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Ev0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Sv0 = [0, [17, 0, 0], r(z)], gv0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Fv0 = r(Xr), Tv0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ov0 = r(tr), Iv0 = r(Z0), Av0 = r(nr), Nv0 = [0, [17, 0, 0], r(z)], Cv0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Pv0 = [0, [15, 0], r(C0)], Dv0 = r(Yr), Lv0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Rv0 = r("Flow_ast.Class.Decorator.expression"), jv0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Gv0 = [0, [17, 0, 0], r(z)], Mv0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Bv0 = r(Xr), qv0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Uv0 = r(tr), Hv0 = r(Z0), Xv0 = r(nr), Yv0 = [0, [17, 0, 0], r(z)], Vv0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], zv0 = [0, [15, 0], r(C0)], Kv0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Wv0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Jv0 = [0, [17, 0, [12, 41, 0]], r(h0)], $v0 = [0, [15, 0], r(C0)], Zv0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Class.Body.Method"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Class.Body.Method@ ")], Qv0 = [0, [17, 0, [12, 41, 0]], r(h0)], r20 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Class.Body.Property"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Class.Body.Property@ ")], e20 = [0, [17, 0, [12, 41, 0]], r(h0)], n20 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Class.Body.PrivateField"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Class.Body.PrivateField@ ")], t20 = [0, [17, 0, [12, 41, 0]], r(h0)], u20 = [0, [15, 0], r(C0)], i20 = r(Yr), f20 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], x20 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], a20 = r("Flow_ast.Class.Body.body"), o20 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], c20 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], s20 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], v20 = [0, [17, 0, 0], r(z)], l20 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], b20 = r(Xr), p20 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], m20 = r(tr), _20 = r(Z0), y20 = r(nr), d20 = [0, [17, 0, 0], r(z)], h20 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], k20 = [0, [15, 0], r(C0)], w20 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], E20 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], S20 = [0, [17, 0, [12, 41, 0]], r(h0)], g20 = [0, [15, 0], r(C0)], F20 = r(Yr), T20 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], O20 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], I20 = r("Flow_ast.Class.Implements.interfaces"), A20 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], N20 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], C20 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], P20 = [0, [17, 0, 0], r(z)], D20 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], L20 = r(Xr), R20 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], j20 = r(tr), G20 = r(Z0), M20 = r(nr), B20 = [0, [17, 0, 0], r(z)], q20 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], U20 = [0, [15, 0], r(C0)], H20 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], X20 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Y20 = [0, [17, 0, [12, 41, 0]], r(h0)], V20 = [0, [15, 0], r(C0)], z20 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], K20 = r("Flow_ast.Class.Implements.Interface.id"), W20 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], J20 = [0, [17, 0, 0], r(z)], $20 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Z20 = r(Z2), Q20 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], rl0 = r(tr), el0 = r(Z0), nl0 = r(nr), tl0 = [0, [17, 0, 0], r(z)], ul0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], il0 = [0, [15, 0], r(C0)], fl0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], xl0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], al0 = [0, [17, 0, [12, 41, 0]], r(h0)], ol0 = [0, [15, 0], r(C0)], cl0 = r(Yr), sl0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], vl0 = r("Flow_ast.Class.Extends.expr"), ll0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], bl0 = [0, [17, 0, 0], r(z)], pl0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ml0 = r(Z2), _l0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], yl0 = r(tr), dl0 = r(Z0), hl0 = r(nr), kl0 = [0, [17, 0, 0], r(z)], wl0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], El0 = r(Xr), Sl0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], gl0 = r(tr), Fl0 = r(Z0), Tl0 = r(nr), Ol0 = [0, [17, 0, 0], r(z)], Il0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Al0 = [0, [15, 0], r(C0)], Nl0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Cl0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Pl0 = [0, [17, 0, [12, 41, 0]], r(h0)], Dl0 = [0, [15, 0], r(C0)], Ll0 = r(Yr), Rl0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], jl0 = r("Flow_ast.Class.PrivateField.key"), Gl0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ml0 = [0, [17, 0, 0], r(z)], Bl0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ql0 = r(Bn), Ul0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Hl0 = [0, [17, 0, 0], r(z)], Xl0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Yl0 = r(rs), Vl0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], zl0 = [0, [17, 0, 0], r(z)], Kl0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Wl0 = r(eu), Jl0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $l0 = [0, [9, 0, 0], r(An)], Zl0 = [0, [17, 0, 0], r(z)], Ql0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], rb0 = r(ou), eb0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], nb0 = r(tr), tb0 = r(Z0), ub0 = r(nr), ib0 = [0, [17, 0, 0], r(z)], fb0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], xb0 = r(Xr), ab0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ob0 = r(tr), cb0 = r(Z0), sb0 = r(nr), vb0 = [0, [17, 0, 0], r(z)], lb0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], bb0 = [0, [15, 0], r(C0)], pb0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], mb0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], _b0 = [0, [17, 0, [12, 41, 0]], r(h0)], yb0 = [0, [15, 0], r(C0)], db0 = r("Flow_ast.Class.Property.Uninitialized"), hb0 = r("Flow_ast.Class.Property.Declared"), kb0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Class.Property.Initialized"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Class.Property.Initialized@ ")], wb0 = [0, [17, 0, [12, 41, 0]], r(h0)], Eb0 = [0, [15, 0], r(C0)], Sb0 = r(Yr), gb0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Fb0 = r("Flow_ast.Class.Property.key"), Tb0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ob0 = [0, [17, 0, 0], r(z)], Ib0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ab0 = r(Bn), Nb0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Cb0 = [0, [17, 0, 0], r(z)], Pb0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Db0 = r(rs), Lb0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Rb0 = [0, [17, 0, 0], r(z)], jb0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Gb0 = r(eu), Mb0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Bb0 = [0, [9, 0, 0], r(An)], qb0 = [0, [17, 0, 0], r(z)], Ub0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Hb0 = r(ou), Xb0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Yb0 = r(tr), Vb0 = r(Z0), zb0 = r(nr), Kb0 = [0, [17, 0, 0], r(z)], Wb0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Jb0 = r(Xr), $b0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Zb0 = r(tr), Qb0 = r(Z0), r40 = r(nr), e40 = [0, [17, 0, 0], r(z)], n40 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], t40 = [0, [15, 0], r(C0)], u40 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], i40 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], f40 = [0, [17, 0, [12, 41, 0]], r(h0)], x40 = [0, [15, 0], r(C0)], a40 = r(Yr), o40 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], c40 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], s40 = r("Flow_ast.Class.Method.kind"), v40 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], l40 = [0, [17, 0, 0], r(z)], b40 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], p40 = r(ui), m40 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _40 = [0, [17, 0, 0], r(z)], y40 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], d40 = r(Bn), h40 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], k40 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], w40 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], E40 = [0, [17, 0, [12, 41, 0]], r(h0)], S40 = [0, [17, 0, 0], r(z)], g40 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], F40 = r(eu), T40 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], O40 = [0, [9, 0, 0], r(An)], I40 = [0, [17, 0, 0], r(z)], A40 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], N40 = r(B_), C40 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], P40 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], D40 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], L40 = [0, [17, 0, 0], r(z)], R40 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], j40 = r(Xr), G40 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], M40 = r(tr), B40 = r(Z0), q40 = r(nr), U40 = [0, [17, 0, 0], r(z)], H40 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], X40 = [0, [15, 0], r(C0)], Y40 = r("Flow_ast.Class.Method.Constructor"), V40 = r("Flow_ast.Class.Method.Method"), z40 = r("Flow_ast.Class.Method.Get"), K40 = r("Flow_ast.Class.Method.Set"), W40 = [0, [15, 0], r(C0)], J40 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], $40 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Z40 = [0, [17, 0, [12, 41, 0]], r(h0)], Q40 = [0, [15, 0], r(C0)], r80 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], e80 = r("Flow_ast.Comment.kind"), n80 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], t80 = [0, [17, 0, 0], r(z)], u80 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], i80 = r("text"), f80 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], x80 = [0, [3, 0, 0], r(Yt)], a80 = [0, [17, 0, 0], r(z)], o80 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], c80 = r("on_newline"), s80 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], v80 = [0, [9, 0, 0], r(An)], l80 = [0, [17, 0, 0], r(z)], b80 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], p80 = [0, [15, 0], r(C0)], m80 = r("Flow_ast.Comment.Line"), _80 = r("Flow_ast.Comment.Block"), y80 = [0, [15, 0], r(C0)], d80 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], h80 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], k80 = [0, [17, 0, [12, 41, 0]], r(h0)], w80 = [0, [15, 0], r(C0)], E80 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Object"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Object@ ")], S80 = [0, [17, 0, [12, 41, 0]], r(h0)], g80 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Array"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Array@ ")], F80 = [0, [17, 0, [12, 41, 0]], r(h0)], T80 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Identifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Identifier@ ")], O80 = [0, [17, 0, [12, 41, 0]], r(h0)], I80 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Expression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Expression@ ")], A80 = [0, [17, 0, [12, 41, 0]], r(h0)], N80 = [0, [15, 0], r(C0)], C80 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], P80 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], D80 = [0, [17, 0, [12, 41, 0]], r(h0)], L80 = [0, [15, 0], r(C0)], R80 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], j80 = r("Flow_ast.Pattern.Identifier.name"), G80 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], M80 = [0, [17, 0, 0], r(z)], B80 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], q80 = r(rs), U80 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], H80 = [0, [17, 0, 0], r(z)], X80 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Y80 = r(Bu), V80 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], z80 = [0, [9, 0, 0], r(An)], K80 = [0, [17, 0, 0], r(z)], W80 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], J80 = [0, [15, 0], r(C0)], $80 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Z80 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Q80 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], r30 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], e30 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], n30 = r("Flow_ast.Pattern.Array.elements"), t30 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], u30 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], i30 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], f30 = [0, [17, 0, 0], r(z)], x30 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], a30 = r(rs), o30 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], c30 = [0, [17, 0, 0], r(z)], s30 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], v30 = r(Xr), l30 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], b30 = r(tr), p30 = r(Z0), m30 = r(nr), _30 = [0, [17, 0, 0], r(z)], y30 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], d30 = [0, [15, 0], r(C0)], h30 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Array.Element"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Array.Element@ ")], k30 = [0, [17, 0, [12, 41, 0]], r(h0)], w30 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Array.RestElement"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Array.RestElement@ ")], E30 = [0, [17, 0, [12, 41, 0]], r(h0)], S30 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Array.Hole"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Array.Hole@ ")], g30 = [0, [17, 0, [12, 41, 0]], r(h0)], F30 = [0, [15, 0], r(C0)], T30 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], O30 = r("Flow_ast.Pattern.Array.Element.argument"), I30 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], A30 = [0, [17, 0, 0], r(z)], N30 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], C30 = r(mi), P30 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], D30 = r(tr), L30 = r(Z0), R30 = r(nr), j30 = [0, [17, 0, 0], r(z)], G30 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], M30 = [0, [15, 0], r(C0)], B30 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], q30 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], U30 = [0, [17, 0, [12, 41, 0]], r(h0)], H30 = [0, [15, 0], r(C0)], X30 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Y30 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], V30 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], z30 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], K30 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], W30 = r("Flow_ast.Pattern.Object.properties"), J30 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $30 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Z30 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Q30 = [0, [17, 0, 0], r(z)], r60 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], e60 = r(rs), n60 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], t60 = [0, [17, 0, 0], r(z)], u60 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], i60 = r(Xr), f60 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], x60 = r(tr), a60 = r(Z0), o60 = r(nr), c60 = [0, [17, 0, 0], r(z)], s60 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], v60 = [0, [15, 0], r(C0)], l60 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Object.Property"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Object.Property@ ")], b60 = [0, [17, 0, [12, 41, 0]], r(h0)], p60 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Object.RestElement"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Object.RestElement@ ")], m60 = [0, [17, 0, [12, 41, 0]], r(h0)], _60 = [0, [15, 0], r(C0)], y60 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], d60 = r("Flow_ast.Pattern.Object.Property.key"), h60 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], k60 = [0, [17, 0, 0], r(z)], w60 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], E60 = r(pi), S60 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], g60 = [0, [17, 0, 0], r(z)], F60 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], T60 = r(mi), O60 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], I60 = r(tr), A60 = r(Z0), N60 = r(nr), C60 = [0, [17, 0, 0], r(z)], P60 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], D60 = r(x6), L60 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], R60 = [0, [9, 0, 0], r(An)], j60 = [0, [17, 0, 0], r(z)], G60 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], M60 = [0, [15, 0], r(C0)], B60 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], q60 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], U60 = [0, [17, 0, [12, 41, 0]], r(h0)], H60 = [0, [15, 0], r(C0)], X60 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Object.Property.Literal"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Object.Property.Literal@ ")], Y60 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], V60 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], z60 = [0, [17, 0, [12, 41, 0]], r(h0)], K60 = [0, [17, 0, [12, 41, 0]], r(h0)], W60 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Object.Property.Identifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Object.Property.Identifier@ ")], J60 = [0, [17, 0, [12, 41, 0]], r(h0)], $60 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Pattern.Object.Property.Computed"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Pattern.Object.Property.Computed@ ")], Z60 = [0, [17, 0, [12, 41, 0]], r(h0)], Q60 = [0, [15, 0], r(C0)], rp0 = r(Yr), ep0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], np0 = r("Flow_ast.Pattern.RestElement.argument"), tp0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], up0 = [0, [17, 0, 0], r(z)], ip0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], fp0 = r(Xr), xp0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ap0 = r(tr), op0 = r(Z0), cp0 = r(nr), sp0 = [0, [17, 0, 0], r(z)], vp0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], lp0 = [0, [15, 0], r(C0)], bp0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], pp0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], mp0 = [0, [17, 0, [12, 41, 0]], r(h0)], _p0 = [0, [15, 0], r(C0)], yp0 = r(Yr), dp0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hp0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], kp0 = r("Flow_ast.JSX.frag_opening_element"), wp0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ep0 = [0, [17, 0, 0], r(z)], Sp0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], gp0 = r("frag_closing_element"), Fp0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Tp0 = [0, [17, 0, 0], r(z)], Op0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ip0 = r("frag_children"), Ap0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Np0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Cp0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Pp0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Dp0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Lp0 = [0, [17, 0, [12, 41, 0]], r(h0)], Rp0 = [0, [17, 0, 0], r(z)], jp0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Gp0 = r("frag_comments"), Mp0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Bp0 = r(tr), qp0 = r(Z0), Up0 = r(nr), Hp0 = [0, [17, 0, 0], r(z)], Xp0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Yp0 = [0, [15, 0], r(C0)], Vp0 = r(Yr), zp0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Kp0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Wp0 = r("Flow_ast.JSX.opening_element"), Jp0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $p0 = [0, [17, 0, 0], r(z)], Zp0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Qp0 = r("closing_element"), r50 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], e50 = r(tr), n50 = r(Z0), t50 = r(nr), u50 = [0, [17, 0, 0], r(z)], i50 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], f50 = r(Ve), x50 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], a50 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], o50 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], c50 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], s50 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], v50 = [0, [17, 0, [12, 41, 0]], r(h0)], l50 = [0, [17, 0, 0], r(z)], b50 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], p50 = r(Xr), m50 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _50 = r(tr), y50 = r(Z0), d50 = r(nr), h50 = [0, [17, 0, 0], r(z)], k50 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], w50 = [0, [15, 0], r(C0)], E50 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Element"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Element@ ")], S50 = [0, [17, 0, [12, 41, 0]], r(h0)], g50 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Fragment"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Fragment@ ")], F50 = [0, [17, 0, [12, 41, 0]], r(h0)], T50 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.ExpressionContainer"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.ExpressionContainer@ ")], O50 = [0, [17, 0, [12, 41, 0]], r(h0)], I50 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.SpreadChild"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.SpreadChild@ ")], A50 = [0, [17, 0, [12, 41, 0]], r(h0)], N50 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Text"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Text@ ")], C50 = [0, [17, 0, [12, 41, 0]], r(h0)], P50 = [0, [15, 0], r(C0)], D50 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], L50 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], R50 = [0, [17, 0, [12, 41, 0]], r(h0)], j50 = [0, [15, 0], r(C0)], G50 = r(Yr), M50 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], B50 = r("Flow_ast.JSX.SpreadChild.expression"), q50 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], U50 = [0, [17, 0, 0], r(z)], H50 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], X50 = r(Xr), Y50 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], V50 = r(tr), z50 = r(Z0), K50 = r(nr), W50 = [0, [17, 0, 0], r(z)], J50 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], $50 = [0, [15, 0], r(C0)], Z50 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Q50 = r("Flow_ast.JSX.Closing.name"), rm0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], em0 = [0, [17, 0, 0], r(z)], nm0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], tm0 = [0, [15, 0], r(C0)], um0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], im0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], fm0 = [0, [17, 0, [12, 41, 0]], r(h0)], xm0 = [0, [15, 0], r(C0)], am0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], om0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], cm0 = r("Flow_ast.JSX.Opening.name"), sm0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], vm0 = [0, [17, 0, 0], r(z)], lm0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], bm0 = r("self_closing"), pm0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], mm0 = [0, [9, 0, 0], r(An)], _m0 = [0, [17, 0, 0], r(z)], ym0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], dm0 = r(kY), hm0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], km0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], wm0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Em0 = [0, [17, 0, 0], r(z)], Sm0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], gm0 = [0, [15, 0], r(C0)], Fm0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Opening.Attribute"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Opening.Attribute@ ")], Tm0 = [0, [17, 0, [12, 41, 0]], r(h0)], Om0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Opening.SpreadAttribute"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Opening.SpreadAttribute@ ")], Im0 = [0, [17, 0, [12, 41, 0]], r(h0)], Am0 = [0, [15, 0], r(C0)], Nm0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Cm0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Pm0 = [0, [17, 0, [12, 41, 0]], r(h0)], Dm0 = [0, [15, 0], r(C0)], Lm0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Identifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Identifier@ ")], Rm0 = [0, [17, 0, [12, 41, 0]], r(h0)], jm0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.NamespacedName"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.NamespacedName@ ")], Gm0 = [0, [17, 0, [12, 41, 0]], r(h0)], Mm0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.MemberExpression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.MemberExpression@ ")], Bm0 = [0, [17, 0, [12, 41, 0]], r(h0)], qm0 = [0, [15, 0], r(C0)], Um0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Hm0 = r("Flow_ast.JSX.MemberExpression._object"), Xm0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ym0 = [0, [17, 0, 0], r(z)], Vm0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], zm0 = r(Iv), Km0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Wm0 = [0, [17, 0, 0], r(z)], Jm0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], $m0 = [0, [15, 0], r(C0)], Zm0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.MemberExpression.Identifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.MemberExpression.Identifier@ ")], Qm0 = [0, [17, 0, [12, 41, 0]], r(h0)], r90 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.MemberExpression.MemberExpression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.MemberExpression.MemberExpression@ ")], e90 = [0, [17, 0, [12, 41, 0]], r(h0)], n90 = [0, [15, 0], r(C0)], t90 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], u90 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], i90 = [0, [17, 0, [12, 41, 0]], r(h0)], f90 = [0, [15, 0], r(C0)], x90 = r(Yr), a90 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], o90 = r("Flow_ast.JSX.SpreadAttribute.argument"), c90 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], s90 = [0, [17, 0, 0], r(z)], v90 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], l90 = r(Xr), b90 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], p90 = r(tr), m90 = r(Z0), _90 = r(nr), y90 = [0, [17, 0, 0], r(z)], d90 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], h90 = [0, [15, 0], r(C0)], k90 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], w90 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], E90 = [0, [17, 0, [12, 41, 0]], r(h0)], S90 = [0, [15, 0], r(C0)], g90 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], F90 = r("Flow_ast.JSX.Attribute.name"), T90 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], O90 = [0, [17, 0, 0], r(z)], I90 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], A90 = r(Bn), N90 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], C90 = r(tr), P90 = r(Z0), D90 = r(nr), L90 = [0, [17, 0, 0], r(z)], R90 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], j90 = [0, [15, 0], r(C0)], G90 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Attribute.Literal ("), [17, [0, r(Pe), 0, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Attribute.Literal (@,")], M90 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], B90 = [0, [17, [0, r(Pe), 0, 0], [11, r(OX), [17, 0, 0]]], r(qU)], q90 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Attribute.ExpressionContainer ("), [17, [0, r(Pe), 0, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Attribute.ExpressionContainer (@,")], U90 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], H90 = [0, [17, [0, r(Pe), 0, 0], [11, r(OX), [17, 0, 0]]], r(qU)], X90 = [0, [15, 0], r(C0)], Y90 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Attribute.Identifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Attribute.Identifier@ ")], V90 = [0, [17, 0, [12, 41, 0]], r(h0)], z90 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.Attribute.NamespacedName"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.Attribute.NamespacedName@ ")], K90 = [0, [17, 0, [12, 41, 0]], r(h0)], W90 = [0, [15, 0], r(C0)], J90 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], $90 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Z90 = [0, [17, 0, [12, 41, 0]], r(h0)], Q90 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], r_0 = r("Flow_ast.JSX.Text.value"), e_0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], n_0 = [0, [3, 0, 0], r(Yt)], t_0 = [0, [17, 0, 0], r(z)], u_0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], i_0 = r(o7), f_0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], x_0 = [0, [3, 0, 0], r(Yt)], a_0 = [0, [17, 0, 0], r(z)], o_0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], c_0 = [0, [15, 0], r(C0)], s_0 = [0, [15, 0], r(C0)], v_0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.JSX.ExpressionContainer.Expression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.JSX.ExpressionContainer.Expression@ ")], l_0 = [0, [17, 0, [12, 41, 0]], r(h0)], b_0 = r("Flow_ast.JSX.ExpressionContainer.EmptyExpression"), p_0 = [0, [15, 0], r(C0)], m_0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], __0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], y_0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], d_0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], h_0 = r("Flow_ast.JSX.ExpressionContainer.expression"), k_0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], w_0 = [0, [17, 0, 0], r(z)], E_0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], S_0 = r(Xr), g_0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], F_0 = r(tr), T_0 = r(Z0), O_0 = r(nr), I_0 = [0, [17, 0, 0], r(z)], A_0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], N_0 = [0, [15, 0], r(C0)], C_0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], P_0 = r("Flow_ast.JSX.NamespacedName.namespace"), D_0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], L_0 = [0, [17, 0, 0], r(z)], R_0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], j_0 = r(ti), G_0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], M_0 = [0, [17, 0, 0], r(z)], B_0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], q_0 = [0, [15, 0], r(C0)], U_0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], H_0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], X_0 = [0, [17, 0, [12, 41, 0]], r(h0)], Y_0 = [0, [15, 0], r(C0)], V_0 = r(Yr), z_0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], K_0 = r("Flow_ast.JSX.Identifier.name"), W_0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], J_0 = [0, [3, 0, 0], r(Yt)], $_0 = [0, [17, 0, 0], r(z)], Z_0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Q_0 = r(Xr), ry0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ey0 = r(tr), ny0 = r(Z0), ty0 = r(nr), uy0 = [0, [17, 0, 0], r(z)], iy0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], fy0 = [0, [15, 0], r(C0)], xy0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], ay0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], oy0 = [0, [17, 0, [12, 41, 0]], r(h0)], cy0 = [0, [15, 0], r(C0)], sy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Array"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Array@ ")], vy0 = [0, [17, 0, [12, 41, 0]], r(h0)], ly0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.ArrowFunction"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.ArrowFunction@ ")], by0 = [0, [17, 0, [12, 41, 0]], r(h0)], py0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Assignment"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Assignment@ ")], my0 = [0, [17, 0, [12, 41, 0]], r(h0)], _y0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Binary"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Binary@ ")], yy0 = [0, [17, 0, [12, 41, 0]], r(h0)], dy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Call"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Call@ ")], hy0 = [0, [17, 0, [12, 41, 0]], r(h0)], ky0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Class"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Class@ ")], wy0 = [0, [17, 0, [12, 41, 0]], r(h0)], Ey0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Comprehension"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Comprehension@ ")], Sy0 = [0, [17, 0, [12, 41, 0]], r(h0)], gy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Conditional"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Conditional@ ")], Fy0 = [0, [17, 0, [12, 41, 0]], r(h0)], Ty0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Function"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Function@ ")], Oy0 = [0, [17, 0, [12, 41, 0]], r(h0)], Iy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Generator"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Generator@ ")], Ay0 = [0, [17, 0, [12, 41, 0]], r(h0)], Ny0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Identifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Identifier@ ")], Cy0 = [0, [17, 0, [12, 41, 0]], r(h0)], Py0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Import"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Import@ ")], Dy0 = [0, [17, 0, [12, 41, 0]], r(h0)], Ly0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.JSXElement"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.JSXElement@ ")], Ry0 = [0, [17, 0, [12, 41, 0]], r(h0)], jy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.JSXFragment"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.JSXFragment@ ")], Gy0 = [0, [17, 0, [12, 41, 0]], r(h0)], My0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Literal"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Literal@ ")], By0 = [0, [17, 0, [12, 41, 0]], r(h0)], qy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Logical"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Logical@ ")], Uy0 = [0, [17, 0, [12, 41, 0]], r(h0)], Hy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Member"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Member@ ")], Xy0 = [0, [17, 0, [12, 41, 0]], r(h0)], Yy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.MetaProperty"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.MetaProperty@ ")], Vy0 = [0, [17, 0, [12, 41, 0]], r(h0)], zy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.New"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.New@ ")], Ky0 = [0, [17, 0, [12, 41, 0]], r(h0)], Wy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Object@ ")], Jy0 = [0, [17, 0, [12, 41, 0]], r(h0)], $y0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.OptionalCall"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.OptionalCall@ ")], Zy0 = [0, [17, 0, [12, 41, 0]], r(h0)], Qy0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.OptionalMember"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.OptionalMember@ ")], rd0 = [0, [17, 0, [12, 41, 0]], r(h0)], ed0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Sequence"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Sequence@ ")], nd0 = [0, [17, 0, [12, 41, 0]], r(h0)], td0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Super"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Super@ ")], ud0 = [0, [17, 0, [12, 41, 0]], r(h0)], id0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.TaggedTemplate"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.TaggedTemplate@ ")], fd0 = [0, [17, 0, [12, 41, 0]], r(h0)], xd0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.TemplateLiteral"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.TemplateLiteral@ ")], ad0 = [0, [17, 0, [12, 41, 0]], r(h0)], od0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.This"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.This@ ")], cd0 = [0, [17, 0, [12, 41, 0]], r(h0)], sd0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.TypeCast"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.TypeCast@ ")], vd0 = [0, [17, 0, [12, 41, 0]], r(h0)], ld0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Unary"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Unary@ ")], bd0 = [0, [17, 0, [12, 41, 0]], r(h0)], pd0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Update"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Update@ ")], md0 = [0, [17, 0, [12, 41, 0]], r(h0)], _d0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Yield"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Yield@ ")], yd0 = [0, [17, 0, [12, 41, 0]], r(h0)], dd0 = [0, [15, 0], r(C0)], hd0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], kd0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], wd0 = [0, [17, 0, [12, 41, 0]], r(h0)], Ed0 = [0, [15, 0], r(C0)], Sd0 = r(Yr), gd0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Fd0 = r("Flow_ast.Expression.Import.argument"), Td0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Od0 = [0, [17, 0, 0], r(z)], Id0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ad0 = r(Xr), Nd0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Cd0 = r(tr), Pd0 = r(Z0), Dd0 = r(nr), Ld0 = [0, [17, 0, 0], r(z)], Rd0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], jd0 = [0, [15, 0], r(C0)], Gd0 = r(Yr), Md0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Bd0 = r("Flow_ast.Expression.Super.comments"), qd0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ud0 = r(tr), Hd0 = r(Z0), Xd0 = r(nr), Yd0 = [0, [17, 0, 0], r(z)], Vd0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], zd0 = [0, [15, 0], r(C0)], Kd0 = r(Yr), Wd0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Jd0 = r("Flow_ast.Expression.This.comments"), $d0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Zd0 = r(tr), Qd0 = r(Z0), rh0 = r(nr), eh0 = [0, [17, 0, 0], r(z)], nh0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], th0 = [0, [15, 0], r(C0)], uh0 = r(Yr), ih0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], fh0 = r("Flow_ast.Expression.MetaProperty.meta"), xh0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ah0 = [0, [17, 0, 0], r(z)], oh0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ch0 = r(Iv), sh0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], vh0 = [0, [17, 0, 0], r(z)], lh0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], bh0 = r(Xr), ph0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], mh0 = r(tr), _h0 = r(Z0), yh0 = r(nr), dh0 = [0, [17, 0, 0], r(z)], hh0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], kh0 = [0, [15, 0], r(C0)], wh0 = r(Yr), Eh0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Sh0 = r("Flow_ast.Expression.TypeCast.expression"), gh0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Fh0 = [0, [17, 0, 0], r(z)], Th0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Oh0 = r(rs), Ih0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ah0 = [0, [17, 0, 0], r(z)], Nh0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ch0 = r(Xr), Ph0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Dh0 = r(tr), Lh0 = r(Z0), Rh0 = r(nr), jh0 = [0, [17, 0, 0], r(z)], Gh0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Mh0 = [0, [15, 0], r(C0)], Bh0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], qh0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Uh0 = r("Flow_ast.Expression.Generator.blocks"), Hh0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Xh0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Yh0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Vh0 = [0, [17, 0, 0], r(z)], zh0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Kh0 = r(O4), Wh0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Jh0 = r(tr), $h0 = r(Z0), Zh0 = r(nr), Qh0 = [0, [17, 0, 0], r(z)], rk0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], ek0 = [0, [15, 0], r(C0)], nk0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], tk0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], uk0 = r("Flow_ast.Expression.Comprehension.blocks"), ik0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], fk0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], xk0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], ak0 = [0, [17, 0, 0], r(z)], ok0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ck0 = r(O4), sk0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], vk0 = r(tr), lk0 = r(Z0), bk0 = r(nr), pk0 = [0, [17, 0, 0], r(z)], mk0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], _k0 = [0, [15, 0], r(C0)], yk0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], dk0 = r("Flow_ast.Expression.Comprehension.Block.left"), hk0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], kk0 = [0, [17, 0, 0], r(z)], wk0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ek0 = r(Nu), Sk0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], gk0 = [0, [17, 0, 0], r(z)], Fk0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Tk0 = r(j8), Ok0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ik0 = [0, [9, 0, 0], r(An)], Ak0 = [0, [17, 0, 0], r(z)], Nk0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Ck0 = [0, [15, 0], r(C0)], Pk0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Dk0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Lk0 = [0, [17, 0, [12, 41, 0]], r(h0)], Rk0 = [0, [15, 0], r(C0)], jk0 = r(Yr), Gk0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Mk0 = r("Flow_ast.Expression.Yield.argument"), Bk0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], qk0 = r(tr), Uk0 = r(Z0), Hk0 = r(nr), Xk0 = [0, [17, 0, 0], r(z)], Yk0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Vk0 = r(Xr), zk0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Kk0 = r(tr), Wk0 = r(Z0), Jk0 = r(nr), $k0 = [0, [17, 0, 0], r(z)], Zk0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Qk0 = r(yY), rw0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ew0 = [0, [9, 0, 0], r(An)], nw0 = [0, [17, 0, 0], r(z)], tw0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], uw0 = r("result_out"), iw0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], fw0 = [0, [17, 0, 0], r(z)], xw0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], aw0 = [0, [15, 0], r(C0)], ow0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], cw0 = r("Flow_ast.Expression.OptionalMember.member"), sw0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], vw0 = [0, [17, 0, 0], r(z)], lw0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], bw0 = r(yU), pw0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], mw0 = [0, [17, 0, 0], r(z)], _w0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], yw0 = r(Bu), dw0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], hw0 = [0, [9, 0, 0], r(An)], kw0 = [0, [17, 0, 0], r(z)], ww0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Ew0 = [0, [15, 0], r(C0)], Sw0 = r(Yr), gw0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Fw0 = r("Flow_ast.Expression.Member._object"), Tw0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ow0 = [0, [17, 0, 0], r(z)], Iw0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Aw0 = r(Iv), Nw0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Cw0 = [0, [17, 0, 0], r(z)], Pw0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Dw0 = r(Xr), Lw0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Rw0 = r(tr), jw0 = r(Z0), Gw0 = r(nr), Mw0 = [0, [17, 0, 0], r(z)], Bw0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], qw0 = [0, [15, 0], r(C0)], Uw0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Member.PropertyIdentifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Member.PropertyIdentifier@ ")], Hw0 = [0, [17, 0, [12, 41, 0]], r(h0)], Xw0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Member.PropertyPrivateName"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Member.PropertyPrivateName@ ")], Yw0 = [0, [17, 0, [12, 41, 0]], r(h0)], Vw0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Member.PropertyExpression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Member.PropertyExpression@ ")], zw0 = [0, [17, 0, [12, 41, 0]], r(h0)], Kw0 = [0, [15, 0], r(C0)], Ww0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Jw0 = r("Flow_ast.Expression.OptionalCall.call"), $w0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Zw0 = [0, [17, 0, 0], r(z)], Qw0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], rE0 = r(yU), eE0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], nE0 = [0, [17, 0, 0], r(z)], tE0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], uE0 = r(Bu), iE0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], fE0 = [0, [9, 0, 0], r(An)], xE0 = [0, [17, 0, 0], r(z)], aE0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], oE0 = [0, [15, 0], r(C0)], cE0 = r(Yr), sE0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], vE0 = r("Flow_ast.Expression.Call.callee"), lE0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], bE0 = [0, [17, 0, 0], r(z)], pE0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], mE0 = r(Z2), _E0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], yE0 = r(tr), dE0 = r(Z0), hE0 = r(nr), kE0 = [0, [17, 0, 0], r(z)], wE0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], EE0 = r(C2), SE0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], gE0 = [0, [17, 0, 0], r(z)], FE0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], TE0 = r(Xr), OE0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], IE0 = r(tr), AE0 = r(Z0), NE0 = r(nr), CE0 = [0, [17, 0, 0], r(z)], PE0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], DE0 = [0, [15, 0], r(C0)], LE0 = r(Yr), RE0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], jE0 = r("Flow_ast.Expression.New.callee"), GE0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ME0 = [0, [17, 0, 0], r(z)], BE0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], qE0 = r(Z2), UE0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], HE0 = r(tr), XE0 = r(Z0), YE0 = r(nr), VE0 = [0, [17, 0, 0], r(z)], zE0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], KE0 = r(C2), WE0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], JE0 = r(tr), $E0 = r(Z0), ZE0 = r(nr), QE0 = [0, [17, 0, 0], r(z)], rS0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], eS0 = r(Xr), nS0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], tS0 = r(tr), uS0 = r(Z0), iS0 = r(nr), fS0 = [0, [17, 0, 0], r(z)], xS0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], aS0 = [0, [15, 0], r(C0)], oS0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], cS0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], sS0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], vS0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], lS0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], bS0 = r("Flow_ast.Expression.ArgList.arguments"), pS0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], mS0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], _S0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], yS0 = [0, [17, 0, 0], r(z)], dS0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hS0 = r(Xr), kS0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], wS0 = r(tr), ES0 = r(Z0), SS0 = r(nr), gS0 = [0, [17, 0, 0], r(z)], FS0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], TS0 = [0, [15, 0], r(C0)], OS0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], IS0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], AS0 = [0, [17, 0, [12, 41, 0]], r(h0)], NS0 = [0, [15, 0], r(C0)], CS0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Expression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Expression@ ")], PS0 = [0, [17, 0, [12, 41, 0]], r(h0)], DS0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Spread"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Spread@ ")], LS0 = [0, [17, 0, [12, 41, 0]], r(h0)], RS0 = [0, [15, 0], r(C0)], jS0 = r(Yr), GS0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], MS0 = r("Flow_ast.Expression.Conditional.test"), BS0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], qS0 = [0, [17, 0, 0], r(z)], US0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], HS0 = r(kv), XS0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], YS0 = [0, [17, 0, 0], r(z)], VS0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], zS0 = r(_3), KS0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], WS0 = [0, [17, 0, 0], r(z)], JS0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], $S0 = r(Xr), ZS0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], QS0 = r(tr), rg0 = r(Z0), eg0 = r(nr), ng0 = [0, [17, 0, 0], r(z)], tg0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], ug0 = [0, [15, 0], r(C0)], ig0 = r(Yr), fg0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], xg0 = r("Flow_ast.Expression.Logical.operator"), ag0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], og0 = [0, [17, 0, 0], r(z)], cg0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], sg0 = r(li), vg0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], lg0 = [0, [17, 0, 0], r(z)], bg0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], pg0 = r(Nu), mg0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _g0 = [0, [17, 0, 0], r(z)], yg0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], dg0 = r(Xr), hg0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], kg0 = r(tr), wg0 = r(Z0), Eg0 = r(nr), Sg0 = [0, [17, 0, 0], r(z)], gg0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Fg0 = [0, [15, 0], r(C0)], Tg0 = r("Flow_ast.Expression.Logical.Or"), Og0 = r("Flow_ast.Expression.Logical.And"), Ig0 = r("Flow_ast.Expression.Logical.NullishCoalesce"), Ag0 = [0, [15, 0], r(C0)], Ng0 = r(Yr), Cg0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Pg0 = r("Flow_ast.Expression.Update.operator"), Dg0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Lg0 = [0, [17, 0, 0], r(z)], Rg0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], jg0 = r(v7), Gg0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Mg0 = [0, [17, 0, 0], r(z)], Bg0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], qg0 = r(XE), Ug0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Hg0 = [0, [9, 0, 0], r(An)], Xg0 = [0, [17, 0, 0], r(z)], Yg0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Vg0 = r(Xr), zg0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Kg0 = r(tr), Wg0 = r(Z0), Jg0 = r(nr), $g0 = [0, [17, 0, 0], r(z)], Zg0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Qg0 = [0, [15, 0], r(C0)], rF0 = r("Flow_ast.Expression.Update.Decrement"), eF0 = r("Flow_ast.Expression.Update.Increment"), nF0 = [0, [15, 0], r(C0)], tF0 = r(Yr), uF0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], iF0 = r("Flow_ast.Expression.Assignment.operator"), fF0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], xF0 = r(tr), aF0 = r(Z0), oF0 = r(nr), cF0 = [0, [17, 0, 0], r(z)], sF0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], vF0 = r(li), lF0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], bF0 = [0, [17, 0, 0], r(z)], pF0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], mF0 = r(Nu), _F0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], yF0 = [0, [17, 0, 0], r(z)], dF0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hF0 = r(Xr), kF0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], wF0 = r(tr), EF0 = r(Z0), SF0 = r(nr), gF0 = [0, [17, 0, 0], r(z)], FF0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], TF0 = [0, [15, 0], r(C0)], OF0 = r("Flow_ast.Expression.Assignment.PlusAssign"), IF0 = r("Flow_ast.Expression.Assignment.MinusAssign"), AF0 = r("Flow_ast.Expression.Assignment.MultAssign"), NF0 = r("Flow_ast.Expression.Assignment.ExpAssign"), CF0 = r("Flow_ast.Expression.Assignment.DivAssign"), PF0 = r("Flow_ast.Expression.Assignment.ModAssign"), DF0 = r("Flow_ast.Expression.Assignment.LShiftAssign"), LF0 = r("Flow_ast.Expression.Assignment.RShiftAssign"), RF0 = r("Flow_ast.Expression.Assignment.RShift3Assign"), jF0 = r("Flow_ast.Expression.Assignment.BitOrAssign"), GF0 = r("Flow_ast.Expression.Assignment.BitXorAssign"), MF0 = r("Flow_ast.Expression.Assignment.BitAndAssign"), BF0 = r("Flow_ast.Expression.Assignment.NullishAssign"), qF0 = r("Flow_ast.Expression.Assignment.AndAssign"), UF0 = r("Flow_ast.Expression.Assignment.OrAssign"), HF0 = [0, [15, 0], r(C0)], XF0 = r(Yr), YF0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], VF0 = r("Flow_ast.Expression.Binary.operator"), zF0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], KF0 = [0, [17, 0, 0], r(z)], WF0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], JF0 = r(li), $F0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ZF0 = [0, [17, 0, 0], r(z)], QF0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], rT0 = r(Nu), eT0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], nT0 = [0, [17, 0, 0], r(z)], tT0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], uT0 = r(Xr), iT0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], fT0 = r(tr), xT0 = r(Z0), aT0 = r(nr), oT0 = [0, [17, 0, 0], r(z)], cT0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], sT0 = [0, [15, 0], r(C0)], vT0 = r("Flow_ast.Expression.Binary.Equal"), lT0 = r("Flow_ast.Expression.Binary.NotEqual"), bT0 = r("Flow_ast.Expression.Binary.StrictEqual"), pT0 = r("Flow_ast.Expression.Binary.StrictNotEqual"), mT0 = r("Flow_ast.Expression.Binary.LessThan"), _T0 = r("Flow_ast.Expression.Binary.LessThanEqual"), yT0 = r("Flow_ast.Expression.Binary.GreaterThan"), dT0 = r("Flow_ast.Expression.Binary.GreaterThanEqual"), hT0 = r("Flow_ast.Expression.Binary.LShift"), kT0 = r("Flow_ast.Expression.Binary.RShift"), wT0 = r("Flow_ast.Expression.Binary.RShift3"), ET0 = r("Flow_ast.Expression.Binary.Plus"), ST0 = r("Flow_ast.Expression.Binary.Minus"), gT0 = r("Flow_ast.Expression.Binary.Mult"), FT0 = r("Flow_ast.Expression.Binary.Exp"), TT0 = r("Flow_ast.Expression.Binary.Div"), OT0 = r("Flow_ast.Expression.Binary.Mod"), IT0 = r("Flow_ast.Expression.Binary.BitOr"), AT0 = r("Flow_ast.Expression.Binary.Xor"), NT0 = r("Flow_ast.Expression.Binary.BitAnd"), CT0 = r("Flow_ast.Expression.Binary.In"), PT0 = r("Flow_ast.Expression.Binary.Instanceof"), DT0 = [0, [15, 0], r(C0)], LT0 = r(Yr), RT0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], jT0 = r("Flow_ast.Expression.Unary.operator"), GT0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], MT0 = [0, [17, 0, 0], r(z)], BT0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], qT0 = r(v7), UT0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], HT0 = [0, [17, 0, 0], r(z)], XT0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], YT0 = r(Xr), VT0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], zT0 = r(tr), KT0 = r(Z0), WT0 = r(nr), JT0 = [0, [17, 0, 0], r(z)], $T0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], ZT0 = [0, [15, 0], r(C0)], QT0 = r("Flow_ast.Expression.Unary.Minus"), rO0 = r("Flow_ast.Expression.Unary.Plus"), eO0 = r("Flow_ast.Expression.Unary.Not"), nO0 = r("Flow_ast.Expression.Unary.BitNot"), tO0 = r("Flow_ast.Expression.Unary.Typeof"), uO0 = r("Flow_ast.Expression.Unary.Void"), iO0 = r("Flow_ast.Expression.Unary.Delete"), fO0 = r("Flow_ast.Expression.Unary.Await"), xO0 = [0, [15, 0], r(C0)], aO0 = r(Yr), oO0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], cO0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], sO0 = r("Flow_ast.Expression.Sequence.expressions"), vO0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], lO0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], bO0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], pO0 = [0, [17, 0, 0], r(z)], mO0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], _O0 = r(Xr), yO0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], dO0 = r(tr), hO0 = r(Z0), kO0 = r(nr), wO0 = [0, [17, 0, 0], r(z)], EO0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], SO0 = [0, [15, 0], r(C0)], gO0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], FO0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], TO0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], OO0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], IO0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], AO0 = r("Flow_ast.Expression.Object.properties"), NO0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], CO0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], PO0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], DO0 = [0, [17, 0, 0], r(z)], LO0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], RO0 = r(Xr), jO0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], GO0 = r(tr), MO0 = r(Z0), BO0 = r(nr), qO0 = [0, [17, 0, 0], r(z)], UO0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], HO0 = [0, [15, 0], r(C0)], XO0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.Property"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Object.Property@ ")], YO0 = [0, [17, 0, [12, 41, 0]], r(h0)], VO0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.SpreadProperty"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Object.SpreadProperty@ ")], zO0 = [0, [17, 0, [12, 41, 0]], r(h0)], KO0 = [0, [15, 0], r(C0)], WO0 = r(Yr), JO0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], $O0 = r("Flow_ast.Expression.Object.SpreadProperty.argument"), ZO0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], QO0 = [0, [17, 0, 0], r(z)], rI0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], eI0 = r(Xr), nI0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], tI0 = r(tr), uI0 = r(Z0), iI0 = r(nr), fI0 = [0, [17, 0, 0], r(z)], xI0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], aI0 = [0, [15, 0], r(C0)], oI0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], cI0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], sI0 = [0, [17, 0, [12, 41, 0]], r(h0)], vI0 = [0, [15, 0], r(C0)], lI0 = r(Yr), bI0 = r(Yr), pI0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.Property.Init {"), [17, [0, r(Pe), 0, 0], 0]]], r("@[<2>Flow_ast.Expression.Object.Property.Init {@,")], mI0 = r(ui), _I0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], yI0 = [0, [17, 0, 0], r(z)], dI0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hI0 = r(Bn), kI0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], wI0 = [0, [17, 0, 0], r(z)], EI0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], SI0 = r(x6), gI0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], FI0 = [0, [9, 0, 0], r(An)], TI0 = [0, [17, 0, 0], r(z)], OI0 = [0, [17, 0, [12, br, 0]], r(V6)], II0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.Property.Method {"), [17, [0, r(Pe), 0, 0], 0]]], r("@[<2>Flow_ast.Expression.Object.Property.Method {@,")], AI0 = r(ui), NI0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], CI0 = [0, [17, 0, 0], r(z)], PI0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], DI0 = r(Bn), LI0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], RI0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], jI0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], GI0 = [0, [17, 0, [12, 41, 0]], r(h0)], MI0 = [0, [17, 0, 0], r(z)], BI0 = [0, [17, 0, [12, br, 0]], r(V6)], qI0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.Property.Get {"), [17, [0, r(Pe), 0, 0], 0]]], r("@[<2>Flow_ast.Expression.Object.Property.Get {@,")], UI0 = r(ui), HI0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], XI0 = [0, [17, 0, 0], r(z)], YI0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], VI0 = r(Bn), zI0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], KI0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], WI0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], JI0 = [0, [17, 0, [12, 41, 0]], r(h0)], $I0 = [0, [17, 0, 0], r(z)], ZI0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], QI0 = r(Xr), rA0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], eA0 = r(tr), nA0 = r(Z0), tA0 = r(nr), uA0 = [0, [17, 0, 0], r(z)], iA0 = [0, [17, 0, [12, br, 0]], r(V6)], fA0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.Property.Set {"), [17, [0, r(Pe), 0, 0], 0]]], r("@[<2>Flow_ast.Expression.Object.Property.Set {@,")], xA0 = r(ui), aA0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], oA0 = [0, [17, 0, 0], r(z)], cA0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], sA0 = r(Bn), vA0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], lA0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], bA0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], pA0 = [0, [17, 0, [12, 41, 0]], r(h0)], mA0 = [0, [17, 0, 0], r(z)], _A0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], yA0 = r(Xr), dA0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], hA0 = r(tr), kA0 = r(Z0), wA0 = r(nr), EA0 = [0, [17, 0, 0], r(z)], SA0 = [0, [17, 0, [12, br, 0]], r(V6)], gA0 = [0, [15, 0], r(C0)], FA0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], TA0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], OA0 = [0, [17, 0, [12, 41, 0]], r(h0)], IA0 = [0, [15, 0], r(C0)], AA0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.Property.Literal"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Object.Property.Literal@ ")], NA0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], CA0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], PA0 = [0, [17, 0, [12, 41, 0]], r(h0)], DA0 = [0, [17, 0, [12, 41, 0]], r(h0)], LA0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.Property.Identifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Object.Property.Identifier@ ")], RA0 = [0, [17, 0, [12, 41, 0]], r(h0)], jA0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.Property.PrivateName"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Object.Property.PrivateName@ ")], GA0 = [0, [17, 0, [12, 41, 0]], r(h0)], MA0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Object.Property.Computed"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Object.Property.Computed@ ")], BA0 = [0, [17, 0, [12, 41, 0]], r(h0)], qA0 = [0, [15, 0], r(C0)], UA0 = r(Yr), HA0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], XA0 = r("Flow_ast.Expression.TaggedTemplate.tag"), YA0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], VA0 = [0, [17, 0, 0], r(z)], zA0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], KA0 = r(OY), WA0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], JA0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], $A0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], ZA0 = [0, [17, 0, [12, 41, 0]], r(h0)], QA0 = [0, [17, 0, 0], r(z)], rN0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], eN0 = r(Xr), nN0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], tN0 = r(tr), uN0 = r(Z0), iN0 = r(nr), fN0 = [0, [17, 0, 0], r(z)], xN0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], aN0 = [0, [15, 0], r(C0)], oN0 = r(Yr), cN0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], sN0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], vN0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], lN0 = r("Flow_ast.Expression.TemplateLiteral.quasis"), bN0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], pN0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], mN0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], _N0 = [0, [17, 0, 0], r(z)], yN0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], dN0 = r(Ug), hN0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], kN0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], wN0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], EN0 = [0, [17, 0, 0], r(z)], SN0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], gN0 = r(Xr), FN0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], TN0 = r(tr), ON0 = r(Z0), IN0 = r(nr), AN0 = [0, [17, 0, 0], r(z)], NN0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], CN0 = [0, [15, 0], r(C0)], PN0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], DN0 = r("Flow_ast.Expression.TemplateLiteral.Element.value"), LN0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], RN0 = [0, [17, 0, 0], r(z)], jN0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], GN0 = r(bU), MN0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], BN0 = [0, [9, 0, 0], r(An)], qN0 = [0, [17, 0, 0], r(z)], UN0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], HN0 = [0, [15, 0], r(C0)], XN0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], YN0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], VN0 = [0, [17, 0, [12, 41, 0]], r(h0)], zN0 = [0, [15, 0], r(C0)], KN0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], WN0 = r("Flow_ast.Expression.TemplateLiteral.Element.raw"), JN0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $N0 = [0, [3, 0, 0], r(Yt)], ZN0 = [0, [17, 0, 0], r(z)], QN0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], rC0 = r(GY), eC0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], nC0 = [0, [3, 0, 0], r(Yt)], tC0 = [0, [17, 0, 0], r(z)], uC0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], iC0 = [0, [15, 0], r(C0)], fC0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], xC0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], aC0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], oC0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], cC0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], sC0 = r("Flow_ast.Expression.Array.elements"), vC0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], lC0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], bC0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], pC0 = [0, [17, 0, 0], r(z)], mC0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], _C0 = r(Xr), yC0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], dC0 = r(tr), hC0 = r(Z0), kC0 = r(nr), wC0 = [0, [17, 0, 0], r(z)], EC0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], SC0 = [0, [15, 0], r(C0)], gC0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Array.Expression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Array.Expression@ ")], FC0 = [0, [17, 0, [12, 41, 0]], r(h0)], TC0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Array.Spread"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Array.Spread@ ")], OC0 = [0, [17, 0, [12, 41, 0]], r(h0)], IC0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.Array.Hole"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.Array.Hole@ ")], AC0 = [0, [17, 0, [12, 41, 0]], r(h0)], NC0 = [0, [15, 0], r(C0)], CC0 = r(Yr), PC0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], DC0 = r("Flow_ast.Expression.SpreadElement.argument"), LC0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], RC0 = [0, [17, 0, 0], r(z)], jC0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], GC0 = r(Xr), MC0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], BC0 = r(tr), qC0 = r(Z0), UC0 = r(nr), HC0 = [0, [17, 0, 0], r(z)], XC0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], YC0 = [0, [15, 0], r(C0)], VC0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], zC0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], KC0 = [0, [17, 0, [12, 41, 0]], r(h0)], WC0 = [0, [15, 0], r(C0)], JC0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], $C0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], ZC0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], QC0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], rP0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], eP0 = r("Flow_ast.Expression.CallTypeArgs.arguments"), nP0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], tP0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], uP0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], iP0 = [0, [17, 0, 0], r(z)], fP0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], xP0 = r(Xr), aP0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], oP0 = r(tr), cP0 = r(Z0), sP0 = r(nr), vP0 = [0, [17, 0, 0], r(z)], lP0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], bP0 = [0, [15, 0], r(C0)], pP0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], mP0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], _P0 = [0, [17, 0, [12, 41, 0]], r(h0)], yP0 = [0, [15, 0], r(C0)], dP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.CallTypeArg.Explicit"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.CallTypeArg.Explicit@ ")], hP0 = [0, [17, 0, [12, 41, 0]], r(h0)], kP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Expression.CallTypeArg.Implicit"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Expression.CallTypeArg.Implicit@ ")], wP0 = [0, [17, 0, [12, 41, 0]], r(h0)], EP0 = [0, [15, 0], r(C0)], SP0 = r(Yr), gP0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], FP0 = r("Flow_ast.Expression.CallTypeArg.Implicit.comments"), TP0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], OP0 = r(tr), IP0 = r(Z0), AP0 = r(nr), NP0 = [0, [17, 0, 0], r(z)], CP0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], PP0 = [0, [15, 0], r(C0)], DP0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], LP0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], RP0 = [0, [17, 0, [12, 41, 0]], r(h0)], jP0 = [0, [15, 0], r(C0)], GP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Block"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Block@ ")], MP0 = [0, [17, 0, [12, 41, 0]], r(h0)], BP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Break"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Break@ ")], qP0 = [0, [17, 0, [12, 41, 0]], r(h0)], UP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ClassDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ClassDeclaration@ ")], HP0 = [0, [17, 0, [12, 41, 0]], r(h0)], XP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Continue"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Continue@ ")], YP0 = [0, [17, 0, [12, 41, 0]], r(h0)], VP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Debugger"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Debugger@ ")], zP0 = [0, [17, 0, [12, 41, 0]], r(h0)], KP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareClass"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareClass@ ")], WP0 = [0, [17, 0, [12, 41, 0]], r(h0)], JP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareExportDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareExportDeclaration@ ")], $P0 = [0, [17, 0, [12, 41, 0]], r(h0)], ZP0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareFunction"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareFunction@ ")], QP0 = [0, [17, 0, [12, 41, 0]], r(h0)], rD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareInterface"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareInterface@ ")], eD0 = [0, [17, 0, [12, 41, 0]], r(h0)], nD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareModule"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareModule@ ")], tD0 = [0, [17, 0, [12, 41, 0]], r(h0)], uD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareModuleExports"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareModuleExports@ ")], iD0 = [0, [17, 0, [12, 41, 0]], r(h0)], fD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareTypeAlias"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareTypeAlias@ ")], xD0 = [0, [17, 0, [12, 41, 0]], r(h0)], aD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareOpaqueType"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareOpaqueType@ ")], oD0 = [0, [17, 0, [12, 41, 0]], r(h0)], cD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareVariable"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareVariable@ ")], sD0 = [0, [17, 0, [12, 41, 0]], r(h0)], vD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DoWhile"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DoWhile@ ")], lD0 = [0, [17, 0, [12, 41, 0]], r(h0)], bD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Empty"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Empty@ ")], pD0 = [0, [17, 0, [12, 41, 0]], r(h0)], mD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.EnumDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.EnumDeclaration@ ")], _D0 = [0, [17, 0, [12, 41, 0]], r(h0)], yD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ExportDefaultDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ExportDefaultDeclaration@ ")], dD0 = [0, [17, 0, [12, 41, 0]], r(h0)], hD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ExportNamedDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ExportNamedDeclaration@ ")], kD0 = [0, [17, 0, [12, 41, 0]], r(h0)], wD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Expression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Expression@ ")], ED0 = [0, [17, 0, [12, 41, 0]], r(h0)], SD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.For"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.For@ ")], gD0 = [0, [17, 0, [12, 41, 0]], r(h0)], FD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ForIn"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ForIn@ ")], TD0 = [0, [17, 0, [12, 41, 0]], r(h0)], OD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ForOf"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ForOf@ ")], ID0 = [0, [17, 0, [12, 41, 0]], r(h0)], AD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.FunctionDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.FunctionDeclaration@ ")], ND0 = [0, [17, 0, [12, 41, 0]], r(h0)], CD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.If"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.If@ ")], PD0 = [0, [17, 0, [12, 41, 0]], r(h0)], DD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ImportDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ImportDeclaration@ ")], LD0 = [0, [17, 0, [12, 41, 0]], r(h0)], RD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.InterfaceDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.InterfaceDeclaration@ ")], jD0 = [0, [17, 0, [12, 41, 0]], r(h0)], GD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Labeled"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Labeled@ ")], MD0 = [0, [17, 0, [12, 41, 0]], r(h0)], BD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Return"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Return@ ")], qD0 = [0, [17, 0, [12, 41, 0]], r(h0)], UD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Switch"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Switch@ ")], HD0 = [0, [17, 0, [12, 41, 0]], r(h0)], XD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Throw"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Throw@ ")], YD0 = [0, [17, 0, [12, 41, 0]], r(h0)], VD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.Try"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.Try@ ")], zD0 = [0, [17, 0, [12, 41, 0]], r(h0)], KD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.TypeAlias"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.TypeAlias@ ")], WD0 = [0, [17, 0, [12, 41, 0]], r(h0)], JD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.OpaqueType"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.OpaqueType@ ")], $D0 = [0, [17, 0, [12, 41, 0]], r(h0)], ZD0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.VariableDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.VariableDeclaration@ ")], QD0 = [0, [17, 0, [12, 41, 0]], r(h0)], rL0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.While"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.While@ ")], eL0 = [0, [17, 0, [12, 41, 0]], r(h0)], nL0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.With"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.With@ ")], tL0 = [0, [17, 0, [12, 41, 0]], r(h0)], uL0 = [0, [15, 0], r(C0)], iL0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], fL0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], xL0 = [0, [17, 0, [12, 41, 0]], r(h0)], aL0 = [0, [15, 0], r(C0)], oL0 = r("Flow_ast.Statement.ExportValue"), cL0 = r("Flow_ast.Statement.ExportType"), sL0 = [0, [15, 0], r(C0)], vL0 = r(Yr), lL0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], bL0 = r("Flow_ast.Statement.Empty.comments"), pL0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], mL0 = r(tr), _L0 = r(Z0), yL0 = r(nr), dL0 = [0, [17, 0, 0], r(z)], hL0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], kL0 = [0, [15, 0], r(C0)], wL0 = r(Yr), EL0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], SL0 = r("Flow_ast.Statement.Expression.expression"), gL0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], FL0 = [0, [17, 0, 0], r(z)], TL0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], OL0 = r(hn), IL0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], AL0 = r(tr), NL0 = [0, [3, 0, 0], r(Yt)], CL0 = r(Z0), PL0 = r(nr), DL0 = [0, [17, 0, 0], r(z)], LL0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], RL0 = r(Xr), jL0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], GL0 = r(tr), ML0 = r(Z0), BL0 = r(nr), qL0 = [0, [17, 0, 0], r(z)], UL0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], HL0 = [0, [15, 0], r(C0)], XL0 = r(Yr), YL0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], VL0 = r("Flow_ast.Statement.ImportDeclaration.import_kind"), zL0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], KL0 = [0, [17, 0, 0], r(z)], WL0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], JL0 = r(vc), $L0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ZL0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], QL0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], rR0 = [0, [17, 0, [12, 41, 0]], r(h0)], eR0 = [0, [17, 0, 0], r(z)], nR0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], tR0 = r(mi), uR0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], iR0 = r(tr), fR0 = r(Z0), xR0 = r(nr), aR0 = [0, [17, 0, 0], r(z)], oR0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], cR0 = r(Cv), sR0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], vR0 = r(tr), lR0 = r(Z0), bR0 = r(nr), pR0 = [0, [17, 0, 0], r(z)], mR0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], _R0 = r(Xr), yR0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], dR0 = r(tr), hR0 = r(Z0), kR0 = r(nr), wR0 = [0, [17, 0, 0], r(z)], ER0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], SR0 = [0, [15, 0], r(C0)], gR0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], FR0 = r("Flow_ast.Statement.ImportDeclaration.kind"), TR0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], OR0 = r(tr), IR0 = r(Z0), AR0 = r(nr), NR0 = [0, [17, 0, 0], r(z)], CR0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], PR0 = r(B2), DR0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], LR0 = r(tr), RR0 = r(Z0), jR0 = r(nr), GR0 = [0, [17, 0, 0], r(z)], MR0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], BR0 = r("remote"), qR0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], UR0 = [0, [17, 0, 0], r(z)], HR0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], XR0 = [0, [15, 0], r(C0)], YR0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], VR0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ImportDeclaration.ImportNamedSpecifiers"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ImportDeclaration.ImportNamedSpecifiers@ ")], zR0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], KR0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], WR0 = [0, [17, 0, [12, 41, 0]], r(h0)], JR0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ImportDeclaration.ImportNamespaceSpecifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ImportDeclaration.ImportNamespaceSpecifier@ ")], $R0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], ZR0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], QR0 = [0, [17, 0, [12, 41, 0]], r(h0)], rj0 = [0, [17, 0, [12, 41, 0]], r(h0)], ej0 = [0, [15, 0], r(C0)], nj0 = r("Flow_ast.Statement.ImportDeclaration.ImportType"), tj0 = r("Flow_ast.Statement.ImportDeclaration.ImportTypeof"), uj0 = r("Flow_ast.Statement.ImportDeclaration.ImportValue"), ij0 = [0, [15, 0], r(C0)], fj0 = r(Yr), xj0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], aj0 = r("Flow_ast.Statement.DeclareExportDeclaration.default"), oj0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], cj0 = r(tr), sj0 = r(Z0), vj0 = r(nr), lj0 = [0, [17, 0, 0], r(z)], bj0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], pj0 = r(P2), mj0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _j0 = r(tr), yj0 = r(Z0), dj0 = r(nr), hj0 = [0, [17, 0, 0], r(z)], kj0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], wj0 = r(Cv), Ej0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Sj0 = r(tr), gj0 = r(Z0), Fj0 = r(nr), Tj0 = [0, [17, 0, 0], r(z)], Oj0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ij0 = r(vc), Aj0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Nj0 = r(tr), Cj0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Pj0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Dj0 = [0, [17, 0, [12, 41, 0]], r(h0)], Lj0 = r(Z0), Rj0 = r(nr), jj0 = [0, [17, 0, 0], r(z)], Gj0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Mj0 = r(Xr), Bj0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], qj0 = r(tr), Uj0 = r(Z0), Hj0 = r(nr), Xj0 = [0, [17, 0, 0], r(z)], Yj0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Vj0 = [0, [15, 0], r(C0)], zj0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareExportDeclaration.Variable"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareExportDeclaration.Variable@ ")], Kj0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Wj0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Jj0 = [0, [17, 0, [12, 41, 0]], r(h0)], $j0 = [0, [17, 0, [12, 41, 0]], r(h0)], Zj0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareExportDeclaration.Function"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareExportDeclaration.Function@ ")], Qj0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], rG0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], eG0 = [0, [17, 0, [12, 41, 0]], r(h0)], nG0 = [0, [17, 0, [12, 41, 0]], r(h0)], tG0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareExportDeclaration.Class"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareExportDeclaration.Class@ ")], uG0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], iG0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], fG0 = [0, [17, 0, [12, 41, 0]], r(h0)], xG0 = [0, [17, 0, [12, 41, 0]], r(h0)], aG0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareExportDeclaration.DefaultType"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareExportDeclaration.DefaultType@ ")], oG0 = [0, [17, 0, [12, 41, 0]], r(h0)], cG0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareExportDeclaration.NamedType"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareExportDeclaration.NamedType@ ")], sG0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], vG0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], lG0 = [0, [17, 0, [12, 41, 0]], r(h0)], bG0 = [0, [17, 0, [12, 41, 0]], r(h0)], pG0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareExportDeclaration.NamedOpaqueType"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareExportDeclaration.NamedOpaqueType@ ")], mG0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], _G0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], yG0 = [0, [17, 0, [12, 41, 0]], r(h0)], dG0 = [0, [17, 0, [12, 41, 0]], r(h0)], hG0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareExportDeclaration.Interface"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareExportDeclaration.Interface@ ")], kG0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], wG0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], EG0 = [0, [17, 0, [12, 41, 0]], r(h0)], SG0 = [0, [17, 0, [12, 41, 0]], r(h0)], gG0 = [0, [15, 0], r(C0)], FG0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ExportDefaultDeclaration.Declaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ExportDefaultDeclaration.Declaration@ ")], TG0 = [0, [17, 0, [12, 41, 0]], r(h0)], OG0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ExportDefaultDeclaration.Expression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ExportDefaultDeclaration.Expression@ ")], IG0 = [0, [17, 0, [12, 41, 0]], r(h0)], AG0 = [0, [15, 0], r(C0)], NG0 = r(Yr), CG0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], PG0 = r("Flow_ast.Statement.ExportDefaultDeclaration.default"), DG0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], LG0 = [0, [17, 0, 0], r(z)], RG0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], jG0 = r(P2), GG0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], MG0 = [0, [17, 0, 0], r(z)], BG0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], qG0 = r(Xr), UG0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], HG0 = r(tr), XG0 = r(Z0), YG0 = r(nr), VG0 = [0, [17, 0, 0], r(z)], zG0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], KG0 = [0, [15, 0], r(C0)], WG0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], JG0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ExportNamedDeclaration.ExportSpecifiers"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ExportNamedDeclaration.ExportSpecifiers@ ")], $G0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], ZG0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], QG0 = [0, [17, 0, [12, 41, 0]], r(h0)], rM0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ExportNamedDeclaration.ExportBatchSpecifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ExportNamedDeclaration.ExportBatchSpecifier@ ")], eM0 = [0, [17, 0, [12, 41, 0]], r(h0)], nM0 = [0, [15, 0], r(C0)], tM0 = r(Yr), uM0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], iM0 = r("Flow_ast.Statement.ExportNamedDeclaration.declaration"), fM0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], xM0 = r(tr), aM0 = r(Z0), oM0 = r(nr), cM0 = [0, [17, 0, 0], r(z)], sM0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], vM0 = r(Cv), lM0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], bM0 = r(tr), pM0 = r(Z0), mM0 = r(nr), _M0 = [0, [17, 0, 0], r(z)], yM0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], dM0 = r(vc), hM0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], kM0 = r(tr), wM0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], EM0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], SM0 = [0, [17, 0, [12, 41, 0]], r(h0)], gM0 = r(Z0), FM0 = r(nr), TM0 = [0, [17, 0, 0], r(z)], OM0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], IM0 = r("export_kind"), AM0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], NM0 = [0, [17, 0, 0], r(z)], CM0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], PM0 = r(Xr), DM0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], LM0 = r(tr), RM0 = r(Z0), jM0 = r(nr), GM0 = [0, [17, 0, 0], r(z)], MM0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], BM0 = [0, [15, 0], r(C0)], qM0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], UM0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], HM0 = r(tr), XM0 = r(Z0), YM0 = r(nr), VM0 = [0, [17, 0, [12, 41, 0]], r(h0)], zM0 = [0, [15, 0], r(C0)], KM0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], WM0 = r("Flow_ast.Statement.ExportNamedDeclaration.ExportSpecifier.local"), JM0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $M0 = [0, [17, 0, 0], r(z)], ZM0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], QM0 = r(A4), rB0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], eB0 = r(tr), nB0 = r(Z0), tB0 = r(nr), uB0 = [0, [17, 0, 0], r(z)], iB0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], fB0 = [0, [15, 0], r(C0)], xB0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], aB0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], oB0 = [0, [17, 0, [12, 41, 0]], r(h0)], cB0 = [0, [15, 0], r(C0)], sB0 = r(Yr), vB0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], lB0 = r("Flow_ast.Statement.DeclareModuleExports.annot"), bB0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], pB0 = [0, [17, 0, 0], r(z)], mB0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], _B0 = r(Xr), yB0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], dB0 = r(tr), hB0 = r(Z0), kB0 = r(nr), wB0 = [0, [17, 0, 0], r(z)], EB0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], SB0 = [0, [15, 0], r(C0)], gB0 = r(Yr), FB0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], TB0 = r("Flow_ast.Statement.DeclareModule.id"), OB0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], IB0 = [0, [17, 0, 0], r(z)], AB0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], NB0 = r(Wn), CB0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], PB0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], DB0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], LB0 = [0, [17, 0, [12, 41, 0]], r(h0)], RB0 = [0, [17, 0, 0], r(z)], jB0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], GB0 = r(Zc), MB0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], BB0 = [0, [17, 0, 0], r(z)], qB0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], UB0 = r(Xr), HB0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], XB0 = r(tr), YB0 = r(Z0), VB0 = r(nr), zB0 = [0, [17, 0, 0], r(z)], KB0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], WB0 = [0, [15, 0], r(C0)], JB0 = r("Flow_ast.Statement.DeclareModule.ES"), $B0 = r("Flow_ast.Statement.DeclareModule.CommonJS"), ZB0 = [0, [15, 0], r(C0)], QB0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareModule.Identifier"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareModule.Identifier@ ")], rq0 = [0, [17, 0, [12, 41, 0]], r(h0)], eq0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.DeclareModule.Literal"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.DeclareModule.Literal@ ")], nq0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], tq0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], uq0 = [0, [17, 0, [12, 41, 0]], r(h0)], iq0 = [0, [17, 0, [12, 41, 0]], r(h0)], fq0 = [0, [15, 0], r(C0)], xq0 = r(Yr), aq0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], oq0 = r("Flow_ast.Statement.DeclareFunction.id"), cq0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], sq0 = [0, [17, 0, 0], r(z)], vq0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], lq0 = r(rs), bq0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], pq0 = [0, [17, 0, 0], r(z)], mq0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], _q0 = r(Qu), yq0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], dq0 = r(tr), hq0 = r(Z0), kq0 = r(nr), wq0 = [0, [17, 0, 0], r(z)], Eq0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Sq0 = r(Xr), gq0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Fq0 = r(tr), Tq0 = r(Z0), Oq0 = r(nr), Iq0 = [0, [17, 0, 0], r(z)], Aq0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Nq0 = [0, [15, 0], r(C0)], Cq0 = r(Yr), Pq0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Dq0 = r("Flow_ast.Statement.DeclareVariable.id"), Lq0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Rq0 = [0, [17, 0, 0], r(z)], jq0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Gq0 = r(rs), Mq0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Bq0 = [0, [17, 0, 0], r(z)], qq0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Uq0 = r(Xr), Hq0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Xq0 = r(tr), Yq0 = r(Z0), Vq0 = r(nr), zq0 = [0, [17, 0, 0], r(z)], Kq0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Wq0 = [0, [15, 0], r(C0)], Jq0 = r(Yr), $q0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Zq0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Qq0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], rU0 = [0, [17, 0, [12, 41, 0]], r(h0)], eU0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], nU0 = r("Flow_ast.Statement.DeclareClass.id"), tU0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], uU0 = [0, [17, 0, 0], r(z)], iU0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], fU0 = r(sv), xU0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], aU0 = r(tr), oU0 = r(Z0), cU0 = r(nr), sU0 = [0, [17, 0, 0], r(z)], vU0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], lU0 = r(Wn), bU0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], pU0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], mU0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], _U0 = [0, [17, 0, [12, 41, 0]], r(h0)], yU0 = [0, [17, 0, 0], r(z)], dU0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hU0 = r(C7), kU0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], wU0 = r(tr), EU0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], SU0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], gU0 = [0, [17, 0, [12, 41, 0]], r(h0)], FU0 = r(Z0), TU0 = r(nr), OU0 = [0, [17, 0, 0], r(z)], IU0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], AU0 = r(Vy), NU0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], CU0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], PU0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], DU0 = [0, [17, 0, 0], r(z)], LU0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], RU0 = r(gs), jU0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], GU0 = r(tr), MU0 = r(Z0), BU0 = r(nr), qU0 = [0, [17, 0, 0], r(z)], UU0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], HU0 = r(Xr), XU0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], YU0 = r(tr), VU0 = r(Z0), zU0 = r(nr), KU0 = [0, [17, 0, 0], r(z)], WU0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], JU0 = [0, [15, 0], r(C0)], $U0 = r(Yr), ZU0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], QU0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], rH0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], eH0 = [0, [17, 0, [12, 41, 0]], r(h0)], nH0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], tH0 = r("Flow_ast.Statement.Interface.id"), uH0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], iH0 = [0, [17, 0, 0], r(z)], fH0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], xH0 = r(sv), aH0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], oH0 = r(tr), cH0 = r(Z0), sH0 = r(nr), vH0 = [0, [17, 0, 0], r(z)], lH0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], bH0 = r(C7), pH0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], mH0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], _H0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], yH0 = [0, [17, 0, 0], r(z)], dH0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hH0 = r(Wn), kH0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], wH0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], EH0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], SH0 = [0, [17, 0, [12, 41, 0]], r(h0)], gH0 = [0, [17, 0, 0], r(z)], FH0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], TH0 = r(Xr), OH0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], IH0 = r(tr), AH0 = r(Z0), NH0 = r(nr), CH0 = [0, [17, 0, 0], r(z)], PH0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], DH0 = [0, [15, 0], r(C0)], LH0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.EnumDeclaration.BooleanBody"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.EnumDeclaration.BooleanBody@ ")], RH0 = [0, [17, 0, [12, 41, 0]], r(h0)], jH0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.EnumDeclaration.NumberBody"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.EnumDeclaration.NumberBody@ ")], GH0 = [0, [17, 0, [12, 41, 0]], r(h0)], MH0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.EnumDeclaration.StringBody"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.EnumDeclaration.StringBody@ ")], BH0 = [0, [17, 0, [12, 41, 0]], r(h0)], qH0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.EnumDeclaration.SymbolBody"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.EnumDeclaration.SymbolBody@ ")], UH0 = [0, [17, 0, [12, 41, 0]], r(h0)], HH0 = [0, [15, 0], r(C0)], XH0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], YH0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], VH0 = [0, [17, 0, [12, 41, 0]], r(h0)], zH0 = [0, [15, 0], r(C0)], KH0 = r(Yr), WH0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], JH0 = r("Flow_ast.Statement.EnumDeclaration.id"), $H0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ZH0 = [0, [17, 0, 0], r(z)], QH0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], rX0 = r(Wn), eX0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], nX0 = [0, [17, 0, 0], r(z)], tX0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], uX0 = r(Xr), iX0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], fX0 = r(tr), xX0 = r(Z0), aX0 = r(nr), oX0 = [0, [17, 0, 0], r(z)], cX0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], sX0 = [0, [15, 0], r(C0)], vX0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], lX0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], bX0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], pX0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], mX0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], _X0 = r("Flow_ast.Statement.EnumDeclaration.SymbolBody.members"), yX0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], dX0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], hX0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], kX0 = [0, [17, 0, 0], r(z)], wX0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], EX0 = r(E4), SX0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], gX0 = [0, [9, 0, 0], r(An)], FX0 = [0, [17, 0, 0], r(z)], TX0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], OX0 = r(Xr), IX0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], AX0 = r(tr), NX0 = r(Z0), CX0 = r(nr), PX0 = [0, [17, 0, 0], r(z)], DX0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], LX0 = [0, [15, 0], r(C0)], RX0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], jX0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], GX0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.EnumDeclaration.StringBody.Defaulted"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.EnumDeclaration.StringBody.Defaulted@ ")], MX0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], BX0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], qX0 = [0, [17, 0, [12, 41, 0]], r(h0)], UX0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.EnumDeclaration.StringBody.Initialized"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.EnumDeclaration.StringBody.Initialized@ ")], HX0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], XX0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], YX0 = [0, [17, 0, [12, 41, 0]], r(h0)], VX0 = [0, [15, 0], r(C0)], zX0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], KX0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], WX0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], JX0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], $X0 = r("Flow_ast.Statement.EnumDeclaration.StringBody.members"), ZX0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], QX0 = [0, [17, 0, 0], r(z)], rY0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], eY0 = r(Ik), nY0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], tY0 = [0, [9, 0, 0], r(An)], uY0 = [0, [17, 0, 0], r(z)], iY0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], fY0 = r(E4), xY0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], aY0 = [0, [9, 0, 0], r(An)], oY0 = [0, [17, 0, 0], r(z)], cY0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], sY0 = r(Xr), vY0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], lY0 = r(tr), bY0 = r(Z0), pY0 = r(nr), mY0 = [0, [17, 0, 0], r(z)], _Y0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], yY0 = [0, [15, 0], r(C0)], dY0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hY0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], kY0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], wY0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], EY0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], SY0 = r("Flow_ast.Statement.EnumDeclaration.NumberBody.members"), gY0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], FY0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], TY0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], OY0 = [0, [17, 0, 0], r(z)], IY0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], AY0 = r(Ik), NY0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], CY0 = [0, [9, 0, 0], r(An)], PY0 = [0, [17, 0, 0], r(z)], DY0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], LY0 = r(E4), RY0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], jY0 = [0, [9, 0, 0], r(An)], GY0 = [0, [17, 0, 0], r(z)], MY0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], BY0 = r(Xr), qY0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], UY0 = r(tr), HY0 = r(Z0), XY0 = r(nr), YY0 = [0, [17, 0, 0], r(z)], VY0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], zY0 = [0, [15, 0], r(C0)], KY0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], WY0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], JY0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], $Y0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ZY0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], QY0 = r("Flow_ast.Statement.EnumDeclaration.BooleanBody.members"), rV0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], eV0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], nV0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], tV0 = [0, [17, 0, 0], r(z)], uV0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], iV0 = r(Ik), fV0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], xV0 = [0, [9, 0, 0], r(An)], aV0 = [0, [17, 0, 0], r(z)], oV0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], cV0 = r(E4), sV0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], vV0 = [0, [9, 0, 0], r(An)], lV0 = [0, [17, 0, 0], r(z)], bV0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], pV0 = r(Xr), mV0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _V0 = r(tr), yV0 = r(Z0), dV0 = r(nr), hV0 = [0, [17, 0, 0], r(z)], kV0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], wV0 = [0, [15, 0], r(C0)], EV0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], SV0 = r("Flow_ast.Statement.EnumDeclaration.InitializedMember.id"), gV0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], FV0 = [0, [17, 0, 0], r(z)], TV0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], OV0 = r(ji), IV0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], AV0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], NV0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], CV0 = [0, [17, 0, [12, 41, 0]], r(h0)], PV0 = [0, [17, 0, 0], r(z)], DV0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], LV0 = [0, [15, 0], r(C0)], RV0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], jV0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], GV0 = [0, [17, 0, [12, 41, 0]], r(h0)], MV0 = [0, [15, 0], r(C0)], BV0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], qV0 = r("Flow_ast.Statement.EnumDeclaration.DefaultedMember.id"), UV0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], HV0 = [0, [17, 0, 0], r(z)], XV0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], YV0 = [0, [15, 0], r(C0)], VV0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], zV0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], KV0 = [0, [17, 0, [12, 41, 0]], r(h0)], WV0 = [0, [15, 0], r(C0)], JV0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ForOf.LeftDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ForOf.LeftDeclaration@ ")], $V0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], ZV0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], QV0 = [0, [17, 0, [12, 41, 0]], r(h0)], rz0 = [0, [17, 0, [12, 41, 0]], r(h0)], ez0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ForOf.LeftPattern"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ForOf.LeftPattern@ ")], nz0 = [0, [17, 0, [12, 41, 0]], r(h0)], tz0 = [0, [15, 0], r(C0)], uz0 = r(Yr), iz0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], fz0 = r("Flow_ast.Statement.ForOf.left"), xz0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], az0 = [0, [17, 0, 0], r(z)], oz0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], cz0 = r(Nu), sz0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], vz0 = [0, [17, 0, 0], r(z)], lz0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], bz0 = r(Wn), pz0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], mz0 = [0, [17, 0, 0], r(z)], _z0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], yz0 = r(wx), dz0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], hz0 = [0, [9, 0, 0], r(An)], kz0 = [0, [17, 0, 0], r(z)], wz0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ez0 = r(Xr), Sz0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], gz0 = r(tr), Fz0 = r(Z0), Tz0 = r(nr), Oz0 = [0, [17, 0, 0], r(z)], Iz0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Az0 = [0, [15, 0], r(C0)], Nz0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ForIn.LeftDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ForIn.LeftDeclaration@ ")], Cz0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Pz0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Dz0 = [0, [17, 0, [12, 41, 0]], r(h0)], Lz0 = [0, [17, 0, [12, 41, 0]], r(h0)], Rz0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.ForIn.LeftPattern"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.ForIn.LeftPattern@ ")], jz0 = [0, [17, 0, [12, 41, 0]], r(h0)], Gz0 = [0, [15, 0], r(C0)], Mz0 = r(Yr), Bz0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], qz0 = r("Flow_ast.Statement.ForIn.left"), Uz0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Hz0 = [0, [17, 0, 0], r(z)], Xz0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Yz0 = r(Nu), Vz0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], zz0 = [0, [17, 0, 0], r(z)], Kz0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Wz0 = r(Wn), Jz0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $z0 = [0, [17, 0, 0], r(z)], Zz0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Qz0 = r(j8), rK0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], eK0 = [0, [9, 0, 0], r(An)], nK0 = [0, [17, 0, 0], r(z)], tK0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], uK0 = r(Xr), iK0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], fK0 = r(tr), xK0 = r(Z0), aK0 = r(nr), oK0 = [0, [17, 0, 0], r(z)], cK0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], sK0 = [0, [15, 0], r(C0)], vK0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.For.InitDeclaration"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.For.InitDeclaration@ ")], lK0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], bK0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], pK0 = [0, [17, 0, [12, 41, 0]], r(h0)], mK0 = [0, [17, 0, [12, 41, 0]], r(h0)], _K0 = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Statement.For.InitExpression"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Statement.For.InitExpression@ ")], yK0 = [0, [17, 0, [12, 41, 0]], r(h0)], dK0 = [0, [15, 0], r(C0)], hK0 = r(Yr), kK0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], wK0 = r("Flow_ast.Statement.For.init"), EK0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], SK0 = r(tr), gK0 = r(Z0), FK0 = r(nr), TK0 = [0, [17, 0, 0], r(z)], OK0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], IK0 = r(Ts), AK0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], NK0 = r(tr), CK0 = r(Z0), PK0 = r(nr), DK0 = [0, [17, 0, 0], r(z)], LK0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], RK0 = r(sU), jK0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], GK0 = r(tr), MK0 = r(Z0), BK0 = r(nr), qK0 = [0, [17, 0, 0], r(z)], UK0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], HK0 = r(Wn), XK0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], YK0 = [0, [17, 0, 0], r(z)], VK0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], zK0 = r(Xr), KK0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], WK0 = r(tr), JK0 = r(Z0), $K0 = r(nr), ZK0 = [0, [17, 0, 0], r(z)], QK0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], rW0 = [0, [15, 0], r(C0)], eW0 = r(Yr), nW0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], tW0 = r("Flow_ast.Statement.DoWhile.body"), uW0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], iW0 = [0, [17, 0, 0], r(z)], fW0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], xW0 = r(Ts), aW0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], oW0 = [0, [17, 0, 0], r(z)], cW0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], sW0 = r(Xr), vW0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], lW0 = r(tr), bW0 = r(Z0), pW0 = r(nr), mW0 = [0, [17, 0, 0], r(z)], _W0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], yW0 = [0, [15, 0], r(C0)], dW0 = r(Yr), hW0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], kW0 = r("Flow_ast.Statement.While.test"), wW0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], EW0 = [0, [17, 0, 0], r(z)], SW0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], gW0 = r(Wn), FW0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], TW0 = [0, [17, 0, 0], r(z)], OW0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], IW0 = r(Xr), AW0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], NW0 = r(tr), CW0 = r(Z0), PW0 = r(nr), DW0 = [0, [17, 0, 0], r(z)], LW0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], RW0 = [0, [15, 0], r(C0)], jW0 = r("Flow_ast.Statement.VariableDeclaration.Var"), GW0 = r("Flow_ast.Statement.VariableDeclaration.Let"), MW0 = r("Flow_ast.Statement.VariableDeclaration.Const"), BW0 = [0, [15, 0], r(C0)], qW0 = r(Yr), UW0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], HW0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], XW0 = r("Flow_ast.Statement.VariableDeclaration.declarations"), YW0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], VW0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], zW0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], KW0 = [0, [17, 0, 0], r(z)], WW0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], JW0 = r(Zc), $W0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ZW0 = [0, [17, 0, 0], r(z)], QW0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], rJ0 = r(Xr), eJ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], nJ0 = r(tr), tJ0 = r(Z0), uJ0 = r(nr), iJ0 = [0, [17, 0, 0], r(z)], fJ0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], xJ0 = [0, [15, 0], r(C0)], aJ0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], oJ0 = r("Flow_ast.Statement.VariableDeclaration.Declarator.id"), cJ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], sJ0 = [0, [17, 0, 0], r(z)], vJ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], lJ0 = r(ji), bJ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], pJ0 = r(tr), mJ0 = r(Z0), _J0 = r(nr), yJ0 = [0, [17, 0, 0], r(z)], dJ0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], hJ0 = [0, [15, 0], r(C0)], kJ0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], wJ0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], EJ0 = [0, [17, 0, [12, 41, 0]], r(h0)], SJ0 = [0, [15, 0], r(C0)], gJ0 = r(Yr), FJ0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], TJ0 = r("Flow_ast.Statement.Try.block"), OJ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], IJ0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], AJ0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], NJ0 = [0, [17, 0, [12, 41, 0]], r(h0)], CJ0 = [0, [17, 0, 0], r(z)], PJ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], DJ0 = r(XU), LJ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], RJ0 = r(tr), jJ0 = r(Z0), GJ0 = r(nr), MJ0 = [0, [17, 0, 0], r(z)], BJ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], qJ0 = r(jH), UJ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], HJ0 = r(tr), XJ0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], YJ0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], VJ0 = [0, [17, 0, [12, 41, 0]], r(h0)], zJ0 = r(Z0), KJ0 = r(nr), WJ0 = [0, [17, 0, 0], r(z)], JJ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], $J0 = r(Xr), ZJ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], QJ0 = r(tr), r$0 = r(Z0), e$0 = r(nr), n$0 = [0, [17, 0, 0], r(z)], t$0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], u$0 = [0, [15, 0], r(C0)], i$0 = r(Yr), f$0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], x$0 = r("Flow_ast.Statement.Try.CatchClause.param"), a$0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], o$0 = r(tr), c$0 = r(Z0), s$0 = r(nr), v$0 = [0, [17, 0, 0], r(z)], l$0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], b$0 = r(Wn), p$0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], m$0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], _$0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], y$0 = [0, [17, 0, [12, 41, 0]], r(h0)], d$0 = [0, [17, 0, 0], r(z)], h$0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], k$0 = r(Xr), w$0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], E$0 = r(tr), S$0 = r(Z0), g$0 = r(nr), F$0 = [0, [17, 0, 0], r(z)], T$0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], O$0 = [0, [15, 0], r(C0)], I$0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], A$0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], N$0 = [0, [17, 0, [12, 41, 0]], r(h0)], C$0 = [0, [15, 0], r(C0)], P$0 = r(Yr), D$0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], L$0 = r("Flow_ast.Statement.Throw.argument"), R$0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], j$0 = [0, [17, 0, 0], r(z)], G$0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], M$0 = r(Xr), B$0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], q$0 = r(tr), U$0 = r(Z0), H$0 = r(nr), X$0 = [0, [17, 0, 0], r(z)], Y$0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], V$0 = [0, [15, 0], r(C0)], z$0 = r(Yr), K$0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], W$0 = r("Flow_ast.Statement.Return.argument"), J$0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $$0 = r(tr), Z$0 = r(Z0), Q$0 = r(nr), rZ0 = [0, [17, 0, 0], r(z)], eZ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], nZ0 = r(Xr), tZ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], uZ0 = r(tr), iZ0 = r(Z0), fZ0 = r(nr), xZ0 = [0, [17, 0, 0], r(z)], aZ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], oZ0 = r("return_out"), cZ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], sZ0 = [0, [17, 0, 0], r(z)], vZ0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], lZ0 = [0, [15, 0], r(C0)], bZ0 = r(Yr), pZ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], mZ0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], _Z0 = r("Flow_ast.Statement.Switch.discriminant"), yZ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], dZ0 = [0, [17, 0, 0], r(z)], hZ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], kZ0 = r(uY), wZ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], EZ0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], SZ0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], gZ0 = [0, [17, 0, 0], r(z)], FZ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], TZ0 = r(Xr), OZ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], IZ0 = r(tr), AZ0 = r(Z0), NZ0 = r(nr), CZ0 = [0, [17, 0, 0], r(z)], PZ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], DZ0 = r("exhaustive_out"), LZ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], RZ0 = [0, [17, 0, 0], r(z)], jZ0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], GZ0 = [0, [15, 0], r(C0)], MZ0 = r(Yr), BZ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], qZ0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], UZ0 = r("Flow_ast.Statement.Switch.Case.test"), HZ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], XZ0 = r(tr), YZ0 = r(Z0), VZ0 = r(nr), zZ0 = [0, [17, 0, 0], r(z)], KZ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], WZ0 = r(kv), JZ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $Z0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], ZZ0 = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], QZ0 = [0, [17, 0, 0], r(z)], rQ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], eQ0 = r(Xr), nQ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], tQ0 = r(tr), uQ0 = r(Z0), iQ0 = r(nr), fQ0 = [0, [17, 0, 0], r(z)], xQ0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], aQ0 = [0, [15, 0], r(C0)], oQ0 = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], cQ0 = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], sQ0 = [0, [17, 0, [12, 41, 0]], r(h0)], vQ0 = [0, [15, 0], r(C0)], lQ0 = r(Yr), bQ0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], pQ0 = r("Flow_ast.Statement.OpaqueType.id"), mQ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _Q0 = [0, [17, 0, 0], r(z)], yQ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], dQ0 = r(sv), hQ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], kQ0 = r(tr), wQ0 = r(Z0), EQ0 = r(nr), SQ0 = [0, [17, 0, 0], r(z)], gQ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], FQ0 = r(kX), TQ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], OQ0 = r(tr), IQ0 = r(Z0), AQ0 = r(nr), NQ0 = [0, [17, 0, 0], r(z)], CQ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], PQ0 = r(IX), DQ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], LQ0 = r(tr), RQ0 = r(Z0), jQ0 = r(nr), GQ0 = [0, [17, 0, 0], r(z)], MQ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], BQ0 = r(Xr), qQ0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], UQ0 = r(tr), HQ0 = r(Z0), XQ0 = r(nr), YQ0 = [0, [17, 0, 0], r(z)], VQ0 = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], zQ0 = [0, [15, 0], r(C0)], KQ0 = r(Yr), WQ0 = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], JQ0 = r("Flow_ast.Statement.TypeAlias.id"), $Q0 = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ZQ0 = [0, [17, 0, 0], r(z)], QQ0 = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], r0r = r(sv), e0r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], n0r = r(tr), t0r = r(Z0), u0r = r(nr), i0r = [0, [17, 0, 0], r(z)], f0r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], x0r = r(Nu), a0r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], o0r = [0, [17, 0, 0], r(z)], c0r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], s0r = r(Xr), v0r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], l0r = r(tr), b0r = r(Z0), p0r = r(nr), m0r = [0, [17, 0, 0], r(z)], _0r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], y0r = [0, [15, 0], r(C0)], d0r = r(Yr), h0r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], k0r = r("Flow_ast.Statement.With._object"), w0r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], E0r = [0, [17, 0, 0], r(z)], S0r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], g0r = r(Wn), F0r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], T0r = [0, [17, 0, 0], r(z)], O0r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], I0r = r(Xr), A0r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], N0r = r(tr), C0r = r(Z0), P0r = r(nr), D0r = [0, [17, 0, 0], r(z)], L0r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], R0r = [0, [15, 0], r(C0)], j0r = r(Yr), G0r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], M0r = r("Flow_ast.Statement.Debugger.comments"), B0r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], q0r = r(tr), U0r = r(Z0), H0r = r(nr), X0r = [0, [17, 0, 0], r(z)], Y0r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], V0r = [0, [15, 0], r(C0)], z0r = r(Yr), K0r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], W0r = r("Flow_ast.Statement.Continue.label"), J0r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $0r = r(tr), Z0r = r(Z0), Q0r = r(nr), rrr = [0, [17, 0, 0], r(z)], err = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], nrr = r(Xr), trr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], urr = r(tr), irr = r(Z0), frr = r(nr), xrr = [0, [17, 0, 0], r(z)], arr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], orr = [0, [15, 0], r(C0)], crr = r(Yr), srr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], vrr = r("Flow_ast.Statement.Break.label"), lrr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], brr = r(tr), prr = r(Z0), mrr = r(nr), _rr = [0, [17, 0, 0], r(z)], yrr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], drr = r(Xr), hrr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], krr = r(tr), wrr = r(Z0), Err = r(nr), Srr = [0, [17, 0, 0], r(z)], grr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Frr = [0, [15, 0], r(C0)], Trr = r(Yr), Orr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Irr = r("Flow_ast.Statement.Labeled.label"), Arr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Nrr = [0, [17, 0, 0], r(z)], Crr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Prr = r(Wn), Drr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Lrr = [0, [17, 0, 0], r(z)], Rrr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], jrr = r(Xr), Grr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Mrr = r(tr), Brr = r(Z0), qrr = r(nr), Urr = [0, [17, 0, 0], r(z)], Hrr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Xrr = [0, [15, 0], r(C0)], Yrr = r(Yr), Vrr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], zrr = r("Flow_ast.Statement.If.test"), Krr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Wrr = [0, [17, 0, 0], r(z)], Jrr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], $rr = r(kv), Zrr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Qrr = [0, [17, 0, 0], r(z)], rer = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], eer = r(_3), ner = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ter = r(tr), uer = r(Z0), ier = r(nr), fer = [0, [17, 0, 0], r(z)], xer = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], aer = r(Xr), oer = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], cer = r(tr), ser = r(Z0), ver = r(nr), ler = [0, [17, 0, 0], r(z)], ber = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], per = [0, [15, 0], r(C0)], mer = r(Yr), _er = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], yer = r("Flow_ast.Statement.If.Alternate.body"), der = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], her = [0, [17, 0, 0], r(z)], ker = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], wer = r(Xr), Eer = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ser = r(tr), ger = r(Z0), Fer = r(nr), Ter = [0, [17, 0, 0], r(z)], Oer = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Ier = [0, [15, 0], r(C0)], Aer = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Ner = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Cer = [0, [17, 0, [12, 41, 0]], r(h0)], Per = [0, [15, 0], r(C0)], Der = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ler = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Rer = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], jer = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ger = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Mer = r("Flow_ast.Statement.Block.body"), Ber = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], qer = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Uer = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Her = [0, [17, 0, 0], r(z)], Xer = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Yer = r(Xr), Ver = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], zer = r(tr), Ker = r(Z0), Wer = r(nr), Jer = [0, [17, 0, 0], r(z)], $er = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Zer = [0, [15, 0], r(C0)], Qer = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Predicate.Declared"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Predicate.Declared@ ")], rnr = [0, [17, 0, [12, 41, 0]], r(h0)], enr = r("Flow_ast.Type.Predicate.Inferred"), nnr = [0, [15, 0], r(C0)], tnr = r(Yr), unr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], inr = r("Flow_ast.Type.Predicate.kind"), fnr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], xnr = [0, [17, 0, 0], r(z)], anr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], onr = r(Xr), cnr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], snr = r(tr), vnr = r(Z0), lnr = r(nr), bnr = [0, [17, 0, 0], r(z)], pnr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], mnr = [0, [15, 0], r(C0)], _nr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], ynr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], dnr = [0, [17, 0, [12, 41, 0]], r(h0)], hnr = [0, [15, 0], r(C0)], knr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], wnr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Enr = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Snr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], gnr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Fnr = r("Flow_ast.Type.TypeArgs.arguments"), Tnr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Onr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Inr = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Anr = [0, [17, 0, 0], r(z)], Nnr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Cnr = r(Xr), Pnr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Dnr = r(tr), Lnr = r(Z0), Rnr = r(nr), jnr = [0, [17, 0, 0], r(z)], Gnr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Mnr = [0, [15, 0], r(C0)], Bnr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], qnr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Unr = [0, [17, 0, [12, 41, 0]], r(h0)], Hnr = [0, [15, 0], r(C0)], Xnr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ynr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Vnr = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], znr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Knr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Wnr = r("Flow_ast.Type.TypeParams.params"), Jnr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $nr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Znr = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Qnr = [0, [17, 0, 0], r(z)], rtr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], etr = r(Xr), ntr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ttr = r(tr), utr = r(Z0), itr = r(nr), ftr = [0, [17, 0, 0], r(z)], xtr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], atr = [0, [15, 0], r(C0)], otr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], ctr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], str = [0, [17, 0, [12, 41, 0]], r(h0)], vtr = [0, [15, 0], r(C0)], ltr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], btr = r("Flow_ast.Type.TypeParam.name"), ptr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], mtr = [0, [17, 0, 0], r(z)], _tr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ytr = r(MU), dtr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], htr = [0, [17, 0, 0], r(z)], ktr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], wtr = r(ou), Etr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Str = r(tr), gtr = r(Z0), Ftr = r(nr), Ttr = [0, [17, 0, 0], r(z)], Otr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Itr = r(mi), Atr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ntr = r(tr), Ctr = r(Z0), Ptr = r(nr), Dtr = [0, [17, 0, 0], r(z)], Ltr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Rtr = [0, [15, 0], r(C0)], jtr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Gtr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Mtr = [0, [17, 0, [12, 41, 0]], r(h0)], Btr = [0, [15, 0], r(C0)], qtr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Missing"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Missing@ ")], Utr = [0, [17, 0, [12, 41, 0]], r(h0)], Htr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Available"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Available@ ")], Xtr = [0, [17, 0, [12, 41, 0]], r(h0)], Ytr = [0, [15, 0], r(C0)], Vtr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], ztr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Ktr = [0, [17, 0, [12, 41, 0]], r(h0)], Wtr = [0, [15, 0], r(C0)], Jtr = r(Yr), $tr = r(Yr), Ztr = r(Yr), Qtr = r(Yr), rur = r(Yr), eur = r(Yr), nur = r(Yr), tur = r(Yr), uur = r(Yr), iur = r(Yr), fur = r(Yr), xur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Any"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Any@ ")], aur = r(tr), our = r(Z0), cur = r(nr), sur = [0, [17, 0, [12, 41, 0]], r(h0)], vur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Mixed"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Mixed@ ")], lur = r(tr), bur = r(Z0), pur = r(nr), mur = [0, [17, 0, [12, 41, 0]], r(h0)], _ur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Empty"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Empty@ ")], yur = r(tr), dur = r(Z0), hur = r(nr), kur = [0, [17, 0, [12, 41, 0]], r(h0)], wur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Void"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Void@ ")], Eur = r(tr), Sur = r(Z0), gur = r(nr), Fur = [0, [17, 0, [12, 41, 0]], r(h0)], Tur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Null"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Null@ ")], Our = r(tr), Iur = r(Z0), Aur = r(nr), Nur = [0, [17, 0, [12, 41, 0]], r(h0)], Cur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Number"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Number@ ")], Pur = r(tr), Dur = r(Z0), Lur = r(nr), Rur = [0, [17, 0, [12, 41, 0]], r(h0)], jur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.BigInt"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.BigInt@ ")], Gur = r(tr), Mur = r(Z0), Bur = r(nr), qur = [0, [17, 0, [12, 41, 0]], r(h0)], Uur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.String"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.String@ ")], Hur = r(tr), Xur = r(Z0), Yur = r(nr), Vur = [0, [17, 0, [12, 41, 0]], r(h0)], zur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Boolean"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Boolean@ ")], Kur = r(tr), Wur = r(Z0), Jur = r(nr), $ur = [0, [17, 0, [12, 41, 0]], r(h0)], Zur = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Symbol"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Symbol@ ")], Qur = r(tr), r7r = r(Z0), e7r = r(nr), n7r = [0, [17, 0, [12, 41, 0]], r(h0)], t7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Exists"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Exists@ ")], u7r = r(tr), i7r = r(Z0), f7r = r(nr), x7r = [0, [17, 0, [12, 41, 0]], r(h0)], a7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Nullable"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Nullable@ ")], o7r = [0, [17, 0, [12, 41, 0]], r(h0)], c7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Function"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Function@ ")], s7r = [0, [17, 0, [12, 41, 0]], r(h0)], v7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Object"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Object@ ")], l7r = [0, [17, 0, [12, 41, 0]], r(h0)], b7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Interface"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Interface@ ")], p7r = [0, [17, 0, [12, 41, 0]], r(h0)], m7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Array"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Array@ ")], _7r = [0, [17, 0, [12, 41, 0]], r(h0)], y7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Generic"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Generic@ ")], d7r = [0, [17, 0, [12, 41, 0]], r(h0)], h7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.IndexedAccess"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.IndexedAccess@ ")], k7r = [0, [17, 0, [12, 41, 0]], r(h0)], w7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.OptionalIndexedAccess"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.OptionalIndexedAccess@ ")], E7r = [0, [17, 0, [12, 41, 0]], r(h0)], S7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Union"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Union@ ")], g7r = [0, [17, 0, [12, 41, 0]], r(h0)], F7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Intersection"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Intersection@ ")], T7r = [0, [17, 0, [12, 41, 0]], r(h0)], O7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Typeof"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Typeof@ ")], I7r = [0, [17, 0, [12, 41, 0]], r(h0)], A7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Tuple"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Tuple@ ")], N7r = [0, [17, 0, [12, 41, 0]], r(h0)], C7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.StringLiteral"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.StringLiteral@ ")], P7r = [0, [17, 0, [12, 41, 0]], r(h0)], D7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.NumberLiteral"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.NumberLiteral@ ")], L7r = [0, [17, 0, [12, 41, 0]], r(h0)], R7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.BigIntLiteral"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.BigIntLiteral@ ")], j7r = [0, [17, 0, [12, 41, 0]], r(h0)], G7r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.BooleanLiteral"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.BooleanLiteral@ ")], M7r = [0, [17, 0, [12, 41, 0]], r(h0)], B7r = [0, [15, 0], r(C0)], q7r = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], U7r = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], H7r = [0, [17, 0, [12, 41, 0]], r(h0)], X7r = [0, [15, 0], r(C0)], Y7r = r(Yr), V7r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], z7r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], K7r = r("Flow_ast.Type.Intersection.types"), W7r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], J7r = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], $7r = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Z7r = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Q7r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], rir = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], eir = [0, [17, 0, [12, 41, 0]], r(h0)], nir = [0, [17, 0, 0], r(z)], tir = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], uir = r(Xr), iir = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], fir = r(tr), xir = r(Z0), air = r(nr), oir = [0, [17, 0, 0], r(z)], cir = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], sir = [0, [15, 0], r(C0)], vir = r(Yr), lir = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], bir = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], pir = r("Flow_ast.Type.Union.types"), mir = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _ir = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], yir = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], dir = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], hir = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], kir = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], wir = [0, [17, 0, [12, 41, 0]], r(h0)], Eir = [0, [17, 0, 0], r(z)], Sir = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], gir = r(Xr), Fir = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Tir = r(tr), Oir = r(Z0), Iir = r(nr), Air = [0, [17, 0, 0], r(z)], Nir = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Cir = [0, [15, 0], r(C0)], Pir = r(Yr), Dir = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Lir = r("Flow_ast.Type.Array.argument"), Rir = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], jir = [0, [17, 0, 0], r(z)], Gir = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Mir = r(Xr), Bir = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], qir = r(tr), Uir = r(Z0), Hir = r(nr), Xir = [0, [17, 0, 0], r(z)], Yir = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Vir = [0, [15, 0], r(C0)], zir = r(Yr), Kir = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Wir = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Jir = r("Flow_ast.Type.Tuple.types"), $ir = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Zir = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Qir = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], rfr = [0, [17, 0, 0], r(z)], efr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], nfr = r(Xr), tfr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ufr = r(tr), ifr = r(Z0), ffr = r(nr), xfr = [0, [17, 0, 0], r(z)], afr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], ofr = [0, [15, 0], r(C0)], cfr = r(Yr), sfr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], vfr = r("Flow_ast.Type.Typeof.argument"), lfr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], bfr = [0, [17, 0, 0], r(z)], pfr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], mfr = r(Xr), _fr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], yfr = r(tr), dfr = r(Z0), hfr = r(nr), kfr = [0, [17, 0, 0], r(z)], wfr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Efr = [0, [15, 0], r(C0)], Sfr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], gfr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Ffr = [0, [17, 0, [12, 41, 0]], r(h0)], Tfr = [0, [15, 0], r(C0)], Ofr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Ifr = r("Flow_ast.Type.Typeof.Target.qualification"), Afr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Nfr = [0, [17, 0, 0], r(z)], Cfr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Pfr = r(mt), Dfr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Lfr = [0, [17, 0, 0], r(z)], Rfr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], jfr = [0, [15, 0], r(C0)], Gfr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Typeof.Target.Unqualified"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Typeof.Target.Unqualified@ ")], Mfr = [0, [17, 0, [12, 41, 0]], r(h0)], Bfr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Typeof.Target.Qualified"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Typeof.Target.Qualified@ ")], qfr = [0, [17, 0, [12, 41, 0]], r(h0)], Ufr = [0, [15, 0], r(C0)], Hfr = r(Yr), Xfr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Yfr = r("Flow_ast.Type.Nullable.argument"), Vfr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], zfr = [0, [17, 0, 0], r(z)], Kfr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Wfr = r(Xr), Jfr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $fr = r(tr), Zfr = r(Z0), Qfr = r(nr), rxr = [0, [17, 0, 0], r(z)], exr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], nxr = [0, [15, 0], r(C0)], txr = r(Yr), uxr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ixr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], fxr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], xxr = [0, [17, 0, [12, 41, 0]], r(h0)], axr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], oxr = r("Flow_ast.Type.Interface.body"), cxr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], sxr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], vxr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], lxr = [0, [17, 0, [12, 41, 0]], r(h0)], bxr = [0, [17, 0, 0], r(z)], pxr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], mxr = r(C7), _xr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], yxr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], dxr = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], hxr = [0, [17, 0, 0], r(z)], kxr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], wxr = r(Xr), Exr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Sxr = r(tr), gxr = r(Z0), Fxr = r(nr), Txr = [0, [17, 0, 0], r(z)], Oxr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Ixr = [0, [15, 0], r(C0)], Axr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Object.Property"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Object.Property@ ")], Nxr = [0, [17, 0, [12, 41, 0]], r(h0)], Cxr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Object.SpreadProperty"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Object.SpreadProperty@ ")], Pxr = [0, [17, 0, [12, 41, 0]], r(h0)], Dxr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Object.Indexer"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Object.Indexer@ ")], Lxr = [0, [17, 0, [12, 41, 0]], r(h0)], Rxr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Object.CallProperty"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Object.CallProperty@ ")], jxr = [0, [17, 0, [12, 41, 0]], r(h0)], Gxr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Object.InternalSlot"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Object.InternalSlot@ ")], Mxr = [0, [17, 0, [12, 41, 0]], r(h0)], Bxr = [0, [15, 0], r(C0)], qxr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Uxr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Hxr = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Xxr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Yxr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Vxr = r("Flow_ast.Type.Object.exact"), zxr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Kxr = [0, [9, 0, 0], r(An)], Wxr = [0, [17, 0, 0], r(z)], Jxr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], $xr = r(HY), Zxr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Qxr = [0, [9, 0, 0], r(An)], rar = [0, [17, 0, 0], r(z)], ear = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], nar = r(X4), tar = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], uar = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], iar = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], far = [0, [17, 0, 0], r(z)], xar = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], aar = r(Xr), oar = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], car = r(tr), sar = r(Z0), lar = r(nr), bar = [0, [17, 0, 0], r(z)], par = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], mar = [0, [15, 0], r(C0)], _ar = r(Yr), yar = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], dar = r("Flow_ast.Type.Object.InternalSlot.id"), har = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], kar = [0, [17, 0, 0], r(z)], war = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ear = r(Bn), Sar = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], gar = [0, [17, 0, 0], r(z)], Far = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Tar = r(Bu), Oar = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Iar = [0, [9, 0, 0], r(An)], Aar = [0, [17, 0, 0], r(z)], Nar = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Car = r(eu), Par = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Dar = [0, [9, 0, 0], r(An)], Lar = [0, [17, 0, 0], r(z)], Rar = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], jar = r(xU), Gar = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Mar = [0, [9, 0, 0], r(An)], Bar = [0, [17, 0, 0], r(z)], qar = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Uar = r(Xr), Har = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Xar = r(tr), Yar = r(Z0), Var = r(nr), zar = [0, [17, 0, 0], r(z)], Kar = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], War = [0, [15, 0], r(C0)], Jar = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], $ar = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Zar = [0, [17, 0, [12, 41, 0]], r(h0)], Qar = [0, [15, 0], r(C0)], ror = r(Yr), eor = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], nor = r("Flow_ast.Type.Object.CallProperty.value"), tor = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], uor = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], ior = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], xor = [0, [17, 0, [12, 41, 0]], r(h0)], aor = [0, [17, 0, 0], r(z)], oor = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], cor = r(eu), sor = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], vor = [0, [9, 0, 0], r(An)], lor = [0, [17, 0, 0], r(z)], bor = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], por = r(Xr), mor = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _or = r(tr), yor = r(Z0), dor = r(nr), hor = [0, [17, 0, 0], r(z)], kor = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], wor = [0, [15, 0], r(C0)], Eor = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Sor = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], gor = [0, [17, 0, [12, 41, 0]], r(h0)], For = [0, [15, 0], r(C0)], Tor = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Oor = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Ior = [0, [17, 0, [12, 41, 0]], r(h0)], Aor = [0, [15, 0], r(C0)], Nor = r(Yr), Cor = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Por = r("Flow_ast.Type.Object.Indexer.id"), Dor = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Lor = r(tr), Ror = r(Z0), jor = r(nr), Gor = [0, [17, 0, 0], r(z)], Mor = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Bor = r(ui), qor = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Uor = [0, [17, 0, 0], r(z)], Hor = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Xor = r(Bn), Yor = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Vor = [0, [17, 0, 0], r(z)], zor = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Kor = r(eu), Wor = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Jor = [0, [9, 0, 0], r(An)], $or = [0, [17, 0, 0], r(z)], Zor = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Qor = r(ou), rcr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ecr = r(tr), ncr = r(Z0), tcr = r(nr), ucr = [0, [17, 0, 0], r(z)], icr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], fcr = r(Xr), xcr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], acr = r(tr), ocr = r(Z0), ccr = r(nr), scr = [0, [17, 0, 0], r(z)], vcr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], lcr = [0, [15, 0], r(C0)], bcr = r(Yr), pcr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], mcr = r("Flow_ast.Type.Object.SpreadProperty.argument"), _cr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ycr = [0, [17, 0, 0], r(z)], dcr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hcr = r(Xr), kcr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], wcr = r(tr), Ecr = r(Z0), Scr = r(nr), gcr = [0, [17, 0, 0], r(z)], Fcr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Tcr = [0, [15, 0], r(C0)], Ocr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Icr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Acr = [0, [17, 0, [12, 41, 0]], r(h0)], Ncr = [0, [15, 0], r(C0)], Ccr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Object.Property.Init"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Object.Property.Init@ ")], Pcr = [0, [17, 0, [12, 41, 0]], r(h0)], Dcr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Object.Property.Get"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Object.Property.Get@ ")], Lcr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Rcr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], jcr = [0, [17, 0, [12, 41, 0]], r(h0)], Gcr = [0, [17, 0, [12, 41, 0]], r(h0)], Mcr = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Object.Property.Set"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Object.Property.Set@ ")], Bcr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], qcr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Ucr = [0, [17, 0, [12, 41, 0]], r(h0)], Hcr = [0, [17, 0, [12, 41, 0]], r(h0)], Xcr = [0, [15, 0], r(C0)], Ycr = r(Yr), Vcr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], zcr = r("Flow_ast.Type.Object.Property.key"), Kcr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Wcr = [0, [17, 0, 0], r(z)], Jcr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], $cr = r(Bn), Zcr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Qcr = [0, [17, 0, 0], r(z)], rsr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], esr = r(Bu), nsr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], tsr = [0, [9, 0, 0], r(An)], usr = [0, [17, 0, 0], r(z)], isr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], fsr = r(eu), xsr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], asr = [0, [9, 0, 0], r(An)], osr = [0, [17, 0, 0], r(z)], csr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ssr = r(Y3), vsr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], lsr = [0, [9, 0, 0], r(An)], bsr = [0, [17, 0, 0], r(z)], psr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], msr = r(xU), _sr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ysr = [0, [9, 0, 0], r(An)], dsr = [0, [17, 0, 0], r(z)], hsr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ksr = r(ou), wsr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Esr = r(tr), Ssr = r(Z0), gsr = r(nr), Fsr = [0, [17, 0, 0], r(z)], Tsr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Osr = r(Xr), Isr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Asr = r(tr), Nsr = r(Z0), Csr = r(nr), Psr = [0, [17, 0, 0], r(z)], Dsr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Lsr = [0, [15, 0], r(C0)], Rsr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], jsr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Gsr = [0, [17, 0, [12, 41, 0]], r(h0)], Msr = [0, [15, 0], r(C0)], Bsr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], qsr = r("Flow_ast.Type.OptionalIndexedAccess.indexed_access"), Usr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Hsr = [0, [17, 0, 0], r(z)], Xsr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ysr = r(Bu), Vsr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], zsr = [0, [9, 0, 0], r(An)], Ksr = [0, [17, 0, 0], r(z)], Wsr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Jsr = [0, [15, 0], r(C0)], $sr = r(Yr), Zsr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Qsr = r("Flow_ast.Type.IndexedAccess._object"), r1r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], e1r = [0, [17, 0, 0], r(z)], n1r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], t1r = r("index"), u1r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], i1r = [0, [17, 0, 0], r(z)], f1r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], x1r = r(Xr), a1r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], o1r = r(tr), c1r = r(Z0), s1r = r(nr), v1r = [0, [17, 0, 0], r(z)], l1r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], b1r = [0, [15, 0], r(C0)], p1r = r(Yr), m1r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], _1r = r("Flow_ast.Type.Generic.id"), y1r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], d1r = [0, [17, 0, 0], r(z)], h1r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], k1r = r(Z2), w1r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], E1r = r(tr), S1r = r(Z0), g1r = r(nr), F1r = [0, [17, 0, 0], r(z)], T1r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], O1r = r(Xr), I1r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], A1r = r(tr), N1r = r(Z0), C1r = r(nr), P1r = [0, [17, 0, 0], r(z)], D1r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], L1r = [0, [15, 0], r(C0)], R1r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], j1r = r("Flow_ast.Type.Generic.Identifier.qualification"), G1r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], M1r = [0, [17, 0, 0], r(z)], B1r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], q1r = r(mt), U1r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], H1r = [0, [17, 0, 0], r(z)], X1r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Y1r = [0, [15, 0], r(C0)], V1r = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], z1r = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], K1r = [0, [17, 0, [12, 41, 0]], r(h0)], W1r = [0, [15, 0], r(C0)], J1r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Generic.Identifier.Unqualified"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Generic.Identifier.Unqualified@ ")], $1r = [0, [17, 0, [12, 41, 0]], r(h0)], Z1r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Type.Generic.Identifier.Qualified"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Type.Generic.Identifier.Qualified@ ")], Q1r = [0, [17, 0, [12, 41, 0]], r(h0)], rvr = [0, [15, 0], r(C0)], evr = r(Yr), nvr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], tvr = r("Flow_ast.Type.Function.tparams"), uvr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ivr = r(tr), fvr = r(Z0), xvr = r(nr), avr = [0, [17, 0, 0], r(z)], ovr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], cvr = r(Ct), svr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], vvr = [0, [17, 0, 0], r(z)], lvr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], bvr = r(Wu), pvr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], mvr = [0, [17, 0, 0], r(z)], _vr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], yvr = r(Xr), dvr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], hvr = r(tr), kvr = r(Z0), wvr = r(nr), Evr = [0, [17, 0, 0], r(z)], Svr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], gvr = [0, [15, 0], r(C0)], Fvr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Tvr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], Ovr = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Ivr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Avr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Nvr = r("Flow_ast.Type.Function.Params.this_"), Cvr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Pvr = r(tr), Dvr = r(Z0), Lvr = r(nr), Rvr = [0, [17, 0, 0], r(z)], jvr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Gvr = r(Ct), Mvr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Bvr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], qvr = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], Uvr = [0, [17, 0, 0], r(z)], Hvr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Xvr = r(ch), Yvr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Vvr = r(tr), zvr = r(Z0), Kvr = r(nr), Wvr = [0, [17, 0, 0], r(z)], Jvr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], $vr = r(Xr), Zvr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Qvr = r(tr), r2r = r(Z0), e2r = r(nr), n2r = [0, [17, 0, 0], r(z)], t2r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], u2r = [0, [15, 0], r(C0)], i2r = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], f2r = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], x2r = [0, [17, 0, [12, 41, 0]], r(h0)], a2r = [0, [15, 0], r(C0)], o2r = r(Yr), c2r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], s2r = r("Flow_ast.Type.Function.ThisParam.annot"), v2r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], l2r = [0, [17, 0, 0], r(z)], b2r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], p2r = r(Xr), m2r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _2r = r(tr), y2r = r(Z0), d2r = r(nr), h2r = [0, [17, 0, 0], r(z)], k2r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], w2r = [0, [15, 0], r(C0)], E2r = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], S2r = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], g2r = [0, [17, 0, [12, 41, 0]], r(h0)], F2r = [0, [15, 0], r(C0)], T2r = r(Yr), O2r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], I2r = r("Flow_ast.Type.Function.RestParam.argument"), A2r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], N2r = [0, [17, 0, 0], r(z)], C2r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], P2r = r(Xr), D2r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], L2r = r(tr), R2r = r(Z0), j2r = r(nr), G2r = [0, [17, 0, 0], r(z)], M2r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], B2r = [0, [15, 0], r(C0)], q2r = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], U2r = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], H2r = [0, [17, 0, [12, 41, 0]], r(h0)], X2r = [0, [15, 0], r(C0)], Y2r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], V2r = r("Flow_ast.Type.Function.Param.name"), z2r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], K2r = r(tr), W2r = r(Z0), J2r = r(nr), $2r = [0, [17, 0, 0], r(z)], Z2r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Q2r = r(rs), rlr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], elr = [0, [17, 0, 0], r(z)], nlr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], tlr = r(Bu), ulr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ilr = [0, [9, 0, 0], r(An)], flr = [0, [17, 0, 0], r(z)], xlr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], alr = [0, [15, 0], r(C0)], olr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], clr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], slr = [0, [17, 0, [12, 41, 0]], r(h0)], vlr = [0, [15, 0], r(C0)], llr = r(Yr), blr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], plr = r("Flow_ast.ComputedKey.expression"), mlr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _lr = [0, [17, 0, 0], r(z)], ylr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], dlr = r(Xr), hlr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], klr = r(tr), wlr = r(Z0), Elr = r(nr), Slr = [0, [17, 0, 0], r(z)], glr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Flr = [0, [15, 0], r(C0)], Tlr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Olr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Ilr = [0, [17, 0, [12, 41, 0]], r(h0)], Alr = [0, [15, 0], r(C0)], Nlr = r(Yr), Clr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Plr = r("Flow_ast.Variance.kind"), Dlr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Llr = [0, [17, 0, 0], r(z)], Rlr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], jlr = r(Xr), Glr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Mlr = r(tr), Blr = r(Z0), qlr = r(nr), Ulr = [0, [17, 0, 0], r(z)], Hlr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Xlr = [0, [15, 0], r(C0)], Ylr = r("Flow_ast.Variance.Minus"), Vlr = r("Flow_ast.Variance.Plus"), zlr = [0, [15, 0], r(C0)], Klr = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], Wlr = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], Jlr = [0, [17, 0, [12, 41, 0]], r(h0)], $lr = [0, [15, 0], r(C0)], Zlr = r(Yr), Qlr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], rbr = r("Flow_ast.BooleanLiteral.value"), ebr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], nbr = [0, [9, 0, 0], r(An)], tbr = [0, [17, 0, 0], r(z)], ubr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], ibr = r(Xr), fbr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], xbr = r(tr), abr = r(Z0), obr = r(nr), cbr = [0, [17, 0, 0], r(z)], sbr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], vbr = [0, [15, 0], r(C0)], lbr = r(Yr), bbr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], pbr = r("Flow_ast.BigIntLiteral.approx_value"), mbr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], _br = [0, [8, [0, 0, 5], 0, 0, 0], r(e8)], ybr = [0, [17, 0, 0], r(z)], dbr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], hbr = r(a1), kbr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], wbr = [0, [3, 0, 0], r(Yt)], Ebr = [0, [17, 0, 0], r(z)], Sbr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], gbr = r(Xr), Fbr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Tbr = r(tr), Obr = r(Z0), Ibr = r(nr), Abr = [0, [17, 0, 0], r(z)], Nbr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Cbr = [0, [15, 0], r(C0)], Pbr = r(Yr), Dbr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Lbr = r("Flow_ast.NumberLiteral.value"), Rbr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], jbr = [0, [8, [0, 0, 5], 0, 0, 0], r(e8)], Gbr = [0, [17, 0, 0], r(z)], Mbr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Bbr = r(o7), qbr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], Ubr = [0, [3, 0, 0], r(Yt)], Hbr = [0, [17, 0, 0], r(z)], Xbr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Ybr = r(Xr), Vbr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], zbr = r(tr), Kbr = r(Z0), Wbr = r(nr), Jbr = [0, [17, 0, 0], r(z)], $br = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Zbr = [0, [15, 0], r(C0)], Qbr = r(Yr), r4r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], e4r = r("Flow_ast.StringLiteral.value"), n4r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], t4r = [0, [3, 0, 0], r(Yt)], u4r = [0, [17, 0, 0], r(z)], i4r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], f4r = r(o7), x4r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], a4r = [0, [3, 0, 0], r(Yt)], o4r = [0, [17, 0, 0], r(z)], c4r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], s4r = r(Xr), v4r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], l4r = r(tr), b4r = r(Z0), p4r = r(nr), m4r = [0, [17, 0, 0], r(z)], _4r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], y4r = [0, [15, 0], r(C0)], d4r = r("Flow_ast.Literal.Null"), h4r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Literal.String"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Literal.String@ ")], k4r = [0, [3, 0, 0], r(Yt)], w4r = [0, [17, 0, [12, 41, 0]], r(h0)], E4r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Literal.Boolean"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Literal.Boolean@ ")], S4r = [0, [9, 0, 0], r(An)], g4r = [0, [17, 0, [12, 41, 0]], r(h0)], F4r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Literal.Number"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Literal.Number@ ")], T4r = [0, [8, [0, 0, 5], 0, 0, 0], r(e8)], O4r = [0, [17, 0, [12, 41, 0]], r(h0)], I4r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Literal.BigInt"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Literal.BigInt@ ")], A4r = [0, [8, [0, 0, 5], 0, 0, 0], r(e8)], N4r = [0, [17, 0, [12, 41, 0]], r(h0)], C4r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("Flow_ast.Literal.RegExp"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>Flow_ast.Literal.RegExp@ ")], P4r = [0, [17, 0, [12, 41, 0]], r(h0)], D4r = [0, [15, 0], r(C0)], L4r = r(Yr), R4r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], j4r = r("Flow_ast.Literal.value"), G4r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], M4r = [0, [17, 0, 0], r(z)], B4r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], q4r = r(o7), U4r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], H4r = [0, [3, 0, 0], r(Yt)], X4r = [0, [17, 0, 0], r(z)], Y4r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], V4r = r(Xr), z4r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], K4r = r(tr), W4r = r(Z0), J4r = r(nr), $4r = [0, [17, 0, 0], r(z)], Z4r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Q4r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], r8r = r("Flow_ast.Literal.RegExp.pattern"), e8r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], n8r = [0, [3, 0, 0], r(Yt)], t8r = [0, [17, 0, 0], r(z)], u8r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], i8r = r(UX), f8r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], x8r = [0, [3, 0, 0], r(Yt)], a8r = [0, [17, 0, 0], r(z)], o8r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], c8r = [0, [15, 0], r(C0)], s8r = [0, [15, 0], r(C0)], v8r = r(Yr), l8r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], b8r = r("Flow_ast.PrivateName.name"), p8r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], m8r = [0, [3, 0, 0], r(Yt)], _8r = [0, [17, 0, 0], r(z)], y8r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], d8r = r(Xr), h8r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], k8r = r(tr), w8r = r(Z0), E8r = r(nr), S8r = [0, [17, 0, 0], r(z)], g8r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], F8r = [0, [15, 0], r(C0)], T8r = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], O8r = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], I8r = [0, [17, 0, [12, 41, 0]], r(h0)], A8r = [0, [15, 0], r(C0)], N8r = r(Yr), C8r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], P8r = r("Flow_ast.Identifier.name"), D8r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], L8r = [0, [3, 0, 0], r(Yt)], R8r = [0, [17, 0, 0], r(z)], j8r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], G8r = r(Xr), M8r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], B8r = r(tr), q8r = r(Z0), U8r = r(nr), H8r = [0, [17, 0, 0], r(z)], X8r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], Y8r = [0, [15, 0], r(C0)], V8r = [0, [12, 40, [18, [1, [0, 0, r(C)]], 0]], r(Zr)], z8r = [0, [12, 44, [17, [0, r(v), 1, 0], 0]], r(zr)], K8r = [0, [17, 0, [12, 41, 0]], r(h0)], W8r = [0, [15, 0], r(C0)], J8r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], $8r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], Z8r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], Q8r = r("Flow_ast.Syntax.leading"), r3r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], e3r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], n3r = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], t3r = [0, [17, 0, 0], r(z)], u3r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], i3r = r("trailing"), f3r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], x3r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, 91, 0]], r(Ye)], a3r = [0, [17, [0, r(Pe), 0, 0], [12, 93, [17, 0, 0]]], r(Xe)], o3r = [0, [17, 0, 0], r(z)], c3r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], s3r = r("internal"), v3r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], l3r = [0, [17, 0, 0], r(z)], b3r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], p3r = [0, [0, 0, 0]], m3r = [0, r(Eu), 21, 2], _3r = [0, [0, 0, 0, 0, 0]], y3r = [0, r(Eu), 32, 2], d3r = [0, [0, 0, 0, 0, 0]], h3r = [0, r(Eu), 43, 2], k3r = [0, [0, [0, [0, 0, 0]], 0, 0, 0, 0]], w3r = [0, r(Eu), 70, 2], E3r = [0, [0, 0, 0]], S3r = [0, r(Eu), 80, 2], g3r = [0, [0, 0, 0]], F3r = [0, r(Eu), 90, 2], T3r = [0, [0, 0, 0]], O3r = [0, r(Eu), L7, 2], I3r = [0, [0, 0, 0]], A3r = [0, r(Eu), Ht, 2], N3r = [0, [0, 0, 0, 0, 0, 0, 0]], C3r = [0, r(Eu), br, 2], P3r = [0, [0, 0, 0, 0, 0]], D3r = [0, r(Eu), QH, 2], L3r = [0, [0, [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0, 0, 0, 0, 0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]]]], R3r = [0, r(Eu), 485, 2], j3r = [0, [0, [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0, 0, 0]], G3r = [0, r(Eu), YX, 2], M3r = [0, [0, [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0]], B3r = [0, r(Eu), 1460, 2], q3r = [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0, [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0, 0, 0, 0, 0]], U3r = [0, r(Eu), 1604, 2], H3r = [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0]], X3r = [0, r(Eu), 1689, 2], Y3r = [0, [0, 0, 0, 0, 0, 0, 0]], V3r = [0, r(Eu), 1705, 2], z3r = [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0]], K3r = [0, r(Eu), 1828, 2], W3r = [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], J3r = [0, r(Eu), 1895, 2], $3r = [0, [0, 0, 0, 0, 0]], Z3r = [0, r(Eu), 1907, 2], Q3r = [0, [0, 0, 0]], r6r = [0, [0, 0, 0, 0, 0]], e6r = [0, [0, 0, 0, 0, 0]], n6r = [0, [0, [0, [0, 0, 0]], 0, 0, 0, 0]], t6r = [0, [0, 0, 0]], u6r = [0, [0, 0, 0]], i6r = [0, [0, 0, 0]], f6r = [0, [0, 0, 0]], x6r = [0, [0, 0, 0, 0, 0, 0, 0]], a6r = [0, [0, 0, 0, 0, 0]], o6r = [0, [0, [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0, 0, 0, 0, 0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]]]], c6r = [0, [0, [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0, 0, 0]], s6r = [0, [0, [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0]], v6r = [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0, [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0, 0, 0, 0, 0]], l6r = [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0]], 0, 0, 0, 0]], b6r = [0, [0, 0, 0, 0, 0, 0, 0]], p6r = [0, [0, [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0]], m6r = [0, [0, [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], [0, [0, 0, 0, 0, 0]], 0, 0, 0, 0]], _6r = [0, [0, 0, 0, 0, 0]], y6r = [0, 1], d6r = [0, 0], h6r = [0, 2], k6r = [0, 0], w6r = [0, 1], E6r = [0, 1], S6r = [0, 1], g6r = [0, 1], F6r = [0, 1], T6r = [0, 0, 0], O6r = [0, 0, 0], I6r = [0, r(wu), r(zx), r(ia), r(Ia), r(ou), r(wc), r(fx), r(cc), r(Bf), r(Oa), r(Da), r(tc), r(Ca), r(Sf), r(Zo), r(fa), r(Dx), r(Of), r(sx), r(Ao), r(Hf), r(ja), r(Jo), r(Vx), r(Zx), r(dx), r(oa), r(xo), r(ua), r(g7), r(Aa), r(Lf), r(Ea), r(Qx), r(Zf), r(Ha), r(ha), r(P7), r(hc), r(Po), r(Fx), r(Kx), r(df), r(Fc), r(qf), r(vx), r(Wu), r(_x), r(mo), r(Le), r(Qu), r(Qo), r(Va), r(So), r(ra), r(lc), r(co), r(uo), r(Df), r(qa), r(zf), r($f), r(Ec), r(bc), r(lo), r(mf), r(Ka), r(rx), r(nx), r(pi), r(ro), r(Ba), r(wf), r(Ko), r(Ya), r(pc), r(ac), r(Qa), r(ca), r(xx), r(Pf), r(xf), r(Vf), r(tx), r(_o), r(wo), r(Jf), r(uc), r(Tf), r(jx), r(Ua), r(mx), r(hf), r(Af), r(yc), r(ur), r(dc), r($x), r(Xx), r(Lo), r(Wx), r(ux), r(Rx), r(ko), r(Lx), r(kc), r(bx), r($a), r(Sc), r(Ro), r(Wa), r(nc), r(nf), r(Px), r(sa), r(Ax), r(xa), r(Ga), r(Na), r(_c), r(Cx), r(af), r(cf), r(ex), r(Ja), r(ho), r(sc), r(Gf), r(Wo), r(na), r(wa), r(sf), r(r7), r(Co), r(Ox), r(Do), r(Yx), r(ma), r(gc), r(qu), r(zo), r(fo), r(_f), r(_i), r(Kf), r(mc), r($o), r(j7), r(yx), r(po), r(bf), r(Uo), r(Ex), r(Ma), r(yo), r(ix), r(uf), r(jf), r(Tc), r(Nx), r(kx), r(No), r(Ic), r(pa), r(ga), r(vi), r(xc), r(hx), r(jo), r(Vo), r(Oc), r(qx), r(Eo), r(Uf), r(Ff), r(ta), r(Ix), r(Au), r(no), r(io), r(ec), r(lf), r(Fo), r(ba), r(Cf), r(Mx), r(rc), r(Nf), r(Mf), r(Ux), r(Xa), r(Hx), r(vo), r(eo), r(bo), r(s7), r(ka), r(Go), r(Sx), r(Ta), r(la), r(to), r(Wf), r(Mo), r(Io), r(ox), r(O7), r(A7), r(Za), r(ao), r(Qf), r(da), r(kf), r(Fa), r(ax), r(Tx), r(Xf), r(Bo), r(Ef), r(ff), r(To), r(Rf), r(ic), r(yf), r(Ho), r(oo), r(Xo), r(gf), r(ef), r(lx), r(_a), r(px), r(If), r(I7), r(Yo), r(ut), r(Bx), r(of), r(pf), r(Jx), r(Yf), r(za), r(so), r(go), r(va), r(Gx), r(J4)], A6r = [0, r(df), r(ex), r(yo), r(Kf), r(If), r(zf), r(Ua), r(tx), r(Px), r(wa), r(Jo), r(P7), r(Ya), r(no), r(ic), r(_c), r(mx), r(af), r(eo), r(Ux), r(zx), r(vi), r(kc), r(jx), r($o), r(vo), r(Af), r(_i), r(Ia), r(qx), r(uo), r(Wf), r(lx), r(ix), r(ef), r(Ga), r(Cf), r(po), r(bc), r(xc), r(ha), r(Jx), r(_o), r(fo), r(Fx), r(bo), r(Lx), r(hf), r(ff), r(Fa), r(ro), r(So), r(Vf), r(Va), r(Wa), r(Xf), r(ac), r(Qu), r(Pf), r(Uo), r(yc), r(sa), r(Na), r(mc), r(ux), r(Za), r(Zx), r(Nf), r(xf), r(nc), r(Qf), r(Rx), r(Ma), r(co), r(go), r(la), r(Fo), r($x), r(nx), r(va), r(_a), r(vx), r(ou), r(Qo), r(fa), r(zo), r(pf), r(ga), r(ua), r(sc), r(Rf), r(uc), r(Ha), r(s7), r(Vo), r(Vx), r(wu), r(xo), r(Io), r(tc), r(Ka), r(_x), r(Da), r(kf), r(Mo), r(cc), r(Cx), r(ra), r(na), r(Xa), r(Ff), r(pc), r(io), r(ko), r(mf), r(Eo), r(Of), r(oa), r(wc), r(Fc), r(Dx), r(Oa), r(Bo), r(hx), r(ax), r(Lo), r(Ex), r(Bf), r(da), r(Tf), r($a), r(Yf), r(Xx), r(oo), r(To), r(Co), r(lo), r(Ba), r(Sc), r(dc), r(qu), r(Wu), r(Yo), r(Zo), r(sx), r(hc), r(Ec), r(g7), r(O7), r(_f), r(Ko), r(Ix), r(cf), r(pi), r(Nx), r(Hx), r(Ox), r(Tx), r(uf), r(Wx), r(Ja), r(j7), r(bf), r(Sf), r(Mf), r(Le), r(Ic), r(ma), r(rc), r(lf), r(Jf), r(qf), r(Do), r(ca), r(Df), r(dx), r(xx), r(Ao), r(px), r(Ta), r(Xo), r(to), r(Bx), r(Gf), r(Zf), r(yx), r(mo), r(gc), r(Ho), r(wo), r(xa), r(Ef), r(sf), r(ka), r(ja), r(Gx), r(fx), r(gf), r(Hf), r(Go), r(Ax), r(ho), r(ao), r(bx), r(qa), r(Wo), r(Uf), r(Ro), r(Ea), r(za), r($f), r(of), r(Au), r(rx), r(ta), r(kx), r(No), r(Kx), r(A7), r(jf), r(lc), r(ba), r(Sx), r(Lf), r(Qx), r(Po), r(pa), r(ec), r(Ca), r(jo), r(wf), r(ut), r(Yx), r(yf), r(nf), r(Qa), r(Tc), r(ox), r(Mx), r(I7), r(so), r(r7), r(ia), r(Oc), r(Aa), r(ur)], N6r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("File_key.LibFile"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>File_key.LibFile@ ")], C6r = [0, [3, 0, 0], r(Yt)], P6r = [0, [17, 0, [12, 41, 0]], r(h0)], D6r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("File_key.SourceFile"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>File_key.SourceFile@ ")], L6r = [0, [3, 0, 0], r(Yt)], R6r = [0, [17, 0, [12, 41, 0]], r(h0)], j6r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("File_key.JsonFile"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>File_key.JsonFile@ ")], G6r = [0, [3, 0, 0], r(Yt)], M6r = [0, [17, 0, [12, 41, 0]], r(h0)], B6r = [0, [12, 40, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r("File_key.ResourceFile"), [17, [0, r(v), 1, 0], 0]]]], r("(@[<2>File_key.ResourceFile@ ")], q6r = [0, [3, 0, 0], r(Yt)], U6r = [0, [17, 0, [12, 41, 0]], r(h0)], H6r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], X6r = r("Loc.line"), Y6r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], V6r = [0, [4, 0, 0, 0, 0], r(N2)], z6r = [0, [17, 0, 0], r(z)], K6r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], W6r = r(I2), J6r = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], $6r = [0, [4, 0, 0, 0, 0], r(N2)], Z6r = [0, [17, 0, 0], r(z)], Q6r = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], rpr = [0, [15, 0], r(C0)], epr = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [11, r(wr), 0]], r(kr)], npr = r("Loc.source"), tpr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], upr = r(tr), ipr = r(Z0), fpr = r(nr), xpr = [0, [17, 0, 0], r(z)], apr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], opr = r(S7), cpr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], spr = [0, [17, 0, 0], r(z)], vpr = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], lpr = r("_end"), bpr = [0, [18, [1, [0, 0, r(C)]], [2, 0, [11, r(J), [17, [0, r(v), 1, 0], 0]]]], r(W)], ppr = [0, [17, 0, 0], r(z)], mpr = [0, [17, [0, r(v), 1, 0], [12, br, [17, 0, 0]]], r(Er)], _pr = [0, r(Gx), r(va), r(go), r(so), r(za), r(Yf), r(Jx), r(pf), r(of), r(Bx), r(ut), r(Yo), r(I7), r(If), r(px), r(_a), r(lx), r(ef), r(gf), r(Xo), r(oo), r(Ho), r(yf), r(ic), r(Rf), r(To), r(ff), r(Ef), r(Bo), r(Xf), r(Tx), r(ax), r(Fa), r(kf), r(da), r(Qf), r(ao), r(Za), r(A7), r(O7), r(ox), r(Io), r(Mo), r(Wf), r(to), r(la), r(Ta), r(Sx), r(Go), r(ka), r(s7), r(bo), r(eo), r(vo), r(Hx), r(Xa), r(Ux), r(Mf), r(Nf), r(rc), r(Mx), r(Cf), r(ba), r(Fo), r(lf), r(ec), r(io), r(no), r(Au), r(Ix), r(ta), r(Ff), r(Uf), r(Eo), r(qx), r(Oc), r(Vo), r(jo), r(hx), r(xc), r(vi), r(ga), r(pa), r(Ic), r(No), r(kx), r(Nx), r(Tc), r(jf), r(uf), r(ix), r(yo), r(Ma), r(Ex), r(Uo), r(bf), r(po), r(yx), r(j7), r($o), r(mc), r(Kf), r(_i), r(_f), r(fo), r(zo), r(qu), r(gc), r(ma), r(Yx), r(Do), r(Ox), r(Co), r(r7), r(sf), r(wa), r(na), r(Wo), r(Gf), r(sc), r(ho), r(Ja), r(ex), r(cf), r(af), r(Cx), r(_c), r(Na), r(Ga), r(xa), r(Ax), r(sa), r(Px), r(nf), r(nc), r(Wa), r(Ro), r(Sc), r($a), r(bx), r(kc), r(Lx), r(ko), r(Rx), r(ux), r(Wx), r(Lo), r(Xx), r($x), r(dc), r(ur), r(yc), r(Af), r(hf), r(mx), r(Ua), r(jx), r(Tf), r(uc), r(Jf), r(wo), r(_o), r(tx), r(Vf), r(xf), r(Pf), r(xx), r(ca), r(Qa), r(ac), r(pc), r(Ya), r(Ko), r(wf), r(Ba), r(ro), r(pi), r(nx), r(rx), r(Ka), r(mf), r(lo), r(bc), r(Ec), r($f), r(zf), r(qa), r(Df), r(uo), r(co), r(lc), r(ra), r(So), r(Va), r(Qo), r(Qu), r(Le), r(mo), r(_x), r(Wu), r(vx), r(qf), r(Fc), r(df), r(Kx), r(Fx), r(Po), r(hc), r(P7), r(ha), r(Ha), r(Zf), r(Qx), r(Ea), r(Lf), r(Aa), r(g7), r(ua), r(xo), r(oa), r(dx), r(Zx), r(Vx), r(Jo), r(ja), r(Hf), r(Ao), r(sx), r(Of), r(Dx), r(fa), r(Zo), r(Sf), r(Ca), r(tc), r(Da), r(Oa), r(Bf), r(cc), r(fx), r(wc), r(ou), r(Ia), r(ia), r(zx), r(wu)], ypr = [0, r(wu), r(zx), r(ia), r(Ia), r(ou), r(wc), r(fx), r(cc), r(Bf), r(Oa), r(Da), r(tc), r(Ca), r(Sf), r(Zo), r(fa), r(Dx), r(Of), r(sx), r(Ao), r(Hf), r(ja), r(Jo), r(Vx), r(Zx), r(dx), r(oa), r(xo), r(ua), r(g7), r(Aa), r(Lf), r(Ea), r(Qx), r(Zf), r(Ha), r(ha), r(P7), r(hc), r(Po), r(Fx), r(Kx), r(df), r(Fc), r(qf), r(vx), r(Wu), r(_x), r(mo), r(Le), r(Qu), r(Qo), r(Va), r(So), r(ra), r(lc), r(co), r(uo), r(Df), r(qa), r(zf), r($f), r(Ec), r(bc), r(lo), r(mf), r(Ka), r(rx), r(nx), r(pi), r(ro), r(Ba), r(wf), r(Ko), r(Ya), r(pc), r(ac), r(Qa), r(ca), r(xx), r(Pf), r(xf), r(Vf), r(tx), r(_o), r(wo), r(Jf), r(uc), r(Tf), r(jx), r(Ua), r(mx), r(hf), r(Af), r(yc), r(ur), r(dc), r($x), r(Xx), r(Lo), r(Wx), r(ux), r(Rx), r(ko), r(Lx), r(kc), r(bx), r($a), r(Sc), r(Ro), r(Wa), r(nc), r(nf), r(Px), r(sa), r(Ax), r(xa), r(Ga), r(Na), r(_c), r(Cx), r(af), r(cf), r(ex), r(Ja), r(ho), r(sc), r(Gf), r(Wo), r(na), r(wa), r(sf), r(r7), r(Co), r(Ox), r(Do), r(Yx), r(ma), r(gc), r(qu), r(zo), r(fo), r(_f), r(_i), r(Kf), r(mc), r($o), r(j7), r(yx), r(po), r(bf), r(Uo), r(Ex), r(Ma), r(yo), r(ix), r(uf), r(jf), r(Tc), r(Nx), r(kx), r(No), r(Ic), r(pa), r(ga), r(vi), r(xc), r(hx), r(jo), r(Vo), r(Oc), r(qx), r(Eo), r(Uf), r(Ff), r(ta), r(Ix), r(Au), r(no), r(io), r(ec), r(lf), r(Fo), r(ba), r(Cf), r(Mx), r(rc), r(Nf), r(Mf), r(Ux), r(Xa), r(Hx), r(vo), r(eo), r(bo), r(s7), r(ka), r(Go), r(Sx), r(Ta), r(la), r(to), r(Wf), r(Mo), r(Io), r(ox), r(O7), r(A7), r(Za), r(ao), r(Qf), r(da), r(kf), r(Fa), r(ax), r(Tx), r(Xf), r(Bo), r(Ef), r(ff), r(To), r(Rf), r(ic), r(yf), r(Ho), r(oo), r(Xo), r(gf), r(ef), r(lx), r(_a), r(px), r(If), r(I7), r(Yo), r(ut), r(Bx), r(of), r(pf), r(Jx), r(Yf), r(za), r(so), r(go), r(va), r(Gx), r(J4)], dpr = [0, r(df), r(ex), r(yo), r(Kf), r(If), r(zf), r(Ua), r(tx), r(Px), r(wa), r(Jo), r(P7), r(Ya), r(no), r(ic), r(_c), r(mx), r(af), r(eo), r(Ux), r(zx), r(vi), r(kc), r(jx), r($o), r(vo), r(Af), r(_i), r(Ia), r(qx), r(uo), r(Wf), r(lx), r(ix), r(ef), r(Ga), r(Cf), r(po), r(bc), r(xc), r(ha), r(Jx), r(_o), r(fo), r(Fx), r(bo), r(Lx), r(hf), r(ff), r(Fa), r(ro), r(So), r(Vf), r(Va), r(Wa), r(Xf), r(ac), r(Qu), r(Pf), r(Uo), r(yc), r(sa), r(Na), r(mc), r(ux), r(Za), r(Zx), r(Nf), r(xf), r(nc), r(Qf), r(Rx), r(Ma), r(co), r(go), r(la), r(Fo), r($x), r(nx), r(va), r(_a), r(vx), r(ou), r(Qo), r(fa), r(zo), r(pf), r(ga), r(ua), r(sc), r(Rf), r(uc), r(Ha), r(s7), r(Vo), r(Vx), r(wu), r(xo), r(Io), r(tc), r(Ka), r(_x), r(Da), r(kf), r(Mo), r(cc), r(Cx), r(ra), r(na), r(Xa), r(Ff), r(pc), r(io), r(ko), r(mf), r(Eo), r(Of), r(oa), r(wc), r(Fc), r(Dx), r(Oa), r(Bo), r(hx), r(ax), r(Lo), r(Ex), r(Bf), r(da), r(Tf), r($a), r(Yf), r(Xx), r(oo), r(To), r(Co), r(lo), r(Ba), r(Sc), r(dc), r(qu), r(Wu), r(Yo), r(Zo), r(sx), r(hc), r(Ec), r(g7), r(O7), r(_f), r(Ko), r(Ix), r(cf), r(pi), r(Nx), r(Hx), r(Ox), r(Tx), r(uf), r(Wx), r(Ja), r(j7), r(bf), r(Sf), r(Mf), r(Le), r(Ic), r(ma), r(rc), r(lf), r(Jf), r(qf), r(Do), r(ca), r(Df), r(dx), r(xx), r(Ao), r(px), r(Ta), r(Xo), r(to), r(Bx), r(Gf), r(Zf), r(yx), r(mo), r(gc), r(Ho), r(wo), r(xa), r(Ef), r(sf), r(ka), r(ja), r(Gx), r(fx), r(gf), r(Hf), r(Go), r(Ax), r(ho), r(ao), r(bx), r(qa), r(Wo), r(Uf), r(Ro), r(Ea), r(za), r($f), r(of), r(Au), r(rx), r(ta), r(kx), r(No), r(Kx), r(A7), r(jf), r(lc), r(ba), r(Sx), r(Lf), r(Qx), r(Po), r(pa), r(ec), r(Ca), r(jo), r(wf), r(ut), r(Yx), r(yf), r(nf), r(Qa), r(Tc), r(ox), r(Mx), r(I7), r(so), r(r7), r(ia), r(Oc), r(Aa), r(ur)], hpr = r(yV), kpr = r(UY), wpr = r(GX), Epr = r(ZY), Spr = r(g3), gpr = r(tX), Fpr = r(cv), Tpr = r(PU), Opr = r(_Y), Ipr = r(wX), Apr = r(mX), Npr = r(as), Cpr = r(Oo), Ppr = r(zU), Dpr = r(rX), Lpr = r(Zu), Rpr = r(WY), jpr = r(PH), Gpr = r(A6), Mpr = r(Bh), Bpr = r(R2), qpr = r(j2), Upr = r(rH), Hpr = r(YU), Xpr = r(xY), Ypr = r(vX), Vpr = r(yH), zpr = r(SX), Kpr = r(vU), Wpr = r(ZX), Jpr = r(bX), $pr = r(dH), Zpr = r(TH), Qpr = r(WH), r5r = r(iV), e5r = r(LU), n5r = r(aX), t5r = r("Set.remove_min_elt"), u5r = [0, [12, 59, [17, [0, r(v), 1, 0], 0]], r(o0)], i5r = [0, [18, [1, [0, [11, r(d), 0], r(d)]], [12, us, 0]], r("@[<2>{")], f5r = [0, [12, 32, 0], r(bi)], x5r = [0, [12, 32, 0], r(bi)], a5r = [0, [17, [0, r(Pe), 0, 0], [12, br, [17, 0, 0]]], r("@,}@]")], o5r = [0, r("src/hack_forked/utils/collections/flow_set.ml"), 363, 14], c5r = [0, [0, 36, 37], [0, 48, 58], [0, 65, 91], [0, 95, 96], [0, 97, us], [0, Xg, yg], [0, Ai, Kg], [0, mS, wk], [0, dh, iw], [0, at, cT], [0, d6, jw], [0, wt, 706], [0, iX, 722], [0, 736, 741], [0, 748, 749], [0, 750, 751], [0, 768, 885], [0, 886, 888], [0, 890, 894], [0, 895, 896], [0, 902, 907], [0, 908, tY], [0, 910, 930], [0, zX, 1014], [0, 1015, 1154], [0, 1155, 1160], [0, 1162, jU], [0, 1329, 1367], [0, 1369, 1370], [0, 1376, 1417], [0, 1425, 1470], [0, 1471, 1472], [0, 1473, 1475], [0, 1476, 1478], [0, 1479, 1480], [0, 1488, 1515], [0, 1519, 1523], [0, 1552, 1563], [0, 1568, 1642], [0, 1646, 1748], [0, 1749, 1757], [0, 1759, 1769], [0, 1770, 1789], [0, 1791, 1792], [0, 1808, 1867], [0, 1869, 1970], [0, 1984, 2038], [0, 2042, 2043], [0, 2045, 2046], [0, Vd, 2094], [0, 2112, 2140], [0, 2144, 2155], [0, 2208, 2229], [0, 2230, 2238], [0, 2259, 2274], [0, 2275, 2404], [0, 2406, 2416], [0, 2417, 2436], [0, 2437, 2445], [0, 2447, 2449], [0, 2451, 2473], [0, 2474, 2481], [0, 2482, 2483], [0, 2486, 2490], [0, 2492, 2501], [0, 2503, 2505], [0, 2507, 2511], [0, 2519, 2520], [0, 2524, 2526], [0, 2527, 2532], [0, 2534, 2546], [0, 2556, 2557], [0, 2558, 2559], [0, 2561, 2564], [0, 2565, 2571], [0, 2575, 2577], [0, 2579, 2601], [0, 2602, 2609], [0, 2610, 2612], [0, 2613, 2615], [0, 2616, 2618], [0, 2620, 2621], [0, 2622, 2627], [0, 2631, 2633], [0, 2635, 2638], [0, 2641, 2642], [0, 2649, 2653], [0, 2654, 2655], [0, 2662, 2678], [0, 2689, 2692], [0, 2693, 2702], [0, 2703, 2706], [0, 2707, 2729], [0, 2730, 2737], [0, 2738, 2740], [0, 2741, 2746], [0, 2748, 2758], [0, 2759, 2762], [0, 2763, 2766], [0, 2768, 2769], [0, 2784, 2788], [0, 2790, 2800], [0, 2809, 2816], [0, 2817, 2820], [0, 2821, 2829], [0, 2831, 2833], [0, 2835, 2857], [0, 2858, 2865], [0, 2866, 2868], [0, 2869, 2874], [0, 2876, 2885], [0, 2887, 2889], [0, 2891, 2894], [0, 2902, 2904], [0, 2908, 2910], [0, 2911, 2916], [0, 2918, 2928], [0, 2929, 2930], [0, 2946, 2948], [0, 2949, 2955], [0, 2958, 2961], [0, 2962, 2966], [0, 2969, 2971], [0, 2972, 2973], [0, 2974, 2976], [0, 2979, 2981], [0, 2984, 2987], [0, 2990, 3002], [0, 3006, 3011], [0, 3014, 3017], [0, 3018, 3022], [0, 3024, 3025], [0, 3031, 3032], [0, 3046, 3056], [0, 3072, 3085], [0, 3086, 3089], [0, 3090, 3113], [0, 3114, 3130], [0, 3133, 3141], [0, 3142, 3145], [0, 3146, 3150], [0, 3157, 3159], [0, 3160, 3163], [0, 3168, 3172], [0, 3174, 3184], [0, 3200, 3204], [0, 3205, 3213], [0, 3214, 3217], [0, 3218, 3241], [0, 3242, 3252], [0, 3253, 3258], [0, 3260, 3269], [0, 3270, 3273], [0, 3274, 3278], [0, 3285, 3287], [0, 3294, 3295], [0, 3296, 3300], [0, 3302, 3312], [0, 3313, 3315], [0, 3328, 3332], [0, 3333, 3341], [0, 3342, 3345], [0, 3346, 3397], [0, 3398, 3401], [0, 3402, 3407], [0, 3412, 3416], [0, 3423, 3428], [0, 3430, 3440], [0, 3450, 3456], [0, 3458, 3460], [0, 3461, 3479], [0, 3482, 3506], [0, 3507, 3516], [0, 3517, 3518], [0, 3520, 3527], [0, 3530, 3531], [0, 3535, 3541], [0, 3542, 3543], [0, 3544, 3552], [0, 3558, 3568], [0, 3570, 3572], [0, 3585, 3643], [0, 3648, 3663], [0, 3664, 3674], [0, 3713, 3715], [0, 3716, 3717], [0, 3718, 3723], [0, 3724, 3748], [0, 3749, 3750], [0, 3751, 3774], [0, 3776, 3781], [0, 3782, 3783], [0, 3784, 3790], [0, 3792, 3802], [0, 3804, 3808], [0, 3840, 3841], [0, 3864, 3866], [0, 3872, 3882], [0, 3893, 3894], [0, 3895, 3896], [0, 3897, 3898], [0, 3902, 3912], [0, 3913, 3949], [0, 3953, 3973], [0, 3974, 3992], [0, 3993, 4029], [0, 4038, 4039], [0, _X, 4170], [0, 4176, 4254], [0, 4256, 4294], [0, 4295, 4296], [0, 4301, 4302], [0, 4304, 4347], [0, 4348, 4681], [0, 4682, 4686], [0, 4688, 4695], [0, 4696, 4697], [0, 4698, 4702], [0, 4704, 4745], [0, 4746, 4750], [0, 4752, 4785], [0, 4786, 4790], [0, 4792, 4799], [0, 4800, 4801], [0, 4802, 4806], [0, 4808, 4823], [0, 4824, 4881], [0, 4882, 4886], [0, 4888, 4955], [0, 4957, 4960], [0, 4969, 4978], [0, 4992, 5008], [0, 5024, 5110], [0, 5112, 5118], [0, 5121, 5741], [0, 5743, Ev], [0, 5761, 5787], [0, 5792, 5867], [0, 5870, 5881], [0, 5888, 5901], [0, 5902, 5909], [0, 5920, 5941], [0, 5952, 5972], [0, 5984, 5997], [0, 5998, 6001], [0, 6002, 6004], [0, 6016, 6100], [0, 6103, 6104], [0, 6108, 6110], [0, 6112, 6122], [0, 6155, 6158], [0, 6160, 6170], [0, 6176, 6265], [0, 6272, 6315], [0, 6320, 6390], [0, 6400, 6431], [0, 6432, 6444], [0, 6448, 6460], [0, 6470, 6510], [0, 6512, 6517], [0, 6528, 6572], [0, 6576, 6602], [0, 6608, 6619], [0, 6656, 6684], [0, 6688, 6751], [0, 6752, 6781], [0, 6783, 6794], [0, 6800, 6810], [0, 6823, 6824], [0, 6832, 6846], [0, 6912, 6988], [0, 6992, 7002], [0, 7019, 7028], [0, 7040, 7156], [0, 7168, 7224], [0, 7232, 7242], [0, 7245, 7294], [0, 7296, 7305], [0, 7312, 7355], [0, 7357, 7360], [0, 7376, 7379], [0, 7380, 7419], [0, 7424, 7674], [0, 7675, 7958], [0, 7960, 7966], [0, 7968, 8006], [0, 8008, 8014], [0, 8016, 8024], [0, 8025, 8026], [0, 8027, 8028], [0, 8029, 8030], [0, 8031, 8062], [0, 8064, 8117], [0, 8118, 8125], [0, 8126, 8127], [0, 8130, 8133], [0, 8134, 8141], [0, 8144, 8148], [0, 8150, 8156], [0, 8160, 8173], [0, 8178, 8181], [0, 8182, 8189], [0, FY, _U], [0, 8255, 8257], [0, 8276, 8277], [0, np, 8306], [0, I3, 8320], [0, 8336, 8349], [0, 8400, 8413], [0, 8417, 8418], [0, 8421, 8433], [0, a3, 8451], [0, j3, 8456], [0, 8458, F4], [0, _6, 8470], [0, cU, 8478], [0, u8, Z3], [0, r3, vp], [0, D8, C8], [0, 8490, 8506], [0, 8508, 8512], [0, 8517, 8522], [0, v8, 8527], [0, 8544, 8585], [0, 11264, 11311], [0, 11312, 11359], [0, 11360, 11493], [0, 11499, 11508], [0, 11520, M4], [0, q8, 11560], [0, C3, 11566], [0, 11568, 11624], [0, m4, 11632], [0, D6, 11671], [0, 11680, G4], [0, 11688, K8], [0, 11696, o8], [0, 11704, W8], [0, 11712, K6], [0, 11720, G8], [0, 11728, T6], [0, 11736, 11743], [0, 11744, 11776], [0, 12293, 12296], [0, 12321, O3], [0, 12337, 12342], [0, 12344, 12349], [0, 12353, 12439], [0, 12441, S3], [0, 12449, U4], [0, 12540, 12544], [0, 12549, S8], [0, 12593, 12687], [0, 12704, 12731], [0, 12784, 12800], [0, 13312, 19894], [0, 19968, 40944], [0, 40960, 42125], [0, 42192, 42238], [0, 42240, 42509], [0, 42512, 42540], [0, 42560, 42608], [0, 42612, H3], [0, 42623, 42738], [0, 42775, 42784], [0, 42786, 42889], [0, 42891, 42944], [0, 42946, 42951], [0, T8, 43048], [0, 43072, 43124], [0, 43136, 43206], [0, 43216, 43226], [0, 43232, 43256], [0, t3, y8], [0, 43261, 43310], [0, 43312, 43348], [0, 43360, 43389], [0, 43392, 43457], [0, w8, 43482], [0, 43488, l6], [0, 43520, 43575], [0, 43584, 43598], [0, 43600, 43610], [0, 43616, 43639], [0, bp, 43715], [0, 43739, 43742], [0, 43744, 43760], [0, 43762, 43767], [0, 43777, 43783], [0, 43785, 43791], [0, 43793, 43799], [0, 43808, w6], [0, 43816, X3], [0, 43824, ov], [0, 43868, o3], [0, 43888, 44011], [0, 44012, 44014], [0, 44016, 44026], [0, 44032, 55204], [0, 55216, 55239], [0, 55243, 55292], [0, 63744, 64110], [0, 64112, 64218], [0, 64256, 64263], [0, 64275, 64280], [0, n3, fp], [0, 64298, et], [0, 64312, K3], [0, R6, j4], [0, 64320, U3], [0, 64323, L8], [0, 64326, 64434], [0, 64467, 64830], [0, 64848, 64912], [0, 64914, 64968], [0, 65008, 65020], [0, 65024, 65040], [0, 65056, 65072], [0, 65075, 65077], [0, 65101, 65104], [0, 65136, u3], [0, 65142, 65277], [0, 65296, 65306], [0, 65313, 65339], [0, 65343, r8], [0, 65345, 65371], [0, 65382, 65471], [0, 65474, 65480], [0, 65482, 65488], [0, 65490, 65496], [0, 65498, 65501], [0, ow, ep], [0, 65549, Z8], [0, 65576, K4], [0, 65596, g6], [0, 65599, 65614], [0, 65616, 65630], [0, 65664, 65787], [0, 65856, 65909], [0, 66045, 66046], [0, 66176, 66205], [0, 66208, 66257], [0, 66272, 66273], [0, 66304, 66336], [0, 66349, 66379], [0, 66384, 66427], [0, 66432, 66462], [0, 66464, 66500], [0, 66504, Q3], [0, 66513, 66518], [0, 66560, 66718], [0, 66720, 66730], [0, 66736, 66772], [0, 66776, 66812], [0, 66816, 66856], [0, 66864, 66916], [0, 67072, 67383], [0, 67392, 67414], [0, 67424, 67432], [0, 67584, 67590], [0, op, $4], [0, 67594, m8], [0, 67639, 67641], [0, B6, 67645], [0, 67647, 67670], [0, 67680, 67703], [0, 67712, 67743], [0, 67808, Y8], [0, 67828, 67830], [0, 67840, 67862], [0, 67872, 67898], [0, 67968, 68024], [0, 68030, 68032], [0, E7, 68100], [0, 68101, 68103], [0, 68108, p4], [0, 68117, Q8], [0, 68121, 68150], [0, 68152, 68155], [0, 68159, 68160], [0, 68192, 68221], [0, 68224, 68253], [0, 68288, $6], [0, 68297, 68327], [0, 68352, 68406], [0, 68416, 68438], [0, 68448, 68467], [0, 68480, 68498], [0, 68608, 68681], [0, 68736, 68787], [0, 68800, 68851], [0, 68864, 68904], [0, 68912, 68922], [0, 69376, 69405], [0, $8, 69416], [0, 69424, 69457], [0, 69600, 69623], [0, 69632, 69703], [0, 69734, q3], [0, 69759, 69819], [0, 69840, 69865], [0, 69872, 69882], [0, 69888, 69941], [0, 69942, 69952], [0, _4, T3], [0, 69968, 70004], [0, Y6, 70007], [0, 70016, 70085], [0, 70089, 70093], [0, 70096, h8], [0, f3, 70109], [0, 70144, N8], [0, 70163, 70200], [0, 70206, 70207], [0, 70272, d3], [0, A8, xp], [0, 70282, I8], [0, 70287, s8], [0, 70303, 70313], [0, 70320, 70379], [0, 70384, 70394], [0, 70400, i6], [0, 70405, 70413], [0, 70415, 70417], [0, 70419, x3], [0, 70442, c8], [0, 70450, P4], [0, 70453, 70458], [0, 70459, 70469], [0, 70471, 70473], [0, 70475, 70478], [0, G6, 70481], [0, 70487, 70488], [0, 70493, 70500], [0, 70502, 70509], [0, 70512, 70517], [0, 70656, 70731], [0, 70736, 70746], [0, J6, 70752], [0, 70784, r6], [0, Q6, 70856], [0, 70864, 70874], [0, 71040, 71094], [0, 71096, 71105], [0, 71128, 71134], [0, 71168, 71233], [0, i8, 71237], [0, 71248, 71258], [0, 71296, 71353], [0, 71360, 71370], [0, 71424, 71451], [0, 71453, 71468], [0, 71472, 71482], [0, 71680, 71739], [0, 71840, 71914], [0, 71935, 71936], [0, 72096, 72104], [0, 72106, 72152], [0, 72154, ip], [0, m3, 72165], [0, B8, 72255], [0, 72263, 72264], [0, i3, 72346], [0, D4, 72350], [0, 72384, 72441], [0, 72704, J3], [0, 72714, 72759], [0, 72760, 72769], [0, 72784, 72794], [0, 72818, 72848], [0, 72850, 72872], [0, 72873, 72887], [0, 72960, L3], [0, 72968, h4], [0, 72971, 73015], [0, 73018, 73019], [0, 73020, 73022], [0, 73023, 73032], [0, 73040, 73050], [0, 73056, j6], [0, 73063, h3], [0, 73066, 73103], [0, 73104, 73106], [0, 73107, 73113], [0, 73120, 73130], [0, 73440, 73463], [0, 73728, 74650], [0, 74752, 74863], [0, 74880, 75076], [0, 77824, 78895], [0, 82944, 83527], [0, 92160, 92729], [0, 92736, 92767], [0, 92768, 92778], [0, 92880, 92910], [0, 92912, 92917], [0, 92928, 92983], [0, 92992, 92996], [0, 93008, 93018], [0, 93027, 93048], [0, 93053, 93072], [0, 93760, 93824], [0, 93952, 94027], [0, Q4, 94088], [0, 94095, 94112], [0, 94176, p6], [0, h6, 94180], [0, 94208, 100344], [0, 100352, 101107], [0, 110592, 110879], [0, 110928, 110931], [0, 110948, 110952], [0, 110960, 111356], [0, 113664, 113771], [0, 113776, 113789], [0, 113792, 113801], [0, 113808, 113818], [0, 113821, 113823], [0, 119141, 119146], [0, 119149, 119155], [0, 119163, 119171], [0, 119173, 119180], [0, 119210, 119214], [0, 119362, 119365], [0, 119808, O6], [0, 119894, B3], [0, 119966, 119968], [0, k3, 119971], [0, 119973, 119975], [0, 119977, rp], [0, 119982, b8], [0, b4, M6], [0, 119997, A3], [0, 120005, R4], [0, 120071, 120075], [0, 120077, C6], [0, 120086, lp], [0, 120094, P3], [0, 120123, e6], [0, 120128, q4], [0, M3, 120135], [0, 120138, L6], [0, 120146, 120486], [0, 120488, L4], [0, 120514, z3], [0, 120540, s6], [0, 120572, Y4], [0, 120598, s3], [0, 120630, z4], [0, 120656, E6], [0, 120688, l4], [0, 120714, b6], [0, 120746, w3], [0, 120772, 120780], [0, 120782, 120832], [0, 121344, 121399], [0, 121403, 121453], [0, 121461, 121462], [0, 121476, 121477], [0, 121499, 121504], [0, 121505, 121520], [0, 122880, 122887], [0, 122888, 122905], [0, 122907, 122914], [0, 122915, 122917], [0, 122918, 122923], [0, 123136, 123181], [0, 123184, 123198], [0, 123200, 123210], [0, cp, 123215], [0, 123584, 123642], [0, 124928, 125125], [0, 125136, 125143], [0, 125184, 125260], [0, 125264, 125274], [0, 126464, P6], [0, 126469, $3], [0, 126497, c3], [0, F8, 126501], [0, n8, _8], [0, 126505, v6], [0, 126516, x8], [0, y6, a8], [0, E3, 126524], [0, W3, 126531], [0, R8, H6], [0, g8, t8], [0, v3, B4], [0, 126541, T4], [0, 126545, F6], [0, k8, 126549], [0, f8, S4], [0, On, q6], [0, g4, M8], [0, U6, v4], [0, u6, I4], [0, 126561, ap], [0, z6, 126565], [0, 126567, b3], [0, 126572, a6], [0, 126580, J8], [0, 126585, R3], [0, Z4, E8], [0, 126592, z8], [0, 126603, 126620], [0, 126625, G3], [0, 126629, e3], [0, 126635, 126652], [0, 131072, 173783], [0, 173824, 177973], [0, 177984, 178206], [0, 178208, 183970], [0, 183984, 191457], [0, 194560, 195102], [0, 917760, 918e3]], s5r = r(O2), v5r = r(hv), l5r = r(Tv), b5r = r(W4), p5r = r("Cannot export an enum with `export type`, try `export enum E {}` or `module.exports = E;` instead."), m5r = r("Enum members are separated with `,`. Replace `;` with `,`."), _5r = r("Unexpected reserved word"), y5r = r("Unexpected reserved type"), d5r = r("Unexpected `super` outside of a class method"), h5r = r("`super()` is only valid in a class constructor"), k5r = r("Unexpected end of input"), w5r = r("Unexpected variance sigil"), E5r = r("Unexpected static modifier"), S5r = r("Unexpected proto modifier"), g5r = r("Type aliases are not allowed in untyped mode"), F5r = r("Opaque type aliases are not allowed in untyped mode"), T5r = r("Type annotations are not allowed in untyped mode"), O5r = r("Type declarations are not allowed in untyped mode"), I5r = r("Type imports are not allowed in untyped mode"), A5r = r("Type exports are not allowed in untyped mode"), N5r = r("Interfaces are not allowed in untyped mode"), C5r = r("Spreading a type is only allowed inside an object type"), P5r = r("Explicit inexact syntax must come at the end of an object type"), D5r = r("Explicit inexact syntax cannot appear inside an explicit exact object type"), L5r = r("Explicit inexact syntax can only appear inside an object type"), R5r = r("Illegal newline after throw"), j5r = r("A bigint literal must be an integer"), G5r = r("A bigint literal cannot use exponential notation"), M5r = r("Invalid regular expression"), B5r = r("Invalid regular expression: missing /"), q5r = r("Invalid left-hand side in assignment"), U5r = r("Invalid left-hand side in exponentiation expression"), H5r = r("Invalid left-hand side in for-in"), X5r = r("Invalid left-hand side in for-of"), Y5r = r("Invalid optional indexed access. Indexed access uses bracket notation. Use the format `T?.[K]`."), V5r = r("found an expression instead"), z5r = r("Expected an object pattern, array pattern, or an identifier but "), K5r = r("More than one default clause in switch statement"), W5r = r("Missing catch or finally after try"), J5r = r("Illegal continue statement"), $5r = r("Illegal break statement"), Z5r = r("Illegal return statement"), Q5r = r("Illegal Unicode escape"), rmr = r("Strict mode code may not include a with statement"), emr = r("Catch variable may not be eval or arguments in strict mode"), nmr = r("Variable name may not be eval or arguments in strict mode"), tmr = r("Parameter name eval or arguments is not allowed in strict mode"), umr = r("Strict mode function may not have duplicate parameter names"), imr = r('Illegal "use strict" directive in function with non-simple parameter list'), fmr = r("Function name may not be eval or arguments in strict mode"), xmr = r("Octal literals are not allowed in strict mode."), amr = r("Number literals with leading zeros are not allowed in strict mode."), omr = r("Delete of an unqualified identifier in strict mode."), cmr = r("Duplicate data property in object literal not allowed in strict mode"), smr = r("Object literal may not have data and accessor property with the same name"), vmr = r("Object literal may not have multiple get/set accessors with the same name"), lmr = r("`typeof` can only be used to get the type of variables."), bmr = r("Assignment to eval or arguments is not allowed in strict mode"), pmr = r("Postfix increment/decrement may not have eval or arguments operand in strict mode"), mmr = r("Prefix increment/decrement may not have eval or arguments operand in strict mode"), _mr = r("Use of future reserved word in strict mode"), ymr = r("JSX attributes must only be assigned a non-empty expression"), dmr = r("JSX value should be either an expression or a quoted JSX text"), hmr = r("Const must be initialized"), kmr = r("Destructuring assignment must be initialized"), wmr = r("Illegal newline before arrow"), Emr = r(vF), Smr = r("Async functions can only be declared at top level or "), gmr = r(vF), Fmr = r("Generators can only be declared at top level or "), Tmr = r("elements must be wrapped in an enclosing parent tag"), Omr = r("Unexpected token <. Remember, adjacent JSX "), Imr = r("Rest parameter must be final parameter of an argument list"), Amr = r("Rest element must be final element of an array pattern"), Nmr = r("Rest property must be final property of an object pattern"), Cmr = r("async is an implementation detail and isn't necessary for your declare function statement. It is sufficient for your declare function to just have a Promise return type."), Pmr = r("`declare` modifier can only appear on class fields."), Dmr = r("Unexpected token `=`. Initializers are not allowed in a `declare`."), Lmr = r("Unexpected token `=`. Initializers are not allowed in a `declare opaque type`."), Rmr = r("`declare export let` is not supported. Use `declare export var` instead."), jmr = r("`declare export const` is not supported. Use `declare export var` instead."), Gmr = r("`declare export type` is not supported. Use `export type` instead."), Mmr = r("`declare export interface` is not supported. Use `export interface` instead."), Bmr = r("`export * as` is an early-stage proposal and is not enabled by default. To enable support in the parser, use the `esproposal_export_star_as` option"), qmr = r("Found a decorator in an unsupported position."), Umr = r("Type parameter declaration needs a default, since a preceding type parameter declaration has a default."), Hmr = r("Duplicate `declare module.exports` statement!"), Xmr = r("Found both `declare module.exports` and `declare export` in the same module. Modules can only have 1 since they are either an ES module xor they are a CommonJS module."), Ymr = r("Getter should have zero parameters"), Vmr = r("Setter should have exactly one parameter"), zmr = r("`import type` or `import typeof`!"), Kmr = r("Imports within a `declare module` body must always be "), Wmr = r("The `type` and `typeof` keywords on named imports can only be used on regular `import` statements. It cannot be used with `import type` or `import typeof` statements"), Jmr = r("Missing comma between import specifiers"), $mr = r("Missing comma between export specifiers"), Zmr = r("Malformed unicode"), Qmr = r("Classes may only have one constructor"), r9r = r("Private fields may not be deleted."), e9r = r("Private fields can only be referenced from within a class."), n9r = r("You may not access a private field through the `super` keyword."), t9r = r("Yield expression not allowed in formal parameter"), u9r = r("`await` is an invalid identifier in async functions"), i9r = r("`yield` is an invalid identifier in generators"), f9r = r("either a `let` binding pattern, or a member expression."), x9r = r("`let [` is ambiguous in this position because it is "), a9r = r("Literals cannot be used as shorthand properties."), o9r = r("Computed properties must have a value."), c9r = r("Object pattern can't contain methods"), s9r = r("A trailing comma is not permitted after the rest element"), v9r = r("An optional chain may not be used in a `new` expression."), l9r = r("Template literals may not be used in an optional chain."), b9r = r("Unexpected whitespace between `#` and identifier"), p9r = r("A type annotation is required for the `this` parameter."), m9r = r("The `this` parameter must be the first function parameter."), _9r = r("The `this` parameter cannot be optional."), y9r = r("A getter cannot have a `this` parameter."), d9r = r("A setter cannot have a `this` parameter."), h9r = r("Arrow functions cannot have a `this` parameter; arrow functions automatically bind `this` when declared."), k9r = r("Constructors cannot have a `this` parameter; constructors don't bind `this` like other functions."), w9r = [0, [11, r("Boolean enum members need to be initialized. Use either `"), [2, 0, [11, r(" = true,` or `"), [2, 0, [11, r(" = false,` in enum `"), [2, 0, [11, r(Fs), 0]]]]]]], r("Boolean enum members need to be initialized. Use either `%s = true,` or `%s = false,` in enum `%s`.")], E9r = [0, [11, r("Enum member names need to be unique, but the name `"), [2, 0, [11, r("` has already been used before in enum `"), [2, 0, [11, r(Fs), 0]]]]], r("Enum member names need to be unique, but the name `%s` has already been used before in enum `%s`.")], S9r = [0, [11, r(DU), [2, 0, [11, r("` has inconsistent member initializers. Either use no initializers, or consistently use literals (either booleans, numbers, or strings) for all member initializers."), 0]]], r("Enum `%s` has inconsistent member initializers. Either use no initializers, or consistently use literals (either booleans, numbers, or strings) for all member initializers.")], g9r = [0, [11, r("Use one of `boolean`, `number`, `string`, or `symbol` in enum `"), [2, 0, [11, r(Fs), 0]]], r("Use one of `boolean`, `number`, `string`, or `symbol` in enum `%s`.")], F9r = [0, [11, r("Enum type `"), [2, 0, [11, r("` is not valid. "), [2, 0, 0]]]], r("Enum type `%s` is not valid. %s")], T9r = [0, [11, r("Supplied enum type is not valid. "), [2, 0, 0]], r("Supplied enum type is not valid. %s")], O9r = [0, [11, r("Enum member names and initializers are separated with `=`. Replace `"), [2, 0, [11, r(":` with `"), [2, 0, [11, r(" =`."), 0]]]]], r("Enum member names and initializers are separated with `=`. Replace `%s:` with `%s =`.")], I9r = [0, [11, r("Symbol enum members cannot be initialized. Use `"), [2, 0, [11, r(",` in enum `"), [2, 0, [11, r(Fs), 0]]]]], r("Symbol enum members cannot be initialized. Use `%s,` in enum `%s`.")], A9r = [0, [11, r(DU), [2, 0, [11, r("` has type `"), [2, 0, [11, r("`, so the initializer of `"), [2, 0, [11, r("` needs to be a "), [2, 0, [11, r(" literal."), 0]]]]]]]]], r("Enum `%s` has type `%s`, so the initializer of `%s` needs to be a %s literal.")], N9r = [0, [11, r("The enum member initializer for `"), [2, 0, [11, r("` needs to be a literal (either a boolean, number, or string) in enum `"), [2, 0, [11, r(Fs), 0]]]]], r("The enum member initializer for `%s` needs to be a literal (either a boolean, number, or string) in enum `%s`.")], C9r = [0, [11, r("Enum member names cannot start with lowercase 'a' through 'z'. Instead of using `"), [2, 0, [11, r("`, consider using `"), [2, 0, [11, r("`, in enum `"), [2, 0, [11, r(Fs), 0]]]]]]], r("Enum member names cannot start with lowercase 'a' through 'z'. Instead of using `%s`, consider using `%s`, in enum `%s`.")], P9r = r("The `...` must come at the end of the enum body. Remove the trailing comma."), D9r = r("The `...` must come after all enum members. Move it to the end of the enum body."), L9r = [0, [11, r("Number enum members need to be initialized, e.g. `"), [2, 0, [11, r(" = 1,` in enum `"), [2, 0, [11, r(Fs), 0]]]]], r("Number enum members need to be initialized, e.g. `%s = 1,` in enum `%s`.")], R9r = [0, [11, r("String enum members need to consistently either all use initializers, or use no initializers, in enum "), [2, 0, [12, 46, 0]]], r("String enum members need to consistently either all use initializers, or use no initializers, in enum %s.")], j9r = [0, [11, r(zH), [2, 0, 0]], r("Unexpected %s")], G9r = [0, [11, r(zH), [2, 0, [11, r(", expected "), [2, 0, 0]]]], r("Unexpected %s, expected %s")], M9r = [0, [11, r(dV), [2, 0, [11, r("`. Did you mean `"), [2, 0, [11, r("`?"), 0]]]]], r("Unexpected token `%s`. Did you mean `%s`?")], B9r = r(D3), q9r = r("Invalid flags supplied to RegExp constructor '"), U9r = r("Remove the period."), H9r = r("Indexed access uses bracket notation."), X9r = [0, [11, r("Invalid indexed access. "), [2, 0, [11, r(" Use the format `T[K]`."), 0]]], r("Invalid indexed access. %s Use the format `T[K]`.")], Y9r = r(D3), V9r = r("Undefined label '"), z9r = r("' has already been declared"), K9r = r(" '"), W9r = r("Expected corresponding JSX closing tag for "), J9r = r(vF), $9r = r("In strict mode code, functions can only be declared at top level or "), Z9r = r("inside a block, or as the body of an if statement."), Q9r = r("In non-strict mode code, functions can only be declared at top level, "), r_r = [0, [11, r("Duplicate export for `"), [2, 0, [12, 96, 0]]], r("Duplicate export for `%s`")], e_r = r("` is declared more than once."), n_r = r("Private fields may only be declared once. `#"), t_r = r("static "), u_r = r(C), i_r = r(JY), f_r = r("methods"), x_r = r("fields"), a_r = r(Fs), o_r = r(" named `"), c_r = r("Classes may not have "), s_r = r("` has not been declared."), v_r = r("Private fields must be declared before they can be referenced. `#"), l_r = [0, [11, r(dV), [2, 0, [11, r("`. Parentheses are required to combine `??` with `&&` or `||` expressions."), 0]]], r("Unexpected token `%s`. Parentheses are required to combine `??` with `&&` or `||` expressions.")], b_r = r("Parse_error.Error"), p_r = [0, r("src/third-party/sedlex/flow_sedlexing.ml"), v1, 4], m_r = r("Flow_sedlexing.MalFormed"), __r = [0, 1, 0], y_r = [0, 0, [0, 1, 0], [0, 1, 0]], d_r = r(JU), h_r = r("end of input"), k_r = r(rl), w_r = r("template literal part"), E_r = r(rl), S_r = r(XH), g_r = r(JU), F_r = r(rl), T_r = r(hv), O_r = r(rl), I_r = r(a1), A_r = r(rl), N_r = r(Tv), C_r = r("an"), P_r = r(_i), D_r = r(bi), L_r = [0, [11, r("token `"), [2, 0, [12, 96, 0]]], r("token `%s`")], R_r = r(SH), j_r = r(p3), G_r = r("{|"), M_r = r("|}"), B_r = r(KX), q_r = r(Z0), U_r = r("["), H_r = r("]"), X_r = r($Y), Y_r = r(","), V_r = r(Ra), z_r = r("=>"), K_r = r("..."), W_r = r(AX), J_r = r(JY), $_r = r(M2), Z_r = r(N3), Q_r = r(R2), ryr = r(j2), eyr = r(Wu), nyr = r(P7), tyr = r(f1), uyr = r(g7), iyr = r(k4), fyr = r(U2), xyr = r(W6), ayr = r(P8), oyr = r(D2), cyr = r(G2), syr = r(xs), vyr = r(Ci), lyr = r(Gi), byr = r(I7), pyr = r(k6), myr = r(o6), _yr = r(A7), yyr = r(mi), dyr = r(y4), hyr = r(U8), kyr = r(tp), wyr = r(q2), Eyr = r(C7), Syr = r(eu), gyr = r(H4), Fyr = r(i1), Tyr = r(J2), Oyr = r(es), Iyr = r(ns), Ayr = r(p8), Nyr = r(y3), Cyr = r(qu), Pyr = r(yv), Dyr = r(gs), Lyr = r(r7), Ryr = r(d4), jyr = r(w4), Gyr = r(c6), Myr = r(S6), Byr = r(wu), qyr = r(O7), Uyr = r(T2), Hyr = r($c), Xyr = r(ud), Yyr = r(LS), Vyr = r(Os), zyr = r(wx), Kyr = r("%checks"), Wyr = r(bX), Jyr = r(ZX), $yr = r(vU), Zyr = r(TH), Qyr = r(dH), rdr = r(WH), edr = r(SX), ndr = r(yH), tdr = r(xY), udr = r(vX), idr = r(YU), fdr = r(rH), xdr = r(iV), adr = r(LU), odr = r(aX), cdr = r(zO), sdr = r("?."), vdr = r(Fn), ldr = r("?"), bdr = r(o1), pdr = r(ZH), mdr = r(XX), _dr = r(PH), ydr = r(A6), ddr = r(Bh), hdr = r(yV), kdr = r(UY), wdr = r(GX), Edr = r(ZY), Sdr = r(tX), gdr = r(PU), Fdr = r(g3), Tdr = r(cv), Odr = r(_Y), Idr = r(wX), Adr = r(mX), Ndr = r(as), Cdr = r(Oo), Pdr = r(Zu), Ddr = r(zU), Ldr = r(rX), Rdr = r(WY), jdr = r(rf), Gdr = r(tV), Mdr = r(mH), Bdr = r(lV), qdr = r(C), Udr = r(t6), Hdr = r(X8), Xdr = r(s7), Ydr = r(hv), Vdr = r(a1), zdr = r(Tv), Kdr = r(ns), Wdr = r(W4), Jdr = r(Zu), $dr = r(Zu), Zdr = r(O2), Qdr = r(I6), rhr = r("T_LCURLY"), ehr = r("T_RCURLY"), nhr = r("T_LCURLYBAR"), thr = r("T_RCURLYBAR"), uhr = r("T_LPAREN"), ihr = r("T_RPAREN"), fhr = r("T_LBRACKET"), xhr = r("T_RBRACKET"), ahr = r("T_SEMICOLON"), ohr = r("T_COMMA"), chr = r("T_PERIOD"), shr = r("T_ARROW"), vhr = r("T_ELLIPSIS"), lhr = r("T_AT"), bhr = r("T_POUND"), phr = r("T_FUNCTION"), mhr = r("T_IF"), _hr = r("T_IN"), yhr = r("T_INSTANCEOF"), dhr = r("T_RETURN"), hhr = r("T_SWITCH"), khr = r("T_THIS"), whr = r("T_THROW"), Ehr = r("T_TRY"), Shr = r("T_VAR"), ghr = r("T_WHILE"), Fhr = r("T_WITH"), Thr = r("T_CONST"), Ohr = r("T_LET"), Ihr = r("T_NULL"), Ahr = r("T_FALSE"), Nhr = r("T_TRUE"), Chr = r("T_BREAK"), Phr = r("T_CASE"), Dhr = r("T_CATCH"), Lhr = r("T_CONTINUE"), Rhr = r("T_DEFAULT"), jhr = r("T_DO"), Ghr = r("T_FINALLY"), Mhr = r("T_FOR"), Bhr = r("T_CLASS"), qhr = r("T_EXTENDS"), Uhr = r("T_STATIC"), Hhr = r("T_ELSE"), Xhr = r("T_NEW"), Yhr = r("T_DELETE"), Vhr = r("T_TYPEOF"), zhr = r("T_VOID"), Khr = r("T_ENUM"), Whr = r("T_EXPORT"), Jhr = r("T_IMPORT"), $hr = r("T_SUPER"), Zhr = r("T_IMPLEMENTS"), Qhr = r("T_INTERFACE"), rkr = r("T_PACKAGE"), ekr = r("T_PRIVATE"), nkr = r("T_PROTECTED"), tkr = r("T_PUBLIC"), ukr = r("T_YIELD"), ikr = r("T_DEBUGGER"), fkr = r("T_DECLARE"), xkr = r("T_TYPE"), akr = r("T_OPAQUE"), okr = r("T_OF"), ckr = r("T_ASYNC"), skr = r("T_AWAIT"), vkr = r("T_CHECKS"), lkr = r("T_RSHIFT3_ASSIGN"), bkr = r("T_RSHIFT_ASSIGN"), pkr = r("T_LSHIFT_ASSIGN"), mkr = r("T_BIT_XOR_ASSIGN"), _kr = r("T_BIT_OR_ASSIGN"), ykr = r("T_BIT_AND_ASSIGN"), dkr = r("T_MOD_ASSIGN"), hkr = r("T_DIV_ASSIGN"), kkr = r("T_MULT_ASSIGN"), wkr = r("T_EXP_ASSIGN"), Ekr = r("T_MINUS_ASSIGN"), Skr = r("T_PLUS_ASSIGN"), gkr = r("T_NULLISH_ASSIGN"), Fkr = r("T_AND_ASSIGN"), Tkr = r("T_OR_ASSIGN"), Okr = r("T_ASSIGN"), Ikr = r("T_PLING_PERIOD"), Akr = r("T_PLING_PLING"), Nkr = r("T_PLING"), Ckr = r("T_COLON"), Pkr = r("T_OR"), Dkr = r("T_AND"), Lkr = r("T_BIT_OR"), Rkr = r("T_BIT_XOR"), jkr = r("T_BIT_AND"), Gkr = r("T_EQUAL"), Mkr = r("T_NOT_EQUAL"), Bkr = r("T_STRICT_EQUAL"), qkr = r("T_STRICT_NOT_EQUAL"), Ukr = r("T_LESS_THAN_EQUAL"), Hkr = r("T_GREATER_THAN_EQUAL"), Xkr = r("T_LESS_THAN"), Ykr = r("T_GREATER_THAN"), Vkr = r("T_LSHIFT"), zkr = r("T_RSHIFT"), Kkr = r("T_RSHIFT3"), Wkr = r("T_PLUS"), Jkr = r("T_MINUS"), $kr = r("T_DIV"), Zkr = r("T_MULT"), Qkr = r("T_EXP"), rwr = r("T_MOD"), ewr = r("T_NOT"), nwr = r("T_BIT_NOT"), twr = r("T_INCR"), uwr = r("T_DECR"), iwr = r("T_EOF"), fwr = r("T_ANY_TYPE"), xwr = r("T_MIXED_TYPE"), awr = r("T_EMPTY_TYPE"), owr = r("T_NUMBER_TYPE"), cwr = r("T_BIGINT_TYPE"), swr = r("T_STRING_TYPE"), vwr = r("T_VOID_TYPE"), lwr = r("T_SYMBOL_TYPE"), bwr = r("T_NUMBER"), pwr = r("T_BIGINT"), mwr = r("T_STRING"), _wr = r("T_TEMPLATE_PART"), ywr = r("T_IDENTIFIER"), dwr = r("T_REGEXP"), hwr = r("T_ERROR"), kwr = r("T_JSX_IDENTIFIER"), wwr = r("T_JSX_TEXT"), Ewr = r("T_BOOLEAN_TYPE"), Swr = r("T_NUMBER_SINGLETON_TYPE"), gwr = r("T_BIGINT_SINGLETON_TYPE"), Fwr = [0, r(FX), VT, 9], Twr = [0, r(FX), N6, 9], Owr = r(HH), Iwr = r("*/"), Awr = r(HH), Nwr = r("unreachable line_comment"), Cwr = r("unreachable string_quote"), Pwr = r("\\"), Dwr = r("unreachable template_part"), Lwr = r("${"), Rwr = r(zY), jwr = r(zY), Gwr = r(UI), Mwr = r("unreachable regexp_class"), Bwr = r(oY), qwr = r("unreachable regexp_body"), Uwr = r(C), Hwr = r(C), Xwr = r(C), Ywr = r(C), Vwr = r("unreachable jsxtext"), zwr = r(D3), Kwr = r(V2), Wwr = r(g3), Jwr = r(cv), $wr = r(SH), Zwr = r(p3), Qwr = r("{'}'}"), rEr = r(p3), eEr = r("{'>'}"), nEr = r(cv), tEr = r(b1), uEr = r("iexcl"), iEr = r("aelig"), fEr = r("Nu"), xEr = r("Eacute"), aEr = r("Atilde"), oEr = r("'int'"), cEr = r("AElig"), sEr = r("Aacute"), vEr = r("Acirc"), lEr = r("Agrave"), bEr = r("Alpha"), pEr = r("Aring"), mEr = [0, SY], _Er = [0, 913], yEr = [0, at], dEr = [0, iI], hEr = [0, VT], kEr = [0, _H], wEr = [0, 8747], EEr = r("Auml"), SEr = r("Beta"), gEr = r("Ccedil"), FEr = r("Chi"), TEr = r("Dagger"), OEr = r("Delta"), IEr = r("ETH"), AEr = [0, wH], NEr = [0, 916], CEr = [0, 8225], PEr = [0, 935], DEr = [0, uX], LEr = [0, 914], REr = [0, WX], jEr = [0, vY], GEr = r("Icirc"), MEr = r("Ecirc"), BEr = r("Egrave"), qEr = r("Epsilon"), UEr = r("Eta"), HEr = r("Euml"), XEr = r("Gamma"), YEr = r("Iacute"), VEr = [0, $H], zEr = [0, 915], KEr = [0, fV], WEr = [0, 919], JEr = [0, 917], $Er = [0, RU], ZEr = [0, xH], QEr = r("Igrave"), rSr = r("Iota"), eSr = r("Iuml"), nSr = r("Kappa"), tSr = r("Lambda"), uSr = r("Mu"), iSr = r("Ntilde"), fSr = [0, Zg], xSr = [0, 924], aSr = [0, 923], oSr = [0, 922], cSr = [0, LX], sSr = [0, 921], vSr = [0, rY], lSr = [0, CH], bSr = [0, mY], pSr = r("Sigma"), mSr = r("Otilde"), _Sr = r("OElig"), ySr = r("Oacute"), dSr = r("Ocirc"), hSr = r("Ograve"), kSr = r("Omega"), wSr = r("Omicron"), ESr = r("Oslash"), SSr = [0, d6], gSr = [0, 927], FSr = [0, 937], TSr = [0, N6], OSr = [0, EY], ISr = [0, EU], ASr = [0, 338], NSr = r("Ouml"), CSr = r("Phi"), PSr = r("Pi"), DSr = r("Prime"), LSr = r("Psi"), RSr = r("Rho"), jSr = r("Scaron"), GSr = [0, 352], MSr = [0, 929], BSr = [0, 936], qSr = [0, 8243], USr = [0, 928], HSr = [0, 934], XSr = [0, dT], YSr = [0, qX], VSr = r("Uuml"), zSr = r("THORN"), KSr = r("Tau"), WSr = r("Theta"), JSr = r("Uacute"), $Sr = r("Ucirc"), ZSr = r("Ugrave"), QSr = r("Upsilon"), rgr = [0, 933], egr = [0, sp], ngr = [0, NU], tgr = [0, Lw], ugr = [0, 920], igr = [0, 932], fgr = [0, NX], xgr = r("Xi"), agr = r("Yacute"), ogr = r("Yuml"), cgr = r("Zeta"), sgr = r("aacute"), vgr = r("acirc"), lgr = r("acute"), bgr = [0, mU], pgr = [0, tk], mgr = [0, HO], _gr = [0, 918], ygr = [0, 376], dgr = [0, HX], hgr = [0, 926], kgr = [0, aA], wgr = [0, zX], Egr = [0, 925], Sgr = r("delta"), ggr = r("cap"), Fgr = r("aring"), Tgr = r("agrave"), Ogr = r("alefsym"), Igr = r("alpha"), Agr = r("amp"), Ngr = r("and"), Cgr = r("ang"), Pgr = r("apos"), Dgr = [0, 39], Lgr = [0, 8736], Rgr = [0, 8743], jgr = [0, 38], Ggr = [0, 945], Mgr = [0, 8501], Bgr = [0, dv], qgr = r("asymp"), Ugr = r("atilde"), Hgr = r("auml"), Xgr = r("bdquo"), Ygr = r("beta"), Vgr = r("brvbar"), zgr = r("bull"), Kgr = [0, 8226], Wgr = [0, MY], Jgr = [0, 946], $gr = [0, 8222], Zgr = [0, sV], Qgr = [0, eX], rFr = [0, 8776], eFr = [0, dU], nFr = r("copy"), tFr = r("ccedil"), uFr = r("cedil"), iFr = r("cent"), fFr = r("chi"), xFr = r("circ"), aFr = r("clubs"), oFr = r("cong"), cFr = [0, 8773], sFr = [0, 9827], vFr = [0, iX], lFr = [0, 967], bFr = [0, Sd], pFr = [0, wk], mFr = [0, VX], _Fr = r("crarr"), yFr = r("cup"), dFr = r("curren"), hFr = r("dArr"), kFr = r("dagger"), wFr = r("darr"), EFr = r("deg"), SFr = [0, kV], gFr = [0, 8595], FFr = [0, 8224], TFr = [0, 8659], OFr = [0, PF], IFr = [0, 8746], AFr = [0, 8629], NFr = [0, RX], CFr = [0, 8745], PFr = r("fnof"), DFr = r("ensp"), LFr = r("diams"), RFr = r("divide"), jFr = r("eacute"), GFr = r("ecirc"), MFr = r("egrave"), BFr = r(s7), qFr = r("emsp"), UFr = [0, 8195], HFr = [0, 8709], XFr = [0, eT], YFr = [0, aH], VFr = [0, wT], zFr = [0, jw], KFr = [0, 9830], WFr = r("epsilon"), JFr = r("equiv"), $Fr = r("eta"), ZFr = r("eth"), QFr = r("euml"), rTr = r("euro"), eTr = r("exist"), nTr = [0, 8707], tTr = [0, 8364], uTr = [0, eH], iTr = [0, v1], fTr = [0, 951], xTr = [0, 8801], aTr = [0, 949], oTr = [0, 8194], cTr = r("gt"), sTr = r("forall"), vTr = r("frac12"), lTr = r("frac14"), bTr = r("frac34"), pTr = r("frasl"), mTr = r("gamma"), _Tr = r("ge"), yTr = [0, 8805], dTr = [0, 947], hTr = [0, 8260], kTr = [0, PY], wTr = [0, cY], ETr = [0, sX], STr = [0, 8704], gTr = r("hArr"), FTr = r("harr"), TTr = r("hearts"), OTr = r("hellip"), ITr = r("iacute"), ATr = r("icirc"), NTr = [0, pH], CTr = [0, YY], PTr = [0, 8230], DTr = [0, 9829], LTr = [0, 8596], RTr = [0, 8660], jTr = [0, 62], GTr = [0, 402], MTr = [0, 948], BTr = [0, Bd], qTr = r("prime"), UTr = r("ndash"), HTr = r("le"), XTr = r("kappa"), YTr = r("igrave"), VTr = r("image"), zTr = r("infin"), KTr = r("iota"), WTr = r("iquest"), JTr = r("isin"), $Tr = r("iuml"), ZTr = [0, f6], QTr = [0, 8712], rOr = [0, yX], eOr = [0, 953], nOr = [0, 8734], tOr = [0, 8465], uOr = [0, mO], iOr = r("lArr"), fOr = r("lambda"), xOr = r("lang"), aOr = r("laquo"), oOr = r("larr"), cOr = r("lceil"), sOr = r("ldquo"), vOr = [0, 8220], lOr = [0, 8968], bOr = [0, 8592], pOr = [0, yg], mOr = [0, 10216], _Or = [0, 955], yOr = [0, 8656], dOr = [0, 954], hOr = r("macr"), kOr = r("lfloor"), wOr = r("lowast"), EOr = r("loz"), SOr = r("lrm"), gOr = r("lsaquo"), FOr = r("lsquo"), TOr = r("lt"), OOr = [0, 60], IOr = [0, 8216], AOr = [0, 8249], NOr = [0, _U], COr = [0, 9674], POr = [0, 8727], DOr = [0, 8970], LOr = r("mdash"), ROr = r("micro"), jOr = r("middot"), GOr = r(pY), MOr = r("mu"), BOr = r("nabla"), qOr = r("nbsp"), UOr = [0, sY], HOr = [0, 8711], XOr = [0, 956], YOr = [0, 8722], VOr = [0, mS], zOr = [0, Ai], KOr = [0, 8212], WOr = [0, dX], JOr = [0, 8804], $Or = r("or"), ZOr = r("oacute"), QOr = r("ne"), rIr = r("ni"), eIr = r("not"), nIr = r("notin"), tIr = r("nsub"), uIr = r("ntilde"), iIr = r("nu"), fIr = [0, 957], xIr = [0, Wy], aIr = [0, 8836], oIr = [0, 8713], cIr = [0, BU], sIr = [0, 8715], vIr = [0, 8800], lIr = r("ocirc"), bIr = r("oelig"), pIr = r("ograve"), mIr = r("oline"), _Ir = r("omega"), yIr = r("omicron"), dIr = r("oplus"), hIr = [0, 8853], kIr = [0, 959], wIr = [0, 969], EIr = [0, 8254], SIr = [0, TT], gIr = [0, 339], FIr = [0, l8], TIr = [0, uH], OIr = r("part"), IIr = r("ordf"), AIr = r("ordm"), NIr = r("oslash"), CIr = r("otilde"), PIr = r("otimes"), DIr = r("ouml"), LIr = r("para"), RIr = [0, Kg], jIr = [0, $2], GIr = [0, 8855], MIr = [0, rV], BIr = [0, wt], qIr = [0, dh], UIr = [0, Xg], HIr = r("permil"), XIr = r("perp"), YIr = r("phi"), VIr = r("pi"), zIr = r("piv"), KIr = r("plusmn"), WIr = r("pound"), JIr = [0, Mn], $Ir = [0, oV], ZIr = [0, 982], QIr = [0, 960], rAr = [0, 966], eAr = [0, 8869], nAr = [0, 8240], tAr = [0, 8706], uAr = [0, 8744], iAr = [0, 8211], fAr = r("sup1"), xAr = r("rlm"), aAr = r("raquo"), oAr = r("prod"), cAr = r("prop"), sAr = r("psi"), vAr = r("quot"), lAr = r("rArr"), bAr = r("radic"), pAr = r("rang"), mAr = [0, 10217], _Ar = [0, 8730], yAr = [0, 8658], dAr = [0, 34], hAr = [0, 968], kAr = [0, 8733], wAr = [0, 8719], EAr = r("rarr"), SAr = r("rceil"), gAr = r("rdquo"), FAr = r("real"), TAr = r("reg"), OAr = r("rfloor"), IAr = r("rho"), AAr = [0, 961], NAr = [0, 8971], CAr = [0, nH], PAr = [0, 8476], DAr = [0, 8221], LAr = [0, 8969], RAr = [0, 8594], jAr = [0, iw], GAr = r("sigma"), MAr = r("rsaquo"), BAr = r("rsquo"), qAr = r("sbquo"), UAr = r("scaron"), HAr = r("sdot"), XAr = r("sect"), YAr = r("shy"), VAr = [0, wY], zAr = [0, DT], KAr = [0, 8901], WAr = [0, 353], JAr = [0, 8218], $Ar = [0, 8217], ZAr = [0, 8250], QAr = r("sigmaf"), rNr = r("sim"), eNr = r("spades"), nNr = r("sub"), tNr = r("sube"), uNr = r("sum"), iNr = r("sup"), fNr = [0, 8835], xNr = [0, 8721], aNr = [0, 8838], oNr = [0, 8834], cNr = [0, 9824], sNr = [0, 8764], vNr = [0, 962], lNr = [0, 963], bNr = [0, 8207], pNr = r("uarr"), mNr = r("thetasym"), _Nr = r("sup2"), yNr = r("sup3"), dNr = r("supe"), hNr = r("szlig"), kNr = r("tau"), wNr = r("there4"), ENr = r("theta"), SNr = [0, 952], gNr = [0, 8756], FNr = [0, 964], TNr = [0, d8], ONr = [0, 8839], INr = [0, qY], ANr = [0, OO], NNr = r("thinsp"), CNr = r("thorn"), PNr = r("tilde"), DNr = r("times"), LNr = r("trade"), RNr = r("uArr"), jNr = r("uacute"), GNr = [0, nl], MNr = [0, 8657], BNr = [0, 8482], qNr = [0, cT], UNr = [0, 732], HNr = [0, gv], XNr = [0, 8201], YNr = [0, 977], VNr = r("xi"), zNr = r("ucirc"), KNr = r("ugrave"), WNr = r("uml"), JNr = r("upsih"), $Nr = r("upsilon"), ZNr = r("uuml"), QNr = r("weierp"), rCr = [0, cU], eCr = [0, Y2], nCr = [0, 965], tCr = [0, 978], uCr = [0, DY], iCr = [0, 249], fCr = [0, 251], xCr = r("yacute"), aCr = r("yen"), oCr = r("yuml"), cCr = r("zeta"), sCr = r("zwj"), vCr = r("zwnj"), lCr = [0, FY], bCr = [0, 8205], pCr = [0, 950], mCr = [0, Ow], _Cr = [0, nY], yCr = [0, ih], dCr = [0, 958], hCr = [0, 8593], kCr = [0, AU], wCr = [0, 8242], ECr = [0, WU], SCr = r($Y), gCr = r(Bh), FCr = r("unreachable jsx_child"), TCr = r("unreachable type_token wholenumber"), OCr = r("unreachable type_token wholebigint"), ICr = r("unreachable type_token floatbigint"), ACr = r("unreachable type_token scinumber"), NCr = r("unreachable type_token scibigint"), CCr = r("unreachable type_token hexnumber"), PCr = r("unreachable type_token hexbigint"), DCr = r("unreachable type_token legacyoctnumber"), LCr = r("unreachable type_token octnumber"), RCr = r("unreachable type_token octbigint"), jCr = r("unreachable type_token binnumber"), GCr = r("unreachable type_token bigbigint"), MCr = r("unreachable type_token"), BCr = r(o1), qCr = r(o1), UCr = r(FU), HCr = r(X8), XCr = r(t6), YCr = r(a1), VCr = r(I6), zCr = r(O2), KCr = r(s7), WCr = r(C7), JCr = r(Ci), $Cr = r(r7), ZCr = [9, 1], QCr = [9, 0], rPr = r(xs), ePr = r(hv), nPr = r(eu), tPr = r(Tv), uPr = r(W4), iPr = r(Gi), fPr = r(es), xPr = r(ns), aPr = r("unreachable template_tail"), oPr = r(p3), cPr = [0, r(C), r(C), r(C)], sPr = r("unreachable jsx_tag"), vPr = r(D3), lPr = r("unreachable regexp"), bPr = r("unreachable token wholenumber"), pPr = r("unreachable token wholebigint"), mPr = r("unreachable token floatbigint"), _Pr = r("unreachable token scinumber"), yPr = r("unreachable token scibigint"), dPr = r("unreachable token hexnumber"), hPr = r("unreachable token hexbigint"), kPr = r("unreachable token legacyoctnumber"), wPr = r("unreachable token legacynonoctnumber"), EPr = r("unreachable token octnumber"), SPr = r("unreachable token octbigint"), gPr = r("unreachable token bignumber"), FPr = r("unreachable token bigint"), TPr = r("unreachable token"), OPr = r(o1), IPr = r(o1), APr = r(FU), NPr = [6, r("#!")], CPr = r("expected ?"), PPr = r(j2), DPr = r(y4), LPr = r(D2), RPr = r(Os), jPr = r(wx), GPr = r(I7), MPr = r(k6), BPr = r(o6), qPr = r(q2), UPr = r(A7), HPr = r(O7), XPr = r(T2), YPr = r(mi), VPr = r(J2), zPr = r(tp), KPr = r(H4), WPr = r(p8), JPr = r(y3), $Pr = r(C7), ZPr = r(Ci), QPr = r(U8), rDr = r(M2), eDr = r(N3), nDr = r(gs), tDr = r(qu), uDr = r(R2), iDr = r(yv), fDr = r(d4), xDr = r(r7), aDr = r(G2), oDr = r(i1), cDr = r(xs), sDr = r(LS), vDr = r(ud), lDr = r(w4), bDr = r(c6), pDr = r(S6), mDr = r(Wu), _Dr = r(eu), yDr = r(es), dDr = r(P7), hDr = r(f1), kDr = r(g7), wDr = r(Gi), EDr = r(k4), SDr = r($c), gDr = r(U2), FDr = r(ns), TDr = r(W6), ODr = r(P8), IDr = r(wu), ADr = r("unreachable string_escape"), NDr = r($u), CDr = r(H2), PDr = r(H2), DDr = r($u), LDr = r(gX), RDr = r(lY), jDr = r("n"), GDr = r("r"), MDr = r("t"), BDr = r(hV), qDr = r(H2), UDr = r(b1), HDr = r(b1), XDr = r("unreachable id_char"), YDr = r(b1), VDr = r(b1), zDr = r("Invalid (lexer) bigint "), KDr = r("Invalid (lexer) bigint binary/octal "), WDr = r(H2), JDr = r(hH), $Dr = r(lU), ZDr = r(Dd), QDr = [10, r("token ILLEGAL")], rLr = r("\0"), eLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), nLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), tLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), uLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), iLr = r("\0\0"), fLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), xLr = r(""), aLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), oLr = r("\0"), cLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), sLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), vLr = r("\0\0\0\0"), lLr = r("\0\0\0"), bLr = r("\x07\x07"), pLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), mLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), _Lr = r(`\x07\b
\v\f\r`), yLr = r(""), dLr = r("\0\0\0"), hLr = r("\0"), kLr = r("\0\0\0\0\0\0"), wLr = r(""), ELr = r(""), SLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), gLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), FLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), TLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), OLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), ILr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), ALr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), NLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), CLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), PLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), DLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), LLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\x07\b\0\0\0\0\0\0 \x07\b"), RLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), jLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), GLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), MLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), BLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), qLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), ULr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), HLr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), XLr = r(`\x07\b
\v\x07\f\r\x1B ! "#$% `), YLr = r(""), VLr = r(""), zLr = r("\0\0\0\0"), KLr = r(`\x07\b
\v\f\r\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x1B\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07`), WLr = r(`\x07\b
\v\f\r\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07\x07`), JLr = r("\0\0"), $Lr = r(""), ZLr = r(""), QLr = r("\x07"), rRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), eRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), nRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), tRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), uRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), iRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), fRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), xRr = r("\0\0\0\0\0\0\0"), aRr = r("\x07"), oRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), cRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), sRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), vRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), lRr = r("\0"), bRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), pRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), mRr = r("\0\0"), _Rr = r("\0"), yRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), dRr = r(""), hRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), kRr = r(""), wRr = r(""), ERr = r(""), SRr = r("\0"), gRr = r("\0\0\0"), FRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), TRr = r(""), ORr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), IRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), ARr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), NRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), CRr = r("\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0\0"), PRr = [0, [11, r("the identifier `"), [2, 0, [12, 96, 0]]], r("the identifier `%s`")], DRr = [0, 1], LRr = [0, 1], RRr = r("@flow"), jRr = r(EX), GRr = r(EX), MRr = [0, [11, r("an identifier. When exporting a "), [2, 0, [11, r(" as a named export, you must specify a "), [2, 0, [11, r(" name. Did you mean `export default "), [2, 0, [11, r(" ...`?"), 0]]]]]]], r("an identifier. When exporting a %s as a named export, you must specify a %s name. Did you mean `export default %s ...`?")], BRr = r(F3), qRr = r("Peeking current location when not available"), URr = r(r7), HRr = r(bv), XRr = r(t6), YRr = r(a1), VRr = r(I6), zRr = r(O2), KRr = r(s7), WRr = r(C7), JRr = r(Ci), $Rr = r(X8), ZRr = r(xs), QRr = r(hv), rjr = r(eu), ejr = r(Tv), njr = r(Gi), tjr = r(es), ujr = r(ns), ijr = r(Ci), fjr = r(xs), xjr = r(Gi), ajr = r(Ci), ojr = r(xs), cjr = r(Gi), sjr = r(C2), vjr = r("eval"), ljr = r(gs), bjr = r(r7), pjr = r(d4), mjr = r(w4), _jr = r(c6), yjr = r(S6), djr = r(eu), hjr = r(wu), kjr = r(p8), wjr = r(N3), Ejr = r(mi), Sjr = r(wx), gjr = r(I7), Fjr = r(k6), Tjr = r(o6), Ojr = r(q2), Ijr = r(D2), Ajr = r(A7), Njr = r(O7), Cjr = r(J2), Pjr = r(y4), Djr = r(H4), Ljr = r(y3), Rjr = r(C7), jjr = r(U8), Gjr = r(tp), Mjr = r(M2), Bjr = r(g7), qjr = r(qu), Ujr = r(R2), Hjr = r(j2), Xjr = r(i1), Yjr = r(Wu), Vjr = r(yv), zjr = r(P7), Kjr = r(f1), Wjr = r(k4), Jjr = r(es), $jr = r(U2), Zjr = r(ns), Qjr = r(W6), rGr = r(P8), eGr = r(wu), nGr = [0, r("src/parser/parser_env.ml"), 343, 9], tGr = r("Internal Error: Tried to add_declared_private with outside of class scope."), uGr = r("Internal Error: `exit_class` called before a matching `enter_class`"), iGr = r(C), fGr = [0, 0, 0], xGr = [0, 0, 0], aGr = r("Parser_env.Try.Rollback"), oGr = r(C), cGr = r(C), sGr = [0, r(wu), r(zx), r(ia), r(gU), r(AY), r(Ia), r(ou), r(wc), r(fx), r(cc), r(Bf), r(Oa), r(Da), r(tc), r(Ca), r(Sf), r(Zo), r(fa), r(Dx), r(Of), r(sx), r(Ao), r(Hf), r(ja), r(Jo), r(Vx), r(Zx), r(dx), r(oa), r(xo), r(ua), r(g7), r(Aa), r(Lf), r(Ea), r(Qx), r(Zf), r(Ha), r(ha), r(P7), r(hc), r(Po), r(Fx), r(Kx), r(df), r(Fc), r(qf), r(vx), r(Wu), r(_x), r(mo), r(Le), r(Qu), r(Qo), r(Va), r(So), r(ra), r(lc), r(co), r(uo), r(Df), r(qa), r(zf), r($f), r(Ec), r(bc), r(lo), r(mf), r(Ka), r(rx), r(nx), r(pi), r(ro), r(Ba), r(wf), r(Ko), r(Ya), r(pc), r(ac), r(Qa), r(ca), r(xx), r(Pf), r(xf), r(Vf), r(tx), r(_o), r(wo), r(Jf), r(uc), r(Tf), r(jx), r(Ua), r(mx), r(hf), r(Af), r(yc), r(ur), r(dc), r($x), r(Xx), r(Lo), r(Wx), r(ux), r(Rx), r(ko), r(Lx), r(kc), r(bx), r($a), r(Sc), r(Ro), r(Wa), r(nc), r(nf), r(Px), r(sa), r(Ax), r(xa), r(Ga), r(Na), r(_c), r(Cx), r(af), r(cf), r(ex), r(Ja), r(ho), r(sc), r(Gf), r(Wo), r(na), r(wa), r(sf), r(r7), r(Co), r(Ox), r(Do), r(Yx), r(ma), r(gc), r(qu), r(zo), r(fo), r(_f), r(_i), r(Kf), r(mc), r($o), r(j7), r(yx), r(po), r(bf), r(Uo), r(Ex), r(Ma), r(yo), r(ix), r(uf), r(jf), r(Tc), r(Nx), r(kx), r(No), r(Ic), r(pa), r(ga), r(vi), r(xc), r(hx), r(jo), r(Vo), r(Oc), r(qx), r(Eo), r(Uf), r(Ff), r(ta), r(Ix), r(Au), r(no), r(io), r(ec), r(lf), r(Fo), r(ba), r(Cf), r(Mx), r(rc), r(Nf), r(Mf), r(Ux), r(Xa), r(Hx), r(vo), r(eo), r(bo), r(s7), r(ka), r(Go), r(Sx), r(Ta), r(la), r(to), r(Wf), r(Mo), r(Io), r(ox), r(O7), r(A7), r(Za), r(ao), r(Qf), r(cH), r(da), r(oH), r(VU), r(kf), r(Fa), r(ax), r(Tx), r(Xf), r(Bo), r(Ef), r(ff), r(To), r(Rf), r(ic), r(yf), r(Ho), r(oo), r(Xo), r(gf), r(ef), r(lx), r(_a), r(px), r(If), r(I7), r(Yo), r(ut), r(Bx), r(of), r(pf), r(Jx), r(Yf), r(za), r(so), r(go), r(va), r(Gx), r(J4)], vGr = [0, r(wu), r(zx), r(ia), r(Ia), r(ou), r(wc), r(fx), r(cc), r(Bf), r(Oa), r(Da), r(tc), r(Ca), r(Sf), r(Zo), r(fa), r(Dx), r(Of), r(sx), r(Ao), r(Hf), r(ja), r(Jo), r(Vx), r(Zx), r(dx), r(oa), r(xo), r(ua), r(g7), r(Aa), r(Lf), r(Ea), r(Qx), r(Zf), r(Ha), r(ha), r(P7), r(hc), r(Po), r(Fx), r(Kx), r(df), r(Fc), r(qf), r(vx), r(Wu), r(_x), r(mo), r(Le), r(Qu), r(Qo), r(Va), r(So), r(ra), r(lc), r(co), r(uo), r(Df), r(qa), r(zf), r($f), r(Ec), r(bc), r(lo), r(mf), r(Ka), r(rx), r(nx), r(pi), r(ro), r(Ba), r(wf), r(Ko), r(Ya), r(pc), r(ac), r(Qa), r(ca), r(xx), r(Pf), r(xf), r(Vf), r(tx), r(_o), r(wo), r(Jf), r(uc), r(Tf), r(jx), r(Ua), r(mx), r(hf), r(Af), r(yc), r(ur), r(dc), r($x), r(Xx), r(Lo), r(Wx), r(ux), r(Rx), r(ko), r(Lx), r(kc), r(bx), r($a), r(Sc), r(Ro), r(Wa), r(nc), r(nf), r(Px), r(sa), r(Ax), r(xa), r(Ga), r(Na), r(_c), r(Cx), r(af), r(cf), r(ex), r(Ja), r(ho), r(sc), r(Gf), r(Wo), r(na), r(wa), r(sf), r(r7), r(Co), r(Ox), r(Do), r(Yx), r(ma), r(gc), r(qu), r(zo), r(fo), r(_f), r(_i), r(Kf), r(mc), r($o), r(j7), r(yx), r(po), r(bf), r(Uo), r(Ex), r(Ma), r(yo), r(ix), r(uf), r(jf), r(Tc), r(Nx), r(kx), r(No), r(Ic), r(pa), r(ga), r(vi), r(xc), r(hx), r(jo), r(Vo), r(Oc), r(qx), r(Eo), r(Uf), r(Ff), r(ta), r(Ix), r(Au), r(no), r(io), r(ec), r(lf), r(Fo), r(ba), r(Cf), r(Mx), r(rc), r(Nf), r(Mf), r(Ux), r(Xa), r(Hx), r(vo), r(eo), r(bo), r(s7), r(ka), r(Go), r(Sx), r(Ta), r(la), r(to), r(Wf), r(Mo), r(Io), r(ox), r(O7), r(A7), r(Za), r(ao), r(Qf), r(da), r(kf), r(Fa), r(ax), r(Tx), r(Xf), r(Bo), r(Ef), r(ff), r(To), r(Rf), r(ic), r(yf), r(Ho), r(oo), r(Xo), r(gf), r(ef), r(lx), r(_a), r(px), r(If), r(I7), r(Yo), r(ut), r(Bx), r(of), r(pf), r(Jx), r(Yf), r(za), r(so), r(go), r(va), r(Gx), r(J4)], lGr = [0, r(df), r(ex), r(yo), r(Kf), r(If), r(zf), r(Ua), r(tx), r(Px), r(wa), r(Jo), r(P7), r(Ya), r(no), r(ic), r(_c), r(mx), r(af), r(eo), r(Ux), r(zx), r(vi), r(kc), r(jx), r($o), r(vo), r(Af), r(_i), r(Ia), r(qx), r(uo), r(Wf), r(lx), r(ix), r(ef), r(Ga), r(Cf), r(po), r(bc), r(xc), r(ha), r(Jx), r(_o), r(fo), r(Fx), r(bo), r(Lx), r(hf), r(ff), r(Fa), r(ro), r(So), r(Vf), r(Va), r(Wa), r(Xf), r(ac), r(Qu), r(Pf), r(Uo), r(yc), r(sa), r(Na), r(mc), r(ux), r(Za), r(Zx), r(Nf), r(xf), r(nc), r(Qf), r(Rx), r(Ma), r(co), r(go), r(la), r(Fo), r($x), r(nx), r(va), r(_a), r(vx), r(ou), r(Qo), r(fa), r(zo), r(pf), r(ga), r(ua), r(sc), r(Rf), r(uc), r(Ha), r(s7), r(Vo), r(Vx), r(wu), r(xo), r(Io), r(tc), r(Ka), r(_x), r(Da), r(kf), r(Mo), r(cc), r(Cx), r(ra), r(na), r(Xa), r(Ff), r(pc), r(io), r(ko), r(mf), r(Eo), r(Of), r(oa), r(wc), r(Fc), r(Dx), r(Oa), r(Bo), r(hx), r(ax), r(Lo), r(Ex), r(Bf), r(da), r(Tf), r($a), r(Yf), r(Xx), r(oo), r(To), r(Co), r(lo), r(Ba), r(Sc), r(dc), r(qu), r(Wu), r(Yo), r(Zo), r(sx), r(hc), r(Ec), r(g7), r(O7), r(_f), r(Ko), r(Ix), r(cf), r(pi), r(Nx), r(Hx), r(Ox), r(Tx), r(uf), r(Wx), r(Ja), r(j7), r(bf), r(Sf), r(Mf), r(Le), r(Ic), r(ma), r(rc), r(lf), r(Jf), r(qf), r(Do), r(ca), r(Df), r(dx), r(xx), r(Ao), r(px), r(Ta), r(Xo), r(to), r(Bx), r(Gf), r(Zf), r(yx), r(mo), r(gc), r(Ho), r(wo), r(xa), r(Ef), r(sf), r(ka), r(ja), r(Gx), r(fx), r(gf), r(Hf), r(Go), r(Ax), r(ho), r(ao), r(bx), r(qa), r(Wo), r(Uf), r(Ro), r(Ea), r(za), r($f), r(of), r(Au), r(rx), r(ta), r(kx), r(No), r(Kx), r(A7), r(jf), r(lc), r(ba), r(Sx), r(Lf), r(Qx), r(Po), r(pa), r(ec), r(Ca), r(jo), r(wf), r(ut), r(Yx), r(yf), r(nf), r(Qa), r(Tc), r(ox), r(Mx), r(I7), r(so), r(r7), r(ia), r(Oc), r(Aa), r(ur)], bGr = [0, r(df), r(ex), r(yo), r(Kf), r(If), r(zf), r(Ua), r(tx), r(Px), r(wa), r(Jo), r(P7), r(Ya), r(no), r(ic), r(_c), r(mx), r(af), r(eo), r(Ux), r(zx), r(vi), r(kc), r(jx), r($o), r(vo), r(Af), r(_i), r(Ia), r(AY), r(qx), r(uo), r(Wf), r(lx), r(ix), r(ef), r(Ga), r(Cf), r(po), r(bc), r(xc), r(ha), r(Jx), r(_o), r(fo), r(Fx), r(bo), r(Lx), r(hf), r(ff), r(Fa), r(ro), r(So), r(oH), r(Vf), r(Va), r(Wa), r(Xf), r(ac), r(Qu), r(Pf), r(Uo), r(yc), r(sa), r(Na), r(mc), r(ux), r(Za), r(Zx), r(Nf), r(xf), r(nc), r(Qf), r(Rx), r(Ma), r(co), r(go), r(la), r(Fo), r($x), r(nx), r(va), r(_a), r(vx), r(ou), r(Qo), r(fa), r(zo), r(pf), r(ga), r(ua), r(sc), r(Rf), r(uc), r(Ha), r(s7), r(Vo), r(Vx), r(wu), r(xo), r(Io), r(tc), r(Ka), r(_x), r(Da), r(kf), r(Mo), r(cc), r(Cx), r(ra), r(na), r(Xa), r(Ff), r(pc), r(io), r(ko), r(mf), r(Eo), r(Of), r(oa), r(wc), r(Fc), r(Dx), r(Oa), r(Bo), r(hx), r(ax), r(Lo), r(Ex), r(Bf), r(da), r(Tf), r($a), r(Yf), r(Xx), r(oo), r(To), r(Co), r(lo), r(Ba), r(Sc), r(dc), r(qu), r(Wu), r(Yo), r(Zo), r(sx), r(hc), r(Ec), r(g7), r(O7), r(_f), r(Ko), r(Ix), r(cf), r(pi), r(Nx), r(Hx), r(Ox), r(Tx), r(uf), r(Wx), r(Ja), r(j7), r(bf), r(Sf), r(Mf), r(Le), r(Ic), r(ma), r(rc), r(lf), r(Jf), r(qf), r(Do), r(ca), r(Df), r(dx), r(xx), r(Ao), r(px), r(Ta), r(Xo), r(to), r(Bx), r(Gf), r(VU), r(Zf), r(yx), r(mo), r(gc), r(Ho), r(wo), r(xa), r(Ef), r(sf), r(ka), r(ja), r(cH), r(Gx), r(fx), r(gf), r(Hf), r(gU), r(Go), r(Ax), r(ho), r(ao), r(bx), r(qa), r(Wo), r(Uf), r(Ro), r(Ea), r(za), r($f), r(of), r(Au), r(rx), r(ta), r(kx), r(No), r(Kx), r(A7), r(jf), r(lc), r(ba), r(Sx), r(Lf), r(Qx), r(Po), r(pa), r(ec), r(Ca), r(jo), r(wf), r(ut), r(Yx), r(yf), r(nf), r(Qa), r(Tc), r(ox), r(Mx), r(I7), r(so), r(r7), r(ia), r(Oc), r(Aa), r(ur)], pGr = r(V4), mGr = r(I2), _Gr = [0, [11, r("Failure while looking up "), [2, 0, [11, r(". Index: "), [4, 0, 0, 0, [11, r(". Length: "), [4, 0, 0, 0, [12, 46, 0]]]]]]], r("Failure while looking up %s. Index: %d. Length: %d.")], yGr = [0, 0, 0, 0], dGr = r("Offset_utils.Offset_lookup_failed"), hGr = r(QY), kGr = r(wE), wGr = r(jY), EGr = r($X), SGr = r($X), gGr = r(jY), FGr = r($c), TGr = r(Xr), OGr = r(Wn), IGr = r("Program"), AGr = r(Yh), NGr = r("BreakStatement"), CGr = r(Yh), PGr = r("ContinueStatement"), DGr = r("DebuggerStatement"), LGr = r(vc), RGr = r("DeclareExportAllDeclaration"), jGr = r(vc), GGr = r(Cv), MGr = r(P2), BGr = r(mi), qGr = r("DeclareExportDeclaration"), UGr = r(Zc), HGr = r(Wn), XGr = r(mt), YGr = r("DeclareModule"), VGr = r(N7), zGr = r("DeclareModuleExports"), KGr = r(Ts), WGr = r(Wn), JGr = r("DoWhileStatement"), $Gr = r("EmptyStatement"), ZGr = r(_O), QGr = r(P2), rMr = r("ExportDefaultDeclaration"), eMr = r(_O), nMr = r(A4), tMr = r(vc), uMr = r("ExportAllDeclaration"), iMr = r(_O), fMr = r(vc), xMr = r(Cv), aMr = r(P2), oMr = r("ExportNamedDeclaration"), cMr = r(hn), sMr = r(Au), vMr = r("ExpressionStatement"), lMr = r(Wn), bMr = r(sU), pMr = r(Ts), mMr = r(ji), _Mr = r("ForStatement"), yMr = r(j8), dMr = r(Wn), hMr = r(Nu), kMr = r(li), wMr = r("ForInStatement"), EMr = r(wx), SMr = r(Wn), gMr = r(Nu), FMr = r(li), TMr = r("ForOfStatement"), OMr = r(_3), IMr = r(kv), AMr = r(Ts), NMr = r("IfStatement"), CMr = r($c), PMr = r(es), DMr = r(Bn), LMr = r(pX), RMr = r(vc), jMr = r(Cv), GMr = r("ImportDeclaration"), MMr = r(Wn), BMr = r(Yh), qMr = r("LabeledStatement"), UMr = r(v7), HMr = r("ReturnStatement"), XMr = r(uY), YMr = r("discriminant"), VMr = r("SwitchStatement"), zMr = r(v7), KMr = r("ThrowStatement"), WMr = r(jH), JMr = r(XU), $Mr = r(ut), ZMr = r("TryStatement"), QMr = r(Wn), rBr = r(Ts), eBr = r("WhileStatement"), nBr = r(Wn), tBr = r(ck), uBr = r("WithStatement"), iBr = r(GH), fBr = r("ArrayExpression"), xBr = r(T7), aBr = r(m6), oBr = r(Au), cBr = r(Qu), sBr = r(j7), vBr = r(Os), lBr = r(Wn), bBr = r(Ct), pBr = r(mt), mBr = r("ArrowFunctionExpression"), _Br = r(zO), yBr = r(Nu), dBr = r(li), hBr = r(ul), kBr = r("AssignmentExpression"), wBr = r(Nu), EBr = r(li), SBr = r(ul), gBr = r("BinaryExpression"), FBr = r("CallExpression"), TBr = r(O4), OBr = r(bY), IBr = r("ComprehensionExpression"), ABr = r(_3), NBr = r(kv), CBr = r(Ts), PBr = r("ConditionalExpression"), DBr = r(O4), LBr = r(bY), RBr = r("GeneratorExpression"), jBr = r(vc), GBr = r("ImportExpression"), MBr = r(ZH), BBr = r(XX), qBr = r(Fn), UBr = r(Nu), HBr = r(li), XBr = r(ul), YBr = r("LogicalExpression"), VBr = r("MemberExpression"), zBr = r(Iv), KBr = r(el), WBr = r("MetaProperty"), JBr = r(C2), $Br = r(CX), ZBr = r(UH), QBr = r("NewExpression"), rqr = r(X4), eqr = r("ObjectExpression"), nqr = r(Bu), tqr = r("OptionalCallExpression"), uqr = r(Bu), iqr = r("OptionalMemberExpression"), fqr = r(Ug), xqr = r("SequenceExpression"), aqr = r("Super"), oqr = r("ThisExpression"), cqr = r(N7), sqr = r(Au), vqr = r("TypeCastExpression"), lqr = r(v7), bqr = r("AwaitExpression"), pqr = r(Oo), mqr = r(as), _qr = r(rf), yqr = r(tV), dqr = r(es), hqr = r(ns), kqr = r(J2), wqr = r("matched above"), Eqr = r(v7), Sqr = r(XE), gqr = r(ul), Fqr = r("UnaryExpression"), Tqr = r(lV), Oqr = r(mH), Iqr = r(XE), Aqr = r(v7), Nqr = r(ul), Cqr = r("UpdateExpression"), Pqr = r(yY), Dqr = r(v7), Lqr = r("YieldExpression"), Rqr = r("Unexpected FunctionDeclaration with BodyExpression"), jqr = r(T7), Gqr = r(m6), Mqr = r(Au), Bqr = r(Qu), qqr = r(j7), Uqr = r(Os), Hqr = r(Wn), Xqr = r(Ct), Yqr = r(mt), Vqr = r("FunctionDeclaration"), zqr = r("Unexpected FunctionExpression with BodyExpression"), Kqr = r(T7), Wqr = r(m6), Jqr = r(Au), $qr = r(Qu), Zqr = r(j7), Qqr = r(Os), rUr = r(Wn), eUr = r(Ct), nUr = r(mt), tUr = r("FunctionExpression"), uUr = r(Bu), iUr = r(N7), fUr = r(ti), xUr = r(gn), aUr = r(Bu), oUr = r(N7), cUr = r(ti), sUr = r("PrivateIdentifier"), vUr = r(Bu), lUr = r(N7), bUr = r(ti), pUr = r(gn), mUr = r(kv), _Ur = r(Ts), yUr = r("SwitchCase"), dUr = r(Wn), hUr = r("param"), kUr = r("CatchClause"), wUr = r(Wn), EUr = r("BlockStatement"), SUr = r(mt), gUr = r("DeclareVariable"), FUr = r(Qu), TUr = r(mt), OUr = r("DeclareFunction"), IUr = r(Vy), AUr = r(gs), NUr = r(C7), CUr = r(Wn), PUr = r(T7), DUr = r(mt), LUr = r("DeclareClass"), RUr = r(C7), jUr = r(Wn), GUr = r(T7), MUr = r(mt), BUr = r("DeclareInterface"), qUr = r(Bn), UUr = r($c), HUr = r(A4), XUr = r("ExportNamespaceSpecifier"), YUr = r(Nu), VUr = r(T7), zUr = r(mt), KUr = r("DeclareTypeAlias"), WUr = r(Nu), JUr = r(T7), $Ur = r(mt), ZUr = r("TypeAlias"), QUr = r("DeclareOpaqueType"), rHr = r("OpaqueType"), eHr = r(IX), nHr = r(kX), tHr = r(T7), uHr = r(mt), iHr = r("ClassDeclaration"), fHr = r("ClassExpression"), xHr = r(B_), aHr = r(gs), oHr = r("superTypeParameters"), cHr = r("superClass"), sHr = r(T7), vHr = r(Wn), lHr = r(mt), bHr = r(Au), pHr = r("Decorator"), mHr = r(T7), _Hr = r(mt), yHr = r("ClassImplements"), dHr = r(Wn), hHr = r("ClassBody"), kHr = r(wv), wHr = r(F2), EHr = r(t1), SHr = r(lv), gHr = r(B_), FHr = r(pv), THr = r(eu), OHr = r(Zc), IHr = r(Bn), AHr = r(ui), NHr = r("MethodDefinition"), CHr = r(T2), PHr = r(ou), DHr = r(eu), LHr = r(pv), RHr = r(N7), jHr = r(Bn), GHr = r(ui), MHr = r(vV), BHr = r("Internal Error: Private name found in class prop"), qHr = r(T2), UHr = r(ou), HHr = r(eu), XHr = r(pv), YHr = r(N7), VHr = r(Bn), zHr = r(ui), KHr = r(vV), WHr = r(mt), JHr = r(PX), $Hr = r(ji), ZHr = r(mt), QHr = r("EnumStringMember"), rXr = r(mt), eXr = r(PX), nXr = r(ji), tXr = r(mt), uXr = r("EnumNumberMember"), iXr = r(ji), fXr = r(mt), xXr = r("EnumBooleanMember"), aXr = r(O8), oXr = r(jT), cXr = r(N4), sXr = r("EnumBooleanBody"), vXr = r(O8), lXr = r(jT), bXr = r(N4), pXr = r("EnumNumberBody"), mXr = r(O8), _Xr = r(jT), yXr = r(N4), dXr = r("EnumStringBody"), hXr = r(O8), kXr = r(N4), wXr = r("EnumSymbolBody"), EXr = r(Wn), SXr = r(mt), gXr = r("EnumDeclaration"), FXr = r(C7), TXr = r(Wn), OXr = r(T7), IXr = r(mt), AXr = r("InterfaceDeclaration"), NXr = r(T7), CXr = r(mt), PXr = r("InterfaceExtends"), DXr = r(N7), LXr = r(X4), RXr = r("ObjectPattern"), jXr = r(N7), GXr = r(GH), MXr = r("ArrayPattern"), BXr = r(Nu), qXr = r(li), UXr = r(jF), HXr = r(N7), XXr = r(ti), YXr = r(gn), VXr = r(v7), zXr = r(cX), KXr = r(v7), WXr = r(cX), JXr = r(Nu), $Xr = r(li), ZXr = r(jF), QXr = r(ji), rYr = r(ji), eYr = r(t1), nYr = r(lv), tYr = r(bH), uYr = r(pv), iYr = r(x6), fYr = r(F2), xYr = r(Zc), aYr = r(Bn), oYr = r(ui), cYr = r(wU), sYr = r(v7), vYr = r("SpreadProperty"), lYr = r(Nu), bYr = r(li), pYr = r(jF), mYr = r(pv), _Yr = r(x6), yYr = r(F2), dYr = r(Zc), hYr = r(Bn), kYr = r(ui), wYr = r(wU), EYr = r(v7), SYr = r("SpreadElement"), gYr = r(j8), FYr = r(Nu), TYr = r(li), OYr = r("ComprehensionBlock"), IYr = r("We should not create Literal nodes for bigints"), AYr = r(UX), NYr = r(pi), CYr = r("regex"), PYr = r(o7), DYr = r(Bn), LYr = r(o7), RYr = r(Bn), jYr = r(X6), GYr = r(o7), MYr = r(Bn), BYr = r(X6), qYr = r(a1), UYr = r(Bn), HYr = r("BigIntLiteral"), XYr = r(o7), YYr = r(Bn), VYr = r(X6), zYr = r(Gi), KYr = r(Ci), WYr = r(o7), JYr = r(Bn), $Yr = r(X6), ZYr = r(Ug), QYr = r("quasis"), rVr = r("TemplateLiteral"), eVr = r(GY), nVr = r(o7), tVr = r(bU), uVr = r(Bn), iVr = r("TemplateElement"), fVr = r(OY), xVr = r("tag"), aVr = r("TaggedTemplateExpression"), oVr = r(U2), cVr = r(G2), sVr = r(D2), vVr = r(Zc), lVr = r("declarations"), bVr = r("VariableDeclaration"), pVr = r(ji), mVr = r(mt), _Vr = r("VariableDeclarator"), yVr = r(Zc), dVr = r("Variance"), hVr = r("AnyTypeAnnotation"), kVr = r("MixedTypeAnnotation"), wVr = r("EmptyTypeAnnotation"), EVr = r("VoidTypeAnnotation"), SVr = r("NullLiteralTypeAnnotation"), gVr = r("SymbolTypeAnnotation"), FVr = r("NumberTypeAnnotation"), TVr = r("BigIntTypeAnnotation"), OVr = r("StringTypeAnnotation"), IVr = r("BooleanTypeAnnotation"), AVr = r(N7), NVr = r("NullableTypeAnnotation"), CVr = r(T7), PVr = r(ch), DVr = r(m6), LVr = r(f1), RVr = r(Ct), jVr = r("FunctionTypeAnnotation"), GVr = r(Bu), MVr = r(N7), BVr = r(ti), qVr = r(qH), UVr = r(Bu), HVr = r(N7), XVr = r(ti), YVr = r(qH), VVr = [0, 0, 0, 0, 0], zVr = r("internalSlots"), KVr = r("callProperties"), WVr = r("indexers"), JVr = r(X4), $Vr = r("exact"), ZVr = r(HY), QVr = r("ObjectTypeAnnotation"), rzr = r(bH), ezr = r("There should not be computed object type property keys"), nzr = r(ji), tzr = r(t1), uzr = r(lv), izr = r(Zc), fzr = r(ou), xzr = r(Y3), azr = r(eu), ozr = r(Bu), czr = r(F2), szr = r(Bn), vzr = r(ui), lzr = r("ObjectTypeProperty"), bzr = r(v7), pzr = r("ObjectTypeSpreadProperty"), mzr = r(ou), _zr = r(eu), yzr = r(Bn), dzr = r(ui), hzr = r(mt), kzr = r("ObjectTypeIndexer"), wzr = r(eu), Ezr = r(Bn), Szr = r("ObjectTypeCallProperty"), gzr = r(Bn), Fzr = r(F2), Tzr = r(eu), Ozr = r(Bu), Izr = r(mt), Azr = r("ObjectTypeInternalSlot"), Nzr = r(Wn), Czr = r(C7), Pzr = r("InterfaceTypeAnnotation"), Dzr = r("elementType"), Lzr = r("ArrayTypeAnnotation"), Rzr = r(mt), jzr = r(fY), Gzr = r("QualifiedTypeIdentifier"), Mzr = r(T7), Bzr = r(mt), qzr = r("GenericTypeAnnotation"), Uzr = r("indexType"), Hzr = r("objectType"), Xzr = r("IndexedAccessType"), Yzr = r(Bu), Vzr = r("OptionalIndexedAccessType"), zzr = r(Z6), Kzr = r("UnionTypeAnnotation"), Wzr = r(Z6), Jzr = r("IntersectionTypeAnnotation"), $zr = r(v7), Zzr = r("TypeofTypeAnnotation"), Qzr = r(mt), rKr = r(fY), eKr = r("QualifiedTypeofIdentifier"), nKr = r(Z6), tKr = r("TupleTypeAnnotation"), uKr = r(o7), iKr = r(Bn), fKr = r("StringLiteralTypeAnnotation"), xKr = r(o7), aKr = r(Bn), oKr = r("NumberLiteralTypeAnnotation"), cKr = r(o7), sKr = r(Bn), vKr = r("BigIntLiteralTypeAnnotation"), lKr = r(Gi), bKr = r(Ci), pKr = r(o7), mKr = r(Bn), _Kr = r("BooleanLiteralTypeAnnotation"), yKr = r("ExistsTypeAnnotation"), dKr = r(N7), hKr = r("TypeAnnotation"), kKr = r(Ct), wKr = r("TypeParameterDeclaration"), EKr = r(mi), SKr = r(ou), gKr = r(MU), FKr = r(ti), TKr = r("TypeParameter"), OKr = r(Ct), IKr = r(AH), AKr = r(Ct), NKr = r(AH), CKr = r(bv), PKr = r(Ve), DKr = r("closingElement"), LKr = r("openingElement"), RKr = r("JSXElement"), jKr = r("closingFragment"), GKr = r(Ve), MKr = r("openingFragment"), BKr = r("JSXFragment"), qKr = r("selfClosing"), UKr = r(kY), HKr = r(ti), XKr = r("JSXOpeningElement"), YKr = r("JSXOpeningFragment"), VKr = r(ti), zKr = r("JSXClosingElement"), KKr = r("JSXClosingFragment"), WKr = r(Bn), JKr = r(ti), $Kr = r("JSXAttribute"), ZKr = r(v7), QKr = r("JSXSpreadAttribute"), rWr = r("JSXEmptyExpression"), eWr = r(Au), nWr = r("JSXExpressionContainer"), tWr = r(Au), uWr = r("JSXSpreadChild"), iWr = r(o7), fWr = r(Bn), xWr = r("JSXText"), aWr = r(Iv), oWr = r(ck), cWr = r("JSXMemberExpression"), sWr = r(ti), vWr = r("namespace"), lWr = r("JSXNamespacedName"), bWr = r(ti), pWr = r("JSXIdentifier"), mWr = r(A4), _Wr = r(B2), yWr = r("ExportSpecifier"), dWr = r(B2), hWr = r("ImportDefaultSpecifier"), kWr = r(B2), wWr = r("ImportNamespaceSpecifier"), EWr = r(pX), SWr = r(B2), gWr = r("imported"), FWr = r("ImportSpecifier"), TWr = r("Line"), OWr = r("Block"), IWr = r(Bn), AWr = r(Bn), NWr = r("DeclaredPredicate"), CWr = r("InferredPredicate"), PWr = r(C2), DWr = r(CX), LWr = r(UH), RWr = r(pv), jWr = r(Iv), GWr = r(ck), MWr = r("message"), BWr = r(wE), qWr = r(KH), UWr = r(S7), HWr = r(vc), XWr = r(I2), YWr = r(V4), VWr = [0, [3, 0, 0], r(Yt)], zWr = r(M2), KWr = r(N3), WWr = r(R2), JWr = r(j2), $Wr = r(Wu), ZWr = r(P7), QWr = r(f1), rJr = r(g7), eJr = r(k4), nJr = r(U2), tJr = r(W6), uJr = r(P8), iJr = r(D2), fJr = r(G2), xJr = r(xs), aJr = r(Ci), oJr = r(Gi), cJr = r(I7), sJr = r(k6), vJr = r(o6), lJr = r(A7), bJr = r(mi), pJr = r(y4), mJr = r(U8), _Jr = r(tp), yJr = r(q2), dJr = r(C7), hJr = r(eu), kJr = r(H4), wJr = r(i1), EJr = r(J2), SJr = r(es), gJr = r(ns), FJr = r(p8), TJr = r(y3), OJr = r(qu), IJr = r(yv), AJr = r(gs), NJr = r(r7), CJr = r(d4), PJr = r(w4), DJr = r(c6), LJr = r(S6), RJr = r(wu), jJr = r(O7), GJr = r(T2), MJr = r($c), BJr = r(ud), qJr = r(LS), UJr = r(Os), HJr = r(wx), XJr = r(t6), YJr = r(X8), VJr = r(s7), zJr = r(hv), KJr = r(a1), WJr = r(Tv), JJr = r(ns), $Jr = r(W4), ZJr = r(O2), QJr = r(I6), r$r = [0, r(F3)], e$r = r(C), n$r = [7, 0], t$r = r(C), u$r = [0, 1], i$r = [0, 2], f$r = [0, 3], x$r = [0, 0], a$r = [0, 0], o$r = [0, 0, 0, 0, 0], c$r = [0, r(vv), 906, 6], s$r = [0, r(vv), tY, 6], v$r = [0, 0], l$r = [0, r(vv), 1012, 8], b$r = r(Y3), p$r = [0, r(vv), 1029, 8], m$r = r("Can not have both `static` and `proto`"), _$r = r(eu), y$r = r(Y3), d$r = r(t1), h$r = r(lv), k$r = r(t1), w$r = r(wv), E$r = r(lH), S$r = [0, 0, 0, 0], g$r = [0, [0, 0, 0, 0, 0]], F$r = r(f1), T$r = [0, r("a type")], O$r = [0, 0], I$r = [0, 0], A$r = [14, 1], N$r = [14, 0], C$r = [0, r(vv), OH, 15], P$r = [0, r(vv), D7, 15], D$r = [0, 44], L$r = [0, 44], R$r = r(M2), j$r = [0, r(C), 0], G$r = [0, 0, 0], M$r = [0, 0, 0], B$r = [0, 0, 0], q$r = [0, 41], U$r = r(Zu), H$r = r(Zu), X$r = [0, r("a regular expression")], Y$r = r(C), V$r = r(C), z$r = r(C), K$r = [0, r("src/parser/expression_parser.ml"), jU, 17], W$r = [0, r("a template literal part")], J$r = [0, [0, r(C), r(C)], 1], $$r = r(xs), Z$r = r(xs), Q$r = r(Gi), rZr = r(Ci), eZr = r("Invalid bigint "), nZr = r("Invalid bigint binary/octal "), tZr = r(H2), uZr = r(hH), iZr = r(Dd), fZr = r(Dd), xZr = r(lU), aZr = [0, 44], oZr = [0, 1], cZr = [0, 1], sZr = [0, 1], vZr = [0, 1], lZr = [0, 0], bZr = r(bv), pZr = r(bv), mZr = r(i1), _Zr = r(OS), yZr = [0, r("the identifier `target`")], dZr = [0, 0], hZr = r(qu), kZr = r(el), wZr = r(el), EZr = r(yv), SZr = [0, 0], gZr = [0, r("either a call or access of `super`")], FZr = r(yv), TZr = [0, 0], OZr = [0, 1], IZr = [0, 0], AZr = [0, 1], NZr = [0, 0], CZr = [0, 1], PZr = [0, 0], DZr = [0, 2], LZr = [0, 3], RZr = [0, 7], jZr = [0, 6], GZr = [0, 4], MZr = [0, 5], BZr = [0, [0, 17, [0, 2]]], qZr = [0, [0, 18, [0, 3]]], UZr = [0, [0, 19, [0, 4]]], HZr = [0, [0, 0, [0, 5]]], XZr = [0, [0, 1, [0, 5]]], YZr = [0, [0, 2, [0, 5]]], VZr = [0, [0, 3, [0, 5]]], zZr = [0, [0, 5, [0, 6]]], KZr = [0, [0, 7, [0, 6]]], WZr = [0, [0, 4, [0, 6]]], JZr = [0, [0, 6, [0, 6]]], $Zr = [0, [0, 8, [0, 7]]], ZZr = [0, [0, 9, [0, 7]]], QZr = [0, [0, 10, [0, 7]]], rQr = [0, [0, 11, [0, 8]]], eQr = [0, [0, 12, [0, 8]]], nQr = [0, [0, 15, [0, 9]]], tQr = [0, [0, 13, [0, 9]]], uQr = [0, [0, 14, [1, 10]]], iQr = [0, [0, 16, [0, 9]]], fQr = [0, [0, 21, [0, 6]]], xQr = [0, [0, 20, [0, 6]]], aQr = [23, r(Fn)], oQr = [0, [0, 8]], cQr = [0, [0, 7]], sQr = [0, [0, 6]], vQr = [0, [0, 10]], lQr = [0, [0, 9]], bQr = [0, [0, 11]], pQr = [0, [0, 5]], mQr = [0, [0, 4]], _Qr = [0, [0, 2]], yQr = [0, [0, 3]], dQr = [0, [0, 1]], hQr = [0, [0, 0]], kQr = [0, [0, 12]], wQr = [0, [0, 13]], EQr = [0, [0, 14]], SQr = [0, 0], gQr = r(qu), FQr = r(i1), TQr = r(OS), OQr = r(el), IQr = r(Os), AQr = r(qu), NQr = r(i1), CQr = r(OS), PQr = r(el), DQr = r(o1), LQr = r(Ra), RQr = [17, r("JSX fragment")], jQr = [0, Ni], GQr = [1, Ni], MQr = r(C), BQr = [0, r(C)], qQr = [0, r(F3)], UQr = r(C), HQr = [0, 0, 0, 0], XQr = [0, r("src/hack_forked/utils/collections/flow_map.ml"), 717, 36], YQr = [0, 0, 0], VQr = r(q2), zQr = [0, r(C), 0], KQr = r("unexpected PrivateName in Property, expected a PrivateField"), WQr = r(wv), JQr = r(lH), $Qr = [0, 0, 0], ZQr = r(wv), QQr = r(wv), r0e = r(t1), e0e = r(lv), n0e = [0, 1], t0e = [0, 1], u0e = [0, 1], i0e = r(wv), f0e = r(t1), x0e = r(lv), a0e = r(zO), o0e = r(wu), c0e = r(wx), s0e = r("Internal Error: private name found in object props"), v0e = r(pV), l0e = [0, r(F3)], b0e = r(wu), p0e = r(wx), m0e = r(wu), _0e = r(wx), y0e = r(pV), d0e = [10, r(_i)], h0e = [0, 1], k0e = r(c1), w0e = r(K2), E0e = [0, r(GS), 1763, 21], S0e = r(K2), g0e = r(c1), F0e = [0, r("a declaration, statement or export specifiers")], T0e = [0, 40], O0e = r(c1), I0e = r(K2), A0e = [0, r(C), r(C), 0], N0e = [0, r(OU)], C0e = r(hU), P0e = r("exports"), D0e = [0, 1], L0e = [0, 1], R0e = [0, 0], j0e = r(hU), G0e = [0, 40], M0e = r(Vy), B0e = [0, 0], q0e = [0, 1], U0e = [0, 83], H0e = [0, 0], X0e = [0, 1], Y0e = r(c1), V0e = r(c1), z0e = r(K2), K0e = r(c1), W0e = [0, r("the keyword `as`")], J0e = r(c1), $0e = r(K2), Z0e = [0, r(OU)], Q0e = [0, r("the keyword `from`")], rre = [0, r(C), r(C), 0], ere = [0, r(aU)], nre = r("Label"), tre = [0, r(aU)], ure = [0, 0, 0], ire = [0, 29], fre = [0, r(GS), 431, 22], xre = [0, 28], are = [0, r(GS), 450, 22], ore = [0, 0], cre = r("the token `;`"), sre = [0, 0], vre = [0, 0], lre = r(wx), bre = r(G2), pre = r(wu), mre = [0, r(KU)], _re = [15, [0, 0]], yre = [0, r(KU)], dre = r("use strict"), hre = [0, 0, 0, 0], kre = r(UI), wre = r("Nooo: "), Ere = r(mi), Sre = r("Parser error: No such thing as an expression pattern!"), gre = r(C), Fre = [0, [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]], Tre = [0, r("src/parser/parser_flow.ml"), DT, 28], Ore = [0, [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]], Ire = r(Bn), Are = r(QY), Nre = r(I2), Cre = r(V4), Pre = r(KH), Dre = r(I2), Lre = r(V4), Rre = r(S7), jre = r(wE), Gre = r("normal"), Mre = r($c), Bre = r("jsxTag"), qre = r("jsxChild"), Ure = r("template"), Hre = r(XH), Xre = r("context"), Yre = r($c), Vre = r("use_strict"), zre = r(Z6), Kre = r("esproposal_export_star_as"), Wre = r("esproposal_decorators"), Jre = r("enums"), $re = r("Internal error: ");
function jt(t) {
if (typeof t == "number")
return 0;
switch (t[0]) {
case 0:
return [0, jt(t[1])];
case 1:
return [1, jt(t[1])];
case 2:
return [2, jt(t[1])];
case 3:
return [3, jt(t[1])];
case 4:
return [4, jt(t[1])];
case 5:
return [5, jt(t[1])];
case 6:
return [6, jt(t[1])];
case 7:
return [7, jt(t[1])];
case 8:
var n4 = t[1];
return [8, n4, jt(t[2])];
case 9:
var e = t[1];
return [9, e, e, jt(t[3])];
case 10:
return [10, jt(t[1])];
case 11:
return [11, jt(t[1])];
case 12:
return [12, jt(t[1])];
case 13:
return [13, jt(t[1])];
default:
return [14, jt(t[1])];
}
}
function t7(t, n4) {
if (typeof t == "number")
return n4;
switch (t[0]) {
case 0:
return [0, t7(t[1], n4)];
case 1:
return [1, t7(t[1], n4)];
case 2:
return [2, t7(t[1], n4)];
case 3:
return [3, t7(t[1], n4)];
case 4:
return [4, t7(t[1], n4)];
case 5:
return [5, t7(t[1], n4)];
case 6:
return [6, t7(t[1], n4)];
case 7:
return [7, t7(t[1], n4)];
case 8:
var e = t[1];
return [8, e, t7(t[2], n4)];
case 9:
var i = t[2], x = t[1];
return [9, x, i, t7(t[3], n4)];
case 10:
return [10, t7(t[1], n4)];
case 11:
return [11, t7(t[1], n4)];
case 12:
return [12, t7(t[1], n4)];
case 13:
return [13, t7(t[1], n4)];
default:
return [14, t7(t[1], n4)];
}
}
function It(t, n4) {
if (typeof t == "number")
return n4;
switch (t[0]) {
case 0:
return [0, It(t[1], n4)];
case 1:
return [1, It(t[1], n4)];
case 2:
var e = t[1];
return [2, e, It(t[2], n4)];
case 3:
var i = t[1];
return [3, i, It(t[2], n4)];
case 4:
var x = t[3], c = t[2], s = t[1];
return [4, s, c, x, It(t[4], n4)];
case 5:
var p = t[3], y = t[2], T = t[1];
return [5, T, y, p, It(t[4], n4)];
case 6:
var E = t[3], h = t[2], w = t[1];
return [6, w, h, E, It(t[4], n4)];
case 7:
var G = t[3], A = t[2], S = t[1];
return [7, S, A, G, It(t[4], n4)];
case 8:
var M = t[3], K = t[2], V = t[1];
return [8, V, K, M, It(t[4], n4)];
case 9:
var f0 = t[1];
return [9, f0, It(t[2], n4)];
case 10:
return [10, It(t[1], n4)];
case 11:
var m0 = t[1];
return [11, m0, It(t[2], n4)];
case 12:
var k0 = t[1];
return [12, k0, It(t[2], n4)];
case 13:
var g0 = t[2], e0 = t[1];
return [13, e0, g0, It(t[3], n4)];
case 14:
var x0 = t[2], l = t[1];
return [14, l, x0, It(t[3], n4)];
case 15:
return [15, It(t[1], n4)];
case 16:
return [16, It(t[1], n4)];
case 17:
var c0 = t[1];
return [17, c0, It(t[2], n4)];
case 18:
var t0 = t[1];
return [18, t0, It(t[2], n4)];
case 19:
return [19, It(t[1], n4)];
case 20:
var a0 = t[2], w0 = t[1];
return [20, w0, a0, It(t[3], n4)];
case 21:
var _0 = t[1];
return [21, _0, It(t[2], n4)];
case 22:
return [22, It(t[1], n4)];
case 23:
var E0 = t[1];
return [23, E0, It(t[2], n4)];
default:
var X0 = t[2], b = t[1];
return [24, b, X0, It(t[3], n4)];
}
}
function iN(t, n4, e) {
return t[1] === n4 ? (t[1] = e, 1) : 0;
}
function ke(t) {
throw [0, B7, t];
}
function Cu(t) {
throw [0, eN, t];
}
G7(0);
function Fp(t) {
return 0 <= t ? t : -t | 0;
}
var Zre = kH;
function Te(t, n4) {
var e = nn(t), i = nn(n4), x = Pt(e + i | 0);
return As(t, 0, x, 0, e), As(n4, 0, x, e, i), x;
}
function Qre(t) {
return t ? ki0 : wi0;
}
function un(t, n4) {
if (t) {
var e = t[1];
return [0, e, un(t[2], n4)];
}
return n4;
}
ri0(0);
var ree = ZV(1), Lc = ZV(2);
function eee(t) {
function n4(e) {
for (var i = e; ; ) {
if (i) {
var x = i[2], c = i[1];
try {
m1(c);
} catch (y) {
if (y = Et(y), y[1] !== nz)
throw y;
var s = y;
}
var i = x;
continue;
}
return 0;
}
}
return n4(ei0(0));
}
function vl(t, n4) {
return JA(t, n4, 0, nn(n4));
}
function cz(t) {
return vl(Lc, t), QV(Lc, 10), m1(Lc);
}
var fN = [0, eee];
function sz(t) {
for (; ; ) {
var n4 = fN[1], e = [0, 1], i = 1 - iN(fN, n4, function(x, c) {
return function(s) {
return iN(x, 1, 0) && u(t, 0), u(c, 0);
};
}(e, n4));
if (!i)
return i;
}
}
function xN(t) {
return u(fN[1], 0);
}
ZA(r(mV), xN), oi0(0) && sz(function(t) {
return O70(t);
});
function vz(t) {
return 25 < (t + V3 | 0) >>> 0 ? t : t + SU | 0;
}
var lz = si0(0)[1], ll = (4 * ai0(0) | 0) - 1 | 0;
G7(0);
var nee = xi0(0);
function Rc(t) {
for (var n4 = 0, e = t; ; ) {
if (e) {
var n4 = n4 + 1 | 0, e = e[2];
continue;
}
return n4;
}
}
function bl(t) {
return t ? t[1] : ke(Ni0);
}
function bz(t) {
return t ? t[2] : ke(Ai0);
}
function jc(t, n4) {
for (var e = t, i = n4; ; ) {
if (e) {
var x = [0, e[1], i], e = e[2], i = x;
continue;
}
return i;
}
}
function de(t) {
return jc(t, 0);
}
function pl(t) {
if (t) {
var n4 = t[1];
return un(n4, pl(t[2]));
}
return 0;
}
function k1(t, n4) {
if (n4) {
var e = n4[2], i = u(t, n4[1]);
return [0, i, k1(t, e)];
}
return 0;
}
function Tp(t, n4) {
for (var e = 0, i = n4; ; ) {
if (i) {
var x = i[2], e = [0, u(t, i[1]), e], i = x;
continue;
}
return e;
}
}
function Pu(t, n4) {
for (var e = n4; ; ) {
if (e) {
var i = e[2];
u(t, e[1]);
var e = i;
continue;
}
return 0;
}
}
function be(t, n4, e) {
for (var i = n4, x = e; ; ) {
if (x) {
var c = x[2], i = a(t, i, x[1]), x = c;
continue;
}
return i;
}
}
function aN(t, n4, e) {
if (n4) {
var i = n4[1];
return a(t, i, aN(t, n4[2], e));
}
return e;
}
function pz(t, n4, e) {
for (var i = n4, x = e; ; ) {
if (i) {
if (x) {
var c = x[2], s = i[2];
a(t, i[1], x[1]);
var i = s, x = c;
continue;
}
} else if (!x)
return 0;
return Cu(Ii0);
}
}
function oN(t, n4) {
for (var e = n4; ; ) {
if (e) {
var i = e[2], x = BV(e[1], t) === 0 ? 1 : 0;
if (x)
return x;
var e = i;
continue;
}
return 0;
}
}
function tee(t, n4) {
for (var e = n4; ; ) {
if (e) {
var i = e[1], x = e[2], c = i[2];
if (BV(i[1], t) === 0)
return c;
var e = x;
continue;
}
throw Kt;
}
}
function ml(t) {
var n4 = 0;
return function(e) {
for (var i = n4, x = e; ; ) {
if (x) {
var c = x[2], s = x[1];
if (u(t, s)) {
var i = [0, s, i], x = c;
continue;
}
var x = c;
continue;
}
return de(i);
}
};
}
function w1(t, n4) {
var e = Pt(t);
return T70(e, 0, t, n4), e;
}
function mz(t) {
var n4 = l7(t), e = Pt(n4);
return Is(t, 0, e, 0, n4), e;
}
function _z(t, n4, e) {
if (0 <= n4 && 0 <= e && !((l7(t) - e | 0) < n4)) {
var i = Pt(e);
return Is(t, n4, i, 0, e), i;
}
return Cu(Di0);
}
function qv(t, n4, e) {
return _z(t, n4, e);
}
function yz(t, n4, e, i, x) {
return 0 <= x && 0 <= n4 && !((l7(t) - x | 0) < n4) && 0 <= i && !((l7(e) - x | 0) < i) ? Is(t, n4, e, i, x) : Cu(Pi0);
}
function ss(t, n4, e, i, x) {
return 0 <= x && 0 <= n4 && !((nn(t) - x | 0) < n4) && 0 <= i && !((l7(e) - x | 0) < i) ? As(t, n4, e, i, x) : Cu(Ci0);
}
function Op(t, n4) {
return w1(t, n4);
}
function p7(t, n4, e) {
return _z(t, n4, e);
}
var dz = Ee;
function hz(t, n4) {
var e = n4.length - 1 - 1 | 0, i = 0;
if (!(e < 0))
for (var x = i; ; ) {
u(t, n4[1 + x]);
var c = x + 1 | 0;
if (e !== x) {
var x = c;
continue;
}
break;
}
return 0;
}
function Ip(t, n4) {
var e = n4.length - 1;
if (e === 0)
return [0];
var i = Gv(e, u(t, n4[1])), x = e - 1 | 0, c = 1;
if (!(x < 1))
for (var s = c; ; ) {
i[1 + s] = u(t, n4[1 + s]);
var p = s + 1 | 0;
if (x !== s) {
var s = p;
continue;
}
break;
}
return i;
}
function _l(t) {
if (t)
for (var n4 = 0, e = t, i = t[2], x = t[1]; ; ) {
if (e) {
var n4 = n4 + 1 | 0, e = e[2];
continue;
}
for (var c = Gv(n4, x), s = 1, p = i; ; ) {
if (p) {
var y = p[2];
c[1 + s] = p[1];
var s = s + 1 | 0, p = y;
continue;
}
return c;
}
}
return [0];
}
G7(0);
function cN(t) {
function n4(v0) {
return v0 ? v0[5] : 0;
}
function e(v0, P, L, Q) {
var i0 = n4(v0), l0 = n4(Q), S0 = l0 <= i0 ? i0 + 1 | 0 : l0 + 1 | 0;
return [0, v0, P, L, Q, S0];
}
function i(v0, P) {
return [0, 0, v0, P, 0, 1];
}
function x(v0, P, L, Q) {
var i0 = v0 ? v0[5] : 0, l0 = Q ? Q[5] : 0;
if ((l0 + 2 | 0) < i0) {
if (v0) {
var S0 = v0[4], T0 = v0[3], rr = v0[2], R0 = v0[1], B = n4(S0);
if (B <= n4(R0))
return e(R0, rr, T0, e(S0, P, L, Q));
if (S0) {
var Z = S0[3], p0 = S0[2], b0 = S0[1], O0 = e(S0[4], P, L, Q);
return e(e(R0, rr, T0, b0), p0, Z, O0);
}
return Cu(qi0);
}
return Cu(Ui0);
}
if ((i0 + 2 | 0) < l0) {
if (Q) {
var q0 = Q[4], er = Q[3], yr = Q[2], vr = Q[1], $0 = n4(vr);
if ($0 <= n4(q0))
return e(e(v0, P, L, vr), yr, er, q0);
if (vr) {
var Sr = vr[3], Mr = vr[2], Br = vr[1], qr = e(vr[4], yr, er, q0);
return e(e(v0, P, L, Br), Mr, Sr, qr);
}
return Cu(Hi0);
}
return Cu(Xi0);
}
var jr = l0 <= i0 ? i0 + 1 | 0 : l0 + 1 | 0;
return [0, v0, P, L, Q, jr];
}
var c = 0;
function s(v0) {
return v0 ? 0 : 1;
}
function p(v0, P, L) {
if (L) {
var Q = L[4], i0 = L[3], l0 = L[2], S0 = L[1], T0 = L[5], rr = a(t[1], v0, l0);
if (rr === 0)
return i0 === P ? L : [0, S0, v0, P, Q, T0];
if (0 <= rr) {
var R0 = p(v0, P, Q);
return Q === R0 ? L : x(S0, l0, i0, R0);
}
var B = p(v0, P, S0);
return S0 === B ? L : x(B, l0, i0, Q);
}
return [0, 0, v0, P, 0, 1];
}
function y(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[4], i0 = L[3], l0 = L[1], S0 = a(t[1], v0, L[2]);
if (S0 === 0)
return i0;
var T0 = 0 <= S0 ? Q : l0, L = T0;
continue;
}
throw Kt;
}
}
function T(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[2], i0 = L[4], l0 = L[3], S0 = L[1];
if (u(v0, Q))
for (var T0 = Q, rr = l0, R0 = S0; ; ) {
if (R0) {
var B = R0[2], Z = R0[4], p0 = R0[3], b0 = R0[1];
if (u(v0, B)) {
var T0 = B, rr = p0, R0 = b0;
continue;
}
var R0 = Z;
continue;
}
return [0, T0, rr];
}
var L = i0;
continue;
}
throw Kt;
}
}
function E(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[2], i0 = L[4], l0 = L[3], S0 = L[1];
if (u(v0, Q))
for (var T0 = Q, rr = l0, R0 = S0; ; ) {
if (R0) {
var B = R0[2], Z = R0[4], p0 = R0[3], b0 = R0[1];
if (u(v0, B)) {
var T0 = B, rr = p0, R0 = b0;
continue;
}
var R0 = Z;
continue;
}
return [0, [0, T0, rr]];
}
var L = i0;
continue;
}
return 0;
}
}
function h(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[2], i0 = L[4], l0 = L[3], S0 = L[1];
if (u(v0, Q))
for (var T0 = Q, rr = l0, R0 = i0; ; ) {
if (R0) {
var B = R0[2], Z = R0[4], p0 = R0[3], b0 = R0[1];
if (u(v0, B)) {
var T0 = B, rr = p0, R0 = Z;
continue;
}
var R0 = b0;
continue;
}
return [0, T0, rr];
}
var L = S0;
continue;
}
throw Kt;
}
}
function w(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[2], i0 = L[4], l0 = L[3], S0 = L[1];
if (u(v0, Q))
for (var T0 = Q, rr = l0, R0 = i0; ; ) {
if (R0) {
var B = R0[2], Z = R0[4], p0 = R0[3], b0 = R0[1];
if (u(v0, B)) {
var T0 = B, rr = p0, R0 = Z;
continue;
}
var R0 = b0;
continue;
}
return [0, [0, T0, rr]];
}
var L = S0;
continue;
}
return 0;
}
}
function G(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[4], i0 = L[3], l0 = L[1], S0 = a(t[1], v0, L[2]);
if (S0 === 0)
return [0, i0];
var T0 = 0 <= S0 ? Q : l0, L = T0;
continue;
}
return 0;
}
}
function A(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[4], i0 = L[1], l0 = a(t[1], v0, L[2]), S0 = l0 === 0 ? 1 : 0;
if (S0)
return S0;
var T0 = 0 <= l0 ? Q : i0, L = T0;
continue;
}
return 0;
}
}
function S(v0) {
for (var P = v0; ; ) {
if (P) {
var L = P[1];
if (L) {
var P = L;
continue;
}
return [0, P[2], P[3]];
}
throw Kt;
}
}
function M(v0) {
for (var P = v0; ; ) {
if (P) {
var L = P[1];
if (L) {
var P = L;
continue;
}
return [0, [0, P[2], P[3]]];
}
return 0;
}
}
function K(v0) {
for (var P = v0; ; ) {
if (P) {
if (P[4]) {
var P = P[4];
continue;
}
return [0, P[2], P[3]];
}
throw Kt;
}
}
function V(v0) {
for (var P = v0; ; ) {
if (P) {
if (P[4]) {
var P = P[4];
continue;
}
return [0, [0, P[2], P[3]]];
}
return 0;
}
}
function f0(v0) {
if (v0) {
var P = v0[1];
if (P) {
var L = v0[4], Q = v0[3], i0 = v0[2];
return x(f0(P), i0, Q, L);
}
return v0[4];
}
return Cu(ji0);
}
function m0(v0, P) {
if (v0) {
if (P) {
var L = S(P), Q = L[2], i0 = L[1];
return x(v0, i0, Q, f0(P));
}
return v0;
}
return P;
}
function k0(v0, P) {
if (P) {
var L = P[4], Q = P[3], i0 = P[2], l0 = P[1], S0 = a(t[1], v0, i0);
if (S0 === 0)
return m0(l0, L);
if (0 <= S0) {
var T0 = k0(v0, L);
return L === T0 ? P : x(l0, i0, Q, T0);
}
var rr = k0(v0, l0);
return l0 === rr ? P : x(rr, i0, Q, L);
}
return 0;
}
function g0(v0, P, L) {
if (L) {
var Q = L[4], i0 = L[3], l0 = L[2], S0 = L[1], T0 = L[5], rr = a(t[1], v0, l0);
if (rr === 0) {
var R0 = u(P, [0, i0]);
if (R0) {
var B = R0[1];
return i0 === B ? L : [0, S0, v0, B, Q, T0];
}
return m0(S0, Q);
}
if (0 <= rr) {
var Z = g0(v0, P, Q);
return Q === Z ? L : x(S0, l0, i0, Z);
}
var p0 = g0(v0, P, S0);
return S0 === p0 ? L : x(p0, l0, i0, Q);
}
var b0 = u(P, 0);
return b0 ? [0, 0, v0, b0[1], 0, 1] : 0;
}
function e0(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[4], i0 = L[3], l0 = L[2];
e0(v0, L[1]), a(v0, l0, i0);
var L = Q;
continue;
}
return 0;
}
}
function x0(v0, P) {
if (P) {
var L = P[5], Q = P[4], i0 = P[3], l0 = P[2], S0 = x0(v0, P[1]), T0 = u(v0, i0);
return [0, S0, l0, T0, x0(v0, Q), L];
}
return 0;
}
function l(v0, P) {
if (P) {
var L = P[2], Q = P[5], i0 = P[4], l0 = P[3], S0 = l(v0, P[1]), T0 = a(v0, L, l0);
return [0, S0, L, T0, l(v0, i0), Q];
}
return 0;
}
function c0(v0, P, L) {
for (var Q = P, i0 = L; ; ) {
if (Q) {
var l0 = Q[4], S0 = Q[3], T0 = Q[2], rr = ir(v0, T0, S0, c0(v0, Q[1], i0)), Q = l0, i0 = rr;
continue;
}
return i0;
}
}
function t0(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[4], i0 = L[1], l0 = a(v0, L[2], L[3]);
if (l0) {
var S0 = t0(v0, i0);
if (S0) {
var L = Q;
continue;
}
var T0 = S0;
} else
var T0 = l0;
return T0;
}
return 1;
}
}
function a0(v0, P) {
for (var L = P; ; ) {
if (L) {
var Q = L[4], i0 = L[1], l0 = a(v0, L[2], L[3]);
if (l0)
var S0 = l0;
else {
var T0 = a0(v0, i0);
if (!T0) {
var L = Q;
continue;
}
var S0 = T0;
}
return S0;
}
return 0;
}
}
function w0(v0, P, L) {
if (L) {
var Q = L[4], i0 = L[3], l0 = L[2];
return x(w0(v0, P, L[1]), l0, i0, Q);
}
return i(v0, P);
}
function _0(v0, P, L) {
if (L) {
var Q = L[3], i0 = L[2], l0 = L[1];
return x(l0, i0, Q, _0(v0, P, L[4]));
}
return i(v0, P);
}
function E0(v0, P, L, Q) {
if (v0) {
if (Q) {
var i0 = Q[5], l0 = v0[5], S0 = Q[4], T0 = Q[3], rr = Q[2], R0 = Q[1], B = v0[4], Z = v0[3], p0 = v0[2], b0 = v0[1];
return (i0 + 2 | 0) < l0 ? x(b0, p0, Z, E0(B, P, L, Q)) : (l0 + 2 | 0) < i0 ? x(E0(v0, P, L, R0), rr, T0, S0) : e(v0, P, L, Q);
}
return _0(P, L, v0);
}
return w0(P, L, Q);
}
function X0(v0, P) {
if (v0) {
if (P) {
var L = S(P), Q = L[2], i0 = L[1];
return E0(v0, i0, Q, f0(P));
}
return v0;
}
return P;
}
function b(v0, P, L, Q) {
return L ? E0(v0, P, L[1], Q) : X0(v0, Q);
}
function G0(v0, P) {
if (P) {
var L = P[4], Q = P[3], i0 = P[2], l0 = P[1], S0 = a(t[1], v0, i0);
if (S0 === 0)
return [0, l0, [0, Q], L];
if (0 <= S0) {
var T0 = G0(v0, L), rr = T0[3], R0 = T0[2];
return [0, E0(l0, i0, Q, T0[1]), R0, rr];
}
var B = G0(v0, l0), Z = B[2], p0 = B[1];
return [0, p0, Z, E0(B[3], i0, Q, L)];
}
return Gi0;
}
function X(v0, P, L) {
if (P) {
var Q = P[2], i0 = P[5], l0 = P[4], S0 = P[3], T0 = P[1];
if (n4(L) <= i0) {
var rr = G0(Q, L), R0 = rr[2], B = rr[1], Z = X(v0, l0, rr[3]), p0 = ir(v0, Q, [0, S0], R0);
return b(X(v0, T0, B), Q, p0, Z);
}
} else if (!L)
return 0;
if (L) {
var b0 = L[2], O0 = L[4], q0 = L[3], er = L[1], yr = G0(b0, P), vr = yr[2], $0 = yr[1], Sr = X(v0, yr[3], O0), Mr = ir(v0, b0, vr, [0, q0]);
return b(X(v0, $0, er), b0, Mr, Sr);
}
throw [0, wn, Mi0];
}
function s0(v0, P, L) {
if (P) {
if (L) {
var Q = L[3], i0 = L[2], l0 = P[3], S0 = P[2], T0 = L[4], rr = L[1], R0 = P[4], B = P[1];
if (L[5] <= P[5]) {
var Z = G0(S0, L), p0 = Z[2], b0 = Z[3], O0 = s0(v0, B, Z[1]), q0 = s0(v0, R0, b0);
return p0 ? b(O0, S0, ir(v0, S0, l0, p0[1]), q0) : E0(O0, S0, l0, q0);
}
var er = G0(i0, P), yr = er[2], vr = er[3], $0 = s0(v0, er[1], rr), Sr = s0(v0, vr, T0);
return yr ? b($0, i0, ir(v0, i0, yr[1], Q), Sr) : E0($0, i0, Q, Sr);
}
var Mr = P;
} else
var Mr = L;
return Mr;
}
function dr(v0, P) {
if (P) {
var L = P[4], Q = P[3], i0 = P[2], l0 = P[1], S0 = dr(v0, l0), T0 = a(v0, i0, Q), rr = dr(v0, L);
return T0 ? l0 === S0 && L === rr ? P : E0(S0, i0, Q, rr) : X0(S0, rr);
}
return 0;
}
function Ar(v0, P) {
if (P) {
var L = P[2], Q = P[4], i0 = P[3], l0 = Ar(v0, P[1]), S0 = a(v0, L, i0), T0 = Ar(v0, Q);
return S0 ? E0(l0, L, S0[1], T0) : X0(l0, T0);
}
return 0;
}
function ar(v0, P) {
if (P) {
var L = P[3], Q = P[2], i0 = P[4], l0 = ar(v0, P[1]), S0 = l0[2], T0 = l0[1], rr = a(v0, Q, L), R0 = ar(v0, i0), B = R0[2], Z = R0[1];
if (rr) {
var p0 = X0(S0, B);
return [0, E0(T0, Q, L, Z), p0];
}
var b0 = E0(S0, Q, L, B);
return [0, X0(T0, Z), b0];
}
return Bi0;
}
function W0(v0, P) {
for (var L = v0, Q = P; ; ) {
if (L) {
var i0 = [0, L[2], L[3], L[4], Q], L = L[1], Q = i0;
continue;
}
return Q;
}
}
function Lr(v0, P, L) {
for (var Q = W0(L, 0), i0 = W0(P, 0), l0 = Q; ; ) {
if (i0) {
if (l0) {
var S0 = l0[4], T0 = l0[3], rr = l0[2], R0 = i0[4], B = i0[3], Z = i0[2], p0 = a(t[1], i0[1], l0[1]);
if (p0 === 0) {
var b0 = a(v0, Z, rr);
if (b0 === 0) {
var O0 = W0(T0, S0), i0 = W0(B, R0), l0 = O0;
continue;
}
return b0;
}
return p0;
}
return 1;
}
return l0 ? -1 : 0;
}
}
function Tr(v0, P, L) {
for (var Q = W0(L, 0), i0 = W0(P, 0), l0 = Q; ; ) {
if (i0) {
if (l0) {
var S0 = l0[4], T0 = l0[3], rr = l0[2], R0 = i0[4], B = i0[3], Z = i0[2], p0 = a(t[1], i0[1], l0[1]) === 0 ? 1 : 0;
if (p0) {
var b0 = a(v0, Z, rr);
if (b0) {
var O0 = W0(T0, S0), i0 = W0(B, R0), l0 = O0;
continue;
}
var q0 = b0;
} else
var q0 = p0;
return q0;
}
return 0;
}
return l0 ? 0 : 1;
}
}
function Hr(v0) {
if (v0) {
var P = v0[1], L = Hr(v0[4]);
return (Hr(P) + 1 | 0) + L | 0;
}
return 0;
}
function Or(v0, P) {
for (var L = v0, Q = P; ; ) {
if (Q) {
var i0 = Q[3], l0 = Q[2], S0 = Q[1], L = [0, [0, l0, i0], Or(L, Q[4])], Q = S0;
continue;
}
return L;
}
}
function xr(v0) {
return Or(0, v0);
}
function Rr(v0, P) {
for (var L = P, Q = v0; ; ) {
var i0 = u(Q, 0);
if (i0) {
var l0 = i0[1], S0 = i0[2], L = p(l0[1], l0[2], L), Q = S0;
continue;
}
return L;
}
}
function Wr(v0) {
return Rr(v0, c);
}
function Jr(v0, P) {
if (v0) {
var L = v0[2], Q = v0[1], i0 = W0(v0[3], v0[4]);
return [0, [0, Q, L], function(l0) {
return Jr(i0, l0);
}];
}
return 0;
}
function or(v0) {
var P = W0(v0, 0);
return function(L) {
return Jr(P, L);
};
}
function _r(v0, P) {
for (var L = v0, Q = P; ; ) {
if (L) {
var i0 = [0, L[2], L[3], L[1], Q], L = L[4], Q = i0;
continue;
}
return Q;
}
}
function Ir(v0, P) {
if (v0) {
var L = v0[2], Q = v0[1], i0 = _r(v0[3], v0[4]);
return [0, [0, Q, L], function(l0) {
return Ir(i0, l0);
}];
}
return 0;
}
function fe(v0) {
var P = _r(v0, 0);
return function(L) {
return Ir(P, L);
};
}
return [0, c, s, A, p, g0, i, k0, X, s0, Lr, Tr, e0, c0, t0, a0, dr, Ar, ar, Hr, xr, S, M, K, V, S, M, G0, y, G, T, E, h, w, x0, l, or, fe, function(v0, P) {
for (var L = P, Q = 0; ; ) {
if (L) {
var i0 = L[4], l0 = L[3], S0 = L[2], T0 = L[1], rr = a(t[1], S0, v0);
if (rr !== 0) {
if (0 <= rr) {
var L = T0, Q = [0, S0, l0, i0, Q];
continue;
}
var L = i0;
continue;
}
var R0 = [0, S0, l0, i0, Q];
} else
var R0 = Q;
return function(B) {
return Jr(R0, B);
};
}
}, Rr, Wr];
}
G7(0);
function yl(t) {
return [0, 0, 0];
}
function dl(t) {
return t[1] = 0, t[2] = 0, 0;
}
function E1(t, n4) {
return n4[1] = [0, t, n4[1]], n4[2] = n4[2] + 1 | 0, 0;
}
function Uv(t) {
var n4 = t[1];
if (n4) {
var e = n4[1];
return t[1] = n4[2], t[2] = t[2] - 1 | 0, [0, e];
}
return 0;
}
function Hv(t) {
var n4 = t[1];
return n4 ? [0, n4[1]] : 0;
}
var uee = [wt, Yi0, G7(0)];
function kz(t) {
return [0, 0, 0, 0];
}
function sN(t) {
return t[1] = 0, t[2] = 0, t[3] = 0, 0;
}
function vN(t, n4) {
var e = [0, t, 0], i = n4[3];
return i ? (n4[1] = n4[1] + 1 | 0, i[2] = e, n4[3] = e, 0) : (n4[1] = 1, n4[2] = e, n4[3] = e, 0);
}
var iee = [wt, Vi0, G7(0)];
function fee(t) {
throw iee;
}
function xee(t) {
var n4 = t[1];
t[1] = fee;
try {
var e = u(n4, 0);
return ti0(t, e), e;
} catch (i) {
throw i = Et(i), t[1] = function(x) {
throw i;
}, i;
}
}
function $n(t) {
var n4 = 1 <= t ? t : 1, e = ll < n4 ? ll : n4, i = Pt(e);
return [0, i, 0, e, i];
}
function Gt(t) {
return qv(t[1], 0, t[2]);
}
function lN(t, n4) {
for (var e = t[2], i = [0, t[3]]; ; ) {
if (i[1] < (e + n4 | 0)) {
i[1] = 2 * i[1] | 0;
continue;
}
ll < i[1] && ((e + n4 | 0) <= ll ? i[1] = ll : ke(Ki0));
var x = Pt(i[1]);
if (yz(t[1], 0, x, 0, t[2]), t[1] = x, t[3] = i[1], (t[2] + n4 | 0) <= t[3]) {
if ((e + n4 | 0) <= t[3])
return 0;
throw [0, wn, Ji0];
}
throw [0, wn, Wi0];
}
}
function qi(t, n4) {
var e = t[2];
return t[3] <= e && lN(t, 1), Jn(t[1], e, n4), t[2] = e + 1 | 0, 0;
}
function wz(t, n4, e, i) {
var x = e < 0 ? 1 : 0;
if (x)
var s = x;
else
var c = i < 0 ? 1 : 0, s = c || ((nn(n4) - i | 0) < e ? 1 : 0);
s && Cu(zi0);
var p = t[2] + i | 0;
return t[3] < p && lN(t, i), As(n4, e, t[1], t[2], i), t[2] = p, 0;
}
function bN(t, n4, e, i) {
return wz(t, n4, e, i);
}
function mn(t, n4) {
var e = nn(n4), i = t[2] + e | 0;
return t[3] < i && lN(t, e), As(n4, 0, t[1], t[2], e), t[2] = i, 0;
}
function pN(t) {
return t[2] === 5 ? 12 : -6;
}
function Ez(t) {
return [0, 0, Pt(t)];
}
function Sz(t, n4) {
var e = l7(t[2]), i = t[1] + n4 | 0, x = e < i ? 1 : 0;
if (x) {
var c = e * 2 | 0, s = i <= c ? c : i, p = Pt(s);
yz(t[2], 0, p, 0, e), t[2] = p;
var y = 0;
} else
var y = x;
return y;
}
function Xv(t, n4) {
return Sz(t, 1), p1(t[2], t[1], n4), t[1] = t[1] + 1 | 0, 0;
}
function Du(t, n4) {
var e = nn(n4);
return Sz(t, e), ss(n4, 0, t[2], t[1], e), t[1] = t[1] + e | 0, 0;
}
function gz(t) {
return qv(t[2], 0, t[1]);
}
function Fz(t) {
if (typeof t == "number")
switch (t) {
case 0:
return Qx0;
case 1:
return ra0;
case 2:
return ea0;
case 3:
return na0;
case 4:
return ta0;
case 5:
return ua0;
default:
return ia0;
}
else
switch (t[0]) {
case 0:
return t[1];
case 1:
return t[1];
default:
return Te(fa0, Op(1, t[1]));
}
}
function mN(t, n4) {
for (var e = n4; ; ) {
if (typeof e == "number")
return 0;
switch (e[0]) {
case 0:
var i = e[1];
Du(t, Zi0);
var e = i;
continue;
case 1:
var x = e[1];
Du(t, Qi0);
var e = x;
continue;
case 2:
var c = e[1];
Du(t, rf0);
var e = c;
continue;
case 3:
var s = e[1];
Du(t, ef0);
var e = s;
continue;
case 4:
var p = e[1];
Du(t, nf0);
var e = p;
continue;
case 5:
var y = e[1];
Du(t, tf0);
var e = y;
continue;
case 6:
var T = e[1];
Du(t, uf0);
var e = T;
continue;
case 7:
var E = e[1];
Du(t, if0);
var e = E;
continue;
case 8:
var h = e[2], w = e[1];
Du(t, ff0), mN(t, w), Du(t, xf0);
var e = h;
continue;
case 9:
var G = e[3], A = e[1];
Du(t, af0), mN(t, A), Du(t, of0);
var e = G;
continue;
case 10:
var S = e[1];
Du(t, cf0);
var e = S;
continue;
case 11:
var M = e[1];
Du(t, sf0);
var e = M;
continue;
case 12:
var K = e[1];
Du(t, vf0);
var e = K;
continue;
case 13:
var V = e[1];
Du(t, lf0);
var e = V;
continue;
default:
var f0 = e[1];
Du(t, bf0);
var e = f0;
continue;
}
}
}
function tu(t) {
if (typeof t == "number")
return 0;
switch (t[0]) {
case 0:
return [0, tu(t[1])];
case 1:
return [1, tu(t[1])];
case 2:
return [2, tu(t[1])];
case 3:
return [3, tu(t[1])];
case 4:
return [4, tu(t[1])];
case 5:
return [5, tu(t[1])];
case 6:
return [6, tu(t[1])];
case 7:
return [7, tu(t[1])];
case 8:
var n4 = t[1];
return [8, n4, tu(t[2])];
case 9:
var e = t[2], i = t[1];
return [9, e, i, tu(t[3])];
case 10:
return [10, tu(t[1])];
case 11:
return [11, tu(t[1])];
case 12:
return [12, tu(t[1])];
case 13:
return [13, tu(t[1])];
default:
return [14, tu(t[1])];
}
}
function Lu(t) {
if (typeof t == "number") {
var n4 = function(sr) {
return 0;
}, e = function(sr) {
return 0;
}, i = function(sr) {
return 0;
};
return [0, function(sr) {
return 0;
}, i, e, n4];
} else
switch (t[0]) {
case 0:
var x = Lu(t[1]), c = x[4], s = x[3], p = x[2], y = x[1], T = function(sr) {
return u(p, 0), 0;
};
return [0, function(sr) {
return u(y, 0), 0;
}, T, s, c];
case 1:
var E = Lu(t[1]), h = E[4], w = E[3], G = E[2], A = E[1], S = function(sr) {
return u(G, 0), 0;
};
return [0, function(sr) {
return u(A, 0), 0;
}, S, w, h];
case 2:
var M = Lu(t[1]), K = M[4], V = M[3], f0 = M[2], m0 = M[1], k0 = function(sr) {
return u(f0, 0), 0;
};
return [0, function(sr) {
return u(m0, 0), 0;
}, k0, V, K];
case 3:
var g0 = Lu(t[1]), e0 = g0[4], x0 = g0[3], l = g0[2], c0 = g0[1], t0 = function(sr) {
return u(l, 0), 0;
};
return [0, function(sr) {
return u(c0, 0), 0;
}, t0, x0, e0];
case 4:
var a0 = Lu(t[1]), w0 = a0[4], _0 = a0[3], E0 = a0[2], X0 = a0[1], b = function(sr) {
return u(E0, 0), 0;
};
return [0, function(sr) {
return u(X0, 0), 0;
}, b, _0, w0];
case 5:
var G0 = Lu(t[1]), X = G0[4], s0 = G0[3], dr = G0[2], Ar = G0[1], ar = function(sr) {
return u(dr, 0), 0;
};
return [0, function(sr) {
return u(Ar, 0), 0;
}, ar, s0, X];
case 6:
var W0 = Lu(t[1]), Lr = W0[4], Tr = W0[3], Hr = W0[2], Or = W0[1], xr = function(sr) {
return u(Hr, 0), 0;
};
return [0, function(sr) {
return u(Or, 0), 0;
}, xr, Tr, Lr];
case 7:
var Rr = Lu(t[1]), Wr = Rr[4], Jr = Rr[3], or = Rr[2], _r = Rr[1], Ir = function(sr) {
return u(or, 0), 0;
};
return [0, function(sr) {
return u(_r, 0), 0;
}, Ir, Jr, Wr];
case 8:
var fe = Lu(t[2]), v0 = fe[4], P = fe[3], L = fe[2], Q = fe[1], i0 = function(sr) {
return u(L, 0), 0;
};
return [0, function(sr) {
return u(Q, 0), 0;
}, i0, P, v0];
case 9:
var l0 = t[2], S0 = t[1], T0 = Lu(t[3]), rr = T0[4], R0 = T0[3], B = T0[2], Z = T0[1], p0 = Lu(lu(tu(S0), l0)), b0 = p0[4], O0 = p0[3], q0 = p0[2], er = p0[1], yr = function(sr) {
return u(b0, 0), u(rr, 0), 0;
}, vr = function(sr) {
return u(R0, 0), u(O0, 0), 0;
}, $0 = function(sr) {
return u(q0, 0), u(B, 0), 0;
};
return [0, function(sr) {
return u(Z, 0), u(er, 0), 0;
}, $0, vr, yr];
case 10:
var Sr = Lu(t[1]), Mr = Sr[4], Br = Sr[3], qr = Sr[2], jr = Sr[1], $r = function(sr) {
return u(qr, 0), 0;
};
return [0, function(sr) {
return u(jr, 0), 0;
}, $r, Br, Mr];
case 11:
var ne = Lu(t[1]), Qr = ne[4], pe = ne[3], oe = ne[2], me = ne[1], ae = function(sr) {
return u(oe, 0), 0;
};
return [0, function(sr) {
return u(me, 0), 0;
}, ae, pe, Qr];
case 12:
var ce = Lu(t[1]), ge = ce[4], H0 = ce[3], Fr = ce[2], _ = ce[1], k = function(sr) {
return u(Fr, 0), 0;
};
return [0, function(sr) {
return u(_, 0), 0;
}, k, H0, ge];
case 13:
var I = Lu(t[1]), U = I[4], Y = I[3], y0 = I[2], D0 = I[1], I0 = function(sr) {
return u(U, 0), 0;
}, D = function(sr) {
return u(Y, 0), 0;
}, u0 = function(sr) {
return u(y0, 0), 0;
};
return [0, function(sr) {
return u(D0, 0), 0;
}, u0, D, I0];
default:
var Y0 = Lu(t[1]), J0 = Y0[4], fr = Y0[3], Q0 = Y0[2], F0 = Y0[1], gr = function(sr) {
return u(J0, 0), 0;
}, mr = function(sr) {
return u(fr, 0), 0;
}, Cr = function(sr) {
return u(Q0, 0), 0;
};
return [0, function(sr) {
return u(F0, 0), 0;
}, Cr, mr, gr];
}
}
function lu(t, n4) {
var e = 0;
if (typeof t == "number") {
if (typeof n4 == "number")
return 0;
switch (n4[0]) {
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
e = 4;
break;
case 8:
e = 5;
break;
case 9:
e = 6;
break;
default:
throw [0, wn, pf0];
}
} else
switch (t[0]) {
case 0:
var i = 0, x = t[1];
if (typeof n4 != "number")
switch (n4[0]) {
case 0:
return [0, lu(x, n4[1])];
case 8:
e = 5, i = 1;
break;
case 9:
e = 6, i = 1;
break;
case 10:
i = 1;
break;
case 11:
e = 1, i = 1;
break;
case 12:
e = 2, i = 1;
break;
case 13:
e = 3, i = 1;
break;
case 14:
e = 4, i = 1;
break;
}
i || (e = 7);
break;
case 1:
var c = 0, s = t[1];
if (typeof n4 != "number")
switch (n4[0]) {
case 1:
return [1, lu(s, n4[1])];
case 8:
e = 5, c = 1;
break;
case 9:
e = 6, c = 1;
break;
case 10:
c = 1;
break;
case 11:
e = 1, c = 1;
break;
case 12:
e = 2, c = 1;
break;
case 13:
e = 3, c = 1;
break;
case 14:
e = 4, c = 1;
break;
}
c || (e = 7);
break;
case 2:
var p = 0, y = t[1];
if (typeof n4 == "number")
p = 1;
else
switch (n4[0]) {
case 2:
return [2, lu(y, n4[1])];
case 8:
e = 5;
break;
case 9:
e = 6;
break;
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
e = 4;
break;
default:
p = 1;
}
p && (e = 7);
break;
case 3:
var T = 0, E = t[1];
if (typeof n4 == "number")
T = 1;
else
switch (n4[0]) {
case 3:
return [3, lu(E, n4[1])];
case 8:
e = 5;
break;
case 9:
e = 6;
break;
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
e = 4;
break;
default:
T = 1;
}
T && (e = 7);
break;
case 4:
var h = 0, w = t[1];
if (typeof n4 == "number")
h = 1;
else
switch (n4[0]) {
case 4:
return [4, lu(w, n4[1])];
case 8:
e = 5;
break;
case 9:
e = 6;
break;
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
e = 4;
break;
default:
h = 1;
}
h && (e = 7);
break;
case 5:
var G = 0, A = t[1];
if (typeof n4 == "number")
G = 1;
else
switch (n4[0]) {
case 5:
return [5, lu(A, n4[1])];
case 8:
e = 5;
break;
case 9:
e = 6;
break;
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
e = 4;
break;
default:
G = 1;
}
G && (e = 7);
break;
case 6:
var S = 0, M = t[1];
if (typeof n4 == "number")
S = 1;
else
switch (n4[0]) {
case 6:
return [6, lu(M, n4[1])];
case 8:
e = 5;
break;
case 9:
e = 6;
break;
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
e = 4;
break;
default:
S = 1;
}
S && (e = 7);
break;
case 7:
var K = 0, V = t[1];
if (typeof n4 == "number")
K = 1;
else
switch (n4[0]) {
case 7:
return [7, lu(V, n4[1])];
case 8:
e = 5;
break;
case 9:
e = 6;
break;
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
e = 4;
break;
default:
K = 1;
}
K && (e = 7);
break;
case 8:
var f0 = 0, m0 = t[2], k0 = t[1];
if (typeof n4 == "number")
f0 = 1;
else
switch (n4[0]) {
case 8:
var g0 = n4[1], e0 = lu(m0, n4[2]);
return [8, lu(k0, g0), e0];
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
e = 4;
break;
default:
f0 = 1;
}
if (f0)
throw [0, wn, Ff0];
break;
case 9:
var x0 = 0, l = t[3], c0 = t[2], t0 = t[1];
if (typeof n4 == "number")
x0 = 1;
else
switch (n4[0]) {
case 8:
e = 5;
break;
case 9:
var a0 = n4[3], w0 = n4[2], _0 = n4[1], E0 = Lu(lu(tu(c0), _0)), X0 = E0[4];
return u(E0[2], 0), u(X0, 0), [9, t0, w0, lu(l, a0)];
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
e = 4;
break;
default:
x0 = 1;
}
if (x0)
throw [0, wn, Of0];
break;
case 10:
var b = t[1];
if (typeof n4 != "number" && n4[0] === 10)
return [10, lu(b, n4[1])];
throw [0, wn, mf0];
case 11:
var G0 = 0, X = t[1];
if (typeof n4 == "number")
G0 = 1;
else
switch (n4[0]) {
case 10:
break;
case 11:
return [11, lu(X, n4[1])];
default:
G0 = 1;
}
if (G0)
throw [0, wn, yf0];
break;
case 12:
var s0 = 0, dr = t[1];
if (typeof n4 == "number")
s0 = 1;
else
switch (n4[0]) {
case 10:
break;
case 11:
e = 1;
break;
case 12:
return [12, lu(dr, n4[1])];
default:
s0 = 1;
}
if (s0)
throw [0, wn, hf0];
break;
case 13:
var Ar = 0, ar = t[1];
if (typeof n4 == "number")
Ar = 1;
else
switch (n4[0]) {
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
return [13, lu(ar, n4[1])];
default:
Ar = 1;
}
if (Ar)
throw [0, wn, wf0];
break;
default:
var W0 = 0, Lr = t[1];
if (typeof n4 == "number")
W0 = 1;
else
switch (n4[0]) {
case 10:
break;
case 11:
e = 1;
break;
case 12:
e = 2;
break;
case 13:
e = 3;
break;
case 14:
return [14, lu(Lr, n4[1])];
default:
W0 = 1;
}
if (W0)
throw [0, wn, Sf0];
}
switch (e) {
case 0:
throw [0, wn, _f0];
case 1:
throw [0, wn, df0];
case 2:
throw [0, wn, kf0];
case 3:
throw [0, wn, Ef0];
case 4:
throw [0, wn, gf0];
case 5:
throw [0, wn, Tf0];
case 6:
throw [0, wn, If0];
default:
throw [0, wn, Af0];
}
}
var Tu = [wt, xa0, G7(0)];
function Ap(t, n4) {
if (typeof t == "number")
return [0, 0, n4];
if (t[0] === 0)
return [0, [0, t[1], t[2]], n4];
if (typeof n4 != "number" && n4[0] === 2)
return [0, [1, t[1]], n4[1]];
throw Tu;
}
function hl(t, n4, e) {
var i = Ap(t, e);
if (typeof n4 == "number") {
if (n4) {
var x = i[2];
if (typeof x != "number" && x[0] === 2)
return [0, i[1], 1, x[1]];
throw Tu;
}
return [0, i[1], 0, i[2]];
}
return [0, i[1], [0, n4[1]], i[2]];
}
function m7(t, n4, e) {
if (typeof t == "number")
return [0, 0, _t(n4, e)];
switch (t[0]) {
case 0:
if (typeof e != "number" && e[0] === 0) {
var i = m7(t[1], n4, e[1]);
return [0, [0, i[1]], i[2]];
}
break;
case 1:
if (typeof e != "number" && e[0] === 1) {
var x = m7(t[1], n4, e[1]);
return [0, [1, x[1]], x[2]];
}
break;
case 2:
if (typeof e != "number" && e[0] === 2) {
var c = m7(t[1], n4, e[1]);
return [0, [2, c[1]], c[2]];
}
break;
case 3:
if (typeof e != "number" && e[0] === 3) {
var s = m7(t[1], n4, e[1]);
return [0, [3, s[1]], s[2]];
}
break;
case 4:
if (typeof e != "number" && e[0] === 4) {
var p = m7(t[1], n4, e[1]);
return [0, [4, p[1]], p[2]];
}
break;
case 5:
if (typeof e != "number" && e[0] === 5) {
var y = m7(t[1], n4, e[1]);
return [0, [5, y[1]], y[2]];
}
break;
case 6:
if (typeof e != "number" && e[0] === 6) {
var T = m7(t[1], n4, e[1]);
return [0, [6, T[1]], T[2]];
}
break;
case 7:
if (typeof e != "number" && e[0] === 7) {
var E = m7(t[1], n4, e[1]);
return [0, [7, E[1]], E[2]];
}
break;
case 8:
if (typeof e != "number" && e[0] === 8) {
var h = e[1], w = e[2], G = t[2];
if (cl([0, t[1]], [0, h]))
throw Tu;
var A = m7(G, n4, w);
return [0, [8, h, A[1]], A[2]];
}
break;
case 9:
if (typeof e != "number" && e[0] === 9) {
var S = e[2], M = e[1], K = e[3], V = t[3], f0 = t[2], m0 = t[1], k0 = [0, jt(M)];
if (cl([0, jt(m0)], k0))
throw Tu;
var g0 = [0, jt(S)];
if (cl([0, jt(f0)], g0))
throw Tu;
var e0 = Lu(lu(tu(M), S)), x0 = e0[4];
u(e0[2], 0), u(x0, 0);
var l = m7(jt(V), n4, K), c0 = l[2];
return [0, [9, M, S, tu(l[1])], c0];
}
break;
case 10:
if (typeof e != "number" && e[0] === 10) {
var t0 = m7(t[1], n4, e[1]);
return [0, [10, t0[1]], t0[2]];
}
break;
case 11:
if (typeof e != "number" && e[0] === 11) {
var a0 = m7(t[1], n4, e[1]);
return [0, [11, a0[1]], a0[2]];
}
break;
case 13:
if (typeof e != "number" && e[0] === 13) {
var w0 = m7(t[1], n4, e[1]);
return [0, [13, w0[1]], w0[2]];
}
break;
case 14:
if (typeof e != "number" && e[0] === 14) {
var _0 = m7(t[1], n4, e[1]);
return [0, [14, _0[1]], _0[2]];
}
break;
}
throw Tu;
}
function _t(t, n4) {
if (typeof t == "number")
return [0, 0, n4];
switch (t[0]) {
case 0:
if (typeof n4 != "number" && n4[0] === 0) {
var e = _t(t[1], n4[1]);
return [0, [0, e[1]], e[2]];
}
break;
case 1:
if (typeof n4 != "number" && n4[0] === 0) {
var i = _t(t[1], n4[1]);
return [0, [1, i[1]], i[2]];
}
break;
case 2:
var x = t[2], c = Ap(t[1], n4), s = c[2], p = c[1];
if (typeof s != "number" && s[0] === 1) {
var y = _t(x, s[1]);
return [0, [2, p, y[1]], y[2]];
}
throw Tu;
case 3:
var T = t[2], E = Ap(t[1], n4), h = E[2], w = E[1];
if (typeof h != "number" && h[0] === 1) {
var G = _t(T, h[1]);
return [0, [3, w, G[1]], G[2]];
}
throw Tu;
case 4:
var A = t[4], S = t[1], M = hl(t[2], t[3], n4), K = M[3], V = M[1];
if (typeof K != "number" && K[0] === 2) {
var f0 = M[2], m0 = _t(A, K[1]);
return [0, [4, S, V, f0, m0[1]], m0[2]];
}
throw Tu;
case 5:
var k0 = t[4], g0 = t[1], e0 = hl(t[2], t[3], n4), x0 = e0[3], l = e0[1];
if (typeof x0 != "number" && x0[0] === 3) {
var c0 = e0[2], t0 = _t(k0, x0[1]);
return [0, [5, g0, l, c0, t0[1]], t0[2]];
}
throw Tu;
case 6:
var a0 = t[4], w0 = t[1], _0 = hl(t[2], t[3], n4), E0 = _0[3], X0 = _0[1];
if (typeof E0 != "number" && E0[0] === 4) {
var b = _0[2], G0 = _t(a0, E0[1]);
return [0, [6, w0, X0, b, G0[1]], G0[2]];
}
throw Tu;
case 7:
var X = t[4], s0 = t[1], dr = hl(t[2], t[3], n4), Ar = dr[3], ar = dr[1];
if (typeof Ar != "number" && Ar[0] === 5) {
var W0 = dr[2], Lr = _t(X, Ar[1]);
return [0, [7, s0, ar, W0, Lr[1]], Lr[2]];
}
throw Tu;
case 8:
var Tr = t[4], Hr = t[1], Or = hl(t[2], t[3], n4), xr = Or[3], Rr = Or[1];
if (typeof xr != "number" && xr[0] === 6) {
var Wr = Or[2], Jr = _t(Tr, xr[1]);
return [0, [8, Hr, Rr, Wr, Jr[1]], Jr[2]];
}
throw Tu;
case 9:
var or = t[2], _r = Ap(t[1], n4), Ir = _r[2], fe = _r[1];
if (typeof Ir != "number" && Ir[0] === 7) {
var v0 = _t(or, Ir[1]);
return [0, [9, fe, v0[1]], v0[2]];
}
throw Tu;
case 10:
var P = _t(t[1], n4);
return [0, [10, P[1]], P[2]];
case 11:
var L = t[1], Q = _t(t[2], n4);
return [0, [11, L, Q[1]], Q[2]];
case 12:
var i0 = t[1], l0 = _t(t[2], n4);
return [0, [12, i0, l0[1]], l0[2]];
case 13:
if (typeof n4 != "number" && n4[0] === 8) {
var S0 = n4[1], T0 = n4[2], rr = t[3], R0 = t[1];
if (cl([0, t[2]], [0, S0]))
throw Tu;
var B = _t(rr, T0);
return [0, [13, R0, S0, B[1]], B[2]];
}
break;
case 14:
if (typeof n4 != "number" && n4[0] === 9) {
var Z = n4[1], p0 = n4[3], b0 = t[3], O0 = t[2], q0 = t[1], er = [0, jt(Z)];
if (cl([0, jt(O0)], er))
throw Tu;
var yr = _t(b0, jt(p0));
return [0, [14, q0, Z, yr[1]], yr[2]];
}
break;
case 15:
if (typeof n4 != "number" && n4[0] === 10) {
var vr = _t(t[1], n4[1]);
return [0, [15, vr[1]], vr[2]];
}
break;
case 16:
if (typeof n4 != "number" && n4[0] === 11) {
var $0 = _t(t[1], n4[1]);
return [0, [16, $0[1]], $0[2]];
}
break;
case 17:
var Sr = t[1], Mr = _t(t[2], n4);
return [0, [17, Sr, Mr[1]], Mr[2]];
case 18:
var Br = t[2], qr = t[1];
if (qr[0] === 0) {
var jr = qr[1], $r = jr[2], ne = _t(jr[1], n4), Qr = ne[1], pe = _t(Br, ne[2]);
return [0, [18, [0, [0, Qr, $r]], pe[1]], pe[2]];
}
var oe = qr[1], me = oe[2], ae = _t(oe[1], n4), ce = ae[1], ge = _t(Br, ae[2]);
return [0, [18, [1, [0, ce, me]], ge[1]], ge[2]];
case 19:
if (typeof n4 != "number" && n4[0] === 13) {
var H0 = _t(t[1], n4[1]);
return [0, [19, H0[1]], H0[2]];
}
break;
case 20:
if (typeof n4 != "number" && n4[0] === 1) {
var Fr = t[2], _ = t[1], k = _t(t[3], n4[1]);
return [0, [20, _, Fr, k[1]], k[2]];
}
break;
case 21:
if (typeof n4 != "number" && n4[0] === 2) {
var I = t[1], U = _t(t[2], n4[1]);
return [0, [21, I, U[1]], U[2]];
}
break;
case 23:
var Y = t[2], y0 = t[1];
if (typeof y0 == "number")
switch (y0) {
case 0:
return q7(y0, Y, n4);
case 1:
return q7(y0, Y, n4);
case 2:
if (typeof n4 != "number" && n4[0] === 14) {
var D0 = _t(Y, n4[1]);
return [0, [23, 2, D0[1]], D0[2]];
}
throw Tu;
default:
return q7(y0, Y, n4);
}
else
switch (y0[0]) {
case 0:
return q7(y0, Y, n4);
case 1:
return q7(y0, Y, n4);
case 2:
return q7(y0, Y, n4);
case 3:
return q7(y0, Y, n4);
case 4:
return q7(y0, Y, n4);
case 5:
return q7(y0, Y, n4);
case 6:
return q7(y0, Y, n4);
case 7:
return q7(y0, Y, n4);
case 8:
return q7([8, y0[1], y0[2]], Y, n4);
case 9:
var I0 = y0[1], D = m7(y0[2], Y, n4), u0 = D[2];
return [0, [23, [9, I0, D[1]], u0[1]], u0[2]];
case 10:
return q7(y0, Y, n4);
default:
return q7(y0, Y, n4);
}
}
throw Tu;
}
function q7(t, n4, e) {
var i = _t(n4, e);
return [0, [23, t, i[1]], i[2]];
}
function U7(t, n4, e) {
var i = nn(e), x = 0 <= n4 ? t : 0, c = Fp(n4);
if (c <= i)
return e;
var s = x === 2 ? 48 : 32, p = w1(c, s);
switch (x) {
case 0:
ss(e, 0, p, 0, i);
break;
case 1:
ss(e, 0, p, c - i | 0, i);
break;
default:
var y = 0;
if (0 < i) {
var T = 0;
Ot(e, 0) !== 43 && Ot(e, 0) !== 45 && Ot(e, 0) !== 32 && (y = 1, T = 1), T || (p1(p, 0, Ot(e, 0)), ss(e, 1, p, (c - i | 0) + 1 | 0, i - 1 | 0));
} else
y = 1;
if (y) {
var E = 0;
if (1 < i && Ot(e, 0) === 48) {
var h = 0;
fc !== Ot(e, 1) && Ot(e, 1) !== 88 && (E = 1, h = 1), h || (p1(p, 1, Ot(e, 1)), ss(e, 2, p, (c - i | 0) + 2 | 0, i - 2 | 0));
} else
E = 1;
E && ss(e, 0, p, c - i | 0, i);
}
}
return p;
}
function Yv(t, n4) {
var e = Fp(t), i = nn(n4), x = Ot(n4, 0), c = 0;
if (58 <= x)
71 <= x ? 5 < (x + V3 | 0) >>> 0 || (c = 1) : 65 <= x && (c = 1);
else {
var s = 0;
if (x !== 32)
if (43 <= x)
switch (x + cy | 0) {
case 5:
if (i < (e + 2 | 0) && 1 < i) {
var p = 0;
if ((fc === Ot(n4, 1) || Ot(n4, 1) === 88) && (p = 1), p) {
var y = w1(e + 2 | 0, 48);
return p1(y, 1, Ot(n4, 1)), ss(n4, 2, y, (e - i | 0) + 4 | 0, i - 2 | 0), y;
}
}
c = 1, s = 1;
break;
case 0:
case 2:
break;
case 1:
case 3:
case 4:
s = 1;
break;
default:
c = 1, s = 1;
}
else
s = 1;
if (!s && i < (e + 1 | 0)) {
var T = w1(e + 1 | 0, 48);
return p1(T, 0, x), ss(n4, 1, T, (e - i | 0) + 2 | 0, i - 1 | 0), T;
}
}
if (c && i < e) {
var E = w1(e, 48);
return ss(n4, 0, E, e - i | 0, i), E;
}
return n4;
}
function aee(t) {
for (var n4 = 0, e = nn(t); ; ) {
if (e <= n4)
var i = t;
else {
var x = Vr(t, n4) + SU | 0, c = 0;
if (59 < x >>> 0 ? 33 < (x + TS | 0) >>> 0 && (c = 1) : x === 2 && (c = 1), !c) {
var n4 = n4 + 1 | 0;
continue;
}
var s = t, p = [0, 0], y = l7(s) - 1 | 0, T = 0;
if (!(y < 0))
for (var E = T; ; ) {
var h = Hu(s, E), w = 0;
if (32 <= h) {
var G = h - 34 | 0, A = 0;
if (58 < G >>> 0 ? 93 <= G && (A = 1) : 56 < (G - 1 | 0) >>> 0 && (w = 1, A = 1), !A) {
var S = 1;
w = 2;
}
} else
11 <= h ? h === 13 && (w = 1) : 8 <= h && (w = 1);
switch (w) {
case 0:
var S = 4;
break;
case 1:
var S = 2;
break;
}
p[1] = p[1] + S | 0;
var M = E + 1 | 0;
if (y !== E) {
var E = M;
continue;
}
break;
}
if (p[1] === l7(s))
var K = mz(s);
else {
var V = Pt(p[1]);
p[1] = 0;
var f0 = l7(s) - 1 | 0, m0 = 0;
if (!(f0 < 0))
for (var k0 = m0; ; ) {
var g0 = Hu(s, k0), e0 = 0;
if (35 <= g0)
g0 === 92 ? e0 = 2 : zn <= g0 ? e0 = 1 : e0 = 3;
else if (32 <= g0)
34 <= g0 ? e0 = 2 : e0 = 3;
else if (14 <= g0)
e0 = 1;
else
switch (g0) {
case 8:
Jn(V, p[1], 92), p[1]++, Jn(V, p[1], 98);
break;
case 9:
Jn(V, p[1], 92), p[1]++, Jn(V, p[1], x1);
break;
case 10:
Jn(V, p[1], 92), p[1]++, Jn(V, p[1], Ht);
break;
case 13:
Jn(V, p[1], 92), p[1]++, Jn(V, p[1], u1);
break;
default:
e0 = 1;
}
switch (e0) {
case 1:
Jn(V, p[1], 92), p[1]++, Jn(V, p[1], 48 + (g0 / ni | 0) | 0), p[1]++, Jn(V, p[1], 48 + ((g0 / 10 | 0) % 10 | 0) | 0), p[1]++, Jn(V, p[1], 48 + (g0 % 10 | 0) | 0);
break;
case 2:
Jn(V, p[1], 92), p[1]++, Jn(V, p[1], g0);
break;
case 3:
Jn(V, p[1], g0);
break;
}
p[1]++;
var x0 = k0 + 1 | 0;
if (f0 !== k0) {
var k0 = x0;
continue;
}
break;
}
var K = V;
}
var i = K;
}
var l = nn(i), c0 = w1(l + 2 | 0, 34);
return As(i, 0, c0, 1, l), c0;
}
}
function Tz(t, n4) {
var e = Fp(n4), i = iz ? iz[1] : 70;
switch (t[2]) {
case 0:
var x = Ri;
break;
case 1:
var x = L7;
break;
case 2:
var x = 69;
break;
case 3:
var x = c7;
break;
case 4:
var x = 71;
break;
case 5:
var x = i;
break;
case 6:
var x = D7;
break;
case 7:
var x = 72;
break;
default:
var x = 70;
}
var c = Ez(16);
switch (Xv(c, 37), t[1]) {
case 0:
break;
case 1:
Xv(c, 43);
break;
default:
Xv(c, 32);
}
return 8 <= t[2] && Xv(c, 35), Xv(c, 46), Du(c, r(C + e)), Xv(c, x), gz(c);
}
function Np(t, n4) {
if (13 <= t) {
var e = [0, 0], i = nn(n4) - 1 | 0, x = 0;
if (!(i < 0))
for (var c = x; ; ) {
9 < (Vr(n4, c) + zt | 0) >>> 0 || e[1]++;
var s = c + 1 | 0;
if (i !== c) {
var c = s;
continue;
}
break;
}
var p = e[1], y = Pt(nn(n4) + ((p - 1 | 0) / 3 | 0) | 0), T = [0, 0], E = function(K) {
return p1(y, T[1], K), T[1]++, 0;
}, h = [0, ((p - 1 | 0) % 3 | 0) + 1 | 0], w = nn(n4) - 1 | 0, G = 0;
if (!(w < 0))
for (var A = G; ; ) {
var S = Vr(n4, A);
9 < (S + zt | 0) >>> 0 || (h[1] === 0 && (E(95), h[1] = 3), h[1] += -1), E(S);
var M = A + 1 | 0;
if (w !== A) {
var A = M;
continue;
}
break;
}
return y;
}
return n4;
}
function oee(t, n4) {
switch (t) {
case 1:
var e = Bx0;
break;
case 2:
var e = qx0;
break;
case 4:
var e = Ux0;
break;
case 5:
var e = Hx0;
break;
case 6:
var e = Xx0;
break;
case 7:
var e = Yx0;
break;
case 8:
var e = Vx0;
break;
case 9:
var e = zx0;
break;
case 10:
var e = Kx0;
break;
case 11:
var e = Wx0;
break;
case 0:
case 13:
var e = Jx0;
break;
case 3:
case 14:
var e = $x0;
break;
default:
var e = Zx0;
}
return Np(t, hp(e, n4));
}
function cee(t, n4) {
switch (t) {
case 1:
var e = bx0;
break;
case 2:
var e = px0;
break;
case 4:
var e = mx0;
break;
case 5:
var e = _x0;
break;
case 6:
var e = yx0;
break;
case 7:
var e = dx0;
break;
case 8:
var e = hx0;
break;
case 9:
var e = kx0;
break;
case 10:
var e = wx0;
break;
case 11:
var e = Ex0;
break;
case 0:
case 13:
var e = Sx0;
break;
case 3:
case 14:
var e = gx0;
break;
default:
var e = Fx0;
}
return Np(t, hp(e, n4));
}
function see(t, n4) {
switch (t) {
case 1:
var e = ex0;
break;
case 2:
var e = nx0;
break;
case 4:
var e = tx0;
break;
case 5:
var e = ux0;
break;
case 6:
var e = ix0;
break;
case 7:
var e = fx0;
break;
case 8:
var e = xx0;
break;
case 9:
var e = ax0;
break;
case 10:
var e = ox0;
break;
case 11:
var e = cx0;
break;
case 0:
case 13:
var e = sx0;
break;
case 3:
case 14:
var e = vx0;
break;
default:
var e = lx0;
}
return Np(t, hp(e, n4));
}
function vee(t, n4) {
switch (t) {
case 1:
var e = Tx0;
break;
case 2:
var e = Ox0;
break;
case 4:
var e = Ix0;
break;
case 5:
var e = Ax0;
break;
case 6:
var e = Nx0;
break;
case 7:
var e = Cx0;
break;
case 8:
var e = Px0;
break;
case 9:
var e = Dx0;
break;
case 10:
var e = Lx0;
break;
case 11:
var e = Rx0;
break;
case 0:
case 13:
var e = jx0;
break;
case 3:
case 14:
var e = Gx0;
break;
default:
var e = Mx0;
}
return Np(t, L70(e, n4));
}
function vs(t, n4, e) {
function i(m0) {
switch (t[1]) {
case 0:
var k0 = 45;
break;
case 1:
var k0 = 43;
break;
default:
var k0 = 32;
}
return N70(e, n4, k0);
}
function x(m0) {
var k0 = l70(e);
return k0 === 3 ? e < 0 ? Zf0 : Qf0 : 4 <= k0 ? $f0 : m0;
}
switch (t[2]) {
case 5:
for (var c = zA(Tz(t, n4), e), s = 0, p = nn(c); ; ) {
if (s === p)
var y = 0;
else {
var T = Ot(c, s) + l1 | 0, E = 0;
if (23 < T >>> 0 ? T === 55 && (E = 1) : 21 < (T - 1 | 0) >>> 0 && (E = 1), !E) {
var s = s + 1 | 0;
continue;
}
var y = 1;
}
var h = y ? c : Te(c, rx0);
return x(h);
}
case 6:
return i(0);
case 7:
var w = i(0), G = l7(w);
if (G === 0)
var A = w;
else {
var S = Pt(G), M = G - 1 | 0, K = 0;
if (!(M < 0))
for (var V = K; ; ) {
Jn(S, V, vz(Hu(w, V)));
var f0 = V + 1 | 0;
if (M !== V) {
var V = f0;
continue;
}
break;
}
var A = S;
}
return A;
case 8:
return x(i(0));
default:
return zA(Tz(t, n4), e);
}
}
function kl(t, n4, e, i) {
for (var x = n4, c = e, s = i; ; ) {
if (typeof s == "number")
return u(x, c);
switch (s[0]) {
case 0:
var p = s[1];
return function(or) {
return Xn(x, [5, c, or], p);
};
case 1:
var y = s[1];
return function(or) {
var _r = 0;
if (40 <= or)
if (or === 92)
var Ir = Ei0;
else
zn <= or ? _r = 1 : _r = 2;
else if (32 <= or)
if (39 <= or)
var Ir = Si0;
else
_r = 2;
else if (14 <= or)
_r = 1;
else
switch (or) {
case 8:
var Ir = gi0;
break;
case 9:
var Ir = Fi0;
break;
case 10:
var Ir = Ti0;
break;
case 13:
var Ir = Oi0;
break;
default:
_r = 1;
}
switch (_r) {
case 1:
var fe = Pt(4);
Jn(fe, 0, 92), Jn(fe, 1, 48 + (or / ni | 0) | 0), Jn(fe, 2, 48 + ((or / 10 | 0) % 10 | 0) | 0), Jn(fe, 3, 48 + (or % 10 | 0) | 0);
var Ir = fe;
break;
case 2:
var v0 = Pt(1);
Jn(v0, 0, or);
var Ir = v0;
break;
}
var P = nn(Ir), L = w1(P + 2 | 0, 39);
return As(Ir, 0, L, 1, P), Xn(x, [4, c, L], y);
};
case 2:
var T = s[2], E = s[1];
return dN(x, c, T, E, function(or) {
return or;
});
case 3:
return dN(x, c, s[2], s[1], aee);
case 4:
return Cp(x, c, s[4], s[2], s[3], oee, s[1]);
case 5:
return Cp(x, c, s[4], s[2], s[3], cee, s[1]);
case 6:
return Cp(x, c, s[4], s[2], s[3], see, s[1]);
case 7:
return Cp(x, c, s[4], s[2], s[3], vee, s[1]);
case 8:
var h = s[4], w = s[3], G = s[2], A = s[1];
if (typeof G == "number") {
if (typeof w == "number")
return w ? function(or, _r) {
return Xn(x, [4, c, vs(A, or, _r)], h);
} : function(or) {
return Xn(x, [4, c, vs(A, pN(A), or)], h);
};
var S = w[1];
return function(or) {
return Xn(x, [4, c, vs(A, S, or)], h);
};
} else {
if (G[0] === 0) {
var M = G[2], K = G[1];
if (typeof w == "number")
return w ? function(or, _r) {
return Xn(x, [4, c, U7(K, M, vs(A, or, _r))], h);
} : function(or) {
return Xn(x, [4, c, U7(K, M, vs(A, pN(A), or))], h);
};
var V = w[1];
return function(or) {
return Xn(x, [4, c, U7(K, M, vs(A, V, or))], h);
};
}
var f0 = G[1];
if (typeof w == "number")
return w ? function(or, _r, Ir) {
return Xn(x, [4, c, U7(f0, or, vs(A, _r, Ir))], h);
} : function(or, _r) {
return Xn(x, [4, c, U7(f0, or, vs(A, pN(A), _r))], h);
};
var m0 = w[1];
return function(or, _r) {
return Xn(x, [4, c, U7(f0, or, vs(A, m0, _r))], h);
};
}
case 9:
return dN(x, c, s[2], s[1], Qre);
case 10:
var c = [7, c], s = s[1];
continue;
case 11:
var c = [2, c, s[1]], s = s[2];
continue;
case 12:
var c = [3, c, s[1]], s = s[2];
continue;
case 13:
var k0 = s[3], g0 = s[2], e0 = Ez(16);
mN(e0, g0);
var x0 = gz(e0);
return function(or) {
return Xn(x, [4, c, x0], k0);
};
case 14:
var l = s[3], c0 = s[2];
return function(or) {
var _r = or[1], Ir = _t(_r, jt(tu(c0)));
if (typeof Ir[2] == "number")
return Xn(x, c, It(Ir[1], l));
throw Tu;
};
case 15:
var t0 = s[1];
return function(or, _r) {
return Xn(x, [6, c, function(Ir) {
return a(or, Ir, _r);
}], t0);
};
case 16:
var a0 = s[1];
return function(or) {
return Xn(x, [6, c, or], a0);
};
case 17:
var c = [0, c, s[1]], s = s[2];
continue;
case 18:
var w0 = s[1];
if (w0[0] === 0) {
var _0 = s[2], E0 = w0[1][1], X0 = 0, x = function(fe, v0, P) {
return function(L) {
return Xn(v0, [1, fe, [0, L]], P);
};
}(c, x, _0), c = X0, s = E0;
continue;
}
var b = s[2], G0 = w0[1][1], X = 0, x = function(or, _r, Ir) {
return function(fe) {
return Xn(_r, [1, or, [1, fe]], Ir);
};
}(c, x, b), c = X, s = G0;
continue;
case 19:
throw [0, wn, Cf0];
case 20:
var s0 = s[3], dr = [8, c, Pf0];
return function(or) {
return Xn(x, dr, s0);
};
case 21:
var Ar = s[2];
return function(or) {
return Xn(x, [4, c, hp(Nf0, or)], Ar);
};
case 22:
var ar = s[1];
return function(or) {
return Xn(x, [5, c, or], ar);
};
case 23:
var W0 = s[2], Lr = s[1];
if (typeof Lr == "number")
switch (Lr) {
case 0:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 1:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 2:
throw [0, wn, Df0];
default:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
}
else
switch (Lr[0]) {
case 0:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 1:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 2:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 3:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 4:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 5:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 6:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 7:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 8:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
case 9:
var Tr = Lr[2];
return t < 50 ? _N(t + 1 | 0, x, c, Tr, W0) : Fu(_N, [0, x, c, Tr, W0]);
case 10:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
default:
return t < 50 ? ct(t + 1 | 0, x, c, W0) : Fu(ct, [0, x, c, W0]);
}
default:
var Hr = s[3], Or = s[1], xr = u(s[2], 0);
return t < 50 ? yN(t + 1 | 0, x, c, Hr, Or, xr) : Fu(yN, [0, x, c, Hr, Or, xr]);
}
}
}
function _N(t, n4, e, i, x) {
if (typeof i == "number")
return t < 50 ? ct(t + 1 | 0, n4, e, x) : Fu(ct, [0, n4, e, x]);
switch (i[0]) {
case 0:
var c = i[1];
return function(m0) {
return ii(n4, e, c, x);
};
case 1:
var s = i[1];
return function(m0) {
return ii(n4, e, s, x);
};
case 2:
var p = i[1];
return function(m0) {
return ii(n4, e, p, x);
};
case 3:
var y = i[1];
return function(m0) {
return ii(n4, e, y, x);
};
case 4:
var T = i[1];
return function(m0) {
return ii(n4, e, T, x);
};
case 5:
var E = i[1];
return function(m0) {
return ii(n4, e, E, x);
};
case 6:
var h = i[1];
return function(m0) {
return ii(n4, e, h, x);
};
case 7:
var w = i[1];
return function(m0) {
return ii(n4, e, w, x);
};
case 8:
var G = i[2];
return function(m0) {
return ii(n4, e, G, x);
};
case 9:
var A = i[3], S = i[2], M = lu(tu(i[1]), S);
return function(m0) {
return ii(n4, e, t7(M, A), x);
};
case 10:
var K = i[1];
return function(m0, k0) {
return ii(n4, e, K, x);
};
case 11:
var V = i[1];
return function(m0) {
return ii(n4, e, V, x);
};
case 12:
var f0 = i[1];
return function(m0) {
return ii(n4, e, f0, x);
};
case 13:
throw [0, wn, Lf0];
default:
throw [0, wn, Rf0];
}
}
function ct(t, n4, e, i) {
var x = [8, e, jf0];
return t < 50 ? kl(t + 1 | 0, n4, x, i) : Fu(kl, [0, n4, x, i]);
}
function yN(t, n4, e, i, x, c) {
if (x) {
var s = x[1];
return function(y) {
return lee(n4, e, i, s, u(c, y));
};
}
var p = [4, e, c];
return t < 50 ? kl(t + 1 | 0, n4, p, i) : Fu(kl, [0, n4, p, i]);
}
function Xn(t, n4, e) {
return QA(kl(0, t, n4, e));
}
function ii(t, n4, e, i) {
return QA(_N(0, t, n4, e, i));
}
function lee(t, n4, e, i, x) {
return QA(yN(0, t, n4, e, i, x));
}
function dN(t, n4, e, i, x) {
if (typeof i == "number")
return function(y) {
return Xn(t, [4, n4, u(x, y)], e);
};
if (i[0] === 0) {
var c = i[2], s = i[1];
return function(y) {
return Xn(t, [4, n4, U7(s, c, u(x, y))], e);
};
}
var p = i[1];
return function(y, T) {
return Xn(t, [4, n4, U7(p, y, u(x, T))], e);
};
}
function Cp(t, n4, e, i, x, c, s) {
if (typeof i == "number") {
if (typeof x == "number")
return x ? function(G, A) {
return Xn(t, [4, n4, Yv(G, a(c, s, A))], e);
} : function(G) {
return Xn(t, [4, n4, a(c, s, G)], e);
};
var p = x[1];
return function(G) {
return Xn(t, [4, n4, Yv(p, a(c, s, G))], e);
};
} else {
if (i[0] === 0) {
var y = i[2], T = i[1];
if (typeof x == "number")
return x ? function(G, A) {
return Xn(t, [4, n4, U7(T, y, Yv(G, a(c, s, A)))], e);
} : function(G) {
return Xn(t, [4, n4, U7(T, y, a(c, s, G))], e);
};
var E = x[1];
return function(G) {
return Xn(t, [4, n4, U7(T, y, Yv(E, a(c, s, G)))], e);
};
}
var h = i[1];
if (typeof x == "number")
return x ? function(G, A, S) {
return Xn(t, [4, n4, U7(h, G, Yv(A, a(c, s, S)))], e);
} : function(G, A) {
return Xn(t, [4, n4, U7(h, G, a(c, s, A))], e);
};
var w = x[1];
return function(G, A) {
return Xn(t, [4, n4, U7(h, G, Yv(w, a(c, s, A)))], e);
};
}
}
function ls(t, n4) {
for (var e = n4; ; ) {
if (typeof e == "number")
return 0;
switch (e[0]) {
case 0:
var i = e[1], x = Fz(e[2]);
return ls(t, i), vl(t, x);
case 1:
var c = e[2], s = e[1];
if (c[0] === 0) {
var p = c[1];
ls(t, s), vl(t, Gf0);
var e = p;
continue;
}
var y = c[1];
ls(t, s), vl(t, Mf0);
var e = y;
continue;
case 6:
var T = e[2];
return ls(t, e[1]), u(T, t);
case 7:
return ls(t, e[1]), m1(t);
case 8:
var E = e[2];
return ls(t, e[1]), Cu(E);
case 2:
case 4:
var h = e[2];
return ls(t, e[1]), vl(t, h);
default:
var w = e[2];
return ls(t, e[1]), QV(t, w);
}
}
}
function bs(t, n4) {
for (var e = n4; ; ) {
if (typeof e == "number")
return 0;
switch (e[0]) {
case 0:
var i = e[1], x = Fz(e[2]);
return bs(t, i), mn(t, x);
case 1:
var c = e[2], s = e[1];
if (c[0] === 0) {
var p = c[1];
bs(t, s), mn(t, Bf0);
var e = p;
continue;
}
var y = c[1];
bs(t, s), mn(t, qf0);
var e = y;
continue;
case 6:
var T = e[2];
return bs(t, e[1]), mn(t, u(T, 0));
case 7:
var e = e[1];
continue;
case 8:
var E = e[2];
return bs(t, e[1]), Cu(E);
case 2:
case 4:
var h = e[2];
return bs(t, e[1]), mn(t, h);
default:
var w = e[2];
return bs(t, e[1]), qi(t, w);
}
}
}
function bee(t) {
if (qn(t, Hf0))
return Xf0;
var n4 = nn(t);
function e(S) {
var M = Uf0[1], K = $n(C4);
return u(Xn(function(V) {
return bs(K, V), ke(Gt(K));
}, 0, M), t);
}
function i(S) {
for (var M = S; ; ) {
if (M === n4)
return M;
var K = Ot(t, M);
if (K !== 9 && K !== 32)
return M;
var M = M + 1 | 0;
}
}
function x(S, M) {
for (var K = M; ; ) {
if (K === n4 || 25 < (Ot(t, K) + V3 | 0) >>> 0)
return K;
var K = K + 1 | 0;
}
}
function c(S, M) {
for (var K = M; ; ) {
if (K === n4)
return K;
var V = Ot(t, K), f0 = 0;
if (48 <= V ? 58 <= V || (f0 = 1) : V === 45 && (f0 = 1), f0) {
var K = K + 1 | 0;
continue;
}
return K;
}
}
var s = i(0), p = x(s, s), y = p7(t, s, p - s | 0), T = i(p), E = c(T, T);
if (T === E)
var h = 0;
else
try {
var w = Bi(p7(t, T, E - T | 0)), h = w;
} catch (S) {
if (S = Et(S), S[1] !== B7)
throw S;
var h = e(0);
}
i(E) !== n4 && e(0);
var G = 0;
if (n0(y, Yf0) && n0(y, Vf0))
var A = n0(y, zf0) ? n0(y, Kf0) ? n0(y, Wf0) ? n0(y, Jf0) ? e(0) : 1 : 2 : 3 : 0;
else
G = 1;
if (G)
var A = 4;
return [0, h, A];
}
function hN(t, n4) {
var e = n4[1], i = 0;
return Xn(function(x) {
return ls(t, x), 0;
}, i, e);
}
function kN(t) {
return hN(Lc, t);
}
function Qn(t) {
var n4 = t[1];
return Xn(function(e) {
var i = $n(64);
return bs(i, e), Gt(i);
}, 0, n4);
}
var wN = [0, 0];
function EN(t, n4) {
var e = t[1 + n4];
if (1 - (typeof e == "number" ? 1 : 0)) {
if (h1(e) === Y2)
return u(Qn(Da0), e);
if (h1(e) === ih)
for (var i = zA(di0, e), x = 0, c = nn(i); ; ) {
if (c <= x)
return Te(i, hi0);
var s = Ot(i, x), p = 0;
if (48 <= s ? 58 <= s || (p = 1) : s === 45 && (p = 1), p) {
var x = x + 1 | 0;
continue;
}
return i;
}
return La0;
}
return u(Qn(Pa0), e);
}
function Oz(t, n4) {
if (t.length - 1 <= n4)
return aa0;
var e = Oz(t, n4 + 1 | 0), i = EN(t, n4);
return a(Qn(oa0), i, e);
}
function Pp(t) {
function n4(k0) {
for (var g0 = k0; ; ) {
if (g0) {
var e0 = g0[2], x0 = g0[1];
try {
var l = 0, c0 = u(x0, t);
l = 1;
} catch {
}
if (l && c0)
return [0, c0[1]];
var g0 = e0;
continue;
}
return 0;
}
}
var e = n4(wN[1]);
if (e)
return e[1];
if (t === rN)
return Sa0;
if (t === uz)
return ga0;
if (t[1] === tz) {
var i = t[2], x = i[3], c = i[2], s = i[1];
return b7(Qn(nN), s, c, x, x + 5 | 0, Fa0);
}
if (t[1] === wn) {
var p = t[2], y = p[3], T = p[2], E = p[1];
return b7(Qn(nN), E, T, y, y + 6 | 0, Ta0);
}
if (t[1] === sl) {
var h = t[2], w = h[3], G = h[2], A = h[1];
return b7(Qn(nN), A, G, w, w + 6 | 0, Oa0);
}
if (h1(t) === 0) {
var S = t.length - 1, M = t[1][1];
if (2 < S >>> 0)
var K = Oz(t, 2), V = EN(t, 1), f0 = a(Qn(Ia0), V, K);
else
switch (S) {
case 0:
var f0 = Aa0;
break;
case 1:
var f0 = Na0;
break;
default:
var m0 = EN(t, 1), f0 = u(Qn(Ca0), m0);
}
return Te(M, f0);
}
return t[1];
}
function SN(t, n4) {
var e = F70(n4), i = e.length - 1 - 1 | 0, x = 0;
if (!(i < 0))
for (var c = x; ; ) {
var s = nu(e, c)[1 + c], p = function(f0) {
return function(m0) {
return m0 ? f0 === 0 ? ma0 : _a0 : f0 === 0 ? ya0 : da0;
};
}(c);
if (s[0] === 0)
var y = s[5], T = s[4], E = s[3], h = s[6] ? ha0 : ka0, w = s[2], G = s[7], A = p(s[1]), M = [0, mi0(Qn(wa0), A, G, w, h, E, T, y)];
else if (s[1])
var M = 0;
else
var S = p(0), M = [0, u(Qn(Ea0), S)];
if (M) {
var K = M[1];
u(hN(t, ba0), K);
}
var V = c + 1 | 0;
if (i !== c) {
var c = V;
continue;
}
break;
}
return 0;
}
function Iz(t) {
for (; ; ) {
var n4 = wN[1], e = 1 - iN(wN, n4, [0, t, n4]);
if (!e)
return e;
}
}
var pee = Ra0.slice();
function mee(t, n4) {
var e = Pp(t);
u(kN(la0), e), SN(Lc, n4);
var i = U70(0);
if (i < 0) {
var x = Fp(i);
cz(nu(pee, x)[1 + x]);
}
return m1(Lc);
}
var _ee = [0];
ZA(r(BH), function(t, n4) {
try {
try {
var e = n4 ? _ee : HV(0);
try {
xN(0);
} catch {
}
try {
var i = mee(t, e), x = i;
} catch (y) {
y = Et(y);
var c = Pp(t);
u(kN(ca0), c), SN(Lc, e);
var s = Pp(y);
u(kN(sa0), s), SN(Lc, HV(0));
var x = m1(Lc);
}
var p = x;
} catch (y) {
if (y = Et(y), y !== rN)
throw y;
var p = cz(va0);
}
return p;
} catch {
return 0;
}
});
var gN = [wt, to0, G7(0)], Dp = 0, Az = -1;
function wl(t, n4) {
return t[13] = t[13] + n4[3] | 0, vN(n4, t[28]);
}
var Nz = 1000000010;
function FN(t, n4) {
return ir(t[17], n4, 0, nn(n4));
}
function Lp(t) {
return u(t[19], 0);
}
function Cz(t, n4, e) {
return t[9] = t[9] - n4 | 0, FN(t, e), t[11] = 0, 0;
}
function Rp(t, n4) {
var e = n0(n4, no0);
return e && Cz(t, nn(n4), n4);
}
function Vv(t, n4, e) {
var i = n4[3], x = n4[2];
Rp(t, n4[1]), Lp(t), t[11] = 1;
var c = (t[6] - e | 0) + x | 0, s = t[8], p = s <= c ? s : c;
return t[10] = p, t[9] = t[6] - t[10] | 0, u(t[21], t[10]), Rp(t, i);
}
function Pz(t, n4) {
return Vv(t, eo0, n4);
}
function El(t, n4) {
var e = n4[2], i = n4[3];
return Rp(t, n4[1]), t[9] = t[9] - e | 0, u(t[20], e), Rp(t, i);
}
function Dz(t) {
for (; ; ) {
var n4 = t[28][2], e = n4 ? [0, n4[1]] : 0;
if (e) {
var i = e[1], x = i[1], c = i[2], s = 0 <= x ? 1 : 0, p = i[3], y = t[13] - t[12] | 0, T = s || (t[9] <= y ? 1 : 0);
if (T) {
var E = t[28], h = E[2];
if (h) {
if (h[2]) {
var w = h[2];
E[1] = E[1] - 1 | 0, E[2] = w;
} else
sN(E);
var G = 0 <= x ? x : Nz;
if (typeof c == "number")
switch (c) {
case 0:
var A = Hv(t[3]);
if (A) {
var S = A[1][1], M = function(L, Q) {
if (Q) {
var i0 = Q[1], l0 = Q[2];
return q70(L, i0) ? [0, L, Q] : [0, i0, M(L, l0)];
}
return [0, L, 0];
};
S[1] = M(t[6] - t[9] | 0, S[1]);
}
break;
case 1:
Uv(t[2]);
break;
case 2:
Uv(t[3]);
break;
case 3:
var K = Hv(t[2]);
K ? Pz(t, K[1][2]) : Lp(t);
break;
case 4:
if (t[10] !== (t[6] - t[9] | 0)) {
var V = t[28], f0 = V[2];
if (f0) {
var m0 = f0[1];
if (f0[2]) {
var k0 = f0[2];
V[1] = V[1] - 1 | 0, V[2] = k0;
var g0 = [0, m0];
} else {
sN(V);
var g0 = [0, m0];
}
} else
var g0 = 0;
if (g0) {
var e0 = g0[1], x0 = e0[1];
t[12] = t[12] - e0[3] | 0, t[9] = t[9] + x0 | 0;
}
}
break;
default:
var l = Uv(t[5]);
l && FN(t, u(t[25], l[1]));
}
else
switch (c[0]) {
case 0:
Cz(t, G, c[1]);
break;
case 1:
var c0 = c[2], t0 = c[1], a0 = c0[1], w0 = c0[2], _0 = Hv(t[2]);
if (_0) {
var E0 = _0[1], X0 = E0[2];
switch (E0[1]) {
case 0:
El(t, t0);
break;
case 1:
Vv(t, c0, X0);
break;
case 2:
Vv(t, c0, X0);
break;
case 3:
t[9] < (G + nn(a0) | 0) ? Vv(t, c0, X0) : El(t, t0);
break;
case 4:
t[11] || !(t[9] < (G + nn(a0) | 0) || ((t[6] - X0 | 0) + w0 | 0) < t[10]) ? El(t, t0) : Vv(t, c0, X0);
break;
default:
El(t, t0);
}
}
break;
case 2:
var b = t[6] - t[9] | 0, G0 = c[2], X = c[1], s0 = Hv(t[3]);
if (s0) {
var dr = s0[1][1], Ar = dr[1];
if (Ar)
for (var ar = dr[1], W0 = Ar[1]; ; ) {
if (ar) {
var Lr = ar[1], Tr = ar[2];
if (!(b <= Lr)) {
var ar = Tr;
continue;
}
var Hr = Lr;
} else
var Hr = W0;
var Or = Hr;
break;
}
else
var Or = b;
var xr = Or - b | 0;
0 <= xr ? El(t, [0, Za0, xr + X | 0, $a0]) : Vv(t, [0, ro0, Or + G0 | 0, Qa0], t[6]);
}
break;
case 3:
var Rr = c[2], Wr = c[1];
if (t[8] < (t[6] - t[9] | 0)) {
var Jr = Hv(t[2]);
if (Jr) {
var or = Jr[1], _r = or[2], Ir = or[1];
t[9] < _r && !(3 < (Ir - 1 | 0) >>> 0) && Pz(t, _r);
} else
Lp(t);
}
var fe = t[9] - Wr | 0, v0 = Rr === 1 ? 1 : t[9] < G ? Rr : 5;
E1([0, v0, fe], t[2]);
break;
case 4:
E1(c[1], t[3]);
break;
default:
var P = c[1];
FN(t, u(t[24], P)), E1(P, t[5]);
}
t[12] = p + t[12] | 0;
continue;
}
throw uee;
}
return T;
}
return 0;
}
}
function Lz(t, n4) {
return wl(t, n4), Dz(t);
}
function Rz(t, n4, e) {
return Lz(t, [0, n4, [0, e], n4]);
}
function TN(t) {
return dl(t), E1([0, -1, [0, Az, Ja0, 0]], t);
}
function ON(t, n4) {
var e = Hv(t[1]);
if (e) {
var i = e[1], x = i[2], c = x[1];
if (i[1] < t[12])
return TN(t[1]);
var s = x[2];
if (typeof s != "number")
switch (s[0]) {
case 3:
var p = 1 - n4, y = p && (x[1] = t[13] + c | 0, Uv(t[1]), 0);
return y;
case 1:
case 2:
var T = n4 && (x[1] = t[13] + c | 0, Uv(t[1]), 0);
return T;
}
return 0;
}
return 0;
}
function jz(t, n4, e) {
return wl(t, e), n4 && ON(t, 1), E1([0, t[13], e], t[1]);
}
function Gz(t, n4, e) {
if (t[14] = t[14] + 1 | 0, t[14] < t[15])
return jz(t, 0, [0, -t[13] | 0, [3, n4, e], 0]);
var i = t[14] === t[15] ? 1 : 0;
if (i) {
var x = t[16];
return Rz(t, nn(x), x);
}
return i;
}
function Mz(t, n4) {
var e = 1 < t[14] ? 1 : 0;
if (e) {
t[14] < t[15] && (wl(t, [0, Dp, 1, 0]), ON(t, 1), ON(t, 0)), t[14] = t[14] - 1 | 0;
var i = 0;
} else
var i = e;
return i;
}
function Bz(t, n4) {
t[23] && wl(t, [0, Dp, 5, 0]);
var e = t[22];
if (e) {
var i = Uv(t[4]);
if (i)
return u(t[27], i[1]);
var x = 0;
} else
var x = e;
return x;
}
function IN(t, n4) {
var e = t[4];
function i(x) {
return Bz(t, 0);
}
for (Pu(i, e[1]); ; ) {
if (1 < t[14]) {
Mz(t, 0);
continue;
}
return t[13] = Nz, Dz(t), n4 && Lp(t), t[12] = 1, t[13] = 1, sN(t[28]), TN(t[1]), dl(t[2]), dl(t[3]), dl(t[4]), dl(t[5]), t[10] = 0, t[14] = 0, t[9] = t[6], Gz(t, 0, 3);
}
}
function AN(t, n4, e) {
var i = t[14] < t[15] ? 1 : 0;
return i && Rz(t, n4, e);
}
function qz(t, n4, e) {
return AN(t, n4, e);
}
function g(t, n4) {
return qz(t, nn(n4), n4);
}
function Sl(t, n4) {
return qz(t, 1, Op(1, n4));
}
function gl(t, n4) {
return IN(t, 0), u(t[18], 0);
}
var Uz = Op(80, 32);
function Hz(t, n4) {
for (var e = n4; ; ) {
var i = 0 < e ? 1 : 0;
if (i) {
if (80 < e) {
ir(t[17], Uz, 0, 80);
var e = e + fX | 0;
continue;
}
return ir(t[17], Uz, 0, e);
}
return i;
}
}
function yee(t) {
return t[1] === gN ? Te(Ha0, Te(t[2], Ua0)) : Xa0;
}
function dee(t) {
return t[1] === gN ? Te(Ba0, Te(t[2], Ma0)) : qa0;
}
function hee(t) {
return 0;
}
function kee(t) {
return 0;
}
function Xz(t, n4) {
function e(w) {
return 0;
}
function i(w) {
return 0;
}
function x(w) {
return 0;
}
var c = kz(0), s = [0, Az, ja0, 0];
vN(s, c);
var p = yl(0);
TN(p), E1([0, 1, s], p);
var y = yl(0), T = yl(0), E = yl(0), h = [0, p, yl(0), E, T, y, 78, 10, 68, 78, 0, 1, 1, 1, 1, Zre, Ga0, t, n4, x, i, e, 0, 0, yee, dee, hee, kee, c];
return h[19] = function(w) {
return ir(h[17], Ya0, 0, 1);
}, h[20] = function(w) {
return Hz(h, w);
}, h[21] = function(w) {
return Hz(h, w);
}, h;
}
function Yz(t) {
function n4(e) {
return m1(t);
}
return Xz(function(e, i, x) {
return 0 <= i && 0 <= x && !((nn(e) - x | 0) < i) ? JA(t, e, i, x) : Cu(yi0);
}, n4);
}
function NN(t) {
function n4(e) {
return 0;
}
return Xz(function(e, i, x) {
return wz(t, e, i, x);
}, n4);
}
var wee = CI;
function Vz(t) {
return $n(wee);
}
var Eee = Vz(0), See = Yz(ree), gee = Yz(Lc);
NN(Eee);
function zz(t, n4) {
var e = $n(16), i = NN(e);
a(t, i, n4), gl(i, 0);
var x = e[2];
if (2 <= x) {
var c = x - 2 | 0, s = 1;
return 0 <= c && !((e[2] - c | 0) < 1) ? qv(e[1], s, c) : Cu($i0);
}
return Gt(e);
}
function H7(t, n4) {
var e = 0;
if (typeof n4 == "number")
return 0;
switch (n4[0]) {
case 0:
var i = n4[2];
if (H7(t, n4[1]), typeof i == "number")
switch (i) {
case 0:
return Mz(t, 0);
case 1:
return Bz(t, 0);
case 2:
return gl(t, 0);
case 3:
var x = t[14] < t[15] ? 1 : 0;
return x && Lz(t, [0, Dp, 3, 0]);
case 4:
return IN(t, 1), u(t[18], 0);
case 5:
return Sl(t, 64);
default:
return Sl(t, 37);
}
else
switch (i[0]) {
case 0:
var c = [0, Wa0, i[2], Ka0], s = t[14] < t[15] ? 1 : 0, p = [0, za0, i[3], Va0], y = c[3], T = c[2], E = c[1];
return s && jz(t, 1, [0, -t[13] | 0, [1, c, p], (nn(E) + T | 0) + nn(y) | 0]);
case 1:
return 0;
default:
var h = i[1];
return Sl(t, 64), Sl(t, h);
}
case 1:
var w = n4[2], G = n4[1];
if (w[0] === 0) {
var A = w[1];
H7(t, G);
var S = [0, gN, zz(H7, A)];
t[22] && (E1(S, t[4]), u(t[26], S));
var M = t[23];
return M && wl(t, [0, Dp, [5, S], 0]);
}
var K = w[1];
H7(t, G);
var V = bee(zz(H7, K));
return Gz(t, V[1], V[2]);
case 2:
var f0 = n4[1], m0 = 0;
if (typeof f0 != "number" && f0[0] === 0) {
var k0 = f0[2], g0 = 0;
if (typeof k0 != "number" && k0[0] === 1) {
var e0 = n4[2], x0 = k0[2], l = f0[1];
m0 = 1, g0 = 1;
}
}
if (!m0) {
var c0 = n4[2], t0 = f0;
e = 2;
}
break;
case 3:
var a0 = n4[1], w0 = 0;
if (typeof a0 != "number" && a0[0] === 0) {
var _0 = a0[2], E0 = 0;
if (typeof _0 != "number" && _0[0] === 1) {
var X0 = n4[2], b = _0[2], G0 = a0[1];
e = 1, w0 = 1, E0 = 1;
}
}
if (!w0) {
var X = n4[2], s0 = a0;
e = 3;
}
break;
case 4:
var dr = n4[1], Ar = 0;
if (typeof dr != "number" && dr[0] === 0) {
var ar = dr[2], W0 = 0;
if (typeof ar != "number" && ar[0] === 1) {
var e0 = n4[2], x0 = ar[2], l = dr[1];
Ar = 1, W0 = 1;
}
}
if (!Ar) {
var c0 = n4[2], t0 = dr;
e = 2;
}
break;
case 5:
var Lr = n4[1], Tr = 0;
if (typeof Lr == "number" || Lr[0] !== 0)
Tr = 1;
else {
var Hr = Lr[2], Or = 0;
if (typeof Hr != "number" && Hr[0] === 1) {
var X0 = n4[2], b = Hr[2], G0 = Lr[1];
e = 1, Or = 1;
}
Or || (Tr = 1);
}
if (Tr) {
var X = n4[2], s0 = Lr;
e = 3;
}
break;
case 6:
var xr = n4[2];
return H7(t, n4[1]), u(xr, t);
case 7:
return H7(t, n4[1]), gl(t, 0);
default:
var Rr = n4[2];
return H7(t, n4[1]), Cu(Rr);
}
switch (e) {
case 0:
return H7(t, l), AN(t, x0, e0);
case 1:
return H7(t, G0), AN(t, b, Op(1, X0));
case 2:
return H7(t, t0), g(t, c0);
default:
return H7(t, s0), Sl(t, X);
}
}
function f(t) {
return function(n4) {
var e = n4[1], i = 0;
return Xn(function(x) {
return H7(t, x), 0;
}, i, e);
};
}
function P0(t) {
var n4 = t[1], e = Vz(0), i = NN(e);
return Xn(function(x) {
H7(i, x), IN(i, 0);
var c = Gt(e);
return e[2] = 0, e[1] = e[4], e[3] = l7(e[1]), c;
}, 0, n4);
}
sz(function(t) {
return gl(See, 0), gl(gee, 0);
});
function Fee(t, n4) {
var e = h1(n4) === wt ? n4 : n4[1];
return ZA(t, e);
}
var Tee = 2;
function Oee(t) {
var n4 = [0, 0], e = nn(t) - 1 | 0, i = 0;
if (!(e < 0))
for (var x = i; ; ) {
var c = Ot(t, x);
n4[1] = (d8 * n4[1] | 0) + c | 0;
var s = x + 1 | 0;
if (e !== x) {
var x = s;
continue;
}
break;
}
n4[1] = n4[1] & kH;
var p = 1073741823 < n4[1] ? n4[1] + 2147483648 | 0 : n4[1];
return p;
}
var ps = cN([0, Ee]), S1 = cN([0, Ee]), Cs = cN([0, Cc]), Kz = $A(0, 0), Iee = [0, 0];
function Wz(t) {
return 2 < t ? Wz((t + 1 | 0) / 2 | 0) * 2 | 0 : t;
}
function Jz(t) {
Iee[1]++;
var n4 = t.length - 1, e = Gv((n4 * 2 | 0) + 2 | 0, Kz);
nu(e, 0)[1] = n4;
var i = ((Wz(n4) * 32 | 0) / 8 | 0) - 1 | 0;
nu(e, 1)[2] = i;
var x = n4 - 1 | 0, c = 0;
if (!(x < 0))
for (var s = c; ; ) {
var p = (s * 2 | 0) + 3 | 0, y = nu(t, s)[1 + s];
nu(e, p)[1 + p] = y;
var T = s + 1 | 0;
if (x !== s) {
var s = T;
continue;
}
break;
}
return [0, Tee, e, S1[1], Cs[1], 0, 0, ps[1], 0];
}
function CN(t, n4) {
var e = t[2].length - 1, i = e < n4 ? 1 : 0;
if (i) {
var x = Gv(n4, Kz), c = t[2], s = 0;
0 <= e && !((c.length - 1 - e | 0) < 0) && !((x.length - 1 - e | 0) < 0) && (o70(c, 0, x, 0, e), s = 1), s || Cu(Li0), t[2] = x;
var p = 0;
} else
var p = i;
return p;
}
var $z = [0, 0], Aee = [0, 0];
function PN(t) {
var n4 = t[2].length - 1;
return CN(t, n4 + 1 | 0), n4;
}
function Fl(t, n4) {
try {
var e = a(S1[28], n4, t[3]);
return e;
} catch (x) {
if (x = Et(x), x === Kt) {
var i = PN(t);
return t[3] = ir(S1[4], n4, i, t[3]), t[4] = ir(Cs[4], i, 1, t[4]), i;
}
throw x;
}
}
function DN(t, n4) {
return Ip(function(e) {
return Fl(t, e);
}, n4);
}
function Zz(t, n4, e) {
return Aee[1]++, a(Cs[28], n4, t[4]) ? (CN(t, n4 + 1 | 0), nu(t[2], n4)[1 + n4] = e, 0) : (t[6] = [0, [0, n4, e], t[6]], 0);
}
function Nee(t, n4) {
try {
var e = tee(n4, t[6]);
return e;
} catch (i) {
if (i = Et(i), i === Kt)
return nu(t[2], n4)[1 + n4];
throw i;
}
}
function LN(t) {
if (t === 0)
return 0;
for (var n4 = t.length - 1 - 1 | 0, e = 0; ; ) {
if (0 <= n4) {
var i = [0, t[1 + n4], e], n4 = n4 - 1 | 0, e = i;
continue;
}
return e;
}
}
function Cee(t) {
var n4 = t[1];
return t[1] = n4 + 1 | 0, n4;
}
function RN(t, n4) {
try {
var e = a(ps[28], n4, t[7]);
return e;
} catch (x) {
if (x = Et(x), x === Kt) {
var i = Cee(t);
return n0(n4, io0) && (t[7] = ir(ps[4], n4, i, t[7])), i;
}
throw x;
}
}
function jN(t) {
return qV(t, 0) ? [0] : t;
}
function Pee(t, n4) {
try {
var e = a(ps[28], n4, t[7]);
return e;
} catch (i) {
throw i = Et(i), i === Kt ? [0, wn, uo0] : i;
}
}
function GN(t, n4, e, i, x, c) {
var s = x[2], p = x[4], y = LN(n4), T = LN(e), E = LN(i), h = k1(function(b) {
return Fl(t, b);
}, T), w = k1(function(b) {
return Fl(t, b);
}, E);
t[5] = [0, [0, t[3], t[4], t[6], t[7], h, y], t[5]];
var G = ps[1], A = t[7];
function S(b, G0, X) {
return oN(b, y) ? ir(ps[4], b, G0, X) : X;
}
t[7] = ir(ps[13], S, A, G);
var M = [0, S1[1]], K = [0, Cs[1]];
pz(function(b, G0) {
M[1] = ir(S1[4], b, G0, M[1]);
var X = K[1];
try {
var s0 = a(Cs[28], G0, t[4]), dr = s0;
} catch (Ar) {
if (Ar = Et(Ar), Ar !== Kt)
throw Ar;
var dr = 1;
}
return K[1] = ir(Cs[4], G0, dr, X), 0;
}, E, w), pz(function(b, G0) {
return M[1] = ir(S1[4], b, G0, M[1]), K[1] = ir(Cs[4], G0, 0, K[1]), 0;
}, T, h), t[3] = M[1], t[4] = K[1];
var V = 0, f0 = t[6];
t[6] = aN(function(b, G0) {
return oN(b[1], h) ? G0 : [0, b, G0];
}, f0, V);
var m0 = c ? a(s, t, p) : u(s, t), k0 = bl(t[5]), g0 = k0[6], e0 = k0[5], x0 = k0[4], l = k0[3], c0 = k0[2], t0 = k0[1];
t[5] = bz(t[5]), t[7] = be(function(b, G0) {
var X = a(ps[28], G0, t[7]);
return ir(ps[4], G0, X, b);
}, x0, g0), t[3] = t0, t[4] = c0;
var a0 = t[6];
t[6] = aN(function(b, G0) {
return oN(b[1], e0) ? G0 : [0, b, G0];
}, a0, l);
var w0 = 0, _0 = jN(i), E0 = [0, Ip(function(b) {
return Nee(t, Fl(t, b));
}, _0), w0], X0 = jN(n4);
return c70([0, [0, m0], [0, Ip(function(b) {
return Pee(t, b);
}, X0), E0]]);
}
function jp(t, n4) {
if (t === 0)
var e = Jz([0]);
else {
var i = Jz(Ip(Oee, t)), x = t.length - 1 - 1 | 0, c = 0;
if (!(x < 0))
for (var s = c; ; ) {
var p = (s * 2 | 0) + 2 | 0;
i[3] = ir(S1[4], t[1 + s], p, i[3]), i[4] = ir(Cs[4], p, 1, i[4]);
var y = s + 1 | 0;
if (x !== s) {
var s = y;
continue;
}
break;
}
var e = i;
}
var T = u(n4, e);
return $z[1] = ($z[1] + e[1] | 0) - 1 | 0, e[8] = de(e[8]), CN(e, 3 + ((nu(e[2], 1)[2] * 16 | 0) / 32 | 0) | 0), [0, u(T, 0), n4, T, 0];
}
function Gp(t, n4) {
if (t)
return t;
var e = $A(wt, n4[1]);
return e[1] = n4[2], ui0(e);
}
function MN(t, n4, e) {
if (t)
return n4;
var i = e[8];
if (i !== 0)
for (var x = i; ; ) {
if (x) {
var c = x[2];
u(x[1], n4);
var x = c;
continue;
}
break;
}
return n4;
}
function Mp(t) {
var n4 = PN(t), e = 0;
if (n4 % 2 | 0 && !((2 + ((nu(t[2], 1)[2] * 16 | 0) / 32 | 0) | 0) < n4)) {
var i = PN(t);
e = 1;
}
if (!e)
var i = n4;
return nu(t[2], i)[1 + i] = 0, i;
}
function BN(t, n4) {
for (var e = [0, 0], i = n4.length - 1; ; ) {
if (e[1] < i) {
var x = e[1], c = nu(n4, x)[1 + x], s = function(p0) {
e[1]++;
var b0 = e[1];
return nu(n4, b0)[1 + b0];
}, p = s(0);
if (typeof p == "number")
switch (p) {
case 0:
var y = s(0), Z = function(b0) {
return function(O0) {
return b0;
};
}(y);
break;
case 1:
var T = s(0), Z = function(b0) {
return function(O0) {
return O0[1 + b0];
};
}(T);
break;
case 2:
var E = s(0), h = s(0), Z = function(b0, O0) {
return function(q0) {
return q0[1 + b0][1 + O0];
};
}(E, h);
break;
case 3:
var w = s(0), Z = function(b0) {
return function(O0) {
return u(O0[1][1 + b0], O0);
};
}(w);
break;
case 4:
var G = s(0), Z = function(b0) {
return function(O0, q0) {
return O0[1 + b0] = q0, 0;
};
}(G);
break;
case 5:
var A = s(0), S = s(0), Z = function(b0, O0) {
return function(q0) {
return u(b0, O0);
};
}(A, S);
break;
case 6:
var M = s(0), K = s(0), Z = function(b0, O0) {
return function(q0) {
return u(b0, q0[1 + O0]);
};
}(M, K);
break;
case 7:
var V = s(0), f0 = s(0), m0 = s(0), Z = function(b0, O0, q0) {
return function(er) {
return u(b0, er[1 + O0][1 + q0]);
};
}(V, f0, m0);
break;
case 8:
var k0 = s(0), g0 = s(0), Z = function(b0, O0) {
return function(q0) {
return u(b0, u(q0[1][1 + O0], q0));
};
}(k0, g0);
break;
case 9:
var e0 = s(0), x0 = s(0), l = s(0), Z = function(b0, O0, q0) {
return function(er) {
return a(b0, O0, q0);
};
}(e0, x0, l);
break;
case 10:
var c0 = s(0), t0 = s(0), a0 = s(0), Z = function(b0, O0, q0) {
return function(er) {
return a(b0, O0, er[1 + q0]);
};
}(c0, t0, a0);
break;
case 11:
var w0 = s(0), _0 = s(0), E0 = s(0), X0 = s(0), Z = function(b0, O0, q0, er) {
return function(yr) {
return a(b0, O0, yr[1 + q0][1 + er]);
};
}(w0, _0, E0, X0);
break;
case 12:
var b = s(0), G0 = s(0), X = s(0), Z = function(b0, O0, q0) {
return function(er) {
return a(b0, O0, u(er[1][1 + q0], er));
};
}(b, G0, X);
break;
case 13:
var s0 = s(0), dr = s(0), Ar = s(0), Z = function(b0, O0, q0) {
return function(er) {
return a(b0, er[1 + O0], q0);
};
}(s0, dr, Ar);
break;
case 14:
var ar = s(0), W0 = s(0), Lr = s(0), Tr = s(0), Z = function(b0, O0, q0, er) {
return function(yr) {
return a(b0, yr[1 + O0][1 + q0], er);
};
}(ar, W0, Lr, Tr);
break;
case 15:
var Hr = s(0), Or = s(0), xr = s(0), Z = function(b0, O0, q0) {
return function(er) {
return a(b0, u(er[1][1 + O0], er), q0);
};
}(Hr, Or, xr);
break;
case 16:
var Rr = s(0), Wr = s(0), Z = function(b0, O0) {
return function(q0) {
return a(q0[1][1 + b0], q0, O0);
};
}(Rr, Wr);
break;
case 17:
var Jr = s(0), or = s(0), Z = function(b0, O0) {
return function(q0) {
return a(q0[1][1 + b0], q0, q0[1 + O0]);
};
}(Jr, or);
break;
case 18:
var _r = s(0), Ir = s(0), fe = s(0), Z = function(b0, O0, q0) {
return function(er) {
return a(er[1][1 + b0], er, er[1 + O0][1 + q0]);
};
}(_r, Ir, fe);
break;
case 19:
var v0 = s(0), P = s(0), Z = function(b0, O0) {
return function(q0) {
var er = u(q0[1][1 + O0], q0);
return a(q0[1][1 + b0], q0, er);
};
}(v0, P);
break;
case 20:
var L = s(0), Q = s(0);
Mp(t);
var Z = function(b0, O0) {
return function(q0) {
return u(Ze(O0, b0, 0), O0);
};
}(L, Q);
break;
case 21:
var i0 = s(0), l0 = s(0);
Mp(t);
var Z = function(b0, O0) {
return function(q0) {
var er = q0[1 + O0];
return u(Ze(er, b0, 0), er);
};
}(i0, l0);
break;
case 22:
var S0 = s(0), T0 = s(0), rr = s(0);
Mp(t);
var Z = function(b0, O0, q0) {
return function(er) {
var yr = er[1 + O0][1 + q0];
return u(Ze(yr, b0, 0), yr);
};
}(S0, T0, rr);
break;
default:
var R0 = s(0), B = s(0);
Mp(t);
var Z = function(b0, O0) {
return function(q0) {
var er = u(q0[1][1 + O0], q0);
return u(Ze(er, b0, 0), er);
};
}(R0, B);
}
else
var Z = p;
Zz(t, c, Z), e[1]++;
continue;
}
return 0;
}
}
function Qz(t, n4) {
var e = n4.length - 1, i = $A(0, e), x = e - 1 | 0, c = 0;
if (!(x < 0))
for (var s = c; ; ) {
var p = nu(n4, s)[1 + s];
if (typeof p == "number")
switch (p) {
case 0:
var E = function(G) {
function A(S) {
var M = i[1 + G];
if (A === M)
throw [0, sl, t];
return u(M, S);
}
return A;
}(s);
break;
case 1:
var y = [];
N(y, [$2, function(G, A) {
return function(S) {
var M = i[1 + A];
if (G === M)
throw [0, sl, t];
var K = h1(M);
return nl === K ? M[1] : $2 === K ? xee(M) : M;
};
}(y, s)]);
var E = y;
break;
default:
var T = function(G) {
throw [0, sl, t];
}, E = [0, T, T, T, 0];
}
else
var E = p[0] === 0 ? Qz(t, p[1]) : p[1];
i[1 + s] = E;
var h = s + 1 | 0;
if (x !== s) {
var s = h;
continue;
}
break;
}
return i;
}
function bu(t, n4) {
return typeof n4 != "number" && n4[0] === 0 ? Qz(t, n4[1]) : ke(oo0);
}
function rK(t, n4, e) {
if (h1(e) === 0 && t.length - 1 <= e.length - 1) {
var i = t.length - 1 - 1 | 0, x = 0;
if (!(i < 0))
for (var c = x; ; ) {
var s = e[1 + c], p = nu(t, c)[1 + c];
if (typeof p == "number")
if (p === 2) {
var y = 0;
if (h1(s) === 0 && s.length - 1 === 4)
for (var T = 0, E = n4[1 + c]; ; ) {
E[1 + T] = s[1 + T];
var h = T + 1 | 0;
if (T !== 3) {
var T = h;
continue;
}
break;
}
else
y = 1;
if (y)
throw [0, wn, fo0];
} else
n4[1 + c] = s;
else
p[0] === 0 && rK(p[1], n4[1 + c], s);
var w = c + 1 | 0;
if (i !== c) {
var c = w;
continue;
}
break;
}
return 0;
}
throw [0, wn, xo0];
}
function pu(t, n4, e) {
return typeof t != "number" && t[0] === 0 ? rK(t[1], n4, e) : ke(ao0);
}
try {
rz(co0);
} catch (t) {
if (t = Et(t), t !== Kt)
throw t;
}
try {
rz(so0);
} catch (t) {
if (t = Et(t), t !== Kt)
throw t;
}
n0(lz, vo0) && n0(lz, lo0);
function g1(t, n4) {
function e(i) {
return qi(t, i);
}
return ow <= n4 ? (e(v1 | n4 >>> 18 | 0), e(Rt | (n4 >>> 12 | 0) & 63), e(Rt | (n4 >>> 6 | 0) & 63), e(Rt | n4 & 63)) : Vd <= n4 ? (e(dv | n4 >>> 12 | 0), e(Rt | (n4 >>> 6 | 0) & 63), e(Rt | n4 & 63)) : Rt <= n4 ? (e(at | n4 >>> 6 | 0), e(Rt | n4 & 63)) : e(n4);
}
var qN = j0, u7 = null, eK = void 0;
function Bp(t) {
return t !== eK ? 1 : 0;
}
var Dee = qN.Array, UN = [wt, po0, G7(0)], Lee = qN.Error;
Fee(mo0, [0, UN, {}]);
function nK(t) {
throw t;
}
Iz(function(t) {
return t[1] === UN ? [0, M7(t[2].toString())] : 0;
}), Iz(function(t) {
return t instanceof Dee ? 0 : [0, M7(t.toString())];
});
var Dr = bu(m3r, p3r), Ln = bu(y3r, _3r), qp = bu(h3r, d3r), Tl = bu(w3r, k3r), F1 = bu(S3r, E3r), HN = bu(F3r, g3r), tK = bu(O3r, T3r), XN = bu(A3r, I3r), zv = bu(C3r, N3r), Up = bu(D3r, P3r), Je = bu(R3r, L3r), Xu = bu(G3r, j3r), qe = bu(B3r, M3r), YN = bu(U3r, q3r), di = bu(X3r, H3r), uu = bu(V3r, Y3r), T1 = bu(K3r, z3r), Ps = bu(J3r, W3r), VN = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, uK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Ree = bu(Z3r, $3r);
N(VN, function(t, n4, e, i) {
u(f(e), Z8r), a(f(e), r3r, Q8r);
var x = i[1];
u(f(e), e3r);
var c = 0;
be(function(y, T) {
y && u(f(e), $8r);
function E(h) {
return u(t, h);
}
return ir(uu[1], E, e, T), 1;
}, c, x), u(f(e), n3r), u(f(e), t3r), u(f(e), u3r), a(f(e), f3r, i3r);
var s = i[2];
u(f(e), x3r);
var p = 0;
return be(function(y, T) {
y && u(f(e), J8r);
function E(h) {
return u(t, h);
}
return ir(uu[1], E, e, T), 1;
}, p, s), u(f(e), a3r), u(f(e), o3r), u(f(e), c3r), a(f(e), v3r, s3r), a(n4, e, i[3]), u(f(e), l3r), u(f(e), b3r);
}), N(uK, function(t, n4, e) {
var i = a(VN, t, n4);
return a(P0(W8r), i, e);
}), pu(Q3r, Dr, [0, VN, uK]);
var zN = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, iK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Hp = function t(n4, e, i) {
return t.fun(n4, e, i);
}, fK = function t(n4, e) {
return t.fun(n4, e);
};
N(zN, function(t, n4, e, i) {
u(f(e), V8r), a(n4, e, i[1]), u(f(e), z8r);
var x = i[2];
return ir(Hp, function(c) {
return u(t, c);
}, e, x), u(f(e), K8r);
}), N(iK, function(t, n4, e) {
var i = a(zN, t, n4);
return a(P0(Y8r), i, e);
}), N(Hp, function(t, n4, e) {
u(f(n4), C8r), a(f(n4), D8r, P8r);
var i = e[1];
a(f(n4), L8r, i), u(f(n4), R8r), u(f(n4), j8r), a(f(n4), M8r, G8r);
var x = e[2];
if (x) {
g(n4, B8r);
var c = x[1], s = function(y, T) {
return g(y, N8r);
}, p = function(y) {
return u(t, y);
};
R(Dr[1], p, s, n4, c), g(n4, q8r);
} else
g(n4, U8r);
return u(f(n4), H8r), u(f(n4), X8r);
}), N(fK, function(t, n4) {
var e = u(Hp, t);
return a(P0(A8r), e, n4);
}), pu(r6r, Ln, [0, zN, iK, Hp, fK]);
var KN = function t(n4, e, i) {
return t.fun(n4, e, i);
}, xK = function t(n4, e) {
return t.fun(n4, e);
}, Xp = function t(n4, e, i) {
return t.fun(n4, e, i);
}, aK = function t(n4, e) {
return t.fun(n4, e);
};
N(KN, function(t, n4, e) {
u(f(n4), T8r), a(t, n4, e[1]), u(f(n4), O8r);
var i = e[2];
return ir(Xp, function(x) {
return u(t, x);
}, n4, i), u(f(n4), I8r);
}), N(xK, function(t, n4) {
var e = u(KN, t);
return a(P0(F8r), e, n4);
}), N(Xp, function(t, n4, e) {
u(f(n4), l8r), a(f(n4), p8r, b8r);
var i = e[1];
a(f(n4), m8r, i), u(f(n4), _8r), u(f(n4), y8r), a(f(n4), h8r, d8r);
var x = e[2];
if (x) {
g(n4, k8r);
var c = x[1], s = function(y, T) {
return g(y, v8r);
}, p = function(y) {
return u(t, y);
};
R(Dr[1], p, s, n4, c), g(n4, w8r);
} else
g(n4, E8r);
return u(f(n4), S8r), u(f(n4), g8r);
}), N(aK, function(t, n4) {
var e = u(Xp, t);
return a(P0(s8r), e, n4);
}), pu(e6r, qp, [0, KN, xK, Xp, aK]);
function oK(t, n4) {
u(f(t), Q4r), a(f(t), e8r, r8r);
var e = n4[1];
a(f(t), n8r, e), u(f(t), t8r), u(f(t), u8r), a(f(t), f8r, i8r);
var i = n4[2];
return a(f(t), x8r, i), u(f(t), a8r), u(f(t), o8r);
}
var cK = [0, oK, function(t) {
return a(P0(c8r), oK, t);
}], WN = function t(n4, e, i) {
return t.fun(n4, e, i);
}, sK = function t(n4, e) {
return t.fun(n4, e);
}, Yp = function t(n4, e) {
return t.fun(n4, e);
}, vK = function t(n4) {
return t.fun(n4);
};
N(WN, function(t, n4, e) {
u(f(n4), R4r), a(f(n4), G4r, j4r), a(Yp, n4, e[1]), u(f(n4), M4r), u(f(n4), B4r), a(f(n4), U4r, q4r);
var i = e[2];
a(f(n4), H4r, i), u(f(n4), X4r), u(f(n4), Y4r), a(f(n4), z4r, V4r);
var x = e[3];
if (x) {
g(n4, K4r);
var c = x[1], s = function(y, T) {
return g(y, L4r);
}, p = function(y) {
return u(t, y);
};
R(Dr[1], p, s, n4, c), g(n4, W4r);
} else
g(n4, J4r);
return u(f(n4), $4r), u(f(n4), Z4r);
}), N(sK, function(t, n4) {
var e = u(WN, t);
return a(P0(D4r), e, n4);
}), N(Yp, function(t, n4) {
if (typeof n4 == "number")
return g(t, d4r);
switch (n4[0]) {
case 0:
u(f(t), h4r);
var e = n4[1];
return a(f(t), k4r, e), u(f(t), w4r);
case 1:
u(f(t), E4r);
var i = n4[1];
return a(f(t), S4r, i), u(f(t), g4r);
case 2:
u(f(t), F4r);
var x = n4[1];
return a(f(t), T4r, x), u(f(t), O4r);
case 3:
u(f(t), I4r);
var c = n4[1];
return a(f(t), A4r, c), u(f(t), N4r);
default:
return u(f(t), C4r), a(cK[1], t, n4[1]), u(f(t), P4r);
}
}), N(vK, function(t) {
return a(P0(y4r), Yp, t);
}), pu(n6r, Tl, [0, cK, WN, sK, Yp, vK]);
var JN = function t(n4, e, i) {
return t.fun(n4, e, i);
}, lK = function t(n4, e) {
return t.fun(n4, e);
};
N(JN, function(t, n4, e) {
u(f(n4), r4r), a(f(n4), n4r, e4r);
var i = e[1];
a(f(n4), t4r, i), u(f(n4), u4r), u(f(n4), i4r), a(f(n4), x4r, f4r);
var x = e[2];
a(f(n4), a4r, x), u(f(n4), o4r), u(f(n4), c4r), a(f(n4), v4r, s4r);
var c = e[3];
if (c) {
g(n4, l4r);
var s = c[1], p = function(T, E) {
return g(T, Qbr);
}, y = function(T) {
return u(t, T);
};
R(Dr[1], y, p, n4, s), g(n4, b4r);
} else
g(n4, p4r);
return u(f(n4), m4r), u(f(n4), _4r);
}), N(lK, function(t, n4) {
var e = u(JN, t);
return a(P0(Zbr), e, n4);
}), pu(t6r, F1, [0, JN, lK]);
var $N = function t(n4, e, i) {
return t.fun(n4, e, i);
}, bK = function t(n4, e) {
return t.fun(n4, e);
};
N($N, function(t, n4, e) {
u(f(n4), Dbr), a(f(n4), Rbr, Lbr);
var i = e[1];
a(f(n4), jbr, i), u(f(n4), Gbr), u(f(n4), Mbr), a(f(n4), qbr, Bbr);
var x = e[2];
a(f(n4), Ubr, x), u(f(n4), Hbr), u(f(n4), Xbr), a(f(n4), Vbr, Ybr);
var c = e[3];
if (c) {
g(n4, zbr);
var s = c[1], p = function(T, E) {
return g(T, Pbr);
}, y = function(T) {
return u(t, T);
};
R(Dr[1], y, p, n4, s), g(n4, Kbr);
} else
g(n4, Wbr);
return u(f(n4), Jbr), u(f(n4), $br);
}), N(bK, function(t, n4) {
var e = u($N, t);
return a(P0(Cbr), e, n4);
}), pu(u6r, HN, [0, $N, bK]);
var ZN = function t(n4, e, i) {
return t.fun(n4, e, i);
}, pK = function t(n4, e) {
return t.fun(n4, e);
};
N(ZN, function(t, n4, e) {
u(f(n4), bbr), a(f(n4), mbr, pbr);
var i = e[1];
a(f(n4), _br, i), u(f(n4), ybr), u(f(n4), dbr), a(f(n4), kbr, hbr);
var x = e[2];
a(f(n4), wbr, x), u(f(n4), Ebr), u(f(n4), Sbr), a(f(n4), Fbr, gbr);
var c = e[3];
if (c) {
g(n4, Tbr);
var s = c[1], p = function(T, E) {
return g(T, lbr);
}, y = function(T) {
return u(t, T);
};
R(Dr[1], y, p, n4, s), g(n4, Obr);
} else
g(n4, Ibr);
return u(f(n4), Abr), u(f(n4), Nbr);
}), N(pK, function(t, n4) {
var e = u(ZN, t);
return a(P0(vbr), e, n4);
}), pu(i6r, tK, [0, ZN, pK]);
var QN = function t(n4, e, i) {
return t.fun(n4, e, i);
}, mK = function t(n4, e) {
return t.fun(n4, e);
};
N(QN, function(t, n4, e) {
u(f(n4), Qlr), a(f(n4), ebr, rbr);
var i = e[1];
a(f(n4), nbr, i), u(f(n4), tbr), u(f(n4), ubr), a(f(n4), fbr, ibr);
var x = e[2];
if (x) {
g(n4, xbr);
var c = x[1], s = function(y, T) {
return g(y, Zlr);
}, p = function(y) {
return u(t, y);
};
R(Dr[1], p, s, n4, c), g(n4, abr);
} else
g(n4, obr);
return u(f(n4), cbr), u(f(n4), sbr);
}), N(mK, function(t, n4) {
var e = u(QN, t);
return a(P0($lr), e, n4);
}), pu(f6r, XN, [0, QN, mK]);
var rC = function t(n4, e, i) {
return t.fun(n4, e, i);
}, _K = function t(n4, e) {
return t.fun(n4, e);
}, Vp = function t(n4, e) {
return t.fun(n4, e);
}, yK = function t(n4) {
return t.fun(n4);
}, zp = function t(n4, e, i) {
return t.fun(n4, e, i);
}, dK = function t(n4, e) {
return t.fun(n4, e);
};
N(rC, function(t, n4, e) {
u(f(n4), Klr), a(t, n4, e[1]), u(f(n4), Wlr);
var i = e[2];
return ir(zp, function(x) {
return u(t, x);
}, n4, i), u(f(n4), Jlr);
}), N(_K, function(t, n4) {
var e = u(rC, t);
return a(P0(zlr), e, n4);
}), N(Vp, function(t, n4) {
return n4 ? g(t, Ylr) : g(t, Vlr);
}), N(yK, function(t) {
return a(P0(Xlr), Vp, t);
}), N(zp, function(t, n4, e) {
u(f(n4), Clr), a(f(n4), Dlr, Plr), a(Vp, n4, e[1]), u(f(n4), Llr), u(f(n4), Rlr), a(f(n4), Glr, jlr);
var i = e[2];
if (i) {
g(n4, Mlr);
var x = i[1], c = function(p, y) {
return g(p, Nlr);
}, s = function(p) {
return u(t, p);
};
R(Dr[1], s, c, n4, x), g(n4, Blr);
} else
g(n4, qlr);
return u(f(n4), Ulr), u(f(n4), Hlr);
}), N(dK, function(t, n4) {
var e = u(zp, t);
return a(P0(Alr), e, n4);
}), pu(x6r, zv, [0, rC, _K, Vp, yK, zp, dK]);
var eC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, hK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, nC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, kK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(eC, function(t, n4, e, i) {
u(f(e), Tlr), a(t, e, i[1]), u(f(e), Olr);
var x = i[2];
function c(p) {
return u(n4, p);
}
function s(p) {
return u(t, p);
}
return R(Up[3], s, c, e, x), u(f(e), Ilr);
}), N(hK, function(t, n4, e) {
var i = a(eC, t, n4);
return a(P0(Flr), i, e);
}), N(nC, function(t, n4, e, i) {
u(f(e), blr), a(f(e), mlr, plr);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(qe[31], s, c, e, x), u(f(e), _lr), u(f(e), ylr), a(f(e), hlr, dlr);
var p = i[2];
if (p) {
g(e, klr);
var y = p[1], T = function(h, w) {
return g(h, llr);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, wlr);
} else
g(e, Elr);
return u(f(e), Slr), u(f(e), glr);
}), N(kK, function(t, n4, e) {
var i = a(nC, t, n4);
return a(P0(vlr), i, e);
}), pu(a6r, Up, [0, eC, hK, nC, kK]);
var tC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, wK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Kp = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, EK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(tC, function(t, n4, e, i) {
u(f(e), olr), a(t, e, i[1]), u(f(e), clr);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Kp, function(s) {
return u(t, s);
}, c, e, x), u(f(e), slr);
}), N(wK, function(t, n4, e) {
var i = a(tC, t, n4);
return a(P0(alr), i, e);
}), N(Kp, function(t, n4, e, i) {
u(f(e), Y2r), a(f(e), z2r, V2r);
var x = i[1];
if (x) {
g(e, K2r);
var c = x[1], s = function(w) {
return u(n4, w);
}, p = function(w) {
return u(t, w);
};
R(Ln[1], p, s, e, c), g(e, W2r);
} else
g(e, J2r);
u(f(e), $2r), u(f(e), Z2r), a(f(e), rlr, Q2r);
var y = i[2];
function T(w) {
return u(n4, w);
}
function E(w) {
return u(t, w);
}
R(Je[13], E, T, e, y), u(f(e), elr), u(f(e), nlr), a(f(e), ulr, tlr);
var h = i[3];
return a(f(e), ilr, h), u(f(e), flr), u(f(e), xlr);
}), N(EK, function(t, n4, e) {
var i = a(Kp, t, n4);
return a(P0(X2r), i, e);
});
var uC = [0, tC, wK, Kp, EK], iC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, SK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Wp = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, gK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(iC, function(t, n4, e, i) {
u(f(e), q2r), a(t, e, i[1]), u(f(e), U2r);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Wp, function(s) {
return u(t, s);
}, c, e, x), u(f(e), H2r);
}), N(SK, function(t, n4, e) {
var i = a(iC, t, n4);
return a(P0(B2r), i, e);
}), N(Wp, function(t, n4, e, i) {
u(f(e), O2r), a(f(e), A2r, I2r);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(uC[1], s, c, e, x), u(f(e), N2r), u(f(e), C2r), a(f(e), D2r, P2r);
var p = i[2];
if (p) {
g(e, L2r);
var y = p[1], T = function(h, w) {
return g(h, T2r);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, R2r);
} else
g(e, j2r);
return u(f(e), G2r), u(f(e), M2r);
}), N(gK, function(t, n4, e) {
var i = a(Wp, t, n4);
return a(P0(F2r), i, e);
});
var FK = [0, iC, SK, Wp, gK], fC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, TK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Jp = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, OK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(fC, function(t, n4, e, i) {
u(f(e), E2r), a(t, e, i[1]), u(f(e), S2r);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Jp, function(s) {
return u(t, s);
}, c, e, x), u(f(e), g2r);
}), N(TK, function(t, n4, e) {
var i = a(fC, t, n4);
return a(P0(w2r), i, e);
}), N(Jp, function(t, n4, e, i) {
u(f(e), c2r), a(f(e), v2r, s2r);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(Je[17], s, c, e, x), u(f(e), l2r), u(f(e), b2r), a(f(e), m2r, p2r);
var p = i[2];
if (p) {
g(e, _2r);
var y = p[1], T = function(h, w) {
return g(h, o2r);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, y2r);
} else
g(e, d2r);
return u(f(e), h2r), u(f(e), k2r);
}), N(OK, function(t, n4, e) {
var i = a(Jp, t, n4);
return a(P0(a2r), i, e);
});
var IK = [0, fC, TK, Jp, OK], xC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, AK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, $p = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, NK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(xC, function(t, n4, e, i) {
u(f(e), i2r), a(t, e, i[1]), u(f(e), f2r);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R($p, function(s) {
return u(t, s);
}, c, e, x), u(f(e), x2r);
}), N(AK, function(t, n4, e) {
var i = a(xC, t, n4);
return a(P0(u2r), i, e);
}), N($p, function(t, n4, e, i) {
u(f(e), Avr), a(f(e), Cvr, Nvr);
var x = i[1];
if (x) {
g(e, Pvr);
var c = x[1], s = function(V) {
return u(n4, V);
}, p = function(V) {
return u(t, V);
};
R(IK[1], p, s, e, c), g(e, Dvr);
} else
g(e, Lvr);
u(f(e), Rvr), u(f(e), jvr), a(f(e), Mvr, Gvr);
var y = i[2];
u(f(e), Bvr);
var T = 0;
be(function(V, f0) {
V && u(f(e), Ivr);
function m0(g0) {
return u(n4, g0);
}
function k0(g0) {
return u(t, g0);
}
return R(uC[1], k0, m0, e, f0), 1;
}, T, y), u(f(e), qvr), u(f(e), Uvr), u(f(e), Hvr), a(f(e), Yvr, Xvr);
var E = i[3];
if (E) {
g(e, Vvr);
var h = E[1], w = function(V) {
return u(n4, V);
}, G = function(V) {
return u(t, V);
};
R(FK[1], G, w, e, h), g(e, zvr);
} else
g(e, Kvr);
u(f(e), Wvr), u(f(e), Jvr), a(f(e), Zvr, $vr);
var A = i[4];
if (A) {
g(e, Qvr);
var S = A[1], M = function(V, f0) {
u(f(V), Tvr);
var m0 = 0;
return be(function(k0, g0) {
k0 && u(f(V), Fvr);
function e0(x0) {
return u(t, x0);
}
return ir(uu[1], e0, V, g0), 1;
}, m0, f0), u(f(V), Ovr);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, r2r);
} else
g(e, e2r);
return u(f(e), n2r), u(f(e), t2r);
}), N(NK, function(t, n4, e) {
var i = a($p, t, n4);
return a(P0(gvr), i, e);
});
var CK = [0, xC, AK, $p, NK], aC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, PK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(aC, function(t, n4, e, i) {
u(f(e), nvr), a(f(e), uvr, tvr);
var x = i[1];
if (x) {
g(e, ivr);
var c = x[1], s = function(V) {
return u(n4, V);
}, p = function(V) {
return u(t, V);
};
R(Je[22][1], p, s, e, c), g(e, fvr);
} else
g(e, xvr);
u(f(e), avr), u(f(e), ovr), a(f(e), svr, cvr);
var y = i[2];
function T(V) {
return u(n4, V);
}
function E(V) {
return u(t, V);
}
R(CK[1], E, T, e, y), u(f(e), vvr), u(f(e), lvr), a(f(e), pvr, bvr);
var h = i[3];
function w(V) {
return u(n4, V);
}
function G(V) {
return u(t, V);
}
R(Je[13], G, w, e, h), u(f(e), mvr), u(f(e), _vr), a(f(e), dvr, yvr);
var A = i[4];
if (A) {
g(e, hvr);
var S = A[1], M = function(V, f0) {
return g(V, evr);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, kvr);
} else
g(e, wvr);
return u(f(e), Evr), u(f(e), Svr);
}), N(PK, function(t, n4, e) {
var i = a(aC, t, n4);
return a(P0(rvr), i, e);
});
var Ol = [0, uC, FK, IK, CK, aC, PK], Zp = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, DK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Qp = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, LK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, r5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, RK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(Zp, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), J1r);
var x = i[1], c = function(T) {
return u(n4, T);
}, s = function(T) {
return u(t, T);
};
return R(Ln[1], s, c, e, x), u(f(e), $1r);
}
u(f(e), Z1r);
var p = i[1];
function y(T) {
return u(n4, T);
}
return R(Qp, function(T) {
return u(t, T);
}, y, e, p), u(f(e), Q1r);
}), N(DK, function(t, n4, e) {
var i = a(Zp, t, n4);
return a(P0(W1r), i, e);
}), N(Qp, function(t, n4, e, i) {
u(f(e), V1r), a(t, e, i[1]), u(f(e), z1r);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(r5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), K1r);
}), N(LK, function(t, n4, e) {
var i = a(Qp, t, n4);
return a(P0(Y1r), i, e);
}), N(r5, function(t, n4, e, i) {
u(f(e), R1r), a(f(e), G1r, j1r);
var x = i[1];
function c(T) {
return u(n4, T);
}
R(Zp, function(T) {
return u(t, T);
}, c, e, x), u(f(e), M1r), u(f(e), B1r), a(f(e), U1r, q1r);
var s = i[2];
function p(T) {
return u(n4, T);
}
function y(T) {
return u(t, T);
}
return R(Ln[1], y, p, e, s), u(f(e), H1r), u(f(e), X1r);
}), N(RK, function(t, n4, e) {
var i = a(r5, t, n4);
return a(P0(L1r), i, e);
});
var jK = [0, Zp, DK, Qp, LK, r5, RK], oC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, GK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(oC, function(t, n4, e, i) {
u(f(e), m1r), a(f(e), y1r, _1r);
var x = i[1];
function c(S) {
return u(n4, S);
}
function s(S) {
return u(t, S);
}
R(jK[1], s, c, e, x), u(f(e), d1r), u(f(e), h1r), a(f(e), w1r, k1r);
var p = i[2];
if (p) {
g(e, E1r);
var y = p[1], T = function(S) {
return u(n4, S);
}, E = function(S) {
return u(t, S);
};
R(Je[23][1], E, T, e, y), g(e, S1r);
} else
g(e, g1r);
u(f(e), F1r), u(f(e), T1r), a(f(e), I1r, O1r);
var h = i[3];
if (h) {
g(e, A1r);
var w = h[1], G = function(S, M) {
return g(S, p1r);
}, A = function(S) {
return u(t, S);
};
R(Dr[1], A, G, e, w), g(e, N1r);
} else
g(e, C1r);
return u(f(e), P1r), u(f(e), D1r);
}), N(GK, function(t, n4, e) {
var i = a(oC, t, n4);
return a(P0(b1r), i, e);
});
var cC = [0, jK, oC, GK], sC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, MK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(sC, function(t, n4, e, i) {
u(f(e), Zsr), a(f(e), r1r, Qsr);
var x = i[1];
function c(A) {
return u(n4, A);
}
function s(A) {
return u(t, A);
}
R(Je[13], s, c, e, x), u(f(e), e1r), u(f(e), n1r), a(f(e), u1r, t1r);
var p = i[2];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(Je[13], T, y, e, p), u(f(e), i1r), u(f(e), f1r), a(f(e), a1r, x1r);
var E = i[3];
if (E) {
g(e, o1r);
var h = E[1], w = function(A, S) {
return g(A, $sr);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, c1r);
} else
g(e, s1r);
return u(f(e), v1r), u(f(e), l1r);
}), N(MK, function(t, n4, e) {
var i = a(sC, t, n4);
return a(P0(Jsr), i, e);
});
var vC = [0, sC, MK], lC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, BK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(lC, function(t, n4, e, i) {
u(f(e), Bsr), a(f(e), Usr, qsr);
var x = i[1];
function c(y) {
return u(n4, y);
}
function s(y) {
return u(t, y);
}
R(vC[1], s, c, e, x), u(f(e), Hsr), u(f(e), Xsr), a(f(e), Vsr, Ysr);
var p = i[2];
return a(f(e), zsr, p), u(f(e), Ksr), u(f(e), Wsr);
}), N(BK, function(t, n4, e) {
var i = a(lC, t, n4);
return a(P0(Msr), i, e);
});
var qK = [0, lC, BK], bC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, UK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, e5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, HK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, n5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, XK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(bC, function(t, n4, e, i) {
u(f(e), Rsr), a(t, e, i[1]), u(f(e), jsr);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(e5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Gsr);
}), N(UK, function(t, n4, e) {
var i = a(bC, t, n4);
return a(P0(Lsr), i, e);
}), N(e5, function(t, n4, e, i) {
u(f(e), Vcr), a(f(e), Kcr, zcr);
var x = i[1];
function c(m0) {
return u(n4, m0);
}
function s(m0) {
return u(t, m0);
}
R(qe[7][1][1], s, c, e, x), u(f(e), Wcr), u(f(e), Jcr), a(f(e), Zcr, $cr);
var p = i[2];
function y(m0) {
return u(n4, m0);
}
R(n5, function(m0) {
return u(t, m0);
}, y, e, p), u(f(e), Qcr), u(f(e), rsr), a(f(e), nsr, esr);
var T = i[3];
a(f(e), tsr, T), u(f(e), usr), u(f(e), isr), a(f(e), xsr, fsr);
var E = i[4];
a(f(e), asr, E), u(f(e), osr), u(f(e), csr), a(f(e), vsr, ssr);
var h = i[5];
a(f(e), lsr, h), u(f(e), bsr), u(f(e), psr), a(f(e), _sr, msr);
var w = i[6];
a(f(e), ysr, w), u(f(e), dsr), u(f(e), hsr), a(f(e), wsr, ksr);
var G = i[7];
if (G) {
g(e, Esr);
var A = G[1], S = function(m0) {
return u(t, m0);
};
ir(zv[1], S, e, A), g(e, Ssr);
} else
g(e, gsr);
u(f(e), Fsr), u(f(e), Tsr), a(f(e), Isr, Osr);
var M = i[8];
if (M) {
g(e, Asr);
var K = M[1], V = function(m0, k0) {
return g(m0, Ycr);
}, f0 = function(m0) {
return u(t, m0);
};
R(Dr[1], f0, V, e, K), g(e, Nsr);
} else
g(e, Csr);
return u(f(e), Psr), u(f(e), Dsr);
}), N(HK, function(t, n4, e) {
var i = a(e5, t, n4);
return a(P0(Xcr), i, e);
}), N(n5, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), Ccr);
var x = i[1], c = function(S) {
return u(n4, S);
}, s = function(S) {
return u(t, S);
};
return R(Je[13], s, c, e, x), u(f(e), Pcr);
case 1:
var p = i[1];
u(f(e), Dcr), u(f(e), Lcr), a(t, e, p[1]), u(f(e), Rcr);
var y = p[2], T = function(S) {
return u(n4, S);
}, E = function(S) {
return u(t, S);
};
return R(Ol[5], E, T, e, y), u(f(e), jcr), u(f(e), Gcr);
default:
var h = i[1];
u(f(e), Mcr), u(f(e), Bcr), a(t, e, h[1]), u(f(e), qcr);
var w = h[2], G = function(S) {
return u(n4, S);
}, A = function(S) {
return u(t, S);
};
return R(Ol[5], A, G, e, w), u(f(e), Ucr), u(f(e), Hcr);
}
}), N(XK, function(t, n4, e) {
var i = a(n5, t, n4);
return a(P0(Ncr), i, e);
});
var YK = [0, bC, UK, e5, HK, n5, XK], pC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, VK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, t5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, zK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(pC, function(t, n4, e, i) {
u(f(e), Ocr), a(t, e, i[1]), u(f(e), Icr);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(t5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Acr);
}), N(VK, function(t, n4, e) {
var i = a(pC, t, n4);
return a(P0(Tcr), i, e);
}), N(t5, function(t, n4, e, i) {
u(f(e), pcr), a(f(e), _cr, mcr);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(Je[13], s, c, e, x), u(f(e), ycr), u(f(e), dcr), a(f(e), kcr, hcr);
var p = i[2];
if (p) {
g(e, wcr);
var y = p[1], T = function(h, w) {
return g(h, bcr);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, Ecr);
} else
g(e, Scr);
return u(f(e), gcr), u(f(e), Fcr);
}), N(zK, function(t, n4, e) {
var i = a(t5, t, n4);
return a(P0(lcr), i, e);
});
var KK = [0, pC, VK, t5, zK], u5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, WK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, mC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, JK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(u5, function(t, n4, e, i) {
u(f(e), Cor), a(f(e), Dor, Por);
var x = i[1];
if (x) {
g(e, Lor);
var c = x[1], s = function(g0) {
return u(t, g0);
}, p = function(g0) {
return u(t, g0);
};
R(Ln[1], p, s, e, c), g(e, Ror);
} else
g(e, jor);
u(f(e), Gor), u(f(e), Mor), a(f(e), qor, Bor);
var y = i[2];
function T(g0) {
return u(n4, g0);
}
function E(g0) {
return u(t, g0);
}
R(Je[13], E, T, e, y), u(f(e), Uor), u(f(e), Hor), a(f(e), Yor, Xor);
var h = i[3];
function w(g0) {
return u(n4, g0);
}
function G(g0) {
return u(t, g0);
}
R(Je[13], G, w, e, h), u(f(e), Vor), u(f(e), zor), a(f(e), Wor, Kor);
var A = i[4];
a(f(e), Jor, A), u(f(e), $or), u(f(e), Zor), a(f(e), rcr, Qor);
var S = i[5];
if (S) {
g(e, ecr);
var M = S[1], K = function(g0) {
return u(t, g0);
};
ir(zv[1], K, e, M), g(e, ncr);
} else
g(e, tcr);
u(f(e), ucr), u(f(e), icr), a(f(e), xcr, fcr);
var V = i[6];
if (V) {
g(e, acr);
var f0 = V[1], m0 = function(g0, e0) {
return g(g0, Nor);
}, k0 = function(g0) {
return u(t, g0);
};
R(Dr[1], k0, m0, e, f0), g(e, ocr);
} else
g(e, ccr);
return u(f(e), scr), u(f(e), vcr);
}), N(WK, function(t, n4, e) {
var i = a(u5, t, n4);
return a(P0(Aor), i, e);
}), N(mC, function(t, n4, e, i) {
u(f(e), Tor), a(t, e, i[1]), u(f(e), Oor);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(u5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Ior);
}), N(JK, function(t, n4, e) {
var i = a(mC, t, n4);
return a(P0(For), i, e);
});
var $K = [0, u5, WK, mC, JK], _C = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, ZK = function t(n4, e, i) {
return t.fun(n4, e, i);
}, i5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, QK = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(_C, function(t, n4, e, i) {
u(f(e), Eor), a(t, e, i[1]), u(f(e), Sor);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(i5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), gor);
}), N(ZK, function(t, n4, e) {
var i = a(_C, t, n4);
return a(P0(wor), i, e);
}), N(i5, function(t, n4, e, i) {
u(f(e), eor), a(f(e), tor, nor);
var x = i[1];
u(f(e), uor), a(t, e, x[1]), u(f(e), ior);
var c = x[2];
function s(G) {
return u(n4, G);
}
function p(G) {
return u(t, G);
}
R(Ol[5], p, s, e, c), u(f(e), xor), u(f(e), aor), u(f(e), oor), a(f(e), sor, cor);
var y = i[2];
a(f(e), vor, y), u(f(e), lor), u(f(e), bor), a(f(e), mor, por);
var T = i[3];
if (T) {
g(e, _or);
var E = T[1], h = function(G, A) {
return g(G, ror);
}, w = function(G) {
return u(t, G);
};
R(Dr[1], w, h, e, E), g(e, yor);
} else
g(e, dor);
return u(f(e), hor), u(f(e), kor);
}), N(QK, function(t, n4, e) {
var i = a(i5, t, n4);
return a(P0(Qar), i, e);
});
var rW = [0, _C, ZK, i5, QK], yC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, eW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, f5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, nW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(yC, function(t, n4, e, i) {
u(f(e), Jar), a(t, e, i[1]), u(f(e), $ar);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(f5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Zar);
}), N(eW, function(t, n4, e) {
var i = a(yC, t, n4);
return a(P0(War), i, e);
}), N(f5, function(t, n4, e, i) {
u(f(e), yar), a(f(e), har, dar);
var x = i[1];
function c(K) {
return u(t, K);
}
function s(K) {
return u(t, K);
}
R(Ln[1], s, c, e, x), u(f(e), kar), u(f(e), war), a(f(e), Sar, Ear);
var p = i[2];
function y(K) {
return u(n4, K);
}
function T(K) {
return u(t, K);
}
R(Je[13], T, y, e, p), u(f(e), gar), u(f(e), Far), a(f(e), Oar, Tar);
var E = i[3];
a(f(e), Iar, E), u(f(e), Aar), u(f(e), Nar), a(f(e), Par, Car);
var h = i[4];
a(f(e), Dar, h), u(f(e), Lar), u(f(e), Rar), a(f(e), Gar, jar);
var w = i[5];
a(f(e), Mar, w), u(f(e), Bar), u(f(e), qar), a(f(e), Har, Uar);
var G = i[6];
if (G) {
g(e, Xar);
var A = G[1], S = function(K, V) {
return g(K, _ar);
}, M = function(K) {
return u(t, K);
};
R(Dr[1], M, S, e, A), g(e, Yar);
} else
g(e, Var);
return u(f(e), zar), u(f(e), Kar);
}), N(nW, function(t, n4, e) {
var i = a(f5, t, n4);
return a(P0(mar), i, e);
});
var tW = [0, yC, eW, f5, nW], dC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, uW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, x5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, iW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(dC, function(t, n4, e, i) {
u(f(e), Yxr), a(f(e), zxr, Vxr);
var x = i[1];
a(f(e), Kxr, x), u(f(e), Wxr), u(f(e), Jxr), a(f(e), Zxr, $xr);
var c = i[2];
a(f(e), Qxr, c), u(f(e), rar), u(f(e), ear), a(f(e), tar, nar);
var s = i[3];
u(f(e), uar);
var p = 0;
be(function(w, G) {
w && u(f(e), Xxr);
function A(S) {
return u(n4, S);
}
return R(x5, function(S) {
return u(t, S);
}, A, e, G), 1;
}, p, s), u(f(e), iar), u(f(e), far), u(f(e), xar), a(f(e), oar, aar);
var y = i[4];
if (y) {
g(e, car);
var T = y[1], E = function(w, G) {
u(f(w), Uxr);
var A = 0;
return be(function(S, M) {
S && u(f(w), qxr);
function K(V) {
return u(t, V);
}
return ir(uu[1], K, w, M), 1;
}, A, G), u(f(w), Hxr);
}, h = function(w) {
return u(t, w);
};
R(Dr[1], h, E, e, T), g(e, sar);
} else
g(e, lar);
return u(f(e), bar), u(f(e), par);
}), N(uW, function(t, n4, e) {
var i = a(dC, t, n4);
return a(P0(Bxr), i, e);
}), N(x5, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), Axr);
var x = i[1], c = function(f0) {
return u(n4, f0);
}, s = function(f0) {
return u(t, f0);
};
return R(YK[1], s, c, e, x), u(f(e), Nxr);
case 1:
u(f(e), Cxr);
var p = i[1], y = function(f0) {
return u(n4, f0);
}, T = function(f0) {
return u(t, f0);
};
return R(KK[1], T, y, e, p), u(f(e), Pxr);
case 2:
u(f(e), Dxr);
var E = i[1], h = function(f0) {
return u(n4, f0);
}, w = function(f0) {
return u(t, f0);
};
return R($K[3], w, h, e, E), u(f(e), Lxr);
case 3:
u(f(e), Rxr);
var G = i[1], A = function(f0) {
return u(n4, f0);
}, S = function(f0) {
return u(t, f0);
};
return R(rW[1], S, A, e, G), u(f(e), jxr);
default:
u(f(e), Gxr);
var M = i[1], K = function(f0) {
return u(n4, f0);
}, V = function(f0) {
return u(t, f0);
};
return R(tW[1], V, K, e, M), u(f(e), Mxr);
}
}), N(iW, function(t, n4, e) {
var i = a(x5, t, n4);
return a(P0(Ixr), i, e);
});
var hC = [0, YK, KK, $K, rW, tW, dC, uW, x5, iW], kC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, fW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(kC, function(t, n4, e, i) {
u(f(e), axr), a(f(e), cxr, oxr);
var x = i[1];
u(f(e), sxr), a(t, e, x[1]), u(f(e), vxr);
var c = x[2];
function s(A) {
return u(n4, A);
}
function p(A) {
return u(t, A);
}
R(hC[6], p, s, e, c), u(f(e), lxr), u(f(e), bxr), u(f(e), pxr), a(f(e), _xr, mxr);
var y = i[2];
u(f(e), yxr);
var T = 0;
be(function(A, S) {
A && u(f(e), uxr), u(f(e), ixr), a(t, e, S[1]), u(f(e), fxr);
var M = S[2];
function K(f0) {
return u(n4, f0);
}
function V(f0) {
return u(t, f0);
}
return R(cC[2], V, K, e, M), u(f(e), xxr), 1;
}, T, y), u(f(e), dxr), u(f(e), hxr), u(f(e), kxr), a(f(e), Exr, wxr);
var E = i[3];
if (E) {
g(e, Sxr);
var h = E[1], w = function(A, S) {
return g(A, txr);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, gxr);
} else
g(e, Fxr);
return u(f(e), Txr), u(f(e), Oxr);
}), N(fW, function(t, n4, e) {
var i = a(kC, t, n4);
return a(P0(nxr), i, e);
});
var xW = [0, kC, fW], wC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, aW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(wC, function(t, n4, e, i) {
u(f(e), Xfr), a(f(e), Vfr, Yfr);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(Je[13], s, c, e, x), u(f(e), zfr), u(f(e), Kfr), a(f(e), Jfr, Wfr);
var p = i[2];
if (p) {
g(e, $fr);
var y = p[1], T = function(h, w) {
return g(h, Hfr);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, Zfr);
} else
g(e, Qfr);
return u(f(e), rxr), u(f(e), exr);
}), N(aW, function(t, n4, e) {
var i = a(wC, t, n4);
return a(P0(Ufr), i, e);
});
var oW = [0, wC, aW], a5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, cW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, o5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, sW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, c5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, vW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(a5, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), Gfr);
var x = i[1], c = function(T) {
return u(n4, T);
}, s = function(T) {
return u(t, T);
};
return R(Ln[1], s, c, e, x), u(f(e), Mfr);
}
u(f(e), Bfr);
var p = i[1];
function y(T) {
return u(n4, T);
}
return R(c5, function(T) {
return u(t, T);
}, y, e, p), u(f(e), qfr);
}), N(cW, function(t, n4, e) {
var i = a(a5, t, n4);
return a(P0(jfr), i, e);
}), N(o5, function(t, n4, e, i) {
u(f(e), Ofr), a(f(e), Afr, Ifr);
var x = i[1];
function c(T) {
return u(n4, T);
}
R(a5, function(T) {
return u(t, T);
}, c, e, x), u(f(e), Nfr), u(f(e), Cfr), a(f(e), Dfr, Pfr);
var s = i[2];
function p(T) {
return u(n4, T);
}
function y(T) {
return u(t, T);
}
return R(Ln[1], y, p, e, s), u(f(e), Lfr), u(f(e), Rfr);
}), N(sW, function(t, n4, e) {
var i = a(o5, t, n4);
return a(P0(Tfr), i, e);
}), N(c5, function(t, n4, e, i) {
u(f(e), Sfr), a(n4, e, i[1]), u(f(e), gfr);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(o5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Ffr);
}), N(vW, function(t, n4, e) {
var i = a(c5, t, n4);
return a(P0(Efr), i, e);
});
var lW = [0, a5, cW, o5, sW, c5, vW], EC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, bW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(EC, function(t, n4, e, i) {
u(f(e), sfr), a(f(e), lfr, vfr);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(lW[1], s, c, e, x), u(f(e), bfr), u(f(e), pfr), a(f(e), _fr, mfr);
var p = i[2];
if (p) {
g(e, yfr);
var y = p[1], T = function(h, w) {
return g(h, cfr);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, dfr);
} else
g(e, hfr);
return u(f(e), kfr), u(f(e), wfr);
}), N(bW, function(t, n4, e) {
var i = a(EC, t, n4);
return a(P0(ofr), i, e);
});
var pW = [0, lW, EC, bW], SC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, mW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(SC, function(t, n4, e, i) {
u(f(e), Wir), a(f(e), $ir, Jir);
var x = i[1];
u(f(e), Zir);
var c = 0;
be(function(E, h) {
E && u(f(e), Kir);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(Je[13], G, w, e, h), 1;
}, c, x), u(f(e), Qir), u(f(e), rfr), u(f(e), efr), a(f(e), tfr, nfr);
var s = i[2];
if (s) {
g(e, ufr);
var p = s[1], y = function(E, h) {
return g(E, zir);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, ifr);
} else
g(e, ffr);
return u(f(e), xfr), u(f(e), afr);
}), N(mW, function(t, n4, e) {
var i = a(SC, t, n4);
return a(P0(Vir), i, e);
});
var _W = [0, SC, mW], gC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, yW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(gC, function(t, n4, e, i) {
u(f(e), Dir), a(f(e), Rir, Lir);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(Je[13], s, c, e, x), u(f(e), jir), u(f(e), Gir), a(f(e), Bir, Mir);
var p = i[2];
if (p) {
g(e, qir);
var y = p[1], T = function(h, w) {
return g(h, Pir);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, Uir);
} else
g(e, Hir);
return u(f(e), Xir), u(f(e), Yir);
}), N(yW, function(t, n4, e) {
var i = a(gC, t, n4);
return a(P0(Cir), i, e);
});
var dW = [0, gC, yW], FC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, hW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(FC, function(t, n4, e, i) {
u(f(e), bir), a(f(e), mir, pir);
var x = i[1];
u(f(e), _ir);
var c = x[1];
function s(K) {
return u(n4, K);
}
function p(K) {
return u(t, K);
}
R(Je[13], p, s, e, c), u(f(e), yir);
var y = x[2];
function T(K) {
return u(n4, K);
}
function E(K) {
return u(t, K);
}
R(Je[13], E, T, e, y), u(f(e), dir), u(f(e), hir);
var h = x[3], w = 0;
be(function(K, V) {
K && u(f(e), lir);
function f0(k0) {
return u(n4, k0);
}
function m0(k0) {
return u(t, k0);
}
return R(Je[13], m0, f0, e, V), 1;
}, w, h), u(f(e), kir), u(f(e), wir), u(f(e), Eir), u(f(e), Sir), a(f(e), Fir, gir);
var G = i[2];
if (G) {
g(e, Tir);
var A = G[1], S = function(K, V) {
return g(K, vir);
}, M = function(K) {
return u(t, K);
};
R(Dr[1], M, S, e, A), g(e, Oir);
} else
g(e, Iir);
return u(f(e), Air), u(f(e), Nir);
}), N(hW, function(t, n4, e) {
var i = a(FC, t, n4);
return a(P0(sir), i, e);
});
var kW = [0, FC, hW], TC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, wW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(TC, function(t, n4, e, i) {
u(f(e), z7r), a(f(e), W7r, K7r);
var x = i[1];
u(f(e), J7r);
var c = x[1];
function s(K) {
return u(n4, K);
}
function p(K) {
return u(t, K);
}
R(Je[13], p, s, e, c), u(f(e), $7r);
var y = x[2];
function T(K) {
return u(n4, K);
}
function E(K) {
return u(t, K);
}
R(Je[13], E, T, e, y), u(f(e), Z7r), u(f(e), Q7r);
var h = x[3], w = 0;
be(function(K, V) {
K && u(f(e), V7r);
function f0(k0) {
return u(n4, k0);
}
function m0(k0) {
return u(t, k0);
}
return R(Je[13], m0, f0, e, V), 1;
}, w, h), u(f(e), rir), u(f(e), eir), u(f(e), nir), u(f(e), tir), a(f(e), iir, uir);
var G = i[2];
if (G) {
g(e, fir);
var A = G[1], S = function(K, V) {
return g(K, Y7r);
}, M = function(K) {
return u(t, K);
};
R(Dr[1], M, S, e, A), g(e, xir);
} else
g(e, air);
return u(f(e), oir), u(f(e), cir);
}), N(wW, function(t, n4, e) {
var i = a(TC, t, n4);
return a(P0(X7r), i, e);
});
var EW = [0, TC, wW], s5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, SW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, v5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, gW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, OC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, FW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, IC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, TW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(s5, function(t, n4, e, i) {
u(f(e), q7r), a(n4, e, i[1]), u(f(e), U7r);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(v5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), H7r);
}), N(SW, function(t, n4, e) {
var i = a(s5, t, n4);
return a(P0(B7r), i, e);
}), N(v5, function(t, n4, e, i) {
switch (i[0]) {
case 0:
var x = i[1];
if (u(f(e), xur), x) {
g(e, aur);
var c = x[1], s = function(U, Y) {
return g(U, fur);
}, p = function(U) {
return u(t, U);
};
R(Dr[1], p, s, e, c), g(e, our);
} else
g(e, cur);
return u(f(e), sur);
case 1:
var y = i[1];
if (u(f(e), vur), y) {
g(e, lur);
var T = y[1], E = function(U, Y) {
return g(U, iur);
}, h = function(U) {
return u(t, U);
};
R(Dr[1], h, E, e, T), g(e, bur);
} else
g(e, pur);
return u(f(e), mur);
case 2:
var w = i[1];
if (u(f(e), _ur), w) {
g(e, yur);
var G = w[1], A = function(U, Y) {
return g(U, uur);
}, S = function(U) {
return u(t, U);
};
R(Dr[1], S, A, e, G), g(e, dur);
} else
g(e, hur);
return u(f(e), kur);
case 3:
var M = i[1];
if (u(f(e), wur), M) {
g(e, Eur);
var K = M[1], V = function(U, Y) {
return g(U, tur);
}, f0 = function(U) {
return u(t, U);
};
R(Dr[1], f0, V, e, K), g(e, Sur);
} else
g(e, gur);
return u(f(e), Fur);
case 4:
var m0 = i[1];
if (u(f(e), Tur), m0) {
g(e, Our);
var k0 = m0[1], g0 = function(U, Y) {
return g(U, nur);
}, e0 = function(U) {
return u(t, U);
};
R(Dr[1], e0, g0, e, k0), g(e, Iur);
} else
g(e, Aur);
return u(f(e), Nur);
case 5:
var x0 = i[1];
if (u(f(e), Cur), x0) {
g(e, Pur);
var l = x0[1], c0 = function(U, Y) {
return g(U, eur);
}, t0 = function(U) {
return u(t, U);
};
R(Dr[1], t0, c0, e, l), g(e, Dur);
} else
g(e, Lur);
return u(f(e), Rur);
case 6:
var a0 = i[1];
if (u(f(e), jur), a0) {
g(e, Gur);
var w0 = a0[1], _0 = function(U, Y) {
return g(U, rur);
}, E0 = function(U) {
return u(t, U);
};
R(Dr[1], E0, _0, e, w0), g(e, Mur);
} else
g(e, Bur);
return u(f(e), qur);
case 7:
var X0 = i[1];
if (u(f(e), Uur), X0) {
g(e, Hur);
var b = X0[1], G0 = function(U, Y) {
return g(U, Qtr);
}, X = function(U) {
return u(t, U);
};
R(Dr[1], X, G0, e, b), g(e, Xur);
} else
g(e, Yur);
return u(f(e), Vur);
case 8:
var s0 = i[1];
if (u(f(e), zur), s0) {
g(e, Kur);
var dr = s0[1], Ar = function(U, Y) {
return g(U, Ztr);
}, ar = function(U) {
return u(t, U);
};
R(Dr[1], ar, Ar, e, dr), g(e, Wur);
} else
g(e, Jur);
return u(f(e), $ur);
case 9:
var W0 = i[1];
if (u(f(e), Zur), W0) {
g(e, Qur);
var Lr = W0[1], Tr = function(U, Y) {
return g(U, $tr);
}, Hr = function(U) {
return u(t, U);
};
R(Dr[1], Hr, Tr, e, Lr), g(e, r7r);
} else
g(e, e7r);
return u(f(e), n7r);
case 10:
var Or = i[1];
if (u(f(e), t7r), Or) {
g(e, u7r);
var xr = Or[1], Rr = function(U, Y) {
return g(U, Jtr);
}, Wr = function(U) {
return u(t, U);
};
R(Dr[1], Wr, Rr, e, xr), g(e, i7r);
} else
g(e, f7r);
return u(f(e), x7r);
case 11:
u(f(e), a7r);
var Jr = i[1], or = function(U) {
return u(n4, U);
}, _r = function(U) {
return u(t, U);
};
return R(oW[1], _r, or, e, Jr), u(f(e), o7r);
case 12:
u(f(e), c7r);
var Ir = i[1], fe = function(U) {
return u(n4, U);
}, v0 = function(U) {
return u(t, U);
};
return R(Ol[5], v0, fe, e, Ir), u(f(e), s7r);
case 13:
u(f(e), v7r);
var P = i[1], L = function(U) {
return u(n4, U);
}, Q = function(U) {
return u(t, U);
};
return R(hC[6], Q, L, e, P), u(f(e), l7r);
case 14:
u(f(e), b7r);
var i0 = i[1], l0 = function(U) {
return u(n4, U);
}, S0 = function(U) {
return u(t, U);
};
return R(xW[1], S0, l0, e, i0), u(f(e), p7r);
case 15:
u(f(e), m7r);
var T0 = i[1], rr = function(U) {
return u(n4, U);
}, R0 = function(U) {
return u(t, U);
};
return R(dW[1], R0, rr, e, T0), u(f(e), _7r);
case 16:
u(f(e), y7r);
var B = i[1], Z = function(U) {
return u(n4, U);
}, p0 = function(U) {
return u(t, U);
};
return R(cC[2], p0, Z, e, B), u(f(e), d7r);
case 17:
u(f(e), h7r);
var b0 = i[1], O0 = function(U) {
return u(n4, U);
}, q0 = function(U) {
return u(t, U);
};
return R(vC[1], q0, O0, e, b0), u(f(e), k7r);
case 18:
u(f(e), w7r);
var er = i[1], yr = function(U) {
return u(n4, U);
}, vr = function(U) {
return u(t, U);
};
return R(qK[1], vr, yr, e, er), u(f(e), E7r);
case 19:
u(f(e), S7r);
var $0 = i[1], Sr = function(U) {
return u(n4, U);
}, Mr = function(U) {
return u(t, U);
};
return R(kW[1], Mr, Sr, e, $0), u(f(e), g7r);
case 20:
u(f(e), F7r);
var Br = i[1], qr = function(U) {
return u(n4, U);
}, jr = function(U) {
return u(t, U);
};
return R(EW[1], jr, qr, e, Br), u(f(e), T7r);
case 21:
u(f(e), O7r);
var $r = i[1], ne = function(U) {
return u(n4, U);
}, Qr = function(U) {
return u(t, U);
};
return R(pW[2], Qr, ne, e, $r), u(f(e), I7r);
case 22:
u(f(e), A7r);
var pe = i[1], oe = function(U) {
return u(n4, U);
}, me = function(U) {
return u(t, U);
};
return R(_W[1], me, oe, e, pe), u(f(e), N7r);
case 23:
u(f(e), C7r);
var ae = i[1], ce = function(U) {
return u(t, U);
};
return ir(F1[1], ce, e, ae), u(f(e), P7r);
case 24:
u(f(e), D7r);
var ge = i[1], H0 = function(U) {
return u(t, U);
};
return ir(HN[1], H0, e, ge), u(f(e), L7r);
case 25:
u(f(e), R7r);
var Fr = i[1], _ = function(U) {
return u(t, U);
};
return ir(tK[1], _, e, Fr), u(f(e), j7r);
default:
u(f(e), G7r);
var k = i[1], I = function(U) {
return u(t, U);
};
return ir(XN[1], I, e, k), u(f(e), M7r);
}
}), N(gW, function(t, n4, e) {
var i = a(v5, t, n4);
return a(P0(Wtr), i, e);
}), N(OC, function(t, n4, e, i) {
u(f(e), Vtr), a(t, e, i[1]), u(f(e), ztr);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(s5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Ktr);
}), N(FW, function(t, n4, e) {
var i = a(OC, t, n4);
return a(P0(Ytr), i, e);
}), N(IC, function(t, n4, e, i) {
if (i[0] === 0)
return u(f(e), qtr), a(n4, e, i[1]), u(f(e), Utr);
u(f(e), Htr);
var x = i[1];
function c(p) {
return u(n4, p);
}
function s(p) {
return u(t, p);
}
return R(Je[17], s, c, e, x), u(f(e), Xtr);
}), N(TW, function(t, n4, e) {
var i = a(IC, t, n4);
return a(P0(Btr), i, e);
});
var AC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, OW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, l5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, IW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(AC, function(t, n4, e, i) {
u(f(e), jtr), a(t, e, i[1]), u(f(e), Gtr);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(l5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Mtr);
}), N(OW, function(t, n4, e) {
var i = a(AC, t, n4);
return a(P0(Rtr), i, e);
}), N(l5, function(t, n4, e, i) {
u(f(e), ltr), a(f(e), ptr, btr);
var x = i[1];
function c(K) {
return u(t, K);
}
function s(K) {
return u(t, K);
}
R(Ln[1], s, c, e, x), u(f(e), mtr), u(f(e), _tr), a(f(e), dtr, ytr);
var p = i[2];
function y(K) {
return u(n4, K);
}
function T(K) {
return u(t, K);
}
R(Je[19], T, y, e, p), u(f(e), htr), u(f(e), ktr), a(f(e), Etr, wtr);
var E = i[3];
if (E) {
g(e, Str);
var h = E[1], w = function(K) {
return u(t, K);
};
ir(zv[1], w, e, h), g(e, gtr);
} else
g(e, Ftr);
u(f(e), Ttr), u(f(e), Otr), a(f(e), Atr, Itr);
var G = i[4];
if (G) {
g(e, Ntr);
var A = G[1], S = function(K) {
return u(n4, K);
}, M = function(K) {
return u(t, K);
};
R(Je[13], M, S, e, A), g(e, Ctr);
} else
g(e, Ptr);
return u(f(e), Dtr), u(f(e), Ltr);
}), N(IW, function(t, n4, e) {
var i = a(l5, t, n4);
return a(P0(vtr), i, e);
});
var AW = [0, AC, OW, l5, IW], NC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, NW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, b5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, CW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(NC, function(t, n4, e, i) {
u(f(e), otr), a(t, e, i[1]), u(f(e), ctr);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(b5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), str);
}), N(NW, function(t, n4, e) {
var i = a(NC, t, n4);
return a(P0(atr), i, e);
}), N(b5, function(t, n4, e, i) {
u(f(e), Knr), a(f(e), Jnr, Wnr);
var x = i[1];
u(f(e), $nr);
var c = 0;
be(function(E, h) {
E && u(f(e), znr);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(AW[1], G, w, e, h), 1;
}, c, x), u(f(e), Znr), u(f(e), Qnr), u(f(e), rtr), a(f(e), ntr, etr);
var s = i[2];
if (s) {
g(e, ttr);
var p = s[1], y = function(E, h) {
u(f(E), Ynr);
var w = 0;
return be(function(G, A) {
G && u(f(E), Xnr);
function S(M) {
return u(t, M);
}
return ir(uu[1], S, E, A), 1;
}, w, h), u(f(E), Vnr);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, utr);
} else
g(e, itr);
return u(f(e), ftr), u(f(e), xtr);
}), N(CW, function(t, n4, e) {
var i = a(b5, t, n4);
return a(P0(Hnr), i, e);
});
var CC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, PW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, p5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, DW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, jee = [0, NC, NW, b5, CW];
N(CC, function(t, n4, e, i) {
u(f(e), Bnr), a(t, e, i[1]), u(f(e), qnr);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(p5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Unr);
}), N(PW, function(t, n4, e) {
var i = a(CC, t, n4);
return a(P0(Mnr), i, e);
}), N(p5, function(t, n4, e, i) {
u(f(e), gnr), a(f(e), Tnr, Fnr);
var x = i[1];
u(f(e), Onr);
var c = 0;
be(function(E, h) {
E && u(f(e), Snr);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(Je[13], G, w, e, h), 1;
}, c, x), u(f(e), Inr), u(f(e), Anr), u(f(e), Nnr), a(f(e), Pnr, Cnr);
var s = i[2];
if (s) {
g(e, Dnr);
var p = s[1], y = function(E, h) {
u(f(E), wnr);
var w = 0;
return be(function(G, A) {
G && u(f(E), knr);
function S(M) {
return u(t, M);
}
return ir(uu[1], S, E, A), 1;
}, w, h), u(f(E), Enr);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, Lnr);
} else
g(e, Rnr);
return u(f(e), jnr), u(f(e), Gnr);
}), N(DW, function(t, n4, e) {
var i = a(p5, t, n4);
return a(P0(hnr), i, e);
});
var PC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, LW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, m5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, RW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, _5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, jW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Gee = [0, CC, PW, p5, DW];
N(PC, function(t, n4, e, i) {
u(f(e), _nr), a(t, e, i[1]), u(f(e), ynr);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(m5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), dnr);
}), N(LW, function(t, n4, e) {
var i = a(PC, t, n4);
return a(P0(mnr), i, e);
}), N(m5, function(t, n4, e, i) {
u(f(e), unr), a(f(e), fnr, inr);
var x = i[1];
function c(E) {
return u(n4, E);
}
R(_5, function(E) {
return u(t, E);
}, c, e, x), u(f(e), xnr), u(f(e), anr), a(f(e), cnr, onr);
var s = i[2];
if (s) {
g(e, snr);
var p = s[1], y = function(E, h) {
return g(E, tnr);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, vnr);
} else
g(e, lnr);
return u(f(e), bnr), u(f(e), pnr);
}), N(RW, function(t, n4, e) {
var i = a(m5, t, n4);
return a(P0(nnr), i, e);
}), N(_5, function(t, n4, e, i) {
if (i) {
u(f(e), Qer);
var x = i[1], c = function(p) {
return u(n4, p);
}, s = function(p) {
return u(t, p);
};
return R(qe[31], s, c, e, x), u(f(e), rnr);
}
return g(e, enr);
}), N(jW, function(t, n4, e) {
var i = a(_5, t, n4);
return a(P0(Zer), i, e);
}), pu(o6r, Je, [0, Ol, cC, vC, qK, hC, xW, oW, pW, _W, dW, kW, EW, s5, SW, v5, gW, OC, FW, IC, TW, AW, jee, Gee, [0, PC, LW, m5, RW, _5, jW]]);
var DC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, GW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(DC, function(t, n4, e, i) {
u(f(e), Ger), a(f(e), Ber, Mer);
var x = i[1];
u(f(e), qer);
var c = 0;
be(function(E, h) {
E && u(f(e), jer);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(Xu[35], G, w, e, h), 1;
}, c, x), u(f(e), Uer), u(f(e), Her), u(f(e), Xer), a(f(e), Ver, Yer);
var s = i[2];
if (s) {
g(e, zer);
var p = s[1], y = function(E, h) {
u(f(E), Ler);
var w = 0;
return be(function(G, A) {
G && u(f(E), Der);
function S(M) {
return u(t, M);
}
return ir(uu[1], S, E, A), 1;
}, w, h), u(f(E), Rer);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, Ker);
} else
g(e, Wer);
return u(f(e), Jer), u(f(e), $er);
}), N(GW, function(t, n4, e) {
var i = a(DC, t, n4);
return a(P0(Per), i, e);
});
var Kv = [0, DC, GW], LC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, MW = function t(n4, e, i) {
return t.fun(n4, e, i);
}, y5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, BW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(LC, function(t, n4, e, i) {
u(f(e), Aer), a(t, e, i[1]), u(f(e), Ner);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(y5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Cer);
}), N(MW, function(t, n4, e) {
var i = a(LC, t, n4);
return a(P0(Ier), i, e);
}), N(y5, function(t, n4, e, i) {
u(f(e), _er), a(f(e), der, yer);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(Xu[35], s, c, e, x), u(f(e), her), u(f(e), ker), a(f(e), Eer, wer);
var p = i[2];
if (p) {
g(e, Ser);
var y = p[1], T = function(h, w) {
return g(h, mer);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, ger);
} else
g(e, Fer);
return u(f(e), Ter), u(f(e), Oer);
}), N(BW, function(t, n4, e) {
var i = a(y5, t, n4);
return a(P0(per), i, e);
});
var qW = [0, LC, MW, y5, BW], RC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, UW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(RC, function(t, n4, e, i) {
u(f(e), Vrr), a(f(e), Krr, zrr);
var x = i[1];
function c(V) {
return u(n4, V);
}
function s(V) {
return u(t, V);
}
R(qe[31], s, c, e, x), u(f(e), Wrr), u(f(e), Jrr), a(f(e), Zrr, $rr);
var p = i[2];
function y(V) {
return u(n4, V);
}
function T(V) {
return u(t, V);
}
R(Xu[35], T, y, e, p), u(f(e), Qrr), u(f(e), rer), a(f(e), ner, eer);
var E = i[3];
if (E) {
g(e, ter);
var h = E[1], w = function(V) {
return u(n4, V);
}, G = function(V) {
return u(t, V);
};
R(qW[1], G, w, e, h), g(e, uer);
} else
g(e, ier);
u(f(e), fer), u(f(e), xer), a(f(e), oer, aer);
var A = i[4];
if (A) {
g(e, cer);
var S = A[1], M = function(V, f0) {
return g(V, Yrr);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, ser);
} else
g(e, ver);
return u(f(e), ler), u(f(e), ber);
}), N(UW, function(t, n4, e) {
var i = a(RC, t, n4);
return a(P0(Xrr), i, e);
});
var HW = [0, qW, RC, UW], jC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, XW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(jC, function(t, n4, e, i) {
u(f(e), Orr), a(f(e), Arr, Irr);
var x = i[1];
function c(A) {
return u(t, A);
}
function s(A) {
return u(t, A);
}
R(Ln[1], s, c, e, x), u(f(e), Nrr), u(f(e), Crr), a(f(e), Drr, Prr);
var p = i[2];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(Xu[35], T, y, e, p), u(f(e), Lrr), u(f(e), Rrr), a(f(e), Grr, jrr);
var E = i[3];
if (E) {
g(e, Mrr);
var h = E[1], w = function(A, S) {
return g(A, Trr);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, Brr);
} else
g(e, qrr);
return u(f(e), Urr), u(f(e), Hrr);
}), N(XW, function(t, n4, e) {
var i = a(jC, t, n4);
return a(P0(Frr), i, e);
});
var YW = [0, jC, XW], GC = function t(n4, e, i) {
return t.fun(n4, e, i);
}, VW = function t(n4, e) {
return t.fun(n4, e);
};
N(GC, function(t, n4, e) {
u(f(n4), srr), a(f(n4), lrr, vrr);
var i = e[1];
if (i) {
g(n4, brr);
var x = i[1], c = function(h) {
return u(t, h);
}, s = function(h) {
return u(t, h);
};
R(Ln[1], s, c, n4, x), g(n4, prr);
} else
g(n4, mrr);
u(f(n4), _rr), u(f(n4), yrr), a(f(n4), hrr, drr);
var p = e[2];
if (p) {
g(n4, krr);
var y = p[1], T = function(h, w) {
return g(h, crr);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, n4, y), g(n4, wrr);
} else
g(n4, Err);
return u(f(n4), Srr), u(f(n4), grr);
}), N(VW, function(t, n4) {
var e = u(GC, t);
return a(P0(orr), e, n4);
});
var zW = [0, GC, VW], MC = function t(n4, e, i) {
return t.fun(n4, e, i);
}, KW = function t(n4, e) {
return t.fun(n4, e);
};
N(MC, function(t, n4, e) {
u(f(n4), K0r), a(f(n4), J0r, W0r);
var i = e[1];
if (i) {
g(n4, $0r);
var x = i[1], c = function(h) {
return u(t, h);
}, s = function(h) {
return u(t, h);
};
R(Ln[1], s, c, n4, x), g(n4, Z0r);
} else
g(n4, Q0r);
u(f(n4), rrr), u(f(n4), err), a(f(n4), trr, nrr);
var p = e[2];
if (p) {
g(n4, urr);
var y = p[1], T = function(h, w) {
return g(h, z0r);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, n4, y), g(n4, irr);
} else
g(n4, frr);
return u(f(n4), xrr), u(f(n4), arr);
}), N(KW, function(t, n4) {
var e = u(MC, t);
return a(P0(V0r), e, n4);
});
var WW = [0, MC, KW], BC = function t(n4, e, i) {
return t.fun(n4, e, i);
}, JW = function t(n4, e) {
return t.fun(n4, e);
};
N(BC, function(t, n4, e) {
u(f(n4), G0r), a(f(n4), B0r, M0r);
var i = e[1];
if (i) {
g(n4, q0r);
var x = i[1], c = function(p, y) {
return g(p, j0r);
}, s = function(p) {
return u(t, p);
};
R(Dr[1], s, c, n4, x), g(n4, U0r);
} else
g(n4, H0r);
return u(f(n4), X0r), u(f(n4), Y0r);
}), N(JW, function(t, n4) {
var e = u(BC, t);
return a(P0(R0r), e, n4);
});
var $W = [0, BC, JW], qC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, ZW = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(qC, function(t, n4, e, i) {
u(f(e), h0r), a(f(e), w0r, k0r);
var x = i[1];
function c(A) {
return u(n4, A);
}
function s(A) {
return u(t, A);
}
R(qe[31], s, c, e, x), u(f(e), E0r), u(f(e), S0r), a(f(e), F0r, g0r);
var p = i[2];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(Xu[35], T, y, e, p), u(f(e), T0r), u(f(e), O0r), a(f(e), A0r, I0r);
var E = i[3];
if (E) {
g(e, N0r);
var h = E[1], w = function(A, S) {
return g(A, d0r);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, C0r);
} else
g(e, P0r);
return u(f(e), D0r), u(f(e), L0r);
}), N(ZW, function(t, n4, e) {
var i = a(qC, t, n4);
return a(P0(y0r), i, e);
});
var QW = [0, qC, ZW], UC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, rJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(UC, function(t, n4, e, i) {
u(f(e), WQ0), a(f(e), $Q0, JQ0);
var x = i[1];
function c(V) {
return u(n4, V);
}
function s(V) {
return u(t, V);
}
R(Ln[1], s, c, e, x), u(f(e), ZQ0), u(f(e), QQ0), a(f(e), e0r, r0r);
var p = i[2];
if (p) {
g(e, n0r);
var y = p[1], T = function(V) {
return u(n4, V);
}, E = function(V) {
return u(t, V);
};
R(Je[22][1], E, T, e, y), g(e, t0r);
} else
g(e, u0r);
u(f(e), i0r), u(f(e), f0r), a(f(e), a0r, x0r);
var h = i[3];
function w(V) {
return u(n4, V);
}
function G(V) {
return u(t, V);
}
R(Je[13], G, w, e, h), u(f(e), o0r), u(f(e), c0r), a(f(e), v0r, s0r);
var A = i[4];
if (A) {
g(e, l0r);
var S = A[1], M = function(V, f0) {
return g(V, KQ0);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, b0r);
} else
g(e, p0r);
return u(f(e), m0r), u(f(e), _0r);
}), N(rJ, function(t, n4, e) {
var i = a(UC, t, n4);
return a(P0(zQ0), i, e);
});
var d5 = [0, UC, rJ], HC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, eJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(HC, function(t, n4, e, i) {
u(f(e), bQ0), a(f(e), mQ0, pQ0);
var x = i[1];
function c(e0) {
return u(n4, e0);
}
function s(e0) {
return u(t, e0);
}
R(Ln[1], s, c, e, x), u(f(e), _Q0), u(f(e), yQ0), a(f(e), hQ0, dQ0);
var p = i[2];
if (p) {
g(e, kQ0);
var y = p[1], T = function(e0) {
return u(n4, e0);
}, E = function(e0) {
return u(t, e0);
};
R(Je[22][1], E, T, e, y), g(e, wQ0);
} else
g(e, EQ0);
u(f(e), SQ0), u(f(e), gQ0), a(f(e), TQ0, FQ0);
var h = i[3];
if (h) {
g(e, OQ0);
var w = h[1], G = function(e0) {
return u(n4, e0);
}, A = function(e0) {
return u(t, e0);
};
R(Je[13], A, G, e, w), g(e, IQ0);
} else
g(e, AQ0);
u(f(e), NQ0), u(f(e), CQ0), a(f(e), DQ0, PQ0);
var S = i[4];
if (S) {
g(e, LQ0);
var M = S[1], K = function(e0) {
return u(n4, e0);
}, V = function(e0) {
return u(t, e0);
};
R(Je[13], V, K, e, M), g(e, RQ0);
} else
g(e, jQ0);
u(f(e), GQ0), u(f(e), MQ0), a(f(e), qQ0, BQ0);
var f0 = i[5];
if (f0) {
g(e, UQ0);
var m0 = f0[1], k0 = function(e0, x0) {
return g(e0, lQ0);
}, g0 = function(e0) {
return u(t, e0);
};
R(Dr[1], g0, k0, e, m0), g(e, HQ0);
} else
g(e, XQ0);
return u(f(e), YQ0), u(f(e), VQ0);
}), N(eJ, function(t, n4, e) {
var i = a(HC, t, n4);
return a(P0(vQ0), i, e);
});
var h5 = [0, HC, eJ], XC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, nJ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, k5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, tJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(XC, function(t, n4, e, i) {
u(f(e), oQ0), a(t, e, i[1]), u(f(e), cQ0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(k5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), sQ0);
}), N(nJ, function(t, n4, e) {
var i = a(XC, t, n4);
return a(P0(aQ0), i, e);
}), N(k5, function(t, n4, e, i) {
u(f(e), qZ0), a(f(e), HZ0, UZ0);
var x = i[1];
if (x) {
g(e, XZ0);
var c = x[1], s = function(A) {
return u(n4, A);
}, p = function(A) {
return u(t, A);
};
R(qe[31], p, s, e, c), g(e, YZ0);
} else
g(e, VZ0);
u(f(e), zZ0), u(f(e), KZ0), a(f(e), JZ0, WZ0);
var y = i[2];
u(f(e), $Z0);
var T = 0;
be(function(A, S) {
A && u(f(e), BZ0);
function M(V) {
return u(n4, V);
}
function K(V) {
return u(t, V);
}
return R(Xu[35], K, M, e, S), 1;
}, T, y), u(f(e), ZZ0), u(f(e), QZ0), u(f(e), rQ0), a(f(e), nQ0, eQ0);
var E = i[3];
if (E) {
g(e, tQ0);
var h = E[1], w = function(A, S) {
return g(A, MZ0);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, uQ0);
} else
g(e, iQ0);
return u(f(e), fQ0), u(f(e), xQ0);
}), N(tJ, function(t, n4, e) {
var i = a(k5, t, n4);
return a(P0(GZ0), i, e);
});
var uJ = [0, XC, nJ, k5, tJ], YC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, iJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(YC, function(t, n4, e, i) {
u(f(e), mZ0), a(f(e), yZ0, _Z0);
var x = i[1];
function c(G) {
return u(n4, G);
}
function s(G) {
return u(t, G);
}
R(qe[31], s, c, e, x), u(f(e), dZ0), u(f(e), hZ0), a(f(e), wZ0, kZ0);
var p = i[2];
u(f(e), EZ0);
var y = 0;
be(function(G, A) {
G && u(f(e), pZ0);
function S(K) {
return u(n4, K);
}
function M(K) {
return u(t, K);
}
return R(uJ[1], M, S, e, A), 1;
}, y, p), u(f(e), SZ0), u(f(e), gZ0), u(f(e), FZ0), a(f(e), OZ0, TZ0);
var T = i[3];
if (T) {
g(e, IZ0);
var E = T[1], h = function(G, A) {
return g(G, bZ0);
}, w = function(G) {
return u(t, G);
};
R(Dr[1], w, h, e, E), g(e, AZ0);
} else
g(e, NZ0);
return u(f(e), CZ0), u(f(e), PZ0), a(f(e), LZ0, DZ0), a(n4, e, i[4]), u(f(e), RZ0), u(f(e), jZ0);
}), N(iJ, function(t, n4, e) {
var i = a(YC, t, n4);
return a(P0(lZ0), i, e);
});
var fJ = [0, uJ, YC, iJ], VC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, xJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(VC, function(t, n4, e, i) {
u(f(e), K$0), a(f(e), J$0, W$0);
var x = i[1];
if (x) {
g(e, $$0);
var c = x[1], s = function(w) {
return u(n4, w);
}, p = function(w) {
return u(t, w);
};
R(qe[31], p, s, e, c), g(e, Z$0);
} else
g(e, Q$0);
u(f(e), rZ0), u(f(e), eZ0), a(f(e), tZ0, nZ0);
var y = i[2];
if (y) {
g(e, uZ0);
var T = y[1], E = function(w, G) {
return g(w, z$0);
}, h = function(w) {
return u(t, w);
};
R(Dr[1], h, E, e, T), g(e, iZ0);
} else
g(e, fZ0);
return u(f(e), xZ0), u(f(e), aZ0), a(f(e), cZ0, oZ0), a(n4, e, i[3]), u(f(e), sZ0), u(f(e), vZ0);
}), N(xJ, function(t, n4, e) {
var i = a(VC, t, n4);
return a(P0(V$0), i, e);
});
var aJ = [0, VC, xJ], zC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, oJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(zC, function(t, n4, e, i) {
u(f(e), D$0), a(f(e), R$0, L$0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(qe[31], s, c, e, x), u(f(e), j$0), u(f(e), G$0), a(f(e), B$0, M$0);
var p = i[2];
if (p) {
g(e, q$0);
var y = p[1], T = function(h, w) {
return g(h, P$0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, U$0);
} else
g(e, H$0);
return u(f(e), X$0), u(f(e), Y$0);
}), N(oJ, function(t, n4, e) {
var i = a(zC, t, n4);
return a(P0(C$0), i, e);
});
var cJ = [0, zC, oJ], KC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, sJ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, w5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, vJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(KC, function(t, n4, e, i) {
u(f(e), I$0), a(t, e, i[1]), u(f(e), A$0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(w5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), N$0);
}), N(sJ, function(t, n4, e) {
var i = a(KC, t, n4);
return a(P0(O$0), i, e);
}), N(w5, function(t, n4, e, i) {
u(f(e), f$0), a(f(e), a$0, x$0);
var x = i[1];
if (x) {
g(e, o$0);
var c = x[1], s = function(M) {
return u(n4, M);
}, p = function(M) {
return u(t, M);
};
R(di[5], p, s, e, c), g(e, c$0);
} else
g(e, s$0);
u(f(e), v$0), u(f(e), l$0), a(f(e), p$0, b$0);
var y = i[2];
u(f(e), m$0), a(t, e, y[1]), u(f(e), _$0);
var T = y[2];
function E(M) {
return u(n4, M);
}
function h(M) {
return u(t, M);
}
R(Kv[1], h, E, e, T), u(f(e), y$0), u(f(e), d$0), u(f(e), h$0), a(f(e), w$0, k$0);
var w = i[3];
if (w) {
g(e, E$0);
var G = w[1], A = function(M, K) {
return g(M, i$0);
}, S = function(M) {
return u(t, M);
};
R(Dr[1], S, A, e, G), g(e, S$0);
} else
g(e, g$0);
return u(f(e), F$0), u(f(e), T$0);
}), N(vJ, function(t, n4, e) {
var i = a(w5, t, n4);
return a(P0(u$0), i, e);
});
var lJ = [0, KC, sJ, w5, vJ], WC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, bJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(WC, function(t, n4, e, i) {
u(f(e), FJ0), a(f(e), OJ0, TJ0);
var x = i[1];
u(f(e), IJ0), a(t, e, x[1]), u(f(e), AJ0);
var c = x[2];
function s(k0) {
return u(n4, k0);
}
function p(k0) {
return u(t, k0);
}
R(Kv[1], p, s, e, c), u(f(e), NJ0), u(f(e), CJ0), u(f(e), PJ0), a(f(e), LJ0, DJ0);
var y = i[2];
if (y) {
g(e, RJ0);
var T = y[1], E = function(k0) {
return u(n4, k0);
}, h = function(k0) {
return u(t, k0);
};
R(lJ[1], h, E, e, T), g(e, jJ0);
} else
g(e, GJ0);
u(f(e), MJ0), u(f(e), BJ0), a(f(e), UJ0, qJ0);
var w = i[3];
if (w) {
var G = w[1];
g(e, HJ0), u(f(e), XJ0), a(t, e, G[1]), u(f(e), YJ0);
var A = G[2], S = function(k0) {
return u(n4, k0);
}, M = function(k0) {
return u(t, k0);
};
R(Kv[1], M, S, e, A), u(f(e), VJ0), g(e, zJ0);
} else
g(e, KJ0);
u(f(e), WJ0), u(f(e), JJ0), a(f(e), ZJ0, $J0);
var K = i[4];
if (K) {
g(e, QJ0);
var V = K[1], f0 = function(k0, g0) {
return g(k0, gJ0);
}, m0 = function(k0) {
return u(t, k0);
};
R(Dr[1], m0, f0, e, V), g(e, r$0);
} else
g(e, e$0);
return u(f(e), n$0), u(f(e), t$0);
}), N(bJ, function(t, n4, e) {
var i = a(WC, t, n4);
return a(P0(SJ0), i, e);
});
var pJ = [0, lJ, WC, bJ], JC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, mJ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, E5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, _J = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(JC, function(t, n4, e, i) {
u(f(e), kJ0), a(t, e, i[1]), u(f(e), wJ0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(E5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), EJ0);
}), N(mJ, function(t, n4, e) {
var i = a(JC, t, n4);
return a(P0(hJ0), i, e);
}), N(E5, function(t, n4, e, i) {
u(f(e), aJ0), a(f(e), cJ0, oJ0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(di[5], s, c, e, x), u(f(e), sJ0), u(f(e), vJ0), a(f(e), bJ0, lJ0);
var p = i[2];
if (p) {
g(e, pJ0);
var y = p[1], T = function(h) {
return u(n4, h);
}, E = function(h) {
return u(t, h);
};
R(qe[31], E, T, e, y), g(e, mJ0);
} else
g(e, _J0);
return u(f(e), yJ0), u(f(e), dJ0);
}), N(_J, function(t, n4, e) {
var i = a(E5, t, n4);
return a(P0(xJ0), i, e);
});
var yJ = [0, JC, mJ, E5, _J], $C = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, dJ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, S5 = function t(n4, e) {
return t.fun(n4, e);
}, hJ = function t(n4) {
return t.fun(n4);
};
N($C, function(t, n4, e, i) {
u(f(e), HW0), a(f(e), YW0, XW0);
var x = i[1];
u(f(e), VW0);
var c = 0;
be(function(E, h) {
E && u(f(e), UW0);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(yJ[1], G, w, e, h), 1;
}, c, x), u(f(e), zW0), u(f(e), KW0), u(f(e), WW0), a(f(e), $W0, JW0), a(S5, e, i[2]), u(f(e), ZW0), u(f(e), QW0), a(f(e), eJ0, rJ0);
var s = i[3];
if (s) {
g(e, nJ0);
var p = s[1], y = function(E, h) {
return g(E, qW0);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, tJ0);
} else
g(e, uJ0);
return u(f(e), iJ0), u(f(e), fJ0);
}), N(dJ, function(t, n4, e) {
var i = a($C, t, n4);
return a(P0(BW0), i, e);
}), N(S5, function(t, n4) {
switch (n4) {
case 0:
return g(t, jW0);
case 1:
return g(t, GW0);
default:
return g(t, MW0);
}
}), N(hJ, function(t) {
return a(P0(RW0), S5, t);
});
var Il = [0, yJ, $C, dJ, S5, hJ], ZC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, kJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(ZC, function(t, n4, e, i) {
u(f(e), hW0), a(f(e), wW0, kW0);
var x = i[1];
function c(A) {
return u(n4, A);
}
function s(A) {
return u(t, A);
}
R(qe[31], s, c, e, x), u(f(e), EW0), u(f(e), SW0), a(f(e), FW0, gW0);
var p = i[2];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(Xu[35], T, y, e, p), u(f(e), TW0), u(f(e), OW0), a(f(e), AW0, IW0);
var E = i[3];
if (E) {
g(e, NW0);
var h = E[1], w = function(A, S) {
return g(A, dW0);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, CW0);
} else
g(e, PW0);
return u(f(e), DW0), u(f(e), LW0);
}), N(kJ, function(t, n4, e) {
var i = a(ZC, t, n4);
return a(P0(yW0), i, e);
});
var wJ = [0, ZC, kJ], QC = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, EJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(QC, function(t, n4, e, i) {
u(f(e), nW0), a(f(e), uW0, tW0);
var x = i[1];
function c(A) {
return u(n4, A);
}
function s(A) {
return u(t, A);
}
R(Xu[35], s, c, e, x), u(f(e), iW0), u(f(e), fW0), a(f(e), aW0, xW0);
var p = i[2];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(qe[31], T, y, e, p), u(f(e), oW0), u(f(e), cW0), a(f(e), vW0, sW0);
var E = i[3];
if (E) {
g(e, lW0);
var h = E[1], w = function(A, S) {
return g(A, eW0);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, bW0);
} else
g(e, pW0);
return u(f(e), mW0), u(f(e), _W0);
}), N(EJ, function(t, n4, e) {
var i = a(QC, t, n4);
return a(P0(rW0), i, e);
});
var SJ = [0, QC, EJ], rP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, gJ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, g5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, FJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(rP, function(t, n4, e, i) {
u(f(e), kK0), a(f(e), EK0, wK0);
var x = i[1];
if (x) {
g(e, SK0);
var c = x[1], s = function(g0) {
return u(n4, g0);
};
R(g5, function(g0) {
return u(t, g0);
}, s, e, c), g(e, gK0);
} else
g(e, FK0);
u(f(e), TK0), u(f(e), OK0), a(f(e), AK0, IK0);
var p = i[2];
if (p) {
g(e, NK0);
var y = p[1], T = function(g0) {
return u(n4, g0);
}, E = function(g0) {
return u(t, g0);
};
R(qe[31], E, T, e, y), g(e, CK0);
} else
g(e, PK0);
u(f(e), DK0), u(f(e), LK0), a(f(e), jK0, RK0);
var h = i[3];
if (h) {
g(e, GK0);
var w = h[1], G = function(g0) {
return u(n4, g0);
}, A = function(g0) {
return u(t, g0);
};
R(qe[31], A, G, e, w), g(e, MK0);
} else
g(e, BK0);
u(f(e), qK0), u(f(e), UK0), a(f(e), XK0, HK0);
var S = i[4];
function M(g0) {
return u(n4, g0);
}
function K(g0) {
return u(t, g0);
}
R(Xu[35], K, M, e, S), u(f(e), YK0), u(f(e), VK0), a(f(e), KK0, zK0);
var V = i[5];
if (V) {
g(e, WK0);
var f0 = V[1], m0 = function(g0, e0) {
return g(g0, hK0);
}, k0 = function(g0) {
return u(t, g0);
};
R(Dr[1], k0, m0, e, f0), g(e, JK0);
} else
g(e, $K0);
return u(f(e), ZK0), u(f(e), QK0);
}), N(gJ, function(t, n4, e) {
var i = a(rP, t, n4);
return a(P0(dK0), i, e);
}), N(g5, function(t, n4, e, i) {
if (i[0] === 0) {
var x = i[1];
u(f(e), vK0), u(f(e), lK0), a(t, e, x[1]), u(f(e), bK0);
var c = x[2], s = function(h) {
return u(n4, h);
}, p = function(h) {
return u(t, h);
};
return R(Il[2], p, s, e, c), u(f(e), pK0), u(f(e), mK0);
}
u(f(e), _K0);
var y = i[1];
function T(h) {
return u(n4, h);
}
function E(h) {
return u(t, h);
}
return R(qe[31], E, T, e, y), u(f(e), yK0);
}), N(FJ, function(t, n4, e) {
var i = a(g5, t, n4);
return a(P0(sK0), i, e);
});
var TJ = [0, rP, gJ, g5, FJ], eP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, OJ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, F5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, IJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(eP, function(t, n4, e, i) {
u(f(e), Bz0), a(f(e), Uz0, qz0);
var x = i[1];
function c(K) {
return u(n4, K);
}
R(F5, function(K) {
return u(t, K);
}, c, e, x), u(f(e), Hz0), u(f(e), Xz0), a(f(e), Vz0, Yz0);
var s = i[2];
function p(K) {
return u(n4, K);
}
function y(K) {
return u(t, K);
}
R(qe[31], y, p, e, s), u(f(e), zz0), u(f(e), Kz0), a(f(e), Jz0, Wz0);
var T = i[3];
function E(K) {
return u(n4, K);
}
function h(K) {
return u(t, K);
}
R(Xu[35], h, E, e, T), u(f(e), $z0), u(f(e), Zz0), a(f(e), rK0, Qz0);
var w = i[4];
a(f(e), eK0, w), u(f(e), nK0), u(f(e), tK0), a(f(e), iK0, uK0);
var G = i[5];
if (G) {
g(e, fK0);
var A = G[1], S = function(K, V) {
return g(K, Mz0);
}, M = function(K) {
return u(t, K);
};
R(Dr[1], M, S, e, A), g(e, xK0);
} else
g(e, aK0);
return u(f(e), oK0), u(f(e), cK0);
}), N(OJ, function(t, n4, e) {
var i = a(eP, t, n4);
return a(P0(Gz0), i, e);
}), N(F5, function(t, n4, e, i) {
if (i[0] === 0) {
var x = i[1];
u(f(e), Nz0), u(f(e), Cz0), a(t, e, x[1]), u(f(e), Pz0);
var c = x[2], s = function(h) {
return u(n4, h);
}, p = function(h) {
return u(t, h);
};
return R(Il[2], p, s, e, c), u(f(e), Dz0), u(f(e), Lz0);
}
u(f(e), Rz0);
var y = i[1];
function T(h) {
return u(n4, h);
}
function E(h) {
return u(t, h);
}
return R(di[5], E, T, e, y), u(f(e), jz0);
}), N(IJ, function(t, n4, e) {
var i = a(F5, t, n4);
return a(P0(Az0), i, e);
});
var AJ = [0, eP, OJ, F5, IJ], nP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, NJ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, T5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, CJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(nP, function(t, n4, e, i) {
u(f(e), iz0), a(f(e), xz0, fz0);
var x = i[1];
function c(K) {
return u(n4, K);
}
R(T5, function(K) {
return u(t, K);
}, c, e, x), u(f(e), az0), u(f(e), oz0), a(f(e), sz0, cz0);
var s = i[2];
function p(K) {
return u(n4, K);
}
function y(K) {
return u(t, K);
}
R(qe[31], y, p, e, s), u(f(e), vz0), u(f(e), lz0), a(f(e), pz0, bz0);
var T = i[3];
function E(K) {
return u(n4, K);
}
function h(K) {
return u(t, K);
}
R(Xu[35], h, E, e, T), u(f(e), mz0), u(f(e), _z0), a(f(e), dz0, yz0);
var w = i[4];
a(f(e), hz0, w), u(f(e), kz0), u(f(e), wz0), a(f(e), Sz0, Ez0);
var G = i[5];
if (G) {
g(e, gz0);
var A = G[1], S = function(K, V) {
return g(K, uz0);
}, M = function(K) {
return u(t, K);
};
R(Dr[1], M, S, e, A), g(e, Fz0);
} else
g(e, Tz0);
return u(f(e), Oz0), u(f(e), Iz0);
}), N(NJ, function(t, n4, e) {
var i = a(nP, t, n4);
return a(P0(tz0), i, e);
}), N(T5, function(t, n4, e, i) {
if (i[0] === 0) {
var x = i[1];
u(f(e), JV0), u(f(e), $V0), a(t, e, x[1]), u(f(e), ZV0);
var c = x[2], s = function(h) {
return u(n4, h);
}, p = function(h) {
return u(t, h);
};
return R(Il[2], p, s, e, c), u(f(e), QV0), u(f(e), rz0);
}
u(f(e), ez0);
var y = i[1];
function T(h) {
return u(n4, h);
}
function E(h) {
return u(t, h);
}
return R(di[5], E, T, e, y), u(f(e), nz0);
}), N(CJ, function(t, n4, e) {
var i = a(T5, t, n4);
return a(P0(WV0), i, e);
});
var PJ = [0, nP, NJ, T5, CJ], tP = function t(n4, e, i) {
return t.fun(n4, e, i);
}, DJ = function t(n4, e) {
return t.fun(n4, e);
}, O5 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, LJ = function t(n4, e) {
return t.fun(n4, e);
};
N(tP, function(t, n4, e) {
u(f(n4), VV0), a(t, n4, e[1]), u(f(n4), zV0);
var i = e[2];
return ir(O5, function(x) {
return u(t, x);
}, n4, i), u(f(n4), KV0);
}), N(DJ, function(t, n4) {
var e = u(tP, t);
return a(P0(YV0), e, n4);
}), N(O5, function(t, n4, e) {
u(f(n4), BV0), a(f(n4), UV0, qV0);
var i = e[1];
function x(s) {
return u(t, s);
}
function c(s) {
return u(t, s);
}
return R(Ln[1], c, x, n4, i), u(f(n4), HV0), u(f(n4), XV0);
}), N(LJ, function(t, n4) {
var e = u(O5, t);
return a(P0(MV0), e, n4);
});
var uP = [0, tP, DJ, O5, LJ], iP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, RJ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, I5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, jJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(iP, function(t, n4, e, i) {
u(f(e), RV0), a(n4, e, i[1]), u(f(e), jV0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(I5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), GV0);
}), N(RJ, function(t, n4, e) {
var i = a(iP, t, n4);
return a(P0(LV0), i, e);
}), N(I5, function(t, n4, e, i) {
u(f(e), EV0), a(f(e), gV0, SV0);
var x = i[1];
function c(y) {
return u(n4, y);
}
function s(y) {
return u(n4, y);
}
R(Ln[1], s, c, e, x), u(f(e), FV0), u(f(e), TV0), a(f(e), IV0, OV0);
var p = i[2];
return u(f(e), AV0), a(n4, e, p[1]), u(f(e), NV0), a(t, e, p[2]), u(f(e), CV0), u(f(e), PV0), u(f(e), DV0);
}), N(jJ, function(t, n4, e) {
var i = a(I5, t, n4);
return a(P0(wV0), i, e);
});
var A5 = [0, iP, RJ, I5, jJ], fP = function t(n4, e, i) {
return t.fun(n4, e, i);
}, GJ = function t(n4, e) {
return t.fun(n4, e);
};
N(fP, function(t, n4, e) {
u(f(n4), ZY0), a(f(n4), rV0, QY0);
var i = e[1];
u(f(n4), eV0);
var x = 0;
be(function(h, w) {
h && u(f(n4), $Y0);
function G(S) {
return u(t, S);
}
function A(S) {
function M(K) {
return u(t, K);
}
return a(XN[1], M, S);
}
return R(A5[1], A, G, n4, w), 1;
}, x, i), u(f(n4), nV0), u(f(n4), tV0), u(f(n4), uV0), a(f(n4), fV0, iV0);
var c = e[2];
a(f(n4), xV0, c), u(f(n4), aV0), u(f(n4), oV0), a(f(n4), sV0, cV0);
var s = e[3];
a(f(n4), vV0, s), u(f(n4), lV0), u(f(n4), bV0), a(f(n4), mV0, pV0);
var p = e[4];
if (p) {
g(n4, _V0);
var y = p[1], T = function(h, w) {
u(f(h), WY0);
var G = 0;
return be(function(A, S) {
A && u(f(h), KY0);
function M(K) {
return u(t, K);
}
return ir(uu[1], M, h, S), 1;
}, G, w), u(f(h), JY0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, n4, y), g(n4, yV0);
} else
g(n4, dV0);
return u(f(n4), hV0), u(f(n4), kV0);
}), N(GJ, function(t, n4) {
var e = u(fP, t);
return a(P0(zY0), e, n4);
});
var MJ = [0, fP, GJ], xP = function t(n4, e, i) {
return t.fun(n4, e, i);
}, BJ = function t(n4, e) {
return t.fun(n4, e);
};
N(xP, function(t, n4, e) {
u(f(n4), EY0), a(f(n4), gY0, SY0);
var i = e[1];
u(f(n4), FY0);
var x = 0;
be(function(h, w) {
h && u(f(n4), wY0);
function G(S) {
return u(t, S);
}
function A(S) {
function M(K) {
return u(t, K);
}
return a(HN[1], M, S);
}
return R(A5[1], A, G, n4, w), 1;
}, x, i), u(f(n4), TY0), u(f(n4), OY0), u(f(n4), IY0), a(f(n4), NY0, AY0);
var c = e[2];
a(f(n4), CY0, c), u(f(n4), PY0), u(f(n4), DY0), a(f(n4), RY0, LY0);
var s = e[3];
a(f(n4), jY0, s), u(f(n4), GY0), u(f(n4), MY0), a(f(n4), qY0, BY0);
var p = e[4];
if (p) {
g(n4, UY0);
var y = p[1], T = function(h, w) {
u(f(h), hY0);
var G = 0;
return be(function(A, S) {
A && u(f(h), dY0);
function M(K) {
return u(t, K);
}
return ir(uu[1], M, h, S), 1;
}, G, w), u(f(h), kY0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, n4, y), g(n4, HY0);
} else
g(n4, XY0);
return u(f(n4), YY0), u(f(n4), VY0);
}), N(BJ, function(t, n4) {
var e = u(xP, t);
return a(P0(yY0), e, n4);
});
var qJ = [0, xP, BJ], aP = function t(n4, e, i) {
return t.fun(n4, e, i);
}, UJ = function t(n4, e) {
return t.fun(n4, e);
}, N5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, HJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(aP, function(t, n4, e) {
u(f(n4), JX0), a(f(n4), ZX0, $X0);
var i = e[1];
function x(h) {
return u(t, h);
}
R(N5, function(h) {
function w(G) {
return u(t, G);
}
return a(F1[1], w, h);
}, x, n4, i), u(f(n4), QX0), u(f(n4), rY0), a(f(n4), nY0, eY0);
var c = e[2];
a(f(n4), tY0, c), u(f(n4), uY0), u(f(n4), iY0), a(f(n4), xY0, fY0);
var s = e[3];
a(f(n4), aY0, s), u(f(n4), oY0), u(f(n4), cY0), a(f(n4), vY0, sY0);
var p = e[4];
if (p) {
g(n4, lY0);
var y = p[1], T = function(h, w) {
u(f(h), KX0);
var G = 0;
return be(function(A, S) {
A && u(f(h), zX0);
function M(K) {
return u(t, K);
}
return ir(uu[1], M, h, S), 1;
}, G, w), u(f(h), WX0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, n4, y), g(n4, bY0);
} else
g(n4, pY0);
return u(f(n4), mY0), u(f(n4), _Y0);
}), N(UJ, function(t, n4) {
var e = u(aP, t);
return a(P0(VX0), e, n4);
}), N(N5, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), GX0), u(f(e), MX0);
var x = i[1], c = 0;
return be(function(y, T) {
y && u(f(e), jX0);
function E(h) {
return u(n4, h);
}
return ir(uP[1], E, e, T), 1;
}, c, x), u(f(e), BX0), u(f(e), qX0);
}
u(f(e), UX0), u(f(e), HX0);
var s = i[1], p = 0;
return be(function(y, T) {
y && u(f(e), RX0);
function E(w) {
return u(n4, w);
}
function h(w) {
return u(t, w);
}
return R(A5[1], h, E, e, T), 1;
}, p, s), u(f(e), XX0), u(f(e), YX0);
}), N(HJ, function(t, n4, e) {
var i = a(N5, t, n4);
return a(P0(LX0), i, e);
});
var XJ = [0, aP, UJ, N5, HJ], oP = function t(n4, e, i) {
return t.fun(n4, e, i);
}, YJ = function t(n4, e) {
return t.fun(n4, e);
};
N(oP, function(t, n4, e) {
u(f(n4), mX0), a(f(n4), yX0, _X0);
var i = e[1];
u(f(n4), dX0);
var x = 0;
be(function(E, h) {
E && u(f(n4), pX0);
function w(G) {
return u(t, G);
}
return ir(uP[1], w, n4, h), 1;
}, x, i), u(f(n4), hX0), u(f(n4), kX0), u(f(n4), wX0), a(f(n4), SX0, EX0);
var c = e[2];
a(f(n4), gX0, c), u(f(n4), FX0), u(f(n4), TX0), a(f(n4), IX0, OX0);
var s = e[3];
if (s) {
g(n4, AX0);
var p = s[1], y = function(E, h) {
u(f(E), lX0);
var w = 0;
return be(function(G, A) {
G && u(f(E), vX0);
function S(M) {
return u(t, M);
}
return ir(uu[1], S, E, A), 1;
}, w, h), u(f(E), bX0);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, n4, p), g(n4, NX0);
} else
g(n4, CX0);
return u(f(n4), PX0), u(f(n4), DX0);
}), N(YJ, function(t, n4) {
var e = u(oP, t);
return a(P0(sX0), e, n4);
});
var VJ = [0, oP, YJ], cP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, zJ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, C5 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, KJ = function t(n4, e) {
return t.fun(n4, e);
}, P5 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, WJ = function t(n4, e) {
return t.fun(n4, e);
};
N(cP, function(t, n4, e, i) {
u(f(e), WH0), a(f(e), $H0, JH0);
var x = i[1];
function c(w) {
return u(n4, w);
}
function s(w) {
return u(t, w);
}
R(Ln[1], s, c, e, x), u(f(e), ZH0), u(f(e), QH0), a(f(e), eX0, rX0);
var p = i[2];
ir(C5, function(w) {
return u(t, w);
}, e, p), u(f(e), nX0), u(f(e), tX0), a(f(e), iX0, uX0);
var y = i[3];
if (y) {
g(e, fX0);
var T = y[1], E = function(w, G) {
return g(w, KH0);
}, h = function(w) {
return u(t, w);
};
R(Dr[1], h, E, e, T), g(e, xX0);
} else
g(e, aX0);
return u(f(e), oX0), u(f(e), cX0);
}), N(zJ, function(t, n4, e) {
var i = a(cP, t, n4);
return a(P0(zH0), i, e);
}), N(C5, function(t, n4, e) {
u(f(n4), XH0), a(t, n4, e[1]), u(f(n4), YH0);
var i = e[2];
return ir(P5, function(x) {
return u(t, x);
}, n4, i), u(f(n4), VH0);
}), N(KJ, function(t, n4) {
var e = u(C5, t);
return a(P0(HH0), e, n4);
}), N(P5, function(t, n4, e) {
switch (e[0]) {
case 0:
u(f(n4), LH0);
var i = e[1], x = function(h) {
return u(t, h);
};
return ir(MJ[1], x, n4, i), u(f(n4), RH0);
case 1:
u(f(n4), jH0);
var c = e[1], s = function(h) {
return u(t, h);
};
return ir(qJ[1], s, n4, c), u(f(n4), GH0);
case 2:
u(f(n4), MH0);
var p = e[1], y = function(h) {
return u(t, h);
};
return ir(XJ[1], y, n4, p), u(f(n4), BH0);
default:
u(f(n4), qH0);
var T = e[1], E = function(h) {
return u(t, h);
};
return ir(VJ[1], E, n4, T), u(f(n4), UH0);
}
}), N(WJ, function(t, n4) {
var e = u(P5, t);
return a(P0(DH0), e, n4);
});
var JJ = [0, uP, A5, MJ, qJ, XJ, VJ, cP, zJ, C5, KJ, P5, WJ], sP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, $J = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(sP, function(t, n4, e, i) {
u(f(e), nH0), a(f(e), uH0, tH0);
var x = i[1];
function c(k0) {
return u(n4, k0);
}
function s(k0) {
return u(t, k0);
}
R(Ln[1], s, c, e, x), u(f(e), iH0), u(f(e), fH0), a(f(e), aH0, xH0);
var p = i[2];
if (p) {
g(e, oH0);
var y = p[1], T = function(k0) {
return u(n4, k0);
}, E = function(k0) {
return u(t, k0);
};
R(Je[22][1], E, T, e, y), g(e, cH0);
} else
g(e, sH0);
u(f(e), vH0), u(f(e), lH0), a(f(e), pH0, bH0);
var h = i[3];
u(f(e), mH0);
var w = 0;
be(function(k0, g0) {
k0 && u(f(e), ZU0), u(f(e), QU0), a(t, e, g0[1]), u(f(e), rH0);
var e0 = g0[2];
function x0(c0) {
return u(n4, c0);
}
function l(c0) {
return u(t, c0);
}
return R(Je[2][2], l, x0, e, e0), u(f(e), eH0), 1;
}, w, h), u(f(e), _H0), u(f(e), yH0), u(f(e), dH0), a(f(e), kH0, hH0);
var G = i[4];
u(f(e), wH0), a(t, e, G[1]), u(f(e), EH0);
var A = G[2];
function S(k0) {
return u(n4, k0);
}
function M(k0) {
return u(t, k0);
}
R(Je[5][6], M, S, e, A), u(f(e), SH0), u(f(e), gH0), u(f(e), FH0), a(f(e), OH0, TH0);
var K = i[5];
if (K) {
g(e, IH0);
var V = K[1], f0 = function(k0, g0) {
return g(k0, $U0);
}, m0 = function(k0) {
return u(t, k0);
};
R(Dr[1], m0, f0, e, V), g(e, AH0);
} else
g(e, NH0);
return u(f(e), CH0), u(f(e), PH0);
}), N($J, function(t, n4, e) {
var i = a(sP, t, n4);
return a(P0(JU0), i, e);
});
var D5 = [0, sP, $J], vP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, ZJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(vP, function(t, n4, e, i) {
u(f(e), eU0), a(f(e), tU0, nU0);
var x = i[1];
function c(_0) {
return u(n4, _0);
}
function s(_0) {
return u(t, _0);
}
R(Ln[1], s, c, e, x), u(f(e), uU0), u(f(e), iU0), a(f(e), xU0, fU0);
var p = i[2];
if (p) {
g(e, aU0);
var y = p[1], T = function(_0) {
return u(n4, _0);
}, E = function(_0) {
return u(t, _0);
};
R(Je[22][1], E, T, e, y), g(e, oU0);
} else
g(e, cU0);
u(f(e), sU0), u(f(e), vU0), a(f(e), bU0, lU0);
var h = i[3];
u(f(e), pU0), a(t, e, h[1]), u(f(e), mU0);
var w = h[2];
function G(_0) {
return u(n4, _0);
}
function A(_0) {
return u(t, _0);
}
R(Je[5][6], A, G, e, w), u(f(e), _U0), u(f(e), yU0), u(f(e), dU0), a(f(e), kU0, hU0);
var S = i[4];
if (S) {
var M = S[1];
g(e, wU0), u(f(e), EU0), a(t, e, M[1]), u(f(e), SU0);
var K = M[2], V = function(_0) {
return u(n4, _0);
}, f0 = function(_0) {
return u(t, _0);
};
R(Je[2][2], f0, V, e, K), u(f(e), gU0), g(e, FU0);
} else
g(e, TU0);
u(f(e), OU0), u(f(e), IU0), a(f(e), NU0, AU0);
var m0 = i[5];
u(f(e), CU0);
var k0 = 0;
be(function(_0, E0) {
_0 && u(f(e), $q0), u(f(e), Zq0), a(t, e, E0[1]), u(f(e), Qq0);
var X0 = E0[2];
function b(X) {
return u(n4, X);
}
function G0(X) {
return u(t, X);
}
return R(Je[2][2], G0, b, e, X0), u(f(e), rU0), 1;
}, k0, m0), u(f(e), PU0), u(f(e), DU0), u(f(e), LU0), a(f(e), jU0, RU0);
var g0 = i[6];
if (g0) {
g(e, GU0);
var e0 = g0[1], x0 = function(_0) {
return u(n4, _0);
}, l = function(_0) {
return u(t, _0);
};
R(T1[5][2], l, x0, e, e0), g(e, MU0);
} else
g(e, BU0);
u(f(e), qU0), u(f(e), UU0), a(f(e), XU0, HU0);
var c0 = i[7];
if (c0) {
g(e, YU0);
var t0 = c0[1], a0 = function(_0, E0) {
return g(_0, Jq0);
}, w0 = function(_0) {
return u(t, _0);
};
R(Dr[1], w0, a0, e, t0), g(e, VU0);
} else
g(e, zU0);
return u(f(e), KU0), u(f(e), WU0);
}), N(ZJ, function(t, n4, e) {
var i = a(vP, t, n4);
return a(P0(Wq0), i, e);
});
var lP = [0, vP, ZJ], bP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, QJ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(bP, function(t, n4, e, i) {
u(f(e), Pq0), a(f(e), Lq0, Dq0);
var x = i[1];
function c(A) {
return u(n4, A);
}
function s(A) {
return u(t, A);
}
R(Ln[1], s, c, e, x), u(f(e), Rq0), u(f(e), jq0), a(f(e), Mq0, Gq0);
var p = i[2];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(Je[17], T, y, e, p), u(f(e), Bq0), u(f(e), qq0), a(f(e), Hq0, Uq0);
var E = i[3];
if (E) {
g(e, Xq0);
var h = E[1], w = function(A, S) {
return g(A, Cq0);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, Yq0);
} else
g(e, Vq0);
return u(f(e), zq0), u(f(e), Kq0);
}), N(QJ, function(t, n4, e) {
var i = a(bP, t, n4);
return a(P0(Nq0), i, e);
});
var pP = [0, bP, QJ], mP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, r$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(mP, function(t, n4, e, i) {
u(f(e), aq0), a(f(e), cq0, oq0);
var x = i[1];
function c(V) {
return u(n4, V);
}
function s(V) {
return u(t, V);
}
R(Ln[1], s, c, e, x), u(f(e), sq0), u(f(e), vq0), a(f(e), bq0, lq0);
var p = i[2];
function y(V) {
return u(n4, V);
}
function T(V) {
return u(t, V);
}
R(Je[17], T, y, e, p), u(f(e), pq0), u(f(e), mq0), a(f(e), yq0, _q0);
var E = i[3];
if (E) {
g(e, dq0);
var h = E[1], w = function(V) {
return u(n4, V);
}, G = function(V) {
return u(t, V);
};
R(Je[24][1], G, w, e, h), g(e, hq0);
} else
g(e, kq0);
u(f(e), wq0), u(f(e), Eq0), a(f(e), gq0, Sq0);
var A = i[4];
if (A) {
g(e, Fq0);
var S = A[1], M = function(V, f0) {
return g(V, xq0);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, Tq0);
} else
g(e, Oq0);
return u(f(e), Iq0), u(f(e), Aq0);
}), N(r$, function(t, n4, e) {
var i = a(mP, t, n4);
return a(P0(fq0), i, e);
});
var _P = [0, mP, r$], L5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, e$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, R5 = function t(n4, e) {
return t.fun(n4, e);
}, n$ = function t(n4) {
return t.fun(n4);
}, yP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, t$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(L5, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), QB0);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(Ln[1], s, c, e, x), u(f(e), rq0);
}
var p = i[1];
u(f(e), eq0), u(f(e), nq0), a(n4, e, p[1]), u(f(e), tq0);
var y = p[2];
function T(E) {
return u(t, E);
}
return ir(F1[1], T, e, y), u(f(e), uq0), u(f(e), iq0);
}), N(e$, function(t, n4, e) {
var i = a(L5, t, n4);
return a(P0(ZB0), i, e);
}), N(R5, function(t, n4) {
return n4 ? g(t, JB0) : g(t, $B0);
}), N(n$, function(t) {
return a(P0(WB0), R5, t);
}), N(yP, function(t, n4, e, i) {
u(f(e), FB0), a(f(e), OB0, TB0);
var x = i[1];
function c(A) {
return u(n4, A);
}
R(L5, function(A) {
return u(t, A);
}, c, e, x), u(f(e), IB0), u(f(e), AB0), a(f(e), CB0, NB0);
var s = i[2];
u(f(e), PB0), a(t, e, s[1]), u(f(e), DB0);
var p = s[2];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(Kv[1], T, y, e, p), u(f(e), LB0), u(f(e), RB0), u(f(e), jB0), a(f(e), MB0, GB0), a(R5, e, i[3]), u(f(e), BB0), u(f(e), qB0), a(f(e), HB0, UB0);
var E = i[4];
if (E) {
g(e, XB0);
var h = E[1], w = function(A, S) {
return g(A, gB0);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, YB0);
} else
g(e, VB0);
return u(f(e), zB0), u(f(e), KB0);
}), N(t$, function(t, n4, e) {
var i = a(yP, t, n4);
return a(P0(SB0), i, e);
});
var u$ = [0, L5, e$, R5, n$, yP, t$], dP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, i$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(dP, function(t, n4, e, i) {
u(f(e), vB0), a(f(e), bB0, lB0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(Je[17], s, c, e, x), u(f(e), pB0), u(f(e), mB0), a(f(e), yB0, _B0);
var p = i[2];
if (p) {
g(e, dB0);
var y = p[1], T = function(h, w) {
return g(h, sB0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, hB0);
} else
g(e, kB0);
return u(f(e), wB0), u(f(e), EB0);
}), N(i$, function(t, n4, e) {
var i = a(dP, t, n4);
return a(P0(cB0), i, e);
});
var f$ = [0, dP, i$], hP = function t(n4, e, i) {
return t.fun(n4, e, i);
}, x$ = function t(n4, e) {
return t.fun(n4, e);
}, j5 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, a$ = function t(n4, e) {
return t.fun(n4, e);
};
N(hP, function(t, n4, e) {
u(f(n4), xB0), a(t, n4, e[1]), u(f(n4), aB0);
var i = e[2];
return ir(j5, function(x) {
return u(t, x);
}, n4, i), u(f(n4), oB0);
}), N(x$, function(t, n4) {
var e = u(hP, t);
return a(P0(fB0), e, n4);
}), N(j5, function(t, n4, e) {
u(f(n4), KM0), a(f(n4), JM0, WM0);
var i = e[1];
function x(E) {
return u(t, E);
}
function c(E) {
return u(t, E);
}
R(Ln[1], c, x, n4, i), u(f(n4), $M0), u(f(n4), ZM0), a(f(n4), rB0, QM0);
var s = e[2];
if (s) {
g(n4, eB0);
var p = s[1], y = function(E) {
return u(t, E);
}, T = function(E) {
return u(t, E);
};
R(Ln[1], T, y, n4, p), g(n4, nB0);
} else
g(n4, tB0);
return u(f(n4), uB0), u(f(n4), iB0);
}), N(a$, function(t, n4) {
var e = u(j5, t);
return a(P0(zM0), e, n4);
});
var o$ = [0, hP, x$, j5, a$], kP = function t(n4, e, i) {
return t.fun(n4, e, i);
}, c$ = function t(n4, e) {
return t.fun(n4, e);
};
N(kP, function(t, n4, e) {
var i = e[2];
if (u(f(n4), qM0), a(t, n4, e[1]), u(f(n4), UM0), i) {
g(n4, HM0);
var x = i[1], c = function(p) {
return u(t, p);
}, s = function(p) {
return u(t, p);
};
R(Ln[1], s, c, n4, x), g(n4, XM0);
} else
g(n4, YM0);
return u(f(n4), VM0);
}), N(c$, function(t, n4) {
var e = u(kP, t);
return a(P0(BM0), e, n4);
});
var s$ = [0, kP, c$], wP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, v$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, G5 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, l$ = function t(n4, e) {
return t.fun(n4, e);
};
N(wP, function(t, n4, e, i) {
u(f(e), uM0), a(f(e), fM0, iM0);
var x = i[1];
if (x) {
g(e, xM0);
var c = x[1], s = function(V) {
return u(n4, V);
}, p = function(V) {
return u(t, V);
};
R(Xu[35], p, s, e, c), g(e, aM0);
} else
g(e, oM0);
u(f(e), cM0), u(f(e), sM0), a(f(e), lM0, vM0);
var y = i[2];
if (y) {
g(e, bM0);
var T = y[1];
ir(G5, function(V) {
return u(t, V);
}, e, T), g(e, pM0);
} else
g(e, mM0);
u(f(e), _M0), u(f(e), yM0), a(f(e), hM0, dM0);
var E = i[3];
if (E) {
var h = E[1];
g(e, kM0), u(f(e), wM0), a(t, e, h[1]), u(f(e), EM0);
var w = h[2], G = function(V) {
return u(t, V);
};
ir(F1[1], G, e, w), u(f(e), SM0), g(e, gM0);
} else
g(e, FM0);
u(f(e), TM0), u(f(e), OM0), a(f(e), AM0, IM0), a(Xu[33], e, i[4]), u(f(e), NM0), u(f(e), CM0), a(f(e), DM0, PM0);
var A = i[5];
if (A) {
g(e, LM0);
var S = A[1], M = function(V, f0) {
return g(V, tM0);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, RM0);
} else
g(e, jM0);
return u(f(e), GM0), u(f(e), MM0);
}), N(v$, function(t, n4, e) {
var i = a(wP, t, n4);
return a(P0(nM0), i, e);
}), N(G5, function(t, n4, e) {
if (e[0] === 0) {
u(f(n4), JG0), u(f(n4), $G0);
var i = e[1], x = 0;
return be(function(p, y) {
p && u(f(n4), WG0);
function T(E) {
return u(t, E);
}
return ir(o$[1], T, n4, y), 1;
}, x, i), u(f(n4), ZG0), u(f(n4), QG0);
}
u(f(n4), rM0);
var c = e[1];
function s(p) {
return u(t, p);
}
return ir(s$[1], s, n4, c), u(f(n4), eM0);
}), N(l$, function(t, n4) {
var e = u(G5, t);
return a(P0(KG0), e, n4);
});
var EP = [0, o$, s$, wP, v$, G5, l$], SP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, b$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, M5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, p$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(SP, function(t, n4, e, i) {
u(f(e), CG0), a(f(e), DG0, PG0), a(t, e, i[1]), u(f(e), LG0), u(f(e), RG0), a(f(e), GG0, jG0);
var x = i[2];
function c(E) {
return u(n4, E);
}
R(M5, function(E) {
return u(t, E);
}, c, e, x), u(f(e), MG0), u(f(e), BG0), a(f(e), UG0, qG0);
var s = i[3];
if (s) {
g(e, HG0);
var p = s[1], y = function(E, h) {
return g(E, NG0);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, XG0);
} else
g(e, YG0);
return u(f(e), VG0), u(f(e), zG0);
}), N(b$, function(t, n4, e) {
var i = a(SP, t, n4);
return a(P0(AG0), i, e);
}), N(M5, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), FG0);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(Xu[35], s, c, e, x), u(f(e), TG0);
}
u(f(e), OG0);
var p = i[1];
function y(E) {
return u(n4, E);
}
function T(E) {
return u(t, E);
}
return R(qe[31], T, y, e, p), u(f(e), IG0);
}), N(p$, function(t, n4, e) {
var i = a(M5, t, n4);
return a(P0(gG0), i, e);
});
var m$ = [0, SP, b$, M5, p$], B5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, _$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, gP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, y$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(B5, function(t, n4, e, i) {
switch (i[0]) {
case 0:
var x = i[1];
u(f(e), zj0), u(f(e), Kj0), a(t, e, x[1]), u(f(e), Wj0);
var c = x[2], s = function(E0) {
return u(n4, E0);
}, p = function(E0) {
return u(t, E0);
};
return R(pP[1], p, s, e, c), u(f(e), Jj0), u(f(e), $j0);
case 1:
var y = i[1];
u(f(e), Zj0), u(f(e), Qj0), a(t, e, y[1]), u(f(e), rG0);
var T = y[2], E = function(E0) {
return u(n4, E0);
}, h = function(E0) {
return u(t, E0);
};
return R(_P[1], h, E, e, T), u(f(e), eG0), u(f(e), nG0);
case 2:
var w = i[1];
u(f(e), tG0), u(f(e), uG0), a(t, e, w[1]), u(f(e), iG0);
var G = w[2], A = function(E0) {
return u(n4, E0);
}, S = function(E0) {
return u(t, E0);
};
return R(lP[1], S, A, e, G), u(f(e), fG0), u(f(e), xG0);
case 3:
u(f(e), aG0);
var M = i[1], K = function(E0) {
return u(n4, E0);
}, V = function(E0) {
return u(t, E0);
};
return R(Je[13], V, K, e, M), u(f(e), oG0);
case 4:
var f0 = i[1];
u(f(e), cG0), u(f(e), sG0), a(t, e, f0[1]), u(f(e), vG0);
var m0 = f0[2], k0 = function(E0) {
return u(n4, E0);
}, g0 = function(E0) {
return u(t, E0);
};
return R(d5[1], g0, k0, e, m0), u(f(e), lG0), u(f(e), bG0);
case 5:
var e0 = i[1];
u(f(e), pG0), u(f(e), mG0), a(t, e, e0[1]), u(f(e), _G0);
var x0 = e0[2], l = function(E0) {
return u(n4, E0);
}, c0 = function(E0) {
return u(t, E0);
};
return R(h5[1], c0, l, e, x0), u(f(e), yG0), u(f(e), dG0);
default:
var t0 = i[1];
u(f(e), hG0), u(f(e), kG0), a(t, e, t0[1]), u(f(e), wG0);
var a0 = t0[2], w0 = function(E0) {
return u(n4, E0);
}, _0 = function(E0) {
return u(t, E0);
};
return R(D5[1], _0, w0, e, a0), u(f(e), EG0), u(f(e), SG0);
}
}), N(_$, function(t, n4, e) {
var i = a(B5, t, n4);
return a(P0(Vj0), i, e);
}), N(gP, function(t, n4, e, i) {
u(f(e), xj0), a(f(e), oj0, aj0);
var x = i[1];
x ? (g(e, cj0), a(t, e, x[1]), g(e, sj0)) : g(e, vj0), u(f(e), lj0), u(f(e), bj0), a(f(e), mj0, pj0);
var c = i[2];
if (c) {
g(e, _j0);
var s = c[1], p = function(f0) {
return u(n4, f0);
};
R(B5, function(f0) {
return u(t, f0);
}, p, e, s), g(e, yj0);
} else
g(e, dj0);
u(f(e), hj0), u(f(e), kj0), a(f(e), Ej0, wj0);
var y = i[3];
if (y) {
g(e, Sj0);
var T = y[1], E = function(f0) {
return u(t, f0);
};
ir(EP[5], E, e, T), g(e, gj0);
} else
g(e, Fj0);
u(f(e), Tj0), u(f(e), Oj0), a(f(e), Aj0, Ij0);
var h = i[4];
if (h) {
var w = h[1];
g(e, Nj0), u(f(e), Cj0), a(t, e, w[1]), u(f(e), Pj0);
var G = w[2], A = function(f0) {
return u(t, f0);
};
ir(F1[1], A, e, G), u(f(e), Dj0), g(e, Lj0);
} else
g(e, Rj0);
u(f(e), jj0), u(f(e), Gj0), a(f(e), Bj0, Mj0);
var S = i[5];
if (S) {
g(e, qj0);
var M = S[1], K = function(f0, m0) {
return g(f0, fj0);
}, V = function(f0) {
return u(t, f0);
};
R(Dr[1], V, K, e, M), g(e, Uj0);
} else
g(e, Hj0);
return u(f(e), Xj0), u(f(e), Yj0);
}), N(y$, function(t, n4, e) {
var i = a(gP, t, n4);
return a(P0(ij0), i, e);
});
var d$ = [0, B5, _$, gP, y$], Al = function t(n4, e) {
return t.fun(n4, e);
}, h$ = function t(n4) {
return t.fun(n4);
}, q5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, k$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, U5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, w$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, FP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, E$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(Al, function(t, n4) {
switch (n4) {
case 0:
return g(t, nj0);
case 1:
return g(t, tj0);
default:
return g(t, uj0);
}
}), N(h$, function(t) {
return a(P0(ej0), Al, t);
}), N(q5, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), VR0), u(f(e), zR0);
var x = i[1], c = 0;
return be(function(E, h) {
E && u(f(e), YR0);
function w(G) {
return u(n4, G);
}
return R(U5, function(G) {
return u(t, G);
}, w, e, h), 1;
}, c, x), u(f(e), KR0), u(f(e), WR0);
}
var s = i[1];
u(f(e), JR0), u(f(e), $R0), a(t, e, s[1]), u(f(e), ZR0);
var p = s[2];
function y(E) {
return u(n4, E);
}
function T(E) {
return u(t, E);
}
return R(Ln[1], T, y, e, p), u(f(e), QR0), u(f(e), rj0);
}), N(k$, function(t, n4, e) {
var i = a(q5, t, n4);
return a(P0(XR0), i, e);
}), N(U5, function(t, n4, e, i) {
u(f(e), gR0), a(f(e), TR0, FR0);
var x = i[1];
x ? (g(e, OR0), a(Al, e, x[1]), g(e, IR0)) : g(e, AR0), u(f(e), NR0), u(f(e), CR0), a(f(e), DR0, PR0);
var c = i[2];
if (c) {
g(e, LR0);
var s = c[1], p = function(w) {
return u(n4, w);
}, y = function(w) {
return u(t, w);
};
R(Ln[1], y, p, e, s), g(e, RR0);
} else
g(e, jR0);
u(f(e), GR0), u(f(e), MR0), a(f(e), qR0, BR0);
var T = i[3];
function E(w) {
return u(n4, w);
}
function h(w) {
return u(t, w);
}
return R(Ln[1], h, E, e, T), u(f(e), UR0), u(f(e), HR0);
}), N(w$, function(t, n4, e) {
var i = a(U5, t, n4);
return a(P0(SR0), i, e);
}), N(FP, function(t, n4, e, i) {
u(f(e), YL0), a(f(e), zL0, VL0), a(Al, e, i[1]), u(f(e), KL0), u(f(e), WL0), a(f(e), $L0, JL0);
var x = i[2];
u(f(e), ZL0), a(t, e, x[1]), u(f(e), QL0);
var c = x[2];
function s(V) {
return u(t, V);
}
ir(F1[1], s, e, c), u(f(e), rR0), u(f(e), eR0), u(f(e), nR0), a(f(e), uR0, tR0);
var p = i[3];
if (p) {
g(e, iR0);
var y = p[1], T = function(V) {
return u(n4, V);
}, E = function(V) {
return u(t, V);
};
R(Ln[1], E, T, e, y), g(e, fR0);
} else
g(e, xR0);
u(f(e), aR0), u(f(e), oR0), a(f(e), sR0, cR0);
var h = i[4];
if (h) {
g(e, vR0);
var w = h[1], G = function(V) {
return u(n4, V);
};
R(q5, function(V) {
return u(t, V);
}, G, e, w), g(e, lR0);
} else
g(e, bR0);
u(f(e), pR0), u(f(e), mR0), a(f(e), yR0, _R0);
var A = i[5];
if (A) {
g(e, dR0);
var S = A[1], M = function(V, f0) {
return g(V, XL0);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, hR0);
} else
g(e, kR0);
return u(f(e), wR0), u(f(e), ER0);
}), N(E$, function(t, n4, e) {
var i = a(FP, t, n4);
return a(P0(HL0), i, e);
});
var S$ = [0, Al, h$, q5, k$, U5, w$, FP, E$], TP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, g$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(TP, function(t, n4, e, i) {
u(f(e), EL0), a(f(e), gL0, SL0);
var x = i[1];
function c(G) {
return u(n4, G);
}
function s(G) {
return u(t, G);
}
R(qe[31], s, c, e, x), u(f(e), FL0), u(f(e), TL0), a(f(e), IL0, OL0);
var p = i[2];
if (p) {
g(e, AL0);
var y = p[1];
a(f(e), NL0, y), g(e, CL0);
} else
g(e, PL0);
u(f(e), DL0), u(f(e), LL0), a(f(e), jL0, RL0);
var T = i[3];
if (T) {
g(e, GL0);
var E = T[1], h = function(G, A) {
return g(G, wL0);
}, w = function(G) {
return u(t, G);
};
R(Dr[1], w, h, e, E), g(e, ML0);
} else
g(e, BL0);
return u(f(e), qL0), u(f(e), UL0);
}), N(g$, function(t, n4, e) {
var i = a(TP, t, n4);
return a(P0(kL0), i, e);
});
var F$ = [0, TP, g$], OP = function t(n4, e, i) {
return t.fun(n4, e, i);
}, T$ = function t(n4, e) {
return t.fun(n4, e);
};
N(OP, function(t, n4, e) {
u(f(n4), lL0), a(f(n4), pL0, bL0);
var i = e[1];
if (i) {
g(n4, mL0);
var x = i[1], c = function(p, y) {
return g(p, vL0);
}, s = function(p) {
return u(t, p);
};
R(Dr[1], s, c, n4, x), g(n4, _L0);
} else
g(n4, yL0);
return u(f(n4), dL0), u(f(n4), hL0);
}), N(T$, function(t, n4) {
var e = u(OP, t);
return a(P0(sL0), e, n4);
});
var O$ = [0, OP, T$], IP = function t(n4, e) {
return t.fun(n4, e);
}, I$ = function t(n4) {
return t.fun(n4);
}, AP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, A$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, H5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, N$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(IP, function(t, n4) {
return n4 ? g(t, oL0) : g(t, cL0);
}), N(I$, function(t) {
return a(P0(aL0), IP, t);
}), N(AP, function(t, n4, e, i) {
u(f(e), iL0), a(t, e, i[1]), u(f(e), fL0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(H5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), xL0);
}), N(A$, function(t, n4, e) {
var i = a(AP, t, n4);
return a(P0(uL0), i, e);
}), N(H5, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), GP0);
var x = i[1], c = function(d0) {
return u(n4, d0);
}, s = function(d0) {
return u(t, d0);
};
return R(Kv[1], s, c, e, x), u(f(e), MP0);
case 1:
u(f(e), BP0);
var p = i[1], y = function(d0) {
return u(t, d0);
};
return ir(zW[1], y, e, p), u(f(e), qP0);
case 2:
u(f(e), UP0);
var T = i[1], E = function(d0) {
return u(n4, d0);
}, h = function(d0) {
return u(t, d0);
};
return R(T1[8], h, E, e, T), u(f(e), HP0);
case 3:
u(f(e), XP0);
var w = i[1], G = function(d0) {
return u(t, d0);
};
return ir(WW[1], G, e, w), u(f(e), YP0);
case 4:
u(f(e), VP0);
var A = i[1], S = function(d0) {
return u(t, d0);
};
return ir($W[1], S, e, A), u(f(e), zP0);
case 5:
u(f(e), KP0);
var M = i[1], K = function(d0) {
return u(n4, d0);
}, V = function(d0) {
return u(t, d0);
};
return R(lP[1], V, K, e, M), u(f(e), WP0);
case 6:
u(f(e), JP0);
var f0 = i[1], m0 = function(d0) {
return u(n4, d0);
}, k0 = function(d0) {
return u(t, d0);
};
return R(d$[3], k0, m0, e, f0), u(f(e), $P0);
case 7:
u(f(e), ZP0);
var g0 = i[1], e0 = function(d0) {
return u(n4, d0);
}, x0 = function(d0) {
return u(t, d0);
};
return R(_P[1], x0, e0, e, g0), u(f(e), QP0);
case 8:
u(f(e), rD0);
var l = i[1], c0 = function(d0) {
return u(n4, d0);
}, t0 = function(d0) {
return u(t, d0);
};
return R(D5[1], t0, c0, e, l), u(f(e), eD0);
case 9:
u(f(e), nD0);
var a0 = i[1], w0 = function(d0) {
return u(n4, d0);
}, _0 = function(d0) {
return u(t, d0);
};
return R(u$[5], _0, w0, e, a0), u(f(e), tD0);
case 10:
u(f(e), uD0);
var E0 = i[1], X0 = function(d0) {
return u(n4, d0);
}, b = function(d0) {
return u(t, d0);
};
return R(f$[1], b, X0, e, E0), u(f(e), iD0);
case 11:
u(f(e), fD0);
var G0 = i[1], X = function(d0) {
return u(n4, d0);
}, s0 = function(d0) {
return u(t, d0);
};
return R(d5[1], s0, X, e, G0), u(f(e), xD0);
case 12:
u(f(e), aD0);
var dr = i[1], Ar = function(d0) {
return u(n4, d0);
}, ar = function(d0) {
return u(t, d0);
};
return R(h5[1], ar, Ar, e, dr), u(f(e), oD0);
case 13:
u(f(e), cD0);
var W0 = i[1], Lr = function(d0) {
return u(n4, d0);
}, Tr = function(d0) {
return u(t, d0);
};
return R(pP[1], Tr, Lr, e, W0), u(f(e), sD0);
case 14:
u(f(e), vD0);
var Hr = i[1], Or = function(d0) {
return u(n4, d0);
}, xr = function(d0) {
return u(t, d0);
};
return R(SJ[1], xr, Or, e, Hr), u(f(e), lD0);
case 15:
u(f(e), bD0);
var Rr = i[1], Wr = function(d0) {
return u(t, d0);
};
return ir(O$[1], Wr, e, Rr), u(f(e), pD0);
case 16:
u(f(e), mD0);
var Jr = i[1], or = function(d0) {
return u(n4, d0);
}, _r = function(d0) {
return u(t, d0);
};
return R(JJ[7], _r, or, e, Jr), u(f(e), _D0);
case 17:
u(f(e), yD0);
var Ir = i[1], fe = function(d0) {
return u(n4, d0);
}, v0 = function(d0) {
return u(t, d0);
};
return R(m$[1], v0, fe, e, Ir), u(f(e), dD0);
case 18:
u(f(e), hD0);
var P = i[1], L = function(d0) {
return u(n4, d0);
}, Q = function(d0) {
return u(t, d0);
};
return R(EP[3], Q, L, e, P), u(f(e), kD0);
case 19:
u(f(e), wD0);
var i0 = i[1], l0 = function(d0) {
return u(n4, d0);
}, S0 = function(d0) {
return u(t, d0);
};
return R(F$[1], S0, l0, e, i0), u(f(e), ED0);
case 20:
u(f(e), SD0);
var T0 = i[1], rr = function(d0) {
return u(n4, d0);
}, R0 = function(d0) {
return u(t, d0);
};
return R(TJ[1], R0, rr, e, T0), u(f(e), gD0);
case 21:
u(f(e), FD0);
var B = i[1], Z = function(d0) {
return u(n4, d0);
}, p0 = function(d0) {
return u(t, d0);
};
return R(AJ[1], p0, Z, e, B), u(f(e), TD0);
case 22:
u(f(e), OD0);
var b0 = i[1], O0 = function(d0) {
return u(n4, d0);
}, q0 = function(d0) {
return u(t, d0);
};
return R(PJ[1], q0, O0, e, b0), u(f(e), ID0);
case 23:
u(f(e), AD0);
var er = i[1], yr = function(d0) {
return u(n4, d0);
}, vr = function(d0) {
return u(t, d0);
};
return R(Ps[5], vr, yr, e, er), u(f(e), ND0);
case 24:
u(f(e), CD0);
var $0 = i[1], Sr = function(d0) {
return u(n4, d0);
}, Mr = function(d0) {
return u(t, d0);
};
return R(HW[2], Mr, Sr, e, $0), u(f(e), PD0);
case 25:
u(f(e), DD0);
var Br = i[1], qr = function(d0) {
return u(n4, d0);
}, jr = function(d0) {
return u(t, d0);
};
return R(S$[7], jr, qr, e, Br), u(f(e), LD0);
case 26:
u(f(e), RD0);
var $r = i[1], ne = function(d0) {
return u(n4, d0);
}, Qr = function(d0) {
return u(t, d0);
};
return R(D5[1], Qr, ne, e, $r), u(f(e), jD0);
case 27:
u(f(e), GD0);
var pe = i[1], oe = function(d0) {
return u(n4, d0);
}, me = function(d0) {
return u(t, d0);
};
return R(YW[1], me, oe, e, pe), u(f(e), MD0);
case 28:
u(f(e), BD0);
var ae = i[1], ce = function(d0) {
return u(n4, d0);
}, ge = function(d0) {
return u(t, d0);
};
return R(aJ[1], ge, ce, e, ae), u(f(e), qD0);
case 29:
u(f(e), UD0);
var H0 = i[1], Fr = function(d0) {
return u(n4, d0);
}, _ = function(d0) {
return u(t, d0);
};
return R(fJ[2], _, Fr, e, H0), u(f(e), HD0);
case 30:
u(f(e), XD0);
var k = i[1], I = function(d0) {
return u(n4, d0);
}, U = function(d0) {
return u(t, d0);
};
return R(cJ[1], U, I, e, k), u(f(e), YD0);
case 31:
u(f(e), VD0);
var Y = i[1], y0 = function(d0) {
return u(n4, d0);
}, D0 = function(d0) {
return u(t, d0);
};
return R(pJ[2], D0, y0, e, Y), u(f(e), zD0);
case 32:
u(f(e), KD0);
var I0 = i[1], D = function(d0) {
return u(n4, d0);
}, u0 = function(d0) {
return u(t, d0);
};
return R(d5[1], u0, D, e, I0), u(f(e), WD0);
case 33:
u(f(e), JD0);
var Y0 = i[1], J0 = function(d0) {
return u(n4, d0);
}, fr = function(d0) {
return u(t, d0);
};
return R(h5[1], fr, J0, e, Y0), u(f(e), $D0);
case 34:
u(f(e), ZD0);
var Q0 = i[1], F0 = function(d0) {
return u(n4, d0);
}, gr = function(d0) {
return u(t, d0);
};
return R(Il[2], gr, F0, e, Q0), u(f(e), QD0);
case 35:
u(f(e), rL0);
var mr = i[1], Cr = function(d0) {
return u(n4, d0);
}, sr = function(d0) {
return u(t, d0);
};
return R(wJ[1], sr, Cr, e, mr), u(f(e), eL0);
default:
u(f(e), nL0);
var Pr = i[1], K0 = function(d0) {
return u(n4, d0);
}, Ur = function(d0) {
return u(t, d0);
};
return R(QW[1], Ur, K0, e, Pr), u(f(e), tL0);
}
}), N(N$, function(t, n4, e) {
var i = a(H5, t, n4);
return a(P0(jP0), i, e);
}), pu(c6r, Xu, [0, Kv, HW, YW, zW, WW, $W, QW, d5, h5, fJ, aJ, cJ, pJ, Il, wJ, SJ, TJ, AJ, PJ, JJ, D5, lP, pP, _P, u$, f$, EP, m$, d$, S$, F$, O$, IP, I$, AP, A$, H5, N$]);
var NP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, C$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, X5 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, P$ = function t(n4, e) {
return t.fun(n4, e);
};
N(NP, function(t, n4, e, i) {
u(f(e), DP0), a(n4, e, i[1]), u(f(e), LP0);
var x = i[2];
return ir(X5, function(c) {
return u(t, c);
}, e, x), u(f(e), RP0);
}), N(C$, function(t, n4, e) {
var i = a(NP, t, n4);
return a(P0(PP0), i, e);
}), N(X5, function(t, n4, e) {
u(f(n4), gP0), a(f(n4), TP0, FP0);
var i = e[1];
if (i) {
g(n4, OP0);
var x = i[1], c = function(p, y) {
return g(p, SP0);
}, s = function(p) {
return u(t, p);
};
R(Dr[1], s, c, n4, x), g(n4, IP0);
} else
g(n4, AP0);
return u(f(n4), NP0), u(f(n4), CP0);
}), N(P$, function(t, n4) {
var e = u(X5, t);
return a(P0(EP0), e, n4);
});
var D$ = [0, NP, C$, X5, P$], CP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, L$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(CP, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), dP0);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(Je[13], s, c, e, x), u(f(e), hP0);
}
u(f(e), kP0);
var p = i[1];
function y(E) {
return u(n4, E);
}
function T(E) {
return u(t, E);
}
return R(D$[1], T, y, e, p), u(f(e), wP0);
}), N(L$, function(t, n4, e) {
var i = a(CP, t, n4);
return a(P0(yP0), i, e);
});
var R$ = [0, D$, CP, L$], PP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, j$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Y5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, G$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(PP, function(t, n4, e, i) {
u(f(e), pP0), a(t, e, i[1]), u(f(e), mP0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Y5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), _P0);
}), N(j$, function(t, n4, e) {
var i = a(PP, t, n4);
return a(P0(bP0), i, e);
}), N(Y5, function(t, n4, e, i) {
u(f(e), rP0), a(f(e), nP0, eP0);
var x = i[1];
u(f(e), tP0);
var c = 0;
be(function(E, h) {
E && u(f(e), QC0);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(R$[2], G, w, e, h), 1;
}, c, x), u(f(e), uP0), u(f(e), iP0), u(f(e), fP0), a(f(e), aP0, xP0);
var s = i[2];
if (s) {
g(e, oP0);
var p = s[1], y = function(E, h) {
u(f(E), $C0);
var w = 0;
return be(function(G, A) {
G && u(f(E), JC0);
function S(M) {
return u(t, M);
}
return ir(uu[1], S, E, A), 1;
}, w, h), u(f(E), ZC0);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, cP0);
} else
g(e, sP0);
return u(f(e), vP0), u(f(e), lP0);
}), N(G$, function(t, n4, e) {
var i = a(Y5, t, n4);
return a(P0(WC0), i, e);
});
var DP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, M$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, V5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, B$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Mee = [0, PP, j$, Y5, G$];
N(DP, function(t, n4, e, i) {
u(f(e), VC0), a(t, e, i[1]), u(f(e), zC0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(V5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), KC0);
}), N(M$, function(t, n4, e) {
var i = a(DP, t, n4);
return a(P0(YC0), i, e);
}), N(V5, function(t, n4, e, i) {
u(f(e), PC0), a(f(e), LC0, DC0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(qe[31], s, c, e, x), u(f(e), RC0), u(f(e), jC0), a(f(e), MC0, GC0);
var p = i[2];
if (p) {
g(e, BC0);
var y = p[1], T = function(h, w) {
return g(h, CC0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, qC0);
} else
g(e, UC0);
return u(f(e), HC0), u(f(e), XC0);
}), N(B$, function(t, n4, e) {
var i = a(V5, t, n4);
return a(P0(NC0), i, e);
});
var LP = [0, DP, M$, V5, B$], z5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, q$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(z5, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), gC0);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(qe[31], s, c, e, x), u(f(e), FC0);
case 1:
u(f(e), TC0);
var p = i[1], y = function(E) {
return u(n4, E);
}, T = function(E) {
return u(t, E);
};
return R(LP[1], T, y, e, p), u(f(e), OC0);
default:
return u(f(e), IC0), a(t, e, i[1]), u(f(e), AC0);
}
}), N(q$, function(t, n4, e) {
var i = a(z5, t, n4);
return a(P0(SC0), i, e);
});
var RP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, U$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(RP, function(t, n4, e, i) {
u(f(e), cC0), a(f(e), vC0, sC0);
var x = i[1];
u(f(e), lC0);
var c = 0;
be(function(E, h) {
E && u(f(e), oC0);
function w(G) {
return u(n4, G);
}
return R(z5, function(G) {
return u(t, G);
}, w, e, h), 1;
}, c, x), u(f(e), bC0), u(f(e), pC0), u(f(e), mC0), a(f(e), yC0, _C0);
var s = i[2];
if (s) {
g(e, dC0);
var p = s[1], y = function(E, h) {
u(f(E), xC0);
var w = 0;
return be(function(G, A) {
G && u(f(E), fC0);
function S(M) {
return u(t, M);
}
return ir(uu[1], S, E, A), 1;
}, w, h), u(f(E), aC0);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, hC0);
} else
g(e, kC0);
return u(f(e), wC0), u(f(e), EC0);
}), N(U$, function(t, n4, e) {
var i = a(RP, t, n4);
return a(P0(iC0), i, e);
});
var H$ = [0, z5, q$, RP, U$], K5 = function t(n4, e) {
return t.fun(n4, e);
}, X$ = function t(n4) {
return t.fun(n4);
}, jP = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Y$ = function t(n4, e) {
return t.fun(n4, e);
}, W5 = function t(n4, e) {
return t.fun(n4, e);
}, V$ = function t(n4) {
return t.fun(n4);
};
N(K5, function(t, n4) {
u(f(t), KN0), a(f(t), JN0, WN0);
var e = n4[1];
a(f(t), $N0, e), u(f(t), ZN0), u(f(t), QN0), a(f(t), eC0, rC0);
var i = n4[2];
return a(f(t), nC0, i), u(f(t), tC0), u(f(t), uC0);
}), N(X$, function(t) {
return a(P0(zN0), K5, t);
}), N(jP, function(t, n4, e) {
return u(f(n4), XN0), a(t, n4, e[1]), u(f(n4), YN0), a(W5, n4, e[2]), u(f(n4), VN0);
}), N(Y$, function(t, n4) {
var e = u(jP, t);
return a(P0(HN0), e, n4);
}), N(W5, function(t, n4) {
u(f(t), PN0), a(f(t), LN0, DN0), a(K5, t, n4[1]), u(f(t), RN0), u(f(t), jN0), a(f(t), MN0, GN0);
var e = n4[2];
return a(f(t), BN0, e), u(f(t), qN0), u(f(t), UN0);
}), N(V$, function(t) {
return a(P0(CN0), W5, t);
});
var z$ = [0, K5, X$, jP, Y$, W5, V$], GP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, K$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(GP, function(t, n4, e, i) {
u(f(e), vN0), a(f(e), bN0, lN0);
var x = i[1];
u(f(e), pN0);
var c = 0;
be(function(w, G) {
w && u(f(e), sN0);
function A(S) {
return u(t, S);
}
return ir(z$[3], A, e, G), 1;
}, c, x), u(f(e), mN0), u(f(e), _N0), u(f(e), yN0), a(f(e), hN0, dN0);
var s = i[2];
u(f(e), kN0);
var p = 0;
be(function(w, G) {
w && u(f(e), cN0);
function A(M) {
return u(n4, M);
}
function S(M) {
return u(t, M);
}
return R(qe[31], S, A, e, G), 1;
}, p, s), u(f(e), wN0), u(f(e), EN0), u(f(e), SN0), a(f(e), FN0, gN0);
var y = i[3];
if (y) {
g(e, TN0);
var T = y[1], E = function(w, G) {
return g(w, oN0);
}, h = function(w) {
return u(t, w);
};
R(Dr[1], h, E, e, T), g(e, ON0);
} else
g(e, IN0);
return u(f(e), AN0), u(f(e), NN0);
}), N(K$, function(t, n4, e) {
var i = a(GP, t, n4);
return a(P0(aN0), i, e);
});
var MP = [0, z$, GP, K$], BP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, W$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(BP, function(t, n4, e, i) {
u(f(e), HA0), a(f(e), YA0, XA0);
var x = i[1];
function c(S) {
return u(n4, S);
}
function s(S) {
return u(t, S);
}
R(qe[31], s, c, e, x), u(f(e), VA0), u(f(e), zA0), a(f(e), WA0, KA0);
var p = i[2];
u(f(e), JA0), a(t, e, p[1]), u(f(e), $A0);
var y = p[2];
function T(S) {
return u(n4, S);
}
function E(S) {
return u(t, S);
}
R(MP[2], E, T, e, y), u(f(e), ZA0), u(f(e), QA0), u(f(e), rN0), a(f(e), nN0, eN0);
var h = i[3];
if (h) {
g(e, tN0);
var w = h[1], G = function(S, M) {
return g(S, UA0);
}, A = function(S) {
return u(t, S);
};
R(Dr[1], A, G, e, w), g(e, uN0);
} else
g(e, iN0);
return u(f(e), fN0), u(f(e), xN0);
}), N(W$, function(t, n4, e) {
var i = a(BP, t, n4);
return a(P0(qA0), i, e);
});
var J$ = [0, BP, W$], O1 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, $$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, qP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, Z$ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, J5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, Q$ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(O1, function(t, n4, e, i) {
switch (i[0]) {
case 0:
var x = i[1];
u(f(e), AA0), u(f(e), NA0), a(n4, e, x[1]), u(f(e), CA0);
var c = x[2], s = function(S) {
return u(t, S);
};
return ir(Tl[2], s, e, c), u(f(e), PA0), u(f(e), DA0);
case 1:
u(f(e), LA0);
var p = i[1], y = function(S) {
return u(n4, S);
}, T = function(S) {
return u(t, S);
};
return R(Ln[1], T, y, e, p), u(f(e), RA0);
case 2:
u(f(e), jA0);
var E = i[1], h = function(S) {
return u(t, S);
};
return ir(qp[1], h, e, E), u(f(e), GA0);
default:
u(f(e), MA0);
var w = i[1], G = function(S) {
return u(n4, S);
}, A = function(S) {
return u(t, S);
};
return R(Up[1], A, G, e, w), u(f(e), BA0);
}
}), N($$, function(t, n4, e) {
var i = a(O1, t, n4);
return a(P0(IA0), i, e);
}), N(qP, function(t, n4, e, i) {
u(f(e), FA0), a(t, e, i[1]), u(f(e), TA0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(J5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), OA0);
}), N(Z$, function(t, n4, e) {
var i = a(qP, t, n4);
return a(P0(gA0), i, e);
}), N(J5, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), pI0), a(f(e), _I0, mI0);
var x = i[1], c = function(s0) {
return u(n4, s0);
};
R(O1, function(s0) {
return u(t, s0);
}, c, e, x), u(f(e), yI0), u(f(e), dI0), a(f(e), kI0, hI0);
var s = i[2], p = function(s0) {
return u(n4, s0);
}, y = function(s0) {
return u(t, s0);
};
R(qe[31], y, p, e, s), u(f(e), wI0), u(f(e), EI0), a(f(e), gI0, SI0);
var T = i[3];
return a(f(e), FI0, T), u(f(e), TI0), u(f(e), OI0);
case 1:
var E = i[2];
u(f(e), II0), a(f(e), NI0, AI0);
var h = i[1], w = function(s0) {
return u(n4, s0);
};
R(O1, function(s0) {
return u(t, s0);
}, w, e, h), u(f(e), CI0), u(f(e), PI0), a(f(e), LI0, DI0), u(f(e), RI0), a(t, e, E[1]), u(f(e), jI0);
var G = E[2], A = function(s0) {
return u(n4, s0);
}, S = function(s0) {
return u(t, s0);
};
return R(Ps[5], S, A, e, G), u(f(e), GI0), u(f(e), MI0), u(f(e), BI0);
case 2:
var M = i[3], K = i[2];
u(f(e), qI0), a(f(e), HI0, UI0);
var V = i[1], f0 = function(s0) {
return u(n4, s0);
};
R(O1, function(s0) {
return u(t, s0);
}, f0, e, V), u(f(e), XI0), u(f(e), YI0), a(f(e), zI0, VI0), u(f(e), KI0), a(t, e, K[1]), u(f(e), WI0);
var m0 = K[2], k0 = function(s0) {
return u(n4, s0);
}, g0 = function(s0) {
return u(t, s0);
};
if (R(Ps[5], g0, k0, e, m0), u(f(e), JI0), u(f(e), $I0), u(f(e), ZI0), a(f(e), rA0, QI0), M) {
g(e, eA0);
var e0 = M[1], x0 = function(s0, dr) {
return g(s0, bI0);
}, l = function(s0) {
return u(t, s0);
};
R(Dr[1], l, x0, e, e0), g(e, nA0);
} else
g(e, tA0);
return u(f(e), uA0), u(f(e), iA0);
default:
var c0 = i[3], t0 = i[2];
u(f(e), fA0), a(f(e), aA0, xA0);
var a0 = i[1], w0 = function(s0) {
return u(n4, s0);
};
R(O1, function(s0) {
return u(t, s0);
}, w0, e, a0), u(f(e), oA0), u(f(e), cA0), a(f(e), vA0, sA0), u(f(e), lA0), a(t, e, t0[1]), u(f(e), bA0);
var _0 = t0[2], E0 = function(s0) {
return u(n4, s0);
}, X0 = function(s0) {
return u(t, s0);
};
if (R(Ps[5], X0, E0, e, _0), u(f(e), pA0), u(f(e), mA0), u(f(e), _A0), a(f(e), dA0, yA0), c0) {
g(e, hA0);
var b = c0[1], G0 = function(s0, dr) {
return g(s0, lI0);
}, X = function(s0) {
return u(t, s0);
};
R(Dr[1], X, G0, e, b), g(e, kA0);
} else
g(e, wA0);
return u(f(e), EA0), u(f(e), SA0);
}
}), N(Q$, function(t, n4, e) {
var i = a(J5, t, n4);
return a(P0(vI0), i, e);
});
var rZ = [0, O1, $$, qP, Z$, J5, Q$], UP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, eZ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, $5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, nZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(UP, function(t, n4, e, i) {
u(f(e), oI0), a(t, e, i[1]), u(f(e), cI0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R($5, function(s) {
return u(t, s);
}, c, e, x), u(f(e), sI0);
}), N(eZ, function(t, n4, e) {
var i = a(UP, t, n4);
return a(P0(aI0), i, e);
}), N($5, function(t, n4, e, i) {
u(f(e), JO0), a(f(e), ZO0, $O0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(qe[31], s, c, e, x), u(f(e), QO0), u(f(e), rI0), a(f(e), nI0, eI0);
var p = i[2];
if (p) {
g(e, tI0);
var y = p[1], T = function(h, w) {
return g(h, WO0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, uI0);
} else
g(e, iI0);
return u(f(e), fI0), u(f(e), xI0);
}), N(nZ, function(t, n4, e) {
var i = a($5, t, n4);
return a(P0(KO0), i, e);
});
var tZ = [0, UP, eZ, $5, nZ], Z5 = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, uZ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, HP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, iZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(Z5, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), XO0);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(rZ[3], s, c, e, x), u(f(e), YO0);
}
u(f(e), VO0);
var p = i[1];
function y(E) {
return u(n4, E);
}
function T(E) {
return u(t, E);
}
return R(tZ[1], T, y, e, p), u(f(e), zO0);
}), N(uZ, function(t, n4, e) {
var i = a(Z5, t, n4);
return a(P0(HO0), i, e);
}), N(HP, function(t, n4, e, i) {
u(f(e), IO0), a(f(e), NO0, AO0);
var x = i[1];
u(f(e), CO0);
var c = 0;
be(function(E, h) {
E && u(f(e), OO0);
function w(G) {
return u(n4, G);
}
return R(Z5, function(G) {
return u(t, G);
}, w, e, h), 1;
}, c, x), u(f(e), PO0), u(f(e), DO0), u(f(e), LO0), a(f(e), jO0, RO0);
var s = i[2];
if (s) {
g(e, GO0);
var p = s[1], y = function(E, h) {
u(f(E), FO0);
var w = 0;
return be(function(G, A) {
G && u(f(E), gO0);
function S(M) {
return u(t, M);
}
return ir(uu[1], S, E, A), 1;
}, w, h), u(f(E), TO0);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, MO0);
} else
g(e, BO0);
return u(f(e), qO0), u(f(e), UO0);
}), N(iZ, function(t, n4, e) {
var i = a(HP, t, n4);
return a(P0(SO0), i, e);
});
var fZ = [0, rZ, tZ, Z5, uZ, HP, iZ], XP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, xZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(XP, function(t, n4, e, i) {
u(f(e), cO0), a(f(e), vO0, sO0);
var x = i[1];
u(f(e), lO0);
var c = 0;
be(function(E, h) {
E && u(f(e), oO0);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(qe[31], G, w, e, h), 1;
}, c, x), u(f(e), bO0), u(f(e), pO0), u(f(e), mO0), a(f(e), yO0, _O0);
var s = i[2];
if (s) {
g(e, dO0);
var p = s[1], y = function(E, h) {
return g(E, aO0);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, hO0);
} else
g(e, kO0);
return u(f(e), wO0), u(f(e), EO0);
}), N(xZ, function(t, n4, e) {
var i = a(XP, t, n4);
return a(P0(xO0), i, e);
});
var aZ = [0, XP, xZ], Q5 = function t(n4, e) {
return t.fun(n4, e);
}, oZ = function t(n4) {
return t.fun(n4);
}, YP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, cZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(Q5, function(t, n4) {
switch (n4) {
case 0:
return g(t, QT0);
case 1:
return g(t, rO0);
case 2:
return g(t, eO0);
case 3:
return g(t, nO0);
case 4:
return g(t, tO0);
case 5:
return g(t, uO0);
case 6:
return g(t, iO0);
default:
return g(t, fO0);
}
}), N(oZ, function(t) {
return a(P0(ZT0), Q5, t);
}), N(YP, function(t, n4, e, i) {
u(f(e), RT0), a(f(e), GT0, jT0), a(Q5, e, i[1]), u(f(e), MT0), u(f(e), BT0), a(f(e), UT0, qT0);
var x = i[2];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(qe[31], s, c, e, x), u(f(e), HT0), u(f(e), XT0), a(f(e), VT0, YT0);
var p = i[3];
if (p) {
g(e, zT0);
var y = p[1], T = function(h, w) {
return g(h, LT0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, KT0);
} else
g(e, WT0);
return u(f(e), JT0), u(f(e), $T0);
}), N(cZ, function(t, n4, e) {
var i = a(YP, t, n4);
return a(P0(DT0), i, e);
});
var sZ = [0, Q5, oZ, YP, cZ], rm = function t(n4, e) {
return t.fun(n4, e);
}, vZ = function t(n4) {
return t.fun(n4);
}, VP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, lZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(rm, function(t, n4) {
switch (n4) {
case 0:
return g(t, vT0);
case 1:
return g(t, lT0);
case 2:
return g(t, bT0);
case 3:
return g(t, pT0);
case 4:
return g(t, mT0);
case 5:
return g(t, _T0);
case 6:
return g(t, yT0);
case 7:
return g(t, dT0);
case 8:
return g(t, hT0);
case 9:
return g(t, kT0);
case 10:
return g(t, wT0);
case 11:
return g(t, ET0);
case 12:
return g(t, ST0);
case 13:
return g(t, gT0);
case 14:
return g(t, FT0);
case 15:
return g(t, TT0);
case 16:
return g(t, OT0);
case 17:
return g(t, IT0);
case 18:
return g(t, AT0);
case 19:
return g(t, NT0);
case 20:
return g(t, CT0);
default:
return g(t, PT0);
}
}), N(vZ, function(t) {
return a(P0(sT0), rm, t);
}), N(VP, function(t, n4, e, i) {
u(f(e), YF0), a(f(e), zF0, VF0), a(rm, e, i[1]), u(f(e), KF0), u(f(e), WF0), a(f(e), $F0, JF0);
var x = i[2];
function c(A) {
return u(n4, A);
}
function s(A) {
return u(t, A);
}
R(qe[31], s, c, e, x), u(f(e), ZF0), u(f(e), QF0), a(f(e), eT0, rT0);
var p = i[3];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(qe[31], T, y, e, p), u(f(e), nT0), u(f(e), tT0), a(f(e), iT0, uT0);
var E = i[4];
if (E) {
g(e, fT0);
var h = E[1], w = function(A, S) {
return g(A, XF0);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, xT0);
} else
g(e, aT0);
return u(f(e), oT0), u(f(e), cT0);
}), N(lZ, function(t, n4, e) {
var i = a(VP, t, n4);
return a(P0(HF0), i, e);
});
var bZ = [0, rm, vZ, VP, lZ], em = function t(n4, e) {
return t.fun(n4, e);
}, pZ = function t(n4) {
return t.fun(n4);
}, zP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, mZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(em, function(t, n4) {
switch (n4) {
case 0:
return g(t, OF0);
case 1:
return g(t, IF0);
case 2:
return g(t, AF0);
case 3:
return g(t, NF0);
case 4:
return g(t, CF0);
case 5:
return g(t, PF0);
case 6:
return g(t, DF0);
case 7:
return g(t, LF0);
case 8:
return g(t, RF0);
case 9:
return g(t, jF0);
case 10:
return g(t, GF0);
case 11:
return g(t, MF0);
case 12:
return g(t, BF0);
case 13:
return g(t, qF0);
default:
return g(t, UF0);
}
}), N(pZ, function(t) {
return a(P0(TF0), em, t);
}), N(zP, function(t, n4, e, i) {
u(f(e), uF0), a(f(e), fF0, iF0);
var x = i[1];
x ? (g(e, xF0), a(em, e, x[1]), g(e, aF0)) : g(e, oF0), u(f(e), cF0), u(f(e), sF0), a(f(e), lF0, vF0);
var c = i[2];
function s(S) {
return u(n4, S);
}
function p(S) {
return u(t, S);
}
R(di[5], p, s, e, c), u(f(e), bF0), u(f(e), pF0), a(f(e), _F0, mF0);
var y = i[3];
function T(S) {
return u(n4, S);
}
function E(S) {
return u(t, S);
}
R(qe[31], E, T, e, y), u(f(e), yF0), u(f(e), dF0), a(f(e), kF0, hF0);
var h = i[4];
if (h) {
g(e, wF0);
var w = h[1], G = function(S, M) {
return g(S, tF0);
}, A = function(S) {
return u(t, S);
};
R(Dr[1], A, G, e, w), g(e, EF0);
} else
g(e, SF0);
return u(f(e), gF0), u(f(e), FF0);
}), N(mZ, function(t, n4, e) {
var i = a(zP, t, n4);
return a(P0(nF0), i, e);
});
var _Z = [0, em, pZ, zP, mZ], nm = function t(n4, e) {
return t.fun(n4, e);
}, yZ = function t(n4) {
return t.fun(n4);
}, KP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, dZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(nm, function(t, n4) {
return n4 ? g(t, rF0) : g(t, eF0);
}), N(yZ, function(t) {
return a(P0(Qg0), nm, t);
}), N(KP, function(t, n4, e, i) {
u(f(e), Cg0), a(f(e), Dg0, Pg0), a(nm, e, i[1]), u(f(e), Lg0), u(f(e), Rg0), a(f(e), Gg0, jg0);
var x = i[2];
function c(w) {
return u(n4, w);
}
function s(w) {
return u(t, w);
}
R(qe[31], s, c, e, x), u(f(e), Mg0), u(f(e), Bg0), a(f(e), Ug0, qg0);
var p = i[3];
a(f(e), Hg0, p), u(f(e), Xg0), u(f(e), Yg0), a(f(e), zg0, Vg0);
var y = i[4];
if (y) {
g(e, Kg0);
var T = y[1], E = function(w, G) {
return g(w, Ng0);
}, h = function(w) {
return u(t, w);
};
R(Dr[1], h, E, e, T), g(e, Wg0);
} else
g(e, Jg0);
return u(f(e), $g0), u(f(e), Zg0);
}), N(dZ, function(t, n4, e) {
var i = a(KP, t, n4);
return a(P0(Ag0), i, e);
});
var hZ = [0, nm, yZ, KP, dZ], tm = function t(n4, e) {
return t.fun(n4, e);
}, kZ = function t(n4) {
return t.fun(n4);
}, WP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, wZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(tm, function(t, n4) {
switch (n4) {
case 0:
return g(t, Tg0);
case 1:
return g(t, Og0);
default:
return g(t, Ig0);
}
}), N(kZ, function(t) {
return a(P0(Fg0), tm, t);
}), N(WP, function(t, n4, e, i) {
u(f(e), fg0), a(f(e), ag0, xg0), a(tm, e, i[1]), u(f(e), og0), u(f(e), cg0), a(f(e), vg0, sg0);
var x = i[2];
function c(A) {
return u(n4, A);
}
function s(A) {
return u(t, A);
}
R(qe[31], s, c, e, x), u(f(e), lg0), u(f(e), bg0), a(f(e), mg0, pg0);
var p = i[3];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(qe[31], T, y, e, p), u(f(e), _g0), u(f(e), yg0), a(f(e), hg0, dg0);
var E = i[4];
if (E) {
g(e, kg0);
var h = E[1], w = function(A, S) {
return g(A, ig0);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, wg0);
} else
g(e, Eg0);
return u(f(e), Sg0), u(f(e), gg0);
}), N(wZ, function(t, n4, e) {
var i = a(WP, t, n4);
return a(P0(ug0), i, e);
});
var EZ = [0, tm, kZ, WP, wZ], JP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, SZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(JP, function(t, n4, e, i) {
u(f(e), GS0), a(f(e), BS0, MS0);
var x = i[1];
function c(K) {
return u(n4, K);
}
function s(K) {
return u(t, K);
}
R(qe[31], s, c, e, x), u(f(e), qS0), u(f(e), US0), a(f(e), XS0, HS0);
var p = i[2];
function y(K) {
return u(n4, K);
}
function T(K) {
return u(t, K);
}
R(qe[31], T, y, e, p), u(f(e), YS0), u(f(e), VS0), a(f(e), KS0, zS0);
var E = i[3];
function h(K) {
return u(n4, K);
}
function w(K) {
return u(t, K);
}
R(qe[31], w, h, e, E), u(f(e), WS0), u(f(e), JS0), a(f(e), ZS0, $S0);
var G = i[4];
if (G) {
g(e, QS0);
var A = G[1], S = function(K, V) {
return g(K, jS0);
}, M = function(K) {
return u(t, K);
};
R(Dr[1], M, S, e, A), g(e, rg0);
} else
g(e, eg0);
return u(f(e), ng0), u(f(e), tg0);
}), N(SZ, function(t, n4, e) {
var i = a(JP, t, n4);
return a(P0(RS0), i, e);
});
var gZ = [0, JP, SZ], um = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, FZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(um, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), CS0);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(qe[31], s, c, e, x), u(f(e), PS0);
}
u(f(e), DS0);
var p = i[1];
function y(E) {
return u(n4, E);
}
function T(E) {
return u(t, E);
}
return R(LP[1], T, y, e, p), u(f(e), LS0);
}), N(FZ, function(t, n4, e) {
var i = a(um, t, n4);
return a(P0(NS0), i, e);
});
var $P = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, TZ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, im = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, OZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N($P, function(t, n4, e, i) {
u(f(e), OS0), a(t, e, i[1]), u(f(e), IS0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(im, function(s) {
return u(t, s);
}, c, e, x), u(f(e), AS0);
}), N(TZ, function(t, n4, e) {
var i = a($P, t, n4);
return a(P0(TS0), i, e);
}), N(im, function(t, n4, e, i) {
u(f(e), lS0), a(f(e), pS0, bS0);
var x = i[1];
u(f(e), mS0);
var c = 0;
be(function(E, h) {
E && u(f(e), vS0);
function w(G) {
return u(n4, G);
}
return R(um, function(G) {
return u(t, G);
}, w, e, h), 1;
}, c, x), u(f(e), _S0), u(f(e), yS0), u(f(e), dS0), a(f(e), kS0, hS0);
var s = i[2];
if (s) {
g(e, wS0);
var p = s[1], y = function(E, h) {
u(f(E), cS0);
var w = 0;
return be(function(G, A) {
G && u(f(E), oS0);
function S(M) {
return u(t, M);
}
return ir(uu[1], S, E, A), 1;
}, w, h), u(f(E), sS0);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, ES0);
} else
g(e, SS0);
return u(f(e), gS0), u(f(e), FS0);
}), N(OZ, function(t, n4, e) {
var i = a(im, t, n4);
return a(P0(aS0), i, e);
});
var ZP = [0, $P, TZ, im, OZ], QP = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, IZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(QP, function(t, n4, e, i) {
u(f(e), RE0), a(f(e), GE0, jE0);
var x = i[1];
function c(f0) {
return u(n4, f0);
}
function s(f0) {
return u(t, f0);
}
R(qe[31], s, c, e, x), u(f(e), ME0), u(f(e), BE0), a(f(e), UE0, qE0);
var p = i[2];
if (p) {
g(e, HE0);
var y = p[1], T = function(f0) {
return u(n4, f0);
}, E = function(f0) {
return u(t, f0);
};
R(qe[2][1], E, T, e, y), g(e, XE0);
} else
g(e, YE0);
u(f(e), VE0), u(f(e), zE0), a(f(e), WE0, KE0);
var h = i[3];
if (h) {
g(e, JE0);
var w = h[1], G = function(f0) {
return u(n4, f0);
}, A = function(f0) {
return u(t, f0);
};
R(ZP[1], A, G, e, w), g(e, $E0);
} else
g(e, ZE0);
u(f(e), QE0), u(f(e), rS0), a(f(e), nS0, eS0);
var S = i[4];
if (S) {
g(e, tS0);
var M = S[1], K = function(f0, m0) {
return g(f0, LE0);
}, V = function(f0) {
return u(t, f0);
};
R(Dr[1], V, K, e, M), g(e, uS0);
} else
g(e, iS0);
return u(f(e), fS0), u(f(e), xS0);
}), N(IZ, function(t, n4, e) {
var i = a(QP, t, n4);
return a(P0(DE0), i, e);
});
var AZ = [0, QP, IZ], rD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, NZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(rD, function(t, n4, e, i) {
u(f(e), sE0), a(f(e), lE0, vE0);
var x = i[1];
function c(V) {
return u(n4, V);
}
function s(V) {
return u(t, V);
}
R(qe[31], s, c, e, x), u(f(e), bE0), u(f(e), pE0), a(f(e), _E0, mE0);
var p = i[2];
if (p) {
g(e, yE0);
var y = p[1], T = function(V) {
return u(n4, V);
}, E = function(V) {
return u(t, V);
};
R(qe[2][1], E, T, e, y), g(e, dE0);
} else
g(e, hE0);
u(f(e), kE0), u(f(e), wE0), a(f(e), SE0, EE0);
var h = i[3];
function w(V) {
return u(n4, V);
}
function G(V) {
return u(t, V);
}
R(ZP[1], G, w, e, h), u(f(e), gE0), u(f(e), FE0), a(f(e), OE0, TE0);
var A = i[4];
if (A) {
g(e, IE0);
var S = A[1], M = function(V, f0) {
return g(V, cE0);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, AE0);
} else
g(e, NE0);
return u(f(e), CE0), u(f(e), PE0);
}), N(NZ, function(t, n4, e) {
var i = a(rD, t, n4);
return a(P0(oE0), i, e);
});
var eD = [0, rD, NZ], nD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, CZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(nD, function(t, n4, e, i) {
u(f(e), Ww0), a(f(e), $w0, Jw0);
var x = i[1];
function c(y) {
return u(n4, y);
}
function s(y) {
return u(t, y);
}
R(eD[1], s, c, e, x), u(f(e), Zw0), u(f(e), Qw0), a(f(e), eE0, rE0), a(n4, e, i[2]), u(f(e), nE0), u(f(e), tE0), a(f(e), iE0, uE0);
var p = i[3];
return a(f(e), fE0, p), u(f(e), xE0), u(f(e), aE0);
}), N(CZ, function(t, n4, e) {
var i = a(nD, t, n4);
return a(P0(Kw0), i, e);
});
var PZ = [0, nD, CZ], fm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, DZ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, tD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, LZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(fm, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), Uw0);
var x = i[1], c = function(w) {
return u(n4, w);
}, s = function(w) {
return u(t, w);
};
return R(Ln[1], s, c, e, x), u(f(e), Hw0);
case 1:
u(f(e), Xw0);
var p = i[1], y = function(w) {
return u(t, w);
};
return ir(qp[1], y, e, p), u(f(e), Yw0);
default:
u(f(e), Vw0);
var T = i[1], E = function(w) {
return u(n4, w);
}, h = function(w) {
return u(t, w);
};
return R(qe[31], h, E, e, T), u(f(e), zw0);
}
}), N(DZ, function(t, n4, e) {
var i = a(fm, t, n4);
return a(P0(qw0), i, e);
}), N(tD, function(t, n4, e, i) {
u(f(e), gw0), a(f(e), Tw0, Fw0);
var x = i[1];
function c(G) {
return u(n4, G);
}
function s(G) {
return u(t, G);
}
R(qe[31], s, c, e, x), u(f(e), Ow0), u(f(e), Iw0), a(f(e), Nw0, Aw0);
var p = i[2];
function y(G) {
return u(n4, G);
}
R(fm, function(G) {
return u(t, G);
}, y, e, p), u(f(e), Cw0), u(f(e), Pw0), a(f(e), Lw0, Dw0);
var T = i[3];
if (T) {
g(e, Rw0);
var E = T[1], h = function(G, A) {
return g(G, Sw0);
}, w = function(G) {
return u(t, G);
};
R(Dr[1], w, h, e, E), g(e, jw0);
} else
g(e, Gw0);
return u(f(e), Mw0), u(f(e), Bw0);
}), N(LZ, function(t, n4, e) {
var i = a(tD, t, n4);
return a(P0(Ew0), i, e);
});
var uD = [0, fm, DZ, tD, LZ], iD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, RZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(iD, function(t, n4, e, i) {
u(f(e), ow0), a(f(e), sw0, cw0);
var x = i[1];
function c(y) {
return u(n4, y);
}
function s(y) {
return u(t, y);
}
R(uD[3], s, c, e, x), u(f(e), vw0), u(f(e), lw0), a(f(e), pw0, bw0), a(n4, e, i[2]), u(f(e), mw0), u(f(e), _w0), a(f(e), dw0, yw0);
var p = i[3];
return a(f(e), hw0, p), u(f(e), kw0), u(f(e), ww0);
}), N(RZ, function(t, n4, e) {
var i = a(iD, t, n4);
return a(P0(aw0), i, e);
});
var jZ = [0, iD, RZ], fD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, GZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(fD, function(t, n4, e, i) {
u(f(e), Gk0), a(f(e), Bk0, Mk0);
var x = i[1];
if (x) {
g(e, qk0);
var c = x[1], s = function(G) {
return u(n4, G);
}, p = function(G) {
return u(t, G);
};
R(qe[31], p, s, e, c), g(e, Uk0);
} else
g(e, Hk0);
u(f(e), Xk0), u(f(e), Yk0), a(f(e), zk0, Vk0);
var y = i[2];
if (y) {
g(e, Kk0);
var T = y[1], E = function(G, A) {
return g(G, jk0);
}, h = function(G) {
return u(t, G);
};
R(Dr[1], h, E, e, T), g(e, Wk0);
} else
g(e, Jk0);
u(f(e), $k0), u(f(e), Zk0), a(f(e), rw0, Qk0);
var w = i[3];
return a(f(e), ew0, w), u(f(e), nw0), u(f(e), tw0), a(f(e), iw0, uw0), a(n4, e, i[4]), u(f(e), fw0), u(f(e), xw0);
}), N(GZ, function(t, n4, e) {
var i = a(fD, t, n4);
return a(P0(Rk0), i, e);
});
var MZ = [0, fD, GZ], xD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, BZ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, xm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, qZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(xD, function(t, n4, e, i) {
u(f(e), Pk0), a(t, e, i[1]), u(f(e), Dk0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(xm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Lk0);
}), N(BZ, function(t, n4, e) {
var i = a(xD, t, n4);
return a(P0(Ck0), i, e);
}), N(xm, function(t, n4, e, i) {
u(f(e), yk0), a(f(e), hk0, dk0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(di[5], s, c, e, x), u(f(e), kk0), u(f(e), wk0), a(f(e), Sk0, Ek0);
var p = i[2];
function y(h) {
return u(n4, h);
}
function T(h) {
return u(t, h);
}
R(qe[31], T, y, e, p), u(f(e), gk0), u(f(e), Fk0), a(f(e), Ok0, Tk0);
var E = i[3];
return a(f(e), Ik0, E), u(f(e), Ak0), u(f(e), Nk0);
}), N(qZ, function(t, n4, e) {
var i = a(xm, t, n4);
return a(P0(_k0), i, e);
});
var UZ = [0, xD, BZ, xm, qZ], aD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, HZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(aD, function(t, n4, e, i) {
u(f(e), tk0), a(f(e), ik0, uk0);
var x = i[1];
u(f(e), fk0);
var c = 0;
be(function(E, h) {
E && u(f(e), nk0);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(UZ[1], G, w, e, h), 1;
}, c, x), u(f(e), xk0), u(f(e), ak0), u(f(e), ok0), a(f(e), sk0, ck0);
var s = i[2];
if (s) {
g(e, vk0);
var p = s[1], y = function(E) {
return u(n4, E);
}, T = function(E) {
return u(t, E);
};
R(qe[31], T, y, e, p), g(e, lk0);
} else
g(e, bk0);
return u(f(e), pk0), u(f(e), mk0);
}), N(HZ, function(t, n4, e) {
var i = a(aD, t, n4);
return a(P0(ek0), i, e);
});
var oD = [0, UZ, aD, HZ], cD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, XZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(cD, function(t, n4, e, i) {
u(f(e), qh0), a(f(e), Hh0, Uh0);
var x = i[1];
u(f(e), Xh0);
var c = 0;
be(function(E, h) {
E && u(f(e), Bh0);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(oD[1][1], G, w, e, h), 1;
}, c, x), u(f(e), Yh0), u(f(e), Vh0), u(f(e), zh0), a(f(e), Wh0, Kh0);
var s = i[2];
if (s) {
g(e, Jh0);
var p = s[1], y = function(E) {
return u(n4, E);
}, T = function(E) {
return u(t, E);
};
R(qe[31], T, y, e, p), g(e, $h0);
} else
g(e, Zh0);
return u(f(e), Qh0), u(f(e), rk0);
}), N(XZ, function(t, n4, e) {
var i = a(cD, t, n4);
return a(P0(Mh0), i, e);
});
var YZ = [0, cD, XZ], sD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, VZ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(sD, function(t, n4, e, i) {
u(f(e), Eh0), a(f(e), gh0, Sh0);
var x = i[1];
function c(A) {
return u(n4, A);
}
function s(A) {
return u(t, A);
}
R(qe[31], s, c, e, x), u(f(e), Fh0), u(f(e), Th0), a(f(e), Ih0, Oh0);
var p = i[2];
function y(A) {
return u(n4, A);
}
function T(A) {
return u(t, A);
}
R(Je[17], T, y, e, p), u(f(e), Ah0), u(f(e), Nh0), a(f(e), Ph0, Ch0);
var E = i[3];
if (E) {
g(e, Dh0);
var h = E[1], w = function(A, S) {
return g(A, wh0);
}, G = function(A) {
return u(t, A);
};
R(Dr[1], G, w, e, h), g(e, Lh0);
} else
g(e, Rh0);
return u(f(e), jh0), u(f(e), Gh0);
}), N(VZ, function(t, n4, e) {
var i = a(sD, t, n4);
return a(P0(kh0), i, e);
});
var zZ = [0, sD, VZ], vD = function t(n4, e, i) {
return t.fun(n4, e, i);
}, KZ = function t(n4, e) {
return t.fun(n4, e);
};
N(vD, function(t, n4, e) {
u(f(n4), ih0), a(f(n4), xh0, fh0);
var i = e[1];
function x(G) {
return u(t, G);
}
function c(G) {
return u(t, G);
}
R(Ln[1], c, x, n4, i), u(f(n4), ah0), u(f(n4), oh0), a(f(n4), sh0, ch0);
var s = e[2];
function p(G) {
return u(t, G);
}
function y(G) {
return u(t, G);
}
R(Ln[1], y, p, n4, s), u(f(n4), vh0), u(f(n4), lh0), a(f(n4), ph0, bh0);
var T = e[3];
if (T) {
g(n4, mh0);
var E = T[1], h = function(G, A) {
return g(G, uh0);
}, w = function(G) {
return u(t, G);
};
R(Dr[1], w, h, n4, E), g(n4, _h0);
} else
g(n4, yh0);
return u(f(n4), dh0), u(f(n4), hh0);
}), N(KZ, function(t, n4) {
var e = u(vD, t);
return a(P0(th0), e, n4);
});
var WZ = [0, vD, KZ], lD = function t(n4, e, i) {
return t.fun(n4, e, i);
}, JZ = function t(n4, e) {
return t.fun(n4, e);
};
N(lD, function(t, n4, e) {
u(f(n4), Wd0), a(f(n4), $d0, Jd0);
var i = e[1];
if (i) {
g(n4, Zd0);
var x = i[1], c = function(p, y) {
return g(p, Kd0);
}, s = function(p) {
return u(t, p);
};
R(Dr[1], s, c, n4, x), g(n4, Qd0);
} else
g(n4, rh0);
return u(f(n4), eh0), u(f(n4), nh0);
}), N(JZ, function(t, n4) {
var e = u(lD, t);
return a(P0(zd0), e, n4);
});
var $Z = [0, lD, JZ], bD = function t(n4, e, i) {
return t.fun(n4, e, i);
}, ZZ = function t(n4, e) {
return t.fun(n4, e);
};
N(bD, function(t, n4, e) {
u(f(n4), Md0), a(f(n4), qd0, Bd0);
var i = e[1];
if (i) {
g(n4, Ud0);
var x = i[1], c = function(p, y) {
return g(p, Gd0);
}, s = function(p) {
return u(t, p);
};
R(Dr[1], s, c, n4, x), g(n4, Hd0);
} else
g(n4, Xd0);
return u(f(n4), Yd0), u(f(n4), Vd0);
}), N(ZZ, function(t, n4) {
var e = u(bD, t);
return a(P0(jd0), e, n4);
});
var QZ = [0, bD, ZZ], pD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, rQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(pD, function(t, n4, e, i) {
u(f(e), gd0), a(f(e), Td0, Fd0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(qe[31], s, c, e, x), u(f(e), Od0), u(f(e), Id0), a(f(e), Nd0, Ad0);
var p = i[2];
if (p) {
g(e, Cd0);
var y = p[1], T = function(h, w) {
return g(h, Sd0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, Pd0);
} else
g(e, Dd0);
return u(f(e), Ld0), u(f(e), Rd0);
}), N(rQ, function(t, n4, e) {
var i = a(pD, t, n4);
return a(P0(Ed0), i, e);
});
var eQ = [0, pD, rQ], mD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, nQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, am = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, tQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(mD, function(t, n4, e, i) {
u(f(e), hd0), a(n4, e, i[1]), u(f(e), kd0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(am, function(s) {
return u(t, s);
}, c, e, x), u(f(e), wd0);
}), N(nQ, function(t, n4, e) {
var i = a(mD, t, n4);
return a(P0(dd0), i, e);
}), N(am, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), sy0);
var x = i[1], c = function(Y) {
return u(n4, Y);
}, s = function(Y) {
return u(t, Y);
};
return R(H$[3], s, c, e, x), u(f(e), vy0);
case 1:
u(f(e), ly0);
var p = i[1], y = function(Y) {
return u(n4, Y);
}, T = function(Y) {
return u(t, Y);
};
return R(Ps[5], T, y, e, p), u(f(e), by0);
case 2:
u(f(e), py0);
var E = i[1], h = function(Y) {
return u(n4, Y);
}, w = function(Y) {
return u(t, Y);
};
return R(_Z[3], w, h, e, E), u(f(e), my0);
case 3:
u(f(e), _y0);
var G = i[1], A = function(Y) {
return u(n4, Y);
}, S = function(Y) {
return u(t, Y);
};
return R(bZ[3], S, A, e, G), u(f(e), yy0);
case 4:
u(f(e), dy0);
var M = i[1], K = function(Y) {
return u(n4, Y);
}, V = function(Y) {
return u(t, Y);
};
return R(eD[1], V, K, e, M), u(f(e), hy0);
case 5:
u(f(e), ky0);
var f0 = i[1], m0 = function(Y) {
return u(n4, Y);
}, k0 = function(Y) {
return u(t, Y);
};
return R(T1[8], k0, m0, e, f0), u(f(e), wy0);
case 6:
u(f(e), Ey0);
var g0 = i[1], e0 = function(Y) {
return u(n4, Y);
}, x0 = function(Y) {
return u(t, Y);
};
return R(oD[2], x0, e0, e, g0), u(f(e), Sy0);
case 7:
u(f(e), gy0);
var l = i[1], c0 = function(Y) {
return u(n4, Y);
}, t0 = function(Y) {
return u(t, Y);
};
return R(gZ[1], t0, c0, e, l), u(f(e), Fy0);
case 8:
u(f(e), Ty0);
var a0 = i[1], w0 = function(Y) {
return u(n4, Y);
}, _0 = function(Y) {
return u(t, Y);
};
return R(Ps[5], _0, w0, e, a0), u(f(e), Oy0);
case 9:
u(f(e), Iy0);
var E0 = i[1], X0 = function(Y) {
return u(n4, Y);
}, b = function(Y) {
return u(t, Y);
};
return R(YZ[1], b, X0, e, E0), u(f(e), Ay0);
case 10:
u(f(e), Ny0);
var G0 = i[1], X = function(Y) {
return u(n4, Y);
}, s0 = function(Y) {
return u(t, Y);
};
return R(Ln[1], s0, X, e, G0), u(f(e), Cy0);
case 11:
u(f(e), Py0);
var dr = i[1], Ar = function(Y) {
return u(n4, Y);
}, ar = function(Y) {
return u(t, Y);
};
return R(eQ[1], ar, Ar, e, dr), u(f(e), Dy0);
case 12:
u(f(e), Ly0);
var W0 = i[1], Lr = function(Y) {
return u(n4, Y);
}, Tr = function(Y) {
return u(t, Y);
};
return R(YN[17], Tr, Lr, e, W0), u(f(e), Ry0);
case 13:
u(f(e), jy0);
var Hr = i[1], Or = function(Y) {
return u(n4, Y);
}, xr = function(Y) {
return u(t, Y);
};
return R(YN[19], xr, Or, e, Hr), u(f(e), Gy0);
case 14:
u(f(e), My0);
var Rr = i[1], Wr = function(Y) {
return u(t, Y);
};
return ir(Tl[2], Wr, e, Rr), u(f(e), By0);
case 15:
u(f(e), qy0);
var Jr = i[1], or = function(Y) {
return u(n4, Y);
}, _r = function(Y) {
return u(t, Y);
};
return R(EZ[3], _r, or, e, Jr), u(f(e), Uy0);
case 16:
u(f(e), Hy0);
var Ir = i[1], fe = function(Y) {
return u(n4, Y);
}, v0 = function(Y) {
return u(t, Y);
};
return R(uD[3], v0, fe, e, Ir), u(f(e), Xy0);
case 17:
u(f(e), Yy0);
var P = i[1], L = function(Y) {
return u(t, Y);
};
return ir(WZ[1], L, e, P), u(f(e), Vy0);
case 18:
u(f(e), zy0);
var Q = i[1], i0 = function(Y) {
return u(n4, Y);
}, l0 = function(Y) {
return u(t, Y);
};
return R(AZ[1], l0, i0, e, Q), u(f(e), Ky0);
case 19:
u(f(e), Wy0);
var S0 = i[1], T0 = function(Y) {
return u(n4, Y);
}, rr = function(Y) {
return u(t, Y);
};
return R(fZ[5], rr, T0, e, S0), u(f(e), Jy0);
case 20:
u(f(e), $y0);
var R0 = i[1], B = function(Y) {
return u(n4, Y);
}, Z = function(Y) {
return u(t, Y);
};
return R(PZ[1], Z, B, e, R0), u(f(e), Zy0);
case 21:
u(f(e), Qy0);
var p0 = i[1], b0 = function(Y) {
return u(n4, Y);
}, O0 = function(Y) {
return u(t, Y);
};
return R(jZ[1], O0, b0, e, p0), u(f(e), rd0);
case 22:
u(f(e), ed0);
var q0 = i[1], er = function(Y) {
return u(n4, Y);
}, yr = function(Y) {
return u(t, Y);
};
return R(aZ[1], yr, er, e, q0), u(f(e), nd0);
case 23:
u(f(e), td0);
var vr = i[1], $0 = function(Y) {
return u(t, Y);
};
return ir(QZ[1], $0, e, vr), u(f(e), ud0);
case 24:
u(f(e), id0);
var Sr = i[1], Mr = function(Y) {
return u(n4, Y);
}, Br = function(Y) {
return u(t, Y);
};
return R(J$[1], Br, Mr, e, Sr), u(f(e), fd0);
case 25:
u(f(e), xd0);
var qr = i[1], jr = function(Y) {
return u(n4, Y);
}, $r = function(Y) {
return u(t, Y);
};
return R(MP[2], $r, jr, e, qr), u(f(e), ad0);
case 26:
u(f(e), od0);
var ne = i[1], Qr = function(Y) {
return u(t, Y);
};
return ir($Z[1], Qr, e, ne), u(f(e), cd0);
case 27:
u(f(e), sd0);
var pe = i[1], oe = function(Y) {
return u(n4, Y);
}, me = function(Y) {
return u(t, Y);
};
return R(zZ[1], me, oe, e, pe), u(f(e), vd0);
case 28:
u(f(e), ld0);
var ae = i[1], ce = function(Y) {
return u(n4, Y);
}, ge = function(Y) {
return u(t, Y);
};
return R(sZ[3], ge, ce, e, ae), u(f(e), bd0);
case 29:
u(f(e), pd0);
var H0 = i[1], Fr = function(Y) {
return u(n4, Y);
}, _ = function(Y) {
return u(t, Y);
};
return R(hZ[3], _, Fr, e, H0), u(f(e), md0);
default:
u(f(e), _d0);
var k = i[1], I = function(Y) {
return u(n4, Y);
}, U = function(Y) {
return u(t, Y);
};
return R(MZ[1], U, I, e, k), u(f(e), yd0);
}
}), N(tQ, function(t, n4, e) {
var i = a(am, t, n4);
return a(P0(cy0), i, e);
}), pu(s6r, qe, [0, R$, Mee, LP, H$, MP, J$, fZ, aZ, sZ, bZ, _Z, hZ, EZ, gZ, um, FZ, ZP, AZ, eD, PZ, uD, jZ, MZ, oD, YZ, zZ, WZ, $Z, QZ, eQ, mD, nQ, am, tQ]);
var _D = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, uQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, om = function t(n4, e, i) {
return t.fun(n4, e, i);
}, iQ = function t(n4, e) {
return t.fun(n4, e);
};
N(_D, function(t, n4, e, i) {
u(f(e), xy0), a(n4, e, i[1]), u(f(e), ay0);
var x = i[2];
return ir(om, function(c) {
return u(t, c);
}, e, x), u(f(e), oy0);
}), N(uQ, function(t, n4, e) {
var i = a(_D, t, n4);
return a(P0(fy0), i, e);
}), N(om, function(t, n4, e) {
u(f(n4), z_0), a(f(n4), W_0, K_0);
var i = e[1];
a(f(n4), J_0, i), u(f(n4), $_0), u(f(n4), Z_0), a(f(n4), ry0, Q_0);
var x = e[2];
if (x) {
g(n4, ey0);
var c = x[1], s = function(y, T) {
return g(y, V_0);
}, p = function(y) {
return u(t, y);
};
R(Dr[1], p, s, n4, c), g(n4, ny0);
} else
g(n4, ty0);
return u(f(n4), uy0), u(f(n4), iy0);
}), N(iQ, function(t, n4) {
var e = u(om, t);
return a(P0(Y_0), e, n4);
});
var I1 = [0, _D, uQ, om, iQ], yD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, fQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, cm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, xQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(yD, function(t, n4, e, i) {
u(f(e), U_0), a(t, e, i[1]), u(f(e), H_0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(cm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), X_0);
}), N(fQ, function(t, n4, e) {
var i = a(yD, t, n4);
return a(P0(q_0), i, e);
}), N(cm, function(t, n4, e, i) {
u(f(e), C_0), a(f(e), D_0, P_0);
var x = i[1];
function c(E) {
return u(n4, E);
}
function s(E) {
return u(t, E);
}
R(I1[1], s, c, e, x), u(f(e), L_0), u(f(e), R_0), a(f(e), G_0, j_0);
var p = i[2];
function y(E) {
return u(n4, E);
}
function T(E) {
return u(t, E);
}
return R(I1[1], T, y, e, p), u(f(e), M_0), u(f(e), B_0);
}), N(xQ, function(t, n4, e) {
var i = a(cm, t, n4);
return a(P0(N_0), i, e);
});
var dD = [0, yD, fQ, cm, xQ], hD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, aQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, sm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, oQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(hD, function(t, n4, e, i) {
u(f(e), d_0), a(f(e), k_0, h_0);
var x = i[1];
function c(E) {
return u(n4, E);
}
R(sm, function(E) {
return u(t, E);
}, c, e, x), u(f(e), w_0), u(f(e), E_0), a(f(e), g_0, S_0);
var s = i[2];
if (s) {
g(e, F_0);
var p = s[1], y = function(E, h) {
u(f(E), __0);
var w = 0;
return be(function(G, A) {
G && u(f(E), m_0);
function S(M) {
return u(t, M);
}
return ir(uu[1], S, E, A), 1;
}, w, h), u(f(E), y_0);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, T_0);
} else
g(e, O_0);
return u(f(e), I_0), u(f(e), A_0);
}), N(aQ, function(t, n4, e) {
var i = a(hD, t, n4);
return a(P0(p_0), i, e);
}), N(sm, function(t, n4, e, i) {
if (i) {
u(f(e), v_0);
var x = i[1], c = function(p) {
return u(n4, p);
}, s = function(p) {
return u(t, p);
};
return R(qe[31], s, c, e, x), u(f(e), l_0);
}
return g(e, b_0);
}), N(oQ, function(t, n4, e) {
var i = a(sm, t, n4);
return a(P0(s_0), i, e);
});
var kD = [0, hD, aQ, sm, oQ];
function cQ(t, n4) {
u(f(t), Q90), a(f(t), e_0, r_0);
var e = n4[1];
a(f(t), n_0, e), u(f(t), t_0), u(f(t), u_0), a(f(t), f_0, i_0);
var i = n4[2];
return a(f(t), x_0, i), u(f(t), a_0), u(f(t), o_0);
}
var sQ = [0, cQ, function(t) {
return a(P0(c_0), cQ, t);
}], wD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, vQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, vm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, lQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, lm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, bQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, bm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, pQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(wD, function(t, n4, e, i) {
u(f(e), J90), a(t, e, i[1]), u(f(e), $90);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(bm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Z90);
}), N(vQ, function(t, n4, e) {
var i = a(wD, t, n4);
return a(P0(W90), i, e);
}), N(vm, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), Y90);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(I1[1], s, c, e, x), u(f(e), V90);
}
u(f(e), z90);
var p = i[1];
function y(E) {
return u(n4, E);
}
function T(E) {
return u(t, E);
}
return R(dD[1], T, y, e, p), u(f(e), K90);
}), N(lQ, function(t, n4, e) {
var i = a(vm, t, n4);
return a(P0(X90), i, e);
}), N(lm, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), G90), a(n4, e, i[1]), u(f(e), M90);
var x = i[2], c = function(T) {
return u(t, T);
};
return ir(Tl[2], c, e, x), u(f(e), B90);
}
u(f(e), q90), a(n4, e, i[1]), u(f(e), U90);
var s = i[2];
function p(T) {
return u(n4, T);
}
function y(T) {
return u(t, T);
}
return R(kD[1], y, p, e, s), u(f(e), H90);
}), N(bQ, function(t, n4, e) {
var i = a(lm, t, n4);
return a(P0(j90), i, e);
}), N(bm, function(t, n4, e, i) {
u(f(e), g90), a(f(e), T90, F90);
var x = i[1];
function c(T) {
return u(n4, T);
}
R(vm, function(T) {
return u(t, T);
}, c, e, x), u(f(e), O90), u(f(e), I90), a(f(e), N90, A90);
var s = i[2];
if (s) {
g(e, C90);
var p = s[1], y = function(T) {
return u(n4, T);
};
R(lm, function(T) {
return u(t, T);
}, y, e, p), g(e, P90);
} else
g(e, D90);
return u(f(e), L90), u(f(e), R90);
}), N(pQ, function(t, n4, e) {
var i = a(bm, t, n4);
return a(P0(S90), i, e);
});
var mQ = [0, wD, vQ, vm, lQ, lm, bQ, bm, pQ], ED = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, _Q = function t(n4, e, i) {
return t.fun(n4, e, i);
}, pm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, yQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(ED, function(t, n4, e, i) {
u(f(e), k90), a(t, e, i[1]), u(f(e), w90);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(pm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), E90);
}), N(_Q, function(t, n4, e) {
var i = a(ED, t, n4);
return a(P0(h90), i, e);
}), N(pm, function(t, n4, e, i) {
u(f(e), a90), a(f(e), c90, o90);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(qe[31], s, c, e, x), u(f(e), s90), u(f(e), v90), a(f(e), b90, l90);
var p = i[2];
if (p) {
g(e, p90);
var y = p[1], T = function(h, w) {
return g(h, x90);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, m90);
} else
g(e, _90);
return u(f(e), y90), u(f(e), d90);
}), N(yQ, function(t, n4, e) {
var i = a(pm, t, n4);
return a(P0(f90), i, e);
});
var dQ = [0, ED, _Q, pm, yQ], mm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, hQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, _m = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, kQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, ym = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, wQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(mm, function(t, n4, e, i) {
u(f(e), t90), a(t, e, i[1]), u(f(e), u90);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(ym, function(s) {
return u(t, s);
}, c, e, x), u(f(e), i90);
}), N(hQ, function(t, n4, e) {
var i = a(mm, t, n4);
return a(P0(n90), i, e);
}), N(_m, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), Zm0);
var x = i[1], c = function(T) {
return u(n4, T);
}, s = function(T) {
return u(t, T);
};
return R(I1[1], s, c, e, x), u(f(e), Qm0);
}
u(f(e), r90);
var p = i[1];
function y(T) {
return u(n4, T);
}
return R(mm, function(T) {
return u(t, T);
}, y, e, p), u(f(e), e90);
}), N(kQ, function(t, n4, e) {
var i = a(_m, t, n4);
return a(P0($m0), i, e);
}), N(ym, function(t, n4, e, i) {
u(f(e), Um0), a(f(e), Xm0, Hm0);
var x = i[1];
function c(T) {
return u(n4, T);
}
R(_m, function(T) {
return u(t, T);
}, c, e, x), u(f(e), Ym0), u(f(e), Vm0), a(f(e), Km0, zm0);
var s = i[2];
function p(T) {
return u(n4, T);
}
function y(T) {
return u(t, T);
}
return R(I1[1], y, p, e, s), u(f(e), Wm0), u(f(e), Jm0);
}), N(wQ, function(t, n4, e) {
var i = a(ym, t, n4);
return a(P0(qm0), i, e);
});
var EQ = [0, mm, hQ, _m, kQ, ym, wQ], Nl = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, SQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(Nl, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), Lm0);
var x = i[1], c = function(G) {
return u(n4, G);
}, s = function(G) {
return u(t, G);
};
return R(I1[1], s, c, e, x), u(f(e), Rm0);
case 1:
u(f(e), jm0);
var p = i[1], y = function(G) {
return u(n4, G);
}, T = function(G) {
return u(t, G);
};
return R(dD[1], T, y, e, p), u(f(e), Gm0);
default:
u(f(e), Mm0);
var E = i[1], h = function(G) {
return u(n4, G);
}, w = function(G) {
return u(t, G);
};
return R(EQ[1], w, h, e, E), u(f(e), Bm0);
}
}), N(SQ, function(t, n4, e) {
var i = a(Nl, t, n4);
return a(P0(Dm0), i, e);
});
var SD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, gQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, dm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, FQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, hm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, TQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(SD, function(t, n4, e, i) {
u(f(e), Nm0), a(t, e, i[1]), u(f(e), Cm0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(hm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Pm0);
}), N(gQ, function(t, n4, e) {
var i = a(SD, t, n4);
return a(P0(Am0), i, e);
}), N(dm, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), Fm0);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(mQ[1], s, c, e, x), u(f(e), Tm0);
}
u(f(e), Om0);
var p = i[1];
function y(E) {
return u(n4, E);
}
function T(E) {
return u(t, E);
}
return R(dQ[1], T, y, e, p), u(f(e), Im0);
}), N(FQ, function(t, n4, e) {
var i = a(dm, t, n4);
return a(P0(gm0), i, e);
}), N(hm, function(t, n4, e, i) {
u(f(e), om0), a(f(e), sm0, cm0);
var x = i[1];
function c(T) {
return u(n4, T);
}
R(Nl, function(T) {
return u(t, T);
}, c, e, x), u(f(e), vm0), u(f(e), lm0), a(f(e), pm0, bm0);
var s = i[2];
a(f(e), mm0, s), u(f(e), _m0), u(f(e), ym0), a(f(e), hm0, dm0);
var p = i[3];
u(f(e), km0);
var y = 0;
return be(function(T, E) {
T && u(f(e), am0);
function h(w) {
return u(n4, w);
}
return R(dm, function(w) {
return u(t, w);
}, h, e, E), 1;
}, y, p), u(f(e), wm0), u(f(e), Em0), u(f(e), Sm0);
}), N(TQ, function(t, n4, e) {
var i = a(hm, t, n4);
return a(P0(xm0), i, e);
});
var OQ = [0, SD, gQ, dm, FQ, hm, TQ], gD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, IQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, km = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, AQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(gD, function(t, n4, e, i) {
u(f(e), um0), a(t, e, i[1]), u(f(e), im0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(km, function(s) {
return u(t, s);
}, c, e, x), u(f(e), fm0);
}), N(IQ, function(t, n4, e) {
var i = a(gD, t, n4);
return a(P0(tm0), i, e);
}), N(km, function(t, n4, e, i) {
u(f(e), Z50), a(f(e), rm0, Q50);
var x = i[1];
function c(s) {
return u(n4, s);
}
return R(Nl, function(s) {
return u(t, s);
}, c, e, x), u(f(e), em0), u(f(e), nm0);
}), N(AQ, function(t, n4, e) {
var i = a(km, t, n4);
return a(P0($50), i, e);
});
var NQ = [0, gD, IQ, km, AQ], FD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, CQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(FD, function(t, n4, e, i) {
u(f(e), M50), a(f(e), q50, B50);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(qe[31], s, c, e, x), u(f(e), U50), u(f(e), H50), a(f(e), Y50, X50);
var p = i[2];
if (p) {
g(e, V50);
var y = p[1], T = function(h, w) {
return g(h, G50);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, z50);
} else
g(e, K50);
return u(f(e), W50), u(f(e), J50);
}), N(CQ, function(t, n4, e) {
var i = a(FD, t, n4);
return a(P0(j50), i, e);
});
var PQ = [0, FD, CQ], Cl = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, DQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, wm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, LQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Em = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, RQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Sm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, jQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(Cl, function(t, n4, e, i) {
u(f(e), D50), a(t, e, i[1]), u(f(e), L50);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(wm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), R50);
}), N(DQ, function(t, n4, e) {
var i = a(Cl, t, n4);
return a(P0(P50), i, e);
}), N(wm, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), E50);
var x = i[1], c = function(A) {
return u(n4, A);
};
return R(Em, function(A) {
return u(t, A);
}, c, e, x), u(f(e), S50);
case 1:
u(f(e), g50);
var s = i[1], p = function(A) {
return u(n4, A);
};
return R(Sm, function(A) {
return u(t, A);
}, p, e, s), u(f(e), F50);
case 2:
u(f(e), T50);
var y = i[1], T = function(A) {
return u(n4, A);
}, E = function(A) {
return u(t, A);
};
return R(kD[1], E, T, e, y), u(f(e), O50);
case 3:
u(f(e), I50);
var h = i[1], w = function(A) {
return u(n4, A);
}, G = function(A) {
return u(t, A);
};
return R(PQ[1], G, w, e, h), u(f(e), A50);
default:
return u(f(e), N50), a(sQ[1], e, i[1]), u(f(e), C50);
}
}), N(LQ, function(t, n4, e) {
var i = a(wm, t, n4);
return a(P0(w50), i, e);
}), N(Em, function(t, n4, e, i) {
u(f(e), Kp0), a(f(e), Jp0, Wp0);
var x = i[1];
function c(V) {
return u(n4, V);
}
function s(V) {
return u(t, V);
}
R(OQ[1], s, c, e, x), u(f(e), $p0), u(f(e), Zp0), a(f(e), r50, Qp0);
var p = i[2];
if (p) {
g(e, e50);
var y = p[1], T = function(V) {
return u(n4, V);
}, E = function(V) {
return u(t, V);
};
R(NQ[1], E, T, e, y), g(e, n50);
} else
g(e, t50);
u(f(e), u50), u(f(e), i50), a(f(e), x50, f50);
var h = i[3];
u(f(e), a50), a(t, e, h[1]), u(f(e), o50), u(f(e), c50);
var w = h[2], G = 0;
be(function(V, f0) {
V && u(f(e), zp0);
function m0(k0) {
return u(n4, k0);
}
return R(Cl, function(k0) {
return u(t, k0);
}, m0, e, f0), 1;
}, G, w), u(f(e), s50), u(f(e), v50), u(f(e), l50), u(f(e), b50), a(f(e), m50, p50);
var A = i[4];
if (A) {
g(e, _50);
var S = A[1], M = function(V, f0) {
return g(V, Vp0);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, y50);
} else
g(e, d50);
return u(f(e), h50), u(f(e), k50);
}), N(RQ, function(t, n4, e) {
var i = a(Em, t, n4);
return a(P0(Yp0), i, e);
}), N(Sm, function(t, n4, e, i) {
u(f(e), hp0), a(f(e), wp0, kp0), a(t, e, i[1]), u(f(e), Ep0), u(f(e), Sp0), a(f(e), Fp0, gp0), a(t, e, i[2]), u(f(e), Tp0), u(f(e), Op0), a(f(e), Ap0, Ip0);
var x = i[3];
u(f(e), Np0), a(t, e, x[1]), u(f(e), Cp0), u(f(e), Pp0);
var c = x[2], s = 0;
be(function(h, w) {
h && u(f(e), dp0);
function G(A) {
return u(n4, A);
}
return R(Cl, function(A) {
return u(t, A);
}, G, e, w), 1;
}, s, c), u(f(e), Dp0), u(f(e), Lp0), u(f(e), Rp0), u(f(e), jp0), a(f(e), Mp0, Gp0);
var p = i[4];
if (p) {
g(e, Bp0);
var y = p[1], T = function(h, w) {
return g(h, yp0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, qp0);
} else
g(e, Up0);
return u(f(e), Hp0), u(f(e), Xp0);
}), N(jQ, function(t, n4, e) {
var i = a(Sm, t, n4);
return a(P0(_p0), i, e);
}), pu(v6r, YN, [0, I1, dD, kD, sQ, mQ, dQ, EQ, Nl, SQ, OQ, NQ, PQ, Cl, DQ, wm, LQ, Em, RQ, Sm, jQ]);
var TD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, GQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, gm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, MQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(TD, function(t, n4, e, i) {
u(f(e), bp0), a(t, e, i[1]), u(f(e), pp0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(gm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), mp0);
}), N(GQ, function(t, n4, e) {
var i = a(TD, t, n4);
return a(P0(lp0), i, e);
}), N(gm, function(t, n4, e, i) {
u(f(e), ep0), a(f(e), tp0, np0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(di[5], s, c, e, x), u(f(e), up0), u(f(e), ip0), a(f(e), xp0, fp0);
var p = i[2];
if (p) {
g(e, ap0);
var y = p[1], T = function(h, w) {
return g(h, rp0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, op0);
} else
g(e, cp0);
return u(f(e), sp0), u(f(e), vp0);
}), N(MQ, function(t, n4, e) {
var i = a(gm, t, n4);
return a(P0(Q60), i, e);
});
var OD = [0, TD, GQ, gm, MQ], Fm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, BQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, ID = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, qQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Tm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, UQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(Fm, function(t, n4, e, i) {
switch (i[0]) {
case 0:
var x = i[1];
u(f(e), X60), u(f(e), Y60), a(t, e, x[1]), u(f(e), V60);
var c = x[2], s = function(G) {
return u(t, G);
};
return ir(Tl[2], s, e, c), u(f(e), z60), u(f(e), K60);
case 1:
u(f(e), W60);
var p = i[1], y = function(G) {
return u(n4, G);
}, T = function(G) {
return u(t, G);
};
return R(Ln[1], T, y, e, p), u(f(e), J60);
default:
u(f(e), $60);
var E = i[1], h = function(G) {
return u(n4, G);
}, w = function(G) {
return u(t, G);
};
return R(Up[1], w, h, e, E), u(f(e), Z60);
}
}), N(BQ, function(t, n4, e) {
var i = a(Fm, t, n4);
return a(P0(H60), i, e);
}), N(ID, function(t, n4, e, i) {
u(f(e), B60), a(t, e, i[1]), u(f(e), q60);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Tm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), U60);
}), N(qQ, function(t, n4, e) {
var i = a(ID, t, n4);
return a(P0(M60), i, e);
}), N(Tm, function(t, n4, e, i) {
u(f(e), y60), a(f(e), h60, d60);
var x = i[1];
function c(A) {
return u(n4, A);
}
R(Fm, function(A) {
return u(t, A);
}, c, e, x), u(f(e), k60), u(f(e), w60), a(f(e), S60, E60);
var s = i[2];
function p(A) {
return u(n4, A);
}
function y(A) {
return u(t, A);
}
R(di[5], y, p, e, s), u(f(e), g60), u(f(e), F60), a(f(e), O60, T60);
var T = i[3];
if (T) {
g(e, I60);
var E = T[1], h = function(A) {
return u(n4, A);
}, w = function(A) {
return u(t, A);
};
R(qe[31], w, h, e, E), g(e, A60);
} else
g(e, N60);
u(f(e), C60), u(f(e), P60), a(f(e), L60, D60);
var G = i[4];
return a(f(e), R60, G), u(f(e), j60), u(f(e), G60);
}), N(UQ, function(t, n4, e) {
var i = a(Tm, t, n4);
return a(P0(_60), i, e);
});
var HQ = [0, Fm, BQ, ID, qQ, Tm, UQ], Om = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, XQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, AD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, YQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(Om, function(t, n4, e, i) {
if (i[0] === 0) {
u(f(e), l60);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(HQ[3], s, c, e, x), u(f(e), b60);
}
u(f(e), p60);
var p = i[1];
function y(E) {
return u(n4, E);
}
function T(E) {
return u(t, E);
}
return R(OD[1], T, y, e, p), u(f(e), m60);
}), N(XQ, function(t, n4, e) {
var i = a(Om, t, n4);
return a(P0(v60), i, e);
}), N(AD, function(t, n4, e, i) {
u(f(e), K30), a(f(e), J30, W30);
var x = i[1];
u(f(e), $30);
var c = 0;
be(function(G, A) {
G && u(f(e), z30);
function S(M) {
return u(n4, M);
}
return R(Om, function(M) {
return u(t, M);
}, S, e, A), 1;
}, c, x), u(f(e), Z30), u(f(e), Q30), u(f(e), r60), a(f(e), n60, e60);
var s = i[2];
function p(G) {
return u(n4, G);
}
function y(G) {
return u(t, G);
}
R(Je[19], y, p, e, s), u(f(e), t60), u(f(e), u60), a(f(e), f60, i60);
var T = i[3];
if (T) {
g(e, x60);
var E = T[1], h = function(G, A) {
u(f(G), Y30);
var S = 0;
return be(function(M, K) {
M && u(f(G), X30);
function V(f0) {
return u(t, f0);
}
return ir(uu[1], V, G, K), 1;
}, S, A), u(f(G), V30);
}, w = function(G) {
return u(t, G);
};
R(Dr[1], w, h, e, E), g(e, a60);
} else
g(e, o60);
return u(f(e), c60), u(f(e), s60);
}), N(YQ, function(t, n4, e) {
var i = a(AD, t, n4);
return a(P0(H30), i, e);
});
var VQ = [0, HQ, Om, XQ, AD, YQ], ND = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, zQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Im = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, KQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(ND, function(t, n4, e, i) {
u(f(e), B30), a(t, e, i[1]), u(f(e), q30);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Im, function(s) {
return u(t, s);
}, c, e, x), u(f(e), U30);
}), N(zQ, function(t, n4, e) {
var i = a(ND, t, n4);
return a(P0(M30), i, e);
}), N(Im, function(t, n4, e, i) {
u(f(e), T30), a(f(e), I30, O30);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(di[5], s, c, e, x), u(f(e), A30), u(f(e), N30), a(f(e), P30, C30);
var p = i[2];
if (p) {
g(e, D30);
var y = p[1], T = function(h) {
return u(n4, h);
}, E = function(h) {
return u(t, h);
};
R(qe[31], E, T, e, y), g(e, L30);
} else
g(e, R30);
return u(f(e), j30), u(f(e), G30);
}), N(KQ, function(t, n4, e) {
var i = a(Im, t, n4);
return a(P0(F30), i, e);
});
var WQ = [0, ND, zQ, Im, KQ], Am = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, JQ = function t(n4, e, i) {
return t.fun(n4, e, i);
}, CD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, $Q = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(Am, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), h30);
var x = i[1], c = function(E) {
return u(n4, E);
}, s = function(E) {
return u(t, E);
};
return R(WQ[1], s, c, e, x), u(f(e), k30);
case 1:
u(f(e), w30);
var p = i[1], y = function(E) {
return u(n4, E);
}, T = function(E) {
return u(t, E);
};
return R(OD[1], T, y, e, p), u(f(e), E30);
default:
return u(f(e), S30), a(t, e, i[1]), u(f(e), g30);
}
}), N(JQ, function(t, n4, e) {
var i = a(Am, t, n4);
return a(P0(d30), i, e);
}), N(CD, function(t, n4, e, i) {
u(f(e), e30), a(f(e), t30, n30);
var x = i[1];
u(f(e), u30);
var c = 0;
be(function(G, A) {
G && u(f(e), r30);
function S(M) {
return u(n4, M);
}
return R(Am, function(M) {
return u(t, M);
}, S, e, A), 1;
}, c, x), u(f(e), i30), u(f(e), f30), u(f(e), x30), a(f(e), o30, a30);
var s = i[2];
function p(G) {
return u(n4, G);
}
function y(G) {
return u(t, G);
}
R(Je[19], y, p, e, s), u(f(e), c30), u(f(e), s30), a(f(e), l30, v30);
var T = i[3];
if (T) {
g(e, b30);
var E = T[1], h = function(G, A) {
u(f(G), Z80);
var S = 0;
return be(function(M, K) {
M && u(f(G), $80);
function V(f0) {
return u(t, f0);
}
return ir(uu[1], V, G, K), 1;
}, S, A), u(f(G), Q80);
}, w = function(G) {
return u(t, G);
};
R(Dr[1], w, h, e, E), g(e, p30);
} else
g(e, m30);
return u(f(e), _30), u(f(e), y30);
}), N($Q, function(t, n4, e) {
var i = a(CD, t, n4);
return a(P0(J80), i, e);
});
var ZQ = [0, WQ, Am, JQ, CD, $Q], PD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, QQ = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(PD, function(t, n4, e, i) {
u(f(e), R80), a(f(e), G80, j80);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(Ln[1], s, c, e, x), u(f(e), M80), u(f(e), B80), a(f(e), U80, q80);
var p = i[2];
function y(h) {
return u(n4, h);
}
function T(h) {
return u(t, h);
}
R(Je[19], T, y, e, p), u(f(e), H80), u(f(e), X80), a(f(e), V80, Y80);
var E = i[3];
return a(f(e), z80, E), u(f(e), K80), u(f(e), W80);
}), N(QQ, function(t, n4, e) {
var i = a(PD, t, n4);
return a(P0(L80), i, e);
});
var r00 = [0, PD, QQ], DD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, e00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Nm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, n00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(DD, function(t, n4, e, i) {
u(f(e), C80), a(n4, e, i[1]), u(f(e), P80);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Nm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), D80);
}), N(e00, function(t, n4, e) {
var i = a(DD, t, n4);
return a(P0(N80), i, e);
}), N(Nm, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), E80);
var x = i[1], c = function(M) {
return u(n4, M);
}, s = function(M) {
return u(t, M);
};
return R(VQ[4], s, c, e, x), u(f(e), S80);
case 1:
u(f(e), g80);
var p = i[1], y = function(M) {
return u(n4, M);
}, T = function(M) {
return u(t, M);
};
return R(ZQ[4], T, y, e, p), u(f(e), F80);
case 2:
u(f(e), T80);
var E = i[1], h = function(M) {
return u(n4, M);
}, w = function(M) {
return u(t, M);
};
return R(r00[1], w, h, e, E), u(f(e), O80);
default:
u(f(e), I80);
var G = i[1], A = function(M) {
return u(n4, M);
}, S = function(M) {
return u(t, M);
};
return R(qe[31], S, A, e, G), u(f(e), A80);
}
}), N(n00, function(t, n4, e) {
var i = a(Nm, t, n4);
return a(P0(w80), i, e);
}), pu(l6r, di, [0, OD, VQ, ZQ, r00, DD, e00, Nm, n00]);
var LD = function t(n4, e, i) {
return t.fun(n4, e, i);
}, t00 = function t(n4, e) {
return t.fun(n4, e);
}, Cm = function t(n4, e) {
return t.fun(n4, e);
}, u00 = function t(n4) {
return t.fun(n4);
}, Pm = function t(n4, e) {
return t.fun(n4, e);
}, i00 = function t(n4) {
return t.fun(n4);
};
N(LD, function(t, n4, e) {
return u(f(n4), d80), a(t, n4, e[1]), u(f(n4), h80), a(Pm, n4, e[2]), u(f(n4), k80);
}), N(t00, function(t, n4) {
var e = u(LD, t);
return a(P0(y80), e, n4);
}), N(Cm, function(t, n4) {
return n4 ? g(t, m80) : g(t, _80);
}), N(u00, function(t) {
return a(P0(p80), Cm, t);
}), N(Pm, function(t, n4) {
u(f(t), r80), a(f(t), n80, e80), a(Cm, t, n4[1]), u(f(t), t80), u(f(t), u80), a(f(t), f80, i80);
var e = n4[2];
a(f(t), x80, e), u(f(t), a80), u(f(t), o80), a(f(t), s80, c80);
var i = n4[3];
return a(f(t), v80, i), u(f(t), l80), u(f(t), b80);
}), N(i00, function(t) {
return a(P0(Q40), Pm, t);
}), pu(b6r, uu, [0, LD, t00, Cm, u00, Pm, i00]);
var RD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, f00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Dm = function t(n4, e) {
return t.fun(n4, e);
}, x00 = function t(n4) {
return t.fun(n4);
}, Lm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, a00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(RD, function(t, n4, e, i) {
u(f(e), J40), a(n4, e, i[1]), u(f(e), $40);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Lm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Z40);
}), N(f00, function(t, n4, e) {
var i = a(RD, t, n4);
return a(P0(W40), i, e);
}), N(Dm, function(t, n4) {
switch (n4) {
case 0:
return g(t, Y40);
case 1:
return g(t, V40);
case 2:
return g(t, z40);
default:
return g(t, K40);
}
}), N(x00, function(t) {
return a(P0(X40), Dm, t);
}), N(Lm, function(t, n4, e, i) {
u(f(e), c40), a(f(e), v40, s40), a(Dm, e, i[1]), u(f(e), l40), u(f(e), b40), a(f(e), m40, p40);
var x = i[2];
function c(V) {
return u(n4, V);
}
function s(V) {
return u(t, V);
}
R(qe[7][1][1], s, c, e, x), u(f(e), _40), u(f(e), y40), a(f(e), h40, d40);
var p = i[3];
u(f(e), k40), a(t, e, p[1]), u(f(e), w40);
var y = p[2];
function T(V) {
return u(n4, V);
}
function E(V) {
return u(t, V);
}
R(Ps[5], E, T, e, y), u(f(e), E40), u(f(e), S40), u(f(e), g40), a(f(e), T40, F40);
var h = i[4];
a(f(e), O40, h), u(f(e), I40), u(f(e), A40), a(f(e), C40, N40);
var w = i[5];
u(f(e), P40);
var G = 0;
be(function(V, f0) {
V && u(f(e), o40);
function m0(g0) {
return u(n4, g0);
}
function k0(g0) {
return u(t, g0);
}
return R(T1[7][1], k0, m0, e, f0), 1;
}, G, w), u(f(e), D40), u(f(e), L40), u(f(e), R40), a(f(e), G40, j40);
var A = i[6];
if (A) {
g(e, M40);
var S = A[1], M = function(V, f0) {
return g(V, a40);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, B40);
} else
g(e, q40);
return u(f(e), U40), u(f(e), H40);
}), N(a00, function(t, n4, e) {
var i = a(Lm, t, n4);
return a(P0(x40), i, e);
});
var o00 = [0, RD, f00, Dm, x00, Lm, a00], jD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, c00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Rm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, s00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, jm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, v00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(jD, function(t, n4, e, i) {
u(f(e), u40), a(n4, e, i[1]), u(f(e), i40);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Rm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), f40);
}), N(c00, function(t, n4, e) {
var i = a(jD, t, n4);
return a(P0(t40), i, e);
}), N(Rm, function(t, n4, e, i) {
u(f(e), gb0), a(f(e), Tb0, Fb0);
var x = i[1];
function c(m0) {
return u(n4, m0);
}
function s(m0) {
return u(t, m0);
}
R(qe[7][1][1], s, c, e, x), u(f(e), Ob0), u(f(e), Ib0), a(f(e), Nb0, Ab0);
var p = i[2];
function y(m0) {
return u(n4, m0);
}
R(jm, function(m0) {
return u(t, m0);
}, y, e, p), u(f(e), Cb0), u(f(e), Pb0), a(f(e), Lb0, Db0);
var T = i[3];
function E(m0) {
return u(n4, m0);
}
function h(m0) {
return u(t, m0);
}
R(Je[19], h, E, e, T), u(f(e), Rb0), u(f(e), jb0), a(f(e), Mb0, Gb0);
var w = i[4];
a(f(e), Bb0, w), u(f(e), qb0), u(f(e), Ub0), a(f(e), Xb0, Hb0);
var G = i[5];
if (G) {
g(e, Yb0);
var A = G[1], S = function(m0) {
return u(t, m0);
};
ir(zv[1], S, e, A), g(e, Vb0);
} else
g(e, zb0);
u(f(e), Kb0), u(f(e), Wb0), a(f(e), $b0, Jb0);
var M = i[6];
if (M) {
g(e, Zb0);
var K = M[1], V = function(m0, k0) {
return g(m0, Sb0);
}, f0 = function(m0) {
return u(t, m0);
};
R(Dr[1], f0, V, e, K), g(e, Qb0);
} else
g(e, r40);
return u(f(e), e40), u(f(e), n40);
}), N(s00, function(t, n4, e) {
var i = a(Rm, t, n4);
return a(P0(Eb0), i, e);
}), N(jm, function(t, n4, e, i) {
if (typeof i == "number")
return i ? g(e, db0) : g(e, hb0);
u(f(e), kb0);
var x = i[1];
function c(p) {
return u(n4, p);
}
function s(p) {
return u(t, p);
}
return R(qe[31], s, c, e, x), u(f(e), wb0);
}), N(v00, function(t, n4, e) {
var i = a(jm, t, n4);
return a(P0(yb0), i, e);
});
var l00 = [0, jD, c00, Rm, s00, jm, v00], GD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, b00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Gm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, p00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(GD, function(t, n4, e, i) {
u(f(e), pb0), a(n4, e, i[1]), u(f(e), mb0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Gm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), _b0);
}), N(b00, function(t, n4, e) {
var i = a(GD, t, n4);
return a(P0(bb0), i, e);
}), N(Gm, function(t, n4, e, i) {
u(f(e), Rl0), a(f(e), Gl0, jl0);
var x = i[1];
function c(m0) {
return u(t, m0);
}
ir(qp[1], c, e, x), u(f(e), Ml0), u(f(e), Bl0), a(f(e), Ul0, ql0);
var s = i[2];
function p(m0) {
return u(n4, m0);
}
function y(m0) {
return u(t, m0);
}
R(T1[2][5], y, p, e, s), u(f(e), Hl0), u(f(e), Xl0), a(f(e), Vl0, Yl0);
var T = i[3];
function E(m0) {
return u(n4, m0);
}
function h(m0) {
return u(t, m0);
}
R(Je[19], h, E, e, T), u(f(e), zl0), u(f(e), Kl0), a(f(e), Jl0, Wl0);
var w = i[4];
a(f(e), $l0, w), u(f(e), Zl0), u(f(e), Ql0), a(f(e), eb0, rb0);
var G = i[5];
if (G) {
g(e, nb0);
var A = G[1], S = function(m0) {
return u(t, m0);
};
ir(zv[1], S, e, A), g(e, tb0);
} else
g(e, ub0);
u(f(e), ib0), u(f(e), fb0), a(f(e), ab0, xb0);
var M = i[6];
if (M) {
g(e, ob0);
var K = M[1], V = function(m0, k0) {
return g(m0, Ll0);
}, f0 = function(m0) {
return u(t, m0);
};
R(Dr[1], f0, V, e, K), g(e, cb0);
} else
g(e, sb0);
return u(f(e), vb0), u(f(e), lb0);
}), N(p00, function(t, n4, e) {
var i = a(Gm, t, n4);
return a(P0(Dl0), i, e);
});
var m00 = [0, GD, b00, Gm, p00], MD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, _00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Mm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, y00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(MD, function(t, n4, e, i) {
u(f(e), Nl0), a(t, e, i[1]), u(f(e), Cl0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Mm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Pl0);
}), N(_00, function(t, n4, e) {
var i = a(MD, t, n4);
return a(P0(Al0), i, e);
}), N(Mm, function(t, n4, e, i) {
u(f(e), sl0), a(f(e), ll0, vl0);
var x = i[1];
function c(S) {
return u(n4, S);
}
function s(S) {
return u(t, S);
}
R(qe[31], s, c, e, x), u(f(e), bl0), u(f(e), pl0), a(f(e), _l0, ml0);
var p = i[2];
if (p) {
g(e, yl0);
var y = p[1], T = function(S) {
return u(n4, S);
}, E = function(S) {
return u(t, S);
};
R(Je[23][1], E, T, e, y), g(e, dl0);
} else
g(e, hl0);
u(f(e), kl0), u(f(e), wl0), a(f(e), Sl0, El0);
var h = i[3];
if (h) {
g(e, gl0);
var w = h[1], G = function(S, M) {
return g(S, cl0);
}, A = function(S) {
return u(t, S);
};
R(Dr[1], A, G, e, w), g(e, Fl0);
} else
g(e, Tl0);
return u(f(e), Ol0), u(f(e), Il0);
}), N(y00, function(t, n4, e) {
var i = a(Mm, t, n4);
return a(P0(ol0), i, e);
});
var d00 = [0, MD, _00, Mm, y00], BD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, h00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Bm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, k00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(BD, function(t, n4, e, i) {
u(f(e), fl0), a(t, e, i[1]), u(f(e), xl0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Bm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), al0);
}), N(h00, function(t, n4, e) {
var i = a(BD, t, n4);
return a(P0(il0), i, e);
}), N(Bm, function(t, n4, e, i) {
u(f(e), z20), a(f(e), W20, K20);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(Ln[1], s, c, e, x), u(f(e), J20), u(f(e), $20), a(f(e), Q20, Z20);
var p = i[2];
if (p) {
g(e, rl0);
var y = p[1], T = function(h) {
return u(n4, h);
}, E = function(h) {
return u(t, h);
};
R(Je[23][1], E, T, e, y), g(e, el0);
} else
g(e, nl0);
return u(f(e), tl0), u(f(e), ul0);
}), N(k00, function(t, n4, e) {
var i = a(Bm, t, n4);
return a(P0(V20), i, e);
});
var w00 = [0, BD, h00, Bm, k00], qD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, E00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, qm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, S00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(qD, function(t, n4, e, i) {
u(f(e), H20), a(t, e, i[1]), u(f(e), X20);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(qm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Y20);
}), N(E00, function(t, n4, e) {
var i = a(qD, t, n4);
return a(P0(U20), i, e);
}), N(qm, function(t, n4, e, i) {
u(f(e), O20), a(f(e), A20, I20);
var x = i[1];
u(f(e), N20);
var c = 0;
be(function(E, h) {
E && u(f(e), T20);
function w(A) {
return u(n4, A);
}
function G(A) {
return u(t, A);
}
return R(w00[1], G, w, e, h), 1;
}, c, x), u(f(e), C20), u(f(e), P20), u(f(e), D20), a(f(e), R20, L20);
var s = i[2];
if (s) {
g(e, j20);
var p = s[1], y = function(E, h) {
return g(E, F20);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, G20);
} else
g(e, M20);
return u(f(e), B20), u(f(e), q20);
}), N(S00, function(t, n4, e) {
var i = a(qm, t, n4);
return a(P0(g20), i, e);
});
var g00 = [0, w00, qD, E00, qm, S00], UD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, F00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Um = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, T00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Hm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, O00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(UD, function(t, n4, e, i) {
u(f(e), w20), a(t, e, i[1]), u(f(e), E20);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Um, function(s) {
return u(t, s);
}, c, e, x), u(f(e), S20);
}), N(F00, function(t, n4, e) {
var i = a(UD, t, n4);
return a(P0(k20), i, e);
}), N(Um, function(t, n4, e, i) {
u(f(e), x20), a(f(e), o20, a20);
var x = i[1];
u(f(e), c20);
var c = 0;
be(function(E, h) {
E && u(f(e), f20);
function w(G) {
return u(n4, G);
}
return R(Hm, function(G) {
return u(t, G);
}, w, e, h), 1;
}, c, x), u(f(e), s20), u(f(e), v20), u(f(e), l20), a(f(e), p20, b20);
var s = i[2];
if (s) {
g(e, m20);
var p = s[1], y = function(E, h) {
return g(E, i20);
}, T = function(E) {
return u(t, E);
};
R(Dr[1], T, y, e, p), g(e, _20);
} else
g(e, y20);
return u(f(e), d20), u(f(e), h20);
}), N(T00, function(t, n4, e) {
var i = a(Um, t, n4);
return a(P0(u20), i, e);
}), N(Hm, function(t, n4, e, i) {
switch (i[0]) {
case 0:
u(f(e), Zv0);
var x = i[1], c = function(G) {
return u(n4, G);
}, s = function(G) {
return u(t, G);
};
return R(o00[1], s, c, e, x), u(f(e), Qv0);
case 1:
u(f(e), r20);
var p = i[1], y = function(G) {
return u(n4, G);
}, T = function(G) {
return u(t, G);
};
return R(l00[1], T, y, e, p), u(f(e), e20);
default:
u(f(e), n20);
var E = i[1], h = function(G) {
return u(n4, G);
}, w = function(G) {
return u(t, G);
};
return R(m00[1], w, h, e, E), u(f(e), t20);
}
}), N(O00, function(t, n4, e) {
var i = a(Hm, t, n4);
return a(P0($v0), i, e);
});
var HD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, I00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Xm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, A00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Bee = [0, UD, F00, Um, T00, Hm, O00];
N(HD, function(t, n4, e, i) {
u(f(e), Kv0), a(t, e, i[1]), u(f(e), Wv0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Xm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Jv0);
}), N(I00, function(t, n4, e) {
var i = a(HD, t, n4);
return a(P0(zv0), i, e);
}), N(Xm, function(t, n4, e, i) {
u(f(e), Lv0), a(f(e), jv0, Rv0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(qe[31], s, c, e, x), u(f(e), Gv0), u(f(e), Mv0), a(f(e), qv0, Bv0);
var p = i[2];
if (p) {
g(e, Uv0);
var y = p[1], T = function(h, w) {
return g(h, Dv0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, Hv0);
} else
g(e, Xv0);
return u(f(e), Yv0), u(f(e), Vv0);
}), N(A00, function(t, n4, e) {
var i = a(Xm, t, n4);
return a(P0(Pv0), i, e);
});
var N00 = [0, HD, I00, Xm, A00], XD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, C00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(XD, function(t, n4, e, i) {
u(f(e), q10), a(f(e), H10, U10);
var x = i[1];
if (x) {
g(e, X10);
var c = x[1], s = function(w0) {
return u(n4, w0);
}, p = function(w0) {
return u(t, w0);
};
R(Ln[1], p, s, e, c), g(e, Y10);
} else
g(e, V10);
u(f(e), z10), u(f(e), K10), a(f(e), J10, W10);
var y = i[2];
function T(w0) {
return u(n4, w0);
}
function E(w0) {
return u(t, w0);
}
R(T1[6][1], E, T, e, y), u(f(e), $10), u(f(e), Z10), a(f(e), rv0, Q10);
var h = i[3];
if (h) {
g(e, ev0);
var w = h[1], G = function(w0) {
return u(n4, w0);
}, A = function(w0) {
return u(t, w0);
};
R(Je[22][1], A, G, e, w), g(e, nv0);
} else
g(e, tv0);
u(f(e), uv0), u(f(e), iv0), a(f(e), xv0, fv0);
var S = i[4];
if (S) {
g(e, av0);
var M = S[1], K = function(w0) {
return u(n4, w0);
}, V = function(w0) {
return u(t, w0);
};
R(d00[1], V, K, e, M), g(e, ov0);
} else
g(e, cv0);
u(f(e), sv0), u(f(e), vv0), a(f(e), bv0, lv0);
var f0 = i[5];
if (f0) {
g(e, pv0);
var m0 = f0[1], k0 = function(w0) {
return u(n4, w0);
}, g0 = function(w0) {
return u(t, w0);
};
R(g00[2], g0, k0, e, m0), g(e, mv0);
} else
g(e, _v0);
u(f(e), yv0), u(f(e), dv0), a(f(e), kv0, hv0);
var e0 = i[6];
u(f(e), wv0);
var x0 = 0;
be(function(w0, _0) {
w0 && u(f(e), B10);
function E0(b) {
return u(n4, b);
}
function X0(b) {
return u(t, b);
}
return R(N00[1], X0, E0, e, _0), 1;
}, x0, e0), u(f(e), Ev0), u(f(e), Sv0), u(f(e), gv0), a(f(e), Tv0, Fv0);
var l = i[7];
if (l) {
g(e, Ov0);
var c0 = l[1], t0 = function(w0, _0) {
return g(w0, M10);
}, a0 = function(w0) {
return u(t, w0);
};
R(Dr[1], a0, t0, e, c0), g(e, Iv0);
} else
g(e, Av0);
return u(f(e), Nv0), u(f(e), Cv0);
}), N(C00, function(t, n4, e) {
var i = a(XD, t, n4);
return a(P0(G10), i, e);
}), pu(p6r, T1, [0, o00, l00, m00, d00, g00, Bee, N00, XD, C00]);
var YD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, P00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Ym = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, D00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(YD, function(t, n4, e, i) {
u(f(e), L10), a(t, e, i[1]), u(f(e), R10);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Ym, function(s) {
return u(t, s);
}, c, e, x), u(f(e), j10);
}), N(P00, function(t, n4, e) {
var i = a(YD, t, n4);
return a(P0(D10), i, e);
}), N(Ym, function(t, n4, e, i) {
u(f(e), w10), a(f(e), S10, E10);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(di[5], s, c, e, x), u(f(e), g10), u(f(e), F10), a(f(e), O10, T10);
var p = i[2];
if (p) {
g(e, I10);
var y = p[1], T = function(h, w) {
return g(h, k10);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, A10);
} else
g(e, N10);
return u(f(e), C10), u(f(e), P10);
}), N(D00, function(t, n4, e) {
var i = a(Ym, t, n4);
return a(P0(h10), i, e);
});
var L00 = [0, YD, P00, Ym, D00], VD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, R00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Vm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, j00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(VD, function(t, n4, e, i) {
u(f(e), _10), a(t, e, i[1]), u(f(e), y10);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Vm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), d10);
}), N(R00, function(t, n4, e) {
var i = a(VD, t, n4);
return a(P0(m10), i, e);
}), N(Vm, function(t, n4, e, i) {
u(f(e), u10), a(f(e), f10, i10);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(di[5], s, c, e, x), u(f(e), x10), u(f(e), a10), a(f(e), c10, o10);
var p = i[2];
if (p) {
g(e, s10);
var y = p[1], T = function(h) {
return u(n4, h);
}, E = function(h) {
return u(t, h);
};
R(qe[31], E, T, e, y), g(e, v10);
} else
g(e, l10);
return u(f(e), b10), u(f(e), p10);
}), N(j00, function(t, n4, e) {
var i = a(Vm, t, n4);
return a(P0(t10), i, e);
});
var G00 = [0, VD, R00, Vm, j00], zD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, M00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, zm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, B00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(zD, function(t, n4, e, i) {
u(f(e), r10), a(t, e, i[1]), u(f(e), e10);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(zm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), n10);
}), N(M00, function(t, n4, e) {
var i = a(zD, t, n4);
return a(P0(Qs0), i, e);
}), N(zm, function(t, n4, e, i) {
u(f(e), qs0), a(f(e), Hs0, Us0);
var x = i[1];
function c(h) {
return u(n4, h);
}
function s(h) {
return u(t, h);
}
R(Je[17], s, c, e, x), u(f(e), Xs0), u(f(e), Ys0), a(f(e), zs0, Vs0);
var p = i[2];
if (p) {
g(e, Ks0);
var y = p[1], T = function(h, w) {
return g(h, Bs0);
}, E = function(h) {
return u(t, h);
};
R(Dr[1], E, T, e, y), g(e, Ws0);
} else
g(e, Js0);
return u(f(e), $s0), u(f(e), Zs0);
}), N(B00, function(t, n4, e) {
var i = a(zm, t, n4);
return a(P0(Ms0), i, e);
});
var q00 = [0, zD, M00, zm, B00], KD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, U00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Km = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, H00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(KD, function(t, n4, e, i) {
u(f(e), Rs0), a(t, e, i[1]), u(f(e), js0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Km, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Gs0);
}), N(U00, function(t, n4, e) {
var i = a(KD, t, n4);
return a(P0(Ls0), i, e);
}), N(Km, function(t, n4, e, i) {
u(f(e), xs0), a(f(e), os0, as0);
var x = i[1];
if (x) {
g(e, cs0);
var c = x[1], s = function(V) {
return u(n4, V);
}, p = function(V) {
return u(t, V);
};
R(q00[1], p, s, e, c), g(e, ss0);
} else
g(e, vs0);
u(f(e), ls0), u(f(e), bs0), a(f(e), ms0, ps0);
var y = i[2];
u(f(e), _s0);
var T = 0;
be(function(V, f0) {
V && u(f(e), fs0);
function m0(g0) {
return u(n4, g0);
}
function k0(g0) {
return u(t, g0);
}
return R(G00[1], k0, m0, e, f0), 1;
}, T, y), u(f(e), ys0), u(f(e), ds0), u(f(e), hs0), a(f(e), ws0, ks0);
var E = i[3];
if (E) {
g(e, Es0);
var h = E[1], w = function(V) {
return u(n4, V);
}, G = function(V) {
return u(t, V);
};
R(L00[1], G, w, e, h), g(e, Ss0);
} else
g(e, gs0);
u(f(e), Fs0), u(f(e), Ts0), a(f(e), Is0, Os0);
var A = i[4];
if (A) {
g(e, As0);
var S = A[1], M = function(V, f0) {
u(f(V), us0);
var m0 = 0;
return be(function(k0, g0) {
k0 && u(f(V), ts0);
function e0(x0) {
return u(t, x0);
}
return ir(uu[1], e0, V, g0), 1;
}, m0, f0), u(f(V), is0);
}, K = function(V) {
return u(t, V);
};
R(Dr[1], K, M, e, S), g(e, Ns0);
} else
g(e, Cs0);
return u(f(e), Ps0), u(f(e), Ds0);
}), N(H00, function(t, n4, e) {
var i = a(Km, t, n4);
return a(P0(ns0), i, e);
});
var X00 = [0, KD, U00, Km, H00], WD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, Y00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Wm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, V00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(WD, function(t, n4, e, i) {
u(f(e), ec0), a(f(e), tc0, nc0);
var x = i[1];
if (x) {
g(e, uc0);
var c = x[1], s = function(_0) {
return u(n4, _0);
}, p = function(_0) {
return u(t, _0);
};
R(Ln[1], p, s, e, c), g(e, ic0);
} else
g(e, fc0);
u(f(e), xc0), u(f(e), ac0), a(f(e), cc0, oc0);
var y = i[2];
function T(_0) {
return u(n4, _0);
}
function E(_0) {
return u(t, _0);
}
R(X00[1], E, T, e, y), u(f(e), sc0), u(f(e), vc0), a(f(e), bc0, lc0);
var h = i[3];
function w(_0) {
return u(n4, _0);
}
R(Wm, function(_0) {
return u(t, _0);
}, w, e, h), u(f(e), pc0), u(f(e), mc0), a(f(e), yc0, _c0);
var G = i[4];
a(f(e), dc0, G), u(f(e), hc0), u(f(e), kc0), a(f(e), Ec0, wc0);
var A = i[5];
a(f(e), Sc0, A), u(f(e), gc0), u(f(e), Fc0), a(f(e), Oc0, Tc0);
var S = i[6];
if (S) {
g(e, Ic0);
var M = S[1], K = function(_0) {
return u(n4, _0);
}, V = function(_0) {
return u(t, _0);
};
R(Je[24][1], V, K, e, M), g(e, Ac0);
} else
g(e, Nc0);
u(f(e), Cc0), u(f(e), Pc0), a(f(e), Lc0, Dc0);
var f0 = i[7];
function m0(_0) {
return u(n4, _0);
}
function k0(_0) {
return u(t, _0);
}
R(Je[19], k0, m0, e, f0), u(f(e), Rc0), u(f(e), jc0), a(f(e), Mc0, Gc0);
var g0 = i[8];
if (g0) {
g(e, Bc0);
var e0 = g0[1], x0 = function(_0) {
return u(n4, _0);
}, l = function(_0) {
return u(t, _0);
};
R(Je[22][1], l, x0, e, e0), g(e, qc0);
} else
g(e, Uc0);
u(f(e), Hc0), u(f(e), Xc0), a(f(e), Vc0, Yc0);
var c0 = i[9];
if (c0) {
g(e, zc0);
var t0 = c0[1], a0 = function(_0, E0) {
return g(_0, rc0);
}, w0 = function(_0) {
return u(t, _0);
};
R(Dr[1], w0, a0, e, t0), g(e, Kc0);
} else
g(e, Wc0);
return u(f(e), Jc0), u(f(e), $c0), a(f(e), Qc0, Zc0), a(t, e, i[10]), u(f(e), rs0), u(f(e), es0);
}), N(Y00, function(t, n4, e) {
var i = a(WD, t, n4);
return a(P0(Qo0), i, e);
}), N(Wm, function(t, n4, e, i) {
if (i[0] === 0) {
var x = i[1];
u(f(e), Vo0), u(f(e), zo0), a(t, e, x[1]), u(f(e), Ko0);
var c = x[2], s = function(h) {
return u(n4, h);
}, p = function(h) {
return u(t, h);
};
return R(Xu[1][1], p, s, e, c), u(f(e), Wo0), u(f(e), Jo0);
}
u(f(e), $o0);
var y = i[1];
function T(h) {
return u(n4, h);
}
function E(h) {
return u(t, h);
}
return R(qe[31], E, T, e, y), u(f(e), Zo0);
}), N(V00, function(t, n4, e) {
var i = a(Wm, t, n4);
return a(P0(Yo0), i, e);
}), pu(m6r, Ps, [0, L00, G00, q00, X00, WD, Y00, Wm, V00]);
var JD = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, z00 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Jm = function t(n4, e, i, x) {
return t.fun(n4, e, i, x);
}, K00 = function t(n4, e, i) {
return t.fun(n4, e, i);
};
N(JD, function(t, n4, e, i) {
u(f(e), Uo0), a(t, e, i[1]), u(f(e), Ho0);
var x = i[2];
function c(s) {
return u(n4, s);
}
return R(Jm, function(s) {
return u(t, s);
}, c, e, x), u(f(e), Xo0);
}), N(z00, function(t, n4, e) {
var i = a(JD, t, n4);
return a(P0(qo0), i, e);
}), N(Jm, function(t, n4, e, i) {
u(f(e), ko0), a(f(e), Eo0, wo0);
var x = i[1];
u(f(e), So0);
var c = 0;
be(function(w, G) {
w && u(f(e), ho0);
function A(M) {
return u(n4, M);
}
function S(M) {
return u(t, M);
}
return R(Xu[35], S, A, e, G), 1;
}, c, x), u(f(e), go0), u(f(e), Fo0), u(f(e), To0), a(f(e), Io0, Oo0);
var s = i[2];
if (s) {
g(e, Ao0);
var p = s[1], y = function(w, G) {
return g(w, do0);
}, T = function(w) {
return u(t, w);
};
R(Dr[1], T, y, e, p), g(e, No0);
} else
g(e, Co0);
u(f(e), Po0), u(f(e), Do0), a(f(e), Ro0, Lo0);
var E = i[3];
u(f(e), jo0);
var h = 0;
return be(function(w, G) {
w && u(f(e), yo0);
function A(S) {
return u(t, S);
}
return ir(uu[1], A, e, G), 1;
}, h, E), u(f(e), Go0), u(f(e), Mo0), u(f(e), Bo0);
}), N(K00, function(t, n4, e) {
var i = a(Jm, t, n4);
return a(P0(_o0), i, e);
}), pu(_6r, Ree, [0, JD, z00, Jm, K00]);
function ze(t, n4) {
if (n4) {
var e = n4[1], i = u(t, e);
return e === i ? n4 : [0, i];
}
return n4;
}
function te(t, n4, e, i, x) {
var c = a(t, n4, e);
return e === c ? i : u(x, c);
}
function ee(t, n4, e, i) {
var x = u(t, n4);
return n4 === x ? e : u(i, x);
}
function mu(t, n4) {
var e = n4[1];
function i(x) {
return [0, e, x];
}
return te(t, e, n4[2], n4, i);
}
function Un(t, n4) {
var e = be(function(i, x) {
var c = u(t, x), s = i[2], p = s || (c !== x ? 1 : 0);
return [0, [0, c, i[1]], p];
}, O6r, n4);
return e[2] ? de(e[1]) : n4;
}
var $D = jp(A6r, function(t) {
var n4 = DN(t, I6r), e = n4[1], i = n4[2], x = n4[3], c = n4[4], s = n4[5], p = n4[6], y = n4[7], T = n4[8], E = n4[9], h = n4[10], w = n4[11], G = n4[12], A = n4[13], S = n4[14], M = n4[15], K = n4[16], V = n4[17], f0 = n4[18], m0 = n4[19], k0 = n4[20], g0 = n4[21], e0 = n4[22], x0 = n4[23], l = n4[24], c0 = n4[25], t0 = n4[26], a0 = n4[27], w0 = n4[28], _0 = n4[29], E0 = n4[30], X0 = n4[31], b = n4[32], G0 = n4[33], X = n4[34], s0 = n4[35], dr = n4[36], Ar = n4[37], ar = n4[38], W0 = n4[39], Lr = n4[40], Tr = n4[41], Hr = n4[42], Or = n4[43], xr = n4[44], Rr = n4[45], Wr = n4[46], Jr = n4[47], or = n4[49], _r = n4[50], Ir = n4[51], fe = n4[52], v0 = n4[53], P = n4[54], L = n4[55], Q = n4[56], i0 = n4[57], l0 = n4[58], S0 = n4[59], T0 = n4[60], rr = n4[61], R0 = n4[62], B = n4[63], Z = n4[65], p0 = n4[66], b0 = n4[67], O0 = n4[68], q0 = n4[69], er = n4[70], yr = n4[71], vr = n4[72], $0 = n4[73], Sr = n4[74], Mr = n4[75], Br = n4[76], qr = n4[77], jr = n4[78], $r = n4[79], ne = n4[80], Qr = n4[81], pe = n4[82], oe = n4[83], me = n4[84], ae = n4[85], ce = n4[86], ge = n4[87], H0 = n4[88], Fr = n4[89], _ = n4[90], k = n4[91], I = n4[92], U = n4[93], Y = n4[94], y0 = n4[95], D0 = n4[96], I0 = n4[97], D = n4[98], u0 = n4[99], Y0 = n4[ni], J0 = n4[L7], fr = n4[Ri], Q0 = n4[c7], F0 = n4[D7], gr = n4[R7], mr = n4[Xt], Cr = n4[Qc], sr = n4[fs2], Pr = n4[Fv], K0 = n4[Ht], Ur = n4[vf], d0 = n4[F7], Kr = n4[Pn], re = n4[u1], xe = n4[Av], je = n4[x1], ve = n4[A2], Ie = n4[z2], Me = n4[Sv], Be = n4[fc], fn = n4[tl], Ke = n4[In], Ae = n4[us], xn = n4[X2], Qe = n4[br], yn = n4[DX], on = n4[zn], Ce = n4[Rt], We = n4[eV], rn = n4[Jw], bn = n4[Qg], Cn = n4[YH], Hn = n4[133], Sn = n4[134], vt = n4[135], At = n4[QH], gt = n4[137], Jt = n4[OH], Bt = n4[139], Ft = n4[gH], Nt = n4[141], du = n4[142], Ku = n4[143], lt = n4[cV], xu = n4[145], Mu = n4[146], z7 = n4[MX], Yi = n4[148], a7 = n4[fH], Yc = n4[150], K7 = n4[151], qt = n4[152], bt = n4[153], U0 = n4[NH], L0 = n4[155], Re = n4[156], He = n4[157], he = n4[158], _e = n4[159], Zn = n4[sY], dn = n4[WU], it = n4[Sd], ft = n4[Mn], Rn = n4[PF], nt = n4[nY], ht = n4[MY], tn = n4[DT], cn = n4[DY], tt = n4[RX], Tt = n4[Xg], Fi = n4[yg], hs = n4[BU], Iu = n4[wY], Vs = n4[nH], Vi = n4[dX], zs = n4[kV], Ks = n4[oV], en = n4[OO], ci = n4[qY], Ws = n4[mU], c2 = n4[Ai], B9 = n4[Kg], q9 = n4[mS], U9 = n4[wk], Js = n4[AU], s2 = n4[dh], H9 = n4[iw], X9 = n4[cY], Y9 = n4[sX], X1 = n4[PY], si = n4[yX], ob = n4[at], cb = n4[VT], sb = n4[iI], V9 = n4[vY], z9 = n4[WX], K9 = n4[SY], vb = n4[_H], W9 = n4[uX], J9 = n4[RU], $9 = n4[mY], Z9 = n4[xH], lb = n4[fV], Q9 = n4[rY], Y1 = n4[$H], v2 = n4[CH], bb = n4[LX], pb = n4[wH], mb = n4[Zg], Tn = n4[N6], jn = n4[EU], V1 = n4[EY], _b = n4[qX], yb = n4[dT], r_ = n4[cT], Vc = n4[d6], e_ = n4[sp], l2 = n4[Lw], db = n4[NU], zc = n4[aA], n_ = n4[HX], $s = n4[NX], hb = n4[d8], z1 = n4[dv], t_ = n4[HO], ks = n4[tk], u_ = n4[eX], K1 = n4[sV], i_ = n4[dU], b2 = n4[Bd], f_ = n4[VX], Zs = n4[eT], kb = n4[wT], Qs = n4[aH], x_ = n4[eH], zi = n4[mO], Kc = n4[YY], r1 = n4[pH], a_ = n4[f6], p2 = n4[v1], m2 = n4[Wy], _2 = n4[TT], o_ = n4[uH], e1 = n4[l8], c_ = n4[rV], y2 = n4[$2], XL = n4[48], W1 = n4[64];
function YL(o, F, m) {
var O = m[2], H = m[1], $ = ze(u(o[1][1 + en], o), H), r0 = a(o[1][1 + s0], o, O);
return O === r0 && H === $ ? m : [0, $, r0, m[3], m[4]];
}
function J1(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + Kc], o, r0), z0 = ze(u(o[1][1 + V], o), $), Nr = a(o[1][1 + t0], o, H), Gr = a(o[1][1 + s0], o, O);
return r0 === M0 && H === Nr && $ === z0 && O === Gr ? m : [0, M0, z0, Nr, Gr];
}
function VL(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + en], o, $), M0 = a(o[1][1 + Or], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function $1(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + _r], o, $), M0 = a(o[1][1 + Or], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function zL(o, F, m) {
var O = m[2], H = O[2], $ = O[1], r0 = ir(o[1][1 + p], o, F, $), M0 = ze(u(o[1][1 + en], o), H);
return $ === r0 && H === M0 ? m : [0, m[1], [0, r0, M0]];
}
function Ti(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = Un(a(o[1][1 + y], o, H), $), M0 = a(o[1][1 + s0], o, O);
return $ === r0 && O === M0 ? m : [0, r0, H, M0];
}
function KL(o, F, m) {
var O = m[4], H = m[2], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, m[1], $, m[3], r0];
}
function WL(o, F, m) {
var O = m[3], H = m[2], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, m[1], $, r0];
}
function d2(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + en], o, $), M0 = a(o[1][1 + l], o, H), z0 = a(o[1][1 + s0], o, O);
return r0 === $ && M0 === H && z0 === O ? m : [0, r0, M0, z0];
}
function JL(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = mu(u(o[1][1 + zi], o), r0);
if ($)
var z0 = $[1], Nr = z0[1], Gr = function($t) {
return [0, [0, Nr, $t]];
}, Fe = z0[2], ye = te(u(o[1][1 + K1], o), Nr, Fe, $, Gr);
else
var ye = $;
if (H)
var Dn = H[1], pn = Dn[1], xt = function($t) {
return [0, [0, pn, $t]];
}, pt = Dn[2], kt = te(u(o[1][1 + zi], o), pn, pt, H, xt);
else
var kt = H;
var Kn = a(o[1][1 + s0], o, O);
return r0 === M0 && $ === ye && H === kt && O === Kn ? m : [0, M0, ye, kt, Kn];
}
function Z1(o, F, m) {
var O = m[2], H = m[1], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function $L(o, F, m) {
var O = m[1], H = a(o[1][1 + s0], o, O);
return O === H ? m : [0, H];
}
function Q1(o, F) {
return F;
}
function ZL(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = Un(u(o[1][1 + b], o), $), M0 = Un(u(o[1][1 + en], o), H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function wb(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + en], o, $), M0 = mu(u(o[1][1 + G0], o), H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function QL(o, F) {
var m = F[2], O = m[3], H = m[2], $ = m[1], r0 = ze(u(o[1][1 + en], o), $), M0 = a(o[1][1 + Tr], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? F : [0, F[1], [0, r0, M0, z0]];
}
function Eb(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + en], o, $), M0 = Un(u(o[1][1 + Ar], o), H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0, m[4]];
}
function rR(o, F, m) {
var O = m[1], H = a(o[1][1 + s0], o, O);
return O === H ? m : [0, H];
}
function eR(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function h2(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function nR(o, F) {
return [0, a(o[1][1 + Or], o, F), 0];
}
function tR(o, F) {
var m = u(o[1][1 + Hr], o), O = be(function(H, $) {
var r0 = H[1], M0 = u(m, $);
if (M0) {
if (M0[2])
return [0, jc(M0, r0), 1];
var z0 = M0[1], Nr = H[2], Gr = Nr || ($ !== z0 ? 1 : 0);
return [0, [0, z0, r0], Gr];
}
return [0, r0, 1];
}, T6r, F);
return O[2] ? de(O[1]) : F;
}
function s_(o, F) {
return a(o[1][1 + Tr], o, F);
}
function uR(o, F, m) {
var O = m[2], H = m[1], $ = Un(u(o[1][1 + en], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function k2(o, F, m) {
var O = m[2], H = m[1], $ = ze(u(o[1][1 + en], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0, m[3]];
}
function iR(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + Re], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function w2(o, F) {
return a(o[1][1 + en], o, F);
}
function fR(o, F) {
var m = F[2], O = m[2], H = m[1];
if (H)
var $ = function(Nr) {
return [0, Nr];
}, r0 = H[1], M0 = ee(u(o[1][1 + en], o), r0, H, $);
else
var M0 = H;
var z0 = a(o[1][1 + s0], o, O);
return H === M0 && O === z0 ? F : [0, F[1], [0, M0, z0]];
}
function rv(o, F) {
return a(o[1][1 + en], o, F);
}
function xR(o, F, m) {
return ir(o[1][1 + er], o, F, m);
}
function Sb(o, F, m) {
return ir(o[1][1 + er], o, F, m);
}
function aR(o, F, m) {
var O = m[2], H = O[2], $ = O[1], r0 = ir(o[1][1 + Z], o, F, $), M0 = a(o[1][1 + s0], o, H);
return r0 === $ && H === M0 ? m : [0, m[1], [0, r0, M0]];
}
function gb(o, F, m) {
return ir(o[1][1 + er], o, F, m);
}
function oR(o, F, m) {
var O = m[2], H = O[2], $ = O[1], r0 = ir(o[1][1 + b0], o, F, $), M0 = ze(u(o[1][1 + en], o), H);
return $ === r0 && H === M0 ? m : [0, m[1], [0, r0, M0]];
}
function Fb(o, F, m) {
switch (m[0]) {
case 0:
var O = function(M0) {
return [0, M0];
}, H = m[1];
return ee(a(o[1][1 + O0], o, F), H, m, O);
case 1:
var $ = function(M0) {
return [1, M0];
}, r0 = m[1];
return ee(a(o[1][1 + p0], o, F), r0, m, $);
default:
return m;
}
}
function cR(o, F, m) {
return ir(o[1][1 + er], o, F, m);
}
function Gn(o, F, m) {
return ir(o[1][1 + er], o, F, m);
}
function v_(o, F, m) {
var O = m[2], H = O[2], $ = O[1], r0 = ir(o[1][1 + fe], o, F, $), M0 = a(o[1][1 + s0], o, H);
return r0 === $ && H === M0 ? m : [0, m[1], [0, r0, M0]];
}
function sR(o, F, m) {
return a(o[1][1 + Tn], o, m);
}
function vR(o, F, m) {
return ir(o[1][1 + R0], o, F, m);
}
function ev(o, F, m) {
var O = m[1];
function H(r0) {
return [0, O, r0];
}
var $ = m[2];
return te(a(o[1][1 + rr], o, F), O, $, m, H);
}
function Tb(o, F, m) {
switch (m[0]) {
case 0:
var O = function(Nr) {
return [0, Nr];
}, H = m[1];
return ee(a(o[1][1 + L], o, F), H, m, O);
case 1:
var $ = function(Nr) {
return [1, Nr];
}, r0 = m[1];
return ee(a(o[1][1 + i0], o, F), r0, m, $);
default:
var M0 = function(Nr) {
return [2, Nr];
}, z0 = m[1];
return ee(a(o[1][1 + l0], o, F), z0, m, M0);
}
}
function l_(o, F, m) {
var O = m[2], H = O[4], $ = O[3], r0 = O[2], M0 = O[1], z0 = ir(o[1][1 + Q], o, F, M0), Nr = ir(o[1][1 + P], o, F, r0), Gr = ze(u(o[1][1 + en], o), $);
if (H) {
var Fe = 0;
if (z0[0] === 1) {
var ye = Nr[2];
if (ye[0] === 2)
var pn = qn(z0[1][2][1], ye[1][1][2][1]);
else
Fe = 1;
} else
Fe = 1;
if (Fe)
var Dn = M0 === z0 ? 1 : 0, pn = Dn && (r0 === Nr ? 1 : 0);
} else
var pn = H;
return z0 === M0 && Nr === r0 && Gr === $ && H === pn ? m : [0, m[1], [0, z0, Nr, Gr, pn]];
}
function Ob(o, F, m) {
if (m[0] === 0) {
var O = function(M0) {
return [0, M0];
}, H = m[1];
return ee(a(o[1][1 + S0], o, F), H, m, O);
}
function $(M0) {
return [1, M0];
}
var r0 = m[1];
return ee(a(o[1][1 + v0], o, F), r0, m, $);
}
function lR(o, F, m, O) {
return ir(o[1][1 + J0], o, m, O);
}
function b_(o, F, m) {
return a(o[1][1 + lt], o, m);
}
function bR(o, F, m) {
var O = m[2];
switch (O[0]) {
case 0:
var H = O[1], $ = H[3], r0 = H[2], M0 = H[1], z0 = Un(a(o[1][1 + T0], o, F), M0), Nr = a(o[1][1 + x0], o, r0), Gr = a(o[1][1 + s0], o, $), Fe = 0;
if (z0 === M0 && Nr === r0 && Gr === $) {
var ye = O;
Fe = 1;
}
if (!Fe)
var ye = [0, [0, z0, Nr, Gr]];
var Ji = ye;
break;
case 1:
var Dn = O[1], pn = Dn[3], xt = Dn[2], pt = Dn[1], kt = Un(a(o[1][1 + q0], o, F), pt), Kn = a(o[1][1 + x0], o, xt), $t = a(o[1][1 + s0], o, pn), W7 = 0;
if (pn === $t && kt === pt && Kn === xt) {
var J7 = O;
W7 = 1;
}
if (!W7)
var J7 = [1, [0, kt, Kn, $t]];
var Ji = J7;
break;
case 2:
var w7 = O[1], $7 = w7[2], Z7 = w7[1], Q7 = ir(o[1][1 + R0], o, F, Z7), ri = a(o[1][1 + x0], o, $7), ei = 0;
if (Z7 === Q7 && $7 === ri) {
var Wi = O;
ei = 1;
}
if (!ei)
var Wi = [2, [0, Q7, ri, w7[3]]];
var Ji = Wi;
break;
default:
var uv = function(fv) {
return [3, fv];
}, iv = O[1], Ji = ee(u(o[1][1 + B], o), iv, O, uv);
}
return O === Ji ? m : [0, m[1], Ji];
}
function p_(o, F) {
return ir(o[1][1 + er], o, 0, F);
}
function Ib(o, F, m) {
var O = F && F[1];
return ir(o[1][1 + er], o, [0, O], m);
}
function m_(o, F) {
return a(o[1][1 + m2], o, F);
}
function pR(o, F) {
return a(o[1][1 + m2], o, F);
}
function __(o, F) {
return ir(o[1][1 + r1], o, F6r, F);
}
function Ab(o, F, m) {
return ir(o[1][1 + r1], o, [0, F], m);
}
function mR(o, F) {
return ir(o[1][1 + r1], o, g6r, F);
}
function _R(o, F, m) {
var O = m[5], H = m[4], $ = m[3], r0 = m[2], M0 = m[1], z0 = a(o[1][1 + Kc], o, M0), Nr = ze(u(o[1][1 + V], o), r0), Gr = ze(u(o[1][1 + t0], o), $), Fe = ze(u(o[1][1 + t0], o), H), ye = a(o[1][1 + s0], o, O);
return M0 === z0 && $ === Gr && r0 === Nr && $ === Gr && H === Fe && O === ye ? m : [0, z0, Nr, Gr, Fe, ye];
}
function yR(o, F) {
return a(o[1][1 + Tn], o, F);
}
function Nb(o, F) {
return a(o[1][1 + lt], o, F);
}
function dR(o, F) {
var m = F[1];
function O($) {
return [0, m, $];
}
var H = F[2];
return te(u(o[1][1 + J0], o), m, H, F, O);
}
function hR(o, F) {
switch (F[0]) {
case 0:
var m = function(Gr) {
return [0, Gr];
}, O = F[1];
return ee(u(o[1][1 + pe], o), O, F, m);
case 1:
var H = function(Gr) {
return [1, Gr];
}, $ = F[1];
return ee(u(o[1][1 + oe], o), $, F, H);
case 2:
var r0 = function(Gr) {
return [2, Gr];
}, M0 = F[1];
return ee(u(o[1][1 + or], o), M0, F, r0);
default:
var z0 = function(Gr) {
return [3, Gr];
}, Nr = F[1];
return ee(u(o[1][1 + me], o), Nr, F, z0);
}
}
function y_(o, F) {
var m = F[2], O = F[1];
switch (m[0]) {
case 0:
var H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + ae], o, r0), z0 = a(o[1][1 + en], o, $);
if (H) {
var Nr = 0;
if (M0[0] === 1) {
var Gr = z0[2];
if (Gr[0] === 10)
var ye = qn(M0[1][2][1], Gr[1][2][1]);
else
Nr = 1;
} else
Nr = 1;
if (Nr)
var Fe = r0 === M0 ? 1 : 0, ye = Fe && ($ === z0 ? 1 : 0);
} else
var ye = H;
return r0 === M0 && $ === z0 && H === ye ? F : [0, O, [0, M0, z0, ye]];
case 1:
var Dn = m[2], pn = m[1], xt = a(o[1][1 + ae], o, pn), pt = mu(u(o[1][1 + _e], o), Dn);
return pn === xt && Dn === pt ? F : [0, O, [1, xt, pt]];
case 2:
var kt = m[3], Kn = m[2], $t = m[1], W7 = a(o[1][1 + ae], o, $t), J7 = mu(u(o[1][1 + _e], o), Kn), w7 = a(o[1][1 + s0], o, kt);
return $t === W7 && Kn === J7 && kt === w7 ? F : [0, O, [2, W7, J7, w7]];
default:
var $7 = m[3], Z7 = m[2], Q7 = m[1], ri = a(o[1][1 + ae], o, Q7), ei = mu(u(o[1][1 + _e], o), Z7), Wi = a(o[1][1 + s0], o, $7);
return Q7 === ri && Z7 === ei && $7 === Wi ? F : [0, O, [3, ri, ei, Wi]];
}
}
function kR(o, F, m) {
var O = m[2], H = m[1], $ = Un(function(M0) {
if (M0[0] === 0) {
var z0 = M0[1], Nr = a(o[1][1 + Qr], o, z0);
return z0 === Nr ? M0 : [0, Nr];
}
var Gr = M0[1], Fe = a(o[1][1 + xr], o, Gr);
return Gr === Fe ? M0 : [1, Fe];
}, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function Cb(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + en], o, r0), z0 = ze(u(o[1][1 + b2], o), $), Nr = ze(u(o[1][1 + Zs], o), H), Gr = a(o[1][1 + s0], o, O);
return r0 === M0 && $ === z0 && H === Nr && O === Gr ? m : [0, M0, z0, Nr, Gr];
}
function wR(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + lt], o, $), M0 = a(o[1][1 + lt], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function ER(o, F) {
return a(o[1][1 + en], o, F);
}
function d_(o, F) {
return a(o[1][1 + or], o, F);
}
function SR(o, F) {
return a(o[1][1 + lt], o, F);
}
function E2(o, F) {
switch (F[0]) {
case 0:
var m = function(z0) {
return [0, z0];
}, O = F[1];
return ee(u(o[1][1 + y0], o), O, F, m);
case 1:
var H = function(z0) {
return [1, z0];
}, $ = F[1];
return ee(u(o[1][1 + D], o), $, F, H);
default:
var r0 = function(z0) {
return [2, z0];
}, M0 = F[1];
return ee(u(o[1][1 + D0], o), M0, F, r0);
}
}
function gR(o, F, m) {
var O = m[1], H = ir(o[1][1 + u0], o, F, O);
return O === H ? m : [0, H, m[2], m[3]];
}
function FR(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + en], o, $), M0 = a(o[1][1 + I0], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function TR(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = a(o[1][1 + en], o, $), M0 = a(o[1][1 + en], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, m[1], r0, M0, z0];
}
function Pb(o, F, m) {
var O = m[3], H = a(o[1][1 + s0], o, O);
return O === H ? m : [0, m[1], m[2], H];
}
function OR(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + Q0], o, $), M0 = a(o[1][1 + Or], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function IR(o, F) {
var m = F[2], O = m[2], H = a(o[1][1 + s0], o, O);
return O === H ? F : [0, F[1], [0, m[1], H]];
}
function Db(o, F) {
return a(o[1][1 + ve], o, F);
}
function AR(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + K0], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + Ur], o), $, F, H);
}
function NR(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + Pr], o, H), r0 = a(o[1][1 + d0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function hu(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + d0], o, H), r0 = a(o[1][1 + d0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function ku(o, F) {
return a(o[1][1 + Ur], o, F);
}
function Oi(o, F) {
return a(o[1][1 + sr], o, F);
}
function k7(o, F) {
return a(o[1][1 + d0], o, F);
}
function Ki(o, F) {
switch (F[0]) {
case 0:
var m = function(z0) {
return [0, z0];
}, O = F[1];
return ee(u(o[1][1 + ve], o), O, F, m);
case 1:
var H = function(z0) {
return [1, z0];
}, $ = F[1];
return ee(u(o[1][1 + xe], o), $, F, H);
default:
var r0 = function(z0) {
return [2, z0];
}, M0 = F[1];
return ee(u(o[1][1 + je], o), M0, F, r0);
}
}
function nv(o, F) {
var m = F[2], O = F[1], H = a(o[1][1 + en], o, O), $ = a(o[1][1 + s0], o, m);
return O === H && m === $ ? F : [0, H, $];
}
function Lb(o, F, m) {
var O = m[2], H = m[1], $ = a(o[1][1 + s0], o, O);
if (H) {
var r0 = H[1], M0 = a(o[1][1 + en], o, r0);
return r0 === M0 && O === $ ? m : [0, [0, M0], $];
}
return O === $ ? m : [0, 0, $];
}
function tv(o, F) {
var m = F[2], O = F[1];
switch (m[0]) {
case 0:
var H = function(ye) {
return [0, O, [0, ye]];
}, $ = m[1];
return te(u(o[1][1 + Me], o), O, $, F, H);
case 1:
var r0 = function(ye) {
return [0, O, [1, ye]];
}, M0 = m[1];
return te(u(o[1][1 + Kr], o), O, M0, F, r0);
case 2:
var z0 = function(ye) {
return [0, O, [2, ye]];
}, Nr = m[1];
return te(u(o[1][1 + re], o), O, Nr, F, z0);
case 3:
var Gr = function(ye) {
return [0, O, [3, ye]];
}, Fe = m[1];
return ee(u(o[1][1 + F0], o), Fe, F, Gr);
default:
return F;
}
}
function Rb(o, F) {
var m = F[2], O = Un(u(o[1][1 + Ke], o), m);
return m === O ? F : [0, F[1], O];
}
function jb(o, F, m) {
return ir(o[1][1 + J0], o, F, m);
}
function CR(o, F, m) {
return ir(o[1][1 + re], o, F, m);
}
function Mne(o, F) {
if (F[0] === 0) {
var m = F[1], O = function(z0) {
return [0, m, z0];
}, H = F[2];
return te(u(o[1][1 + Ae], o), m, H, F, O);
}
var $ = F[1];
function r0(z0) {
return [1, $, z0];
}
var M0 = F[2];
return te(u(o[1][1 + xn], o), $, M0, F, r0);
}
function Bne(o, F) {
return a(o[1][1 + sr], o, F);
}
function qne(o, F) {
return a(o[1][1 + d0], o, F);
}
function Une(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + on], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + yn], o), $, F, H);
}
function Hne(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + Ce], o, H), r0 = ze(u(o[1][1 + Qe], o), O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function Xne(o, F, m) {
var O = m[2], H = m[1], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function Yne(o, F) {
if (F[0] === 0) {
var m = function(z0) {
return [0, z0];
}, O = F[1];
return ee(u(o[1][1 + We], o), O, F, m);
}
var H = F[1], $ = H[1];
function r0(z0) {
return [1, [0, $, z0]];
}
var M0 = H[2];
return te(u(o[1][1 + gr], o), $, M0, F, r0);
}
function Vne(o, F) {
var m = F[2][1], O = a(o[1][1 + Ie], o, m);
return m === O ? F : [0, F[1], [0, O]];
}
function zne(o, F) {
var m = F[2], O = m[3], H = m[1], $ = a(o[1][1 + Ie], o, H), r0 = Un(u(o[1][1 + Cr], o), O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, m[2], r0]];
}
function Kne(o, F, m) {
var O = m[4], H = m[3], $ = a(o[1][1 + fn], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, m[1], m[2], $, r0];
}
function Wne(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + mr], o, r0), z0 = ze(u(o[1][1 + Be], o), $), Nr = a(o[1][1 + fn], o, H), Gr = a(o[1][1 + s0], o, O);
return r0 === M0 && $ === z0 && H === Nr && O === Gr ? m : [0, M0, z0, Nr, Gr];
}
function Jne(o, F, m, O) {
var H = 2 <= F ? a(o[1][1 + R0], o, S6r) : u(o[1][1 + Kc], o);
return u(H, O);
}
function $ne(o, F, m) {
var O = 2 <= F ? a(o[1][1 + R0], o, E6r) : u(o[1][1 + Kc], o);
return u(O, m);
}
function Zne(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = 0;
if (F) {
var M0 = 0;
if ($)
switch ($[1]) {
case 2:
break;
case 0:
r0 = 1, M0 = 2;
break;
default:
M0 = 1;
}
var z0 = 0;
switch (M0) {
case 2:
z0 = 1;
break;
case 0:
if (2 <= F) {
var Nr = 0, Gr = 0;
z0 = 1;
}
break;
}
if (!z0)
var Nr = 1, Gr = 0;
} else
r0 = 1;
if (r0)
var Nr = 1, Gr = 1;
var Fe = a(Gr ? o[1][1 + m0] : o[1][1 + lt], o, O);
if (H)
var ye = Nr ? u(o[1][1 + Kc], o) : a(o[1][1 + R0], o, w6r), Dn = function(xt) {
return [0, xt];
}, pn = ee(ye, H[1], H, Dn);
else
var pn = H;
return H === pn && O === Fe ? m : [0, $, pn, Fe];
}
function Qne(o, F, m) {
if (m[0] === 0) {
var O = m[1], H = Un(a(o[1][1 + gt], o, F), O);
return O === H ? m : [0, H];
}
var $ = m[1], r0 = $[1];
function M0(Nr) {
return [1, [0, r0, Nr]];
}
var z0 = $[2];
return te(a(o[1][1 + At], o, F), r0, z0, m, M0);
}
function rte(o, F, m) {
var O = m[5], H = m[4], $ = m[3], r0 = m[1], M0 = ze(a(o[1][1 + vt], o, r0), H), z0 = ze(a(o[1][1 + Jt], o, r0), $), Nr = a(o[1][1 + s0], o, O);
return H === M0 && $ === z0 && O === Nr ? m : [0, r0, m[2], z0, M0, Nr];
}
function ete(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + _r], o, r0), z0 = ir(o[1][1 + du], o, H !== 0 ? 1 : 0, $), Nr = u(o[1][1 + Ku], o), Gr = ze(function(ye) {
return mu(Nr, ye);
}, H), Fe = a(o[1][1 + s0], o, O);
return r0 === M0 && $ === z0 && H === Gr && O === Fe ? m : [0, M0, z0, Gr, Fe];
}
function nte(o, F, m) {
var O = m[2], H = m[1], $ = a(o[1][1 + Or], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function tte(o, F, m) {
return a(o[1][1 + Or], o, m);
}
function ute(o, F, m) {
var O = m[2], H = m[1], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function ite(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function fte(o, F) {
var m = F[2], O = m[2], H = a(o[1][1 + s0], o, O);
return O === H ? F : [0, F[1], [0, m[1], H]];
}
function xte(o, F, m) {
return ir(o[1][1 + Hn], o, F, m);
}
function ate(o, F, m) {
var O = m[5], H = m[4], $ = m[3], r0 = m[2], M0 = m[1], z0 = a(o[1][1 + Kc], o, M0), Nr = ze(u(o[1][1 + V], o), r0), Gr = u(o[1][1 + xu], o), Fe = Un(function(pn) {
return mu(Gr, pn);
}, $), ye = mu(u(o[1][1 + qr], o), H), Dn = a(o[1][1 + s0], o, O);
return z0 === M0 && Nr === r0 && Fe === $ && ye === H && Dn === O ? m : [0, z0, Nr, Fe, ye, Dn];
}
function ote(o, F) {
return a(o[1][1 + k0], o, F);
}
function cte(o, F) {
return a(o[1][1 + k0], o, F);
}
function ste(o, F) {
return a(o[1][1 + lt], o, F);
}
function vte(o, F) {
var m = F[2], O = m[2], H = a(o[1][1 + s0], o, O);
return O === H ? F : [0, F[1], [0, m[1], H]];
}
function lte(o, F, m) {
return m;
}
function bte(o, F) {
return ir(o[1][1 + R0], o, k6r, F);
}
function pte(o, F) {
var m = F[1];
function O($) {
return [0, m, $];
}
var H = F[2];
return te(u(o[1][1 + zi], o), m, H, F, O);
}
function mte(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + ft], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + en], o), $, F, H);
}
function _te(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + Re], o, H), r0 = ze(u(o[1][1 + en], o), O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function yte(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + l], o, H), r0 = a(o[1][1 + s0], o, O);
return $ === H && r0 === O ? F : [0, F[1], [0, $, r0]];
}
function dte(o, F) {
var m = F[2], O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = Un(u(o[1][1 + He], o), $), z0 = ze(u(o[1][1 + bt], o), H), Nr = ze(u(o[1][1 + K7], o), r0), Gr = a(o[1][1 + s0], o, O);
return $ === M0 && H === z0 && O === Gr && r0 === Nr ? F : [0, F[1], [0, Nr, M0, z0, Gr]];
}
function hte(o, F, m) {
var O = m[9], H = m[8], $ = m[7], r0 = m[6], M0 = m[3], z0 = m[2], Nr = m[1], Gr = ze(u(o[1][1 + he], o), Nr), Fe = a(o[1][1 + U0], o, z0), ye = a(o[1][1 + x0], o, $), Dn = a(o[1][1 + it], o, M0), pn = ze(u(o[1][1 + Ir], o), r0), xt = ze(u(o[1][1 + V], o), H), pt = a(o[1][1 + s0], o, O);
return Nr === Gr && z0 === Fe && M0 === Dn && r0 === pn && $ === ye && H === xt && O === pt ? m : [0, Gr, Fe, Dn, m[4], m[5], pn, ye, xt, pt, m[10]];
}
function kte(o, F, m) {
return ir(o[1][1 + Rn], o, F, m);
}
function wte(o, F, m) {
return ir(o[1][1 + _e], o, F, m);
}
function Ete(o, F, m) {
return ir(o[1][1 + Rn], o, F, m);
}
function Ste(o, F) {
if (F[0] === 0)
return F;
var m = F[1], O = a(o[1][1 + l], o, m);
return O === m ? F : [1, O];
}
function gte(o, F) {
var m = F[1];
function O($) {
return [0, m, $];
}
var H = F[2];
return ee(u(o[1][1 + t0], o), H, F, O);
}
function Fte(o, F) {
var m = F[2], O = F[1];
switch (m[0]) {
case 0:
var H = function($e) {
return [0, O, [0, $e]];
}, $ = m[1];
return ee(u(o[1][1 + s0], o), $, F, H);
case 1:
var r0 = function($e) {
return [0, O, [1, $e]];
}, M0 = m[1];
return ee(u(o[1][1 + s0], o), M0, F, r0);
case 2:
var z0 = function($e) {
return [0, O, [2, $e]];
}, Nr = m[1];
return ee(u(o[1][1 + s0], o), Nr, F, z0);
case 3:
var Gr = function($e) {
return [0, O, [3, $e]];
}, Fe = m[1];
return ee(u(o[1][1 + s0], o), Fe, F, Gr);
case 4:
var ye = function($e) {
return [0, O, [4, $e]];
}, Dn = m[1];
return ee(u(o[1][1 + s0], o), Dn, F, ye);
case 5:
var pn = function($e) {
return [0, O, [5, $e]];
}, xt = m[1];
return ee(u(o[1][1 + s0], o), xt, F, pn);
case 6:
var pt = function($e) {
return [0, O, [6, $e]];
}, kt = m[1];
return ee(u(o[1][1 + s0], o), kt, F, pt);
case 7:
var Kn = function($e) {
return [0, O, [7, $e]];
}, $t = m[1];
return ee(u(o[1][1 + s0], o), $t, F, Kn);
case 8:
var W7 = function($e) {
return [0, O, [8, $e]];
}, J7 = m[1];
return ee(u(o[1][1 + s0], o), J7, F, W7);
case 9:
var w7 = function($e) {
return [0, O, [9, $e]];
}, $7 = m[1];
return ee(u(o[1][1 + s0], o), $7, F, w7);
case 10:
var Z7 = function($e) {
return [0, O, [10, $e]];
}, Q7 = m[1];
return ee(u(o[1][1 + s0], o), Q7, F, Z7);
case 11:
var ri = function($e) {
return [0, O, [11, $e]];
}, ei = m[1];
return ee(u(o[1][1 + k], o), ei, F, ri);
case 12:
var Wi = function($e) {
return [0, O, [12, $e]];
}, uv = m[1];
return te(u(o[1][1 + a7], o), O, uv, F, Wi);
case 13:
var iv = function($e) {
return [0, O, [13, $e]];
}, Ji = m[1];
return te(u(o[1][1 + qr], o), O, Ji, F, iv);
case 14:
var fv = function($e) {
return [0, O, [14, $e]];
}, Gb = m[1];
return te(u(o[1][1 + bn], o), O, Gb, F, fv);
case 15:
var Mb = function($e) {
return [0, O, [15, $e]];
}, Bb = m[1];
return ee(u(o[1][1 + e1], o), Bb, F, Mb);
case 16:
var qb = function($e) {
return [0, O, [16, $e]];
}, Ub = m[1];
return te(u(o[1][1 + xu], o), O, Ub, F, qb);
case 17:
var Hb = function($e) {
return [0, O, [17, $e]];
}, Xb = m[1];
return te(u(o[1][1 + Sn], o), O, Xb, F, Hb);
case 18:
var Yb = function($e) {
return [0, O, [18, $e]];
}, Vb = m[1];
return te(u(o[1][1 + vr], o), O, Vb, F, Yb);
case 19:
var zb = function($e) {
return [0, O, [19, $e]];
}, Kb = m[1];
return te(u(o[1][1 + h], o), O, Kb, F, zb);
case 20:
var Wb = function($e) {
return [0, O, [20, $e]];
}, Jb = m[1];
return te(u(o[1][1 + rn], o), O, Jb, F, Wb);
case 21:
var $b = function($e) {
return [0, O, [21, $e]];
}, Zb = m[1];
return ee(u(o[1][1 + G], o), Zb, F, $b);
case 22:
var Qb = function($e) {
return [0, O, [22, $e]];
}, r4 = m[1];
return ee(u(o[1][1 + a0], o), r4, F, Qb);
case 23:
var e4 = function($e) {
return [0, O, [23, $e]];
}, n42 = m[1];
return te(u(o[1][1 + Lr], o), O, n42, F, e4);
case 24:
var t4 = function($e) {
return [0, O, [24, $e]];
}, u4 = m[1];
return te(u(o[1][1 + _], o), O, u4, F, t4);
case 25:
var i4 = function($e) {
return [0, O, [25, $e]];
}, f4 = m[1];
return te(u(o[1][1 + p2], o), O, f4, F, i4);
default:
var x4 = function($e) {
return [0, O, [26, $e]];
}, a4 = m[1];
return te(u(o[1][1 + x_], o), O, a4, F, x4);
}
}
function Tte(o, F, m) {
var O = m[2], H = m[1], $ = H[3], r0 = H[2], M0 = H[1], z0 = a(o[1][1 + t0], o, M0), Nr = a(o[1][1 + t0], o, r0), Gr = Un(u(o[1][1 + t0], o), $), Fe = a(o[1][1 + s0], o, O);
return z0 === M0 && Nr === r0 && Gr === $ && Fe === O ? m : [0, [0, z0, Nr, Gr], Fe];
}
function Ote(o, F, m) {
var O = m[2], H = m[1], $ = H[3], r0 = H[2], M0 = H[1], z0 = a(o[1][1 + t0], o, M0), Nr = a(o[1][1 + t0], o, r0), Gr = Un(u(o[1][1 + t0], o), $), Fe = a(o[1][1 + s0], o, O);
return z0 === M0 && Nr === r0 && Gr === $ && Fe === O ? m : [0, [0, z0, Nr, Gr], Fe];
}
function Ite(o, F) {
var m = F[2], O = F[1], H = a(o[1][1 + t0], o, O), $ = a(o[1][1 + s0], o, m);
return O === H && m === $ ? F : [0, H, $];
}
function Ate(o, F) {
var m = F[2], O = F[1], H = Un(u(o[1][1 + t0], o), O), $ = a(o[1][1 + s0], o, m);
return O === H && m === $ ? F : [0, H, $];
}
function Nte(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + K], o, H), r0 = a(o[1][1 + S], o, O);
return $ === H && r0 === O ? F : [0, F[1], [0, $, r0]];
}
function Cte(o, F) {
return a(o[1][1 + lt], o, F);
}
function Pte(o, F) {
return a(o[1][1 + lt], o, F);
}
function Dte(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + M], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + A], o), $, F, H);
}
function Lte(o, F) {
var m = F[2], O = F[1], H = a(o[1][1 + K], o, O), $ = a(o[1][1 + s0], o, m);
return O === H && m === $ ? F : [0, H, $];
}
function Rte(o, F) {
var m = F[2], O = F[1], H = a(o[1][1 + t0], o, O), $ = a(o[1][1 + s0], o, m);
return O === H && m === $ ? F : [0, H, $];
}
function jte(o, F, m) {
var O = m[2], H = a(o[1][1 + s0], o, O);
return O === H ? m : [0, m[1], H];
}
function Gte(o, F, m) {
var O = m[3], H = a(o[1][1 + s0], o, O);
return O === H ? m : [0, m[1], m[2], H];
}
function Mte(o, F, m) {
var O = m[3], H = a(o[1][1 + s0], o, O);
return O === H ? m : [0, m[1], m[2], H];
}
function Bte(o, F, m) {
var O = m[3], H = a(o[1][1 + s0], o, O);
return O === H ? m : [0, m[1], m[2], H];
}
function qte(o, F, m) {
var O = m[1], H = ir(o[1][1 + Sn], o, F, O);
return H === O ? m : [0, H, m[2]];
}
function Ute(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + t0], o, $), M0 = a(o[1][1 + t0], o, H), z0 = a(o[1][1 + s0], o, O);
return r0 === $ && M0 === H && z0 === O ? m : [0, r0, M0, z0];
}
function Hte(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + z7], o, $), M0 = ze(u(o[1][1 + e0], o), H), z0 = a(o[1][1 + s0], o, O);
return r0 === $ && M0 === H && z0 === O ? m : [0, r0, M0, z0];
}
function Xte(o, F) {
var m = F[2], O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + x0], o, $), z0 = a(o[1][1 + c], o, H), Nr = ze(u(o[1][1 + t0], o), O), Gr = a(o[1][1 + Kc], o, r0);
return Gr === r0 && M0 === $ && z0 === H && Nr === O ? F : [0, F[1], [0, Gr, M0, z0, Nr]];
}
function Yte(o, F) {
var m = F[2], O = m[2], H = m[1], $ = Un(u(o[1][1 + f0], o), H), r0 = a(o[1][1 + s0], o, O);
return $ === H && r0 === O ? F : [0, F[1], [0, $, r0]];
}
function Vte(o, F) {
var m = F[2], O = m[2], H = m[1], $ = Un(u(o[1][1 + t0], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function zte(o, F) {
return ze(u(o[1][1 + s], o), F);
}
function Kte(o, F) {
var m = F[2], O = m[2], H = a(o[1][1 + s0], o, O);
return O === H ? F : [0, F[1], [0, m[1], H]];
}
function Wte(o, F) {
return a(o[1][1 + lt], o, F);
}
function Jte(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + z7], o, H), r0 = a(o[1][1 + Y], o, O);
return $ === H && r0 === O ? F : [0, F[1], [0, $, r0]];
}
function $te(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + m0], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + Mu], o), $, F, H);
}
function Zte(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = u(o[1][1 + xu], o), M0 = Un(function(Gr) {
return mu(r0, Gr);
}, H), z0 = mu(u(o[1][1 + qr], o), $), Nr = a(o[1][1 + s0], o, O);
return M0 === H && z0 === $ && O === Nr ? m : [0, z0, M0, Nr];
}
function Qte(o, F, m) {
var O = m[4], H = m[3], $ = Un(function(M0) {
switch (M0[0]) {
case 0:
var z0 = function(Kn) {
return [0, Kn];
}, Nr = M0[1];
return ee(u(o[1][1 + ne], o), Nr, M0, z0);
case 1:
var Gr = function(Kn) {
return [1, Kn];
}, Fe = M0[1];
return ee(u(o[1][1 + jr], o), Fe, M0, Gr);
case 2:
var ye = function(Kn) {
return [2, Kn];
}, Dn = M0[1];
return ee(u(o[1][1 + ge], o), Dn, M0, ye);
case 3:
var pn = function(Kn) {
return [3, Kn];
}, xt = M0[1];
return ee(u(o[1][1 + H0], o), xt, M0, pn);
default:
var pt = function(Kn) {
return [4, Kn];
}, kt = M0[1];
return ee(u(o[1][1 + ce], o), kt, M0, pt);
}
}, H), r0 = a(o[1][1 + s0], o, O);
return $ === H && O === r0 ? m : [0, m[1], m[2], $, r0];
}
function rue(o, F) {
var m = F[2], O = m[3], H = m[1], $ = H[2], r0 = H[1], M0 = ir(o[1][1 + a7], o, r0, $), z0 = a(o[1][1 + s0], o, O);
return $ === M0 && O === z0 ? F : [0, F[1], [0, [0, r0, M0], m[2], z0]];
}
function eue(o, F) {
var m = F[2], O = m[6], H = m[2], $ = m[1], r0 = a(o[1][1 + lt], o, $), M0 = a(o[1][1 + t0], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? F : [0, F[1], [0, r0, M0, m[3], m[4], m[5], z0]];
}
function nue(o, F) {
var m = F[2], O = m[6], H = m[5], $ = m[3], r0 = m[2], M0 = a(o[1][1 + t0], o, r0), z0 = a(o[1][1 + t0], o, $), Nr = a(o[1][1 + c], o, H), Gr = a(o[1][1 + s0], o, O);
return M0 === r0 && z0 === $ && Nr === H && Gr === O ? F : [0, F[1], [0, m[1], M0, z0, m[4], Nr, Gr]];
}
function tue(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + t0], o, H), r0 = a(o[1][1 + s0], o, O);
return $ === H && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function uue(o, F) {
var m = F[2], O = m[8], H = m[7], $ = m[2], r0 = m[1], M0 = a(o[1][1 + ae], o, r0), z0 = a(o[1][1 + $r], o, $), Nr = a(o[1][1 + c], o, H), Gr = a(o[1][1 + s0], o, O);
return M0 === r0 && z0 === $ && Nr === H && Gr === O ? F : [0, F[1], [0, M0, z0, m[3], m[4], m[5], m[6], Nr, Gr]];
}
function iue(o, F) {
var m = F[1];
function O($) {
return [0, m, $];
}
var H = F[2];
return te(u(o[1][1 + a7], o), m, H, F, O);
}
function fue(o, F) {
var m = F[1];
function O($) {
return [0, m, $];
}
var H = F[2];
return te(u(o[1][1 + a7], o), m, H, F, O);
}
function xue(o, F) {
switch (F[0]) {
case 0:
var m = function(z0) {
return [0, z0];
}, O = F[1];
return ee(u(o[1][1 + t0], o), O, F, m);
case 1:
var H = function(z0) {
return [1, z0];
}, $ = F[1];
return ee(u(o[1][1 + Br], o), $, F, H);
default:
var r0 = function(z0) {
return [2, z0];
}, M0 = F[1];
return ee(u(o[1][1 + Mr], o), M0, F, r0);
}
}
function aue(o, F) {
return a(o[1][1 + lt], o, F);
}
function oue(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = $[2], M0 = r0[4], z0 = r0[3], Nr = r0[2], Gr = r0[1], Fe = m[1], ye = ze(u(o[1][1 + Yc], o), Gr), Dn = Un(u(o[1][1 + L0], o), Nr), pn = ze(u(o[1][1 + qt], o), z0), xt = a(o[1][1 + t0], o, H), pt = ze(u(o[1][1 + V], o), Fe), kt = a(o[1][1 + s0], o, O), Kn = a(o[1][1 + s0], o, M0);
return Dn === Nr && pn === z0 && xt === H && pt === Fe && kt === O && Kn === M0 && ye === Gr ? m : [0, pt, [0, $[1], [0, ye, Dn, pn, Kn]], xt, kt];
}
function cue(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + l], o, H), r0 = a(o[1][1 + s0], o, O);
return $ === H && r0 === O ? F : [0, F[1], [0, $, r0]];
}
function sue(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + L0], o, H), r0 = a(o[1][1 + s0], o, O);
return $ === H && r0 === O ? F : [0, F[1], [0, $, r0]];
}
function vue(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + t0], o, O), r0 = ze(u(o[1][1 + lt], o), H);
return $ === O && r0 === H ? F : [0, F[1], [0, r0, $, m[3]]];
}
function lue(o, F) {
var m = F[1];
function O($) {
return [0, m, $];
}
var H = F[2];
return te(u(o[1][1 + T], o), m, H, F, O);
}
function bue(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + Fi], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + en], o), $, F, H);
}
function pue(o, F, m) {
var O = m[5], H = m[4], $ = m[3], r0 = m[2], M0 = m[1], z0 = ze(u(o[1][1 + nt], o), M0), Nr = ze(u(o[1][1 + _r], o), r0), Gr = ze(u(o[1][1 + en], o), $), Fe = a(o[1][1 + Or], o, H), ye = a(o[1][1 + s0], o, O);
return M0 === z0 && r0 === Nr && $ === Gr && H === Fe && O === ye ? m : [0, z0, Nr, Gr, Fe, ye];
}
function mue(o, F) {
var m = F[1];
function O($) {
return [0, m, $];
}
var H = F[2];
return te(u(o[1][1 + T], o), m, H, F, O);
}
function _ue(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + tt], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + Tt], o), $, F, H);
}
function yue(o, F, m) {
var O = m[5], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + tn], o, r0), z0 = a(o[1][1 + en], o, $), Nr = a(o[1][1 + Or], o, H), Gr = a(o[1][1 + s0], o, O);
return r0 === M0 && $ === z0 && H === Nr && O === Gr ? m : [0, M0, z0, Nr, m[4], Gr];
}
function due(o, F) {
var m = F[1];
function O($) {
return [0, m, $];
}
var H = F[2];
return te(u(o[1][1 + T], o), m, H, F, O);
}
function hue(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + Vs], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + Vi], o), $, F, H);
}
function kue(o, F, m) {
var O = m[5], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + hs], o, r0), z0 = a(o[1][1 + en], o, $), Nr = a(o[1][1 + Or], o, H), Gr = a(o[1][1 + s0], o, O);
return r0 === M0 && $ === z0 && H === Nr && O === Gr ? m : [0, M0, z0, Nr, m[4], Gr];
}
function wue(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + en], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + Rr], o), $, F, H);
}
function Eue(o, F, m) {
var O = m[3], H = m[1], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, m[2], r0];
}
function Sue(o, F) {
if (F[0] === 0) {
var m = F[1], O = Un(u(o[1][1 + Ws], o), m);
return m === O ? F : [0, O];
}
var H = F[1], $ = a(o[1][1 + U9], o, H);
return H === $ ? F : [1, $];
}
function gue(o, F) {
var m = F[2], O = ze(u(o[1][1 + lt], o), m);
return m === O ? F : [0, F[1], O];
}
function Fue(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + lt], o, H), r0 = ze(u(o[1][1 + lt], o), O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function Tue(o, F, m) {
var O = m[5], H = m[2], $ = m[1], r0 = ze(u(o[1][1 + ci], o), H), M0 = ze(u(o[1][1 + Or], o), $), z0 = a(o[1][1 + s0], o, O);
return H === r0 && $ === M0 && O === z0 ? m : [0, M0, r0, m[3], m[4], z0];
}
function Oue(o, F) {
if (F[0] === 0) {
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + Or], o), O, F, m);
}
function H(r0) {
return [1, r0];
}
var $ = F[1];
return ee(u(o[1][1 + en], o), $, F, H);
}
function Iue(o, F, m) {
var O = m[3], H = m[2], $ = a(o[1][1 + B9], o, H), r0 = a(o[1][1 + s0], o, O);
return $ === H && r0 === O ? m : [0, m[1], $, r0];
}
function Aue(o, F) {
return a(o[1][1 + lt], o, F);
}
function Nue(o, F) {
var m = F[2], O = m[1], H = a(o[1][1 + X1], o, O);
return O === H ? F : [0, F[1], [0, H, m[2]]];
}
function Cue(o, F) {
var m = F[2], O = m[1], H = a(o[1][1 + X1], o, O);
return O === H ? F : [0, F[1], [0, H, m[2]]];
}
function Pue(o, F) {
var m = F[2], O = m[1], H = a(o[1][1 + X1], o, O);
return O === H ? F : [0, F[1], [0, H, m[2]]];
}
function Due(o, F) {
var m = F[2][1], O = a(o[1][1 + X1], o, m);
return m === O ? F : [0, F[1], [0, O]];
}
function Lue(o, F) {
var m = F[3], O = F[1], H = Un(u(o[1][1 + si], o), O), $ = a(o[1][1 + s0], o, m);
return O === H && m === $ ? F : [0, H, F[2], $];
}
function Rue(o, F) {
var m = F[4], O = F[1];
if (O[0] === 0)
var H = function(ye) {
return [0, ye];
}, $ = O[1], r0 = u(o[1][1 + si], o), Gr = ee(function(ye) {
return Un(r0, ye);
}, $, O, H);
else
var M0 = function(ye) {
return [1, ye];
}, z0 = O[1], Nr = u(o[1][1 + s2], o), Gr = ee(function(ye) {
return Un(Nr, ye);
}, z0, O, M0);
var Fe = a(o[1][1 + s0], o, m);
return O === Gr && m === Fe ? F : [0, Gr, F[2], F[3], Fe];
}
function jue(o, F) {
var m = F[4], O = F[1], H = Un(u(o[1][1 + X9], o), O), $ = a(o[1][1 + s0], o, m);
return O === H && m === $ ? F : [0, H, F[2], F[3], $];
}
function Gue(o, F) {
var m = F[4], O = F[1], H = Un(u(o[1][1 + cb], o), O), $ = a(o[1][1 + s0], o, m);
return O === H && m === $ ? F : [0, H, F[2], F[3], $];
}
function Mue(o, F) {
var m = F[2], O = F[1];
switch (m[0]) {
case 0:
var H = function(ye) {
return [0, O, [0, ye]];
}, $ = m[1];
return ee(u(o[1][1 + sb], o), $, F, H);
case 1:
var r0 = function(ye) {
return [0, O, [1, ye]];
}, M0 = m[1];
return ee(u(o[1][1 + Y9], o), M0, F, r0);
case 2:
var z0 = function(ye) {
return [0, O, [2, ye]];
}, Nr = m[1];
return ee(u(o[1][1 + H9], o), Nr, F, z0);
default:
var Gr = function(ye) {
return [0, O, [3, ye]];
}, Fe = m[1];
return ee(u(o[1][1 + Js], o), Fe, F, Gr);
}
}
function Bue(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = ir(o[1][1 + R0], o, h6r, $), M0 = a(o[1][1 + V9], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function que(o, F, m) {
var O = m[1], H = a(o[1][1 + s0], o, O);
return O === H ? m : [0, H];
}
function Uue(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + Or], o, $), M0 = a(o[1][1 + _r], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function Hue(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = ir(o[1][1 + R0], o, d6r, $), M0 = a(o[1][1 + l], o, H), z0 = a(o[1][1 + s0], o, O);
return r0 === $ && M0 === H && z0 === O ? m : [0, r0, M0, z0];
}
function Xue(o, F, m) {
return ir(o[1][1 + c0], o, F, m);
}
function Yue(o, F, m) {
var O = m[2], H = m[1], $ = a(o[1][1 + l], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function Vue(o, F, m) {
var O = m[4], H = m[2], $ = mu(u(o[1][1 + zi], o), H), r0 = a(o[1][1 + s0], o, O);
return $ === H && O === r0 ? m : [0, m[1], $, m[3], r0];
}
function zue(o, F, m) {
return ir(o[1][1 + Hn], o, F, m);
}
function Kue(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + he], o, r0), z0 = a(o[1][1 + l], o, $), Nr = ze(u(o[1][1 + Ir], o), H), Gr = a(o[1][1 + s0], o, O);
return M0 === r0 && z0 === $ && Nr === H && Gr === O ? m : [0, M0, z0, Nr, Gr];
}
function Wue(o, F) {
switch (F[0]) {
case 0:
var m = F[1], O = m[2], H = m[1], $ = ir(o[1][1 + vb], o, H, O);
return $ === O ? F : [0, [0, H, $]];
case 1:
var r0 = F[1], M0 = r0[2], z0 = r0[1], Nr = ir(o[1][1 + lb], o, z0, M0);
return Nr === M0 ? F : [1, [0, z0, Nr]];
case 2:
var Gr = F[1], Fe = Gr[2], ye = Gr[1], Dn = ir(o[1][1 + v2], o, ye, Fe);
return Dn === Fe ? F : [2, [0, ye, Dn]];
case 3:
var pn = F[1], xt = a(o[1][1 + t0], o, pn);
return xt === pn ? F : [3, xt];
case 4:
var pt = F[1], kt = pt[2], Kn = pt[1], $t = ir(o[1][1 + c0], o, Kn, kt);
return $t === kt ? F : [4, [0, Kn, $t]];
case 5:
var W7 = F[1], J7 = W7[2], w7 = W7[1], $7 = ir(o[1][1 + Sr], o, w7, J7);
return $7 === J7 ? F : [5, [0, w7, $7]];
default:
var Z7 = F[1], Q7 = Z7[2], ri = Z7[1], ei = ir(o[1][1 + Hn], o, ri, Q7);
return ei === Q7 ? F : [6, [0, ri, ei]];
}
}
function Jue(o, F, m) {
var O = m[5], H = m[3], $ = m[2], r0 = ze(u(o[1][1 + ci], o), H), M0 = ze(u(o[1][1 + Q9], o), $), z0 = a(o[1][1 + s0], o, O);
return H === r0 && $ === M0 && O === z0 ? m : [0, m[1], M0, r0, m[4], z0];
}
function $ue(o, F, m) {
var O = m[7], H = m[6], $ = m[5], r0 = m[4], M0 = m[3], z0 = m[2], Nr = m[1], Gr = a(o[1][1 + db], o, Nr), Fe = ze(u(o[1][1 + V], o), z0), ye = mu(u(o[1][1 + qr], o), M0), Dn = u(o[1][1 + xu], o), pn = ze(function($t) {
return mu(Dn, $t);
}, r0), xt = u(o[1][1 + xu], o), pt = Un(function($t) {
return mu(xt, $t);
}, $), kt = ze(u(o[1][1 + l2], o), H), Kn = a(o[1][1 + s0], o, O);
return Gr === Nr && Fe === z0 && ye === M0 && pn === r0 && pt === $ && kt === H && Kn === O ? m : [0, Gr, Fe, ye, pn, pt, kt, Kn];
}
function Zue(o, F, m) {
var O = m[1], H = a(o[1][1 + s0], o, O);
return O === H ? m : [0, H];
}
function Que(o, F, m) {
var O = m[2], H = m[1], $ = ze(u(o[1][1 + Q0], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function r7e(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + _r], o, r0), z0 = a(o[1][1 + en], o, $), Nr = a(o[1][1 + en], o, H), Gr = a(o[1][1 + s0], o, O);
return r0 === M0 && $ === z0 && H === Nr && O === Gr ? m : [0, M0, z0, Nr, Gr];
}
function e7e(o, F, m) {
return m;
}
function n7e(o, F, m) {
var O = m[6], H = m[5], $ = m[3], r0 = m[2], M0 = m[1], z0 = a(o[1][1 + or], o, M0), Nr = a(o[1][1 + _b], o, r0), Gr = a(o[1][1 + x0], o, $), Fe = a(o[1][1 + c], o, H), ye = a(o[1][1 + s0], o, O);
return M0 === z0 && r0 === Nr && Gr === $ && Fe === H && ye === O ? m : [0, z0, Nr, Gr, m[4], Fe, ye];
}
function t7e(o, F) {
if (typeof F == "number")
return F;
var m = F[1], O = a(o[1][1 + en], o, m);
return m === O ? F : [0, O];
}
function u7e(o, F, m) {
var O = m[6], H = m[5], $ = m[3], r0 = m[2], M0 = m[1], z0 = a(o[1][1 + ae], o, M0), Nr = a(o[1][1 + _b], o, r0), Gr = a(o[1][1 + x0], o, $), Fe = a(o[1][1 + c], o, H), ye = a(o[1][1 + s0], o, O);
return M0 === z0 && r0 === Nr && Gr === $ && Fe === H && ye === O ? m : [0, z0, Nr, Gr, m[4], Fe, ye];
}
function i7e(o, F, m) {
var O = m[6], H = m[5], $ = m[3], r0 = m[2], M0 = a(o[1][1 + ae], o, r0), z0 = mu(u(o[1][1 + _e], o), $), Nr = Un(u(o[1][1 + hb], o), H), Gr = a(o[1][1 + s0], o, O);
return r0 === M0 && $ === z0 && H === Nr && O === Gr ? m : [0, m[1], M0, z0, m[4], Nr, Gr];
}
function f7e(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + m0], o, H), r0 = ze(u(o[1][1 + e0], o), O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function x7e(o, F) {
var m = F[2], O = m[2], H = m[1], $ = Un(u(o[1][1 + e_], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function a7e(o, F) {
switch (F[0]) {
case 0:
var m = F[1], O = m[1], H = function(pn) {
return [0, [0, O, pn]];
}, $ = m[2];
return te(u(o[1][1 + Vc], o), O, $, F, H);
case 1:
var r0 = F[1], M0 = r0[1], z0 = function(pn) {
return [1, [0, M0, pn]];
}, Nr = r0[2];
return te(u(o[1][1 + yb], o), M0, Nr, F, z0);
default:
var Gr = F[1], Fe = Gr[1], ye = function(pn) {
return [2, [0, Fe, pn]];
}, Dn = Gr[2];
return te(u(o[1][1 + r_], o), Fe, Dn, F, ye);
}
}
function o7e(o, F) {
var m = F[2], O = m[2], H = m[1], $ = a(o[1][1 + en], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function c7e(o, F) {
var m = F[2], O = m[2], H = m[1], $ = Un(u(o[1][1 + $s], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function s7e(o, F) {
return ir(o[1][1 + R0], o, y6r, F);
}
function v7e(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + en], o, $), M0 = ze(u(o[1][1 + e0], o), H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function l7e(o, F, m) {
var O = m[7], H = m[6], $ = m[5], r0 = m[4], M0 = m[3], z0 = m[2], Nr = m[1], Gr = ze(u(o[1][1 + db], o), Nr), Fe = a(o[1][1 + t_], o, z0), ye = ze(u(o[1][1 + V], o), M0), Dn = u(o[1][1 + zc], o), pn = ze(function(Kn) {
return mu(Dn, Kn);
}, r0), xt = ze(u(o[1][1 + l2], o), $), pt = Un(u(o[1][1 + hb], o), H), kt = a(o[1][1 + s0], o, O);
return Nr === Gr && z0 === Fe && r0 === pn && $ === xt && H === pt && O === kt && M0 === ye ? m : [0, Gr, Fe, ye, pn, xt, pt, kt];
}
function b7e(o, F, m) {
return ir(o[1][1 + ks], o, F, m);
}
function p7e(o, F, m) {
return ir(o[1][1 + ks], o, F, m);
}
function m7e(o, F, m) {
var O = m[3], H = m[2], $ = m[1], r0 = ze(u(o[1][1 + u_], o), $), M0 = a(o[1][1 + i_], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, r0, M0, z0];
}
function _7e(o, F) {
return mu(u(o[1][1 + zi], o), F);
}
function y7e(o, F) {
if (F[0] === 0) {
var m = F[1], O = a(o[1][1 + t0], o, m);
return O === m ? F : [0, O];
}
var H = F[1], $ = H[2][1], r0 = a(o[1][1 + s0], o, $);
return $ === r0 ? F : [1, [0, H[1], [0, r0]]];
}
function d7e(o, F) {
var m = F[2], O = m[2], H = m[1], $ = Un(u(o[1][1 + f_], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function h7e(o, F, m) {
var O = m[1], H = ir(o[1][1 + kb], o, F, O);
return O === H ? m : [0, H, m[2], m[3]];
}
function k7e(o, F) {
var m = F[2], O = m[2], H = m[1], $ = Un(u(o[1][1 + Ks], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? F : [0, F[1], [0, $, r0]];
}
function w7e(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = m[1], M0 = a(o[1][1 + en], o, r0), z0 = ze(u(o[1][1 + b2], o), $), Nr = a(o[1][1 + Zs], o, H), Gr = a(o[1][1 + s0], o, O);
return r0 === M0 && $ === z0 && H === Nr && O === Gr ? m : [0, M0, z0, Nr, Gr];
}
function E7e(o, F, m) {
var O = m[2], H = m[1], $ = ze(u(o[1][1 + Q0], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function S7e(o, F, m) {
var O = m[2], H = m[1], $ = a(o[1][1 + Tr], o, H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function g7e(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = a(o[1][1 + en], o, $), M0 = a(o[1][1 + en], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, m[1], r0, M0, z0];
}
function F7e(o, F, m) {
var O = m[4], H = m[3], $ = m[2], r0 = a(o[1][1 + m2], o, $), M0 = a(o[1][1 + en], o, H), z0 = a(o[1][1 + s0], o, O);
return $ === r0 && H === M0 && O === z0 ? m : [0, m[1], r0, M0, z0];
}
function T7e(o, F, m) {
return ir(o[1][1 + Rn], o, F, m);
}
function O7e(o, F) {
switch (F[0]) {
case 0:
var m = function(r0) {
return [0, r0];
}, O = F[1];
return ee(u(o[1][1 + en], o), O, F, m);
case 1:
var H = function(r0) {
return [1, r0];
}, $ = F[1];
return ee(u(o[1][1 + Rr], o), $, F, H);
default:
return F;
}
}
function I7e(o, F, m) {
var O = m[2], H = m[1], $ = Un(u(o[1][1 + c_], o), H), r0 = a(o[1][1 + s0], o, O);
return H === $ && O === r0 ? m : [0, $, r0];
}
function A7e(o, F) {
var m = F[2], O = F[1];
switch (m[0]) {
case 0:
var H = function(Ue) {
return [0, O, [0, Ue]];
}, $ = m[1];
return te(u(o[1][1 + y2], o), O, $, F, H);
case 1:
var r0 = function(Ue) {
return [0, O, [1, Ue]];
}, M0 = m[1];
return te(u(o[1][1 + o_], o), O, M0, F, r0);
case 2:
var z0 = function(Ue) {
return [0, O, [2, Ue]];
}, Nr = m[1];
return te(u(o[1][1 + _2], o), O, Nr, F, z0);
case 3:
var Gr = function(Ue) {
return [0, O, [3, Ue]];
}, Fe = m[1];
return te(u(o[1][1 + a_], o), O, Fe, F, Gr);
case 4:
var ye = function(Ue) {
return [0, O, [4, Ue]];
}, Dn = m[1];
return te(u(o[1][1 + kb], o), O, Dn, F, ye);
case 5:
var pn = function(Ue) {
return [0, O, [5, Ue]];
}, xt = m[1];
return te(u(o[1][1 + n_], o), O, xt, F, pn);
case 6:
var pt = function(Ue) {
return [0, O, [6, Ue]];
}, kt = m[1];
return te(u(o[1][1 + jn], o), O, kt, F, pt);
case 7:
var Kn = function(Ue) {
return [0, O, [7, Ue]];
}, $t = m[1];
return te(u(o[1][1 + mb], o), O, $t, F, Kn);
case 8:
var W7 = function(Ue) {
return [0, O, [8, Ue]];
}, J7 = m[1];
return te(u(o[1][1 + Zn], o), O, J7, F, W7);
case 9:
var w7 = function(Ue) {
return [0, O, [9, Ue]];
}, $7 = m[1];
return te(u(o[1][1 + Yi], o), O, $7, F, w7);
case 10:
var Z7 = function(Ue) {
return [0, O, [10, Ue]];
}, Q7 = m[1];
return ee(u(o[1][1 + lt], o), Q7, F, Z7);
case 11:
var ri = function(Ue) {
return [0, O, [11, Ue]];
}, ei = m[1];
return ee(a(o[1][1 + Ft], o, O), ei, F, ri);
case 12:
var Wi = function(Ue) {
return [0, O, [12, Ue]];
}, uv = m[1];
return te(u(o[1][1 + Me], o), O, uv, F, Wi);
case 13:
var iv = function(Ue) {
return [0, O, [13, Ue]];
}, Ji = m[1];
return te(u(o[1][1 + Kr], o), O, Ji, F, iv);
case 14:
var fv = function(Ue) {
return [0, O, [14, Ue]];
}, Gb = m[1];
return te(u(o[1][1 + J0], o), O, Gb, F, fv);
case 15:
var Mb = function(Ue) {
return [0, O, [15, Ue]];
}, Bb = m[1];
return te(u(o[1][1 + Y0], o), O, Bb, F, Mb);
case 16:
var qb = function(Ue) {
return [0, O, [16, Ue]];
}, Ub = m[1];
return te(u(o[1][1 + u0], o), O, Ub, F, qb);
case 17:
var Hb = function(Ue) {
return [0, O, [17, Ue]];
}, Xb = m[1];
return te(u(o[1][1 + U], o), O, Xb, F, Hb);
case 18:
var Yb = function(Ue) {
return [0, O, [18, Ue]];
}, Vb = m[1];
return te(u(o[1][1 + I], o), O, Vb, F, Yb);
case 19:
var zb = function(Ue) {
return [0, O, [19, Ue]];
}, Kb = m[1];
return te(u(o[1][1 + Fr], o), O, Kb, F, zb);
case 20:
var Wb = function(Ue) {
return [0, O, [20, Ue]];
}, Jb = m[1];
return ee(a(o[1][1 + $0], o, O), Jb, F, Wb);
case 21:
var $b = function(Ue) {
return [0, O, [21, Ue]];
}, Zb = m[1];
return te(u(o[1][1 + yr], o), O, Zb, F, $b);
case 22:
var Qb = function(Ue) {
return [0, O, [22, Ue]];
}, r4 = m[1];
return te(u(o[1][1 + Wr], o), O, r4, F, Qb);
case 23:
var e4 = function(Ue) {
return [0, O, [23, Ue]];
}, n42 = m[1];
return te(u(o[1][1 + W0], o), O, n42, F, e4);
case 24:
var t4 = function(Ue) {
return [0, O, [24, Ue]];
}, u4 = m[1];
return te(u(o[1][1 + X], o), O, u4, F, t4);
case 25:
var i4 = function(Ue) {
return [0, O, [25, Ue]];
}, f4 = m[1];
return te(u(o[1][1 + G0], o), O, f4, F, i4);
case 26:
var x4 = function(Ue) {
return [0, O, [26, Ue]];
}, a4 = m[1];
return te(u(o[1][1 + X0], o), O, a4, F, x4);
case 27:
var $e = function(Ue) {
return [0, O, [27, Ue]];
}, PR = m[1];
return te(u(o[1][1 + g0], o), O, PR, F, $e);
case 28:
var DR = function(Ue) {
return [0, O, [28, Ue]];
}, LR = m[1];
return te(u(o[1][1 + w], o), O, LR, F, DR);
case 29:
var RR = function(Ue) {
return [0, O, [29, Ue]];
}, jR = m[1];
return te(u(o[1][1 + E], o), O, jR, F, RR);
default:
var GR = function(Ue) {
return [0, O, [30, Ue]];
}, MR = m[1];
return te(u(o[1][1 + e], o), O, MR, F, GR);
}
}
function N7e(o, F) {
var m = F[2], O = F[1], H = Un(u(o[1][1 + V1], o), O), $ = Un(u(o[1][1 + V1], o), m);
return O === H && m === $ ? F : [0, H, $, F[3]];
}
var C7e = 8;
function P7e(o, F) {
return F;
}
function D7e(o, F) {
var m = F[2], O = F[1];
switch (m[0]) {
case 0:
var H = function(Oe) {
return [0, O, [0, Oe]];
}, $ = m[1];
return te(u(o[1][1 + zi], o), O, $, F, H);
case 1:
var r0 = function(Oe) {
return [0, O, [1, Oe]];
}, M0 = m[1];
return te(u(o[1][1 + Qs], o), O, M0, F, r0);
case 2:
var z0 = function(Oe) {
return [0, O, [2, Oe]];
}, Nr = m[1];
return te(u(o[1][1 + z1], o), O, Nr, F, z0);
case 3:
var Gr = function(Oe) {
return [0, O, [3, Oe]];
}, Fe = m[1];
return te(u(o[1][1 + pb], o), O, Fe, F, Gr);
case 4:
var ye = function(Oe) {
return [0, O, [4, Oe]];
}, Dn = m[1];
return te(u(o[1][1 + bb], o), O, Dn, F, ye);
case 5:
var pn = function(Oe) {
return [0, O, [5, Oe]];
}, xt = m[1];
return te(u(o[1][1 + v2], o), O, xt, F, pn);
case 6:
var pt = function(Oe) {
return [0, O, [6, Oe]];
}, kt = m[1];
return te(u(o[1][1 + Y1], o), O, kt, F, pt);
case 7:
var Kn = function(Oe) {
return [0, O, [7, Oe]];
}, $t = m[1];
return te(u(o[1][1 + lb], o), O, $t, F, Kn);
case 8:
var W7 = function(Oe) {
return [0, O, [8, Oe]];
}, J7 = m[1];
return te(u(o[1][1 + Z9], o), O, J7, F, W7);
case 9:
var w7 = function(Oe) {
return [0, O, [9, Oe]];
}, $7 = m[1];
return te(u(o[1][1 + $9], o), O, $7, F, w7);
case 10:
var Z7 = function(Oe) {
return [0, O, [10, Oe]];
}, Q7 = m[1];
return te(u(o[1][1 + J9], o), O, Q7, F, Z7);
case 11:
var ri = function(Oe) {
return [0, O, [11, Oe]];
}, ei = m[1];
return te(u(o[1][1 + W9], o), O, ei, F, ri);
case 12:
var Wi = function(Oe) {
return [0, O, [33, Oe]];
}, uv = m[1];
return te(u(o[1][1 + Sr], o), O, uv, F, Wi);
case 13:
var iv = function(Oe) {
return [0, O, [13, Oe]];
}, Ji = m[1];
return te(u(o[1][1 + vb], o), O, Ji, F, iv);
case 14:
var fv = function(Oe) {
return [0, O, [14, Oe]];
}, Gb = m[1];
return te(u(o[1][1 + K9], o), O, Gb, F, fv);
case 15:
var Mb = function(Oe) {
return [0, O, [15, Oe]];
}, Bb = m[1];
return te(u(o[1][1 + z9], o), O, Bb, F, Mb);
case 16:
var qb = function(Oe) {
return [0, O, [16, Oe]];
}, Ub = m[1];
return te(u(o[1][1 + ob], o), O, Ub, F, qb);
case 17:
var Hb = function(Oe) {
return [0, O, [17, Oe]];
}, Xb = m[1];
return te(u(o[1][1 + q9], o), O, Xb, F, Hb);
case 18:
var Yb = function(Oe) {
return [0, O, [18, Oe]];
}, Vb = m[1];
return te(u(o[1][1 + c2], o), O, Vb, F, Yb);
case 19:
var zb = function(Oe) {
return [0, O, [19, Oe]];
}, Kb = m[1];
return te(u(o[1][1 + zs], o), O, Kb, F, zb);
case 20:
var Wb = function(Oe) {
return [0, O, [20, Oe]];
}, Jb = m[1];
return te(u(o[1][1 + ht], o), O, Jb, F, Wb);
case 21:
var $b = function(Oe) {
return [0, O, [21, Oe]];
}, Zb = m[1];
return te(u(o[1][1 + Iu], o), O, Zb, F, $b);
case 22:
var Qb = function(Oe) {
return [0, O, [22, Oe]];
}, r4 = m[1];
return te(u(o[1][1 + cn], o), O, r4, F, Qb);
case 23:
var e4 = function(Oe) {
return [0, O, [23, Oe]];
}, n42 = m[1];
return te(u(o[1][1 + dn], o), O, n42, F, e4);
case 24:
var t4 = function(Oe) {
return [0, O, [24, Oe]];
}, u4 = m[1];
return te(u(o[1][1 + Nt], o), O, u4, F, t4);
case 25:
var i4 = function(Oe) {
return [0, O, [25, Oe]];
}, f4 = m[1];
return te(u(o[1][1 + Bt], o), O, f4, F, i4);
case 26:
var x4 = function(Oe) {
return [0, O, [26, Oe]];
}, a4 = m[1];
return te(u(o[1][1 + Cn], o), O, a4, F, x4);
case 27:
var $e = function(Oe) {
return [0, O, [27, Oe]];
}, PR = m[1];
return te(u(o[1][1 + fr], o), O, PR, F, $e);
case 28:
var DR = function(Oe) {
return [0, O, [28, Oe]];
}, LR = m[1];
return te(u(o[1][1 + Jr], o), O, LR, F, DR);
case 29:
var RR = function(Oe) {
return [0, O, [29, Oe]];
}, jR = m[1];
return te(u(o[1][1 + ar], o), O, jR, F, RR);
case 30:
var GR = function(Oe) {
return [0, O, [30, Oe]];
}, MR = m[1];
return te(u(o[1][1 + E0], o), O, MR, F, GR);
case 31:
var Ue = function(Oe) {
return [0, O, [31, Oe]];
}, L7e = m[1];
return te(u(o[1][1 + w0], o), O, L7e, F, Ue);
case 32:
var R7e = function(Oe) {
return [0, O, [32, Oe]];
}, j7e = m[1];
return te(u(o[1][1 + c0], o), O, j7e, F, R7e);
case 33:
var G7e = function(Oe) {
return [0, O, [33, Oe]];
}, M7e = m[1];
return te(u(o[1][1 + Sr], o), O, M7e, F, G7e);
case 34:
var B7e = function(Oe) {
return [0, O, [34, Oe]];
}, q7e = m[1];
return te(u(o[1][1 + T], o), O, q7e, F, B7e);
case 35:
var U7e = function(Oe) {
return [0, O, [35, Oe]];
}, H7e = m[1];
return te(u(o[1][1 + x], o), O, H7e, F, U7e);
default:
var X7e = function(Oe) {
return [0, O, [36, Oe]];
}, Y7e = m[1];
return te(u(o[1][1 + i], o), O, Y7e, F, X7e);
}
}
return BN(t, [0, XL, function(o, F) {
var m = F[2], O = m[3], H = m[2], $ = m[1], r0 = a(o[1][1 + _0], o, $), M0 = a(o[1][1 + s0], o, H), z0 = Un(u(o[1][1 + V1], o), O);
return $ === r0 && H === M0 && O === z0 ? F : [0, F[1], [0, r0, M0, z0]];
}, Or, D7e, V1, P7e, s0, C7e, ze, dr, dr, N7e, en, A7e, y2, I7e, c_, O7e, o_, T7e, _2, F7e, a_, g7e, zi, S7e, Qs, E7e, kb, w7e, Zs, k7e, $0, h7e, b2, d7e, f_, y7e, i_, _7e, K1, m7e, z1, p7e, n_, b7e, ks, l7e, zc, v7e, db, s7e, t_, c7e, hb, o7e, $s, a7e, l2, x7e, e_, f7e, Vc, i7e, yb, u7e, _b, t7e, r_, n7e, jn, e7e, mb, r7e, pb, Que, bb, Zue, v2, $ue, Y1, Jue, Q9, Wue, lb, Kue, Z9, zue, $9, Vue, J9, Yue, W9, Xue, vb, Hue, K9, Uue, z9, que, ob, Bue, V9, Mue, sb, Gue, Y9, jue, H9, Rue, Js, Lue, si, Due, cb, Pue, X9, Cue, s2, Nue, X1, Aue, q9, Iue, B9, Oue, c2, Tue, Ws, Fue, U9, gue, ci, Sue, zs, Eue, Ks, wue, Iu, kue, hs, hue, Vs, due, cn, yue, tn, _ue, tt, mue, ht, pue, nt, bue, Fi, lue, L0, vue, qt, sue, Yc, cue, a7, oue, Q0, aue, $r, xue, Br, fue, Mr, iue, ne, uue, jr, tue, ge, nue, ce, eue, H0, rue, qr, Qte, bn, Zte, z7, $te, Mu, Jte, Y, Wte, s, Kte, c, zte, e0, Vte, V, Yte, f0, Xte, xu, Hte, Sn, Ute, vr, qte, Lr, Bte, _, Mte, p2, Gte, x_, jte, k, Rte, G, Lte, K, Dte, M, Pte, S, Cte, A, Nte, a0, Ate, e1, Ite, h, Ote, rn, Tte, t0, Fte, l, gte, x0, Ste, dn, Ete, Zn, wte, _e, kte, Rn, hte, U0, dte, K7, yte, He, _te, it, mte, ft, pte, he, bte, Yi, lte, lt, vte, k0, ste, m0, cte, Kc, ote, Hn, ate, Cn, xte, or, fte, Tn, ite, Ft, ute, du, tte, Ku, nte, Nt, ete, Bt, rte, vt, Qne, gt, Zne, Jt, $ne, At, Jne, Me, Wne, Kr, Kne, mr, zne, Be, Vne, Cr, Yne, gr, Xne, We, Hne, Ce, Une, on, qne, yn, Bne, Qe, Mne, xn, CR, Ae, jb, fn, Rb, Ke, tv, re, Lb, F0, nv, Ie, Ki, ve, k7, xe, Oi, je, ku, sr, hu, Ur, NR, Pr, AR, K0, Db, d0, IR, fr, OR, J0, Pb, Y0, TR, u0, FR, yr, gR, I0, E2, y0, SR, D, d_, D0, ER, U, wR, I, Cb, Fr, kR, Qr, y_, ae, hR, pe, dR, oe, Nb, me, yR, Sr, _R, Re, mR, p, Ab, u_, __, Vi, pR, Tt, m_, r1, Ib, m2, p_, er, bR, R0, b_, rr, lR, T0, Ob, S0, l_, Q, Tb, L, ev, i0, vR, l0, sR, v0, v_, P, Gn, fe, cR, q0, Fb, O0, oR, b0, gb, p0, aR, Z, Sb, W1, xR, B, rv, Ir, fR, _r, w2, bt, iR, Jr, k2, Wr, uR, _0, s_, Tr, tR, Hr, nR, Rr, h2, xr, eR, W0, rR, ar, Eb, Ar, QL, X, wb, G0, ZL, b, Q1, X0, $L, E0, Z1, w0, JL, g0, d2, w, WL, E, KL, T, Ti, y, zL, x, $1, i, VL, c0, J1, e, YL]), function(o, F) {
return Gp(F, t);
};
});
function W00(t) {
switch (t[0]) {
case 0:
return 1;
case 3:
return 3;
default:
return 2;
}
}
function J00(t, n4) {
u(f(t), H6r), a(f(t), Y6r, X6r);
var e = n4[1];
a(f(t), V6r, e), u(f(t), z6r), u(f(t), K6r), a(f(t), J6r, W6r);
var i = n4[2];
return a(f(t), $6r, i), u(f(t), Z6r), u(f(t), Q6r);
}
var $00 = function t(n4, e) {
return t.fun(n4, e);
}, qee = function t(n4) {
return t.fun(n4);
};
N($00, function(t, n4) {
u(f(t), epr), a(f(t), tpr, npr);
var e = n4[1];
if (e) {
g(t, upr);
var i = e[1];
switch (i[0]) {
case 0:
u(f(t), N6r);
var x = i[1];
a(f(t), C6r, x), u(f(t), P6r);
break;
case 1:
u(f(t), D6r);
var c = i[1];
a(f(t), L6r, c), u(f(t), R6r);
break;
case 2:
u(f(t), j6r);
var s = i[1];
a(f(t), G6r, s), u(f(t), M6r);
break;
default:
u(f(t), B6r);
var p = i[1];
a(f(t), q6r, p), u(f(t), U6r);
}
g(t, ipr);
} else
g(t, fpr);
return u(f(t), xpr), u(f(t), apr), a(f(t), cpr, opr), J00(t, n4[2]), u(f(t), spr), u(f(t), vpr), a(f(t), bpr, lpr), J00(t, n4[3]), u(f(t), ppr), u(f(t), mpr);
}), N(qee, function(t) {
return a(P0(rpr), $00, t);
});
function yt(t, n4) {
return [0, t[1], t[2], n4[3]];
}
function ms(t, n4) {
var e = t[1] - n4[1] | 0;
return e === 0 ? t[2] - n4[2] | 0 : e;
}
function Z00(t, n4) {
var e = n4[1], i = t[1];
if (i)
if (e)
var x = e[1], c = i[1], s = W00(x), p = W00(c) - s | 0, T = p === 0 ? Ee(c[1], x[1]) : p;
else
var T = -1;
else
var y = e && 1, T = y;
if (T === 0) {
var E = ms(t[2], n4[2]);
return E === 0 ? ms(t[3], n4[3]) : E;
}
return T;
}
function Wv(t, n4) {
return Z00(t, n4) === 0 ? 1 : 0;
}
var ZD = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Uee = jp(dpr, function(t) {
var n4 = DN(t, ypr)[35], e = GN(t, 0, 0, _pr, $D, 1)[1];
return Zz(t, n4, function(i, x) {
return 0;
}), function(i, x) {
var c = Gp(x, t);
return u(e, c), MN(x, c, t);
};
});
N(ZD, function(t, n4, e) {
var i = e[2];
switch (i[0]) {
case 0:
var x = i[1][1];
return be(function(s, p) {
var y = p[0] === 0 ? p[1][2][2] : p[1][2][1];
return ir(ZD, t, s, y);
}, n4, x);
case 1:
var c = i[1][1];
return be(function(s, p) {
return p[0] === 2 ? s : ir(ZD, t, s, p[1][2][1]);
}, n4, c);
case 2:
return a(t, n4, i[1][1]);
default:
return n4;
}
});
function Gc(t, n4) {
return [0, n4[1], [0, n4[2], t]];
}
function Q00(t, n4, e) {
var i = t && t[1], x = n4 && n4[1];
return [0, i, x, e];
}
function lr(t, n4, e) {
var i = t && t[1], x = n4 && n4[1];
return !i && !x ? x : [0, Q00([0, i], [0, x], 0)];
}
function _u(t, n4, e, i) {
var x = t && t[1], c = n4 && n4[1];
return !x && !c && !e ? e : [0, Q00([0, x], [0, c], e)];
}
function _7(t, n4) {
if (t) {
if (n4) {
var e = n4[1], i = t[1], x = [0, un(i[2], e[2])];
return lr([0, un(e[1], i[1])], x, 0);
}
var c = t;
} else
var c = n4;
return c;
}
function QD(t, n4) {
if (n4) {
if (t) {
var e = n4[1], i = t[1], x = i[3], c = [0, un(i[2], e[2])];
return _u([0, un(e[1], i[1])], c, x, 0);
}
var s = n4[1];
return _u([0, s[1]], [0, s[2]], 0, 0);
}
return t;
}
function Jv(t, n4) {
for (var e = t, i = n4; ; ) {
if (typeof e == "number")
return i;
if (e[0] === 0)
return [0, e[1], 0, i];
var x = [0, e[2], e[4], i], e = e[3], i = x;
}
}
function rr0(t, n4) {
if (t)
var e = Jv(t[2], t[3]), i = function(c) {
return rr0(e, c);
}, x = [0, t[1], i];
else
var x = t;
return x;
}
function Hee(t) {
var n4 = Jv(t, 0);
return function(e) {
return rr0(n4, e);
};
}
function _s(t) {
return typeof t == "number" ? 0 : t[0] === 0 ? 1 : t[1];
}
function Xee(t) {
return [0, t];
}
function X7(t, n4, e) {
var i = 0;
if (typeof t == "number") {
if (typeof e == "number")
return [0, n4];
e[0] === 1 && (i = 1);
} else if (t[0] === 0)
typeof e != "number" && e[0] === 1 && (i = 1);
else {
var x = t[1];
if (typeof e != "number" && e[0] === 1) {
var c = e[1], s = c <= x ? x + 1 | 0 : c + 1 | 0;
return [1, s, n4, t, e];
}
var p = x;
i = 2;
}
switch (i) {
case 1:
var p = e[1];
break;
case 0:
return [1, 2, n4, t, e];
}
return [1, p + 1 | 0, n4, t, e];
}
function Ds(t, n4, e) {
var i = _s(t), x = _s(e), c = x <= i ? i + 1 | 0 : x + 1 | 0;
return [1, c, n4, t, e];
}
function rL(t, n4) {
var e = n4 !== 0 ? 1 : 0;
if (e) {
if (n4 !== 1) {
var i = n4 >>> 1 | 0, x = rL(t, i), c = u(t, 0), s = rL(t, (n4 - i | 0) - 1 | 0);
return [1, _s(x) + 1 | 0, c, x, s];
}
var p = [0, u(t, 0)];
} else
var p = e;
return p;
}
function hi(t, n4, e) {
var i = _s(t), x = _s(e);
if ((x + 2 | 0) < i) {
var c = t[4], s = t[3], p = t[2], y = _s(c);
if (y <= _s(s))
return Ds(s, p, X7(c, n4, e));
var T = X7(c[4], n4, e), E = c[2];
return Ds(X7(s, p, c[3]), E, T);
}
if ((i + 2 | 0) < x) {
var h = e[4], w = e[3], G = e[2], A = _s(w);
if (A <= _s(h))
return Ds(X7(t, n4, w), G, h);
var S = X7(w[4], G, h), M = w[2];
return Ds(X7(t, n4, w[3]), M, S);
}
return X7(t, n4, e);
}
function er0(t, n4) {
if (typeof n4 == "number")
return [0, t];
if (n4[0] === 0)
return X7([0, t], n4[1], 0);
var e = n4[4], i = n4[2];
return hi(er0(t, n4[3]), i, e);
}
function nr0(t, n4) {
if (typeof n4 == "number")
return [0, t];
if (n4[0] === 0)
return X7(0, n4[1], [0, t]);
var e = nr0(t, n4[4]);
return hi(n4[3], n4[2], e);
}
function Yu(t, n4, e) {
if (typeof t == "number")
return er0(n4, e);
if (t[0] === 0) {
if (typeof e != "number") {
if (e[0] === 0)
return X7(t, n4, e);
if (3 < e[1]) {
var i = e[4], x = e[2];
return hi(Yu(t, n4, e[3]), x, i);
}
return Ds(t, n4, e);
}
} else {
var c = t[4], s = t[3], p = t[2], y = t[1];
if (typeof e != "number") {
if (e[0] === 0)
return 3 < y ? hi(s, p, Yu(c, n4, e)) : Ds(t, n4, e);
var T = e[1];
if ((T + 2 | 0) < y)
return hi(s, p, Yu(c, n4, e));
if ((y + 2 | 0) < T) {
var E = e[4], h = e[2];
return hi(Yu(t, n4, e[3]), h, E);
}
return Ds(t, n4, e);
}
}
return nr0(n4, t);
}
function Pl(t) {
for (var n4 = t; ; ) {
if (typeof n4 == "number")
throw Kt;
if (n4[0] === 0)
return n4[1];
if (typeof n4[3] == "number")
return n4[2];
var n4 = n4[3];
}
}
function tr0(t) {
for (var n4 = t; ; ) {
if (typeof n4 == "number")
return 0;
if (n4[0] === 0)
return [0, n4[1]];
if (typeof n4[3] == "number")
return [0, n4[2]];
var n4 = n4[3];
}
}
function ur0(t) {
for (var n4 = t; ; ) {
if (typeof n4 == "number")
throw Kt;
if (n4[0] === 0)
return n4[1];
if (typeof n4[4] == "number")
return n4[2];
var n4 = n4[4];
}
}
function Yee(t) {
for (var n4 = t; ; ) {
if (typeof n4 == "number")
return 0;
if (n4[0] === 0)
return [0, n4[1]];
if (typeof n4[4] == "number")
return [0, n4[2]];
var n4 = n4[4];
}
}
function eL(t) {
if (typeof t == "number")
return Cu(t5r);
if (t[0] === 0)
return 0;
if (typeof t[3] == "number")
return t[4];
var n4 = t[4], e = t[2];
return hi(eL(t[3]), e, n4);
}
function Dl(t, n4) {
if (typeof t == "number")
return n4;
if (typeof n4 == "number")
return t;
var e = eL(n4);
return Yu(t, Pl(n4), e);
}
function nL(t) {
if (typeof t == "number")
return 0;
if (t[0] === 0)
return 1;
var n4 = nL(t[4]);
return (nL(t[3]) + 1 | 0) + n4 | 0;
}
function ir0(t, n4) {
for (var e = t, i = n4; ; ) {
if (typeof i == "number")
return e;
if (i[0] === 0)
return [0, i[1], e];
var x = i[3], c = ir0(e, i[4]), e = [0, i[2], c], i = x;
}
}
function fr0(t) {
return ir0(0, t);
}
var st = 0;
function tL(t) {
var n4 = typeof t == "number" ? 1 : 0, e = n4 && 1;
return e;
}
function uL(t) {
function n4(e0, x0) {
if (typeof x0 == "number")
return [0, e0];
if (x0[0] === 0) {
var l = x0[1], c0 = a(t[1], e0, l);
return c0 === 0 ? x0 : 0 <= c0 ? X7(x0, e0, st) : X7([0, e0], l, st);
}
var t0 = x0[4], a0 = x0[3], w0 = x0[2], _0 = a(t[1], e0, w0);
if (_0 === 0)
return x0;
if (0 <= _0) {
var E0 = n4(e0, t0);
return t0 === E0 ? x0 : hi(a0, w0, E0);
}
var X0 = n4(e0, a0);
return a0 === X0 ? x0 : hi(X0, w0, t0);
}
function e(e0, x0) {
if (typeof x0 == "number")
return [0, st, 0, st];
if (x0[0] === 0) {
var l = a(t[1], e0, x0[1]);
return l === 0 ? [0, st, 1, st] : 0 <= l ? [0, x0, 0, st] : [0, st, 0, x0];
}
var c0 = x0[4], t0 = x0[3], a0 = x0[2], w0 = a(t[1], e0, a0);
if (w0 === 0)
return [0, t0, 1, c0];
if (0 <= w0) {
var _0 = e(e0, c0), E0 = _0[3], X0 = _0[2];
return [0, Yu(t0, a0, _0[1]), X0, E0];
}
var b = e(e0, t0), G0 = Yu(b[3], a0, c0);
return [0, b[1], b[2], G0];
}
function i(e0, x0) {
for (var l = x0; ; ) {
if (typeof l == "number")
return 0;
if (l[0] === 0)
return a(t[1], e0, l[1]) === 0 ? 1 : 0;
var c0 = a(t[1], e0, l[2]), t0 = c0 === 0 ? 1 : 0;
if (t0)
return t0;
var a0 = 0 <= c0 ? l[4] : l[3], l = a0;
}
}
function x(e0, x0) {
if (typeof x0 == "number")
return st;
if (x0[0] === 0)
return a(t[1], e0, x0[1]) === 0 ? st : x0;
var l = x0[4], c0 = x0[3], t0 = x0[2], a0 = a(t[1], e0, t0);
if (a0 === 0) {
if (typeof c0 == "number")
return l;
if (typeof l == "number")
return c0;
var w0 = eL(l);
return hi(c0, Pl(l), w0);
}
if (0 <= a0) {
var _0 = x(e0, l);
return l === _0 ? x0 : hi(c0, t0, _0);
}
var E0 = x(e0, c0);
return c0 === E0 ? x0 : hi(E0, t0, l);
}
function c(e0, x0) {
if (typeof e0 == "number")
return x0;
if (e0[0] === 1) {
var l = e0[2], c0 = e0[1];
if (typeof x0 != "number") {
if (x0[0] === 0)
return n4(x0[1], e0);
var t0 = x0[2], a0 = x0[1];
if (a0 <= c0) {
if (a0 === 1)
return n4(t0, e0);
var w0 = e(l, x0), _0 = c(e0[4], w0[3]);
return Yu(c(e0[3], w0[1]), l, _0);
}
if (c0 === 1)
return n4(l, x0);
var E0 = e(t0, e0), X0 = c(E0[3], x0[4]);
return Yu(c(E0[1], x0[3]), t0, X0);
}
}
return typeof x0 == "number" ? e0 : n4(e0[1], x0);
}
function s(e0, x0) {
if (typeof e0 == "number" || typeof x0 == "number")
return st;
if (typeof e0 != "number" && e0[0] !== 0) {
var l = e0[4], c0 = e0[3], t0 = e0[2], a0 = e(t0, x0), w0 = a0[1];
if (a0[2]) {
var _0 = s(l, a0[3]);
return Yu(s(c0, w0), t0, _0);
}
var E0 = s(l, a0[3]);
return Dl(s(c0, w0), E0);
}
return i(e0[1], x0) ? e0 : st;
}
function p(e0, x0) {
if (typeof x0 == "number")
return [0, st, function(s0) {
return st;
}];
if (x0[0] === 0)
return a(t[1], e0, x0[1]) === 0 ? 0 : [0, st, function(s0) {
return st;
}];
var l = x0[4], c0 = x0[3], t0 = x0[2], a0 = a(t[1], e0, t0);
if (a0 === 0)
return 0;
if (0 <= a0) {
var w0 = p(e0, l);
if (w0)
var _0 = w0[2], E0 = [0, Yu(c0, t0, w0[1]), _0];
else
var E0 = w0;
return E0;
}
var X0 = p(e0, c0);
if (X0)
var b = X0[2], G0 = function(s0) {
return Yu(u(b, 0), t0, l);
}, X = [0, X0[1], G0];
else
var X = X0;
return X;
}
function y(e0, x0) {
for (var l = e0, c0 = x0; ; ) {
if (typeof l != "number" && typeof c0 != "number") {
var t0 = 0;
if (typeof l != "number" && l[0] !== 0) {
if (typeof c0 != "number" && c0[0] !== 0) {
if (l === c0)
return 0;
var a0 = p(l[2], c0);
if (a0) {
var w0 = y(l[3], a0[1]);
if (w0) {
var _0 = u(a0[2], 0), l = l[4], c0 = _0;
continue;
}
var E0 = w0;
} else
var E0 = a0;
return E0;
}
var X0 = l, b = c0[1];
t0 = 1;
}
if (!t0)
var X0 = c0, b = l[1];
return 1 - i(b, X0);
}
return 1;
}
}
function T(e0, x0) {
if (typeof e0 == "number")
return st;
if (typeof x0 == "number")
return e0;
if (typeof e0 != "number" && e0[0] !== 0) {
var l = e0[4], c0 = e0[3], t0 = e0[2], a0 = e(t0, x0), w0 = a0[1];
if (a0[2]) {
var _0 = T(l, a0[3]);
return Dl(T(c0, w0), _0);
}
var E0 = T(l, a0[3]);
return Yu(T(c0, w0), t0, E0);
}
return i(e0[1], x0) ? st : e0;
}
function E(e0, x0) {
for (var l = Jv(x0, 0), c0 = Jv(e0, 0), t0 = l; ; ) {
if (c0) {
if (t0) {
var a0 = a(t[1], c0[1], t0[1]);
if (a0 === 0) {
var w0 = Jv(t0[2], t0[3]), c0 = Jv(c0[2], c0[3]), t0 = w0;
continue;
}
return a0;
}
return 1;
}
var _0 = t0 && -1;
return _0;
}
}
function h(e0, x0) {
return E(e0, x0) === 0 ? 1 : 0;
}
function w(e0, x0) {
for (var l = e0, c0 = x0; ; ) {
if (typeof l == "number")
return 1;
if (l[0] === 0) {
var t0 = l[1];
if (typeof c0 != "number") {
if (c0[0] === 0) {
var a0 = a(t[1], t0, c0[1]) === 0 ? 1 : 0, w0 = a0 && 1;
return w0;
}
var _0 = a(t[1], t0, c0[2]);
if (_0 === 0)
return 1;
if (0 <= _0) {
var c0 = c0[4];
continue;
}
var c0 = c0[3];
continue;
}
} else {
var E0 = l[4], X0 = l[3], b = l[2];
if (typeof c0 != "number") {
if (c0[0] === 0) {
var G0 = l[1] === 1 ? 1 : 0, X = G0 && (a(t[1], b, c0[1]) === 0 ? 1 : 0);
return X;
}
var s0 = c0[4], dr = c0[3], Ar = a(t[1], b, c0[2]);
if (Ar === 0) {
var ar = w(X0, dr);
if (ar) {
var l = E0, c0 = s0;
continue;
}
return ar;
}
if (0 <= Ar) {
var W0 = w(X7(st, b, E0), s0);
if (W0) {
var l = X0;
continue;
}
return W0;
}
var Lr = w(X7(X0, b, st), dr);
if (Lr) {
var l = E0;
continue;
}
return Lr;
}
}
return 0;
}
}
function G(e0, x0) {
for (var l = x0; ; ) {
if (typeof l == "number")
return 0;
if (l[0] === 0)
return u(e0, l[1]);
G(e0, l[3]), u(e0, l[2]);
var l = l[4];
}
}
function A(e0, x0, l) {
for (var c0 = x0, t0 = l; ; ) {
if (typeof c0 == "number")
return t0;
if (c0[0] === 0)
return a(e0, c0[1], t0);
var a0 = A(e0, c0[3], t0), w0 = a(e0, c0[2], a0), c0 = c0[4], t0 = w0;
}
}
function S(e0, x0) {
for (var l = x0; ; ) {
if (typeof l == "number")
return 1;
if (l[0] === 0)
return u(e0, l[1]);
var c0 = u(e0, l[2]);
if (c0) {
var t0 = S(e0, l[3]);
if (t0) {
var l = l[4];
continue;
}
var a0 = t0;
} else
var a0 = c0;
return a0;
}
}
function M(e0, x0) {
for (var l = x0; ; ) {
if (typeof l == "number")
return 0;
if (l[0] === 0)
return u(e0, l[1]);
var c0 = u(e0, l[2]);
if (c0)
var t0 = c0;
else {
var a0 = M(e0, l[3]);
if (!a0) {
var l = l[4];
continue;
}
var t0 = a0;
}
return t0;
}
}
function K(e0, x0) {
if (typeof x0 == "number")
return st;
if (x0[0] === 0)
return u(e0, x0[1]) ? x0 : st;
var l = x0[4], c0 = x0[3], t0 = x0[2], a0 = K(e0, c0), w0 = u(e0, t0), _0 = K(e0, l);
return w0 ? c0 === a0 && l === _0 ? x0 : Yu(a0, t0, _0) : Dl(a0, _0);
}
function V(e0, x0) {
if (typeof x0 == "number")
return [0, st, st];
if (x0[0] === 0)
return u(e0, x0[1]) ? [0, x0, st] : [0, st, x0];
var l = x0[2], c0 = V(e0, x0[3]), t0 = c0[2], a0 = c0[1], w0 = u(e0, l), _0 = V(e0, x0[4]), E0 = _0[2], X0 = _0[1];
if (w0) {
var b = Dl(t0, E0);
return [0, Yu(a0, l, X0), b];
}
var G0 = Yu(t0, l, E0);
return [0, Dl(a0, X0), G0];
}
function f0(e0, x0) {
for (var l = x0; ; ) {
if (typeof l == "number")
throw Kt;
if (l[0] === 0) {
var c0 = l[1];
if (a(t[1], e0, c0) === 0)
return c0;
throw Kt;
}
var t0 = l[2], a0 = a(t[1], e0, t0);
if (a0 === 0)
return t0;
var w0 = 0 <= a0 ? l[4] : l[3], l = w0;
}
}
function m0(e0, x0) {
for (var l = x0; ; ) {
if (typeof l == "number")
return 0;
if (l[0] === 0) {
var c0 = l[1], t0 = a(t[1], e0, c0) === 0 ? 1 : 0, a0 = t0 && [0, c0];
return a0;
}
var w0 = l[2], _0 = a(t[1], e0, w0);
if (_0 === 0)
return [0, w0];
var E0 = 0 <= _0 ? l[4] : l[3], l = E0;
}
}
function k0(e0, x0) {
if (typeof x0 == "number")
return st;
if (x0[0] === 0) {
var l = x0[1], c0 = u(e0, l);
return l === c0 ? x0 : [0, c0];
}
var t0 = x0[4], a0 = x0[3], w0 = x0[2], _0 = k0(e0, a0), E0 = u(e0, w0), X0 = k0(e0, t0);
if (a0 === _0 && w0 === E0 && t0 === X0)
return x0;
var b = 0;
if (!tL(_0)) {
var G0 = ur0(_0);
0 <= a(t[1], G0, E0) && (b = 1);
}
if (!b) {
var X = 0;
if (!tL(X0)) {
var s0 = Pl(X0);
0 <= a(t[1], E0, s0) && (X = 1);
}
if (!X)
return Yu(_0, E0, X0);
}
return c(_0, n4(E0, X0));
}
function g0(e0) {
if (e0) {
var x0 = e0[2], l = e0[1];
if (x0) {
var c0 = x0[2], t0 = x0[1];
if (c0) {
var a0 = c0[2], w0 = c0[1];
if (a0) {
var _0 = a0[2], E0 = a0[1];
if (_0) {
if (_0[2]) {
var X0 = t[1], b = function(ar, W0) {
if (ar === 2) {
if (W0) {
var Lr = W0[2];
if (Lr) {
var Tr = Lr[1], Hr = W0[1], Or = Lr[2], xr = a(X0, Hr, Tr), Rr = xr === 0 ? [0, Hr, 0] : 0 < xr ? [0, Hr, [0, Tr, 0]] : [0, Tr, [0, Hr, 0]];
return [0, Rr, Or];
}
}
} else if (ar === 3 && W0) {
var Wr = W0[2];
if (Wr) {
var Jr = Wr[2];
if (Jr) {
var or = Jr[1], _r = Wr[1], Ir = W0[1], fe = Jr[2], v0 = a(X0, Ir, _r);
if (v0 === 0)
var P = a(X0, _r, or), L = P === 0 ? [0, _r, 0] : 0 < P ? [0, _r, [0, or, 0]] : [0, or, [0, _r, 0]], Q = L;
else if (0 < v0) {
var i0 = a(X0, _r, or);
if (i0 === 0)
var T0 = [0, Ir, [0, _r, 0]];
else if (0 < i0)
var T0 = [0, Ir, [0, _r, [0, or, 0]]];
else
var l0 = a(X0, Ir, or), S0 = l0 === 0 ? [0, Ir, [0, _r, 0]] : 0 < l0 ? [0, Ir, [0, or, [0, _r, 0]]] : [0, or, [0, Ir, [0, _r, 0]]], T0 = S0;
var Q = T0;
} else {
var rr = a(X0, Ir, or);
if (rr === 0)
var Z = [0, _r, [0, Ir, 0]];
else if (0 < rr)
var Z = [0, _r, [0, Ir, [0, or, 0]]];
else
var R0 = a(X0, _r, or), B = R0 === 0 ? [0, _r, [0, Ir, 0]] : 0 < R0 ? [0, _r, [0, or, [0, Ir, 0]]] : [0, or, [0, _r, [0, Ir, 0]]], Z = B;
var Q = Z;
}
return [0, Q, fe];
}
}
}
for (var p0 = ar >> 1, b0 = G0(p0, W0), O0 = b0[1], q0 = G0(ar - p0 | 0, b0[2]), er = O0, yr = q0[1], vr = 0, $0 = q0[2]; ; ) {
if (er) {
if (yr) {
var Sr = yr[2], Mr = yr[1], Br = er[2], qr = er[1], jr = a(X0, qr, Mr);
if (jr === 0) {
var er = Br, yr = Sr, vr = [0, qr, vr];
continue;
}
if (0 <= jr) {
var yr = Sr, vr = [0, Mr, vr];
continue;
}
var er = Br, vr = [0, qr, vr];
continue;
}
var $r = jc(er, vr);
} else
var $r = jc(yr, vr);
return [0, $r, $0];
}
}, G0 = function(ar, W0) {
if (ar === 2) {
if (W0) {
var Lr = W0[2];
if (Lr) {
var Tr = Lr[1], Hr = W0[1], Or = Lr[2], xr = a(X0, Hr, Tr), Rr = xr === 0 ? [0, Hr, 0] : 0 <= xr ? [0, Tr, [0, Hr, 0]] : [0, Hr, [0, Tr, 0]];
return [0, Rr, Or];
}
}
} else if (ar === 3 && W0) {
var Wr = W0[2];
if (Wr) {
var Jr = Wr[2];
if (Jr) {
var or = Jr[1], _r = Wr[1], Ir = W0[1], fe = Jr[2], v0 = a(X0, Ir, _r);
if (v0 === 0)
var P = a(X0, _r, or), L = P === 0 ? [0, _r, 0] : 0 <= P ? [0, or, [0, _r, 0]] : [0, _r, [0, or, 0]], Q = L;
else if (0 <= v0) {
var i0 = a(X0, Ir, or);
if (i0 === 0)
var T0 = [0, _r, [0, Ir, 0]];
else if (0 <= i0)
var l0 = a(X0, _r, or), S0 = l0 === 0 ? [0, _r, [0, Ir, 0]] : 0 <= l0 ? [0, or, [0, _r, [0, Ir, 0]]] : [0, _r, [0, or, [0, Ir, 0]]], T0 = S0;
else
var T0 = [0, _r, [0, Ir, [0, or, 0]]];
var Q = T0;
} else {
var rr = a(X0, _r, or);
if (rr === 0)
var Z = [0, Ir, [0, _r, 0]];
else if (0 <= rr)
var R0 = a(X0, Ir, or), B = R0 === 0 ? [0, Ir, [0, _r, 0]] : 0 <= R0 ? [0, or, [0, Ir, [0, _r, 0]]] : [0, Ir, [0, or, [0, _r, 0]]], Z = B;
else
var Z = [0, Ir, [0, _r, [0, or, 0]]];
var Q = Z;
}
return [0, Q, fe];
}
}
}
for (var p0 = ar >> 1, b0 = b(p0, W0), O0 = b0[1], q0 = b(ar - p0 | 0, b0[2]), er = O0, yr = q0[1], vr = 0, $0 = q0[2]; ; ) {
if (er) {
if (yr) {
var Sr = yr[2], Mr = yr[1], Br = er[2], qr = er[1], jr = a(X0, qr, Mr);
if (jr === 0) {
var er = Br, yr = Sr, vr = [0, qr, vr];
continue;
}
if (0 < jr) {
var er = Br, vr = [0, qr, vr];
continue;
}
var yr = Sr, vr = [0, Mr, vr];
continue;
}
var $r = jc(er, vr);
} else
var $r = jc(yr, vr);
return [0, $r, $0];
}
}, X = Rc(e0), s0 = 2 <= X ? G0(X, e0)[1] : e0, dr = function(ar, W0) {
if (!(3 < ar >>> 0))
switch (ar) {
case 0:
return [0, 0, W0];
case 1:
if (W0)
return [0, [0, W0[1]], W0[2]];
break;
case 2:
if (W0) {
var Lr = W0[2];
if (Lr)
return [0, [1, 2, Lr[1], [0, W0[1]], 0], Lr[2]];
}
break;
default:
if (W0) {
var Tr = W0[2];
if (Tr) {
var Hr = Tr[2];
if (Hr)
return [0, [1, 2, Tr[1], [0, W0[1]], [0, Hr[1]]], Hr[2]];
}
}
}
var Or = ar / 2 | 0, xr = dr(Or, W0), Rr = xr[2];
if (Rr) {
var Wr = dr((ar - Or | 0) - 1 | 0, Rr[2]), Jr = Wr[2];
return [0, Ds(xr[1], Rr[1], Wr[1]), Jr];
}
throw [0, wn, o5r];
};
return dr(Rc(s0), s0)[1];
}
var Ar = n4(E0, n4(w0, n4(t0, [0, l])));
return n4(_0[1], Ar);
}
return n4(E0, n4(w0, n4(t0, [0, l])));
}
return n4(w0, n4(t0, [0, l]));
}
return n4(t0, [0, l]);
}
return [0, l];
}
return st;
}
return [0, st, tL, i, n4, Xee, x, c, s, y, T, E, h, w, G, k0, A, S, M, K, V, nL, fr0, Pl, tr0, ur0, Yee, Pl, tr0, f0, m0, Hee, g0, function(e0, x0, l) {
u(f(x0), i5r);
var c0 = fr0(l);
c0 && u(f(x0), f5r);
var t0 = 0;
return be(function(a0, w0) {
return a0 && u(f(x0), u5r), a(e0, x0, w0), 1;
}, t0, c0), c0 && u(f(x0), x5r), u(f(x0), a5r);
}, rL];
}
var xr0 = c5r.slice();
function iL(t) {
for (var n4 = 0, e = xr0.length - 1 - 1 | 0; ; ) {
if (e < n4)
return 0;
var i = n4 + ((e - n4 | 0) / 2 | 0) | 0, x = xr0[1 + i];
if (t < x[1]) {
var e = i - 1 | 0;
continue;
}
if (x[2] <= t) {
var n4 = i + 1 | 0;
continue;
}
return 1;
}
}
var ar0 = function t(n4, e) {
return t.fun(n4, e);
};
N(ar0, function(t, n4) {
if (typeof t == "number") {
var e = t;
if (55 <= e)
switch (e) {
case 55:
if (typeof n4 == "number") {
var i = n4 !== 55 ? 1 : 0;
if (!i)
return i;
}
break;
case 56:
if (typeof n4 == "number") {
var x = n4 !== 56 ? 1 : 0;
if (!x)
return x;
}
break;
case 57:
if (typeof n4 == "number") {
var c = n4 !== 57 ? 1 : 0;
if (!c)
return c;
}
break;
case 58:
if (typeof n4 == "number") {
var s = n4 !== 58 ? 1 : 0;
if (!s)
return s;
}
break;
case 59:
if (typeof n4 == "number") {
var p = n4 !== 59 ? 1 : 0;
if (!p)
return p;
}
break;
case 60:
if (typeof n4 == "number") {
var y = n4 !== 60 ? 1 : 0;
if (!y)
return y;
}
break;
case 61:
if (typeof n4 == "number") {
var T = n4 !== 61 ? 1 : 0;
if (!T)
return T;
}
break;
case 62:
if (typeof n4 == "number") {
var E = n4 !== 62 ? 1 : 0;
if (!E)
return E;
}
break;
case 63:
if (typeof n4 == "number") {
var h = n4 !== 63 ? 1 : 0;
if (!h)
return h;
}
break;
case 64:
if (typeof n4 == "number") {
var w = n4 !== 64 ? 1 : 0;
if (!w)
return w;
}
break;
case 65:
if (typeof n4 == "number") {
var G = n4 !== 65 ? 1 : 0;
if (!G)
return G;
}
break;
case 66:
if (typeof n4 == "number") {
var A = n4 !== 66 ? 1 : 0;
if (!A)
return A;
}
break;
case 67:
if (typeof n4 == "number") {
var S = n4 !== 67 ? 1 : 0;
if (!S)
return S;
}
break;
case 68:
if (typeof n4 == "number") {
var M = n4 !== 68 ? 1 : 0;
if (!M)
return M;
}
break;
case 69:
if (typeof n4 == "number") {
var K = n4 !== 69 ? 1 : 0;
if (!K)
return K;
}
break;
case 70:
if (typeof n4 == "number") {
var V = n4 !== 70 ? 1 : 0;
if (!V)
return V;
}
break;
case 71:
if (typeof n4 == "number") {
var f0 = n4 !== 71 ? 1 : 0;
if (!f0)
return f0;
}
break;
case 72:
if (typeof n4 == "number") {
var m0 = n4 !== 72 ? 1 : 0;
if (!m0)
return m0;
}
break;
case 73:
if (typeof n4 == "number") {
var k0 = n4 !== 73 ? 1 : 0;
if (!k0)
return k0;
}
break;
case 74:
if (typeof n4 == "number") {
var g0 = n4 !== 74 ? 1 : 0;
if (!g0)
return g0;
}
break;
case 75:
if (typeof n4 == "number") {
var e0 = n4 !== 75 ? 1 : 0;
if (!e0)
return e0;
}
break;
case 76:
if (typeof n4 == "number") {
var x0 = n4 !== 76 ? 1 : 0;
if (!x0)
return x0;
}
break;
case 77:
if (typeof n4 == "number") {
var l = n4 !== 77 ? 1 : 0;
if (!l)
return l;
}
break;
case 78:
if (typeof n4 == "number") {
var c0 = n4 !== 78 ? 1 : 0;
if (!c0)
return c0;
}
break;
case 79:
if (typeof n4 == "number") {
var t0 = n4 !== 79 ? 1 : 0;
if (!t0)
return t0;
}
break;
case 80:
if (typeof n4 == "number") {
var a0 = n4 !== 80 ? 1 : 0;
if (!a0)
return a0;
}
break;
case 81:
if (typeof n4 == "number") {
var w0 = n4 !== 81 ? 1 : 0;
if (!w0)
return w0;
}
break;
case 82:
if (typeof n4 == "number") {
var _0 = n4 !== 82 ? 1 : 0;
if (!_0)
return _0;
}
break;
case 83:
if (typeof n4 == "number") {
var E0 = n4 !== 83 ? 1 : 0;
if (!E0)
return E0;
}
break;
case 84:
if (typeof n4 == "number") {
var X0 = n4 !== 84 ? 1 : 0;
if (!X0)
return X0;
}
break;
case 85:
if (typeof n4 == "number") {
var b = n4 !== 85 ? 1 : 0;
if (!b)
return b;
}
break;
case 86:
if (typeof n4 == "number") {
var G0 = n4 !== 86 ? 1 : 0;
if (!G0)
return G0;
}
break;
case 87:
if (typeof n4 == "number") {
var X = n4 !== 87 ? 1 : 0;
if (!X)
return X;
}
break;
case 88:
if (typeof n4 == "number") {
var s0 = n4 !== 88 ? 1 : 0;
if (!s0)
return s0;
}
break;
case 89:
if (typeof n4 == "number") {
var dr = n4 !== 89 ? 1 : 0;
if (!dr)
return dr;
}
break;
case 90:
if (typeof n4 == "number") {
var Ar = n4 !== 90 ? 1 : 0;
if (!Ar)
return Ar;
}
break;
case 91:
if (typeof n4 == "number") {
var ar = n4 !== 91 ? 1 : 0;
if (!ar)
return ar;
}
break;
case 92:
if (typeof n4 == "number") {
var W0 = n4 !== 92 ? 1 : 0;
if (!W0)
return W0;
}
break;
case 93:
if (typeof n4 == "number") {
var Lr = n4 !== 93 ? 1 : 0;
if (!Lr)
return Lr;
}
break;
case 94:
if (typeof n4 == "number") {
var Tr = n4 !== 94 ? 1 : 0;
if (!Tr)
return Tr;
}
break;
case 95:
if (typeof n4 == "number") {
var Hr = n4 !== 95 ? 1 : 0;
if (!Hr)
return Hr;
}
break;
case 96:
if (typeof n4 == "number") {
var Or = n4 !== 96 ? 1 : 0;
if (!Or)
return Or;
}
break;
case 97:
if (typeof n4 == "number") {
var xr = n4 !== 97 ? 1 : 0;
if (!xr)
return xr;
}
break;
case 98:
if (typeof n4 == "number") {
var Rr = n4 !== 98 ? 1 : 0;
if (!Rr)
return Rr;
}
break;
case 99:
if (typeof n4 == "number") {
var Wr = n4 !== 99 ? 1 : 0;
if (!Wr)
return Wr;
}
break;
case 100:
if (typeof n4 == "number") {
var Jr = ni !== n4 ? 1 : 0;
if (!Jr)
return Jr;
}
break;
case 101:
if (typeof n4 == "number") {
var or = L7 !== n4 ? 1 : 0;
if (!or)
return or;
}
break;
case 102:
if (typeof n4 == "number") {
var _r = Ri !== n4 ? 1 : 0;
if (!_r)
return _r;
}
break;
case 103:
if (typeof n4 == "number") {
var Ir = c7 !== n4 ? 1 : 0;
if (!Ir)
return Ir;
}
break;
case 104:
if (typeof n4 == "number") {
var fe = D7 !== n4 ? 1 : 0;
if (!fe)
return fe;
}
break;
case 105:
if (typeof n4 == "number") {
var v0 = R7 !== n4 ? 1 : 0;
if (!v0)
return v0;
}
break;
case 106:
if (typeof n4 == "number") {
var P = Xt !== n4 ? 1 : 0;
if (!P)
return P;
}
break;
case 107:
if (typeof n4 == "number") {
var L = Qc !== n4 ? 1 : 0;
if (!L)
return L;
}
break;
default:
if (typeof n4 == "number" && fs2 <= n4)
return 0;
}
else
switch (e) {
case 0:
if (typeof n4 == "number" && !n4)
return n4;
break;
case 1:
if (typeof n4 == "number") {
var Q = n4 !== 1 ? 1 : 0;
if (!Q)
return Q;
}
break;
case 2:
if (typeof n4 == "number") {
var i0 = n4 !== 2 ? 1 : 0;
if (!i0)
return i0;
}
break;
case 3:
if (typeof n4 == "number") {
var l0 = n4 !== 3 ? 1 : 0;
if (!l0)
return l0;
}
break;
case 4:
if (typeof n4 == "number") {
var S0 = n4 !== 4 ? 1 : 0;
if (!S0)
return S0;
}
break;
case 5:
if (typeof n4 == "number") {
var T0 = n4 !== 5 ? 1 : 0;
if (!T0)
return T0;
}
break;
case 6:
if (typeof n4 == "number") {
var rr = n4 !== 6 ? 1 : 0;
if (!rr)
return rr;
}
break;
case 7:
if (typeof n4 == "number") {
var R0 = n4 !== 7 ? 1 : 0;
if (!R0)
return R0;
}
break;
case 8:
if (typeof n4 == "number") {
var B = n4 !== 8 ? 1 : 0;
if (!B)
return B;
}
break;
case 9:
if (typeof n4 == "number") {
var Z = n4 !== 9 ? 1 : 0;
if (!Z)
return Z;
}
break;
case 10:
if (typeof n4 == "number") {
var p0 = n4 !== 10 ? 1 : 0;
if (!p0)
return p0;
}
break;
case 11:
if (typeof n4 == "number") {
var b0 = n4 !== 11 ? 1 : 0;
if (!b0)
return b0;
}
break;
case 12:
if (typeof n4 == "number") {
var O0 = n4 !== 12 ? 1 : 0;
if (!O0)
return O0;
}
break;
case 13:
if (typeof n4 == "number") {
var q0 = n4 !== 13 ? 1 : 0;
if (!q0)
return q0;
}
break;
case 14:
if (typeof n4 == "number") {
var er = n4 !== 14 ? 1 : 0;
if (!er)
return er;
}
break;
case 15:
if (typeof n4 == "number") {
var yr = n4 !== 15 ? 1 : 0;
if (!yr)
return yr;
}
break;
case 16:
if (typeof n4 == "number") {
var vr = n4 !== 16 ? 1 : 0;
if (!vr)
return vr;
}
break;
case 17:
if (typeof n4 == "number") {
var $0 = n4 !== 17 ? 1 : 0;
if (!$0)
return $0;
}
break;
case 18:
if (typeof n4 == "number") {
var Sr = n4 !== 18 ? 1 : 0;
if (!Sr)
return Sr;
}
break;
case 19:
if (typeof n4 == "number") {
var Mr = n4 !== 19 ? 1 : 0;
if (!Mr)
return Mr;
}
break;
case 20:
if (typeof n4 == "number") {
var Br = n4 !== 20 ? 1 : 0;
if (!Br)
return Br;
}
break;
case 21:
if (typeof n4 == "number") {
var qr = n4 !== 21 ? 1 : 0;
if (!qr)
return qr;
}
break;
case 22:
if (typeof n4 == "number") {
var jr = n4 !== 22 ? 1 : 0;
if (!jr)
return jr;
}
break;
case 23:
if (typeof n4 == "number") {
var $r = n4 !== 23 ? 1 : 0;
if (!$r)
return $r;
}
break;
case 24:
if (typeof n4 == "number") {
var ne = n4 !== 24 ? 1 : 0;
if (!ne)
return ne;
}
break;
case 25:
if (typeof n4 == "number") {
var Qr = n4 !== 25 ? 1 : 0;
if (!Qr)
return Qr;
}
break;
case 26:
if (typeof n4 == "number") {
var pe = n4 !== 26 ? 1 : 0;
if (!pe)
return pe;
}
break;
case 27:
if (typeof n4 == "number") {
var oe = n4 !== 27 ? 1 : 0;
if (!oe)
return oe;
}
break;
case 28:
if (typeof n4 == "number") {
var me = n4 !== 28 ? 1 : 0;
if (!me)
return me;
}
break;
case 29:
if (typeof n4 == "number") {
var ae = n4 !== 29 ? 1 : 0;
if (!ae)
return ae;
}
break;
case 30:
if (typeof n4 == "number") {
var ce = n4 !== 30 ? 1 : 0;
if (!ce)
return ce;
}
break;
case 31:
if (typeof n4 == "number") {
var ge = n4 !== 31 ? 1 : 0;
if (!ge)
return ge;
}
break;
case 32:
if (typeof n4 == "number") {
var H0 = n4 !== 32 ? 1 : 0;
if (!H0)
return H0;
}
break;
case 33:
if (typeof n4 == "number") {
var Fr = n4 !== 33 ? 1 : 0;
if (!Fr)
return Fr;
}
break;
case 34:
if (typeof n4 == "number") {
var _ = n4 !== 34 ? 1 : 0;
if (!_)
return _;
}
break;
case 35:
if (typeof n4 == "number") {
var k = n4 !== 35 ? 1 : 0;
if (!k)
return k;
}
break;
case 36:
if (typeof n4 == "number") {
var I = n4 !== 36 ? 1 : 0;
if (!I)
return I;
}
break;
case 37:
if (typeof n4 == "number") {
var U = n4 !== 37 ? 1 : 0;
if (!U)
return U;
}
break;
case 38:
if (typeof n4 == "number") {
var Y = n4 !== 38 ? 1 : 0;
if (!Y)
return Y;
}
break;
case 39:
if (typeof n4 == "number") {
var y0 = n4 !== 39 ? 1 : 0;
if (!y0)
return y0;
}
break;
case 40:
if (typeof n4 == "number") {
var D0 = n4 !== 40 ? 1 : 0;
if (!D0)
return D0;
}
break;
case 41:
if (typeof n4 == "number") {
var I0 = n4 !== 41 ? 1 : 0;
if (!I0)
return I0;
}
break;
case 42:
if (typeof n4 == "number") {
var D = n4 !== 42 ? 1 : 0;
if (!D)
return D;
}
break;
case 43:
if (typeof n4 == "number") {
var u0 = n4 !== 43 ? 1 : 0;
if (!u0)
return u0;
}
break;
case 44:
if (typeof n4 == "number") {
var Y0 = n4 !== 44 ? 1 : 0;
if (!Y0)
return Y0;
}
break;
case 45:
if (typeof n4 == "number") {
var J0 = n4 !== 45 ? 1 : 0;
if (!J0)
return J0;
}
break;
case 46:
if (typeof n4 == "number") {
var fr = n4 !== 46 ? 1 : 0;
if (!fr)
return fr;
}
break;
case 47:
if (typeof n4 == "number") {
var Q0 = n4 !== 47 ? 1 : 0;
if (!Q0)
return Q0;
}
break;
case 48:
if (typeof n4 == "number") {
var F0 = n4 !== 48 ? 1 : 0;
if (!F0)
return F0;
}
break;
case 49:
if (typeof n4 == "number") {
var gr = n4 !== 49 ? 1 : 0;
if (!gr)
return gr;
}
break;
case 50:
if (typeof n4 == "number") {
var mr = n4 !== 50 ? 1 : 0;
if (!mr)
return mr;
}
break;
case 51:
if (typeof n4 == "number") {
var Cr = n4 !== 51 ? 1 : 0;
if (!Cr)
return Cr;
}
break;
case 52:
if (typeof n4 == "number") {
var sr = n4 !== 52 ? 1 : 0;
if (!sr)
return sr;
}
break;
case 53:
if (typeof n4 == "number") {
var Pr = n4 !== 53 ? 1 : 0;
if (!Pr)
return Pr;
}
break;
default:
if (typeof n4 == "number") {
var K0 = n4 !== 54 ? 1 : 0;
if (!K0)
return K0;
}
}
} else
switch (t[0]) {
case 0:
if (typeof n4 != "number" && n4[0] === 0) {
var Ur = Ee(t[1], n4[1]);
return Ur === 0 ? Ee(t[2], n4[2]) : Ur;
}
break;
case 1:
if (typeof n4 != "number" && n4[0] === 1) {
var d0 = Ee(t[1], n4[1]);
return d0 === 0 ? Ee(t[2], n4[2]) : d0;
}
break;
case 2:
if (typeof n4 != "number" && n4[0] === 2)
return Ee(t[1], n4[1]);
break;
case 3:
if (typeof n4 != "number" && n4[0] === 3) {
var Kr = n4[2], re = t[2], xe = Ee(t[1], n4[1]);
if (xe === 0) {
if (re)
return Kr ? Ee(re[1], Kr[1]) : 1;
var je = Kr && -1;
return je;
}
return xe;
}
break;
case 4:
if (typeof n4 != "number" && n4[0] === 4)
return Ee(t[1], n4[1]);
break;
case 5:
if (typeof n4 != "number" && n4[0] === 5) {
var ve = n4[2], Ie = t[2], Me = Ee(t[1], n4[1]);
if (Me === 0) {
if (Ie)
if (ve) {
var Be = ve[1], fn = Ie[1], Ke = 0;
switch (fn) {
case 0:
if (Be)
Ke = 1;
else
var on = Be;
break;
case 1:
var Ae = Be !== 1 ? 1 : 0;
if (Ae)
Ke = 1;
else
var on = Ae;
break;
case 2:
var xn = Be !== 2 ? 1 : 0;
if (xn)
Ke = 1;
else
var on = xn;
break;
default:
if (3 <= Be)
var on = 0;
else
Ke = 1;
}
if (Ke)
var Qe = function(Nt) {
switch (Nt) {
case 0:
return 0;
case 1:
return 1;
case 2:
return 2;
default:
return 3;
}
}, yn = Qe(Be), on = Cc(Qe(fn), yn);
var Ce = on;
} else
var Ce = 1;
else
var Ce = ve && -1;
return Ce === 0 ? Ee(t[3], n4[3]) : Ce;
}
return Me;
}
break;
case 6:
if (typeof n4 != "number" && n4[0] === 6) {
var We = Ee(t[1], n4[1]);
return We === 0 ? Ee(t[2], n4[2]) : We;
}
break;
case 7:
if (typeof n4 != "number" && n4[0] === 7)
return Cc(t[1], n4[1]);
break;
case 8:
if (typeof n4 != "number" && n4[0] === 8) {
var rn = Ee(t[1], n4[1]);
return rn === 0 ? Ee(t[2], n4[2]) : rn;
}
break;
case 9:
if (typeof n4 != "number" && n4[0] === 9)
return Ee(t[1], n4[1]);
break;
case 10:
if (typeof n4 != "number" && n4[0] === 10)
return Ee(t[1], n4[1]);
break;
case 11:
if (typeof n4 != "number" && n4[0] === 11) {
var bn = Ee(t[1], n4[1]);
return bn === 0 ? Ee(t[2], n4[2]) : bn;
}
break;
case 12:
if (typeof n4 != "number" && n4[0] === 12) {
var Cn = Ee(t[1], n4[1]);
return Cn === 0 ? Ee(t[2], n4[2]) : Cn;
}
break;
case 13:
if (typeof n4 != "number" && n4[0] === 13)
return Ee(t[1], n4[1]);
break;
case 14:
if (typeof n4 != "number" && n4[0] === 14)
return Cc(t[1], n4[1]);
break;
case 15:
if (typeof n4 != "number" && n4[0] === 15)
return Ee(t[1], n4[1]);
break;
case 16:
if (typeof n4 != "number" && n4[0] === 16) {
var Hn = Ee(t[1], n4[1]);
return Hn === 0 ? Ee(t[2], n4[2]) : Hn;
}
break;
case 17:
if (typeof n4 != "number" && n4[0] === 17)
return Ee(t[1], n4[1]);
break;
case 18:
if (typeof n4 != "number" && n4[0] === 18)
return Cc(t[1], n4[1]);
break;
case 19:
if (typeof n4 != "number" && n4[0] === 19)
return Ee(t[1], n4[1]);
break;
case 20:
if (typeof n4 != "number" && n4[0] === 20)
return Ee(t[1], n4[1]);
break;
case 21:
if (typeof n4 != "number" && n4[0] === 21) {
var Sn = Ee(t[1], n4[1]);
if (Sn === 0) {
var vt = Cc(t[2], n4[2]);
if (vt === 0) {
var At = Cc(t[3], n4[3]);
return At === 0 ? Cc(t[4], n4[4]) : At;
}
return vt;
}
return Sn;
}
break;
case 22:
if (typeof n4 != "number" && n4[0] === 22)
return Ee(t[1], n4[1]);
break;
default:
if (typeof n4 != "number" && n4[0] === 23)
return Ee(t[1], n4[1]);
}
function gt(Bt) {
if (typeof Bt == "number") {
var Ft = Bt;
if (55 <= Ft)
switch (Ft) {
case 55:
return 72;
case 56:
return 73;
case 57:
return 74;
case 58:
return 76;
case 59:
return 77;
case 60:
return 78;
case 61:
return 80;
case 62:
return 81;
case 63:
return 82;
case 64:
return 83;
case 65:
return 84;
case 66:
return 85;
case 67:
return 86;
case 68:
return 87;
case 69:
return 88;
case 70:
return 89;
case 71:
return 90;
case 72:
return 91;
case 73:
return 92;
case 74:
return 93;
case 75:
return 94;
case 76:
return 96;
case 77:
return 97;
case 78:
return 98;
case 79:
return 99;
case 80:
return ni;
case 81:
return L7;
case 82:
return Ri;
case 83:
return c7;
case 84:
return D7;
case 85:
return R7;
case 86:
return Xt;
case 87:
return Qc;
case 88:
return Ht;
case 89:
return F7;
case 90:
return Pn;
case 91:
return u1;
case 92:
return Av;
case 93:
return x1;
case 94:
return A2;
case 95:
return z2;
case 96:
return Sv;
case 97:
return fc;
case 98:
return tl;
case 99:
return In;
case 100:
return us;
case 101:
return br;
case 102:
return DX;
case 103:
return zn;
case 104:
return Rt;
case 105:
return eV;
case 106:
return Jw;
case 107:
return Qg;
default:
return YH;
}
switch (Ft) {
case 0:
return 4;
case 1:
return 8;
case 2:
return 15;
case 3:
return 16;
case 4:
return 17;
case 5:
return 18;
case 6:
return 19;
case 7:
return 20;
case 8:
return 21;
case 9:
return 22;
case 10:
return 23;
case 11:
return 24;
case 12:
return 25;
case 13:
return 26;
case 14:
return 27;
case 15:
return 28;
case 16:
return 29;
case 17:
return 30;
case 18:
return 31;
case 19:
return 32;
case 20:
return 33;
case 21:
return 34;
case 22:
return 35;
case 23:
return 36;
case 24:
return 37;
case 25:
return 39;
case 26:
return 40;
case 27:
return 41;
case 28:
return 42;
case 29:
return 43;
case 30:
return 45;
case 31:
return 46;
case 32:
return 47;
case 33:
return 48;
case 34:
return 51;
case 35:
return 52;
case 36:
return 53;
case 37:
return 54;
case 38:
return 55;
case 39:
return 56;
case 40:
return 57;
case 41:
return 58;
case 42:
return 59;
case 43:
return 60;
case 44:
return 61;
case 45:
return 62;
case 46:
return 63;
case 47:
return 64;
case 48:
return 65;
case 49:
return 66;
case 50:
return 67;
case 51:
return 68;
case 52:
return 69;
case 53:
return 70;
default:
return 71;
}
} else
switch (Bt[0]) {
case 0:
return 0;
case 1:
return 1;
case 2:
return 2;
case 3:
return 3;
case 4:
return 5;
case 5:
return 6;
case 6:
return 7;
case 7:
return 9;
case 8:
return 10;
case 9:
return 11;
case 10:
return 12;
case 11:
return 13;
case 12:
return 14;
case 13:
return 38;
case 14:
return 44;
case 15:
return 49;
case 16:
return 50;
case 17:
return 75;
case 18:
return 79;
case 19:
return 95;
case 20:
return fs2;
case 21:
return Fv;
case 22:
return vf;
default:
return X2;
}
}
var Jt = gt(n4);
return Cc(gt(t), Jt);
});
var Vee = [wt, b_r, G7(0)];
G7(0);
var A1 = [wt, m_r, G7(0)], or0 = 0, cr0 = 0, sr0 = 0, vr0 = 0, lr0 = 0, br0 = 0, pr0 = 0, mr0 = 0, _r0 = 0, yr0 = 0;
function j(t) {
if (t[3] === t[2])
return -1;
var n4 = t[1][1 + t[3]];
return t[3] = t[3] + 1 | 0, n4 === 10 && (t[5] !== 0 && (t[5] = t[5] + 1 | 0), t[4] = t[3]), n4;
}
function B0(t, n4) {
return t[9] = t[3], t[10] = t[4], t[11] = t[5], t[12] = n4, 0;
}
function En(t) {
return t[6] = t[3], t[7] = t[4], t[8] = t[5], B0(t, -1);
}
function q(t) {
return t[3] = t[9], t[4] = t[10], t[5] = t[11], t[12];
}
function $v(t) {
return t[3] = t[6], t[4] = t[7], t[5] = t[8], 0;
}
function fL(t, n4) {
return t[6] = n4, 0;
}
function $m(t) {
return t[3] - t[6] | 0;
}
function Ll(t) {
var n4 = t[3] - t[6] | 0, e = t[6], i = t[1];
return 0 <= e && 0 <= n4 && !((i.length - 1 - n4 | 0) < e) ? s70(i, e, n4) : Cu(Ri0);
}
function dr0(t) {
var n4 = t[6];
return nu(t[1], n4)[1 + n4];
}
function Rl(t, n4, e, i) {
for (var x = 0, c = e, s = n4; ; ) {
if (0 < c) {
var p = t[1 + s];
if (0 <= p) {
if (zn < p)
if (xX < p)
if (H8 < p) {
if (mI < p)
throw A1;
Jn(i, x, v1 | p >>> 18 | 0), Jn(i, x + 1 | 0, Rt | (p >>> 12 | 0) & 63), Jn(i, x + 2 | 0, Rt | (p >>> 6 | 0) & 63), Jn(i, x + 3 | 0, Rt | p & 63);
var y = x + 4 | 0;
} else {
Jn(i, x, dv | p >>> 12 | 0), Jn(i, x + 1 | 0, Rt | (p >>> 6 | 0) & 63), Jn(i, x + 2 | 0, Rt | p & 63);
var y = x + 3 | 0;
}
else {
Jn(i, x, at | p >>> 6 | 0), Jn(i, x + 1 | 0, Rt | p & 63);
var y = x + 2 | 0;
}
else {
Jn(i, x, p);
var y = x + 1 | 0;
}
var x = y, c = c - 1 | 0, s = s + 1 | 0;
continue;
}
throw A1;
}
return x;
}
}
function hr0(t) {
for (var n4 = nn(t), e = Gv(n4, 0), i = 0, x = 0; ; ) {
if (x < n4) {
var c = Vr(t, x), s = 0;
if (at <= c)
if (v1 <= c)
if (wt <= c)
s = 1;
else {
var p = Vr(t, x + 1 | 0), y = Vr(t, x + 2 | 0), T = Vr(t, x + 3 | 0), E = (p >>> 6 | 0) !== 2 ? 1 : 0;
if (E)
var w = E;
else
var h = (y >>> 6 | 0) !== 2 ? 1 : 0, w = h || ((T >>> 6 | 0) !== 2 ? 1 : 0);
if (w)
throw A1;
e[1 + i] = (c & 7) << 18 | (p & 63) << 12 | (y & 63) << 6 | T & 63;
var G = x + 4 | 0;
}
else if (dv <= c) {
var A = Vr(t, x + 1 | 0), S = Vr(t, x + 2 | 0), M = (c & 15) << 12 | (A & 63) << 6 | S & 63, K = (A >>> 6 | 0) !== 2 ? 1 : 0, V = K || ((S >>> 6 | 0) !== 2 ? 1 : 0);
if (V)
var m0 = V;
else
var f0 = 55296 <= M ? 1 : 0, m0 = f0 && (M <= 57088 ? 1 : 0);
if (m0)
throw A1;
e[1 + i] = M;
var G = x + 3 | 0;
} else {
var k0 = Vr(t, x + 1 | 0);
if ((k0 >>> 6 | 0) !== 2)
throw A1;
e[1 + i] = (c & 31) << 6 | k0 & 63;
var G = x + 2 | 0;
}
else if (Rt <= c)
s = 1;
else {
e[1 + i] = c;
var G = x + 1 | 0;
}
if (s)
throw A1;
var i = i + 1 | 0, x = G;
continue;
}
return [0, e, i, yr0, _r0, mr0, pr0, br0, lr0, vr0, sr0, cr0, or0];
}
}
function jl(t, n4, e) {
var i = t[6] + n4 | 0, x = Pt(e * 4 | 0), c = t[1];
if ((i + e | 0) <= c.length - 1)
return qv(x, 0, Rl(c, i, e, x));
throw [0, wn, p_r];
}
function Se(t) {
var n4 = t[6], e = t[3] - n4 | 0, i = Pt(e * 4 | 0);
return qv(i, 0, Rl(t[1], n4, e, i));
}
function Gl(t, n4) {
var e = t[6], i = t[3] - e | 0, x = Pt(i * 4 | 0);
return bN(n4, x, 0, Rl(t[1], e, i, x));
}
function xL(t) {
var n4 = t.length - 1, e = Pt(n4 * 4 | 0);
return qv(e, 0, Rl(t, 0, n4, e));
}
function kr0(t, n4) {
return t[3] = t[3] - n4 | 0, 0;
}
var wr0 = 0;
function zee(t, n4, e) {
return [0, t, n4, __r, 0, e, wr0, y_r];
}
function Er0(t) {
var n4 = t[2];
return [0, t[1], [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12]], t[3], t[4], t[5], t[6], t[7]];
}
function Sr0(t) {
return t[3][1];
}
function Zm(t, n4) {
return t !== n4[4] ? [0, n4[1], n4[2], n4[3], t, n4[5], n4[6], n4[7]] : n4;
}
var aL = function t(n4, e) {
return t.fun(n4, e);
}, gr0 = function t(n4, e) {
return t.fun(n4, e);
}, oL = function t(n4, e) {
return t.fun(n4, e);
}, cL = function t(n4, e) {
return t.fun(n4, e);
}, Fr0 = function t(n4, e) {
return t.fun(n4, e);
};
N(aL, function(t, n4) {
if (typeof t == "number") {
var e = t;
if (61 <= e)
if (92 <= e)
switch (e) {
case 92:
if (typeof n4 == "number" && n4 === 92)
return 1;
break;
case 93:
if (typeof n4 == "number" && n4 === 93)
return 1;
break;
case 94:
if (typeof n4 == "number" && n4 === 94)
return 1;
break;
case 95:
if (typeof n4 == "number" && n4 === 95)
return 1;
break;
case 96:
if (typeof n4 == "number" && n4 === 96)
return 1;
break;
case 97:
if (typeof n4 == "number" && n4 === 97)
return 1;
break;
case 98:
if (typeof n4 == "number" && n4 === 98)
return 1;
break;
case 99:
if (typeof n4 == "number" && n4 === 99)
return 1;
break;
case 100:
if (typeof n4 == "number" && ni === n4)
return 1;
break;
case 101:
if (typeof n4 == "number" && L7 === n4)
return 1;
break;
case 102:
if (typeof n4 == "number" && Ri === n4)
return 1;
break;
case 103:
if (typeof n4 == "number" && c7 === n4)
return 1;
break;
case 104:
if (typeof n4 == "number" && D7 === n4)
return 1;
break;
case 105:
if (typeof n4 == "number" && R7 === n4)
return 1;
break;
case 106:
if (typeof n4 == "number" && Xt === n4)
return 1;
break;
case 107:
if (typeof n4 == "number" && Qc === n4)
return 1;
break;
case 108:
if (typeof n4 == "number" && fs2 === n4)
return 1;
break;
case 109:
if (typeof n4 == "number" && Fv === n4)
return 1;
break;
case 110:
if (typeof n4 == "number" && Ht === n4)
return 1;
break;
case 111:
if (typeof n4 == "number" && vf === n4)
return 1;
break;
case 112:
if (typeof n4 == "number" && F7 === n4)
return 1;
break;
case 113:
if (typeof n4 == "number" && Pn === n4)
return 1;
break;
case 114:
if (typeof n4 == "number" && u1 === n4)
return 1;
break;
case 115:
if (typeof n4 == "number" && Av === n4)
return 1;
break;
case 116:
if (typeof n4 == "number" && x1 === n4)
return 1;
break;
case 117:
if (typeof n4 == "number" && A2 === n4)
return 1;
break;
case 118:
if (typeof n4 == "number" && z2 === n4)
return 1;
break;
case 119:
if (typeof n4 == "number" && Sv === n4)
return 1;
break;
case 120:
if (typeof n4 == "number" && fc === n4)
return 1;
break;
default:
if (typeof n4 == "number" && tl <= n4)
return 1;
}
else
switch (e) {
case 61:
if (typeof n4 == "number" && n4 === 61)
return 1;
break;
case 62:
if (typeof n4 == "number" && n4 === 62)
return 1;
break;
case 63:
if (typeof n4 == "number" && n4 === 63)
return 1;
break;
case 64:
if (typeof n4 == "number" && n4 === 64)
return 1;
break;
case 65:
if (typeof n4 == "number" && n4 === 65)
return 1;
break;
case 66:
if (typeof n4 == "number" && n4 === 66)
return 1;
break;
case 67:
if (typeof n4 == "number" && n4 === 67)
return 1;
break;
case 68:
if (typeof n4 == "number" && n4 === 68)
return 1;
break;
case 69:
if (typeof n4 == "number" && n4 === 69)
return 1;
break;
case 70:
if (typeof n4 == "number" && n4 === 70)
return 1;
break;
case 71:
if (typeof n4 == "number" && n4 === 71)
return 1;
break;
case 72:
if (typeof n4 == "number" && n4 === 72)
return 1;
break;
case 73:
if (typeof n4 == "number" && n4 === 73)
return 1;
break;
case 74:
if (typeof n4 == "number" && n4 === 74)
return 1;
break;
case 75:
if (typeof n4 == "number" && n4 === 75)
return 1;
break;
case 76:
if (typeof n4 == "number" && n4 === 76)
return 1;
break;
case 77:
if (typeof n4 == "number" && n4 === 77)
return 1;
break;
case 78:
if (typeof n4 == "number" && n4 === 78)
return 1;
break;
case 79:
if (typeof n4 == "number" && n4 === 79)
return 1;
break;
case 80:
if (typeof n4 == "number" && n4 === 80)
return 1;
break;
case 81:
if (typeof n4 == "number" && n4 === 81)
return 1;
break;
case 82:
if (typeof n4 == "number" && n4 === 82)
return 1;
break;
case 83:
if (typeof n4 == "number" && n4 === 83)
return 1;
break;
case 84:
if (typeof n4 == "number" && n4 === 84)
return 1;
break;
case 85:
if (typeof n4 == "number" && n4 === 85)
return 1;
break;
case 86:
if (typeof n4 == "number" && n4 === 86)
return 1;
break;
case 87:
if (typeof n4 == "number" && n4 === 87)
return 1;
break;
case 88:
if (typeof n4 == "number" && n4 === 88)
return 1;
break;
case 89:
if (typeof n4 == "number" && n4 === 89)
return 1;
break;
case 90:
if (typeof n4 == "number" && n4 === 90)
return 1;
break;
default:
if (typeof n4 == "number" && n4 === 91)
return 1;
}
else if (31 <= e)
switch (e) {
case 31:
if (typeof n4 == "number" && n4 === 31)
return 1;
break;
case 32:
if (typeof n4 == "number" && n4 === 32)
return 1;
break;
case 33:
if (typeof n4 == "number" && n4 === 33)
return 1;
break;
case 34:
if (typeof n4 == "number" && n4 === 34)
return 1;
break;
case 35:
if (typeof n4 == "number" && n4 === 35)
return 1;
break;
case 36:
if (typeof n4 == "number" && n4 === 36)
return 1;
break;
case 37:
if (typeof n4 == "number" && n4 === 37)
return 1;
break;
case 38:
if (typeof n4 == "number" && n4 === 38)
return 1;
break;
case 39:
if (typeof n4 == "number" && n4 === 39)
return 1;
break;
case 40:
if (typeof n4 == "number" && n4 === 40)
return 1;
break;
case 41:
if (typeof n4 == "number" && n4 === 41)
return 1;
break;
case 42:
if (typeof n4 == "number" && n4 === 42)
return 1;
break;
case 43:
if (typeof n4 == "number" && n4 === 43)
return 1;
break;
case 44:
if (typeof n4 == "number" && n4 === 44)
return 1;
break;
case 45:
if (typeof n4 == "number" && n4 === 45)
return 1;
break;
case 46:
if (typeof n4 == "number" && n4 === 46)
return 1;
break;
case 47:
if (typeof n4 == "number" && n4 === 47)
return 1;
break;
case 48:
if (typeof n4 == "number" && n4 === 48)
return 1;
break;
case 49:
if (typeof n4 == "number" && n4 === 49)
return 1;
break;
case 50:
if (typeof n4 == "number" && n4 === 50)
return 1;
break;
case 51:
if (typeof n4 == "number" && n4 === 51)
return 1;
break;
case 52:
if (typeof n4 == "number" && n4 === 52)
return 1;
break;
case 53:
if (typeof n4 == "number" && n4 === 53)
return 1;
break;
case 54:
if (typeof n4 == "number" && n4 === 54)
return 1;
break;
case 55:
if (typeof n4 == "number" && n4 === 55)
return 1;
break;
case 56:
if (typeof n4 == "number" && n4 === 56)
return 1;
break;
case 57:
if (typeof n4 == "number" && n4 === 57)
return 1;
break;
case 58:
if (typeof n4 == "number" && n4 === 58)
return 1;
break;
case 59:
if (typeof n4 == "number" && n4 === 59)
return 1;
break;
default:
if (typeof n4 == "number" && n4 === 60)
return 1;
}
else
switch (e) {
case 0:
if (typeof n4 == "number" && !n4)
return 1;
break;
case 1:
if (typeof n4 == "number" && n4 === 1)
return 1;
break;
case 2:
if (typeof n4 == "number" && n4 === 2)
return 1;
break;
case 3:
if (typeof n4 == "number" && n4 === 3)
return 1;
break;
case 4:
if (typeof n4 == "number" && n4 === 4)
return 1;
break;
case 5:
if (typeof n4 == "number" && n4 === 5)
return 1;
break;
case 6:
if (typeof n4 == "number" && n4 === 6)
return 1;
break;
case 7:
if (typeof n4 == "number" && n4 === 7)
return 1;
break;
case 8:
if (typeof n4 == "number" && n4 === 8)
return 1;
break;
case 9:
if (typeof n4 == "number" && n4 === 9)
return 1;
break;
case 10:
if (typeof n4 == "number" && n4 === 10)
return 1;
break;
case 11:
if (typeof n4 == "number" && n4 === 11)
return 1;
break;
case 12:
if (typeof n4 == "number" && n4 === 12)
return 1;
break;
case 13:
if (typeof n4 == "number" && n4 === 13)
return 1;
break;
case 14:
if (typeof n4 == "number" && n4 === 14)
return 1;
break;
case 15:
if (typeof n4 == "number" && n4 === 15)
return 1;
break;
case 16:
if (typeof n4 == "number" && n4 === 16)
return 1;
break;
case 17:
if (typeof n4 == "number" && n4 === 17)
return 1;
break;
case 18:
if (typeof n4 == "number" && n4 === 18)
return 1;
break;
case 19:
if (typeof n4 == "number" && n4 === 19)
return 1;
break;
case 20:
if (typeof n4 == "number" && n4 === 20)
return 1;
break;
case 21:
if (typeof n4 == "number" && n4 === 21)
return 1;
break;
case 22:
if (typeof n4 == "number" && n4 === 22)
return 1;
break;
case 23:
if (typeof n4 == "number" && n4 === 23)
return 1;
break;
case 24:
if (typeof n4 == "number" && n4 === 24)
return 1;
break;
case 25:
if (typeof n4 == "number" && n4 === 25)
return 1;
break;
case 26:
if (typeof n4 == "number" && n4 === 26)
return 1;
break;
case 27:
if (typeof n4 == "number" && n4 === 27)
return 1;
break;
case 28:
if (typeof n4 == "number" && n4 === 28)
return 1;
break;
case 29:
if (typeof n4 == "number" && n4 === 29)
return 1;
break;
default:
if (typeof n4 == "number" && n4 === 30)
return 1;
}
} else
switch (t[0]) {
case 0:
if (typeof n4 != "number" && n4[0] === 0) {
var i = n4[1], x = u(u(oL, t[1]), i), c = x && qn(t[2], n4[2]);
return c;
}
break;
case 1:
if (typeof n4 != "number" && n4[0] === 1) {
var s = n4[1], p = u(u(cL, t[1]), s), y = p && qn(t[2], n4[2]);
return y;
}
break;
case 2:
if (typeof n4 != "number" && n4[0] === 2) {
var T = n4[1], E = t[1], h = Wv(E[1], T[1]), w = h && qn(E[2], T[2]), G = w && qn(E[3], T[3]), A = G && (E[4] === T[4] ? 1 : 0);
return A;
}
break;
case 3:
if (typeof n4 != "number" && n4[0] === 3) {
var S = n4[1], M = t[1], K = Wv(M[1], S[1]);
if (K)
var V = S[2], f0 = u(u(Fr0, M[2]), V);
else
var f0 = K;
var m0 = f0 && (M[3] === S[3] ? 1 : 0);
return m0;
}
break;
case 4:
if (typeof n4 != "number" && n4[0] === 4) {
var k0 = Wv(t[1], n4[1]), g0 = k0 && qn(t[2], n4[2]), e0 = g0 && qn(t[3], n4[3]);
return e0;
}
break;
case 5:
if (typeof n4 != "number" && n4[0] === 5) {
var x0 = Wv(t[1], n4[1]), l = x0 && qn(t[2], n4[2]), c0 = l && qn(t[3], n4[3]);
return c0;
}
break;
case 6:
if (typeof n4 != "number" && n4[0] === 6)
return qn(t[1], n4[1]);
break;
case 7:
if (typeof n4 != "number" && n4[0] === 7) {
var t0 = qn(t[1], n4[1]);
return t0 && Wv(t[2], n4[2]);
}
break;
case 8:
if (typeof n4 != "number" && n4[0] === 8) {
var a0 = Wv(t[1], n4[1]), w0 = a0 && qn(t[2], n4[2]), _0 = w0 && qn(t[3], n4[3]);
return _0;
}
break;
case 9:
if (typeof n4 != "number" && n4[0] === 9) {
var E0 = n4[1];
return u(u(gr0, t[1]), E0);
}
break;
case 10:
if (typeof n4 != "number" && n4[0] === 10) {
var X0 = n4[1], b = u(u(oL, t[1]), X0), G0 = b && (t[2] == n4[2] ? 1 : 0), X = G0 && qn(t[3], n4[3]);
return X;
}
break;
default:
if (typeof n4 != "number" && n4[0] === 11) {
var s0 = n4[1], dr = u(u(cL, t[1]), s0), Ar = dr && (t[2] == n4[2] ? 1 : 0), ar = Ar && qn(t[3], n4[3]);
return ar;
}
}
return 0;
}), N(gr0, function(t, n4) {
if (t) {
if (n4)
return 1;
} else if (!n4)
return 1;
return 0;
}), N(oL, function(t, n4) {
switch (t) {
case 0:
if (!n4)
return 1;
break;
case 1:
if (n4 === 1)
return 1;
break;
case 2:
if (n4 === 2)
return 1;
break;
case 3:
if (n4 === 3)
return 1;
break;
default:
if (4 <= n4)
return 1;
}
return 0;
}), N(cL, function(t, n4) {
switch (t) {
case 0:
if (!n4)
return 1;
break;
case 1:
if (n4 === 1)
return 1;
break;
default:
if (2 <= n4)
return 1;
}
return 0;
}), N(Fr0, function(t, n4) {
var e = qn(t[1], n4[1]), i = e && qn(t[2], n4[2]), x = i && qn(t[3], n4[3]);
return x;
});
function Tr0(t) {
if (typeof t == "number") {
var n4 = t;
if (61 <= n4) {
if (92 <= n4)
switch (n4) {
case 92:
return Gkr;
case 93:
return Mkr;
case 94:
return Bkr;
case 95:
return qkr;
case 96:
return Ukr;
case 97:
return Hkr;
case 98:
return Xkr;
case 99:
return Ykr;
case 100:
return Vkr;
case 101:
return zkr;
case 102:
return Kkr;
case 103:
return Wkr;
case 104:
return Jkr;
case 105:
return $kr;
case 106:
return Zkr;
case 107:
return Qkr;
case 108:
return rwr;
case 109:
return ewr;
case 110:
return nwr;
case 111:
return twr;
case 112:
return uwr;
case 113:
return iwr;
case 114:
return fwr;
case 115:
return xwr;
case 116:
return awr;
case 117:
return owr;
case 118:
return cwr;
case 119:
return swr;
case 120:
return vwr;
default:
return lwr;
}
switch (n4) {
case 61:
return xkr;
case 62:
return akr;
case 63:
return okr;
case 64:
return ckr;
case 65:
return skr;
case 66:
return vkr;
case 67:
return lkr;
case 68:
return bkr;
case 69:
return pkr;
case 70:
return mkr;
case 71:
return _kr;
case 72:
return ykr;
case 73:
return dkr;
case 74:
return hkr;
case 75:
return kkr;
case 76:
return wkr;
case 77:
return Ekr;
case 78:
return Skr;
case 79:
return gkr;
case 80:
return Fkr;
case 81:
return Tkr;
case 82:
return Okr;
case 83:
return Ikr;
case 84:
return Akr;
case 85:
return Nkr;
case 86:
return Ckr;
case 87:
return Pkr;
case 88:
return Dkr;
case 89:
return Lkr;
case 90:
return Rkr;
default:
return jkr;
}
}
if (31 <= n4)
switch (n4) {
case 31:
return Nhr;
case 32:
return Chr;
case 33:
return Phr;
case 34:
return Dhr;
case 35:
return Lhr;
case 36:
return Rhr;
case 37:
return jhr;
case 38:
return Ghr;
case 39:
return Mhr;
case 40:
return Bhr;
case 41:
return qhr;
case 42:
return Uhr;
case 43:
return Hhr;
case 44:
return Xhr;
case 45:
return Yhr;
case 46:
return Vhr;
case 47:
return zhr;
case 48:
return Khr;
case 49:
return Whr;
case 50:
return Jhr;
case 51:
return $hr;
case 52:
return Zhr;
case 53:
return Qhr;
case 54:
return rkr;
case 55:
return ekr;
case 56:
return nkr;
case 57:
return tkr;
case 58:
return ukr;
case 59:
return ikr;
default:
return fkr;
}
switch (n4) {
case 0:
return rhr;
case 1:
return ehr;
case 2:
return nhr;
case 3:
return thr;
case 4:
return uhr;
case 5:
return ihr;
case 6:
return fhr;
case 7:
return xhr;
case 8:
return ahr;
case 9:
return ohr;
case 10:
return chr;
case 11:
return shr;
case 12:
return vhr;
case 13:
return lhr;
case 14:
return bhr;
case 15:
return phr;
case 16:
return mhr;
case 17:
return _hr;
case 18:
return yhr;
case 19:
return dhr;
case 20:
return hhr;
case 21:
return khr;
case 22:
return whr;
case 23:
return Ehr;
case 24:
return Shr;
case 25:
return ghr;
case 26:
return Fhr;
case 27:
return Thr;
case 28:
return Ohr;
case 29:
return Ihr;
default:
return Ahr;
}
} else
switch (t[0]) {
case 0:
return bwr;
case 1:
return pwr;
case 2:
return mwr;
case 3:
return _wr;
case 4:
return ywr;
case 5:
return dwr;
case 6:
return hwr;
case 7:
return kwr;
case 8:
return wwr;
case 9:
return Ewr;
case 10:
return Swr;
default:
return gwr;
}
}
function sL(t) {
if (typeof t == "number") {
var n4 = t;
if (61 <= n4) {
if (92 <= n4)
switch (n4) {
case 92:
return hdr;
case 93:
return kdr;
case 94:
return wdr;
case 95:
return Edr;
case 96:
return Sdr;
case 97:
return gdr;
case 98:
return Fdr;
case 99:
return Tdr;
case 100:
return Odr;
case 101:
return Idr;
case 102:
return Adr;
case 103:
return Ndr;
case 104:
return Cdr;
case 105:
return Pdr;
case 106:
return Ddr;
case 107:
return Ldr;
case 108:
return Rdr;
case 109:
return jdr;
case 110:
return Gdr;
case 111:
return Mdr;
case 112:
return Bdr;
case 113:
return qdr;
case 114:
return Udr;
case 115:
return Hdr;
case 116:
return Xdr;
case 117:
return Ydr;
case 118:
return Vdr;
case 119:
return zdr;
case 120:
return Kdr;
default:
return Wdr;
}
switch (n4) {
case 61:
return Hyr;
case 62:
return Xyr;
case 63:
return Yyr;
case 64:
return Vyr;
case 65:
return zyr;
case 66:
return Kyr;
case 67:
return Wyr;
case 68:
return Jyr;
case 69:
return $yr;
case 70:
return Zyr;
case 71:
return Qyr;
case 72:
return rdr;
case 73:
return edr;
case 74:
return ndr;
case 75:
return tdr;
case 76:
return udr;
case 77:
return idr;
case 78:
return fdr;
case 79:
return xdr;
case 80:
return adr;
case 81:
return odr;
case 82:
return cdr;
case 83:
return sdr;
case 84:
return vdr;
case 85:
return ldr;
case 86:
return bdr;
case 87:
return pdr;
case 88:
return mdr;
case 89:
return _dr;
case 90:
return ydr;
default:
return ddr;
}
}
if (31 <= n4)
switch (n4) {
case 31:
return lyr;
case 32:
return byr;
case 33:
return pyr;
case 34:
return myr;
case 35:
return _yr;
case 36:
return yyr;
case 37:
return dyr;
case 38:
return hyr;
case 39:
return kyr;
case 40:
return wyr;
case 41:
return Eyr;
case 42:
return Syr;
case 43:
return gyr;
case 44:
return Fyr;
case 45:
return Tyr;
case 46:
return Oyr;
case 47:
return Iyr;
case 48:
return Ayr;
case 49:
return Nyr;
case 50:
return Cyr;
case 51:
return Pyr;
case 52:
return Dyr;
case 53:
return Lyr;
case 54:
return Ryr;
case 55:
return jyr;
case 56:
return Gyr;
case 57:
return Myr;
case 58:
return Byr;
case 59:
return qyr;
default:
return Uyr;
}
switch (n4) {
case 0:
return R_r;
case 1:
return j_r;
case 2:
return G_r;
case 3:
return M_r;
case 4:
return B_r;
case 5:
return q_r;
case 6:
return U_r;
case 7:
return H_r;
case 8:
return X_r;
case 9:
return Y_r;
case 10:
return V_r;
case 11:
return z_r;
case 12:
return K_r;
case 13:
return W_r;
case 14:
return J_r;
case 15:
return $_r;
case 16:
return Z_r;
case 17:
return Q_r;
case 18:
return ryr;
case 19:
return eyr;
case 20:
return nyr;
case 21:
return tyr;
case 22:
return uyr;
case 23:
return iyr;
case 24:
return fyr;
case 25:
return xyr;
case 26:
return ayr;
case 27:
return oyr;
case 28:
return cyr;
case 29:
return syr;
default:
return vyr;
}
} else
switch (t[0]) {
case 2:
return t[1][3];
case 3:
return t[1][2][3];
case 5:
var e = Te(Jdr, t[3]);
return Te($dr, Te(t[2], e));
case 9:
return t[1] ? Zdr : Qdr;
case 0:
case 1:
return t[2];
case 6:
case 7:
return t[1];
default:
return t[3];
}
}
function Ml(t) {
return u(Qn(L_r), t);
}
function vL(t, n4) {
var e = t && t[1], i = 0;
if (typeof n4 == "number")
if (Pn === n4)
var x = d_r, c = h_r;
else
i = 1;
else
switch (n4[0]) {
case 3:
var x = k_r, c = w_r;
break;
case 5:
var x = E_r, c = S_r;
break;
case 6:
case 9:
i = 1;
break;
case 0:
case 10:
var x = F_r, c = T_r;
break;
case 1:
case 11:
var x = O_r, c = I_r;
break;
case 2:
case 8:
var x = A_r, c = N_r;
break;
default:
var x = C_r, c = P_r;
}
if (i)
var x = g_r, c = Ml(sL(n4));
return e ? Te(x, Te(D_r, c)) : c;
}
function lL(t) {
return 45 < t ? 46 < t ? -1 : 0 : -1;
}
function Mc(t) {
if (8 < t) {
if (Ev < t) {
if (tf < t) {
if (La < t)
return -1;
if (ea < t) {
var n4 = t <= qo ? 1 : 0, e = n4 && -1;
return e;
}
if (aa < t) {
if (oc < t) {
if (cx < t) {
var i = t <= Sa ? 1 : 0, x = i && -1;
return x;
}
return 0;
}
return -1;
}
if (Pa < t) {
var c = t <= ya ? 1 : 0, s = c && -1;
return s;
}
return 0;
}
return -1;
}
return Vr(eLr, t - 9 | 0) - 1 | 0;
}
return -1;
}
function vn(t) {
return 47 < t ? 57 < t ? -1 : 0 : -1;
}
function Nn(t) {
return 47 < t ? Ri < t ? -1 : Vr(vRr, t + zt | 0) - 1 | 0 : -1;
}
function ki(t) {
return 47 < t ? Ht < t ? -1 : Vr(tRr, t + zt | 0) - 1 | 0 : -1;
}
function Zv(t) {
return 35 < t ? In < t ? -1 : Vr(gLr, t + su | 0) - 1 | 0 : -1;
}
function Qm(t) {
return 87 < t ? fc < t ? -1 : Vr(uN, t - 88 | 0) - 1 | 0 : -1;
}
function r9(t) {
return 45 < t ? 57 < t ? -1 : Vr(lRr, t + l1 | 0) - 1 | 0 : -1;
}
function Or0(t) {
return 35 < t ? In < t ? -1 : Vr(FLr, t + su | 0) - 1 | 0 : -1;
}
function N1(t) {
return 47 < t ? br < t ? -1 : Vr(rRr, t + zt | 0) - 1 | 0 : -1;
}
function Qv(t) {
return 35 < t ? In < t ? -1 : Vr(TLr, t + su | 0) - 1 | 0 : -1;
}
function C1(t) {
if (8 < t) {
if (Ev < t) {
if (tf < t) {
if (La < t)
return -1;
if (ea < t) {
var n4 = t <= qo ? 1 : 0, e = n4 && -1;
return e;
}
if (aa < t) {
if (oc < t) {
if (cx < t) {
var i = t <= Sa ? 1 : 0, x = i && -1;
return x;
}
return 0;
}
return -1;
}
if (Pa < t) {
var c = t <= ya ? 1 : 0, s = c && -1;
return s;
}
return 0;
}
return -1;
}
return Vr(nLr, t - 9 | 0) - 1 | 0;
}
return -1;
}
function e9(t) {
return 45 < t ? L7 < t ? -1 : Vr(pLr, t + l1 | 0) - 1 | 0 : -1;
}
function Ir0(t) {
return 35 < t ? In < t ? -1 : Vr(OLr, t + su | 0) - 1 | 0 : -1;
}
function n9(t) {
return 47 < t ? 95 < t ? -1 : Vr(FRr, t + zt | 0) - 1 | 0 : -1;
}
function t9(t) {
return 47 < t ? Ht < t ? -1 : Vr(ORr, t + zt | 0) - 1 | 0 : -1;
}
function u9(t) {
return 47 < t ? Ht < t ? -1 : Vr(uRr, t + zt | 0) - 1 | 0 : -1;
}
function i9(t) {
return 35 < t ? In < t ? -1 : Vr(ILr, t + su | 0) - 1 | 0 : -1;
}
function f9(t) {
if (8 < t) {
if (Ev < t) {
if (tf < t) {
if (La < t)
return -1;
if (ea < t) {
var n4 = t <= qo ? 1 : 0, e = n4 && -1;
return e;
}
if (aa < t) {
if (oc < t) {
if (cx < t) {
var i = t <= Sa ? 1 : 0, x = i && -1;
return x;
}
return 0;
}
return -1;
}
if (Pa < t) {
var c = t <= ya ? 1 : 0, s = c && -1;
return s;
}
return 0;
}
return -1;
}
return Vr(tLr, t - 9 | 0) - 1 | 0;
}
return -1;
}
function x9(t) {
return 44 < t ? 57 < t ? -1 : Vr(oLr, t + mv | 0) - 1 | 0 : -1;
}
function Bc(t) {
return 47 < t ? 49 < t ? -1 : 0 : -1;
}
function a9(t) {
return 47 < t ? 95 < t ? -1 : Vr(cLr, t + zt | 0) - 1 | 0 : -1;
}
function P1(t) {
return 47 < t ? 57 < t ? -1 : Vr(TRr, t + zt | 0) - 1 | 0 : -1;
}
function o9(t) {
return 35 < t ? In < t ? -1 : Vr(ALr, t + su | 0) - 1 | 0 : -1;
}
function Ar0(t) {
return u1 < t ? Av < t ? -1 : 0 : -1;
}
function Ui(t) {
return 60 < t ? 61 < t ? -1 : 0 : -1;
}
function Nr0(t) {
if (-1 < t) {
if (In < t) {
if (us < t) {
if (Ju < t) {
var n4 = t <= Uu ? 1 : 0, e = n4 && -1;
return e;
}
return 0;
}
return -1;
}
return Vr(kLr, t) - 1 | 0;
}
return -1;
}
function r2(t) {
return 47 < t ? Ht < t ? -1 : Vr(iRr, t + zt | 0) - 1 | 0 : -1;
}
function c9(t) {
return 47 < t ? Ht < t ? -1 : Vr(sRr, t + zt | 0) - 1 | 0 : -1;
}
function bL(t) {
return 60 < t ? 62 < t ? -1 : Vr(tN, t + TS | 0) - 1 | 0 : -1;
}
function s9(t) {
return 65 < t ? 98 < t ? -1 : Vr(uN, t - 66 | 0) - 1 | 0 : -1;
}
function Mt(t) {
return 35 < t ? In < t ? -1 : Vr(NLr, t + su | 0) - 1 | 0 : -1;
}
function v9(t) {
return Av < t ? x1 < t ? -1 : 0 : -1;
}
function Vu(t) {
return 47 < t ? 55 < t ? -1 : 0 : -1;
}
function pL(t) {
return Fv < t ? Ht < t ? -1 : 0 : -1;
}
function l9(t) {
return Ht < t ? vf < t ? -1 : 0 : -1;
}
function Bl(t) {
return 98 < t ? 99 < t ? -1 : 0 : -1;
}
function Ls(t) {
return 47 < t ? 48 < t ? -1 : 0 : -1;
}
function ql(t) {
if (8 < t) {
if (Ev < t) {
if (tf < t) {
if (La < t)
return -1;
if (ea < t) {
var n4 = t <= qo ? 1 : 0, e = n4 && -1;
return e;
}
if (aa < t) {
if (oc < t) {
if (cx < t) {
var i = t <= Sa ? 1 : 0, x = i && -1;
return x;
}
return 0;
}
return -1;
}
if (Pa < t) {
var c = t <= ya ? 1 : 0, s = c && -1;
return s;
}
return 0;
}
return -1;
}
return Vr(uLr, t - 9 | 0) - 1 | 0;
}
return -1;
}
function b9(t) {
return 45 < t ? L7 < t ? -1 : Vr(sLr, t + l1 | 0) - 1 | 0 : -1;
}
function p9(t) {
return 78 < t ? vf < t ? -1 : Vr(uN, t - 79 | 0) - 1 | 0 : -1;
}
function Cr0(t) {
return 41 < t ? 42 < t ? -1 : 0 : -1;
}
function Pr0(t) {
return 35 < t ? In < t ? -1 : Vr(CLr, t + su | 0) - 1 | 0 : -1;
}
function m9(t) {
return 47 < t ? L7 < t ? -1 : Vr(eRr, t + zt | 0) - 1 | 0 : -1;
}
function Rs(t) {
return 35 < t ? In < t ? -1 : Vr(PLr, t + su | 0) - 1 | 0 : -1;
}
function Dr0(t) {
return 41 < t ? 61 < t ? -1 : Vr(oRr, t + hy | 0) - 1 | 0 : -1;
}
function D1(t) {
return 44 < t ? 48 < t ? -1 : Vr(JLr, t + mv | 0) - 1 | 0 : -1;
}
function Lr0(t) {
return 44 < t ? 45 < t ? -1 : 0 : -1;
}
function Rr0(t) {
return D7 < t ? R7 < t ? -1 : 0 : -1;
}
function _9(t) {
return Qc < t ? fs2 < t ? -1 : 0 : -1;
}
function jr0(t) {
return 99 < t ? ni < t ? -1 : 0 : -1;
}
function y9(t) {
return 47 < t ? Ri < t ? -1 : Vr(bRr, t + zt | 0) - 1 | 0 : -1;
}
function d9(t) {
return Pn < t ? u1 < t ? -1 : 0 : -1;
}
function L1(t) {
return 45 < t ? 57 < t ? -1 : Vr(_Rr, t + l1 | 0) - 1 | 0 : -1;
}
function Gr0(t) {
return 35 < t ? In < t ? -1 : Vr(DLr, t + su | 0) - 1 | 0 : -1;
}
function R1(t) {
return 47 < t ? us < t ? -1 : Vr(IRr, t + zt | 0) - 1 | 0 : -1;
}
function mL(t) {
return 35 < t ? In < t ? -1 : Vr(LLr, t + su | 0) - 1 | 0 : -1;
}
function fi(t) {
return 9 < t ? 10 < t ? -1 : 0 : -1;
}
function Mr0(t) {
return 35 < t ? In < t ? -1 : Vr(RLr, t + su | 0) - 1 | 0 : -1;
}
function Br0(t) {
return 96 < t ? 97 < t ? -1 : 0 : -1;
}
function qc(t) {
return 35 < t ? In < t ? -1 : Vr(jLr, t + su | 0) - 1 | 0 : -1;
}
function h9(t) {
return 35 < t ? In < t ? -1 : Vr(GLr, t + su | 0) - 1 | 0 : -1;
}
function js(t) {
return 47 < t ? 95 < t ? -1 : Vr(fRr, t + zt | 0) - 1 | 0 : -1;
}
function qr0(t) {
return 35 < t ? In < t ? -1 : Vr(MLr, t + su | 0) - 1 | 0 : -1;
}
function Ul(t) {
return ni < t ? L7 < t ? -1 : 0 : -1;
}
function Ur0(t) {
if (8 < t) {
if (Ev < t) {
if (tf < t) {
if (La < t)
return -1;
if (ea < t) {
var n4 = t <= qo ? 1 : 0, e = n4 && -1;
return e;
}
if (aa < t) {
if (oc < t) {
if (cx < t) {
var i = t <= Sa ? 1 : 0, x = i && -1;
return x;
}
return 0;
}
return -1;
}
if (Pa < t) {
var c = t <= ya ? 1 : 0, s = c && -1;
return s;
}
return 0;
}
return -1;
}
return Vr(fLr, t - 9 | 0) - 1 | 0;
}
return -1;
}
function Hr0(t) {
return 35 < t ? In < t ? -1 : Vr(yRr, t + su | 0) - 1 | 0 : -1;
}
function k9(t) {
return 41 < t ? 47 < t ? -1 : Vr(zLr, t + hy | 0) - 1 | 0 : -1;
}
function w9(t) {
return 35 < t ? In < t ? -1 : Vr(BLr, t + su | 0) - 1 | 0 : -1;
}
function Xr0(t) {
return 35 < t ? In < t ? -1 : Vr(qLr, t + su | 0) - 1 | 0 : -1;
}
function Yr0(t) {
return z2 < t ? Sv < t ? -1 : 0 : -1;
}
function E9(t) {
return 35 < t ? In < t ? -1 : Vr(ULr, t + su | 0) - 1 | 0 : -1;
}
function i7(t) {
return 47 < t ? L7 < t ? -1 : Vr(nRr, t + zt | 0) - 1 | 0 : -1;
}
function S9(t) {
return 42 < t ? 57 < t ? -1 : Vr(dLr, t + cy | 0) - 1 | 0 : -1;
}
function Uc(t) {
return 45 < t ? 95 < t ? -1 : Vr(SLr, t + l1 | 0) - 1 | 0 : -1;
}
function Gs(t) {
return x1 < t ? A2 < t ? -1 : 0 : -1;
}
function Vr0(t) {
return 46 < t ? 47 < t ? -1 : 0 : -1;
}
function zr0(t) {
return 57 < t ? 58 < t ? -1 : 0 : -1;
}
function _n(t) {
return 35 < t ? In < t ? -1 : Vr(HLr, t + su | 0) - 1 | 0 : -1;
}
function Kr0(t) {
for (; ; ) {
En(t);
var n4 = j(t), e = In < n4 ? 1 : Vr(wRr, n4 + 1 | 0) - 1 | 0;
if (3 < e >>> 0)
var i = q(t);
else
switch (e) {
case 0:
var i = 1;
break;
case 1:
var i = 2;
break;
case 2:
var i = 0;
break;
default:
if (B0(t, 2), Gs(j(t)) === 0) {
var x = R1(j(t));
if (x === 0)
if (Nn(j(t)) === 0 && Nn(j(t)) === 0)
var c = Nn(j(t)) !== 0 ? 1 : 0, i = c && q(t);
else
var i = q(t);
else if (x === 1 && Nn(j(t)) === 0)
for (; ; ) {
var s = N1(j(t));
if (s !== 0) {
var p = s !== 1 ? 1 : 0, i = p && q(t);
break;
}
}
else
var i = q(t);
} else
var i = q(t);
}
if (2 < i >>> 0)
throw [0, wn, Fwr];
switch (i) {
case 0:
continue;
case 1:
return 1;
default:
if (iL(dr0(t)))
continue;
return kr0(t, 1), 0;
}
}
}
function g9(t, n4) {
var e = n4 - t[3][2] | 0;
return [0, Sr0(t), e];
}
function Hl(t, n4, e) {
var i = g9(t, e), x = g9(t, n4);
return [0, t[1], x, i];
}
function Ru(t, n4) {
return g9(t, n4[6]);
}
function y7(t, n4) {
return g9(t, n4[3]);
}
function rt(t, n4) {
return Hl(t, n4[6], n4[3]);
}
function Wr0(t, n4) {
var e = 0;
if (typeof n4 == "number")
e = 1;
else
switch (n4[0]) {
case 2:
var i = n4[1][1];
break;
case 3:
return n4[1][1];
case 4:
var i = n4[1];
break;
case 7:
var i = n4[2];
break;
case 5:
case 8:
return n4[1];
default:
e = 1;
}
return e ? rt(t, t[2]) : i;
}
function ju(t, n4, e) {
return [0, t[1], t[2], t[3], t[4], t[5], [0, [0, n4, e], t[6]], t[7]];
}
function Jr0(t, n4, e) {
return ju(t, n4, [10, Ml(e)]);
}
function _L(t, n4, e, i) {
return ju(t, n4, [12, e, i]);
}
function wi(t, n4) {
return ju(t, n4, QDr);
}
function d7(t, n4) {
var e = n4[3], i = [0, Sr0(t) + 1 | 0, e];
return [0, t[1], t[2], i, t[4], t[5], t[6], t[7]];
}
function $r0(t) {
var n4 = nn(t);
return n4 !== 0 && Ht === Ot(t, n4 - 1 | 0) ? p7(t, 0, n4 - 1 | 0) : t;
}
function Ei(t, n4, e, i, x) {
var c = [0, t[1], n4, e], s = Gt(i), p = x ? 0 : 1;
return [0, c, [0, p, s, t[7][3][1] < c[2][1] ? 1 : 0]];
}
function Hc(t, n4) {
if (Ot(n4, 0) === 45)
var e = p7(n4, 1, nn(n4) - 1 | 0), i = 1;
else
var e = n4, i = 0;
switch (t) {
case 1:
try {
var x = jv(Rv(Te(WDr, e))), c = x;
} catch (T) {
if (T = Et(T), T[1] !== B7)
throw T;
var c = ke(Te(JDr, e));
}
break;
case 0:
case 3:
try {
var s = jv(Rv(e)), c = s;
} catch (T) {
if (T = Et(T), T[1] !== B7)
throw T;
var c = ke(Te($Dr, e));
}
break;
default:
try {
var p = al(e), c = p;
} catch (T) {
if (T = Et(T), T[1] !== B7)
throw T;
var c = ke(Te(ZDr, e));
}
}
var y = i ? -c : c;
return [10, t, y, n4];
}
function Hi(t, n4) {
if (Ot(n4, 0) === 45)
var e = p7(n4, 1, nn(n4) - 1 | 0), i = 1;
else
var e = n4, i = 0;
if (2 <= t) {
var x = $r0(e);
try {
var c = al(x), s = c;
} catch (w) {
if (w = Et(w), w[1] !== B7)
throw w;
var s = ke(Te(zDr, x));
}
var p = s;
} else {
var y = $r0(e);
try {
var T = jv(Rv(y)), E = T;
} catch (G) {
if (G = Et(G), G[1] !== B7)
throw G;
var E = ke(Te(KDr, y));
}
var p = E;
}
var h = i ? -p : p;
return [11, t, h, n4];
}
function Zr0(t, n4, e) {
return iL(e) ? t : ju(t, n4, 37);
}
function Qr0(t, n4, e, i, x) {
var c = Hl(t, n4 + e[6] | 0, n4 + e[3] | 0);
return [0, c, jl(e, i, ($m(e) - i | 0) - x | 0)];
}
function re0(t, n4) {
for (var e = t[2][6], i = [0, n4, n4.length - 1, yr0, _r0, mr0, pr0, br0, lr0, vr0, sr0, cr0, or0], x = $n(n4.length - 1), c = t; ; ) {
En(i);
var s = j(i), p = 92 < s ? 1 : Vr(ZLr, s + 1 | 0) - 1 | 0;
if (2 < p >>> 0)
var y = q(i);
else
switch (p) {
case 0:
var y = 2;
break;
case 1:
for (; ; ) {
B0(i, 3);
var T = j(i);
if (-1 < T)
if (91 < T)
var E = T <= 92 ? 1 : 0, h = E && -1;
else
var h = 0;
else
var h = -1;
if (h !== 0) {
var y = q(i);
break;
}
}
break;
default:
if (B0(i, 3), Gs(j(i)) === 0) {
var w = R1(j(i));
if (w === 0)
if (Nn(j(i)) === 0 && Nn(j(i)) === 0)
var G = Nn(j(i)) !== 0 ? 1 : 0, y = G && q(i);
else
var y = q(i);
else if (w === 1 && Nn(j(i)) === 0)
for (; ; ) {
var A = N1(j(i));
if (A !== 0) {
var y = A === 1 ? 1 : q(i);
break;
}
}
else
var y = q(i);
} else
var y = q(i);
}
if (3 < y >>> 0)
return ke(XDr);
switch (y) {
case 0:
var S = Qr0(c, e, i, 2, 0), M = S[1], K = Bi(Te(YDr, S[2])), V = 0 <= K ? 1 : 0, f0 = V && (K <= 55295 ? 1 : 0);
if (f0)
var k0 = f0;
else
var m0 = 57344 <= K ? 1 : 0, k0 = m0 && (K <= mI ? 1 : 0);
var g0 = k0 ? Zr0(c, M, K) : ju(c, M, 37);
g1(x, K);
var c = g0;
continue;
case 1:
var e0 = Qr0(c, e, i, 3, 1), x0 = Bi(Te(VDr, e0[2])), l = Zr0(c, e0[1], x0);
g1(x, x0);
var c = l;
continue;
case 2:
return [0, c, Gt(x)];
default:
Gl(i, x);
continue;
}
}
}
function Dt(t, n4, e) {
var i = wi(t, rt(t, n4));
return $v(n4), a(e, i, n4);
}
function j1(t, n4, e) {
for (var i = t; ; ) {
En(e);
var x = j(e);
if (-1 < x)
if (42 < x)
var c = x <= Uu ? 1 : 0, s = c && (Ju < x ? 1 : 0);
else
var s = Vr(xLr, x) - 1 | 0;
else
var s = -1;
if (3 < s >>> 0)
var p = q(e);
else
switch (s) {
case 0:
for (; ; ) {
B0(e, 3);
var y = j(e);
if (-1 < y)
if (41 < y)
if (42 < y)
if (Ju < y)
var T = y <= Uu ? 1 : 0, E = T && -1;
else
var E = 0;
else
var E = -1;
else
var E = Vr(mRr, y) - 1 | 0;
else
var E = -1;
if (E !== 0) {
var p = q(e);
break;
}
}
break;
case 1:
var p = 0;
break;
case 2:
B0(e, 0);
var h = fi(j(e)) !== 0 ? 1 : 0, p = h && q(e);
break;
default:
B0(e, 3);
var w = j(e), G = 44 < w ? 47 < w ? -1 : Vr(SRr, w + mv | 0) - 1 | 0 : -1, p = G === 0 ? Vr0(j(e)) === 0 ? 2 : q(e) : G === 1 ? 1 : q(e);
}
if (3 < p >>> 0) {
var A = wi(i, rt(i, e));
return [0, A, y7(A, e)];
}
switch (p) {
case 0:
var S = d7(i, e);
Gl(e, n4);
var i = S;
continue;
case 1:
var M = i[4] ? _L(i, rt(i, e), Iwr, Owr) : i;
return [0, M, y7(M, e)];
case 2:
if (i[4])
return [0, i, y7(i, e)];
mn(n4, Awr);
continue;
default:
Gl(e, n4);
continue;
}
}
}
function e2(t, n4, e) {
for (; ; ) {
En(e);
var i = j(e), x = 13 < i ? Uu < i ? 1 : Ju < i ? 2 : 1 : Vr(VLr, i + 1 | 0) - 1 | 0;
if (3 < x >>> 0)
var c = q(e);
else
switch (x) {
case 0:
var c = 0;
break;
case 1:
for (; ; ) {
B0(e, 2);
var s = j(e);
if (-1 < s)
if (12 < s)
if (13 < s)
if (Ju < s)
var p = s <= Uu ? 1 : 0, y = p && -1;
else
var y = 0;
else
var y = -1;
else
var y = Vr(rLr, s) - 1 | 0;
else
var y = -1;
if (y !== 0) {
var c = q(e);
break;
}
}
break;
case 2:
var c = 1;
break;
default:
B0(e, 1);
var c = fi(j(e)) === 0 ? 1 : q(e);
}
if (2 < c >>> 0)
return ke(Nwr);
switch (c) {
case 0:
return [0, t, y7(t, e)];
case 1:
var T = y7(t, e), E = d7(t, e), h = $m(e);
return [0, E, [0, T[1], T[2] - h | 0]];
default:
Gl(e, n4);
continue;
}
}
}
function ee0(t, n4) {
function e(k0) {
return B0(k0, 3), Vu(j(k0)) === 0 ? 2 : q(k0);
}
En(n4);
var i = j(n4), x = fc < i ? Uu < i ? 1 : Ju < i ? 2 : 1 : Vr(_Lr, i + 1 | 0) - 1 | 0;
if (14 < x >>> 0)
var c = q(n4);
else
switch (x) {
case 1:
var c = 16;
break;
case 2:
var c = 15;
break;
case 3:
B0(n4, 15);
var c = fi(j(n4)) === 0 ? 15 : q(n4);
break;
case 4:
B0(n4, 4);
var c = Vu(j(n4)) === 0 ? e(n4) : q(n4);
break;
case 5:
B0(n4, 11);
var c = Vu(j(n4)) === 0 ? e(n4) : q(n4);
break;
case 7:
var c = 5;
break;
case 8:
var c = 6;
break;
case 9:
var c = 7;
break;
case 10:
var c = 8;
break;
case 11:
var c = 9;
break;
case 12:
B0(n4, 14);
var s = R1(j(n4));
if (s === 0)
var c = Nn(j(n4)) === 0 && Nn(j(n4)) === 0 && Nn(j(n4)) === 0 ? 12 : q(n4);
else if (s === 1 && Nn(j(n4)) === 0)
for (; ; ) {
var p = N1(j(n4));
if (p !== 0) {
var c = p === 1 ? 13 : q(n4);
break;
}
}
else
var c = q(n4);
break;
case 13:
var c = 10;
break;
case 14:
B0(n4, 14);
var c = Nn(j(n4)) === 0 && Nn(j(n4)) === 0 ? 1 : q(n4);
break;
default:
var c = 0;
}
if (16 < c >>> 0)
return ke(ADr);
switch (c) {
case 1:
var y = Se(n4);
return [0, t, y, [0, Bi(Te(NDr, y))], 0];
case 2:
var T = Se(n4), E = Bi(Te(CDr, T));
return C4 <= E ? [0, t, T, [0, E >>> 3 | 0, 48 + (E & 7) | 0], 1] : [0, t, T, [0, E], 1];
case 3:
var h = Se(n4);
return [0, t, h, [0, Bi(Te(PDr, h))], 1];
case 4:
return [0, t, DDr, [0, 0], 0];
case 5:
return [0, t, LDr, [0, 8], 0];
case 6:
return [0, t, RDr, [0, 12], 0];
case 7:
return [0, t, jDr, [0, 10], 0];
case 8:
return [0, t, GDr, [0, 13], 0];
case 9:
return [0, t, MDr, [0, 9], 0];
case 10:
return [0, t, BDr, [0, 11], 0];
case 11:
var w = Se(n4);
return [0, t, w, [0, Bi(Te(qDr, w))], 1];
case 12:
var G = Se(n4);
return [0, t, G, [0, Bi(Te(UDr, p7(G, 1, nn(G) - 1 | 0)))], 0];
case 13:
var A = Se(n4), S = Bi(Te(HDr, p7(A, 2, nn(A) - 3 | 0))), M = mI < S ? wi(t, rt(t, n4)) : t;
return [0, M, A, [0, S], 0];
case 14:
var K = Se(n4), V = Ll(n4);
return [0, wi(t, rt(t, n4)), K, V, 0];
case 15:
var f0 = Se(n4);
return [0, d7(t, n4), f0, [0], 0];
default:
var m0 = Se(n4);
return [0, t, m0, Ll(n4), 0];
}
}
function ne0(t, n4, e, i, x, c) {
for (var s = t, p = x; ; ) {
En(c);
var y = j(c), T = 92 < y ? 1 : Vr($Lr, y + 1 | 0) - 1 | 0;
if (4 < T >>> 0)
var E = q(c);
else
switch (T) {
case 0:
var E = 3;
break;
case 1:
for (; ; ) {
B0(c, 4);
var h = j(c);
if (-1 < h)
if (91 < h)
var w = h <= 92 ? 1 : 0, G = w && -1;
else
var G = Vr(lLr, h) - 1 | 0;
else
var G = -1;
if (G !== 0) {
var E = q(c);
break;
}
}
break;
case 2:
var E = 2;
break;
case 3:
var E = 0;
break;
default:
var E = 1;
}
if (4 < E >>> 0)
return ke(Cwr);
switch (E) {
case 0:
var A = Se(c);
if (mn(i, A), qn(n4, A))
return [0, s, y7(s, c), p];
mn(e, A);
continue;
case 1:
mn(i, Pwr);
var S = ee0(s, c), M = S[4], K = M || p;
mn(i, S[2]);
var V = S[3];
hz(function(w0) {
return g1(e, w0);
}, V);
var s = S[1], p = K;
continue;
case 2:
var f0 = Se(c);
mn(i, f0);
var m0 = d7(wi(s, rt(s, c)), c);
return mn(e, f0), [0, m0, y7(m0, c), p];
case 3:
var k0 = Se(c);
mn(i, k0);
var g0 = wi(s, rt(s, c));
return mn(e, k0), [0, g0, y7(g0, c), p];
default:
var e0 = c[6], x0 = c[3] - e0 | 0, l = Pt(x0 * 4 | 0), c0 = Rl(c[1], e0, x0, l);
bN(i, l, 0, c0), bN(e, l, 0, c0);
continue;
}
}
}
function te0(t, n4, e, i, x) {
for (var c = t; ; ) {
En(x);
var s = j(x), p = 96 < s ? 1 : Vr(aRr, s + 1 | 0) - 1 | 0;
if (6 < p >>> 0)
var y = q(x);
else
switch (p) {
case 0:
var y = 0;
break;
case 1:
for (; ; ) {
B0(x, 6);
var T = j(x);
if (-1 < T)
if (95 < T)
var E = T <= 96 ? 1 : 0, h = E && -1;
else
var h = Vr(vLr, T) - 1 | 0;
else
var h = -1;
if (h !== 0) {
var y = q(x);
break;
}
}
break;
case 2:
var y = 5;
break;
case 3:
B0(x, 5);
var y = fi(j(x)) === 0 ? 4 : q(x);
break;
case 4:
B0(x, 6);
var w = j(x), G = In < w ? us < w ? -1 : 0 : -1, y = G === 0 ? 2 : q(x);
break;
case 5:
var y = 3;
break;
default:
var y = 1;
}
if (6 < y >>> 0)
return ke(Dwr);
switch (y) {
case 0:
return [0, wi(c, rt(c, x)), 1];
case 1:
return qi(i, 96), [0, c, 1];
case 2:
return mn(i, Lwr), [0, c, 0];
case 3:
qi(e, 92), qi(i, 92);
var A = ee0(c, x), S = A[2];
mn(e, S), mn(i, S);
var M = A[3];
hz(function(m0) {
return g1(n4, m0);
}, M);
var c = A[1];
continue;
case 4:
mn(e, Rwr), mn(i, jwr), mn(n4, Gwr);
var c = d7(c, x);
continue;
case 5:
var K = Se(x);
mn(e, K), mn(i, K), qi(n4, 10);
var c = d7(c, x);
continue;
default:
var V = Se(x);
mn(e, V), mn(i, V), mn(n4, V);
continue;
}
}
}
function Kee(t, n4) {
function e(U0) {
for (; ; )
if (B0(U0, 33), _n(j(U0)) !== 0)
return q(U0);
}
function i(U0) {
for (; ; )
if (B0(U0, 27), _n(j(U0)) !== 0)
return q(U0);
}
function x(U0) {
B0(U0, 26);
var L0 = Mt(j(U0));
if (L0 === 0) {
for (; ; )
if (B0(U0, 25), _n(j(U0)) !== 0)
return q(U0);
}
return L0 === 1 ? i(U0) : q(U0);
}
function c(U0) {
for (; ; )
if (B0(U0, 27), _n(j(U0)) !== 0)
return q(U0);
}
function s(U0) {
B0(U0, 26);
var L0 = Mt(j(U0));
if (L0 === 0) {
for (; ; )
if (B0(U0, 25), _n(j(U0)) !== 0)
return q(U0);
}
return L0 === 1 ? c(U0) : q(U0);
}
function p(U0) {
r:
for (; ; ) {
if (vn(j(U0)) === 0)
for (; ; ) {
B0(U0, 28);
var L0 = qc(j(U0));
if (3 < L0 >>> 0)
return q(U0);
switch (L0) {
case 0:
return c(U0);
case 1:
continue;
case 2:
continue r;
default:
return s(U0);
}
}
return q(U0);
}
}
function y(U0) {
B0(U0, 33);
var L0 = Hr0(j(U0));
if (3 < L0 >>> 0)
return q(U0);
switch (L0) {
case 0:
return e(U0);
case 1:
var Re = P1(j(U0));
if (Re === 0)
for (; ; ) {
B0(U0, 28);
var He = Qv(j(U0));
if (2 < He >>> 0)
return q(U0);
switch (He) {
case 0:
return c(U0);
case 1:
continue;
default:
return s(U0);
}
}
if (Re === 1)
for (; ; ) {
B0(U0, 28);
var he = qc(j(U0));
if (3 < he >>> 0)
return q(U0);
switch (he) {
case 0:
return c(U0);
case 1:
continue;
case 2:
return p(U0);
default:
return s(U0);
}
}
return q(U0);
case 2:
for (; ; ) {
B0(U0, 28);
var _e = Qv(j(U0));
if (2 < _e >>> 0)
return q(U0);
switch (_e) {
case 0:
return i(U0);
case 1:
continue;
default:
return x(U0);
}
}
default:
for (; ; ) {
B0(U0, 28);
var Zn = qc(j(U0));
if (3 < Zn >>> 0)
return q(U0);
switch (Zn) {
case 0:
return i(U0);
case 1:
continue;
case 2:
return p(U0);
default:
return x(U0);
}
}
}
}
function T(U0) {
B0(U0, 31);
var L0 = Mt(j(U0));
if (L0 === 0) {
for (; ; )
if (B0(U0, 29), _n(j(U0)) !== 0)
return q(U0);
}
return L0 === 1 ? e(U0) : q(U0);
}
function E(U0) {
return B0(U0, 3), zr0(j(U0)) === 0 ? 3 : q(U0);
}
function h(U0) {
return _9(j(U0)) === 0 && l9(j(U0)) === 0 && Yr0(j(U0)) === 0 && Lr0(j(U0)) === 0 && Rr0(j(U0)) === 0 && pL(j(U0)) === 0 && Bl(j(U0)) === 0 && _9(j(U0)) === 0 && Gs(j(U0)) === 0 && jr0(j(U0)) === 0 && Ul(j(U0)) === 0 ? 3 : q(U0);
}
function w(U0) {
B0(U0, 34);
var L0 = Pr0(j(U0));
if (3 < L0 >>> 0)
return q(U0);
switch (L0) {
case 0:
return e(U0);
case 1:
for (; ; ) {
B0(U0, 34);
var Re = Rs(j(U0));
if (4 < Re >>> 0)
return q(U0);
switch (Re) {
case 0:
return e(U0);
case 1:
continue;
case 2:
return y(U0);
case 3:
r:
for (; ; ) {
if (vn(j(U0)) === 0)
for (; ; ) {
B0(U0, 34);
var He = Rs(j(U0));
if (4 < He >>> 0)
return q(U0);
switch (He) {
case 0:
return e(U0);
case 1:
continue;
case 2:
return y(U0);
case 3:
continue r;
default:
return T(U0);
}
}
return q(U0);
}
default:
return T(U0);
}
}
case 2:
return y(U0);
default:
return T(U0);
}
}
function G(U0) {
for (; ; )
if (B0(U0, 19), _n(j(U0)) !== 0)
return q(U0);
}
function A(U0) {
B0(U0, 34);
var L0 = Qv(j(U0));
if (2 < L0 >>> 0)
return q(U0);
switch (L0) {
case 0:
return e(U0);
case 1:
for (; ; ) {
B0(U0, 34);
var Re = qc(j(U0));
if (3 < Re >>> 0)
return q(U0);
switch (Re) {
case 0:
return e(U0);
case 1:
continue;
case 2:
r:
for (; ; ) {
if (vn(j(U0)) === 0)
for (; ; ) {
B0(U0, 34);
var He = qc(j(U0));
if (3 < He >>> 0)
return q(U0);
switch (He) {
case 0:
return e(U0);
case 1:
continue;
case 2:
continue r;
default:
return T(U0);
}
}
return q(U0);
}
default:
return T(U0);
}
}
default:
return T(U0);
}
}
function S(U0) {
for (; ; )
if (B0(U0, 17), _n(j(U0)) !== 0)
return q(U0);
}
function M(U0) {
for (; ; )
if (B0(U0, 17), _n(j(U0)) !== 0)
return q(U0);
}
function K(U0) {
for (; ; )
if (B0(U0, 11), _n(j(U0)) !== 0)
return q(U0);
}
function V(U0) {
for (; ; )
if (B0(U0, 11), _n(j(U0)) !== 0)
return q(U0);
}
function f0(U0) {
for (; ; )
if (B0(U0, 15), _n(j(U0)) !== 0)
return q(U0);
}
function m0(U0) {
for (; ; )
if (B0(U0, 15), _n(j(U0)) !== 0)
return q(U0);
}
function k0(U0) {
for (; ; )
if (B0(U0, 23), _n(j(U0)) !== 0)
return q(U0);
}
function g0(U0) {
for (; ; )
if (B0(U0, 23), _n(j(U0)) !== 0)
return q(U0);
}
function e0(U0) {
B0(U0, 32);
var L0 = Mt(j(U0));
if (L0 === 0) {
for (; ; )
if (B0(U0, 30), _n(j(U0)) !== 0)
return q(U0);
}
return L0 === 1 ? e(U0) : q(U0);
}
function x0(U0) {
r:
for (; ; ) {
if (vn(j(U0)) === 0)
for (; ; ) {
B0(U0, 34);
var L0 = qr0(j(U0));
if (4 < L0 >>> 0)
return q(U0);
switch (L0) {
case 0:
return e(U0);
case 1:
return A(U0);
case 2:
continue;
case 3:
continue r;
default:
return e0(U0);
}
}
return q(U0);
}
}
En(n4);
var l = j(n4), c0 = tf < l ? pw < l ? -1 : Yk < l ? P3 < l ? Hw < l ? FE < l ? Zy < l ? 1 : 8 : Qh < l ? mE < l ? xF < l ? 1 : 8 : eO < l ? 1 : 8 : ZF < l ? _A < l ? 1 : 8 : bI < l ? 1 : 8 : P6 < l ? S4 < l ? a6 < l ? z8 < l ? G3 < l ? e3 < l ? LO < l ? 1 : 8 : GI < l ? 1 : 8 : Cy < l ? wg < l ? 1 : 8 : Uw < l ? 1 : 8 : R3 < l ? E8 < l ? Bg < l ? 1 : 8 : Z4 < l ? 1 : 8 : J8 < l ? JT < l ? 1 : 8 : Sk < l ? 1 : 8 : I4 < l ? KO < l ? b3 < l ? nw < l ? 1 : 8 : ok < l ? 1 : 8 : ap < l ? z6 < l ? 1 : 8 : Ah < l ? 1 : 8 : M8 < l ? v4 < l ? u6 < l ? 1 : 8 : U6 < l ? 1 : 8 : q6 < l ? g4 < l ? 1 : 8 : On < l ? 1 : 8 : GE < l ? B4 < l ? F6 < l ? TF < l ? f8 < l ? 1 : 8 : k8 < l ? 1 : 8 : T4 < l ? QF < l ? 1 : 8 : dd < l ? 1 : 8 : H6 < l ? t8 < l ? v3 < l ? 1 : 8 : g8 < l ? 1 : 8 : HS < l ? R8 < l ? 1 : 8 : W3 < l ? 1 : 8 : _8 < l ? x8 < l ? a8 < l ? E3 < l ? 1 : 8 : y6 < l ? 1 : 8 : v6 < l ? sF < l ? 1 : 8 : eI < l ? 1 : 8 : c3 < l ? jS < l ? n8 < l ? 1 : 8 : F8 < l ? 1 : 8 : $3 < l ? bF < l ? 1 : 8 : ag < l ? 1 : 8 : aw < l ? Fk < l ? gk < l ? Xk < l ? IT < l ? Wk < l ? 1 : 8 : xA < l ? 1 : 8 : Ey < l ? WS < l ? 1 : 8 : hh < l ? 1 : 8 : Ad < l ? yF < l ? IE < l ? 1 : 8 : eE < l ? 1 : 8 : Ty < l ? G_ < l ? 1 : 8 : LE < l ? 1 : 8 : GF < l ? Ww < l ? Uy < l ? tw < l ? 1 : 8 : zI < l ? 1 : 8 : BO < l ? cp < l ? 1 : 8 : BT < l ? 1 : 8 : Ut < l ? NA < l ? OA < l ? 1 : 8 : 1 : 8 : s6 < l ? E6 < l ? b6 < l ? w3 < l ? WE < l ? 1 : 8 : Xw < l ? 1 : 8 : l4 < l ? iE < l ? 1 : 8 : Ay < l ? 1 : 8 : s3 < l ? z4 < l ? ug < l ? 1 : 8 : XF < l ? 1 : 8 : Y4 < l ? hT < l ? 1 : 8 : Ny < l ? 1 : 8 : L6 < l ? L4 < l ? z3 < l ? US < l ? 1 : 8 : Zw < l ? 1 : 8 : ly < l ? By < l ? 1 : 8 : WT < l ? 1 : 8 : q4 < l ? _d < l ? QT < l ? 1 : 8 : M3 < l ? 1 : 8 : e6 < l ? bO < l ? 1 : 8 : eS < l ? 1 : 8 : xT < l ? Q4 < l ? Kh < l ? rp < l ? R4 < l ? C6 < l ? lp < l ? j_ < l ? 1 : 8 : Rh < l ? 1 : 8 : yh < l ? Mg < l ? 1 : 8 : NS < l ? 1 : 8 : M6 < l ? A3 < l ? xk < l ? 1 : 8 : lE < l ? 1 : 8 : b8 < l ? b4 < l ? 1 : 8 : td < l ? 1 : 8 : B3 < l ? IS < l ? DE < l ? vT < l ? 1 : 8 : ST < l ? 1 : 8 : xS < l ? k3 < l ? 1 : 8 : Jh < l ? 1 : 8 : my < l ? O6 < l ? Rg < l ? 1 : 8 : py < l ? 1 : 8 : GO < l ? kF < l ? 1 : 8 : KF < l ? 1 : 8 : jI < l ? kn < l ? BF < l ? Pd < l ? PO < l ? 1 : 8 : Lh < l ? 1 : 8 : Xd < l ? PS < l ? 1 : 8 : mA < l ? 1 : 8 : lO < l ? PI < l ? cI < l ? 1 : 8 : H_ < l ? 1 : 8 : wS < l ? oT < l ? 1 : 8 : dg < l ? 1 : 8 : DO < l ? Dw < l ? Kd < l ? mw < l ? 1 : 8 : nF < l ? 1 : 8 : Wg < l ? Gk < l ? 1 : 8 : XO < l ? 1 : 8 : aS < l ? p6 < l ? h6 < l ? 1 : 8 : rT < l ? 1 : 8 : yd < l ? tT < l ? 1 : 8 : VS < l ? 1 : 8 : JO < l ? Lk < l ? kS < l ? Cw < l ? LI < l ? $g < l ? 1 : 8 : WO < l ? 1 : 8 : Uk < l ? Ss < l ? 1 : 8 : RF < l ? 1 : 8 : Sh < l ? AS < l ? gI < l ? 1 : 8 : cg < l ? 1 : 8 : Mh < l ? Ed < l ? 1 : 8 : sI < l ? 1 : 8 : sT < l ? mg < l ? fF < l ? $I < l ? 1 : 8 : yk < l ? 1 : 8 : q_ < l ? Sw < l ? 1 : 8 : nT < l ? 1 : 8 : Bk < l ? IO < l ? dA < l ? 1 : 8 : hg < l ? 1 : 8 : ZE < l ? mF < l ? 1 : 8 : UF < l ? 1 : 8 : h4 < l ? h3 < l ? pT < l ? BI < l ? dS < l ? 1 : 8 : lA < l ? 1 : 8 : jE < l ? Jk < l ? 1 : 8 : Gg < l ? 1 : 8 : PT < l ? j6 < l ? _k < l ? 1 : 8 : aT < l ? 1 : 8 : YS < l ? HT < l ? 1 : 8 : pE < l ? 1 : 8 : Jc < l ? Tk < l ? L3 < l ? zg < l ? 1 : 8 : oh < l ? 1 : 8 : CF < l ? mh < l ? 1 : 8 : iA < l ? 1 : 8 : HE < l ? J3 < l ? by < l ? 1 : 8 : SI < l ? 1 : 8 : WF < l ? oS < l ? 1 : 8 : D4 < l ? 1 : 8 : pS < l ? Bw < l ? ak < l ? wO < l ? aI < l ? _g < l ? Dh < l ? 1 : 8 : i3 < l ? 1 : 8 : bE < l ? Gh < l ? 1 : 8 : zk < l ? 1 : 8 : ip < l ? wd < l ? B8 < l ? 1 : 8 : m3 < l ? 1 : 8 : Fw < l ? yE < l ? 1 : 8 : Hg < l ? 1 : 8 : _y < l ? ew < l ? rO < l ? JF < l ? 1 : 8 : Hh < l ? 1 : 8 : rw < l ? AI < l ? 1 : 8 : yO < l ? 1 : 8 : bS < l ? MO < l ? SA < l ? 1 : 8 : gA < l ? 1 : 8 : og < l ? XI < l ? 1 : 8 : ES < l ? 1 : 8 : wI < l ? DI < l ? iS < l ? zy < l ? Q_ < l ? 1 : 8 : _T < l ? 1 : 8 : rI < l ? Fg < l ? 1 : 8 : sA < l ? 1 : 8 : xE < l ? FT < l ? Eg < l ? 1 : 8 : AT < l ? 1 : 8 : Py < l ? i8 < l ? 1 : 8 : eg < l ? 1 : 8 : qd < l ? U_ < l ? TO < l ? md < l ? 1 : 8 : zE < l ? 1 : 8 : r6 < l ? Q6 < l ? 1 : 8 : Nh < l ? 1 : 8 : J6 < l ? MF < l ? Xy < l ? 1 : 8 : Dk < l ? 1 : 8 : RE < l ? Ag < l ? 1 : 8 : NI < l ? 1 : 8 : N8 < l ? R_ < l ? P4 < l ? rF < l ? Yd < l ? ZS < l ? 1 : 8 : G6 < l ? 1 : 8 : hE < l ? oI < l ? 1 : 8 : Rw < l ? 1 : 8 : x3 < l ? c8 < l ? YF < l ? 1 : 8 : Gw < l ? 1 : 8 : dk < l ? vI < l ? 1 : 8 : ky < l ? 1 : 8 : I8 < l ? xI < l ? i6 < l ? Sy < l ? 1 : 8 : qI < l ? 1 : 8 : s8 < l ? Nw < l ? 1 : 8 : _E < l ? 1 : 8 : d3 < l ? xp < l ? uE < l ? 1 : 8 : A8 < l ? 1 : 8 : lw < l ? $F < l ? 1 : 8 : WI < l ? 1 : 8 : Jd < l ? nI < l ? h8 < l ? wy < l ? uw < l ? 1 : 8 : f3 < l ? 1 : 8 : yI < l ? EA < l ? 1 : 8 : Xh < l ? 1 : 8 : Sg < l ? aE < l ? AO < l ? 1 : 8 : Y6 < l ? 1 : 8 : Mw < l ? vw < l ? 1 : 8 : T3 < l ? 1 : 8 : Ck < l ? NF < l ? Gy < l ? _4 < l ? 1 : 8 : lh < l ? 1 : 8 : Jg < l ? Qk < l ? 1 : 8 : vS < l ? 1 : 8 : q3 < l ? CO < l ? ww < l ? 1 : 8 : dE < l ? 1 : 8 : rg < l ? nA < l ? 1 : 8 : IA < l ? 1 : 8 : sh < l ? $E < l ? Mk < l ? $6 < l ? Uh < l ? cF < l ? eF < l ? bd < l ? uT < l ? 1 : 8 : KI < l ? 1 : 8 : ME < l ? NT < l ? 1 : 8 : $8 < l ? 1 : 8 : CA < l ? EE < l ? XT < l ? 1 : 8 : wh < l ? 1 : 8 : Ch < l ? Ud < l ? 1 : 8 : hS < l ? 1 : 8 : FA < l ? RO < l ? Vg < l ? bg < l ? 1 : 8 : Yg < l ? 1 : 8 : zw < l ? fh < l ? 1 : 8 : jg < l ? 1 : 8 : Pg < l ? _S < l ? Zh < l ? 1 : 8 : Ig < l ? 1 : 8 : fA < l ? gT < l ? 1 : 8 : jk < l ? 1 : 8 : tS < l ? Q8 < l ? QI < l ? PE < l ? UE < l ? 1 : 8 : yA < l ? 1 : 8 : FO < l ? qy < l ? 1 : 8 : oA < l ? 1 : 8 : cA < l ? p4 < l ? hk < l ? 1 : 8 : sO < l ? 1 : 8 : gh < l ? E7 < l ? 1 : 8 : rk < l ? 1 : 8 : Y8 < l ? J_ < l ? Ih < l ? Rd < l ? 1 : 8 : ET < l ? 1 : 8 : jh < l ? Nk < l ? 1 : 8 : vd < l ? 1 : 8 : id < l ? vg < l ? rE < l ? 1 : 8 : ZT < l ? 1 : 8 : ng < l ? Iw < l ? 1 : 8 : GT < l ? 1 : 8 : Cd < l ? pk < l ? Qt < l ? m8 < l ? OT < l ? B6 < l ? 1 : 8 : Od < l ? 1 : 8 : $4 < l ? ny < l ? 1 : 8 : op < l ? 1 : 8 : pO < l ? qE < l ? ld < l ? 1 : 8 : X_ < l ? 1 : 8 : FS < l ? YT < l ? 1 : 8 : BS < l ? 1 : 8 : Yw < l ? fy < l ? bA < l ? Wd < l ? 1 : 8 : bT < l ? 1 : 8 : aF < l ? ek < l ? 1 : 8 : II < l ? 1 : 8 : cO < l ? fd < l ? ig < l ? 1 : 8 : _h < l ? 1 : 8 : aO < l ? hO < l ? 1 : 8 : uO < l ? 1 : 8 : CS < l ? JE < l ? oO < l ? lk < l ? cd < l ? 1 : 8 : xh < l ? 1 : 8 : QS < l ? hw < l ? 1 : 8 : Iy < l ? 1 : 8 : pA < l ? rS < l ? $T < l ? 1 : 8 : tI < l ? 1 : 8 : xy < l ? Aw < l ? 1 : 8 : 1 : iT < l ? Q3 < l ? uk < l ? 8 : rd < l ? 1 : 8 : qF < l ? nE < l ? 1 : 8 : bk < l ? 1 : 8 : pg < l ? iO < l ? fI < l ? 1 : 8 : qO < l ? 1 : 8 : My < l ? 1 : 8 : L8 < l ? Fh < l ? cw < l ? Nd < l ? tA < l ? 8 : rh < l ? fT < l ? 1 : 8 : pF < l ? 1 : 8 : nd < l ? JI < l ? ty < l ? 1 : 8 : kd < l ? 1 : 8 : VE < l ? Qd < l ? 1 : 8 : wF < l ? 1 : 8 : ep < l ? K4 < l ? g6 < l ? yy < l ? 1 : 8 : tg < l ? 1 : 8 : Z8 < l ? kO < l ? 1 : 8 : Hk < l ? 1 : 8 : Ok < l ? H8 < l ? FF < l ? 1 : 8 : gy < l ? 1 : 8 : sg < l ? Ak < l ? 1 : 8 : gE < l ? 1 : 8 : qw < l ? ry < l ? uy < l ? yS < l ? sE < l ? 1 : 8 : 1 : 8 : Ew < l ? 8 : r8 < l ? CT < l ? 1 : 8 : kg < l ? 1 : 8 : uI < l ? u3 < l ? qo < l ? La < l ? 1 : 2 : HI < l ? 1 : 8 : sw < l ? UT < l ? 1 : 8 : KS < l ? 1 : 8 : kI < l ? fS < l ? eh < l ? 1 : 8 : LT < l ? 1 : 8 : qT < l ? $w < l ? 1 : 8 : TI < l ? 1 : 8 : YI < l ? fk < l ? et < l ? j4 < l ? U3 < l ? ah < l ? 1 : 8 : hF < l ? 1 : 8 : K3 < l ? R6 < l ? 1 : 8 : od < l ? 1 : 8 : RT < l ? fp < l ? xw < l ? 1 : 8 : uS < l ? 1 : 8 : fO < l ? n3 < l ? 1 : 8 : sd < l ? 1 : 8 : Ek < l ? JS < l ? nh < l ? xg < l ? 1 : 8 : ay < l ? 1 : 8 : Zk < l ? $h < l ? 1 : 8 : AE < l ? 1 : 8 : Eh < l ? dy < l ? lF < l ? 1 : 8 : hA < l ? 1 : 8 : Dy < l ? 1 : 8 : zh < l ? X3 < l ? o3 < l ? Qw < l ? 1 : 8 : ov < l ? 8 : MT < l ? 1 : 8 : OF < l ? w6 < l ? DF < l ? 1 : 8 : Zd < l ? 1 : 8 : qk < l ? jd < l ? 1 : 8 : eA < l ? 1 : 8 : Wh < l ? lS < l ? mT < l ? lI < l ? 1 : 8 : 1 : jy < l ? 8 : Qy < l ? 1 : 8 : IF < l ? bh < l ? 1 : 8 : zT < l ? NO < l ? 1 : 8 : vO < l ? 1 : 8 : gd < l ? T8 < l ? Z_ < l ? xd < l ? SO < l ? uh < l ? FI < l ? Pw < l ? 1 : 8 : kA < l ? 1 : 8 : Hd < l ? oy < l ? 1 : 8 : vk < l ? 1 : 8 : LF < l ? AA < l ? bp < l ? 1 : 8 : 1 : 8 : kT < l ? Vh < l ? SS < l ? Jy < l ? 1 : 8 : gS < l ? 1 : 8 : l6 < l ? Vk < l ? 1 : 8 : SE < l ? 1 : 8 : Tw < l ? yw < l ? 1 : 8 : hd < l ? ZO < l ? 1 : 8 : w8 < l ? 1 : 8 : SF < l ? jO < l ? zF < l ? Oy < l ? Th < l ? 1 : 8 : uA < l ? 1 : 8 : ey < l ? QE < l ? 1 : 8 : vy < l ? 1 : 8 : $y < l ? y8 < l ? Yy < l ? 1 : 8 : t3 < l ? 1 : 8 : tE < l ? sy < l ? 1 : 8 : lg < l ? 1 : 8 : Ky < l ? TA < l ? cS < l ? tF < l ? 1 : 8 : zd < l ? 1 : 8 : dI < l ? mk < l ? 1 : 8 : xO < l ? 1 : 8 : RS < l && vA < l ? 1 : 8 : kk < l ? Md < l ? fg < l ? ik < l ? 8 : ed < l ? _I < l ? 1 : 8 : Ld < l ? 1 : 8 : cE < l ? sk < l ? W_ < l ? 1 : 8 : 1 : 8 : Ng < l ? YO < l && sS < l ? 1 : 8 : _w < l ? tO < l ? Gd < l ? 1 : 8 : 1 : EF < l ? 8 : 1 : Fd < l ? OE < l ? H3 < l ? 8 : fw < l ? 1 : 8 : EI < l ? nk < l ? gO < l ? 1 : 8 : M_ < l ? 1 : 8 : Td < l ? 1 : 8 : HF < l ? Og < l ? Ph < l ? 1 : 8 : zS < l ? 1 : 8 : $k < l ? KE < l ? 8 : ad < l ? 1 : 8 : Y_ < l ? kE < l ? 1 : 8 : ru < l ? 1 : 8 : qS < l ? BE < l ? EO < l ? U4 < l ? Fy < l ? S8 < l ? MI < l ? 1 : 8 : iF < l ? 1 : 8 : VF < l ? 1 : 8 : gw < l ? S3 < l ? le < l ? 1 : 8 : 1 : 8 : Cg < l ? qh < l ? z_ < l ? V_ < l ? 1 : 8 : 1 : 8 : Vw < l ? O3 < l ? ph < l ? 1 : 8 : oF < l ? 1 : 8 : gg < l ? 1 : 8 : K8 < l ? G8 < l ? Sa < l ? RI < l ? 8 : ea < l ? 1 : 2 : T6 < l ? Hy < l ? 1 : 8 : CE < l ? 1 : 8 : W8 < l ? K6 < l ? Lg < l ? 1 : 8 : lT < l ? 1 : 8 : o8 < l ? Zt < l ? 1 : 8 : Pk < l ? 1 : 8 : DS < l ? fE < l ? G4 < l ? Dg < l ? 1 : 8 : rA < l ? 1 : 8 : D6 < l ? AF < l ? 1 : 8 : m4 < l ? 1 : 8 : hI < l ? oE < l ? OI < l ? 1 : 8 : C3 < l ? 1 : 8 : M4 < l ? q8 < l ? 1 : 8 : Tg < l ? 1 : 8 : C8 < l ? nS < l ? vE < l ? Kk < l ? yT < l ? $_ < l ? 1 : 8 : gF < l ? 1 : 8 : YE < l ? 1 : 8 : th < l ? pI < l ? 8 : 1 : 8 : UO < l ? dw < l ? KT < l ? v8 < l ? 1 : 8 : PA < l ? 1 : 8 : Kw < l ? K_ < l ? 1 : 8 : 1 : 8 : qg < l ? QO < l ? Z3 < l ? vp < l ? D8 < l ? 1 : 8 : r3 < l ? 1 : 8 : hr < l ? u8 < l ? 1 : 8 : 1 : F4 < l ? $S < l ? 8 : _6 < l ? 1 : 8 : dF < l ? Ly < l ? 1 : 8 : j3 < l ? 1 : 8 : Rk < l ? VI < l ? nO < l ? a3 < l ? 1 : 8 : dO < l ? 1 : 8 : Ry < l ? I3 < l ? 1 : 8 : np < l ? 1 : 8 : ya < l ? oc < l ? cx < l ? 1 : 2 : aa < l ? 1 : 2 : Ju < l ? Uu < l ? 1 : 3 : Pa < l ? 1 : 2 : Vr(XLr, l + 1 | 0) - 1 | 0;
if (36 < c0 >>> 0)
var t0 = q(n4);
else
switch (c0) {
case 0:
var t0 = 98;
break;
case 1:
var t0 = 99;
break;
case 2:
if (B0(n4, 1), Mc(j(n4)) === 0) {
for (; ; )
if (B0(n4, 1), Mc(j(n4)) !== 0) {
var t0 = q(n4);
break;
}
} else
var t0 = q(n4);
break;
case 3:
var t0 = 0;
break;
case 4:
B0(n4, 0);
var a0 = fi(j(n4)) !== 0 ? 1 : 0, t0 = a0 && q(n4);
break;
case 5:
B0(n4, 88);
var t0 = Ui(j(n4)) === 0 ? (B0(n4, 58), Ui(j(n4)) === 0 ? 54 : q(n4)) : q(n4);
break;
case 6:
var t0 = 7;
break;
case 7:
B0(n4, 95);
var w0 = j(n4), _0 = 32 < w0 ? 33 < w0 ? -1 : 0 : -1, t0 = _0 === 0 ? 6 : q(n4);
break;
case 8:
var t0 = 97;
break;
case 9:
B0(n4, 84);
var t0 = Ui(j(n4)) === 0 ? 71 : q(n4);
break;
case 10:
B0(n4, 86);
var E0 = j(n4), X0 = 37 < E0 ? 61 < E0 ? -1 : Vr(ARr, E0 - 38 | 0) - 1 | 0 : -1, t0 = X0 === 0 ? (B0(n4, 51), Ui(j(n4)) === 0 ? 76 : q(n4)) : X0 === 1 ? 72 : q(n4);
break;
case 11:
var t0 = 38;
break;
case 12:
var t0 = 39;
break;
case 13:
B0(n4, 82);
var b = Dr0(j(n4));
if (2 < b >>> 0)
var t0 = q(n4);
else
switch (b) {
case 0:
B0(n4, 83);
var t0 = Ui(j(n4)) === 0 ? 70 : q(n4);
break;
case 1:
var t0 = 4;
break;
default:
var t0 = 69;
}
break;
case 14:
B0(n4, 80);
var G0 = j(n4), X = 42 < G0 ? 61 < G0 ? -1 : Vr(NRr, G0 + cy | 0) - 1 | 0 : -1, t0 = X === 0 ? 59 : X === 1 ? 67 : q(n4);
break;
case 15:
var t0 = 45;
break;
case 16:
B0(n4, 81);
var s0 = j(n4), dr = 44 < s0 ? 61 < s0 ? -1 : Vr(mLr, s0 + mv | 0) - 1 | 0 : -1, t0 = dr === 0 ? 60 : dr === 1 ? 68 : q(n4);
break;
case 17:
B0(n4, 43);
var Ar = L1(j(n4));
if (Ar === 0)
var t0 = lL(j(n4)) === 0 ? 42 : q(n4);
else if (Ar === 1)
for (; ; ) {
B0(n4, 34);
var ar = Rs(j(n4));
if (4 < ar >>> 0)
var t0 = q(n4);
else
switch (ar) {
case 0:
var t0 = e(n4);
break;
case 1:
continue;
case 2:
var t0 = y(n4);
break;
case 3:
r:
for (; ; ) {
if (vn(j(n4)) === 0)
for (; ; ) {
B0(n4, 34);
var W0 = Rs(j(n4));
if (4 < W0 >>> 0)
var Lr = q(n4);
else
switch (W0) {
case 0:
var Lr = e(n4);
break;
case 1:
continue;
case 2:
var Lr = y(n4);
break;
case 3:
continue r;
default:
var Lr = T(n4);
}
break;
}
else
var Lr = q(n4);
var t0 = Lr;
break;
}
break;
default:
var t0 = T(n4);
}
break;
}
else
var t0 = q(n4);
break;
case 18:
B0(n4, 93);
var Tr = Dr0(j(n4));
if (2 < Tr >>> 0)
var t0 = q(n4);
else
switch (Tr) {
case 0:
B0(n4, 2);
var Hr = f9(j(n4));
if (2 < Hr >>> 0)
var t0 = q(n4);
else
switch (Hr) {
case 0:
for (; ; ) {
var Or = f9(j(n4));
if (2 < Or >>> 0)
var t0 = q(n4);
else
switch (Or) {
case 0:
continue;
case 1:
var t0 = E(n4);
break;
default:
var t0 = h(n4);
}
break;
}
break;
case 1:
var t0 = E(n4);
break;
default:
var t0 = h(n4);
}
break;
case 1:
var t0 = 5;
break;
default:
var t0 = 92;
}
break;
case 19:
B0(n4, 34);
var xr = mL(j(n4));
if (8 < xr >>> 0)
var t0 = q(n4);
else
switch (xr) {
case 0:
var t0 = e(n4);
break;
case 1:
var t0 = w(n4);
break;
case 2:
for (; ; ) {
B0(n4, 20);
var Rr = Xr0(j(n4));
if (4 < Rr >>> 0)
var t0 = q(n4);
else
switch (Rr) {
case 0:
var t0 = G(n4);
break;
case 1:
var t0 = A(n4);
break;
case 2:
continue;
case 3:
for (; ; ) {
B0(n4, 18);
var Wr = i9(j(n4));
if (3 < Wr >>> 0)
var t0 = q(n4);
else
switch (Wr) {
case 0:
var t0 = S(n4);
break;
case 1:
var t0 = A(n4);
break;
case 2:
continue;
default:
B0(n4, 17);
var Jr = Mt(j(n4));
if (Jr === 0) {
for (; ; )
if (B0(n4, 17), _n(j(n4)) !== 0) {
var t0 = q(n4);
break;
}
} else
var t0 = Jr === 1 ? S(n4) : q(n4);
}
break;
}
break;
default:
B0(n4, 19);
var or = Mt(j(n4));
if (or === 0) {
for (; ; )
if (B0(n4, 19), _n(j(n4)) !== 0) {
var t0 = q(n4);
break;
}
} else
var t0 = or === 1 ? G(n4) : q(n4);
}
break;
}
break;
case 3:
for (; ; ) {
B0(n4, 18);
var _r = i9(j(n4));
if (3 < _r >>> 0)
var t0 = q(n4);
else
switch (_r) {
case 0:
var t0 = M(n4);
break;
case 1:
var t0 = A(n4);
break;
case 2:
continue;
default:
B0(n4, 17);
var Ir = Mt(j(n4));
if (Ir === 0) {
for (; ; )
if (B0(n4, 17), _n(j(n4)) !== 0) {
var t0 = q(n4);
break;
}
} else
var t0 = Ir === 1 ? M(n4) : q(n4);
}
break;
}
break;
case 4:
B0(n4, 33);
var fe = Gr0(j(n4));
if (fe === 0)
var t0 = e(n4);
else if (fe === 1)
for (; ; ) {
B0(n4, 12);
var v0 = w9(j(n4));
if (3 < v0 >>> 0)
var t0 = q(n4);
else
switch (v0) {
case 0:
var t0 = K(n4);
break;
case 1:
continue;
case 2:
r:
for (; ; ) {
if (Bc(j(n4)) === 0)
for (; ; ) {
B0(n4, 12);
var P = w9(j(n4));
if (3 < P >>> 0)
var Q = q(n4);
else
switch (P) {
case 0:
var Q = V(n4);
break;
case 1:
continue;
case 2:
continue r;
default:
B0(n4, 10);
var L = Mt(j(n4));
if (L === 0) {
for (; ; )
if (B0(n4, 9), _n(j(n4)) !== 0) {
var Q = q(n4);
break;
}
} else
var Q = L === 1 ? V(n4) : q(n4);
}
break;
}
else
var Q = q(n4);
var t0 = Q;
break;
}
break;
default:
B0(n4, 10);
var i0 = Mt(j(n4));
if (i0 === 0) {
for (; ; )
if (B0(n4, 9), _n(j(n4)) !== 0) {
var t0 = q(n4);
break;
}
} else
var t0 = i0 === 1 ? K(n4) : q(n4);
}
break;
}
else
var t0 = q(n4);
break;
case 5:
var t0 = y(n4);
break;
case 6:
B0(n4, 33);
var l0 = Mr0(j(n4));
if (l0 === 0)
var t0 = e(n4);
else if (l0 === 1)
for (; ; ) {
B0(n4, 16);
var S0 = h9(j(n4));
if (3 < S0 >>> 0)
var t0 = q(n4);
else
switch (S0) {
case 0:
var t0 = f0(n4);
break;
case 1:
continue;
case 2:
r:
for (; ; ) {
if (Vu(j(n4)) === 0)
for (; ; ) {
B0(n4, 16);
var T0 = h9(j(n4));
if (3 < T0 >>> 0)
var R0 = q(n4);
else
switch (T0) {
case 0:
var R0 = m0(n4);
break;
case 1:
continue;
case 2:
continue r;
default:
B0(n4, 14);
var rr = Mt(j(n4));
if (rr === 0) {
for (; ; )
if (B0(n4, 13), _n(j(n4)) !== 0) {
var R0 = q(n4);
break;
}
} else
var R0 = rr === 1 ? m0(n4) : q(n4);
}
break;
}
else
var R0 = q(n4);
var t0 = R0;
break;
}
break;
default:
B0(n4, 14);
var B = Mt(j(n4));
if (B === 0) {
for (; ; )
if (B0(n4, 13), _n(j(n4)) !== 0) {
var t0 = q(n4);
break;
}
} else
var t0 = B === 1 ? f0(n4) : q(n4);
}
break;
}
else
var t0 = q(n4);
break;
case 7:
B0(n4, 33);
var Z = Or0(j(n4));
if (Z === 0)
var t0 = e(n4);
else if (Z === 1)
for (; ; ) {
B0(n4, 24);
var p0 = E9(j(n4));
if (3 < p0 >>> 0)
var t0 = q(n4);
else
switch (p0) {
case 0:
var t0 = k0(n4);
break;
case 1:
continue;
case 2:
r:
for (; ; ) {
if (Nn(j(n4)) === 0)
for (; ; ) {
B0(n4, 24);
var b0 = E9(j(n4));
if (3 < b0 >>> 0)
var q0 = q(n4);
else
switch (b0) {
case 0:
var q0 = g0(n4);
break;
case 1:
continue;
case 2:
continue r;
default:
B0(n4, 22);
var O0 = Mt(j(n4));
if (O0 === 0) {
for (; ; )
if (B0(n4, 21), _n(j(n4)) !== 0) {
var q0 = q(n4);
break;
}
} else
var q0 = O0 === 1 ? g0(n4) : q(n4);
}
break;
}
else
var q0 = q(n4);
var t0 = q0;
break;
}
break;
default:
B0(n4, 22);
var er = Mt(j(n4));
if (er === 0) {
for (; ; )
if (B0(n4, 21), _n(j(n4)) !== 0) {
var t0 = q(n4);
break;
}
} else
var t0 = er === 1 ? k0(n4) : q(n4);
}
break;
}
else
var t0 = q(n4);
break;
default:
var t0 = e0(n4);
}
break;
case 20:
B0(n4, 34);
var yr = o9(j(n4));
if (5 < yr >>> 0)
var t0 = q(n4);
else
switch (yr) {
case 0:
var t0 = e(n4);
break;
case 1:
var t0 = w(n4);
break;
case 2:
for (; ; ) {
B0(n4, 34);
var vr = o9(j(n4));
if (5 < vr >>> 0)
var t0 = q(n4);
else
switch (vr) {
case 0:
var t0 = e(n4);
break;
case 1:
var t0 = w(n4);
break;
case 2:
continue;
case 3:
var t0 = y(n4);
break;
case 4:
var t0 = x0(n4);
break;
default:
var t0 = e0(n4);
}
break;
}
break;
case 3:
var t0 = y(n4);
break;
case 4:
var t0 = x0(n4);
break;
default:
var t0 = e0(n4);
}
break;
case 21:
var t0 = 46;
break;
case 22:
var t0 = 44;
break;
case 23:
B0(n4, 78);
var $0 = j(n4), Sr = 59 < $0 ? 61 < $0 ? -1 : Vr(tN, $0 - 60 | 0) - 1 | 0 : -1, t0 = Sr === 0 ? (B0(n4, 62), Ui(j(n4)) === 0 ? 61 : q(n4)) : Sr === 1 ? 55 : q(n4);
break;
case 24:
B0(n4, 90);
var Mr = bL(j(n4)), t0 = Mr === 0 ? (B0(n4, 57), Ui(j(n4)) === 0 ? 53 : q(n4)) : Mr === 1 ? 91 : q(n4);
break;
case 25:
B0(n4, 79);
var Br = bL(j(n4));
if (Br === 0)
var t0 = 56;
else if (Br === 1) {
B0(n4, 66);
var qr = bL(j(n4)), t0 = qr === 0 ? 63 : qr === 1 ? (B0(n4, 65), Ui(j(n4)) === 0 ? 64 : q(n4)) : q(n4);
} else
var t0 = q(n4);
break;
case 26:
B0(n4, 50);
var jr = j(n4), $r = 45 < jr ? 63 < jr ? -1 : Vr(aLr, jr + l1 | 0) - 1 | 0 : -1, t0 = $r === 0 ? (B0(n4, 48), vn(j(n4)) === 0 ? 47 : q(n4)) : $r === 1 ? (B0(n4, 49), Ui(j(n4)) === 0 ? 75 : q(n4)) : q(n4);
break;
case 27:
B0(n4, 94);
var ne = j(n4), Qr = 63 < ne ? 64 < ne ? -1 : 0 : -1;
if (Qr === 0) {
var pe = j(n4), oe = 96 < pe ? R7 < pe ? -1 : Vr(xRr, pe + V3 | 0) - 1 | 0 : -1;
if (oe === 0)
if (Ar0(j(n4)) === 0) {
var me = j(n4), ae = fc < me ? tl < me ? -1 : 0 : -1;
if (ae === 0 && pL(j(n4)) === 0 && Bl(j(n4)) === 0)
var ce = j(n4), ge = 72 < ce ? 73 < ce ? -1 : 0 : -1, t0 = ge === 0 && v9(j(n4)) === 0 && Ul(j(n4)) === 0 && d9(j(n4)) === 0 && Br0(j(n4)) === 0 && v9(j(n4)) === 0 && l9(j(n4)) === 0 && d9(j(n4)) === 0 ? 35 : q(n4);
else
var t0 = q(n4);
} else
var t0 = q(n4);
else
var t0 = oe === 1 && v9(j(n4)) === 0 && Ul(j(n4)) === 0 && d9(j(n4)) === 0 && Br0(j(n4)) === 0 && v9(j(n4)) === 0 && l9(j(n4)) === 0 && d9(j(n4)) === 0 ? 35 : q(n4);
} else
var t0 = q(n4);
break;
case 28:
var t0 = 40;
break;
case 29:
if (B0(n4, 96), Gs(j(n4)) === 0) {
var H0 = R1(j(n4));
if (H0 === 0)
var t0 = Nn(j(n4)) === 0 && Nn(j(n4)) === 0 && Nn(j(n4)) === 0 ? 97 : q(n4);
else if (H0 === 1 && Nn(j(n4)) === 0)
for (; ; ) {
var Fr = N1(j(n4));
if (Fr !== 0) {
var t0 = Fr === 1 ? 97 : q(n4);
break;
}
}
else
var t0 = q(n4);
} else
var t0 = q(n4);
break;
case 30:
var t0 = 41;
break;
case 31:
B0(n4, 87);
var t0 = Ui(j(n4)) === 0 ? 74 : q(n4);
break;
case 32:
var t0 = 8;
break;
case 33:
var t0 = 36;
break;
case 34:
B0(n4, 85);
var _ = j(n4), k = 60 < _ ? X2 < _ ? -1 : Vr(CRr, _ + TS | 0) - 1 | 0 : -1, t0 = k === 0 ? 73 : k === 1 ? (B0(n4, 52), Ui(j(n4)) === 0 ? 77 : q(n4)) : q(n4);
break;
case 35:
var t0 = 37;
break;
default:
var t0 = 89;
}
if (99 < t0 >>> 0)
return ke(TPr);
var I = t0;
if (50 <= I)
switch (I) {
case 50:
return [0, t, 85];
case 51:
return [0, t, 88];
case 52:
return [0, t, 87];
case 53:
return [0, t, 94];
case 54:
return [0, t, 95];
case 55:
return [0, t, 96];
case 56:
return [0, t, 97];
case 57:
return [0, t, 92];
case 58:
return [0, t, 93];
case 59:
return [0, t, vf];
case 60:
return [0, t, F7];
case 61:
return [0, t, 69];
case 62:
return [0, t, ni];
case 63:
return [0, t, 68];
case 64:
return [0, t, 67];
case 65:
return [0, t, Ri];
case 66:
return [0, t, L7];
case 67:
return [0, t, 78];
case 68:
return [0, t, 77];
case 69:
return [0, t, 75];
case 70:
return [0, t, 76];
case 71:
return [0, t, 73];
case 72:
return [0, t, 72];
case 73:
return [0, t, 71];
case 74:
return [0, t, 70];
case 75:
return [0, t, 79];
case 76:
return [0, t, 80];
case 77:
return [0, t, 81];
case 78:
return [0, t, 98];
case 79:
return [0, t, 99];
case 80:
return [0, t, c7];
case 81:
return [0, t, D7];
case 82:
return [0, t, Xt];
case 83:
return [0, t, Qc];
case 84:
return [0, t, fs2];
case 85:
return [0, t, 89];
case 86:
return [0, t, 91];
case 87:
return [0, t, 90];
case 88:
return [0, t, Fv];
case 89:
return [0, t, Ht];
case 90:
return [0, t, 82];
case 91:
return [0, t, 11];
case 92:
return [0, t, 74];
case 93:
return [0, t, R7];
case 94:
return [0, t, 13];
case 95:
return [0, t, 14];
case 96:
return [2, wi(t, rt(t, n4))];
case 97:
var U = n4[6];
Kr0(n4);
var Y = Hl(t, U, n4[3]);
fL(n4, U);
var y0 = Ll(n4), D0 = re0(t, y0), I0 = D0[2], D = Ee(I0, PPr);
if (0 <= D) {
if (!(0 < D))
return [0, t, 18];
var u0 = Ee(I0, iDr);
if (0 <= u0) {
if (!(0 < u0))
return [0, t, 51];
var Y0 = Ee(I0, yDr);
if (0 <= Y0) {
if (!(0 < Y0))
return [0, t, 46];
if (!n0(I0, gDr))
return [0, t, 24];
if (!n0(I0, FDr))
return [0, t, 47];
if (!n0(I0, TDr))
return [0, t, 25];
if (!n0(I0, ODr))
return [0, t, 26];
if (!n0(I0, IDr))
return [0, t, 58];
} else {
if (!n0(I0, dDr))
return [0, t, 20];
if (!n0(I0, hDr))
return [0, t, 21];
if (!n0(I0, kDr))
return [0, t, 22];
if (!n0(I0, wDr))
return [0, t, 31];
if (!n0(I0, EDr))
return [0, t, 23];
if (!n0(I0, SDr))
return [0, t, 61];
}
} else {
var J0 = Ee(I0, fDr);
if (0 <= J0) {
if (!(0 < J0))
return [0, t, 54];
if (!n0(I0, lDr))
return [0, t, 55];
if (!n0(I0, bDr))
return [0, t, 56];
if (!n0(I0, pDr))
return [0, t, 57];
if (!n0(I0, mDr))
return [0, t, 19];
if (!n0(I0, _Dr))
return [0, t, 42];
} else {
if (!n0(I0, xDr))
return [0, t, 53];
if (!n0(I0, aDr))
return [0, t, 28];
if (!n0(I0, oDr))
return [0, t, 44];
if (!n0(I0, cDr))
return [0, t, 29];
if (!n0(I0, sDr))
return [0, t, 63];
if (!n0(I0, vDr))
return [0, t, 62];
}
}
} else {
var fr = Ee(I0, DPr);
if (0 <= fr) {
if (!(0 < fr))
return [0, t, 37];
var Q0 = Ee(I0, zPr);
if (0 <= Q0) {
if (!(0 < Q0))
return [0, t, 39];
if (!n0(I0, rDr))
return [0, t, 15];
if (!n0(I0, eDr))
return [0, t, 16];
if (!n0(I0, nDr))
return [0, t, 52];
if (!n0(I0, tDr))
return [0, t, 50];
if (!n0(I0, uDr))
return [0, t, 17];
} else {
if (!n0(I0, KPr))
return [0, t, 43];
if (!n0(I0, WPr))
return [0, t, 48];
if (!n0(I0, JPr))
return [0, t, 49];
if (!n0(I0, $Pr))
return [0, t, 41];
if (!n0(I0, ZPr))
return [0, t, 30];
if (!n0(I0, QPr))
return [0, t, 38];
}
} else {
var F0 = Ee(I0, LPr);
if (0 <= F0) {
if (!(0 < F0))
return [0, t, 27];
if (!n0(I0, UPr))
return [0, t, 35];
if (!n0(I0, HPr))
return [0, t, 59];
if (!n0(I0, XPr))
return [0, t, 60];
if (!n0(I0, YPr))
return [0, t, 36];
if (!n0(I0, VPr))
return [0, t, 45];
} else {
if (!n0(I0, RPr))
return [0, t, 64];
if (!n0(I0, jPr))
return [0, t, 65];
if (!n0(I0, GPr))
return [0, t, 32];
if (!n0(I0, MPr))
return [0, t, 33];
if (!n0(I0, BPr))
return [0, t, 34];
if (!n0(I0, qPr))
return [0, t, 40];
}
}
}
var gr = [4, Y, I0, xL(y0)];
return [0, D0[1], gr];
case 98:
var mr = t[4] ? ju(t, rt(t, n4), 6) : t;
return [0, mr, Pn];
default:
var Cr = wi(t, rt(t, n4));
return [0, Cr, [6, Se(n4)]];
}
switch (I) {
case 0:
return [2, d7(t, n4)];
case 1:
return [2, t];
case 2:
var sr = Ru(t, n4), Pr = $n(zn), K0 = j1(t, Pr, n4), Ur = K0[1];
return [1, Ur, Ei(Ur, sr, K0[2], Pr, 1)];
case 3:
var d0 = Se(n4);
if (t[5]) {
var Kr = t[4] ? Jr0(t, rt(t, n4), d0) : t, re = Zm(1, Kr), xe = $m(n4);
return qn(jl(n4, xe - 1 | 0, 1), OPr) && n0(jl(n4, xe - 2 | 0, 1), IPr) ? [0, re, 86] : [2, re];
}
var je = Ru(t, n4), ve = $n(zn);
mn(ve, p7(d0, 2, nn(d0) - 2 | 0));
var Ie = j1(t, ve, n4), Me = Ie[1];
return [1, Me, Ei(Me, je, Ie[2], ve, 1)];
case 4:
if (t[4])
return [2, Zm(0, t)];
$v(n4), En(n4);
var Be = Cr0(j(n4)) !== 0 ? 1 : 0, fn = Be && q(n4);
return fn === 0 ? [0, t, Xt] : ke(APr);
case 5:
var Ke = Ru(t, n4), Ae = $n(zn), xn = e2(t, Ae, n4), Qe = xn[1];
return [1, Qe, Ei(Qe, Ke, xn[2], Ae, 0)];
case 6:
return n4[6] === 0 ? [2, e2(t, $n(zn), n4)[1]] : [0, t, NPr];
case 7:
var yn = Se(n4), on = Ru(t, n4), Ce = $n(zn), We = $n(zn);
mn(We, yn);
var rn = ne0(t, yn, Ce, We, 0, n4), bn = rn[1], Cn = [0, bn[1], on, rn[2]], Hn = rn[3], Sn = Gt(We);
return [0, bn, [2, [0, Cn, Gt(Ce), Sn, Hn]]];
case 8:
var vt = $n(zn), At = $n(zn), gt = $n(zn);
Gl(n4, gt);
var Jt = Ru(t, n4), Bt = te0(t, vt, At, gt, n4), Ft = Bt[1], Nt = y7(Ft, n4), du = [0, Ft[1], Jt, Nt], Ku = Bt[2], lt = Gt(gt), xu = Gt(At);
return [0, Ft, [3, [0, du, [0, Gt(vt), xu, lt], Ku]]];
case 9:
return Dt(t, n4, function(U0, L0) {
if (En(L0), Ls(j(L0)) === 0 && s9(j(L0)) === 0 && Bc(j(L0)) === 0)
for (; ; ) {
var Re = t9(j(L0));
if (2 < Re >>> 0)
var _e = q(L0);
else
switch (Re) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (Bc(j(L0)) === 0)
for (; ; ) {
var He = t9(j(L0));
if (2 < He >>> 0)
var he = q(L0);
else
switch (He) {
case 0:
continue;
case 1:
continue r;
default:
var he = 0;
}
break;
}
else
var he = q(L0);
var _e = he;
break;
}
break;
default:
var _e = 0;
}
break;
}
else
var _e = q(L0);
return _e === 0 ? [0, U0, [1, 0, Se(L0)]] : ke(FPr);
});
case 10:
return [0, t, [1, 0, Se(n4)]];
case 11:
return Dt(t, n4, function(U0, L0) {
if (En(L0), Ls(j(L0)) === 0 && s9(j(L0)) === 0 && Bc(j(L0)) === 0)
for (; ; ) {
B0(L0, 0);
var Re = n9(j(L0));
if (Re !== 0) {
if (Re === 1)
r:
for (; ; ) {
if (Bc(j(L0)) === 0)
for (; ; ) {
B0(L0, 0);
var He = n9(j(L0));
if (He !== 0) {
if (He === 1)
continue r;
var he = q(L0);
break;
}
}
else
var he = q(L0);
var _e = he;
break;
}
else
var _e = q(L0);
break;
}
}
else
var _e = q(L0);
return _e === 0 ? [0, U0, [0, 0, Se(L0)]] : ke(gPr);
});
case 12:
return [0, t, [0, 0, Se(n4)]];
case 13:
return Dt(t, n4, function(U0, L0) {
if (En(L0), Ls(j(L0)) === 0 && p9(j(L0)) === 0 && Vu(j(L0)) === 0)
for (; ; ) {
var Re = c9(j(L0));
if (2 < Re >>> 0)
var _e = q(L0);
else
switch (Re) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (Vu(j(L0)) === 0)
for (; ; ) {
var He = c9(j(L0));
if (2 < He >>> 0)
var he = q(L0);
else
switch (He) {
case 0:
continue;
case 1:
continue r;
default:
var he = 0;
}
break;
}
else
var he = q(L0);
var _e = he;
break;
}
break;
default:
var _e = 0;
}
break;
}
else
var _e = q(L0);
return _e === 0 ? [0, U0, [1, 1, Se(L0)]] : ke(SPr);
});
case 14:
return [0, t, [1, 1, Se(n4)]];
case 15:
return Dt(t, n4, function(U0, L0) {
if (En(L0), Ls(j(L0)) === 0 && p9(j(L0)) === 0 && Vu(j(L0)) === 0)
for (; ; ) {
B0(L0, 0);
var Re = a9(j(L0));
if (Re !== 0) {
if (Re === 1)
r:
for (; ; ) {
if (Vu(j(L0)) === 0)
for (; ; ) {
B0(L0, 0);
var He = a9(j(L0));
if (He !== 0) {
if (He === 1)
continue r;
var he = q(L0);
break;
}
}
else
var he = q(L0);
var _e = he;
break;
}
else
var _e = q(L0);
break;
}
}
else
var _e = q(L0);
return _e === 0 ? [0, U0, [0, 3, Se(L0)]] : ke(EPr);
});
case 16:
return [0, t, [0, 3, Se(n4)]];
case 17:
return Dt(t, n4, function(U0, L0) {
if (En(L0), Ls(j(L0)) === 0)
for (; ; ) {
var Re = j(L0), He = 47 < Re ? 57 < Re ? -1 : Vr(dRr, Re + zt | 0) - 1 | 0 : -1;
if (He !== 0) {
if (He === 1) {
for (; ; )
if (B0(L0, 0), vn(j(L0)) !== 0) {
var he = q(L0);
break;
}
} else
var he = q(L0);
break;
}
}
else
var he = q(L0);
return he === 0 ? [0, U0, [0, 2, Se(L0)]] : ke(wPr);
});
case 18:
return [0, t, [0, 2, Se(n4)]];
case 19:
return Dt(t, n4, function(U0, L0) {
if (En(L0), Ls(j(L0)) === 0 && Vu(j(L0)) === 0) {
for (; ; )
if (B0(L0, 0), Vu(j(L0)) !== 0) {
var Re = q(L0);
break;
}
} else
var Re = q(L0);
return Re === 0 ? [0, U0, [0, 1, Se(L0)]] : ke(kPr);
});
case 20:
return [0, t, [0, 1, Se(n4)]];
case 21:
return Dt(t, n4, function(U0, L0) {
if (En(L0), Ls(j(L0)) === 0 && Qm(j(L0)) === 0 && Nn(j(L0)) === 0)
for (; ; ) {
var Re = u9(j(L0));
if (2 < Re >>> 0)
var _e = q(L0);
else
switch (Re) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (Nn(j(L0)) === 0)
for (; ; ) {
var He = u9(j(L0));
if (2 < He >>> 0)
var he = q(L0);
else
switch (He) {
case 0:
continue;
case 1:
continue r;
default:
var he = 0;
}
break;
}
else
var he = q(L0);
var _e = he;
break;
}
break;
default:
var _e = 0;
}
break;
}
else
var _e = q(L0);
return _e === 0 ? [0, U0, [1, 2, Se(L0)]] : ke(hPr);
});
case 23:
return Dt(t, n4, function(U0, L0) {
if (En(L0), Ls(j(L0)) === 0 && Qm(j(L0)) === 0 && Nn(j(L0)) === 0)
for (; ; ) {
B0(L0, 0);
var Re = y9(j(L0));
if (Re !== 0) {
if (Re === 1)
r:
for (; ; ) {
if (Nn(j(L0)) === 0)
for (; ; ) {
B0(L0, 0);
var He = y9(j(L0));
if (He !== 0) {
if (He === 1)
continue r;
var he = q(L0);
break;
}
}
else
var he = q(L0);
var _e = he;
break;
}
else
var _e = q(L0);
break;
}
}
else
var _e = q(L0);
return _e === 0 ? [0, U0, [0, 4, Se(L0)]] : ke(dPr);
});
case 25:
return Dt(t, n4, function(U0, L0) {
function Re(cn) {
for (; ; ) {
var tt = ki(j(cn));
if (2 < tt >>> 0)
return q(cn);
switch (tt) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (vn(j(cn)) === 0)
for (; ; ) {
var Tt = ki(j(cn));
if (2 < Tt >>> 0)
return q(cn);
switch (Tt) {
case 0:
continue;
case 1:
continue r;
default:
return 0;
}
}
return q(cn);
}
default:
return 0;
}
}
}
function He(cn) {
for (; ; ) {
var tt = r2(j(cn));
if (tt !== 0) {
var Tt = tt !== 1 ? 1 : 0;
return Tt && q(cn);
}
}
}
function he(cn) {
var tt = S9(j(cn));
if (2 < tt >>> 0)
return q(cn);
switch (tt) {
case 0:
var Tt = P1(j(cn));
return Tt === 0 ? He(cn) : Tt === 1 ? Re(cn) : q(cn);
case 1:
return He(cn);
default:
return Re(cn);
}
}
function _e(cn) {
var tt = m9(j(cn));
if (tt === 0)
for (; ; ) {
var Tt = i7(j(cn));
if (2 < Tt >>> 0)
return q(cn);
switch (Tt) {
case 0:
continue;
case 1:
return he(cn);
default:
r:
for (; ; ) {
if (vn(j(cn)) === 0)
for (; ; ) {
var Fi = i7(j(cn));
if (2 < Fi >>> 0)
return q(cn);
switch (Fi) {
case 0:
continue;
case 1:
return he(cn);
default:
continue r;
}
}
return q(cn);
}
}
}
return tt === 1 ? he(cn) : q(cn);
}
En(L0);
var Zn = r9(j(L0));
if (2 < Zn >>> 0)
var dn = q(L0);
else
switch (Zn) {
case 0:
if (vn(j(L0)) === 0)
for (; ; ) {
var it = i7(j(L0));
if (2 < it >>> 0)
var dn = q(L0);
else
switch (it) {
case 0:
continue;
case 1:
var dn = he(L0);
break;
default:
r:
for (; ; ) {
if (vn(j(L0)) === 0)
for (; ; ) {
var ft = i7(j(L0));
if (2 < ft >>> 0)
var Rn = q(L0);
else
switch (ft) {
case 0:
continue;
case 1:
var Rn = he(L0);
break;
default:
continue r;
}
break;
}
else
var Rn = q(L0);
var dn = Rn;
break;
}
}
break;
}
else
var dn = q(L0);
break;
case 1:
var nt = e9(j(L0)), dn = nt === 0 ? _e(L0) : nt === 1 ? he(L0) : q(L0);
break;
default:
for (; ; ) {
var ht = b9(j(L0));
if (2 < ht >>> 0)
var dn = q(L0);
else
switch (ht) {
case 0:
var dn = _e(L0);
break;
case 1:
continue;
default:
var dn = he(L0);
}
break;
}
}
if (dn === 0) {
var tn = ju(U0, rt(U0, L0), 23);
return [0, tn, [1, 2, Se(L0)]];
}
return ke(yPr);
});
case 26:
var Mu = ju(t, rt(t, n4), 23);
return [0, Mu, [1, 2, Se(n4)]];
case 27:
return Dt(t, n4, function(U0, L0) {
function Re(tn) {
for (; ; ) {
B0(tn, 0);
var cn = js(j(tn));
if (cn !== 0) {
if (cn === 1)
r:
for (; ; ) {
if (vn(j(tn)) === 0)
for (; ; ) {
B0(tn, 0);
var tt = js(j(tn));
if (tt !== 0) {
if (tt === 1)
continue r;
return q(tn);
}
}
return q(tn);
}
return q(tn);
}
}
}
function He(tn) {
for (; ; )
if (B0(tn, 0), vn(j(tn)) !== 0)
return q(tn);
}
function he(tn) {
var cn = S9(j(tn));
if (2 < cn >>> 0)
return q(tn);
switch (cn) {
case 0:
var tt = P1(j(tn));
return tt === 0 ? He(tn) : tt === 1 ? Re(tn) : q(tn);
case 1:
return He(tn);
default:
return Re(tn);
}
}
function _e(tn) {
var cn = m9(j(tn));
if (cn === 0)
for (; ; ) {
var tt = i7(j(tn));
if (2 < tt >>> 0)
return q(tn);
switch (tt) {
case 0:
continue;
case 1:
return he(tn);
default:
r:
for (; ; ) {
if (vn(j(tn)) === 0)
for (; ; ) {
var Tt = i7(j(tn));
if (2 < Tt >>> 0)
return q(tn);
switch (Tt) {
case 0:
continue;
case 1:
return he(tn);
default:
continue r;
}
}
return q(tn);
}
}
}
return cn === 1 ? he(tn) : q(tn);
}
En(L0);
var Zn = r9(j(L0));
if (2 < Zn >>> 0)
var dn = q(L0);
else
switch (Zn) {
case 0:
if (vn(j(L0)) === 0)
for (; ; ) {
var it = i7(j(L0));
if (2 < it >>> 0)
var dn = q(L0);
else
switch (it) {
case 0:
continue;
case 1:
var dn = he(L0);
break;
default:
r:
for (; ; ) {
if (vn(j(L0)) === 0)
for (; ; ) {
var ft = i7(j(L0));
if (2 < ft >>> 0)
var Rn = q(L0);
else
switch (ft) {
case 0:
continue;
case 1:
var Rn = he(L0);
break;
default:
continue r;
}
break;
}
else
var Rn = q(L0);
var dn = Rn;
break;
}
}
break;
}
else
var dn = q(L0);
break;
case 1:
var nt = e9(j(L0)), dn = nt === 0 ? _e(L0) : nt === 1 ? he(L0) : q(L0);
break;
default:
for (; ; ) {
var ht = b9(j(L0));
if (2 < ht >>> 0)
var dn = q(L0);
else
switch (ht) {
case 0:
var dn = _e(L0);
break;
case 1:
continue;
default:
var dn = he(L0);
}
break;
}
}
return dn === 0 ? [0, U0, [0, 4, Se(L0)]] : ke(_Pr);
});
case 29:
return Dt(t, n4, function(U0, L0) {
function Re(nt) {
for (; ; ) {
var ht = ki(j(nt));
if (2 < ht >>> 0)
return q(nt);
switch (ht) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (vn(j(nt)) === 0)
for (; ; ) {
var tn = ki(j(nt));
if (2 < tn >>> 0)
return q(nt);
switch (tn) {
case 0:
continue;
case 1:
continue r;
default:
return 0;
}
}
return q(nt);
}
default:
return 0;
}
}
}
function He(nt) {
var ht = r2(j(nt));
if (ht === 0)
return Re(nt);
var tn = ht !== 1 ? 1 : 0;
return tn && q(nt);
}
En(L0);
var he = r9(j(L0));
if (2 < he >>> 0)
var _e = q(L0);
else
switch (he) {
case 0:
var _e = vn(j(L0)) === 0 ? Re(L0) : q(L0);
break;
case 1:
for (; ; ) {
var Zn = L1(j(L0));
if (Zn === 0)
var _e = He(L0);
else {
if (Zn === 1)
continue;
var _e = q(L0);
}
break;
}
break;
default:
for (; ; ) {
var dn = Uc(j(L0));
if (2 < dn >>> 0)
var _e = q(L0);
else
switch (dn) {
case 0:
var _e = He(L0);
break;
case 1:
continue;
default:
r:
for (; ; ) {
if (vn(j(L0)) === 0)
for (; ; ) {
var it = Uc(j(L0));
if (2 < it >>> 0)
var ft = q(L0);
else
switch (it) {
case 0:
var ft = He(L0);
break;
case 1:
continue;
default:
continue r;
}
break;
}
else
var ft = q(L0);
var _e = ft;
break;
}
}
break;
}
}
if (_e === 0) {
var Rn = ju(U0, rt(U0, L0), 22);
return [0, Rn, [1, 2, Se(L0)]];
}
return ke(mPr);
});
case 30:
return Dt(t, n4, function(U0, L0) {
En(L0);
var Re = P1(j(L0));
if (Re === 0)
for (; ; ) {
var He = r2(j(L0));
if (He !== 0) {
var he = He !== 1 ? 1 : 0, it = he && q(L0);
break;
}
}
else if (Re === 1)
for (; ; ) {
var _e = ki(j(L0));
if (2 < _e >>> 0)
var it = q(L0);
else
switch (_e) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (vn(j(L0)) === 0)
for (; ; ) {
var Zn = ki(j(L0));
if (2 < Zn >>> 0)
var dn = q(L0);
else
switch (Zn) {
case 0:
continue;
case 1:
continue r;
default:
var dn = 0;
}
break;
}
else
var dn = q(L0);
var it = dn;
break;
}
break;
default:
var it = 0;
}
break;
}
else
var it = q(L0);
return it === 0 ? [0, U0, [1, 2, Se(L0)]] : ke(pPr);
});
case 31:
var z7 = ju(t, rt(t, n4), 22);
return [0, z7, [1, 2, Se(n4)]];
case 33:
return Dt(t, n4, function(U0, L0) {
function Re(Rn) {
for (; ; ) {
B0(Rn, 0);
var nt = js(j(Rn));
if (nt !== 0) {
if (nt === 1)
r:
for (; ; ) {
if (vn(j(Rn)) === 0)
for (; ; ) {
B0(Rn, 0);
var ht = js(j(Rn));
if (ht !== 0) {
if (ht === 1)
continue r;
return q(Rn);
}
}
return q(Rn);
}
return q(Rn);
}
}
}
function He(Rn) {
return B0(Rn, 0), vn(j(Rn)) === 0 ? Re(Rn) : q(Rn);
}
En(L0);
var he = r9(j(L0));
if (2 < he >>> 0)
var _e = q(L0);
else
switch (he) {
case 0:
var _e = vn(j(L0)) === 0 ? Re(L0) : q(L0);
break;
case 1:
for (; ; ) {
B0(L0, 0);
var Zn = L1(j(L0));
if (Zn === 0)
var _e = He(L0);
else {
if (Zn === 1)
continue;
var _e = q(L0);
}
break;
}
break;
default:
for (; ; ) {
B0(L0, 0);
var dn = Uc(j(L0));
if (2 < dn >>> 0)
var _e = q(L0);
else
switch (dn) {
case 0:
var _e = He(L0);
break;
case 1:
continue;
default:
r:
for (; ; ) {
if (vn(j(L0)) === 0)
for (; ; ) {
B0(L0, 0);
var it = Uc(j(L0));
if (2 < it >>> 0)
var ft = q(L0);
else
switch (it) {
case 0:
var ft = He(L0);
break;
case 1:
continue;
default:
continue r;
}
break;
}
else
var ft = q(L0);
var _e = ft;
break;
}
}
break;
}
}
return _e === 0 ? [0, U0, [0, 4, Se(L0)]] : ke(bPr);
});
case 35:
var Yi = rt(t, n4), a7 = Se(n4);
return [0, t, [4, Yi, a7, a7]];
case 36:
return [0, t, 0];
case 37:
return [0, t, 1];
case 38:
return [0, t, 4];
case 39:
return [0, t, 5];
case 40:
return [0, t, 6];
case 41:
return [0, t, 7];
case 42:
return [0, t, 12];
case 43:
return [0, t, 10];
case 44:
return [0, t, 8];
case 45:
return [0, t, 9];
case 46:
return [0, t, 86];
case 47:
$v(n4), En(n4);
var Yc = j(n4), K7 = 62 < Yc ? 63 < Yc ? -1 : 0 : -1, qt = K7 !== 0 ? 1 : 0, bt = qt && q(n4);
return bt === 0 ? [0, t, 85] : ke(CPr);
case 48:
return [0, t, 83];
case 49:
return [0, t, 84];
case 22:
case 32:
return [0, t, [1, 2, Se(n4)]];
default:
return [0, t, [0, 4, Se(n4)]];
}
}
function Wee(t, n4) {
En(n4);
var e = j(n4), i = tf < e ? Sa < e ? qo < e ? La < e ? 1 : 2 : ea < e ? 1 : 2 : ya < e ? oc < e ? cx < e ? 1 : 2 : aa < e ? 1 : 2 : Ju < e ? Uu < e ? 1 : 3 : Pa < e ? 1 : 2 : Vr(YLr, e + 1 | 0) - 1 | 0;
if (5 < i >>> 0)
var x = q(n4);
else
switch (i) {
case 0:
var x = 0;
break;
case 1:
var x = 6;
break;
case 2:
if (B0(n4, 2), Mc(j(n4)) === 0) {
for (; ; )
if (B0(n4, 2), Mc(j(n4)) !== 0) {
var x = q(n4);
break;
}
} else
var x = q(n4);
break;
case 3:
var x = 1;
break;
case 4:
B0(n4, 1);
var x = fi(j(n4)) === 0 ? 1 : q(n4);
break;
default:
B0(n4, 5);
var c = k9(j(n4)), x = c === 0 ? 4 : c === 1 ? 3 : q(n4);
}
if (6 < x >>> 0)
return ke(lPr);
switch (x) {
case 0:
return [0, t, Pn];
case 1:
return [2, d7(t, n4)];
case 2:
return [2, t];
case 3:
var s = Ru(t, n4), p = $n(zn), y = e2(t, p, n4), T = y[1];
return [1, T, Ei(T, s, y[2], p, 0)];
case 4:
var E = Ru(t, n4), h = $n(zn), w = j1(t, h, n4), G = w[1];
return [1, G, Ei(G, E, w[2], h, 1)];
case 5:
var A = Ru(t, n4), S = $n(zn), M = t;
r:
for (; ; ) {
En(n4);
var K = j(n4), V = 92 < K ? Uu < K ? 1 : Ju < K ? 2 : 1 : Vr(QLr, K + 1 | 0) - 1 | 0;
if (6 < V >>> 0)
var f0 = q(n4);
else
switch (V) {
case 0:
var f0 = 0;
break;
case 1:
for (; ; ) {
B0(n4, 7);
var m0 = j(n4);
if (-1 < m0)
if (90 < m0)
if (92 < m0)
if (Ju < m0)
var k0 = m0 <= Uu ? 1 : 0, g0 = k0 && -1;
else
var g0 = 0;
else
var g0 = -1;
else
var g0 = Vr(gRr, m0) - 1 | 0;
else
var g0 = -1;
if (g0 !== 0) {
var f0 = q(n4);
break;
}
}
break;
case 2:
var f0 = 6;
break;
case 3:
B0(n4, 6);
var f0 = fi(j(n4)) === 0 ? 6 : q(n4);
break;
case 4:
if (B0(n4, 4), Ir0(j(n4)) === 0) {
for (; ; )
if (B0(n4, 3), Ir0(j(n4)) !== 0) {
var f0 = q(n4);
break;
}
} else
var f0 = q(n4);
break;
case 5:
var f0 = 5;
break;
default:
B0(n4, 7);
var e0 = j(n4);
if (-1 < e0)
if (13 < e0)
var x0 = e0 <= Uu ? 1 : 0, l = x0 && (Ju < e0 ? 1 : 0);
else
var l = Vr(wLr, e0) - 1 | 0;
else
var l = -1;
if (2 < l >>> 0)
var f0 = q(n4);
else
switch (l) {
case 0:
var f0 = 2;
break;
case 1:
var f0 = 1;
break;
default:
B0(n4, 1);
var f0 = fi(j(n4)) === 0 ? 1 : q(n4);
}
}
if (7 < f0 >>> 0)
var c0 = ke(qwr);
else
switch (f0) {
case 0:
var c0 = [0, ju(M, rt(M, n4), 25), Uwr];
break;
case 1:
var c0 = [0, d7(ju(M, rt(M, n4), 25), n4), Hwr];
break;
case 3:
var t0 = Se(n4), c0 = [0, M, p7(t0, 1, nn(t0) - 1 | 0)];
break;
case 4:
var c0 = [0, M, Xwr];
break;
case 5:
for (qi(S, 91); ; ) {
En(n4);
var a0 = j(n4), w0 = 93 < a0 ? 1 : Vr(ELr, a0 + 1 | 0) - 1 | 0;
if (3 < w0 >>> 0)
var _0 = q(n4);
else
switch (w0) {
case 0:
var _0 = 0;
break;
case 1:
for (; ; ) {
B0(n4, 4);
var E0 = j(n4);
if (-1 < E0)
if (91 < E0)
var X0 = E0 <= 93 ? 1 : 0, b = X0 && -1;
else
var b = 0;
else
var b = -1;
if (b !== 0) {
var _0 = q(n4);
break;
}
}
break;
case 2:
B0(n4, 4);
var G0 = j(n4), X = 91 < G0 ? 93 < G0 ? -1 : Vr(tN, G0 - 92 | 0) - 1 | 0 : -1, _0 = X === 0 ? 1 : X === 1 ? 2 : q(n4);
break;
default:
var _0 = 3;
}
if (4 < _0 >>> 0)
var s0 = ke(Mwr);
else
switch (_0) {
case 0:
var s0 = M;
break;
case 1:
mn(S, Bwr);
continue;
case 2:
qi(S, 92), qi(S, 93);
continue;
case 3:
qi(S, 93);
var s0 = M;
break;
default:
mn(S, Se(n4));
continue;
}
var M = s0;
continue r;
}
case 6:
var c0 = [0, d7(ju(M, rt(M, n4), 25), n4), Ywr];
break;
default:
mn(S, Se(n4));
continue;
}
var dr = c0[1], Ar = y7(dr, n4), ar = [0, dr[1], A, Ar], W0 = c0[2];
return [0, dr, [5, ar, Gt(S), W0]];
}
default:
var Lr = wi(t, rt(t, n4));
return [0, Lr, [6, Se(n4)]];
}
}
function yL(t, n4, e, i, x) {
for (var c = t; ; ) {
var s = function(Cn) {
for (; ; )
if (B0(Cn, 6), Nr0(j(Cn)) !== 0)
return q(Cn);
};
En(x);
var p = j(x), y = br < p ? Uu < p ? 1 : Ju < p ? 2 : 1 : Vr(bLr, p + 1 | 0) - 1 | 0;
if (6 < y >>> 0)
var T = q(x);
else
switch (y) {
case 0:
var T = 1;
break;
case 1:
var T = s(x);
break;
case 2:
var T = 2;
break;
case 3:
B0(x, 2);
var T = fi(j(x)) === 0 ? 2 : q(x);
break;
case 4:
var T = 0;
break;
case 5:
B0(x, 6);
var E = j(x), h = 34 < E ? In < E ? -1 : Vr(cRr, E - 35 | 0) - 1 | 0 : -1;
if (h === 0) {
var w = j(x), G = 47 < w ? fc < w ? -1 : Vr(hRr, w + zt | 0) - 1 | 0 : -1;
if (G === 0)
for (; ; ) {
var A = j(x), S = 47 < A ? 59 < A ? -1 : Vr(hLr, A + zt | 0) - 1 | 0 : -1;
if (S !== 0) {
var T = S === 1 ? 4 : q(x);
break;
}
}
else if (G === 1 && Nn(j(x)) === 0)
for (; ; ) {
var M = j(x), K = 47 < M ? Ri < M ? -1 : Vr(pRr, M + zt | 0) - 1 | 0 : -1;
if (K !== 0) {
var T = K === 1 ? 3 : q(x);
break;
}
}
else
var T = q(x);
} else if (h === 1 && _n(j(x)) === 0) {
var V = Zv(j(x));
if (V === 0) {
var f0 = Zv(j(x));
if (f0 === 0) {
var m0 = Zv(j(x));
if (m0 === 0) {
var k0 = Zv(j(x));
if (k0 === 0) {
var g0 = Zv(j(x));
if (g0 === 0) {
var e0 = Zv(j(x));
if (e0 === 0)
var x0 = j(x), l = 58 < x0 ? 59 < x0 ? -1 : 0 : -1, T = l === 0 ? 5 : q(x);
else
var T = e0 === 1 ? 5 : q(x);
} else
var T = g0 === 1 ? 5 : q(x);
} else
var T = k0 === 1 ? 5 : q(x);
} else
var T = m0 === 1 ? 5 : q(x);
} else
var T = f0 === 1 ? 5 : q(x);
} else
var T = V === 1 ? 5 : q(x);
} else
var T = q(x);
break;
default:
B0(x, 0);
var T = Nr0(j(x)) === 0 ? s(x) : q(x);
}
if (6 < T >>> 0)
return ke(Vwr);
switch (T) {
case 0:
var c0 = Se(x), t0 = 0;
switch (n4) {
case 0:
n0(c0, zwr) || (t0 = 1);
break;
case 1:
n0(c0, Kwr) || (t0 = 1);
break;
default:
var a0 = 0;
if (n0(c0, Wwr)) {
if (!n0(c0, Jwr))
return _L(c, rt(c, x), nEr, eEr);
if (n0(c0, $wr)) {
if (!n0(c0, Zwr))
return _L(c, rt(c, x), rEr, Qwr);
a0 = 1;
}
}
if (!a0)
return $v(x), c;
}
if (t0)
return c;
mn(i, c0), mn(e, c0);
continue;
case 1:
return wi(c, rt(c, x));
case 2:
var w0 = Se(x);
mn(i, w0), mn(e, w0);
var c = d7(c, x);
continue;
case 3:
var _0 = Se(x), E0 = p7(_0, 3, nn(_0) - 4 | 0);
mn(i, _0), g1(e, Bi(Te(tEr, E0)));
continue;
case 4:
var X0 = Se(x), b = p7(X0, 2, nn(X0) - 3 | 0);
mn(i, X0), g1(e, Bi(b));
continue;
case 5:
var G0 = Se(x), X = p7(G0, 1, nn(G0) - 2 | 0);
mn(i, G0);
var s0 = Ee(X, uEr), dr = 0;
if (0 <= s0)
if (0 < s0) {
var Ar = Ee(X, qTr), ar = 0;
if (0 <= Ar)
if (0 < Ar) {
var W0 = Ee(X, fAr), Lr = 0;
if (0 <= W0)
if (0 < W0) {
var Tr = Ee(X, pNr), Hr = 0;
if (0 <= Tr)
if (0 < Tr) {
var Or = Ee(X, VNr), xr = 0;
if (0 <= Or)
if (0 < Or)
if (n0(X, xCr))
if (n0(X, aCr))
if (n0(X, oCr))
if (n0(X, cCr))
if (n0(X, sCr))
if (n0(X, vCr))
ar = 1, Lr = 1, Hr = 1, xr = 1;
else
var Rr = lCr;
else
var Rr = bCr;
else
var Rr = pCr;
else
var Rr = mCr;
else
var Rr = _Cr;
else
var Rr = yCr;
else
var Rr = dCr;
else if (n0(X, zNr))
if (n0(X, KNr))
if (n0(X, WNr))
if (n0(X, JNr))
if (n0(X, $Nr))
if (n0(X, ZNr))
if (n0(X, QNr))
ar = 1, Lr = 1, Hr = 1, xr = 1;
else
var Rr = rCr;
else
var Rr = eCr;
else
var Rr = nCr;
else
var Rr = tCr;
else
var Rr = uCr;
else
var Rr = iCr;
else
var Rr = fCr;
if (!xr)
var Wr = Rr;
} else
var Wr = hCr;
else {
var Jr = Ee(X, mNr), or = 0;
if (0 <= Jr)
if (0 < Jr)
if (n0(X, NNr))
if (n0(X, CNr))
if (n0(X, PNr))
if (n0(X, DNr))
if (n0(X, LNr))
if (n0(X, RNr))
if (n0(X, jNr))
ar = 1, Lr = 1, Hr = 1, or = 1;
else
var _r = GNr;
else
var _r = MNr;
else
var _r = BNr;
else
var _r = qNr;
else
var _r = UNr;
else
var _r = HNr;
else
var _r = XNr;
else
var _r = YNr;
else if (n0(X, _Nr))
if (n0(X, yNr))
if (n0(X, dNr))
if (n0(X, hNr))
if (n0(X, kNr))
if (n0(X, wNr))
if (n0(X, ENr))
ar = 1, Lr = 1, Hr = 1, or = 1;
else
var _r = SNr;
else
var _r = gNr;
else
var _r = FNr;
else
var _r = TNr;
else
var _r = ONr;
else
var _r = INr;
else
var _r = ANr;
if (!or)
var Wr = _r;
}
if (!Hr)
var Ir = Wr;
} else
var Ir = kCr;
else {
var fe = Ee(X, xAr), v0 = 0;
if (0 <= fe)
if (0 < fe) {
var P = Ee(X, GAr), L = 0;
if (0 <= P)
if (0 < P)
if (n0(X, QAr))
if (n0(X, rNr))
if (n0(X, eNr))
if (n0(X, nNr))
if (n0(X, tNr))
if (n0(X, uNr))
if (n0(X, iNr))
ar = 1, Lr = 1, v0 = 1, L = 1;
else
var Q = fNr;
else
var Q = xNr;
else
var Q = aNr;
else
var Q = oNr;
else
var Q = cNr;
else
var Q = sNr;
else
var Q = vNr;
else
var Q = lNr;
else if (n0(X, MAr))
if (n0(X, BAr))
if (n0(X, qAr))
if (n0(X, UAr))
if (n0(X, HAr))
if (n0(X, XAr))
if (n0(X, YAr))
ar = 1, Lr = 1, v0 = 1, L = 1;
else
var Q = VAr;
else
var Q = zAr;
else
var Q = KAr;
else
var Q = WAr;
else
var Q = JAr;
else
var Q = $Ar;
else
var Q = ZAr;
if (!L)
var i0 = Q;
} else
var i0 = bNr;
else {
var l0 = Ee(X, aAr), S0 = 0;
if (0 <= l0)
if (0 < l0)
if (n0(X, EAr))
if (n0(X, SAr))
if (n0(X, gAr))
if (n0(X, FAr))
if (n0(X, TAr))
if (n0(X, OAr))
if (n0(X, IAr))
ar = 1, Lr = 1, v0 = 1, S0 = 1;
else
var T0 = AAr;
else
var T0 = NAr;
else
var T0 = CAr;
else
var T0 = PAr;
else
var T0 = DAr;
else
var T0 = LAr;
else
var T0 = RAr;
else
var T0 = jAr;
else if (n0(X, oAr))
if (n0(X, cAr))
if (n0(X, sAr))
if (n0(X, vAr))
if (n0(X, lAr))
if (n0(X, bAr))
if (n0(X, pAr))
ar = 1, Lr = 1, v0 = 1, S0 = 1;
else
var T0 = mAr;
else
var T0 = _Ar;
else
var T0 = yAr;
else
var T0 = dAr;
else
var T0 = hAr;
else
var T0 = kAr;
else
var T0 = wAr;
if (!S0)
var i0 = T0;
}
if (!v0)
var Ir = i0;
}
if (!Lr)
var rr = Ir;
} else
var rr = wCr;
else {
var R0 = Ee(X, UTr), B = 0;
if (0 <= R0)
if (0 < R0) {
var Z = Ee(X, $Or), p0 = 0;
if (0 <= Z)
if (0 < Z) {
var b0 = Ee(X, OIr), O0 = 0;
if (0 <= b0)
if (0 < b0)
if (n0(X, HIr))
if (n0(X, XIr))
if (n0(X, YIr))
if (n0(X, VIr))
if (n0(X, zIr))
if (n0(X, KIr))
if (n0(X, WIr))
ar = 1, B = 1, p0 = 1, O0 = 1;
else
var q0 = JIr;
else
var q0 = $Ir;
else
var q0 = ZIr;
else
var q0 = QIr;
else
var q0 = rAr;
else
var q0 = eAr;
else
var q0 = nAr;
else
var q0 = tAr;
else if (n0(X, IIr))
if (n0(X, AIr))
if (n0(X, NIr))
if (n0(X, CIr))
if (n0(X, PIr))
if (n0(X, DIr))
if (n0(X, LIr))
ar = 1, B = 1, p0 = 1, O0 = 1;
else
var q0 = RIr;
else
var q0 = jIr;
else
var q0 = GIr;
else
var q0 = MIr;
else
var q0 = BIr;
else
var q0 = qIr;
else
var q0 = UIr;
if (!O0)
var er = q0;
} else
var er = uAr;
else {
var yr = Ee(X, ZOr), vr = 0;
if (0 <= yr)
if (0 < yr)
if (n0(X, lIr))
if (n0(X, bIr))
if (n0(X, pIr))
if (n0(X, mIr))
if (n0(X, _Ir))
if (n0(X, yIr))
if (n0(X, dIr))
ar = 1, B = 1, p0 = 1, vr = 1;
else
var $0 = hIr;
else
var $0 = kIr;
else
var $0 = wIr;
else
var $0 = EIr;
else
var $0 = SIr;
else
var $0 = gIr;
else
var $0 = FIr;
else
var $0 = TIr;
else if (n0(X, QOr))
if (n0(X, rIr))
if (n0(X, eIr))
if (n0(X, nIr))
if (n0(X, tIr))
if (n0(X, uIr))
if (n0(X, iIr))
ar = 1, B = 1, p0 = 1, vr = 1;
else
var $0 = fIr;
else
var $0 = xIr;
else
var $0 = aIr;
else
var $0 = oIr;
else
var $0 = cIr;
else
var $0 = sIr;
else
var $0 = vIr;
if (!vr)
var er = $0;
}
if (!p0)
var Sr = er;
} else
var Sr = iAr;
else {
var Mr = Ee(X, HTr), Br = 0;
if (0 <= Mr)
if (0 < Mr) {
var qr = Ee(X, hOr), jr = 0;
if (0 <= qr)
if (0 < qr)
if (n0(X, LOr))
if (n0(X, ROr))
if (n0(X, jOr))
if (n0(X, GOr))
if (n0(X, MOr))
if (n0(X, BOr))
if (n0(X, qOr))
ar = 1, B = 1, Br = 1, jr = 1;
else
var $r = UOr;
else
var $r = HOr;
else
var $r = XOr;
else
var $r = YOr;
else
var $r = VOr;
else
var $r = zOr;
else
var $r = KOr;
else
var $r = WOr;
else if (n0(X, kOr))
if (n0(X, wOr))
if (n0(X, EOr))
if (n0(X, SOr))
if (n0(X, gOr))
if (n0(X, FOr))
if (n0(X, TOr))
ar = 1, B = 1, Br = 1, jr = 1;
else
var $r = OOr;
else
var $r = IOr;
else
var $r = AOr;
else
var $r = NOr;
else
var $r = COr;
else
var $r = POr;
else
var $r = DOr;
if (!jr)
var ne = $r;
} else
var ne = JOr;
else {
var Qr = Ee(X, XTr), pe = 0;
if (0 <= Qr)
if (0 < Qr)
if (n0(X, iOr))
if (n0(X, fOr))
if (n0(X, xOr))
if (n0(X, aOr))
if (n0(X, oOr))
if (n0(X, cOr))
if (n0(X, sOr))
ar = 1, B = 1, Br = 1, pe = 1;
else
var oe = vOr;
else
var oe = lOr;
else
var oe = bOr;
else
var oe = pOr;
else
var oe = mOr;
else
var oe = _Or;
else
var oe = yOr;
else
var oe = dOr;
else if (n0(X, YTr))
if (n0(X, VTr))
if (n0(X, zTr))
if (n0(X, KTr))
if (n0(X, WTr))
if (n0(X, JTr))
if (n0(X, $Tr))
ar = 1, B = 1, Br = 1, pe = 1;
else
var oe = ZTr;
else
var oe = QTr;
else
var oe = rOr;
else
var oe = eOr;
else
var oe = nOr;
else
var oe = tOr;
else
var oe = uOr;
if (!pe)
var ne = oe;
}
if (!Br)
var Sr = ne;
}
if (!B)
var rr = Sr;
}
if (!ar) {
var me = rr;
dr = 1;
}
} else {
var me = ECr;
dr = 1;
}
else {
var ae = Ee(X, iEr), ce = 0;
if (0 <= ae)
if (0 < ae) {
var ge = Ee(X, Sgr), H0 = 0;
if (0 <= ge)
if (0 < ge) {
var Fr = Ee(X, PFr), _ = 0;
if (0 <= Fr)
if (0 < Fr) {
var k = Ee(X, cTr), I = 0;
if (0 <= k)
if (0 < k)
if (n0(X, gTr))
if (n0(X, FTr))
if (n0(X, TTr))
if (n0(X, OTr))
if (n0(X, ITr))
if (n0(X, ATr))
ce = 1, H0 = 1, _ = 1, I = 1;
else
var U = NTr;
else
var U = CTr;
else
var U = PTr;
else
var U = DTr;
else
var U = LTr;
else
var U = RTr;
else
var U = jTr;
else if (n0(X, sTr))
if (n0(X, vTr))
if (n0(X, lTr))
if (n0(X, bTr))
if (n0(X, pTr))
if (n0(X, mTr))
if (n0(X, _Tr))
ce = 1, H0 = 1, _ = 1, I = 1;
else
var U = yTr;
else
var U = dTr;
else
var U = hTr;
else
var U = kTr;
else
var U = wTr;
else
var U = ETr;
else
var U = STr;
if (!I)
var Y = U;
} else
var Y = GTr;
else {
var y0 = Ee(X, DFr), D0 = 0;
if (0 <= y0)
if (0 < y0)
if (n0(X, WFr))
if (n0(X, JFr))
if (n0(X, $Fr))
if (n0(X, ZFr))
if (n0(X, QFr))
if (n0(X, rTr))
if (n0(X, eTr))
ce = 1, H0 = 1, _ = 1, D0 = 1;
else
var I0 = nTr;
else
var I0 = tTr;
else
var I0 = uTr;
else
var I0 = iTr;
else
var I0 = fTr;
else
var I0 = xTr;
else
var I0 = aTr;
else
var I0 = oTr;
else if (n0(X, LFr))
if (n0(X, RFr))
if (n0(X, jFr))
if (n0(X, GFr))
if (n0(X, MFr))
if (n0(X, BFr))
if (n0(X, qFr))
ce = 1, H0 = 1, _ = 1, D0 = 1;
else
var I0 = UFr;
else
var I0 = HFr;
else
var I0 = XFr;
else
var I0 = YFr;
else
var I0 = VFr;
else
var I0 = zFr;
else
var I0 = KFr;
if (!D0)
var Y = I0;
}
if (!_)
var D = Y;
} else
var D = MTr;
else {
var u0 = Ee(X, ggr), Y0 = 0;
if (0 <= u0)
if (0 < u0) {
var J0 = Ee(X, nFr), fr = 0;
if (0 <= J0)
if (0 < J0)
if (n0(X, _Fr))
if (n0(X, yFr))
if (n0(X, dFr))
if (n0(X, hFr))
if (n0(X, kFr))
if (n0(X, wFr))
if (n0(X, EFr))
ce = 1, H0 = 1, Y0 = 1, fr = 1;
else
var Q0 = SFr;
else
var Q0 = gFr;
else
var Q0 = FFr;
else
var Q0 = TFr;
else
var Q0 = OFr;
else
var Q0 = IFr;
else
var Q0 = AFr;
else
var Q0 = NFr;
else if (n0(X, tFr))
if (n0(X, uFr))
if (n0(X, iFr))
if (n0(X, fFr))
if (n0(X, xFr))
if (n0(X, aFr))
if (n0(X, oFr))
ce = 1, H0 = 1, Y0 = 1, fr = 1;
else
var Q0 = cFr;
else
var Q0 = sFr;
else
var Q0 = vFr;
else
var Q0 = lFr;
else
var Q0 = bFr;
else
var Q0 = pFr;
else
var Q0 = mFr;
if (!fr)
var F0 = Q0;
} else
var F0 = CFr;
else {
var gr = Ee(X, Fgr), mr = 0;
if (0 <= gr)
if (0 < gr)
if (n0(X, qgr))
if (n0(X, Ugr))
if (n0(X, Hgr))
if (n0(X, Xgr))
if (n0(X, Ygr))
if (n0(X, Vgr))
if (n0(X, zgr))
ce = 1, H0 = 1, Y0 = 1, mr = 1;
else
var Cr = Kgr;
else
var Cr = Wgr;
else
var Cr = Jgr;
else
var Cr = $gr;
else
var Cr = Zgr;
else
var Cr = Qgr;
else
var Cr = rFr;
else
var Cr = eFr;
else if (n0(X, Tgr))
if (n0(X, Ogr))
if (n0(X, Igr))
if (n0(X, Agr))
if (n0(X, Ngr))
if (n0(X, Cgr))
if (n0(X, Pgr))
ce = 1, H0 = 1, Y0 = 1, mr = 1;
else
var Cr = Dgr;
else
var Cr = Lgr;
else
var Cr = Rgr;
else
var Cr = jgr;
else
var Cr = Ggr;
else
var Cr = Mgr;
else
var Cr = Bgr;
if (!mr)
var F0 = Cr;
}
if (!Y0)
var D = F0;
}
if (!H0)
var sr = D;
} else
var sr = BTr;
else {
var Pr = Ee(X, fEr), K0 = 0;
if (0 <= Pr)
if (0 < Pr) {
var Ur = Ee(X, pSr), d0 = 0;
if (0 <= Ur)
if (0 < Ur) {
var Kr = Ee(X, VSr), re = 0;
if (0 <= Kr)
if (0 < Kr)
if (n0(X, xgr))
if (n0(X, agr))
if (n0(X, ogr))
if (n0(X, cgr))
if (n0(X, sgr))
if (n0(X, vgr))
if (n0(X, lgr))
ce = 1, K0 = 1, d0 = 1, re = 1;
else
var xe = bgr;
else
var xe = pgr;
else
var xe = mgr;
else
var xe = _gr;
else
var xe = ygr;
else
var xe = dgr;
else
var xe = hgr;
else
var xe = kgr;
else if (n0(X, zSr))
if (n0(X, KSr))
if (n0(X, WSr))
if (n0(X, JSr))
if (n0(X, $Sr))
if (n0(X, ZSr))
if (n0(X, QSr))
ce = 1, K0 = 1, d0 = 1, re = 1;
else
var xe = rgr;
else
var xe = egr;
else
var xe = ngr;
else
var xe = tgr;
else
var xe = ugr;
else
var xe = igr;
else
var xe = fgr;
if (!re)
var je = xe;
} else
var je = wgr;
else {
var ve = Ee(X, mSr), Ie = 0;
if (0 <= ve)
if (0 < ve)
if (n0(X, NSr))
if (n0(X, CSr))
if (n0(X, PSr))
if (n0(X, DSr))
if (n0(X, LSr))
if (n0(X, RSr))
if (n0(X, jSr))
ce = 1, K0 = 1, d0 = 1, Ie = 1;
else
var Me = GSr;
else
var Me = MSr;
else
var Me = BSr;
else
var Me = qSr;
else
var Me = USr;
else
var Me = HSr;
else
var Me = XSr;
else
var Me = YSr;
else if (n0(X, _Sr))
if (n0(X, ySr))
if (n0(X, dSr))
if (n0(X, hSr))
if (n0(X, kSr))
if (n0(X, wSr))
if (n0(X, ESr))
ce = 1, K0 = 1, d0 = 1, Ie = 1;
else
var Me = SSr;
else
var Me = gSr;
else
var Me = FSr;
else
var Me = TSr;
else
var Me = OSr;
else
var Me = ISr;
else
var Me = ASr;
if (!Ie)
var je = Me;
}
if (!d0)
var Be = je;
} else
var Be = Egr;
else {
var fn = Ee(X, xEr), Ke = 0;
if (0 <= fn)
if (0 < fn) {
var Ae = Ee(X, GEr), xn = 0;
if (0 <= Ae)
if (0 < Ae)
if (n0(X, QEr))
if (n0(X, rSr))
if (n0(X, eSr))
if (n0(X, nSr))
if (n0(X, tSr))
if (n0(X, uSr))
if (n0(X, iSr))
ce = 1, K0 = 1, Ke = 1, xn = 1;
else
var Qe = fSr;
else
var Qe = xSr;
else
var Qe = aSr;
else
var Qe = oSr;
else
var Qe = cSr;
else
var Qe = sSr;
else
var Qe = vSr;
else
var Qe = lSr;
else if (n0(X, MEr))
if (n0(X, BEr))
if (n0(X, qEr))
if (n0(X, UEr))
if (n0(X, HEr))
if (n0(X, XEr))
if (n0(X, YEr))
ce = 1, K0 = 1, Ke = 1, xn = 1;
else
var Qe = VEr;
else
var Qe = zEr;
else
var Qe = KEr;
else
var Qe = WEr;
else
var Qe = JEr;
else
var Qe = $Er;
else
var Qe = ZEr;
if (!xn)
var yn = Qe;
} else
var yn = bSr;
else {
var on = Ee(X, aEr), Ce = 0;
if (0 <= on)
if (0 < on)
if (n0(X, EEr))
if (n0(X, SEr))
if (n0(X, gEr))
if (n0(X, FEr))
if (n0(X, TEr))
if (n0(X, OEr))
if (n0(X, IEr))
ce = 1, K0 = 1, Ke = 1, Ce = 1;
else
var We = AEr;
else
var We = NEr;
else
var We = CEr;
else
var We = PEr;
else
var We = DEr;
else
var We = LEr;
else
var We = REr;
else
var We = jEr;
else if (n0(X, oEr))
if (n0(X, cEr))
if (n0(X, sEr))
if (n0(X, vEr))
if (n0(X, lEr))
if (n0(X, bEr))
if (n0(X, pEr))
ce = 1, K0 = 1, Ke = 1, Ce = 1;
else
var We = mEr;
else
var We = _Er;
else
var We = yEr;
else
var We = dEr;
else
var We = hEr;
else
var We = kEr;
else
var We = wEr;
if (!Ce)
var yn = We;
}
if (!Ke)
var Be = yn;
}
if (!K0)
var sr = Be;
}
if (!ce) {
var me = sr;
dr = 1;
}
}
var rn = dr ? me : 0;
rn ? g1(e, rn[1]) : mn(e, Te(gCr, Te(X, SCr)));
continue;
default:
var bn = Se(x);
mn(i, bn), mn(e, bn);
continue;
}
}
}
function Jee(t, n4) {
En(n4);
var e = j(n4), i = tf < e ? pw < e ? -1 : Yk < e ? P3 < e ? Hw < e ? FE < e ? Zy < e ? 1 : 6 : Qh < e ? mE < e ? xF < e ? 1 : 6 : eO < e ? 1 : 6 : ZF < e ? _A < e ? 1 : 6 : bI < e ? 1 : 6 : P6 < e ? S4 < e ? a6 < e ? z8 < e ? G3 < e ? e3 < e ? LO < e ? 1 : 6 : GI < e ? 1 : 6 : Cy < e ? wg < e ? 1 : 6 : Uw < e ? 1 : 6 : R3 < e ? E8 < e ? Bg < e ? 1 : 6 : Z4 < e ? 1 : 6 : J8 < e ? JT < e ? 1 : 6 : Sk < e ? 1 : 6 : I4 < e ? KO < e ? b3 < e ? nw < e ? 1 : 6 : ok < e ? 1 : 6 : ap < e ? z6 < e ? 1 : 6 : Ah < e ? 1 : 6 : M8 < e ? v4 < e ? u6 < e ? 1 : 6 : U6 < e ? 1 : 6 : q6 < e ? g4 < e ? 1 : 6 : On < e ? 1 : 6 : GE < e ? B4 < e ? F6 < e ? TF < e ? f8 < e ? 1 : 6 : k8 < e ? 1 : 6 : T4 < e ? QF < e ? 1 : 6 : dd < e ? 1 : 6 : H6 < e ? t8 < e ? v3 < e ? 1 : 6 : g8 < e ? 1 : 6 : HS < e ? R8 < e ? 1 : 6 : W3 < e ? 1 : 6 : _8 < e ? x8 < e ? a8 < e ? E3 < e ? 1 : 6 : y6 < e ? 1 : 6 : v6 < e ? sF < e ? 1 : 6 : eI < e ? 1 : 6 : c3 < e ? jS < e ? n8 < e ? 1 : 6 : F8 < e ? 1 : 6 : $3 < e ? bF < e ? 1 : 6 : ag < e ? 1 : 6 : aw < e ? Fk < e ? gk < e ? Xk < e ? IT < e ? Wk < e ? 1 : 6 : xA < e ? 1 : 6 : Ey < e ? WS < e ? 1 : 6 : hh < e ? 1 : 6 : Ad < e ? yF < e ? IE < e ? 1 : 6 : eE < e ? 1 : 6 : Ty < e ? G_ < e ? 1 : 6 : LE < e ? 1 : 6 : GF < e ? Ww < e ? Uy < e ? tw < e ? 1 : 6 : zI < e ? 1 : 6 : BO < e ? cp < e ? 1 : 6 : BT < e ? 1 : 6 : Ut < e ? NA < e ? OA < e ? 1 : 6 : 1 : 6 : s6 < e ? E6 < e ? b6 < e ? w3 < e ? WE < e ? 1 : 6 : Xw < e ? 1 : 6 : l4 < e ? iE < e ? 1 : 6 : Ay < e ? 1 : 6 : s3 < e ? z4 < e ? ug < e ? 1 : 6 : XF < e ? 1 : 6 : Y4 < e ? hT < e ? 1 : 6 : Ny < e ? 1 : 6 : L6 < e ? L4 < e ? z3 < e ? US < e ? 1 : 6 : Zw < e ? 1 : 6 : ly < e ? By < e ? 1 : 6 : WT < e ? 1 : 6 : q4 < e ? _d < e ? QT < e ? 1 : 6 : M3 < e ? 1 : 6 : e6 < e ? bO < e ? 1 : 6 : eS < e ? 1 : 6 : xT < e ? Q4 < e ? Kh < e ? rp < e ? R4 < e ? C6 < e ? lp < e ? j_ < e ? 1 : 6 : Rh < e ? 1 : 6 : yh < e ? Mg < e ? 1 : 6 : NS < e ? 1 : 6 : M6 < e ? A3 < e ? xk < e ? 1 : 6 : lE < e ? 1 : 6 : b8 < e ? b4 < e ? 1 : 6 : td < e ? 1 : 6 : B3 < e ? IS < e ? DE < e ? vT < e ? 1 : 6 : ST < e ? 1 : 6 : xS < e ? k3 < e ? 1 : 6 : Jh < e ? 1 : 6 : my < e ? O6 < e ? Rg < e ? 1 : 6 : py < e ? 1 : 6 : GO < e ? kF < e ? 1 : 6 : KF < e ? 1 : 6 : jI < e ? kn < e ? BF < e ? Pd < e ? PO < e ? 1 : 6 : Lh < e ? 1 : 6 : Xd < e ? PS < e ? 1 : 6 : mA < e ? 1 : 6 : lO < e ? PI < e ? cI < e ? 1 : 6 : H_ < e ? 1 : 6 : wS < e ? oT < e ? 1 : 6 : dg < e ? 1 : 6 : DO < e ? Dw < e ? Kd < e ? mw < e ? 1 : 6 : nF < e ? 1 : 6 : Wg < e ? Gk < e ? 1 : 6 : XO < e ? 1 : 6 : aS < e ? p6 < e ? h6 < e ? 1 : 6 : rT < e ? 1 : 6 : yd < e ? tT < e ? 1 : 6 : VS < e ? 1 : 6 : JO < e ? Lk < e ? kS < e ? Cw < e ? LI < e ? $g < e ? 1 : 6 : WO < e ? 1 : 6 : Uk < e ? Ss < e ? 1 : 6 : RF < e ? 1 : 6 : Sh < e ? AS < e ? gI < e ? 1 : 6 : cg < e ? 1 : 6 : Mh < e ? Ed < e ? 1 : 6 : sI < e ? 1 : 6 : sT < e ? mg < e ? fF < e ? $I < e ? 1 : 6 : yk < e ? 1 : 6 : q_ < e ? Sw < e ? 1 : 6 : nT < e ? 1 : 6 : Bk < e ? IO < e ? dA < e ? 1 : 6 : hg < e ? 1 : 6 : ZE < e ? mF < e ? 1 : 6 : UF < e ? 1 : 6 : h4 < e ? h3 < e ? pT < e ? BI < e ? dS < e ? 1 : 6 : lA < e ? 1 : 6 : jE < e ? Jk < e ? 1 : 6 : Gg < e ? 1 : 6 : PT < e ? j6 < e ? _k < e ? 1 : 6 : aT < e ? 1 : 6 : YS < e ? HT < e ? 1 : 6 : pE < e ? 1 : 6 : Jc < e ? Tk < e ? L3 < e ? zg < e ? 1 : 6 : oh < e ? 1 : 6 : CF < e ? mh < e ? 1 : 6 : iA < e ? 1 : 6 : HE < e ? J3 < e ? by < e ? 1 : 6 : SI < e ? 1 : 6 : WF < e ? oS < e ? 1 : 6 : D4 < e ? 1 : 6 : pS < e ? Bw < e ? ak < e ? wO < e ? aI < e ? _g < e ? Dh < e ? 1 : 6 : i3 < e ? 1 : 6 : bE < e ? Gh < e ? 1 : 6 : zk < e ? 1 : 6 : ip < e ? wd < e ? B8 < e ? 1 : 6 : m3 < e ? 1 : 6 : Fw < e ? yE < e ? 1 : 6 : Hg < e ? 1 : 6 : _y < e ? ew < e ? rO < e ? JF < e ? 1 : 6 : Hh < e ? 1 : 6 : rw < e ? AI < e ? 1 : 6 : yO < e ? 1 : 6 : bS < e ? MO < e ? SA < e ? 1 : 6 : gA < e ? 1 : 6 : og < e ? XI < e ? 1 : 6 : ES < e ? 1 : 6 : wI < e ? DI < e ? iS < e ? zy < e ? Q_ < e ? 1 : 6 : _T < e ? 1 : 6 : rI < e ? Fg < e ? 1 : 6 : sA < e ? 1 : 6 : xE < e ? FT < e ? Eg < e ? 1 : 6 : AT < e ? 1 : 6 : Py < e ? i8 < e ? 1 : 6 : eg < e ? 1 : 6 : qd < e ? U_ < e ? TO < e ? md < e ? 1 : 6 : zE < e ? 1 : 6 : r6 < e ? Q6 < e ? 1 : 6 : Nh < e ? 1 : 6 : J6 < e ? MF < e ? Xy < e ? 1 : 6 : Dk < e ? 1 : 6 : RE < e ? Ag < e ? 1 : 6 : NI < e ? 1 : 6 : N8 < e ? R_ < e ? P4 < e ? rF < e ? Yd < e ? ZS < e ? 1 : 6 : G6 < e ? 1 : 6 : hE < e ? oI < e ? 1 : 6 : Rw < e ? 1 : 6 : x3 < e ? c8 < e ? YF < e ? 1 : 6 : Gw < e ? 1 : 6 : dk < e ? vI < e ? 1 : 6 : ky < e ? 1 : 6 : I8 < e ? xI < e ? i6 < e ? Sy < e ? 1 : 6 : qI < e ? 1 : 6 : s8 < e ? Nw < e ? 1 : 6 : _E < e ? 1 : 6 : d3 < e ? xp < e ? uE < e ? 1 : 6 : A8 < e ? 1 : 6 : lw < e ? $F < e ? 1 : 6 : WI < e ? 1 : 6 : Jd < e ? nI < e ? h8 < e ? wy < e ? uw < e ? 1 : 6 : f3 < e ? 1 : 6 : yI < e ? EA < e ? 1 : 6 : Xh < e ? 1 : 6 : Sg < e ? aE < e ? AO < e ? 1 : 6 : Y6 < e ? 1 : 6 : Mw < e ? vw < e ? 1 : 6 : T3 < e ? 1 : 6 : Ck < e ? NF < e ? Gy < e ? _4 < e ? 1 : 6 : lh < e ? 1 : 6 : Jg < e ? Qk < e ? 1 : 6 : vS < e ? 1 : 6 : q3 < e ? CO < e ? ww < e ? 1 : 6 : dE < e ? 1 : 6 : rg < e ? nA < e ? 1 : 6 : IA < e ? 1 : 6 : sh < e ? $E < e ? Mk < e ? $6 < e ? Uh < e ? cF < e ? eF < e ? bd < e ? uT < e ? 1 : 6 : KI < e ? 1 : 6 : ME < e ? NT < e ? 1 : 6 : $8 < e ? 1 : 6 : CA < e ? EE < e ? XT < e ? 1 : 6 : wh < e ? 1 : 6 : Ch < e ? Ud < e ? 1 : 6 : hS < e ? 1 : 6 : FA < e ? RO < e ? Vg < e ? bg < e ? 1 : 6 : Yg < e ? 1 : 6 : zw < e ? fh < e ? 1 : 6 : jg < e ? 1 : 6 : Pg < e ? _S < e ? Zh < e ? 1 : 6 : Ig < e ? 1 : 6 : fA < e ? gT < e ? 1 : 6 : jk < e ? 1 : 6 : tS < e ? Q8 < e ? QI < e ? PE < e ? UE < e ? 1 : 6 : yA < e ? 1 : 6 : FO < e ? qy < e ? 1 : 6 : oA < e ? 1 : 6 : cA < e ? p4 < e ? hk < e ? 1 : 6 : sO < e ? 1 : 6 : gh < e ? E7 < e ? 1 : 6 : rk < e ? 1 : 6 : Y8 < e ? J_ < e ? Ih < e ? Rd < e ? 1 : 6 : ET < e ? 1 : 6 : jh < e ? Nk < e ? 1 : 6 : vd < e ? 1 : 6 : id < e ? vg < e ? rE < e ? 1 : 6 : ZT < e ? 1 : 6 : ng < e ? Iw < e ? 1 : 6 : GT < e ? 1 : 6 : Cd < e ? pk < e ? Qt < e ? m8 < e ? OT < e ? B6 < e ? 1 : 6 : Od < e ? 1 : 6 : $4 < e ? ny < e ? 1 : 6 : op < e ? 1 : 6 : pO < e ? qE < e ? ld < e ? 1 : 6 : X_ < e ? 1 : 6 : FS < e ? YT < e ? 1 : 6 : BS < e ? 1 : 6 : Yw < e ? fy < e ? bA < e ? Wd < e ? 1 : 6 : bT < e ? 1 : 6 : aF < e ? ek < e ? 1 : 6 : II < e ? 1 : 6 : cO < e ? fd < e ? ig < e ? 1 : 6 : _h < e ? 1 : 6 : aO < e ? hO < e ? 1 : 6 : uO < e ? 1 : 6 : CS < e ? JE < e ? oO < e ? lk < e ? cd < e ? 1 : 6 : xh < e ? 1 : 6 : QS < e ? hw < e ? 1 : 6 : Iy < e ? 1 : 6 : pA < e ? rS < e ? $T < e ? 1 : 6 : tI < e ? 1 : 6 : xy < e ? Aw < e ? 1 : 6 : 1 : iT < e ? Q3 < e ? uk < e ? 6 : rd < e ? 1 : 6 : qF < e ? nE < e ? 1 : 6 : bk < e ? 1 : 6 : pg < e ? iO < e ? fI < e ? 1 : 6 : qO < e ? 1 : 6 : My < e ? 1 : 6 : L8 < e ? Fh < e ? cw < e ? Nd < e ? tA < e ? 6 : rh < e ? fT < e ? 1 : 6 : pF < e ? 1 : 6 : nd < e ? JI < e ? ty < e ? 1 : 6 : kd < e ? 1 : 6 : VE < e ? Qd < e ? 1 : 6 : wF < e ? 1 : 6 : ep < e ? K4 < e ? g6 < e ? yy < e ? 1 : 6 : tg < e ? 1 : 6 : Z8 < e ? kO < e ? 1 : 6 : Hk < e ? 1 : 6 : Ok < e ? H8 < e ? FF < e ? 1 : 6 : gy < e ? 1 : 6 : sg < e ? Ak < e ? 1 : 6 : gE < e ? 1 : 6 : qw < e ? ry < e ? uy < e ? yS < e ? sE < e ? 1 : 6 : 1 : 6 : Ew < e ? 6 : r8 < e ? CT < e ? 1 : 6 : kg < e ? 1 : 6 : uI < e ? u3 < e ? qo < e ? La < e ? 1 : 2 : HI < e ? 1 : 6 : sw < e ? UT < e ? 1 : 6 : KS < e ? 1 : 6 : kI < e ? fS < e ? eh < e ? 1 : 6 : LT < e ? 1 : 6 : qT < e ? $w < e ? 1 : 6 : TI < e ? 1 : 6 : YI < e ? fk < e ? et < e ? j4 < e ? U3 < e ? ah < e ? 1 : 6 : hF < e ? 1 : 6 : K3 < e ? R6 < e ? 1 : 6 : od < e ? 1 : 6 : RT < e ? fp < e ? xw < e ? 1 : 6 : uS < e ? 1 : 6 : fO < e ? n3 < e ? 1 : 6 : sd < e ? 1 : 6 : Ek < e ? JS < e ? nh < e ? xg < e ? 1 : 6 : ay < e ? 1 : 6 : Zk < e ? $h < e ? 1 : 6 : AE < e ? 1 : 6 : Eh < e ? dy < e ? lF < e ? 1 : 6 : hA < e ? 1 : 6 : Dy < e ? 1 : 6 : zh < e ? X3 < e ? o3 < e ? Qw < e ? 1 : 6 : ov < e ? 6 : MT < e ? 1 : 6 : OF < e ? w6 < e ? DF < e ? 1 : 6 : Zd < e ? 1 : 6 : qk < e ? jd < e ? 1 : 6 : eA < e ? 1 : 6 : Wh < e ? lS < e ? mT < e ? lI < e ? 1 : 6 : 1 : jy < e ? 6 : Qy < e ? 1 : 6 : IF < e ? bh < e ? 1 : 6 : zT < e ? NO < e ? 1 : 6 : vO < e ? 1 : 6 : gd < e ? T8 < e ? Z_ < e ? xd < e ? SO < e ? uh < e ? FI < e ? Pw < e ? 1 : 6 : kA < e ? 1 : 6 : Hd < e ? oy < e ? 1 : 6 : vk < e ? 1 : 6 : LF < e ? AA < e ? bp < e ? 1 : 6 : 1 : 6 : kT < e ? Vh < e ? SS < e ? Jy < e ? 1 : 6 : gS < e ? 1 : 6 : l6 < e ? Vk < e ? 1 : 6 : SE < e ? 1 : 6 : Tw < e ? yw < e ? 1 : 6 : hd < e ? ZO < e ? 1 : 6 : w8 < e ? 1 : 6 : SF < e ? jO < e ? zF < e ? Oy < e ? Th < e ? 1 : 6 : uA < e ? 1 : 6 : ey < e ? QE < e ? 1 : 6 : vy < e ? 1 : 6 : $y < e ? y8 < e ? Yy < e ? 1 : 6 : t3 < e ? 1 : 6 : tE < e ? sy < e ? 1 : 6 : lg < e ? 1 : 6 : Ky < e ? TA < e ? cS < e ? tF < e ? 1 : 6 : zd < e ? 1 : 6 : dI < e ? mk < e ? 1 : 6 : xO < e ? 1 : 6 : RS < e && vA < e ? 1 : 6 : kk < e ? Md < e ? fg < e ? ik < e ? 6 : ed < e ? _I < e ? 1 : 6 : Ld < e ? 1 : 6 : cE < e ? sk < e ? W_ < e ? 1 : 6 : 1 : 6 : Ng < e ? YO < e && sS < e ? 1 : 6 : _w < e ? tO < e ? Gd < e ? 1 : 6 : 1 : EF < e ? 6 : 1 : Fd < e ? OE < e ? H3 < e ? 6 : fw < e ? 1 : 6 : EI < e ? nk < e ? gO < e ? 1 : 6 : M_ < e ? 1 : 6 : Td < e ? 1 : 6 : HF < e ? Og < e ? Ph < e ? 1 : 6 : zS < e ? 1 : 6 : $k < e ? KE < e ? 6 : ad < e ? 1 : 6 : Y_ < e ? kE < e ? 1 : 6 : ru < e ? 1 : 6 : qS < e ? BE < e ? EO < e ? U4 < e ? Fy < e ? S8 < e ? MI < e ? 1 : 6 : iF < e ? 1 : 6 : VF < e ? 1 : 6 : gw < e ? S3 < e ? le < e ? 1 : 6 : 1 : 6 : Cg < e ? qh < e ? z_ < e ? V_ < e ? 1 : 6 : 1 : 6 : Vw < e ? O3 < e ? ph < e ? 1 : 6 : oF < e ? 1 : 6 : gg < e ? 1 : 6 : K8 < e ? G8 < e ? Sa < e ? RI < e ? 6 : ea < e ? 1 : 2 : T6 < e ? Hy < e ? 1 : 6 : CE < e ? 1 : 6 : W8 < e ? K6 < e ? Lg < e ? 1 : 6 : lT < e ? 1 : 6 : o8 < e ? Zt < e ? 1 : 6 : Pk < e ? 1 : 6 : DS < e ? fE < e ? G4 < e ? Dg < e ? 1 : 6 : rA < e ? 1 : 6 : D6 < e ? AF < e ? 1 : 6 : m4 < e ? 1 : 6 : hI < e ? oE < e ? OI < e ? 1 : 6 : C3 < e ? 1 : 6 : M4 < e ? q8 < e ? 1 : 6 : Tg < e ? 1 : 6 : C8 < e ? nS < e ? vE < e ? Kk < e ? yT < e ? $_ < e ? 1 : 6 : gF < e ? 1 : 6 : YE < e ? 1 : 6 : th < e ? pI < e ? 6 : 1 : 6 : UO < e ? dw < e ? KT < e ? v8 < e ? 1 : 6 : PA < e ? 1 : 6 : Kw < e ? K_ < e ? 1 : 6 : 1 : 6 : qg < e ? QO < e ? Z3 < e ? vp < e ? D8 < e ? 1 : 6 : r3 < e ? 1 : 6 : hr < e ? u8 < e ? 1 : 6 : 1 : F4 < e ? $S < e ? 6 : _6 < e ? 1 : 6 : dF < e ? Ly < e ? 1 : 6 : j3 < e ? 1 : 6 : Rk < e ? VI < e ? nO < e ? a3 < e ? 1 : 6 : dO < e ? 1 : 6 : Ry < e ? I3 < e ? 1 : 6 : np < e ? 1 : 6 : ya < e ? oc < e ? cx < e ? 1 : 2 : aa < e ? 1 : 2 : Ju < e ? Uu < e ? 1 : 3 : Pa < e ? 1 : 2 : Vr(WLr, e + 1 | 0) - 1 | 0;
if (14 < i >>> 0)
var x = q(n4);
else
switch (i) {
case 0:
var x = 0;
break;
case 1:
var x = 14;
break;
case 2:
if (B0(n4, 2), Mc(j(n4)) === 0) {
for (; ; )
if (B0(n4, 2), Mc(j(n4)) !== 0) {
var x = q(n4);
break;
}
} else
var x = q(n4);
break;
case 3:
var x = 1;
break;
case 4:
B0(n4, 1);
var x = fi(j(n4)) === 0 ? 1 : q(n4);
break;
case 5:
var x = 12;
break;
case 6:
var x = 13;
break;
case 7:
var x = 10;
break;
case 8:
B0(n4, 6);
var c = k9(j(n4)), x = c === 0 ? 4 : c === 1 ? 3 : q(n4);
break;
case 9:
var x = 9;
break;
case 10:
var x = 5;
break;
case 11:
var x = 11;
break;
case 12:
var x = 7;
break;
case 13:
if (B0(n4, 14), Gs(j(n4)) === 0) {
var s = R1(j(n4));
if (s === 0)
var x = Nn(j(n4)) === 0 && Nn(j(n4)) === 0 && Nn(j(n4)) === 0 ? 13 : q(n4);
else if (s === 1 && Nn(j(n4)) === 0)
for (; ; ) {
var p = N1(j(n4));
if (p !== 0) {
var x = p === 1 ? 13 : q(n4);
break;
}
}
else
var x = q(n4);
} else
var x = q(n4);
break;
default:
var x = 8;
}
if (14 < x >>> 0)
return ke(sPr);
switch (x) {
case 0:
return [0, t, Pn];
case 1:
return [2, d7(t, n4)];
case 2:
return [2, t];
case 3:
var y = Ru(t, n4), T = $n(zn), E = e2(t, T, n4), h = E[1];
return [1, h, Ei(h, y, E[2], T, 0)];
case 4:
var w = Ru(t, n4), G = $n(zn), A = j1(t, G, n4), S = A[1];
return [1, S, Ei(S, w, A[2], G, 1)];
case 5:
return [0, t, 98];
case 6:
return [0, t, R7];
case 7:
return [0, t, 99];
case 8:
return [0, t, 0];
case 9:
return [0, t, 86];
case 10:
return [0, t, 10];
case 11:
return [0, t, 82];
case 12:
var M = Se(n4), K = Ru(t, n4), V = $n(zn), f0 = $n(zn);
mn(f0, M);
var m0 = qn(M, vPr) ? 0 : 1, k0 = yL(t, m0, V, f0, n4), g0 = y7(k0, n4);
mn(f0, M);
var e0 = Gt(V), x0 = Gt(f0);
return [0, k0, [8, [0, k0[1], K, g0], e0, x0]];
case 13:
for (var l = n4[6]; ; ) {
En(n4);
var c0 = j(n4), t0 = In < c0 ? 1 : Vr(ERr, c0 + 1 | 0) - 1 | 0;
if (3 < t0 >>> 0)
var a0 = q(n4);
else
switch (t0) {
case 0:
var a0 = 1;
break;
case 1:
var a0 = 2;
break;
case 2:
var a0 = 0;
break;
default:
if (B0(n4, 2), Gs(j(n4)) === 0) {
var w0 = R1(j(n4));
if (w0 === 0)
if (Nn(j(n4)) === 0 && Nn(j(n4)) === 0)
var _0 = Nn(j(n4)) !== 0 ? 1 : 0, a0 = _0 && q(n4);
else
var a0 = q(n4);
else if (w0 === 1 && Nn(j(n4)) === 0)
for (; ; ) {
var E0 = N1(j(n4));
if (E0 !== 0) {
var X0 = E0 !== 1 ? 1 : 0, a0 = X0 && q(n4);
break;
}
}
else
var a0 = q(n4);
} else
var a0 = q(n4);
}
if (2 < a0 >>> 0)
throw [0, wn, Twr];
switch (a0) {
case 0:
continue;
case 1:
break;
default:
if (iL(dr0(n4)))
continue;
kr0(n4, 1);
}
var b = n4[3];
fL(n4, l);
var G0 = Ll(n4), X = Hl(t, l, b);
return [0, t, [7, xL(G0), X]];
}
default:
return [0, t, [6, Se(n4)]];
}
}
function $ee(t, n4) {
En(n4);
var e = j(n4);
if (-1 < e)
if (tf < e)
if (Sa < e)
if (qo < e)
var i = e <= La ? 1 : 0, T = i && 1;
else
var x = e <= ea ? 1 : 0, T = x && 1;
else if (ya < e)
if (oc < e)
var c = e <= cx ? 1 : 0, T = c && 1;
else
var s = e <= aa ? 1 : 0, T = s && 1;
else if (Ju < e)
var p = e <= Uu ? 1 : 0, T = p && 2;
else
var y = e <= Pa ? 1 : 0, T = y && 1;
else
var T = Vr(yLr, e) - 1 | 0;
else
var T = -1;
if (5 < T >>> 0)
var E = q(n4);
else
switch (T) {
case 0:
var E = 5;
break;
case 1:
if (B0(n4, 1), Mc(j(n4)) === 0) {
for (; ; )
if (B0(n4, 1), Mc(j(n4)) !== 0) {
var E = q(n4);
break;
}
} else
var E = q(n4);
break;
case 2:
var E = 0;
break;
case 3:
B0(n4, 0);
var h = fi(j(n4)) !== 0 ? 1 : 0, E = h && q(n4);
break;
case 4:
B0(n4, 5);
var w = k9(j(n4)), E = w === 0 ? 3 : w === 1 ? 2 : q(n4);
break;
default:
var E = 4;
}
if (5 < E >>> 0)
return ke(aPr);
switch (E) {
case 0:
return [2, d7(t, n4)];
case 1:
return [2, t];
case 2:
var G = Ru(t, n4), A = $n(zn), S = e2(t, A, n4), M = S[1];
return [1, M, Ei(M, G, S[2], A, 0)];
case 3:
var K = Ru(t, n4), V = $n(zn), f0 = j1(t, V, n4), m0 = f0[1];
return [1, m0, Ei(m0, K, f0[2], V, 1)];
case 4:
var k0 = Ru(t, n4), g0 = $n(zn), e0 = $n(zn), x0 = $n(zn);
mn(x0, oPr);
var l = te0(t, g0, e0, x0, n4), c0 = l[1], t0 = y7(c0, n4), a0 = [0, c0[1], k0, t0], w0 = l[2], _0 = Gt(x0), E0 = Gt(e0);
return [0, c0, [3, [0, a0, [0, Gt(g0), E0, _0], w0]]];
default:
var X0 = wi(t, rt(t, n4));
return [0, X0, [3, [0, rt(X0, n4), cPr, 1]]];
}
}
function Zee(t, n4) {
function e(D) {
for (; ; )
if (B0(D, 29), _n(j(D)) !== 0)
return q(D);
}
function i(D) {
B0(D, 27);
var u0 = Mt(j(D));
if (u0 === 0) {
for (; ; )
if (B0(D, 25), _n(j(D)) !== 0)
return q(D);
}
return u0 === 1 ? e(D) : q(D);
}
function x(D) {
for (; ; )
if (B0(D, 23), _n(j(D)) !== 0)
return q(D);
}
function c(D) {
B0(D, 22);
var u0 = Mt(j(D));
if (u0 === 0) {
for (; ; )
if (B0(D, 21), _n(j(D)) !== 0)
return q(D);
}
return u0 === 1 ? x(D) : q(D);
}
function s(D) {
for (; ; )
if (B0(D, 23), _n(j(D)) !== 0)
return q(D);
}
function p(D) {
B0(D, 22);
var u0 = Mt(j(D));
if (u0 === 0) {
for (; ; )
if (B0(D, 21), _n(j(D)) !== 0)
return q(D);
}
return u0 === 1 ? s(D) : q(D);
}
function y(D) {
r:
for (; ; ) {
if (vn(j(D)) === 0)
for (; ; ) {
B0(D, 24);
var u0 = qc(j(D));
if (3 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return s(D);
case 1:
continue;
case 2:
continue r;
default:
return p(D);
}
}
return q(D);
}
}
function T(D) {
B0(D, 29);
var u0 = Hr0(j(D));
if (3 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return e(D);
case 1:
var Y0 = P1(j(D));
if (Y0 === 0)
for (; ; ) {
B0(D, 24);
var J0 = Qv(j(D));
if (2 < J0 >>> 0)
return q(D);
switch (J0) {
case 0:
return s(D);
case 1:
continue;
default:
return p(D);
}
}
if (Y0 === 1)
for (; ; ) {
B0(D, 24);
var fr = qc(j(D));
if (3 < fr >>> 0)
return q(D);
switch (fr) {
case 0:
return s(D);
case 1:
continue;
case 2:
return y(D);
default:
return p(D);
}
}
return q(D);
case 2:
for (; ; ) {
B0(D, 24);
var Q0 = Qv(j(D));
if (2 < Q0 >>> 0)
return q(D);
switch (Q0) {
case 0:
return x(D);
case 1:
continue;
default:
return c(D);
}
}
default:
for (; ; ) {
B0(D, 24);
var F0 = qc(j(D));
if (3 < F0 >>> 0)
return q(D);
switch (F0) {
case 0:
return x(D);
case 1:
continue;
case 2:
return y(D);
default:
return c(D);
}
}
}
}
function E(D) {
for (; ; ) {
B0(D, 30);
var u0 = Rs(j(D));
if (4 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return e(D);
case 1:
continue;
case 2:
return T(D);
case 3:
r:
for (; ; ) {
if (vn(j(D)) === 0)
for (; ; ) {
B0(D, 30);
var Y0 = Rs(j(D));
if (4 < Y0 >>> 0)
return q(D);
switch (Y0) {
case 0:
return e(D);
case 1:
continue;
case 2:
return T(D);
case 3:
continue r;
default:
return i(D);
}
}
return q(D);
}
default:
return i(D);
}
}
}
function h(D) {
return vn(j(D)) === 0 ? E(D) : q(D);
}
function w(D) {
for (; ; )
if (B0(D, 19), _n(j(D)) !== 0)
return q(D);
}
function G(D) {
for (; ; )
if (B0(D, 19), _n(j(D)) !== 0)
return q(D);
}
function A(D) {
B0(D, 29);
var u0 = Or0(j(D));
if (u0 === 0)
return e(D);
if (u0 === 1)
for (; ; ) {
B0(D, 20);
var Y0 = E9(j(D));
if (3 < Y0 >>> 0)
return q(D);
switch (Y0) {
case 0:
return G(D);
case 1:
continue;
case 2:
r:
for (; ; ) {
if (Nn(j(D)) === 0)
for (; ; ) {
B0(D, 20);
var J0 = E9(j(D));
if (3 < J0 >>> 0)
return q(D);
switch (J0) {
case 0:
return w(D);
case 1:
continue;
case 2:
continue r;
default:
B0(D, 18);
var fr = Mt(j(D));
if (fr === 0) {
for (; ; )
if (B0(D, 17), _n(j(D)) !== 0)
return q(D);
}
return fr === 1 ? w(D) : q(D);
}
}
return q(D);
}
default:
B0(D, 18);
var Q0 = Mt(j(D));
if (Q0 === 0) {
for (; ; )
if (B0(D, 17), _n(j(D)) !== 0)
return q(D);
}
return Q0 === 1 ? G(D) : q(D);
}
}
return q(D);
}
function S(D) {
for (; ; )
if (B0(D, 13), _n(j(D)) !== 0)
return q(D);
}
function M(D) {
for (; ; )
if (B0(D, 13), _n(j(D)) !== 0)
return q(D);
}
function K(D) {
B0(D, 29);
var u0 = Mr0(j(D));
if (u0 === 0)
return e(D);
if (u0 === 1)
for (; ; ) {
B0(D, 14);
var Y0 = h9(j(D));
if (3 < Y0 >>> 0)
return q(D);
switch (Y0) {
case 0:
return M(D);
case 1:
continue;
case 2:
r:
for (; ; ) {
if (Vu(j(D)) === 0)
for (; ; ) {
B0(D, 14);
var J0 = h9(j(D));
if (3 < J0 >>> 0)
return q(D);
switch (J0) {
case 0:
return S(D);
case 1:
continue;
case 2:
continue r;
default:
B0(D, 12);
var fr = Mt(j(D));
if (fr === 0) {
for (; ; )
if (B0(D, 11), _n(j(D)) !== 0)
return q(D);
}
return fr === 1 ? S(D) : q(D);
}
}
return q(D);
}
default:
B0(D, 12);
var Q0 = Mt(j(D));
if (Q0 === 0) {
for (; ; )
if (B0(D, 11), _n(j(D)) !== 0)
return q(D);
}
return Q0 === 1 ? M(D) : q(D);
}
}
return q(D);
}
function V(D) {
for (; ; )
if (B0(D, 9), _n(j(D)) !== 0)
return q(D);
}
function f0(D) {
for (; ; )
if (B0(D, 9), _n(j(D)) !== 0)
return q(D);
}
function m0(D) {
B0(D, 29);
var u0 = Gr0(j(D));
if (u0 === 0)
return e(D);
if (u0 === 1)
for (; ; ) {
B0(D, 10);
var Y0 = w9(j(D));
if (3 < Y0 >>> 0)
return q(D);
switch (Y0) {
case 0:
return f0(D);
case 1:
continue;
case 2:
r:
for (; ; ) {
if (Bc(j(D)) === 0)
for (; ; ) {
B0(D, 10);
var J0 = w9(j(D));
if (3 < J0 >>> 0)
return q(D);
switch (J0) {
case 0:
return V(D);
case 1:
continue;
case 2:
continue r;
default:
B0(D, 8);
var fr = Mt(j(D));
if (fr === 0) {
for (; ; )
if (B0(D, 7), _n(j(D)) !== 0)
return q(D);
}
return fr === 1 ? V(D) : q(D);
}
}
return q(D);
}
default:
B0(D, 8);
var Q0 = Mt(j(D));
if (Q0 === 0) {
for (; ; )
if (B0(D, 7), _n(j(D)) !== 0)
return q(D);
}
return Q0 === 1 ? f0(D) : q(D);
}
}
return q(D);
}
function k0(D) {
B0(D, 28);
var u0 = Mt(j(D));
if (u0 === 0) {
for (; ; )
if (B0(D, 26), _n(j(D)) !== 0)
return q(D);
}
return u0 === 1 ? e(D) : q(D);
}
function g0(D) {
B0(D, 30);
var u0 = Qv(j(D));
if (2 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return e(D);
case 1:
for (; ; ) {
B0(D, 30);
var Y0 = qc(j(D));
if (3 < Y0 >>> 0)
return q(D);
switch (Y0) {
case 0:
return e(D);
case 1:
continue;
case 2:
r:
for (; ; ) {
if (vn(j(D)) === 0)
for (; ; ) {
B0(D, 30);
var J0 = qc(j(D));
if (3 < J0 >>> 0)
return q(D);
switch (J0) {
case 0:
return e(D);
case 1:
continue;
case 2:
continue r;
default:
return i(D);
}
}
return q(D);
}
default:
return i(D);
}
}
default:
return i(D);
}
}
function e0(D) {
for (; ; ) {
B0(D, 30);
var u0 = i9(j(D));
if (3 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return e(D);
case 1:
return g0(D);
case 2:
continue;
default:
return k0(D);
}
}
}
function x0(D) {
for (; ; )
if (B0(D, 15), _n(j(D)) !== 0)
return q(D);
}
function l(D) {
B0(D, 15);
var u0 = Mt(j(D));
if (u0 === 0) {
for (; ; )
if (B0(D, 15), _n(j(D)) !== 0)
return q(D);
}
return u0 === 1 ? x0(D) : q(D);
}
function c0(D) {
for (; ; ) {
B0(D, 16);
var u0 = Xr0(j(D));
if (4 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return x0(D);
case 1:
return g0(D);
case 2:
continue;
case 3:
for (; ; ) {
B0(D, 15);
var Y0 = i9(j(D));
if (3 < Y0 >>> 0)
return q(D);
switch (Y0) {
case 0:
return x0(D);
case 1:
return g0(D);
case 2:
continue;
default:
return l(D);
}
}
default:
return l(D);
}
}
}
function t0(D) {
B0(D, 30);
var u0 = Pr0(j(D));
if (3 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return e(D);
case 1:
for (; ; ) {
B0(D, 30);
var Y0 = Rs(j(D));
if (4 < Y0 >>> 0)
return q(D);
switch (Y0) {
case 0:
return e(D);
case 1:
continue;
case 2:
return T(D);
case 3:
r:
for (; ; ) {
if (vn(j(D)) === 0)
for (; ; ) {
B0(D, 30);
var J0 = Rs(j(D));
if (4 < J0 >>> 0)
return q(D);
switch (J0) {
case 0:
return e(D);
case 1:
continue;
case 2:
return T(D);
case 3:
continue r;
default:
return i(D);
}
}
return q(D);
}
default:
return i(D);
}
}
case 2:
return T(D);
default:
return i(D);
}
}
function a0(D) {
B0(D, 30);
var u0 = mL(j(D));
if (8 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return e(D);
case 1:
return t0(D);
case 2:
return c0(D);
case 3:
return e0(D);
case 4:
return m0(D);
case 5:
return T(D);
case 6:
return K(D);
case 7:
return A(D);
default:
return k0(D);
}
}
function w0(D) {
r:
for (; ; ) {
if (vn(j(D)) === 0)
for (; ; ) {
B0(D, 30);
var u0 = qr0(j(D));
if (4 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return e(D);
case 1:
return g0(D);
case 2:
continue;
case 3:
continue r;
default:
return k0(D);
}
}
return q(D);
}
}
function _0(D) {
for (; ; ) {
B0(D, 30);
var u0 = o9(j(D));
if (5 < u0 >>> 0)
return q(D);
switch (u0) {
case 0:
return e(D);
case 1:
return t0(D);
case 2:
continue;
case 3:
return T(D);
case 4:
return w0(D);
default:
return k0(D);
}
}
}
function E0(D) {
return B0(D, 3), zr0(j(D)) === 0 ? 3 : q(D);
}
function X0(D) {
return _9(j(D)) === 0 && l9(j(D)) === 0 && Yr0(j(D)) === 0 && Lr0(j(D)) === 0 && Rr0(j(D)) === 0 && pL(j(D)) === 0 && Bl(j(D)) === 0 && _9(j(D)) === 0 && Gs(j(D)) === 0 && jr0(j(D)) === 0 && Ul(j(D)) === 0 ? 3 : q(D);
}
En(n4);
var b = j(n4), G0 = tf < b ? pw < b ? -1 : Yk < b ? P3 < b ? Hw < b ? FE < b ? Zy < b ? 1 : 6 : Qh < b ? mE < b ? xF < b ? 1 : 6 : eO < b ? 1 : 6 : ZF < b ? _A < b ? 1 : 6 : bI < b ? 1 : 6 : P6 < b ? S4 < b ? a6 < b ? z8 < b ? G3 < b ? e3 < b ? LO < b ? 1 : 6 : GI < b ? 1 : 6 : Cy < b ? wg < b ? 1 : 6 : Uw < b ? 1 : 6 : R3 < b ? E8 < b ? Bg < b ? 1 : 6 : Z4 < b ? 1 : 6 : J8 < b ? JT < b ? 1 : 6 : Sk < b ? 1 : 6 : I4 < b ? KO < b ? b3 < b ? nw < b ? 1 : 6 : ok < b ? 1 : 6 : ap < b ? z6 < b ? 1 : 6 : Ah < b ? 1 : 6 : M8 < b ? v4 < b ? u6 < b ? 1 : 6 : U6 < b ? 1 : 6 : q6 < b ? g4 < b ? 1 : 6 : On < b ? 1 : 6 : GE < b ? B4 < b ? F6 < b ? TF < b ? f8 < b ? 1 : 6 : k8 < b ? 1 : 6 : T4 < b ? QF < b ? 1 : 6 : dd < b ? 1 : 6 : H6 < b ? t8 < b ? v3 < b ? 1 : 6 : g8 < b ? 1 : 6 : HS < b ? R8 < b ? 1 : 6 : W3 < b ? 1 : 6 : _8 < b ? x8 < b ? a8 < b ? E3 < b ? 1 : 6 : y6 < b ? 1 : 6 : v6 < b ? sF < b ? 1 : 6 : eI < b ? 1 : 6 : c3 < b ? jS < b ? n8 < b ? 1 : 6 : F8 < b ? 1 : 6 : $3 < b ? bF < b ? 1 : 6 : ag < b ? 1 : 6 : aw < b ? Fk < b ? gk < b ? Xk < b ? IT < b ? Wk < b ? 1 : 6 : xA < b ? 1 : 6 : Ey < b ? WS < b ? 1 : 6 : hh < b ? 1 : 6 : Ad < b ? yF < b ? IE < b ? 1 : 6 : eE < b ? 1 : 6 : Ty < b ? G_ < b ? 1 : 6 : LE < b ? 1 : 6 : GF < b ? Ww < b ? Uy < b ? tw < b ? 1 : 6 : zI < b ? 1 : 6 : BO < b ? cp < b ? 1 : 6 : BT < b ? 1 : 6 : Ut < b ? NA < b ? OA < b ? 1 : 6 : 1 : 6 : s6 < b ? E6 < b ? b6 < b ? w3 < b ? WE < b ? 1 : 6 : Xw < b ? 1 : 6 : l4 < b ? iE < b ? 1 : 6 : Ay < b ? 1 : 6 : s3 < b ? z4 < b ? ug < b ? 1 : 6 : XF < b ? 1 : 6 : Y4 < b ? hT < b ? 1 : 6 : Ny < b ? 1 : 6 : L6 < b ? L4 < b ? z3 < b ? US < b ? 1 : 6 : Zw < b ? 1 : 6 : ly < b ? By < b ? 1 : 6 : WT < b ? 1 : 6 : q4 < b ? _d < b ? QT < b ? 1 : 6 : M3 < b ? 1 : 6 : e6 < b ? bO < b ? 1 : 6 : eS < b ? 1 : 6 : xT < b ? Q4 < b ? Kh < b ? rp < b ? R4 < b ? C6 < b ? lp < b ? j_ < b ? 1 : 6 : Rh < b ? 1 : 6 : yh < b ? Mg < b ? 1 : 6 : NS < b ? 1 : 6 : M6 < b ? A3 < b ? xk < b ? 1 : 6 : lE < b ? 1 : 6 : b8 < b ? b4 < b ? 1 : 6 : td < b ? 1 : 6 : B3 < b ? IS < b ? DE < b ? vT < b ? 1 : 6 : ST < b ? 1 : 6 : xS < b ? k3 < b ? 1 : 6 : Jh < b ? 1 : 6 : my < b ? O6 < b ? Rg < b ? 1 : 6 : py < b ? 1 : 6 : GO < b ? kF < b ? 1 : 6 : KF < b ? 1 : 6 : jI < b ? kn < b ? BF < b ? Pd < b ? PO < b ? 1 : 6 : Lh < b ? 1 : 6 : Xd < b ? PS < b ? 1 : 6 : mA < b ? 1 : 6 : lO < b ? PI < b ? cI < b ? 1 : 6 : H_ < b ? 1 : 6 : wS < b ? oT < b ? 1 : 6 : dg < b ? 1 : 6 : DO < b ? Dw < b ? Kd < b ? mw < b ? 1 : 6 : nF < b ? 1 : 6 : Wg < b ? Gk < b ? 1 : 6 : XO < b ? 1 : 6 : aS < b ? p6 < b ? h6 < b ? 1 : 6 : rT < b ? 1 : 6 : yd < b ? tT < b ? 1 : 6 : VS < b ? 1 : 6 : JO < b ? Lk < b ? kS < b ? Cw < b ? LI < b ? $g < b ? 1 : 6 : WO < b ? 1 : 6 : Uk < b ? Ss < b ? 1 : 6 : RF < b ? 1 : 6 : Sh < b ? AS < b ? gI < b ? 1 : 6 : cg < b ? 1 : 6 : Mh < b ? Ed < b ? 1 : 6 : sI < b ? 1 : 6 : sT < b ? mg < b ? fF < b ? $I < b ? 1 : 6 : yk < b ? 1 : 6 : q_ < b ? Sw < b ? 1 : 6 : nT < b ? 1 : 6 : Bk < b ? IO < b ? dA < b ? 1 : 6 : hg < b ? 1 : 6 : ZE < b ? mF < b ? 1 : 6 : UF < b ? 1 : 6 : h4 < b ? h3 < b ? pT < b ? BI < b ? dS < b ? 1 : 6 : lA < b ? 1 : 6 : jE < b ? Jk < b ? 1 : 6 : Gg < b ? 1 : 6 : PT < b ? j6 < b ? _k < b ? 1 : 6 : aT < b ? 1 : 6 : YS < b ? HT < b ? 1 : 6 : pE < b ? 1 : 6 : Jc < b ? Tk < b ? L3 < b ? zg < b ? 1 : 6 : oh < b ? 1 : 6 : CF < b ? mh < b ? 1 : 6 : iA < b ? 1 : 6 : HE < b ? J3 < b ? by < b ? 1 : 6 : SI < b ? 1 : 6 : WF < b ? oS < b ? 1 : 6 : D4 < b ? 1 : 6 : pS < b ? Bw < b ? ak < b ? wO < b ? aI < b ? _g < b ? Dh < b ? 1 : 6 : i3 < b ? 1 : 6 : bE < b ? Gh < b ? 1 : 6 : zk < b ? 1 : 6 : ip < b ? wd < b ? B8 < b ? 1 : 6 : m3 < b ? 1 : 6 : Fw < b ? yE < b ? 1 : 6 : Hg < b ? 1 : 6 : _y < b ? ew < b ? rO < b ? JF < b ? 1 : 6 : Hh < b ? 1 : 6 : rw < b ? AI < b ? 1 : 6 : yO < b ? 1 : 6 : bS < b ? MO < b ? SA < b ? 1 : 6 : gA < b ? 1 : 6 : og < b ? XI < b ? 1 : 6 : ES < b ? 1 : 6 : wI < b ? DI < b ? iS < b ? zy < b ? Q_ < b ? 1 : 6 : _T < b ? 1 : 6 : rI < b ? Fg < b ? 1 : 6 : sA < b ? 1 : 6 : xE < b ? FT < b ? Eg < b ? 1 : 6 : AT < b ? 1 : 6 : Py < b ? i8 < b ? 1 : 6 : eg < b ? 1 : 6 : qd < b ? U_ < b ? TO < b ? md < b ? 1 : 6 : zE < b ? 1 : 6 : r6 < b ? Q6 < b ? 1 : 6 : Nh < b ? 1 : 6 : J6 < b ? MF < b ? Xy < b ? 1 : 6 : Dk < b ? 1 : 6 : RE < b ? Ag < b ? 1 : 6 : NI < b ? 1 : 6 : N8 < b ? R_ < b ? P4 < b ? rF < b ? Yd < b ? ZS < b ? 1 : 6 : G6 < b ? 1 : 6 : hE < b ? oI < b ? 1 : 6 : Rw < b ? 1 : 6 : x3 < b ? c8 < b ? YF < b ? 1 : 6 : Gw < b ? 1 : 6 : dk < b ? vI < b ? 1 : 6 : ky < b ? 1 : 6 : I8 < b ? xI < b ? i6 < b ? Sy < b ? 1 : 6 : qI < b ? 1 : 6 : s8 < b ? Nw < b ? 1 : 6 : _E < b ? 1 : 6 : d3 < b ? xp < b ? uE < b ? 1 : 6 : A8 < b ? 1 : 6 : lw < b ? $F < b ? 1 : 6 : WI < b ? 1 : 6 : Jd < b ? nI < b ? h8 < b ? wy < b ? uw < b ? 1 : 6 : f3 < b ? 1 : 6 : yI < b ? EA < b ? 1 : 6 : Xh < b ? 1 : 6 : Sg < b ? aE < b ? AO < b ? 1 : 6 : Y6 < b ? 1 : 6 : Mw < b ? vw < b ? 1 : 6 : T3 < b ? 1 : 6 : Ck < b ? NF < b ? Gy < b ? _4 < b ? 1 : 6 : lh < b ? 1 : 6 : Jg < b ? Qk < b ? 1 : 6 : vS < b ? 1 : 6 : q3 < b ? CO < b ? ww < b ? 1 : 6 : dE < b ? 1 : 6 : rg < b ? nA < b ? 1 : 6 : IA < b ? 1 : 6 : sh < b ? $E < b ? Mk < b ? $6 < b ? Uh < b ? cF < b ? eF < b ? bd < b ? uT < b ? 1 : 6 : KI < b ? 1 : 6 : ME < b ? NT < b ? 1 : 6 : $8 < b ? 1 : 6 : CA < b ? EE < b ? XT < b ? 1 : 6 : wh < b ? 1 : 6 : Ch < b ? Ud < b ? 1 : 6 : hS < b ? 1 : 6 : FA < b ? RO < b ? Vg < b ? bg < b ? 1 : 6 : Yg < b ? 1 : 6 : zw < b ? fh < b ? 1 : 6 : jg < b ? 1 : 6 : Pg < b ? _S < b ? Zh < b ? 1 : 6 : Ig < b ? 1 : 6 : fA < b ? gT < b ? 1 : 6 : jk < b ? 1 : 6 : tS < b ? Q8 < b ? QI < b ? PE < b ? UE < b ? 1 : 6 : yA < b ? 1 : 6 : FO < b ? qy < b ? 1 : 6 : oA < b ? 1 : 6 : cA < b ? p4 < b ? hk < b ? 1 : 6 : sO < b ? 1 : 6 : gh < b ? E7 < b ? 1 : 6 : rk < b ? 1 : 6 : Y8 < b ? J_ < b ? Ih < b ? Rd < b ? 1 : 6 : ET < b ? 1 : 6 : jh < b ? Nk < b ? 1 : 6 : vd < b ? 1 : 6 : id < b ? vg < b ? rE < b ? 1 : 6 : ZT < b ? 1 : 6 : ng < b ? Iw < b ? 1 : 6 : GT < b ? 1 : 6 : Cd < b ? pk < b ? Qt < b ? m8 < b ? OT < b ? B6 < b ? 1 : 6 : Od < b ? 1 : 6 : $4 < b ? ny < b ? 1 : 6 : op < b ? 1 : 6 : pO < b ? qE < b ? ld < b ? 1 : 6 : X_ < b ? 1 : 6 : FS < b ? YT < b ? 1 : 6 : BS < b ? 1 : 6 : Yw < b ? fy < b ? bA < b ? Wd < b ? 1 : 6 : bT < b ? 1 : 6 : aF < b ? ek < b ? 1 : 6 : II < b ? 1 : 6 : cO < b ? fd < b ? ig < b ? 1 : 6 : _h < b ? 1 : 6 : aO < b ? hO < b ? 1 : 6 : uO < b ? 1 : 6 : CS < b ? JE < b ? oO < b ? lk < b ? cd < b ? 1 : 6 : xh < b ? 1 : 6 : QS < b ? hw < b ? 1 : 6 : Iy < b ? 1 : 6 : pA < b ? rS < b ? $T < b ? 1 : 6 : tI < b ? 1 : 6 : xy < b ? Aw < b ? 1 : 6 : 1 : iT < b ? Q3 < b ? uk < b ? 6 : rd < b ? 1 : 6 : qF < b ? nE < b ? 1 : 6 : bk < b ? 1 : 6 : pg < b ? iO < b ? fI < b ? 1 : 6 : qO < b ? 1 : 6 : My < b ? 1 : 6 : L8 < b ? Fh < b ? cw < b ? Nd < b ? tA < b ? 6 : rh < b ? fT < b ? 1 : 6 : pF < b ? 1 : 6 : nd < b ? JI < b ? ty < b ? 1 : 6 : kd < b ? 1 : 6 : VE < b ? Qd < b ? 1 : 6 : wF < b ? 1 : 6 : ep < b ? K4 < b ? g6 < b ? yy < b ? 1 : 6 : tg < b ? 1 : 6 : Z8 < b ? kO < b ? 1 : 6 : Hk < b ? 1 : 6 : Ok < b ? H8 < b ? FF < b ? 1 : 6 : gy < b ? 1 : 6 : sg < b ? Ak < b ? 1 : 6 : gE < b ? 1 : 6 : qw < b ? ry < b ? uy < b ? yS < b ? sE < b ? 1 : 6 : 1 : 6 : Ew < b ? 6 : r8 < b ? CT < b ? 1 : 6 : kg < b ? 1 : 6 : uI < b ? u3 < b ? qo < b ? La < b ? 1 : 2 : HI < b ? 1 : 6 : sw < b ? UT < b ? 1 : 6 : KS < b ? 1 : 6 : kI < b ? fS < b ? eh < b ? 1 : 6 : LT < b ? 1 : 6 : qT < b ? $w < b ? 1 : 6 : TI < b ? 1 : 6 : YI < b ? fk < b ? et < b ? j4 < b ? U3 < b ? ah < b ? 1 : 6 : hF < b ? 1 : 6 : K3 < b ? R6 < b ? 1 : 6 : od < b ? 1 : 6 : RT < b ? fp < b ? xw < b ? 1 : 6 : uS < b ? 1 : 6 : fO < b ? n3 < b ? 1 : 6 : sd < b ? 1 : 6 : Ek < b ? JS < b ? nh < b ? xg < b ? 1 : 6 : ay < b ? 1 : 6 : Zk < b ? $h < b ? 1 : 6 : AE < b ? 1 : 6 : Eh < b ? dy < b ? lF < b ? 1 : 6 : hA < b ? 1 : 6 : Dy < b ? 1 : 6 : zh < b ? X3 < b ? o3 < b ? Qw < b ? 1 : 6 : ov < b ? 6 : MT < b ? 1 : 6 : OF < b ? w6 < b ? DF < b ? 1 : 6 : Zd < b ? 1 : 6 : qk < b ? jd < b ? 1 : 6 : eA < b ? 1 : 6 : Wh < b ? lS < b ? mT < b ? lI < b ? 1 : 6 : 1 : jy < b ? 6 : Qy < b ? 1 : 6 : IF < b ? bh < b ? 1 : 6 : zT < b ? NO < b ? 1 : 6 : vO < b ? 1 : 6 : gd < b ? T8 < b ? Z_ < b ? xd < b ? SO < b ? uh < b ? FI < b ? Pw < b ? 1 : 6 : kA < b ? 1 : 6 : Hd < b ? oy < b ? 1 : 6 : vk < b ? 1 : 6 : LF < b ? AA < b ? bp < b ? 1 : 6 : 1 : 6 : kT < b ? Vh < b ? SS < b ? Jy < b ? 1 : 6 : gS < b ? 1 : 6 : l6 < b ? Vk < b ? 1 : 6 : SE < b ? 1 : 6 : Tw < b ? yw < b ? 1 : 6 : hd < b ? ZO < b ? 1 : 6 : w8 < b ? 1 : 6 : SF < b ? jO < b ? zF < b ? Oy < b ? Th < b ? 1 : 6 : uA < b ? 1 : 6 : ey < b ? QE < b ? 1 : 6 : vy < b ? 1 : 6 : $y < b ? y8 < b ? Yy < b ? 1 : 6 : t3 < b ? 1 : 6 : tE < b ? sy < b ? 1 : 6 : lg < b ? 1 : 6 : Ky < b ? TA < b ? cS < b ? tF < b ? 1 : 6 : zd < b ? 1 : 6 : dI < b ? mk < b ? 1 : 6 : xO < b ? 1 : 6 : RS < b && vA < b ? 1 : 6 : kk < b ? Md < b ? fg < b ? ik < b ? 6 : ed < b ? _I < b ? 1 : 6 : Ld < b ? 1 : 6 : cE < b ? sk < b ? W_ < b ? 1 : 6 : 1 : 6 : Ng < b ? YO < b && sS < b ? 1 : 6 : _w < b ? tO < b ? Gd < b ? 1 : 6 : 1 : EF < b ? 6 : 1 : Fd < b ? OE < b ? H3 < b ? 6 : fw < b ? 1 : 6 : EI < b ? nk < b ? gO < b ? 1 : 6 : M_ < b ? 1 : 6 : Td < b ? 1 : 6 : HF < b ? Og < b ? Ph < b ? 1 : 6 : zS < b ? 1 : 6 : $k < b ? KE < b ? 6 : ad < b ? 1 : 6 : Y_ < b ? kE < b ? 1 : 6 : ru < b ? 1 : 6 : qS < b ? BE < b ? EO < b ? U4 < b ? Fy < b ? S8 < b ? MI < b ? 1 : 6 : iF < b ? 1 : 6 : VF < b ? 1 : 6 : gw < b ? S3 < b ? le < b ? 1 : 6 : 1 : 6 : Cg < b ? qh < b ? z_ < b ? V_ < b ? 1 : 6 : 1 : 6 : Vw < b ? O3 < b ? ph < b ? 1 : 6 : oF < b ? 1 : 6 : gg < b ? 1 : 6 : K8 < b ? G8 < b ? Sa < b ? RI < b ? 6 : ea < b ? 1 : 2 : T6 < b ? Hy < b ? 1 : 6 : CE < b ? 1 : 6 : W8 < b ? K6 < b ? Lg < b ? 1 : 6 : lT < b ? 1 : 6 : o8 < b ? Zt < b ? 1 : 6 : Pk < b ? 1 : 6 : DS < b ? fE < b ? G4 < b ? Dg < b ? 1 : 6 : rA < b ? 1 : 6 : D6 < b ? AF < b ? 1 : 6 : m4 < b ? 1 : 6 : hI < b ? oE < b ? OI < b ? 1 : 6 : C3 < b ? 1 : 6 : M4 < b ? q8 < b ? 1 : 6 : Tg < b ? 1 : 6 : C8 < b ? nS < b ? vE < b ? Kk < b ? yT < b ? $_ < b ? 1 : 6 : gF < b ? 1 : 6 : YE < b ? 1 : 6 : th < b ? pI < b ? 6 : 1 : 6 : UO < b ? dw < b ? KT < b ? v8 < b ? 1 : 6 : PA < b ? 1 : 6 : Kw < b ? K_ < b ? 1 : 6 : 1 : 6 : qg < b ? QO < b ? Z3 < b ? vp < b ? D8 < b ? 1 : 6 : r3 < b ? 1 : 6 : hr < b ? u8 < b ? 1 : 6 : 1 : F4 < b ? $S < b ? 6 : _6 < b ? 1 : 6 : dF < b ? Ly < b ? 1 : 6 : j3 < b ? 1 : 6 : Rk < b ? VI < b ? nO < b ? a3 < b ? 1 : 6 : dO < b ? 1 : 6 : Ry < b ? I3 < b ? 1 : 6 : np < b ? 1 : 6 : ya < b ? oc < b ? cx < b ? 1 : 2 : aa < b ? 1 : 2 : Ju < b ? Uu < b ? 1 : 3 : Pa < b ? 1 : 2 : Vr(KLr, b + 1 | 0) - 1 | 0;
if (30 < G0 >>> 0)
var X = q(n4);
else
switch (G0) {
case 0:
var X = 62;
break;
case 1:
var X = 63;
break;
case 2:
if (B0(n4, 1), Mc(j(n4)) === 0) {
for (; ; )
if (B0(n4, 1), Mc(j(n4)) !== 0) {
var X = q(n4);
break;
}
} else
var X = q(n4);
break;
case 3:
var X = 0;
break;
case 4:
B0(n4, 0);
var s0 = fi(j(n4)) !== 0 ? 1 : 0, X = s0 && q(n4);
break;
case 5:
var X = 6;
break;
case 6:
var X = 61;
break;
case 7:
if (B0(n4, 63), Bl(j(n4)) === 0) {
var dr = j(n4), Ar = c7 < dr ? D7 < dr ? -1 : 0 : -1;
if (Ar === 0 && Ul(j(n4)) === 0 && Bl(j(n4)) === 0)
var ar = j(n4), W0 = Xt < ar ? Qc < ar ? -1 : 0 : -1, X = W0 === 0 && Ar0(j(n4)) === 0 ? 31 : q(n4);
else
var X = q(n4);
} else
var X = q(n4);
break;
case 8:
var X = 56;
break;
case 9:
var X = 38;
break;
case 10:
var X = 39;
break;
case 11:
B0(n4, 53);
var X = Vr0(j(n4)) === 0 ? 4 : q(n4);
break;
case 12:
var X = 59;
break;
case 13:
var X = 43;
break;
case 14:
B0(n4, 60);
var Lr = ql(j(n4));
if (3 < Lr >>> 0)
var X = q(n4);
else
switch (Lr) {
case 0:
for (; ; ) {
var Tr = ql(j(n4));
if (3 < Tr >>> 0)
var X = q(n4);
else
switch (Tr) {
case 0:
continue;
case 1:
var X = h(n4);
break;
case 2:
var X = a0(n4);
break;
default:
var X = _0(n4);
}
break;
}
break;
case 1:
var X = h(n4);
break;
case 2:
var X = a0(n4);
break;
default:
var X = _0(n4);
}
break;
case 15:
B0(n4, 41);
var Hr = L1(j(n4)), X = Hr === 0 ? lL(j(n4)) === 0 ? 40 : q(n4) : Hr === 1 ? E(n4) : q(n4);
break;
case 16:
B0(n4, 63);
var Or = k9(j(n4));
if (Or === 0) {
B0(n4, 2);
var xr = f9(j(n4));
if (2 < xr >>> 0)
var X = q(n4);
else
switch (xr) {
case 0:
for (; ; ) {
var Rr = f9(j(n4));
if (2 < Rr >>> 0)
var X = q(n4);
else
switch (Rr) {
case 0:
continue;
case 1:
var X = E0(n4);
break;
default:
var X = X0(n4);
}
break;
}
break;
case 1:
var X = E0(n4);
break;
default:
var X = X0(n4);
}
} else
var X = Or === 1 ? 5 : q(n4);
break;
case 17:
B0(n4, 30);
var Wr = mL(j(n4));
if (8 < Wr >>> 0)
var X = q(n4);
else
switch (Wr) {
case 0:
var X = e(n4);
break;
case 1:
var X = t0(n4);
break;
case 2:
var X = c0(n4);
break;
case 3:
var X = e0(n4);
break;
case 4:
var X = m0(n4);
break;
case 5:
var X = T(n4);
break;
case 6:
var X = K(n4);
break;
case 7:
var X = A(n4);
break;
default:
var X = k0(n4);
}
break;
case 18:
B0(n4, 30);
var Jr = o9(j(n4));
if (5 < Jr >>> 0)
var X = q(n4);
else
switch (Jr) {
case 0:
var X = e(n4);
break;
case 1:
var X = t0(n4);
break;
case 2:
var X = _0(n4);
break;
case 3:
var X = T(n4);
break;
case 4:
var X = w0(n4);
break;
default:
var X = k0(n4);
}
break;
case 19:
var X = 44;
break;
case 20:
var X = 42;
break;
case 21:
var X = 49;
break;
case 22:
B0(n4, 51);
var or = j(n4), _r = 61 < or ? 62 < or ? -1 : 0 : -1, X = _r === 0 ? 57 : q(n4);
break;
case 23:
var X = 50;
break;
case 24:
B0(n4, 46);
var X = lL(j(n4)) === 0 ? 45 : q(n4);
break;
case 25:
var X = 32;
break;
case 26:
if (B0(n4, 63), Gs(j(n4)) === 0) {
var Ir = R1(j(n4));
if (Ir === 0)
var X = Nn(j(n4)) === 0 && Nn(j(n4)) === 0 && Nn(j(n4)) === 0 ? 61 : q(n4);
else if (Ir === 1 && Nn(j(n4)) === 0)
for (; ; ) {
var fe = N1(j(n4));
if (fe !== 0) {
var X = fe === 1 ? 61 : q(n4);
break;
}
}
else
var X = q(n4);
} else
var X = q(n4);
break;
case 27:
var X = 33;
break;
case 28:
B0(n4, 34);
var v0 = j(n4), P = us < v0 ? X2 < v0 ? -1 : 0 : -1, X = P === 0 ? 36 : q(n4);
break;
case 29:
B0(n4, 55);
var L = j(n4), Q = X2 < L ? br < L ? -1 : 0 : -1, X = Q === 0 ? 37 : q(n4);
break;
default:
var X = 35;
}
if (63 < X >>> 0)
return ke(MCr);
var i0 = X;
if (32 <= i0)
switch (i0) {
case 34:
return [0, t, 0];
case 35:
return [0, t, 1];
case 36:
return [0, t, 2];
case 37:
return [0, t, 3];
case 38:
return [0, t, 4];
case 39:
return [0, t, 5];
case 40:
return [0, t, 12];
case 41:
return [0, t, 10];
case 42:
return [0, t, 8];
case 43:
return [0, t, 9];
case 45:
return [0, t, 83];
case 49:
return [0, t, 98];
case 50:
return [0, t, 99];
case 53:
return [0, t, Xt];
case 55:
return [0, t, 89];
case 56:
return [0, t, 91];
case 57:
return [0, t, 11];
case 59:
return [0, t, c7];
case 60:
return [0, t, D7];
case 61:
var l0 = n4[6];
Kr0(n4);
var S0 = Hl(t, l0, n4[3]);
fL(n4, l0);
var T0 = Ll(n4), rr = re0(t, T0), R0 = rr[2], B = rr[1], Z = Ee(R0, HCr);
if (0 <= Z) {
if (!(0 < Z))
return [0, B, Av];
if (!n0(R0, rPr))
return [0, B, 29];
if (!n0(R0, ePr))
return [0, B, A2];
if (!n0(R0, nPr))
return [0, B, 42];
if (!n0(R0, tPr))
return [0, B, Sv];
if (!n0(R0, uPr))
return [0, B, tl];
if (!n0(R0, iPr))
return [0, B, 31];
if (!n0(R0, fPr))
return [0, B, 46];
if (!n0(R0, xPr))
return [0, B, fc];
} else {
if (!n0(R0, XCr))
return [0, B, u1];
if (!n0(R0, YCr))
return [0, B, z2];
if (!n0(R0, VCr))
return [0, B, QCr];
if (!n0(R0, zCr))
return [0, B, ZCr];
if (!n0(R0, KCr))
return [0, B, x1];
if (!n0(R0, WCr))
return [0, B, 41];
if (!n0(R0, JCr))
return [0, B, 30];
if (!n0(R0, $Cr))
return [0, B, 53];
}
return [0, B, [4, S0, R0, xL(T0)]];
case 62:
var p0 = t[4] ? ju(t, rt(t, n4), 6) : t;
return [0, p0, Pn];
case 63:
return [0, t, [6, Se(n4)]];
case 32:
case 47:
return [0, t, 6];
case 33:
case 48:
return [0, t, 7];
case 44:
case 54:
return [0, t, 86];
case 46:
case 52:
return [0, t, 85];
default:
return [0, t, 82];
}
switch (i0) {
case 0:
return [2, d7(t, n4)];
case 1:
return [2, t];
case 2:
var b0 = Ru(t, n4), O0 = $n(zn), q0 = j1(t, O0, n4), er = q0[1];
return [1, er, Ei(er, b0, q0[2], O0, 1)];
case 3:
var yr = Se(n4);
if (t[5]) {
var vr = t[4] ? Jr0(t, rt(t, n4), yr) : t, $0 = Zm(1, vr), Sr = $m(n4);
return qn(jl(n4, Sr - 1 | 0, 1), BCr) && n0(jl(n4, Sr - 2 | 0, 1), qCr) ? [0, $0, 86] : [2, $0];
}
var Mr = Ru(t, n4), Br = $n(zn);
mn(Br, yr);
var qr = j1(t, Br, n4), jr = qr[1];
return [1, jr, Ei(jr, Mr, qr[2], Br, 1)];
case 4:
if (t[4])
return [2, Zm(0, t)];
$v(n4), En(n4);
var $r = Cr0(j(n4)) !== 0 ? 1 : 0, ne = $r && q(n4);
return ne === 0 ? [0, t, Xt] : ke(UCr);
case 5:
var Qr = Ru(t, n4), pe = $n(zn), oe = e2(t, pe, n4), me = oe[1];
return [1, me, Ei(me, Qr, oe[2], pe, 0)];
case 6:
var ae = Se(n4), ce = Ru(t, n4), ge = $n(zn), H0 = $n(zn);
mn(H0, ae);
var Fr = ne0(t, ae, ge, H0, 0, n4), _ = Fr[1], k = [0, _[1], ce, Fr[2]], I = Fr[3], U = Gt(H0);
return [0, _, [2, [0, k, Gt(ge), U, I]]];
case 7:
return Dt(t, n4, function(D, u0) {
function Y0(F0) {
if (s9(j(F0)) === 0) {
if (Bc(j(F0)) === 0)
for (; ; ) {
var gr = t9(j(F0));
if (2 < gr >>> 0)
return q(F0);
switch (gr) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (Bc(j(F0)) === 0)
for (; ; ) {
var mr = t9(j(F0));
if (2 < mr >>> 0)
return q(F0);
switch (mr) {
case 0:
continue;
case 1:
continue r;
default:
return 0;
}
}
return q(F0);
}
default:
return 0;
}
}
return q(F0);
}
return q(F0);
}
En(u0);
var J0 = D1(j(u0));
if (J0 === 0)
for (; ; ) {
var fr = C1(j(u0));
if (fr !== 0) {
var Q0 = fr === 1 ? Y0(u0) : q(u0);
break;
}
}
else
var Q0 = J0 === 1 ? Y0(u0) : q(u0);
return Q0 === 0 ? [0, D, Hi(0, Se(u0))] : ke(GCr);
});
case 8:
return [0, t, Hi(0, Se(n4))];
case 9:
return Dt(t, n4, function(D, u0) {
function Y0(F0) {
if (s9(j(F0)) === 0) {
if (Bc(j(F0)) === 0)
for (; ; ) {
B0(F0, 0);
var gr = n9(j(F0));
if (gr !== 0) {
if (gr === 1)
r:
for (; ; ) {
if (Bc(j(F0)) === 0)
for (; ; ) {
B0(F0, 0);
var mr = n9(j(F0));
if (mr !== 0) {
if (mr === 1)
continue r;
return q(F0);
}
}
return q(F0);
}
return q(F0);
}
}
return q(F0);
}
return q(F0);
}
En(u0);
var J0 = D1(j(u0));
if (J0 === 0)
for (; ; ) {
var fr = C1(j(u0));
if (fr !== 0) {
var Q0 = fr === 1 ? Y0(u0) : q(u0);
break;
}
}
else
var Q0 = J0 === 1 ? Y0(u0) : q(u0);
return Q0 === 0 ? [0, D, Hc(0, Se(u0))] : ke(jCr);
});
case 10:
return [0, t, Hc(0, Se(n4))];
case 11:
return Dt(t, n4, function(D, u0) {
function Y0(F0) {
if (p9(j(F0)) === 0) {
if (Vu(j(F0)) === 0)
for (; ; ) {
var gr = c9(j(F0));
if (2 < gr >>> 0)
return q(F0);
switch (gr) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (Vu(j(F0)) === 0)
for (; ; ) {
var mr = c9(j(F0));
if (2 < mr >>> 0)
return q(F0);
switch (mr) {
case 0:
continue;
case 1:
continue r;
default:
return 0;
}
}
return q(F0);
}
default:
return 0;
}
}
return q(F0);
}
return q(F0);
}
En(u0);
var J0 = D1(j(u0));
if (J0 === 0)
for (; ; ) {
var fr = C1(j(u0));
if (fr !== 0) {
var Q0 = fr === 1 ? Y0(u0) : q(u0);
break;
}
}
else
var Q0 = J0 === 1 ? Y0(u0) : q(u0);
return Q0 === 0 ? [0, D, Hi(1, Se(u0))] : ke(RCr);
});
case 12:
return [0, t, Hi(1, Se(n4))];
case 13:
return Dt(t, n4, function(D, u0) {
function Y0(F0) {
if (p9(j(F0)) === 0) {
if (Vu(j(F0)) === 0)
for (; ; ) {
B0(F0, 0);
var gr = a9(j(F0));
if (gr !== 0) {
if (gr === 1)
r:
for (; ; ) {
if (Vu(j(F0)) === 0)
for (; ; ) {
B0(F0, 0);
var mr = a9(j(F0));
if (mr !== 0) {
if (mr === 1)
continue r;
return q(F0);
}
}
return q(F0);
}
return q(F0);
}
}
return q(F0);
}
return q(F0);
}
En(u0);
var J0 = D1(j(u0));
if (J0 === 0)
for (; ; ) {
var fr = C1(j(u0));
if (fr !== 0) {
var Q0 = fr === 1 ? Y0(u0) : q(u0);
break;
}
}
else
var Q0 = J0 === 1 ? Y0(u0) : q(u0);
return Q0 === 0 ? [0, D, Hc(3, Se(u0))] : ke(LCr);
});
case 14:
return [0, t, Hc(3, Se(n4))];
case 15:
return Dt(t, n4, function(D, u0) {
function Y0(F0) {
if (Vu(j(F0)) === 0) {
for (; ; )
if (B0(F0, 0), Vu(j(F0)) !== 0)
return q(F0);
}
return q(F0);
}
En(u0);
var J0 = D1(j(u0));
if (J0 === 0)
for (; ; ) {
var fr = C1(j(u0));
if (fr !== 0) {
var Q0 = fr === 1 ? Y0(u0) : q(u0);
break;
}
}
else
var Q0 = J0 === 1 ? Y0(u0) : q(u0);
return Q0 === 0 ? [0, D, Hc(1, Se(u0))] : ke(DCr);
});
case 16:
return [0, t, Hc(1, Se(n4))];
case 17:
return Dt(t, n4, function(D, u0) {
function Y0(F0) {
if (Qm(j(F0)) === 0) {
if (Nn(j(F0)) === 0)
for (; ; ) {
var gr = u9(j(F0));
if (2 < gr >>> 0)
return q(F0);
switch (gr) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (Nn(j(F0)) === 0)
for (; ; ) {
var mr = u9(j(F0));
if (2 < mr >>> 0)
return q(F0);
switch (mr) {
case 0:
continue;
case 1:
continue r;
default:
return 0;
}
}
return q(F0);
}
default:
return 0;
}
}
return q(F0);
}
return q(F0);
}
En(u0);
var J0 = D1(j(u0));
if (J0 === 0)
for (; ; ) {
var fr = C1(j(u0));
if (fr !== 0) {
var Q0 = fr === 1 ? Y0(u0) : q(u0);
break;
}
}
else
var Q0 = J0 === 1 ? Y0(u0) : q(u0);
return Q0 === 0 ? [0, D, Hi(2, Se(u0))] : ke(PCr);
});
case 19:
return Dt(t, n4, function(D, u0) {
function Y0(F0) {
if (Qm(j(F0)) === 0) {
if (Nn(j(F0)) === 0)
for (; ; ) {
B0(F0, 0);
var gr = y9(j(F0));
if (gr !== 0) {
if (gr === 1)
r:
for (; ; ) {
if (Nn(j(F0)) === 0)
for (; ; ) {
B0(F0, 0);
var mr = y9(j(F0));
if (mr !== 0) {
if (mr === 1)
continue r;
return q(F0);
}
}
return q(F0);
}
return q(F0);
}
}
return q(F0);
}
return q(F0);
}
En(u0);
var J0 = D1(j(u0));
if (J0 === 0)
for (; ; ) {
var fr = C1(j(u0));
if (fr !== 0) {
var Q0 = fr === 1 ? Y0(u0) : q(u0);
break;
}
}
else
var Q0 = J0 === 1 ? Y0(u0) : q(u0);
return Q0 === 0 ? [0, D, Hc(4, Se(u0))] : ke(CCr);
});
case 21:
return Dt(t, n4, function(D, u0) {
function Y0(d0) {
for (; ; ) {
var Kr = ki(j(d0));
if (2 < Kr >>> 0)
return q(d0);
switch (Kr) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (vn(j(d0)) === 0)
for (; ; ) {
var re = ki(j(d0));
if (2 < re >>> 0)
return q(d0);
switch (re) {
case 0:
continue;
case 1:
continue r;
default:
return 0;
}
}
return q(d0);
}
default:
return 0;
}
}
}
function J0(d0) {
for (; ; ) {
var Kr = r2(j(d0));
if (Kr !== 0) {
var re = Kr !== 1 ? 1 : 0;
return re && q(d0);
}
}
}
function fr(d0) {
var Kr = S9(j(d0));
if (2 < Kr >>> 0)
return q(d0);
switch (Kr) {
case 0:
var re = P1(j(d0));
return re === 0 ? J0(d0) : re === 1 ? Y0(d0) : q(d0);
case 1:
return J0(d0);
default:
return Y0(d0);
}
}
function Q0(d0) {
if (vn(j(d0)) === 0)
for (; ; ) {
var Kr = i7(j(d0));
if (2 < Kr >>> 0)
return q(d0);
switch (Kr) {
case 0:
continue;
case 1:
return fr(d0);
default:
r:
for (; ; ) {
if (vn(j(d0)) === 0)
for (; ; ) {
var re = i7(j(d0));
if (2 < re >>> 0)
return q(d0);
switch (re) {
case 0:
continue;
case 1:
return fr(d0);
default:
continue r;
}
}
return q(d0);
}
}
}
return q(d0);
}
function F0(d0) {
var Kr = m9(j(d0));
if (Kr === 0)
for (; ; ) {
var re = i7(j(d0));
if (2 < re >>> 0)
return q(d0);
switch (re) {
case 0:
continue;
case 1:
return fr(d0);
default:
r:
for (; ; ) {
if (vn(j(d0)) === 0)
for (; ; ) {
var xe = i7(j(d0));
if (2 < xe >>> 0)
return q(d0);
switch (xe) {
case 0:
continue;
case 1:
return fr(d0);
default:
continue r;
}
}
return q(d0);
}
}
}
return Kr === 1 ? fr(d0) : q(d0);
}
function gr(d0) {
var Kr = e9(j(d0));
return Kr === 0 ? F0(d0) : Kr === 1 ? fr(d0) : q(d0);
}
function mr(d0) {
for (; ; ) {
var Kr = b9(j(d0));
if (2 < Kr >>> 0)
return q(d0);
switch (Kr) {
case 0:
return F0(d0);
case 1:
continue;
default:
return fr(d0);
}
}
}
En(u0);
var Cr = x9(j(u0));
if (3 < Cr >>> 0)
var sr = q(u0);
else
switch (Cr) {
case 0:
for (; ; ) {
var Pr = ql(j(u0));
if (3 < Pr >>> 0)
var sr = q(u0);
else
switch (Pr) {
case 0:
continue;
case 1:
var sr = Q0(u0);
break;
case 2:
var sr = gr(u0);
break;
default:
var sr = mr(u0);
}
break;
}
break;
case 1:
var sr = Q0(u0);
break;
case 2:
var sr = gr(u0);
break;
default:
var sr = mr(u0);
}
if (sr === 0) {
var K0 = Se(u0), Ur = ju(D, rt(D, u0), 23);
return [0, Ur, Hi(2, K0)];
}
return ke(NCr);
});
case 22:
var Y = Se(n4), y0 = ju(t, rt(t, n4), 23);
return [0, y0, Hi(2, Y)];
case 23:
return Dt(t, n4, function(D, u0) {
function Y0(K0) {
for (; ; ) {
B0(K0, 0);
var Ur = js(j(K0));
if (Ur !== 0) {
if (Ur === 1)
r:
for (; ; ) {
if (vn(j(K0)) === 0)
for (; ; ) {
B0(K0, 0);
var d0 = js(j(K0));
if (d0 !== 0) {
if (d0 === 1)
continue r;
return q(K0);
}
}
return q(K0);
}
return q(K0);
}
}
}
function J0(K0) {
for (; ; )
if (B0(K0, 0), vn(j(K0)) !== 0)
return q(K0);
}
function fr(K0) {
var Ur = S9(j(K0));
if (2 < Ur >>> 0)
return q(K0);
switch (Ur) {
case 0:
var d0 = P1(j(K0));
return d0 === 0 ? J0(K0) : d0 === 1 ? Y0(K0) : q(K0);
case 1:
return J0(K0);
default:
return Y0(K0);
}
}
function Q0(K0) {
if (vn(j(K0)) === 0)
for (; ; ) {
var Ur = i7(j(K0));
if (2 < Ur >>> 0)
return q(K0);
switch (Ur) {
case 0:
continue;
case 1:
return fr(K0);
default:
r:
for (; ; ) {
if (vn(j(K0)) === 0)
for (; ; ) {
var d0 = i7(j(K0));
if (2 < d0 >>> 0)
return q(K0);
switch (d0) {
case 0:
continue;
case 1:
return fr(K0);
default:
continue r;
}
}
return q(K0);
}
}
}
return q(K0);
}
function F0(K0) {
var Ur = m9(j(K0));
if (Ur === 0)
for (; ; ) {
var d0 = i7(j(K0));
if (2 < d0 >>> 0)
return q(K0);
switch (d0) {
case 0:
continue;
case 1:
return fr(K0);
default:
r:
for (; ; ) {
if (vn(j(K0)) === 0)
for (; ; ) {
var Kr = i7(j(K0));
if (2 < Kr >>> 0)
return q(K0);
switch (Kr) {
case 0:
continue;
case 1:
return fr(K0);
default:
continue r;
}
}
return q(K0);
}
}
}
return Ur === 1 ? fr(K0) : q(K0);
}
function gr(K0) {
var Ur = e9(j(K0));
return Ur === 0 ? F0(K0) : Ur === 1 ? fr(K0) : q(K0);
}
function mr(K0) {
for (; ; ) {
var Ur = b9(j(K0));
if (2 < Ur >>> 0)
return q(K0);
switch (Ur) {
case 0:
return F0(K0);
case 1:
continue;
default:
return fr(K0);
}
}
}
En(u0);
var Cr = x9(j(u0));
if (3 < Cr >>> 0)
var sr = q(u0);
else
switch (Cr) {
case 0:
for (; ; ) {
var Pr = ql(j(u0));
if (3 < Pr >>> 0)
var sr = q(u0);
else
switch (Pr) {
case 0:
continue;
case 1:
var sr = Q0(u0);
break;
case 2:
var sr = gr(u0);
break;
default:
var sr = mr(u0);
}
break;
}
break;
case 1:
var sr = Q0(u0);
break;
case 2:
var sr = gr(u0);
break;
default:
var sr = mr(u0);
}
return sr === 0 ? [0, D, Hc(4, Se(u0))] : ke(ACr);
});
case 25:
return Dt(t, n4, function(D, u0) {
function Y0(K0) {
for (; ; ) {
var Ur = ki(j(K0));
if (2 < Ur >>> 0)
return q(K0);
switch (Ur) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (vn(j(K0)) === 0)
for (; ; ) {
var d0 = ki(j(K0));
if (2 < d0 >>> 0)
return q(K0);
switch (d0) {
case 0:
continue;
case 1:
continue r;
default:
return 0;
}
}
return q(K0);
}
default:
return 0;
}
}
}
function J0(K0) {
return vn(j(K0)) === 0 ? Y0(K0) : q(K0);
}
function fr(K0) {
var Ur = r2(j(K0));
if (Ur === 0)
return Y0(K0);
var d0 = Ur !== 1 ? 1 : 0;
return d0 && q(K0);
}
function Q0(K0) {
for (; ; ) {
var Ur = L1(j(K0));
if (Ur === 0)
return fr(K0);
if (Ur !== 1)
return q(K0);
}
}
function F0(K0) {
for (; ; ) {
var Ur = Uc(j(K0));
if (2 < Ur >>> 0)
return q(K0);
switch (Ur) {
case 0:
return fr(K0);
case 1:
continue;
default:
r:
for (; ; ) {
if (vn(j(K0)) === 0)
for (; ; ) {
var d0 = Uc(j(K0));
if (2 < d0 >>> 0)
return q(K0);
switch (d0) {
case 0:
return fr(K0);
case 1:
continue;
default:
continue r;
}
}
return q(K0);
}
}
}
}
En(u0);
var gr = x9(j(u0));
if (3 < gr >>> 0)
var mr = q(u0);
else
switch (gr) {
case 0:
for (; ; ) {
var Cr = ql(j(u0));
if (3 < Cr >>> 0)
var mr = q(u0);
else
switch (Cr) {
case 0:
continue;
case 1:
var mr = J0(u0);
break;
case 2:
var mr = Q0(u0);
break;
default:
var mr = F0(u0);
}
break;
}
break;
case 1:
var mr = J0(u0);
break;
case 2:
var mr = Q0(u0);
break;
default:
var mr = F0(u0);
}
if (mr === 0) {
var sr = Se(u0), Pr = ju(D, rt(D, u0), 22);
return [0, Pr, Hi(2, sr)];
}
return ke(ICr);
});
case 26:
return Dt(t, n4, function(D, u0) {
function Y0(mr) {
for (; ; ) {
var Cr = r2(j(mr));
if (Cr !== 0) {
var sr = Cr !== 1 ? 1 : 0;
return sr && q(mr);
}
}
}
function J0(mr) {
for (; ; ) {
var Cr = ki(j(mr));
if (2 < Cr >>> 0)
return q(mr);
switch (Cr) {
case 0:
continue;
case 1:
r:
for (; ; ) {
if (vn(j(mr)) === 0)
for (; ; ) {
var sr = ki(j(mr));
if (2 < sr >>> 0)
return q(mr);
switch (sr) {
case 0:
continue;
case 1:
continue r;
default:
return 0;
}
}
return q(mr);
}
default:
return 0;
}
}
}
En(u0);
var fr = j(u0), Q0 = 44 < fr ? 57 < fr ? -1 : Vr(iLr, fr + mv | 0) - 1 | 0 : -1;
if (2 < Q0 >>> 0)
var F0 = q(u0);
else
switch (Q0) {
case 0:
for (; ; ) {
var gr = Ur0(j(u0));
if (2 < gr >>> 0)
var F0 = q(u0);
else
switch (gr) {
case 0:
continue;
case 1:
var F0 = Y0(u0);
break;
default:
var F0 = J0(u0);
}
break;
}
break;
case 1:
var F0 = Y0(u0);
break;
default:
var F0 = J0(u0);
}
return F0 === 0 ? [0, D, Hi(2, Se(u0))] : ke(OCr);
});
case 27:
var D0 = Se(n4), I0 = ju(t, rt(t, n4), 22);
return [0, I0, Hi(2, D0)];
case 29:
return Dt(t, n4, function(D, u0) {
function Y0(re) {
for (; ; ) {
B0(re, 0);
var xe = js(j(re));
if (xe !== 0) {
if (xe === 1)
r:
for (; ; ) {
if (vn(j(re)) === 0)
for (; ; ) {
B0(re, 0);
var je = js(j(re));
if (je !== 0) {
if (je === 1)
continue r;
return q(re);
}
}
return q(re);
}
return q(re);
}
}
}
function J0(re) {
return B0(re, 0), vn(j(re)) === 0 ? Y0(re) : q(re);
}
En(u0);
var fr = x9(j(u0));
if (3 < fr >>> 0)
var Q0 = q(u0);
else
switch (fr) {
case 0:
for (; ; ) {
var F0 = Ur0(j(u0));
if (2 < F0 >>> 0)
var Q0 = q(u0);
else
switch (F0) {
case 0:
continue;
case 1:
for (; ; ) {
B0(u0, 0);
var gr = L1(j(u0)), mr = gr !== 0 ? 1 : 0;
if (mr) {
if (gr === 1)
continue;
var Q0 = q(u0);
} else
var Q0 = mr;
break;
}
break;
default:
for (; ; ) {
B0(u0, 0);
var Cr = Uc(j(u0));
if (2 < Cr >>> 0)
var Q0 = q(u0);
else
switch (Cr) {
case 0:
var Q0 = 0;
break;
case 1:
continue;
default:
r:
for (; ; ) {
if (vn(j(u0)) === 0)
for (; ; ) {
B0(u0, 0);
var sr = Uc(j(u0));
if (2 < sr >>> 0)
var Pr = q(u0);
else
switch (sr) {
case 0:
var Pr = 0;
break;
case 1:
continue;
default:
continue r;
}
break;
}
else
var Pr = q(u0);
var Q0 = Pr;
break;
}
}
break;
}
}
break;
}
break;
case 1:
var Q0 = vn(j(u0)) === 0 ? Y0(u0) : q(u0);
break;
case 2:
for (; ; ) {
B0(u0, 0);
var K0 = L1(j(u0));
if (K0 === 0)
var Q0 = J0(u0);
else {
if (K0 === 1)
continue;
var Q0 = q(u0);
}
break;
}
break;
default:
for (; ; ) {
B0(u0, 0);
var Ur = Uc(j(u0));
if (2 < Ur >>> 0)
var Q0 = q(u0);
else
switch (Ur) {
case 0:
var Q0 = J0(u0);
break;
case 1:
continue;
default:
r:
for (; ; ) {
if (vn(j(u0)) === 0)
for (; ; ) {
B0(u0, 0);
var d0 = Uc(j(u0));
if (2 < d0 >>> 0)
var Kr = q(u0);
else
switch (d0) {
case 0:
var Kr = J0(u0);
break;
case 1:
continue;
default:
continue r;
}
break;
}
else
var Kr = q(u0);
var Q0 = Kr;
break;
}
}
break;
}
}
return Q0 === 0 ? [0, D, Hc(4, Se(u0))] : ke(TCr);
});
case 31:
return [0, t, 66];
case 18:
case 28:
return [0, t, Hi(2, Se(n4))];
default:
return [0, t, Hc(4, Se(n4))];
}
}
function Xl(t) {
return function(n4) {
for (var e = 0, i = n4; ; ) {
var x = a(t, i, i[2]);
switch (x[0]) {
case 0:
var c = x[2], s = x[1], p = Wr0(s, c), y = e === 0 ? 0 : de(e), T = s[6];
if (T === 0)
return [0, [0, s[1], s[2], s[3], s[4], s[5], s[6], p], [0, c, p, 0, y]];
var E = [0, c, p, de(T), y];
return [0, [0, s[1], s[2], s[3], s[4], s[5], wr0, p], E];
case 1:
var h = x[2], w = x[1], e = [0, h, e], i = [0, w[1], w[2], w[3], w[4], w[5], w[6], h[1]];
continue;
default:
var i = x[1];
continue;
}
}
};
}
var Qee = Xl(Wee), rne = Xl(Jee), ene = Xl($ee), nne = Xl(Zee), tne = Xl(Kee), Gu = uL([0, dz]);
function Yl(t, n4) {
return [0, 0, 0, n4, Er0(t)];
}
function F9(t) {
var n4 = t[4];
switch (t[3]) {
case 0:
var c0 = u(tne, n4);
break;
case 1:
var c0 = u(nne, n4);
break;
case 2:
var c0 = u(rne, n4);
break;
case 3:
var e = y7(n4, n4[2]), i = $n(zn), x = $n(zn), c = n4[2];
En(c);
var s = j(c), p = us < s ? Uu < s ? 1 : Ju < s ? 2 : 1 : Vr(kRr, s + 1 | 0) - 1 | 0;
if (5 < p >>> 0)
var y = q(c);
else
switch (p) {
case 0:
var y = 1;
break;
case 1:
var y = 4;
break;
case 2:
var y = 0;
break;
case 3:
B0(c, 0);
var T = fi(j(c)) !== 0 ? 1 : 0, y = T && q(c);
break;
case 4:
var y = 2;
break;
default:
var y = 3;
}
if (4 < y >>> 0)
var E = ke(FCr);
else
switch (y) {
case 0:
var h = Se(c);
mn(x, h), mn(i, h);
var w = yL(d7(n4, c), 2, i, x, c), G = y7(w, c), A = Gt(i), S = Gt(x), E = [0, w, [8, [0, w[1], e, G], A, S]];
break;
case 1:
var E = [0, n4, Pn];
break;
case 2:
var E = [0, n4, 98];
break;
case 3:
var E = [0, n4, 0];
break;
default:
$v(c);
var M = yL(n4, 2, i, x, c), K = y7(M, c), V = Gt(i), f0 = Gt(x), E = [0, M, [8, [0, M[1], e, K], V, f0]];
}
var m0 = E[2], k0 = E[1], g0 = Wr0(k0, m0), e0 = k0[6];
if (e0 === 0)
var l = [0, k0, [0, m0, g0, 0, 0]];
else
var x0 = [0, m0, g0, de(e0), 0], l = [0, [0, k0[1], k0[2], k0[3], k0[4], k0[5], 0, k0[7]], x0];
var c0 = l;
break;
case 4:
var c0 = u(ene, n4);
break;
default:
var c0 = u(Qee, n4);
}
var t0 = c0[1], a0 = Er0(t0), w0 = [0, a0, c0[2]];
return t[4] = t0, t[1] ? t[2] = [0, w0] : t[1] = [0, w0], w0;
}
function ue0(t) {
var n4 = t[1];
return n4 ? n4[1][2] : F9(t)[2];
}
function une(t, n4, e, i) {
var x = t && t[1], c = n4 && n4[1];
try {
var s = 0, p = hr0(i), y = s, T = p;
} catch (A) {
if (A = Et(A), A !== A1)
throw A;
var E = [0, [0, [0, e, fz[2], fz[3]], 86], 0], y = E, T = hr0(iGr);
}
var h = c ? c[1] : Bv, w = zee(e, T, h[4]), G = [0, Yl(w, 0)];
return [0, [0, y], [0, 0], Gu[1], [0, 0], h[5], 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, [0, xGr], [0, w], G, [0, x], h, e, [0, 0], [0, fGr]];
}
function n2(t) {
return bl(t[23][1]);
}
function iu(t) {
return t[27][4];
}
function ue(t, n4) {
var e = n4[2];
t[1][1] = [0, [0, n4[1], e], t[1][1]];
var i = t[22];
return i && a(i[1], t, e);
}
function Vl(t, n4) {
return t[30][1] = n4, 0;
}
function Ms(t, n4) {
if (t === 0)
return ue0(n4[25][1]);
if (t === 1) {
var e = n4[25][1];
e[1] || F9(e);
var i = e[2];
return i ? i[1][2] : F9(e)[2];
}
throw [0, wn, nGr];
}
function ys(t, n4) {
return t === n4[5] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], t, n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], n4[15], n4[16], n4[17], n4[18], n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function dL(t, n4) {
return t === n4[17] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], n4[15], n4[16], t, n4[18], n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function ie0(t, n4) {
return t === n4[18] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], n4[15], n4[16], n4[17], t, n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function fe0(t, n4) {
return t === n4[19] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], n4[15], n4[16], n4[17], n4[18], t, n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function t2(t, n4) {
return t === n4[21] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], n4[15], n4[16], n4[17], n4[18], n4[19], n4[20], t, n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function T9(t, n4) {
return t === n4[14] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], t, n4[15], n4[16], n4[17], n4[18], n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function zl(t, n4) {
return t === n4[8] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], t, n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], n4[15], n4[16], n4[17], n4[18], n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function Kl(t, n4) {
return t === n4[12] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], t, n4[13], n4[14], n4[15], n4[16], n4[17], n4[18], n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function u2(t, n4) {
return t === n4[15] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], t, n4[16], n4[17], n4[18], n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function xe0(t, n4) {
return t === n4[6] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], t, n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], n4[15], n4[16], n4[17], n4[18], n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function ae0(t, n4) {
return t === n4[7] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], t, n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], n4[15], n4[16], n4[17], n4[18], n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function hL(t, n4) {
return t === n4[13] ? n4 : [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], t, n4[14], n4[15], n4[16], n4[17], n4[18], n4[19], n4[20], n4[21], n4[22], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function O9(t, n4) {
return [0, n4[1], n4[2], n4[3], n4[4], n4[5], n4[6], n4[7], n4[8], n4[9], n4[10], n4[11], n4[12], n4[13], n4[14], n4[15], n4[16], n4[17], n4[18], n4[19], n4[20], n4[21], [0, t], n4[23], n4[24], n4[25], n4[26], n4[27], n4[28], n4[29], n4[30]];
}
function kL(t) {
function n4(e) {
return ue(t, e);
}
return function(e) {
return Pu(n4, e);
};
}
function i2(t) {
var n4 = t[4][1], e = n4 && [0, n4[1][2]];
return e;
}
function oe0(t) {
var n4 = t[4][1], e = n4 && [0, n4[1][1]];
return e;
}
function ce0(t) {
return [0, t[1], t[2], t[3], t[4], t[5], t[6], t[7], t[8], t[9], t[10], t[11], t[12], t[13], t[14], t[15], t[16], t[17], t[18], t[19], t[20], t[21], 0, t[23], t[24], t[25], t[26], t[27], t[28], t[29], t[30]];
}
function se0(t, n4, e, i) {
return [0, t[1], t[2], Gu[1], t[4], t[5], 0, 0, 0, 0, 0, 1, t[12], t[13], t[14], t[15], t[16], e, n4, t[19], i, t[21], t[22], t[23], t[24], t[25], t[26], t[27], t[28], t[29], t[30]];
}
function ve0(t) {
var n4 = Ee(t, wjr), e = 0;
if (0 <= n4) {
if (0 < n4) {
var i = Ee(t, Bjr);
0 <= i ? 0 < i && n0(t, Wjr) && n0(t, Jjr) && n0(t, $jr) && n0(t, Zjr) && n0(t, Qjr) && n0(t, rGr) && n0(t, eGr) && (e = 1) : n0(t, qjr) && n0(t, Ujr) && n0(t, Hjr) && n0(t, Xjr) && n0(t, Yjr) && n0(t, Vjr) && n0(t, zjr) && n0(t, Kjr) && (e = 1);
}
} else {
var x = Ee(t, Ejr);
0 <= x ? 0 < x && n0(t, Cjr) && n0(t, Pjr) && n0(t, Djr) && n0(t, Ljr) && n0(t, Rjr) && n0(t, jjr) && n0(t, Gjr) && n0(t, Mjr) && (e = 1) : n0(t, Sjr) && n0(t, gjr) && n0(t, Fjr) && n0(t, Tjr) && n0(t, Ojr) && n0(t, Ijr) && n0(t, Ajr) && n0(t, Njr) && (e = 1);
}
return e ? 0 : 1;
}
function I9(t) {
return n0(t, kjr) ? 0 : 1;
}
function wL(t) {
if (typeof t == "number") {
if (t === 48)
return 1;
} else if (t[0] === 4 && I9(t[3]))
return 1;
return 0;
}
function f2(t) {
return n0(t, ljr) && n0(t, bjr) && n0(t, pjr) && n0(t, mjr) && n0(t, _jr) && n0(t, yjr) && n0(t, djr) && n0(t, hjr) ? 0 : 1;
}
function EL(t) {
if (typeof t == "number")
switch (t) {
case 42:
case 52:
case 53:
case 54:
case 55:
case 56:
case 57:
case 58:
return 1;
}
else if (t[0] === 4 && f2(t[3]))
return 1;
return 0;
}
function Bs(t) {
return n0(t, sjr) && n0(t, vjr) ? 0 : 1;
}
function le0(t) {
return typeof t != "number" && t[0] === 4 && Bs(t[3]) ? 1 : 0;
}
function SL(t) {
var n4 = ve0(t);
if (n4)
var e = n4;
else {
var i = I9(t);
if (i)
var e = i;
else {
if (n0(t, ajr) && n0(t, ojr) && n0(t, cjr))
return 0;
var e = 1;
}
}
return e;
}
function be0(t) {
var n4 = Ee(t, URr), e = 0;
return 0 <= n4 ? 0 < n4 && n0(t, $Rr) && n0(t, ZRr) && n0(t, QRr) && n0(t, rjr) && n0(t, ejr) && n0(t, njr) && n0(t, tjr) && n0(t, ujr) && (e = 1) : n0(t, HRr) && n0(t, XRr) && n0(t, YRr) && n0(t, VRr) && n0(t, zRr) && n0(t, KRr) && n0(t, WRr) && n0(t, JRr) && (e = 1), e ? 0 : 1;
}
function Yn(t, n4) {
return Ms(t, n4)[1];
}
function Wl(t, n4) {
return Ms(t, n4)[2];
}
function N0(t) {
return Yn(0, t);
}
function De(t) {
return Wl(0, t);
}
function G1(t) {
var n4 = i2(t), e = n4 ? n4[1] : ke(qRr);
return [0, e[1], e[3], e[3]];
}
function gL(t) {
return Ms(0, t)[3];
}
function pr(t) {
var n4 = Ms(0, t)[4];
return n4 && u(ml(function(e) {
return ms(t[30][1], e[1][2]) <= 0 ? 1 : 0;
}), n4);
}
function pe0(t) {
for (var n4 = Ms(0, t)[4]; ; ) {
if (n4) {
var e = n4[2], i = ms(n4[1][1][2], t[30][1]) < 0 ? 1 : 0;
if (i)
return i;
var n4 = e;
continue;
}
return 0;
}
}
function Jl(t, n4) {
var e = 0 < t ? [0, Wl(t - 1 | 0, n4)] : i2(n4);
if (e)
var i = e[1][2][1], x = i < Wl(t, n4)[2][1] ? 1 : 0;
else
var x = e;
return x;
}
function f7(t) {
return Jl(0, t);
}
function me0(t, n4) {
var e = Yn(t, n4);
if (typeof e == "number") {
var i = e - 2 | 0;
if (Ht < i >>> 0) {
if (!(F7 < (i + 1 | 0) >>> 0))
return 1;
} else {
var x = i !== 6 ? 1 : 0;
if (!x)
return x;
}
}
return Jl(t, n4);
}
function x2(t) {
return me0(0, t);
}
function A9(t, n4) {
var e = Yn(t, n4);
if (EL(e) || wL(e) || le0(e))
return 1;
var i = 0;
if (typeof e == "number")
switch (e) {
case 14:
case 28:
case 60:
case 61:
case 62:
case 63:
case 64:
case 65:
i = 1;
break;
}
else
e[0] === 4 && (i = 1);
return i ? 1 : 0;
}
function _e0(t, n4) {
var e = n2(n4);
if (e === 1) {
var i = Yn(t, n4);
return typeof i != "number" && i[0] === 4 ? 1 : 0;
}
if (e)
return 0;
var x = Yn(t, n4);
if (typeof x == "number")
switch (x) {
case 42:
case 46:
case 47:
return 0;
case 15:
case 16:
case 17:
case 18:
case 19:
case 20:
case 21:
case 22:
case 23:
case 24:
case 25:
case 26:
case 27:
case 28:
case 29:
case 30:
case 31:
case 32:
case 33:
case 34:
case 35:
case 36:
case 37:
case 38:
case 39:
case 40:
case 41:
case 43:
case 44:
case 45:
case 48:
case 49:
case 50:
case 51:
case 52:
case 53:
case 54:
case 55:
case 56:
case 57:
case 58:
case 59:
case 60:
case 61:
case 62:
case 63:
case 64:
case 65:
case 114:
case 115:
case 116:
case 117:
case 118:
case 119:
case 120:
case 121:
break;
default:
return 0;
}
else
switch (x[0]) {
case 4:
if (be0(x[3]))
return 0;
break;
case 9:
case 10:
case 11:
break;
default:
return 0;
}
return 1;
}
function M1(t) {
return A9(0, t);
}
function qs(t) {
var n4 = N0(t) === 15 ? 1 : 0;
if (n4)
var e = n4;
else {
var i = N0(t) === 64 ? 1 : 0;
if (i) {
var x = Yn(1, t) === 15 ? 1 : 0;
if (x)
var c = Wl(1, t)[2][1], e = De(t)[3][1] === c ? 1 : 0;
else
var e = x;
} else
var e = i;
}
return e;
}
function $l(t) {
var n4 = N0(t);
if (typeof n4 == "number") {
var e = 0;
if ((n4 === 13 || n4 === 40) && (e = 1), e)
return 1;
}
return 0;
}
function Ge(t, n4) {
return ue(t, [0, De(t), n4]);
}
function ye0(t, n4) {
if (wL(n4))
return 2;
if (EL(n4))
return 55;
var e = vL(0, n4);
return t ? [11, e, t[1]] : [10, e];
}
function St(t, n4) {
var e = gL(n4);
return u(kL(n4), e), Ge(n4, ye0(t, N0(n4)));
}
function N9(t) {
function n4(e) {
return ue(t, [0, e[1], 76]);
}
return function(e) {
return Pu(n4, e);
};
}
function de0(t, n4) {
var e = t[6] ? ir(Qn(MRr), n4, n4, n4) : BRr;
return St([0, e], t);
}
function Si(t, n4) {
var e = t[5];
return e && Ge(t, n4);
}
function Y7(t, n4) {
var e = t[5];
return e && ue(t, [0, n4[1], n4[2]]);
}
function B1(t, n4) {
return ue(t, [0, n4, [18, t[5]]]);
}
function ie(t) {
var n4 = t[26][1];
if (n4) {
var e = n2(t), i = N0(t), x = [0, De(t), i, e];
u(n4[1], x);
}
var c = t[25][1], s = c[1], p = s ? s[1][1] : F9(c)[1];
t[24][1] = p;
var y = gL(t);
u(kL(t), y);
var T = t[2][1], E = jc(Ms(0, t)[4], T);
t[2][1] = E;
var h = [0, Ms(0, t)];
t[4][1] = h;
var w = t[25][1];
return w[2] ? (w[1] = w[2], w[2] = 0, 0) : (ue0(w), w[1] = 0, 0);
}
function fu(t, n4) {
var e = a(aL, N0(t), n4);
return e && ie(t), e;
}
function zu(t, n4) {
t[23][1] = [0, n4, t[23][1]];
var e = n2(t), i = Yl(t[24][1], e);
return t[25][1] = i, 0;
}
function h7(t) {
var n4 = t[23][1], e = n4 ? n4[2] : ke(GRr);
t[23][1] = e;
var i = n2(t), x = Yl(t[24][1], i);
return t[25][1] = x, 0;
}
function we(t) {
var n4 = De(t);
if (N0(t) === 9 && Jl(1, t)) {
var e = pr(t), i = Ms(1, t)[4], x = un(e, u(ml(function(s) {
return s[1][2][1] <= n4[3][1] ? 1 : 0;
}), i));
return Vl(t, [0, n4[3][1] + 1 | 0, 0]), x;
}
var c = pr(t);
return Vl(t, n4[3]), c;
}
function Us(t) {
var n4 = t[4][1];
if (n4) {
var e = n4[1][2], i = pr(t), x = u(ml(function(p) {
return p[1][2][1] <= e[3][1] ? 1 : 0;
}), i);
Vl(t, [0, e[3][1] + 1 | 0, 0]);
var c = x;
} else
var c = n4;
return c;
}
function q1(t, n4) {
return St([0, vL(DRr, n4)], t);
}
function V0(t, n4) {
return 1 - a(aL, N0(t), n4) && q1(t, n4), ie(t);
}
function he0(t, n4) {
var e = fu(t, n4);
return 1 - e && q1(t, n4), e;
}
function Zl(t, n4) {
var e = N0(t), i = 0;
return typeof e != "number" && e[0] === 4 && qn(e[3], n4) && (i = 1), i || St([0, u(Qn(PRr), n4)], t), ie(t);
}
var Hs = [wt, aGr, G7(0)];
function ine(t) {
var n4 = t[26][1];
if (n4) {
var e = kz(0), i = [0, function(s) {
return vN(s, e);
}];
t[26][1] = i;
var x = [0, [0, n4[1], e]];
} else
var x = n4;
return [0, t[1][1], t[2][1], t[4][1], t[23][1], t[24][1], t[30][1], x];
}
function ke0(t, n4, e) {
if (e) {
var i = e[1], x = i[1];
if (n4[26][1] = [0, x], t)
for (var c = i[2][2]; ; ) {
if (c) {
var s = c[2];
u(x, c[1]);
var c = s;
continue;
}
return 0;
}
var p = t;
} else
var p = e;
return p;
}
function fne(t, n4) {
ke0(0, t, n4[7]), t[1][1] = n4[1], t[2][1] = n4[2], t[4][1] = n4[3], t[23][1] = n4[4], t[24][1] = n4[5], t[30][1] = n4[6];
var e = n2(t), i = Yl(t[24][1], e);
return t[25][1] = i, 0;
}
function xne(t, n4, e) {
return ke0(1, t, n4[7]), [0, e];
}
function FL(t, n4) {
var e = ine(t);
try {
var i = xne(t, e, u(n4, t));
return i;
} catch (x) {
if (x = Et(x), x === Hs)
return fne(t, e);
throw x;
}
}
function we0(t, n4, e) {
var i = FL(t, e);
return i ? i[1] : n4;
}
function Ql(t, n4) {
var e = de(n4);
if (e) {
var i = e[1], x = u(t, i);
return i === x ? n4 : de([0, x, e[2]]);
}
return n4;
}
var Ee0 = jp(lGr, function(t) {
var n4 = RN(t, cGr), e = DN(t, vGr), i = e[22], x = e[26], c = e[35], s = e[77], p = e[cV], y = e[OO], T = e[sp], E = e[HO], h = e[Bd], w = e[eT], G = e[6], A = e[7], S = e[10], M = e[17], K = e[21], V = e[27], f0 = e[33], m0 = e[36], k0 = e[46], g0 = e[51], e0 = e[89], x0 = e[92], l = e[97], c0 = e[99], t0 = e[ni], a0 = e[Pn], w0 = e[Sv], _0 = e[Jw], E0 = e[Qg], X0 = e[gH], b = e[MX], G0 = e[fH], X = e[NH], s0 = e[Sd], dr = e[PF], Ar = e[Zg], ar = e[N6], W0 = e[Lw], Lr = e[aA], Tr = e[tk], Hr = e[wT], Or = e[mO], xr = e[f6], Rr = e[TT], Wr = e[l8], Jr = e[$2], or = GN(t, 0, 0, xz, $D, 1)[1];
function _r(H0, Fr, _) {
var k = _[2], I = k[2], U = k[1], Y = _[1];
if (I) {
var y0 = function(D) {
return [0, Y, [0, U, [0, D]]];
}, D0 = I[1];
return ee(u(H0[1][1 + y], H0), D0, _, y0);
}
function I0(D) {
return [0, Y, [0, D, I]];
}
return ee(a(H0[1][1 + G], H0, Fr), U, _, I0);
}
function Ir(H0, Fr, _) {
var k = _[2], I = _[1], U = I[3], Y = I[2];
if (U)
var y0 = Ql(u(H0[1][1 + x], H0), U), D0 = Y;
else
var y0 = 0, D0 = a(H0[1][1 + x], H0, Y);
var I0 = a(H0[1][1 + c], H0, k);
return Y === D0 && U === y0 && k === I0 ? _ : [0, [0, I[1], D0, y0], I0];
}
function fe(H0, Fr) {
var _ = Fr[2], k = _[1], I = Fr[1];
function U(y0) {
return [0, I, [0, k, y0]];
}
var Y = _[2];
return ee(u(H0[1][1 + c], H0), Y, Fr, U);
}
function v0(H0, Fr, _) {
function k(U) {
return [0, _[1], _[2], U];
}
var I = _[3];
return ee(u(H0[1][1 + c], H0), I, _, k);
}
function P(H0, Fr) {
function _(I) {
return [0, Fr[1], I];
}
var k = Fr[2];
return ee(u(H0[1][1 + c], H0), k, Fr, _);
}
function L(H0, Fr, _) {
function k(U) {
return [0, _[1], _[2], U];
}
var I = _[3];
return ee(u(H0[1][1 + c], H0), I, _, k);
}
function Q(H0, Fr, _) {
var k = _[2], I = _[1], U = Ql(u(H0[1][1 + y], H0), I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, U, Y];
}
function i0(H0, Fr) {
var _ = Fr[2], k = _[1], I = Fr[1];
function U(y0) {
return [0, I, [0, k, y0]];
}
var Y = _[2];
return ee(u(H0[1][1 + c], H0), Y, Fr, U);
}
function l0(H0, Fr, _) {
function k(U) {
return [0, _[1], _[2], _[3], U];
}
var I = _[4];
return ee(u(H0[1][1 + c], H0), I, _, k);
}
function S0(H0, Fr, _) {
function k(U) {
return [0, _[1], U];
}
var I = _[2];
return ee(u(H0[1][1 + c], H0), I, _, k);
}
function T0(H0, Fr, _) {
var k = _[3], I = _[2], U = a(H0[1][1 + l], H0, I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, _[1], U, Y];
}
function rr(H0, Fr, _) {
var k = _[4], I = _[3], U = _[2], Y = _[1], y0 = a(H0[1][1 + c], H0, k);
if (I) {
var D0 = ze(u(H0[1][1 + w], H0), I);
return I === D0 && k === y0 ? _ : [0, _[1], _[2], D0, y0];
}
if (U) {
var I0 = ze(u(H0[1][1 + h], H0), U);
return U === I0 && k === y0 ? _ : [0, _[1], I0, _[3], y0];
}
var D = a(H0[1][1 + y], H0, Y);
return Y === D && k === y0 ? _ : [0, D, _[2], _[3], y0];
}
function R0(H0, Fr, _) {
var k = _[4], I = _[3], U = a(H0[1][1 + y], H0, I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, _[1], _[2], U, Y];
}
function B(H0, Fr, _) {
function k(U) {
return [0, _[1], _[2], _[3], U];
}
var I = _[4];
return ee(u(H0[1][1 + c], H0), I, _, k);
}
function Z(H0, Fr, _) {
function k(U) {
return [0, _[1], _[2], _[3], U];
}
var I = _[4];
return ee(u(H0[1][1 + c], H0), I, _, k);
}
function p0(H0, Fr, _) {
var k = _[2], I = _[1], U = I[3], Y = I[2];
if (U)
var y0 = Ql(u(H0[1][1 + x], H0), U), D0 = Y;
else
var y0 = 0, D0 = a(H0[1][1 + x], H0, Y);
var I0 = a(H0[1][1 + c], H0, k);
return Y === D0 && U === y0 && k === I0 ? _ : [0, [0, I[1], D0, y0], I0];
}
function b0(H0, Fr, _) {
var k = _[3], I = _[1], U = mu(u(H0[1][1 + s], H0), I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, U, _[2], Y];
}
function O0(H0, Fr, _) {
function k(U) {
return [0, _[1], U];
}
var I = _[2];
return ee(u(H0[1][1 + c], H0), I, _, k);
}
function q0(H0, Fr) {
if (Fr[0] === 0) {
var _ = function(D0) {
return [0, D0];
}, k = Fr[1];
return ee(u(H0[1][1 + p], H0), k, Fr, _);
}
var I = Fr[1], U = I[2], Y = U[2], y0 = a(H0[1][1 + p], H0, Y);
return Y === y0 ? Fr : [1, [0, I[1], [0, U[1], y0]]];
}
function er(H0, Fr, _) {
var k = _[4], I = _[3], U = a(H0[1][1 + x], H0, I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, _[1], _[2], U, Y];
}
function yr(H0, Fr) {
var _ = Fr[2], k = Fr[1];
function I(Y) {
return [0, k, [0, _[1], _[2], _[3], Y]];
}
var U = _[4];
return ee(u(H0[1][1 + c], H0), U, [0, k, _], I);
}
function vr(H0, Fr, _) {
var k = _[9], I = _[3], U = a(H0[1][1 + s0], H0, I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, _[1], _[2], U, _[4], _[5], _[6], _[7], _[8], Y, _[10]];
}
function $0(H0, Fr, _) {
var k = _[4], I = _[3], U = a(H0[1][1 + y], H0, I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, _[1], _[2], U, Y];
}
function Sr(H0, Fr) {
var _ = Fr[2], k = _[1], I = Fr[1];
function U(y0) {
return [0, I, [0, k, y0]];
}
var Y = _[2];
return ee(u(H0[1][1 + c], H0), Y, Fr, U);
}
function Mr(H0, Fr) {
var _ = Fr[2], k = _[2], I = _[1], U = Fr[1];
if (k === 0) {
var Y = function(I0) {
return [0, U, [0, I0, k]];
};
return ee(u(H0[1][1 + p], H0), I, Fr, Y);
}
function y0(I0) {
return [0, U, [0, I, I0]];
}
var D0 = u(H0[1][1 + i], H0);
return ee(function(I0) {
return ze(D0, I0);
}, k, Fr, y0);
}
function Br(H0, Fr) {
var _ = Fr[2], k = _[2], I = Fr[1];
function U(D0) {
return [0, I, [0, D0, k]];
}
var Y = _[1], y0 = u(H0[1][1 + T], H0);
return ee(function(D0) {
return Ql(y0, D0);
}, Y, Fr, U);
}
function qr(H0, Fr, _) {
var k = _[2];
if (k === 0) {
var I = function(D0) {
return [0, D0, _[2], _[3]];
}, U = _[1];
return ee(u(H0[1][1 + y], H0), U, _, I);
}
function Y(D0) {
return [0, _[1], D0, _[3]];
}
var y0 = u(H0[1][1 + i], H0);
return ee(function(D0) {
return ze(y0, D0);
}, k, _, Y);
}
function jr(H0, Fr) {
var _ = Fr[2], k = _[1], I = Fr[1];
function U(y0) {
return [0, I, [0, k, y0]];
}
var Y = _[2];
return ee(u(H0[1][1 + c], H0), Y, Fr, U);
}
function $r(H0, Fr, _) {
var k = _[7], I = _[2], U = a(H0[1][1 + E], H0, I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, _[1], U, _[3], _[4], _[5], _[6], Y];
}
function ne(H0, Fr) {
var _ = Fr[2], k = _[1], I = Fr[1];
function U(y0) {
return [0, I, [0, k, y0]];
}
var Y = _[2];
return ee(u(H0[1][1 + c], H0), Y, Fr, U);
}
function Qr(H0, Fr) {
var _ = Fr[2], k = _[1], I = Fr[1];
function U(y0) {
return [0, I, [0, k, y0]];
}
var Y = _[2];
return ee(u(H0[1][1 + c], H0), Y, Fr, U);
}
function pe(H0, Fr, _) {
var k = _[4], I = _[3], U = a(H0[1][1 + w], H0, I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, _[1], _[2], U, Y];
}
function oe(H0, Fr, _) {
function k(U) {
return [0, _[1], U];
}
var I = _[2];
return ee(u(H0[1][1 + c], H0), I, _, k);
}
function me(H0, Fr, _) {
var k = _[4], I = _[3], U = a(H0[1][1 + y], H0, I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, _[1], _[2], U, Y];
}
function ae(H0, Fr, _) {
var k = _[4], I = _[3], U = a(H0[1][1 + y], H0, I), Y = a(H0[1][1 + c], H0, k);
return I === U && k === Y ? _ : [0, _[1], _[2], U, Y];
}
function ce(H0, Fr) {
function _(I) {
return [0, Fr[1], I];
}
var k = Fr[2];
return ee(u(H0[1][1 + c], H0), k, Fr, _);
}
function ge(H0, Fr, _) {
function k(U) {
return [0, _[1], U];
}
var I = _[2];
return ee(u(H0[1][1 + c], H0), I, _, k);
}
return BN(t, [0, m0, function(H0, Fr) {
var _ = Fr[2], k = u(ml(function(U) {
return ms(U[1][2], H0[1 + n4]) < 0 ? 1 : 0;
}), _), I = Rc(k);
return Rc(_) === I ? Fr : [0, Fr[1], k, Fr[3]];
}, Jr, ge, Wr, ce, Rr, ae, xr, me, Or, oe, Hr, pe, w, Qr, h, ne, Tr, $r, E, jr, Lr, qr, W0, Br, T, Mr, ar, Sr, Ar, $0, dr, vr, X, yr, G0, er, b, q0, X0, O0, E0, b0, _0, p0, w0, Z, a0, B, t0, R0, x0, rr, c0, T0, e0, S0, s, l0, g0, i0, k0, Q, f0, L, V, P, K, v0, M, fe, S, Ir, A, _r]), function(H0, Fr, _) {
var k = Gp(Fr, t);
return k[1 + n4] = _, u(or, k), MN(Fr, k, t);
};
});
function C9(t) {
var n4 = i2(t);
if (n4)
var e = n4[1], i = pe0(t) ? (Vl(t, e[3]), [0, a(Ee0[1], 0, e[3])]) : 0, x = i;
else
var x = n4;
return [0, 0, function(c, s) {
return x ? a(s, x[1], c) : c;
}];
}
function rb(t) {
var n4 = i2(t);
if (n4) {
var e = n4[1];
if (pe0(t)) {
Vl(t, e[3]);
var i = Us(t), x = [0, a(Ee0[1], 0, [0, e[3][1] + 1 | 0, 0])], c = i;
} else
var x = 0, c = Us(t);
} else
var x = 0, c = 0;
return [0, c, function(s, p) {
return x ? a(p, x[1], s) : s;
}];
}
function Wt(t) {
return f7(t) ? rb(t) : C9(t);
}
function ds(t, n4) {
var e = Wt(t);
function i(x, c) {
return a(Ze(x, Nv, 27), x, c);
}
return a(e[2], n4, i);
}
function xi(t, n4) {
if (n4)
var e = Wt(t), i = function(c, s) {
return a(Ze(c, _F, 30), c, s);
}, x = [0, a(e[2], n4[1], i)];
else
var x = n4;
return x;
}
function a2(t, n4) {
var e = Wt(t);
function i(x, c) {
return a(Ze(x, -983660142, 32), x, c);
}
return a(e[2], n4, i);
}
function eb(t, n4) {
var e = Wt(t);
function i(x, c) {
return a(Ze(x, -455772979, 33), x, c);
}
return a(e[2], n4, i);
}
function Se0(t, n4) {
if (n4)
var e = Wt(t), i = function(c, s) {
return a(Ze(c, FH, 34), c, s);
}, x = [0, a(e[2], n4[1], i)];
else
var x = n4;
return x;
}
function Xi(t, n4) {
var e = Wt(t);
function i(x, c) {
return a(Ze(x, VY, 35), x, c);
}
return a(e[2], n4, i);
}
function ge0(t, n4) {
var e = Wt(t);
function i(x, c) {
var s = u(Ze(x, tH, 37), x);
return Ql(function(p) {
return mu(s, p);
}, c);
}
return a(e[2], n4, i);
}
function Fe0(t, n4) {
var e = Wt(t);
function i(x, c) {
return a(Ze(x, -21476009, 38), x, c);
}
return a(e[2], n4, i);
}
jp(bGr, function(t) {
var n4 = RN(t, oGr), e = jN(sGr), i = e.length - 1, x = az.length - 1, c = Gv(i + x | 0, 0), s = i - 1 | 0, p = 0;
if (!(s < 0))
for (var y = p; ; ) {
var T = Fl(t, nu(e, y)[1 + y]);
nu(c, y)[1 + y] = T;
var E = y + 1 | 0;
if (s !== y) {
var y = E;
continue;
}
break;
}
var h = x - 1 | 0, w = 0;
if (!(h < 0))
for (var G = w; ; ) {
var A = G + i | 0, S = RN(t, nu(az, G)[1 + G]);
nu(c, A)[1 + A] = S;
var M = G + 1 | 0;
if (h !== G) {
var G = M;
continue;
}
break;
}
var K = c[4], V = c[5], f0 = c[d6], m0 = c[sp], k0 = c[ih], g0 = c[gv], e0 = c[38], x0 = c[dT], l = c[Wy], c0 = GN(t, 0, 0, xz, $D, 1)[1];
function t0(b, G0, X) {
return a(b[1][1 + f0], b, X[2]), X;
}
function a0(b, G0) {
return a(b[1][1 + m0], b, G0), G0;
}
function w0(b, G0) {
var X = G0[1], s0 = b[1 + g0];
if (s0) {
var dr = ms(s0[1][1][2], X[2]) < 0 ? 1 : 0, Ar = dr && (b[1 + g0] = [0, G0], 0);
return Ar;
}
var ar = 0 <= ms(X[2], b[1 + n4][3]) ? 1 : 0, W0 = ar && (b[1 + g0] = [0, G0], 0);
return W0;
}
function _0(b, G0) {
var X = G0[1], s0 = b[1 + k0];
if (s0) {
var dr = ms(X[2], s0[1][1][2]) < 0 ? 1 : 0, Ar = dr && (b[1 + k0] = [0, G0], 0);
return Ar;
}
var ar = ms(X[2], b[1 + n4][2]) < 0 ? 1 : 0, W0 = ar && (b[1 + k0] = [0, G0], 0);
return W0;
}
function E0(b, G0) {
return G0 && a(b[1][1 + m0], b, G0[1]);
}
function X0(b, G0) {
var X = G0[1];
Pu(u(b[1][1 + V], b), X);
var s0 = G0[2];
return Pu(u(b[1][1 + K], b), s0);
}
return BN(t, [0, x0, function(b) {
return [0, b[1 + k0], b[1 + g0]];
}, m0, X0, f0, E0, V, _0, K, w0, e0, a0, l, t0]), function(b, G0, X) {
var s0 = Gp(G0, t);
return s0[1 + n4] = X, u(c0, s0), s0[1 + k0] = 0, s0[1 + g0] = 0, MN(G0, s0, t);
};
});
function Te0(t) {
return t === 3 ? 2 : (4 <= t, 1);
}
function TL(t, n4, e) {
if (e) {
var i = e[1], x = 0;
if (i === 8232 || Uu === i)
x = 1;
else if (i === 10)
var s = 6;
else if (i === 13)
var s = 5;
else if (ow <= i)
var s = 3;
else if (Vd <= i)
var s = 2;
else
var c = Rt <= i ? 1 : 0, s = c && 1;
if (x)
var s = 7;
var p = s;
} else
var p = 4;
return [0, p, t];
}
var ane = [wt, dGr, G7(0)];
function Oe0(t, n4, e, i) {
try {
var x = nu(t, n4)[1 + n4];
return x;
} catch (c) {
throw c = Et(c), c[1] === eN ? [0, ane, e, ir(Qn(_Gr), i, n4, t.length - 1)] : c;
}
}
function P9(t, n4) {
if (n4[1] === 0 && n4[2] === 0)
return 0;
var e = Oe0(t, n4[1] - 1 | 0, n4, pGr);
return Oe0(e, n4[2], n4, mGr);
}
var one = Ee;
function cne(t, n4) {
return a(f(t), VWr, n4);
}
u(uL([0, one])[33], cne);
function Ie0(t) {
var n4 = N0(t), e = 0;
if (typeof n4 == "number")
switch (n4) {
case 15:
var i = zWr;
break;
case 16:
var i = KWr;
break;
case 17:
var i = WWr;
break;
case 18:
var i = JWr;
break;
case 19:
var i = $Wr;
break;
case 20:
var i = ZWr;
break;
case 21:
var i = QWr;
break;
case 22:
var i = rJr;
break;
case 23:
var i = eJr;
break;
case 24:
var i = nJr;
break;
case 25:
var i = tJr;
break;
case 26:
var i = uJr;
break;
case 27:
var i = iJr;
break;
case 28:
var i = fJr;
break;
case 29:
var i = xJr;
break;
case 30:
var i = aJr;
break;
case 31:
var i = oJr;
break;
case 32:
var i = cJr;
break;
case 33:
var i = sJr;
break;
case 34:
var i = vJr;
break;
case 35:
var i = lJr;
break;
case 36:
var i = bJr;
break;
case 37:
var i = pJr;
break;
case 38:
var i = mJr;
break;
case 39:
var i = _Jr;
break;
case 40:
var i = yJr;
break;
case 41:
var i = dJr;
break;
case 42:
var i = hJr;
break;
case 43:
var i = kJr;
break;
case 44:
var i = wJr;
break;
case 45:
var i = EJr;
break;
case 46:
var i = SJr;
break;
case 47:
var i = gJr;
break;
case 48:
var i = FJr;
break;
case 49:
var i = TJr;
break;
case 50:
var i = OJr;
break;
case 51:
var i = IJr;
break;
case 52:
var i = AJr;
break;
case 53:
var i = NJr;
break;
case 54:
var i = CJr;
break;
case 55:
var i = PJr;
break;
case 56:
var i = DJr;
break;
case 57:
var i = LJr;
break;
case 58:
var i = RJr;
break;
case 59:
var i = jJr;
break;
case 60:
var i = GJr;
break;
case 61:
var i = MJr;
break;
case 62:
var i = BJr;
break;
case 63:
var i = qJr;
break;
case 64:
var i = UJr;
break;
case 65:
var i = HJr;
break;
case 114:
var i = XJr;
break;
case 115:
var i = YJr;
break;
case 116:
var i = VJr;
break;
case 117:
var i = zJr;
break;
case 118:
var i = KJr;
break;
case 119:
var i = WJr;
break;
case 120:
var i = JJr;
break;
case 121:
var i = $Jr;
break;
default:
e = 1;
}
else
switch (n4[0]) {
case 4:
var i = n4[2];
break;
case 9:
var i = n4[1] ? ZJr : QJr;
break;
default:
e = 1;
}
if (e) {
St(r$r, t);
var i = e$r;
}
return ie(t), i;
}
function V7(t) {
var n4 = De(t), e = pr(t), i = Ie0(t);
return [0, n4, [0, i, lr([0, e], [0, we(t)], 0)]];
}
function Ae0(t) {
var n4 = De(t), e = pr(t);
V0(t, 14);
var i = De(t), x = Ie0(t), c = lr([0, e], [0, we(t)], 0), s = yt(n4, i), p = i[2], y = n4[3], T = y[1] === p[1] ? 1 : 0, E = T && (y[2] === p[2] ? 1 : 0);
return 1 - E && ue(t, [0, s, L7]), [0, s, [0, x, c]];
}
function U1(t) {
var n4 = t[2], e = n4[3] === 0 ? 1 : 0;
if (e)
for (var i = n4[2]; ; ) {
if (i) {
var x = i[1][2], c = 0, s = i[2];
if (x[1][2][0] === 2 && !x[2]) {
var p = 1;
c = 1;
}
if (!c)
var p = 0;
if (p) {
var i = s;
continue;
}
return p;
}
return 1;
}
return e;
}
function nb(t) {
for (var n4 = t; ; ) {
var e = n4[2];
if (e[0] === 27) {
var i = e[1][2];
if (i[2][0] === 23)
return 1;
var n4 = i;
continue;
}
return 0;
}
}
function cr(t, n4, e) {
var i = t ? t[1] : De(e), x = u(n4, e), c = i2(e), s = c ? yt(i, c[1]) : i;
return [0, s, x];
}
function OL(t, n4, e) {
var i = cr(t, n4, e), x = i[2];
return [0, [0, i[1], x[1]], x[2]];
}
function sne(t) {
function n4(B) {
var Z = De(B), p0 = N0(B);
if (typeof p0 == "number") {
if (c7 === p0) {
var b0 = pr(B);
return ie(B), [0, [0, Z, [0, 0, lr([0, b0], 0, 0)]]];
}
if (D7 === p0) {
var O0 = pr(B);
return ie(B), [0, [0, Z, [0, 1, lr([0, O0], 0, 0)]]];
}
}
return 0;
}
var e = function B(Z) {
return B.fun(Z);
}, i = function B(Z) {
return B.fun(Z);
}, x = function B(Z) {
return B.fun(Z);
}, c = function B(Z, p0, b0) {
return B.fun(Z, p0, b0);
}, s = function B(Z) {
return B.fun(Z);
}, p = function B(Z, p0, b0) {
return B.fun(Z, p0, b0);
}, y = function B(Z) {
return B.fun(Z);
}, T = function B(Z, p0) {
return B.fun(Z, p0);
}, E = function B(Z) {
return B.fun(Z);
}, h = function B(Z) {
return B.fun(Z);
}, w = function B(Z, p0, b0) {
return B.fun(Z, p0, b0);
}, G = function B(Z, p0, b0, O0) {
return B.fun(Z, p0, b0, O0);
}, A = function B(Z) {
return B.fun(Z);
}, S = function B(Z, p0) {
return B.fun(Z, p0);
}, M = function B(Z) {
return B.fun(Z);
}, K = function B(Z) {
return B.fun(Z);
}, V = function B(Z) {
return B.fun(Z);
}, f0 = function B(Z) {
return B.fun(Z);
}, m0 = function B(Z) {
return B.fun(Z);
}, k0 = function B(Z) {
return B.fun(Z);
}, g0 = function B(Z, p0) {
return B.fun(Z, p0);
}, e0 = function B(Z) {
return B.fun(Z);
}, x0 = function B(Z) {
return B.fun(Z);
}, l = function B(Z) {
return B.fun(Z);
}, c0 = function B(Z) {
return B.fun(Z);
}, t0 = function B(Z) {
return B.fun(Z);
}, a0 = function B(Z) {
return B.fun(Z);
}, w0 = function B(Z) {
return B.fun(Z);
}, _0 = function B(Z, p0, b0, O0) {
return B.fun(Z, p0, b0, O0);
}, E0 = function B(Z, p0, b0, O0) {
return B.fun(Z, p0, b0, O0);
}, X0 = function B(Z) {
return B.fun(Z);
}, b = function B(Z) {
return B.fun(Z);
}, G0 = function B(Z) {
return B.fun(Z);
}, X = function B(Z) {
return B.fun(Z);
}, s0 = function B(Z) {
return B.fun(Z);
}, dr = function B(Z) {
return B.fun(Z);
}, Ar = function B(Z, p0) {
return B.fun(Z, p0);
}, ar = function B(Z, p0) {
return B.fun(Z, p0);
}, W0 = function B(Z) {
return B.fun(Z);
}, Lr = function B(Z, p0, b0) {
return B.fun(Z, p0, b0);
};
N(e, function(B) {
return u(x, B);
}), N(i, function(B) {
return 1 - iu(B) && Ge(B, 12), cr(0, function(Z) {
return V0(Z, 86), u(e, Z);
}, B);
}), N(x, function(B) {
var Z = N0(B) === 89 ? 1 : 0;
if (Z) {
var p0 = pr(B);
ie(B);
var b0 = p0;
} else
var b0 = Z;
return ir(c, B, [0, b0], u(s, B));
}), N(c, function(B, Z, p0) {
var b0 = Z && Z[1];
if (N0(B) === 89) {
var O0 = [0, p0, 0], q0 = function(er) {
for (var yr = O0; ; ) {
var vr = N0(er);
if (typeof vr == "number" && vr === 89) {
V0(er, 89);
var yr = [0, u(s, er), yr];
continue;
}
var $0 = de(yr);
if ($0) {
var Sr = $0[2];
if (Sr) {
var Mr = lr([0, b0], 0, 0);
return [19, [0, [0, $0[1], Sr[1], Sr[2]], Mr]];
}
}
throw [0, wn, P$r];
}
};
return cr([0, p0[1]], q0, B);
}
return p0;
}), N(s, function(B) {
var Z = N0(B) === 91 ? 1 : 0;
if (Z) {
var p0 = pr(B);
ie(B);
var b0 = p0;
} else
var b0 = Z;
return ir(p, B, [0, b0], u(y, B));
}), N(p, function(B, Z, p0) {
var b0 = Z && Z[1];
if (N0(B) === 91) {
var O0 = [0, p0, 0], q0 = function(er) {
for (var yr = O0; ; ) {
var vr = N0(er);
if (typeof vr == "number" && vr === 91) {
V0(er, 91);
var yr = [0, u(y, er), yr];
continue;
}
var $0 = de(yr);
if ($0) {
var Sr = $0[2];
if (Sr) {
var Mr = lr([0, b0], 0, 0);
return [20, [0, [0, $0[1], Sr[1], Sr[2]], Mr]];
}
}
throw [0, wn, C$r];
}
};
return cr([0, p0[1]], q0, B);
}
return p0;
}), N(y, function(B) {
return a(T, B, u(E, B));
}), N(T, function(B, Z) {
var p0 = N0(B);
if (typeof p0 == "number" && p0 === 11 && !B[15]) {
var b0 = a(g0, B, Z);
return R(_0, B, b0[1], 0, [0, b0[1], [0, 0, [0, b0, 0], 0, 0]]);
}
return Z;
}), N(E, function(B) {
var Z = N0(B);
return typeof Z == "number" && Z === 85 ? cr(0, function(p0) {
var b0 = pr(p0);
V0(p0, 85);
var O0 = lr([0, b0], 0, 0);
return [11, [0, u(E, p0), O0]];
}, B) : u(h, B);
}), N(h, function(B) {
return ir(w, 0, B, u(V, B));
}), N(w, function(B, Z, p0) {
var b0 = B && B[1];
if (f7(Z))
return p0;
var O0 = N0(Z);
if (typeof O0 == "number") {
if (O0 === 6)
return ie(Z), R(G, b0, 0, Z, p0);
if (O0 === 10) {
var q0 = Yn(1, Z);
return typeof q0 == "number" && q0 === 6 ? (Ge(Z, A$r), V0(Z, 10), V0(Z, 6), R(G, b0, 0, Z, p0)) : (Ge(Z, N$r), p0);
}
if (O0 === 83)
return ie(Z), N0(Z) !== 6 && Ge(Z, 30), V0(Z, 6), R(G, 1, 1, Z, p0);
}
return p0;
}), N(G, function(B, Z, p0, b0) {
function O0(q0) {
if (!Z && fu(q0, 7))
return [15, [0, b0, lr(0, [0, we(q0)], 0)]];
var er = u(e, q0);
V0(q0, 7);
var yr = [0, b0, er, lr(0, [0, we(q0)], 0)];
return B ? [18, [0, yr, Z]] : [17, yr];
}
return ir(w, [0, B], p0, cr([0, b0[1]], O0, p0));
}), N(A, function(B) {
return a(S, B, a(t[13], 0, B));
}), N(S, function(B, Z) {
for (var p0 = [0, Z[1], [0, Z]]; ; ) {
var b0 = p0[2];
if (N0(B) === 10 && A9(1, B)) {
var O0 = function(vr) {
return function($0) {
return V0($0, 10), [0, vr, V7($0)];
};
}(b0), q0 = cr([0, p0[1]], O0, B), er = q0[1], p0 = [0, er, [1, [0, er, q0[2]]]];
continue;
}
return b0;
}
}), N(M, function(B) {
var Z = N0(B);
if (typeof Z == "number") {
if (Z === 4) {
ie(B);
var p0 = u(M, B);
return V0(B, 5), p0;
}
} else if (Z[0] === 4)
return [0, u(A, B)];
return Ge(B, 51), 0;
}), N(K, function(B) {
return cr(0, function(Z) {
var p0 = pr(Z);
V0(Z, 46);
var b0 = u(M, Z);
if (b0) {
var O0 = lr([0, p0], 0, 0);
return [21, [0, b0[1], O0]];
}
return I$r;
}, B);
}), N(V, function(B) {
var Z = De(B), p0 = N0(B), b0 = 0;
if (typeof p0 == "number")
switch (p0) {
case 4:
return u(a0, B);
case 6:
return u(k0, B);
case 46:
return u(K, B);
case 53:
return cr(0, function(ge) {
var H0 = pr(ge);
V0(ge, 53);
var Fr = u(X0, ge), _ = lr([0, H0], 0, 0);
return [14, [0, Fr[2], Fr[1], _]];
}, B);
case 98:
return u(w0, B);
case 106:
var O0 = pr(B);
return V0(B, Xt), [0, Z, [10, lr([0, O0], [0, we(B)], 0)]];
case 42:
b0 = 1;
break;
case 0:
case 2:
var q0 = R(E0, 0, 1, 1, B);
return [0, q0[1], [13, q0[2]]];
case 30:
case 31:
var er = pr(B);
return V0(B, p0), [0, Z, [26, [0, p0 === 31 ? 1 : 0, lr([0, er], [0, we(B)], 0)]]];
}
else
switch (p0[0]) {
case 2:
var yr = p0[1], vr = yr[4], $0 = yr[3], Sr = yr[2], Mr = yr[1];
vr && Si(B, 45);
var Br = pr(B);
return V0(B, [2, [0, Mr, Sr, $0, vr]]), [0, Mr, [23, [0, Sr, $0, lr([0, Br], [0, we(B)], 0)]]];
case 10:
var qr = p0[3], jr = p0[2], $r = p0[1], ne = pr(B);
V0(B, [10, $r, jr, qr]);
var Qr = we(B);
return $r === 1 && Si(B, 45), [0, Z, [24, [0, jr, qr, lr([0, ne], [0, Qr], 0)]]];
case 11:
var pe = p0[3], oe = p0[2], me = pr(B);
return V0(B, [11, p0[1], oe, pe]), [0, Z, [25, [0, oe, pe, lr([0, me], [0, we(B)], 0)]]];
case 4:
b0 = 1;
break;
}
if (b0) {
var ae = u(dr, B);
return [0, ae[1], [16, ae[2]]];
}
var ce = u(m0, B);
return ce ? [0, Z, ce[1]] : (St(T$r, B), [0, Z, O$r]);
}), N(f0, function(B) {
var Z = 0;
if (typeof B == "number")
switch (B) {
case 29:
case 114:
case 115:
case 116:
case 117:
case 118:
case 119:
case 120:
case 121:
Z = 1;
break;
}
else
B[0] === 9 && (Z = 1);
return Z ? 1 : 0;
}), N(m0, function(B) {
var Z = pr(B), p0 = N0(B);
if (typeof p0 == "number")
switch (p0) {
case 29:
return ie(B), [0, [4, lr([0, Z], [0, we(B)], 0)]];
case 114:
return ie(B), [0, [0, lr([0, Z], [0, we(B)], 0)]];
case 115:
return ie(B), [0, [1, lr([0, Z], [0, we(B)], 0)]];
case 116:
return ie(B), [0, [2, lr([0, Z], [0, we(B)], 0)]];
case 117:
return ie(B), [0, [5, lr([0, Z], [0, we(B)], 0)]];
case 118:
return ie(B), [0, [6, lr([0, Z], [0, we(B)], 0)]];
case 119:
return ie(B), [0, [7, lr([0, Z], [0, we(B)], 0)]];
case 120:
return ie(B), [0, [3, lr([0, Z], [0, we(B)], 0)]];
case 121:
return ie(B), [0, [9, lr([0, Z], [0, we(B)], 0)]];
}
else if (p0[0] === 9)
return ie(B), [0, [8, lr([0, Z], [0, we(B)], 0)]];
return 0;
}), N(k0, function(B) {
return cr(0, function(Z) {
var p0 = pr(Z);
V0(Z, 6);
for (var b0 = u2(0, Z), O0 = 0; ; ) {
var q0 = N0(b0);
if (typeof q0 == "number") {
var er = 0;
if ((q0 === 7 || Pn === q0) && (er = 1), er) {
var yr = de(O0);
return V0(Z, 7), [22, [0, yr, lr([0, p0], [0, we(Z)], 0)]];
}
}
var vr = [0, u(e, b0), O0];
N0(b0) !== 7 && V0(b0, 9);
var O0 = vr;
}
}, B);
}), N(g0, function(B, Z) {
return [0, Z[1], [0, 0, Z, 0]];
}), N(e0, function(B) {
return cr(0, function(Z) {
zu(Z, 0);
var p0 = a(t[13], 0, Z);
h7(Z), 1 - iu(Z) && Ge(Z, 12);
var b0 = fu(Z, 85);
return V0(Z, 86), [0, [0, p0], u(e, Z), b0];
}, B);
});
function Tr(B) {
var Z = Yn(1, B);
return typeof Z == "number" && !(1 < (Z + W2 | 0) >>> 0) ? u(e0, B) : a(g0, B, u(e, B));
}
N(x0, function(B) {
var Z = 0;
return function(p0) {
for (var b0 = Z, O0 = p0; ; ) {
var q0 = N0(B);
if (typeof q0 == "number")
switch (q0) {
case 5:
case 12:
case 113:
var er = q0 === 12 ? 1 : 0, yr = er && [0, cr(0, function(jr) {
var $r = pr(jr);
V0(jr, 12);
var ne = lr([0, $r], 0, 0);
return [0, Tr(jr), ne];
}, B)];
return [0, b0, de(O0), yr, 0];
}
else if (q0[0] === 4 && !n0(q0[3], F$r)) {
var vr = 0;
if ((Yn(1, B) === 86 || Yn(1, B) === 85) && (vr = 1), vr) {
var $0 = b0 !== 0 ? 1 : 0, Sr = $0 || (O0 !== 0 ? 1 : 0);
Sr && Ge(B, c7);
var Mr = cr(0, function($r) {
var ne = pr($r);
ie($r), N0($r) === 85 && Ge($r, D7);
var Qr = lr([0, ne], 0, 0);
return [0, u(i, $r), Qr];
}, B);
N0(B) !== 5 && V0(B, 9);
var b0 = [0, Mr];
continue;
}
}
var Br = [0, Tr(B), O0];
N0(B) !== 5 && V0(B, 9);
var O0 = Br;
}
};
}), N(l, function(B) {
return cr(0, function(Z) {
var p0 = pr(Z);
V0(Z, 4);
var b0 = a(x0, Z, 0), O0 = pr(Z);
V0(Z, 5);
var q0 = _u([0, p0], [0, we(Z)], O0, 0);
return [0, b0[1], b0[2], b0[3], q0];
}, B);
}), N(c0, function(B) {
var Z = pr(B);
V0(B, 4);
var p0 = u2(0, B), b0 = N0(p0), O0 = 0;
if (typeof b0 == "number")
switch (b0) {
case 5:
var q0 = g$r;
break;
case 42:
O0 = 2;
break;
case 12:
case 113:
var q0 = [0, a(x0, p0, 0)];
break;
default:
O0 = 1;
}
else
b0[0] === 4 ? O0 = 2 : O0 = 1;
switch (O0) {
case 1:
if (u(f0, b0)) {
var er = Yn(1, p0), yr = 0;
if (typeof er == "number" && !(1 < (er + W2 | 0) >>> 0)) {
var vr = [0, a(x0, p0, 0)];
yr = 1;
}
if (!yr)
var vr = [1, u(e, p0)];
var q0 = vr;
} else
var q0 = [1, u(e, p0)];
break;
case 2:
var q0 = u(t0, p0);
break;
}
if (q0[0] === 0)
var $0 = q0;
else {
var Sr = q0[1];
if (B[15])
var Mr = q0;
else {
var Br = N0(B), qr = 0;
if (typeof Br == "number")
if (Br === 5)
var jr = Yn(1, B) === 11 ? [0, a(x0, B, [0, a(g0, B, Sr), 0])] : [1, Sr];
else if (Br === 9) {
V0(B, 9);
var jr = [0, a(x0, B, [0, a(g0, B, Sr), 0])];
} else
qr = 1;
else
qr = 1;
if (qr)
var jr = q0;
var Mr = jr;
}
var $0 = Mr;
}
var $r = pr(B);
V0(B, 5);
var ne = we(B);
if ($0[0] === 0) {
var Qr = $0[1], pe = _u([0, Z], [0, ne], $r, 0);
return [0, [0, Qr[1], Qr[2], Qr[3], pe]];
}
return [1, ir(Lr, $0[1], Z, ne)];
}), N(t0, function(B) {
var Z = Yn(1, B);
return typeof Z == "number" && !(1 < (Z + W2 | 0) >>> 0) ? [0, a(x0, B, 0)] : [1, ir(c, B, 0, ir(p, B, 0, a(T, B, ir(w, 0, B, a(ar, B, u(b, B))))))];
}), N(a0, function(B) {
var Z = De(B), p0 = cr(0, c0, B), b0 = p0[2];
return b0[0] === 0 ? R(_0, B, Z, 0, [0, p0[1], b0[1]]) : b0[1];
}), N(w0, function(B) {
var Z = De(B), p0 = xi(B, u(X, B));
return R(_0, B, Z, p0, u(l, B));
}), N(_0, function(B, Z, p0, b0) {
return cr([0, Z], function(O0) {
return V0(O0, 11), [12, [0, p0, b0, u(e, O0), 0]];
}, B);
});
function Hr(B, Z, p0) {
return cr([0, Z], function(b0) {
var O0 = u(l, b0);
return V0(b0, 86), [0, p0, O0, u(e, b0), 0];
}, B);
}
function Or(B, Z) {
var p0 = N0(Z);
if (typeof p0 == "number" && !(10 <= p0))
switch (p0) {
case 1:
if (!B)
return 0;
break;
case 3:
if (B)
return 0;
break;
case 8:
case 9:
return ie(Z);
}
return q1(Z, 9);
}
function xr(B, Z) {
return Z && ue(B, [0, Z[1][1], 7]);
}
function Rr(B, Z) {
return Z && ue(B, [0, Z[1], 9]);
}
N(E0, function(B, Z, p0, b0) {
var O0 = Z && (N0(b0) === 2 ? 1 : 0), q0 = Z && 1 - O0;
return cr(0, function(er) {
var yr = pr(er), vr = O0 && 2;
V0(er, vr);
var $0 = u2(0, er), Sr = S$r;
r:
for (; ; ) {
var Mr = Sr[3], Br = Sr[2], qr = Sr[1];
if (B && p0)
throw [0, wn, c$r];
if (q0 && !p0)
throw [0, wn, s$r];
var jr = De($0), $r = N0($0);
if (typeof $r == "number") {
var ne = 0;
if (13 <= $r) {
if (Pn === $r) {
var Qr = [0, de(qr), Br, Mr];
ne = 1;
}
} else if ($r)
switch ($r - 1 | 0) {
case 0:
if (!O0) {
var Qr = [0, de(qr), Br, Mr];
ne = 1;
}
break;
case 2:
if (O0) {
var Qr = [0, de(qr), Br, Mr];
ne = 1;
}
break;
case 11:
if (!p0) {
ie($0);
var pe = N0($0);
if (typeof pe == "number" && !(10 <= pe))
switch (pe) {
case 1:
case 3:
case 8:
case 9:
ue($0, [0, jr, 20]), Or(O0, $0);
continue;
}
var oe = gL($0);
u(kL($0), oe), ue($0, [0, jr, 17]), ie($0), Or(O0, $0);
continue;
}
var me = pr($0);
ie($0);
var ae = N0($0), ce = 0;
if (typeof ae == "number" && !(10 <= ae))
switch (ae) {
case 1:
case 3:
case 8:
case 9:
Or(O0, $0);
var ge = N0($0), H0 = 0;
if (typeof ge == "number") {
var Fr = ge - 1 | 0;
if (!(2 < Fr >>> 0))
switch (Fr) {
case 0:
if (q0) {
var Qr = [0, de(qr), 1, me];
ne = 1, ce = 1, H0 = 1;
}
break;
case 1:
break;
default:
ue($0, [0, jr, 19]);
var Qr = [0, de(qr), Br, Mr];
ne = 1, ce = 1, H0 = 1;
}
}
if (!H0) {
ue($0, [0, jr, 18]);
continue;
}
break;
}
if (!ce) {
var _ = [1, cr([0, jr], function(K7) {
return function(qt) {
var bt = lr([0, K7], 0, 0);
return [0, u(e, qt), bt];
};
}(me), $0)];
Or(O0, $0);
var Sr = [0, [0, _, qr], Br, Mr];
continue;
}
break;
}
if (ne) {
var k = pr(er), I = un(Qr[3], k), U = O0 ? 3 : 1;
V0(er, U);
var Y = _u([0, yr], [0, we(er)], I, 0);
return [0, O0, Qr[2], Qr[1], Y];
}
}
for (var y0 = B, D0 = B, I0 = 0, D = 0, u0 = 0, Y0 = 0; ; ) {
var J0 = N0($0), fr = 0;
if (typeof J0 == "number")
switch (J0) {
case 6:
Rr($0, u0);
var Q0 = Yn(1, $0), F0 = 0;
if (typeof Q0 == "number" && Q0 === 6) {
xr($0, I0);
var Cr = [4, cr([0, jr], function(qt, bt, U0) {
return function(L0) {
var Re = un(bt, pr(L0));
V0(L0, 6), V0(L0, 6);
var He = V7(L0);
V0(L0, 7), V0(L0, 7);
var he = N0(L0), _e = 0;
if (typeof he == "number") {
var Zn = 0;
if (he !== 4 && he !== 98 && (Zn = 1), !Zn) {
var dn = Hr(L0, qt, xi(L0, u(X, L0))), it = 0, ft = [0, dn[1], [12, dn[2]]], Rn = 1, nt = 0;
_e = 1;
}
}
if (!_e) {
var ht = fu(L0, 85), tn = we(L0);
V0(L0, 86);
var it = tn, ft = u(e, L0), Rn = 0, nt = ht;
}
return [0, He, ft, nt, U0 !== 0 ? 1 : 0, Rn, lr([0, Re], [0, it], 0)];
};
}(jr, Y0, D), $0)];
F0 = 1;
}
if (!F0)
var Cr = [2, cr([0, jr], function(K7, qt, bt) {
return function(U0) {
var L0 = un(K7, pr(U0));
V0(U0, 6);
var Re = Yn(1, U0) === 86 ? 1 : 0;
if (Re) {
var He = V7(U0);
V0(U0, 86);
var he = [0, He];
} else
var he = Re;
var _e = u(e, U0);
V0(U0, 7);
var Zn = we(U0);
V0(U0, 86);
var dn = u(e, U0);
return [0, he, _e, dn, qt !== 0 ? 1 : 0, bt, lr([0, L0], [0, Zn], 0)];
};
}(Y0, D, I0), $0)];
break;
case 42:
if (y0) {
if (I0 === 0) {
var gr = [0, De($0)], mr = un(Y0, pr($0));
ie($0);
var y0 = 0, D0 = 0, D = gr, Y0 = mr;
continue;
}
throw [0, wn, l$r];
}
fr = 1;
break;
case 103:
case 104:
if (I0 === 0) {
var y0 = 0, D0 = 0, I0 = n4($0);
continue;
}
fr = 1;
break;
case 4:
case 98:
Rr($0, u0), xr($0, I0);
var Cr = [3, cr([0, jr], function(K7, qt) {
return function(bt) {
var U0 = De(bt), L0 = Hr(bt, U0, xi(bt, u(X, bt)));
return [0, L0, qt !== 0 ? 1 : 0, lr([0, K7], 0, 0)];
};
}(Y0, D), $0)];
break;
default:
fr = 1;
}
else if (J0[0] === 4 && !n0(J0[3], b$r)) {
if (D0) {
if (I0 === 0) {
var sr = [0, De($0)], Pr = un(Y0, pr($0));
ie($0);
var y0 = 0, D0 = 0, u0 = sr, Y0 = Pr;
continue;
}
throw [0, wn, p$r];
}
fr = 1;
} else
fr = 1;
if (fr) {
var K0 = 0;
if (D) {
var Ur = D[1];
if (u0) {
var Cr = ke(m$r);
K0 = 1;
} else if (typeof J0 == "number" && !(1 < (J0 + W2 | 0) >>> 0)) {
var d0 = [0, Ur, [1, Gc(lr([0, Y0], 0, 0), [0, Ur, _$r])]], Kr = 0, re = u0, xe = 0;
K0 = 2;
}
} else if (u0) {
var je = u0[1];
if (typeof J0 == "number" && !(1 < (J0 + W2 | 0) >>> 0)) {
var d0 = [0, je, [1, Gc(lr([0, Y0], 0, 0), [0, je, y$r])]], Kr = 0, re = 0, xe = D;
K0 = 2;
}
}
var ve = 0;
switch (K0) {
case 0:
var Ie = function(qt) {
zu(qt, 0);
var bt = a(t[20], 0, qt);
return h7(qt), bt;
}, Me = pr($0), Be = Ie($0), fn = Be[1], Ke = Be[2], Ae = 0;
if (Ke[0] === 1) {
var xn = Ke[1][2][1], Qe = 0;
if (n0(xn, d$r) && n0(xn, h$r) && (Qe = 1), !Qe) {
var yn = N0($0), on = 0;
if (typeof yn == "number") {
var Ce = yn - 5 | 0;
if (92 < Ce >>> 0) {
if (!(94 < (Ce + 1 | 0) >>> 0)) {
Rr($0, u0), xr($0, I0);
var We = Ke;
Ae = 1, on = 1;
}
} else if (!(1 < (Ce + fX | 0) >>> 0)) {
var d0 = [0, fn, Ke], Kr = Y0, re = u0, xe = D;
ve = 1, Ae = 2, on = 1;
}
}
if (!on) {
Xi($0, Ke);
var rn = Ie($0), bn = qn(xn, k$r), Cn = un(Y0, Me);
Rr($0, u0), xr($0, I0);
var Cr = [0, cr([0, jr], function(bt, U0, L0, Re, He) {
return function(he) {
var _e = L0[1], Zn = Xi(he, L0[2]), dn = Hr(he, bt, 0), it = dn[2][2];
if (Re) {
var ft = it[2], Rn = 0;
if (ft[1])
ue(he, [0, _e, R7]), Rn = 1;
else {
var nt = 0;
!ft[2] && !ft[3] && (Rn = 1, nt = 1), nt || ue(he, [0, _e, 80]);
}
} else {
var ht = it[2], tn = 0;
if (ht[1])
ue(he, [0, _e, Xt]), tn = 1;
else {
var cn = ht[2], tt = 0;
if (ht[3])
ue(he, [0, _e, 81]);
else {
var Tt = 0;
cn && !cn[2] && (Tt = 1), Tt || (ue(he, [0, _e, 81]), tt = 1);
}
tt || (tn = 1);
}
}
var Fi = lr([0, He], 0, 0), hs = 0, Iu = 0, Vs = 0, Vi = U0 !== 0 ? 1 : 0, zs = 0, Ks = Re ? [1, dn] : [2, dn];
return [0, Zn, Ks, zs, Vi, Vs, Iu, hs, Fi];
};
}(jr, D, rn, bn, Cn), $0)];
Ae = 2;
}
}
}
var Hn = 0;
switch (Ae) {
case 2:
Hn = 1;
break;
case 0:
var Sn = Be[2], vt = N0($0), At = 0;
if (typeof vt == "number") {
var gt = 0;
if (vt !== 4 && vt !== 98 && (gt = 1), !gt) {
Rr($0, u0), xr($0, I0);
var We = Sn;
At = 1;
}
}
if (!At) {
var Jt = D !== 0 ? 1 : 0, Bt = 0;
if (Sn[0] === 1) {
var Ft = Sn[1], Nt = Ft[2][1], du = 0;
if (B) {
var Ku = 0;
!qn(w$r, Nt) && (!Jt || !qn(E$r, Nt)) && (Ku = 1), Ku || (ue($0, [0, Ft[1], [21, Nt, Jt, 0, 0]]), Bt = 1, du = 1);
}
}
var d0 = [0, fn, Sn], Kr = Y0, re = u0, xe = D;
ve = 1, Hn = 1;
}
break;
}
if (!Hn)
var lt = Xi($0, We), xu = Hr($0, jr, xi($0, u(X, $0))), Mu = [0, xu[1], [12, xu[2]]], z7 = [0, lt, [0, Mu], 0, D !== 0 ? 1 : 0, 0, 1, 0, lr([0, Y0], 0, 0)], Cr = [0, [0, Mu[1], z7]];
break;
case 2:
ve = 1;
break;
}
if (ve) {
var Yi = d0[2], a7 = d0[1];
1 - iu($0) && Ge($0, 12);
var Cr = [0, cr([0, jr], function(qt, bt, U0, L0, Re, He) {
return function(he) {
var _e = fu(he, 85), Zn = he0(he, 86) ? u(e, he) : [0, He, v$r];
return [0, Re, [0, Zn], _e, bt !== 0 ? 1 : 0, U0 !== 0 ? 1 : 0, 0, qt, lr([0, L0], 0, 0)];
};
}(I0, xe, re, Kr, Yi, a7), $0)];
}
}
Or(O0, $0);
var Sr = [0, [0, Cr, qr], Br, Mr];
continue r;
}
}
}, b0);
}), N(X0, function(B) {
var Z = N0(B) === 41 ? 1 : 0;
if (Z) {
V0(B, 41);
for (var p0 = 0; ; ) {
var b0 = [0, u(dr, B), p0], O0 = N0(B);
if (typeof O0 == "number" && O0 === 9) {
V0(B, 9);
var p0 = b0;
continue;
}
var q0 = ge0(B, de(b0));
break;
}
} else
var q0 = Z;
return [0, q0, R(E0, 0, 0, 0, B)];
}), N(b, function(B) {
var Z = V7(B), p0 = Z[2], b0 = p0[1], O0 = Z[1];
return be0(b0) && ue(B, [0, O0, 3]), [0, O0, [0, b0, p0[2]]];
}), N(G0, function(B) {
return cr(0, function(Z) {
var p0 = u(b, Z), b0 = N0(Z) === 86 ? [1, u(i, Z)] : [0, G1(Z)];
return [0, p0, b0];
}, B);
}), N(X, function(B) {
var Z = N0(B) === 98 ? 1 : 0;
if (Z) {
1 - iu(B) && Ge(B, 12);
var p0 = [0, cr(0, function(O0) {
var q0 = pr(O0);
V0(O0, 98);
for (var er = 0, yr = 0; ; ) {
var vr = cr(0, function(ne) {
return function(Qr) {
var pe = n4(Qr), oe = u(G0, Qr), me = oe[2], ae = N0(Qr), ce = 0;
if (typeof ae == "number" && ae === 82) {
ie(Qr);
var ge = 1, H0 = [0, u(e, Qr)];
ce = 1;
}
if (!ce) {
ne && ue(Qr, [0, oe[1], 77]);
var ge = ne, H0 = 0;
}
return [0, pe, me[1], me[2], H0, ge];
};
}(er), O0), $0 = vr[2], Sr = [0, [0, vr[1], [0, $0[2], $0[3], $0[1], $0[4]]], yr], Mr = N0(O0), Br = 0;
if (typeof Mr == "number") {
var qr = 0;
if (Mr !== 99 && Pn !== Mr && (qr = 1), !qr) {
var jr = de(Sr);
Br = 1;
}
}
if (!Br) {
if (V0(O0, 9), N0(O0) !== 99) {
var er = $0[5], yr = Sr;
continue;
}
var jr = de(Sr);
}
var $r = pr(O0);
return V0(O0, 99), [0, jr, _u([0, q0], [0, we(O0)], $r, 0)];
}
}, B)];
} else
var p0 = Z;
return p0;
}), N(s0, function(B) {
var Z = N0(B) === 98 ? 1 : 0, p0 = Z && [0, cr(0, function(b0) {
var O0 = pr(b0);
V0(b0, 98);
for (var q0 = u2(0, b0), er = 0; ; ) {
var yr = N0(q0);
if (typeof yr == "number") {
var vr = 0;
if ((yr === 99 || Pn === yr) && (vr = 1), vr) {
var $0 = de(er), Sr = pr(q0);
return V0(q0, 99), [0, $0, _u([0, O0], [0, we(q0)], Sr, 0)];
}
}
var Mr = [0, u(e, q0), er];
N0(q0) !== 99 && V0(q0, 9);
var er = Mr;
}
}, B)];
return p0;
}), N(dr, function(B) {
return a(Ar, B, u(b, B));
}), N(Ar, function(B, Z) {
function p0(b0) {
for (var O0 = [0, Z[1], [0, Z]]; ; ) {
var q0 = O0[2], er = O0[1];
if (N0(b0) === 10 && _e0(1, b0)) {
var yr = cr([0, er], function(qr) {
return function(jr) {
return V0(jr, 10), [0, qr, u(b, jr)];
};
}(q0), b0), vr = yr[1], O0 = [0, vr, [1, [0, vr, yr[2]]]];
continue;
}
if (N0(b0) === 98)
var $0 = Wt(b0), Sr = function(Br, qr) {
return a(Ze(Br, -860373976, 77), Br, qr);
}, Mr = a($0[2], q0, Sr);
else
var Mr = q0;
return [0, Mr, u(s0, b0), 0];
}
}
return cr([0, Z[1]], p0, B);
}), N(ar, function(B, Z) {
var p0 = a(Ar, B, Z);
return [0, p0[1], [16, p0[2]]];
}), N(W0, function(B) {
var Z = N0(B);
return typeof Z == "number" && Z === 86 ? [1, u(i, B)] : [0, G1(B)];
}), N(Lr, function(B, Z, p0) {
var b0 = B[2];
function O0(gr) {
return _7(gr, lr([0, Z], [0, p0], 0));
}
switch (b0[0]) {
case 0:
var F0 = [0, O0(b0[1])];
break;
case 1:
var F0 = [1, O0(b0[1])];
break;
case 2:
var F0 = [2, O0(b0[1])];
break;
case 3:
var F0 = [3, O0(b0[1])];
break;
case 4:
var F0 = [4, O0(b0[1])];
break;
case 5:
var F0 = [5, O0(b0[1])];
break;
case 6:
var F0 = [6, O0(b0[1])];
break;
case 7:
var F0 = [7, O0(b0[1])];
break;
case 8:
var F0 = [8, O0(b0[1])];
break;
case 9:
var F0 = [9, O0(b0[1])];
break;
case 10:
var F0 = [10, O0(b0[1])];
break;
case 11:
var q0 = b0[1], er = O0(q0[2]), F0 = [11, [0, q0[1], er]];
break;
case 12:
var yr = b0[1], vr = O0(yr[4]), F0 = [12, [0, yr[1], yr[2], yr[3], vr]];
break;
case 13:
var $0 = b0[1], Sr = lr([0, Z], [0, p0], 0), Mr = QD($0[4], Sr), F0 = [13, [0, $0[1], $0[2], $0[3], Mr]];
break;
case 14:
var Br = b0[1], qr = O0(Br[3]), F0 = [14, [0, Br[1], Br[2], qr]];
break;
case 15:
var jr = b0[1], $r = O0(jr[2]), F0 = [15, [0, jr[1], $r]];
break;
case 16:
var ne = b0[1], Qr = O0(ne[3]), F0 = [16, [0, ne[1], ne[2], Qr]];
break;
case 17:
var pe = b0[1], oe = O0(pe[3]), F0 = [17, [0, pe[1], pe[2], oe]];
break;
case 18:
var me = b0[1], ae = me[1], ce = me[2], ge = O0(ae[3]), F0 = [18, [0, [0, ae[1], ae[2], ge], ce]];
break;
case 19:
var H0 = b0[1], Fr = O0(H0[2]), F0 = [19, [0, H0[1], Fr]];
break;
case 20:
var _ = b0[1], k = O0(_[2]), F0 = [20, [0, _[1], k]];
break;
case 21:
var I = b0[1], U = O0(I[2]), F0 = [21, [0, I[1], U]];
break;
case 22:
var Y = b0[1], y0 = O0(Y[2]), F0 = [22, [0, Y[1], y0]];
break;
case 23:
var D0 = b0[1], I0 = O0(D0[3]), F0 = [23, [0, D0[1], D0[2], I0]];
break;
case 24:
var D = b0[1], u0 = O0(D[3]), F0 = [24, [0, D[1], D[2], u0]];
break;
case 25:
var Y0 = b0[1], J0 = O0(Y0[3]), F0 = [25, [0, Y0[1], Y0[2], J0]];
break;
default:
var fr = b0[1], Q0 = O0(fr[2]), F0 = [26, [0, fr[1], Q0]];
}
return [0, B[1], F0];
});
function Wr(B) {
var Z = pr(B);
if (V0(B, 66), N0(B) === 4) {
var p0 = un(Z, pr(B));
V0(B, 4), zu(B, 0);
var b0 = u(t[9], B);
return h7(B), V0(B, 5), [0, [0, b0], lr([0, p0], [0, we(B)], 0)];
}
return [0, 0, lr([0, Z], [0, we(B)], 0)];
}
var Jr = 0;
function or(B) {
var Z = u2(0, B), p0 = N0(Z);
return typeof p0 == "number" && p0 === 66 ? [0, cr(Jr, Wr, Z)] : 0;
}
function _r(B) {
var Z = N0(B), p0 = Yn(1, B);
if (typeof Z == "number" && Z === 86) {
if (typeof p0 == "number" && p0 === 66) {
V0(B, 86);
var b0 = or(B);
return [0, [0, G1(B)], b0];
}
var O0 = u(W0, B), q0 = N0(B) === 66 ? a2(B, O0) : O0;
return [0, q0, or(B)];
}
return [0, [0, G1(B)], 0];
}
function Ir(B, Z) {
var p0 = ys(1, Z);
zu(p0, 1);
var b0 = u(B, p0);
return h7(p0), b0;
}
function fe(B) {
return Ir(e, B);
}
function v0(B) {
return Ir(b, B);
}
function P(B) {
return Ir(X, B);
}
function L(B) {
return Ir(s0, B);
}
function Q(B, Z) {
return Ir(ir(E0, B, 0, 0), Z);
}
function i0(B) {
return Ir(X0, B);
}
function l0(B) {
return Ir(l, B);
}
function S0(B) {
return Ir(i, B);
}
function T0(B) {
return Ir(W0, B);
}
function rr(B) {
return Ir(or, B);
}
function R0(B) {
return Ir(_r, B);
}
return [0, fe, v0, P, L, function(B) {
return Ir(dr, B);
}, Q, i0, l0, S0, T0, rr, R0];
}
function vne(t) {
function n4(c, s) {
if (s[0] === 0)
return s[1];
var p = s[2][1];
return Pu(function(y) {
return ue(c, y);
}, p), s[1];
}
function e(c, s, p) {
var y = c ? c[1] : 26;
if (p[0] === 0)
var T = p[1];
else {
var E = p[2][2];
Pu(function(A) {
return ue(s, A);
}, E);
var T = p[1];
}
1 - u(t[23], T) && ue(s, [0, T[1], y]);
var h = T[2], w = 0;
return h[0] === 10 && Bs(h[1][2][1]) && (Y7(s, [0, T[1], 52]), w = 1), a(t[19], s, T);
}
function i(c, s) {
return [0, [0, c, s[1]], [0, c, s[2]]];
}
function x(c, s) {
var p = jc(c[2], s[2]);
return [0, jc(c[1], s[1]), p];
}
return [0, n4, e, B$r, i, x, function(c) {
var s = de(c[2]);
return [0, de(c[1]), s];
}];
}
function lne(t) {
function n4(S) {
var M = N0(S);
if (typeof M == "number") {
var K = M - 99 | 0, V = 0;
if (6 < K >>> 0 ? K === 14 && (V = 1) : 4 < (K - 1 | 0) >>> 0 && (V = 1), V)
return we(S);
}
var f0 = f7(S);
return f0 && Us(S);
}
function e(S) {
var M = pr(S);
zu(S, 0);
var K = cr(0, function(f0) {
V0(f0, 0), V0(f0, 12);
var m0 = u(t[10], f0);
return V0(f0, 1), m0;
}, S);
h7(S);
var V = lr([0, M], [0, n4(S)], 0);
return [0, K[1], [0, K[2], V]];
}
function i(S) {
return N0(S) === 1 ? 0 : [0, u(t[7], S)];
}
function x(S) {
var M = pr(S);
zu(S, 0);
var K = cr(0, function(f0) {
V0(f0, 0);
var m0 = i(f0);
return V0(f0, 1), m0;
}, S);
h7(S);
var V = _u([0, M], [0, n4(S)], 0, 0);
return [0, K[1], [0, K[2], V]];
}
function c(S) {
zu(S, 0);
var M = cr(0, function(K) {
V0(K, 0);
var V = N0(K), f0 = 0;
if (typeof V == "number" && V === 12) {
var m0 = pr(K);
V0(K, 12);
var k0 = u(t[10], K), x0 = [3, [0, k0, lr([0, m0], 0, 0)]];
f0 = 1;
}
if (!f0)
var g0 = i(K), e0 = g0 ? 0 : pr(K), x0 = [2, [0, g0, _u(0, 0, e0, 0)]];
return V0(K, 1), x0;
}, S);
return h7(S), [0, M[1], M[2]];
}
function s(S) {
var M = De(S), K = N0(S), V = 0;
if (typeof K != "number" && K[0] === 7) {
var f0 = K[1];
V = 1;
}
if (!V) {
St(qQr, S);
var f0 = UQr;
}
var m0 = pr(S);
ie(S);
var k0 = N0(S), g0 = 0;
if (typeof k0 == "number") {
var e0 = k0 + jX | 0, x0 = 0;
if (72 < e0 >>> 0 ? e0 !== 76 && (x0 = 1) : 70 < (e0 - 1 | 0) >>> 0 || (x0 = 1), !x0) {
var l = we(S);
g0 = 1;
}
}
if (!g0)
var l = n4(S);
return [0, M, [0, f0, lr([0, m0], [0, l], 0)]];
}
function p(S) {
var M = Yn(1, S);
if (typeof M == "number") {
if (M === 10)
for (var K = cr(0, function(m0) {
var k0 = [0, s(m0)];
return V0(m0, 10), [0, k0, s(m0)];
}, S); ; ) {
var V = N0(S);
if (typeof V == "number" && V === 10) {
var f0 = function(k0) {
return function(g0) {
return V0(g0, 10), [0, [1, k0], s(g0)];
};
}(K), K = cr([0, K[1]], f0, S);
continue;
}
return [2, K];
}
if (M === 86)
return [1, cr(0, function(m0) {
var k0 = s(m0);
return V0(m0, 86), [0, k0, s(m0)];
}, S)];
}
return [0, s(S)];
}
function y(S) {
return cr(0, function(M) {
var K = Yn(1, M), V = 0;
if (typeof K == "number" && K === 86) {
var f0 = [1, cr(0, function(b) {
var G0 = s(b);
return V0(b, 86), [0, G0, s(b)];
}, M)];
V = 1;
}
if (!V)
var f0 = [0, s(M)];
var m0 = N0(M), k0 = 0;
if (typeof m0 == "number" && m0 === 82) {
V0(M, 82);
var g0 = pr(M), e0 = N0(M), x0 = 0;
if (typeof e0 == "number")
if (e0 === 0) {
var l = x(M), c0 = l[2], t0 = l[1];
c0[1] || ue(M, [0, t0, 56]);
var a0 = [0, [1, t0, c0]];
} else
x0 = 1;
else if (e0[0] === 8) {
V0(M, e0);
var w0 = [0, e0[2]], _0 = lr([0, g0], [0, n4(M)], 0), a0 = [0, [0, e0[1], [0, w0, e0[3], _0]]];
} else
x0 = 1;
if (x0) {
Ge(M, 57);
var a0 = [0, [0, De(M), [0, BQr, MQr, 0]]];
}
var E0 = a0;
k0 = 1;
}
if (!k0)
var E0 = 0;
return [0, f0, E0];
}, S);
}
function T(S) {
return cr(0, function(M) {
V0(M, 98);
var K = N0(M);
if (typeof K == "number") {
if (K === 99)
return ie(M), jQr;
} else if (K[0] === 7)
for (var V = 0, f0 = p(M); ; ) {
var m0 = N0(M);
if (typeof m0 == "number") {
if (m0 === 0) {
var V = [0, [1, e(M)], V];
continue;
}
} else if (m0[0] === 7) {
var V = [0, [0, y(M)], V];
continue;
}
var k0 = de(V), g0 = [0, s1, [0, f0, fu(M, R7), k0]];
return fu(M, 99) ? [0, g0] : (q1(M, 99), [1, g0]);
}
return q1(M, 99), GQr;
}, S);
}
function E(S) {
return cr(0, function(M) {
V0(M, 98), V0(M, R7);
var K = N0(M);
if (typeof K == "number") {
if (K === 99)
return ie(M), Ni;
} else if (K[0] === 7) {
var V = p(M);
return he0(M, 99), [0, s1, [0, V]];
}
return q1(M, 99), Ni;
}, S);
}
var h = function S(M) {
return S.fun(M);
}, w = function S(M) {
return S.fun(M);
}, G = function S(M) {
return S.fun(M);
};
N(h, function(S) {
var M = N0(S);
if (typeof M == "number") {
if (M === 0)
return c(S);
} else if (M[0] === 8)
return V0(S, M), [0, M[1], [4, [0, M[2], M[3]]]];
var K = u(G, S), V = K[2], f0 = K[1];
return Ni <= V[1] ? [0, f0, [1, V[2]]] : [0, f0, [0, V[2]]];
});
function A(S) {
switch (S[0]) {
case 0:
return S[1][2][1];
case 1:
var M = S[1][2], K = Te(DQr, M[2][2][1]);
return Te(M[1][2][1], K);
default:
var V = S[1][2], f0 = V[1], m0 = f0[0] === 0 ? f0[1][2][1] : A([2, f0[1]]);
return Te(m0, Te(LQr, V[2][2][1]));
}
}
return N(w, function(S) {
var M = pr(S), K = T(S);
h7(S);
var V = K[2];
if (V[0] === 0)
var f0 = V[1], m0 = typeof f0 == "number" ? 0 : f0[2][2], k0 = m0;
else
var k0 = 1;
if (k0)
var g0 = IU, e0 = g0, x0 = cr(0, function(qr) {
return 0;
}, S);
else {
zu(S, 3);
for (var l = De(S), c0 = 0; ; ) {
var t0 = i2(S), a0 = N0(S), w0 = 0;
if (typeof a0 == "number") {
var _0 = 0;
if (a0 === 98) {
zu(S, 2);
var E0 = N0(S), X0 = Yn(1, S), b = 0;
if (typeof E0 == "number" && E0 === 98 && typeof X0 == "number") {
var G0 = 0;
if (R7 !== X0 && Pn !== X0 && (G0 = 1), !G0) {
var X = E(S), s0 = X[2], dr = X[1], Ar = typeof s0 == "number" ? [0, Ni, dr] : [0, s1, [0, dr, s0[2]]], ar = S[23][1], W0 = 0;
if (ar) {
var Lr = ar[2];
if (Lr) {
var Tr = Lr[2];
W0 = 1;
}
}
if (!W0)
var Tr = ke(jRr);
S[23][1] = Tr;
var Hr = n2(S), Or = Yl(S[24][1], Hr);
S[25][1] = Or;
var xr = [0, de(c0), t0, Ar];
b = 1;
}
}
if (!b) {
var Rr = u(w, S), Wr = Rr[2], Jr = Rr[1], or = Ni <= Wr[1] ? [0, Jr, [1, Wr[2]]] : [0, Jr, [0, Wr[2]]], c0 = [0, or, c0];
continue;
}
} else if (Pn === a0) {
St(0, S);
var xr = [0, de(c0), t0, IU];
} else
w0 = 1, _0 = 1;
if (!_0)
var _r = t0 ? t0[1] : l, Ir = yt(l, _r), e0 = xr[3], x0 = [0, Ir, xr[1]];
} else
w0 = 1;
if (w0) {
var c0 = [0, u(h, S), c0];
continue;
}
break;
}
}
var fe = we(S), v0 = 0;
if (typeof e0 != "number") {
var P = e0[1], L = 0;
if (s1 === P) {
var Q = e0[2], i0 = K[2];
if (i0[0] === 0) {
var l0 = i0[1];
if (typeof l0 == "number")
Ge(S, RQr);
else {
var S0 = A(l0[2][1]);
n0(A(Q[2][1]), S0) && Ge(S, [17, S0]);
}
}
var T0 = Q[1];
} else if (Ni === P) {
var rr = K[2];
if (rr[0] === 0) {
var R0 = rr[1];
typeof R0 != "number" && Ge(S, [17, A(R0[2][1])]);
}
var T0 = e0[2];
} else
L = 1;
if (!L) {
var B = T0;
v0 = 1;
}
}
if (!v0)
var B = K[1];
var Z = K[2][1], p0 = K[1];
if (typeof Z == "number") {
var b0 = 0, O0 = lr([0, M], [0, fe], 0);
if (typeof e0 != "number") {
var q0 = e0[1], er = 0;
if (s1 === q0)
var yr = e0[2][1];
else if (Ni === q0)
var yr = e0[2];
else
er = 1;
if (!er) {
var vr = yr;
b0 = 1;
}
}
if (!b0)
var vr = B;
var $0 = [0, Ni, [0, p0, vr, x0, O0]];
} else {
var Sr = 0, Mr = lr([0, M], [0, fe], 0);
if (typeof e0 != "number" && s1 === e0[1]) {
var Br = [0, e0[2]];
Sr = 1;
}
if (!Sr)
var Br = 0;
var $0 = [0, s1, [0, [0, p0, Z[2]], Br, x0, Mr]];
}
return [0, yt(K[1], B), $0];
}), N(G, function(S) {
return zu(S, 2), u(w, S);
}), [0, n4, e, i, x, c, s, p, y, T, E, h, w, G];
}
function gi(t) {
return typeof t == "number" ? 0 : t[0] === 0 ? 1 : t[1];
}
function bne(t, n4) {
return [0, t, n4];
}
function tb(t, n4, e) {
return [1, 2, n4, e, t, 0];
}
function ub(t, n4, e) {
return [1, 2, t, n4, 0, e];
}
function Xc(t, n4, e, i) {
var x = gi(t), c = gi(i), s = c <= x ? x + 1 | 0 : c + 1 | 0;
return s === 1 ? [0, n4, e] : [1, s, n4, e, t, i];
}
function IL(t, n4) {
var e = n4 !== 0 ? 1 : 0;
if (e) {
if (n4 !== 1) {
var i = n4 >>> 1 | 0, x = IL(t, i), c = u(t, 0), s = IL(t, (n4 - i | 0) - 1 | 0), p = c[2], y = c[1];
return [1, gi(x) + 1 | 0, y, p, x, s];
}
var T = u(t, 0), E = [0, T[1], T[2]];
} else
var E = e;
return E;
}
function D9(t, n4, e, i) {
var x = gi(t), c = gi(i), s = c <= x ? x + 1 | 0 : c + 1 | 0;
return [1, s, n4, e, t, i];
}
function Ou(t, n4, e, i) {
var x = gi(t), c = gi(i);
if ((c + 2 | 0) < x) {
var s = t[5], p = t[4], y = t[3], T = t[2], E = gi(s);
if (E <= gi(p))
return D9(p, T, y, Xc(s, n4, e, i));
var h = Xc(s[5], n4, e, i), w = s[3], G = s[2];
return D9(Xc(p, T, y, s[4]), G, w, h);
}
if ((x + 2 | 0) < c) {
var A = i[5], S = i[4], M = i[3], K = i[2], V = gi(S);
if (V <= gi(A))
return D9(Xc(t, n4, e, S), K, M, A);
var f0 = Xc(S[5], K, M, A), m0 = S[3], k0 = S[2];
return D9(Xc(t, n4, e, S[4]), k0, m0, f0);
}
return Xc(t, n4, e, i);
}
var x7 = 0;
function pne(t) {
var n4 = typeof t == "number" ? 1 : 0, e = n4 && 1;
return e;
}
function Xs(t, n4) {
for (var e = t, i = n4; ; ) {
if (typeof e == "number")
return i;
if (e[0] === 0)
return [0, e[1], e[2], x7, i];
var x = [0, e[2], e[3], e[5], i], e = e[4], i = x;
}
}
function Ne0(t) {
for (var n4 = t; ; ) {
if (typeof n4 == "number")
throw Kt;
if (n4[0] === 0)
return n4;
if (typeof n4[4] == "number")
return [0, n4[2], n4[3]];
var n4 = n4[4];
}
}
function Ce0(t) {
for (var n4 = t; ; ) {
var e = n4[4];
if (typeof e == "number")
return [0, n4[2], n4[3]];
if (e[0] === 0)
return e;
var n4 = e;
}
}
function Pe0(t) {
for (var n4 = t; ; ) {
if (typeof n4 == "number")
return 0;
if (n4[0] === 0)
return [0, [0, n4[1], n4[2]]];
if (typeof n4[4] == "number")
return [0, [0, n4[2], n4[3]]];
var n4 = n4[4];
}
}
function mne(t) {
for (var n4 = t; ; ) {
if (typeof n4 == "number")
throw Kt;
if (n4[0] === 0)
return n4;
if (typeof n4[5] == "number")
return [0, n4[2], n4[3]];
var n4 = n4[5];
}
}
function _ne(t) {
for (var n4 = t; ; ) {
if (typeof n4 == "number")
return 0;
if (n4[0] === 0)
return [0, [0, n4[1], n4[2]]];
if (typeof n4[5] == "number")
return [0, [0, n4[2], n4[3]]];
var n4 = n4[5];
}
}
function AL(t) {
var n4 = t[5], e = t[4], i = t[3], x = t[2];
return typeof e == "number" ? n4 : e[0] === 0 ? Ou(0, x, i, n4) : Ou(AL(e), x, i, n4);
}
function NL(t, n4) {
if (typeof n4 == "number")
return t;
if (n4[0] === 0)
return tb(t, n4[1], n4[2]);
var e = n4[5], i = n4[3], x = n4[2];
return Ou(NL(t, n4[4]), x, i, e);
}
function De0(t, n4, e) {
if (typeof e == "number")
return [0, t, n4];
if (e[0] === 0)
return ub(t, n4, e);
var i = e[5], x = e[3], c = e[2];
return Ou(De0(t, n4, e[4]), c, x, i);
}
function CL(t, n4) {
if (typeof n4 == "number")
return t;
if (n4[0] === 0)
return ub(n4[1], n4[2], t);
var e = CL(t, n4[5]);
return Ou(n4[4], n4[2], n4[3], e);
}
function Le0(t, n4, e) {
if (typeof e == "number")
return [0, t, n4];
if (e[0] === 0)
return tb(e, t, n4);
var i = Le0(t, n4, e[5]);
return Ou(e[4], e[2], e[3], i);
}
function Re0(t, n4) {
if (typeof t == "number")
return n4;
if (t[0] === 1 && typeof n4 != "number") {
if (n4[0] === 0)
return CL(n4, t);
var e = Ce0(n4), i = AL(n4);
return Ou(t, e[1], e[2], i);
}
return typeof n4 == "number" ? t : NL(t, n4);
}
function ai(t, n4, e, i) {
if (typeof t == "number")
return De0(n4, e, i);
if (t[0] === 0) {
if (typeof i != "number") {
if (i[0] === 0)
return [1, 2, n4, e, t, i];
if (3 < i[1]) {
var x = i[5], c = i[3], s = i[2];
return Ou(ai(t, n4, e, i[4]), s, c, x);
}
return Xc(t, n4, e, i);
}
} else {
var p = t[5], y = t[4], T = t[3], E = t[2], h = t[1];
if (typeof i != "number") {
if (i[0] === 0)
return 3 < h ? Ou(y, E, T, ai(p, n4, e, i)) : Xc(t, n4, e, i);
var w = i[1];
if ((w + 2 | 0) < h)
return Ou(y, E, T, ai(p, n4, e, i));
if ((h + 2 | 0) < w) {
var G = i[5], A = i[3], S = i[2];
return Ou(ai(t, n4, e, i[4]), S, A, G);
}
return Xc(t, n4, e, i);
}
}
return Le0(n4, e, t);
}
function L9(t, n4) {
if (typeof t == "number")
return n4;
if (t[0] === 1 && typeof n4 != "number") {
if (n4[0] === 0)
return CL(n4, t);
var e = Ce0(n4), i = AL(n4);
return ai(t, e[1], e[2], i);
}
return typeof n4 == "number" ? t : NL(t, n4);
}
function ib(t, n4, e, i) {
return e ? ai(t, n4, e[1], i) : L9(t, i);
}
function je0(t, n4) {
for (var e = n4; ; ) {
if (typeof e == "number")
return 0;
if (e[0] === 0)
return a(t, e[1], e[2]);
je0(t, e[4]), a(t, e[2], e[3]);
var e = e[5];
}
}
function PL(t, n4) {
if (typeof n4 == "number")
return 0;
if (n4[0] === 0) {
var e = u(t, n4[2]);
return [0, n4[1], e];
}
var i = PL(t, n4[4]), x = u(t, n4[3]), c = PL(t, n4[5]);
return [1, n4[1], n4[2], x, i, c];
}
function DL(t, n4) {
if (typeof n4 == "number")
return 0;
if (n4[0] === 0) {
var e = n4[1];
return [0, e, a(t, e, n4[2])];
}
var i = n4[2], x = DL(t, n4[4]), c = a(t, i, n4[3]), s = DL(t, n4[5]);
return [1, n4[1], i, c, x, s];
}
function LL(t, n4, e) {
for (var i = n4, x = e; ; ) {
if (typeof i == "number")
return x;
if (i[0] === 0)
return ir(t, i[1], i[2], x);
var c = LL(t, i[4], x), s = ir(t, i[2], i[3], c), i = i[5], x = s;
}
}
function Ge0(t, n4) {
for (var e = t, i = n4; ; ) {
if (typeof i == "number")
return e;
if (i[0] === 0)
return [0, i[1], e];
var x = i[4], c = Ge0(e, i[5]), e = [0, i[2], c], i = x;
}
}
function Me0(t) {
return Ge0(0, t);
}
function Be0(t, n4) {
for (var e = n4; ; ) {
if (typeof e == "number")
return 1;
if (e[0] === 0)
return a(t, e[1], e[2]);
var i = a(t, e[2], e[3]);
if (i) {
var x = Be0(t, e[4]);
if (x) {
var e = e[5];
continue;
}
var c = x;
} else
var c = i;
return c;
}
}
function qe0(t, n4) {
for (var e = n4; ; ) {
if (typeof e == "number")
return 0;
if (e[0] === 0)
return a(t, e[1], e[2]);
var i = a(t, e[2], e[3]);
if (i)
var x = i;
else {
var c = qe0(t, e[4]);
if (!c) {
var e = e[5];
continue;
}
var x = c;
}
return x;
}
}
function RL(t, n4) {
if (typeof n4 == "number")
return 0;
if (n4[0] === 0)
return a(t, n4[1], n4[2]) ? n4 : x7;
var e = n4[5], i = n4[4], x = n4[3], c = n4[2], s = RL(t, i), p = a(t, c, x), y = RL(t, e);
return p ? i === s && e === y ? n4 : ai(s, c, x, y) : L9(s, y);
}
function jL(t) {
if (typeof t == "number")
return 0;
if (t[0] === 0)
return 1;
var n4 = jL(t[5]);
return (jL(t[4]) + 1 | 0) + n4 | 0;
}
function Ue0(t, n4) {
for (var e = t, i = n4; ; ) {
if (typeof i == "number")
return e;
if (i[0] === 0)
return [0, i, e];
var x = i[4], c = Ue0(e, i[5]), e = [0, [0, i[2], i[3]], c], i = x;
}
}
function yne(t) {
return Ue0(0, t);
}
var R9 = function(t) {
function n4(A, S, M) {
if (typeof M == "number")
return [0, A, S];
if (M[0] === 0) {
var K = M[1], V = a(t[1], A, K);
return V === 0 ? M[2] === S ? M : [0, K, S] : 0 <= V ? tb(M, A, S) : ub(A, S, M);
}
var f0 = M[5], m0 = M[4], k0 = M[3], g0 = M[2], e0 = a(t[1], A, g0);
if (e0 === 0)
return k0 === S ? M : [1, M[1], A, S, m0, f0];
if (0 <= e0) {
var x0 = n4(A, S, f0);
return f0 === x0 ? M : Ou(m0, g0, k0, x0);
}
var l = n4(A, S, m0);
return m0 === l ? M : Ou(l, g0, k0, f0);
}
function e(A, S) {
for (var M = S; ; ) {
if (typeof M == "number")
throw Kt;
if (M[0] === 0) {
if (a(t[1], A, M[1]) === 0)
return M[2];
throw Kt;
}
var K = a(t[1], A, M[2]);
if (K === 0)
return M[3];
var V = 0 <= K ? M[5] : M[4], M = V;
}
}
function i(A, S) {
for (var M = S; ; ) {
if (typeof M == "number")
return 0;
if (M[0] === 0) {
var K = a(t[1], A, M[1]) === 0 ? 1 : 0, V = K && [0, M[2]];
return V;
}
var f0 = a(t[1], A, M[2]);
if (f0 === 0)
return [0, M[3]];
var m0 = 0 <= f0 ? M[5] : M[4], M = m0;
}
}
function x(A, S) {
for (var M = S; ; ) {
if (typeof M == "number")
return 0;
if (M[0] === 0)
return a(t[1], A, M[1]) === 0 ? 1 : 0;
var K = a(t[1], A, M[2]), V = K === 0 ? 1 : 0;
if (V)
return V;
var f0 = 0 <= K ? M[5] : M[4], M = f0;
}
}
function c(A, S) {
if (typeof S == "number")
return S;
if (S[0] === 0)
return a(t[1], A, S[1]) === 0 ? x7 : S;
var M = S[5], K = S[4], V = S[3], f0 = S[2], m0 = a(t[1], A, f0);
if (m0 === 0)
return Re0(K, M);
if (0 <= m0) {
var k0 = c(A, M);
return M === k0 ? S : Ou(K, f0, V, k0);
}
var g0 = c(A, K);
return K === g0 ? S : Ou(g0, f0, V, M);
}
function s(A, S, M) {
if (typeof M == "number")
return [0, A, u(S, 0)];
if (M[0] === 0) {
var K = M[2], V = M[1], f0 = a(t[1], A, V);
if (f0 === 0) {
var m0 = u(S, [0, K]);
return K === m0 ? M : [0, V, m0];
}
var k0 = u(S, 0);
return 0 <= f0 ? tb(M, A, k0) : ub(A, k0, M);
}
var g0 = M[5], e0 = M[4], x0 = M[3], l = M[2], c0 = a(t[1], A, l);
if (c0 === 0) {
var t0 = u(S, [0, x0]);
return x0 === t0 ? M : [1, M[1], A, t0, e0, g0];
}
if (0 <= c0) {
var a0 = s(A, S, g0);
return g0 === a0 ? M : Ou(e0, l, x0, a0);
}
var w0 = s(A, S, e0);
return e0 === w0 ? M : Ou(w0, l, x0, g0);
}
function p(A, S, M) {
if (typeof M == "number") {
var K = u(S, 0);
return K && [0, A, K[1]];
} else {
if (M[0] === 0) {
var V = M[2], f0 = M[1], m0 = a(t[1], A, f0);
if (m0 === 0) {
var k0 = u(S, [0, V]);
if (k0) {
var g0 = k0[1];
return V === g0 ? M : [0, f0, g0];
}
return x7;
}
var e0 = u(S, 0);
if (e0) {
var x0 = e0[1];
return 0 <= m0 ? tb(M, A, x0) : ub(A, x0, M);
}
return M;
}
var l = M[5], c0 = M[4], t0 = M[3], a0 = M[2], w0 = a(t[1], A, a0);
if (w0 === 0) {
var _0 = u(S, [0, t0]);
if (_0) {
var E0 = _0[1];
return t0 === E0 ? M : [1, M[1], A, E0, c0, l];
}
return Re0(c0, l);
}
if (0 <= w0) {
var X0 = p(A, S, l);
return l === X0 ? M : Ou(c0, a0, t0, X0);
}
var b = p(A, S, c0);
return c0 === b ? M : Ou(b, a0, t0, l);
}
}
function y(A, S) {
if (typeof S == "number")
return HQr;
if (S[0] === 0) {
var M = a(t[1], A, S[1]);
return M === 0 ? [0, x7, [0, S[2]], x7] : 0 <= M ? [0, S, 0, x7] : [0, x7, 0, S];
}
var K = S[5], V = S[4], f0 = S[3], m0 = S[2], k0 = a(t[1], A, m0);
if (k0 === 0)
return [0, V, [0, f0], K];
if (0 <= k0) {
var g0 = y(A, K), e0 = g0[3], x0 = g0[2];
return [0, ai(V, m0, f0, g0[1]), x0, e0];
}
var l = y(A, V), c0 = ai(l[3], m0, f0, K);
return [0, l[1], l[2], c0];
}
function T(A, S, M) {
if (typeof S == "number") {
if (typeof M == "number")
return 0;
if (M[0] === 0) {
var K = M[1], V = ir(A, K, 0, [0, M[2]]);
return V ? [0, K, V[1]] : x7;
}
} else if (S[0] === 0) {
var f0 = S[2], m0 = S[1];
if (typeof M == "number") {
var k0 = ir(A, m0, [0, f0], 0);
return k0 ? [0, m0, k0[1]] : x7;
} else if (M[0] === 0) {
var g0 = y(m0, M), e0 = T(A, x7, g0[3]), x0 = ir(A, m0, [0, f0], g0[2]);
return ib(T(A, x7, g0[1]), m0, x0, e0);
}
} else {
var l = S[2], c0 = gi(M);
if (c0 <= S[1]) {
var t0 = y(l, M), a0 = T(A, S[5], t0[3]), w0 = ir(A, l, [0, S[3]], t0[2]);
return ib(T(A, S[4], t0[1]), l, w0, a0);
}
var _0 = 0;
if ((typeof M == "number" || M[0] !== 1) && (_0 = 1), _0)
throw [0, wn, XQr];
}
var E0 = M[2], X0 = y(E0, S), b = T(A, X0[3], M[5]), G0 = ir(A, E0, X0[2], [0, M[3]]);
return ib(T(A, X0[1], M[4]), E0, G0, b);
}
function E(A, S, M) {
var K = 0;
if (typeof S == "number") {
var _0 = M;
K = 1;
} else if (S[0] === 1) {
var V = S[3], f0 = S[2];
if (typeof M != "number" && M[0] !== 0) {
var m0 = M[3], k0 = M[2];
if (M[1] <= S[1]) {
var g0 = y(f0, M), e0 = g0[2], x0 = E(A, S[4], g0[1]), l = E(A, S[5], g0[3]);
return e0 ? ib(x0, f0, ir(A, f0, V, e0[1]), l) : ai(x0, f0, V, l);
}
var c0 = y(k0, S), t0 = c0[2], a0 = E(A, c0[1], M[4]), w0 = E(A, c0[3], M[5]);
return t0 ? ib(a0, k0, ir(A, k0, t0[1], m0), w0) : ai(a0, k0, m0, w0);
}
}
if (!K)
if (typeof M == "number")
var _0 = S;
else {
if (M[0] === 0) {
var E0 = M[2], X0 = M[1];
return p(X0, function(X) {
return X ? ir(A, X0, X[1], E0) : [0, E0];
}, S);
}
var b = S[2], G0 = S[1];
return p(G0, function(X) {
return X ? ir(A, G0, b, X[1]) : [0, b];
}, M);
}
return _0;
}
function h(A, S) {
if (typeof S == "number")
return YQr;
if (S[0] === 0)
return a(A, S[1], S[2]) ? [0, S, x7] : [0, x7, S];
var M = S[3], K = S[2], V = h(A, S[4]), f0 = V[2], m0 = V[1], k0 = a(A, K, M), g0 = h(A, S[5]), e0 = g0[2], x0 = g0[1];
if (k0) {
var l = L9(f0, e0);
return [0, ai(m0, K, M, x0), l];
}
var c0 = ai(f0, K, M, e0);
return [0, L9(m0, x0), c0];
}
function w(A, S, M) {
for (var K = Xs(M, 0), V = Xs(S, 0), f0 = K; ; ) {
if (V) {
if (f0) {
var m0 = a(t[1], V[1], f0[1]);
if (m0 === 0) {
var k0 = a(A, V[2], f0[2]);
if (k0 === 0) {
var g0 = Xs(f0[3], f0[4]), V = Xs(V[3], V[4]), f0 = g0;
continue;
}
return k0;
}
return m0;
}
return 1;
}
var e0 = f0 && -1;
return e0;
}
}
function G(A, S, M) {
for (var K = Xs(M, 0), V = Xs(S, 0), f0 = K; ; ) {
if (V) {
if (f0) {
var m0 = a(t[1], V[1], f0[1]) === 0 ? 1 : 0;
if (m0) {
var k0 = a(A, V[2], f0[2]);
if (k0) {
var g0 = Xs(f0[3], f0[4]), V = Xs(V[3], V[4]), f0 = g0;
continue;
}
var e0 = k0;
} else
var e0 = m0;
} else
var e0 = f0;
return e0;
}
return f0 ? 0 : 1;
}
}
return [0, x7, pne, x, n4, p, s, bne, c, T, E, w, G, je0, LL, Be0, qe0, RL, h, jL, yne, Ne0, Pe0, mne, _ne, Me0, Me0, function(A, S, M) {
var K = [0, x7, 0], V = LL(function(f0, m0, k0) {
var g0 = k0[1], e0 = u(S, f0), x0 = k0[2], l = x0 || (e0 !== f0 ? 1 : 0);
if (A)
var c0 = A[1], t0 = s(e0, function(a0) {
return a0 ? a(c0, a0[1], m0) : m0;
}, g0);
else
var t0 = n4(e0, m0, g0);
return [0, t0, l];
}, M, K);
return V[2] ? V[1] : M;
}, Ne0, Pe0, y, e, i, PL, DL, IL];
}([0, dz]);
function o2(t) {
return [0, G1(t)];
}
var GL = uL([0, function(t, n4) {
var e = Z00(t[1], n4[1]);
return e === 0 ? a(ar0, t[2], n4[2]) : e;
}]);
function fb(t, n4, e) {
var i = e[2][1];
return qn(i, gre) ? n4 : a(Gu[3], i, n4) ? (ue(t, [0, e[1], [19, i]]), n4) : a(Gu[4], i, n4);
}
function ML(t, n4) {
var e = n4[2];
switch (e[0]) {
case 0:
var i = e[1][1];
return be(function(c, s) {
var p = s[0] === 0 ? s[1][2][2] : s[1][2][1];
return ML(c, p);
}, t, i);
case 1:
var x = e[1][1];
return be(function(c, s) {
return s[0] === 2 ? c : ML(c, s[1][2][1]);
}, t, x);
case 2:
return [0, e[1][1], t];
default:
return ke(Sre);
}
}
var se = bu(Tre, Fre), ln = sne(se), Vn = function(t) {
var n4 = [0, o$r, Gu[1], 0, 0];
function e(a0) {
var w0 = N0(a0);
if (typeof w0 == "number") {
var _0 = 0;
if (8 <= w0 ? 10 <= w0 || (_0 = 1) : w0 === 1 && (_0 = 1), _0)
return 1;
}
return 0;
}
function i(a0) {
var w0 = V7(a0), _0 = N0(a0), E0 = 0;
if (typeof _0 == "number") {
var X0 = 0;
if (_0 === 82 ? V0(a0, 82) : _0 === 86 ? (Ge(a0, [4, w0[2][1]]), V0(a0, 86)) : X0 = 1, !X0) {
var b = De(a0), G0 = pr(a0), X = N0(a0), s0 = 0;
if (typeof X == "number")
switch (X) {
case 30:
case 31:
ie(a0);
var dr = we(a0), Ar = e(a0) ? [1, b, [0, X === 31 ? 1 : 0, lr([0, G0], [0, dr], 0)]] : [0, b], ar = Ar;
break;
default:
s0 = 1;
}
else
switch (X[0]) {
case 0:
var W0 = X[2], Lr = ir(se[24], a0, X[1], W0), Tr = we(a0), Hr = e(a0) ? [2, b, [0, Lr, W0, lr([0, G0], [0, Tr], 0)]] : [0, b], ar = Hr;
break;
case 2:
var Or = X[1], xr = Or[1];
Or[4] && Si(a0, 45), ie(a0);
var Rr = we(a0);
if (e(a0))
var Wr = lr([0, G0], [0, Rr], 0), Jr = [3, xr, [0, Or[2], Or[3], Wr]];
else
var Jr = [0, xr];
var ar = Jr;
break;
default:
s0 = 1;
}
if (s0) {
ie(a0);
var ar = [0, b];
}
var or = ar;
E0 = 1;
}
}
if (!E0)
var or = 0;
return [0, w0, or];
}
var x = 0;
function c(a0) {
var w0 = pr(a0);
V0(a0, 48);
var _0 = a(se[13], 0, a0), E0 = _0[2][1], X0 = _0[1], b = cr(0, function(G0) {
var X = fu(G0, 63);
if (X) {
zu(G0, 1);
var s0 = N0(G0), dr = 0;
if (typeof s0 == "number")
switch (s0) {
case 117:
var Ar = u$r;
break;
case 119:
var Ar = i$r;
break;
case 121:
var Ar = f$r;
break;
default:
dr = 1;
}
else
switch (s0[0]) {
case 4:
Ge(G0, [3, E0, [0, s0[2]]]);
var Ar = 0;
break;
case 9:
if (s0[1])
var Ar = x$r;
else
dr = 1;
break;
default:
dr = 1;
}
if (dr) {
Ge(G0, [3, E0, 0]);
var Ar = 0;
}
ie(G0), h7(G0);
var ar = Ar;
} else
var ar = X;
var W0 = ar !== 0 ? 1 : 0, Lr = W0 && pr(G0);
V0(G0, 0);
for (var Tr = n4; ; ) {
var Hr = N0(G0);
if (typeof Hr == "number") {
var Or = Hr - 2 | 0;
if (Ht < Or >>> 0) {
if (!(F7 < (Or + 1 | 0) >>> 0)) {
var xr = Tr[3], Rr = Tr[4], Wr = de(Tr[1][4]), Jr = de(Tr[1][3]), or = de(Tr[1][2]), _r = de(Tr[1][1]), Ir = un(Rr, pr(G0));
V0(G0, 1);
var fe = N0(G0), v0 = 0;
if (typeof fe == "number") {
var P = 0;
if (fe !== 1 && Pn !== fe && (v0 = 1, P = 1), !P)
var Q = we(G0);
} else
v0 = 1;
if (v0)
var L = f7(G0), Q = L && Us(G0);
var i0 = _u([0, Lr], [0, Q], Ir, 0);
if (ar)
switch (ar[1]) {
case 0:
return [0, [0, _r, 1, xr, i0]];
case 1:
return [1, [0, or, 1, xr, i0]];
case 2:
var l0 = 1;
break;
default:
return [3, [0, Wr, xr, i0]];
}
else {
var S0 = Rc(_r), T0 = Rc(or), rr = Rc(Jr), R0 = Rc(Wr), B = 0;
if (S0 === 0 && T0 === 0) {
var Z = 0;
if (rr === 0 && R0 === 0 && (B = 1, Z = 1), !Z) {
var l0 = 0;
B = 2;
}
}
var p0 = 0;
switch (B) {
case 0:
if (T0 === 0 && rr === 0 && R0 <= S0)
return Pu(function(K0) {
return ue(G0, [0, K0[1], [0, E0, K0[2][1][2][1]]]);
}, Wr), [0, [0, _r, 0, xr, i0]];
if (S0 === 0 && rr === 0 && R0 <= T0)
return Pu(function(K0) {
return ue(G0, [0, K0[1], [8, E0, K0[2][1][2][1]]]);
}, Wr), [1, [0, or, 0, xr, i0]];
ue(G0, [0, X0, [2, E0]]);
break;
case 1:
break;
default:
p0 = 1;
}
if (!p0)
return [2, [0, a$r, 0, xr, i0]];
}
var b0 = Rc(Jr), O0 = Rc(Wr);
if (b0 !== 0) {
var q0 = 0;
if (O0 !== 0 && (b0 < O0 ? (Pu(function(K0) {
return ue(G0, [0, K0[1], [9, E0]]);
}, Jr), q0 = 1) : Pu(function(K0) {
return ue(G0, [0, K0[1], [9, E0]]);
}, Wr)), !q0)
return [2, [0, [1, Jr], l0, xr, i0]];
}
return [2, [0, [0, Wr], l0, xr, i0]];
}
} else if (Or === 10) {
var er = De(G0), yr = pr(G0);
ie(G0);
var vr = N0(G0), $0 = 0;
if (typeof vr == "number") {
var Sr = vr - 2 | 0, Mr = 0;
if (Ht < Sr >>> 0)
F7 < (Sr + 1 | 0) >>> 0 && (Mr = 1);
else if (Sr === 7) {
V0(G0, 9);
var Br = N0(G0), qr = 0;
if (typeof Br == "number") {
var jr = 0;
if (Br !== 1 && Pn !== Br && (jr = 1), !jr) {
var $r = 1;
qr = 1;
}
}
if (!qr)
var $r = 0;
ue(G0, [0, er, [7, $r]]);
} else
Mr = 1;
Mr || ($0 = 1);
}
$0 || ue(G0, [0, er, n$r]);
var Tr = [0, Tr[1], Tr[2], 1, yr];
continue;
}
}
var ne = Tr[2], Qr = Tr[1], pe = cr(x, i, G0), oe = pe[2], me = oe[1], ae = me[2][1];
if (qn(ae, t$r))
var ce = Tr;
else {
var ge = me[1], H0 = oe[2], Fr = pe[1], _ = Ot(ae, 0), k = 97 <= _ ? 1 : 0, I = k && (_ <= In ? 1 : 0);
I && ue(G0, [0, ge, [6, E0, ae]]), a(Gu[3], ae, ne) && ue(G0, [0, ge, [1, E0, ae]]);
var U = Tr[4], Y = Tr[3], y0 = a(Gu[4], ae, ne), D0 = [0, Tr[1], y0, Y, U], I0 = function(Ur) {
return function(d0, Kr) {
return ar && ar[1] !== d0 ? ue(G0, [0, Kr, [5, E0, ar, Ur]]) : 0;
};
}(ae);
if (typeof H0 == "number") {
var D = 0;
if (ar) {
var u0 = ar[1], Y0 = 0;
if (u0 === 1 ? ue(G0, [0, Fr, [8, E0, ae]]) : u0 ? (D = 1, Y0 = 1) : ue(G0, [0, Fr, [0, E0, ae]]), !Y0)
var J0 = D0;
} else
D = 1;
if (D)
var J0 = [0, [0, Qr[1], Qr[2], Qr[3], [0, [0, Fr, [0, me]], Qr[4]]], y0, Y, U];
} else
switch (H0[0]) {
case 0:
ue(G0, [0, H0[1], [5, E0, ar, ae]]);
var J0 = D0;
break;
case 1:
var fr = H0[1];
I0(0, fr);
var J0 = [0, [0, [0, [0, Fr, [0, me, [0, fr, H0[2]]]], Qr[1]], Qr[2], Qr[3], Qr[4]], y0, Y, U];
break;
case 2:
var Q0 = H0[1];
I0(1, Q0);
var J0 = [0, [0, Qr[1], [0, [0, Fr, [0, me, [0, Q0, H0[2]]]], Qr[2]], Qr[3], Qr[4]], y0, Y, U];
break;
default:
var F0 = H0[1];
I0(2, F0);
var J0 = [0, [0, Qr[1], Qr[2], [0, [0, Fr, [0, me, [0, F0, H0[2]]]], Qr[3]], Qr[4]], y0, Y, U];
}
var ce = J0;
}
var gr = N0(G0), mr = 0;
if (typeof gr == "number") {
var Cr = gr - 2 | 0, sr = 0;
Ht < Cr >>> 0 ? F7 < (Cr + 1 | 0) >>> 0 && (sr = 1) : Cr === 6 ? (Ge(G0, 1), V0(G0, 8)) : sr = 1, sr || (mr = 1);
}
mr || V0(G0, 9);
var Tr = ce;
}
}, a0);
return [16, [0, _0, b, lr([0, w0], 0, 0)]];
}
var s = 0;
function p(a0) {
return cr(s, c, a0);
}
function y(a0, w0) {
var _0 = w0[2][1], E0 = w0[1], X0 = a0[1];
Bs(_0) && Y7(X0, [0, E0, 41]);
var b = I9(_0), G0 = b || f2(_0);
return G0 && Y7(X0, [0, E0, 55]), [0, X0, a0[2]];
}
function T(a0, w0) {
var _0 = w0[2];
switch (_0[0]) {
case 0:
return be(E, a0, _0[1][1]);
case 1:
return be(h, a0, _0[1][1]);
case 2:
var E0 = _0[1][1], X0 = E0[2][1], b = a0[2], G0 = a0[1];
a(Gu[3], X0, b) && ue(G0, [0, E0[1], 42]);
var X = y([0, G0, b], E0), s0 = a(Gu[4], X0, X[2]);
return [0, X[1], s0];
default:
return ue(a0[1], [0, w0[1], 31]), a0;
}
}
function E(a0, w0) {
if (w0[0] === 0) {
var _0 = w0[1][2], E0 = _0[1], X0 = E0[0] === 1 ? y(a0, E0[1]) : a0;
return T(X0, _0[2]);
}
return T(a0, w0[1][2][1]);
}
function h(a0, w0) {
return w0[0] === 2 ? a0 : T(a0, w0[1][2][1]);
}
function w(a0, w0, _0, E0) {
var X0 = a0[5], b = U1(E0), G0 = E0[2], X = G0[3], s0 = ys(X0 ? 0 : w0, a0), dr = w0 || X0 || 1 - b;
if (dr) {
if (_0) {
var Ar = _0[1], ar = Ar[2][1], W0 = Ar[1];
Bs(ar) && Y7(s0, [0, W0, 44]);
var Lr = I9(ar), Tr = Lr || f2(ar);
Tr && Y7(s0, [0, W0, 55]);
}
var Hr = G0[2], Or = [0, s0, Gu[1]], xr = be(function(or, _r) {
return T(or, _r[2][1]);
}, Or, Hr), Rr = X && (T(xr, X[1][2][1]), 0), Wr = Rr;
} else
var Wr = dr;
return Wr;
}
var G = function a0(w0, _0) {
return a0.fun(w0, _0);
};
function A(a0) {
N0(a0) === 21 && Ge(a0, c7);
var w0 = a(se[18], a0, 41), _0 = N0(a0) === 82 ? 1 : 0, E0 = _0 && (V0(a0, 82), [0, u(se[10], a0)]);
return [0, w0, E0];
}
var S = 0;
N(G, function(a0, w0) {
var _0 = N0(a0);
if (typeof _0 == "number") {
var E0 = _0 - 5 | 0, X0 = 0;
if (7 < E0 >>> 0 ? fs2 === E0 && (X0 = 1) : 5 < (E0 - 1 | 0) >>> 0 && (X0 = 1), X0) {
var b = _0 === 12 ? 1 : 0;
if (b)
var G0 = pr(a0), X = cr(0, function(ar) {
return V0(ar, 12), a(se[18], ar, 41);
}, a0), s0 = lr([0, G0], 0, 0), dr = [0, [0, X[1], [0, X[2], s0]]];
else
var dr = b;
return N0(a0) !== 5 && Ge(a0, 64), [0, de(w0), dr];
}
}
var Ar = cr(S, A, a0);
return N0(a0) !== 5 && V0(a0, 9), a(G, a0, [0, Ar, w0]);
});
function M(a0, w0) {
function _0(X0) {
var b = dL(w0, ie0(a0, X0)), G0 = 1, X = b[10] === 1 ? b : [0, b[1], b[2], b[3], b[4], b[5], b[6], b[7], b[8], b[9], G0, b[11], b[12], b[13], b[14], b[15], b[16], b[17], b[18], b[19], b[20], b[21], b[22], b[23], b[24], b[25], b[26], b[27], b[28], b[29], b[30]], s0 = pr(X);
V0(X, 4);
var dr = iu(X), Ar = dr && (N0(X) === 21 ? 1 : 0);
if (Ar) {
var ar = pr(X), W0 = cr(0, function(or) {
return V0(or, 21), N0(or) === 86 ? [0, u(t[9], or)] : (Ge(or, Ri), 0);
}, X), Lr = W0[2];
if (Lr) {
N0(X) === 9 && ie(X);
var Tr = lr([0, ar], 0, 0), Hr = [0, [0, W0[1], [0, Lr[1], Tr]]];
} else
var Hr = Lr;
var Or = Hr;
} else
var Or = Ar;
var xr = a(G, X, 0), Rr = pr(X);
V0(X, 5);
var Wr = _u([0, s0], [0, we(X)], Rr, 0);
return [0, Or, xr[1], xr[2], Wr];
}
var E0 = 0;
return function(X0) {
return cr(E0, _0, X0);
};
}
function K(a0, w0, _0, E0, X0) {
var b = se0(a0, w0, _0, X0), G0 = a(se[16], E0, b);
return [0, [0, G0[1]], G0[2]];
}
function V(a0, w0, _0) {
var E0 = De(a0), X0 = N0(a0), b = 0;
if (typeof X0 == "number")
if (c7 === X0) {
var G0 = pr(a0);
ie(a0);
var s0 = [0, [0, E0, [0, 0, lr([0, G0], 0, 0)]]];
} else if (D7 === X0) {
var X = pr(a0);
ie(a0);
var s0 = [0, [0, E0, [0, 1, lr([0, X], 0, 0)]]];
} else
b = 1;
else
b = 1;
if (b)
var s0 = 0;
if (s0) {
var dr = 0;
if (!w0 && !_0 && (dr = 1), !dr)
return ue(a0, [0, s0[1][1], 7]), 0;
}
return s0;
}
function f0(a0) {
if (Xt === N0(a0)) {
var w0 = pr(a0);
return ie(a0), [0, 1, w0];
}
return M$r;
}
function m0(a0) {
if (N0(a0) === 64 && !Jl(1, a0)) {
var w0 = pr(a0);
return ie(a0), [0, 1, w0];
}
return G$r;
}
function k0(a0) {
var w0 = m0(a0), _0 = w0[1], E0 = w0[2], X0 = cr(0, function(W0) {
var Lr = pr(W0);
V0(W0, 15);
var Tr = f0(W0), Hr = Tr[1], Or = pl([0, E0, [0, Lr, [0, Tr[2], 0]]]), xr = W0[7], Rr = N0(W0), Wr = 0;
if (xr && typeof Rr == "number") {
if (Rr === 4) {
var Ir = 0, fe = 0;
Wr = 1;
} else if (Rr === 98) {
var Jr = xi(W0, u(t[3], W0)), or = N0(W0) === 4 ? 0 : [0, ds(W0, a(se[13], D$r, W0))], Ir = or, fe = Jr;
Wr = 1;
}
}
if (!Wr)
var _r = M1(W0) ? ds(W0, a(se[13], L$r, W0)) : (de0(W0, R$r), [0, De(W0), j$r]), Ir = [0, _r], fe = xi(W0, u(t[3], W0));
var v0 = u(M(_0, Hr), W0), P = N0(W0) === 86 ? v0 : eb(W0, v0), L = u(t[12], W0), Q = L[2], i0 = L[1];
if (Q)
var l0 = Se0(W0, Q), S0 = i0;
else
var l0 = Q, S0 = a2(W0, i0);
return [0, Hr, fe, Ir, P, S0, l0, Or];
}, a0), b = X0[2], G0 = b[4], X = b[3], s0 = b[1], dr = K(a0, _0, s0, 0, U1(G0));
w(a0, dr[2], X, G0);
var Ar = X0[1], ar = lr([0, b[7]], 0, 0);
return [23, [0, X, G0, dr[1], _0, s0, b[6], b[5], b[2], ar, Ar]];
}
var g0 = 0;
function e0(a0) {
return cr(g0, k0, a0);
}
function x0(a0, w0) {
var _0 = pr(w0);
V0(w0, a0);
for (var E0 = 0, X0 = 0; ; ) {
var b = cr(0, function(ar) {
var W0 = a(se[18], ar, 40);
if (fu(ar, 82))
var Lr = 0, Tr = [0, u(se[10], ar)];
else if (W0[2][0] === 2)
var Lr = 0, Tr = 0;
else
var Lr = [0, [0, W0[1], 59]], Tr = 0;
return [0, [0, W0, Tr], Lr];
}, w0), G0 = b[2], X = G0[2], s0 = [0, [0, b[1], G0[1]], E0], dr = X ? [0, X[1], X0] : X0;
if (fu(w0, 9)) {
var E0 = s0, X0 = dr;
continue;
}
var Ar = de(dr);
return [0, de(s0), _0, Ar];
}
}
var l = 24;
function c0(a0) {
return x0(l, a0);
}
function t0(a0) {
var w0 = x0(27, T9(1, a0)), _0 = w0[1], E0 = w0[3], X0 = de(be(function(b, G0) {
return G0[2][2] ? b : [0, [0, G0[1], 58], b];
}, E0, _0));
return [0, _0, w0[2], X0];
}
return [0, m0, f0, V, M, K, w, function(a0) {
return x0(28, T9(1, a0));
}, t0, c0, e0, p];
}(ln), j9 = vne(se), oi = function(t) {
function n4(P) {
var L = P[2];
switch (L[0]) {
case 17:
var Q = L[1], i0 = Q[1][2][1];
if (n0(i0, AQr)) {
if (!n0(i0, NQr)) {
var l0 = n0(Q[2][2][1], CQr);
if (!l0)
return l0;
}
} else {
var S0 = n0(Q[2][2][1], PQr);
if (!S0)
return S0;
}
break;
case 0:
case 10:
case 16:
case 19:
break;
default:
return 0;
}
return 1;
}
var e = t[1], i = function P(L) {
return P.fun(L);
}, x = function P(L) {
return P.fun(L);
}, c = function P(L) {
return P.fun(L);
}, s = function P(L) {
return P.fun(L);
}, p = function P(L) {
return P.fun(L);
}, y = function P(L) {
return P.fun(L);
}, T = function P(L) {
return P.fun(L);
}, E = function P(L) {
return P.fun(L);
}, h = function P(L) {
return P.fun(L);
}, w = function P(L) {
return P.fun(L);
}, G = function P(L) {
return P.fun(L);
}, A = function P(L) {
return P.fun(L);
}, S = function P(L) {
return P.fun(L);
}, M = function P(L) {
return P.fun(L);
}, K = function P(L) {
return P.fun(L);
}, V = function P(L) {
return P.fun(L);
}, f0 = function P(L) {
return P.fun(L);
}, m0 = function P(L, Q, i0, l0, S0) {
return P.fun(L, Q, i0, l0, S0);
}, k0 = function P(L, Q, i0, l0) {
return P.fun(L, Q, i0, l0);
}, g0 = function P(L) {
return P.fun(L);
}, e0 = function P(L) {
return P.fun(L);
}, x0 = function P(L) {
return P.fun(L);
}, l = function P(L, Q, i0, l0, S0) {
return P.fun(L, Q, i0, l0, S0);
}, c0 = function P(L, Q, i0, l0) {
return P.fun(L, Q, i0, l0);
}, t0 = function P(L) {
return P.fun(L);
}, a0 = function P(L, Q, i0) {
return P.fun(L, Q, i0);
}, w0 = function P(L) {
return P.fun(L);
}, _0 = function P(L, Q, i0) {
return P.fun(L, Q, i0);
}, E0 = function P(L) {
return P.fun(L);
}, X0 = function P(L) {
return P.fun(L);
}, b = function P(L, Q) {
return P.fun(L, Q);
}, G0 = function P(L, Q, i0, l0) {
return P.fun(L, Q, i0, l0);
}, X = function P(L) {
return P.fun(L);
}, s0 = function P(L, Q, i0) {
return P.fun(L, Q, i0);
}, dr = function P(L) {
return P.fun(L);
}, Ar = function P(L) {
return P.fun(L);
}, ar = function P(L) {
return P.fun(L);
}, W0 = function P(L, Q, i0) {
return P.fun(L, Q, i0);
}, Lr = t[2];
function Tr(P) {
var L = De(P), Q = u(y, P), i0 = u(p, P);
if (i0) {
var l0 = i0[1];
return [0, cr([0, L], function(S0) {
var T0 = ir(Lr, 0, S0, Q);
return [2, [0, l0, T0, u(x, S0), 0]];
}, P)];
}
return Q;
}
function Hr(P, L) {
if (typeof L == "number") {
var Q = L !== 55 ? 1 : 0;
if (!Q)
return Q;
}
throw Hs;
}
function Or(P) {
var L = O9(Hr, P), Q = Tr(L), i0 = N0(L);
if (typeof i0 == "number") {
if (i0 === 11)
throw Hs;
if (i0 === 86) {
var l0 = oe0(L), S0 = 0;
if (l0) {
var T0 = l0[1];
if (typeof T0 == "number" && T0 === 5) {
var rr = 1;
S0 = 1;
}
}
if (!S0)
var rr = 0;
if (rr)
throw Hs;
}
}
if (M1(L)) {
if (Q[0] === 0) {
var R0 = Q[1][2];
if (R0[0] === 10 && !n0(R0[1][2][1], IQr) && !f7(L))
throw Hs;
}
return Q;
}
return Q;
}
N(i, function(P) {
var L = N0(P), Q = 0, i0 = M1(P);
if (typeof L == "number") {
var l0 = 0;
if (22 <= L)
if (L === 58) {
if (P[17])
return [0, u(c, P)];
l0 = 1;
} else
L !== 98 && (l0 = 1);
else
L !== 4 && !(21 <= L) && (l0 = 1);
l0 || (Q = 1);
}
if (!Q && !i0)
return Tr(P);
var S0 = 0;
if (L === 64 && iu(P) && Yn(1, P) === 98) {
var T0 = Or, rr = ar;
S0 = 1;
}
if (!S0)
var T0 = ar, rr = Or;
var R0 = FL(P, rr);
if (R0)
return R0[1];
var B = FL(P, T0);
return B ? B[1] : Tr(P);
}), N(x, function(P) {
return a(e, P, u(i, P));
}), N(c, function(P) {
return cr(0, function(L) {
L[10] && Ge(L, 91);
var Q = pr(L), i0 = De(L);
V0(L, 58);
var l0 = De(L);
if (x2(L))
var S0 = 0, T0 = 0;
else {
var rr = fu(L, Xt), R0 = N0(L), B = 0;
if (typeof R0 == "number") {
var Z = 0;
if (R0 !== 86)
if (10 <= R0)
Z = 1;
else
switch (R0) {
case 0:
case 2:
case 3:
case 4:
case 6:
Z = 1;
break;
}
if (!Z) {
var p0 = 0;
B = 1;
}
}
if (!B)
var p0 = 1;
var b0 = rr || p0, O0 = b0 && [0, u(x, L)], S0 = rr, T0 = O0;
}
var q0 = T0 ? 0 : we(L), er = yt(i0, l0);
return [30, [0, T0, lr([0, Q], [0, q0], 0), S0, er]];
}, P);
}), N(s, function(P) {
var L = P[2];
switch (L[0]) {
case 17:
var Q = L[1], i0 = Q[1][2][1];
if (n0(i0, gQr)) {
if (!n0(i0, FQr)) {
var l0 = n0(Q[2][2][1], TQr);
if (!l0)
return l0;
}
} else {
var S0 = n0(Q[2][2][1], OQr);
if (!S0)
return S0;
}
break;
case 10:
case 16:
break;
default:
return 0;
}
return 1;
}), N(p, function(P) {
var L = N0(P), Q = 0;
if (typeof L == "number") {
var i0 = L - 67 | 0;
if (!(15 < i0 >>> 0)) {
switch (i0) {
case 0:
var l0 = oQr;
break;
case 1:
var l0 = cQr;
break;
case 2:
var l0 = sQr;
break;
case 3:
var l0 = vQr;
break;
case 4:
var l0 = lQr;
break;
case 5:
var l0 = bQr;
break;
case 6:
var l0 = pQr;
break;
case 7:
var l0 = mQr;
break;
case 8:
var l0 = _Qr;
break;
case 9:
var l0 = yQr;
break;
case 10:
var l0 = dQr;
break;
case 11:
var l0 = hQr;
break;
case 12:
var l0 = kQr;
break;
case 13:
var l0 = wQr;
break;
case 14:
var l0 = EQr;
break;
default:
var l0 = SQr;
}
var S0 = l0;
Q = 1;
}
}
if (!Q)
var S0 = 0;
return S0 !== 0 && ie(P), S0;
}), N(y, function(P) {
var L = De(P), Q = u(E, P);
if (N0(P) === 85) {
ie(P);
var i0 = u(x, Kl(0, P));
V0(P, 86);
var l0 = cr(0, x, P), S0 = yt(L, l0[1]), T0 = l0[2];
return [0, [0, S0, [7, [0, a(e, P, Q), i0, T0, 0]]]];
}
return Q;
}), N(T, function(P) {
return a(e, P, u(y, P));
});
function xr(P, L, Q, i0, l0) {
var S0 = a(e, P, L);
return [0, [0, l0, [15, [0, i0, S0, a(e, P, Q), 0]]]];
}
function Rr(P, L, Q, i0) {
for (var l0 = P, S0 = Q, T0 = i0; ; ) {
var rr = N0(L);
if (typeof rr == "number" && rr === 84) {
1 - l0 && Ge(L, aQr), V0(L, 84);
var R0 = cr(0, h, L), B = R0[2], Z = R0[1], p0 = N0(L), b0 = 0;
if (typeof p0 == "number" && !(1 < (p0 - 87 | 0) >>> 0)) {
Ge(L, [23, sL(p0)]);
var O0 = Jr(L, B, Z), q0 = Wr(L, O0[2], O0[1]), er = q0[2], yr = q0[1];
b0 = 1;
}
if (!b0)
var er = B, yr = Z;
var vr = yt(T0, yr), l0 = 1, S0 = xr(L, S0, er, 2, vr), T0 = vr;
continue;
}
return [0, T0, S0];
}
}
function Wr(P, L, Q) {
for (var i0 = L, l0 = Q; ; ) {
var S0 = N0(P);
if (typeof S0 == "number" && S0 === 87) {
ie(P);
var T0 = cr(0, h, P), rr = Jr(P, T0[2], T0[1]), R0 = yt(l0, rr[1]), B = Rr(0, P, xr(P, i0, rr[2], 0, R0), R0), i0 = B[2], l0 = B[1];
continue;
}
return [0, l0, i0];
}
}
function Jr(P, L, Q) {
for (var i0 = L, l0 = Q; ; ) {
var S0 = N0(P);
if (typeof S0 == "number" && S0 === 88) {
ie(P);
var T0 = cr(0, h, P), rr = yt(l0, T0[1]), R0 = Rr(0, P, xr(P, i0, T0[2], 1, rr), rr), i0 = R0[2], l0 = R0[1];
continue;
}
return [0, l0, i0];
}
}
N(E, function(P) {
var L = cr(0, h, P), Q = L[2], i0 = L[1], l0 = N0(P), S0 = 0;
if (typeof l0 == "number" && l0 === 84) {
var rr = Rr(1, P, Q, i0);
S0 = 1;
}
if (!S0)
var T0 = Jr(P, Q, i0), rr = Wr(P, T0[2], T0[1]);
return rr[2];
});
function or(P, L, Q, i0) {
return [0, i0, [3, [0, Q, P, L, 0]]];
}
N(h, function(P) {
var L = 0;
r:
for (; ; ) {
var Q = cr(0, function(k) {
var I = u(w, k) !== 0 ? 1 : 0;
return [0, I, u(G, Kl(0, k))];
}, P), i0 = Q[2], l0 = i0[2], S0 = Q[1];
if (N0(P) === 98) {
var T0 = 0;
l0[0] === 0 && l0[1][2][0] === 12 ? Ge(P, 63) : T0 = 1;
}
var rr = N0(P), R0 = 0;
if (typeof rr == "number") {
var B = rr - 17 | 0, Z = 0;
if (1 < B >>> 0)
if (72 <= B)
switch (B - 72 | 0) {
case 0:
var p0 = BZr;
break;
case 1:
var p0 = qZr;
break;
case 2:
var p0 = UZr;
break;
case 3:
var p0 = HZr;
break;
case 4:
var p0 = XZr;
break;
case 5:
var p0 = YZr;
break;
case 6:
var p0 = VZr;
break;
case 7:
var p0 = zZr;
break;
case 8:
var p0 = KZr;
break;
case 9:
var p0 = WZr;
break;
case 10:
var p0 = JZr;
break;
case 11:
var p0 = $Zr;
break;
case 12:
var p0 = ZZr;
break;
case 13:
var p0 = QZr;
break;
case 14:
var p0 = rQr;
break;
case 15:
var p0 = eQr;
break;
case 16:
var p0 = nQr;
break;
case 17:
var p0 = tQr;
break;
case 18:
var p0 = uQr;
break;
case 19:
var p0 = iQr;
break;
default:
Z = 1;
}
else
Z = 1;
else
var p0 = B ? fQr : P[12] ? 0 : xQr;
if (!Z) {
var b0 = p0;
R0 = 1;
}
}
if (!R0)
var b0 = 0;
if (b0 !== 0 && ie(P), !L && !b0)
return l0;
if (b0) {
var O0 = b0[1], q0 = O0[1], er = i0[1], yr = er && (q0 === 14 ? 1 : 0);
yr && ue(P, [0, S0, 27]);
for (var vr = a(e, P, l0), $0 = vr, Sr = [0, q0, O0[2]], Mr = S0, Br = L; ; ) {
var qr = Sr[2], jr = Sr[1];
if (Br) {
var $r = Br[1], ne = $r[2], Qr = ne[2], pe = Qr[0] === 0 ? Qr[1] : Qr[1] - 1 | 0;
if (qr[1] <= pe) {
var oe = yt($r[3], Mr), me = or($r[1], $0, ne[1], oe), $0 = me, Sr = [0, jr, qr], Mr = oe, Br = Br[2];
continue;
}
}
var L = [0, [0, $0, [0, jr, qr], Mr], Br];
continue r;
}
}
for (var ae = a(e, P, l0), ce = S0, ge = L; ; ) {
if (ge) {
var H0 = ge[1], Fr = yt(H0[3], ce), _ = ge[2], ae = or(H0[1], ae, H0[2][1], Fr), ce = Fr, ge = _;
continue;
}
return [0, ae];
}
}
}), N(w, function(P) {
var L = N0(P);
if (typeof L == "number") {
if (48 <= L) {
if (c7 <= L) {
if (!(vf <= L))
switch (L - 103 | 0) {
case 0:
return CZr;
case 1:
return PZr;
case 6:
return DZr;
case 7:
return LZr;
}
} else if (L === 65 && P[18])
return RZr;
} else if (45 <= L)
switch (L + mv | 0) {
case 0:
return jZr;
case 1:
return GZr;
default:
return MZr;
}
}
return 0;
}), N(G, function(P) {
var L = De(P), Q = pr(P), i0 = u(w, P);
if (i0) {
var l0 = i0[1];
ie(P);
var S0 = cr(0, A, P), T0 = S0[2], rr = yt(L, S0[1]), R0 = 0;
if (l0 === 6) {
var B = T0[2], Z = 0;
switch (B[0]) {
case 10:
Y7(P, [0, rr, 47]);
break;
case 16:
B[1][2][0] === 1 && ue(P, [0, rr, 88]);
break;
default:
Z = 1;
}
Z || (R0 = 1);
}
return [0, [0, rr, [28, [0, l0, T0, lr([0, Q], 0, 0)]]]];
}
var p0 = N0(P), b0 = 0;
if (typeof p0 == "number")
if (vf === p0)
var O0 = NZr;
else if (F7 === p0)
var O0 = AZr;
else
b0 = 1;
else
b0 = 1;
if (b0)
var O0 = 0;
if (O0) {
ie(P);
var q0 = cr(0, A, P), er = q0[2];
1 - u(s, er) && ue(P, [0, er[1], 26]);
var yr = er[2], vr = 0;
yr[0] === 10 && Bs(yr[1][2][1]) && (Si(P, 54), vr = 1);
var $0 = yt(L, q0[1]), Sr = lr([0, Q], 0, 0);
return [0, [0, $0, [29, [0, O0[1], er, 1, Sr]]]];
}
return u(S, P);
}), N(A, function(P) {
return a(e, P, u(G, P));
}), N(S, function(P) {
var L = u(M, P);
if (f7(P))
return L;
var Q = N0(P), i0 = 0;
if (typeof Q == "number")
if (vf === Q)
var l0 = IZr;
else if (F7 === Q)
var l0 = OZr;
else
i0 = 1;
else
i0 = 1;
if (i0)
var l0 = 0;
if (l0) {
var S0 = a(e, P, L);
1 - u(s, S0) && ue(P, [0, S0[1], 26]);
var T0 = S0[2], rr = 0;
T0[0] === 10 && Bs(T0[1][2][1]) && (Si(P, 53), rr = 1);
var R0 = De(P);
ie(P);
var B = we(P), Z = yt(S0[1], R0), p0 = lr(0, [0, B], 0);
return [0, [0, Z, [29, [0, l0[1], S0, 0, p0]]]];
}
return L;
}), N(M, function(P) {
var L = De(P), Q = 1 - P[16], i0 = 0, l0 = P[16] === 0 ? P : [0, P[1], P[2], P[3], P[4], P[5], P[6], P[7], P[8], P[9], P[10], P[11], P[12], P[13], P[14], P[15], i0, P[17], P[18], P[19], P[20], P[21], P[22], P[23], P[24], P[25], P[26], P[27], P[28], P[29], P[30]], S0 = N0(l0), T0 = 0;
if (typeof S0 == "number") {
var rr = S0 - 44 | 0;
if (!(7 < rr >>> 0)) {
var R0 = 0;
switch (rr) {
case 0:
if (Q)
var B = [0, u(g0, l0)];
else
R0 = 1;
break;
case 6:
var B = [0, u(f0, l0)];
break;
case 7:
var B = [0, u(V, l0)];
break;
default:
R0 = 1;
}
if (!R0) {
var Z = B;
T0 = 1;
}
}
}
if (!T0)
var Z = qs(l0) ? [0, u(t0, l0)] : u(E0, l0);
return b7(m0, 0, 0, l0, L, Z);
}), N(K, function(P) {
return a(e, P, u(M, P));
}), N(V, function(P) {
switch (P[21]) {
case 0:
var L = 0, Q = 0;
break;
case 1:
var L = 0, Q = 1;
break;
default:
var L = 1, Q = 1;
}
var i0 = De(P), l0 = pr(P);
V0(P, 51);
var S0 = [0, i0, [23, [0, lr([0, l0], [0, we(P)], 0)]]], T0 = N0(P);
if (typeof T0 == "number" && !(11 <= T0))
switch (T0) {
case 4:
var rr = L ? S0 : (ue(P, [0, i0, 5]), [0, i0, [10, Gc(0, [0, i0, EZr])]]);
return R(k0, SZr, P, i0, rr);
case 6:
case 10:
var R0 = Q ? S0 : (ue(P, [0, i0, 4]), [0, i0, [10, Gc(0, [0, i0, FZr])]]);
return R(k0, TZr, P, i0, R0);
}
return Q ? St(gZr, P) : ue(P, [0, i0, 4]), S0;
}), N(f0, function(P) {
return cr(0, function(L) {
var Q = pr(L), i0 = De(L);
if (V0(L, 50), fu(L, 10)) {
var l0 = Gc(0, [0, i0, hZr]), S0 = De(L);
Zl(L, kZr);
var T0 = Gc(0, [0, S0, wZr]);
return [17, [0, l0, T0, lr([0, Q], [0, we(L)], 0)]];
}
var rr = pr(L);
V0(L, 4);
var R0 = ir(s0, [0, rr], 0, u(x, Kl(0, L)));
return V0(L, 5), [11, [0, R0, lr([0, Q], [0, we(L)], 0)]];
}, P);
}), N(m0, function(P, L, Q, i0, l0) {
var S0 = P ? P[1] : 1, T0 = L && L[1], rr = b7(l, [0, S0], [0, T0], Q, i0, l0), R0 = oe0(Q), B = 0;
if (R0) {
var Z = R0[1];
if (typeof Z == "number" && Z === 83) {
var p0 = 1;
B = 1;
}
}
if (!B)
var p0 = 0;
function b0(vr) {
var $0 = Wt(vr);
function Sr(Br, qr) {
return a(Ze(Br, Di, 78), Br, qr);
}
var Mr = a(e, vr, rr);
return a($0[2], Mr, Sr);
}
function O0(vr, $0, Sr) {
var Mr = u(x0, $0), Br = Mr[1], qr = yt(i0, Br), jr = [0, Sr, vr, [0, Br, Mr[2]], 0], $r = 0;
if (!p0 && !T0) {
var ne = [4, jr];
$r = 1;
}
if (!$r)
var ne = [20, [0, jr, qr, p0]];
var Qr = T0 || p0;
return b7(m0, [0, S0], [0, Qr], $0, i0, [0, [0, qr, ne]]);
}
if (Q[13])
return rr;
var q0 = N0(Q);
if (typeof q0 == "number") {
var er = q0 - 98 | 0;
if (2 < er >>> 0) {
if (er === -94)
return O0(0, Q, b0(Q));
} else if (er !== 1 && iu(Q)) {
var yr = O9(function(vr, $0) {
throw Hs;
}, Q);
return we0(yr, rr, function(vr) {
var $0 = b0(vr);
return O0(u(e0, vr), vr, $0);
});
}
}
return rr;
}), N(k0, function(P, L, Q, i0) {
var l0 = P ? P[1] : 1;
return a(e, L, b7(m0, [0, l0], 0, L, Q, [0, i0]));
}), N(g0, function(P) {
return cr(0, function(L) {
var Q = De(L), i0 = pr(L);
if (V0(L, 44), L[11] && N0(L) === 10) {
var l0 = we(L);
ie(L);
var S0 = Gc(lr([0, i0], [0, l0], 0), [0, Q, mZr]), T0 = N0(L);
return typeof T0 != "number" && T0[0] === 4 && !n0(T0[3], _Zr) ? [17, [0, S0, a(se[13], 0, L), 0]] : (St(yZr, L), ie(L), [10, S0]);
}
var rr = De(L), R0 = N0(L), B = 0;
if (typeof R0 == "number")
if (R0 === 44)
var Z = u(g0, L);
else if (R0 === 51)
var Z = u(V, hL(1, L));
else
B = 1;
else
B = 1;
if (B)
var Z = qs(L) ? u(t0, L) : u(X0, L);
var p0 = R(c0, dZr, hL(1, L), rr, Z), b0 = N0(L), O0 = 0;
if (typeof b0 != "number" && b0[0] === 3) {
var q0 = R(G0, L, rr, p0, b0[1]);
O0 = 1;
}
if (!O0)
var q0 = p0;
var er = 0;
if (N0(L) !== 4) {
var yr = 0;
if (iu(L) && N0(L) === 98 && (yr = 1), !yr) {
var Sr = q0;
er = 1;
}
}
if (!er)
var vr = Wt(L), $0 = function(ne, Qr) {
return a(Ze(ne, Di, 79), ne, Qr);
}, Sr = a(vr[2], q0, $0);
var Mr = iu(L), Br = Mr && we0(O9(function(ne, Qr) {
throw Hs;
}, L), 0, e0), qr = N0(L), jr = 0;
if (typeof qr == "number" && qr === 4) {
var $r = [0, u(x0, L)];
jr = 1;
}
if (!jr)
var $r = 0;
return [18, [0, Sr, Br, $r, lr([0, i0], 0, 0)]];
}, P);
});
function _r(P) {
var L = pr(P);
V0(P, 98);
for (var Q = 0; ; ) {
var i0 = N0(P);
if (typeof i0 == "number") {
var l0 = 0;
if ((i0 === 99 || Pn === i0) && (l0 = 1), l0) {
var S0 = de(Q), T0 = pr(P);
V0(P, 99);
var rr = N0(P) === 4 ? Wt(P)[1] : we(P);
return [0, S0, _u([0, L], [0, rr], T0, 0)];
}
}
var R0 = N0(P), B = 0;
if (typeof R0 != "number" && R0[0] === 4 && !n0(R0[2], bZr)) {
var Z = De(P), p0 = pr(P);
Zl(P, pZr);
var b0 = [1, [0, Z, [0, lr([0, p0], [0, we(P)], 0)]]];
B = 1;
}
if (!B)
var b0 = [0, u(ln[1], P)];
var O0 = [0, b0, Q];
N0(P) !== 99 && V0(P, 9);
var Q = O0;
}
}
N(e0, function(P) {
zu(P, 1);
var L = N0(P) === 98 ? 1 : 0, Q = L && [0, cr(0, _r, P)];
return h7(P), Q;
});
function Ir(P) {
var L = pr(P);
V0(P, 12);
var Q = u(x, P);
return [0, Q, lr([0, L], 0, 0)];
}
N(x0, function(P) {
return cr(0, function(L) {
var Q = pr(L);
V0(L, 4);
for (var i0 = 0; ; ) {
var l0 = N0(L);
if (typeof l0 == "number") {
var S0 = 0;
if ((l0 === 5 || Pn === l0) && (S0 = 1), S0) {
var T0 = de(i0), rr = pr(L);
return V0(L, 5), [0, T0, _u([0, Q], [0, we(L)], rr, 0)];
}
}
var R0 = N0(L), B = 0;
if (typeof R0 == "number" && R0 === 12) {
var Z = [1, cr(0, Ir, L)];
B = 1;
}
if (!B)
var Z = [0, u(x, L)];
var p0 = [0, Z, i0];
N0(L) !== 5 && V0(L, 9);
var i0 = p0;
}
}, P);
}), N(l, function(P, L, Q, i0, l0) {
var S0 = P ? P[1] : 1, T0 = L && L[1], rr = N0(Q), R0 = 0;
if (typeof rr == "number")
switch (rr) {
case 6:
ie(Q);
var B = 0, Z = [0, T0], p0 = [0, S0];
R0 = 2;
break;
case 10:
ie(Q);
var b0 = 0, O0 = [0, T0], q0 = [0, S0];
R0 = 1;
break;
case 83:
1 - S0 && Ge(Q, 99), V0(Q, 83);
var er = 0, yr = N0(Q);
if (typeof yr == "number")
switch (yr) {
case 4:
return l0;
case 6:
ie(Q);
var B = oZr, Z = cZr, p0 = [0, S0];
R0 = 2, er = 1;
break;
case 98:
if (iu(Q))
return l0;
break;
}
else if (yr[0] === 3)
return Ge(Q, ni), l0;
if (!er) {
var b0 = sZr, O0 = vZr, q0 = [0, S0];
R0 = 1;
}
break;
}
else if (rr[0] === 3) {
T0 && Ge(Q, ni);
var vr = rr[1];
return b7(m0, lZr, 0, Q, i0, [0, R(G0, Q, i0, a(e, Q, l0), vr)]);
}
switch (R0) {
case 0:
return l0;
case 1:
var $0 = q0 ? S0 : 1, Sr = O0 && O0[1], Mr = b0 && b0[1], Br = N0(Q), qr = 0;
if (typeof Br == "number" && Br === 14) {
var jr = Ae0(Q), $r = jr[1], ne = Q[29][1], Qr = jr[2][1];
if (ne) {
var pe = ne[1];
Q[29][1] = [0, [0, pe[1], [0, [0, Qr, $r], pe[2]]], ne[2]];
} else
ue(Q, [0, $r, 89]);
var me = [1, jr], ae = $r;
qr = 1;
}
if (!qr)
var oe = V7(Q), me = [0, oe], ae = oe[1];
var ce = yt(i0, ae), ge = 0;
l0[0] === 0 && l0[1][2][0] === 23 && me[0] === 1 && (ue(Q, [0, ce, 90]), ge = 1);
var H0 = [0, a(e, Q, l0), me, 0], Fr = Sr ? [21, [0, H0, ce, Mr]] : [16, H0];
return b7(m0, [0, $0], [0, Sr], Q, i0, [0, [0, ce, Fr]]);
default:
var _ = p0 ? S0 : 1, k = Z && Z[1], I = B && B[1], U = hL(0, Q), Y = u(se[7], U), y0 = De(Q);
V0(Q, 7);
var D0 = we(Q), I0 = yt(i0, y0), D = lr(0, [0, D0], 0), u0 = [0, a(e, Q, l0), [2, Y], D], Y0 = k ? [21, [0, u0, I0, I]] : [16, u0];
return b7(m0, [0, _], [0, k], Q, i0, [0, [0, I0, Y0]]);
}
}), N(c0, function(P, L, Q, i0) {
var l0 = P ? P[1] : 1;
return a(e, L, b7(l, [0, l0], 0, L, Q, [0, i0]));
}), N(t0, function(P) {
return cr(0, function(L) {
var Q = u(Vn[1], L), i0 = Q[1], l0 = Q[2], S0 = cr(0, function(q0) {
var er = pr(q0);
V0(q0, 15);
var yr = u(Vn[2], q0), vr = yr[1], $0 = pl([0, l0, [0, er, [0, yr[2], 0]]]);
if (N0(q0) === 4)
var Sr = 0, Mr = 0;
else {
var Br = N0(q0), qr = 0;
if (typeof Br == "number") {
var jr = Br !== 98 ? 1 : 0;
if (!jr) {
var ne = jr;
qr = 1;
}
}
if (!qr)
var $r = dL(vr, ie0(i0, q0)), ne = [0, ds($r, a(se[13], aZr, $r))];
var Sr = xi(q0, u(ln[3], q0)), Mr = ne;
}
var Qr = t2(0, q0), pe = ir(Vn[4], i0, vr, Qr), oe = N0(Qr) === 86 ? pe : eb(Qr, pe), me = u(ln[12], Qr), ae = me[2], ce = me[1];
if (ae)
var ge = Se0(Qr, ae), H0 = ce;
else
var ge = ae, H0 = a2(Qr, ce);
return [0, Mr, oe, vr, ge, H0, Sr, $0];
}, L), T0 = S0[2], rr = T0[3], R0 = T0[2], B = T0[1], Z = U1(R0), p0 = b7(Vn[5], L, i0, rr, 1, Z);
R(Vn[6], L, p0[2], B, R0);
var b0 = S0[1], O0 = lr([0, T0[7]], 0, 0);
return [8, [0, B, R0, p0[1], i0, rr, T0[4], T0[5], T0[6], O0, b0]];
}, P);
}), N(a0, function(P, L, Q) {
switch (L) {
case 1:
Si(P, 45);
try {
var i0 = jv(Rv(Te(tZr, Q))), l0 = i0;
} catch (R0) {
if (R0 = Et(R0), R0[1] !== B7)
throw R0;
var l0 = ke(Te(uZr, Q));
}
break;
case 2:
Si(P, 46);
try {
var S0 = al(Q), l0 = S0;
} catch (R0) {
if (R0 = Et(R0), R0[1] !== B7)
throw R0;
var l0 = ke(Te(iZr, Q));
}
break;
case 4:
try {
var T0 = al(Q), l0 = T0;
} catch (R0) {
if (R0 = Et(R0), R0[1] !== B7)
throw R0;
var l0 = ke(Te(fZr, Q));
}
break;
default:
try {
var rr = jv(Rv(Q)), l0 = rr;
} catch (R0) {
if (R0 = Et(R0), R0[1] !== B7)
throw R0;
var l0 = ke(Te(xZr, Q));
}
}
return V0(P, [0, L, Q]), l0;
}), N(w0, function(P) {
var L = nn(P);
return L !== 0 && Ht === Ot(P, L - 1 | 0) ? p7(P, 0, L - 1 | 0) : P;
}), N(_0, function(P, L, Q) {
if (2 <= L) {
var i0 = u(w0, Q);
try {
var l0 = al(i0), S0 = l0;
} catch (Z) {
if (Z = Et(Z), Z[1] !== B7)
throw Z;
var S0 = ke(Te(eZr, i0));
}
var T0 = S0;
} else {
var rr = u(w0, Q);
try {
var R0 = jv(Rv(rr)), B = R0;
} catch (p0) {
if (p0 = Et(p0), p0[1] !== B7)
throw p0;
var B = ke(Te(nZr, rr));
}
var T0 = B;
}
return V0(P, [1, L, Q]), T0;
}), N(E0, function(P) {
var L = De(P), Q = pr(P), i0 = N0(P);
if (typeof i0 == "number")
switch (i0) {
case 0:
var l0 = u(se[12], P);
return [1, [0, l0[1], [19, l0[2]]], l0[3]];
case 4:
return [0, u(X, P)];
case 6:
var S0 = cr(0, dr, P), T0 = S0[2];
return [1, [0, S0[1], [0, T0[1]]], T0[2]];
case 21:
return ie(P), [0, [0, L, [26, [0, lr([0, Q], [0, we(P)], 0)]]]];
case 29:
return ie(P), [0, [0, L, [14, [0, 0, $$r, lr([0, Q], [0, we(P)], 0)]]]];
case 40:
return [0, u(se[22], P)];
case 98:
var rr = u(se[17], P), R0 = rr[2], B = rr[1], Z = Ni <= R0[1] ? [13, R0[2]] : [12, R0[2]];
return [0, [0, B, Z]];
case 30:
case 31:
ie(P);
var p0 = i0 === 31 ? 1 : 0, b0 = p0 ? Q$r : rZr;
return [0, [0, L, [14, [0, [1, p0], b0, lr([0, Q], [0, we(P)], 0)]]]];
case 74:
case 105:
return [0, u(Ar, P)];
}
else
switch (i0[0]) {
case 0:
var O0 = i0[2], q0 = [2, ir(a0, P, i0[1], O0)];
return [0, [0, L, [14, [0, q0, O0, lr([0, Q], [0, we(P)], 0)]]]];
case 1:
var er = i0[2], yr = [3, ir(_0, P, i0[1], er)];
return [0, [0, L, [14, [0, yr, er, lr([0, Q], [0, we(P)], 0)]]]];
case 2:
var vr = i0[1];
vr[4] && Si(P, 45), ie(P);
var $0 = [0, vr[2]], Sr = lr([0, Q], [0, we(P)], 0);
return [0, [0, vr[1], [14, [0, $0, vr[3], Sr]]]];
case 3:
var Mr = a(b, P, i0[1]);
return [0, [0, Mr[1], [25, Mr[2]]]];
}
if (M1(P)) {
var Br = a(se[13], 0, P);
return [0, [0, Br[1], [10, Br]]];
}
St(0, P);
var qr = 0;
return typeof i0 != "number" && i0[0] === 6 && (ie(P), qr = 1), [0, [0, L, [14, [0, 0, Z$r, lr([0, Q], [0, 0], 0)]]]];
}), N(X0, function(P) {
return a(e, P, u(E0, P));
}), N(b, function(P, L) {
var Q = L[3], i0 = L[2], l0 = L[1], S0 = pr(P);
V0(P, [3, L]);
var T0 = [0, l0, [0, [0, i0[2], i0[1]], Q]];
if (Q)
var rr = 0, R0 = [0, T0, 0], B = l0;
else
for (var Z = [0, T0, 0], p0 = 0; ; ) {
var b0 = u(se[7], P), O0 = [0, b0, p0], q0 = N0(P), er = 0;
if (typeof q0 == "number" && q0 === 1) {
zu(P, 4);
var yr = N0(P), vr = 0;
if (typeof yr != "number" && yr[0] === 3) {
var $0 = yr[1], Sr = $0[3], Mr = $0[2], Br = $0[1];
ie(P);
var qr = [0, [0, Mr[2], Mr[1]], Sr];
h7(P);
var jr = [0, [0, Br, qr], Z];
if (!Sr) {
var Z = jr, p0 = O0;
continue;
}
var $r = de(O0), ne = [0, Br, de(jr), $r];
er = 1, vr = 1;
}
if (!vr)
throw [0, wn, K$r];
}
if (!er) {
St(W$r, P);
var Qr = [0, b0[1], J$r], pe = de(O0), oe = de([0, Qr, Z]), ne = [0, b0[1], oe, pe];
}
var rr = ne[3], R0 = ne[2], B = ne[1];
break;
}
var me = we(P), ae = yt(l0, B);
return [0, ae, [0, R0, rr, lr([0, S0], [0, me], 0)]];
}), N(G0, function(P, L, Q, i0) {
var l0 = Wt(P);
function S0(R0, B) {
return a(Ze(R0, Di, 28), R0, B);
}
var T0 = a(l0[2], Q, S0), rr = a(b, P, i0);
return [0, yt(L, rr[1]), [24, [0, T0, rr, 0]]];
}), N(X, function(P) {
var L = pr(P), Q = cr(0, function(T0) {
V0(T0, 4);
var rr = De(T0), R0 = u(x, T0), B = N0(T0), Z = 0;
if (typeof B == "number")
if (B === 9)
var p0 = [0, ir(W0, T0, rr, [0, R0, 0])];
else if (B === 86)
var p0 = [1, [0, R0, u(ln[9], T0), 0]];
else
Z = 1;
else
Z = 1;
if (Z)
var p0 = [0, R0];
return V0(T0, 5), p0;
}, P), i0 = Q[2], l0 = we(P), S0 = i0[0] === 0 ? i0[1] : [0, Q[1], [27, i0[1]]];
return ir(s0, [0, L], [0, l0], S0);
}), N(s0, function(P, L, Q) {
var i0 = Q[2], l0 = P && P[1], S0 = L && L[1];
function T0(We) {
return _7(We, lr([0, l0], [0, S0], 0));
}
function rr(We) {
return QD(We, lr([0, l0], [0, S0], 0));
}
switch (i0[0]) {
case 0:
var R0 = i0[1], B = rr(R0[2]), Ce = [0, [0, R0[1], B]];
break;
case 1:
var Z = i0[1], p0 = Z[10], b0 = T0(Z[9]), Ce = [1, [0, Z[1], Z[2], Z[3], Z[4], Z[5], Z[6], Z[7], Z[8], b0, p0]];
break;
case 2:
var O0 = i0[1], q0 = T0(O0[4]), Ce = [2, [0, O0[1], O0[2], O0[3], q0]];
break;
case 3:
var er = i0[1], yr = T0(er[4]), Ce = [3, [0, er[1], er[2], er[3], yr]];
break;
case 4:
var vr = i0[1], $0 = T0(vr[4]), Ce = [4, [0, vr[1], vr[2], vr[3], $0]];
break;
case 5:
var Sr = i0[1], Mr = T0(Sr[7]), Ce = [5, [0, Sr[1], Sr[2], Sr[3], Sr[4], Sr[5], Sr[6], Mr]];
break;
case 7:
var Br = i0[1], qr = T0(Br[4]), Ce = [7, [0, Br[1], Br[2], Br[3], qr]];
break;
case 8:
var jr = i0[1], $r = jr[10], ne = T0(jr[9]), Ce = [8, [0, jr[1], jr[2], jr[3], jr[4], jr[5], jr[6], jr[7], jr[8], ne, $r]];
break;
case 10:
var Qr = i0[1], pe = Qr[2], oe = T0(pe[2]), Ce = [10, [0, Qr[1], [0, pe[1], oe]]];
break;
case 11:
var me = i0[1], ae = T0(me[2]), Ce = [11, [0, me[1], ae]];
break;
case 12:
var ce = i0[1], ge = T0(ce[4]), Ce = [12, [0, ce[1], ce[2], ce[3], ge]];
break;
case 13:
var H0 = i0[1], Fr = T0(H0[4]), Ce = [13, [0, H0[1], H0[2], H0[3], Fr]];
break;
case 14:
var _ = i0[1], k = T0(_[3]), Ce = [14, [0, _[1], _[2], k]];
break;
case 15:
var I = i0[1], U = T0(I[4]), Ce = [15, [0, I[1], I[2], I[3], U]];
break;
case 16:
var Y = i0[1], y0 = T0(Y[3]), Ce = [16, [0, Y[1], Y[2], y0]];
break;
case 17:
var D0 = i0[1], I0 = T0(D0[3]), Ce = [17, [0, D0[1], D0[2], I0]];
break;
case 18:
var D = i0[1], u0 = T0(D[4]), Ce = [18, [0, D[1], D[2], D[3], u0]];
break;
case 19:
var Y0 = i0[1], J0 = rr(Y0[2]), Ce = [19, [0, Y0[1], J0]];
break;
case 20:
var fr = i0[1], Q0 = fr[1], F0 = fr[3], gr = fr[2], mr = T0(Q0[4]), Ce = [20, [0, [0, Q0[1], Q0[2], Q0[3], mr], gr, F0]];
break;
case 21:
var Cr = i0[1], sr = Cr[1], Pr = Cr[3], K0 = Cr[2], Ur = T0(sr[3]), Ce = [21, [0, [0, sr[1], sr[2], Ur], K0, Pr]];
break;
case 22:
var d0 = i0[1], Kr = T0(d0[2]), Ce = [22, [0, d0[1], Kr]];
break;
case 23:
var Ce = [23, [0, T0(i0[1][1])]];
break;
case 24:
var re = i0[1], xe = T0(re[3]), Ce = [24, [0, re[1], re[2], xe]];
break;
case 25:
var je = i0[1], ve = T0(je[3]), Ce = [25, [0, je[1], je[2], ve]];
break;
case 26:
var Ce = [26, [0, T0(i0[1][1])]];
break;
case 27:
var Ie = i0[1], Me = T0(Ie[3]), Ce = [27, [0, Ie[1], Ie[2], Me]];
break;
case 28:
var Be = i0[1], fn = T0(Be[3]), Ce = [28, [0, Be[1], Be[2], fn]];
break;
case 29:
var Ke = i0[1], Ae = T0(Ke[4]), Ce = [29, [0, Ke[1], Ke[2], Ke[3], Ae]];
break;
case 30:
var xn = i0[1], Qe = xn[4], yn = xn[3], on = T0(xn[2]), Ce = [30, [0, xn[1], on, yn, Qe]];
break;
default:
var Ce = i0;
}
return [0, Q[1], Ce];
}), N(dr, function(P) {
var L = pr(P);
V0(P, 6);
for (var Q = [0, 0, t[3]]; ; ) {
var i0 = Q[2], l0 = Q[1], S0 = N0(P);
if (typeof S0 == "number") {
var T0 = 0;
if (13 <= S0)
Pn === S0 && (T0 = 1);
else if (7 <= S0)
switch (S0 - 7 | 0) {
case 2:
var rr = De(P);
ie(P);
var Q = [0, [0, [2, rr], l0], i0];
continue;
case 5:
var R0 = pr(P), B = cr(0, function(Qr) {
ie(Qr);
var pe = u(i, Qr);
return pe[0] === 0 ? [0, pe[1], t[3]] : [0, pe[1], pe[2]];
}, P), Z = B[2], p0 = Z[2], b0 = B[1], O0 = lr([0, R0], 0, 0), q0 = [1, [0, b0, [0, Z[1], O0]]], er = N0(P) === 7 ? 1 : 0, yr = 0;
if (!er && Yn(1, P) === 7) {
var vr = [0, p0[1], [0, [0, b0, 65], p0[2]]];
yr = 1;
}
if (!yr)
var vr = p0;
1 - er && V0(P, 9);
var Q = [0, [0, q0, l0], a(t[5], vr, i0)];
continue;
case 0:
T0 = 1;
break;
}
if (T0) {
var $0 = u(t[6], i0), Sr = de(l0), Mr = pr(P);
return V0(P, 7), [0, [0, Sr, _u([0, L], [0, we(P)], Mr, 0)], $0];
}
}
var Br = u(i, P);
if (Br[0] === 0)
var qr = t[3], jr = Br[1];
else
var qr = Br[2], jr = Br[1];
N0(P) !== 7 && V0(P, 9);
var Q = [0, [0, [0, jr], l0], a(t[5], qr, i0)];
}
}), N(Ar, function(P) {
zu(P, 5);
var L = De(P), Q = pr(P), i0 = N0(P), l0 = 0;
if (typeof i0 != "number" && i0[0] === 5) {
var S0 = i0[3], T0 = i0[2];
ie(P);
var rr = we(P), R0 = rr, B = S0, Z = T0, p0 = Te(H$r, Te(T0, Te(U$r, S0)));
l0 = 1;
}
if (!l0) {
St(X$r, P);
var R0 = 0, B = Y$r, Z = V$r, p0 = z$r;
}
h7(P);
var b0 = $n(nn(B)), O0 = nn(B) - 1 | 0, q0 = 0;
if (!(O0 < 0))
for (var er = q0; ; ) {
var yr = Vr(B, er), vr = yr - 100 | 0, $0 = 0;
if (!(21 < vr >>> 0))
switch (vr) {
case 0:
case 3:
case 5:
case 9:
case 15:
case 17:
case 21:
qi(b0, yr), $0 = 1;
break;
}
var Sr = er + 1 | 0;
if (O0 !== er) {
var er = Sr;
continue;
}
break;
}
var Mr = Gt(b0);
return n0(Mr, B) && Ge(P, [13, B]), [0, L, [14, [0, [4, [0, Z, Mr]], p0, lr([0, Q], [0, R0], 0)]]];
});
function fe(P, L) {
if (typeof L == "number") {
var Q = 0;
if (61 <= L) {
var i0 = L - 64 | 0;
27 < i0 >>> 0 ? i0 === 43 && (Q = 1) : 25 < (i0 - 1 | 0) >>> 0 && (Q = 1);
} else {
var l0 = L + hy | 0;
17 < l0 >>> 0 ? -1 <= l0 && (Q = 1) : l0 === 13 && (Q = 1);
}
if (Q)
return 0;
}
throw Hs;
}
function v0(P) {
var L = N0(P);
if (typeof L == "number" && !L) {
var Q = a(se[16], 1, P);
return [0, [0, Q[1]], Q[2]];
}
return [0, [1, u(se[10], P)], 0];
}
return N(ar, function(P) {
var L = O9(fe, P), Q = De(L);
if (Yn(1, L) === 11)
var l0 = 0, S0 = 0;
else
var i0 = u(Vn[1], L), l0 = i0[2], S0 = i0[1];
var T0 = cr(0, function(ne) {
var Qr = xi(ne, u(ln[3], ne));
if (M1(ne) && Qr === 0) {
var pe = a(se[13], q$r, ne), oe = pe[1], me = [0, oe, [0, [0, oe, [2, [0, pe, [0, G1(ne)], 0]]], 0]];
return [0, Qr, [0, oe, [0, 0, [0, me, 0], 0, 0]], [0, [0, oe[1], oe[3], oe[3]]], 0];
}
var ae = ir(Vn[4], ne[18], ne[17], ne), ce = u2(1, ne), ge = u(ln[12], ce);
return [0, Qr, ae, ge[1], ge[2]];
}, L), rr = T0[2], R0 = rr[2], B = R0[2], Z = 0;
if (!B[1]) {
var p0 = 0;
if (!B[3] && B[2] && (p0 = 1), !p0) {
var b0 = ce0(L);
Z = 1;
}
}
if (!Z)
var b0 = L;
var O0 = R0[2], q0 = O0[1], er = q0 ? (ue(b0, [0, q0[1][1], Qc]), [0, R0[1], [0, 0, O0[2], O0[3], O0[4]]]) : R0, yr = U1(er), vr = f7(b0), $0 = vr && (N0(b0) === 11 ? 1 : 0);
$0 && Ge(b0, 60), V0(b0, 11);
var Sr = se0(ce0(b0), S0, 0, yr), Mr = cr(0, v0, Sr), Br = Mr[2];
R(Vn[6], Sr, Br[2], 0, er);
var qr = yt(Q, Mr[1]), jr = T0[1], $r = lr([0, l0], 0, 0);
return [0, [0, qr, [1, [0, 0, er, Br[1], S0, 0, rr[4], rr[3], rr[1], $r, jr]]]];
}), N(W0, function(P, L, Q) {
return cr([0, L], function(i0) {
for (var l0 = Q; ; ) {
var S0 = N0(i0);
if (typeof S0 == "number" && S0 === 9) {
ie(i0);
var l0 = [0, u(x, i0), l0];
continue;
}
return [22, [0, de(l0), 0]];
}
}, P);
}), [0, x, i, T, n4, K, a0, W0];
}(j9), Ys = function(t) {
function n4(e0) {
var x0 = pr(e0);
ie(e0);
var l = lr([0, x0], 0, 0), c0 = u(oi[5], e0), t0 = f7(e0) ? rb(e0) : C9(e0);
function a0(w0, _0) {
return a(Ze(w0, Di, 80), w0, _0);
}
return [0, a(t0[2], c0, a0), l];
}
function e(e0) {
var x0 = e0[27][2];
if (x0)
for (var l = 0; ; ) {
var c0 = N0(e0);
if (typeof c0 == "number" && c0 === 13) {
var l = [0, cr(0, n4, e0), l];
continue;
}
return de(l);
}
return x0;
}
function i(e0, x0) {
var l = e0 && e0[1], c0 = pr(x0), t0 = N0(x0);
if (typeof t0 == "number")
switch (t0) {
case 6:
var a0 = cr(0, function(Jr) {
var or = pr(Jr);
V0(Jr, 6);
var _r = Kl(0, Jr), Ir = u(se[10], _r);
return V0(Jr, 7), [0, Ir, lr([0, or], [0, we(Jr)], 0)];
}, x0), w0 = a0[1];
return [0, w0, [3, [0, w0, a0[2]]]];
case 14:
if (l) {
var _0 = Ae0(x0), E0 = x0[29][1], X0 = _0[2][1];
if (E0) {
var b = E0[1], G0 = E0[2], X = b[2], s0 = [0, [0, a(Gu[4], X0, b[1]), X], G0];
x0[29][1] = s0;
} else
ke(tGr);
return [0, _0[1], [2, _0]];
}
var dr = cr(0, function(Jr) {
return ie(Jr), [1, V7(Jr)];
}, x0), Ar = dr[1];
return ue(x0, [0, Ar, 89]), [0, Ar, dr[2]];
}
else
switch (t0[0]) {
case 0:
var ar = t0[2], W0 = De(x0), Lr = [2, ir(oi[6], x0, t0[1], ar)];
return [0, W0, [0, [0, W0, [0, Lr, ar, lr([0, c0], [0, we(x0)], 0)]]]];
case 2:
var Tr = t0[1], Hr = Tr[4], Or = Tr[3], xr = Tr[2], Rr = Tr[1];
return Hr && Si(x0, 45), V0(x0, [2, [0, Rr, xr, Or, Hr]]), [0, Rr, [0, [0, Rr, [0, [0, xr], Or, lr([0, c0], [0, we(x0)], 0)]]]];
}
var Wr = V7(x0);
return [0, Wr[1], [1, Wr]];
}
function x(e0, x0, l) {
var c0 = u(Vn[2], e0), t0 = c0[1], a0 = c0[2], w0 = i([0, x0], e0), _0 = w0[1], E0 = 0, X0 = Xi(e0, w0[2]);
return [0, X0, cr(0, function(b) {
var G0 = t2(1, b), X = cr(0, function(Tr) {
var Hr = ir(Vn[4], 0, 0, Tr), Or = 0, xr = N0(Tr) === 86 ? Hr : eb(Tr, Hr);
if (l) {
var Rr = xr[2], Wr = 0;
if (Rr[1])
ue(Tr, [0, _0, R7]), Wr = 1;
else {
var Jr = 0;
!Rr[2] && !Rr[3] && (Wr = 1, Jr = 1), Jr || ue(Tr, [0, _0, 80]);
}
} else {
var or = xr[2];
if (or[1])
ue(Tr, [0, _0, Xt]);
else {
var _r = or[2], Ir = 0;
(!_r || _r[2] || or[3]) && (Ir = 1), Ir && (or[3] ? ue(Tr, [0, _0, 81]) : ue(Tr, [0, _0, 81]));
}
}
return [0, Or, xr, a2(Tr, u(ln[10], Tr))];
}, G0), s0 = X[2], dr = s0[2], Ar = U1(dr), ar = b7(Vn[5], G0, E0, t0, 0, Ar);
R(Vn[6], G0, ar[2], 0, dr);
var W0 = X[1], Lr = lr([0, a0], 0, 0);
return [0, 0, dr, ar[1], E0, t0, 0, s0[3], s0[1], Lr, W0];
}, e0)];
}
function c(e0) {
var x0 = u(oi[2], e0);
return x0[0] === 0 ? [0, x0[1], t[3]] : [0, x0[1], x0[2]];
}
function s(e0, x0) {
switch (x0[0]) {
case 0:
var l = x0[1], c0 = l[1];
return ue(e0, [0, c0, 95]), [0, c0, [14, l[2]]];
case 1:
var t0 = x0[1], a0 = t0[2][1], w0 = t0[1], _0 = 0;
return SL(a0) && n0(a0, o0e) && n0(a0, c0e) && (ue(e0, [0, w0, 2]), _0 = 1), !_0 && f2(a0) && Y7(e0, [0, w0, 55]), [0, w0, [10, t0]];
case 2:
return ke(s0e);
default:
var E0 = x0[1][2][1];
return ue(e0, [0, E0[1], 96]), E0;
}
}
function p(e0, x0, l) {
function c0(a0) {
var w0 = t2(1, a0), _0 = cr(0, function(dr) {
var Ar = xi(dr, u(ln[3], dr));
if (e0)
if (x0)
var ar = 1, W0 = 1;
else
var ar = dr[18], W0 = 0;
else if (x0)
var ar = 0, W0 = 1;
else
var ar = 0, W0 = 0;
var Lr = ir(Vn[4], ar, W0, dr), Tr = N0(dr) === 86 ? Lr : eb(dr, Lr);
return [0, Ar, Tr, a2(dr, u(ln[10], dr))];
}, w0), E0 = _0[2], X0 = E0[2], b = U1(X0), G0 = b7(Vn[5], w0, e0, x0, 0, b);
R(Vn[6], w0, G0[2], 0, X0);
var X = _0[1], s0 = lr([0, l], 0, 0);
return [0, 0, X0, G0[1], e0, x0, 0, E0[3], E0[1], s0, X];
}
var t0 = 0;
return function(a0) {
return cr(t0, c0, a0);
};
}
function y(e0) {
return V0(e0, 86), c(e0);
}
function T(e0, x0, l, c0, t0, a0) {
var w0 = cr([0, x0], function(E0) {
if (!c0 && !t0) {
var X0 = N0(E0);
if (typeof X0 == "number") {
var b = 0;
if (86 <= X0) {
if (X0 === 98)
b = 1;
else if (!(87 <= X0)) {
var G0 = y(E0);
return [0, [0, l, G0[1], 0], G0[2]];
}
} else {
if (X0 === 82) {
if (l[0] === 1)
var X = l[1], s0 = De(E0), dr = function(Rr) {
var Wr = pr(Rr);
V0(Rr, 82);
var Jr = we(Rr), or = a(se[19], Rr, [0, X[1], [10, X]]), _r = u(se[10], Rr);
return [2, [0, 0, or, _r, lr([0, Wr], [0, Jr], 0)]];
}, Ar = cr([0, X[1]], dr, E0), ar = [0, Ar, [0, [0, [0, s0, [10, Ml(a0e)]], 0], 0]];
else
var ar = y(E0);
return [0, [0, l, ar[1], 1], ar[2]];
}
if (!(10 <= X0))
switch (X0) {
case 4:
b = 1;
break;
case 1:
case 9:
var W0 = [0, l, s(E0, l), 1];
return [0, W0, t[3]];
}
}
if (b) {
var Lr = Xi(E0, l), Tr = [1, Lr, u(p(c0, t0, a0), E0)];
return [0, Tr, t[3]];
}
}
var Hr = [0, l, s(E0, l), 1];
return [0, Hr, t[3]];
}
var Or = Xi(E0, l), xr = [1, Or, u(p(c0, t0, a0), E0)];
return [0, xr, t[3]];
}, e0), _0 = w0[2];
return [0, [0, [0, w0[1], _0[1]]], _0[2]];
}
function E(e0) {
var x0 = cr(0, function(c0) {
var t0 = pr(c0);
V0(c0, 0);
for (var a0 = 0, w0 = [0, 0, t[3]]; ; ) {
var _0 = w0[2], E0 = w0[1], X0 = N0(c0);
if (typeof X0 == "number") {
var b = 0;
if ((X0 === 1 || Pn === X0) && (b = 1), b) {
var G0 = a0 ? [0, _0[1], [0, [0, a0[1], 98], _0[2]]] : _0, X = u(t[6], G0), s0 = de(E0), dr = pr(c0);
return V0(c0, 1), [0, [0, s0, _u([0, t0], [0, we(c0)], dr, 0)], X];
}
}
if (N0(c0) === 12)
var Ar = pr(c0), ar = cr(0, function(y0) {
return V0(y0, 12), c(y0);
}, c0), W0 = ar[2], Lr = W0[2], Tr = lr([0, Ar], 0, 0), Hr = [0, [1, [0, ar[1], [0, W0[1], Tr]]], Lr];
else {
var Or = De(c0), xr = Yn(1, c0), Rr = 0;
if (typeof xr == "number") {
var Wr = 0;
if (86 <= xr)
xr !== 98 && 87 <= xr && (Wr = 1);
else if (xr !== 82)
if (10 <= xr)
Wr = 1;
else
switch (xr) {
case 1:
case 4:
case 9:
break;
default:
Wr = 1;
}
if (!Wr) {
var or = 0, _r = 0;
Rr = 1;
}
}
if (!Rr)
var Jr = u(Vn[1], c0), or = Jr[2], _r = Jr[1];
var Ir = u(Vn[2], c0), fe = Ir[1], v0 = un(or, Ir[2]), P = N0(c0), L = 0;
if (!_r && !fe && typeof P != "number" && P[0] === 4) {
var Q = P[3], i0 = 0;
if (n0(Q, f0e))
if (n0(Q, x0e))
i0 = 1;
else {
var l0 = pr(c0), S0 = i(0, c0)[2], T0 = N0(c0), rr = 0;
if (typeof T0 == "number") {
var R0 = 0;
if (86 <= T0)
T0 !== 98 && 87 <= T0 && (R0 = 1);
else if (T0 !== 82)
if (10 <= T0)
R0 = 1;
else
switch (T0) {
case 1:
case 4:
case 9:
break;
default:
R0 = 1;
}
if (!R0) {
var B = T(c0, Or, S0, 0, 0, 0);
rr = 1;
}
}
if (!rr) {
Xi(c0, S0);
var Z = t[3], p0 = cr([0, Or], function(I0) {
return x(I0, 0, 0);
}, c0), b0 = p0[2], O0 = lr([0, l0], 0, 0), B = [0, [0, [0, p0[1], [3, b0[1], b0[2], O0]]], Z];
}
var q0 = B;
}
else {
var er = pr(c0), yr = i(0, c0)[2], vr = N0(c0), $0 = 0;
if (typeof vr == "number") {
var Sr = 0;
if (86 <= vr)
vr !== 98 && 87 <= vr && (Sr = 1);
else if (vr !== 82)
if (10 <= vr)
Sr = 1;
else
switch (vr) {
case 1:
case 4:
case 9:
break;
default:
Sr = 1;
}
if (!Sr) {
var Mr = T(c0, Or, yr, 0, 0, 0);
$0 = 1;
}
}
if (!$0) {
Xi(c0, yr);
var Br = t[3], qr = cr([0, Or], function(D) {
return x(D, 0, 1);
}, c0), jr = qr[2], $r = lr([0, er], 0, 0), Mr = [0, [0, [0, qr[1], [2, jr[1], jr[2], $r]]], Br];
}
var q0 = Mr;
}
if (!i0) {
var ne = q0;
L = 1;
}
}
if (!L)
var ne = T(c0, Or, i(0, c0)[2], _r, fe, v0);
var Hr = ne;
}
var Qr = Hr[1], pe = 0;
if (Qr[0] === 1 && N0(c0) === 9) {
var oe = [0, De(c0)];
pe = 1;
}
if (!pe)
var oe = 0;
var me = a(t[5], Hr[2], _0), ae = N0(c0), ce = 0;
if (typeof ae == "number") {
var ge = ae - 2 | 0, H0 = 0;
if (Ht < ge >>> 0 ? F7 < (ge + 1 | 0) >>> 0 && (H0 = 1) : ge === 7 ? ie(c0) : H0 = 1, !H0) {
var Fr = me;
ce = 1;
}
}
if (!ce) {
var _ = vL(LRr, 9), k = ye0([0, _], N0(c0)), I = [0, De(c0), k];
fu(c0, 8);
var Fr = a(t[4], I, me);
}
var a0 = oe, w0 = [0, [0, Qr, E0], Fr];
}
}, e0), l = x0[2];
return [0, x0[1], l[1], l[2]];
}
function h(e0, x0, l, c0) {
var t0 = l[2][1], a0 = l[1];
if (qn(t0, i0e))
return ue(e0, [0, a0, [21, t0, 0, uV === c0 ? 1 : 0, 1]]), x0;
var w0 = a(R9[32], t0, x0);
if (w0) {
var _0 = w0[1], E0 = 0;
return TE === c0 ? Id === _0 && (E0 = 1) : Id === c0 && TE === _0 && (E0 = 1), E0 || ue(e0, [0, a0, [20, t0]]), ir(R9[4], t0, QX, x0);
}
return ir(R9[4], t0, c0, x0);
}
function w(e0, x0) {
return cr(0, function(l) {
var c0 = x0 && pr(l);
V0(l, 52);
for (var t0 = 0; ; ) {
var a0 = [0, cr(0, function(E0) {
var X0 = u(ln[2], E0);
if (N0(E0) === 98)
var b = Wt(E0), G0 = function(s0, dr) {
return a(Ze(s0, Nv, 81), s0, dr);
}, X = a(b[2], X0, G0);
else
var X = X0;
return [0, X, u(ln[4], E0)];
}, l), t0], w0 = N0(l);
if (typeof w0 == "number" && w0 === 9) {
V0(l, 9);
var t0 = a0;
continue;
}
var _0 = de(a0);
return [0, _0, lr([0, c0], 0, 0)];
}
}, e0);
}
function G(e0, x0) {
return x0 && ue(e0, [0, x0[1][1], 7]);
}
function A(e0, x0) {
return x0 && ue(e0, [0, x0[1], 68]);
}
function S(e0, x0, l, c0, t0, a0, w0, _0, E0, X0) {
for (; ; ) {
var b = N0(e0), G0 = 0;
if (typeof b == "number") {
var X = b - 1 | 0, s0 = 0;
if (7 < X >>> 0) {
var dr = X - 81 | 0;
if (4 < dr >>> 0)
s0 = 1;
else
switch (dr) {
case 3:
St(0, e0), ie(e0);
continue;
case 0:
case 4:
break;
default:
s0 = 1;
}
} else
5 < (X - 1 | 0) >>> 0 || (s0 = 1);
!s0 && !t0 && !a0 && (G0 = 1);
}
if (!G0) {
var Ar = N0(e0), ar = 0;
if (typeof Ar == "number") {
var W0 = 0;
if (Ar !== 4 && Ar !== 98 && (ar = 1, W0 = 1), !W0)
var Tr = 0;
} else
ar = 1;
if (ar)
var Lr = x2(e0), Tr = Lr && 1;
if (!Tr) {
A(e0, _0), G(e0, E0);
var Hr = 0;
if (!w0) {
var Or = 0;
switch (c0[0]) {
case 0:
var xr = c0[1][2][1], Rr = 0;
typeof xr != "number" && xr[0] === 0 && (n0(xr[1], ZQr) && (Or = 1), Rr = 1), Rr || (Or = 1);
break;
case 1:
n0(c0[1][2][1], QQr) && (Or = 1);
break;
default:
Or = 1;
}
if (!Or) {
var Wr = t2(2, e0), Jr = 0;
Hr = 1;
}
}
if (!Hr)
var Wr = t2(1, e0), Jr = 1;
var or = Xi(Wr, c0), _r = cr(0, function(S0) {
var T0 = cr(0, function(p0) {
var b0 = xi(p0, u(ln[3], p0));
if (t0)
if (a0)
var O0 = 1, q0 = 1;
else
var O0 = p0[18], q0 = 0;
else if (a0)
var O0 = 0, q0 = 1;
else
var O0 = 0, q0 = 0;
var er = ir(Vn[4], O0, q0, p0), yr = N0(p0) === 86 ? er : eb(p0, er), vr = yr[2], $0 = vr[1], Sr = 0;
if ($0 && Jr === 0) {
ue(p0, [0, $0[1][1], fs2]);
var Mr = [0, yr[1], [0, 0, vr[2], vr[3], vr[4]]];
Sr = 1;
}
if (!Sr)
var Mr = yr;
return [0, b0, Mr, a2(p0, u(ln[10], p0))];
}, S0), rr = T0[2], R0 = rr[2], B = U1(R0), Z = b7(Vn[5], S0, t0, a0, 0, B);
return R(Vn[6], S0, Z[2], 0, R0), [0, 0, R0, Z[1], t0, a0, 0, rr[3], rr[1], 0, T0[1]];
}, Wr), Ir = [0, Jr, or, _r, w0, l, lr([0, X0], 0, 0)];
return [0, [0, yt(x0, _r[1]), Ir]];
}
}
var fe = cr([0, x0], function(S0) {
var T0 = u(ln[10], S0), rr = N0(S0);
if (_0) {
var R0 = 0;
if (typeof rr == "number" && rr === 82) {
Ge(S0, 69), ie(S0);
var B = 0;
} else
R0 = 1;
if (R0)
var B = 0;
} else {
var Z = 0;
if (typeof rr == "number" && rr === 82) {
ie(S0);
var p0 = t2(1, S0), B = [0, u(se[7], p0)];
} else
Z = 1;
if (Z)
var B = 1;
}
var b0 = N0(S0), O0 = 0;
if (typeof b0 == "number" && !(9 <= b0))
switch (b0) {
case 8:
ie(S0);
var q0 = N0(S0), er = 0;
if (typeof q0 == "number") {
var yr = 0;
if (q0 !== 1 && Pn !== q0 && (er = 1, yr = 1), !yr)
var $0 = we(S0);
} else
er = 1;
if (er)
var vr = f7(S0), $0 = vr && Us(S0);
var Sr = [0, c0, T0, B, $0];
O0 = 1;
break;
case 4:
case 6:
St(0, S0);
var Sr = [0, c0, T0, B, 0];
O0 = 1;
break;
}
if (!O0) {
var Mr = N0(S0), Br = 0;
if (typeof Mr == "number") {
var qr = 0;
if (Mr !== 1 && Pn !== Mr && (Br = 1, qr = 1), !qr)
var jr = [0, 0, function(H0, Fr) {
return H0;
}];
} else
Br = 1;
if (Br)
var jr = f7(S0) ? rb(S0) : C9(S0);
if (typeof B == "number")
if (T0[0] === 0)
var $r = function(_, k) {
return a(Ze(_, VY, 83), _, k);
}, pe = B, oe = T0, me = a(jr[2], c0, $r);
else
var ne = function(_, k) {
return a(Ze(_, NE, 84), _, k);
}, pe = B, oe = [1, a(jr[2], T0[1], ne)], me = c0;
else
var Qr = function(ge, H0) {
return a(Ze(ge, Di, 85), ge, H0);
}, pe = [0, a(jr[2], B[1], Qr)], oe = T0, me = c0;
var Sr = [0, me, oe, pe, 0];
}
var ae = lr([0, X0], [0, Sr[4]], 0);
return [0, Sr[1], Sr[2], Sr[3], ae];
}, e0), v0 = fe[2], P = v0[4], L = v0[3], Q = v0[2], i0 = v0[1], l0 = fe[1];
return i0[0] === 2 ? [2, [0, l0, [0, i0[1], L, Q, w0, E0, P]]] : [1, [0, l0, [0, i0, L, Q, w0, E0, P]]];
}
}
function M(e0, x0) {
var l = Yn(e0, x0);
if (typeof l == "number") {
var c0 = 0;
if (86 <= l)
(l === 98 || !(87 <= l)) && (c0 = 1);
else if (l === 82)
c0 = 1;
else if (!(9 <= l))
switch (l) {
case 1:
case 4:
case 8:
c0 = 1;
break;
}
if (c0)
return 1;
}
return 0;
}
var K = 0;
function V(e0) {
return M(K, e0);
}
function f0(e0, x0, l, c0) {
var t0 = e0 && e0[1], a0 = ys(1, x0), w0 = un(t0, e(a0)), _0 = pr(a0);
V0(a0, 40);
var E0 = T9(1, a0), X0 = N0(E0), b = 0;
if (l && typeof X0 == "number") {
var G0 = 0;
if (52 <= X0 ? X0 !== 98 && 53 <= X0 && (G0 = 1) : X0 !== 41 && X0 && (G0 = 1), !G0) {
var Ar = 0;
b = 1;
}
}
if (!b)
if (M1(a0))
var X = a(se[13], 0, E0), s0 = Wt(a0), dr = function(v0, P) {
return a(Ze(v0, Nv, 88), v0, P);
}, Ar = [0, a(s0[2], X, dr)];
else {
de0(a0, VQr);
var Ar = [0, [0, De(a0), zQr]];
}
var ar = u(ln[3], a0);
if (ar)
var W0 = Wt(a0), Lr = function(v0, P) {
return a(Ze(v0, _F, 86), v0, P);
}, Tr = [0, a(W0[2], ar[1], Lr)];
else
var Tr = ar;
var Hr = pr(a0), Or = fu(a0, 41);
if (Or)
var xr = cr(0, function(v0) {
var P = dL(0, v0), L = u(oi[5], P);
if (N0(v0) === 98)
var Q = Wt(v0), i0 = function(T0, rr) {
return a(Ze(T0, Di, 82), T0, rr);
}, l0 = a(Q[2], L, i0);
else
var l0 = L;
var S0 = u(ln[4], v0);
return [0, l0, S0, lr([0, Hr], 0, 0)];
}, a0), Rr = xr[1], Wr = Wt(a0), Jr = function(v0, P) {
return ir(Ze(v0, -663447790, 87), v0, Rr, P);
}, or = [0, [0, Rr, a(Wr[2], xr[2], Jr)]];
else
var or = Or;
var _r = N0(a0) === 52 ? 1 : 0;
if (_r) {
1 - iu(a0) && Ge(a0, 16);
var Ir = [0, Fe0(a0, w(a0, 1))];
} else
var Ir = _r;
var fe = cr(0, function(v0) {
var P = pr(v0);
if (fu(v0, 0)) {
v0[29][1] = [0, [0, Gu[1], 0], v0[29][1]];
for (var L = 0, Q = R9[1], i0 = 0; ; ) {
var l0 = N0(v0);
if (typeof l0 == "number") {
var S0 = l0 - 2 | 0;
if (Ht < S0 >>> 0) {
if (!(F7 < (S0 + 1 | 0) >>> 0)) {
var T0 = de(i0), rr = function(xu, Mu) {
return u(ml(function(z7) {
return 1 - a(Gu[3], z7[1], xu);
}), Mu);
}, R0 = v0[29][1];
if (R0) {
var B = R0[1], Z = B[1];
if (R0[2]) {
var p0 = R0[2], b0 = rr(Z, B[2]), O0 = bl(p0), q0 = bz(p0), er = un(O0[2], b0);
v0[29][1] = [0, [0, O0[1], er], q0];
} else {
var yr = rr(Z, B[2]);
Pu(function(xu) {
return ue(v0, [0, xu[2], [22, xu[1]]]);
}, yr), v0[29][1] = 0;
}
} else
ke(uGr);
V0(v0, 1);
var vr = N0(v0), $0 = 0;
if (!c0) {
var Sr = 0;
if (typeof vr == "number" && (vr === 1 || Pn === vr) && (Sr = 1), !Sr) {
var Mr = f7(v0);
if (Mr) {
var Br = Us(v0);
$0 = 1;
} else {
var Br = Mr;
$0 = 1;
}
}
}
if (!$0)
var Br = we(v0);
return [0, T0, lr([0, P], [0, Br], 0)];
}
} else if (S0 === 6) {
V0(v0, 8);
continue;
}
}
var qr = De(v0), jr = e(v0), $r = N0(v0), ne = 0;
if (typeof $r == "number" && $r === 60 && !M(1, v0)) {
var Qr = [0, De(v0)], pe = pr(v0);
ie(v0);
var oe = pe, me = Qr;
ne = 1;
}
if (!ne)
var oe = 0, me = 0;
var ae = Yn(1, v0) !== 4 ? 1 : 0;
if (ae)
var ce = Yn(1, v0) !== 98 ? 1 : 0, ge = ce && (N0(v0) === 42 ? 1 : 0);
else
var ge = ae;
if (ge) {
var H0 = pr(v0);
ie(v0);
var Fr = H0;
} else
var Fr = ge;
var _ = N0(v0) === 64 ? 1 : 0;
if (_)
var k = 1 - M(1, v0), I = k && 1 - Jl(1, v0);
else
var I = _;
if (I) {
var U = pr(v0);
ie(v0);
var Y = U;
} else
var Y = I;
var y0 = u(Vn[2], v0), D0 = y0[1], I0 = ir(Vn[3], v0, I, D0), D = 0;
if (!D0 && I0) {
var u0 = u(Vn[2], v0), Y0 = u0[2], J0 = u0[1];
D = 1;
}
if (!D)
var Y0 = y0[2], J0 = D0;
var fr = pl([0, oe, [0, Fr, [0, Y, [0, Y0, 0]]]]), Q0 = N0(v0), F0 = 0;
if (!I && !J0 && typeof Q0 != "number" && Q0[0] === 4) {
var gr = Q0[3];
if (n0(gr, r0e)) {
if (!n0(gr, e0e)) {
var mr = pr(v0), Cr = i(n0e, v0)[2];
if (V(v0)) {
var Ie = S(v0, qr, jr, Cr, I, J0, ge, me, I0, fr);
F0 = 1;
} else {
A(v0, me), G(v0, I0), Xi(v0, Cr);
var sr = un(fr, mr), Pr = cr([0, qr], function(Mu) {
return x(Mu, 1, 0);
}, v0), K0 = Pr[2], Ur = lr([0, sr], 0, 0), Ie = [0, [0, Pr[1], [0, 3, K0[1], K0[2], ge, jr, Ur]]];
F0 = 1;
}
}
} else {
var d0 = pr(v0), Kr = i(t0e, v0)[2];
if (V(v0)) {
var Ie = S(v0, qr, jr, Kr, I, J0, ge, me, I0, fr);
F0 = 1;
} else {
A(v0, me), G(v0, I0), Xi(v0, Kr);
var re = un(fr, d0), xe = cr([0, qr], function(Mu) {
return x(Mu, 1, 1);
}, v0), je = xe[2], ve = lr([0, re], 0, 0), Ie = [0, [0, xe[1], [0, 2, je[1], je[2], ge, jr, ve]]];
F0 = 1;
}
}
}
if (!F0)
var Ie = S(v0, qr, jr, i(u0e, v0)[2], I, J0, ge, me, I0, fr);
switch (Ie[0]) {
case 0:
var Me = Ie[1], Be = Me[2];
switch (Be[1]) {
case 0:
if (Be[4])
var Ft = Q, Nt = L;
else {
L && ue(v0, [0, Me[1], 87]);
var Ft = Q, Nt = 1;
}
break;
case 1:
var fn = Be[2], Ke = fn[0] === 2 ? h(v0, Q, fn[1], uV) : Q, Ft = Ke, Nt = L;
break;
case 2:
var Ae = Be[2], xn = Ae[0] === 2 ? h(v0, Q, Ae[1], TE) : Q, Ft = xn, Nt = L;
break;
default:
var Qe = Be[2], yn = Qe[0] === 2 ? h(v0, Q, Qe[1], Id) : Q, Ft = yn, Nt = L;
}
break;
case 1:
var on = Ie[1][2], Ce = on[4], We = on[1], rn = 0;
switch (We[0]) {
case 0:
var bn = We[1], Cn = bn[2][1], Hn = 0;
if (typeof Cn != "number" && Cn[0] === 0) {
var vt = Cn[1], At = bn[1];
rn = 1, Hn = 1;
}
Hn || (rn = 2);
break;
case 1:
var Sn = We[1], vt = Sn[2][1], At = Sn[1];
rn = 1;
break;
case 2:
ke(KQr);
break;
default:
rn = 2;
}
switch (rn) {
case 1:
var gt = qn(vt, WQr);
if (gt)
var Bt = gt;
else
var Jt = qn(vt, JQr), Bt = Jt && Ce;
Bt && ue(v0, [0, At, [21, vt, Ce, 0, 0]]);
break;
case 2:
break;
}
var Ft = Q, Nt = L;
break;
default:
var Ft = h(v0, Q, Ie[1][2][1], QX), Nt = L;
}
var L = Nt, Q = Ft, i0 = [0, Ie, i0];
}
}
return q1(v0, 0), $Qr;
}, a0);
return [0, Ar, fe, Tr, or, Ir, w0, lr([0, _0], 0, 0)];
}
function m0(e0, x0) {
return cr(0, function(l) {
return [2, f0([0, x0], l, l[7], 0)];
}, e0);
}
function k0(e0) {
return [5, f0(0, e0, 1, 1)];
}
var g0 = 0;
return [0, i, E, m0, function(e0) {
return cr(g0, k0, e0);
}, w, e];
}(j9), dt = function(t) {
function n4(_) {
var k = u(Vn[10], _);
if (_[5])
B1(_, k[1]);
else {
var I = k[2], U = 0;
if (I[0] === 23) {
var Y = I[1], y0 = k[1], D0 = 0;
Y[4] ? ue(_, [0, y0, 61]) : Y[5] ? ue(_, [0, y0, 62]) : (U = 1, D0 = 1);
} else
U = 1;
}
return k;
}
function e(_, k, I) {
var U = I[2][1], Y = I[1];
if (n0(U, lre)) {
if (n0(U, bre))
return n0(U, pre) ? f2(U) ? Y7(k, [0, Y, 55]) : SL(U) ? ue(k, [0, Y, [10, Ml(U)]]) : _ && Bs(U) ? Y7(k, [0, Y, _[1]]) : 0 : k[17] ? ue(k, [0, Y, 2]) : Y7(k, [0, Y, 55]);
if (k[5])
return Y7(k, [0, Y, 55]);
var y0 = k[14];
return y0 && ue(k, [0, Y, [10, Ml(U)]]);
}
var D0 = k[18];
return D0 && ue(k, [0, Y, 2]);
}
function i(_, k) {
var I = k[4], U = k[3], Y = k[2], y0 = k[1];
I && Si(_, 45);
var D0 = pr(_);
return V0(_, [2, [0, y0, Y, U, I]]), [0, y0, [0, Y, U, lr([0, D0], [0, we(_)], 0)]];
}
function x(_, k, I) {
var U = _ ? _[1] : cre, Y = k ? k[1] : 1, y0 = N0(I);
if (typeof y0 == "number") {
var D0 = y0 - 2 | 0;
if (Ht < D0 >>> 0) {
if (!(F7 < (D0 + 1 | 0) >>> 0)) {
var I0 = function(Y0, J0) {
return Y0;
};
return [1, [0, we(I), I0]];
}
} else if (D0 === 6) {
ie(I);
var D = N0(I);
if (typeof D == "number") {
var u0 = 0;
if ((D === 1 || Pn === D) && (u0 = 1), u0)
return [0, we(I)];
}
return f7(I) ? [0, Us(I)] : sre;
}
}
return f7(I) ? [1, rb(I)] : (Y && St([0, U], I), vre);
}
function c(_) {
var k = N0(_);
if (typeof k == "number") {
var I = 0;
if ((k === 1 || Pn === k) && (I = 1), I) {
var U = function(Y, y0) {
return Y;
};
return [0, we(_), U];
}
}
return f7(_) ? rb(_) : C9(_);
}
function s(_, k, I) {
var U = x(0, 0, k);
if (U[0] === 0)
return [0, U[1], I];
var Y = de(I);
if (Y)
var y0 = function(D, u0) {
return ir(Ze(D, 634872468, 89), D, _, u0);
}, D0 = a(U[1][2], Y[1], y0), I0 = de([0, D0, Y[2]]);
else
var I0 = Y;
return [0, 0, I0];
}
var p = function _(k) {
return _.fun(k);
}, y = function _(k) {
return _.fun(k);
}, T = function _(k) {
return _.fun(k);
}, E = function _(k) {
return _.fun(k);
}, h = function _(k) {
return _.fun(k);
}, w = function _(k, I) {
return _.fun(k, I);
}, G = function _(k) {
return _.fun(k);
}, A = function _(k) {
return _.fun(k);
}, S = function _(k, I, U) {
return _.fun(k, I, U);
}, M = function _(k) {
return _.fun(k);
}, K = function _(k) {
return _.fun(k);
}, V = function _(k, I) {
return _.fun(k, I);
}, f0 = function _(k) {
return _.fun(k);
}, m0 = function _(k) {
return _.fun(k);
}, k0 = function _(k, I) {
return _.fun(k, I);
}, g0 = function _(k) {
return _.fun(k);
}, e0 = function _(k, I) {
return _.fun(k, I);
}, x0 = function _(k) {
return _.fun(k);
}, l = function _(k, I) {
return _.fun(k, I);
}, c0 = function _(k) {
return _.fun(k);
}, t0 = function _(k, I) {
return _.fun(k, I);
}, a0 = function _(k, I) {
return _.fun(k, I);
}, w0 = function _(k, I) {
return _.fun(k, I);
}, _0 = function _(k) {
return _.fun(k);
}, E0 = function _(k) {
return _.fun(k);
}, X0 = function _(k, I, U) {
return _.fun(k, I, U);
}, b = function _(k, I) {
return _.fun(k, I);
}, G0 = function _(k, I) {
return _.fun(k, I);
}, X = function _(k) {
return _.fun(k);
};
function s0(_) {
var k = pr(_);
V0(_, 59);
var I = N0(_) === 8 ? 1 : 0, U = I && we(_), Y = x(0, 0, _), y0 = Y[0] === 0 ? Y[1] : Y[1][1];
return [4, [0, lr([0, k], [0, un(U, y0)], 0)]];
}
var dr = 0;
function Ar(_) {
return cr(dr, s0, _);
}
function ar(_) {
var k = pr(_);
V0(_, 37);
var I = zl(1, _), U = u(se[2], I), Y = 1 - _[5], y0 = Y && nb(U);
y0 && B1(_, U[1]);
var D0 = we(_);
V0(_, 25);
var I0 = we(_);
V0(_, 4);
var D = u(se[7], _);
V0(_, 5);
var u0 = N0(_) === 8 ? 1 : 0, Y0 = u0 && we(_), J0 = x(0, ore, _), fr = J0[0] === 0 ? un(Y0, J0[1]) : J0[1][1];
return [14, [0, U, D, lr([0, k], [0, un(D0, un(I0, fr))], 0)]];
}
var W0 = 0;
function Lr(_) {
return cr(W0, ar, _);
}
function Tr(_, k, I) {
var U = I[2][1];
if (U && !U[1][2][2]) {
var Y = U[2];
if (!Y)
return Y;
}
return ue(_, [0, I[1], k]);
}
function Hr(_, k) {
var I = 1 - _[5], U = I && nb(k);
return U && B1(_, k[1]);
}
function Or(_) {
var k = pr(_);
V0(_, 39);
var I = _[18], U = I && fu(_, 65), Y = un(k, pr(_));
V0(_, 4);
var y0 = lr([0, Y], 0, 0), D0 = Kl(1, _), I0 = N0(D0), D = 0;
if (typeof I0 == "number")
if (24 <= I0)
if (29 <= I0)
D = 1;
else
switch (I0 - 24 | 0) {
case 0:
var u0 = cr(0, Vn[9], D0), Y0 = u0[2], J0 = lr([0, Y0[2]], 0, 0), Pr = Y0[3], K0 = [0, [1, [0, u0[1], [0, Y0[1], 0, J0]]]];
break;
case 3:
var fr = cr(0, Vn[8], D0), Q0 = fr[2], F0 = lr([0, Q0[2]], 0, 0), Pr = Q0[3], K0 = [0, [1, [0, fr[1], [0, Q0[1], 2, F0]]]];
break;
case 4:
var gr = cr(0, Vn[7], D0), mr = gr[2], Cr = lr([0, mr[2]], 0, 0), Pr = mr[3], K0 = [0, [1, [0, gr[1], [0, mr[1], 1, Cr]]]];
break;
default:
D = 1;
}
else if (I0 === 8)
var Pr = 0, K0 = 0;
else
D = 1;
else
D = 1;
if (D)
var sr = T9(1, D0), Pr = 0, K0 = [0, [0, u(se[8], sr)]];
var Ur = N0(_);
if (typeof Ur == "number") {
if (Ur === 17) {
if (K0) {
var d0 = K0[1];
if (d0[0] === 0)
var Kr = [1, ir(t[2], xre, _, d0[1])];
else {
var re = d0[1];
Tr(_, 28, re);
var Kr = [0, re];
}
U ? V0(_, 63) : V0(_, 17);
var xe = u(se[7], _);
V0(_, 5);
var je = zl(1, _), ve = u(se[2], je);
return Hr(_, ve), [21, [0, Kr, xe, ve, 0, y0]];
}
throw [0, wn, are];
}
if (Ur === 63) {
if (K0) {
var Ie = K0[1];
if (Ie[0] === 0)
var Me = [1, ir(t[2], ire, _, Ie[1])];
else {
var Be = Ie[1];
Tr(_, 29, Be);
var Me = [0, Be];
}
V0(_, 63);
var fn = u(se[10], _);
V0(_, 5);
var Ke = zl(1, _), Ae = u(se[2], Ke);
return Hr(_, Ae), [22, [0, Me, fn, Ae, U, y0]];
}
throw [0, wn, fre];
}
}
if (Pu(function(gt) {
return ue(_, gt);
}, Pr), U ? V0(_, 63) : V0(_, 8), K0)
var xn = K0[1], Qe = xn[0] === 0 ? [0, [1, a(t[1], _, xn[1])]] : [0, [0, xn[1]]], yn = Qe;
else
var yn = K0;
var on = N0(_), Ce = 0;
if (typeof on == "number") {
var We = on !== 8 ? 1 : 0;
if (!We) {
var rn = We;
Ce = 1;
}
}
if (!Ce)
var rn = [0, u(se[7], _)];
V0(_, 8);
var bn = N0(_), Cn = 0;
if (typeof bn == "number") {
var Hn = bn !== 5 ? 1 : 0;
if (!Hn) {
var Sn = Hn;
Cn = 1;
}
}
if (!Cn)
var Sn = [0, u(se[7], _)];
V0(_, 5);
var vt = zl(1, _), At = u(se[2], vt);
return Hr(_, At), [20, [0, yn, rn, Sn, At, y0]];
}
var xr = 0;
function Rr(_) {
return cr(xr, Or, _);
}
function Wr(_) {
var k = qs(_) ? n4(_) : u(se[2], _), I = 1 - _[5], U = I && nb(k);
return U && B1(_, k[1]), k;
}
function Jr(_) {
var k = pr(_);
V0(_, 43);
var I = Wr(_);
return [0, I, lr([0, k], 0, 0)];
}
function or(_) {
var k = pr(_);
V0(_, 16);
var I = un(k, pr(_));
V0(_, 4);
var U = u(se[7], _);
V0(_, 5);
var Y = Wr(_), y0 = N0(_) === 43 ? 1 : 0, D0 = y0 && [0, cr(0, Jr, _)];
return [24, [0, U, Y, D0, lr([0, I], 0, 0)]];
}
var _r = 0;
function Ir(_) {
return cr(_r, or, _);
}
function fe(_) {
1 - _[11] && Ge(_, 36);
var k = pr(_), I = De(_);
V0(_, 19);
var U = N0(_) === 8 ? 1 : 0, Y = U && we(_), y0 = 0;
if (N0(_) !== 8 && !x2(_)) {
var D0 = [0, u(se[7], _)];
y0 = 1;
}
if (!y0)
var D0 = 0;
var I0 = yt(I, De(_)), D = x(0, 0, _), u0 = 0;
if (D[0] === 0)
var Y0 = D[1];
else {
var J0 = D[1];
if (D0) {
var fr = function(sr, Pr) {
return a(Ze(sr, Di, 90), sr, Pr);
}, Q0 = [0, a(J0[2], D0[1], fr)], F0 = Y;
u0 = 1;
} else
var Y0 = J0[1];
}
if (!u0)
var Q0 = D0, F0 = un(Y, Y0);
return [28, [0, Q0, lr([0, k], [0, F0], 0), I0]];
}
var v0 = 0;
function P(_) {
return cr(v0, fe, _);
}
function L(_) {
var k = pr(_);
V0(_, 20), V0(_, 4);
var I = u(se[7], _);
V0(_, 5), V0(_, 0);
for (var U = ure; ; ) {
var Y = U[2], y0 = N0(_);
if (typeof y0 == "number") {
var D0 = 0;
if ((y0 === 1 || Pn === y0) && (D0 = 1), D0) {
var I0 = de(Y);
V0(_, 1);
var D = c(_), u0 = I[1];
return [29, [0, I, I0, lr([0, k], [0, D[1]], 0), u0]];
}
}
var Y0 = U[1], J0 = OL(0, function(Q0) {
return function(F0) {
var gr = pr(F0), mr = N0(F0), Cr = 0;
if (typeof mr == "number" && mr === 36) {
Q0 && Ge(F0, 32), V0(F0, 36);
var sr = we(F0), Pr = 0;
Cr = 1;
}
if (!Cr) {
V0(F0, 33);
var sr = 0, Pr = [0, u(se[7], F0)];
}
var K0 = Q0 || (Pr === 0 ? 1 : 0);
V0(F0, 86);
var Ur = un(sr, c(F0)[1]);
function d0(je) {
if (typeof je == "number") {
var ve = je - 1 | 0, Ie = 0;
if (32 < ve >>> 0 ? ve === 35 && (Ie = 1) : 30 < (ve - 1 | 0) >>> 0 && (Ie = 1), Ie)
return 1;
}
return 0;
}
var Kr = 1, re = F0[9] === 1 ? F0 : [0, F0[1], F0[2], F0[3], F0[4], F0[5], F0[6], F0[7], F0[8], Kr, F0[10], F0[11], F0[12], F0[13], F0[14], F0[15], F0[16], F0[17], F0[18], F0[19], F0[20], F0[21], F0[22], F0[23], F0[24], F0[25], F0[26], F0[27], F0[28], F0[29], F0[30]], xe = a(se[4], d0, re);
return [0, [0, Pr, xe, lr([0, gr], [0, Ur], 0)], K0];
};
}(Y0), _), U = [0, J0[2], [0, J0[1], Y]];
}
}
var Q = 0;
function i0(_) {
return cr(Q, L, _);
}
function l0(_) {
var k = pr(_), I = De(_);
V0(_, 22), f7(_) && ue(_, [0, I, 21]);
var U = u(se[7], _), Y = x(0, 0, _);
if (Y[0] === 0)
var D0 = U, I0 = Y[1];
else
var y0 = function(D, u0) {
return a(Ze(D, Di, 91), D, u0);
}, D0 = a(Y[1][2], U, y0), I0 = 0;
return [30, [0, D0, lr([0, k], [0, I0], 0)]];
}
var S0 = 0;
function T0(_) {
return cr(S0, l0, _);
}
function rr(_) {
var k = pr(_);
V0(_, 23);
var I = u(se[15], _);
if (N0(_) === 34)
var U = Wt(_), Y = function(sr, Pr) {
var K0 = Pr[1];
return [0, K0, ir(Ze(sr, V8, 29), sr, K0, Pr[2])];
}, y0 = a(U[2], I, Y);
else
var y0 = I;
var D0 = N0(_), I0 = 0;
if (typeof D0 == "number" && D0 === 34) {
var D = [0, cr(0, function(Pr) {
var K0 = pr(Pr);
V0(Pr, 34);
var Ur = we(Pr), d0 = N0(Pr) === 4 ? 1 : 0;
if (d0) {
V0(Pr, 4);
var Kr = [0, a(se[18], Pr, 39)];
V0(Pr, 5);
var re = Kr;
} else
var re = d0;
var xe = u(se[15], Pr);
if (N0(Pr) === 38)
var Ie = xe;
else
var je = c(Pr), ve = function(Me, Be) {
var fn = Be[1];
return [0, fn, ir(Ze(Me, V8, 92), Me, fn, Be[2])];
}, Ie = a(je[2], xe, ve);
return [0, re, Ie, lr([0, K0], [0, Ur], 0)];
}, _)];
I0 = 1;
}
if (!I0)
var D = 0;
var u0 = N0(_), Y0 = 0;
if (typeof u0 == "number" && u0 === 38) {
V0(_, 38);
var J0 = u(se[15], _), fr = J0[1], Q0 = c(_), F0 = function(Pr, K0) {
return ir(Ze(Pr, V8, 93), Pr, fr, K0);
}, gr = [0, [0, fr, a(Q0[2], J0[2], F0)]];
Y0 = 1;
}
if (!Y0)
var gr = 0;
var mr = D === 0 ? 1 : 0, Cr = mr && (gr === 0 ? 1 : 0);
return Cr && ue(_, [0, y0[1], 33]), [31, [0, y0, D, gr, lr([0, k], 0, 0)]];
}
var R0 = 0;
function B(_) {
return cr(R0, rr, _);
}
function Z(_) {
var k = u(Vn[9], _), I = s(0, _, k[1]), U = 0, Y = k[3];
Pu(function(D0) {
return ue(_, D0);
}, Y);
var y0 = lr([0, k[2]], [0, I[1]], 0);
return [34, [0, I[2], U, y0]];
}
var p0 = 0;
function b0(_) {
return cr(p0, Z, _);
}
function O0(_) {
var k = u(Vn[8], _), I = s(2, _, k[1]), U = 2, Y = k[3];
Pu(function(D0) {
return ue(_, D0);
}, Y);
var y0 = lr([0, k[2]], [0, I[1]], 0);
return [34, [0, I[2], U, y0]];
}
var q0 = 0;
function er(_) {
return cr(q0, O0, _);
}
function yr(_) {
var k = u(Vn[7], _), I = s(1, _, k[1]), U = 1, Y = k[3];
Pu(function(D0) {
return ue(_, D0);
}, Y);
var y0 = lr([0, k[2]], [0, I[1]], 0);
return [34, [0, I[2], U, y0]];
}
var vr = 0;
function $0(_) {
return cr(vr, yr, _);
}
function Sr(_) {
var k = pr(_);
V0(_, 25);
var I = un(k, pr(_));
V0(_, 4);
var U = u(se[7], _);
V0(_, 5);
var Y = zl(1, _), y0 = u(se[2], Y), D0 = 1 - _[5], I0 = D0 && nb(y0);
return I0 && B1(_, y0[1]), [35, [0, U, y0, lr([0, I], 0, 0)]];
}
var Mr = 0;
function Br(_) {
return cr(Mr, Sr, _);
}
function qr(_) {
var k = pr(_), I = u(se[7], _), U = N0(_), Y = I[2];
if (Y[0] === 10 && typeof U == "number" && U === 86) {
var y0 = Y[1], D0 = y0[2][1];
V0(_, 86), a(Gu[3], D0, _[3]) && ue(_, [0, I[1], [16, nre, D0]]);
var I0 = _[30], D = _[29], u0 = _[28], Y0 = _[27], J0 = _[26], fr = _[25], Q0 = _[24], F0 = _[23], gr = _[22], mr = _[21], Cr = _[20], sr = _[19], Pr = _[18], K0 = _[17], Ur = _[16], d0 = _[15], Kr = _[14], re = _[13], xe = _[12], je = _[11], ve = _[10], Ie = _[9], Me = _[8], Be = _[7], fn = _[6], Ke = _[5], Ae = _[4], xn = a(Gu[4], D0, _[3]), Qe = [0, _[1], _[2], xn, Ae, Ke, fn, Be, Me, Ie, ve, je, xe, re, Kr, d0, Ur, K0, Pr, sr, Cr, mr, gr, F0, Q0, fr, J0, Y0, u0, D, I0], yn = qs(Qe) ? n4(Qe) : u(se[2], Qe);
return [27, [0, y0, yn, lr([0, k], 0, 0)]];
}
var on = x(tre, 0, _);
if (on[0] === 0)
var We = I, rn = on[1];
else
var Ce = function(bn, Cn) {
return a(Ze(bn, Di, 94), bn, Cn);
}, We = a(on[1][2], I, Ce), rn = 0;
return [19, [0, We, 0, lr(0, [0, rn], 0)]];
}
var jr = 0;
function $r(_) {
return cr(jr, qr, _);
}
function ne(_) {
var k = u(se[7], _), I = x(ere, 0, _);
if (I[0] === 0)
var Y = k, y0 = I[1];
else
var U = function(sr, Pr) {
return a(Ze(sr, Di, 95), sr, Pr);
}, Y = a(I[1][2], k, U), y0 = 0;
var D0 = _[19];
if (D0) {
var I0 = Y[2], D = 0;
if (I0[0] === 14) {
var u0 = I0[1], Y0 = 0, J0 = u0[1];
if (typeof J0 != "number" && J0[0] === 0) {
var fr = u0[2], Q0 = 1 < nn(fr) ? 1 : 0;
if (Q0)
var F0 = Ot(fr, nn(fr) - 1 | 0), gr = Ot(fr, 0) === F0 ? 1 : 0;
else
var gr = Q0;
var mr = gr && [0, p7(fr, 1, nn(fr) - 2 | 0)], Cr = mr;
Y0 = 1;
}
Y0 || (D = 1);
} else
D = 1;
if (D)
var Cr = 0;
} else
var Cr = D0;
return [19, [0, Y, Cr, lr(0, [0, y0], 0)]];
}
var Qr = 0;
function pe(_) {
return cr(Qr, ne, _);
}
function oe(_) {
var k = N0(_), I = 0;
if (typeof k != "number" && k[0] === 4 && !n0(k[3], $0e)) {
ie(_);
var U = N0(_);
if (typeof U != "number" && U[0] === 2)
return i(_, U[1]);
St(Z0e, _), I = 1;
}
return I || St(Q0e, _), [0, G1(_), rre];
}
function me(_, k, I) {
function U(D) {
return _ ? u(ln[2], D) : a(se[13], 0, D);
}
var Y = Yn(1, I);
if (typeof Y == "number")
switch (Y) {
case 1:
case 9:
case 113:
return [0, U(I), 0];
}
else if (Y[0] === 4 && !n0(Y[3], J0e)) {
var y0 = V7(I);
return ie(I), [0, y0, [0, U(I)]];
}
var D0 = N0(I);
if (k && typeof D0 == "number") {
var I0 = 0;
if ((D0 === 46 || D0 === 61) && (I0 = 1), I0)
return Ge(I, k[1]), ie(I), [0, u(ln[2], I), 0];
}
return [0, U(I), 0];
}
function ae(_, k) {
var I = N0(_);
if (typeof I == "number" && Xt === I) {
var U = cr(0, function(Ae) {
ie(Ae);
var xn = N0(Ae);
return typeof xn != "number" && xn[0] === 4 && !n0(xn[3], K0e) ? (ie(Ae), 2 <= k ? [0, a(se[13], 0, Ae)] : [0, u(ln[2], Ae)]) : (St(W0e, Ae), 0);
}, _), Y = U[2], y0 = Y && [0, [0, U[1], Y[1]]], D0 = y0 && [0, [1, y0[1]]];
return D0;
}
V0(_, 0);
for (var I0 = 0, D = 0; ; ) {
var u0 = I0 ? I0[1] : 1, Y0 = N0(_);
if (typeof Y0 == "number") {
var J0 = 0;
if ((Y0 === 1 || Pn === Y0) && (J0 = 1), J0) {
var fr = de(D);
return V0(_, 1), [0, [0, fr]];
}
}
if (1 - u0 && Ge(_, 84), k === 2) {
var Q0 = N0(_), F0 = 0;
if (typeof Q0 == "number")
if (Q0 === 46)
var gr = X0e;
else if (Q0 === 61)
var gr = H0e;
else
F0 = 1;
else
F0 = 1;
if (F0)
var gr = 0;
var mr = N0(_), Cr = 0;
if (typeof mr == "number") {
var sr = 0;
if (mr !== 46 && mr !== 61 && (sr = 1), !sr) {
var Pr = 1;
Cr = 1;
}
}
if (!Cr)
var Pr = 0;
if (Pr) {
var K0 = V7(_), Ur = N0(_), d0 = 0;
if (typeof Ur == "number")
switch (Ur) {
case 1:
case 9:
case 113:
e(0, _, K0);
var Ie = [0, 0, 0, K0];
d0 = 1;
break;
}
else if (Ur[0] === 4 && !n0(Ur[3], Y0e)) {
var Kr = Yn(1, _), re = 0;
if (typeof Kr == "number")
switch (Kr) {
case 1:
case 9:
case 113:
var xe = [0, gr, 0, u(ln[2], _)];
re = 1;
break;
}
else if (Kr[0] === 4 && !n0(Kr[3], V0e)) {
var je = V7(_);
ie(_);
var xe = [0, gr, [0, u(ln[2], _)], je];
re = 1;
}
if (!re) {
e(0, _, K0), ie(_);
var xe = [0, 0, [0, a(se[13], 0, _)], K0];
}
var Ie = xe;
d0 = 1;
}
if (!d0)
var ve = me(1, 0, _), Ie = [0, gr, ve[2], ve[1]];
var Be = Ie;
} else
var Me = me(0, 0, _), Be = [0, 0, Me[2], Me[1]];
var Ke = Be;
} else
var fn = me(1, U0e, _), Ke = [0, 0, fn[2], fn[1]];
var I0 = [0, fu(_, 9)], D = [0, Ke, D];
}
}
function ce(_, k) {
var I = x(0, 0, _);
if (I[0] === 0)
return [0, I[1], k];
function U(Y, y0) {
var D0 = y0[1];
return [0, D0, ir(Ze(Y, wA, 96), Y, D0, y0[2])];
}
return [0, 0, a(I[1][2], k, U)];
}
function ge(_) {
var k = ys(1, _), I = pr(k);
V0(k, 50);
var U = N0(k), Y = 0;
if (typeof U == "number")
switch (U) {
case 46:
if (iu(k)) {
V0(k, 46);
var y0 = N0(k), D0 = 0;
if (typeof y0 == "number") {
var I0 = 0;
if (Xt !== y0 && y0 && (I0 = 1), !I0) {
var J0 = 1;
Y = 2, D0 = 1;
}
}
if (!D0) {
var D = 1;
Y = 1;
}
}
break;
case 61:
if (iu(k)) {
var u0 = Yn(1, k), Y0 = 0;
if (typeof u0 == "number")
switch (u0) {
case 0:
ie(k);
var J0 = 0;
Y = 2, Y0 = 2;
break;
case 106:
ie(k), St(0, k);
var J0 = 0;
Y = 2, Y0 = 2;
break;
case 9:
Y0 = 1;
break;
}
else
u0[0] === 4 && !n0(u0[3], z0e) && (Y0 = 1);
switch (Y0) {
case 2:
break;
case 0:
ie(k);
var D = 0;
Y = 1;
break;
default:
var D = 2;
Y = 1;
}
}
break;
case 0:
case 106:
var J0 = 2;
Y = 2;
break;
}
else if (U[0] === 2) {
var fr = ce(k, i(k, U[1])), Q0 = lr([0, I], [0, fr[1]], 0);
return [25, [0, 2, fr[2], 0, 0, Q0]];
}
switch (Y) {
case 0:
var D = 2;
break;
case 1:
break;
default:
var F0 = ae(k, J0), gr = ce(k, oe(k)), mr = lr([0, I], [0, gr[1]], 0);
return [25, [0, J0, gr[2], 0, F0, mr]];
}
var Cr = 2 <= D ? a(se[13], 0, k) : u(ln[2], k), sr = N0(k), Pr = 0;
if (typeof sr == "number" && sr === 9) {
V0(k, 9);
var K0 = ae(k, D);
Pr = 1;
}
if (!Pr)
var K0 = 0;
var Ur = ce(k, oe(k)), d0 = lr([0, I], [0, Ur[1]], 0);
return [25, [0, D, Ur[2], [0, Cr], K0, d0]];
}
var H0 = 0;
function Fr(_) {
return cr(H0, ge, _);
}
return N(p, function(_) {
var k = De(_), I = pr(_);
return V0(_, 8), [0, k, [15, [0, lr([0, I], [0, c(_)[1]], 0)]]];
}), N(y, function(_) {
var k = pr(_), I = cr(0, function(Y0) {
V0(Y0, 32);
var J0 = 0;
if (N0(Y0) !== 8 && !x2(Y0)) {
var fr = a(se[13], 0, Y0), Q0 = fr[2][1];
1 - a(Gu[3], Q0, Y0[3]) && Ge(Y0, [15, Q0]);
var F0 = [0, fr];
J0 = 1;
}
if (!J0)
var F0 = 0;
var gr = x(0, 0, Y0), mr = 0;
if (gr[0] === 0)
var Cr = gr[1];
else {
var sr = gr[1];
if (F0) {
var Pr = function(xe, je) {
return a(Ze(xe, Nv, 97), xe, je);
}, K0 = [0, a(sr[2], F0[1], Pr)], Ur = 0;
mr = 1;
} else
var Cr = sr[1];
}
if (!mr)
var K0 = F0, Ur = Cr;
return [0, K0, Ur];
}, _), U = I[2], Y = U[1], y0 = I[1], D0 = Y === 0 ? 1 : 0;
if (D0)
var I0 = _[8], D = I0 || _[9], u0 = 1 - D;
else
var u0 = D0;
return u0 && ue(_, [0, y0, 35]), [0, y0, [1, [0, Y, lr([0, k], [0, U[2]], 0)]]];
}), N(T, function(_) {
var k = pr(_), I = cr(0, function(D0) {
V0(D0, 35);
var I0 = 0;
if (N0(D0) !== 8 && !x2(D0)) {
var D = a(se[13], 0, D0), u0 = D[2][1];
1 - a(Gu[3], u0, D0[3]) && Ge(D0, [15, u0]);
var Y0 = [0, D];
I0 = 1;
}
if (!I0)
var Y0 = 0;
var J0 = x(0, 0, D0), fr = 0;
if (J0[0] === 0)
var Q0 = J0[1];
else {
var F0 = J0[1];
if (Y0) {
var gr = function(Ur, d0) {
return a(Ze(Ur, Nv, 98), Ur, d0);
}, mr = [0, a(F0[2], Y0[1], gr)], Cr = 0;
fr = 1;
} else
var Q0 = F0[1];
}
if (!fr)
var mr = Y0, Cr = Q0;
return [0, mr, Cr];
}, _), U = I[2], Y = I[1];
1 - _[8] && ue(_, [0, Y, 34]);
var y0 = lr([0, k], [0, U[2]], 0);
return [0, Y, [3, [0, U[1], y0]]];
}), N(E, function(_) {
var k = cr(0, function(U) {
var Y = pr(U);
V0(U, 26);
var y0 = un(Y, pr(U));
V0(U, 4);
var D0 = u(se[7], U);
V0(U, 5);
var I0 = u(se[2], U), D = 1 - U[5], u0 = D && nb(I0);
return u0 && B1(U, I0[1]), [36, [0, D0, I0, lr([0, y0], 0, 0)]];
}, _), I = k[1];
return Y7(_, [0, I, 38]), [0, I, k[2]];
}), N(h, function(_) {
var k = u(se[15], _), I = k[1], U = c(_);
function Y(y0, D0) {
return ir(Ze(y0, V8, 99), y0, I, D0);
}
return [0, I, [0, a(U[2], k[2], Y)]];
}), N(w, function(_, k) {
1 - iu(k) && Ge(k, 10);
var I = un(_, pr(k));
V0(k, 61), zu(k, 1);
var U = u(ln[2], k), Y = N0(k) === 98 ? ds(k, U) : U, y0 = u(ln[3], k);
V0(k, 82);
var D0 = u(ln[1], k);
h7(k);
var I0 = x(0, 0, k);
if (I0[0] === 0)
var u0 = D0, Y0 = I0[1];
else
var D = function(J0, fr) {
return a(Ze(J0, _v, ni), J0, fr);
}, u0 = a(I0[1][2], D0, D), Y0 = 0;
return [0, Y, y0, u0, lr([0, I], [0, Y0], 0)];
}), N(G, function(_) {
return cr(0, function(k) {
var I = pr(k);
return V0(k, 60), [11, a(w, I, k)];
}, _);
}), N(A, function(_) {
if (A9(1, _) && !me0(1, _)) {
var k = cr(0, u(w, 0), _);
return [0, k[1], [32, k[2]]];
}
return u(se[2], _);
}), N(S, function(_, k, I) {
var U = _ && _[1];
1 - iu(I) && Ge(I, 11);
var Y = un(k, pr(I));
V0(I, 62);
var y0 = pr(I);
V0(I, 61);
var D0 = un(Y, y0);
zu(I, 1);
var I0 = u(ln[2], I), D = N0(I) === 98 ? ds(I, I0) : I0, u0 = u(ln[3], I), Y0 = N0(I), J0 = 0;
if (typeof Y0 == "number" && Y0 === 86) {
V0(I, 86);
var fr = [0, u(ln[1], I)];
J0 = 1;
}
if (!J0)
var fr = 0;
if (U) {
var Q0 = N0(I), F0 = 0;
if (typeof Q0 == "number" && Q0 === 82) {
Ge(I, 70), ie(I);
var gr = 0;
if (N0(I) !== 8 && !x2(I)) {
var mr = [0, u(ln[1], I)];
gr = 1;
}
if (!gr)
var mr = 0;
} else
F0 = 1;
if (F0)
var mr = 0;
var Cr = mr;
} else {
V0(I, 82);
var Cr = [0, u(ln[1], I)];
}
h7(I);
var sr = x(0, 0, I);
if (sr[0] === 0)
var Pr = Cr, K0 = fr, Ur = u0, d0 = D, Kr = sr[1];
else {
var re = sr[1][2];
if (Cr)
var xe = function(rn, bn) {
return a(Ze(rn, _v, L7), rn, bn);
}, Pr = [0, a(re, Cr[1], xe)], K0 = fr, Ur = u0, d0 = D, Kr = 0;
else if (fr)
var je = 0, ve = function(rn, bn) {
return a(Ze(rn, _v, Ri), rn, bn);
}, Pr = je, K0 = [0, a(re, fr[1], ve)], Ur = u0, d0 = D, Kr = 0;
else if (u0)
var Ie = 0, Me = 0, Be = function(rn, bn) {
return a(Ze(rn, _F, c7), rn, bn);
}, Pr = Ie, K0 = Me, Ur = [0, a(re, u0[1], Be)], d0 = D, Kr = 0;
else
var fn = 0, Ke = 0, Ae = 0, Pr = fn, K0 = Ke, Ur = Ae, xn = 0, d0 = a(re, D, function(rn, bn) {
return a(Ze(rn, Nv, D7), rn, bn);
}), Kr = xn;
}
return [0, d0, Ur, Pr, K0, lr([0, D0], [0, Kr], 0)];
}), N(M, function(_) {
return cr(0, function(k) {
var I = pr(k);
return V0(k, 60), [12, ir(S, q0e, I, k)];
}, _);
}), N(K, function(_) {
var k = Yn(1, _);
if (typeof k == "number" && k === 61) {
var I = cr(0, a(S, B0e, 0), _);
return [0, I[1], [33, I[2]]];
}
return u(se[2], _);
}), N(V, function(_, k) {
1 - iu(k) && Ge(k, 16);
var I = un(_, pr(k));
V0(k, 53);
var U = u(ln[2], k), Y = N0(k) === 41 ? U : ds(k, U), y0 = u(ln[3], k), D0 = N0(k) === 41 ? y0 : xi(k, y0), I0 = u(ln[7], k), D = c(k);
function u0(fr, Q0) {
var F0 = Q0[1];
return [0, F0, ir(Ze(fr, VH, R7), fr, F0, Q0[2])];
}
var Y0 = a(D[2], I0[2], u0), J0 = lr([0, I], 0, 0);
return [0, Y, D0, I0[1], Y0, J0];
}), N(f0, function(_) {
return cr(0, function(k) {
var I = pr(k);
return V0(k, 60), [8, a(V, I, k)];
}, _);
}), N(m0, function(_) {
var k = A9(1, _), I = 1, U = k || _e0(I, _);
if (U) {
var Y = cr(0, u(V, 0), _);
return [0, Y[1], [26, Y[2]]];
}
return pe(_);
}), N(k0, function(_, k) {
var I = ys(1, k), U = un(_, pr(I));
V0(I, 40);
var Y = a(se[13], 0, I), y0 = N0(I), D0 = 0;
if (typeof y0 == "number") {
var I0 = 0;
if (y0 !== 98 && y0 && (I0 = 1), !I0) {
var D = ds(I, Y);
D0 = 1;
}
}
if (!D0)
var D = Y;
var u0 = u(ln[3], I), Y0 = N0(I), J0 = 0;
if (typeof Y0 == "number" && !Y0) {
var fr = xi(I, u0);
J0 = 1;
}
if (!J0)
var fr = u0;
var Q0 = fu(I, 41);
if (Q0) {
var F0 = u(ln[5], I), gr = N0(I), mr = 0;
if (typeof gr == "number" && !gr) {
var Cr = Wt(I), sr = function(Sn, vt) {
return mu(u(Ze(Sn, tH, 36), Sn), vt);
}, Pr = [0, a(Cr[2], F0, sr)];
mr = 1;
}
if (!mr)
var Pr = [0, F0];
var K0 = Pr;
} else
var K0 = Q0;
var Ur = N0(I), d0 = 0;
if (typeof Ur != "number" && Ur[0] === 4 && !n0(Ur[3], M0e)) {
ie(I);
for (var Kr = 0; ; ) {
var re = [0, u(ln[5], I), Kr], xe = N0(I);
if (typeof xe == "number" && xe === 9) {
V0(I, 9);
var Kr = re;
continue;
}
var je = de(re), ve = N0(I), Ie = 0;
if (typeof ve == "number" && !ve) {
var Me = ge0(I, je);
Ie = 1;
}
if (!Ie)
var Me = je;
var Be = Me;
d0 = 1;
break;
}
}
if (!d0)
var Be = 0;
var fn = N0(I), Ke = 0;
if (typeof fn == "number" && fn === 52) {
var Ae = a(Ys[5], I, 0), xn = N0(I), Qe = 0;
if (typeof xn == "number" && !xn) {
var yn = [0, Fe0(I, Ae)];
Qe = 1;
}
if (!Qe)
var yn = [0, Ae];
var on = yn;
Ke = 1;
}
if (!Ke)
var on = 0;
var Ce = a(ln[6], 1, I), We = c(I);
function rn(Cn, Hn) {
var Sn = Hn[1];
return [0, Sn, ir(Ze(Cn, VH, Xt), Cn, Sn, Hn[2])];
}
var bn = a(We[2], Ce, rn);
return [0, D, fr, bn, K0, Be, on, lr([0, U], 0, 0)];
}), N(g0, function(_) {
return cr(0, function(k) {
var I = pr(k);
return V0(k, 60), [5, a(k0, I, k)];
}, _);
}), N(e0, function(_, k) {
var I = _ && _[1], U = un(I, pr(k));
V0(k, 15);
var Y = ds(k, a(se[13], 0, k)), y0 = cr(0, function(mr) {
var Cr = xi(mr, u(ln[3], mr)), sr = u(ln[8], mr);
V0(mr, 86);
var Pr = u(ln[1], mr);
zu(mr, 1);
var K0 = N0(mr);
if (h7(mr), K0 === 66)
var Ur = Wt(mr), d0 = function(re, xe) {
return a(Ze(re, _v, 31), re, xe);
}, Kr = a(Ur[2], Pr, d0);
else
var Kr = Pr;
return [12, [0, Cr, sr, Kr, 0]];
}, k), D0 = u(ln[11], k), I0 = x(0, 0, k);
if (I0[0] === 0)
var D = D0, u0 = y0, Y0 = I0[1];
else {
var J0 = I0[1][2];
if (D0)
var fr = function(Pr, K0) {
return a(Ze(Pr, FH, Qc), Pr, K0);
}, D = [0, a(J0, D0[1], fr)], u0 = y0, Y0 = 0;
else
var Q0 = 0, D = Q0, F0 = 0, u0 = a(J0, y0, function(Pr, K0) {
return a(Ze(Pr, _v, fs2), Pr, K0);
}), Y0 = F0;
}
var gr = [0, u0[1], u0];
return [0, Y, gr, D, lr([0, U], [0, Y0], 0)];
}), N(x0, function(_) {
return cr(0, function(k) {
var I = pr(k);
V0(k, 60);
var U = N0(k), Y = 0;
return typeof U == "number" && U === 64 && (Ge(k, 67), V0(k, 64), Y = 1), [7, a(e0, [0, I], k)];
}, _);
}), N(l, function(_, k) {
var I = un(k, pr(_));
V0(_, 24);
var U = a(se[13], G0e, _), Y = u(ln[9], _), y0 = x(0, 0, _);
if (y0[0] === 0)
var I0 = Y, D = y0[1];
else
var D0 = function(u0, Y0) {
return a(Ze(u0, NE, Fv), u0, Y0);
}, I0 = a(y0[1][2], Y, D0), D = 0;
return [0, U, I0, lr([0, I], [0, D], 0)];
}), N(c0, function(_) {
return cr(0, function(k) {
var I = pr(k);
return V0(k, 60), [13, a(l, k, I)];
}, _);
}), N(t0, function(_, k) {
var I = De(k), U = pr(k);
V0(k, 60);
var Y = un(U, pr(k));
return Zl(k, j0e), !_ && N0(k) !== 10 ? cr([0, I], function(y0) {
var D0 = N0(y0), I0 = 0;
if (typeof D0 != "number" && D0[0] === 2) {
var D = i(y0, D0[1]), u0 = Wt(y0), Y0 = function(Cr, sr) {
var Pr = sr[1];
return [0, Pr, ir(Ze(Cr, wA, 39), Cr, Pr, sr[2])];
}, J0 = [1, a(u0[2], D, Y0)];
I0 = 1;
}
if (!I0)
var J0 = [0, ds(y0, a(se[13], 0, y0))];
var fr = OL(0, function(mr) {
var Cr = pr(mr);
V0(mr, 0);
for (var sr = 0, Pr = 0; ; ) {
var K0 = N0(mr);
if (typeof K0 == "number") {
var Ur = 0;
if ((K0 === 1 || Pn === K0) && (Ur = 1), Ur) {
var d0 = de(Pr), Kr = d0 === 0 ? 1 : 0, re = Kr && pr(mr);
return V0(mr, 1), [0, [0, d0, _u([0, Cr], [0, c(mr)[1]], re, 0)], sr];
}
}
var xe = a(w0, D0e, mr), je = xe[2], ve = 0;
if (sr)
if (sr[1])
if (je[0] === 10) {
Ge(mr, 79);
var Ae = sr;
} else
ve = 1;
else
switch (je[0]) {
case 6:
var Ie = je[1][2], Me = 0;
if (Ie)
switch (Ie[1][0]) {
case 4:
case 6:
Me = 1;
break;
}
Me || Ge(mr, 79);
var Ae = sr;
break;
case 10:
Ge(mr, 78);
var Ae = sr;
break;
default:
ve = 1;
}
else
switch (je[0]) {
case 6:
var Be = je[1][2], fn = 0;
if (Be)
switch (Be[1][0]) {
case 4:
case 6:
var Ke = sr;
fn = 1;
break;
}
if (!fn)
var Ke = L0e;
var Ae = Ke;
break;
case 10:
var Ae = R0e;
break;
default:
ve = 1;
}
if (ve)
var Ae = sr;
var sr = Ae, Pr = [0, xe, Pr];
}
}, y0), Q0 = fr[2], F0 = Q0 && Q0[1], gr = lr([0, Y], 0, 0);
return [9, [0, J0, fr[1], F0, gr]];
}, k) : cr([0, I], u(a0, Y), k);
}), N(a0, function(_, k) {
var I = pr(k);
V0(k, 10);
var U = pr(k);
Zl(k, P0e);
var Y = pl([0, _, [0, I, [0, U, [0, pr(k), 0]]]]), y0 = u(ln[9], k), D0 = x(0, 0, k);
if (D0[0] === 0)
var u0 = D0[1], Y0 = y0;
else
var I0 = 0, D = function(J0, fr) {
return a(Ze(J0, NE, Ht), J0, fr);
}, u0 = I0, Y0 = a(D0[1][2], y0, D);
return [10, [0, Y0, lr([0, Y], [0, u0], 0)]];
}), N(w0, function(_, k) {
var I = _ && _[1];
1 - iu(k) && Ge(k, 13);
var U = Yn(1, k);
if (typeof U == "number")
switch (U) {
case 24:
return u(c0, k);
case 40:
return u(g0, k);
case 46:
if (N0(k) === 50)
return Fr(k);
break;
case 49:
if (I)
return a(X, [0, I], k);
break;
case 53:
return u(f0, k);
case 61:
var Y = N0(k);
return typeof Y == "number" && Y === 50 && I ? Fr(k) : u(G, k);
case 62:
return u(M, k);
case 15:
case 64:
return u(x0, k);
}
else if (U[0] === 4 && !n0(U[3], C0e))
return a(t0, I, k);
if (I) {
var y0 = N0(k);
return typeof y0 == "number" && y0 === 50 ? (Ge(k, 82), u(se[2], k)) : u(c0, k);
}
return u(se[2], k);
}), N(_0, function(_) {
Zl(_, I0e);
var k = N0(_);
if (typeof k != "number" && k[0] === 2)
return i(_, k[1]);
var I = [0, De(_), A0e];
return St(N0e, _), I;
}), N(E0, function(_) {
var k = u(_0, _), I = k[2], U = k[1], Y = x(0, 0, _);
if (Y[0] === 0)
return [0, [0, U, I], Y[1]];
var y0 = 0;
function D0(I0, D) {
return ir(Ze(I0, wA, vf), I0, U, D);
}
return [0, [0, U, a(Y[1][2], I, D0)], y0];
}), N(X0, function(_, k, I) {
var U = _ ? _[1] : 1, Y = N0(k);
if (typeof Y == "number") {
var y0 = 0;
if ((Y === 1 || Pn === Y) && (y0 = 1), y0)
return de(I);
}
1 - U && Ge(k, 85);
var D0 = cr(0, function(I0) {
var D = V7(I0), u0 = N0(I0), Y0 = 0;
if (typeof u0 != "number" && u0[0] === 4 && !n0(u0[3], O0e)) {
ie(I0);
var J0 = [0, V7(I0)];
Y0 = 1;
}
if (!Y0)
var J0 = 0;
return [0, D, J0];
}, k);
return ir(X0, [0, fu(k, 9)], k, [0, D0, I]);
}), N(b, function(_, k) {
return Pu(function(I) {
var U = I[2];
return U[2] ? 0 : e(T0e, _, U[1]);
}, k);
}), N(G0, function(_, k) {
var I = xe0(1, ys(1, k)), U = pr(I), Y = De(I);
V0(I, 49);
var y0 = N0(I);
if (typeof y0 == "number") {
if (53 <= y0) {
if (!(63 <= y0))
switch (y0 + pU | 0) {
case 0:
return cr([0, Y], function(D) {
1 - iu(D) && Ge(D, 15);
var u0 = cr(0, u(V, 0), D), Y0 = [0, u0[1], [26, u0[2]]];
return [18, [0, [0, Y0], 0, 0, 0, lr([0, U], 0, 0)]];
}, I);
case 8:
if (Yn(1, I) !== 0)
return cr([0, Y], function(D) {
1 - iu(D) && Ge(D, 15);
var u0 = Yn(1, D);
if (typeof u0 == "number") {
if (u0 === 48)
return Ge(D, 0), V0(D, 61), [18, [0, 0, 0, 0, 0, lr([0, U], 0, 0)]];
if (Xt === u0) {
V0(D, 61);
var Y0 = De(D);
V0(D, Xt);
var J0 = u(E0, D), fr = lr([0, U], [0, J0[2]], 0);
return [18, [0, 0, [0, [1, [0, Y0, 0]]], [0, J0[1]], 0, fr]];
}
}
var Q0 = cr(0, u(w, 0), D), F0 = [0, Q0[1], [32, Q0[2]]];
return [18, [0, [0, F0], 0, 0, 0, lr([0, U], 0, 0)]];
}, I);
break;
case 9:
return cr([0, Y], function(D) {
var u0 = cr(0, function(J0) {
return u(a(S, 0, 0), J0);
}, D), Y0 = [0, u0[1], [33, u0[2]]];
return [18, [0, [0, Y0], 0, 0, 0, lr([0, U], 0, 0)]];
}, I);
}
} else if (y0 === 36)
return cr([0, Y], function(D) {
var u0 = un(U, pr(D)), Y0 = cr(0, function(Ur) {
return V0(Ur, 36);
}, D), J0 = ae0(1, D);
if (qs(J0))
var fr = 0, Q0 = [0, u(Vn[10], J0)];
else if ($l(J0))
var fr = 0, Q0 = [0, a(Ys[3], J0, _)];
else if (N0(J0) === 48)
var fr = 0, Q0 = [0, u(Vn[11], J0)];
else {
var F0 = u(se[10], J0), gr = x(0, 0, J0);
if (gr[0] === 0)
var sr = gr[1], Pr = F0;
else
var mr = 0, Cr = function(Kr, re) {
return a(Ze(Kr, Di, F7), Kr, re);
}, sr = mr, Pr = a(gr[1][2], F0, Cr);
var fr = sr, Q0 = [1, Pr];
}
var K0 = lr([0, u0], [0, fr], 0);
return [17, [0, Y0[1], Q0, K0]];
}, I);
}
if ($l(I))
return cr([0, Y], function(D) {
var u0 = a(Ys[3], D, _);
return [18, [0, [0, u0], 0, 0, 1, lr([0, U], 0, 0)]];
}, I);
if (qs(I))
return cr([0, Y], function(D) {
u(N9(D), _);
var u0 = u(Vn[10], D);
return [18, [0, [0, u0], 0, 0, 1, lr([0, U], 0, 0)]];
}, I);
if (typeof y0 == "number")
if (29 <= y0) {
if (y0 === 48) {
if (I[27][1])
return cr([0, Y], function(D) {
var u0 = a(se[3], [0, _], D);
return [18, [0, [0, u0], 0, 0, 1, lr([0, U], 0, 0)]];
}, I);
} else if (Xt === y0)
return cr([0, Y], function(D) {
var u0 = De(D);
V0(D, Xt);
var Y0 = D[27][3], J0 = N0(D), fr = 0;
if (typeof J0 != "number" && J0[0] === 4 && !n0(J0[3], g0e)) {
ie(D);
var Q0 = Y0 ? [0, a(se[13], 0, D)] : (Ge(D, 13), 0), F0 = Q0;
fr = 1;
}
if (!fr)
var F0 = 0;
var gr = u(E0, D), mr = lr([0, U], [0, gr[2]], 0);
return [18, [0, 0, [0, [1, [0, u0, F0]]], [0, gr[1]], 1, mr]];
}, I);
} else {
var D0 = 0;
if ((y0 === 24 || 27 <= y0) && (D0 = 1), D0)
return cr([0, Y], function(D) {
var u0 = a(se[3], [0, _], D);
return [18, [0, [0, u0], 0, 0, 1, lr([0, U], 0, 0)]];
}, I);
}
var I0 = fu(I, 61) ? 0 : 1;
return fu(I, 0) ? cr([0, Y], function(D) {
var u0 = ir(X0, 0, D, 0);
V0(D, 1);
var Y0 = N0(D), J0 = 0;
if (typeof Y0 != "number" && Y0[0] === 4 && !n0(Y0[3], S0e)) {
var fr = u(E0, D), Q0 = fr[2], F0 = [0, fr[1]];
J0 = 1;
}
if (!J0) {
a(b, D, u0);
var gr = x(0, 0, D), mr = gr[0] === 0 ? gr[1] : gr[1][1], Q0 = mr, F0 = 0;
}
return [18, [0, 0, [0, [0, u0]], F0, I0, lr([0, U], [0, Q0], 0)]];
}, I) : (St(F0e, I), a(se[3], [0, _], I));
}), N(X, function(_) {
var k = _ && _[1];
function I(Y) {
1 - iu(Y) && Ge(Y, 13);
var y0 = pr(Y);
V0(Y, 60);
var D0 = xe0(1, ys(1, Y)), I0 = un(y0, pr(D0));
V0(D0, 49);
var D = N0(D0);
if (typeof D == "number")
if (53 <= D) {
if (Xt === D) {
var u0 = De(D0);
V0(D0, Xt);
var Y0 = D0[27][3], J0 = N0(D0), fr = 0;
if (typeof J0 != "number" && J0[0] === 4 && !n0(J0[3], k0e)) {
ie(D0);
var Q0 = Y0 ? [0, a(se[13], 0, D0)] : (Ge(D0, 13), 0), F0 = Q0;
fr = 1;
}
if (!fr)
var F0 = 0;
var gr = u(E0, D0), mr = lr([0, I0], [0, gr[2]], 0);
return [6, [0, 0, 0, [0, [1, [0, u0, F0]]], [0, gr[1]], mr]];
}
if (!(63 <= D))
switch (D + pU | 0) {
case 0:
if (k) {
var Cr = cr(0, u(V, 0), D0);
return [6, [0, 0, [0, [6, Cr]], 0, 0, lr([0, I0], 0, 0)]];
}
break;
case 8:
if (k) {
var sr = cr(0, u(w, 0), D0);
return [6, [0, 0, [0, [4, sr]], 0, 0, lr([0, I0], 0, 0)]];
}
break;
case 9:
var Pr = cr(0, a(S, h0e, 0), D0);
return [6, [0, 0, [0, [5, Pr]], 0, 0, lr([0, I0], 0, 0)]];
}
} else {
var K0 = D - 15 | 0;
if (!(25 < K0 >>> 0))
switch (K0) {
case 21:
var Ur = un(I0, pr(D0)), d0 = cr(0, function(Nt) {
return V0(Nt, 36);
}, D0), Kr = ae0(1, D0), re = N0(Kr), xe = 0;
if (typeof re == "number")
if (re === 15)
var je = 0, ve = je, Ie = [0, [1, cr(0, function(Nt) {
return a(e0, 0, Nt);
}, Kr)]];
else if (re === 40)
var ve = 0, Ie = [0, [2, cr(0, u(k0, 0), Kr)]];
else
xe = 1;
else
xe = 1;
if (xe) {
var Me = u(ln[1], Kr), Be = x(0, 0, Kr);
if (Be[0] === 0)
var Ae = Be[1], xn = Me;
else
var fn = 0, Ke = function(Ku, lt) {
return a(Ze(Ku, _v, Pn), Ku, lt);
}, Ae = fn, xn = a(Be[1][2], Me, Ke);
var ve = Ae, Ie = [0, [3, xn]];
}
var Qe = lr([0, Ur], [0, ve], 0);
return [6, [0, [0, d0[1]], Ie, 0, 0, Qe]];
case 0:
case 9:
case 12:
case 13:
case 25:
var yn = N0(D0);
if (typeof yn == "number") {
var on = 0;
if (25 <= yn)
if (29 <= yn) {
if (yn === 40) {
var Ce = [0, [2, cr(0, u(k0, 0), D0)]];
on = 1;
}
} else
27 <= yn && (on = 2);
else if (yn === 15) {
var Ce = [0, [1, cr(0, function(du) {
return a(e0, 0, du);
}, D0)]];
on = 1;
} else
24 <= yn && (on = 2);
var We = 0;
switch (on) {
case 0:
break;
case 2:
var rn = 0;
typeof yn == "number" ? yn === 27 ? Ge(D0, 72) : yn === 28 ? Ge(D0, 71) : rn = 1 : rn = 1;
var Ce = [0, [0, cr(0, function(du) {
return a(l, du, 0);
}, D0)]];
We = 1;
break;
default:
We = 1;
}
if (We)
return [6, [0, 0, Ce, 0, 0, lr([0, I0], 0, 0)]];
}
throw [0, wn, E0e];
}
}
var bn = N0(D0), Cn = 0;
typeof bn == "number" ? bn === 53 ? Ge(D0, 74) : bn === 61 ? Ge(D0, 73) : Cn = 1 : Cn = 1, V0(D0, 0);
var Hn = ir(X0, 0, D0, 0);
V0(D0, 1);
var Sn = N0(D0), vt = 0;
if (typeof Sn != "number" && Sn[0] === 4 && !n0(Sn[3], w0e)) {
var At = u(E0, D0), gt = At[2], Jt = [0, At[1]];
vt = 1;
}
if (!vt) {
a(b, D0, Hn);
var Bt = x(0, 0, D0), Ft = Bt[0] === 0 ? Bt[1] : Bt[1][1], gt = Ft, Jt = 0;
}
return [6, [0, 0, 0, [0, [0, Hn]], Jt, lr([0, I0], [0, gt], 0)]];
}
var U = 0;
return function(Y) {
return cr(U, I, Y);
};
}), [0, Rr, Ir, $0, B, Br, E, h, y, T, Ar, w0, X, M, Lr, p, G0, pe, Fr, m0, $r, K, P, i0, T0, A, b0, er];
}(j9), He0 = function(t) {
var n4 = function y(T, E) {
return y.fun(T, E);
}, e = function y(T, E) {
return y.fun(T, E);
}, i = function y(T, E) {
return y.fun(T, E);
};
N(n4, function(y, T) {
for (var E = T[2], h = E[2], w = o2(y), G = 0, A = E[1]; ; ) {
if (A) {
var S = A[1];
if (S[0] === 0) {
var M = S[1], K = M[2];
switch (K[0]) {
case 0:
var V = K[2], f0 = K[1];
switch (f0[0]) {
case 0:
var m0 = [0, f0[1]];
break;
case 1:
var m0 = [1, f0[1]];
break;
case 2:
var m0 = ke(y0e);
break;
default:
var m0 = [2, f0[1]];
}
var k0 = V[2], g0 = 0;
if (k0[0] === 2) {
var e0 = k0[1];
if (!e0[1]) {
var x0 = [0, e0[3]], l = e0[2];
g0 = 1;
}
}
if (!g0)
var x0 = 0, l = a(i, y, V);
var c0 = [0, [0, [0, M[1], [0, m0, l, x0, K[3]]]], G];
break;
case 1:
ue(y, [0, K[2][1], 97]);
var c0 = G;
break;
default:
ue(y, [0, K[2][1], d0e]);
var c0 = G;
}
var G = c0, A = A[2];
continue;
}
var t0 = S[1], a0 = t0[1];
if (A[2]) {
ue(y, [0, a0, 66]);
var A = A[2];
continue;
}
var w0 = t0[2], _0 = w0[2], G = [0, [1, [0, a0, [0, a(i, y, w0[1]), _0]]], G], A = 0;
continue;
}
var E0 = [0, [0, de(G), w, h]];
return [0, T[1], E0];
}
});
function x(y, T) {
return u(se[23], T) ? [0, a(i, y, T)] : (ue(y, [0, T[1], 26]), 0);
}
N(e, function(y, T) {
for (var E = T[2], h = E[2], w = o2(y), G = 0, A = E[1]; ; ) {
if (A) {
var S = A[1];
switch (S[0]) {
case 0:
var M = S[1], K = M[2];
if (K[0] === 2) {
var V = K[1];
if (!V[1]) {
var G = [0, [0, [0, M[1], [0, V[2], [0, V[3]]]]], G], A = A[2];
continue;
}
}
var f0 = x(y, M);
if (f0)
var m0 = f0[1], k0 = [0, [0, [0, m0[1], [0, m0, 0]]], G];
else
var k0 = G;
var G = k0, A = A[2];
continue;
case 1:
var g0 = S[1], e0 = g0[1];
if (A[2]) {
ue(y, [0, e0, 65]);
var A = A[2];
continue;
}
var x0 = g0[2], l = x(y, x0[1]), c0 = l ? [0, [1, [0, e0, [0, l[1], x0[2]]]], G] : G, G = c0, A = 0;
continue;
default:
var G = [0, [2, S[1]], G], A = A[2];
continue;
}
}
var t0 = [1, [0, de(G), w, h]];
return [0, T[1], t0];
}
}), N(i, function(y, T) {
var E = T[2], h = T[1];
switch (E[0]) {
case 0:
return a(e, y, [0, h, E[1]]);
case 10:
var w = E[1], G = w[2][1], A = w[1], S = 0;
if (y[5] && Bs(G) ? ue(y, [0, A, 52]) : S = 1, S && 1 - y[5]) {
var M = 0;
if (y[17] && qn(G, m0e) ? ue(y, [0, A, 93]) : M = 1, M) {
var K = y[18], V = K && qn(G, _0e);
V && ue(y, [0, A, 92]);
}
}
return [0, h, [2, [0, w, o2(y), 0]]];
case 19:
return a(n4, y, [0, h, E[1]]);
default:
return [0, h, [3, [0, h, E]]];
}
});
function c(y) {
function T(w) {
var G = N0(w);
return typeof G == "number" && G === 82 ? (V0(w, 82), [0, u(se[10], w)]) : 0;
}
function E(w) {
var G = pr(w);
V0(w, 0);
for (var A = 0, S = 0, M = 0; ; ) {
var K = N0(w);
if (typeof K == "number") {
var V = 0;
if ((K === 1 || Pn === K) && (V = 1), V) {
S && ue(w, [0, S[1], 98]);
var f0 = de(M), m0 = pr(w);
V0(w, 1);
var k0 = we(w), g0 = N0(w) === 86 ? [1, u(t[9], w)] : o2(w);
return [0, [0, f0, g0, _u([0, G], [0, k0], m0, 0)]];
}
}
if (N0(w) === 12)
var e0 = pr(w), x0 = cr(0, function(Jr) {
return V0(Jr, 12), p(Jr, y);
}, w), l = lr([0, e0], 0, 0), c0 = [0, [1, [0, x0[1], [0, x0[2], l]]]];
else {
var t0 = De(w), a0 = a(se[20], 0, w), w0 = N0(w), _0 = 0;
if (typeof w0 == "number" && w0 === 86) {
V0(w, 86);
var E0 = cr([0, t0], function(or) {
var _r = p(or, y);
return [0, _r, T(or)];
}, w), X0 = E0[2], b = a0[2];
switch (b[0]) {
case 0:
var G0 = [0, b[1]];
break;
case 1:
var G0 = [1, b[1]];
break;
case 2:
var G0 = ke(v0e);
break;
default:
var G0 = [2, b[1]];
}
var c0 = [0, [0, [0, E0[1], [0, G0, X0[1], X0[2], 0]]]];
} else
_0 = 1;
if (_0) {
var X = a0[2];
if (X[0] === 1) {
var s0 = X[1], dr = s0[2][1], Ar = s0[1], ar = 0;
SL(dr) && n0(dr, b0e) && n0(dr, p0e) && (ue(w, [0, Ar, 2]), ar = 1), !ar && f2(dr) && Y7(w, [0, Ar, 55]);
var W0 = cr([0, t0], function(or, _r) {
return function(Ir) {
var fe = [0, _r, [2, [0, or, o2(Ir), 0]]];
return [0, fe, T(Ir)];
};
}(s0, Ar), w), Lr = W0[2], c0 = [0, [0, [0, W0[1], [0, [1, s0], Lr[1], Lr[2], 1]]]];
} else {
St(l0e, w);
var c0 = 0;
}
}
}
if (c0) {
var Tr = c0[1], Hr = A ? (ue(w, [0, Tr[1][1], 66]), 0) : S;
if (Tr[0] === 0)
var Rr = Hr, Wr = A;
else
var Or = N0(w) === 9 ? 1 : 0, xr = Or && [0, De(w)], Rr = xr, Wr = 1;
N0(w) !== 1 && V0(w, 9);
var A = Wr, S = Rr, M = [0, Tr, M];
continue;
}
}
}
var h = 0;
return function(w) {
return cr(h, E, w);
};
}
function s(y) {
function T(h) {
var w = pr(h);
V0(h, 6);
for (var G = 0; ; ) {
var A = N0(h);
if (typeof A == "number") {
var S = 0;
if (13 <= A)
Pn === A && (S = 1);
else if (7 <= A)
switch (A - 7 | 0) {
case 2:
var M = De(h);
V0(h, 9);
var G = [0, [2, M], G];
continue;
case 5:
var K = pr(h), V = cr(0, function(_0) {
return V0(_0, 12), p(_0, y);
}, h), f0 = V[1], m0 = lr([0, K], 0, 0), k0 = [1, [0, f0, [0, V[2], m0]]];
N0(h) !== 7 && (ue(h, [0, f0, 65]), N0(h) === 9 && ie(h));
var G = [0, k0, G];
continue;
case 0:
S = 1;
break;
}
if (S) {
var g0 = de(G), e0 = pr(h);
V0(h, 7);
var x0 = N0(h) === 86 ? [1, u(t[9], h)] : o2(h);
return [1, [0, g0, x0, _u([0, w], [0, we(h)], e0, 0)]];
}
}
var l = cr(0, function(w0) {
var _0 = p(w0, y), E0 = N0(w0), X0 = 0;
if (typeof E0 == "number" && E0 === 82) {
V0(w0, 82);
var b = [0, u(se[10], w0)];
X0 = 1;
}
if (!X0)
var b = 0;
return [0, _0, b];
}, h), c0 = l[2], t0 = [0, [0, l[1], [0, c0[1], c0[2]]]];
N0(h) !== 7 && V0(h, 9);
var G = [0, t0, G];
}
}
var E = 0;
return function(h) {
return cr(E, T, h);
};
}
function p(y, T) {
var E = N0(y);
if (typeof E == "number") {
if (E === 6)
return u(s(T), y);
if (!E)
return u(c(T), y);
}
var h = ir(se[14], y, 0, T);
return [0, h[1], [2, h[2]]];
}
return [0, n4, e, i, c, s, p];
}(ln), dne = lne(se), hne = ln[9];
function Xe0(t, n4) {
var e = N0(n4), i = 0;
if (typeof e == "number" ? e === 28 ? n4[5] ? Ge(n4, 55) : n4[14] && St(0, n4) : e === 58 ? n4[17] ? Ge(n4, 2) : n4[5] && Ge(n4, 55) : e === 65 ? n4[18] && Ge(n4, 2) : i = 1 : i = 1, i)
if (EL(e))
Si(n4, 55);
else {
var x = 0;
if (typeof e == "number")
switch (e) {
case 15:
case 16:
case 17:
case 18:
case 19:
case 20:
case 21:
case 22:
case 23:
case 24:
case 25:
case 26:
case 27:
case 32:
case 33:
case 34:
case 35:
case 36:
case 37:
case 38:
case 39:
case 40:
case 41:
case 43:
case 44:
case 45:
case 46:
case 47:
case 49:
case 50:
case 51:
case 58:
case 59:
case 65:
var c = 1;
x = 1;
break;
}
else if (e[0] === 4 && ve0(e[3])) {
var c = 1;
x = 1;
}
if (!x)
var c = 0;
var s = 0;
if (c)
var p = c;
else {
var y = wL(e);
if (y)
var p = y;
else {
var T = 0;
if (typeof e == "number")
switch (e) {
case 29:
case 30:
case 31:
break;
default:
T = 1;
}
else if (e[0] === 4) {
var E = e[3];
n0(E, ijr) && n0(E, fjr) && n0(E, xjr) && (T = 1);
} else
T = 1;
if (T) {
var h = 0;
s = 1;
} else
var p = 1;
}
}
if (!s)
var h = p;
if (h)
St(0, n4);
else {
var w = 0;
t && le0(e) ? Si(n4, t[1]) : w = 1;
}
}
return V7(n4);
}
var Ye0 = function t(n4) {
return t.fun(n4);
}, BL = function t(n4, e, i) {
return t.fun(n4, e, i);
}, qL = function t(n4) {
return t.fun(n4);
}, Ve0 = function t(n4, e) {
return t.fun(n4, e);
}, UL = function t(n4, e) {
return t.fun(n4, e);
}, HL = function t(n4, e) {
return t.fun(n4, e);
}, G9 = function t(n4, e) {
return t.fun(n4, e);
}, xb = function t(n4, e) {
return t.fun(n4, e);
}, M9 = function t(n4) {
return t.fun(n4);
}, ze0 = function t(n4) {
return t.fun(n4);
}, Ke0 = function t(n4) {
return t.fun(n4);
}, We0 = function t(n4, e, i) {
return t.fun(n4, e, i);
}, Je0 = function t(n4) {
return t.fun(n4);
}, $e0 = function t(n4) {
return t.fun(n4);
}, Ze0 = Ys[3], kne = oi[3], wne = oi[1], Ene = oi[5], Sne = Ys[2], gne = Ys[1], Fne = Ys[4], Tne = oi[4], One = oi[6], Ine = dne[13], Ane = He0[6], Nne = He0[3];
N(Ye0, function(t) {
var n4 = pr(t), e = de(n4), i = 5;
r:
for (; ; ) {
if (e)
for (var x = e[2], c = e[1], s = c[2], p = c[1], y = s[2], T = 0, E = nn(y); ; ) {
if (E < (T + 5 | 0))
var h = 0;
else {
var w = qn(p7(y, T, i), RRr);
if (!w) {
var T = T + 1 | 0;
continue;
}
var h = w;
}
if (!h) {
var e = x;
continue r;
}
t[30][1] = p[3];
var G = de([0, [0, p, s], x]);
break;
}
else
var G = e;
if (G === 0) {
var A = 0;
if (n4) {
var S = n4[1], M = S[2];
if (!M[1]) {
var K = M[2], V = 0;
if (1 <= nn(K) && Ot(K, 0) === 42) {
t[30][1] = S[1][3];
var f0 = [0, S, 0];
A = 1, V = 1;
}
}
}
if (!A)
var f0 = 0;
} else
var f0 = G;
var m0 = a(Ve0, t, function(c0) {
return 0;
}), k0 = De(t);
V0(t, Pn);
var g0 = Gu[1];
if (be(function(c0, t0) {
var a0 = t0[2];
switch (a0[0]) {
case 17:
return fb(t, c0, Gc(0, [0, a0[1][1], Ere]));
case 18:
var w0 = a0[1], _0 = w0[1];
if (_0) {
if (!w0[2]) {
var E0 = _0[1], X0 = E0[2], b = 0;
switch (X0[0]) {
case 34:
var G0 = X0[1][1], X = 0, s0 = be(function(Tr, Hr) {
return be(ML, Tr, [0, Hr[2][1], 0]);
}, X, G0);
return be(function(Tr, Hr) {
return fb(t, Tr, Hr);
}, c0, s0);
case 2:
case 23:
var dr = X0[1][1];
if (dr)
var Ar = dr[1];
else
b = 1;
break;
case 16:
case 26:
case 32:
case 33:
var Ar = X0[1][1];
break;
default:
b = 1;
}
return b ? c0 : fb(t, c0, Gc(0, [0, E0[1], Ar[2][1]]));
}
} else {
var ar = w0[2];
if (ar) {
var W0 = ar[1];
if (W0[0] === 0) {
var Lr = W0[1];
return be(function(Tr, Hr) {
var Or = Hr[2], xr = Or[2];
return xr ? fb(t, Tr, xr[1]) : fb(t, Tr, Or[1]);
}, c0, Lr);
}
return c0;
}
}
return c0;
default:
return c0;
}
}, g0, m0), m0)
var e0 = bl(de(m0))[1], x0 = yt(bl(m0)[1], e0);
else
var x0 = k0;
var l = de(t[2][1]);
return [0, x0, [0, m0, lr([0, f0], 0, 0), l]];
}
}), N(BL, function(t, n4, e) {
for (var i = fe0(1, t), x = hre; ; ) {
var c = x[3], s = x[2], p = x[1], y = N0(i), T = 0;
if (typeof y == "number" && Pn === y)
var E = [0, i, p, s, c];
else
T = 1;
if (T)
if (u(n4, y))
var E = [0, i, p, s, c];
else {
var h = 0;
if (typeof y == "number" || y[0] !== 2)
h = 1;
else {
var w = u(e, i), G = [0, w, s], A = w[2];
if (A[0] === 19) {
var S = A[1][2];
if (S) {
var M = qn(S[1], dre), K = M && 1 - i[20];
K && ue(i, [0, w[1], 43]);
var V = M ? ys(1, i) : i, f0 = [0, y, p], m0 = c || M, i = V, x = [0, f0, G, m0];
continue;
}
}
var E = [0, i, p, G, c];
}
if (h)
var E = [0, i, p, s, c];
}
var k0 = fe0(0, i), g0 = de(p);
return Pu(function(e0) {
if (typeof e0 != "number" && e0[0] === 2) {
var x0 = e0[1], l = x0[4];
return l && Y7(k0, [0, x0[1], 45]);
}
return ke(Te(wre, Te(Tr0(e0), kre)));
}, g0), [0, k0, E[3], c];
}
}), N(qL, function(t) {
var n4 = u(Ys[6], t), e = N0(t);
if (typeof e == "number") {
var i = e - 49 | 0;
if (!(11 < i >>> 0))
switch (i) {
case 0:
return a(dt[16], n4, t);
case 1:
u(N9(t), n4);
var x = Yn(1, t);
if (typeof x == "number") {
var c = 0;
if ((x === 4 || x === 10) && (c = 1), c)
return u(dt[17], t);
}
return u(dt[18], t);
case 11:
if (Yn(1, t) === 49)
return u(N9(t), n4), a(dt[12], 0, t);
break;
}
}
return a(xb, [0, n4], t);
}), N(Ve0, function(t, n4) {
var e = ir(BL, t, n4, qL), i = a(UL, n4, e[1]), x = e[2];
return be(function(c, s) {
return [0, s, c];
}, i, x);
}), N(UL, function(t, n4) {
for (var e = 0; ; ) {
var i = N0(n4);
if (typeof i == "number" && Pn === i || u(t, i))
return de(e);
var e = [0, u(qL, n4), e];
}
}), N(HL, function(t, n4) {
var e = ir(BL, n4, t, function(s) {
return a(xb, 0, s);
}), i = a(G9, t, e[1]), x = e[2], c = be(function(s, p) {
return [0, p, s];
}, i, x);
return [0, c, e[3]];
}), N(G9, function(t, n4) {
for (var e = 0; ; ) {
var i = N0(n4);
if (typeof i == "number" && Pn === i || u(t, i))
return de(e);
var e = [0, a(xb, 0, n4), e];
}
}), N(xb, function(t, n4) {
var e = t && t[1];
1 - $l(n4) && u(N9(n4), e);
var i = N0(n4);
if (typeof i == "number") {
if (i === 27)
return u(dt[27], n4);
if (i === 28)
return u(dt[3], n4);
}
if (qs(n4))
return u(Vn[10], n4);
if ($l(n4))
return a(Ze0, n4, e);
if (typeof i == "number") {
var x = i + zt | 0;
if (!(14 < x >>> 0))
switch (x) {
case 0:
if (n4[27][1])
return u(Vn[11], n4);
break;
case 5:
return u(dt[19], n4);
case 12:
return a(dt[11], 0, n4);
case 13:
return u(dt[25], n4);
case 14:
return u(dt[21], n4);
}
}
return u(M9, n4);
}), N(M9, function(t) {
var n4 = N0(t);
if (typeof n4 == "number")
switch (n4) {
case 0:
return u(dt[7], t);
case 8:
return u(dt[15], t);
case 19:
return u(dt[22], t);
case 20:
return u(dt[23], t);
case 22:
return u(dt[24], t);
case 23:
return u(dt[4], t);
case 24:
return u(dt[26], t);
case 25:
return u(dt[5], t);
case 26:
return u(dt[6], t);
case 32:
return u(dt[8], t);
case 35:
return u(dt[9], t);
case 37:
return u(dt[14], t);
case 39:
return u(dt[1], t);
case 59:
return u(dt[10], t);
case 113:
return St(mre, t), [0, De(t), _re];
case 16:
case 43:
return u(dt[2], t);
case 1:
case 5:
case 7:
case 9:
case 10:
case 11:
case 12:
case 17:
case 18:
case 33:
case 34:
case 36:
case 38:
case 41:
case 42:
case 49:
case 83:
case 86:
return St(yre, t), ie(t), u(M9, t);
}
if (qs(t)) {
var e = u(Vn[10], t);
return B1(t, e[1]), e;
}
if (typeof n4 == "number" && n4 === 28 && Yn(1, t) === 6) {
var i = Wl(1, t);
return ue(t, [0, yt(De(t), i), 94]), u(dt[17], t);
}
return M1(t) ? u(dt[20], t) : ($l(t) && (St(0, t), ie(t)), u(dt[17], t));
}), N(ze0, function(t) {
var n4 = De(t), e = u(oi[1], t), i = N0(t);
return typeof i == "number" && i === 9 ? ir(oi[7], t, n4, [0, e, 0]) : e;
}), N(Ke0, function(t) {
var n4 = De(t), e = u(oi[2], t), i = N0(t);
if (typeof i == "number" && i === 9) {
var x = [0, a(j9[1], t, e), 0];
return [0, ir(oi[7], t, n4, x)];
}
return e;
}), N(We0, function(t, n4, e) {
var i = n4 && n4[1];
return cr(0, function(x) {
var c = 1 - i, s = Xe0([0, e], x), p = c && (N0(x) === 85 ? 1 : 0);
return p && (1 - iu(x) && Ge(x, 12), V0(x, 85)), [0, s, u(ln[10], x), p];
}, t);
}), N(Je0, function(t) {
var n4 = De(t), e = pr(t);
V0(t, 0);
var i = a(G9, function(y) {
return y === 1 ? 1 : 0;
}, t), x = i === 0 ? 1 : 0, c = De(t), s = x && pr(t);
V0(t, 1);
var p = [0, i, _u([0, e], [0, we(t)], s, 0)];
return [0, yt(n4, c), p];
}), N($e0, function(t) {
function n4(i) {
var x = pr(i);
V0(i, 0);
var c = a(HL, function(S) {
return S === 1 ? 1 : 0;
}, i), s = c[1], p = s === 0 ? 1 : 0, y = p && pr(i);
V0(i, 1);
var T = N0(i), E = 0;
if (!t) {
var h = 0;
if (typeof T == "number" && (T === 1 || Pn === T) && (h = 1), !h) {
var w = f7(i);
if (w) {
var G = Us(i);
E = 1;
} else {
var G = w;
E = 1;
}
}
}
if (!E)
var G = we(i);
var A = _u([0, x], [0, G], y, 0);
return [0, [0, s, A], c[2]];
}
var e = 0;
return function(i) {
return OL(e, n4, i);
};
}), pu(Ore, se, [0, Ye0, M9, xb, G9, HL, UL, ze0, Ke0, kne, wne, Ene, Sne, Xe0, We0, Je0, $e0, Ine, Ane, Nne, gne, Ze0, Fne, Tne, One, hne]);
var Qe0 = [0, 0], rn0 = sn;
function Cne(t) {
function n4(e, i) {
var x = i[2], c = i[1], s = sL(x), p = [0, [0, Ire, u(t[1], s)], 0], y = P9(e, c[3]), T = [0, u(t[5], y), 0], E = P9(e, c[2]), h = [0, u(t[5], E), T], w = [0, [0, Are, u(t[4], h)], p], G = [0, [0, Nre, u(t[5], c[3][2])], 0], A = [0, [0, Cre, u(t[5], c[3][1])], G], S = [0, [0, Pre, u(t[3], A)], 0], M = [0, [0, Dre, u(t[5], c[2][2])], 0], K = [0, [0, Lre, u(t[5], c[2][1])], M], V = [0, [0, Rre, u(t[3], K)], S], f0 = [0, [0, jre, u(t[3], V)], w];
switch (i[3]) {
case 0:
var m0 = Gre;
break;
case 1:
var m0 = Mre;
break;
case 2:
var m0 = Bre;
break;
case 3:
var m0 = qre;
break;
case 4:
var m0 = Ure;
break;
default:
var m0 = Hre;
}
var k0 = [0, [0, Xre, u(t[1], m0)], f0], g0 = Tr0(x), e0 = [0, [0, Yre, u(t[1], g0)], k0];
return u(t[3], e0);
}
return [0, n4, function(e, i) {
var x = de(Tp(function(c) {
return n4(e, c);
}, i));
return u(t[4], x);
}];
}
var Pne = M70;
function H1(t) {
return B70(_l(t));
}
function yu(t) {
return G70(_l(t));
}
function Dne(t) {
return t;
}
function Lne(t) {
return t;
}
function en0(t, n4, e) {
try {
var i = new RegExp(sn(n4), sn(e));
return i;
} catch {
return u7;
}
}
var Rne = Cne([0, rn0, Pne, H1, yu, Dne, Lne, u7, en0]), jne = [0, 1], nn0 = function(t) {
function n4(E, h) {
return yu(de(Tp(E, h)));
}
function e(E, h) {
return h ? u(E, h[1]) : u7;
}
function i(E, h) {
return h[0] === 0 ? u7 : u(E, h[1]);
}
function x(E) {
return H1([0, [0, YWr, E[1]], [0, [0, XWr, E[2]], 0]]);
}
function c(E) {
var h = E[1], w = h ? sn(h[1][1]) : u7, G = [0, [0, qWr, x(E[3])], 0];
return H1([0, [0, HWr, w], [0, [0, UWr, x(E[2])], G]]);
}
function s(E) {
return n4(function(h) {
var w = h[2], G = 0;
if (typeof w == "number") {
var A = w;
if (55 <= A)
switch (A) {
case 55:
var S = _mr;
break;
case 56:
var S = ymr;
break;
case 57:
var S = dmr;
break;
case 58:
var S = hmr;
break;
case 59:
var S = kmr;
break;
case 60:
var S = wmr;
break;
case 61:
var S = Te(Smr, Emr);
break;
case 62:
var S = Te(Fmr, gmr);
break;
case 63:
var S = Te(Omr, Tmr);
break;
case 64:
var S = Imr;
break;
case 65:
var S = Amr;
break;
case 66:
var S = Nmr;
break;
case 67:
var S = Cmr;
break;
case 68:
var S = Pmr;
break;
case 69:
var S = Dmr;
break;
case 70:
var S = Lmr;
break;
case 71:
var S = Rmr;
break;
case 72:
var S = jmr;
break;
case 73:
var S = Gmr;
break;
case 74:
var S = Mmr;
break;
case 75:
var S = Bmr;
break;
case 76:
var S = qmr;
break;
case 77:
var S = Umr;
break;
case 78:
var S = Hmr;
break;
case 79:
var S = Xmr;
break;
case 80:
var S = Ymr;
break;
case 81:
var S = Vmr;
break;
case 82:
var S = Te(Kmr, zmr);
break;
case 83:
var S = Wmr;
break;
case 84:
var S = Jmr;
break;
case 85:
var S = $mr;
break;
case 86:
var S = Zmr;
break;
case 87:
var S = Qmr;
break;
case 88:
var S = r9r;
break;
case 89:
var S = e9r;
break;
case 90:
var S = n9r;
break;
case 91:
var S = t9r;
break;
case 92:
var S = u9r;
break;
case 93:
var S = i9r;
break;
case 94:
var S = Te(x9r, f9r);
break;
case 95:
var S = a9r;
break;
case 96:
var S = o9r;
break;
case 97:
var S = c9r;
break;
case 98:
var S = s9r;
break;
case 99:
var S = v9r;
break;
case 100:
var S = l9r;
break;
case 101:
var S = b9r;
break;
case 102:
var S = p9r;
break;
case 103:
var S = m9r;
break;
case 104:
var S = _9r;
break;
case 105:
var S = y9r;
break;
case 106:
var S = d9r;
break;
case 107:
var S = h9r;
break;
default:
var S = k9r;
}
else
switch (A) {
case 0:
var S = p5r;
break;
case 1:
var S = m5r;
break;
case 2:
var S = _5r;
break;
case 3:
var S = y5r;
break;
case 4:
var S = d5r;
break;
case 5:
var S = h5r;
break;
case 6:
var S = k5r;
break;
case 7:
var S = w5r;
break;
case 8:
var S = E5r;
break;
case 9:
var S = S5r;
break;
case 10:
var S = g5r;
break;
case 11:
var S = F5r;
break;
case 12:
var S = T5r;
break;
case 13:
var S = O5r;
break;
case 14:
var S = I5r;
break;
case 15:
var S = A5r;
break;
case 16:
var S = N5r;
break;
case 17:
var S = C5r;
break;
case 18:
var S = P5r;
break;
case 19:
var S = D5r;
break;
case 20:
var S = L5r;
break;
case 21:
var S = R5r;
break;
case 22:
var S = j5r;
break;
case 23:
var S = G5r;
break;
case 24:
var S = M5r;
break;
case 25:
var S = B5r;
break;
case 26:
var S = q5r;
break;
case 27:
var S = U5r;
break;
case 28:
var S = H5r;
break;
case 29:
var S = X5r;
break;
case 30:
var S = Y5r;
break;
case 31:
var S = Te(z5r, V5r);
break;
case 32:
var S = K5r;
break;
case 33:
var S = W5r;
break;
case 34:
var S = J5r;
break;
case 35:
var S = $5r;
break;
case 36:
var S = Z5r;
break;
case 37:
var S = Q5r;
break;
case 38:
var S = rmr;
break;
case 39:
var S = emr;
break;
case 40:
var S = nmr;
break;
case 41:
var S = tmr;
break;
case 42:
var S = umr;
break;
case 43:
var S = imr;
break;
case 44:
var S = fmr;
break;
case 45:
var S = xmr;
break;
case 46:
var S = amr;
break;
case 47:
var S = omr;
break;
case 48:
var S = cmr;
break;
case 49:
var S = smr;
break;
case 50:
var S = vmr;
break;
case 51:
var S = lmr;
break;
case 52:
var S = bmr;
break;
case 53:
var S = pmr;
break;
default:
var S = mmr;
}
} else
switch (w[0]) {
case 0:
var M = w[2], K = w[1], S = ir(Qn(w9r), M, M, K);
break;
case 1:
var V = w[1], f0 = w[2], S = a(Qn(E9r), f0, V);
break;
case 2:
var m0 = w[1], S = u(Qn(S9r), m0);
break;
case 3:
var k0 = w[2], g0 = w[1], e0 = u(Qn(g9r), g0);
if (k0)
var x0 = k0[1], S = a(Qn(F9r), x0, e0);
else
var S = u(Qn(T9r), e0);
break;
case 4:
var l = w[1], S = a(Qn(O9r), l, l);
break;
case 5:
var c0 = w[3], t0 = w[2], a0 = w[1];
if (t0) {
var w0 = t0[1];
if (3 <= w0)
var S = a(Qn(I9r), c0, a0);
else {
switch (w0) {
case 0:
var _0 = s5r;
break;
case 1:
var _0 = v5r;
break;
case 2:
var _0 = l5r;
break;
default:
var _0 = b5r;
}
var S = R(Qn(A9r), a0, _0, c0, _0);
}
} else
var S = a(Qn(N9r), c0, a0);
break;
case 6:
var E0 = w[2], X0 = E0;
if (l7(X0) === 0)
var b = X0;
else {
var G0 = mz(X0);
Jn(G0, 0, vz(Hu(X0, 0)));
var b = G0;
}
var X = b, s0 = w[1], S = ir(Qn(C9r), E0, X, s0);
break;
case 7:
var S = w[1] ? P9r : D9r;
break;
case 8:
var dr = w[1], Ar = w[2], S = a(Qn(L9r), Ar, dr);
break;
case 9:
var ar = w[1], S = u(Qn(R9r), ar);
break;
case 10:
var W0 = w[1], S = u(Qn(j9r), W0);
break;
case 11:
var Lr = w[2], Tr = w[1], S = a(Qn(G9r), Tr, Lr);
break;
case 12:
var Hr = w[2], Or = w[1], S = a(Qn(M9r), Or, Hr);
break;
case 13:
var S = Te(q9r, Te(w[1], B9r));
break;
case 14:
var xr = w[1] ? U9r : H9r, S = u(Qn(X9r), xr);
break;
case 15:
var S = Te(V9r, Te(w[1], Y9r));
break;
case 16:
var Rr = Te(K9r, Te(w[2], z9r)), S = Te(w[1], Rr);
break;
case 17:
var S = Te(W9r, w[1]);
break;
case 18:
var S = w[1] ? Te($9r, J9r) : Te(Q9r, Z9r);
break;
case 19:
var Wr = w[1], S = u(Qn(r_r), Wr);
break;
case 20:
var S = Te(n_r, Te(w[1], e_r));
break;
case 21:
var Jr = w[1], or = w[2] ? t_r : u_r, _r = w[4] ? Te(i_r, Jr) : Jr, Ir = w[3] ? f_r : x_r, S = Te(c_r, Te(or, Te(Ir, Te(o_r, Te(_r, a_r)))));
break;
case 22:
var S = Te(v_r, Te(w[1], s_r));
break;
default:
var fe = w[1], S = u(Qn(l_r), fe);
}
var v0 = [0, [0, MWr, sn(S)], G];
return H1([0, [0, BWr, c(h[1])], v0]);
}, E);
}
function p(E) {
if (E) {
var h = E[1], w = [0, un(h[3], h[2])];
return lr([0, h[1]], w, 0);
}
return E;
}
function y(E) {
function h(_) {
return n4(H0, _);
}
function w(_, k, I, U) {
var Y = t[1];
if (Y) {
if (E)
var y0 = E[1], D0 = [0, P9(y0, k[3]), 0], I0 = [0, [0, hGr, yu([0, P9(y0, k[2]), D0])], 0];
else
var I0 = E;
var D = un(I0, [0, [0, kGr, c(k)], 0]);
} else
var D = Y;
if (I) {
var u0 = I[1], Y0 = u0[1];
if (Y0) {
var J0 = u0[2];
if (J0)
var fr = [0, [0, wGr, h(J0)], 0], Q0 = [0, [0, EGr, h(Y0)], fr];
else
var Q0 = [0, [0, SGr, h(Y0)], 0];
var mr = Q0;
} else
var F0 = u0[2], gr = F0 && [0, [0, gGr, h(F0)], 0], mr = gr;
var Cr = mr;
} else
var Cr = I;
return H1(jc(un(D, un(Cr, [0, [0, FGr, sn(_)], 0])), U));
}
function G(_) {
return n4(Q, _);
}
function A(_) {
var k = _[2], I = G(k[1]), U = [0, [0, OGr, I], [0, [0, TGr, h(k[3])], 0]];
return w(IGr, _[1], k[2], U);
}
function S(_) {
var k = _[2];
return w(xUr, _[1], k[2], [0, [0, fUr, sn(k[1])], [0, [0, iUr, u7], [0, [0, uUr, false], 0]]]);
}
function M(_) {
if (_[0] === 0)
return S(_[1]);
var k = _[1], I = k[2], U = M(I[1]), Y = [0, [0, rKr, U], [0, [0, Qzr, S(I[2])], 0]];
return w(eKr, k[1], 0, Y);
}
function K(_) {
var k = _[2], I = k[1], U = I[0] === 0 ? S(I[1]) : K(I[1]), Y = [0, [0, jzr, U], [0, [0, Rzr, S(k[2])], 0]];
return w(Gzr, _[1], 0, Y);
}
function V(_) {
var k = _[2], I = k[1], U = I[0] === 0 ? S(I[1]) : K(I[1]), Y = [0, [0, Bzr, U], [0, [0, Mzr, e($r, k[2])], 0]];
return w(qzr, _[1], k[3], Y);
}
function f0(_) {
var k = _[2], I = k[2], U = k[1], Y = _[1];
if (typeof U == "number")
var y0 = u7;
else
switch (U[0]) {
case 0:
var y0 = sn(U[1]);
break;
case 1:
var y0 = !!U[1];
break;
case 2:
var y0 = U[1];
break;
case 3:
var y0 = ke(IYr);
break;
default:
var D0 = U[1], y0 = en0(Y, D0[1], D0[2]);
}
var I0 = 0;
if (typeof U != "number" && U[0] === 4) {
var D = U[1], u0 = [0, [0, CYr, H1([0, [0, NYr, sn(D[1])], [0, [0, AYr, sn(D[2])], 0]])], 0], Y0 = [0, [0, DYr, y0], [0, [0, PYr, sn(I)], u0]];
I0 = 1;
}
if (!I0)
var Y0 = [0, [0, RYr, y0], [0, [0, LYr, sn(I)], 0]];
return w(jYr, Y, k[3], Y0);
}
function m0(_) {
var k = [0, [0, Uzr, g0(_[2])], 0];
return [0, [0, Hzr, g0(_[1])], k];
}
function k0(_, k) {
var I = k[2], U = [0, [0, GVr, !!I[3]], 0], Y = [0, [0, MVr, g0(I[2])], U], y0 = [0, [0, BVr, e(S, I[1])], Y];
return w(qVr, k[1], _, y0);
}
function g0(_) {
var k = _[2], I = _[1];
switch (k[0]) {
case 0:
return w(hVr, I, k[1], 0);
case 1:
return w(kVr, I, k[1], 0);
case 2:
return w(wVr, I, k[1], 0);
case 3:
return w(EVr, I, k[1], 0);
case 4:
return w(SVr, I, k[1], 0);
case 5:
return w(FVr, I, k[1], 0);
case 6:
return w(TVr, I, k[1], 0);
case 7:
return w(OVr, I, k[1], 0);
case 8:
return w(IVr, I, k[1], 0);
case 9:
return w(gVr, I, k[1], 0);
case 10:
return w(yKr, I, k[1], 0);
case 11:
var U = k[1], Y = [0, [0, AVr, g0(U[1])], 0];
return w(NVr, I, U[2], Y);
case 12:
return e0([0, I, k[1]]);
case 13:
return x0(1, [0, I, k[1]]);
case 14:
var y0 = k[1], D0 = [0, [0, Nzr, x0(0, y0[1])], 0], I0 = [0, [0, Czr, n4(fe, y0[2])], D0];
return w(Pzr, I, y0[3], I0);
case 15:
var D = k[1], u0 = [0, [0, Dzr, g0(D[1])], 0];
return w(Lzr, I, D[2], u0);
case 16:
return V([0, I, k[1]]);
case 17:
var Y0 = k[1], J0 = m0(Y0);
return w(Xzr, I, Y0[3], J0);
case 18:
var fr = k[1], Q0 = fr[1], F0 = [0, [0, Yzr, !!fr[2]], 0], gr = un(m0(Q0), F0);
return w(Vzr, I, Q0[3], gr);
case 19:
var mr = k[1], Cr = mr[1], sr = [0, [0, zzr, n4(g0, [0, Cr[1], [0, Cr[2], Cr[3]]])], 0];
return w(Kzr, I, mr[2], sr);
case 20:
var Pr = k[1], K0 = Pr[1], Ur = [0, [0, Wzr, n4(g0, [0, K0[1], [0, K0[2], K0[3]]])], 0];
return w(Jzr, I, Pr[2], Ur);
case 21:
var d0 = k[1], Kr = [0, [0, $zr, M(d0[1])], 0];
return w(Zzr, I, d0[2], Kr);
case 22:
var re = k[1], xe = [0, [0, nKr, n4(g0, re[1])], 0];
return w(tKr, I, re[2], xe);
case 23:
var je = k[1];
return w(fKr, I, je[3], [0, [0, iKr, sn(je[1])], [0, [0, uKr, sn(je[2])], 0]]);
case 24:
var ve = k[1];
return w(oKr, I, ve[3], [0, [0, aKr, ve[1]], [0, [0, xKr, sn(ve[2])], 0]]);
case 25:
var Ie = k[1];
return w(vKr, I, Ie[3], [0, [0, sKr, u7], [0, [0, cKr, sn(Ie[2])], 0]]);
default:
var Me = k[1], Be = Me[1], fn = 0, Ke = Be ? lKr : bKr;
return w(_Kr, I, Me[2], [0, [0, mKr, !!Be], [0, [0, pKr, sn(Ke)], fn]]);
}
}
function e0(_) {
var k = _[2], I = k[2][2], U = k[4], Y = _7(p(I[4]), U), y0 = [0, [0, CVr, e(qr, k[1])], 0], D0 = [0, [0, PVr, e(Mr, I[3])], y0], I0 = [0, [0, DVr, g0(k[3])], D0], D = [0, [0, LVr, e(Br, I[1])], I0], u0 = I[2], Y0 = [0, [0, RVr, n4(function(J0) {
return k0(0, J0);
}, u0)], D];
return w(jVr, _[1], Y, Y0);
}
function x0(_, k) {
var I = k[2], U = I[3], Y = be(function(fr, Q0) {
var F0 = fr[4], gr = fr[3], mr = fr[2], Cr = fr[1];
switch (Q0[0]) {
case 0:
var sr = Q0[1], Pr = sr[2], K0 = Pr[2], Ur = Pr[1];
switch (Ur[0]) {
case 0:
var d0 = f0(Ur[1]);
break;
case 1:
var d0 = S(Ur[1]);
break;
case 2:
var d0 = ke(rzr);
break;
default:
var d0 = ke(ezr);
}
switch (K0[0]) {
case 0:
var xe = nzr, je = g0(K0[1]);
break;
case 1:
var Kr = K0[1], xe = tzr, je = e0([0, Kr[1], Kr[2]]);
break;
default:
var re = K0[1], xe = uzr, je = e0([0, re[1], re[2]]);
}
var ve = [0, [0, izr, sn(xe)], 0], Ie = [0, [0, fzr, e(Sr, Pr[7])], ve];
return [0, [0, w(lzr, sr[1], Pr[8], [0, [0, vzr, d0], [0, [0, szr, je], [0, [0, czr, !!Pr[6]], [0, [0, ozr, !!Pr[3]], [0, [0, azr, !!Pr[4]], [0, [0, xzr, !!Pr[5]], Ie]]]]]]), Cr], mr, gr, F0];
case 1:
var Me = Q0[1], Be = Me[2], fn = [0, [0, bzr, g0(Be[1])], 0];
return [0, [0, w(pzr, Me[1], Be[2], fn), Cr], mr, gr, F0];
case 2:
var Ke = Q0[1], Ae = Ke[2], xn = [0, [0, mzr, e(Sr, Ae[5])], 0], Qe = [0, [0, _zr, !!Ae[4]], xn], yn = [0, [0, yzr, g0(Ae[3])], Qe], on = [0, [0, dzr, g0(Ae[2])], yn], Ce = [0, [0, hzr, e(S, Ae[1])], on];
return [0, Cr, [0, w(kzr, Ke[1], Ae[6], Ce), mr], gr, F0];
case 3:
var We = Q0[1], rn = We[2], bn = [0, [0, wzr, !!rn[2]], 0], Cn = [0, [0, Ezr, e0(rn[1])], bn];
return [0, Cr, mr, [0, w(Szr, We[1], rn[3], Cn), gr], F0];
default:
var Hn = Q0[1], Sn = Hn[2], vt = [0, [0, gzr, g0(Sn[2])], 0], At = [0, [0, Ozr, !!Sn[3]], [0, [0, Tzr, !!Sn[4]], [0, [0, Fzr, !!Sn[5]], vt]]], gt = [0, [0, Izr, S(Sn[1])], At];
return [0, Cr, mr, gr, [0, w(Azr, Hn[1], Sn[6], gt), F0]];
}
}, VVr, U), y0 = [0, [0, zVr, yu(de(Y[4]))], 0], D0 = [0, [0, KVr, yu(de(Y[3]))], y0], I0 = [0, [0, WVr, yu(de(Y[2]))], D0], D = [0, [0, JVr, yu(de(Y[1]))], I0], u0 = [0, [0, $Vr, !!I[1]], D], Y0 = _ ? [0, [0, ZVr, !!I[2]], u0] : u0, J0 = p(I[4]);
return w(QVr, k[1], J0, Y0);
}
function l(_) {
var k = [0, [0, dKr, g0(_[2])], 0];
return w(hKr, _[1], 0, k);
}
function c0(_) {
var k = _[2];
switch (k[2]) {
case 0:
var I = oVr;
break;
case 1:
var I = cVr;
break;
default:
var I = sVr;
}
var U = [0, [0, vVr, sn(I)], 0], Y = [0, [0, lVr, n4($0, k[1])], U];
return w(bVr, _[1], k[3], Y);
}
function t0(_) {
var k = _[2];
return w(VYr, _[1], k[3], [0, [0, YYr, sn(k[1])], [0, [0, XYr, sn(k[2])], 0]]);
}
function a0(_) {
var k = _[2], I = [0, [0, XXr, f1], [0, [0, HXr, l(k[1])], 0]];
return w(YXr, _[1], k[2], I);
}
function w0(_, k) {
var I = k[1][2], U = [0, [0, vUr, !!k[3]], 0], Y = [0, [0, lUr, i(l, k[2])], U];
return w(pUr, _, I[2], [0, [0, bUr, sn(I[1])], Y]);
}
function _0(_) {
var k = _[2];
return w(sUr, _[1], k[2], [0, [0, cUr, sn(k[1])], [0, [0, oUr, u7], [0, [0, aUr, false], 0]]]);
}
function E0(_) {
return n4(q0, _[2][1]);
}
function X0(_) {
var k = _[2], I = [0, [0, jKr, w(KKr, k[2], 0, 0)], 0], U = [0, [0, GKr, n4(ae, k[3][2])], I], Y = [0, [0, MKr, w(YKr, k[1], 0, 0)], U];
return w(BKr, _[1], k[4], Y);
}
function b(_) {
var k = _[2];
return w(pWr, _[1], k[2], [0, [0, bWr, sn(k[1])], 0]);
}
function G0(_) {
var k = _[2], I = [0, [0, sWr, b(k[2])], 0], U = [0, [0, vWr, b(k[1])], I];
return w(lWr, _[1], 0, U);
}
function X(_) {
var k = _[2], I = k[1], U = I[0] === 0 ? b(I[1]) : X(I[1]), Y = [0, [0, oWr, U], [0, [0, aWr, b(k[2])], 0]];
return w(cWr, _[1], 0, Y);
}
function s0(_) {
switch (_[0]) {
case 0:
return b(_[1]);
case 1:
return G0(_[1]);
default:
return X(_[1]);
}
}
function dr(_) {
var k = _[2], I = [0, [0, PKr, n4(ae, k[3][2])], 0], U = [0, [0, DKr, e(oe, k[2])], I], Y = k[1], y0 = Y[2], D0 = [0, [0, qKr, !!y0[2]], 0], I0 = [0, [0, UKr, n4(pe, y0[3])], D0], D = [0, [0, HKr, s0(y0[1])], I0], u0 = [0, [0, LKr, w(XKr, Y[1], 0, D)], U];
return w(RKr, _[1], k[4], u0);
}
function Ar(_) {
var k = _[2], I = [0, [0, ZYr, n4(xr, k[2])], 0], U = [0, [0, QYr, n4(vr, k[1])], I];
return w(rVr, _[1], k[3], U);
}
function ar(_, k) {
var I = k[2], U = I[7], Y = I[5], y0 = I[4];
if (y0)
var D0 = y0[1][2], I0 = _7(D0[3], U), D = I0, u0 = D0[2], Y0 = [0, D0[1]];
else
var D = U, u0 = 0, Y0 = 0;
if (Y)
var J0 = Y[1][2], fr = _7(J0[2], D), Q0 = fr, F0 = n4(T0, J0[1]);
else
var Q0 = D, F0 = yu(0);
var gr = [0, [0, aHr, F0], [0, [0, xHr, n4(S0, I[6])], 0]], mr = [0, [0, oHr, e($r, u0)], gr], Cr = [0, [0, cHr, e(xr, Y0)], mr], sr = [0, [0, sHr, e(qr, I[3])], Cr], Pr = I[2], K0 = Pr[2], Ur = [0, [0, dHr, n4(rr, K0[1])], 0], d0 = [0, [0, vHr, w(hHr, Pr[1], K0[2], Ur)], sr], Kr = [0, [0, lHr, e(S, I[1])], d0];
return w(_, k[1], Q0, Kr);
}
function W0(_) {
var k = _[2], I = [0, [0, wUr, G(k[1])], 0], U = p(k[2]);
return w(EUr, _[1], U, I);
}
function Lr(_) {
var k = _[2];
switch (k[0]) {
case 0:
var I = 0, U = S(k[1]);
break;
case 1:
var I = 0, U = _0(k[1]);
break;
default:
var I = 1, U = xr(k[1]);
}
return [0, [0, GWr, xr(_[1])], [0, [0, jWr, U], [0, [0, RWr, !!I], 0]]];
}
function Tr(_) {
var k = [0, [0, PWr, E0(_[3])], 0], I = [0, [0, DWr, e(ne, _[2])], k];
return [0, [0, LWr, xr(_[1])], I];
}
function Hr(_) {
var k = _[2], I = k[3], U = k[2], Y = k[1];
if (I) {
var y0 = I[1], D0 = y0[2], I0 = [0, [0, VXr, Or(D0[1])], 0], D = w(zXr, y0[1], D0[2], I0), u0 = de([0, D, Tp(R0, U)]), Y0 = Y ? [0, a0(Y[1]), u0] : u0;
return yu(Y0);
}
var J0 = k1(R0, U), fr = Y ? [0, a0(Y[1]), J0] : J0;
return yu(fr);
}
function Or(_) {
var k = _[2], I = _[1];
switch (k[0]) {
case 0:
var U = k[1], Y = [0, [0, DXr, i(l, U[2])], 0], y0 = [0, [0, LXr, n4(b0, U[1])], Y];
return w(RXr, I, p(U[3]), y0);
case 1:
var D0 = k[1], I0 = [0, [0, jXr, i(l, D0[2])], 0], D = [0, [0, GXr, n4(Z, D0[1])], I0];
return w(MXr, I, p(D0[3]), D);
case 2:
return w0(I, k[1]);
default:
return xr(k[1]);
}
}
function xr(_) {
var k = _[2], I = _[1];
switch (k[0]) {
case 0:
var U = k[1], Y = [0, [0, iBr, n4(er, U[1])], 0];
return w(fBr, I, p(U[2]), Y);
case 1:
var y0 = k[1], D0 = y0[7], I0 = y0[3], D = y0[2];
if (I0[0] === 0)
var u0 = 0, Y0 = W0(I0[1]);
else
var u0 = 1, Y0 = xr(I0[1]);
var J0 = D0[0] === 0 ? 0 : [0, D0[1]], fr = y0[9], Q0 = _7(p(D[2][4]), fr), F0 = [0, [0, xBr, e(qr, y0[8])], 0], gr = [0, [0, oBr, !!u0], [0, [0, aBr, e(l, J0)], F0]], mr = [0, [0, sBr, false], [0, [0, cBr, e(Fr, y0[6])], gr]], Cr = [0, [0, lBr, Y0], [0, [0, vBr, !!y0[4]], mr]];
return w(mBr, I, Q0, [0, [0, pBr, u7], [0, [0, bBr, Hr(D)], Cr]]);
case 2:
var sr = k[1], Pr = sr[1];
if (Pr) {
switch (Pr[1]) {
case 0:
var K0 = Upr;
break;
case 1:
var K0 = Hpr;
break;
case 2:
var K0 = Xpr;
break;
case 3:
var K0 = Ypr;
break;
case 4:
var K0 = Vpr;
break;
case 5:
var K0 = zpr;
break;
case 6:
var K0 = Kpr;
break;
case 7:
var K0 = Wpr;
break;
case 8:
var K0 = Jpr;
break;
case 9:
var K0 = $pr;
break;
case 10:
var K0 = Zpr;
break;
case 11:
var K0 = Qpr;
break;
case 12:
var K0 = r5r;
break;
case 13:
var K0 = e5r;
break;
default:
var K0 = n5r;
}
var Ur = K0;
} else
var Ur = _Br;
var d0 = [0, [0, yBr, xr(sr[3])], 0], Kr = [0, [0, dBr, Or(sr[2])], d0];
return w(kBr, I, sr[4], [0, [0, hBr, sn(Ur)], Kr]);
case 3:
var re = k[1], xe = [0, [0, wBr, xr(re[3])], 0], je = [0, [0, EBr, xr(re[2])], xe];
switch (re[1]) {
case 0:
var ve = hpr;
break;
case 1:
var ve = kpr;
break;
case 2:
var ve = wpr;
break;
case 3:
var ve = Epr;
break;
case 4:
var ve = Spr;
break;
case 5:
var ve = gpr;
break;
case 6:
var ve = Fpr;
break;
case 7:
var ve = Tpr;
break;
case 8:
var ve = Opr;
break;
case 9:
var ve = Ipr;
break;
case 10:
var ve = Apr;
break;
case 11:
var ve = Npr;
break;
case 12:
var ve = Cpr;
break;
case 13:
var ve = Ppr;
break;
case 14:
var ve = Dpr;
break;
case 15:
var ve = Lpr;
break;
case 16:
var ve = Rpr;
break;
case 17:
var ve = jpr;
break;
case 18:
var ve = Gpr;
break;
case 19:
var ve = Mpr;
break;
case 20:
var ve = Bpr;
break;
default:
var ve = qpr;
}
return w(gBr, I, re[4], [0, [0, SBr, sn(ve)], je]);
case 4:
var Ie = k[1], Me = Ie[4], Be = _7(p(Ie[3][2][2]), Me);
return w(FBr, I, Be, Tr(Ie));
case 5:
return ar(fHr, [0, I, k[1]]);
case 6:
var fn = k[1], Ke = [0, [0, TBr, e(xr, fn[2])], 0];
return w(IBr, I, 0, [0, [0, OBr, n4(yr, fn[1])], Ke]);
case 7:
var Ae = k[1], xn = [0, [0, ABr, xr(Ae[3])], 0], Qe = [0, [0, NBr, xr(Ae[2])], xn], yn = [0, [0, CBr, xr(Ae[1])], Qe];
return w(PBr, I, Ae[4], yn);
case 8:
return Rr([0, I, k[1]]);
case 9:
var on = k[1], Ce = [0, [0, DBr, e(xr, on[2])], 0];
return w(RBr, I, 0, [0, [0, LBr, n4(yr, on[1])], Ce]);
case 10:
return S(k[1]);
case 11:
var We = k[1], rn = [0, [0, jBr, xr(We[1])], 0];
return w(GBr, I, We[2], rn);
case 12:
return dr([0, I, k[1]]);
case 13:
return X0([0, I, k[1]]);
case 14:
var bn = k[1], Cn = bn[1];
return typeof Cn != "number" && Cn[0] === 3 ? w(HYr, I, bn[3], [0, [0, UYr, u7], [0, [0, qYr, sn(bn[2])], 0]]) : f0([0, I, bn]);
case 15:
var Hn = k[1];
switch (Hn[1]) {
case 0:
var Sn = MBr;
break;
case 1:
var Sn = BBr;
break;
default:
var Sn = qBr;
}
var vt = [0, [0, UBr, xr(Hn[3])], 0], At = [0, [0, HBr, xr(Hn[2])], vt];
return w(YBr, I, Hn[4], [0, [0, XBr, sn(Sn)], At]);
case 16:
var gt = k[1], Jt = Lr(gt);
return w(VBr, I, gt[3], Jt);
case 17:
var Bt = k[1], Ft = [0, [0, zBr, S(Bt[2])], 0], Nt = [0, [0, KBr, S(Bt[1])], Ft];
return w(WBr, I, Bt[3], Nt);
case 18:
var du = k[1], Ku = du[4], lt = du[3];
if (lt)
var xu = lt[1], Mu = _7(p(xu[2][2]), Ku), z7 = Mu, Yi = E0(xu);
else
var z7 = Ku, Yi = yu(0);
var a7 = [0, [0, $Br, e(ne, du[2])], [0, [0, JBr, Yi], 0]];
return w(QBr, I, z7, [0, [0, ZBr, xr(du[1])], a7]);
case 19:
var Yc = k[1], K7 = [0, [0, rqr, n4(p0, Yc[1])], 0];
return w(eqr, I, p(Yc[2]), K7);
case 20:
var qt = k[1], bt = qt[1], U0 = bt[4], L0 = _7(p(bt[3][2][2]), U0), Re = [0, [0, nqr, !!qt[3]], 0];
return w(tqr, I, L0, un(Tr(bt), Re));
case 21:
var He = k[1], he = He[1], _e = [0, [0, uqr, !!He[3]], 0], Zn = un(Lr(he), _e);
return w(iqr, I, he[3], Zn);
case 22:
var dn = k[1], it = [0, [0, fqr, n4(xr, dn[1])], 0];
return w(xqr, I, dn[2], it);
case 23:
return w(aqr, I, k[1][1], 0);
case 24:
var ft = k[1], Rn = [0, [0, fVr, Ar(ft[2])], 0], nt = [0, [0, xVr, xr(ft[1])], Rn];
return w(aVr, I, ft[3], nt);
case 25:
return Ar([0, I, k[1]]);
case 26:
return w(oqr, I, k[1][1], 0);
case 27:
var ht = k[1], tn = [0, [0, cqr, l(ht[2])], 0], cn = [0, [0, sqr, xr(ht[1])], tn];
return w(vqr, I, ht[3], cn);
case 28:
var tt = k[1], Tt = tt[3], Fi = tt[2], hs = tt[1];
if (7 <= hs)
return w(bqr, I, Tt, [0, [0, lqr, xr(Fi)], 0]);
switch (hs) {
case 0:
var Iu = pqr;
break;
case 1:
var Iu = mqr;
break;
case 2:
var Iu = _qr;
break;
case 3:
var Iu = yqr;
break;
case 4:
var Iu = dqr;
break;
case 5:
var Iu = hqr;
break;
case 6:
var Iu = kqr;
break;
default:
var Iu = ke(wqr);
}
var Vs = [0, [0, Sqr, true], [0, [0, Eqr, xr(Fi)], 0]];
return w(Fqr, I, Tt, [0, [0, gqr, sn(Iu)], Vs]);
case 29:
var Vi = k[1], zs = Vi[1] ? Tqr : Oqr, Ks = [0, [0, Iqr, !!Vi[3]], 0], en = [0, [0, Aqr, xr(Vi[2])], Ks];
return w(Cqr, I, Vi[4], [0, [0, Nqr, sn(zs)], en]);
default:
var ci = k[1], Ws = [0, [0, Pqr, !!ci[3]], 0], c2 = [0, [0, Dqr, e(xr, ci[1])], Ws];
return w(Lqr, I, ci[2], c2);
}
}
function Rr(_) {
var k = _[2], I = k[7], U = k[3], Y = k[2], y0 = U[0] === 0 ? U[1] : ke(zqr), D0 = I[0] === 0 ? 0 : [0, I[1]], I0 = k[9], D = _7(p(Y[2][4]), I0), u0 = [0, [0, Kqr, e(qr, k[8])], 0], Y0 = [0, [0, Jqr, false], [0, [0, Wqr, e(l, D0)], u0]], J0 = [0, [0, $qr, e(Fr, k[6])], Y0], fr = [0, [0, Qqr, !!k[4]], [0, [0, Zqr, !!k[5]], J0]], Q0 = [0, [0, rUr, W0(y0)], fr], F0 = [0, [0, eUr, Hr(Y)], Q0], gr = [0, [0, nUr, e(S, k[1])], F0];
return w(tUr, _[1], D, gr);
}
function Wr(_) {
var k = _[2], I = [0, [0, FXr, n4(fe, k[3])], 0], U = [0, [0, TXr, x0(0, k[4])], I], Y = [0, [0, OXr, e(qr, k[2])], U], y0 = [0, [0, IXr, S(k[1])], Y];
return w(AXr, _[1], k[5], y0);
}
function Jr(_, k) {
var I = k[2], U = _ ? QUr : rHr, Y = [0, [0, eHr, e(g0, I[4])], 0], y0 = [0, [0, nHr, e(g0, I[3])], Y], D0 = [0, [0, tHr, e(qr, I[2])], y0], I0 = [0, [0, uHr, S(I[1])], D0];
return w(U, k[1], I[5], I0);
}
function or(_) {
var k = _[2], I = [0, [0, WUr, g0(k[3])], 0], U = [0, [0, JUr, e(qr, k[2])], I], Y = [0, [0, $Ur, S(k[1])], U];
return w(ZUr, _[1], k[4], Y);
}
function _r(_) {
if (_) {
var k = _[1];
if (k[0] === 0)
return n4(ge, k[1]);
var I = k[1], U = I[2];
if (U) {
var Y = [0, [0, HUr, S(U[1])], 0];
return yu([0, w(XUr, I[1], 0, Y), 0]);
}
return yu(0);
}
return yu(0);
}
function Ir(_) {
return _ ? qUr : UUr;
}
function fe(_) {
var k = _[2], I = k[1], U = I[0] === 0 ? S(I[1]) : K(I[1]), Y = [0, [0, CXr, U], [0, [0, NXr, e($r, k[2])], 0]];
return w(PXr, _[1], k[3], Y);
}
function v0(_) {
var k = _[2], I = k[6], U = k[4], Y = yu(U ? [0, fe(U[1]), 0] : 0), y0 = I ? n4(T0, I[1][2][1]) : yu(0), D0 = [0, [0, NUr, Y], [0, [0, AUr, y0], [0, [0, IUr, n4(fe, k[5])], 0]]], I0 = [0, [0, CUr, x0(0, k[3])], D0], D = [0, [0, PUr, e(qr, k[2])], I0], u0 = [0, [0, DUr, S(k[1])], D];
return w(LUr, _[1], k[7], u0);
}
function P(_) {
var k = _[2], I = k[2], U = k[1], Y = yt(U[1], I[1]), y0 = [0, [0, FUr, e(Fr, k[3])], 0], D0 = [0, [0, TUr, w0(Y, [0, U, [1, I], 0])], y0];
return w(OUr, _[1], k[4], D0);
}
function L(_) {
var k = _[2], I = k[2], U = k[1], Y = [0, [0, SUr, w0(yt(U[1], I[1]), [0, U, [1, I], 0])], 0];
return w(gUr, _[1], k[3], Y);
}
function Q(_) {
var k = _[2], I = _[1];
switch (k[0]) {
case 0:
return W0([0, I, k[1]]);
case 1:
var U = k[1], Y = [0, [0, AGr, e(S, U[1])], 0];
return w(NGr, I, U[2], Y);
case 2:
return ar(iHr, [0, I, k[1]]);
case 3:
var y0 = k[1], D0 = [0, [0, CGr, e(S, y0[1])], 0];
return w(PGr, I, y0[2], D0);
case 4:
return w(DGr, I, k[1][1], 0);
case 5:
return v0([0, I, k[1]]);
case 6:
var I0 = k[1], D = I0[5], u0 = I0[4], Y0 = I0[3], J0 = I0[2];
if (Y0) {
var fr = Y0[1];
if (fr[0] !== 0 && !fr[1][2])
return w(RGr, I, D, [0, [0, LGr, e(t0, u0)], 0]);
}
if (J0) {
var Q0 = J0[1];
switch (Q0[0]) {
case 0:
var F0 = L(Q0[1]);
break;
case 1:
var F0 = P(Q0[1]);
break;
case 2:
var F0 = v0(Q0[1]);
break;
case 3:
var F0 = g0(Q0[1]);
break;
case 4:
var F0 = or(Q0[1]);
break;
case 5:
var F0 = Jr(1, Q0[1]);
break;
default:
var F0 = Wr(Q0[1]);
}
var gr = F0;
} else
var gr = u7;
var mr = [0, [0, jGr, e(t0, u0)], 0], Cr = [0, [0, MGr, gr], [0, [0, GGr, _r(Y0)], mr]], sr = I0[1], Pr = sr && 1;
return w(qGr, I, D, [0, [0, BGr, !!Pr], Cr]);
case 7:
return P([0, I, k[1]]);
case 8:
var K0 = k[1], Ur = [0, [0, RUr, n4(fe, K0[3])], 0], d0 = [0, [0, jUr, x0(0, K0[4])], Ur], Kr = [0, [0, GUr, e(qr, K0[2])], d0], re = [0, [0, MUr, S(K0[1])], Kr];
return w(BUr, I, K0[5], re);
case 9:
var xe = k[1], je = xe[1], ve = je[0] === 0 ? S(je[1]) : t0(je[1]), Ie = 0, Me = xe[3] ? "ES" : "CommonJS", Be = [0, [0, XGr, ve], [0, [0, HGr, W0(xe[2])], [0, [0, UGr, Me], Ie]]];
return w(YGr, I, xe[4], Be);
case 10:
var fn = k[1], Ke = [0, [0, VGr, l(fn[1])], 0];
return w(zGr, I, fn[2], Ke);
case 11:
var Ae = k[1], xn = [0, [0, YUr, g0(Ae[3])], 0], Qe = [0, [0, VUr, e(qr, Ae[2])], xn], yn = [0, [0, zUr, S(Ae[1])], Qe];
return w(KUr, I, Ae[4], yn);
case 12:
return Jr(1, [0, I, k[1]]);
case 13:
return L([0, I, k[1]]);
case 14:
var on = k[1], Ce = [0, [0, KGr, xr(on[2])], 0], We = [0, [0, WGr, Q(on[1])], Ce];
return w(JGr, I, on[3], We);
case 15:
return w($Gr, I, k[1][1], 0);
case 16:
var rn = k[1], bn = rn[2], Cn = bn[2], Hn = bn[1];
switch (Cn[0]) {
case 0:
var Sn = Cn[1], vt = [0, [0, oXr, !!Sn[2]], [0, [0, aXr, !!Sn[3]], 0]], At = Sn[1], gt = [0, [0, cXr, n4(function(hu) {
var ku = hu[2], Oi = ku[2], k7 = Oi[2], Ki = k7[1], nv = 0, Lb = Ki ? zYr : KYr, tv = [0, [0, iXr, w($Yr, Oi[1], k7[2], [0, [0, JYr, !!Ki], [0, [0, WYr, sn(Lb)], 0]])], nv], Rb = [0, [0, fXr, S(ku[1])], tv];
return w(xXr, hu[1], 0, Rb);
}, At)], vt], bt = w(sXr, Hn, p(Sn[4]), gt);
break;
case 1:
var Jt = Cn[1], Bt = [0, [0, lXr, !!Jt[2]], [0, [0, vXr, !!Jt[3]], 0]], Ft = Jt[1], Nt = [0, [0, bXr, n4(function(hu) {
var ku = hu[2], Oi = ku[2], k7 = Oi[2], Ki = [0, [0, nXr, w(BYr, Oi[1], k7[3], [0, [0, MYr, k7[1]], [0, [0, GYr, sn(k7[2])], 0]])], 0], nv = [0, [0, tXr, S(ku[1])], Ki];
return w(uXr, hu[1], 0, nv);
}, Ft)], Bt], bt = w(pXr, Hn, p(Jt[4]), Nt);
break;
case 2:
var du = Cn[1], Ku = du[1];
if (Ku[0] === 0)
var lt = Ku[1], Mu = k1(function(hu) {
var ku = [0, [0, rXr, S(hu[2][1])], 0];
return w(eXr, hu[1], 0, ku);
}, lt);
else
var xu = Ku[1], Mu = k1(function(hu) {
var ku = hu[2], Oi = [0, [0, $Hr, t0(ku[2])], 0], k7 = [0, [0, ZHr, S(ku[1])], Oi];
return w(QHr, hu[1], 0, k7);
}, xu);
var z7 = [0, [0, _Xr, !!du[2]], [0, [0, mXr, !!du[3]], 0]], Yi = [0, [0, yXr, yu(Mu)], z7], bt = w(dXr, Hn, p(du[4]), Yi);
break;
default:
var a7 = Cn[1], Yc = [0, [0, hXr, !!a7[2]], 0], K7 = a7[1], qt = [0, [0, kXr, n4(function(hu) {
var ku = [0, [0, WHr, S(hu[2][1])], 0];
return w(JHr, hu[1], 0, ku);
}, K7)], Yc], bt = w(wXr, Hn, p(a7[3]), qt);
}
var U0 = [0, [0, SXr, S(rn[1])], [0, [0, EXr, bt], 0]];
return w(gXr, I, rn[3], U0);
case 17:
var L0 = k[1], Re = L0[2], He = Re[0] === 0 ? Q(Re[1]) : xr(Re[1]), he = [0, [0, QGr, He], [0, [0, ZGr, sn(Ir(1))], 0]];
return w(rMr, I, L0[3], he);
case 18:
var _e = k[1], Zn = _e[5], dn = _e[4], it = _e[3], ft = _e[2];
if (ft) {
var Rn = ft[1];
if (Rn[0] !== 0) {
var nt = [0, [0, eMr, sn(Ir(dn))], 0], ht = [0, [0, nMr, e(S, Rn[1][2])], nt];
return w(uMr, I, Zn, [0, [0, tMr, e(t0, it)], ht]);
}
}
var tn = [0, [0, iMr, sn(Ir(dn))], 0], cn = [0, [0, fMr, e(t0, it)], tn], tt = [0, [0, xMr, _r(ft)], cn];
return w(oMr, I, Zn, [0, [0, aMr, e(Q, _e[1])], tt]);
case 19:
var Tt = k[1], Fi = [0, [0, cMr, e(rn0, Tt[2])], 0], hs = [0, [0, sMr, xr(Tt[1])], Fi];
return w(vMr, I, Tt[3], hs);
case 20:
var Iu = k[1], Vs = function(hu) {
return hu[0] === 0 ? c0(hu[1]) : xr(hu[1]);
}, Vi = [0, [0, lMr, Q(Iu[4])], 0], zs = [0, [0, bMr, e(xr, Iu[3])], Vi], Ks = [0, [0, pMr, e(xr, Iu[2])], zs], en = [0, [0, mMr, e(Vs, Iu[1])], Ks];
return w(_Mr, I, Iu[5], en);
case 21:
var ci = k[1], Ws = ci[1], c2 = Ws[0] === 0 ? c0(Ws[1]) : Or(Ws[1]), B9 = [0, [0, yMr, !!ci[4]], 0], q9 = [0, [0, dMr, Q(ci[3])], B9], U9 = [0, [0, kMr, c2], [0, [0, hMr, xr(ci[2])], q9]];
return w(wMr, I, ci[5], U9);
case 22:
var Js = k[1], s2 = Js[1], H9 = s2[0] === 0 ? c0(s2[1]) : Or(s2[1]), X9 = [0, [0, EMr, !!Js[4]], 0], Y9 = [0, [0, SMr, Q(Js[3])], X9], X1 = [0, [0, FMr, H9], [0, [0, gMr, xr(Js[2])], Y9]];
return w(TMr, I, Js[5], X1);
case 23:
var si = k[1], ob = si[7], cb = si[3], sb = si[2], V9 = cb[0] === 0 ? cb[1] : ke(Rqr), z9 = ob[0] === 0 ? 0 : [0, ob[1]], K9 = si[9], vb = _7(p(sb[2][4]), K9), W9 = [0, [0, jqr, e(qr, si[8])], 0], J9 = [0, [0, Mqr, false], [0, [0, Gqr, e(l, z9)], W9]], $9 = [0, [0, Bqr, e(Fr, si[6])], J9], Z9 = [0, [0, Uqr, !!si[4]], [0, [0, qqr, !!si[5]], $9]], lb = [0, [0, Hqr, W0(V9)], Z9], Q9 = [0, [0, Xqr, Hr(sb)], lb];
return w(Vqr, I, vb, [0, [0, Yqr, e(S, si[1])], Q9]);
case 24:
var Y1 = k[1], v2 = Y1[3];
if (v2) {
var bb = v2[1][2], pb = bb[2], mb = bb[1], Tn = mb[2], jn = function(ku) {
return _7(ku, pb);
};
switch (Tn[0]) {
case 0:
var V1 = Tn[1], _b = QD(V1[2], pb), Gn = [0, [0, V1[1], _b]];
break;
case 1:
var yb = Tn[1], r_ = jn(yb[2]), Gn = [1, [0, yb[1], r_]];
break;
case 2:
var Vc = Tn[1], e_ = jn(Vc[7]), Gn = [2, [0, Vc[1], Vc[2], Vc[3], Vc[4], Vc[5], Vc[6], e_]];
break;
case 3:
var l2 = Tn[1], db = jn(l2[2]), Gn = [3, [0, l2[1], db]];
break;
case 4:
var Gn = [4, [0, jn(Tn[1][1])]];
break;
case 5:
var zc = Tn[1], n_ = jn(zc[7]), Gn = [5, [0, zc[1], zc[2], zc[3], zc[4], zc[5], zc[6], n_]];
break;
case 6:
var $s = Tn[1], hb = jn($s[5]), Gn = [6, [0, $s[1], $s[2], $s[3], $s[4], hb]];
break;
case 7:
var z1 = Tn[1], t_ = jn(z1[4]), Gn = [7, [0, z1[1], z1[2], z1[3], t_]];
break;
case 8:
var ks = Tn[1], u_ = jn(ks[5]), Gn = [8, [0, ks[1], ks[2], ks[3], ks[4], u_]];
break;
case 9:
var K1 = Tn[1], i_ = jn(K1[4]), Gn = [9, [0, K1[1], K1[2], K1[3], i_]];
break;
case 10:
var b2 = Tn[1], f_ = jn(b2[2]), Gn = [10, [0, b2[1], f_]];
break;
case 11:
var Zs = Tn[1], kb = jn(Zs[4]), Gn = [11, [0, Zs[1], Zs[2], Zs[3], kb]];
break;
case 12:
var Qs = Tn[1], x_ = jn(Qs[5]), Gn = [12, [0, Qs[1], Qs[2], Qs[3], Qs[4], x_]];
break;
case 13:
var zi = Tn[1], Kc = jn(zi[3]), Gn = [13, [0, zi[1], zi[2], Kc]];
break;
case 14:
var r1 = Tn[1], a_ = jn(r1[3]), Gn = [14, [0, r1[1], r1[2], a_]];
break;
case 15:
var Gn = [15, [0, jn(Tn[1][1])]];
break;
case 16:
var p2 = Tn[1], m2 = jn(p2[3]), Gn = [16, [0, p2[1], p2[2], m2]];
break;
case 17:
var _2 = Tn[1], o_ = jn(_2[3]), Gn = [17, [0, _2[1], _2[2], o_]];
break;
case 18:
var e1 = Tn[1], c_ = jn(e1[5]), Gn = [18, [0, e1[1], e1[2], e1[3], e1[4], c_]];
break;
case 19:
var y2 = Tn[1], XL = jn(y2[3]), Gn = [19, [0, y2[1], y2[2], XL]];
break;
case 20:
var W1 = Tn[1], YL = jn(W1[5]), Gn = [20, [0, W1[1], W1[2], W1[3], W1[4], YL]];
break;
case 21:
var J1 = Tn[1], VL = jn(J1[5]), Gn = [21, [0, J1[1], J1[2], J1[3], J1[4], VL]];
break;
case 22:
var $1 = Tn[1], zL = jn($1[5]), Gn = [22, [0, $1[1], $1[2], $1[3], $1[4], zL]];
break;
case 23:
var Ti = Tn[1], KL = Ti[10], WL = jn(Ti[9]), Gn = [23, [0, Ti[1], Ti[2], Ti[3], Ti[4], Ti[5], Ti[6], Ti[7], Ti[8], WL, KL]];
break;
case 24:
var d2 = Tn[1], JL = jn(d2[4]), Gn = [24, [0, d2[1], d2[2], d2[3], JL]];
break;
case 25:
var Z1 = Tn[1], $L = jn(Z1[5]), Gn = [25, [0, Z1[1], Z1[2], Z1[3], Z1[4], $L]];
break;
case 26:
var Q1 = Tn[1], ZL = jn(Q1[5]), Gn = [26, [0, Q1[1], Q1[2], Q1[3], Q1[4], ZL]];
break;
case 27:
var wb = Tn[1], QL = jn(wb[3]), Gn = [27, [0, wb[1], wb[2], QL]];
break;
case 28:
var Eb = Tn[1], rR = Eb[3], eR = jn(Eb[2]), Gn = [28, [0, Eb[1], eR, rR]];
break;
case 29:
var h2 = Tn[1], nR = h2[4], tR = jn(h2[3]), Gn = [29, [0, h2[1], h2[2], tR, nR]];
break;
case 30:
var s_ = Tn[1], uR = jn(s_[2]), Gn = [30, [0, s_[1], uR]];
break;
case 31:
var k2 = Tn[1], iR = jn(k2[4]), Gn = [31, [0, k2[1], k2[2], k2[3], iR]];
break;
case 32:
var w2 = Tn[1], fR = jn(w2[4]), Gn = [32, [0, w2[1], w2[2], w2[3], fR]];
break;
case 33:
var rv = Tn[1], xR = jn(rv[5]), Gn = [33, [0, rv[1], rv[2], rv[3], rv[4], xR]];
break;
case 34:
var Sb = Tn[1], aR = jn(Sb[3]), Gn = [34, [0, Sb[1], Sb[2], aR]];
break;
case 35:
var gb = Tn[1], oR = jn(gb[3]), Gn = [35, [0, gb[1], gb[2], oR]];
break;
default:
var Fb = Tn[1], cR = jn(Fb[3]), Gn = [36, [0, Fb[1], Fb[2], cR]];
}
var v_ = Q([0, mb[1], Gn]);
} else
var v_ = u7;
var sR = [0, [0, IMr, Q(Y1[2])], [0, [0, OMr, v_], 0]], vR = [0, [0, AMr, xr(Y1[1])], sR];
return w(NMr, I, Y1[4], vR);
case 25:
var ev = k[1], Tb = ev[4], l_ = ev[3];
if (Tb) {
var Ob = Tb[1];
if (Ob[0] === 0)
var lR = Ob[1], p_ = k1(function(ku) {
var Oi = ku[1], k7 = ku[3], Ki = ku[2], nv = Ki ? yt(k7[1], Ki[1][1]) : k7[1], Lb = Ki ? Ki[1] : k7, tv = 0, Rb = 0;
if (Oi)
switch (Oi[1]) {
case 0:
var jb = $c;
break;
case 1:
var jb = es;
break;
default:
tv = 1;
}
else
tv = 1;
if (tv)
var jb = u7;
var CR = [0, [0, SWr, S(Lb)], [0, [0, EWr, jb], Rb]];
return w(FWr, nv, 0, [0, [0, gWr, S(k7)], CR]);
}, lR);
else
var b_ = Ob[1], bR = [0, [0, kWr, S(b_[2])], 0], p_ = [0, w(wWr, b_[1], 0, bR), 0];
var Ib = p_;
} else
var Ib = Tb;
if (l_)
var m_ = l_[1], pR = [0, [0, dWr, S(m_)], 0], __ = [0, w(hWr, m_[1], 0, pR), Ib];
else
var __ = Ib;
switch (ev[1]) {
case 0:
var Ab = CMr;
break;
case 1:
var Ab = PMr;
break;
default:
var Ab = DMr;
}
var mR = [0, [0, LMr, sn(Ab)], 0], _R = [0, [0, RMr, t0(ev[2])], mR], yR = [0, [0, jMr, yu(__)], _R];
return w(GMr, I, ev[5], yR);
case 26:
return Wr([0, I, k[1]]);
case 27:
var Nb = k[1], dR = [0, [0, MMr, Q(Nb[2])], 0], hR = [0, [0, BMr, S(Nb[1])], dR];
return w(qMr, I, Nb[3], hR);
case 28:
var y_ = k[1], kR = [0, [0, UMr, e(xr, y_[1])], 0];
return w(HMr, I, y_[2], kR);
case 29:
var Cb = k[1], wR = [0, [0, XMr, n4(i0, Cb[2])], 0], ER = [0, [0, YMr, xr(Cb[1])], wR];
return w(VMr, I, Cb[3], ER);
case 30:
var d_ = k[1], SR = [0, [0, zMr, xr(d_[1])], 0];
return w(KMr, I, d_[2], SR);
case 31:
var E2 = k[1], gR = [0, [0, WMr, e(W0, E2[3])], 0], FR = [0, [0, JMr, e(l0, E2[2])], gR], TR = [0, [0, $Mr, W0(E2[1])], FR];
return w(ZMr, I, E2[4], TR);
case 32:
return or([0, I, k[1]]);
case 33:
return Jr(0, [0, I, k[1]]);
case 34:
return c0([0, I, k[1]]);
case 35:
var Pb = k[1], OR = [0, [0, QMr, Q(Pb[2])], 0], IR = [0, [0, rBr, xr(Pb[1])], OR];
return w(eBr, I, Pb[3], IR);
default:
var Db = k[1], AR = [0, [0, nBr, Q(Db[2])], 0], NR = [0, [0, tBr, xr(Db[1])], AR];
return w(uBr, I, Db[3], NR);
}
}
function i0(_) {
var k = _[2], I = [0, [0, mUr, n4(Q, k[2])], 0], U = [0, [0, _Ur, e(xr, k[1])], I];
return w(yUr, _[1], k[3], U);
}
function l0(_) {
var k = _[2], I = [0, [0, dUr, W0(k[2])], 0], U = [0, [0, hUr, e(Or, k[1])], I];
return w(kUr, _[1], k[3], U);
}
function S0(_) {
var k = _[2], I = [0, [0, bHr, xr(k[1])], 0];
return w(pHr, _[1], k[2], I);
}
function T0(_) {
var k = _[2], I = [0, [0, mHr, e($r, k[2])], 0], U = [0, [0, _Hr, S(k[1])], I];
return w(yHr, _[1], 0, U);
}
function rr(_) {
switch (_[0]) {
case 0:
var k = _[1], I = k[2], U = I[6], Y = I[2];
switch (Y[0]) {
case 0:
var I0 = U, D = 0, u0 = f0(Y[1]);
break;
case 1:
var I0 = U, D = 0, u0 = S(Y[1]);
break;
case 2:
var I0 = U, D = 0, u0 = _0(Y[1]);
break;
default:
var y0 = Y[1][2], D0 = _7(y0[2], U), I0 = D0, D = 1, u0 = xr(y0[1]);
}
switch (I[1]) {
case 0:
var Y0 = kHr;
break;
case 1:
var Y0 = wHr;
break;
case 2:
var Y0 = EHr;
break;
default:
var Y0 = SHr;
}
var J0 = [0, [0, FHr, !!D], [0, [0, gHr, n4(S0, I[5])], 0]], fr = [0, [0, OHr, sn(Y0)], [0, [0, THr, !!I[4]], J0]], Q0 = [0, [0, AHr, u0], [0, [0, IHr, Rr(I[3])], fr]];
return w(NHr, k[1], I0, Q0);
case 1:
var F0 = _[1], gr = F0[2], mr = gr[6], Cr = gr[2], sr = gr[1];
switch (sr[0]) {
case 0:
var d0 = mr, Kr = 0, re = f0(sr[1]);
break;
case 1:
var d0 = mr, Kr = 0, re = S(sr[1]);
break;
case 2:
var Pr = ke(BHr), d0 = Pr[3], Kr = Pr[2], re = Pr[1];
break;
default:
var K0 = sr[1][2], Ur = _7(K0[2], mr), d0 = Ur, Kr = 1, re = xr(K0[1]);
}
if (typeof Cr == "number")
if (Cr)
var xe = 0, je = 0;
else
var xe = 1, je = 0;
else
var xe = 0, je = [0, Cr[1]];
var ve = xe && [0, [0, qHr, !!xe], 0], Ie = [0, [0, UHr, e(Sr, gr[5])], 0], Me = [0, [0, XHr, !!Kr], [0, [0, HHr, !!gr[4]], Ie]], Be = [0, [0, YHr, i(l, gr[3])], Me], fn = un([0, [0, zHr, re], [0, [0, VHr, e(xr, je)], Be]], ve);
return w(KHr, F0[1], d0, fn);
default:
var Ke = _[1], Ae = Ke[2], xn = Ae[2];
if (typeof xn == "number")
if (xn)
var Qe = 0, yn = 0;
else
var Qe = 1, yn = 0;
else
var Qe = 0, yn = [0, xn[1]];
var on = Qe && [0, [0, CHr, !!Qe], 0], Ce = [0, [0, PHr, e(Sr, Ae[5])], 0], We = [0, [0, LHr, false], [0, [0, DHr, !!Ae[4]], Ce]], rn = [0, [0, RHr, i(l, Ae[3])], We], bn = [0, [0, jHr, e(xr, yn)], rn], Cn = un([0, [0, GHr, _0(Ae[1])], bn], on);
return w(MHr, Ke[1], Ae[6], Cn);
}
}
function R0(_) {
var k = _[2], I = k[2], U = k[1];
if (I) {
var Y = [0, [0, BXr, xr(I[1])], 0], y0 = [0, [0, qXr, Or(U)], Y];
return w(UXr, _[1], 0, y0);
}
return Or(U);
}
function B(_, k) {
var I = [0, [0, KXr, Or(k[1])], 0];
return w(WXr, _, k[2], I);
}
function Z(_) {
switch (_[0]) {
case 0:
var k = _[1], I = k[2], U = I[2], Y = I[1];
if (U) {
var y0 = [0, [0, JXr, xr(U[1])], 0], D0 = [0, [0, $Xr, Or(Y)], y0];
return w(ZXr, k[1], 0, D0);
}
return Or(Y);
case 1:
var I0 = _[1];
return B(I0[1], I0[2]);
default:
return u7;
}
}
function p0(_) {
if (_[0] === 0) {
var k = _[1], I = k[2];
switch (I[0]) {
case 0:
var U = xr(I[2]), Y0 = 0, J0 = I[3], fr = 0, Q0 = QXr, F0 = U, gr = I[1];
break;
case 1:
var Y = I[2], y0 = Rr([0, Y[1], Y[2]]), Y0 = 0, J0 = 0, fr = 1, Q0 = rYr, F0 = y0, gr = I[1];
break;
case 2:
var D0 = I[2], I0 = Rr([0, D0[1], D0[2]]), Y0 = I[3], J0 = 0, fr = 0, Q0 = eYr, F0 = I0, gr = I[1];
break;
default:
var D = I[2], u0 = Rr([0, D[1], D[2]]), Y0 = I[3], J0 = 0, fr = 0, Q0 = nYr, F0 = u0, gr = I[1];
}
switch (gr[0]) {
case 0:
var Pr = Y0, K0 = 0, Ur = f0(gr[1]);
break;
case 1:
var Pr = Y0, K0 = 0, Ur = S(gr[1]);
break;
case 2:
var mr = ke(tYr), Pr = mr[3], K0 = mr[2], Ur = mr[1];
break;
default:
var Cr = gr[1][2], sr = _7(Cr[2], Y0), Pr = sr, K0 = 1, Ur = xr(Cr[1]);
}
return w(cYr, k[1], Pr, [0, [0, oYr, Ur], [0, [0, aYr, F0], [0, [0, xYr, sn(Q0)], [0, [0, fYr, !!fr], [0, [0, iYr, !!J0], [0, [0, uYr, !!K0], 0]]]]]]);
}
var d0 = _[1], Kr = d0[2], re = [0, [0, sYr, xr(Kr[1])], 0];
return w(vYr, d0[1], Kr[2], re);
}
function b0(_) {
if (_[0] === 0) {
var k = _[1], I = k[2], U = I[3], Y = I[2], y0 = I[1];
switch (y0[0]) {
case 0:
var D = 0, u0 = 0, Y0 = f0(y0[1]);
break;
case 1:
var D = 0, u0 = 0, Y0 = S(y0[1]);
break;
default:
var D0 = y0[1][2], I0 = xr(D0[1]), D = D0[2], u0 = 1, Y0 = I0;
}
if (U)
var J0 = U[1], fr = yt(Y[1], J0[1]), Q0 = [0, [0, lYr, xr(J0)], 0], F0 = w(pYr, fr, 0, [0, [0, bYr, Or(Y)], Q0]);
else
var F0 = Or(Y);
return w(wYr, k[1], D, [0, [0, kYr, Y0], [0, [0, hYr, F0], [0, [0, dYr, ji], [0, [0, yYr, false], [0, [0, _Yr, !!I[4]], [0, [0, mYr, !!u0], 0]]]]]]);
}
var gr = _[1];
return B(gr[1], gr[2]);
}
function O0(_) {
var k = _[2], I = [0, [0, EYr, xr(k[1])], 0];
return w(SYr, _[1], k[2], I);
}
function q0(_) {
return _[0] === 0 ? xr(_[1]) : O0(_[1]);
}
function er(_) {
switch (_[0]) {
case 0:
return xr(_[1]);
case 1:
return O0(_[1]);
default:
return u7;
}
}
function yr(_) {
var k = _[2], I = [0, [0, gYr, !!k[3]], 0], U = [0, [0, FYr, xr(k[2])], I], Y = [0, [0, TYr, Or(k[1])], U];
return w(OYr, _[1], 0, Y);
}
function vr(_) {
var k = _[2], I = k[1], U = H1([0, [0, nVr, sn(I[1])], [0, [0, eVr, sn(I[2])], 0]]);
return w(iVr, _[1], 0, [0, [0, uVr, U], [0, [0, tVr, !!k[2]], 0]]);
}
function $0(_) {
var k = _[2], I = [0, [0, pVr, e(xr, k[2])], 0], U = [0, [0, mVr, Or(k[1])], I];
return w(_Vr, _[1], 0, U);
}
function Sr(_) {
var k = _[2], I = k[1] ? pY : "plus";
return w(dVr, _[1], k[2], [0, [0, yVr, I], 0]);
}
function Mr(_) {
var k = _[2];
return k0(k[2], k[1]);
}
function Br(_) {
var k = _[2], I = [0, [0, HVr, g0(k[1][2])], [0, [0, UVr, false], 0]], U = [0, [0, XVr, e(S, 0)], I];
return w(YVr, _[1], k[2], U);
}
function qr(_) {
var k = _[2], I = [0, [0, kKr, n4(jr, k[1])], 0], U = p(k[2]);
return w(wKr, _[1], U, I);
}
function jr(_) {
var k = _[2], I = k[1][2], U = [0, [0, EKr, e(g0, k[4])], 0], Y = [0, [0, SKr, e(Sr, k[3])], U], y0 = [0, [0, gKr, i(l, k[2])], Y];
return w(TKr, _[1], I[2], [0, [0, FKr, sn(I[1])], y0]);
}
function $r(_) {
var k = _[2], I = [0, [0, OKr, n4(g0, k[1])], 0], U = p(k[2]);
return w(IKr, _[1], U, I);
}
function ne(_) {
var k = _[2], I = [0, [0, AKr, n4(Qr, k[1])], 0], U = p(k[2]);
return w(NKr, _[1], U, I);
}
function Qr(_) {
if (_[0] === 0)
return g0(_[1]);
var k = _[1], I = k[1], U = k[2][1];
return V([0, I, [0, [0, Gc(0, [0, I, CKr])], 0, U]]);
}
function pe(_) {
if (_[0] === 0) {
var k = _[1], I = k[2], U = I[1], Y = U[0] === 0 ? b(U[1]) : G0(U[1]), y0 = [0, [0, JKr, Y], [0, [0, WKr, e(ce, I[2])], 0]];
return w($Kr, k[1], 0, y0);
}
var D0 = _[1], I0 = D0[2], D = [0, [0, ZKr, xr(I0[1])], 0];
return w(QKr, D0[1], I0[2], D);
}
function oe(_) {
var k = [0, [0, VKr, s0(_[2][1])], 0];
return w(zKr, _[1], 0, k);
}
function me(_) {
var k = _[2], I = k[1], U = _[1], Y = I ? xr(I[1]) : w(rWr, [0, U[1], [0, U[2][1], U[2][2] + 1 | 0], [0, U[3][1], U[3][2] - 1 | 0]], 0, 0);
return w(nWr, U, p(k[2]), [0, [0, eWr, Y], 0]);
}
function ae(_) {
var k = _[2], I = _[1];
switch (k[0]) {
case 0:
return dr([0, I, k[1]]);
case 1:
return X0([0, I, k[1]]);
case 2:
return me([0, I, k[1]]);
case 3:
var U = k[1], Y = [0, [0, tWr, xr(U[1])], 0];
return w(uWr, I, U[2], Y);
default:
var y0 = k[1];
return w(xWr, I, 0, [0, [0, fWr, sn(y0[1])], [0, [0, iWr, sn(y0[2])], 0]]);
}
}
function ce(_) {
return _[0] === 0 ? f0([0, _[1], _[2]]) : me([0, _[1], _[2]]);
}
function ge(_) {
var k = _[2], I = k[2], U = k[1], Y = S(I ? I[1] : U), y0 = [0, [0, _Wr, S(U)], [0, [0, mWr, Y], 0]];
return w(yWr, _[1], 0, y0);
}
function H0(_) {
var k = _[2];
if (k[1])
var I = k[2], U = TWr;
else
var I = k[2], U = OWr;
return w(U, _[1], 0, [0, [0, IWr, sn(I)], 0]);
}
function Fr(_) {
var k = _[2], I = k[1];
if (I)
var U = [0, [0, AWr, xr(I[1])], 0], Y = NWr;
else
var U = 0, Y = CWr;
return w(Y, _[1], k[2], U);
}
return [0, A, xr];
}
function T(E) {
return y(E)[1];
}
return [0, T, function(E) {
return y(E)[2];
}, s];
}(jne);
function ab(t, n4, e) {
var i = n4[e];
return Bp(i) ? i | 0 : t;
}
function Gne(t, n4) {
var e = qV(n4, eK) ? {} : n4, i = M7(t), x = ab(Bv[5], e, Vre), c = ab(Bv[4], e, zre), s = ab(Bv[3], e, Kre), p = ab(Bv[2], e, Wre), y = [0, [0, ab(Bv[1], e, Jre), p, s, c, x]], T = e.tokens, E = Bp(T), h = E && T | 0, w = e.comments, G = Bp(w) ? w | 0 : 1, A = e.all_comments, S = Bp(A) ? A | 0 : 1, M = [0, 0], K = h && [0, function(b0) {
return M[1] = [0, b0, M[1]], 0;
}], V = [0, y], f0 = [0, K], m0 = oz ? oz[1] : 1, k0 = f0 && f0[1], g0 = V && V[1], e0 = [0, g0], x0 = [0, k0], l = 0, c0 = x0 && x0[1], t0 = e0 && e0[1], a0 = une([0, c0], [0, t0], l, i), w0 = u(se[1], a0), _0 = de(a0[1][1]), E0 = [0, GL[1], 0], X0 = de(be(function(b0, O0) {
var q0 = b0[2], er = b0[1];
return a(GL[3], O0, er) ? [0, er, q0] : [0, a(GL[4], O0, er), [0, O0, q0]];
}, E0, _0)[2]);
if (X0 && m0)
throw [0, Vee, X0[1], X0[2]];
Qe0[1] = 0;
for (var b = nn(i) - 0 | 0, G0 = i, X = 0, s0 = 0; ; ) {
if (s0 === b)
var dr = X;
else {
var Ar = Hu(G0, s0), ar = 0;
if (0 <= Ar && !(zn < Ar))
var W0 = 1;
else
ar = 1;
if (ar) {
var Lr = 0;
if (iI <= Ar && !(d8 < Ar))
var W0 = 2;
else
Lr = 1;
if (Lr) {
var Tr = 0;
if (dv <= Ar && !(f6 < Ar))
var W0 = 3;
else
Tr = 1;
if (Tr) {
var Hr = 0;
if (v1 <= Ar && !(l8 < Ar))
var W0 = 4;
else
Hr = 1;
if (Hr)
var W0 = 0;
}
}
}
if (W0 === 0) {
var X = TL(X, s0, 0), s0 = s0 + 1 | 0;
continue;
}
if (!((b - s0 | 0) < W0)) {
var Or = W0 - 1 | 0, xr = s0 + W0 | 0;
if (3 < Or >>> 0)
throw [0, wn, bo0];
switch (Or) {
case 0:
var Rr = Hu(G0, s0);
break;
case 1:
var Rr = (Hu(G0, s0) & 31) << 6 | Hu(G0, s0 + 1 | 0) & 63;
break;
case 2:
var Rr = (Hu(G0, s0) & 15) << 12 | (Hu(G0, s0 + 1 | 0) & 63) << 6 | Hu(G0, s0 + 2 | 0) & 63;
break;
default:
var Rr = (Hu(G0, s0) & 7) << 18 | (Hu(G0, s0 + 1 | 0) & 63) << 12 | (Hu(G0, s0 + 2 | 0) & 63) << 6 | Hu(G0, s0 + 3 | 0) & 63;
}
var X = TL(X, s0, [0, Rr]), s0 = xr;
continue;
}
var dr = TL(X, s0, 0);
}
for (var Wr = yGr, Jr = de([0, 6, dr]); ; ) {
var or = Wr[3], _r = Wr[2], Ir = Wr[1];
if (Jr) {
var fe = Jr[1];
if (fe === 5) {
var v0 = Jr[2];
if (v0 && v0[1] === 6) {
var P = _l(de([0, Ir, _r])), Wr = [0, Ir + 2 | 0, 0, [0, P, or]], Jr = v0[2];
continue;
}
} else if (!(6 <= fe)) {
var L = Jr[2], Wr = [0, Ir + Te0(fe) | 0, [0, Ir, _r], or], Jr = L;
continue;
}
var Q = _l(de([0, Ir, _r])), i0 = Jr[2], Wr = [0, Ir + Te0(fe) | 0, 0, [0, Q, or]], Jr = i0;
continue;
}
var l0 = _l(de(or));
if (G)
var T0 = w0;
else
var S0 = u(Uee[1], 0), T0 = a(Ze(S0, -201766268, 25), S0, w0);
if (S)
var R0 = T0;
else
var rr = T0[2], R0 = [0, T0[1], [0, rr[1], rr[2], 0]];
var B = a(nn0[1], [0, l0], R0), Z = un(X0, Qe0[1]);
if (B.errors = u(nn0[3], Z), h) {
var p0 = M[1];
B.tokens = yu(Tp(u(Rne[1], l0), p0));
}
return B;
}
}
}
if (typeof A0 < "u")
var tn0 = A0;
else {
var un0 = {};
qN.flow = un0;
var tn0 = un0;
}
tn0.parse = function(t, n4) {
try {
var e = Gne(t, n4);
return e;
} catch (i) {
return i = Et(i), i[1] === UN ? u(nK, i[2]) : u(nK, new Lee(sn(Te($re, Pp(i)))));
}
}, xN(0);
}(globalThis);
} });
Lt();
var Fae = Hu0(), Tae = lae(), Oae = bae(), Iae = kae(), Aae = { comments: false, enums: true, esproposal_decorators: true, esproposal_export_star_as: true, tokens: true };
function Nae(A0) {
let { message: j0, loc: { start: ur, end: hr } } = A0;
return Fae(j0, { start: { line: ur.line, column: ur.column + 1 }, end: { line: hr.line, column: hr.column + 1 } });
}
function Cae(A0, j0) {
let ur = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, { parse: hr } = gae(), le = hr(Oae(A0), Aae), [Ve] = le.errors;
if (Ve)
throw Nae(Ve);
return ur.originalText = A0, Iae(le, ur);
}
a70.exports = { parsers: { flow: Tae(Cae) } };
});
return Pae();
});
}
});
// node_modules/prettier/parser-typescript.js
var require_parser_typescript = __commonJS({
"node_modules/prettier/parser-typescript.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.typescript = e();
}
})(function() {
"use strict";
var dt = (a, _) => () => (_ || a((_ = { exports: {} }).exports, _), _.exports);
var Mi = dt((dH, J7) => {
var Yh = function(a) {
return a && a.Math == Math && a;
};
J7.exports = Yh(typeof globalThis == "object" && globalThis) || Yh(typeof window == "object" && window) || Yh(typeof self == "object" && self) || Yh(typeof global == "object" && global) || function() {
return this;
}() || Function("return this")();
});
var Ha = dt((mH, F7) => {
F7.exports = function(a) {
try {
return !!a();
} catch {
return true;
}
};
});
var As = dt((hH, B7) => {
var tq = Ha();
B7.exports = !tq(function() {
return Object.defineProperty({}, 1, { get: function() {
return 7;
} })[1] != 7;
});
});
var p6 = dt((gH, q7) => {
var rq = Ha();
q7.exports = !rq(function() {
var a = function() {
}.bind();
return typeof a != "function" || a.hasOwnProperty("prototype");
});
});
var Zh = dt((yH, U7) => {
var nq = p6(), Qh = Function.prototype.call;
U7.exports = nq ? Qh.bind(Qh) : function() {
return Qh.apply(Qh, arguments);
};
});
var H7 = dt((V7) => {
"use strict";
var z7 = {}.propertyIsEnumerable, W7 = Object.getOwnPropertyDescriptor, iq = W7 && !z7.call({ 1: 2 }, 1);
V7.f = iq ? function(_) {
var v = W7(this, _);
return !!v && v.enumerable;
} : z7;
});
var f6 = dt((bH, G7) => {
G7.exports = function(a, _) {
return { enumerable: !(a & 1), configurable: !(a & 2), writable: !(a & 4), value: _ };
};
});
var Ps = dt((TH, X7) => {
var $7 = p6(), K7 = Function.prototype, d6 = K7.call, aq = $7 && K7.bind.bind(d6, d6);
X7.exports = $7 ? aq : function(a) {
return function() {
return d6.apply(a, arguments);
};
};
});
var Z7 = dt((SH, Q7) => {
var Y7 = Ps(), sq = Y7({}.toString), oq = Y7("".slice);
Q7.exports = function(a) {
return oq(sq(a), 8, -1);
};
});
var tw = dt((xH, ew) => {
var _q = Ps(), cq = Ha(), lq = Z7(), m6 = Object, uq = _q("".split);
ew.exports = cq(function() {
return !m6("z").propertyIsEnumerable(0);
}) ? function(a) {
return lq(a) == "String" ? uq(a, "") : m6(a);
} : m6;
});
var h6 = dt((EH, rw) => {
rw.exports = function(a) {
return a == null;
};
});
var g6 = dt((wH, nw) => {
var pq = h6(), fq = TypeError;
nw.exports = function(a) {
if (pq(a))
throw fq("Can't call method on " + a);
return a;
};
});
var e1 = dt((CH, iw) => {
var dq = tw(), mq = g6();
iw.exports = function(a) {
return dq(mq(a));
};
});
var v6 = dt((AH, aw) => {
var y6 = typeof document == "object" && document.all, hq = typeof y6 > "u" && y6 !== void 0;
aw.exports = { all: y6, IS_HTMLDDA: hq };
});
var aa = dt((PH, ow) => {
var sw = v6(), gq = sw.all;
ow.exports = sw.IS_HTMLDDA ? function(a) {
return typeof a == "function" || a === gq;
} : function(a) {
return typeof a == "function";
};
});
var Jc = dt((DH, lw) => {
var _w = aa(), cw = v6(), yq = cw.all;
lw.exports = cw.IS_HTMLDDA ? function(a) {
return typeof a == "object" ? a !== null : _w(a) || a === yq;
} : function(a) {
return typeof a == "object" ? a !== null : _w(a);
};
});
var t1 = dt((kH, uw) => {
var b6 = Mi(), vq = aa(), bq = function(a) {
return vq(a) ? a : void 0;
};
uw.exports = function(a, _) {
return arguments.length < 2 ? bq(b6[a]) : b6[a] && b6[a][_];
};
});
var fw = dt((IH, pw) => {
var Tq = Ps();
pw.exports = Tq({}.isPrototypeOf);
});
var mw = dt((NH, dw) => {
var Sq = t1();
dw.exports = Sq("navigator", "userAgent") || "";
});
var Sw = dt((OH, Tw) => {
var bw = Mi(), T6 = mw(), hw = bw.process, gw = bw.Deno, yw = hw && hw.versions || gw && gw.version, vw = yw && yw.v8, sa, r1;
vw && (sa = vw.split("."), r1 = sa[0] > 0 && sa[0] < 4 ? 1 : +(sa[0] + sa[1]));
!r1 && T6 && (sa = T6.match(/Edge\/(\d+)/), (!sa || sa[1] >= 74) && (sa = T6.match(/Chrome\/(\d+)/), sa && (r1 = +sa[1])));
Tw.exports = r1;
});
var S6 = dt((MH, Ew) => {
var xw = Sw(), xq = Ha();
Ew.exports = !!Object.getOwnPropertySymbols && !xq(function() {
var a = Symbol();
return !String(a) || !(Object(a) instanceof Symbol) || !Symbol.sham && xw && xw < 41;
});
});
var x6 = dt((LH, ww) => {
var Eq = S6();
ww.exports = Eq && !Symbol.sham && typeof Symbol.iterator == "symbol";
});
var E6 = dt((RH, Cw) => {
var wq = t1(), Cq = aa(), Aq = fw(), Pq = x6(), Dq = Object;
Cw.exports = Pq ? function(a) {
return typeof a == "symbol";
} : function(a) {
var _ = wq("Symbol");
return Cq(_) && Aq(_.prototype, Dq(a));
};
});
var Pw = dt((jH, Aw) => {
var kq = String;
Aw.exports = function(a) {
try {
return kq(a);
} catch {
return "Object";
}
};
});
var kw = dt((JH, Dw) => {
var Iq = aa(), Nq = Pw(), Oq = TypeError;
Dw.exports = function(a) {
if (Iq(a))
return a;
throw Oq(Nq(a) + " is not a function");
};
});
var Nw = dt((FH, Iw) => {
var Mq = kw(), Lq = h6();
Iw.exports = function(a, _) {
var v = a[_];
return Lq(v) ? void 0 : Mq(v);
};
});
var Mw = dt((BH, Ow) => {
var w6 = Zh(), C6 = aa(), A6 = Jc(), Rq = TypeError;
Ow.exports = function(a, _) {
var v, h;
if (_ === "string" && C6(v = a.toString) && !A6(h = w6(v, a)) || C6(v = a.valueOf) && !A6(h = w6(v, a)) || _ !== "string" && C6(v = a.toString) && !A6(h = w6(v, a)))
return h;
throw Rq("Can't convert object to primitive value");
};
});
var Rw = dt((qH, Lw) => {
Lw.exports = false;
});
var n1 = dt((UH, Jw) => {
var jw = Mi(), jq = Object.defineProperty;
Jw.exports = function(a, _) {
try {
jq(jw, a, { value: _, configurable: true, writable: true });
} catch {
jw[a] = _;
}
return _;
};
});
var i1 = dt((zH, Bw) => {
var Jq = Mi(), Fq = n1(), Fw = "__core-js_shared__", Bq = Jq[Fw] || Fq(Fw, {});
Bw.exports = Bq;
});
var P6 = dt((WH, Uw) => {
var qq = Rw(), qw = i1();
(Uw.exports = function(a, _) {
return qw[a] || (qw[a] = _ !== void 0 ? _ : {});
})("versions", []).push({ version: "3.26.1", mode: qq ? "pure" : "global", copyright: "\xA9 2014-2022 Denis Pushkarev (zloirock.ru)", license: "https://github.com/zloirock/core-js/blob/v3.26.1/LICENSE", source: "https://github.com/zloirock/core-js" });
});
var Ww = dt((VH, zw) => {
var Uq = g6(), zq = Object;
zw.exports = function(a) {
return zq(Uq(a));
};
});
var oo = dt((HH, Vw) => {
var Wq = Ps(), Vq = Ww(), Hq = Wq({}.hasOwnProperty);
Vw.exports = Object.hasOwn || function(_, v) {
return Hq(Vq(_), v);
};
});
var D6 = dt((GH, Hw) => {
var Gq = Ps(), $q = 0, Kq = Math.random(), Xq = Gq(1 .toString);
Hw.exports = function(a) {
return "Symbol(" + (a === void 0 ? "" : a) + ")_" + Xq(++$q + Kq, 36);
};
});
var Qw = dt(($H, Yw) => {
var Yq = Mi(), Qq = P6(), Gw = oo(), Zq = D6(), $w = S6(), Xw = x6(), Fc = Qq("wks"), p_ = Yq.Symbol, Kw = p_ && p_.for, eU = Xw ? p_ : p_ && p_.withoutSetter || Zq;
Yw.exports = function(a) {
if (!Gw(Fc, a) || !($w || typeof Fc[a] == "string")) {
var _ = "Symbol." + a;
$w && Gw(p_, a) ? Fc[a] = p_[a] : Xw && Kw ? Fc[a] = Kw(_) : Fc[a] = eU(_);
}
return Fc[a];
};
});
var rC = dt((KH, tC) => {
var tU = Zh(), Zw = Jc(), eC = E6(), rU = Nw(), nU = Mw(), iU = Qw(), aU = TypeError, sU = iU("toPrimitive");
tC.exports = function(a, _) {
if (!Zw(a) || eC(a))
return a;
var v = rU(a, sU), h;
if (v) {
if (_ === void 0 && (_ = "default"), h = tU(v, a, _), !Zw(h) || eC(h))
return h;
throw aU("Can't convert object to primitive value");
}
return _ === void 0 && (_ = "number"), nU(a, _);
};
});
var k6 = dt((XH, nC) => {
var oU = rC(), _U = E6();
nC.exports = function(a) {
var _ = oU(a, "string");
return _U(_) ? _ : _ + "";
};
});
var sC = dt((YH, aC) => {
var cU = Mi(), iC = Jc(), I6 = cU.document, lU = iC(I6) && iC(I6.createElement);
aC.exports = function(a) {
return lU ? I6.createElement(a) : {};
};
});
var N6 = dt((QH, oC) => {
var uU = As(), pU = Ha(), fU = sC();
oC.exports = !uU && !pU(function() {
return Object.defineProperty(fU("div"), "a", { get: function() {
return 7;
} }).a != 7;
});
});
var O6 = dt((cC) => {
var dU = As(), mU = Zh(), hU = H7(), gU = f6(), yU = e1(), vU = k6(), bU = oo(), TU = N6(), _C = Object.getOwnPropertyDescriptor;
cC.f = dU ? _C : function(_, v) {
if (_ = yU(_), v = vU(v), TU)
try {
return _C(_, v);
} catch {
}
if (bU(_, v))
return gU(!mU(hU.f, _, v), _[v]);
};
});
var uC = dt((eG, lC) => {
var SU = As(), xU = Ha();
lC.exports = SU && xU(function() {
return Object.defineProperty(function() {
}, "prototype", { value: 42, writable: false }).prototype != 42;
});
});
var a1 = dt((tG, pC) => {
var EU = Jc(), wU = String, CU = TypeError;
pC.exports = function(a) {
if (EU(a))
return a;
throw CU(wU(a) + " is not an object");
};
});
var dp = dt((dC) => {
var AU = As(), PU = N6(), DU = uC(), s1 = a1(), fC = k6(), kU = TypeError, M6 = Object.defineProperty, IU = Object.getOwnPropertyDescriptor, L6 = "enumerable", R6 = "configurable", j6 = "writable";
dC.f = AU ? DU ? function(_, v, h) {
if (s1(_), v = fC(v), s1(h), typeof _ == "function" && v === "prototype" && "value" in h && j6 in h && !h[j6]) {
var D = IU(_, v);
D && D[j6] && (_[v] = h.value, h = { configurable: R6 in h ? h[R6] : D[R6], enumerable: L6 in h ? h[L6] : D[L6], writable: false });
}
return M6(_, v, h);
} : M6 : function(_, v, h) {
if (s1(_), v = fC(v), s1(h), PU)
try {
return M6(_, v, h);
} catch {
}
if ("get" in h || "set" in h)
throw kU("Accessors not supported");
return "value" in h && (_[v] = h.value), _;
};
});
var J6 = dt((nG, mC) => {
var NU = As(), OU = dp(), MU = f6();
mC.exports = NU ? function(a, _, v) {
return OU.f(a, _, MU(1, v));
} : function(a, _, v) {
return a[_] = v, a;
};
});
var yC = dt((iG, gC) => {
var F6 = As(), LU = oo(), hC = Function.prototype, RU = F6 && Object.getOwnPropertyDescriptor, B6 = LU(hC, "name"), jU = B6 && function() {
}.name === "something", JU = B6 && (!F6 || F6 && RU(hC, "name").configurable);
gC.exports = { EXISTS: B6, PROPER: jU, CONFIGURABLE: JU };
});
var bC = dt((aG, vC) => {
var FU = Ps(), BU = aa(), q6 = i1(), qU = FU(Function.toString);
BU(q6.inspectSource) || (q6.inspectSource = function(a) {
return qU(a);
});
vC.exports = q6.inspectSource;
});
var xC = dt((sG, SC) => {
var UU = Mi(), zU = aa(), TC = UU.WeakMap;
SC.exports = zU(TC) && /native code/.test(String(TC));
});
var CC = dt((oG, wC) => {
var WU = P6(), VU = D6(), EC = WU("keys");
wC.exports = function(a) {
return EC[a] || (EC[a] = VU(a));
};
});
var U6 = dt((_G, AC) => {
AC.exports = {};
});
var IC = dt((cG, kC) => {
var HU = xC(), DC = Mi(), GU = Jc(), $U = J6(), z6 = oo(), W6 = i1(), KU = CC(), XU = U6(), PC = "Object already initialized", V6 = DC.TypeError, YU = DC.WeakMap, o1, mp, _1, QU = function(a) {
return _1(a) ? mp(a) : o1(a, {});
}, ZU = function(a) {
return function(_) {
var v;
if (!GU(_) || (v = mp(_)).type !== a)
throw V6("Incompatible receiver, " + a + " required");
return v;
};
};
HU || W6.state ? (oa = W6.state || (W6.state = new YU()), oa.get = oa.get, oa.has = oa.has, oa.set = oa.set, o1 = function(a, _) {
if (oa.has(a))
throw V6(PC);
return _.facade = a, oa.set(a, _), _;
}, mp = function(a) {
return oa.get(a) || {};
}, _1 = function(a) {
return oa.has(a);
}) : (f_ = KU("state"), XU[f_] = true, o1 = function(a, _) {
if (z6(a, f_))
throw V6(PC);
return _.facade = a, $U(a, f_, _), _;
}, mp = function(a) {
return z6(a, f_) ? a[f_] : {};
}, _1 = function(a) {
return z6(a, f_);
});
var oa, f_;
kC.exports = { set: o1, get: mp, has: _1, enforce: QU, getterFor: ZU };
});
var G6 = dt((lG, OC) => {
var ez = Ha(), tz = aa(), c1 = oo(), H6 = As(), rz = yC().CONFIGURABLE, nz = bC(), NC = IC(), iz = NC.enforce, az = NC.get, l1 = Object.defineProperty, sz = H6 && !ez(function() {
return l1(function() {
}, "length", { value: 8 }).length !== 8;
}), oz = String(String).split("String"), _z = OC.exports = function(a, _, v) {
String(_).slice(0, 7) === "Symbol(" && (_ = "[" + String(_).replace(/^Symbol\(([^)]*)\)/, "$1") + "]"), v && v.getter && (_ = "get " + _), v && v.setter && (_ = "set " + _), (!c1(a, "name") || rz && a.name !== _) && (H6 ? l1(a, "name", { value: _, configurable: true }) : a.name = _), sz && v && c1(v, "arity") && a.length !== v.arity && l1(a, "length", { value: v.arity });
try {
v && c1(v, "constructor") && v.constructor ? H6 && l1(a, "prototype", { writable: false }) : a.prototype && (a.prototype = void 0);
} catch {
}
var h = iz(a);
return c1(h, "source") || (h.source = oz.join(typeof _ == "string" ? _ : "")), a;
};
Function.prototype.toString = _z(function() {
return tz(this) && az(this).source || nz(this);
}, "toString");
});
var LC = dt((uG, MC) => {
var cz = aa(), lz = dp(), uz = G6(), pz = n1();
MC.exports = function(a, _, v, h) {
h || (h = {});
var D = h.enumerable, P = h.name !== void 0 ? h.name : _;
if (cz(v) && uz(v, P, h), h.global)
D ? a[_] = v : pz(_, v);
else {
try {
h.unsafe ? a[_] && (D = true) : delete a[_];
} catch {
}
D ? a[_] = v : lz.f(a, _, { value: v, enumerable: false, configurable: !h.nonConfigurable, writable: !h.nonWritable });
}
return a;
};
});
var jC = dt((pG, RC) => {
var fz = Math.ceil, dz = Math.floor;
RC.exports = Math.trunc || function(_) {
var v = +_;
return (v > 0 ? dz : fz)(v);
};
});
var $6 = dt((fG, JC) => {
var mz = jC();
JC.exports = function(a) {
var _ = +a;
return _ !== _ || _ === 0 ? 0 : mz(_);
};
});
var BC = dt((dG, FC) => {
var hz = $6(), gz = Math.max, yz = Math.min;
FC.exports = function(a, _) {
var v = hz(a);
return v < 0 ? gz(v + _, 0) : yz(v, _);
};
});
var UC = dt((mG, qC) => {
var vz = $6(), bz = Math.min;
qC.exports = function(a) {
return a > 0 ? bz(vz(a), 9007199254740991) : 0;
};
});
var WC = dt((hG, zC) => {
var Tz = UC();
zC.exports = function(a) {
return Tz(a.length);
};
});
var GC = dt((gG, HC) => {
var Sz = e1(), xz = BC(), Ez = WC(), VC = function(a) {
return function(_, v, h) {
var D = Sz(_), P = Ez(D), y = xz(h, P), m;
if (a && v != v) {
for (; P > y; )
if (m = D[y++], m != m)
return true;
} else
for (; P > y; y++)
if ((a || y in D) && D[y] === v)
return a || y || 0;
return !a && -1;
};
};
HC.exports = { includes: VC(true), indexOf: VC(false) };
});
var XC = dt((yG, KC) => {
var wz = Ps(), K6 = oo(), Cz = e1(), Az = GC().indexOf, Pz = U6(), $C = wz([].push);
KC.exports = function(a, _) {
var v = Cz(a), h = 0, D = [], P;
for (P in v)
!K6(Pz, P) && K6(v, P) && $C(D, P);
for (; _.length > h; )
K6(v, P = _[h++]) && (~Az(D, P) || $C(D, P));
return D;
};
});
var QC = dt((vG, YC) => {
YC.exports = ["constructor", "hasOwnProperty", "isPrototypeOf", "propertyIsEnumerable", "toLocaleString", "toString", "valueOf"];
});
var e9 = dt((ZC) => {
var Dz = XC(), kz = QC(), Iz = kz.concat("length", "prototype");
ZC.f = Object.getOwnPropertyNames || function(_) {
return Dz(_, Iz);
};
});
var r9 = dt((t9) => {
t9.f = Object.getOwnPropertySymbols;
});
var i9 = dt((SG, n9) => {
var Nz = t1(), Oz = Ps(), Mz = e9(), Lz = r9(), Rz = a1(), jz = Oz([].concat);
n9.exports = Nz("Reflect", "ownKeys") || function(_) {
var v = Mz.f(Rz(_)), h = Lz.f;
return h ? jz(v, h(_)) : v;
};
});
var o9 = dt((xG, s9) => {
var a9 = oo(), Jz = i9(), Fz = O6(), Bz = dp();
s9.exports = function(a, _, v) {
for (var h = Jz(_), D = Bz.f, P = Fz.f, y = 0; y < h.length; y++) {
var m = h[y];
!a9(a, m) && !(v && a9(v, m)) && D(a, m, P(_, m));
}
};
});
var c9 = dt((EG, _9) => {
var qz = Ha(), Uz = aa(), zz = /#|\.prototype\./, hp = function(a, _) {
var v = Vz[Wz(a)];
return v == Gz ? true : v == Hz ? false : Uz(_) ? qz(_) : !!_;
}, Wz = hp.normalize = function(a) {
return String(a).replace(zz, ".").toLowerCase();
}, Vz = hp.data = {}, Hz = hp.NATIVE = "N", Gz = hp.POLYFILL = "P";
_9.exports = hp;
});
var u9 = dt((wG, l9) => {
var X6 = Mi(), $z = O6().f, Kz = J6(), Xz = LC(), Yz = n1(), Qz = o9(), Zz = c9();
l9.exports = function(a, _) {
var v = a.target, h = a.global, D = a.stat, P, y, m, C, d, E;
if (h ? y = X6 : D ? y = X6[v] || Yz(v, {}) : y = (X6[v] || {}).prototype, y)
for (m in _) {
if (d = _[m], a.dontCallGetSet ? (E = $z(y, m), C = E && E.value) : C = y[m], P = Zz(h ? m : v + (D ? "." : "#") + m, a.forced), !P && C !== void 0) {
if (typeof d == typeof C)
continue;
Qz(d, C);
}
(a.sham || C && C.sham) && Kz(d, "sham", true), Xz(y, m, d, a);
}
};
});
var p9 = dt(() => {
var eW = u9(), Y6 = Mi();
eW({ global: true, forced: Y6.globalThis !== Y6 }, { globalThis: Y6 });
});
var f9 = dt(() => {
p9();
});
var h9 = dt((kG, m9) => {
var d9 = G6(), tW = dp();
m9.exports = function(a, _, v) {
return v.get && d9(v.get, _, { getter: true }), v.set && d9(v.set, _, { setter: true }), tW.f(a, _, v);
};
});
var y9 = dt((IG, g9) => {
"use strict";
var rW = a1();
g9.exports = function() {
var a = rW(this), _ = "";
return a.hasIndices && (_ += "d"), a.global && (_ += "g"), a.ignoreCase && (_ += "i"), a.multiline && (_ += "m"), a.dotAll && (_ += "s"), a.unicode && (_ += "u"), a.unicodeSets && (_ += "v"), a.sticky && (_ += "y"), _;
};
});
var T9 = dt(() => {
var nW = Mi(), iW = As(), aW = h9(), sW = y9(), oW = Ha(), v9 = nW.RegExp, b9 = v9.prototype, _W = iW && oW(function() {
var a = true;
try {
v9(".", "d");
} catch {
a = false;
}
var _ = {}, v = "", h = a ? "dgimsy" : "gimsy", D = function(C, d) {
Object.defineProperty(_, C, { get: function() {
return v += d, true;
} });
}, P = { dotAll: "s", global: "g", ignoreCase: "i", multiline: "m", sticky: "y" };
a && (P.hasIndices = "d");
for (var y in P)
D(y, P[y]);
var m = Object.getOwnPropertyDescriptor(b9, "flags").get.call(_);
return m !== h || v !== h;
});
_W && aW(b9, "flags", { configurable: true, get: sW });
});
var uH = dt((MG, v5) => {
f9();
T9();
var iT = Object.defineProperty, cW = Object.getOwnPropertyDescriptor, aT = Object.getOwnPropertyNames, lW = Object.prototype.hasOwnProperty, yp = (a, _) => function() {
return a && (_ = (0, a[aT(a)[0]])(a = 0)), _;
}, Oe = (a, _) => function() {
return _ || (0, a[aT(a)[0]])((_ = { exports: {} }).exports, _), _.exports;
}, m1 = (a, _) => {
for (var v in _)
iT(a, v, { get: _[v], enumerable: true });
}, uW = (a, _, v, h) => {
if (_ && typeof _ == "object" || typeof _ == "function")
for (let D of aT(_))
!lW.call(a, D) && D !== v && iT(a, D, { get: () => _[D], enumerable: !(h = cW(_, D)) || h.enumerable });
return a;
}, Li = (a) => uW(iT({}, "__esModule", { value: true }), a), cn, De = yp({ ""() {
cn = { env: {}, argv: [] };
} }), w9 = Oe({ "src/common/parser-create-error.js"(a, _) {
"use strict";
De();
function v(h, D) {
let P = new SyntaxError(h + " (" + D.start.line + ":" + D.start.column + ")");
return P.loc = D, P;
}
_.exports = v;
} }), pW = Oe({ "src/utils/try-combinations.js"(a, _) {
"use strict";
De();
function v() {
let h;
for (var D = arguments.length, P = new Array(D), y = 0; y < D; y++)
P[y] = arguments[y];
for (let [m, C] of P.entries())
try {
return { result: C() };
} catch (d) {
m === 0 && (h = d);
}
return { error: h };
}
_.exports = v;
} }), C9 = {};
m1(C9, { EOL: () => eT, arch: () => fW, cpus: () => O9, default: () => J9, endianness: () => A9, freemem: () => I9, getNetworkInterfaces: () => j9, hostname: () => P9, loadavg: () => D9, networkInterfaces: () => R9, platform: () => dW, release: () => L9, tmpDir: () => Q6, tmpdir: () => Z6, totalmem: () => N9, type: () => M9, uptime: () => k9 });
function A9() {
if (typeof u1 > "u") {
var a = new ArrayBuffer(2), _ = new Uint8Array(a), v = new Uint16Array(a);
if (_[0] = 1, _[1] = 2, v[0] === 258)
u1 = "BE";
else if (v[0] === 513)
u1 = "LE";
else
throw new Error("unable to figure out endianess");
}
return u1;
}
function P9() {
return typeof globalThis.location < "u" ? globalThis.location.hostname : "";
}
function D9() {
return [];
}
function k9() {
return 0;
}
function I9() {
return Number.MAX_VALUE;
}
function N9() {
return Number.MAX_VALUE;
}
function O9() {
return [];
}
function M9() {
return "Browser";
}
function L9() {
return typeof globalThis.navigator < "u" ? globalThis.navigator.appVersion : "";
}
function R9() {
}
function j9() {
}
function fW() {
return "javascript";
}
function dW() {
return "browser";
}
function Q6() {
return "/tmp";
}
var u1, Z6, eT, J9, mW = yp({ "node-modules-polyfills:os"() {
De(), Z6 = Q6, eT = `
`, J9 = { EOL: eT, tmpdir: Z6, tmpDir: Q6, networkInterfaces: R9, getNetworkInterfaces: j9, release: L9, type: M9, cpus: O9, totalmem: N9, freemem: I9, uptime: k9, loadavg: D9, hostname: P9, endianness: A9 };
} }), hW = Oe({ "node-modules-polyfills-commonjs:os"(a, _) {
De();
var v = (mW(), Li(C9));
if (v && v.default) {
_.exports = v.default;
for (let h in v)
_.exports[h] = v[h];
} else
v && (_.exports = v);
} }), gW = Oe({ "node_modules/detect-newline/index.js"(a, _) {
"use strict";
De();
var v = (h) => {
if (typeof h != "string")
throw new TypeError("Expected a string");
let D = h.match(/(?:\r?\n)/g) || [];
if (D.length === 0)
return;
let P = D.filter((m) => m === `\r
`).length, y = D.length - P;
return P > y ? `\r
` : `
`;
};
_.exports = v, _.exports.graceful = (h) => typeof h == "string" && v(h) || `
`;
} }), yW = Oe({ "node_modules/jest-docblock/build/index.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.extract = M, a.parse = W, a.parseWithComments = K, a.print = ce, a.strip = q;
function _() {
let me = hW();
return _ = function() {
return me;
}, me;
}
function v() {
let me = h(gW());
return v = function() {
return me;
}, me;
}
function h(me) {
return me && me.__esModule ? me : { default: me };
}
var D = /\*\/$/, P = /^\/\*\*?/, y = /^\s*(\/\*\*?(.|\r?\n)*?\*\/)/, m = /(^|\s+)\/\/([^\r\n]*)/g, C = /^(\r?\n)+/, d = /(?:^|\r?\n) *(@[^\r\n]*?) *\r?\n *(?![^@\r\n]*\/\/[^]*)([^@\r\n\s][^@\r\n]+?) *\r?\n/g, E = /(?:^|\r?\n) *@(\S+) *([^\r\n]*)/g, I = /(\r?\n|^) *\* ?/g, c = [];
function M(me) {
let Ae = me.match(y);
return Ae ? Ae[0].trimLeft() : "";
}
function q(me) {
let Ae = me.match(y);
return Ae && Ae[0] ? me.substring(Ae[0].length) : me;
}
function W(me) {
return K(me).pragmas;
}
function K(me) {
let Ae = (0, v().default)(me) || _().EOL;
me = me.replace(P, "").replace(D, "").replace(I, "$1");
let te = "";
for (; te !== me; )
te = me, me = me.replace(d, `${Ae}$1 $2${Ae}`);
me = me.replace(C, "").trimRight();
let he = /* @__PURE__ */ Object.create(null), Pe = me.replace(E, "").replace(C, "").trimRight(), R;
for (; R = E.exec(me); ) {
let pe = R[2].replace(m, "");
typeof he[R[1]] == "string" || Array.isArray(he[R[1]]) ? he[R[1]] = c.concat(he[R[1]], pe) : he[R[1]] = pe;
}
return { comments: Pe, pragmas: he };
}
function ce(me) {
let { comments: Ae = "", pragmas: te = {} } = me, he = (0, v().default)(Ae) || _().EOL, Pe = "/**", R = " *", pe = " */", ke = Object.keys(te), Je = ke.map((ee) => Ie(ee, te[ee])).reduce((ee, je) => ee.concat(je), []).map((ee) => `${R} ${ee}${he}`).join("");
if (!Ae) {
if (ke.length === 0)
return "";
if (ke.length === 1 && !Array.isArray(te[ke[0]])) {
let ee = te[ke[0]];
return `${Pe} ${Ie(ke[0], ee)[0]}${pe}`;
}
}
let Xe = Ae.split(he).map((ee) => `${R} ${ee}`).join(he) + he;
return Pe + he + (Ae ? Xe : "") + (Ae && ke.length ? R + he : "") + Je + pe;
}
function Ie(me, Ae) {
return c.concat(Ae).map((te) => `@${me} ${te}`.trim());
}
} }), vW = Oe({ "src/common/end-of-line.js"(a, _) {
"use strict";
De();
function v(y) {
let m = y.indexOf("\r");
return m >= 0 ? y.charAt(m + 1) === `
` ? "crlf" : "cr" : "lf";
}
function h(y) {
switch (y) {
case "cr":
return "\r";
case "crlf":
return `\r
`;
default:
return `
`;
}
}
function D(y, m) {
let C;
switch (m) {
case `
`:
C = /\n/g;
break;
case "\r":
C = /\r/g;
break;
case `\r
`:
C = /\r\n/g;
break;
default:
throw new Error(`Unexpected "eol" ${JSON.stringify(m)}.`);
}
let d = y.match(C);
return d ? d.length : 0;
}
function P(y) {
return y.replace(/\r\n?/g, `
`);
}
_.exports = { guessEndOfLine: v, convertEndOfLineToChars: h, countEndOfLineChars: D, normalizeEndOfLine: P };
} }), bW = Oe({ "src/language-js/utils/get-shebang.js"(a, _) {
"use strict";
De();
function v(h) {
if (!h.startsWith("#!"))
return "";
let D = h.indexOf(`
`);
return D === -1 ? h : h.slice(0, D);
}
_.exports = v;
} }), TW = Oe({ "src/language-js/pragma.js"(a, _) {
"use strict";
De();
var { parseWithComments: v, strip: h, extract: D, print: P } = yW(), { normalizeEndOfLine: y } = vW(), m = bW();
function C(I) {
let c = m(I);
c && (I = I.slice(c.length + 1));
let M = D(I), { pragmas: q, comments: W } = v(M);
return { shebang: c, text: I, pragmas: q, comments: W };
}
function d(I) {
let c = Object.keys(C(I).pragmas);
return c.includes("prettier") || c.includes("format");
}
function E(I) {
let { shebang: c, text: M, pragmas: q, comments: W } = C(I), K = h(M), ce = P({ pragmas: Object.assign({ format: "" }, q), comments: W.trimStart() });
return (c ? `${c}
` : "") + y(ce) + (K.startsWith(`
`) ? `
` : `
`) + K;
}
_.exports = { hasPragma: d, insertPragma: E };
} }), F9 = Oe({ "src/utils/is-non-empty-array.js"(a, _) {
"use strict";
De();
function v(h) {
return Array.isArray(h) && h.length > 0;
}
_.exports = v;
} }), B9 = Oe({ "src/language-js/loc.js"(a, _) {
"use strict";
De();
var v = F9();
function h(C) {
var d, E;
let I = C.range ? C.range[0] : C.start, c = (d = (E = C.declaration) === null || E === void 0 ? void 0 : E.decorators) !== null && d !== void 0 ? d : C.decorators;
return v(c) ? Math.min(h(c[0]), I) : I;
}
function D(C) {
return C.range ? C.range[1] : C.end;
}
function P(C, d) {
let E = h(C);
return Number.isInteger(E) && E === h(d);
}
function y(C, d) {
let E = D(C);
return Number.isInteger(E) && E === D(d);
}
function m(C, d) {
return P(C, d) && y(C, d);
}
_.exports = { locStart: h, locEnd: D, hasSameLocStart: P, hasSameLoc: m };
} }), SW = Oe({ "src/language-js/parse/utils/create-parser.js"(a, _) {
"use strict";
De();
var { hasPragma: v } = TW(), { locStart: h, locEnd: D } = B9();
function P(y) {
return y = typeof y == "function" ? { parse: y } : y, Object.assign({ astFormat: "estree", hasPragma: v, locStart: h, locEnd: D }, y);
}
_.exports = P;
} }), xW = Oe({ "src/language-js/parse/utils/replace-hashbang.js"(a, _) {
"use strict";
De();
function v(h) {
return h.charAt(0) === "#" && h.charAt(1) === "!" ? "//" + h.slice(2) : h;
}
_.exports = v;
} }), EW = Oe({ "src/language-js/utils/is-ts-keyword-type.js"(a, _) {
"use strict";
De();
function v(h) {
let { type: D } = h;
return D.startsWith("TS") && D.endsWith("Keyword");
}
_.exports = v;
} }), wW = Oe({ "src/language-js/utils/is-block-comment.js"(a, _) {
"use strict";
De();
var v = /* @__PURE__ */ new Set(["Block", "CommentBlock", "MultiLine"]), h = (D) => v.has(D == null ? void 0 : D.type);
_.exports = h;
} }), CW = Oe({ "src/language-js/utils/is-type-cast-comment.js"(a, _) {
"use strict";
De();
var v = wW();
function h(D) {
return v(D) && D.value[0] === "*" && /@(?:type|satisfies)\b/.test(D.value);
}
_.exports = h;
} }), AW = Oe({ "src/utils/get-last.js"(a, _) {
"use strict";
De();
var v = (h) => h[h.length - 1];
_.exports = v;
} }), q9 = Oe({ "src/language-js/parse/postprocess/visit-node.js"(a, _) {
"use strict";
De();
function v(h, D) {
if (Array.isArray(h)) {
for (let P = 0; P < h.length; P++)
h[P] = v(h[P], D);
return h;
}
if (h && typeof h == "object" && typeof h.type == "string") {
let P = Object.keys(h);
for (let y = 0; y < P.length; y++)
h[P[y]] = v(h[P[y]], D);
return D(h) || h;
}
return h;
}
_.exports = v;
} }), U9 = Oe({ "src/language-js/parse/postprocess/throw-syntax-error.js"(a, _) {
"use strict";
De();
var v = w9();
function h(D, P) {
let { start: y, end: m } = D.loc;
throw v(P, { start: { line: y.line, column: y.column + 1 }, end: { line: m.line, column: m.column + 1 } });
}
_.exports = h;
} }), PW = Oe({ "src/language-js/parse/postprocess/index.js"(a, _) {
"use strict";
De();
var { locStart: v, locEnd: h } = B9(), D = EW(), P = CW(), y = AW(), m = q9(), C = U9();
function d(M, q) {
if (q.parser !== "typescript" && q.parser !== "flow" && q.parser !== "acorn" && q.parser !== "espree" && q.parser !== "meriyah") {
let K = /* @__PURE__ */ new Set();
M = m(M, (ce) => {
ce.leadingComments && ce.leadingComments.some(P) && K.add(v(ce));
}), M = m(M, (ce) => {
if (ce.type === "ParenthesizedExpression") {
let { expression: Ie } = ce;
if (Ie.type === "TypeCastExpression")
return Ie.range = ce.range, Ie;
let me = v(ce);
if (!K.has(me))
return Ie.extra = Object.assign(Object.assign({}, Ie.extra), {}, { parenthesized: true }), Ie;
}
});
}
return M = m(M, (K) => {
switch (K.type) {
case "ChainExpression":
return E(K.expression);
case "LogicalExpression": {
if (I(K))
return c(K);
break;
}
case "VariableDeclaration": {
let ce = y(K.declarations);
ce && ce.init && W(K, ce);
break;
}
case "TSParenthesizedType":
return D(K.typeAnnotation) || K.typeAnnotation.type === "TSThisType" || (K.typeAnnotation.range = [v(K), h(K)]), K.typeAnnotation;
case "TSTypeParameter":
if (typeof K.name == "string") {
let ce = v(K);
K.name = { type: "Identifier", name: K.name, range: [ce, ce + K.name.length] };
}
break;
case "ObjectExpression":
if (q.parser === "typescript") {
let ce = K.properties.find((Ie) => Ie.type === "Property" && Ie.value.type === "TSEmptyBodyFunctionExpression");
ce && C(ce.value, "Unexpected token.");
}
break;
case "SequenceExpression": {
let ce = y(K.expressions);
K.range = [v(K), Math.min(h(ce), h(K))];
break;
}
case "TopicReference":
q.__isUsingHackPipeline = true;
break;
case "ExportAllDeclaration": {
let { exported: ce } = K;
if (q.parser === "meriyah" && ce && ce.type === "Identifier") {
let Ie = q.originalText.slice(v(ce), h(ce));
(Ie.startsWith('"') || Ie.startsWith("'")) && (K.exported = Object.assign(Object.assign({}, K.exported), {}, { type: "Literal", value: K.exported.name, raw: Ie }));
}
break;
}
case "PropertyDefinition":
if (q.parser === "meriyah" && K.static && !K.computed && !K.key) {
let ce = "static", Ie = v(K);
Object.assign(K, { static: false, key: { type: "Identifier", name: ce, range: [Ie, Ie + ce.length] } });
}
break;
}
}), M;
function W(K, ce) {
q.originalText[h(ce)] !== ";" && (K.range = [v(K), h(ce)]);
}
}
function E(M) {
switch (M.type) {
case "CallExpression":
M.type = "OptionalCallExpression", M.callee = E(M.callee);
break;
case "MemberExpression":
M.type = "OptionalMemberExpression", M.object = E(M.object);
break;
case "TSNonNullExpression":
M.expression = E(M.expression);
break;
}
return M;
}
function I(M) {
return M.type === "LogicalExpression" && M.right.type === "LogicalExpression" && M.operator === M.right.operator;
}
function c(M) {
return I(M) ? c({ type: "LogicalExpression", operator: M.operator, left: c({ type: "LogicalExpression", operator: M.operator, left: M.left, right: M.right.left, range: [v(M.left), h(M.right.left)] }), right: M.right.right, range: [v(M), h(M)] }) : M;
}
_.exports = d;
} }), vr = Oe({ "node_modules/typescript/lib/typescript.js"(a, _) {
De();
var v = Object.defineProperty, h = Object.getOwnPropertyNames, D = (e, t) => function() {
return e && (t = (0, e[h(e)[0]])(e = 0)), t;
}, P = (e, t) => function() {
return t || (0, e[h(e)[0]])((t = { exports: {} }).exports, t), t.exports;
}, y = (e, t) => {
for (var r in t)
v(e, r, { get: t[r], enumerable: true });
}, m, C, d, E = D({ "src/compiler/corePublic.ts"() {
"use strict";
m = "5.0", C = "5.0.2", d = ((e) => (e[e.LessThan = -1] = "LessThan", e[e.EqualTo = 0] = "EqualTo", e[e.GreaterThan = 1] = "GreaterThan", e))(d || {});
} });
function I(e) {
return e ? e.length : 0;
}
function c(e, t) {
if (e)
for (let r = 0; r < e.length; r++) {
let s = t(e[r], r);
if (s)
return s;
}
}
function M(e, t) {
if (e)
for (let r = e.length - 1; r >= 0; r--) {
let s = t(e[r], r);
if (s)
return s;
}
}
function q(e, t) {
if (e !== void 0)
for (let r = 0; r < e.length; r++) {
let s = t(e[r], r);
if (s !== void 0)
return s;
}
}
function W(e, t) {
for (let r of e) {
let s = t(r);
if (s !== void 0)
return s;
}
}
function K(e, t, r) {
let s = r;
if (e) {
let f = 0;
for (let x of e)
s = t(s, x, f), f++;
}
return s;
}
function ce(e, t, r) {
let s = [];
Y.assertEqual(e.length, t.length);
for (let f = 0; f < e.length; f++)
s.push(r(e[f], t[f], f));
return s;
}
function Ie(e, t) {
if (e.length <= 1)
return e;
let r = [];
for (let s = 0, f = e.length; s < f; s++)
s && r.push(t), r.push(e[s]);
return r;
}
function me(e, t) {
if (e) {
for (let r = 0; r < e.length; r++)
if (!t(e[r], r))
return false;
}
return true;
}
function Ae(e, t, r) {
if (e !== void 0)
for (let s = r != null ? r : 0; s < e.length; s++) {
let f = e[s];
if (t(f, s))
return f;
}
}
function te(e, t, r) {
if (e !== void 0)
for (let s = r != null ? r : e.length - 1; s >= 0; s--) {
let f = e[s];
if (t(f, s))
return f;
}
}
function he(e, t, r) {
if (e === void 0)
return -1;
for (let s = r != null ? r : 0; s < e.length; s++)
if (t(e[s], s))
return s;
return -1;
}
function Pe(e, t, r) {
if (e === void 0)
return -1;
for (let s = r != null ? r : e.length - 1; s >= 0; s--)
if (t(e[s], s))
return s;
return -1;
}
function R(e, t) {
for (let r = 0; r < e.length; r++) {
let s = t(e[r], r);
if (s)
return s;
}
return Y.fail();
}
function pe(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : fa;
if (e) {
for (let s of e)
if (r(s, t))
return true;
}
return false;
}
function ke(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : fa;
return e.length === t.length && e.every((s, f) => r(s, t[f]));
}
function Je(e, t, r) {
for (let s = r || 0; s < e.length; s++)
if (pe(t, e.charCodeAt(s)))
return s;
return -1;
}
function Xe(e, t) {
let r = 0;
if (e)
for (let s = 0; s < e.length; s++) {
let f = e[s];
t(f, s) && r++;
}
return r;
}
function ee(e, t) {
if (e) {
let r = e.length, s = 0;
for (; s < r && t(e[s]); )
s++;
if (s < r) {
let f = e.slice(0, s);
for (s++; s < r; ) {
let x = e[s];
t(x) && f.push(x), s++;
}
return f;
}
}
return e;
}
function je(e, t) {
let r = 0;
for (let s = 0; s < e.length; s++)
t(e[s], s, e) && (e[r] = e[s], r++);
e.length = r;
}
function nt(e) {
e.length = 0;
}
function Ze(e, t) {
let r;
if (e) {
r = [];
for (let s = 0; s < e.length; s++)
r.push(t(e[s], s));
}
return r;
}
function* st(e, t) {
for (let r of e)
yield t(r);
}
function tt(e, t) {
if (e)
for (let r = 0; r < e.length; r++) {
let s = e[r], f = t(s, r);
if (s !== f) {
let x = e.slice(0, r);
for (x.push(f), r++; r < e.length; r++)
x.push(t(e[r], r));
return x;
}
}
return e;
}
function ct(e) {
let t = [];
for (let r of e)
r && (ir(r) ? jr(t, r) : t.push(r));
return t;
}
function ne(e, t) {
let r;
if (e)
for (let s = 0; s < e.length; s++) {
let f = t(e[s], s);
f && (ir(f) ? r = jr(r, f) : r = tr(r, f));
}
return r || Bt;
}
function ge(e, t) {
let r = [];
if (e)
for (let s = 0; s < e.length; s++) {
let f = t(e[s], s);
f && (ir(f) ? jr(r, f) : r.push(f));
}
return r;
}
function* Fe(e, t) {
for (let r of e) {
let s = t(r);
s && (yield* s);
}
}
function at(e, t) {
let r;
if (e)
for (let s = 0; s < e.length; s++) {
let f = e[s], x = t(f, s);
(r || f !== x || ir(x)) && (r || (r = e.slice(0, s)), ir(x) ? jr(r, x) : r.push(x));
}
return r || e;
}
function Pt(e, t) {
let r = [];
for (let s = 0; s < e.length; s++) {
let f = t(e[s], s);
if (f === void 0)
return;
r.push(f);
}
return r;
}
function qt(e, t) {
let r = [];
if (e)
for (let s = 0; s < e.length; s++) {
let f = t(e[s], s);
f !== void 0 && r.push(f);
}
return r;
}
function* Zr(e, t) {
for (let r of e) {
let s = t(r);
s !== void 0 && (yield s);
}
}
function Ri(e, t) {
if (!e)
return;
let r = /* @__PURE__ */ new Map();
return e.forEach((s, f) => {
let x = t(f, s);
if (x !== void 0) {
let [w, A] = x;
w !== void 0 && A !== void 0 && r.set(w, A);
}
}), r;
}
function la(e, t, r) {
if (e.has(t))
return e.get(t);
let s = r();
return e.set(t, s), s;
}
function ua(e, t) {
return e.has(t) ? false : (e.add(t), true);
}
function* Ka(e) {
yield e;
}
function co(e, t, r) {
let s;
if (e) {
s = [];
let f = e.length, x, w, A = 0, g = 0;
for (; A < f; ) {
for (; g < f; ) {
let B = e[g];
if (w = t(B, g), g === 0)
x = w;
else if (w !== x)
break;
g++;
}
if (A < g) {
let B = r(e.slice(A, g), x, A, g);
B && s.push(B), A = g;
}
x = w, g++;
}
}
return s;
}
function be(e, t) {
if (!e)
return;
let r = /* @__PURE__ */ new Map();
return e.forEach((s, f) => {
let [x, w] = t(f, s);
r.set(x, w);
}), r;
}
function Ke(e, t) {
if (e)
if (t) {
for (let r of e)
if (t(r))
return true;
} else
return e.length > 0;
return false;
}
function Et(e, t, r) {
let s;
for (let f = 0; f < e.length; f++)
t(e[f]) ? s = s === void 0 ? f : s : s !== void 0 && (r(s, f), s = void 0);
s !== void 0 && r(s, e.length);
}
function Ft(e, t) {
return Ke(t) ? Ke(e) ? [...e, ...t] : t : e;
}
function or(e, t) {
return t;
}
function Wr(e) {
return e.map(or);
}
function m_(e, t, r) {
let s = Wr(e);
ks(e, s, r);
let f = e[s[0]], x = [s[0]];
for (let w = 1; w < s.length; w++) {
let A = s[w], g = e[A];
t(f, g) || (x.push(A), f = g);
}
return x.sort(), x.map((w) => e[w]);
}
function Uc(e, t) {
let r = [];
for (let s of e)
qn(r, s, t);
return r;
}
function ji(e, t, r) {
return e.length === 0 ? [] : e.length === 1 ? e.slice() : r ? m_(e, t, r) : Uc(e, t);
}
function lo(e, t) {
if (e.length === 0)
return Bt;
let r = e[0], s = [r];
for (let f = 1; f < e.length; f++) {
let x = e[f];
switch (t(x, r)) {
case true:
case 0:
continue;
case -1:
return Y.fail("Array is unsorted.");
}
s.push(r = x);
}
return s;
}
function zc() {
return [];
}
function Qn(e, t, r, s) {
if (e.length === 0)
return e.push(t), true;
let f = Ya(e, t, rr, r);
return f < 0 ? (e.splice(~f, 0, t), true) : s ? (e.splice(f, 0, t), true) : false;
}
function uo(e, t, r) {
return lo(Is(e, t), r || t || ri);
}
function Wc(e, t) {
if (e.length < 2)
return true;
for (let r = 1, s = e.length; r < s; r++)
if (t(e[r - 1], e[r]) === 1)
return false;
return true;
}
function Vc(e, t, r, s) {
let f = 3;
if (e.length < 2)
return f;
let x = t(e[0]);
for (let w = 1, A = e.length; w < A && f !== 0; w++) {
let g = t(e[w]);
f & 1 && r(x, g) > 0 && (f &= -2), f & 2 && s(x, g) > 0 && (f &= -3), x = g;
}
return f;
}
function Hc(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : fa;
if (!e || !t)
return e === t;
if (e.length !== t.length)
return false;
for (let s = 0; s < e.length; s++)
if (!r(e[s], t[s], s))
return false;
return true;
}
function Gc(e) {
let t;
if (e)
for (let r = 0; r < e.length; r++) {
let s = e[r];
(t || !s) && (t || (t = e.slice(0, r)), s && t.push(s));
}
return t || e;
}
function h_(e, t, r) {
if (!t || !e || t.length === 0 || e.length === 0)
return t;
let s = [];
e:
for (let f = 0, x = 0; x < t.length; x++) {
x > 0 && Y.assertGreaterThanOrEqual(r(t[x], t[x - 1]), 0);
t:
for (let w = f; f < e.length; f++)
switch (f > w && Y.assertGreaterThanOrEqual(r(e[f], e[f - 1]), 0), r(t[x], e[f])) {
case -1:
s.push(t[x]);
continue e;
case 0:
continue e;
case 1:
continue t;
}
}
return s;
}
function tr(e, t) {
return t === void 0 ? e : e === void 0 ? [t] : (e.push(t), e);
}
function $c(e, t) {
return e === void 0 ? t : t === void 0 ? e : ir(e) ? ir(t) ? Ft(e, t) : tr(e, t) : ir(t) ? tr(t, e) : [e, t];
}
function po(e, t) {
return t < 0 ? e.length + t : t;
}
function jr(e, t, r, s) {
if (t === void 0 || t.length === 0)
return e;
if (e === void 0)
return t.slice(r, s);
r = r === void 0 ? 0 : po(t, r), s = s === void 0 ? t.length : po(t, s);
for (let f = r; f < s && f < t.length; f++)
t[f] !== void 0 && e.push(t[f]);
return e;
}
function qn(e, t, r) {
return pe(e, t, r) ? false : (e.push(t), true);
}
function g_(e, t, r) {
return e ? (qn(e, t, r), e) : [t];
}
function ks(e, t, r) {
t.sort((s, f) => r(e[s], e[f]) || Vr(s, f));
}
function Is(e, t) {
return e.length === 0 ? e : e.slice().sort(t);
}
function* y_(e) {
for (let t = e.length - 1; t >= 0; t--)
yield e[t];
}
function Ns(e, t) {
let r = Wr(e);
return ks(e, r, t), r.map((s) => e[s]);
}
function Kc(e, t, r, s) {
for (; r < s; ) {
if (e[r] !== t[r])
return false;
r++;
}
return true;
}
function pa(e) {
return e === void 0 || e.length === 0 ? void 0 : e[0];
}
function Xc(e) {
if (e)
for (let t of e)
return t;
}
function fo(e) {
return Y.assert(e.length !== 0), e[0];
}
function v_(e) {
for (let t of e)
return t;
Y.fail("iterator is empty");
}
function Cn(e) {
return e === void 0 || e.length === 0 ? void 0 : e[e.length - 1];
}
function Zn(e) {
return Y.assert(e.length !== 0), e[e.length - 1];
}
function Xa(e) {
return e && e.length === 1 ? e[0] : void 0;
}
function Yc(e) {
return Y.checkDefined(Xa(e));
}
function mo(e) {
return e && e.length === 1 ? e[0] : e;
}
function ei(e, t, r) {
let s = e.slice(0);
return s[t] = r, s;
}
function Ya(e, t, r, s, f) {
return b_(e, r(t), r, s, f);
}
function b_(e, t, r, s, f) {
if (!Ke(e))
return -1;
let x = f || 0, w = e.length - 1;
for (; x <= w; ) {
let A = x + (w - x >> 1), g = r(e[A], A);
switch (s(g, t)) {
case -1:
x = A + 1;
break;
case 0:
return A;
case 1:
w = A - 1;
break;
}
}
return ~x;
}
function Qa(e, t, r, s, f) {
if (e && e.length > 0) {
let x = e.length;
if (x > 0) {
let w = s === void 0 || s < 0 ? 0 : s, A = f === void 0 || w + f > x - 1 ? x - 1 : w + f, g;
for (arguments.length <= 2 ? (g = e[w], w++) : g = r; w <= A; )
g = t(g, e[w], w), w++;
return g;
}
}
return r;
}
function Jr(e, t) {
return ni.call(e, t);
}
function Qc(e, t) {
return ni.call(e, t) ? e[t] : void 0;
}
function ho(e) {
let t = [];
for (let r in e)
ni.call(e, r) && t.push(r);
return t;
}
function T_(e) {
let t = [];
do {
let r = Object.getOwnPropertyNames(e);
for (let s of r)
qn(t, s);
} while (e = Object.getPrototypeOf(e));
return t;
}
function go(e) {
let t = [];
for (let r in e)
ni.call(e, r) && t.push(e[r]);
return t;
}
function yo(e, t) {
let r = new Array(e);
for (let s = 0; s < e; s++)
r[s] = t(s);
return r;
}
function Za(e, t) {
let r = [];
for (let s of e)
r.push(t ? t(s) : s);
return r;
}
function vo(e) {
for (var t = arguments.length, r = new Array(t > 1 ? t - 1 : 0), s = 1; s < t; s++)
r[s - 1] = arguments[s];
for (let f of r)
if (f !== void 0)
for (let x in f)
Jr(f, x) && (e[x] = f[x]);
return e;
}
function S_(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : fa;
if (e === t)
return true;
if (!e || !t)
return false;
for (let s in e)
if (ni.call(e, s) && (!ni.call(t, s) || !r(e[s], t[s])))
return false;
for (let s in t)
if (ni.call(t, s) && !ni.call(e, s))
return false;
return true;
}
function Zc(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : rr, s = /* @__PURE__ */ new Map();
for (let f of e) {
let x = t(f);
x !== void 0 && s.set(x, r(f));
}
return s;
}
function Os(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : rr, s = [];
for (let f of e)
s[t(f)] = r(f);
return s;
}
function bo(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : rr, s = Be();
for (let f of e)
s.add(t(f), r(f));
return s;
}
function el(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : rr;
return Za(bo(e, t).values(), r);
}
function x_(e, t) {
var r;
let s = {};
if (e)
for (let f of e) {
let x = `${t(f)}`;
((r = s[x]) != null ? r : s[x] = []).push(f);
}
return s;
}
function E_(e) {
let t = {};
for (let r in e)
ni.call(e, r) && (t[r] = e[r]);
return t;
}
function S(e, t) {
let r = {};
for (let s in t)
ni.call(t, s) && (r[s] = t[s]);
for (let s in e)
ni.call(e, s) && (r[s] = e[s]);
return r;
}
function H(e, t) {
for (let r in t)
ni.call(t, r) && (e[r] = t[r]);
}
function le(e, t) {
return t ? t.bind(e) : void 0;
}
function Be() {
let e = /* @__PURE__ */ new Map();
return e.add = rt, e.remove = ut, e;
}
function rt(e, t) {
let r = this.get(e);
return r ? r.push(t) : this.set(e, r = [t]), r;
}
function ut(e, t) {
let r = this.get(e);
r && (bT(r, t), r.length || this.delete(e));
}
function Ht() {
return Be();
}
function Fr(e) {
let t = (e == null ? void 0 : e.slice()) || [], r = 0;
function s() {
return r === t.length;
}
function f() {
t.push(...arguments);
}
function x() {
if (s())
throw new Error("Queue is empty");
let w = t[r];
if (t[r] = void 0, r++, r > 100 && r > t.length >> 1) {
let A = t.length - r;
t.copyWithin(0, r), t.length = A, r = 0;
}
return w;
}
return { enqueue: f, dequeue: x, isEmpty: s };
}
function Cr(e, t) {
let r = /* @__PURE__ */ new Map(), s = 0;
function* f() {
for (let w of r.values())
ir(w) ? yield* w : yield w;
}
let x = { has(w) {
let A = e(w);
if (!r.has(A))
return false;
let g = r.get(A);
if (!ir(g))
return t(g, w);
for (let B of g)
if (t(B, w))
return true;
return false;
}, add(w) {
let A = e(w);
if (r.has(A)) {
let g = r.get(A);
if (ir(g))
pe(g, w, t) || (g.push(w), s++);
else {
let B = g;
t(B, w) || (r.set(A, [B, w]), s++);
}
} else
r.set(A, w), s++;
return this;
}, delete(w) {
let A = e(w);
if (!r.has(A))
return false;
let g = r.get(A);
if (ir(g)) {
for (let B = 0; B < g.length; B++)
if (t(g[B], w))
return g.length === 1 ? r.delete(A) : g.length === 2 ? r.set(A, g[1 - B]) : U1(g, B), s--, true;
} else if (t(g, w))
return r.delete(A), s--, true;
return false;
}, clear() {
r.clear(), s = 0;
}, get size() {
return s;
}, forEach(w) {
for (let A of Za(r.values()))
if (ir(A))
for (let g of A)
w(g, g, x);
else {
let g = A;
w(g, g, x);
}
}, keys() {
return f();
}, values() {
return f();
}, *entries() {
for (let w of f())
yield [w, w];
}, [Symbol.iterator]: () => f(), [Symbol.toStringTag]: r[Symbol.toStringTag] };
return x;
}
function ir(e) {
return Array.isArray(e);
}
function en(e) {
return ir(e) ? e : [e];
}
function Ji(e) {
return typeof e == "string";
}
function gi(e) {
return typeof e == "number";
}
function ln(e, t) {
return e !== void 0 && t(e) ? e : void 0;
}
function ti(e, t) {
return e !== void 0 && t(e) ? e : Y.fail(`Invalid cast. The supplied value ${e} did not pass the test '${Y.getFunctionName(t)}'.`);
}
function yn(e) {
}
function w_() {
return false;
}
function vp() {
return true;
}
function C1() {
}
function rr(e) {
return e;
}
function bp(e) {
return e.toLowerCase();
}
function Tp(e) {
return G1.test(e) ? e.replace(G1, bp) : e;
}
function A1() {
throw new Error("Not implemented");
}
function tl(e) {
let t;
return () => (e && (t = e(), e = void 0), t);
}
function An(e) {
let t = /* @__PURE__ */ new Map();
return (r) => {
let s = `${typeof r}:${r}`, f = t.get(s);
return f === void 0 && !t.has(s) && (f = e(r), t.set(s, f)), f;
};
}
function P1(e) {
let t = /* @__PURE__ */ new WeakMap();
return (r) => {
let s = t.get(r);
return s === void 0 && !t.has(r) && (s = e(r), t.set(r, s)), s;
};
}
function D1(e, t) {
return function() {
for (var r = arguments.length, s = new Array(r), f = 0; f < r; f++)
s[f] = arguments[f];
let x = t.get(s);
return x === void 0 && !t.has(s) && (x = e(...s), t.set(s, x)), x;
};
}
function k1(e, t, r, s, f) {
if (f) {
let x = [];
for (let w = 0; w < arguments.length; w++)
x[w] = arguments[w];
return (w) => Qa(x, (A, g) => g(A), w);
} else
return s ? (x) => s(r(t(e(x)))) : r ? (x) => r(t(e(x))) : t ? (x) => t(e(x)) : e ? (x) => e(x) : (x) => x;
}
function fa(e, t) {
return e === t;
}
function Ms(e, t) {
return e === t || e !== void 0 && t !== void 0 && e.toUpperCase() === t.toUpperCase();
}
function To(e, t) {
return fa(e, t);
}
function Sp(e, t) {
return e === t ? 0 : e === void 0 ? -1 : t === void 0 ? 1 : e < t ? -1 : 1;
}
function Vr(e, t) {
return Sp(e, t);
}
function I1(e, t) {
return Vr(e == null ? void 0 : e.start, t == null ? void 0 : t.start) || Vr(e == null ? void 0 : e.length, t == null ? void 0 : t.length);
}
function N1(e, t) {
return Qa(e, (r, s) => t(r, s) === -1 ? r : s);
}
function C_(e, t) {
return e === t ? 0 : e === void 0 ? -1 : t === void 0 ? 1 : (e = e.toUpperCase(), t = t.toUpperCase(), e < t ? -1 : e > t ? 1 : 0);
}
function O1(e, t) {
return e === t ? 0 : e === void 0 ? -1 : t === void 0 ? 1 : (e = e.toLowerCase(), t = t.toLowerCase(), e < t ? -1 : e > t ? 1 : 0);
}
function ri(e, t) {
return Sp(e, t);
}
function rl(e) {
return e ? C_ : ri;
}
function M1() {
return Ap;
}
function xp(e) {
Ap !== e && (Ap = e, K1 = void 0);
}
function L1(e, t) {
return (K1 || (K1 = AT(Ap)))(e, t);
}
function R1(e, t, r, s) {
return e === t ? 0 : e === void 0 ? -1 : t === void 0 ? 1 : s(e[r], t[r]);
}
function j1(e, t) {
return Vr(e ? 1 : 0, t ? 1 : 0);
}
function Ep(e, t, r) {
let s = Math.max(2, Math.floor(e.length * 0.34)), f = Math.floor(e.length * 0.4) + 1, x;
for (let w of t) {
let A = r(w);
if (A !== void 0 && Math.abs(A.length - e.length) <= s) {
if (A === e || A.length < 3 && A.toLowerCase() !== e.toLowerCase())
continue;
let g = J1(e, A, f - 0.1);
if (g === void 0)
continue;
Y.assert(g < f), f = g, x = w;
}
}
return x;
}
function J1(e, t, r) {
let s = new Array(t.length + 1), f = new Array(t.length + 1), x = r + 0.01;
for (let A = 0; A <= t.length; A++)
s[A] = A;
for (let A = 1; A <= e.length; A++) {
let g = e.charCodeAt(A - 1), B = Math.ceil(A > r ? A - r : 1), N = Math.floor(t.length > r + A ? r + A : t.length);
f[0] = A;
let X = A;
for (let $ = 1; $ < B; $++)
f[$] = x;
for (let $ = B; $ <= N; $++) {
let ae = e[A - 1].toLowerCase() === t[$ - 1].toLowerCase() ? s[$ - 1] + 0.1 : s[$ - 1] + 2, Te = g === t.charCodeAt($ - 1) ? s[$ - 1] : Math.min(s[$] + 1, f[$ - 1] + 1, ae);
f[$] = Te, X = Math.min(X, Te);
}
for (let $ = N + 1; $ <= t.length; $++)
f[$] = x;
if (X > r)
return;
let F = s;
s = f, f = F;
}
let w = s[t.length];
return w > r ? void 0 : w;
}
function es(e, t) {
let r = e.length - t.length;
return r >= 0 && e.indexOf(t, r) === r;
}
function F1(e, t) {
return es(e, t) ? e.slice(0, e.length - t.length) : e;
}
function B1(e, t) {
return es(e, t) ? e.slice(0, e.length - t.length) : void 0;
}
function Fi(e, t) {
return e.indexOf(t) !== -1;
}
function q1(e) {
let t = e.length;
for (let r = t - 1; r > 0; r--) {
let s = e.charCodeAt(r);
if (s >= 48 && s <= 57)
do
--r, s = e.charCodeAt(r);
while (r > 0 && s >= 48 && s <= 57);
else if (r > 4 && (s === 110 || s === 78)) {
if (--r, s = e.charCodeAt(r), s !== 105 && s !== 73 || (--r, s = e.charCodeAt(r), s !== 109 && s !== 77))
break;
--r, s = e.charCodeAt(r);
} else
break;
if (s !== 45 && s !== 46)
break;
t = r;
}
return t === e.length ? e : e.slice(0, t);
}
function J(e, t) {
for (let r = 0; r < e.length; r++)
if (e[r] === t)
return vT(e, r), true;
return false;
}
function vT(e, t) {
for (let r = t; r < e.length - 1; r++)
e[r] = e[r + 1];
e.pop();
}
function U1(e, t) {
e[t] = e[e.length - 1], e.pop();
}
function bT(e, t) {
return b5(e, (r) => r === t);
}
function b5(e, t) {
for (let r = 0; r < e.length; r++)
if (t(e[r]))
return U1(e, r), true;
return false;
}
function wp(e) {
return e ? rr : Tp;
}
function T5(e) {
let { prefix: t, suffix: r } = e;
return `${t}*${r}`;
}
function S5(e, t) {
return Y.assert(z1(e, t)), t.substring(e.prefix.length, t.length - e.suffix.length);
}
function TT(e, t, r) {
let s, f = -1;
for (let x of e) {
let w = t(x);
z1(w, r) && w.prefix.length > f && (f = w.prefix.length, s = x);
}
return s;
}
function Pn(e, t) {
return e.lastIndexOf(t, 0) === 0;
}
function x5(e, t) {
return Pn(e, t) ? e.substr(t.length) : e;
}
function ST(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : rr;
return Pn(r(e), r(t)) ? e.substring(t.length) : void 0;
}
function z1(e, t) {
let { prefix: r, suffix: s } = e;
return t.length >= r.length + s.length && Pn(t, r) && es(t, s);
}
function E5(e, t) {
return (r) => e(r) && t(r);
}
function W1() {
for (var e = arguments.length, t = new Array(e), r = 0; r < e; r++)
t[r] = arguments[r];
return function() {
let s;
for (let f of t)
if (s = f(...arguments), s)
return s;
return s;
};
}
function w5(e) {
return function() {
return !e(...arguments);
};
}
function C5(e) {
}
function Cp(e) {
return e === void 0 ? void 0 : [e];
}
function A5(e, t, r, s, f, x) {
x = x || yn;
let w = 0, A = 0, g = e.length, B = t.length, N = false;
for (; w < g && A < B; ) {
let X = e[w], F = t[A], $ = r(X, F);
$ === -1 ? (s(X), w++, N = true) : $ === 1 ? (f(F), A++, N = true) : (x(F, X), w++, A++);
}
for (; w < g; )
s(e[w++]), N = true;
for (; A < B; )
f(t[A++]), N = true;
return N;
}
function P5(e) {
let t = [];
return xT(e, t, void 0, 0), t;
}
function xT(e, t, r, s) {
for (let f of e[s]) {
let x;
r ? (x = r.slice(), x.push(f)) : x = [f], s === e.length - 1 ? t.push(x) : xT(e, t, x, s + 1);
}
}
function D5(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : " ";
return t <= e.length ? e : r.repeat(t - e.length) + e;
}
function k5(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : " ";
return t <= e.length ? e : e + r.repeat(t - e.length);
}
function I5(e, t) {
if (e) {
let r = e.length, s = 0;
for (; s < r && t(e[s]); )
s++;
return e.slice(0, s);
}
}
function N5(e, t) {
if (e) {
let r = e.length, s = 0;
for (; s < r && t(e[s]); )
s++;
return e.slice(s);
}
}
function O5(e) {
let t = e.length - 1;
for (; t >= 0 && os(e.charCodeAt(t)); )
t--;
return e.slice(0, t + 1);
}
function M5() {
return typeof cn < "u" && cn.nextTick && !cn.browser && typeof _ == "object";
}
var Bt, V1, ET, H1, wT, ni, CT, G1, $1, AT, K1, Ap, Pp, X1, nl, L5 = D({ "src/compiler/core.ts"() {
"use strict";
nn(), Bt = [], V1 = /* @__PURE__ */ new Map(), ET = /* @__PURE__ */ new Set(), H1 = ((e) => (e[e.None = 0] = "None", e[e.CaseSensitive = 1] = "CaseSensitive", e[e.CaseInsensitive = 2] = "CaseInsensitive", e[e.Both = 3] = "Both", e))(H1 || {}), wT = Array.prototype.at ? (e, t) => e == null ? void 0 : e.at(t) : (e, t) => {
if (e && (t = po(e, t), t < e.length))
return e[t];
}, ni = Object.prototype.hasOwnProperty, CT = { push: yn, length: 0 }, G1 = /[^\u0130\u0131\u00DFa-z0-9\\/:\-_\. ]+/g, $1 = ((e) => (e[e.None = 0] = "None", e[e.Normal = 1] = "Normal", e[e.Aggressive = 2] = "Aggressive", e[e.VeryAggressive = 3] = "VeryAggressive", e))($1 || {}), AT = (() => {
let e, t, r = A();
return g;
function s(B, N, X) {
if (B === N)
return 0;
if (B === void 0)
return -1;
if (N === void 0)
return 1;
let F = X(B, N);
return F < 0 ? -1 : F > 0 ? 1 : 0;
}
function f(B) {
let N = new Intl.Collator(B, { usage: "sort", sensitivity: "variant" }).compare;
return (X, F) => s(X, F, N);
}
function x(B) {
if (B !== void 0)
return w();
return (X, F) => s(X, F, N);
function N(X, F) {
return X.localeCompare(F);
}
}
function w() {
return (X, F) => s(X, F, B);
function B(X, F) {
return N(X.toUpperCase(), F.toUpperCase()) || N(X, F);
}
function N(X, F) {
return X < F ? -1 : X > F ? 1 : 0;
}
}
function A() {
return typeof Intl == "object" && typeof Intl.Collator == "function" ? f : typeof String.prototype.localeCompare == "function" && typeof String.prototype.toLocaleUpperCase == "function" && "a".localeCompare("B") < 0 ? x : w;
}
function g(B) {
return B === void 0 ? e || (e = r(B)) : B === "en-US" ? t || (t = r(B)) : r(B);
}
})(), Pp = String.prototype.trim ? (e) => e.trim() : (e) => X1(nl(e)), X1 = String.prototype.trimEnd ? (e) => e.trimEnd() : O5, nl = String.prototype.trimStart ? (e) => e.trimStart() : (e) => e.replace(/^\s+/g, "");
} }), Y1, Y, PT = D({ "src/compiler/debug.ts"() {
"use strict";
nn(), nn(), Y1 = ((e) => (e[e.Off = 0] = "Off", e[e.Error = 1] = "Error", e[e.Warning = 2] = "Warning", e[e.Info = 3] = "Info", e[e.Verbose = 4] = "Verbose", e))(Y1 || {}), ((e) => {
let t = 0;
e.currentLogLevel = 2, e.isDebugging = false;
function r(ue) {
return e.currentLogLevel <= ue;
}
e.shouldLog = r;
function s(ue, He) {
e.loggingHost && r(ue) && e.loggingHost.log(ue, He);
}
function f(ue) {
s(3, ue);
}
e.log = f, ((ue) => {
function He(zt) {
s(1, zt);
}
ue.error = He;
function _t(zt) {
s(2, zt);
}
ue.warn = _t;
function ft(zt) {
s(3, zt);
}
ue.log = ft;
function Kt(zt) {
s(4, zt);
}
ue.trace = Kt;
})(f = e.log || (e.log = {}));
let x = {};
function w() {
return t;
}
e.getAssertionLevel = w;
function A(ue) {
let He = t;
if (t = ue, ue > He)
for (let _t of ho(x)) {
let ft = x[_t];
ft !== void 0 && e[_t] !== ft.assertion && ue >= ft.level && (e[_t] = ft, x[_t] = void 0);
}
}
e.setAssertionLevel = A;
function g(ue) {
return t >= ue;
}
e.shouldAssert = g;
function B(ue, He) {
return g(ue) ? true : (x[He] = { level: ue, assertion: e[He] }, e[He] = yn, false);
}
function N(ue, He) {
debugger;
let _t = new Error(ue ? `Debug Failure. ${ue}` : "Debug Failure.");
throw Error.captureStackTrace && Error.captureStackTrace(_t, He || N), _t;
}
e.fail = N;
function X(ue, He, _t) {
return N(`${He || "Unexpected node."}\r
Node ${mr(ue.kind)} was unexpected.`, _t || X);
}
e.failBadSyntaxKind = X;
function F(ue, He, _t, ft) {
ue || (He = He ? `False expression: ${He}` : "False expression.", _t && (He += `\r
Verbose Debug Information: ` + (typeof _t == "string" ? _t : _t())), N(He, ft || F));
}
e.assert = F;
function $(ue, He, _t, ft, Kt) {
if (ue !== He) {
let zt = _t ? ft ? `${_t} ${ft}` : _t : "";
N(`Expected ${ue} === ${He}. ${zt}`, Kt || $);
}
}
e.assertEqual = $;
function ae(ue, He, _t, ft) {
ue >= He && N(`Expected ${ue} < ${He}. ${_t || ""}`, ft || ae);
}
e.assertLessThan = ae;
function Te(ue, He, _t) {
ue > He && N(`Expected ${ue} <= ${He}`, _t || Te);
}
e.assertLessThanOrEqual = Te;
function Se(ue, He, _t) {
ue < He && N(`Expected ${ue} >= ${He}`, _t || Se);
}
e.assertGreaterThanOrEqual = Se;
function Ye(ue, He, _t) {
ue == null && N(He, _t || Ye);
}
e.assertIsDefined = Ye;
function Ne(ue, He, _t) {
return Ye(ue, He, _t || Ne), ue;
}
e.checkDefined = Ne;
function oe(ue, He, _t) {
for (let ft of ue)
Ye(ft, He, _t || oe);
}
e.assertEachIsDefined = oe;
function Ve(ue, He, _t) {
return oe(ue, He, _t || Ve), ue;
}
e.checkEachDefined = Ve;
function pt(ue) {
let He = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : "Illegal value:", _t = arguments.length > 2 ? arguments[2] : void 0, ft = typeof ue == "object" && Jr(ue, "kind") && Jr(ue, "pos") ? "SyntaxKind: " + mr(ue.kind) : JSON.stringify(ue);
return N(`${He} ${ft}`, _t || pt);
}
e.assertNever = pt;
function Gt(ue, He, _t, ft) {
B(1, "assertEachNode") && F(He === void 0 || me(ue, He), _t || "Unexpected node.", () => `Node array did not pass test '${pn(He)}'.`, ft || Gt);
}
e.assertEachNode = Gt;
function Nt(ue, He, _t, ft) {
B(1, "assertNode") && F(ue !== void 0 && (He === void 0 || He(ue)), _t || "Unexpected node.", () => `Node ${mr(ue == null ? void 0 : ue.kind)} did not pass test '${pn(He)}'.`, ft || Nt);
}
e.assertNode = Nt;
function Xt(ue, He, _t, ft) {
B(1, "assertNotNode") && F(ue === void 0 || He === void 0 || !He(ue), _t || "Unexpected node.", () => `Node ${mr(ue.kind)} should not have passed test '${pn(He)}'.`, ft || Xt);
}
e.assertNotNode = Xt;
function er(ue, He, _t, ft) {
B(1, "assertOptionalNode") && F(He === void 0 || ue === void 0 || He(ue), _t || "Unexpected node.", () => `Node ${mr(ue == null ? void 0 : ue.kind)} did not pass test '${pn(He)}'.`, ft || er);
}
e.assertOptionalNode = er;
function Tn(ue, He, _t, ft) {
B(1, "assertOptionalToken") && F(He === void 0 || ue === void 0 || ue.kind === He, _t || "Unexpected node.", () => `Node ${mr(ue == null ? void 0 : ue.kind)} was not a '${mr(He)}' token.`, ft || Tn);
}
e.assertOptionalToken = Tn;
function Hr(ue, He, _t) {
B(1, "assertMissingNode") && F(ue === void 0, He || "Unexpected node.", () => `Node ${mr(ue.kind)} was unexpected'.`, _t || Hr);
}
e.assertMissingNode = Hr;
function Gi(ue) {
}
e.type = Gi;
function pn(ue) {
if (typeof ue != "function")
return "";
if (Jr(ue, "name"))
return ue.name;
{
let He = Function.prototype.toString.call(ue), _t = /^function\s+([\w\$]+)\s*\(/.exec(He);
return _t ? _t[1] : "";
}
}
e.getFunctionName = pn;
function fn(ue) {
return `{ name: ${dl(ue.escapedName)}; flags: ${Sn(ue.flags)}; declarations: ${Ze(ue.declarations, (He) => mr(He.kind))} }`;
}
e.formatSymbol = fn;
function Ut() {
let ue = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : 0, He = arguments.length > 1 ? arguments[1] : void 0, _t = arguments.length > 2 ? arguments[2] : void 0, ft = an(He);
if (ue === 0)
return ft.length > 0 && ft[0][0] === 0 ? ft[0][1] : "0";
if (_t) {
let Kt = [], zt = ue;
for (let [xe, Le] of ft) {
if (xe > ue)
break;
xe !== 0 && xe & ue && (Kt.push(Le), zt &= ~xe);
}
if (zt === 0)
return Kt.join("|");
} else
for (let [Kt, zt] of ft)
if (Kt === ue)
return zt;
return ue.toString();
}
e.formatEnum = Ut;
let kn = /* @__PURE__ */ new Map();
function an(ue) {
let He = kn.get(ue);
if (He)
return He;
let _t = [];
for (let Kt in ue) {
let zt = ue[Kt];
typeof zt == "number" && _t.push([zt, Kt]);
}
let ft = Ns(_t, (Kt, zt) => Vr(Kt[0], zt[0]));
return kn.set(ue, ft), ft;
}
function mr(ue) {
return Ut(ue, Np, false);
}
e.formatSyntaxKind = mr;
function $i(ue) {
return Ut(ue, zp, false);
}
e.formatSnippetKind = $i;
function dn(ue) {
return Ut(ue, Op, true);
}
e.formatNodeFlags = dn;
function Ur(ue) {
return Ut(ue, Mp, true);
}
e.formatModifierFlags = Ur;
function Gr(ue) {
return Ut(ue, Up, true);
}
e.formatTransformFlags = Gr;
function _r(ue) {
return Ut(ue, Wp, true);
}
e.formatEmitFlags = _r;
function Sn(ue) {
return Ut(ue, jp, true);
}
e.formatSymbolFlags = Sn;
function In(ue) {
return Ut(ue, Jp, true);
}
e.formatTypeFlags = In;
function pr(ue) {
return Ut(ue, Bp, true);
}
e.formatSignatureFlags = pr;
function Zt(ue) {
return Ut(ue, Fp, true);
}
e.formatObjectFlags = Zt;
function Or(ue) {
return Ut(ue, il, true);
}
e.formatFlowFlags = Or;
function Nn(ue) {
return Ut(ue, Lp, true);
}
e.formatRelationComparisonResult = Nn;
function ar(ue) {
return Ut(ue, CheckMode, true);
}
e.formatCheckMode = ar;
function oi(ue) {
return Ut(ue, SignatureCheckMode, true);
}
e.formatSignatureCheckMode = oi;
function cr(ue) {
return Ut(ue, TypeFacts, true);
}
e.formatTypeFacts = cr;
let $r = false, hr;
function On(ue) {
"__debugFlowFlags" in ue || Object.defineProperties(ue, { __tsDebuggerDisplay: { value() {
let He = this.flags & 2 ? "FlowStart" : this.flags & 4 ? "FlowBranchLabel" : this.flags & 8 ? "FlowLoopLabel" : this.flags & 16 ? "FlowAssignment" : this.flags & 32 ? "FlowTrueCondition" : this.flags & 64 ? "FlowFalseCondition" : this.flags & 128 ? "FlowSwitchClause" : this.flags & 256 ? "FlowArrayMutation" : this.flags & 512 ? "FlowCall" : this.flags & 1024 ? "FlowReduceLabel" : this.flags & 1 ? "FlowUnreachable" : "UnknownFlow", _t = this.flags & ~(2048 - 1);
return `${He}${_t ? ` (${Or(_t)})` : ""}`;
} }, __debugFlowFlags: { get() {
return Ut(this.flags, il, true);
} }, __debugToString: { value() {
return St(this);
} } });
}
function nr(ue) {
$r && (typeof Object.setPrototypeOf == "function" ? (hr || (hr = Object.create(Object.prototype), On(hr)), Object.setPrototypeOf(ue, hr)) : On(ue));
}
e.attachFlowNodeDebugInfo = nr;
let br;
function Kr(ue) {
"__tsDebuggerDisplay" in ue || Object.defineProperties(ue, { __tsDebuggerDisplay: { value(He) {
return He = String(He).replace(/(?:,[\s\w\d_]+:[^,]+)+\]$/, "]"), `NodeArray ${He}`;
} } });
}
function wa(ue) {
$r && (typeof Object.setPrototypeOf == "function" ? (br || (br = Object.create(Array.prototype), Kr(br)), Object.setPrototypeOf(ue, br)) : Kr(ue));
}
e.attachNodeArrayDebugInfo = wa;
function $n() {
if ($r)
return;
let ue = /* @__PURE__ */ new WeakMap(), He = /* @__PURE__ */ new WeakMap();
Object.defineProperties(lr.getSymbolConstructor().prototype, { __tsDebuggerDisplay: { value() {
let ft = this.flags & 33554432 ? "TransientSymbol" : "Symbol", Kt = this.flags & -33554433;
return `${ft} '${rf(this)}'${Kt ? ` (${Sn(Kt)})` : ""}`;
} }, __debugFlags: { get() {
return Sn(this.flags);
} } }), Object.defineProperties(lr.getTypeConstructor().prototype, { __tsDebuggerDisplay: { value() {
let ft = this.flags & 98304 ? "NullableType" : this.flags & 384 ? `LiteralType ${JSON.stringify(this.value)}` : this.flags & 2048 ? `LiteralType ${this.value.negative ? "-" : ""}${this.value.base10Value}n` : this.flags & 8192 ? "UniqueESSymbolType" : this.flags & 32 ? "EnumType" : this.flags & 67359327 ? `IntrinsicType ${this.intrinsicName}` : this.flags & 1048576 ? "UnionType" : this.flags & 2097152 ? "IntersectionType" : this.flags & 4194304 ? "IndexType" : this.flags & 8388608 ? "IndexedAccessType" : this.flags & 16777216 ? "ConditionalType" : this.flags & 33554432 ? "SubstitutionType" : this.flags & 262144 ? "TypeParameter" : this.flags & 524288 ? this.objectFlags & 3 ? "InterfaceType" : this.objectFlags & 4 ? "TypeReference" : this.objectFlags & 8 ? "TupleType" : this.objectFlags & 16 ? "AnonymousType" : this.objectFlags & 32 ? "MappedType" : this.objectFlags & 1024 ? "ReverseMappedType" : this.objectFlags & 256 ? "EvolvingArrayType" : "ObjectType" : "Type", Kt = this.flags & 524288 ? this.objectFlags & -1344 : 0;
return `${ft}${this.symbol ? ` '${rf(this.symbol)}'` : ""}${Kt ? ` (${Zt(Kt)})` : ""}`;
} }, __debugFlags: { get() {
return In(this.flags);
} }, __debugObjectFlags: { get() {
return this.flags & 524288 ? Zt(this.objectFlags) : "";
} }, __debugTypeToString: { value() {
let ft = ue.get(this);
return ft === void 0 && (ft = this.checker.typeToString(this), ue.set(this, ft)), ft;
} } }), Object.defineProperties(lr.getSignatureConstructor().prototype, { __debugFlags: { get() {
return pr(this.flags);
} }, __debugSignatureToString: { value() {
var ft;
return (ft = this.checker) == null ? void 0 : ft.signatureToString(this);
} } });
let _t = [lr.getNodeConstructor(), lr.getIdentifierConstructor(), lr.getTokenConstructor(), lr.getSourceFileConstructor()];
for (let ft of _t)
Jr(ft.prototype, "__debugKind") || Object.defineProperties(ft.prototype, { __tsDebuggerDisplay: { value() {
return `${cs(this) ? "GeneratedIdentifier" : yt(this) ? `Identifier '${qr(this)}'` : vn(this) ? `PrivateIdentifier '${qr(this)}'` : Gn(this) ? `StringLiteral ${JSON.stringify(this.text.length < 10 ? this.text : this.text.slice(10) + "...")}` : zs(this) ? `NumericLiteral ${this.text}` : Uv(this) ? `BigIntLiteral ${this.text}n` : Fo(this) ? "TypeParameterDeclaration" : Vs(this) ? "ParameterDeclaration" : nc(this) ? "ConstructorDeclaration" : Gl(this) ? "GetAccessorDeclaration" : ic(this) ? "SetAccessorDeclaration" : Vv(this) ? "CallSignatureDeclaration" : R8(this) ? "ConstructSignatureDeclaration" : Hv(this) ? "IndexSignatureDeclaration" : j8(this) ? "TypePredicateNode" : ac(this) ? "TypeReferenceNode" : $l(this) ? "FunctionTypeNode" : Gv(this) ? "ConstructorTypeNode" : J8(this) ? "TypeQueryNode" : id(this) ? "TypeLiteralNode" : F8(this) ? "ArrayTypeNode" : B8(this) ? "TupleTypeNode" : q8(this) ? "OptionalTypeNode" : U8(this) ? "RestTypeNode" : z8(this) ? "UnionTypeNode" : W8(this) ? "IntersectionTypeNode" : V8(this) ? "ConditionalTypeNode" : H8(this) ? "InferTypeNode" : Kv(this) ? "ParenthesizedTypeNode" : Xv(this) ? "ThisTypeNode" : G8(this) ? "TypeOperatorNode" : $8(this) ? "IndexedAccessTypeNode" : K8(this) ? "MappedTypeNode" : Yv(this) ? "LiteralTypeNode" : $v(this) ? "NamedTupleMember" : Kl(this) ? "ImportTypeNode" : mr(this.kind)}${this.flags ? ` (${dn(this.flags)})` : ""}`;
} }, __debugKind: { get() {
return mr(this.kind);
} }, __debugNodeFlags: { get() {
return dn(this.flags);
} }, __debugModifierFlags: { get() {
return Ur(Y4(this));
} }, __debugTransformFlags: { get() {
return Gr(this.transformFlags);
} }, __debugIsParseTreeNode: { get() {
return pl(this);
} }, __debugEmitFlags: { get() {
return _r(xi(this));
} }, __debugGetText: { value(Kt) {
if (fs2(this))
return "";
let zt = He.get(this);
if (zt === void 0) {
let xe = fl(this), Le = xe && Si(xe);
zt = Le ? No(Le, xe, Kt) : "", He.set(this, zt);
}
return zt;
} } });
$r = true;
}
e.enableDebugInfo = $n;
function Ki(ue) {
let He = ue & 7, _t = He === 0 ? "in out" : He === 3 ? "[bivariant]" : He === 2 ? "in" : He === 1 ? "out" : He === 4 ? "[independent]" : "";
return ue & 8 ? _t += " (unmeasurable)" : ue & 16 && (_t += " (unreliable)"), _t;
}
e.formatVariance = Ki;
class Mn {
__debugToString() {
var He;
switch (this.kind) {
case 3:
return ((He = this.debugInfo) == null ? void 0 : He.call(this)) || "(function mapper)";
case 0:
return `${this.source.__debugTypeToString()} -> ${this.target.__debugTypeToString()}`;
case 1:
return ce(this.sources, this.targets || Ze(this.sources, () => "any"), (_t, ft) => `${_t.__debugTypeToString()} -> ${typeof ft == "string" ? ft : ft.__debugTypeToString()}`).join(", ");
case 2:
return ce(this.sources, this.targets, (_t, ft) => `${_t.__debugTypeToString()} -> ${ft().__debugTypeToString()}`).join(", ");
case 5:
case 4:
return `m1: ${this.mapper1.__debugToString().split(`
`).join(`
`)}
m2: ${this.mapper2.__debugToString().split(`
`).join(`
`)}`;
default:
return pt(this);
}
}
}
e.DebugTypeMapper = Mn;
function _i(ue) {
return e.isDebugging ? Object.setPrototypeOf(ue, Mn.prototype) : ue;
}
e.attachDebugPrototypeIfDebug = _i;
function Ca(ue) {
return console.log(St(ue));
}
e.printControlFlowGraph = Ca;
function St(ue) {
let He = -1;
function _t(U) {
return U.id || (U.id = He, He--), U.id;
}
let ft;
((U) => {
U.lr = "\u2500", U.ud = "\u2502", U.dr = "\u256D", U.dl = "\u256E", U.ul = "\u256F", U.ur = "\u2570", U.udr = "\u251C", U.udl = "\u2524", U.dlr = "\u252C", U.ulr = "\u2534", U.udlr = "\u256B";
})(ft || (ft = {}));
let Kt;
((U) => {
U[U.None = 0] = "None", U[U.Up = 1] = "Up", U[U.Down = 2] = "Down", U[U.Left = 4] = "Left", U[U.Right = 8] = "Right", U[U.UpDown = 3] = "UpDown", U[U.LeftRight = 12] = "LeftRight", U[U.UpLeft = 5] = "UpLeft", U[U.UpRight = 9] = "UpRight", U[U.DownLeft = 6] = "DownLeft", U[U.DownRight = 10] = "DownRight", U[U.UpDownLeft = 7] = "UpDownLeft", U[U.UpDownRight = 11] = "UpDownRight", U[U.UpLeftRight = 13] = "UpLeftRight", U[U.DownLeftRight = 14] = "DownLeftRight", U[U.UpDownLeftRight = 15] = "UpDownLeftRight", U[U.NoChildren = 16] = "NoChildren";
})(Kt || (Kt = {}));
let zt = 2032, xe = 882, Le = /* @__PURE__ */ Object.create(null), Re = [], ot = [], Ct = Aa(ue, /* @__PURE__ */ new Set());
for (let U of Re)
U.text = xn(U.flowNode, U.circular), $s(U);
let Mt = li(Ct), It = Yi(Mt);
return Qi(Ct, 0), Dt();
function Mr(U) {
return !!(U.flags & 128);
}
function gr(U) {
return !!(U.flags & 12) && !!U.antecedents;
}
function Ln(U) {
return !!(U.flags & zt);
}
function ys(U) {
return !!(U.flags & xe);
}
function ci(U) {
let L = [];
for (let fe of U.edges)
fe.source === U && L.push(fe.target);
return L;
}
function Xi(U) {
let L = [];
for (let fe of U.edges)
fe.target === U && L.push(fe.source);
return L;
}
function Aa(U, L) {
let fe = _t(U), T = Le[fe];
if (T && L.has(U))
return T.circular = true, T = { id: -1, flowNode: U, edges: [], text: "", lane: -1, endLane: -1, level: -1, circular: "circularity" }, Re.push(T), T;
if (L.add(U), !T)
if (Le[fe] = T = { id: fe, flowNode: U, edges: [], text: "", lane: -1, endLane: -1, level: -1, circular: false }, Re.push(T), gr(U))
for (let it of U.antecedents)
vs(T, it, L);
else
Ln(U) && vs(T, U.antecedent, L);
return L.delete(U), T;
}
function vs(U, L, fe) {
let T = Aa(L, fe), it = { source: U, target: T };
ot.push(it), U.edges.push(it), T.edges.push(it);
}
function $s(U) {
if (U.level !== -1)
return U.level;
let L = 0;
for (let fe of Xi(U))
L = Math.max(L, $s(fe) + 1);
return U.level = L;
}
function li(U) {
let L = 0;
for (let fe of ci(U))
L = Math.max(L, li(fe));
return L + 1;
}
function Yi(U) {
let L = Z(Array(U), 0);
for (let fe of Re)
L[fe.level] = Math.max(L[fe.level], fe.text.length);
return L;
}
function Qi(U, L) {
if (U.lane === -1) {
U.lane = L, U.endLane = L;
let fe = ci(U);
for (let T = 0; T < fe.length; T++) {
T > 0 && L++;
let it = fe[T];
Qi(it, L), it.endLane > U.endLane && (L = it.endLane);
}
U.endLane = L;
}
}
function bs(U) {
if (U & 2)
return "Start";
if (U & 4)
return "Branch";
if (U & 8)
return "Loop";
if (U & 16)
return "Assignment";
if (U & 32)
return "True";
if (U & 64)
return "False";
if (U & 128)
return "SwitchClause";
if (U & 256)
return "ArrayMutation";
if (U & 512)
return "Call";
if (U & 1024)
return "ReduceLabel";
if (U & 1)
return "Unreachable";
throw new Error();
}
function Ai(U) {
let L = Si(U);
return No(L, U, false);
}
function xn(U, L) {
let fe = bs(U.flags);
if (L && (fe = `${fe}#${_t(U)}`), ys(U))
U.node && (fe += ` (${Ai(U.node)})`);
else if (Mr(U)) {
let T = [];
for (let it = U.clauseStart; it < U.clauseEnd; it++) {
let mt = U.switchStatement.caseBlock.clauses[it];
oE(mt) ? T.push("default") : T.push(Ai(mt.expression));
}
fe += ` (${T.join(", ")})`;
}
return L === "circularity" ? `Circular(${fe})` : fe;
}
function Dt() {
let U = It.length, L = Re.reduce((_e, Ge) => Math.max(_e, Ge.lane), 0) + 1, fe = Z(Array(L), ""), T = It.map(() => Array(L)), it = It.map(() => Z(Array(L), 0));
for (let _e of Re) {
T[_e.level][_e.lane] = _e;
let Ge = ci(_e);
for (let jt = 0; jt < Ge.length; jt++) {
let Yt = Ge[jt], $t = 8;
Yt.lane === _e.lane && ($t |= 4), jt > 0 && ($t |= 1), jt < Ge.length - 1 && ($t |= 2), it[_e.level][Yt.lane] |= $t;
}
Ge.length === 0 && (it[_e.level][_e.lane] |= 16);
let bt = Xi(_e);
for (let jt = 0; jt < bt.length; jt++) {
let Yt = bt[jt], $t = 4;
jt > 0 && ($t |= 1), jt < bt.length - 1 && ($t |= 2), it[_e.level - 1][Yt.lane] |= $t;
}
}
for (let _e = 0; _e < U; _e++)
for (let Ge = 0; Ge < L; Ge++) {
let bt = _e > 0 ? it[_e - 1][Ge] : 0, jt = Ge > 0 ? it[_e][Ge - 1] : 0, Yt = it[_e][Ge];
Yt || (bt & 8 && (Yt |= 12), jt & 2 && (Yt |= 3), it[_e][Ge] = Yt);
}
for (let _e = 0; _e < U; _e++)
for (let Ge = 0; Ge < fe.length; Ge++) {
let bt = it[_e][Ge], jt = bt & 4 ? "\u2500" : " ", Yt = T[_e][Ge];
Yt ? (mt(Ge, Yt.text), _e < U - 1 && (mt(Ge, " "), mt(Ge, ie(jt, It[_e] - Yt.text.length)))) : _e < U - 1 && mt(Ge, ie(jt, It[_e] + 1)), mt(Ge, Pi(bt)), mt(Ge, bt & 8 && _e < U - 1 && !T[_e + 1][Ge] ? "\u2500" : " ");
}
return `
${fe.join(`
`)}
`;
function mt(_e, Ge) {
fe[_e] += Ge;
}
}
function Pi(U) {
switch (U) {
case 3:
return "\u2502";
case 12:
return "\u2500";
case 5:
return "\u256F";
case 9:
return "\u2570";
case 6:
return "\u256E";
case 10:
return "\u256D";
case 7:
return "\u2524";
case 11:
return "\u251C";
case 13:
return "\u2534";
case 14:
return "\u252C";
case 15:
return "\u256B";
}
return " ";
}
function Z(U, L) {
if (U.fill)
U.fill(L);
else
for (let fe = 0; fe < U.length; fe++)
U[fe] = L;
return U;
}
function ie(U, L) {
if (U.repeat)
return L > 0 ? U.repeat(L) : "";
let fe = "";
for (; fe.length < L; )
fe += U;
return fe;
}
}
e.formatControlFlowGraph = St;
})(Y || (Y = {}));
} }), R5 = () => {
}, j5 = () => {
}, J5 = () => {
}, ts = Date.now, F5 = () => {
}, Dp = new Proxy(() => {
}, { get: () => Dp });
function DT(e) {
var t;
if (Q1) {
let r = (t = Z1.get(e)) != null ? t : 0;
Z1.set(e, r + 1), Ip.set(e, ts()), kp == null || kp.mark(e), typeof onProfilerEvent == "function" && onProfilerEvent(e);
}
}
function B5(e, t, r) {
var s, f;
if (Q1) {
let x = (s = r !== void 0 ? Ip.get(r) : void 0) != null ? s : ts(), w = (f = t !== void 0 ? Ip.get(t) : void 0) != null ? f : kT, A = eg.get(e) || 0;
eg.set(e, A + (x - w)), kp == null || kp.measure(e, t, r);
}
}
var kp, q5, Q1, kT, Ip, Z1, eg, pH = D({ "src/compiler/performance.ts"() {
"use strict";
nn(), q5 = { enter: yn, exit: yn }, Q1 = false, kT = ts(), Ip = /* @__PURE__ */ new Map(), Z1 = /* @__PURE__ */ new Map(), eg = /* @__PURE__ */ new Map();
} }), IT = () => {
}, U5 = () => {
}, rs;
function z5(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : true, r = qp[e.category];
return t ? r.toLowerCase() : r;
}
var Np, Op, Mp, tg, Lp, rg, ng, il, ig, Rp, ag, sg, og, _g, cg, lg, ug, pg, fg, dg, mg, hg, gg, yg, vg, jp, bg, Tg, Sg, xg, Jp, Fp, Eg, wg, Cg, Ag, Pg, Bp, Dg, kg, Ig, Ng, Og, Mg, qp, Lg, Rg, jg, Jg, Fg, Bg, qg, Ug, zg, Wg, Vg, Hg, Gg, $g, Kg, Up, zp, Wp, Xg, Yg, Qg, Zg, ey, ty, ry, ny, Vp, NT = D({ "src/compiler/types.ts"() {
"use strict";
Np = ((e) => (e[e.Unknown = 0] = "Unknown", e[e.EndOfFileToken = 1] = "EndOfFileToken", e[e.SingleLineCommentTrivia = 2] = "SingleLineCommentTrivia", e[e.MultiLineCommentTrivia = 3] = "MultiLineCommentTrivia", e[e.NewLineTrivia = 4] = "NewLineTrivia", e[e.WhitespaceTrivia = 5] = "WhitespaceTrivia", e[e.ShebangTrivia = 6] = "ShebangTrivia", e[e.ConflictMarkerTrivia = 7] = "ConflictMarkerTrivia", e[e.NumericLiteral = 8] = "NumericLiteral", e[e.BigIntLiteral = 9] = "BigIntLiteral", e[e.StringLiteral = 10] = "StringLiteral", e[e.JsxText = 11] = "JsxText", e[e.JsxTextAllWhiteSpaces = 12] = "JsxTextAllWhiteSpaces", e[e.RegularExpressionLiteral = 13] = "RegularExpressionLiteral", e[e.NoSubstitutionTemplateLiteral = 14] = "NoSubstitutionTemplateLiteral", e[e.TemplateHead = 15] = "TemplateHead", e[e.TemplateMiddle = 16] = "TemplateMiddle", e[e.TemplateTail = 17] = "TemplateTail", e[e.OpenBraceToken = 18] = "OpenBraceToken", e[e.CloseBraceToken = 19] = "CloseBraceToken", e[e.OpenParenToken = 20] = "OpenParenToken", e[e.CloseParenToken = 21] = "CloseParenToken", e[e.OpenBracketToken = 22] = "OpenBracketToken", e[e.CloseBracketToken = 23] = "CloseBracketToken", e[e.DotToken = 24] = "DotToken", e[e.DotDotDotToken = 25] = "DotDotDotToken", e[e.SemicolonToken = 26] = "SemicolonToken", e[e.CommaToken = 27] = "CommaToken", e[e.QuestionDotToken = 28] = "QuestionDotToken", e[e.LessThanToken = 29] = "LessThanToken", e[e.LessThanSlashToken = 30] = "LessThanSlashToken", e[e.GreaterThanToken = 31] = "GreaterThanToken", e[e.LessThanEqualsToken = 32] = "LessThanEqualsToken", e[e.GreaterThanEqualsToken = 33] = "GreaterThanEqualsToken", e[e.EqualsEqualsToken = 34] = "EqualsEqualsToken", e[e.ExclamationEqualsToken = 35] = "ExclamationEqualsToken", e[e.EqualsEqualsEqualsToken = 36] = "EqualsEqualsEqualsToken", e[e.ExclamationEqualsEqualsToken = 37] = "ExclamationEqualsEqualsToken", e[e.EqualsGreaterThanToken = 38] = "EqualsGreaterThanToken", e[e.PlusToken = 39] = "PlusToken", e[e.MinusToken = 40] = "MinusToken", e[e.AsteriskToken = 41] = "AsteriskToken", e[e.AsteriskAsteriskToken = 42] = "AsteriskAsteriskToken", e[e.SlashToken = 43] = "SlashToken", e[e.PercentToken = 44] = "PercentToken", e[e.PlusPlusToken = 45] = "PlusPlusToken", e[e.MinusMinusToken = 46] = "MinusMinusToken", e[e.LessThanLessThanToken = 47] = "LessThanLessThanToken", e[e.GreaterThanGreaterThanToken = 48] = "GreaterThanGreaterThanToken", e[e.GreaterThanGreaterThanGreaterThanToken = 49] = "GreaterThanGreaterThanGreaterThanToken", e[e.AmpersandToken = 50] = "AmpersandToken", e[e.BarToken = 51] = "BarToken", e[e.CaretToken = 52] = "CaretToken", e[e.ExclamationToken = 53] = "ExclamationToken", e[e.TildeToken = 54] = "TildeToken", e[e.AmpersandAmpersandToken = 55] = "AmpersandAmpersandToken", e[e.BarBarToken = 56] = "BarBarToken", e[e.QuestionToken = 57] = "QuestionToken", e[e.ColonToken = 58] = "ColonToken", e[e.AtToken = 59] = "AtToken", e[e.QuestionQuestionToken = 60] = "QuestionQuestionToken", e[e.BacktickToken = 61] = "BacktickToken", e[e.HashToken = 62] = "HashToken", e[e.EqualsToken = 63] = "EqualsToken", e[e.PlusEqualsToken = 64] = "PlusEqualsToken", e[e.MinusEqualsToken = 65] = "MinusEqualsToken", e[e.AsteriskEqualsToken = 66] = "AsteriskEqualsToken", e[e.AsteriskAsteriskEqualsToken = 67] = "AsteriskAsteriskEqualsToken", e[e.SlashEqualsToken = 68] = "SlashEqualsToken", e[e.PercentEqualsToken = 69] = "PercentEqualsToken", e[e.LessThanLessThanEqualsToken = 70] = "LessThanLessThanEqualsToken", e[e.GreaterThanGreaterThanEqualsToken = 71] = "GreaterThanGreaterThanEqualsToken", e[e.GreaterThanGreaterThanGreaterThanEqualsToken = 72] = "GreaterThanGreaterThanGreaterThanEqualsToken", e[e.AmpersandEqualsToken = 73] = "AmpersandEqualsToken", e[e.BarEqualsToken = 74] = "BarEqualsToken", e[e.BarBarEqualsToken = 75] = "BarBarEqualsToken", e[e.AmpersandAmpersandEqualsToken = 76] = "AmpersandAmpersandEqualsToken", e[e.QuestionQuestionEqualsToken = 77] = "QuestionQuestionEqualsToken", e[e.CaretEqualsToken = 78] = "CaretEqualsToken", e[e.Identifier = 79] = "Identifier", e[e.PrivateIdentifier = 80] = "PrivateIdentifier", e[e.BreakKeyword = 81] = "BreakKeyword", e[e.CaseKeyword = 82] = "CaseKeyword", e[e.CatchKeyword = 83] = "CatchKeyword", e[e.ClassKeyword = 84] = "ClassKeyword", e[e.ConstKeyword = 85] = "ConstKeyword", e[e.ContinueKeyword = 86] = "ContinueKeyword", e[e.DebuggerKeyword = 87] = "DebuggerKeyword", e[e.DefaultKeyword = 88] = "DefaultKeyword", e[e.DeleteKeyword = 89] = "DeleteKeyword", e[e.DoKeyword = 90] = "DoKeyword", e[e.ElseKeyword = 91] = "ElseKeyword", e[e.EnumKeyword = 92] = "EnumKeyword", e[e.ExportKeyword = 93] = "ExportKeyword", e[e.ExtendsKeyword = 94] = "ExtendsKeyword", e[e.FalseKeyword = 95] = "FalseKeyword", e[e.FinallyKeyword = 96] = "FinallyKeyword", e[e.ForKeyword = 97] = "ForKeyword", e[e.FunctionKeyword = 98] = "FunctionKeyword", e[e.IfKeyword = 99] = "IfKeyword", e[e.ImportKeyword = 100] = "ImportKeyword", e[e.InKeyword = 101] = "InKeyword", e[e.InstanceOfKeyword = 102] = "InstanceOfKeyword", e[e.NewKeyword = 103] = "NewKeyword", e[e.NullKeyword = 104] = "NullKeyword", e[e.ReturnKeyword = 105] = "ReturnKeyword", e[e.SuperKeyword = 106] = "SuperKeyword", e[e.SwitchKeyword = 107] = "SwitchKeyword", e[e.ThisKeyword = 108] = "ThisKeyword", e[e.ThrowKeyword = 109] = "ThrowKeyword", e[e.TrueKeyword = 110] = "TrueKeyword", e[e.TryKeyword = 111] = "TryKeyword", e[e.TypeOfKeyword = 112] = "TypeOfKeyword", e[e.VarKeyword = 113] = "VarKeyword", e[e.VoidKeyword = 114] = "VoidKeyword", e[e.WhileKeyword = 115] = "WhileKeyword", e[e.WithKeyword = 116] = "WithKeyword", e[e.ImplementsKeyword = 117] = "ImplementsKeyword", e[e.InterfaceKeyword = 118] = "InterfaceKeyword", e[e.LetKeyword = 119] = "LetKeyword", e[e.PackageKeyword = 120] = "PackageKeyword", e[e.PrivateKeyword = 121] = "PrivateKeyword", e[e.ProtectedKeyword = 122] = "ProtectedKeyword", e[e.PublicKeyword = 123] = "PublicKeyword", e[e.StaticKeyword = 124] = "StaticKeyword", e[e.YieldKeyword = 125] = "YieldKeyword", e[e.AbstractKeyword = 126] = "AbstractKeyword", e[e.AccessorKeyword = 127] = "AccessorKeyword", e[e.AsKeyword = 128] = "AsKeyword", e[e.AssertsKeyword = 129] = "AssertsKeyword", e[e.AssertKeyword = 130] = "AssertKeyword", e[e.AnyKeyword = 131] = "AnyKeyword", e[e.AsyncKeyword = 132] = "AsyncKeyword", e[e.AwaitKeyword = 133] = "AwaitKeyword", e[e.BooleanKeyword = 134] = "BooleanKeyword", e[e.ConstructorKeyword = 135] = "ConstructorKeyword", e[e.DeclareKeyword = 136] = "DeclareKeyword", e[e.GetKeyword = 137] = "GetKeyword", e[e.InferKeyword = 138] = "InferKeyword", e[e.IntrinsicKeyword = 139] = "IntrinsicKeyword", e[e.IsKeyword = 140] = "IsKeyword", e[e.KeyOfKeyword = 141] = "KeyOfKeyword", e[e.ModuleKeyword = 142] = "ModuleKeyword", e[e.NamespaceKeyword = 143] = "NamespaceKeyword", e[e.NeverKeyword = 144] = "NeverKeyword", e[e.OutKeyword = 145] = "OutKeyword", e[e.ReadonlyKeyword = 146] = "ReadonlyKeyword", e[e.RequireKeyword = 147] = "RequireKeyword", e[e.NumberKeyword = 148] = "NumberKeyword", e[e.ObjectKeyword = 149] = "ObjectKeyword", e[e.SatisfiesKeyword = 150] = "SatisfiesKeyword", e[e.SetKeyword = 151] = "SetKeyword", e[e.StringKeyword = 152] = "StringKeyword", e[e.SymbolKeyword = 153] = "SymbolKeyword", e[e.TypeKeyword = 154] = "TypeKeyword", e[e.UndefinedKeyword = 155] = "UndefinedKeyword", e[e.UniqueKeyword = 156] = "UniqueKeyword", e[e.UnknownKeyword = 157] = "UnknownKeyword", e[e.FromKeyword = 158] = "FromKeyword", e[e.GlobalKeyword = 159] = "GlobalKeyword", e[e.BigIntKeyword = 160] = "BigIntKeyword", e[e.OverrideKeyword = 161] = "OverrideKeyword", e[e.OfKeyword = 162] = "OfKeyword", e[e.QualifiedName = 163] = "QualifiedName", e[e.ComputedPropertyName = 164] = "ComputedPropertyName", e[e.TypeParameter = 165] = "TypeParameter", e[e.Parameter = 166] = "Parameter", e[e.Decorator = 167] = "Decorator", e[e.PropertySignature = 168] = "PropertySignature", e[e.PropertyDeclaration = 169] = "PropertyDeclaration", e[e.MethodSignature = 170] = "MethodSignature", e[e.MethodDeclaration = 171] = "MethodDeclaration", e[e.ClassStaticBlockDeclaration = 172] = "ClassStaticBlockDeclaration", e[e.Constructor = 173] = "Constructor", e[e.GetAccessor = 174] = "GetAccessor", e[e.SetAccessor = 175] = "SetAccessor", e[e.CallSignature = 176] = "CallSignature", e[e.ConstructSignature = 177] = "ConstructSignature", e[e.IndexSignature = 178] = "IndexSignature", e[e.TypePredicate = 179] = "TypePredicate", e[e.TypeReference = 180] = "TypeReference", e[e.FunctionType = 181] = "FunctionType", e[e.ConstructorType = 182] = "ConstructorType", e[e.TypeQuery = 183] = "TypeQuery", e[e.TypeLiteral = 184] = "TypeLiteral", e[e.ArrayType = 185] = "ArrayType", e[e.TupleType = 186] = "TupleType", e[e.OptionalType = 187] = "OptionalType", e[e.RestType = 188] = "RestType", e[e.UnionType = 189] = "UnionType", e[e.IntersectionType = 190] = "IntersectionType", e[e.ConditionalType = 191] = "ConditionalType", e[e.InferType = 192] = "InferType", e[e.ParenthesizedType = 193] = "ParenthesizedType", e[e.ThisType = 194] = "ThisType", e[e.TypeOperator = 195] = "TypeOperator", e[e.IndexedAccessType = 196] = "IndexedAccessType", e[e.MappedType = 197] = "MappedType", e[e.LiteralType = 198] = "LiteralType", e[e.NamedTupleMember = 199] = "NamedTupleMember", e[e.TemplateLiteralType = 200] = "TemplateLiteralType", e[e.TemplateLiteralTypeSpan = 201] = "TemplateLiteralTypeSpan", e[e.ImportType = 202] = "ImportType", e[e.ObjectBindingPattern = 203] = "ObjectBindingPattern", e[e.ArrayBindingPattern = 204] = "ArrayBindingPattern", e[e.BindingElement = 205] = "BindingElement", e[e.ArrayLiteralExpression = 206] = "ArrayLiteralExpression", e[e.ObjectLiteralExpression = 207] = "ObjectLiteralExpression", e[e.PropertyAccessExpression = 208] = "PropertyAccessExpression", e[e.ElementAccessExpression = 209] = "ElementAccessExpression", e[e.CallExpression = 210] = "CallExpression", e[e.NewExpression = 211] = "NewExpression", e[e.TaggedTemplateExpression = 212] = "TaggedTemplateExpression", e[e.TypeAssertionExpression = 213] = "TypeAssertionExpression", e[e.ParenthesizedExpression = 214] = "ParenthesizedExpression", e[e.FunctionExpression = 215] = "FunctionExpression", e[e.ArrowFunction = 216] = "ArrowFunction", e[e.DeleteExpression = 217] = "DeleteExpression", e[e.TypeOfExpression = 218] = "TypeOfExpression", e[e.VoidExpression = 219] = "VoidExpression", e[e.AwaitExpression = 220] = "AwaitExpression", e[e.PrefixUnaryExpression = 221] = "PrefixUnaryExpression", e[e.PostfixUnaryExpression = 222] = "PostfixUnaryExpression", e[e.BinaryExpression = 223] = "BinaryExpression", e[e.ConditionalExpression = 224] = "ConditionalExpression", e[e.TemplateExpression = 225] = "TemplateExpression", e[e.YieldExpression = 226] = "YieldExpression", e[e.SpreadElement = 227] = "SpreadElement", e[e.ClassExpression = 228] = "ClassExpression", e[e.OmittedExpression = 229] = "OmittedExpression", e[e.ExpressionWithTypeArguments = 230] = "ExpressionWithTypeArguments", e[e.AsExpression = 231] = "AsExpression", e[e.NonNullExpression = 232] = "NonNullExpression", e[e.MetaProperty = 233] = "MetaProperty", e[e.SyntheticExpression = 234] = "SyntheticExpression", e[e.SatisfiesExpression = 235] = "SatisfiesExpression", e[e.TemplateSpan = 236] = "TemplateSpan", e[e.SemicolonClassElement = 237] = "SemicolonClassElement", e[e.Block = 238] = "Block", e[e.EmptyStatement = 239] = "EmptyStatement", e[e.VariableStatement = 240] = "VariableStatement", e[e.ExpressionStatement = 241] = "ExpressionStatement", e[e.IfStatement = 242] = "IfStatement", e[e.DoStatement = 243] = "DoStatement", e[e.WhileStatement = 244] = "WhileStatement", e[e.ForStatement = 245] = "ForStatement", e[e.ForInStatement = 246] = "ForInStatement", e[e.ForOfStatement = 247] = "ForOfStatement", e[e.ContinueStatement = 248] = "ContinueStatement", e[e.BreakStatement = 249] = "BreakStatement", e[e.ReturnStatement = 250] = "ReturnStatement", e[e.WithStatement = 251] = "WithStatement", e[e.SwitchStatement = 252] = "SwitchStatement", e[e.LabeledStatement = 253] = "LabeledStatement", e[e.ThrowStatement = 254] = "ThrowStatement", e[e.TryStatement = 255] = "TryStatement", e[e.DebuggerStatement = 256] = "DebuggerStatement", e[e.VariableDeclaration = 257] = "VariableDeclaration", e[e.VariableDeclarationList = 258] = "VariableDeclarationList", e[e.FunctionDeclaration = 259] = "FunctionDeclaration", e[e.ClassDeclaration = 260] = "ClassDeclaration", e[e.InterfaceDeclaration = 261] = "InterfaceDeclaration", e[e.TypeAliasDeclaration = 262] = "TypeAliasDeclaration", e[e.EnumDeclaration = 263] = "EnumDeclaration", e[e.ModuleDeclaration = 264] = "ModuleDeclaration", e[e.ModuleBlock = 265] = "ModuleBlock", e[e.CaseBlock = 266] = "CaseBlock", e[e.NamespaceExportDeclaration = 267] = "NamespaceExportDeclaration", e[e.ImportEqualsDeclaration = 268] = "ImportEqualsDeclaration", e[e.ImportDeclaration = 269] = "ImportDeclaration", e[e.ImportClause = 270] = "ImportClause", e[e.NamespaceImport = 271] = "NamespaceImport", e[e.NamedImports = 272] = "NamedImports", e[e.ImportSpecifier = 273] = "ImportSpecifier", e[e.ExportAssignment = 274] = "ExportAssignment", e[e.ExportDeclaration = 275] = "ExportDeclaration", e[e.NamedExports = 276] = "NamedExports", e[e.NamespaceExport = 277] = "NamespaceExport", e[e.ExportSpecifier = 278] = "ExportSpecifier", e[e.MissingDeclaration = 279] = "MissingDeclaration", e[e.ExternalModuleReference = 280] = "ExternalModuleReference", e[e.JsxElement = 281] = "JsxElement", e[e.JsxSelfClosingElement = 282] = "JsxSelfClosingElement", e[e.JsxOpeningElement = 283] = "JsxOpeningElement", e[e.JsxClosingElement = 284] = "JsxClosingElement", e[e.JsxFragment = 285] = "JsxFragment", e[e.JsxOpeningFragment = 286] = "JsxOpeningFragment", e[e.JsxClosingFragment = 287] = "JsxClosingFragment", e[e.JsxAttribute = 288] = "JsxAttribute", e[e.JsxAttributes = 289] = "JsxAttributes", e[e.JsxSpreadAttribute = 290] = "JsxSpreadAttribute", e[e.JsxExpression = 291] = "JsxExpression", e[e.CaseClause = 292] = "CaseClause", e[e.DefaultClause = 293] = "DefaultClause", e[e.HeritageClause = 294] = "HeritageClause", e[e.CatchClause = 295] = "CatchClause", e[e.AssertClause = 296] = "AssertClause", e[e.AssertEntry = 297] = "AssertEntry", e[e.ImportTypeAssertionContainer = 298] = "ImportTypeAssertionContainer", e[e.PropertyAssignment = 299] = "PropertyAssignment", e[e.ShorthandPropertyAssignment = 300] = "ShorthandPropertyAssignment", e[e.SpreadAssignment = 301] = "SpreadAssignment", e[e.EnumMember = 302] = "EnumMember", e[e.UnparsedPrologue = 303] = "UnparsedPrologue", e[e.UnparsedPrepend = 304] = "UnparsedPrepend", e[e.UnparsedText = 305] = "UnparsedText", e[e.UnparsedInternalText = 306] = "UnparsedInternalText", e[e.UnparsedSyntheticReference = 307] = "UnparsedSyntheticReference", e[e.SourceFile = 308] = "SourceFile", e[e.Bundle = 309] = "Bundle", e[e.UnparsedSource = 310] = "UnparsedSource", e[e.InputFiles = 311] = "InputFiles", e[e.JSDocTypeExpression = 312] = "JSDocTypeExpression", e[e.JSDocNameReference = 313] = "JSDocNameReference", e[e.JSDocMemberName = 314] = "JSDocMemberName", e[e.JSDocAllType = 315] = "JSDocAllType", e[e.JSDocUnknownType = 316] = "JSDocUnknownType", e[e.JSDocNullableType = 317] = "JSDocNullableType", e[e.JSDocNonNullableType = 318] = "JSDocNonNullableType", e[e.JSDocOptionalType = 319] = "JSDocOptionalType", e[e.JSDocFunctionType = 320] = "JSDocFunctionType", e[e.JSDocVariadicType = 321] = "JSDocVariadicType", e[e.JSDocNamepathType = 322] = "JSDocNamepathType", e[e.JSDoc = 323] = "JSDoc", e[e.JSDocComment = 323] = "JSDocComment", e[e.JSDocText = 324] = "JSDocText", e[e.JSDocTypeLiteral = 325] = "JSDocTypeLiteral", e[e.JSDocSignature = 326] = "JSDocSignature", e[e.JSDocLink = 327] = "JSDocLink", e[e.JSDocLinkCode = 328] = "JSDocLinkCode", e[e.JSDocLinkPlain = 329] = "JSDocLinkPlain", e[e.JSDocTag = 330] = "JSDocTag", e[e.JSDocAugmentsTag = 331] = "JSDocAugmentsTag", e[e.JSDocImplementsTag = 332] = "JSDocImplementsTag", e[e.JSDocAuthorTag = 333] = "JSDocAuthorTag", e[e.JSDocDeprecatedTag = 334] = "JSDocDeprecatedTag", e[e.JSDocClassTag = 335] = "JSDocClassTag", e[e.JSDocPublicTag = 336] = "JSDocPublicTag", e[e.JSDocPrivateTag = 337] = "JSDocPrivateTag", e[e.JSDocProtectedTag = 338] = "JSDocProtectedTag", e[e.JSDocReadonlyTag = 339] = "JSDocReadonlyTag", e[e.JSDocOverrideTag = 340] = "JSDocOverrideTag", e[e.JSDocCallbackTag = 341] = "JSDocCallbackTag", e[e.JSDocOverloadTag = 342] = "JSDocOverloadTag", e[e.JSDocEnumTag = 343] = "JSDocEnumTag", e[e.JSDocParameterTag = 344] = "JSDocParameterTag", e[e.JSDocReturnTag = 345] = "JSDocReturnTag", e[e.JSDocThisTag = 346] = "JSDocThisTag", e[e.JSDocTypeTag = 347] = "JSDocTypeTag", e[e.JSDocTemplateTag = 348] = "JSDocTemplateTag", e[e.JSDocTypedefTag = 349] = "JSDocTypedefTag", e[e.JSDocSeeTag = 350] = "JSDocSeeTag", e[e.JSDocPropertyTag = 351] = "JSDocPropertyTag", e[e.JSDocThrowsTag = 352] = "JSDocThrowsTag", e[e.JSDocSatisfiesTag = 353] = "JSDocSatisfiesTag", e[e.SyntaxList = 354] = "SyntaxList", e[e.NotEmittedStatement = 355] = "NotEmittedStatement", e[e.PartiallyEmittedExpression = 356] = "PartiallyEmittedExpression", e[e.CommaListExpression = 357] = "CommaListExpression", e[e.MergeDeclarationMarker = 358] = "MergeDeclarationMarker", e[e.EndOfDeclarationMarker = 359] = "EndOfDeclarationMarker", e[e.SyntheticReferenceExpression = 360] = "SyntheticReferenceExpression", e[e.Count = 361] = "Count", e[e.FirstAssignment = 63] = "FirstAssignment", e[e.LastAssignment = 78] = "LastAssignment", e[e.FirstCompoundAssignment = 64] = "FirstCompoundAssignment", e[e.LastCompoundAssignment = 78] = "LastCompoundAssignment", e[e.FirstReservedWord = 81] = "FirstReservedWord", e[e.LastReservedWord = 116] = "LastReservedWord", e[e.FirstKeyword = 81] = "FirstKeyword", e[e.LastKeyword = 162] = "LastKeyword", e[e.FirstFutureReservedWord = 117] = "FirstFutureReservedWord", e[e.LastFutureReservedWord = 125] = "LastFutureReservedWord", e[e.FirstTypeNode = 179] = "FirstTypeNode", e[e.LastTypeNode = 202] = "LastTypeNode", e[e.FirstPunctuation = 18] = "FirstPunctuation", e[e.LastPunctuation = 78] = "LastPunctuation", e[e.FirstToken = 0] = "FirstToken", e[e.LastToken = 162] = "LastToken", e[e.FirstTriviaToken = 2] = "FirstTriviaToken", e[e.LastTriviaToken = 7] = "LastTriviaToken", e[e.FirstLiteralToken = 8] = "FirstLiteralToken", e[e.LastLiteralToken = 14] = "LastLiteralToken", e[e.FirstTemplateToken = 14] = "FirstTemplateToken", e[e.LastTemplateToken = 17] = "LastTemplateToken", e[e.FirstBinaryOperator = 29] = "FirstBinaryOperator", e[e.LastBinaryOperator = 78] = "LastBinaryOperator", e[e.FirstStatement = 240] = "FirstStatement", e[e.LastStatement = 256] = "LastStatement", e[e.FirstNode = 163] = "FirstNode", e[e.FirstJSDocNode = 312] = "FirstJSDocNode", e[e.LastJSDocNode = 353] = "LastJSDocNode", e[e.FirstJSDocTagNode = 330] = "FirstJSDocTagNode", e[e.LastJSDocTagNode = 353] = "LastJSDocTagNode", e[e.FirstContextualKeyword = 126] = "FirstContextualKeyword", e[e.LastContextualKeyword = 162] = "LastContextualKeyword", e))(Np || {}), Op = ((e) => (e[e.None = 0] = "None", e[e.Let = 1] = "Let", e[e.Const = 2] = "Const", e[e.NestedNamespace = 4] = "NestedNamespace", e[e.Synthesized = 8] = "Synthesized", e[e.Namespace = 16] = "Namespace", e[e.OptionalChain = 32] = "OptionalChain", e[e.ExportContext = 64] = "ExportContext", e[e.ContainsThis = 128] = "ContainsThis", e[e.HasImplicitReturn = 256] = "HasImplicitReturn", e[e.HasExplicitReturn = 512] = "HasExplicitReturn", e[e.GlobalAugmentation = 1024] = "GlobalAugmentation", e[e.HasAsyncFunctions = 2048] = "HasAsyncFunctions", e[e.DisallowInContext = 4096] = "DisallowInContext", e[e.YieldContext = 8192] = "YieldContext", e[e.DecoratorContext = 16384] = "DecoratorContext", e[e.AwaitContext = 32768] = "AwaitContext", e[e.DisallowConditionalTypesContext = 65536] = "DisallowConditionalTypesContext", e[e.ThisNodeHasError = 131072] = "ThisNodeHasError", e[e.JavaScriptFile = 262144] = "JavaScriptFile", e[e.ThisNodeOrAnySubNodesHasError = 524288] = "ThisNodeOrAnySubNodesHasError", e[e.HasAggregatedChildData = 1048576] = "HasAggregatedChildData", e[e.PossiblyContainsDynamicImport = 2097152] = "PossiblyContainsDynamicImport", e[e.PossiblyContainsImportMeta = 4194304] = "PossiblyContainsImportMeta", e[e.JSDoc = 8388608] = "JSDoc", e[e.Ambient = 16777216] = "Ambient", e[e.InWithStatement = 33554432] = "InWithStatement", e[e.JsonFile = 67108864] = "JsonFile", e[e.TypeCached = 134217728] = "TypeCached", e[e.Deprecated = 268435456] = "Deprecated", e[e.BlockScoped = 3] = "BlockScoped", e[e.ReachabilityCheckFlags = 768] = "ReachabilityCheckFlags", e[e.ReachabilityAndEmitFlags = 2816] = "ReachabilityAndEmitFlags", e[e.ContextFlags = 50720768] = "ContextFlags", e[e.TypeExcludesFlags = 40960] = "TypeExcludesFlags", e[e.PermanentlySetIncrementalFlags = 6291456] = "PermanentlySetIncrementalFlags", e[e.IdentifierHasExtendedUnicodeEscape = 128] = "IdentifierHasExtendedUnicodeEscape", e[e.IdentifierIsInJSDocNamespace = 2048] = "IdentifierIsInJSDocNamespace", e))(Op || {}), Mp = ((e) => (e[e.None = 0] = "None", e[e.Export = 1] = "Export", e[e.Ambient = 2] = "Ambient", e[e.Public = 4] = "Public", e[e.Private = 8] = "Private", e[e.Protected = 16] = "Protected", e[e.Static = 32] = "Static", e[e.Readonly = 64] = "Readonly", e[e.Accessor = 128] = "Accessor", e[e.Abstract = 256] = "Abstract", e[e.Async = 512] = "Async", e[e.Default = 1024] = "Default", e[e.Const = 2048] = "Const", e[e.HasComputedJSDocModifiers = 4096] = "HasComputedJSDocModifiers", e[e.Deprecated = 8192] = "Deprecated", e[e.Override = 16384] = "Override", e[e.In = 32768] = "In", e[e.Out = 65536] = "Out", e[e.Decorator = 131072] = "Decorator", e[e.HasComputedFlags = 536870912] = "HasComputedFlags", e[e.AccessibilityModifier = 28] = "AccessibilityModifier", e[e.ParameterPropertyModifier = 16476] = "ParameterPropertyModifier", e[e.NonPublicAccessibilityModifier = 24] = "NonPublicAccessibilityModifier", e[e.TypeScriptModifier = 117086] = "TypeScriptModifier", e[e.ExportDefault = 1025] = "ExportDefault", e[e.All = 258047] = "All", e[e.Modifier = 126975] = "Modifier", e))(Mp || {}), tg = ((e) => (e[e.None = 0] = "None", e[e.IntrinsicNamedElement = 1] = "IntrinsicNamedElement", e[e.IntrinsicIndexedElement = 2] = "IntrinsicIndexedElement", e[e.IntrinsicElement = 3] = "IntrinsicElement", e))(tg || {}), Lp = ((e) => (e[e.Succeeded = 1] = "Succeeded", e[e.Failed = 2] = "Failed", e[e.Reported = 4] = "Reported", e[e.ReportsUnmeasurable = 8] = "ReportsUnmeasurable", e[e.ReportsUnreliable = 16] = "ReportsUnreliable", e[e.ReportsMask = 24] = "ReportsMask", e))(Lp || {}), rg = ((e) => (e[e.None = 0] = "None", e[e.Auto = 1] = "Auto", e[e.Loop = 2] = "Loop", e[e.Unique = 3] = "Unique", e[e.Node = 4] = "Node", e[e.KindMask = 7] = "KindMask", e[e.ReservedInNestedScopes = 8] = "ReservedInNestedScopes", e[e.Optimistic = 16] = "Optimistic", e[e.FileLevel = 32] = "FileLevel", e[e.AllowNameSubstitution = 64] = "AllowNameSubstitution", e))(rg || {}), ng = ((e) => (e[e.None = 0] = "None", e[e.PrecedingLineBreak = 1] = "PrecedingLineBreak", e[e.PrecedingJSDocComment = 2] = "PrecedingJSDocComment", e[e.Unterminated = 4] = "Unterminated", e[e.ExtendedUnicodeEscape = 8] = "ExtendedUnicodeEscape", e[e.Scientific = 16] = "Scientific", e[e.Octal = 32] = "Octal", e[e.HexSpecifier = 64] = "HexSpecifier", e[e.BinarySpecifier = 128] = "BinarySpecifier", e[e.OctalSpecifier = 256] = "OctalSpecifier", e[e.ContainsSeparator = 512] = "ContainsSeparator", e[e.UnicodeEscape = 1024] = "UnicodeEscape", e[e.ContainsInvalidEscape = 2048] = "ContainsInvalidEscape", e[e.BinaryOrOctalSpecifier = 384] = "BinaryOrOctalSpecifier", e[e.NumericLiteralFlags = 1008] = "NumericLiteralFlags", e[e.TemplateLiteralLikeFlags = 2048] = "TemplateLiteralLikeFlags", e))(ng || {}), il = ((e) => (e[e.Unreachable = 1] = "Unreachable", e[e.Start = 2] = "Start", e[e.BranchLabel = 4] = "BranchLabel", e[e.LoopLabel = 8] = "LoopLabel", e[e.Assignment = 16] = "Assignment", e[e.TrueCondition = 32] = "TrueCondition", e[e.FalseCondition = 64] = "FalseCondition", e[e.SwitchClause = 128] = "SwitchClause", e[e.ArrayMutation = 256] = "ArrayMutation", e[e.Call = 512] = "Call", e[e.ReduceLabel = 1024] = "ReduceLabel", e[e.Referenced = 2048] = "Referenced", e[e.Shared = 4096] = "Shared", e[e.Label = 12] = "Label", e[e.Condition = 96] = "Condition", e))(il || {}), ig = ((e) => (e[e.ExpectError = 0] = "ExpectError", e[e.Ignore = 1] = "Ignore", e))(ig || {}), Rp = class {
}, ag = ((e) => (e[e.RootFile = 0] = "RootFile", e[e.SourceFromProjectReference = 1] = "SourceFromProjectReference", e[e.OutputFromProjectReference = 2] = "OutputFromProjectReference", e[e.Import = 3] = "Import", e[e.ReferenceFile = 4] = "ReferenceFile", e[e.TypeReferenceDirective = 5] = "TypeReferenceDirective", e[e.LibFile = 6] = "LibFile", e[e.LibReferenceDirective = 7] = "LibReferenceDirective", e[e.AutomaticTypeDirectiveFile = 8] = "AutomaticTypeDirectiveFile", e))(ag || {}), sg = ((e) => (e[e.FilePreprocessingReferencedDiagnostic = 0] = "FilePreprocessingReferencedDiagnostic", e[e.FilePreprocessingFileExplainingDiagnostic = 1] = "FilePreprocessingFileExplainingDiagnostic", e[e.ResolutionDiagnostics = 2] = "ResolutionDiagnostics", e))(sg || {}), og = ((e) => (e[e.Js = 0] = "Js", e[e.Dts = 1] = "Dts", e))(og || {}), _g = ((e) => (e[e.Not = 0] = "Not", e[e.SafeModules = 1] = "SafeModules", e[e.Completely = 2] = "Completely", e))(_g || {}), cg = ((e) => (e[e.Success = 0] = "Success", e[e.DiagnosticsPresent_OutputsSkipped = 1] = "DiagnosticsPresent_OutputsSkipped", e[e.DiagnosticsPresent_OutputsGenerated = 2] = "DiagnosticsPresent_OutputsGenerated", e[e.InvalidProject_OutputsSkipped = 3] = "InvalidProject_OutputsSkipped", e[e.ProjectReferenceCycle_OutputsSkipped = 4] = "ProjectReferenceCycle_OutputsSkipped", e))(cg || {}), lg = ((e) => (e[e.Ok = 0] = "Ok", e[e.NeedsOverride = 1] = "NeedsOverride", e[e.HasInvalidOverride = 2] = "HasInvalidOverride", e))(lg || {}), ug = ((e) => (e[e.None = 0] = "None", e[e.Literal = 1] = "Literal", e[e.Subtype = 2] = "Subtype", e))(ug || {}), pg = ((e) => (e[e.None = 0] = "None", e[e.Signature = 1] = "Signature", e[e.NoConstraints = 2] = "NoConstraints", e[e.Completions = 4] = "Completions", e[e.SkipBindingPatterns = 8] = "SkipBindingPatterns", e))(pg || {}), fg = ((e) => (e[e.None = 0] = "None", e[e.NoTruncation = 1] = "NoTruncation", e[e.WriteArrayAsGenericType = 2] = "WriteArrayAsGenericType", e[e.GenerateNamesForShadowedTypeParams = 4] = "GenerateNamesForShadowedTypeParams", e[e.UseStructuralFallback = 8] = "UseStructuralFallback", e[e.ForbidIndexedAccessSymbolReferences = 16] = "ForbidIndexedAccessSymbolReferences", e[e.WriteTypeArgumentsOfSignature = 32] = "WriteTypeArgumentsOfSignature", e[e.UseFullyQualifiedType = 64] = "UseFullyQualifiedType", e[e.UseOnlyExternalAliasing = 128] = "UseOnlyExternalAliasing", e[e.SuppressAnyReturnType = 256] = "SuppressAnyReturnType", e[e.WriteTypeParametersInQualifiedName = 512] = "WriteTypeParametersInQualifiedName", e[e.MultilineObjectLiterals = 1024] = "MultilineObjectLiterals", e[e.WriteClassExpressionAsTypeLiteral = 2048] = "WriteClassExpressionAsTypeLiteral", e[e.UseTypeOfFunction = 4096] = "UseTypeOfFunction", e[e.OmitParameterModifiers = 8192] = "OmitParameterModifiers", e[e.UseAliasDefinedOutsideCurrentScope = 16384] = "UseAliasDefinedOutsideCurrentScope", e[e.UseSingleQuotesForStringLiteralType = 268435456] = "UseSingleQuotesForStringLiteralType", e[e.NoTypeReduction = 536870912] = "NoTypeReduction", e[e.OmitThisParameter = 33554432] = "OmitThisParameter", e[e.AllowThisInObjectLiteral = 32768] = "AllowThisInObjectLiteral", e[e.AllowQualifiedNameInPlaceOfIdentifier = 65536] = "AllowQualifiedNameInPlaceOfIdentifier", e[e.AllowAnonymousIdentifier = 131072] = "AllowAnonymousIdentifier", e[e.AllowEmptyUnionOrIntersection = 262144] = "AllowEmptyUnionOrIntersection", e[e.AllowEmptyTuple = 524288] = "AllowEmptyTuple", e[e.AllowUniqueESSymbolType = 1048576] = "AllowUniqueESSymbolType", e[e.AllowEmptyIndexInfoType = 2097152] = "AllowEmptyIndexInfoType", e[e.WriteComputedProps = 1073741824] = "WriteComputedProps", e[e.AllowNodeModulesRelativePaths = 67108864] = "AllowNodeModulesRelativePaths", e[e.DoNotIncludeSymbolChain = 134217728] = "DoNotIncludeSymbolChain", e[e.IgnoreErrors = 70221824] = "IgnoreErrors", e[e.InObjectTypeLiteral = 4194304] = "InObjectTypeLiteral", e[e.InTypeAlias = 8388608] = "InTypeAlias", e[e.InInitialEntityName = 16777216] = "InInitialEntityName", e))(fg || {}), dg = ((e) => (e[e.None = 0] = "None", e[e.NoTruncation = 1] = "NoTruncation", e[e.WriteArrayAsGenericType = 2] = "WriteArrayAsGenericType", e[e.UseStructuralFallback = 8] = "UseStructuralFallback", e[e.WriteTypeArgumentsOfSignature = 32] = "WriteTypeArgumentsOfSignature", e[e.UseFullyQualifiedType = 64] = "UseFullyQualifiedType", e[e.SuppressAnyReturnType = 256] = "SuppressAnyReturnType", e[e.MultilineObjectLiterals = 1024] = "MultilineObjectLiterals", e[e.WriteClassExpressionAsTypeLiteral = 2048] = "WriteClassExpressionAsTypeLiteral", e[e.UseTypeOfFunction = 4096] = "UseTypeOfFunction", e[e.OmitParameterModifiers = 8192] = "OmitParameterModifiers", e[e.UseAliasDefinedOutsideCurrentScope = 16384] = "UseAliasDefinedOutsideCurrentScope", e[e.UseSingleQuotesForStringLiteralType = 268435456] = "UseSingleQuotesForStringLiteralType", e[e.NoTypeReduction = 536870912] = "NoTypeReduction", e[e.OmitThisParameter = 33554432] = "OmitThisParameter", e[e.AllowUniqueESSymbolType = 1048576] = "AllowUniqueESSymbolType", e[e.AddUndefined = 131072] = "AddUndefined", e[e.WriteArrowStyleSignature = 262144] = "WriteArrowStyleSignature", e[e.InArrayType = 524288] = "InArrayType", e[e.InElementType = 2097152] = "InElementType", e[e.InFirstTypeArgument = 4194304] = "InFirstTypeArgument", e[e.InTypeAlias = 8388608] = "InTypeAlias", e[e.NodeBuilderFlagsMask = 848330091] = "NodeBuilderFlagsMask", e))(dg || {}), mg = ((e) => (e[e.None = 0] = "None", e[e.WriteTypeParametersOrArguments = 1] = "WriteTypeParametersOrArguments", e[e.UseOnlyExternalAliasing = 2] = "UseOnlyExternalAliasing", e[e.AllowAnyNodeKind = 4] = "AllowAnyNodeKind", e[e.UseAliasDefinedOutsideCurrentScope = 8] = "UseAliasDefinedOutsideCurrentScope", e[e.WriteComputedProps = 16] = "WriteComputedProps", e[e.DoNotIncludeSymbolChain = 32] = "DoNotIncludeSymbolChain", e))(mg || {}), hg = ((e) => (e[e.Accessible = 0] = "Accessible", e[e.NotAccessible = 1] = "NotAccessible", e[e.CannotBeNamed = 2] = "CannotBeNamed", e))(hg || {}), gg = ((e) => (e[e.UnionOrIntersection = 0] = "UnionOrIntersection", e[e.Spread = 1] = "Spread", e))(gg || {}), yg = ((e) => (e[e.This = 0] = "This", e[e.Identifier = 1] = "Identifier", e[e.AssertsThis = 2] = "AssertsThis", e[e.AssertsIdentifier = 3] = "AssertsIdentifier", e))(yg || {}), vg = ((e) => (e[e.Unknown = 0] = "Unknown", e[e.TypeWithConstructSignatureAndValue = 1] = "TypeWithConstructSignatureAndValue", e[e.VoidNullableOrNeverType = 2] = "VoidNullableOrNeverType", e[e.NumberLikeType = 3] = "NumberLikeType", e[e.BigIntLikeType = 4] = "BigIntLikeType", e[e.StringLikeType = 5] = "StringLikeType", e[e.BooleanType = 6] = "BooleanType", e[e.ArrayLikeType = 7] = "ArrayLikeType", e[e.ESSymbolType = 8] = "ESSymbolType", e[e.Promise = 9] = "Promise", e[e.TypeWithCallSignature = 10] = "TypeWithCallSignature", e[e.ObjectType = 11] = "ObjectType", e))(vg || {}), jp = ((e) => (e[e.None = 0] = "None", e[e.FunctionScopedVariable = 1] = "FunctionScopedVariable", e[e.BlockScopedVariable = 2] = "BlockScopedVariable", e[e.Property = 4] = "Property", e[e.EnumMember = 8] = "EnumMember", e[e.Function = 16] = "Function", e[e.Class = 32] = "Class", e[e.Interface = 64] = "Interface", e[e.ConstEnum = 128] = "ConstEnum", e[e.RegularEnum = 256] = "RegularEnum", e[e.ValueModule = 512] = "ValueModule", e[e.NamespaceModule = 1024] = "NamespaceModule", e[e.TypeLiteral = 2048] = "TypeLiteral", e[e.ObjectLiteral = 4096] = "ObjectLiteral", e[e.Method = 8192] = "Method", e[e.Constructor = 16384] = "Constructor", e[e.GetAccessor = 32768] = "GetAccessor", e[e.SetAccessor = 65536] = "SetAccessor", e[e.Signature = 131072] = "Signature", e[e.TypeParameter = 262144] = "TypeParameter", e[e.TypeAlias = 524288] = "TypeAlias", e[e.ExportValue = 1048576] = "ExportValue", e[e.Alias = 2097152] = "Alias", e[e.Prototype = 4194304] = "Prototype", e[e.ExportStar = 8388608] = "ExportStar", e[e.Optional = 16777216] = "Optional", e[e.Transient = 33554432] = "Transient", e[e.Assignment = 67108864] = "Assignment", e[e.ModuleExports = 134217728] = "ModuleExports", e[e.All = 67108863] = "All", e[e.Enum = 384] = "Enum", e[e.Variable = 3] = "Variable", e[e.Value = 111551] = "Value", e[e.Type = 788968] = "Type", e[e.Namespace = 1920] = "Namespace", e[e.Module = 1536] = "Module", e[e.Accessor = 98304] = "Accessor", e[e.FunctionScopedVariableExcludes = 111550] = "FunctionScopedVariableExcludes", e[e.BlockScopedVariableExcludes = 111551] = "BlockScopedVariableExcludes", e[e.ParameterExcludes = 111551] = "ParameterExcludes", e[e.PropertyExcludes = 0] = "PropertyExcludes", e[e.EnumMemberExcludes = 900095] = "EnumMemberExcludes", e[e.FunctionExcludes = 110991] = "FunctionExcludes", e[e.ClassExcludes = 899503] = "ClassExcludes", e[e.InterfaceExcludes = 788872] = "InterfaceExcludes", e[e.RegularEnumExcludes = 899327] = "RegularEnumExcludes", e[e.ConstEnumExcludes = 899967] = "ConstEnumExcludes", e[e.ValueModuleExcludes = 110735] = "ValueModuleExcludes", e[e.NamespaceModuleExcludes = 0] = "NamespaceModuleExcludes", e[e.MethodExcludes = 103359] = "MethodExcludes", e[e.GetAccessorExcludes = 46015] = "GetAccessorExcludes", e[e.SetAccessorExcludes = 78783] = "SetAccessorExcludes", e[e.AccessorExcludes = 13247] = "AccessorExcludes", e[e.TypeParameterExcludes = 526824] = "TypeParameterExcludes", e[e.TypeAliasExcludes = 788968] = "TypeAliasExcludes", e[e.AliasExcludes = 2097152] = "AliasExcludes", e[e.ModuleMember = 2623475] = "ModuleMember", e[e.ExportHasLocal = 944] = "ExportHasLocal", e[e.BlockScoped = 418] = "BlockScoped", e[e.PropertyOrAccessor = 98308] = "PropertyOrAccessor", e[e.ClassMember = 106500] = "ClassMember", e[e.ExportSupportsDefaultModifier = 112] = "ExportSupportsDefaultModifier", e[e.ExportDoesNotSupportDefaultModifier = -113] = "ExportDoesNotSupportDefaultModifier", e[e.Classifiable = 2885600] = "Classifiable", e[e.LateBindingContainer = 6256] = "LateBindingContainer", e))(jp || {}), bg = ((e) => (e[e.Numeric = 0] = "Numeric", e[e.Literal = 1] = "Literal", e))(bg || {}), Tg = ((e) => (e[e.None = 0] = "None", e[e.Instantiated = 1] = "Instantiated", e[e.SyntheticProperty = 2] = "SyntheticProperty", e[e.SyntheticMethod = 4] = "SyntheticMethod", e[e.Readonly = 8] = "Readonly", e[e.ReadPartial = 16] = "ReadPartial", e[e.WritePartial = 32] = "WritePartial", e[e.HasNonUniformType = 64] = "HasNonUniformType", e[e.HasLiteralType = 128] = "HasLiteralType", e[e.ContainsPublic = 256] = "ContainsPublic", e[e.ContainsProtected = 512] = "ContainsProtected", e[e.ContainsPrivate = 1024] = "ContainsPrivate", e[e.ContainsStatic = 2048] = "ContainsStatic", e[e.Late = 4096] = "Late", e[e.ReverseMapped = 8192] = "ReverseMapped", e[e.OptionalParameter = 16384] = "OptionalParameter", e[e.RestParameter = 32768] = "RestParameter", e[e.DeferredType = 65536] = "DeferredType", e[e.HasNeverType = 131072] = "HasNeverType", e[e.Mapped = 262144] = "Mapped", e[e.StripOptional = 524288] = "StripOptional", e[e.Unresolved = 1048576] = "Unresolved", e[e.Synthetic = 6] = "Synthetic", e[e.Discriminant = 192] = "Discriminant", e[e.Partial = 48] = "Partial", e))(Tg || {}), Sg = ((e) => (e.Call = "__call", e.Constructor = "__constructor", e.New = "__new", e.Index = "__index", e.ExportStar = "__export", e.Global = "__global", e.Missing = "__missing", e.Type = "__type", e.Object = "__object", e.JSXAttributes = "__jsxAttributes", e.Class = "__class", e.Function = "__function", e.Computed = "__computed", e.Resolving = "__resolving__", e.ExportEquals = "export=", e.Default = "default", e.This = "this", e))(Sg || {}), xg = ((e) => (e[e.None = 0] = "None", e[e.TypeChecked = 1] = "TypeChecked", e[e.LexicalThis = 2] = "LexicalThis", e[e.CaptureThis = 4] = "CaptureThis", e[e.CaptureNewTarget = 8] = "CaptureNewTarget", e[e.SuperInstance = 16] = "SuperInstance", e[e.SuperStatic = 32] = "SuperStatic", e[e.ContextChecked = 64] = "ContextChecked", e[e.MethodWithSuperPropertyAccessInAsync = 128] = "MethodWithSuperPropertyAccessInAsync", e[e.MethodWithSuperPropertyAssignmentInAsync = 256] = "MethodWithSuperPropertyAssignmentInAsync", e[e.CaptureArguments = 512] = "CaptureArguments", e[e.EnumValuesComputed = 1024] = "EnumValuesComputed", e[e.LexicalModuleMergesWithClass = 2048] = "LexicalModuleMergesWithClass", e[e.LoopWithCapturedBlockScopedBinding = 4096] = "LoopWithCapturedBlockScopedBinding", e[e.ContainsCapturedBlockScopeBinding = 8192] = "ContainsCapturedBlockScopeBinding", e[e.CapturedBlockScopedBinding = 16384] = "CapturedBlockScopedBinding", e[e.BlockScopedBindingInLoop = 32768] = "BlockScopedBindingInLoop", e[e.ClassWithBodyScopedClassBinding = 65536] = "ClassWithBodyScopedClassBinding", e[e.BodyScopedClassBinding = 131072] = "BodyScopedClassBinding", e[e.NeedsLoopOutParameter = 262144] = "NeedsLoopOutParameter", e[e.AssignmentsMarked = 524288] = "AssignmentsMarked", e[e.ClassWithConstructorReference = 1048576] = "ClassWithConstructorReference", e[e.ConstructorReferenceInClass = 2097152] = "ConstructorReferenceInClass", e[e.ContainsClassWithPrivateIdentifiers = 4194304] = "ContainsClassWithPrivateIdentifiers", e[e.ContainsSuperPropertyInStaticInitializer = 8388608] = "ContainsSuperPropertyInStaticInitializer", e[e.InCheckIdentifier = 16777216] = "InCheckIdentifier", e))(xg || {}), Jp = ((e) => (e[e.Any = 1] = "Any", e[e.Unknown = 2] = "Unknown", e[e.String = 4] = "String", e[e.Number = 8] = "Number", e[e.Boolean = 16] = "Boolean", e[e.Enum = 32] = "Enum", e[e.BigInt = 64] = "BigInt", e[e.StringLiteral = 128] = "StringLiteral", e[e.NumberLiteral = 256] = "NumberLiteral", e[e.BooleanLiteral = 512] = "BooleanLiteral", e[e.EnumLiteral = 1024] = "EnumLiteral", e[e.BigIntLiteral = 2048] = "BigIntLiteral", e[e.ESSymbol = 4096] = "ESSymbol", e[e.UniqueESSymbol = 8192] = "UniqueESSymbol", e[e.Void = 16384] = "Void", e[e.Undefined = 32768] = "Undefined", e[e.Null = 65536] = "Null", e[e.Never = 131072] = "Never", e[e.TypeParameter = 262144] = "TypeParameter", e[e.Object = 524288] = "Object", e[e.Union = 1048576] = "Union", e[e.Intersection = 2097152] = "Intersection", e[e.Index = 4194304] = "Index", e[e.IndexedAccess = 8388608] = "IndexedAccess", e[e.Conditional = 16777216] = "Conditional", e[e.Substitution = 33554432] = "Substitution", e[e.NonPrimitive = 67108864] = "NonPrimitive", e[e.TemplateLiteral = 134217728] = "TemplateLiteral", e[e.StringMapping = 268435456] = "StringMapping", e[e.AnyOrUnknown = 3] = "AnyOrUnknown", e[e.Nullable = 98304] = "Nullable", e[e.Literal = 2944] = "Literal", e[e.Unit = 109472] = "Unit", e[e.Freshable = 2976] = "Freshable", e[e.StringOrNumberLiteral = 384] = "StringOrNumberLiteral", e[e.StringOrNumberLiteralOrUnique = 8576] = "StringOrNumberLiteralOrUnique", e[e.DefinitelyFalsy = 117632] = "DefinitelyFalsy", e[e.PossiblyFalsy = 117724] = "PossiblyFalsy", e[e.Intrinsic = 67359327] = "Intrinsic", e[e.Primitive = 134348796] = "Primitive", e[e.StringLike = 402653316] = "StringLike", e[e.NumberLike = 296] = "NumberLike", e[e.BigIntLike = 2112] = "BigIntLike", e[e.BooleanLike = 528] = "BooleanLike", e[e.EnumLike = 1056] = "EnumLike", e[e.ESSymbolLike = 12288] = "ESSymbolLike", e[e.VoidLike = 49152] = "VoidLike", e[e.DefinitelyNonNullable = 470302716] = "DefinitelyNonNullable", e[e.DisjointDomains = 469892092] = "DisjointDomains", e[e.UnionOrIntersection = 3145728] = "UnionOrIntersection", e[e.StructuredType = 3670016] = "StructuredType", e[e.TypeVariable = 8650752] = "TypeVariable", e[e.InstantiableNonPrimitive = 58982400] = "InstantiableNonPrimitive", e[e.InstantiablePrimitive = 406847488] = "InstantiablePrimitive", e[e.Instantiable = 465829888] = "Instantiable", e[e.StructuredOrInstantiable = 469499904] = "StructuredOrInstantiable", e[e.ObjectFlagsType = 3899393] = "ObjectFlagsType", e[e.Simplifiable = 25165824] = "Simplifiable", e[e.Singleton = 67358815] = "Singleton", e[e.Narrowable = 536624127] = "Narrowable", e[e.IncludesMask = 205258751] = "IncludesMask", e[e.IncludesMissingType = 262144] = "IncludesMissingType", e[e.IncludesNonWideningType = 4194304] = "IncludesNonWideningType", e[e.IncludesWildcard = 8388608] = "IncludesWildcard", e[e.IncludesEmptyObject = 16777216] = "IncludesEmptyObject", e[e.IncludesInstantiable = 33554432] = "IncludesInstantiable", e[e.NotPrimitiveUnion = 36323363] = "NotPrimitiveUnion", e))(Jp || {}), Fp = ((e) => (e[e.None = 0] = "None", e[e.Class = 1] = "Class", e[e.Interface = 2] = "Interface", e[e.Reference = 4] = "Reference", e[e.Tuple = 8] = "Tuple", e[e.Anonymous = 16] = "Anonymous", e[e.Mapped = 32] = "Mapped", e[e.Instantiated = 64] = "Instantiated", e[e.ObjectLiteral = 128] = "ObjectLiteral", e[e.EvolvingArray = 256] = "EvolvingArray", e[e.ObjectLiteralPatternWithComputedProperties = 512] = "ObjectLiteralPatternWithComputedProperties", e[e.ReverseMapped = 1024] = "ReverseMapped", e[e.JsxAttributes = 2048] = "JsxAttributes", e[e.JSLiteral = 4096] = "JSLiteral", e[e.FreshLiteral = 8192] = "FreshLiteral", e[e.ArrayLiteral = 16384] = "ArrayLiteral", e[e.PrimitiveUnion = 32768] = "PrimitiveUnion", e[e.ContainsWideningType = 65536] = "ContainsWideningType", e[e.ContainsObjectOrArrayLiteral = 131072] = "ContainsObjectOrArrayLiteral", e[e.NonInferrableType = 262144] = "NonInferrableType", e[e.CouldContainTypeVariablesComputed = 524288] = "CouldContainTypeVariablesComputed", e[e.CouldContainTypeVariables = 1048576] = "CouldContainTypeVariables", e[e.ClassOrInterface = 3] = "ClassOrInterface", e[e.RequiresWidening = 196608] = "RequiresWidening", e[e.PropagatingFlags = 458752] = "PropagatingFlags", e[e.ObjectTypeKindMask = 1343] = "ObjectTypeKindMask", e[e.ContainsSpread = 2097152] = "ContainsSpread", e[e.ObjectRestType = 4194304] = "ObjectRestType", e[e.InstantiationExpressionType = 8388608] = "InstantiationExpressionType", e[e.IsClassInstanceClone = 16777216] = "IsClassInstanceClone", e[e.IdenticalBaseTypeCalculated = 33554432] = "IdenticalBaseTypeCalculated", e[e.IdenticalBaseTypeExists = 67108864] = "IdenticalBaseTypeExists", e[e.IsGenericTypeComputed = 2097152] = "IsGenericTypeComputed", e[e.IsGenericObjectType = 4194304] = "IsGenericObjectType", e[e.IsGenericIndexType = 8388608] = "IsGenericIndexType", e[e.IsGenericType = 12582912] = "IsGenericType", e[e.ContainsIntersections = 16777216] = "ContainsIntersections", e[e.IsUnknownLikeUnionComputed = 33554432] = "IsUnknownLikeUnionComputed", e[e.IsUnknownLikeUnion = 67108864] = "IsUnknownLikeUnion", e[e.IsNeverIntersectionComputed = 16777216] = "IsNeverIntersectionComputed", e[e.IsNeverIntersection = 33554432] = "IsNeverIntersection", e))(Fp || {}), Eg = ((e) => (e[e.Invariant = 0] = "Invariant", e[e.Covariant = 1] = "Covariant", e[e.Contravariant = 2] = "Contravariant", e[e.Bivariant = 3] = "Bivariant", e[e.Independent = 4] = "Independent", e[e.VarianceMask = 7] = "VarianceMask", e[e.Unmeasurable = 8] = "Unmeasurable", e[e.Unreliable = 16] = "Unreliable", e[e.AllowsStructuralFallback = 24] = "AllowsStructuralFallback", e))(Eg || {}), wg = ((e) => (e[e.Required = 1] = "Required", e[e.Optional = 2] = "Optional", e[e.Rest = 4] = "Rest", e[e.Variadic = 8] = "Variadic", e[e.Fixed = 3] = "Fixed", e[e.Variable = 12] = "Variable", e[e.NonRequired = 14] = "NonRequired", e[e.NonRest = 11] = "NonRest", e))(wg || {}), Cg = ((e) => (e[e.None = 0] = "None", e[e.IncludeUndefined = 1] = "IncludeUndefined", e[e.NoIndexSignatures = 2] = "NoIndexSignatures", e[e.Writing = 4] = "Writing", e[e.CacheSymbol = 8] = "CacheSymbol", e[e.NoTupleBoundsCheck = 16] = "NoTupleBoundsCheck", e[e.ExpressionPosition = 32] = "ExpressionPosition", e[e.ReportDeprecated = 64] = "ReportDeprecated", e[e.SuppressNoImplicitAnyError = 128] = "SuppressNoImplicitAnyError", e[e.Contextual = 256] = "Contextual", e[e.Persistent = 1] = "Persistent", e))(Cg || {}), Ag = ((e) => (e[e.Component = 0] = "Component", e[e.Function = 1] = "Function", e[e.Mixed = 2] = "Mixed", e))(Ag || {}), Pg = ((e) => (e[e.Call = 0] = "Call", e[e.Construct = 1] = "Construct", e))(Pg || {}), Bp = ((e) => (e[e.None = 0] = "None", e[e.HasRestParameter = 1] = "HasRestParameter", e[e.HasLiteralTypes = 2] = "HasLiteralTypes", e[e.Abstract = 4] = "Abstract", e[e.IsInnerCallChain = 8] = "IsInnerCallChain", e[e.IsOuterCallChain = 16] = "IsOuterCallChain", e[e.IsUntypedSignatureInJSFile = 32] = "IsUntypedSignatureInJSFile", e[e.PropagatingFlags = 39] = "PropagatingFlags", e[e.CallChainFlags = 24] = "CallChainFlags", e))(Bp || {}), Dg = ((e) => (e[e.String = 0] = "String", e[e.Number = 1] = "Number", e))(Dg || {}), kg = ((e) => (e[e.Simple = 0] = "Simple", e[e.Array = 1] = "Array", e[e.Deferred = 2] = "Deferred", e[e.Function = 3] = "Function", e[e.Composite = 4] = "Composite", e[e.Merged = 5] = "Merged", e))(kg || {}), Ig = ((e) => (e[e.None = 0] = "None", e[e.NakedTypeVariable = 1] = "NakedTypeVariable", e[e.SpeculativeTuple = 2] = "SpeculativeTuple", e[e.SubstituteSource = 4] = "SubstituteSource", e[e.HomomorphicMappedType = 8] = "HomomorphicMappedType", e[e.PartialHomomorphicMappedType = 16] = "PartialHomomorphicMappedType", e[e.MappedTypeConstraint = 32] = "MappedTypeConstraint", e[e.ContravariantConditional = 64] = "ContravariantConditional", e[e.ReturnType = 128] = "ReturnType", e[e.LiteralKeyof = 256] = "LiteralKeyof", e[e.NoConstraints = 512] = "NoConstraints", e[e.AlwaysStrict = 1024] = "AlwaysStrict", e[e.MaxValue = 2048] = "MaxValue", e[e.PriorityImpliesCombination = 416] = "PriorityImpliesCombination", e[e.Circularity = -1] = "Circularity", e))(Ig || {}), Ng = ((e) => (e[e.None = 0] = "None", e[e.NoDefault = 1] = "NoDefault", e[e.AnyDefault = 2] = "AnyDefault", e[e.SkippedGenericFunction = 4] = "SkippedGenericFunction", e))(Ng || {}), Og = ((e) => (e[e.False = 0] = "False", e[e.Unknown = 1] = "Unknown", e[e.Maybe = 3] = "Maybe", e[e.True = -1] = "True", e))(Og || {}), Mg = ((e) => (e[e.None = 0] = "None", e[e.ExportsProperty = 1] = "ExportsProperty", e[e.ModuleExports = 2] = "ModuleExports", e[e.PrototypeProperty = 3] = "PrototypeProperty", e[e.ThisProperty = 4] = "ThisProperty", e[e.Property = 5] = "Property", e[e.Prototype = 6] = "Prototype", e[e.ObjectDefinePropertyValue = 7] = "ObjectDefinePropertyValue", e[e.ObjectDefinePropertyExports = 8] = "ObjectDefinePropertyExports", e[e.ObjectDefinePrototypeProperty = 9] = "ObjectDefinePrototypeProperty", e))(Mg || {}), qp = ((e) => (e[e.Warning = 0] = "Warning", e[e.Error = 1] = "Error", e[e.Suggestion = 2] = "Suggestion", e[e.Message = 3] = "Message", e))(qp || {}), Lg = ((e) => (e[e.Classic = 1] = "Classic", e[e.NodeJs = 2] = "NodeJs", e[e.Node10 = 2] = "Node10", e[e.Node16 = 3] = "Node16", e[e.NodeNext = 99] = "NodeNext", e[e.Bundler = 100] = "Bundler", e))(Lg || {}), Rg = ((e) => (e[e.Legacy = 1] = "Legacy", e[e.Auto = 2] = "Auto", e[e.Force = 3] = "Force", e))(Rg || {}), jg = ((e) => (e[e.FixedPollingInterval = 0] = "FixedPollingInterval", e[e.PriorityPollingInterval = 1] = "PriorityPollingInterval", e[e.DynamicPriorityPolling = 2] = "DynamicPriorityPolling", e[e.FixedChunkSizePolling = 3] = "FixedChunkSizePolling", e[e.UseFsEvents = 4] = "UseFsEvents", e[e.UseFsEventsOnParentDirectory = 5] = "UseFsEventsOnParentDirectory", e))(jg || {}), Jg = ((e) => (e[e.UseFsEvents = 0] = "UseFsEvents", e[e.FixedPollingInterval = 1] = "FixedPollingInterval", e[e.DynamicPriorityPolling = 2] = "DynamicPriorityPolling", e[e.FixedChunkSizePolling = 3] = "FixedChunkSizePolling", e))(Jg || {}), Fg = ((e) => (e[e.FixedInterval = 0] = "FixedInterval", e[e.PriorityInterval = 1] = "PriorityInterval", e[e.DynamicPriority = 2] = "DynamicPriority", e[e.FixedChunkSize = 3] = "FixedChunkSize", e))(Fg || {}), Bg = ((e) => (e[e.None = 0] = "None", e[e.CommonJS = 1] = "CommonJS", e[e.AMD = 2] = "AMD", e[e.UMD = 3] = "UMD", e[e.System = 4] = "System", e[e.ES2015 = 5] = "ES2015", e[e.ES2020 = 6] = "ES2020", e[e.ES2022 = 7] = "ES2022", e[e.ESNext = 99] = "ESNext", e[e.Node16 = 100] = "Node16", e[e.NodeNext = 199] = "NodeNext", e))(Bg || {}), qg = ((e) => (e[e.None = 0] = "None", e[e.Preserve = 1] = "Preserve", e[e.React = 2] = "React", e[e.ReactNative = 3] = "ReactNative", e[e.ReactJSX = 4] = "ReactJSX", e[e.ReactJSXDev = 5] = "ReactJSXDev", e))(qg || {}), Ug = ((e) => (e[e.Remove = 0] = "Remove", e[e.Preserve = 1] = "Preserve", e[e.Error = 2] = "Error", e))(Ug || {}), zg = ((e) => (e[e.CarriageReturnLineFeed = 0] = "CarriageReturnLineFeed", e[e.LineFeed = 1] = "LineFeed", e))(zg || {}), Wg = ((e) => (e[e.Unknown = 0] = "Unknown", e[e.JS = 1] = "JS", e[e.JSX = 2] = "JSX", e[e.TS = 3] = "TS", e[e.TSX = 4] = "TSX", e[e.External = 5] = "External", e[e.JSON = 6] = "JSON", e[e.Deferred = 7] = "Deferred", e))(Wg || {}), Vg = ((e) => (e[e.ES3 = 0] = "ES3", e[e.ES5 = 1] = "ES5", e[e.ES2015 = 2] = "ES2015", e[e.ES2016 = 3] = "ES2016", e[e.ES2017 = 4] = "ES2017", e[e.ES2018 = 5] = "ES2018", e[e.ES2019 = 6] = "ES2019", e[e.ES2020 = 7] = "ES2020", e[e.ES2021 = 8] = "ES2021", e[e.ES2022 = 9] = "ES2022", e[e.ESNext = 99] = "ESNext", e[e.JSON = 100] = "JSON", e[e.Latest = 99] = "Latest", e))(Vg || {}), Hg = ((e) => (e[e.Standard = 0] = "Standard", e[e.JSX = 1] = "JSX", e))(Hg || {}), Gg = ((e) => (e[e.None = 0] = "None", e[e.Recursive = 1] = "Recursive", e))(Gg || {}), $g = ((e) => (e[e.nullCharacter = 0] = "nullCharacter", e[e.maxAsciiCharacter = 127] = "maxAsciiCharacter", e[e.lineFeed = 10] = "lineFeed", e[e.carriageReturn = 13] = "carriageReturn", e[e.lineSeparator = 8232] = "lineSeparator", e[e.paragraphSeparator = 8233] = "paragraphSeparator", e[e.nextLine = 133] = "nextLine", e[e.space = 32] = "space", e[e.nonBreakingSpace = 160] = "nonBreakingSpace", e[e.enQuad = 8192] = "enQuad", e[e.emQuad = 8193] = "emQuad", e[e.enSpace = 8194] = "enSpace", e[e.emSpace = 8195] = "emSpace", e[e.threePerEmSpace = 8196] = "threePerEmSpace", e[e.fourPerEmSpace = 8197] = "fourPerEmSpace", e[e.sixPerEmSpace = 8198] = "sixPerEmSpace", e[e.figureSpace = 8199] = "figureSpace", e[e.punctuationSpace = 8200] = "punctuationSpace", e[e.thinSpace = 8201] = "thinSpace", e[e.hairSpace = 8202] = "hairSpace", e[e.zeroWidthSpace = 8203] = "zeroWidthSpace", e[e.narrowNoBreakSpace = 8239] = "narrowNoBreakSpace", e[e.ideographicSpace = 12288] = "ideographicSpace", e[e.mathematicalSpace = 8287] = "mathematicalSpace", e[e.ogham = 5760] = "ogham", e[e._ = 95] = "_", e[e.$ = 36] = "$", e[e._0 = 48] = "_0", e[e._1 = 49] = "_1", e[e._2 = 50] = "_2", e[e._3 = 51] = "_3", e[e._4 = 52] = "_4", e[e._5 = 53] = "_5", e[e._6 = 54] = "_6", e[e._7 = 55] = "_7", e[e._8 = 56] = "_8", e[e._9 = 57] = "_9", e[e.a = 97] = "a", e[e.b = 98] = "b", e[e.c = 99] = "c", e[e.d = 100] = "d", e[e.e = 101] = "e", e[e.f = 102] = "f", e[e.g = 103] = "g", e[e.h = 104] = "h", e[e.i = 105] = "i", e[e.j = 106] = "j", e[e.k = 107] = "k", e[e.l = 108] = "l", e[e.m = 109] = "m", e[e.n = 110] = "n", e[e.o = 111] = "o", e[e.p = 112] = "p", e[e.q = 113] = "q", e[e.r = 114] = "r", e[e.s = 115] = "s", e[e.t = 116] = "t", e[e.u = 117] = "u", e[e.v = 118] = "v", e[e.w = 119] = "w", e[e.x = 120] = "x", e[e.y = 121] = "y", e[e.z = 122] = "z", e[e.A = 65] = "A", e[e.B = 66] = "B", e[e.C = 67] = "C", e[e.D = 68] = "D", e[e.E = 69] = "E", e[e.F = 70] = "F", e[e.G = 71] = "G", e[e.H = 72] = "H", e[e.I = 73] = "I", e[e.J = 74] = "J", e[e.K = 75] = "K", e[e.L = 76] = "L", e[e.M = 77] = "M", e[e.N = 78] = "N", e[e.O = 79] = "O", e[e.P = 80] = "P", e[e.Q = 81] = "Q", e[e.R = 82] = "R", e[e.S = 83] = "S", e[e.T = 84] = "T", e[e.U = 85] = "U", e[e.V = 86] = "V", e[e.W = 87] = "W", e[e.X = 88] = "X", e[e.Y = 89] = "Y", e[e.Z = 90] = "Z", e[e.ampersand = 38] = "ampersand", e[e.asterisk = 42] = "asterisk", e[e.at = 64] = "at", e[e.backslash = 92] = "backslash", e[e.backtick = 96] = "backtick", e[e.bar = 124] = "bar", e[e.caret = 94] = "caret", e[e.closeBrace = 125] = "closeBrace", e[e.closeBracket = 93] = "closeBracket", e[e.closeParen = 41] = "closeParen", e[e.colon = 58] = "colon", e[e.comma = 44] = "comma", e[e.dot = 46] = "dot", e[e.doubleQuote = 34] = "doubleQuote", e[e.equals = 61] = "equals", e[e.exclamation = 33] = "exclamation", e[e.greaterThan = 62] = "greaterThan", e[e.hash = 35] = "hash", e[e.lessThan = 60] = "lessThan", e[e.minus = 45] = "minus", e[e.openBrace = 123] = "openBrace", e[e.openBracket = 91] = "openBracket", e[e.openParen = 40] = "openParen", e[e.percent = 37] = "percent", e[e.plus = 43] = "plus", e[e.question = 63] = "question", e[e.semicolon = 59] = "semicolon", e[e.singleQuote = 39] = "singleQuote", e[e.slash = 47] = "slash", e[e.tilde = 126] = "tilde", e[e.backspace = 8] = "backspace", e[e.formFeed = 12] = "formFeed", e[e.byteOrderMark = 65279] = "byteOrderMark", e[e.tab = 9] = "tab", e[e.verticalTab = 11] = "verticalTab", e))($g || {}), Kg = ((e) => (e.Ts = ".ts", e.Tsx = ".tsx", e.Dts = ".d.ts", e.Js = ".js", e.Jsx = ".jsx", e.Json = ".json", e.TsBuildInfo = ".tsbuildinfo", e.Mjs = ".mjs", e.Mts = ".mts", e.Dmts = ".d.mts", e.Cjs = ".cjs", e.Cts = ".cts", e.Dcts = ".d.cts", e))(Kg || {}), Up = ((e) => (e[e.None = 0] = "None", e[e.ContainsTypeScript = 1] = "ContainsTypeScript", e[e.ContainsJsx = 2] = "ContainsJsx", e[e.ContainsESNext = 4] = "ContainsESNext", e[e.ContainsES2022 = 8] = "ContainsES2022", e[e.ContainsES2021 = 16] = "ContainsES2021", e[e.ContainsES2020 = 32] = "ContainsES2020", e[e.ContainsES2019 = 64] = "ContainsES2019", e[e.ContainsES2018 = 128] = "ContainsES2018", e[e.ContainsES2017 = 256] = "ContainsES2017", e[e.ContainsES2016 = 512] = "ContainsES2016", e[e.ContainsES2015 = 1024] = "ContainsES2015", e[e.ContainsGenerator = 2048] = "ContainsGenerator", e[e.ContainsDestructuringAssignment = 4096] = "ContainsDestructuringAssignment", e[e.ContainsTypeScriptClassSyntax = 8192] = "ContainsTypeScriptClassSyntax", e[e.ContainsLexicalThis = 16384] = "ContainsLexicalThis", e[e.ContainsRestOrSpread = 32768] = "ContainsRestOrSpread", e[e.ContainsObjectRestOrSpread = 65536] = "ContainsObjectRestOrSpread", e[e.ContainsComputedPropertyName = 131072] = "ContainsComputedPropertyName", e[e.ContainsBlockScopedBinding = 262144] = "ContainsBlockScopedBinding", e[e.ContainsBindingPattern = 524288] = "ContainsBindingPattern", e[e.ContainsYield = 1048576] = "ContainsYield", e[e.ContainsAwait = 2097152] = "ContainsAwait", e[e.ContainsHoistedDeclarationOrCompletion = 4194304] = "ContainsHoistedDeclarationOrCompletion", e[e.ContainsDynamicImport = 8388608] = "ContainsDynamicImport", e[e.ContainsClassFields = 16777216] = "ContainsClassFields", e[e.ContainsDecorators = 33554432] = "ContainsDecorators", e[e.ContainsPossibleTopLevelAwait = 67108864] = "ContainsPossibleTopLevelAwait", e[e.ContainsLexicalSuper = 134217728] = "ContainsLexicalSuper", e[e.ContainsUpdateExpressionForIdentifier = 268435456] = "ContainsUpdateExpressionForIdentifier", e[e.ContainsPrivateIdentifierInExpression = 536870912] = "ContainsPrivateIdentifierInExpression", e[e.HasComputedFlags = -2147483648] = "HasComputedFlags", e[e.AssertTypeScript = 1] = "AssertTypeScript", e[e.AssertJsx = 2] = "AssertJsx", e[e.AssertESNext = 4] = "AssertESNext", e[e.AssertES2022 = 8] = "AssertES2022", e[e.AssertES2021 = 16] = "AssertES2021", e[e.AssertES2020 = 32] = "AssertES2020", e[e.AssertES2019 = 64] = "AssertES2019", e[e.AssertES2018 = 128] = "AssertES2018", e[e.AssertES2017 = 256] = "AssertES2017", e[e.AssertES2016 = 512] = "AssertES2016", e[e.AssertES2015 = 1024] = "AssertES2015", e[e.AssertGenerator = 2048] = "AssertGenerator", e[e.AssertDestructuringAssignment = 4096] = "AssertDestructuringAssignment", e[e.OuterExpressionExcludes = -2147483648] = "OuterExpressionExcludes", e[e.PropertyAccessExcludes = -2147483648] = "PropertyAccessExcludes", e[e.NodeExcludes = -2147483648] = "NodeExcludes", e[e.ArrowFunctionExcludes = -2072174592] = "ArrowFunctionExcludes", e[e.FunctionExcludes = -1937940480] = "FunctionExcludes", e[e.ConstructorExcludes = -1937948672] = "ConstructorExcludes", e[e.MethodOrAccessorExcludes = -2005057536] = "MethodOrAccessorExcludes", e[e.PropertyExcludes = -2013249536] = "PropertyExcludes", e[e.ClassExcludes = -2147344384] = "ClassExcludes", e[e.ModuleExcludes = -1941676032] = "ModuleExcludes", e[e.TypeExcludes = -2] = "TypeExcludes", e[e.ObjectLiteralExcludes = -2147278848] = "ObjectLiteralExcludes", e[e.ArrayLiteralOrCallOrNewExcludes = -2147450880] = "ArrayLiteralOrCallOrNewExcludes", e[e.VariableDeclarationListExcludes = -2146893824] = "VariableDeclarationListExcludes", e[e.ParameterExcludes = -2147483648] = "ParameterExcludes", e[e.CatchClauseExcludes = -2147418112] = "CatchClauseExcludes", e[e.BindingPatternExcludes = -2147450880] = "BindingPatternExcludes", e[e.ContainsLexicalThisOrSuper = 134234112] = "ContainsLexicalThisOrSuper", e[e.PropertyNamePropagatingFlags = 134234112] = "PropertyNamePropagatingFlags", e))(Up || {}), zp = ((e) => (e[e.TabStop = 0] = "TabStop", e[e.Placeholder = 1] = "Placeholder", e[e.Choice = 2] = "Choice", e[e.Variable = 3] = "Variable", e))(zp || {}), Wp = ((e) => (e[e.None = 0] = "None", e[e.SingleLine = 1] = "SingleLine", e[e.MultiLine = 2] = "MultiLine", e[e.AdviseOnEmitNode = 4] = "AdviseOnEmitNode", e[e.NoSubstitution = 8] = "NoSubstitution", e[e.CapturesThis = 16] = "CapturesThis", e[e.NoLeadingSourceMap = 32] = "NoLeadingSourceMap", e[e.NoTrailingSourceMap = 64] = "NoTrailingSourceMap", e[e.NoSourceMap = 96] = "NoSourceMap", e[e.NoNestedSourceMaps = 128] = "NoNestedSourceMaps", e[e.NoTokenLeadingSourceMaps = 256] = "NoTokenLeadingSourceMaps", e[e.NoTokenTrailingSourceMaps = 512] = "NoTokenTrailingSourceMaps", e[e.NoTokenSourceMaps = 768] = "NoTokenSourceMaps", e[e.NoLeadingComments = 1024] = "NoLeadingComments", e[e.NoTrailingComments = 2048] = "NoTrailingComments", e[e.NoComments = 3072] = "NoComments", e[e.NoNestedComments = 4096] = "NoNestedComments", e[e.HelperName = 8192] = "HelperName", e[e.ExportName = 16384] = "ExportName", e[e.LocalName = 32768] = "LocalName", e[e.InternalName = 65536] = "InternalName", e[e.Indented = 131072] = "Indented", e[e.NoIndentation = 262144] = "NoIndentation", e[e.AsyncFunctionBody = 524288] = "AsyncFunctionBody", e[e.ReuseTempVariableScope = 1048576] = "ReuseTempVariableScope", e[e.CustomPrologue = 2097152] = "CustomPrologue", e[e.NoHoisting = 4194304] = "NoHoisting", e[e.HasEndOfDeclarationMarker = 8388608] = "HasEndOfDeclarationMarker", e[e.Iterator = 16777216] = "Iterator", e[e.NoAsciiEscaping = 33554432] = "NoAsciiEscaping", e))(Wp || {}), Xg = ((e) => (e[e.None = 0] = "None", e[e.TypeScriptClassWrapper = 1] = "TypeScriptClassWrapper", e[e.NeverApplyImportHelper = 2] = "NeverApplyImportHelper", e[e.IgnoreSourceNewlines = 4] = "IgnoreSourceNewlines", e[e.Immutable = 8] = "Immutable", e[e.IndirectCall = 16] = "IndirectCall", e[e.TransformPrivateStaticElements = 32] = "TransformPrivateStaticElements", e))(Xg || {}), Yg = ((e) => (e[e.Extends = 1] = "Extends", e[e.Assign = 2] = "Assign", e[e.Rest = 4] = "Rest", e[e.Decorate = 8] = "Decorate", e[e.ESDecorateAndRunInitializers = 8] = "ESDecorateAndRunInitializers", e[e.Metadata = 16] = "Metadata", e[e.Param = 32] = "Param", e[e.Awaiter = 64] = "Awaiter", e[e.Generator = 128] = "Generator", e[e.Values = 256] = "Values", e[e.Read = 512] = "Read", e[e.SpreadArray = 1024] = "SpreadArray", e[e.Await = 2048] = "Await", e[e.AsyncGenerator = 4096] = "AsyncGenerator", e[e.AsyncDelegator = 8192] = "AsyncDelegator", e[e.AsyncValues = 16384] = "AsyncValues", e[e.ExportStar = 32768] = "ExportStar", e[e.ImportStar = 65536] = "ImportStar", e[e.ImportDefault = 131072] = "ImportDefault", e[e.MakeTemplateObject = 262144] = "MakeTemplateObject", e[e.ClassPrivateFieldGet = 524288] = "ClassPrivateFieldGet", e[e.ClassPrivateFieldSet = 1048576] = "ClassPrivateFieldSet", e[e.ClassPrivateFieldIn = 2097152] = "ClassPrivateFieldIn", e[e.CreateBinding = 4194304] = "CreateBinding", e[e.SetFunctionName = 8388608] = "SetFunctionName", e[e.PropKey = 16777216] = "PropKey", e[e.FirstEmitHelper = 1] = "FirstEmitHelper", e[e.LastEmitHelper = 16777216] = "LastEmitHelper", e[e.ForOfIncludes = 256] = "ForOfIncludes", e[e.ForAwaitOfIncludes = 16384] = "ForAwaitOfIncludes", e[e.AsyncGeneratorIncludes = 6144] = "AsyncGeneratorIncludes", e[e.AsyncDelegatorIncludes = 26624] = "AsyncDelegatorIncludes", e[e.SpreadIncludes = 1536] = "SpreadIncludes", e))(Yg || {}), Qg = ((e) => (e[e.SourceFile = 0] = "SourceFile", e[e.Expression = 1] = "Expression", e[e.IdentifierName = 2] = "IdentifierName", e[e.MappedTypeParameter = 3] = "MappedTypeParameter", e[e.Unspecified = 4] = "Unspecified", e[e.EmbeddedStatement = 5] = "EmbeddedStatement", e[e.JsxAttributeValue = 6] = "JsxAttributeValue", e))(Qg || {}), Zg = ((e) => (e[e.Parentheses = 1] = "Parentheses", e[e.TypeAssertions = 2] = "TypeAssertions", e[e.NonNullAssertions = 4] = "NonNullAssertions", e[e.PartiallyEmittedExpressions = 8] = "PartiallyEmittedExpressions", e[e.Assertions = 6] = "Assertions", e[e.All = 15] = "All", e[e.ExcludeJSDocTypeAssertion = 16] = "ExcludeJSDocTypeAssertion", e))(Zg || {}), ey = ((e) => (e[e.None = 0] = "None", e[e.InParameters = 1] = "InParameters", e[e.VariablesHoistedInParameters = 2] = "VariablesHoistedInParameters", e))(ey || {}), ty = ((e) => (e.Prologue = "prologue", e.EmitHelpers = "emitHelpers", e.NoDefaultLib = "no-default-lib", e.Reference = "reference", e.Type = "type", e.TypeResolutionModeRequire = "type-require", e.TypeResolutionModeImport = "type-import", e.Lib = "lib", e.Prepend = "prepend", e.Text = "text", e.Internal = "internal", e))(ty || {}), ry = ((e) => (e[e.None = 0] = "None", e[e.SingleLine = 0] = "SingleLine", e[e.MultiLine = 1] = "MultiLine", e[e.PreserveLines = 2] = "PreserveLines", e[e.LinesMask = 3] = "LinesMask", e[e.NotDelimited = 0] = "NotDelimited", e[e.BarDelimited = 4] = "BarDelimited", e[e.AmpersandDelimited = 8] = "AmpersandDelimited", e[e.CommaDelimited = 16] = "CommaDelimited", e[e.AsteriskDelimited = 32] = "AsteriskDelimited", e[e.DelimitersMask = 60] = "DelimitersMask", e[e.AllowTrailingComma = 64] = "AllowTrailingComma", e[e.Indented = 128] = "Indented", e[e.SpaceBetweenBraces = 256] = "SpaceBetweenBraces", e[e.SpaceBetweenSiblings = 512] = "SpaceBetweenSiblings", e[e.Braces = 1024] = "Braces", e[e.Parenthesis = 2048] = "Parenthesis", e[e.AngleBrackets = 4096] = "AngleBrackets", e[e.SquareBrackets = 8192] = "SquareBrackets", e[e.BracketsMask = 15360] = "BracketsMask", e[e.OptionalIfUndefined = 16384] = "OptionalIfUndefined", e[e.OptionalIfEmpty = 32768] = "OptionalIfEmpty", e[e.Optional = 49152] = "Optional", e[e.PreferNewLine = 65536] = "PreferNewLine", e[e.NoTrailingNewLine = 131072] = "NoTrailingNewLine", e[e.NoInterveningComments = 262144] = "NoInterveningComments", e[e.NoSpaceIfEmpty = 524288] = "NoSpaceIfEmpty", e[e.SingleElement = 1048576] = "SingleElement", e[e.SpaceAfterList = 2097152] = "SpaceAfterList", e[e.Modifiers = 2359808] = "Modifiers", e[e.HeritageClauses = 512] = "HeritageClauses", e[e.SingleLineTypeLiteralMembers = 768] = "SingleLineTypeLiteralMembers", e[e.MultiLineTypeLiteralMembers = 32897] = "MultiLineTypeLiteralMembers", e[e.SingleLineTupleTypeElements = 528] = "SingleLineTupleTypeElements", e[e.MultiLineTupleTypeElements = 657] = "MultiLineTupleTypeElements", e[e.UnionTypeConstituents = 516] = "UnionTypeConstituents", e[e.IntersectionTypeConstituents = 520] = "IntersectionTypeConstituents", e[e.ObjectBindingPatternElements = 525136] = "ObjectBindingPatternElements", e[e.ArrayBindingPatternElements = 524880] = "ArrayBindingPatternElements", e[e.ObjectLiteralExpressionProperties = 526226] = "ObjectLiteralExpressionProperties", e[e.ImportClauseEntries = 526226] = "ImportClauseEntries", e[e.ArrayLiteralExpressionElements = 8914] = "ArrayLiteralExpressionElements", e[e.CommaListElements = 528] = "CommaListElements", e[e.CallExpressionArguments = 2576] = "CallExpressionArguments", e[e.NewExpressionArguments = 18960] = "NewExpressionArguments", e[e.TemplateExpressionSpans = 262144] = "TemplateExpressionSpans", e[e.SingleLineBlockStatements = 768] = "SingleLineBlockStatements", e[e.MultiLineBlockStatements = 129] = "MultiLineBlockStatements", e[e.VariableDeclarationList = 528] = "VariableDeclarationList", e[e.SingleLineFunctionBodyStatements = 768] = "SingleLineFunctionBodyStatements", e[e.MultiLineFunctionBodyStatements = 1] = "MultiLineFunctionBodyStatements", e[e.ClassHeritageClauses = 0] = "ClassHeritageClauses", e[e.ClassMembers = 129] = "ClassMembers", e[e.InterfaceMembers = 129] = "InterfaceMembers", e[e.EnumMembers = 145] = "EnumMembers", e[e.CaseBlockClauses = 129] = "CaseBlockClauses", e[e.NamedImportsOrExportsElements = 525136] = "NamedImportsOrExportsElements", e[e.JsxElementOrFragmentChildren = 262144] = "JsxElementOrFragmentChildren", e[e.JsxElementAttributes = 262656] = "JsxElementAttributes", e[e.CaseOrDefaultClauseStatements = 163969] = "CaseOrDefaultClauseStatements", e[e.HeritageClauseTypes = 528] = "HeritageClauseTypes", e[e.SourceFileStatements = 131073] = "SourceFileStatements", e[e.Decorators = 2146305] = "Decorators", e[e.TypeArguments = 53776] = "TypeArguments", e[e.TypeParameters = 53776] = "TypeParameters", e[e.Parameters = 2576] = "Parameters", e[e.IndexSignatureParameters = 8848] = "IndexSignatureParameters", e[e.JSDocComment = 33] = "JSDocComment", e))(ry || {}), ny = ((e) => (e[e.None = 0] = "None", e[e.TripleSlashXML = 1] = "TripleSlashXML", e[e.SingleLine = 2] = "SingleLine", e[e.MultiLine = 4] = "MultiLine", e[e.All = 7] = "All", e[e.Default = 7] = "Default", e))(ny || {}), Vp = { reference: { args: [{ name: "types", optional: true, captureSpan: true }, { name: "lib", optional: true, captureSpan: true }, { name: "path", optional: true, captureSpan: true }, { name: "no-default-lib", optional: true }, { name: "resolution-mode", optional: true }], kind: 1 }, "amd-dependency": { args: [{ name: "path" }, { name: "name", optional: true }], kind: 1 }, "amd-module": { args: [{ name: "name" }], kind: 1 }, "ts-check": { kind: 2 }, "ts-nocheck": { kind: 2 }, jsx: { args: [{ name: "factory" }], kind: 4 }, jsxfrag: { args: [{ name: "factory" }], kind: 4 }, jsximportsource: { args: [{ name: "factory" }], kind: 4 }, jsxruntime: { args: [{ name: "factory" }], kind: 4 } };
} }), W5 = () => {
}, iy;
function ay(e) {
return e === 47 || e === 92;
}
function V5(e) {
return al(e) < 0;
}
function A_(e) {
return al(e) > 0;
}
function H5(e) {
let t = al(e);
return t > 0 && t === e.length;
}
function sy(e) {
return al(e) !== 0;
}
function So(e) {
return /^\.\.?($|[\\/])/.test(e);
}
function G5(e) {
return !sy(e) && !So(e);
}
function OT(e) {
return Fi(sl(e), ".");
}
function ns(e, t) {
return e.length > t.length && es(e, t);
}
function da(e, t) {
for (let r of t)
if (ns(e, r))
return true;
return false;
}
function Hp(e) {
return e.length > 0 && ay(e.charCodeAt(e.length - 1));
}
function MT(e) {
return e >= 97 && e <= 122 || e >= 65 && e <= 90;
}
function $5(e, t) {
let r = e.charCodeAt(t);
if (r === 58)
return t + 1;
if (r === 37 && e.charCodeAt(t + 1) === 51) {
let s = e.charCodeAt(t + 2);
if (s === 97 || s === 65)
return t + 3;
}
return -1;
}
function al(e) {
if (!e)
return 0;
let t = e.charCodeAt(0);
if (t === 47 || t === 92) {
if (e.charCodeAt(1) !== t)
return 1;
let s = e.indexOf(t === 47 ? zn : py, 2);
return s < 0 ? e.length : s + 1;
}
if (MT(t) && e.charCodeAt(1) === 58) {
let s = e.charCodeAt(2);
if (s === 47 || s === 92)
return 3;
if (e.length === 2)
return 2;
}
let r = e.indexOf(fy);
if (r !== -1) {
let s = r + fy.length, f = e.indexOf(zn, s);
if (f !== -1) {
let x = e.slice(0, r), w = e.slice(s, f);
if (x === "file" && (w === "" || w === "localhost") && MT(e.charCodeAt(f + 1))) {
let A = $5(e, f + 2);
if (A !== -1) {
if (e.charCodeAt(A) === 47)
return ~(A + 1);
if (A === e.length)
return ~A;
}
}
return ~(f + 1);
}
return ~e.length;
}
return 0;
}
function Bi(e) {
let t = al(e);
return t < 0 ? ~t : t;
}
function ma(e) {
e = Eo(e);
let t = Bi(e);
return t === e.length ? e : (e = P_(e), e.slice(0, Math.max(t, e.lastIndexOf(zn))));
}
function sl(e, t, r) {
if (e = Eo(e), Bi(e) === e.length)
return "";
e = P_(e);
let f = e.slice(Math.max(Bi(e), e.lastIndexOf(zn) + 1)), x = t !== void 0 && r !== void 0 ? Gp(f, t, r) : void 0;
return x ? f.slice(0, f.length - x.length) : f;
}
function LT(e, t, r) {
if (Pn(t, ".") || (t = "." + t), e.length >= t.length && e.charCodeAt(e.length - t.length) === 46) {
let s = e.slice(e.length - t.length);
if (r(s, t))
return s;
}
}
function K5(e, t, r) {
if (typeof t == "string")
return LT(e, t, r) || "";
for (let s of t) {
let f = LT(e, s, r);
if (f)
return f;
}
return "";
}
function Gp(e, t, r) {
if (t)
return K5(P_(e), t, r ? Ms : To);
let s = sl(e), f = s.lastIndexOf(".");
return f >= 0 ? s.substring(f) : "";
}
function X5(e, t) {
let r = e.substring(0, t), s = e.substring(t).split(zn);
return s.length && !Cn(s) && s.pop(), [r, ...s];
}
function qi(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : "";
return e = tn(t, e), X5(e, Bi(e));
}
function xo(e) {
return e.length === 0 ? "" : (e[0] && wo(e[0])) + e.slice(1).join(zn);
}
function Eo(e) {
return e.indexOf("\\") !== -1 ? e.replace(BT, zn) : e;
}
function is(e) {
if (!Ke(e))
return [];
let t = [e[0]];
for (let r = 1; r < e.length; r++) {
let s = e[r];
if (s && s !== ".") {
if (s === "..") {
if (t.length > 1) {
if (t[t.length - 1] !== "..") {
t.pop();
continue;
}
} else if (t[0])
continue;
}
t.push(s);
}
}
return t;
}
function tn(e) {
e && (e = Eo(e));
for (var t = arguments.length, r = new Array(t > 1 ? t - 1 : 0), s = 1; s < t; s++)
r[s - 1] = arguments[s];
for (let f of r)
f && (f = Eo(f), !e || Bi(f) !== 0 ? e = f : e = wo(e) + f);
return e;
}
function oy(e) {
for (var t = arguments.length, r = new Array(t > 1 ? t - 1 : 0), s = 1; s < t; s++)
r[s - 1] = arguments[s];
return Un(Ke(r) ? tn(e, ...r) : Eo(e));
}
function $p(e, t) {
return is(qi(e, t));
}
function as(e, t) {
return xo($p(e, t));
}
function Un(e) {
if (e = Eo(e), !ol.test(e))
return e;
let t = e.replace(/\/\.\//g, "/").replace(/^\.\//, "");
if (t !== e && (e = t, !ol.test(e)))
return e;
let r = xo(is(qi(e)));
return r && Hp(e) ? wo(r) : r;
}
function Y5(e) {
return e.length === 0 ? "" : e.slice(1).join(zn);
}
function Q5(e, t) {
return Y5($p(e, t));
}
function Ui(e, t, r) {
let s = A_(e) ? Un(e) : as(e, t);
return r(s);
}
function P_(e) {
return Hp(e) ? e.substr(0, e.length - 1) : e;
}
function wo(e) {
return Hp(e) ? e : e + zn;
}
function _y(e) {
return !sy(e) && !So(e) ? "./" + e : e;
}
function RT(e, t, r, s) {
let f = r !== void 0 && s !== void 0 ? Gp(e, r, s) : Gp(e);
return f ? e.slice(0, e.length - f.length) + (Pn(t, ".") ? t : "." + t) : e;
}
function cy(e, t, r) {
if (e === t)
return 0;
if (e === void 0)
return -1;
if (t === void 0)
return 1;
let s = e.substring(0, Bi(e)), f = t.substring(0, Bi(t)), x = C_(s, f);
if (x !== 0)
return x;
let w = e.substring(s.length), A = t.substring(f.length);
if (!ol.test(w) && !ol.test(A))
return r(w, A);
let g = is(qi(e)), B = is(qi(t)), N = Math.min(g.length, B.length);
for (let X = 1; X < N; X++) {
let F = r(g[X], B[X]);
if (F !== 0)
return F;
}
return Vr(g.length, B.length);
}
function Z5(e, t) {
return cy(e, t, ri);
}
function eA(e, t) {
return cy(e, t, C_);
}
function tA(e, t, r, s) {
return typeof r == "string" ? (e = tn(r, e), t = tn(r, t)) : typeof r == "boolean" && (s = r), cy(e, t, rl(s));
}
function jT(e, t, r, s) {
if (typeof r == "string" ? (e = tn(r, e), t = tn(r, t)) : typeof r == "boolean" && (s = r), e === void 0 || t === void 0)
return false;
if (e === t)
return true;
let f = is(qi(e)), x = is(qi(t));
if (x.length < f.length)
return false;
let w = s ? Ms : To;
for (let A = 0; A < f.length; A++)
if (!(A === 0 ? Ms : w)(f[A], x[A]))
return false;
return true;
}
function rA(e, t, r) {
let s = r(e), f = r(t);
return Pn(s, f + "/") || Pn(s, f + "\\");
}
function ly(e, t, r, s) {
let f = is(qi(e)), x = is(qi(t)), w;
for (w = 0; w < f.length && w < x.length; w++) {
let B = s(f[w]), N = s(x[w]);
if (!(w === 0 ? Ms : r)(B, N))
break;
}
if (w === 0)
return x;
let A = x.slice(w), g = [];
for (; w < f.length; w++)
g.push("..");
return ["", ...g, ...A];
}
function JT(e, t, r) {
Y.assert(Bi(e) > 0 == Bi(t) > 0, "Paths must either both be absolute or both be relative");
let x = ly(e, t, (typeof r == "boolean" ? r : false) ? Ms : To, typeof r == "function" ? r : rr);
return xo(x);
}
function nA(e, t, r) {
return A_(e) ? uy(t, e, t, r, false) : e;
}
function iA(e, t, r) {
return _y(JT(ma(e), t, r));
}
function uy(e, t, r, s, f) {
let x = ly(oy(r, e), oy(r, t), To, s), w = x[0];
if (f && A_(w)) {
let A = w.charAt(0) === zn ? "file://" : "file:///";
x[0] = A + w;
}
return xo(x);
}
function FT(e, t) {
for (; ; ) {
let r = t(e);
if (r !== void 0)
return r;
let s = ma(e);
if (s === e)
return;
e = s;
}
}
function aA(e) {
return es(e, "/node_modules");
}
var zn, py, fy, BT, ol, sA = D({ "src/compiler/path.ts"() {
"use strict";
nn(), zn = "/", py = "\\", fy = "://", BT = /\\/g, ol = /(?:\/\/)|(?:^|\/)\.\.?(?:$|\/)/;
} });
function i(e, t, r, s, f, x, w) {
return { code: e, category: t, key: r, message: s, reportsUnnecessary: f, elidedInCompatabilityPyramid: x, reportsDeprecated: w };
}
var ve, oA = D({ "src/compiler/diagnosticInformationMap.generated.ts"() {
"use strict";
NT(), ve = { Unterminated_string_literal: i(1002, 1, "Unterminated_string_literal_1002", "Unterminated string literal."), Identifier_expected: i(1003, 1, "Identifier_expected_1003", "Identifier expected."), _0_expected: i(1005, 1, "_0_expected_1005", "'{0}' expected."), A_file_cannot_have_a_reference_to_itself: i(1006, 1, "A_file_cannot_have_a_reference_to_itself_1006", "A file cannot have a reference to itself."), The_parser_expected_to_find_a_1_to_match_the_0_token_here: i(1007, 1, "The_parser_expected_to_find_a_1_to_match_the_0_token_here_1007", "The parser expected to find a '{1}' to match the '{0}' token here."), Trailing_comma_not_allowed: i(1009, 1, "Trailing_comma_not_allowed_1009", "Trailing comma not allowed."), Asterisk_Slash_expected: i(1010, 1, "Asterisk_Slash_expected_1010", "'*/' expected."), An_element_access_expression_should_take_an_argument: i(1011, 1, "An_element_access_expression_should_take_an_argument_1011", "An element access expression should take an argument."), Unexpected_token: i(1012, 1, "Unexpected_token_1012", "Unexpected token."), A_rest_parameter_or_binding_pattern_may_not_have_a_trailing_comma: i(1013, 1, "A_rest_parameter_or_binding_pattern_may_not_have_a_trailing_comma_1013", "A rest parameter or binding pattern may not have a trailing comma."), A_rest_parameter_must_be_last_in_a_parameter_list: i(1014, 1, "A_rest_parameter_must_be_last_in_a_parameter_list_1014", "A rest parameter must be last in a parameter list."), Parameter_cannot_have_question_mark_and_initializer: i(1015, 1, "Parameter_cannot_have_question_mark_and_initializer_1015", "Parameter cannot have question mark and initializer."), A_required_parameter_cannot_follow_an_optional_parameter: i(1016, 1, "A_required_parameter_cannot_follow_an_optional_parameter_1016", "A required parameter cannot follow an optional parameter."), An_index_signature_cannot_have_a_rest_parameter: i(1017, 1, "An_index_signature_cannot_have_a_rest_parameter_1017", "An index signature cannot have a rest parameter."), An_index_signature_parameter_cannot_have_an_accessibility_modifier: i(1018, 1, "An_index_signature_parameter_cannot_have_an_accessibility_modifier_1018", "An index signature parameter cannot have an accessibility modifier."), An_index_signature_parameter_cannot_have_a_question_mark: i(1019, 1, "An_index_signature_parameter_cannot_have_a_question_mark_1019", "An index signature parameter cannot have a question mark."), An_index_signature_parameter_cannot_have_an_initializer: i(1020, 1, "An_index_signature_parameter_cannot_have_an_initializer_1020", "An index signature parameter cannot have an initializer."), An_index_signature_must_have_a_type_annotation: i(1021, 1, "An_index_signature_must_have_a_type_annotation_1021", "An index signature must have a type annotation."), An_index_signature_parameter_must_have_a_type_annotation: i(1022, 1, "An_index_signature_parameter_must_have_a_type_annotation_1022", "An index signature parameter must have a type annotation."), readonly_modifier_can_only_appear_on_a_property_declaration_or_index_signature: i(1024, 1, "readonly_modifier_can_only_appear_on_a_property_declaration_or_index_signature_1024", "'readonly' modifier can only appear on a property declaration or index signature."), An_index_signature_cannot_have_a_trailing_comma: i(1025, 1, "An_index_signature_cannot_have_a_trailing_comma_1025", "An index signature cannot have a trailing comma."), Accessibility_modifier_already_seen: i(1028, 1, "Accessibility_modifier_already_seen_1028", "Accessibility modifier already seen."), _0_modifier_must_precede_1_modifier: i(1029, 1, "_0_modifier_must_precede_1_modifier_1029", "'{0}' modifier must precede '{1}' modifier."), _0_modifier_already_seen: i(1030, 1, "_0_modifier_already_seen_1030", "'{0}' modifier already seen."), _0_modifier_cannot_appear_on_class_elements_of_this_kind: i(1031, 1, "_0_modifier_cannot_appear_on_class_elements_of_this_kind_1031", "'{0}' modifier cannot appear on class elements of this kind."), super_must_be_followed_by_an_argument_list_or_member_access: i(1034, 1, "super_must_be_followed_by_an_argument_list_or_member_access_1034", "'super' must be followed by an argument list or member access."), Only_ambient_modules_can_use_quoted_names: i(1035, 1, "Only_ambient_modules_can_use_quoted_names_1035", "Only ambient modules can use quoted names."), Statements_are_not_allowed_in_ambient_contexts: i(1036, 1, "Statements_are_not_allowed_in_ambient_contexts_1036", "Statements are not allowed in ambient contexts."), A_declare_modifier_cannot_be_used_in_an_already_ambient_context: i(1038, 1, "A_declare_modifier_cannot_be_used_in_an_already_ambient_context_1038", "A 'declare' modifier cannot be used in an already ambient context."), Initializers_are_not_allowed_in_ambient_contexts: i(1039, 1, "Initializers_are_not_allowed_in_ambient_contexts_1039", "Initializers are not allowed in ambient contexts."), _0_modifier_cannot_be_used_in_an_ambient_context: i(1040, 1, "_0_modifier_cannot_be_used_in_an_ambient_context_1040", "'{0}' modifier cannot be used in an ambient context."), _0_modifier_cannot_be_used_here: i(1042, 1, "_0_modifier_cannot_be_used_here_1042", "'{0}' modifier cannot be used here."), _0_modifier_cannot_appear_on_a_module_or_namespace_element: i(1044, 1, "_0_modifier_cannot_appear_on_a_module_or_namespace_element_1044", "'{0}' modifier cannot appear on a module or namespace element."), Top_level_declarations_in_d_ts_files_must_start_with_either_a_declare_or_export_modifier: i(1046, 1, "Top_level_declarations_in_d_ts_files_must_start_with_either_a_declare_or_export_modifier_1046", "Top-level declarations in .d.ts files must start with either a 'declare' or 'export' modifier."), A_rest_parameter_cannot_be_optional: i(1047, 1, "A_rest_parameter_cannot_be_optional_1047", "A rest parameter cannot be optional."), A_rest_parameter_cannot_have_an_initializer: i(1048, 1, "A_rest_parameter_cannot_have_an_initializer_1048", "A rest parameter cannot have an initializer."), A_set_accessor_must_have_exactly_one_parameter: i(1049, 1, "A_set_accessor_must_have_exactly_one_parameter_1049", "A 'set' accessor must have exactly one parameter."), A_set_accessor_cannot_have_an_optional_parameter: i(1051, 1, "A_set_accessor_cannot_have_an_optional_parameter_1051", "A 'set' accessor cannot have an optional parameter."), A_set_accessor_parameter_cannot_have_an_initializer: i(1052, 1, "A_set_accessor_parameter_cannot_have_an_initializer_1052", "A 'set' accessor parameter cannot have an initializer."), A_set_accessor_cannot_have_rest_parameter: i(1053, 1, "A_set_accessor_cannot_have_rest_parameter_1053", "A 'set' accessor cannot have rest parameter."), A_get_accessor_cannot_have_parameters: i(1054, 1, "A_get_accessor_cannot_have_parameters_1054", "A 'get' accessor cannot have parameters."), Type_0_is_not_a_valid_async_function_return_type_in_ES5_SlashES3_because_it_does_not_refer_to_a_Promise_compatible_constructor_value: i(1055, 1, "Type_0_is_not_a_valid_async_function_return_type_in_ES5_SlashES3_because_it_does_not_refer_to_a_Prom_1055", "Type '{0}' is not a valid async function return type in ES5/ES3 because it does not refer to a Promise-compatible constructor value."), Accessors_are_only_available_when_targeting_ECMAScript_5_and_higher: i(1056, 1, "Accessors_are_only_available_when_targeting_ECMAScript_5_and_higher_1056", "Accessors are only available when targeting ECMAScript 5 and higher."), The_return_type_of_an_async_function_must_either_be_a_valid_promise_or_must_not_contain_a_callable_then_member: i(1058, 1, "The_return_type_of_an_async_function_must_either_be_a_valid_promise_or_must_not_contain_a_callable_t_1058", "The return type of an async function must either be a valid promise or must not contain a callable 'then' member."), A_promise_must_have_a_then_method: i(1059, 1, "A_promise_must_have_a_then_method_1059", "A promise must have a 'then' method."), The_first_parameter_of_the_then_method_of_a_promise_must_be_a_callback: i(1060, 1, "The_first_parameter_of_the_then_method_of_a_promise_must_be_a_callback_1060", "The first parameter of the 'then' method of a promise must be a callback."), Enum_member_must_have_initializer: i(1061, 1, "Enum_member_must_have_initializer_1061", "Enum member must have initializer."), Type_is_referenced_directly_or_indirectly_in_the_fulfillment_callback_of_its_own_then_method: i(1062, 1, "Type_is_referenced_directly_or_indirectly_in_the_fulfillment_callback_of_its_own_then_method_1062", "Type is referenced directly or indirectly in the fulfillment callback of its own 'then' method."), An_export_assignment_cannot_be_used_in_a_namespace: i(1063, 1, "An_export_assignment_cannot_be_used_in_a_namespace_1063", "An export assignment cannot be used in a namespace."), The_return_type_of_an_async_function_or_method_must_be_the_global_Promise_T_type_Did_you_mean_to_write_Promise_0: i(1064, 1, "The_return_type_of_an_async_function_or_method_must_be_the_global_Promise_T_type_Did_you_mean_to_wri_1064", "The return type of an async function or method must be the global Promise type. Did you mean to write 'Promise<{0}>'?"), In_ambient_enum_declarations_member_initializer_must_be_constant_expression: i(1066, 1, "In_ambient_enum_declarations_member_initializer_must_be_constant_expression_1066", "In ambient enum declarations member initializer must be constant expression."), Unexpected_token_A_constructor_method_accessor_or_property_was_expected: i(1068, 1, "Unexpected_token_A_constructor_method_accessor_or_property_was_expected_1068", "Unexpected token. A constructor, method, accessor, or property was expected."), Unexpected_token_A_type_parameter_name_was_expected_without_curly_braces: i(1069, 1, "Unexpected_token_A_type_parameter_name_was_expected_without_curly_braces_1069", "Unexpected token. A type parameter name was expected without curly braces."), _0_modifier_cannot_appear_on_a_type_member: i(1070, 1, "_0_modifier_cannot_appear_on_a_type_member_1070", "'{0}' modifier cannot appear on a type member."), _0_modifier_cannot_appear_on_an_index_signature: i(1071, 1, "_0_modifier_cannot_appear_on_an_index_signature_1071", "'{0}' modifier cannot appear on an index signature."), A_0_modifier_cannot_be_used_with_an_import_declaration: i(1079, 1, "A_0_modifier_cannot_be_used_with_an_import_declaration_1079", "A '{0}' modifier cannot be used with an import declaration."), Invalid_reference_directive_syntax: i(1084, 1, "Invalid_reference_directive_syntax_1084", "Invalid 'reference' directive syntax."), Octal_literals_are_not_available_when_targeting_ECMAScript_5_and_higher_Use_the_syntax_0: i(1085, 1, "Octal_literals_are_not_available_when_targeting_ECMAScript_5_and_higher_Use_the_syntax_0_1085", "Octal literals are not available when targeting ECMAScript 5 and higher. Use the syntax '{0}'."), _0_modifier_cannot_appear_on_a_constructor_declaration: i(1089, 1, "_0_modifier_cannot_appear_on_a_constructor_declaration_1089", "'{0}' modifier cannot appear on a constructor declaration."), _0_modifier_cannot_appear_on_a_parameter: i(1090, 1, "_0_modifier_cannot_appear_on_a_parameter_1090", "'{0}' modifier cannot appear on a parameter."), Only_a_single_variable_declaration_is_allowed_in_a_for_in_statement: i(1091, 1, "Only_a_single_variable_declaration_is_allowed_in_a_for_in_statement_1091", "Only a single variable declaration is allowed in a 'for...in' statement."), Type_parameters_cannot_appear_on_a_constructor_declaration: i(1092, 1, "Type_parameters_cannot_appear_on_a_constructor_declaration_1092", "Type parameters cannot appear on a constructor declaration."), Type_annotation_cannot_appear_on_a_constructor_declaration: i(1093, 1, "Type_annotation_cannot_appear_on_a_constructor_declaration_1093", "Type annotation cannot appear on a constructor declaration."), An_accessor_cannot_have_type_parameters: i(1094, 1, "An_accessor_cannot_have_type_parameters_1094", "An accessor cannot have type parameters."), A_set_accessor_cannot_have_a_return_type_annotation: i(1095, 1, "A_set_accessor_cannot_have_a_return_type_annotation_1095", "A 'set' accessor cannot have a return type annotation."), An_index_signature_must_have_exactly_one_parameter: i(1096, 1, "An_index_signature_must_have_exactly_one_parameter_1096", "An index signature must have exactly one parameter."), _0_list_cannot_be_empty: i(1097, 1, "_0_list_cannot_be_empty_1097", "'{0}' list cannot be empty."), Type_parameter_list_cannot_be_empty: i(1098, 1, "Type_parameter_list_cannot_be_empty_1098", "Type parameter list cannot be empty."), Type_argument_list_cannot_be_empty: i(1099, 1, "Type_argument_list_cannot_be_empty_1099", "Type argument list cannot be empty."), Invalid_use_of_0_in_strict_mode: i(1100, 1, "Invalid_use_of_0_in_strict_mode_1100", "Invalid use of '{0}' in strict mode."), with_statements_are_not_allowed_in_strict_mode: i(1101, 1, "with_statements_are_not_allowed_in_strict_mode_1101", "'with' statements are not allowed in strict mode."), delete_cannot_be_called_on_an_identifier_in_strict_mode: i(1102, 1, "delete_cannot_be_called_on_an_identifier_in_strict_mode_1102", "'delete' cannot be called on an identifier in strict mode."), for_await_loops_are_only_allowed_within_async_functions_and_at_the_top_levels_of_modules: i(1103, 1, "for_await_loops_are_only_allowed_within_async_functions_and_at_the_top_levels_of_modules_1103", "'for await' loops are only allowed within async functions and at the top levels of modules."), A_continue_statement_can_only_be_used_within_an_enclosing_iteration_statement: i(1104, 1, "A_continue_statement_can_only_be_used_within_an_enclosing_iteration_statement_1104", "A 'continue' statement can only be used within an enclosing iteration statement."), A_break_statement_can_only_be_used_within_an_enclosing_iteration_or_switch_statement: i(1105, 1, "A_break_statement_can_only_be_used_within_an_enclosing_iteration_or_switch_statement_1105", "A 'break' statement can only be used within an enclosing iteration or switch statement."), The_left_hand_side_of_a_for_of_statement_may_not_be_async: i(1106, 1, "The_left_hand_side_of_a_for_of_statement_may_not_be_async_1106", "The left-hand side of a 'for...of' statement may not be 'async'."), Jump_target_cannot_cross_function_boundary: i(1107, 1, "Jump_target_cannot_cross_function_boundary_1107", "Jump target cannot cross function boundary."), A_return_statement_can_only_be_used_within_a_function_body: i(1108, 1, "A_return_statement_can_only_be_used_within_a_function_body_1108", "A 'return' statement can only be used within a function body."), Expression_expected: i(1109, 1, "Expression_expected_1109", "Expression expected."), Type_expected: i(1110, 1, "Type_expected_1110", "Type expected."), A_default_clause_cannot_appear_more_than_once_in_a_switch_statement: i(1113, 1, "A_default_clause_cannot_appear_more_than_once_in_a_switch_statement_1113", "A 'default' clause cannot appear more than once in a 'switch' statement."), Duplicate_label_0: i(1114, 1, "Duplicate_label_0_1114", "Duplicate label '{0}'."), A_continue_statement_can_only_jump_to_a_label_of_an_enclosing_iteration_statement: i(1115, 1, "A_continue_statement_can_only_jump_to_a_label_of_an_enclosing_iteration_statement_1115", "A 'continue' statement can only jump to a label of an enclosing iteration statement."), A_break_statement_can_only_jump_to_a_label_of_an_enclosing_statement: i(1116, 1, "A_break_statement_can_only_jump_to_a_label_of_an_enclosing_statement_1116", "A 'break' statement can only jump to a label of an enclosing statement."), An_object_literal_cannot_have_multiple_properties_with_the_same_name: i(1117, 1, "An_object_literal_cannot_have_multiple_properties_with_the_same_name_1117", "An object literal cannot have multiple properties with the same name."), An_object_literal_cannot_have_multiple_get_Slashset_accessors_with_the_same_name: i(1118, 1, "An_object_literal_cannot_have_multiple_get_Slashset_accessors_with_the_same_name_1118", "An object literal cannot have multiple get/set accessors with the same name."), An_object_literal_cannot_have_property_and_accessor_with_the_same_name: i(1119, 1, "An_object_literal_cannot_have_property_and_accessor_with_the_same_name_1119", "An object literal cannot have property and accessor with the same name."), An_export_assignment_cannot_have_modifiers: i(1120, 1, "An_export_assignment_cannot_have_modifiers_1120", "An export assignment cannot have modifiers."), Octal_literals_are_not_allowed_in_strict_mode: i(1121, 1, "Octal_literals_are_not_allowed_in_strict_mode_1121", "Octal literals are not allowed in strict mode."), Variable_declaration_list_cannot_be_empty: i(1123, 1, "Variable_declaration_list_cannot_be_empty_1123", "Variable declaration list cannot be empty."), Digit_expected: i(1124, 1, "Digit_expected_1124", "Digit expected."), Hexadecimal_digit_expected: i(1125, 1, "Hexadecimal_digit_expected_1125", "Hexadecimal digit expected."), Unexpected_end_of_text: i(1126, 1, "Unexpected_end_of_text_1126", "Unexpected end of text."), Invalid_character: i(1127, 1, "Invalid_character_1127", "Invalid character."), Declaration_or_statement_expected: i(1128, 1, "Declaration_or_statement_expected_1128", "Declaration or statement expected."), Statement_expected: i(1129, 1, "Statement_expected_1129", "Statement expected."), case_or_default_expected: i(1130, 1, "case_or_default_expected_1130", "'case' or 'default' expected."), Property_or_signature_expected: i(1131, 1, "Property_or_signature_expected_1131", "Property or signature expected."), Enum_member_expected: i(1132, 1, "Enum_member_expected_1132", "Enum member expected."), Variable_declaration_expected: i(1134, 1, "Variable_declaration_expected_1134", "Variable declaration expected."), Argument_expression_expected: i(1135, 1, "Argument_expression_expected_1135", "Argument expression expected."), Property_assignment_expected: i(1136, 1, "Property_assignment_expected_1136", "Property assignment expected."), Expression_or_comma_expected: i(1137, 1, "Expression_or_comma_expected_1137", "Expression or comma expected."), Parameter_declaration_expected: i(1138, 1, "Parameter_declaration_expected_1138", "Parameter declaration expected."), Type_parameter_declaration_expected: i(1139, 1, "Type_parameter_declaration_expected_1139", "Type parameter declaration expected."), Type_argument_expected: i(1140, 1, "Type_argument_expected_1140", "Type argument expected."), String_literal_expected: i(1141, 1, "String_literal_expected_1141", "String literal expected."), Line_break_not_permitted_here: i(1142, 1, "Line_break_not_permitted_here_1142", "Line break not permitted here."), or_expected: i(1144, 1, "or_expected_1144", "'{' or ';' expected."), or_JSX_element_expected: i(1145, 1, "or_JSX_element_expected_1145", "'{' or JSX element expected."), Declaration_expected: i(1146, 1, "Declaration_expected_1146", "Declaration expected."), Import_declarations_in_a_namespace_cannot_reference_a_module: i(1147, 1, "Import_declarations_in_a_namespace_cannot_reference_a_module_1147", "Import declarations in a namespace cannot reference a module."), Cannot_use_imports_exports_or_module_augmentations_when_module_is_none: i(1148, 1, "Cannot_use_imports_exports_or_module_augmentations_when_module_is_none_1148", "Cannot use imports, exports, or module augmentations when '--module' is 'none'."), File_name_0_differs_from_already_included_file_name_1_only_in_casing: i(1149, 1, "File_name_0_differs_from_already_included_file_name_1_only_in_casing_1149", "File name '{0}' differs from already included file name '{1}' only in casing."), const_declarations_must_be_initialized: i(1155, 1, "const_declarations_must_be_initialized_1155", "'const' declarations must be initialized."), const_declarations_can_only_be_declared_inside_a_block: i(1156, 1, "const_declarations_can_only_be_declared_inside_a_block_1156", "'const' declarations can only be declared inside a block."), let_declarations_can_only_be_declared_inside_a_block: i(1157, 1, "let_declarations_can_only_be_declared_inside_a_block_1157", "'let' declarations can only be declared inside a block."), Unterminated_template_literal: i(1160, 1, "Unterminated_template_literal_1160", "Unterminated template literal."), Unterminated_regular_expression_literal: i(1161, 1, "Unterminated_regular_expression_literal_1161", "Unterminated regular expression literal."), An_object_member_cannot_be_declared_optional: i(1162, 1, "An_object_member_cannot_be_declared_optional_1162", "An object member cannot be declared optional."), A_yield_expression_is_only_allowed_in_a_generator_body: i(1163, 1, "A_yield_expression_is_only_allowed_in_a_generator_body_1163", "A 'yield' expression is only allowed in a generator body."), Computed_property_names_are_not_allowed_in_enums: i(1164, 1, "Computed_property_names_are_not_allowed_in_enums_1164", "Computed property names are not allowed in enums."), A_computed_property_name_in_an_ambient_context_must_refer_to_an_expression_whose_type_is_a_literal_type_or_a_unique_symbol_type: i(1165, 1, "A_computed_property_name_in_an_ambient_context_must_refer_to_an_expression_whose_type_is_a_literal_t_1165", "A computed property name in an ambient context must refer to an expression whose type is a literal type or a 'unique symbol' type."), A_computed_property_name_in_a_class_property_declaration_must_have_a_simple_literal_type_or_a_unique_symbol_type: i(1166, 1, "A_computed_property_name_in_a_class_property_declaration_must_have_a_simple_literal_type_or_a_unique_1166", "A computed property name in a class property declaration must have a simple literal type or a 'unique symbol' type."), A_computed_property_name_in_a_method_overload_must_refer_to_an_expression_whose_type_is_a_literal_type_or_a_unique_symbol_type: i(1168, 1, "A_computed_property_name_in_a_method_overload_must_refer_to_an_expression_whose_type_is_a_literal_ty_1168", "A computed property name in a method overload must refer to an expression whose type is a literal type or a 'unique symbol' type."), A_computed_property_name_in_an_interface_must_refer_to_an_expression_whose_type_is_a_literal_type_or_a_unique_symbol_type: i(1169, 1, "A_computed_property_name_in_an_interface_must_refer_to_an_expression_whose_type_is_a_literal_type_or_1169", "A computed property name in an interface must refer to an expression whose type is a literal type or a 'unique symbol' type."), A_computed_property_name_in_a_type_literal_must_refer_to_an_expression_whose_type_is_a_literal_type_or_a_unique_symbol_type: i(1170, 1, "A_computed_property_name_in_a_type_literal_must_refer_to_an_expression_whose_type_is_a_literal_type__1170", "A computed property name in a type literal must refer to an expression whose type is a literal type or a 'unique symbol' type."), A_comma_expression_is_not_allowed_in_a_computed_property_name: i(1171, 1, "A_comma_expression_is_not_allowed_in_a_computed_property_name_1171", "A comma expression is not allowed in a computed property name."), extends_clause_already_seen: i(1172, 1, "extends_clause_already_seen_1172", "'extends' clause already seen."), extends_clause_must_precede_implements_clause: i(1173, 1, "extends_clause_must_precede_implements_clause_1173", "'extends' clause must precede 'implements' clause."), Classes_can_only_extend_a_single_class: i(1174, 1, "Classes_can_only_extend_a_single_class_1174", "Classes can only extend a single class."), implements_clause_already_seen: i(1175, 1, "implements_clause_already_seen_1175", "'implements' clause already seen."), Interface_declaration_cannot_have_implements_clause: i(1176, 1, "Interface_declaration_cannot_have_implements_clause_1176", "Interface declaration cannot have 'implements' clause."), Binary_digit_expected: i(1177, 1, "Binary_digit_expected_1177", "Binary digit expected."), Octal_digit_expected: i(1178, 1, "Octal_digit_expected_1178", "Octal digit expected."), Unexpected_token_expected: i(1179, 1, "Unexpected_token_expected_1179", "Unexpected token. '{' expected."), Property_destructuring_pattern_expected: i(1180, 1, "Property_destructuring_pattern_expected_1180", "Property destructuring pattern expected."), Array_element_destructuring_pattern_expected: i(1181, 1, "Array_element_destructuring_pattern_expected_1181", "Array element destructuring pattern expected."), A_destructuring_declaration_must_have_an_initializer: i(1182, 1, "A_destructuring_declaration_must_have_an_initializer_1182", "A destructuring declaration must have an initializer."), An_implementation_cannot_be_declared_in_ambient_contexts: i(1183, 1, "An_implementation_cannot_be_declared_in_ambient_contexts_1183", "An implementation cannot be declared in ambient contexts."), Modifiers_cannot_appear_here: i(1184, 1, "Modifiers_cannot_appear_here_1184", "Modifiers cannot appear here."), Merge_conflict_marker_encountered: i(1185, 1, "Merge_conflict_marker_encountered_1185", "Merge conflict marker encountered."), A_rest_element_cannot_have_an_initializer: i(1186, 1, "A_rest_element_cannot_have_an_initializer_1186", "A rest element cannot have an initializer."), A_parameter_property_may_not_be_declared_using_a_binding_pattern: i(1187, 1, "A_parameter_property_may_not_be_declared_using_a_binding_pattern_1187", "A parameter property may not be declared using a binding pattern."), Only_a_single_variable_declaration_is_allowed_in_a_for_of_statement: i(1188, 1, "Only_a_single_variable_declaration_is_allowed_in_a_for_of_statement_1188", "Only a single variable declaration is allowed in a 'for...of' statement."), The_variable_declaration_of_a_for_in_statement_cannot_have_an_initializer: i(1189, 1, "The_variable_declaration_of_a_for_in_statement_cannot_have_an_initializer_1189", "The variable declaration of a 'for...in' statement cannot have an initializer."), The_variable_declaration_of_a_for_of_statement_cannot_have_an_initializer: i(1190, 1, "The_variable_declaration_of_a_for_of_statement_cannot_have_an_initializer_1190", "The variable declaration of a 'for...of' statement cannot have an initializer."), An_import_declaration_cannot_have_modifiers: i(1191, 1, "An_import_declaration_cannot_have_modifiers_1191", "An import declaration cannot have modifiers."), Module_0_has_no_default_export: i(1192, 1, "Module_0_has_no_default_export_1192", "Module '{0}' has no default export."), An_export_declaration_cannot_have_modifiers: i(1193, 1, "An_export_declaration_cannot_have_modifiers_1193", "An export declaration cannot have modifiers."), Export_declarations_are_not_permitted_in_a_namespace: i(1194, 1, "Export_declarations_are_not_permitted_in_a_namespace_1194", "Export declarations are not permitted in a namespace."), export_Asterisk_does_not_re_export_a_default: i(1195, 1, "export_Asterisk_does_not_re_export_a_default_1195", "'export *' does not re-export a default."), Catch_clause_variable_type_annotation_must_be_any_or_unknown_if_specified: i(1196, 1, "Catch_clause_variable_type_annotation_must_be_any_or_unknown_if_specified_1196", "Catch clause variable type annotation must be 'any' or 'unknown' if specified."), Catch_clause_variable_cannot_have_an_initializer: i(1197, 1, "Catch_clause_variable_cannot_have_an_initializer_1197", "Catch clause variable cannot have an initializer."), An_extended_Unicode_escape_value_must_be_between_0x0_and_0x10FFFF_inclusive: i(1198, 1, "An_extended_Unicode_escape_value_must_be_between_0x0_and_0x10FFFF_inclusive_1198", "An extended Unicode escape value must be between 0x0 and 0x10FFFF inclusive."), Unterminated_Unicode_escape_sequence: i(1199, 1, "Unterminated_Unicode_escape_sequence_1199", "Unterminated Unicode escape sequence."), Line_terminator_not_permitted_before_arrow: i(1200, 1, "Line_terminator_not_permitted_before_arrow_1200", "Line terminator not permitted before arrow."), Import_assignment_cannot_be_used_when_targeting_ECMAScript_modules_Consider_using_import_Asterisk_as_ns_from_mod_import_a_from_mod_import_d_from_mod_or_another_module_format_instead: i(1202, 1, "Import_assignment_cannot_be_used_when_targeting_ECMAScript_modules_Consider_using_import_Asterisk_as_1202", `Import assignment cannot be used when targeting ECMAScript modules. Consider using 'import * as ns from "mod"', 'import {a} from "mod"', 'import d from "mod"', or another module format instead.`), Export_assignment_cannot_be_used_when_targeting_ECMAScript_modules_Consider_using_export_default_or_another_module_format_instead: i(1203, 1, "Export_assignment_cannot_be_used_when_targeting_ECMAScript_modules_Consider_using_export_default_or__1203", "Export assignment cannot be used when targeting ECMAScript modules. Consider using 'export default' or another module format instead."), Re_exporting_a_type_when_0_is_enabled_requires_using_export_type: i(1205, 1, "Re_exporting_a_type_when_0_is_enabled_requires_using_export_type_1205", "Re-exporting a type when '{0}' is enabled requires using 'export type'."), Decorators_are_not_valid_here: i(1206, 1, "Decorators_are_not_valid_here_1206", "Decorators are not valid here."), Decorators_cannot_be_applied_to_multiple_get_Slashset_accessors_of_the_same_name: i(1207, 1, "Decorators_cannot_be_applied_to_multiple_get_Slashset_accessors_of_the_same_name_1207", "Decorators cannot be applied to multiple get/set accessors of the same name."), Invalid_optional_chain_from_new_expression_Did_you_mean_to_call_0: i(1209, 1, "Invalid_optional_chain_from_new_expression_Did_you_mean_to_call_0_1209", "Invalid optional chain from new expression. Did you mean to call '{0}()'?"), Code_contained_in_a_class_is_evaluated_in_JavaScript_s_strict_mode_which_does_not_allow_this_use_of_0_For_more_information_see_https_Colon_Slash_Slashdeveloper_mozilla_org_Slashen_US_Slashdocs_SlashWeb_SlashJavaScript_SlashReference_SlashStrict_mode: i(1210, 1, "Code_contained_in_a_class_is_evaluated_in_JavaScript_s_strict_mode_which_does_not_allow_this_use_of__1210", "Code contained in a class is evaluated in JavaScript's strict mode which does not allow this use of '{0}'. For more information, see https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Strict_mode."), A_class_declaration_without_the_default_modifier_must_have_a_name: i(1211, 1, "A_class_declaration_without_the_default_modifier_must_have_a_name_1211", "A class declaration without the 'default' modifier must have a name."), Identifier_expected_0_is_a_reserved_word_in_strict_mode: i(1212, 1, "Identifier_expected_0_is_a_reserved_word_in_strict_mode_1212", "Identifier expected. '{0}' is a reserved word in strict mode."), Identifier_expected_0_is_a_reserved_word_in_strict_mode_Class_definitions_are_automatically_in_strict_mode: i(1213, 1, "Identifier_expected_0_is_a_reserved_word_in_strict_mode_Class_definitions_are_automatically_in_stric_1213", "Identifier expected. '{0}' is a reserved word in strict mode. Class definitions are automatically in strict mode."), Identifier_expected_0_is_a_reserved_word_in_strict_mode_Modules_are_automatically_in_strict_mode: i(1214, 1, "Identifier_expected_0_is_a_reserved_word_in_strict_mode_Modules_are_automatically_in_strict_mode_1214", "Identifier expected. '{0}' is a reserved word in strict mode. Modules are automatically in strict mode."), Invalid_use_of_0_Modules_are_automatically_in_strict_mode: i(1215, 1, "Invalid_use_of_0_Modules_are_automatically_in_strict_mode_1215", "Invalid use of '{0}'. Modules are automatically in strict mode."), Identifier_expected_esModule_is_reserved_as_an_exported_marker_when_transforming_ECMAScript_modules: i(1216, 1, "Identifier_expected_esModule_is_reserved_as_an_exported_marker_when_transforming_ECMAScript_modules_1216", "Identifier expected. '__esModule' is reserved as an exported marker when transforming ECMAScript modules."), Export_assignment_is_not_supported_when_module_flag_is_system: i(1218, 1, "Export_assignment_is_not_supported_when_module_flag_is_system_1218", "Export assignment is not supported when '--module' flag is 'system'."), Generators_are_not_allowed_in_an_ambient_context: i(1221, 1, "Generators_are_not_allowed_in_an_ambient_context_1221", "Generators are not allowed in an ambient context."), An_overload_signature_cannot_be_declared_as_a_generator: i(1222, 1, "An_overload_signature_cannot_be_declared_as_a_generator_1222", "An overload signature cannot be declared as a generator."), _0_tag_already_specified: i(1223, 1, "_0_tag_already_specified_1223", "'{0}' tag already specified."), Signature_0_must_be_a_type_predicate: i(1224, 1, "Signature_0_must_be_a_type_predicate_1224", "Signature '{0}' must be a type predicate."), Cannot_find_parameter_0: i(1225, 1, "Cannot_find_parameter_0_1225", "Cannot find parameter '{0}'."), Type_predicate_0_is_not_assignable_to_1: i(1226, 1, "Type_predicate_0_is_not_assignable_to_1_1226", "Type predicate '{0}' is not assignable to '{1}'."), Parameter_0_is_not_in_the_same_position_as_parameter_1: i(1227, 1, "Parameter_0_is_not_in_the_same_position_as_parameter_1_1227", "Parameter '{0}' is not in the same position as parameter '{1}'."), A_type_predicate_is_only_allowed_in_return_type_position_for_functions_and_methods: i(1228, 1, "A_type_predicate_is_only_allowed_in_return_type_position_for_functions_and_methods_1228", "A type predicate is only allowed in return type position for functions and methods."), A_type_predicate_cannot_reference_a_rest_parameter: i(1229, 1, "A_type_predicate_cannot_reference_a_rest_parameter_1229", "A type predicate cannot reference a rest parameter."), A_type_predicate_cannot_reference_element_0_in_a_binding_pattern: i(1230, 1, "A_type_predicate_cannot_reference_element_0_in_a_binding_pattern_1230", "A type predicate cannot reference element '{0}' in a binding pattern."), An_export_assignment_must_be_at_the_top_level_of_a_file_or_module_declaration: i(1231, 1, "An_export_assignment_must_be_at_the_top_level_of_a_file_or_module_declaration_1231", "An export assignment must be at the top level of a file or module declaration."), An_import_declaration_can_only_be_used_at_the_top_level_of_a_namespace_or_module: i(1232, 1, "An_import_declaration_can_only_be_used_at_the_top_level_of_a_namespace_or_module_1232", "An import declaration can only be used at the top level of a namespace or module."), An_export_declaration_can_only_be_used_at_the_top_level_of_a_namespace_or_module: i(1233, 1, "An_export_declaration_can_only_be_used_at_the_top_level_of_a_namespace_or_module_1233", "An export declaration can only be used at the top level of a namespace or module."), An_ambient_module_declaration_is_only_allowed_at_the_top_level_in_a_file: i(1234, 1, "An_ambient_module_declaration_is_only_allowed_at_the_top_level_in_a_file_1234", "An ambient module declaration is only allowed at the top level in a file."), A_namespace_declaration_is_only_allowed_at_the_top_level_of_a_namespace_or_module: i(1235, 1, "A_namespace_declaration_is_only_allowed_at_the_top_level_of_a_namespace_or_module_1235", "A namespace declaration is only allowed at the top level of a namespace or module."), The_return_type_of_a_property_decorator_function_must_be_either_void_or_any: i(1236, 1, "The_return_type_of_a_property_decorator_function_must_be_either_void_or_any_1236", "The return type of a property decorator function must be either 'void' or 'any'."), The_return_type_of_a_parameter_decorator_function_must_be_either_void_or_any: i(1237, 1, "The_return_type_of_a_parameter_decorator_function_must_be_either_void_or_any_1237", "The return type of a parameter decorator function must be either 'void' or 'any'."), Unable_to_resolve_signature_of_class_decorator_when_called_as_an_expression: i(1238, 1, "Unable_to_resolve_signature_of_class_decorator_when_called_as_an_expression_1238", "Unable to resolve signature of class decorator when called as an expression."), Unable_to_resolve_signature_of_parameter_decorator_when_called_as_an_expression: i(1239, 1, "Unable_to_resolve_signature_of_parameter_decorator_when_called_as_an_expression_1239", "Unable to resolve signature of parameter decorator when called as an expression."), Unable_to_resolve_signature_of_property_decorator_when_called_as_an_expression: i(1240, 1, "Unable_to_resolve_signature_of_property_decorator_when_called_as_an_expression_1240", "Unable to resolve signature of property decorator when called as an expression."), Unable_to_resolve_signature_of_method_decorator_when_called_as_an_expression: i(1241, 1, "Unable_to_resolve_signature_of_method_decorator_when_called_as_an_expression_1241", "Unable to resolve signature of method decorator when called as an expression."), abstract_modifier_can_only_appear_on_a_class_method_or_property_declaration: i(1242, 1, "abstract_modifier_can_only_appear_on_a_class_method_or_property_declaration_1242", "'abstract' modifier can only appear on a class, method, or property declaration."), _0_modifier_cannot_be_used_with_1_modifier: i(1243, 1, "_0_modifier_cannot_be_used_with_1_modifier_1243", "'{0}' modifier cannot be used with '{1}' modifier."), Abstract_methods_can_only_appear_within_an_abstract_class: i(1244, 1, "Abstract_methods_can_only_appear_within_an_abstract_class_1244", "Abstract methods can only appear within an abstract class."), Method_0_cannot_have_an_implementation_because_it_is_marked_abstract: i(1245, 1, "Method_0_cannot_have_an_implementation_because_it_is_marked_abstract_1245", "Method '{0}' cannot have an implementation because it is marked abstract."), An_interface_property_cannot_have_an_initializer: i(1246, 1, "An_interface_property_cannot_have_an_initializer_1246", "An interface property cannot have an initializer."), A_type_literal_property_cannot_have_an_initializer: i(1247, 1, "A_type_literal_property_cannot_have_an_initializer_1247", "A type literal property cannot have an initializer."), A_class_member_cannot_have_the_0_keyword: i(1248, 1, "A_class_member_cannot_have_the_0_keyword_1248", "A class member cannot have the '{0}' keyword."), A_decorator_can_only_decorate_a_method_implementation_not_an_overload: i(1249, 1, "A_decorator_can_only_decorate_a_method_implementation_not_an_overload_1249", "A decorator can only decorate a method implementation, not an overload."), Function_declarations_are_not_allowed_inside_blocks_in_strict_mode_when_targeting_ES3_or_ES5: i(1250, 1, "Function_declarations_are_not_allowed_inside_blocks_in_strict_mode_when_targeting_ES3_or_ES5_1250", "Function declarations are not allowed inside blocks in strict mode when targeting 'ES3' or 'ES5'."), Function_declarations_are_not_allowed_inside_blocks_in_strict_mode_when_targeting_ES3_or_ES5_Class_definitions_are_automatically_in_strict_mode: i(1251, 1, "Function_declarations_are_not_allowed_inside_blocks_in_strict_mode_when_targeting_ES3_or_ES5_Class_d_1251", "Function declarations are not allowed inside blocks in strict mode when targeting 'ES3' or 'ES5'. Class definitions are automatically in strict mode."), Function_declarations_are_not_allowed_inside_blocks_in_strict_mode_when_targeting_ES3_or_ES5_Modules_are_automatically_in_strict_mode: i(1252, 1, "Function_declarations_are_not_allowed_inside_blocks_in_strict_mode_when_targeting_ES3_or_ES5_Modules_1252", "Function declarations are not allowed inside blocks in strict mode when targeting 'ES3' or 'ES5'. Modules are automatically in strict mode."), A_const_initializer_in_an_ambient_context_must_be_a_string_or_numeric_literal_or_literal_enum_reference: i(1254, 1, "A_const_initializer_in_an_ambient_context_must_be_a_string_or_numeric_literal_or_literal_enum_refere_1254", "A 'const' initializer in an ambient context must be a string or numeric literal or literal enum reference."), A_definite_assignment_assertion_is_not_permitted_in_this_context: i(1255, 1, "A_definite_assignment_assertion_is_not_permitted_in_this_context_1255", "A definite assignment assertion '!' is not permitted in this context."), A_required_element_cannot_follow_an_optional_element: i(1257, 1, "A_required_element_cannot_follow_an_optional_element_1257", "A required element cannot follow an optional element."), A_default_export_must_be_at_the_top_level_of_a_file_or_module_declaration: i(1258, 1, "A_default_export_must_be_at_the_top_level_of_a_file_or_module_declaration_1258", "A default export must be at the top level of a file or module declaration."), Module_0_can_only_be_default_imported_using_the_1_flag: i(1259, 1, "Module_0_can_only_be_default_imported_using_the_1_flag_1259", "Module '{0}' can only be default-imported using the '{1}' flag"), Keywords_cannot_contain_escape_characters: i(1260, 1, "Keywords_cannot_contain_escape_characters_1260", "Keywords cannot contain escape characters."), Already_included_file_name_0_differs_from_file_name_1_only_in_casing: i(1261, 1, "Already_included_file_name_0_differs_from_file_name_1_only_in_casing_1261", "Already included file name '{0}' differs from file name '{1}' only in casing."), Identifier_expected_0_is_a_reserved_word_at_the_top_level_of_a_module: i(1262, 1, "Identifier_expected_0_is_a_reserved_word_at_the_top_level_of_a_module_1262", "Identifier expected. '{0}' is a reserved word at the top-level of a module."), Declarations_with_initializers_cannot_also_have_definite_assignment_assertions: i(1263, 1, "Declarations_with_initializers_cannot_also_have_definite_assignment_assertions_1263", "Declarations with initializers cannot also have definite assignment assertions."), Declarations_with_definite_assignment_assertions_must_also_have_type_annotations: i(1264, 1, "Declarations_with_definite_assignment_assertions_must_also_have_type_annotations_1264", "Declarations with definite assignment assertions must also have type annotations."), A_rest_element_cannot_follow_another_rest_element: i(1265, 1, "A_rest_element_cannot_follow_another_rest_element_1265", "A rest element cannot follow another rest element."), An_optional_element_cannot_follow_a_rest_element: i(1266, 1, "An_optional_element_cannot_follow_a_rest_element_1266", "An optional element cannot follow a rest element."), Property_0_cannot_have_an_initializer_because_it_is_marked_abstract: i(1267, 1, "Property_0_cannot_have_an_initializer_because_it_is_marked_abstract_1267", "Property '{0}' cannot have an initializer because it is marked abstract."), An_index_signature_parameter_type_must_be_string_number_symbol_or_a_template_literal_type: i(1268, 1, "An_index_signature_parameter_type_must_be_string_number_symbol_or_a_template_literal_type_1268", "An index signature parameter type must be 'string', 'number', 'symbol', or a template literal type."), Cannot_use_export_import_on_a_type_or_type_only_namespace_when_0_is_enabled: i(1269, 1, "Cannot_use_export_import_on_a_type_or_type_only_namespace_when_0_is_enabled_1269", "Cannot use 'export import' on a type or type-only namespace when '{0}' is enabled."), Decorator_function_return_type_0_is_not_assignable_to_type_1: i(1270, 1, "Decorator_function_return_type_0_is_not_assignable_to_type_1_1270", "Decorator function return type '{0}' is not assignable to type '{1}'."), Decorator_function_return_type_is_0_but_is_expected_to_be_void_or_any: i(1271, 1, "Decorator_function_return_type_is_0_but_is_expected_to_be_void_or_any_1271", "Decorator function return type is '{0}' but is expected to be 'void' or 'any'."), A_type_referenced_in_a_decorated_signature_must_be_imported_with_import_type_or_a_namespace_import_when_isolatedModules_and_emitDecoratorMetadata_are_enabled: i(1272, 1, "A_type_referenced_in_a_decorated_signature_must_be_imported_with_import_type_or_a_namespace_import_w_1272", "A type referenced in a decorated signature must be imported with 'import type' or a namespace import when 'isolatedModules' and 'emitDecoratorMetadata' are enabled."), _0_modifier_cannot_appear_on_a_type_parameter: i(1273, 1, "_0_modifier_cannot_appear_on_a_type_parameter_1273", "'{0}' modifier cannot appear on a type parameter"), _0_modifier_can_only_appear_on_a_type_parameter_of_a_class_interface_or_type_alias: i(1274, 1, "_0_modifier_can_only_appear_on_a_type_parameter_of_a_class_interface_or_type_alias_1274", "'{0}' modifier can only appear on a type parameter of a class, interface or type alias"), accessor_modifier_can_only_appear_on_a_property_declaration: i(1275, 1, "accessor_modifier_can_only_appear_on_a_property_declaration_1275", "'accessor' modifier can only appear on a property declaration."), An_accessor_property_cannot_be_declared_optional: i(1276, 1, "An_accessor_property_cannot_be_declared_optional_1276", "An 'accessor' property cannot be declared optional."), _0_modifier_can_only_appear_on_a_type_parameter_of_a_function_method_or_class: i(1277, 1, "_0_modifier_can_only_appear_on_a_type_parameter_of_a_function_method_or_class_1277", "'{0}' modifier can only appear on a type parameter of a function, method or class"), The_runtime_will_invoke_the_decorator_with_1_arguments_but_the_decorator_expects_0: i(1278, 1, "The_runtime_will_invoke_the_decorator_with_1_arguments_but_the_decorator_expects_0_1278", "The runtime will invoke the decorator with {1} arguments, but the decorator expects {0}."), The_runtime_will_invoke_the_decorator_with_1_arguments_but_the_decorator_expects_at_least_0: i(1279, 1, "The_runtime_will_invoke_the_decorator_with_1_arguments_but_the_decorator_expects_at_least_0_1279", "The runtime will invoke the decorator with {1} arguments, but the decorator expects at least {0}."), Namespaces_are_not_allowed_in_global_script_files_when_0_is_enabled_If_this_file_is_not_intended_to_be_a_global_script_set_moduleDetection_to_force_or_add_an_empty_export_statement: i(1280, 1, "Namespaces_are_not_allowed_in_global_script_files_when_0_is_enabled_If_this_file_is_not_intended_to__1280", "Namespaces are not allowed in global script files when '{0}' is enabled. If this file is not intended to be a global script, set 'moduleDetection' to 'force' or add an empty 'export {}' statement."), Cannot_access_0_from_another_file_without_qualification_when_1_is_enabled_Use_2_instead: i(1281, 1, "Cannot_access_0_from_another_file_without_qualification_when_1_is_enabled_Use_2_instead_1281", "Cannot access '{0}' from another file without qualification when '{1}' is enabled. Use '{2}' instead."), An_export_declaration_must_reference_a_value_when_verbatimModuleSyntax_is_enabled_but_0_only_refers_to_a_type: i(1282, 1, "An_export_declaration_must_reference_a_value_when_verbatimModuleSyntax_is_enabled_but_0_only_refers__1282", "An 'export =' declaration must reference a value when 'verbatimModuleSyntax' is enabled, but '{0}' only refers to a type."), An_export_declaration_must_reference_a_real_value_when_verbatimModuleSyntax_is_enabled_but_0_resolves_to_a_type_only_declaration: i(1283, 1, "An_export_declaration_must_reference_a_real_value_when_verbatimModuleSyntax_is_enabled_but_0_resolve_1283", "An 'export =' declaration must reference a real value when 'verbatimModuleSyntax' is enabled, but '{0}' resolves to a type-only declaration."), An_export_default_must_reference_a_value_when_verbatimModuleSyntax_is_enabled_but_0_only_refers_to_a_type: i(1284, 1, "An_export_default_must_reference_a_value_when_verbatimModuleSyntax_is_enabled_but_0_only_refers_to_a_1284", "An 'export default' must reference a value when 'verbatimModuleSyntax' is enabled, but '{0}' only refers to a type."), An_export_default_must_reference_a_real_value_when_verbatimModuleSyntax_is_enabled_but_0_resolves_to_a_type_only_declaration: i(1285, 1, "An_export_default_must_reference_a_real_value_when_verbatimModuleSyntax_is_enabled_but_0_resolves_to_1285", "An 'export default' must reference a real value when 'verbatimModuleSyntax' is enabled, but '{0}' resolves to a type-only declaration."), ESM_syntax_is_not_allowed_in_a_CommonJS_module_when_verbatimModuleSyntax_is_enabled: i(1286, 1, "ESM_syntax_is_not_allowed_in_a_CommonJS_module_when_verbatimModuleSyntax_is_enabled_1286", "ESM syntax is not allowed in a CommonJS module when 'verbatimModuleSyntax' is enabled."), A_top_level_export_modifier_cannot_be_used_on_value_declarations_in_a_CommonJS_module_when_verbatimModuleSyntax_is_enabled: i(1287, 1, "A_top_level_export_modifier_cannot_be_used_on_value_declarations_in_a_CommonJS_module_when_verbatimM_1287", "A top-level 'export' modifier cannot be used on value declarations in a CommonJS module when 'verbatimModuleSyntax' is enabled."), An_import_alias_cannot_resolve_to_a_type_or_type_only_declaration_when_verbatimModuleSyntax_is_enabled: i(1288, 1, "An_import_alias_cannot_resolve_to_a_type_or_type_only_declaration_when_verbatimModuleSyntax_is_enabl_1288", "An import alias cannot resolve to a type or type-only declaration when 'verbatimModuleSyntax' is enabled."), with_statements_are_not_allowed_in_an_async_function_block: i(1300, 1, "with_statements_are_not_allowed_in_an_async_function_block_1300", "'with' statements are not allowed in an async function block."), await_expressions_are_only_allowed_within_async_functions_and_at_the_top_levels_of_modules: i(1308, 1, "await_expressions_are_only_allowed_within_async_functions_and_at_the_top_levels_of_modules_1308", "'await' expressions are only allowed within async functions and at the top levels of modules."), The_current_file_is_a_CommonJS_module_and_cannot_use_await_at_the_top_level: i(1309, 1, "The_current_file_is_a_CommonJS_module_and_cannot_use_await_at_the_top_level_1309", "The current file is a CommonJS module and cannot use 'await' at the top level."), Did_you_mean_to_use_a_Colon_An_can_only_follow_a_property_name_when_the_containing_object_literal_is_part_of_a_destructuring_pattern: i(1312, 1, "Did_you_mean_to_use_a_Colon_An_can_only_follow_a_property_name_when_the_containing_object_literal_is_1312", "Did you mean to use a ':'? An '=' can only follow a property name when the containing object literal is part of a destructuring pattern."), The_body_of_an_if_statement_cannot_be_the_empty_statement: i(1313, 1, "The_body_of_an_if_statement_cannot_be_the_empty_statement_1313", "The body of an 'if' statement cannot be the empty statement."), Global_module_exports_may_only_appear_in_module_files: i(1314, 1, "Global_module_exports_may_only_appear_in_module_files_1314", "Global module exports may only appear in module files."), Global_module_exports_may_only_appear_in_declaration_files: i(1315, 1, "Global_module_exports_may_only_appear_in_declaration_files_1315", "Global module exports may only appear in declaration files."), Global_module_exports_may_only_appear_at_top_level: i(1316, 1, "Global_module_exports_may_only_appear_at_top_level_1316", "Global module exports may only appear at top level."), A_parameter_property_cannot_be_declared_using_a_rest_parameter: i(1317, 1, "A_parameter_property_cannot_be_declared_using_a_rest_parameter_1317", "A parameter property cannot be declared using a rest parameter."), An_abstract_accessor_cannot_have_an_implementation: i(1318, 1, "An_abstract_accessor_cannot_have_an_implementation_1318", "An abstract accessor cannot have an implementation."), A_default_export_can_only_be_used_in_an_ECMAScript_style_module: i(1319, 1, "A_default_export_can_only_be_used_in_an_ECMAScript_style_module_1319", "A default export can only be used in an ECMAScript-style module."), Type_of_await_operand_must_either_be_a_valid_promise_or_must_not_contain_a_callable_then_member: i(1320, 1, "Type_of_await_operand_must_either_be_a_valid_promise_or_must_not_contain_a_callable_then_member_1320", "Type of 'await' operand must either be a valid promise or must not contain a callable 'then' member."), Type_of_yield_operand_in_an_async_generator_must_either_be_a_valid_promise_or_must_not_contain_a_callable_then_member: i(1321, 1, "Type_of_yield_operand_in_an_async_generator_must_either_be_a_valid_promise_or_must_not_contain_a_cal_1321", "Type of 'yield' operand in an async generator must either be a valid promise or must not contain a callable 'then' member."), Type_of_iterated_elements_of_a_yield_Asterisk_operand_must_either_be_a_valid_promise_or_must_not_contain_a_callable_then_member: i(1322, 1, "Type_of_iterated_elements_of_a_yield_Asterisk_operand_must_either_be_a_valid_promise_or_must_not_con_1322", "Type of iterated elements of a 'yield*' operand must either be a valid promise or must not contain a callable 'then' member."), Dynamic_imports_are_only_supported_when_the_module_flag_is_set_to_es2020_es2022_esnext_commonjs_amd_system_umd_node16_or_nodenext: i(1323, 1, "Dynamic_imports_are_only_supported_when_the_module_flag_is_set_to_es2020_es2022_esnext_commonjs_amd__1323", "Dynamic imports are only supported when the '--module' flag is set to 'es2020', 'es2022', 'esnext', 'commonjs', 'amd', 'system', 'umd', 'node16', or 'nodenext'."), Dynamic_imports_only_support_a_second_argument_when_the_module_option_is_set_to_esnext_node16_or_nodenext: i(1324, 1, "Dynamic_imports_only_support_a_second_argument_when_the_module_option_is_set_to_esnext_node16_or_nod_1324", "Dynamic imports only support a second argument when the '--module' option is set to 'esnext', 'node16', or 'nodenext'."), Argument_of_dynamic_import_cannot_be_spread_element: i(1325, 1, "Argument_of_dynamic_import_cannot_be_spread_element_1325", "Argument of dynamic import cannot be spread element."), This_use_of_import_is_invalid_import_calls_can_be_written_but_they_must_have_parentheses_and_cannot_have_type_arguments: i(1326, 1, "This_use_of_import_is_invalid_import_calls_can_be_written_but_they_must_have_parentheses_and_cannot__1326", "This use of 'import' is invalid. 'import()' calls can be written, but they must have parentheses and cannot have type arguments."), String_literal_with_double_quotes_expected: i(1327, 1, "String_literal_with_double_quotes_expected_1327", "String literal with double quotes expected."), Property_value_can_only_be_string_literal_numeric_literal_true_false_null_object_literal_or_array_literal: i(1328, 1, "Property_value_can_only_be_string_literal_numeric_literal_true_false_null_object_literal_or_array_li_1328", "Property value can only be string literal, numeric literal, 'true', 'false', 'null', object literal or array literal."), _0_accepts_too_few_arguments_to_be_used_as_a_decorator_here_Did_you_mean_to_call_it_first_and_write_0: i(1329, 1, "_0_accepts_too_few_arguments_to_be_used_as_a_decorator_here_Did_you_mean_to_call_it_first_and_write__1329", "'{0}' accepts too few arguments to be used as a decorator here. Did you mean to call it first and write '@{0}()'?"), A_property_of_an_interface_or_type_literal_whose_type_is_a_unique_symbol_type_must_be_readonly: i(1330, 1, "A_property_of_an_interface_or_type_literal_whose_type_is_a_unique_symbol_type_must_be_readonly_1330", "A property of an interface or type literal whose type is a 'unique symbol' type must be 'readonly'."), A_property_of_a_class_whose_type_is_a_unique_symbol_type_must_be_both_static_and_readonly: i(1331, 1, "A_property_of_a_class_whose_type_is_a_unique_symbol_type_must_be_both_static_and_readonly_1331", "A property of a class whose type is a 'unique symbol' type must be both 'static' and 'readonly'."), A_variable_whose_type_is_a_unique_symbol_type_must_be_const: i(1332, 1, "A_variable_whose_type_is_a_unique_symbol_type_must_be_const_1332", "A variable whose type is a 'unique symbol' type must be 'const'."), unique_symbol_types_may_not_be_used_on_a_variable_declaration_with_a_binding_name: i(1333, 1, "unique_symbol_types_may_not_be_used_on_a_variable_declaration_with_a_binding_name_1333", "'unique symbol' types may not be used on a variable declaration with a binding name."), unique_symbol_types_are_only_allowed_on_variables_in_a_variable_statement: i(1334, 1, "unique_symbol_types_are_only_allowed_on_variables_in_a_variable_statement_1334", "'unique symbol' types are only allowed on variables in a variable statement."), unique_symbol_types_are_not_allowed_here: i(1335, 1, "unique_symbol_types_are_not_allowed_here_1335", "'unique symbol' types are not allowed here."), An_index_signature_parameter_type_cannot_be_a_literal_type_or_generic_type_Consider_using_a_mapped_object_type_instead: i(1337, 1, "An_index_signature_parameter_type_cannot_be_a_literal_type_or_generic_type_Consider_using_a_mapped_o_1337", "An index signature parameter type cannot be a literal type or generic type. Consider using a mapped object type instead."), infer_declarations_are_only_permitted_in_the_extends_clause_of_a_conditional_type: i(1338, 1, "infer_declarations_are_only_permitted_in_the_extends_clause_of_a_conditional_type_1338", "'infer' declarations are only permitted in the 'extends' clause of a conditional type."), Module_0_does_not_refer_to_a_value_but_is_used_as_a_value_here: i(1339, 1, "Module_0_does_not_refer_to_a_value_but_is_used_as_a_value_here_1339", "Module '{0}' does not refer to a value, but is used as a value here."), Module_0_does_not_refer_to_a_type_but_is_used_as_a_type_here_Did_you_mean_typeof_import_0: i(1340, 1, "Module_0_does_not_refer_to_a_type_but_is_used_as_a_type_here_Did_you_mean_typeof_import_0_1340", "Module '{0}' does not refer to a type, but is used as a type here. Did you mean 'typeof import('{0}')'?"), Class_constructor_may_not_be_an_accessor: i(1341, 1, "Class_constructor_may_not_be_an_accessor_1341", "Class constructor may not be an accessor."), The_import_meta_meta_property_is_only_allowed_when_the_module_option_is_es2020_es2022_esnext_system_node16_or_nodenext: i(1343, 1, "The_import_meta_meta_property_is_only_allowed_when_the_module_option_is_es2020_es2022_esnext_system__1343", "The 'import.meta' meta-property is only allowed when the '--module' option is 'es2020', 'es2022', 'esnext', 'system', 'node16', or 'nodenext'."), A_label_is_not_allowed_here: i(1344, 1, "A_label_is_not_allowed_here_1344", "'A label is not allowed here."), An_expression_of_type_void_cannot_be_tested_for_truthiness: i(1345, 1, "An_expression_of_type_void_cannot_be_tested_for_truthiness_1345", "An expression of type 'void' cannot be tested for truthiness."), This_parameter_is_not_allowed_with_use_strict_directive: i(1346, 1, "This_parameter_is_not_allowed_with_use_strict_directive_1346", "This parameter is not allowed with 'use strict' directive."), use_strict_directive_cannot_be_used_with_non_simple_parameter_list: i(1347, 1, "use_strict_directive_cannot_be_used_with_non_simple_parameter_list_1347", "'use strict' directive cannot be used with non-simple parameter list."), Non_simple_parameter_declared_here: i(1348, 1, "Non_simple_parameter_declared_here_1348", "Non-simple parameter declared here."), use_strict_directive_used_here: i(1349, 1, "use_strict_directive_used_here_1349", "'use strict' directive used here."), Print_the_final_configuration_instead_of_building: i(1350, 3, "Print_the_final_configuration_instead_of_building_1350", "Print the final configuration instead of building."), An_identifier_or_keyword_cannot_immediately_follow_a_numeric_literal: i(1351, 1, "An_identifier_or_keyword_cannot_immediately_follow_a_numeric_literal_1351", "An identifier or keyword cannot immediately follow a numeric literal."), A_bigint_literal_cannot_use_exponential_notation: i(1352, 1, "A_bigint_literal_cannot_use_exponential_notation_1352", "A bigint literal cannot use exponential notation."), A_bigint_literal_must_be_an_integer: i(1353, 1, "A_bigint_literal_must_be_an_integer_1353", "A bigint literal must be an integer."), readonly_type_modifier_is_only_permitted_on_array_and_tuple_literal_types: i(1354, 1, "readonly_type_modifier_is_only_permitted_on_array_and_tuple_literal_types_1354", "'readonly' type modifier is only permitted on array and tuple literal types."), A_const_assertions_can_only_be_applied_to_references_to_enum_members_or_string_number_boolean_array_or_object_literals: i(1355, 1, "A_const_assertions_can_only_be_applied_to_references_to_enum_members_or_string_number_boolean_array__1355", "A 'const' assertions can only be applied to references to enum members, or string, number, boolean, array, or object literals."), Did_you_mean_to_mark_this_function_as_async: i(1356, 1, "Did_you_mean_to_mark_this_function_as_async_1356", "Did you mean to mark this function as 'async'?"), An_enum_member_name_must_be_followed_by_a_or: i(1357, 1, "An_enum_member_name_must_be_followed_by_a_or_1357", "An enum member name must be followed by a ',', '=', or '}'."), Tagged_template_expressions_are_not_permitted_in_an_optional_chain: i(1358, 1, "Tagged_template_expressions_are_not_permitted_in_an_optional_chain_1358", "Tagged template expressions are not permitted in an optional chain."), Identifier_expected_0_is_a_reserved_word_that_cannot_be_used_here: i(1359, 1, "Identifier_expected_0_is_a_reserved_word_that_cannot_be_used_here_1359", "Identifier expected. '{0}' is a reserved word that cannot be used here."), Type_0_does_not_satisfy_the_expected_type_1: i(1360, 1, "Type_0_does_not_satisfy_the_expected_type_1_1360", "Type '{0}' does not satisfy the expected type '{1}'."), _0_cannot_be_used_as_a_value_because_it_was_imported_using_import_type: i(1361, 1, "_0_cannot_be_used_as_a_value_because_it_was_imported_using_import_type_1361", "'{0}' cannot be used as a value because it was imported using 'import type'."), _0_cannot_be_used_as_a_value_because_it_was_exported_using_export_type: i(1362, 1, "_0_cannot_be_used_as_a_value_because_it_was_exported_using_export_type_1362", "'{0}' cannot be used as a value because it was exported using 'export type'."), A_type_only_import_can_specify_a_default_import_or_named_bindings_but_not_both: i(1363, 1, "A_type_only_import_can_specify_a_default_import_or_named_bindings_but_not_both_1363", "A type-only import can specify a default import or named bindings, but not both."), Convert_to_type_only_export: i(1364, 3, "Convert_to_type_only_export_1364", "Convert to type-only export"), Convert_all_re_exported_types_to_type_only_exports: i(1365, 3, "Convert_all_re_exported_types_to_type_only_exports_1365", "Convert all re-exported types to type-only exports"), Split_into_two_separate_import_declarations: i(1366, 3, "Split_into_two_separate_import_declarations_1366", "Split into two separate import declarations"), Split_all_invalid_type_only_imports: i(1367, 3, "Split_all_invalid_type_only_imports_1367", "Split all invalid type-only imports"), Class_constructor_may_not_be_a_generator: i(1368, 1, "Class_constructor_may_not_be_a_generator_1368", "Class constructor may not be a generator."), Did_you_mean_0: i(1369, 3, "Did_you_mean_0_1369", "Did you mean '{0}'?"), This_import_is_never_used_as_a_value_and_must_use_import_type_because_importsNotUsedAsValues_is_set_to_error: i(1371, 1, "This_import_is_never_used_as_a_value_and_must_use_import_type_because_importsNotUsedAsValues_is_set__1371", "This import is never used as a value and must use 'import type' because 'importsNotUsedAsValues' is set to 'error'."), Convert_to_type_only_import: i(1373, 3, "Convert_to_type_only_import_1373", "Convert to type-only import"), Convert_all_imports_not_used_as_a_value_to_type_only_imports: i(1374, 3, "Convert_all_imports_not_used_as_a_value_to_type_only_imports_1374", "Convert all imports not used as a value to type-only imports"), await_expressions_are_only_allowed_at_the_top_level_of_a_file_when_that_file_is_a_module_but_this_file_has_no_imports_or_exports_Consider_adding_an_empty_export_to_make_this_file_a_module: i(1375, 1, "await_expressions_are_only_allowed_at_the_top_level_of_a_file_when_that_file_is_a_module_but_this_fi_1375", "'await' expressions are only allowed at the top level of a file when that file is a module, but this file has no imports or exports. Consider adding an empty 'export {}' to make this file a module."), _0_was_imported_here: i(1376, 3, "_0_was_imported_here_1376", "'{0}' was imported here."), _0_was_exported_here: i(1377, 3, "_0_was_exported_here_1377", "'{0}' was exported here."), Top_level_await_expressions_are_only_allowed_when_the_module_option_is_set_to_es2022_esnext_system_node16_or_nodenext_and_the_target_option_is_set_to_es2017_or_higher: i(1378, 1, "Top_level_await_expressions_are_only_allowed_when_the_module_option_is_set_to_es2022_esnext_system_n_1378", "Top-level 'await' expressions are only allowed when the 'module' option is set to 'es2022', 'esnext', 'system', 'node16', or 'nodenext', and the 'target' option is set to 'es2017' or higher."), An_import_alias_cannot_reference_a_declaration_that_was_exported_using_export_type: i(1379, 1, "An_import_alias_cannot_reference_a_declaration_that_was_exported_using_export_type_1379", "An import alias cannot reference a declaration that was exported using 'export type'."), An_import_alias_cannot_reference_a_declaration_that_was_imported_using_import_type: i(1380, 1, "An_import_alias_cannot_reference_a_declaration_that_was_imported_using_import_type_1380", "An import alias cannot reference a declaration that was imported using 'import type'."), Unexpected_token_Did_you_mean_or_rbrace: i(1381, 1, "Unexpected_token_Did_you_mean_or_rbrace_1381", "Unexpected token. Did you mean `{'}'}` or `}`?"), Unexpected_token_Did_you_mean_or_gt: i(1382, 1, "Unexpected_token_Did_you_mean_or_gt_1382", "Unexpected token. Did you mean `{'>'}` or `>`?"), Function_type_notation_must_be_parenthesized_when_used_in_a_union_type: i(1385, 1, "Function_type_notation_must_be_parenthesized_when_used_in_a_union_type_1385", "Function type notation must be parenthesized when used in a union type."), Constructor_type_notation_must_be_parenthesized_when_used_in_a_union_type: i(1386, 1, "Constructor_type_notation_must_be_parenthesized_when_used_in_a_union_type_1386", "Constructor type notation must be parenthesized when used in a union type."), Function_type_notation_must_be_parenthesized_when_used_in_an_intersection_type: i(1387, 1, "Function_type_notation_must_be_parenthesized_when_used_in_an_intersection_type_1387", "Function type notation must be parenthesized when used in an intersection type."), Constructor_type_notation_must_be_parenthesized_when_used_in_an_intersection_type: i(1388, 1, "Constructor_type_notation_must_be_parenthesized_when_used_in_an_intersection_type_1388", "Constructor type notation must be parenthesized when used in an intersection type."), _0_is_not_allowed_as_a_variable_declaration_name: i(1389, 1, "_0_is_not_allowed_as_a_variable_declaration_name_1389", "'{0}' is not allowed as a variable declaration name."), _0_is_not_allowed_as_a_parameter_name: i(1390, 1, "_0_is_not_allowed_as_a_parameter_name_1390", "'{0}' is not allowed as a parameter name."), An_import_alias_cannot_use_import_type: i(1392, 1, "An_import_alias_cannot_use_import_type_1392", "An import alias cannot use 'import type'"), Imported_via_0_from_file_1: i(1393, 3, "Imported_via_0_from_file_1_1393", "Imported via {0} from file '{1}'"), Imported_via_0_from_file_1_with_packageId_2: i(1394, 3, "Imported_via_0_from_file_1_with_packageId_2_1394", "Imported via {0} from file '{1}' with packageId '{2}'"), Imported_via_0_from_file_1_to_import_importHelpers_as_specified_in_compilerOptions: i(1395, 3, "Imported_via_0_from_file_1_to_import_importHelpers_as_specified_in_compilerOptions_1395", "Imported via {0} from file '{1}' to import 'importHelpers' as specified in compilerOptions"), Imported_via_0_from_file_1_with_packageId_2_to_import_importHelpers_as_specified_in_compilerOptions: i(1396, 3, "Imported_via_0_from_file_1_with_packageId_2_to_import_importHelpers_as_specified_in_compilerOptions_1396", "Imported via {0} from file '{1}' with packageId '{2}' to import 'importHelpers' as specified in compilerOptions"), Imported_via_0_from_file_1_to_import_jsx_and_jsxs_factory_functions: i(1397, 3, "Imported_via_0_from_file_1_to_import_jsx_and_jsxs_factory_functions_1397", "Imported via {0} from file '{1}' to import 'jsx' and 'jsxs' factory functions"), Imported_via_0_from_file_1_with_packageId_2_to_import_jsx_and_jsxs_factory_functions: i(1398, 3, "Imported_via_0_from_file_1_with_packageId_2_to_import_jsx_and_jsxs_factory_functions_1398", "Imported via {0} from file '{1}' with packageId '{2}' to import 'jsx' and 'jsxs' factory functions"), File_is_included_via_import_here: i(1399, 3, "File_is_included_via_import_here_1399", "File is included via import here."), Referenced_via_0_from_file_1: i(1400, 3, "Referenced_via_0_from_file_1_1400", "Referenced via '{0}' from file '{1}'"), File_is_included_via_reference_here: i(1401, 3, "File_is_included_via_reference_here_1401", "File is included via reference here."), Type_library_referenced_via_0_from_file_1: i(1402, 3, "Type_library_referenced_via_0_from_file_1_1402", "Type library referenced via '{0}' from file '{1}'"), Type_library_referenced_via_0_from_file_1_with_packageId_2: i(1403, 3, "Type_library_referenced_via_0_from_file_1_with_packageId_2_1403", "Type library referenced via '{0}' from file '{1}' with packageId '{2}'"), File_is_included_via_type_library_reference_here: i(1404, 3, "File_is_included_via_type_library_reference_here_1404", "File is included via type library reference here."), Library_referenced_via_0_from_file_1: i(1405, 3, "Library_referenced_via_0_from_file_1_1405", "Library referenced via '{0}' from file '{1}'"), File_is_included_via_library_reference_here: i(1406, 3, "File_is_included_via_library_reference_here_1406", "File is included via library reference here."), Matched_by_include_pattern_0_in_1: i(1407, 3, "Matched_by_include_pattern_0_in_1_1407", "Matched by include pattern '{0}' in '{1}'"), File_is_matched_by_include_pattern_specified_here: i(1408, 3, "File_is_matched_by_include_pattern_specified_here_1408", "File is matched by include pattern specified here."), Part_of_files_list_in_tsconfig_json: i(1409, 3, "Part_of_files_list_in_tsconfig_json_1409", "Part of 'files' list in tsconfig.json"), File_is_matched_by_files_list_specified_here: i(1410, 3, "File_is_matched_by_files_list_specified_here_1410", "File is matched by 'files' list specified here."), Output_from_referenced_project_0_included_because_1_specified: i(1411, 3, "Output_from_referenced_project_0_included_because_1_specified_1411", "Output from referenced project '{0}' included because '{1}' specified"), Output_from_referenced_project_0_included_because_module_is_specified_as_none: i(1412, 3, "Output_from_referenced_project_0_included_because_module_is_specified_as_none_1412", "Output from referenced project '{0}' included because '--module' is specified as 'none'"), File_is_output_from_referenced_project_specified_here: i(1413, 3, "File_is_output_from_referenced_project_specified_here_1413", "File is output from referenced project specified here."), Source_from_referenced_project_0_included_because_1_specified: i(1414, 3, "Source_from_referenced_project_0_included_because_1_specified_1414", "Source from referenced project '{0}' included because '{1}' specified"), Source_from_referenced_project_0_included_because_module_is_specified_as_none: i(1415, 3, "Source_from_referenced_project_0_included_because_module_is_specified_as_none_1415", "Source from referenced project '{0}' included because '--module' is specified as 'none'"), File_is_source_from_referenced_project_specified_here: i(1416, 3, "File_is_source_from_referenced_project_specified_here_1416", "File is source from referenced project specified here."), Entry_point_of_type_library_0_specified_in_compilerOptions: i(1417, 3, "Entry_point_of_type_library_0_specified_in_compilerOptions_1417", "Entry point of type library '{0}' specified in compilerOptions"), Entry_point_of_type_library_0_specified_in_compilerOptions_with_packageId_1: i(1418, 3, "Entry_point_of_type_library_0_specified_in_compilerOptions_with_packageId_1_1418", "Entry point of type library '{0}' specified in compilerOptions with packageId '{1}'"), File_is_entry_point_of_type_library_specified_here: i(1419, 3, "File_is_entry_point_of_type_library_specified_here_1419", "File is entry point of type library specified here."), Entry_point_for_implicit_type_library_0: i(1420, 3, "Entry_point_for_implicit_type_library_0_1420", "Entry point for implicit type library '{0}'"), Entry_point_for_implicit_type_library_0_with_packageId_1: i(1421, 3, "Entry_point_for_implicit_type_library_0_with_packageId_1_1421", "Entry point for implicit type library '{0}' with packageId '{1}'"), Library_0_specified_in_compilerOptions: i(1422, 3, "Library_0_specified_in_compilerOptions_1422", "Library '{0}' specified in compilerOptions"), File_is_library_specified_here: i(1423, 3, "File_is_library_specified_here_1423", "File is library specified here."), Default_library: i(1424, 3, "Default_library_1424", "Default library"), Default_library_for_target_0: i(1425, 3, "Default_library_for_target_0_1425", "Default library for target '{0}'"), File_is_default_library_for_target_specified_here: i(1426, 3, "File_is_default_library_for_target_specified_here_1426", "File is default library for target specified here."), Root_file_specified_for_compilation: i(1427, 3, "Root_file_specified_for_compilation_1427", "Root file specified for compilation"), File_is_output_of_project_reference_source_0: i(1428, 3, "File_is_output_of_project_reference_source_0_1428", "File is output of project reference source '{0}'"), File_redirects_to_file_0: i(1429, 3, "File_redirects_to_file_0_1429", "File redirects to file '{0}'"), The_file_is_in_the_program_because_Colon: i(1430, 3, "The_file_is_in_the_program_because_Colon_1430", "The file is in the program because:"), for_await_loops_are_only_allowed_at_the_top_level_of_a_file_when_that_file_is_a_module_but_this_file_has_no_imports_or_exports_Consider_adding_an_empty_export_to_make_this_file_a_module: i(1431, 1, "for_await_loops_are_only_allowed_at_the_top_level_of_a_file_when_that_file_is_a_module_but_this_file_1431", "'for await' loops are only allowed at the top level of a file when that file is a module, but this file has no imports or exports. Consider adding an empty 'export {}' to make this file a module."), Top_level_for_await_loops_are_only_allowed_when_the_module_option_is_set_to_es2022_esnext_system_node16_or_nodenext_and_the_target_option_is_set_to_es2017_or_higher: i(1432, 1, "Top_level_for_await_loops_are_only_allowed_when_the_module_option_is_set_to_es2022_esnext_system_nod_1432", "Top-level 'for await' loops are only allowed when the 'module' option is set to 'es2022', 'esnext', 'system', 'node16', or 'nodenext', and the 'target' option is set to 'es2017' or higher."), Neither_decorators_nor_modifiers_may_be_applied_to_this_parameters: i(1433, 1, "Neither_decorators_nor_modifiers_may_be_applied_to_this_parameters_1433", "Neither decorators nor modifiers may be applied to 'this' parameters."), Unexpected_keyword_or_identifier: i(1434, 1, "Unexpected_keyword_or_identifier_1434", "Unexpected keyword or identifier."), Unknown_keyword_or_identifier_Did_you_mean_0: i(1435, 1, "Unknown_keyword_or_identifier_Did_you_mean_0_1435", "Unknown keyword or identifier. Did you mean '{0}'?"), Decorators_must_precede_the_name_and_all_keywords_of_property_declarations: i(1436, 1, "Decorators_must_precede_the_name_and_all_keywords_of_property_declarations_1436", "Decorators must precede the name and all keywords of property declarations."), Namespace_must_be_given_a_name: i(1437, 1, "Namespace_must_be_given_a_name_1437", "Namespace must be given a name."), Interface_must_be_given_a_name: i(1438, 1, "Interface_must_be_given_a_name_1438", "Interface must be given a name."), Type_alias_must_be_given_a_name: i(1439, 1, "Type_alias_must_be_given_a_name_1439", "Type alias must be given a name."), Variable_declaration_not_allowed_at_this_location: i(1440, 1, "Variable_declaration_not_allowed_at_this_location_1440", "Variable declaration not allowed at this location."), Cannot_start_a_function_call_in_a_type_annotation: i(1441, 1, "Cannot_start_a_function_call_in_a_type_annotation_1441", "Cannot start a function call in a type annotation."), Expected_for_property_initializer: i(1442, 1, "Expected_for_property_initializer_1442", "Expected '=' for property initializer."), Module_declaration_names_may_only_use_or_quoted_strings: i(1443, 1, "Module_declaration_names_may_only_use_or_quoted_strings_1443", `Module declaration names may only use ' or " quoted strings.`), _0_is_a_type_and_must_be_imported_using_a_type_only_import_when_preserveValueImports_and_isolatedModules_are_both_enabled: i(1444, 1, "_0_is_a_type_and_must_be_imported_using_a_type_only_import_when_preserveValueImports_and_isolatedMod_1444", "'{0}' is a type and must be imported using a type-only import when 'preserveValueImports' and 'isolatedModules' are both enabled."), _0_resolves_to_a_type_only_declaration_and_must_be_imported_using_a_type_only_import_when_preserveValueImports_and_isolatedModules_are_both_enabled: i(1446, 1, "_0_resolves_to_a_type_only_declaration_and_must_be_imported_using_a_type_only_import_when_preserveVa_1446", "'{0}' resolves to a type-only declaration and must be imported using a type-only import when 'preserveValueImports' and 'isolatedModules' are both enabled."), _0_resolves_to_a_type_only_declaration_and_must_be_re_exported_using_a_type_only_re_export_when_1_is_enabled: i(1448, 1, "_0_resolves_to_a_type_only_declaration_and_must_be_re_exported_using_a_type_only_re_export_when_1_is_1448", "'{0}' resolves to a type-only declaration and must be re-exported using a type-only re-export when '{1}' is enabled."), Preserve_unused_imported_values_in_the_JavaScript_output_that_would_otherwise_be_removed: i(1449, 3, "Preserve_unused_imported_values_in_the_JavaScript_output_that_would_otherwise_be_removed_1449", "Preserve unused imported values in the JavaScript output that would otherwise be removed."), Dynamic_imports_can_only_accept_a_module_specifier_and_an_optional_assertion_as_arguments: i(1450, 3, "Dynamic_imports_can_only_accept_a_module_specifier_and_an_optional_assertion_as_arguments_1450", "Dynamic imports can only accept a module specifier and an optional assertion as arguments"), Private_identifiers_are_only_allowed_in_class_bodies_and_may_only_be_used_as_part_of_a_class_member_declaration_property_access_or_on_the_left_hand_side_of_an_in_expression: i(1451, 1, "Private_identifiers_are_only_allowed_in_class_bodies_and_may_only_be_used_as_part_of_a_class_member__1451", "Private identifiers are only allowed in class bodies and may only be used as part of a class member declaration, property access, or on the left-hand-side of an 'in' expression"), resolution_mode_assertions_are_only_supported_when_moduleResolution_is_node16_or_nodenext: i(1452, 1, "resolution_mode_assertions_are_only_supported_when_moduleResolution_is_node16_or_nodenext_1452", "'resolution-mode' assertions are only supported when `moduleResolution` is `node16` or `nodenext`."), resolution_mode_should_be_either_require_or_import: i(1453, 1, "resolution_mode_should_be_either_require_or_import_1453", "`resolution-mode` should be either `require` or `import`."), resolution_mode_can_only_be_set_for_type_only_imports: i(1454, 1, "resolution_mode_can_only_be_set_for_type_only_imports_1454", "`resolution-mode` can only be set for type-only imports."), resolution_mode_is_the_only_valid_key_for_type_import_assertions: i(1455, 1, "resolution_mode_is_the_only_valid_key_for_type_import_assertions_1455", "`resolution-mode` is the only valid key for type import assertions."), Type_import_assertions_should_have_exactly_one_key_resolution_mode_with_value_import_or_require: i(1456, 1, "Type_import_assertions_should_have_exactly_one_key_resolution_mode_with_value_import_or_require_1456", "Type import assertions should have exactly one key - `resolution-mode` - with value `import` or `require`."), Matched_by_default_include_pattern_Asterisk_Asterisk_Slash_Asterisk: i(1457, 3, "Matched_by_default_include_pattern_Asterisk_Asterisk_Slash_Asterisk_1457", "Matched by default include pattern '**/*'"), File_is_ECMAScript_module_because_0_has_field_type_with_value_module: i(1458, 3, "File_is_ECMAScript_module_because_0_has_field_type_with_value_module_1458", `File is ECMAScript module because '{0}' has field "type" with value "module"`), File_is_CommonJS_module_because_0_has_field_type_whose_value_is_not_module: i(1459, 3, "File_is_CommonJS_module_because_0_has_field_type_whose_value_is_not_module_1459", `File is CommonJS module because '{0}' has field "type" whose value is not "module"`), File_is_CommonJS_module_because_0_does_not_have_field_type: i(1460, 3, "File_is_CommonJS_module_because_0_does_not_have_field_type_1460", `File is CommonJS module because '{0}' does not have field "type"`), File_is_CommonJS_module_because_package_json_was_not_found: i(1461, 3, "File_is_CommonJS_module_because_package_json_was_not_found_1461", "File is CommonJS module because 'package.json' was not found"), The_import_meta_meta_property_is_not_allowed_in_files_which_will_build_into_CommonJS_output: i(1470, 1, "The_import_meta_meta_property_is_not_allowed_in_files_which_will_build_into_CommonJS_output_1470", "The 'import.meta' meta-property is not allowed in files which will build into CommonJS output."), Module_0_cannot_be_imported_using_this_construct_The_specifier_only_resolves_to_an_ES_module_which_cannot_be_imported_with_require_Use_an_ECMAScript_import_instead: i(1471, 1, "Module_0_cannot_be_imported_using_this_construct_The_specifier_only_resolves_to_an_ES_module_which_c_1471", "Module '{0}' cannot be imported using this construct. The specifier only resolves to an ES module, which cannot be imported with 'require'. Use an ECMAScript import instead."), catch_or_finally_expected: i(1472, 1, "catch_or_finally_expected_1472", "'catch' or 'finally' expected."), An_import_declaration_can_only_be_used_at_the_top_level_of_a_module: i(1473, 1, "An_import_declaration_can_only_be_used_at_the_top_level_of_a_module_1473", "An import declaration can only be used at the top level of a module."), An_export_declaration_can_only_be_used_at_the_top_level_of_a_module: i(1474, 1, "An_export_declaration_can_only_be_used_at_the_top_level_of_a_module_1474", "An export declaration can only be used at the top level of a module."), Control_what_method_is_used_to_detect_module_format_JS_files: i(1475, 3, "Control_what_method_is_used_to_detect_module_format_JS_files_1475", "Control what method is used to detect module-format JS files."), auto_Colon_Treat_files_with_imports_exports_import_meta_jsx_with_jsx_Colon_react_jsx_or_esm_format_with_module_Colon_node16_as_modules: i(1476, 3, "auto_Colon_Treat_files_with_imports_exports_import_meta_jsx_with_jsx_Colon_react_jsx_or_esm_format_w_1476", '"auto": Treat files with imports, exports, import.meta, jsx (with jsx: react-jsx), or esm format (with module: node16+) as modules.'), An_instantiation_expression_cannot_be_followed_by_a_property_access: i(1477, 1, "An_instantiation_expression_cannot_be_followed_by_a_property_access_1477", "An instantiation expression cannot be followed by a property access."), Identifier_or_string_literal_expected: i(1478, 1, "Identifier_or_string_literal_expected_1478", "Identifier or string literal expected."), The_current_file_is_a_CommonJS_module_whose_imports_will_produce_require_calls_however_the_referenced_file_is_an_ECMAScript_module_and_cannot_be_imported_with_require_Consider_writing_a_dynamic_import_0_call_instead: i(1479, 1, "The_current_file_is_a_CommonJS_module_whose_imports_will_produce_require_calls_however_the_reference_1479", `The current file is a CommonJS module whose imports will produce 'require' calls; however, the referenced file is an ECMAScript module and cannot be imported with 'require'. Consider writing a dynamic 'import("{0}")' call instead.`), To_convert_this_file_to_an_ECMAScript_module_change_its_file_extension_to_0_or_create_a_local_package_json_file_with_type_Colon_module: i(1480, 3, "To_convert_this_file_to_an_ECMAScript_module_change_its_file_extension_to_0_or_create_a_local_packag_1480", 'To convert this file to an ECMAScript module, change its file extension to \'{0}\' or create a local package.json file with `{ "type": "module" }`.'), To_convert_this_file_to_an_ECMAScript_module_change_its_file_extension_to_0_or_add_the_field_type_Colon_module_to_1: i(1481, 3, "To_convert_this_file_to_an_ECMAScript_module_change_its_file_extension_to_0_or_add_the_field_type_Co_1481", `To convert this file to an ECMAScript module, change its file extension to '{0}', or add the field \`"type": "module"\` to '{1}'.`), To_convert_this_file_to_an_ECMAScript_module_add_the_field_type_Colon_module_to_0: i(1482, 3, "To_convert_this_file_to_an_ECMAScript_module_add_the_field_type_Colon_module_to_0_1482", 'To convert this file to an ECMAScript module, add the field `"type": "module"` to \'{0}\'.'), To_convert_this_file_to_an_ECMAScript_module_create_a_local_package_json_file_with_type_Colon_module: i(1483, 3, "To_convert_this_file_to_an_ECMAScript_module_create_a_local_package_json_file_with_type_Colon_module_1483", 'To convert this file to an ECMAScript module, create a local package.json file with `{ "type": "module" }`.'), _0_is_a_type_and_must_be_imported_using_a_type_only_import_when_verbatimModuleSyntax_is_enabled: i(1484, 1, "_0_is_a_type_and_must_be_imported_using_a_type_only_import_when_verbatimModuleSyntax_is_enabled_1484", "'{0}' is a type and must be imported using a type-only import when 'verbatimModuleSyntax' is enabled."), _0_resolves_to_a_type_only_declaration_and_must_be_imported_using_a_type_only_import_when_verbatimModuleSyntax_is_enabled: i(1485, 1, "_0_resolves_to_a_type_only_declaration_and_must_be_imported_using_a_type_only_import_when_verbatimMo_1485", "'{0}' resolves to a type-only declaration and must be imported using a type-only import when 'verbatimModuleSyntax' is enabled."), Decorator_used_before_export_here: i(1486, 1, "Decorator_used_before_export_here_1486", "Decorator used before 'export' here."), The_types_of_0_are_incompatible_between_these_types: i(2200, 1, "The_types_of_0_are_incompatible_between_these_types_2200", "The types of '{0}' are incompatible between these types."), The_types_returned_by_0_are_incompatible_between_these_types: i(2201, 1, "The_types_returned_by_0_are_incompatible_between_these_types_2201", "The types returned by '{0}' are incompatible between these types."), Call_signature_return_types_0_and_1_are_incompatible: i(2202, 1, "Call_signature_return_types_0_and_1_are_incompatible_2202", "Call signature return types '{0}' and '{1}' are incompatible.", void 0, true), Construct_signature_return_types_0_and_1_are_incompatible: i(2203, 1, "Construct_signature_return_types_0_and_1_are_incompatible_2203", "Construct signature return types '{0}' and '{1}' are incompatible.", void 0, true), Call_signatures_with_no_arguments_have_incompatible_return_types_0_and_1: i(2204, 1, "Call_signatures_with_no_arguments_have_incompatible_return_types_0_and_1_2204", "Call signatures with no arguments have incompatible return types '{0}' and '{1}'.", void 0, true), Construct_signatures_with_no_arguments_have_incompatible_return_types_0_and_1: i(2205, 1, "Construct_signatures_with_no_arguments_have_incompatible_return_types_0_and_1_2205", "Construct signatures with no arguments have incompatible return types '{0}' and '{1}'.", void 0, true), The_type_modifier_cannot_be_used_on_a_named_import_when_import_type_is_used_on_its_import_statement: i(2206, 1, "The_type_modifier_cannot_be_used_on_a_named_import_when_import_type_is_used_on_its_import_statement_2206", "The 'type' modifier cannot be used on a named import when 'import type' is used on its import statement."), The_type_modifier_cannot_be_used_on_a_named_export_when_export_type_is_used_on_its_export_statement: i(2207, 1, "The_type_modifier_cannot_be_used_on_a_named_export_when_export_type_is_used_on_its_export_statement_2207", "The 'type' modifier cannot be used on a named export when 'export type' is used on its export statement."), This_type_parameter_might_need_an_extends_0_constraint: i(2208, 1, "This_type_parameter_might_need_an_extends_0_constraint_2208", "This type parameter might need an `extends {0}` constraint."), The_project_root_is_ambiguous_but_is_required_to_resolve_export_map_entry_0_in_file_1_Supply_the_rootDir_compiler_option_to_disambiguate: i(2209, 1, "The_project_root_is_ambiguous_but_is_required_to_resolve_export_map_entry_0_in_file_1_Supply_the_roo_2209", "The project root is ambiguous, but is required to resolve export map entry '{0}' in file '{1}'. Supply the `rootDir` compiler option to disambiguate."), The_project_root_is_ambiguous_but_is_required_to_resolve_import_map_entry_0_in_file_1_Supply_the_rootDir_compiler_option_to_disambiguate: i(2210, 1, "The_project_root_is_ambiguous_but_is_required_to_resolve_import_map_entry_0_in_file_1_Supply_the_roo_2210", "The project root is ambiguous, but is required to resolve import map entry '{0}' in file '{1}'. Supply the `rootDir` compiler option to disambiguate."), Add_extends_constraint: i(2211, 3, "Add_extends_constraint_2211", "Add `extends` constraint."), Add_extends_constraint_to_all_type_parameters: i(2212, 3, "Add_extends_constraint_to_all_type_parameters_2212", "Add `extends` constraint to all type parameters"), Duplicate_identifier_0: i(2300, 1, "Duplicate_identifier_0_2300", "Duplicate identifier '{0}'."), Initializer_of_instance_member_variable_0_cannot_reference_identifier_1_declared_in_the_constructor: i(2301, 1, "Initializer_of_instance_member_variable_0_cannot_reference_identifier_1_declared_in_the_constructor_2301", "Initializer of instance member variable '{0}' cannot reference identifier '{1}' declared in the constructor."), Static_members_cannot_reference_class_type_parameters: i(2302, 1, "Static_members_cannot_reference_class_type_parameters_2302", "Static members cannot reference class type parameters."), Circular_definition_of_import_alias_0: i(2303, 1, "Circular_definition_of_import_alias_0_2303", "Circular definition of import alias '{0}'."), Cannot_find_name_0: i(2304, 1, "Cannot_find_name_0_2304", "Cannot find name '{0}'."), Module_0_has_no_exported_member_1: i(2305, 1, "Module_0_has_no_exported_member_1_2305", "Module '{0}' has no exported member '{1}'."), File_0_is_not_a_module: i(2306, 1, "File_0_is_not_a_module_2306", "File '{0}' is not a module."), Cannot_find_module_0_or_its_corresponding_type_declarations: i(2307, 1, "Cannot_find_module_0_or_its_corresponding_type_declarations_2307", "Cannot find module '{0}' or its corresponding type declarations."), Module_0_has_already_exported_a_member_named_1_Consider_explicitly_re_exporting_to_resolve_the_ambiguity: i(2308, 1, "Module_0_has_already_exported_a_member_named_1_Consider_explicitly_re_exporting_to_resolve_the_ambig_2308", "Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity."), An_export_assignment_cannot_be_used_in_a_module_with_other_exported_elements: i(2309, 1, "An_export_assignment_cannot_be_used_in_a_module_with_other_exported_elements_2309", "An export assignment cannot be used in a module with other exported elements."), Type_0_recursively_references_itself_as_a_base_type: i(2310, 1, "Type_0_recursively_references_itself_as_a_base_type_2310", "Type '{0}' recursively references itself as a base type."), Cannot_find_name_0_Did_you_mean_to_write_this_in_an_async_function: i(2311, 1, "Cannot_find_name_0_Did_you_mean_to_write_this_in_an_async_function_2311", "Cannot find name '{0}'. Did you mean to write this in an async function?"), An_interface_can_only_extend_an_object_type_or_intersection_of_object_types_with_statically_known_members: i(2312, 1, "An_interface_can_only_extend_an_object_type_or_intersection_of_object_types_with_statically_known_me_2312", "An interface can only extend an object type or intersection of object types with statically known members."), Type_parameter_0_has_a_circular_constraint: i(2313, 1, "Type_parameter_0_has_a_circular_constraint_2313", "Type parameter '{0}' has a circular constraint."), Generic_type_0_requires_1_type_argument_s: i(2314, 1, "Generic_type_0_requires_1_type_argument_s_2314", "Generic type '{0}' requires {1} type argument(s)."), Type_0_is_not_generic: i(2315, 1, "Type_0_is_not_generic_2315", "Type '{0}' is not generic."), Global_type_0_must_be_a_class_or_interface_type: i(2316, 1, "Global_type_0_must_be_a_class_or_interface_type_2316", "Global type '{0}' must be a class or interface type."), Global_type_0_must_have_1_type_parameter_s: i(2317, 1, "Global_type_0_must_have_1_type_parameter_s_2317", "Global type '{0}' must have {1} type parameter(s)."), Cannot_find_global_type_0: i(2318, 1, "Cannot_find_global_type_0_2318", "Cannot find global type '{0}'."), Named_property_0_of_types_1_and_2_are_not_identical: i(2319, 1, "Named_property_0_of_types_1_and_2_are_not_identical_2319", "Named property '{0}' of types '{1}' and '{2}' are not identical."), Interface_0_cannot_simultaneously_extend_types_1_and_2: i(2320, 1, "Interface_0_cannot_simultaneously_extend_types_1_and_2_2320", "Interface '{0}' cannot simultaneously extend types '{1}' and '{2}'."), Excessive_stack_depth_comparing_types_0_and_1: i(2321, 1, "Excessive_stack_depth_comparing_types_0_and_1_2321", "Excessive stack depth comparing types '{0}' and '{1}'."), Type_0_is_not_assignable_to_type_1: i(2322, 1, "Type_0_is_not_assignable_to_type_1_2322", "Type '{0}' is not assignable to type '{1}'."), Cannot_redeclare_exported_variable_0: i(2323, 1, "Cannot_redeclare_exported_variable_0_2323", "Cannot redeclare exported variable '{0}'."), Property_0_is_missing_in_type_1: i(2324, 1, "Property_0_is_missing_in_type_1_2324", "Property '{0}' is missing in type '{1}'."), Property_0_is_private_in_type_1_but_not_in_type_2: i(2325, 1, "Property_0_is_private_in_type_1_but_not_in_type_2_2325", "Property '{0}' is private in type '{1}' but not in type '{2}'."), Types_of_property_0_are_incompatible: i(2326, 1, "Types_of_property_0_are_incompatible_2326", "Types of property '{0}' are incompatible."), Property_0_is_optional_in_type_1_but_required_in_type_2: i(2327, 1, "Property_0_is_optional_in_type_1_but_required_in_type_2_2327", "Property '{0}' is optional in type '{1}' but required in type '{2}'."), Types_of_parameters_0_and_1_are_incompatible: i(2328, 1, "Types_of_parameters_0_and_1_are_incompatible_2328", "Types of parameters '{0}' and '{1}' are incompatible."), Index_signature_for_type_0_is_missing_in_type_1: i(2329, 1, "Index_signature_for_type_0_is_missing_in_type_1_2329", "Index signature for type '{0}' is missing in type '{1}'."), _0_and_1_index_signatures_are_incompatible: i(2330, 1, "_0_and_1_index_signatures_are_incompatible_2330", "'{0}' and '{1}' index signatures are incompatible."), this_cannot_be_referenced_in_a_module_or_namespace_body: i(2331, 1, "this_cannot_be_referenced_in_a_module_or_namespace_body_2331", "'this' cannot be referenced in a module or namespace body."), this_cannot_be_referenced_in_current_location: i(2332, 1, "this_cannot_be_referenced_in_current_location_2332", "'this' cannot be referenced in current location."), this_cannot_be_referenced_in_constructor_arguments: i(2333, 1, "this_cannot_be_referenced_in_constructor_arguments_2333", "'this' cannot be referenced in constructor arguments."), this_cannot_be_referenced_in_a_static_property_initializer: i(2334, 1, "this_cannot_be_referenced_in_a_static_property_initializer_2334", "'this' cannot be referenced in a static property initializer."), super_can_only_be_referenced_in_a_derived_class: i(2335, 1, "super_can_only_be_referenced_in_a_derived_class_2335", "'super' can only be referenced in a derived class."), super_cannot_be_referenced_in_constructor_arguments: i(2336, 1, "super_cannot_be_referenced_in_constructor_arguments_2336", "'super' cannot be referenced in constructor arguments."), Super_calls_are_not_permitted_outside_constructors_or_in_nested_functions_inside_constructors: i(2337, 1, "Super_calls_are_not_permitted_outside_constructors_or_in_nested_functions_inside_constructors_2337", "Super calls are not permitted outside constructors or in nested functions inside constructors."), super_property_access_is_permitted_only_in_a_constructor_member_function_or_member_accessor_of_a_derived_class: i(2338, 1, "super_property_access_is_permitted_only_in_a_constructor_member_function_or_member_accessor_of_a_der_2338", "'super' property access is permitted only in a constructor, member function, or member accessor of a derived class."), Property_0_does_not_exist_on_type_1: i(2339, 1, "Property_0_does_not_exist_on_type_1_2339", "Property '{0}' does not exist on type '{1}'."), Only_public_and_protected_methods_of_the_base_class_are_accessible_via_the_super_keyword: i(2340, 1, "Only_public_and_protected_methods_of_the_base_class_are_accessible_via_the_super_keyword_2340", "Only public and protected methods of the base class are accessible via the 'super' keyword."), Property_0_is_private_and_only_accessible_within_class_1: i(2341, 1, "Property_0_is_private_and_only_accessible_within_class_1_2341", "Property '{0}' is private and only accessible within class '{1}'."), This_syntax_requires_an_imported_helper_named_1_which_does_not_exist_in_0_Consider_upgrading_your_version_of_0: i(2343, 1, "This_syntax_requires_an_imported_helper_named_1_which_does_not_exist_in_0_Consider_upgrading_your_ve_2343", "This syntax requires an imported helper named '{1}' which does not exist in '{0}'. Consider upgrading your version of '{0}'."), Type_0_does_not_satisfy_the_constraint_1: i(2344, 1, "Type_0_does_not_satisfy_the_constraint_1_2344", "Type '{0}' does not satisfy the constraint '{1}'."), Argument_of_type_0_is_not_assignable_to_parameter_of_type_1: i(2345, 1, "Argument_of_type_0_is_not_assignable_to_parameter_of_type_1_2345", "Argument of type '{0}' is not assignable to parameter of type '{1}'."), Call_target_does_not_contain_any_signatures: i(2346, 1, "Call_target_does_not_contain_any_signatures_2346", "Call target does not contain any signatures."), Untyped_function_calls_may_not_accept_type_arguments: i(2347, 1, "Untyped_function_calls_may_not_accept_type_arguments_2347", "Untyped function calls may not accept type arguments."), Value_of_type_0_is_not_callable_Did_you_mean_to_include_new: i(2348, 1, "Value_of_type_0_is_not_callable_Did_you_mean_to_include_new_2348", "Value of type '{0}' is not callable. Did you mean to include 'new'?"), This_expression_is_not_callable: i(2349, 1, "This_expression_is_not_callable_2349", "This expression is not callable."), Only_a_void_function_can_be_called_with_the_new_keyword: i(2350, 1, "Only_a_void_function_can_be_called_with_the_new_keyword_2350", "Only a void function can be called with the 'new' keyword."), This_expression_is_not_constructable: i(2351, 1, "This_expression_is_not_constructable_2351", "This expression is not constructable."), Conversion_of_type_0_to_type_1_may_be_a_mistake_because_neither_type_sufficiently_overlaps_with_the_other_If_this_was_intentional_convert_the_expression_to_unknown_first: i(2352, 1, "Conversion_of_type_0_to_type_1_may_be_a_mistake_because_neither_type_sufficiently_overlaps_with_the__2352", "Conversion of type '{0}' to type '{1}' may be a mistake because neither type sufficiently overlaps with the other. If this was intentional, convert the expression to 'unknown' first."), Object_literal_may_only_specify_known_properties_and_0_does_not_exist_in_type_1: i(2353, 1, "Object_literal_may_only_specify_known_properties_and_0_does_not_exist_in_type_1_2353", "Object literal may only specify known properties, and '{0}' does not exist in type '{1}'."), This_syntax_requires_an_imported_helper_but_module_0_cannot_be_found: i(2354, 1, "This_syntax_requires_an_imported_helper_but_module_0_cannot_be_found_2354", "This syntax requires an imported helper but module '{0}' cannot be found."), A_function_whose_declared_type_is_neither_void_nor_any_must_return_a_value: i(2355, 1, "A_function_whose_declared_type_is_neither_void_nor_any_must_return_a_value_2355", "A function whose declared type is neither 'void' nor 'any' must return a value."), An_arithmetic_operand_must_be_of_type_any_number_bigint_or_an_enum_type: i(2356, 1, "An_arithmetic_operand_must_be_of_type_any_number_bigint_or_an_enum_type_2356", "An arithmetic operand must be of type 'any', 'number', 'bigint' or an enum type."), The_operand_of_an_increment_or_decrement_operator_must_be_a_variable_or_a_property_access: i(2357, 1, "The_operand_of_an_increment_or_decrement_operator_must_be_a_variable_or_a_property_access_2357", "The operand of an increment or decrement operator must be a variable or a property access."), The_left_hand_side_of_an_instanceof_expression_must_be_of_type_any_an_object_type_or_a_type_parameter: i(2358, 1, "The_left_hand_side_of_an_instanceof_expression_must_be_of_type_any_an_object_type_or_a_type_paramete_2358", "The left-hand side of an 'instanceof' expression must be of type 'any', an object type or a type parameter."), The_right_hand_side_of_an_instanceof_expression_must_be_of_type_any_or_of_a_type_assignable_to_the_Function_interface_type: i(2359, 1, "The_right_hand_side_of_an_instanceof_expression_must_be_of_type_any_or_of_a_type_assignable_to_the_F_2359", "The right-hand side of an 'instanceof' expression must be of type 'any' or of a type assignable to the 'Function' interface type."), The_left_hand_side_of_an_arithmetic_operation_must_be_of_type_any_number_bigint_or_an_enum_type: i(2362, 1, "The_left_hand_side_of_an_arithmetic_operation_must_be_of_type_any_number_bigint_or_an_enum_type_2362", "The left-hand side of an arithmetic operation must be of type 'any', 'number', 'bigint' or an enum type."), The_right_hand_side_of_an_arithmetic_operation_must_be_of_type_any_number_bigint_or_an_enum_type: i(2363, 1, "The_right_hand_side_of_an_arithmetic_operation_must_be_of_type_any_number_bigint_or_an_enum_type_2363", "The right-hand side of an arithmetic operation must be of type 'any', 'number', 'bigint' or an enum type."), The_left_hand_side_of_an_assignment_expression_must_be_a_variable_or_a_property_access: i(2364, 1, "The_left_hand_side_of_an_assignment_expression_must_be_a_variable_or_a_property_access_2364", "The left-hand side of an assignment expression must be a variable or a property access."), Operator_0_cannot_be_applied_to_types_1_and_2: i(2365, 1, "Operator_0_cannot_be_applied_to_types_1_and_2_2365", "Operator '{0}' cannot be applied to types '{1}' and '{2}'."), Function_lacks_ending_return_statement_and_return_type_does_not_include_undefined: i(2366, 1, "Function_lacks_ending_return_statement_and_return_type_does_not_include_undefined_2366", "Function lacks ending return statement and return type does not include 'undefined'."), This_comparison_appears_to_be_unintentional_because_the_types_0_and_1_have_no_overlap: i(2367, 1, "This_comparison_appears_to_be_unintentional_because_the_types_0_and_1_have_no_overlap_2367", "This comparison appears to be unintentional because the types '{0}' and '{1}' have no overlap."), Type_parameter_name_cannot_be_0: i(2368, 1, "Type_parameter_name_cannot_be_0_2368", "Type parameter name cannot be '{0}'."), A_parameter_property_is_only_allowed_in_a_constructor_implementation: i(2369, 1, "A_parameter_property_is_only_allowed_in_a_constructor_implementation_2369", "A parameter property is only allowed in a constructor implementation."), A_rest_parameter_must_be_of_an_array_type: i(2370, 1, "A_rest_parameter_must_be_of_an_array_type_2370", "A rest parameter must be of an array type."), A_parameter_initializer_is_only_allowed_in_a_function_or_constructor_implementation: i(2371, 1, "A_parameter_initializer_is_only_allowed_in_a_function_or_constructor_implementation_2371", "A parameter initializer is only allowed in a function or constructor implementation."), Parameter_0_cannot_reference_itself: i(2372, 1, "Parameter_0_cannot_reference_itself_2372", "Parameter '{0}' cannot reference itself."), Parameter_0_cannot_reference_identifier_1_declared_after_it: i(2373, 1, "Parameter_0_cannot_reference_identifier_1_declared_after_it_2373", "Parameter '{0}' cannot reference identifier '{1}' declared after it."), Duplicate_index_signature_for_type_0: i(2374, 1, "Duplicate_index_signature_for_type_0_2374", "Duplicate index signature for type '{0}'."), Type_0_is_not_assignable_to_type_1_with_exactOptionalPropertyTypes_Colon_true_Consider_adding_undefined_to_the_types_of_the_target_s_properties: i(2375, 1, "Type_0_is_not_assignable_to_type_1_with_exactOptionalPropertyTypes_Colon_true_Consider_adding_undefi_2375", "Type '{0}' is not assignable to type '{1}' with 'exactOptionalPropertyTypes: true'. Consider adding 'undefined' to the types of the target's properties."), A_super_call_must_be_the_first_statement_in_the_constructor_to_refer_to_super_or_this_when_a_derived_class_contains_initialized_properties_parameter_properties_or_private_identifiers: i(2376, 1, "A_super_call_must_be_the_first_statement_in_the_constructor_to_refer_to_super_or_this_when_a_derived_2376", "A 'super' call must be the first statement in the constructor to refer to 'super' or 'this' when a derived class contains initialized properties, parameter properties, or private identifiers."), Constructors_for_derived_classes_must_contain_a_super_call: i(2377, 1, "Constructors_for_derived_classes_must_contain_a_super_call_2377", "Constructors for derived classes must contain a 'super' call."), A_get_accessor_must_return_a_value: i(2378, 1, "A_get_accessor_must_return_a_value_2378", "A 'get' accessor must return a value."), Argument_of_type_0_is_not_assignable_to_parameter_of_type_1_with_exactOptionalPropertyTypes_Colon_true_Consider_adding_undefined_to_the_types_of_the_target_s_properties: i(2379, 1, "Argument_of_type_0_is_not_assignable_to_parameter_of_type_1_with_exactOptionalPropertyTypes_Colon_tr_2379", "Argument of type '{0}' is not assignable to parameter of type '{1}' with 'exactOptionalPropertyTypes: true'. Consider adding 'undefined' to the types of the target's properties."), The_return_type_of_a_get_accessor_must_be_assignable_to_its_set_accessor_type: i(2380, 1, "The_return_type_of_a_get_accessor_must_be_assignable_to_its_set_accessor_type_2380", "The return type of a 'get' accessor must be assignable to its 'set' accessor type"), Overload_signatures_must_all_be_exported_or_non_exported: i(2383, 1, "Overload_signatures_must_all_be_exported_or_non_exported_2383", "Overload signatures must all be exported or non-exported."), Overload_signatures_must_all_be_ambient_or_non_ambient: i(2384, 1, "Overload_signatures_must_all_be_ambient_or_non_ambient_2384", "Overload signatures must all be ambient or non-ambient."), Overload_signatures_must_all_be_public_private_or_protected: i(2385, 1, "Overload_signatures_must_all_be_public_private_or_protected_2385", "Overload signatures must all be public, private or protected."), Overload_signatures_must_all_be_optional_or_required: i(2386, 1, "Overload_signatures_must_all_be_optional_or_required_2386", "Overload signatures must all be optional or required."), Function_overload_must_be_static: i(2387, 1, "Function_overload_must_be_static_2387", "Function overload must be static."), Function_overload_must_not_be_static: i(2388, 1, "Function_overload_must_not_be_static_2388", "Function overload must not be static."), Function_implementation_name_must_be_0: i(2389, 1, "Function_implementation_name_must_be_0_2389", "Function implementation name must be '{0}'."), Constructor_implementation_is_missing: i(2390, 1, "Constructor_implementation_is_missing_2390", "Constructor implementation is missing."), Function_implementation_is_missing_or_not_immediately_following_the_declaration: i(2391, 1, "Function_implementation_is_missing_or_not_immediately_following_the_declaration_2391", "Function implementation is missing or not immediately following the declaration."), Multiple_constructor_implementations_are_not_allowed: i(2392, 1, "Multiple_constructor_implementations_are_not_allowed_2392", "Multiple constructor implementations are not allowed."), Duplicate_function_implementation: i(2393, 1, "Duplicate_function_implementation_2393", "Duplicate function implementation."), This_overload_signature_is_not_compatible_with_its_implementation_signature: i(2394, 1, "This_overload_signature_is_not_compatible_with_its_implementation_signature_2394", "This overload signature is not compatible with its implementation signature."), Individual_declarations_in_merged_declaration_0_must_be_all_exported_or_all_local: i(2395, 1, "Individual_declarations_in_merged_declaration_0_must_be_all_exported_or_all_local_2395", "Individual declarations in merged declaration '{0}' must be all exported or all local."), Duplicate_identifier_arguments_Compiler_uses_arguments_to_initialize_rest_parameters: i(2396, 1, "Duplicate_identifier_arguments_Compiler_uses_arguments_to_initialize_rest_parameters_2396", "Duplicate identifier 'arguments'. Compiler uses 'arguments' to initialize rest parameters."), Declaration_name_conflicts_with_built_in_global_identifier_0: i(2397, 1, "Declaration_name_conflicts_with_built_in_global_identifier_0_2397", "Declaration name conflicts with built-in global identifier '{0}'."), constructor_cannot_be_used_as_a_parameter_property_name: i(2398, 1, "constructor_cannot_be_used_as_a_parameter_property_name_2398", "'constructor' cannot be used as a parameter property name."), Duplicate_identifier_this_Compiler_uses_variable_declaration_this_to_capture_this_reference: i(2399, 1, "Duplicate_identifier_this_Compiler_uses_variable_declaration_this_to_capture_this_reference_2399", "Duplicate identifier '_this'. Compiler uses variable declaration '_this' to capture 'this' reference."), Expression_resolves_to_variable_declaration_this_that_compiler_uses_to_capture_this_reference: i(2400, 1, "Expression_resolves_to_variable_declaration_this_that_compiler_uses_to_capture_this_reference_2400", "Expression resolves to variable declaration '_this' that compiler uses to capture 'this' reference."), A_super_call_must_be_a_root_level_statement_within_a_constructor_of_a_derived_class_that_contains_initialized_properties_parameter_properties_or_private_identifiers: i(2401, 1, "A_super_call_must_be_a_root_level_statement_within_a_constructor_of_a_derived_class_that_contains_in_2401", "A 'super' call must be a root-level statement within a constructor of a derived class that contains initialized properties, parameter properties, or private identifiers."), Expression_resolves_to_super_that_compiler_uses_to_capture_base_class_reference: i(2402, 1, "Expression_resolves_to_super_that_compiler_uses_to_capture_base_class_reference_2402", "Expression resolves to '_super' that compiler uses to capture base class reference."), Subsequent_variable_declarations_must_have_the_same_type_Variable_0_must_be_of_type_1_but_here_has_type_2: i(2403, 1, "Subsequent_variable_declarations_must_have_the_same_type_Variable_0_must_be_of_type_1_but_here_has_t_2403", "Subsequent variable declarations must have the same type. Variable '{0}' must be of type '{1}', but here has type '{2}'."), The_left_hand_side_of_a_for_in_statement_cannot_use_a_type_annotation: i(2404, 1, "The_left_hand_side_of_a_for_in_statement_cannot_use_a_type_annotation_2404", "The left-hand side of a 'for...in' statement cannot use a type annotation."), The_left_hand_side_of_a_for_in_statement_must_be_of_type_string_or_any: i(2405, 1, "The_left_hand_side_of_a_for_in_statement_must_be_of_type_string_or_any_2405", "The left-hand side of a 'for...in' statement must be of type 'string' or 'any'."), The_left_hand_side_of_a_for_in_statement_must_be_a_variable_or_a_property_access: i(2406, 1, "The_left_hand_side_of_a_for_in_statement_must_be_a_variable_or_a_property_access_2406", "The left-hand side of a 'for...in' statement must be a variable or a property access."), The_right_hand_side_of_a_for_in_statement_must_be_of_type_any_an_object_type_or_a_type_parameter_but_here_has_type_0: i(2407, 1, "The_right_hand_side_of_a_for_in_statement_must_be_of_type_any_an_object_type_or_a_type_parameter_but_2407", "The right-hand side of a 'for...in' statement must be of type 'any', an object type or a type parameter, but here has type '{0}'."), Setters_cannot_return_a_value: i(2408, 1, "Setters_cannot_return_a_value_2408", "Setters cannot return a value."), Return_type_of_constructor_signature_must_be_assignable_to_the_instance_type_of_the_class: i(2409, 1, "Return_type_of_constructor_signature_must_be_assignable_to_the_instance_type_of_the_class_2409", "Return type of constructor signature must be assignable to the instance type of the class."), The_with_statement_is_not_supported_All_symbols_in_a_with_block_will_have_type_any: i(2410, 1, "The_with_statement_is_not_supported_All_symbols_in_a_with_block_will_have_type_any_2410", "The 'with' statement is not supported. All symbols in a 'with' block will have type 'any'."), Type_0_is_not_assignable_to_type_1_with_exactOptionalPropertyTypes_Colon_true_Consider_adding_undefined_to_the_type_of_the_target: i(2412, 1, "Type_0_is_not_assignable_to_type_1_with_exactOptionalPropertyTypes_Colon_true_Consider_adding_undefi_2412", "Type '{0}' is not assignable to type '{1}' with 'exactOptionalPropertyTypes: true'. Consider adding 'undefined' to the type of the target."), Property_0_of_type_1_is_not_assignable_to_2_index_type_3: i(2411, 1, "Property_0_of_type_1_is_not_assignable_to_2_index_type_3_2411", "Property '{0}' of type '{1}' is not assignable to '{2}' index type '{3}'."), _0_index_type_1_is_not_assignable_to_2_index_type_3: i(2413, 1, "_0_index_type_1_is_not_assignable_to_2_index_type_3_2413", "'{0}' index type '{1}' is not assignable to '{2}' index type '{3}'."), Class_name_cannot_be_0: i(2414, 1, "Class_name_cannot_be_0_2414", "Class name cannot be '{0}'."), Class_0_incorrectly_extends_base_class_1: i(2415, 1, "Class_0_incorrectly_extends_base_class_1_2415", "Class '{0}' incorrectly extends base class '{1}'."), Property_0_in_type_1_is_not_assignable_to_the_same_property_in_base_type_2: i(2416, 1, "Property_0_in_type_1_is_not_assignable_to_the_same_property_in_base_type_2_2416", "Property '{0}' in type '{1}' is not assignable to the same property in base type '{2}'."), Class_static_side_0_incorrectly_extends_base_class_static_side_1: i(2417, 1, "Class_static_side_0_incorrectly_extends_base_class_static_side_1_2417", "Class static side '{0}' incorrectly extends base class static side '{1}'."), Type_of_computed_property_s_value_is_0_which_is_not_assignable_to_type_1: i(2418, 1, "Type_of_computed_property_s_value_is_0_which_is_not_assignable_to_type_1_2418", "Type of computed property's value is '{0}', which is not assignable to type '{1}'."), Types_of_construct_signatures_are_incompatible: i(2419, 1, "Types_of_construct_signatures_are_incompatible_2419", "Types of construct signatures are incompatible."), Class_0_incorrectly_implements_interface_1: i(2420, 1, "Class_0_incorrectly_implements_interface_1_2420", "Class '{0}' incorrectly implements interface '{1}'."), A_class_can_only_implement_an_object_type_or_intersection_of_object_types_with_statically_known_members: i(2422, 1, "A_class_can_only_implement_an_object_type_or_intersection_of_object_types_with_statically_known_memb_2422", "A class can only implement an object type or intersection of object types with statically known members."), Class_0_defines_instance_member_function_1_but_extended_class_2_defines_it_as_instance_member_accessor: i(2423, 1, "Class_0_defines_instance_member_function_1_but_extended_class_2_defines_it_as_instance_member_access_2423", "Class '{0}' defines instance member function '{1}', but extended class '{2}' defines it as instance member accessor."), Class_0_defines_instance_member_property_1_but_extended_class_2_defines_it_as_instance_member_function: i(2425, 1, "Class_0_defines_instance_member_property_1_but_extended_class_2_defines_it_as_instance_member_functi_2425", "Class '{0}' defines instance member property '{1}', but extended class '{2}' defines it as instance member function."), Class_0_defines_instance_member_accessor_1_but_extended_class_2_defines_it_as_instance_member_function: i(2426, 1, "Class_0_defines_instance_member_accessor_1_but_extended_class_2_defines_it_as_instance_member_functi_2426", "Class '{0}' defines instance member accessor '{1}', but extended class '{2}' defines it as instance member function."), Interface_name_cannot_be_0: i(2427, 1, "Interface_name_cannot_be_0_2427", "Interface name cannot be '{0}'."), All_declarations_of_0_must_have_identical_type_parameters: i(2428, 1, "All_declarations_of_0_must_have_identical_type_parameters_2428", "All declarations of '{0}' must have identical type parameters."), Interface_0_incorrectly_extends_interface_1: i(2430, 1, "Interface_0_incorrectly_extends_interface_1_2430", "Interface '{0}' incorrectly extends interface '{1}'."), Enum_name_cannot_be_0: i(2431, 1, "Enum_name_cannot_be_0_2431", "Enum name cannot be '{0}'."), In_an_enum_with_multiple_declarations_only_one_declaration_can_omit_an_initializer_for_its_first_enum_element: i(2432, 1, "In_an_enum_with_multiple_declarations_only_one_declaration_can_omit_an_initializer_for_its_first_enu_2432", "In an enum with multiple declarations, only one declaration can omit an initializer for its first enum element."), A_namespace_declaration_cannot_be_in_a_different_file_from_a_class_or_function_with_which_it_is_merged: i(2433, 1, "A_namespace_declaration_cannot_be_in_a_different_file_from_a_class_or_function_with_which_it_is_merg_2433", "A namespace declaration cannot be in a different file from a class or function with which it is merged."), A_namespace_declaration_cannot_be_located_prior_to_a_class_or_function_with_which_it_is_merged: i(2434, 1, "A_namespace_declaration_cannot_be_located_prior_to_a_class_or_function_with_which_it_is_merged_2434", "A namespace declaration cannot be located prior to a class or function with which it is merged."), Ambient_modules_cannot_be_nested_in_other_modules_or_namespaces: i(2435, 1, "Ambient_modules_cannot_be_nested_in_other_modules_or_namespaces_2435", "Ambient modules cannot be nested in other modules or namespaces."), Ambient_module_declaration_cannot_specify_relative_module_name: i(2436, 1, "Ambient_module_declaration_cannot_specify_relative_module_name_2436", "Ambient module declaration cannot specify relative module name."), Module_0_is_hidden_by_a_local_declaration_with_the_same_name: i(2437, 1, "Module_0_is_hidden_by_a_local_declaration_with_the_same_name_2437", "Module '{0}' is hidden by a local declaration with the same name."), Import_name_cannot_be_0: i(2438, 1, "Import_name_cannot_be_0_2438", "Import name cannot be '{0}'."), Import_or_export_declaration_in_an_ambient_module_declaration_cannot_reference_module_through_relative_module_name: i(2439, 1, "Import_or_export_declaration_in_an_ambient_module_declaration_cannot_reference_module_through_relati_2439", "Import or export declaration in an ambient module declaration cannot reference module through relative module name."), Import_declaration_conflicts_with_local_declaration_of_0: i(2440, 1, "Import_declaration_conflicts_with_local_declaration_of_0_2440", "Import declaration conflicts with local declaration of '{0}'."), Duplicate_identifier_0_Compiler_reserves_name_1_in_top_level_scope_of_a_module: i(2441, 1, "Duplicate_identifier_0_Compiler_reserves_name_1_in_top_level_scope_of_a_module_2441", "Duplicate identifier '{0}'. Compiler reserves name '{1}' in top level scope of a module."), Types_have_separate_declarations_of_a_private_property_0: i(2442, 1, "Types_have_separate_declarations_of_a_private_property_0_2442", "Types have separate declarations of a private property '{0}'."), Property_0_is_protected_but_type_1_is_not_a_class_derived_from_2: i(2443, 1, "Property_0_is_protected_but_type_1_is_not_a_class_derived_from_2_2443", "Property '{0}' is protected but type '{1}' is not a class derived from '{2}'."), Property_0_is_protected_in_type_1_but_public_in_type_2: i(2444, 1, "Property_0_is_protected_in_type_1_but_public_in_type_2_2444", "Property '{0}' is protected in type '{1}' but public in type '{2}'."), Property_0_is_protected_and_only_accessible_within_class_1_and_its_subclasses: i(2445, 1, "Property_0_is_protected_and_only_accessible_within_class_1_and_its_subclasses_2445", "Property '{0}' is protected and only accessible within class '{1}' and its subclasses."), Property_0_is_protected_and_only_accessible_through_an_instance_of_class_1_This_is_an_instance_of_class_2: i(2446, 1, "Property_0_is_protected_and_only_accessible_through_an_instance_of_class_1_This_is_an_instance_of_cl_2446", "Property '{0}' is protected and only accessible through an instance of class '{1}'. This is an instance of class '{2}'."), The_0_operator_is_not_allowed_for_boolean_types_Consider_using_1_instead: i(2447, 1, "The_0_operator_is_not_allowed_for_boolean_types_Consider_using_1_instead_2447", "The '{0}' operator is not allowed for boolean types. Consider using '{1}' instead."), Block_scoped_variable_0_used_before_its_declaration: i(2448, 1, "Block_scoped_variable_0_used_before_its_declaration_2448", "Block-scoped variable '{0}' used before its declaration."), Class_0_used_before_its_declaration: i(2449, 1, "Class_0_used_before_its_declaration_2449", "Class '{0}' used before its declaration."), Enum_0_used_before_its_declaration: i(2450, 1, "Enum_0_used_before_its_declaration_2450", "Enum '{0}' used before its declaration."), Cannot_redeclare_block_scoped_variable_0: i(2451, 1, "Cannot_redeclare_block_scoped_variable_0_2451", "Cannot redeclare block-scoped variable '{0}'."), An_enum_member_cannot_have_a_numeric_name: i(2452, 1, "An_enum_member_cannot_have_a_numeric_name_2452", "An enum member cannot have a numeric name."), Variable_0_is_used_before_being_assigned: i(2454, 1, "Variable_0_is_used_before_being_assigned_2454", "Variable '{0}' is used before being assigned."), Type_alias_0_circularly_references_itself: i(2456, 1, "Type_alias_0_circularly_references_itself_2456", "Type alias '{0}' circularly references itself."), Type_alias_name_cannot_be_0: i(2457, 1, "Type_alias_name_cannot_be_0_2457", "Type alias name cannot be '{0}'."), An_AMD_module_cannot_have_multiple_name_assignments: i(2458, 1, "An_AMD_module_cannot_have_multiple_name_assignments_2458", "An AMD module cannot have multiple name assignments."), Module_0_declares_1_locally_but_it_is_not_exported: i(2459, 1, "Module_0_declares_1_locally_but_it_is_not_exported_2459", "Module '{0}' declares '{1}' locally, but it is not exported."), Module_0_declares_1_locally_but_it_is_exported_as_2: i(2460, 1, "Module_0_declares_1_locally_but_it_is_exported_as_2_2460", "Module '{0}' declares '{1}' locally, but it is exported as '{2}'."), Type_0_is_not_an_array_type: i(2461, 1, "Type_0_is_not_an_array_type_2461", "Type '{0}' is not an array type."), A_rest_element_must_be_last_in_a_destructuring_pattern: i(2462, 1, "A_rest_element_must_be_last_in_a_destructuring_pattern_2462", "A rest element must be last in a destructuring pattern."), A_binding_pattern_parameter_cannot_be_optional_in_an_implementation_signature: i(2463, 1, "A_binding_pattern_parameter_cannot_be_optional_in_an_implementation_signature_2463", "A binding pattern parameter cannot be optional in an implementation signature."), A_computed_property_name_must_be_of_type_string_number_symbol_or_any: i(2464, 1, "A_computed_property_name_must_be_of_type_string_number_symbol_or_any_2464", "A computed property name must be of type 'string', 'number', 'symbol', or 'any'."), this_cannot_be_referenced_in_a_computed_property_name: i(2465, 1, "this_cannot_be_referenced_in_a_computed_property_name_2465", "'this' cannot be referenced in a computed property name."), super_cannot_be_referenced_in_a_computed_property_name: i(2466, 1, "super_cannot_be_referenced_in_a_computed_property_name_2466", "'super' cannot be referenced in a computed property name."), A_computed_property_name_cannot_reference_a_type_parameter_from_its_containing_type: i(2467, 1, "A_computed_property_name_cannot_reference_a_type_parameter_from_its_containing_type_2467", "A computed property name cannot reference a type parameter from its containing type."), Cannot_find_global_value_0: i(2468, 1, "Cannot_find_global_value_0_2468", "Cannot find global value '{0}'."), The_0_operator_cannot_be_applied_to_type_symbol: i(2469, 1, "The_0_operator_cannot_be_applied_to_type_symbol_2469", "The '{0}' operator cannot be applied to type 'symbol'."), Spread_operator_in_new_expressions_is_only_available_when_targeting_ECMAScript_5_and_higher: i(2472, 1, "Spread_operator_in_new_expressions_is_only_available_when_targeting_ECMAScript_5_and_higher_2472", "Spread operator in 'new' expressions is only available when targeting ECMAScript 5 and higher."), Enum_declarations_must_all_be_const_or_non_const: i(2473, 1, "Enum_declarations_must_all_be_const_or_non_const_2473", "Enum declarations must all be const or non-const."), const_enum_member_initializers_must_be_constant_expressions: i(2474, 1, "const_enum_member_initializers_must_be_constant_expressions_2474", "const enum member initializers must be constant expressions."), const_enums_can_only_be_used_in_property_or_index_access_expressions_or_the_right_hand_side_of_an_import_declaration_or_export_assignment_or_type_query: i(2475, 1, "const_enums_can_only_be_used_in_property_or_index_access_expressions_or_the_right_hand_side_of_an_im_2475", "'const' enums can only be used in property or index access expressions or the right hand side of an import declaration or export assignment or type query."), A_const_enum_member_can_only_be_accessed_using_a_string_literal: i(2476, 1, "A_const_enum_member_can_only_be_accessed_using_a_string_literal_2476", "A const enum member can only be accessed using a string literal."), const_enum_member_initializer_was_evaluated_to_a_non_finite_value: i(2477, 1, "const_enum_member_initializer_was_evaluated_to_a_non_finite_value_2477", "'const' enum member initializer was evaluated to a non-finite value."), const_enum_member_initializer_was_evaluated_to_disallowed_value_NaN: i(2478, 1, "const_enum_member_initializer_was_evaluated_to_disallowed_value_NaN_2478", "'const' enum member initializer was evaluated to disallowed value 'NaN'."), let_is_not_allowed_to_be_used_as_a_name_in_let_or_const_declarations: i(2480, 1, "let_is_not_allowed_to_be_used_as_a_name_in_let_or_const_declarations_2480", "'let' is not allowed to be used as a name in 'let' or 'const' declarations."), Cannot_initialize_outer_scoped_variable_0_in_the_same_scope_as_block_scoped_declaration_1: i(2481, 1, "Cannot_initialize_outer_scoped_variable_0_in_the_same_scope_as_block_scoped_declaration_1_2481", "Cannot initialize outer scoped variable '{0}' in the same scope as block scoped declaration '{1}'."), The_left_hand_side_of_a_for_of_statement_cannot_use_a_type_annotation: i(2483, 1, "The_left_hand_side_of_a_for_of_statement_cannot_use_a_type_annotation_2483", "The left-hand side of a 'for...of' statement cannot use a type annotation."), Export_declaration_conflicts_with_exported_declaration_of_0: i(2484, 1, "Export_declaration_conflicts_with_exported_declaration_of_0_2484", "Export declaration conflicts with exported declaration of '{0}'."), The_left_hand_side_of_a_for_of_statement_must_be_a_variable_or_a_property_access: i(2487, 1, "The_left_hand_side_of_a_for_of_statement_must_be_a_variable_or_a_property_access_2487", "The left-hand side of a 'for...of' statement must be a variable or a property access."), Type_0_must_have_a_Symbol_iterator_method_that_returns_an_iterator: i(2488, 1, "Type_0_must_have_a_Symbol_iterator_method_that_returns_an_iterator_2488", "Type '{0}' must have a '[Symbol.iterator]()' method that returns an iterator."), An_iterator_must_have_a_next_method: i(2489, 1, "An_iterator_must_have_a_next_method_2489", "An iterator must have a 'next()' method."), The_type_returned_by_the_0_method_of_an_iterator_must_have_a_value_property: i(2490, 1, "The_type_returned_by_the_0_method_of_an_iterator_must_have_a_value_property_2490", "The type returned by the '{0}()' method of an iterator must have a 'value' property."), The_left_hand_side_of_a_for_in_statement_cannot_be_a_destructuring_pattern: i(2491, 1, "The_left_hand_side_of_a_for_in_statement_cannot_be_a_destructuring_pattern_2491", "The left-hand side of a 'for...in' statement cannot be a destructuring pattern."), Cannot_redeclare_identifier_0_in_catch_clause: i(2492, 1, "Cannot_redeclare_identifier_0_in_catch_clause_2492", "Cannot redeclare identifier '{0}' in catch clause."), Tuple_type_0_of_length_1_has_no_element_at_index_2: i(2493, 1, "Tuple_type_0_of_length_1_has_no_element_at_index_2_2493", "Tuple type '{0}' of length '{1}' has no element at index '{2}'."), Using_a_string_in_a_for_of_statement_is_only_supported_in_ECMAScript_5_and_higher: i(2494, 1, "Using_a_string_in_a_for_of_statement_is_only_supported_in_ECMAScript_5_and_higher_2494", "Using a string in a 'for...of' statement is only supported in ECMAScript 5 and higher."), Type_0_is_not_an_array_type_or_a_string_type: i(2495, 1, "Type_0_is_not_an_array_type_or_a_string_type_2495", "Type '{0}' is not an array type or a string type."), The_arguments_object_cannot_be_referenced_in_an_arrow_function_in_ES3_and_ES5_Consider_using_a_standard_function_expression: i(2496, 1, "The_arguments_object_cannot_be_referenced_in_an_arrow_function_in_ES3_and_ES5_Consider_using_a_stand_2496", "The 'arguments' object cannot be referenced in an arrow function in ES3 and ES5. Consider using a standard function expression."), This_module_can_only_be_referenced_with_ECMAScript_imports_Slashexports_by_turning_on_the_0_flag_and_referencing_its_default_export: i(2497, 1, "This_module_can_only_be_referenced_with_ECMAScript_imports_Slashexports_by_turning_on_the_0_flag_and_2497", "This module can only be referenced with ECMAScript imports/exports by turning on the '{0}' flag and referencing its default export."), Module_0_uses_export_and_cannot_be_used_with_export_Asterisk: i(2498, 1, "Module_0_uses_export_and_cannot_be_used_with_export_Asterisk_2498", "Module '{0}' uses 'export =' and cannot be used with 'export *'."), An_interface_can_only_extend_an_identifier_Slashqualified_name_with_optional_type_arguments: i(2499, 1, "An_interface_can_only_extend_an_identifier_Slashqualified_name_with_optional_type_arguments_2499", "An interface can only extend an identifier/qualified-name with optional type arguments."), A_class_can_only_implement_an_identifier_Slashqualified_name_with_optional_type_arguments: i(2500, 1, "A_class_can_only_implement_an_identifier_Slashqualified_name_with_optional_type_arguments_2500", "A class can only implement an identifier/qualified-name with optional type arguments."), A_rest_element_cannot_contain_a_binding_pattern: i(2501, 1, "A_rest_element_cannot_contain_a_binding_pattern_2501", "A rest element cannot contain a binding pattern."), _0_is_referenced_directly_or_indirectly_in_its_own_type_annotation: i(2502, 1, "_0_is_referenced_directly_or_indirectly_in_its_own_type_annotation_2502", "'{0}' is referenced directly or indirectly in its own type annotation."), Cannot_find_namespace_0: i(2503, 1, "Cannot_find_namespace_0_2503", "Cannot find namespace '{0}'."), Type_0_must_have_a_Symbol_asyncIterator_method_that_returns_an_async_iterator: i(2504, 1, "Type_0_must_have_a_Symbol_asyncIterator_method_that_returns_an_async_iterator_2504", "Type '{0}' must have a '[Symbol.asyncIterator]()' method that returns an async iterator."), A_generator_cannot_have_a_void_type_annotation: i(2505, 1, "A_generator_cannot_have_a_void_type_annotation_2505", "A generator cannot have a 'void' type annotation."), _0_is_referenced_directly_or_indirectly_in_its_own_base_expression: i(2506, 1, "_0_is_referenced_directly_or_indirectly_in_its_own_base_expression_2506", "'{0}' is referenced directly or indirectly in its own base expression."), Type_0_is_not_a_constructor_function_type: i(2507, 1, "Type_0_is_not_a_constructor_function_type_2507", "Type '{0}' is not a constructor function type."), No_base_constructor_has_the_specified_number_of_type_arguments: i(2508, 1, "No_base_constructor_has_the_specified_number_of_type_arguments_2508", "No base constructor has the specified number of type arguments."), Base_constructor_return_type_0_is_not_an_object_type_or_intersection_of_object_types_with_statically_known_members: i(2509, 1, "Base_constructor_return_type_0_is_not_an_object_type_or_intersection_of_object_types_with_statically_2509", "Base constructor return type '{0}' is not an object type or intersection of object types with statically known members."), Base_constructors_must_all_have_the_same_return_type: i(2510, 1, "Base_constructors_must_all_have_the_same_return_type_2510", "Base constructors must all have the same return type."), Cannot_create_an_instance_of_an_abstract_class: i(2511, 1, "Cannot_create_an_instance_of_an_abstract_class_2511", "Cannot create an instance of an abstract class."), Overload_signatures_must_all_be_abstract_or_non_abstract: i(2512, 1, "Overload_signatures_must_all_be_abstract_or_non_abstract_2512", "Overload signatures must all be abstract or non-abstract."), Abstract_method_0_in_class_1_cannot_be_accessed_via_super_expression: i(2513, 1, "Abstract_method_0_in_class_1_cannot_be_accessed_via_super_expression_2513", "Abstract method '{0}' in class '{1}' cannot be accessed via super expression."), A_tuple_type_cannot_be_indexed_with_a_negative_value: i(2514, 1, "A_tuple_type_cannot_be_indexed_with_a_negative_value_2514", "A tuple type cannot be indexed with a negative value."), Non_abstract_class_0_does_not_implement_inherited_abstract_member_1_from_class_2: i(2515, 1, "Non_abstract_class_0_does_not_implement_inherited_abstract_member_1_from_class_2_2515", "Non-abstract class '{0}' does not implement inherited abstract member '{1}' from class '{2}'."), All_declarations_of_an_abstract_method_must_be_consecutive: i(2516, 1, "All_declarations_of_an_abstract_method_must_be_consecutive_2516", "All declarations of an abstract method must be consecutive."), Cannot_assign_an_abstract_constructor_type_to_a_non_abstract_constructor_type: i(2517, 1, "Cannot_assign_an_abstract_constructor_type_to_a_non_abstract_constructor_type_2517", "Cannot assign an abstract constructor type to a non-abstract constructor type."), A_this_based_type_guard_is_not_compatible_with_a_parameter_based_type_guard: i(2518, 1, "A_this_based_type_guard_is_not_compatible_with_a_parameter_based_type_guard_2518", "A 'this'-based type guard is not compatible with a parameter-based type guard."), An_async_iterator_must_have_a_next_method: i(2519, 1, "An_async_iterator_must_have_a_next_method_2519", "An async iterator must have a 'next()' method."), Duplicate_identifier_0_Compiler_uses_declaration_1_to_support_async_functions: i(2520, 1, "Duplicate_identifier_0_Compiler_uses_declaration_1_to_support_async_functions_2520", "Duplicate identifier '{0}'. Compiler uses declaration '{1}' to support async functions."), The_arguments_object_cannot_be_referenced_in_an_async_function_or_method_in_ES3_and_ES5_Consider_using_a_standard_function_or_method: i(2522, 1, "The_arguments_object_cannot_be_referenced_in_an_async_function_or_method_in_ES3_and_ES5_Consider_usi_2522", "The 'arguments' object cannot be referenced in an async function or method in ES3 and ES5. Consider using a standard function or method."), yield_expressions_cannot_be_used_in_a_parameter_initializer: i(2523, 1, "yield_expressions_cannot_be_used_in_a_parameter_initializer_2523", "'yield' expressions cannot be used in a parameter initializer."), await_expressions_cannot_be_used_in_a_parameter_initializer: i(2524, 1, "await_expressions_cannot_be_used_in_a_parameter_initializer_2524", "'await' expressions cannot be used in a parameter initializer."), Initializer_provides_no_value_for_this_binding_element_and_the_binding_element_has_no_default_value: i(2525, 1, "Initializer_provides_no_value_for_this_binding_element_and_the_binding_element_has_no_default_value_2525", "Initializer provides no value for this binding element and the binding element has no default value."), A_this_type_is_available_only_in_a_non_static_member_of_a_class_or_interface: i(2526, 1, "A_this_type_is_available_only_in_a_non_static_member_of_a_class_or_interface_2526", "A 'this' type is available only in a non-static member of a class or interface."), The_inferred_type_of_0_references_an_inaccessible_1_type_A_type_annotation_is_necessary: i(2527, 1, "The_inferred_type_of_0_references_an_inaccessible_1_type_A_type_annotation_is_necessary_2527", "The inferred type of '{0}' references an inaccessible '{1}' type. A type annotation is necessary."), A_module_cannot_have_multiple_default_exports: i(2528, 1, "A_module_cannot_have_multiple_default_exports_2528", "A module cannot have multiple default exports."), Duplicate_identifier_0_Compiler_reserves_name_1_in_top_level_scope_of_a_module_containing_async_functions: i(2529, 1, "Duplicate_identifier_0_Compiler_reserves_name_1_in_top_level_scope_of_a_module_containing_async_func_2529", "Duplicate identifier '{0}'. Compiler reserves name '{1}' in top level scope of a module containing async functions."), Property_0_is_incompatible_with_index_signature: i(2530, 1, "Property_0_is_incompatible_with_index_signature_2530", "Property '{0}' is incompatible with index signature."), Object_is_possibly_null: i(2531, 1, "Object_is_possibly_null_2531", "Object is possibly 'null'."), Object_is_possibly_undefined: i(2532, 1, "Object_is_possibly_undefined_2532", "Object is possibly 'undefined'."), Object_is_possibly_null_or_undefined: i(2533, 1, "Object_is_possibly_null_or_undefined_2533", "Object is possibly 'null' or 'undefined'."), A_function_returning_never_cannot_have_a_reachable_end_point: i(2534, 1, "A_function_returning_never_cannot_have_a_reachable_end_point_2534", "A function returning 'never' cannot have a reachable end point."), Type_0_cannot_be_used_to_index_type_1: i(2536, 1, "Type_0_cannot_be_used_to_index_type_1_2536", "Type '{0}' cannot be used to index type '{1}'."), Type_0_has_no_matching_index_signature_for_type_1: i(2537, 1, "Type_0_has_no_matching_index_signature_for_type_1_2537", "Type '{0}' has no matching index signature for type '{1}'."), Type_0_cannot_be_used_as_an_index_type: i(2538, 1, "Type_0_cannot_be_used_as_an_index_type_2538", "Type '{0}' cannot be used as an index type."), Cannot_assign_to_0_because_it_is_not_a_variable: i(2539, 1, "Cannot_assign_to_0_because_it_is_not_a_variable_2539", "Cannot assign to '{0}' because it is not a variable."), Cannot_assign_to_0_because_it_is_a_read_only_property: i(2540, 1, "Cannot_assign_to_0_because_it_is_a_read_only_property_2540", "Cannot assign to '{0}' because it is a read-only property."), Index_signature_in_type_0_only_permits_reading: i(2542, 1, "Index_signature_in_type_0_only_permits_reading_2542", "Index signature in type '{0}' only permits reading."), Duplicate_identifier_newTarget_Compiler_uses_variable_declaration_newTarget_to_capture_new_target_meta_property_reference: i(2543, 1, "Duplicate_identifier_newTarget_Compiler_uses_variable_declaration_newTarget_to_capture_new_target_me_2543", "Duplicate identifier '_newTarget'. Compiler uses variable declaration '_newTarget' to capture 'new.target' meta-property reference."), Expression_resolves_to_variable_declaration_newTarget_that_compiler_uses_to_capture_new_target_meta_property_reference: i(2544, 1, "Expression_resolves_to_variable_declaration_newTarget_that_compiler_uses_to_capture_new_target_meta__2544", "Expression resolves to variable declaration '_newTarget' that compiler uses to capture 'new.target' meta-property reference."), A_mixin_class_must_have_a_constructor_with_a_single_rest_parameter_of_type_any: i(2545, 1, "A_mixin_class_must_have_a_constructor_with_a_single_rest_parameter_of_type_any_2545", "A mixin class must have a constructor with a single rest parameter of type 'any[]'."), The_type_returned_by_the_0_method_of_an_async_iterator_must_be_a_promise_for_a_type_with_a_value_property: i(2547, 1, "The_type_returned_by_the_0_method_of_an_async_iterator_must_be_a_promise_for_a_type_with_a_value_pro_2547", "The type returned by the '{0}()' method of an async iterator must be a promise for a type with a 'value' property."), Type_0_is_not_an_array_type_or_does_not_have_a_Symbol_iterator_method_that_returns_an_iterator: i(2548, 1, "Type_0_is_not_an_array_type_or_does_not_have_a_Symbol_iterator_method_that_returns_an_iterator_2548", "Type '{0}' is not an array type or does not have a '[Symbol.iterator]()' method that returns an iterator."), Type_0_is_not_an_array_type_or_a_string_type_or_does_not_have_a_Symbol_iterator_method_that_returns_an_iterator: i(2549, 1, "Type_0_is_not_an_array_type_or_a_string_type_or_does_not_have_a_Symbol_iterator_method_that_returns__2549", "Type '{0}' is not an array type or a string type or does not have a '[Symbol.iterator]()' method that returns an iterator."), Property_0_does_not_exist_on_type_1_Do_you_need_to_change_your_target_library_Try_changing_the_lib_compiler_option_to_2_or_later: i(2550, 1, "Property_0_does_not_exist_on_type_1_Do_you_need_to_change_your_target_library_Try_changing_the_lib_c_2550", "Property '{0}' does not exist on type '{1}'. Do you need to change your target library? Try changing the 'lib' compiler option to '{2}' or later."), Property_0_does_not_exist_on_type_1_Did_you_mean_2: i(2551, 1, "Property_0_does_not_exist_on_type_1_Did_you_mean_2_2551", "Property '{0}' does not exist on type '{1}'. Did you mean '{2}'?"), Cannot_find_name_0_Did_you_mean_1: i(2552, 1, "Cannot_find_name_0_Did_you_mean_1_2552", "Cannot find name '{0}'. Did you mean '{1}'?"), Computed_values_are_not_permitted_in_an_enum_with_string_valued_members: i(2553, 1, "Computed_values_are_not_permitted_in_an_enum_with_string_valued_members_2553", "Computed values are not permitted in an enum with string valued members."), Expected_0_arguments_but_got_1: i(2554, 1, "Expected_0_arguments_but_got_1_2554", "Expected {0} arguments, but got {1}."), Expected_at_least_0_arguments_but_got_1: i(2555, 1, "Expected_at_least_0_arguments_but_got_1_2555", "Expected at least {0} arguments, but got {1}."), A_spread_argument_must_either_have_a_tuple_type_or_be_passed_to_a_rest_parameter: i(2556, 1, "A_spread_argument_must_either_have_a_tuple_type_or_be_passed_to_a_rest_parameter_2556", "A spread argument must either have a tuple type or be passed to a rest parameter."), Expected_0_type_arguments_but_got_1: i(2558, 1, "Expected_0_type_arguments_but_got_1_2558", "Expected {0} type arguments, but got {1}."), Type_0_has_no_properties_in_common_with_type_1: i(2559, 1, "Type_0_has_no_properties_in_common_with_type_1_2559", "Type '{0}' has no properties in common with type '{1}'."), Value_of_type_0_has_no_properties_in_common_with_type_1_Did_you_mean_to_call_it: i(2560, 1, "Value_of_type_0_has_no_properties_in_common_with_type_1_Did_you_mean_to_call_it_2560", "Value of type '{0}' has no properties in common with type '{1}'. Did you mean to call it?"), Object_literal_may_only_specify_known_properties_but_0_does_not_exist_in_type_1_Did_you_mean_to_write_2: i(2561, 1, "Object_literal_may_only_specify_known_properties_but_0_does_not_exist_in_type_1_Did_you_mean_to_writ_2561", "Object literal may only specify known properties, but '{0}' does not exist in type '{1}'. Did you mean to write '{2}'?"), Base_class_expressions_cannot_reference_class_type_parameters: i(2562, 1, "Base_class_expressions_cannot_reference_class_type_parameters_2562", "Base class expressions cannot reference class type parameters."), The_containing_function_or_module_body_is_too_large_for_control_flow_analysis: i(2563, 1, "The_containing_function_or_module_body_is_too_large_for_control_flow_analysis_2563", "The containing function or module body is too large for control flow analysis."), Property_0_has_no_initializer_and_is_not_definitely_assigned_in_the_constructor: i(2564, 1, "Property_0_has_no_initializer_and_is_not_definitely_assigned_in_the_constructor_2564", "Property '{0}' has no initializer and is not definitely assigned in the constructor."), Property_0_is_used_before_being_assigned: i(2565, 1, "Property_0_is_used_before_being_assigned_2565", "Property '{0}' is used before being assigned."), A_rest_element_cannot_have_a_property_name: i(2566, 1, "A_rest_element_cannot_have_a_property_name_2566", "A rest element cannot have a property name."), Enum_declarations_can_only_merge_with_namespace_or_other_enum_declarations: i(2567, 1, "Enum_declarations_can_only_merge_with_namespace_or_other_enum_declarations_2567", "Enum declarations can only merge with namespace or other enum declarations."), Property_0_may_not_exist_on_type_1_Did_you_mean_2: i(2568, 1, "Property_0_may_not_exist_on_type_1_Did_you_mean_2_2568", "Property '{0}' may not exist on type '{1}'. Did you mean '{2}'?"), Could_not_find_name_0_Did_you_mean_1: i(2570, 1, "Could_not_find_name_0_Did_you_mean_1_2570", "Could not find name '{0}'. Did you mean '{1}'?"), Object_is_of_type_unknown: i(2571, 1, "Object_is_of_type_unknown_2571", "Object is of type 'unknown'."), A_rest_element_type_must_be_an_array_type: i(2574, 1, "A_rest_element_type_must_be_an_array_type_2574", "A rest element type must be an array type."), No_overload_expects_0_arguments_but_overloads_do_exist_that_expect_either_1_or_2_arguments: i(2575, 1, "No_overload_expects_0_arguments_but_overloads_do_exist_that_expect_either_1_or_2_arguments_2575", "No overload expects {0} arguments, but overloads do exist that expect either {1} or {2} arguments."), Property_0_does_not_exist_on_type_1_Did_you_mean_to_access_the_static_member_2_instead: i(2576, 1, "Property_0_does_not_exist_on_type_1_Did_you_mean_to_access_the_static_member_2_instead_2576", "Property '{0}' does not exist on type '{1}'. Did you mean to access the static member '{2}' instead?"), Return_type_annotation_circularly_references_itself: i(2577, 1, "Return_type_annotation_circularly_references_itself_2577", "Return type annotation circularly references itself."), Unused_ts_expect_error_directive: i(2578, 1, "Unused_ts_expect_error_directive_2578", "Unused '@ts-expect-error' directive."), Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_node_Try_npm_i_save_dev_types_Slashnode: i(2580, 1, "Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_node_Try_npm_i_save_dev_types_Slashno_2580", "Cannot find name '{0}'. Do you need to install type definitions for node? Try `npm i --save-dev @types/node`."), Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_jQuery_Try_npm_i_save_dev_types_Slashjquery: i(2581, 1, "Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_jQuery_Try_npm_i_save_dev_types_Slash_2581", "Cannot find name '{0}'. Do you need to install type definitions for jQuery? Try `npm i --save-dev @types/jquery`."), Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_a_test_runner_Try_npm_i_save_dev_types_Slashjest_or_npm_i_save_dev_types_Slashmocha: i(2582, 1, "Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_a_test_runner_Try_npm_i_save_dev_type_2582", "Cannot find name '{0}'. Do you need to install type definitions for a test runner? Try `npm i --save-dev @types/jest` or `npm i --save-dev @types/mocha`."), Cannot_find_name_0_Do_you_need_to_change_your_target_library_Try_changing_the_lib_compiler_option_to_1_or_later: i(2583, 1, "Cannot_find_name_0_Do_you_need_to_change_your_target_library_Try_changing_the_lib_compiler_option_to_2583", "Cannot find name '{0}'. Do you need to change your target library? Try changing the 'lib' compiler option to '{1}' or later."), Cannot_find_name_0_Do_you_need_to_change_your_target_library_Try_changing_the_lib_compiler_option_to_include_dom: i(2584, 1, "Cannot_find_name_0_Do_you_need_to_change_your_target_library_Try_changing_the_lib_compiler_option_to_2584", "Cannot find name '{0}'. Do you need to change your target library? Try changing the 'lib' compiler option to include 'dom'."), _0_only_refers_to_a_type_but_is_being_used_as_a_value_here_Do_you_need_to_change_your_target_library_Try_changing_the_lib_compiler_option_to_es2015_or_later: i(2585, 1, "_0_only_refers_to_a_type_but_is_being_used_as_a_value_here_Do_you_need_to_change_your_target_library_2585", "'{0}' only refers to a type, but is being used as a value here. Do you need to change your target library? Try changing the 'lib' compiler option to es2015 or later."), Cannot_assign_to_0_because_it_is_a_constant: i(2588, 1, "Cannot_assign_to_0_because_it_is_a_constant_2588", "Cannot assign to '{0}' because it is a constant."), Type_instantiation_is_excessively_deep_and_possibly_infinite: i(2589, 1, "Type_instantiation_is_excessively_deep_and_possibly_infinite_2589", "Type instantiation is excessively deep and possibly infinite."), Expression_produces_a_union_type_that_is_too_complex_to_represent: i(2590, 1, "Expression_produces_a_union_type_that_is_too_complex_to_represent_2590", "Expression produces a union type that is too complex to represent."), Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_node_Try_npm_i_save_dev_types_Slashnode_and_then_add_node_to_the_types_field_in_your_tsconfig: i(2591, 1, "Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_node_Try_npm_i_save_dev_types_Slashno_2591", "Cannot find name '{0}'. Do you need to install type definitions for node? Try `npm i --save-dev @types/node` and then add 'node' to the types field in your tsconfig."), Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_jQuery_Try_npm_i_save_dev_types_Slashjquery_and_then_add_jquery_to_the_types_field_in_your_tsconfig: i(2592, 1, "Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_jQuery_Try_npm_i_save_dev_types_Slash_2592", "Cannot find name '{0}'. Do you need to install type definitions for jQuery? Try `npm i --save-dev @types/jquery` and then add 'jquery' to the types field in your tsconfig."), Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_a_test_runner_Try_npm_i_save_dev_types_Slashjest_or_npm_i_save_dev_types_Slashmocha_and_then_add_jest_or_mocha_to_the_types_field_in_your_tsconfig: i(2593, 1, "Cannot_find_name_0_Do_you_need_to_install_type_definitions_for_a_test_runner_Try_npm_i_save_dev_type_2593", "Cannot find name '{0}'. Do you need to install type definitions for a test runner? Try `npm i --save-dev @types/jest` or `npm i --save-dev @types/mocha` and then add 'jest' or 'mocha' to the types field in your tsconfig."), This_module_is_declared_with_export_and_can_only_be_used_with_a_default_import_when_using_the_0_flag: i(2594, 1, "This_module_is_declared_with_export_and_can_only_be_used_with_a_default_import_when_using_the_0_flag_2594", "This module is declared with 'export =', and can only be used with a default import when using the '{0}' flag."), _0_can_only_be_imported_by_using_a_default_import: i(2595, 1, "_0_can_only_be_imported_by_using_a_default_import_2595", "'{0}' can only be imported by using a default import."), _0_can_only_be_imported_by_turning_on_the_esModuleInterop_flag_and_using_a_default_import: i(2596, 1, "_0_can_only_be_imported_by_turning_on_the_esModuleInterop_flag_and_using_a_default_import_2596", "'{0}' can only be imported by turning on the 'esModuleInterop' flag and using a default import."), _0_can_only_be_imported_by_using_a_require_call_or_by_using_a_default_import: i(2597, 1, "_0_can_only_be_imported_by_using_a_require_call_or_by_using_a_default_import_2597", "'{0}' can only be imported by using a 'require' call or by using a default import."), _0_can_only_be_imported_by_using_a_require_call_or_by_turning_on_the_esModuleInterop_flag_and_using_a_default_import: i(2598, 1, "_0_can_only_be_imported_by_using_a_require_call_or_by_turning_on_the_esModuleInterop_flag_and_using__2598", "'{0}' can only be imported by using a 'require' call or by turning on the 'esModuleInterop' flag and using a default import."), JSX_element_implicitly_has_type_any_because_the_global_type_JSX_Element_does_not_exist: i(2602, 1, "JSX_element_implicitly_has_type_any_because_the_global_type_JSX_Element_does_not_exist_2602", "JSX element implicitly has type 'any' because the global type 'JSX.Element' does not exist."), Property_0_in_type_1_is_not_assignable_to_type_2: i(2603, 1, "Property_0_in_type_1_is_not_assignable_to_type_2_2603", "Property '{0}' in type '{1}' is not assignable to type '{2}'."), JSX_element_type_0_does_not_have_any_construct_or_call_signatures: i(2604, 1, "JSX_element_type_0_does_not_have_any_construct_or_call_signatures_2604", "JSX element type '{0}' does not have any construct or call signatures."), Property_0_of_JSX_spread_attribute_is_not_assignable_to_target_property: i(2606, 1, "Property_0_of_JSX_spread_attribute_is_not_assignable_to_target_property_2606", "Property '{0}' of JSX spread attribute is not assignable to target property."), JSX_element_class_does_not_support_attributes_because_it_does_not_have_a_0_property: i(2607, 1, "JSX_element_class_does_not_support_attributes_because_it_does_not_have_a_0_property_2607", "JSX element class does not support attributes because it does not have a '{0}' property."), The_global_type_JSX_0_may_not_have_more_than_one_property: i(2608, 1, "The_global_type_JSX_0_may_not_have_more_than_one_property_2608", "The global type 'JSX.{0}' may not have more than one property."), JSX_spread_child_must_be_an_array_type: i(2609, 1, "JSX_spread_child_must_be_an_array_type_2609", "JSX spread child must be an array type."), _0_is_defined_as_an_accessor_in_class_1_but_is_overridden_here_in_2_as_an_instance_property: i(2610, 1, "_0_is_defined_as_an_accessor_in_class_1_but_is_overridden_here_in_2_as_an_instance_property_2610", "'{0}' is defined as an accessor in class '{1}', but is overridden here in '{2}' as an instance property."), _0_is_defined_as_a_property_in_class_1_but_is_overridden_here_in_2_as_an_accessor: i(2611, 1, "_0_is_defined_as_a_property_in_class_1_but_is_overridden_here_in_2_as_an_accessor_2611", "'{0}' is defined as a property in class '{1}', but is overridden here in '{2}' as an accessor."), Property_0_will_overwrite_the_base_property_in_1_If_this_is_intentional_add_an_initializer_Otherwise_add_a_declare_modifier_or_remove_the_redundant_declaration: i(2612, 1, "Property_0_will_overwrite_the_base_property_in_1_If_this_is_intentional_add_an_initializer_Otherwise_2612", "Property '{0}' will overwrite the base property in '{1}'. If this is intentional, add an initializer. Otherwise, add a 'declare' modifier or remove the redundant declaration."), Module_0_has_no_default_export_Did_you_mean_to_use_import_1_from_0_instead: i(2613, 1, "Module_0_has_no_default_export_Did_you_mean_to_use_import_1_from_0_instead_2613", "Module '{0}' has no default export. Did you mean to use 'import { {1} } from {0}' instead?"), Module_0_has_no_exported_member_1_Did_you_mean_to_use_import_1_from_0_instead: i(2614, 1, "Module_0_has_no_exported_member_1_Did_you_mean_to_use_import_1_from_0_instead_2614", "Module '{0}' has no exported member '{1}'. Did you mean to use 'import {1} from {0}' instead?"), Type_of_property_0_circularly_references_itself_in_mapped_type_1: i(2615, 1, "Type_of_property_0_circularly_references_itself_in_mapped_type_1_2615", "Type of property '{0}' circularly references itself in mapped type '{1}'."), _0_can_only_be_imported_by_using_import_1_require_2_or_a_default_import: i(2616, 1, "_0_can_only_be_imported_by_using_import_1_require_2_or_a_default_import_2616", "'{0}' can only be imported by using 'import {1} = require({2})' or a default import."), _0_can_only_be_imported_by_using_import_1_require_2_or_by_turning_on_the_esModuleInterop_flag_and_using_a_default_import: i(2617, 1, "_0_can_only_be_imported_by_using_import_1_require_2_or_by_turning_on_the_esModuleInterop_flag_and_us_2617", "'{0}' can only be imported by using 'import {1} = require({2})' or by turning on the 'esModuleInterop' flag and using a default import."), Source_has_0_element_s_but_target_requires_1: i(2618, 1, "Source_has_0_element_s_but_target_requires_1_2618", "Source has {0} element(s) but target requires {1}."), Source_has_0_element_s_but_target_allows_only_1: i(2619, 1, "Source_has_0_element_s_but_target_allows_only_1_2619", "Source has {0} element(s) but target allows only {1}."), Target_requires_0_element_s_but_source_may_have_fewer: i(2620, 1, "Target_requires_0_element_s_but_source_may_have_fewer_2620", "Target requires {0} element(s) but source may have fewer."), Target_allows_only_0_element_s_but_source_may_have_more: i(2621, 1, "Target_allows_only_0_element_s_but_source_may_have_more_2621", "Target allows only {0} element(s) but source may have more."), Source_provides_no_match_for_required_element_at_position_0_in_target: i(2623, 1, "Source_provides_no_match_for_required_element_at_position_0_in_target_2623", "Source provides no match for required element at position {0} in target."), Source_provides_no_match_for_variadic_element_at_position_0_in_target: i(2624, 1, "Source_provides_no_match_for_variadic_element_at_position_0_in_target_2624", "Source provides no match for variadic element at position {0} in target."), Variadic_element_at_position_0_in_source_does_not_match_element_at_position_1_in_target: i(2625, 1, "Variadic_element_at_position_0_in_source_does_not_match_element_at_position_1_in_target_2625", "Variadic element at position {0} in source does not match element at position {1} in target."), Type_at_position_0_in_source_is_not_compatible_with_type_at_position_1_in_target: i(2626, 1, "Type_at_position_0_in_source_is_not_compatible_with_type_at_position_1_in_target_2626", "Type at position {0} in source is not compatible with type at position {1} in target."), Type_at_positions_0_through_1_in_source_is_not_compatible_with_type_at_position_2_in_target: i(2627, 1, "Type_at_positions_0_through_1_in_source_is_not_compatible_with_type_at_position_2_in_target_2627", "Type at positions {0} through {1} in source is not compatible with type at position {2} in target."), Cannot_assign_to_0_because_it_is_an_enum: i(2628, 1, "Cannot_assign_to_0_because_it_is_an_enum_2628", "Cannot assign to '{0}' because it is an enum."), Cannot_assign_to_0_because_it_is_a_class: i(2629, 1, "Cannot_assign_to_0_because_it_is_a_class_2629", "Cannot assign to '{0}' because it is a class."), Cannot_assign_to_0_because_it_is_a_function: i(2630, 1, "Cannot_assign_to_0_because_it_is_a_function_2630", "Cannot assign to '{0}' because it is a function."), Cannot_assign_to_0_because_it_is_a_namespace: i(2631, 1, "Cannot_assign_to_0_because_it_is_a_namespace_2631", "Cannot assign to '{0}' because it is a namespace."), Cannot_assign_to_0_because_it_is_an_import: i(2632, 1, "Cannot_assign_to_0_because_it_is_an_import_2632", "Cannot assign to '{0}' because it is an import."), JSX_property_access_expressions_cannot_include_JSX_namespace_names: i(2633, 1, "JSX_property_access_expressions_cannot_include_JSX_namespace_names_2633", "JSX property access expressions cannot include JSX namespace names"), _0_index_signatures_are_incompatible: i(2634, 1, "_0_index_signatures_are_incompatible_2634", "'{0}' index signatures are incompatible."), Type_0_has_no_signatures_for_which_the_type_argument_list_is_applicable: i(2635, 1, "Type_0_has_no_signatures_for_which_the_type_argument_list_is_applicable_2635", "Type '{0}' has no signatures for which the type argument list is applicable."), Type_0_is_not_assignable_to_type_1_as_implied_by_variance_annotation: i(2636, 1, "Type_0_is_not_assignable_to_type_1_as_implied_by_variance_annotation_2636", "Type '{0}' is not assignable to type '{1}' as implied by variance annotation."), Variance_annotations_are_only_supported_in_type_aliases_for_object_function_constructor_and_mapped_types: i(2637, 1, "Variance_annotations_are_only_supported_in_type_aliases_for_object_function_constructor_and_mapped_t_2637", "Variance annotations are only supported in type aliases for object, function, constructor, and mapped types."), Type_0_may_represent_a_primitive_value_which_is_not_permitted_as_the_right_operand_of_the_in_operator: i(2638, 1, "Type_0_may_represent_a_primitive_value_which_is_not_permitted_as_the_right_operand_of_the_in_operato_2638", "Type '{0}' may represent a primitive value, which is not permitted as the right operand of the 'in' operator."), Cannot_augment_module_0_with_value_exports_because_it_resolves_to_a_non_module_entity: i(2649, 1, "Cannot_augment_module_0_with_value_exports_because_it_resolves_to_a_non_module_entity_2649", "Cannot augment module '{0}' with value exports because it resolves to a non-module entity."), A_member_initializer_in_a_enum_declaration_cannot_reference_members_declared_after_it_including_members_defined_in_other_enums: i(2651, 1, "A_member_initializer_in_a_enum_declaration_cannot_reference_members_declared_after_it_including_memb_2651", "A member initializer in a enum declaration cannot reference members declared after it, including members defined in other enums."), Merged_declaration_0_cannot_include_a_default_export_declaration_Consider_adding_a_separate_export_default_0_declaration_instead: i(2652, 1, "Merged_declaration_0_cannot_include_a_default_export_declaration_Consider_adding_a_separate_export_d_2652", "Merged declaration '{0}' cannot include a default export declaration. Consider adding a separate 'export default {0}' declaration instead."), Non_abstract_class_expression_does_not_implement_inherited_abstract_member_0_from_class_1: i(2653, 1, "Non_abstract_class_expression_does_not_implement_inherited_abstract_member_0_from_class_1_2653", "Non-abstract class expression does not implement inherited abstract member '{0}' from class '{1}'."), JSX_expressions_must_have_one_parent_element: i(2657, 1, "JSX_expressions_must_have_one_parent_element_2657", "JSX expressions must have one parent element."), Type_0_provides_no_match_for_the_signature_1: i(2658, 1, "Type_0_provides_no_match_for_the_signature_1_2658", "Type '{0}' provides no match for the signature '{1}'."), super_is_only_allowed_in_members_of_object_literal_expressions_when_option_target_is_ES2015_or_higher: i(2659, 1, "super_is_only_allowed_in_members_of_object_literal_expressions_when_option_target_is_ES2015_or_highe_2659", "'super' is only allowed in members of object literal expressions when option 'target' is 'ES2015' or higher."), super_can_only_be_referenced_in_members_of_derived_classes_or_object_literal_expressions: i(2660, 1, "super_can_only_be_referenced_in_members_of_derived_classes_or_object_literal_expressions_2660", "'super' can only be referenced in members of derived classes or object literal expressions."), Cannot_export_0_Only_local_declarations_can_be_exported_from_a_module: i(2661, 1, "Cannot_export_0_Only_local_declarations_can_be_exported_from_a_module_2661", "Cannot export '{0}'. Only local declarations can be exported from a module."), Cannot_find_name_0_Did_you_mean_the_static_member_1_0: i(2662, 1, "Cannot_find_name_0_Did_you_mean_the_static_member_1_0_2662", "Cannot find name '{0}'. Did you mean the static member '{1}.{0}'?"), Cannot_find_name_0_Did_you_mean_the_instance_member_this_0: i(2663, 1, "Cannot_find_name_0_Did_you_mean_the_instance_member_this_0_2663", "Cannot find name '{0}'. Did you mean the instance member 'this.{0}'?"), Invalid_module_name_in_augmentation_module_0_cannot_be_found: i(2664, 1, "Invalid_module_name_in_augmentation_module_0_cannot_be_found_2664", "Invalid module name in augmentation, module '{0}' cannot be found."), Invalid_module_name_in_augmentation_Module_0_resolves_to_an_untyped_module_at_1_which_cannot_be_augmented: i(2665, 1, "Invalid_module_name_in_augmentation_Module_0_resolves_to_an_untyped_module_at_1_which_cannot_be_augm_2665", "Invalid module name in augmentation. Module '{0}' resolves to an untyped module at '{1}', which cannot be augmented."), Exports_and_export_assignments_are_not_permitted_in_module_augmentations: i(2666, 1, "Exports_and_export_assignments_are_not_permitted_in_module_augmentations_2666", "Exports and export assignments are not permitted in module augmentations."), Imports_are_not_permitted_in_module_augmentations_Consider_moving_them_to_the_enclosing_external_module: i(2667, 1, "Imports_are_not_permitted_in_module_augmentations_Consider_moving_them_to_the_enclosing_external_mod_2667", "Imports are not permitted in module augmentations. Consider moving them to the enclosing external module."), export_modifier_cannot_be_applied_to_ambient_modules_and_module_augmentations_since_they_are_always_visible: i(2668, 1, "export_modifier_cannot_be_applied_to_ambient_modules_and_module_augmentations_since_they_are_always__2668", "'export' modifier cannot be applied to ambient modules and module augmentations since they are always visible."), Augmentations_for_the_global_scope_can_only_be_directly_nested_in_external_modules_or_ambient_module_declarations: i(2669, 1, "Augmentations_for_the_global_scope_can_only_be_directly_nested_in_external_modules_or_ambient_module_2669", "Augmentations for the global scope can only be directly nested in external modules or ambient module declarations."), Augmentations_for_the_global_scope_should_have_declare_modifier_unless_they_appear_in_already_ambient_context: i(2670, 1, "Augmentations_for_the_global_scope_should_have_declare_modifier_unless_they_appear_in_already_ambien_2670", "Augmentations for the global scope should have 'declare' modifier unless they appear in already ambient context."), Cannot_augment_module_0_because_it_resolves_to_a_non_module_entity: i(2671, 1, "Cannot_augment_module_0_because_it_resolves_to_a_non_module_entity_2671", "Cannot augment module '{0}' because it resolves to a non-module entity."), Cannot_assign_a_0_constructor_type_to_a_1_constructor_type: i(2672, 1, "Cannot_assign_a_0_constructor_type_to_a_1_constructor_type_2672", "Cannot assign a '{0}' constructor type to a '{1}' constructor type."), Constructor_of_class_0_is_private_and_only_accessible_within_the_class_declaration: i(2673, 1, "Constructor_of_class_0_is_private_and_only_accessible_within_the_class_declaration_2673", "Constructor of class '{0}' is private and only accessible within the class declaration."), Constructor_of_class_0_is_protected_and_only_accessible_within_the_class_declaration: i(2674, 1, "Constructor_of_class_0_is_protected_and_only_accessible_within_the_class_declaration_2674", "Constructor of class '{0}' is protected and only accessible within the class declaration."), Cannot_extend_a_class_0_Class_constructor_is_marked_as_private: i(2675, 1, "Cannot_extend_a_class_0_Class_constructor_is_marked_as_private_2675", "Cannot extend a class '{0}'. Class constructor is marked as private."), Accessors_must_both_be_abstract_or_non_abstract: i(2676, 1, "Accessors_must_both_be_abstract_or_non_abstract_2676", "Accessors must both be abstract or non-abstract."), A_type_predicate_s_type_must_be_assignable_to_its_parameter_s_type: i(2677, 1, "A_type_predicate_s_type_must_be_assignable_to_its_parameter_s_type_2677", "A type predicate's type must be assignable to its parameter's type."), Type_0_is_not_comparable_to_type_1: i(2678, 1, "Type_0_is_not_comparable_to_type_1_2678", "Type '{0}' is not comparable to type '{1}'."), A_function_that_is_called_with_the_new_keyword_cannot_have_a_this_type_that_is_void: i(2679, 1, "A_function_that_is_called_with_the_new_keyword_cannot_have_a_this_type_that_is_void_2679", "A function that is called with the 'new' keyword cannot have a 'this' type that is 'void'."), A_0_parameter_must_be_the_first_parameter: i(2680, 1, "A_0_parameter_must_be_the_first_parameter_2680", "A '{0}' parameter must be the first parameter."), A_constructor_cannot_have_a_this_parameter: i(2681, 1, "A_constructor_cannot_have_a_this_parameter_2681", "A constructor cannot have a 'this' parameter."), this_implicitly_has_type_any_because_it_does_not_have_a_type_annotation: i(2683, 1, "this_implicitly_has_type_any_because_it_does_not_have_a_type_annotation_2683", "'this' implicitly has type 'any' because it does not have a type annotation."), The_this_context_of_type_0_is_not_assignable_to_method_s_this_of_type_1: i(2684, 1, "The_this_context_of_type_0_is_not_assignable_to_method_s_this_of_type_1_2684", "The 'this' context of type '{0}' is not assignable to method's 'this' of type '{1}'."), The_this_types_of_each_signature_are_incompatible: i(2685, 1, "The_this_types_of_each_signature_are_incompatible_2685", "The 'this' types of each signature are incompatible."), _0_refers_to_a_UMD_global_but_the_current_file_is_a_module_Consider_adding_an_import_instead: i(2686, 1, "_0_refers_to_a_UMD_global_but_the_current_file_is_a_module_Consider_adding_an_import_instead_2686", "'{0}' refers to a UMD global, but the current file is a module. Consider adding an import instead."), All_declarations_of_0_must_have_identical_modifiers: i(2687, 1, "All_declarations_of_0_must_have_identical_modifiers_2687", "All declarations of '{0}' must have identical modifiers."), Cannot_find_type_definition_file_for_0: i(2688, 1, "Cannot_find_type_definition_file_for_0_2688", "Cannot find type definition file for '{0}'."), Cannot_extend_an_interface_0_Did_you_mean_implements: i(2689, 1, "Cannot_extend_an_interface_0_Did_you_mean_implements_2689", "Cannot extend an interface '{0}'. Did you mean 'implements'?"), _0_only_refers_to_a_type_but_is_being_used_as_a_value_here_Did_you_mean_to_use_1_in_0: i(2690, 1, "_0_only_refers_to_a_type_but_is_being_used_as_a_value_here_Did_you_mean_to_use_1_in_0_2690", "'{0}' only refers to a type, but is being used as a value here. Did you mean to use '{1} in {0}'?"), _0_is_a_primitive_but_1_is_a_wrapper_object_Prefer_using_0_when_possible: i(2692, 1, "_0_is_a_primitive_but_1_is_a_wrapper_object_Prefer_using_0_when_possible_2692", "'{0}' is a primitive, but '{1}' is a wrapper object. Prefer using '{0}' when possible."), _0_only_refers_to_a_type_but_is_being_used_as_a_value_here: i(2693, 1, "_0_only_refers_to_a_type_but_is_being_used_as_a_value_here_2693", "'{0}' only refers to a type, but is being used as a value here."), Namespace_0_has_no_exported_member_1: i(2694, 1, "Namespace_0_has_no_exported_member_1_2694", "Namespace '{0}' has no exported member '{1}'."), Left_side_of_comma_operator_is_unused_and_has_no_side_effects: i(2695, 1, "Left_side_of_comma_operator_is_unused_and_has_no_side_effects_2695", "Left side of comma operator is unused and has no side effects.", true), The_Object_type_is_assignable_to_very_few_other_types_Did_you_mean_to_use_the_any_type_instead: i(2696, 1, "The_Object_type_is_assignable_to_very_few_other_types_Did_you_mean_to_use_the_any_type_instead_2696", "The 'Object' type is assignable to very few other types. Did you mean to use the 'any' type instead?"), An_async_function_or_method_must_return_a_Promise_Make_sure_you_have_a_declaration_for_Promise_or_include_ES2015_in_your_lib_option: i(2697, 1, "An_async_function_or_method_must_return_a_Promise_Make_sure_you_have_a_declaration_for_Promise_or_in_2697", "An async function or method must return a 'Promise'. Make sure you have a declaration for 'Promise' or include 'ES2015' in your '--lib' option."), Spread_types_may_only_be_created_from_object_types: i(2698, 1, "Spread_types_may_only_be_created_from_object_types_2698", "Spread types may only be created from object types."), Static_property_0_conflicts_with_built_in_property_Function_0_of_constructor_function_1: i(2699, 1, "Static_property_0_conflicts_with_built_in_property_Function_0_of_constructor_function_1_2699", "Static property '{0}' conflicts with built-in property 'Function.{0}' of constructor function '{1}'."), Rest_types_may_only_be_created_from_object_types: i(2700, 1, "Rest_types_may_only_be_created_from_object_types_2700", "Rest types may only be created from object types."), The_target_of_an_object_rest_assignment_must_be_a_variable_or_a_property_access: i(2701, 1, "The_target_of_an_object_rest_assignment_must_be_a_variable_or_a_property_access_2701", "The target of an object rest assignment must be a variable or a property access."), _0_only_refers_to_a_type_but_is_being_used_as_a_namespace_here: i(2702, 1, "_0_only_refers_to_a_type_but_is_being_used_as_a_namespace_here_2702", "'{0}' only refers to a type, but is being used as a namespace here."), The_operand_of_a_delete_operator_must_be_a_property_reference: i(2703, 1, "The_operand_of_a_delete_operator_must_be_a_property_reference_2703", "The operand of a 'delete' operator must be a property reference."), The_operand_of_a_delete_operator_cannot_be_a_read_only_property: i(2704, 1, "The_operand_of_a_delete_operator_cannot_be_a_read_only_property_2704", "The operand of a 'delete' operator cannot be a read-only property."), An_async_function_or_method_in_ES5_SlashES3_requires_the_Promise_constructor_Make_sure_you_have_a_declaration_for_the_Promise_constructor_or_include_ES2015_in_your_lib_option: i(2705, 1, "An_async_function_or_method_in_ES5_SlashES3_requires_the_Promise_constructor_Make_sure_you_have_a_de_2705", "An async function or method in ES5/ES3 requires the 'Promise' constructor. Make sure you have a declaration for the 'Promise' constructor or include 'ES2015' in your '--lib' option."), Required_type_parameters_may_not_follow_optional_type_parameters: i(2706, 1, "Required_type_parameters_may_not_follow_optional_type_parameters_2706", "Required type parameters may not follow optional type parameters."), Generic_type_0_requires_between_1_and_2_type_arguments: i(2707, 1, "Generic_type_0_requires_between_1_and_2_type_arguments_2707", "Generic type '{0}' requires between {1} and {2} type arguments."), Cannot_use_namespace_0_as_a_value: i(2708, 1, "Cannot_use_namespace_0_as_a_value_2708", "Cannot use namespace '{0}' as a value."), Cannot_use_namespace_0_as_a_type: i(2709, 1, "Cannot_use_namespace_0_as_a_type_2709", "Cannot use namespace '{0}' as a type."), _0_are_specified_twice_The_attribute_named_0_will_be_overwritten: i(2710, 1, "_0_are_specified_twice_The_attribute_named_0_will_be_overwritten_2710", "'{0}' are specified twice. The attribute named '{0}' will be overwritten."), A_dynamic_import_call_returns_a_Promise_Make_sure_you_have_a_declaration_for_Promise_or_include_ES2015_in_your_lib_option: i(2711, 1, "A_dynamic_import_call_returns_a_Promise_Make_sure_you_have_a_declaration_for_Promise_or_include_ES20_2711", "A dynamic import call returns a 'Promise'. Make sure you have a declaration for 'Promise' or include 'ES2015' in your '--lib' option."), A_dynamic_import_call_in_ES5_SlashES3_requires_the_Promise_constructor_Make_sure_you_have_a_declaration_for_the_Promise_constructor_or_include_ES2015_in_your_lib_option: i(2712, 1, "A_dynamic_import_call_in_ES5_SlashES3_requires_the_Promise_constructor_Make_sure_you_have_a_declarat_2712", "A dynamic import call in ES5/ES3 requires the 'Promise' constructor. Make sure you have a declaration for the 'Promise' constructor or include 'ES2015' in your '--lib' option."), Cannot_access_0_1_because_0_is_a_type_but_not_a_namespace_Did_you_mean_to_retrieve_the_type_of_the_property_1_in_0_with_0_1: i(2713, 1, "Cannot_access_0_1_because_0_is_a_type_but_not_a_namespace_Did_you_mean_to_retrieve_the_type_of_the_p_2713", `Cannot access '{0}.{1}' because '{0}' is a type, but not a namespace. Did you mean to retrieve the type of the property '{1}' in '{0}' with '{0}["{1}"]'?`), The_expression_of_an_export_assignment_must_be_an_identifier_or_qualified_name_in_an_ambient_context: i(2714, 1, "The_expression_of_an_export_assignment_must_be_an_identifier_or_qualified_name_in_an_ambient_context_2714", "The expression of an export assignment must be an identifier or qualified name in an ambient context."), Abstract_property_0_in_class_1_cannot_be_accessed_in_the_constructor: i(2715, 1, "Abstract_property_0_in_class_1_cannot_be_accessed_in_the_constructor_2715", "Abstract property '{0}' in class '{1}' cannot be accessed in the constructor."), Type_parameter_0_has_a_circular_default: i(2716, 1, "Type_parameter_0_has_a_circular_default_2716", "Type parameter '{0}' has a circular default."), Subsequent_property_declarations_must_have_the_same_type_Property_0_must_be_of_type_1_but_here_has_type_2: i(2717, 1, "Subsequent_property_declarations_must_have_the_same_type_Property_0_must_be_of_type_1_but_here_has_t_2717", "Subsequent property declarations must have the same type. Property '{0}' must be of type '{1}', but here has type '{2}'."), Duplicate_property_0: i(2718, 1, "Duplicate_property_0_2718", "Duplicate property '{0}'."), Type_0_is_not_assignable_to_type_1_Two_different_types_with_this_name_exist_but_they_are_unrelated: i(2719, 1, "Type_0_is_not_assignable_to_type_1_Two_different_types_with_this_name_exist_but_they_are_unrelated_2719", "Type '{0}' is not assignable to type '{1}'. Two different types with this name exist, but they are unrelated."), Class_0_incorrectly_implements_class_1_Did_you_mean_to_extend_1_and_inherit_its_members_as_a_subclass: i(2720, 1, "Class_0_incorrectly_implements_class_1_Did_you_mean_to_extend_1_and_inherit_its_members_as_a_subclas_2720", "Class '{0}' incorrectly implements class '{1}'. Did you mean to extend '{1}' and inherit its members as a subclass?"), Cannot_invoke_an_object_which_is_possibly_null: i(2721, 1, "Cannot_invoke_an_object_which_is_possibly_null_2721", "Cannot invoke an object which is possibly 'null'."), Cannot_invoke_an_object_which_is_possibly_undefined: i(2722, 1, "Cannot_invoke_an_object_which_is_possibly_undefined_2722", "Cannot invoke an object which is possibly 'undefined'."), Cannot_invoke_an_object_which_is_possibly_null_or_undefined: i(2723, 1, "Cannot_invoke_an_object_which_is_possibly_null_or_undefined_2723", "Cannot invoke an object which is possibly 'null' or 'undefined'."), _0_has_no_exported_member_named_1_Did_you_mean_2: i(2724, 1, "_0_has_no_exported_member_named_1_Did_you_mean_2_2724", "'{0}' has no exported member named '{1}'. Did you mean '{2}'?"), Class_name_cannot_be_Object_when_targeting_ES5_with_module_0: i(2725, 1, "Class_name_cannot_be_Object_when_targeting_ES5_with_module_0_2725", "Class name cannot be 'Object' when targeting ES5 with module {0}."), Cannot_find_lib_definition_for_0: i(2726, 1, "Cannot_find_lib_definition_for_0_2726", "Cannot find lib definition for '{0}'."), Cannot_find_lib_definition_for_0_Did_you_mean_1: i(2727, 1, "Cannot_find_lib_definition_for_0_Did_you_mean_1_2727", "Cannot find lib definition for '{0}'. Did you mean '{1}'?"), _0_is_declared_here: i(2728, 3, "_0_is_declared_here_2728", "'{0}' is declared here."), Property_0_is_used_before_its_initialization: i(2729, 1, "Property_0_is_used_before_its_initialization_2729", "Property '{0}' is used before its initialization."), An_arrow_function_cannot_have_a_this_parameter: i(2730, 1, "An_arrow_function_cannot_have_a_this_parameter_2730", "An arrow function cannot have a 'this' parameter."), Implicit_conversion_of_a_symbol_to_a_string_will_fail_at_runtime_Consider_wrapping_this_expression_in_String: i(2731, 1, "Implicit_conversion_of_a_symbol_to_a_string_will_fail_at_runtime_Consider_wrapping_this_expression_i_2731", "Implicit conversion of a 'symbol' to a 'string' will fail at runtime. Consider wrapping this expression in 'String(...)'."), Cannot_find_module_0_Consider_using_resolveJsonModule_to_import_module_with_json_extension: i(2732, 1, "Cannot_find_module_0_Consider_using_resolveJsonModule_to_import_module_with_json_extension_2732", "Cannot find module '{0}'. Consider using '--resolveJsonModule' to import module with '.json' extension."), Property_0_was_also_declared_here: i(2733, 1, "Property_0_was_also_declared_here_2733", "Property '{0}' was also declared here."), Are_you_missing_a_semicolon: i(2734, 1, "Are_you_missing_a_semicolon_2734", "Are you missing a semicolon?"), Did_you_mean_for_0_to_be_constrained_to_type_new_args_Colon_any_1: i(2735, 1, "Did_you_mean_for_0_to_be_constrained_to_type_new_args_Colon_any_1_2735", "Did you mean for '{0}' to be constrained to type 'new (...args: any[]) => {1}'?"), Operator_0_cannot_be_applied_to_type_1: i(2736, 1, "Operator_0_cannot_be_applied_to_type_1_2736", "Operator '{0}' cannot be applied to type '{1}'."), BigInt_literals_are_not_available_when_targeting_lower_than_ES2020: i(2737, 1, "BigInt_literals_are_not_available_when_targeting_lower_than_ES2020_2737", "BigInt literals are not available when targeting lower than ES2020."), An_outer_value_of_this_is_shadowed_by_this_container: i(2738, 3, "An_outer_value_of_this_is_shadowed_by_this_container_2738", "An outer value of 'this' is shadowed by this container."), Type_0_is_missing_the_following_properties_from_type_1_Colon_2: i(2739, 1, "Type_0_is_missing_the_following_properties_from_type_1_Colon_2_2739", "Type '{0}' is missing the following properties from type '{1}': {2}"), Type_0_is_missing_the_following_properties_from_type_1_Colon_2_and_3_more: i(2740, 1, "Type_0_is_missing_the_following_properties_from_type_1_Colon_2_and_3_more_2740", "Type '{0}' is missing the following properties from type '{1}': {2}, and {3} more."), Property_0_is_missing_in_type_1_but_required_in_type_2: i(2741, 1, "Property_0_is_missing_in_type_1_but_required_in_type_2_2741", "Property '{0}' is missing in type '{1}' but required in type '{2}'."), The_inferred_type_of_0_cannot_be_named_without_a_reference_to_1_This_is_likely_not_portable_A_type_annotation_is_necessary: i(2742, 1, "The_inferred_type_of_0_cannot_be_named_without_a_reference_to_1_This_is_likely_not_portable_A_type_a_2742", "The inferred type of '{0}' cannot be named without a reference to '{1}'. This is likely not portable. A type annotation is necessary."), No_overload_expects_0_type_arguments_but_overloads_do_exist_that_expect_either_1_or_2_type_arguments: i(2743, 1, "No_overload_expects_0_type_arguments_but_overloads_do_exist_that_expect_either_1_or_2_type_arguments_2743", "No overload expects {0} type arguments, but overloads do exist that expect either {1} or {2} type arguments."), Type_parameter_defaults_can_only_reference_previously_declared_type_parameters: i(2744, 1, "Type_parameter_defaults_can_only_reference_previously_declared_type_parameters_2744", "Type parameter defaults can only reference previously declared type parameters."), This_JSX_tag_s_0_prop_expects_type_1_which_requires_multiple_children_but_only_a_single_child_was_provided: i(2745, 1, "This_JSX_tag_s_0_prop_expects_type_1_which_requires_multiple_children_but_only_a_single_child_was_pr_2745", "This JSX tag's '{0}' prop expects type '{1}' which requires multiple children, but only a single child was provided."), This_JSX_tag_s_0_prop_expects_a_single_child_of_type_1_but_multiple_children_were_provided: i(2746, 1, "This_JSX_tag_s_0_prop_expects_a_single_child_of_type_1_but_multiple_children_were_provided_2746", "This JSX tag's '{0}' prop expects a single child of type '{1}', but multiple children were provided."), _0_components_don_t_accept_text_as_child_elements_Text_in_JSX_has_the_type_string_but_the_expected_type_of_1_is_2: i(2747, 1, "_0_components_don_t_accept_text_as_child_elements_Text_in_JSX_has_the_type_string_but_the_expected_t_2747", "'{0}' components don't accept text as child elements. Text in JSX has the type 'string', but the expected type of '{1}' is '{2}'."), Cannot_access_ambient_const_enums_when_0_is_enabled: i(2748, 1, "Cannot_access_ambient_const_enums_when_0_is_enabled_2748", "Cannot access ambient const enums when '{0}' is enabled."), _0_refers_to_a_value_but_is_being_used_as_a_type_here_Did_you_mean_typeof_0: i(2749, 1, "_0_refers_to_a_value_but_is_being_used_as_a_type_here_Did_you_mean_typeof_0_2749", "'{0}' refers to a value, but is being used as a type here. Did you mean 'typeof {0}'?"), The_implementation_signature_is_declared_here: i(2750, 1, "The_implementation_signature_is_declared_here_2750", "The implementation signature is declared here."), Circularity_originates_in_type_at_this_location: i(2751, 1, "Circularity_originates_in_type_at_this_location_2751", "Circularity originates in type at this location."), The_first_export_default_is_here: i(2752, 1, "The_first_export_default_is_here_2752", "The first export default is here."), Another_export_default_is_here: i(2753, 1, "Another_export_default_is_here_2753", "Another export default is here."), super_may_not_use_type_arguments: i(2754, 1, "super_may_not_use_type_arguments_2754", "'super' may not use type arguments."), No_constituent_of_type_0_is_callable: i(2755, 1, "No_constituent_of_type_0_is_callable_2755", "No constituent of type '{0}' is callable."), Not_all_constituents_of_type_0_are_callable: i(2756, 1, "Not_all_constituents_of_type_0_are_callable_2756", "Not all constituents of type '{0}' are callable."), Type_0_has_no_call_signatures: i(2757, 1, "Type_0_has_no_call_signatures_2757", "Type '{0}' has no call signatures."), Each_member_of_the_union_type_0_has_signatures_but_none_of_those_signatures_are_compatible_with_each_other: i(2758, 1, "Each_member_of_the_union_type_0_has_signatures_but_none_of_those_signatures_are_compatible_with_each_2758", "Each member of the union type '{0}' has signatures, but none of those signatures are compatible with each other."), No_constituent_of_type_0_is_constructable: i(2759, 1, "No_constituent_of_type_0_is_constructable_2759", "No constituent of type '{0}' is constructable."), Not_all_constituents_of_type_0_are_constructable: i(2760, 1, "Not_all_constituents_of_type_0_are_constructable_2760", "Not all constituents of type '{0}' are constructable."), Type_0_has_no_construct_signatures: i(2761, 1, "Type_0_has_no_construct_signatures_2761", "Type '{0}' has no construct signatures."), Each_member_of_the_union_type_0_has_construct_signatures_but_none_of_those_signatures_are_compatible_with_each_other: i(2762, 1, "Each_member_of_the_union_type_0_has_construct_signatures_but_none_of_those_signatures_are_compatible_2762", "Each member of the union type '{0}' has construct signatures, but none of those signatures are compatible with each other."), Cannot_iterate_value_because_the_next_method_of_its_iterator_expects_type_1_but_for_of_will_always_send_0: i(2763, 1, "Cannot_iterate_value_because_the_next_method_of_its_iterator_expects_type_1_but_for_of_will_always_s_2763", "Cannot iterate value because the 'next' method of its iterator expects type '{1}', but for-of will always send '{0}'."), Cannot_iterate_value_because_the_next_method_of_its_iterator_expects_type_1_but_array_spread_will_always_send_0: i(2764, 1, "Cannot_iterate_value_because_the_next_method_of_its_iterator_expects_type_1_but_array_spread_will_al_2764", "Cannot iterate value because the 'next' method of its iterator expects type '{1}', but array spread will always send '{0}'."), Cannot_iterate_value_because_the_next_method_of_its_iterator_expects_type_1_but_array_destructuring_will_always_send_0: i(2765, 1, "Cannot_iterate_value_because_the_next_method_of_its_iterator_expects_type_1_but_array_destructuring__2765", "Cannot iterate value because the 'next' method of its iterator expects type '{1}', but array destructuring will always send '{0}'."), Cannot_delegate_iteration_to_value_because_the_next_method_of_its_iterator_expects_type_1_but_the_containing_generator_will_always_send_0: i(2766, 1, "Cannot_delegate_iteration_to_value_because_the_next_method_of_its_iterator_expects_type_1_but_the_co_2766", "Cannot delegate iteration to value because the 'next' method of its iterator expects type '{1}', but the containing generator will always send '{0}'."), The_0_property_of_an_iterator_must_be_a_method: i(2767, 1, "The_0_property_of_an_iterator_must_be_a_method_2767", "The '{0}' property of an iterator must be a method."), The_0_property_of_an_async_iterator_must_be_a_method: i(2768, 1, "The_0_property_of_an_async_iterator_must_be_a_method_2768", "The '{0}' property of an async iterator must be a method."), No_overload_matches_this_call: i(2769, 1, "No_overload_matches_this_call_2769", "No overload matches this call."), The_last_overload_gave_the_following_error: i(2770, 1, "The_last_overload_gave_the_following_error_2770", "The last overload gave the following error."), The_last_overload_is_declared_here: i(2771, 1, "The_last_overload_is_declared_here_2771", "The last overload is declared here."), Overload_0_of_1_2_gave_the_following_error: i(2772, 1, "Overload_0_of_1_2_gave_the_following_error_2772", "Overload {0} of {1}, '{2}', gave the following error."), Did_you_forget_to_use_await: i(2773, 1, "Did_you_forget_to_use_await_2773", "Did you forget to use 'await'?"), This_condition_will_always_return_true_since_this_function_is_always_defined_Did_you_mean_to_call_it_instead: i(2774, 1, "This_condition_will_always_return_true_since_this_function_is_always_defined_Did_you_mean_to_call_it_2774", "This condition will always return true since this function is always defined. Did you mean to call it instead?"), Assertions_require_every_name_in_the_call_target_to_be_declared_with_an_explicit_type_annotation: i(2775, 1, "Assertions_require_every_name_in_the_call_target_to_be_declared_with_an_explicit_type_annotation_2775", "Assertions require every name in the call target to be declared with an explicit type annotation."), Assertions_require_the_call_target_to_be_an_identifier_or_qualified_name: i(2776, 1, "Assertions_require_the_call_target_to_be_an_identifier_or_qualified_name_2776", "Assertions require the call target to be an identifier or qualified name."), The_operand_of_an_increment_or_decrement_operator_may_not_be_an_optional_property_access: i(2777, 1, "The_operand_of_an_increment_or_decrement_operator_may_not_be_an_optional_property_access_2777", "The operand of an increment or decrement operator may not be an optional property access."), The_target_of_an_object_rest_assignment_may_not_be_an_optional_property_access: i(2778, 1, "The_target_of_an_object_rest_assignment_may_not_be_an_optional_property_access_2778", "The target of an object rest assignment may not be an optional property access."), The_left_hand_side_of_an_assignment_expression_may_not_be_an_optional_property_access: i(2779, 1, "The_left_hand_side_of_an_assignment_expression_may_not_be_an_optional_property_access_2779", "The left-hand side of an assignment expression may not be an optional property access."), The_left_hand_side_of_a_for_in_statement_may_not_be_an_optional_property_access: i(2780, 1, "The_left_hand_side_of_a_for_in_statement_may_not_be_an_optional_property_access_2780", "The left-hand side of a 'for...in' statement may not be an optional property access."), The_left_hand_side_of_a_for_of_statement_may_not_be_an_optional_property_access: i(2781, 1, "The_left_hand_side_of_a_for_of_statement_may_not_be_an_optional_property_access_2781", "The left-hand side of a 'for...of' statement may not be an optional property access."), _0_needs_an_explicit_type_annotation: i(2782, 3, "_0_needs_an_explicit_type_annotation_2782", "'{0}' needs an explicit type annotation."), _0_is_specified_more_than_once_so_this_usage_will_be_overwritten: i(2783, 1, "_0_is_specified_more_than_once_so_this_usage_will_be_overwritten_2783", "'{0}' is specified more than once, so this usage will be overwritten."), get_and_set_accessors_cannot_declare_this_parameters: i(2784, 1, "get_and_set_accessors_cannot_declare_this_parameters_2784", "'get' and 'set' accessors cannot declare 'this' parameters."), This_spread_always_overwrites_this_property: i(2785, 1, "This_spread_always_overwrites_this_property_2785", "This spread always overwrites this property."), _0_cannot_be_used_as_a_JSX_component: i(2786, 1, "_0_cannot_be_used_as_a_JSX_component_2786", "'{0}' cannot be used as a JSX component."), Its_return_type_0_is_not_a_valid_JSX_element: i(2787, 1, "Its_return_type_0_is_not_a_valid_JSX_element_2787", "Its return type '{0}' is not a valid JSX element."), Its_instance_type_0_is_not_a_valid_JSX_element: i(2788, 1, "Its_instance_type_0_is_not_a_valid_JSX_element_2788", "Its instance type '{0}' is not a valid JSX element."), Its_element_type_0_is_not_a_valid_JSX_element: i(2789, 1, "Its_element_type_0_is_not_a_valid_JSX_element_2789", "Its element type '{0}' is not a valid JSX element."), The_operand_of_a_delete_operator_must_be_optional: i(2790, 1, "The_operand_of_a_delete_operator_must_be_optional_2790", "The operand of a 'delete' operator must be optional."), Exponentiation_cannot_be_performed_on_bigint_values_unless_the_target_option_is_set_to_es2016_or_later: i(2791, 1, "Exponentiation_cannot_be_performed_on_bigint_values_unless_the_target_option_is_set_to_es2016_or_lat_2791", "Exponentiation cannot be performed on 'bigint' values unless the 'target' option is set to 'es2016' or later."), Cannot_find_module_0_Did_you_mean_to_set_the_moduleResolution_option_to_nodenext_or_to_add_aliases_to_the_paths_option: i(2792, 1, "Cannot_find_module_0_Did_you_mean_to_set_the_moduleResolution_option_to_nodenext_or_to_add_aliases_t_2792", "Cannot find module '{0}'. Did you mean to set the 'moduleResolution' option to 'nodenext', or to add aliases to the 'paths' option?"), The_call_would_have_succeeded_against_this_implementation_but_implementation_signatures_of_overloads_are_not_externally_visible: i(2793, 1, "The_call_would_have_succeeded_against_this_implementation_but_implementation_signatures_of_overloads_2793", "The call would have succeeded against this implementation, but implementation signatures of overloads are not externally visible."), Expected_0_arguments_but_got_1_Did_you_forget_to_include_void_in_your_type_argument_to_Promise: i(2794, 1, "Expected_0_arguments_but_got_1_Did_you_forget_to_include_void_in_your_type_argument_to_Promise_2794", "Expected {0} arguments, but got {1}. Did you forget to include 'void' in your type argument to 'Promise'?"), The_intrinsic_keyword_can_only_be_used_to_declare_compiler_provided_intrinsic_types: i(2795, 1, "The_intrinsic_keyword_can_only_be_used_to_declare_compiler_provided_intrinsic_types_2795", "The 'intrinsic' keyword can only be used to declare compiler provided intrinsic types."), It_is_likely_that_you_are_missing_a_comma_to_separate_these_two_template_expressions_They_form_a_tagged_template_expression_which_cannot_be_invoked: i(2796, 1, "It_is_likely_that_you_are_missing_a_comma_to_separate_these_two_template_expressions_They_form_a_tag_2796", "It is likely that you are missing a comma to separate these two template expressions. They form a tagged template expression which cannot be invoked."), A_mixin_class_that_extends_from_a_type_variable_containing_an_abstract_construct_signature_must_also_be_declared_abstract: i(2797, 1, "A_mixin_class_that_extends_from_a_type_variable_containing_an_abstract_construct_signature_must_also_2797", "A mixin class that extends from a type variable containing an abstract construct signature must also be declared 'abstract'."), The_declaration_was_marked_as_deprecated_here: i(2798, 1, "The_declaration_was_marked_as_deprecated_here_2798", "The declaration was marked as deprecated here."), Type_produces_a_tuple_type_that_is_too_large_to_represent: i(2799, 1, "Type_produces_a_tuple_type_that_is_too_large_to_represent_2799", "Type produces a tuple type that is too large to represent."), Expression_produces_a_tuple_type_that_is_too_large_to_represent: i(2800, 1, "Expression_produces_a_tuple_type_that_is_too_large_to_represent_2800", "Expression produces a tuple type that is too large to represent."), This_condition_will_always_return_true_since_this_0_is_always_defined: i(2801, 1, "This_condition_will_always_return_true_since_this_0_is_always_defined_2801", "This condition will always return true since this '{0}' is always defined."), Type_0_can_only_be_iterated_through_when_using_the_downlevelIteration_flag_or_with_a_target_of_es2015_or_higher: i(2802, 1, "Type_0_can_only_be_iterated_through_when_using_the_downlevelIteration_flag_or_with_a_target_of_es201_2802", "Type '{0}' can only be iterated through when using the '--downlevelIteration' flag or with a '--target' of 'es2015' or higher."), Cannot_assign_to_private_method_0_Private_methods_are_not_writable: i(2803, 1, "Cannot_assign_to_private_method_0_Private_methods_are_not_writable_2803", "Cannot assign to private method '{0}'. Private methods are not writable."), Duplicate_identifier_0_Static_and_instance_elements_cannot_share_the_same_private_name: i(2804, 1, "Duplicate_identifier_0_Static_and_instance_elements_cannot_share_the_same_private_name_2804", "Duplicate identifier '{0}'. Static and instance elements cannot share the same private name."), Private_accessor_was_defined_without_a_getter: i(2806, 1, "Private_accessor_was_defined_without_a_getter_2806", "Private accessor was defined without a getter."), This_syntax_requires_an_imported_helper_named_1_with_2_parameters_which_is_not_compatible_with_the_one_in_0_Consider_upgrading_your_version_of_0: i(2807, 1, "This_syntax_requires_an_imported_helper_named_1_with_2_parameters_which_is_not_compatible_with_the_o_2807", "This syntax requires an imported helper named '{1}' with {2} parameters, which is not compatible with the one in '{0}'. Consider upgrading your version of '{0}'."), A_get_accessor_must_be_at_least_as_accessible_as_the_setter: i(2808, 1, "A_get_accessor_must_be_at_least_as_accessible_as_the_setter_2808", "A get accessor must be at least as accessible as the setter"), Declaration_or_statement_expected_This_follows_a_block_of_statements_so_if_you_intended_to_write_a_destructuring_assignment_you_might_need_to_wrap_the_whole_assignment_in_parentheses: i(2809, 1, "Declaration_or_statement_expected_This_follows_a_block_of_statements_so_if_you_intended_to_write_a_d_2809", "Declaration or statement expected. This '=' follows a block of statements, so if you intended to write a destructuring assignment, you might need to wrap the whole assignment in parentheses."), Expected_1_argument_but_got_0_new_Promise_needs_a_JSDoc_hint_to_produce_a_resolve_that_can_be_called_without_arguments: i(2810, 1, "Expected_1_argument_but_got_0_new_Promise_needs_a_JSDoc_hint_to_produce_a_resolve_that_can_be_called_2810", "Expected 1 argument, but got 0. 'new Promise()' needs a JSDoc hint to produce a 'resolve' that can be called without arguments."), Initializer_for_property_0: i(2811, 1, "Initializer_for_property_0_2811", "Initializer for property '{0}'"), Property_0_does_not_exist_on_type_1_Try_changing_the_lib_compiler_option_to_include_dom: i(2812, 1, "Property_0_does_not_exist_on_type_1_Try_changing_the_lib_compiler_option_to_include_dom_2812", "Property '{0}' does not exist on type '{1}'. Try changing the 'lib' compiler option to include 'dom'."), Class_declaration_cannot_implement_overload_list_for_0: i(2813, 1, "Class_declaration_cannot_implement_overload_list_for_0_2813", "Class declaration cannot implement overload list for '{0}'."), Function_with_bodies_can_only_merge_with_classes_that_are_ambient: i(2814, 1, "Function_with_bodies_can_only_merge_with_classes_that_are_ambient_2814", "Function with bodies can only merge with classes that are ambient."), arguments_cannot_be_referenced_in_property_initializers: i(2815, 1, "arguments_cannot_be_referenced_in_property_initializers_2815", "'arguments' cannot be referenced in property initializers."), Cannot_use_this_in_a_static_property_initializer_of_a_decorated_class: i(2816, 1, "Cannot_use_this_in_a_static_property_initializer_of_a_decorated_class_2816", "Cannot use 'this' in a static property initializer of a decorated class."), Property_0_has_no_initializer_and_is_not_definitely_assigned_in_a_class_static_block: i(2817, 1, "Property_0_has_no_initializer_and_is_not_definitely_assigned_in_a_class_static_block_2817", "Property '{0}' has no initializer and is not definitely assigned in a class static block."), Duplicate_identifier_0_Compiler_reserves_name_1_when_emitting_super_references_in_static_initializers: i(2818, 1, "Duplicate_identifier_0_Compiler_reserves_name_1_when_emitting_super_references_in_static_initializer_2818", "Duplicate identifier '{0}'. Compiler reserves name '{1}' when emitting 'super' references in static initializers."), Namespace_name_cannot_be_0: i(2819, 1, "Namespace_name_cannot_be_0_2819", "Namespace name cannot be '{0}'."), Type_0_is_not_assignable_to_type_1_Did_you_mean_2: i(2820, 1, "Type_0_is_not_assignable_to_type_1_Did_you_mean_2_2820", "Type '{0}' is not assignable to type '{1}'. Did you mean '{2}'?"), Import_assertions_are_only_supported_when_the_module_option_is_set_to_esnext_or_nodenext: i(2821, 1, "Import_assertions_are_only_supported_when_the_module_option_is_set_to_esnext_or_nodenext_2821", "Import assertions are only supported when the '--module' option is set to 'esnext' or 'nodenext'."), Import_assertions_cannot_be_used_with_type_only_imports_or_exports: i(2822, 1, "Import_assertions_cannot_be_used_with_type_only_imports_or_exports_2822", "Import assertions cannot be used with type-only imports or exports."), Cannot_find_namespace_0_Did_you_mean_1: i(2833, 1, "Cannot_find_namespace_0_Did_you_mean_1_2833", "Cannot find namespace '{0}'. Did you mean '{1}'?"), Relative_import_paths_need_explicit_file_extensions_in_EcmaScript_imports_when_moduleResolution_is_node16_or_nodenext_Consider_adding_an_extension_to_the_import_path: i(2834, 1, "Relative_import_paths_need_explicit_file_extensions_in_EcmaScript_imports_when_moduleResolution_is_n_2834", "Relative import paths need explicit file extensions in EcmaScript imports when '--moduleResolution' is 'node16' or 'nodenext'. Consider adding an extension to the import path."), Relative_import_paths_need_explicit_file_extensions_in_EcmaScript_imports_when_moduleResolution_is_node16_or_nodenext_Did_you_mean_0: i(2835, 1, "Relative_import_paths_need_explicit_file_extensions_in_EcmaScript_imports_when_moduleResolution_is_n_2835", "Relative import paths need explicit file extensions in EcmaScript imports when '--moduleResolution' is 'node16' or 'nodenext'. Did you mean '{0}'?"), Import_assertions_are_not_allowed_on_statements_that_transpile_to_commonjs_require_calls: i(2836, 1, "Import_assertions_are_not_allowed_on_statements_that_transpile_to_commonjs_require_calls_2836", "Import assertions are not allowed on statements that transpile to commonjs 'require' calls."), Import_assertion_values_must_be_string_literal_expressions: i(2837, 1, "Import_assertion_values_must_be_string_literal_expressions_2837", "Import assertion values must be string literal expressions."), All_declarations_of_0_must_have_identical_constraints: i(2838, 1, "All_declarations_of_0_must_have_identical_constraints_2838", "All declarations of '{0}' must have identical constraints."), This_condition_will_always_return_0_since_JavaScript_compares_objects_by_reference_not_value: i(2839, 1, "This_condition_will_always_return_0_since_JavaScript_compares_objects_by_reference_not_value_2839", "This condition will always return '{0}' since JavaScript compares objects by reference, not value."), An_interface_cannot_extend_a_primitive_type_like_0_an_interface_can_only_extend_named_types_and_classes: i(2840, 1, "An_interface_cannot_extend_a_primitive_type_like_0_an_interface_can_only_extend_named_types_and_clas_2840", "An interface cannot extend a primitive type like '{0}'; an interface can only extend named types and classes"), The_type_of_this_expression_cannot_be_named_without_a_resolution_mode_assertion_which_is_an_unstable_feature_Use_nightly_TypeScript_to_silence_this_error_Try_updating_with_npm_install_D_typescript_next: i(2841, 1, "The_type_of_this_expression_cannot_be_named_without_a_resolution_mode_assertion_which_is_an_unstable_2841", "The type of this expression cannot be named without a 'resolution-mode' assertion, which is an unstable feature. Use nightly TypeScript to silence this error. Try updating with 'npm install -D typescript@next'."), _0_is_an_unused_renaming_of_1_Did_you_intend_to_use_it_as_a_type_annotation: i(2842, 1, "_0_is_an_unused_renaming_of_1_Did_you_intend_to_use_it_as_a_type_annotation_2842", "'{0}' is an unused renaming of '{1}'. Did you intend to use it as a type annotation?"), We_can_only_write_a_type_for_0_by_adding_a_type_for_the_entire_parameter_here: i(2843, 1, "We_can_only_write_a_type_for_0_by_adding_a_type_for_the_entire_parameter_here_2843", "We can only write a type for '{0}' by adding a type for the entire parameter here."), Type_of_instance_member_variable_0_cannot_reference_identifier_1_declared_in_the_constructor: i(2844, 1, "Type_of_instance_member_variable_0_cannot_reference_identifier_1_declared_in_the_constructor_2844", "Type of instance member variable '{0}' cannot reference identifier '{1}' declared in the constructor."), This_condition_will_always_return_0: i(2845, 1, "This_condition_will_always_return_0_2845", "This condition will always return '{0}'."), A_declaration_file_cannot_be_imported_without_import_type_Did_you_mean_to_import_an_implementation_file_0_instead: i(2846, 1, "A_declaration_file_cannot_be_imported_without_import_type_Did_you_mean_to_import_an_implementation_f_2846", "A declaration file cannot be imported without 'import type'. Did you mean to import an implementation file '{0}' instead?"), Import_declaration_0_is_using_private_name_1: i(4e3, 1, "Import_declaration_0_is_using_private_name_1_4000", "Import declaration '{0}' is using private name '{1}'."), Type_parameter_0_of_exported_class_has_or_is_using_private_name_1: i(4002, 1, "Type_parameter_0_of_exported_class_has_or_is_using_private_name_1_4002", "Type parameter '{0}' of exported class has or is using private name '{1}'."), Type_parameter_0_of_exported_interface_has_or_is_using_private_name_1: i(4004, 1, "Type_parameter_0_of_exported_interface_has_or_is_using_private_name_1_4004", "Type parameter '{0}' of exported interface has or is using private name '{1}'."), Type_parameter_0_of_constructor_signature_from_exported_interface_has_or_is_using_private_name_1: i(4006, 1, "Type_parameter_0_of_constructor_signature_from_exported_interface_has_or_is_using_private_name_1_4006", "Type parameter '{0}' of constructor signature from exported interface has or is using private name '{1}'."), Type_parameter_0_of_call_signature_from_exported_interface_has_or_is_using_private_name_1: i(4008, 1, "Type_parameter_0_of_call_signature_from_exported_interface_has_or_is_using_private_name_1_4008", "Type parameter '{0}' of call signature from exported interface has or is using private name '{1}'."), Type_parameter_0_of_public_static_method_from_exported_class_has_or_is_using_private_name_1: i(4010, 1, "Type_parameter_0_of_public_static_method_from_exported_class_has_or_is_using_private_name_1_4010", "Type parameter '{0}' of public static method from exported class has or is using private name '{1}'."), Type_parameter_0_of_public_method_from_exported_class_has_or_is_using_private_name_1: i(4012, 1, "Type_parameter_0_of_public_method_from_exported_class_has_or_is_using_private_name_1_4012", "Type parameter '{0}' of public method from exported class has or is using private name '{1}'."), Type_parameter_0_of_method_from_exported_interface_has_or_is_using_private_name_1: i(4014, 1, "Type_parameter_0_of_method_from_exported_interface_has_or_is_using_private_name_1_4014", "Type parameter '{0}' of method from exported interface has or is using private name '{1}'."), Type_parameter_0_of_exported_function_has_or_is_using_private_name_1: i(4016, 1, "Type_parameter_0_of_exported_function_has_or_is_using_private_name_1_4016", "Type parameter '{0}' of exported function has or is using private name '{1}'."), Implements_clause_of_exported_class_0_has_or_is_using_private_name_1: i(4019, 1, "Implements_clause_of_exported_class_0_has_or_is_using_private_name_1_4019", "Implements clause of exported class '{0}' has or is using private name '{1}'."), extends_clause_of_exported_class_0_has_or_is_using_private_name_1: i(4020, 1, "extends_clause_of_exported_class_0_has_or_is_using_private_name_1_4020", "'extends' clause of exported class '{0}' has or is using private name '{1}'."), extends_clause_of_exported_class_has_or_is_using_private_name_0: i(4021, 1, "extends_clause_of_exported_class_has_or_is_using_private_name_0_4021", "'extends' clause of exported class has or is using private name '{0}'."), extends_clause_of_exported_interface_0_has_or_is_using_private_name_1: i(4022, 1, "extends_clause_of_exported_interface_0_has_or_is_using_private_name_1_4022", "'extends' clause of exported interface '{0}' has or is using private name '{1}'."), Exported_variable_0_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4023, 1, "Exported_variable_0_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named_4023", "Exported variable '{0}' has or is using name '{1}' from external module {2} but cannot be named."), Exported_variable_0_has_or_is_using_name_1_from_private_module_2: i(4024, 1, "Exported_variable_0_has_or_is_using_name_1_from_private_module_2_4024", "Exported variable '{0}' has or is using name '{1}' from private module '{2}'."), Exported_variable_0_has_or_is_using_private_name_1: i(4025, 1, "Exported_variable_0_has_or_is_using_private_name_1_4025", "Exported variable '{0}' has or is using private name '{1}'."), Public_static_property_0_of_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4026, 1, "Public_static_property_0_of_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot__4026", "Public static property '{0}' of exported class has or is using name '{1}' from external module {2} but cannot be named."), Public_static_property_0_of_exported_class_has_or_is_using_name_1_from_private_module_2: i(4027, 1, "Public_static_property_0_of_exported_class_has_or_is_using_name_1_from_private_module_2_4027", "Public static property '{0}' of exported class has or is using name '{1}' from private module '{2}'."), Public_static_property_0_of_exported_class_has_or_is_using_private_name_1: i(4028, 1, "Public_static_property_0_of_exported_class_has_or_is_using_private_name_1_4028", "Public static property '{0}' of exported class has or is using private name '{1}'."), Public_property_0_of_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4029, 1, "Public_property_0_of_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_name_4029", "Public property '{0}' of exported class has or is using name '{1}' from external module {2} but cannot be named."), Public_property_0_of_exported_class_has_or_is_using_name_1_from_private_module_2: i(4030, 1, "Public_property_0_of_exported_class_has_or_is_using_name_1_from_private_module_2_4030", "Public property '{0}' of exported class has or is using name '{1}' from private module '{2}'."), Public_property_0_of_exported_class_has_or_is_using_private_name_1: i(4031, 1, "Public_property_0_of_exported_class_has_or_is_using_private_name_1_4031", "Public property '{0}' of exported class has or is using private name '{1}'."), Property_0_of_exported_interface_has_or_is_using_name_1_from_private_module_2: i(4032, 1, "Property_0_of_exported_interface_has_or_is_using_name_1_from_private_module_2_4032", "Property '{0}' of exported interface has or is using name '{1}' from private module '{2}'."), Property_0_of_exported_interface_has_or_is_using_private_name_1: i(4033, 1, "Property_0_of_exported_interface_has_or_is_using_private_name_1_4033", "Property '{0}' of exported interface has or is using private name '{1}'."), Parameter_type_of_public_static_setter_0_from_exported_class_has_or_is_using_name_1_from_private_module_2: i(4034, 1, "Parameter_type_of_public_static_setter_0_from_exported_class_has_or_is_using_name_1_from_private_mod_4034", "Parameter type of public static setter '{0}' from exported class has or is using name '{1}' from private module '{2}'."), Parameter_type_of_public_static_setter_0_from_exported_class_has_or_is_using_private_name_1: i(4035, 1, "Parameter_type_of_public_static_setter_0_from_exported_class_has_or_is_using_private_name_1_4035", "Parameter type of public static setter '{0}' from exported class has or is using private name '{1}'."), Parameter_type_of_public_setter_0_from_exported_class_has_or_is_using_name_1_from_private_module_2: i(4036, 1, "Parameter_type_of_public_setter_0_from_exported_class_has_or_is_using_name_1_from_private_module_2_4036", "Parameter type of public setter '{0}' from exported class has or is using name '{1}' from private module '{2}'."), Parameter_type_of_public_setter_0_from_exported_class_has_or_is_using_private_name_1: i(4037, 1, "Parameter_type_of_public_setter_0_from_exported_class_has_or_is_using_private_name_1_4037", "Parameter type of public setter '{0}' from exported class has or is using private name '{1}'."), Return_type_of_public_static_getter_0_from_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4038, 1, "Return_type_of_public_static_getter_0_from_exported_class_has_or_is_using_name_1_from_external_modul_4038", "Return type of public static getter '{0}' from exported class has or is using name '{1}' from external module {2} but cannot be named."), Return_type_of_public_static_getter_0_from_exported_class_has_or_is_using_name_1_from_private_module_2: i(4039, 1, "Return_type_of_public_static_getter_0_from_exported_class_has_or_is_using_name_1_from_private_module_4039", "Return type of public static getter '{0}' from exported class has or is using name '{1}' from private module '{2}'."), Return_type_of_public_static_getter_0_from_exported_class_has_or_is_using_private_name_1: i(4040, 1, "Return_type_of_public_static_getter_0_from_exported_class_has_or_is_using_private_name_1_4040", "Return type of public static getter '{0}' from exported class has or is using private name '{1}'."), Return_type_of_public_getter_0_from_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4041, 1, "Return_type_of_public_getter_0_from_exported_class_has_or_is_using_name_1_from_external_module_2_but_4041", "Return type of public getter '{0}' from exported class has or is using name '{1}' from external module {2} but cannot be named."), Return_type_of_public_getter_0_from_exported_class_has_or_is_using_name_1_from_private_module_2: i(4042, 1, "Return_type_of_public_getter_0_from_exported_class_has_or_is_using_name_1_from_private_module_2_4042", "Return type of public getter '{0}' from exported class has or is using name '{1}' from private module '{2}'."), Return_type_of_public_getter_0_from_exported_class_has_or_is_using_private_name_1: i(4043, 1, "Return_type_of_public_getter_0_from_exported_class_has_or_is_using_private_name_1_4043", "Return type of public getter '{0}' from exported class has or is using private name '{1}'."), Return_type_of_constructor_signature_from_exported_interface_has_or_is_using_name_0_from_private_module_1: i(4044, 1, "Return_type_of_constructor_signature_from_exported_interface_has_or_is_using_name_0_from_private_mod_4044", "Return type of constructor signature from exported interface has or is using name '{0}' from private module '{1}'."), Return_type_of_constructor_signature_from_exported_interface_has_or_is_using_private_name_0: i(4045, 1, "Return_type_of_constructor_signature_from_exported_interface_has_or_is_using_private_name_0_4045", "Return type of constructor signature from exported interface has or is using private name '{0}'."), Return_type_of_call_signature_from_exported_interface_has_or_is_using_name_0_from_private_module_1: i(4046, 1, "Return_type_of_call_signature_from_exported_interface_has_or_is_using_name_0_from_private_module_1_4046", "Return type of call signature from exported interface has or is using name '{0}' from private module '{1}'."), Return_type_of_call_signature_from_exported_interface_has_or_is_using_private_name_0: i(4047, 1, "Return_type_of_call_signature_from_exported_interface_has_or_is_using_private_name_0_4047", "Return type of call signature from exported interface has or is using private name '{0}'."), Return_type_of_index_signature_from_exported_interface_has_or_is_using_name_0_from_private_module_1: i(4048, 1, "Return_type_of_index_signature_from_exported_interface_has_or_is_using_name_0_from_private_module_1_4048", "Return type of index signature from exported interface has or is using name '{0}' from private module '{1}'."), Return_type_of_index_signature_from_exported_interface_has_or_is_using_private_name_0: i(4049, 1, "Return_type_of_index_signature_from_exported_interface_has_or_is_using_private_name_0_4049", "Return type of index signature from exported interface has or is using private name '{0}'."), Return_type_of_public_static_method_from_exported_class_has_or_is_using_name_0_from_external_module_1_but_cannot_be_named: i(4050, 1, "Return_type_of_public_static_method_from_exported_class_has_or_is_using_name_0_from_external_module__4050", "Return type of public static method from exported class has or is using name '{0}' from external module {1} but cannot be named."), Return_type_of_public_static_method_from_exported_class_has_or_is_using_name_0_from_private_module_1: i(4051, 1, "Return_type_of_public_static_method_from_exported_class_has_or_is_using_name_0_from_private_module_1_4051", "Return type of public static method from exported class has or is using name '{0}' from private module '{1}'."), Return_type_of_public_static_method_from_exported_class_has_or_is_using_private_name_0: i(4052, 1, "Return_type_of_public_static_method_from_exported_class_has_or_is_using_private_name_0_4052", "Return type of public static method from exported class has or is using private name '{0}'."), Return_type_of_public_method_from_exported_class_has_or_is_using_name_0_from_external_module_1_but_cannot_be_named: i(4053, 1, "Return_type_of_public_method_from_exported_class_has_or_is_using_name_0_from_external_module_1_but_c_4053", "Return type of public method from exported class has or is using name '{0}' from external module {1} but cannot be named."), Return_type_of_public_method_from_exported_class_has_or_is_using_name_0_from_private_module_1: i(4054, 1, "Return_type_of_public_method_from_exported_class_has_or_is_using_name_0_from_private_module_1_4054", "Return type of public method from exported class has or is using name '{0}' from private module '{1}'."), Return_type_of_public_method_from_exported_class_has_or_is_using_private_name_0: i(4055, 1, "Return_type_of_public_method_from_exported_class_has_or_is_using_private_name_0_4055", "Return type of public method from exported class has or is using private name '{0}'."), Return_type_of_method_from_exported_interface_has_or_is_using_name_0_from_private_module_1: i(4056, 1, "Return_type_of_method_from_exported_interface_has_or_is_using_name_0_from_private_module_1_4056", "Return type of method from exported interface has or is using name '{0}' from private module '{1}'."), Return_type_of_method_from_exported_interface_has_or_is_using_private_name_0: i(4057, 1, "Return_type_of_method_from_exported_interface_has_or_is_using_private_name_0_4057", "Return type of method from exported interface has or is using private name '{0}'."), Return_type_of_exported_function_has_or_is_using_name_0_from_external_module_1_but_cannot_be_named: i(4058, 1, "Return_type_of_exported_function_has_or_is_using_name_0_from_external_module_1_but_cannot_be_named_4058", "Return type of exported function has or is using name '{0}' from external module {1} but cannot be named."), Return_type_of_exported_function_has_or_is_using_name_0_from_private_module_1: i(4059, 1, "Return_type_of_exported_function_has_or_is_using_name_0_from_private_module_1_4059", "Return type of exported function has or is using name '{0}' from private module '{1}'."), Return_type_of_exported_function_has_or_is_using_private_name_0: i(4060, 1, "Return_type_of_exported_function_has_or_is_using_private_name_0_4060", "Return type of exported function has or is using private name '{0}'."), Parameter_0_of_constructor_from_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4061, 1, "Parameter_0_of_constructor_from_exported_class_has_or_is_using_name_1_from_external_module_2_but_can_4061", "Parameter '{0}' of constructor from exported class has or is using name '{1}' from external module {2} but cannot be named."), Parameter_0_of_constructor_from_exported_class_has_or_is_using_name_1_from_private_module_2: i(4062, 1, "Parameter_0_of_constructor_from_exported_class_has_or_is_using_name_1_from_private_module_2_4062", "Parameter '{0}' of constructor from exported class has or is using name '{1}' from private module '{2}'."), Parameter_0_of_constructor_from_exported_class_has_or_is_using_private_name_1: i(4063, 1, "Parameter_0_of_constructor_from_exported_class_has_or_is_using_private_name_1_4063", "Parameter '{0}' of constructor from exported class has or is using private name '{1}'."), Parameter_0_of_constructor_signature_from_exported_interface_has_or_is_using_name_1_from_private_module_2: i(4064, 1, "Parameter_0_of_constructor_signature_from_exported_interface_has_or_is_using_name_1_from_private_mod_4064", "Parameter '{0}' of constructor signature from exported interface has or is using name '{1}' from private module '{2}'."), Parameter_0_of_constructor_signature_from_exported_interface_has_or_is_using_private_name_1: i(4065, 1, "Parameter_0_of_constructor_signature_from_exported_interface_has_or_is_using_private_name_1_4065", "Parameter '{0}' of constructor signature from exported interface has or is using private name '{1}'."), Parameter_0_of_call_signature_from_exported_interface_has_or_is_using_name_1_from_private_module_2: i(4066, 1, "Parameter_0_of_call_signature_from_exported_interface_has_or_is_using_name_1_from_private_module_2_4066", "Parameter '{0}' of call signature from exported interface has or is using name '{1}' from private module '{2}'."), Parameter_0_of_call_signature_from_exported_interface_has_or_is_using_private_name_1: i(4067, 1, "Parameter_0_of_call_signature_from_exported_interface_has_or_is_using_private_name_1_4067", "Parameter '{0}' of call signature from exported interface has or is using private name '{1}'."), Parameter_0_of_public_static_method_from_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4068, 1, "Parameter_0_of_public_static_method_from_exported_class_has_or_is_using_name_1_from_external_module__4068", "Parameter '{0}' of public static method from exported class has or is using name '{1}' from external module {2} but cannot be named."), Parameter_0_of_public_static_method_from_exported_class_has_or_is_using_name_1_from_private_module_2: i(4069, 1, "Parameter_0_of_public_static_method_from_exported_class_has_or_is_using_name_1_from_private_module_2_4069", "Parameter '{0}' of public static method from exported class has or is using name '{1}' from private module '{2}'."), Parameter_0_of_public_static_method_from_exported_class_has_or_is_using_private_name_1: i(4070, 1, "Parameter_0_of_public_static_method_from_exported_class_has_or_is_using_private_name_1_4070", "Parameter '{0}' of public static method from exported class has or is using private name '{1}'."), Parameter_0_of_public_method_from_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4071, 1, "Parameter_0_of_public_method_from_exported_class_has_or_is_using_name_1_from_external_module_2_but_c_4071", "Parameter '{0}' of public method from exported class has or is using name '{1}' from external module {2} but cannot be named."), Parameter_0_of_public_method_from_exported_class_has_or_is_using_name_1_from_private_module_2: i(4072, 1, "Parameter_0_of_public_method_from_exported_class_has_or_is_using_name_1_from_private_module_2_4072", "Parameter '{0}' of public method from exported class has or is using name '{1}' from private module '{2}'."), Parameter_0_of_public_method_from_exported_class_has_or_is_using_private_name_1: i(4073, 1, "Parameter_0_of_public_method_from_exported_class_has_or_is_using_private_name_1_4073", "Parameter '{0}' of public method from exported class has or is using private name '{1}'."), Parameter_0_of_method_from_exported_interface_has_or_is_using_name_1_from_private_module_2: i(4074, 1, "Parameter_0_of_method_from_exported_interface_has_or_is_using_name_1_from_private_module_2_4074", "Parameter '{0}' of method from exported interface has or is using name '{1}' from private module '{2}'."), Parameter_0_of_method_from_exported_interface_has_or_is_using_private_name_1: i(4075, 1, "Parameter_0_of_method_from_exported_interface_has_or_is_using_private_name_1_4075", "Parameter '{0}' of method from exported interface has or is using private name '{1}'."), Parameter_0_of_exported_function_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4076, 1, "Parameter_0_of_exported_function_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named_4076", "Parameter '{0}' of exported function has or is using name '{1}' from external module {2} but cannot be named."), Parameter_0_of_exported_function_has_or_is_using_name_1_from_private_module_2: i(4077, 1, "Parameter_0_of_exported_function_has_or_is_using_name_1_from_private_module_2_4077", "Parameter '{0}' of exported function has or is using name '{1}' from private module '{2}'."), Parameter_0_of_exported_function_has_or_is_using_private_name_1: i(4078, 1, "Parameter_0_of_exported_function_has_or_is_using_private_name_1_4078", "Parameter '{0}' of exported function has or is using private name '{1}'."), Exported_type_alias_0_has_or_is_using_private_name_1: i(4081, 1, "Exported_type_alias_0_has_or_is_using_private_name_1_4081", "Exported type alias '{0}' has or is using private name '{1}'."), Default_export_of_the_module_has_or_is_using_private_name_0: i(4082, 1, "Default_export_of_the_module_has_or_is_using_private_name_0_4082", "Default export of the module has or is using private name '{0}'."), Type_parameter_0_of_exported_type_alias_has_or_is_using_private_name_1: i(4083, 1, "Type_parameter_0_of_exported_type_alias_has_or_is_using_private_name_1_4083", "Type parameter '{0}' of exported type alias has or is using private name '{1}'."), Exported_type_alias_0_has_or_is_using_private_name_1_from_module_2: i(4084, 1, "Exported_type_alias_0_has_or_is_using_private_name_1_from_module_2_4084", "Exported type alias '{0}' has or is using private name '{1}' from module {2}."), Extends_clause_for_inferred_type_0_has_or_is_using_private_name_1: i(4085, 1, "Extends_clause_for_inferred_type_0_has_or_is_using_private_name_1_4085", "Extends clause for inferred type '{0}' has or is using private name '{1}'."), Conflicting_definitions_for_0_found_at_1_and_2_Consider_installing_a_specific_version_of_this_library_to_resolve_the_conflict: i(4090, 1, "Conflicting_definitions_for_0_found_at_1_and_2_Consider_installing_a_specific_version_of_this_librar_4090", "Conflicting definitions for '{0}' found at '{1}' and '{2}'. Consider installing a specific version of this library to resolve the conflict."), Parameter_0_of_index_signature_from_exported_interface_has_or_is_using_name_1_from_private_module_2: i(4091, 1, "Parameter_0_of_index_signature_from_exported_interface_has_or_is_using_name_1_from_private_module_2_4091", "Parameter '{0}' of index signature from exported interface has or is using name '{1}' from private module '{2}'."), Parameter_0_of_index_signature_from_exported_interface_has_or_is_using_private_name_1: i(4092, 1, "Parameter_0_of_index_signature_from_exported_interface_has_or_is_using_private_name_1_4092", "Parameter '{0}' of index signature from exported interface has or is using private name '{1}'."), Property_0_of_exported_class_expression_may_not_be_private_or_protected: i(4094, 1, "Property_0_of_exported_class_expression_may_not_be_private_or_protected_4094", "Property '{0}' of exported class expression may not be private or protected."), Public_static_method_0_of_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4095, 1, "Public_static_method_0_of_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_4095", "Public static method '{0}' of exported class has or is using name '{1}' from external module {2} but cannot be named."), Public_static_method_0_of_exported_class_has_or_is_using_name_1_from_private_module_2: i(4096, 1, "Public_static_method_0_of_exported_class_has_or_is_using_name_1_from_private_module_2_4096", "Public static method '{0}' of exported class has or is using name '{1}' from private module '{2}'."), Public_static_method_0_of_exported_class_has_or_is_using_private_name_1: i(4097, 1, "Public_static_method_0_of_exported_class_has_or_is_using_private_name_1_4097", "Public static method '{0}' of exported class has or is using private name '{1}'."), Public_method_0_of_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4098, 1, "Public_method_0_of_exported_class_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named_4098", "Public method '{0}' of exported class has or is using name '{1}' from external module {2} but cannot be named."), Public_method_0_of_exported_class_has_or_is_using_name_1_from_private_module_2: i(4099, 1, "Public_method_0_of_exported_class_has_or_is_using_name_1_from_private_module_2_4099", "Public method '{0}' of exported class has or is using name '{1}' from private module '{2}'."), Public_method_0_of_exported_class_has_or_is_using_private_name_1: i(4100, 1, "Public_method_0_of_exported_class_has_or_is_using_private_name_1_4100", "Public method '{0}' of exported class has or is using private name '{1}'."), Method_0_of_exported_interface_has_or_is_using_name_1_from_private_module_2: i(4101, 1, "Method_0_of_exported_interface_has_or_is_using_name_1_from_private_module_2_4101", "Method '{0}' of exported interface has or is using name '{1}' from private module '{2}'."), Method_0_of_exported_interface_has_or_is_using_private_name_1: i(4102, 1, "Method_0_of_exported_interface_has_or_is_using_private_name_1_4102", "Method '{0}' of exported interface has or is using private name '{1}'."), Type_parameter_0_of_exported_mapped_object_type_is_using_private_name_1: i(4103, 1, "Type_parameter_0_of_exported_mapped_object_type_is_using_private_name_1_4103", "Type parameter '{0}' of exported mapped object type is using private name '{1}'."), The_type_0_is_readonly_and_cannot_be_assigned_to_the_mutable_type_1: i(4104, 1, "The_type_0_is_readonly_and_cannot_be_assigned_to_the_mutable_type_1_4104", "The type '{0}' is 'readonly' and cannot be assigned to the mutable type '{1}'."), Private_or_protected_member_0_cannot_be_accessed_on_a_type_parameter: i(4105, 1, "Private_or_protected_member_0_cannot_be_accessed_on_a_type_parameter_4105", "Private or protected member '{0}' cannot be accessed on a type parameter."), Parameter_0_of_accessor_has_or_is_using_private_name_1: i(4106, 1, "Parameter_0_of_accessor_has_or_is_using_private_name_1_4106", "Parameter '{0}' of accessor has or is using private name '{1}'."), Parameter_0_of_accessor_has_or_is_using_name_1_from_private_module_2: i(4107, 1, "Parameter_0_of_accessor_has_or_is_using_name_1_from_private_module_2_4107", "Parameter '{0}' of accessor has or is using name '{1}' from private module '{2}'."), Parameter_0_of_accessor_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named: i(4108, 1, "Parameter_0_of_accessor_has_or_is_using_name_1_from_external_module_2_but_cannot_be_named_4108", "Parameter '{0}' of accessor has or is using name '{1}' from external module '{2}' but cannot be named."), Type_arguments_for_0_circularly_reference_themselves: i(4109, 1, "Type_arguments_for_0_circularly_reference_themselves_4109", "Type arguments for '{0}' circularly reference themselves."), Tuple_type_arguments_circularly_reference_themselves: i(4110, 1, "Tuple_type_arguments_circularly_reference_themselves_4110", "Tuple type arguments circularly reference themselves."), Property_0_comes_from_an_index_signature_so_it_must_be_accessed_with_0: i(4111, 1, "Property_0_comes_from_an_index_signature_so_it_must_be_accessed_with_0_4111", "Property '{0}' comes from an index signature, so it must be accessed with ['{0}']."), This_member_cannot_have_an_override_modifier_because_its_containing_class_0_does_not_extend_another_class: i(4112, 1, "This_member_cannot_have_an_override_modifier_because_its_containing_class_0_does_not_extend_another__4112", "This member cannot have an 'override' modifier because its containing class '{0}' does not extend another class."), This_member_cannot_have_an_override_modifier_because_it_is_not_declared_in_the_base_class_0: i(4113, 1, "This_member_cannot_have_an_override_modifier_because_it_is_not_declared_in_the_base_class_0_4113", "This member cannot have an 'override' modifier because it is not declared in the base class '{0}'."), This_member_must_have_an_override_modifier_because_it_overrides_a_member_in_the_base_class_0: i(4114, 1, "This_member_must_have_an_override_modifier_because_it_overrides_a_member_in_the_base_class_0_4114", "This member must have an 'override' modifier because it overrides a member in the base class '{0}'."), This_parameter_property_must_have_an_override_modifier_because_it_overrides_a_member_in_base_class_0: i(4115, 1, "This_parameter_property_must_have_an_override_modifier_because_it_overrides_a_member_in_base_class_0_4115", "This parameter property must have an 'override' modifier because it overrides a member in base class '{0}'."), This_member_must_have_an_override_modifier_because_it_overrides_an_abstract_method_that_is_declared_in_the_base_class_0: i(4116, 1, "This_member_must_have_an_override_modifier_because_it_overrides_an_abstract_method_that_is_declared__4116", "This member must have an 'override' modifier because it overrides an abstract method that is declared in the base class '{0}'."), This_member_cannot_have_an_override_modifier_because_it_is_not_declared_in_the_base_class_0_Did_you_mean_1: i(4117, 1, "This_member_cannot_have_an_override_modifier_because_it_is_not_declared_in_the_base_class_0_Did_you__4117", "This member cannot have an 'override' modifier because it is not declared in the base class '{0}'. Did you mean '{1}'?"), The_type_of_this_node_cannot_be_serialized_because_its_property_0_cannot_be_serialized: i(4118, 1, "The_type_of_this_node_cannot_be_serialized_because_its_property_0_cannot_be_serialized_4118", "The type of this node cannot be serialized because its property '{0}' cannot be serialized."), This_member_must_have_a_JSDoc_comment_with_an_override_tag_because_it_overrides_a_member_in_the_base_class_0: i(4119, 1, "This_member_must_have_a_JSDoc_comment_with_an_override_tag_because_it_overrides_a_member_in_the_base_4119", "This member must have a JSDoc comment with an '@override' tag because it overrides a member in the base class '{0}'."), This_parameter_property_must_have_a_JSDoc_comment_with_an_override_tag_because_it_overrides_a_member_in_the_base_class_0: i(4120, 1, "This_parameter_property_must_have_a_JSDoc_comment_with_an_override_tag_because_it_overrides_a_member_4120", "This parameter property must have a JSDoc comment with an '@override' tag because it overrides a member in the base class '{0}'."), This_member_cannot_have_a_JSDoc_comment_with_an_override_tag_because_its_containing_class_0_does_not_extend_another_class: i(4121, 1, "This_member_cannot_have_a_JSDoc_comment_with_an_override_tag_because_its_containing_class_0_does_not_4121", "This member cannot have a JSDoc comment with an '@override' tag because its containing class '{0}' does not extend another class."), This_member_cannot_have_a_JSDoc_comment_with_an_override_tag_because_it_is_not_declared_in_the_base_class_0: i(4122, 1, "This_member_cannot_have_a_JSDoc_comment_with_an_override_tag_because_it_is_not_declared_in_the_base__4122", "This member cannot have a JSDoc comment with an '@override' tag because it is not declared in the base class '{0}'."), This_member_cannot_have_a_JSDoc_comment_with_an_override_tag_because_it_is_not_declared_in_the_base_class_0_Did_you_mean_1: i(4123, 1, "This_member_cannot_have_a_JSDoc_comment_with_an_override_tag_because_it_is_not_declared_in_the_base__4123", "This member cannot have a JSDoc comment with an 'override' tag because it is not declared in the base class '{0}'. Did you mean '{1}'?"), Compiler_option_0_of_value_1_is_unstable_Use_nightly_TypeScript_to_silence_this_error_Try_updating_with_npm_install_D_typescript_next: i(4124, 1, "Compiler_option_0_of_value_1_is_unstable_Use_nightly_TypeScript_to_silence_this_error_Try_updating_w_4124", "Compiler option '{0}' of value '{1}' is unstable. Use nightly TypeScript to silence this error. Try updating with 'npm install -D typescript@next'."), resolution_mode_assertions_are_unstable_Use_nightly_TypeScript_to_silence_this_error_Try_updating_with_npm_install_D_typescript_next: i(4125, 1, "resolution_mode_assertions_are_unstable_Use_nightly_TypeScript_to_silence_this_error_Try_updating_wi_4125", "'resolution-mode' assertions are unstable. Use nightly TypeScript to silence this error. Try updating with 'npm install -D typescript@next'."), The_current_host_does_not_support_the_0_option: i(5001, 1, "The_current_host_does_not_support_the_0_option_5001", "The current host does not support the '{0}' option."), Cannot_find_the_common_subdirectory_path_for_the_input_files: i(5009, 1, "Cannot_find_the_common_subdirectory_path_for_the_input_files_5009", "Cannot find the common subdirectory path for the input files."), File_specification_cannot_end_in_a_recursive_directory_wildcard_Asterisk_Asterisk_Colon_0: i(5010, 1, "File_specification_cannot_end_in_a_recursive_directory_wildcard_Asterisk_Asterisk_Colon_0_5010", "File specification cannot end in a recursive directory wildcard ('**'): '{0}'."), Cannot_read_file_0_Colon_1: i(5012, 1, "Cannot_read_file_0_Colon_1_5012", "Cannot read file '{0}': {1}."), Failed_to_parse_file_0_Colon_1: i(5014, 1, "Failed_to_parse_file_0_Colon_1_5014", "Failed to parse file '{0}': {1}."), Unknown_compiler_option_0: i(5023, 1, "Unknown_compiler_option_0_5023", "Unknown compiler option '{0}'."), Compiler_option_0_requires_a_value_of_type_1: i(5024, 1, "Compiler_option_0_requires_a_value_of_type_1_5024", "Compiler option '{0}' requires a value of type {1}."), Unknown_compiler_option_0_Did_you_mean_1: i(5025, 1, "Unknown_compiler_option_0_Did_you_mean_1_5025", "Unknown compiler option '{0}'. Did you mean '{1}'?"), Could_not_write_file_0_Colon_1: i(5033, 1, "Could_not_write_file_0_Colon_1_5033", "Could not write file '{0}': {1}."), Option_project_cannot_be_mixed_with_source_files_on_a_command_line: i(5042, 1, "Option_project_cannot_be_mixed_with_source_files_on_a_command_line_5042", "Option 'project' cannot be mixed with source files on a command line."), Option_isolatedModules_can_only_be_used_when_either_option_module_is_provided_or_option_target_is_ES2015_or_higher: i(5047, 1, "Option_isolatedModules_can_only_be_used_when_either_option_module_is_provided_or_option_target_is_ES_5047", "Option 'isolatedModules' can only be used when either option '--module' is provided or option 'target' is 'ES2015' or higher."), Option_0_cannot_be_specified_when_option_target_is_ES3: i(5048, 1, "Option_0_cannot_be_specified_when_option_target_is_ES3_5048", "Option '{0}' cannot be specified when option 'target' is 'ES3'."), Option_0_can_only_be_used_when_either_option_inlineSourceMap_or_option_sourceMap_is_provided: i(5051, 1, "Option_0_can_only_be_used_when_either_option_inlineSourceMap_or_option_sourceMap_is_provided_5051", "Option '{0} can only be used when either option '--inlineSourceMap' or option '--sourceMap' is provided."), Option_0_cannot_be_specified_without_specifying_option_1: i(5052, 1, "Option_0_cannot_be_specified_without_specifying_option_1_5052", "Option '{0}' cannot be specified without specifying option '{1}'."), Option_0_cannot_be_specified_with_option_1: i(5053, 1, "Option_0_cannot_be_specified_with_option_1_5053", "Option '{0}' cannot be specified with option '{1}'."), A_tsconfig_json_file_is_already_defined_at_Colon_0: i(5054, 1, "A_tsconfig_json_file_is_already_defined_at_Colon_0_5054", "A 'tsconfig.json' file is already defined at: '{0}'."), Cannot_write_file_0_because_it_would_overwrite_input_file: i(5055, 1, "Cannot_write_file_0_because_it_would_overwrite_input_file_5055", "Cannot write file '{0}' because it would overwrite input file."), Cannot_write_file_0_because_it_would_be_overwritten_by_multiple_input_files: i(5056, 1, "Cannot_write_file_0_because_it_would_be_overwritten_by_multiple_input_files_5056", "Cannot write file '{0}' because it would be overwritten by multiple input files."), Cannot_find_a_tsconfig_json_file_at_the_specified_directory_Colon_0: i(5057, 1, "Cannot_find_a_tsconfig_json_file_at_the_specified_directory_Colon_0_5057", "Cannot find a tsconfig.json file at the specified directory: '{0}'."), The_specified_path_does_not_exist_Colon_0: i(5058, 1, "The_specified_path_does_not_exist_Colon_0_5058", "The specified path does not exist: '{0}'."), Invalid_value_for_reactNamespace_0_is_not_a_valid_identifier: i(5059, 1, "Invalid_value_for_reactNamespace_0_is_not_a_valid_identifier_5059", "Invalid value for '--reactNamespace'. '{0}' is not a valid identifier."), Pattern_0_can_have_at_most_one_Asterisk_character: i(5061, 1, "Pattern_0_can_have_at_most_one_Asterisk_character_5061", "Pattern '{0}' can have at most one '*' character."), Substitution_0_in_pattern_1_can_have_at_most_one_Asterisk_character: i(5062, 1, "Substitution_0_in_pattern_1_can_have_at_most_one_Asterisk_character_5062", "Substitution '{0}' in pattern '{1}' can have at most one '*' character."), Substitutions_for_pattern_0_should_be_an_array: i(5063, 1, "Substitutions_for_pattern_0_should_be_an_array_5063", "Substitutions for pattern '{0}' should be an array."), Substitution_0_for_pattern_1_has_incorrect_type_expected_string_got_2: i(5064, 1, "Substitution_0_for_pattern_1_has_incorrect_type_expected_string_got_2_5064", "Substitution '{0}' for pattern '{1}' has incorrect type, expected 'string', got '{2}'."), File_specification_cannot_contain_a_parent_directory_that_appears_after_a_recursive_directory_wildcard_Asterisk_Asterisk_Colon_0: i(5065, 1, "File_specification_cannot_contain_a_parent_directory_that_appears_after_a_recursive_directory_wildca_5065", "File specification cannot contain a parent directory ('..') that appears after a recursive directory wildcard ('**'): '{0}'."), Substitutions_for_pattern_0_shouldn_t_be_an_empty_array: i(5066, 1, "Substitutions_for_pattern_0_shouldn_t_be_an_empty_array_5066", "Substitutions for pattern '{0}' shouldn't be an empty array."), Invalid_value_for_jsxFactory_0_is_not_a_valid_identifier_or_qualified_name: i(5067, 1, "Invalid_value_for_jsxFactory_0_is_not_a_valid_identifier_or_qualified_name_5067", "Invalid value for 'jsxFactory'. '{0}' is not a valid identifier or qualified-name."), Adding_a_tsconfig_json_file_will_help_organize_projects_that_contain_both_TypeScript_and_JavaScript_files_Learn_more_at_https_Colon_Slash_Slashaka_ms_Slashtsconfig: i(5068, 1, "Adding_a_tsconfig_json_file_will_help_organize_projects_that_contain_both_TypeScript_and_JavaScript__5068", "Adding a tsconfig.json file will help organize projects that contain both TypeScript and JavaScript files. Learn more at https://aka.ms/tsconfig."), Option_0_cannot_be_specified_without_specifying_option_1_or_option_2: i(5069, 1, "Option_0_cannot_be_specified_without_specifying_option_1_or_option_2_5069", "Option '{0}' cannot be specified without specifying option '{1}' or option '{2}'."), Option_resolveJsonModule_cannot_be_specified_when_moduleResolution_is_set_to_classic: i(5070, 1, "Option_resolveJsonModule_cannot_be_specified_when_moduleResolution_is_set_to_classic_5070", "Option '--resolveJsonModule' cannot be specified when 'moduleResolution' is set to 'classic'."), Option_resolveJsonModule_can_only_be_specified_when_module_code_generation_is_commonjs_amd_es2015_or_esNext: i(5071, 1, "Option_resolveJsonModule_can_only_be_specified_when_module_code_generation_is_commonjs_amd_es2015_or_5071", "Option '--resolveJsonModule' can only be specified when module code generation is 'commonjs', 'amd', 'es2015' or 'esNext'."), Unknown_build_option_0: i(5072, 1, "Unknown_build_option_0_5072", "Unknown build option '{0}'."), Build_option_0_requires_a_value_of_type_1: i(5073, 1, "Build_option_0_requires_a_value_of_type_1_5073", "Build option '{0}' requires a value of type {1}."), Option_incremental_can_only_be_specified_using_tsconfig_emitting_to_single_file_or_when_option_tsBuildInfoFile_is_specified: i(5074, 1, "Option_incremental_can_only_be_specified_using_tsconfig_emitting_to_single_file_or_when_option_tsBui_5074", "Option '--incremental' can only be specified using tsconfig, emitting to single file or when option '--tsBuildInfoFile' is specified."), _0_is_assignable_to_the_constraint_of_type_1_but_1_could_be_instantiated_with_a_different_subtype_of_constraint_2: i(5075, 1, "_0_is_assignable_to_the_constraint_of_type_1_but_1_could_be_instantiated_with_a_different_subtype_of_5075", "'{0}' is assignable to the constraint of type '{1}', but '{1}' could be instantiated with a different subtype of constraint '{2}'."), _0_and_1_operations_cannot_be_mixed_without_parentheses: i(5076, 1, "_0_and_1_operations_cannot_be_mixed_without_parentheses_5076", "'{0}' and '{1}' operations cannot be mixed without parentheses."), Unknown_build_option_0_Did_you_mean_1: i(5077, 1, "Unknown_build_option_0_Did_you_mean_1_5077", "Unknown build option '{0}'. Did you mean '{1}'?"), Unknown_watch_option_0: i(5078, 1, "Unknown_watch_option_0_5078", "Unknown watch option '{0}'."), Unknown_watch_option_0_Did_you_mean_1: i(5079, 1, "Unknown_watch_option_0_Did_you_mean_1_5079", "Unknown watch option '{0}'. Did you mean '{1}'?"), Watch_option_0_requires_a_value_of_type_1: i(5080, 1, "Watch_option_0_requires_a_value_of_type_1_5080", "Watch option '{0}' requires a value of type {1}."), Cannot_find_a_tsconfig_json_file_at_the_current_directory_Colon_0: i(5081, 1, "Cannot_find_a_tsconfig_json_file_at_the_current_directory_Colon_0_5081", "Cannot find a tsconfig.json file at the current directory: {0}."), _0_could_be_instantiated_with_an_arbitrary_type_which_could_be_unrelated_to_1: i(5082, 1, "_0_could_be_instantiated_with_an_arbitrary_type_which_could_be_unrelated_to_1_5082", "'{0}' could be instantiated with an arbitrary type which could be unrelated to '{1}'."), Cannot_read_file_0: i(5083, 1, "Cannot_read_file_0_5083", "Cannot read file '{0}'."), Tuple_members_must_all_have_names_or_all_not_have_names: i(5084, 1, "Tuple_members_must_all_have_names_or_all_not_have_names_5084", "Tuple members must all have names or all not have names."), A_tuple_member_cannot_be_both_optional_and_rest: i(5085, 1, "A_tuple_member_cannot_be_both_optional_and_rest_5085", "A tuple member cannot be both optional and rest."), A_labeled_tuple_element_is_declared_as_optional_with_a_question_mark_after_the_name_and_before_the_colon_rather_than_after_the_type: i(5086, 1, "A_labeled_tuple_element_is_declared_as_optional_with_a_question_mark_after_the_name_and_before_the_c_5086", "A labeled tuple element is declared as optional with a question mark after the name and before the colon, rather than after the type."), A_labeled_tuple_element_is_declared_as_rest_with_a_before_the_name_rather_than_before_the_type: i(5087, 1, "A_labeled_tuple_element_is_declared_as_rest_with_a_before_the_name_rather_than_before_the_type_5087", "A labeled tuple element is declared as rest with a '...' before the name, rather than before the type."), The_inferred_type_of_0_references_a_type_with_a_cyclic_structure_which_cannot_be_trivially_serialized_A_type_annotation_is_necessary: i(5088, 1, "The_inferred_type_of_0_references_a_type_with_a_cyclic_structure_which_cannot_be_trivially_serialize_5088", "The inferred type of '{0}' references a type with a cyclic structure which cannot be trivially serialized. A type annotation is necessary."), Option_0_cannot_be_specified_when_option_jsx_is_1: i(5089, 1, "Option_0_cannot_be_specified_when_option_jsx_is_1_5089", "Option '{0}' cannot be specified when option 'jsx' is '{1}'."), Non_relative_paths_are_not_allowed_when_baseUrl_is_not_set_Did_you_forget_a_leading_Slash: i(5090, 1, "Non_relative_paths_are_not_allowed_when_baseUrl_is_not_set_Did_you_forget_a_leading_Slash_5090", "Non-relative paths are not allowed when 'baseUrl' is not set. Did you forget a leading './'?"), Option_preserveConstEnums_cannot_be_disabled_when_0_is_enabled: i(5091, 1, "Option_preserveConstEnums_cannot_be_disabled_when_0_is_enabled_5091", "Option 'preserveConstEnums' cannot be disabled when '{0}' is enabled."), The_root_value_of_a_0_file_must_be_an_object: i(5092, 1, "The_root_value_of_a_0_file_must_be_an_object_5092", "The root value of a '{0}' file must be an object."), Compiler_option_0_may_only_be_used_with_build: i(5093, 1, "Compiler_option_0_may_only_be_used_with_build_5093", "Compiler option '--{0}' may only be used with '--build'."), Compiler_option_0_may_not_be_used_with_build: i(5094, 1, "Compiler_option_0_may_not_be_used_with_build_5094", "Compiler option '--{0}' may not be used with '--build'."), Option_0_can_only_be_used_when_module_is_set_to_es2015_or_later: i(5095, 1, "Option_0_can_only_be_used_when_module_is_set_to_es2015_or_later_5095", "Option '{0}' can only be used when 'module' is set to 'es2015' or later."), Option_allowImportingTsExtensions_can_only_be_used_when_either_noEmit_or_emitDeclarationOnly_is_set: i(5096, 1, "Option_allowImportingTsExtensions_can_only_be_used_when_either_noEmit_or_emitDeclarationOnly_is_set_5096", "Option 'allowImportingTsExtensions' can only be used when either 'noEmit' or 'emitDeclarationOnly' is set."), An_import_path_can_only_end_with_a_0_extension_when_allowImportingTsExtensions_is_enabled: i(5097, 1, "An_import_path_can_only_end_with_a_0_extension_when_allowImportingTsExtensions_is_enabled_5097", "An import path can only end with a '{0}' extension when 'allowImportingTsExtensions' is enabled."), Option_0_can_only_be_used_when_moduleResolution_is_set_to_node16_nodenext_or_bundler: i(5098, 1, "Option_0_can_only_be_used_when_moduleResolution_is_set_to_node16_nodenext_or_bundler_5098", "Option '{0}' can only be used when 'moduleResolution' is set to 'node16', 'nodenext', or 'bundler'."), Option_0_is_deprecated_and_will_stop_functioning_in_TypeScript_1_Specify_compilerOption_ignoreDeprecations_Colon_2_to_silence_this_error: i(5101, 1, "Option_0_is_deprecated_and_will_stop_functioning_in_TypeScript_1_Specify_compilerOption_ignoreDeprec_5101", `Option '{0}' is deprecated and will stop functioning in TypeScript {1}. Specify compilerOption '"ignoreDeprecations": "{2}"' to silence this error.`), Option_0_has_been_removed_Please_remove_it_from_your_configuration: i(5102, 1, "Option_0_has_been_removed_Please_remove_it_from_your_configuration_5102", "Option '{0}' has been removed. Please remove it from your configuration."), Invalid_value_for_ignoreDeprecations: i(5103, 1, "Invalid_value_for_ignoreDeprecations_5103", "Invalid value for '--ignoreDeprecations'."), Option_0_is_redundant_and_cannot_be_specified_with_option_1: i(5104, 1, "Option_0_is_redundant_and_cannot_be_specified_with_option_1_5104", "Option '{0}' is redundant and cannot be specified with option '{1}'."), Option_verbatimModuleSyntax_cannot_be_used_when_module_is_set_to_UMD_AMD_or_System: i(5105, 1, "Option_verbatimModuleSyntax_cannot_be_used_when_module_is_set_to_UMD_AMD_or_System_5105", "Option 'verbatimModuleSyntax' cannot be used when 'module' is set to 'UMD', 'AMD', or 'System'."), Use_0_instead: i(5106, 3, "Use_0_instead_5106", "Use '{0}' instead."), Option_0_1_is_deprecated_and_will_stop_functioning_in_TypeScript_2_Specify_compilerOption_ignoreDeprecations_Colon_3_to_silence_this_error: i(5107, 1, "Option_0_1_is_deprecated_and_will_stop_functioning_in_TypeScript_2_Specify_compilerOption_ignoreDepr_5107", `Option '{0}={1}' is deprecated and will stop functioning in TypeScript {2}. Specify compilerOption '"ignoreDeprecations": "{3}"' to silence this error.`), Option_0_1_has_been_removed_Please_remove_it_from_your_configuration: i(5108, 1, "Option_0_1_has_been_removed_Please_remove_it_from_your_configuration_5108", "Option '{0}={1}' has been removed. Please remove it from your configuration."), Generates_a_sourcemap_for_each_corresponding_d_ts_file: i(6e3, 3, "Generates_a_sourcemap_for_each_corresponding_d_ts_file_6000", "Generates a sourcemap for each corresponding '.d.ts' file."), Concatenate_and_emit_output_to_single_file: i(6001, 3, "Concatenate_and_emit_output_to_single_file_6001", "Concatenate and emit output to single file."), Generates_corresponding_d_ts_file: i(6002, 3, "Generates_corresponding_d_ts_file_6002", "Generates corresponding '.d.ts' file."), Specify_the_location_where_debugger_should_locate_TypeScript_files_instead_of_source_locations: i(6004, 3, "Specify_the_location_where_debugger_should_locate_TypeScript_files_instead_of_source_locations_6004", "Specify the location where debugger should locate TypeScript files instead of source locations."), Watch_input_files: i(6005, 3, "Watch_input_files_6005", "Watch input files."), Redirect_output_structure_to_the_directory: i(6006, 3, "Redirect_output_structure_to_the_directory_6006", "Redirect output structure to the directory."), Do_not_erase_const_enum_declarations_in_generated_code: i(6007, 3, "Do_not_erase_const_enum_declarations_in_generated_code_6007", "Do not erase const enum declarations in generated code."), Do_not_emit_outputs_if_any_errors_were_reported: i(6008, 3, "Do_not_emit_outputs_if_any_errors_were_reported_6008", "Do not emit outputs if any errors were reported."), Do_not_emit_comments_to_output: i(6009, 3, "Do_not_emit_comments_to_output_6009", "Do not emit comments to output."), Do_not_emit_outputs: i(6010, 3, "Do_not_emit_outputs_6010", "Do not emit outputs."), Allow_default_imports_from_modules_with_no_default_export_This_does_not_affect_code_emit_just_typechecking: i(6011, 3, "Allow_default_imports_from_modules_with_no_default_export_This_does_not_affect_code_emit_just_typech_6011", "Allow default imports from modules with no default export. This does not affect code emit, just typechecking."), Skip_type_checking_of_declaration_files: i(6012, 3, "Skip_type_checking_of_declaration_files_6012", "Skip type checking of declaration files."), Do_not_resolve_the_real_path_of_symlinks: i(6013, 3, "Do_not_resolve_the_real_path_of_symlinks_6013", "Do not resolve the real path of symlinks."), Only_emit_d_ts_declaration_files: i(6014, 3, "Only_emit_d_ts_declaration_files_6014", "Only emit '.d.ts' declaration files."), Specify_ECMAScript_target_version: i(6015, 3, "Specify_ECMAScript_target_version_6015", "Specify ECMAScript target version."), Specify_module_code_generation: i(6016, 3, "Specify_module_code_generation_6016", "Specify module code generation."), Print_this_message: i(6017, 3, "Print_this_message_6017", "Print this message."), Print_the_compiler_s_version: i(6019, 3, "Print_the_compiler_s_version_6019", "Print the compiler's version."), Compile_the_project_given_the_path_to_its_configuration_file_or_to_a_folder_with_a_tsconfig_json: i(6020, 3, "Compile_the_project_given_the_path_to_its_configuration_file_or_to_a_folder_with_a_tsconfig_json_6020", "Compile the project given the path to its configuration file, or to a folder with a 'tsconfig.json'."), Syntax_Colon_0: i(6023, 3, "Syntax_Colon_0_6023", "Syntax: {0}"), options: i(6024, 3, "options_6024", "options"), file: i(6025, 3, "file_6025", "file"), Examples_Colon_0: i(6026, 3, "Examples_Colon_0_6026", "Examples: {0}"), Options_Colon: i(6027, 3, "Options_Colon_6027", "Options:"), Version_0: i(6029, 3, "Version_0_6029", "Version {0}"), Insert_command_line_options_and_files_from_a_file: i(6030, 3, "Insert_command_line_options_and_files_from_a_file_6030", "Insert command line options and files from a file."), Starting_compilation_in_watch_mode: i(6031, 3, "Starting_compilation_in_watch_mode_6031", "Starting compilation in watch mode..."), File_change_detected_Starting_incremental_compilation: i(6032, 3, "File_change_detected_Starting_incremental_compilation_6032", "File change detected. Starting incremental compilation..."), KIND: i(6034, 3, "KIND_6034", "KIND"), FILE: i(6035, 3, "FILE_6035", "FILE"), VERSION: i(6036, 3, "VERSION_6036", "VERSION"), LOCATION: i(6037, 3, "LOCATION_6037", "LOCATION"), DIRECTORY: i(6038, 3, "DIRECTORY_6038", "DIRECTORY"), STRATEGY: i(6039, 3, "STRATEGY_6039", "STRATEGY"), FILE_OR_DIRECTORY: i(6040, 3, "FILE_OR_DIRECTORY_6040", "FILE OR DIRECTORY"), Errors_Files: i(6041, 3, "Errors_Files_6041", "Errors Files"), Generates_corresponding_map_file: i(6043, 3, "Generates_corresponding_map_file_6043", "Generates corresponding '.map' file."), Compiler_option_0_expects_an_argument: i(6044, 1, "Compiler_option_0_expects_an_argument_6044", "Compiler option '{0}' expects an argument."), Unterminated_quoted_string_in_response_file_0: i(6045, 1, "Unterminated_quoted_string_in_response_file_0_6045", "Unterminated quoted string in response file '{0}'."), Argument_for_0_option_must_be_Colon_1: i(6046, 1, "Argument_for_0_option_must_be_Colon_1_6046", "Argument for '{0}' option must be: {1}."), Locale_must_be_of_the_form_language_or_language_territory_For_example_0_or_1: i(6048, 1, "Locale_must_be_of_the_form_language_or_language_territory_For_example_0_or_1_6048", "Locale must be of the form or -. For example '{0}' or '{1}'."), Unable_to_open_file_0: i(6050, 1, "Unable_to_open_file_0_6050", "Unable to open file '{0}'."), Corrupted_locale_file_0: i(6051, 1, "Corrupted_locale_file_0_6051", "Corrupted locale file {0}."), Raise_error_on_expressions_and_declarations_with_an_implied_any_type: i(6052, 3, "Raise_error_on_expressions_and_declarations_with_an_implied_any_type_6052", "Raise error on expressions and declarations with an implied 'any' type."), File_0_not_found: i(6053, 1, "File_0_not_found_6053", "File '{0}' not found."), File_0_has_an_unsupported_extension_The_only_supported_extensions_are_1: i(6054, 1, "File_0_has_an_unsupported_extension_The_only_supported_extensions_are_1_6054", "File '{0}' has an unsupported extension. The only supported extensions are {1}."), Suppress_noImplicitAny_errors_for_indexing_objects_lacking_index_signatures: i(6055, 3, "Suppress_noImplicitAny_errors_for_indexing_objects_lacking_index_signatures_6055", "Suppress noImplicitAny errors for indexing objects lacking index signatures."), Do_not_emit_declarations_for_code_that_has_an_internal_annotation: i(6056, 3, "Do_not_emit_declarations_for_code_that_has_an_internal_annotation_6056", "Do not emit declarations for code that has an '@internal' annotation."), Specify_the_root_directory_of_input_files_Use_to_control_the_output_directory_structure_with_outDir: i(6058, 3, "Specify_the_root_directory_of_input_files_Use_to_control_the_output_directory_structure_with_outDir_6058", "Specify the root directory of input files. Use to control the output directory structure with --outDir."), File_0_is_not_under_rootDir_1_rootDir_is_expected_to_contain_all_source_files: i(6059, 1, "File_0_is_not_under_rootDir_1_rootDir_is_expected_to_contain_all_source_files_6059", "File '{0}' is not under 'rootDir' '{1}'. 'rootDir' is expected to contain all source files."), Specify_the_end_of_line_sequence_to_be_used_when_emitting_files_Colon_CRLF_dos_or_LF_unix: i(6060, 3, "Specify_the_end_of_line_sequence_to_be_used_when_emitting_files_Colon_CRLF_dos_or_LF_unix_6060", "Specify the end of line sequence to be used when emitting files: 'CRLF' (dos) or 'LF' (unix)."), NEWLINE: i(6061, 3, "NEWLINE_6061", "NEWLINE"), Option_0_can_only_be_specified_in_tsconfig_json_file_or_set_to_null_on_command_line: i(6064, 1, "Option_0_can_only_be_specified_in_tsconfig_json_file_or_set_to_null_on_command_line_6064", "Option '{0}' can only be specified in 'tsconfig.json' file or set to 'null' on command line."), Enables_experimental_support_for_ES7_decorators: i(6065, 3, "Enables_experimental_support_for_ES7_decorators_6065", "Enables experimental support for ES7 decorators."), Enables_experimental_support_for_emitting_type_metadata_for_decorators: i(6066, 3, "Enables_experimental_support_for_emitting_type_metadata_for_decorators_6066", "Enables experimental support for emitting type metadata for decorators."), Initializes_a_TypeScript_project_and_creates_a_tsconfig_json_file: i(6070, 3, "Initializes_a_TypeScript_project_and_creates_a_tsconfig_json_file_6070", "Initializes a TypeScript project and creates a tsconfig.json file."), Successfully_created_a_tsconfig_json_file: i(6071, 3, "Successfully_created_a_tsconfig_json_file_6071", "Successfully created a tsconfig.json file."), Suppress_excess_property_checks_for_object_literals: i(6072, 3, "Suppress_excess_property_checks_for_object_literals_6072", "Suppress excess property checks for object literals."), Stylize_errors_and_messages_using_color_and_context_experimental: i(6073, 3, "Stylize_errors_and_messages_using_color_and_context_experimental_6073", "Stylize errors and messages using color and context (experimental)."), Do_not_report_errors_on_unused_labels: i(6074, 3, "Do_not_report_errors_on_unused_labels_6074", "Do not report errors on unused labels."), Report_error_when_not_all_code_paths_in_function_return_a_value: i(6075, 3, "Report_error_when_not_all_code_paths_in_function_return_a_value_6075", "Report error when not all code paths in function return a value."), Report_errors_for_fallthrough_cases_in_switch_statement: i(6076, 3, "Report_errors_for_fallthrough_cases_in_switch_statement_6076", "Report errors for fallthrough cases in switch statement."), Do_not_report_errors_on_unreachable_code: i(6077, 3, "Do_not_report_errors_on_unreachable_code_6077", "Do not report errors on unreachable code."), Disallow_inconsistently_cased_references_to_the_same_file: i(6078, 3, "Disallow_inconsistently_cased_references_to_the_same_file_6078", "Disallow inconsistently-cased references to the same file."), Specify_library_files_to_be_included_in_the_compilation: i(6079, 3, "Specify_library_files_to_be_included_in_the_compilation_6079", "Specify library files to be included in the compilation."), Specify_JSX_code_generation: i(6080, 3, "Specify_JSX_code_generation_6080", "Specify JSX code generation."), File_0_has_an_unsupported_extension_so_skipping_it: i(6081, 3, "File_0_has_an_unsupported_extension_so_skipping_it_6081", "File '{0}' has an unsupported extension, so skipping it."), Only_amd_and_system_modules_are_supported_alongside_0: i(6082, 1, "Only_amd_and_system_modules_are_supported_alongside_0_6082", "Only 'amd' and 'system' modules are supported alongside --{0}."), Base_directory_to_resolve_non_absolute_module_names: i(6083, 3, "Base_directory_to_resolve_non_absolute_module_names_6083", "Base directory to resolve non-absolute module names."), Deprecated_Use_jsxFactory_instead_Specify_the_object_invoked_for_createElement_when_targeting_react_JSX_emit: i(6084, 3, "Deprecated_Use_jsxFactory_instead_Specify_the_object_invoked_for_createElement_when_targeting_react__6084", "[Deprecated] Use '--jsxFactory' instead. Specify the object invoked for createElement when targeting 'react' JSX emit"), Enable_tracing_of_the_name_resolution_process: i(6085, 3, "Enable_tracing_of_the_name_resolution_process_6085", "Enable tracing of the name resolution process."), Resolving_module_0_from_1: i(6086, 3, "Resolving_module_0_from_1_6086", "======== Resolving module '{0}' from '{1}'. ========"), Explicitly_specified_module_resolution_kind_Colon_0: i(6087, 3, "Explicitly_specified_module_resolution_kind_Colon_0_6087", "Explicitly specified module resolution kind: '{0}'."), Module_resolution_kind_is_not_specified_using_0: i(6088, 3, "Module_resolution_kind_is_not_specified_using_0_6088", "Module resolution kind is not specified, using '{0}'."), Module_name_0_was_successfully_resolved_to_1: i(6089, 3, "Module_name_0_was_successfully_resolved_to_1_6089", "======== Module name '{0}' was successfully resolved to '{1}'. ========"), Module_name_0_was_not_resolved: i(6090, 3, "Module_name_0_was_not_resolved_6090", "======== Module name '{0}' was not resolved. ========"), paths_option_is_specified_looking_for_a_pattern_to_match_module_name_0: i(6091, 3, "paths_option_is_specified_looking_for_a_pattern_to_match_module_name_0_6091", "'paths' option is specified, looking for a pattern to match module name '{0}'."), Module_name_0_matched_pattern_1: i(6092, 3, "Module_name_0_matched_pattern_1_6092", "Module name '{0}', matched pattern '{1}'."), Trying_substitution_0_candidate_module_location_Colon_1: i(6093, 3, "Trying_substitution_0_candidate_module_location_Colon_1_6093", "Trying substitution '{0}', candidate module location: '{1}'."), Resolving_module_name_0_relative_to_base_url_1_2: i(6094, 3, "Resolving_module_name_0_relative_to_base_url_1_2_6094", "Resolving module name '{0}' relative to base url '{1}' - '{2}'."), Loading_module_as_file_Slash_folder_candidate_module_location_0_target_file_types_Colon_1: i(6095, 3, "Loading_module_as_file_Slash_folder_candidate_module_location_0_target_file_types_Colon_1_6095", "Loading module as file / folder, candidate module location '{0}', target file types: {1}."), File_0_does_not_exist: i(6096, 3, "File_0_does_not_exist_6096", "File '{0}' does not exist."), File_0_exists_use_it_as_a_name_resolution_result: i(6097, 3, "File_0_exists_use_it_as_a_name_resolution_result_6097", "File '{0}' exists - use it as a name resolution result."), Loading_module_0_from_node_modules_folder_target_file_types_Colon_1: i(6098, 3, "Loading_module_0_from_node_modules_folder_target_file_types_Colon_1_6098", "Loading module '{0}' from 'node_modules' folder, target file types: {1}."), Found_package_json_at_0: i(6099, 3, "Found_package_json_at_0_6099", "Found 'package.json' at '{0}'."), package_json_does_not_have_a_0_field: i(6100, 3, "package_json_does_not_have_a_0_field_6100", "'package.json' does not have a '{0}' field."), package_json_has_0_field_1_that_references_2: i(6101, 3, "package_json_has_0_field_1_that_references_2_6101", "'package.json' has '{0}' field '{1}' that references '{2}'."), Allow_javascript_files_to_be_compiled: i(6102, 3, "Allow_javascript_files_to_be_compiled_6102", "Allow javascript files to be compiled."), Checking_if_0_is_the_longest_matching_prefix_for_1_2: i(6104, 3, "Checking_if_0_is_the_longest_matching_prefix_for_1_2_6104", "Checking if '{0}' is the longest matching prefix for '{1}' - '{2}'."), Expected_type_of_0_field_in_package_json_to_be_1_got_2: i(6105, 3, "Expected_type_of_0_field_in_package_json_to_be_1_got_2_6105", "Expected type of '{0}' field in 'package.json' to be '{1}', got '{2}'."), baseUrl_option_is_set_to_0_using_this_value_to_resolve_non_relative_module_name_1: i(6106, 3, "baseUrl_option_is_set_to_0_using_this_value_to_resolve_non_relative_module_name_1_6106", "'baseUrl' option is set to '{0}', using this value to resolve non-relative module name '{1}'."), rootDirs_option_is_set_using_it_to_resolve_relative_module_name_0: i(6107, 3, "rootDirs_option_is_set_using_it_to_resolve_relative_module_name_0_6107", "'rootDirs' option is set, using it to resolve relative module name '{0}'."), Longest_matching_prefix_for_0_is_1: i(6108, 3, "Longest_matching_prefix_for_0_is_1_6108", "Longest matching prefix for '{0}' is '{1}'."), Loading_0_from_the_root_dir_1_candidate_location_2: i(6109, 3, "Loading_0_from_the_root_dir_1_candidate_location_2_6109", "Loading '{0}' from the root dir '{1}', candidate location '{2}'."), Trying_other_entries_in_rootDirs: i(6110, 3, "Trying_other_entries_in_rootDirs_6110", "Trying other entries in 'rootDirs'."), Module_resolution_using_rootDirs_has_failed: i(6111, 3, "Module_resolution_using_rootDirs_has_failed_6111", "Module resolution using 'rootDirs' has failed."), Do_not_emit_use_strict_directives_in_module_output: i(6112, 3, "Do_not_emit_use_strict_directives_in_module_output_6112", "Do not emit 'use strict' directives in module output."), Enable_strict_null_checks: i(6113, 3, "Enable_strict_null_checks_6113", "Enable strict null checks."), Unknown_option_excludes_Did_you_mean_exclude: i(6114, 1, "Unknown_option_excludes_Did_you_mean_exclude_6114", "Unknown option 'excludes'. Did you mean 'exclude'?"), Raise_error_on_this_expressions_with_an_implied_any_type: i(6115, 3, "Raise_error_on_this_expressions_with_an_implied_any_type_6115", "Raise error on 'this' expressions with an implied 'any' type."), Resolving_type_reference_directive_0_containing_file_1_root_directory_2: i(6116, 3, "Resolving_type_reference_directive_0_containing_file_1_root_directory_2_6116", "======== Resolving type reference directive '{0}', containing file '{1}', root directory '{2}'. ========"), Type_reference_directive_0_was_successfully_resolved_to_1_primary_Colon_2: i(6119, 3, "Type_reference_directive_0_was_successfully_resolved_to_1_primary_Colon_2_6119", "======== Type reference directive '{0}' was successfully resolved to '{1}', primary: {2}. ========"), Type_reference_directive_0_was_not_resolved: i(6120, 3, "Type_reference_directive_0_was_not_resolved_6120", "======== Type reference directive '{0}' was not resolved. ========"), Resolving_with_primary_search_path_0: i(6121, 3, "Resolving_with_primary_search_path_0_6121", "Resolving with primary search path '{0}'."), Root_directory_cannot_be_determined_skipping_primary_search_paths: i(6122, 3, "Root_directory_cannot_be_determined_skipping_primary_search_paths_6122", "Root directory cannot be determined, skipping primary search paths."), Resolving_type_reference_directive_0_containing_file_1_root_directory_not_set: i(6123, 3, "Resolving_type_reference_directive_0_containing_file_1_root_directory_not_set_6123", "======== Resolving type reference directive '{0}', containing file '{1}', root directory not set. ========"), Type_declaration_files_to_be_included_in_compilation: i(6124, 3, "Type_declaration_files_to_be_included_in_compilation_6124", "Type declaration files to be included in compilation."), Looking_up_in_node_modules_folder_initial_location_0: i(6125, 3, "Looking_up_in_node_modules_folder_initial_location_0_6125", "Looking up in 'node_modules' folder, initial location '{0}'."), Containing_file_is_not_specified_and_root_directory_cannot_be_determined_skipping_lookup_in_node_modules_folder: i(6126, 3, "Containing_file_is_not_specified_and_root_directory_cannot_be_determined_skipping_lookup_in_node_mod_6126", "Containing file is not specified and root directory cannot be determined, skipping lookup in 'node_modules' folder."), Resolving_type_reference_directive_0_containing_file_not_set_root_directory_1: i(6127, 3, "Resolving_type_reference_directive_0_containing_file_not_set_root_directory_1_6127", "======== Resolving type reference directive '{0}', containing file not set, root directory '{1}'. ========"), Resolving_type_reference_directive_0_containing_file_not_set_root_directory_not_set: i(6128, 3, "Resolving_type_reference_directive_0_containing_file_not_set_root_directory_not_set_6128", "======== Resolving type reference directive '{0}', containing file not set, root directory not set. ========"), Resolving_real_path_for_0_result_1: i(6130, 3, "Resolving_real_path_for_0_result_1_6130", "Resolving real path for '{0}', result '{1}'."), Cannot_compile_modules_using_option_0_unless_the_module_flag_is_amd_or_system: i(6131, 1, "Cannot_compile_modules_using_option_0_unless_the_module_flag_is_amd_or_system_6131", "Cannot compile modules using option '{0}' unless the '--module' flag is 'amd' or 'system'."), File_name_0_has_a_1_extension_stripping_it: i(6132, 3, "File_name_0_has_a_1_extension_stripping_it_6132", "File name '{0}' has a '{1}' extension - stripping it."), _0_is_declared_but_its_value_is_never_read: i(6133, 1, "_0_is_declared_but_its_value_is_never_read_6133", "'{0}' is declared but its value is never read.", true), Report_errors_on_unused_locals: i(6134, 3, "Report_errors_on_unused_locals_6134", "Report errors on unused locals."), Report_errors_on_unused_parameters: i(6135, 3, "Report_errors_on_unused_parameters_6135", "Report errors on unused parameters."), The_maximum_dependency_depth_to_search_under_node_modules_and_load_JavaScript_files: i(6136, 3, "The_maximum_dependency_depth_to_search_under_node_modules_and_load_JavaScript_files_6136", "The maximum dependency depth to search under node_modules and load JavaScript files."), Cannot_import_type_declaration_files_Consider_importing_0_instead_of_1: i(6137, 1, "Cannot_import_type_declaration_files_Consider_importing_0_instead_of_1_6137", "Cannot import type declaration files. Consider importing '{0}' instead of '{1}'."), Property_0_is_declared_but_its_value_is_never_read: i(6138, 1, "Property_0_is_declared_but_its_value_is_never_read_6138", "Property '{0}' is declared but its value is never read.", true), Import_emit_helpers_from_tslib: i(6139, 3, "Import_emit_helpers_from_tslib_6139", "Import emit helpers from 'tslib'."), Auto_discovery_for_typings_is_enabled_in_project_0_Running_extra_resolution_pass_for_module_1_using_cache_location_2: i(6140, 1, "Auto_discovery_for_typings_is_enabled_in_project_0_Running_extra_resolution_pass_for_module_1_using__6140", "Auto discovery for typings is enabled in project '{0}'. Running extra resolution pass for module '{1}' using cache location '{2}'."), Parse_in_strict_mode_and_emit_use_strict_for_each_source_file: i(6141, 3, "Parse_in_strict_mode_and_emit_use_strict_for_each_source_file_6141", 'Parse in strict mode and emit "use strict" for each source file.'), Module_0_was_resolved_to_1_but_jsx_is_not_set: i(6142, 1, "Module_0_was_resolved_to_1_but_jsx_is_not_set_6142", "Module '{0}' was resolved to '{1}', but '--jsx' is not set."), Module_0_was_resolved_as_locally_declared_ambient_module_in_file_1: i(6144, 3, "Module_0_was_resolved_as_locally_declared_ambient_module_in_file_1_6144", "Module '{0}' was resolved as locally declared ambient module in file '{1}'."), Module_0_was_resolved_as_ambient_module_declared_in_1_since_this_file_was_not_modified: i(6145, 3, "Module_0_was_resolved_as_ambient_module_declared_in_1_since_this_file_was_not_modified_6145", "Module '{0}' was resolved as ambient module declared in '{1}' since this file was not modified."), Specify_the_JSX_factory_function_to_use_when_targeting_react_JSX_emit_e_g_React_createElement_or_h: i(6146, 3, "Specify_the_JSX_factory_function_to_use_when_targeting_react_JSX_emit_e_g_React_createElement_or_h_6146", "Specify the JSX factory function to use when targeting 'react' JSX emit, e.g. 'React.createElement' or 'h'."), Resolution_for_module_0_was_found_in_cache_from_location_1: i(6147, 3, "Resolution_for_module_0_was_found_in_cache_from_location_1_6147", "Resolution for module '{0}' was found in cache from location '{1}'."), Directory_0_does_not_exist_skipping_all_lookups_in_it: i(6148, 3, "Directory_0_does_not_exist_skipping_all_lookups_in_it_6148", "Directory '{0}' does not exist, skipping all lookups in it."), Show_diagnostic_information: i(6149, 3, "Show_diagnostic_information_6149", "Show diagnostic information."), Show_verbose_diagnostic_information: i(6150, 3, "Show_verbose_diagnostic_information_6150", "Show verbose diagnostic information."), Emit_a_single_file_with_source_maps_instead_of_having_a_separate_file: i(6151, 3, "Emit_a_single_file_with_source_maps_instead_of_having_a_separate_file_6151", "Emit a single file with source maps instead of having a separate file."), Emit_the_source_alongside_the_sourcemaps_within_a_single_file_requires_inlineSourceMap_or_sourceMap_to_be_set: i(6152, 3, "Emit_the_source_alongside_the_sourcemaps_within_a_single_file_requires_inlineSourceMap_or_sourceMap__6152", "Emit the source alongside the sourcemaps within a single file; requires '--inlineSourceMap' or '--sourceMap' to be set."), Transpile_each_file_as_a_separate_module_similar_to_ts_transpileModule: i(6153, 3, "Transpile_each_file_as_a_separate_module_similar_to_ts_transpileModule_6153", "Transpile each file as a separate module (similar to 'ts.transpileModule')."), Print_names_of_generated_files_part_of_the_compilation: i(6154, 3, "Print_names_of_generated_files_part_of_the_compilation_6154", "Print names of generated files part of the compilation."), Print_names_of_files_part_of_the_compilation: i(6155, 3, "Print_names_of_files_part_of_the_compilation_6155", "Print names of files part of the compilation."), The_locale_used_when_displaying_messages_to_the_user_e_g_en_us: i(6156, 3, "The_locale_used_when_displaying_messages_to_the_user_e_g_en_us_6156", "The locale used when displaying messages to the user (e.g. 'en-us')"), Do_not_generate_custom_helper_functions_like_extends_in_compiled_output: i(6157, 3, "Do_not_generate_custom_helper_functions_like_extends_in_compiled_output_6157", "Do not generate custom helper functions like '__extends' in compiled output."), Do_not_include_the_default_library_file_lib_d_ts: i(6158, 3, "Do_not_include_the_default_library_file_lib_d_ts_6158", "Do not include the default library file (lib.d.ts)."), Do_not_add_triple_slash_references_or_imported_modules_to_the_list_of_compiled_files: i(6159, 3, "Do_not_add_triple_slash_references_or_imported_modules_to_the_list_of_compiled_files_6159", "Do not add triple-slash references or imported modules to the list of compiled files."), Deprecated_Use_skipLibCheck_instead_Skip_type_checking_of_default_library_declaration_files: i(6160, 3, "Deprecated_Use_skipLibCheck_instead_Skip_type_checking_of_default_library_declaration_files_6160", "[Deprecated] Use '--skipLibCheck' instead. Skip type checking of default library declaration files."), List_of_folders_to_include_type_definitions_from: i(6161, 3, "List_of_folders_to_include_type_definitions_from_6161", "List of folders to include type definitions from."), Disable_size_limitations_on_JavaScript_projects: i(6162, 3, "Disable_size_limitations_on_JavaScript_projects_6162", "Disable size limitations on JavaScript projects."), The_character_set_of_the_input_files: i(6163, 3, "The_character_set_of_the_input_files_6163", "The character set of the input files."), Do_not_truncate_error_messages: i(6165, 3, "Do_not_truncate_error_messages_6165", "Do not truncate error messages."), Output_directory_for_generated_declaration_files: i(6166, 3, "Output_directory_for_generated_declaration_files_6166", "Output directory for generated declaration files."), A_series_of_entries_which_re_map_imports_to_lookup_locations_relative_to_the_baseUrl: i(6167, 3, "A_series_of_entries_which_re_map_imports_to_lookup_locations_relative_to_the_baseUrl_6167", "A series of entries which re-map imports to lookup locations relative to the 'baseUrl'."), List_of_root_folders_whose_combined_content_represents_the_structure_of_the_project_at_runtime: i(6168, 3, "List_of_root_folders_whose_combined_content_represents_the_structure_of_the_project_at_runtime_6168", "List of root folders whose combined content represents the structure of the project at runtime."), Show_all_compiler_options: i(6169, 3, "Show_all_compiler_options_6169", "Show all compiler options."), Deprecated_Use_outFile_instead_Concatenate_and_emit_output_to_single_file: i(6170, 3, "Deprecated_Use_outFile_instead_Concatenate_and_emit_output_to_single_file_6170", "[Deprecated] Use '--outFile' instead. Concatenate and emit output to single file"), Command_line_Options: i(6171, 3, "Command_line_Options_6171", "Command-line Options"), Provide_full_support_for_iterables_in_for_of_spread_and_destructuring_when_targeting_ES5_or_ES3: i(6179, 3, "Provide_full_support_for_iterables_in_for_of_spread_and_destructuring_when_targeting_ES5_or_ES3_6179", "Provide full support for iterables in 'for-of', spread, and destructuring when targeting 'ES5' or 'ES3'."), Enable_all_strict_type_checking_options: i(6180, 3, "Enable_all_strict_type_checking_options_6180", "Enable all strict type-checking options."), Scoped_package_detected_looking_in_0: i(6182, 3, "Scoped_package_detected_looking_in_0_6182", "Scoped package detected, looking in '{0}'"), Reusing_resolution_of_module_0_from_1_of_old_program_it_was_successfully_resolved_to_2: i(6183, 3, "Reusing_resolution_of_module_0_from_1_of_old_program_it_was_successfully_resolved_to_2_6183", "Reusing resolution of module '{0}' from '{1}' of old program, it was successfully resolved to '{2}'."), Reusing_resolution_of_module_0_from_1_of_old_program_it_was_successfully_resolved_to_2_with_Package_ID_3: i(6184, 3, "Reusing_resolution_of_module_0_from_1_of_old_program_it_was_successfully_resolved_to_2_with_Package__6184", "Reusing resolution of module '{0}' from '{1}' of old program, it was successfully resolved to '{2}' with Package ID '{3}'."), Enable_strict_checking_of_function_types: i(6186, 3, "Enable_strict_checking_of_function_types_6186", "Enable strict checking of function types."), Enable_strict_checking_of_property_initialization_in_classes: i(6187, 3, "Enable_strict_checking_of_property_initialization_in_classes_6187", "Enable strict checking of property initialization in classes."), Numeric_separators_are_not_allowed_here: i(6188, 1, "Numeric_separators_are_not_allowed_here_6188", "Numeric separators are not allowed here."), Multiple_consecutive_numeric_separators_are_not_permitted: i(6189, 1, "Multiple_consecutive_numeric_separators_are_not_permitted_6189", "Multiple consecutive numeric separators are not permitted."), Whether_to_keep_outdated_console_output_in_watch_mode_instead_of_clearing_the_screen: i(6191, 3, "Whether_to_keep_outdated_console_output_in_watch_mode_instead_of_clearing_the_screen_6191", "Whether to keep outdated console output in watch mode instead of clearing the screen."), All_imports_in_import_declaration_are_unused: i(6192, 1, "All_imports_in_import_declaration_are_unused_6192", "All imports in import declaration are unused.", true), Found_1_error_Watching_for_file_changes: i(6193, 3, "Found_1_error_Watching_for_file_changes_6193", "Found 1 error. Watching for file changes."), Found_0_errors_Watching_for_file_changes: i(6194, 3, "Found_0_errors_Watching_for_file_changes_6194", "Found {0} errors. Watching for file changes."), Resolve_keyof_to_string_valued_property_names_only_no_numbers_or_symbols: i(6195, 3, "Resolve_keyof_to_string_valued_property_names_only_no_numbers_or_symbols_6195", "Resolve 'keyof' to string valued property names only (no numbers or symbols)."), _0_is_declared_but_never_used: i(6196, 1, "_0_is_declared_but_never_used_6196", "'{0}' is declared but never used.", true), Include_modules_imported_with_json_extension: i(6197, 3, "Include_modules_imported_with_json_extension_6197", "Include modules imported with '.json' extension"), All_destructured_elements_are_unused: i(6198, 1, "All_destructured_elements_are_unused_6198", "All destructured elements are unused.", true), All_variables_are_unused: i(6199, 1, "All_variables_are_unused_6199", "All variables are unused.", true), Definitions_of_the_following_identifiers_conflict_with_those_in_another_file_Colon_0: i(6200, 1, "Definitions_of_the_following_identifiers_conflict_with_those_in_another_file_Colon_0_6200", "Definitions of the following identifiers conflict with those in another file: {0}"), Conflicts_are_in_this_file: i(6201, 3, "Conflicts_are_in_this_file_6201", "Conflicts are in this file."), Project_references_may_not_form_a_circular_graph_Cycle_detected_Colon_0: i(6202, 1, "Project_references_may_not_form_a_circular_graph_Cycle_detected_Colon_0_6202", "Project references may not form a circular graph. Cycle detected: {0}"), _0_was_also_declared_here: i(6203, 3, "_0_was_also_declared_here_6203", "'{0}' was also declared here."), and_here: i(6204, 3, "and_here_6204", "and here."), All_type_parameters_are_unused: i(6205, 1, "All_type_parameters_are_unused_6205", "All type parameters are unused."), package_json_has_a_typesVersions_field_with_version_specific_path_mappings: i(6206, 3, "package_json_has_a_typesVersions_field_with_version_specific_path_mappings_6206", "'package.json' has a 'typesVersions' field with version-specific path mappings."), package_json_does_not_have_a_typesVersions_entry_that_matches_version_0: i(6207, 3, "package_json_does_not_have_a_typesVersions_entry_that_matches_version_0_6207", "'package.json' does not have a 'typesVersions' entry that matches version '{0}'."), package_json_has_a_typesVersions_entry_0_that_matches_compiler_version_1_looking_for_a_pattern_to_match_module_name_2: i(6208, 3, "package_json_has_a_typesVersions_entry_0_that_matches_compiler_version_1_looking_for_a_pattern_to_ma_6208", "'package.json' has a 'typesVersions' entry '{0}' that matches compiler version '{1}', looking for a pattern to match module name '{2}'."), package_json_has_a_typesVersions_entry_0_that_is_not_a_valid_semver_range: i(6209, 3, "package_json_has_a_typesVersions_entry_0_that_is_not_a_valid_semver_range_6209", "'package.json' has a 'typesVersions' entry '{0}' that is not a valid semver range."), An_argument_for_0_was_not_provided: i(6210, 3, "An_argument_for_0_was_not_provided_6210", "An argument for '{0}' was not provided."), An_argument_matching_this_binding_pattern_was_not_provided: i(6211, 3, "An_argument_matching_this_binding_pattern_was_not_provided_6211", "An argument matching this binding pattern was not provided."), Did_you_mean_to_call_this_expression: i(6212, 3, "Did_you_mean_to_call_this_expression_6212", "Did you mean to call this expression?"), Did_you_mean_to_use_new_with_this_expression: i(6213, 3, "Did_you_mean_to_use_new_with_this_expression_6213", "Did you mean to use 'new' with this expression?"), Enable_strict_bind_call_and_apply_methods_on_functions: i(6214, 3, "Enable_strict_bind_call_and_apply_methods_on_functions_6214", "Enable strict 'bind', 'call', and 'apply' methods on functions."), Using_compiler_options_of_project_reference_redirect_0: i(6215, 3, "Using_compiler_options_of_project_reference_redirect_0_6215", "Using compiler options of project reference redirect '{0}'."), Found_1_error: i(6216, 3, "Found_1_error_6216", "Found 1 error."), Found_0_errors: i(6217, 3, "Found_0_errors_6217", "Found {0} errors."), Module_name_0_was_successfully_resolved_to_1_with_Package_ID_2: i(6218, 3, "Module_name_0_was_successfully_resolved_to_1_with_Package_ID_2_6218", "======== Module name '{0}' was successfully resolved to '{1}' with Package ID '{2}'. ========"), Type_reference_directive_0_was_successfully_resolved_to_1_with_Package_ID_2_primary_Colon_3: i(6219, 3, "Type_reference_directive_0_was_successfully_resolved_to_1_with_Package_ID_2_primary_Colon_3_6219", "======== Type reference directive '{0}' was successfully resolved to '{1}' with Package ID '{2}', primary: {3}. ========"), package_json_had_a_falsy_0_field: i(6220, 3, "package_json_had_a_falsy_0_field_6220", "'package.json' had a falsy '{0}' field."), Disable_use_of_source_files_instead_of_declaration_files_from_referenced_projects: i(6221, 3, "Disable_use_of_source_files_instead_of_declaration_files_from_referenced_projects_6221", "Disable use of source files instead of declaration files from referenced projects."), Emit_class_fields_with_Define_instead_of_Set: i(6222, 3, "Emit_class_fields_with_Define_instead_of_Set_6222", "Emit class fields with Define instead of Set."), Generates_a_CPU_profile: i(6223, 3, "Generates_a_CPU_profile_6223", "Generates a CPU profile."), Disable_solution_searching_for_this_project: i(6224, 3, "Disable_solution_searching_for_this_project_6224", "Disable solution searching for this project."), Specify_strategy_for_watching_file_Colon_FixedPollingInterval_default_PriorityPollingInterval_DynamicPriorityPolling_FixedChunkSizePolling_UseFsEvents_UseFsEventsOnParentDirectory: i(6225, 3, "Specify_strategy_for_watching_file_Colon_FixedPollingInterval_default_PriorityPollingInterval_Dynami_6225", "Specify strategy for watching file: 'FixedPollingInterval' (default), 'PriorityPollingInterval', 'DynamicPriorityPolling', 'FixedChunkSizePolling', 'UseFsEvents', 'UseFsEventsOnParentDirectory'."), Specify_strategy_for_watching_directory_on_platforms_that_don_t_support_recursive_watching_natively_Colon_UseFsEvents_default_FixedPollingInterval_DynamicPriorityPolling_FixedChunkSizePolling: i(6226, 3, "Specify_strategy_for_watching_directory_on_platforms_that_don_t_support_recursive_watching_natively__6226", "Specify strategy for watching directory on platforms that don't support recursive watching natively: 'UseFsEvents' (default), 'FixedPollingInterval', 'DynamicPriorityPolling', 'FixedChunkSizePolling'."), Specify_strategy_for_creating_a_polling_watch_when_it_fails_to_create_using_file_system_events_Colon_FixedInterval_default_PriorityInterval_DynamicPriority_FixedChunkSize: i(6227, 3, "Specify_strategy_for_creating_a_polling_watch_when_it_fails_to_create_using_file_system_events_Colon_6227", "Specify strategy for creating a polling watch when it fails to create using file system events: 'FixedInterval' (default), 'PriorityInterval', 'DynamicPriority', 'FixedChunkSize'."), Tag_0_expects_at_least_1_arguments_but_the_JSX_factory_2_provides_at_most_3: i(6229, 1, "Tag_0_expects_at_least_1_arguments_but_the_JSX_factory_2_provides_at_most_3_6229", "Tag '{0}' expects at least '{1}' arguments, but the JSX factory '{2}' provides at most '{3}'."), Option_0_can_only_be_specified_in_tsconfig_json_file_or_set_to_false_or_null_on_command_line: i(6230, 1, "Option_0_can_only_be_specified_in_tsconfig_json_file_or_set_to_false_or_null_on_command_line_6230", "Option '{0}' can only be specified in 'tsconfig.json' file or set to 'false' or 'null' on command line."), Could_not_resolve_the_path_0_with_the_extensions_Colon_1: i(6231, 1, "Could_not_resolve_the_path_0_with_the_extensions_Colon_1_6231", "Could not resolve the path '{0}' with the extensions: {1}."), Declaration_augments_declaration_in_another_file_This_cannot_be_serialized: i(6232, 1, "Declaration_augments_declaration_in_another_file_This_cannot_be_serialized_6232", "Declaration augments declaration in another file. This cannot be serialized."), This_is_the_declaration_being_augmented_Consider_moving_the_augmenting_declaration_into_the_same_file: i(6233, 1, "This_is_the_declaration_being_augmented_Consider_moving_the_augmenting_declaration_into_the_same_fil_6233", "This is the declaration being augmented. Consider moving the augmenting declaration into the same file."), This_expression_is_not_callable_because_it_is_a_get_accessor_Did_you_mean_to_use_it_without: i(6234, 1, "This_expression_is_not_callable_because_it_is_a_get_accessor_Did_you_mean_to_use_it_without_6234", "This expression is not callable because it is a 'get' accessor. Did you mean to use it without '()'?"), Disable_loading_referenced_projects: i(6235, 3, "Disable_loading_referenced_projects_6235", "Disable loading referenced projects."), Arguments_for_the_rest_parameter_0_were_not_provided: i(6236, 1, "Arguments_for_the_rest_parameter_0_were_not_provided_6236", "Arguments for the rest parameter '{0}' were not provided."), Generates_an_event_trace_and_a_list_of_types: i(6237, 3, "Generates_an_event_trace_and_a_list_of_types_6237", "Generates an event trace and a list of types."), Specify_the_module_specifier_to_be_used_to_import_the_jsx_and_jsxs_factory_functions_from_eg_react: i(6238, 1, "Specify_the_module_specifier_to_be_used_to_import_the_jsx_and_jsxs_factory_functions_from_eg_react_6238", "Specify the module specifier to be used to import the 'jsx' and 'jsxs' factory functions from. eg, react"), File_0_exists_according_to_earlier_cached_lookups: i(6239, 3, "File_0_exists_according_to_earlier_cached_lookups_6239", "File '{0}' exists according to earlier cached lookups."), File_0_does_not_exist_according_to_earlier_cached_lookups: i(6240, 3, "File_0_does_not_exist_according_to_earlier_cached_lookups_6240", "File '{0}' does not exist according to earlier cached lookups."), Resolution_for_type_reference_directive_0_was_found_in_cache_from_location_1: i(6241, 3, "Resolution_for_type_reference_directive_0_was_found_in_cache_from_location_1_6241", "Resolution for type reference directive '{0}' was found in cache from location '{1}'."), Resolving_type_reference_directive_0_containing_file_1: i(6242, 3, "Resolving_type_reference_directive_0_containing_file_1_6242", "======== Resolving type reference directive '{0}', containing file '{1}'. ========"), Interpret_optional_property_types_as_written_rather_than_adding_undefined: i(6243, 3, "Interpret_optional_property_types_as_written_rather_than_adding_undefined_6243", "Interpret optional property types as written, rather than adding 'undefined'."), Modules: i(6244, 3, "Modules_6244", "Modules"), File_Management: i(6245, 3, "File_Management_6245", "File Management"), Emit: i(6246, 3, "Emit_6246", "Emit"), JavaScript_Support: i(6247, 3, "JavaScript_Support_6247", "JavaScript Support"), Type_Checking: i(6248, 3, "Type_Checking_6248", "Type Checking"), Editor_Support: i(6249, 3, "Editor_Support_6249", "Editor Support"), Watch_and_Build_Modes: i(6250, 3, "Watch_and_Build_Modes_6250", "Watch and Build Modes"), Compiler_Diagnostics: i(6251, 3, "Compiler_Diagnostics_6251", "Compiler Diagnostics"), Interop_Constraints: i(6252, 3, "Interop_Constraints_6252", "Interop Constraints"), Backwards_Compatibility: i(6253, 3, "Backwards_Compatibility_6253", "Backwards Compatibility"), Language_and_Environment: i(6254, 3, "Language_and_Environment_6254", "Language and Environment"), Projects: i(6255, 3, "Projects_6255", "Projects"), Output_Formatting: i(6256, 3, "Output_Formatting_6256", "Output Formatting"), Completeness: i(6257, 3, "Completeness_6257", "Completeness"), _0_should_be_set_inside_the_compilerOptions_object_of_the_config_json_file: i(6258, 1, "_0_should_be_set_inside_the_compilerOptions_object_of_the_config_json_file_6258", "'{0}' should be set inside the 'compilerOptions' object of the config json file"), Found_1_error_in_1: i(6259, 3, "Found_1_error_in_1_6259", "Found 1 error in {1}"), Found_0_errors_in_the_same_file_starting_at_Colon_1: i(6260, 3, "Found_0_errors_in_the_same_file_starting_at_Colon_1_6260", "Found {0} errors in the same file, starting at: {1}"), Found_0_errors_in_1_files: i(6261, 3, "Found_0_errors_in_1_files_6261", "Found {0} errors in {1} files."), File_name_0_has_a_1_extension_looking_up_2_instead: i(6262, 3, "File_name_0_has_a_1_extension_looking_up_2_instead_6262", "File name '{0}' has a '{1}' extension - looking up '{2}' instead."), Module_0_was_resolved_to_1_but_allowArbitraryExtensions_is_not_set: i(6263, 1, "Module_0_was_resolved_to_1_but_allowArbitraryExtensions_is_not_set_6263", "Module '{0}' was resolved to '{1}', but '--allowArbitraryExtensions' is not set."), Enable_importing_files_with_any_extension_provided_a_declaration_file_is_present: i(6264, 3, "Enable_importing_files_with_any_extension_provided_a_declaration_file_is_present_6264", "Enable importing files with any extension, provided a declaration file is present."), Directory_0_has_no_containing_package_json_scope_Imports_will_not_resolve: i(6270, 3, "Directory_0_has_no_containing_package_json_scope_Imports_will_not_resolve_6270", "Directory '{0}' has no containing package.json scope. Imports will not resolve."), Import_specifier_0_does_not_exist_in_package_json_scope_at_path_1: i(6271, 3, "Import_specifier_0_does_not_exist_in_package_json_scope_at_path_1_6271", "Import specifier '{0}' does not exist in package.json scope at path '{1}'."), Invalid_import_specifier_0_has_no_possible_resolutions: i(6272, 3, "Invalid_import_specifier_0_has_no_possible_resolutions_6272", "Invalid import specifier '{0}' has no possible resolutions."), package_json_scope_0_has_no_imports_defined: i(6273, 3, "package_json_scope_0_has_no_imports_defined_6273", "package.json scope '{0}' has no imports defined."), package_json_scope_0_explicitly_maps_specifier_1_to_null: i(6274, 3, "package_json_scope_0_explicitly_maps_specifier_1_to_null_6274", "package.json scope '{0}' explicitly maps specifier '{1}' to null."), package_json_scope_0_has_invalid_type_for_target_of_specifier_1: i(6275, 3, "package_json_scope_0_has_invalid_type_for_target_of_specifier_1_6275", "package.json scope '{0}' has invalid type for target of specifier '{1}'"), Export_specifier_0_does_not_exist_in_package_json_scope_at_path_1: i(6276, 3, "Export_specifier_0_does_not_exist_in_package_json_scope_at_path_1_6276", "Export specifier '{0}' does not exist in package.json scope at path '{1}'."), Resolution_of_non_relative_name_failed_trying_with_modern_Node_resolution_features_disabled_to_see_if_npm_library_needs_configuration_update: i(6277, 3, "Resolution_of_non_relative_name_failed_trying_with_modern_Node_resolution_features_disabled_to_see_i_6277", "Resolution of non-relative name failed; trying with modern Node resolution features disabled to see if npm library needs configuration update."), There_are_types_at_0_but_this_result_could_not_be_resolved_when_respecting_package_json_exports_The_1_library_may_need_to_update_its_package_json_or_typings: i(6278, 3, "There_are_types_at_0_but_this_result_could_not_be_resolved_when_respecting_package_json_exports_The__6278", `There are types at '{0}', but this result could not be resolved when respecting package.json "exports". The '{1}' library may need to update its package.json or typings.`), Enable_project_compilation: i(6302, 3, "Enable_project_compilation_6302", "Enable project compilation"), Composite_projects_may_not_disable_declaration_emit: i(6304, 1, "Composite_projects_may_not_disable_declaration_emit_6304", "Composite projects may not disable declaration emit."), Output_file_0_has_not_been_built_from_source_file_1: i(6305, 1, "Output_file_0_has_not_been_built_from_source_file_1_6305", "Output file '{0}' has not been built from source file '{1}'."), Referenced_project_0_must_have_setting_composite_Colon_true: i(6306, 1, "Referenced_project_0_must_have_setting_composite_Colon_true_6306", `Referenced project '{0}' must have setting "composite": true.`), File_0_is_not_listed_within_the_file_list_of_project_1_Projects_must_list_all_files_or_use_an_include_pattern: i(6307, 1, "File_0_is_not_listed_within_the_file_list_of_project_1_Projects_must_list_all_files_or_use_an_includ_6307", "File '{0}' is not listed within the file list of project '{1}'. Projects must list all files or use an 'include' pattern."), Cannot_prepend_project_0_because_it_does_not_have_outFile_set: i(6308, 1, "Cannot_prepend_project_0_because_it_does_not_have_outFile_set_6308", "Cannot prepend project '{0}' because it does not have 'outFile' set"), Output_file_0_from_project_1_does_not_exist: i(6309, 1, "Output_file_0_from_project_1_does_not_exist_6309", "Output file '{0}' from project '{1}' does not exist"), Referenced_project_0_may_not_disable_emit: i(6310, 1, "Referenced_project_0_may_not_disable_emit_6310", "Referenced project '{0}' may not disable emit."), Project_0_is_out_of_date_because_output_1_is_older_than_input_2: i(6350, 3, "Project_0_is_out_of_date_because_output_1_is_older_than_input_2_6350", "Project '{0}' is out of date because output '{1}' is older than input '{2}'"), Project_0_is_up_to_date_because_newest_input_1_is_older_than_output_2: i(6351, 3, "Project_0_is_up_to_date_because_newest_input_1_is_older_than_output_2_6351", "Project '{0}' is up to date because newest input '{1}' is older than output '{2}'"), Project_0_is_out_of_date_because_output_file_1_does_not_exist: i(6352, 3, "Project_0_is_out_of_date_because_output_file_1_does_not_exist_6352", "Project '{0}' is out of date because output file '{1}' does not exist"), Project_0_is_out_of_date_because_its_dependency_1_is_out_of_date: i(6353, 3, "Project_0_is_out_of_date_because_its_dependency_1_is_out_of_date_6353", "Project '{0}' is out of date because its dependency '{1}' is out of date"), Project_0_is_up_to_date_with_d_ts_files_from_its_dependencies: i(6354, 3, "Project_0_is_up_to_date_with_d_ts_files_from_its_dependencies_6354", "Project '{0}' is up to date with .d.ts files from its dependencies"), Projects_in_this_build_Colon_0: i(6355, 3, "Projects_in_this_build_Colon_0_6355", "Projects in this build: {0}"), A_non_dry_build_would_delete_the_following_files_Colon_0: i(6356, 3, "A_non_dry_build_would_delete_the_following_files_Colon_0_6356", "A non-dry build would delete the following files: {0}"), A_non_dry_build_would_build_project_0: i(6357, 3, "A_non_dry_build_would_build_project_0_6357", "A non-dry build would build project '{0}'"), Building_project_0: i(6358, 3, "Building_project_0_6358", "Building project '{0}'..."), Updating_output_timestamps_of_project_0: i(6359, 3, "Updating_output_timestamps_of_project_0_6359", "Updating output timestamps of project '{0}'..."), Project_0_is_up_to_date: i(6361, 3, "Project_0_is_up_to_date_6361", "Project '{0}' is up to date"), Skipping_build_of_project_0_because_its_dependency_1_has_errors: i(6362, 3, "Skipping_build_of_project_0_because_its_dependency_1_has_errors_6362", "Skipping build of project '{0}' because its dependency '{1}' has errors"), Project_0_can_t_be_built_because_its_dependency_1_has_errors: i(6363, 3, "Project_0_can_t_be_built_because_its_dependency_1_has_errors_6363", "Project '{0}' can't be built because its dependency '{1}' has errors"), Build_one_or_more_projects_and_their_dependencies_if_out_of_date: i(6364, 3, "Build_one_or_more_projects_and_their_dependencies_if_out_of_date_6364", "Build one or more projects and their dependencies, if out of date"), Delete_the_outputs_of_all_projects: i(6365, 3, "Delete_the_outputs_of_all_projects_6365", "Delete the outputs of all projects."), Show_what_would_be_built_or_deleted_if_specified_with_clean: i(6367, 3, "Show_what_would_be_built_or_deleted_if_specified_with_clean_6367", "Show what would be built (or deleted, if specified with '--clean')"), Option_build_must_be_the_first_command_line_argument: i(6369, 1, "Option_build_must_be_the_first_command_line_argument_6369", "Option '--build' must be the first command line argument."), Options_0_and_1_cannot_be_combined: i(6370, 1, "Options_0_and_1_cannot_be_combined_6370", "Options '{0}' and '{1}' cannot be combined."), Updating_unchanged_output_timestamps_of_project_0: i(6371, 3, "Updating_unchanged_output_timestamps_of_project_0_6371", "Updating unchanged output timestamps of project '{0}'..."), Project_0_is_out_of_date_because_output_of_its_dependency_1_has_changed: i(6372, 3, "Project_0_is_out_of_date_because_output_of_its_dependency_1_has_changed_6372", "Project '{0}' is out of date because output of its dependency '{1}' has changed"), Updating_output_of_project_0: i(6373, 3, "Updating_output_of_project_0_6373", "Updating output of project '{0}'..."), A_non_dry_build_would_update_timestamps_for_output_of_project_0: i(6374, 3, "A_non_dry_build_would_update_timestamps_for_output_of_project_0_6374", "A non-dry build would update timestamps for output of project '{0}'"), A_non_dry_build_would_update_output_of_project_0: i(6375, 3, "A_non_dry_build_would_update_output_of_project_0_6375", "A non-dry build would update output of project '{0}'"), Cannot_update_output_of_project_0_because_there_was_error_reading_file_1: i(6376, 3, "Cannot_update_output_of_project_0_because_there_was_error_reading_file_1_6376", "Cannot update output of project '{0}' because there was error reading file '{1}'"), Cannot_write_file_0_because_it_will_overwrite_tsbuildinfo_file_generated_by_referenced_project_1: i(6377, 1, "Cannot_write_file_0_because_it_will_overwrite_tsbuildinfo_file_generated_by_referenced_project_1_6377", "Cannot write file '{0}' because it will overwrite '.tsbuildinfo' file generated by referenced project '{1}'"), Composite_projects_may_not_disable_incremental_compilation: i(6379, 1, "Composite_projects_may_not_disable_incremental_compilation_6379", "Composite projects may not disable incremental compilation."), Specify_file_to_store_incremental_compilation_information: i(6380, 3, "Specify_file_to_store_incremental_compilation_information_6380", "Specify file to store incremental compilation information"), Project_0_is_out_of_date_because_output_for_it_was_generated_with_version_1_that_differs_with_current_version_2: i(6381, 3, "Project_0_is_out_of_date_because_output_for_it_was_generated_with_version_1_that_differs_with_curren_6381", "Project '{0}' is out of date because output for it was generated with version '{1}' that differs with current version '{2}'"), Skipping_build_of_project_0_because_its_dependency_1_was_not_built: i(6382, 3, "Skipping_build_of_project_0_because_its_dependency_1_was_not_built_6382", "Skipping build of project '{0}' because its dependency '{1}' was not built"), Project_0_can_t_be_built_because_its_dependency_1_was_not_built: i(6383, 3, "Project_0_can_t_be_built_because_its_dependency_1_was_not_built_6383", "Project '{0}' can't be built because its dependency '{1}' was not built"), Have_recompiles_in_incremental_and_watch_assume_that_changes_within_a_file_will_only_affect_files_directly_depending_on_it: i(6384, 3, "Have_recompiles_in_incremental_and_watch_assume_that_changes_within_a_file_will_only_affect_files_di_6384", "Have recompiles in '--incremental' and '--watch' assume that changes within a file will only affect files directly depending on it."), _0_is_deprecated: i(6385, 2, "_0_is_deprecated_6385", "'{0}' is deprecated.", void 0, void 0, true), Performance_timings_for_diagnostics_or_extendedDiagnostics_are_not_available_in_this_session_A_native_implementation_of_the_Web_Performance_API_could_not_be_found: i(6386, 3, "Performance_timings_for_diagnostics_or_extendedDiagnostics_are_not_available_in_this_session_A_nativ_6386", "Performance timings for '--diagnostics' or '--extendedDiagnostics' are not available in this session. A native implementation of the Web Performance API could not be found."), The_signature_0_of_1_is_deprecated: i(6387, 2, "The_signature_0_of_1_is_deprecated_6387", "The signature '{0}' of '{1}' is deprecated.", void 0, void 0, true), Project_0_is_being_forcibly_rebuilt: i(6388, 3, "Project_0_is_being_forcibly_rebuilt_6388", "Project '{0}' is being forcibly rebuilt"), Reusing_resolution_of_module_0_from_1_of_old_program_it_was_not_resolved: i(6389, 3, "Reusing_resolution_of_module_0_from_1_of_old_program_it_was_not_resolved_6389", "Reusing resolution of module '{0}' from '{1}' of old program, it was not resolved."), Reusing_resolution_of_type_reference_directive_0_from_1_of_old_program_it_was_successfully_resolved_to_2: i(6390, 3, "Reusing_resolution_of_type_reference_directive_0_from_1_of_old_program_it_was_successfully_resolved__6390", "Reusing resolution of type reference directive '{0}' from '{1}' of old program, it was successfully resolved to '{2}'."), Reusing_resolution_of_type_reference_directive_0_from_1_of_old_program_it_was_successfully_resolved_to_2_with_Package_ID_3: i(6391, 3, "Reusing_resolution_of_type_reference_directive_0_from_1_of_old_program_it_was_successfully_resolved__6391", "Reusing resolution of type reference directive '{0}' from '{1}' of old program, it was successfully resolved to '{2}' with Package ID '{3}'."), Reusing_resolution_of_type_reference_directive_0_from_1_of_old_program_it_was_not_resolved: i(6392, 3, "Reusing_resolution_of_type_reference_directive_0_from_1_of_old_program_it_was_not_resolved_6392", "Reusing resolution of type reference directive '{0}' from '{1}' of old program, it was not resolved."), Reusing_resolution_of_module_0_from_1_found_in_cache_from_location_2_it_was_successfully_resolved_to_3: i(6393, 3, "Reusing_resolution_of_module_0_from_1_found_in_cache_from_location_2_it_was_successfully_resolved_to_6393", "Reusing resolution of module '{0}' from '{1}' found in cache from location '{2}', it was successfully resolved to '{3}'."), Reusing_resolution_of_module_0_from_1_found_in_cache_from_location_2_it_was_successfully_resolved_to_3_with_Package_ID_4: i(6394, 3, "Reusing_resolution_of_module_0_from_1_found_in_cache_from_location_2_it_was_successfully_resolved_to_6394", "Reusing resolution of module '{0}' from '{1}' found in cache from location '{2}', it was successfully resolved to '{3}' with Package ID '{4}'."), Reusing_resolution_of_module_0_from_1_found_in_cache_from_location_2_it_was_not_resolved: i(6395, 3, "Reusing_resolution_of_module_0_from_1_found_in_cache_from_location_2_it_was_not_resolved_6395", "Reusing resolution of module '{0}' from '{1}' found in cache from location '{2}', it was not resolved."), Reusing_resolution_of_type_reference_directive_0_from_1_found_in_cache_from_location_2_it_was_successfully_resolved_to_3: i(6396, 3, "Reusing_resolution_of_type_reference_directive_0_from_1_found_in_cache_from_location_2_it_was_succes_6396", "Reusing resolution of type reference directive '{0}' from '{1}' found in cache from location '{2}', it was successfully resolved to '{3}'."), Reusing_resolution_of_type_reference_directive_0_from_1_found_in_cache_from_location_2_it_was_successfully_resolved_to_3_with_Package_ID_4: i(6397, 3, "Reusing_resolution_of_type_reference_directive_0_from_1_found_in_cache_from_location_2_it_was_succes_6397", "Reusing resolution of type reference directive '{0}' from '{1}' found in cache from location '{2}', it was successfully resolved to '{3}' with Package ID '{4}'."), Reusing_resolution_of_type_reference_directive_0_from_1_found_in_cache_from_location_2_it_was_not_resolved: i(6398, 3, "Reusing_resolution_of_type_reference_directive_0_from_1_found_in_cache_from_location_2_it_was_not_re_6398", "Reusing resolution of type reference directive '{0}' from '{1}' found in cache from location '{2}', it was not resolved."), Project_0_is_out_of_date_because_buildinfo_file_1_indicates_that_some_of_the_changes_were_not_emitted: i(6399, 3, "Project_0_is_out_of_date_because_buildinfo_file_1_indicates_that_some_of_the_changes_were_not_emitte_6399", "Project '{0}' is out of date because buildinfo file '{1}' indicates that some of the changes were not emitted"), Project_0_is_up_to_date_but_needs_to_update_timestamps_of_output_files_that_are_older_than_input_files: i(6400, 3, "Project_0_is_up_to_date_but_needs_to_update_timestamps_of_output_files_that_are_older_than_input_fil_6400", "Project '{0}' is up to date but needs to update timestamps of output files that are older than input files"), Project_0_is_out_of_date_because_there_was_error_reading_file_1: i(6401, 3, "Project_0_is_out_of_date_because_there_was_error_reading_file_1_6401", "Project '{0}' is out of date because there was error reading file '{1}'"), Resolving_in_0_mode_with_conditions_1: i(6402, 3, "Resolving_in_0_mode_with_conditions_1_6402", "Resolving in {0} mode with conditions {1}."), Matched_0_condition_1: i(6403, 3, "Matched_0_condition_1_6403", "Matched '{0}' condition '{1}'."), Using_0_subpath_1_with_target_2: i(6404, 3, "Using_0_subpath_1_with_target_2_6404", "Using '{0}' subpath '{1}' with target '{2}'."), Saw_non_matching_condition_0: i(6405, 3, "Saw_non_matching_condition_0_6405", "Saw non-matching condition '{0}'."), Project_0_is_out_of_date_because_buildinfo_file_1_indicates_there_is_change_in_compilerOptions: i(6406, 3, "Project_0_is_out_of_date_because_buildinfo_file_1_indicates_there_is_change_in_compilerOptions_6406", "Project '{0}' is out of date because buildinfo file '{1}' indicates there is change in compilerOptions"), Allow_imports_to_include_TypeScript_file_extensions_Requires_moduleResolution_bundler_and_either_noEmit_or_emitDeclarationOnly_to_be_set: i(6407, 3, "Allow_imports_to_include_TypeScript_file_extensions_Requires_moduleResolution_bundler_and_either_noE_6407", "Allow imports to include TypeScript file extensions. Requires '--moduleResolution bundler' and either '--noEmit' or '--emitDeclarationOnly' to be set."), Use_the_package_json_exports_field_when_resolving_package_imports: i(6408, 3, "Use_the_package_json_exports_field_when_resolving_package_imports_6408", "Use the package.json 'exports' field when resolving package imports."), Use_the_package_json_imports_field_when_resolving_imports: i(6409, 3, "Use_the_package_json_imports_field_when_resolving_imports_6409", "Use the package.json 'imports' field when resolving imports."), Conditions_to_set_in_addition_to_the_resolver_specific_defaults_when_resolving_imports: i(6410, 3, "Conditions_to_set_in_addition_to_the_resolver_specific_defaults_when_resolving_imports_6410", "Conditions to set in addition to the resolver-specific defaults when resolving imports."), true_when_moduleResolution_is_node16_nodenext_or_bundler_otherwise_false: i(6411, 3, "true_when_moduleResolution_is_node16_nodenext_or_bundler_otherwise_false_6411", "`true` when 'moduleResolution' is 'node16', 'nodenext', or 'bundler'; otherwise `false`."), Project_0_is_out_of_date_because_buildinfo_file_1_indicates_that_file_2_was_root_file_of_compilation_but_not_any_more: i(6412, 3, "Project_0_is_out_of_date_because_buildinfo_file_1_indicates_that_file_2_was_root_file_of_compilation_6412", "Project '{0}' is out of date because buildinfo file '{1}' indicates that file '{2}' was root file of compilation but not any more."), Entering_conditional_exports: i(6413, 3, "Entering_conditional_exports_6413", "Entering conditional exports."), Resolved_under_condition_0: i(6414, 3, "Resolved_under_condition_0_6414", "Resolved under condition '{0}'."), Failed_to_resolve_under_condition_0: i(6415, 3, "Failed_to_resolve_under_condition_0_6415", "Failed to resolve under condition '{0}'."), Exiting_conditional_exports: i(6416, 3, "Exiting_conditional_exports_6416", "Exiting conditional exports."), The_expected_type_comes_from_property_0_which_is_declared_here_on_type_1: i(6500, 3, "The_expected_type_comes_from_property_0_which_is_declared_here_on_type_1_6500", "The expected type comes from property '{0}' which is declared here on type '{1}'"), The_expected_type_comes_from_this_index_signature: i(6501, 3, "The_expected_type_comes_from_this_index_signature_6501", "The expected type comes from this index signature."), The_expected_type_comes_from_the_return_type_of_this_signature: i(6502, 3, "The_expected_type_comes_from_the_return_type_of_this_signature_6502", "The expected type comes from the return type of this signature."), Print_names_of_files_that_are_part_of_the_compilation_and_then_stop_processing: i(6503, 3, "Print_names_of_files_that_are_part_of_the_compilation_and_then_stop_processing_6503", "Print names of files that are part of the compilation and then stop processing."), File_0_is_a_JavaScript_file_Did_you_mean_to_enable_the_allowJs_option: i(6504, 1, "File_0_is_a_JavaScript_file_Did_you_mean_to_enable_the_allowJs_option_6504", "File '{0}' is a JavaScript file. Did you mean to enable the 'allowJs' option?"), Print_names_of_files_and_the_reason_they_are_part_of_the_compilation: i(6505, 3, "Print_names_of_files_and_the_reason_they_are_part_of_the_compilation_6505", "Print names of files and the reason they are part of the compilation."), Consider_adding_a_declare_modifier_to_this_class: i(6506, 3, "Consider_adding_a_declare_modifier_to_this_class_6506", "Consider adding a 'declare' modifier to this class."), Allow_JavaScript_files_to_be_a_part_of_your_program_Use_the_checkJS_option_to_get_errors_from_these_files: i(6600, 3, "Allow_JavaScript_files_to_be_a_part_of_your_program_Use_the_checkJS_option_to_get_errors_from_these__6600", "Allow JavaScript files to be a part of your program. Use the 'checkJS' option to get errors from these files."), Allow_import_x_from_y_when_a_module_doesn_t_have_a_default_export: i(6601, 3, "Allow_import_x_from_y_when_a_module_doesn_t_have_a_default_export_6601", "Allow 'import x from y' when a module doesn't have a default export."), Allow_accessing_UMD_globals_from_modules: i(6602, 3, "Allow_accessing_UMD_globals_from_modules_6602", "Allow accessing UMD globals from modules."), Disable_error_reporting_for_unreachable_code: i(6603, 3, "Disable_error_reporting_for_unreachable_code_6603", "Disable error reporting for unreachable code."), Disable_error_reporting_for_unused_labels: i(6604, 3, "Disable_error_reporting_for_unused_labels_6604", "Disable error reporting for unused labels."), Ensure_use_strict_is_always_emitted: i(6605, 3, "Ensure_use_strict_is_always_emitted_6605", "Ensure 'use strict' is always emitted."), Have_recompiles_in_projects_that_use_incremental_and_watch_mode_assume_that_changes_within_a_file_will_only_affect_files_directly_depending_on_it: i(6606, 3, "Have_recompiles_in_projects_that_use_incremental_and_watch_mode_assume_that_changes_within_a_file_wi_6606", "Have recompiles in projects that use 'incremental' and 'watch' mode assume that changes within a file will only affect files directly depending on it."), Specify_the_base_directory_to_resolve_non_relative_module_names: i(6607, 3, "Specify_the_base_directory_to_resolve_non_relative_module_names_6607", "Specify the base directory to resolve non-relative module names."), No_longer_supported_In_early_versions_manually_set_the_text_encoding_for_reading_files: i(6608, 3, "No_longer_supported_In_early_versions_manually_set_the_text_encoding_for_reading_files_6608", "No longer supported. In early versions, manually set the text encoding for reading files."), Enable_error_reporting_in_type_checked_JavaScript_files: i(6609, 3, "Enable_error_reporting_in_type_checked_JavaScript_files_6609", "Enable error reporting in type-checked JavaScript files."), Enable_constraints_that_allow_a_TypeScript_project_to_be_used_with_project_references: i(6611, 3, "Enable_constraints_that_allow_a_TypeScript_project_to_be_used_with_project_references_6611", "Enable constraints that allow a TypeScript project to be used with project references."), Generate_d_ts_files_from_TypeScript_and_JavaScript_files_in_your_project: i(6612, 3, "Generate_d_ts_files_from_TypeScript_and_JavaScript_files_in_your_project_6612", "Generate .d.ts files from TypeScript and JavaScript files in your project."), Specify_the_output_directory_for_generated_declaration_files: i(6613, 3, "Specify_the_output_directory_for_generated_declaration_files_6613", "Specify the output directory for generated declaration files."), Create_sourcemaps_for_d_ts_files: i(6614, 3, "Create_sourcemaps_for_d_ts_files_6614", "Create sourcemaps for d.ts files."), Output_compiler_performance_information_after_building: i(6615, 3, "Output_compiler_performance_information_after_building_6615", "Output compiler performance information after building."), Disables_inference_for_type_acquisition_by_looking_at_filenames_in_a_project: i(6616, 3, "Disables_inference_for_type_acquisition_by_looking_at_filenames_in_a_project_6616", "Disables inference for type acquisition by looking at filenames in a project."), Reduce_the_number_of_projects_loaded_automatically_by_TypeScript: i(6617, 3, "Reduce_the_number_of_projects_loaded_automatically_by_TypeScript_6617", "Reduce the number of projects loaded automatically by TypeScript."), Remove_the_20mb_cap_on_total_source_code_size_for_JavaScript_files_in_the_TypeScript_language_server: i(6618, 3, "Remove_the_20mb_cap_on_total_source_code_size_for_JavaScript_files_in_the_TypeScript_language_server_6618", "Remove the 20mb cap on total source code size for JavaScript files in the TypeScript language server."), Opt_a_project_out_of_multi_project_reference_checking_when_editing: i(6619, 3, "Opt_a_project_out_of_multi_project_reference_checking_when_editing_6619", "Opt a project out of multi-project reference checking when editing."), Disable_preferring_source_files_instead_of_declaration_files_when_referencing_composite_projects: i(6620, 3, "Disable_preferring_source_files_instead_of_declaration_files_when_referencing_composite_projects_6620", "Disable preferring source files instead of declaration files when referencing composite projects."), Emit_more_compliant_but_verbose_and_less_performant_JavaScript_for_iteration: i(6621, 3, "Emit_more_compliant_but_verbose_and_less_performant_JavaScript_for_iteration_6621", "Emit more compliant, but verbose and less performant JavaScript for iteration."), Emit_a_UTF_8_Byte_Order_Mark_BOM_in_the_beginning_of_output_files: i(6622, 3, "Emit_a_UTF_8_Byte_Order_Mark_BOM_in_the_beginning_of_output_files_6622", "Emit a UTF-8 Byte Order Mark (BOM) in the beginning of output files."), Only_output_d_ts_files_and_not_JavaScript_files: i(6623, 3, "Only_output_d_ts_files_and_not_JavaScript_files_6623", "Only output d.ts files and not JavaScript files."), Emit_design_type_metadata_for_decorated_declarations_in_source_files: i(6624, 3, "Emit_design_type_metadata_for_decorated_declarations_in_source_files_6624", "Emit design-type metadata for decorated declarations in source files."), Disable_the_type_acquisition_for_JavaScript_projects: i(6625, 3, "Disable_the_type_acquisition_for_JavaScript_projects_6625", "Disable the type acquisition for JavaScript projects"), Emit_additional_JavaScript_to_ease_support_for_importing_CommonJS_modules_This_enables_allowSyntheticDefaultImports_for_type_compatibility: i(6626, 3, "Emit_additional_JavaScript_to_ease_support_for_importing_CommonJS_modules_This_enables_allowSyntheti_6626", "Emit additional JavaScript to ease support for importing CommonJS modules. This enables 'allowSyntheticDefaultImports' for type compatibility."), Filters_results_from_the_include_option: i(6627, 3, "Filters_results_from_the_include_option_6627", "Filters results from the `include` option."), Remove_a_list_of_directories_from_the_watch_process: i(6628, 3, "Remove_a_list_of_directories_from_the_watch_process_6628", "Remove a list of directories from the watch process."), Remove_a_list_of_files_from_the_watch_mode_s_processing: i(6629, 3, "Remove_a_list_of_files_from_the_watch_mode_s_processing_6629", "Remove a list of files from the watch mode's processing."), Enable_experimental_support_for_legacy_experimental_decorators: i(6630, 3, "Enable_experimental_support_for_legacy_experimental_decorators_6630", "Enable experimental support for legacy experimental decorators."), Print_files_read_during_the_compilation_including_why_it_was_included: i(6631, 3, "Print_files_read_during_the_compilation_including_why_it_was_included_6631", "Print files read during the compilation including why it was included."), Output_more_detailed_compiler_performance_information_after_building: i(6632, 3, "Output_more_detailed_compiler_performance_information_after_building_6632", "Output more detailed compiler performance information after building."), Specify_one_or_more_path_or_node_module_references_to_base_configuration_files_from_which_settings_are_inherited: i(6633, 3, "Specify_one_or_more_path_or_node_module_references_to_base_configuration_files_from_which_settings_a_6633", "Specify one or more path or node module references to base configuration files from which settings are inherited."), Specify_what_approach_the_watcher_should_use_if_the_system_runs_out_of_native_file_watchers: i(6634, 3, "Specify_what_approach_the_watcher_should_use_if_the_system_runs_out_of_native_file_watchers_6634", "Specify what approach the watcher should use if the system runs out of native file watchers."), Include_a_list_of_files_This_does_not_support_glob_patterns_as_opposed_to_include: i(6635, 3, "Include_a_list_of_files_This_does_not_support_glob_patterns_as_opposed_to_include_6635", "Include a list of files. This does not support glob patterns, as opposed to `include`."), Build_all_projects_including_those_that_appear_to_be_up_to_date: i(6636, 3, "Build_all_projects_including_those_that_appear_to_be_up_to_date_6636", "Build all projects, including those that appear to be up to date."), Ensure_that_casing_is_correct_in_imports: i(6637, 3, "Ensure_that_casing_is_correct_in_imports_6637", "Ensure that casing is correct in imports."), Emit_a_v8_CPU_profile_of_the_compiler_run_for_debugging: i(6638, 3, "Emit_a_v8_CPU_profile_of_the_compiler_run_for_debugging_6638", "Emit a v8 CPU profile of the compiler run for debugging."), Allow_importing_helper_functions_from_tslib_once_per_project_instead_of_including_them_per_file: i(6639, 3, "Allow_importing_helper_functions_from_tslib_once_per_project_instead_of_including_them_per_file_6639", "Allow importing helper functions from tslib once per project, instead of including them per-file."), Specify_a_list_of_glob_patterns_that_match_files_to_be_included_in_compilation: i(6641, 3, "Specify_a_list_of_glob_patterns_that_match_files_to_be_included_in_compilation_6641", "Specify a list of glob patterns that match files to be included in compilation."), Save_tsbuildinfo_files_to_allow_for_incremental_compilation_of_projects: i(6642, 3, "Save_tsbuildinfo_files_to_allow_for_incremental_compilation_of_projects_6642", "Save .tsbuildinfo files to allow for incremental compilation of projects."), Include_sourcemap_files_inside_the_emitted_JavaScript: i(6643, 3, "Include_sourcemap_files_inside_the_emitted_JavaScript_6643", "Include sourcemap files inside the emitted JavaScript."), Include_source_code_in_the_sourcemaps_inside_the_emitted_JavaScript: i(6644, 3, "Include_source_code_in_the_sourcemaps_inside_the_emitted_JavaScript_6644", "Include source code in the sourcemaps inside the emitted JavaScript."), Ensure_that_each_file_can_be_safely_transpiled_without_relying_on_other_imports: i(6645, 3, "Ensure_that_each_file_can_be_safely_transpiled_without_relying_on_other_imports_6645", "Ensure that each file can be safely transpiled without relying on other imports."), Specify_what_JSX_code_is_generated: i(6646, 3, "Specify_what_JSX_code_is_generated_6646", "Specify what JSX code is generated."), Specify_the_JSX_factory_function_used_when_targeting_React_JSX_emit_e_g_React_createElement_or_h: i(6647, 3, "Specify_the_JSX_factory_function_used_when_targeting_React_JSX_emit_e_g_React_createElement_or_h_6647", "Specify the JSX factory function used when targeting React JSX emit, e.g. 'React.createElement' or 'h'."), Specify_the_JSX_Fragment_reference_used_for_fragments_when_targeting_React_JSX_emit_e_g_React_Fragment_or_Fragment: i(6648, 3, "Specify_the_JSX_Fragment_reference_used_for_fragments_when_targeting_React_JSX_emit_e_g_React_Fragme_6648", "Specify the JSX Fragment reference used for fragments when targeting React JSX emit e.g. 'React.Fragment' or 'Fragment'."), Specify_module_specifier_used_to_import_the_JSX_factory_functions_when_using_jsx_Colon_react_jsx_Asterisk: i(6649, 3, "Specify_module_specifier_used_to_import_the_JSX_factory_functions_when_using_jsx_Colon_react_jsx_Ast_6649", "Specify module specifier used to import the JSX factory functions when using 'jsx: react-jsx*'."), Make_keyof_only_return_strings_instead_of_string_numbers_or_symbols_Legacy_option: i(6650, 3, "Make_keyof_only_return_strings_instead_of_string_numbers_or_symbols_Legacy_option_6650", "Make keyof only return strings instead of string, numbers or symbols. Legacy option."), Specify_a_set_of_bundled_library_declaration_files_that_describe_the_target_runtime_environment: i(6651, 3, "Specify_a_set_of_bundled_library_declaration_files_that_describe_the_target_runtime_environment_6651", "Specify a set of bundled library declaration files that describe the target runtime environment."), Print_the_names_of_emitted_files_after_a_compilation: i(6652, 3, "Print_the_names_of_emitted_files_after_a_compilation_6652", "Print the names of emitted files after a compilation."), Print_all_of_the_files_read_during_the_compilation: i(6653, 3, "Print_all_of_the_files_read_during_the_compilation_6653", "Print all of the files read during the compilation."), Set_the_language_of_the_messaging_from_TypeScript_This_does_not_affect_emit: i(6654, 3, "Set_the_language_of_the_messaging_from_TypeScript_This_does_not_affect_emit_6654", "Set the language of the messaging from TypeScript. This does not affect emit."), Specify_the_location_where_debugger_should_locate_map_files_instead_of_generated_locations: i(6655, 3, "Specify_the_location_where_debugger_should_locate_map_files_instead_of_generated_locations_6655", "Specify the location where debugger should locate map files instead of generated locations."), Specify_the_maximum_folder_depth_used_for_checking_JavaScript_files_from_node_modules_Only_applicable_with_allowJs: i(6656, 3, "Specify_the_maximum_folder_depth_used_for_checking_JavaScript_files_from_node_modules_Only_applicabl_6656", "Specify the maximum folder depth used for checking JavaScript files from 'node_modules'. Only applicable with 'allowJs'."), Specify_what_module_code_is_generated: i(6657, 3, "Specify_what_module_code_is_generated_6657", "Specify what module code is generated."), Specify_how_TypeScript_looks_up_a_file_from_a_given_module_specifier: i(6658, 3, "Specify_how_TypeScript_looks_up_a_file_from_a_given_module_specifier_6658", "Specify how TypeScript looks up a file from a given module specifier."), Set_the_newline_character_for_emitting_files: i(6659, 3, "Set_the_newline_character_for_emitting_files_6659", "Set the newline character for emitting files."), Disable_emitting_files_from_a_compilation: i(6660, 3, "Disable_emitting_files_from_a_compilation_6660", "Disable emitting files from a compilation."), Disable_generating_custom_helper_functions_like_extends_in_compiled_output: i(6661, 3, "Disable_generating_custom_helper_functions_like_extends_in_compiled_output_6661", "Disable generating custom helper functions like '__extends' in compiled output."), Disable_emitting_files_if_any_type_checking_errors_are_reported: i(6662, 3, "Disable_emitting_files_if_any_type_checking_errors_are_reported_6662", "Disable emitting files if any type checking errors are reported."), Disable_truncating_types_in_error_messages: i(6663, 3, "Disable_truncating_types_in_error_messages_6663", "Disable truncating types in error messages."), Enable_error_reporting_for_fallthrough_cases_in_switch_statements: i(6664, 3, "Enable_error_reporting_for_fallthrough_cases_in_switch_statements_6664", "Enable error reporting for fallthrough cases in switch statements."), Enable_error_reporting_for_expressions_and_declarations_with_an_implied_any_type: i(6665, 3, "Enable_error_reporting_for_expressions_and_declarations_with_an_implied_any_type_6665", "Enable error reporting for expressions and declarations with an implied 'any' type."), Ensure_overriding_members_in_derived_classes_are_marked_with_an_override_modifier: i(6666, 3, "Ensure_overriding_members_in_derived_classes_are_marked_with_an_override_modifier_6666", "Ensure overriding members in derived classes are marked with an override modifier."), Enable_error_reporting_for_codepaths_that_do_not_explicitly_return_in_a_function: i(6667, 3, "Enable_error_reporting_for_codepaths_that_do_not_explicitly_return_in_a_function_6667", "Enable error reporting for codepaths that do not explicitly return in a function."), Enable_error_reporting_when_this_is_given_the_type_any: i(6668, 3, "Enable_error_reporting_when_this_is_given_the_type_any_6668", "Enable error reporting when 'this' is given the type 'any'."), Disable_adding_use_strict_directives_in_emitted_JavaScript_files: i(6669, 3, "Disable_adding_use_strict_directives_in_emitted_JavaScript_files_6669", "Disable adding 'use strict' directives in emitted JavaScript files."), Disable_including_any_library_files_including_the_default_lib_d_ts: i(6670, 3, "Disable_including_any_library_files_including_the_default_lib_d_ts_6670", "Disable including any library files, including the default lib.d.ts."), Enforces_using_indexed_accessors_for_keys_declared_using_an_indexed_type: i(6671, 3, "Enforces_using_indexed_accessors_for_keys_declared_using_an_indexed_type_6671", "Enforces using indexed accessors for keys declared using an indexed type."), Disallow_import_s_require_s_or_reference_s_from_expanding_the_number_of_files_TypeScript_should_add_to_a_project: i(6672, 3, "Disallow_import_s_require_s_or_reference_s_from_expanding_the_number_of_files_TypeScript_should_add__6672", "Disallow 'import's, 'require's or ''s from expanding the number of files TypeScript should add to a project."), Disable_strict_checking_of_generic_signatures_in_function_types: i(6673, 3, "Disable_strict_checking_of_generic_signatures_in_function_types_6673", "Disable strict checking of generic signatures in function types."), Add_undefined_to_a_type_when_accessed_using_an_index: i(6674, 3, "Add_undefined_to_a_type_when_accessed_using_an_index_6674", "Add 'undefined' to a type when accessed using an index."), Enable_error_reporting_when_local_variables_aren_t_read: i(6675, 3, "Enable_error_reporting_when_local_variables_aren_t_read_6675", "Enable error reporting when local variables aren't read."), Raise_an_error_when_a_function_parameter_isn_t_read: i(6676, 3, "Raise_an_error_when_a_function_parameter_isn_t_read_6676", "Raise an error when a function parameter isn't read."), Deprecated_setting_Use_outFile_instead: i(6677, 3, "Deprecated_setting_Use_outFile_instead_6677", "Deprecated setting. Use 'outFile' instead."), Specify_an_output_folder_for_all_emitted_files: i(6678, 3, "Specify_an_output_folder_for_all_emitted_files_6678", "Specify an output folder for all emitted files."), Specify_a_file_that_bundles_all_outputs_into_one_JavaScript_file_If_declaration_is_true_also_designates_a_file_that_bundles_all_d_ts_output: i(6679, 3, "Specify_a_file_that_bundles_all_outputs_into_one_JavaScript_file_If_declaration_is_true_also_designa_6679", "Specify a file that bundles all outputs into one JavaScript file. If 'declaration' is true, also designates a file that bundles all .d.ts output."), Specify_a_set_of_entries_that_re_map_imports_to_additional_lookup_locations: i(6680, 3, "Specify_a_set_of_entries_that_re_map_imports_to_additional_lookup_locations_6680", "Specify a set of entries that re-map imports to additional lookup locations."), Specify_a_list_of_language_service_plugins_to_include: i(6681, 3, "Specify_a_list_of_language_service_plugins_to_include_6681", "Specify a list of language service plugins to include."), Disable_erasing_const_enum_declarations_in_generated_code: i(6682, 3, "Disable_erasing_const_enum_declarations_in_generated_code_6682", "Disable erasing 'const enum' declarations in generated code."), Disable_resolving_symlinks_to_their_realpath_This_correlates_to_the_same_flag_in_node: i(6683, 3, "Disable_resolving_symlinks_to_their_realpath_This_correlates_to_the_same_flag_in_node_6683", "Disable resolving symlinks to their realpath. This correlates to the same flag in node."), Disable_wiping_the_console_in_watch_mode: i(6684, 3, "Disable_wiping_the_console_in_watch_mode_6684", "Disable wiping the console in watch mode."), Enable_color_and_formatting_in_TypeScript_s_output_to_make_compiler_errors_easier_to_read: i(6685, 3, "Enable_color_and_formatting_in_TypeScript_s_output_to_make_compiler_errors_easier_to_read_6685", "Enable color and formatting in TypeScript's output to make compiler errors easier to read."), Specify_the_object_invoked_for_createElement_This_only_applies_when_targeting_react_JSX_emit: i(6686, 3, "Specify_the_object_invoked_for_createElement_This_only_applies_when_targeting_react_JSX_emit_6686", "Specify the object invoked for 'createElement'. This only applies when targeting 'react' JSX emit."), Specify_an_array_of_objects_that_specify_paths_for_projects_Used_in_project_references: i(6687, 3, "Specify_an_array_of_objects_that_specify_paths_for_projects_Used_in_project_references_6687", "Specify an array of objects that specify paths for projects. Used in project references."), Disable_emitting_comments: i(6688, 3, "Disable_emitting_comments_6688", "Disable emitting comments."), Enable_importing_json_files: i(6689, 3, "Enable_importing_json_files_6689", "Enable importing .json files."), Specify_the_root_folder_within_your_source_files: i(6690, 3, "Specify_the_root_folder_within_your_source_files_6690", "Specify the root folder within your source files."), Allow_multiple_folders_to_be_treated_as_one_when_resolving_modules: i(6691, 3, "Allow_multiple_folders_to_be_treated_as_one_when_resolving_modules_6691", "Allow multiple folders to be treated as one when resolving modules."), Skip_type_checking_d_ts_files_that_are_included_with_TypeScript: i(6692, 3, "Skip_type_checking_d_ts_files_that_are_included_with_TypeScript_6692", "Skip type checking .d.ts files that are included with TypeScript."), Skip_type_checking_all_d_ts_files: i(6693, 3, "Skip_type_checking_all_d_ts_files_6693", "Skip type checking all .d.ts files."), Create_source_map_files_for_emitted_JavaScript_files: i(6694, 3, "Create_source_map_files_for_emitted_JavaScript_files_6694", "Create source map files for emitted JavaScript files."), Specify_the_root_path_for_debuggers_to_find_the_reference_source_code: i(6695, 3, "Specify_the_root_path_for_debuggers_to_find_the_reference_source_code_6695", "Specify the root path for debuggers to find the reference source code."), Check_that_the_arguments_for_bind_call_and_apply_methods_match_the_original_function: i(6697, 3, "Check_that_the_arguments_for_bind_call_and_apply_methods_match_the_original_function_6697", "Check that the arguments for 'bind', 'call', and 'apply' methods match the original function."), When_assigning_functions_check_to_ensure_parameters_and_the_return_values_are_subtype_compatible: i(6698, 3, "When_assigning_functions_check_to_ensure_parameters_and_the_return_values_are_subtype_compatible_6698", "When assigning functions, check to ensure parameters and the return values are subtype-compatible."), When_type_checking_take_into_account_null_and_undefined: i(6699, 3, "When_type_checking_take_into_account_null_and_undefined_6699", "When type checking, take into account 'null' and 'undefined'."), Check_for_class_properties_that_are_declared_but_not_set_in_the_constructor: i(6700, 3, "Check_for_class_properties_that_are_declared_but_not_set_in_the_constructor_6700", "Check for class properties that are declared but not set in the constructor."), Disable_emitting_declarations_that_have_internal_in_their_JSDoc_comments: i(6701, 3, "Disable_emitting_declarations_that_have_internal_in_their_JSDoc_comments_6701", "Disable emitting declarations that have '@internal' in their JSDoc comments."), Disable_reporting_of_excess_property_errors_during_the_creation_of_object_literals: i(6702, 3, "Disable_reporting_of_excess_property_errors_during_the_creation_of_object_literals_6702", "Disable reporting of excess property errors during the creation of object literals."), Suppress_noImplicitAny_errors_when_indexing_objects_that_lack_index_signatures: i(6703, 3, "Suppress_noImplicitAny_errors_when_indexing_objects_that_lack_index_signatures_6703", "Suppress 'noImplicitAny' errors when indexing objects that lack index signatures."), Synchronously_call_callbacks_and_update_the_state_of_directory_watchers_on_platforms_that_don_t_support_recursive_watching_natively: i(6704, 3, "Synchronously_call_callbacks_and_update_the_state_of_directory_watchers_on_platforms_that_don_t_supp_6704", "Synchronously call callbacks and update the state of directory watchers on platforms that don`t support recursive watching natively."), Set_the_JavaScript_language_version_for_emitted_JavaScript_and_include_compatible_library_declarations: i(6705, 3, "Set_the_JavaScript_language_version_for_emitted_JavaScript_and_include_compatible_library_declaratio_6705", "Set the JavaScript language version for emitted JavaScript and include compatible library declarations."), Log_paths_used_during_the_moduleResolution_process: i(6706, 3, "Log_paths_used_during_the_moduleResolution_process_6706", "Log paths used during the 'moduleResolution' process."), Specify_the_path_to_tsbuildinfo_incremental_compilation_file: i(6707, 3, "Specify_the_path_to_tsbuildinfo_incremental_compilation_file_6707", "Specify the path to .tsbuildinfo incremental compilation file."), Specify_options_for_automatic_acquisition_of_declaration_files: i(6709, 3, "Specify_options_for_automatic_acquisition_of_declaration_files_6709", "Specify options for automatic acquisition of declaration files."), Specify_multiple_folders_that_act_like_Slashnode_modules_Slash_types: i(6710, 3, "Specify_multiple_folders_that_act_like_Slashnode_modules_Slash_types_6710", "Specify multiple folders that act like './node_modules/@types'."), Specify_type_package_names_to_be_included_without_being_referenced_in_a_source_file: i(6711, 3, "Specify_type_package_names_to_be_included_without_being_referenced_in_a_source_file_6711", "Specify type package names to be included without being referenced in a source file."), Emit_ECMAScript_standard_compliant_class_fields: i(6712, 3, "Emit_ECMAScript_standard_compliant_class_fields_6712", "Emit ECMAScript-standard-compliant class fields."), Enable_verbose_logging: i(6713, 3, "Enable_verbose_logging_6713", "Enable verbose logging."), Specify_how_directories_are_watched_on_systems_that_lack_recursive_file_watching_functionality: i(6714, 3, "Specify_how_directories_are_watched_on_systems_that_lack_recursive_file_watching_functionality_6714", "Specify how directories are watched on systems that lack recursive file-watching functionality."), Specify_how_the_TypeScript_watch_mode_works: i(6715, 3, "Specify_how_the_TypeScript_watch_mode_works_6715", "Specify how the TypeScript watch mode works."), Require_undeclared_properties_from_index_signatures_to_use_element_accesses: i(6717, 3, "Require_undeclared_properties_from_index_signatures_to_use_element_accesses_6717", "Require undeclared properties from index signatures to use element accesses."), Specify_emit_Slashchecking_behavior_for_imports_that_are_only_used_for_types: i(6718, 3, "Specify_emit_Slashchecking_behavior_for_imports_that_are_only_used_for_types_6718", "Specify emit/checking behavior for imports that are only used for types."), Default_catch_clause_variables_as_unknown_instead_of_any: i(6803, 3, "Default_catch_clause_variables_as_unknown_instead_of_any_6803", "Default catch clause variables as 'unknown' instead of 'any'."), Do_not_transform_or_elide_any_imports_or_exports_not_marked_as_type_only_ensuring_they_are_written_in_the_output_file_s_format_based_on_the_module_setting: i(6804, 3, "Do_not_transform_or_elide_any_imports_or_exports_not_marked_as_type_only_ensuring_they_are_written_i_6804", "Do not transform or elide any imports or exports not marked as type-only, ensuring they are written in the output file's format based on the 'module' setting."), one_of_Colon: i(6900, 3, "one_of_Colon_6900", "one of:"), one_or_more_Colon: i(6901, 3, "one_or_more_Colon_6901", "one or more:"), type_Colon: i(6902, 3, "type_Colon_6902", "type:"), default_Colon: i(6903, 3, "default_Colon_6903", "default:"), module_system_or_esModuleInterop: i(6904, 3, "module_system_or_esModuleInterop_6904", 'module === "system" or esModuleInterop'), false_unless_strict_is_set: i(6905, 3, "false_unless_strict_is_set_6905", "`false`, unless `strict` is set"), false_unless_composite_is_set: i(6906, 3, "false_unless_composite_is_set_6906", "`false`, unless `composite` is set"), node_modules_bower_components_jspm_packages_plus_the_value_of_outDir_if_one_is_specified: i(6907, 3, "node_modules_bower_components_jspm_packages_plus_the_value_of_outDir_if_one_is_specified_6907", '`["node_modules", "bower_components", "jspm_packages"]`, plus the value of `outDir` if one is specified.'), if_files_is_specified_otherwise_Asterisk_Asterisk_Slash_Asterisk: i(6908, 3, "if_files_is_specified_otherwise_Asterisk_Asterisk_Slash_Asterisk_6908", '`[]` if `files` is specified, otherwise `["**/*"]`'), true_if_composite_false_otherwise: i(6909, 3, "true_if_composite_false_otherwise_6909", "`true` if `composite`, `false` otherwise"), module_AMD_or_UMD_or_System_or_ES6_then_Classic_Otherwise_Node: i(69010, 3, "module_AMD_or_UMD_or_System_or_ES6_then_Classic_Otherwise_Node_69010", "module === `AMD` or `UMD` or `System` or `ES6`, then `Classic`, Otherwise `Node`"), Computed_from_the_list_of_input_files: i(6911, 3, "Computed_from_the_list_of_input_files_6911", "Computed from the list of input files"), Platform_specific: i(6912, 3, "Platform_specific_6912", "Platform specific"), You_can_learn_about_all_of_the_compiler_options_at_0: i(6913, 3, "You_can_learn_about_all_of_the_compiler_options_at_0_6913", "You can learn about all of the compiler options at {0}"), Including_watch_w_will_start_watching_the_current_project_for_the_file_changes_Once_set_you_can_config_watch_mode_with_Colon: i(6914, 3, "Including_watch_w_will_start_watching_the_current_project_for_the_file_changes_Once_set_you_can_conf_6914", "Including --watch, -w will start watching the current project for the file changes. Once set, you can config watch mode with:"), Using_build_b_will_make_tsc_behave_more_like_a_build_orchestrator_than_a_compiler_This_is_used_to_trigger_building_composite_projects_which_you_can_learn_more_about_at_0: i(6915, 3, "Using_build_b_will_make_tsc_behave_more_like_a_build_orchestrator_than_a_compiler_This_is_used_to_tr_6915", "Using --build, -b will make tsc behave more like a build orchestrator than a compiler. This is used to trigger building composite projects which you can learn more about at {0}"), COMMON_COMMANDS: i(6916, 3, "COMMON_COMMANDS_6916", "COMMON COMMANDS"), ALL_COMPILER_OPTIONS: i(6917, 3, "ALL_COMPILER_OPTIONS_6917", "ALL COMPILER OPTIONS"), WATCH_OPTIONS: i(6918, 3, "WATCH_OPTIONS_6918", "WATCH OPTIONS"), BUILD_OPTIONS: i(6919, 3, "BUILD_OPTIONS_6919", "BUILD OPTIONS"), COMMON_COMPILER_OPTIONS: i(6920, 3, "COMMON_COMPILER_OPTIONS_6920", "COMMON COMPILER OPTIONS"), COMMAND_LINE_FLAGS: i(6921, 3, "COMMAND_LINE_FLAGS_6921", "COMMAND LINE FLAGS"), tsc_Colon_The_TypeScript_Compiler: i(6922, 3, "tsc_Colon_The_TypeScript_Compiler_6922", "tsc: The TypeScript Compiler"), Compiles_the_current_project_tsconfig_json_in_the_working_directory: i(6923, 3, "Compiles_the_current_project_tsconfig_json_in_the_working_directory_6923", "Compiles the current project (tsconfig.json in the working directory.)"), Ignoring_tsconfig_json_compiles_the_specified_files_with_default_compiler_options: i(6924, 3, "Ignoring_tsconfig_json_compiles_the_specified_files_with_default_compiler_options_6924", "Ignoring tsconfig.json, compiles the specified files with default compiler options."), Build_a_composite_project_in_the_working_directory: i(6925, 3, "Build_a_composite_project_in_the_working_directory_6925", "Build a composite project in the working directory."), Creates_a_tsconfig_json_with_the_recommended_settings_in_the_working_directory: i(6926, 3, "Creates_a_tsconfig_json_with_the_recommended_settings_in_the_working_directory_6926", "Creates a tsconfig.json with the recommended settings in the working directory."), Compiles_the_TypeScript_project_located_at_the_specified_path: i(6927, 3, "Compiles_the_TypeScript_project_located_at_the_specified_path_6927", "Compiles the TypeScript project located at the specified path."), An_expanded_version_of_this_information_showing_all_possible_compiler_options: i(6928, 3, "An_expanded_version_of_this_information_showing_all_possible_compiler_options_6928", "An expanded version of this information, showing all possible compiler options"), Compiles_the_current_project_with_additional_settings: i(6929, 3, "Compiles_the_current_project_with_additional_settings_6929", "Compiles the current project, with additional settings."), true_for_ES2022_and_above_including_ESNext: i(6930, 3, "true_for_ES2022_and_above_including_ESNext_6930", "`true` for ES2022 and above, including ESNext."), List_of_file_name_suffixes_to_search_when_resolving_a_module: i(6931, 1, "List_of_file_name_suffixes_to_search_when_resolving_a_module_6931", "List of file name suffixes to search when resolving a module."), Variable_0_implicitly_has_an_1_type: i(7005, 1, "Variable_0_implicitly_has_an_1_type_7005", "Variable '{0}' implicitly has an '{1}' type."), Parameter_0_implicitly_has_an_1_type: i(7006, 1, "Parameter_0_implicitly_has_an_1_type_7006", "Parameter '{0}' implicitly has an '{1}' type."), Member_0_implicitly_has_an_1_type: i(7008, 1, "Member_0_implicitly_has_an_1_type_7008", "Member '{0}' implicitly has an '{1}' type."), new_expression_whose_target_lacks_a_construct_signature_implicitly_has_an_any_type: i(7009, 1, "new_expression_whose_target_lacks_a_construct_signature_implicitly_has_an_any_type_7009", "'new' expression, whose target lacks a construct signature, implicitly has an 'any' type."), _0_which_lacks_return_type_annotation_implicitly_has_an_1_return_type: i(7010, 1, "_0_which_lacks_return_type_annotation_implicitly_has_an_1_return_type_7010", "'{0}', which lacks return-type annotation, implicitly has an '{1}' return type."), Function_expression_which_lacks_return_type_annotation_implicitly_has_an_0_return_type: i(7011, 1, "Function_expression_which_lacks_return_type_annotation_implicitly_has_an_0_return_type_7011", "Function expression, which lacks return-type annotation, implicitly has an '{0}' return type."), This_overload_implicitly_returns_the_type_0_because_it_lacks_a_return_type_annotation: i(7012, 1, "This_overload_implicitly_returns_the_type_0_because_it_lacks_a_return_type_annotation_7012", "This overload implicitly returns the type '{0}' because it lacks a return type annotation."), Construct_signature_which_lacks_return_type_annotation_implicitly_has_an_any_return_type: i(7013, 1, "Construct_signature_which_lacks_return_type_annotation_implicitly_has_an_any_return_type_7013", "Construct signature, which lacks return-type annotation, implicitly has an 'any' return type."), Function_type_which_lacks_return_type_annotation_implicitly_has_an_0_return_type: i(7014, 1, "Function_type_which_lacks_return_type_annotation_implicitly_has_an_0_return_type_7014", "Function type, which lacks return-type annotation, implicitly has an '{0}' return type."), Element_implicitly_has_an_any_type_because_index_expression_is_not_of_type_number: i(7015, 1, "Element_implicitly_has_an_any_type_because_index_expression_is_not_of_type_number_7015", "Element implicitly has an 'any' type because index expression is not of type 'number'."), Could_not_find_a_declaration_file_for_module_0_1_implicitly_has_an_any_type: i(7016, 1, "Could_not_find_a_declaration_file_for_module_0_1_implicitly_has_an_any_type_7016", "Could not find a declaration file for module '{0}'. '{1}' implicitly has an 'any' type."), Element_implicitly_has_an_any_type_because_type_0_has_no_index_signature: i(7017, 1, "Element_implicitly_has_an_any_type_because_type_0_has_no_index_signature_7017", "Element implicitly has an 'any' type because type '{0}' has no index signature."), Object_literal_s_property_0_implicitly_has_an_1_type: i(7018, 1, "Object_literal_s_property_0_implicitly_has_an_1_type_7018", "Object literal's property '{0}' implicitly has an '{1}' type."), Rest_parameter_0_implicitly_has_an_any_type: i(7019, 1, "Rest_parameter_0_implicitly_has_an_any_type_7019", "Rest parameter '{0}' implicitly has an 'any[]' type."), Call_signature_which_lacks_return_type_annotation_implicitly_has_an_any_return_type: i(7020, 1, "Call_signature_which_lacks_return_type_annotation_implicitly_has_an_any_return_type_7020", "Call signature, which lacks return-type annotation, implicitly has an 'any' return type."), _0_implicitly_has_type_any_because_it_does_not_have_a_type_annotation_and_is_referenced_directly_or_indirectly_in_its_own_initializer: i(7022, 1, "_0_implicitly_has_type_any_because_it_does_not_have_a_type_annotation_and_is_referenced_directly_or__7022", "'{0}' implicitly has type 'any' because it does not have a type annotation and is referenced directly or indirectly in its own initializer."), _0_implicitly_has_return_type_any_because_it_does_not_have_a_return_type_annotation_and_is_referenced_directly_or_indirectly_in_one_of_its_return_expressions: i(7023, 1, "_0_implicitly_has_return_type_any_because_it_does_not_have_a_return_type_annotation_and_is_reference_7023", "'{0}' implicitly has return type 'any' because it does not have a return type annotation and is referenced directly or indirectly in one of its return expressions."), Function_implicitly_has_return_type_any_because_it_does_not_have_a_return_type_annotation_and_is_referenced_directly_or_indirectly_in_one_of_its_return_expressions: i(7024, 1, "Function_implicitly_has_return_type_any_because_it_does_not_have_a_return_type_annotation_and_is_ref_7024", "Function implicitly has return type 'any' because it does not have a return type annotation and is referenced directly or indirectly in one of its return expressions."), Generator_implicitly_has_yield_type_0_because_it_does_not_yield_any_values_Consider_supplying_a_return_type_annotation: i(7025, 1, "Generator_implicitly_has_yield_type_0_because_it_does_not_yield_any_values_Consider_supplying_a_retu_7025", "Generator implicitly has yield type '{0}' because it does not yield any values. Consider supplying a return type annotation."), JSX_element_implicitly_has_type_any_because_no_interface_JSX_0_exists: i(7026, 1, "JSX_element_implicitly_has_type_any_because_no_interface_JSX_0_exists_7026", "JSX element implicitly has type 'any' because no interface 'JSX.{0}' exists."), Unreachable_code_detected: i(7027, 1, "Unreachable_code_detected_7027", "Unreachable code detected.", true), Unused_label: i(7028, 1, "Unused_label_7028", "Unused label.", true), Fallthrough_case_in_switch: i(7029, 1, "Fallthrough_case_in_switch_7029", "Fallthrough case in switch."), Not_all_code_paths_return_a_value: i(7030, 1, "Not_all_code_paths_return_a_value_7030", "Not all code paths return a value."), Binding_element_0_implicitly_has_an_1_type: i(7031, 1, "Binding_element_0_implicitly_has_an_1_type_7031", "Binding element '{0}' implicitly has an '{1}' type."), Property_0_implicitly_has_type_any_because_its_set_accessor_lacks_a_parameter_type_annotation: i(7032, 1, "Property_0_implicitly_has_type_any_because_its_set_accessor_lacks_a_parameter_type_annotation_7032", "Property '{0}' implicitly has type 'any', because its set accessor lacks a parameter type annotation."), Property_0_implicitly_has_type_any_because_its_get_accessor_lacks_a_return_type_annotation: i(7033, 1, "Property_0_implicitly_has_type_any_because_its_get_accessor_lacks_a_return_type_annotation_7033", "Property '{0}' implicitly has type 'any', because its get accessor lacks a return type annotation."), Variable_0_implicitly_has_type_1_in_some_locations_where_its_type_cannot_be_determined: i(7034, 1, "Variable_0_implicitly_has_type_1_in_some_locations_where_its_type_cannot_be_determined_7034", "Variable '{0}' implicitly has type '{1}' in some locations where its type cannot be determined."), Try_npm_i_save_dev_types_Slash_1_if_it_exists_or_add_a_new_declaration_d_ts_file_containing_declare_module_0: i(7035, 1, "Try_npm_i_save_dev_types_Slash_1_if_it_exists_or_add_a_new_declaration_d_ts_file_containing_declare__7035", "Try `npm i --save-dev @types/{1}` if it exists or add a new declaration (.d.ts) file containing `declare module '{0}';`"), Dynamic_import_s_specifier_must_be_of_type_string_but_here_has_type_0: i(7036, 1, "Dynamic_import_s_specifier_must_be_of_type_string_but_here_has_type_0_7036", "Dynamic import's specifier must be of type 'string', but here has type '{0}'."), Enables_emit_interoperability_between_CommonJS_and_ES_Modules_via_creation_of_namespace_objects_for_all_imports_Implies_allowSyntheticDefaultImports: i(7037, 3, "Enables_emit_interoperability_between_CommonJS_and_ES_Modules_via_creation_of_namespace_objects_for__7037", "Enables emit interoperability between CommonJS and ES Modules via creation of namespace objects for all imports. Implies 'allowSyntheticDefaultImports'."), Type_originates_at_this_import_A_namespace_style_import_cannot_be_called_or_constructed_and_will_cause_a_failure_at_runtime_Consider_using_a_default_import_or_import_require_here_instead: i(7038, 3, "Type_originates_at_this_import_A_namespace_style_import_cannot_be_called_or_constructed_and_will_cau_7038", "Type originates at this import. A namespace-style import cannot be called or constructed, and will cause a failure at runtime. Consider using a default import or import require here instead."), Mapped_object_type_implicitly_has_an_any_template_type: i(7039, 1, "Mapped_object_type_implicitly_has_an_any_template_type_7039", "Mapped object type implicitly has an 'any' template type."), If_the_0_package_actually_exposes_this_module_consider_sending_a_pull_request_to_amend_https_Colon_Slash_Slashgithub_com_SlashDefinitelyTyped_SlashDefinitelyTyped_Slashtree_Slashmaster_Slashtypes_Slash_1: i(7040, 1, "If_the_0_package_actually_exposes_this_module_consider_sending_a_pull_request_to_amend_https_Colon_S_7040", "If the '{0}' package actually exposes this module, consider sending a pull request to amend 'https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/{1}'"), The_containing_arrow_function_captures_the_global_value_of_this: i(7041, 1, "The_containing_arrow_function_captures_the_global_value_of_this_7041", "The containing arrow function captures the global value of 'this'."), Module_0_was_resolved_to_1_but_resolveJsonModule_is_not_used: i(7042, 1, "Module_0_was_resolved_to_1_but_resolveJsonModule_is_not_used_7042", "Module '{0}' was resolved to '{1}', but '--resolveJsonModule' is not used."), Variable_0_implicitly_has_an_1_type_but_a_better_type_may_be_inferred_from_usage: i(7043, 2, "Variable_0_implicitly_has_an_1_type_but_a_better_type_may_be_inferred_from_usage_7043", "Variable '{0}' implicitly has an '{1}' type, but a better type may be inferred from usage."), Parameter_0_implicitly_has_an_1_type_but_a_better_type_may_be_inferred_from_usage: i(7044, 2, "Parameter_0_implicitly_has_an_1_type_but_a_better_type_may_be_inferred_from_usage_7044", "Parameter '{0}' implicitly has an '{1}' type, but a better type may be inferred from usage."), Member_0_implicitly_has_an_1_type_but_a_better_type_may_be_inferred_from_usage: i(7045, 2, "Member_0_implicitly_has_an_1_type_but_a_better_type_may_be_inferred_from_usage_7045", "Member '{0}' implicitly has an '{1}' type, but a better type may be inferred from usage."), Variable_0_implicitly_has_type_1_in_some_locations_but_a_better_type_may_be_inferred_from_usage: i(7046, 2, "Variable_0_implicitly_has_type_1_in_some_locations_but_a_better_type_may_be_inferred_from_usage_7046", "Variable '{0}' implicitly has type '{1}' in some locations, but a better type may be inferred from usage."), Rest_parameter_0_implicitly_has_an_any_type_but_a_better_type_may_be_inferred_from_usage: i(7047, 2, "Rest_parameter_0_implicitly_has_an_any_type_but_a_better_type_may_be_inferred_from_usage_7047", "Rest parameter '{0}' implicitly has an 'any[]' type, but a better type may be inferred from usage."), Property_0_implicitly_has_type_any_but_a_better_type_for_its_get_accessor_may_be_inferred_from_usage: i(7048, 2, "Property_0_implicitly_has_type_any_but_a_better_type_for_its_get_accessor_may_be_inferred_from_usage_7048", "Property '{0}' implicitly has type 'any', but a better type for its get accessor may be inferred from usage."), Property_0_implicitly_has_type_any_but_a_better_type_for_its_set_accessor_may_be_inferred_from_usage: i(7049, 2, "Property_0_implicitly_has_type_any_but_a_better_type_for_its_set_accessor_may_be_inferred_from_usage_7049", "Property '{0}' implicitly has type 'any', but a better type for its set accessor may be inferred from usage."), _0_implicitly_has_an_1_return_type_but_a_better_type_may_be_inferred_from_usage: i(7050, 2, "_0_implicitly_has_an_1_return_type_but_a_better_type_may_be_inferred_from_usage_7050", "'{0}' implicitly has an '{1}' return type, but a better type may be inferred from usage."), Parameter_has_a_name_but_no_type_Did_you_mean_0_Colon_1: i(7051, 1, "Parameter_has_a_name_but_no_type_Did_you_mean_0_Colon_1_7051", "Parameter has a name but no type. Did you mean '{0}: {1}'?"), Element_implicitly_has_an_any_type_because_type_0_has_no_index_signature_Did_you_mean_to_call_1: i(7052, 1, "Element_implicitly_has_an_any_type_because_type_0_has_no_index_signature_Did_you_mean_to_call_1_7052", "Element implicitly has an 'any' type because type '{0}' has no index signature. Did you mean to call '{1}'?"), Element_implicitly_has_an_any_type_because_expression_of_type_0_can_t_be_used_to_index_type_1: i(7053, 1, "Element_implicitly_has_an_any_type_because_expression_of_type_0_can_t_be_used_to_index_type_1_7053", "Element implicitly has an 'any' type because expression of type '{0}' can't be used to index type '{1}'."), No_index_signature_with_a_parameter_of_type_0_was_found_on_type_1: i(7054, 1, "No_index_signature_with_a_parameter_of_type_0_was_found_on_type_1_7054", "No index signature with a parameter of type '{0}' was found on type '{1}'."), _0_which_lacks_return_type_annotation_implicitly_has_an_1_yield_type: i(7055, 1, "_0_which_lacks_return_type_annotation_implicitly_has_an_1_yield_type_7055", "'{0}', which lacks return-type annotation, implicitly has an '{1}' yield type."), The_inferred_type_of_this_node_exceeds_the_maximum_length_the_compiler_will_serialize_An_explicit_type_annotation_is_needed: i(7056, 1, "The_inferred_type_of_this_node_exceeds_the_maximum_length_the_compiler_will_serialize_An_explicit_ty_7056", "The inferred type of this node exceeds the maximum length the compiler will serialize. An explicit type annotation is needed."), yield_expression_implicitly_results_in_an_any_type_because_its_containing_generator_lacks_a_return_type_annotation: i(7057, 1, "yield_expression_implicitly_results_in_an_any_type_because_its_containing_generator_lacks_a_return_t_7057", "'yield' expression implicitly results in an 'any' type because its containing generator lacks a return-type annotation."), If_the_0_package_actually_exposes_this_module_try_adding_a_new_declaration_d_ts_file_containing_declare_module_1: i(7058, 1, "If_the_0_package_actually_exposes_this_module_try_adding_a_new_declaration_d_ts_file_containing_decl_7058", "If the '{0}' package actually exposes this module, try adding a new declaration (.d.ts) file containing `declare module '{1}';`"), This_syntax_is_reserved_in_files_with_the_mts_or_cts_extension_Use_an_as_expression_instead: i(7059, 1, "This_syntax_is_reserved_in_files_with_the_mts_or_cts_extension_Use_an_as_expression_instead_7059", "This syntax is reserved in files with the .mts or .cts extension. Use an `as` expression instead."), This_syntax_is_reserved_in_files_with_the_mts_or_cts_extension_Add_a_trailing_comma_or_explicit_constraint: i(7060, 1, "This_syntax_is_reserved_in_files_with_the_mts_or_cts_extension_Add_a_trailing_comma_or_explicit_cons_7060", "This syntax is reserved in files with the .mts or .cts extension. Add a trailing comma or explicit constraint."), A_mapped_type_may_not_declare_properties_or_methods: i(7061, 1, "A_mapped_type_may_not_declare_properties_or_methods_7061", "A mapped type may not declare properties or methods."), You_cannot_rename_this_element: i(8e3, 1, "You_cannot_rename_this_element_8000", "You cannot rename this element."), You_cannot_rename_elements_that_are_defined_in_the_standard_TypeScript_library: i(8001, 1, "You_cannot_rename_elements_that_are_defined_in_the_standard_TypeScript_library_8001", "You cannot rename elements that are defined in the standard TypeScript library."), import_can_only_be_used_in_TypeScript_files: i(8002, 1, "import_can_only_be_used_in_TypeScript_files_8002", "'import ... =' can only be used in TypeScript files."), export_can_only_be_used_in_TypeScript_files: i(8003, 1, "export_can_only_be_used_in_TypeScript_files_8003", "'export =' can only be used in TypeScript files."), Type_parameter_declarations_can_only_be_used_in_TypeScript_files: i(8004, 1, "Type_parameter_declarations_can_only_be_used_in_TypeScript_files_8004", "Type parameter declarations can only be used in TypeScript files."), implements_clauses_can_only_be_used_in_TypeScript_files: i(8005, 1, "implements_clauses_can_only_be_used_in_TypeScript_files_8005", "'implements' clauses can only be used in TypeScript files."), _0_declarations_can_only_be_used_in_TypeScript_files: i(8006, 1, "_0_declarations_can_only_be_used_in_TypeScript_files_8006", "'{0}' declarations can only be used in TypeScript files."), Type_aliases_can_only_be_used_in_TypeScript_files: i(8008, 1, "Type_aliases_can_only_be_used_in_TypeScript_files_8008", "Type aliases can only be used in TypeScript files."), The_0_modifier_can_only_be_used_in_TypeScript_files: i(8009, 1, "The_0_modifier_can_only_be_used_in_TypeScript_files_8009", "The '{0}' modifier can only be used in TypeScript files."), Type_annotations_can_only_be_used_in_TypeScript_files: i(8010, 1, "Type_annotations_can_only_be_used_in_TypeScript_files_8010", "Type annotations can only be used in TypeScript files."), Type_arguments_can_only_be_used_in_TypeScript_files: i(8011, 1, "Type_arguments_can_only_be_used_in_TypeScript_files_8011", "Type arguments can only be used in TypeScript files."), Parameter_modifiers_can_only_be_used_in_TypeScript_files: i(8012, 1, "Parameter_modifiers_can_only_be_used_in_TypeScript_files_8012", "Parameter modifiers can only be used in TypeScript files."), Non_null_assertions_can_only_be_used_in_TypeScript_files: i(8013, 1, "Non_null_assertions_can_only_be_used_in_TypeScript_files_8013", "Non-null assertions can only be used in TypeScript files."), Type_assertion_expressions_can_only_be_used_in_TypeScript_files: i(8016, 1, "Type_assertion_expressions_can_only_be_used_in_TypeScript_files_8016", "Type assertion expressions can only be used in TypeScript files."), Octal_literal_types_must_use_ES2015_syntax_Use_the_syntax_0: i(8017, 1, "Octal_literal_types_must_use_ES2015_syntax_Use_the_syntax_0_8017", "Octal literal types must use ES2015 syntax. Use the syntax '{0}'."), Octal_literals_are_not_allowed_in_enums_members_initializer_Use_the_syntax_0: i(8018, 1, "Octal_literals_are_not_allowed_in_enums_members_initializer_Use_the_syntax_0_8018", "Octal literals are not allowed in enums members initializer. Use the syntax '{0}'."), Report_errors_in_js_files: i(8019, 3, "Report_errors_in_js_files_8019", "Report errors in .js files."), JSDoc_types_can_only_be_used_inside_documentation_comments: i(8020, 1, "JSDoc_types_can_only_be_used_inside_documentation_comments_8020", "JSDoc types can only be used inside documentation comments."), JSDoc_typedef_tag_should_either_have_a_type_annotation_or_be_followed_by_property_or_member_tags: i(8021, 1, "JSDoc_typedef_tag_should_either_have_a_type_annotation_or_be_followed_by_property_or_member_tags_8021", "JSDoc '@typedef' tag should either have a type annotation or be followed by '@property' or '@member' tags."), JSDoc_0_is_not_attached_to_a_class: i(8022, 1, "JSDoc_0_is_not_attached_to_a_class_8022", "JSDoc '@{0}' is not attached to a class."), JSDoc_0_1_does_not_match_the_extends_2_clause: i(8023, 1, "JSDoc_0_1_does_not_match_the_extends_2_clause_8023", "JSDoc '@{0} {1}' does not match the 'extends {2}' clause."), JSDoc_param_tag_has_name_0_but_there_is_no_parameter_with_that_name: i(8024, 1, "JSDoc_param_tag_has_name_0_but_there_is_no_parameter_with_that_name_8024", "JSDoc '@param' tag has name '{0}', but there is no parameter with that name."), Class_declarations_cannot_have_more_than_one_augments_or_extends_tag: i(8025, 1, "Class_declarations_cannot_have_more_than_one_augments_or_extends_tag_8025", "Class declarations cannot have more than one '@augments' or '@extends' tag."), Expected_0_type_arguments_provide_these_with_an_extends_tag: i(8026, 1, "Expected_0_type_arguments_provide_these_with_an_extends_tag_8026", "Expected {0} type arguments; provide these with an '@extends' tag."), Expected_0_1_type_arguments_provide_these_with_an_extends_tag: i(8027, 1, "Expected_0_1_type_arguments_provide_these_with_an_extends_tag_8027", "Expected {0}-{1} type arguments; provide these with an '@extends' tag."), JSDoc_may_only_appear_in_the_last_parameter_of_a_signature: i(8028, 1, "JSDoc_may_only_appear_in_the_last_parameter_of_a_signature_8028", "JSDoc '...' may only appear in the last parameter of a signature."), JSDoc_param_tag_has_name_0_but_there_is_no_parameter_with_that_name_It_would_match_arguments_if_it_had_an_array_type: i(8029, 1, "JSDoc_param_tag_has_name_0_but_there_is_no_parameter_with_that_name_It_would_match_arguments_if_it_h_8029", "JSDoc '@param' tag has name '{0}', but there is no parameter with that name. It would match 'arguments' if it had an array type."), The_type_of_a_function_declaration_must_match_the_function_s_signature: i(8030, 1, "The_type_of_a_function_declaration_must_match_the_function_s_signature_8030", "The type of a function declaration must match the function's signature."), You_cannot_rename_a_module_via_a_global_import: i(8031, 1, "You_cannot_rename_a_module_via_a_global_import_8031", "You cannot rename a module via a global import."), Qualified_name_0_is_not_allowed_without_a_leading_param_object_1: i(8032, 1, "Qualified_name_0_is_not_allowed_without_a_leading_param_object_1_8032", "Qualified name '{0}' is not allowed without a leading '@param {object} {1}'."), A_JSDoc_typedef_comment_may_not_contain_multiple_type_tags: i(8033, 1, "A_JSDoc_typedef_comment_may_not_contain_multiple_type_tags_8033", "A JSDoc '@typedef' comment may not contain multiple '@type' tags."), The_tag_was_first_specified_here: i(8034, 1, "The_tag_was_first_specified_here_8034", "The tag was first specified here."), You_cannot_rename_elements_that_are_defined_in_a_node_modules_folder: i(8035, 1, "You_cannot_rename_elements_that_are_defined_in_a_node_modules_folder_8035", "You cannot rename elements that are defined in a 'node_modules' folder."), You_cannot_rename_elements_that_are_defined_in_another_node_modules_folder: i(8036, 1, "You_cannot_rename_elements_that_are_defined_in_another_node_modules_folder_8036", "You cannot rename elements that are defined in another 'node_modules' folder."), Type_satisfaction_expressions_can_only_be_used_in_TypeScript_files: i(8037, 1, "Type_satisfaction_expressions_can_only_be_used_in_TypeScript_files_8037", "Type satisfaction expressions can only be used in TypeScript files."), Decorators_may_not_appear_after_export_or_export_default_if_they_also_appear_before_export: i(8038, 1, "Decorators_may_not_appear_after_export_or_export_default_if_they_also_appear_before_export_8038", "Decorators may not appear after 'export' or 'export default' if they also appear before 'export'."), Declaration_emit_for_this_file_requires_using_private_name_0_An_explicit_type_annotation_may_unblock_declaration_emit: i(9005, 1, "Declaration_emit_for_this_file_requires_using_private_name_0_An_explicit_type_annotation_may_unblock_9005", "Declaration emit for this file requires using private name '{0}'. An explicit type annotation may unblock declaration emit."), Declaration_emit_for_this_file_requires_using_private_name_0_from_module_1_An_explicit_type_annotation_may_unblock_declaration_emit: i(9006, 1, "Declaration_emit_for_this_file_requires_using_private_name_0_from_module_1_An_explicit_type_annotati_9006", "Declaration emit for this file requires using private name '{0}' from module '{1}'. An explicit type annotation may unblock declaration emit."), JSX_attributes_must_only_be_assigned_a_non_empty_expression: i(17e3, 1, "JSX_attributes_must_only_be_assigned_a_non_empty_expression_17000", "JSX attributes must only be assigned a non-empty 'expression'."), JSX_elements_cannot_have_multiple_attributes_with_the_same_name: i(17001, 1, "JSX_elements_cannot_have_multiple_attributes_with_the_same_name_17001", "JSX elements cannot have multiple attributes with the same name."), Expected_corresponding_JSX_closing_tag_for_0: i(17002, 1, "Expected_corresponding_JSX_closing_tag_for_0_17002", "Expected corresponding JSX closing tag for '{0}'."), Cannot_use_JSX_unless_the_jsx_flag_is_provided: i(17004, 1, "Cannot_use_JSX_unless_the_jsx_flag_is_provided_17004", "Cannot use JSX unless the '--jsx' flag is provided."), A_constructor_cannot_contain_a_super_call_when_its_class_extends_null: i(17005, 1, "A_constructor_cannot_contain_a_super_call_when_its_class_extends_null_17005", "A constructor cannot contain a 'super' call when its class extends 'null'."), An_unary_expression_with_the_0_operator_is_not_allowed_in_the_left_hand_side_of_an_exponentiation_expression_Consider_enclosing_the_expression_in_parentheses: i(17006, 1, "An_unary_expression_with_the_0_operator_is_not_allowed_in_the_left_hand_side_of_an_exponentiation_ex_17006", "An unary expression with the '{0}' operator is not allowed in the left-hand side of an exponentiation expression. Consider enclosing the expression in parentheses."), A_type_assertion_expression_is_not_allowed_in_the_left_hand_side_of_an_exponentiation_expression_Consider_enclosing_the_expression_in_parentheses: i(17007, 1, "A_type_assertion_expression_is_not_allowed_in_the_left_hand_side_of_an_exponentiation_expression_Con_17007", "A type assertion expression is not allowed in the left-hand side of an exponentiation expression. Consider enclosing the expression in parentheses."), JSX_element_0_has_no_corresponding_closing_tag: i(17008, 1, "JSX_element_0_has_no_corresponding_closing_tag_17008", "JSX element '{0}' has no corresponding closing tag."), super_must_be_called_before_accessing_this_in_the_constructor_of_a_derived_class: i(17009, 1, "super_must_be_called_before_accessing_this_in_the_constructor_of_a_derived_class_17009", "'super' must be called before accessing 'this' in the constructor of a derived class."), Unknown_type_acquisition_option_0: i(17010, 1, "Unknown_type_acquisition_option_0_17010", "Unknown type acquisition option '{0}'."), super_must_be_called_before_accessing_a_property_of_super_in_the_constructor_of_a_derived_class: i(17011, 1, "super_must_be_called_before_accessing_a_property_of_super_in_the_constructor_of_a_derived_class_17011", "'super' must be called before accessing a property of 'super' in the constructor of a derived class."), _0_is_not_a_valid_meta_property_for_keyword_1_Did_you_mean_2: i(17012, 1, "_0_is_not_a_valid_meta_property_for_keyword_1_Did_you_mean_2_17012", "'{0}' is not a valid meta-property for keyword '{1}'. Did you mean '{2}'?"), Meta_property_0_is_only_allowed_in_the_body_of_a_function_declaration_function_expression_or_constructor: i(17013, 1, "Meta_property_0_is_only_allowed_in_the_body_of_a_function_declaration_function_expression_or_constru_17013", "Meta-property '{0}' is only allowed in the body of a function declaration, function expression, or constructor."), JSX_fragment_has_no_corresponding_closing_tag: i(17014, 1, "JSX_fragment_has_no_corresponding_closing_tag_17014", "JSX fragment has no corresponding closing tag."), Expected_corresponding_closing_tag_for_JSX_fragment: i(17015, 1, "Expected_corresponding_closing_tag_for_JSX_fragment_17015", "Expected corresponding closing tag for JSX fragment."), The_jsxFragmentFactory_compiler_option_must_be_provided_to_use_JSX_fragments_with_the_jsxFactory_compiler_option: i(17016, 1, "The_jsxFragmentFactory_compiler_option_must_be_provided_to_use_JSX_fragments_with_the_jsxFactory_com_17016", "The 'jsxFragmentFactory' compiler option must be provided to use JSX fragments with the 'jsxFactory' compiler option."), An_jsxFrag_pragma_is_required_when_using_an_jsx_pragma_with_JSX_fragments: i(17017, 1, "An_jsxFrag_pragma_is_required_when_using_an_jsx_pragma_with_JSX_fragments_17017", "An @jsxFrag pragma is required when using an @jsx pragma with JSX fragments."), Unknown_type_acquisition_option_0_Did_you_mean_1: i(17018, 1, "Unknown_type_acquisition_option_0_Did_you_mean_1_17018", "Unknown type acquisition option '{0}'. Did you mean '{1}'?"), _0_at_the_end_of_a_type_is_not_valid_TypeScript_syntax_Did_you_mean_to_write_1: i(17019, 1, "_0_at_the_end_of_a_type_is_not_valid_TypeScript_syntax_Did_you_mean_to_write_1_17019", "'{0}' at the end of a type is not valid TypeScript syntax. Did you mean to write '{1}'?"), _0_at_the_start_of_a_type_is_not_valid_TypeScript_syntax_Did_you_mean_to_write_1: i(17020, 1, "_0_at_the_start_of_a_type_is_not_valid_TypeScript_syntax_Did_you_mean_to_write_1_17020", "'{0}' at the start of a type is not valid TypeScript syntax. Did you mean to write '{1}'?"), Circularity_detected_while_resolving_configuration_Colon_0: i(18e3, 1, "Circularity_detected_while_resolving_configuration_Colon_0_18000", "Circularity detected while resolving configuration: {0}"), The_files_list_in_config_file_0_is_empty: i(18002, 1, "The_files_list_in_config_file_0_is_empty_18002", "The 'files' list in config file '{0}' is empty."), No_inputs_were_found_in_config_file_0_Specified_include_paths_were_1_and_exclude_paths_were_2: i(18003, 1, "No_inputs_were_found_in_config_file_0_Specified_include_paths_were_1_and_exclude_paths_were_2_18003", "No inputs were found in config file '{0}'. Specified 'include' paths were '{1}' and 'exclude' paths were '{2}'."), File_is_a_CommonJS_module_it_may_be_converted_to_an_ES_module: i(80001, 2, "File_is_a_CommonJS_module_it_may_be_converted_to_an_ES_module_80001", "File is a CommonJS module; it may be converted to an ES module."), This_constructor_function_may_be_converted_to_a_class_declaration: i(80002, 2, "This_constructor_function_may_be_converted_to_a_class_declaration_80002", "This constructor function may be converted to a class declaration."), Import_may_be_converted_to_a_default_import: i(80003, 2, "Import_may_be_converted_to_a_default_import_80003", "Import may be converted to a default import."), JSDoc_types_may_be_moved_to_TypeScript_types: i(80004, 2, "JSDoc_types_may_be_moved_to_TypeScript_types_80004", "JSDoc types may be moved to TypeScript types."), require_call_may_be_converted_to_an_import: i(80005, 2, "require_call_may_be_converted_to_an_import_80005", "'require' call may be converted to an import."), This_may_be_converted_to_an_async_function: i(80006, 2, "This_may_be_converted_to_an_async_function_80006", "This may be converted to an async function."), await_has_no_effect_on_the_type_of_this_expression: i(80007, 2, "await_has_no_effect_on_the_type_of_this_expression_80007", "'await' has no effect on the type of this expression."), Numeric_literals_with_absolute_values_equal_to_2_53_or_greater_are_too_large_to_be_represented_accurately_as_integers: i(80008, 2, "Numeric_literals_with_absolute_values_equal_to_2_53_or_greater_are_too_large_to_be_represented_accur_80008", "Numeric literals with absolute values equal to 2^53 or greater are too large to be represented accurately as integers."), Add_missing_super_call: i(90001, 3, "Add_missing_super_call_90001", "Add missing 'super()' call"), Make_super_call_the_first_statement_in_the_constructor: i(90002, 3, "Make_super_call_the_first_statement_in_the_constructor_90002", "Make 'super()' call the first statement in the constructor"), Change_extends_to_implements: i(90003, 3, "Change_extends_to_implements_90003", "Change 'extends' to 'implements'"), Remove_unused_declaration_for_Colon_0: i(90004, 3, "Remove_unused_declaration_for_Colon_0_90004", "Remove unused declaration for: '{0}'"), Remove_import_from_0: i(90005, 3, "Remove_import_from_0_90005", "Remove import from '{0}'"), Implement_interface_0: i(90006, 3, "Implement_interface_0_90006", "Implement interface '{0}'"), Implement_inherited_abstract_class: i(90007, 3, "Implement_inherited_abstract_class_90007", "Implement inherited abstract class"), Add_0_to_unresolved_variable: i(90008, 3, "Add_0_to_unresolved_variable_90008", "Add '{0}.' to unresolved variable"), Remove_variable_statement: i(90010, 3, "Remove_variable_statement_90010", "Remove variable statement"), Remove_template_tag: i(90011, 3, "Remove_template_tag_90011", "Remove template tag"), Remove_type_parameters: i(90012, 3, "Remove_type_parameters_90012", "Remove type parameters"), Import_0_from_1: i(90013, 3, "Import_0_from_1_90013", `Import '{0}' from "{1}"`), Change_0_to_1: i(90014, 3, "Change_0_to_1_90014", "Change '{0}' to '{1}'"), Declare_property_0: i(90016, 3, "Declare_property_0_90016", "Declare property '{0}'"), Add_index_signature_for_property_0: i(90017, 3, "Add_index_signature_for_property_0_90017", "Add index signature for property '{0}'"), Disable_checking_for_this_file: i(90018, 3, "Disable_checking_for_this_file_90018", "Disable checking for this file"), Ignore_this_error_message: i(90019, 3, "Ignore_this_error_message_90019", "Ignore this error message"), Initialize_property_0_in_the_constructor: i(90020, 3, "Initialize_property_0_in_the_constructor_90020", "Initialize property '{0}' in the constructor"), Initialize_static_property_0: i(90021, 3, "Initialize_static_property_0_90021", "Initialize static property '{0}'"), Change_spelling_to_0: i(90022, 3, "Change_spelling_to_0_90022", "Change spelling to '{0}'"), Declare_method_0: i(90023, 3, "Declare_method_0_90023", "Declare method '{0}'"), Declare_static_method_0: i(90024, 3, "Declare_static_method_0_90024", "Declare static method '{0}'"), Prefix_0_with_an_underscore: i(90025, 3, "Prefix_0_with_an_underscore_90025", "Prefix '{0}' with an underscore"), Rewrite_as_the_indexed_access_type_0: i(90026, 3, "Rewrite_as_the_indexed_access_type_0_90026", "Rewrite as the indexed access type '{0}'"), Declare_static_property_0: i(90027, 3, "Declare_static_property_0_90027", "Declare static property '{0}'"), Call_decorator_expression: i(90028, 3, "Call_decorator_expression_90028", "Call decorator expression"), Add_async_modifier_to_containing_function: i(90029, 3, "Add_async_modifier_to_containing_function_90029", "Add async modifier to containing function"), Replace_infer_0_with_unknown: i(90030, 3, "Replace_infer_0_with_unknown_90030", "Replace 'infer {0}' with 'unknown'"), Replace_all_unused_infer_with_unknown: i(90031, 3, "Replace_all_unused_infer_with_unknown_90031", "Replace all unused 'infer' with 'unknown'"), Add_parameter_name: i(90034, 3, "Add_parameter_name_90034", "Add parameter name"), Declare_private_property_0: i(90035, 3, "Declare_private_property_0_90035", "Declare private property '{0}'"), Replace_0_with_Promise_1: i(90036, 3, "Replace_0_with_Promise_1_90036", "Replace '{0}' with 'Promise<{1}>'"), Fix_all_incorrect_return_type_of_an_async_functions: i(90037, 3, "Fix_all_incorrect_return_type_of_an_async_functions_90037", "Fix all incorrect return type of an async functions"), Declare_private_method_0: i(90038, 3, "Declare_private_method_0_90038", "Declare private method '{0}'"), Remove_unused_destructuring_declaration: i(90039, 3, "Remove_unused_destructuring_declaration_90039", "Remove unused destructuring declaration"), Remove_unused_declarations_for_Colon_0: i(90041, 3, "Remove_unused_declarations_for_Colon_0_90041", "Remove unused declarations for: '{0}'"), Declare_a_private_field_named_0: i(90053, 3, "Declare_a_private_field_named_0_90053", "Declare a private field named '{0}'."), Includes_imports_of_types_referenced_by_0: i(90054, 3, "Includes_imports_of_types_referenced_by_0_90054", "Includes imports of types referenced by '{0}'"), Remove_type_from_import_declaration_from_0: i(90055, 3, "Remove_type_from_import_declaration_from_0_90055", `Remove 'type' from import declaration from "{0}"`), Remove_type_from_import_of_0_from_1: i(90056, 3, "Remove_type_from_import_of_0_from_1_90056", `Remove 'type' from import of '{0}' from "{1}"`), Add_import_from_0: i(90057, 3, "Add_import_from_0_90057", 'Add import from "{0}"'), Update_import_from_0: i(90058, 3, "Update_import_from_0_90058", 'Update import from "{0}"'), Export_0_from_module_1: i(90059, 3, "Export_0_from_module_1_90059", "Export '{0}' from module '{1}'"), Export_all_referenced_locals: i(90060, 3, "Export_all_referenced_locals_90060", "Export all referenced locals"), Convert_function_to_an_ES2015_class: i(95001, 3, "Convert_function_to_an_ES2015_class_95001", "Convert function to an ES2015 class"), Convert_0_to_1_in_0: i(95003, 3, "Convert_0_to_1_in_0_95003", "Convert '{0}' to '{1} in {0}'"), Extract_to_0_in_1: i(95004, 3, "Extract_to_0_in_1_95004", "Extract to {0} in {1}"), Extract_function: i(95005, 3, "Extract_function_95005", "Extract function"), Extract_constant: i(95006, 3, "Extract_constant_95006", "Extract constant"), Extract_to_0_in_enclosing_scope: i(95007, 3, "Extract_to_0_in_enclosing_scope_95007", "Extract to {0} in enclosing scope"), Extract_to_0_in_1_scope: i(95008, 3, "Extract_to_0_in_1_scope_95008", "Extract to {0} in {1} scope"), Annotate_with_type_from_JSDoc: i(95009, 3, "Annotate_with_type_from_JSDoc_95009", "Annotate with type from JSDoc"), Infer_type_of_0_from_usage: i(95011, 3, "Infer_type_of_0_from_usage_95011", "Infer type of '{0}' from usage"), Infer_parameter_types_from_usage: i(95012, 3, "Infer_parameter_types_from_usage_95012", "Infer parameter types from usage"), Convert_to_default_import: i(95013, 3, "Convert_to_default_import_95013", "Convert to default import"), Install_0: i(95014, 3, "Install_0_95014", "Install '{0}'"), Replace_import_with_0: i(95015, 3, "Replace_import_with_0_95015", "Replace import with '{0}'."), Use_synthetic_default_member: i(95016, 3, "Use_synthetic_default_member_95016", "Use synthetic 'default' member."), Convert_to_ES_module: i(95017, 3, "Convert_to_ES_module_95017", "Convert to ES module"), Add_undefined_type_to_property_0: i(95018, 3, "Add_undefined_type_to_property_0_95018", "Add 'undefined' type to property '{0}'"), Add_initializer_to_property_0: i(95019, 3, "Add_initializer_to_property_0_95019", "Add initializer to property '{0}'"), Add_definite_assignment_assertion_to_property_0: i(95020, 3, "Add_definite_assignment_assertion_to_property_0_95020", "Add definite assignment assertion to property '{0}'"), Convert_all_type_literals_to_mapped_type: i(95021, 3, "Convert_all_type_literals_to_mapped_type_95021", "Convert all type literals to mapped type"), Add_all_missing_members: i(95022, 3, "Add_all_missing_members_95022", "Add all missing members"), Infer_all_types_from_usage: i(95023, 3, "Infer_all_types_from_usage_95023", "Infer all types from usage"), Delete_all_unused_declarations: i(95024, 3, "Delete_all_unused_declarations_95024", "Delete all unused declarations"), Prefix_all_unused_declarations_with_where_possible: i(95025, 3, "Prefix_all_unused_declarations_with_where_possible_95025", "Prefix all unused declarations with '_' where possible"), Fix_all_detected_spelling_errors: i(95026, 3, "Fix_all_detected_spelling_errors_95026", "Fix all detected spelling errors"), Add_initializers_to_all_uninitialized_properties: i(95027, 3, "Add_initializers_to_all_uninitialized_properties_95027", "Add initializers to all uninitialized properties"), Add_definite_assignment_assertions_to_all_uninitialized_properties: i(95028, 3, "Add_definite_assignment_assertions_to_all_uninitialized_properties_95028", "Add definite assignment assertions to all uninitialized properties"), Add_undefined_type_to_all_uninitialized_properties: i(95029, 3, "Add_undefined_type_to_all_uninitialized_properties_95029", "Add undefined type to all uninitialized properties"), Change_all_jsdoc_style_types_to_TypeScript: i(95030, 3, "Change_all_jsdoc_style_types_to_TypeScript_95030", "Change all jsdoc-style types to TypeScript"), Change_all_jsdoc_style_types_to_TypeScript_and_add_undefined_to_nullable_types: i(95031, 3, "Change_all_jsdoc_style_types_to_TypeScript_and_add_undefined_to_nullable_types_95031", "Change all jsdoc-style types to TypeScript (and add '| undefined' to nullable types)"), Implement_all_unimplemented_interfaces: i(95032, 3, "Implement_all_unimplemented_interfaces_95032", "Implement all unimplemented interfaces"), Install_all_missing_types_packages: i(95033, 3, "Install_all_missing_types_packages_95033", "Install all missing types packages"), Rewrite_all_as_indexed_access_types: i(95034, 3, "Rewrite_all_as_indexed_access_types_95034", "Rewrite all as indexed access types"), Convert_all_to_default_imports: i(95035, 3, "Convert_all_to_default_imports_95035", "Convert all to default imports"), Make_all_super_calls_the_first_statement_in_their_constructor: i(95036, 3, "Make_all_super_calls_the_first_statement_in_their_constructor_95036", "Make all 'super()' calls the first statement in their constructor"), Add_qualifier_to_all_unresolved_variables_matching_a_member_name: i(95037, 3, "Add_qualifier_to_all_unresolved_variables_matching_a_member_name_95037", "Add qualifier to all unresolved variables matching a member name"), Change_all_extended_interfaces_to_implements: i(95038, 3, "Change_all_extended_interfaces_to_implements_95038", "Change all extended interfaces to 'implements'"), Add_all_missing_super_calls: i(95039, 3, "Add_all_missing_super_calls_95039", "Add all missing super calls"), Implement_all_inherited_abstract_classes: i(95040, 3, "Implement_all_inherited_abstract_classes_95040", "Implement all inherited abstract classes"), Add_all_missing_async_modifiers: i(95041, 3, "Add_all_missing_async_modifiers_95041", "Add all missing 'async' modifiers"), Add_ts_ignore_to_all_error_messages: i(95042, 3, "Add_ts_ignore_to_all_error_messages_95042", "Add '@ts-ignore' to all error messages"), Annotate_everything_with_types_from_JSDoc: i(95043, 3, "Annotate_everything_with_types_from_JSDoc_95043", "Annotate everything with types from JSDoc"), Add_to_all_uncalled_decorators: i(95044, 3, "Add_to_all_uncalled_decorators_95044", "Add '()' to all uncalled decorators"), Convert_all_constructor_functions_to_classes: i(95045, 3, "Convert_all_constructor_functions_to_classes_95045", "Convert all constructor functions to classes"), Generate_get_and_set_accessors: i(95046, 3, "Generate_get_and_set_accessors_95046", "Generate 'get' and 'set' accessors"), Convert_require_to_import: i(95047, 3, "Convert_require_to_import_95047", "Convert 'require' to 'import'"), Convert_all_require_to_import: i(95048, 3, "Convert_all_require_to_import_95048", "Convert all 'require' to 'import'"), Move_to_a_new_file: i(95049, 3, "Move_to_a_new_file_95049", "Move to a new file"), Remove_unreachable_code: i(95050, 3, "Remove_unreachable_code_95050", "Remove unreachable code"), Remove_all_unreachable_code: i(95051, 3, "Remove_all_unreachable_code_95051", "Remove all unreachable code"), Add_missing_typeof: i(95052, 3, "Add_missing_typeof_95052", "Add missing 'typeof'"), Remove_unused_label: i(95053, 3, "Remove_unused_label_95053", "Remove unused label"), Remove_all_unused_labels: i(95054, 3, "Remove_all_unused_labels_95054", "Remove all unused labels"), Convert_0_to_mapped_object_type: i(95055, 3, "Convert_0_to_mapped_object_type_95055", "Convert '{0}' to mapped object type"), Convert_namespace_import_to_named_imports: i(95056, 3, "Convert_namespace_import_to_named_imports_95056", "Convert namespace import to named imports"), Convert_named_imports_to_namespace_import: i(95057, 3, "Convert_named_imports_to_namespace_import_95057", "Convert named imports to namespace import"), Add_or_remove_braces_in_an_arrow_function: i(95058, 3, "Add_or_remove_braces_in_an_arrow_function_95058", "Add or remove braces in an arrow function"), Add_braces_to_arrow_function: i(95059, 3, "Add_braces_to_arrow_function_95059", "Add braces to arrow function"), Remove_braces_from_arrow_function: i(95060, 3, "Remove_braces_from_arrow_function_95060", "Remove braces from arrow function"), Convert_default_export_to_named_export: i(95061, 3, "Convert_default_export_to_named_export_95061", "Convert default export to named export"), Convert_named_export_to_default_export: i(95062, 3, "Convert_named_export_to_default_export_95062", "Convert named export to default export"), Add_missing_enum_member_0: i(95063, 3, "Add_missing_enum_member_0_95063", "Add missing enum member '{0}'"), Add_all_missing_imports: i(95064, 3, "Add_all_missing_imports_95064", "Add all missing imports"), Convert_to_async_function: i(95065, 3, "Convert_to_async_function_95065", "Convert to async function"), Convert_all_to_async_functions: i(95066, 3, "Convert_all_to_async_functions_95066", "Convert all to async functions"), Add_missing_call_parentheses: i(95067, 3, "Add_missing_call_parentheses_95067", "Add missing call parentheses"), Add_all_missing_call_parentheses: i(95068, 3, "Add_all_missing_call_parentheses_95068", "Add all missing call parentheses"), Add_unknown_conversion_for_non_overlapping_types: i(95069, 3, "Add_unknown_conversion_for_non_overlapping_types_95069", "Add 'unknown' conversion for non-overlapping types"), Add_unknown_to_all_conversions_of_non_overlapping_types: i(95070, 3, "Add_unknown_to_all_conversions_of_non_overlapping_types_95070", "Add 'unknown' to all conversions of non-overlapping types"), Add_missing_new_operator_to_call: i(95071, 3, "Add_missing_new_operator_to_call_95071", "Add missing 'new' operator to call"), Add_missing_new_operator_to_all_calls: i(95072, 3, "Add_missing_new_operator_to_all_calls_95072", "Add missing 'new' operator to all calls"), Add_names_to_all_parameters_without_names: i(95073, 3, "Add_names_to_all_parameters_without_names_95073", "Add names to all parameters without names"), Enable_the_experimentalDecorators_option_in_your_configuration_file: i(95074, 3, "Enable_the_experimentalDecorators_option_in_your_configuration_file_95074", "Enable the 'experimentalDecorators' option in your configuration file"), Convert_parameters_to_destructured_object: i(95075, 3, "Convert_parameters_to_destructured_object_95075", "Convert parameters to destructured object"), Extract_type: i(95077, 3, "Extract_type_95077", "Extract type"), Extract_to_type_alias: i(95078, 3, "Extract_to_type_alias_95078", "Extract to type alias"), Extract_to_typedef: i(95079, 3, "Extract_to_typedef_95079", "Extract to typedef"), Infer_this_type_of_0_from_usage: i(95080, 3, "Infer_this_type_of_0_from_usage_95080", "Infer 'this' type of '{0}' from usage"), Add_const_to_unresolved_variable: i(95081, 3, "Add_const_to_unresolved_variable_95081", "Add 'const' to unresolved variable"), Add_const_to_all_unresolved_variables: i(95082, 3, "Add_const_to_all_unresolved_variables_95082", "Add 'const' to all unresolved variables"), Add_await: i(95083, 3, "Add_await_95083", "Add 'await'"), Add_await_to_initializer_for_0: i(95084, 3, "Add_await_to_initializer_for_0_95084", "Add 'await' to initializer for '{0}'"), Fix_all_expressions_possibly_missing_await: i(95085, 3, "Fix_all_expressions_possibly_missing_await_95085", "Fix all expressions possibly missing 'await'"), Remove_unnecessary_await: i(95086, 3, "Remove_unnecessary_await_95086", "Remove unnecessary 'await'"), Remove_all_unnecessary_uses_of_await: i(95087, 3, "Remove_all_unnecessary_uses_of_await_95087", "Remove all unnecessary uses of 'await'"), Enable_the_jsx_flag_in_your_configuration_file: i(95088, 3, "Enable_the_jsx_flag_in_your_configuration_file_95088", "Enable the '--jsx' flag in your configuration file"), Add_await_to_initializers: i(95089, 3, "Add_await_to_initializers_95089", "Add 'await' to initializers"), Extract_to_interface: i(95090, 3, "Extract_to_interface_95090", "Extract to interface"), Convert_to_a_bigint_numeric_literal: i(95091, 3, "Convert_to_a_bigint_numeric_literal_95091", "Convert to a bigint numeric literal"), Convert_all_to_bigint_numeric_literals: i(95092, 3, "Convert_all_to_bigint_numeric_literals_95092", "Convert all to bigint numeric literals"), Convert_const_to_let: i(95093, 3, "Convert_const_to_let_95093", "Convert 'const' to 'let'"), Prefix_with_declare: i(95094, 3, "Prefix_with_declare_95094", "Prefix with 'declare'"), Prefix_all_incorrect_property_declarations_with_declare: i(95095, 3, "Prefix_all_incorrect_property_declarations_with_declare_95095", "Prefix all incorrect property declarations with 'declare'"), Convert_to_template_string: i(95096, 3, "Convert_to_template_string_95096", "Convert to template string"), Add_export_to_make_this_file_into_a_module: i(95097, 3, "Add_export_to_make_this_file_into_a_module_95097", "Add 'export {}' to make this file into a module"), Set_the_target_option_in_your_configuration_file_to_0: i(95098, 3, "Set_the_target_option_in_your_configuration_file_to_0_95098", "Set the 'target' option in your configuration file to '{0}'"), Set_the_module_option_in_your_configuration_file_to_0: i(95099, 3, "Set_the_module_option_in_your_configuration_file_to_0_95099", "Set the 'module' option in your configuration file to '{0}'"), Convert_invalid_character_to_its_html_entity_code: i(95100, 3, "Convert_invalid_character_to_its_html_entity_code_95100", "Convert invalid character to its html entity code"), Convert_all_invalid_characters_to_HTML_entity_code: i(95101, 3, "Convert_all_invalid_characters_to_HTML_entity_code_95101", "Convert all invalid characters to HTML entity code"), Convert_all_const_to_let: i(95102, 3, "Convert_all_const_to_let_95102", "Convert all 'const' to 'let'"), Convert_function_expression_0_to_arrow_function: i(95105, 3, "Convert_function_expression_0_to_arrow_function_95105", "Convert function expression '{0}' to arrow function"), Convert_function_declaration_0_to_arrow_function: i(95106, 3, "Convert_function_declaration_0_to_arrow_function_95106", "Convert function declaration '{0}' to arrow function"), Fix_all_implicit_this_errors: i(95107, 3, "Fix_all_implicit_this_errors_95107", "Fix all implicit-'this' errors"), Wrap_invalid_character_in_an_expression_container: i(95108, 3, "Wrap_invalid_character_in_an_expression_container_95108", "Wrap invalid character in an expression container"), Wrap_all_invalid_characters_in_an_expression_container: i(95109, 3, "Wrap_all_invalid_characters_in_an_expression_container_95109", "Wrap all invalid characters in an expression container"), Visit_https_Colon_Slash_Slashaka_ms_Slashtsconfig_to_read_more_about_this_file: i(95110, 3, "Visit_https_Colon_Slash_Slashaka_ms_Slashtsconfig_to_read_more_about_this_file_95110", "Visit https://aka.ms/tsconfig to read more about this file"), Add_a_return_statement: i(95111, 3, "Add_a_return_statement_95111", "Add a return statement"), Remove_braces_from_arrow_function_body: i(95112, 3, "Remove_braces_from_arrow_function_body_95112", "Remove braces from arrow function body"), Wrap_the_following_body_with_parentheses_which_should_be_an_object_literal: i(95113, 3, "Wrap_the_following_body_with_parentheses_which_should_be_an_object_literal_95113", "Wrap the following body with parentheses which should be an object literal"), Add_all_missing_return_statement: i(95114, 3, "Add_all_missing_return_statement_95114", "Add all missing return statement"), Remove_braces_from_all_arrow_function_bodies_with_relevant_issues: i(95115, 3, "Remove_braces_from_all_arrow_function_bodies_with_relevant_issues_95115", "Remove braces from all arrow function bodies with relevant issues"), Wrap_all_object_literal_with_parentheses: i(95116, 3, "Wrap_all_object_literal_with_parentheses_95116", "Wrap all object literal with parentheses"), Move_labeled_tuple_element_modifiers_to_labels: i(95117, 3, "Move_labeled_tuple_element_modifiers_to_labels_95117", "Move labeled tuple element modifiers to labels"), Convert_overload_list_to_single_signature: i(95118, 3, "Convert_overload_list_to_single_signature_95118", "Convert overload list to single signature"), Generate_get_and_set_accessors_for_all_overriding_properties: i(95119, 3, "Generate_get_and_set_accessors_for_all_overriding_properties_95119", "Generate 'get' and 'set' accessors for all overriding properties"), Wrap_in_JSX_fragment: i(95120, 3, "Wrap_in_JSX_fragment_95120", "Wrap in JSX fragment"), Wrap_all_unparented_JSX_in_JSX_fragment: i(95121, 3, "Wrap_all_unparented_JSX_in_JSX_fragment_95121", "Wrap all unparented JSX in JSX fragment"), Convert_arrow_function_or_function_expression: i(95122, 3, "Convert_arrow_function_or_function_expression_95122", "Convert arrow function or function expression"), Convert_to_anonymous_function: i(95123, 3, "Convert_to_anonymous_function_95123", "Convert to anonymous function"), Convert_to_named_function: i(95124, 3, "Convert_to_named_function_95124", "Convert to named function"), Convert_to_arrow_function: i(95125, 3, "Convert_to_arrow_function_95125", "Convert to arrow function"), Remove_parentheses: i(95126, 3, "Remove_parentheses_95126", "Remove parentheses"), Could_not_find_a_containing_arrow_function: i(95127, 3, "Could_not_find_a_containing_arrow_function_95127", "Could not find a containing arrow function"), Containing_function_is_not_an_arrow_function: i(95128, 3, "Containing_function_is_not_an_arrow_function_95128", "Containing function is not an arrow function"), Could_not_find_export_statement: i(95129, 3, "Could_not_find_export_statement_95129", "Could not find export statement"), This_file_already_has_a_default_export: i(95130, 3, "This_file_already_has_a_default_export_95130", "This file already has a default export"), Could_not_find_import_clause: i(95131, 3, "Could_not_find_import_clause_95131", "Could not find import clause"), Could_not_find_namespace_import_or_named_imports: i(95132, 3, "Could_not_find_namespace_import_or_named_imports_95132", "Could not find namespace import or named imports"), Selection_is_not_a_valid_type_node: i(95133, 3, "Selection_is_not_a_valid_type_node_95133", "Selection is not a valid type node"), No_type_could_be_extracted_from_this_type_node: i(95134, 3, "No_type_could_be_extracted_from_this_type_node_95134", "No type could be extracted from this type node"), Could_not_find_property_for_which_to_generate_accessor: i(95135, 3, "Could_not_find_property_for_which_to_generate_accessor_95135", "Could not find property for which to generate accessor"), Name_is_not_valid: i(95136, 3, "Name_is_not_valid_95136", "Name is not valid"), Can_only_convert_property_with_modifier: i(95137, 3, "Can_only_convert_property_with_modifier_95137", "Can only convert property with modifier"), Switch_each_misused_0_to_1: i(95138, 3, "Switch_each_misused_0_to_1_95138", "Switch each misused '{0}' to '{1}'"), Convert_to_optional_chain_expression: i(95139, 3, "Convert_to_optional_chain_expression_95139", "Convert to optional chain expression"), Could_not_find_convertible_access_expression: i(95140, 3, "Could_not_find_convertible_access_expression_95140", "Could not find convertible access expression"), Could_not_find_matching_access_expressions: i(95141, 3, "Could_not_find_matching_access_expressions_95141", "Could not find matching access expressions"), Can_only_convert_logical_AND_access_chains: i(95142, 3, "Can_only_convert_logical_AND_access_chains_95142", "Can only convert logical AND access chains"), Add_void_to_Promise_resolved_without_a_value: i(95143, 3, "Add_void_to_Promise_resolved_without_a_value_95143", "Add 'void' to Promise resolved without a value"), Add_void_to_all_Promises_resolved_without_a_value: i(95144, 3, "Add_void_to_all_Promises_resolved_without_a_value_95144", "Add 'void' to all Promises resolved without a value"), Use_element_access_for_0: i(95145, 3, "Use_element_access_for_0_95145", "Use element access for '{0}'"), Use_element_access_for_all_undeclared_properties: i(95146, 3, "Use_element_access_for_all_undeclared_properties_95146", "Use element access for all undeclared properties."), Delete_all_unused_imports: i(95147, 3, "Delete_all_unused_imports_95147", "Delete all unused imports"), Infer_function_return_type: i(95148, 3, "Infer_function_return_type_95148", "Infer function return type"), Return_type_must_be_inferred_from_a_function: i(95149, 3, "Return_type_must_be_inferred_from_a_function_95149", "Return type must be inferred from a function"), Could_not_determine_function_return_type: i(95150, 3, "Could_not_determine_function_return_type_95150", "Could not determine function return type"), Could_not_convert_to_arrow_function: i(95151, 3, "Could_not_convert_to_arrow_function_95151", "Could not convert to arrow function"), Could_not_convert_to_named_function: i(95152, 3, "Could_not_convert_to_named_function_95152", "Could not convert to named function"), Could_not_convert_to_anonymous_function: i(95153, 3, "Could_not_convert_to_anonymous_function_95153", "Could not convert to anonymous function"), Can_only_convert_string_concatenation: i(95154, 3, "Can_only_convert_string_concatenation_95154", "Can only convert string concatenation"), Selection_is_not_a_valid_statement_or_statements: i(95155, 3, "Selection_is_not_a_valid_statement_or_statements_95155", "Selection is not a valid statement or statements"), Add_missing_function_declaration_0: i(95156, 3, "Add_missing_function_declaration_0_95156", "Add missing function declaration '{0}'"), Add_all_missing_function_declarations: i(95157, 3, "Add_all_missing_function_declarations_95157", "Add all missing function declarations"), Method_not_implemented: i(95158, 3, "Method_not_implemented_95158", "Method not implemented."), Function_not_implemented: i(95159, 3, "Function_not_implemented_95159", "Function not implemented."), Add_override_modifier: i(95160, 3, "Add_override_modifier_95160", "Add 'override' modifier"), Remove_override_modifier: i(95161, 3, "Remove_override_modifier_95161", "Remove 'override' modifier"), Add_all_missing_override_modifiers: i(95162, 3, "Add_all_missing_override_modifiers_95162", "Add all missing 'override' modifiers"), Remove_all_unnecessary_override_modifiers: i(95163, 3, "Remove_all_unnecessary_override_modifiers_95163", "Remove all unnecessary 'override' modifiers"), Can_only_convert_named_export: i(95164, 3, "Can_only_convert_named_export_95164", "Can only convert named export"), Add_missing_properties: i(95165, 3, "Add_missing_properties_95165", "Add missing properties"), Add_all_missing_properties: i(95166, 3, "Add_all_missing_properties_95166", "Add all missing properties"), Add_missing_attributes: i(95167, 3, "Add_missing_attributes_95167", "Add missing attributes"), Add_all_missing_attributes: i(95168, 3, "Add_all_missing_attributes_95168", "Add all missing attributes"), Add_undefined_to_optional_property_type: i(95169, 3, "Add_undefined_to_optional_property_type_95169", "Add 'undefined' to optional property type"), Convert_named_imports_to_default_import: i(95170, 3, "Convert_named_imports_to_default_import_95170", "Convert named imports to default import"), Delete_unused_param_tag_0: i(95171, 3, "Delete_unused_param_tag_0_95171", "Delete unused '@param' tag '{0}'"), Delete_all_unused_param_tags: i(95172, 3, "Delete_all_unused_param_tags_95172", "Delete all unused '@param' tags"), Rename_param_tag_name_0_to_1: i(95173, 3, "Rename_param_tag_name_0_to_1_95173", "Rename '@param' tag name '{0}' to '{1}'"), Use_0: i(95174, 3, "Use_0_95174", "Use `{0}`."), Use_Number_isNaN_in_all_conditions: i(95175, 3, "Use_Number_isNaN_in_all_conditions_95175", "Use `Number.isNaN` in all conditions."), No_value_exists_in_scope_for_the_shorthand_property_0_Either_declare_one_or_provide_an_initializer: i(18004, 1, "No_value_exists_in_scope_for_the_shorthand_property_0_Either_declare_one_or_provide_an_initializer_18004", "No value exists in scope for the shorthand property '{0}'. Either declare one or provide an initializer."), Classes_may_not_have_a_field_named_constructor: i(18006, 1, "Classes_may_not_have_a_field_named_constructor_18006", "Classes may not have a field named 'constructor'."), JSX_expressions_may_not_use_the_comma_operator_Did_you_mean_to_write_an_array: i(18007, 1, "JSX_expressions_may_not_use_the_comma_operator_Did_you_mean_to_write_an_array_18007", "JSX expressions may not use the comma operator. Did you mean to write an array?"), Private_identifiers_cannot_be_used_as_parameters: i(18009, 1, "Private_identifiers_cannot_be_used_as_parameters_18009", "Private identifiers cannot be used as parameters."), An_accessibility_modifier_cannot_be_used_with_a_private_identifier: i(18010, 1, "An_accessibility_modifier_cannot_be_used_with_a_private_identifier_18010", "An accessibility modifier cannot be used with a private identifier."), The_operand_of_a_delete_operator_cannot_be_a_private_identifier: i(18011, 1, "The_operand_of_a_delete_operator_cannot_be_a_private_identifier_18011", "The operand of a 'delete' operator cannot be a private identifier."), constructor_is_a_reserved_word: i(18012, 1, "constructor_is_a_reserved_word_18012", "'#constructor' is a reserved word."), Property_0_is_not_accessible_outside_class_1_because_it_has_a_private_identifier: i(18013, 1, "Property_0_is_not_accessible_outside_class_1_because_it_has_a_private_identifier_18013", "Property '{0}' is not accessible outside class '{1}' because it has a private identifier."), The_property_0_cannot_be_accessed_on_type_1_within_this_class_because_it_is_shadowed_by_another_private_identifier_with_the_same_spelling: i(18014, 1, "The_property_0_cannot_be_accessed_on_type_1_within_this_class_because_it_is_shadowed_by_another_priv_18014", "The property '{0}' cannot be accessed on type '{1}' within this class because it is shadowed by another private identifier with the same spelling."), Property_0_in_type_1_refers_to_a_different_member_that_cannot_be_accessed_from_within_type_2: i(18015, 1, "Property_0_in_type_1_refers_to_a_different_member_that_cannot_be_accessed_from_within_type_2_18015", "Property '{0}' in type '{1}' refers to a different member that cannot be accessed from within type '{2}'."), Private_identifiers_are_not_allowed_outside_class_bodies: i(18016, 1, "Private_identifiers_are_not_allowed_outside_class_bodies_18016", "Private identifiers are not allowed outside class bodies."), The_shadowing_declaration_of_0_is_defined_here: i(18017, 1, "The_shadowing_declaration_of_0_is_defined_here_18017", "The shadowing declaration of '{0}' is defined here"), The_declaration_of_0_that_you_probably_intended_to_use_is_defined_here: i(18018, 1, "The_declaration_of_0_that_you_probably_intended_to_use_is_defined_here_18018", "The declaration of '{0}' that you probably intended to use is defined here"), _0_modifier_cannot_be_used_with_a_private_identifier: i(18019, 1, "_0_modifier_cannot_be_used_with_a_private_identifier_18019", "'{0}' modifier cannot be used with a private identifier."), An_enum_member_cannot_be_named_with_a_private_identifier: i(18024, 1, "An_enum_member_cannot_be_named_with_a_private_identifier_18024", "An enum member cannot be named with a private identifier."), can_only_be_used_at_the_start_of_a_file: i(18026, 1, "can_only_be_used_at_the_start_of_a_file_18026", "'#!' can only be used at the start of a file."), Compiler_reserves_name_0_when_emitting_private_identifier_downlevel: i(18027, 1, "Compiler_reserves_name_0_when_emitting_private_identifier_downlevel_18027", "Compiler reserves name '{0}' when emitting private identifier downlevel."), Private_identifiers_are_only_available_when_targeting_ECMAScript_2015_and_higher: i(18028, 1, "Private_identifiers_are_only_available_when_targeting_ECMAScript_2015_and_higher_18028", "Private identifiers are only available when targeting ECMAScript 2015 and higher."), Private_identifiers_are_not_allowed_in_variable_declarations: i(18029, 1, "Private_identifiers_are_not_allowed_in_variable_declarations_18029", "Private identifiers are not allowed in variable declarations."), An_optional_chain_cannot_contain_private_identifiers: i(18030, 1, "An_optional_chain_cannot_contain_private_identifiers_18030", "An optional chain cannot contain private identifiers."), The_intersection_0_was_reduced_to_never_because_property_1_has_conflicting_types_in_some_constituents: i(18031, 1, "The_intersection_0_was_reduced_to_never_because_property_1_has_conflicting_types_in_some_constituent_18031", "The intersection '{0}' was reduced to 'never' because property '{1}' has conflicting types in some constituents."), The_intersection_0_was_reduced_to_never_because_property_1_exists_in_multiple_constituents_and_is_private_in_some: i(18032, 1, "The_intersection_0_was_reduced_to_never_because_property_1_exists_in_multiple_constituents_and_is_pr_18032", "The intersection '{0}' was reduced to 'never' because property '{1}' exists in multiple constituents and is private in some."), Type_0_is_not_assignable_to_type_1_as_required_for_computed_enum_member_values: i(18033, 1, "Type_0_is_not_assignable_to_type_1_as_required_for_computed_enum_member_values_18033", "Type '{0}' is not assignable to type '{1}' as required for computed enum member values."), Specify_the_JSX_fragment_factory_function_to_use_when_targeting_react_JSX_emit_with_jsxFactory_compiler_option_is_specified_e_g_Fragment: i(18034, 3, "Specify_the_JSX_fragment_factory_function_to_use_when_targeting_react_JSX_emit_with_jsxFactory_compi_18034", "Specify the JSX fragment factory function to use when targeting 'react' JSX emit with 'jsxFactory' compiler option is specified, e.g. 'Fragment'."), Invalid_value_for_jsxFragmentFactory_0_is_not_a_valid_identifier_or_qualified_name: i(18035, 1, "Invalid_value_for_jsxFragmentFactory_0_is_not_a_valid_identifier_or_qualified_name_18035", "Invalid value for 'jsxFragmentFactory'. '{0}' is not a valid identifier or qualified-name."), Class_decorators_can_t_be_used_with_static_private_identifier_Consider_removing_the_experimental_decorator: i(18036, 1, "Class_decorators_can_t_be_used_with_static_private_identifier_Consider_removing_the_experimental_dec_18036", "Class decorators can't be used with static private identifier. Consider removing the experimental decorator."), Await_expression_cannot_be_used_inside_a_class_static_block: i(18037, 1, "Await_expression_cannot_be_used_inside_a_class_static_block_18037", "Await expression cannot be used inside a class static block."), For_await_loops_cannot_be_used_inside_a_class_static_block: i(18038, 1, "For_await_loops_cannot_be_used_inside_a_class_static_block_18038", "'For await' loops cannot be used inside a class static block."), Invalid_use_of_0_It_cannot_be_used_inside_a_class_static_block: i(18039, 1, "Invalid_use_of_0_It_cannot_be_used_inside_a_class_static_block_18039", "Invalid use of '{0}'. It cannot be used inside a class static block."), A_return_statement_cannot_be_used_inside_a_class_static_block: i(18041, 1, "A_return_statement_cannot_be_used_inside_a_class_static_block_18041", "A 'return' statement cannot be used inside a class static block."), _0_is_a_type_and_cannot_be_imported_in_JavaScript_files_Use_1_in_a_JSDoc_type_annotation: i(18042, 1, "_0_is_a_type_and_cannot_be_imported_in_JavaScript_files_Use_1_in_a_JSDoc_type_annotation_18042", "'{0}' is a type and cannot be imported in JavaScript files. Use '{1}' in a JSDoc type annotation."), Types_cannot_appear_in_export_declarations_in_JavaScript_files: i(18043, 1, "Types_cannot_appear_in_export_declarations_in_JavaScript_files_18043", "Types cannot appear in export declarations in JavaScript files."), _0_is_automatically_exported_here: i(18044, 3, "_0_is_automatically_exported_here_18044", "'{0}' is automatically exported here."), Properties_with_the_accessor_modifier_are_only_available_when_targeting_ECMAScript_2015_and_higher: i(18045, 1, "Properties_with_the_accessor_modifier_are_only_available_when_targeting_ECMAScript_2015_and_higher_18045", "Properties with the 'accessor' modifier are only available when targeting ECMAScript 2015 and higher."), _0_is_of_type_unknown: i(18046, 1, "_0_is_of_type_unknown_18046", "'{0}' is of type 'unknown'."), _0_is_possibly_null: i(18047, 1, "_0_is_possibly_null_18047", "'{0}' is possibly 'null'."), _0_is_possibly_undefined: i(18048, 1, "_0_is_possibly_undefined_18048", "'{0}' is possibly 'undefined'."), _0_is_possibly_null_or_undefined: i(18049, 1, "_0_is_possibly_null_or_undefined_18049", "'{0}' is possibly 'null' or 'undefined'."), The_value_0_cannot_be_used_here: i(18050, 1, "The_value_0_cannot_be_used_here_18050", "The value '{0}' cannot be used here."), Compiler_option_0_cannot_be_given_an_empty_string: i(18051, 1, "Compiler_option_0_cannot_be_given_an_empty_string_18051", "Compiler option '{0}' cannot be given an empty string.") };
} });
function fr(e) {
return e >= 79;
}
function qT(e) {
return e === 31 || fr(e);
}
function D_(e, t) {
if (e < t[0])
return false;
let r = 0, s = t.length, f;
for (; r + 1 < s; ) {
if (f = r + (s - r) / 2, f -= f % 2, t[f] <= e && e <= t[f + 1])
return true;
e < t[f] ? s = f : r = f + 2;
}
return false;
}
function UT(e, t) {
return t >= 2 ? D_(e, ZT) : t === 1 ? D_(e, YT) : D_(e, KT);
}
function _A(e, t) {
return t >= 2 ? D_(e, eS) : t === 1 ? D_(e, QT) : D_(e, XT);
}
function cA(e) {
let t = [];
return e.forEach((r, s) => {
t[r] = s;
}), t;
}
function Br(e) {
return nS[e];
}
function _l(e) {
return Ty.get(e);
}
function Kp(e) {
let t = [], r = 0, s = 0;
for (; r < e.length; ) {
let f = e.charCodeAt(r);
switch (r++, f) {
case 13:
e.charCodeAt(r) === 10 && r++;
case 10:
t.push(s), s = r;
break;
default:
f > 127 && un(f) && (t.push(s), s = r);
break;
}
}
return t.push(s), t;
}
function lA(e, t, r, s) {
return e.getPositionOfLineAndCharacter ? e.getPositionOfLineAndCharacter(t, r, s) : dy(ss(e), t, r, e.text, s);
}
function dy(e, t, r, s, f) {
(t < 0 || t >= e.length) && (f ? t = t < 0 ? 0 : t >= e.length ? e.length - 1 : t : Y.fail(`Bad line number. Line: ${t}, lineStarts.length: ${e.length} , line map is correct? ${s !== void 0 ? ke(e, Kp(s)) : "unknown"}`));
let x = e[t] + r;
return f ? x > e[t + 1] ? e[t + 1] : typeof s == "string" && x > s.length ? s.length : x : (t < e.length - 1 ? Y.assert(x < e[t + 1]) : s !== void 0 && Y.assert(x <= s.length), x);
}
function ss(e) {
return e.lineMap || (e.lineMap = Kp(e.text));
}
function my(e, t) {
let r = k_(e, t);
return { line: r, character: t - e[r] };
}
function k_(e, t, r) {
let s = Ya(e, t, rr, Vr, r);
return s < 0 && (s = ~s - 1, Y.assert(s !== -1, "position cannot precede the beginning of the file")), s;
}
function I_(e, t, r) {
if (t === r)
return 0;
let s = ss(e), f = Math.min(t, r), x = f === r, w = x ? t : r, A = k_(s, f), g = k_(s, w, A);
return x ? A - g : g - A;
}
function Ls(e, t) {
return my(ss(e), t);
}
function os(e) {
return N_(e) || un(e);
}
function N_(e) {
return e === 32 || e === 9 || e === 11 || e === 12 || e === 160 || e === 133 || e === 5760 || e >= 8192 && e <= 8203 || e === 8239 || e === 8287 || e === 12288 || e === 65279;
}
function un(e) {
return e === 10 || e === 13 || e === 8232 || e === 8233;
}
function O_(e) {
return e >= 48 && e <= 57;
}
function Xp(e) {
return O_(e) || e >= 65 && e <= 70 || e >= 97 && e <= 102;
}
function uA(e) {
return e <= 1114111;
}
function hy(e) {
return e >= 48 && e <= 55;
}
function pA(e, t) {
let r = e.charCodeAt(t);
switch (r) {
case 13:
case 10:
case 9:
case 11:
case 12:
case 32:
case 47:
case 60:
case 124:
case 61:
case 62:
return true;
case 35:
return t === 0;
default:
return r > 127;
}
}
function Ar(e, t, r, s, f) {
if (hs(t))
return t;
let x = false;
for (; ; ) {
let w = e.charCodeAt(t);
switch (w) {
case 13:
e.charCodeAt(t + 1) === 10 && t++;
case 10:
if (t++, r)
return t;
x = !!f;
continue;
case 9:
case 11:
case 12:
case 32:
t++;
continue;
case 47:
if (s)
break;
if (e.charCodeAt(t + 1) === 47) {
for (t += 2; t < e.length && !un(e.charCodeAt(t)); )
t++;
x = false;
continue;
}
if (e.charCodeAt(t + 1) === 42) {
for (t += 2; t < e.length; ) {
if (e.charCodeAt(t) === 42 && e.charCodeAt(t + 1) === 47) {
t += 2;
break;
}
t++;
}
x = false;
continue;
}
break;
case 60:
case 124:
case 61:
case 62:
if (Co(e, t)) {
t = M_(e, t), x = false;
continue;
}
break;
case 35:
if (t === 0 && gy(e, t)) {
t = yy(e, t), x = false;
continue;
}
break;
case 42:
if (x) {
t++, x = false;
continue;
}
break;
default:
if (w > 127 && os(w)) {
t++;
continue;
}
break;
}
return t;
}
}
function Co(e, t) {
if (Y.assert(t >= 0), t === 0 || un(e.charCodeAt(t - 1))) {
let r = e.charCodeAt(t);
if (t + ll < e.length) {
for (let s = 0; s < ll; s++)
if (e.charCodeAt(t + s) !== r)
return false;
return r === 61 || e.charCodeAt(t + ll) === 32;
}
}
return false;
}
function M_(e, t, r) {
r && r(ve.Merge_conflict_marker_encountered, t, ll);
let s = e.charCodeAt(t), f = e.length;
if (s === 60 || s === 62)
for (; t < f && !un(e.charCodeAt(t)); )
t++;
else
for (Y.assert(s === 124 || s === 61); t < f; ) {
let x = e.charCodeAt(t);
if ((x === 61 || x === 62) && x !== s && Co(e, t))
break;
t++;
}
return t;
}
function gy(e, t) {
return Y.assert(t === 0), Qp.test(e);
}
function yy(e, t) {
let r = Qp.exec(e)[0];
return t = t + r.length, t;
}
function Yp(e, t, r, s, f, x, w) {
let A, g, B, N, X = false, F = s, $ = w;
if (r === 0) {
F = true;
let ae = GT(t);
ae && (r = ae.length);
}
e:
for (; r >= 0 && r < t.length; ) {
let ae = t.charCodeAt(r);
switch (ae) {
case 13:
t.charCodeAt(r + 1) === 10 && r++;
case 10:
if (r++, s)
break e;
F = true, X && (N = true);
continue;
case 9:
case 11:
case 12:
case 32:
r++;
continue;
case 47:
let Te = t.charCodeAt(r + 1), Se = false;
if (Te === 47 || Te === 42) {
let Ye = Te === 47 ? 2 : 3, Ne = r;
if (r += 2, Te === 47)
for (; r < t.length; ) {
if (un(t.charCodeAt(r))) {
Se = true;
break;
}
r++;
}
else
for (; r < t.length; ) {
if (t.charCodeAt(r) === 42 && t.charCodeAt(r + 1) === 47) {
r += 2;
break;
}
r++;
}
if (F) {
if (X && ($ = f(A, g, B, N, x, $), !e && $))
return $;
A = Ne, g = r, B = Ye, N = Se, X = true;
}
continue;
}
break e;
default:
if (ae > 127 && os(ae)) {
X && un(ae) && (N = true), r++;
continue;
}
break e;
}
}
return X && ($ = f(A, g, B, N, x, $)), $;
}
function fA(e, t, r, s) {
return Yp(false, e, t, false, r, s);
}
function dA(e, t, r, s) {
return Yp(false, e, t, true, r, s);
}
function zT(e, t, r, s, f) {
return Yp(true, e, t, false, r, s, f);
}
function WT(e, t, r, s, f) {
return Yp(true, e, t, true, r, s, f);
}
function VT(e, t, r, s, f) {
let x = arguments.length > 5 && arguments[5] !== void 0 ? arguments[5] : [];
return x.push({ kind: r, pos: e, end: t, hasTrailingNewLine: s }), x;
}
function Ao(e, t) {
return zT(e, t, VT, void 0, void 0);
}
function HT(e, t) {
return WT(e, t, VT, void 0, void 0);
}
function GT(e) {
let t = Qp.exec(e);
if (t)
return t[0];
}
function Wn(e, t) {
return e >= 65 && e <= 90 || e >= 97 && e <= 122 || e === 36 || e === 95 || e > 127 && UT(e, t);
}
function Rs(e, t, r) {
return e >= 65 && e <= 90 || e >= 97 && e <= 122 || e >= 48 && e <= 57 || e === 36 || e === 95 || (r === 1 ? e === 45 || e === 58 : false) || e > 127 && _A(e, t);
}
function vy(e, t, r) {
let s = ii(e, 0);
if (!Wn(s, t))
return false;
for (let f = yi(s); f < e.length; f += yi(s))
if (!Rs(s = ii(e, f), t, r))
return false;
return true;
}
function Po(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : 0, s = arguments.length > 3 ? arguments[3] : void 0, f = arguments.length > 4 ? arguments[4] : void 0, x = arguments.length > 5 ? arguments[5] : void 0, w = arguments.length > 6 ? arguments[6] : void 0;
var A = s, g, B, N, X, F, $, ae, Te, Se = 0;
ue(A, x, w);
var Ye = { getStartPos: () => N, getTextPos: () => g, getToken: () => F, getTokenPos: () => X, getTokenText: () => A.substring(X, g), getTokenValue: () => $, hasUnicodeEscape: () => (ae & 1024) !== 0, hasExtendedUnicodeEscape: () => (ae & 8) !== 0, hasPrecedingLineBreak: () => (ae & 1) !== 0, hasPrecedingJSDocComment: () => (ae & 2) !== 0, isIdentifier: () => F === 79 || F > 116, isReservedWord: () => F >= 81 && F <= 116, isUnterminated: () => (ae & 4) !== 0, getCommentDirectives: () => Te, getNumericLiteralFlags: () => ae & 1008, getTokenFlags: () => ae, reScanGreaterToken: Sn, reScanAsteriskEqualsToken: In, reScanSlashToken: pr, reScanTemplateToken: Nn, reScanTemplateHeadOrNoSubstitutionTemplate: ar, scanJsxIdentifier: nr, scanJsxAttributeValue: br, reScanJsxAttributeValue: Kr, reScanJsxToken: oi, reScanLessThanToken: cr, reScanHashToken: $r, reScanQuestionToken: hr, reScanInvalidIdentifier: Gr, scanJsxToken: On, scanJsDocToken: wa, scan: Ur, getText: Ca, clearCommentDirectives: St, setText: ue, setScriptTarget: _t, setLanguageVariant: ft, setOnError: He, setTextPos: Kt, setInJSDocType: zt, tryScan: _i, lookAhead: Mn, scanRange: Ki };
return Y.isDebugging && Object.defineProperty(Ye, "__debugShowCurrentPositionInText", { get: () => {
let xe = Ye.getText();
return xe.slice(0, Ye.getStartPos()) + "\u2551" + xe.slice(Ye.getStartPos());
} }), Ye;
function Ne(xe) {
let Le = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : g, Re = arguments.length > 2 ? arguments[2] : void 0;
if (f) {
let ot = g;
g = Le, f(xe, Re || 0), g = ot;
}
}
function oe() {
let xe = g, Le = false, Re = false, ot = "";
for (; ; ) {
let Ct = A.charCodeAt(g);
if (Ct === 95) {
ae |= 512, Le ? (Le = false, Re = true, ot += A.substring(xe, g)) : Ne(Re ? ve.Multiple_consecutive_numeric_separators_are_not_permitted : ve.Numeric_separators_are_not_allowed_here, g, 1), g++, xe = g;
continue;
}
if (O_(Ct)) {
Le = true, Re = false, g++;
continue;
}
break;
}
return A.charCodeAt(g - 1) === 95 && Ne(ve.Numeric_separators_are_not_allowed_here, g - 1, 1), ot + A.substring(xe, g);
}
function Ve() {
let xe = g, Le = oe(), Re, ot;
A.charCodeAt(g) === 46 && (g++, Re = oe());
let Ct = g;
if (A.charCodeAt(g) === 69 || A.charCodeAt(g) === 101) {
g++, ae |= 16, (A.charCodeAt(g) === 43 || A.charCodeAt(g) === 45) && g++;
let It = g, Mr = oe();
Mr ? (ot = A.substring(Ct, It) + Mr, Ct = g) : Ne(ve.Digit_expected);
}
let Mt;
if (ae & 512 ? (Mt = Le, Re && (Mt += "." + Re), ot && (Mt += ot)) : Mt = A.substring(xe, Ct), Re !== void 0 || ae & 16)
return pt(xe, Re === void 0 && !!(ae & 16)), { type: 8, value: "" + +Mt };
{
$ = Mt;
let It = dn();
return pt(xe), { type: It, value: $ };
}
}
function pt(xe, Le) {
if (!Wn(ii(A, g), e))
return;
let Re = g, { length: ot } = an();
ot === 1 && A[Re] === "n" ? Ne(Le ? ve.A_bigint_literal_cannot_use_exponential_notation : ve.A_bigint_literal_must_be_an_integer, xe, Re - xe + 1) : (Ne(ve.An_identifier_or_keyword_cannot_immediately_follow_a_numeric_literal, Re, ot), g = Re);
}
function Gt() {
let xe = g;
for (; hy(A.charCodeAt(g)); )
g++;
return +A.substring(xe, g);
}
function Nt(xe, Le) {
let Re = er(xe, false, Le);
return Re ? parseInt(Re, 16) : -1;
}
function Xt(xe, Le) {
return er(xe, true, Le);
}
function er(xe, Le, Re) {
let ot = [], Ct = false, Mt = false;
for (; ot.length < xe || Le; ) {
let It = A.charCodeAt(g);
if (Re && It === 95) {
ae |= 512, Ct ? (Ct = false, Mt = true) : Ne(Mt ? ve.Multiple_consecutive_numeric_separators_are_not_permitted : ve.Numeric_separators_are_not_allowed_here, g, 1), g++;
continue;
}
if (Ct = Re, It >= 65 && It <= 70)
It += 97 - 65;
else if (!(It >= 48 && It <= 57 || It >= 97 && It <= 102))
break;
ot.push(It), g++, Mt = false;
}
return ot.length < xe && (ot = []), A.charCodeAt(g - 1) === 95 && Ne(ve.Numeric_separators_are_not_allowed_here, g - 1, 1), String.fromCharCode(...ot);
}
function Tn() {
let xe = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : false, Le = A.charCodeAt(g);
g++;
let Re = "", ot = g;
for (; ; ) {
if (g >= B) {
Re += A.substring(ot, g), ae |= 4, Ne(ve.Unterminated_string_literal);
break;
}
let Ct = A.charCodeAt(g);
if (Ct === Le) {
Re += A.substring(ot, g), g++;
break;
}
if (Ct === 92 && !xe) {
Re += A.substring(ot, g), Re += Gi(), ot = g;
continue;
}
if (un(Ct) && !xe) {
Re += A.substring(ot, g), ae |= 4, Ne(ve.Unterminated_string_literal);
break;
}
g++;
}
return Re;
}
function Hr(xe) {
let Le = A.charCodeAt(g) === 96;
g++;
let Re = g, ot = "", Ct;
for (; ; ) {
if (g >= B) {
ot += A.substring(Re, g), ae |= 4, Ne(ve.Unterminated_template_literal), Ct = Le ? 14 : 17;
break;
}
let Mt = A.charCodeAt(g);
if (Mt === 96) {
ot += A.substring(Re, g), g++, Ct = Le ? 14 : 17;
break;
}
if (Mt === 36 && g + 1 < B && A.charCodeAt(g + 1) === 123) {
ot += A.substring(Re, g), g += 2, Ct = Le ? 15 : 16;
break;
}
if (Mt === 92) {
ot += A.substring(Re, g), ot += Gi(xe), Re = g;
continue;
}
if (Mt === 13) {
ot += A.substring(Re, g), g++, g < B && A.charCodeAt(g) === 10 && g++, ot += `
`, Re = g;
continue;
}
g++;
}
return Y.assert(Ct !== void 0), $ = ot, Ct;
}
function Gi(xe) {
let Le = g;
if (g++, g >= B)
return Ne(ve.Unexpected_end_of_text), "";
let Re = A.charCodeAt(g);
switch (g++, Re) {
case 48:
return xe && g < B && O_(A.charCodeAt(g)) ? (g++, ae |= 2048, A.substring(Le, g)) : "\0";
case 98:
return "\b";
case 116:
return " ";
case 110:
return `
`;
case 118:
return "\v";
case 102:
return "\f";
case 114:
return "\r";
case 39:
return "'";
case 34:
return '"';
case 117:
if (xe) {
for (let ot = g; ot < g + 4; ot++)
if (ot < B && !Xp(A.charCodeAt(ot)) && A.charCodeAt(ot) !== 123)
return g = ot, ae |= 2048, A.substring(Le, g);
}
if (g < B && A.charCodeAt(g) === 123) {
if (g++, xe && !Xp(A.charCodeAt(g)))
return ae |= 2048, A.substring(Le, g);
if (xe) {
let ot = g, Ct = Xt(1, false), Mt = Ct ? parseInt(Ct, 16) : -1;
if (!uA(Mt) || A.charCodeAt(g) !== 125)
return ae |= 2048, A.substring(Le, g);
g = ot;
}
return ae |= 8, fn();
}
return ae |= 1024, pn(4);
case 120:
if (xe)
if (Xp(A.charCodeAt(g))) {
if (!Xp(A.charCodeAt(g + 1)))
return g++, ae |= 2048, A.substring(Le, g);
} else
return ae |= 2048, A.substring(Le, g);
return pn(2);
case 13:
g < B && A.charCodeAt(g) === 10 && g++;
case 10:
case 8232:
case 8233:
return "";
default:
return String.fromCharCode(Re);
}
}
function pn(xe) {
let Le = Nt(xe, false);
return Le >= 0 ? String.fromCharCode(Le) : (Ne(ve.Hexadecimal_digit_expected), "");
}
function fn() {
let xe = Xt(1, false), Le = xe ? parseInt(xe, 16) : -1, Re = false;
return Le < 0 ? (Ne(ve.Hexadecimal_digit_expected), Re = true) : Le > 1114111 && (Ne(ve.An_extended_Unicode_escape_value_must_be_between_0x0_and_0x10FFFF_inclusive), Re = true), g >= B ? (Ne(ve.Unexpected_end_of_text), Re = true) : A.charCodeAt(g) === 125 ? g++ : (Ne(ve.Unterminated_Unicode_escape_sequence), Re = true), Re ? "" : by(Le);
}
function Ut() {
if (g + 5 < B && A.charCodeAt(g + 1) === 117) {
let xe = g;
g += 2;
let Le = Nt(4, false);
return g = xe, Le;
}
return -1;
}
function kn() {
if (ii(A, g + 1) === 117 && ii(A, g + 2) === 123) {
let xe = g;
g += 3;
let Le = Xt(1, false), Re = Le ? parseInt(Le, 16) : -1;
return g = xe, Re;
}
return -1;
}
function an() {
let xe = "", Le = g;
for (; g < B; ) {
let Re = ii(A, g);
if (Rs(Re, e))
g += yi(Re);
else if (Re === 92) {
if (Re = kn(), Re >= 0 && Rs(Re, e)) {
g += 3, ae |= 8, xe += fn(), Le = g;
continue;
}
if (Re = Ut(), !(Re >= 0 && Rs(Re, e)))
break;
ae |= 1024, xe += A.substring(Le, g), xe += by(Re), g += 6, Le = g;
} else
break;
}
return xe += A.substring(Le, g), xe;
}
function mr() {
let xe = $.length;
if (xe >= 2 && xe <= 12) {
let Le = $.charCodeAt(0);
if (Le >= 97 && Le <= 122) {
let Re = $T.get($);
if (Re !== void 0)
return F = Re;
}
}
return F = 79;
}
function $i(xe) {
let Le = "", Re = false, ot = false;
for (; ; ) {
let Ct = A.charCodeAt(g);
if (Ct === 95) {
ae |= 512, Re ? (Re = false, ot = true) : Ne(ot ? ve.Multiple_consecutive_numeric_separators_are_not_permitted : ve.Numeric_separators_are_not_allowed_here, g, 1), g++;
continue;
}
if (Re = true, !O_(Ct) || Ct - 48 >= xe)
break;
Le += A[g], g++, ot = false;
}
return A.charCodeAt(g - 1) === 95 && Ne(ve.Numeric_separators_are_not_allowed_here, g - 1, 1), Le;
}
function dn() {
return A.charCodeAt(g) === 110 ? ($ += "n", ae & 384 && ($ = Hf($) + "n"), g++, 9) : ($ = "" + (ae & 128 ? parseInt($.slice(2), 2) : ae & 256 ? parseInt($.slice(2), 8) : +$), 8);
}
function Ur() {
N = g, ae = 0;
let xe = false;
for (; ; ) {
if (X = g, g >= B)
return F = 1;
let Le = ii(A, g);
if (Le === 35 && g === 0 && gy(A, g)) {
if (g = yy(A, g), t)
continue;
return F = 6;
}
switch (Le) {
case 10:
case 13:
if (ae |= 1, t) {
g++;
continue;
} else
return Le === 13 && g + 1 < B && A.charCodeAt(g + 1) === 10 ? g += 2 : g++, F = 4;
case 9:
case 11:
case 12:
case 32:
case 160:
case 5760:
case 8192:
case 8193:
case 8194:
case 8195:
case 8196:
case 8197:
case 8198:
case 8199:
case 8200:
case 8201:
case 8202:
case 8203:
case 8239:
case 8287:
case 12288:
case 65279:
if (t) {
g++;
continue;
} else {
for (; g < B && N_(A.charCodeAt(g)); )
g++;
return F = 5;
}
case 33:
return A.charCodeAt(g + 1) === 61 ? A.charCodeAt(g + 2) === 61 ? (g += 3, F = 37) : (g += 2, F = 35) : (g++, F = 53);
case 34:
case 39:
return $ = Tn(), F = 10;
case 96:
return F = Hr(false);
case 37:
return A.charCodeAt(g + 1) === 61 ? (g += 2, F = 69) : (g++, F = 44);
case 38:
return A.charCodeAt(g + 1) === 38 ? A.charCodeAt(g + 2) === 61 ? (g += 3, F = 76) : (g += 2, F = 55) : A.charCodeAt(g + 1) === 61 ? (g += 2, F = 73) : (g++, F = 50);
case 40:
return g++, F = 20;
case 41:
return g++, F = 21;
case 42:
if (A.charCodeAt(g + 1) === 61)
return g += 2, F = 66;
if (A.charCodeAt(g + 1) === 42)
return A.charCodeAt(g + 2) === 61 ? (g += 3, F = 67) : (g += 2, F = 42);
if (g++, Se && !xe && ae & 1) {
xe = true;
continue;
}
return F = 41;
case 43:
return A.charCodeAt(g + 1) === 43 ? (g += 2, F = 45) : A.charCodeAt(g + 1) === 61 ? (g += 2, F = 64) : (g++, F = 39);
case 44:
return g++, F = 27;
case 45:
return A.charCodeAt(g + 1) === 45 ? (g += 2, F = 46) : A.charCodeAt(g + 1) === 61 ? (g += 2, F = 65) : (g++, F = 40);
case 46:
return O_(A.charCodeAt(g + 1)) ? ($ = Ve().value, F = 8) : A.charCodeAt(g + 1) === 46 && A.charCodeAt(g + 2) === 46 ? (g += 3, F = 25) : (g++, F = 24);
case 47:
if (A.charCodeAt(g + 1) === 47) {
for (g += 2; g < B && !un(A.charCodeAt(g)); )
g++;
if (Te = Zt(Te, A.slice(X, g), tS, X), t)
continue;
return F = 2;
}
if (A.charCodeAt(g + 1) === 42) {
g += 2, A.charCodeAt(g) === 42 && A.charCodeAt(g + 1) !== 47 && (ae |= 2);
let Mr = false, gr = X;
for (; g < B; ) {
let Ln = A.charCodeAt(g);
if (Ln === 42 && A.charCodeAt(g + 1) === 47) {
g += 2, Mr = true;
break;
}
g++, un(Ln) && (gr = g, ae |= 1);
}
if (Te = Zt(Te, A.slice(gr, g), rS, gr), Mr || Ne(ve.Asterisk_Slash_expected), t)
continue;
return Mr || (ae |= 4), F = 3;
}
return A.charCodeAt(g + 1) === 61 ? (g += 2, F = 68) : (g++, F = 43);
case 48:
if (g + 2 < B && (A.charCodeAt(g + 1) === 88 || A.charCodeAt(g + 1) === 120))
return g += 2, $ = Xt(1, true), $ || (Ne(ve.Hexadecimal_digit_expected), $ = "0"), $ = "0x" + $, ae |= 64, F = dn();
if (g + 2 < B && (A.charCodeAt(g + 1) === 66 || A.charCodeAt(g + 1) === 98))
return g += 2, $ = $i(2), $ || (Ne(ve.Binary_digit_expected), $ = "0"), $ = "0b" + $, ae |= 128, F = dn();
if (g + 2 < B && (A.charCodeAt(g + 1) === 79 || A.charCodeAt(g + 1) === 111))
return g += 2, $ = $i(8), $ || (Ne(ve.Octal_digit_expected), $ = "0"), $ = "0o" + $, ae |= 256, F = dn();
if (g + 1 < B && hy(A.charCodeAt(g + 1)))
return $ = "" + Gt(), ae |= 32, F = 8;
case 49:
case 50:
case 51:
case 52:
case 53:
case 54:
case 55:
case 56:
case 57:
return { type: F, value: $ } = Ve(), F;
case 58:
return g++, F = 58;
case 59:
return g++, F = 26;
case 60:
if (Co(A, g)) {
if (g = M_(A, g, Ne), t)
continue;
return F = 7;
}
return A.charCodeAt(g + 1) === 60 ? A.charCodeAt(g + 2) === 61 ? (g += 3, F = 70) : (g += 2, F = 47) : A.charCodeAt(g + 1) === 61 ? (g += 2, F = 32) : r === 1 && A.charCodeAt(g + 1) === 47 && A.charCodeAt(g + 2) !== 42 ? (g += 2, F = 30) : (g++, F = 29);
case 61:
if (Co(A, g)) {
if (g = M_(A, g, Ne), t)
continue;
return F = 7;
}
return A.charCodeAt(g + 1) === 61 ? A.charCodeAt(g + 2) === 61 ? (g += 3, F = 36) : (g += 2, F = 34) : A.charCodeAt(g + 1) === 62 ? (g += 2, F = 38) : (g++, F = 63);
case 62:
if (Co(A, g)) {
if (g = M_(A, g, Ne), t)
continue;
return F = 7;
}
return g++, F = 31;
case 63:
return A.charCodeAt(g + 1) === 46 && !O_(A.charCodeAt(g + 2)) ? (g += 2, F = 28) : A.charCodeAt(g + 1) === 63 ? A.charCodeAt(g + 2) === 61 ? (g += 3, F = 77) : (g += 2, F = 60) : (g++, F = 57);
case 91:
return g++, F = 22;
case 93:
return g++, F = 23;
case 94:
return A.charCodeAt(g + 1) === 61 ? (g += 2, F = 78) : (g++, F = 52);
case 123:
return g++, F = 18;
case 124:
if (Co(A, g)) {
if (g = M_(A, g, Ne), t)
continue;
return F = 7;
}
return A.charCodeAt(g + 1) === 124 ? A.charCodeAt(g + 2) === 61 ? (g += 3, F = 75) : (g += 2, F = 56) : A.charCodeAt(g + 1) === 61 ? (g += 2, F = 74) : (g++, F = 51);
case 125:
return g++, F = 19;
case 126:
return g++, F = 54;
case 64:
return g++, F = 59;
case 92:
let Re = kn();
if (Re >= 0 && Wn(Re, e))
return g += 3, ae |= 8, $ = fn() + an(), F = mr();
let ot = Ut();
return ot >= 0 && Wn(ot, e) ? (g += 6, ae |= 1024, $ = String.fromCharCode(ot) + an(), F = mr()) : (Ne(ve.Invalid_character), g++, F = 0);
case 35:
if (g !== 0 && A[g + 1] === "!")
return Ne(ve.can_only_be_used_at_the_start_of_a_file), g++, F = 0;
let Ct = ii(A, g + 1);
if (Ct === 92) {
g++;
let Mr = kn();
if (Mr >= 0 && Wn(Mr, e))
return g += 3, ae |= 8, $ = "#" + fn() + an(), F = 80;
let gr = Ut();
if (gr >= 0 && Wn(gr, e))
return g += 6, ae |= 1024, $ = "#" + String.fromCharCode(gr) + an(), F = 80;
g--;
}
return Wn(Ct, e) ? (g++, _r(Ct, e)) : ($ = "#", Ne(ve.Invalid_character, g++, yi(Le))), F = 80;
default:
let Mt = _r(Le, e);
if (Mt)
return F = Mt;
if (N_(Le)) {
g += yi(Le);
continue;
} else if (un(Le)) {
ae |= 1, g += yi(Le);
continue;
}
let It = yi(Le);
return Ne(ve.Invalid_character, g, It), g += It, F = 0;
}
}
}
function Gr() {
Y.assert(F === 0, "'reScanInvalidIdentifier' should only be called when the current token is 'SyntaxKind.Unknown'."), g = X = N, ae = 0;
let xe = ii(A, g), Le = _r(xe, 99);
return Le ? F = Le : (g += yi(xe), F);
}
function _r(xe, Le) {
let Re = xe;
if (Wn(Re, Le)) {
for (g += yi(Re); g < B && Rs(Re = ii(A, g), Le); )
g += yi(Re);
return $ = A.substring(X, g), Re === 92 && ($ += an()), mr();
}
}
function Sn() {
if (F === 31) {
if (A.charCodeAt(g) === 62)
return A.charCodeAt(g + 1) === 62 ? A.charCodeAt(g + 2) === 61 ? (g += 3, F = 72) : (g += 2, F = 49) : A.charCodeAt(g + 1) === 61 ? (g += 2, F = 71) : (g++, F = 48);
if (A.charCodeAt(g) === 61)
return g++, F = 33;
}
return F;
}
function In() {
return Y.assert(F === 66, "'reScanAsteriskEqualsToken' should only be called on a '*='"), g = X + 1, F = 63;
}
function pr() {
if (F === 43 || F === 68) {
let xe = X + 1, Le = false, Re = false;
for (; ; ) {
if (xe >= B) {
ae |= 4, Ne(ve.Unterminated_regular_expression_literal);
break;
}
let ot = A.charCodeAt(xe);
if (un(ot)) {
ae |= 4, Ne(ve.Unterminated_regular_expression_literal);
break;
}
if (Le)
Le = false;
else if (ot === 47 && !Re) {
xe++;
break;
} else
ot === 91 ? Re = true : ot === 92 ? Le = true : ot === 93 && (Re = false);
xe++;
}
for (; xe < B && Rs(A.charCodeAt(xe), e); )
xe++;
g = xe, $ = A.substring(X, g), F = 13;
}
return F;
}
function Zt(xe, Le, Re, ot) {
let Ct = Or(nl(Le), Re);
return Ct === void 0 ? xe : tr(xe, { range: { pos: ot, end: g }, type: Ct });
}
function Or(xe, Le) {
let Re = Le.exec(xe);
if (Re)
switch (Re[1]) {
case "ts-expect-error":
return 0;
case "ts-ignore":
return 1;
}
}
function Nn(xe) {
return Y.assert(F === 19, "'reScanTemplateToken' should only be called on a '}'"), g = X, F = Hr(xe);
}
function ar() {
return g = X, F = Hr(true);
}
function oi() {
let xe = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : true;
return g = X = N, F = On(xe);
}
function cr() {
return F === 47 ? (g = X + 1, F = 29) : F;
}
function $r() {
return F === 80 ? (g = X + 1, F = 62) : F;
}
function hr() {
return Y.assert(F === 60, "'reScanQuestionToken' should only be called on a '??'"), g = X + 1, F = 57;
}
function On() {
let xe = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : true;
if (N = X = g, g >= B)
return F = 1;
let Le = A.charCodeAt(g);
if (Le === 60)
return A.charCodeAt(g + 1) === 47 ? (g += 2, F = 30) : (g++, F = 29);
if (Le === 123)
return g++, F = 18;
let Re = 0;
for (; g < B && (Le = A.charCodeAt(g), Le !== 123); ) {
if (Le === 60) {
if (Co(A, g))
return g = M_(A, g, Ne), F = 7;
break;
}
if (Le === 62 && Ne(ve.Unexpected_token_Did_you_mean_or_gt, g, 1), Le === 125 && Ne(ve.Unexpected_token_Did_you_mean_or_rbrace, g, 1), un(Le) && Re === 0)
Re = -1;
else {
if (!xe && un(Le) && Re > 0)
break;
os(Le) || (Re = g);
}
g++;
}
return $ = A.substring(N, g), Re === -1 ? 12 : 11;
}
function nr() {
if (fr(F)) {
let xe = false;
for (; g < B; ) {
let Le = A.charCodeAt(g);
if (Le === 45) {
$ += "-", g++;
continue;
} else if (Le === 58 && !xe) {
$ += ":", g++, xe = true, F = 79;
continue;
}
let Re = g;
if ($ += an(), g === Re)
break;
}
return $.slice(-1) === ":" && ($ = $.slice(0, -1), g--), mr();
}
return F;
}
function br() {
switch (N = g, A.charCodeAt(g)) {
case 34:
case 39:
return $ = Tn(true), F = 10;
default:
return Ur();
}
}
function Kr() {
return g = X = N, br();
}
function wa() {
if (N = X = g, ae = 0, g >= B)
return F = 1;
let xe = ii(A, g);
switch (g += yi(xe), xe) {
case 9:
case 11:
case 12:
case 32:
for (; g < B && N_(A.charCodeAt(g)); )
g++;
return F = 5;
case 64:
return F = 59;
case 13:
A.charCodeAt(g) === 10 && g++;
case 10:
return ae |= 1, F = 4;
case 42:
return F = 41;
case 123:
return F = 18;
case 125:
return F = 19;
case 91:
return F = 22;
case 93:
return F = 23;
case 60:
return F = 29;
case 62:
return F = 31;
case 61:
return F = 63;
case 44:
return F = 27;
case 46:
return F = 24;
case 96:
return F = 61;
case 35:
return F = 62;
case 92:
g--;
let Le = kn();
if (Le >= 0 && Wn(Le, e))
return g += 3, ae |= 8, $ = fn() + an(), F = mr();
let Re = Ut();
return Re >= 0 && Wn(Re, e) ? (g += 6, ae |= 1024, $ = String.fromCharCode(Re) + an(), F = mr()) : (g++, F = 0);
}
if (Wn(xe, e)) {
let Le = xe;
for (; g < B && Rs(Le = ii(A, g), e) || A.charCodeAt(g) === 45; )
g += yi(Le);
return $ = A.substring(X, g), Le === 92 && ($ += an()), F = mr();
} else
return F = 0;
}
function $n(xe, Le) {
let Re = g, ot = N, Ct = X, Mt = F, It = $, Mr = ae, gr = xe();
return (!gr || Le) && (g = Re, N = ot, X = Ct, F = Mt, $ = It, ae = Mr), gr;
}
function Ki(xe, Le, Re) {
let ot = B, Ct = g, Mt = N, It = X, Mr = F, gr = $, Ln = ae, ys = Te;
ue(A, xe, Le);
let ci = Re();
return B = ot, g = Ct, N = Mt, X = It, F = Mr, $ = gr, ae = Ln, Te = ys, ci;
}
function Mn(xe) {
return $n(xe, true);
}
function _i(xe) {
return $n(xe, false);
}
function Ca() {
return A;
}
function St() {
Te = void 0;
}
function ue(xe, Le, Re) {
A = xe || "", B = Re === void 0 ? A.length : Le + Re, Kt(Le || 0);
}
function He(xe) {
f = xe;
}
function _t(xe) {
e = xe;
}
function ft(xe) {
r = xe;
}
function Kt(xe) {
Y.assert(xe >= 0), g = xe, N = xe, X = xe, F = 0, $ = void 0, ae = 0;
}
function zt(xe) {
Se += xe ? 1 : -1;
}
}
function yi(e) {
return e >= 65536 ? 2 : 1;
}
function mA(e) {
if (Y.assert(0 <= e && e <= 1114111), e <= 65535)
return String.fromCharCode(e);
let t = Math.floor((e - 65536) / 1024) + 55296, r = (e - 65536) % 1024 + 56320;
return String.fromCharCode(t, r);
}
function by(e) {
return iS(e);
}
var cl, $T, Ty, KT, XT, YT, QT, ZT, eS, tS, rS, nS, ll, Qp, ii, iS, hA = D({ "src/compiler/scanner.ts"() {
"use strict";
nn(), cl = { abstract: 126, accessor: 127, any: 131, as: 128, asserts: 129, assert: 130, bigint: 160, boolean: 134, break: 81, case: 82, catch: 83, class: 84, continue: 86, const: 85, constructor: 135, debugger: 87, declare: 136, default: 88, delete: 89, do: 90, else: 91, enum: 92, export: 93, extends: 94, false: 95, finally: 96, for: 97, from: 158, function: 98, get: 137, if: 99, implements: 117, import: 100, in: 101, infer: 138, instanceof: 102, interface: 118, intrinsic: 139, is: 140, keyof: 141, let: 119, module: 142, namespace: 143, never: 144, new: 103, null: 104, number: 148, object: 149, package: 120, private: 121, protected: 122, public: 123, override: 161, out: 145, readonly: 146, require: 147, global: 159, return: 105, satisfies: 150, set: 151, static: 124, string: 152, super: 106, switch: 107, symbol: 153, this: 108, throw: 109, true: 110, try: 111, type: 154, typeof: 112, undefined: 155, unique: 156, unknown: 157, var: 113, void: 114, while: 115, with: 116, yield: 125, async: 132, await: 133, of: 162 }, $T = new Map(Object.entries(cl)), Ty = new Map(Object.entries(Object.assign(Object.assign({}, cl), {}, { "{": 18, "}": 19, "(": 20, ")": 21, "[": 22, "]": 23, ".": 24, "...": 25, ";": 26, ",": 27, "<": 29, ">": 31, "<=": 32, ">=": 33, "==": 34, "!=": 35, "===": 36, "!==": 37, "=>": 38, "+": 39, "-": 40, "**": 42, "*": 41, "/": 43, "%": 44, "++": 45, "--": 46, "<<": 47, "": 30, ">>": 48, ">>>": 49, "&": 50, "|": 51, "^": 52, "!": 53, "~": 54, "&&": 55, "||": 56, "?": 57, "??": 60, "?.": 28, ":": 58, "=": 63, "+=": 64, "-=": 65, "*=": 66, "**=": 67, "/=": 68, "%=": 69, "<<=": 70, ">>=": 71, ">>>=": 72, "&=": 73, "|=": 74, "^=": 78, "||=": 75, "&&=": 76, "??=": 77, "@": 59, "#": 62, "`": 61 }))), KT = [170, 170, 181, 181, 186, 186, 192, 214, 216, 246, 248, 543, 546, 563, 592, 685, 688, 696, 699, 705, 720, 721, 736, 740, 750, 750, 890, 890, 902, 902, 904, 906, 908, 908, 910, 929, 931, 974, 976, 983, 986, 1011, 1024, 1153, 1164, 1220, 1223, 1224, 1227, 1228, 1232, 1269, 1272, 1273, 1329, 1366, 1369, 1369, 1377, 1415, 1488, 1514, 1520, 1522, 1569, 1594, 1600, 1610, 1649, 1747, 1749, 1749, 1765, 1766, 1786, 1788, 1808, 1808, 1810, 1836, 1920, 1957, 2309, 2361, 2365, 2365, 2384, 2384, 2392, 2401, 2437, 2444, 2447, 2448, 2451, 2472, 2474, 2480, 2482, 2482, 2486, 2489, 2524, 2525, 2527, 2529, 2544, 2545, 2565, 2570, 2575, 2576, 2579, 2600, 2602, 2608, 2610, 2611, 2613, 2614, 2616, 2617, 2649, 2652, 2654, 2654, 2674, 2676, 2693, 2699, 2701, 2701, 2703, 2705, 2707, 2728, 2730, 2736, 2738, 2739, 2741, 2745, 2749, 2749, 2768, 2768, 2784, 2784, 2821, 2828, 2831, 2832, 2835, 2856, 2858, 2864, 2866, 2867, 2870, 2873, 2877, 2877, 2908, 2909, 2911, 2913, 2949, 2954, 2958, 2960, 2962, 2965, 2969, 2970, 2972, 2972, 2974, 2975, 2979, 2980, 2984, 2986, 2990, 2997, 2999, 3001, 3077, 3084, 3086, 3088, 3090, 3112, 3114, 3123, 3125, 3129, 3168, 3169, 3205, 3212, 3214, 3216, 3218, 3240, 3242, 3251, 3253, 3257, 3294, 3294, 3296, 3297, 3333, 3340, 3342, 3344, 3346, 3368, 3370, 3385, 3424, 3425, 3461, 3478, 3482, 3505, 3507, 3515, 3517, 3517, 3520, 3526, 3585, 3632, 3634, 3635, 3648, 3654, 3713, 3714, 3716, 3716, 3719, 3720, 3722, 3722, 3725, 3725, 3732, 3735, 3737, 3743, 3745, 3747, 3749, 3749, 3751, 3751, 3754, 3755, 3757, 3760, 3762, 3763, 3773, 3773, 3776, 3780, 3782, 3782, 3804, 3805, 3840, 3840, 3904, 3911, 3913, 3946, 3976, 3979, 4096, 4129, 4131, 4135, 4137, 4138, 4176, 4181, 4256, 4293, 4304, 4342, 4352, 4441, 4447, 4514, 4520, 4601, 4608, 4614, 4616, 4678, 4680, 4680, 4682, 4685, 4688, 4694, 4696, 4696, 4698, 4701, 4704, 4742, 4744, 4744, 4746, 4749, 4752, 4782, 4784, 4784, 4786, 4789, 4792, 4798, 4800, 4800, 4802, 4805, 4808, 4814, 4816, 4822, 4824, 4846, 4848, 4878, 4880, 4880, 4882, 4885, 4888, 4894, 4896, 4934, 4936, 4954, 5024, 5108, 5121, 5740, 5743, 5750, 5761, 5786, 5792, 5866, 6016, 6067, 6176, 6263, 6272, 6312, 7680, 7835, 7840, 7929, 7936, 7957, 7960, 7965, 7968, 8005, 8008, 8013, 8016, 8023, 8025, 8025, 8027, 8027, 8029, 8029, 8031, 8061, 8064, 8116, 8118, 8124, 8126, 8126, 8130, 8132, 8134, 8140, 8144, 8147, 8150, 8155, 8160, 8172, 8178, 8180, 8182, 8188, 8319, 8319, 8450, 8450, 8455, 8455, 8458, 8467, 8469, 8469, 8473, 8477, 8484, 8484, 8486, 8486, 8488, 8488, 8490, 8493, 8495, 8497, 8499, 8505, 8544, 8579, 12293, 12295, 12321, 12329, 12337, 12341, 12344, 12346, 12353, 12436, 12445, 12446, 12449, 12538, 12540, 12542, 12549, 12588, 12593, 12686, 12704, 12727, 13312, 19893, 19968, 40869, 40960, 42124, 44032, 55203, 63744, 64045, 64256, 64262, 64275, 64279, 64285, 64285, 64287, 64296, 64298, 64310, 64312, 64316, 64318, 64318, 64320, 64321, 64323, 64324, 64326, 64433, 64467, 64829, 64848, 64911, 64914, 64967, 65008, 65019, 65136, 65138, 65140, 65140, 65142, 65276, 65313, 65338, 65345, 65370, 65382, 65470, 65474, 65479, 65482, 65487, 65490, 65495, 65498, 65500], XT = [170, 170, 181, 181, 186, 186, 192, 214, 216, 246, 248, 543, 546, 563, 592, 685, 688, 696, 699, 705, 720, 721, 736, 740, 750, 750, 768, 846, 864, 866, 890, 890, 902, 902, 904, 906, 908, 908, 910, 929, 931, 974, 976, 983, 986, 1011, 1024, 1153, 1155, 1158, 1164, 1220, 1223, 1224, 1227, 1228, 1232, 1269, 1272, 1273, 1329, 1366, 1369, 1369, 1377, 1415, 1425, 1441, 1443, 1465, 1467, 1469, 1471, 1471, 1473, 1474, 1476, 1476, 1488, 1514, 1520, 1522, 1569, 1594, 1600, 1621, 1632, 1641, 1648, 1747, 1749, 1756, 1759, 1768, 1770, 1773, 1776, 1788, 1808, 1836, 1840, 1866, 1920, 1968, 2305, 2307, 2309, 2361, 2364, 2381, 2384, 2388, 2392, 2403, 2406, 2415, 2433, 2435, 2437, 2444, 2447, 2448, 2451, 2472, 2474, 2480, 2482, 2482, 2486, 2489, 2492, 2492, 2494, 2500, 2503, 2504, 2507, 2509, 2519, 2519, 2524, 2525, 2527, 2531, 2534, 2545, 2562, 2562, 2565, 2570, 2575, 2576, 2579, 2600, 2602, 2608, 2610, 2611, 2613, 2614, 2616, 2617, 2620, 2620, 2622, 2626, 2631, 2632, 2635, 2637, 2649, 2652, 2654, 2654, 2662, 2676, 2689, 2691, 2693, 2699, 2701, 2701, 2703, 2705, 2707, 2728, 2730, 2736, 2738, 2739, 2741, 2745, 2748, 2757, 2759, 2761, 2763, 2765, 2768, 2768, 2784, 2784, 2790, 2799, 2817, 2819, 2821, 2828, 2831, 2832, 2835, 2856, 2858, 2864, 2866, 2867, 2870, 2873, 2876, 2883, 2887, 2888, 2891, 2893, 2902, 2903, 2908, 2909, 2911, 2913, 2918, 2927, 2946, 2947, 2949, 2954, 2958, 2960, 2962, 2965, 2969, 2970, 2972, 2972, 2974, 2975, 2979, 2980, 2984, 2986, 2990, 2997, 2999, 3001, 3006, 3010, 3014, 3016, 3018, 3021, 3031, 3031, 3047, 3055, 3073, 3075, 3077, 3084, 3086, 3088, 3090, 3112, 3114, 3123, 3125, 3129, 3134, 3140, 3142, 3144, 3146, 3149, 3157, 3158, 3168, 3169, 3174, 3183, 3202, 3203, 3205, 3212, 3214, 3216, 3218, 3240, 3242, 3251, 3253, 3257, 3262, 3268, 3270, 3272, 3274, 3277, 3285, 3286, 3294, 3294, 3296, 3297, 3302, 3311, 3330, 3331, 3333, 3340, 3342, 3344, 3346, 3368, 3370, 3385, 3390, 3395, 3398, 3400, 3402, 3405, 3415, 3415, 3424, 3425, 3430, 3439, 3458, 3459, 3461, 3478, 3482, 3505, 3507, 3515, 3517, 3517, 3520, 3526, 3530, 3530, 3535, 3540, 3542, 3542, 3544, 3551, 3570, 3571, 3585, 3642, 3648, 3662, 3664, 3673, 3713, 3714, 3716, 3716, 3719, 3720, 3722, 3722, 3725, 3725, 3732, 3735, 3737, 3743, 3745, 3747, 3749, 3749, 3751, 3751, 3754, 3755, 3757, 3769, 3771, 3773, 3776, 3780, 3782, 3782, 3784, 3789, 3792, 3801, 3804, 3805, 3840, 3840, 3864, 3865, 3872, 3881, 3893, 3893, 3895, 3895, 3897, 3897, 3902, 3911, 3913, 3946, 3953, 3972, 3974, 3979, 3984, 3991, 3993, 4028, 4038, 4038, 4096, 4129, 4131, 4135, 4137, 4138, 4140, 4146, 4150, 4153, 4160, 4169, 4176, 4185, 4256, 4293, 4304, 4342, 4352, 4441, 4447, 4514, 4520, 4601, 4608, 4614, 4616, 4678, 4680, 4680, 4682, 4685, 4688, 4694, 4696, 4696, 4698, 4701, 4704, 4742, 4744, 4744, 4746, 4749, 4752, 4782, 4784, 4784, 4786, 4789, 4792, 4798, 4800, 4800, 4802, 4805, 4808, 4814, 4816, 4822, 4824, 4846, 4848, 4878, 4880, 4880, 4882, 4885, 4888, 4894, 4896, 4934, 4936, 4954, 4969, 4977, 5024, 5108, 5121, 5740, 5743, 5750, 5761, 5786, 5792, 5866, 6016, 6099, 6112, 6121, 6160, 6169, 6176, 6263, 6272, 6313, 7680, 7835, 7840, 7929, 7936, 7957, 7960, 7965, 7968, 8005, 8008, 8013, 8016, 8023, 8025, 8025, 8027, 8027, 8029, 8029, 8031, 8061, 8064, 8116, 8118, 8124, 8126, 8126, 8130, 8132, 8134, 8140, 8144, 8147, 8150, 8155, 8160, 8172, 8178, 8180, 8182, 8188, 8255, 8256, 8319, 8319, 8400, 8412, 8417, 8417, 8450, 8450, 8455, 8455, 8458, 8467, 8469, 8469, 8473, 8477, 8484, 8484, 8486, 8486, 8488, 8488, 8490, 8493, 8495, 8497, 8499, 8505, 8544, 8579, 12293, 12295, 12321, 12335, 12337, 12341, 12344, 12346, 12353, 12436, 12441, 12442, 12445, 12446, 12449, 12542, 12549, 12588, 12593, 12686, 12704, 12727, 13312, 19893, 19968, 40869, 40960, 42124, 44032, 55203, 63744, 64045, 64256, 64262, 64275, 64279, 64285, 64296, 64298, 64310, 64312, 64316, 64318, 64318, 64320, 64321, 64323, 64324, 64326, 64433, 64467, 64829, 64848, 64911, 64914, 64967, 65008, 65019, 65056, 65059, 65075, 65076, 65101, 65103, 65136, 65138, 65140, 65140, 65142, 65276, 65296, 65305, 65313, 65338, 65343, 65343, 65345, 65370, 65381, 65470, 65474, 65479, 65482, 65487, 65490, 65495, 65498, 65500], YT = [170, 170, 181, 181, 186, 186, 192, 214, 216, 246, 248, 705, 710, 721, 736, 740, 748, 748, 750, 750, 880, 884, 886, 887, 890, 893, 902, 902, 904, 906, 908, 908, 910, 929, 931, 1013, 1015, 1153, 1162, 1319, 1329, 1366, 1369, 1369, 1377, 1415, 1488, 1514, 1520, 1522, 1568, 1610, 1646, 1647, 1649, 1747, 1749, 1749, 1765, 1766, 1774, 1775, 1786, 1788, 1791, 1791, 1808, 1808, 1810, 1839, 1869, 1957, 1969, 1969, 1994, 2026, 2036, 2037, 2042, 2042, 2048, 2069, 2074, 2074, 2084, 2084, 2088, 2088, 2112, 2136, 2208, 2208, 2210, 2220, 2308, 2361, 2365, 2365, 2384, 2384, 2392, 2401, 2417, 2423, 2425, 2431, 2437, 2444, 2447, 2448, 2451, 2472, 2474, 2480, 2482, 2482, 2486, 2489, 2493, 2493, 2510, 2510, 2524, 2525, 2527, 2529, 2544, 2545, 2565, 2570, 2575, 2576, 2579, 2600, 2602, 2608, 2610, 2611, 2613, 2614, 2616, 2617, 2649, 2652, 2654, 2654, 2674, 2676, 2693, 2701, 2703, 2705, 2707, 2728, 2730, 2736, 2738, 2739, 2741, 2745, 2749, 2749, 2768, 2768, 2784, 2785, 2821, 2828, 2831, 2832, 2835, 2856, 2858, 2864, 2866, 2867, 2869, 2873, 2877, 2877, 2908, 2909, 2911, 2913, 2929, 2929, 2947, 2947, 2949, 2954, 2958, 2960, 2962, 2965, 2969, 2970, 2972, 2972, 2974, 2975, 2979, 2980, 2984, 2986, 2990, 3001, 3024, 3024, 3077, 3084, 3086, 3088, 3090, 3112, 3114, 3123, 3125, 3129, 3133, 3133, 3160, 3161, 3168, 3169, 3205, 3212, 3214, 3216, 3218, 3240, 3242, 3251, 3253, 3257, 3261, 3261, 3294, 3294, 3296, 3297, 3313, 3314, 3333, 3340, 3342, 3344, 3346, 3386, 3389, 3389, 3406, 3406, 3424, 3425, 3450, 3455, 3461, 3478, 3482, 3505, 3507, 3515, 3517, 3517, 3520, 3526, 3585, 3632, 3634, 3635, 3648, 3654, 3713, 3714, 3716, 3716, 3719, 3720, 3722, 3722, 3725, 3725, 3732, 3735, 3737, 3743, 3745, 3747, 3749, 3749, 3751, 3751, 3754, 3755, 3757, 3760, 3762, 3763, 3773, 3773, 3776, 3780, 3782, 3782, 3804, 3807, 3840, 3840, 3904, 3911, 3913, 3948, 3976, 3980, 4096, 4138, 4159, 4159, 4176, 4181, 4186, 4189, 4193, 4193, 4197, 4198, 4206, 4208, 4213, 4225, 4238, 4238, 4256, 4293, 4295, 4295, 4301, 4301, 4304, 4346, 4348, 4680, 4682, 4685, 4688, 4694, 4696, 4696, 4698, 4701, 4704, 4744, 4746, 4749, 4752, 4784, 4786, 4789, 4792, 4798, 4800, 4800, 4802, 4805, 4808, 4822, 4824, 4880, 4882, 4885, 4888, 4954, 4992, 5007, 5024, 5108, 5121, 5740, 5743, 5759, 5761, 5786, 5792, 5866, 5870, 5872, 5888, 5900, 5902, 5905, 5920, 5937, 5952, 5969, 5984, 5996, 5998, 6e3, 6016, 6067, 6103, 6103, 6108, 6108, 6176, 6263, 6272, 6312, 6314, 6314, 6320, 6389, 6400, 6428, 6480, 6509, 6512, 6516, 6528, 6571, 6593, 6599, 6656, 6678, 6688, 6740, 6823, 6823, 6917, 6963, 6981, 6987, 7043, 7072, 7086, 7087, 7098, 7141, 7168, 7203, 7245, 7247, 7258, 7293, 7401, 7404, 7406, 7409, 7413, 7414, 7424, 7615, 7680, 7957, 7960, 7965, 7968, 8005, 8008, 8013, 8016, 8023, 8025, 8025, 8027, 8027, 8029, 8029, 8031, 8061, 8064, 8116, 8118, 8124, 8126, 8126, 8130, 8132, 8134, 8140, 8144, 8147, 8150, 8155, 8160, 8172, 8178, 8180, 8182, 8188, 8305, 8305, 8319, 8319, 8336, 8348, 8450, 8450, 8455, 8455, 8458, 8467, 8469, 8469, 8473, 8477, 8484, 8484, 8486, 8486, 8488, 8488, 8490, 8493, 8495, 8505, 8508, 8511, 8517, 8521, 8526, 8526, 8544, 8584, 11264, 11310, 11312, 11358, 11360, 11492, 11499, 11502, 11506, 11507, 11520, 11557, 11559, 11559, 11565, 11565, 11568, 11623, 11631, 11631, 11648, 11670, 11680, 11686, 11688, 11694, 11696, 11702, 11704, 11710, 11712, 11718, 11720, 11726, 11728, 11734, 11736, 11742, 11823, 11823, 12293, 12295, 12321, 12329, 12337, 12341, 12344, 12348, 12353, 12438, 12445, 12447, 12449, 12538, 12540, 12543, 12549, 12589, 12593, 12686, 12704, 12730, 12784, 12799, 13312, 19893, 19968, 40908, 40960, 42124, 42192, 42237, 42240, 42508, 42512, 42527, 42538, 42539, 42560, 42606, 42623, 42647, 42656, 42735, 42775, 42783, 42786, 42888, 42891, 42894, 42896, 42899, 42912, 42922, 43e3, 43009, 43011, 43013, 43015, 43018, 43020, 43042, 43072, 43123, 43138, 43187, 43250, 43255, 43259, 43259, 43274, 43301, 43312, 43334, 43360, 43388, 43396, 43442, 43471, 43471, 43520, 43560, 43584, 43586, 43588, 43595, 43616, 43638, 43642, 43642, 43648, 43695, 43697, 43697, 43701, 43702, 43705, 43709, 43712, 43712, 43714, 43714, 43739, 43741, 43744, 43754, 43762, 43764, 43777, 43782, 43785, 43790, 43793, 43798, 43808, 43814, 43816, 43822, 43968, 44002, 44032, 55203, 55216, 55238, 55243, 55291, 63744, 64109, 64112, 64217, 64256, 64262, 64275, 64279, 64285, 64285, 64287, 64296, 64298, 64310, 64312, 64316, 64318, 64318, 64320, 64321, 64323, 64324, 64326, 64433, 64467, 64829, 64848, 64911, 64914, 64967, 65008, 65019, 65136, 65140, 65142, 65276, 65313, 65338, 65345, 65370, 65382, 65470, 65474, 65479, 65482, 65487, 65490, 65495, 65498, 65500], QT = [170, 170, 181, 181, 186, 186, 192, 214, 216, 246, 248, 705, 710, 721, 736, 740, 748, 748, 750, 750, 768, 884, 886, 887, 890, 893, 902, 902, 904, 906, 908, 908, 910, 929, 931, 1013, 1015, 1153, 1155, 1159, 1162, 1319, 1329, 1366, 1369, 1369, 1377, 1415, 1425, 1469, 1471, 1471, 1473, 1474, 1476, 1477, 1479, 1479, 1488, 1514, 1520, 1522, 1552, 1562, 1568, 1641, 1646, 1747, 1749, 1756, 1759, 1768, 1770, 1788, 1791, 1791, 1808, 1866, 1869, 1969, 1984, 2037, 2042, 2042, 2048, 2093, 2112, 2139, 2208, 2208, 2210, 2220, 2276, 2302, 2304, 2403, 2406, 2415, 2417, 2423, 2425, 2431, 2433, 2435, 2437, 2444, 2447, 2448, 2451, 2472, 2474, 2480, 2482, 2482, 2486, 2489, 2492, 2500, 2503, 2504, 2507, 2510, 2519, 2519, 2524, 2525, 2527, 2531, 2534, 2545, 2561, 2563, 2565, 2570, 2575, 2576, 2579, 2600, 2602, 2608, 2610, 2611, 2613, 2614, 2616, 2617, 2620, 2620, 2622, 2626, 2631, 2632, 2635, 2637, 2641, 2641, 2649, 2652, 2654, 2654, 2662, 2677, 2689, 2691, 2693, 2701, 2703, 2705, 2707, 2728, 2730, 2736, 2738, 2739, 2741, 2745, 2748, 2757, 2759, 2761, 2763, 2765, 2768, 2768, 2784, 2787, 2790, 2799, 2817, 2819, 2821, 2828, 2831, 2832, 2835, 2856, 2858, 2864, 2866, 2867, 2869, 2873, 2876, 2884, 2887, 2888, 2891, 2893, 2902, 2903, 2908, 2909, 2911, 2915, 2918, 2927, 2929, 2929, 2946, 2947, 2949, 2954, 2958, 2960, 2962, 2965, 2969, 2970, 2972, 2972, 2974, 2975, 2979, 2980, 2984, 2986, 2990, 3001, 3006, 3010, 3014, 3016, 3018, 3021, 3024, 3024, 3031, 3031, 3046, 3055, 3073, 3075, 3077, 3084, 3086, 3088, 3090, 3112, 3114, 3123, 3125, 3129, 3133, 3140, 3142, 3144, 3146, 3149, 3157, 3158, 3160, 3161, 3168, 3171, 3174, 3183, 3202, 3203, 3205, 3212, 3214, 3216, 3218, 3240, 3242, 3251, 3253, 3257, 3260, 3268, 3270, 3272, 3274, 3277, 3285, 3286, 3294, 3294, 3296, 3299, 3302, 3311, 3313, 3314, 3330, 3331, 3333, 3340, 3342, 3344, 3346, 3386, 3389, 3396, 3398, 3400, 3402, 3406, 3415, 3415, 3424, 3427, 3430, 3439, 3450, 3455, 3458, 3459, 3461, 3478, 3482, 3505, 3507, 3515, 3517, 3517, 3520, 3526, 3530, 3530, 3535, 3540, 3542, 3542, 3544, 3551, 3570, 3571, 3585, 3642, 3648, 3662, 3664, 3673, 3713, 3714, 3716, 3716, 3719, 3720, 3722, 3722, 3725, 3725, 3732, 3735, 3737, 3743, 3745, 3747, 3749, 3749, 3751, 3751, 3754, 3755, 3757, 3769, 3771, 3773, 3776, 3780, 3782, 3782, 3784, 3789, 3792, 3801, 3804, 3807, 3840, 3840, 3864, 3865, 3872, 3881, 3893, 3893, 3895, 3895, 3897, 3897, 3902, 3911, 3913, 3948, 3953, 3972, 3974, 3991, 3993, 4028, 4038, 4038, 4096, 4169, 4176, 4253, 4256, 4293, 4295, 4295, 4301, 4301, 4304, 4346, 4348, 4680, 4682, 4685, 4688, 4694, 4696, 4696, 4698, 4701, 4704, 4744, 4746, 4749, 4752, 4784, 4786, 4789, 4792, 4798, 4800, 4800, 4802, 4805, 4808, 4822, 4824, 4880, 4882, 4885, 4888, 4954, 4957, 4959, 4992, 5007, 5024, 5108, 5121, 5740, 5743, 5759, 5761, 5786, 5792, 5866, 5870, 5872, 5888, 5900, 5902, 5908, 5920, 5940, 5952, 5971, 5984, 5996, 5998, 6e3, 6002, 6003, 6016, 6099, 6103, 6103, 6108, 6109, 6112, 6121, 6155, 6157, 6160, 6169, 6176, 6263, 6272, 6314, 6320, 6389, 6400, 6428, 6432, 6443, 6448, 6459, 6470, 6509, 6512, 6516, 6528, 6571, 6576, 6601, 6608, 6617, 6656, 6683, 6688, 6750, 6752, 6780, 6783, 6793, 6800, 6809, 6823, 6823, 6912, 6987, 6992, 7001, 7019, 7027, 7040, 7155, 7168, 7223, 7232, 7241, 7245, 7293, 7376, 7378, 7380, 7414, 7424, 7654, 7676, 7957, 7960, 7965, 7968, 8005, 8008, 8013, 8016, 8023, 8025, 8025, 8027, 8027, 8029, 8029, 8031, 8061, 8064, 8116, 8118, 8124, 8126, 8126, 8130, 8132, 8134, 8140, 8144, 8147, 8150, 8155, 8160, 8172, 8178, 8180, 8182, 8188, 8204, 8205, 8255, 8256, 8276, 8276, 8305, 8305, 8319, 8319, 8336, 8348, 8400, 8412, 8417, 8417, 8421, 8432, 8450, 8450, 8455, 8455, 8458, 8467, 8469, 8469, 8473, 8477, 8484, 8484, 8486, 8486, 8488, 8488, 8490, 8493, 8495, 8505, 8508, 8511, 8517, 8521, 8526, 8526, 8544, 8584, 11264, 11310, 11312, 11358, 11360, 11492, 11499, 11507, 11520, 11557, 11559, 11559, 11565, 11565, 11568, 11623, 11631, 11631, 11647, 11670, 11680, 11686, 11688, 11694, 11696, 11702, 11704, 11710, 11712, 11718, 11720, 11726, 11728, 11734, 11736, 11742, 11744, 11775, 11823, 11823, 12293, 12295, 12321, 12335, 12337, 12341, 12344, 12348, 12353, 12438, 12441, 12442, 12445, 12447, 12449, 12538, 12540, 12543, 12549, 12589, 12593, 12686, 12704, 12730, 12784, 12799, 13312, 19893, 19968, 40908, 40960, 42124, 42192, 42237, 42240, 42508, 42512, 42539, 42560, 42607, 42612, 42621, 42623, 42647, 42655, 42737, 42775, 42783, 42786, 42888, 42891, 42894, 42896, 42899, 42912, 42922, 43e3, 43047, 43072, 43123, 43136, 43204, 43216, 43225, 43232, 43255, 43259, 43259, 43264, 43309, 43312, 43347, 43360, 43388, 43392, 43456, 43471, 43481, 43520, 43574, 43584, 43597, 43600, 43609, 43616, 43638, 43642, 43643, 43648, 43714, 43739, 43741, 43744, 43759, 43762, 43766, 43777, 43782, 43785, 43790, 43793, 43798, 43808, 43814, 43816, 43822, 43968, 44010, 44012, 44013, 44016, 44025, 44032, 55203, 55216, 55238, 55243, 55291, 63744, 64109, 64112, 64217, 64256, 64262, 64275, 64279, 64285, 64296, 64298, 64310, 64312, 64316, 64318, 64318, 64320, 64321, 64323, 64324, 64326, 64433, 64467, 64829, 64848, 64911, 64914, 64967, 65008, 65019, 65024, 65039, 65056, 65062, 65075, 65076, 65101, 65103, 65136, 65140, 65142, 65276, 65296, 65305, 65313, 65338, 65343, 65343, 65345, 65370, 65382, 65470, 65474, 65479, 65482, 65487, 65490, 65495, 65498, 65500], ZT = [65, 90, 97, 122, 170, 170, 181, 181, 186, 186, 192, 214, 216, 246, 248, 705, 710, 721, 736, 740, 748, 748, 750, 750, 880, 884, 886, 887, 890, 893, 895, 895, 902, 902, 904, 906, 908, 908, 910, 929, 931, 1013, 1015, 1153, 1162, 1327, 1329, 1366, 1369, 1369, 1376, 1416, 1488, 1514, 1519, 1522, 1568, 1610, 1646, 1647, 1649, 1747, 1749, 1749, 1765, 1766, 1774, 1775, 1786, 1788, 1791, 1791, 1808, 1808, 1810, 1839, 1869, 1957, 1969, 1969, 1994, 2026, 2036, 2037, 2042, 2042, 2048, 2069, 2074, 2074, 2084, 2084, 2088, 2088, 2112, 2136, 2144, 2154, 2208, 2228, 2230, 2237, 2308, 2361, 2365, 2365, 2384, 2384, 2392, 2401, 2417, 2432, 2437, 2444, 2447, 2448, 2451, 2472, 2474, 2480, 2482, 2482, 2486, 2489, 2493, 2493, 2510, 2510, 2524, 2525, 2527, 2529, 2544, 2545, 2556, 2556, 2565, 2570, 2575, 2576, 2579, 2600, 2602, 2608, 2610, 2611, 2613, 2614, 2616, 2617, 2649, 2652, 2654, 2654, 2674, 2676, 2693, 2701, 2703, 2705, 2707, 2728, 2730, 2736, 2738, 2739, 2741, 2745, 2749, 2749, 2768, 2768, 2784, 2785, 2809, 2809, 2821, 2828, 2831, 2832, 2835, 2856, 2858, 2864, 2866, 2867, 2869, 2873, 2877, 2877, 2908, 2909, 2911, 2913, 2929, 2929, 2947, 2947, 2949, 2954, 2958, 2960, 2962, 2965, 2969, 2970, 2972, 2972, 2974, 2975, 2979, 2980, 2984, 2986, 2990, 3001, 3024, 3024, 3077, 3084, 3086, 3088, 3090, 3112, 3114, 3129, 3133, 3133, 3160, 3162, 3168, 3169, 3200, 3200, 3205, 3212, 3214, 3216, 3218, 3240, 3242, 3251, 3253, 3257, 3261, 3261, 3294, 3294, 3296, 3297, 3313, 3314, 3333, 3340, 3342, 3344, 3346, 3386, 3389, 3389, 3406, 3406, 3412, 3414, 3423, 3425, 3450, 3455, 3461, 3478, 3482, 3505, 3507, 3515, 3517, 3517, 3520, 3526, 3585, 3632, 3634, 3635, 3648, 3654, 3713, 3714, 3716, 3716, 3718, 3722, 3724, 3747, 3749, 3749, 3751, 3760, 3762, 3763, 3773, 3773, 3776, 3780, 3782, 3782, 3804, 3807, 3840, 3840, 3904, 3911, 3913, 3948, 3976, 3980, 4096, 4138, 4159, 4159, 4176, 4181, 4186, 4189, 4193, 4193, 4197, 4198, 4206, 4208, 4213, 4225, 4238, 4238, 4256, 4293, 4295, 4295, 4301, 4301, 4304, 4346, 4348, 4680, 4682, 4685, 4688, 4694, 4696, 4696, 4698, 4701, 4704, 4744, 4746, 4749, 4752, 4784, 4786, 4789, 4792, 4798, 4800, 4800, 4802, 4805, 4808, 4822, 4824, 4880, 4882, 4885, 4888, 4954, 4992, 5007, 5024, 5109, 5112, 5117, 5121, 5740, 5743, 5759, 5761, 5786, 5792, 5866, 5870, 5880, 5888, 5900, 5902, 5905, 5920, 5937, 5952, 5969, 5984, 5996, 5998, 6e3, 6016, 6067, 6103, 6103, 6108, 6108, 6176, 6264, 6272, 6312, 6314, 6314, 6320, 6389, 6400, 6430, 6480, 6509, 6512, 6516, 6528, 6571, 6576, 6601, 6656, 6678, 6688, 6740, 6823, 6823, 6917, 6963, 6981, 6987, 7043, 7072, 7086, 7087, 7098, 7141, 7168, 7203, 7245, 7247, 7258, 7293, 7296, 7304, 7312, 7354, 7357, 7359, 7401, 7404, 7406, 7411, 7413, 7414, 7418, 7418, 7424, 7615, 7680, 7957, 7960, 7965, 7968, 8005, 8008, 8013, 8016, 8023, 8025, 8025, 8027, 8027, 8029, 8029, 8031, 8061, 8064, 8116, 8118, 8124, 8126, 8126, 8130, 8132, 8134, 8140, 8144, 8147, 8150, 8155, 8160, 8172, 8178, 8180, 8182, 8188, 8305, 8305, 8319, 8319, 8336, 8348, 8450, 8450, 8455, 8455, 8458, 8467, 8469, 8469, 8472, 8477, 8484, 8484, 8486, 8486, 8488, 8488, 8490, 8505, 8508, 8511, 8517, 8521, 8526, 8526, 8544, 8584, 11264, 11310, 11312, 11358, 11360, 11492, 11499, 11502, 11506, 11507, 11520, 11557, 11559, 11559, 11565, 11565, 11568, 11623, 11631, 11631, 11648, 11670, 11680, 11686, 11688, 11694, 11696, 11702, 11704, 11710, 11712, 11718, 11720, 11726, 11728, 11734, 11736, 11742, 12293, 12295, 12321, 12329, 12337, 12341, 12344, 12348, 12353, 12438, 12443, 12447, 12449, 12538, 12540, 12543, 12549, 12591, 12593, 12686, 12704, 12730, 12784, 12799, 13312, 19893, 19968, 40943, 40960, 42124, 42192, 42237, 42240, 42508, 42512, 42527, 42538, 42539, 42560, 42606, 42623, 42653, 42656, 42735, 42775, 42783, 42786, 42888, 42891, 42943, 42946, 42950, 42999, 43009, 43011, 43013, 43015, 43018, 43020, 43042, 43072, 43123, 43138, 43187, 43250, 43255, 43259, 43259, 43261, 43262, 43274, 43301, 43312, 43334, 43360, 43388, 43396, 43442, 43471, 43471, 43488, 43492, 43494, 43503, 43514, 43518, 43520, 43560, 43584, 43586, 43588, 43595, 43616, 43638, 43642, 43642, 43646, 43695, 43697, 43697, 43701, 43702, 43705, 43709, 43712, 43712, 43714, 43714, 43739, 43741, 43744, 43754, 43762, 43764, 43777, 43782, 43785, 43790, 43793, 43798, 43808, 43814, 43816, 43822, 43824, 43866, 43868, 43879, 43888, 44002, 44032, 55203, 55216, 55238, 55243, 55291, 63744, 64109, 64112, 64217, 64256, 64262, 64275, 64279, 64285, 64285, 64287, 64296, 64298, 64310, 64312, 64316, 64318, 64318, 64320, 64321, 64323, 64324, 64326, 64433, 64467, 64829, 64848, 64911, 64914, 64967, 65008, 65019, 65136, 65140, 65142, 65276, 65313, 65338, 65345, 65370, 65382, 65470, 65474, 65479, 65482, 65487, 65490, 65495, 65498, 65500, 65536, 65547, 65549, 65574, 65576, 65594, 65596, 65597, 65599, 65613, 65616, 65629, 65664, 65786, 65856, 65908, 66176, 66204, 66208, 66256, 66304, 66335, 66349, 66378, 66384, 66421, 66432, 66461, 66464, 66499, 66504, 66511, 66513, 66517, 66560, 66717, 66736, 66771, 66776, 66811, 66816, 66855, 66864, 66915, 67072, 67382, 67392, 67413, 67424, 67431, 67584, 67589, 67592, 67592, 67594, 67637, 67639, 67640, 67644, 67644, 67647, 67669, 67680, 67702, 67712, 67742, 67808, 67826, 67828, 67829, 67840, 67861, 67872, 67897, 67968, 68023, 68030, 68031, 68096, 68096, 68112, 68115, 68117, 68119, 68121, 68149, 68192, 68220, 68224, 68252, 68288, 68295, 68297, 68324, 68352, 68405, 68416, 68437, 68448, 68466, 68480, 68497, 68608, 68680, 68736, 68786, 68800, 68850, 68864, 68899, 69376, 69404, 69415, 69415, 69424, 69445, 69600, 69622, 69635, 69687, 69763, 69807, 69840, 69864, 69891, 69926, 69956, 69956, 69968, 70002, 70006, 70006, 70019, 70066, 70081, 70084, 70106, 70106, 70108, 70108, 70144, 70161, 70163, 70187, 70272, 70278, 70280, 70280, 70282, 70285, 70287, 70301, 70303, 70312, 70320, 70366, 70405, 70412, 70415, 70416, 70419, 70440, 70442, 70448, 70450, 70451, 70453, 70457, 70461, 70461, 70480, 70480, 70493, 70497, 70656, 70708, 70727, 70730, 70751, 70751, 70784, 70831, 70852, 70853, 70855, 70855, 71040, 71086, 71128, 71131, 71168, 71215, 71236, 71236, 71296, 71338, 71352, 71352, 71424, 71450, 71680, 71723, 71840, 71903, 71935, 71935, 72096, 72103, 72106, 72144, 72161, 72161, 72163, 72163, 72192, 72192, 72203, 72242, 72250, 72250, 72272, 72272, 72284, 72329, 72349, 72349, 72384, 72440, 72704, 72712, 72714, 72750, 72768, 72768, 72818, 72847, 72960, 72966, 72968, 72969, 72971, 73008, 73030, 73030, 73056, 73061, 73063, 73064, 73066, 73097, 73112, 73112, 73440, 73458, 73728, 74649, 74752, 74862, 74880, 75075, 77824, 78894, 82944, 83526, 92160, 92728, 92736, 92766, 92880, 92909, 92928, 92975, 92992, 92995, 93027, 93047, 93053, 93071, 93760, 93823, 93952, 94026, 94032, 94032, 94099, 94111, 94176, 94177, 94179, 94179, 94208, 100343, 100352, 101106, 110592, 110878, 110928, 110930, 110948, 110951, 110960, 111355, 113664, 113770, 113776, 113788, 113792, 113800, 113808, 113817, 119808, 119892, 119894, 119964, 119966, 119967, 119970, 119970, 119973, 119974, 119977, 119980, 119982, 119993, 119995, 119995, 119997, 120003, 120005, 120069, 120071, 120074, 120077, 120084, 120086, 120092, 120094, 120121, 120123, 120126, 120128, 120132, 120134, 120134, 120138, 120144, 120146, 120485, 120488, 120512, 120514, 120538, 120540, 120570, 120572, 120596, 120598, 120628, 120630, 120654, 120656, 120686, 120688, 120712, 120714, 120744, 120746, 120770, 120772, 120779, 123136, 123180, 123191, 123197, 123214, 123214, 123584, 123627, 124928, 125124, 125184, 125251, 125259, 125259, 126464, 126467, 126469, 126495, 126497, 126498, 126500, 126500, 126503, 126503, 126505, 126514, 126516, 126519, 126521, 126521, 126523, 126523, 126530, 126530, 126535, 126535, 126537, 126537, 126539, 126539, 126541, 126543, 126545, 126546, 126548, 126548, 126551, 126551, 126553, 126553, 126555, 126555, 126557, 126557, 126559, 126559, 126561, 126562, 126564, 126564, 126567, 126570, 126572, 126578, 126580, 126583, 126585, 126588, 126590, 126590, 126592, 126601, 126603, 126619, 126625, 126627, 126629, 126633, 126635, 126651, 131072, 173782, 173824, 177972, 177984, 178205, 178208, 183969, 183984, 191456, 194560, 195101], eS = [48, 57, 65, 90, 95, 95, 97, 122, 170, 170, 181, 181, 183, 183, 186, 186, 192, 214, 216, 246, 248, 705, 710, 721, 736, 740, 748, 748, 750, 750, 768, 884, 886, 887, 890, 893, 895, 895, 902, 906, 908, 908, 910, 929, 931, 1013, 1015, 1153, 1155, 1159, 1162, 1327, 1329, 1366, 1369, 1369, 1376, 1416, 1425, 1469, 1471, 1471, 1473, 1474, 1476, 1477, 1479, 1479, 1488, 1514, 1519, 1522, 1552, 1562, 1568, 1641, 1646, 1747, 1749, 1756, 1759, 1768, 1770, 1788, 1791, 1791, 1808, 1866, 1869, 1969, 1984, 2037, 2042, 2042, 2045, 2045, 2048, 2093, 2112, 2139, 2144, 2154, 2208, 2228, 2230, 2237, 2259, 2273, 2275, 2403, 2406, 2415, 2417, 2435, 2437, 2444, 2447, 2448, 2451, 2472, 2474, 2480, 2482, 2482, 2486, 2489, 2492, 2500, 2503, 2504, 2507, 2510, 2519, 2519, 2524, 2525, 2527, 2531, 2534, 2545, 2556, 2556, 2558, 2558, 2561, 2563, 2565, 2570, 2575, 2576, 2579, 2600, 2602, 2608, 2610, 2611, 2613, 2614, 2616, 2617, 2620, 2620, 2622, 2626, 2631, 2632, 2635, 2637, 2641, 2641, 2649, 2652, 2654, 2654, 2662, 2677, 2689, 2691, 2693, 2701, 2703, 2705, 2707, 2728, 2730, 2736, 2738, 2739, 2741, 2745, 2748, 2757, 2759, 2761, 2763, 2765, 2768, 2768, 2784, 2787, 2790, 2799, 2809, 2815, 2817, 2819, 2821, 2828, 2831, 2832, 2835, 2856, 2858, 2864, 2866, 2867, 2869, 2873, 2876, 2884, 2887, 2888, 2891, 2893, 2902, 2903, 2908, 2909, 2911, 2915, 2918, 2927, 2929, 2929, 2946, 2947, 2949, 2954, 2958, 2960, 2962, 2965, 2969, 2970, 2972, 2972, 2974, 2975, 2979, 2980, 2984, 2986, 2990, 3001, 3006, 3010, 3014, 3016, 3018, 3021, 3024, 3024, 3031, 3031, 3046, 3055, 3072, 3084, 3086, 3088, 3090, 3112, 3114, 3129, 3133, 3140, 3142, 3144, 3146, 3149, 3157, 3158, 3160, 3162, 3168, 3171, 3174, 3183, 3200, 3203, 3205, 3212, 3214, 3216, 3218, 3240, 3242, 3251, 3253, 3257, 3260, 3268, 3270, 3272, 3274, 3277, 3285, 3286, 3294, 3294, 3296, 3299, 3302, 3311, 3313, 3314, 3328, 3331, 3333, 3340, 3342, 3344, 3346, 3396, 3398, 3400, 3402, 3406, 3412, 3415, 3423, 3427, 3430, 3439, 3450, 3455, 3458, 3459, 3461, 3478, 3482, 3505, 3507, 3515, 3517, 3517, 3520, 3526, 3530, 3530, 3535, 3540, 3542, 3542, 3544, 3551, 3558, 3567, 3570, 3571, 3585, 3642, 3648, 3662, 3664, 3673, 3713, 3714, 3716, 3716, 3718, 3722, 3724, 3747, 3749, 3749, 3751, 3773, 3776, 3780, 3782, 3782, 3784, 3789, 3792, 3801, 3804, 3807, 3840, 3840, 3864, 3865, 3872, 3881, 3893, 3893, 3895, 3895, 3897, 3897, 3902, 3911, 3913, 3948, 3953, 3972, 3974, 3991, 3993, 4028, 4038, 4038, 4096, 4169, 4176, 4253, 4256, 4293, 4295, 4295, 4301, 4301, 4304, 4346, 4348, 4680, 4682, 4685, 4688, 4694, 4696, 4696, 4698, 4701, 4704, 4744, 4746, 4749, 4752, 4784, 4786, 4789, 4792, 4798, 4800, 4800, 4802, 4805, 4808, 4822, 4824, 4880, 4882, 4885, 4888, 4954, 4957, 4959, 4969, 4977, 4992, 5007, 5024, 5109, 5112, 5117, 5121, 5740, 5743, 5759, 5761, 5786, 5792, 5866, 5870, 5880, 5888, 5900, 5902, 5908, 5920, 5940, 5952, 5971, 5984, 5996, 5998, 6e3, 6002, 6003, 6016, 6099, 6103, 6103, 6108, 6109, 6112, 6121, 6155, 6157, 6160, 6169, 6176, 6264, 6272, 6314, 6320, 6389, 6400, 6430, 6432, 6443, 6448, 6459, 6470, 6509, 6512, 6516, 6528, 6571, 6576, 6601, 6608, 6618, 6656, 6683, 6688, 6750, 6752, 6780, 6783, 6793, 6800, 6809, 6823, 6823, 6832, 6845, 6912, 6987, 6992, 7001, 7019, 7027, 7040, 7155, 7168, 7223, 7232, 7241, 7245, 7293, 7296, 7304, 7312, 7354, 7357, 7359, 7376, 7378, 7380, 7418, 7424, 7673, 7675, 7957, 7960, 7965, 7968, 8005, 8008, 8013, 8016, 8023, 8025, 8025, 8027, 8027, 8029, 8029, 8031, 8061, 8064, 8116, 8118, 8124, 8126, 8126, 8130, 8132, 8134, 8140, 8144, 8147, 8150, 8155, 8160, 8172, 8178, 8180, 8182, 8188, 8255, 8256, 8276, 8276, 8305, 8305, 8319, 8319, 8336, 8348, 8400, 8412, 8417, 8417, 8421, 8432, 8450, 8450, 8455, 8455, 8458, 8467, 8469, 8469, 8472, 8477, 8484, 8484, 8486, 8486, 8488, 8488, 8490, 8505, 8508, 8511, 8517, 8521, 8526, 8526, 8544, 8584, 11264, 11310, 11312, 11358, 11360, 11492, 11499, 11507, 11520, 11557, 11559, 11559, 11565, 11565, 11568, 11623, 11631, 11631, 11647, 11670, 11680, 11686, 11688, 11694, 11696, 11702, 11704, 11710, 11712, 11718, 11720, 11726, 11728, 11734, 11736, 11742, 11744, 11775, 12293, 12295, 12321, 12335, 12337, 12341, 12344, 12348, 12353, 12438, 12441, 12447, 12449, 12538, 12540, 12543, 12549, 12591, 12593, 12686, 12704, 12730, 12784, 12799, 13312, 19893, 19968, 40943, 40960, 42124, 42192, 42237, 42240, 42508, 42512, 42539, 42560, 42607, 42612, 42621, 42623, 42737, 42775, 42783, 42786, 42888, 42891, 42943, 42946, 42950, 42999, 43047, 43072, 43123, 43136, 43205, 43216, 43225, 43232, 43255, 43259, 43259, 43261, 43309, 43312, 43347, 43360, 43388, 43392, 43456, 43471, 43481, 43488, 43518, 43520, 43574, 43584, 43597, 43600, 43609, 43616, 43638, 43642, 43714, 43739, 43741, 43744, 43759, 43762, 43766, 43777, 43782, 43785, 43790, 43793, 43798, 43808, 43814, 43816, 43822, 43824, 43866, 43868, 43879, 43888, 44010, 44012, 44013, 44016, 44025, 44032, 55203, 55216, 55238, 55243, 55291, 63744, 64109, 64112, 64217, 64256, 64262, 64275, 64279, 64285, 64296, 64298, 64310, 64312, 64316, 64318, 64318, 64320, 64321, 64323, 64324, 64326, 64433, 64467, 64829, 64848, 64911, 64914, 64967, 65008, 65019, 65024, 65039, 65056, 65071, 65075, 65076, 65101, 65103, 65136, 65140, 65142, 65276, 65296, 65305, 65313, 65338, 65343, 65343, 65345, 65370, 65382, 65470, 65474, 65479, 65482, 65487, 65490, 65495, 65498, 65500, 65536, 65547, 65549, 65574, 65576, 65594, 65596, 65597, 65599, 65613, 65616, 65629, 65664, 65786, 65856, 65908, 66045, 66045, 66176, 66204, 66208, 66256, 66272, 66272, 66304, 66335, 66349, 66378, 66384, 66426, 66432, 66461, 66464, 66499, 66504, 66511, 66513, 66517, 66560, 66717, 66720, 66729, 66736, 66771, 66776, 66811, 66816, 66855, 66864, 66915, 67072, 67382, 67392, 67413, 67424, 67431, 67584, 67589, 67592, 67592, 67594, 67637, 67639, 67640, 67644, 67644, 67647, 67669, 67680, 67702, 67712, 67742, 67808, 67826, 67828, 67829, 67840, 67861, 67872, 67897, 67968, 68023, 68030, 68031, 68096, 68099, 68101, 68102, 68108, 68115, 68117, 68119, 68121, 68149, 68152, 68154, 68159, 68159, 68192, 68220, 68224, 68252, 68288, 68295, 68297, 68326, 68352, 68405, 68416, 68437, 68448, 68466, 68480, 68497, 68608, 68680, 68736, 68786, 68800, 68850, 68864, 68903, 68912, 68921, 69376, 69404, 69415, 69415, 69424, 69456, 69600, 69622, 69632, 69702, 69734, 69743, 69759, 69818, 69840, 69864, 69872, 69881, 69888, 69940, 69942, 69951, 69956, 69958, 69968, 70003, 70006, 70006, 70016, 70084, 70089, 70092, 70096, 70106, 70108, 70108, 70144, 70161, 70163, 70199, 70206, 70206, 70272, 70278, 70280, 70280, 70282, 70285, 70287, 70301, 70303, 70312, 70320, 70378, 70384, 70393, 70400, 70403, 70405, 70412, 70415, 70416, 70419, 70440, 70442, 70448, 70450, 70451, 70453, 70457, 70459, 70468, 70471, 70472, 70475, 70477, 70480, 70480, 70487, 70487, 70493, 70499, 70502, 70508, 70512, 70516, 70656, 70730, 70736, 70745, 70750, 70751, 70784, 70853, 70855, 70855, 70864, 70873, 71040, 71093, 71096, 71104, 71128, 71133, 71168, 71232, 71236, 71236, 71248, 71257, 71296, 71352, 71360, 71369, 71424, 71450, 71453, 71467, 71472, 71481, 71680, 71738, 71840, 71913, 71935, 71935, 72096, 72103, 72106, 72151, 72154, 72161, 72163, 72164, 72192, 72254, 72263, 72263, 72272, 72345, 72349, 72349, 72384, 72440, 72704, 72712, 72714, 72758, 72760, 72768, 72784, 72793, 72818, 72847, 72850, 72871, 72873, 72886, 72960, 72966, 72968, 72969, 72971, 73014, 73018, 73018, 73020, 73021, 73023, 73031, 73040, 73049, 73056, 73061, 73063, 73064, 73066, 73102, 73104, 73105, 73107, 73112, 73120, 73129, 73440, 73462, 73728, 74649, 74752, 74862, 74880, 75075, 77824, 78894, 82944, 83526, 92160, 92728, 92736, 92766, 92768, 92777, 92880, 92909, 92912, 92916, 92928, 92982, 92992, 92995, 93008, 93017, 93027, 93047, 93053, 93071, 93760, 93823, 93952, 94026, 94031, 94087, 94095, 94111, 94176, 94177, 94179, 94179, 94208, 100343, 100352, 101106, 110592, 110878, 110928, 110930, 110948, 110951, 110960, 111355, 113664, 113770, 113776, 113788, 113792, 113800, 113808, 113817, 113821, 113822, 119141, 119145, 119149, 119154, 119163, 119170, 119173, 119179, 119210, 119213, 119362, 119364, 119808, 119892, 119894, 119964, 119966, 119967, 119970, 119970, 119973, 119974, 119977, 119980, 119982, 119993, 119995, 119995, 119997, 120003, 120005, 120069, 120071, 120074, 120077, 120084, 120086, 120092, 120094, 120121, 120123, 120126, 120128, 120132, 120134, 120134, 120138, 120144, 120146, 120485, 120488, 120512, 120514, 120538, 120540, 120570, 120572, 120596, 120598, 120628, 120630, 120654, 120656, 120686, 120688, 120712, 120714, 120744, 120746, 120770, 120772, 120779, 120782, 120831, 121344, 121398, 121403, 121452, 121461, 121461, 121476, 121476, 121499, 121503, 121505, 121519, 122880, 122886, 122888, 122904, 122907, 122913, 122915, 122916, 122918, 122922, 123136, 123180, 123184, 123197, 123200, 123209, 123214, 123214, 123584, 123641, 124928, 125124, 125136, 125142, 125184, 125259, 125264, 125273, 126464, 126467, 126469, 126495, 126497, 126498, 126500, 126500, 126503, 126503, 126505, 126514, 126516, 126519, 126521, 126521, 126523, 126523, 126530, 126530, 126535, 126535, 126537, 126537, 126539, 126539, 126541, 126543, 126545, 126546, 126548, 126548, 126551, 126551, 126553, 126553, 126555, 126555, 126557, 126557, 126559, 126559, 126561, 126562, 126564, 126564, 126567, 126570, 126572, 126578, 126580, 126583, 126585, 126588, 126590, 126590, 126592, 126601, 126603, 126619, 126625, 126627, 126629, 126633, 126635, 126651, 131072, 173782, 173824, 177972, 177984, 178205, 178208, 183969, 183984, 191456, 194560, 195101, 917760, 917999], tS = /^\/\/\/?\s*@(ts-expect-error|ts-ignore)/, rS = /^(?:\/|\*)*\s*@(ts-expect-error|ts-ignore)/, nS = cA(Ty), ll = 7, Qp = /^#!.*/, ii = String.prototype.codePointAt ? (e, t) => e.codePointAt(t) : function(t, r) {
let s = t.length;
if (r < 0 || r >= s)
return;
let f = t.charCodeAt(r);
if (f >= 55296 && f <= 56319 && s > r + 1) {
let x = t.charCodeAt(r + 1);
if (x >= 56320 && x <= 57343)
return (f - 55296) * 1024 + x - 56320 + 65536;
}
return f;
}, iS = String.fromCodePoint ? (e) => String.fromCodePoint(e) : mA;
} });
function gA(e) {
return So(e) || A_(e);
}
function yA(e) {
return uo(e, av);
}
function aS(e) {
switch (Uf(e)) {
case 99:
return "lib.esnext.full.d.ts";
case 9:
return "lib.es2022.full.d.ts";
case 8:
return "lib.es2021.full.d.ts";
case 7:
return "lib.es2020.full.d.ts";
case 6:
return "lib.es2019.full.d.ts";
case 5:
return "lib.es2018.full.d.ts";
case 4:
return "lib.es2017.full.d.ts";
case 3:
return "lib.es2016.full.d.ts";
case 2:
return "lib.es6.d.ts";
default:
return "lib.d.ts";
}
}
function Ir(e) {
return e.start + e.length;
}
function sS(e) {
return e.length === 0;
}
function vA(e, t) {
return t >= e.start && t < Ir(e);
}
function bA(e, t) {
return t >= e.pos && t <= e.end;
}
function TA(e, t) {
return t.start >= e.start && Ir(t) <= Ir(e);
}
function SA(e, t) {
return oS(e, t) !== void 0;
}
function oS(e, t) {
let r = _S(e, t);
return r && r.length === 0 ? void 0 : r;
}
function xA(e, t) {
return Sy(e.start, e.length, t.start, t.length);
}
function EA(e, t, r) {
return Sy(e.start, e.length, t, r);
}
function Sy(e, t, r, s) {
let f = e + t, x = r + s;
return r <= f && x >= e;
}
function wA(e, t) {
return t <= Ir(e) && t >= e.start;
}
function _S(e, t) {
let r = Math.max(e.start, t.start), s = Math.min(Ir(e), Ir(t));
return r <= s ? ha(r, s) : void 0;
}
function L_(e, t) {
if (e < 0)
throw new Error("start < 0");
if (t < 0)
throw new Error("length < 0");
return { start: e, length: t };
}
function ha(e, t) {
return L_(e, t - e);
}
function R_(e) {
return L_(e.span.start, e.newLength);
}
function cS(e) {
return sS(e.span) && e.newLength === 0;
}
function Zp(e, t) {
if (t < 0)
throw new Error("newLength < 0");
return { span: e, newLength: t };
}
function CA(e) {
if (e.length === 0)
return Vy;
if (e.length === 1)
return e[0];
let t = e[0], r = t.span.start, s = Ir(t.span), f = r + t.newLength;
for (let x = 1; x < e.length; x++) {
let w = e[x], A = r, g = s, B = f, N = w.span.start, X = Ir(w.span), F = N + w.newLength;
r = Math.min(A, N), s = Math.max(g, g + (X - B)), f = Math.max(F, F + (B - X));
}
return Zp(ha(r, s), f - r);
}
function AA(e) {
if (e && e.kind === 165) {
for (let t = e; t; t = t.parent)
if (ga(t) || bi(t) || t.kind === 261)
return t;
}
}
function lS(e, t) {
return Vs(e) && rn(e, 16476) && t.kind === 173;
}
function uS(e) {
return df(e) ? me(e.elements, pS) : false;
}
function pS(e) {
return cd(e) ? true : uS(e.name);
}
function fS(e) {
let t = e.parent;
for (; Xl(t.parent); )
t = t.parent.parent;
return t.parent;
}
function xy(e, t) {
Xl(e) && (e = fS(e));
let r = t(e);
return e.kind === 257 && (e = e.parent), e && e.kind === 258 && (r |= t(e), e = e.parent), e && e.kind === 240 && (r |= t(e)), r;
}
function ef(e) {
return xy(e, Rf);
}
function PA(e) {
return xy(e, K4);
}
function tf(e) {
return xy(e, (t) => t.flags);
}
function DA(e, t, r) {
let s = e.toLowerCase(), f = /^([a-z]+)([_\-]([a-z]+))?$/.exec(s);
if (!f) {
r && r.push(Ol(ve.Locale_must_be_of_the_form_language_or_language_territory_For_example_0_or_1, "en", "ja-jp"));
return;
}
let x = f[1], w = f[3];
pe(Hy, s) && !A(x, w, r) && A(x, void 0, r), xp(e);
function A(g, B, N) {
let X = Un(t.getExecutingFilePath()), F = ma(X), $ = tn(F, g);
if (B && ($ = $ + "-" + B), $ = t.resolvePath(tn($, "diagnosticMessages.generated.json")), !t.fileExists($))
return false;
let ae = "";
try {
ae = t.readFile($);
} catch {
return N && N.push(Ol(ve.Unable_to_open_file_0, $)), false;
}
try {
yx(JSON.parse(ae));
} catch {
return N && N.push(Ol(ve.Corrupted_locale_file_0, $)), false;
}
return true;
}
}
function ul(e, t) {
if (e)
for (; e.original !== void 0; )
e = e.original;
return !e || !t || t(e) ? e : void 0;
}
function zi(e, t) {
for (; e; ) {
let r = t(e);
if (r === "quit")
return;
if (r)
return e;
e = e.parent;
}
}
function pl(e) {
return (e.flags & 8) === 0;
}
function fl(e, t) {
if (e === void 0 || pl(e))
return e;
for (e = e.original; e; ) {
if (pl(e))
return !t || t(e) ? e : void 0;
e = e.original;
}
}
function vi(e) {
return e.length >= 2 && e.charCodeAt(0) === 95 && e.charCodeAt(1) === 95 ? "_" + e : e;
}
function dl(e) {
let t = e;
return t.length >= 3 && t.charCodeAt(0) === 95 && t.charCodeAt(1) === 95 && t.charCodeAt(2) === 95 ? t.substr(1) : t;
}
function qr(e) {
return dl(e.escapedText);
}
function dS(e) {
let t = _l(e.escapedText);
return t ? ln(t, ba) : void 0;
}
function rf(e) {
return e.valueDeclaration && zS(e.valueDeclaration) ? qr(e.valueDeclaration.name) : dl(e.escapedName);
}
function mS(e) {
let t = e.parent.parent;
if (t) {
if (ko(t))
return nf(t);
switch (t.kind) {
case 240:
if (t.declarationList && t.declarationList.declarations[0])
return nf(t.declarationList.declarations[0]);
break;
case 241:
let r = t.expression;
switch (r.kind === 223 && r.operatorToken.kind === 63 && (r = r.left), r.kind) {
case 208:
return r.name;
case 209:
let s = r.argumentExpression;
if (yt(s))
return s;
}
break;
case 214:
return nf(t.expression);
case 253: {
if (ko(t.statement) || mf(t.statement))
return nf(t.statement);
break;
}
}
}
}
function nf(e) {
let t = ml(e);
return t && yt(t) ? t : void 0;
}
function hS(e, t) {
return !!(af(e) && yt(e.name) && qr(e.name) === qr(t) || zo(e) && Ke(e.declarationList.declarations, (r) => hS(r, t)));
}
function gS(e) {
return e.name || mS(e);
}
function af(e) {
return !!e.name;
}
function Ey(e) {
switch (e.kind) {
case 79:
return e;
case 351:
case 344: {
let { name: r } = e;
if (r.kind === 163)
return r.right;
break;
}
case 210:
case 223: {
let r = e;
switch (ps(r)) {
case 1:
case 4:
case 5:
case 3:
return Cf(r.left);
case 7:
case 8:
case 9:
return r.arguments[1];
default:
return;
}
}
case 349:
return gS(e);
case 343:
return mS(e);
case 274: {
let { expression: r } = e;
return yt(r) ? r : void 0;
}
case 209:
let t = e;
if (x0(t))
return t.argumentExpression;
}
return e.name;
}
function ml(e) {
if (e !== void 0)
return Ey(e) || (ad(e) || sd(e) || _d(e) ? yS(e) : void 0);
}
function yS(e) {
if (e.parent) {
if (lc(e.parent) || Xl(e.parent))
return e.parent.name;
if (ur(e.parent) && e === e.parent.right) {
if (yt(e.parent.left))
return e.parent.left;
if (Lo(e.parent.left))
return Cf(e.parent.left);
} else if (Vi(e.parent) && yt(e.parent.name))
return e.parent.name;
} else
return;
}
function kA(e) {
if (Il(e))
return ee(e.modifiers, zl);
}
function sf(e) {
if (rn(e, 126975))
return ee(e.modifiers, Oy);
}
function vS(e, t) {
if (e.name)
if (yt(e.name)) {
let r = e.name.escapedText;
return j_(e.parent, t).filter((s) => pc(s) && yt(s.name) && s.name.escapedText === r);
} else {
let r = e.parent.parameters.indexOf(e);
Y.assert(r > -1, "Parameters should always be in their parents' parameter list");
let s = j_(e.parent, t).filter(pc);
if (r < s.length)
return [s[r]];
}
return Bt;
}
function of(e) {
return vS(e, false);
}
function bS(e) {
return vS(e, true);
}
function TS(e, t) {
let r = e.name.escapedText;
return j_(e.parent, t).filter((s) => Go(s) && s.typeParameters.some((f) => f.name.escapedText === r));
}
function SS(e) {
return TS(e, false);
}
function xS(e) {
return TS(e, true);
}
function IA(e) {
return !!Nr(e, pc);
}
function ES(e) {
return Nr(e, md);
}
function wS(e) {
return MS(e, hE);
}
function NA(e) {
return Nr(e, pE);
}
function OA(e) {
return Nr(e, d2);
}
function CS(e) {
return Nr(e, d2, true);
}
function MA(e) {
return Nr(e, m2);
}
function AS(e) {
return Nr(e, m2, true);
}
function LA(e) {
return Nr(e, h2);
}
function PS(e) {
return Nr(e, h2, true);
}
function RA(e) {
return Nr(e, g2);
}
function DS(e) {
return Nr(e, g2, true);
}
function kS(e) {
return Nr(e, fE, true);
}
function jA(e) {
return Nr(e, v2);
}
function IS(e) {
return Nr(e, v2, true);
}
function JA(e) {
return Nr(e, dE);
}
function FA(e) {
return Nr(e, mE);
}
function NS(e) {
return Nr(e, b2);
}
function BA(e) {
return Nr(e, Go);
}
function wy(e) {
return Nr(e, T2);
}
function _f(e) {
let t = Nr(e, au);
if (t && t.typeExpression && t.typeExpression.type)
return t;
}
function cf(e) {
let t = Nr(e, au);
return !t && Vs(e) && (t = Ae(of(e), (r) => !!r.typeExpression)), t && t.typeExpression && t.typeExpression.type;
}
function OS(e) {
let t = NS(e);
if (t && t.typeExpression)
return t.typeExpression.type;
let r = _f(e);
if (r && r.typeExpression) {
let s = r.typeExpression.type;
if (id(s)) {
let f = Ae(s.members, Vv);
return f && f.type;
}
if ($l(s) || dd(s))
return s.type;
}
}
function j_(e, t) {
var r, s;
if (!Af(e))
return Bt;
let f = (r = e.jsDoc) == null ? void 0 : r.jsDocCache;
if (f === void 0 || t) {
let x = r4(e, t);
Y.assert(x.length < 2 || x[0] !== x[1]), f = ne(x, (w) => Ho(w) ? w.tags : w), t || ((s = e.jsDoc) != null || (e.jsDoc = []), e.jsDoc.jsDocCache = f);
}
return f;
}
function hl(e) {
return j_(e, false);
}
function qA(e) {
return j_(e, true);
}
function Nr(e, t, r) {
return Ae(j_(e, r), t);
}
function MS(e, t) {
return hl(e).filter(t);
}
function UA(e, t) {
return hl(e).filter((r) => r.kind === t);
}
function zA(e) {
return typeof e == "string" ? e : e == null ? void 0 : e.map((t) => t.kind === 324 ? t.text : WA(t)).join("");
}
function WA(e) {
let t = e.kind === 327 ? "link" : e.kind === 328 ? "linkcode" : "linkplain", r = e.name ? ls(e.name) : "", s = e.name && e.text.startsWith("://") ? "" : " ";
return `{@${t} ${r}${s}${e.text}}`;
}
function VA(e) {
if (iu(e)) {
if (y2(e.parent)) {
let t = P0(e.parent);
if (t && I(t.tags))
return ne(t.tags, (r) => Go(r) ? r.typeParameters : void 0);
}
return Bt;
}
if (Cl(e))
return Y.assert(e.parent.kind === 323), ne(e.parent.tags, (t) => Go(t) ? t.typeParameters : void 0);
if (e.typeParameters || IE(e) && e.typeParameters)
return e.typeParameters;
if (Pr(e)) {
let t = F4(e);
if (t.length)
return t;
let r = cf(e);
if (r && $l(r) && r.typeParameters)
return r.typeParameters;
}
return Bt;
}
function HA(e) {
return e.constraint ? e.constraint : Go(e.parent) && e === e.parent.typeParameters[0] ? e.parent.constraint : void 0;
}
function js(e) {
return e.kind === 79 || e.kind === 80;
}
function GA(e) {
return e.kind === 175 || e.kind === 174;
}
function LS(e) {
return bn(e) && !!(e.flags & 32);
}
function RS(e) {
return gs(e) && !!(e.flags & 32);
}
function Cy(e) {
return sc(e) && !!(e.flags & 32);
}
function Ay(e) {
let t = e.kind;
return !!(e.flags & 32) && (t === 208 || t === 209 || t === 210 || t === 232);
}
function Py(e) {
return Ay(e) && !Uo(e) && !!e.questionDotToken;
}
function $A(e) {
return Py(e.parent) && e.parent.expression === e;
}
function KA(e) {
return !Ay(e.parent) || Py(e.parent) || e !== e.parent.expression;
}
function XA(e) {
return e.kind === 223 && e.operatorToken.kind === 60;
}
function jS(e) {
return ac(e) && yt(e.typeName) && e.typeName.escapedText === "const" && !e.typeArguments;
}
function lf(e) {
return $o(e, 8);
}
function JS(e) {
return Uo(e) && !!(e.flags & 32);
}
function YA(e) {
return e.kind === 249 || e.kind === 248;
}
function QA(e) {
return e.kind === 277 || e.kind === 276;
}
function FS(e) {
switch (e.kind) {
case 305:
case 306:
return true;
default:
return false;
}
}
function ZA(e) {
return FS(e) || e.kind === 303 || e.kind === 307;
}
function Dy(e) {
return e.kind === 351 || e.kind === 344;
}
function eP(e) {
return gl(e.kind);
}
function gl(e) {
return e >= 163;
}
function BS(e) {
return e >= 0 && e <= 162;
}
function tP(e) {
return BS(e.kind);
}
function _s(e) {
return Jr(e, "pos") && Jr(e, "end");
}
function ky(e) {
return 8 <= e && e <= 14;
}
function Iy(e) {
return ky(e.kind);
}
function rP(e) {
switch (e.kind) {
case 207:
case 206:
case 13:
case 215:
case 228:
return true;
}
return false;
}
function yl(e) {
return 14 <= e && e <= 17;
}
function nP(e) {
return yl(e.kind);
}
function iP(e) {
let t = e.kind;
return t === 16 || t === 17;
}
function aP(e) {
return nE(e) || aE(e);
}
function qS(e) {
switch (e.kind) {
case 273:
return e.isTypeOnly || e.parent.parent.isTypeOnly;
case 271:
return e.parent.isTypeOnly;
case 270:
case 268:
return e.isTypeOnly;
}
return false;
}
function US(e) {
switch (e.kind) {
case 278:
return e.isTypeOnly || e.parent.parent.isTypeOnly;
case 275:
return e.isTypeOnly && !!e.moduleSpecifier && !e.exportClause;
case 277:
return e.parent.isTypeOnly;
}
return false;
}
function sP(e) {
return qS(e) || US(e);
}
function oP(e) {
return Gn(e) || yt(e);
}
function _P(e) {
return e.kind === 10 || yl(e.kind);
}
function cs(e) {
var t;
return yt(e) && ((t = e.emitNode) == null ? void 0 : t.autoGenerate) !== void 0;
}
function Ny(e) {
var t;
return vn(e) && ((t = e.emitNode) == null ? void 0 : t.autoGenerate) !== void 0;
}
function zS(e) {
return (Bo(e) || Ly(e)) && vn(e.name);
}
function cP(e) {
return bn(e) && vn(e.name);
}
function Wi(e) {
switch (e) {
case 126:
case 127:
case 132:
case 85:
case 136:
case 88:
case 93:
case 101:
case 123:
case 121:
case 122:
case 146:
case 124:
case 145:
case 161:
return true;
}
return false;
}
function WS(e) {
return !!(Q0(e) & 16476);
}
function VS(e) {
return WS(e) || e === 124 || e === 161 || e === 127;
}
function Oy(e) {
return Wi(e.kind);
}
function lP(e) {
let t = e.kind;
return t === 163 || t === 79;
}
function vl(e) {
let t = e.kind;
return t === 79 || t === 80 || t === 10 || t === 8 || t === 164;
}
function uP(e) {
let t = e.kind;
return t === 79 || t === 203 || t === 204;
}
function ga(e) {
return !!e && My(e.kind);
}
function uf(e) {
return !!e && (My(e.kind) || Hl(e));
}
function HS(e) {
return e && GS(e.kind);
}
function pP(e) {
return e.kind === 110 || e.kind === 95;
}
function GS(e) {
switch (e) {
case 259:
case 171:
case 173:
case 174:
case 175:
case 215:
case 216:
return true;
default:
return false;
}
}
function My(e) {
switch (e) {
case 170:
case 176:
case 326:
case 177:
case 178:
case 181:
case 320:
case 182:
return true;
default:
return GS(e);
}
}
function fP(e) {
return wi(e) || rE(e) || Ql(e) && ga(e.parent);
}
function Js(e) {
let t = e.kind;
return t === 173 || t === 169 || t === 171 || t === 174 || t === 175 || t === 178 || t === 172 || t === 237;
}
function bi(e) {
return e && (e.kind === 260 || e.kind === 228);
}
function pf(e) {
return e && (e.kind === 174 || e.kind === 175);
}
function $S(e) {
return Bo(e) && H4(e);
}
function Ly(e) {
switch (e.kind) {
case 171:
case 174:
case 175:
return true;
default:
return false;
}
}
function dP(e) {
switch (e.kind) {
case 171:
case 174:
case 175:
case 169:
return true;
default:
return false;
}
}
function ff(e) {
return Oy(e) || zl(e);
}
function Ry(e) {
let t = e.kind;
return t === 177 || t === 176 || t === 168 || t === 170 || t === 178 || t === 174 || t === 175;
}
function mP(e) {
return Ry(e) || Js(e);
}
function jy(e) {
let t = e.kind;
return t === 299 || t === 300 || t === 301 || t === 171 || t === 174 || t === 175;
}
function Jy(e) {
return hx(e.kind);
}
function hP(e) {
switch (e.kind) {
case 181:
case 182:
return true;
}
return false;
}
function df(e) {
if (e) {
let t = e.kind;
return t === 204 || t === 203;
}
return false;
}
function KS(e) {
let t = e.kind;
return t === 206 || t === 207;
}
function gP(e) {
let t = e.kind;
return t === 205 || t === 229;
}
function Fy(e) {
switch (e.kind) {
case 257:
case 166:
case 205:
return true;
}
return false;
}
function yP(e) {
return Vi(e) || Vs(e) || YS(e) || ZS(e);
}
function vP(e) {
return XS(e) || QS(e);
}
function XS(e) {
switch (e.kind) {
case 203:
case 207:
return true;
}
return false;
}
function YS(e) {
switch (e.kind) {
case 205:
case 299:
case 300:
case 301:
return true;
}
return false;
}
function QS(e) {
switch (e.kind) {
case 204:
case 206:
return true;
}
return false;
}
function ZS(e) {
switch (e.kind) {
case 205:
case 229:
case 227:
case 206:
case 207:
case 79:
case 208:
case 209:
return true;
}
return ms(e, true);
}
function bP(e) {
let t = e.kind;
return t === 208 || t === 163 || t === 202;
}
function TP(e) {
let t = e.kind;
return t === 208 || t === 163;
}
function SP(e) {
switch (e.kind) {
case 283:
case 282:
case 210:
case 211:
case 212:
case 167:
return true;
default:
return false;
}
}
function xP(e) {
return e.kind === 210 || e.kind === 211;
}
function EP(e) {
let t = e.kind;
return t === 225 || t === 14;
}
function Do(e) {
return e3(lf(e).kind);
}
function e3(e) {
switch (e) {
case 208:
case 209:
case 211:
case 210:
case 281:
case 282:
case 285:
case 212:
case 206:
case 214:
case 207:
case 228:
case 215:
case 79:
case 80:
case 13:
case 8:
case 9:
case 10:
case 14:
case 225:
case 95:
case 104:
case 108:
case 110:
case 106:
case 232:
case 230:
case 233:
case 100:
case 279:
return true;
default:
return false;
}
}
function t3(e) {
return r3(lf(e).kind);
}
function r3(e) {
switch (e) {
case 221:
case 222:
case 217:
case 218:
case 219:
case 220:
case 213:
return true;
default:
return e3(e);
}
}
function wP(e) {
switch (e.kind) {
case 222:
return true;
case 221:
return e.operator === 45 || e.operator === 46;
default:
return false;
}
}
function CP(e) {
switch (e.kind) {
case 104:
case 110:
case 95:
case 221:
return true;
default:
return Iy(e);
}
}
function mf(e) {
return AP(lf(e).kind);
}
function AP(e) {
switch (e) {
case 224:
case 226:
case 216:
case 223:
case 227:
case 231:
case 229:
case 357:
case 356:
case 235:
return true;
default:
return r3(e);
}
}
function PP(e) {
let t = e.kind;
return t === 213 || t === 231;
}
function DP(e) {
return c2(e) || Z8(e);
}
function n3(e, t) {
switch (e.kind) {
case 245:
case 246:
case 247:
case 243:
case 244:
return true;
case 253:
return t && n3(e.statement, t);
}
return false;
}
function i3(e) {
return Vo(e) || cc(e);
}
function kP(e) {
return Ke(e, i3);
}
function IP(e) {
return !bf(e) && !Vo(e) && !rn(e, 1) && !yf(e);
}
function NP(e) {
return bf(e) || Vo(e) || rn(e, 1);
}
function OP(e) {
return e.kind === 246 || e.kind === 247;
}
function MP(e) {
return Ql(e) || mf(e);
}
function LP(e) {
return Ql(e);
}
function RP(e) {
return r2(e) || mf(e);
}
function jP(e) {
let t = e.kind;
return t === 265 || t === 264 || t === 79;
}
function JP(e) {
let t = e.kind;
return t === 265 || t === 264;
}
function FP(e) {
let t = e.kind;
return t === 79 || t === 264;
}
function BP(e) {
let t = e.kind;
return t === 272 || t === 271;
}
function qP(e) {
return e.kind === 264 || e.kind === 263;
}
function UP(e) {
switch (e.kind) {
case 216:
case 223:
case 205:
case 210:
case 176:
case 260:
case 228:
case 172:
case 173:
case 182:
case 177:
case 209:
case 263:
case 302:
case 274:
case 275:
case 278:
case 259:
case 215:
case 181:
case 174:
case 79:
case 270:
case 268:
case 273:
case 178:
case 261:
case 341:
case 343:
case 320:
case 344:
case 351:
case 326:
case 349:
case 325:
case 288:
case 289:
case 290:
case 197:
case 171:
case 170:
case 264:
case 199:
case 277:
case 267:
case 271:
case 211:
case 14:
case 8:
case 207:
case 166:
case 208:
case 299:
case 169:
case 168:
case 175:
case 300:
case 308:
case 301:
case 10:
case 262:
case 184:
case 165:
case 257:
return true;
default:
return false;
}
}
function zP(e) {
switch (e.kind) {
case 216:
case 238:
case 176:
case 266:
case 295:
case 172:
case 191:
case 173:
case 182:
case 177:
case 245:
case 246:
case 247:
case 259:
case 215:
case 181:
case 174:
case 178:
case 341:
case 343:
case 320:
case 326:
case 349:
case 197:
case 171:
case 170:
case 264:
case 175:
case 308:
case 262:
return true;
default:
return false;
}
}
function WP(e) {
return e === 216 || e === 205 || e === 260 || e === 228 || e === 172 || e === 173 || e === 263 || e === 302 || e === 278 || e === 259 || e === 215 || e === 174 || e === 270 || e === 268 || e === 273 || e === 261 || e === 288 || e === 171 || e === 170 || e === 264 || e === 267 || e === 271 || e === 277 || e === 166 || e === 299 || e === 169 || e === 168 || e === 175 || e === 300 || e === 262 || e === 165 || e === 257 || e === 349 || e === 341 || e === 351;
}
function By(e) {
return e === 259 || e === 279 || e === 260 || e === 261 || e === 262 || e === 263 || e === 264 || e === 269 || e === 268 || e === 275 || e === 274 || e === 267;
}
function qy(e) {
return e === 249 || e === 248 || e === 256 || e === 243 || e === 241 || e === 239 || e === 246 || e === 247 || e === 245 || e === 242 || e === 253 || e === 250 || e === 252 || e === 254 || e === 255 || e === 240 || e === 244 || e === 251 || e === 355 || e === 359 || e === 358;
}
function ko(e) {
return e.kind === 165 ? e.parent && e.parent.kind !== 348 || Pr(e) : WP(e.kind);
}
function VP(e) {
return By(e.kind);
}
function HP(e) {
return qy(e.kind);
}
function a3(e) {
let t = e.kind;
return qy(t) || By(t) || GP(e);
}
function GP(e) {
return e.kind !== 238 || e.parent !== void 0 && (e.parent.kind === 255 || e.parent.kind === 295) ? false : !O3(e);
}
function s3(e) {
let t = e.kind;
return qy(t) || By(t) || t === 238;
}
function $P(e) {
let t = e.kind;
return t === 280 || t === 163 || t === 79;
}
function KP(e) {
let t = e.kind;
return t === 108 || t === 79 || t === 208;
}
function o3(e) {
let t = e.kind;
return t === 281 || t === 291 || t === 282 || t === 11 || t === 285;
}
function XP(e) {
let t = e.kind;
return t === 288 || t === 290;
}
function YP(e) {
let t = e.kind;
return t === 10 || t === 291;
}
function _3(e) {
let t = e.kind;
return t === 283 || t === 282;
}
function QP(e) {
let t = e.kind;
return t === 292 || t === 293;
}
function Uy(e) {
return e.kind >= 312 && e.kind <= 353;
}
function c3(e) {
return e.kind === 323 || e.kind === 322 || e.kind === 324 || Sl(e) || zy(e) || f2(e) || iu(e);
}
function zy(e) {
return e.kind >= 330 && e.kind <= 353;
}
function bl(e) {
return e.kind === 175;
}
function Tl(e) {
return e.kind === 174;
}
function ya(e) {
if (!Af(e))
return false;
let { jsDoc: t } = e;
return !!t && t.length > 0;
}
function ZP(e) {
return !!e.type;
}
function l3(e) {
return !!e.initializer;
}
function eD(e) {
switch (e.kind) {
case 257:
case 166:
case 205:
case 169:
case 299:
case 302:
return true;
default:
return false;
}
}
function Wy(e) {
return e.kind === 288 || e.kind === 290 || jy(e);
}
function tD(e) {
return e.kind === 180 || e.kind === 230;
}
function rD(e) {
let t = Gy;
for (let r of e) {
if (!r.length)
continue;
let s = 0;
for (; s < r.length && s < t && os(r.charCodeAt(s)); s++)
;
if (s < t && (t = s), t === 0)
return 0;
}
return t === Gy ? void 0 : t;
}
function Ti(e) {
return e.kind === 10 || e.kind === 14;
}
function Sl(e) {
return e.kind === 327 || e.kind === 328 || e.kind === 329;
}
function nD(e) {
let t = Cn(e.parameters);
return !!t && u3(t);
}
function u3(e) {
let t = pc(e) ? e.typeExpression && e.typeExpression.type : e.type;
return e.dotDotDotToken !== void 0 || !!t && t.kind === 321;
}
var Vy, Hy, Gy, iD = D({ "src/compiler/utilitiesPublic.ts"() {
"use strict";
nn(), Vy = Zp(L_(0, 0), 0), Hy = ["cs", "de", "es", "fr", "it", "ja", "ko", "pl", "pt-br", "ru", "tr", "zh-cn", "zh-tw"], Gy = 1073741823;
} });
function aD(e, t) {
let r = e.declarations;
if (r) {
for (let s of r)
if (s.kind === t)
return s;
}
}
function sD(e, t) {
return ee(e.declarations || Bt, (r) => r.kind === t);
}
function oD(e) {
let t = /* @__PURE__ */ new Map();
if (e)
for (let r of e)
t.set(r.escapedName, r);
return t;
}
function $y(e) {
return (e.flags & 33554432) !== 0;
}
function _D() {
var e = "";
let t = (r) => e += r;
return { getText: () => e, write: t, rawWrite: t, writeKeyword: t, writeOperator: t, writePunctuation: t, writeSpace: t, writeStringLiteral: t, writeLiteral: t, writeParameter: t, writeProperty: t, writeSymbol: (r, s) => t(r), writeTrailingSemicolon: t, writeComment: t, getTextPos: () => e.length, getLine: () => 0, getColumn: () => 0, getIndent: () => 0, isAtStartOfLine: () => false, hasTrailingComment: () => false, hasTrailingWhitespace: () => !!e.length && os(e.charCodeAt(e.length - 1)), writeLine: () => e += " ", increaseIndent: yn, decreaseIndent: yn, clear: () => e = "" };
}
function cD(e, t) {
return e.configFilePath !== t.configFilePath || p3(e, t);
}
function p3(e, t) {
return J_(e, t, moduleResolutionOptionDeclarations);
}
function lD(e, t) {
return J_(e, t, optionsAffectingProgramStructure);
}
function J_(e, t, r) {
return e !== t && r.some((s) => !gv(uv(e, s), uv(t, s)));
}
function uD(e, t) {
for (; ; ) {
let r = t(e);
if (r === "quit")
return;
if (r !== void 0)
return r;
if (wi(e))
return;
e = e.parent;
}
}
function pD(e, t) {
let r = e.entries();
for (let [s, f] of r) {
let x = t(f, s);
if (x)
return x;
}
}
function fD(e, t) {
let r = e.keys();
for (let s of r) {
let f = t(s);
if (f)
return f;
}
}
function dD(e, t) {
e.forEach((r, s) => {
t.set(s, r);
});
}
function mD(e) {
let t = Z_.getText();
try {
return e(Z_), Z_.getText();
} finally {
Z_.clear(), Z_.writeKeyword(t);
}
}
function hf(e) {
return e.end - e.pos;
}
function hD(e, t, r) {
var s, f;
return (f = (s = e == null ? void 0 : e.resolvedModules) == null ? void 0 : s.get(t, r)) == null ? void 0 : f.resolvedModule;
}
function gD(e, t, r, s) {
e.resolvedModules || (e.resolvedModules = createModeAwareCache()), e.resolvedModules.set(t, s, r);
}
function yD(e, t, r, s) {
e.resolvedTypeReferenceDirectiveNames || (e.resolvedTypeReferenceDirectiveNames = createModeAwareCache()), e.resolvedTypeReferenceDirectiveNames.set(t, s, r);
}
function vD(e, t, r) {
var s, f;
return (f = (s = e == null ? void 0 : e.resolvedTypeReferenceDirectiveNames) == null ? void 0 : s.get(t, r)) == null ? void 0 : f.resolvedTypeReferenceDirective;
}
function bD(e, t) {
return e.path === t.path && !e.prepend == !t.prepend && !e.circular == !t.circular;
}
function TD(e, t) {
return e === t || e.resolvedModule === t.resolvedModule || !!e.resolvedModule && !!t.resolvedModule && e.resolvedModule.isExternalLibraryImport === t.resolvedModule.isExternalLibraryImport && e.resolvedModule.extension === t.resolvedModule.extension && e.resolvedModule.resolvedFileName === t.resolvedModule.resolvedFileName && e.resolvedModule.originalPath === t.resolvedModule.originalPath && SD(e.resolvedModule.packageId, t.resolvedModule.packageId);
}
function SD(e, t) {
return e === t || !!e && !!t && e.name === t.name && e.subModuleName === t.subModuleName && e.version === t.version;
}
function f3(e) {
let { name: t, subModuleName: r } = e;
return r ? `${t}/${r}` : t;
}
function xD(e) {
return `${f3(e)}@${e.version}`;
}
function ED(e, t) {
return e === t || e.resolvedTypeReferenceDirective === t.resolvedTypeReferenceDirective || !!e.resolvedTypeReferenceDirective && !!t.resolvedTypeReferenceDirective && e.resolvedTypeReferenceDirective.resolvedFileName === t.resolvedTypeReferenceDirective.resolvedFileName && !!e.resolvedTypeReferenceDirective.primary == !!t.resolvedTypeReferenceDirective.primary && e.resolvedTypeReferenceDirective.originalPath === t.resolvedTypeReferenceDirective.originalPath;
}
function wD(e, t, r, s, f, x) {
Y.assert(e.length === r.length);
for (let w = 0; w < e.length; w++) {
let A = r[w], g = e[w], B = x.getName(g), N = x.getMode(g, t), X = s && s.get(B, N);
if (X ? !A || !f(X, A) : A)
return true;
}
return false;
}
function Ky(e) {
return CD(e), (e.flags & 524288) !== 0;
}
function CD(e) {
e.flags & 1048576 || ((e.flags & 131072 || xr(e, Ky)) && (e.flags |= 524288), e.flags |= 1048576);
}
function Si(e) {
for (; e && e.kind !== 308; )
e = e.parent;
return e;
}
function AD(e) {
return Si(e.valueDeclaration || E3(e));
}
function PD(e, t) {
return !!e && (e.scriptKind === 1 || e.scriptKind === 2) && !e.checkJsDirective && t === void 0;
}
function DD(e) {
switch (e.kind) {
case 238:
case 266:
case 245:
case 246:
case 247:
return true;
}
return false;
}
function kD(e, t) {
return Y.assert(e >= 0), ss(t)[e];
}
function ID(e) {
let t = Si(e), r = Ls(t, e.pos);
return `${t.fileName}(${r.line + 1},${r.character + 1})`;
}
function d3(e, t) {
Y.assert(e >= 0);
let r = ss(t), s = e, f = t.text;
if (s + 1 === r.length)
return f.length - 1;
{
let x = r[s], w = r[s + 1] - 1;
for (Y.assert(un(f.charCodeAt(w))); x <= w && un(f.charCodeAt(w)); )
w--;
return w;
}
}
function m3(e, t, r) {
return !(r && r(t)) && !e.identifiers.has(t);
}
function va(e) {
return e === void 0 ? true : e.pos === e.end && e.pos >= 0 && e.kind !== 1;
}
function xl(e) {
return !va(e);
}
function ND(e, t) {
return Fo(e) ? t === e.expression : Hl(e) ? t === e.modifiers : Wl(e) ? t === e.initializer : Bo(e) ? t === e.questionToken && $S(e) : lc(e) ? t === e.modifiers || t === e.questionToken || t === e.exclamationToken || F_(e.modifiers, t, ff) : nu(e) ? t === e.equalsToken || t === e.modifiers || t === e.questionToken || t === e.exclamationToken || F_(e.modifiers, t, ff) : Vl(e) ? t === e.exclamationToken : nc(e) ? t === e.typeParameters || t === e.type || F_(e.typeParameters, t, Fo) : Gl(e) ? t === e.typeParameters || F_(e.typeParameters, t, Fo) : ic(e) ? t === e.typeParameters || t === e.type || F_(e.typeParameters, t, Fo) : a2(e) ? t === e.modifiers || F_(e.modifiers, t, ff) : false;
}
function F_(e, t, r) {
return !e || ir(t) || !r(t) ? false : pe(e, t);
}
function h3(e, t, r) {
if (t === void 0 || t.length === 0)
return e;
let s = 0;
for (; s < e.length && r(e[s]); ++s)
;
return e.splice(s, 0, ...t), e;
}
function g3(e, t, r) {
if (t === void 0)
return e;
let s = 0;
for (; s < e.length && r(e[s]); ++s)
;
return e.splice(s, 0, t), e;
}
function y3(e) {
return us(e) || !!(xi(e) & 2097152);
}
function OD(e, t) {
return h3(e, t, us);
}
function MD(e, t) {
return h3(e, t, y3);
}
function LD(e, t) {
return g3(e, t, us);
}
function RD(e, t) {
return g3(e, t, y3);
}
function jD(e, t, r) {
if (e.charCodeAt(t + 1) === 47 && t + 2 < r && e.charCodeAt(t + 2) === 47) {
let s = e.substring(t, r);
return !!(bv.test(s) || Tv.test(s) || i8.test(s) || a8.test(s));
}
return false;
}
function v3(e, t) {
return e.charCodeAt(t + 1) === 42 && e.charCodeAt(t + 2) === 33;
}
function JD(e, t) {
let r = new Map(t.map((w) => [`${Ls(e, w.range.end).line}`, w])), s = /* @__PURE__ */ new Map();
return { getUnusedExpectations: f, markUsed: x };
function f() {
return Za(r.entries()).filter((w) => {
let [A, g] = w;
return g.type === 0 && !s.get(A);
}).map((w) => {
let [A, g] = w;
return g;
});
}
function x(w) {
return r.has(`${w}`) ? (s.set(`${w}`, true), true) : false;
}
}
function Io(e, t, r) {
return va(e) ? e.pos : Uy(e) || e.kind === 11 ? Ar((t || Si(e)).text, e.pos, false, true) : r && ya(e) ? Io(e.jsDoc[0], t) : e.kind === 354 && e._children.length > 0 ? Io(e._children[0], t, r) : Ar((t || Si(e)).text, e.pos, false, false, q3(e));
}
function FD(e, t) {
let r = !va(e) && fc(e) ? te(e.modifiers, zl) : void 0;
return r ? Ar((t || Si(e)).text, r.end) : Io(e, t);
}
function No(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
return B_(e.text, t, r);
}
function BD(e) {
return !!zi(e, lE);
}
function b3(e) {
return !!(cc(e) && e.exportClause && ld(e.exportClause) && e.exportClause.name.escapedText === "default");
}
function B_(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
if (va(t))
return "";
let s = e.substring(r ? t.pos : Ar(e, t.pos), t.end);
return BD(t) && (s = s.split(/\r\n|\n|\r/).map((f) => nl(f.replace(/^\s*\*/, ""))).join(`
`)), s;
}
function gf(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
return No(Si(e), e, t);
}
function qD(e) {
return e.pos;
}
function UD(e, t) {
return Ya(e, t, qD, Vr);
}
function xi(e) {
let t = e.emitNode;
return t && t.flags || 0;
}
function zD(e) {
let t = e.emitNode;
return t && t.internalFlags || 0;
}
function WD(e, t, r) {
var s;
if (t && VD(e, r))
return No(t, e);
switch (e.kind) {
case 10: {
let f = r & 2 ? A4 : r & 1 || xi(e) & 33554432 ? Nf : Of;
return e.singleQuote ? "'" + f(e.text, 39) + "'" : '"' + f(e.text, 34) + '"';
}
case 14:
case 15:
case 16:
case 17: {
let f = r & 1 || xi(e) & 33554432 ? Nf : Of, x = (s = e.rawText) != null ? s : SN(f(e.text, 96));
switch (e.kind) {
case 14:
return "`" + x + "`";
case 15:
return "`" + x + "${";
case 16:
return "}" + x + "${";
case 17:
return "}" + x + "`";
}
break;
}
case 8:
case 9:
return e.text;
case 13:
return r & 4 && e.isUnterminated ? e.text + (e.text.charCodeAt(e.text.length - 1) === 92 ? " /" : "/") : e.text;
}
return Y.fail(`Literal kind '${e.kind}' not accounted for.`);
}
function VD(e, t) {
return fs2(e) || !e.parent || t & 4 && e.isUnterminated ? false : zs(e) && e.numericLiteralFlags & 512 ? !!(t & 8) : !Uv(e);
}
function HD(e) {
return Ji(e) ? '"' + Of(e) + '"' : "" + e;
}
function GD(e) {
return sl(e).replace(/^(\d)/, "_$1").replace(/\W/g, "_");
}
function $D(e) {
return (tf(e) & 3) !== 0 || T3(e);
}
function T3(e) {
let t = If(e);
return t.kind === 257 && t.parent.kind === 295;
}
function yf(e) {
return Ea(e) && (e.name.kind === 10 || vf(e));
}
function KD(e) {
return Ea(e) && e.name.kind === 10;
}
function XD(e) {
return Ea(e) && Gn(e.name);
}
function S3(e) {
return Ea(e) || yt(e);
}
function YD(e) {
return QD(e.valueDeclaration);
}
function QD(e) {
return !!e && e.kind === 264 && !e.body;
}
function ZD(e) {
return e.kind === 308 || e.kind === 264 || uf(e);
}
function vf(e) {
return !!(e.flags & 1024);
}
function Xy(e) {
return yf(e) && x3(e);
}
function x3(e) {
switch (e.parent.kind) {
case 308:
return Qo(e.parent);
case 265:
return yf(e.parent.parent) && wi(e.parent.parent.parent) && !Qo(e.parent.parent.parent);
}
return false;
}
function E3(e) {
var t;
return (t = e.declarations) == null ? void 0 : t.find((r) => !Xy(r) && !(Ea(r) && vf(r)));
}
function ek(e) {
return e === 1 || e === 100 || e === 199;
}
function Yy(e, t) {
return Qo(e) || zf(t) || ek(Ei(t)) && !!e.commonJsModuleIndicator;
}
function tk(e, t) {
switch (e.scriptKind) {
case 1:
case 3:
case 2:
case 4:
break;
default:
return false;
}
return e.isDeclarationFile ? false : lv(t, "alwaysStrict") || SE(e.statements) ? true : Qo(e) || zf(t) ? Ei(t) >= 5 ? true : !t.noImplicitUseStrict : false;
}
function rk(e) {
return !!(e.flags & 16777216) || rn(e, 2);
}
function w3(e, t) {
switch (e.kind) {
case 308:
case 266:
case 295:
case 264:
case 245:
case 246:
case 247:
case 173:
case 171:
case 174:
case 175:
case 259:
case 215:
case 216:
case 169:
case 172:
return true;
case 238:
return !uf(t);
}
return false;
}
function nk(e) {
switch (Y.type(e), e.kind) {
case 341:
case 349:
case 326:
return true;
default:
return C3(e);
}
}
function C3(e) {
switch (Y.type(e), e.kind) {
case 176:
case 177:
case 170:
case 178:
case 181:
case 182:
case 320:
case 260:
case 228:
case 261:
case 262:
case 348:
case 259:
case 171:
case 173:
case 174:
case 175:
case 215:
case 216:
return true;
default:
return false;
}
}
function Qy(e) {
switch (e.kind) {
case 269:
case 268:
return true;
default:
return false;
}
}
function ik(e) {
return Qy(e) || Ef(e);
}
function ak(e) {
switch (e.kind) {
case 269:
case 268:
case 240:
case 260:
case 259:
case 264:
case 262:
case 261:
case 263:
return true;
default:
return false;
}
}
function sk(e) {
return bf(e) || Ea(e) || Kl(e) || s0(e);
}
function bf(e) {
return Qy(e) || cc(e);
}
function Zy(e) {
return zi(e.parent, (t) => w3(t, t.parent));
}
function ok(e, t) {
let r = Zy(e);
for (; r; )
t(r), r = Zy(r);
}
function A3(e) {
return !e || hf(e) === 0 ? "(Missing)" : gf(e);
}
function _k(e) {
return e.declaration ? A3(e.declaration.parameters[0].name) : void 0;
}
function ck(e) {
return e.kind === 164 && !Ta(e.expression);
}
function e0(e) {
var t;
switch (e.kind) {
case 79:
case 80:
return (t = e.emitNode) != null && t.autoGenerate ? void 0 : e.escapedText;
case 10:
case 8:
case 14:
return vi(e.text);
case 164:
return Ta(e.expression) ? vi(e.expression.text) : void 0;
default:
return Y.assertNever(e);
}
}
function lk(e) {
return Y.checkDefined(e0(e));
}
function ls(e) {
switch (e.kind) {
case 108:
return "this";
case 80:
case 79:
return hf(e) === 0 ? qr(e) : gf(e);
case 163:
return ls(e.left) + "." + ls(e.right);
case 208:
return yt(e.name) || vn(e.name) ? ls(e.expression) + "." + ls(e.name) : Y.assertNever(e.name);
case 314:
return ls(e.left) + ls(e.right);
default:
return Y.assertNever(e);
}
}
function uk(e, t, r, s, f, x) {
let w = Si(e);
return P3(w, e, t, r, s, f, x);
}
function pk(e, t, r, s, f, x, w) {
let A = Ar(e.text, t.pos);
return iv(e, A, t.end - A, r, s, f, x, w);
}
function P3(e, t, r, s, f, x, w) {
let A = i0(e, t);
return iv(e, A.start, A.length, r, s, f, x, w);
}
function fk(e, t, r, s) {
let f = i0(e, t);
return r0(e, f.start, f.length, r, s);
}
function dk(e, t, r, s) {
let f = Ar(e.text, t.pos);
return r0(e, f, t.end - f, r, s);
}
function t0(e, t, r) {
Y.assertGreaterThanOrEqual(t, 0), Y.assertGreaterThanOrEqual(r, 0), e && (Y.assertLessThanOrEqual(t, e.text.length), Y.assertLessThanOrEqual(t + r, e.text.length));
}
function r0(e, t, r, s, f) {
return t0(e, t, r), { file: e, start: t, length: r, code: s.code, category: s.category, messageText: s.next ? s : s.messageText, relatedInformation: f };
}
function mk(e, t, r) {
return { file: e, start: 0, length: 0, code: t.code, category: t.category, messageText: t.next ? t : t.messageText, relatedInformation: r };
}
function hk(e) {
return typeof e.messageText == "string" ? { code: e.code, category: e.category, messageText: e.messageText, next: e.next } : e.messageText;
}
function gk(e, t, r) {
return { file: e, start: t.pos, length: t.end - t.pos, code: r.code, category: r.category, messageText: r.message };
}
function n0(e, t) {
let r = Po(e.languageVersion, true, e.languageVariant, e.text, void 0, t);
r.scan();
let s = r.getTokenPos();
return ha(s, r.getTextPos());
}
function yk(e, t) {
let r = Po(e.languageVersion, true, e.languageVariant, e.text, void 0, t);
return r.scan(), r.getToken();
}
function vk(e, t) {
let r = Ar(e.text, t.pos);
if (t.body && t.body.kind === 238) {
let { line: s } = Ls(e, t.body.pos), { line: f } = Ls(e, t.body.end);
if (s < f)
return L_(r, d3(s, e) - r + 1);
}
return ha(r, t.end);
}
function i0(e, t) {
let r = t;
switch (t.kind) {
case 308:
let x = Ar(e.text, 0, false);
return x === e.text.length ? L_(0, 0) : n0(e, x);
case 257:
case 205:
case 260:
case 228:
case 261:
case 264:
case 263:
case 302:
case 259:
case 215:
case 171:
case 174:
case 175:
case 262:
case 169:
case 168:
case 271:
r = t.name;
break;
case 216:
return vk(e, t);
case 292:
case 293:
let w = Ar(e.text, t.pos), A = t.statements.length > 0 ? t.statements[0].pos : t.end;
return ha(w, A);
}
if (r === void 0)
return n0(e, t.pos);
Y.assert(!Ho(r));
let s = va(r), f = s || td(t) ? r.pos : Ar(e.text, r.pos);
return s ? (Y.assert(f === r.pos, "This failure could trigger https://github.com/Microsoft/TypeScript/issues/20809"), Y.assert(f === r.end, "This failure could trigger https://github.com/Microsoft/TypeScript/issues/20809")) : (Y.assert(f >= r.pos, "This failure could trigger https://github.com/Microsoft/TypeScript/issues/20809"), Y.assert(f <= r.end, "This failure could trigger https://github.com/Microsoft/TypeScript/issues/20809")), ha(f, r.end);
}
function bk(e) {
return (e.externalModuleIndicator || e.commonJsModuleIndicator) !== void 0;
}
function a0(e) {
return e.scriptKind === 6;
}
function Tk(e) {
return !!(ef(e) & 2048);
}
function Sk(e) {
return !!(ef(e) & 64 && !lS(e, e.parent));
}
function D3(e) {
return !!(tf(e) & 2);
}
function xk(e) {
return !!(tf(e) & 1);
}
function Ek(e) {
return e.kind === 210 && e.expression.kind === 106;
}
function s0(e) {
return e.kind === 210 && e.expression.kind === 100;
}
function o0(e) {
return t2(e) && e.keywordToken === 100 && e.name.escapedText === "meta";
}
function k3(e) {
return Kl(e) && Yv(e.argument) && Gn(e.argument.literal);
}
function us(e) {
return e.kind === 241 && e.expression.kind === 10;
}
function Tf(e) {
return !!(xi(e) & 2097152);
}
function _0(e) {
return Tf(e) && Wo(e);
}
function wk(e) {
return yt(e.name) && !e.initializer;
}
function c0(e) {
return Tf(e) && zo(e) && me(e.declarationList.declarations, wk);
}
function Ck(e, t) {
return e.kind !== 11 ? Ao(t.text, e.pos) : void 0;
}
function I3(e, t) {
let r = e.kind === 166 || e.kind === 165 || e.kind === 215 || e.kind === 216 || e.kind === 214 || e.kind === 257 || e.kind === 278 ? Ft(HT(t, e.pos), Ao(t, e.pos)) : Ao(t, e.pos);
return ee(r, (s) => t.charCodeAt(s.pos + 1) === 42 && t.charCodeAt(s.pos + 2) === 42 && t.charCodeAt(s.pos + 3) !== 47);
}
function l0(e) {
if (179 <= e.kind && e.kind <= 202)
return true;
switch (e.kind) {
case 131:
case 157:
case 148:
case 160:
case 152:
case 134:
case 153:
case 149:
case 155:
case 144:
return true;
case 114:
return e.parent.kind !== 219;
case 230:
return ru(e.parent) && !Z0(e);
case 165:
return e.parent.kind === 197 || e.parent.kind === 192;
case 79:
(e.parent.kind === 163 && e.parent.right === e || e.parent.kind === 208 && e.parent.name === e) && (e = e.parent), Y.assert(e.kind === 79 || e.kind === 163 || e.kind === 208, "'node' was expected to be a qualified name, identifier or property access in 'isPartOfTypeNode'.");
case 163:
case 208:
case 108: {
let { parent: t } = e;
if (t.kind === 183)
return false;
if (t.kind === 202)
return !t.isTypeOf;
if (179 <= t.kind && t.kind <= 202)
return true;
switch (t.kind) {
case 230:
return ru(t.parent) && !Z0(t);
case 165:
return e === t.constraint;
case 348:
return e === t.constraint;
case 169:
case 168:
case 166:
case 257:
return e === t.type;
case 259:
case 215:
case 216:
case 173:
case 171:
case 170:
case 174:
case 175:
return e === t.type;
case 176:
case 177:
case 178:
return e === t.type;
case 213:
return e === t.type;
case 210:
case 211:
return pe(t.typeArguments, e);
case 212:
return false;
}
}
}
return false;
}
function Ak(e, t) {
for (; e; ) {
if (e.kind === t)
return true;
e = e.parent;
}
return false;
}
function Pk(e, t) {
return r(e);
function r(s) {
switch (s.kind) {
case 250:
return t(s);
case 266:
case 238:
case 242:
case 243:
case 244:
case 245:
case 246:
case 247:
case 251:
case 252:
case 292:
case 293:
case 253:
case 255:
case 295:
return xr(s, r);
}
}
}
function Dk(e, t) {
return r(e);
function r(s) {
switch (s.kind) {
case 226:
t(s);
let f = s.expression;
f && r(f);
return;
case 263:
case 261:
case 264:
case 262:
return;
default:
if (ga(s)) {
if (s.name && s.name.kind === 164) {
r(s.name.expression);
return;
}
} else
l0(s) || xr(s, r);
}
}
}
function kk(e) {
return e && e.kind === 185 ? e.elementType : e && e.kind === 180 ? Xa(e.typeArguments) : void 0;
}
function Ik(e) {
switch (e.kind) {
case 261:
case 260:
case 228:
case 184:
return e.members;
case 207:
return e.properties;
}
}
function u0(e) {
if (e)
switch (e.kind) {
case 205:
case 302:
case 166:
case 299:
case 169:
case 168:
case 300:
case 257:
return true;
}
return false;
}
function Nk(e) {
return u0(e) || pf(e);
}
function N3(e) {
return e.parent.kind === 258 && e.parent.parent.kind === 240;
}
function Ok(e) {
return Pr(e) ? Hs(e.parent) && ur(e.parent.parent) && ps(e.parent.parent) === 2 || p0(e.parent) : false;
}
function p0(e) {
return Pr(e) ? ur(e) && ps(e) === 1 : false;
}
function Mk(e) {
return (Vi(e) ? D3(e) && yt(e.name) && N3(e) : Bo(e) ? $0(e) && Lf(e) : Wl(e) && $0(e)) || p0(e);
}
function Lk(e) {
switch (e.kind) {
case 171:
case 170:
case 173:
case 174:
case 175:
case 259:
case 215:
return true;
}
return false;
}
function Rk(e, t) {
for (; ; ) {
if (t && t(e), e.statement.kind !== 253)
return e.statement;
e = e.statement;
}
}
function O3(e) {
return e && e.kind === 238 && ga(e.parent);
}
function jk(e) {
return e && e.kind === 171 && e.parent.kind === 207;
}
function Jk(e) {
return (e.kind === 171 || e.kind === 174 || e.kind === 175) && (e.parent.kind === 207 || e.parent.kind === 228);
}
function Fk(e) {
return e && e.kind === 1;
}
function Bk(e) {
return e && e.kind === 0;
}
function f0(e, t, r) {
return e.properties.filter((s) => {
if (s.kind === 299) {
let f = e0(s.name);
return t === f || !!r && r === f;
}
return false;
});
}
function qk(e, t, r) {
return q(f0(e, t), (s) => Yl(s.initializer) ? Ae(s.initializer.elements, (f) => Gn(f) && f.text === r) : void 0);
}
function M3(e) {
if (e && e.statements.length) {
let t = e.statements[0].expression;
return ln(t, Hs);
}
}
function Uk(e, t, r) {
return q(L3(e, t), (s) => Yl(s.initializer) ? Ae(s.initializer.elements, (f) => Gn(f) && f.text === r) : void 0);
}
function L3(e, t) {
let r = M3(e);
return r ? f0(r, t) : Bt;
}
function zk(e) {
return zi(e.parent, ga);
}
function Wk(e) {
return zi(e.parent, HS);
}
function Vk(e) {
return zi(e.parent, bi);
}
function Hk(e) {
return zi(e.parent, (t) => bi(t) || ga(t) ? "quit" : Hl(t));
}
function Gk(e) {
return zi(e.parent, uf);
}
function d0(e, t, r) {
for (Y.assert(e.kind !== 308); ; ) {
if (e = e.parent, !e)
return Y.fail();
switch (e.kind) {
case 164:
if (r && bi(e.parent.parent))
return e;
e = e.parent.parent;
break;
case 167:
e.parent.kind === 166 && Js(e.parent.parent) ? e = e.parent.parent : Js(e.parent) && (e = e.parent);
break;
case 216:
if (!t)
continue;
case 259:
case 215:
case 264:
case 172:
case 169:
case 168:
case 171:
case 170:
case 173:
case 174:
case 175:
case 176:
case 177:
case 178:
case 263:
case 308:
return e;
}
}
}
function $k(e) {
switch (e.kind) {
case 216:
case 259:
case 215:
case 169:
return true;
case 238:
switch (e.parent.kind) {
case 173:
case 171:
case 174:
case 175:
return true;
default:
return false;
}
default:
return false;
}
}
function Kk(e) {
yt(e) && (_c(e.parent) || Wo(e.parent)) && e.parent.name === e && (e = e.parent);
let t = d0(e, true, false);
return wi(t);
}
function Xk(e) {
let t = d0(e, false, false);
if (t)
switch (t.kind) {
case 173:
case 259:
case 215:
return t;
}
}
function Yk(e, t) {
for (; ; ) {
if (e = e.parent, !e)
return;
switch (e.kind) {
case 164:
e = e.parent;
break;
case 259:
case 215:
case 216:
if (!t)
continue;
case 169:
case 168:
case 171:
case 170:
case 173:
case 174:
case 175:
case 172:
return e;
case 167:
e.parent.kind === 166 && Js(e.parent.parent) ? e = e.parent.parent : Js(e.parent) && (e = e.parent);
break;
}
}
}
function Qk(e) {
if (e.kind === 215 || e.kind === 216) {
let t = e, r = e.parent;
for (; r.kind === 214; )
t = r, r = r.parent;
if (r.kind === 210 && r.expression === t)
return r;
}
}
function Zk(e) {
return e.kind === 106 || Sf(e);
}
function Sf(e) {
let t = e.kind;
return (t === 208 || t === 209) && e.expression.kind === 106;
}
function eI(e) {
let t = e.kind;
return (t === 208 || t === 209) && e.expression.kind === 108;
}
function tI(e) {
var t;
return !!e && Vi(e) && ((t = e.initializer) == null ? void 0 : t.kind) === 108;
}
function rI(e) {
return !!e && (nu(e) || lc(e)) && ur(e.parent.parent) && e.parent.parent.operatorToken.kind === 63 && e.parent.parent.right.kind === 108;
}
function nI(e) {
switch (e.kind) {
case 180:
return e.typeName;
case 230:
return Bs(e.expression) ? e.expression : void 0;
case 79:
case 163:
return e;
}
}
function iI(e) {
switch (e.kind) {
case 212:
return e.tag;
case 283:
case 282:
return e.tagName;
default:
return e.expression;
}
}
function R3(e, t, r, s) {
if (e && af(t) && vn(t.name))
return false;
switch (t.kind) {
case 260:
return true;
case 228:
return !e;
case 169:
return r !== void 0 && (e ? _c(r) : bi(r) && !W4(t) && !V4(t));
case 174:
case 175:
case 171:
return t.body !== void 0 && r !== void 0 && (e ? _c(r) : bi(r));
case 166:
return e ? r !== void 0 && r.body !== void 0 && (r.kind === 173 || r.kind === 171 || r.kind === 175) && j4(r) !== t && s !== void 0 && s.kind === 260 : false;
}
return false;
}
function q_(e, t, r, s) {
return Il(t) && R3(e, t, r, s);
}
function m0(e, t, r, s) {
return q_(e, t, r, s) || h0(e, t, r);
}
function h0(e, t, r) {
switch (t.kind) {
case 260:
return Ke(t.members, (s) => m0(e, s, t, r));
case 228:
return !e && Ke(t.members, (s) => m0(e, s, t, r));
case 171:
case 175:
case 173:
return Ke(t.parameters, (s) => q_(e, s, t, r));
default:
return false;
}
}
function aI(e, t) {
if (q_(e, t))
return true;
let r = R4(t);
return !!r && h0(e, r, t);
}
function sI(e, t, r) {
let s;
if (pf(t)) {
let { firstAccessor: f, secondAccessor: x, setAccessor: w } = W0(r.members, t), A = Il(f) ? f : x && Il(x) ? x : void 0;
if (!A || t !== A)
return false;
s = w == null ? void 0 : w.parameters;
} else
Vl(t) && (s = t.parameters);
if (q_(e, t, r))
return true;
if (s) {
for (let f of s)
if (!kl(f) && q_(e, f, t, r))
return true;
}
return false;
}
function j3(e) {
if (e.textSourceNode) {
switch (e.textSourceNode.kind) {
case 10:
return j3(e.textSourceNode);
case 14:
return e.text === "";
}
return false;
}
return e.text === "";
}
function xf(e) {
let { parent: t } = e;
return t.kind === 283 || t.kind === 282 || t.kind === 284 ? t.tagName === e : false;
}
function g0(e) {
switch (e.kind) {
case 106:
case 104:
case 110:
case 95:
case 13:
case 206:
case 207:
case 208:
case 209:
case 210:
case 211:
case 212:
case 231:
case 213:
case 235:
case 232:
case 214:
case 215:
case 228:
case 216:
case 219:
case 217:
case 218:
case 221:
case 222:
case 223:
case 224:
case 227:
case 225:
case 229:
case 281:
case 282:
case 285:
case 226:
case 220:
case 233:
return true;
case 230:
return !ru(e.parent) && !md(e.parent);
case 163:
for (; e.parent.kind === 163; )
e = e.parent;
return e.parent.kind === 183 || Sl(e.parent) || fd(e.parent) || uc(e.parent) || xf(e);
case 314:
for (; uc(e.parent); )
e = e.parent;
return e.parent.kind === 183 || Sl(e.parent) || fd(e.parent) || uc(e.parent) || xf(e);
case 80:
return ur(e.parent) && e.parent.left === e && e.parent.operatorToken.kind === 101;
case 79:
if (e.parent.kind === 183 || Sl(e.parent) || fd(e.parent) || uc(e.parent) || xf(e))
return true;
case 8:
case 9:
case 10:
case 14:
case 108:
return J3(e);
default:
return false;
}
}
function J3(e) {
let { parent: t } = e;
switch (t.kind) {
case 257:
case 166:
case 169:
case 168:
case 302:
case 299:
case 205:
return t.initializer === e;
case 241:
case 242:
case 243:
case 244:
case 250:
case 251:
case 252:
case 292:
case 254:
return t.expression === e;
case 245:
let r = t;
return r.initializer === e && r.initializer.kind !== 258 || r.condition === e || r.incrementor === e;
case 246:
case 247:
let s = t;
return s.initializer === e && s.initializer.kind !== 258 || s.expression === e;
case 213:
case 231:
return e === t.expression;
case 236:
return e === t.expression;
case 164:
return e === t.expression;
case 167:
case 291:
case 290:
case 301:
return true;
case 230:
return t.expression === e && !l0(t);
case 300:
return t.objectAssignmentInitializer === e;
case 235:
return e === t.expression;
default:
return g0(t);
}
}
function F3(e) {
for (; e.kind === 163 || e.kind === 79; )
e = e.parent;
return e.kind === 183;
}
function oI(e) {
return ld(e) && !!e.parent.moduleSpecifier;
}
function B3(e) {
return e.kind === 268 && e.moduleReference.kind === 280;
}
function _I(e) {
return Y.assert(B3(e)), e.moduleReference.expression;
}
function cI(e) {
return Ef(e) && rv(e.initializer).arguments[0];
}
function lI(e) {
return e.kind === 268 && e.moduleReference.kind !== 280;
}
function y0(e) {
return Pr(e);
}
function uI(e) {
return !Pr(e);
}
function Pr(e) {
return !!e && !!(e.flags & 262144);
}
function pI(e) {
return !!e && !!(e.flags & 67108864);
}
function fI(e) {
return !a0(e);
}
function q3(e) {
return !!e && !!(e.flags & 8388608);
}
function dI(e) {
return ac(e) && yt(e.typeName) && e.typeName.escapedText === "Object" && e.typeArguments && e.typeArguments.length === 2 && (e.typeArguments[0].kind === 152 || e.typeArguments[0].kind === 148);
}
function El(e, t) {
if (e.kind !== 210)
return false;
let { expression: r, arguments: s } = e;
if (r.kind !== 79 || r.escapedText !== "require" || s.length !== 1)
return false;
let f = s[0];
return !t || Ti(f);
}
function U3(e) {
return z3(e, false);
}
function Ef(e) {
return z3(e, true);
}
function mI(e) {
return Xl(e) && Ef(e.parent.parent);
}
function z3(e, t) {
return Vi(e) && !!e.initializer && El(t ? rv(e.initializer) : e.initializer, true);
}
function W3(e) {
return zo(e) && e.declarationList.declarations.length > 0 && me(e.declarationList.declarations, (t) => U3(t));
}
function hI(e) {
return e === 39 || e === 34;
}
function gI(e, t) {
return No(t, e).charCodeAt(0) === 34;
}
function v0(e) {
return ur(e) || Lo(e) || yt(e) || sc(e);
}
function V3(e) {
return Pr(e) && e.initializer && ur(e.initializer) && (e.initializer.operatorToken.kind === 56 || e.initializer.operatorToken.kind === 60) && e.name && Bs(e.name) && z_(e.name, e.initializer.left) ? e.initializer.right : e.initializer;
}
function yI(e) {
let t = V3(e);
return t && U_(t, Nl(e.name));
}
function vI(e, t) {
return c(e.properties, (r) => lc(r) && yt(r.name) && r.name.escapedText === "value" && r.initializer && U_(r.initializer, t));
}
function bI(e) {
if (e && e.parent && ur(e.parent) && e.parent.operatorToken.kind === 63) {
let t = Nl(e.parent.left);
return U_(e.parent.right, t) || TI(e.parent.left, e.parent.right, t);
}
if (e && sc(e) && S0(e)) {
let t = vI(e.arguments[2], e.arguments[1].text === "prototype");
if (t)
return t;
}
}
function U_(e, t) {
if (sc(e)) {
let r = Pl(e.expression);
return r.kind === 215 || r.kind === 216 ? e : void 0;
}
if (e.kind === 215 || e.kind === 228 || e.kind === 216 || Hs(e) && (e.properties.length === 0 || t))
return e;
}
function TI(e, t, r) {
let s = ur(t) && (t.operatorToken.kind === 56 || t.operatorToken.kind === 60) && U_(t.right, r);
if (s && z_(e, t.left))
return s;
}
function SI(e) {
let t = Vi(e.parent) ? e.parent.name : ur(e.parent) && e.parent.operatorToken.kind === 63 ? e.parent.left : void 0;
return t && U_(e.right, Nl(t)) && Bs(t) && z_(t, e.left);
}
function xI(e) {
if (ur(e.parent)) {
let t = (e.parent.operatorToken.kind === 56 || e.parent.operatorToken.kind === 60) && ur(e.parent.parent) ? e.parent.parent : e.parent;
if (t.operatorToken.kind === 63 && yt(t.left))
return t.left;
} else if (Vi(e.parent))
return e.parent.name;
}
function z_(e, t) {
return L0(e) && L0(t) ? kf(e) === kf(t) : js(e) && wf(t) && (t.expression.kind === 108 || yt(t.expression) && (t.expression.escapedText === "window" || t.expression.escapedText === "self" || t.expression.escapedText === "global")) ? z_(e, $3(t)) : wf(e) && wf(t) ? Fs(e) === Fs(t) && z_(e.expression, t.expression) : false;
}
function b0(e) {
for (; ms(e, true); )
e = e.right;
return e;
}
function H3(e) {
return yt(e) && e.escapedText === "exports";
}
function G3(e) {
return yt(e) && e.escapedText === "module";
}
function T0(e) {
return (bn(e) || wl(e)) && G3(e.expression) && Fs(e) === "exports";
}
function ps(e) {
let t = EI(e);
return t === 5 || Pr(e) ? t : 0;
}
function S0(e) {
return I(e.arguments) === 3 && bn(e.expression) && yt(e.expression.expression) && qr(e.expression.expression) === "Object" && qr(e.expression.name) === "defineProperty" && Ta(e.arguments[1]) && V_(e.arguments[0], true);
}
function wf(e) {
return bn(e) || wl(e);
}
function wl(e) {
return gs(e) && Ta(e.argumentExpression);
}
function W_(e, t) {
return bn(e) && (!t && e.expression.kind === 108 || yt(e.name) && V_(e.expression, true)) || x0(e, t);
}
function x0(e, t) {
return wl(e) && (!t && e.expression.kind === 108 || Bs(e.expression) || W_(e.expression, true));
}
function V_(e, t) {
return Bs(e) || W_(e, t);
}
function $3(e) {
return bn(e) ? e.name : e.argumentExpression;
}
function EI(e) {
if (sc(e)) {
if (!S0(e))
return 0;
let t = e.arguments[0];
return H3(t) || T0(t) ? 8 : W_(t) && Fs(t) === "prototype" ? 9 : 7;
}
return e.operatorToken.kind !== 63 || !Lo(e.left) || wI(b0(e)) ? 0 : V_(e.left.expression, true) && Fs(e.left) === "prototype" && Hs(X3(e)) ? 6 : K3(e.left);
}
function wI(e) {
return Qv(e) && zs(e.expression) && e.expression.text === "0";
}
function Cf(e) {
if (bn(e))
return e.name;
let t = Pl(e.argumentExpression);
return zs(t) || Ti(t) ? t : e;
}
function Fs(e) {
let t = Cf(e);
if (t) {
if (yt(t))
return t.escapedText;
if (Ti(t) || zs(t))
return vi(t.text);
}
}
function K3(e) {
if (e.expression.kind === 108)
return 4;
if (T0(e))
return 2;
if (V_(e.expression, true)) {
if (Nl(e.expression))
return 3;
let t = e;
for (; !yt(t.expression); )
t = t.expression;
let r = t.expression;
if ((r.escapedText === "exports" || r.escapedText === "module" && Fs(t) === "exports") && W_(e))
return 1;
if (V_(e, true) || gs(e) && M0(e))
return 5;
}
return 0;
}
function X3(e) {
for (; ur(e.right); )
e = e.right;
return e.right;
}
function CI(e) {
return ur(e) && ps(e) === 3;
}
function AI(e) {
return Pr(e) && e.parent && e.parent.kind === 241 && (!gs(e) || wl(e)) && !!_f(e.parent);
}
function PI(e, t) {
let { valueDeclaration: r } = e;
(!r || !(t.flags & 16777216 && !Pr(t) && !(r.flags & 16777216)) && v0(r) && !v0(t) || r.kind !== t.kind && S3(r)) && (e.valueDeclaration = t);
}
function DI(e) {
if (!e || !e.valueDeclaration)
return false;
let t = e.valueDeclaration;
return t.kind === 259 || Vi(t) && t.initializer && ga(t.initializer);
}
function kI(e) {
var t, r;
switch (e.kind) {
case 257:
case 205:
return (t = zi(e.initializer, (s) => El(s, true))) == null ? void 0 : t.arguments[0];
case 269:
return ln(e.moduleSpecifier, Ti);
case 268:
return ln((r = ln(e.moduleReference, ud)) == null ? void 0 : r.expression, Ti);
case 270:
case 277:
return ln(e.parent.moduleSpecifier, Ti);
case 271:
case 278:
return ln(e.parent.parent.moduleSpecifier, Ti);
case 273:
return ln(e.parent.parent.parent.moduleSpecifier, Ti);
default:
Y.assertNever(e);
}
}
function II(e) {
return Y3(e) || Y.failBadSyntaxKind(e.parent);
}
function Y3(e) {
switch (e.parent.kind) {
case 269:
case 275:
return e.parent;
case 280:
return e.parent.parent;
case 210:
return s0(e.parent) || El(e.parent, false) ? e.parent : void 0;
case 198:
return Y.assert(Gn(e)), ln(e.parent.parent, Kl);
default:
return;
}
}
function E0(e) {
switch (e.kind) {
case 269:
case 275:
return e.moduleSpecifier;
case 268:
return e.moduleReference.kind === 280 ? e.moduleReference.expression : void 0;
case 202:
return k3(e) ? e.argument.literal : void 0;
case 210:
return e.arguments[0];
case 264:
return e.name.kind === 10 ? e.name : void 0;
default:
return Y.assertNever(e);
}
}
function Q3(e) {
switch (e.kind) {
case 269:
return e.importClause && ln(e.importClause.namedBindings, _2);
case 268:
return e;
case 275:
return e.exportClause && ln(e.exportClause, ld);
default:
return Y.assertNever(e);
}
}
function Z3(e) {
return e.kind === 269 && !!e.importClause && !!e.importClause.name;
}
function NI(e, t) {
if (e.name) {
let r = t(e);
if (r)
return r;
}
if (e.namedBindings) {
let r = _2(e.namedBindings) ? t(e.namedBindings) : c(e.namedBindings.elements, t);
if (r)
return r;
}
}
function OI(e) {
if (e)
switch (e.kind) {
case 166:
case 171:
case 170:
case 300:
case 299:
case 169:
case 168:
return e.questionToken !== void 0;
}
return false;
}
function MI(e) {
let t = dd(e) ? pa(e.parameters) : void 0, r = ln(t && t.name, yt);
return !!r && r.escapedText === "new";
}
function Cl(e) {
return e.kind === 349 || e.kind === 341 || e.kind === 343;
}
function LI(e) {
return Cl(e) || n2(e);
}
function RI(e) {
return Zl(e) && ur(e.expression) && e.expression.operatorToken.kind === 63 ? b0(e.expression) : void 0;
}
function e4(e) {
return Zl(e) && ur(e.expression) && ps(e.expression) !== 0 && ur(e.expression.right) && (e.expression.right.operatorToken.kind === 56 || e.expression.right.operatorToken.kind === 60) ? e.expression.right.right : void 0;
}
function w0(e) {
switch (e.kind) {
case 240:
let t = Al(e);
return t && t.initializer;
case 169:
return e.initializer;
case 299:
return e.initializer;
}
}
function Al(e) {
return zo(e) ? pa(e.declarationList.declarations) : void 0;
}
function t4(e) {
return Ea(e) && e.body && e.body.kind === 264 ? e.body : void 0;
}
function jI(e) {
if (e.kind >= 240 && e.kind <= 256)
return true;
switch (e.kind) {
case 79:
case 108:
case 106:
case 163:
case 233:
case 209:
case 208:
case 205:
case 215:
case 216:
case 171:
case 174:
case 175:
return true;
default:
return false;
}
}
function Af(e) {
switch (e.kind) {
case 216:
case 223:
case 238:
case 249:
case 176:
case 292:
case 260:
case 228:
case 172:
case 173:
case 182:
case 177:
case 248:
case 256:
case 243:
case 209:
case 239:
case 1:
case 263:
case 302:
case 274:
case 275:
case 278:
case 241:
case 246:
case 247:
case 245:
case 259:
case 215:
case 181:
case 174:
case 79:
case 242:
case 269:
case 268:
case 178:
case 261:
case 320:
case 326:
case 253:
case 171:
case 170:
case 264:
case 199:
case 267:
case 207:
case 166:
case 214:
case 208:
case 299:
case 169:
case 168:
case 250:
case 175:
case 300:
case 301:
case 252:
case 254:
case 255:
case 262:
case 165:
case 257:
case 240:
case 244:
case 251:
return true;
default:
return false;
}
}
function r4(e, t) {
let r;
u0(e) && l3(e) && ya(e.initializer) && (r = jr(r, n4(e, Zn(e.initializer.jsDoc))));
let s = e;
for (; s && s.parent; ) {
if (ya(s) && (r = jr(r, n4(e, Zn(s.jsDoc)))), s.kind === 166) {
r = jr(r, (t ? bS : of)(s));
break;
}
if (s.kind === 165) {
r = jr(r, (t ? xS : SS)(s));
break;
}
s = a4(s);
}
return r || Bt;
}
function n4(e, t) {
if (Ho(t)) {
let r = ee(t.tags, (s) => i4(e, s));
return t.tags === r ? [t] : r;
}
return i4(e, t) ? [t] : void 0;
}
function i4(e, t) {
return !(au(t) || T2(t)) || !t.parent || !Ho(t.parent) || !qo(t.parent.parent) || t.parent.parent === e;
}
function a4(e) {
let t = e.parent;
if (t.kind === 299 || t.kind === 274 || t.kind === 169 || t.kind === 241 && e.kind === 208 || t.kind === 250 || t4(t) || ur(e) && e.operatorToken.kind === 63)
return t;
if (t.parent && (Al(t.parent) === e || ur(t) && t.operatorToken.kind === 63))
return t.parent;
if (t.parent && t.parent.parent && (Al(t.parent.parent) || w0(t.parent.parent) === e || e4(t.parent.parent)))
return t.parent.parent;
}
function JI(e) {
if (e.symbol)
return e.symbol;
if (!yt(e.name))
return;
let t = e.name.escapedText, r = C0(e);
if (!r)
return;
let s = Ae(r.parameters, (f) => f.name.kind === 79 && f.name.escapedText === t);
return s && s.symbol;
}
function FI(e) {
if (Ho(e.parent) && e.parent.tags) {
let t = Ae(e.parent.tags, Cl);
if (t)
return t;
}
return C0(e);
}
function C0(e) {
let t = A0(e);
if (t)
return Wl(t) && t.type && ga(t.type) ? t.type : ga(t) ? t : void 0;
}
function A0(e) {
let t = s4(e);
if (t)
return e4(t) || RI(t) || w0(t) || Al(t) || t4(t) || t;
}
function s4(e) {
let t = P0(e);
if (!t)
return;
let r = t.parent;
if (r && r.jsDoc && t === Cn(r.jsDoc))
return r;
}
function P0(e) {
return zi(e.parent, Ho);
}
function BI(e) {
let t = e.name.escapedText, { typeParameters: r } = e.parent.parent.parent;
return r && Ae(r, (s) => s.name.escapedText === t);
}
function qI(e) {
return !!e.typeArguments;
}
function o4(e) {
let t = e.parent;
for (; ; ) {
switch (t.kind) {
case 223:
let r = t.operatorToken.kind;
return G_(r) && t.left === e ? r === 63 || jf(r) ? 1 : 2 : 0;
case 221:
case 222:
let s = t.operator;
return s === 45 || s === 46 ? 2 : 0;
case 246:
case 247:
return t.initializer === e ? 1 : 0;
case 214:
case 206:
case 227:
case 232:
e = t;
break;
case 301:
e = t.parent;
break;
case 300:
if (t.name !== e)
return 0;
e = t.parent;
break;
case 299:
if (t.name === e)
return 0;
e = t.parent;
break;
default:
return 0;
}
t = e.parent;
}
}
function UI(e) {
return o4(e) !== 0;
}
function zI(e) {
switch (e.kind) {
case 238:
case 240:
case 251:
case 242:
case 252:
case 266:
case 292:
case 293:
case 253:
case 245:
case 246:
case 247:
case 243:
case 244:
case 255:
case 295:
return true;
}
return false;
}
function WI(e) {
return ad(e) || sd(e) || Ly(e) || Wo(e) || nc(e);
}
function _4(e, t) {
for (; e && e.kind === t; )
e = e.parent;
return e;
}
function VI(e) {
return _4(e, 193);
}
function D0(e) {
return _4(e, 214);
}
function HI(e) {
let t;
for (; e && e.kind === 193; )
t = e, e = e.parent;
return [t, e];
}
function GI(e) {
for (; Kv(e); )
e = e.type;
return e;
}
function Pl(e, t) {
return $o(e, t ? 17 : 1);
}
function $I(e) {
return e.kind !== 208 && e.kind !== 209 ? false : (e = D0(e.parent), e && e.kind === 217);
}
function KI(e, t) {
for (; e; ) {
if (e === t)
return true;
e = e.parent;
}
return false;
}
function c4(e) {
return !wi(e) && !df(e) && ko(e.parent) && e.parent.name === e;
}
function XI(e) {
let t = e.parent;
switch (e.kind) {
case 10:
case 14:
case 8:
if (Ws(t))
return t.parent;
case 79:
if (ko(t))
return t.name === e ? t : void 0;
if (rc(t)) {
let r = t.parent;
return pc(r) && r.name === t ? r : void 0;
} else {
let r = t.parent;
return ur(r) && ps(r) !== 0 && (r.left.symbol || r.symbol) && ml(r) === e ? r : void 0;
}
case 80:
return ko(t) && t.name === e ? t : void 0;
default:
return;
}
}
function l4(e) {
return Ta(e) && e.parent.kind === 164 && ko(e.parent.parent);
}
function YI(e) {
let t = e.parent;
switch (t.kind) {
case 169:
case 168:
case 171:
case 170:
case 174:
case 175:
case 302:
case 299:
case 208:
return t.name === e;
case 163:
return t.right === e;
case 205:
case 273:
return t.propertyName === e;
case 278:
case 288:
case 282:
case 283:
case 284:
return true;
}
return false;
}
function QI(e) {
return e.kind === 268 || e.kind === 267 || e.kind === 270 && e.name || e.kind === 271 || e.kind === 277 || e.kind === 273 || e.kind === 278 || e.kind === 274 && I0(e) ? true : Pr(e) && (ur(e) && ps(e) === 2 && I0(e) || bn(e) && ur(e.parent) && e.parent.left === e && e.parent.operatorToken.kind === 63 && k0(e.parent.right));
}
function u4(e) {
switch (e.parent.kind) {
case 270:
case 273:
case 271:
case 278:
case 274:
case 268:
case 277:
return e.parent;
case 163:
do
e = e.parent;
while (e.parent.kind === 163);
return u4(e);
}
}
function k0(e) {
return Bs(e) || _d(e);
}
function I0(e) {
let t = p4(e);
return k0(t);
}
function p4(e) {
return Vo(e) ? e.expression : e.right;
}
function ZI(e) {
return e.kind === 300 ? e.name : e.kind === 299 ? e.initializer : e.parent.right;
}
function f4(e) {
let t = d4(e);
if (t && Pr(e)) {
let r = ES(e);
if (r)
return r.class;
}
return t;
}
function d4(e) {
let t = Pf(e.heritageClauses, 94);
return t && t.types.length > 0 ? t.types[0] : void 0;
}
function m4(e) {
if (Pr(e))
return wS(e).map((t) => t.class);
{
let t = Pf(e.heritageClauses, 117);
return t == null ? void 0 : t.types;
}
}
function h4(e) {
return eu(e) ? g4(e) || Bt : bi(e) && Ft(Cp(f4(e)), m4(e)) || Bt;
}
function g4(e) {
let t = Pf(e.heritageClauses, 94);
return t ? t.types : void 0;
}
function Pf(e, t) {
if (e) {
for (let r of e)
if (r.token === t)
return r;
}
}
function eN(e, t) {
for (; e; ) {
if (e.kind === t)
return e;
e = e.parent;
}
}
function ba(e) {
return 81 <= e && e <= 162;
}
function N0(e) {
return 126 <= e && e <= 162;
}
function y4(e) {
return ba(e) && !N0(e);
}
function tN(e) {
return 117 <= e && e <= 125;
}
function rN(e) {
let t = _l(e);
return t !== void 0 && y4(t);
}
function nN(e) {
let t = _l(e);
return t !== void 0 && ba(t);
}
function iN(e) {
let t = dS(e);
return !!t && !N0(t);
}
function aN(e) {
return 2 <= e && e <= 7;
}
function sN(e) {
if (!e)
return 4;
let t = 0;
switch (e.kind) {
case 259:
case 215:
case 171:
e.asteriskToken && (t |= 1);
case 216:
rn(e, 512) && (t |= 2);
break;
}
return e.body || (t |= 4), t;
}
function oN(e) {
switch (e.kind) {
case 259:
case 215:
case 216:
case 171:
return e.body !== void 0 && e.asteriskToken === void 0 && rn(e, 512);
}
return false;
}
function Ta(e) {
return Ti(e) || zs(e);
}
function O0(e) {
return od(e) && (e.operator === 39 || e.operator === 40) && zs(e.operand);
}
function v4(e) {
let t = ml(e);
return !!t && M0(t);
}
function M0(e) {
if (!(e.kind === 164 || e.kind === 209))
return false;
let t = gs(e) ? Pl(e.argumentExpression) : e.expression;
return !Ta(t) && !O0(t);
}
function Df(e) {
switch (e.kind) {
case 79:
case 80:
return e.escapedText;
case 10:
case 8:
return vi(e.text);
case 164:
let t = e.expression;
return Ta(t) ? vi(t.text) : O0(t) ? t.operator === 40 ? Br(t.operator) + t.operand.text : t.operand.text : void 0;
default:
return Y.assertNever(e);
}
}
function L0(e) {
switch (e.kind) {
case 79:
case 10:
case 14:
case 8:
return true;
default:
return false;
}
}
function kf(e) {
return js(e) ? qr(e) : e.text;
}
function b4(e) {
return js(e) ? e.escapedText : vi(e.text);
}
function _N(e) {
return `__@${getSymbolId(e)}@${e.escapedName}`;
}
function cN(e, t) {
return `__#${getSymbolId(e)}@${t}`;
}
function lN(e) {
return Pn(e.escapedName, "__@");
}
function uN(e) {
return Pn(e.escapedName, "__#");
}
function pN(e) {
return e.kind === 79 && e.escapedText === "Symbol";
}
function T4(e) {
return yt(e) ? qr(e) === "__proto__" : Gn(e) && e.text === "__proto__";
}
function H_(e, t) {
switch (e = $o(e), e.kind) {
case 228:
case 215:
if (e.name)
return false;
break;
case 216:
break;
default:
return false;
}
return typeof t == "function" ? t(e) : true;
}
function S4(e) {
switch (e.kind) {
case 299:
return !T4(e.name);
case 300:
return !!e.objectAssignmentInitializer;
case 257:
return yt(e.name) && !!e.initializer;
case 166:
return yt(e.name) && !!e.initializer && !e.dotDotDotToken;
case 205:
return yt(e.name) && !!e.initializer && !e.dotDotDotToken;
case 169:
return !!e.initializer;
case 223:
switch (e.operatorToken.kind) {
case 63:
case 76:
case 75:
case 77:
return yt(e.left);
}
break;
case 274:
return true;
}
return false;
}
function fN(e, t) {
if (!S4(e))
return false;
switch (e.kind) {
case 299:
return H_(e.initializer, t);
case 300:
return H_(e.objectAssignmentInitializer, t);
case 257:
case 166:
case 205:
case 169:
return H_(e.initializer, t);
case 223:
return H_(e.right, t);
case 274:
return H_(e.expression, t);
}
}
function dN(e) {
return e.escapedText === "push" || e.escapedText === "unshift";
}
function mN(e) {
return If(e).kind === 166;
}
function If(e) {
for (; e.kind === 205; )
e = e.parent.parent;
return e;
}
function hN(e) {
let t = e.kind;
return t === 173 || t === 215 || t === 259 || t === 216 || t === 171 || t === 174 || t === 175 || t === 264 || t === 308;
}
function fs2(e) {
return hs(e.pos) || hs(e.end);
}
function gN(e) {
return fl(e, wi) || e;
}
function yN(e) {
let t = R0(e), r = e.kind === 211 && e.arguments !== void 0;
return x4(e.kind, t, r);
}
function x4(e, t, r) {
switch (e) {
case 211:
return r ? 0 : 1;
case 221:
case 218:
case 219:
case 217:
case 220:
case 224:
case 226:
return 1;
case 223:
switch (t) {
case 42:
case 63:
case 64:
case 65:
case 67:
case 66:
case 68:
case 69:
case 70:
case 71:
case 72:
case 73:
case 78:
case 74:
case 75:
case 76:
case 77:
return 1;
}
}
return 0;
}
function vN(e) {
let t = R0(e), r = e.kind === 211 && e.arguments !== void 0;
return E4(e.kind, t, r);
}
function R0(e) {
return e.kind === 223 ? e.operatorToken.kind : e.kind === 221 || e.kind === 222 ? e.operator : e.kind;
}
function E4(e, t, r) {
switch (e) {
case 357:
return 0;
case 227:
return 1;
case 226:
return 2;
case 224:
return 4;
case 223:
switch (t) {
case 27:
return 0;
case 63:
case 64:
case 65:
case 67:
case 66:
case 68:
case 69:
case 70:
case 71:
case 72:
case 73:
case 78:
case 74:
case 75:
case 76:
case 77:
return 3;
default:
return Dl(t);
}
case 213:
case 232:
case 221:
case 218:
case 219:
case 217:
case 220:
return 16;
case 222:
return 17;
case 210:
return 18;
case 211:
return r ? 19 : 18;
case 212:
case 208:
case 209:
case 233:
return 19;
case 231:
case 235:
return 11;
case 108:
case 106:
case 79:
case 80:
case 104:
case 110:
case 95:
case 8:
case 9:
case 10:
case 206:
case 207:
case 215:
case 216:
case 228:
case 13:
case 14:
case 225:
case 214:
case 229:
case 281:
case 282:
case 285:
return 20;
default:
return -1;
}
}
function Dl(e) {
switch (e) {
case 60:
return 4;
case 56:
return 5;
case 55:
return 6;
case 51:
return 7;
case 52:
return 8;
case 50:
return 9;
case 34:
case 35:
case 36:
case 37:
return 10;
case 29:
case 31:
case 32:
case 33:
case 102:
case 101:
case 128:
case 150:
return 11;
case 47:
case 48:
case 49:
return 12;
case 39:
case 40:
return 13;
case 41:
case 43:
case 44:
return 14;
case 42:
return 15;
}
return -1;
}
function bN(e) {
return ee(e, (t) => {
switch (t.kind) {
case 291:
return !!t.expression;
case 11:
return !t.containsOnlyTriviaWhiteSpaces;
default:
return true;
}
});
}
function TN() {
let e = [], t = [], r = /* @__PURE__ */ new Map(), s = false;
return { add: x, lookup: f, getGlobalDiagnostics: w, getDiagnostics: A };
function f(g) {
let B;
if (g.file ? B = r.get(g.file.fileName) : B = e, !B)
return;
let N = Ya(B, g, rr, qf);
if (N >= 0)
return B[N];
}
function x(g) {
let B;
g.file ? (B = r.get(g.file.fileName), B || (B = [], r.set(g.file.fileName, B), Qn(t, g.file.fileName, ri))) : (s && (s = false, e = e.slice()), B = e), Qn(B, g, qf);
}
function w() {
return s = true, e;
}
function A(g) {
if (g)
return r.get(g) || [];
let B = ge(t, (N) => r.get(N));
return e.length && B.unshift(...e), B;
}
}
function SN(e) {
return e.replace(s8, "\\${");
}
function w4(e) {
return e && !!(k8(e) ? e.templateFlags : e.head.templateFlags || Ke(e.templateSpans, (t) => !!t.literal.templateFlags));
}
function C4(e) {
return "\\u" + ("0000" + e.toString(16).toUpperCase()).slice(-4);
}
function xN(e, t, r) {
if (e.charCodeAt(0) === 0) {
let s = r.charCodeAt(t + e.length);
return s >= 48 && s <= 57 ? "\\x00" : "\\0";
}
return l8.get(e) || C4(e.charCodeAt(0));
}
function Nf(e, t) {
let r = t === 96 ? c8 : t === 39 ? _8 : o8;
return e.replace(r, xN);
}
function Of(e, t) {
return e = Nf(e, t), Cv.test(e) ? e.replace(Cv, (r) => C4(r.charCodeAt(0))) : e;
}
function EN(e) {
return "" + e.toString(16).toUpperCase() + ";";
}
function wN(e) {
return e.charCodeAt(0) === 0 ? "" : f8.get(e) || EN(e.charCodeAt(0));
}
function A4(e, t) {
let r = t === 39 ? p8 : u8;
return e.replace(r, wN);
}
function CN(e) {
let t = e.length;
return t >= 2 && e.charCodeAt(0) === e.charCodeAt(t - 1) && AN(e.charCodeAt(0)) ? e.substring(1, t - 1) : e;
}
function AN(e) {
return e === 39 || e === 34 || e === 96;
}
function P4(e) {
let t = e.charCodeAt(0);
return t >= 97 && t <= 122 || Fi(e, "-") || Fi(e, ":");
}
function j0(e) {
let t = jo[1];
for (let r = jo.length; r <= e; r++)
jo.push(jo[r - 1] + t);
return jo[e];
}
function Oo() {
return jo[1].length;
}
function PN() {
return Fi(C, "-dev") || Fi(C, "-insiders");
}
function DN(e) {
var t, r, s, f, x, w = false;
function A(Se) {
let Ye = Kp(Se);
Ye.length > 1 ? (f = f + Ye.length - 1, x = t.length - Se.length + Zn(Ye), s = x - t.length === 0) : s = false;
}
function g(Se) {
Se && Se.length && (s && (Se = j0(r) + Se, s = false), t += Se, A(Se));
}
function B(Se) {
Se && (w = false), g(Se);
}
function N(Se) {
Se && (w = true), g(Se);
}
function X() {
t = "", r = 0, s = true, f = 0, x = 0, w = false;
}
function F(Se) {
Se !== void 0 && (t += Se, A(Se), w = false);
}
function $(Se) {
Se && Se.length && B(Se);
}
function ae(Se) {
(!s || Se) && (t += e, f++, x = t.length, s = true, w = false);
}
function Te() {
return s ? t.length : t.length + e.length;
}
return X(), { write: B, rawWrite: F, writeLiteral: $, writeLine: ae, increaseIndent: () => {
r++;
}, decreaseIndent: () => {
r--;
}, getIndent: () => r, getTextPos: () => t.length, getLine: () => f, getColumn: () => s ? r * Oo() : t.length - x, getText: () => t, isAtStartOfLine: () => s, hasTrailingComment: () => w, hasTrailingWhitespace: () => !!t.length && os(t.charCodeAt(t.length - 1)), clear: X, writeKeyword: B, writeOperator: B, writeParameter: B, writeProperty: B, writePunctuation: B, writeSpace: B, writeStringLiteral: B, writeSymbol: (Se, Ye) => B(Se), writeTrailingSemicolon: B, writeComment: N, getTextPosWithWriteLine: Te };
}
function kN(e) {
let t = false;
function r() {
t && (e.writeTrailingSemicolon(";"), t = false);
}
return Object.assign(Object.assign({}, e), {}, { writeTrailingSemicolon() {
t = true;
}, writeLiteral(s) {
r(), e.writeLiteral(s);
}, writeStringLiteral(s) {
r(), e.writeStringLiteral(s);
}, writeSymbol(s, f) {
r(), e.writeSymbol(s, f);
}, writePunctuation(s) {
r(), e.writePunctuation(s);
}, writeKeyword(s) {
r(), e.writeKeyword(s);
}, writeOperator(s) {
r(), e.writeOperator(s);
}, writeParameter(s) {
r(), e.writeParameter(s);
}, writeSpace(s) {
r(), e.writeSpace(s);
}, writeProperty(s) {
r(), e.writeProperty(s);
}, writeComment(s) {
r(), e.writeComment(s);
}, writeLine() {
r(), e.writeLine();
}, increaseIndent() {
r(), e.increaseIndent();
}, decreaseIndent() {
r(), e.decreaseIndent();
} });
}
function J0(e) {
return e.useCaseSensitiveFileNames ? e.useCaseSensitiveFileNames() : false;
}
function D4(e) {
return wp(J0(e));
}
function k4(e, t, r) {
return t.moduleName || F0(e, t.fileName, r && r.fileName);
}
function I4(e, t) {
return e.getCanonicalFileName(as(t, e.getCurrentDirectory()));
}
function IN(e, t, r) {
let s = t.getExternalModuleFileFromDeclaration(r);
if (!s || s.isDeclarationFile)
return;
let f = E0(r);
if (!(f && Ti(f) && !So(f.text) && I4(e, s.path).indexOf(I4(e, wo(e.getCommonSourceDirectory()))) === -1))
return k4(e, s);
}
function F0(e, t, r) {
let s = (g) => e.getCanonicalFileName(g), f = Ui(r ? ma(r) : e.getCommonSourceDirectory(), e.getCurrentDirectory(), s), x = as(t, e.getCurrentDirectory()), w = uy(f, x, f, s, false), A = Ll(w);
return r ? _y(A) : A;
}
function NN(e, t, r) {
let s = t.getCompilerOptions(), f;
return s.outDir ? f = Ll(M4(e, t, s.outDir)) : f = Ll(e), f + r;
}
function ON(e, t) {
return N4(e, t.getCompilerOptions(), t.getCurrentDirectory(), t.getCommonSourceDirectory(), (r) => t.getCanonicalFileName(r));
}
function N4(e, t, r, s, f) {
let x = t.declarationDir || t.outDir, w = x ? U0(e, x, r, s, f) : e, A = O4(w);
return Ll(w) + A;
}
function O4(e) {
return da(e, [".mjs", ".mts"]) ? ".d.mts" : da(e, [".cjs", ".cts"]) ? ".d.cts" : da(e, [".json"]) ? ".d.json.ts" : ".d.ts";
}
function MN(e) {
return da(e, [".d.mts", ".mjs", ".mts"]) ? [".mts", ".mjs"] : da(e, [".d.cts", ".cjs", ".cts"]) ? [".cts", ".cjs"] : da(e, [".d.json.ts"]) ? [".json"] : [".tsx", ".ts", ".jsx", ".js"];
}
function B0(e) {
return e.outFile || e.out;
}
function LN(e, t) {
var r, s;
if (e.paths)
return (s = e.baseUrl) != null ? s : Y.checkDefined(e.pathsBasePath || ((r = t.getCurrentDirectory) == null ? void 0 : r.call(t)), "Encountered 'paths' without a 'baseUrl', config file, or host 'getCurrentDirectory'.");
}
function RN(e, t, r) {
let s = e.getCompilerOptions();
if (B0(s)) {
let f = Ei(s), x = s.emitDeclarationOnly || f === 2 || f === 4;
return ee(e.getSourceFiles(), (w) => (x || !Qo(w)) && q0(w, e, r));
} else {
let f = t === void 0 ? e.getSourceFiles() : [t];
return ee(f, (x) => q0(x, e, r));
}
}
function q0(e, t, r) {
return !(t.getCompilerOptions().noEmitForJsFiles && y0(e)) && !e.isDeclarationFile && !t.isSourceFileFromExternalLibrary(e) && (r || !(a0(e) && t.getResolvedProjectReferenceToRedirect(e.fileName)) && !t.isSourceOfProjectReferenceRedirect(e.fileName));
}
function M4(e, t, r) {
return U0(e, r, t.getCurrentDirectory(), t.getCommonSourceDirectory(), (s) => t.getCanonicalFileName(s));
}
function U0(e, t, r, s, f) {
let x = as(e, r);
return x = f(x).indexOf(f(s)) === 0 ? x.substring(s.length) : x, tn(t, x);
}
function jN(e, t, r, s, f, x, w) {
e.writeFile(r, s, f, (A) => {
t.add(Ol(ve.Could_not_write_file_0_Colon_1, r, A));
}, x, w);
}
function L4(e, t, r) {
if (e.length > Bi(e) && !r(e)) {
let s = ma(e);
L4(s, t, r), t(e);
}
}
function JN(e, t, r, s, f, x) {
try {
s(e, t, r);
} catch {
L4(ma(Un(e)), f, x), s(e, t, r);
}
}
function FN(e, t) {
let r = ss(e);
return k_(r, t);
}
function ds(e, t) {
return k_(e, t);
}
function R4(e) {
return Ae(e.members, (t) => nc(t) && xl(t.body));
}
function z0(e) {
if (e && e.parameters.length > 0) {
let t = e.parameters.length === 2 && kl(e.parameters[0]);
return e.parameters[t ? 1 : 0];
}
}
function BN(e) {
let t = z0(e);
return t && t.type;
}
function j4(e) {
if (e.parameters.length && !iu(e)) {
let t = e.parameters[0];
if (kl(t))
return t;
}
}
function kl(e) {
return Mf(e.name);
}
function Mf(e) {
return !!e && e.kind === 79 && J4(e);
}
function qN(e) {
if (!Mf(e))
return false;
for (; rc(e.parent) && e.parent.left === e; )
e = e.parent;
return e.parent.kind === 183;
}
function J4(e) {
return e.escapedText === "this";
}
function W0(e, t) {
let r, s, f, x;
return v4(t) ? (r = t, t.kind === 174 ? f = t : t.kind === 175 ? x = t : Y.fail("Accessor has wrong kind")) : c(e, (w) => {
if (pf(w) && G0(w) === G0(t)) {
let A = Df(w.name), g = Df(t.name);
A === g && (r ? s || (s = w) : r = w, w.kind === 174 && !f && (f = w), w.kind === 175 && !x && (x = w));
}
}), { firstAccessor: r, secondAccessor: s, getAccessor: f, setAccessor: x };
}
function V0(e) {
if (!Pr(e) && Wo(e))
return;
let t = e.type;
return t || !Pr(e) ? t : Dy(e) ? e.typeExpression && e.typeExpression.type : cf(e);
}
function UN(e) {
return e.type;
}
function zN(e) {
return iu(e) ? e.type && e.type.typeExpression && e.type.typeExpression.type : e.type || (Pr(e) ? OS(e) : void 0);
}
function F4(e) {
return ne(hl(e), (t) => WN(t) ? t.typeParameters : void 0);
}
function WN(e) {
return Go(e) && !(e.parent.kind === 323 && (e.parent.tags.some(Cl) || e.parent.tags.some(y2)));
}
function VN(e) {
let t = z0(e);
return t && V0(t);
}
function B4(e, t, r, s) {
q4(e, t, r.pos, s);
}
function q4(e, t, r, s) {
s && s.length && r !== s[0].pos && ds(e, r) !== ds(e, s[0].pos) && t.writeLine();
}
function HN(e, t, r, s) {
r !== s && ds(e, r) !== ds(e, s) && t.writeLine();
}
function U4(e, t, r, s, f, x, w, A) {
if (s && s.length > 0) {
f && r.writeSpace(" ");
let g = false;
for (let B of s)
g && (r.writeSpace(" "), g = false), A(e, t, r, B.pos, B.end, w), B.hasTrailingNewLine ? r.writeLine() : g = true;
g && x && r.writeSpace(" ");
}
}
function GN(e, t, r, s, f, x, w) {
let A, g;
if (w ? f.pos === 0 && (A = ee(Ao(e, f.pos), B)) : A = Ao(e, f.pos), A) {
let N = [], X;
for (let F of A) {
if (X) {
let $ = ds(t, X.end);
if (ds(t, F.pos) >= $ + 2)
break;
}
N.push(F), X = F;
}
if (N.length) {
let F = ds(t, Zn(N).end);
ds(t, Ar(e, f.pos)) >= F + 2 && (B4(t, r, f, A), U4(e, t, r, N, false, true, x, s), g = { nodePos: f.pos, detachedCommentEndPos: Zn(N).end });
}
}
return g;
function B(N) {
return v3(e, N.pos);
}
}
function $N(e, t, r, s, f, x) {
if (e.charCodeAt(s + 1) === 42) {
let w = my(t, s), A = t.length, g;
for (let B = s, N = w.line; B < f; N++) {
let X = N + 1 === A ? e.length + 1 : t[N + 1];
if (B !== s) {
g === void 0 && (g = z4(e, t[w.line], s));
let $ = r.getIndent() * Oo() - g + z4(e, B, X);
if ($ > 0) {
let ae = $ % Oo(), Te = j0(($ - ae) / Oo());
for (r.rawWrite(Te); ae; )
r.rawWrite(" "), ae--;
} else
r.rawWrite("");
}
KN(e, f, r, x, B, X), B = X;
}
} else
r.writeComment(e.substring(s, f));
}
function KN(e, t, r, s, f, x) {
let w = Math.min(t, x - 1), A = Pp(e.substring(f, w));
A ? (r.writeComment(A), w !== t && r.writeLine()) : r.rawWrite(s);
}
function z4(e, t, r) {
let s = 0;
for (; t < r && N_(e.charCodeAt(t)); t++)
e.charCodeAt(t) === 9 ? s += Oo() - s % Oo() : s++;
return s;
}
function XN(e) {
return Rf(e) !== 0;
}
function YN(e) {
return X0(e) !== 0;
}
function H0(e, t) {
return !!G4(e, t);
}
function rn(e, t) {
return !!$4(e, t);
}
function G0(e) {
return Js(e) && Lf(e) || Hl(e);
}
function Lf(e) {
return rn(e, 32);
}
function QN(e) {
return H0(e, 16384);
}
function W4(e) {
return rn(e, 256);
}
function V4(e) {
return rn(e, 2);
}
function H4(e) {
return rn(e, 128);
}
function $0(e) {
return H0(e, 64);
}
function Il(e) {
return rn(e, 131072);
}
function G4(e, t) {
return Rf(e) & t;
}
function $4(e, t) {
return X0(e) & t;
}
function K0(e, t, r) {
return e.kind >= 0 && e.kind <= 162 ? 0 : (e.modifierFlagsCache & 536870912 || (e.modifierFlagsCache = Y0(e) | 536870912), t && !(e.modifierFlagsCache & 4096) && (r || Pr(e)) && e.parent && (e.modifierFlagsCache |= X4(e) | 4096), e.modifierFlagsCache & -536875009);
}
function Rf(e) {
return K0(e, true);
}
function K4(e) {
return K0(e, true, true);
}
function X0(e) {
return K0(e, false);
}
function X4(e) {
let t = 0;
return e.parent && !Vs(e) && (Pr(e) && (CS(e) && (t |= 4), AS(e) && (t |= 8), PS(e) && (t |= 16), DS(e) && (t |= 64), kS(e) && (t |= 16384)), IS(e) && (t |= 8192)), t;
}
function Y4(e) {
return Y0(e) | X4(e);
}
function Y0(e) {
let t = fc(e) ? Vn(e.modifiers) : 0;
return (e.flags & 4 || e.kind === 79 && e.flags & 2048) && (t |= 1), t;
}
function Vn(e) {
let t = 0;
if (e)
for (let r of e)
t |= Q0(r.kind);
return t;
}
function Q0(e) {
switch (e) {
case 124:
return 32;
case 123:
return 4;
case 122:
return 16;
case 121:
return 8;
case 126:
return 256;
case 127:
return 128;
case 93:
return 1;
case 136:
return 2;
case 85:
return 2048;
case 88:
return 1024;
case 132:
return 512;
case 146:
return 64;
case 161:
return 16384;
case 101:
return 32768;
case 145:
return 65536;
case 167:
return 131072;
}
return 0;
}
function Q4(e) {
return e === 56 || e === 55;
}
function ZN(e) {
return Q4(e) || e === 53;
}
function jf(e) {
return e === 75 || e === 76 || e === 77;
}
function eO(e) {
return ur(e) && jf(e.operatorToken.kind);
}
function Z4(e) {
return Q4(e) || e === 60;
}
function tO(e) {
return ur(e) && Z4(e.operatorToken.kind);
}
function G_(e) {
return e >= 63 && e <= 78;
}
function ex(e) {
let t = tx(e);
return t && !t.isImplements ? t.class : void 0;
}
function tx(e) {
if (e2(e)) {
if (ru(e.parent) && bi(e.parent.parent))
return { class: e.parent.parent, isImplements: e.parent.token === 117 };
if (md(e.parent)) {
let t = A0(e.parent);
if (t && bi(t))
return { class: t, isImplements: false };
}
}
}
function ms(e, t) {
return ur(e) && (t ? e.operatorToken.kind === 63 : G_(e.operatorToken.kind)) && Do(e.left);
}
function rO(e) {
return ms(e.parent) && e.parent.left === e;
}
function nO(e) {
if (ms(e, true)) {
let t = e.left.kind;
return t === 207 || t === 206;
}
return false;
}
function Z0(e) {
return ex(e) !== void 0;
}
function Bs(e) {
return e.kind === 79 || rx(e);
}
function iO(e) {
switch (e.kind) {
case 79:
return e;
case 163:
do
e = e.left;
while (e.kind !== 79);
return e;
case 208:
do
e = e.expression;
while (e.kind !== 79);
return e;
}
}
function ev(e) {
return e.kind === 79 || e.kind === 108 || e.kind === 106 || e.kind === 233 || e.kind === 208 && ev(e.expression) || e.kind === 214 && ev(e.expression);
}
function rx(e) {
return bn(e) && yt(e.name) && Bs(e.expression);
}
function tv(e) {
if (bn(e)) {
let t = tv(e.expression);
if (t !== void 0)
return t + "." + ls(e.name);
} else if (gs(e)) {
let t = tv(e.expression);
if (t !== void 0 && vl(e.argumentExpression))
return t + "." + Df(e.argumentExpression);
} else if (yt(e))
return dl(e.escapedText);
}
function Nl(e) {
return W_(e) && Fs(e) === "prototype";
}
function aO(e) {
return e.parent.kind === 163 && e.parent.right === e || e.parent.kind === 208 && e.parent.name === e;
}
function nx(e) {
return bn(e.parent) && e.parent.name === e || gs(e.parent) && e.parent.argumentExpression === e;
}
function sO(e) {
return rc(e.parent) && e.parent.right === e || bn(e.parent) && e.parent.name === e || uc(e.parent) && e.parent.right === e;
}
function oO(e) {
return e.kind === 207 && e.properties.length === 0;
}
function _O(e) {
return e.kind === 206 && e.elements.length === 0;
}
function cO(e) {
if (!(!lO(e) || !e.declarations)) {
for (let t of e.declarations)
if (t.localSymbol)
return t.localSymbol;
}
}
function lO(e) {
return e && I(e.declarations) > 0 && rn(e.declarations[0], 1024);
}
function uO(e) {
return Ae(y8, (t) => ns(e, t));
}
function pO(e) {
let t = [], r = e.length;
for (let s = 0; s < r; s++) {
let f = e.charCodeAt(s);
f < 128 ? t.push(f) : f < 2048 ? (t.push(f >> 6 | 192), t.push(f & 63 | 128)) : f < 65536 ? (t.push(f >> 12 | 224), t.push(f >> 6 & 63 | 128), t.push(f & 63 | 128)) : f < 131072 ? (t.push(f >> 18 | 240), t.push(f >> 12 & 63 | 128), t.push(f >> 6 & 63 | 128), t.push(f & 63 | 128)) : Y.assert(false, "Unexpected code point");
}
return t;
}
function ix(e) {
let t = "", r = pO(e), s = 0, f = r.length, x, w, A, g;
for (; s < f; )
x = r[s] >> 2, w = (r[s] & 3) << 4 | r[s + 1] >> 4, A = (r[s + 1] & 15) << 2 | r[s + 2] >> 6, g = r[s + 2] & 63, s + 1 >= f ? A = g = 64 : s + 2 >= f && (g = 64), t += xa.charAt(x) + xa.charAt(w) + xa.charAt(A) + xa.charAt(g), s += 3;
return t;
}
function fO(e) {
let t = "", r = 0, s = e.length;
for (; r < s; ) {
let f = e[r];
if (f < 128)
t += String.fromCharCode(f), r++;
else if ((f & 192) === 192) {
let x = f & 63;
r++;
let w = e[r];
for (; (w & 192) === 128; )
x = x << 6 | w & 63, r++, w = e[r];
t += String.fromCharCode(x);
} else
t += String.fromCharCode(f), r++;
}
return t;
}
function dO(e, t) {
return e && e.base64encode ? e.base64encode(t) : ix(t);
}
function mO(e, t) {
if (e && e.base64decode)
return e.base64decode(t);
let r = t.length, s = [], f = 0;
for (; f < r && t.charCodeAt(f) !== xa.charCodeAt(64); ) {
let x = xa.indexOf(t[f]), w = xa.indexOf(t[f + 1]), A = xa.indexOf(t[f + 2]), g = xa.indexOf(t[f + 3]), B = (x & 63) << 2 | w >> 4 & 3, N = (w & 15) << 4 | A >> 2 & 15, X = (A & 3) << 6 | g & 63;
N === 0 && A !== 0 ? s.push(B) : X === 0 && g !== 0 ? s.push(B, N) : s.push(B, N, X), f += 4;
}
return fO(s);
}
function ax(e, t) {
let r = Ji(t) ? t : t.readFile(e);
if (!r)
return;
let s = parseConfigFileTextToJson(e, r);
return s.error ? void 0 : s.config;
}
function hO(e, t) {
return ax(e, t) || {};
}
function sx(e, t) {
return !t.directoryExists || t.directoryExists(e);
}
function ox(e) {
switch (e.newLine) {
case 0:
return d8;
case 1:
case void 0:
return m8;
}
}
function Jf(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : e;
return Y.assert(t >= e || t === -1), { pos: e, end: t };
}
function gO(e, t) {
return Jf(e.pos, t);
}
function Ff(e, t) {
return Jf(t, e.end);
}
function _x(e) {
let t = fc(e) ? te(e.modifiers, zl) : void 0;
return t && !hs(t.end) ? Ff(e, t.end) : e;
}
function yO(e) {
if (Bo(e) || Vl(e))
return Ff(e, e.name.pos);
let t = fc(e) ? Cn(e.modifiers) : void 0;
return t && !hs(t.end) ? Ff(e, t.end) : _x(e);
}
function vO(e) {
return e.pos === e.end;
}
function bO(e, t) {
return Jf(e, e + Br(t).length);
}
function TO(e, t) {
return cx(e, e, t);
}
function SO(e, t, r) {
return $_(K_(e, r, false), K_(t, r, false), r);
}
function xO(e, t, r) {
return $_(e.end, t.end, r);
}
function cx(e, t, r) {
return $_(K_(e, r, false), t.end, r);
}
function EO(e, t, r) {
return $_(e.end, K_(t, r, false), r);
}
function wO(e, t, r, s) {
let f = K_(t, r, s);
return I_(r, e.end, f);
}
function CO(e, t, r) {
return I_(r, e.end, t.end);
}
function AO(e, t) {
return !$_(e.pos, e.end, t);
}
function $_(e, t, r) {
return I_(r, e, t) === 0;
}
function K_(e, t, r) {
return hs(e.pos) ? -1 : Ar(t.text, e.pos, false, r);
}
function PO(e, t, r, s) {
let f = Ar(r.text, e, false, s), x = kO(f, t, r);
return I_(r, x != null ? x : t, f);
}
function DO(e, t, r, s) {
let f = Ar(r.text, e, false, s);
return I_(r, e, Math.min(t, f));
}
function kO(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 0, r = arguments.length > 2 ? arguments[2] : void 0;
for (; e-- > t; )
if (!os(r.text.charCodeAt(e)))
return e;
}
function IO(e) {
let t = fl(e);
if (t)
switch (t.parent.kind) {
case 263:
case 264:
return t === t.parent.name;
}
return false;
}
function NO(e) {
return ee(e.declarations, lx);
}
function lx(e) {
return Vi(e) && e.initializer !== void 0;
}
function OO(e) {
return e.watch && Jr(e, "watch");
}
function MO(e) {
e.close();
}
function ux(e) {
return e.flags & 33554432 ? e.links.checkFlags : 0;
}
function LO(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
if (e.valueDeclaration) {
let r = t && e.declarations && Ae(e.declarations, ic) || e.flags & 32768 && Ae(e.declarations, Gl) || e.valueDeclaration, s = ef(r);
return e.parent && e.parent.flags & 32 ? s : s & -29;
}
if (ux(e) & 6) {
let r = e.links.checkFlags, s = r & 1024 ? 8 : r & 256 ? 4 : 16, f = r & 2048 ? 32 : 0;
return s | f;
}
return e.flags & 4194304 ? 36 : 0;
}
function RO(e, t) {
return e.flags & 2097152 ? t.getAliasedSymbol(e) : e;
}
function jO(e) {
return e.exportSymbol ? e.exportSymbol.flags | e.flags : e.flags;
}
function JO(e) {
return Mo(e) === 1;
}
function FO(e) {
return Mo(e) !== 0;
}
function Mo(e) {
let { parent: t } = e;
if (!t)
return 0;
switch (t.kind) {
case 214:
return Mo(t);
case 222:
case 221:
let { operator: s } = t;
return s === 45 || s === 46 ? r() : 0;
case 223:
let { left: f, operatorToken: x } = t;
return f === e && G_(x.kind) ? x.kind === 63 ? 1 : r() : 0;
case 208:
return t.name !== e ? 0 : Mo(t);
case 299: {
let w = Mo(t.parent);
return e === t.name ? BO(w) : w;
}
case 300:
return e === t.objectAssignmentInitializer ? 0 : Mo(t.parent);
case 206:
return Mo(t);
default:
return 0;
}
function r() {
return t.parent && D0(t.parent).kind === 241 ? 1 : 2;
}
}
function BO(e) {
switch (e) {
case 0:
return 1;
case 1:
return 0;
case 2:
return 2;
default:
return Y.assertNever(e);
}
}
function px(e, t) {
if (!e || !t || Object.keys(e).length !== Object.keys(t).length)
return false;
for (let r in e)
if (typeof e[r] == "object") {
if (!px(e[r], t[r]))
return false;
} else if (typeof e[r] != "function" && e[r] !== t[r])
return false;
return true;
}
function qO(e, t) {
e.forEach(t), e.clear();
}
function fx(e, t, r) {
let { onDeleteValue: s, onExistingValue: f } = r;
e.forEach((x, w) => {
let A = t.get(w);
A === void 0 ? (e.delete(w), s(x, w)) : f && f(x, A, w);
});
}
function UO(e, t, r) {
fx(e, t, r);
let { createNewValue: s } = r;
t.forEach((f, x) => {
e.has(x) || e.set(x, s(x, f));
});
}
function zO(e) {
if (e.flags & 32) {
let t = dx(e);
return !!t && rn(t, 256);
}
return false;
}
function dx(e) {
var t;
return (t = e.declarations) == null ? void 0 : t.find(bi);
}
function Bf(e) {
return e.flags & 3899393 ? e.objectFlags : 0;
}
function WO(e, t) {
return !!FT(e, (r) => t(r) ? true : void 0);
}
function VO(e) {
return !!e && !!e.declarations && !!e.declarations[0] && a2(e.declarations[0]);
}
function HO(e) {
let { moduleSpecifier: t } = e;
return Gn(t) ? t.text : gf(t);
}
function mx(e) {
let t;
return xr(e, (r) => {
xl(r) && (t = r);
}, (r) => {
for (let s = r.length - 1; s >= 0; s--)
if (xl(r[s])) {
t = r[s];
break;
}
}), t;
}
function GO(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : true;
return e.has(t) ? false : (e.set(t, r), true);
}
function $O(e) {
return bi(e) || eu(e) || id(e);
}
function hx(e) {
return e >= 179 && e <= 202 || e === 131 || e === 157 || e === 148 || e === 160 || e === 149 || e === 134 || e === 152 || e === 153 || e === 114 || e === 155 || e === 144 || e === 139 || e === 230 || e === 315 || e === 316 || e === 317 || e === 318 || e === 319 || e === 320 || e === 321;
}
function Lo(e) {
return e.kind === 208 || e.kind === 209;
}
function KO(e) {
return e.kind === 208 ? e.name : (Y.assert(e.kind === 209), e.argumentExpression);
}
function XO(e) {
switch (e.kind) {
case "text":
case "internal":
return true;
default:
return false;
}
}
function YO(e) {
return e.kind === 272 || e.kind === 276;
}
function rv(e) {
for (; Lo(e); )
e = e.expression;
return e;
}
function QO(e, t) {
if (Lo(e.parent) && nx(e))
return r(e.parent);
function r(s) {
if (s.kind === 208) {
let f = t(s.name);
if (f !== void 0)
return f;
} else if (s.kind === 209)
if (yt(s.argumentExpression) || Ti(s.argumentExpression)) {
let f = t(s.argumentExpression);
if (f !== void 0)
return f;
} else
return;
if (Lo(s.expression))
return r(s.expression);
if (yt(s.expression))
return t(s.expression);
}
}
function ZO(e, t) {
for (; ; ) {
switch (e.kind) {
case 222:
e = e.operand;
continue;
case 223:
e = e.left;
continue;
case 224:
e = e.condition;
continue;
case 212:
e = e.tag;
continue;
case 210:
if (t)
return e;
case 231:
case 209:
case 208:
case 232:
case 356:
case 235:
e = e.expression;
continue;
}
return e;
}
}
function eM(e, t) {
this.flags = e, this.escapedName = t, this.declarations = void 0, this.valueDeclaration = void 0, this.id = 0, this.mergeId = 0, this.parent = void 0, this.members = void 0, this.exports = void 0, this.exportSymbol = void 0, this.constEnumOnlyModule = void 0, this.isReferenced = void 0, this.isAssigned = void 0, this.links = void 0;
}
function tM(e, t) {
this.flags = t, (Y.isDebugging || rs) && (this.checker = e);
}
function rM(e, t) {
this.flags = t, Y.isDebugging && (this.checker = e);
}
function nv(e, t, r) {
this.pos = t, this.end = r, this.kind = e, this.id = 0, this.flags = 0, this.modifierFlagsCache = 0, this.transformFlags = 0, this.parent = void 0, this.original = void 0, this.emitNode = void 0;
}
function nM(e, t, r) {
this.pos = t, this.end = r, this.kind = e, this.id = 0, this.flags = 0, this.transformFlags = 0, this.parent = void 0, this.emitNode = void 0;
}
function iM(e, t, r) {
this.pos = t, this.end = r, this.kind = e, this.id = 0, this.flags = 0, this.transformFlags = 0, this.parent = void 0, this.original = void 0, this.emitNode = void 0;
}
function aM(e, t, r) {
this.fileName = e, this.text = t, this.skipTrivia = r || ((s) => s);
}
function sM(e) {
Av.push(e), e(lr);
}
function gx(e) {
Object.assign(lr, e), c(Av, (t) => t(lr));
}
function X_(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : 0;
return e.replace(/{(\d+)}/g, (s, f) => "" + Y.checkDefined(t[+f + r]));
}
function yx(e) {
jl = e;
}
function vx(e) {
!jl && e && (jl = e());
}
function Y_(e) {
return jl && jl[e.key] || e.message;
}
function Ro(e, t, r, s) {
t0(void 0, t, r);
let f = Y_(s);
return arguments.length > 4 && (f = X_(f, arguments, 4)), { file: void 0, start: t, length: r, messageText: f, category: s.category, code: s.code, reportsUnnecessary: s.reportsUnnecessary, fileName: e };
}
function oM(e) {
return e.file === void 0 && e.start !== void 0 && e.length !== void 0 && typeof e.fileName == "string";
}
function bx(e, t) {
let r = t.fileName || "", s = t.text.length;
Y.assertEqual(e.fileName, r), Y.assertLessThanOrEqual(e.start, s), Y.assertLessThanOrEqual(e.start + e.length, s);
let f = { file: t, start: e.start, length: e.length, messageText: e.messageText, category: e.category, code: e.code, reportsUnnecessary: e.reportsUnnecessary };
if (e.relatedInformation) {
f.relatedInformation = [];
for (let x of e.relatedInformation)
oM(x) && x.fileName === r ? (Y.assertLessThanOrEqual(x.start, s), Y.assertLessThanOrEqual(x.start + x.length, s), f.relatedInformation.push(bx(x, t))) : f.relatedInformation.push(x);
}
return f;
}
function qs(e, t) {
let r = [];
for (let s of e)
r.push(bx(s, t));
return r;
}
function iv(e, t, r, s) {
t0(e, t, r);
let f = Y_(s);
return arguments.length > 4 && (f = X_(f, arguments, 4)), { file: e, start: t, length: r, messageText: f, category: s.category, code: s.code, reportsUnnecessary: s.reportsUnnecessary, reportsDeprecated: s.reportsDeprecated };
}
function _M(e, t) {
let r = Y_(t);
return arguments.length > 2 && (r = X_(r, arguments, 2)), r;
}
function Ol(e) {
let t = Y_(e);
return arguments.length > 1 && (t = X_(t, arguments, 1)), { file: void 0, start: void 0, length: void 0, messageText: t, category: e.category, code: e.code, reportsUnnecessary: e.reportsUnnecessary, reportsDeprecated: e.reportsDeprecated };
}
function cM(e, t) {
return { file: void 0, start: void 0, length: void 0, code: e.code, category: e.category, messageText: e.next ? e : e.messageText, relatedInformation: t };
}
function lM(e, t) {
let r = Y_(t);
return arguments.length > 2 && (r = X_(r, arguments, 2)), { messageText: r, category: t.category, code: t.code, next: e === void 0 || Array.isArray(e) ? e : [e] };
}
function uM(e, t) {
let r = e;
for (; r.next; )
r = r.next[0];
r.next = [t];
}
function Tx(e) {
return e.file ? e.file.path : void 0;
}
function av(e, t) {
return qf(e, t) || pM(e, t) || 0;
}
function qf(e, t) {
return ri(Tx(e), Tx(t)) || Vr(e.start, t.start) || Vr(e.length, t.length) || Vr(e.code, t.code) || Sx(e.messageText, t.messageText) || 0;
}
function pM(e, t) {
return !e.relatedInformation && !t.relatedInformation ? 0 : e.relatedInformation && t.relatedInformation ? Vr(e.relatedInformation.length, t.relatedInformation.length) || c(e.relatedInformation, (r, s) => {
let f = t.relatedInformation[s];
return av(r, f);
}) || 0 : e.relatedInformation ? -1 : 1;
}
function Sx(e, t) {
if (typeof e == "string" && typeof t == "string")
return ri(e, t);
if (typeof e == "string")
return -1;
if (typeof t == "string")
return 1;
let r = ri(e.messageText, t.messageText);
if (r)
return r;
if (!e.next && !t.next)
return 0;
if (!e.next)
return -1;
if (!t.next)
return 1;
let s = Math.min(e.next.length, t.next.length);
for (let f = 0; f < s; f++)
if (r = Sx(e.next[f], t.next[f]), r)
return r;
return e.next.length < t.next.length ? -1 : e.next.length > t.next.length ? 1 : 0;
}
function sv(e) {
return e === 4 || e === 2 || e === 1 || e === 6 ? 1 : 0;
}
function xx(e) {
if (e.transformFlags & 2)
return _3(e) || pd(e) ? e : xr(e, xx);
}
function fM(e) {
return e.isDeclarationFile ? void 0 : xx(e);
}
function dM(e) {
return (e.impliedNodeFormat === 99 || da(e.fileName, [".cjs", ".cts", ".mjs", ".mts"])) && !e.isDeclarationFile ? true : void 0;
}
function Ex(e) {
switch (wx(e)) {
case 3:
return (f) => {
f.externalModuleIndicator = ou(f) || !f.isDeclarationFile || void 0;
};
case 1:
return (f) => {
f.externalModuleIndicator = ou(f);
};
case 2:
let t = [ou];
(e.jsx === 4 || e.jsx === 5) && t.push(fM), t.push(dM);
let r = W1(...t);
return (f) => void (f.externalModuleIndicator = r(f));
}
}
function Uf(e) {
var t;
return (t = e.target) != null ? t : e.module === 100 && 9 || e.module === 199 && 99 || 1;
}
function Ei(e) {
return typeof e.module == "number" ? e.module : Uf(e) >= 2 ? 5 : 1;
}
function mM(e) {
return e >= 5 && e <= 99;
}
function Ml(e) {
let t = e.moduleResolution;
if (t === void 0)
switch (Ei(e)) {
case 1:
t = 2;
break;
case 100:
t = 3;
break;
case 199:
t = 99;
break;
default:
t = 1;
break;
}
return t;
}
function wx(e) {
return e.moduleDetection || (Ei(e) === 100 || Ei(e) === 199 ? 3 : 2);
}
function hM(e) {
switch (Ei(e)) {
case 1:
case 2:
case 5:
case 6:
case 7:
case 99:
case 100:
case 199:
return true;
default:
return false;
}
}
function zf(e) {
return !!(e.isolatedModules || e.verbatimModuleSyntax);
}
function gM(e) {
return e.verbatimModuleSyntax || e.isolatedModules && e.preserveValueImports;
}
function yM(e) {
return e.allowUnreachableCode === false;
}
function vM(e) {
return e.allowUnusedLabels === false;
}
function bM(e) {
return !!(cv(e) && e.declarationMap);
}
function ov(e) {
if (e.esModuleInterop !== void 0)
return e.esModuleInterop;
switch (Ei(e)) {
case 100:
case 199:
return true;
}
}
function TM(e) {
return e.allowSyntheticDefaultImports !== void 0 ? e.allowSyntheticDefaultImports : ov(e) || Ei(e) === 4 || Ml(e) === 100;
}
function _v(e) {
return e >= 3 && e <= 99 || e === 100;
}
function SM(e) {
let t = Ml(e);
if (!_v(t))
return false;
if (e.resolvePackageJsonExports !== void 0)
return e.resolvePackageJsonExports;
switch (t) {
case 3:
case 99:
case 100:
return true;
}
return false;
}
function xM(e) {
let t = Ml(e);
if (!_v(t))
return false;
if (e.resolvePackageJsonExports !== void 0)
return e.resolvePackageJsonExports;
switch (t) {
case 3:
case 99:
case 100:
return true;
}
return false;
}
function Cx(e) {
return e.resolveJsonModule !== void 0 ? e.resolveJsonModule : Ml(e) === 100;
}
function cv(e) {
return !!(e.declaration || e.composite);
}
function EM(e) {
return !!(e.preserveConstEnums || zf(e));
}
function wM(e) {
return !!(e.incremental || e.composite);
}
function lv(e, t) {
return e[t] === void 0 ? !!e.strict : !!e[t];
}
function Ax(e) {
return e.allowJs === void 0 ? !!e.checkJs : e.allowJs;
}
function CM(e) {
return e.useDefineForClassFields === void 0 ? Uf(e) >= 9 : e.useDefineForClassFields;
}
function AM(e, t) {
return J_(t, e, semanticDiagnosticsOptionDeclarations);
}
function PM(e, t) {
return J_(t, e, affectsEmitOptionDeclarations);
}
function DM(e, t) {
return J_(t, e, affectsDeclarationPathOptionDeclarations);
}
function uv(e, t) {
return t.strictFlag ? lv(e, t.name) : e[t.name];
}
function kM(e) {
let t = e.jsx;
return t === 2 || t === 4 || t === 5;
}
function IM(e, t) {
let r = t == null ? void 0 : t.pragmas.get("jsximportsource"), s = ir(r) ? r[r.length - 1] : r;
return e.jsx === 4 || e.jsx === 5 || e.jsxImportSource || s ? (s == null ? void 0 : s.arguments.factory) || e.jsxImportSource || "react" : void 0;
}
function NM(e, t) {
return e ? `${e}/${t.jsx === 5 ? "jsx-dev-runtime" : "jsx-runtime"}` : void 0;
}
function OM(e) {
let t = false;
for (let r = 0; r < e.length; r++)
if (e.charCodeAt(r) === 42)
if (!t)
t = true;
else
return false;
return true;
}
function MM(e, t) {
let r, s, f, x = false;
return { getSymlinkedFiles: () => f, getSymlinkedDirectories: () => r, getSymlinkedDirectoriesByRealpath: () => s, setSymlinkedFile: (A, g) => (f || (f = /* @__PURE__ */ new Map())).set(A, g), setSymlinkedDirectory: (A, g) => {
let B = Ui(A, e, t);
Hx(B) || (B = wo(B), g !== false && !(r != null && r.has(B)) && (s || (s = Be())).add(wo(g.realPath), A), (r || (r = /* @__PURE__ */ new Map())).set(B, g));
}, setSymlinksFromResolutions(A, g) {
var B, N;
Y.assert(!x), x = true;
for (let X of A)
(B = X.resolvedModules) == null || B.forEach((F) => w(this, F.resolvedModule)), (N = X.resolvedTypeReferenceDirectiveNames) == null || N.forEach((F) => w(this, F.resolvedTypeReferenceDirective));
g.forEach((X) => w(this, X.resolvedTypeReferenceDirective));
}, hasProcessedResolutions: () => x };
function w(A, g) {
if (!g || !g.originalPath || !g.resolvedFileName)
return;
let { resolvedFileName: B, originalPath: N } = g;
A.setSymlinkedFile(Ui(N, e, t), B);
let [X, F] = LM(B, N, e, t) || Bt;
X && F && A.setSymlinkedDirectory(F, { real: X, realPath: Ui(X, e, t) });
}
}
function LM(e, t, r, s) {
let f = qi(as(e, r)), x = qi(as(t, r)), w = false;
for (; f.length >= 2 && x.length >= 2 && !Px(f[f.length - 2], s) && !Px(x[x.length - 2], s) && s(f[f.length - 1]) === s(x[x.length - 1]); )
f.pop(), x.pop(), w = true;
return w ? [xo(f), xo(x)] : void 0;
}
function Px(e, t) {
return e !== void 0 && (t(e) === "node_modules" || Pn(e, "@"));
}
function RM(e) {
return ay(e.charCodeAt(0)) ? e.slice(1) : void 0;
}
function jM(e, t, r) {
let s = ST(e, t, r);
return s === void 0 ? void 0 : RM(s);
}
function JM(e) {
return e.replace(Xf, FM);
}
function FM(e) {
return "\\" + e;
}
function Wf(e, t, r) {
let s = pv(e, t, r);
return !s || !s.length ? void 0 : `^(${s.map((w) => `(${w})`).join("|")})${r === "exclude" ? "($|/)" : "$"}`;
}
function pv(e, t, r) {
if (!(e === void 0 || e.length === 0))
return ne(e, (s) => s && kx(s, t, r, Nv[r]));
}
function Dx(e) {
return !/[.*?]/.test(e);
}
function BM(e, t, r) {
let s = e && kx(e, t, r, Nv[r]);
return s && `^(${s})${r === "exclude" ? "($|/)" : "$"}`;
}
function kx(e, t, r, s) {
let { singleAsteriskRegexFragment: f, doubleAsteriskRegexFragment: x, replaceWildcardCharacter: w } = s, A = "", g = false, B = $p(e, t), N = Zn(B);
if (r !== "exclude" && N === "**")
return;
B[0] = P_(B[0]), Dx(N) && B.push("**", "*");
let X = 0;
for (let F of B) {
if (F === "**")
A += x;
else if (r === "directories" && (A += "(", X++), g && (A += zn), r !== "exclude") {
let $ = "";
F.charCodeAt(0) === 42 ? ($ += "([^./]" + f + ")?", F = F.substr(1)) : F.charCodeAt(0) === 63 && ($ += "[^./]", F = F.substr(1)), $ += F.replace(Xf, w), $ !== F && (A += Yf), A += $;
} else
A += F.replace(Xf, w);
g = true;
}
for (; X > 0; )
A += ")?", X--;
return A;
}
function fv(e, t) {
return e === "*" ? t : e === "?" ? "[^/]" : "\\" + e;
}
function Ix(e, t, r, s, f) {
e = Un(e), f = Un(f);
let x = tn(f, e);
return { includeFilePatterns: Ze(pv(r, x, "files"), (w) => `^${w}$`), includeFilePattern: Wf(r, x, "files"), includeDirectoryPattern: Wf(r, x, "directories"), excludePattern: Wf(t, x, "exclude"), basePaths: UM(e, r, s) };
}
function Vf(e, t) {
return new RegExp(e, t ? "" : "i");
}
function qM(e, t, r, s, f, x, w, A, g) {
e = Un(e), x = Un(x);
let B = Ix(e, r, s, f, x), N = B.includeFilePatterns && B.includeFilePatterns.map((Ye) => Vf(Ye, f)), X = B.includeDirectoryPattern && Vf(B.includeDirectoryPattern, f), F = B.excludePattern && Vf(B.excludePattern, f), $ = N ? N.map(() => []) : [[]], ae = /* @__PURE__ */ new Map(), Te = wp(f);
for (let Ye of B.basePaths)
Se(Ye, tn(x, Ye), w);
return ct($);
function Se(Ye, Ne, oe) {
let Ve = Te(g(Ne));
if (ae.has(Ve))
return;
ae.set(Ve, true);
let { files: pt, directories: Gt } = A(Ye);
for (let Nt of Is(pt, ri)) {
let Xt = tn(Ye, Nt), er = tn(Ne, Nt);
if (!(t && !da(Xt, t)) && !(F && F.test(er)))
if (!N)
$[0].push(Xt);
else {
let Tn = he(N, (Hr) => Hr.test(er));
Tn !== -1 && $[Tn].push(Xt);
}
}
if (!(oe !== void 0 && (oe--, oe === 0)))
for (let Nt of Is(Gt, ri)) {
let Xt = tn(Ye, Nt), er = tn(Ne, Nt);
(!X || X.test(er)) && (!F || !F.test(er)) && Se(Xt, er, oe);
}
}
}
function UM(e, t, r) {
let s = [e];
if (t) {
let f = [];
for (let x of t) {
let w = A_(x) ? x : Un(tn(e, x));
f.push(zM(w));
}
f.sort(rl(!r));
for (let x of f)
me(s, (w) => !jT(w, x, e, !r)) && s.push(x);
}
return s;
}
function zM(e) {
let t = Je(e, h8);
return t < 0 ? OT(e) ? P_(ma(e)) : e : e.substring(0, e.lastIndexOf(zn, t));
}
function Nx(e, t) {
return t || Ox(e) || 3;
}
function Ox(e) {
switch (e.substr(e.lastIndexOf(".")).toLowerCase()) {
case ".js":
case ".cjs":
case ".mjs":
return 1;
case ".jsx":
return 2;
case ".ts":
case ".cts":
case ".mts":
return 3;
case ".tsx":
return 4;
case ".json":
return 6;
default:
return 0;
}
}
function Mx(e, t) {
let r = e && Ax(e);
if (!t || t.length === 0)
return r ? Jl : Jo;
let s = r ? Jl : Jo, f = ct(s);
return [...s, ...qt(t, (w) => w.scriptKind === 7 || r && WM(w.scriptKind) && f.indexOf(w.extension) === -1 ? [w.extension] : void 0)];
}
function Lx(e, t) {
return !e || !Cx(e) ? t : t === Jl ? v8 : t === Jo ? g8 : [...t, [".json"]];
}
function WM(e) {
return e === 1 || e === 2;
}
function dv(e) {
return Ke(Lv, (t) => ns(e, t));
}
function mv(e) {
return Ke(Ov, (t) => ns(e, t));
}
function Rx(e) {
let { imports: t } = e, r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : W1(dv, mv);
return q(t, (s) => {
let { text: f } = s;
return So(f) ? r(f) : void 0;
}) || false;
}
function VM(e, t, r, s) {
if (e === "js" || t === 99)
return shouldAllowImportingTsExtension(r) && f() !== 2 ? 3 : 2;
if (e === "minimal")
return 0;
if (e === "index")
return 1;
if (!shouldAllowImportingTsExtension(r))
return Rx(s) ? 2 : 0;
return f();
function f() {
let x = false, w = s.imports.length ? s.imports.map((A) => A.text) : y0(s) ? HM(s).map((A) => A.arguments[0].text) : Bt;
for (let A of w)
if (So(A)) {
if (mv(A))
return 3;
dv(A) && (x = true);
}
return x ? 2 : 0;
}
}
function HM(e) {
let t = 0, r;
for (let s of e.statements) {
if (t > 3)
break;
W3(s) ? r = Ft(r, s.declarationList.declarations.map((f) => f.initializer)) : Zl(s) && El(s.expression, true) ? r = tr(r, s.expression) : t++;
}
return r || Bt;
}
function GM(e, t, r) {
if (!e)
return false;
let s = Mx(t, r);
for (let f of ct(Lx(t, s)))
if (ns(e, f))
return true;
return false;
}
function jx(e) {
let t = e.match(/\//g);
return t ? t.length : 0;
}
function $M(e, t) {
return Vr(jx(e), jx(t));
}
function Ll(e) {
for (let t of Qf) {
let r = Jx(e, t);
if (r !== void 0)
return r;
}
return e;
}
function Jx(e, t) {
return ns(e, t) ? Fx(e, t) : void 0;
}
function Fx(e, t) {
return e.substring(0, e.length - t.length);
}
function KM(e, t) {
return RT(e, t, Qf, false);
}
function Bx(e) {
let t = e.indexOf("*");
return t === -1 ? e : e.indexOf("*", t + 1) !== -1 ? void 0 : { prefix: e.substr(0, t), suffix: e.substr(t + 1) };
}
function XM(e) {
return qt(ho(e), (t) => Bx(t));
}
function hs(e) {
return !(e >= 0);
}
function qx(e) {
return e === ".ts" || e === ".tsx" || e === ".d.ts" || e === ".cts" || e === ".mts" || e === ".d.mts" || e === ".d.cts" || Pn(e, ".d.") && es(e, ".ts");
}
function YM(e) {
return qx(e) || e === ".json";
}
function QM(e) {
let t = hv(e);
return t !== void 0 ? t : Y.fail(`File ${e} has unknown extension.`);
}
function ZM(e) {
return hv(e) !== void 0;
}
function hv(e) {
return Ae(Qf, (t) => ns(e, t));
}
function eL(e, t) {
return e.checkJsDirective ? e.checkJsDirective.enabled : t.checkJs;
}
function tL(e, t) {
let r = [];
for (let s of e) {
if (s === t)
return t;
Ji(s) || r.push(s);
}
return TT(r, (s) => s, t);
}
function rL(e, t) {
let r = e.indexOf(t);
return Y.assert(r !== -1), e.slice(r);
}
function Rl(e) {
for (var t = arguments.length, r = new Array(t > 1 ? t - 1 : 0), s = 1; s < t; s++)
r[s - 1] = arguments[s];
return r.length && (e.relatedInformation || (e.relatedInformation = []), Y.assert(e.relatedInformation !== Bt, "Diagnostic had empty array singleton for related info, but is still being constructed!"), e.relatedInformation.push(...r)), e;
}
function nL(e, t) {
Y.assert(e.length !== 0);
let r = t(e[0]), s = r;
for (let f = 1; f < e.length; f++) {
let x = t(e[f]);
x < r ? r = x : x > s && (s = x);
}
return { min: r, max: s };
}
function iL(e) {
return { pos: Io(e), end: e.end };
}
function aL(e, t) {
let r = t.pos - 1, s = Math.min(e.text.length, Ar(e.text, t.end) + 1);
return { pos: r, end: s };
}
function sL(e, t, r) {
return t.skipLibCheck && e.isDeclarationFile || t.skipDefaultLibCheck && e.hasNoDefaultLib || r.isSourceOfProjectReferenceRedirect(e.fileName);
}
function gv(e, t) {
return e === t || typeof e == "object" && e !== null && typeof t == "object" && t !== null && S_(e, t, gv);
}
function Hf(e) {
let t;
switch (e.charCodeAt(1)) {
case 98:
case 66:
t = 1;
break;
case 111:
case 79:
t = 3;
break;
case 120:
case 88:
t = 4;
break;
default:
let B = e.length - 1, N = 0;
for (; e.charCodeAt(N) === 48; )
N++;
return e.slice(N, B) || "0";
}
let r = 2, s = e.length - 1, f = (s - r) * t, x = new Uint16Array((f >>> 4) + (f & 15 ? 1 : 0));
for (let B = s - 1, N = 0; B >= r; B--, N += t) {
let X = N >>> 4, F = e.charCodeAt(B), ae = (F <= 57 ? F - 48 : 10 + F - (F <= 70 ? 65 : 97)) << (N & 15);
x[X] |= ae;
let Te = ae >>> 16;
Te && (x[X + 1] |= Te);
}
let w = "", A = x.length - 1, g = true;
for (; g; ) {
let B = 0;
g = false;
for (let N = A; N >= 0; N--) {
let X = B << 16 | x[N], F = X / 10 | 0;
x[N] = F, B = X - F * 10, F && !g && (A = N, g = true);
}
w = B + w;
}
return w;
}
function yv(e) {
let { negative: t, base10Value: r } = e;
return (t && r !== "0" ? "-" : "") + r;
}
function oL(e) {
if (zx(e, false))
return Ux(e);
}
function Ux(e) {
let t = e.startsWith("-"), r = Hf(`${t ? e.slice(1) : e}n`);
return { negative: t, base10Value: r };
}
function zx(e, t) {
if (e === "")
return false;
let r = Po(99, false), s = true;
r.setOnError(() => s = false), r.setText(e + "n");
let f = r.scan(), x = f === 40;
x && (f = r.scan());
let w = r.getTokenFlags();
return s && f === 9 && r.getTextPos() === e.length + 1 && !(w & 512) && (!t || e === yv({ negative: x, base10Value: Hf(r.getTokenValue()) }));
}
function _L(e) {
return !!(e.flags & 16777216) || F3(e) || uL(e) || lL(e) || !(g0(e) || cL(e));
}
function cL(e) {
return yt(e) && nu(e.parent) && e.parent.name === e;
}
function lL(e) {
for (; e.kind === 79 || e.kind === 208; )
e = e.parent;
if (e.kind !== 164)
return false;
if (rn(e.parent, 256))
return true;
let t = e.parent.parent.kind;
return t === 261 || t === 184;
}
function uL(e) {
if (e.kind !== 79)
return false;
let t = zi(e.parent, (r) => {
switch (r.kind) {
case 294:
return true;
case 208:
case 230:
return false;
default:
return "quit";
}
});
return (t == null ? void 0 : t.token) === 117 || (t == null ? void 0 : t.parent.kind) === 261;
}
function pL(e) {
return ac(e) && yt(e.typeName);
}
function fL(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fa;
if (e.length < 2)
return true;
let r = e[0];
for (let s = 1, f = e.length; s < f; s++) {
let x = e[s];
if (!t(r, x))
return false;
}
return true;
}
function Gf(e, t) {
return e.pos = t, e;
}
function Wx(e, t) {
return e.end = t, e;
}
function Us(e, t, r) {
return Wx(Gf(e, t), r);
}
function $f(e, t, r) {
return Us(e, t, t + r);
}
function dL(e, t) {
return e && (e.flags = t), e;
}
function Sa(e, t) {
return e && t && (e.parent = t), e;
}
function Q_(e, t) {
if (e)
for (let r of e)
Sa(r, t);
return e;
}
function Vx(e, t) {
if (!e)
return e;
return D2(e, Uy(e) ? r : f), e;
function r(x, w) {
if (t && x.parent === w)
return "skip";
Sa(x, w);
}
function s(x) {
if (ya(x))
for (let w of x.jsDoc)
r(w, x), D2(w, r);
}
function f(x, w) {
return r(x, w) || s(x);
}
}
function mL(e) {
return !cd(e);
}
function hL(e) {
return Yl(e) && me(e.elements, mL);
}
function gL(e) {
for (Y.assertIsDefined(e.parent); ; ) {
let t = e.parent;
if (qo(t)) {
e = t;
continue;
}
if (Zl(t) || Qv(t) || eE(t) && (t.initializer === e || t.incrementor === e))
return true;
if (oc(t)) {
if (e !== Zn(t.elements))
return true;
e = t;
continue;
}
if (ur(t) && t.operatorToken.kind === 27) {
if (e === t.left)
return true;
e = t;
continue;
}
return false;
}
}
function Hx(e) {
return Ke(ignoredPaths, (t) => Fi(e, t));
}
function yL(e) {
if (!e.parent)
return;
switch (e.kind) {
case 165:
let { parent: r } = e;
return r.kind === 192 ? void 0 : r.typeParameters;
case 166:
return e.parent.parameters;
case 201:
return e.parent.templateSpans;
case 236:
return e.parent.templateSpans;
case 167: {
let { parent: s } = e;
return ME(s) ? s.modifiers : void 0;
}
case 294:
return e.parent.heritageClauses;
}
let { parent: t } = e;
if (zy(e))
return f2(e.parent) ? void 0 : e.parent.tags;
switch (t.kind) {
case 184:
case 261:
return Ry(e) ? t.members : void 0;
case 189:
case 190:
return t.types;
case 186:
case 206:
case 357:
case 272:
case 276:
return t.elements;
case 207:
case 289:
return t.properties;
case 210:
case 211:
return Jy(e) ? t.typeArguments : t.expression === e ? void 0 : t.arguments;
case 281:
case 285:
return o3(e) ? t.children : void 0;
case 283:
case 282:
return Jy(e) ? t.typeArguments : void 0;
case 238:
case 292:
case 293:
case 265:
return t.statements;
case 266:
return t.clauses;
case 260:
case 228:
return Js(e) ? t.members : void 0;
case 263:
return cE(e) ? t.members : void 0;
case 308:
return t.statements;
}
}
function vL(e) {
if (!e.typeParameters) {
if (Ke(e.parameters, (t) => !V0(t)))
return true;
if (e.kind !== 216) {
let t = pa(e.parameters);
if (!(t && kl(t)))
return true;
}
}
return false;
}
function bL(e) {
return e === "Infinity" || e === "-Infinity" || e === "NaN";
}
function Gx(e) {
return e.kind === 257 && e.parent.kind === 295;
}
function TL(e) {
let t = e.valueDeclaration && If(e.valueDeclaration);
return !!t && (Vs(t) || Gx(t));
}
function SL(e) {
return e.kind === 215 || e.kind === 216;
}
function xL(e) {
return e.replace(/\$/gm, () => "\\$");
}
function $x(e) {
return (+e).toString() === e;
}
function EL(e, t, r, s) {
return vy(e, t) ? si.createIdentifier(e) : !s && $x(e) && +e >= 0 ? si.createNumericLiteral(+e) : si.createStringLiteral(e, !!r);
}
function Kx(e) {
return !!(e.flags & 262144 && e.isThisType);
}
function wL(e) {
let t = 0, r = 0, s = 0, f = 0, x;
((B) => {
B[B.BeforeNodeModules = 0] = "BeforeNodeModules", B[B.NodeModules = 1] = "NodeModules", B[B.Scope = 2] = "Scope", B[B.PackageContent = 3] = "PackageContent";
})(x || (x = {}));
let w = 0, A = 0, g = 0;
for (; A >= 0; )
switch (w = A, A = e.indexOf("/", w + 1), g) {
case 0:
e.indexOf(nodeModulesPathPart, w) === w && (t = w, r = A, g = 1);
break;
case 1:
case 2:
g === 1 && e.charAt(w + 1) === "@" ? g = 2 : (s = A, g = 3);
break;
case 3:
e.indexOf(nodeModulesPathPart, w) === w ? g = 1 : g = 3;
break;
}
return f = w, g > 1 ? { topLevelNodeModulesIndex: t, topLevelPackageNameIndex: r, packageRootIndex: s, fileNameIndex: f } : void 0;
}
function CL(e) {
var t;
return e.kind === 344 ? (t = e.typeExpression) == null ? void 0 : t.type : e.type;
}
function Xx(e) {
switch (e.kind) {
case 165:
case 260:
case 261:
case 262:
case 263:
case 349:
case 341:
case 343:
return true;
case 270:
return e.isTypeOnly;
case 273:
case 278:
return e.parent.parent.isTypeOnly;
default:
return false;
}
}
function AL(e) {
return i2(e) || zo(e) || Wo(e) || _c(e) || eu(e) || Xx(e) || Ea(e) && !Xy(e) && !vf(e);
}
function Yx(e) {
if (!Dy(e))
return false;
let { isBracketed: t, typeExpression: r } = e;
return t || !!r && r.type.kind === 319;
}
function PL(e, t) {
if (e.length === 0)
return false;
let r = e.charCodeAt(0);
return r === 35 ? e.length > 1 && Wn(e.charCodeAt(1), t) : Wn(r, t);
}
function Qx(e) {
var t;
return ((t = getSnippetElement(e)) == null ? void 0 : t.kind) === 0;
}
function Zx(e) {
return Pr(e) && (e.type && e.type.kind === 319 || of(e).some((t) => {
let { isBracketed: r, typeExpression: s } = t;
return r || !!s && s.type.kind === 319;
}));
}
function DL(e) {
switch (e.kind) {
case 169:
case 168:
return !!e.questionToken;
case 166:
return !!e.questionToken || Zx(e);
case 351:
case 344:
return Yx(e);
default:
return false;
}
}
function kL(e) {
let t = e.kind;
return (t === 208 || t === 209) && Uo(e.expression);
}
function IL(e) {
return Pr(e) && qo(e) && ya(e) && !!wy(e);
}
function NL(e) {
return Y.checkDefined(e8(e));
}
function e8(e) {
let t = wy(e);
return t && t.typeExpression && t.typeExpression.type;
}
var t8, Kf, r8, n8, Z_, vv, bv, i8, Tv, a8, Sv, xv, Ev, wv, s8, o8, _8, c8, l8, Cv, u8, p8, f8, jo, xa, d8, m8, lr, Av, jl, Xf, h8, Pv, Yf, Dv, kv, Iv, Nv, Jo, Ov, g8, y8, Mv, Lv, Jl, v8, Rv, b8, jv, Qf, T8, OL = D({ "src/compiler/utilities.ts"() {
"use strict";
nn(), t8 = [], Kf = "tslib", r8 = 160, n8 = 1e6, Z_ = _D(), vv = ((e) => (e[e.None = 0] = "None", e[e.NeverAsciiEscape = 1] = "NeverAsciiEscape", e[e.JsxAttributeEscape = 2] = "JsxAttributeEscape", e[e.TerminateUnterminatedLiterals = 4] = "TerminateUnterminatedLiterals", e[e.AllowNumericSeparator = 8] = "AllowNumericSeparator", e))(vv || {}), bv = /^(\/\/\/\s* /, i8 = /^(\/\/\/\s* /, Tv = /^(\/\/\/\s* /, a8 = /^(\/\/\/\s* /, Sv = ((e) => (e[e.None = 0] = "None", e[e.Definite = 1] = "Definite", e[e.Compound = 2] = "Compound", e))(Sv || {}), xv = ((e) => (e[e.Normal = 0] = "Normal", e[e.Generator = 1] = "Generator", e[e.Async = 2] = "Async", e[e.Invalid = 4] = "Invalid", e[e.AsyncGenerator = 3] = "AsyncGenerator", e))(xv || {}), Ev = ((e) => (e[e.Left = 0] = "Left", e[e.Right = 1] = "Right", e))(Ev || {}), wv = ((e) => (e[e.Comma = 0] = "Comma", e[e.Spread = 1] = "Spread", e[e.Yield = 2] = "Yield", e[e.Assignment = 3] = "Assignment", e[e.Conditional = 4] = "Conditional", e[e.Coalesce = 4] = "Coalesce", e[e.LogicalOR = 5] = "LogicalOR", e[e.LogicalAND = 6] = "LogicalAND", e[e.BitwiseOR = 7] = "BitwiseOR", e[e.BitwiseXOR = 8] = "BitwiseXOR", e[e.BitwiseAND = 9] = "BitwiseAND", e[e.Equality = 10] = "Equality", e[e.Relational = 11] = "Relational", e[e.Shift = 12] = "Shift", e[e.Additive = 13] = "Additive", e[e.Multiplicative = 14] = "Multiplicative", e[e.Exponentiation = 15] = "Exponentiation", e[e.Unary = 16] = "Unary", e[e.Update = 17] = "Update", e[e.LeftHandSide = 18] = "LeftHandSide", e[e.Member = 19] = "Member", e[e.Primary = 20] = "Primary", e[e.Highest = 20] = "Highest", e[e.Lowest = 0] = "Lowest", e[e.Invalid = -1] = "Invalid", e))(wv || {}), s8 = /\$\{/g, o8 = /[\\\"\u0000-\u001f\t\v\f\b\r\n\u2028\u2029\u0085]/g, _8 = /[\\\'\u0000-\u001f\t\v\f\b\r\n\u2028\u2029\u0085]/g, c8 = /\r\n|[\\\`\u0000-\u001f\t\v\f\b\r\u2028\u2029\u0085]/g, l8 = new Map(Object.entries({ " ": "\\t", "\v": "\\v", "\f": "\\f", "\b": "\\b", "\r": "\\r", "\n": "\\n", "\\": "\\\\", '"': '\\"', "'": "\\'", "`": "\\`", "\u2028": "\\u2028", "\u2029": "\\u2029", "\x85": "\\u0085", "\r\n": "\\r\\n" })), Cv = /[^\u0000-\u007F]/g, u8 = /[\"\u0000-\u001f\u2028\u2029\u0085]/g, p8 = /[\'\u0000-\u001f\u2028\u2029\u0085]/g, f8 = new Map(Object.entries({ '"': """, "'": "'" })), jo = ["", " "], xa = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=", d8 = `\r
`, m8 = `
`, lr = { getNodeConstructor: () => nv, getTokenConstructor: () => nM, getIdentifierConstructor: () => iM, getPrivateIdentifierConstructor: () => nv, getSourceFileConstructor: () => nv, getSymbolConstructor: () => eM, getTypeConstructor: () => tM, getSignatureConstructor: () => rM, getSourceMapSourceConstructor: () => aM }, Av = [], Xf = /[^\w\s\/]/g, h8 = [42, 63], Pv = ["node_modules", "bower_components", "jspm_packages"], Yf = `(?!(${Pv.join("|")})(/|$))`, Dv = { singleAsteriskRegexFragment: "([^./]|(\\.(?!min\\.js$))?)*", doubleAsteriskRegexFragment: `(/${Yf}[^/.][^/]*)*?`, replaceWildcardCharacter: (e) => fv(e, Dv.singleAsteriskRegexFragment) }, kv = { singleAsteriskRegexFragment: "[^/]*", doubleAsteriskRegexFragment: `(/${Yf}[^/.][^/]*)*?`, replaceWildcardCharacter: (e) => fv(e, kv.singleAsteriskRegexFragment) }, Iv = { singleAsteriskRegexFragment: "[^/]*", doubleAsteriskRegexFragment: "(/.+?)?", replaceWildcardCharacter: (e) => fv(e, Iv.singleAsteriskRegexFragment) }, Nv = { files: Dv, directories: kv, exclude: Iv }, Jo = [[".ts", ".tsx", ".d.ts"], [".cts", ".d.cts"], [".mts", ".d.mts"]], Ov = ct(Jo), g8 = [...Jo, [".json"]], y8 = [".d.ts", ".d.cts", ".d.mts", ".cts", ".mts", ".ts", ".tsx", ".cts", ".mts"], Mv = [[".js", ".jsx"], [".mjs"], [".cjs"]], Lv = ct(Mv), Jl = [[".ts", ".tsx", ".d.ts", ".js", ".jsx"], [".cts", ".d.cts", ".cjs"], [".mts", ".d.mts", ".mjs"]], v8 = [...Jl, [".json"]], Rv = [".d.ts", ".d.cts", ".d.mts"], b8 = [".ts", ".cts", ".mts", ".tsx"], jv = ((e) => (e[e.Minimal = 0] = "Minimal", e[e.Index = 1] = "Index", e[e.JsExtension = 2] = "JsExtension", e[e.TsExtension = 3] = "TsExtension", e))(jv || {}), Qf = [".d.ts", ".d.mts", ".d.cts", ".mjs", ".mts", ".cjs", ".cts", ".ts", ".js", ".tsx", ".jsx", ".json"], T8 = { files: Bt, directories: Bt };
} });
function S8() {
let e, t, r, s, f;
return { createBaseSourceFileNode: x, createBaseIdentifierNode: w, createBasePrivateIdentifierNode: A, createBaseTokenNode: g, createBaseNode: B };
function x(N) {
return new (f || (f = lr.getSourceFileConstructor()))(N, -1, -1);
}
function w(N) {
return new (r || (r = lr.getIdentifierConstructor()))(N, -1, -1);
}
function A(N) {
return new (s || (s = lr.getPrivateIdentifierConstructor()))(N, -1, -1);
}
function g(N) {
return new (t || (t = lr.getTokenConstructor()))(N, -1, -1);
}
function B(N) {
return new (e || (e = lr.getNodeConstructor()))(N, -1, -1);
}
}
var ML = D({ "src/compiler/factory/baseNodeFactory.ts"() {
"use strict";
nn();
} }), Jv, LL = D({ "src/compiler/factory/parenthesizerRules.ts"() {
"use strict";
nn(), Jv = { getParenthesizeLeftSideOfBinaryForOperator: (e) => rr, getParenthesizeRightSideOfBinaryForOperator: (e) => rr, parenthesizeLeftSideOfBinary: (e, t) => t, parenthesizeRightSideOfBinary: (e, t, r) => r, parenthesizeExpressionOfComputedPropertyName: rr, parenthesizeConditionOfConditionalExpression: rr, parenthesizeBranchOfConditionalExpression: rr, parenthesizeExpressionOfExportDefault: rr, parenthesizeExpressionOfNew: (e) => ti(e, Do), parenthesizeLeftSideOfAccess: (e) => ti(e, Do), parenthesizeOperandOfPostfixUnary: (e) => ti(e, Do), parenthesizeOperandOfPrefixUnary: (e) => ti(e, t3), parenthesizeExpressionsOfCommaDelimitedList: (e) => ti(e, _s), parenthesizeExpressionForDisallowedComma: rr, parenthesizeExpressionOfExpressionStatement: rr, parenthesizeConciseBodyOfArrowFunction: rr, parenthesizeCheckTypeOfConditionalType: rr, parenthesizeExtendsTypeOfConditionalType: rr, parenthesizeConstituentTypesOfUnionType: (e) => ti(e, _s), parenthesizeConstituentTypeOfUnionType: rr, parenthesizeConstituentTypesOfIntersectionType: (e) => ti(e, _s), parenthesizeConstituentTypeOfIntersectionType: rr, parenthesizeOperandOfTypeOperator: rr, parenthesizeOperandOfReadonlyTypeOperator: rr, parenthesizeNonArrayTypeOfPostfixType: rr, parenthesizeElementTypesOfTupleType: (e) => ti(e, _s), parenthesizeElementTypeOfTupleType: rr, parenthesizeTypeOfOptionalType: rr, parenthesizeTypeArguments: (e) => e && ti(e, _s), parenthesizeLeadingTypeArgument: rr };
} }), RL = () => {
}, x8 = () => new Proxy({}, { get: () => () => {
} });
function jL(e) {
Bv.push(e);
}
function Zf(e, t) {
let r = e & 8 ? JL : FL, s = tl(() => e & 1 ? Jv : createParenthesizerRules(Ye)), f = tl(() => e & 2 ? nullNodeConverters : x8(Ye)), x = An((n9) => (o, l) => xu(o, n9, l)), w = An((n9) => (o) => Tu(n9, o)), A = An((n9) => (o) => Su(o, n9)), g = An((n9) => () => db(n9)), B = An((n9) => (o) => Ac(n9, o)), N = An((n9) => (o, l) => mb(n9, o, l)), X = An((n9) => (o, l) => Km(n9, o, l)), F = An((n9) => (o, l) => Xm(n9, o, l)), $ = An((n9) => (o, l) => ph(n9, o, l)), ae = An((n9) => (o, l, p) => Cb(n9, o, l, p)), Te = An((n9) => (o, l, p) => fh(n9, o, l, p)), Se = An((n9) => (o, l, p, k) => Ab(n9, o, l, p, k)), Ye = { get parenthesizer() {
return s();
}, get converters() {
return f();
}, baseFactory: t, flags: e, createNodeArray: Ne, createNumericLiteral: Gt, createBigIntLiteral: Nt, createStringLiteral: er, createStringLiteralFromNode: Tn, createRegularExpressionLiteral: Hr, createLiteralLikeNode: Gi, createIdentifier: Ut, createTempVariable: kn, createLoopVariable: an, createUniqueName: mr, getGeneratedNameForNode: $i, createPrivateIdentifier: Ur, createUniquePrivateName: _r, getGeneratedPrivateNameForNode: Sn, createToken: pr, createSuper: Zt, createThis: Or, createNull: Nn, createTrue: ar, createFalse: oi, createModifier: cr, createModifiersFromModifierFlags: $r, createQualifiedName: hr, updateQualifiedName: On, createComputedPropertyName: nr, updateComputedPropertyName: br, createTypeParameterDeclaration: Kr, updateTypeParameterDeclaration: wa, createParameterDeclaration: $n, updateParameterDeclaration: Ki, createDecorator: Mn, updateDecorator: _i, createPropertySignature: Ca, updatePropertySignature: St, createPropertyDeclaration: He, updatePropertyDeclaration: _t, createMethodSignature: ft, updateMethodSignature: Kt, createMethodDeclaration: zt, updateMethodDeclaration: xe, createConstructorDeclaration: Mt, updateConstructorDeclaration: It, createGetAccessorDeclaration: gr, updateGetAccessorDeclaration: Ln, createSetAccessorDeclaration: ci, updateSetAccessorDeclaration: Xi, createCallSignature: vs, updateCallSignature: $s, createConstructSignature: li, updateConstructSignature: Yi, createIndexSignature: Qi, updateIndexSignature: bs, createClassStaticBlockDeclaration: Re, updateClassStaticBlockDeclaration: ot, createTemplateLiteralTypeSpan: Ai, updateTemplateLiteralTypeSpan: xn, createKeywordTypeNode: Dt, createTypePredicateNode: Pi, updateTypePredicateNode: Z, createTypeReferenceNode: ie, updateTypeReferenceNode: U, createFunctionTypeNode: L, updateFunctionTypeNode: fe, createConstructorTypeNode: it, updateConstructorTypeNode: Ge, createTypeQueryNode: Yt, updateTypeQueryNode: $t, createTypeLiteralNode: Wt, updateTypeLiteralNode: Xr, createArrayTypeNode: Dr, updateArrayTypeNode: Lr, createTupleTypeNode: yr, updateTupleTypeNode: Rn, createNamedTupleMember: wt, updateNamedTupleMember: Tr, createOptionalTypeNode: Tt, updateOptionalTypeNode: kt, createRestTypeNode: de, updateRestTypeNode: jn, createUnionTypeNode: e_, updateUnionTypeNode: mc, createIntersectionTypeNode: Da, updateIntersectionTypeNode: Ts, createConditionalTypeNode: Ot, updateConditionalTypeNode: dr, createInferTypeNode: Dd, updateInferTypeNode: ea, createImportTypeNode: Id, updateImportTypeNode: ka, createParenthesizedType: t_, updateParenthesizedType: En, createThisTypeNode: Er, createTypeOperatorNode: Q, updateTypeOperatorNode: Jn, createIndexedAccessTypeNode: Ia, updateIndexedAccessTypeNode: Ss, createMappedTypeNode: hc, updateMappedTypeNode: wr, createLiteralTypeNode: zr, updateLiteralTypeNode: xs, createTemplateLiteralType: kd, updateTemplateLiteralType: sn, createObjectBindingPattern: Nd, updateObjectBindingPattern: R2, createArrayBindingPattern: Es, updateArrayBindingPattern: j2, createBindingElement: gc, updateBindingElement: Ks, createArrayLiteralExpression: uu, updateArrayLiteralExpression: Od, createObjectLiteralExpression: r_, updateObjectLiteralExpression: J2, createPropertyAccessExpression: e & 4 ? (n9, o) => setEmitFlags(ta(n9, o), 262144) : ta, updatePropertyAccessExpression: Ld, createPropertyAccessChain: e & 4 ? (n9, o, l) => setEmitFlags(Xs(n9, o, l), 262144) : Xs, updatePropertyAccessChain: Rd, createElementAccessExpression: pu, updateElementAccessExpression: F2, createElementAccessChain: fu, updateElementAccessChain: jd, createCallExpression: Na, updateCallExpression: B2, createCallChain: du, updateCallChain: Kn, createNewExpression: vc, updateNewExpression: mu, createTaggedTemplateExpression: hu, updateTaggedTemplateExpression: q2, createTypeAssertion: Fd, updateTypeAssertion: Bd, createParenthesizedExpression: gu, updateParenthesizedExpression: qd, createFunctionExpression: yu, updateFunctionExpression: Ud, createArrowFunction: vu, updateArrowFunction: zd, createDeleteExpression: bu, updateDeleteExpression: U2, createTypeOfExpression: mn, updateTypeOfExpression: z2, createVoidExpression: ui, updateVoidExpression: W2, createAwaitExpression: Oa, updateAwaitExpression: Ys, createPrefixUnaryExpression: Tu, updatePrefixUnaryExpression: bc, createPostfixUnaryExpression: Su, updatePostfixUnaryExpression: Wd, createBinaryExpression: xu, updateBinaryExpression: V2, createConditionalExpression: Eu, updateConditionalExpression: H2, createTemplateExpression: Di, updateTemplateExpression: Hd, createTemplateHead: Sc, createTemplateMiddle: Cu, createTemplateTail: G2, createNoSubstitutionTemplateLiteral: $d, createTemplateLiteralLikeNode: Qs, createYieldExpression: Kd, updateYieldExpression: $2, createSpreadElement: Xd, updateSpreadElement: K2, createClassExpression: Yd, updateClassExpression: xc, createOmittedExpression: X2, createExpressionWithTypeArguments: Qd, updateExpressionWithTypeArguments: Xn, createAsExpression: Ec, updateAsExpression: Zd, createNonNullExpression: em, updateNonNullExpression: Au, createSatisfiesExpression: tm, updateSatisfiesExpression: Pu, createNonNullChain: pi, updateNonNullChain: rm, createMetaProperty: wc, updateMetaProperty: ra, createTemplateSpan: i_, updateTemplateSpan: nm, createSemicolonClassElement: im, createBlock: Zs, updateBlock: am, createVariableStatement: sm, updateVariableStatement: om, createEmptyStatement: Du, createExpressionStatement: a_, updateExpressionStatement: Y2, createIfStatement: ku, updateIfStatement: Q2, createDoStatement: Iu, updateDoStatement: Z2, createWhileStatement: _m, updateWhileStatement: eb, createForStatement: Nu, updateForStatement: cm, createForInStatement: lm, updateForInStatement: tb, createForOfStatement: um, updateForOfStatement: rb, createContinueStatement: pm, updateContinueStatement: fm, createBreakStatement: Ou, updateBreakStatement: dm, createReturnStatement: mm, updateReturnStatement: nb, createWithStatement: Mu, updateWithStatement: hm, createSwitchStatement: Lu, updateSwitchStatement: eo, createLabeledStatement: gm, updateLabeledStatement: ym, createThrowStatement: vm, updateThrowStatement: ib, createTryStatement: bm, updateTryStatement: ab, createDebuggerStatement: Tm, createVariableDeclaration: Cc, updateVariableDeclaration: Sm, createVariableDeclarationList: Ru, updateVariableDeclarationList: sb, createFunctionDeclaration: xm, updateFunctionDeclaration: ju, createClassDeclaration: Em, updateClassDeclaration: Ju, createInterfaceDeclaration: wm, updateInterfaceDeclaration: Cm, createTypeAliasDeclaration: sr, updateTypeAliasDeclaration: Ma, createEnumDeclaration: Fu, updateEnumDeclaration: La, createModuleDeclaration: Am, updateModuleDeclaration: Sr, createModuleBlock: Ra, updateModuleBlock: Yr, createCaseBlock: Pm, updateCaseBlock: _b, createNamespaceExportDeclaration: Dm, updateNamespaceExportDeclaration: km, createImportEqualsDeclaration: Im, updateImportEqualsDeclaration: Nm, createImportDeclaration: Om, updateImportDeclaration: Mm, createImportClause: Lm, updateImportClause: Rm, createAssertClause: Bu, updateAssertClause: lb, createAssertEntry: s_, updateAssertEntry: jm, createImportTypeAssertionContainer: qu, updateImportTypeAssertionContainer: Jm, createNamespaceImport: Fm, updateNamespaceImport: Uu, createNamespaceExport: Bm, updateNamespaceExport: qm, createNamedImports: Um, updateNamedImports: ub, createImportSpecifier: zm, updateImportSpecifier: pb, createExportAssignment: zu, updateExportAssignment: Wu, createExportDeclaration: na, updateExportDeclaration: Wm, createNamedExports: to, updateNamedExports: Hm, createExportSpecifier: Vu, updateExportSpecifier: o_, createMissingDeclaration: fb, createExternalModuleReference: Gm, updateExternalModuleReference: $m, get createJSDocAllType() {
return g(315);
}, get createJSDocUnknownType() {
return g(316);
}, get createJSDocNonNullableType() {
return X(318);
}, get updateJSDocNonNullableType() {
return F(318);
}, get createJSDocNullableType() {
return X(317);
}, get updateJSDocNullableType() {
return F(317);
}, get createJSDocOptionalType() {
return B(319);
}, get updateJSDocOptionalType() {
return N(319);
}, get createJSDocVariadicType() {
return B(321);
}, get updateJSDocVariadicType() {
return N(321);
}, get createJSDocNamepathType() {
return B(322);
}, get updateJSDocNamepathType() {
return N(322);
}, createJSDocFunctionType: Ym, updateJSDocFunctionType: hb, createJSDocTypeLiteral: Qm, updateJSDocTypeLiteral: gb, createJSDocTypeExpression: Zm, updateJSDocTypeExpression: yb, createJSDocSignature: eh, updateJSDocSignature: Hu, createJSDocTemplateTag: __, updateJSDocTemplateTag: Gu, createJSDocTypedefTag: $u, updateJSDocTypedefTag: th, createJSDocParameterTag: Pc, updateJSDocParameterTag: vb, createJSDocPropertyTag: Ku, updateJSDocPropertyTag: bb, createJSDocCallbackTag: rh, updateJSDocCallbackTag: nh, createJSDocOverloadTag: ih, updateJSDocOverloadTag: ah, createJSDocAugmentsTag: sh, updateJSDocAugmentsTag: Xu, createJSDocImplementsTag: Yu, updateJSDocImplementsTag: wb, createJSDocSeeTag: ro, updateJSDocSeeTag: Tb, createJSDocNameReference: ws, updateJSDocNameReference: Dc, createJSDocMemberName: oh, updateJSDocMemberName: Sb, createJSDocLink: _h, updateJSDocLink: xb, createJSDocLinkCode: ch, updateJSDocLinkCode: lh, createJSDocLinkPlain: uh, updateJSDocLinkPlain: Eb, get createJSDocTypeTag() {
return Te(347);
}, get updateJSDocTypeTag() {
return Se(347);
}, get createJSDocReturnTag() {
return Te(345);
}, get updateJSDocReturnTag() {
return Se(345);
}, get createJSDocThisTag() {
return Te(346);
}, get updateJSDocThisTag() {
return Se(346);
}, get createJSDocAuthorTag() {
return $(333);
}, get updateJSDocAuthorTag() {
return ae(333);
}, get createJSDocClassTag() {
return $(335);
}, get updateJSDocClassTag() {
return ae(335);
}, get createJSDocPublicTag() {
return $(336);
}, get updateJSDocPublicTag() {
return ae(336);
}, get createJSDocPrivateTag() {
return $(337);
}, get updateJSDocPrivateTag() {
return ae(337);
}, get createJSDocProtectedTag() {
return $(338);
}, get updateJSDocProtectedTag() {
return ae(338);
}, get createJSDocReadonlyTag() {
return $(339);
}, get updateJSDocReadonlyTag() {
return ae(339);
}, get createJSDocOverrideTag() {
return $(340);
}, get updateJSDocOverrideTag() {
return ae(340);
}, get createJSDocDeprecatedTag() {
return $(334);
}, get updateJSDocDeprecatedTag() {
return ae(334);
}, get createJSDocThrowsTag() {
return Te(352);
}, get updateJSDocThrowsTag() {
return Se(352);
}, get createJSDocSatisfiesTag() {
return Te(353);
}, get updateJSDocSatisfiesTag() {
return Se(353);
}, createJSDocEnumTag: mh, updateJSDocEnumTag: Db, createJSDocUnknownTag: dh, updateJSDocUnknownTag: Pb, createJSDocText: hh, updateJSDocText: Qu, createJSDocComment: gh, updateJSDocComment: yh, createJsxElement: Zu, updateJsxElement: kb, createJsxSelfClosingElement: c_, updateJsxSelfClosingElement: vh, createJsxOpeningElement: bh, updateJsxOpeningElement: Ib, createJsxClosingElement: on, updateJsxClosingElement: Th, createJsxFragment: ep, createJsxText: l_, updateJsxText: Ob, createJsxOpeningFragment: kc, createJsxJsxClosingFragment: Mb, updateJsxFragment: Nb, createJsxAttribute: Sh, updateJsxAttribute: Lb, createJsxAttributes: xh, updateJsxAttributes: tp, createJsxSpreadAttribute: no, updateJsxSpreadAttribute: Rb, createJsxExpression: Ic, updateJsxExpression: Eh, createCaseClause: wh, updateCaseClause: rp, createDefaultClause: np, updateDefaultClause: jb, createHeritageClause: Ch, updateHeritageClause: Ah, createCatchClause: ip, updateCatchClause: Ph, createPropertyAssignment: Fa, updatePropertyAssignment: Jb, createShorthandPropertyAssignment: Dh, updateShorthandPropertyAssignment: Bb, createSpreadAssignment: ap, updateSpreadAssignment: ki, createEnumMember: sp, updateEnumMember: qb, createSourceFile: Ub, updateSourceFile: Mh, createRedirectedSourceFile: Ih, createBundle: Lh, updateBundle: Wb, createUnparsedSource: Nc, createUnparsedPrologue: Vb, createUnparsedPrepend: Hb, createUnparsedTextLike: Gb, createUnparsedSyntheticReference: $b, createInputFiles: Kb, createSyntheticExpression: Rh, createSyntaxList: jh, createNotEmittedStatement: Jh, createPartiallyEmittedExpression: Fh, updatePartiallyEmittedExpression: Bh, createCommaListExpression: Mc, updateCommaListExpression: Xb, createEndOfDeclarationMarker: Yb, createMergeDeclarationMarker: Qb, createSyntheticReferenceExpression: Uh, updateSyntheticReferenceExpression: _p, cloneNode: cp, get createComma() {
return x(27);
}, get createAssignment() {
return x(63);
}, get createLogicalOr() {
return x(56);
}, get createLogicalAnd() {
return x(55);
}, get createBitwiseOr() {
return x(51);
}, get createBitwiseXor() {
return x(52);
}, get createBitwiseAnd() {
return x(50);
}, get createStrictEquality() {
return x(36);
}, get createStrictInequality() {
return x(37);
}, get createEquality() {
return x(34);
}, get createInequality() {
return x(35);
}, get createLessThan() {
return x(29);
}, get createLessThanEquals() {
return x(32);
}, get createGreaterThan() {
return x(31);
}, get createGreaterThanEquals() {
return x(33);
}, get createLeftShift() {
return x(47);
}, get createRightShift() {
return x(48);
}, get createUnsignedRightShift() {
return x(49);
}, get createAdd() {
return x(39);
}, get createSubtract() {
return x(40);
}, get createMultiply() {
return x(41);
}, get createDivide() {
return x(43);
}, get createModulo() {
return x(44);
}, get createExponent() {
return x(42);
}, get createPrefixPlus() {
return w(39);
}, get createPrefixMinus() {
return w(40);
}, get createPrefixIncrement() {
return w(45);
}, get createPrefixDecrement() {
return w(46);
}, get createBitwiseNot() {
return w(54);
}, get createLogicalNot() {
return w(53);
}, get createPostfixIncrement() {
return A(45);
}, get createPostfixDecrement() {
return A(46);
}, createImmediatelyInvokedFunctionExpression: n6, createImmediatelyInvokedArrowFunction: Lc, createVoidZero: Rc, createExportDefault: zh, createExternalModuleExport: i6, createTypeCheck: a6, createMethodCall: Ba, createGlobalMethodCall: io, createFunctionBindCall: s6, createFunctionCallCall: o6, createFunctionApplyCall: _6, createArraySliceCall: Wh, createArrayConcatCall: Vh, createObjectDefinePropertyCall: u, createObjectGetOwnPropertyDescriptorCall: b, createReflectGetCall: O, createReflectSetCall: j, createPropertyDescriptor: re, createCallBinding: Jt, createAssignmentTargetWrapper: Lt, inlineExpressions: At, getInternalName: Fn, getLocalName: di, getExportName: Ii, getDeclarationName: _n, getNamespaceMemberName: qa, getExternalModuleOrNamespaceExportName: Hh, restoreOuterExpressions: We, restoreEnclosingLabel: $e, createUseStrictPrologue: wn, copyPrologue: lp, copyStandardPrologue: Ua, copyCustomPrologue: up, ensureUseStrict: Qr, liftToBlock: jc, mergeLexicalEnvironment: $h, updateModifiers: Kh };
return c(Bv, (n9) => n9(Ye)), Ye;
function Ne(n9, o) {
if (n9 === void 0 || n9 === Bt)
n9 = [];
else if (_s(n9)) {
if (o === void 0 || n9.hasTrailingComma === o)
return n9.transformFlags === void 0 && E8(n9), Y.attachNodeArrayDebugInfo(n9), n9;
let k = n9.slice();
return k.pos = n9.pos, k.end = n9.end, k.hasTrailingComma = o, k.transformFlags = n9.transformFlags, Y.attachNodeArrayDebugInfo(k), k;
}
let l = n9.length, p = l >= 1 && l <= 4 ? n9.slice() : n9;
return p.pos = -1, p.end = -1, p.hasTrailingComma = !!o, p.transformFlags = 0, E8(p), Y.attachNodeArrayDebugInfo(p), p;
}
function oe(n9) {
return t.createBaseNode(n9);
}
function Ve(n9) {
let o = oe(n9);
return o.symbol = void 0, o.localSymbol = void 0, o;
}
function pt(n9, o) {
return n9 !== o && (n9.typeArguments = o.typeArguments), r(n9, o);
}
function Gt(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 0, l = Ve(8);
return l.text = typeof n9 == "number" ? n9 + "" : n9, l.numericLiteralFlags = o, o & 384 && (l.transformFlags |= 1024), l;
}
function Nt(n9) {
let o = In(9);
return o.text = typeof n9 == "string" ? n9 : yv(n9) + "n", o.transformFlags |= 4, o;
}
function Xt(n9, o) {
let l = Ve(10);
return l.text = n9, l.singleQuote = o, l;
}
function er(n9, o, l) {
let p = Xt(n9, o);
return p.hasExtendedUnicodeEscape = l, l && (p.transformFlags |= 1024), p;
}
function Tn(n9) {
let o = Xt(kf(n9), void 0);
return o.textSourceNode = n9, o;
}
function Hr(n9) {
let o = In(13);
return o.text = n9, o;
}
function Gi(n9, o) {
switch (n9) {
case 8:
return Gt(o, 0);
case 9:
return Nt(o);
case 10:
return er(o, void 0);
case 11:
return l_(o, false);
case 12:
return l_(o, true);
case 13:
return Hr(o);
case 14:
return Qs(n9, o, void 0, 0);
}
}
function pn(n9) {
let o = t.createBaseIdentifierNode(79);
return o.escapedText = n9, o.jsDoc = void 0, o.flowNode = void 0, o.symbol = void 0, o;
}
function fn(n9, o, l, p) {
let k = pn(vi(n9));
return setIdentifierAutoGenerate(k, { flags: o, id: Bl, prefix: l, suffix: p }), Bl++, k;
}
function Ut(n9, o, l) {
o === void 0 && n9 && (o = _l(n9)), o === 79 && (o = void 0);
let p = pn(vi(n9));
return l && (p.flags |= 128), p.escapedText === "await" && (p.transformFlags |= 67108864), p.flags & 128 && (p.transformFlags |= 1024), p;
}
function kn(n9, o, l, p) {
let k = 1;
o && (k |= 8);
let V = fn("", k, l, p);
return n9 && n9(V), V;
}
function an(n9) {
let o = 2;
return n9 && (o |= 8), fn("", o, void 0, void 0);
}
function mr(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 0, l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0;
return Y.assert(!(o & 7), "Argument out of range: flags"), Y.assert((o & 48) !== 32, "GeneratedIdentifierFlags.FileLevel cannot be set without also setting GeneratedIdentifierFlags.Optimistic"), fn(n9, 3 | o, l, p);
}
function $i(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 0, l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0;
Y.assert(!(o & 7), "Argument out of range: flags");
let k = n9 ? js(n9) ? bd(false, l, n9, p, qr) : `generated@${getNodeId(n9)}` : "";
(l || p) && (o |= 16);
let V = fn(k, 4 | o, l, p);
return V.original = n9, V;
}
function dn(n9) {
let o = t.createBasePrivateIdentifierNode(80);
return o.escapedText = n9, o.transformFlags |= 16777216, o;
}
function Ur(n9) {
return Pn(n9, "#") || Y.fail("First character of private identifier must be #: " + n9), dn(vi(n9));
}
function Gr(n9, o, l, p) {
let k = dn(vi(n9));
return setIdentifierAutoGenerate(k, { flags: o, id: Bl, prefix: l, suffix: p }), Bl++, k;
}
function _r(n9, o, l) {
n9 && !Pn(n9, "#") && Y.fail("First character of private identifier must be #: " + n9);
let p = 8 | (n9 ? 3 : 1);
return Gr(n9 != null ? n9 : "", p, o, l);
}
function Sn(n9, o, l) {
let p = js(n9) ? bd(true, o, n9, l, qr) : `#generated@${getNodeId(n9)}`, V = Gr(p, 4 | (o || l ? 16 : 0), o, l);
return V.original = n9, V;
}
function In(n9) {
return t.createBaseTokenNode(n9);
}
function pr(n9) {
Y.assert(n9 >= 0 && n9 <= 162, "Invalid token"), Y.assert(n9 <= 14 || n9 >= 17, "Invalid token. Use 'createTemplateLiteralLikeNode' to create template literals."), Y.assert(n9 <= 8 || n9 >= 14, "Invalid token. Use 'createLiteralLikeNode' to create literals."), Y.assert(n9 !== 79, "Invalid token. Use 'createIdentifier' to create identifiers");
let o = In(n9), l = 0;
switch (n9) {
case 132:
l = 384;
break;
case 123:
case 121:
case 122:
case 146:
case 126:
case 136:
case 85:
case 131:
case 148:
case 160:
case 144:
case 149:
case 101:
case 145:
case 161:
case 152:
case 134:
case 153:
case 114:
case 157:
case 155:
l = 1;
break;
case 106:
l = 134218752, o.flowNode = void 0;
break;
case 124:
l = 1024;
break;
case 127:
l = 16777216;
break;
case 108:
l = 16384, o.flowNode = void 0;
break;
}
return l && (o.transformFlags |= l), o;
}
function Zt() {
return pr(106);
}
function Or() {
return pr(108);
}
function Nn() {
return pr(104);
}
function ar() {
return pr(110);
}
function oi() {
return pr(95);
}
function cr(n9) {
return pr(n9);
}
function $r(n9) {
let o = [];
return n9 & 1 && o.push(cr(93)), n9 & 2 && o.push(cr(136)), n9 & 1024 && o.push(cr(88)), n9 & 2048 && o.push(cr(85)), n9 & 4 && o.push(cr(123)), n9 & 8 && o.push(cr(121)), n9 & 16 && o.push(cr(122)), n9 & 256 && o.push(cr(126)), n9 & 32 && o.push(cr(124)), n9 & 16384 && o.push(cr(161)), n9 & 64 && o.push(cr(146)), n9 & 128 && o.push(cr(127)), n9 & 512 && o.push(cr(132)), n9 & 32768 && o.push(cr(101)), n9 & 65536 && o.push(cr(145)), o.length ? o : void 0;
}
function hr(n9, o) {
let l = oe(163);
return l.left = n9, l.right = Qt(o), l.transformFlags |= ye(l.left) | ec(l.right), l.flowNode = void 0, l;
}
function On(n9, o, l) {
return n9.left !== o || n9.right !== l ? r(hr(o, l), n9) : n9;
}
function nr(n9) {
let o = oe(164);
return o.expression = s().parenthesizeExpressionOfComputedPropertyName(n9), o.transformFlags |= ye(o.expression) | 1024 | 131072, o;
}
function br(n9, o) {
return n9.expression !== o ? r(nr(o), n9) : n9;
}
function Kr(n9, o, l, p) {
let k = Ve(165);
return k.modifiers = xt(n9), k.name = Qt(o), k.constraint = l, k.default = p, k.transformFlags = 1, k.expression = void 0, k.jsDoc = void 0, k;
}
function wa(n9, o, l, p, k) {
return n9.modifiers !== o || n9.name !== l || n9.constraint !== p || n9.default !== k ? r(Kr(o, l, p, k), n9) : n9;
}
function $n(n9, o, l, p, k, V) {
var we, et;
let ht = Ve(166);
return ht.modifiers = xt(n9), ht.dotDotDotToken = o, ht.name = Qt(l), ht.questionToken = p, ht.type = k, ht.initializer = Wa(V), Mf(ht.name) ? ht.transformFlags = 1 : ht.transformFlags = gt(ht.modifiers) | ye(ht.dotDotDotToken) | ai(ht.name) | ye(ht.questionToken) | ye(ht.initializer) | (((we = ht.questionToken) != null ? we : ht.type) ? 1 : 0) | (((et = ht.dotDotDotToken) != null ? et : ht.initializer) ? 1024 : 0) | (Vn(ht.modifiers) & 16476 ? 8192 : 0), ht.jsDoc = void 0, ht;
}
function Ki(n9, o, l, p, k, V, we) {
return n9.modifiers !== o || n9.dotDotDotToken !== l || n9.name !== p || n9.questionToken !== k || n9.type !== V || n9.initializer !== we ? r($n(o, l, p, k, V, we), n9) : n9;
}
function Mn(n9) {
let o = oe(167);
return o.expression = s().parenthesizeLeftSideOfAccess(n9, false), o.transformFlags |= ye(o.expression) | 1 | 8192 | 33554432, o;
}
function _i(n9, o) {
return n9.expression !== o ? r(Mn(o), n9) : n9;
}
function Ca(n9, o, l, p) {
let k = Ve(168);
return k.modifiers = xt(n9), k.name = Qt(o), k.type = p, k.questionToken = l, k.transformFlags = 1, k.initializer = void 0, k.jsDoc = void 0, k;
}
function St(n9, o, l, p, k) {
return n9.modifiers !== o || n9.name !== l || n9.questionToken !== p || n9.type !== k ? ue(Ca(o, l, p, k), n9) : n9;
}
function ue(n9, o) {
return n9 !== o && (n9.initializer = o.initializer), r(n9, o);
}
function He(n9, o, l, p, k) {
let V = Ve(169);
V.modifiers = xt(n9), V.name = Qt(o), V.questionToken = l && ql(l) ? l : void 0, V.exclamationToken = l && rd(l) ? l : void 0, V.type = p, V.initializer = Wa(k);
let we = V.flags & 16777216 || Vn(V.modifiers) & 2;
return V.transformFlags = gt(V.modifiers) | ai(V.name) | ye(V.initializer) | (we || V.questionToken || V.exclamationToken || V.type ? 1 : 0) | (Ws(V.name) || Vn(V.modifiers) & 32 && V.initializer ? 8192 : 0) | 16777216, V.jsDoc = void 0, V;
}
function _t(n9, o, l, p, k, V) {
return n9.modifiers !== o || n9.name !== l || n9.questionToken !== (p !== void 0 && ql(p) ? p : void 0) || n9.exclamationToken !== (p !== void 0 && rd(p) ? p : void 0) || n9.type !== k || n9.initializer !== V ? r(He(o, l, p, k, V), n9) : n9;
}
function ft(n9, o, l, p, k, V) {
let we = Ve(170);
return we.modifiers = xt(n9), we.name = Qt(o), we.questionToken = l, we.typeParameters = xt(p), we.parameters = xt(k), we.type = V, we.transformFlags = 1, we.jsDoc = void 0, we.locals = void 0, we.nextContainer = void 0, we.typeArguments = void 0, we;
}
function Kt(n9, o, l, p, k, V, we) {
return n9.modifiers !== o || n9.name !== l || n9.questionToken !== p || n9.typeParameters !== k || n9.parameters !== V || n9.type !== we ? pt(ft(o, l, p, k, V, we), n9) : n9;
}
function zt(n9, o, l, p, k, V, we, et) {
let ht = Ve(171);
if (ht.modifiers = xt(n9), ht.asteriskToken = o, ht.name = Qt(l), ht.questionToken = p, ht.exclamationToken = void 0, ht.typeParameters = xt(k), ht.parameters = Ne(V), ht.type = we, ht.body = et, !ht.body)
ht.transformFlags = 1;
else {
let hn = Vn(ht.modifiers) & 512, Ni = !!ht.asteriskToken, ia = hn && Ni;
ht.transformFlags = gt(ht.modifiers) | ye(ht.asteriskToken) | ai(ht.name) | ye(ht.questionToken) | gt(ht.typeParameters) | gt(ht.parameters) | ye(ht.type) | ye(ht.body) & -67108865 | (ia ? 128 : hn ? 256 : Ni ? 2048 : 0) | (ht.questionToken || ht.typeParameters || ht.type ? 1 : 0) | 1024;
}
return ht.typeArguments = void 0, ht.jsDoc = void 0, ht.locals = void 0, ht.nextContainer = void 0, ht.flowNode = void 0, ht.endFlowNode = void 0, ht.returnFlowNode = void 0, ht;
}
function xe(n9, o, l, p, k, V, we, et, ht) {
return n9.modifiers !== o || n9.asteriskToken !== l || n9.name !== p || n9.questionToken !== k || n9.typeParameters !== V || n9.parameters !== we || n9.type !== et || n9.body !== ht ? Le(zt(o, l, p, k, V, we, et, ht), n9) : n9;
}
function Le(n9, o) {
return n9 !== o && (n9.exclamationToken = o.exclamationToken), r(n9, o);
}
function Re(n9) {
let o = Ve(172);
return o.body = n9, o.transformFlags = ye(n9) | 16777216, o.modifiers = void 0, o.jsDoc = void 0, o.locals = void 0, o.nextContainer = void 0, o.endFlowNode = void 0, o.returnFlowNode = void 0, o;
}
function ot(n9, o) {
return n9.body !== o ? Ct(Re(o), n9) : n9;
}
function Ct(n9, o) {
return n9 !== o && (n9.modifiers = o.modifiers), r(n9, o);
}
function Mt(n9, o, l) {
let p = Ve(173);
return p.modifiers = xt(n9), p.parameters = Ne(o), p.body = l, p.transformFlags = gt(p.modifiers) | gt(p.parameters) | ye(p.body) & -67108865 | 1024, p.typeParameters = void 0, p.type = void 0, p.typeArguments = void 0, p.jsDoc = void 0, p.locals = void 0, p.nextContainer = void 0, p.endFlowNode = void 0, p.returnFlowNode = void 0, p;
}
function It(n9, o, l, p) {
return n9.modifiers !== o || n9.parameters !== l || n9.body !== p ? Mr(Mt(o, l, p), n9) : n9;
}
function Mr(n9, o) {
return n9 !== o && (n9.typeParameters = o.typeParameters, n9.type = o.type), pt(n9, o);
}
function gr(n9, o, l, p, k) {
let V = Ve(174);
return V.modifiers = xt(n9), V.name = Qt(o), V.parameters = Ne(l), V.type = p, V.body = k, V.body ? V.transformFlags = gt(V.modifiers) | ai(V.name) | gt(V.parameters) | ye(V.type) | ye(V.body) & -67108865 | (V.type ? 1 : 0) : V.transformFlags = 1, V.typeArguments = void 0, V.typeParameters = void 0, V.jsDoc = void 0, V.locals = void 0, V.nextContainer = void 0, V.flowNode = void 0, V.endFlowNode = void 0, V.returnFlowNode = void 0, V;
}
function Ln(n9, o, l, p, k, V) {
return n9.modifiers !== o || n9.name !== l || n9.parameters !== p || n9.type !== k || n9.body !== V ? ys(gr(o, l, p, k, V), n9) : n9;
}
function ys(n9, o) {
return n9 !== o && (n9.typeParameters = o.typeParameters), pt(n9, o);
}
function ci(n9, o, l, p) {
let k = Ve(175);
return k.modifiers = xt(n9), k.name = Qt(o), k.parameters = Ne(l), k.body = p, k.body ? k.transformFlags = gt(k.modifiers) | ai(k.name) | gt(k.parameters) | ye(k.body) & -67108865 | (k.type ? 1 : 0) : k.transformFlags = 1, k.typeArguments = void 0, k.typeParameters = void 0, k.type = void 0, k.jsDoc = void 0, k.locals = void 0, k.nextContainer = void 0, k.flowNode = void 0, k.endFlowNode = void 0, k.returnFlowNode = void 0, k;
}
function Xi(n9, o, l, p, k) {
return n9.modifiers !== o || n9.name !== l || n9.parameters !== p || n9.body !== k ? Aa(ci(o, l, p, k), n9) : n9;
}
function Aa(n9, o) {
return n9 !== o && (n9.typeParameters = o.typeParameters, n9.type = o.type), pt(n9, o);
}
function vs(n9, o, l) {
let p = Ve(176);
return p.typeParameters = xt(n9), p.parameters = xt(o), p.type = l, p.transformFlags = 1, p.jsDoc = void 0, p.locals = void 0, p.nextContainer = void 0, p.typeArguments = void 0, p;
}
function $s(n9, o, l, p) {
return n9.typeParameters !== o || n9.parameters !== l || n9.type !== p ? pt(vs(o, l, p), n9) : n9;
}
function li(n9, o, l) {
let p = Ve(177);
return p.typeParameters = xt(n9), p.parameters = xt(o), p.type = l, p.transformFlags = 1, p.jsDoc = void 0, p.locals = void 0, p.nextContainer = void 0, p.typeArguments = void 0, p;
}
function Yi(n9, o, l, p) {
return n9.typeParameters !== o || n9.parameters !== l || n9.type !== p ? pt(li(o, l, p), n9) : n9;
}
function Qi(n9, o, l) {
let p = Ve(178);
return p.modifiers = xt(n9), p.parameters = xt(o), p.type = l, p.transformFlags = 1, p.jsDoc = void 0, p.locals = void 0, p.nextContainer = void 0, p.typeArguments = void 0, p;
}
function bs(n9, o, l, p) {
return n9.parameters !== l || n9.type !== p || n9.modifiers !== o ? pt(Qi(o, l, p), n9) : n9;
}
function Ai(n9, o) {
let l = oe(201);
return l.type = n9, l.literal = o, l.transformFlags = 1, l;
}
function xn(n9, o, l) {
return n9.type !== o || n9.literal !== l ? r(Ai(o, l), n9) : n9;
}
function Dt(n9) {
return pr(n9);
}
function Pi(n9, o, l) {
let p = oe(179);
return p.assertsModifier = n9, p.parameterName = Qt(o), p.type = l, p.transformFlags = 1, p;
}
function Z(n9, o, l, p) {
return n9.assertsModifier !== o || n9.parameterName !== l || n9.type !== p ? r(Pi(o, l, p), n9) : n9;
}
function ie(n9, o) {
let l = oe(180);
return l.typeName = Qt(n9), l.typeArguments = o && s().parenthesizeTypeArguments(Ne(o)), l.transformFlags = 1, l;
}
function U(n9, o, l) {
return n9.typeName !== o || n9.typeArguments !== l ? r(ie(o, l), n9) : n9;
}
function L(n9, o, l) {
let p = Ve(181);
return p.typeParameters = xt(n9), p.parameters = xt(o), p.type = l, p.transformFlags = 1, p.modifiers = void 0, p.jsDoc = void 0, p.locals = void 0, p.nextContainer = void 0, p.typeArguments = void 0, p;
}
function fe(n9, o, l, p) {
return n9.typeParameters !== o || n9.parameters !== l || n9.type !== p ? T(L(o, l, p), n9) : n9;
}
function T(n9, o) {
return n9 !== o && (n9.modifiers = o.modifiers), pt(n9, o);
}
function it() {
return arguments.length === 4 ? mt(...arguments) : arguments.length === 3 ? _e(...arguments) : Y.fail("Incorrect number of arguments specified.");
}
function mt(n9, o, l, p) {
let k = Ve(182);
return k.modifiers = xt(n9), k.typeParameters = xt(o), k.parameters = xt(l), k.type = p, k.transformFlags = 1, k.jsDoc = void 0, k.locals = void 0, k.nextContainer = void 0, k.typeArguments = void 0, k;
}
function _e(n9, o, l) {
return mt(void 0, n9, o, l);
}
function Ge() {
return arguments.length === 5 ? bt(...arguments) : arguments.length === 4 ? jt(...arguments) : Y.fail("Incorrect number of arguments specified.");
}
function bt(n9, o, l, p, k) {
return n9.modifiers !== o || n9.typeParameters !== l || n9.parameters !== p || n9.type !== k ? pt(it(o, l, p, k), n9) : n9;
}
function jt(n9, o, l, p) {
return bt(n9, n9.modifiers, o, l, p);
}
function Yt(n9, o) {
let l = oe(183);
return l.exprName = n9, l.typeArguments = o && s().parenthesizeTypeArguments(o), l.transformFlags = 1, l;
}
function $t(n9, o, l) {
return n9.exprName !== o || n9.typeArguments !== l ? r(Yt(o, l), n9) : n9;
}
function Wt(n9) {
let o = Ve(184);
return o.members = Ne(n9), o.transformFlags = 1, o;
}
function Xr(n9, o) {
return n9.members !== o ? r(Wt(o), n9) : n9;
}
function Dr(n9) {
let o = oe(185);
return o.elementType = s().parenthesizeNonArrayTypeOfPostfixType(n9), o.transformFlags = 1, o;
}
function Lr(n9, o) {
return n9.elementType !== o ? r(Dr(o), n9) : n9;
}
function yr(n9) {
let o = oe(186);
return o.elements = Ne(s().parenthesizeElementTypesOfTupleType(n9)), o.transformFlags = 1, o;
}
function Rn(n9, o) {
return n9.elements !== o ? r(yr(o), n9) : n9;
}
function wt(n9, o, l, p) {
let k = Ve(199);
return k.dotDotDotToken = n9, k.name = o, k.questionToken = l, k.type = p, k.transformFlags = 1, k.jsDoc = void 0, k;
}
function Tr(n9, o, l, p, k) {
return n9.dotDotDotToken !== o || n9.name !== l || n9.questionToken !== p || n9.type !== k ? r(wt(o, l, p, k), n9) : n9;
}
function Tt(n9) {
let o = oe(187);
return o.type = s().parenthesizeTypeOfOptionalType(n9), o.transformFlags = 1, o;
}
function kt(n9, o) {
return n9.type !== o ? r(Tt(o), n9) : n9;
}
function de(n9) {
let o = oe(188);
return o.type = n9, o.transformFlags = 1, o;
}
function jn(n9, o) {
return n9.type !== o ? r(de(o), n9) : n9;
}
function Zi(n9, o, l) {
let p = oe(n9);
return p.types = Ye.createNodeArray(l(o)), p.transformFlags = 1, p;
}
function Pa(n9, o, l) {
return n9.types !== o ? r(Zi(n9.kind, o, l), n9) : n9;
}
function e_(n9) {
return Zi(189, n9, s().parenthesizeConstituentTypesOfUnionType);
}
function mc(n9, o) {
return Pa(n9, o, s().parenthesizeConstituentTypesOfUnionType);
}
function Da(n9) {
return Zi(190, n9, s().parenthesizeConstituentTypesOfIntersectionType);
}
function Ts(n9, o) {
return Pa(n9, o, s().parenthesizeConstituentTypesOfIntersectionType);
}
function Ot(n9, o, l, p) {
let k = oe(191);
return k.checkType = s().parenthesizeCheckTypeOfConditionalType(n9), k.extendsType = s().parenthesizeExtendsTypeOfConditionalType(o), k.trueType = l, k.falseType = p, k.transformFlags = 1, k.locals = void 0, k.nextContainer = void 0, k;
}
function dr(n9, o, l, p, k) {
return n9.checkType !== o || n9.extendsType !== l || n9.trueType !== p || n9.falseType !== k ? r(Ot(o, l, p, k), n9) : n9;
}
function Dd(n9) {
let o = oe(192);
return o.typeParameter = n9, o.transformFlags = 1, o;
}
function ea(n9, o) {
return n9.typeParameter !== o ? r(Dd(o), n9) : n9;
}
function kd(n9, o) {
let l = oe(200);
return l.head = n9, l.templateSpans = Ne(o), l.transformFlags = 1, l;
}
function sn(n9, o, l) {
return n9.head !== o || n9.templateSpans !== l ? r(kd(o, l), n9) : n9;
}
function Id(n9, o, l, p) {
let k = arguments.length > 4 && arguments[4] !== void 0 ? arguments[4] : false, V = oe(202);
return V.argument = n9, V.assertions = o, V.qualifier = l, V.typeArguments = p && s().parenthesizeTypeArguments(p), V.isTypeOf = k, V.transformFlags = 1, V;
}
function ka(n9, o, l, p, k) {
let V = arguments.length > 5 && arguments[5] !== void 0 ? arguments[5] : n9.isTypeOf;
return n9.argument !== o || n9.assertions !== l || n9.qualifier !== p || n9.typeArguments !== k || n9.isTypeOf !== V ? r(Id(o, l, p, k, V), n9) : n9;
}
function t_(n9) {
let o = oe(193);
return o.type = n9, o.transformFlags = 1, o;
}
function En(n9, o) {
return n9.type !== o ? r(t_(o), n9) : n9;
}
function Er() {
let n9 = oe(194);
return n9.transformFlags = 1, n9;
}
function Q(n9, o) {
let l = oe(195);
return l.operator = n9, l.type = n9 === 146 ? s().parenthesizeOperandOfReadonlyTypeOperator(o) : s().parenthesizeOperandOfTypeOperator(o), l.transformFlags = 1, l;
}
function Jn(n9, o) {
return n9.type !== o ? r(Q(n9.operator, o), n9) : n9;
}
function Ia(n9, o) {
let l = oe(196);
return l.objectType = s().parenthesizeNonArrayTypeOfPostfixType(n9), l.indexType = o, l.transformFlags = 1, l;
}
function Ss(n9, o, l) {
return n9.objectType !== o || n9.indexType !== l ? r(Ia(o, l), n9) : n9;
}
function hc(n9, o, l, p, k, V) {
let we = Ve(197);
return we.readonlyToken = n9, we.typeParameter = o, we.nameType = l, we.questionToken = p, we.type = k, we.members = V && Ne(V), we.transformFlags = 1, we.locals = void 0, we.nextContainer = void 0, we;
}
function wr(n9, o, l, p, k, V, we) {
return n9.readonlyToken !== o || n9.typeParameter !== l || n9.nameType !== p || n9.questionToken !== k || n9.type !== V || n9.members !== we ? r(hc(o, l, p, k, V, we), n9) : n9;
}
function zr(n9) {
let o = oe(198);
return o.literal = n9, o.transformFlags = 1, o;
}
function xs(n9, o) {
return n9.literal !== o ? r(zr(o), n9) : n9;
}
function Nd(n9) {
let o = oe(203);
return o.elements = Ne(n9), o.transformFlags |= gt(o.elements) | 1024 | 524288, o.transformFlags & 32768 && (o.transformFlags |= 65664), o;
}
function R2(n9, o) {
return n9.elements !== o ? r(Nd(o), n9) : n9;
}
function Es(n9) {
let o = oe(204);
return o.elements = Ne(n9), o.transformFlags |= gt(o.elements) | 1024 | 524288, o;
}
function j2(n9, o) {
return n9.elements !== o ? r(Es(o), n9) : n9;
}
function gc(n9, o, l, p) {
let k = Ve(205);
return k.dotDotDotToken = n9, k.propertyName = Qt(o), k.name = Qt(l), k.initializer = Wa(p), k.transformFlags |= ye(k.dotDotDotToken) | ai(k.propertyName) | ai(k.name) | ye(k.initializer) | (k.dotDotDotToken ? 32768 : 0) | 1024, k.flowNode = void 0, k;
}
function Ks(n9, o, l, p, k) {
return n9.propertyName !== l || n9.dotDotDotToken !== o || n9.name !== p || n9.initializer !== k ? r(gc(o, l, p, k), n9) : n9;
}
function uu(n9, o) {
let l = oe(206), p = n9 && Cn(n9), k = Ne(n9, p && cd(p) ? true : void 0);
return l.elements = s().parenthesizeExpressionsOfCommaDelimitedList(k), l.multiLine = o, l.transformFlags |= gt(l.elements), l;
}
function Od(n9, o) {
return n9.elements !== o ? r(uu(o, n9.multiLine), n9) : n9;
}
function r_(n9, o) {
let l = Ve(207);
return l.properties = Ne(n9), l.multiLine = o, l.transformFlags |= gt(l.properties), l.jsDoc = void 0, l;
}
function J2(n9, o) {
return n9.properties !== o ? r(r_(o, n9.multiLine), n9) : n9;
}
function Md(n9, o, l) {
let p = Ve(208);
return p.expression = n9, p.questionDotToken = o, p.name = l, p.transformFlags = ye(p.expression) | ye(p.questionDotToken) | (yt(p.name) ? ec(p.name) : ye(p.name) | 536870912), p.jsDoc = void 0, p.flowNode = void 0, p;
}
function ta(n9, o) {
let l = Md(s().parenthesizeLeftSideOfAccess(n9, false), void 0, Qt(o));
return nd(n9) && (l.transformFlags |= 384), l;
}
function Ld(n9, o, l) {
return LS(n9) ? Rd(n9, o, n9.questionDotToken, ti(l, yt)) : n9.expression !== o || n9.name !== l ? r(ta(o, l), n9) : n9;
}
function Xs(n9, o, l) {
let p = Md(s().parenthesizeLeftSideOfAccess(n9, true), o, Qt(l));
return p.flags |= 32, p.transformFlags |= 32, p;
}
function Rd(n9, o, l, p) {
return Y.assert(!!(n9.flags & 32), "Cannot update a PropertyAccessExpression using updatePropertyAccessChain. Use updatePropertyAccess instead."), n9.expression !== o || n9.questionDotToken !== l || n9.name !== p ? r(Xs(o, l, p), n9) : n9;
}
function yc(n9, o, l) {
let p = Ve(209);
return p.expression = n9, p.questionDotToken = o, p.argumentExpression = l, p.transformFlags |= ye(p.expression) | ye(p.questionDotToken) | ye(p.argumentExpression), p.jsDoc = void 0, p.flowNode = void 0, p;
}
function pu(n9, o) {
let l = yc(s().parenthesizeLeftSideOfAccess(n9, false), void 0, za(o));
return nd(n9) && (l.transformFlags |= 384), l;
}
function F2(n9, o, l) {
return RS(n9) ? jd(n9, o, n9.questionDotToken, l) : n9.expression !== o || n9.argumentExpression !== l ? r(pu(o, l), n9) : n9;
}
function fu(n9, o, l) {
let p = yc(s().parenthesizeLeftSideOfAccess(n9, true), o, za(l));
return p.flags |= 32, p.transformFlags |= 32, p;
}
function jd(n9, o, l, p) {
return Y.assert(!!(n9.flags & 32), "Cannot update a ElementAccessExpression using updateElementAccessChain. Use updateElementAccess instead."), n9.expression !== o || n9.questionDotToken !== l || n9.argumentExpression !== p ? r(fu(o, l, p), n9) : n9;
}
function Jd(n9, o, l, p) {
let k = Ve(210);
return k.expression = n9, k.questionDotToken = o, k.typeArguments = l, k.arguments = p, k.transformFlags |= ye(k.expression) | ye(k.questionDotToken) | gt(k.typeArguments) | gt(k.arguments), k.typeArguments && (k.transformFlags |= 1), Sf(k.expression) && (k.transformFlags |= 16384), k;
}
function Na(n9, o, l) {
let p = Jd(s().parenthesizeLeftSideOfAccess(n9, false), void 0, xt(o), s().parenthesizeExpressionsOfCommaDelimitedList(Ne(l)));
return M8(p.expression) && (p.transformFlags |= 8388608), p;
}
function B2(n9, o, l, p) {
return Cy(n9) ? Kn(n9, o, n9.questionDotToken, l, p) : n9.expression !== o || n9.typeArguments !== l || n9.arguments !== p ? r(Na(o, l, p), n9) : n9;
}
function du(n9, o, l, p) {
let k = Jd(s().parenthesizeLeftSideOfAccess(n9, true), o, xt(l), s().parenthesizeExpressionsOfCommaDelimitedList(Ne(p)));
return k.flags |= 32, k.transformFlags |= 32, k;
}
function Kn(n9, o, l, p, k) {
return Y.assert(!!(n9.flags & 32), "Cannot update a CallExpression using updateCallChain. Use updateCall instead."), n9.expression !== o || n9.questionDotToken !== l || n9.typeArguments !== p || n9.arguments !== k ? r(du(o, l, p, k), n9) : n9;
}
function vc(n9, o, l) {
let p = Ve(211);
return p.expression = s().parenthesizeExpressionOfNew(n9), p.typeArguments = xt(o), p.arguments = l ? s().parenthesizeExpressionsOfCommaDelimitedList(l) : void 0, p.transformFlags |= ye(p.expression) | gt(p.typeArguments) | gt(p.arguments) | 32, p.typeArguments && (p.transformFlags |= 1), p;
}
function mu(n9, o, l, p) {
return n9.expression !== o || n9.typeArguments !== l || n9.arguments !== p ? r(vc(o, l, p), n9) : n9;
}
function hu(n9, o, l) {
let p = oe(212);
return p.tag = s().parenthesizeLeftSideOfAccess(n9, false), p.typeArguments = xt(o), p.template = l, p.transformFlags |= ye(p.tag) | gt(p.typeArguments) | ye(p.template) | 1024, p.typeArguments && (p.transformFlags |= 1), w4(p.template) && (p.transformFlags |= 128), p;
}
function q2(n9, o, l, p) {
return n9.tag !== o || n9.typeArguments !== l || n9.template !== p ? r(hu(o, l, p), n9) : n9;
}
function Fd(n9, o) {
let l = oe(213);
return l.expression = s().parenthesizeOperandOfPrefixUnary(o), l.type = n9, l.transformFlags |= ye(l.expression) | ye(l.type) | 1, l;
}
function Bd(n9, o, l) {
return n9.type !== o || n9.expression !== l ? r(Fd(o, l), n9) : n9;
}
function gu(n9) {
let o = oe(214);
return o.expression = n9, o.transformFlags = ye(o.expression), o.jsDoc = void 0, o;
}
function qd(n9, o) {
return n9.expression !== o ? r(gu(o), n9) : n9;
}
function yu(n9, o, l, p, k, V, we) {
let et = Ve(215);
et.modifiers = xt(n9), et.asteriskToken = o, et.name = Qt(l), et.typeParameters = xt(p), et.parameters = Ne(k), et.type = V, et.body = we;
let ht = Vn(et.modifiers) & 512, hn = !!et.asteriskToken, Ni = ht && hn;
return et.transformFlags = gt(et.modifiers) | ye(et.asteriskToken) | ai(et.name) | gt(et.typeParameters) | gt(et.parameters) | ye(et.type) | ye(et.body) & -67108865 | (Ni ? 128 : ht ? 256 : hn ? 2048 : 0) | (et.typeParameters || et.type ? 1 : 0) | 4194304, et.typeArguments = void 0, et.jsDoc = void 0, et.locals = void 0, et.nextContainer = void 0, et.flowNode = void 0, et.endFlowNode = void 0, et.returnFlowNode = void 0, et;
}
function Ud(n9, o, l, p, k, V, we, et) {
return n9.name !== p || n9.modifiers !== o || n9.asteriskToken !== l || n9.typeParameters !== k || n9.parameters !== V || n9.type !== we || n9.body !== et ? pt(yu(o, l, p, k, V, we, et), n9) : n9;
}
function vu(n9, o, l, p, k, V) {
let we = Ve(216);
we.modifiers = xt(n9), we.typeParameters = xt(o), we.parameters = Ne(l), we.type = p, we.equalsGreaterThanToken = k != null ? k : pr(38), we.body = s().parenthesizeConciseBodyOfArrowFunction(V);
let et = Vn(we.modifiers) & 512;
return we.transformFlags = gt(we.modifiers) | gt(we.typeParameters) | gt(we.parameters) | ye(we.type) | ye(we.equalsGreaterThanToken) | ye(we.body) & -67108865 | (we.typeParameters || we.type ? 1 : 0) | (et ? 16640 : 0) | 1024, we.typeArguments = void 0, we.jsDoc = void 0, we.locals = void 0, we.nextContainer = void 0, we.flowNode = void 0, we.endFlowNode = void 0, we.returnFlowNode = void 0, we;
}
function zd(n9, o, l, p, k, V, we) {
return n9.modifiers !== o || n9.typeParameters !== l || n9.parameters !== p || n9.type !== k || n9.equalsGreaterThanToken !== V || n9.body !== we ? pt(vu(o, l, p, k, V, we), n9) : n9;
}
function bu(n9) {
let o = oe(217);
return o.expression = s().parenthesizeOperandOfPrefixUnary(n9), o.transformFlags |= ye(o.expression), o;
}
function U2(n9, o) {
return n9.expression !== o ? r(bu(o), n9) : n9;
}
function mn(n9) {
let o = oe(218);
return o.expression = s().parenthesizeOperandOfPrefixUnary(n9), o.transformFlags |= ye(o.expression), o;
}
function z2(n9, o) {
return n9.expression !== o ? r(mn(o), n9) : n9;
}
function ui(n9) {
let o = oe(219);
return o.expression = s().parenthesizeOperandOfPrefixUnary(n9), o.transformFlags |= ye(o.expression), o;
}
function W2(n9, o) {
return n9.expression !== o ? r(ui(o), n9) : n9;
}
function Oa(n9) {
let o = oe(220);
return o.expression = s().parenthesizeOperandOfPrefixUnary(n9), o.transformFlags |= ye(o.expression) | 256 | 128 | 2097152, o;
}
function Ys(n9, o) {
return n9.expression !== o ? r(Oa(o), n9) : n9;
}
function Tu(n9, o) {
let l = oe(221);
return l.operator = n9, l.operand = s().parenthesizeOperandOfPrefixUnary(o), l.transformFlags |= ye(l.operand), (n9 === 45 || n9 === 46) && yt(l.operand) && !cs(l.operand) && !E2(l.operand) && (l.transformFlags |= 268435456), l;
}
function bc(n9, o) {
return n9.operand !== o ? r(Tu(n9.operator, o), n9) : n9;
}
function Su(n9, o) {
let l = oe(222);
return l.operator = o, l.operand = s().parenthesizeOperandOfPostfixUnary(n9), l.transformFlags |= ye(l.operand), yt(l.operand) && !cs(l.operand) && !E2(l.operand) && (l.transformFlags |= 268435456), l;
}
function Wd(n9, o) {
return n9.operand !== o ? r(Su(o, n9.operator), n9) : n9;
}
function xu(n9, o, l) {
let p = Ve(223), k = c6(o), V = k.kind;
return p.left = s().parenthesizeLeftSideOfBinary(V, n9), p.operatorToken = k, p.right = s().parenthesizeRightSideOfBinary(V, p.left, l), p.transformFlags |= ye(p.left) | ye(p.operatorToken) | ye(p.right), V === 60 ? p.transformFlags |= 32 : V === 63 ? Hs(p.left) ? p.transformFlags |= 5248 | Vd(p.left) : Yl(p.left) && (p.transformFlags |= 5120 | Vd(p.left)) : V === 42 || V === 67 ? p.transformFlags |= 512 : jf(V) && (p.transformFlags |= 16), V === 101 && vn(p.left) && (p.transformFlags |= 536870912), p.jsDoc = void 0, p;
}
function Vd(n9) {
return A2(n9) ? 65536 : 0;
}
function V2(n9, o, l, p) {
return n9.left !== o || n9.operatorToken !== l || n9.right !== p ? r(xu(o, l, p), n9) : n9;
}
function Eu(n9, o, l, p, k) {
let V = oe(224);
return V.condition = s().parenthesizeConditionOfConditionalExpression(n9), V.questionToken = o != null ? o : pr(57), V.whenTrue = s().parenthesizeBranchOfConditionalExpression(l), V.colonToken = p != null ? p : pr(58), V.whenFalse = s().parenthesizeBranchOfConditionalExpression(k), V.transformFlags |= ye(V.condition) | ye(V.questionToken) | ye(V.whenTrue) | ye(V.colonToken) | ye(V.whenFalse), V;
}
function H2(n9, o, l, p, k, V) {
return n9.condition !== o || n9.questionToken !== l || n9.whenTrue !== p || n9.colonToken !== k || n9.whenFalse !== V ? r(Eu(o, l, p, k, V), n9) : n9;
}
function Di(n9, o) {
let l = oe(225);
return l.head = n9, l.templateSpans = Ne(o), l.transformFlags |= ye(l.head) | gt(l.templateSpans) | 1024, l;
}
function Hd(n9, o, l) {
return n9.head !== o || n9.templateSpans !== l ? r(Di(o, l), n9) : n9;
}
function Tc(n9, o, l) {
let p = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : 0;
Y.assert(!(p & -2049), "Unsupported template flags.");
let k;
if (l !== void 0 && l !== o && (k = BL(n9, l), typeof k == "object"))
return Y.fail("Invalid raw text");
if (o === void 0) {
if (k === void 0)
return Y.fail("Arguments 'text' and 'rawText' may not both be undefined.");
o = k;
} else
k !== void 0 && Y.assert(o === k, "Expected argument 'text' to be the normalized (i.e. 'cooked') version of argument 'rawText'.");
return o;
}
function Gd(n9) {
let o = 1024;
return n9 && (o |= 128), o;
}
function n_(n9, o, l, p) {
let k = In(n9);
return k.text = o, k.rawText = l, k.templateFlags = p & 2048, k.transformFlags = Gd(k.templateFlags), k;
}
function wu(n9, o, l, p) {
let k = Ve(n9);
return k.text = o, k.rawText = l, k.templateFlags = p & 2048, k.transformFlags = Gd(k.templateFlags), k;
}
function Qs(n9, o, l, p) {
return n9 === 14 ? wu(n9, o, l, p) : n_(n9, o, l, p);
}
function Sc(n9, o, l) {
return n9 = Tc(15, n9, o, l), Qs(15, n9, o, l);
}
function Cu(n9, o, l) {
return n9 = Tc(15, n9, o, l), Qs(16, n9, o, l);
}
function G2(n9, o, l) {
return n9 = Tc(15, n9, o, l), Qs(17, n9, o, l);
}
function $d(n9, o, l) {
return n9 = Tc(15, n9, o, l), wu(14, n9, o, l);
}
function Kd(n9, o) {
Y.assert(!n9 || !!o, "A `YieldExpression` with an asteriskToken must have an expression.");
let l = oe(226);
return l.expression = o && s().parenthesizeExpressionForDisallowedComma(o), l.asteriskToken = n9, l.transformFlags |= ye(l.expression) | ye(l.asteriskToken) | 1024 | 128 | 1048576, l;
}
function $2(n9, o, l) {
return n9.expression !== l || n9.asteriskToken !== o ? r(Kd(o, l), n9) : n9;
}
function Xd(n9) {
let o = oe(227);
return o.expression = s().parenthesizeExpressionForDisallowedComma(n9), o.transformFlags |= ye(o.expression) | 1024 | 32768, o;
}
function K2(n9, o) {
return n9.expression !== o ? r(Xd(o), n9) : n9;
}
function Yd(n9, o, l, p, k) {
let V = Ve(228);
return V.modifiers = xt(n9), V.name = Qt(o), V.typeParameters = xt(l), V.heritageClauses = xt(p), V.members = Ne(k), V.transformFlags |= gt(V.modifiers) | ai(V.name) | gt(V.typeParameters) | gt(V.heritageClauses) | gt(V.members) | (V.typeParameters ? 1 : 0) | 1024, V.jsDoc = void 0, V;
}
function xc(n9, o, l, p, k, V) {
return n9.modifiers !== o || n9.name !== l || n9.typeParameters !== p || n9.heritageClauses !== k || n9.members !== V ? r(Yd(o, l, p, k, V), n9) : n9;
}
function X2() {
return oe(229);
}
function Qd(n9, o) {
let l = oe(230);
return l.expression = s().parenthesizeLeftSideOfAccess(n9, false), l.typeArguments = o && s().parenthesizeTypeArguments(o), l.transformFlags |= ye(l.expression) | gt(l.typeArguments) | 1024, l;
}
function Xn(n9, o, l) {
return n9.expression !== o || n9.typeArguments !== l ? r(Qd(o, l), n9) : n9;
}
function Ec(n9, o) {
let l = oe(231);
return l.expression = n9, l.type = o, l.transformFlags |= ye(l.expression) | ye(l.type) | 1, l;
}
function Zd(n9, o, l) {
return n9.expression !== o || n9.type !== l ? r(Ec(o, l), n9) : n9;
}
function em(n9) {
let o = oe(232);
return o.expression = s().parenthesizeLeftSideOfAccess(n9, false), o.transformFlags |= ye(o.expression) | 1, o;
}
function Au(n9, o) {
return JS(n9) ? rm(n9, o) : n9.expression !== o ? r(em(o), n9) : n9;
}
function tm(n9, o) {
let l = oe(235);
return l.expression = n9, l.type = o, l.transformFlags |= ye(l.expression) | ye(l.type) | 1, l;
}
function Pu(n9, o, l) {
return n9.expression !== o || n9.type !== l ? r(tm(o, l), n9) : n9;
}
function pi(n9) {
let o = oe(232);
return o.flags |= 32, o.expression = s().parenthesizeLeftSideOfAccess(n9, true), o.transformFlags |= ye(o.expression) | 1, o;
}
function rm(n9, o) {
return Y.assert(!!(n9.flags & 32), "Cannot update a NonNullExpression using updateNonNullChain. Use updateNonNullExpression instead."), n9.expression !== o ? r(pi(o), n9) : n9;
}
function wc(n9, o) {
let l = oe(233);
switch (l.keywordToken = n9, l.name = o, l.transformFlags |= ye(l.name), n9) {
case 103:
l.transformFlags |= 1024;
break;
case 100:
l.transformFlags |= 4;
break;
default:
return Y.assertNever(n9);
}
return l.flowNode = void 0, l;
}
function ra(n9, o) {
return n9.name !== o ? r(wc(n9.keywordToken, o), n9) : n9;
}
function i_(n9, o) {
let l = oe(236);
return l.expression = n9, l.literal = o, l.transformFlags |= ye(l.expression) | ye(l.literal) | 1024, l;
}
function nm(n9, o, l) {
return n9.expression !== o || n9.literal !== l ? r(i_(o, l), n9) : n9;
}
function im() {
let n9 = oe(237);
return n9.transformFlags |= 1024, n9;
}
function Zs(n9, o) {
let l = oe(238);
return l.statements = Ne(n9), l.multiLine = o, l.transformFlags |= gt(l.statements), l.jsDoc = void 0, l.locals = void 0, l.nextContainer = void 0, l;
}
function am(n9, o) {
return n9.statements !== o ? r(Zs(o, n9.multiLine), n9) : n9;
}
function sm(n9, o) {
let l = oe(240);
return l.modifiers = xt(n9), l.declarationList = ir(o) ? Ru(o) : o, l.transformFlags |= gt(l.modifiers) | ye(l.declarationList), Vn(l.modifiers) & 2 && (l.transformFlags = 1), l.jsDoc = void 0, l.flowNode = void 0, l;
}
function om(n9, o, l) {
return n9.modifiers !== o || n9.declarationList !== l ? r(sm(o, l), n9) : n9;
}
function Du() {
let n9 = oe(239);
return n9.jsDoc = void 0, n9;
}
function a_(n9) {
let o = oe(241);
return o.expression = s().parenthesizeExpressionOfExpressionStatement(n9), o.transformFlags |= ye(o.expression), o.jsDoc = void 0, o.flowNode = void 0, o;
}
function Y2(n9, o) {
return n9.expression !== o ? r(a_(o), n9) : n9;
}
function ku(n9, o, l) {
let p = oe(242);
return p.expression = n9, p.thenStatement = Yn(o), p.elseStatement = Yn(l), p.transformFlags |= ye(p.expression) | ye(p.thenStatement) | ye(p.elseStatement), p.jsDoc = void 0, p.flowNode = void 0, p;
}
function Q2(n9, o, l, p) {
return n9.expression !== o || n9.thenStatement !== l || n9.elseStatement !== p ? r(ku(o, l, p), n9) : n9;
}
function Iu(n9, o) {
let l = oe(243);
return l.statement = Yn(n9), l.expression = o, l.transformFlags |= ye(l.statement) | ye(l.expression), l.jsDoc = void 0, l.flowNode = void 0, l;
}
function Z2(n9, o, l) {
return n9.statement !== o || n9.expression !== l ? r(Iu(o, l), n9) : n9;
}
function _m(n9, o) {
let l = oe(244);
return l.expression = n9, l.statement = Yn(o), l.transformFlags |= ye(l.expression) | ye(l.statement), l.jsDoc = void 0, l.flowNode = void 0, l;
}
function eb(n9, o, l) {
return n9.expression !== o || n9.statement !== l ? r(_m(o, l), n9) : n9;
}
function Nu(n9, o, l, p) {
let k = oe(245);
return k.initializer = n9, k.condition = o, k.incrementor = l, k.statement = Yn(p), k.transformFlags |= ye(k.initializer) | ye(k.condition) | ye(k.incrementor) | ye(k.statement), k.jsDoc = void 0, k.locals = void 0, k.nextContainer = void 0, k.flowNode = void 0, k;
}
function cm(n9, o, l, p, k) {
return n9.initializer !== o || n9.condition !== l || n9.incrementor !== p || n9.statement !== k ? r(Nu(o, l, p, k), n9) : n9;
}
function lm(n9, o, l) {
let p = oe(246);
return p.initializer = n9, p.expression = o, p.statement = Yn(l), p.transformFlags |= ye(p.initializer) | ye(p.expression) | ye(p.statement), p.jsDoc = void 0, p.locals = void 0, p.nextContainer = void 0, p.flowNode = void 0, p;
}
function tb(n9, o, l, p) {
return n9.initializer !== o || n9.expression !== l || n9.statement !== p ? r(lm(o, l, p), n9) : n9;
}
function um(n9, o, l, p) {
let k = oe(247);
return k.awaitModifier = n9, k.initializer = o, k.expression = s().parenthesizeExpressionForDisallowedComma(l), k.statement = Yn(p), k.transformFlags |= ye(k.awaitModifier) | ye(k.initializer) | ye(k.expression) | ye(k.statement) | 1024, n9 && (k.transformFlags |= 128), k.jsDoc = void 0, k.locals = void 0, k.nextContainer = void 0, k.flowNode = void 0, k;
}
function rb(n9, o, l, p, k) {
return n9.awaitModifier !== o || n9.initializer !== l || n9.expression !== p || n9.statement !== k ? r(um(o, l, p, k), n9) : n9;
}
function pm(n9) {
let o = oe(248);
return o.label = Qt(n9), o.transformFlags |= ye(o.label) | 4194304, o.jsDoc = void 0, o.flowNode = void 0, o;
}
function fm(n9, o) {
return n9.label !== o ? r(pm(o), n9) : n9;
}
function Ou(n9) {
let o = oe(249);
return o.label = Qt(n9), o.transformFlags |= ye(o.label) | 4194304, o.jsDoc = void 0, o.flowNode = void 0, o;
}
function dm(n9, o) {
return n9.label !== o ? r(Ou(o), n9) : n9;
}
function mm(n9) {
let o = oe(250);
return o.expression = n9, o.transformFlags |= ye(o.expression) | 128 | 4194304, o.jsDoc = void 0, o.flowNode = void 0, o;
}
function nb(n9, o) {
return n9.expression !== o ? r(mm(o), n9) : n9;
}
function Mu(n9, o) {
let l = oe(251);
return l.expression = n9, l.statement = Yn(o), l.transformFlags |= ye(l.expression) | ye(l.statement), l.jsDoc = void 0, l.flowNode = void 0, l;
}
function hm(n9, o, l) {
return n9.expression !== o || n9.statement !== l ? r(Mu(o, l), n9) : n9;
}
function Lu(n9, o) {
let l = oe(252);
return l.expression = s().parenthesizeExpressionForDisallowedComma(n9), l.caseBlock = o, l.transformFlags |= ye(l.expression) | ye(l.caseBlock), l.jsDoc = void 0, l.flowNode = void 0, l.possiblyExhaustive = false, l;
}
function eo(n9, o, l) {
return n9.expression !== o || n9.caseBlock !== l ? r(Lu(o, l), n9) : n9;
}
function gm(n9, o) {
let l = oe(253);
return l.label = Qt(n9), l.statement = Yn(o), l.transformFlags |= ye(l.label) | ye(l.statement), l.jsDoc = void 0, l.flowNode = void 0, l;
}
function ym(n9, o, l) {
return n9.label !== o || n9.statement !== l ? r(gm(o, l), n9) : n9;
}
function vm(n9) {
let o = oe(254);
return o.expression = n9, o.transformFlags |= ye(o.expression), o.jsDoc = void 0, o.flowNode = void 0, o;
}
function ib(n9, o) {
return n9.expression !== o ? r(vm(o), n9) : n9;
}
function bm(n9, o, l) {
let p = oe(255);
return p.tryBlock = n9, p.catchClause = o, p.finallyBlock = l, p.transformFlags |= ye(p.tryBlock) | ye(p.catchClause) | ye(p.finallyBlock), p.jsDoc = void 0, p.flowNode = void 0, p;
}
function ab(n9, o, l, p) {
return n9.tryBlock !== o || n9.catchClause !== l || n9.finallyBlock !== p ? r(bm(o, l, p), n9) : n9;
}
function Tm() {
let n9 = oe(256);
return n9.jsDoc = void 0, n9.flowNode = void 0, n9;
}
function Cc(n9, o, l, p) {
var k;
let V = Ve(257);
return V.name = Qt(n9), V.exclamationToken = o, V.type = l, V.initializer = Wa(p), V.transformFlags |= ai(V.name) | ye(V.initializer) | (((k = V.exclamationToken) != null ? k : V.type) ? 1 : 0), V.jsDoc = void 0, V;
}
function Sm(n9, o, l, p, k) {
return n9.name !== o || n9.type !== p || n9.exclamationToken !== l || n9.initializer !== k ? r(Cc(o, l, p, k), n9) : n9;
}
function Ru(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 0, l = oe(258);
return l.flags |= o & 3, l.declarations = Ne(n9), l.transformFlags |= gt(l.declarations) | 4194304, o & 3 && (l.transformFlags |= 263168), l;
}
function sb(n9, o) {
return n9.declarations !== o ? r(Ru(o, n9.flags), n9) : n9;
}
function xm(n9, o, l, p, k, V, we) {
let et = Ve(259);
if (et.modifiers = xt(n9), et.asteriskToken = o, et.name = Qt(l), et.typeParameters = xt(p), et.parameters = Ne(k), et.type = V, et.body = we, !et.body || Vn(et.modifiers) & 2)
et.transformFlags = 1;
else {
let ht = Vn(et.modifiers) & 512, hn = !!et.asteriskToken, Ni = ht && hn;
et.transformFlags = gt(et.modifiers) | ye(et.asteriskToken) | ai(et.name) | gt(et.typeParameters) | gt(et.parameters) | ye(et.type) | ye(et.body) & -67108865 | (Ni ? 128 : ht ? 256 : hn ? 2048 : 0) | (et.typeParameters || et.type ? 1 : 0) | 4194304;
}
return et.typeArguments = void 0, et.jsDoc = void 0, et.locals = void 0, et.nextContainer = void 0, et.endFlowNode = void 0, et.returnFlowNode = void 0, et;
}
function ju(n9, o, l, p, k, V, we, et) {
return n9.modifiers !== o || n9.asteriskToken !== l || n9.name !== p || n9.typeParameters !== k || n9.parameters !== V || n9.type !== we || n9.body !== et ? ob(xm(o, l, p, k, V, we, et), n9) : n9;
}
function ob(n9, o) {
return n9 !== o && n9.modifiers === o.modifiers && (n9.modifiers = o.modifiers), pt(n9, o);
}
function Em(n9, o, l, p, k) {
let V = Ve(260);
return V.modifiers = xt(n9), V.name = Qt(o), V.typeParameters = xt(l), V.heritageClauses = xt(p), V.members = Ne(k), Vn(V.modifiers) & 2 ? V.transformFlags = 1 : (V.transformFlags |= gt(V.modifiers) | ai(V.name) | gt(V.typeParameters) | gt(V.heritageClauses) | gt(V.members) | (V.typeParameters ? 1 : 0) | 1024, V.transformFlags & 8192 && (V.transformFlags |= 1)), V.jsDoc = void 0, V;
}
function Ju(n9, o, l, p, k, V) {
return n9.modifiers !== o || n9.name !== l || n9.typeParameters !== p || n9.heritageClauses !== k || n9.members !== V ? r(Em(o, l, p, k, V), n9) : n9;
}
function wm(n9, o, l, p, k) {
let V = Ve(261);
return V.modifiers = xt(n9), V.name = Qt(o), V.typeParameters = xt(l), V.heritageClauses = xt(p), V.members = Ne(k), V.transformFlags = 1, V.jsDoc = void 0, V;
}
function Cm(n9, o, l, p, k, V) {
return n9.modifiers !== o || n9.name !== l || n9.typeParameters !== p || n9.heritageClauses !== k || n9.members !== V ? r(wm(o, l, p, k, V), n9) : n9;
}
function sr(n9, o, l, p) {
let k = Ve(262);
return k.modifiers = xt(n9), k.name = Qt(o), k.typeParameters = xt(l), k.type = p, k.transformFlags = 1, k.jsDoc = void 0, k.locals = void 0, k.nextContainer = void 0, k;
}
function Ma(n9, o, l, p, k) {
return n9.modifiers !== o || n9.name !== l || n9.typeParameters !== p || n9.type !== k ? r(sr(o, l, p, k), n9) : n9;
}
function Fu(n9, o, l) {
let p = Ve(263);
return p.modifiers = xt(n9), p.name = Qt(o), p.members = Ne(l), p.transformFlags |= gt(p.modifiers) | ye(p.name) | gt(p.members) | 1, p.transformFlags &= -67108865, p.jsDoc = void 0, p;
}
function La(n9, o, l, p) {
return n9.modifiers !== o || n9.name !== l || n9.members !== p ? r(Fu(o, l, p), n9) : n9;
}
function Am(n9, o, l) {
let p = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : 0, k = Ve(264);
return k.modifiers = xt(n9), k.flags |= p & 1044, k.name = o, k.body = l, Vn(k.modifiers) & 2 ? k.transformFlags = 1 : k.transformFlags |= gt(k.modifiers) | ye(k.name) | ye(k.body) | 1, k.transformFlags &= -67108865, k.jsDoc = void 0, k.locals = void 0, k.nextContainer = void 0, k;
}
function Sr(n9, o, l, p) {
return n9.modifiers !== o || n9.name !== l || n9.body !== p ? r(Am(o, l, p, n9.flags), n9) : n9;
}
function Ra(n9) {
let o = oe(265);
return o.statements = Ne(n9), o.transformFlags |= gt(o.statements), o.jsDoc = void 0, o;
}
function Yr(n9, o) {
return n9.statements !== o ? r(Ra(o), n9) : n9;
}
function Pm(n9) {
let o = oe(266);
return o.clauses = Ne(n9), o.transformFlags |= gt(o.clauses), o.locals = void 0, o.nextContainer = void 0, o;
}
function _b(n9, o) {
return n9.clauses !== o ? r(Pm(o), n9) : n9;
}
function Dm(n9) {
let o = Ve(267);
return o.name = Qt(n9), o.transformFlags |= ec(o.name) | 1, o.modifiers = void 0, o.jsDoc = void 0, o;
}
function km(n9, o) {
return n9.name !== o ? cb(Dm(o), n9) : n9;
}
function cb(n9, o) {
return n9 !== o && (n9.modifiers = o.modifiers), r(n9, o);
}
function Im(n9, o, l, p) {
let k = Ve(268);
return k.modifiers = xt(n9), k.name = Qt(l), k.isTypeOnly = o, k.moduleReference = p, k.transformFlags |= gt(k.modifiers) | ec(k.name) | ye(k.moduleReference), ud(k.moduleReference) || (k.transformFlags |= 1), k.transformFlags &= -67108865, k.jsDoc = void 0, k;
}
function Nm(n9, o, l, p, k) {
return n9.modifiers !== o || n9.isTypeOnly !== l || n9.name !== p || n9.moduleReference !== k ? r(Im(o, l, p, k), n9) : n9;
}
function Om(n9, o, l, p) {
let k = oe(269);
return k.modifiers = xt(n9), k.importClause = o, k.moduleSpecifier = l, k.assertClause = p, k.transformFlags |= ye(k.importClause) | ye(k.moduleSpecifier), k.transformFlags &= -67108865, k.jsDoc = void 0, k;
}
function Mm(n9, o, l, p, k) {
return n9.modifiers !== o || n9.importClause !== l || n9.moduleSpecifier !== p || n9.assertClause !== k ? r(Om(o, l, p, k), n9) : n9;
}
function Lm(n9, o, l) {
let p = Ve(270);
return p.isTypeOnly = n9, p.name = o, p.namedBindings = l, p.transformFlags |= ye(p.name) | ye(p.namedBindings), n9 && (p.transformFlags |= 1), p.transformFlags &= -67108865, p;
}
function Rm(n9, o, l, p) {
return n9.isTypeOnly !== o || n9.name !== l || n9.namedBindings !== p ? r(Lm(o, l, p), n9) : n9;
}
function Bu(n9, o) {
let l = oe(296);
return l.elements = Ne(n9), l.multiLine = o, l.transformFlags |= 4, l;
}
function lb(n9, o, l) {
return n9.elements !== o || n9.multiLine !== l ? r(Bu(o, l), n9) : n9;
}
function s_(n9, o) {
let l = oe(297);
return l.name = n9, l.value = o, l.transformFlags |= 4, l;
}
function jm(n9, o, l) {
return n9.name !== o || n9.value !== l ? r(s_(o, l), n9) : n9;
}
function qu(n9, o) {
let l = oe(298);
return l.assertClause = n9, l.multiLine = o, l;
}
function Jm(n9, o, l) {
return n9.assertClause !== o || n9.multiLine !== l ? r(qu(o, l), n9) : n9;
}
function Fm(n9) {
let o = Ve(271);
return o.name = n9, o.transformFlags |= ye(o.name), o.transformFlags &= -67108865, o;
}
function Uu(n9, o) {
return n9.name !== o ? r(Fm(o), n9) : n9;
}
function Bm(n9) {
let o = Ve(277);
return o.name = n9, o.transformFlags |= ye(o.name) | 4, o.transformFlags &= -67108865, o;
}
function qm(n9, o) {
return n9.name !== o ? r(Bm(o), n9) : n9;
}
function Um(n9) {
let o = oe(272);
return o.elements = Ne(n9), o.transformFlags |= gt(o.elements), o.transformFlags &= -67108865, o;
}
function ub(n9, o) {
return n9.elements !== o ? r(Um(o), n9) : n9;
}
function zm(n9, o, l) {
let p = Ve(273);
return p.isTypeOnly = n9, p.propertyName = o, p.name = l, p.transformFlags |= ye(p.propertyName) | ye(p.name), p.transformFlags &= -67108865, p;
}
function pb(n9, o, l, p) {
return n9.isTypeOnly !== o || n9.propertyName !== l || n9.name !== p ? r(zm(o, l, p), n9) : n9;
}
function zu(n9, o, l) {
let p = Ve(274);
return p.modifiers = xt(n9), p.isExportEquals = o, p.expression = o ? s().parenthesizeRightSideOfBinary(63, void 0, l) : s().parenthesizeExpressionOfExportDefault(l), p.transformFlags |= gt(p.modifiers) | ye(p.expression), p.transformFlags &= -67108865, p.jsDoc = void 0, p;
}
function Wu(n9, o, l) {
return n9.modifiers !== o || n9.expression !== l ? r(zu(o, n9.isExportEquals, l), n9) : n9;
}
function na(n9, o, l, p, k) {
let V = Ve(275);
return V.modifiers = xt(n9), V.isTypeOnly = o, V.exportClause = l, V.moduleSpecifier = p, V.assertClause = k, V.transformFlags |= gt(V.modifiers) | ye(V.exportClause) | ye(V.moduleSpecifier), V.transformFlags &= -67108865, V.jsDoc = void 0, V;
}
function Wm(n9, o, l, p, k, V) {
return n9.modifiers !== o || n9.isTypeOnly !== l || n9.exportClause !== p || n9.moduleSpecifier !== k || n9.assertClause !== V ? Vm(na(o, l, p, k, V), n9) : n9;
}
function Vm(n9, o) {
return n9 !== o && n9.modifiers === o.modifiers && (n9.modifiers = o.modifiers), r(n9, o);
}
function to(n9) {
let o = oe(276);
return o.elements = Ne(n9), o.transformFlags |= gt(o.elements), o.transformFlags &= -67108865, o;
}
function Hm(n9, o) {
return n9.elements !== o ? r(to(o), n9) : n9;
}
function Vu(n9, o, l) {
let p = oe(278);
return p.isTypeOnly = n9, p.propertyName = Qt(o), p.name = Qt(l), p.transformFlags |= ye(p.propertyName) | ye(p.name), p.transformFlags &= -67108865, p.jsDoc = void 0, p;
}
function o_(n9, o, l, p) {
return n9.isTypeOnly !== o || n9.propertyName !== l || n9.name !== p ? r(Vu(o, l, p), n9) : n9;
}
function fb() {
let n9 = Ve(279);
return n9.jsDoc = void 0, n9;
}
function Gm(n9) {
let o = oe(280);
return o.expression = n9, o.transformFlags |= ye(o.expression), o.transformFlags &= -67108865, o;
}
function $m(n9, o) {
return n9.expression !== o ? r(Gm(o), n9) : n9;
}
function db(n9) {
return oe(n9);
}
function Km(n9, o) {
let l = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false, p = Ac(n9, l ? o && s().parenthesizeNonArrayTypeOfPostfixType(o) : o);
return p.postfix = l, p;
}
function Ac(n9, o) {
let l = oe(n9);
return l.type = o, l;
}
function Xm(n9, o, l) {
return o.type !== l ? r(Km(n9, l, o.postfix), o) : o;
}
function mb(n9, o, l) {
return o.type !== l ? r(Ac(n9, l), o) : o;
}
function Ym(n9, o) {
let l = Ve(320);
return l.parameters = xt(n9), l.type = o, l.transformFlags = gt(l.parameters) | (l.type ? 1 : 0), l.jsDoc = void 0, l.locals = void 0, l.nextContainer = void 0, l.typeArguments = void 0, l;
}
function hb(n9, o, l) {
return n9.parameters !== o || n9.type !== l ? r(Ym(o, l), n9) : n9;
}
function Qm(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false, l = Ve(325);
return l.jsDocPropertyTags = xt(n9), l.isArrayType = o, l;
}
function gb(n9, o, l) {
return n9.jsDocPropertyTags !== o || n9.isArrayType !== l ? r(Qm(o, l), n9) : n9;
}
function Zm(n9) {
let o = oe(312);
return o.type = n9, o;
}
function yb(n9, o) {
return n9.type !== o ? r(Zm(o), n9) : n9;
}
function eh(n9, o, l) {
let p = Ve(326);
return p.typeParameters = xt(n9), p.parameters = Ne(o), p.type = l, p.jsDoc = void 0, p.locals = void 0, p.nextContainer = void 0, p;
}
function Hu(n9, o, l, p) {
return n9.typeParameters !== o || n9.parameters !== l || n9.type !== p ? r(eh(o, l, p), n9) : n9;
}
function fi(n9) {
let o = ed(n9.kind);
return n9.tagName.escapedText === vi(o) ? n9.tagName : Ut(o);
}
function ja(n9, o, l) {
let p = oe(n9);
return p.tagName = o, p.comment = l, p;
}
function Ja(n9, o, l) {
let p = Ve(n9);
return p.tagName = o, p.comment = l, p;
}
function __(n9, o, l, p) {
let k = ja(348, n9 != null ? n9 : Ut("template"), p);
return k.constraint = o, k.typeParameters = Ne(l), k;
}
function Gu(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fi(n9), l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0, k = arguments.length > 4 ? arguments[4] : void 0;
return n9.tagName !== o || n9.constraint !== l || n9.typeParameters !== p || n9.comment !== k ? r(__(o, l, p, k), n9) : n9;
}
function $u(n9, o, l, p) {
let k = Ja(349, n9 != null ? n9 : Ut("typedef"), p);
return k.typeExpression = o, k.fullName = l, k.name = w2(l), k.locals = void 0, k.nextContainer = void 0, k;
}
function th(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fi(n9), l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0, k = arguments.length > 4 ? arguments[4] : void 0;
return n9.tagName !== o || n9.typeExpression !== l || n9.fullName !== p || n9.comment !== k ? r($u(o, l, p, k), n9) : n9;
}
function Pc(n9, o, l, p, k, V) {
let we = Ja(344, n9 != null ? n9 : Ut("param"), V);
return we.typeExpression = p, we.name = o, we.isNameFirst = !!k, we.isBracketed = l, we;
}
function vb(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fi(n9), l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0, k = arguments.length > 4 ? arguments[4] : void 0, V = arguments.length > 5 ? arguments[5] : void 0, we = arguments.length > 6 ? arguments[6] : void 0;
return n9.tagName !== o || n9.name !== l || n9.isBracketed !== p || n9.typeExpression !== k || n9.isNameFirst !== V || n9.comment !== we ? r(Pc(o, l, p, k, V, we), n9) : n9;
}
function Ku(n9, o, l, p, k, V) {
let we = Ja(351, n9 != null ? n9 : Ut("prop"), V);
return we.typeExpression = p, we.name = o, we.isNameFirst = !!k, we.isBracketed = l, we;
}
function bb(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fi(n9), l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0, k = arguments.length > 4 ? arguments[4] : void 0, V = arguments.length > 5 ? arguments[5] : void 0, we = arguments.length > 6 ? arguments[6] : void 0;
return n9.tagName !== o || n9.name !== l || n9.isBracketed !== p || n9.typeExpression !== k || n9.isNameFirst !== V || n9.comment !== we ? r(Ku(o, l, p, k, V, we), n9) : n9;
}
function rh(n9, o, l, p) {
let k = Ja(341, n9 != null ? n9 : Ut("callback"), p);
return k.typeExpression = o, k.fullName = l, k.name = w2(l), k.locals = void 0, k.nextContainer = void 0, k;
}
function nh(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fi(n9), l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0, k = arguments.length > 4 ? arguments[4] : void 0;
return n9.tagName !== o || n9.typeExpression !== l || n9.fullName !== p || n9.comment !== k ? r(rh(o, l, p, k), n9) : n9;
}
function ih(n9, o, l) {
let p = ja(342, n9 != null ? n9 : Ut("overload"), l);
return p.typeExpression = o, p;
}
function ah(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fi(n9), l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0;
return n9.tagName !== o || n9.typeExpression !== l || n9.comment !== p ? r(ih(o, l, p), n9) : n9;
}
function sh(n9, o, l) {
let p = ja(331, n9 != null ? n9 : Ut("augments"), l);
return p.class = o, p;
}
function Xu(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fi(n9), l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0;
return n9.tagName !== o || n9.class !== l || n9.comment !== p ? r(sh(o, l, p), n9) : n9;
}
function Yu(n9, o, l) {
let p = ja(332, n9 != null ? n9 : Ut("implements"), l);
return p.class = o, p;
}
function ro(n9, o, l) {
let p = ja(350, n9 != null ? n9 : Ut("see"), l);
return p.name = o, p;
}
function Tb(n9, o, l, p) {
return n9.tagName !== o || n9.name !== l || n9.comment !== p ? r(ro(o, l, p), n9) : n9;
}
function ws(n9) {
let o = oe(313);
return o.name = n9, o;
}
function Dc(n9, o) {
return n9.name !== o ? r(ws(o), n9) : n9;
}
function oh(n9, o) {
let l = oe(314);
return l.left = n9, l.right = o, l.transformFlags |= ye(l.left) | ye(l.right), l;
}
function Sb(n9, o, l) {
return n9.left !== o || n9.right !== l ? r(oh(o, l), n9) : n9;
}
function _h(n9, o) {
let l = oe(327);
return l.name = n9, l.text = o, l;
}
function xb(n9, o, l) {
return n9.name !== o ? r(_h(o, l), n9) : n9;
}
function ch(n9, o) {
let l = oe(328);
return l.name = n9, l.text = o, l;
}
function lh(n9, o, l) {
return n9.name !== o ? r(ch(o, l), n9) : n9;
}
function uh(n9, o) {
let l = oe(329);
return l.name = n9, l.text = o, l;
}
function Eb(n9, o, l) {
return n9.name !== o ? r(uh(o, l), n9) : n9;
}
function wb(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fi(n9), l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0;
return n9.tagName !== o || n9.class !== l || n9.comment !== p ? r(Yu(o, l, p), n9) : n9;
}
function ph(n9, o, l) {
return ja(n9, o != null ? o : Ut(ed(n9)), l);
}
function Cb(n9, o) {
let l = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : fi(o), p = arguments.length > 3 ? arguments[3] : void 0;
return o.tagName !== l || o.comment !== p ? r(ph(n9, l, p), o) : o;
}
function fh(n9, o, l, p) {
let k = ja(n9, o != null ? o : Ut(ed(n9)), p);
return k.typeExpression = l, k;
}
function Ab(n9, o) {
let l = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : fi(o), p = arguments.length > 3 ? arguments[3] : void 0, k = arguments.length > 4 ? arguments[4] : void 0;
return o.tagName !== l || o.typeExpression !== p || o.comment !== k ? r(fh(n9, l, p, k), o) : o;
}
function dh(n9, o) {
return ja(330, n9, o);
}
function Pb(n9, o, l) {
return n9.tagName !== o || n9.comment !== l ? r(dh(o, l), n9) : n9;
}
function mh(n9, o, l) {
let p = Ja(343, n9 != null ? n9 : Ut(ed(343)), l);
return p.typeExpression = o, p.locals = void 0, p.nextContainer = void 0, p;
}
function Db(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : fi(n9), l = arguments.length > 2 ? arguments[2] : void 0, p = arguments.length > 3 ? arguments[3] : void 0;
return n9.tagName !== o || n9.typeExpression !== l || n9.comment !== p ? r(mh(o, l, p), n9) : n9;
}
function hh(n9) {
let o = oe(324);
return o.text = n9, o;
}
function Qu(n9, o) {
return n9.text !== o ? r(hh(o), n9) : n9;
}
function gh(n9, o) {
let l = oe(323);
return l.comment = n9, l.tags = xt(o), l;
}
function yh(n9, o, l) {
return n9.comment !== o || n9.tags !== l ? r(gh(o, l), n9) : n9;
}
function Zu(n9, o, l) {
let p = oe(281);
return p.openingElement = n9, p.children = Ne(o), p.closingElement = l, p.transformFlags |= ye(p.openingElement) | gt(p.children) | ye(p.closingElement) | 2, p;
}
function kb(n9, o, l, p) {
return n9.openingElement !== o || n9.children !== l || n9.closingElement !== p ? r(Zu(o, l, p), n9) : n9;
}
function c_(n9, o, l) {
let p = oe(282);
return p.tagName = n9, p.typeArguments = xt(o), p.attributes = l, p.transformFlags |= ye(p.tagName) | gt(p.typeArguments) | ye(p.attributes) | 2, p.typeArguments && (p.transformFlags |= 1), p;
}
function vh(n9, o, l, p) {
return n9.tagName !== o || n9.typeArguments !== l || n9.attributes !== p ? r(c_(o, l, p), n9) : n9;
}
function bh(n9, o, l) {
let p = oe(283);
return p.tagName = n9, p.typeArguments = xt(o), p.attributes = l, p.transformFlags |= ye(p.tagName) | gt(p.typeArguments) | ye(p.attributes) | 2, o && (p.transformFlags |= 1), p;
}
function Ib(n9, o, l, p) {
return n9.tagName !== o || n9.typeArguments !== l || n9.attributes !== p ? r(bh(o, l, p), n9) : n9;
}
function on(n9) {
let o = oe(284);
return o.tagName = n9, o.transformFlags |= ye(o.tagName) | 2, o;
}
function Th(n9, o) {
return n9.tagName !== o ? r(on(o), n9) : n9;
}
function ep(n9, o, l) {
let p = oe(285);
return p.openingFragment = n9, p.children = Ne(o), p.closingFragment = l, p.transformFlags |= ye(p.openingFragment) | gt(p.children) | ye(p.closingFragment) | 2, p;
}
function Nb(n9, o, l, p) {
return n9.openingFragment !== o || n9.children !== l || n9.closingFragment !== p ? r(ep(o, l, p), n9) : n9;
}
function l_(n9, o) {
let l = oe(11);
return l.text = n9, l.containsOnlyTriviaWhiteSpaces = !!o, l.transformFlags |= 2, l;
}
function Ob(n9, o, l) {
return n9.text !== o || n9.containsOnlyTriviaWhiteSpaces !== l ? r(l_(o, l), n9) : n9;
}
function kc() {
let n9 = oe(286);
return n9.transformFlags |= 2, n9;
}
function Mb() {
let n9 = oe(287);
return n9.transformFlags |= 2, n9;
}
function Sh(n9, o) {
let l = Ve(288);
return l.name = n9, l.initializer = o, l.transformFlags |= ye(l.name) | ye(l.initializer) | 2, l;
}
function Lb(n9, o, l) {
return n9.name !== o || n9.initializer !== l ? r(Sh(o, l), n9) : n9;
}
function xh(n9) {
let o = Ve(289);
return o.properties = Ne(n9), o.transformFlags |= gt(o.properties) | 2, o;
}
function tp(n9, o) {
return n9.properties !== o ? r(xh(o), n9) : n9;
}
function no(n9) {
let o = oe(290);
return o.expression = n9, o.transformFlags |= ye(o.expression) | 2, o;
}
function Rb(n9, o) {
return n9.expression !== o ? r(no(o), n9) : n9;
}
function Ic(n9, o) {
let l = oe(291);
return l.dotDotDotToken = n9, l.expression = o, l.transformFlags |= ye(l.dotDotDotToken) | ye(l.expression) | 2, l;
}
function Eh(n9, o) {
return n9.expression !== o ? r(Ic(n9.dotDotDotToken, o), n9) : n9;
}
function wh(n9, o) {
let l = oe(292);
return l.expression = s().parenthesizeExpressionForDisallowedComma(n9), l.statements = Ne(o), l.transformFlags |= ye(l.expression) | gt(l.statements), l.jsDoc = void 0, l;
}
function rp(n9, o, l) {
return n9.expression !== o || n9.statements !== l ? r(wh(o, l), n9) : n9;
}
function np(n9) {
let o = oe(293);
return o.statements = Ne(n9), o.transformFlags = gt(o.statements), o;
}
function jb(n9, o) {
return n9.statements !== o ? r(np(o), n9) : n9;
}
function Ch(n9, o) {
let l = oe(294);
switch (l.token = n9, l.types = Ne(o), l.transformFlags |= gt(l.types), n9) {
case 94:
l.transformFlags |= 1024;
break;
case 117:
l.transformFlags |= 1;
break;
default:
return Y.assertNever(n9);
}
return l;
}
function Ah(n9, o) {
return n9.types !== o ? r(Ch(n9.token, o), n9) : n9;
}
function ip(n9, o) {
let l = oe(295);
return l.variableDeclaration = Xh(n9), l.block = o, l.transformFlags |= ye(l.variableDeclaration) | ye(l.block) | (n9 ? 0 : 64), l.locals = void 0, l.nextContainer = void 0, l;
}
function Ph(n9, o, l) {
return n9.variableDeclaration !== o || n9.block !== l ? r(ip(o, l), n9) : n9;
}
function Fa(n9, o) {
let l = Ve(299);
return l.name = Qt(n9), l.initializer = s().parenthesizeExpressionForDisallowedComma(o), l.transformFlags |= ai(l.name) | ye(l.initializer), l.modifiers = void 0, l.questionToken = void 0, l.exclamationToken = void 0, l.jsDoc = void 0, l;
}
function Jb(n9, o, l) {
return n9.name !== o || n9.initializer !== l ? Fb(Fa(o, l), n9) : n9;
}
function Fb(n9, o) {
return n9 !== o && (n9.modifiers = o.modifiers, n9.questionToken = o.questionToken, n9.exclamationToken = o.exclamationToken), r(n9, o);
}
function Dh(n9, o) {
let l = Ve(300);
return l.name = Qt(n9), l.objectAssignmentInitializer = o && s().parenthesizeExpressionForDisallowedComma(o), l.transformFlags |= ec(l.name) | ye(l.objectAssignmentInitializer) | 1024, l.equalsToken = void 0, l.modifiers = void 0, l.questionToken = void 0, l.exclamationToken = void 0, l.jsDoc = void 0, l;
}
function Bb(n9, o, l) {
return n9.name !== o || n9.objectAssignmentInitializer !== l ? kh(Dh(o, l), n9) : n9;
}
function kh(n9, o) {
return n9 !== o && (n9.modifiers = o.modifiers, n9.questionToken = o.questionToken, n9.exclamationToken = o.exclamationToken, n9.equalsToken = o.equalsToken), r(n9, o);
}
function ap(n9) {
let o = Ve(301);
return o.expression = s().parenthesizeExpressionForDisallowedComma(n9), o.transformFlags |= ye(o.expression) | 128 | 65536, o.jsDoc = void 0, o;
}
function ki(n9, o) {
return n9.expression !== o ? r(ap(o), n9) : n9;
}
function sp(n9, o) {
let l = Ve(302);
return l.name = Qt(n9), l.initializer = o && s().parenthesizeExpressionForDisallowedComma(o), l.transformFlags |= ye(l.name) | ye(l.initializer) | 1, l.jsDoc = void 0, l;
}
function qb(n9, o, l) {
return n9.name !== o || n9.initializer !== l ? r(sp(o, l), n9) : n9;
}
function Ub(n9, o, l) {
let p = t.createBaseSourceFileNode(308);
return p.statements = Ne(n9), p.endOfFileToken = o, p.flags |= l, p.text = "", p.fileName = "", p.path = "", p.resolvedPath = "", p.originalFileName = "", p.languageVersion = 0, p.languageVariant = 0, p.scriptKind = 0, p.isDeclarationFile = false, p.hasNoDefaultLib = false, p.transformFlags |= gt(p.statements) | ye(p.endOfFileToken), p.locals = void 0, p.nextContainer = void 0, p.endFlowNode = void 0, p.nodeCount = 0, p.identifierCount = 0, p.symbolCount = 0, p.parseDiagnostics = void 0, p.bindDiagnostics = void 0, p.bindSuggestionDiagnostics = void 0, p.lineMap = void 0, p.externalModuleIndicator = void 0, p.setExternalModuleIndicator = void 0, p.pragmas = void 0, p.checkJsDirective = void 0, p.referencedFiles = void 0, p.typeReferenceDirectives = void 0, p.libReferenceDirectives = void 0, p.amdDependencies = void 0, p.commentDirectives = void 0, p.identifiers = void 0, p.packageJsonLocations = void 0, p.packageJsonScope = void 0, p.imports = void 0, p.moduleAugmentations = void 0, p.ambientModuleNames = void 0, p.resolvedModules = void 0, p.classifiableNames = void 0, p.impliedNodeFormat = void 0, p;
}
function Ih(n9) {
let o = Object.create(n9.redirectTarget);
return Object.defineProperties(o, { id: { get() {
return this.redirectInfo.redirectTarget.id;
}, set(l) {
this.redirectInfo.redirectTarget.id = l;
} }, symbol: { get() {
return this.redirectInfo.redirectTarget.symbol;
}, set(l) {
this.redirectInfo.redirectTarget.symbol = l;
} } }), o.redirectInfo = n9, o;
}
function Nh(n9) {
let o = Ih(n9.redirectInfo);
return o.flags |= n9.flags & -9, o.fileName = n9.fileName, o.path = n9.path, o.resolvedPath = n9.resolvedPath, o.originalFileName = n9.originalFileName, o.packageJsonLocations = n9.packageJsonLocations, o.packageJsonScope = n9.packageJsonScope, o.emitNode = void 0, o;
}
function op(n9) {
let o = t.createBaseSourceFileNode(308);
o.flags |= n9.flags & -9;
for (let l in n9)
if (!(Jr(o, l) || !Jr(n9, l))) {
if (l === "emitNode") {
o.emitNode = void 0;
continue;
}
o[l] = n9[l];
}
return o;
}
function Oh(n9) {
let o = n9.redirectInfo ? Nh(n9) : op(n9);
return Dn(o, n9), o;
}
function zb(n9, o, l, p, k, V, we) {
let et = Oh(n9);
return et.statements = Ne(o), et.isDeclarationFile = l, et.referencedFiles = p, et.typeReferenceDirectives = k, et.hasNoDefaultLib = V, et.libReferenceDirectives = we, et.transformFlags = gt(et.statements) | ye(et.endOfFileToken), et;
}
function Mh(n9, o) {
let l = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : n9.isDeclarationFile, p = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : n9.referencedFiles, k = arguments.length > 4 && arguments[4] !== void 0 ? arguments[4] : n9.typeReferenceDirectives, V = arguments.length > 5 && arguments[5] !== void 0 ? arguments[5] : n9.hasNoDefaultLib, we = arguments.length > 6 && arguments[6] !== void 0 ? arguments[6] : n9.libReferenceDirectives;
return n9.statements !== o || n9.isDeclarationFile !== l || n9.referencedFiles !== p || n9.typeReferenceDirectives !== k || n9.hasNoDefaultLib !== V || n9.libReferenceDirectives !== we ? r(zb(n9, o, l, p, k, V, we), n9) : n9;
}
function Lh(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : Bt, l = oe(309);
return l.prepends = o, l.sourceFiles = n9, l.syntheticFileReferences = void 0, l.syntheticTypeReferences = void 0, l.syntheticLibReferences = void 0, l.hasNoDefaultLib = void 0, l;
}
function Wb(n9, o) {
let l = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : Bt;
return n9.sourceFiles !== o || n9.prepends !== l ? r(Lh(o, l), n9) : n9;
}
function Nc(n9, o, l) {
let p = oe(310);
return p.prologues = n9, p.syntheticReferences = o, p.texts = l, p.fileName = "", p.text = "", p.referencedFiles = Bt, p.libReferenceDirectives = Bt, p.getLineAndCharacterOfPosition = (k) => Ls(p, k), p;
}
function Oc(n9, o) {
let l = oe(n9);
return l.data = o, l;
}
function Vb(n9) {
return Oc(303, n9);
}
function Hb(n9, o) {
let l = Oc(304, n9);
return l.texts = o, l;
}
function Gb(n9, o) {
return Oc(o ? 306 : 305, n9);
}
function $b(n9) {
let o = oe(307);
return o.data = n9.data, o.section = n9, o;
}
function Kb() {
let n9 = oe(311);
return n9.javascriptText = "", n9.declarationText = "", n9;
}
function Rh(n9) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false, l = arguments.length > 2 ? arguments[2] : void 0, p = oe(234);
return p.type = n9, p.isSpread = o, p.tupleNameSource = l, p;
}
function jh(n9) {
let o = oe(354);
return o._children = n9, o;
}
function Jh(n9) {
let o = oe(355);
return o.original = n9, Rt(o, n9), o;
}
function Fh(n9, o) {
let l = oe(356);
return l.expression = n9, l.original = o, l.transformFlags |= ye(l.expression) | 1, Rt(l, o), l;
}
function Bh(n9, o) {
return n9.expression !== o ? r(Fh(o, n9.original), n9) : n9;
}
function qh(n9) {
if (fs2(n9) && !pl(n9) && !n9.original && !n9.emitNode && !n9.id) {
if (oc(n9))
return n9.elements;
if (ur(n9) && I8(n9.operatorToken))
return [n9.left, n9.right];
}
return n9;
}
function Mc(n9) {
let o = oe(357);
return o.elements = Ne(at(n9, qh)), o.transformFlags |= gt(o.elements), o;
}
function Xb(n9, o) {
return n9.elements !== o ? r(Mc(o), n9) : n9;
}
function Yb(n9) {
let o = oe(359);
return o.emitNode = {}, o.original = n9, o;
}
function Qb(n9) {
let o = oe(358);
return o.emitNode = {}, o.original = n9, o;
}
function Uh(n9, o) {
let l = oe(360);
return l.expression = n9, l.thisArg = o, l.transformFlags |= ye(l.expression) | ye(l.thisArg), l;
}
function _p(n9, o, l) {
return n9.expression !== o || n9.thisArg !== l ? r(Uh(o, l), n9) : n9;
}
function Zb(n9) {
let o = pn(n9.escapedText);
return o.flags |= n9.flags & -9, o.transformFlags = n9.transformFlags, Dn(o, n9), setIdentifierAutoGenerate(o, Object.assign({}, n9.emitNode.autoGenerate)), o;
}
function e6(n9) {
let o = pn(n9.escapedText);
o.flags |= n9.flags & -9, o.jsDoc = n9.jsDoc, o.flowNode = n9.flowNode, o.symbol = n9.symbol, o.transformFlags = n9.transformFlags, Dn(o, n9);
let l = getIdentifierTypeArguments(n9);
return l && setIdentifierTypeArguments(o, l), o;
}
function t6(n9) {
let o = dn(n9.escapedText);
return o.flags |= n9.flags & -9, o.transformFlags = n9.transformFlags, Dn(o, n9), setIdentifierAutoGenerate(o, Object.assign({}, n9.emitNode.autoGenerate)), o;
}
function r6(n9) {
let o = dn(n9.escapedText);
return o.flags |= n9.flags & -9, o.transformFlags = n9.transformFlags, Dn(o, n9), o;
}
function cp(n9) {
if (n9 === void 0)
return n9;
if (wi(n9))
return Oh(n9);
if (cs(n9))
return Zb(n9);
if (yt(n9))
return e6(n9);
if (Ny(n9))
return t6(n9);
if (vn(n9))
return r6(n9);
let o = gl(n9.kind) ? t.createBaseNode(n9.kind) : t.createBaseTokenNode(n9.kind);
o.flags |= n9.flags & -9, o.transformFlags = n9.transformFlags, Dn(o, n9);
for (let l in n9)
Jr(o, l) || !Jr(n9, l) || (o[l] = n9[l]);
return o;
}
function n6(n9, o, l) {
return Na(yu(void 0, void 0, void 0, void 0, o ? [o] : [], void 0, Zs(n9, true)), void 0, l ? [l] : []);
}
function Lc(n9, o, l) {
return Na(vu(void 0, void 0, o ? [o] : [], void 0, void 0, Zs(n9, true)), void 0, l ? [l] : []);
}
function Rc() {
return ui(Gt("0"));
}
function zh(n9) {
return zu(void 0, false, n9);
}
function i6(n9) {
return na(void 0, false, to([Vu(false, void 0, n9)]));
}
function a6(n9, o) {
return o === "undefined" ? Ye.createStrictEquality(n9, Rc()) : Ye.createStrictEquality(mn(n9), er(o));
}
function Ba(n9, o, l) {
return Cy(n9) ? du(Xs(n9, void 0, o), void 0, void 0, l) : Na(ta(n9, o), void 0, l);
}
function s6(n9, o, l) {
return Ba(n9, "bind", [o, ...l]);
}
function o6(n9, o, l) {
return Ba(n9, "call", [o, ...l]);
}
function _6(n9, o, l) {
return Ba(n9, "apply", [o, l]);
}
function io(n9, o, l) {
return Ba(Ut(n9), o, l);
}
function Wh(n9, o) {
return Ba(n9, "slice", o === void 0 ? [] : [za(o)]);
}
function Vh(n9, o) {
return Ba(n9, "concat", o);
}
function u(n9, o, l) {
return io("Object", "defineProperty", [n9, za(o), l]);
}
function b(n9, o) {
return io("Object", "getOwnPropertyDescriptor", [n9, za(o)]);
}
function O(n9, o, l) {
return io("Reflect", "get", l ? [n9, o, l] : [n9, o]);
}
function j(n9, o, l, p) {
return io("Reflect", "set", p ? [n9, o, l, p] : [n9, o, l]);
}
function z(n9, o, l) {
return l ? (n9.push(Fa(o, l)), true) : false;
}
function re(n9, o) {
let l = [];
z(l, "enumerable", za(n9.enumerable)), z(l, "configurable", za(n9.configurable));
let p = z(l, "writable", za(n9.writable));
p = z(l, "value", n9.value) || p;
let k = z(l, "get", n9.get);
return k = z(l, "set", n9.set) || k, Y.assert(!(p && k), "A PropertyDescriptor may not be both an accessor descriptor and a data descriptor."), r_(l, !o);
}
function Ee(n9, o) {
switch (n9.kind) {
case 214:
return qd(n9, o);
case 213:
return Bd(n9, n9.type, o);
case 231:
return Zd(n9, o, n9.type);
case 235:
return Pu(n9, o, n9.type);
case 232:
return Au(n9, o);
case 356:
return Bh(n9, o);
}
}
function qe(n9) {
return qo(n9) && fs2(n9) && fs2(getSourceMapRange(n9)) && fs2(getCommentRange(n9)) && !Ke(getSyntheticLeadingComments(n9)) && !Ke(getSyntheticTrailingComments(n9));
}
function We(n9, o) {
let l = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : 15;
return n9 && yd(n9, l) && !qe(n9) ? Ee(n9, We(n9.expression, o)) : o;
}
function $e(n9, o, l) {
if (!o)
return n9;
let p = ym(o, o.label, tE(o.statement) ? $e(n9, o.statement) : n9);
return l && l(o), p;
}
function lt(n9, o) {
let l = Pl(n9);
switch (l.kind) {
case 79:
return o;
case 108:
case 8:
case 9:
case 10:
return false;
case 206:
return l.elements.length !== 0;
case 207:
return l.properties.length > 0;
default:
return true;
}
}
function Jt(n9, o, l) {
let p = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : false, k = $o(n9, 15), V, we;
return Sf(k) ? (V = Or(), we = k) : nd(k) ? (V = Or(), we = l !== void 0 && l < 2 ? Rt(Ut("_super"), k) : k) : xi(k) & 8192 ? (V = Rc(), we = s().parenthesizeLeftSideOfAccess(k, false)) : bn(k) ? lt(k.expression, p) ? (V = kn(o), we = ta(Rt(Ye.createAssignment(V, k.expression), k.expression), k.name), Rt(we, k)) : (V = k.expression, we = k) : gs(k) ? lt(k.expression, p) ? (V = kn(o), we = pu(Rt(Ye.createAssignment(V, k.expression), k.expression), k.argumentExpression), Rt(we, k)) : (V = k.expression, we = k) : (V = Rc(), we = s().parenthesizeLeftSideOfAccess(n9, false)), { target: we, thisArg: V };
}
function Lt(n9, o) {
return ta(gu(r_([ci(void 0, "value", [$n(void 0, void 0, n9, void 0, void 0, void 0)], Zs([a_(o)]))])), "value");
}
function At(n9) {
return n9.length > 10 ? Mc(n9) : Qa(n9, Ye.createComma);
}
function kr(n9, o, l) {
let p = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : 0, k = ml(n9);
if (k && yt(k) && !cs(k)) {
let V = Sa(Rt(cp(k), k), k.parent);
return p |= xi(k), l || (p |= 96), o || (p |= 3072), p && setEmitFlags(V, p), V;
}
return $i(n9);
}
function Fn(n9, o, l) {
return kr(n9, o, l, 98304);
}
function di(n9, o, l) {
return kr(n9, o, l, 32768);
}
function Ii(n9, o, l) {
return kr(n9, o, l, 16384);
}
function _n(n9, o, l) {
return kr(n9, o, l);
}
function qa(n9, o, l, p) {
let k = ta(n9, fs2(o) ? o : cp(o));
Rt(k, o);
let V = 0;
return p || (V |= 96), l || (V |= 3072), V && setEmitFlags(k, V), k;
}
function Hh(n9, o, l, p) {
return n9 && rn(o, 1) ? qa(n9, kr(o), l, p) : Ii(o, l, p);
}
function lp(n9, o, l, p) {
let k = Ua(n9, o, 0, l);
return up(n9, o, k, p);
}
function Gh(n9) {
return Gn(n9.expression) && n9.expression.text === "use strict";
}
function wn() {
return vd(a_(er("use strict")));
}
function Ua(n9, o) {
let l = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : 0, p = arguments.length > 3 ? arguments[3] : void 0;
Y.assert(o.length === 0, "Prologue directives should be at the first statement in the target statements array");
let k = false, V = n9.length;
for (; l < V; ) {
let we = n9[l];
if (us(we))
Gh(we) && (k = true), o.push(we);
else
break;
l++;
}
return p && !k && o.push(wn()), l;
}
function up(n9, o, l, p) {
let k = arguments.length > 4 && arguments[4] !== void 0 ? arguments[4] : vp, V = n9.length;
for (; l !== void 0 && l < V; ) {
let we = n9[l];
if (xi(we) & 2097152 && k(we))
tr(o, p ? visitNode(we, p, a3) : we);
else
break;
l++;
}
return l;
}
function Qr(n9) {
return TE(n9) ? n9 : Rt(Ne([wn(), ...n9]), n9);
}
function jc(n9) {
return Y.assert(me(n9, s3), "Cannot lift nodes to a Block."), Xa(n9) || Zs(n9);
}
function Cs(n9, o, l) {
let p = l;
for (; p < n9.length && o(n9[p]); )
p++;
return p;
}
function $h(n9, o) {
if (!Ke(o))
return n9;
let l = Cs(n9, us, 0), p = Cs(n9, _0, l), k = Cs(n9, c0, p), V = Cs(o, us, 0), we = Cs(o, _0, V), et = Cs(o, c0, we), ht = Cs(o, Tf, et);
Y.assert(ht === o.length, "Expected declarations to be valid standard or custom prologues");
let hn = _s(n9) ? n9.slice() : n9;
if (ht > et && hn.splice(k, 0, ...o.slice(et, ht)), et > we && hn.splice(p, 0, ...o.slice(we, et)), we > V && hn.splice(l, 0, ...o.slice(V, we)), V > 0)
if (l === 0)
hn.splice(0, 0, ...o.slice(0, V));
else {
let Ni = /* @__PURE__ */ new Map();
for (let ia = 0; ia < l; ia++) {
let Oi = n9[ia];
Ni.set(Oi.expression.text, true);
}
for (let ia = V - 1; ia >= 0; ia--) {
let Oi = o[ia];
Ni.has(Oi.expression.text) || hn.unshift(Oi);
}
}
return _s(n9) ? Rt(Ne(hn, n9.hasTrailingComma), n9) : n9;
}
function Kh(n9, o) {
var l;
let p;
return typeof o == "number" ? p = $r(o) : p = o, Fo(n9) ? wa(n9, p, n9.name, n9.constraint, n9.default) : Vs(n9) ? Ki(n9, p, n9.dotDotDotToken, n9.name, n9.questionToken, n9.type, n9.initializer) : Gv(n9) ? bt(n9, p, n9.typeParameters, n9.parameters, n9.type) : Wl(n9) ? St(n9, p, n9.name, n9.questionToken, n9.type) : Bo(n9) ? _t(n9, p, n9.name, (l = n9.questionToken) != null ? l : n9.exclamationToken, n9.type, n9.initializer) : L8(n9) ? Kt(n9, p, n9.name, n9.questionToken, n9.typeParameters, n9.parameters, n9.type) : Vl(n9) ? xe(n9, p, n9.asteriskToken, n9.name, n9.questionToken, n9.typeParameters, n9.parameters, n9.type, n9.body) : nc(n9) ? It(n9, p, n9.parameters, n9.body) : Gl(n9) ? Ln(n9, p, n9.name, n9.parameters, n9.type, n9.body) : ic(n9) ? Xi(n9, p, n9.name, n9.parameters, n9.body) : Hv(n9) ? bs(n9, p, n9.parameters, n9.type) : ad(n9) ? Ud(n9, p, n9.asteriskToken, n9.name, n9.typeParameters, n9.parameters, n9.type, n9.body) : sd(n9) ? zd(n9, p, n9.typeParameters, n9.parameters, n9.type, n9.equalsGreaterThanToken, n9.body) : _d(n9) ? xc(n9, p, n9.name, n9.typeParameters, n9.heritageClauses, n9.members) : zo(n9) ? om(n9, p, n9.declarationList) : Wo(n9) ? ju(n9, p, n9.asteriskToken, n9.name, n9.typeParameters, n9.parameters, n9.type, n9.body) : _c(n9) ? Ju(n9, p, n9.name, n9.typeParameters, n9.heritageClauses, n9.members) : eu(n9) ? Cm(n9, p, n9.name, n9.typeParameters, n9.heritageClauses, n9.members) : n2(n9) ? Ma(n9, p, n9.name, n9.typeParameters, n9.type) : i2(n9) ? La(n9, p, n9.name, n9.members) : Ea(n9) ? Sr(n9, p, n9.name, n9.body) : s2(n9) ? Nm(n9, p, n9.isTypeOnly, n9.name, n9.moduleReference) : o2(n9) ? Mm(n9, p, n9.importClause, n9.moduleSpecifier, n9.assertClause) : Vo(n9) ? Wu(n9, p, n9.expression) : cc(n9) ? Wm(n9, p, n9.isTypeOnly, n9.exportClause, n9.moduleSpecifier, n9.assertClause) : Y.assertNever(n9);
}
function xt(n9) {
return n9 ? Ne(n9) : void 0;
}
function Qt(n9) {
return typeof n9 == "string" ? Ut(n9) : n9;
}
function za(n9) {
return typeof n9 == "string" ? er(n9) : typeof n9 == "number" ? Gt(n9) : typeof n9 == "boolean" ? n9 ? ar() : oi() : n9;
}
function Wa(n9) {
return n9 && s().parenthesizeExpressionForDisallowedComma(n9);
}
function c6(n9) {
return typeof n9 == "number" ? pr(n9) : n9;
}
function Yn(n9) {
return n9 && c2(n9) ? Rt(Dn(Du(), n9), n9) : n9;
}
function Xh(n9) {
return typeof n9 == "string" || n9 && !Vi(n9) ? Cc(n9, void 0, void 0, void 0) : n9;
}
}
function JL(e, t) {
return e !== t && Rt(e, t), e;
}
function FL(e, t) {
return e !== t && (Dn(e, t), Rt(e, t)), e;
}
function ed(e) {
switch (e) {
case 347:
return "type";
case 345:
return "returns";
case 346:
return "this";
case 343:
return "enum";
case 333:
return "author";
case 335:
return "class";
case 336:
return "public";
case 337:
return "private";
case 338:
return "protected";
case 339:
return "readonly";
case 340:
return "override";
case 348:
return "template";
case 349:
return "typedef";
case 344:
return "param";
case 351:
return "prop";
case 341:
return "callback";
case 342:
return "overload";
case 331:
return "augments";
case 332:
return "implements";
default:
return Y.fail(`Unsupported kind: ${Y.formatSyntaxKind(e)}`);
}
}
function BL(e, t) {
switch (Hn || (Hn = Po(99, false, 0)), e) {
case 14:
Hn.setText("`" + t + "`");
break;
case 15:
Hn.setText("`" + t + "${");
break;
case 16:
Hn.setText("}" + t + "${");
break;
case 17:
Hn.setText("}" + t + "`");
break;
}
let r = Hn.scan();
if (r === 19 && (r = Hn.reScanTemplateToken(false)), Hn.isUnterminated())
return Hn.setText(void 0), qv;
let s;
switch (r) {
case 14:
case 15:
case 16:
case 17:
s = Hn.getTokenValue();
break;
}
return s === void 0 || Hn.scan() !== 1 ? (Hn.setText(void 0), qv) : (Hn.setText(void 0), s);
}
function ai(e) {
return e && yt(e) ? ec(e) : ye(e);
}
function ec(e) {
return ye(e) & -67108865;
}
function qL(e, t) {
return t | e.transformFlags & 134234112;
}
function ye(e) {
if (!e)
return 0;
let t = e.transformFlags & ~w8(e.kind);
return af(e) && vl(e.name) ? qL(e.name, t) : t;
}
function gt(e) {
return e ? e.transformFlags : 0;
}
function E8(e) {
let t = 0;
for (let r of e)
t |= ye(r);
e.transformFlags = t;
}
function w8(e) {
if (e >= 179 && e <= 202)
return -2;
switch (e) {
case 210:
case 211:
case 206:
return -2147450880;
case 264:
return -1941676032;
case 166:
return -2147483648;
case 216:
return -2072174592;
case 215:
case 259:
return -1937940480;
case 258:
return -2146893824;
case 260:
case 228:
return -2147344384;
case 173:
return -1937948672;
case 169:
return -2013249536;
case 171:
case 174:
case 175:
return -2005057536;
case 131:
case 148:
case 160:
case 144:
case 152:
case 149:
case 134:
case 153:
case 114:
case 165:
case 168:
case 170:
case 176:
case 177:
case 178:
case 261:
case 262:
return -2;
case 207:
return -2147278848;
case 295:
return -2147418112;
case 203:
case 204:
return -2147450880;
case 213:
case 235:
case 231:
case 356:
case 214:
case 106:
return -2147483648;
case 208:
case 209:
return -2147483648;
default:
return -2147483648;
}
}
function Fl(e) {
return e.flags |= 8, e;
}
function UL(e, t, r) {
let s, f, x, w, A, g, B, N, X, F;
Ji(e) ? (x = "", w = e, A = e.length, g = t, B = r) : (Y.assert(t === "js" || t === "dts"), x = (t === "js" ? e.javascriptPath : e.declarationPath) || "", g = t === "js" ? e.javascriptMapPath : e.declarationMapPath, N = () => t === "js" ? e.javascriptText : e.declarationText, X = () => t === "js" ? e.javascriptMapText : e.declarationMapText, A = () => N().length, e.buildInfo && e.buildInfo.bundle && (Y.assert(r === void 0 || typeof r == "boolean"), s = r, f = t === "js" ? e.buildInfo.bundle.js : e.buildInfo.bundle.dts, F = e.oldFileOfCurrentEmit));
let $ = F ? WL(Y.checkDefined(f)) : zL(f, s, A);
return $.fileName = x, $.sourceMapPath = g, $.oldFileOfCurrentEmit = F, N && X ? (Object.defineProperty($, "text", { get: N }), Object.defineProperty($, "sourceMapText", { get: X })) : (Y.assert(!F), $.text = w != null ? w : "", $.sourceMapText = B), $;
}
function zL(e, t, r) {
let s, f, x, w, A, g, B, N;
for (let F of e ? e.sections : Bt)
switch (F.kind) {
case "prologue":
s = tr(s, Rt(si.createUnparsedPrologue(F.data), F));
break;
case "emitHelpers":
f = tr(f, getAllUnscopedEmitHelpers().get(F.data));
break;
case "no-default-lib":
N = true;
break;
case "reference":
x = tr(x, { pos: -1, end: -1, fileName: F.data });
break;
case "type":
w = tr(w, { pos: -1, end: -1, fileName: F.data });
break;
case "type-import":
w = tr(w, { pos: -1, end: -1, fileName: F.data, resolutionMode: 99 });
break;
case "type-require":
w = tr(w, { pos: -1, end: -1, fileName: F.data, resolutionMode: 1 });
break;
case "lib":
A = tr(A, { pos: -1, end: -1, fileName: F.data });
break;
case "prepend":
let $;
for (let ae of F.texts)
(!t || ae.kind !== "internal") && ($ = tr($, Rt(si.createUnparsedTextLike(ae.data, ae.kind === "internal"), ae)));
g = jr(g, $), B = tr(B, si.createUnparsedPrepend(F.data, $ != null ? $ : Bt));
break;
case "internal":
if (t) {
B || (B = []);
break;
}
case "text":
B = tr(B, Rt(si.createUnparsedTextLike(F.data, F.kind === "internal"), F));
break;
default:
Y.assertNever(F);
}
if (!B) {
let F = si.createUnparsedTextLike(void 0, false);
$f(F, 0, typeof r == "function" ? r() : r), B = [F];
}
let X = dc.createUnparsedSource(s != null ? s : Bt, void 0, B);
return Q_(s, X), Q_(B, X), Q_(g, X), X.hasNoDefaultLib = N, X.helpers = f, X.referencedFiles = x || Bt, X.typeReferenceDirectives = w, X.libReferenceDirectives = A || Bt, X;
}
function WL(e) {
let t, r;
for (let f of e.sections)
switch (f.kind) {
case "internal":
case "text":
t = tr(t, Rt(si.createUnparsedTextLike(f.data, f.kind === "internal"), f));
break;
case "no-default-lib":
case "reference":
case "type":
case "type-import":
case "type-require":
case "lib":
r = tr(r, Rt(si.createUnparsedSyntheticReference(f), f));
break;
case "prologue":
case "emitHelpers":
case "prepend":
break;
default:
Y.assertNever(f);
}
let s = si.createUnparsedSource(Bt, r, t != null ? t : Bt);
return Q_(r, s), Q_(t, s), s.helpers = Ze(e.sources && e.sources.helpers, (f) => getAllUnscopedEmitHelpers().get(f)), s;
}
function VL(e, t, r, s, f, x) {
return Ji(e) ? A8(void 0, e, r, s, void 0, t, f, x) : C8(e, t, r, s, f, x);
}
function C8(e, t, r, s, f, x, w, A) {
let g = dc.createInputFiles();
g.javascriptPath = t, g.javascriptMapPath = r, g.declarationPath = s, g.declarationMapPath = f, g.buildInfoPath = x;
let B = /* @__PURE__ */ new Map(), N = (ae) => {
if (ae === void 0)
return;
let Te = B.get(ae);
return Te === void 0 && (Te = e(ae), B.set(ae, Te !== void 0 ? Te : false)), Te !== false ? Te : void 0;
}, X = (ae) => {
let Te = N(ae);
return Te !== void 0 ? Te : `/* Input file ${ae} was missing */\r
`;
}, F;
return Object.defineProperties(g, { javascriptText: { get: () => X(t) }, javascriptMapText: { get: () => N(r) }, declarationText: { get: () => X(Y.checkDefined(s)) }, declarationMapText: { get: () => N(f) }, buildInfo: { get: () => {
var ae, Te;
if (F === void 0 && x)
if (w != null && w.getBuildInfo)
F = (ae = w.getBuildInfo(x, A.configFilePath)) != null ? ae : false;
else {
let Se = N(x);
F = Se !== void 0 && (Te = getBuildInfo(x, Se)) != null ? Te : false;
}
return F || void 0;
} } }), g;
}
function A8(e, t, r, s, f, x, w, A, g, B, N) {
let X = dc.createInputFiles();
return X.javascriptPath = e, X.javascriptText = t, X.javascriptMapPath = r, X.javascriptMapText = s, X.declarationPath = f, X.declarationText = x, X.declarationMapPath = w, X.declarationMapText = A, X.buildInfoPath = g, X.buildInfo = B, X.oldFileOfCurrentEmit = N, X;
}
function HL(e, t, r) {
return new (D8 || (D8 = lr.getSourceMapSourceConstructor()))(e, t, r);
}
function Dn(e, t) {
if (e.original = t, t) {
let r = t.emitNode;
r && (e.emitNode = GL(r, e.emitNode));
}
return e;
}
function GL(e, t) {
let { flags: r, internalFlags: s, leadingComments: f, trailingComments: x, commentRange: w, sourceMapRange: A, tokenSourceMapRanges: g, constantValue: B, helpers: N, startsOnNewLine: X, snippetElement: F } = e;
if (t || (t = {}), f && (t.leadingComments = jr(f.slice(), t.leadingComments)), x && (t.trailingComments = jr(x.slice(), t.trailingComments)), r && (t.flags = r), s && (t.internalFlags = s & -9), w && (t.commentRange = w), A && (t.sourceMapRange = A), g && (t.tokenSourceMapRanges = $L(g, t.tokenSourceMapRanges)), B !== void 0 && (t.constantValue = B), N)
for (let $ of N)
t.helpers = g_(t.helpers, $);
return X !== void 0 && (t.startsOnNewLine = X), F !== void 0 && (t.snippetElement = F), t;
}
function $L(e, t) {
t || (t = []);
for (let r in e)
t[r] = e[r];
return t;
}
var Bl, Fv, Bv, Hn, qv, tc, P8, si, D8, KL = D({ "src/compiler/factory/nodeFactory.ts"() {
"use strict";
nn(), Bl = 0, Fv = ((e) => (e[e.None = 0] = "None", e[e.NoParenthesizerRules = 1] = "NoParenthesizerRules", e[e.NoNodeConverters = 2] = "NoNodeConverters", e[e.NoIndentationOnFreshPropertyAccess = 4] = "NoIndentationOnFreshPropertyAccess", e[e.NoOriginalNode = 8] = "NoOriginalNode", e))(Fv || {}), Bv = [], qv = {}, tc = S8(), P8 = { createBaseSourceFileNode: (e) => Fl(tc.createBaseSourceFileNode(e)), createBaseIdentifierNode: (e) => Fl(tc.createBaseIdentifierNode(e)), createBasePrivateIdentifierNode: (e) => Fl(tc.createBasePrivateIdentifierNode(e)), createBaseTokenNode: (e) => Fl(tc.createBaseTokenNode(e)), createBaseNode: (e) => Fl(tc.createBaseNode(e)) }, si = Zf(4, P8);
} }), XL = () => {
}, YL = () => {
};
function zs(e) {
return e.kind === 8;
}
function Uv(e) {
return e.kind === 9;
}
function Gn(e) {
return e.kind === 10;
}
function td(e) {
return e.kind === 11;
}
function QL(e) {
return e.kind === 13;
}
function k8(e) {
return e.kind === 14;
}
function ZL(e) {
return e.kind === 15;
}
function eR(e) {
return e.kind === 16;
}
function tR(e) {
return e.kind === 17;
}
function rR(e) {
return e.kind === 25;
}
function I8(e) {
return e.kind === 27;
}
function zv(e) {
return e.kind === 39;
}
function Wv(e) {
return e.kind === 40;
}
function nR(e) {
return e.kind === 41;
}
function rd(e) {
return e.kind === 53;
}
function ql(e) {
return e.kind === 57;
}
function iR(e) {
return e.kind === 58;
}
function aR(e) {
return e.kind === 28;
}
function sR(e) {
return e.kind === 38;
}
function yt(e) {
return e.kind === 79;
}
function vn(e) {
return e.kind === 80;
}
function N8(e) {
return e.kind === 93;
}
function oR(e) {
return e.kind === 88;
}
function Ul(e) {
return e.kind === 132;
}
function _R(e) {
return e.kind === 129;
}
function cR(e) {
return e.kind === 133;
}
function O8(e) {
return e.kind === 146;
}
function lR(e) {
return e.kind === 124;
}
function uR(e) {
return e.kind === 126;
}
function pR(e) {
return e.kind === 161;
}
function fR(e) {
return e.kind === 127;
}
function nd(e) {
return e.kind === 106;
}
function M8(e) {
return e.kind === 100;
}
function dR(e) {
return e.kind === 82;
}
function rc(e) {
return e.kind === 163;
}
function Ws(e) {
return e.kind === 164;
}
function Fo(e) {
return e.kind === 165;
}
function Vs(e) {
return e.kind === 166;
}
function zl(e) {
return e.kind === 167;
}
function Wl(e) {
return e.kind === 168;
}
function Bo(e) {
return e.kind === 169;
}
function L8(e) {
return e.kind === 170;
}
function Vl(e) {
return e.kind === 171;
}
function Hl(e) {
return e.kind === 172;
}
function nc(e) {
return e.kind === 173;
}
function Gl(e) {
return e.kind === 174;
}
function ic(e) {
return e.kind === 175;
}
function Vv(e) {
return e.kind === 176;
}
function R8(e) {
return e.kind === 177;
}
function Hv(e) {
return e.kind === 178;
}
function j8(e) {
return e.kind === 179;
}
function ac(e) {
return e.kind === 180;
}
function $l(e) {
return e.kind === 181;
}
function Gv(e) {
return e.kind === 182;
}
function J8(e) {
return e.kind === 183;
}
function id(e) {
return e.kind === 184;
}
function F8(e) {
return e.kind === 185;
}
function B8(e) {
return e.kind === 186;
}
function $v(e) {
return e.kind === 199;
}
function q8(e) {
return e.kind === 187;
}
function U8(e) {
return e.kind === 188;
}
function z8(e) {
return e.kind === 189;
}
function W8(e) {
return e.kind === 190;
}
function V8(e) {
return e.kind === 191;
}
function H8(e) {
return e.kind === 192;
}
function Kv(e) {
return e.kind === 193;
}
function Xv(e) {
return e.kind === 194;
}
function G8(e) {
return e.kind === 195;
}
function $8(e) {
return e.kind === 196;
}
function K8(e) {
return e.kind === 197;
}
function Yv(e) {
return e.kind === 198;
}
function Kl(e) {
return e.kind === 202;
}
function mR(e) {
return e.kind === 201;
}
function hR(e) {
return e.kind === 200;
}
function gR(e) {
return e.kind === 203;
}
function yR(e) {
return e.kind === 204;
}
function Xl(e) {
return e.kind === 205;
}
function Yl(e) {
return e.kind === 206;
}
function Hs(e) {
return e.kind === 207;
}
function bn(e) {
return e.kind === 208;
}
function gs(e) {
return e.kind === 209;
}
function sc(e) {
return e.kind === 210;
}
function X8(e) {
return e.kind === 211;
}
function Y8(e) {
return e.kind === 212;
}
function vR(e) {
return e.kind === 213;
}
function qo(e) {
return e.kind === 214;
}
function ad(e) {
return e.kind === 215;
}
function sd(e) {
return e.kind === 216;
}
function bR(e) {
return e.kind === 217;
}
function TR(e) {
return e.kind === 218;
}
function Qv(e) {
return e.kind === 219;
}
function SR(e) {
return e.kind === 220;
}
function od(e) {
return e.kind === 221;
}
function Q8(e) {
return e.kind === 222;
}
function ur(e) {
return e.kind === 223;
}
function xR(e) {
return e.kind === 224;
}
function ER(e) {
return e.kind === 225;
}
function wR(e) {
return e.kind === 226;
}
function Zv(e) {
return e.kind === 227;
}
function _d(e) {
return e.kind === 228;
}
function cd(e) {
return e.kind === 229;
}
function e2(e) {
return e.kind === 230;
}
function CR(e) {
return e.kind === 231;
}
function AR(e) {
return e.kind === 235;
}
function Uo(e) {
return e.kind === 232;
}
function t2(e) {
return e.kind === 233;
}
function PR(e) {
return e.kind === 234;
}
function Z8(e) {
return e.kind === 356;
}
function oc(e) {
return e.kind === 357;
}
function DR(e) {
return e.kind === 236;
}
function kR(e) {
return e.kind === 237;
}
function Ql(e) {
return e.kind === 238;
}
function zo(e) {
return e.kind === 240;
}
function IR(e) {
return e.kind === 239;
}
function Zl(e) {
return e.kind === 241;
}
function NR(e) {
return e.kind === 242;
}
function OR(e) {
return e.kind === 243;
}
function MR(e) {
return e.kind === 244;
}
function eE(e) {
return e.kind === 245;
}
function LR(e) {
return e.kind === 246;
}
function RR(e) {
return e.kind === 247;
}
function jR(e) {
return e.kind === 248;
}
function JR(e) {
return e.kind === 249;
}
function FR(e) {
return e.kind === 250;
}
function BR(e) {
return e.kind === 251;
}
function qR(e) {
return e.kind === 252;
}
function tE(e) {
return e.kind === 253;
}
function UR(e) {
return e.kind === 254;
}
function zR(e) {
return e.kind === 255;
}
function WR(e) {
return e.kind === 256;
}
function Vi(e) {
return e.kind === 257;
}
function r2(e) {
return e.kind === 258;
}
function Wo(e) {
return e.kind === 259;
}
function _c(e) {
return e.kind === 260;
}
function eu(e) {
return e.kind === 261;
}
function n2(e) {
return e.kind === 262;
}
function i2(e) {
return e.kind === 263;
}
function Ea(e) {
return e.kind === 264;
}
function rE(e) {
return e.kind === 265;
}
function VR(e) {
return e.kind === 266;
}
function a2(e) {
return e.kind === 267;
}
function s2(e) {
return e.kind === 268;
}
function o2(e) {
return e.kind === 269;
}
function HR(e) {
return e.kind === 270;
}
function GR(e) {
return e.kind === 298;
}
function $R(e) {
return e.kind === 296;
}
function KR(e) {
return e.kind === 297;
}
function _2(e) {
return e.kind === 271;
}
function ld(e) {
return e.kind === 277;
}
function XR(e) {
return e.kind === 272;
}
function nE(e) {
return e.kind === 273;
}
function Vo(e) {
return e.kind === 274;
}
function cc(e) {
return e.kind === 275;
}
function iE(e) {
return e.kind === 276;
}
function aE(e) {
return e.kind === 278;
}
function YR(e) {
return e.kind === 279;
}
function c2(e) {
return e.kind === 355;
}
function QR(e) {
return e.kind === 360;
}
function ZR(e) {
return e.kind === 358;
}
function ej(e) {
return e.kind === 359;
}
function ud(e) {
return e.kind === 280;
}
function l2(e) {
return e.kind === 281;
}
function tj(e) {
return e.kind === 282;
}
function tu(e) {
return e.kind === 283;
}
function sE(e) {
return e.kind === 284;
}
function pd(e) {
return e.kind === 285;
}
function u2(e) {
return e.kind === 286;
}
function rj(e) {
return e.kind === 287;
}
function nj(e) {
return e.kind === 288;
}
function p2(e) {
return e.kind === 289;
}
function ij(e) {
return e.kind === 290;
}
function aj(e) {
return e.kind === 291;
}
function sj(e) {
return e.kind === 292;
}
function oE(e) {
return e.kind === 293;
}
function ru(e) {
return e.kind === 294;
}
function oj(e) {
return e.kind === 295;
}
function lc(e) {
return e.kind === 299;
}
function nu(e) {
return e.kind === 300;
}
function _E(e) {
return e.kind === 301;
}
function cE(e) {
return e.kind === 302;
}
function _j(e) {
return e.kind === 304;
}
function wi(e) {
return e.kind === 308;
}
function cj(e) {
return e.kind === 309;
}
function lj(e) {
return e.kind === 310;
}
function lE(e) {
return e.kind === 312;
}
function fd(e) {
return e.kind === 313;
}
function uc(e) {
return e.kind === 314;
}
function uj(e) {
return e.kind === 327;
}
function pj(e) {
return e.kind === 328;
}
function fj(e) {
return e.kind === 329;
}
function dj(e) {
return e.kind === 315;
}
function mj(e) {
return e.kind === 316;
}
function uE(e) {
return e.kind === 317;
}
function hj(e) {
return e.kind === 318;
}
function gj(e) {
return e.kind === 319;
}
function dd(e) {
return e.kind === 320;
}
function yj(e) {
return e.kind === 321;
}
function vj(e) {
return e.kind === 322;
}
function Ho(e) {
return e.kind === 323;
}
function f2(e) {
return e.kind === 325;
}
function iu(e) {
return e.kind === 326;
}
function md(e) {
return e.kind === 331;
}
function bj(e) {
return e.kind === 333;
}
function pE(e) {
return e.kind === 335;
}
function Tj(e) {
return e.kind === 341;
}
function d2(e) {
return e.kind === 336;
}
function m2(e) {
return e.kind === 337;
}
function h2(e) {
return e.kind === 338;
}
function g2(e) {
return e.kind === 339;
}
function fE(e) {
return e.kind === 340;
}
function y2(e) {
return e.kind === 342;
}
function v2(e) {
return e.kind === 334;
}
function Sj(e) {
return e.kind === 350;
}
function dE(e) {
return e.kind === 343;
}
function pc(e) {
return e.kind === 344;
}
function b2(e) {
return e.kind === 345;
}
function mE(e) {
return e.kind === 346;
}
function au(e) {
return e.kind === 347;
}
function Go(e) {
return e.kind === 348;
}
function xj(e) {
return e.kind === 349;
}
function Ej(e) {
return e.kind === 330;
}
function wj(e) {
return e.kind === 351;
}
function hE(e) {
return e.kind === 332;
}
function T2(e) {
return e.kind === 353;
}
function Cj(e) {
return e.kind === 352;
}
function Aj(e) {
return e.kind === 354;
}
var Pj = D({ "src/compiler/factory/nodeTests.ts"() {
"use strict";
nn();
} });
function Dj(e) {
return e.createExportDeclaration(void 0, false, e.createNamedExports([]), void 0);
}
function hd(e, t, r, s) {
if (Ws(r))
return Rt(e.createElementAccessExpression(t, r.expression), s);
{
let f = Rt(js(r) ? e.createPropertyAccessExpression(t, r) : e.createElementAccessExpression(t, r), r);
return addEmitFlags(f, 128), f;
}
}
function S2(e, t) {
let r = dc.createIdentifier(e || "React");
return Sa(r, fl(t)), r;
}
function x2(e, t, r) {
if (rc(t)) {
let s = x2(e, t.left, r), f = e.createIdentifier(qr(t.right));
return f.escapedText = t.right.escapedText, e.createPropertyAccessExpression(s, f);
} else
return S2(qr(t), r);
}
function gE(e, t, r, s) {
return t ? x2(e, t, s) : e.createPropertyAccessExpression(S2(r, s), "createElement");
}
function kj(e, t, r, s) {
return t ? x2(e, t, s) : e.createPropertyAccessExpression(S2(r, s), "Fragment");
}
function Ij(e, t, r, s, f, x) {
let w = [r];
if (s && w.push(s), f && f.length > 0)
if (s || w.push(e.createNull()), f.length > 1)
for (let A of f)
vd(A), w.push(A);
else
w.push(f[0]);
return Rt(e.createCallExpression(t, void 0, w), x);
}
function Nj(e, t, r, s, f, x, w) {
let g = [kj(e, r, s, x), e.createNull()];
if (f && f.length > 0)
if (f.length > 1)
for (let B of f)
vd(B), g.push(B);
else
g.push(f[0]);
return Rt(e.createCallExpression(gE(e, t, s, x), void 0, g), w);
}
function Oj(e, t, r) {
if (r2(t)) {
let s = fo(t.declarations), f = e.updateVariableDeclaration(s, s.name, void 0, void 0, r);
return Rt(e.createVariableStatement(void 0, e.updateVariableDeclarationList(t, [f])), t);
} else {
let s = Rt(e.createAssignment(t, r), t);
return Rt(e.createExpressionStatement(s), t);
}
}
function Mj(e, t, r) {
return Ql(t) ? e.updateBlock(t, Rt(e.createNodeArray([r, ...t.statements]), t.statements)) : e.createBlock(e.createNodeArray([t, r]), true);
}
function yE(e, t) {
if (rc(t)) {
let r = yE(e, t.left), s = Sa(Rt(e.cloneNode(t.right), t.right), t.right.parent);
return Rt(e.createPropertyAccessExpression(r, s), t);
} else
return Sa(Rt(e.cloneNode(t), t), t.parent);
}
function vE(e, t) {
return yt(t) ? e.createStringLiteralFromNode(t) : Ws(t) ? Sa(Rt(e.cloneNode(t.expression), t.expression), t.expression.parent) : Sa(Rt(e.cloneNode(t), t), t.parent);
}
function Lj(e, t, r, s, f) {
let { firstAccessor: x, getAccessor: w, setAccessor: A } = W0(t, r);
if (r === x)
return Rt(e.createObjectDefinePropertyCall(s, vE(e, r.name), e.createPropertyDescriptor({ enumerable: e.createFalse(), configurable: true, get: w && Rt(Dn(e.createFunctionExpression(sf(w), void 0, void 0, void 0, w.parameters, void 0, w.body), w), w), set: A && Rt(Dn(e.createFunctionExpression(sf(A), void 0, void 0, void 0, A.parameters, void 0, A.body), A), A) }, !f)), x);
}
function Rj(e, t, r) {
return Dn(Rt(e.createAssignment(hd(e, r, t.name, t.name), t.initializer), t), t);
}
function jj(e, t, r) {
return Dn(Rt(e.createAssignment(hd(e, r, t.name, t.name), e.cloneNode(t.name)), t), t);
}
function Jj(e, t, r) {
return Dn(Rt(e.createAssignment(hd(e, r, t.name, t.name), Dn(Rt(e.createFunctionExpression(sf(t), t.asteriskToken, void 0, void 0, t.parameters, void 0, t.body), t), t)), t), t);
}
function Fj(e, t, r, s) {
switch (r.name && vn(r.name) && Y.failBadSyntaxKind(r.name, "Private identifiers are not allowed in object literals."), r.kind) {
case 174:
case 175:
return Lj(e, t.properties, r, s, !!t.multiLine);
case 299:
return Rj(e, r, s);
case 300:
return jj(e, r, s);
case 171:
return Jj(e, r, s);
}
}
function Bj(e, t, r, s, f) {
let x = t.operator;
Y.assert(x === 45 || x === 46, "Expected 'node' to be a pre- or post-increment or pre- or post-decrement expression");
let w = e.createTempVariable(s);
r = e.createAssignment(w, r), Rt(r, t.operand);
let A = od(t) ? e.createPrefixUnaryExpression(x, w) : e.createPostfixUnaryExpression(w, x);
return Rt(A, t), f && (A = e.createAssignment(f, A), Rt(A, t)), r = e.createComma(r, A), Rt(r, t), Q8(t) && (r = e.createComma(r, w), Rt(r, t)), r;
}
function qj(e) {
return (xi(e) & 65536) !== 0;
}
function E2(e) {
return (xi(e) & 32768) !== 0;
}
function Uj(e) {
return (xi(e) & 16384) !== 0;
}
function bE(e) {
return Gn(e.expression) && e.expression.text === "use strict";
}
function TE(e) {
for (let t of e)
if (us(t)) {
if (bE(t))
return t;
} else
break;
}
function SE(e) {
let t = pa(e);
return t !== void 0 && us(t) && bE(t);
}
function gd(e) {
return e.kind === 223 && e.operatorToken.kind === 27;
}
function zj(e) {
return gd(e) || oc(e);
}
function xE(e) {
return qo(e) && Pr(e) && !!_f(e);
}
function Wj(e) {
let t = cf(e);
return Y.assertIsDefined(t), t;
}
function yd(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 15;
switch (e.kind) {
case 214:
return t & 16 && xE(e) ? false : (t & 1) !== 0;
case 213:
case 231:
case 230:
case 235:
return (t & 2) !== 0;
case 232:
return (t & 4) !== 0;
case 356:
return (t & 8) !== 0;
}
return false;
}
function $o(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 15;
for (; yd(e, t); )
e = e.expression;
return e;
}
function Vj(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 15, r = e.parent;
for (; yd(r, t); )
r = r.parent, Y.assert(r);
return r;
}
function Hj(e) {
return $o(e, 6);
}
function vd(e) {
return setStartsOnNewLine(e, true);
}
function EE(e) {
let t = ul(e, wi), r = t && t.emitNode;
return r && r.externalHelpersModuleName;
}
function Gj(e) {
let t = ul(e, wi), r = t && t.emitNode;
return !!r && (!!r.externalHelpersModuleName || !!r.externalHelpers);
}
function $j(e, t, r, s, f, x, w) {
if (s.importHelpers && Yy(r, s)) {
let A, g = Ei(s);
if (g >= 5 && g <= 99 || r.impliedNodeFormat === 99) {
let B = getEmitHelpers(r);
if (B) {
let N = [];
for (let X of B)
if (!X.scoped) {
let F = X.importName;
F && qn(N, F);
}
if (Ke(N)) {
N.sort(ri), A = e.createNamedImports(Ze(N, ($) => m3(r, $) ? e.createImportSpecifier(false, void 0, e.createIdentifier($)) : e.createImportSpecifier(false, e.createIdentifier($), t.getUnscopedHelperName($))));
let X = ul(r, wi), F = getOrCreateEmitNode(X);
F.externalHelpers = true;
}
}
} else {
let B = wE(e, r, s, f, x || w);
B && (A = e.createNamespaceImport(B));
}
if (A) {
let B = e.createImportDeclaration(void 0, e.createImportClause(false, void 0, A), e.createStringLiteral(Kf), void 0);
return addInternalEmitFlags(B, 2), B;
}
}
}
function wE(e, t, r, s, f) {
if (r.importHelpers && Yy(t, r)) {
let x = EE(t);
if (x)
return x;
let w = Ei(r), A = (s || ov(r) && f) && w !== 4 && (w < 5 || t.impliedNodeFormat === 1);
if (!A) {
let g = getEmitHelpers(t);
if (g) {
for (let B of g)
if (!B.scoped) {
A = true;
break;
}
}
}
if (A) {
let g = ul(t, wi), B = getOrCreateEmitNode(g);
return B.externalHelpersModuleName || (B.externalHelpersModuleName = e.createUniqueName(Kf));
}
}
}
function Kj(e, t, r) {
let s = Q3(t);
if (s && !Z3(t) && !b3(t)) {
let f = s.name;
return cs(f) ? f : e.createIdentifier(No(r, f) || qr(f));
}
if (t.kind === 269 && t.importClause || t.kind === 275 && t.moduleSpecifier)
return e.getGeneratedNameForNode(t);
}
function Xj(e, t, r, s, f, x) {
let w = E0(t);
if (w && Gn(w))
return Qj(t, s, e, f, x) || Yj(e, w, r) || e.cloneNode(w);
}
function Yj(e, t, r) {
let s = r.renamedDependencies && r.renamedDependencies.get(t.text);
return s ? e.createStringLiteral(s) : void 0;
}
function CE(e, t, r, s) {
if (t) {
if (t.moduleName)
return e.createStringLiteral(t.moduleName);
if (!t.isDeclarationFile && B0(s))
return e.createStringLiteral(F0(r, t.fileName));
}
}
function Qj(e, t, r, s, f) {
return CE(r, s.getExternalModuleFileFromDeclaration(e), t, f);
}
function AE(e) {
if (Fy(e))
return e.initializer;
if (lc(e)) {
let t = e.initializer;
return ms(t, true) ? t.right : void 0;
}
if (nu(e))
return e.objectAssignmentInitializer;
if (ms(e, true))
return e.right;
if (Zv(e))
return AE(e.expression);
}
function Ko(e) {
if (Fy(e))
return e.name;
if (jy(e)) {
switch (e.kind) {
case 299:
return Ko(e.initializer);
case 300:
return e.name;
case 301:
return Ko(e.expression);
}
return;
}
return ms(e, true) ? Ko(e.left) : Zv(e) ? Ko(e.expression) : e;
}
function Zj(e) {
switch (e.kind) {
case 166:
case 205:
return e.dotDotDotToken;
case 227:
case 301:
return e;
}
}
function eJ(e) {
let t = PE(e);
return Y.assert(!!t || _E(e), "Invalid property name for binding element."), t;
}
function PE(e) {
switch (e.kind) {
case 205:
if (e.propertyName) {
let r = e.propertyName;
return vn(r) ? Y.failBadSyntaxKind(r) : Ws(r) && DE(r.expression) ? r.expression : r;
}
break;
case 299:
if (e.name) {
let r = e.name;
return vn(r) ? Y.failBadSyntaxKind(r) : Ws(r) && DE(r.expression) ? r.expression : r;
}
break;
case 301:
return e.name && vn(e.name) ? Y.failBadSyntaxKind(e.name) : e.name;
}
let t = Ko(e);
if (t && vl(t))
return t;
}
function DE(e) {
let t = e.kind;
return t === 10 || t === 8;
}
function kE(e) {
switch (e.kind) {
case 203:
case 204:
case 206:
return e.elements;
case 207:
return e.properties;
}
}
function w2(e) {
if (e) {
let t = e;
for (; ; ) {
if (yt(t) || !t.body)
return yt(t) ? t : t.name;
t = t.body;
}
}
}
function tJ(e) {
let t = e.kind;
return t === 173 || t === 175;
}
function IE(e) {
let t = e.kind;
return t === 173 || t === 174 || t === 175;
}
function rJ(e) {
let t = e.kind;
return t === 299 || t === 300 || t === 259 || t === 173 || t === 178 || t === 172 || t === 279 || t === 240 || t === 261 || t === 262 || t === 263 || t === 264 || t === 268 || t === 269 || t === 267 || t === 275 || t === 274;
}
function nJ(e) {
let t = e.kind;
return t === 172 || t === 299 || t === 300 || t === 279 || t === 267;
}
function iJ(e) {
return ql(e) || rd(e);
}
function aJ(e) {
return yt(e) || Xv(e);
}
function sJ(e) {
return O8(e) || zv(e) || Wv(e);
}
function oJ(e) {
return ql(e) || zv(e) || Wv(e);
}
function _J(e) {
return yt(e) || Gn(e);
}
function cJ(e) {
let t = e.kind;
return t === 104 || t === 110 || t === 95 || Iy(e) || od(e);
}
function lJ(e) {
return e === 42;
}
function uJ(e) {
return e === 41 || e === 43 || e === 44;
}
function pJ(e) {
return lJ(e) || uJ(e);
}
function fJ(e) {
return e === 39 || e === 40;
}
function dJ(e) {
return fJ(e) || pJ(e);
}
function mJ(e) {
return e === 47 || e === 48 || e === 49;
}
function hJ(e) {
return mJ(e) || dJ(e);
}
function gJ(e) {
return e === 29 || e === 32 || e === 31 || e === 33 || e === 102 || e === 101;
}
function yJ(e) {
return gJ(e) || hJ(e);
}
function vJ(e) {
return e === 34 || e === 36 || e === 35 || e === 37;
}
function bJ(e) {
return vJ(e) || yJ(e);
}
function TJ(e) {
return e === 50 || e === 51 || e === 52;
}
function SJ(e) {
return TJ(e) || bJ(e);
}
function xJ(e) {
return e === 55 || e === 56;
}
function EJ(e) {
return xJ(e) || SJ(e);
}
function wJ(e) {
return e === 60 || EJ(e) || G_(e);
}
function CJ(e) {
return wJ(e) || e === 27;
}
function AJ(e) {
return CJ(e.kind);
}
function PJ(e, t, r, s, f, x) {
let w = new OE(e, t, r, s, f, x);
return A;
function A(g, B) {
let N = { value: void 0 }, X = [Td.enter], F = [g], $ = [void 0], ae = 0;
for (; X[ae] !== Td.done; )
ae = X[ae](w, ae, X, F, $, N, B);
return Y.assertEqual(ae, 0), N.value;
}
}
function NE(e) {
return e === 93 || e === 88;
}
function DJ(e) {
let t = e.kind;
return NE(t);
}
function kJ(e) {
let t = e.kind;
return Wi(t) && !NE(t);
}
function IJ(e, t) {
if (t !== void 0)
return t.length === 0 ? t : Rt(e.createNodeArray([], t.hasTrailingComma), t);
}
function NJ(e) {
var t;
let r = e.emitNode.autoGenerate;
if (r.flags & 4) {
let s = r.id, f = e, x = f.original;
for (; x; ) {
f = x;
let w = (t = f.emitNode) == null ? void 0 : t.autoGenerate;
if (js(f) && (w === void 0 || w.flags & 4 && w.id !== s))
break;
x = f.original;
}
return f;
}
return e;
}
function C2(e, t) {
return typeof e == "object" ? bd(false, e.prefix, e.node, e.suffix, t) : typeof e == "string" ? e.length > 0 && e.charCodeAt(0) === 35 ? e.slice(1) : e : "";
}
function OJ(e, t) {
return typeof e == "string" ? e : MJ(e, Y.checkDefined(t));
}
function MJ(e, t) {
return Ny(e) ? t(e).slice(1) : cs(e) ? t(e) : vn(e) ? e.escapedText.slice(1) : qr(e);
}
function bd(e, t, r, s, f) {
return t = C2(t, f), s = C2(s, f), r = OJ(r, f), `${e ? "#" : ""}${t}${r}${s}`;
}
function LJ(e, t, r, s) {
return e.updatePropertyDeclaration(t, r, e.getGeneratedPrivateNameForNode(t.name, void 0, "_accessor_storage"), void 0, void 0, s);
}
function RJ(e, t, r, s) {
return e.createGetAccessorDeclaration(r, s, [], void 0, e.createBlock([e.createReturnStatement(e.createPropertyAccessExpression(e.createThis(), e.getGeneratedPrivateNameForNode(t.name, void 0, "_accessor_storage")))]));
}
function jJ(e, t, r, s) {
return e.createSetAccessorDeclaration(r, s, [e.createParameterDeclaration(void 0, void 0, "value")], e.createBlock([e.createExpressionStatement(e.createAssignment(e.createPropertyAccessExpression(e.createThis(), e.getGeneratedPrivateNameForNode(t.name, void 0, "_accessor_storage")), e.createIdentifier("value")))]));
}
function JJ(e) {
let t = e.expression;
for (; ; ) {
if (t = $o(t), oc(t)) {
t = Zn(t.elements);
continue;
}
if (gd(t)) {
t = t.right;
continue;
}
if (ms(t, true) && cs(t.left))
return t;
break;
}
}
function FJ(e) {
return qo(e) && fs2(e) && !e.emitNode;
}
function su(e, t) {
if (FJ(e))
su(e.expression, t);
else if (gd(e))
su(e.left, t), su(e.right, t);
else if (oc(e))
for (let r of e.elements)
su(r, t);
else
t.push(e);
}
function BJ(e) {
let t = [];
return su(e, t), t;
}
function A2(e) {
if (e.transformFlags & 65536)
return true;
if (e.transformFlags & 128)
for (let t of kE(e)) {
let r = Ko(t);
if (r && KS(r) && (r.transformFlags & 65536 || r.transformFlags & 128 && A2(r)))
return true;
}
return false;
}
var Td, OE, qJ = D({ "src/compiler/factory/utilities.ts"() {
"use strict";
nn(), ((e) => {
function t(N, X, F, $, ae, Te, Se) {
let Ye = X > 0 ? ae[X - 1] : void 0;
return Y.assertEqual(F[X], t), ae[X] = N.onEnter($[X], Ye, Se), F[X] = A(N, t), X;
}
e.enter = t;
function r(N, X, F, $, ae, Te, Se) {
Y.assertEqual(F[X], r), Y.assertIsDefined(N.onLeft), F[X] = A(N, r);
let Ye = N.onLeft($[X].left, ae[X], $[X]);
return Ye ? (B(X, $, Ye), g(X, F, $, ae, Ye)) : X;
}
e.left = r;
function s(N, X, F, $, ae, Te, Se) {
return Y.assertEqual(F[X], s), Y.assertIsDefined(N.onOperator), F[X] = A(N, s), N.onOperator($[X].operatorToken, ae[X], $[X]), X;
}
e.operator = s;
function f(N, X, F, $, ae, Te, Se) {
Y.assertEqual(F[X], f), Y.assertIsDefined(N.onRight), F[X] = A(N, f);
let Ye = N.onRight($[X].right, ae[X], $[X]);
return Ye ? (B(X, $, Ye), g(X, F, $, ae, Ye)) : X;
}
e.right = f;
function x(N, X, F, $, ae, Te, Se) {
Y.assertEqual(F[X], x), F[X] = A(N, x);
let Ye = N.onExit($[X], ae[X]);
if (X > 0) {
if (X--, N.foldState) {
let Ne = F[X] === x ? "right" : "left";
ae[X] = N.foldState(ae[X], Ye, Ne);
}
} else
Te.value = Ye;
return X;
}
e.exit = x;
function w(N, X, F, $, ae, Te, Se) {
return Y.assertEqual(F[X], w), X;
}
e.done = w;
function A(N, X) {
switch (X) {
case t:
if (N.onLeft)
return r;
case r:
if (N.onOperator)
return s;
case s:
if (N.onRight)
return f;
case f:
return x;
case x:
return w;
case w:
return w;
default:
Y.fail("Invalid state");
}
}
e.nextState = A;
function g(N, X, F, $, ae) {
return N++, X[N] = t, F[N] = ae, $[N] = void 0, N;
}
function B(N, X, F) {
if (Y.shouldAssert(2))
for (; N >= 0; )
Y.assert(X[N] !== F, "Circular traversal detected."), N--;
}
})(Td || (Td = {})), OE = class {
constructor(e, t, r, s, f, x) {
this.onEnter = e, this.onLeft = t, this.onOperator = r, this.onRight = s, this.onExit = f, this.foldState = x;
}
};
} });
function Rt(e, t) {
return t ? Us(e, t.pos, t.end) : e;
}
function fc(e) {
let t = e.kind;
return t === 165 || t === 166 || t === 168 || t === 169 || t === 170 || t === 171 || t === 173 || t === 174 || t === 175 || t === 178 || t === 182 || t === 215 || t === 216 || t === 228 || t === 240 || t === 259 || t === 260 || t === 261 || t === 262 || t === 263 || t === 264 || t === 268 || t === 269 || t === 274 || t === 275;
}
function ME(e) {
let t = e.kind;
return t === 166 || t === 169 || t === 171 || t === 174 || t === 175 || t === 228 || t === 260;
}
var UJ = D({ "src/compiler/factory/utilitiesPublic.ts"() {
"use strict";
nn();
} });
function G(e, t) {
return t && e(t);
}
function ze(e, t, r) {
if (r) {
if (t)
return t(r);
for (let s of r) {
let f = e(s);
if (f)
return f;
}
}
}
function LE(e, t) {
return e.charCodeAt(t + 1) === 42 && e.charCodeAt(t + 2) === 42 && e.charCodeAt(t + 3) !== 47;
}
function ou(e) {
return c(e.statements, zJ) || WJ(e);
}
function zJ(e) {
return fc(e) && VJ(e, 93) || s2(e) && ud(e.moduleReference) || o2(e) || Vo(e) || cc(e) ? e : void 0;
}
function WJ(e) {
return e.flags & 4194304 ? RE(e) : void 0;
}
function RE(e) {
return HJ(e) ? e : xr(e, RE);
}
function VJ(e, t) {
return Ke(e.modifiers, (r) => r.kind === t);
}
function HJ(e) {
return t2(e) && e.keywordToken === 100 && e.name.escapedText === "meta";
}
function jE(e, t, r) {
return ze(t, r, e.typeParameters) || ze(t, r, e.parameters) || G(t, e.type);
}
function JE(e, t, r) {
return ze(t, r, e.types);
}
function FE(e, t, r) {
return G(t, e.type);
}
function BE(e, t, r) {
return ze(t, r, e.elements);
}
function qE(e, t, r) {
return G(t, e.expression) || G(t, e.questionDotToken) || ze(t, r, e.typeArguments) || ze(t, r, e.arguments);
}
function UE(e, t, r) {
return ze(t, r, e.statements);
}
function zE(e, t, r) {
return G(t, e.label);
}
function WE(e, t, r) {
return ze(t, r, e.modifiers) || G(t, e.name) || ze(t, r, e.typeParameters) || ze(t, r, e.heritageClauses) || ze(t, r, e.members);
}
function VE(e, t, r) {
return ze(t, r, e.elements);
}
function HE(e, t, r) {
return G(t, e.propertyName) || G(t, e.name);
}
function GE(e, t, r) {
return G(t, e.tagName) || ze(t, r, e.typeArguments) || G(t, e.attributes);
}
function Xo(e, t, r) {
return G(t, e.type);
}
function $E(e, t, r) {
return G(t, e.tagName) || (e.isNameFirst ? G(t, e.name) || G(t, e.typeExpression) : G(t, e.typeExpression) || G(t, e.name)) || (typeof e.comment == "string" ? void 0 : ze(t, r, e.comment));
}
function Yo(e, t, r) {
return G(t, e.tagName) || G(t, e.typeExpression) || (typeof e.comment == "string" ? void 0 : ze(t, r, e.comment));
}
function P2(e, t, r) {
return G(t, e.name);
}
function Gs(e, t, r) {
return G(t, e.tagName) || (typeof e.comment == "string" ? void 0 : ze(t, r, e.comment));
}
function GJ(e, t, r) {
return G(t, e.expression);
}
function xr(e, t, r) {
if (e === void 0 || e.kind <= 162)
return;
let s = o7[e.kind];
return s === void 0 ? void 0 : s(e, t, r);
}
function D2(e, t, r) {
let s = KE(e), f = [];
for (; f.length < s.length; )
f.push(e);
for (; s.length !== 0; ) {
let x = s.pop(), w = f.pop();
if (ir(x)) {
if (r) {
let A = r(x, w);
if (A) {
if (A === "skip")
continue;
return A;
}
}
for (let A = x.length - 1; A >= 0; --A)
s.push(x[A]), f.push(w);
} else {
let A = t(x, w);
if (A) {
if (A === "skip")
continue;
return A;
}
if (x.kind >= 163)
for (let g of KE(x))
s.push(g), f.push(x);
}
}
}
function KE(e) {
let t = [];
return xr(e, r, r), t;
function r(s) {
t.unshift(s);
}
}
function XE(e) {
e.externalModuleIndicator = ou(e);
}
function YE(e, t, r) {
let s = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : false, f = arguments.length > 4 ? arguments[4] : void 0;
var x, w;
(x = rs) == null || x.push(rs.Phase.Parse, "createSourceFile", { path: e }, true), DT("beforeParse");
let A;
Dp.logStartParseSourceFile(e);
let { languageVersion: g, setExternalModuleIndicator: B, impliedNodeFormat: N } = typeof r == "object" ? r : { languageVersion: r };
if (g === 100)
A = Ci.parseSourceFile(e, t, g, void 0, s, 6, yn);
else {
let X = N === void 0 ? B : (F) => (F.impliedNodeFormat = N, (B || XE)(F));
A = Ci.parseSourceFile(e, t, g, void 0, s, f, X);
}
return Dp.logStopParseSourceFile(), DT("afterParse"), B5("Parse", "beforeParse", "afterParse"), (w = rs) == null || w.pop(), A;
}
function $J(e, t) {
return Ci.parseIsolatedEntityName(e, t);
}
function KJ(e, t) {
return Ci.parseJsonText(e, t);
}
function Qo(e) {
return e.externalModuleIndicator !== void 0;
}
function k2(e, t, r) {
let s = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : false, f = Sd.updateSourceFile(e, t, r, s);
return f.flags |= e.flags & 6291456, f;
}
function XJ(e, t, r) {
let s = Ci.JSDocParser.parseIsolatedJSDocComment(e, t, r);
return s && s.jsDoc && Ci.fixupParentReferences(s.jsDoc), s;
}
function YJ(e, t, r) {
return Ci.JSDocParser.parseJSDocTypeExpressionForTests(e, t, r);
}
function QE(e) {
return da(e, Rv) || ns(e, ".ts") && Fi(sl(e), ".d.");
}
function QJ(e, t, r, s) {
if (e) {
if (e === "import")
return 99;
if (e === "require")
return 1;
s(t, r - t, ve.resolution_mode_should_be_either_require_or_import);
}
}
function ZE(e, t) {
let r = [];
for (let s of Ao(t, 0) || Bt) {
let f = t.substring(s.pos, s.end);
eF(r, s, f);
}
e.pragmas = /* @__PURE__ */ new Map();
for (let s of r) {
if (e.pragmas.has(s.name)) {
let f = e.pragmas.get(s.name);
f instanceof Array ? f.push(s.args) : e.pragmas.set(s.name, [f, s.args]);
continue;
}
e.pragmas.set(s.name, s.args);
}
}
function e7(e, t) {
e.checkJsDirective = void 0, e.referencedFiles = [], e.typeReferenceDirectives = [], e.libReferenceDirectives = [], e.amdDependencies = [], e.hasNoDefaultLib = false, e.pragmas.forEach((r, s) => {
switch (s) {
case "reference": {
let f = e.referencedFiles, x = e.typeReferenceDirectives, w = e.libReferenceDirectives;
c(en(r), (A) => {
let { types: g, lib: B, path: N, ["resolution-mode"]: X } = A.arguments;
if (A.arguments["no-default-lib"])
e.hasNoDefaultLib = true;
else if (g) {
let F = QJ(X, g.pos, g.end, t);
x.push(Object.assign({ pos: g.pos, end: g.end, fileName: g.value }, F ? { resolutionMode: F } : {}));
} else
B ? w.push({ pos: B.pos, end: B.end, fileName: B.value }) : N ? f.push({ pos: N.pos, end: N.end, fileName: N.value }) : t(A.range.pos, A.range.end - A.range.pos, ve.Invalid_reference_directive_syntax);
});
break;
}
case "amd-dependency": {
e.amdDependencies = Ze(en(r), (f) => ({ name: f.arguments.name, path: f.arguments.path }));
break;
}
case "amd-module": {
if (r instanceof Array)
for (let f of r)
e.moduleName && t(f.range.pos, f.range.end - f.range.pos, ve.An_AMD_module_cannot_have_multiple_name_assignments), e.moduleName = f.arguments.name;
else
e.moduleName = r.arguments.name;
break;
}
case "ts-nocheck":
case "ts-check": {
c(en(r), (f) => {
(!e.checkJsDirective || f.range.pos > e.checkJsDirective.pos) && (e.checkJsDirective = { enabled: s === "ts-check", end: f.range.end, pos: f.range.pos });
});
break;
}
case "jsx":
case "jsxfrag":
case "jsximportsource":
case "jsxruntime":
return;
default:
Y.fail("Unhandled pragma kind");
}
});
}
function ZJ(e) {
if (xd.has(e))
return xd.get(e);
let t = new RegExp(`(\\s${e}\\s*=\\s*)(?:(?:'([^']*)')|(?:"([^"]*)"))`, "im");
return xd.set(e, t), t;
}
function eF(e, t, r) {
let s = t.kind === 2 && _7.exec(r);
if (s) {
let x = s[1].toLowerCase(), w = Vp[x];
if (!w || !(w.kind & 1))
return;
if (w.args) {
let A = {};
for (let g of w.args) {
let N = ZJ(g.name).exec(r);
if (!N && !g.optional)
return;
if (N) {
let X = N[2] || N[3];
if (g.captureSpan) {
let F = t.pos + N.index + N[1].length + 1;
A[g.name] = { value: X, pos: F, end: F + X.length };
} else
A[g.name] = X;
}
}
e.push({ name: x, args: { arguments: A, range: t } });
} else
e.push({ name: x, args: { arguments: {}, range: t } });
return;
}
let f = t.kind === 2 && c7.exec(r);
if (f)
return t7(e, t, 2, f);
if (t.kind === 3) {
let x = /@(\S+)(\s+.*)?$/gim, w;
for (; w = x.exec(r); )
t7(e, t, 4, w);
}
}
function t7(e, t, r, s) {
if (!s)
return;
let f = s[1].toLowerCase(), x = Vp[f];
if (!x || !(x.kind & r))
return;
let w = s[2], A = tF(x, w);
A !== "fail" && e.push({ name: f, args: { arguments: A, range: t } });
}
function tF(e, t) {
if (!t)
return {};
if (!e.args)
return {};
let r = Pp(t).split(/\s+/), s = {};
for (let f = 0; f < e.args.length; f++) {
let x = e.args[f];
if (!r[f] && !x.optional)
return "fail";
if (x.captureSpan)
return Y.fail("Capture spans not yet implemented for non-xml pragmas");
s[x.name] = r[f];
}
return s;
}
function Hi(e, t) {
return e.kind !== t.kind ? false : e.kind === 79 ? e.escapedText === t.escapedText : e.kind === 108 ? true : e.name.escapedText === t.name.escapedText && Hi(e.expression, t.expression);
}
var r7, n7, i7, a7, s7, I2, dc, o7, Ci, Sd, xd, _7, c7, rF = D({ "src/compiler/parser.ts"() {
"use strict";
nn(), nn(), IT(), I2 = { createBaseSourceFileNode: (e) => new (s7 || (s7 = lr.getSourceFileConstructor()))(e, -1, -1), createBaseIdentifierNode: (e) => new (i7 || (i7 = lr.getIdentifierConstructor()))(e, -1, -1), createBasePrivateIdentifierNode: (e) => new (a7 || (a7 = lr.getPrivateIdentifierConstructor()))(e, -1, -1), createBaseTokenNode: (e) => new (n7 || (n7 = lr.getTokenConstructor()))(e, -1, -1), createBaseNode: (e) => new (r7 || (r7 = lr.getNodeConstructor()))(e, -1, -1) }, dc = Zf(1, I2), o7 = { [163]: function(t, r, s) {
return G(r, t.left) || G(r, t.right);
}, [165]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || G(r, t.constraint) || G(r, t.default) || G(r, t.expression);
}, [300]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || G(r, t.questionToken) || G(r, t.exclamationToken) || G(r, t.equalsToken) || G(r, t.objectAssignmentInitializer);
}, [301]: function(t, r, s) {
return G(r, t.expression);
}, [166]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.dotDotDotToken) || G(r, t.name) || G(r, t.questionToken) || G(r, t.type) || G(r, t.initializer);
}, [169]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || G(r, t.questionToken) || G(r, t.exclamationToken) || G(r, t.type) || G(r, t.initializer);
}, [168]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || G(r, t.questionToken) || G(r, t.type) || G(r, t.initializer);
}, [299]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || G(r, t.questionToken) || G(r, t.exclamationToken) || G(r, t.initializer);
}, [257]: function(t, r, s) {
return G(r, t.name) || G(r, t.exclamationToken) || G(r, t.type) || G(r, t.initializer);
}, [205]: function(t, r, s) {
return G(r, t.dotDotDotToken) || G(r, t.propertyName) || G(r, t.name) || G(r, t.initializer);
}, [178]: function(t, r, s) {
return ze(r, s, t.modifiers) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type);
}, [182]: function(t, r, s) {
return ze(r, s, t.modifiers) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type);
}, [181]: function(t, r, s) {
return ze(r, s, t.modifiers) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type);
}, [176]: jE, [177]: jE, [171]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.asteriskToken) || G(r, t.name) || G(r, t.questionToken) || G(r, t.exclamationToken) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type) || G(r, t.body);
}, [170]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || G(r, t.questionToken) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type);
}, [173]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type) || G(r, t.body);
}, [174]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type) || G(r, t.body);
}, [175]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type) || G(r, t.body);
}, [259]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.asteriskToken) || G(r, t.name) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type) || G(r, t.body);
}, [215]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.asteriskToken) || G(r, t.name) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type) || G(r, t.body);
}, [216]: function(t, r, s) {
return ze(r, s, t.modifiers) || ze(r, s, t.typeParameters) || ze(r, s, t.parameters) || G(r, t.type) || G(r, t.equalsGreaterThanToken) || G(r, t.body);
}, [172]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.body);
}, [180]: function(t, r, s) {
return G(r, t.typeName) || ze(r, s, t.typeArguments);
}, [179]: function(t, r, s) {
return G(r, t.assertsModifier) || G(r, t.parameterName) || G(r, t.type);
}, [183]: function(t, r, s) {
return G(r, t.exprName) || ze(r, s, t.typeArguments);
}, [184]: function(t, r, s) {
return ze(r, s, t.members);
}, [185]: function(t, r, s) {
return G(r, t.elementType);
}, [186]: function(t, r, s) {
return ze(r, s, t.elements);
}, [189]: JE, [190]: JE, [191]: function(t, r, s) {
return G(r, t.checkType) || G(r, t.extendsType) || G(r, t.trueType) || G(r, t.falseType);
}, [192]: function(t, r, s) {
return G(r, t.typeParameter);
}, [202]: function(t, r, s) {
return G(r, t.argument) || G(r, t.assertions) || G(r, t.qualifier) || ze(r, s, t.typeArguments);
}, [298]: function(t, r, s) {
return G(r, t.assertClause);
}, [193]: FE, [195]: FE, [196]: function(t, r, s) {
return G(r, t.objectType) || G(r, t.indexType);
}, [197]: function(t, r, s) {
return G(r, t.readonlyToken) || G(r, t.typeParameter) || G(r, t.nameType) || G(r, t.questionToken) || G(r, t.type) || ze(r, s, t.members);
}, [198]: function(t, r, s) {
return G(r, t.literal);
}, [199]: function(t, r, s) {
return G(r, t.dotDotDotToken) || G(r, t.name) || G(r, t.questionToken) || G(r, t.type);
}, [203]: BE, [204]: BE, [206]: function(t, r, s) {
return ze(r, s, t.elements);
}, [207]: function(t, r, s) {
return ze(r, s, t.properties);
}, [208]: function(t, r, s) {
return G(r, t.expression) || G(r, t.questionDotToken) || G(r, t.name);
}, [209]: function(t, r, s) {
return G(r, t.expression) || G(r, t.questionDotToken) || G(r, t.argumentExpression);
}, [210]: qE, [211]: qE, [212]: function(t, r, s) {
return G(r, t.tag) || G(r, t.questionDotToken) || ze(r, s, t.typeArguments) || G(r, t.template);
}, [213]: function(t, r, s) {
return G(r, t.type) || G(r, t.expression);
}, [214]: function(t, r, s) {
return G(r, t.expression);
}, [217]: function(t, r, s) {
return G(r, t.expression);
}, [218]: function(t, r, s) {
return G(r, t.expression);
}, [219]: function(t, r, s) {
return G(r, t.expression);
}, [221]: function(t, r, s) {
return G(r, t.operand);
}, [226]: function(t, r, s) {
return G(r, t.asteriskToken) || G(r, t.expression);
}, [220]: function(t, r, s) {
return G(r, t.expression);
}, [222]: function(t, r, s) {
return G(r, t.operand);
}, [223]: function(t, r, s) {
return G(r, t.left) || G(r, t.operatorToken) || G(r, t.right);
}, [231]: function(t, r, s) {
return G(r, t.expression) || G(r, t.type);
}, [232]: function(t, r, s) {
return G(r, t.expression);
}, [235]: function(t, r, s) {
return G(r, t.expression) || G(r, t.type);
}, [233]: function(t, r, s) {
return G(r, t.name);
}, [224]: function(t, r, s) {
return G(r, t.condition) || G(r, t.questionToken) || G(r, t.whenTrue) || G(r, t.colonToken) || G(r, t.whenFalse);
}, [227]: function(t, r, s) {
return G(r, t.expression);
}, [238]: UE, [265]: UE, [308]: function(t, r, s) {
return ze(r, s, t.statements) || G(r, t.endOfFileToken);
}, [240]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.declarationList);
}, [258]: function(t, r, s) {
return ze(r, s, t.declarations);
}, [241]: function(t, r, s) {
return G(r, t.expression);
}, [242]: function(t, r, s) {
return G(r, t.expression) || G(r, t.thenStatement) || G(r, t.elseStatement);
}, [243]: function(t, r, s) {
return G(r, t.statement) || G(r, t.expression);
}, [244]: function(t, r, s) {
return G(r, t.expression) || G(r, t.statement);
}, [245]: function(t, r, s) {
return G(r, t.initializer) || G(r, t.condition) || G(r, t.incrementor) || G(r, t.statement);
}, [246]: function(t, r, s) {
return G(r, t.initializer) || G(r, t.expression) || G(r, t.statement);
}, [247]: function(t, r, s) {
return G(r, t.awaitModifier) || G(r, t.initializer) || G(r, t.expression) || G(r, t.statement);
}, [248]: zE, [249]: zE, [250]: function(t, r, s) {
return G(r, t.expression);
}, [251]: function(t, r, s) {
return G(r, t.expression) || G(r, t.statement);
}, [252]: function(t, r, s) {
return G(r, t.expression) || G(r, t.caseBlock);
}, [266]: function(t, r, s) {
return ze(r, s, t.clauses);
}, [292]: function(t, r, s) {
return G(r, t.expression) || ze(r, s, t.statements);
}, [293]: function(t, r, s) {
return ze(r, s, t.statements);
}, [253]: function(t, r, s) {
return G(r, t.label) || G(r, t.statement);
}, [254]: function(t, r, s) {
return G(r, t.expression);
}, [255]: function(t, r, s) {
return G(r, t.tryBlock) || G(r, t.catchClause) || G(r, t.finallyBlock);
}, [295]: function(t, r, s) {
return G(r, t.variableDeclaration) || G(r, t.block);
}, [167]: function(t, r, s) {
return G(r, t.expression);
}, [260]: WE, [228]: WE, [261]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || ze(r, s, t.typeParameters) || ze(r, s, t.heritageClauses) || ze(r, s, t.members);
}, [262]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || ze(r, s, t.typeParameters) || G(r, t.type);
}, [263]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || ze(r, s, t.members);
}, [302]: function(t, r, s) {
return G(r, t.name) || G(r, t.initializer);
}, [264]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || G(r, t.body);
}, [268]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name) || G(r, t.moduleReference);
}, [269]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.importClause) || G(r, t.moduleSpecifier) || G(r, t.assertClause);
}, [270]: function(t, r, s) {
return G(r, t.name) || G(r, t.namedBindings);
}, [296]: function(t, r, s) {
return ze(r, s, t.elements);
}, [297]: function(t, r, s) {
return G(r, t.name) || G(r, t.value);
}, [267]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.name);
}, [271]: function(t, r, s) {
return G(r, t.name);
}, [277]: function(t, r, s) {
return G(r, t.name);
}, [272]: VE, [276]: VE, [275]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.exportClause) || G(r, t.moduleSpecifier) || G(r, t.assertClause);
}, [273]: HE, [278]: HE, [274]: function(t, r, s) {
return ze(r, s, t.modifiers) || G(r, t.expression);
}, [225]: function(t, r, s) {
return G(r, t.head) || ze(r, s, t.templateSpans);
}, [236]: function(t, r, s) {
return G(r, t.expression) || G(r, t.literal);
}, [200]: function(t, r, s) {
return G(r, t.head) || ze(r, s, t.templateSpans);
}, [201]: function(t, r, s) {
return G(r, t.type) || G(r, t.literal);
}, [164]: function(t, r, s) {
return G(r, t.expression);
}, [294]: function(t, r, s) {
return ze(r, s, t.types);
}, [230]: function(t, r, s) {
return G(r, t.expression) || ze(r, s, t.typeArguments);
}, [280]: function(t, r, s) {
return G(r, t.expression);
}, [279]: function(t, r, s) {
return ze(r, s, t.modifiers);
}, [357]: function(t, r, s) {
return ze(r, s, t.elements);
}, [281]: function(t, r, s) {
return G(r, t.openingElement) || ze(r, s, t.children) || G(r, t.closingElement);
}, [285]: function(t, r, s) {
return G(r, t.openingFragment) || ze(r, s, t.children) || G(r, t.closingFragment);
}, [282]: GE, [283]: GE, [289]: function(t, r, s) {
return ze(r, s, t.properties);
}, [288]: function(t, r, s) {
return G(r, t.name) || G(r, t.initializer);
}, [290]: function(t, r, s) {
return G(r, t.expression);
}, [291]: function(t, r, s) {
return G(r, t.dotDotDotToken) || G(r, t.expression);
}, [284]: function(t, r, s) {
return G(r, t.tagName);
}, [187]: Xo, [188]: Xo, [312]: Xo, [318]: Xo, [317]: Xo, [319]: Xo, [321]: Xo, [320]: function(t, r, s) {
return ze(r, s, t.parameters) || G(r, t.type);
}, [323]: function(t, r, s) {
return (typeof t.comment == "string" ? void 0 : ze(r, s, t.comment)) || ze(r, s, t.tags);
}, [350]: function(t, r, s) {
return G(r, t.tagName) || G(r, t.name) || (typeof t.comment == "string" ? void 0 : ze(r, s, t.comment));
}, [313]: function(t, r, s) {
return G(r, t.name);
}, [314]: function(t, r, s) {
return G(r, t.left) || G(r, t.right);
}, [344]: $E, [351]: $E, [333]: function(t, r, s) {
return G(r, t.tagName) || (typeof t.comment == "string" ? void 0 : ze(r, s, t.comment));
}, [332]: function(t, r, s) {
return G(r, t.tagName) || G(r, t.class) || (typeof t.comment == "string" ? void 0 : ze(r, s, t.comment));
}, [331]: function(t, r, s) {
return G(r, t.tagName) || G(r, t.class) || (typeof t.comment == "string" ? void 0 : ze(r, s, t.comment));
}, [348]: function(t, r, s) {
return G(r, t.tagName) || G(r, t.constraint) || ze(r, s, t.typeParameters) || (typeof t.comment == "string" ? void 0 : ze(r, s, t.comment));
}, [349]: function(t, r, s) {
return G(r, t.tagName) || (t.typeExpression && t.typeExpression.kind === 312 ? G(r, t.typeExpression) || G(r, t.fullName) || (typeof t.comment == "string" ? void 0 : ze(r, s, t.comment)) : G(r, t.fullName) || G(r, t.typeExpression) || (typeof t.comment == "string" ? void 0 : ze(r, s, t.comment)));
}, [341]: function(t, r, s) {
return G(r, t.tagName) || G(r, t.fullName) || G(r, t.typeExpression) || (typeof t.comment == "string" ? void 0 : ze(r, s, t.comment));
}, [345]: Yo, [347]: Yo, [346]: Yo, [343]: Yo, [353]: Yo, [352]: Yo, [342]: Yo, [326]: function(t, r, s) {
return c(t.typeParameters, r) || c(t.parameters, r) || G(r, t.type);
}, [327]: P2, [328]: P2, [329]: P2, [325]: function(t, r, s) {
return c(t.jsDocPropertyTags, r);
}, [330]: Gs, [335]: Gs, [336]: Gs, [337]: Gs, [338]: Gs, [339]: Gs, [334]: Gs, [340]: Gs, [356]: GJ }, ((e) => {
var t = Po(99, true), r = 20480, s, f, x, w, A;
function g(u) {
return oi++, u;
}
var B = { createBaseSourceFileNode: (u) => g(new A(u, 0, 0)), createBaseIdentifierNode: (u) => g(new x(u, 0, 0)), createBasePrivateIdentifierNode: (u) => g(new w(u, 0, 0)), createBaseTokenNode: (u) => g(new f(u, 0, 0)), createBaseNode: (u) => g(new s(u, 0, 0)) }, N = Zf(11, B), { createNodeArray: X, createNumericLiteral: F, createStringLiteral: $, createLiteralLikeNode: ae, createIdentifier: Te, createPrivateIdentifier: Se, createToken: Ye, createArrayLiteralExpression: Ne, createObjectLiteralExpression: oe, createPropertyAccessExpression: Ve, createPropertyAccessChain: pt, createElementAccessExpression: Gt, createElementAccessChain: Nt, createCallExpression: Xt, createCallChain: er, createNewExpression: Tn, createParenthesizedExpression: Hr, createBlock: Gi, createVariableStatement: pn, createExpressionStatement: fn, createIfStatement: Ut, createWhileStatement: kn, createForStatement: an, createForOfStatement: mr, createVariableDeclaration: $i, createVariableDeclarationList: dn } = N, Ur, Gr, _r, Sn, In, pr, Zt, Or, Nn, ar, oi, cr, $r, hr, On, nr, br = true, Kr = false;
function wa(u, b, O, j) {
let z = arguments.length > 4 && arguments[4] !== void 0 ? arguments[4] : false, re = arguments.length > 5 ? arguments[5] : void 0, Ee = arguments.length > 6 ? arguments[6] : void 0;
var qe;
if (re = Nx(u, re), re === 6) {
let $e = Ki(u, b, O, j, z);
return convertToObjectWorker($e, (qe = $e.statements[0]) == null ? void 0 : qe.expression, $e.parseDiagnostics, false, void 0, void 0), $e.referencedFiles = Bt, $e.typeReferenceDirectives = Bt, $e.libReferenceDirectives = Bt, $e.amdDependencies = Bt, $e.hasNoDefaultLib = false, $e.pragmas = V1, $e;
}
Mn(u, b, O, j, re);
let We = Ca(O, z, re, Ee || XE);
return _i(), We;
}
e.parseSourceFile = wa;
function $n(u, b) {
Mn("", u, b, void 0, 1), _e();
let O = Ys(true), j = T() === 1 && !Zt.length;
return _i(), j ? O : void 0;
}
e.parseIsolatedEntityName = $n;
function Ki(u, b) {
let O = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : 2, j = arguments.length > 3 ? arguments[3] : void 0, z = arguments.length > 4 && arguments[4] !== void 0 ? arguments[4] : false;
Mn(u, b, O, j, 6), Gr = nr, _e();
let re = L(), Ee, qe;
if (T() === 1)
Ee = Er([], re, re), qe = sn();
else {
let lt;
for (; T() !== 1; ) {
let At;
switch (T()) {
case 22:
At = ah();
break;
case 110:
case 95:
case 104:
At = sn();
break;
case 40:
wt(() => _e() === 8 && _e() !== 58) ? At = qm() : At = Xu();
break;
case 8:
case 10:
if (wt(() => _e() !== 58)) {
At = Di();
break;
}
default:
At = Xu();
break;
}
lt && ir(lt) ? lt.push(At) : lt ? lt = [lt, At] : (lt = At, T() !== 1 && Dt(ve.Unexpected_token));
}
let Jt = ir(lt) ? Q(Ne(lt), re) : Y.checkDefined(lt), Lt = fn(Jt);
Q(Lt, re), Ee = Er([Lt], re), qe = ea(1, ve.Unexpected_token);
}
let We = Kt(u, 2, 6, false, Ee, qe, Gr, yn);
z && ft(We), We.nodeCount = oi, We.identifierCount = $r, We.identifiers = cr, We.parseDiagnostics = qs(Zt, We), Or && (We.jsDocDiagnostics = qs(Or, We));
let $e = We;
return _i(), $e;
}
e.parseJsonText = Ki;
function Mn(u, b, O, j, z) {
switch (s = lr.getNodeConstructor(), f = lr.getTokenConstructor(), x = lr.getIdentifierConstructor(), w = lr.getPrivateIdentifierConstructor(), A = lr.getSourceFileConstructor(), Ur = Un(u), _r = b, Sn = O, Nn = j, In = z, pr = sv(z), Zt = [], hr = 0, cr = /* @__PURE__ */ new Map(), $r = 0, oi = 0, Gr = 0, br = true, In) {
case 1:
case 2:
nr = 262144;
break;
case 6:
nr = 67371008;
break;
default:
nr = 0;
break;
}
Kr = false, t.setText(_r), t.setOnError(U), t.setScriptTarget(Sn), t.setLanguageVariant(pr);
}
function _i() {
t.clearCommentDirectives(), t.setText(""), t.setOnError(void 0), _r = void 0, Sn = void 0, Nn = void 0, In = void 0, pr = void 0, Gr = 0, Zt = void 0, Or = void 0, hr = 0, cr = void 0, On = void 0, br = true;
}
function Ca(u, b, O, j) {
let z = QE(Ur);
z && (nr |= 16777216), Gr = nr, _e();
let re = Kn(0, on);
Y.assert(T() === 1);
let Ee = He(sn()), qe = Kt(Ur, u, O, z, re, Ee, Gr, j);
return ZE(qe, _r), e7(qe, We), qe.commentDirectives = t.getCommentDirectives(), qe.nodeCount = oi, qe.identifierCount = $r, qe.identifiers = cr, qe.parseDiagnostics = qs(Zt, qe), Or && (qe.jsDocDiagnostics = qs(Or, qe)), b && ft(qe), qe;
function We($e, lt, Jt) {
Zt.push(Ro(Ur, $e, lt, Jt));
}
}
function St(u, b) {
return b ? He(u) : u;
}
let ue = false;
function He(u) {
Y.assert(!u.jsDoc);
let b = qt(I3(u, _r), (O) => Vh.parseJSDocComment(u, O.pos, O.end - O.pos));
return b.length && (u.jsDoc = b), ue && (ue = false, u.flags |= 268435456), u;
}
function _t(u) {
let b = Nn, O = Sd.createSyntaxCursor(u);
Nn = { currentNode: lt };
let j = [], z = Zt;
Zt = [];
let re = 0, Ee = We(u.statements, 0);
for (; Ee !== -1; ) {
let Jt = u.statements[re], Lt = u.statements[Ee];
jr(j, u.statements, re, Ee), re = $e(u.statements, Ee);
let At = he(z, (Fn) => Fn.start >= Jt.pos), kr = At >= 0 ? he(z, (Fn) => Fn.start >= Lt.pos, At) : -1;
At >= 0 && jr(Zt, z, At, kr >= 0 ? kr : void 0), Rn(() => {
let Fn = nr;
for (nr |= 32768, t.setTextPos(Lt.pos), _e(); T() !== 1; ) {
let di = t.getStartPos(), Ii = vc(0, on);
if (j.push(Ii), di === t.getStartPos() && _e(), re >= 0) {
let _n = u.statements[re];
if (Ii.end === _n.pos)
break;
Ii.end > _n.pos && (re = $e(u.statements, re + 1));
}
}
nr = Fn;
}, 2), Ee = re >= 0 ? We(u.statements, re) : -1;
}
if (re >= 0) {
let Jt = u.statements[re];
jr(j, u.statements, re);
let Lt = he(z, (At) => At.start >= Jt.pos);
Lt >= 0 && jr(Zt, z, Lt);
}
return Nn = b, N.updateSourceFile(u, Rt(X(j), u.statements));
function qe(Jt) {
return !(Jt.flags & 32768) && !!(Jt.transformFlags & 67108864);
}
function We(Jt, Lt) {
for (let At = Lt; At < Jt.length; At++)
if (qe(Jt[At]))
return At;
return -1;
}
function $e(Jt, Lt) {
for (let At = Lt; At < Jt.length; At++)
if (!qe(Jt[At]))
return At;
return -1;
}
function lt(Jt) {
let Lt = O.currentNode(Jt);
return br && Lt && qe(Lt) && (Lt.intersectsChange = true), Lt;
}
}
function ft(u) {
Vx(u, true);
}
e.fixupParentReferences = ft;
function Kt(u, b, O, j, z, re, Ee, qe) {
let We = N.createSourceFile(z, re, Ee);
return $f(We, 0, _r.length), $e(We), !j && Qo(We) && We.transformFlags & 67108864 && (We = _t(We), $e(We)), We;
function $e(lt) {
lt.text = _r, lt.bindDiagnostics = [], lt.bindSuggestionDiagnostics = void 0, lt.languageVersion = b, lt.fileName = u, lt.languageVariant = sv(O), lt.isDeclarationFile = j, lt.scriptKind = O, qe(lt), lt.setExternalModuleIndicator = qe;
}
}
function zt(u, b) {
u ? nr |= b : nr &= ~b;
}
function xe(u) {
zt(u, 4096);
}
function Le(u) {
zt(u, 8192);
}
function Re(u) {
zt(u, 16384);
}
function ot(u) {
zt(u, 32768);
}
function Ct(u, b) {
let O = u & nr;
if (O) {
zt(false, O);
let j = b();
return zt(true, O), j;
}
return b();
}
function Mt(u, b) {
let O = u & ~nr;
if (O) {
zt(true, O);
let j = b();
return zt(false, O), j;
}
return b();
}
function It(u) {
return Ct(4096, u);
}
function Mr(u) {
return Mt(4096, u);
}
function gr(u) {
return Ct(65536, u);
}
function Ln(u) {
return Mt(65536, u);
}
function ys(u) {
return Mt(8192, u);
}
function ci(u) {
return Mt(16384, u);
}
function Xi(u) {
return Mt(32768, u);
}
function Aa(u) {
return Ct(32768, u);
}
function vs(u) {
return Mt(40960, u);
}
function $s(u) {
return Ct(40960, u);
}
function li(u) {
return (nr & u) !== 0;
}
function Yi() {
return li(8192);
}
function Qi() {
return li(4096);
}
function bs() {
return li(65536);
}
function Ai() {
return li(16384);
}
function xn() {
return li(32768);
}
function Dt(u, b) {
return Z(t.getTokenPos(), t.getTextPos(), u, b);
}
function Pi(u, b, O, j) {
let z = Cn(Zt), re;
return (!z || u !== z.start) && (re = Ro(Ur, u, b, O, j), Zt.push(re)), Kr = true, re;
}
function Z(u, b, O, j) {
return Pi(u, b - u, O, j);
}
function ie(u, b, O) {
Z(u.pos, u.end, b, O);
}
function U(u, b) {
Pi(t.getTextPos(), b, u);
}
function L() {
return t.getStartPos();
}
function fe() {
return t.hasPrecedingJSDocComment();
}
function T() {
return ar;
}
function it() {
return ar = t.scan();
}
function mt(u) {
return _e(), u();
}
function _e() {
return ba(ar) && (t.hasUnicodeEscape() || t.hasExtendedUnicodeEscape()) && Z(t.getTokenPos(), t.getTextPos(), ve.Keywords_cannot_contain_escape_characters), it();
}
function Ge() {
return ar = t.scanJsDocToken();
}
function bt() {
return ar = t.reScanGreaterToken();
}
function jt() {
return ar = t.reScanSlashToken();
}
function Yt(u) {
return ar = t.reScanTemplateToken(u);
}
function $t() {
return ar = t.reScanTemplateHeadOrNoSubstitutionTemplate();
}
function Wt() {
return ar = t.reScanLessThanToken();
}
function Xr() {
return ar = t.reScanHashToken();
}
function Dr() {
return ar = t.scanJsxIdentifier();
}
function Lr() {
return ar = t.scanJsxToken();
}
function yr() {
return ar = t.scanJsxAttributeValue();
}
function Rn(u, b) {
let O = ar, j = Zt.length, z = Kr, re = nr, Ee = b !== 0 ? t.lookAhead(u) : t.tryScan(u);
return Y.assert(re === nr), (!Ee || b !== 0) && (ar = O, b !== 2 && (Zt.length = j), Kr = z), Ee;
}
function wt(u) {
return Rn(u, 1);
}
function Tr(u) {
return Rn(u, 0);
}
function Tt() {
return T() === 79 ? true : T() > 116;
}
function kt() {
return T() === 79 ? true : T() === 125 && Yi() || T() === 133 && xn() ? false : T() > 116;
}
function de(u, b) {
let O = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : true;
return T() === u ? (O && _e(), true) : (b ? Dt(b) : Dt(ve._0_expected, Br(u)), false);
}
let jn = Object.keys(cl).filter((u) => u.length > 2);
function Zi(u) {
var b;
if (Y8(u)) {
Z(Ar(_r, u.template.pos), u.template.end, ve.Module_declaration_names_may_only_use_or_quoted_strings);
return;
}
let O = yt(u) ? qr(u) : void 0;
if (!O || !vy(O, Sn)) {
Dt(ve._0_expected, Br(26));
return;
}
let j = Ar(_r, u.pos);
switch (O) {
case "const":
case "let":
case "var":
Z(j, u.end, ve.Variable_declaration_not_allowed_at_this_location);
return;
case "declare":
return;
case "interface":
Pa(ve.Interface_name_cannot_be_0, ve.Interface_must_be_given_a_name, 18);
return;
case "is":
Z(j, t.getTextPos(), ve.A_type_predicate_is_only_allowed_in_return_type_position_for_functions_and_methods);
return;
case "module":
case "namespace":
Pa(ve.Namespace_name_cannot_be_0, ve.Namespace_must_be_given_a_name, 18);
return;
case "type":
Pa(ve.Type_alias_name_cannot_be_0, ve.Type_alias_must_be_given_a_name, 63);
return;
}
let z = (b = Ep(O, jn, (re) => re)) != null ? b : e_(O);
if (z) {
Z(j, u.end, ve.Unknown_keyword_or_identifier_Did_you_mean_0, z);
return;
}
T() !== 0 && Z(j, u.end, ve.Unexpected_keyword_or_identifier);
}
function Pa(u, b, O) {
T() === O ? Dt(b) : Dt(u, t.getTokenValue());
}
function e_(u) {
for (let b of jn)
if (u.length > b.length + 2 && Pn(u, b))
return `${b} ${u.slice(b.length)}`;
}
function mc(u, b, O) {
if (T() === 59 && !t.hasPrecedingLineBreak()) {
Dt(ve.Decorators_must_precede_the_name_and_all_keywords_of_property_declarations);
return;
}
if (T() === 20) {
Dt(ve.Cannot_start_a_function_call_in_a_type_annotation), _e();
return;
}
if (b && !ka()) {
O ? Dt(ve._0_expected, Br(26)) : Dt(ve.Expected_for_property_initializer);
return;
}
if (!t_()) {
if (O) {
Dt(ve._0_expected, Br(26));
return;
}
Zi(u);
}
}
function Da(u) {
return T() === u ? (Ge(), true) : (Dt(ve._0_expected, Br(u)), false);
}
function Ts(u, b, O, j) {
if (T() === b) {
_e();
return;
}
let z = Dt(ve._0_expected, Br(b));
O && z && Rl(z, Ro(Ur, j, 1, ve.The_parser_expected_to_find_a_1_to_match_the_0_token_here, Br(u), Br(b)));
}
function Ot(u) {
return T() === u ? (_e(), true) : false;
}
function dr(u) {
if (T() === u)
return sn();
}
function Dd(u) {
if (T() === u)
return Id();
}
function ea(u, b, O) {
return dr(u) || Jn(u, false, b || ve._0_expected, O || Br(u));
}
function kd(u) {
return Dd(u) || Jn(u, false, ve._0_expected, Br(u));
}
function sn() {
let u = L(), b = T();
return _e(), Q(Ye(b), u);
}
function Id() {
let u = L(), b = T();
return Ge(), Q(Ye(b), u);
}
function ka() {
return T() === 26 ? true : T() === 19 || T() === 1 || t.hasPrecedingLineBreak();
}
function t_() {
return ka() ? (T() === 26 && _e(), true) : false;
}
function En() {
return t_() || de(26);
}
function Er(u, b, O, j) {
let z = X(u, j);
return Us(z, b, O != null ? O : t.getStartPos()), z;
}
function Q(u, b, O) {
return Us(u, b, O != null ? O : t.getStartPos()), nr && (u.flags |= nr), Kr && (Kr = false, u.flags |= 131072), u;
}
function Jn(u, b, O, j) {
b ? Pi(t.getStartPos(), 0, O, j) : O && Dt(O, j);
let z = L(), re = u === 79 ? Te("", void 0) : yl(u) ? N.createTemplateLiteralLikeNode(u, "", "", void 0) : u === 8 ? F("", void 0) : u === 10 ? $("", void 0) : u === 279 ? N.createMissingDeclaration() : Ye(u);
return Q(re, z);
}
function Ia(u) {
let b = cr.get(u);
return b === void 0 && cr.set(u, b = u), b;
}
function Ss(u, b, O) {
if (u) {
$r++;
let qe = L(), We = T(), $e = Ia(t.getTokenValue()), lt = t.hasExtendedUnicodeEscape();
return it(), Q(Te($e, We, lt), qe);
}
if (T() === 80)
return Dt(O || ve.Private_identifiers_are_not_allowed_outside_class_bodies), Ss(true);
if (T() === 0 && t.tryScan(() => t.reScanInvalidIdentifier() === 79))
return Ss(true);
$r++;
let j = T() === 1, z = t.isReservedWord(), re = t.getTokenText(), Ee = z ? ve.Identifier_expected_0_is_a_reserved_word_that_cannot_be_used_here : ve.Identifier_expected;
return Jn(79, j, b || Ee, re);
}
function hc(u) {
return Ss(Tt(), void 0, u);
}
function wr(u, b) {
return Ss(kt(), u, b);
}
function zr(u) {
return Ss(fr(T()), u);
}
function xs() {
return fr(T()) || T() === 10 || T() === 8;
}
function Nd() {
return fr(T()) || T() === 10;
}
function R2(u) {
if (T() === 10 || T() === 8) {
let b = Di();
return b.text = Ia(b.text), b;
}
return u && T() === 22 ? j2() : T() === 80 ? gc() : zr();
}
function Es() {
return R2(true);
}
function j2() {
let u = L();
de(22);
let b = It(Sr);
return de(23), Q(N.createComputedPropertyName(b), u);
}
function gc() {
let u = L(), b = Se(Ia(t.getTokenValue()));
return _e(), Q(b, u);
}
function Ks(u) {
return T() === u && Tr(Od);
}
function uu() {
return _e(), t.hasPrecedingLineBreak() ? false : ta();
}
function Od() {
switch (T()) {
case 85:
return _e() === 92;
case 93:
return _e(), T() === 88 ? wt(Ld) : T() === 154 ? wt(J2) : r_();
case 88:
return Ld();
case 124:
case 137:
case 151:
return _e(), ta();
default:
return uu();
}
}
function r_() {
return T() === 59 || T() !== 41 && T() !== 128 && T() !== 18 && ta();
}
function J2() {
return _e(), r_();
}
function Md() {
return Wi(T()) && Tr(Od);
}
function ta() {
return T() === 22 || T() === 18 || T() === 41 || T() === 25 || xs();
}
function Ld() {
return _e(), T() === 84 || T() === 98 || T() === 118 || T() === 59 || T() === 126 && wt(gh) || T() === 132 && wt(yh);
}
function Xs(u, b) {
if (mu(u))
return true;
switch (u) {
case 0:
case 1:
case 3:
return !(T() === 26 && b) && vh();
case 2:
return T() === 82 || T() === 88;
case 4:
return wt(om);
case 5:
return wt(Jb) || T() === 26 && !b;
case 6:
return T() === 22 || xs();
case 12:
switch (T()) {
case 22:
case 41:
case 25:
case 24:
return true;
default:
return xs();
}
case 18:
return xs();
case 9:
return T() === 22 || T() === 25 || xs();
case 24:
return Nd();
case 7:
return T() === 18 ? wt(Rd) : b ? kt() && !fu() : Fu() && !fu();
case 8:
return tp();
case 10:
return T() === 27 || T() === 25 || tp();
case 19:
return T() === 101 || T() === 85 || kt();
case 15:
switch (T()) {
case 27:
case 24:
return true;
}
case 11:
return T() === 25 || La();
case 16:
return Ec(false);
case 17:
return Ec(true);
case 20:
case 21:
return T() === 27 || eo();
case 22:
return Oc();
case 23:
return fr(T());
case 13:
return fr(T()) || T() === 18;
case 14:
return true;
}
return Y.fail("Non-exhaustive case in 'isListElement'.");
}
function Rd() {
if (Y.assert(T() === 18), _e() === 19) {
let u = _e();
return u === 27 || u === 18 || u === 94 || u === 117;
}
return true;
}
function yc() {
return _e(), kt();
}
function pu() {
return _e(), fr(T());
}
function F2() {
return _e(), qT(T());
}
function fu() {
return T() === 117 || T() === 94 ? wt(jd) : false;
}
function jd() {
return _e(), La();
}
function Jd() {
return _e(), eo();
}
function Na(u) {
if (T() === 1)
return true;
switch (u) {
case 1:
case 2:
case 4:
case 5:
case 6:
case 12:
case 9:
case 23:
case 24:
return T() === 19;
case 3:
return T() === 19 || T() === 82 || T() === 88;
case 7:
return T() === 18 || T() === 94 || T() === 117;
case 8:
return B2();
case 19:
return T() === 31 || T() === 20 || T() === 18 || T() === 94 || T() === 117;
case 11:
return T() === 21 || T() === 26;
case 15:
case 21:
case 10:
return T() === 23;
case 17:
case 16:
case 18:
return T() === 21 || T() === 23;
case 20:
return T() !== 27;
case 22:
return T() === 18 || T() === 19;
case 13:
return T() === 31 || T() === 43;
case 14:
return T() === 29 && wt(Xb);
default:
return false;
}
}
function B2() {
return !!(ka() || jm(T()) || T() === 38);
}
function du() {
for (let u = 0; u < 25; u++)
if (hr & 1 << u && (Xs(u, true) || Na(u)))
return true;
return false;
}
function Kn(u, b) {
let O = hr;
hr |= 1 << u;
let j = [], z = L();
for (; !Na(u); ) {
if (Xs(u, false)) {
j.push(vc(u, b));
continue;
}
if (bu(u))
break;
}
return hr = O, Er(j, z);
}
function vc(u, b) {
let O = mu(u);
return O ? hu(O) : b();
}
function mu(u, b) {
var O;
if (!Nn || !q2(u) || Kr)
return;
let j = Nn.currentNode(b != null ? b : t.getStartPos());
if (!(va(j) || j.intersectsChange || Ky(j) || (j.flags & 50720768) !== nr) && Fd(j, u))
return Af(j) && ((O = j.jsDoc) != null && O.jsDocCache) && (j.jsDoc.jsDocCache = void 0), j;
}
function hu(u) {
return t.setTextPos(u.end), _e(), u;
}
function q2(u) {
switch (u) {
case 5:
case 2:
case 0:
case 1:
case 3:
case 6:
case 4:
case 8:
case 17:
case 16:
return true;
}
return false;
}
function Fd(u, b) {
switch (b) {
case 5:
return Bd(u);
case 2:
return gu(u);
case 0:
case 1:
case 3:
return qd(u);
case 6:
return yu(u);
case 4:
return Ud(u);
case 8:
return vu(u);
case 17:
case 16:
return zd(u);
}
return false;
}
function Bd(u) {
if (u)
switch (u.kind) {
case 173:
case 178:
case 174:
case 175:
case 169:
case 237:
return true;
case 171:
let b = u;
return !(b.name.kind === 79 && b.name.escapedText === "constructor");
}
return false;
}
function gu(u) {
if (u)
switch (u.kind) {
case 292:
case 293:
return true;
}
return false;
}
function qd(u) {
if (u)
switch (u.kind) {
case 259:
case 240:
case 238:
case 242:
case 241:
case 254:
case 250:
case 252:
case 249:
case 248:
case 246:
case 247:
case 245:
case 244:
case 251:
case 239:
case 255:
case 253:
case 243:
case 256:
case 269:
case 268:
case 275:
case 274:
case 264:
case 260:
case 261:
case 263:
case 262:
return true;
}
return false;
}
function yu(u) {
return u.kind === 302;
}
function Ud(u) {
if (u)
switch (u.kind) {
case 177:
case 170:
case 178:
case 168:
case 176:
return true;
}
return false;
}
function vu(u) {
return u.kind !== 257 ? false : u.initializer === void 0;
}
function zd(u) {
return u.kind !== 166 ? false : u.initializer === void 0;
}
function bu(u) {
return U2(u), du() ? true : (_e(), false);
}
function U2(u) {
switch (u) {
case 0:
return T() === 88 ? Dt(ve._0_expected, Br(93)) : Dt(ve.Declaration_or_statement_expected);
case 1:
return Dt(ve.Declaration_or_statement_expected);
case 2:
return Dt(ve.case_or_default_expected);
case 3:
return Dt(ve.Statement_expected);
case 18:
case 4:
return Dt(ve.Property_or_signature_expected);
case 5:
return Dt(ve.Unexpected_token_A_constructor_method_accessor_or_property_was_expected);
case 6:
return Dt(ve.Enum_member_expected);
case 7:
return Dt(ve.Expression_expected);
case 8:
return ba(T()) ? Dt(ve._0_is_not_allowed_as_a_variable_declaration_name, Br(T())) : Dt(ve.Variable_declaration_expected);
case 9:
return Dt(ve.Property_destructuring_pattern_expected);
case 10:
return Dt(ve.Array_element_destructuring_pattern_expected);
case 11:
return Dt(ve.Argument_expression_expected);
case 12:
return Dt(ve.Property_assignment_expected);
case 15:
return Dt(ve.Expression_or_comma_expected);
case 17:
return Dt(ve.Parameter_declaration_expected);
case 16:
return ba(T()) ? Dt(ve._0_is_not_allowed_as_a_parameter_name, Br(T())) : Dt(ve.Parameter_declaration_expected);
case 19:
return Dt(ve.Type_parameter_declaration_expected);
case 20:
return Dt(ve.Type_argument_expected);
case 21:
return Dt(ve.Type_expected);
case 22:
return Dt(ve.Unexpected_token_expected);
case 23:
return Dt(ve.Identifier_expected);
case 13:
return Dt(ve.Identifier_expected);
case 14:
return Dt(ve.Identifier_expected);
case 24:
return Dt(ve.Identifier_or_string_literal_expected);
case 25:
return Y.fail("ParsingContext.Count used as a context");
default:
Y.assertNever(u);
}
}
function mn(u, b, O) {
let j = hr;
hr |= 1 << u;
let z = [], re = L(), Ee = -1;
for (; ; ) {
if (Xs(u, false)) {
let qe = t.getStartPos(), We = vc(u, b);
if (!We) {
hr = j;
return;
}
if (z.push(We), Ee = t.getTokenPos(), Ot(27))
continue;
if (Ee = -1, Na(u))
break;
de(27, z2(u)), O && T() === 26 && !t.hasPrecedingLineBreak() && _e(), qe === t.getStartPos() && _e();
continue;
}
if (Na(u) || bu(u))
break;
}
return hr = j, Er(z, re, void 0, Ee >= 0);
}
function z2(u) {
return u === 6 ? ve.An_enum_member_name_must_be_followed_by_a_or : void 0;
}
function ui() {
let u = Er([], L());
return u.isMissingList = true, u;
}
function W2(u) {
return !!u.isMissingList;
}
function Oa(u, b, O, j) {
if (de(O)) {
let z = mn(u, b);
return de(j), z;
}
return ui();
}
function Ys(u, b) {
let O = L(), j = u ? zr(b) : wr(b);
for (; Ot(24) && T() !== 29; )
j = Q(N.createQualifiedName(j, bc(u, false)), O);
return j;
}
function Tu(u, b) {
return Q(N.createQualifiedName(u, b), u.pos);
}
function bc(u, b) {
if (t.hasPrecedingLineBreak() && fr(T()) && wt(Qu))
return Jn(79, true, ve.Identifier_expected);
if (T() === 80) {
let O = gc();
return b ? O : Jn(79, true, ve.Identifier_expected);
}
return u ? zr() : wr();
}
function Su(u) {
let b = L(), O = [], j;
do
j = H2(u), O.push(j);
while (j.literal.kind === 16);
return Er(O, b);
}
function Wd(u) {
let b = L();
return Q(N.createTemplateExpression(Hd(u), Su(u)), b);
}
function xu() {
let u = L();
return Q(N.createTemplateLiteralType(Hd(false), Vd()), u);
}
function Vd() {
let u = L(), b = [], O;
do
O = V2(), b.push(O);
while (O.literal.kind === 16);
return Er(b, u);
}
function V2() {
let u = L();
return Q(N.createTemplateLiteralTypeSpan(sr(), Eu(false)), u);
}
function Eu(u) {
return T() === 19 ? (Yt(u), Tc()) : ea(17, ve._0_expected, Br(19));
}
function H2(u) {
let b = L();
return Q(N.createTemplateSpan(It(Sr), Eu(u)), b);
}
function Di() {
return n_(T());
}
function Hd(u) {
u && $t();
let b = n_(T());
return Y.assert(b.kind === 15, "Template head has wrong token kind"), b;
}
function Tc() {
let u = n_(T());
return Y.assert(u.kind === 16 || u.kind === 17, "Template fragment has wrong token kind"), u;
}
function Gd(u) {
let b = u === 14 || u === 17, O = t.getTokenText();
return O.substring(1, O.length - (t.isUnterminated() ? 0 : b ? 1 : 2));
}
function n_(u) {
let b = L(), O = yl(u) ? N.createTemplateLiteralLikeNode(u, t.getTokenValue(), Gd(u), t.getTokenFlags() & 2048) : u === 8 ? F(t.getTokenValue(), t.getNumericLiteralFlags()) : u === 10 ? $(t.getTokenValue(), void 0, t.hasExtendedUnicodeEscape()) : ky(u) ? ae(u, t.getTokenValue()) : Y.fail();
return t.hasExtendedUnicodeEscape() && (O.hasExtendedUnicodeEscape = true), t.isUnterminated() && (O.isUnterminated = true), _e(), Q(O, b);
}
function wu() {
return Ys(true, ve.Type_expected);
}
function Qs() {
if (!t.hasPrecedingLineBreak() && Wt() === 29)
return Oa(20, sr, 29, 31);
}
function Sc() {
let u = L();
return Q(N.createTypeReferenceNode(wu(), Qs()), u);
}
function Cu(u) {
switch (u.kind) {
case 180:
return va(u.typeName);
case 181:
case 182: {
let { parameters: b, type: O } = u;
return W2(b) || Cu(O);
}
case 193:
return Cu(u.type);
default:
return false;
}
}
function G2(u) {
return _e(), Q(N.createTypePredicateNode(void 0, u, sr()), u.pos);
}
function $d() {
let u = L();
return _e(), Q(N.createThisTypeNode(), u);
}
function Kd() {
let u = L();
return _e(), Q(N.createJSDocAllType(), u);
}
function $2() {
let u = L();
return _e(), Q(N.createJSDocNonNullableType(Lu(), false), u);
}
function Xd() {
let u = L();
return _e(), T() === 27 || T() === 19 || T() === 21 || T() === 31 || T() === 63 || T() === 51 ? Q(N.createJSDocUnknownType(), u) : Q(N.createJSDocNullableType(sr(), false), u);
}
function K2() {
let u = L(), b = fe();
if (wt(qh)) {
_e();
let O = ra(36), j = pi(58, false);
return St(Q(N.createJSDocFunctionType(O, j), u), b);
}
return Q(N.createTypeReferenceNode(zr(), void 0), u);
}
function Yd() {
let u = L(), b;
return (T() === 108 || T() === 103) && (b = zr(), de(58)), Q(N.createParameterDeclaration(void 0, void 0, b, void 0, xc(), void 0), u);
}
function xc() {
t.setInJSDocType(true);
let u = L();
if (Ot(142)) {
let j = N.createJSDocNamepathType(void 0);
e:
for (; ; )
switch (T()) {
case 19:
case 1:
case 27:
case 5:
break e;
default:
Ge();
}
return t.setInJSDocType(false), Q(j, u);
}
let b = Ot(25), O = Ju();
return t.setInJSDocType(false), b && (O = Q(N.createJSDocVariadicType(O), u)), T() === 63 ? (_e(), Q(N.createJSDocOptionalType(O), u)) : O;
}
function X2() {
let u = L();
de(112);
let b = Ys(true), O = t.hasPrecedingLineBreak() ? void 0 : Nc();
return Q(N.createTypeQueryNode(b, O), u);
}
function Qd() {
let u = L(), b = ki(false, true), O = wr(), j, z;
Ot(94) && (eo() || !La() ? j = sr() : z = Wu());
let re = Ot(63) ? sr() : void 0, Ee = N.createTypeParameterDeclaration(b, O, j, re);
return Ee.expression = z, Q(Ee, u);
}
function Xn() {
if (T() === 29)
return Oa(19, Qd, 29, 31);
}
function Ec(u) {
return T() === 25 || tp() || Wi(T()) || T() === 59 || eo(!u);
}
function Zd(u) {
let b = no(ve.Private_identifiers_cannot_be_used_as_parameters);
return hf(b) === 0 && !Ke(u) && Wi(T()) && _e(), b;
}
function em() {
return Tt() || T() === 22 || T() === 18;
}
function Au(u) {
return Pu(u);
}
function tm(u) {
return Pu(u, false);
}
function Pu(u) {
let b = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : true, O = L(), j = fe(), z = u ? Xi(() => ki(true)) : Aa(() => ki(true));
if (T() === 108) {
let We = N.createParameterDeclaration(z, void 0, Ss(true), void 0, Ma(), void 0), $e = pa(z);
return $e && ie($e, ve.Neither_decorators_nor_modifiers_may_be_applied_to_this_parameters), St(Q(We, O), j);
}
let re = br;
br = false;
let Ee = dr(25);
if (!b && !em())
return;
let qe = St(Q(N.createParameterDeclaration(z, Ee, Zd(z), dr(57), Ma(), Ra()), O), j);
return br = re, qe;
}
function pi(u, b) {
if (rm(u, b))
return gr(Ju);
}
function rm(u, b) {
return u === 38 ? (de(u), true) : Ot(58) ? true : b && T() === 38 ? (Dt(ve._0_expected, Br(58)), _e(), true) : false;
}
function wc(u, b) {
let O = Yi(), j = xn();
Le(!!(u & 1)), ot(!!(u & 2));
let z = u & 32 ? mn(17, Yd) : mn(16, () => b ? Au(j) : tm(j));
return Le(O), ot(j), z;
}
function ra(u) {
if (!de(20))
return ui();
let b = wc(u, true);
return de(21), b;
}
function i_() {
Ot(27) || En();
}
function nm(u) {
let b = L(), O = fe();
u === 177 && de(103);
let j = Xn(), z = ra(4), re = pi(58, true);
i_();
let Ee = u === 176 ? N.createCallSignature(j, z, re) : N.createConstructSignature(j, z, re);
return St(Q(Ee, b), O);
}
function im() {
return T() === 22 && wt(Zs);
}
function Zs() {
if (_e(), T() === 25 || T() === 23)
return true;
if (Wi(T())) {
if (_e(), kt())
return true;
} else if (kt())
_e();
else
return false;
return T() === 58 || T() === 27 ? true : T() !== 57 ? false : (_e(), T() === 58 || T() === 27 || T() === 23);
}
function am(u, b, O) {
let j = Oa(16, () => Au(false), 22, 23), z = Ma();
i_();
let re = N.createIndexSignature(O, j, z);
return St(Q(re, u), b);
}
function sm(u, b, O) {
let j = Es(), z = dr(57), re;
if (T() === 20 || T() === 29) {
let Ee = Xn(), qe = ra(4), We = pi(58, true);
re = N.createMethodSignature(O, j, z, Ee, qe, We);
} else {
let Ee = Ma();
re = N.createPropertySignature(O, j, z, Ee), T() === 63 && (re.initializer = Ra());
}
return i_(), St(Q(re, u), b);
}
function om() {
if (T() === 20 || T() === 29 || T() === 137 || T() === 151)
return true;
let u = false;
for (; Wi(T()); )
u = true, _e();
return T() === 22 ? true : (xs() && (u = true, _e()), u ? T() === 20 || T() === 29 || T() === 57 || T() === 58 || T() === 27 || ka() : false);
}
function Du() {
if (T() === 20 || T() === 29)
return nm(176);
if (T() === 103 && wt(a_))
return nm(177);
let u = L(), b = fe(), O = ki(false);
return Ks(137) ? Fa(u, b, O, 174, 4) : Ks(151) ? Fa(u, b, O, 175, 4) : im() ? am(u, b, O) : sm(u, b, O);
}
function a_() {
return _e(), T() === 20 || T() === 29;
}
function Y2() {
return _e() === 24;
}
function ku() {
switch (_e()) {
case 20:
case 29:
case 24:
return true;
}
return false;
}
function Q2() {
let u = L();
return Q(N.createTypeLiteralNode(Iu()), u);
}
function Iu() {
let u;
return de(18) ? (u = Kn(4, Du), de(19)) : u = ui(), u;
}
function Z2() {
return _e(), T() === 39 || T() === 40 ? _e() === 146 : (T() === 146 && _e(), T() === 22 && yc() && _e() === 101);
}
function _m() {
let u = L(), b = zr();
de(101);
let O = sr();
return Q(N.createTypeParameterDeclaration(void 0, b, O, void 0), u);
}
function eb() {
let u = L();
de(18);
let b;
(T() === 146 || T() === 39 || T() === 40) && (b = sn(), b.kind !== 146 && de(146)), de(22);
let O = _m(), j = Ot(128) ? sr() : void 0;
de(23);
let z;
(T() === 57 || T() === 39 || T() === 40) && (z = sn(), z.kind !== 57 && de(57));
let re = Ma();
En();
let Ee = Kn(4, Du);
return de(19), Q(N.createMappedTypeNode(b, O, j, z, re, Ee), u);
}
function Nu() {
let u = L();
if (Ot(25))
return Q(N.createRestTypeNode(sr()), u);
let b = sr();
if (uE(b) && b.pos === b.type.pos) {
let O = N.createOptionalTypeNode(b.type);
return Rt(O, b), O.flags = b.flags, O;
}
return b;
}
function cm() {
return _e() === 58 || T() === 57 && _e() === 58;
}
function lm() {
return T() === 25 ? fr(_e()) && cm() : fr(T()) && cm();
}
function tb() {
if (wt(lm)) {
let u = L(), b = fe(), O = dr(25), j = zr(), z = dr(57);
de(58);
let re = Nu(), Ee = N.createNamedTupleMember(O, j, z, re);
return St(Q(Ee, u), b);
}
return Nu();
}
function um() {
let u = L();
return Q(N.createTupleTypeNode(Oa(21, tb, 22, 23)), u);
}
function rb() {
let u = L();
de(20);
let b = sr();
return de(21), Q(N.createParenthesizedType(b), u);
}
function pm() {
let u;
if (T() === 126) {
let b = L();
_e();
let O = Q(Ye(126), b);
u = Er([O], b);
}
return u;
}
function fm() {
let u = L(), b = fe(), O = pm(), j = Ot(103);
Y.assert(!O || j, "Per isStartOfFunctionOrConstructorType, a function type cannot have modifiers.");
let z = Xn(), re = ra(4), Ee = pi(38, false), qe = j ? N.createConstructorTypeNode(O, z, re, Ee) : N.createFunctionTypeNode(z, re, Ee);
return St(Q(qe, u), b);
}
function Ou() {
let u = sn();
return T() === 24 ? void 0 : u;
}
function dm(u) {
let b = L();
u && _e();
let O = T() === 110 || T() === 95 || T() === 104 ? sn() : n_(T());
return u && (O = Q(N.createPrefixUnaryExpression(40, O), b)), Q(N.createLiteralTypeNode(O), b);
}
function mm() {
return _e(), T() === 100;
}
function nb() {
let u = L(), b = t.getTokenPos();
de(18);
let O = t.hasPrecedingLineBreak();
de(130), de(58);
let j = _p(true);
if (!de(19)) {
let z = Cn(Zt);
z && z.code === ve._0_expected.code && Rl(z, Ro(Ur, b, 1, ve.The_parser_expected_to_find_a_1_to_match_the_0_token_here, "{", "}"));
}
return Q(N.createImportTypeAssertionContainer(j, O), u);
}
function Mu() {
Gr |= 2097152;
let u = L(), b = Ot(112);
de(100), de(20);
let O = sr(), j;
Ot(27) && (j = nb()), de(21);
let z = Ot(24) ? wu() : void 0, re = Qs();
return Q(N.createImportTypeNode(O, j, z, re, b), u);
}
function hm() {
return _e(), T() === 8 || T() === 9;
}
function Lu() {
switch (T()) {
case 131:
case 157:
case 152:
case 148:
case 160:
case 153:
case 134:
case 155:
case 144:
case 149:
return Tr(Ou) || Sc();
case 66:
t.reScanAsteriskEqualsToken();
case 41:
return Kd();
case 60:
t.reScanQuestionToken();
case 57:
return Xd();
case 98:
return K2();
case 53:
return $2();
case 14:
case 10:
case 8:
case 9:
case 110:
case 95:
case 104:
return dm();
case 40:
return wt(hm) ? dm(true) : Sc();
case 114:
return sn();
case 108: {
let u = $d();
return T() === 140 && !t.hasPrecedingLineBreak() ? G2(u) : u;
}
case 112:
return wt(mm) ? Mu() : X2();
case 18:
return wt(Z2) ? eb() : Q2();
case 22:
return um();
case 20:
return rb();
case 100:
return Mu();
case 129:
return wt(Qu) ? Cm() : Sc();
case 15:
return xu();
default:
return Sc();
}
}
function eo(u) {
switch (T()) {
case 131:
case 157:
case 152:
case 148:
case 160:
case 134:
case 146:
case 153:
case 156:
case 114:
case 155:
case 104:
case 108:
case 112:
case 144:
case 18:
case 22:
case 29:
case 51:
case 50:
case 103:
case 10:
case 8:
case 9:
case 110:
case 95:
case 149:
case 41:
case 57:
case 53:
case 25:
case 138:
case 100:
case 129:
case 14:
case 15:
return true;
case 98:
return !u;
case 40:
return !u && wt(hm);
case 20:
return !u && wt(gm);
default:
return kt();
}
}
function gm() {
return _e(), T() === 21 || Ec(false) || eo();
}
function ym() {
let u = L(), b = Lu();
for (; !t.hasPrecedingLineBreak(); )
switch (T()) {
case 53:
_e(), b = Q(N.createJSDocNonNullableType(b, true), u);
break;
case 57:
if (wt(Jd))
return b;
_e(), b = Q(N.createJSDocNullableType(b, true), u);
break;
case 22:
if (de(22), eo()) {
let O = sr();
de(23), b = Q(N.createIndexedAccessTypeNode(b, O), u);
} else
de(23), b = Q(N.createArrayTypeNode(b), u);
break;
default:
return b;
}
return b;
}
function vm(u) {
let b = L();
return de(u), Q(N.createTypeOperatorNode(u, Tm()), b);
}
function ib() {
if (Ot(94)) {
let u = Ln(sr);
if (bs() || T() !== 57)
return u;
}
}
function bm() {
let u = L(), b = wr(), O = Tr(ib), j = N.createTypeParameterDeclaration(void 0, b, O);
return Q(j, u);
}
function ab() {
let u = L();
return de(138), Q(N.createInferTypeNode(bm()), u);
}
function Tm() {
let u = T();
switch (u) {
case 141:
case 156:
case 146:
return vm(u);
case 138:
return ab();
}
return gr(ym);
}
function Cc(u) {
if (ju()) {
let b = fm(), O;
return $l(b) ? O = u ? ve.Function_type_notation_must_be_parenthesized_when_used_in_a_union_type : ve.Function_type_notation_must_be_parenthesized_when_used_in_an_intersection_type : O = u ? ve.Constructor_type_notation_must_be_parenthesized_when_used_in_a_union_type : ve.Constructor_type_notation_must_be_parenthesized_when_used_in_an_intersection_type, ie(b, O), b;
}
}
function Sm(u, b, O) {
let j = L(), z = u === 51, re = Ot(u), Ee = re && Cc(z) || b();
if (T() === u || re) {
let qe = [Ee];
for (; Ot(u); )
qe.push(Cc(z) || b());
Ee = Q(O(Er(qe, j)), j);
}
return Ee;
}
function Ru() {
return Sm(50, Tm, N.createIntersectionTypeNode);
}
function sb() {
return Sm(51, Ru, N.createUnionTypeNode);
}
function xm() {
return _e(), T() === 103;
}
function ju() {
return T() === 29 || T() === 20 && wt(Em) ? true : T() === 103 || T() === 126 && wt(xm);
}
function ob() {
if (Wi(T()) && ki(false), kt() || T() === 108)
return _e(), true;
if (T() === 22 || T() === 18) {
let u = Zt.length;
return no(), u === Zt.length;
}
return false;
}
function Em() {
return _e(), !!(T() === 21 || T() === 25 || ob() && (T() === 58 || T() === 27 || T() === 57 || T() === 63 || T() === 21 && (_e(), T() === 38)));
}
function Ju() {
let u = L(), b = kt() && Tr(wm), O = sr();
return b ? Q(N.createTypePredicateNode(void 0, b, O), u) : O;
}
function wm() {
let u = wr();
if (T() === 140 && !t.hasPrecedingLineBreak())
return _e(), u;
}
function Cm() {
let u = L(), b = ea(129), O = T() === 108 ? $d() : wr(), j = Ot(140) ? sr() : void 0;
return Q(N.createTypePredicateNode(b, O, j), u);
}
function sr() {
if (nr & 40960)
return Ct(40960, sr);
if (ju())
return fm();
let u = L(), b = sb();
if (!bs() && !t.hasPrecedingLineBreak() && Ot(94)) {
let O = Ln(sr);
de(57);
let j = gr(sr);
de(58);
let z = gr(sr);
return Q(N.createConditionalTypeNode(b, O, j, z), u);
}
return b;
}
function Ma() {
return Ot(58) ? sr() : void 0;
}
function Fu() {
switch (T()) {
case 108:
case 106:
case 104:
case 110:
case 95:
case 8:
case 9:
case 10:
case 14:
case 15:
case 20:
case 22:
case 18:
case 98:
case 84:
case 103:
case 43:
case 68:
case 79:
return true;
case 100:
return wt(ku);
default:
return kt();
}
}
function La() {
if (Fu())
return true;
switch (T()) {
case 39:
case 40:
case 54:
case 53:
case 89:
case 112:
case 114:
case 45:
case 46:
case 29:
case 133:
case 125:
case 80:
case 59:
return true;
default:
return Jm() ? true : kt();
}
}
function Am() {
return T() !== 18 && T() !== 98 && T() !== 84 && T() !== 59 && La();
}
function Sr() {
let u = Ai();
u && Re(false);
let b = L(), O = Yr(true), j;
for (; j = dr(27); )
O = Uu(O, j, Yr(true), b);
return u && Re(true), O;
}
function Ra() {
return Ot(63) ? Yr(true) : void 0;
}
function Yr(u) {
if (Pm())
return Dm();
let b = cb(u) || Mm(u);
if (b)
return b;
let O = L(), j = s_(0);
return j.kind === 79 && T() === 38 ? km(O, j, u, void 0) : Do(j) && G_(bt()) ? Uu(j, sn(), Yr(u), O) : lb(j, O, u);
}
function Pm() {
return T() === 125 ? Yi() ? true : wt(Zu) : false;
}
function _b() {
return _e(), !t.hasPrecedingLineBreak() && kt();
}
function Dm() {
let u = L();
return _e(), !t.hasPrecedingLineBreak() && (T() === 41 || La()) ? Q(N.createYieldExpression(dr(41), Yr(true)), u) : Q(N.createYieldExpression(void 0, void 0), u);
}
function km(u, b, O, j) {
Y.assert(T() === 38, "parseSimpleArrowFunctionExpression should only have been called if we had a =>");
let z = N.createParameterDeclaration(void 0, void 0, b, void 0, void 0, void 0);
Q(z, b.pos);
let re = Er([z], z.pos, z.end), Ee = ea(38), qe = Bu(!!j, O), We = N.createArrowFunction(j, void 0, re, void 0, Ee, qe);
return He(Q(We, u));
}
function cb(u) {
let b = Im();
if (b !== 0)
return b === 1 ? Rm(true, true) : Tr(() => Om(u));
}
function Im() {
return T() === 20 || T() === 29 || T() === 132 ? wt(Nm) : T() === 38 ? 1 : 0;
}
function Nm() {
if (T() === 132 && (_e(), t.hasPrecedingLineBreak() || T() !== 20 && T() !== 29))
return 0;
let u = T(), b = _e();
if (u === 20) {
if (b === 21)
switch (_e()) {
case 38:
case 58:
case 18:
return 1;
default:
return 0;
}
if (b === 22 || b === 18)
return 2;
if (b === 25)
return 1;
if (Wi(b) && b !== 132 && wt(yc))
return _e() === 128 ? 0 : 1;
if (!kt() && b !== 108)
return 0;
switch (_e()) {
case 58:
return 1;
case 57:
return _e(), T() === 58 || T() === 27 || T() === 63 || T() === 21 ? 1 : 0;
case 27:
case 63:
case 21:
return 2;
}
return 0;
} else
return Y.assert(u === 29), !kt() && T() !== 85 ? 0 : pr === 1 ? wt(() => {
Ot(85);
let j = _e();
if (j === 94)
switch (_e()) {
case 63:
case 31:
case 43:
return false;
default:
return true;
}
else if (j === 27 || j === 63)
return true;
return false;
}) ? 1 : 0 : 2;
}
function Om(u) {
let b = t.getTokenPos();
if (On != null && On.has(b))
return;
let O = Rm(false, u);
return O || (On || (On = /* @__PURE__ */ new Set())).add(b), O;
}
function Mm(u) {
if (T() === 132 && wt(Lm) === 1) {
let b = L(), O = sp(), j = s_(0);
return km(b, j, u, O);
}
}
function Lm() {
if (T() === 132) {
if (_e(), t.hasPrecedingLineBreak() || T() === 38)
return 0;
let u = s_(0);
if (!t.hasPrecedingLineBreak() && u.kind === 79 && T() === 38)
return 1;
}
return 0;
}
function Rm(u, b) {
let O = L(), j = fe(), z = sp(), re = Ke(z, Ul) ? 2 : 0, Ee = Xn(), qe;
if (de(20)) {
if (u)
qe = wc(re, u);
else {
let di = wc(re, u);
if (!di)
return;
qe = di;
}
if (!de(21) && !u)
return;
} else {
if (!u)
return;
qe = ui();
}
let We = T() === 58, $e = pi(58, false);
if ($e && !u && Cu($e))
return;
let lt = $e;
for (; (lt == null ? void 0 : lt.kind) === 193; )
lt = lt.type;
let Jt = lt && dd(lt);
if (!u && T() !== 38 && (Jt || T() !== 18))
return;
let Lt = T(), At = ea(38), kr = Lt === 38 || Lt === 18 ? Bu(Ke(z, Ul), b) : wr();
if (!b && We && T() !== 58)
return;
let Fn = N.createArrowFunction(z, Ee, qe, $e, At, kr);
return St(Q(Fn, O), j);
}
function Bu(u, b) {
if (T() === 18)
return Dc(u ? 2 : 0);
if (T() !== 26 && T() !== 98 && T() !== 84 && vh() && !Am())
return Dc(16 | (u ? 2 : 0));
let O = br;
br = false;
let j = u ? Xi(() => Yr(b)) : Aa(() => Yr(b));
return br = O, j;
}
function lb(u, b, O) {
let j = dr(57);
if (!j)
return u;
let z;
return Q(N.createConditionalExpression(u, j, Ct(r, () => Yr(false)), z = ea(58), xl(z) ? Yr(O) : Jn(79, false, ve._0_expected, Br(58))), b);
}
function s_(u) {
let b = L(), O = Wu();
return qu(u, O, b);
}
function jm(u) {
return u === 101 || u === 162;
}
function qu(u, b, O) {
for (; ; ) {
bt();
let j = Dl(T());
if (!(T() === 42 ? j >= u : j > u) || T() === 101 && Qi())
break;
if (T() === 128 || T() === 150) {
if (t.hasPrecedingLineBreak())
break;
{
let re = T();
_e(), b = re === 150 ? Fm(b, sr()) : Bm(b, sr());
}
} else
b = Uu(b, sn(), s_(j), O);
}
return b;
}
function Jm() {
return Qi() && T() === 101 ? false : Dl(T()) > 0;
}
function Fm(u, b) {
return Q(N.createSatisfiesExpression(u, b), u.pos);
}
function Uu(u, b, O, j) {
return Q(N.createBinaryExpression(u, b, O), j);
}
function Bm(u, b) {
return Q(N.createAsExpression(u, b), u.pos);
}
function qm() {
let u = L();
return Q(N.createPrefixUnaryExpression(T(), mt(na)), u);
}
function Um() {
let u = L();
return Q(N.createDeleteExpression(mt(na)), u);
}
function ub() {
let u = L();
return Q(N.createTypeOfExpression(mt(na)), u);
}
function zm() {
let u = L();
return Q(N.createVoidExpression(mt(na)), u);
}
function pb() {
return T() === 133 ? xn() ? true : wt(Zu) : false;
}
function zu() {
let u = L();
return Q(N.createAwaitExpression(mt(na)), u);
}
function Wu() {
if (Wm()) {
let O = L(), j = Vm();
return T() === 42 ? qu(Dl(T()), j, O) : j;
}
let u = T(), b = na();
if (T() === 42) {
let O = Ar(_r, b.pos), { end: j } = b;
b.kind === 213 ? Z(O, j, ve.A_type_assertion_expression_is_not_allowed_in_the_left_hand_side_of_an_exponentiation_expression_Consider_enclosing_the_expression_in_parentheses) : Z(O, j, ve.An_unary_expression_with_the_0_operator_is_not_allowed_in_the_left_hand_side_of_an_exponentiation_expression_Consider_enclosing_the_expression_in_parentheses, Br(u));
}
return b;
}
function na() {
switch (T()) {
case 39:
case 40:
case 54:
case 53:
return qm();
case 89:
return Um();
case 112:
return ub();
case 114:
return zm();
case 29:
return pr === 1 ? o_(true) : Zm();
case 133:
if (pb())
return zu();
default:
return Vm();
}
}
function Wm() {
switch (T()) {
case 39:
case 40:
case 54:
case 53:
case 89:
case 112:
case 114:
case 133:
return false;
case 29:
if (pr !== 1)
return false;
default:
return true;
}
}
function Vm() {
if (T() === 45 || T() === 46) {
let b = L();
return Q(N.createPrefixUnaryExpression(T(), mt(to)), b);
} else if (pr === 1 && T() === 29 && wt(F2))
return o_(true);
let u = to();
if (Y.assert(Do(u)), (T() === 45 || T() === 46) && !t.hasPrecedingLineBreak()) {
let b = T();
return _e(), Q(N.createPostfixUnaryExpression(u, b), u.pos);
}
return u;
}
function to() {
let u = L(), b;
return T() === 100 ? wt(a_) ? (Gr |= 2097152, b = sn()) : wt(Y2) ? (_e(), _e(), b = Q(N.createMetaProperty(100, zr()), u), Gr |= 4194304) : b = Hm() : b = T() === 106 ? Vu() : Hm(), $u(u, b);
}
function Hm() {
let u = L(), b = Ku();
return Ja(u, b, true);
}
function Vu() {
let u = L(), b = sn();
if (T() === 29) {
let O = L(), j = Tr(Pc);
j !== void 0 && (Z(O, L(), ve.super_may_not_use_type_arguments), __() || (b = N.createExpressionWithTypeArguments(b, j)));
}
return T() === 20 || T() === 24 || T() === 22 ? b : (ea(24, ve.super_must_be_followed_by_an_argument_list_or_member_access), Q(Ve(b, bc(true, true)), u));
}
function o_(u, b, O) {
let j = L(), z = Km(u), re;
if (z.kind === 283) {
let Ee = $m(z), qe, We = Ee[Ee.length - 1];
if ((We == null ? void 0 : We.kind) === 281 && !Hi(We.openingElement.tagName, We.closingElement.tagName) && Hi(z.tagName, We.closingElement.tagName)) {
let $e = We.children.end, lt = Q(N.createJsxElement(We.openingElement, We.children, Q(N.createJsxClosingElement(Q(Te(""), $e, $e)), $e, $e)), We.openingElement.pos, $e);
Ee = Er([...Ee.slice(0, Ee.length - 1), lt], Ee.pos, $e), qe = We.closingElement;
} else
qe = Qm(z, u), Hi(z.tagName, qe.tagName) || (O && tu(O) && Hi(qe.tagName, O.tagName) ? ie(z.tagName, ve.JSX_element_0_has_no_corresponding_closing_tag, B_(_r, z.tagName)) : ie(qe.tagName, ve.Expected_corresponding_JSX_closing_tag_for_0, B_(_r, z.tagName)));
re = Q(N.createJsxElement(z, Ee, qe), j);
} else
z.kind === 286 ? re = Q(N.createJsxFragment(z, $m(z), gb(u)), j) : (Y.assert(z.kind === 282), re = z);
if (u && T() === 29) {
let Ee = typeof b > "u" ? re.pos : b, qe = Tr(() => o_(true, Ee));
if (qe) {
let We = Jn(27, false);
return $f(We, qe.pos, 0), Z(Ar(_r, Ee), qe.end, ve.JSX_expressions_must_have_one_parent_element), Q(N.createBinaryExpression(re, We, qe), j);
}
}
return re;
}
function fb() {
let u = L(), b = N.createJsxText(t.getTokenValue(), ar === 12);
return ar = t.scanJsxToken(), Q(b, u);
}
function Gm(u, b) {
switch (b) {
case 1:
if (u2(u))
ie(u, ve.JSX_fragment_has_no_corresponding_closing_tag);
else {
let O = u.tagName, j = Ar(_r, O.pos);
Z(j, O.end, ve.JSX_element_0_has_no_corresponding_closing_tag, B_(_r, u.tagName));
}
return;
case 30:
case 7:
return;
case 11:
case 12:
return fb();
case 18:
return Xm(false);
case 29:
return o_(false, void 0, u);
default:
return Y.assertNever(b);
}
}
function $m(u) {
let b = [], O = L(), j = hr;
for (hr |= 1 << 14; ; ) {
let z = Gm(u, ar = t.reScanJsxToken());
if (!z || (b.push(z), tu(u) && (z == null ? void 0 : z.kind) === 281 && !Hi(z.openingElement.tagName, z.closingElement.tagName) && Hi(u.tagName, z.closingElement.tagName)))
break;
}
return hr = j, Er(b, O);
}
function db() {
let u = L();
return Q(N.createJsxAttributes(Kn(13, mb)), u);
}
function Km(u) {
let b = L();
if (de(29), T() === 31)
return Lr(), Q(N.createJsxOpeningFragment(), b);
let O = Ac(), j = nr & 262144 ? void 0 : Nc(), z = db(), re;
return T() === 31 ? (Lr(), re = N.createJsxOpeningElement(O, j, z)) : (de(43), de(31, void 0, false) && (u ? _e() : Lr()), re = N.createJsxSelfClosingElement(O, j, z)), Q(re, b);
}
function Ac() {
let u = L();
Dr();
let b = T() === 108 ? sn() : zr();
for (; Ot(24); )
b = Q(Ve(b, bc(true, false)), u);
return b;
}
function Xm(u) {
let b = L();
if (!de(18))
return;
let O, j;
return T() !== 19 && (O = dr(25), j = Sr()), u ? de(19) : de(19, void 0, false) && Lr(), Q(N.createJsxExpression(O, j), b);
}
function mb() {
if (T() === 18)
return hb();
Dr();
let u = L();
return Q(N.createJsxAttribute(zr(), Ym()), u);
}
function Ym() {
if (T() === 63) {
if (yr() === 10)
return Di();
if (T() === 18)
return Xm(true);
if (T() === 29)
return o_(true);
Dt(ve.or_JSX_element_expected);
}
}
function hb() {
let u = L();
de(18), de(25);
let b = Sr();
return de(19), Q(N.createJsxSpreadAttribute(b), u);
}
function Qm(u, b) {
let O = L();
de(30);
let j = Ac();
return de(31, void 0, false) && (b || !Hi(u.tagName, j) ? _e() : Lr()), Q(N.createJsxClosingElement(j), O);
}
function gb(u) {
let b = L();
return de(30), de(31, ve.Expected_corresponding_closing_tag_for_JSX_fragment, false) && (u ? _e() : Lr()), Q(N.createJsxJsxClosingFragment(), b);
}
function Zm() {
Y.assert(pr !== 1, "Type assertions should never be parsed in JSX; they should be parsed as comparisons or JSX elements/fragments.");
let u = L();
de(29);
let b = sr();
de(31);
let O = na();
return Q(N.createTypeAssertion(b, O), u);
}
function yb() {
return _e(), fr(T()) || T() === 22 || __();
}
function eh() {
return T() === 28 && wt(yb);
}
function Hu(u) {
if (u.flags & 32)
return true;
if (Uo(u)) {
let b = u.expression;
for (; Uo(b) && !(b.flags & 32); )
b = b.expression;
if (b.flags & 32) {
for (; Uo(u); )
u.flags |= 32, u = u.expression;
return true;
}
}
return false;
}
function fi(u, b, O) {
let j = bc(true, true), z = O || Hu(b), re = z ? pt(b, O, j) : Ve(b, j);
if (z && vn(re.name) && ie(re.name, ve.An_optional_chain_cannot_contain_private_identifiers), e2(b) && b.typeArguments) {
let Ee = b.typeArguments.pos - 1, qe = Ar(_r, b.typeArguments.end) + 1;
Z(Ee, qe, ve.An_instantiation_expression_cannot_be_followed_by_a_property_access);
}
return Q(re, u);
}
function ja(u, b, O) {
let j;
if (T() === 23)
j = Jn(79, true, ve.An_element_access_expression_should_take_an_argument);
else {
let re = It(Sr);
Ta(re) && (re.text = Ia(re.text)), j = re;
}
de(23);
let z = O || Hu(b) ? Nt(b, O, j) : Gt(b, j);
return Q(z, u);
}
function Ja(u, b, O) {
for (; ; ) {
let j, z = false;
if (O && eh() ? (j = ea(28), z = fr(T())) : z = Ot(24), z) {
b = fi(u, b, j);
continue;
}
if ((j || !Ai()) && Ot(22)) {
b = ja(u, b, j);
continue;
}
if (__()) {
b = !j && b.kind === 230 ? Gu(u, b.expression, j, b.typeArguments) : Gu(u, b, j, void 0);
continue;
}
if (!j) {
if (T() === 53 && !t.hasPrecedingLineBreak()) {
_e(), b = Q(N.createNonNullExpression(b), u);
continue;
}
let re = Tr(Pc);
if (re) {
b = Q(N.createExpressionWithTypeArguments(b, re), u);
continue;
}
}
return b;
}
}
function __() {
return T() === 14 || T() === 15;
}
function Gu(u, b, O, j) {
let z = N.createTaggedTemplateExpression(b, j, T() === 14 ? ($t(), Di()) : Wd(true));
return (O || b.flags & 32) && (z.flags |= 32), z.questionDotToken = O, Q(z, u);
}
function $u(u, b) {
for (; ; ) {
b = Ja(u, b, true);
let O, j = dr(28);
if (j && (O = Tr(Pc), __())) {
b = Gu(u, b, j, O);
continue;
}
if (O || T() === 20) {
!j && b.kind === 230 && (O = b.typeArguments, b = b.expression);
let z = th(), re = j || Hu(b) ? er(b, j, O, z) : Xt(b, O, z);
b = Q(re, u);
continue;
}
if (j) {
let z = Jn(79, false, ve.Identifier_expected);
b = Q(pt(b, j, z), u);
}
break;
}
return b;
}
function th() {
de(20);
let u = mn(11, ih);
return de(21), u;
}
function Pc() {
if (nr & 262144 || Wt() !== 29)
return;
_e();
let u = mn(20, sr);
if (bt() === 31)
return _e(), u && vb() ? u : void 0;
}
function vb() {
switch (T()) {
case 20:
case 14:
case 15:
return true;
case 29:
case 31:
case 39:
case 40:
return false;
}
return t.hasPrecedingLineBreak() || Jm() || !La();
}
function Ku() {
switch (T()) {
case 8:
case 9:
case 10:
case 14:
return Di();
case 108:
case 106:
case 104:
case 110:
case 95:
return sn();
case 20:
return bb();
case 22:
return ah();
case 18:
return Xu();
case 132:
if (!wt(yh))
break;
return Yu();
case 59:
return Ub();
case 84:
return Ih();
case 98:
return Yu();
case 103:
return Tb();
case 43:
case 68:
if (jt() === 13)
return Di();
break;
case 15:
return Wd(false);
case 80:
return gc();
}
return wr(ve.Expression_expected);
}
function bb() {
let u = L(), b = fe();
de(20);
let O = It(Sr);
return de(21), St(Q(Hr(O), u), b);
}
function rh() {
let u = L();
de(25);
let b = Yr(true);
return Q(N.createSpreadElement(b), u);
}
function nh() {
return T() === 25 ? rh() : T() === 27 ? Q(N.createOmittedExpression(), L()) : Yr(true);
}
function ih() {
return Ct(r, nh);
}
function ah() {
let u = L(), b = t.getTokenPos(), O = de(22), j = t.hasPrecedingLineBreak(), z = mn(15, nh);
return Ts(22, 23, O, b), Q(Ne(z, j), u);
}
function sh() {
let u = L(), b = fe();
if (dr(25)) {
let lt = Yr(true);
return St(Q(N.createSpreadAssignment(lt), u), b);
}
let O = ki(true);
if (Ks(137))
return Fa(u, b, O, 174, 0);
if (Ks(151))
return Fa(u, b, O, 175, 0);
let j = dr(41), z = kt(), re = Es(), Ee = dr(57), qe = dr(53);
if (j || T() === 20 || T() === 29)
return Ah(u, b, O, j, re, Ee, qe);
let We;
if (z && T() !== 58) {
let lt = dr(63), Jt = lt ? It(() => Yr(true)) : void 0;
We = N.createShorthandPropertyAssignment(re, Jt), We.equalsToken = lt;
} else {
de(58);
let lt = It(() => Yr(true));
We = N.createPropertyAssignment(re, lt);
}
return We.modifiers = O, We.questionToken = Ee, We.exclamationToken = qe, St(Q(We, u), b);
}
function Xu() {
let u = L(), b = t.getTokenPos(), O = de(18), j = t.hasPrecedingLineBreak(), z = mn(12, sh, true);
return Ts(18, 19, O, b), Q(oe(z, j), u);
}
function Yu() {
let u = Ai();
Re(false);
let b = L(), O = fe(), j = ki(false);
de(98);
let z = dr(41), re = z ? 1 : 0, Ee = Ke(j, Ul) ? 2 : 0, qe = re && Ee ? vs(ro) : re ? ys(ro) : Ee ? Xi(ro) : ro(), We = Xn(), $e = ra(re | Ee), lt = pi(58, false), Jt = Dc(re | Ee);
Re(u);
let Lt = N.createFunctionExpression(j, z, qe, We, $e, lt, Jt);
return St(Q(Lt, b), O);
}
function ro() {
return Tt() ? hc() : void 0;
}
function Tb() {
let u = L();
if (de(103), Ot(24)) {
let re = zr();
return Q(N.createMetaProperty(103, re), u);
}
let b = L(), O = Ja(b, Ku(), false), j;
O.kind === 230 && (j = O.typeArguments, O = O.expression), T() === 28 && Dt(ve.Invalid_optional_chain_from_new_expression_Did_you_mean_to_call_0, B_(_r, O));
let z = T() === 20 ? th() : void 0;
return Q(Tn(O, j, z), u);
}
function ws(u, b) {
let O = L(), j = fe(), z = t.getTokenPos(), re = de(18, b);
if (re || u) {
let Ee = t.hasPrecedingLineBreak(), qe = Kn(1, on);
Ts(18, 19, re, z);
let We = St(Q(Gi(qe, Ee), O), j);
return T() === 63 && (Dt(ve.Declaration_or_statement_expected_This_follows_a_block_of_statements_so_if_you_intended_to_write_a_destructuring_assignment_you_might_need_to_wrap_the_whole_assignment_in_parentheses), _e()), We;
} else {
let Ee = ui();
return St(Q(Gi(Ee, void 0), O), j);
}
}
function Dc(u, b) {
let O = Yi();
Le(!!(u & 1));
let j = xn();
ot(!!(u & 2));
let z = br;
br = false;
let re = Ai();
re && Re(false);
let Ee = ws(!!(u & 16), b);
return re && Re(true), br = z, Le(O), ot(j), Ee;
}
function oh() {
let u = L(), b = fe();
return de(26), St(Q(N.createEmptyStatement(), u), b);
}
function Sb() {
let u = L(), b = fe();
de(99);
let O = t.getTokenPos(), j = de(20), z = It(Sr);
Ts(20, 21, j, O);
let re = on(), Ee = Ot(91) ? on() : void 0;
return St(Q(Ut(z, re, Ee), u), b);
}
function _h() {
let u = L(), b = fe();
de(90);
let O = on();
de(115);
let j = t.getTokenPos(), z = de(20), re = It(Sr);
return Ts(20, 21, z, j), Ot(26), St(Q(N.createDoStatement(O, re), u), b);
}
function xb() {
let u = L(), b = fe();
de(115);
let O = t.getTokenPos(), j = de(20), z = It(Sr);
Ts(20, 21, j, O);
let re = on();
return St(Q(kn(z, re), u), b);
}
function ch() {
let u = L(), b = fe();
de(97);
let O = dr(133);
de(20);
let j;
T() !== 26 && (T() === 113 || T() === 119 || T() === 85 ? j = Eh(true) : j = Mr(Sr));
let z;
if (O ? de(162) : Ot(162)) {
let re = It(() => Yr(true));
de(21), z = mr(O, j, re, on());
} else if (Ot(101)) {
let re = It(Sr);
de(21), z = N.createForInStatement(j, re, on());
} else {
de(26);
let re = T() !== 26 && T() !== 21 ? It(Sr) : void 0;
de(26);
let Ee = T() !== 21 ? It(Sr) : void 0;
de(21), z = an(j, re, Ee, on());
}
return St(Q(z, u), b);
}
function lh(u) {
let b = L(), O = fe();
de(u === 249 ? 81 : 86);
let j = ka() ? void 0 : wr();
En();
let z = u === 249 ? N.createBreakStatement(j) : N.createContinueStatement(j);
return St(Q(z, b), O);
}
function uh() {
let u = L(), b = fe();
de(105);
let O = ka() ? void 0 : It(Sr);
return En(), St(Q(N.createReturnStatement(O), u), b);
}
function Eb() {
let u = L(), b = fe();
de(116);
let O = t.getTokenPos(), j = de(20), z = It(Sr);
Ts(20, 21, j, O);
let re = Mt(33554432, on);
return St(Q(N.createWithStatement(z, re), u), b);
}
function wb() {
let u = L(), b = fe();
de(82);
let O = It(Sr);
de(58);
let j = Kn(3, on);
return St(Q(N.createCaseClause(O, j), u), b);
}
function ph() {
let u = L();
de(88), de(58);
let b = Kn(3, on);
return Q(N.createDefaultClause(b), u);
}
function Cb() {
return T() === 82 ? wb() : ph();
}
function fh() {
let u = L();
de(18);
let b = Kn(2, Cb);
return de(19), Q(N.createCaseBlock(b), u);
}
function Ab() {
let u = L(), b = fe();
de(107), de(20);
let O = It(Sr);
de(21);
let j = fh();
return St(Q(N.createSwitchStatement(O, j), u), b);
}
function dh() {
let u = L(), b = fe();
de(109);
let O = t.hasPrecedingLineBreak() ? void 0 : It(Sr);
return O === void 0 && ($r++, O = Q(Te(""), L())), t_() || Zi(O), St(Q(N.createThrowStatement(O), u), b);
}
function Pb() {
let u = L(), b = fe();
de(111);
let O = ws(false), j = T() === 83 ? mh() : void 0, z;
return (!j || T() === 96) && (de(96, ve.catch_or_finally_expected), z = ws(false)), St(Q(N.createTryStatement(O, j, z), u), b);
}
function mh() {
let u = L();
de(83);
let b;
Ot(20) ? (b = Ic(), de(21)) : b = void 0;
let O = ws(false);
return Q(N.createCatchClause(b, O), u);
}
function Db() {
let u = L(), b = fe();
return de(87), En(), St(Q(N.createDebuggerStatement(), u), b);
}
function hh() {
let u = L(), b = fe(), O, j = T() === 20, z = It(Sr);
return yt(z) && Ot(58) ? O = N.createLabeledStatement(z, on()) : (t_() || Zi(z), O = fn(z), j && (b = false)), St(Q(O, u), b);
}
function Qu() {
return _e(), fr(T()) && !t.hasPrecedingLineBreak();
}
function gh() {
return _e(), T() === 84 && !t.hasPrecedingLineBreak();
}
function yh() {
return _e(), T() === 98 && !t.hasPrecedingLineBreak();
}
function Zu() {
return _e(), (fr(T()) || T() === 8 || T() === 9 || T() === 10) && !t.hasPrecedingLineBreak();
}
function kb() {
for (; ; )
switch (T()) {
case 113:
case 119:
case 85:
case 98:
case 84:
case 92:
return true;
case 118:
case 154:
return _b();
case 142:
case 143:
return Ob();
case 126:
case 127:
case 132:
case 136:
case 121:
case 122:
case 123:
case 146:
if (_e(), t.hasPrecedingLineBreak())
return false;
continue;
case 159:
return _e(), T() === 18 || T() === 79 || T() === 93;
case 100:
return _e(), T() === 10 || T() === 41 || T() === 18 || fr(T());
case 93:
let u = _e();
if (u === 154 && (u = wt(_e)), u === 63 || u === 41 || u === 18 || u === 88 || u === 128 || u === 59)
return true;
continue;
case 124:
_e();
continue;
default:
return false;
}
}
function c_() {
return wt(kb);
}
function vh() {
switch (T()) {
case 59:
case 26:
case 18:
case 113:
case 119:
case 98:
case 84:
case 92:
case 99:
case 90:
case 115:
case 97:
case 86:
case 81:
case 105:
case 116:
case 107:
case 109:
case 111:
case 87:
case 83:
case 96:
return true;
case 100:
return c_() || wt(ku);
case 85:
case 93:
return c_();
case 132:
case 136:
case 118:
case 142:
case 143:
case 154:
case 159:
return true;
case 127:
case 123:
case 121:
case 122:
case 124:
case 146:
return c_() || !wt(Qu);
default:
return La();
}
}
function bh() {
return _e(), Tt() || T() === 18 || T() === 22;
}
function Ib() {
return wt(bh);
}
function on() {
switch (T()) {
case 26:
return oh();
case 18:
return ws(false);
case 113:
return rp(L(), fe(), void 0);
case 119:
if (Ib())
return rp(L(), fe(), void 0);
break;
case 98:
return np(L(), fe(), void 0);
case 84:
return Nh(L(), fe(), void 0);
case 99:
return Sb();
case 90:
return _h();
case 115:
return xb();
case 97:
return ch();
case 86:
return lh(248);
case 81:
return lh(249);
case 105:
return uh();
case 116:
return Eb();
case 107:
return Ab();
case 109:
return dh();
case 111:
case 83:
case 96:
return Pb();
case 87:
return Db();
case 59:
return ep();
case 132:
case 118:
case 154:
case 142:
case 143:
case 136:
case 85:
case 92:
case 93:
case 100:
case 121:
case 122:
case 123:
case 126:
case 127:
case 124:
case 146:
case 159:
if (c_())
return ep();
break;
}
return hh();
}
function Th(u) {
return u.kind === 136;
}
function ep() {
let u = L(), b = fe(), O = ki(true);
if (Ke(O, Th)) {
let z = Nb(u);
if (z)
return z;
for (let re of O)
re.flags |= 16777216;
return Mt(16777216, () => l_(u, b, O));
} else
return l_(u, b, O);
}
function Nb(u) {
return Mt(16777216, () => {
let b = mu(hr, u);
if (b)
return hu(b);
});
}
function l_(u, b, O) {
switch (T()) {
case 113:
case 119:
case 85:
return rp(u, b, O);
case 98:
return np(u, b, O);
case 84:
return Nh(u, b, O);
case 118:
return Hb(u, b, O);
case 154:
return Gb(u, b, O);
case 92:
return Kb(u, b, O);
case 159:
case 142:
case 143:
return Fh(u, b, O);
case 100:
return Qb(u, b, O);
case 93:
switch (_e(), T()) {
case 88:
case 63:
return _6(u, b, O);
case 128:
return Yb(u, b, O);
default:
return o6(u, b, O);
}
default:
if (O) {
let j = Jn(279, true, ve.Declaration_expected);
return Gf(j, u), j.modifiers = O, j;
}
return;
}
}
function Ob() {
return _e(), !t.hasPrecedingLineBreak() && (kt() || T() === 10);
}
function kc(u, b) {
if (T() !== 18) {
if (u & 4) {
i_();
return;
}
if (ka()) {
En();
return;
}
}
return Dc(u, b);
}
function Mb() {
let u = L();
if (T() === 27)
return Q(N.createOmittedExpression(), u);
let b = dr(25), O = no(), j = Ra();
return Q(N.createBindingElement(b, void 0, O, j), u);
}
function Sh() {
let u = L(), b = dr(25), O = Tt(), j = Es(), z;
O && T() !== 58 ? (z = j, j = void 0) : (de(58), z = no());
let re = Ra();
return Q(N.createBindingElement(b, j, z, re), u);
}
function Lb() {
let u = L();
de(18);
let b = mn(9, Sh);
return de(19), Q(N.createObjectBindingPattern(b), u);
}
function xh() {
let u = L();
de(22);
let b = mn(10, Mb);
return de(23), Q(N.createArrayBindingPattern(b), u);
}
function tp() {
return T() === 18 || T() === 22 || T() === 80 || Tt();
}
function no(u) {
return T() === 22 ? xh() : T() === 18 ? Lb() : hc(u);
}
function Rb() {
return Ic(true);
}
function Ic(u) {
let b = L(), O = fe(), j = no(ve.Private_identifiers_are_not_allowed_in_variable_declarations), z;
u && j.kind === 79 && T() === 53 && !t.hasPrecedingLineBreak() && (z = sn());
let re = Ma(), Ee = jm(T()) ? void 0 : Ra(), qe = $i(j, z, re, Ee);
return St(Q(qe, b), O);
}
function Eh(u) {
let b = L(), O = 0;
switch (T()) {
case 113:
break;
case 119:
O |= 1;
break;
case 85:
O |= 2;
break;
default:
Y.fail();
}
_e();
let j;
if (T() === 162 && wt(wh))
j = ui();
else {
let z = Qi();
xe(u), j = mn(8, u ? Ic : Rb), xe(z);
}
return Q(dn(j, O), b);
}
function wh() {
return yc() && _e() === 21;
}
function rp(u, b, O) {
let j = Eh(false);
En();
let z = pn(O, j);
return St(Q(z, u), b);
}
function np(u, b, O) {
let j = xn(), z = Vn(O);
de(98);
let re = dr(41), Ee = z & 1024 ? ro() : hc(), qe = re ? 1 : 0, We = z & 512 ? 2 : 0, $e = Xn();
z & 1 && ot(true);
let lt = ra(qe | We), Jt = pi(58, false), Lt = kc(qe | We, ve.or_expected);
ot(j);
let At = N.createFunctionDeclaration(O, re, Ee, $e, lt, Jt, Lt);
return St(Q(At, u), b);
}
function jb() {
if (T() === 135)
return de(135);
if (T() === 10 && wt(_e) === 20)
return Tr(() => {
let u = Di();
return u.text === "constructor" ? u : void 0;
});
}
function Ch(u, b, O) {
return Tr(() => {
if (jb()) {
let j = Xn(), z = ra(0), re = pi(58, false), Ee = kc(0, ve.or_expected), qe = N.createConstructorDeclaration(O, z, Ee);
return qe.typeParameters = j, qe.type = re, St(Q(qe, u), b);
}
});
}
function Ah(u, b, O, j, z, re, Ee, qe) {
let We = j ? 1 : 0, $e = Ke(O, Ul) ? 2 : 0, lt = Xn(), Jt = ra(We | $e), Lt = pi(58, false), At = kc(We | $e, qe), kr = N.createMethodDeclaration(O, j, z, re, lt, Jt, Lt, At);
return kr.exclamationToken = Ee, St(Q(kr, u), b);
}
function ip(u, b, O, j, z) {
let re = !z && !t.hasPrecedingLineBreak() ? dr(53) : void 0, Ee = Ma(), qe = Ct(45056, Ra);
mc(j, Ee, qe);
let We = N.createPropertyDeclaration(O, j, z || re, Ee, qe);
return St(Q(We, u), b);
}
function Ph(u, b, O) {
let j = dr(41), z = Es(), re = dr(57);
return j || T() === 20 || T() === 29 ? Ah(u, b, O, j, z, re, void 0, ve.or_expected) : ip(u, b, O, z, re);
}
function Fa(u, b, O, j, z) {
let re = Es(), Ee = Xn(), qe = ra(0), We = pi(58, false), $e = kc(z), lt = j === 174 ? N.createGetAccessorDeclaration(O, re, qe, We, $e) : N.createSetAccessorDeclaration(O, re, qe, $e);
return lt.typeParameters = Ee, ic(lt) && (lt.type = We), St(Q(lt, u), b);
}
function Jb() {
let u;
if (T() === 59)
return true;
for (; Wi(T()); ) {
if (u = T(), VS(u))
return true;
_e();
}
if (T() === 41 || (xs() && (u = T(), _e()), T() === 22))
return true;
if (u !== void 0) {
if (!ba(u) || u === 151 || u === 137)
return true;
switch (T()) {
case 20:
case 29:
case 53:
case 58:
case 63:
case 57:
return true;
default:
return ka();
}
}
return false;
}
function Fb(u, b, O) {
ea(124);
let j = Dh(), z = St(Q(N.createClassStaticBlockDeclaration(j), u), b);
return z.modifiers = O, z;
}
function Dh() {
let u = Yi(), b = xn();
Le(false), ot(true);
let O = ws(false);
return Le(u), ot(b), O;
}
function Bb() {
if (xn() && T() === 133) {
let u = L(), b = wr(ve.Expression_expected);
_e();
let O = Ja(u, b, true);
return $u(u, O);
}
return to();
}
function kh() {
let u = L();
if (!Ot(59))
return;
let b = ci(Bb);
return Q(N.createDecorator(b), u);
}
function ap(u, b, O) {
let j = L(), z = T();
if (T() === 85 && b) {
if (!Tr(uu))
return;
} else {
if (O && T() === 124 && wt(Mc))
return;
if (u && T() === 124)
return;
if (!Md())
return;
}
return Q(Ye(z), j);
}
function ki(u, b, O) {
let j = L(), z, re, Ee, qe = false, We = false, $e = false;
if (u && T() === 59)
for (; re = kh(); )
z = tr(z, re);
for (; Ee = ap(qe, b, O); )
Ee.kind === 124 && (qe = true), z = tr(z, Ee), We = true;
if (We && u && T() === 59)
for (; re = kh(); )
z = tr(z, re), $e = true;
if ($e)
for (; Ee = ap(qe, b, O); )
Ee.kind === 124 && (qe = true), z = tr(z, Ee);
return z && Er(z, j);
}
function sp() {
let u;
if (T() === 132) {
let b = L();
_e();
let O = Q(Ye(132), b);
u = Er([O], b);
}
return u;
}
function qb() {
let u = L();
if (T() === 26)
return _e(), Q(N.createSemicolonClassElement(), u);
let b = fe(), O = ki(true, true, true);
if (T() === 124 && wt(Mc))
return Fb(u, b, O);
if (Ks(137))
return Fa(u, b, O, 174, 0);
if (Ks(151))
return Fa(u, b, O, 175, 0);
if (T() === 135 || T() === 10) {
let j = Ch(u, b, O);
if (j)
return j;
}
if (im())
return am(u, b, O);
if (fr(T()) || T() === 10 || T() === 8 || T() === 41 || T() === 22)
if (Ke(O, Th)) {
for (let z of O)
z.flags |= 16777216;
return Mt(16777216, () => Ph(u, b, O));
} else
return Ph(u, b, O);
if (O) {
let j = Jn(79, true, ve.Declaration_expected);
return ip(u, b, O, j, void 0);
}
return Y.fail("Should not have attempted to parse class member declaration.");
}
function Ub() {
let u = L(), b = fe(), O = ki(true);
if (T() === 84)
return op(u, b, O, 228);
let j = Jn(279, true, ve.Expression_expected);
return Gf(j, u), j.modifiers = O, j;
}
function Ih() {
return op(L(), fe(), void 0, 228);
}
function Nh(u, b, O) {
return op(u, b, O, 260);
}
function op(u, b, O, j) {
let z = xn();
de(84);
let re = Oh(), Ee = Xn();
Ke(O, N8) && ot(true);
let qe = Mh(), We;
de(18) ? (We = Vb(), de(19)) : We = ui(), ot(z);
let $e = j === 260 ? N.createClassDeclaration(O, re, Ee, qe, We) : N.createClassExpression(O, re, Ee, qe, We);
return St(Q($e, u), b);
}
function Oh() {
return Tt() && !zb() ? Ss(Tt()) : void 0;
}
function zb() {
return T() === 117 && wt(pu);
}
function Mh() {
if (Oc())
return Kn(22, Lh);
}
function Lh() {
let u = L(), b = T();
Y.assert(b === 94 || b === 117), _e();
let O = mn(7, Wb);
return Q(N.createHeritageClause(b, O), u);
}
function Wb() {
let u = L(), b = to();
if (b.kind === 230)
return b;
let O = Nc();
return Q(N.createExpressionWithTypeArguments(b, O), u);
}
function Nc() {
return T() === 29 ? Oa(20, sr, 29, 31) : void 0;
}
function Oc() {
return T() === 94 || T() === 117;
}
function Vb() {
return Kn(5, qb);
}
function Hb(u, b, O) {
de(118);
let j = wr(), z = Xn(), re = Mh(), Ee = Iu(), qe = N.createInterfaceDeclaration(O, j, z, re, Ee);
return St(Q(qe, u), b);
}
function Gb(u, b, O) {
de(154);
let j = wr(), z = Xn();
de(63);
let re = T() === 139 && Tr(Ou) || sr();
En();
let Ee = N.createTypeAliasDeclaration(O, j, z, re);
return St(Q(Ee, u), b);
}
function $b() {
let u = L(), b = fe(), O = Es(), j = It(Ra);
return St(Q(N.createEnumMember(O, j), u), b);
}
function Kb(u, b, O) {
de(92);
let j = wr(), z;
de(18) ? (z = $s(() => mn(6, $b)), de(19)) : z = ui();
let re = N.createEnumDeclaration(O, j, z);
return St(Q(re, u), b);
}
function Rh() {
let u = L(), b;
return de(18) ? (b = Kn(1, on), de(19)) : b = ui(), Q(N.createModuleBlock(b), u);
}
function jh(u, b, O, j) {
let z = j & 16, re = wr(), Ee = Ot(24) ? jh(L(), false, void 0, 4 | z) : Rh(), qe = N.createModuleDeclaration(O, re, Ee, j);
return St(Q(qe, u), b);
}
function Jh(u, b, O) {
let j = 0, z;
T() === 159 ? (z = wr(), j |= 1024) : (z = Di(), z.text = Ia(z.text));
let re;
T() === 18 ? re = Rh() : En();
let Ee = N.createModuleDeclaration(O, z, re, j);
return St(Q(Ee, u), b);
}
function Fh(u, b, O) {
let j = 0;
if (T() === 159)
return Jh(u, b, O);
if (Ot(143))
j |= 16;
else if (de(142), T() === 10)
return Jh(u, b, O);
return jh(u, b, O, j);
}
function Bh() {
return T() === 147 && wt(qh);
}
function qh() {
return _e() === 20;
}
function Mc() {
return _e() === 18;
}
function Xb() {
return _e() === 43;
}
function Yb(u, b, O) {
de(128), de(143);
let j = wr();
En();
let z = N.createNamespaceExportDeclaration(j);
return z.modifiers = O, St(Q(z, u), b);
}
function Qb(u, b, O) {
de(100);
let j = t.getStartPos(), z;
kt() && (z = wr());
let re = false;
if (T() !== 158 && (z == null ? void 0 : z.escapedText) === "type" && (kt() || Zb()) && (re = true, z = kt() ? wr() : void 0), z && !e6())
return t6(u, b, O, z, re);
let Ee;
(z || T() === 41 || T() === 18) && (Ee = r6(z, j, re), de(158));
let qe = Lc(), We;
T() === 130 && !t.hasPrecedingLineBreak() && (We = _p()), En();
let $e = N.createImportDeclaration(O, Ee, qe, We);
return St(Q($e, u), b);
}
function Uh() {
let u = L(), b = fr(T()) ? zr() : n_(10);
de(58);
let O = Yr(true);
return Q(N.createAssertEntry(b, O), u);
}
function _p(u) {
let b = L();
u || de(130);
let O = t.getTokenPos();
if (de(18)) {
let j = t.hasPrecedingLineBreak(), z = mn(24, Uh, true);
if (!de(19)) {
let re = Cn(Zt);
re && re.code === ve._0_expected.code && Rl(re, Ro(Ur, O, 1, ve.The_parser_expected_to_find_a_1_to_match_the_0_token_here, "{", "}"));
}
return Q(N.createAssertClause(z, j), b);
} else {
let j = Er([], L(), void 0, false);
return Q(N.createAssertClause(j, false), b);
}
}
function Zb() {
return T() === 41 || T() === 18;
}
function e6() {
return T() === 27 || T() === 158;
}
function t6(u, b, O, j, z) {
de(63);
let re = cp();
En();
let Ee = N.createImportEqualsDeclaration(O, z, j, re);
return St(Q(Ee, u), b);
}
function r6(u, b, O) {
let j;
return (!u || Ot(27)) && (j = T() === 41 ? Rc() : zh(272)), Q(N.createImportClause(O, u, j), b);
}
function cp() {
return Bh() ? n6() : Ys(false);
}
function n6() {
let u = L();
de(147), de(20);
let b = Lc();
return de(21), Q(N.createExternalModuleReference(b), u);
}
function Lc() {
if (T() === 10) {
let u = Di();
return u.text = Ia(u.text), u;
} else
return Sr();
}
function Rc() {
let u = L();
de(41), de(128);
let b = wr();
return Q(N.createNamespaceImport(b), u);
}
function zh(u) {
let b = L(), O = u === 272 ? N.createNamedImports(Oa(23, a6, 18, 19)) : N.createNamedExports(Oa(23, i6, 18, 19));
return Q(O, b);
}
function i6() {
let u = fe();
return St(Ba(278), u);
}
function a6() {
return Ba(273);
}
function Ba(u) {
let b = L(), O = ba(T()) && !kt(), j = t.getTokenPos(), z = t.getTextPos(), re = false, Ee, qe = true, We = zr();
if (We.escapedText === "type")
if (T() === 128) {
let Jt = zr();
if (T() === 128) {
let Lt = zr();
fr(T()) ? (re = true, Ee = Jt, We = lt(), qe = false) : (Ee = We, We = Lt, qe = false);
} else
fr(T()) ? (Ee = We, qe = false, We = lt()) : (re = true, We = Jt);
} else
fr(T()) && (re = true, We = lt());
qe && T() === 128 && (Ee = We, de(128), We = lt()), u === 273 && O && Z(j, z, ve.Identifier_expected);
let $e = u === 273 ? N.createImportSpecifier(re, Ee, We) : N.createExportSpecifier(re, Ee, We);
return Q($e, b);
function lt() {
return O = ba(T()) && !kt(), j = t.getTokenPos(), z = t.getTextPos(), zr();
}
}
function s6(u) {
return Q(N.createNamespaceExport(zr()), u);
}
function o6(u, b, O) {
let j = xn();
ot(true);
let z, re, Ee, qe = Ot(154), We = L();
Ot(41) ? (Ot(128) && (z = s6(We)), de(158), re = Lc()) : (z = zh(276), (T() === 158 || T() === 10 && !t.hasPrecedingLineBreak()) && (de(158), re = Lc())), re && T() === 130 && !t.hasPrecedingLineBreak() && (Ee = _p()), En(), ot(j);
let $e = N.createExportDeclaration(O, qe, z, re, Ee);
return St(Q($e, u), b);
}
function _6(u, b, O) {
let j = xn();
ot(true);
let z;
Ot(63) ? z = true : de(88);
let re = Yr(true);
En(), ot(j);
let Ee = N.createExportAssignment(O, z, re);
return St(Q(Ee, u), b);
}
let io;
((u) => {
u[u.SourceElements = 0] = "SourceElements", u[u.BlockStatements = 1] = "BlockStatements", u[u.SwitchClauses = 2] = "SwitchClauses", u[u.SwitchClauseStatements = 3] = "SwitchClauseStatements", u[u.TypeMembers = 4] = "TypeMembers", u[u.ClassMembers = 5] = "ClassMembers", u[u.EnumMembers = 6] = "EnumMembers", u[u.HeritageClauseElement = 7] = "HeritageClauseElement", u[u.VariableDeclarations = 8] = "VariableDeclarations", u[u.ObjectBindingElements = 9] = "ObjectBindingElements", u[u.ArrayBindingElements = 10] = "ArrayBindingElements", u[u.ArgumentExpressions = 11] = "ArgumentExpressions", u[u.ObjectLiteralMembers = 12] = "ObjectLiteralMembers", u[u.JsxAttributes = 13] = "JsxAttributes", u[u.JsxChildren = 14] = "JsxChildren", u[u.ArrayLiteralMembers = 15] = "ArrayLiteralMembers", u[u.Parameters = 16] = "Parameters", u[u.JSDocParameters = 17] = "JSDocParameters", u[u.RestProperties = 18] = "RestProperties", u[u.TypeParameters = 19] = "TypeParameters", u[u.TypeArguments = 20] = "TypeArguments", u[u.TupleElementTypes = 21] = "TupleElementTypes", u[u.HeritageClauses = 22] = "HeritageClauses", u[u.ImportOrExportSpecifiers = 23] = "ImportOrExportSpecifiers", u[u.AssertEntries = 24] = "AssertEntries", u[u.Count = 25] = "Count";
})(io || (io = {}));
let Wh;
((u) => {
u[u.False = 0] = "False", u[u.True = 1] = "True", u[u.Unknown = 2] = "Unknown";
})(Wh || (Wh = {}));
let Vh;
((u) => {
function b($e, lt, Jt) {
Mn("file.js", $e, 99, void 0, 1), t.setText($e, lt, Jt), ar = t.scan();
let Lt = O(), At = Kt("file.js", 99, 1, false, [], Ye(1), 0, yn), kr = qs(Zt, At);
return Or && (At.jsDocDiagnostics = qs(Or, At)), _i(), Lt ? { jsDocTypeExpression: Lt, diagnostics: kr } : void 0;
}
u.parseJSDocTypeExpressionForTests = b;
function O($e) {
let lt = L(), Jt = ($e ? Ot : de)(18), Lt = Mt(8388608, xc);
(!$e || Jt) && Da(19);
let At = N.createJSDocTypeExpression(Lt);
return ft(At), Q(At, lt);
}
u.parseJSDocTypeExpression = O;
function j() {
let $e = L(), lt = Ot(18), Jt = L(), Lt = Ys(false);
for (; T() === 80; )
Xr(), Ge(), Lt = Q(N.createJSDocMemberName(Lt, wr()), Jt);
lt && Da(19);
let At = N.createJSDocNameReference(Lt);
return ft(At), Q(At, $e);
}
u.parseJSDocNameReference = j;
function z($e, lt, Jt) {
Mn("", $e, 99, void 0, 1);
let Lt = Mt(8388608, () => We(lt, Jt)), kr = qs(Zt, { languageVariant: 0, text: $e });
return _i(), Lt ? { jsDoc: Lt, diagnostics: kr } : void 0;
}
u.parseIsolatedJSDocComment = z;
function re($e, lt, Jt) {
let Lt = ar, At = Zt.length, kr = Kr, Fn = Mt(8388608, () => We(lt, Jt));
return Sa(Fn, $e), nr & 262144 && (Or || (Or = []), Or.push(...Zt)), ar = Lt, Zt.length = At, Kr = kr, Fn;
}
u.parseJSDocComment = re;
let Ee;
(($e) => {
$e[$e.BeginningOfLine = 0] = "BeginningOfLine", $e[$e.SawAsterisk = 1] = "SawAsterisk", $e[$e.SavingComments = 2] = "SavingComments", $e[$e.SavingBackticks = 3] = "SavingBackticks";
})(Ee || (Ee = {}));
let qe;
(($e) => {
$e[$e.Property = 1] = "Property", $e[$e.Parameter = 2] = "Parameter", $e[$e.CallbackParameter = 4] = "CallbackParameter";
})(qe || (qe = {}));
function We() {
let $e = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : 0, lt = arguments.length > 1 ? arguments[1] : void 0, Jt = _r, Lt = lt === void 0 ? Jt.length : $e + lt;
if (lt = Lt - $e, Y.assert($e >= 0), Y.assert($e <= Lt), Y.assert(Lt <= Jt.length), !LE(Jt, $e))
return;
let At, kr, Fn, di, Ii, _n = [], qa = [];
return t.scanRange($e + 3, lt - 5, () => {
let se = 1, Me, Ce = $e - (Jt.lastIndexOf(`
`, $e) + 1) + 4;
function Ue(vt) {
Me || (Me = Ce), _n.push(vt), Ce += vt.length;
}
for (Ge(); u_(5); )
;
u_(4) && (se = 0, Ce = 0);
e:
for (; ; ) {
switch (T()) {
case 59:
se === 0 || se === 1 ? (lp(_n), Ii || (Ii = L()), za(up(Ce)), se = 0, Me = void 0) : Ue(t.getTokenText());
break;
case 4:
_n.push(t.getTokenText()), se = 0, Ce = 0;
break;
case 41:
let vt = t.getTokenText();
se === 1 || se === 2 ? (se = 2, Ue(vt)) : (se = 1, Ce += vt.length);
break;
case 5:
let Vt = t.getTokenText();
se === 2 ? _n.push(Vt) : Me !== void 0 && Ce + Vt.length > Me && _n.push(Vt.slice(Me - Ce)), Ce += Vt.length;
break;
case 1:
break e;
case 18:
se = 2;
let Rr = t.getStartPos(), gn = t.getTextPos() - 1, mi = $h(gn);
if (mi) {
di || Hh(_n), qa.push(Q(N.createJSDocText(_n.join("")), di != null ? di : $e, Rr)), qa.push(mi), _n = [], di = t.getTextPos();
break;
}
default:
se = 2, Ue(t.getTokenText());
break;
}
Ge();
}
lp(_n), qa.length && _n.length && qa.push(Q(N.createJSDocText(_n.join("")), di != null ? di : $e, Ii)), qa.length && At && Y.assertIsDefined(Ii, "having parsed tags implies that the end of the comment span should be set");
let Qe = At && Er(At, kr, Fn);
return Q(N.createJSDocComment(qa.length ? Er(qa, $e, Ii) : _n.length ? _n.join("") : void 0, Qe), $e, Lt);
});
function Hh(se) {
for (; se.length && (se[0] === `
` || se[0] === "\r"); )
se.shift();
}
function lp(se) {
for (; se.length && se[se.length - 1].trim() === ""; )
se.pop();
}
function Gh() {
for (; ; ) {
if (Ge(), T() === 1)
return true;
if (!(T() === 5 || T() === 4))
return false;
}
}
function wn() {
if (!((T() === 5 || T() === 4) && wt(Gh)))
for (; T() === 5 || T() === 4; )
Ge();
}
function Ua() {
if ((T() === 5 || T() === 4) && wt(Gh))
return "";
let se = t.hasPrecedingLineBreak(), Me = false, Ce = "";
for (; se && T() === 41 || T() === 5 || T() === 4; )
Ce += t.getTokenText(), T() === 4 ? (se = true, Me = true, Ce = "") : T() === 41 && (se = false), Ge();
return Me ? Ce : "";
}
function up(se) {
Y.assert(T() === 59);
let Me = t.getTokenPos();
Ge();
let Ce = ao(void 0), Ue = Ua(), Qe;
switch (Ce.escapedText) {
case "author":
Qe = V(Me, Ce, se, Ue);
break;
case "implements":
Qe = et(Me, Ce, se, Ue);
break;
case "augments":
case "extends":
Qe = ht(Me, Ce, se, Ue);
break;
case "class":
case "constructor":
Qe = Oi(Me, N.createJSDocClassTag, Ce, se, Ue);
break;
case "public":
Qe = Oi(Me, N.createJSDocPublicTag, Ce, se, Ue);
break;
case "private":
Qe = Oi(Me, N.createJSDocPrivateTag, Ce, se, Ue);
break;
case "protected":
Qe = Oi(Me, N.createJSDocProtectedTag, Ce, se, Ue);
break;
case "readonly":
Qe = Oi(Me, N.createJSDocReadonlyTag, Ce, se, Ue);
break;
case "override":
Qe = Oi(Me, N.createJSDocOverrideTag, Ce, se, Ue);
break;
case "deprecated":
ue = true, Qe = Oi(Me, N.createJSDocDeprecatedTag, Ce, se, Ue);
break;
case "this":
Qe = qB(Me, Ce, se, Ue);
break;
case "enum":
Qe = UB(Me, Ce, se, Ue);
break;
case "arg":
case "argument":
case "param":
return Xh(Me, Ce, 2, se);
case "return":
case "returns":
Qe = o(Me, Ce, se, Ue);
break;
case "template":
Qe = QB(Me, Ce, se, Ue);
break;
case "type":
Qe = l(Me, Ce, se, Ue);
break;
case "typedef":
Qe = zB(Me, Ce, se, Ue);
break;
case "callback":
Qe = VB(Me, Ce, se, Ue);
break;
case "overload":
Qe = HB(Me, Ce, se, Ue);
break;
case "satisfies":
Qe = hn(Me, Ce, se, Ue);
break;
case "see":
Qe = p(Me, Ce, se, Ue);
break;
case "exception":
case "throws":
Qe = k(Me, Ce, se, Ue);
break;
default:
Qe = Qt(Me, Ce, se, Ue);
break;
}
return Qe;
}
function Qr(se, Me, Ce, Ue) {
return Ue || (Ce += Me - se), jc(Ce, Ue.slice(Ce));
}
function jc(se, Me) {
let Ce = L(), Ue = [], Qe = [], vt, Vt = 0, Rr = true, gn;
function mi(hi) {
gn || (gn = se), Ue.push(hi), se += hi.length;
}
Me !== void 0 && (Me !== "" && mi(Me), Vt = 1);
let Va = T();
e:
for (; ; ) {
switch (Va) {
case 4:
Vt = 0, Ue.push(t.getTokenText()), se = 0;
break;
case 59:
if (Vt === 3 || Vt === 2 && (!Rr || wt(Cs))) {
Ue.push(t.getTokenText());
break;
}
t.setTextPos(t.getTextPos() - 1);
case 1:
break e;
case 5:
if (Vt === 2 || Vt === 3)
mi(t.getTokenText());
else {
let so = t.getTokenText();
gn !== void 0 && se + so.length > gn && Ue.push(so.slice(gn - se)), se += so.length;
}
break;
case 18:
Vt = 2;
let hi = t.getStartPos(), pp = t.getTextPos() - 1, fp = $h(pp);
fp ? (Qe.push(Q(N.createJSDocText(Ue.join("")), vt != null ? vt : Ce, hi)), Qe.push(fp), Ue = [], vt = t.getTextPos()) : mi(t.getTokenText());
break;
case 61:
Vt === 3 ? Vt = 2 : Vt = 3, mi(t.getTokenText());
break;
case 41:
if (Vt === 0) {
Vt = 1, se += 1;
break;
}
default:
Vt !== 3 && (Vt = 2), mi(t.getTokenText());
break;
}
Rr = T() === 5, Va = Ge();
}
if (Hh(Ue), lp(Ue), Qe.length)
return Ue.length && Qe.push(Q(N.createJSDocText(Ue.join("")), vt != null ? vt : Ce)), Er(Qe, Ce, t.getTextPos());
if (Ue.length)
return Ue.join("");
}
function Cs() {
let se = Ge();
return se === 5 || se === 4;
}
function $h(se) {
let Me = Tr(Kh);
if (!Me)
return;
Ge(), wn();
let Ce = L(), Ue = fr(T()) ? Ys(true) : void 0;
if (Ue)
for (; T() === 80; )
Xr(), Ge(), Ue = Q(N.createJSDocMemberName(Ue, wr()), Ce);
let Qe = [];
for (; T() !== 19 && T() !== 4 && T() !== 1; )
Qe.push(t.getTokenText()), Ge();
let vt = Me === "link" ? N.createJSDocLink : Me === "linkcode" ? N.createJSDocLinkCode : N.createJSDocLinkPlain;
return Q(vt(Ue, Qe.join("")), se, t.getTextPos());
}
function Kh() {
if (Ua(), T() === 18 && Ge() === 59 && fr(Ge())) {
let se = t.getTokenValue();
if (xt(se))
return se;
}
}
function xt(se) {
return se === "link" || se === "linkcode" || se === "linkplain";
}
function Qt(se, Me, Ce, Ue) {
return Q(N.createJSDocUnknownTag(Me, Qr(se, L(), Ce, Ue)), se);
}
function za(se) {
se && (At ? At.push(se) : (At = [se], kr = se.pos), Fn = se.end);
}
function Wa() {
return Ua(), T() === 18 ? O() : void 0;
}
function c6() {
let se = u_(22);
se && wn();
let Me = u_(61), Ce = ZB();
return Me && kd(61), se && (wn(), dr(63) && Sr(), de(23)), { name: Ce, isBracketed: se };
}
function Yn(se) {
switch (se.kind) {
case 149:
return true;
case 185:
return Yn(se.elementType);
default:
return ac(se) && yt(se.typeName) && se.typeName.escapedText === "Object" && !se.typeArguments;
}
}
function Xh(se, Me, Ce, Ue) {
let Qe = Wa(), vt = !Qe;
Ua();
let { name: Vt, isBracketed: Rr } = c6(), gn = Ua();
vt && !wt(Kh) && (Qe = Wa());
let mi = Qr(se, L(), Ue, gn), Va = Ce !== 4 && n9(Qe, Vt, Ce, Ue);
Va && (Qe = Va, vt = true);
let hi = Ce === 1 ? N.createJSDocPropertyTag(Me, Vt, Rr, Qe, vt, mi) : N.createJSDocParameterTag(Me, Vt, Rr, Qe, vt, mi);
return Q(hi, se);
}
function n9(se, Me, Ce, Ue) {
if (se && Yn(se.type)) {
let Qe = L(), vt, Vt;
for (; vt = Tr(() => u6(Ce, Ue, Me)); )
(vt.kind === 344 || vt.kind === 351) && (Vt = tr(Vt, vt));
if (Vt) {
let Rr = Q(N.createJSDocTypeLiteral(Vt, se.type.kind === 185), Qe);
return Q(N.createJSDocTypeExpression(Rr), Qe);
}
}
}
function o(se, Me, Ce, Ue) {
Ke(At, b2) && Z(Me.pos, t.getTokenPos(), ve._0_tag_already_specified, Me.escapedText);
let Qe = Wa();
return Q(N.createJSDocReturnTag(Me, Qe, Qr(se, L(), Ce, Ue)), se);
}
function l(se, Me, Ce, Ue) {
Ke(At, au) && Z(Me.pos, t.getTokenPos(), ve._0_tag_already_specified, Me.escapedText);
let Qe = O(true), vt = Ce !== void 0 && Ue !== void 0 ? Qr(se, L(), Ce, Ue) : void 0;
return Q(N.createJSDocTypeTag(Me, Qe, vt), se);
}
function p(se, Me, Ce, Ue) {
let vt = T() === 22 || wt(() => Ge() === 59 && fr(Ge()) && xt(t.getTokenValue())) ? void 0 : j(), Vt = Ce !== void 0 && Ue !== void 0 ? Qr(se, L(), Ce, Ue) : void 0;
return Q(N.createJSDocSeeTag(Me, vt, Vt), se);
}
function k(se, Me, Ce, Ue) {
let Qe = Wa(), vt = Qr(se, L(), Ce, Ue);
return Q(N.createJSDocThrowsTag(Me, Qe, vt), se);
}
function V(se, Me, Ce, Ue) {
let Qe = L(), vt = we(), Vt = t.getStartPos(), Rr = Qr(se, Vt, Ce, Ue);
Rr || (Vt = t.getStartPos());
let gn = typeof Rr != "string" ? Er(Ft([Q(vt, Qe, Vt)], Rr), Qe) : vt.text + Rr;
return Q(N.createJSDocAuthorTag(Me, gn), se);
}
function we() {
let se = [], Me = false, Ce = t.getToken();
for (; Ce !== 1 && Ce !== 4; ) {
if (Ce === 29)
Me = true;
else {
if (Ce === 59 && !Me)
break;
if (Ce === 31 && Me) {
se.push(t.getTokenText()), t.setTextPos(t.getTokenPos() + 1);
break;
}
}
se.push(t.getTokenText()), Ce = Ge();
}
return N.createJSDocText(se.join(""));
}
function et(se, Me, Ce, Ue) {
let Qe = Ni();
return Q(N.createJSDocImplementsTag(Me, Qe, Qr(se, L(), Ce, Ue)), se);
}
function ht(se, Me, Ce, Ue) {
let Qe = Ni();
return Q(N.createJSDocAugmentsTag(Me, Qe, Qr(se, L(), Ce, Ue)), se);
}
function hn(se, Me, Ce, Ue) {
let Qe = O(false), vt = Ce !== void 0 && Ue !== void 0 ? Qr(se, L(), Ce, Ue) : void 0;
return Q(N.createJSDocSatisfiesTag(Me, Qe, vt), se);
}
function Ni() {
let se = Ot(18), Me = L(), Ce = ia(), Ue = Nc(), Qe = N.createExpressionWithTypeArguments(Ce, Ue), vt = Q(Qe, Me);
return se && de(19), vt;
}
function ia() {
let se = L(), Me = ao();
for (; Ot(24); ) {
let Ce = ao();
Me = Q(Ve(Me, Ce), se);
}
return Me;
}
function Oi(se, Me, Ce, Ue, Qe) {
return Q(Me(Ce, Qr(se, L(), Ue, Qe)), se);
}
function qB(se, Me, Ce, Ue) {
let Qe = O(true);
return wn(), Q(N.createJSDocThisTag(Me, Qe, Qr(se, L(), Ce, Ue)), se);
}
function UB(se, Me, Ce, Ue) {
let Qe = O(true);
return wn(), Q(N.createJSDocEnumTag(Me, Qe, Qr(se, L(), Ce, Ue)), se);
}
function zB(se, Me, Ce, Ue) {
var Qe;
let vt = Wa();
Ua();
let Vt = l6();
wn();
let Rr = jc(Ce), gn;
if (!vt || Yn(vt.type)) {
let Va, hi, pp, fp = false;
for (; Va = Tr(() => $B(Ce)); )
if (fp = true, Va.kind === 347)
if (hi) {
let so = Dt(ve.A_JSDoc_typedef_comment_may_not_contain_multiple_type_tags);
so && Rl(so, Ro(Ur, 0, 0, ve.The_tag_was_first_specified_here));
break;
} else
hi = Va;
else
pp = tr(pp, Va);
if (fp) {
let so = vt && vt.type.kind === 185, eq = N.createJSDocTypeLiteral(pp, so);
vt = hi && hi.typeExpression && !Yn(hi.typeExpression.type) ? hi.typeExpression : Q(eq, se), gn = vt.end;
}
}
gn = gn || Rr !== void 0 ? L() : ((Qe = Vt != null ? Vt : vt) != null ? Qe : Me).end, Rr || (Rr = Qr(se, gn, Ce, Ue));
let mi = N.createJSDocTypedefTag(Me, vt, Vt, Rr);
return Q(mi, se, gn);
}
function l6(se) {
let Me = t.getTokenPos();
if (!fr(T()))
return;
let Ce = ao();
if (Ot(24)) {
let Ue = l6(true), Qe = N.createModuleDeclaration(void 0, Ce, Ue, se ? 4 : void 0);
return Q(Qe, Me);
}
return se && (Ce.flags |= 2048), Ce;
}
function WB(se) {
let Me = L(), Ce, Ue;
for (; Ce = Tr(() => u6(4, se)); )
Ue = tr(Ue, Ce);
return Er(Ue || [], Me);
}
function j7(se, Me) {
let Ce = WB(Me), Ue = Tr(() => {
if (u_(59)) {
let Qe = up(Me);
if (Qe && Qe.kind === 345)
return Qe;
}
});
return Q(N.createJSDocSignature(void 0, Ce, Ue), se);
}
function VB(se, Me, Ce, Ue) {
let Qe = l6();
wn();
let vt = jc(Ce), Vt = j7(se, Ce);
vt || (vt = Qr(se, L(), Ce, Ue));
let Rr = vt !== void 0 ? L() : Vt.end;
return Q(N.createJSDocCallbackTag(Me, Vt, Qe, vt), se, Rr);
}
function HB(se, Me, Ce, Ue) {
wn();
let Qe = jc(Ce), vt = j7(se, Ce);
Qe || (Qe = Qr(se, L(), Ce, Ue));
let Vt = Qe !== void 0 ? L() : vt.end;
return Q(N.createJSDocOverloadTag(Me, vt, Qe), se, Vt);
}
function GB(se, Me) {
for (; !yt(se) || !yt(Me); )
if (!yt(se) && !yt(Me) && se.right.escapedText === Me.right.escapedText)
se = se.left, Me = Me.left;
else
return false;
return se.escapedText === Me.escapedText;
}
function $B(se) {
return u6(1, se);
}
function u6(se, Me, Ce) {
let Ue = true, Qe = false;
for (; ; )
switch (Ge()) {
case 59:
if (Ue) {
let vt = KB(se, Me);
return vt && (vt.kind === 344 || vt.kind === 351) && se !== 4 && Ce && (yt(vt.name) || !GB(Ce, vt.name.left)) ? false : vt;
}
Qe = false;
break;
case 4:
Ue = true, Qe = false;
break;
case 41:
Qe && (Ue = false), Qe = true;
break;
case 79:
Ue = false;
break;
case 1:
return false;
}
}
function KB(se, Me) {
Y.assert(T() === 59);
let Ce = t.getStartPos();
Ge();
let Ue = ao();
wn();
let Qe;
switch (Ue.escapedText) {
case "type":
return se === 1 && l(Ce, Ue);
case "prop":
case "property":
Qe = 1;
break;
case "arg":
case "argument":
case "param":
Qe = 6;
break;
default:
return false;
}
return se & Qe ? Xh(Ce, Ue, se, Me) : false;
}
function XB() {
let se = L(), Me = u_(22);
Me && wn();
let Ce = ao(ve.Unexpected_token_A_type_parameter_name_was_expected_without_curly_braces), Ue;
if (Me && (wn(), de(63), Ue = Mt(8388608, xc), de(23)), !va(Ce))
return Q(N.createTypeParameterDeclaration(void 0, Ce, void 0, Ue), se);
}
function YB() {
let se = L(), Me = [];
do {
wn();
let Ce = XB();
Ce !== void 0 && Me.push(Ce), Ua();
} while (u_(27));
return Er(Me, se);
}
function QB(se, Me, Ce, Ue) {
let Qe = T() === 18 ? O() : void 0, vt = YB();
return Q(N.createJSDocTemplateTag(Me, Qe, vt, Qr(se, L(), Ce, Ue)), se);
}
function u_(se) {
return T() === se ? (Ge(), true) : false;
}
function ZB() {
let se = ao();
for (Ot(22) && de(23); Ot(24); ) {
let Me = ao();
Ot(22) && de(23), se = Tu(se, Me);
}
return se;
}
function ao(se) {
if (!fr(T()))
return Jn(79, !se, se || ve.Identifier_expected);
$r++;
let Me = t.getTokenPos(), Ce = t.getTextPos(), Ue = T(), Qe = Ia(t.getTokenValue()), vt = Q(Te(Qe, Ue), Me, Ce);
return Ge(), vt;
}
}
})(Vh = e.JSDocParser || (e.JSDocParser = {}));
})(Ci || (Ci = {})), ((e) => {
function t($, ae, Te, Se) {
if (Se = Se || Y.shouldAssert(2), N($, ae, Te, Se), cS(Te))
return $;
if ($.statements.length === 0)
return Ci.parseSourceFile($.fileName, ae, $.languageVersion, void 0, true, $.scriptKind, $.setExternalModuleIndicator);
let Ye = $;
Y.assert(!Ye.hasBeenIncrementallyParsed), Ye.hasBeenIncrementallyParsed = true, Ci.fixupParentReferences(Ye);
let Ne = $.text, oe = X($), Ve = g($, Te);
N($, ae, Ve, Se), Y.assert(Ve.span.start <= Te.span.start), Y.assert(Ir(Ve.span) === Ir(Te.span)), Y.assert(Ir(R_(Ve)) === Ir(R_(Te)));
let pt = R_(Ve).length - Ve.span.length;
A(Ye, Ve.span.start, Ir(Ve.span), Ir(R_(Ve)), pt, Ne, ae, Se);
let Gt = Ci.parseSourceFile($.fileName, ae, $.languageVersion, oe, true, $.scriptKind, $.setExternalModuleIndicator);
return Gt.commentDirectives = r($.commentDirectives, Gt.commentDirectives, Ve.span.start, Ir(Ve.span), pt, Ne, ae, Se), Gt.impliedNodeFormat = $.impliedNodeFormat, Gt;
}
e.updateSourceFile = t;
function r($, ae, Te, Se, Ye, Ne, oe, Ve) {
if (!$)
return ae;
let pt, Gt = false;
for (let Xt of $) {
let { range: er, type: Tn } = Xt;
if (er.end < Te)
pt = tr(pt, Xt);
else if (er.pos > Se) {
Nt();
let Hr = { range: { pos: er.pos + Ye, end: er.end + Ye }, type: Tn };
pt = tr(pt, Hr), Ve && Y.assert(Ne.substring(er.pos, er.end) === oe.substring(Hr.range.pos, Hr.range.end));
}
}
return Nt(), pt;
function Nt() {
Gt || (Gt = true, pt ? ae && pt.push(...ae) : pt = ae);
}
}
function s($, ae, Te, Se, Ye, Ne) {
ae ? Ve($) : oe($);
return;
function oe(pt) {
let Gt = "";
if (Ne && f(pt) && (Gt = Se.substring(pt.pos, pt.end)), pt._children && (pt._children = void 0), Us(pt, pt.pos + Te, pt.end + Te), Ne && f(pt) && Y.assert(Gt === Ye.substring(pt.pos, pt.end)), xr(pt, oe, Ve), ya(pt))
for (let Nt of pt.jsDoc)
oe(Nt);
w(pt, Ne);
}
function Ve(pt) {
pt._children = void 0, Us(pt, pt.pos + Te, pt.end + Te);
for (let Gt of pt)
oe(Gt);
}
}
function f($) {
switch ($.kind) {
case 10:
case 8:
case 79:
return true;
}
return false;
}
function x($, ae, Te, Se, Ye) {
Y.assert($.end >= ae, "Adjusting an element that was entirely before the change range"), Y.assert($.pos <= Te, "Adjusting an element that was entirely after the change range"), Y.assert($.pos <= $.end);
let Ne = Math.min($.pos, Se), oe = $.end >= Te ? $.end + Ye : Math.min($.end, Se);
Y.assert(Ne <= oe), $.parent && (Y.assertGreaterThanOrEqual(Ne, $.parent.pos), Y.assertLessThanOrEqual(oe, $.parent.end)), Us($, Ne, oe);
}
function w($, ae) {
if (ae) {
let Te = $.pos, Se = (Ye) => {
Y.assert(Ye.pos >= Te), Te = Ye.end;
};
if (ya($))
for (let Ye of $.jsDoc)
Se(Ye);
xr($, Se), Y.assert(Te <= $.end);
}
}
function A($, ae, Te, Se, Ye, Ne, oe, Ve) {
pt($);
return;
function pt(Nt) {
if (Y.assert(Nt.pos <= Nt.end), Nt.pos > Te) {
s(Nt, false, Ye, Ne, oe, Ve);
return;
}
let Xt = Nt.end;
if (Xt >= ae) {
if (Nt.intersectsChange = true, Nt._children = void 0, x(Nt, ae, Te, Se, Ye), xr(Nt, pt, Gt), ya(Nt))
for (let er of Nt.jsDoc)
pt(er);
w(Nt, Ve);
return;
}
Y.assert(Xt < ae);
}
function Gt(Nt) {
if (Y.assert(Nt.pos <= Nt.end), Nt.pos > Te) {
s(Nt, true, Ye, Ne, oe, Ve);
return;
}
let Xt = Nt.end;
if (Xt >= ae) {
Nt.intersectsChange = true, Nt._children = void 0, x(Nt, ae, Te, Se, Ye);
for (let er of Nt)
pt(er);
return;
}
Y.assert(Xt < ae);
}
}
function g($, ae) {
let Se = ae.span.start;
for (let oe = 0; Se > 0 && oe <= 1; oe++) {
let Ve = B($, Se);
Y.assert(Ve.pos <= Se);
let pt = Ve.pos;
Se = Math.max(0, pt - 1);
}
let Ye = ha(Se, Ir(ae.span)), Ne = ae.newLength + (ae.span.start - Se);
return Zp(Ye, Ne);
}
function B($, ae) {
let Te = $, Se;
if (xr($, Ne), Se) {
let oe = Ye(Se);
oe.pos > Te.pos && (Te = oe);
}
return Te;
function Ye(oe) {
for (; ; ) {
let Ve = mx(oe);
if (Ve)
oe = Ve;
else
return oe;
}
}
function Ne(oe) {
if (!va(oe))
if (oe.pos <= ae) {
if (oe.pos >= Te.pos && (Te = oe), ae < oe.end)
return xr(oe, Ne), true;
Y.assert(oe.end <= ae), Se = oe;
} else
return Y.assert(oe.pos > ae), true;
}
}
function N($, ae, Te, Se) {
let Ye = $.text;
if (Te && (Y.assert(Ye.length - Te.span.length + Te.newLength === ae.length), Se || Y.shouldAssert(3))) {
let Ne = Ye.substr(0, Te.span.start), oe = ae.substr(0, Te.span.start);
Y.assert(Ne === oe);
let Ve = Ye.substring(Ir(Te.span), Ye.length), pt = ae.substring(Ir(R_(Te)), ae.length);
Y.assert(Ve === pt);
}
}
function X($) {
let ae = $.statements, Te = 0;
Y.assert(Te < ae.length);
let Se = ae[Te], Ye = -1;
return { currentNode(oe) {
return oe !== Ye && (Se && Se.end === oe && Te < ae.length - 1 && (Te++, Se = ae[Te]), (!Se || Se.pos !== oe) && Ne(oe)), Ye = oe, Y.assert(!Se || Se.pos === oe), Se;
} };
function Ne(oe) {
ae = void 0, Te = -1, Se = void 0, xr($, Ve, pt);
return;
function Ve(Gt) {
return oe >= Gt.pos && oe < Gt.end ? (xr(Gt, Ve, pt), true) : false;
}
function pt(Gt) {
if (oe >= Gt.pos && oe < Gt.end)
for (let Nt = 0; Nt < Gt.length; Nt++) {
let Xt = Gt[Nt];
if (Xt) {
if (Xt.pos === oe)
return ae = Gt, Te = Nt, Se = Xt, true;
if (Xt.pos < oe && oe < Xt.end)
return xr(Xt, Ve, pt), true;
}
}
return false;
}
}
}
e.createSyntaxCursor = X;
let F;
(($) => {
$[$.Value = -1] = "Value";
})(F || (F = {}));
})(Sd || (Sd = {})), xd = /* @__PURE__ */ new Map(), _7 = /^\/\/\/\s*<(\S+)\s.*?\/>/im, c7 = /^\/\/\/?\s*@(\S+)\s*(.*)\s*$/im;
} }), nF = () => {
}, iF = () => {
}, aF = () => {
}, sF = () => {
}, oF = () => {
}, _F = () => {
}, cF = () => {
}, lF = () => {
}, uF = () => {
}, pF = () => {
}, fF = () => {
}, dF = () => {
}, mF = () => {
}, hF = () => {
}, gF = () => {
}, yF = () => {
}, vF = () => {
}, bF = () => {
}, TF = () => {
}, SF = () => {
}, xF = () => {
}, EF = () => {
}, wF = () => {
}, CF = () => {
}, AF = () => {
}, PF = () => {
}, DF = () => {
}, kF = () => {
}, IF = () => {
}, NF = () => {
}, OF = () => {
}, MF = () => {
}, LF = () => {
}, RF = () => {
}, jF = () => {
}, JF = () => {
}, FF = () => {
}, BF = () => {
}, qF = () => {
}, UF = () => {
}, zF = () => {
}, WF = () => {
}, VF = () => {
}, HF = () => {
}, GF = () => {
}, $F = () => {
}, nn = D({ "src/compiler/_namespaces/ts.ts"() {
"use strict";
E(), L5(), PT(), R5(), j5(), F5(), U5(), NT(), W5(), sA(), oA(), hA(), iD(), OL(), ML(), LL(), RL(), KL(), XL(), YL(), Pj(), qJ(), UJ(), rF(), nF(), iF(), aF(), sF(), _F(), cF(), lF(), uF(), pF(), fF(), dF(), mF(), hF(), gF(), yF(), vF(), bF(), TF(), SF(), xF(), EF(), wF(), CF(), AF(), PF(), DF(), kF(), IF(), NF(), OF(), MF(), LF(), RF(), jF(), JF(), FF(), BF(), qF(), UF(), zF(), WF(), VF(), HF(), GF(), $F(), oF(), IT();
} }), l7 = () => {
}, KF = () => {
}, u7 = () => {
}, Zo, u7 = () => {
PT(), Zo = Po(99, true);
}, XF = () => {
}, YF = () => {
}, QF = () => {
}, ZF = () => {
}, eB = () => {
}, tB = () => {
}, rB = () => {
}, nB = () => {
}, iB = () => {
}, aB = () => {
}, p7 = () => {
}, f7 = () => {
};
function d7(e, t, r, s) {
let f = gl(e) ? new wd(e, t, r) : e === 79 ? new Ad(79, t, r) : e === 80 ? new Pd(80, t, r) : new O2(e, t, r);
return f.parent = s, f.flags = s.flags & 50720768, f;
}
function sB(e, t) {
if (!gl(e.kind))
return Bt;
let r = [];
if (c3(e))
return e.forEachChild((w) => {
r.push(w);
}), r;
Zo.setText((t || e.getSourceFile()).text);
let s = e.pos, f = (w) => {
_u(r, s, w.pos, e), r.push(w), s = w.end;
}, x = (w) => {
_u(r, s, w.pos, e), r.push(oB(w, e)), s = w.end;
};
return c(e.jsDoc, f), s = e.pos, e.forEachChild(f, x), _u(r, s, e.end, e), Zo.setText(void 0), r;
}
function _u(e, t, r, s) {
for (Zo.setTextPos(t); t < r; ) {
let f = Zo.scan(), x = Zo.getTextPos();
if (x <= r) {
if (f === 79) {
if (Qx(s))
continue;
Y.fail(`Did not expect ${Y.formatSyntaxKind(s.kind)} to have an Identifier in its trivia`);
}
e.push(d7(f, t, x, s));
}
if (t = x, f === 1)
break;
}
}
function oB(e, t) {
let r = d7(354, e.pos, e.end, t);
r._children = [];
let s = e.pos;
for (let f of e)
_u(r._children, s, f.pos, t), r._children.push(f), s = f.end;
return _u(r._children, s, e.end, t), r;
}
function m7(e) {
return hl(e).some((t) => t.tagName.text === "inheritDoc" || t.tagName.text === "inheritdoc");
}
function Ed(e, t) {
if (!e)
return Bt;
let r = ts_JsDoc_exports.getJsDocTagsFromDeclarations(e, t);
if (t && (r.length === 0 || e.some(m7))) {
let s = /* @__PURE__ */ new Set();
for (let f of e) {
let x = h7(t, f, (w) => {
var A;
if (!s.has(w))
return s.add(w), f.kind === 174 || f.kind === 175 ? w.getContextualJsDocTags(f, t) : ((A = w.declarations) == null ? void 0 : A.length) === 1 ? w.getJsDocTags() : void 0;
});
x && (r = [...x, ...r]);
}
}
return r;
}
function cu(e, t) {
if (!e)
return Bt;
let r = ts_JsDoc_exports.getJsDocCommentsFromDeclarations(e, t);
if (t && (r.length === 0 || e.some(m7))) {
let s = /* @__PURE__ */ new Set();
for (let f of e) {
let x = h7(t, f, (w) => {
if (!s.has(w))
return s.add(w), f.kind === 174 || f.kind === 175 ? w.getContextualDocumentationComment(f, t) : w.getDocumentationComment(t);
});
x && (r = r.length === 0 ? x.slice() : x.concat(lineBreakPart(), r));
}
}
return r;
}
function h7(e, t, r) {
var s;
let f = ((s = t.parent) == null ? void 0 : s.kind) === 173 ? t.parent.parent : t.parent;
if (!f)
return;
let x = Lf(t);
return q(h4(f), (w) => {
let A = e.getTypeAtLocation(w), g = x && A.symbol ? e.getTypeOfSymbol(A.symbol) : A, B = e.getPropertyOfType(g, t.symbol.name);
return B ? r(B) : void 0;
});
}
function _B() {
return { getNodeConstructor: () => wd, getTokenConstructor: () => O2, getIdentifierConstructor: () => Ad, getPrivateIdentifierConstructor: () => Pd, getSourceFileConstructor: () => P7, getSymbolConstructor: () => w7, getTypeConstructor: () => C7, getSignatureConstructor: () => A7, getSourceMapSourceConstructor: () => D7 };
}
function lu(e) {
let t = true;
for (let s in e)
if (Jr(e, s) && !g7(s)) {
t = false;
break;
}
if (t)
return e;
let r = {};
for (let s in e)
if (Jr(e, s)) {
let f = g7(s) ? s : s.charAt(0).toLowerCase() + s.substr(1);
r[f] = e[s];
}
return r;
}
function g7(e) {
return !e.length || e.charAt(0) === e.charAt(0).toLowerCase();
}
function cB(e) {
return e ? Ze(e, (t) => t.text).join("") : "";
}
function y7() {
return { target: 1, jsx: 1 };
}
function v7() {
return ts_codefix_exports.getSupportedErrorCodes();
}
function b7(e, t, r) {
e.version = r, e.scriptSnapshot = t;
}
function N2(e, t, r, s, f, x) {
let w = YE(e, getSnapshotText(t), r, f, x);
return b7(w, t, s), w;
}
function T7(e, t, r, s, f) {
if (s && r !== e.version) {
let w, A = s.span.start !== 0 ? e.text.substr(0, s.span.start) : "", g = Ir(s.span) !== e.text.length ? e.text.substr(Ir(s.span)) : "";
if (s.newLength === 0)
w = A && g ? A + g : A || g;
else {
let N = t.getText(s.span.start, s.span.start + s.newLength);
w = A && g ? A + N + g : A ? A + N : N + g;
}
let B = k2(e, w, s, f);
return b7(B, t, r), B.nameTable = void 0, e !== B && e.scriptSnapshot && (e.scriptSnapshot.dispose && e.scriptSnapshot.dispose(), e.scriptSnapshot = void 0), B;
}
let x = { languageVersion: e.languageVersion, impliedNodeFormat: e.impliedNodeFormat, setExternalModuleIndicator: e.setExternalModuleIndicator };
return N2(e.fileName, t, x, r, true, e.scriptKind);
}
function lB(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : createDocumentRegistry(e.useCaseSensitiveFileNames && e.useCaseSensitiveFileNames(), e.getCurrentDirectory()), r = arguments.length > 2 ? arguments[2] : void 0;
var s;
let f;
r === void 0 ? f = 0 : typeof r == "boolean" ? f = r ? 2 : 0 : f = r;
let x = new k7(e), w, A, g = 0, B = e.getCancellationToken ? new N7(e.getCancellationToken()) : I7, N = e.getCurrentDirectory();
vx((s = e.getLocalizedDiagnosticMessages) == null ? void 0 : s.bind(e));
function X(Z) {
e.log && e.log(Z);
}
let F = J0(e), $ = wp(F), ae = getSourceMapper({ useCaseSensitiveFileNames: () => F, getCurrentDirectory: () => N, getProgram: Ye, fileExists: le(e, e.fileExists), readFile: le(e, e.readFile), getDocumentPositionMapper: le(e, e.getDocumentPositionMapper), getSourceFileLike: le(e, e.getSourceFileLike), log: X });
function Te(Z) {
let ie = w.getSourceFile(Z);
if (!ie) {
let U = new Error(`Could not find source file: '${Z}'.`);
throw U.ProgramFiles = w.getSourceFiles().map((L) => L.fileName), U;
}
return ie;
}
function Se() {
var Z, ie, U;
if (Y.assert(f !== 2), e.getProjectVersion) {
let Tt = e.getProjectVersion();
if (Tt) {
if (A === Tt && !((Z = e.hasChangedAutomaticTypeDirectiveNames) != null && Z.call(e)))
return;
A = Tt;
}
}
let L = e.getTypeRootsVersion ? e.getTypeRootsVersion() : 0;
g !== L && (X("TypeRoots version has changed; provide new program"), w = void 0, g = L);
let fe = e.getScriptFileNames().slice(), T = e.getCompilationSettings() || y7(), it = e.hasInvalidatedResolutions || w_, mt = le(e, e.hasChangedAutomaticTypeDirectiveNames), _e = (ie = e.getProjectReferences) == null ? void 0 : ie.call(e), Ge, bt = { getSourceFile: wt, getSourceFileByPath: Tr, getCancellationToken: () => B, getCanonicalFileName: $, useCaseSensitiveFileNames: () => F, getNewLine: () => ox(T), getDefaultLibFileName: (Tt) => e.getDefaultLibFileName(Tt), writeFile: yn, getCurrentDirectory: () => N, fileExists: (Tt) => e.fileExists(Tt), readFile: (Tt) => e.readFile && e.readFile(Tt), getSymlinkCache: le(e, e.getSymlinkCache), realpath: le(e, e.realpath), directoryExists: (Tt) => sx(Tt, e), getDirectories: (Tt) => e.getDirectories ? e.getDirectories(Tt) : [], readDirectory: (Tt, kt, de, jn, Zi) => (Y.checkDefined(e.readDirectory, "'LanguageServiceHost.readDirectory' must be implemented to correctly process 'projectReferences'"), e.readDirectory(Tt, kt, de, jn, Zi)), onReleaseOldSourceFile: Rn, onReleaseParsedCommandLine: yr, hasInvalidatedResolutions: it, hasChangedAutomaticTypeDirectiveNames: mt, trace: le(e, e.trace), resolveModuleNames: le(e, e.resolveModuleNames), getModuleResolutionCache: le(e, e.getModuleResolutionCache), createHash: le(e, e.createHash), resolveTypeReferenceDirectives: le(e, e.resolveTypeReferenceDirectives), resolveModuleNameLiterals: le(e, e.resolveModuleNameLiterals), resolveTypeReferenceDirectiveReferences: le(e, e.resolveTypeReferenceDirectiveReferences), useSourceOfProjectReferenceRedirect: le(e, e.useSourceOfProjectReferenceRedirect), getParsedCommandLine: Dr }, jt = bt.getSourceFile, { getSourceFileWithCache: Yt } = changeCompilerHostLikeToUseCache(bt, (Tt) => Ui(Tt, N, $), function() {
for (var Tt = arguments.length, kt = new Array(Tt), de = 0; de < Tt; de++)
kt[de] = arguments[de];
return jt.call(bt, ...kt);
});
bt.getSourceFile = Yt, (U = e.setCompilerHost) == null || U.call(e, bt);
let $t = { useCaseSensitiveFileNames: F, fileExists: (Tt) => bt.fileExists(Tt), readFile: (Tt) => bt.readFile(Tt), readDirectory: function() {
return bt.readDirectory(...arguments);
}, trace: bt.trace, getCurrentDirectory: bt.getCurrentDirectory, onUnRecoverableConfigFileDiagnostic: yn }, Wt = t.getKeyForCompilationSettings(T);
if (isProgramUptoDate(w, fe, T, (Tt, kt) => e.getScriptVersion(kt), (Tt) => bt.fileExists(Tt), it, mt, Dr, _e))
return;
let Xr = { rootNames: fe, options: T, host: bt, oldProgram: w, projectReferences: _e };
w = createProgram(Xr), bt = void 0, Ge = void 0, ae.clearCache(), w.getTypeChecker();
return;
function Dr(Tt) {
let kt = Ui(Tt, N, $), de = Ge == null ? void 0 : Ge.get(kt);
if (de !== void 0)
return de || void 0;
let jn = e.getParsedCommandLine ? e.getParsedCommandLine(Tt) : Lr(Tt);
return (Ge || (Ge = /* @__PURE__ */ new Map())).set(kt, jn || false), jn;
}
function Lr(Tt) {
let kt = wt(Tt, 100);
if (kt)
return kt.path = Ui(Tt, N, $), kt.resolvedPath = kt.path, kt.originalFileName = kt.fileName, parseJsonSourceFileConfigFileContent(kt, $t, as(ma(Tt), N), void 0, as(Tt, N));
}
function yr(Tt, kt, de) {
var jn;
e.getParsedCommandLine ? (jn = e.onReleaseParsedCommandLine) == null || jn.call(e, Tt, kt, de) : kt && Rn(kt.sourceFile, de);
}
function Rn(Tt, kt) {
let de = t.getKeyForCompilationSettings(kt);
t.releaseDocumentWithKey(Tt.resolvedPath, de, Tt.scriptKind, Tt.impliedNodeFormat);
}
function wt(Tt, kt, de, jn) {
return Tr(Tt, Ui(Tt, N, $), kt, de, jn);
}
function Tr(Tt, kt, de, jn, Zi) {
Y.assert(bt, "getOrCreateSourceFileByPath called after typical CompilerHost lifetime, check the callstack something with a reference to an old host.");
let Pa = e.getScriptSnapshot(Tt);
if (!Pa)
return;
let e_ = getScriptKind(Tt, e), mc = e.getScriptVersion(Tt);
if (!Zi) {
let Da = w && w.getSourceFileByPath(kt);
if (Da) {
if (e_ === Da.scriptKind)
return t.updateDocumentWithKey(Tt, kt, e, Wt, Pa, mc, e_, de);
t.releaseDocumentWithKey(Da.resolvedPath, t.getKeyForCompilationSettings(w.getCompilerOptions()), Da.scriptKind, Da.impliedNodeFormat);
}
}
return t.acquireDocumentWithKey(Tt, kt, e, Wt, Pa, mc, e_, de);
}
}
function Ye() {
if (f === 2) {
Y.assert(w === void 0);
return;
}
return Se(), w;
}
function Ne() {
var Z;
return (Z = e.getPackageJsonAutoImportProvider) == null ? void 0 : Z.call(e);
}
function oe(Z, ie) {
let U = w.getTypeChecker(), L = fe();
if (!L)
return false;
for (let it of Z)
for (let mt of it.references) {
let _e = T(mt);
if (Y.assertIsDefined(_e), ie.has(mt) || ts_FindAllReferences_exports.isDeclarationOfSymbol(_e, L)) {
ie.add(mt), mt.isDefinition = true;
let Ge = getMappedDocumentSpan(mt, ae, le(e, e.fileExists));
Ge && ie.add(Ge);
} else
mt.isDefinition = false;
}
return true;
function fe() {
for (let it of Z)
for (let mt of it.references) {
if (ie.has(mt)) {
let Ge = T(mt);
return Y.assertIsDefined(Ge), U.getSymbolAtLocation(Ge);
}
let _e = getMappedDocumentSpan(mt, ae, le(e, e.fileExists));
if (_e && ie.has(_e)) {
let Ge = T(_e);
if (Ge)
return U.getSymbolAtLocation(Ge);
}
}
}
function T(it) {
let mt = w.getSourceFile(it.fileName);
if (!mt)
return;
let _e = getTouchingPropertyName(mt, it.textSpan.start);
return ts_FindAllReferences_exports.Core.getAdjustedNode(_e, { use: ts_FindAllReferences_exports.FindReferencesUse.References });
}
}
function Ve() {
w = void 0;
}
function pt() {
if (w) {
let Z = t.getKeyForCompilationSettings(w.getCompilerOptions());
c(w.getSourceFiles(), (ie) => t.releaseDocumentWithKey(ie.resolvedPath, Z, ie.scriptKind, ie.impliedNodeFormat)), w = void 0;
}
e = void 0;
}
function Gt(Z) {
return Se(), w.getSyntacticDiagnostics(Te(Z), B).slice();
}
function Nt(Z) {
Se();
let ie = Te(Z), U = w.getSemanticDiagnostics(ie, B);
if (!cv(w.getCompilerOptions()))
return U.slice();
let L = w.getDeclarationDiagnostics(ie, B);
return [...U, ...L];
}
function Xt(Z) {
return Se(), computeSuggestionDiagnostics(Te(Z), w, B);
}
function er() {
return Se(), [...w.getOptionsDiagnostics(B), ...w.getGlobalDiagnostics(B)];
}
function Tn(Z, ie) {
let U = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : emptyOptions, L = arguments.length > 3 ? arguments[3] : void 0, fe = Object.assign(Object.assign({}, U), {}, { includeCompletionsForModuleExports: U.includeCompletionsForModuleExports || U.includeExternalModuleExports, includeCompletionsWithInsertText: U.includeCompletionsWithInsertText || U.includeInsertTextCompletions });
return Se(), ts_Completions_exports.getCompletionsAtPosition(e, w, X, Te(Z), ie, fe, U.triggerCharacter, U.triggerKind, B, L && ts_formatting_exports.getFormatContext(L, e), U.includeSymbol);
}
function Hr(Z, ie, U, L, fe) {
let T = arguments.length > 5 && arguments[5] !== void 0 ? arguments[5] : emptyOptions, it = arguments.length > 6 ? arguments[6] : void 0;
return Se(), ts_Completions_exports.getCompletionEntryDetails(w, X, Te(Z), ie, { name: U, source: fe, data: it }, e, L && ts_formatting_exports.getFormatContext(L, e), T, B);
}
function Gi(Z, ie, U, L) {
let fe = arguments.length > 4 && arguments[4] !== void 0 ? arguments[4] : emptyOptions;
return Se(), ts_Completions_exports.getCompletionEntrySymbol(w, X, Te(Z), ie, { name: U, source: L }, e, fe);
}
function pn(Z, ie) {
Se();
let U = Te(Z), L = getTouchingPropertyName(U, ie);
if (L === U)
return;
let fe = w.getTypeChecker(), T = fn(L), it = mB(T, fe);
if (!it || fe.isUnknownSymbol(it)) {
let jt = Ut(U, T, ie) ? fe.getTypeAtLocation(T) : void 0;
return jt && { kind: "", kindModifiers: "", textSpan: createTextSpanFromNode(T, U), displayParts: fe.runWithCancellationToken(B, (Yt) => typeToDisplayParts(Yt, jt, getContainerNode(T))), documentation: jt.symbol ? jt.symbol.getDocumentationComment(fe) : void 0, tags: jt.symbol ? jt.symbol.getJsDocTags(fe) : void 0 };
}
let { symbolKind: mt, displayParts: _e, documentation: Ge, tags: bt } = fe.runWithCancellationToken(B, (jt) => ts_SymbolDisplay_exports.getSymbolDisplayPartsDocumentationAndSymbolKind(jt, it, U, getContainerNode(T), T));
return { kind: mt, kindModifiers: ts_SymbolDisplay_exports.getSymbolModifiers(fe, it), textSpan: createTextSpanFromNode(T, U), displayParts: _e, documentation: Ge, tags: bt };
}
function fn(Z) {
return X8(Z.parent) && Z.pos === Z.parent.pos ? Z.parent.expression : $v(Z.parent) && Z.pos === Z.parent.pos || o0(Z.parent) && Z.parent.name === Z ? Z.parent : Z;
}
function Ut(Z, ie, U) {
switch (ie.kind) {
case 79:
return !isLabelName(ie) && !isTagName(ie) && !jS(ie.parent);
case 208:
case 163:
return !isInComment(Z, U);
case 108:
case 194:
case 106:
case 199:
return true;
case 233:
return o0(ie);
default:
return false;
}
}
function kn(Z, ie, U, L) {
return Se(), ts_GoToDefinition_exports.getDefinitionAtPosition(w, Te(Z), ie, U, L);
}
function an(Z, ie) {
return Se(), ts_GoToDefinition_exports.getDefinitionAndBoundSpan(w, Te(Z), ie);
}
function mr(Z, ie) {
return Se(), ts_GoToDefinition_exports.getTypeDefinitionAtPosition(w.getTypeChecker(), Te(Z), ie);
}
function $i(Z, ie) {
return Se(), ts_FindAllReferences_exports.getImplementationsAtPosition(w, B, w.getSourceFiles(), Te(Z), ie);
}
function dn(Z, ie) {
return ne(Ur(Z, ie, [Z]), (U) => U.highlightSpans.map((L) => Object.assign(Object.assign({ fileName: U.fileName, textSpan: L.textSpan, isWriteAccess: L.kind === "writtenReference" }, L.isInString && { isInString: true }), L.contextSpan && { contextSpan: L.contextSpan })));
}
function Ur(Z, ie, U) {
let L = Un(Z);
Y.assert(U.some((it) => Un(it) === L)), Se();
let fe = qt(U, (it) => w.getSourceFile(it)), T = Te(Z);
return DocumentHighlights.getDocumentHighlights(w, B, T, ie, fe);
}
function Gr(Z, ie, U, L, fe) {
Se();
let T = Te(Z), it = getAdjustedRenameLocation(getTouchingPropertyName(T, ie));
if (ts_Rename_exports.nodeIsEligibleForRename(it))
if (yt(it) && (tu(it.parent) || sE(it.parent)) && P4(it.escapedText)) {
let { openingElement: mt, closingElement: _e } = it.parent.parent;
return [mt, _e].map((Ge) => {
let bt = createTextSpanFromNode(Ge.tagName, T);
return Object.assign({ fileName: T.fileName, textSpan: bt }, ts_FindAllReferences_exports.toContextSpan(bt, T, Ge.parent));
});
} else
return Sn(it, ie, { findInStrings: U, findInComments: L, providePrefixAndSuffixTextForRename: fe, use: ts_FindAllReferences_exports.FindReferencesUse.Rename }, (mt, _e, Ge) => ts_FindAllReferences_exports.toRenameLocation(mt, _e, Ge, fe || false));
}
function _r(Z, ie) {
return Se(), Sn(getTouchingPropertyName(Te(Z), ie), ie, { use: ts_FindAllReferences_exports.FindReferencesUse.References }, ts_FindAllReferences_exports.toReferenceEntry);
}
function Sn(Z, ie, U, L) {
Se();
let fe = U && U.use === ts_FindAllReferences_exports.FindReferencesUse.Rename ? w.getSourceFiles().filter((T) => !w.isSourceFileDefaultLibrary(T)) : w.getSourceFiles();
return ts_FindAllReferences_exports.findReferenceOrRenameEntries(w, B, fe, Z, ie, U, L);
}
function In(Z, ie) {
return Se(), ts_FindAllReferences_exports.findReferencedSymbols(w, B, w.getSourceFiles(), Te(Z), ie);
}
function pr(Z) {
return Se(), ts_FindAllReferences_exports.Core.getReferencesForFileName(Z, w, w.getSourceFiles()).map(ts_FindAllReferences_exports.toReferenceEntry);
}
function Zt(Z, ie, U) {
let L = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : false;
Se();
let fe = U ? [Te(U)] : w.getSourceFiles();
return getNavigateToItems(fe, w.getTypeChecker(), B, Z, ie, L);
}
function Or(Z, ie, U) {
Se();
let L = Te(Z), fe = e.getCustomTransformers && e.getCustomTransformers();
return getFileEmitOutput(w, L, !!ie, B, fe, U);
}
function Nn(Z, ie) {
let { triggerReason: U } = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : emptyOptions;
Se();
let L = Te(Z);
return ts_SignatureHelp_exports.getSignatureHelpItems(w, L, ie, U, B);
}
function ar(Z) {
return x.getCurrentSourceFile(Z);
}
function oi(Z, ie, U) {
let L = x.getCurrentSourceFile(Z), fe = getTouchingPropertyName(L, ie);
if (fe === L)
return;
switch (fe.kind) {
case 208:
case 163:
case 10:
case 95:
case 110:
case 104:
case 106:
case 108:
case 194:
case 79:
break;
default:
return;
}
let T = fe;
for (; ; )
if (isRightSideOfPropertyAccess(T) || isRightSideOfQualifiedName(T))
T = T.parent;
else if (isNameOfModuleDeclaration(T))
if (T.parent.parent.kind === 264 && T.parent.parent.body === T.parent)
T = T.parent.parent.name;
else
break;
else
break;
return ha(T.getStart(), fe.getEnd());
}
function cr(Z, ie) {
let U = x.getCurrentSourceFile(Z);
return ts_BreakpointResolver_exports.spanInSourceFileAtLocation(U, ie);
}
function $r(Z) {
return getNavigationBarItems(x.getCurrentSourceFile(Z), B);
}
function hr(Z) {
return getNavigationTree(x.getCurrentSourceFile(Z), B);
}
function On(Z, ie, U) {
return Se(), (U || "original") === "2020" ? ts_classifier_exports.v2020.getSemanticClassifications(w, B, Te(Z), ie) : getSemanticClassifications(w.getTypeChecker(), B, Te(Z), w.getClassifiableNames(), ie);
}
function nr(Z, ie, U) {
return Se(), (U || "original") === "original" ? getEncodedSemanticClassifications(w.getTypeChecker(), B, Te(Z), w.getClassifiableNames(), ie) : ts_classifier_exports.v2020.getEncodedSemanticClassifications(w, B, Te(Z), ie);
}
function br(Z, ie) {
return getSyntacticClassifications(B, x.getCurrentSourceFile(Z), ie);
}
function Kr(Z, ie) {
return getEncodedSyntacticClassifications(B, x.getCurrentSourceFile(Z), ie);
}
function wa(Z) {
let ie = x.getCurrentSourceFile(Z);
return ts_OutliningElementsCollector_exports.collectElements(ie, B);
}
let $n = new Map(Object.entries({ [18]: 19, [20]: 21, [22]: 23, [31]: 29 }));
$n.forEach((Z, ie) => $n.set(Z.toString(), Number(ie)));
function Ki(Z, ie) {
let U = x.getCurrentSourceFile(Z), L = getTouchingToken(U, ie), fe = L.getStart(U) === ie ? $n.get(L.kind.toString()) : void 0, T = fe && findChildOfKind(L.parent, fe, U);
return T ? [createTextSpanFromNode(L, U), createTextSpanFromNode(T, U)].sort((it, mt) => it.start - mt.start) : Bt;
}
function Mn(Z, ie, U) {
let L = ts(), fe = lu(U), T = x.getCurrentSourceFile(Z);
X("getIndentationAtPosition: getCurrentSourceFile: " + (ts() - L)), L = ts();
let it = ts_formatting_exports.SmartIndenter.getIndentation(ie, T, fe);
return X("getIndentationAtPosition: computeIndentation : " + (ts() - L)), it;
}
function _i(Z, ie, U, L) {
let fe = x.getCurrentSourceFile(Z);
return ts_formatting_exports.formatSelection(ie, U, fe, ts_formatting_exports.getFormatContext(lu(L), e));
}
function Ca(Z, ie) {
return ts_formatting_exports.formatDocument(x.getCurrentSourceFile(Z), ts_formatting_exports.getFormatContext(lu(ie), e));
}
function St(Z, ie, U, L) {
let fe = x.getCurrentSourceFile(Z), T = ts_formatting_exports.getFormatContext(lu(L), e);
if (!isInComment(fe, ie))
switch (U) {
case "{":
return ts_formatting_exports.formatOnOpeningCurly(ie, fe, T);
case "}":
return ts_formatting_exports.formatOnClosingCurly(ie, fe, T);
case ";":
return ts_formatting_exports.formatOnSemicolon(ie, fe, T);
case `
`:
return ts_formatting_exports.formatOnEnter(ie, fe, T);
}
return [];
}
function ue(Z, ie, U, L, fe) {
let T = arguments.length > 5 && arguments[5] !== void 0 ? arguments[5] : emptyOptions;
Se();
let it = Te(Z), mt = ha(ie, U), _e = ts_formatting_exports.getFormatContext(fe, e);
return ne(ji(L, fa, Vr), (Ge) => (B.throwIfCancellationRequested(), ts_codefix_exports.getFixes({ errorCode: Ge, sourceFile: it, span: mt, program: w, host: e, cancellationToken: B, formatContext: _e, preferences: T })));
}
function He(Z, ie, U) {
let L = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : emptyOptions;
Se(), Y.assert(Z.type === "file");
let fe = Te(Z.fileName), T = ts_formatting_exports.getFormatContext(U, e);
return ts_codefix_exports.getAllFixes({ fixId: ie, sourceFile: fe, program: w, host: e, cancellationToken: B, formatContext: T, preferences: L });
}
function _t(Z, ie) {
let U = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : emptyOptions;
var L;
Se(), Y.assert(Z.type === "file");
let fe = Te(Z.fileName), T = ts_formatting_exports.getFormatContext(ie, e), it = (L = Z.mode) != null ? L : Z.skipDestructiveCodeActions ? "SortAndCombine" : "All";
return ts_OrganizeImports_exports.organizeImports(fe, T, e, w, U, it);
}
function ft(Z, ie, U) {
let L = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : emptyOptions;
return getEditsForFileRename(Ye(), Z, ie, e, ts_formatting_exports.getFormatContext(U, e), L, ae);
}
function Kt(Z, ie) {
let U = typeof Z == "string" ? ie : Z;
return ir(U) ? Promise.all(U.map((L) => zt(L))) : zt(U);
}
function zt(Z) {
let ie = (U) => Ui(U, N, $);
return Y.assertEqual(Z.type, "install package"), e.installPackage ? e.installPackage({ fileName: ie(Z.file), packageName: Z.packageName }) : Promise.reject("Host does not implement `installPackage`");
}
function xe(Z, ie, U, L) {
let fe = L ? ts_formatting_exports.getFormatContext(L, e).options : void 0;
return ts_JsDoc_exports.getDocCommentTemplateAtPosition(getNewLineOrDefaultFromHost(e, fe), x.getCurrentSourceFile(Z), ie, U);
}
function Le(Z, ie, U) {
if (U === 60)
return false;
let L = x.getCurrentSourceFile(Z);
if (isInString(L, ie))
return false;
if (isInsideJsxElementOrAttribute(L, ie))
return U === 123;
if (isInTemplateString(L, ie))
return false;
switch (U) {
case 39:
case 34:
case 96:
return !isInComment(L, ie);
}
return true;
}
function Re(Z, ie) {
let U = x.getCurrentSourceFile(Z), L = findPrecedingToken(ie, U);
if (!L)
return;
let fe = L.kind === 31 && tu(L.parent) ? L.parent.parent : td(L) && l2(L.parent) ? L.parent : void 0;
if (fe && gr(fe))
return { newText: `${fe.openingElement.tagName.getText(U)}>` };
let T = L.kind === 31 && u2(L.parent) ? L.parent.parent : td(L) && pd(L.parent) ? L.parent : void 0;
if (T && Ln(T))
return { newText: ">" };
}
function ot(Z, ie) {
return { lineStarts: Z.getLineStarts(), firstLine: Z.getLineAndCharacterOfPosition(ie.pos).line, lastLine: Z.getLineAndCharacterOfPosition(ie.end).line };
}
function Ct(Z, ie, U) {
let L = x.getCurrentSourceFile(Z), fe = [], { lineStarts: T, firstLine: it, lastLine: mt } = ot(L, ie), _e = U || false, Ge = Number.MAX_VALUE, bt = /* @__PURE__ */ new Map(), jt = new RegExp(/\S/), Yt = isInsideJsxElement(L, T[it]), $t = Yt ? "{/*" : "//";
for (let Wt = it; Wt <= mt; Wt++) {
let Xr = L.text.substring(T[Wt], L.getLineEndOfPosition(T[Wt])), Dr = jt.exec(Xr);
Dr && (Ge = Math.min(Ge, Dr.index), bt.set(Wt.toString(), Dr.index), Xr.substr(Dr.index, $t.length) !== $t && (_e = U === void 0 || U));
}
for (let Wt = it; Wt <= mt; Wt++) {
if (it !== mt && T[Wt] === ie.end)
continue;
let Xr = bt.get(Wt.toString());
Xr !== void 0 && (Yt ? fe.push.apply(fe, Mt(Z, { pos: T[Wt] + Ge, end: L.getLineEndOfPosition(T[Wt]) }, _e, Yt)) : _e ? fe.push({ newText: $t, span: { length: 0, start: T[Wt] + Ge } }) : L.text.substr(T[Wt] + Xr, $t.length) === $t && fe.push({ newText: "", span: { length: $t.length, start: T[Wt] + Xr } }));
}
return fe;
}
function Mt(Z, ie, U, L) {
var fe;
let T = x.getCurrentSourceFile(Z), it = [], { text: mt } = T, _e = false, Ge = U || false, bt = [], { pos: jt } = ie, Yt = L !== void 0 ? L : isInsideJsxElement(T, jt), $t = Yt ? "{/*" : "/*", Wt = Yt ? "*/}" : "*/", Xr = Yt ? "\\{\\/\\*" : "\\/\\*", Dr = Yt ? "\\*\\/\\}" : "\\*\\/";
for (; jt <= ie.end; ) {
let Lr = mt.substr(jt, $t.length) === $t ? $t.length : 0, yr = isInComment(T, jt + Lr);
if (yr)
Yt && (yr.pos--, yr.end++), bt.push(yr.pos), yr.kind === 3 && bt.push(yr.end), _e = true, jt = yr.end + 1;
else {
let Rn = mt.substring(jt, ie.end).search(`(${Xr})|(${Dr})`);
Ge = U !== void 0 ? U : Ge || !isTextWhiteSpaceLike(mt, jt, Rn === -1 ? ie.end : jt + Rn), jt = Rn === -1 ? ie.end + 1 : jt + Rn + Wt.length;
}
}
if (Ge || !_e) {
((fe = isInComment(T, ie.pos)) == null ? void 0 : fe.kind) !== 2 && Qn(bt, ie.pos, Vr), Qn(bt, ie.end, Vr);
let Lr = bt[0];
mt.substr(Lr, $t.length) !== $t && it.push({ newText: $t, span: { length: 0, start: Lr } });
for (let yr = 1; yr < bt.length - 1; yr++)
mt.substr(bt[yr] - Wt.length, Wt.length) !== Wt && it.push({ newText: Wt, span: { length: 0, start: bt[yr] } }), mt.substr(bt[yr], $t.length) !== $t && it.push({ newText: $t, span: { length: 0, start: bt[yr] } });
it.length % 2 !== 0 && it.push({ newText: Wt, span: { length: 0, start: bt[bt.length - 1] } });
} else
for (let Lr of bt) {
let yr = Lr - Wt.length > 0 ? Lr - Wt.length : 0, Rn = mt.substr(yr, Wt.length) === Wt ? Wt.length : 0;
it.push({ newText: "", span: { length: $t.length, start: Lr - Rn } });
}
return it;
}
function It(Z, ie) {
let U = x.getCurrentSourceFile(Z), { firstLine: L, lastLine: fe } = ot(U, ie);
return L === fe && ie.pos !== ie.end ? Mt(Z, ie, true) : Ct(Z, ie, true);
}
function Mr(Z, ie) {
let U = x.getCurrentSourceFile(Z), L = [], { pos: fe } = ie, { end: T } = ie;
fe === T && (T += isInsideJsxElement(U, fe) ? 2 : 1);
for (let it = fe; it <= T; it++) {
let mt = isInComment(U, it);
if (mt) {
switch (mt.kind) {
case 2:
L.push.apply(L, Ct(Z, { end: mt.end, pos: mt.pos + 1 }, false));
break;
case 3:
L.push.apply(L, Mt(Z, { end: mt.end, pos: mt.pos + 1 }, false));
}
it = mt.end + 1;
}
}
return L;
}
function gr(Z) {
let { openingElement: ie, closingElement: U, parent: L } = Z;
return !Hi(ie.tagName, U.tagName) || l2(L) && Hi(ie.tagName, L.openingElement.tagName) && gr(L);
}
function Ln(Z) {
let { closingFragment: ie, parent: U } = Z;
return !!(ie.flags & 131072) || pd(U) && Ln(U);
}
function ys(Z, ie, U) {
let L = x.getCurrentSourceFile(Z), fe = ts_formatting_exports.getRangeOfEnclosingComment(L, ie);
return fe && (!U || fe.kind === 3) ? createTextSpanFromRange(fe) : void 0;
}
function ci(Z, ie) {
Se();
let U = Te(Z);
B.throwIfCancellationRequested();
let L = U.text, fe = [];
if (ie.length > 0 && !_e(U.fileName)) {
let Ge = it(), bt;
for (; bt = Ge.exec(L); ) {
B.throwIfCancellationRequested();
let jt = 3;
Y.assert(bt.length === ie.length + jt);
let Yt = bt[1], $t = bt.index + Yt.length;
if (!isInComment(U, $t))
continue;
let Wt;
for (let Dr = 0; Dr < ie.length; Dr++)
bt[Dr + jt] && (Wt = ie[Dr]);
if (Wt === void 0)
return Y.fail();
if (mt(L.charCodeAt($t + Wt.text.length)))
continue;
let Xr = bt[2];
fe.push({ descriptor: Wt, message: Xr, position: $t });
}
}
return fe;
function T(Ge) {
return Ge.replace(/[\-\[\]\/\{\}\(\)\*\+\?\.\\\^\$\|]/g, "\\$&");
}
function it() {
let Ge = /(?:\/\/+\s*)/.source, bt = /(?:\/\*+\s*)/.source, Yt = "(" + /(?:^(?:\s|\*)*)/.source + "|" + Ge + "|" + bt + ")", $t = "(?:" + Ze(ie, (yr) => "(" + T(yr.text) + ")").join("|") + ")", Wt = /(?:$|\*\/)/.source, Xr = /(?:.*?)/.source, Dr = "(" + $t + Xr + ")", Lr = Yt + Dr + Wt;
return new RegExp(Lr, "gim");
}
function mt(Ge) {
return Ge >= 97 && Ge <= 122 || Ge >= 65 && Ge <= 90 || Ge >= 48 && Ge <= 57;
}
function _e(Ge) {
return Fi(Ge, "/node_modules/");
}
}
function Xi(Z, ie, U) {
return Se(), ts_Rename_exports.getRenameInfo(w, Te(Z), ie, U || {});
}
function Aa(Z, ie, U, L, fe, T) {
let [it, mt] = typeof ie == "number" ? [ie, void 0] : [ie.pos, ie.end];
return { file: Z, startPosition: it, endPosition: mt, program: Ye(), host: e, formatContext: ts_formatting_exports.getFormatContext(L, e), cancellationToken: B, preferences: U, triggerReason: fe, kind: T };
}
function vs(Z, ie, U) {
return { file: Z, program: Ye(), host: e, span: ie, preferences: U, cancellationToken: B };
}
function $s(Z, ie) {
return ts_SmartSelectionRange_exports.getSmartSelectionRange(ie, x.getCurrentSourceFile(Z));
}
function li(Z, ie) {
let U = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : emptyOptions, L = arguments.length > 3 ? arguments[3] : void 0, fe = arguments.length > 4 ? arguments[4] : void 0;
Se();
let T = Te(Z);
return ts_refactor_exports.getApplicableRefactors(Aa(T, ie, U, emptyOptions, L, fe));
}
function Yi(Z, ie, U, L, fe) {
let T = arguments.length > 5 && arguments[5] !== void 0 ? arguments[5] : emptyOptions;
Se();
let it = Te(Z);
return ts_refactor_exports.getEditsForRefactor(Aa(it, U, T, ie), L, fe);
}
function Qi(Z, ie) {
return ie === 0 ? { line: 0, character: 0 } : ae.toLineColumnOffset(Z, ie);
}
function bs(Z, ie) {
Se();
let U = ts_CallHierarchy_exports.resolveCallHierarchyDeclaration(w, getTouchingPropertyName(Te(Z), ie));
return U && mapOneOrMany(U, (L) => ts_CallHierarchy_exports.createCallHierarchyItem(w, L));
}
function Ai(Z, ie) {
Se();
let U = Te(Z), L = firstOrOnly(ts_CallHierarchy_exports.resolveCallHierarchyDeclaration(w, ie === 0 ? U : getTouchingPropertyName(U, ie)));
return L ? ts_CallHierarchy_exports.getIncomingCalls(w, L, B) : [];
}
function xn(Z, ie) {
Se();
let U = Te(Z), L = firstOrOnly(ts_CallHierarchy_exports.resolveCallHierarchyDeclaration(w, ie === 0 ? U : getTouchingPropertyName(U, ie)));
return L ? ts_CallHierarchy_exports.getOutgoingCalls(w, L) : [];
}
function Dt(Z, ie) {
let U = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : emptyOptions;
Se();
let L = Te(Z);
return ts_InlayHints_exports.provideInlayHints(vs(L, ie, U));
}
let Pi = { dispose: pt, cleanupSemanticCache: Ve, getSyntacticDiagnostics: Gt, getSemanticDiagnostics: Nt, getSuggestionDiagnostics: Xt, getCompilerOptionsDiagnostics: er, getSyntacticClassifications: br, getSemanticClassifications: On, getEncodedSyntacticClassifications: Kr, getEncodedSemanticClassifications: nr, getCompletionsAtPosition: Tn, getCompletionEntryDetails: Hr, getCompletionEntrySymbol: Gi, getSignatureHelpItems: Nn, getQuickInfoAtPosition: pn, getDefinitionAtPosition: kn, getDefinitionAndBoundSpan: an, getImplementationAtPosition: $i, getTypeDefinitionAtPosition: mr, getReferencesAtPosition: _r, findReferences: In, getFileReferences: pr, getOccurrencesAtPosition: dn, getDocumentHighlights: Ur, getNameOrDottedNameSpan: oi, getBreakpointStatementAtPosition: cr, getNavigateToItems: Zt, getRenameInfo: Xi, getSmartSelectionRange: $s, findRenameLocations: Gr, getNavigationBarItems: $r, getNavigationTree: hr, getOutliningSpans: wa, getTodoComments: ci, getBraceMatchingAtPosition: Ki, getIndentationAtPosition: Mn, getFormattingEditsForRange: _i, getFormattingEditsForDocument: Ca, getFormattingEditsAfterKeystroke: St, getDocCommentTemplateAtPosition: xe, isValidBraceCompletionAtPosition: Le, getJsxClosingTagAtPosition: Re, getSpanOfEnclosingComment: ys, getCodeFixesAtPosition: ue, getCombinedCodeFix: He, applyCodeActionCommand: Kt, organizeImports: _t, getEditsForFileRename: ft, getEmitOutput: Or, getNonBoundSourceFile: ar, getProgram: Ye, getCurrentProgram: () => w, getAutoImportProvider: Ne, updateIsDefinitionOfReferencedSymbols: oe, getApplicableRefactors: li, getEditsForRefactor: Yi, toLineColumnOffset: Qi, getSourceMapper: () => ae, clearSourceMapperCache: () => ae.clearCache(), prepareCallHierarchy: bs, provideCallHierarchyIncomingCalls: Ai, provideCallHierarchyOutgoingCalls: xn, toggleLineComment: Ct, toggleMultilineComment: Mt, commentSelection: It, uncommentSelection: Mr, provideInlayHints: Dt, getSupportedCodeFixes: v7 };
switch (f) {
case 0:
break;
case 1:
M2.forEach((Z) => Pi[Z] = () => {
throw new Error(`LanguageService Operation: ${Z} not allowed in LanguageServiceMode.PartialSemantic`);
});
break;
case 2:
M7.forEach((Z) => Pi[Z] = () => {
throw new Error(`LanguageService Operation: ${Z} not allowed in LanguageServiceMode.Syntactic`);
});
break;
default:
Y.assertNever(f);
}
return Pi;
}
function uB(e) {
return e.nameTable || pB(e), e.nameTable;
}
function pB(e) {
let t = e.nameTable = /* @__PURE__ */ new Map();
e.forEachChild(function r(s) {
if (yt(s) && !isTagName(s) && s.escapedText || Ta(s) && fB(s)) {
let f = b4(s);
t.set(f, t.get(f) === void 0 ? s.pos : -1);
} else if (vn(s)) {
let f = s.escapedText;
t.set(f, t.get(f) === void 0 ? s.pos : -1);
}
if (xr(s, r), ya(s))
for (let f of s.jsDoc)
xr(f, r);
});
}
function fB(e) {
return c4(e) || e.parent.kind === 280 || hB(e) || l4(e);
}
function S7(e) {
let t = dB(e);
return t && (Hs(t.parent) || p2(t.parent)) ? t : void 0;
}
function dB(e) {
switch (e.kind) {
case 10:
case 14:
case 8:
if (e.parent.kind === 164)
return Wy(e.parent.parent) ? e.parent.parent : void 0;
case 79:
return Wy(e.parent) && (e.parent.parent.kind === 207 || e.parent.parent.kind === 289) && e.parent.name === e ? e.parent : void 0;
}
}
function mB(e, t) {
let r = S7(e);
if (r) {
let s = t.getContextualType(r.parent), f = s && x7(r, t, s, false);
if (f && f.length === 1)
return fo(f);
}
return t.getSymbolAtLocation(e);
}
function x7(e, t, r, s) {
let f = getNameFromPropertyName(e.name);
if (!f)
return Bt;
if (!r.isUnion()) {
let w = r.getProperty(f);
return w ? [w] : Bt;
}
let x = qt(r.types, (w) => (Hs(e.parent) || p2(e.parent)) && t.isTypeInvalidDueToUnionDiscriminant(w, e.parent) ? void 0 : w.getProperty(f));
if (s && (x.length === 0 || x.length === r.types.length)) {
let w = r.getProperty(f);
if (w)
return [w];
}
return x.length === 0 ? qt(r.types, (w) => w.getProperty(f)) : x;
}
function hB(e) {
return e && e.parent && e.parent.kind === 209 && e.parent.argumentExpression === e;
}
function gB(e) {
if (iy)
return tn(ma(Un(iy.getExecutingFilePath())), aS(e));
throw new Error("getDefaultLibFilePath is only supported when consumed as a node module. ");
}
var E7, wd, Cd, w7, O2, Ad, Pd, C7, A7, P7, D7, k7, I7, N7, O7, M2, M7, yB = D({ "src/services/services.ts"() {
"use strict";
L2(), L2(), p7(), f7(), E7 = "0.8", wd = class {
constructor(e, t, r) {
this.pos = t, this.end = r, this.flags = 0, this.modifierFlagsCache = 0, this.transformFlags = 0, this.parent = void 0, this.kind = e;
}
assertHasRealPosition(e) {
Y.assert(!hs(this.pos) && !hs(this.end), e || "Node must have a real position for this operation");
}
getSourceFile() {
return Si(this);
}
getStart(e, t) {
return this.assertHasRealPosition(), Io(this, e, t);
}
getFullStart() {
return this.assertHasRealPosition(), this.pos;
}
getEnd() {
return this.assertHasRealPosition(), this.end;
}
getWidth(e) {
return this.assertHasRealPosition(), this.getEnd() - this.getStart(e);
}
getFullWidth() {
return this.assertHasRealPosition(), this.end - this.pos;
}
getLeadingTriviaWidth(e) {
return this.assertHasRealPosition(), this.getStart(e) - this.pos;
}
getFullText(e) {
return this.assertHasRealPosition(), (e || this.getSourceFile()).text.substring(this.pos, this.end);
}
getText(e) {
return this.assertHasRealPosition(), e || (e = this.getSourceFile()), e.text.substring(this.getStart(e), this.getEnd());
}
getChildCount(e) {
return this.getChildren(e).length;
}
getChildAt(e, t) {
return this.getChildren(t)[e];
}
getChildren(e) {
return this.assertHasRealPosition("Node without a real position cannot be scanned and thus has no token nodes - use forEachChild and collect the result if that's fine"), this._children || (this._children = sB(this, e));
}
getFirstToken(e) {
this.assertHasRealPosition();
let t = this.getChildren(e);
if (!t.length)
return;
let r = Ae(t, (s) => s.kind < 312 || s.kind > 353);
return r.kind < 163 ? r : r.getFirstToken(e);
}
getLastToken(e) {
this.assertHasRealPosition();
let t = this.getChildren(e), r = Cn(t);
if (r)
return r.kind < 163 ? r : r.getLastToken(e);
}
forEachChild(e, t) {
return xr(this, e, t);
}
}, Cd = class {
constructor(e, t) {
this.pos = e, this.end = t, this.flags = 0, this.modifierFlagsCache = 0, this.transformFlags = 0, this.parent = void 0;
}
getSourceFile() {
return Si(this);
}
getStart(e, t) {
return Io(this, e, t);
}
getFullStart() {
return this.pos;
}
getEnd() {
return this.end;
}
getWidth(e) {
return this.getEnd() - this.getStart(e);
}
getFullWidth() {
return this.end - this.pos;
}
getLeadingTriviaWidth(e) {
return this.getStart(e) - this.pos;
}
getFullText(e) {
return (e || this.getSourceFile()).text.substring(this.pos, this.end);
}
getText(e) {
return e || (e = this.getSourceFile()), e.text.substring(this.getStart(e), this.getEnd());
}
getChildCount() {
return this.getChildren().length;
}
getChildAt(e) {
return this.getChildren()[e];
}
getChildren() {
return this.kind === 1 && this.jsDoc || Bt;
}
getFirstToken() {
}
getLastToken() {
}
forEachChild() {
}
}, w7 = class {
constructor(e, t) {
this.id = 0, this.mergeId = 0, this.flags = e, this.escapedName = t;
}
getFlags() {
return this.flags;
}
get name() {
return rf(this);
}
getEscapedName() {
return this.escapedName;
}
getName() {
return this.name;
}
getDeclarations() {
return this.declarations;
}
getDocumentationComment(e) {
if (!this.documentationComment)
if (this.documentationComment = Bt, !this.declarations && $y(this) && this.links.target && $y(this.links.target) && this.links.target.links.tupleLabelDeclaration) {
let t = this.links.target.links.tupleLabelDeclaration;
this.documentationComment = cu([t], e);
} else
this.documentationComment = cu(this.declarations, e);
return this.documentationComment;
}
getContextualDocumentationComment(e, t) {
if (e) {
if (Tl(e) && (this.contextualGetAccessorDocumentationComment || (this.contextualGetAccessorDocumentationComment = cu(ee(this.declarations, Tl), t)), I(this.contextualGetAccessorDocumentationComment)))
return this.contextualGetAccessorDocumentationComment;
if (bl(e) && (this.contextualSetAccessorDocumentationComment || (this.contextualSetAccessorDocumentationComment = cu(ee(this.declarations, bl), t)), I(this.contextualSetAccessorDocumentationComment)))
return this.contextualSetAccessorDocumentationComment;
}
return this.getDocumentationComment(t);
}
getJsDocTags(e) {
return this.tags === void 0 && (this.tags = Ed(this.declarations, e)), this.tags;
}
getContextualJsDocTags(e, t) {
if (e) {
if (Tl(e) && (this.contextualGetAccessorTags || (this.contextualGetAccessorTags = Ed(ee(this.declarations, Tl), t)), I(this.contextualGetAccessorTags)))
return this.contextualGetAccessorTags;
if (bl(e) && (this.contextualSetAccessorTags || (this.contextualSetAccessorTags = Ed(ee(this.declarations, bl), t)), I(this.contextualSetAccessorTags)))
return this.contextualSetAccessorTags;
}
return this.getJsDocTags(t);
}
}, O2 = class extends Cd {
constructor(e, t, r) {
super(t, r), this.kind = e;
}
}, Ad = class extends Cd {
constructor(e, t, r) {
super(t, r), this.kind = 79;
}
get text() {
return qr(this);
}
}, Ad.prototype.kind = 79, Pd = class extends Cd {
constructor(e, t, r) {
super(t, r), this.kind = 80;
}
get text() {
return qr(this);
}
}, Pd.prototype.kind = 80, C7 = class {
constructor(e, t) {
this.checker = e, this.flags = t;
}
getFlags() {
return this.flags;
}
getSymbol() {
return this.symbol;
}
getProperties() {
return this.checker.getPropertiesOfType(this);
}
getProperty(e) {
return this.checker.getPropertyOfType(this, e);
}
getApparentProperties() {
return this.checker.getAugmentedPropertiesOfType(this);
}
getCallSignatures() {
return this.checker.getSignaturesOfType(this, 0);
}
getConstructSignatures() {
return this.checker.getSignaturesOfType(this, 1);
}
getStringIndexType() {
return this.checker.getIndexTypeOfType(this, 0);
}
getNumberIndexType() {
return this.checker.getIndexTypeOfType(this, 1);
}
getBaseTypes() {
return this.isClassOrInterface() ? this.checker.getBaseTypes(this) : void 0;
}
isNullableType() {
return this.checker.isNullableType(this);
}
getNonNullableType() {
return this.checker.getNonNullableType(this);
}
getNonOptionalType() {
return this.checker.getNonOptionalType(this);
}
getConstraint() {
return this.checker.getBaseConstraintOfType(this);
}
getDefault() {
return this.checker.getDefaultFromTypeParameter(this);
}
isUnion() {
return !!(this.flags & 1048576);
}
isIntersection() {
return !!(this.flags & 2097152);
}
isUnionOrIntersection() {
return !!(this.flags & 3145728);
}
isLiteral() {
return !!(this.flags & 2432);
}
isStringLiteral() {
return !!(this.flags & 128);
}
isNumberLiteral() {
return !!(this.flags & 256);
}
isTypeParameter() {
return !!(this.flags & 262144);
}
isClassOrInterface() {
return !!(Bf(this) & 3);
}
isClass() {
return !!(Bf(this) & 1);
}
isIndexType() {
return !!(this.flags & 4194304);
}
get typeArguments() {
if (Bf(this) & 4)
return this.checker.getTypeArguments(this);
}
}, A7 = class {
constructor(e, t) {
this.checker = e, this.flags = t;
}
getDeclaration() {
return this.declaration;
}
getTypeParameters() {
return this.typeParameters;
}
getParameters() {
return this.parameters;
}
getReturnType() {
return this.checker.getReturnTypeOfSignature(this);
}
getTypeParameterAtPosition(e) {
let t = this.checker.getParameterType(this, e);
if (t.isIndexType() && Kx(t.type)) {
let r = t.type.getConstraint();
if (r)
return this.checker.getIndexType(r);
}
return t;
}
getDocumentationComment() {
return this.documentationComment || (this.documentationComment = cu(Cp(this.declaration), this.checker));
}
getJsDocTags() {
return this.jsDocTags || (this.jsDocTags = Ed(Cp(this.declaration), this.checker));
}
}, P7 = class extends wd {
constructor(e, t, r) {
super(e, t, r), this.kind = 308;
}
update(e, t) {
return k2(this, e, t);
}
getLineAndCharacterOfPosition(e) {
return Ls(this, e);
}
getLineStarts() {
return ss(this);
}
getPositionOfLineAndCharacter(e, t, r) {
return dy(ss(this), e, t, this.text, r);
}
getLineEndOfPosition(e) {
let { line: t } = this.getLineAndCharacterOfPosition(e), r = this.getLineStarts(), s;
t + 1 >= r.length && (s = this.getEnd()), s || (s = r[t + 1] - 1);
let f = this.getFullText();
return f[s] === `
` && f[s - 1] === "\r" ? s - 1 : s;
}
getNamedDeclarations() {
return this.namedDeclarations || (this.namedDeclarations = this.computeNamedDeclarations()), this.namedDeclarations;
}
computeNamedDeclarations() {
let e = Be();
return this.forEachChild(f), e;
function t(x) {
let w = s(x);
w && e.add(w, x);
}
function r(x) {
let w = e.get(x);
return w || e.set(x, w = []), w;
}
function s(x) {
let w = Ey(x);
return w && (Ws(w) && bn(w.expression) ? w.expression.name.text : vl(w) ? getNameFromPropertyName(w) : void 0);
}
function f(x) {
switch (x.kind) {
case 259:
case 215:
case 171:
case 170:
let w = x, A = s(w);
if (A) {
let N = r(A), X = Cn(N);
X && w.parent === X.parent && w.symbol === X.symbol ? w.body && !X.body && (N[N.length - 1] = w) : N.push(w);
}
xr(x, f);
break;
case 260:
case 228:
case 261:
case 262:
case 263:
case 264:
case 268:
case 278:
case 273:
case 270:
case 271:
case 174:
case 175:
case 184:
t(x), xr(x, f);
break;
case 166:
if (!rn(x, 16476))
break;
case 257:
case 205: {
let N = x;
if (df(N.name)) {
xr(N.name, f);
break;
}
N.initializer && f(N.initializer);
}
case 302:
case 169:
case 168:
t(x);
break;
case 275:
let g = x;
g.exportClause && (iE(g.exportClause) ? c(g.exportClause.elements, f) : f(g.exportClause.name));
break;
case 269:
let B = x.importClause;
B && (B.name && t(B.name), B.namedBindings && (B.namedBindings.kind === 271 ? t(B.namedBindings) : c(B.namedBindings.elements, f)));
break;
case 223:
ps(x) !== 0 && t(x);
default:
xr(x, f);
}
}
}
}, D7 = class {
constructor(e, t, r) {
this.fileName = e, this.text = t, this.skipTrivia = r;
}
getLineAndCharacterOfPosition(e) {
return Ls(this, e);
}
}, k7 = class {
constructor(e) {
this.host = e;
}
getCurrentSourceFile(e) {
var t, r, s, f, x, w, A, g;
let B = this.host.getScriptSnapshot(e);
if (!B)
throw new Error("Could not find file: '" + e + "'.");
let N = getScriptKind(e, this.host), X = this.host.getScriptVersion(e), F;
if (this.currentFileName !== e) {
let $ = { languageVersion: 99, impliedNodeFormat: getImpliedNodeFormatForFile(Ui(e, this.host.getCurrentDirectory(), ((s = (r = (t = this.host).getCompilerHost) == null ? void 0 : r.call(t)) == null ? void 0 : s.getCanonicalFileName) || D4(this.host)), (g = (A = (w = (x = (f = this.host).getCompilerHost) == null ? void 0 : x.call(f)) == null ? void 0 : w.getModuleResolutionCache) == null ? void 0 : A.call(w)) == null ? void 0 : g.getPackageJsonInfoCache(), this.host, this.host.getCompilationSettings()), setExternalModuleIndicator: Ex(this.host.getCompilationSettings()) };
F = N2(e, B, $, X, true, N);
} else if (this.currentFileVersion !== X) {
let $ = B.getChangeRange(this.currentFileScriptSnapshot);
F = T7(this.currentSourceFile, B, X, $);
}
return F && (this.currentFileVersion = X, this.currentFileName = e, this.currentFileScriptSnapshot = B, this.currentSourceFile = F), this.currentSourceFile;
}
}, I7 = { isCancellationRequested: w_, throwIfCancellationRequested: yn }, N7 = class {
constructor(e) {
this.cancellationToken = e;
}
isCancellationRequested() {
return this.cancellationToken.isCancellationRequested();
}
throwIfCancellationRequested() {
var e;
if (this.isCancellationRequested())
throw (e = rs) == null || e.instant(rs.Phase.Session, "cancellationThrown", { kind: "CancellationTokenObject" }), new Rp();
}
}, O7 = class {
constructor(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : 20;
this.hostCancellationToken = e, this.throttleWaitMilliseconds = t, this.lastCancellationCheckTime = 0;
}
isCancellationRequested() {
let e = ts();
return Math.abs(e - this.lastCancellationCheckTime) >= this.throttleWaitMilliseconds ? (this.lastCancellationCheckTime = e, this.hostCancellationToken.isCancellationRequested()) : false;
}
throwIfCancellationRequested() {
var e;
if (this.isCancellationRequested())
throw (e = rs) == null || e.instant(rs.Phase.Session, "cancellationThrown", { kind: "ThrottledCancellationToken" }), new Rp();
}
}, M2 = ["getSemanticDiagnostics", "getSuggestionDiagnostics", "getCompilerOptionsDiagnostics", "getSemanticClassifications", "getEncodedSemanticClassifications", "getCodeFixesAtPosition", "getCombinedCodeFix", "applyCodeActionCommand", "organizeImports", "getEditsForFileRename", "getEmitOutput", "getApplicableRefactors", "getEditsForRefactor", "prepareCallHierarchy", "provideCallHierarchyIncomingCalls", "provideCallHierarchyOutgoingCalls", "provideInlayHints", "getSupportedCodeFixes"], M7 = [...M2, "getCompletionsAtPosition", "getCompletionEntryDetails", "getCompletionEntrySymbol", "getSignatureHelpItems", "getQuickInfoAtPosition", "getDefinitionAtPosition", "getDefinitionAndBoundSpan", "getImplementationAtPosition", "getTypeDefinitionAtPosition", "getReferencesAtPosition", "findReferences", "getOccurrencesAtPosition", "getDocumentHighlights", "getNavigateToItems", "getRenameInfo", "findRenameLocations", "getApplicableRefactors"], gx(_B());
} }), vB = () => {
}, bB = () => {
}, TB = () => {
}, SB = () => {
}, xB = () => {
}, EB = () => {
}, wB = () => {
}, CB = () => {
}, AB = () => {
}, PB = () => {
}, DB = () => {
}, kB = () => {
}, IB = () => {
}, NB = () => {
}, OB = () => {
}, MB = () => {
}, LB = () => {
}, RB = () => {
}, jB = () => {
}, JB = () => {
}, L2 = D({ "src/services/_namespaces/ts.ts"() {
"use strict";
nn(), l7(), KF(), u7(), XF(), YF(), QF(), ZF(), eB(), tB(), rB(), nB(), iB(), aB(), yB(), vB(), bB(), TB(), SB(), xB(), EB(), wB(), CB(), AB(), PB(), DB(), p7(), f7(), kB(), IB(), NB(), OB(), MB(), LB(), RB(), jB(), JB();
} }), FB = () => {
}, L7 = {};
y(L7, { ANONYMOUS: () => ANONYMOUS, AccessFlags: () => Cg, AssertionLevel: () => $1, AssignmentDeclarationKind: () => Mg, AssignmentKind: () => Sv, Associativity: () => Ev, BreakpointResolver: () => ts_BreakpointResolver_exports, BuilderFileEmit: () => BuilderFileEmit, BuilderProgramKind: () => BuilderProgramKind, BuilderState: () => BuilderState, BundleFileSectionKind: () => ty, CallHierarchy: () => ts_CallHierarchy_exports, CharacterCodes: () => $g, CheckFlags: () => Tg, CheckMode: () => CheckMode, ClassificationType: () => ClassificationType, ClassificationTypeNames: () => ClassificationTypeNames, CommentDirectiveType: () => ig, Comparison: () => d, CompletionInfoFlags: () => CompletionInfoFlags, CompletionTriggerKind: () => CompletionTriggerKind, Completions: () => ts_Completions_exports, ConfigFileProgramReloadLevel: () => ConfigFileProgramReloadLevel, ContextFlags: () => pg, CoreServicesShimHostAdapter: () => CoreServicesShimHostAdapter, Debug: () => Y, DiagnosticCategory: () => qp, Diagnostics: () => ve, DocumentHighlights: () => DocumentHighlights, ElementFlags: () => wg, EmitFlags: () => Wp, EmitHint: () => Qg, EmitOnly: () => og, EndOfLineState: () => EndOfLineState, EnumKind: () => bg, ExitStatus: () => cg, ExportKind: () => ExportKind, Extension: () => Kg, ExternalEmitHelpers: () => Yg, FileIncludeKind: () => ag, FilePreprocessingDiagnosticsKind: () => sg, FileSystemEntryKind: () => FileSystemEntryKind, FileWatcherEventKind: () => FileWatcherEventKind, FindAllReferences: () => ts_FindAllReferences_exports, FlattenLevel: () => FlattenLevel, FlowFlags: () => il, ForegroundColorEscapeSequences: () => ForegroundColorEscapeSequences, FunctionFlags: () => xv, GeneratedIdentifierFlags: () => rg, GetLiteralTextFlags: () => vv, GoToDefinition: () => ts_GoToDefinition_exports, HighlightSpanKind: () => HighlightSpanKind, ImportKind: () => ImportKind, ImportsNotUsedAsValues: () => Ug, IndentStyle: () => IndentStyle, IndexKind: () => Dg, InferenceFlags: () => Ng, InferencePriority: () => Ig, InlayHintKind: () => InlayHintKind, InlayHints: () => ts_InlayHints_exports, InternalEmitFlags: () => Xg, InternalSymbolName: () => Sg, InvalidatedProjectKind: () => InvalidatedProjectKind, JsDoc: () => ts_JsDoc_exports, JsTyping: () => ts_JsTyping_exports, JsxEmit: () => qg, JsxFlags: () => tg, JsxReferenceKind: () => Ag, LanguageServiceMode: () => LanguageServiceMode, LanguageServiceShimHostAdapter: () => LanguageServiceShimHostAdapter, LanguageVariant: () => Hg, LexicalEnvironmentFlags: () => ey, ListFormat: () => ry, LogLevel: () => Y1, MemberOverrideStatus: () => lg, ModifierFlags: () => Mp, ModuleDetectionKind: () => Rg, ModuleInstanceState: () => ModuleInstanceState, ModuleKind: () => Bg, ModuleResolutionKind: () => Lg, ModuleSpecifierEnding: () => jv, NavigateTo: () => ts_NavigateTo_exports, NavigationBar: () => ts_NavigationBar_exports, NewLineKind: () => zg, NodeBuilderFlags: () => fg, NodeCheckFlags: () => xg, NodeFactoryFlags: () => Fv, NodeFlags: () => Op, NodeResolutionFeatures: () => NodeResolutionFeatures, ObjectFlags: () => Fp, OperationCanceledException: () => Rp, OperatorPrecedence: () => wv, OrganizeImports: () => ts_OrganizeImports_exports, OrganizeImportsMode: () => OrganizeImportsMode, OuterExpressionKinds: () => Zg, OutliningElementsCollector: () => ts_OutliningElementsCollector_exports, OutliningSpanKind: () => OutliningSpanKind, OutputFileType: () => OutputFileType, PackageJsonAutoImportPreference: () => PackageJsonAutoImportPreference, PackageJsonDependencyGroup: () => PackageJsonDependencyGroup, PatternMatchKind: () => PatternMatchKind, PollingInterval: () => PollingInterval, PollingWatchKind: () => Fg, PragmaKindFlags: () => ny, PrivateIdentifierKind: () => PrivateIdentifierKind, ProcessLevel: () => ProcessLevel, QuotePreference: () => QuotePreference, RelationComparisonResult: () => Lp, Rename: () => ts_Rename_exports, ScriptElementKind: () => ScriptElementKind, ScriptElementKindModifier: () => ScriptElementKindModifier, ScriptKind: () => Wg, ScriptSnapshot: () => ScriptSnapshot, ScriptTarget: () => Vg, SemanticClassificationFormat: () => SemanticClassificationFormat, SemanticMeaning: () => SemanticMeaning, SemicolonPreference: () => SemicolonPreference, SignatureCheckMode: () => SignatureCheckMode, SignatureFlags: () => Bp, SignatureHelp: () => ts_SignatureHelp_exports, SignatureKind: () => Pg, SmartSelectionRange: () => ts_SmartSelectionRange_exports, SnippetKind: () => zp, SortKind: () => H1, StructureIsReused: () => _g, SymbolAccessibility: () => hg, SymbolDisplay: () => ts_SymbolDisplay_exports, SymbolDisplayPartKind: () => SymbolDisplayPartKind, SymbolFlags: () => jp, SymbolFormatFlags: () => mg, SyntaxKind: () => Np, SyntheticSymbolKind: () => gg, Ternary: () => Og, ThrottledCancellationToken: () => O7, TokenClass: () => TokenClass, TokenFlags: () => ng, TransformFlags: () => Up, TypeFacts: () => TypeFacts, TypeFlags: () => Jp, TypeFormatFlags: () => dg, TypeMapKind: () => kg, TypePredicateKind: () => yg, TypeReferenceSerializationKind: () => vg, TypeScriptServicesFactory: () => TypeScriptServicesFactory, UnionReduction: () => ug, UpToDateStatusType: () => UpToDateStatusType, VarianceFlags: () => Eg, Version: () => Version, VersionRange: () => VersionRange, WatchDirectoryFlags: () => Gg, WatchDirectoryKind: () => Jg, WatchFileKind: () => jg, WatchLogLevel: () => WatchLogLevel, WatchType: () => WatchType, accessPrivateIdentifier: () => accessPrivateIdentifier, addEmitFlags: () => addEmitFlags, addEmitHelper: () => addEmitHelper, addEmitHelpers: () => addEmitHelpers, addInternalEmitFlags: () => addInternalEmitFlags, addNodeFactoryPatcher: () => jL, addObjectAllocatorPatcher: () => sM, addRange: () => jr, addRelatedInfo: () => Rl, addSyntheticLeadingComment: () => addSyntheticLeadingComment, addSyntheticTrailingComment: () => addSyntheticTrailingComment, addToSeen: () => GO, advancedAsyncSuperHelper: () => advancedAsyncSuperHelper, affectsDeclarationPathOptionDeclarations: () => affectsDeclarationPathOptionDeclarations, affectsEmitOptionDeclarations: () => affectsEmitOptionDeclarations, allKeysStartWithDot: () => allKeysStartWithDot, altDirectorySeparator: () => py, and: () => E5, append: () => tr, appendIfUnique: () => g_, arrayFrom: () => Za, arrayIsEqualTo: () => Hc, arrayIsHomogeneous: () => fL, arrayIsSorted: () => Wc, arrayOf: () => yo, arrayReverseIterator: () => y_, arrayToMap: () => Zc, arrayToMultiMap: () => bo, arrayToNumericMap: () => Os, arraysEqual: () => ke, assertType: () => C5, assign: () => vo, assignHelper: () => assignHelper, asyncDelegator: () => asyncDelegator, asyncGeneratorHelper: () => asyncGeneratorHelper, asyncSuperHelper: () => asyncSuperHelper, asyncValues: () => asyncValues, attachFileToDiagnostics: () => qs, awaitHelper: () => awaitHelper, awaiterHelper: () => awaiterHelper, base64decode: () => mO, base64encode: () => dO, binarySearch: () => Ya, binarySearchKey: () => b_, bindSourceFile: () => bindSourceFile, breakIntoCharacterSpans: () => breakIntoCharacterSpans, breakIntoWordSpans: () => breakIntoWordSpans, buildLinkParts: () => buildLinkParts, buildOpts: () => buildOpts, buildOverload: () => buildOverload, bundlerModuleNameResolver: () => bundlerModuleNameResolver, canBeConvertedToAsync: () => canBeConvertedToAsync, canHaveDecorators: () => ME, canHaveExportModifier: () => AL, canHaveFlowNode: () => jI, canHaveIllegalDecorators: () => rJ, canHaveIllegalModifiers: () => nJ, canHaveIllegalType: () => tJ, canHaveIllegalTypeParameters: () => IE, canHaveJSDoc: () => Af, canHaveLocals: () => zP, canHaveModifiers: () => fc, canHaveSymbol: () => UP, canJsonReportNoInputFiles: () => canJsonReportNoInputFiles, canProduceDiagnostics: () => canProduceDiagnostics, canUsePropertyAccess: () => PL, canWatchDirectoryOrFile: () => canWatchDirectoryOrFile, cartesianProduct: () => P5, cast: () => ti, chainBundle: () => chainBundle, chainDiagnosticMessages: () => lM, changeAnyExtension: () => RT, changeCompilerHostLikeToUseCache: () => changeCompilerHostLikeToUseCache, changeExtension: () => KM, changesAffectModuleResolution: () => cD, changesAffectingProgramStructure: () => lD, childIsDecorated: () => h0, classElementOrClassElementParameterIsDecorated: () => sI, classOrConstructorParameterIsDecorated: () => aI, classPrivateFieldGetHelper: () => classPrivateFieldGetHelper, classPrivateFieldInHelper: () => classPrivateFieldInHelper, classPrivateFieldSetHelper: () => classPrivateFieldSetHelper, classicNameResolver: () => classicNameResolver, classifier: () => ts_classifier_exports, cleanExtendedConfigCache: () => cleanExtendedConfigCache, clear: () => nt, clearMap: () => qO, clearSharedExtendedConfigFileWatcher: () => clearSharedExtendedConfigFileWatcher, climbPastPropertyAccess: () => climbPastPropertyAccess, climbPastPropertyOrElementAccess: () => climbPastPropertyOrElementAccess, clone: () => E_, cloneCompilerOptions: () => cloneCompilerOptions, closeFileWatcher: () => MO, closeFileWatcherOf: () => closeFileWatcherOf, codefix: () => ts_codefix_exports, collapseTextChangeRangesAcrossMultipleVersions: () => CA, collectExternalModuleInfo: () => collectExternalModuleInfo, combine: () => $c, combinePaths: () => tn, commentPragmas: () => Vp, commonOptionsWithBuild: () => commonOptionsWithBuild, commonPackageFolders: () => Pv, compact: () => Gc, compareBooleans: () => j1, compareDataObjects: () => px, compareDiagnostics: () => av, compareDiagnosticsSkipRelatedInformation: () => qf, compareEmitHelpers: () => compareEmitHelpers, compareNumberOfDirectorySeparators: () => $M, comparePaths: () => tA, comparePathsCaseInsensitive: () => eA, comparePathsCaseSensitive: () => Z5, comparePatternKeys: () => comparePatternKeys, compareProperties: () => R1, compareStringsCaseInsensitive: () => C_, compareStringsCaseInsensitiveEslintCompatible: () => O1, compareStringsCaseSensitive: () => ri, compareStringsCaseSensitiveUI: () => L1, compareTextSpans: () => I1, compareValues: () => Vr, compileOnSaveCommandLineOption: () => compileOnSaveCommandLineOption, compilerOptionsAffectDeclarationPath: () => DM, compilerOptionsAffectEmit: () => PM, compilerOptionsAffectSemanticDiagnostics: () => AM, compilerOptionsDidYouMeanDiagnostics: () => compilerOptionsDidYouMeanDiagnostics, compilerOptionsIndicateEsModules: () => compilerOptionsIndicateEsModules, compose: () => k1, computeCommonSourceDirectoryOfFilenames: () => computeCommonSourceDirectoryOfFilenames, computeLineAndCharacterOfPosition: () => my, computeLineOfPosition: () => k_, computeLineStarts: () => Kp, computePositionOfLineAndCharacter: () => dy, computeSignature: () => computeSignature, computeSignatureWithDiagnostics: () => computeSignatureWithDiagnostics, computeSuggestionDiagnostics: () => computeSuggestionDiagnostics, concatenate: () => Ft, concatenateDiagnosticMessageChains: () => uM, consumesNodeCoreModules: () => consumesNodeCoreModules, contains: () => pe, containsIgnoredPath: () => Hx, containsObjectRestOrSpread: () => A2, containsParseError: () => Ky, containsPath: () => jT, convertCompilerOptionsForTelemetry: () => convertCompilerOptionsForTelemetry, convertCompilerOptionsFromJson: () => convertCompilerOptionsFromJson, convertJsonOption: () => convertJsonOption, convertToBase64: () => ix, convertToObject: () => convertToObject, convertToObjectWorker: () => convertToObjectWorker, convertToOptionsWithAbsolutePaths: () => convertToOptionsWithAbsolutePaths, convertToRelativePath: () => nA, convertToTSConfig: () => convertToTSConfig, convertTypeAcquisitionFromJson: () => convertTypeAcquisitionFromJson, copyComments: () => copyComments, copyEntries: () => dD, copyLeadingComments: () => copyLeadingComments, copyProperties: () => H, copyTrailingAsLeadingComments: () => copyTrailingAsLeadingComments, copyTrailingComments: () => copyTrailingComments, couldStartTrivia: () => pA, countWhere: () => Xe, createAbstractBuilder: () => createAbstractBuilder, createAccessorPropertyBackingField: () => LJ, createAccessorPropertyGetRedirector: () => RJ, createAccessorPropertySetRedirector: () => jJ, createBaseNodeFactory: () => S8, createBinaryExpressionTrampoline: () => PJ, createBindingHelper: () => createBindingHelper, createBuildInfo: () => createBuildInfo, createBuilderProgram: () => createBuilderProgram, createBuilderProgramUsingProgramBuildInfo: () => createBuilderProgramUsingProgramBuildInfo, createBuilderStatusReporter: () => createBuilderStatusReporter, createCacheWithRedirects: () => createCacheWithRedirects, createCacheableExportInfoMap: () => createCacheableExportInfoMap, createCachedDirectoryStructureHost: () => createCachedDirectoryStructureHost, createClassifier: () => createClassifier, createCommentDirectivesMap: () => JD, createCompilerDiagnostic: () => Ol, createCompilerDiagnosticForInvalidCustomType: () => createCompilerDiagnosticForInvalidCustomType, createCompilerDiagnosticFromMessageChain: () => cM, createCompilerHost: () => createCompilerHost, createCompilerHostFromProgramHost: () => createCompilerHostFromProgramHost, createCompilerHostWorker: () => createCompilerHostWorker, createDetachedDiagnostic: () => Ro, createDiagnosticCollection: () => TN, createDiagnosticForFileFromMessageChain: () => mk, createDiagnosticForNode: () => uk, createDiagnosticForNodeArray: () => pk, createDiagnosticForNodeArrayFromMessageChain: () => dk, createDiagnosticForNodeFromMessageChain: () => fk, createDiagnosticForNodeInSourceFile: () => P3, createDiagnosticForRange: () => gk, createDiagnosticMessageChainFromDiagnostic: () => hk, createDiagnosticReporter: () => createDiagnosticReporter, createDocumentPositionMapper: () => createDocumentPositionMapper, createDocumentRegistry: () => createDocumentRegistry, createDocumentRegistryInternal: () => createDocumentRegistryInternal, createEmitAndSemanticDiagnosticsBuilderProgram: () => createEmitAndSemanticDiagnosticsBuilderProgram, createEmitHelperFactory: () => createEmitHelperFactory, createEmptyExports: () => Dj, createExpressionForJsxElement: () => Ij, createExpressionForJsxFragment: () => Nj, createExpressionForObjectLiteralElementLike: () => Fj, createExpressionForPropertyName: () => vE, createExpressionFromEntityName: () => yE, createExternalHelpersImportDeclarationIfNeeded: () => $j, createFileDiagnostic: () => iv, createFileDiagnosticFromMessageChain: () => r0, createForOfBindingStatement: () => Oj, createGetCanonicalFileName: () => wp, createGetSourceFile: () => createGetSourceFile, createGetSymbolAccessibilityDiagnosticForNode: () => createGetSymbolAccessibilityDiagnosticForNode, createGetSymbolAccessibilityDiagnosticForNodeName: () => createGetSymbolAccessibilityDiagnosticForNodeName, createGetSymbolWalker: () => createGetSymbolWalker, createIncrementalCompilerHost: () => createIncrementalCompilerHost, createIncrementalProgram: () => createIncrementalProgram, createInputFiles: () => VL, createInputFilesWithFilePaths: () => C8, createInputFilesWithFileTexts: () => A8, createJsxFactoryExpression: () => gE, createLanguageService: () => lB, createLanguageServiceSourceFile: () => N2, createMemberAccessForPropertyName: () => hd, createModeAwareCache: () => createModeAwareCache, createModeAwareCacheKey: () => createModeAwareCacheKey, createModuleResolutionCache: () => createModuleResolutionCache, createModuleResolutionLoader: () => createModuleResolutionLoader, createModuleSpecifierResolutionHost: () => createModuleSpecifierResolutionHost, createMultiMap: () => Be, createNodeConverters: () => x8, createNodeFactory: () => Zf, createOptionNameMap: () => createOptionNameMap, createOverload: () => createOverload, createPackageJsonImportFilter: () => createPackageJsonImportFilter, createPackageJsonInfo: () => createPackageJsonInfo, createParenthesizerRules: () => createParenthesizerRules, createPatternMatcher: () => createPatternMatcher, createPrependNodes: () => createPrependNodes, createPrinter: () => createPrinter, createPrinterWithDefaults: () => createPrinterWithDefaults, createPrinterWithRemoveComments: () => createPrinterWithRemoveComments, createPrinterWithRemoveCommentsNeverAsciiEscape: () => createPrinterWithRemoveCommentsNeverAsciiEscape, createPrinterWithRemoveCommentsOmitTrailingSemicolon: () => createPrinterWithRemoveCommentsOmitTrailingSemicolon, createProgram: () => createProgram, createProgramHost: () => createProgramHost, createPropertyNameNodeForIdentifierOrLiteral: () => EL, createQueue: () => Fr, createRange: () => Jf, createRedirectedBuilderProgram: () => createRedirectedBuilderProgram, createResolutionCache: () => createResolutionCache, createRuntimeTypeSerializer: () => createRuntimeTypeSerializer, createScanner: () => Po, createSemanticDiagnosticsBuilderProgram: () => createSemanticDiagnosticsBuilderProgram, createSet: () => Cr, createSolutionBuilder: () => createSolutionBuilder, createSolutionBuilderHost: () => createSolutionBuilderHost, createSolutionBuilderWithWatch: () => createSolutionBuilderWithWatch, createSolutionBuilderWithWatchHost: () => createSolutionBuilderWithWatchHost, createSortedArray: () => zc, createSourceFile: () => YE, createSourceMapGenerator: () => createSourceMapGenerator, createSourceMapSource: () => HL, createSuperAccessVariableStatement: () => createSuperAccessVariableStatement, createSymbolTable: () => oD, createSymlinkCache: () => MM, createSystemWatchFunctions: () => createSystemWatchFunctions, createTextChange: () => createTextChange, createTextChangeFromStartLength: () => createTextChangeFromStartLength, createTextChangeRange: () => Zp, createTextRangeFromNode: () => createTextRangeFromNode, createTextRangeFromSpan: () => createTextRangeFromSpan, createTextSpan: () => L_, createTextSpanFromBounds: () => ha, createTextSpanFromNode: () => createTextSpanFromNode, createTextSpanFromRange: () => createTextSpanFromRange, createTextSpanFromStringLiteralLikeContent: () => createTextSpanFromStringLiteralLikeContent, createTextWriter: () => DN, createTokenRange: () => bO, createTypeChecker: () => createTypeChecker, createTypeReferenceDirectiveResolutionCache: () => createTypeReferenceDirectiveResolutionCache, createTypeReferenceResolutionLoader: () => createTypeReferenceResolutionLoader, createUnderscoreEscapedMultiMap: () => Ht, createUnparsedSourceFile: () => UL, createWatchCompilerHost: () => createWatchCompilerHost2, createWatchCompilerHostOfConfigFile: () => createWatchCompilerHostOfConfigFile, createWatchCompilerHostOfFilesAndCompilerOptions: () => createWatchCompilerHostOfFilesAndCompilerOptions, createWatchFactory: () => createWatchFactory, createWatchHost: () => createWatchHost, createWatchProgram: () => createWatchProgram, createWatchStatusReporter: () => createWatchStatusReporter, createWriteFileMeasuringIO: () => createWriteFileMeasuringIO, declarationNameToString: () => A3, decodeMappings: () => decodeMappings, decodedTextSpanIntersectsWith: () => Sy, decorateHelper: () => decorateHelper, deduplicate: () => ji, defaultIncludeSpec: () => defaultIncludeSpec, defaultInitCompilerOptions: () => defaultInitCompilerOptions, defaultMaximumTruncationLength: () => r8, detectSortCaseSensitivity: () => Vc, diagnosticCategoryName: () => z5, diagnosticToString: () => diagnosticToString, directoryProbablyExists: () => sx, directorySeparator: () => zn, displayPart: () => displayPart, displayPartsToString: () => cB, disposeEmitNodes: () => disposeEmitNodes, documentSpansEqual: () => documentSpansEqual, dumpTracingLegend: () => dumpTracingLegend, elementAt: () => wT, elideNodes: () => IJ, emitComments: () => U4, emitDetachedComments: () => GN, emitFiles: () => emitFiles, emitFilesAndReportErrors: () => emitFilesAndReportErrors, emitFilesAndReportErrorsAndGetExitStatus: () => emitFilesAndReportErrorsAndGetExitStatus, emitModuleKindIsNonNodeESM: () => mM, emitNewLineBeforeLeadingCommentOfPosition: () => HN, emitNewLineBeforeLeadingComments: () => B4, emitNewLineBeforeLeadingCommentsOfPosition: () => q4, emitSkippedWithNoDiagnostics: () => emitSkippedWithNoDiagnostics, emitUsingBuildInfo: () => emitUsingBuildInfo, emptyArray: () => Bt, emptyFileSystemEntries: () => T8, emptyMap: () => V1, emptyOptions: () => emptyOptions, emptySet: () => ET, endsWith: () => es, ensurePathIsNonModuleName: () => _y, ensureScriptKind: () => Nx, ensureTrailingDirectorySeparator: () => wo, entityNameToString: () => ls, enumerateInsertsAndDeletes: () => A5, equalOwnProperties: () => S_, equateStringsCaseInsensitive: () => Ms, equateStringsCaseSensitive: () => To, equateValues: () => fa, esDecorateHelper: () => esDecorateHelper, escapeJsxAttributeString: () => A4, escapeLeadingUnderscores: () => vi, escapeNonAsciiString: () => Of, escapeSnippetText: () => xL, escapeString: () => Nf, every: () => me, expandPreOrPostfixIncrementOrDecrementExpression: () => Bj, explainFiles: () => explainFiles, explainIfFileIsRedirectAndImpliedFormat: () => explainIfFileIsRedirectAndImpliedFormat, exportAssignmentIsAlias: () => I0, exportStarHelper: () => exportStarHelper, expressionResultIsUnused: () => gL, extend: () => S, extendsHelper: () => extendsHelper, extensionFromPath: () => QM, extensionIsTS: () => qx, externalHelpersModuleNameText: () => Kf, factory: () => si, fileExtensionIs: () => ns, fileExtensionIsOneOf: () => da, fileIncludeReasonToDiagnostics: () => fileIncludeReasonToDiagnostics, filter: () => ee, filterMutate: () => je, filterSemanticDiagnostics: () => filterSemanticDiagnostics, find: () => Ae, findAncestor: () => zi, findBestPatternMatch: () => TT, findChildOfKind: () => findChildOfKind, findComputedPropertyNameCacheAssignment: () => JJ, findConfigFile: () => findConfigFile, findContainingList: () => findContainingList, findDiagnosticForNode: () => findDiagnosticForNode, findFirstNonJsxWhitespaceToken: () => findFirstNonJsxWhitespaceToken, findIndex: () => he, findLast: () => te, findLastIndex: () => Pe, findListItemInfo: () => findListItemInfo, findMap: () => R, findModifier: () => findModifier, findNextToken: () => findNextToken, findPackageJson: () => findPackageJson, findPackageJsons: () => findPackageJsons, findPrecedingMatchingToken: () => findPrecedingMatchingToken, findPrecedingToken: () => findPrecedingToken, findSuperStatementIndex: () => findSuperStatementIndex, findTokenOnLeftOfPosition: () => findTokenOnLeftOfPosition, findUseStrictPrologue: () => TE, first: () => fo, firstDefined: () => q, firstDefinedIterator: () => W, firstIterator: () => v_, firstOrOnly: () => firstOrOnly, firstOrUndefined: () => pa, firstOrUndefinedIterator: () => Xc, fixupCompilerOptions: () => fixupCompilerOptions, flatMap: () => ne, flatMapIterator: () => Fe, flatMapToMutable: () => ge, flatten: () => ct, flattenCommaList: () => BJ, flattenDestructuringAssignment: () => flattenDestructuringAssignment, flattenDestructuringBinding: () => flattenDestructuringBinding, flattenDiagnosticMessageText: () => flattenDiagnosticMessageText, forEach: () => c, forEachAncestor: () => uD, forEachAncestorDirectory: () => FT, forEachChild: () => xr, forEachChildRecursively: () => D2, forEachEmittedFile: () => forEachEmittedFile, forEachEnclosingBlockScopeContainer: () => ok, forEachEntry: () => pD, forEachExternalModuleToImportFrom: () => forEachExternalModuleToImportFrom, forEachImportClauseDeclaration: () => NI, forEachKey: () => fD, forEachLeadingCommentRange: () => fA, forEachNameInAccessChainWalkingLeft: () => QO, forEachResolvedProjectReference: () => forEachResolvedProjectReference, forEachReturnStatement: () => Pk, forEachRight: () => M, forEachTrailingCommentRange: () => dA, forEachUnique: () => forEachUnique, forEachYieldExpression: () => Dk, forSomeAncestorDirectory: () => WO, formatColorAndReset: () => formatColorAndReset, formatDiagnostic: () => formatDiagnostic, formatDiagnostics: () => formatDiagnostics, formatDiagnosticsWithColorAndContext: () => formatDiagnosticsWithColorAndContext, formatGeneratedName: () => bd, formatGeneratedNamePart: () => C2, formatLocation: () => formatLocation, formatMessage: () => _M, formatStringFromArgs: () => X_, formatting: () => ts_formatting_exports, fullTripleSlashAMDReferencePathRegEx: () => Tv, fullTripleSlashReferencePathRegEx: () => bv, generateDjb2Hash: () => generateDjb2Hash, generateTSConfig: () => generateTSConfig, generatorHelper: () => generatorHelper, getAdjustedReferenceLocation: () => getAdjustedReferenceLocation, getAdjustedRenameLocation: () => getAdjustedRenameLocation, getAliasDeclarationFromName: () => u4, getAllAccessorDeclarations: () => W0, getAllDecoratorsOfClass: () => getAllDecoratorsOfClass, getAllDecoratorsOfClassElement: () => getAllDecoratorsOfClassElement, getAllJSDocTags: () => MS, getAllJSDocTagsOfKind: () => UA, getAllKeys: () => T_, getAllProjectOutputs: () => getAllProjectOutputs, getAllSuperTypeNodes: () => h4, getAllUnscopedEmitHelpers: () => getAllUnscopedEmitHelpers, getAllowJSCompilerOption: () => Ax, getAllowSyntheticDefaultImports: () => TM, getAncestor: () => eN, getAnyExtensionFromPath: () => Gp, getAreDeclarationMapsEnabled: () => bM, getAssignedExpandoInitializer: () => bI, getAssignedName: () => yS, getAssignmentDeclarationKind: () => ps, getAssignmentDeclarationPropertyAccessKind: () => K3, getAssignmentTargetKind: () => o4, getAutomaticTypeDirectiveNames: () => getAutomaticTypeDirectiveNames, getBaseFileName: () => sl, getBinaryOperatorPrecedence: () => Dl, getBuildInfo: () => getBuildInfo, getBuildInfoFileVersionMap: () => getBuildInfoFileVersionMap, getBuildInfoText: () => getBuildInfoText, getBuildOrderFromAnyBuildOrder: () => getBuildOrderFromAnyBuildOrder, getBuilderCreationParameters: () => getBuilderCreationParameters, getBuilderFileEmit: () => getBuilderFileEmit, getCheckFlags: () => ux, getClassExtendsHeritageElement: () => d4, getClassLikeDeclarationOfSymbol: () => dx, getCombinedLocalAndExportSymbolFlags: () => jO, getCombinedModifierFlags: () => ef, getCombinedNodeFlags: () => tf, getCombinedNodeFlagsAlwaysIncludeJSDoc: () => PA, getCommentRange: () => getCommentRange, getCommonSourceDirectory: () => getCommonSourceDirectory, getCommonSourceDirectoryOfConfig: () => getCommonSourceDirectoryOfConfig, getCompilerOptionValue: () => uv, getCompilerOptionsDiffValue: () => getCompilerOptionsDiffValue, getConditions: () => getConditions, getConfigFileParsingDiagnostics: () => getConfigFileParsingDiagnostics, getConstantValue: () => getConstantValue, getContainerNode: () => getContainerNode, getContainingClass: () => Vk, getContainingClassStaticBlock: () => Hk, getContainingFunction: () => zk, getContainingFunctionDeclaration: () => Wk, getContainingFunctionOrClassStaticBlock: () => Gk, getContainingNodeArray: () => yL, getContainingObjectLiteralElement: () => S7, getContextualTypeFromParent: () => getContextualTypeFromParent, getContextualTypeFromParentOrAncestorTypeNode: () => getContextualTypeFromParentOrAncestorTypeNode, getCurrentTime: () => getCurrentTime, getDeclarationDiagnostics: () => getDeclarationDiagnostics, getDeclarationEmitExtensionForPath: () => O4, getDeclarationEmitOutputFilePath: () => ON, getDeclarationEmitOutputFilePathWorker: () => N4, getDeclarationFromName: () => XI, getDeclarationModifierFlagsFromSymbol: () => LO, getDeclarationOfKind: () => aD, getDeclarationsOfKind: () => sD, getDeclaredExpandoInitializer: () => yI, getDecorators: () => kA, getDefaultCompilerOptions: () => y7, getDefaultExportInfoWorker: () => getDefaultExportInfoWorker, getDefaultFormatCodeSettings: () => getDefaultFormatCodeSettings, getDefaultLibFileName: () => aS, getDefaultLibFilePath: () => gB, getDefaultLikeExportInfo: () => getDefaultLikeExportInfo, getDiagnosticText: () => getDiagnosticText, getDiagnosticsWithinSpan: () => getDiagnosticsWithinSpan, getDirectoryPath: () => ma, getDocumentPositionMapper: () => getDocumentPositionMapper, getESModuleInterop: () => ov, getEditsForFileRename: () => getEditsForFileRename, getEffectiveBaseTypeNode: () => f4, getEffectiveConstraintOfTypeParameter: () => HA, getEffectiveContainerForJSDocTemplateTag: () => FI, getEffectiveImplementsTypeNodes: () => m4, getEffectiveInitializer: () => V3, getEffectiveJSDocHost: () => A0, getEffectiveModifierFlags: () => Rf, getEffectiveModifierFlagsAlwaysIncludeJSDoc: () => K4, getEffectiveModifierFlagsNoCache: () => Y4, getEffectiveReturnTypeNode: () => zN, getEffectiveSetAccessorTypeAnnotationNode: () => VN, getEffectiveTypeAnnotationNode: () => V0, getEffectiveTypeParameterDeclarations: () => VA, getEffectiveTypeRoots: () => getEffectiveTypeRoots, getElementOrPropertyAccessArgumentExpressionOrName: () => Cf, getElementOrPropertyAccessName: () => Fs, getElementsOfBindingOrAssignmentPattern: () => kE, getEmitDeclarations: () => cv, getEmitFlags: () => xi, getEmitHelpers: () => getEmitHelpers, getEmitModuleDetectionKind: () => wx, getEmitModuleKind: () => Ei, getEmitModuleResolutionKind: () => Ml, getEmitScriptTarget: () => Uf, getEnclosingBlockScopeContainer: () => Zy, getEncodedSemanticClassifications: () => getEncodedSemanticClassifications, getEncodedSyntacticClassifications: () => getEncodedSyntacticClassifications, getEndLinePosition: () => d3, getEntityNameFromTypeNode: () => nI, getEntrypointsFromPackageJsonInfo: () => getEntrypointsFromPackageJsonInfo, getErrorCountForSummary: () => getErrorCountForSummary, getErrorSpanForNode: () => i0, getErrorSummaryText: () => getErrorSummaryText, getEscapedTextOfIdentifierOrLiteral: () => b4, getExpandoInitializer: () => U_, getExportAssignmentExpression: () => p4, getExportInfoMap: () => getExportInfoMap, getExportNeedsImportStarHelper: () => getExportNeedsImportStarHelper, getExpressionAssociativity: () => yN, getExpressionPrecedence: () => vN, getExternalHelpersModuleName: () => EE, getExternalModuleImportEqualsDeclarationExpression: () => _I, getExternalModuleName: () => E0, getExternalModuleNameFromDeclaration: () => IN, getExternalModuleNameFromPath: () => F0, getExternalModuleNameLiteral: () => Xj, getExternalModuleRequireArgument: () => cI, getFallbackOptions: () => getFallbackOptions, getFileEmitOutput: () => getFileEmitOutput, getFileMatcherPatterns: () => Ix, getFileNamesFromConfigSpecs: () => getFileNamesFromConfigSpecs, getFileWatcherEventKind: () => getFileWatcherEventKind, getFilesInErrorForSummary: () => getFilesInErrorForSummary, getFirstConstructorWithBody: () => R4, getFirstIdentifier: () => iO, getFirstNonSpaceCharacterPosition: () => getFirstNonSpaceCharacterPosition, getFirstProjectOutput: () => getFirstProjectOutput, getFixableErrorSpanExpression: () => getFixableErrorSpanExpression, getFormatCodeSettingsForWriting: () => getFormatCodeSettingsForWriting, getFullWidth: () => hf, getFunctionFlags: () => sN, getHeritageClause: () => Pf, getHostSignatureFromJSDoc: () => C0, getIdentifierAutoGenerate: () => getIdentifierAutoGenerate, getIdentifierGeneratedImportReference: () => getIdentifierGeneratedImportReference, getIdentifierTypeArguments: () => getIdentifierTypeArguments, getImmediatelyInvokedFunctionExpression: () => Qk, getImpliedNodeFormatForFile: () => getImpliedNodeFormatForFile, getImpliedNodeFormatForFileWorker: () => getImpliedNodeFormatForFileWorker, getImportNeedsImportDefaultHelper: () => getImportNeedsImportDefaultHelper, getImportNeedsImportStarHelper: () => getImportNeedsImportStarHelper, getIndentSize: () => Oo, getIndentString: () => j0, getInitializedVariables: () => NO, getInitializerOfBinaryExpression: () => X3, getInitializerOfBindingOrAssignmentElement: () => AE, getInterfaceBaseTypeNodes: () => g4, getInternalEmitFlags: () => zD, getInvokedExpression: () => iI, getIsolatedModules: () => zf, getJSDocAugmentsTag: () => ES, getJSDocClassTag: () => NA, getJSDocCommentRanges: () => I3, getJSDocCommentsAndTags: () => r4, getJSDocDeprecatedTag: () => jA, getJSDocDeprecatedTagNoCache: () => IS, getJSDocEnumTag: () => JA, getJSDocHost: () => s4, getJSDocImplementsTags: () => wS, getJSDocOverrideTagNoCache: () => kS, getJSDocParameterTags: () => of, getJSDocParameterTagsNoCache: () => bS, getJSDocPrivateTag: () => MA, getJSDocPrivateTagNoCache: () => AS, getJSDocProtectedTag: () => LA, getJSDocProtectedTagNoCache: () => PS, getJSDocPublicTag: () => OA, getJSDocPublicTagNoCache: () => CS, getJSDocReadonlyTag: () => RA, getJSDocReadonlyTagNoCache: () => DS, getJSDocReturnTag: () => NS, getJSDocReturnType: () => OS, getJSDocRoot: () => P0, getJSDocSatisfiesExpressionType: () => NL, getJSDocSatisfiesTag: () => wy, getJSDocTags: () => hl, getJSDocTagsNoCache: () => qA, getJSDocTemplateTag: () => BA, getJSDocThisTag: () => FA, getJSDocType: () => cf, getJSDocTypeAliasName: () => w2, getJSDocTypeAssertionType: () => Wj, getJSDocTypeParameterDeclarations: () => F4, getJSDocTypeParameterTags: () => SS, getJSDocTypeParameterTagsNoCache: () => xS, getJSDocTypeTag: () => _f, getJSXImplicitImportBase: () => IM, getJSXRuntimeImport: () => NM, getJSXTransformEnabled: () => kM, getKeyForCompilerOptions: () => getKeyForCompilerOptions, getLanguageVariant: () => sv, getLastChild: () => mx, getLeadingCommentRanges: () => Ao, getLeadingCommentRangesOfNode: () => Ck, getLeftmostAccessExpression: () => rv, getLeftmostExpression: () => ZO, getLineAndCharacterOfPosition: () => Ls, getLineInfo: () => getLineInfo, getLineOfLocalPosition: () => FN, getLineOfLocalPositionFromLineMap: () => ds, getLineStartPositionForPosition: () => getLineStartPositionForPosition, getLineStarts: () => ss, getLinesBetweenPositionAndNextNonWhitespaceCharacter: () => DO, getLinesBetweenPositionAndPrecedingNonWhitespaceCharacter: () => PO, getLinesBetweenPositions: () => I_, getLinesBetweenRangeEndAndRangeStart: () => wO, getLinesBetweenRangeEndPositions: () => CO, getLiteralText: () => WD, getLocalNameForExternalImport: () => Kj, getLocalSymbolForExportDefault: () => cO, getLocaleSpecificMessage: () => Y_, getLocaleTimeString: () => getLocaleTimeString, getMappedContextSpan: () => getMappedContextSpan, getMappedDocumentSpan: () => getMappedDocumentSpan, getMappedLocation: () => getMappedLocation, getMatchedFileSpec: () => getMatchedFileSpec, getMatchedIncludeSpec: () => getMatchedIncludeSpec, getMeaningFromDeclaration: () => getMeaningFromDeclaration, getMeaningFromLocation: () => getMeaningFromLocation, getMembersOfDeclaration: () => Ik, getModeForFileReference: () => getModeForFileReference, getModeForResolutionAtIndex: () => getModeForResolutionAtIndex, getModeForUsageLocation: () => getModeForUsageLocation, getModifiedTime: () => getModifiedTime, getModifiers: () => sf, getModuleInstanceState: () => getModuleInstanceState, getModuleNameStringLiteralAt: () => getModuleNameStringLiteralAt, getModuleSpecifierEndingPreference: () => VM, getModuleSpecifierResolverHost: () => getModuleSpecifierResolverHost, getNameForExportedSymbol: () => getNameForExportedSymbol, getNameFromIndexInfo: () => _k, getNameFromPropertyName: () => getNameFromPropertyName, getNameOfAccessExpression: () => KO, getNameOfCompilerOptionValue: () => getNameOfCompilerOptionValue, getNameOfDeclaration: () => ml, getNameOfExpando: () => xI, getNameOfJSDocTypedef: () => gS, getNameOrArgument: () => $3, getNameTable: () => uB, getNamesForExportedSymbol: () => getNamesForExportedSymbol, getNamespaceDeclarationNode: () => Q3, getNewLineCharacter: () => ox, getNewLineKind: () => getNewLineKind, getNewLineOrDefaultFromHost: () => getNewLineOrDefaultFromHost, getNewTargetContainer: () => Xk, getNextJSDocCommentLocation: () => a4, getNodeForGeneratedName: () => NJ, getNodeId: () => getNodeId, getNodeKind: () => getNodeKind, getNodeModifiers: () => getNodeModifiers, getNodeModulePathParts: () => wL, getNonAssignedNameOfDeclaration: () => Ey, getNonAssignmentOperatorForCompoundAssignment: () => getNonAssignmentOperatorForCompoundAssignment, getNonAugmentationDeclaration: () => E3, getNonDecoratorTokenPosOfNode: () => FD, getNormalizedAbsolutePath: () => as, getNormalizedAbsolutePathWithoutRoot: () => Q5, getNormalizedPathComponents: () => $p, getObjectFlags: () => Bf, getOperator: () => R0, getOperatorAssociativity: () => x4, getOperatorPrecedence: () => E4, getOptionFromName: () => getOptionFromName, getOptionsNameMap: () => getOptionsNameMap, getOrCreateEmitNode: () => getOrCreateEmitNode, getOrCreateExternalHelpersModuleNameIfNeeded: () => wE, getOrUpdate: () => la, getOriginalNode: () => ul, getOriginalNodeId: () => getOriginalNodeId, getOriginalSourceFile: () => gN, getOutputDeclarationFileName: () => getOutputDeclarationFileName, getOutputExtension: () => getOutputExtension, getOutputFileNames: () => getOutputFileNames, getOutputPathsFor: () => getOutputPathsFor, getOutputPathsForBundle: () => getOutputPathsForBundle, getOwnEmitOutputFilePath: () => NN, getOwnKeys: () => ho, getOwnValues: () => go, getPackageJsonInfo: () => getPackageJsonInfo, getPackageJsonTypesVersionsPaths: () => getPackageJsonTypesVersionsPaths, getPackageJsonsVisibleToFile: () => getPackageJsonsVisibleToFile, getPackageNameFromTypesPackageName: () => getPackageNameFromTypesPackageName, getPackageScopeForPath: () => getPackageScopeForPath, getParameterSymbolFromJSDoc: () => JI, getParameterTypeNode: () => CL, getParentNodeInSpan: () => getParentNodeInSpan, getParseTreeNode: () => fl, getParsedCommandLineOfConfigFile: () => getParsedCommandLineOfConfigFile, getPathComponents: () => qi, getPathComponentsRelativeTo: () => ly, getPathFromPathComponents: () => xo, getPathUpdater: () => getPathUpdater, getPathsBasePath: () => LN, getPatternFromSpec: () => BM, getPendingEmitKind: () => getPendingEmitKind, getPositionOfLineAndCharacter: () => lA, getPossibleGenericSignatures: () => getPossibleGenericSignatures, getPossibleOriginalInputExtensionForExtension: () => MN, getPossibleTypeArgumentsInfo: () => getPossibleTypeArgumentsInfo, getPreEmitDiagnostics: () => getPreEmitDiagnostics, getPrecedingNonSpaceCharacterPosition: () => getPrecedingNonSpaceCharacterPosition, getPrivateIdentifier: () => getPrivateIdentifier, getProperties: () => getProperties, getProperty: () => Qc, getPropertyArrayElementValue: () => qk, getPropertyAssignment: () => f0, getPropertyAssignmentAliasLikeExpression: () => ZI, getPropertyNameForPropertyNameNode: () => Df, getPropertyNameForUniqueESSymbol: () => _N, getPropertyNameOfBindingOrAssignmentElement: () => eJ, getPropertySymbolFromBindingElement: () => getPropertySymbolFromBindingElement, getPropertySymbolsFromContextualType: () => x7, getQuoteFromPreference: () => getQuoteFromPreference, getQuotePreference: () => getQuotePreference, getRangesWhere: () => Et, getRefactorContextSpan: () => getRefactorContextSpan, getReferencedFileLocation: () => getReferencedFileLocation, getRegexFromPattern: () => Vf, getRegularExpressionForWildcard: () => Wf, getRegularExpressionsForWildcards: () => pv, getRelativePathFromDirectory: () => JT, getRelativePathFromFile: () => iA, getRelativePathToDirectoryOrUrl: () => uy, getRenameLocation: () => getRenameLocation, getReplacementSpanForContextToken: () => getReplacementSpanForContextToken, getResolutionDiagnostic: () => getResolutionDiagnostic, getResolutionModeOverrideForClause: () => getResolutionModeOverrideForClause, getResolveJsonModule: () => Cx, getResolvePackageJsonExports: () => SM, getResolvePackageJsonImports: () => xM, getResolvedExternalModuleName: () => k4, getResolvedModule: () => hD, getResolvedTypeReferenceDirective: () => vD, getRestIndicatorOfBindingOrAssignmentElement: () => Zj, getRestParameterElementType: () => kk, getRightMostAssignedExpression: () => b0, getRootDeclaration: () => If, getRootLength: () => Bi, getScriptKind: () => getScriptKind, getScriptKindFromFileName: () => Ox, getScriptTargetFeatures: () => getScriptTargetFeatures, getSelectedEffectiveModifierFlags: () => G4, getSelectedSyntacticModifierFlags: () => $4, getSemanticClassifications: () => getSemanticClassifications, getSemanticJsxChildren: () => bN, getSetAccessorTypeAnnotationNode: () => BN, getSetAccessorValueParameter: () => z0, getSetExternalModuleIndicator: () => Ex, getShebang: () => GT, getSingleInitializerOfVariableStatementOrPropertyDeclaration: () => w0, getSingleVariableOfVariableStatement: () => Al, getSnapshotText: () => getSnapshotText, getSnippetElement: () => getSnippetElement, getSourceFileOfModule: () => AD, getSourceFileOfNode: () => Si, getSourceFilePathInNewDir: () => M4, getSourceFilePathInNewDirWorker: () => U0, getSourceFileVersionAsHashFromText: () => getSourceFileVersionAsHashFromText, getSourceFilesToEmit: () => RN, getSourceMapRange: () => getSourceMapRange, getSourceMapper: () => getSourceMapper, getSourceTextOfNodeFromSourceFile: () => No, getSpanOfTokenAtPosition: () => n0, getSpellingSuggestion: () => Ep, getStartPositionOfLine: () => kD, getStartPositionOfRange: () => K_, getStartsOnNewLine: () => getStartsOnNewLine, getStaticPropertiesAndClassStaticBlock: () => getStaticPropertiesAndClassStaticBlock, getStrictOptionValue: () => lv, getStringComparer: () => rl, getSuperCallFromStatement: () => getSuperCallFromStatement, getSuperContainer: () => Yk, getSupportedCodeFixes: () => v7, getSupportedExtensions: () => Mx, getSupportedExtensionsWithJsonIfResolveJsonModule: () => Lx, getSwitchedType: () => getSwitchedType, getSymbolId: () => getSymbolId, getSymbolNameForPrivateIdentifier: () => cN, getSymbolTarget: () => getSymbolTarget, getSyntacticClassifications: () => getSyntacticClassifications, getSyntacticModifierFlags: () => X0, getSyntacticModifierFlagsNoCache: () => Y0, getSynthesizedDeepClone: () => getSynthesizedDeepClone, getSynthesizedDeepCloneWithReplacements: () => getSynthesizedDeepCloneWithReplacements, getSynthesizedDeepClones: () => getSynthesizedDeepClones, getSynthesizedDeepClonesWithReplacements: () => getSynthesizedDeepClonesWithReplacements, getSyntheticLeadingComments: () => getSyntheticLeadingComments, getSyntheticTrailingComments: () => getSyntheticTrailingComments, getTargetLabel: () => getTargetLabel, getTargetOfBindingOrAssignmentElement: () => Ko, getTemporaryModuleResolutionState: () => getTemporaryModuleResolutionState, getTextOfConstantValue: () => HD, getTextOfIdentifierOrLiteral: () => kf, getTextOfJSDocComment: () => zA, getTextOfNode: () => gf, getTextOfNodeFromSourceText: () => B_, getTextOfPropertyName: () => lk, getThisContainer: () => d0, getThisParameter: () => j4, getTokenAtPosition: () => getTokenAtPosition, getTokenPosOfNode: () => Io, getTokenSourceMapRange: () => getTokenSourceMapRange, getTouchingPropertyName: () => getTouchingPropertyName, getTouchingToken: () => getTouchingToken, getTrailingCommentRanges: () => HT, getTrailingSemicolonDeferringWriter: () => kN, getTransformFlagsSubtreeExclusions: () => w8, getTransformers: () => getTransformers, getTsBuildInfoEmitOutputFilePath: () => getTsBuildInfoEmitOutputFilePath, getTsConfigObjectLiteralExpression: () => M3, getTsConfigPropArray: () => L3, getTsConfigPropArrayElementValue: () => Uk, getTypeAnnotationNode: () => UN, getTypeArgumentOrTypeParameterList: () => getTypeArgumentOrTypeParameterList, getTypeKeywordOfTypeOnlyImport: () => getTypeKeywordOfTypeOnlyImport, getTypeNode: () => getTypeNode, getTypeNodeIfAccessible: () => getTypeNodeIfAccessible, getTypeParameterFromJsDoc: () => BI, getTypeParameterOwner: () => AA, getTypesPackageName: () => getTypesPackageName, getUILocale: () => M1, getUniqueName: () => getUniqueName, getUniqueSymbolId: () => getUniqueSymbolId, getUseDefineForClassFields: () => CM, getWatchErrorSummaryDiagnosticMessage: () => getWatchErrorSummaryDiagnosticMessage, getWatchFactory: () => getWatchFactory, group: () => el, groupBy: () => x_, guessIndentation: () => rD, handleNoEmitOptions: () => handleNoEmitOptions, hasAbstractModifier: () => W4, hasAccessorModifier: () => H4, hasAmbientModifier: () => V4, hasChangesInResolutions: () => wD, hasChildOfKind: () => hasChildOfKind, hasContextSensitiveParameters: () => vL, hasDecorators: () => Il, hasDocComment: () => hasDocComment, hasDynamicName: () => v4, hasEffectiveModifier: () => H0, hasEffectiveModifiers: () => XN, hasEffectiveReadonlyModifier: () => $0, hasExtension: () => OT, hasIndexSignature: () => hasIndexSignature, hasInitializer: () => l3, hasInvalidEscape: () => w4, hasJSDocNodes: () => ya, hasJSDocParameterTags: () => IA, hasJSFileExtension: () => dv, hasJsonModuleEmitEnabled: () => hM, hasOnlyExpressionInitializer: () => eD, hasOverrideModifier: () => QN, hasPossibleExternalModuleReference: () => sk, hasProperty: () => Jr, hasPropertyAccessExpressionWithName: () => hasPropertyAccessExpressionWithName, hasQuestionToken: () => OI, hasRecordedExternalHelpers: () => Gj, hasRestParameter: () => nD, hasScopeMarker: () => kP, hasStaticModifier: () => Lf, hasSyntacticModifier: () => rn, hasSyntacticModifiers: () => YN, hasTSFileExtension: () => mv, hasTabstop: () => Qx, hasTrailingDirectorySeparator: () => Hp, hasType: () => ZP, hasTypeArguments: () => qI, hasZeroOrOneAsteriskCharacter: () => OM, helperString: () => helperString, hostGetCanonicalFileName: () => D4, hostUsesCaseSensitiveFileNames: () => J0, idText: () => qr, identifierIsThisKeyword: () => J4, identifierToKeywordKind: () => dS, identity: () => rr, identitySourceMapConsumer: () => identitySourceMapConsumer, ignoreSourceNewlines: () => ignoreSourceNewlines, ignoredPaths: () => ignoredPaths, importDefaultHelper: () => importDefaultHelper, importFromModuleSpecifier: () => II, importNameElisionDisabled: () => gM, importStarHelper: () => importStarHelper, indexOfAnyCharCode: () => Je, indexOfNode: () => UD, indicesOf: () => Wr, inferredTypesContainingFile: () => inferredTypesContainingFile, insertImports: () => insertImports, insertLeadingStatement: () => Mj, insertSorted: () => Qn, insertStatementAfterCustomPrologue: () => RD, insertStatementAfterStandardPrologue: () => LD, insertStatementsAfterCustomPrologue: () => MD, insertStatementsAfterStandardPrologue: () => OD, intersperse: () => Ie, introducesArgumentsExoticObject: () => Lk, inverseJsxOptionMap: () => inverseJsxOptionMap, isAbstractConstructorSymbol: () => zO, isAbstractModifier: () => uR, isAccessExpression: () => Lo, isAccessibilityModifier: () => isAccessibilityModifier, isAccessor: () => pf, isAccessorModifier: () => fR, isAliasSymbolDeclaration: () => QI, isAliasableExpression: () => k0, isAmbientModule: () => yf, isAmbientPropertyDeclaration: () => rk, isAnonymousFunctionDefinition: () => H_, isAnyDirectorySeparator: () => ay, isAnyImportOrBareOrAccessedRequire: () => ik, isAnyImportOrReExport: () => bf, isAnyImportSyntax: () => Qy, isAnySupportedFileExtension: () => ZM, isApplicableVersionedTypesKey: () => isApplicableVersionedTypesKey, isArgumentExpressionOfElementAccess: () => isArgumentExpressionOfElementAccess, isArray: () => ir, isArrayBindingElement: () => gP, isArrayBindingOrAssignmentElement: () => ZS, isArrayBindingOrAssignmentPattern: () => QS, isArrayBindingPattern: () => yR, isArrayLiteralExpression: () => Yl, isArrayLiteralOrObjectLiteralDestructuringPattern: () => isArrayLiteralOrObjectLiteralDestructuringPattern, isArrayTypeNode: () => F8, isArrowFunction: () => sd, isAsExpression: () => CR, isAssertClause: () => $R, isAssertEntry: () => KR, isAssertionExpression: () => PP, isAssertionKey: () => oP, isAssertsKeyword: () => _R, isAssignmentDeclaration: () => v0, isAssignmentExpression: () => ms, isAssignmentOperator: () => G_, isAssignmentPattern: () => KS, isAssignmentTarget: () => UI, isAsteriskToken: () => nR, isAsyncFunction: () => oN, isAsyncModifier: () => Ul, isAutoAccessorPropertyDeclaration: () => $S, isAwaitExpression: () => SR, isAwaitKeyword: () => cR, isBigIntLiteral: () => Uv, isBinaryExpression: () => ur, isBinaryOperatorToken: () => AJ, isBindableObjectDefinePropertyCall: () => S0, isBindableStaticAccessExpression: () => W_, isBindableStaticElementAccessExpression: () => x0, isBindableStaticNameExpression: () => V_, isBindingElement: () => Xl, isBindingElementOfBareOrAccessedRequire: () => mI, isBindingName: () => uP, isBindingOrAssignmentElement: () => yP, isBindingOrAssignmentPattern: () => vP, isBindingPattern: () => df, isBlock: () => Ql, isBlockOrCatchScoped: () => $D, isBlockScope: () => w3, isBlockScopedContainerTopLevel: () => ZD, isBooleanLiteral: () => pP, isBreakOrContinueStatement: () => YA, isBreakStatement: () => JR, isBuildInfoFile: () => isBuildInfoFile, isBuilderProgram: () => isBuilderProgram2, isBundle: () => cj, isBundleFileTextLike: () => XO, isCallChain: () => Cy, isCallExpression: () => sc, isCallExpressionTarget: () => isCallExpressionTarget, isCallLikeExpression: () => SP, isCallOrNewExpression: () => xP, isCallOrNewExpressionTarget: () => isCallOrNewExpressionTarget, isCallSignatureDeclaration: () => Vv, isCallToHelper: () => isCallToHelper, isCaseBlock: () => VR, isCaseClause: () => sj, isCaseKeyword: () => dR, isCaseOrDefaultClause: () => QP, isCatchClause: () => oj, isCatchClauseVariableDeclaration: () => Gx, isCatchClauseVariableDeclarationOrBindingElement: () => T3, isCheckJsEnabledForFile: () => eL, isChildOfNodeWithKind: () => Ak, isCircularBuildOrder: () => isCircularBuildOrder, isClassDeclaration: () => _c, isClassElement: () => Js, isClassExpression: () => _d, isClassLike: () => bi, isClassMemberModifier: () => VS, isClassOrTypeElement: () => mP, isClassStaticBlockDeclaration: () => Hl, isCollapsedRange: () => vO, isColonToken: () => iR, isCommaExpression: () => gd, isCommaListExpression: () => oc, isCommaSequence: () => zj, isCommaToken: () => I8, isComment: () => isComment, isCommonJsExportPropertyAssignment: () => p0, isCommonJsExportedExpression: () => Ok, isCompoundAssignment: () => isCompoundAssignment, isComputedNonLiteralName: () => ck, isComputedPropertyName: () => Ws, isConciseBody: () => MP, isConditionalExpression: () => xR, isConditionalTypeNode: () => V8, isConstTypeReference: () => jS, isConstructSignatureDeclaration: () => R8, isConstructorDeclaration: () => nc, isConstructorTypeNode: () => Gv, isContextualKeyword: () => N0, isContinueStatement: () => jR, isCustomPrologue: () => Tf, isDebuggerStatement: () => WR, isDeclaration: () => ko, isDeclarationBindingElement: () => Fy, isDeclarationFileName: () => QE, isDeclarationName: () => c4, isDeclarationNameOfEnumOrNamespace: () => IO, isDeclarationReadonly: () => Sk, isDeclarationStatement: () => VP, isDeclarationWithTypeParameterChildren: () => C3, isDeclarationWithTypeParameters: () => nk, isDecorator: () => zl, isDecoratorTarget: () => isDecoratorTarget, isDefaultClause: () => oE, isDefaultImport: () => Z3, isDefaultModifier: () => oR, isDefaultedExpandoInitializer: () => SI, isDeleteExpression: () => bR, isDeleteTarget: () => $I, isDeprecatedDeclaration: () => isDeprecatedDeclaration, isDestructuringAssignment: () => nO, isDiagnosticWithLocation: () => isDiagnosticWithLocation, isDiskPathRoot: () => H5, isDoStatement: () => OR, isDotDotDotToken: () => rR, isDottedName: () => ev, isDynamicName: () => M0, isESSymbolIdentifier: () => pN, isEffectiveExternalModule: () => Yy, isEffectiveModuleDeclaration: () => S3, isEffectiveStrictModeSourceFile: () => tk, isElementAccessChain: () => RS, isElementAccessExpression: () => gs, isEmittedFileOfProgram: () => isEmittedFileOfProgram, isEmptyArrayLiteral: () => _O, isEmptyBindingElement: () => pS, isEmptyBindingPattern: () => uS, isEmptyObjectLiteral: () => oO, isEmptyStatement: () => IR, isEmptyStringLiteral: () => j3, isEndOfDeclarationMarker: () => ej, isEntityName: () => lP, isEntityNameExpression: () => Bs, isEnumConst: () => Tk, isEnumDeclaration: () => i2, isEnumMember: () => cE, isEqualityOperatorKind: () => isEqualityOperatorKind, isEqualsGreaterThanToken: () => sR, isExclamationToken: () => rd, isExcludedFile: () => isExcludedFile, isExclusivelyTypeOnlyImportOrExport: () => isExclusivelyTypeOnlyImportOrExport, isExportAssignment: () => Vo, isExportDeclaration: () => cc, isExportModifier: () => N8, isExportName: () => Uj, isExportNamespaceAsDefaultDeclaration: () => b3, isExportOrDefaultModifier: () => DJ, isExportSpecifier: () => aE, isExportsIdentifier: () => H3, isExportsOrModuleExportsOrAlias: () => isExportsOrModuleExportsOrAlias, isExpression: () => mf, isExpressionNode: () => g0, isExpressionOfExternalModuleImportEqualsDeclaration: () => isExpressionOfExternalModuleImportEqualsDeclaration, isExpressionOfOptionalChainRoot: () => $A, isExpressionStatement: () => Zl, isExpressionWithTypeArguments: () => e2, isExpressionWithTypeArgumentsInClassExtendsClause: () => Z0, isExternalModule: () => Qo, isExternalModuleAugmentation: () => Xy, isExternalModuleImportEqualsDeclaration: () => B3, isExternalModuleIndicator: () => NP, isExternalModuleNameRelative: () => gA, isExternalModuleReference: () => ud, isExternalModuleSymbol: () => isExternalModuleSymbol, isExternalOrCommonJsModule: () => bk, isFileLevelUniqueName: () => m3, isFileProbablyExternalModule: () => ou, isFirstDeclarationOfSymbolParameter: () => isFirstDeclarationOfSymbolParameter, isFixablePromiseHandler: () => isFixablePromiseHandler, isForInOrOfStatement: () => OP, isForInStatement: () => LR, isForInitializer: () => RP, isForOfStatement: () => RR, isForStatement: () => eE, isFunctionBlock: () => O3, isFunctionBody: () => LP, isFunctionDeclaration: () => Wo, isFunctionExpression: () => ad, isFunctionExpressionOrArrowFunction: () => SL, isFunctionLike: () => ga, isFunctionLikeDeclaration: () => HS, isFunctionLikeKind: () => My, isFunctionLikeOrClassStaticBlockDeclaration: () => uf, isFunctionOrConstructorTypeNode: () => hP, isFunctionOrModuleBlock: () => fP, isFunctionSymbol: () => DI, isFunctionTypeNode: () => $l, isFutureReservedKeyword: () => tN, isGeneratedIdentifier: () => cs, isGeneratedPrivateIdentifier: () => Ny, isGetAccessor: () => Tl, isGetAccessorDeclaration: () => Gl, isGetOrSetAccessorDeclaration: () => GA, isGlobalDeclaration: () => isGlobalDeclaration, isGlobalScopeAugmentation: () => vf, isGrammarError: () => ND, isHeritageClause: () => ru, isHoistedFunction: () => _0, isHoistedVariableStatement: () => c0, isIdentifier: () => yt, isIdentifierANonContextualKeyword: () => iN, isIdentifierName: () => YI, isIdentifierOrThisTypeNode: () => aJ, isIdentifierPart: () => Rs, isIdentifierStart: () => Wn, isIdentifierText: () => vy, isIdentifierTypePredicate: () => Fk, isIdentifierTypeReference: () => pL, isIfStatement: () => NR, isIgnoredFileFromWildCardWatching: () => isIgnoredFileFromWildCardWatching, isImplicitGlob: () => Dx, isImportCall: () => s0, isImportClause: () => HR, isImportDeclaration: () => o2, isImportEqualsDeclaration: () => s2, isImportKeyword: () => M8, isImportMeta: () => o0, isImportOrExportSpecifier: () => aP, isImportOrExportSpecifierName: () => isImportOrExportSpecifierName, isImportSpecifier: () => nE, isImportTypeAssertionContainer: () => GR, isImportTypeNode: () => Kl, isImportableFile: () => isImportableFile, isInComment: () => isInComment, isInExpressionContext: () => J3, isInJSDoc: () => q3, isInJSFile: () => Pr, isInJSXText: () => isInJSXText, isInJsonFile: () => pI, isInNonReferenceComment: () => isInNonReferenceComment, isInReferenceComment: () => isInReferenceComment, isInRightSideOfInternalImportEqualsDeclaration: () => isInRightSideOfInternalImportEqualsDeclaration, isInString: () => isInString, isInTemplateString: () => isInTemplateString, isInTopLevelContext: () => Kk, isIncrementalCompilation: () => wM, isIndexSignatureDeclaration: () => Hv, isIndexedAccessTypeNode: () => $8, isInferTypeNode: () => H8, isInfinityOrNaNString: () => bL, isInitializedProperty: () => isInitializedProperty, isInitializedVariable: () => lx, isInsideJsxElement: () => isInsideJsxElement, isInsideJsxElementOrAttribute: () => isInsideJsxElementOrAttribute, isInsideNodeModules: () => isInsideNodeModules, isInsideTemplateLiteral: () => isInsideTemplateLiteral, isInstantiatedModule: () => isInstantiatedModule, isInterfaceDeclaration: () => eu, isInternalDeclaration: () => isInternalDeclaration, isInternalModuleImportEqualsDeclaration: () => lI, isInternalName: () => qj, isIntersectionTypeNode: () => W8, isIntrinsicJsxName: () => P4, isIterationStatement: () => n3, isJSDoc: () => Ho, isJSDocAllType: () => dj, isJSDocAugmentsTag: () => md, isJSDocAuthorTag: () => bj, isJSDocCallbackTag: () => Tj, isJSDocClassTag: () => pE, isJSDocCommentContainingNode: () => c3, isJSDocConstructSignature: () => MI, isJSDocDeprecatedTag: () => v2, isJSDocEnumTag: () => dE, isJSDocFunctionType: () => dd, isJSDocImplementsTag: () => hE, isJSDocIndexSignature: () => dI, isJSDocLikeText: () => LE, isJSDocLink: () => uj, isJSDocLinkCode: () => pj, isJSDocLinkLike: () => Sl, isJSDocLinkPlain: () => fj, isJSDocMemberName: () => uc, isJSDocNameReference: () => fd, isJSDocNamepathType: () => vj, isJSDocNamespaceBody: () => FP, isJSDocNode: () => Uy, isJSDocNonNullableType: () => hj, isJSDocNullableType: () => uE, isJSDocOptionalParameter: () => Zx, isJSDocOptionalType: () => gj, isJSDocOverloadTag: () => y2, isJSDocOverrideTag: () => fE, isJSDocParameterTag: () => pc, isJSDocPrivateTag: () => m2, isJSDocPropertyLikeTag: () => Dy, isJSDocPropertyTag: () => wj, isJSDocProtectedTag: () => h2, isJSDocPublicTag: () => d2, isJSDocReadonlyTag: () => g2, isJSDocReturnTag: () => b2, isJSDocSatisfiesExpression: () => IL, isJSDocSatisfiesTag: () => T2, isJSDocSeeTag: () => Sj, isJSDocSignature: () => iu, isJSDocTag: () => zy, isJSDocTemplateTag: () => Go, isJSDocThisTag: () => mE, isJSDocThrowsTag: () => Cj, isJSDocTypeAlias: () => Cl, isJSDocTypeAssertion: () => xE, isJSDocTypeExpression: () => lE, isJSDocTypeLiteral: () => f2, isJSDocTypeTag: () => au, isJSDocTypedefTag: () => xj, isJSDocUnknownTag: () => Ej, isJSDocUnknownType: () => mj, isJSDocVariadicType: () => yj, isJSXTagName: () => xf, isJsonEqual: () => gv, isJsonSourceFile: () => a0, isJsxAttribute: () => nj, isJsxAttributeLike: () => XP, isJsxAttributes: () => p2, isJsxChild: () => o3, isJsxClosingElement: () => sE, isJsxClosingFragment: () => rj, isJsxElement: () => l2, isJsxExpression: () => aj, isJsxFragment: () => pd, isJsxOpeningElement: () => tu, isJsxOpeningFragment: () => u2, isJsxOpeningLikeElement: () => _3, isJsxOpeningLikeElementTagName: () => isJsxOpeningLikeElementTagName, isJsxSelfClosingElement: () => tj, isJsxSpreadAttribute: () => ij, isJsxTagNameExpression: () => KP, isJsxText: () => td, isJumpStatementTarget: () => isJumpStatementTarget, isKeyword: () => ba, isKnownSymbol: () => lN, isLabelName: () => isLabelName, isLabelOfLabeledStatement: () => isLabelOfLabeledStatement, isLabeledStatement: () => tE, isLateVisibilityPaintedStatement: () => ak, isLeftHandSideExpression: () => Do, isLeftHandSideOfAssignment: () => rO, isLet: () => xk, isLineBreak: () => un, isLiteralComputedPropertyDeclarationName: () => l4, isLiteralExpression: () => Iy, isLiteralExpressionOfObject: () => rP, isLiteralImportTypeNode: () => k3, isLiteralKind: () => ky, isLiteralLikeAccess: () => wf, isLiteralLikeElementAccess: () => wl, isLiteralNameOfPropertyDeclarationOrIndexAccess: () => isLiteralNameOfPropertyDeclarationOrIndexAccess, isLiteralTypeLikeExpression: () => cJ, isLiteralTypeLiteral: () => CP, isLiteralTypeNode: () => Yv, isLocalName: () => E2, isLogicalOperator: () => ZN, isLogicalOrCoalescingAssignmentExpression: () => eO, isLogicalOrCoalescingAssignmentOperator: () => jf, isLogicalOrCoalescingBinaryExpression: () => tO, isLogicalOrCoalescingBinaryOperator: () => Z4, isMappedTypeNode: () => K8, isMemberName: () => js, isMergeDeclarationMarker: () => ZR, isMetaProperty: () => t2, isMethodDeclaration: () => Vl, isMethodOrAccessor: () => Ly, isMethodSignature: () => L8, isMinusToken: () => Wv, isMissingDeclaration: () => YR, isModifier: () => Oy, isModifierKind: () => Wi, isModifierLike: () => ff, isModuleAugmentationExternal: () => x3, isModuleBlock: () => rE, isModuleBody: () => jP, isModuleDeclaration: () => Ea, isModuleExportsAccessExpression: () => T0, isModuleIdentifier: () => G3, isModuleName: () => _J, isModuleOrEnumDeclaration: () => qP, isModuleReference: () => $P, isModuleSpecifierLike: () => isModuleSpecifierLike, isModuleWithStringLiteralName: () => KD, isNameOfFunctionDeclaration: () => isNameOfFunctionDeclaration, isNameOfModuleDeclaration: () => isNameOfModuleDeclaration, isNamedClassElement: () => dP, isNamedDeclaration: () => af, isNamedEvaluation: () => fN, isNamedEvaluationSource: () => S4, isNamedExportBindings: () => QA, isNamedExports: () => iE, isNamedImportBindings: () => BP, isNamedImports: () => XR, isNamedImportsOrExports: () => YO, isNamedTupleMember: () => $v, isNamespaceBody: () => JP, isNamespaceExport: () => ld, isNamespaceExportDeclaration: () => a2, isNamespaceImport: () => _2, isNamespaceReexportDeclaration: () => oI, isNewExpression: () => X8, isNewExpressionTarget: () => isNewExpressionTarget, isNightly: () => PN, isNoSubstitutionTemplateLiteral: () => k8, isNode: () => eP, isNodeArray: () => _s, isNodeArrayMultiLine: () => AO, isNodeDescendantOf: () => KI, isNodeKind: () => gl, isNodeLikeSystem: () => M5, isNodeModulesDirectory: () => aA, isNodeWithPossibleHoistedDeclaration: () => zI, isNonContextualKeyword: () => y4, isNonExportDefaultModifier: () => kJ, isNonGlobalAmbientModule: () => XD, isNonGlobalDeclaration: () => isNonGlobalDeclaration, isNonNullAccess: () => kL, isNonNullChain: () => JS, isNonNullExpression: () => Uo, isNonStaticMethodOrAccessorWithPrivateName: () => isNonStaticMethodOrAccessorWithPrivateName, isNotEmittedOrPartiallyEmittedNode: () => DP, isNotEmittedStatement: () => c2, isNullishCoalesce: () => XA, isNumber: () => gi, isNumericLiteral: () => zs, isNumericLiteralName: () => $x, isObjectBindingElementWithoutPropertyName: () => isObjectBindingElementWithoutPropertyName, isObjectBindingOrAssignmentElement: () => YS, isObjectBindingOrAssignmentPattern: () => XS, isObjectBindingPattern: () => gR, isObjectLiteralElement: () => Wy, isObjectLiteralElementLike: () => jy, isObjectLiteralExpression: () => Hs, isObjectLiteralMethod: () => jk, isObjectLiteralOrClassExpressionMethodOrAccessor: () => Jk, isObjectTypeDeclaration: () => $O, isOctalDigit: () => hy, isOmittedExpression: () => cd, isOptionalChain: () => Ay, isOptionalChainRoot: () => Py, isOptionalDeclaration: () => DL, isOptionalJSDocPropertyLikeTag: () => Yx, isOptionalTypeNode: () => q8, isOuterExpression: () => yd, isOutermostOptionalChain: () => KA, isOverrideModifier: () => pR, isPackedArrayLiteral: () => hL, isParameter: () => Vs, isParameterDeclaration: () => mN, isParameterOrCatchClauseVariable: () => TL, isParameterPropertyDeclaration: () => lS, isParameterPropertyModifier: () => WS, isParenthesizedExpression: () => qo, isParenthesizedTypeNode: () => Kv, isParseTreeNode: () => pl, isPartOfTypeNode: () => l0, isPartOfTypeQuery: () => F3, isPartiallyEmittedExpression: () => Z8, isPatternMatch: () => z1, isPinnedComment: () => v3, isPlainJsFile: () => PD, isPlusToken: () => zv, isPossiblyTypeArgumentPosition: () => isPossiblyTypeArgumentPosition, isPostfixUnaryExpression: () => Q8, isPrefixUnaryExpression: () => od, isPrivateIdentifier: () => vn, isPrivateIdentifierClassElementDeclaration: () => zS, isPrivateIdentifierPropertyAccessExpression: () => cP, isPrivateIdentifierSymbol: () => uN, isProgramBundleEmitBuildInfo: () => isProgramBundleEmitBuildInfo, isProgramUptoDate: () => isProgramUptoDate, isPrologueDirective: () => us, isPropertyAccessChain: () => LS, isPropertyAccessEntityNameExpression: () => rx, isPropertyAccessExpression: () => bn, isPropertyAccessOrQualifiedName: () => TP, isPropertyAccessOrQualifiedNameOrImportTypeNode: () => bP, isPropertyAssignment: () => lc, isPropertyDeclaration: () => Bo, isPropertyName: () => vl, isPropertyNameLiteral: () => L0, isPropertySignature: () => Wl, isProtoSetter: () => T4, isPrototypeAccess: () => Nl, isPrototypePropertyAssignment: () => CI, isPunctuation: () => isPunctuation, isPushOrUnshiftIdentifier: () => dN, isQualifiedName: () => rc, isQuestionDotToken: () => aR, isQuestionOrExclamationToken: () => iJ, isQuestionOrPlusOrMinusToken: () => oJ, isQuestionToken: () => ql, isRawSourceMap: () => isRawSourceMap, isReadonlyKeyword: () => O8, isReadonlyKeywordOrPlusOrMinusToken: () => sJ, isRecognizedTripleSlashComment: () => jD, isReferenceFileLocation: () => isReferenceFileLocation, isReferencedFile: () => isReferencedFile, isRegularExpressionLiteral: () => QL, isRequireCall: () => El, isRequireVariableStatement: () => W3, isRestParameter: () => u3, isRestTypeNode: () => U8, isReturnStatement: () => FR, isReturnStatementWithFixablePromiseHandler: () => isReturnStatementWithFixablePromiseHandler, isRightSideOfAccessExpression: () => nx, isRightSideOfPropertyAccess: () => isRightSideOfPropertyAccess, isRightSideOfQualifiedName: () => isRightSideOfQualifiedName, isRightSideOfQualifiedNameOrPropertyAccess: () => aO, isRightSideOfQualifiedNameOrPropertyAccessOrJSDocMemberName: () => sO, isRootedDiskPath: () => A_, isSameEntityName: () => z_, isSatisfiesExpression: () => AR, isScopeMarker: () => i3, isSemicolonClassElement: () => kR, isSetAccessor: () => bl, isSetAccessorDeclaration: () => ic, isShebangTrivia: () => gy, isShorthandAmbientModuleSymbol: () => YD, isShorthandPropertyAssignment: () => nu, isSignedNumericLiteral: () => O0, isSimpleCopiableExpression: () => isSimpleCopiableExpression, isSimpleInlineableExpression: () => isSimpleInlineableExpression, isSingleOrDoubleQuote: () => hI, isSourceFile: () => wi, isSourceFileFromLibrary: () => isSourceFileFromLibrary, isSourceFileJS: () => y0, isSourceFileNotJS: () => uI, isSourceFileNotJson: () => fI, isSourceMapping: () => isSourceMapping, isSpecialPropertyDeclaration: () => AI, isSpreadAssignment: () => _E, isSpreadElement: () => Zv, isStatement: () => a3, isStatementButNotDeclaration: () => HP, isStatementOrBlock: () => s3, isStatementWithLocals: () => DD, isStatic: () => G0, isStaticModifier: () => lR, isString: () => Ji, isStringAKeyword: () => nN, isStringANonContextualKeyword: () => rN, isStringAndEmptyAnonymousObjectIntersection: () => isStringAndEmptyAnonymousObjectIntersection, isStringDoubleQuoted: () => gI, isStringLiteral: () => Gn, isStringLiteralLike: () => Ti, isStringLiteralOrJsxExpression: () => YP, isStringLiteralOrTemplate: () => isStringLiteralOrTemplate, isStringOrNumericLiteralLike: () => Ta, isStringOrRegularExpressionOrTemplateLiteral: () => isStringOrRegularExpressionOrTemplateLiteral, isStringTextContainingNode: () => _P, isSuperCall: () => Ek, isSuperKeyword: () => nd, isSuperOrSuperProperty: () => Zk, isSuperProperty: () => Sf, isSupportedSourceFileName: () => GM, isSwitchStatement: () => qR, isSyntaxList: () => Aj, isSyntheticExpression: () => PR, isSyntheticReference: () => QR, isTagName: () => isTagName, isTaggedTemplateExpression: () => Y8, isTaggedTemplateTag: () => isTaggedTemplateTag, isTemplateExpression: () => ER, isTemplateHead: () => ZL, isTemplateLiteral: () => EP, isTemplateLiteralKind: () => yl, isTemplateLiteralToken: () => nP, isTemplateLiteralTypeNode: () => hR, isTemplateLiteralTypeSpan: () => mR, isTemplateMiddle: () => eR, isTemplateMiddleOrTemplateTail: () => iP, isTemplateSpan: () => DR, isTemplateTail: () => tR, isTextWhiteSpaceLike: () => isTextWhiteSpaceLike, isThis: () => isThis, isThisContainerOrFunctionBlock: () => $k, isThisIdentifier: () => Mf, isThisInTypeQuery: () => qN, isThisInitializedDeclaration: () => tI, isThisInitializedObjectBindingExpression: () => rI, isThisProperty: () => eI, isThisTypeNode: () => Xv, isThisTypeParameter: () => Kx, isThisTypePredicate: () => Bk, isThrowStatement: () => UR, isToken: () => tP, isTokenKind: () => BS, isTraceEnabled: () => isTraceEnabled, isTransientSymbol: () => $y, isTrivia: () => aN, isTryStatement: () => zR, isTupleTypeNode: () => B8, isTypeAlias: () => LI, isTypeAliasDeclaration: () => n2, isTypeAssertionExpression: () => vR, isTypeDeclaration: () => Xx, isTypeElement: () => Ry, isTypeKeyword: () => isTypeKeyword, isTypeKeywordToken: () => isTypeKeywordToken, isTypeKeywordTokenOrIdentifier: () => isTypeKeywordTokenOrIdentifier, isTypeLiteralNode: () => id, isTypeNode: () => Jy, isTypeNodeKind: () => hx, isTypeOfExpression: () => TR, isTypeOnlyExportDeclaration: () => US, isTypeOnlyImportDeclaration: () => qS, isTypeOnlyImportOrExportDeclaration: () => sP, isTypeOperatorNode: () => G8, isTypeParameterDeclaration: () => Fo, isTypePredicateNode: () => j8, isTypeQueryNode: () => J8, isTypeReferenceNode: () => ac, isTypeReferenceType: () => tD, isUMDExportSymbol: () => VO, isUnaryExpression: () => t3, isUnaryExpressionWithWrite: () => wP, isUnicodeIdentifierStart: () => UT, isUnionTypeNode: () => z8, isUnparsedNode: () => ZA, isUnparsedPrepend: () => _j, isUnparsedSource: () => lj, isUnparsedTextLike: () => FS, isUrl: () => V5, isValidBigIntString: () => zx, isValidESSymbolDeclaration: () => Mk, isValidTypeOnlyAliasUseSite: () => _L, isValueSignatureDeclaration: () => WI, isVarConst: () => D3, isVariableDeclaration: () => Vi, isVariableDeclarationInVariableStatement: () => N3, isVariableDeclarationInitializedToBareOrAccessedRequire: () => Ef, isVariableDeclarationInitializedToRequire: () => U3, isVariableDeclarationList: () => r2, isVariableLike: () => u0, isVariableLikeOrAccessor: () => Nk, isVariableStatement: () => zo, isVoidExpression: () => Qv, isWatchSet: () => OO, isWhileStatement: () => MR, isWhiteSpaceLike: () => os, isWhiteSpaceSingleLine: () => N_, isWithStatement: () => BR, isWriteAccess: () => FO, isWriteOnlyAccess: () => JO, isYieldExpression: () => wR, jsxModeNeedsExplicitImport: () => jsxModeNeedsExplicitImport, keywordPart: () => keywordPart, last: () => Zn, lastOrUndefined: () => Cn, length: () => I, libMap: () => libMap, libs: () => libs, lineBreakPart: () => lineBreakPart, linkNamePart: () => linkNamePart, linkPart: () => linkPart, linkTextPart: () => linkTextPart, listFiles: () => listFiles, loadModuleFromGlobalCache: () => loadModuleFromGlobalCache, loadWithModeAwareCache: () => loadWithModeAwareCache, makeIdentifierFromModuleName: () => GD, makeImport: () => makeImport, makeImportIfNecessary: () => makeImportIfNecessary, makeStringLiteral: () => makeStringLiteral, mangleScopedPackageName: () => mangleScopedPackageName, map: () => Ze, mapAllOrFail: () => Pt, mapDefined: () => qt, mapDefinedEntries: () => Ri, mapDefinedIterator: () => Zr, mapEntries: () => be, mapIterator: () => st, mapOneOrMany: () => mapOneOrMany, mapToDisplayParts: () => mapToDisplayParts, matchFiles: () => qM, matchPatternOrExact: () => tL, matchedText: () => S5, matchesExclude: () => matchesExclude, maybeBind: () => le, maybeSetLocalizedDiagnosticMessages: () => vx, memoize: () => tl, memoizeCached: () => D1, memoizeOne: () => An, memoizeWeak: () => P1, metadataHelper: () => metadataHelper, min: () => N1, minAndMax: () => nL, missingFileModifiedTime: () => missingFileModifiedTime, modifierToFlag: () => Q0, modifiersToFlags: () => Vn, moduleOptionDeclaration: () => moduleOptionDeclaration, moduleResolutionIsEqualTo: () => TD, moduleResolutionNameAndModeGetter: () => moduleResolutionNameAndModeGetter, moduleResolutionOptionDeclarations: () => moduleResolutionOptionDeclarations, moduleResolutionSupportsPackageJsonExportsAndImports: () => _v, moduleResolutionUsesNodeModules: () => moduleResolutionUsesNodeModules, moduleSpecifiers: () => ts_moduleSpecifiers_exports, moveEmitHelpers: () => moveEmitHelpers, moveRangeEnd: () => gO, moveRangePastDecorators: () => _x, moveRangePastModifiers: () => yO, moveRangePos: () => Ff, moveSyntheticComments: () => moveSyntheticComments, mutateMap: () => UO, mutateMapSkippingNewValues: () => fx, needsParentheses: () => needsParentheses, needsScopeMarker: () => IP, newCaseClauseTracker: () => newCaseClauseTracker, newPrivateEnvironment: () => newPrivateEnvironment, noEmitNotification: () => noEmitNotification, noEmitSubstitution: () => noEmitSubstitution, noTransformers: () => noTransformers, noTruncationMaximumTruncationLength: () => n8, nodeCanBeDecorated: () => R3, nodeHasName: () => hS, nodeIsDecorated: () => q_, nodeIsMissing: () => va, nodeIsPresent: () => xl, nodeIsSynthesized: () => fs2, nodeModuleNameResolver: () => nodeModuleNameResolver, nodeModulesPathPart: () => nodeModulesPathPart, nodeNextJsonConfigResolver: () => nodeNextJsonConfigResolver, nodeOrChildIsDecorated: () => m0, nodeOverlapsWithStartEnd: () => nodeOverlapsWithStartEnd, nodePosToString: () => ID, nodeSeenTracker: () => nodeSeenTracker, nodeStartsNewLexicalEnvironment: () => hN, nodeToDisplayParts: () => nodeToDisplayParts, noop: () => yn, noopFileWatcher: () => noopFileWatcher, noopPush: () => CT, normalizePath: () => Un, normalizeSlashes: () => Eo, not: () => w5, notImplemented: () => A1, notImplementedResolver: () => notImplementedResolver, nullNodeConverters: () => nullNodeConverters, nullParenthesizerRules: () => Jv, nullTransformationContext: () => nullTransformationContext, objectAllocator: () => lr, operatorPart: () => operatorPart, optionDeclarations: () => optionDeclarations, optionMapToObject: () => optionMapToObject, optionsAffectingProgramStructure: () => optionsAffectingProgramStructure, optionsForBuild: () => optionsForBuild, optionsForWatch: () => optionsForWatch, optionsHaveChanges: () => J_, optionsHaveModuleResolutionChanges: () => p3, or: () => W1, orderedRemoveItem: () => J, orderedRemoveItemAt: () => vT, outFile: () => B0, packageIdToPackageName: () => f3, packageIdToString: () => xD, padLeft: () => D5, padRight: () => k5, paramHelper: () => paramHelper, parameterIsThisKeyword: () => kl, parameterNamePart: () => parameterNamePart, parseBaseNodeFactory: () => I2, parseBigInt: () => oL, parseBuildCommand: () => parseBuildCommand, parseCommandLine: () => parseCommandLine, parseCommandLineWorker: () => parseCommandLineWorker, parseConfigFileTextToJson: () => parseConfigFileTextToJson, parseConfigFileWithSystem: () => parseConfigFileWithSystem, parseConfigHostFromCompilerHostLike: () => parseConfigHostFromCompilerHostLike, parseCustomTypeOption: () => parseCustomTypeOption, parseIsolatedEntityName: () => $J, parseIsolatedJSDocComment: () => XJ, parseJSDocTypeExpressionForTests: () => YJ, parseJsonConfigFileContent: () => parseJsonConfigFileContent, parseJsonSourceFileConfigFileContent: () => parseJsonSourceFileConfigFileContent, parseJsonText: () => KJ, parseListTypeOption: () => parseListTypeOption, parseNodeFactory: () => dc, parseNodeModuleFromPath: () => parseNodeModuleFromPath, parsePackageName: () => parsePackageName, parsePseudoBigInt: () => Hf, parseValidBigInt: () => Ux, patchWriteFileEnsuringDirectory: () => patchWriteFileEnsuringDirectory, pathContainsNodeModules: () => pathContainsNodeModules, pathIsAbsolute: () => sy, pathIsBareSpecifier: () => G5, pathIsRelative: () => So, patternText: () => T5, perfLogger: () => Dp, performIncrementalCompilation: () => performIncrementalCompilation, performance: () => ts_performance_exports, plainJSErrors: () => plainJSErrors, positionBelongsToNode: () => positionBelongsToNode, positionIsASICandidate: () => positionIsASICandidate, positionIsSynthesized: () => hs, positionsAreOnSameLine: () => $_, preProcessFile: () => preProcessFile, probablyUsesSemicolons: () => probablyUsesSemicolons, processCommentPragmas: () => ZE, processPragmasIntoFields: () => e7, processTaggedTemplateExpression: () => processTaggedTemplateExpression, programContainsEsModules: () => programContainsEsModules, programContainsModules: () => programContainsModules, projectReferenceIsEqualTo: () => bD, propKeyHelper: () => propKeyHelper, propertyNamePart: () => propertyNamePart, pseudoBigIntToString: () => yv, punctuationPart: () => punctuationPart, pushIfUnique: () => qn, quote: () => quote, quotePreferenceFromString: () => quotePreferenceFromString, rangeContainsPosition: () => rangeContainsPosition, rangeContainsPositionExclusive: () => rangeContainsPositionExclusive, rangeContainsRange: () => rangeContainsRange, rangeContainsRangeExclusive: () => rangeContainsRangeExclusive, rangeContainsStartEnd: () => rangeContainsStartEnd, rangeEndIsOnSameLineAsRangeStart: () => EO, rangeEndPositionsAreOnSameLine: () => xO, rangeEquals: () => Kc, rangeIsOnSingleLine: () => TO, rangeOfNode: () => iL, rangeOfTypeParameters: () => aL, rangeOverlapsWithStartEnd: () => rangeOverlapsWithStartEnd, rangeStartIsOnSameLineAsRangeEnd: () => cx, rangeStartPositionsAreOnSameLine: () => SO, readBuilderProgram: () => readBuilderProgram, readConfigFile: () => readConfigFile, readHelper: () => readHelper, readJson: () => hO, readJsonConfigFile: () => readJsonConfigFile, readJsonOrUndefined: () => ax, realizeDiagnostics: () => realizeDiagnostics, reduceEachLeadingCommentRange: () => zT, reduceEachTrailingCommentRange: () => WT, reduceLeft: () => Qa, reduceLeftIterator: () => K, reducePathComponents: () => is, refactor: () => ts_refactor_exports, regExpEscape: () => JM, relativeComplement: () => h_, removeAllComments: () => removeAllComments, removeEmitHelper: () => removeEmitHelper, removeExtension: () => Fx, removeFileExtension: () => Ll, removeIgnoredPath: () => removeIgnoredPath, removeMinAndVersionNumbers: () => q1, removeOptionality: () => removeOptionality, removePrefix: () => x5, removeSuffix: () => F1, removeTrailingDirectorySeparator: () => P_, repeatString: () => repeatString, replaceElement: () => ei, resolutionExtensionIsTSOrJson: () => YM, resolveConfigFileProjectName: () => resolveConfigFileProjectName, resolveJSModule: () => resolveJSModule, resolveModuleName: () => resolveModuleName, resolveModuleNameFromCache: () => resolveModuleNameFromCache, resolvePackageNameToPackageJson: () => resolvePackageNameToPackageJson, resolvePath: () => oy, resolveProjectReferencePath: () => resolveProjectReferencePath, resolveTripleslashReference: () => resolveTripleslashReference, resolveTypeReferenceDirective: () => resolveTypeReferenceDirective, resolvingEmptyArray: () => t8, restHelper: () => restHelper, returnFalse: () => w_, returnNoopFileWatcher: () => returnNoopFileWatcher, returnTrue: () => vp, returnUndefined: () => C1, returnsPromise: () => returnsPromise, runInitializersHelper: () => runInitializersHelper, sameFlatMap: () => at, sameMap: () => tt, sameMapping: () => sameMapping, scanShebangTrivia: () => yy, scanTokenAtPosition: () => yk, scanner: () => Zo, screenStartingMessageCodes: () => screenStartingMessageCodes, semanticDiagnosticsOptionDeclarations: () => semanticDiagnosticsOptionDeclarations, serializeCompilerOptions: () => serializeCompilerOptions, server: () => ts_server_exports, servicesVersion: () => E7, setCommentRange: () => setCommentRange, setConfigFileInOptions: () => setConfigFileInOptions, setConstantValue: () => setConstantValue, setEachParent: () => Q_, setEmitFlags: () => setEmitFlags, setFunctionNameHelper: () => setFunctionNameHelper, setGetSourceFileAsHashVersioned: () => setGetSourceFileAsHashVersioned, setIdentifierAutoGenerate: () => setIdentifierAutoGenerate, setIdentifierGeneratedImportReference: () => setIdentifierGeneratedImportReference, setIdentifierTypeArguments: () => setIdentifierTypeArguments, setInternalEmitFlags: () => setInternalEmitFlags, setLocalizedDiagnosticMessages: () => yx, setModuleDefaultHelper: () => setModuleDefaultHelper, setNodeFlags: () => dL, setObjectAllocator: () => gx, setOriginalNode: () => Dn, setParent: () => Sa, setParentRecursive: () => Vx, setPrivateIdentifier: () => setPrivateIdentifier, setResolvedModule: () => gD, setResolvedTypeReferenceDirective: () => yD, setSnippetElement: () => setSnippetElement, setSourceMapRange: () => setSourceMapRange, setStackTraceLimit: () => setStackTraceLimit, setStartsOnNewLine: () => setStartsOnNewLine, setSyntheticLeadingComments: () => setSyntheticLeadingComments, setSyntheticTrailingComments: () => setSyntheticTrailingComments, setSys: () => setSys, setSysLog: () => setSysLog, setTextRange: () => Rt, setTextRangeEnd: () => Wx, setTextRangePos: () => Gf, setTextRangePosEnd: () => Us, setTextRangePosWidth: () => $f, setTokenSourceMapRange: () => setTokenSourceMapRange, setTypeNode: () => setTypeNode, setUILocale: () => xp, setValueDeclaration: () => PI, shouldAllowImportingTsExtension: () => shouldAllowImportingTsExtension, shouldPreserveConstEnums: () => EM, shouldUseUriStyleNodeCoreModules: () => shouldUseUriStyleNodeCoreModules, showModuleSpecifier: () => HO, signatureHasLiteralTypes: () => signatureHasLiteralTypes, signatureHasRestParameter: () => signatureHasRestParameter, signatureToDisplayParts: () => signatureToDisplayParts, single: () => Yc, singleElementArray: () => Cp, singleIterator: () => Ka, singleOrMany: () => mo, singleOrUndefined: () => Xa, skipAlias: () => RO, skipAssertions: () => Hj, skipConstraint: () => skipConstraint, skipOuterExpressions: () => $o, skipParentheses: () => Pl, skipPartiallyEmittedExpressions: () => lf, skipTrivia: () => Ar, skipTypeChecking: () => sL, skipTypeParentheses: () => GI, skipWhile: () => N5, sliceAfter: () => rL, some: () => Ke, sort: () => Is, sortAndDeduplicate: () => uo, sortAndDeduplicateDiagnostics: () => yA, sourceFileAffectingCompilerOptions: () => sourceFileAffectingCompilerOptions, sourceFileMayBeEmitted: () => q0, sourceMapCommentRegExp: () => sourceMapCommentRegExp, sourceMapCommentRegExpDontCareLineStart: () => sourceMapCommentRegExpDontCareLineStart, spacePart: () => spacePart, spanMap: () => co, spreadArrayHelper: () => spreadArrayHelper, stableSort: () => Ns, startEndContainsRange: () => startEndContainsRange, startEndOverlapsWithStartEnd: () => startEndOverlapsWithStartEnd, startOnNewLine: () => vd, startTracing: () => startTracing, startsWith: () => Pn, startsWithDirectory: () => rA, startsWithUnderscore: () => startsWithUnderscore, startsWithUseStrict: () => SE, stringContains: () => Fi, stringContainsAt: () => stringContainsAt, stringToToken: () => _l, stripQuotes: () => CN, supportedDeclarationExtensions: () => Rv, supportedJSExtensions: () => Mv, supportedJSExtensionsFlat: () => Lv, supportedLocaleDirectories: () => Hy, supportedTSExtensions: () => Jo, supportedTSExtensionsFlat: () => Ov, supportedTSImplementationExtensions: () => b8, suppressLeadingAndTrailingTrivia: () => suppressLeadingAndTrailingTrivia, suppressLeadingTrivia: () => suppressLeadingTrivia, suppressTrailingTrivia: () => suppressTrailingTrivia, symbolEscapedNameNoDefault: () => symbolEscapedNameNoDefault, symbolName: () => rf, symbolNameNoDefault: () => symbolNameNoDefault, symbolPart: () => symbolPart, symbolToDisplayParts: () => symbolToDisplayParts, syntaxMayBeASICandidate: () => syntaxMayBeASICandidate, syntaxRequiresTrailingSemicolonOrASI: () => syntaxRequiresTrailingSemicolonOrASI, sys: () => iy, sysLog: () => sysLog, tagNamesAreEquivalent: () => Hi, takeWhile: () => I5, targetOptionDeclaration: () => targetOptionDeclaration, templateObjectHelper: () => templateObjectHelper, testFormatSettings: () => testFormatSettings, textChangeRangeIsUnchanged: () => cS, textChangeRangeNewSpan: () => R_, textChanges: () => ts_textChanges_exports, textOrKeywordPart: () => textOrKeywordPart, textPart: () => textPart, textRangeContainsPositionInclusive: () => bA, textSpanContainsPosition: () => vA, textSpanContainsTextSpan: () => TA, textSpanEnd: () => Ir, textSpanIntersection: () => _S, textSpanIntersectsWith: () => EA, textSpanIntersectsWithPosition: () => wA, textSpanIntersectsWithTextSpan: () => xA, textSpanIsEmpty: () => sS, textSpanOverlap: () => oS, textSpanOverlapsWith: () => SA, textSpansEqual: () => textSpansEqual, textToKeywordObj: () => cl, timestamp: () => ts, toArray: () => en, toBuilderFileEmit: () => toBuilderFileEmit, toBuilderStateFileInfoForMultiEmit: () => toBuilderStateFileInfoForMultiEmit, toEditorSettings: () => lu, toFileNameLowerCase: () => Tp, toLowerCase: () => bp, toPath: () => Ui, toProgramEmitPending: () => toProgramEmitPending, tokenIsIdentifierOrKeyword: () => fr, tokenIsIdentifierOrKeywordOrGreaterThan: () => qT, tokenToString: () => Br, trace: () => trace, tracing: () => rs, tracingEnabled: () => tracingEnabled, transform: () => transform, transformClassFields: () => transformClassFields, transformDeclarations: () => transformDeclarations, transformECMAScriptModule: () => transformECMAScriptModule, transformES2015: () => transformES2015, transformES2016: () => transformES2016, transformES2017: () => transformES2017, transformES2018: () => transformES2018, transformES2019: () => transformES2019, transformES2020: () => transformES2020, transformES2021: () => transformES2021, transformES5: () => transformES5, transformESDecorators: () => transformESDecorators, transformESNext: () => transformESNext, transformGenerators: () => transformGenerators, transformJsx: () => transformJsx, transformLegacyDecorators: () => transformLegacyDecorators, transformModule: () => transformModule, transformNodeModule: () => transformNodeModule, transformNodes: () => transformNodes, transformSystemModule: () => transformSystemModule, transformTypeScript: () => transformTypeScript, transpile: () => transpile, transpileModule: () => transpileModule, transpileOptionValueCompilerOptions: () => transpileOptionValueCompilerOptions, trimString: () => Pp, trimStringEnd: () => X1, trimStringStart: () => nl, tryAddToSet: () => ua, tryAndIgnoreErrors: () => tryAndIgnoreErrors, tryCast: () => ln, tryDirectoryExists: () => tryDirectoryExists, tryExtractTSExtension: () => uO, tryFileExists: () => tryFileExists, tryGetClassExtendingExpressionWithTypeArguments: () => ex, tryGetClassImplementingOrExtendingExpressionWithTypeArguments: () => tx, tryGetDirectories: () => tryGetDirectories, tryGetExtensionFromPath: () => hv, tryGetImportFromModuleSpecifier: () => Y3, tryGetJSDocSatisfiesTypeNode: () => e8, tryGetModuleNameFromFile: () => CE, tryGetModuleSpecifierFromDeclaration: () => kI, tryGetNativePerformanceHooks: () => J5, tryGetPropertyAccessOrIdentifierToString: () => tv, tryGetPropertyNameOfBindingOrAssignmentElement: () => PE, tryGetSourceMappingURL: () => tryGetSourceMappingURL, tryGetTextOfPropertyName: () => e0, tryIOAndConsumeErrors: () => tryIOAndConsumeErrors, tryParsePattern: () => Bx, tryParsePatterns: () => XM, tryParseRawSourceMap: () => tryParseRawSourceMap, tryReadDirectory: () => tryReadDirectory, tryReadFile: () => tryReadFile, tryRemoveDirectoryPrefix: () => jM, tryRemoveExtension: () => Jx, tryRemovePrefix: () => ST, tryRemoveSuffix: () => B1, typeAcquisitionDeclarations: () => typeAcquisitionDeclarations, typeAliasNamePart: () => typeAliasNamePart, typeDirectiveIsEqualTo: () => ED, typeKeywords: () => typeKeywords, typeParameterNamePart: () => typeParameterNamePart, typeReferenceResolutionNameAndModeGetter: () => typeReferenceResolutionNameAndModeGetter, typeToDisplayParts: () => typeToDisplayParts, unchangedPollThresholds: () => unchangedPollThresholds, unchangedTextChangeRange: () => Vy, unescapeLeadingUnderscores: () => dl, unmangleScopedPackageName: () => unmangleScopedPackageName, unorderedRemoveItem: () => bT, unorderedRemoveItemAt: () => U1, unreachableCodeIsError: () => yM, unusedLabelIsError: () => vM, unwrapInnermostStatementOfLabel: () => Rk, updateErrorForNoInputFiles: () => updateErrorForNoInputFiles, updateLanguageServiceSourceFile: () => T7, updateMissingFilePathsWatch: () => updateMissingFilePathsWatch, updatePackageJsonWatch: () => updatePackageJsonWatch, updateResolutionField: () => updateResolutionField, updateSharedExtendedConfigFileWatcher: () => updateSharedExtendedConfigFileWatcher, updateSourceFile: () => k2, updateWatchingWildcardDirectories: () => updateWatchingWildcardDirectories, usesExtensionsOnImports: () => Rx, usingSingleLineStringWriter: () => mD, utf16EncodeAsString: () => by, validateLocaleAndSetLanguage: () => DA, valuesHelper: () => valuesHelper, version: () => C, versionMajorMinor: () => m, visitArray: () => visitArray, visitCommaListElements: () => visitCommaListElements, visitEachChild: () => visitEachChild, visitFunctionBody: () => visitFunctionBody, visitIterationBody: () => visitIterationBody, visitLexicalEnvironment: () => visitLexicalEnvironment, visitNode: () => visitNode, visitNodes: () => visitNodes2, visitParameterList: () => visitParameterList, walkUpBindingElementsAndPatterns: () => fS, walkUpLexicalEnvironments: () => walkUpLexicalEnvironments, walkUpOuterExpressions: () => Vj, walkUpParenthesizedExpressions: () => D0, walkUpParenthesizedTypes: () => VI, walkUpParenthesizedTypesAndGetParentAndChild: () => HI, whitespaceOrMapCommentRegExp: () => whitespaceOrMapCommentRegExp, writeCommentRange: () => $N, writeFile: () => jN, writeFileEnsuringDirectories: () => JN, zipToModeAwareCache: () => zipToModeAwareCache, zipWith: () => ce });
var R7 = D({ "src/typescript/_namespaces/ts.ts"() {
"use strict";
nn(), l7(), L2(), FB();
} }), BB = P({ "src/typescript/typescript.ts"(e, t) {
R7(), R7(), typeof console < "u" && (Y.loggingHost = { log(r, s) {
switch (r) {
case 1:
return console.error(s);
case 2:
return console.warn(s);
case 3:
return console.log(s);
case 4:
return console.log(s);
}
} }), t.exports = L7;
} });
_.exports = BB();
} }), DW = Oe({ "src/language-js/parse/postprocess/typescript.js"(a, _) {
"use strict";
De();
var v = F9(), h = q9(), D = U9(), P = { AbstractKeyword: 126, SourceFile: 308, PropertyDeclaration: 169 };
function y(c) {
for (; c && c.kind !== P.SourceFile; )
c = c.parent;
return c;
}
function m(c, M) {
let q = y(c), [W, K] = [c.getStart(), c.end].map((ce) => {
let { line: Ie, character: me } = q.getLineAndCharacterOfPosition(ce);
return { line: Ie + 1, column: me };
});
D({ loc: { start: W, end: K } }, M);
}
function C(c) {
let M = vr();
return [true, false].some((q) => M.nodeCanBeDecorated(q, c, c.parent, c.parent.parent));
}
function d(c) {
let { modifiers: M } = c;
if (!v(M))
return;
let q = vr(), { SyntaxKind: W } = q;
for (let K of M)
q.isDecorator(K) && !C(c) && (c.kind === W.MethodDeclaration && !q.nodeIsPresent(c.body) && m(K, "A decorator can only decorate a method implementation, not an overload."), m(K, "Decorators are not valid here."));
}
function E(c, M) {
c.kind !== P.PropertyDeclaration || c.modifiers && !c.modifiers.some((q) => q.kind === P.AbstractKeyword) || c.initializer && M.value === null && D(M, "Abstract property cannot have an initializer");
}
function I(c, M) {
if (!/@|abstract/.test(M.originalText))
return;
let { esTreeNodeToTSNodeMap: q, tsNodeToESTreeNodeMap: W } = c;
h(c.ast, (K) => {
let ce = q.get(K);
if (!ce)
return;
let Ie = W.get(ce);
Ie === K && (d(ce), E(ce, Ie));
});
}
_.exports = { throwErrorForInvalidNodes: I };
} }), Ga = Oe({ "scripts/build/shims/debug.cjs"(a, _) {
"use strict";
De(), _.exports = () => () => {
};
} }), h1 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/internal/constants.js"(a, _) {
De();
var v = "2.0.0", h = 256, D = Number.MAX_SAFE_INTEGER || 9007199254740991, P = 16;
_.exports = { SEMVER_SPEC_VERSION: v, MAX_LENGTH: h, MAX_SAFE_INTEGER: D, MAX_SAFE_COMPONENT_LENGTH: P };
} }), g1 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/internal/debug.js"(a, _) {
De();
var v = typeof cn == "object" && cn.env && cn.env.NODE_DEBUG && /\bsemver\b/i.test(cn.env.NODE_DEBUG) ? function() {
for (var h = arguments.length, D = new Array(h), P = 0; P < h; P++)
D[P] = arguments[P];
return console.error("SEMVER", ...D);
} : () => {
};
_.exports = v;
} }), Bc = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/internal/re.js"(a, _) {
De();
var { MAX_SAFE_COMPONENT_LENGTH: v } = h1(), h = g1();
a = _.exports = {};
var D = a.re = [], P = a.src = [], y = a.t = {}, m = 0, C = (d, E, I) => {
let c = m++;
h(d, c, E), y[d] = c, P[c] = E, D[c] = new RegExp(E, I ? "g" : void 0);
};
C("NUMERICIDENTIFIER", "0|[1-9]\\d*"), C("NUMERICIDENTIFIERLOOSE", "[0-9]+"), C("NONNUMERICIDENTIFIER", "\\d*[a-zA-Z-][a-zA-Z0-9-]*"), C("MAINVERSION", `(${P[y.NUMERICIDENTIFIER]})\\.(${P[y.NUMERICIDENTIFIER]})\\.(${P[y.NUMERICIDENTIFIER]})`), C("MAINVERSIONLOOSE", `(${P[y.NUMERICIDENTIFIERLOOSE]})\\.(${P[y.NUMERICIDENTIFIERLOOSE]})\\.(${P[y.NUMERICIDENTIFIERLOOSE]})`), C("PRERELEASEIDENTIFIER", `(?:${P[y.NUMERICIDENTIFIER]}|${P[y.NONNUMERICIDENTIFIER]})`), C("PRERELEASEIDENTIFIERLOOSE", `(?:${P[y.NUMERICIDENTIFIERLOOSE]}|${P[y.NONNUMERICIDENTIFIER]})`), C("PRERELEASE", `(?:-(${P[y.PRERELEASEIDENTIFIER]}(?:\\.${P[y.PRERELEASEIDENTIFIER]})*))`), C("PRERELEASELOOSE", `(?:-?(${P[y.PRERELEASEIDENTIFIERLOOSE]}(?:\\.${P[y.PRERELEASEIDENTIFIERLOOSE]})*))`), C("BUILDIDENTIFIER", "[0-9A-Za-z-]+"), C("BUILD", `(?:\\+(${P[y.BUILDIDENTIFIER]}(?:\\.${P[y.BUILDIDENTIFIER]})*))`), C("FULLPLAIN", `v?${P[y.MAINVERSION]}${P[y.PRERELEASE]}?${P[y.BUILD]}?`), C("FULL", `^${P[y.FULLPLAIN]}$`), C("LOOSEPLAIN", `[v=\\s]*${P[y.MAINVERSIONLOOSE]}${P[y.PRERELEASELOOSE]}?${P[y.BUILD]}?`), C("LOOSE", `^${P[y.LOOSEPLAIN]}$`), C("GTLT", "((?:<|>)?=?)"), C("XRANGEIDENTIFIERLOOSE", `${P[y.NUMERICIDENTIFIERLOOSE]}|x|X|\\*`), C("XRANGEIDENTIFIER", `${P[y.NUMERICIDENTIFIER]}|x|X|\\*`), C("XRANGEPLAIN", `[v=\\s]*(${P[y.XRANGEIDENTIFIER]})(?:\\.(${P[y.XRANGEIDENTIFIER]})(?:\\.(${P[y.XRANGEIDENTIFIER]})(?:${P[y.PRERELEASE]})?${P[y.BUILD]}?)?)?`), C("XRANGEPLAINLOOSE", `[v=\\s]*(${P[y.XRANGEIDENTIFIERLOOSE]})(?:\\.(${P[y.XRANGEIDENTIFIERLOOSE]})(?:\\.(${P[y.XRANGEIDENTIFIERLOOSE]})(?:${P[y.PRERELEASELOOSE]})?${P[y.BUILD]}?)?)?`), C("XRANGE", `^${P[y.GTLT]}\\s*${P[y.XRANGEPLAIN]}$`), C("XRANGELOOSE", `^${P[y.GTLT]}\\s*${P[y.XRANGEPLAINLOOSE]}$`), C("COERCE", `(^|[^\\d])(\\d{1,${v}})(?:\\.(\\d{1,${v}}))?(?:\\.(\\d{1,${v}}))?(?:$|[^\\d])`), C("COERCERTL", P[y.COERCE], true), C("LONETILDE", "(?:~>?)"), C("TILDETRIM", `(\\s*)${P[y.LONETILDE]}\\s+`, true), a.tildeTrimReplace = "$1~", C("TILDE", `^${P[y.LONETILDE]}${P[y.XRANGEPLAIN]}$`), C("TILDELOOSE", `^${P[y.LONETILDE]}${P[y.XRANGEPLAINLOOSE]}$`), C("LONECARET", "(?:\\^)"), C("CARETTRIM", `(\\s*)${P[y.LONECARET]}\\s+`, true), a.caretTrimReplace = "$1^", C("CARET", `^${P[y.LONECARET]}${P[y.XRANGEPLAIN]}$`), C("CARETLOOSE", `^${P[y.LONECARET]}${P[y.XRANGEPLAINLOOSE]}$`), C("COMPARATORLOOSE", `^${P[y.GTLT]}\\s*(${P[y.LOOSEPLAIN]})$|^$`), C("COMPARATOR", `^${P[y.GTLT]}\\s*(${P[y.FULLPLAIN]})$|^$`), C("COMPARATORTRIM", `(\\s*)${P[y.GTLT]}\\s*(${P[y.LOOSEPLAIN]}|${P[y.XRANGEPLAIN]})`, true), a.comparatorTrimReplace = "$1$2$3", C("HYPHENRANGE", `^\\s*(${P[y.XRANGEPLAIN]})\\s+-\\s+(${P[y.XRANGEPLAIN]})\\s*$`), C("HYPHENRANGELOOSE", `^\\s*(${P[y.XRANGEPLAINLOOSE]})\\s+-\\s+(${P[y.XRANGEPLAINLOOSE]})\\s*$`), C("STAR", "(<|>)?=?\\s*\\*"), C("GTE0", "^\\s*>=\\s*0\\.0\\.0\\s*$"), C("GTE0PRE", "^\\s*>=\\s*0\\.0\\.0-0\\s*$");
} }), y1 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/internal/parse-options.js"(a, _) {
De();
var v = ["includePrerelease", "loose", "rtl"], h = (D) => D ? typeof D != "object" ? { loose: true } : v.filter((P) => D[P]).reduce((P, y) => (P[y] = true, P), {}) : {};
_.exports = h;
} }), z9 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/internal/identifiers.js"(a, _) {
De();
var v = /^[0-9]+$/, h = (P, y) => {
let m = v.test(P), C = v.test(y);
return m && C && (P = +P, y = +y), P === y ? 0 : m && !C ? -1 : C && !m ? 1 : P < y ? -1 : 1;
}, D = (P, y) => h(y, P);
_.exports = { compareIdentifiers: h, rcompareIdentifiers: D };
} }), Bn = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/classes/semver.js"(a, _) {
De();
var v = g1(), { MAX_LENGTH: h, MAX_SAFE_INTEGER: D } = h1(), { re: P, t: y } = Bc(), m = y1(), { compareIdentifiers: C } = z9(), d = class {
constructor(E, I) {
if (I = m(I), E instanceof d) {
if (E.loose === !!I.loose && E.includePrerelease === !!I.includePrerelease)
return E;
E = E.version;
} else if (typeof E != "string")
throw new TypeError(`Invalid Version: ${E}`);
if (E.length > h)
throw new TypeError(`version is longer than ${h} characters`);
v("SemVer", E, I), this.options = I, this.loose = !!I.loose, this.includePrerelease = !!I.includePrerelease;
let c = E.trim().match(I.loose ? P[y.LOOSE] : P[y.FULL]);
if (!c)
throw new TypeError(`Invalid Version: ${E}`);
if (this.raw = E, this.major = +c[1], this.minor = +c[2], this.patch = +c[3], this.major > D || this.major < 0)
throw new TypeError("Invalid major version");
if (this.minor > D || this.minor < 0)
throw new TypeError("Invalid minor version");
if (this.patch > D || this.patch < 0)
throw new TypeError("Invalid patch version");
c[4] ? this.prerelease = c[4].split(".").map((M) => {
if (/^[0-9]+$/.test(M)) {
let q = +M;
if (q >= 0 && q < D)
return q;
}
return M;
}) : this.prerelease = [], this.build = c[5] ? c[5].split(".") : [], this.format();
}
format() {
return this.version = `${this.major}.${this.minor}.${this.patch}`, this.prerelease.length && (this.version += `-${this.prerelease.join(".")}`), this.version;
}
toString() {
return this.version;
}
compare(E) {
if (v("SemVer.compare", this.version, this.options, E), !(E instanceof d)) {
if (typeof E == "string" && E === this.version)
return 0;
E = new d(E, this.options);
}
return E.version === this.version ? 0 : this.compareMain(E) || this.comparePre(E);
}
compareMain(E) {
return E instanceof d || (E = new d(E, this.options)), C(this.major, E.major) || C(this.minor, E.minor) || C(this.patch, E.patch);
}
comparePre(E) {
if (E instanceof d || (E = new d(E, this.options)), this.prerelease.length && !E.prerelease.length)
return -1;
if (!this.prerelease.length && E.prerelease.length)
return 1;
if (!this.prerelease.length && !E.prerelease.length)
return 0;
let I = 0;
do {
let c = this.prerelease[I], M = E.prerelease[I];
if (v("prerelease compare", I, c, M), c === void 0 && M === void 0)
return 0;
if (M === void 0)
return 1;
if (c === void 0)
return -1;
if (c === M)
continue;
return C(c, M);
} while (++I);
}
compareBuild(E) {
E instanceof d || (E = new d(E, this.options));
let I = 0;
do {
let c = this.build[I], M = E.build[I];
if (v("prerelease compare", I, c, M), c === void 0 && M === void 0)
return 0;
if (M === void 0)
return 1;
if (c === void 0)
return -1;
if (c === M)
continue;
return C(c, M);
} while (++I);
}
inc(E, I) {
switch (E) {
case "premajor":
this.prerelease.length = 0, this.patch = 0, this.minor = 0, this.major++, this.inc("pre", I);
break;
case "preminor":
this.prerelease.length = 0, this.patch = 0, this.minor++, this.inc("pre", I);
break;
case "prepatch":
this.prerelease.length = 0, this.inc("patch", I), this.inc("pre", I);
break;
case "prerelease":
this.prerelease.length === 0 && this.inc("patch", I), this.inc("pre", I);
break;
case "major":
(this.minor !== 0 || this.patch !== 0 || this.prerelease.length === 0) && this.major++, this.minor = 0, this.patch = 0, this.prerelease = [];
break;
case "minor":
(this.patch !== 0 || this.prerelease.length === 0) && this.minor++, this.patch = 0, this.prerelease = [];
break;
case "patch":
this.prerelease.length === 0 && this.patch++, this.prerelease = [];
break;
case "pre":
if (this.prerelease.length === 0)
this.prerelease = [0];
else {
let c = this.prerelease.length;
for (; --c >= 0; )
typeof this.prerelease[c] == "number" && (this.prerelease[c]++, c = -2);
c === -1 && this.prerelease.push(0);
}
I && (C(this.prerelease[0], I) === 0 ? isNaN(this.prerelease[1]) && (this.prerelease = [I, 0]) : this.prerelease = [I, 0]);
break;
default:
throw new Error(`invalid increment argument: ${E}`);
}
return this.format(), this.raw = this.version, this;
}
};
_.exports = d;
} }), qc = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/parse.js"(a, _) {
De();
var { MAX_LENGTH: v } = h1(), { re: h, t: D } = Bc(), P = Bn(), y = y1(), m = (C, d) => {
if (d = y(d), C instanceof P)
return C;
if (typeof C != "string" || C.length > v || !(d.loose ? h[D.LOOSE] : h[D.FULL]).test(C))
return null;
try {
return new P(C, d);
} catch {
return null;
}
};
_.exports = m;
} }), kW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/valid.js"(a, _) {
De();
var v = qc(), h = (D, P) => {
let y = v(D, P);
return y ? y.version : null;
};
_.exports = h;
} }), IW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/clean.js"(a, _) {
De();
var v = qc(), h = (D, P) => {
let y = v(D.trim().replace(/^[=v]+/, ""), P);
return y ? y.version : null;
};
_.exports = h;
} }), NW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/inc.js"(a, _) {
De();
var v = Bn(), h = (D, P, y, m) => {
typeof y == "string" && (m = y, y = void 0);
try {
return new v(D instanceof v ? D.version : D, y).inc(P, m).version;
} catch {
return null;
}
};
_.exports = h;
} }), _a = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/compare.js"(a, _) {
De();
var v = Bn(), h = (D, P, y) => new v(D, y).compare(new v(P, y));
_.exports = h;
} }), sT = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/eq.js"(a, _) {
De();
var v = _a(), h = (D, P, y) => v(D, P, y) === 0;
_.exports = h;
} }), OW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/diff.js"(a, _) {
De();
var v = qc(), h = sT(), D = (P, y) => {
if (h(P, y))
return null;
{
let m = v(P), C = v(y), d = m.prerelease.length || C.prerelease.length, E = d ? "pre" : "", I = d ? "prerelease" : "";
for (let c in m)
if ((c === "major" || c === "minor" || c === "patch") && m[c] !== C[c])
return E + c;
return I;
}
};
_.exports = D;
} }), MW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/major.js"(a, _) {
De();
var v = Bn(), h = (D, P) => new v(D, P).major;
_.exports = h;
} }), LW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/minor.js"(a, _) {
De();
var v = Bn(), h = (D, P) => new v(D, P).minor;
_.exports = h;
} }), RW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/patch.js"(a, _) {
De();
var v = Bn(), h = (D, P) => new v(D, P).patch;
_.exports = h;
} }), jW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/prerelease.js"(a, _) {
De();
var v = qc(), h = (D, P) => {
let y = v(D, P);
return y && y.prerelease.length ? y.prerelease : null;
};
_.exports = h;
} }), JW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/rcompare.js"(a, _) {
De();
var v = _a(), h = (D, P, y) => v(P, D, y);
_.exports = h;
} }), FW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/compare-loose.js"(a, _) {
De();
var v = _a(), h = (D, P) => v(D, P, true);
_.exports = h;
} }), oT = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/compare-build.js"(a, _) {
De();
var v = Bn(), h = (D, P, y) => {
let m = new v(D, y), C = new v(P, y);
return m.compare(C) || m.compareBuild(C);
};
_.exports = h;
} }), BW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/sort.js"(a, _) {
De();
var v = oT(), h = (D, P) => D.sort((y, m) => v(y, m, P));
_.exports = h;
} }), qW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/rsort.js"(a, _) {
De();
var v = oT(), h = (D, P) => D.sort((y, m) => v(m, y, P));
_.exports = h;
} }), v1 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/gt.js"(a, _) {
De();
var v = _a(), h = (D, P, y) => v(D, P, y) > 0;
_.exports = h;
} }), _T = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/lt.js"(a, _) {
De();
var v = _a(), h = (D, P, y) => v(D, P, y) < 0;
_.exports = h;
} }), W9 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/neq.js"(a, _) {
De();
var v = _a(), h = (D, P, y) => v(D, P, y) !== 0;
_.exports = h;
} }), cT = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/gte.js"(a, _) {
De();
var v = _a(), h = (D, P, y) => v(D, P, y) >= 0;
_.exports = h;
} }), lT = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/lte.js"(a, _) {
De();
var v = _a(), h = (D, P, y) => v(D, P, y) <= 0;
_.exports = h;
} }), V9 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/cmp.js"(a, _) {
De();
var v = sT(), h = W9(), D = v1(), P = cT(), y = _T(), m = lT(), C = (d, E, I, c) => {
switch (E) {
case "===":
return typeof d == "object" && (d = d.version), typeof I == "object" && (I = I.version), d === I;
case "!==":
return typeof d == "object" && (d = d.version), typeof I == "object" && (I = I.version), d !== I;
case "":
case "=":
case "==":
return v(d, I, c);
case "!=":
return h(d, I, c);
case ">":
return D(d, I, c);
case ">=":
return P(d, I, c);
case "<":
return y(d, I, c);
case "<=":
return m(d, I, c);
default:
throw new TypeError(`Invalid operator: ${E}`);
}
};
_.exports = C;
} }), UW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/coerce.js"(a, _) {
De();
var v = Bn(), h = qc(), { re: D, t: P } = Bc(), y = (m, C) => {
if (m instanceof v)
return m;
if (typeof m == "number" && (m = String(m)), typeof m != "string")
return null;
C = C || {};
let d = null;
if (!C.rtl)
d = m.match(D[P.COERCE]);
else {
let E;
for (; (E = D[P.COERCERTL].exec(m)) && (!d || d.index + d[0].length !== m.length); )
(!d || E.index + E[0].length !== d.index + d[0].length) && (d = E), D[P.COERCERTL].lastIndex = E.index + E[1].length + E[2].length;
D[P.COERCERTL].lastIndex = -1;
}
return d === null ? null : h(`${d[2]}.${d[3] || "0"}.${d[4] || "0"}`, C);
};
_.exports = y;
} }), zW = Oe({ "node_modules/yallist/iterator.js"(a, _) {
"use strict";
De(), _.exports = function(v) {
v.prototype[Symbol.iterator] = function* () {
for (let h = this.head; h; h = h.next)
yield h.value;
};
};
} }), WW = Oe({ "node_modules/yallist/yallist.js"(a, _) {
"use strict";
De(), _.exports = v, v.Node = y, v.create = v;
function v(m) {
var C = this;
if (C instanceof v || (C = new v()), C.tail = null, C.head = null, C.length = 0, m && typeof m.forEach == "function")
m.forEach(function(I) {
C.push(I);
});
else if (arguments.length > 0)
for (var d = 0, E = arguments.length; d < E; d++)
C.push(arguments[d]);
return C;
}
v.prototype.removeNode = function(m) {
if (m.list !== this)
throw new Error("removing node which does not belong to this list");
var C = m.next, d = m.prev;
return C && (C.prev = d), d && (d.next = C), m === this.head && (this.head = C), m === this.tail && (this.tail = d), m.list.length--, m.next = null, m.prev = null, m.list = null, C;
}, v.prototype.unshiftNode = function(m) {
if (m !== this.head) {
m.list && m.list.removeNode(m);
var C = this.head;
m.list = this, m.next = C, C && (C.prev = m), this.head = m, this.tail || (this.tail = m), this.length++;
}
}, v.prototype.pushNode = function(m) {
if (m !== this.tail) {
m.list && m.list.removeNode(m);
var C = this.tail;
m.list = this, m.prev = C, C && (C.next = m), this.tail = m, this.head || (this.head = m), this.length++;
}
}, v.prototype.push = function() {
for (var m = 0, C = arguments.length; m < C; m++)
D(this, arguments[m]);
return this.length;
}, v.prototype.unshift = function() {
for (var m = 0, C = arguments.length; m < C; m++)
P(this, arguments[m]);
return this.length;
}, v.prototype.pop = function() {
if (this.tail) {
var m = this.tail.value;
return this.tail = this.tail.prev, this.tail ? this.tail.next = null : this.head = null, this.length--, m;
}
}, v.prototype.shift = function() {
if (this.head) {
var m = this.head.value;
return this.head = this.head.next, this.head ? this.head.prev = null : this.tail = null, this.length--, m;
}
}, v.prototype.forEach = function(m, C) {
C = C || this;
for (var d = this.head, E = 0; d !== null; E++)
m.call(C, d.value, E, this), d = d.next;
}, v.prototype.forEachReverse = function(m, C) {
C = C || this;
for (var d = this.tail, E = this.length - 1; d !== null; E--)
m.call(C, d.value, E, this), d = d.prev;
}, v.prototype.get = function(m) {
for (var C = 0, d = this.head; d !== null && C < m; C++)
d = d.next;
if (C === m && d !== null)
return d.value;
}, v.prototype.getReverse = function(m) {
for (var C = 0, d = this.tail; d !== null && C < m; C++)
d = d.prev;
if (C === m && d !== null)
return d.value;
}, v.prototype.map = function(m, C) {
C = C || this;
for (var d = new v(), E = this.head; E !== null; )
d.push(m.call(C, E.value, this)), E = E.next;
return d;
}, v.prototype.mapReverse = function(m, C) {
C = C || this;
for (var d = new v(), E = this.tail; E !== null; )
d.push(m.call(C, E.value, this)), E = E.prev;
return d;
}, v.prototype.reduce = function(m, C) {
var d, E = this.head;
if (arguments.length > 1)
d = C;
else if (this.head)
E = this.head.next, d = this.head.value;
else
throw new TypeError("Reduce of empty list with no initial value");
for (var I = 0; E !== null; I++)
d = m(d, E.value, I), E = E.next;
return d;
}, v.prototype.reduceReverse = function(m, C) {
var d, E = this.tail;
if (arguments.length > 1)
d = C;
else if (this.tail)
E = this.tail.prev, d = this.tail.value;
else
throw new TypeError("Reduce of empty list with no initial value");
for (var I = this.length - 1; E !== null; I--)
d = m(d, E.value, I), E = E.prev;
return d;
}, v.prototype.toArray = function() {
for (var m = new Array(this.length), C = 0, d = this.head; d !== null; C++)
m[C] = d.value, d = d.next;
return m;
}, v.prototype.toArrayReverse = function() {
for (var m = new Array(this.length), C = 0, d = this.tail; d !== null; C++)
m[C] = d.value, d = d.prev;
return m;
}, v.prototype.slice = function(m, C) {
C = C || this.length, C < 0 && (C += this.length), m = m || 0, m < 0 && (m += this.length);
var d = new v();
if (C < m || C < 0)
return d;
m < 0 && (m = 0), C > this.length && (C = this.length);
for (var E = 0, I = this.head; I !== null && E < m; E++)
I = I.next;
for (; I !== null && E < C; E++, I = I.next)
d.push(I.value);
return d;
}, v.prototype.sliceReverse = function(m, C) {
C = C || this.length, C < 0 && (C += this.length), m = m || 0, m < 0 && (m += this.length);
var d = new v();
if (C < m || C < 0)
return d;
m < 0 && (m = 0), C > this.length && (C = this.length);
for (var E = this.length, I = this.tail; I !== null && E > C; E--)
I = I.prev;
for (; I !== null && E > m; E--, I = I.prev)
d.push(I.value);
return d;
}, v.prototype.splice = function(m, C) {
m > this.length && (m = this.length - 1), m < 0 && (m = this.length + m);
for (var d = 0, E = this.head; E !== null && d < m; d++)
E = E.next;
for (var I = [], d = 0; E && d < C; d++)
I.push(E.value), E = this.removeNode(E);
E === null && (E = this.tail), E !== this.head && E !== this.tail && (E = E.prev);
for (var d = 0; d < (arguments.length <= 2 ? 0 : arguments.length - 2); d++)
E = h(this, E, d + 2 < 2 || arguments.length <= d + 2 ? void 0 : arguments[d + 2]);
return I;
}, v.prototype.reverse = function() {
for (var m = this.head, C = this.tail, d = m; d !== null; d = d.prev) {
var E = d.prev;
d.prev = d.next, d.next = E;
}
return this.head = C, this.tail = m, this;
};
function h(m, C, d) {
var E = C === m.head ? new y(d, null, C, m) : new y(d, C, C.next, m);
return E.next === null && (m.tail = E), E.prev === null && (m.head = E), m.length++, E;
}
function D(m, C) {
m.tail = new y(C, m.tail, null, m), m.head || (m.head = m.tail), m.length++;
}
function P(m, C) {
m.head = new y(C, null, m.head, m), m.tail || (m.tail = m.head), m.length++;
}
function y(m, C, d, E) {
if (!(this instanceof y))
return new y(m, C, d, E);
this.list = E, this.value = m, C ? (C.next = this, this.prev = C) : this.prev = null, d ? (d.prev = this, this.next = d) : this.next = null;
}
try {
zW()(v);
} catch {
}
} }), VW = Oe({ "node_modules/lru-cache/index.js"(a, _) {
"use strict";
De();
var v = WW(), h = Symbol("max"), D = Symbol("length"), P = Symbol("lengthCalculator"), y = Symbol("allowStale"), m = Symbol("maxAge"), C = Symbol("dispose"), d = Symbol("noDisposeOnSet"), E = Symbol("lruList"), I = Symbol("cache"), c = Symbol("updateAgeOnGet"), M = () => 1, q = class {
constructor(te) {
if (typeof te == "number" && (te = { max: te }), te || (te = {}), te.max && (typeof te.max != "number" || te.max < 0))
throw new TypeError("max must be a non-negative number");
let he = this[h] = te.max || 1 / 0, Pe = te.length || M;
if (this[P] = typeof Pe != "function" ? M : Pe, this[y] = te.stale || false, te.maxAge && typeof te.maxAge != "number")
throw new TypeError("maxAge must be a number");
this[m] = te.maxAge || 0, this[C] = te.dispose, this[d] = te.noDisposeOnSet || false, this[c] = te.updateAgeOnGet || false, this.reset();
}
set max(te) {
if (typeof te != "number" || te < 0)
throw new TypeError("max must be a non-negative number");
this[h] = te || 1 / 0, ce(this);
}
get max() {
return this[h];
}
set allowStale(te) {
this[y] = !!te;
}
get allowStale() {
return this[y];
}
set maxAge(te) {
if (typeof te != "number")
throw new TypeError("maxAge must be a non-negative number");
this[m] = te, ce(this);
}
get maxAge() {
return this[m];
}
set lengthCalculator(te) {
typeof te != "function" && (te = M), te !== this[P] && (this[P] = te, this[D] = 0, this[E].forEach((he) => {
he.length = this[P](he.value, he.key), this[D] += he.length;
})), ce(this);
}
get lengthCalculator() {
return this[P];
}
get length() {
return this[D];
}
get itemCount() {
return this[E].length;
}
rforEach(te, he) {
he = he || this;
for (let Pe = this[E].tail; Pe !== null; ) {
let R = Pe.prev;
Ae(this, te, Pe, he), Pe = R;
}
}
forEach(te, he) {
he = he || this;
for (let Pe = this[E].head; Pe !== null; ) {
let R = Pe.next;
Ae(this, te, Pe, he), Pe = R;
}
}
keys() {
return this[E].toArray().map((te) => te.key);
}
values() {
return this[E].toArray().map((te) => te.value);
}
reset() {
this[C] && this[E] && this[E].length && this[E].forEach((te) => this[C](te.key, te.value)), this[I] = /* @__PURE__ */ new Map(), this[E] = new v(), this[D] = 0;
}
dump() {
return this[E].map((te) => K(this, te) ? false : { k: te.key, v: te.value, e: te.now + (te.maxAge || 0) }).toArray().filter((te) => te);
}
dumpLru() {
return this[E];
}
set(te, he, Pe) {
if (Pe = Pe || this[m], Pe && typeof Pe != "number")
throw new TypeError("maxAge must be a number");
let R = Pe ? Date.now() : 0, pe = this[P](he, te);
if (this[I].has(te)) {
if (pe > this[h])
return Ie(this, this[I].get(te)), false;
let Xe = this[I].get(te).value;
return this[C] && (this[d] || this[C](te, Xe.value)), Xe.now = R, Xe.maxAge = Pe, Xe.value = he, this[D] += pe - Xe.length, Xe.length = pe, this.get(te), ce(this), true;
}
let ke = new me(te, he, pe, R, Pe);
return ke.length > this[h] ? (this[C] && this[C](te, he), false) : (this[D] += ke.length, this[E].unshift(ke), this[I].set(te, this[E].head), ce(this), true);
}
has(te) {
if (!this[I].has(te))
return false;
let he = this[I].get(te).value;
return !K(this, he);
}
get(te) {
return W(this, te, true);
}
peek(te) {
return W(this, te, false);
}
pop() {
let te = this[E].tail;
return te ? (Ie(this, te), te.value) : null;
}
del(te) {
Ie(this, this[I].get(te));
}
load(te) {
this.reset();
let he = Date.now();
for (let Pe = te.length - 1; Pe >= 0; Pe--) {
let R = te[Pe], pe = R.e || 0;
if (pe === 0)
this.set(R.k, R.v);
else {
let ke = pe - he;
ke > 0 && this.set(R.k, R.v, ke);
}
}
}
prune() {
this[I].forEach((te, he) => W(this, he, false));
}
}, W = (te, he, Pe) => {
let R = te[I].get(he);
if (R) {
let pe = R.value;
if (K(te, pe)) {
if (Ie(te, R), !te[y])
return;
} else
Pe && (te[c] && (R.value.now = Date.now()), te[E].unshiftNode(R));
return pe.value;
}
}, K = (te, he) => {
if (!he || !he.maxAge && !te[m])
return false;
let Pe = Date.now() - he.now;
return he.maxAge ? Pe > he.maxAge : te[m] && Pe > te[m];
}, ce = (te) => {
if (te[D] > te[h])
for (let he = te[E].tail; te[D] > te[h] && he !== null; ) {
let Pe = he.prev;
Ie(te, he), he = Pe;
}
}, Ie = (te, he) => {
if (he) {
let Pe = he.value;
te[C] && te[C](Pe.key, Pe.value), te[D] -= Pe.length, te[I].delete(Pe.key), te[E].removeNode(he);
}
}, me = class {
constructor(te, he, Pe, R, pe) {
this.key = te, this.value = he, this.length = Pe, this.now = R, this.maxAge = pe || 0;
}
}, Ae = (te, he, Pe, R) => {
let pe = Pe.value;
K(te, pe) && (Ie(te, Pe), te[y] || (pe = void 0)), pe && he.call(R, pe.value, pe.key, te);
};
_.exports = q;
} }), ca = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/classes/range.js"(a, _) {
De();
var v = class {
constructor(ee, je) {
if (je = P(je), ee instanceof v)
return ee.loose === !!je.loose && ee.includePrerelease === !!je.includePrerelease ? ee : new v(ee.raw, je);
if (ee instanceof y)
return this.raw = ee.value, this.set = [[ee]], this.format(), this;
if (this.options = je, this.loose = !!je.loose, this.includePrerelease = !!je.includePrerelease, this.raw = ee, this.set = ee.split("||").map((nt) => this.parseRange(nt.trim())).filter((nt) => nt.length), !this.set.length)
throw new TypeError(`Invalid SemVer Range: ${ee}`);
if (this.set.length > 1) {
let nt = this.set[0];
if (this.set = this.set.filter((Ze) => !q(Ze[0])), this.set.length === 0)
this.set = [nt];
else if (this.set.length > 1) {
for (let Ze of this.set)
if (Ze.length === 1 && W(Ze[0])) {
this.set = [Ze];
break;
}
}
}
this.format();
}
format() {
return this.range = this.set.map((ee) => ee.join(" ").trim()).join("||").trim(), this.range;
}
toString() {
return this.range;
}
parseRange(ee) {
ee = ee.trim();
let nt = `parseRange:${Object.keys(this.options).join(",")}:${ee}`, Ze = D.get(nt);
if (Ze)
return Ze;
let st = this.options.loose, tt = st ? d[E.HYPHENRANGELOOSE] : d[E.HYPHENRANGE];
ee = ee.replace(tt, Je(this.options.includePrerelease)), m("hyphen replace", ee), ee = ee.replace(d[E.COMPARATORTRIM], I), m("comparator trim", ee), ee = ee.replace(d[E.TILDETRIM], c), ee = ee.replace(d[E.CARETTRIM], M), ee = ee.split(/\s+/).join(" ");
let ct = ee.split(" ").map((at) => ce(at, this.options)).join(" ").split(/\s+/).map((at) => ke(at, this.options));
st && (ct = ct.filter((at) => (m("loose invalid filter", at, this.options), !!at.match(d[E.COMPARATORLOOSE])))), m("range list", ct);
let ne = /* @__PURE__ */ new Map(), ge = ct.map((at) => new y(at, this.options));
for (let at of ge) {
if (q(at))
return [at];
ne.set(at.value, at);
}
ne.size > 1 && ne.has("") && ne.delete("");
let Fe = [...ne.values()];
return D.set(nt, Fe), Fe;
}
intersects(ee, je) {
if (!(ee instanceof v))
throw new TypeError("a Range is required");
return this.set.some((nt) => K(nt, je) && ee.set.some((Ze) => K(Ze, je) && nt.every((st) => Ze.every((tt) => st.intersects(tt, je)))));
}
test(ee) {
if (!ee)
return false;
if (typeof ee == "string")
try {
ee = new C(ee, this.options);
} catch {
return false;
}
for (let je = 0; je < this.set.length; je++)
if (Xe(this.set[je], ee, this.options))
return true;
return false;
}
};
_.exports = v;
var h = VW(), D = new h({ max: 1e3 }), P = y1(), y = b1(), m = g1(), C = Bn(), { re: d, t: E, comparatorTrimReplace: I, tildeTrimReplace: c, caretTrimReplace: M } = Bc(), q = (ee) => ee.value === "<0.0.0-0", W = (ee) => ee.value === "", K = (ee, je) => {
let nt = true, Ze = ee.slice(), st = Ze.pop();
for (; nt && Ze.length; )
nt = Ze.every((tt) => st.intersects(tt, je)), st = Ze.pop();
return nt;
}, ce = (ee, je) => (m("comp", ee, je), ee = te(ee, je), m("caret", ee), ee = me(ee, je), m("tildes", ee), ee = Pe(ee, je), m("xrange", ee), ee = pe(ee, je), m("stars", ee), ee), Ie = (ee) => !ee || ee.toLowerCase() === "x" || ee === "*", me = (ee, je) => ee.trim().split(/\s+/).map((nt) => Ae(nt, je)).join(" "), Ae = (ee, je) => {
let nt = je.loose ? d[E.TILDELOOSE] : d[E.TILDE];
return ee.replace(nt, (Ze, st, tt, ct, ne) => {
m("tilde", ee, Ze, st, tt, ct, ne);
let ge;
return Ie(st) ? ge = "" : Ie(tt) ? ge = `>=${st}.0.0 <${+st + 1}.0.0-0` : Ie(ct) ? ge = `>=${st}.${tt}.0 <${st}.${+tt + 1}.0-0` : ne ? (m("replaceTilde pr", ne), ge = `>=${st}.${tt}.${ct}-${ne} <${st}.${+tt + 1}.0-0`) : ge = `>=${st}.${tt}.${ct} <${st}.${+tt + 1}.0-0`, m("tilde return", ge), ge;
});
}, te = (ee, je) => ee.trim().split(/\s+/).map((nt) => he(nt, je)).join(" "), he = (ee, je) => {
m("caret", ee, je);
let nt = je.loose ? d[E.CARETLOOSE] : d[E.CARET], Ze = je.includePrerelease ? "-0" : "";
return ee.replace(nt, (st, tt, ct, ne, ge) => {
m("caret", ee, st, tt, ct, ne, ge);
let Fe;
return Ie(tt) ? Fe = "" : Ie(ct) ? Fe = `>=${tt}.0.0${Ze} <${+tt + 1}.0.0-0` : Ie(ne) ? tt === "0" ? Fe = `>=${tt}.${ct}.0${Ze} <${tt}.${+ct + 1}.0-0` : Fe = `>=${tt}.${ct}.0${Ze} <${+tt + 1}.0.0-0` : ge ? (m("replaceCaret pr", ge), tt === "0" ? ct === "0" ? Fe = `>=${tt}.${ct}.${ne}-${ge} <${tt}.${ct}.${+ne + 1}-0` : Fe = `>=${tt}.${ct}.${ne}-${ge} <${tt}.${+ct + 1}.0-0` : Fe = `>=${tt}.${ct}.${ne}-${ge} <${+tt + 1}.0.0-0`) : (m("no pr"), tt === "0" ? ct === "0" ? Fe = `>=${tt}.${ct}.${ne}${Ze} <${tt}.${ct}.${+ne + 1}-0` : Fe = `>=${tt}.${ct}.${ne}${Ze} <${tt}.${+ct + 1}.0-0` : Fe = `>=${tt}.${ct}.${ne} <${+tt + 1}.0.0-0`), m("caret return", Fe), Fe;
});
}, Pe = (ee, je) => (m("replaceXRanges", ee, je), ee.split(/\s+/).map((nt) => R(nt, je)).join(" ")), R = (ee, je) => {
ee = ee.trim();
let nt = je.loose ? d[E.XRANGELOOSE] : d[E.XRANGE];
return ee.replace(nt, (Ze, st, tt, ct, ne, ge) => {
m("xRange", ee, Ze, st, tt, ct, ne, ge);
let Fe = Ie(tt), at = Fe || Ie(ct), Pt = at || Ie(ne), qt = Pt;
return st === "=" && qt && (st = ""), ge = je.includePrerelease ? "-0" : "", Fe ? st === ">" || st === "<" ? Ze = "<0.0.0-0" : Ze = "*" : st && qt ? (at && (ct = 0), ne = 0, st === ">" ? (st = ">=", at ? (tt = +tt + 1, ct = 0, ne = 0) : (ct = +ct + 1, ne = 0)) : st === "<=" && (st = "<", at ? tt = +tt + 1 : ct = +ct + 1), st === "<" && (ge = "-0"), Ze = `${st + tt}.${ct}.${ne}${ge}`) : at ? Ze = `>=${tt}.0.0${ge} <${+tt + 1}.0.0-0` : Pt && (Ze = `>=${tt}.${ct}.0${ge} <${tt}.${+ct + 1}.0-0`), m("xRange return", Ze), Ze;
});
}, pe = (ee, je) => (m("replaceStars", ee, je), ee.trim().replace(d[E.STAR], "")), ke = (ee, je) => (m("replaceGTE0", ee, je), ee.trim().replace(d[je.includePrerelease ? E.GTE0PRE : E.GTE0], "")), Je = (ee) => (je, nt, Ze, st, tt, ct, ne, ge, Fe, at, Pt, qt, Zr) => (Ie(Ze) ? nt = "" : Ie(st) ? nt = `>=${Ze}.0.0${ee ? "-0" : ""}` : Ie(tt) ? nt = `>=${Ze}.${st}.0${ee ? "-0" : ""}` : ct ? nt = `>=${nt}` : nt = `>=${nt}${ee ? "-0" : ""}`, Ie(Fe) ? ge = "" : Ie(at) ? ge = `<${+Fe + 1}.0.0-0` : Ie(Pt) ? ge = `<${Fe}.${+at + 1}.0-0` : qt ? ge = `<=${Fe}.${at}.${Pt}-${qt}` : ee ? ge = `<${Fe}.${at}.${+Pt + 1}-0` : ge = `<=${ge}`, `${nt} ${ge}`.trim()), Xe = (ee, je, nt) => {
for (let Ze = 0; Ze < ee.length; Ze++)
if (!ee[Ze].test(je))
return false;
if (je.prerelease.length && !nt.includePrerelease) {
for (let Ze = 0; Ze < ee.length; Ze++)
if (m(ee[Ze].semver), ee[Ze].semver !== y.ANY && ee[Ze].semver.prerelease.length > 0) {
let st = ee[Ze].semver;
if (st.major === je.major && st.minor === je.minor && st.patch === je.patch)
return true;
}
return false;
}
return true;
};
} }), b1 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/classes/comparator.js"(a, _) {
De();
var v = Symbol("SemVer ANY"), h = class {
static get ANY() {
return v;
}
constructor(I, c) {
if (c = D(c), I instanceof h) {
if (I.loose === !!c.loose)
return I;
I = I.value;
}
C("comparator", I, c), this.options = c, this.loose = !!c.loose, this.parse(I), this.semver === v ? this.value = "" : this.value = this.operator + this.semver.version, C("comp", this);
}
parse(I) {
let c = this.options.loose ? P[y.COMPARATORLOOSE] : P[y.COMPARATOR], M = I.match(c);
if (!M)
throw new TypeError(`Invalid comparator: ${I}`);
this.operator = M[1] !== void 0 ? M[1] : "", this.operator === "=" && (this.operator = ""), M[2] ? this.semver = new d(M[2], this.options.loose) : this.semver = v;
}
toString() {
return this.value;
}
test(I) {
if (C("Comparator.test", I, this.options.loose), this.semver === v || I === v)
return true;
if (typeof I == "string")
try {
I = new d(I, this.options);
} catch {
return false;
}
return m(I, this.operator, this.semver, this.options);
}
intersects(I, c) {
if (!(I instanceof h))
throw new TypeError("a Comparator is required");
if ((!c || typeof c != "object") && (c = { loose: !!c, includePrerelease: false }), this.operator === "")
return this.value === "" ? true : new E(I.value, c).test(this.value);
if (I.operator === "")
return I.value === "" ? true : new E(this.value, c).test(I.semver);
let M = (this.operator === ">=" || this.operator === ">") && (I.operator === ">=" || I.operator === ">"), q = (this.operator === "<=" || this.operator === "<") && (I.operator === "<=" || I.operator === "<"), W = this.semver.version === I.semver.version, K = (this.operator === ">=" || this.operator === "<=") && (I.operator === ">=" || I.operator === "<="), ce = m(this.semver, "<", I.semver, c) && (this.operator === ">=" || this.operator === ">") && (I.operator === "<=" || I.operator === "<"), Ie = m(this.semver, ">", I.semver, c) && (this.operator === "<=" || this.operator === "<") && (I.operator === ">=" || I.operator === ">");
return M || q || W && K || ce || Ie;
}
};
_.exports = h;
var D = y1(), { re: P, t: y } = Bc(), m = V9(), C = g1(), d = Bn(), E = ca();
} }), T1 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/functions/satisfies.js"(a, _) {
De();
var v = ca(), h = (D, P, y) => {
try {
P = new v(P, y);
} catch {
return false;
}
return P.test(D);
};
_.exports = h;
} }), HW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/to-comparators.js"(a, _) {
De();
var v = ca(), h = (D, P) => new v(D, P).set.map((y) => y.map((m) => m.value).join(" ").trim().split(" "));
_.exports = h;
} }), GW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/max-satisfying.js"(a, _) {
De();
var v = Bn(), h = ca(), D = (P, y, m) => {
let C = null, d = null, E = null;
try {
E = new h(y, m);
} catch {
return null;
}
return P.forEach((I) => {
E.test(I) && (!C || d.compare(I) === -1) && (C = I, d = new v(C, m));
}), C;
};
_.exports = D;
} }), $W = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/min-satisfying.js"(a, _) {
De();
var v = Bn(), h = ca(), D = (P, y, m) => {
let C = null, d = null, E = null;
try {
E = new h(y, m);
} catch {
return null;
}
return P.forEach((I) => {
E.test(I) && (!C || d.compare(I) === 1) && (C = I, d = new v(C, m));
}), C;
};
_.exports = D;
} }), KW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/min-version.js"(a, _) {
De();
var v = Bn(), h = ca(), D = v1(), P = (y, m) => {
y = new h(y, m);
let C = new v("0.0.0");
if (y.test(C) || (C = new v("0.0.0-0"), y.test(C)))
return C;
C = null;
for (let d = 0; d < y.set.length; ++d) {
let E = y.set[d], I = null;
E.forEach((c) => {
let M = new v(c.semver.version);
switch (c.operator) {
case ">":
M.prerelease.length === 0 ? M.patch++ : M.prerelease.push(0), M.raw = M.format();
case "":
case ">=":
(!I || D(M, I)) && (I = M);
break;
case "<":
case "<=":
break;
default:
throw new Error(`Unexpected operation: ${c.operator}`);
}
}), I && (!C || D(C, I)) && (C = I);
}
return C && y.test(C) ? C : null;
};
_.exports = P;
} }), XW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/valid.js"(a, _) {
De();
var v = ca(), h = (D, P) => {
try {
return new v(D, P).range || "*";
} catch {
return null;
}
};
_.exports = h;
} }), uT = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/outside.js"(a, _) {
De();
var v = Bn(), h = b1(), { ANY: D } = h, P = ca(), y = T1(), m = v1(), C = _T(), d = lT(), E = cT(), I = (c, M, q, W) => {
c = new v(c, W), M = new P(M, W);
let K, ce, Ie, me, Ae;
switch (q) {
case ">":
K = m, ce = d, Ie = C, me = ">", Ae = ">=";
break;
case "<":
K = C, ce = E, Ie = m, me = "<", Ae = "<=";
break;
default:
throw new TypeError('Must provide a hilo val of "<" or ">"');
}
if (y(c, M, W))
return false;
for (let te = 0; te < M.set.length; ++te) {
let he = M.set[te], Pe = null, R = null;
if (he.forEach((pe) => {
pe.semver === D && (pe = new h(">=0.0.0")), Pe = Pe || pe, R = R || pe, K(pe.semver, Pe.semver, W) ? Pe = pe : Ie(pe.semver, R.semver, W) && (R = pe);
}), Pe.operator === me || Pe.operator === Ae || (!R.operator || R.operator === me) && ce(c, R.semver))
return false;
if (R.operator === Ae && Ie(c, R.semver))
return false;
}
return true;
};
_.exports = I;
} }), YW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/gtr.js"(a, _) {
De();
var v = uT(), h = (D, P, y) => v(D, P, ">", y);
_.exports = h;
} }), QW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/ltr.js"(a, _) {
De();
var v = uT(), h = (D, P, y) => v(D, P, "<", y);
_.exports = h;
} }), ZW = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/intersects.js"(a, _) {
De();
var v = ca(), h = (D, P, y) => (D = new v(D, y), P = new v(P, y), D.intersects(P));
_.exports = h;
} }), eV = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/simplify.js"(a, _) {
De();
var v = T1(), h = _a();
_.exports = (D, P, y) => {
let m = [], C = null, d = null, E = D.sort((q, W) => h(q, W, y));
for (let q of E)
v(q, P, y) ? (d = q, C || (C = q)) : (d && m.push([C, d]), d = null, C = null);
C && m.push([C, null]);
let I = [];
for (let [q, W] of m)
q === W ? I.push(q) : !W && q === E[0] ? I.push("*") : W ? q === E[0] ? I.push(`<=${W}`) : I.push(`${q} - ${W}`) : I.push(`>=${q}`);
let c = I.join(" || "), M = typeof P.raw == "string" ? P.raw : String(P);
return c.length < M.length ? c : P;
};
} }), tV = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/ranges/subset.js"(a, _) {
De();
var v = ca(), h = b1(), { ANY: D } = h, P = T1(), y = _a(), m = function(I, c) {
let M = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {};
if (I === c)
return true;
I = new v(I, M), c = new v(c, M);
let q = false;
e:
for (let W of I.set) {
for (let K of c.set) {
let ce = C(W, K, M);
if (q = q || ce !== null, ce)
continue e;
}
if (q)
return false;
}
return true;
}, C = (I, c, M) => {
if (I === c)
return true;
if (I.length === 1 && I[0].semver === D) {
if (c.length === 1 && c[0].semver === D)
return true;
M.includePrerelease ? I = [new h(">=0.0.0-0")] : I = [new h(">=0.0.0")];
}
if (c.length === 1 && c[0].semver === D) {
if (M.includePrerelease)
return true;
c = [new h(">=0.0.0")];
}
let q = /* @__PURE__ */ new Set(), W, K;
for (let R of I)
R.operator === ">" || R.operator === ">=" ? W = d(W, R, M) : R.operator === "<" || R.operator === "<=" ? K = E(K, R, M) : q.add(R.semver);
if (q.size > 1)
return null;
let ce;
if (W && K) {
if (ce = y(W.semver, K.semver, M), ce > 0)
return null;
if (ce === 0 && (W.operator !== ">=" || K.operator !== "<="))
return null;
}
for (let R of q) {
if (W && !P(R, String(W), M) || K && !P(R, String(K), M))
return null;
for (let pe of c)
if (!P(R, String(pe), M))
return false;
return true;
}
let Ie, me, Ae, te, he = K && !M.includePrerelease && K.semver.prerelease.length ? K.semver : false, Pe = W && !M.includePrerelease && W.semver.prerelease.length ? W.semver : false;
he && he.prerelease.length === 1 && K.operator === "<" && he.prerelease[0] === 0 && (he = false);
for (let R of c) {
if (te = te || R.operator === ">" || R.operator === ">=", Ae = Ae || R.operator === "<" || R.operator === "<=", W) {
if (Pe && R.semver.prerelease && R.semver.prerelease.length && R.semver.major === Pe.major && R.semver.minor === Pe.minor && R.semver.patch === Pe.patch && (Pe = false), R.operator === ">" || R.operator === ">=") {
if (Ie = d(W, R, M), Ie === R && Ie !== W)
return false;
} else if (W.operator === ">=" && !P(W.semver, String(R), M))
return false;
}
if (K) {
if (he && R.semver.prerelease && R.semver.prerelease.length && R.semver.major === he.major && R.semver.minor === he.minor && R.semver.patch === he.patch && (he = false), R.operator === "<" || R.operator === "<=") {
if (me = E(K, R, M), me === R && me !== K)
return false;
} else if (K.operator === "<=" && !P(K.semver, String(R), M))
return false;
}
if (!R.operator && (K || W) && ce !== 0)
return false;
}
return !(W && Ae && !K && ce !== 0 || K && te && !W && ce !== 0 || Pe || he);
}, d = (I, c, M) => {
if (!I)
return c;
let q = y(I.semver, c.semver, M);
return q > 0 ? I : q < 0 || c.operator === ">" && I.operator === ">=" ? c : I;
}, E = (I, c, M) => {
if (!I)
return c;
let q = y(I.semver, c.semver, M);
return q < 0 ? I : q > 0 || c.operator === "<" && I.operator === "<=" ? c : I;
};
_.exports = m;
} }), pT = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/semver/index.js"(a, _) {
De();
var v = Bc(), h = h1(), D = Bn(), P = z9(), y = qc(), m = kW(), C = IW(), d = NW(), E = OW(), I = MW(), c = LW(), M = RW(), q = jW(), W = _a(), K = JW(), ce = FW(), Ie = oT(), me = BW(), Ae = qW(), te = v1(), he = _T(), Pe = sT(), R = W9(), pe = cT(), ke = lT(), Je = V9(), Xe = UW(), ee = b1(), je = ca(), nt = T1(), Ze = HW(), st = GW(), tt = $W(), ct = KW(), ne = XW(), ge = uT(), Fe = YW(), at = QW(), Pt = ZW(), qt = eV(), Zr = tV();
_.exports = { parse: y, valid: m, clean: C, inc: d, diff: E, major: I, minor: c, patch: M, prerelease: q, compare: W, rcompare: K, compareLoose: ce, compareBuild: Ie, sort: me, rsort: Ae, gt: te, lt: he, eq: Pe, neq: R, gte: pe, lte: ke, cmp: Je, coerce: Xe, Comparator: ee, Range: je, satisfies: nt, toComparators: Ze, maxSatisfying: st, minSatisfying: tt, minVersion: ct, validRange: ne, outside: ge, gtr: Fe, ltr: at, intersects: Pt, simplifyRange: qt, subset: Zr, SemVer: D, re: v.re, src: v.src, tokens: v.t, SEMVER_SPEC_VERSION: h.SEMVER_SPEC_VERSION, compareIdentifiers: P.compareIdentifiers, rcompareIdentifiers: P.rcompareIdentifiers };
} }), S1 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/version-check.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(C, d, E, I) {
I === void 0 && (I = E);
var c = Object.getOwnPropertyDescriptor(d, E);
(!c || ("get" in c ? !d.__esModule : c.writable || c.configurable)) && (c = { enumerable: true, get: function() {
return d[E];
} }), Object.defineProperty(C, I, c);
} : function(C, d, E, I) {
I === void 0 && (I = E), C[I] = d[E];
}), v = a && a.__setModuleDefault || (Object.create ? function(C, d) {
Object.defineProperty(C, "default", { enumerable: true, value: d });
} : function(C, d) {
C.default = d;
}), h = a && a.__importStar || function(C) {
if (C && C.__esModule)
return C;
var d = {};
if (C != null)
for (var E in C)
E !== "default" && Object.prototype.hasOwnProperty.call(C, E) && _(d, C, E);
return v(d, C), d;
};
Object.defineProperty(a, "__esModule", { value: true }), a.typescriptVersionIsAtLeast = void 0;
var D = h(pT()), P = h(vr()), y = ["3.7", "3.8", "3.9", "4.0", "4.1", "4.2", "4.3", "4.4", "4.5", "4.6", "4.7", "4.8", "4.9", "5.0"], m = {};
a.typescriptVersionIsAtLeast = m;
for (let C of y)
m[C] = true;
} }), fT = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/getModifiers.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(d, E, I, c) {
c === void 0 && (c = I);
var M = Object.getOwnPropertyDescriptor(E, I);
(!M || ("get" in M ? !E.__esModule : M.writable || M.configurable)) && (M = { enumerable: true, get: function() {
return E[I];
} }), Object.defineProperty(d, c, M);
} : function(d, E, I, c) {
c === void 0 && (c = I), d[c] = E[I];
}), v = a && a.__setModuleDefault || (Object.create ? function(d, E) {
Object.defineProperty(d, "default", { enumerable: true, value: E });
} : function(d, E) {
d.default = E;
}), h = a && a.__importStar || function(d) {
if (d && d.__esModule)
return d;
var E = {};
if (d != null)
for (var I in d)
I !== "default" && Object.prototype.hasOwnProperty.call(d, I) && _(E, d, I);
return v(E, d), E;
};
Object.defineProperty(a, "__esModule", { value: true }), a.getDecorators = a.getModifiers = void 0;
var D = h(vr()), P = S1(), y = P.typescriptVersionIsAtLeast["4.8"];
function m(d) {
var E;
if (d != null) {
if (y) {
if (D.canHaveModifiers(d)) {
let I = D.getModifiers(d);
return I ? Array.from(I) : void 0;
}
return;
}
return (E = d.modifiers) === null || E === void 0 ? void 0 : E.filter((I) => !D.isDecorator(I));
}
}
a.getModifiers = m;
function C(d) {
var E;
if (d != null) {
if (y) {
if (D.canHaveDecorators(d)) {
let I = D.getDecorators(d);
return I ? Array.from(I) : void 0;
}
return;
}
return (E = d.decorators) === null || E === void 0 ? void 0 : E.filter(D.isDecorator);
}
}
a.getDecorators = C;
} }), rV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/jsx/xhtml-entities.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.xhtmlEntities = void 0, a.xhtmlEntities = { quot: '"', amp: "&", apos: "'", lt: "<", gt: ">", nbsp: "\xA0", iexcl: "\xA1", cent: "\xA2", pound: "\xA3", curren: "\xA4", yen: "\xA5", brvbar: "\xA6", sect: "\xA7", uml: "\xA8", copy: "\xA9", ordf: "\xAA", laquo: "\xAB", not: "\xAC", shy: "\xAD", reg: "\xAE", macr: "\xAF", deg: "\xB0", plusmn: "\xB1", sup2: "\xB2", sup3: "\xB3", acute: "\xB4", micro: "\xB5", para: "\xB6", middot: "\xB7", cedil: "\xB8", sup1: "\xB9", ordm: "\xBA", raquo: "\xBB", frac14: "\xBC", frac12: "\xBD", frac34: "\xBE", iquest: "\xBF", Agrave: "\xC0", Aacute: "\xC1", Acirc: "\xC2", Atilde: "\xC3", Auml: "\xC4", Aring: "\xC5", AElig: "\xC6", Ccedil: "\xC7", Egrave: "\xC8", Eacute: "\xC9", Ecirc: "\xCA", Euml: "\xCB", Igrave: "\xCC", Iacute: "\xCD", Icirc: "\xCE", Iuml: "\xCF", ETH: "\xD0", Ntilde: "\xD1", Ograve: "\xD2", Oacute: "\xD3", Ocirc: "\xD4", Otilde: "\xD5", Ouml: "\xD6", times: "\xD7", Oslash: "\xD8", Ugrave: "\xD9", Uacute: "\xDA", Ucirc: "\xDB", Uuml: "\xDC", Yacute: "\xDD", THORN: "\xDE", szlig: "\xDF", agrave: "\xE0", aacute: "\xE1", acirc: "\xE2", atilde: "\xE3", auml: "\xE4", aring: "\xE5", aelig: "\xE6", ccedil: "\xE7", egrave: "\xE8", eacute: "\xE9", ecirc: "\xEA", euml: "\xEB", igrave: "\xEC", iacute: "\xED", icirc: "\xEE", iuml: "\xEF", eth: "\xF0", ntilde: "\xF1", ograve: "\xF2", oacute: "\xF3", ocirc: "\xF4", otilde: "\xF5", ouml: "\xF6", divide: "\xF7", oslash: "\xF8", ugrave: "\xF9", uacute: "\xFA", ucirc: "\xFB", uuml: "\xFC", yacute: "\xFD", thorn: "\xFE", yuml: "\xFF", OElig: "\u0152", oelig: "\u0153", Scaron: "\u0160", scaron: "\u0161", Yuml: "\u0178", fnof: "\u0192", circ: "\u02C6", tilde: "\u02DC", Alpha: "\u0391", Beta: "\u0392", Gamma: "\u0393", Delta: "\u0394", Epsilon: "\u0395", Zeta: "\u0396", Eta: "\u0397", Theta: "\u0398", Iota: "\u0399", Kappa: "\u039A", Lambda: "\u039B", Mu: "\u039C", Nu: "\u039D", Xi: "\u039E", Omicron: "\u039F", Pi: "\u03A0", Rho: "\u03A1", Sigma: "\u03A3", Tau: "\u03A4", Upsilon: "\u03A5", Phi: "\u03A6", Chi: "\u03A7", Psi: "\u03A8", Omega: "\u03A9", alpha: "\u03B1", beta: "\u03B2", gamma: "\u03B3", delta: "\u03B4", epsilon: "\u03B5", zeta: "\u03B6", eta: "\u03B7", theta: "\u03B8", iota: "\u03B9", kappa: "\u03BA", lambda: "\u03BB", mu: "\u03BC", nu: "\u03BD", xi: "\u03BE", omicron: "\u03BF", pi: "\u03C0", rho: "\u03C1", sigmaf: "\u03C2", sigma: "\u03C3", tau: "\u03C4", upsilon: "\u03C5", phi: "\u03C6", chi: "\u03C7", psi: "\u03C8", omega: "\u03C9", thetasym: "\u03D1", upsih: "\u03D2", piv: "\u03D6", ensp: "\u2002", emsp: "\u2003", thinsp: "\u2009", zwnj: "\u200C", zwj: "\u200D", lrm: "\u200E", rlm: "\u200F", ndash: "\u2013", mdash: "\u2014", lsquo: "\u2018", rsquo: "\u2019", sbquo: "\u201A", ldquo: "\u201C", rdquo: "\u201D", bdquo: "\u201E", dagger: "\u2020", Dagger: "\u2021", bull: "\u2022", hellip: "\u2026", permil: "\u2030", prime: "\u2032", Prime: "\u2033", lsaquo: "\u2039", rsaquo: "\u203A", oline: "\u203E", frasl: "\u2044", euro: "\u20AC", image: "\u2111", weierp: "\u2118", real: "\u211C", trade: "\u2122", alefsym: "\u2135", larr: "\u2190", uarr: "\u2191", rarr: "\u2192", darr: "\u2193", harr: "\u2194", crarr: "\u21B5", lArr: "\u21D0", uArr: "\u21D1", rArr: "\u21D2", dArr: "\u21D3", hArr: "\u21D4", forall: "\u2200", part: "\u2202", exist: "\u2203", empty: "\u2205", nabla: "\u2207", isin: "\u2208", notin: "\u2209", ni: "\u220B", prod: "\u220F", sum: "\u2211", minus: "\u2212", lowast: "\u2217", radic: "\u221A", prop: "\u221D", infin: "\u221E", ang: "\u2220", and: "\u2227", or: "\u2228", cap: "\u2229", cup: "\u222A", int: "\u222B", there4: "\u2234", sim: "\u223C", cong: "\u2245", asymp: "\u2248", ne: "\u2260", equiv: "\u2261", le: "\u2264", ge: "\u2265", sub: "\u2282", sup: "\u2283", nsub: "\u2284", sube: "\u2286", supe: "\u2287", oplus: "\u2295", otimes: "\u2297", perp: "\u22A5", sdot: "\u22C5", lceil: "\u2308", rceil: "\u2309", lfloor: "\u230A", rfloor: "\u230B", lang: "\u2329", rang: "\u232A", loz: "\u25CA", spades: "\u2660", clubs: "\u2663", hearts: "\u2665", diams: "\u2666" };
} }), H9 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/@typescript-eslint/types/dist/generated/ast-spec.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.AST_TOKEN_TYPES = a.AST_NODE_TYPES = void 0;
var _;
(function(h) {
h.AccessorProperty = "AccessorProperty", h.ArrayExpression = "ArrayExpression", h.ArrayPattern = "ArrayPattern", h.ArrowFunctionExpression = "ArrowFunctionExpression", h.AssignmentExpression = "AssignmentExpression", h.AssignmentPattern = "AssignmentPattern", h.AwaitExpression = "AwaitExpression", h.BinaryExpression = "BinaryExpression", h.BlockStatement = "BlockStatement", h.BreakStatement = "BreakStatement", h.CallExpression = "CallExpression", h.CatchClause = "CatchClause", h.ChainExpression = "ChainExpression", h.ClassBody = "ClassBody", h.ClassDeclaration = "ClassDeclaration", h.ClassExpression = "ClassExpression", h.ConditionalExpression = "ConditionalExpression", h.ContinueStatement = "ContinueStatement", h.DebuggerStatement = "DebuggerStatement", h.Decorator = "Decorator", h.DoWhileStatement = "DoWhileStatement", h.EmptyStatement = "EmptyStatement", h.ExportAllDeclaration = "ExportAllDeclaration", h.ExportDefaultDeclaration = "ExportDefaultDeclaration", h.ExportNamedDeclaration = "ExportNamedDeclaration", h.ExportSpecifier = "ExportSpecifier", h.ExpressionStatement = "ExpressionStatement", h.ForInStatement = "ForInStatement", h.ForOfStatement = "ForOfStatement", h.ForStatement = "ForStatement", h.FunctionDeclaration = "FunctionDeclaration", h.FunctionExpression = "FunctionExpression", h.Identifier = "Identifier", h.IfStatement = "IfStatement", h.ImportAttribute = "ImportAttribute", h.ImportDeclaration = "ImportDeclaration", h.ImportDefaultSpecifier = "ImportDefaultSpecifier", h.ImportExpression = "ImportExpression", h.ImportNamespaceSpecifier = "ImportNamespaceSpecifier", h.ImportSpecifier = "ImportSpecifier", h.JSXAttribute = "JSXAttribute", h.JSXClosingElement = "JSXClosingElement", h.JSXClosingFragment = "JSXClosingFragment", h.JSXElement = "JSXElement", h.JSXEmptyExpression = "JSXEmptyExpression", h.JSXExpressionContainer = "JSXExpressionContainer", h.JSXFragment = "JSXFragment", h.JSXIdentifier = "JSXIdentifier", h.JSXMemberExpression = "JSXMemberExpression", h.JSXNamespacedName = "JSXNamespacedName", h.JSXOpeningElement = "JSXOpeningElement", h.JSXOpeningFragment = "JSXOpeningFragment", h.JSXSpreadAttribute = "JSXSpreadAttribute", h.JSXSpreadChild = "JSXSpreadChild", h.JSXText = "JSXText", h.LabeledStatement = "LabeledStatement", h.Literal = "Literal", h.LogicalExpression = "LogicalExpression", h.MemberExpression = "MemberExpression", h.MetaProperty = "MetaProperty", h.MethodDefinition = "MethodDefinition", h.NewExpression = "NewExpression", h.ObjectExpression = "ObjectExpression", h.ObjectPattern = "ObjectPattern", h.PrivateIdentifier = "PrivateIdentifier", h.Program = "Program", h.Property = "Property", h.PropertyDefinition = "PropertyDefinition", h.RestElement = "RestElement", h.ReturnStatement = "ReturnStatement", h.SequenceExpression = "SequenceExpression", h.SpreadElement = "SpreadElement", h.StaticBlock = "StaticBlock", h.Super = "Super", h.SwitchCase = "SwitchCase", h.SwitchStatement = "SwitchStatement", h.TaggedTemplateExpression = "TaggedTemplateExpression", h.TemplateElement = "TemplateElement", h.TemplateLiteral = "TemplateLiteral", h.ThisExpression = "ThisExpression", h.ThrowStatement = "ThrowStatement", h.TryStatement = "TryStatement", h.UnaryExpression = "UnaryExpression", h.UpdateExpression = "UpdateExpression", h.VariableDeclaration = "VariableDeclaration", h.VariableDeclarator = "VariableDeclarator", h.WhileStatement = "WhileStatement", h.WithStatement = "WithStatement", h.YieldExpression = "YieldExpression", h.TSAbstractAccessorProperty = "TSAbstractAccessorProperty", h.TSAbstractKeyword = "TSAbstractKeyword", h.TSAbstractMethodDefinition = "TSAbstractMethodDefinition", h.TSAbstractPropertyDefinition = "TSAbstractPropertyDefinition", h.TSAnyKeyword = "TSAnyKeyword", h.TSArrayType = "TSArrayType", h.TSAsExpression = "TSAsExpression", h.TSAsyncKeyword = "TSAsyncKeyword", h.TSBigIntKeyword = "TSBigIntKeyword", h.TSBooleanKeyword = "TSBooleanKeyword", h.TSCallSignatureDeclaration = "TSCallSignatureDeclaration", h.TSClassImplements = "TSClassImplements", h.TSConditionalType = "TSConditionalType", h.TSConstructorType = "TSConstructorType", h.TSConstructSignatureDeclaration = "TSConstructSignatureDeclaration", h.TSDeclareFunction = "TSDeclareFunction", h.TSDeclareKeyword = "TSDeclareKeyword", h.TSEmptyBodyFunctionExpression = "TSEmptyBodyFunctionExpression", h.TSEnumDeclaration = "TSEnumDeclaration", h.TSEnumMember = "TSEnumMember", h.TSExportAssignment = "TSExportAssignment", h.TSExportKeyword = "TSExportKeyword", h.TSExternalModuleReference = "TSExternalModuleReference", h.TSFunctionType = "TSFunctionType", h.TSInstantiationExpression = "TSInstantiationExpression", h.TSImportEqualsDeclaration = "TSImportEqualsDeclaration", h.TSImportType = "TSImportType", h.TSIndexedAccessType = "TSIndexedAccessType", h.TSIndexSignature = "TSIndexSignature", h.TSInferType = "TSInferType", h.TSInterfaceBody = "TSInterfaceBody", h.TSInterfaceDeclaration = "TSInterfaceDeclaration", h.TSInterfaceHeritage = "TSInterfaceHeritage", h.TSIntersectionType = "TSIntersectionType", h.TSIntrinsicKeyword = "TSIntrinsicKeyword", h.TSLiteralType = "TSLiteralType", h.TSMappedType = "TSMappedType", h.TSMethodSignature = "TSMethodSignature", h.TSModuleBlock = "TSModuleBlock", h.TSModuleDeclaration = "TSModuleDeclaration", h.TSNamedTupleMember = "TSNamedTupleMember", h.TSNamespaceExportDeclaration = "TSNamespaceExportDeclaration", h.TSNeverKeyword = "TSNeverKeyword", h.TSNonNullExpression = "TSNonNullExpression", h.TSNullKeyword = "TSNullKeyword", h.TSNumberKeyword = "TSNumberKeyword", h.TSObjectKeyword = "TSObjectKeyword", h.TSOptionalType = "TSOptionalType", h.TSParameterProperty = "TSParameterProperty", h.TSPrivateKeyword = "TSPrivateKeyword", h.TSPropertySignature = "TSPropertySignature", h.TSProtectedKeyword = "TSProtectedKeyword", h.TSPublicKeyword = "TSPublicKeyword", h.TSQualifiedName = "TSQualifiedName", h.TSReadonlyKeyword = "TSReadonlyKeyword", h.TSRestType = "TSRestType", h.TSSatisfiesExpression = "TSSatisfiesExpression", h.TSStaticKeyword = "TSStaticKeyword", h.TSStringKeyword = "TSStringKeyword", h.TSSymbolKeyword = "TSSymbolKeyword", h.TSTemplateLiteralType = "TSTemplateLiteralType", h.TSThisType = "TSThisType", h.TSTupleType = "TSTupleType", h.TSTypeAliasDeclaration = "TSTypeAliasDeclaration", h.TSTypeAnnotation = "TSTypeAnnotation", h.TSTypeAssertion = "TSTypeAssertion", h.TSTypeLiteral = "TSTypeLiteral", h.TSTypeOperator = "TSTypeOperator", h.TSTypeParameter = "TSTypeParameter", h.TSTypeParameterDeclaration = "TSTypeParameterDeclaration", h.TSTypeParameterInstantiation = "TSTypeParameterInstantiation", h.TSTypePredicate = "TSTypePredicate", h.TSTypeQuery = "TSTypeQuery", h.TSTypeReference = "TSTypeReference", h.TSUndefinedKeyword = "TSUndefinedKeyword", h.TSUnionType = "TSUnionType", h.TSUnknownKeyword = "TSUnknownKeyword", h.TSVoidKeyword = "TSVoidKeyword";
})(_ = a.AST_NODE_TYPES || (a.AST_NODE_TYPES = {}));
var v;
(function(h) {
h.Boolean = "Boolean", h.Identifier = "Identifier", h.JSXIdentifier = "JSXIdentifier", h.JSXText = "JSXText", h.Keyword = "Keyword", h.Null = "Null", h.Numeric = "Numeric", h.Punctuator = "Punctuator", h.RegularExpression = "RegularExpression", h.String = "String", h.Template = "Template", h.Block = "Block", h.Line = "Line";
})(v = a.AST_TOKEN_TYPES || (a.AST_TOKEN_TYPES = {}));
} }), nV = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/@typescript-eslint/types/dist/lib.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
} }), iV = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/@typescript-eslint/types/dist/parser-options.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
} }), aV = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/@typescript-eslint/types/dist/ts-estree.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(D, P, y, m) {
m === void 0 && (m = y);
var C = Object.getOwnPropertyDescriptor(P, y);
(!C || ("get" in C ? !P.__esModule : C.writable || C.configurable)) && (C = { enumerable: true, get: function() {
return P[y];
} }), Object.defineProperty(D, m, C);
} : function(D, P, y, m) {
m === void 0 && (m = y), D[m] = P[y];
}), v = a && a.__setModuleDefault || (Object.create ? function(D, P) {
Object.defineProperty(D, "default", { enumerable: true, value: P });
} : function(D, P) {
D.default = P;
}), h = a && a.__importStar || function(D) {
if (D && D.__esModule)
return D;
var P = {};
if (D != null)
for (var y in D)
y !== "default" && Object.prototype.hasOwnProperty.call(D, y) && _(P, D, y);
return v(P, D), P;
};
Object.defineProperty(a, "__esModule", { value: true }), a.TSESTree = void 0, a.TSESTree = h(H9());
} }), sV = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/@typescript-eslint/types/dist/index.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(D, P, y, m) {
m === void 0 && (m = y);
var C = Object.getOwnPropertyDescriptor(P, y);
(!C || ("get" in C ? !P.__esModule : C.writable || C.configurable)) && (C = { enumerable: true, get: function() {
return P[y];
} }), Object.defineProperty(D, m, C);
} : function(D, P, y, m) {
m === void 0 && (m = y), D[m] = P[y];
}), v = a && a.__exportStar || function(D, P) {
for (var y in D)
y !== "default" && !Object.prototype.hasOwnProperty.call(P, y) && _(P, D, y);
};
Object.defineProperty(a, "__esModule", { value: true }), a.AST_TOKEN_TYPES = a.AST_NODE_TYPES = void 0;
var h = H9();
Object.defineProperty(a, "AST_NODE_TYPES", { enumerable: true, get: function() {
return h.AST_NODE_TYPES;
} }), Object.defineProperty(a, "AST_TOKEN_TYPES", { enumerable: true, get: function() {
return h.AST_TOKEN_TYPES;
} }), v(nV(), a), v(iV(), a), v(aV(), a);
} }), oV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/ts-estree/ts-nodes.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
} }), _V = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/ts-estree/estree-to-ts-node-types.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
} }), x1 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/ts-estree/index.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(D, P, y, m) {
m === void 0 && (m = y);
var C = Object.getOwnPropertyDescriptor(P, y);
(!C || ("get" in C ? !P.__esModule : C.writable || C.configurable)) && (C = { enumerable: true, get: function() {
return P[y];
} }), Object.defineProperty(D, m, C);
} : function(D, P, y, m) {
m === void 0 && (m = y), D[m] = P[y];
}), v = a && a.__exportStar || function(D, P) {
for (var y in D)
y !== "default" && !Object.prototype.hasOwnProperty.call(P, y) && _(P, D, y);
};
Object.defineProperty(a, "__esModule", { value: true }), a.TSESTree = a.AST_TOKEN_TYPES = a.AST_NODE_TYPES = void 0;
var h = sV();
Object.defineProperty(a, "AST_NODE_TYPES", { enumerable: true, get: function() {
return h.AST_NODE_TYPES;
} }), Object.defineProperty(a, "AST_TOKEN_TYPES", { enumerable: true, get: function() {
return h.AST_TOKEN_TYPES;
} }), Object.defineProperty(a, "TSESTree", { enumerable: true, get: function() {
return h.TSESTree;
} }), v(oV(), a), v(_V(), a);
} }), E1 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/node-utils.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(be, Ke, Et, Ft) {
Ft === void 0 && (Ft = Et);
var or = Object.getOwnPropertyDescriptor(Ke, Et);
(!or || ("get" in or ? !Ke.__esModule : or.writable || or.configurable)) && (or = { enumerable: true, get: function() {
return Ke[Et];
} }), Object.defineProperty(be, Ft, or);
} : function(be, Ke, Et, Ft) {
Ft === void 0 && (Ft = Et), be[Ft] = Ke[Et];
}), v = a && a.__setModuleDefault || (Object.create ? function(be, Ke) {
Object.defineProperty(be, "default", { enumerable: true, value: Ke });
} : function(be, Ke) {
be.default = Ke;
}), h = a && a.__importStar || function(be) {
if (be && be.__esModule)
return be;
var Ke = {};
if (be != null)
for (var Et in be)
Et !== "default" && Object.prototype.hasOwnProperty.call(be, Et) && _(Ke, be, Et);
return v(Ke, be), Ke;
};
Object.defineProperty(a, "__esModule", { value: true }), a.isThisInTypeQuery = a.isThisIdentifier = a.identifierIsThisKeyword = a.firstDefined = a.nodeHasTokens = a.createError = a.TSError = a.convertTokens = a.convertToken = a.getTokenType = a.isChildUnwrappableOptionalChain = a.isChainExpression = a.isOptional = a.isComputedProperty = a.unescapeStringLiteralText = a.hasJSXAncestor = a.findFirstMatchingAncestor = a.findNextToken = a.getTSNodeAccessibility = a.getDeclarationKind = a.isJSXToken = a.isToken = a.getRange = a.canContainDirective = a.getLocFor = a.getLineAndCharacterFor = a.getBinaryExpressionType = a.isJSDocComment = a.isComment = a.isComma = a.getLastModifier = a.hasModifier = a.isESTreeClassMember = a.getTextForTokenKind = a.isLogicalOperator = a.isAssignmentOperator = void 0;
var D = h(vr()), P = fT(), y = rV(), m = x1(), C = S1(), d = C.typescriptVersionIsAtLeast["5.0"], E = D.SyntaxKind, I = [E.BarBarToken, E.AmpersandAmpersandToken, E.QuestionQuestionToken];
function c(be) {
return be.kind >= E.FirstAssignment && be.kind <= E.LastAssignment;
}
a.isAssignmentOperator = c;
function M(be) {
return I.includes(be.kind);
}
a.isLogicalOperator = M;
function q(be) {
return D.tokenToString(be);
}
a.getTextForTokenKind = q;
function W(be) {
return be.kind !== E.SemicolonClassElement;
}
a.isESTreeClassMember = W;
function K(be, Ke) {
let Et = (0, P.getModifiers)(Ke);
return (Et == null ? void 0 : Et.some((Ft) => Ft.kind === be)) === true;
}
a.hasModifier = K;
function ce(be) {
var Ke;
let Et = (0, P.getModifiers)(be);
return Et == null ? null : (Ke = Et[Et.length - 1]) !== null && Ke !== void 0 ? Ke : null;
}
a.getLastModifier = ce;
function Ie(be) {
return be.kind === E.CommaToken;
}
a.isComma = Ie;
function me(be) {
return be.kind === E.SingleLineCommentTrivia || be.kind === E.MultiLineCommentTrivia;
}
a.isComment = me;
function Ae(be) {
return be.kind === E.JSDocComment;
}
a.isJSDocComment = Ae;
function te(be) {
return c(be) ? m.AST_NODE_TYPES.AssignmentExpression : M(be) ? m.AST_NODE_TYPES.LogicalExpression : m.AST_NODE_TYPES.BinaryExpression;
}
a.getBinaryExpressionType = te;
function he(be, Ke) {
let Et = Ke.getLineAndCharacterOfPosition(be);
return { line: Et.line + 1, column: Et.character };
}
a.getLineAndCharacterFor = he;
function Pe(be, Ke, Et) {
return { start: he(be, Et), end: he(Ke, Et) };
}
a.getLocFor = Pe;
function R(be) {
if (be.kind === D.SyntaxKind.Block)
switch (be.parent.kind) {
case D.SyntaxKind.Constructor:
case D.SyntaxKind.GetAccessor:
case D.SyntaxKind.SetAccessor:
case D.SyntaxKind.ArrowFunction:
case D.SyntaxKind.FunctionExpression:
case D.SyntaxKind.FunctionDeclaration:
case D.SyntaxKind.MethodDeclaration:
return true;
default:
return false;
}
return true;
}
a.canContainDirective = R;
function pe(be, Ke) {
return [be.getStart(Ke), be.getEnd()];
}
a.getRange = pe;
function ke(be) {
return be.kind >= E.FirstToken && be.kind <= E.LastToken;
}
a.isToken = ke;
function Je(be) {
return be.kind >= E.JsxElement && be.kind <= E.JsxAttribute;
}
a.isJSXToken = Je;
function Xe(be) {
return be.flags & D.NodeFlags.Let ? "let" : be.flags & D.NodeFlags.Const ? "const" : "var";
}
a.getDeclarationKind = Xe;
function ee(be) {
let Ke = (0, P.getModifiers)(be);
if (Ke == null)
return null;
for (let Et of Ke)
switch (Et.kind) {
case E.PublicKeyword:
return "public";
case E.ProtectedKeyword:
return "protected";
case E.PrivateKeyword:
return "private";
default:
break;
}
return null;
}
a.getTSNodeAccessibility = ee;
function je(be, Ke, Et) {
return Ft(Ke);
function Ft(or) {
return D.isToken(or) && or.pos === be.end ? or : la(or.getChildren(Et), (Wr) => (Wr.pos <= be.pos && Wr.end > be.end || Wr.pos === be.end) && Ri(Wr, Et) ? Ft(Wr) : void 0);
}
}
a.findNextToken = je;
function nt(be, Ke) {
for (; be; ) {
if (Ke(be))
return be;
be = be.parent;
}
}
a.findFirstMatchingAncestor = nt;
function Ze(be) {
return !!nt(be, Je);
}
a.hasJSXAncestor = Ze;
function st(be) {
return be.replace(/&(?:#\d+|#x[\da-fA-F]+|[0-9a-zA-Z]+);/g, (Ke) => {
let Et = Ke.slice(1, -1);
if (Et[0] === "#") {
let Ft = Et[1] === "x" ? parseInt(Et.slice(2), 16) : parseInt(Et.slice(1), 10);
return Ft > 1114111 ? Ke : String.fromCodePoint(Ft);
}
return y.xhtmlEntities[Et] || Ke;
});
}
a.unescapeStringLiteralText = st;
function tt(be) {
return be.kind === E.ComputedPropertyName;
}
a.isComputedProperty = tt;
function ct(be) {
return be.questionToken ? be.questionToken.kind === E.QuestionToken : false;
}
a.isOptional = ct;
function ne(be) {
return be.type === m.AST_NODE_TYPES.ChainExpression;
}
a.isChainExpression = ne;
function ge(be, Ke) {
return ne(Ke) && be.expression.kind !== D.SyntaxKind.ParenthesizedExpression;
}
a.isChildUnwrappableOptionalChain = ge;
function Fe(be) {
let Ke;
if (d && be.kind === E.Identifier ? Ke = D.identifierToKeywordKind(be) : "originalKeywordKind" in be && (Ke = be.originalKeywordKind), Ke)
return Ke === E.NullKeyword ? m.AST_TOKEN_TYPES.Null : Ke >= E.FirstFutureReservedWord && Ke <= E.LastKeyword ? m.AST_TOKEN_TYPES.Identifier : m.AST_TOKEN_TYPES.Keyword;
if (be.kind >= E.FirstKeyword && be.kind <= E.LastFutureReservedWord)
return be.kind === E.FalseKeyword || be.kind === E.TrueKeyword ? m.AST_TOKEN_TYPES.Boolean : m.AST_TOKEN_TYPES.Keyword;
if (be.kind >= E.FirstPunctuation && be.kind <= E.LastPunctuation)
return m.AST_TOKEN_TYPES.Punctuator;
if (be.kind >= E.NoSubstitutionTemplateLiteral && be.kind <= E.TemplateTail)
return m.AST_TOKEN_TYPES.Template;
switch (be.kind) {
case E.NumericLiteral:
return m.AST_TOKEN_TYPES.Numeric;
case E.JsxText:
return m.AST_TOKEN_TYPES.JSXText;
case E.StringLiteral:
return be.parent && (be.parent.kind === E.JsxAttribute || be.parent.kind === E.JsxElement) ? m.AST_TOKEN_TYPES.JSXText : m.AST_TOKEN_TYPES.String;
case E.RegularExpressionLiteral:
return m.AST_TOKEN_TYPES.RegularExpression;
case E.Identifier:
case E.ConstructorKeyword:
case E.GetKeyword:
case E.SetKeyword:
default:
}
return be.parent && be.kind === E.Identifier && (Je(be.parent) || be.parent.kind === E.PropertyAccessExpression && Ze(be)) ? m.AST_TOKEN_TYPES.JSXIdentifier : m.AST_TOKEN_TYPES.Identifier;
}
a.getTokenType = Fe;
function at(be, Ke) {
let Et = be.kind === E.JsxText ? be.getFullStart() : be.getStart(Ke), Ft = be.getEnd(), or = Ke.text.slice(Et, Ft), Wr = Fe(be);
return Wr === m.AST_TOKEN_TYPES.RegularExpression ? { type: Wr, value: or, range: [Et, Ft], loc: Pe(Et, Ft, Ke), regex: { pattern: or.slice(1, or.lastIndexOf("/")), flags: or.slice(or.lastIndexOf("/") + 1) } } : { type: Wr, value: or, range: [Et, Ft], loc: Pe(Et, Ft, Ke) };
}
a.convertToken = at;
function Pt(be) {
let Ke = [];
function Et(Ft) {
if (!(me(Ft) || Ae(Ft)))
if (ke(Ft) && Ft.kind !== E.EndOfFileToken) {
let or = at(Ft, be);
or && Ke.push(or);
} else
Ft.getChildren(be).forEach(Et);
}
return Et(be), Ke;
}
a.convertTokens = Pt;
var qt = class extends Error {
constructor(be, Ke, Et, Ft, or) {
super(be), this.fileName = Ke, this.index = Et, this.lineNumber = Ft, this.column = or, Object.defineProperty(this, "name", { value: new.target.name, enumerable: false, configurable: true });
}
};
a.TSError = qt;
function Zr(be, Ke, Et) {
let Ft = be.getLineAndCharacterOfPosition(Ke);
return new qt(Et, be.fileName, Ke, Ft.line + 1, Ft.character);
}
a.createError = Zr;
function Ri(be, Ke) {
return be.kind === E.EndOfFileToken ? !!be.jsDoc : be.getWidth(Ke) !== 0;
}
a.nodeHasTokens = Ri;
function la(be, Ke) {
if (be !== void 0)
for (let Et = 0; Et < be.length; Et++) {
let Ft = Ke(be[Et], Et);
if (Ft !== void 0)
return Ft;
}
}
a.firstDefined = la;
function ua(be) {
return (d ? D.identifierToKeywordKind(be) : be.originalKeywordKind) === E.ThisKeyword;
}
a.identifierIsThisKeyword = ua;
function Ka(be) {
return !!be && be.kind === E.Identifier && ua(be);
}
a.isThisIdentifier = Ka;
function co(be) {
if (!Ka(be))
return false;
for (; D.isQualifiedName(be.parent) && be.parent.left === be; )
be = be.parent;
return be.parent.kind === E.TypeQuery;
}
a.isThisInTypeQuery = co;
} }), G9 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/convert.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(c, M, q, W) {
W === void 0 && (W = q);
var K = Object.getOwnPropertyDescriptor(M, q);
(!K || ("get" in K ? !M.__esModule : K.writable || K.configurable)) && (K = { enumerable: true, get: function() {
return M[q];
} }), Object.defineProperty(c, W, K);
} : function(c, M, q, W) {
W === void 0 && (W = q), c[W] = M[q];
}), v = a && a.__setModuleDefault || (Object.create ? function(c, M) {
Object.defineProperty(c, "default", { enumerable: true, value: M });
} : function(c, M) {
c.default = M;
}), h = a && a.__importStar || function(c) {
if (c && c.__esModule)
return c;
var M = {};
if (c != null)
for (var q in c)
q !== "default" && Object.prototype.hasOwnProperty.call(c, q) && _(M, c, q);
return v(M, c), M;
};
Object.defineProperty(a, "__esModule", { value: true }), a.Converter = a.convertError = void 0;
var D = h(vr()), P = fT(), y = E1(), m = x1(), C = S1(), d = D.SyntaxKind;
function E(c) {
return (0, y.createError)(c.file, c.start, "message" in c && c.message || c.messageText);
}
a.convertError = E;
var I = class {
constructor(c, M) {
this.esTreeNodeToTSNodeMap = /* @__PURE__ */ new WeakMap(), this.tsNodeToESTreeNodeMap = /* @__PURE__ */ new WeakMap(), this.allowPattern = false, this.inTypeMode = false, this.ast = c, this.options = Object.assign({}, M);
}
getASTMaps() {
return { esTreeNodeToTSNodeMap: this.esTreeNodeToTSNodeMap, tsNodeToESTreeNodeMap: this.tsNodeToESTreeNodeMap };
}
convertProgram() {
return this.converter(this.ast);
}
converter(c, M, q, W) {
if (!c)
return null;
let K = this.inTypeMode, ce = this.allowPattern;
q !== void 0 && (this.inTypeMode = q), W !== void 0 && (this.allowPattern = W);
let Ie = this.convertNode(c, M != null ? M : c.parent);
return this.registerTSNodeInNodeMap(c, Ie), this.inTypeMode = K, this.allowPattern = ce, Ie;
}
fixExports(c, M) {
let q = (0, P.getModifiers)(c);
if ((q == null ? void 0 : q[0].kind) === d.ExportKeyword) {
this.registerTSNodeInNodeMap(c, M);
let W = q[0], K = q[1], ce = K && K.kind === d.DefaultKeyword, Ie = ce ? (0, y.findNextToken)(K, this.ast, this.ast) : (0, y.findNextToken)(W, this.ast, this.ast);
if (M.range[0] = Ie.getStart(this.ast), M.loc = (0, y.getLocFor)(M.range[0], M.range[1], this.ast), ce)
return this.createNode(c, { type: m.AST_NODE_TYPES.ExportDefaultDeclaration, declaration: M, range: [W.getStart(this.ast), M.range[1]], exportKind: "value" });
{
let me = M.type === m.AST_NODE_TYPES.TSInterfaceDeclaration || M.type === m.AST_NODE_TYPES.TSTypeAliasDeclaration, Ae = "declare" in M && M.declare === true;
return this.createNode(c, { type: m.AST_NODE_TYPES.ExportNamedDeclaration, declaration: M, specifiers: [], source: null, exportKind: me || Ae ? "type" : "value", range: [W.getStart(this.ast), M.range[1]], assertions: [] });
}
}
return M;
}
registerTSNodeInNodeMap(c, M) {
M && this.options.shouldPreserveNodeMaps && (this.tsNodeToESTreeNodeMap.has(c) || this.tsNodeToESTreeNodeMap.set(c, M));
}
convertPattern(c, M) {
return this.converter(c, M, this.inTypeMode, true);
}
convertChild(c, M) {
return this.converter(c, M, this.inTypeMode, false);
}
convertType(c, M) {
return this.converter(c, M, true, false);
}
createNode(c, M) {
let q = M;
return q.range || (q.range = (0, y.getRange)(c, this.ast)), q.loc || (q.loc = (0, y.getLocFor)(q.range[0], q.range[1], this.ast)), q && this.options.shouldPreserveNodeMaps && this.esTreeNodeToTSNodeMap.set(q, c), q;
}
convertBindingNameWithTypeAnnotation(c, M, q) {
let W = this.convertPattern(c);
return M && (W.typeAnnotation = this.convertTypeAnnotation(M, q), this.fixParentLocation(W, W.typeAnnotation.range)), W;
}
convertTypeAnnotation(c, M) {
let q = (M == null ? void 0 : M.kind) === d.FunctionType || (M == null ? void 0 : M.kind) === d.ConstructorType ? 2 : 1, W = c.getFullStart() - q, K = (0, y.getLocFor)(W, c.end, this.ast);
return { type: m.AST_NODE_TYPES.TSTypeAnnotation, loc: K, range: [W, c.end], typeAnnotation: this.convertType(c) };
}
convertBodyExpressions(c, M) {
let q = (0, y.canContainDirective)(M);
return c.map((W) => {
let K = this.convertChild(W);
if (q)
if (K != null && K.expression && D.isExpressionStatement(W) && D.isStringLiteral(W.expression)) {
let ce = K.expression.raw;
return K.directive = ce.slice(1, -1), K;
} else
q = false;
return K;
}).filter((W) => W);
}
convertTypeArgumentsToTypeParameters(c, M) {
let q = (0, y.findNextToken)(c, this.ast, this.ast);
return this.createNode(M, { type: m.AST_NODE_TYPES.TSTypeParameterInstantiation, range: [c.pos - 1, q.end], params: c.map((W) => this.convertType(W)) });
}
convertTSTypeParametersToTypeParametersDeclaration(c) {
let M = (0, y.findNextToken)(c, this.ast, this.ast);
return { type: m.AST_NODE_TYPES.TSTypeParameterDeclaration, range: [c.pos - 1, M.end], loc: (0, y.getLocFor)(c.pos - 1, M.end, this.ast), params: c.map((q) => this.convertType(q)) };
}
convertParameters(c) {
return c != null && c.length ? c.map((M) => {
let q = this.convertChild(M), W = (0, P.getDecorators)(M);
return W != null && W.length && (q.decorators = W.map((K) => this.convertChild(K))), q;
}) : [];
}
convertChainExpression(c, M) {
let { child: q, isOptional: W } = (() => c.type === m.AST_NODE_TYPES.MemberExpression ? { child: c.object, isOptional: c.optional } : c.type === m.AST_NODE_TYPES.CallExpression ? { child: c.callee, isOptional: c.optional } : { child: c.expression, isOptional: false })(), K = (0, y.isChildUnwrappableOptionalChain)(M, q);
if (!K && !W)
return c;
if (K && (0, y.isChainExpression)(q)) {
let ce = q.expression;
c.type === m.AST_NODE_TYPES.MemberExpression ? c.object = ce : c.type === m.AST_NODE_TYPES.CallExpression ? c.callee = ce : c.expression = ce;
}
return this.createNode(M, { type: m.AST_NODE_TYPES.ChainExpression, expression: c });
}
deeplyCopy(c) {
if (c.kind === D.SyntaxKind.JSDocFunctionType)
throw (0, y.createError)(this.ast, c.pos, "JSDoc types can only be used inside documentation comments.");
let M = `TS${d[c.kind]}`;
if (this.options.errorOnUnknownASTType && !m.AST_NODE_TYPES[M])
throw new Error(`Unknown AST_NODE_TYPE: "${M}"`);
let q = this.createNode(c, { type: M });
"type" in c && (q.typeAnnotation = c.type && "kind" in c.type && D.isTypeNode(c.type) ? this.convertTypeAnnotation(c.type, c) : null), "typeArguments" in c && (q.typeParameters = c.typeArguments && "pos" in c.typeArguments ? this.convertTypeArgumentsToTypeParameters(c.typeArguments, c) : null), "typeParameters" in c && (q.typeParameters = c.typeParameters && "pos" in c.typeParameters ? this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters) : null);
let W = (0, P.getDecorators)(c);
W != null && W.length && (q.decorators = W.map((ce) => this.convertChild(ce)));
let K = /* @__PURE__ */ new Set(["_children", "decorators", "end", "flags", "illegalDecorators", "heritageClauses", "locals", "localSymbol", "jsDoc", "kind", "modifierFlagsCache", "modifiers", "nextContainer", "parent", "pos", "symbol", "transformFlags", "type", "typeArguments", "typeParameters"]);
return Object.entries(c).filter((ce) => {
let [Ie] = ce;
return !K.has(Ie);
}).forEach((ce) => {
let [Ie, me] = ce;
Array.isArray(me) ? q[Ie] = me.map((Ae) => this.convertChild(Ae)) : me && typeof me == "object" && me.kind ? q[Ie] = this.convertChild(me) : q[Ie] = me;
}), q;
}
convertJSXIdentifier(c) {
let M = this.createNode(c, { type: m.AST_NODE_TYPES.JSXIdentifier, name: c.getText() });
return this.registerTSNodeInNodeMap(c, M), M;
}
convertJSXNamespaceOrIdentifier(c) {
let M = c.getText(), q = M.indexOf(":");
if (q > 0) {
let W = (0, y.getRange)(c, this.ast), K = this.createNode(c, { type: m.AST_NODE_TYPES.JSXNamespacedName, namespace: this.createNode(c, { type: m.AST_NODE_TYPES.JSXIdentifier, name: M.slice(0, q), range: [W[0], W[0] + q] }), name: this.createNode(c, { type: m.AST_NODE_TYPES.JSXIdentifier, name: M.slice(q + 1), range: [W[0] + q + 1, W[1]] }), range: W });
return this.registerTSNodeInNodeMap(c, K), K;
}
return this.convertJSXIdentifier(c);
}
convertJSXTagName(c, M) {
let q;
switch (c.kind) {
case d.PropertyAccessExpression:
if (c.name.kind === d.PrivateIdentifier)
throw new Error("Non-private identifier expected.");
q = this.createNode(c, { type: m.AST_NODE_TYPES.JSXMemberExpression, object: this.convertJSXTagName(c.expression, M), property: this.convertJSXIdentifier(c.name) });
break;
case d.ThisKeyword:
case d.Identifier:
default:
return this.convertJSXNamespaceOrIdentifier(c);
}
return this.registerTSNodeInNodeMap(c, q), q;
}
convertMethodSignature(c) {
let M = this.createNode(c, { type: m.AST_NODE_TYPES.TSMethodSignature, computed: (0, y.isComputedProperty)(c.name), key: this.convertChild(c.name), params: this.convertParameters(c.parameters), kind: (() => {
switch (c.kind) {
case d.GetAccessor:
return "get";
case d.SetAccessor:
return "set";
case d.MethodSignature:
return "method";
}
})() });
(0, y.isOptional)(c) && (M.optional = true), c.type && (M.returnType = this.convertTypeAnnotation(c.type, c)), (0, y.hasModifier)(d.ReadonlyKeyword, c) && (M.readonly = true), c.typeParameters && (M.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters));
let q = (0, y.getTSNodeAccessibility)(c);
return q && (M.accessibility = q), (0, y.hasModifier)(d.ExportKeyword, c) && (M.export = true), (0, y.hasModifier)(d.StaticKeyword, c) && (M.static = true), M;
}
convertAssertClasue(c) {
return c === void 0 ? [] : c.elements.map((M) => this.convertChild(M));
}
applyModifiersToResult(c, M) {
if (!M)
return;
let q = [];
for (let W of M)
switch (W.kind) {
case d.ExportKeyword:
case d.DefaultKeyword:
break;
case d.ConstKeyword:
c.const = true;
break;
case d.DeclareKeyword:
c.declare = true;
break;
default:
q.push(this.convertChild(W));
break;
}
q.length > 0 && (c.modifiers = q);
}
fixParentLocation(c, M) {
M[0] < c.range[0] && (c.range[0] = M[0], c.loc.start = (0, y.getLineAndCharacterFor)(c.range[0], this.ast)), M[1] > c.range[1] && (c.range[1] = M[1], c.loc.end = (0, y.getLineAndCharacterFor)(c.range[1], this.ast));
}
assertModuleSpecifier(c, M) {
var q;
if (!M && c.moduleSpecifier == null)
throw (0, y.createError)(this.ast, c.pos, "Module specifier must be a string literal.");
if (c.moduleSpecifier && ((q = c.moduleSpecifier) === null || q === void 0 ? void 0 : q.kind) !== d.StringLiteral)
throw (0, y.createError)(this.ast, c.moduleSpecifier.pos, "Module specifier must be a string literal.");
}
convertNode(c, M) {
var q, W, K, ce, Ie, me, Ae, te, he, Pe;
switch (c.kind) {
case d.SourceFile:
return this.createNode(c, { type: m.AST_NODE_TYPES.Program, body: this.convertBodyExpressions(c.statements, c), sourceType: c.externalModuleIndicator ? "module" : "script", range: [c.getStart(this.ast), c.endOfFileToken.end] });
case d.Block:
return this.createNode(c, { type: m.AST_NODE_TYPES.BlockStatement, body: this.convertBodyExpressions(c.statements, c) });
case d.Identifier:
return (0, y.isThisInTypeQuery)(c) ? this.createNode(c, { type: m.AST_NODE_TYPES.ThisExpression }) : this.createNode(c, { type: m.AST_NODE_TYPES.Identifier, name: c.text });
case d.PrivateIdentifier:
return this.createNode(c, { type: m.AST_NODE_TYPES.PrivateIdentifier, name: c.text.slice(1) });
case d.WithStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.WithStatement, object: this.convertChild(c.expression), body: this.convertChild(c.statement) });
case d.ReturnStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.ReturnStatement, argument: this.convertChild(c.expression) });
case d.LabeledStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.LabeledStatement, label: this.convertChild(c.label), body: this.convertChild(c.statement) });
case d.ContinueStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.ContinueStatement, label: this.convertChild(c.label) });
case d.BreakStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.BreakStatement, label: this.convertChild(c.label) });
case d.IfStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.IfStatement, test: this.convertChild(c.expression), consequent: this.convertChild(c.thenStatement), alternate: this.convertChild(c.elseStatement) });
case d.SwitchStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.SwitchStatement, discriminant: this.convertChild(c.expression), cases: c.caseBlock.clauses.map((R) => this.convertChild(R)) });
case d.CaseClause:
case d.DefaultClause:
return this.createNode(c, { type: m.AST_NODE_TYPES.SwitchCase, test: c.kind === d.CaseClause ? this.convertChild(c.expression) : null, consequent: c.statements.map((R) => this.convertChild(R)) });
case d.ThrowStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.ThrowStatement, argument: this.convertChild(c.expression) });
case d.TryStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.TryStatement, block: this.convertChild(c.tryBlock), handler: this.convertChild(c.catchClause), finalizer: this.convertChild(c.finallyBlock) });
case d.CatchClause:
return this.createNode(c, { type: m.AST_NODE_TYPES.CatchClause, param: c.variableDeclaration ? this.convertBindingNameWithTypeAnnotation(c.variableDeclaration.name, c.variableDeclaration.type) : null, body: this.convertChild(c.block) });
case d.WhileStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.WhileStatement, test: this.convertChild(c.expression), body: this.convertChild(c.statement) });
case d.DoStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.DoWhileStatement, test: this.convertChild(c.expression), body: this.convertChild(c.statement) });
case d.ForStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.ForStatement, init: this.convertChild(c.initializer), test: this.convertChild(c.condition), update: this.convertChild(c.incrementor), body: this.convertChild(c.statement) });
case d.ForInStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.ForInStatement, left: this.convertPattern(c.initializer), right: this.convertChild(c.expression), body: this.convertChild(c.statement) });
case d.ForOfStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.ForOfStatement, left: this.convertPattern(c.initializer), right: this.convertChild(c.expression), body: this.convertChild(c.statement), await: Boolean(c.awaitModifier && c.awaitModifier.kind === d.AwaitKeyword) });
case d.FunctionDeclaration: {
let R = (0, y.hasModifier)(d.DeclareKeyword, c), pe = this.createNode(c, { type: R || !c.body ? m.AST_NODE_TYPES.TSDeclareFunction : m.AST_NODE_TYPES.FunctionDeclaration, id: this.convertChild(c.name), generator: !!c.asteriskToken, expression: false, async: (0, y.hasModifier)(d.AsyncKeyword, c), params: this.convertParameters(c.parameters), body: this.convertChild(c.body) || void 0 });
return c.type && (pe.returnType = this.convertTypeAnnotation(c.type, c)), c.typeParameters && (pe.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters)), R && (pe.declare = true), this.fixExports(c, pe);
}
case d.VariableDeclaration: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.VariableDeclarator, id: this.convertBindingNameWithTypeAnnotation(c.name, c.type, c), init: this.convertChild(c.initializer) });
return c.exclamationToken && (R.definite = true), R;
}
case d.VariableStatement: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.VariableDeclaration, declarations: c.declarationList.declarations.map((pe) => this.convertChild(pe)), kind: (0, y.getDeclarationKind)(c.declarationList) });
return (0, y.hasModifier)(d.DeclareKeyword, c) && (R.declare = true), this.fixExports(c, R);
}
case d.VariableDeclarationList:
return this.createNode(c, { type: m.AST_NODE_TYPES.VariableDeclaration, declarations: c.declarations.map((R) => this.convertChild(R)), kind: (0, y.getDeclarationKind)(c) });
case d.ExpressionStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.ExpressionStatement, expression: this.convertChild(c.expression) });
case d.ThisKeyword:
return this.createNode(c, { type: m.AST_NODE_TYPES.ThisExpression });
case d.ArrayLiteralExpression:
return this.allowPattern ? this.createNode(c, { type: m.AST_NODE_TYPES.ArrayPattern, elements: c.elements.map((R) => this.convertPattern(R)) }) : this.createNode(c, { type: m.AST_NODE_TYPES.ArrayExpression, elements: c.elements.map((R) => this.convertChild(R)) });
case d.ObjectLiteralExpression:
return this.allowPattern ? this.createNode(c, { type: m.AST_NODE_TYPES.ObjectPattern, properties: c.properties.map((R) => this.convertPattern(R)) }) : this.createNode(c, { type: m.AST_NODE_TYPES.ObjectExpression, properties: c.properties.map((R) => this.convertChild(R)) });
case d.PropertyAssignment:
return this.createNode(c, { type: m.AST_NODE_TYPES.Property, key: this.convertChild(c.name), value: this.converter(c.initializer, c, this.inTypeMode, this.allowPattern), computed: (0, y.isComputedProperty)(c.name), method: false, shorthand: false, kind: "init" });
case d.ShorthandPropertyAssignment:
return c.objectAssignmentInitializer ? this.createNode(c, { type: m.AST_NODE_TYPES.Property, key: this.convertChild(c.name), value: this.createNode(c, { type: m.AST_NODE_TYPES.AssignmentPattern, left: this.convertPattern(c.name), right: this.convertChild(c.objectAssignmentInitializer) }), computed: false, method: false, shorthand: true, kind: "init" }) : this.createNode(c, { type: m.AST_NODE_TYPES.Property, key: this.convertChild(c.name), value: this.convertChild(c.name), computed: false, method: false, shorthand: true, kind: "init" });
case d.ComputedPropertyName:
return this.convertChild(c.expression);
case d.PropertyDeclaration: {
let R = (0, y.hasModifier)(d.AbstractKeyword, c), pe = (0, y.hasModifier)(d.AccessorKeyword, c), ke = (() => pe ? R ? m.AST_NODE_TYPES.TSAbstractAccessorProperty : m.AST_NODE_TYPES.AccessorProperty : R ? m.AST_NODE_TYPES.TSAbstractPropertyDefinition : m.AST_NODE_TYPES.PropertyDefinition)(), Je = this.createNode(c, { type: ke, key: this.convertChild(c.name), value: R ? null : this.convertChild(c.initializer), computed: (0, y.isComputedProperty)(c.name), static: (0, y.hasModifier)(d.StaticKeyword, c), readonly: (0, y.hasModifier)(d.ReadonlyKeyword, c) || void 0, declare: (0, y.hasModifier)(d.DeclareKeyword, c), override: (0, y.hasModifier)(d.OverrideKeyword, c) });
c.type && (Je.typeAnnotation = this.convertTypeAnnotation(c.type, c));
let Xe = (0, P.getDecorators)(c);
Xe && (Je.decorators = Xe.map((je) => this.convertChild(je)));
let ee = (0, y.getTSNodeAccessibility)(c);
return ee && (Je.accessibility = ee), (c.name.kind === d.Identifier || c.name.kind === d.ComputedPropertyName || c.name.kind === d.PrivateIdentifier) && c.questionToken && (Je.optional = true), c.exclamationToken && (Je.definite = true), Je.key.type === m.AST_NODE_TYPES.Literal && c.questionToken && (Je.optional = true), Je;
}
case d.GetAccessor:
case d.SetAccessor:
if (c.parent.kind === d.InterfaceDeclaration || c.parent.kind === d.TypeLiteral)
return this.convertMethodSignature(c);
case d.MethodDeclaration: {
let R = this.createNode(c, { type: c.body ? m.AST_NODE_TYPES.FunctionExpression : m.AST_NODE_TYPES.TSEmptyBodyFunctionExpression, id: null, generator: !!c.asteriskToken, expression: false, async: (0, y.hasModifier)(d.AsyncKeyword, c), body: this.convertChild(c.body), range: [c.parameters.pos - 1, c.end], params: [] });
c.type && (R.returnType = this.convertTypeAnnotation(c.type, c)), c.typeParameters && (R.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters), this.fixParentLocation(R, R.typeParameters.range));
let pe;
if (M.kind === d.ObjectLiteralExpression)
R.params = c.parameters.map((ke) => this.convertChild(ke)), pe = this.createNode(c, { type: m.AST_NODE_TYPES.Property, key: this.convertChild(c.name), value: R, computed: (0, y.isComputedProperty)(c.name), method: c.kind === d.MethodDeclaration, shorthand: false, kind: "init" });
else {
R.params = this.convertParameters(c.parameters);
let ke = (0, y.hasModifier)(d.AbstractKeyword, c) ? m.AST_NODE_TYPES.TSAbstractMethodDefinition : m.AST_NODE_TYPES.MethodDefinition;
pe = this.createNode(c, { type: ke, key: this.convertChild(c.name), value: R, computed: (0, y.isComputedProperty)(c.name), static: (0, y.hasModifier)(d.StaticKeyword, c), kind: "method", override: (0, y.hasModifier)(d.OverrideKeyword, c) });
let Je = (0, P.getDecorators)(c);
Je && (pe.decorators = Je.map((ee) => this.convertChild(ee)));
let Xe = (0, y.getTSNodeAccessibility)(c);
Xe && (pe.accessibility = Xe);
}
return c.questionToken && (pe.optional = true), c.kind === d.GetAccessor ? pe.kind = "get" : c.kind === d.SetAccessor ? pe.kind = "set" : !pe.static && c.name.kind === d.StringLiteral && c.name.text === "constructor" && pe.type !== m.AST_NODE_TYPES.Property && (pe.kind = "constructor"), pe;
}
case d.Constructor: {
let R = (0, y.getLastModifier)(c), pe = R && (0, y.findNextToken)(R, c, this.ast) || c.getFirstToken(), ke = this.createNode(c, { type: c.body ? m.AST_NODE_TYPES.FunctionExpression : m.AST_NODE_TYPES.TSEmptyBodyFunctionExpression, id: null, params: this.convertParameters(c.parameters), generator: false, expression: false, async: false, body: this.convertChild(c.body), range: [c.parameters.pos - 1, c.end] });
c.typeParameters && (ke.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters), this.fixParentLocation(ke, ke.typeParameters.range)), c.type && (ke.returnType = this.convertTypeAnnotation(c.type, c));
let Je = this.createNode(c, { type: m.AST_NODE_TYPES.Identifier, name: "constructor", range: [pe.getStart(this.ast), pe.end] }), Xe = (0, y.hasModifier)(d.StaticKeyword, c), ee = this.createNode(c, { type: (0, y.hasModifier)(d.AbstractKeyword, c) ? m.AST_NODE_TYPES.TSAbstractMethodDefinition : m.AST_NODE_TYPES.MethodDefinition, key: Je, value: ke, computed: false, static: Xe, kind: Xe ? "method" : "constructor", override: false }), je = (0, y.getTSNodeAccessibility)(c);
return je && (ee.accessibility = je), ee;
}
case d.FunctionExpression: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.FunctionExpression, id: this.convertChild(c.name), generator: !!c.asteriskToken, params: this.convertParameters(c.parameters), body: this.convertChild(c.body), async: (0, y.hasModifier)(d.AsyncKeyword, c), expression: false });
return c.type && (R.returnType = this.convertTypeAnnotation(c.type, c)), c.typeParameters && (R.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters)), R;
}
case d.SuperKeyword:
return this.createNode(c, { type: m.AST_NODE_TYPES.Super });
case d.ArrayBindingPattern:
return this.createNode(c, { type: m.AST_NODE_TYPES.ArrayPattern, elements: c.elements.map((R) => this.convertPattern(R)) });
case d.OmittedExpression:
return null;
case d.ObjectBindingPattern:
return this.createNode(c, { type: m.AST_NODE_TYPES.ObjectPattern, properties: c.elements.map((R) => this.convertPattern(R)) });
case d.BindingElement:
if (M.kind === d.ArrayBindingPattern) {
let R = this.convertChild(c.name, M);
return c.initializer ? this.createNode(c, { type: m.AST_NODE_TYPES.AssignmentPattern, left: R, right: this.convertChild(c.initializer) }) : c.dotDotDotToken ? this.createNode(c, { type: m.AST_NODE_TYPES.RestElement, argument: R }) : R;
} else {
let R;
return c.dotDotDotToken ? R = this.createNode(c, { type: m.AST_NODE_TYPES.RestElement, argument: this.convertChild((q = c.propertyName) !== null && q !== void 0 ? q : c.name) }) : R = this.createNode(c, { type: m.AST_NODE_TYPES.Property, key: this.convertChild((W = c.propertyName) !== null && W !== void 0 ? W : c.name), value: this.convertChild(c.name), computed: Boolean(c.propertyName && c.propertyName.kind === d.ComputedPropertyName), method: false, shorthand: !c.propertyName, kind: "init" }), c.initializer && (R.value = this.createNode(c, { type: m.AST_NODE_TYPES.AssignmentPattern, left: this.convertChild(c.name), right: this.convertChild(c.initializer), range: [c.name.getStart(this.ast), c.initializer.end] })), R;
}
case d.ArrowFunction: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.ArrowFunctionExpression, generator: false, id: null, params: this.convertParameters(c.parameters), body: this.convertChild(c.body), async: (0, y.hasModifier)(d.AsyncKeyword, c), expression: c.body.kind !== d.Block });
return c.type && (R.returnType = this.convertTypeAnnotation(c.type, c)), c.typeParameters && (R.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters)), R;
}
case d.YieldExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.YieldExpression, delegate: !!c.asteriskToken, argument: this.convertChild(c.expression) });
case d.AwaitExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.AwaitExpression, argument: this.convertChild(c.expression) });
case d.NoSubstitutionTemplateLiteral:
return this.createNode(c, { type: m.AST_NODE_TYPES.TemplateLiteral, quasis: [this.createNode(c, { type: m.AST_NODE_TYPES.TemplateElement, value: { raw: this.ast.text.slice(c.getStart(this.ast) + 1, c.end - 1), cooked: c.text }, tail: true })], expressions: [] });
case d.TemplateExpression: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TemplateLiteral, quasis: [this.convertChild(c.head)], expressions: [] });
return c.templateSpans.forEach((pe) => {
R.expressions.push(this.convertChild(pe.expression)), R.quasis.push(this.convertChild(pe.literal));
}), R;
}
case d.TaggedTemplateExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.TaggedTemplateExpression, typeParameters: c.typeArguments ? this.convertTypeArgumentsToTypeParameters(c.typeArguments, c) : void 0, tag: this.convertChild(c.tag), quasi: this.convertChild(c.template) });
case d.TemplateHead:
case d.TemplateMiddle:
case d.TemplateTail: {
let R = c.kind === d.TemplateTail;
return this.createNode(c, { type: m.AST_NODE_TYPES.TemplateElement, value: { raw: this.ast.text.slice(c.getStart(this.ast) + 1, c.end - (R ? 1 : 2)), cooked: c.text }, tail: R });
}
case d.SpreadAssignment:
case d.SpreadElement:
return this.allowPattern ? this.createNode(c, { type: m.AST_NODE_TYPES.RestElement, argument: this.convertPattern(c.expression) }) : this.createNode(c, { type: m.AST_NODE_TYPES.SpreadElement, argument: this.convertChild(c.expression) });
case d.Parameter: {
let R, pe;
return c.dotDotDotToken ? R = pe = this.createNode(c, { type: m.AST_NODE_TYPES.RestElement, argument: this.convertChild(c.name) }) : c.initializer ? (R = this.convertChild(c.name), pe = this.createNode(c, { type: m.AST_NODE_TYPES.AssignmentPattern, left: R, right: this.convertChild(c.initializer) }), (0, P.getModifiers)(c) && (pe.range[0] = R.range[0], pe.loc = (0, y.getLocFor)(pe.range[0], pe.range[1], this.ast))) : R = pe = this.convertChild(c.name, M), c.type && (R.typeAnnotation = this.convertTypeAnnotation(c.type, c), this.fixParentLocation(R, R.typeAnnotation.range)), c.questionToken && (c.questionToken.end > R.range[1] && (R.range[1] = c.questionToken.end, R.loc.end = (0, y.getLineAndCharacterFor)(R.range[1], this.ast)), R.optional = true), (0, P.getModifiers)(c) ? this.createNode(c, { type: m.AST_NODE_TYPES.TSParameterProperty, accessibility: (K = (0, y.getTSNodeAccessibility)(c)) !== null && K !== void 0 ? K : void 0, readonly: (0, y.hasModifier)(d.ReadonlyKeyword, c) || void 0, static: (0, y.hasModifier)(d.StaticKeyword, c) || void 0, export: (0, y.hasModifier)(d.ExportKeyword, c) || void 0, override: (0, y.hasModifier)(d.OverrideKeyword, c) || void 0, parameter: pe }) : pe;
}
case d.ClassDeclaration:
case d.ClassExpression: {
let R = (ce = c.heritageClauses) !== null && ce !== void 0 ? ce : [], pe = c.kind === d.ClassDeclaration ? m.AST_NODE_TYPES.ClassDeclaration : m.AST_NODE_TYPES.ClassExpression, ke = R.find((nt) => nt.token === d.ExtendsKeyword), Je = R.find((nt) => nt.token === d.ImplementsKeyword), Xe = this.createNode(c, { type: pe, id: this.convertChild(c.name), body: this.createNode(c, { type: m.AST_NODE_TYPES.ClassBody, body: [], range: [c.members.pos - 1, c.end] }), superClass: ke != null && ke.types[0] ? this.convertChild(ke.types[0].expression) : null });
if (ke) {
if (ke.types.length > 1)
throw (0, y.createError)(this.ast, ke.types[1].pos, "Classes can only extend a single class.");
!((Ie = ke.types[0]) === null || Ie === void 0) && Ie.typeArguments && (Xe.superTypeParameters = this.convertTypeArgumentsToTypeParameters(ke.types[0].typeArguments, ke.types[0]));
}
c.typeParameters && (Xe.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters)), Je && (Xe.implements = Je.types.map((nt) => this.convertChild(nt))), (0, y.hasModifier)(d.AbstractKeyword, c) && (Xe.abstract = true), (0, y.hasModifier)(d.DeclareKeyword, c) && (Xe.declare = true);
let ee = (0, P.getDecorators)(c);
ee && (Xe.decorators = ee.map((nt) => this.convertChild(nt)));
let je = c.members.filter(y.isESTreeClassMember);
return je.length && (Xe.body.body = je.map((nt) => this.convertChild(nt))), this.fixExports(c, Xe);
}
case d.ModuleBlock:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSModuleBlock, body: this.convertBodyExpressions(c.statements, c) });
case d.ImportDeclaration: {
this.assertModuleSpecifier(c, false);
let R = this.createNode(c, { type: m.AST_NODE_TYPES.ImportDeclaration, source: this.convertChild(c.moduleSpecifier), specifiers: [], importKind: "value", assertions: this.convertAssertClasue(c.assertClause) });
if (c.importClause && (c.importClause.isTypeOnly && (R.importKind = "type"), c.importClause.name && R.specifiers.push(this.convertChild(c.importClause)), c.importClause.namedBindings))
switch (c.importClause.namedBindings.kind) {
case d.NamespaceImport:
R.specifiers.push(this.convertChild(c.importClause.namedBindings));
break;
case d.NamedImports:
R.specifiers = R.specifiers.concat(c.importClause.namedBindings.elements.map((pe) => this.convertChild(pe)));
break;
}
return R;
}
case d.NamespaceImport:
return this.createNode(c, { type: m.AST_NODE_TYPES.ImportNamespaceSpecifier, local: this.convertChild(c.name) });
case d.ImportSpecifier:
return this.createNode(c, { type: m.AST_NODE_TYPES.ImportSpecifier, local: this.convertChild(c.name), imported: this.convertChild((me = c.propertyName) !== null && me !== void 0 ? me : c.name), importKind: c.isTypeOnly ? "type" : "value" });
case d.ImportClause: {
let R = this.convertChild(c.name);
return this.createNode(c, { type: m.AST_NODE_TYPES.ImportDefaultSpecifier, local: R, range: R.range });
}
case d.ExportDeclaration:
return ((Ae = c.exportClause) === null || Ae === void 0 ? void 0 : Ae.kind) === d.NamedExports ? (this.assertModuleSpecifier(c, true), this.createNode(c, { type: m.AST_NODE_TYPES.ExportNamedDeclaration, source: this.convertChild(c.moduleSpecifier), specifiers: c.exportClause.elements.map((R) => this.convertChild(R)), exportKind: c.isTypeOnly ? "type" : "value", declaration: null, assertions: this.convertAssertClasue(c.assertClause) })) : (this.assertModuleSpecifier(c, false), this.createNode(c, { type: m.AST_NODE_TYPES.ExportAllDeclaration, source: this.convertChild(c.moduleSpecifier), exportKind: c.isTypeOnly ? "type" : "value", exported: c.exportClause && c.exportClause.kind === d.NamespaceExport ? this.convertChild(c.exportClause.name) : null, assertions: this.convertAssertClasue(c.assertClause) }));
case d.ExportSpecifier:
return this.createNode(c, { type: m.AST_NODE_TYPES.ExportSpecifier, local: this.convertChild((te = c.propertyName) !== null && te !== void 0 ? te : c.name), exported: this.convertChild(c.name), exportKind: c.isTypeOnly ? "type" : "value" });
case d.ExportAssignment:
return c.isExportEquals ? this.createNode(c, { type: m.AST_NODE_TYPES.TSExportAssignment, expression: this.convertChild(c.expression) }) : this.createNode(c, { type: m.AST_NODE_TYPES.ExportDefaultDeclaration, declaration: this.convertChild(c.expression), exportKind: "value" });
case d.PrefixUnaryExpression:
case d.PostfixUnaryExpression: {
let R = (0, y.getTextForTokenKind)(c.operator);
return R === "++" || R === "--" ? this.createNode(c, { type: m.AST_NODE_TYPES.UpdateExpression, operator: R, prefix: c.kind === d.PrefixUnaryExpression, argument: this.convertChild(c.operand) }) : this.createNode(c, { type: m.AST_NODE_TYPES.UnaryExpression, operator: R, prefix: c.kind === d.PrefixUnaryExpression, argument: this.convertChild(c.operand) });
}
case d.DeleteExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.UnaryExpression, operator: "delete", prefix: true, argument: this.convertChild(c.expression) });
case d.VoidExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.UnaryExpression, operator: "void", prefix: true, argument: this.convertChild(c.expression) });
case d.TypeOfExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.UnaryExpression, operator: "typeof", prefix: true, argument: this.convertChild(c.expression) });
case d.TypeOperator:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSTypeOperator, operator: (0, y.getTextForTokenKind)(c.operator), typeAnnotation: this.convertChild(c.type) });
case d.BinaryExpression:
if ((0, y.isComma)(c.operatorToken)) {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.SequenceExpression, expressions: [] }), pe = this.convertChild(c.left);
return pe.type === m.AST_NODE_TYPES.SequenceExpression && c.left.kind !== d.ParenthesizedExpression ? R.expressions = R.expressions.concat(pe.expressions) : R.expressions.push(pe), R.expressions.push(this.convertChild(c.right)), R;
} else {
let R = (0, y.getBinaryExpressionType)(c.operatorToken);
return this.allowPattern && R === m.AST_NODE_TYPES.AssignmentExpression ? this.createNode(c, { type: m.AST_NODE_TYPES.AssignmentPattern, left: this.convertPattern(c.left, c), right: this.convertChild(c.right) }) : this.createNode(c, { type: R, operator: (0, y.getTextForTokenKind)(c.operatorToken.kind), left: this.converter(c.left, c, this.inTypeMode, R === m.AST_NODE_TYPES.AssignmentExpression), right: this.convertChild(c.right) });
}
case d.PropertyAccessExpression: {
let R = this.convertChild(c.expression), pe = this.convertChild(c.name), ke = false, Je = this.createNode(c, { type: m.AST_NODE_TYPES.MemberExpression, object: R, property: pe, computed: ke, optional: c.questionDotToken !== void 0 });
return this.convertChainExpression(Je, c);
}
case d.ElementAccessExpression: {
let R = this.convertChild(c.expression), pe = this.convertChild(c.argumentExpression), ke = true, Je = this.createNode(c, { type: m.AST_NODE_TYPES.MemberExpression, object: R, property: pe, computed: ke, optional: c.questionDotToken !== void 0 });
return this.convertChainExpression(Je, c);
}
case d.CallExpression: {
if (c.expression.kind === d.ImportKeyword) {
if (c.arguments.length !== 1 && c.arguments.length !== 2)
throw (0, y.createError)(this.ast, c.arguments.pos, "Dynamic import requires exactly one or two arguments.");
return this.createNode(c, { type: m.AST_NODE_TYPES.ImportExpression, source: this.convertChild(c.arguments[0]), attributes: c.arguments[1] ? this.convertChild(c.arguments[1]) : null });
}
let R = this.convertChild(c.expression), pe = c.arguments.map((Je) => this.convertChild(Je)), ke = this.createNode(c, { type: m.AST_NODE_TYPES.CallExpression, callee: R, arguments: pe, optional: c.questionDotToken !== void 0 });
return c.typeArguments && (ke.typeParameters = this.convertTypeArgumentsToTypeParameters(c.typeArguments, c)), this.convertChainExpression(ke, c);
}
case d.NewExpression: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.NewExpression, callee: this.convertChild(c.expression), arguments: c.arguments ? c.arguments.map((pe) => this.convertChild(pe)) : [] });
return c.typeArguments && (R.typeParameters = this.convertTypeArgumentsToTypeParameters(c.typeArguments, c)), R;
}
case d.ConditionalExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.ConditionalExpression, test: this.convertChild(c.condition), consequent: this.convertChild(c.whenTrue), alternate: this.convertChild(c.whenFalse) });
case d.MetaProperty:
return this.createNode(c, { type: m.AST_NODE_TYPES.MetaProperty, meta: this.createNode(c.getFirstToken(), { type: m.AST_NODE_TYPES.Identifier, name: (0, y.getTextForTokenKind)(c.keywordToken) }), property: this.convertChild(c.name) });
case d.Decorator:
return this.createNode(c, { type: m.AST_NODE_TYPES.Decorator, expression: this.convertChild(c.expression) });
case d.StringLiteral:
return this.createNode(c, { type: m.AST_NODE_TYPES.Literal, value: M.kind === d.JsxAttribute ? (0, y.unescapeStringLiteralText)(c.text) : c.text, raw: c.getText() });
case d.NumericLiteral:
return this.createNode(c, { type: m.AST_NODE_TYPES.Literal, value: Number(c.text), raw: c.getText() });
case d.BigIntLiteral: {
let R = (0, y.getRange)(c, this.ast), pe = this.ast.text.slice(R[0], R[1]), ke = pe.slice(0, -1).replace(/_/g, ""), Je = typeof BigInt < "u" ? BigInt(ke) : null;
return this.createNode(c, { type: m.AST_NODE_TYPES.Literal, raw: pe, value: Je, bigint: Je == null ? ke : String(Je), range: R });
}
case d.RegularExpressionLiteral: {
let R = c.text.slice(1, c.text.lastIndexOf("/")), pe = c.text.slice(c.text.lastIndexOf("/") + 1), ke = null;
try {
ke = new RegExp(R, pe);
} catch {
ke = null;
}
return this.createNode(c, { type: m.AST_NODE_TYPES.Literal, value: ke, raw: c.text, regex: { pattern: R, flags: pe } });
}
case d.TrueKeyword:
return this.createNode(c, { type: m.AST_NODE_TYPES.Literal, value: true, raw: "true" });
case d.FalseKeyword:
return this.createNode(c, { type: m.AST_NODE_TYPES.Literal, value: false, raw: "false" });
case d.NullKeyword:
return !C.typescriptVersionIsAtLeast["4.0"] && this.inTypeMode ? this.createNode(c, { type: m.AST_NODE_TYPES.TSNullKeyword }) : this.createNode(c, { type: m.AST_NODE_TYPES.Literal, value: null, raw: "null" });
case d.EmptyStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.EmptyStatement });
case d.DebuggerStatement:
return this.createNode(c, { type: m.AST_NODE_TYPES.DebuggerStatement });
case d.JsxElement:
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXElement, openingElement: this.convertChild(c.openingElement), closingElement: this.convertChild(c.closingElement), children: c.children.map((R) => this.convertChild(R)) });
case d.JsxFragment:
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXFragment, openingFragment: this.convertChild(c.openingFragment), closingFragment: this.convertChild(c.closingFragment), children: c.children.map((R) => this.convertChild(R)) });
case d.JsxSelfClosingElement:
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXElement, openingElement: this.createNode(c, { type: m.AST_NODE_TYPES.JSXOpeningElement, typeParameters: c.typeArguments ? this.convertTypeArgumentsToTypeParameters(c.typeArguments, c) : void 0, selfClosing: true, name: this.convertJSXTagName(c.tagName, c), attributes: c.attributes.properties.map((R) => this.convertChild(R)), range: (0, y.getRange)(c, this.ast) }), closingElement: null, children: [] });
case d.JsxOpeningElement:
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXOpeningElement, typeParameters: c.typeArguments ? this.convertTypeArgumentsToTypeParameters(c.typeArguments, c) : void 0, selfClosing: false, name: this.convertJSXTagName(c.tagName, c), attributes: c.attributes.properties.map((R) => this.convertChild(R)) });
case d.JsxClosingElement:
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXClosingElement, name: this.convertJSXTagName(c.tagName, c) });
case d.JsxOpeningFragment:
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXOpeningFragment });
case d.JsxClosingFragment:
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXClosingFragment });
case d.JsxExpression: {
let R = c.expression ? this.convertChild(c.expression) : this.createNode(c, { type: m.AST_NODE_TYPES.JSXEmptyExpression, range: [c.getStart(this.ast) + 1, c.getEnd() - 1] });
return c.dotDotDotToken ? this.createNode(c, { type: m.AST_NODE_TYPES.JSXSpreadChild, expression: R }) : this.createNode(c, { type: m.AST_NODE_TYPES.JSXExpressionContainer, expression: R });
}
case d.JsxAttribute:
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXAttribute, name: this.convertJSXNamespaceOrIdentifier(c.name), value: this.convertChild(c.initializer) });
case d.JsxText: {
let R = c.getFullStart(), pe = c.getEnd(), ke = this.ast.text.slice(R, pe);
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXText, value: (0, y.unescapeStringLiteralText)(ke), raw: ke, range: [R, pe] });
}
case d.JsxSpreadAttribute:
return this.createNode(c, { type: m.AST_NODE_TYPES.JSXSpreadAttribute, argument: this.convertChild(c.expression) });
case d.QualifiedName:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSQualifiedName, left: this.convertChild(c.left), right: this.convertChild(c.right) });
case d.TypeReference:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSTypeReference, typeName: this.convertType(c.typeName), typeParameters: c.typeArguments ? this.convertTypeArgumentsToTypeParameters(c.typeArguments, c) : void 0 });
case d.TypeParameter:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSTypeParameter, name: this.convertType(c.name), constraint: c.constraint ? this.convertType(c.constraint) : void 0, default: c.default ? this.convertType(c.default) : void 0, in: (0, y.hasModifier)(d.InKeyword, c), out: (0, y.hasModifier)(d.OutKeyword, c), const: (0, y.hasModifier)(d.ConstKeyword, c) });
case d.ThisType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSThisType });
case d.AnyKeyword:
case d.BigIntKeyword:
case d.BooleanKeyword:
case d.NeverKeyword:
case d.NumberKeyword:
case d.ObjectKeyword:
case d.StringKeyword:
case d.SymbolKeyword:
case d.UnknownKeyword:
case d.VoidKeyword:
case d.UndefinedKeyword:
case d.IntrinsicKeyword:
return this.createNode(c, { type: m.AST_NODE_TYPES[`TS${d[c.kind]}`] });
case d.NonNullExpression: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSNonNullExpression, expression: this.convertChild(c.expression) });
return this.convertChainExpression(R, c);
}
case d.TypeLiteral:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSTypeLiteral, members: c.members.map((R) => this.convertChild(R)) });
case d.ArrayType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSArrayType, elementType: this.convertType(c.elementType) });
case d.IndexedAccessType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSIndexedAccessType, objectType: this.convertType(c.objectType), indexType: this.convertType(c.indexType) });
case d.ConditionalType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSConditionalType, checkType: this.convertType(c.checkType), extendsType: this.convertType(c.extendsType), trueType: this.convertType(c.trueType), falseType: this.convertType(c.falseType) });
case d.TypeQuery:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSTypeQuery, exprName: this.convertType(c.exprName), typeParameters: c.typeArguments && this.convertTypeArgumentsToTypeParameters(c.typeArguments, c) });
case d.MappedType: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSMappedType, typeParameter: this.convertType(c.typeParameter), nameType: (he = this.convertType(c.nameType)) !== null && he !== void 0 ? he : null });
return c.readonlyToken && (c.readonlyToken.kind === d.ReadonlyKeyword ? R.readonly = true : R.readonly = (0, y.getTextForTokenKind)(c.readonlyToken.kind)), c.questionToken && (c.questionToken.kind === d.QuestionToken ? R.optional = true : R.optional = (0, y.getTextForTokenKind)(c.questionToken.kind)), c.type && (R.typeAnnotation = this.convertType(c.type)), R;
}
case d.ParenthesizedExpression:
return this.convertChild(c.expression, M);
case d.TypeAliasDeclaration: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSTypeAliasDeclaration, id: this.convertChild(c.name), typeAnnotation: this.convertType(c.type) });
return (0, y.hasModifier)(d.DeclareKeyword, c) && (R.declare = true), c.typeParameters && (R.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters)), this.fixExports(c, R);
}
case d.MethodSignature:
return this.convertMethodSignature(c);
case d.PropertySignature: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSPropertySignature, optional: (0, y.isOptional)(c) || void 0, computed: (0, y.isComputedProperty)(c.name), key: this.convertChild(c.name), typeAnnotation: c.type ? this.convertTypeAnnotation(c.type, c) : void 0, initializer: this.convertChild(c.initializer) || void 0, readonly: (0, y.hasModifier)(d.ReadonlyKeyword, c) || void 0, static: (0, y.hasModifier)(d.StaticKeyword, c) || void 0, export: (0, y.hasModifier)(d.ExportKeyword, c) || void 0 }), pe = (0, y.getTSNodeAccessibility)(c);
return pe && (R.accessibility = pe), R;
}
case d.IndexSignature: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSIndexSignature, parameters: c.parameters.map((ke) => this.convertChild(ke)) });
c.type && (R.typeAnnotation = this.convertTypeAnnotation(c.type, c)), (0, y.hasModifier)(d.ReadonlyKeyword, c) && (R.readonly = true);
let pe = (0, y.getTSNodeAccessibility)(c);
return pe && (R.accessibility = pe), (0, y.hasModifier)(d.ExportKeyword, c) && (R.export = true), (0, y.hasModifier)(d.StaticKeyword, c) && (R.static = true), R;
}
case d.ConstructorType: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSConstructorType, params: this.convertParameters(c.parameters), abstract: (0, y.hasModifier)(d.AbstractKeyword, c) });
return c.type && (R.returnType = this.convertTypeAnnotation(c.type, c)), c.typeParameters && (R.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters)), R;
}
case d.FunctionType:
case d.ConstructSignature:
case d.CallSignature: {
let R = c.kind === d.ConstructSignature ? m.AST_NODE_TYPES.TSConstructSignatureDeclaration : c.kind === d.CallSignature ? m.AST_NODE_TYPES.TSCallSignatureDeclaration : m.AST_NODE_TYPES.TSFunctionType, pe = this.createNode(c, { type: R, params: this.convertParameters(c.parameters) });
return c.type && (pe.returnType = this.convertTypeAnnotation(c.type, c)), c.typeParameters && (pe.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters)), pe;
}
case d.ExpressionWithTypeArguments: {
let R = M.kind, pe = R === d.InterfaceDeclaration ? m.AST_NODE_TYPES.TSInterfaceHeritage : R === d.HeritageClause ? m.AST_NODE_TYPES.TSClassImplements : m.AST_NODE_TYPES.TSInstantiationExpression, ke = this.createNode(c, { type: pe, expression: this.convertChild(c.expression) });
return c.typeArguments && (ke.typeParameters = this.convertTypeArgumentsToTypeParameters(c.typeArguments, c)), ke;
}
case d.InterfaceDeclaration: {
let R = (Pe = c.heritageClauses) !== null && Pe !== void 0 ? Pe : [], pe = this.createNode(c, { type: m.AST_NODE_TYPES.TSInterfaceDeclaration, body: this.createNode(c, { type: m.AST_NODE_TYPES.TSInterfaceBody, body: c.members.map((ke) => this.convertChild(ke)), range: [c.members.pos - 1, c.end] }), id: this.convertChild(c.name) });
if (c.typeParameters && (pe.typeParameters = this.convertTSTypeParametersToTypeParametersDeclaration(c.typeParameters)), R.length > 0) {
let ke = [], Je = [];
for (let Xe of R)
if (Xe.token === d.ExtendsKeyword)
for (let ee of Xe.types)
ke.push(this.convertChild(ee, c));
else
for (let ee of Xe.types)
Je.push(this.convertChild(ee, c));
ke.length && (pe.extends = ke), Je.length && (pe.implements = Je);
}
return (0, y.hasModifier)(d.AbstractKeyword, c) && (pe.abstract = true), (0, y.hasModifier)(d.DeclareKeyword, c) && (pe.declare = true), this.fixExports(c, pe);
}
case d.TypePredicate: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSTypePredicate, asserts: c.assertsModifier !== void 0, parameterName: this.convertChild(c.parameterName), typeAnnotation: null });
return c.type && (R.typeAnnotation = this.convertTypeAnnotation(c.type, c), R.typeAnnotation.loc = R.typeAnnotation.typeAnnotation.loc, R.typeAnnotation.range = R.typeAnnotation.typeAnnotation.range), R;
}
case d.ImportType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSImportType, isTypeOf: !!c.isTypeOf, parameter: this.convertChild(c.argument), qualifier: this.convertChild(c.qualifier), typeParameters: c.typeArguments ? this.convertTypeArgumentsToTypeParameters(c.typeArguments, c) : null });
case d.EnumDeclaration: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSEnumDeclaration, id: this.convertChild(c.name), members: c.members.map((pe) => this.convertChild(pe)) });
return this.applyModifiersToResult(R, (0, P.getModifiers)(c)), this.fixExports(c, R);
}
case d.EnumMember: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSEnumMember, id: this.convertChild(c.name) });
return c.initializer && (R.initializer = this.convertChild(c.initializer)), c.name.kind === D.SyntaxKind.ComputedPropertyName && (R.computed = true), R;
}
case d.ModuleDeclaration: {
let R = this.createNode(c, Object.assign({ type: m.AST_NODE_TYPES.TSModuleDeclaration }, (() => {
let pe = this.convertChild(c.name), ke = this.convertChild(c.body);
if (c.flags & D.NodeFlags.GlobalAugmentation) {
if (ke == null || ke.type === m.AST_NODE_TYPES.TSModuleDeclaration)
throw new Error("Expected a valid module body");
if (pe.type !== m.AST_NODE_TYPES.Identifier)
throw new Error("global module augmentation must have an Identifier id");
return { kind: "global", id: pe, body: ke, global: true };
} else if (c.flags & D.NodeFlags.Namespace) {
if (ke == null)
throw new Error("Expected a module body");
if (pe.type !== m.AST_NODE_TYPES.Identifier)
throw new Error("`namespace`s must have an Identifier id");
return { kind: "namespace", id: pe, body: ke };
} else
return Object.assign({ kind: "module", id: pe }, ke != null ? { body: ke } : {});
})()));
return this.applyModifiersToResult(R, (0, P.getModifiers)(c)), this.fixExports(c, R);
}
case d.ParenthesizedType:
return this.convertType(c.type);
case d.UnionType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSUnionType, types: c.types.map((R) => this.convertType(R)) });
case d.IntersectionType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSIntersectionType, types: c.types.map((R) => this.convertType(R)) });
case d.AsExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSAsExpression, expression: this.convertChild(c.expression), typeAnnotation: this.convertType(c.type) });
case d.InferType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSInferType, typeParameter: this.convertType(c.typeParameter) });
case d.LiteralType:
return C.typescriptVersionIsAtLeast["4.0"] && c.literal.kind === d.NullKeyword ? this.createNode(c.literal, { type: m.AST_NODE_TYPES.TSNullKeyword }) : this.createNode(c, { type: m.AST_NODE_TYPES.TSLiteralType, literal: this.convertType(c.literal) });
case d.TypeAssertionExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSTypeAssertion, typeAnnotation: this.convertType(c.type), expression: this.convertChild(c.expression) });
case d.ImportEqualsDeclaration:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSImportEqualsDeclaration, id: this.convertChild(c.name), moduleReference: this.convertChild(c.moduleReference), importKind: c.isTypeOnly ? "type" : "value", isExport: (0, y.hasModifier)(d.ExportKeyword, c) });
case d.ExternalModuleReference:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSExternalModuleReference, expression: this.convertChild(c.expression) });
case d.NamespaceExportDeclaration:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSNamespaceExportDeclaration, id: this.convertChild(c.name) });
case d.AbstractKeyword:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSAbstractKeyword });
case d.TupleType: {
let R = "elementTypes" in c ? c.elementTypes.map((pe) => this.convertType(pe)) : c.elements.map((pe) => this.convertType(pe));
return this.createNode(c, { type: m.AST_NODE_TYPES.TSTupleType, elementTypes: R });
}
case d.NamedTupleMember: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSNamedTupleMember, elementType: this.convertType(c.type, c), label: this.convertChild(c.name, c), optional: c.questionToken != null });
return c.dotDotDotToken ? (R.range[0] = R.label.range[0], R.loc.start = R.label.loc.start, this.createNode(c, { type: m.AST_NODE_TYPES.TSRestType, typeAnnotation: R })) : R;
}
case d.OptionalType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSOptionalType, typeAnnotation: this.convertType(c.type) });
case d.RestType:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSRestType, typeAnnotation: this.convertType(c.type) });
case d.TemplateLiteralType: {
let R = this.createNode(c, { type: m.AST_NODE_TYPES.TSTemplateLiteralType, quasis: [this.convertChild(c.head)], types: [] });
return c.templateSpans.forEach((pe) => {
R.types.push(this.convertChild(pe.type)), R.quasis.push(this.convertChild(pe.literal));
}), R;
}
case d.ClassStaticBlockDeclaration:
return this.createNode(c, { type: m.AST_NODE_TYPES.StaticBlock, body: this.convertBodyExpressions(c.body.statements, c) });
case d.AssertEntry:
return this.createNode(c, { type: m.AST_NODE_TYPES.ImportAttribute, key: this.convertChild(c.name), value: this.convertChild(c.value) });
case d.SatisfiesExpression:
return this.createNode(c, { type: m.AST_NODE_TYPES.TSSatisfiesExpression, expression: this.convertChild(c.expression), typeAnnotation: this.convertChild(c.type) });
default:
return this.deeplyCopy(c);
}
}
};
a.Converter = I;
} }), $a = {};
m1($a, { __assign: () => f1, __asyncDelegator: () => TV, __asyncGenerator: () => bV, __asyncValues: () => SV, __await: () => gp, __awaiter: () => dV, __classPrivateFieldGet: () => CV, __classPrivateFieldSet: () => AV, __createBinding: () => hV, __decorate: () => uV, __exportStar: () => gV, __extends: () => cV, __generator: () => mV, __importDefault: () => wV, __importStar: () => EV, __makeTemplateObject: () => xV, __metadata: () => fV, __param: () => pV, __read: () => $9, __rest: () => lV, __spread: () => yV, __spreadArrays: () => vV, __values: () => tT });
function cV(a, _) {
p1(a, _);
function v() {
this.constructor = a;
}
a.prototype = _ === null ? Object.create(_) : (v.prototype = _.prototype, new v());
}
function lV(a, _) {
var v = {};
for (var h in a)
Object.prototype.hasOwnProperty.call(a, h) && _.indexOf(h) < 0 && (v[h] = a[h]);
if (a != null && typeof Object.getOwnPropertySymbols == "function")
for (var D = 0, h = Object.getOwnPropertySymbols(a); D < h.length; D++)
_.indexOf(h[D]) < 0 && Object.prototype.propertyIsEnumerable.call(a, h[D]) && (v[h[D]] = a[h[D]]);
return v;
}
function uV(a, _, v, h) {
var D = arguments.length, P = D < 3 ? _ : h === null ? h = Object.getOwnPropertyDescriptor(_, v) : h, y;
if (typeof Reflect == "object" && typeof Reflect.decorate == "function")
P = Reflect.decorate(a, _, v, h);
else
for (var m = a.length - 1; m >= 0; m--)
(y = a[m]) && (P = (D < 3 ? y(P) : D > 3 ? y(_, v, P) : y(_, v)) || P);
return D > 3 && P && Object.defineProperty(_, v, P), P;
}
function pV(a, _) {
return function(v, h) {
_(v, h, a);
};
}
function fV(a, _) {
if (typeof Reflect == "object" && typeof Reflect.metadata == "function")
return Reflect.metadata(a, _);
}
function dV(a, _, v, h) {
function D(P) {
return P instanceof v ? P : new v(function(y) {
y(P);
});
}
return new (v || (v = Promise))(function(P, y) {
function m(E) {
try {
d(h.next(E));
} catch (I) {
y(I);
}
}
function C(E) {
try {
d(h.throw(E));
} catch (I) {
y(I);
}
}
function d(E) {
E.done ? P(E.value) : D(E.value).then(m, C);
}
d((h = h.apply(a, _ || [])).next());
});
}
function mV(a, _) {
var v = { label: 0, sent: function() {
if (P[0] & 1)
throw P[1];
return P[1];
}, trys: [], ops: [] }, h, D, P, y;
return y = { next: m(0), throw: m(1), return: m(2) }, typeof Symbol == "function" && (y[Symbol.iterator] = function() {
return this;
}), y;
function m(d) {
return function(E) {
return C([d, E]);
};
}
function C(d) {
if (h)
throw new TypeError("Generator is already executing.");
for (; v; )
try {
if (h = 1, D && (P = d[0] & 2 ? D.return : d[0] ? D.throw || ((P = D.return) && P.call(D), 0) : D.next) && !(P = P.call(D, d[1])).done)
return P;
switch (D = 0, P && (d = [d[0] & 2, P.value]), d[0]) {
case 0:
case 1:
P = d;
break;
case 4:
return v.label++, { value: d[1], done: false };
case 5:
v.label++, D = d[1], d = [0];
continue;
case 7:
d = v.ops.pop(), v.trys.pop();
continue;
default:
if (P = v.trys, !(P = P.length > 0 && P[P.length - 1]) && (d[0] === 6 || d[0] === 2)) {
v = 0;
continue;
}
if (d[0] === 3 && (!P || d[1] > P[0] && d[1] < P[3])) {
v.label = d[1];
break;
}
if (d[0] === 6 && v.label < P[1]) {
v.label = P[1], P = d;
break;
}
if (P && v.label < P[2]) {
v.label = P[2], v.ops.push(d);
break;
}
P[2] && v.ops.pop(), v.trys.pop();
continue;
}
d = _.call(a, v);
} catch (E) {
d = [6, E], D = 0;
} finally {
h = P = 0;
}
if (d[0] & 5)
throw d[1];
return { value: d[0] ? d[1] : void 0, done: true };
}
}
function hV(a, _, v, h) {
h === void 0 && (h = v), a[h] = _[v];
}
function gV(a, _) {
for (var v in a)
v !== "default" && !_.hasOwnProperty(v) && (_[v] = a[v]);
}
function tT(a) {
var _ = typeof Symbol == "function" && Symbol.iterator, v = _ && a[_], h = 0;
if (v)
return v.call(a);
if (a && typeof a.length == "number")
return { next: function() {
return a && h >= a.length && (a = void 0), { value: a && a[h++], done: !a };
} };
throw new TypeError(_ ? "Object is not iterable." : "Symbol.iterator is not defined.");
}
function $9(a, _) {
var v = typeof Symbol == "function" && a[Symbol.iterator];
if (!v)
return a;
var h = v.call(a), D, P = [], y;
try {
for (; (_ === void 0 || _-- > 0) && !(D = h.next()).done; )
P.push(D.value);
} catch (m) {
y = { error: m };
} finally {
try {
D && !D.done && (v = h.return) && v.call(h);
} finally {
if (y)
throw y.error;
}
}
return P;
}
function yV() {
for (var a = [], _ = 0; _ < arguments.length; _++)
a = a.concat($9(arguments[_]));
return a;
}
function vV() {
for (var a = 0, _ = 0, v = arguments.length; _ < v; _++)
a += arguments[_].length;
for (var h = Array(a), D = 0, _ = 0; _ < v; _++)
for (var P = arguments[_], y = 0, m = P.length; y < m; y++, D++)
h[D] = P[y];
return h;
}
function gp(a) {
return this instanceof gp ? (this.v = a, this) : new gp(a);
}
function bV(a, _, v) {
if (!Symbol.asyncIterator)
throw new TypeError("Symbol.asyncIterator is not defined.");
var h = v.apply(a, _ || []), D, P = [];
return D = {}, y("next"), y("throw"), y("return"), D[Symbol.asyncIterator] = function() {
return this;
}, D;
function y(c) {
h[c] && (D[c] = function(M) {
return new Promise(function(q, W) {
P.push([c, M, q, W]) > 1 || m(c, M);
});
});
}
function m(c, M) {
try {
C(h[c](M));
} catch (q) {
I(P[0][3], q);
}
}
function C(c) {
c.value instanceof gp ? Promise.resolve(c.value.v).then(d, E) : I(P[0][2], c);
}
function d(c) {
m("next", c);
}
function E(c) {
m("throw", c);
}
function I(c, M) {
c(M), P.shift(), P.length && m(P[0][0], P[0][1]);
}
}
function TV(a) {
var _, v;
return _ = {}, h("next"), h("throw", function(D) {
throw D;
}), h("return"), _[Symbol.iterator] = function() {
return this;
}, _;
function h(D, P) {
_[D] = a[D] ? function(y) {
return (v = !v) ? { value: gp(a[D](y)), done: D === "return" } : P ? P(y) : y;
} : P;
}
}
function SV(a) {
if (!Symbol.asyncIterator)
throw new TypeError("Symbol.asyncIterator is not defined.");
var _ = a[Symbol.asyncIterator], v;
return _ ? _.call(a) : (a = typeof tT == "function" ? tT(a) : a[Symbol.iterator](), v = {}, h("next"), h("throw"), h("return"), v[Symbol.asyncIterator] = function() {
return this;
}, v);
function h(P) {
v[P] = a[P] && function(y) {
return new Promise(function(m, C) {
y = a[P](y), D(m, C, y.done, y.value);
});
};
}
function D(P, y, m, C) {
Promise.resolve(C).then(function(d) {
P({ value: d, done: m });
}, y);
}
}
function xV(a, _) {
return Object.defineProperty ? Object.defineProperty(a, "raw", { value: _ }) : a.raw = _, a;
}
function EV(a) {
if (a && a.__esModule)
return a;
var _ = {};
if (a != null)
for (var v in a)
Object.hasOwnProperty.call(a, v) && (_[v] = a[v]);
return _.default = a, _;
}
function wV(a) {
return a && a.__esModule ? a : { default: a };
}
function CV(a, _) {
if (!_.has(a))
throw new TypeError("attempted to get private field on non-instance");
return _.get(a);
}
function AV(a, _, v) {
if (!_.has(a))
throw new TypeError("attempted to set private field on non-instance");
return _.set(a, v), v;
}
var p1, f1, Ds = yp({ "node_modules/tslib/tslib.es6.js"() {
De(), p1 = function(a, _) {
return p1 = Object.setPrototypeOf || { __proto__: [] } instanceof Array && function(v, h) {
v.__proto__ = h;
} || function(v, h) {
for (var D in h)
h.hasOwnProperty(D) && (v[D] = h[D]);
}, p1(a, _);
}, f1 = function() {
return f1 = Object.assign || function(_) {
for (var v, h = 1, D = arguments.length; h < D; h++) {
v = arguments[h];
for (var P in v)
Object.prototype.hasOwnProperty.call(v, P) && (_[P] = v[P]);
}
return _;
}, f1.apply(this, arguments);
};
} }), PV = Oe({ "node_modules/tsutils/typeguard/2.8/node.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.isExpressionStatement = a.isExpression = a.isExportSpecifier = a.isExportDeclaration = a.isExportAssignment = a.isEnumMember = a.isEnumDeclaration = a.isEntityNameExpression = a.isEntityName = a.isEmptyStatement = a.isElementAccessExpression = a.isDoStatement = a.isDeleteExpression = a.isDefaultClause = a.isDecorator = a.isDebuggerStatement = a.isComputedPropertyName = a.isContinueStatement = a.isConstructSignatureDeclaration = a.isConstructorTypeNode = a.isConstructorDeclaration = a.isConditionalTypeNode = a.isConditionalExpression = a.isCommaListExpression = a.isClassLikeDeclaration = a.isClassExpression = a.isClassDeclaration = a.isCatchClause = a.isCaseOrDefaultClause = a.isCaseClause = a.isCaseBlock = a.isCallSignatureDeclaration = a.isCallLikeExpression = a.isCallExpression = a.isBreakStatement = a.isBreakOrContinueStatement = a.isBooleanLiteral = a.isBlockLike = a.isBlock = a.isBindingPattern = a.isBindingElement = a.isBinaryExpression = a.isAwaitExpression = a.isAssertionExpression = a.isAsExpression = a.isArrowFunction = a.isArrayTypeNode = a.isArrayLiteralExpression = a.isArrayBindingPattern = a.isAccessorDeclaration = void 0, a.isNamespaceImport = a.isNamespaceDeclaration = a.isNamedImports = a.isNamedExports = a.isModuleDeclaration = a.isModuleBlock = a.isMethodSignature = a.isMethodDeclaration = a.isMetaProperty = a.isMappedTypeNode = a.isLiteralTypeNode = a.isLiteralExpression = a.isLabeledStatement = a.isJsxText = a.isJsxSpreadAttribute = a.isJsxSelfClosingElement = a.isJsxOpeningLikeElement = a.isJsxOpeningFragment = a.isJsxOpeningElement = a.isJsxFragment = a.isJsxExpression = a.isJsxElement = a.isJsxClosingFragment = a.isJsxClosingElement = a.isJsxAttributes = a.isJsxAttributeLike = a.isJsxAttribute = a.isJsDoc = a.isIterationStatement = a.isIntersectionTypeNode = a.isInterfaceDeclaration = a.isInferTypeNode = a.isIndexSignatureDeclaration = a.isIndexedAccessTypeNode = a.isImportSpecifier = a.isImportEqualsDeclaration = a.isImportDeclaration = a.isImportClause = a.isIfStatement = a.isIdentifier = a.isGetAccessorDeclaration = a.isFunctionTypeNode = a.isFunctionExpression = a.isFunctionDeclaration = a.isForStatement = a.isForOfStatement = a.isForInOrOfStatement = a.isForInStatement = a.isExternalModuleReference = a.isExpressionWithTypeArguments = void 0, a.isVariableStatement = a.isVariableDeclaration = a.isUnionTypeNode = a.isTypeQueryNode = a.isTypeReferenceNode = a.isTypePredicateNode = a.isTypeParameterDeclaration = a.isTypeOperatorNode = a.isTypeOfExpression = a.isTypeLiteralNode = a.isTypeAssertion = a.isTypeAliasDeclaration = a.isTupleTypeNode = a.isTryStatement = a.isThrowStatement = a.isTextualLiteral = a.isTemplateLiteral = a.isTemplateExpression = a.isTaggedTemplateExpression = a.isSyntaxList = a.isSwitchStatement = a.isStringLiteral = a.isSpreadElement = a.isSpreadAssignment = a.isSourceFile = a.isSignatureDeclaration = a.isShorthandPropertyAssignment = a.isSetAccessorDeclaration = a.isReturnStatement = a.isRegularExpressionLiteral = a.isQualifiedName = a.isPropertySignature = a.isPropertyDeclaration = a.isPropertyAssignment = a.isPropertyAccessExpression = a.isPrefixUnaryExpression = a.isPostfixUnaryExpression = a.isParenthesizedTypeNode = a.isParenthesizedExpression = a.isParameterDeclaration = a.isOmittedExpression = a.isObjectLiteralExpression = a.isObjectBindingPattern = a.isNumericOrStringLikeLiteral = a.isNumericLiteral = a.isNullLiteral = a.isNoSubstitutionTemplateLiteral = a.isNonNullExpression = a.isNewExpression = a.isNamespaceExportDeclaration = void 0, a.isWithStatement = a.isWhileStatement = a.isVoidExpression = a.isVariableDeclarationList = void 0;
var _ = vr();
function v(J) {
return J.kind === _.SyntaxKind.GetAccessor || J.kind === _.SyntaxKind.SetAccessor;
}
a.isAccessorDeclaration = v;
function h(J) {
return J.kind === _.SyntaxKind.ArrayBindingPattern;
}
a.isArrayBindingPattern = h;
function D(J) {
return J.kind === _.SyntaxKind.ArrayLiteralExpression;
}
a.isArrayLiteralExpression = D;
function P(J) {
return J.kind === _.SyntaxKind.ArrayType;
}
a.isArrayTypeNode = P;
function y(J) {
return J.kind === _.SyntaxKind.ArrowFunction;
}
a.isArrowFunction = y;
function m(J) {
return J.kind === _.SyntaxKind.AsExpression;
}
a.isAsExpression = m;
function C(J) {
return J.kind === _.SyntaxKind.AsExpression || J.kind === _.SyntaxKind.TypeAssertionExpression;
}
a.isAssertionExpression = C;
function d(J) {
return J.kind === _.SyntaxKind.AwaitExpression;
}
a.isAwaitExpression = d;
function E(J) {
return J.kind === _.SyntaxKind.BinaryExpression;
}
a.isBinaryExpression = E;
function I(J) {
return J.kind === _.SyntaxKind.BindingElement;
}
a.isBindingElement = I;
function c(J) {
return J.kind === _.SyntaxKind.ArrayBindingPattern || J.kind === _.SyntaxKind.ObjectBindingPattern;
}
a.isBindingPattern = c;
function M(J) {
return J.kind === _.SyntaxKind.Block;
}
a.isBlock = M;
function q(J) {
return J.statements !== void 0;
}
a.isBlockLike = q;
function W(J) {
return J.kind === _.SyntaxKind.TrueKeyword || J.kind === _.SyntaxKind.FalseKeyword;
}
a.isBooleanLiteral = W;
function K(J) {
return J.kind === _.SyntaxKind.BreakStatement || J.kind === _.SyntaxKind.ContinueStatement;
}
a.isBreakOrContinueStatement = K;
function ce(J) {
return J.kind === _.SyntaxKind.BreakStatement;
}
a.isBreakStatement = ce;
function Ie(J) {
return J.kind === _.SyntaxKind.CallExpression;
}
a.isCallExpression = Ie;
function me(J) {
switch (J.kind) {
case _.SyntaxKind.CallExpression:
case _.SyntaxKind.Decorator:
case _.SyntaxKind.JsxOpeningElement:
case _.SyntaxKind.JsxSelfClosingElement:
case _.SyntaxKind.NewExpression:
case _.SyntaxKind.TaggedTemplateExpression:
return true;
default:
return false;
}
}
a.isCallLikeExpression = me;
function Ae(J) {
return J.kind === _.SyntaxKind.CallSignature;
}
a.isCallSignatureDeclaration = Ae;
function te(J) {
return J.kind === _.SyntaxKind.CaseBlock;
}
a.isCaseBlock = te;
function he(J) {
return J.kind === _.SyntaxKind.CaseClause;
}
a.isCaseClause = he;
function Pe(J) {
return J.kind === _.SyntaxKind.CaseClause || J.kind === _.SyntaxKind.DefaultClause;
}
a.isCaseOrDefaultClause = Pe;
function R(J) {
return J.kind === _.SyntaxKind.CatchClause;
}
a.isCatchClause = R;
function pe(J) {
return J.kind === _.SyntaxKind.ClassDeclaration;
}
a.isClassDeclaration = pe;
function ke(J) {
return J.kind === _.SyntaxKind.ClassExpression;
}
a.isClassExpression = ke;
function Je(J) {
return J.kind === _.SyntaxKind.ClassDeclaration || J.kind === _.SyntaxKind.ClassExpression;
}
a.isClassLikeDeclaration = Je;
function Xe(J) {
return J.kind === _.SyntaxKind.CommaListExpression;
}
a.isCommaListExpression = Xe;
function ee(J) {
return J.kind === _.SyntaxKind.ConditionalExpression;
}
a.isConditionalExpression = ee;
function je(J) {
return J.kind === _.SyntaxKind.ConditionalType;
}
a.isConditionalTypeNode = je;
function nt(J) {
return J.kind === _.SyntaxKind.Constructor;
}
a.isConstructorDeclaration = nt;
function Ze(J) {
return J.kind === _.SyntaxKind.ConstructorType;
}
a.isConstructorTypeNode = Ze;
function st(J) {
return J.kind === _.SyntaxKind.ConstructSignature;
}
a.isConstructSignatureDeclaration = st;
function tt(J) {
return J.kind === _.SyntaxKind.ContinueStatement;
}
a.isContinueStatement = tt;
function ct(J) {
return J.kind === _.SyntaxKind.ComputedPropertyName;
}
a.isComputedPropertyName = ct;
function ne(J) {
return J.kind === _.SyntaxKind.DebuggerStatement;
}
a.isDebuggerStatement = ne;
function ge(J) {
return J.kind === _.SyntaxKind.Decorator;
}
a.isDecorator = ge;
function Fe(J) {
return J.kind === _.SyntaxKind.DefaultClause;
}
a.isDefaultClause = Fe;
function at(J) {
return J.kind === _.SyntaxKind.DeleteExpression;
}
a.isDeleteExpression = at;
function Pt(J) {
return J.kind === _.SyntaxKind.DoStatement;
}
a.isDoStatement = Pt;
function qt(J) {
return J.kind === _.SyntaxKind.ElementAccessExpression;
}
a.isElementAccessExpression = qt;
function Zr(J) {
return J.kind === _.SyntaxKind.EmptyStatement;
}
a.isEmptyStatement = Zr;
function Ri(J) {
return J.kind === _.SyntaxKind.Identifier || w_(J);
}
a.isEntityName = Ri;
function la(J) {
return J.kind === _.SyntaxKind.Identifier || gi(J) && la(J.expression);
}
a.isEntityNameExpression = la;
function ua(J) {
return J.kind === _.SyntaxKind.EnumDeclaration;
}
a.isEnumDeclaration = ua;
function Ka(J) {
return J.kind === _.SyntaxKind.EnumMember;
}
a.isEnumMember = Ka;
function co(J) {
return J.kind === _.SyntaxKind.ExportAssignment;
}
a.isExportAssignment = co;
function be(J) {
return J.kind === _.SyntaxKind.ExportDeclaration;
}
a.isExportDeclaration = be;
function Ke(J) {
return J.kind === _.SyntaxKind.ExportSpecifier;
}
a.isExportSpecifier = Ke;
function Et(J) {
switch (J.kind) {
case _.SyntaxKind.ArrayLiteralExpression:
case _.SyntaxKind.ArrowFunction:
case _.SyntaxKind.AsExpression:
case _.SyntaxKind.AwaitExpression:
case _.SyntaxKind.BinaryExpression:
case _.SyntaxKind.CallExpression:
case _.SyntaxKind.ClassExpression:
case _.SyntaxKind.CommaListExpression:
case _.SyntaxKind.ConditionalExpression:
case _.SyntaxKind.DeleteExpression:
case _.SyntaxKind.ElementAccessExpression:
case _.SyntaxKind.FalseKeyword:
case _.SyntaxKind.FunctionExpression:
case _.SyntaxKind.Identifier:
case _.SyntaxKind.JsxElement:
case _.SyntaxKind.JsxFragment:
case _.SyntaxKind.JsxExpression:
case _.SyntaxKind.JsxOpeningElement:
case _.SyntaxKind.JsxOpeningFragment:
case _.SyntaxKind.JsxSelfClosingElement:
case _.SyntaxKind.MetaProperty:
case _.SyntaxKind.NewExpression:
case _.SyntaxKind.NonNullExpression:
case _.SyntaxKind.NoSubstitutionTemplateLiteral:
case _.SyntaxKind.NullKeyword:
case _.SyntaxKind.NumericLiteral:
case _.SyntaxKind.ObjectLiteralExpression:
case _.SyntaxKind.OmittedExpression:
case _.SyntaxKind.ParenthesizedExpression:
case _.SyntaxKind.PostfixUnaryExpression:
case _.SyntaxKind.PrefixUnaryExpression:
case _.SyntaxKind.PropertyAccessExpression:
case _.SyntaxKind.RegularExpressionLiteral:
case _.SyntaxKind.SpreadElement:
case _.SyntaxKind.StringLiteral:
case _.SyntaxKind.SuperKeyword:
case _.SyntaxKind.TaggedTemplateExpression:
case _.SyntaxKind.TemplateExpression:
case _.SyntaxKind.ThisKeyword:
case _.SyntaxKind.TrueKeyword:
case _.SyntaxKind.TypeAssertionExpression:
case _.SyntaxKind.TypeOfExpression:
case _.SyntaxKind.VoidExpression:
case _.SyntaxKind.YieldExpression:
return true;
default:
return false;
}
}
a.isExpression = Et;
function Ft(J) {
return J.kind === _.SyntaxKind.ExpressionStatement;
}
a.isExpressionStatement = Ft;
function or(J) {
return J.kind === _.SyntaxKind.ExpressionWithTypeArguments;
}
a.isExpressionWithTypeArguments = or;
function Wr(J) {
return J.kind === _.SyntaxKind.ExternalModuleReference;
}
a.isExternalModuleReference = Wr;
function m_(J) {
return J.kind === _.SyntaxKind.ForInStatement;
}
a.isForInStatement = m_;
function Uc(J) {
return J.kind === _.SyntaxKind.ForOfStatement || J.kind === _.SyntaxKind.ForInStatement;
}
a.isForInOrOfStatement = Uc;
function ji(J) {
return J.kind === _.SyntaxKind.ForOfStatement;
}
a.isForOfStatement = ji;
function lo(J) {
return J.kind === _.SyntaxKind.ForStatement;
}
a.isForStatement = lo;
function zc(J) {
return J.kind === _.SyntaxKind.FunctionDeclaration;
}
a.isFunctionDeclaration = zc;
function Qn(J) {
return J.kind === _.SyntaxKind.FunctionExpression;
}
a.isFunctionExpression = Qn;
function uo(J) {
return J.kind === _.SyntaxKind.FunctionType;
}
a.isFunctionTypeNode = uo;
function Wc(J) {
return J.kind === _.SyntaxKind.GetAccessor;
}
a.isGetAccessorDeclaration = Wc;
function Vc(J) {
return J.kind === _.SyntaxKind.Identifier;
}
a.isIdentifier = Vc;
function Hc(J) {
return J.kind === _.SyntaxKind.IfStatement;
}
a.isIfStatement = Hc;
function Gc(J) {
return J.kind === _.SyntaxKind.ImportClause;
}
a.isImportClause = Gc;
function h_(J) {
return J.kind === _.SyntaxKind.ImportDeclaration;
}
a.isImportDeclaration = h_;
function tr(J) {
return J.kind === _.SyntaxKind.ImportEqualsDeclaration;
}
a.isImportEqualsDeclaration = tr;
function $c(J) {
return J.kind === _.SyntaxKind.ImportSpecifier;
}
a.isImportSpecifier = $c;
function po(J) {
return J.kind === _.SyntaxKind.IndexedAccessType;
}
a.isIndexedAccessTypeNode = po;
function jr(J) {
return J.kind === _.SyntaxKind.IndexSignature;
}
a.isIndexSignatureDeclaration = jr;
function qn(J) {
return J.kind === _.SyntaxKind.InferType;
}
a.isInferTypeNode = qn;
function g_(J) {
return J.kind === _.SyntaxKind.InterfaceDeclaration;
}
a.isInterfaceDeclaration = g_;
function ks(J) {
return J.kind === _.SyntaxKind.IntersectionType;
}
a.isIntersectionTypeNode = ks;
function Is(J) {
switch (J.kind) {
case _.SyntaxKind.ForStatement:
case _.SyntaxKind.ForOfStatement:
case _.SyntaxKind.ForInStatement:
case _.SyntaxKind.WhileStatement:
case _.SyntaxKind.DoStatement:
return true;
default:
return false;
}
}
a.isIterationStatement = Is;
function y_(J) {
return J.kind === _.SyntaxKind.JSDocComment;
}
a.isJsDoc = y_;
function Ns(J) {
return J.kind === _.SyntaxKind.JsxAttribute;
}
a.isJsxAttribute = Ns;
function Kc(J) {
return J.kind === _.SyntaxKind.JsxAttribute || J.kind === _.SyntaxKind.JsxSpreadAttribute;
}
a.isJsxAttributeLike = Kc;
function pa(J) {
return J.kind === _.SyntaxKind.JsxAttributes;
}
a.isJsxAttributes = pa;
function Xc(J) {
return J.kind === _.SyntaxKind.JsxClosingElement;
}
a.isJsxClosingElement = Xc;
function fo(J) {
return J.kind === _.SyntaxKind.JsxClosingFragment;
}
a.isJsxClosingFragment = fo;
function v_(J) {
return J.kind === _.SyntaxKind.JsxElement;
}
a.isJsxElement = v_;
function Cn(J) {
return J.kind === _.SyntaxKind.JsxExpression;
}
a.isJsxExpression = Cn;
function Zn(J) {
return J.kind === _.SyntaxKind.JsxFragment;
}
a.isJsxFragment = Zn;
function Xa(J) {
return J.kind === _.SyntaxKind.JsxOpeningElement;
}
a.isJsxOpeningElement = Xa;
function Yc(J) {
return J.kind === _.SyntaxKind.JsxOpeningFragment;
}
a.isJsxOpeningFragment = Yc;
function mo(J) {
return J.kind === _.SyntaxKind.JsxOpeningElement || J.kind === _.SyntaxKind.JsxSelfClosingElement;
}
a.isJsxOpeningLikeElement = mo;
function ei(J) {
return J.kind === _.SyntaxKind.JsxSelfClosingElement;
}
a.isJsxSelfClosingElement = ei;
function Ya(J) {
return J.kind === _.SyntaxKind.JsxSpreadAttribute;
}
a.isJsxSpreadAttribute = Ya;
function b_(J) {
return J.kind === _.SyntaxKind.JsxText;
}
a.isJsxText = b_;
function Qa(J) {
return J.kind === _.SyntaxKind.LabeledStatement;
}
a.isLabeledStatement = Qa;
function Jr(J) {
return J.kind >= _.SyntaxKind.FirstLiteralToken && J.kind <= _.SyntaxKind.LastLiteralToken;
}
a.isLiteralExpression = Jr;
function Qc(J) {
return J.kind === _.SyntaxKind.LiteralType;
}
a.isLiteralTypeNode = Qc;
function ho(J) {
return J.kind === _.SyntaxKind.MappedType;
}
a.isMappedTypeNode = ho;
function T_(J) {
return J.kind === _.SyntaxKind.MetaProperty;
}
a.isMetaProperty = T_;
function go(J) {
return J.kind === _.SyntaxKind.MethodDeclaration;
}
a.isMethodDeclaration = go;
function yo(J) {
return J.kind === _.SyntaxKind.MethodSignature;
}
a.isMethodSignature = yo;
function Za(J) {
return J.kind === _.SyntaxKind.ModuleBlock;
}
a.isModuleBlock = Za;
function vo(J) {
return J.kind === _.SyntaxKind.ModuleDeclaration;
}
a.isModuleDeclaration = vo;
function S_(J) {
return J.kind === _.SyntaxKind.NamedExports;
}
a.isNamedExports = S_;
function Zc(J) {
return J.kind === _.SyntaxKind.NamedImports;
}
a.isNamedImports = Zc;
function Os(J) {
return vo(J) && J.name.kind === _.SyntaxKind.Identifier && J.body !== void 0 && (J.body.kind === _.SyntaxKind.ModuleBlock || Os(J.body));
}
a.isNamespaceDeclaration = Os;
function bo(J) {
return J.kind === _.SyntaxKind.NamespaceImport;
}
a.isNamespaceImport = bo;
function el(J) {
return J.kind === _.SyntaxKind.NamespaceExportDeclaration;
}
a.isNamespaceExportDeclaration = el;
function x_(J) {
return J.kind === _.SyntaxKind.NewExpression;
}
a.isNewExpression = x_;
function E_(J) {
return J.kind === _.SyntaxKind.NonNullExpression;
}
a.isNonNullExpression = E_;
function S(J) {
return J.kind === _.SyntaxKind.NoSubstitutionTemplateLiteral;
}
a.isNoSubstitutionTemplateLiteral = S;
function H(J) {
return J.kind === _.SyntaxKind.NullKeyword;
}
a.isNullLiteral = H;
function le(J) {
return J.kind === _.SyntaxKind.NumericLiteral;
}
a.isNumericLiteral = le;
function Be(J) {
switch (J.kind) {
case _.SyntaxKind.StringLiteral:
case _.SyntaxKind.NumericLiteral:
case _.SyntaxKind.NoSubstitutionTemplateLiteral:
return true;
default:
return false;
}
}
a.isNumericOrStringLikeLiteral = Be;
function rt(J) {
return J.kind === _.SyntaxKind.ObjectBindingPattern;
}
a.isObjectBindingPattern = rt;
function ut(J) {
return J.kind === _.SyntaxKind.ObjectLiteralExpression;
}
a.isObjectLiteralExpression = ut;
function Ht(J) {
return J.kind === _.SyntaxKind.OmittedExpression;
}
a.isOmittedExpression = Ht;
function Fr(J) {
return J.kind === _.SyntaxKind.Parameter;
}
a.isParameterDeclaration = Fr;
function Cr(J) {
return J.kind === _.SyntaxKind.ParenthesizedExpression;
}
a.isParenthesizedExpression = Cr;
function ir(J) {
return J.kind === _.SyntaxKind.ParenthesizedType;
}
a.isParenthesizedTypeNode = ir;
function en(J) {
return J.kind === _.SyntaxKind.PostfixUnaryExpression;
}
a.isPostfixUnaryExpression = en;
function Ji(J) {
return J.kind === _.SyntaxKind.PrefixUnaryExpression;
}
a.isPrefixUnaryExpression = Ji;
function gi(J) {
return J.kind === _.SyntaxKind.PropertyAccessExpression;
}
a.isPropertyAccessExpression = gi;
function ln(J) {
return J.kind === _.SyntaxKind.PropertyAssignment;
}
a.isPropertyAssignment = ln;
function ti(J) {
return J.kind === _.SyntaxKind.PropertyDeclaration;
}
a.isPropertyDeclaration = ti;
function yn(J) {
return J.kind === _.SyntaxKind.PropertySignature;
}
a.isPropertySignature = yn;
function w_(J) {
return J.kind === _.SyntaxKind.QualifiedName;
}
a.isQualifiedName = w_;
function vp(J) {
return J.kind === _.SyntaxKind.RegularExpressionLiteral;
}
a.isRegularExpressionLiteral = vp;
function C1(J) {
return J.kind === _.SyntaxKind.ReturnStatement;
}
a.isReturnStatement = C1;
function rr(J) {
return J.kind === _.SyntaxKind.SetAccessor;
}
a.isSetAccessorDeclaration = rr;
function bp(J) {
return J.kind === _.SyntaxKind.ShorthandPropertyAssignment;
}
a.isShorthandPropertyAssignment = bp;
function Tp(J) {
return J.parameters !== void 0;
}
a.isSignatureDeclaration = Tp;
function A1(J) {
return J.kind === _.SyntaxKind.SourceFile;
}
a.isSourceFile = A1;
function tl(J) {
return J.kind === _.SyntaxKind.SpreadAssignment;
}
a.isSpreadAssignment = tl;
function An(J) {
return J.kind === _.SyntaxKind.SpreadElement;
}
a.isSpreadElement = An;
function P1(J) {
return J.kind === _.SyntaxKind.StringLiteral;
}
a.isStringLiteral = P1;
function D1(J) {
return J.kind === _.SyntaxKind.SwitchStatement;
}
a.isSwitchStatement = D1;
function k1(J) {
return J.kind === _.SyntaxKind.SyntaxList;
}
a.isSyntaxList = k1;
function fa(J) {
return J.kind === _.SyntaxKind.TaggedTemplateExpression;
}
a.isTaggedTemplateExpression = fa;
function Ms(J) {
return J.kind === _.SyntaxKind.TemplateExpression;
}
a.isTemplateExpression = Ms;
function To(J) {
return J.kind === _.SyntaxKind.TemplateExpression || J.kind === _.SyntaxKind.NoSubstitutionTemplateLiteral;
}
a.isTemplateLiteral = To;
function Sp(J) {
return J.kind === _.SyntaxKind.StringLiteral || J.kind === _.SyntaxKind.NoSubstitutionTemplateLiteral;
}
a.isTextualLiteral = Sp;
function Vr(J) {
return J.kind === _.SyntaxKind.ThrowStatement;
}
a.isThrowStatement = Vr;
function I1(J) {
return J.kind === _.SyntaxKind.TryStatement;
}
a.isTryStatement = I1;
function N1(J) {
return J.kind === _.SyntaxKind.TupleType;
}
a.isTupleTypeNode = N1;
function C_(J) {
return J.kind === _.SyntaxKind.TypeAliasDeclaration;
}
a.isTypeAliasDeclaration = C_;
function O1(J) {
return J.kind === _.SyntaxKind.TypeAssertionExpression;
}
a.isTypeAssertion = O1;
function ri(J) {
return J.kind === _.SyntaxKind.TypeLiteral;
}
a.isTypeLiteralNode = ri;
function rl(J) {
return J.kind === _.SyntaxKind.TypeOfExpression;
}
a.isTypeOfExpression = rl;
function M1(J) {
return J.kind === _.SyntaxKind.TypeOperator;
}
a.isTypeOperatorNode = M1;
function xp(J) {
return J.kind === _.SyntaxKind.TypeParameter;
}
a.isTypeParameterDeclaration = xp;
function L1(J) {
return J.kind === _.SyntaxKind.TypePredicate;
}
a.isTypePredicateNode = L1;
function R1(J) {
return J.kind === _.SyntaxKind.TypeReference;
}
a.isTypeReferenceNode = R1;
function j1(J) {
return J.kind === _.SyntaxKind.TypeQuery;
}
a.isTypeQueryNode = j1;
function Ep(J) {
return J.kind === _.SyntaxKind.UnionType;
}
a.isUnionTypeNode = Ep;
function J1(J) {
return J.kind === _.SyntaxKind.VariableDeclaration;
}
a.isVariableDeclaration = J1;
function es(J) {
return J.kind === _.SyntaxKind.VariableStatement;
}
a.isVariableStatement = es;
function F1(J) {
return J.kind === _.SyntaxKind.VariableDeclarationList;
}
a.isVariableDeclarationList = F1;
function B1(J) {
return J.kind === _.SyntaxKind.VoidExpression;
}
a.isVoidExpression = B1;
function Fi(J) {
return J.kind === _.SyntaxKind.WhileStatement;
}
a.isWhileStatement = Fi;
function q1(J) {
return J.kind === _.SyntaxKind.WithStatement;
}
a.isWithStatement = q1;
} }), DV = Oe({ "node_modules/tsutils/typeguard/2.9/node.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.isImportTypeNode = void 0;
var _ = (Ds(), Li($a));
_.__exportStar(PV(), a);
var v = vr();
function h(D) {
return D.kind === v.SyntaxKind.ImportType;
}
a.isImportTypeNode = h;
} }), kV = Oe({ "node_modules/tsutils/typeguard/3.0/node.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.isSyntheticExpression = a.isRestTypeNode = a.isOptionalTypeNode = void 0;
var _ = (Ds(), Li($a));
_.__exportStar(DV(), a);
var v = vr();
function h(y) {
return y.kind === v.SyntaxKind.OptionalType;
}
a.isOptionalTypeNode = h;
function D(y) {
return y.kind === v.SyntaxKind.RestType;
}
a.isRestTypeNode = D;
function P(y) {
return y.kind === v.SyntaxKind.SyntheticExpression;
}
a.isSyntheticExpression = P;
} }), K9 = Oe({ "node_modules/tsutils/typeguard/3.2/node.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.isBigIntLiteral = void 0;
var _ = (Ds(), Li($a));
_.__exportStar(kV(), a);
var v = vr();
function h(D) {
return D.kind === v.SyntaxKind.BigIntLiteral;
}
a.isBigIntLiteral = h;
} }), X9 = Oe({ "node_modules/tsutils/typeguard/node.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
var _ = (Ds(), Li($a));
_.__exportStar(K9(), a);
} }), IV = Oe({ "node_modules/tsutils/typeguard/2.8/type.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.isUniqueESSymbolType = a.isUnionType = a.isUnionOrIntersectionType = a.isTypeVariable = a.isTypeReference = a.isTypeParameter = a.isSubstitutionType = a.isObjectType = a.isLiteralType = a.isIntersectionType = a.isInterfaceType = a.isInstantiableType = a.isIndexedAccessype = a.isIndexedAccessType = a.isGenericType = a.isEnumType = a.isConditionalType = void 0;
var _ = vr();
function v(me) {
return (me.flags & _.TypeFlags.Conditional) !== 0;
}
a.isConditionalType = v;
function h(me) {
return (me.flags & _.TypeFlags.Enum) !== 0;
}
a.isEnumType = h;
function D(me) {
return (me.flags & _.TypeFlags.Object) !== 0 && (me.objectFlags & _.ObjectFlags.ClassOrInterface) !== 0 && (me.objectFlags & _.ObjectFlags.Reference) !== 0;
}
a.isGenericType = D;
function P(me) {
return (me.flags & _.TypeFlags.IndexedAccess) !== 0;
}
a.isIndexedAccessType = P;
function y(me) {
return (me.flags & _.TypeFlags.Index) !== 0;
}
a.isIndexedAccessype = y;
function m(me) {
return (me.flags & _.TypeFlags.Instantiable) !== 0;
}
a.isInstantiableType = m;
function C(me) {
return (me.flags & _.TypeFlags.Object) !== 0 && (me.objectFlags & _.ObjectFlags.ClassOrInterface) !== 0;
}
a.isInterfaceType = C;
function d(me) {
return (me.flags & _.TypeFlags.Intersection) !== 0;
}
a.isIntersectionType = d;
function E(me) {
return (me.flags & (_.TypeFlags.StringOrNumberLiteral | _.TypeFlags.BigIntLiteral)) !== 0;
}
a.isLiteralType = E;
function I(me) {
return (me.flags & _.TypeFlags.Object) !== 0;
}
a.isObjectType = I;
function c(me) {
return (me.flags & _.TypeFlags.Substitution) !== 0;
}
a.isSubstitutionType = c;
function M(me) {
return (me.flags & _.TypeFlags.TypeParameter) !== 0;
}
a.isTypeParameter = M;
function q(me) {
return (me.flags & _.TypeFlags.Object) !== 0 && (me.objectFlags & _.ObjectFlags.Reference) !== 0;
}
a.isTypeReference = q;
function W(me) {
return (me.flags & _.TypeFlags.TypeVariable) !== 0;
}
a.isTypeVariable = W;
function K(me) {
return (me.flags & _.TypeFlags.UnionOrIntersection) !== 0;
}
a.isUnionOrIntersectionType = K;
function ce(me) {
return (me.flags & _.TypeFlags.Union) !== 0;
}
a.isUnionType = ce;
function Ie(me) {
return (me.flags & _.TypeFlags.UniqueESSymbol) !== 0;
}
a.isUniqueESSymbolType = Ie;
} }), S9 = Oe({ "node_modules/tsutils/typeguard/2.9/type.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
var _ = (Ds(), Li($a));
_.__exportStar(IV(), a);
} }), NV = Oe({ "node_modules/tsutils/typeguard/3.0/type.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.isTupleTypeReference = a.isTupleType = void 0;
var _ = (Ds(), Li($a));
_.__exportStar(S9(), a);
var v = vr(), h = S9();
function D(y) {
return (y.flags & v.TypeFlags.Object && y.objectFlags & v.ObjectFlags.Tuple) !== 0;
}
a.isTupleType = D;
function P(y) {
return h.isTypeReference(y) && D(y.target);
}
a.isTupleTypeReference = P;
} }), Y9 = Oe({ "node_modules/tsutils/typeguard/3.2/type.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
var _ = (Ds(), Li($a));
_.__exportStar(NV(), a);
} }), OV = Oe({ "node_modules/tsutils/typeguard/3.2/index.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
var _ = (Ds(), Li($a));
_.__exportStar(K9(), a), _.__exportStar(Y9(), a);
} }), MV = Oe({ "node_modules/tsutils/typeguard/type.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
var _ = (Ds(), Li($a));
_.__exportStar(Y9(), a);
} }), LV = Oe({ "node_modules/tsutils/util/type.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.getBaseClassMemberOfClassElement = a.getIteratorYieldResultFromIteratorResult = a.getInstanceTypeOfClassLikeDeclaration = a.getConstructorTypeOfClassLikeDeclaration = a.getSymbolOfClassLikeDeclaration = a.getPropertyNameFromType = a.symbolHasReadonlyDeclaration = a.isPropertyReadonlyInType = a.getWellKnownSymbolPropertyOfType = a.getPropertyOfType = a.isBooleanLiteralType = a.isFalsyType = a.isThenableType = a.someTypePart = a.intersectionTypeParts = a.unionTypeParts = a.getCallSignaturesOfType = a.isTypeAssignableToString = a.isTypeAssignableToNumber = a.isOptionalChainingUndefinedMarkerType = a.removeOptionalChainingUndefinedMarkerType = a.removeOptionalityFromType = a.isEmptyObjectType = void 0;
var _ = vr(), v = MV(), h = Q9(), D = X9();
function P(ne) {
if (v.isObjectType(ne) && ne.objectFlags & _.ObjectFlags.Anonymous && ne.getProperties().length === 0 && ne.getCallSignatures().length === 0 && ne.getConstructSignatures().length === 0 && ne.getStringIndexType() === void 0 && ne.getNumberIndexType() === void 0) {
let ge = ne.getBaseTypes();
return ge === void 0 || ge.every(P);
}
return false;
}
a.isEmptyObjectType = P;
function y(ne, ge) {
if (!m(ge, _.TypeFlags.Undefined))
return ge;
let Fe = m(ge, _.TypeFlags.Null);
return ge = ne.getNonNullableType(ge), Fe ? ne.getNullableType(ge, _.TypeFlags.Null) : ge;
}
a.removeOptionalityFromType = y;
function m(ne, ge) {
for (let Fe of q(ne))
if (h.isTypeFlagSet(Fe, ge))
return true;
return false;
}
function C(ne, ge) {
if (!v.isUnionType(ge))
return d(ne, ge) ? ge.getNonNullableType() : ge;
let Fe = 0, at = false;
for (let Pt of ge.types)
d(ne, Pt) ? at = true : Fe |= Pt.flags;
return at ? ne.getNullableType(ge.getNonNullableType(), Fe) : ge;
}
a.removeOptionalChainingUndefinedMarkerType = C;
function d(ne, ge) {
return h.isTypeFlagSet(ge, _.TypeFlags.Undefined) && ne.getNullableType(ge.getNonNullableType(), _.TypeFlags.Undefined) !== ge;
}
a.isOptionalChainingUndefinedMarkerType = d;
function E(ne, ge) {
return c(ne, ge, _.TypeFlags.NumberLike);
}
a.isTypeAssignableToNumber = E;
function I(ne, ge) {
return c(ne, ge, _.TypeFlags.StringLike);
}
a.isTypeAssignableToString = I;
function c(ne, ge, Fe) {
Fe |= _.TypeFlags.Any;
let at;
return function Pt(qt) {
if (v.isTypeParameter(qt) && qt.symbol !== void 0 && qt.symbol.declarations !== void 0) {
if (at === void 0)
at = /* @__PURE__ */ new Set([qt]);
else if (!at.has(qt))
at.add(qt);
else
return false;
let Zr = qt.symbol.declarations[0];
return Zr.constraint === void 0 ? true : Pt(ne.getTypeFromTypeNode(Zr.constraint));
}
return v.isUnionType(qt) ? qt.types.every(Pt) : v.isIntersectionType(qt) ? qt.types.some(Pt) : h.isTypeFlagSet(qt, Fe);
}(ge);
}
function M(ne) {
if (v.isUnionType(ne)) {
let ge = [];
for (let Fe of ne.types)
ge.push(...M(Fe));
return ge;
}
if (v.isIntersectionType(ne)) {
let ge;
for (let Fe of ne.types) {
let at = M(Fe);
if (at.length !== 0) {
if (ge !== void 0)
return [];
ge = at;
}
}
return ge === void 0 ? [] : ge;
}
return ne.getCallSignatures();
}
a.getCallSignaturesOfType = M;
function q(ne) {
return v.isUnionType(ne) ? ne.types : [ne];
}
a.unionTypeParts = q;
function W(ne) {
return v.isIntersectionType(ne) ? ne.types : [ne];
}
a.intersectionTypeParts = W;
function K(ne, ge, Fe) {
return ge(ne) ? ne.types.some(Fe) : Fe(ne);
}
a.someTypePart = K;
function ce(ne, ge) {
let Fe = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : ne.getTypeAtLocation(ge);
for (let at of q(ne.getApparentType(Fe))) {
let Pt = at.getProperty("then");
if (Pt === void 0)
continue;
let qt = ne.getTypeOfSymbolAtLocation(Pt, ge);
for (let Zr of q(qt))
for (let Ri of Zr.getCallSignatures())
if (Ri.parameters.length !== 0 && Ie(ne, Ri.parameters[0], ge))
return true;
}
return false;
}
a.isThenableType = ce;
function Ie(ne, ge, Fe) {
let at = ne.getApparentType(ne.getTypeOfSymbolAtLocation(ge, Fe));
if (ge.valueDeclaration.dotDotDotToken && (at = at.getNumberIndexType(), at === void 0))
return false;
for (let Pt of q(at))
if (Pt.getCallSignatures().length !== 0)
return true;
return false;
}
function me(ne) {
return ne.flags & (_.TypeFlags.Undefined | _.TypeFlags.Null | _.TypeFlags.Void) ? true : v.isLiteralType(ne) ? !ne.value : Ae(ne, false);
}
a.isFalsyType = me;
function Ae(ne, ge) {
return h.isTypeFlagSet(ne, _.TypeFlags.BooleanLiteral) && ne.intrinsicName === (ge ? "true" : "false");
}
a.isBooleanLiteralType = Ae;
function te(ne, ge) {
return ge.startsWith("__") ? ne.getProperties().find((Fe) => Fe.escapedName === ge) : ne.getProperty(ge);
}
a.getPropertyOfType = te;
function he(ne, ge, Fe) {
let at = "__@" + ge;
for (let Pt of ne.getProperties()) {
if (!Pt.name.startsWith(at))
continue;
let qt = Fe.getApparentType(Fe.getTypeAtLocation(Pt.valueDeclaration.name.expression)).symbol;
if (Pt.escapedName === Pe(Fe, qt, ge))
return Pt;
}
}
a.getWellKnownSymbolPropertyOfType = he;
function Pe(ne, ge, Fe) {
let at = ge && ne.getTypeOfSymbolAtLocation(ge, ge.valueDeclaration).getProperty(Fe), Pt = at && ne.getTypeOfSymbolAtLocation(at, at.valueDeclaration);
return Pt && v.isUniqueESSymbolType(Pt) ? Pt.escapedName : "__@" + Fe;
}
function R(ne, ge, Fe) {
let at = false, Pt = false;
for (let qt of q(ne))
if (te(qt, ge) === void 0) {
let Zr = (h.isNumericPropertyName(ge) ? Fe.getIndexInfoOfType(qt, _.IndexKind.Number) : void 0) || Fe.getIndexInfoOfType(qt, _.IndexKind.String);
if (Zr !== void 0 && Zr.isReadonly) {
if (at)
return true;
Pt = true;
}
} else {
if (Pt || pe(qt, ge, Fe))
return true;
at = true;
}
return false;
}
a.isPropertyReadonlyInType = R;
function pe(ne, ge, Fe) {
return K(ne, v.isIntersectionType, (at) => {
let Pt = te(at, ge);
if (Pt === void 0)
return false;
if (Pt.flags & _.SymbolFlags.Transient) {
if (/^(?:[1-9]\d*|0)$/.test(ge) && v.isTupleTypeReference(at))
return at.target.readonly;
switch (ke(at, ge, Fe)) {
case true:
return true;
case false:
return false;
default:
}
}
return h.isSymbolFlagSet(Pt, _.SymbolFlags.ValueModule) || Je(Pt, Fe);
});
}
function ke(ne, ge, Fe) {
if (!v.isObjectType(ne) || !h.isObjectFlagSet(ne, _.ObjectFlags.Mapped))
return;
let at = ne.symbol.declarations[0];
return at.readonlyToken !== void 0 && !/^__@[^@]+$/.test(ge) ? at.readonlyToken.kind !== _.SyntaxKind.MinusToken : R(ne.modifiersType, ge, Fe);
}
function Je(ne, ge) {
return (ne.flags & _.SymbolFlags.Accessor) === _.SymbolFlags.GetAccessor || ne.declarations !== void 0 && ne.declarations.some((Fe) => h.isModifierFlagSet(Fe, _.ModifierFlags.Readonly) || D.isVariableDeclaration(Fe) && h.isNodeFlagSet(Fe.parent, _.NodeFlags.Const) || D.isCallExpression(Fe) && h.isReadonlyAssignmentDeclaration(Fe, ge) || D.isEnumMember(Fe) || (D.isPropertyAssignment(Fe) || D.isShorthandPropertyAssignment(Fe)) && h.isInConstContext(Fe.parent));
}
a.symbolHasReadonlyDeclaration = Je;
function Xe(ne) {
if (ne.flags & (_.TypeFlags.StringLiteral | _.TypeFlags.NumberLiteral)) {
let ge = String(ne.value);
return { displayName: ge, symbolName: _.escapeLeadingUnderscores(ge) };
}
if (v.isUniqueESSymbolType(ne))
return { displayName: `[${ne.symbol ? `${ee(ne.symbol) ? "Symbol." : ""}${ne.symbol.name}` : ne.escapedName.replace(/^__@|@\d+$/g, "")}]`, symbolName: ne.escapedName };
}
a.getPropertyNameFromType = Xe;
function ee(ne) {
return h.isSymbolFlagSet(ne, _.SymbolFlags.Property) && ne.valueDeclaration !== void 0 && D.isInterfaceDeclaration(ne.valueDeclaration.parent) && ne.valueDeclaration.parent.name.text === "SymbolConstructor" && je(ne.valueDeclaration.parent);
}
function je(ne) {
return h.isNodeFlagSet(ne.parent, _.NodeFlags.GlobalAugmentation) || D.isSourceFile(ne.parent) && !_.isExternalModule(ne.parent);
}
function nt(ne, ge) {
var Fe;
return ge.getSymbolAtLocation((Fe = ne.name) !== null && Fe !== void 0 ? Fe : h.getChildOfKind(ne, _.SyntaxKind.ClassKeyword));
}
a.getSymbolOfClassLikeDeclaration = nt;
function Ze(ne, ge) {
return ne.kind === _.SyntaxKind.ClassExpression ? ge.getTypeAtLocation(ne) : ge.getTypeOfSymbolAtLocation(nt(ne, ge), ne);
}
a.getConstructorTypeOfClassLikeDeclaration = Ze;
function st(ne, ge) {
return ne.kind === _.SyntaxKind.ClassDeclaration ? ge.getTypeAtLocation(ne) : ge.getDeclaredTypeOfSymbol(nt(ne, ge));
}
a.getInstanceTypeOfClassLikeDeclaration = st;
function tt(ne, ge, Fe) {
return v.isUnionType(ne) && ne.types.find((at) => {
let Pt = at.getProperty("done");
return Pt !== void 0 && Ae(y(Fe, Fe.getTypeOfSymbolAtLocation(Pt, ge)), false);
}) || ne;
}
a.getIteratorYieldResultFromIteratorResult = tt;
function ct(ne, ge) {
if (!D.isClassLikeDeclaration(ne.parent))
return;
let Fe = h.getBaseOfClassLikeExpression(ne.parent);
if (Fe === void 0)
return;
let at = h.getSingleLateBoundPropertyNameOfPropertyName(ne.name, ge);
if (at === void 0)
return;
let Pt = ge.getTypeAtLocation(h.hasModifier(ne.modifiers, _.SyntaxKind.StaticKeyword) ? Fe.expression : Fe);
return te(Pt, at.symbolName);
}
a.getBaseClassMemberOfClassElement = ct;
} }), Q9 = Oe({ "node_modules/tsutils/util/util.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.isValidIdentifier = a.getLineBreakStyle = a.getLineRanges = a.forEachComment = a.forEachTokenWithTrivia = a.forEachToken = a.isFunctionWithBody = a.hasOwnThisReference = a.isBlockScopeBoundary = a.isFunctionScopeBoundary = a.isTypeScopeBoundary = a.isScopeBoundary = a.ScopeBoundarySelector = a.ScopeBoundary = a.isInSingleStatementContext = a.isBlockScopedDeclarationStatement = a.isBlockScopedVariableDeclaration = a.isBlockScopedVariableDeclarationList = a.getVariableDeclarationKind = a.VariableDeclarationKind = a.forEachDeclaredVariable = a.forEachDestructuringIdentifier = a.getPropertyName = a.getWrappedNodeAtPosition = a.getAstNodeAtPosition = a.commentText = a.isPositionInComment = a.getCommentAtPosition = a.getTokenAtPosition = a.getNextToken = a.getPreviousToken = a.getNextStatement = a.getPreviousStatement = a.isModifierFlagSet = a.isObjectFlagSet = a.isSymbolFlagSet = a.isTypeFlagSet = a.isNodeFlagSet = a.hasAccessModifier = a.isParameterProperty = a.hasModifier = a.getModifier = a.isThisParameter = a.isKeywordKind = a.isJsDocKind = a.isTypeNodeKind = a.isAssignmentKind = a.isNodeKind = a.isTokenKind = a.getChildOfKind = void 0, a.getBaseOfClassLikeExpression = a.hasExhaustiveCaseClauses = a.formatPseudoBigInt = a.unwrapParentheses = a.getSingleLateBoundPropertyNameOfPropertyName = a.getLateBoundPropertyNamesOfPropertyName = a.getLateBoundPropertyNames = a.getPropertyNameOfWellKnownSymbol = a.isWellKnownSymbolLiterally = a.isBindableObjectDefinePropertyCall = a.isReadonlyAssignmentDeclaration = a.isInConstContext = a.isConstAssertion = a.getTsCheckDirective = a.getCheckJsDirective = a.isAmbientModule = a.isCompilerOptionEnabled = a.isStrictCompilerOptionEnabled = a.getIIFE = a.isAmbientModuleBlock = a.isStatementInAmbientContext = a.findImportLikeNodes = a.findImports = a.ImportKind = a.parseJsDocOfNode = a.getJsDoc = a.canHaveJsDoc = a.isReassignmentTarget = a.getAccessKind = a.AccessKind = a.isExpressionValueUsed = a.getDeclarationOfBindingElement = a.hasSideEffects = a.SideEffectOptions = a.isSameLine = a.isNumericPropertyName = a.isValidJsxIdentifier = a.isValidNumericLiteral = a.isValidPropertyName = a.isValidPropertyAccess = void 0;
var _ = vr(), v = X9(), h = OV(), D = LV();
function P(S, H, le) {
for (let Be of S.getChildren(le))
if (Be.kind === H)
return Be;
}
a.getChildOfKind = P;
function y(S) {
return S >= _.SyntaxKind.FirstToken && S <= _.SyntaxKind.LastToken;
}
a.isTokenKind = y;
function m(S) {
return S >= _.SyntaxKind.FirstNode;
}
a.isNodeKind = m;
function C(S) {
return S >= _.SyntaxKind.FirstAssignment && S <= _.SyntaxKind.LastAssignment;
}
a.isAssignmentKind = C;
function d(S) {
return S >= _.SyntaxKind.FirstTypeNode && S <= _.SyntaxKind.LastTypeNode;
}
a.isTypeNodeKind = d;
function E(S) {
return S >= _.SyntaxKind.FirstJSDocNode && S <= _.SyntaxKind.LastJSDocNode;
}
a.isJsDocKind = E;
function I(S) {
return S >= _.SyntaxKind.FirstKeyword && S <= _.SyntaxKind.LastKeyword;
}
a.isKeywordKind = I;
function c(S) {
return S.name.kind === _.SyntaxKind.Identifier && S.name.originalKeywordKind === _.SyntaxKind.ThisKeyword;
}
a.isThisParameter = c;
function M(S, H) {
if (S.modifiers !== void 0) {
for (let le of S.modifiers)
if (le.kind === H)
return le;
}
}
a.getModifier = M;
function q(S) {
if (S === void 0)
return false;
for (var H = arguments.length, le = new Array(H > 1 ? H - 1 : 0), Be = 1; Be < H; Be++)
le[Be - 1] = arguments[Be];
for (let rt of S)
if (le.includes(rt.kind))
return true;
return false;
}
a.hasModifier = q;
function W(S) {
return q(S.modifiers, _.SyntaxKind.PublicKeyword, _.SyntaxKind.ProtectedKeyword, _.SyntaxKind.PrivateKeyword, _.SyntaxKind.ReadonlyKeyword);
}
a.isParameterProperty = W;
function K(S) {
return me(S, _.ModifierFlags.AccessibilityModifier);
}
a.hasAccessModifier = K;
function ce(S, H) {
return (S.flags & H) !== 0;
}
a.isNodeFlagSet = ce, a.isTypeFlagSet = ce, a.isSymbolFlagSet = ce;
function Ie(S, H) {
return (S.objectFlags & H) !== 0;
}
a.isObjectFlagSet = Ie;
function me(S, H) {
return (_.getCombinedModifierFlags(S) & H) !== 0;
}
a.isModifierFlagSet = me;
function Ae(S) {
let H = S.parent;
if (v.isBlockLike(H)) {
let le = H.statements.indexOf(S);
if (le > 0)
return H.statements[le - 1];
}
}
a.getPreviousStatement = Ae;
function te(S) {
let H = S.parent;
if (v.isBlockLike(H)) {
let le = H.statements.indexOf(S);
if (le < H.statements.length)
return H.statements[le + 1];
}
}
a.getNextStatement = te;
function he(S, H) {
let { pos: le } = S;
if (le !== 0) {
do
S = S.parent;
while (S.pos === le);
return pe(S, le - 1, H != null ? H : S.getSourceFile(), false);
}
}
a.getPreviousToken = he;
function Pe(S, H) {
if (S.kind === _.SyntaxKind.SourceFile || S.kind === _.SyntaxKind.EndOfFileToken)
return;
let le = S.end;
for (S = S.parent; S.end === le; ) {
if (S.parent === void 0)
return S.endOfFileToken;
S = S.parent;
}
return pe(S, le, H != null ? H : S.getSourceFile(), false);
}
a.getNextToken = Pe;
function R(S, H, le, Be) {
if (!(H < S.pos || H >= S.end))
return y(S.kind) ? S : pe(S, H, le != null ? le : S.getSourceFile(), Be === true);
}
a.getTokenAtPosition = R;
function pe(S, H, le, Be) {
if (!Be && (S = je(S, H), y(S.kind)))
return S;
e:
for (; ; ) {
for (let rt of S.getChildren(le))
if (rt.end > H && (Be || rt.kind !== _.SyntaxKind.JSDocComment)) {
if (y(rt.kind))
return rt;
S = rt;
continue e;
}
return;
}
}
function ke(S, H) {
let le = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : S, Be = R(le, H, S);
if (Be === void 0 || Be.kind === _.SyntaxKind.JsxText || H >= Be.end - (_.tokenToString(Be.kind) || "").length)
return;
let rt = Be.pos === 0 ? (_.getShebang(S.text) || "").length : Be.pos;
return rt !== 0 && _.forEachTrailingCommentRange(S.text, rt, Je, H) || _.forEachLeadingCommentRange(S.text, rt, Je, H);
}
a.getCommentAtPosition = ke;
function Je(S, H, le, Be, rt) {
return rt >= S && rt < H ? { pos: S, end: H, kind: le } : void 0;
}
function Xe(S, H, le) {
return ke(S, H, le) !== void 0;
}
a.isPositionInComment = Xe;
function ee(S, H) {
return S.substring(H.pos + 2, H.kind === _.SyntaxKind.SingleLineCommentTrivia ? H.end : H.end - 2);
}
a.commentText = ee;
function je(S, H) {
if (!(S.pos > H || S.end <= H)) {
for (; m(S.kind); ) {
let le = _.forEachChild(S, (Be) => Be.pos <= H && Be.end > H ? Be : void 0);
if (le === void 0)
break;
S = le;
}
return S;
}
}
a.getAstNodeAtPosition = je;
function nt(S, H) {
if (S.node.pos > H || S.node.end <= H)
return;
e:
for (; ; ) {
for (let le of S.children) {
if (le.node.pos > H)
return S;
if (le.node.end > H) {
S = le;
continue e;
}
}
return S;
}
}
a.getWrappedNodeAtPosition = nt;
function Ze(S) {
if (S.kind === _.SyntaxKind.ComputedPropertyName) {
let H = Os(S.expression);
if (v.isPrefixUnaryExpression(H)) {
let le = false;
switch (H.operator) {
case _.SyntaxKind.MinusToken:
le = true;
case _.SyntaxKind.PlusToken:
return v.isNumericLiteral(H.operand) ? `${le ? "-" : ""}${H.operand.text}` : h.isBigIntLiteral(H.operand) ? `${le ? "-" : ""}${H.operand.text.slice(0, -1)}` : void 0;
default:
return;
}
}
return h.isBigIntLiteral(H) ? H.text.slice(0, -1) : v.isNumericOrStringLikeLiteral(H) ? H.text : void 0;
}
return S.kind === _.SyntaxKind.PrivateIdentifier ? void 0 : S.text;
}
a.getPropertyName = Ze;
function st(S, H) {
for (let le of S.elements) {
if (le.kind !== _.SyntaxKind.BindingElement)
continue;
let Be;
if (le.name.kind === _.SyntaxKind.Identifier ? Be = H(le) : Be = st(le.name, H), Be)
return Be;
}
}
a.forEachDestructuringIdentifier = st;
function tt(S, H) {
for (let le of S.declarations) {
let Be;
if (le.name.kind === _.SyntaxKind.Identifier ? Be = H(le) : Be = st(le.name, H), Be)
return Be;
}
}
a.forEachDeclaredVariable = tt;
var ct;
(function(S) {
S[S.Var = 0] = "Var", S[S.Let = 1] = "Let", S[S.Const = 2] = "Const";
})(ct = a.VariableDeclarationKind || (a.VariableDeclarationKind = {}));
function ne(S) {
return S.flags & _.NodeFlags.Let ? 1 : S.flags & _.NodeFlags.Const ? 2 : 0;
}
a.getVariableDeclarationKind = ne;
function ge(S) {
return (S.flags & _.NodeFlags.BlockScoped) !== 0;
}
a.isBlockScopedVariableDeclarationList = ge;
function Fe(S) {
let H = S.parent;
return H.kind === _.SyntaxKind.CatchClause || ge(H);
}
a.isBlockScopedVariableDeclaration = Fe;
function at(S) {
switch (S.kind) {
case _.SyntaxKind.VariableStatement:
return ge(S.declarationList);
case _.SyntaxKind.ClassDeclaration:
case _.SyntaxKind.EnumDeclaration:
case _.SyntaxKind.InterfaceDeclaration:
case _.SyntaxKind.TypeAliasDeclaration:
return true;
default:
return false;
}
}
a.isBlockScopedDeclarationStatement = at;
function Pt(S) {
switch (S.parent.kind) {
case _.SyntaxKind.ForStatement:
case _.SyntaxKind.ForInStatement:
case _.SyntaxKind.ForOfStatement:
case _.SyntaxKind.WhileStatement:
case _.SyntaxKind.DoStatement:
case _.SyntaxKind.IfStatement:
case _.SyntaxKind.WithStatement:
case _.SyntaxKind.LabeledStatement:
return true;
default:
return false;
}
}
a.isInSingleStatementContext = Pt;
var qt;
(function(S) {
S[S.None = 0] = "None", S[S.Function = 1] = "Function", S[S.Block = 2] = "Block", S[S.Type = 4] = "Type", S[S.ConditionalType = 8] = "ConditionalType";
})(qt = a.ScopeBoundary || (a.ScopeBoundary = {}));
var Zr;
(function(S) {
S[S.Function = 1] = "Function", S[S.Block = 3] = "Block", S[S.Type = 7] = "Type", S[S.InferType = 8] = "InferType";
})(Zr = a.ScopeBoundarySelector || (a.ScopeBoundarySelector = {}));
function Ri(S) {
return ua(S) || Ka(S) || la(S);
}
a.isScopeBoundary = Ri;
function la(S) {
switch (S.kind) {
case _.SyntaxKind.InterfaceDeclaration:
case _.SyntaxKind.TypeAliasDeclaration:
case _.SyntaxKind.MappedType:
return 4;
case _.SyntaxKind.ConditionalType:
return 8;
default:
return 0;
}
}
a.isTypeScopeBoundary = la;
function ua(S) {
switch (S.kind) {
case _.SyntaxKind.FunctionExpression:
case _.SyntaxKind.ArrowFunction:
case _.SyntaxKind.Constructor:
case _.SyntaxKind.ModuleDeclaration:
case _.SyntaxKind.ClassDeclaration:
case _.SyntaxKind.ClassExpression:
case _.SyntaxKind.EnumDeclaration:
case _.SyntaxKind.MethodDeclaration:
case _.SyntaxKind.FunctionDeclaration:
case _.SyntaxKind.GetAccessor:
case _.SyntaxKind.SetAccessor:
case _.SyntaxKind.MethodSignature:
case _.SyntaxKind.CallSignature:
case _.SyntaxKind.ConstructSignature:
case _.SyntaxKind.ConstructorType:
case _.SyntaxKind.FunctionType:
return 1;
case _.SyntaxKind.SourceFile:
return _.isExternalModule(S) ? 1 : 0;
default:
return 0;
}
}
a.isFunctionScopeBoundary = ua;
function Ka(S) {
switch (S.kind) {
case _.SyntaxKind.Block:
let H = S.parent;
return H.kind !== _.SyntaxKind.CatchClause && (H.kind === _.SyntaxKind.SourceFile || !ua(H)) ? 2 : 0;
case _.SyntaxKind.ForStatement:
case _.SyntaxKind.ForInStatement:
case _.SyntaxKind.ForOfStatement:
case _.SyntaxKind.CaseBlock:
case _.SyntaxKind.CatchClause:
case _.SyntaxKind.WithStatement:
return 2;
default:
return 0;
}
}
a.isBlockScopeBoundary = Ka;
function co(S) {
switch (S.kind) {
case _.SyntaxKind.ClassDeclaration:
case _.SyntaxKind.ClassExpression:
case _.SyntaxKind.FunctionExpression:
return true;
case _.SyntaxKind.FunctionDeclaration:
return S.body !== void 0;
case _.SyntaxKind.MethodDeclaration:
case _.SyntaxKind.GetAccessor:
case _.SyntaxKind.SetAccessor:
return S.parent.kind === _.SyntaxKind.ObjectLiteralExpression;
default:
return false;
}
}
a.hasOwnThisReference = co;
function be(S) {
switch (S.kind) {
case _.SyntaxKind.GetAccessor:
case _.SyntaxKind.SetAccessor:
case _.SyntaxKind.FunctionDeclaration:
case _.SyntaxKind.MethodDeclaration:
case _.SyntaxKind.Constructor:
return S.body !== void 0;
case _.SyntaxKind.FunctionExpression:
case _.SyntaxKind.ArrowFunction:
return true;
default:
return false;
}
}
a.isFunctionWithBody = be;
function Ke(S, H) {
let le = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : S.getSourceFile(), Be = [];
for (; ; ) {
if (y(S.kind))
H(S);
else if (S.kind !== _.SyntaxKind.JSDocComment) {
let rt = S.getChildren(le);
if (rt.length === 1) {
S = rt[0];
continue;
}
for (let ut = rt.length - 1; ut >= 0; --ut)
Be.push(rt[ut]);
}
if (Be.length === 0)
break;
S = Be.pop();
}
}
a.forEachToken = Ke;
function Et(S, H) {
let le = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : S.getSourceFile(), Be = le.text, rt = _.createScanner(le.languageVersion, false, le.languageVariant, Be);
return Ke(S, (ut) => {
let Ht = ut.kind === _.SyntaxKind.JsxText || ut.pos === ut.end ? ut.pos : ut.getStart(le);
if (Ht !== ut.pos) {
rt.setTextPos(ut.pos);
let Fr = rt.scan(), Cr = rt.getTokenPos();
for (; Cr < Ht; ) {
let ir = rt.getTextPos();
if (H(Be, Fr, { pos: Cr, end: ir }, ut.parent), ir === Ht)
break;
Fr = rt.scan(), Cr = rt.getTokenPos();
}
}
return H(Be, ut.kind, { end: ut.end, pos: Ht }, ut.parent);
}, le);
}
a.forEachTokenWithTrivia = Et;
function Ft(S, H) {
let le = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : S.getSourceFile(), Be = le.text, rt = le.languageVariant !== _.LanguageVariant.JSX;
return Ke(S, (Ht) => {
if (Ht.pos !== Ht.end && (Ht.kind !== _.SyntaxKind.JsxText && _.forEachLeadingCommentRange(Be, Ht.pos === 0 ? (_.getShebang(Be) || "").length : Ht.pos, ut), rt || or(Ht)))
return _.forEachTrailingCommentRange(Be, Ht.end, ut);
}, le);
function ut(Ht, Fr, Cr) {
H(Be, { pos: Ht, end: Fr, kind: Cr });
}
}
a.forEachComment = Ft;
function or(S) {
switch (S.kind) {
case _.SyntaxKind.CloseBraceToken:
return S.parent.kind !== _.SyntaxKind.JsxExpression || !Wr(S.parent.parent);
case _.SyntaxKind.GreaterThanToken:
switch (S.parent.kind) {
case _.SyntaxKind.JsxOpeningElement:
return S.end !== S.parent.end;
case _.SyntaxKind.JsxOpeningFragment:
return false;
case _.SyntaxKind.JsxSelfClosingElement:
return S.end !== S.parent.end || !Wr(S.parent.parent);
case _.SyntaxKind.JsxClosingElement:
case _.SyntaxKind.JsxClosingFragment:
return !Wr(S.parent.parent.parent);
}
}
return true;
}
function Wr(S) {
return S.kind === _.SyntaxKind.JsxElement || S.kind === _.SyntaxKind.JsxFragment;
}
function m_(S) {
let H = S.getLineStarts(), le = [], Be = H.length, rt = S.text, ut = 0;
for (let Ht = 1; Ht < Be; ++Ht) {
let Fr = H[Ht], Cr = Fr;
for (; Cr > ut && _.isLineBreak(rt.charCodeAt(Cr - 1)); --Cr)
;
le.push({ pos: ut, end: Fr, contentLength: Cr - ut }), ut = Fr;
}
return le.push({ pos: ut, end: S.end, contentLength: S.end - ut }), le;
}
a.getLineRanges = m_;
function Uc(S) {
let H = S.getLineStarts();
return H.length === 1 || H[1] < 2 || S.text[H[1] - 2] !== "\r" ? `
` : `\r
`;
}
a.getLineBreakStyle = Uc;
var ji;
function lo(S, H) {
return ji === void 0 ? ji = _.createScanner(H, false, void 0, S) : (ji.setScriptTarget(H), ji.setText(S)), ji.scan(), ji;
}
function zc(S) {
let H = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : _.ScriptTarget.Latest, le = lo(S, H);
return le.isIdentifier() && le.getTextPos() === S.length && le.getTokenPos() === 0;
}
a.isValidIdentifier = zc;
function Qn(S) {
return S >= 65536 ? 2 : 1;
}
function uo(S) {
let H = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : _.ScriptTarget.Latest;
if (S.length === 0)
return false;
let le = S.codePointAt(0);
if (!_.isIdentifierStart(le, H))
return false;
for (let Be = Qn(le); Be < S.length; Be += Qn(le))
if (le = S.codePointAt(Be), !_.isIdentifierPart(le, H))
return false;
return true;
}
a.isValidPropertyAccess = uo;
function Wc(S) {
let H = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : _.ScriptTarget.Latest;
if (uo(S, H))
return true;
let le = lo(S, H);
return le.getTextPos() === S.length && le.getToken() === _.SyntaxKind.NumericLiteral && le.getTokenValue() === S;
}
a.isValidPropertyName = Wc;
function Vc(S) {
let H = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : _.ScriptTarget.Latest, le = lo(S, H);
return le.getToken() === _.SyntaxKind.NumericLiteral && le.getTextPos() === S.length && le.getTokenPos() === 0;
}
a.isValidNumericLiteral = Vc;
function Hc(S) {
let H = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : _.ScriptTarget.Latest;
if (S.length === 0)
return false;
let le = false, Be = S.codePointAt(0);
if (!_.isIdentifierStart(Be, H))
return false;
for (let rt = Qn(Be); rt < S.length; rt += Qn(Be))
if (Be = S.codePointAt(rt), !_.isIdentifierPart(Be, H) && Be !== 45)
if (!le && Be === 58 && rt + Qn(Be) !== S.length)
le = true;
else
return false;
return true;
}
a.isValidJsxIdentifier = Hc;
function Gc(S) {
return String(+S) === S;
}
a.isNumericPropertyName = Gc;
function h_(S, H, le) {
return _.getLineAndCharacterOfPosition(S, H).line === _.getLineAndCharacterOfPosition(S, le).line;
}
a.isSameLine = h_;
var tr;
(function(S) {
S[S.None = 0] = "None", S[S.TaggedTemplate = 1] = "TaggedTemplate", S[S.Constructor = 2] = "Constructor", S[S.JsxElement = 4] = "JsxElement";
})(tr = a.SideEffectOptions || (a.SideEffectOptions = {}));
function $c(S, H) {
var le, Be;
let rt = [];
for (; ; ) {
switch (S.kind) {
case _.SyntaxKind.CallExpression:
case _.SyntaxKind.PostfixUnaryExpression:
case _.SyntaxKind.AwaitExpression:
case _.SyntaxKind.YieldExpression:
case _.SyntaxKind.DeleteExpression:
return true;
case _.SyntaxKind.TypeAssertionExpression:
case _.SyntaxKind.AsExpression:
case _.SyntaxKind.ParenthesizedExpression:
case _.SyntaxKind.NonNullExpression:
case _.SyntaxKind.VoidExpression:
case _.SyntaxKind.TypeOfExpression:
case _.SyntaxKind.PropertyAccessExpression:
case _.SyntaxKind.SpreadElement:
case _.SyntaxKind.PartiallyEmittedExpression:
S = S.expression;
continue;
case _.SyntaxKind.BinaryExpression:
if (C(S.operatorToken.kind))
return true;
rt.push(S.right), S = S.left;
continue;
case _.SyntaxKind.PrefixUnaryExpression:
switch (S.operator) {
case _.SyntaxKind.PlusPlusToken:
case _.SyntaxKind.MinusMinusToken:
return true;
default:
S = S.operand;
continue;
}
case _.SyntaxKind.ElementAccessExpression:
S.argumentExpression !== void 0 && rt.push(S.argumentExpression), S = S.expression;
continue;
case _.SyntaxKind.ConditionalExpression:
rt.push(S.whenTrue, S.whenFalse), S = S.condition;
continue;
case _.SyntaxKind.NewExpression:
if (H & 2)
return true;
S.arguments !== void 0 && rt.push(...S.arguments), S = S.expression;
continue;
case _.SyntaxKind.TaggedTemplateExpression:
if (H & 1)
return true;
if (rt.push(S.tag), S = S.template, S.kind === _.SyntaxKind.NoSubstitutionTemplateLiteral)
break;
case _.SyntaxKind.TemplateExpression:
for (let ut of S.templateSpans)
rt.push(ut.expression);
break;
case _.SyntaxKind.ClassExpression: {
if (S.decorators !== void 0)
return true;
for (let Ht of S.members) {
if (Ht.decorators !== void 0)
return true;
if (!q(Ht.modifiers, _.SyntaxKind.DeclareKeyword))
if (((le = Ht.name) === null || le === void 0 ? void 0 : le.kind) === _.SyntaxKind.ComputedPropertyName && rt.push(Ht.name.expression), v.isMethodDeclaration(Ht)) {
for (let Fr of Ht.parameters)
if (Fr.decorators !== void 0)
return true;
} else
v.isPropertyDeclaration(Ht) && Ht.initializer !== void 0 && q(Ht.modifiers, _.SyntaxKind.StaticKeyword) && rt.push(Ht.initializer);
}
let ut = E_(S);
if (ut === void 0)
break;
S = ut.expression;
continue;
}
case _.SyntaxKind.ArrayLiteralExpression:
rt.push(...S.elements);
break;
case _.SyntaxKind.ObjectLiteralExpression:
for (let ut of S.properties)
switch (((Be = ut.name) === null || Be === void 0 ? void 0 : Be.kind) === _.SyntaxKind.ComputedPropertyName && rt.push(ut.name.expression), ut.kind) {
case _.SyntaxKind.PropertyAssignment:
rt.push(ut.initializer);
break;
case _.SyntaxKind.SpreadAssignment:
rt.push(ut.expression);
}
break;
case _.SyntaxKind.JsxExpression:
if (S.expression === void 0)
break;
S = S.expression;
continue;
case _.SyntaxKind.JsxElement:
case _.SyntaxKind.JsxFragment:
for (let ut of S.children)
ut.kind !== _.SyntaxKind.JsxText && rt.push(ut);
if (S.kind === _.SyntaxKind.JsxFragment)
break;
S = S.openingElement;
case _.SyntaxKind.JsxSelfClosingElement:
case _.SyntaxKind.JsxOpeningElement:
if (H & 4)
return true;
for (let ut of S.attributes.properties)
ut.kind === _.SyntaxKind.JsxSpreadAttribute ? rt.push(ut.expression) : ut.initializer !== void 0 && rt.push(ut.initializer);
break;
case _.SyntaxKind.CommaListExpression:
rt.push(...S.elements);
}
if (rt.length === 0)
return false;
S = rt.pop();
}
}
a.hasSideEffects = $c;
function po(S) {
let H = S.parent.parent;
for (; H.kind === _.SyntaxKind.BindingElement; )
H = H.parent.parent;
return H;
}
a.getDeclarationOfBindingElement = po;
function jr(S) {
for (; ; ) {
let H = S.parent;
switch (H.kind) {
case _.SyntaxKind.CallExpression:
case _.SyntaxKind.NewExpression:
case _.SyntaxKind.ElementAccessExpression:
case _.SyntaxKind.WhileStatement:
case _.SyntaxKind.DoStatement:
case _.SyntaxKind.WithStatement:
case _.SyntaxKind.ThrowStatement:
case _.SyntaxKind.ReturnStatement:
case _.SyntaxKind.JsxExpression:
case _.SyntaxKind.JsxSpreadAttribute:
case _.SyntaxKind.JsxElement:
case _.SyntaxKind.JsxFragment:
case _.SyntaxKind.JsxSelfClosingElement:
case _.SyntaxKind.ComputedPropertyName:
case _.SyntaxKind.ArrowFunction:
case _.SyntaxKind.ExportSpecifier:
case _.SyntaxKind.ExportAssignment:
case _.SyntaxKind.ImportDeclaration:
case _.SyntaxKind.ExternalModuleReference:
case _.SyntaxKind.Decorator:
case _.SyntaxKind.TaggedTemplateExpression:
case _.SyntaxKind.TemplateSpan:
case _.SyntaxKind.ExpressionWithTypeArguments:
case _.SyntaxKind.TypeOfExpression:
case _.SyntaxKind.AwaitExpression:
case _.SyntaxKind.YieldExpression:
case _.SyntaxKind.LiteralType:
case _.SyntaxKind.JsxAttributes:
case _.SyntaxKind.JsxOpeningElement:
case _.SyntaxKind.JsxClosingElement:
case _.SyntaxKind.IfStatement:
case _.SyntaxKind.CaseClause:
case _.SyntaxKind.SwitchStatement:
return true;
case _.SyntaxKind.PropertyAccessExpression:
return H.expression === S;
case _.SyntaxKind.QualifiedName:
return H.left === S;
case _.SyntaxKind.ShorthandPropertyAssignment:
return H.objectAssignmentInitializer === S || !qn(H);
case _.SyntaxKind.PropertyAssignment:
return H.initializer === S && !qn(H);
case _.SyntaxKind.SpreadAssignment:
case _.SyntaxKind.SpreadElement:
case _.SyntaxKind.ArrayLiteralExpression:
return !qn(H);
case _.SyntaxKind.ParenthesizedExpression:
case _.SyntaxKind.AsExpression:
case _.SyntaxKind.TypeAssertionExpression:
case _.SyntaxKind.PostfixUnaryExpression:
case _.SyntaxKind.PrefixUnaryExpression:
case _.SyntaxKind.NonNullExpression:
S = H;
continue;
case _.SyntaxKind.ForStatement:
return H.condition === S;
case _.SyntaxKind.ForInStatement:
case _.SyntaxKind.ForOfStatement:
return H.expression === S;
case _.SyntaxKind.ConditionalExpression:
if (H.condition === S)
return true;
S = H;
break;
case _.SyntaxKind.PropertyDeclaration:
case _.SyntaxKind.BindingElement:
case _.SyntaxKind.VariableDeclaration:
case _.SyntaxKind.Parameter:
case _.SyntaxKind.EnumMember:
return H.initializer === S;
case _.SyntaxKind.ImportEqualsDeclaration:
return H.moduleReference === S;
case _.SyntaxKind.CommaListExpression:
if (H.elements[H.elements.length - 1] !== S)
return false;
S = H;
break;
case _.SyntaxKind.BinaryExpression:
if (H.right === S) {
if (H.operatorToken.kind === _.SyntaxKind.CommaToken) {
S = H;
break;
}
return true;
}
switch (H.operatorToken.kind) {
case _.SyntaxKind.CommaToken:
case _.SyntaxKind.EqualsToken:
return false;
case _.SyntaxKind.EqualsEqualsEqualsToken:
case _.SyntaxKind.EqualsEqualsToken:
case _.SyntaxKind.ExclamationEqualsEqualsToken:
case _.SyntaxKind.ExclamationEqualsToken:
case _.SyntaxKind.InstanceOfKeyword:
case _.SyntaxKind.PlusToken:
case _.SyntaxKind.MinusToken:
case _.SyntaxKind.AsteriskToken:
case _.SyntaxKind.SlashToken:
case _.SyntaxKind.PercentToken:
case _.SyntaxKind.AsteriskAsteriskToken:
case _.SyntaxKind.GreaterThanToken:
case _.SyntaxKind.GreaterThanGreaterThanToken:
case _.SyntaxKind.GreaterThanGreaterThanGreaterThanToken:
case _.SyntaxKind.GreaterThanEqualsToken:
case _.SyntaxKind.LessThanToken:
case _.SyntaxKind.LessThanLessThanToken:
case _.SyntaxKind.LessThanEqualsToken:
case _.SyntaxKind.AmpersandToken:
case _.SyntaxKind.BarToken:
case _.SyntaxKind.CaretToken:
case _.SyntaxKind.BarBarToken:
case _.SyntaxKind.AmpersandAmpersandToken:
case _.SyntaxKind.QuestionQuestionToken:
case _.SyntaxKind.InKeyword:
case _.SyntaxKind.QuestionQuestionEqualsToken:
case _.SyntaxKind.AmpersandAmpersandEqualsToken:
case _.SyntaxKind.BarBarEqualsToken:
return true;
default:
S = H;
}
break;
default:
return false;
}
}
}
a.isExpressionValueUsed = jr;
function qn(S) {
switch (S.kind) {
case _.SyntaxKind.ShorthandPropertyAssignment:
if (S.objectAssignmentInitializer !== void 0)
return true;
case _.SyntaxKind.PropertyAssignment:
case _.SyntaxKind.SpreadAssignment:
S = S.parent;
break;
case _.SyntaxKind.SpreadElement:
if (S.parent.kind !== _.SyntaxKind.ArrayLiteralExpression)
return false;
S = S.parent;
}
for (; ; )
switch (S.parent.kind) {
case _.SyntaxKind.BinaryExpression:
return S.parent.left === S && S.parent.operatorToken.kind === _.SyntaxKind.EqualsToken;
case _.SyntaxKind.ForOfStatement:
return S.parent.initializer === S;
case _.SyntaxKind.ArrayLiteralExpression:
case _.SyntaxKind.ObjectLiteralExpression:
S = S.parent;
break;
case _.SyntaxKind.SpreadAssignment:
case _.SyntaxKind.PropertyAssignment:
S = S.parent.parent;
break;
case _.SyntaxKind.SpreadElement:
if (S.parent.parent.kind !== _.SyntaxKind.ArrayLiteralExpression)
return false;
S = S.parent.parent;
break;
default:
return false;
}
}
var g_;
(function(S) {
S[S.None = 0] = "None", S[S.Read = 1] = "Read", S[S.Write = 2] = "Write", S[S.Delete = 4] = "Delete", S[S.ReadWrite = 3] = "ReadWrite", S[S.Modification = 6] = "Modification";
})(g_ = a.AccessKind || (a.AccessKind = {}));
function ks(S) {
let H = S.parent;
switch (H.kind) {
case _.SyntaxKind.DeleteExpression:
return 4;
case _.SyntaxKind.PostfixUnaryExpression:
return 3;
case _.SyntaxKind.PrefixUnaryExpression:
return H.operator === _.SyntaxKind.PlusPlusToken || H.operator === _.SyntaxKind.MinusMinusToken ? 3 : 1;
case _.SyntaxKind.BinaryExpression:
return H.right === S ? 1 : C(H.operatorToken.kind) ? H.operatorToken.kind === _.SyntaxKind.EqualsToken ? 2 : 3 : 1;
case _.SyntaxKind.ShorthandPropertyAssignment:
return H.objectAssignmentInitializer === S ? 1 : qn(H) ? 2 : 1;
case _.SyntaxKind.PropertyAssignment:
return H.name === S ? 0 : qn(H) ? 2 : 1;
case _.SyntaxKind.ArrayLiteralExpression:
case _.SyntaxKind.SpreadElement:
case _.SyntaxKind.SpreadAssignment:
return qn(H) ? 2 : 1;
case _.SyntaxKind.ParenthesizedExpression:
case _.SyntaxKind.NonNullExpression:
case _.SyntaxKind.TypeAssertionExpression:
case _.SyntaxKind.AsExpression:
return ks(H);
case _.SyntaxKind.ForOfStatement:
case _.SyntaxKind.ForInStatement:
return H.initializer === S ? 2 : 1;
case _.SyntaxKind.ExpressionWithTypeArguments:
return H.parent.token === _.SyntaxKind.ExtendsKeyword && H.parent.parent.kind !== _.SyntaxKind.InterfaceDeclaration ? 1 : 0;
case _.SyntaxKind.ComputedPropertyName:
case _.SyntaxKind.ExpressionStatement:
case _.SyntaxKind.TypeOfExpression:
case _.SyntaxKind.ElementAccessExpression:
case _.SyntaxKind.ForStatement:
case _.SyntaxKind.IfStatement:
case _.SyntaxKind.DoStatement:
case _.SyntaxKind.WhileStatement:
case _.SyntaxKind.SwitchStatement:
case _.SyntaxKind.WithStatement:
case _.SyntaxKind.ThrowStatement:
case _.SyntaxKind.CallExpression:
case _.SyntaxKind.NewExpression:
case _.SyntaxKind.TaggedTemplateExpression:
case _.SyntaxKind.JsxExpression:
case _.SyntaxKind.Decorator:
case _.SyntaxKind.TemplateSpan:
case _.SyntaxKind.JsxOpeningElement:
case _.SyntaxKind.JsxSelfClosingElement:
case _.SyntaxKind.JsxSpreadAttribute:
case _.SyntaxKind.VoidExpression:
case _.SyntaxKind.ReturnStatement:
case _.SyntaxKind.AwaitExpression:
case _.SyntaxKind.YieldExpression:
case _.SyntaxKind.ConditionalExpression:
case _.SyntaxKind.CaseClause:
case _.SyntaxKind.JsxElement:
return 1;
case _.SyntaxKind.ArrowFunction:
return H.body === S ? 1 : 2;
case _.SyntaxKind.PropertyDeclaration:
case _.SyntaxKind.VariableDeclaration:
case _.SyntaxKind.Parameter:
case _.SyntaxKind.EnumMember:
case _.SyntaxKind.BindingElement:
case _.SyntaxKind.JsxAttribute:
return H.initializer === S ? 1 : 0;
case _.SyntaxKind.PropertyAccessExpression:
return H.expression === S ? 1 : 0;
case _.SyntaxKind.ExportAssignment:
return H.isExportEquals ? 1 : 0;
}
return 0;
}
a.getAccessKind = ks;
function Is(S) {
return (ks(S) & 2) !== 0;
}
a.isReassignmentTarget = Is;
function y_(S) {
switch (S.kind) {
case _.SyntaxKind.Parameter:
case _.SyntaxKind.CallSignature:
case _.SyntaxKind.ConstructSignature:
case _.SyntaxKind.MethodSignature:
case _.SyntaxKind.PropertySignature:
case _.SyntaxKind.ArrowFunction:
case _.SyntaxKind.ParenthesizedExpression:
case _.SyntaxKind.SpreadAssignment:
case _.SyntaxKind.ShorthandPropertyAssignment:
case _.SyntaxKind.PropertyAssignment:
case _.SyntaxKind.FunctionExpression:
case _.SyntaxKind.LabeledStatement:
case _.SyntaxKind.ExpressionStatement:
case _.SyntaxKind.VariableStatement:
case _.SyntaxKind.FunctionDeclaration:
case _.SyntaxKind.Constructor:
case _.SyntaxKind.MethodDeclaration:
case _.SyntaxKind.PropertyDeclaration:
case _.SyntaxKind.GetAccessor:
case _.SyntaxKind.SetAccessor:
case _.SyntaxKind.ClassDeclaration:
case _.SyntaxKind.ClassExpression:
case _.SyntaxKind.InterfaceDeclaration:
case _.SyntaxKind.TypeAliasDeclaration:
case _.SyntaxKind.EnumMember:
case _.SyntaxKind.EnumDeclaration:
case _.SyntaxKind.ModuleDeclaration:
case _.SyntaxKind.ImportEqualsDeclaration:
case _.SyntaxKind.ImportDeclaration:
case _.SyntaxKind.NamespaceExportDeclaration:
case _.SyntaxKind.ExportAssignment:
case _.SyntaxKind.IndexSignature:
case _.SyntaxKind.FunctionType:
case _.SyntaxKind.ConstructorType:
case _.SyntaxKind.JSDocFunctionType:
case _.SyntaxKind.ExportDeclaration:
case _.SyntaxKind.NamedTupleMember:
case _.SyntaxKind.EndOfFileToken:
return true;
default:
return false;
}
}
a.canHaveJsDoc = y_;
function Ns(S, H) {
let le = [];
for (let Be of S.getChildren(H)) {
if (!v.isJsDoc(Be))
break;
le.push(Be);
}
return le;
}
a.getJsDoc = Ns;
function Kc(S, H) {
let le = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : S.getSourceFile();
if (y_(S) && S.kind !== _.SyntaxKind.EndOfFileToken) {
let Be = Ns(S, le);
if (Be.length !== 0 || !H)
return Be;
}
return pa(S, S.getStart(le), le, H);
}
a.parseJsDocOfNode = Kc;
function pa(S, H, le, Be) {
let rt = _[Be && h_(le, S.pos, H) ? "forEachTrailingCommentRange" : "forEachLeadingCommentRange"](le.text, S.pos, (en, Ji, gi) => gi === _.SyntaxKind.MultiLineCommentTrivia && le.text[en + 2] === "*" ? { pos: en } : void 0);
if (rt === void 0)
return [];
let ut = rt.pos, Ht = le.text.slice(ut, H), Fr = _.createSourceFile("jsdoc.ts", `${Ht}var a;`, le.languageVersion), Cr = Ns(Fr.statements[0], Fr);
for (let en of Cr)
ir(en, S);
return Cr;
function ir(en, Ji) {
return en.pos += ut, en.end += ut, en.parent = Ji, _.forEachChild(en, (gi) => ir(gi, en), (gi) => {
gi.pos += ut, gi.end += ut;
for (let ln of gi)
ir(ln, en);
});
}
}
var Xc;
(function(S) {
S[S.ImportDeclaration = 1] = "ImportDeclaration", S[S.ImportEquals = 2] = "ImportEquals", S[S.ExportFrom = 4] = "ExportFrom", S[S.DynamicImport = 8] = "DynamicImport", S[S.Require = 16] = "Require", S[S.ImportType = 32] = "ImportType", S[S.All = 63] = "All", S[S.AllImports = 59] = "AllImports", S[S.AllStaticImports = 3] = "AllStaticImports", S[S.AllImportExpressions = 24] = "AllImportExpressions", S[S.AllRequireLike = 18] = "AllRequireLike", S[S.AllNestedImports = 56] = "AllNestedImports", S[S.AllTopLevelImports = 7] = "AllTopLevelImports";
})(Xc = a.ImportKind || (a.ImportKind = {}));
function fo(S, H) {
let le = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : true, Be = [];
for (let ut of v_(S, H, le))
switch (ut.kind) {
case _.SyntaxKind.ImportDeclaration:
rt(ut.moduleSpecifier);
break;
case _.SyntaxKind.ImportEqualsDeclaration:
rt(ut.moduleReference.expression);
break;
case _.SyntaxKind.ExportDeclaration:
rt(ut.moduleSpecifier);
break;
case _.SyntaxKind.CallExpression:
rt(ut.arguments[0]);
break;
case _.SyntaxKind.ImportType:
v.isLiteralTypeNode(ut.argument) && rt(ut.argument.literal);
break;
default:
throw new Error("unexpected node");
}
return Be;
function rt(ut) {
v.isTextualLiteral(ut) && Be.push(ut);
}
}
a.findImports = fo;
function v_(S, H) {
let le = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : true;
return new Cn(S, H, le).find();
}
a.findImportLikeNodes = v_;
var Cn = class {
constructor(S, H, le) {
this._sourceFile = S, this._options = H, this._ignoreFileName = le, this._result = [];
}
find() {
return this._sourceFile.isDeclarationFile && (this._options &= -25), this._options & 7 && this._findImports(this._sourceFile.statements), this._options & 56 && this._findNestedImports(), this._result;
}
_findImports(S) {
for (let H of S)
v.isImportDeclaration(H) ? this._options & 1 && this._result.push(H) : v.isImportEqualsDeclaration(H) ? this._options & 2 && H.moduleReference.kind === _.SyntaxKind.ExternalModuleReference && this._result.push(H) : v.isExportDeclaration(H) ? H.moduleSpecifier !== void 0 && this._options & 4 && this._result.push(H) : v.isModuleDeclaration(H) && this._findImportsInModule(H);
}
_findImportsInModule(S) {
if (S.body !== void 0) {
if (S.body.kind === _.SyntaxKind.ModuleDeclaration)
return this._findImportsInModule(S.body);
this._findImports(S.body.statements);
}
}
_findNestedImports() {
let S = this._ignoreFileName || (this._sourceFile.flags & _.NodeFlags.JavaScriptFile) !== 0, H, le;
if ((this._options & 56) === 16) {
if (!S)
return;
H = /\brequire\s*[(]/g, le = false;
} else
this._options & 16 && S ? (H = /\b(?:import|require)\s*[(]/g, le = (this._options & 32) !== 0) : (H = /\bimport\s*[(]/g, le = S && (this._options & 32) !== 0);
for (let Be = H.exec(this._sourceFile.text); Be !== null; Be = H.exec(this._sourceFile.text)) {
let rt = pe(this._sourceFile, Be.index, this._sourceFile, Be[0][0] === "i" && le);
if (rt.kind === _.SyntaxKind.ImportKeyword) {
if (rt.end - 6 !== Be.index)
continue;
switch (rt.parent.kind) {
case _.SyntaxKind.ImportType:
this._result.push(rt.parent);
break;
case _.SyntaxKind.CallExpression:
rt.parent.arguments.length > 1 && this._result.push(rt.parent);
}
} else
rt.kind === _.SyntaxKind.Identifier && rt.end - 7 === Be.index && rt.parent.kind === _.SyntaxKind.CallExpression && rt.parent.expression === rt && rt.parent.arguments.length === 1 && this._result.push(rt.parent);
}
}
};
function Zn(S) {
for (; S.flags & _.NodeFlags.NestedNamespace; )
S = S.parent;
return q(S.modifiers, _.SyntaxKind.DeclareKeyword) || Xa(S.parent);
}
a.isStatementInAmbientContext = Zn;
function Xa(S) {
for (; S.kind === _.SyntaxKind.ModuleBlock; ) {
do
S = S.parent;
while (S.flags & _.NodeFlags.NestedNamespace);
if (q(S.modifiers, _.SyntaxKind.DeclareKeyword))
return true;
S = S.parent;
}
return false;
}
a.isAmbientModuleBlock = Xa;
function Yc(S) {
let H = S.parent;
for (; H.kind === _.SyntaxKind.ParenthesizedExpression; )
H = H.parent;
return v.isCallExpression(H) && S.end <= H.expression.end ? H : void 0;
}
a.getIIFE = Yc;
function mo(S, H) {
return (S.strict ? S[H] !== false : S[H] === true) && (H !== "strictPropertyInitialization" || mo(S, "strictNullChecks"));
}
a.isStrictCompilerOptionEnabled = mo;
function ei(S, H) {
switch (H) {
case "stripInternal":
case "declarationMap":
case "emitDeclarationOnly":
return S[H] === true && ei(S, "declaration");
case "declaration":
return S.declaration || ei(S, "composite");
case "incremental":
return S.incremental === void 0 ? ei(S, "composite") : S.incremental;
case "skipDefaultLibCheck":
return S.skipDefaultLibCheck || ei(S, "skipLibCheck");
case "suppressImplicitAnyIndexErrors":
return S.suppressImplicitAnyIndexErrors === true && ei(S, "noImplicitAny");
case "allowSyntheticDefaultImports":
return S.allowSyntheticDefaultImports !== void 0 ? S.allowSyntheticDefaultImports : ei(S, "esModuleInterop") || S.module === _.ModuleKind.System;
case "noUncheckedIndexedAccess":
return S.noUncheckedIndexedAccess === true && ei(S, "strictNullChecks");
case "allowJs":
return S.allowJs === void 0 ? ei(S, "checkJs") : S.allowJs;
case "noImplicitAny":
case "noImplicitThis":
case "strictNullChecks":
case "strictFunctionTypes":
case "strictPropertyInitialization":
case "alwaysStrict":
case "strictBindCallApply":
return mo(S, H);
}
return S[H] === true;
}
a.isCompilerOptionEnabled = ei;
function Ya(S) {
return S.name.kind === _.SyntaxKind.StringLiteral || (S.flags & _.NodeFlags.GlobalAugmentation) !== 0;
}
a.isAmbientModule = Ya;
function b_(S) {
return Qa(S);
}
a.getCheckJsDirective = b_;
function Qa(S) {
let H;
return _.forEachLeadingCommentRange(S, (_.getShebang(S) || "").length, (le, Be, rt) => {
if (rt === _.SyntaxKind.SingleLineCommentTrivia) {
let ut = S.slice(le, Be), Ht = /^\/{2,3}\s*@ts-(no)?check(?:\s|$)/i.exec(ut);
Ht !== null && (H = { pos: le, end: Be, enabled: Ht[1] === void 0 });
}
}), H;
}
a.getTsCheckDirective = Qa;
function Jr(S) {
return v.isTypeReferenceNode(S.type) && S.type.typeName.kind === _.SyntaxKind.Identifier && S.type.typeName.escapedText === "const";
}
a.isConstAssertion = Jr;
function Qc(S) {
let H = S;
for (; ; ) {
let le = H.parent;
e:
switch (le.kind) {
case _.SyntaxKind.TypeAssertionExpression:
case _.SyntaxKind.AsExpression:
return Jr(le);
case _.SyntaxKind.PrefixUnaryExpression:
if (H.kind !== _.SyntaxKind.NumericLiteral)
return false;
switch (le.operator) {
case _.SyntaxKind.PlusToken:
case _.SyntaxKind.MinusToken:
H = le;
break e;
default:
return false;
}
case _.SyntaxKind.PropertyAssignment:
if (le.initializer !== H)
return false;
H = le.parent;
break;
case _.SyntaxKind.ShorthandPropertyAssignment:
H = le.parent;
break;
case _.SyntaxKind.ParenthesizedExpression:
case _.SyntaxKind.ArrayLiteralExpression:
case _.SyntaxKind.ObjectLiteralExpression:
case _.SyntaxKind.TemplateExpression:
H = le;
break;
default:
return false;
}
}
}
a.isInConstContext = Qc;
function ho(S, H) {
if (!T_(S))
return false;
let le = H.getTypeAtLocation(S.arguments[2]);
if (le.getProperty("value") === void 0)
return le.getProperty("set") === void 0;
let Be = le.getProperty("writable");
if (Be === void 0)
return false;
let rt = Be.valueDeclaration !== void 0 && v.isPropertyAssignment(Be.valueDeclaration) ? H.getTypeAtLocation(Be.valueDeclaration.initializer) : H.getTypeOfSymbolAtLocation(Be, S.arguments[2]);
return D.isBooleanLiteralType(rt, false);
}
a.isReadonlyAssignmentDeclaration = ho;
function T_(S) {
return S.arguments.length === 3 && v.isEntityNameExpression(S.arguments[0]) && v.isNumericOrStringLikeLiteral(S.arguments[1]) && v.isPropertyAccessExpression(S.expression) && S.expression.name.escapedText === "defineProperty" && v.isIdentifier(S.expression.expression) && S.expression.expression.escapedText === "Object";
}
a.isBindableObjectDefinePropertyCall = T_;
function go(S) {
return _.isPropertyAccessExpression(S) && _.isIdentifier(S.expression) && S.expression.escapedText === "Symbol";
}
a.isWellKnownSymbolLiterally = go;
function yo(S) {
return { displayName: `[Symbol.${S.name.text}]`, symbolName: "__@" + S.name.text };
}
a.getPropertyNameOfWellKnownSymbol = yo;
var Za = ((S) => {
let [H, le] = S;
return H < "4" || H === "4" && le < "3";
})(_.versionMajorMinor.split("."));
function vo(S, H) {
let le = { known: true, names: [] };
if (S = Os(S), Za && go(S))
le.names.push(yo(S));
else {
let Be = H.getTypeAtLocation(S);
for (let rt of D.unionTypeParts(H.getBaseConstraintOfType(Be) || Be)) {
let ut = D.getPropertyNameFromType(rt);
ut ? le.names.push(ut) : le.known = false;
}
}
return le;
}
a.getLateBoundPropertyNames = vo;
function S_(S, H) {
let le = Ze(S);
return le !== void 0 ? { known: true, names: [{ displayName: le, symbolName: _.escapeLeadingUnderscores(le) }] } : S.kind === _.SyntaxKind.PrivateIdentifier ? { known: true, names: [{ displayName: S.text, symbolName: H.getSymbolAtLocation(S).escapedName }] } : vo(S.expression, H);
}
a.getLateBoundPropertyNamesOfPropertyName = S_;
function Zc(S, H) {
let le = Ze(S);
if (le !== void 0)
return { displayName: le, symbolName: _.escapeLeadingUnderscores(le) };
if (S.kind === _.SyntaxKind.PrivateIdentifier)
return { displayName: S.text, symbolName: H.getSymbolAtLocation(S).escapedName };
let { expression: Be } = S;
return Za && go(Be) ? yo(Be) : D.getPropertyNameFromType(H.getTypeAtLocation(Be));
}
a.getSingleLateBoundPropertyNameOfPropertyName = Zc;
function Os(S) {
for (; S.kind === _.SyntaxKind.ParenthesizedExpression; )
S = S.expression;
return S;
}
a.unwrapParentheses = Os;
function bo(S) {
return `${S.negative ? "-" : ""}${S.base10Value}n`;
}
a.formatPseudoBigInt = bo;
function el(S, H) {
let le = S.caseBlock.clauses.filter(v.isCaseClause);
if (le.length === 0)
return false;
let Be = D.unionTypeParts(H.getTypeAtLocation(S.expression));
if (Be.length > le.length)
return false;
let rt = new Set(Be.map(x_));
if (rt.has(void 0))
return false;
let ut = /* @__PURE__ */ new Set();
for (let Ht of le) {
let Fr = H.getTypeAtLocation(Ht.expression);
if (a.isTypeFlagSet(Fr, _.TypeFlags.Never))
continue;
let Cr = x_(Fr);
if (rt.has(Cr))
ut.add(Cr);
else if (Cr !== "null" && Cr !== "undefined")
return false;
}
return rt.size === ut.size;
}
a.hasExhaustiveCaseClauses = el;
function x_(S) {
if (a.isTypeFlagSet(S, _.TypeFlags.Null))
return "null";
if (a.isTypeFlagSet(S, _.TypeFlags.Undefined))
return "undefined";
if (a.isTypeFlagSet(S, _.TypeFlags.NumberLiteral))
return `${a.isTypeFlagSet(S, _.TypeFlags.EnumLiteral) ? "enum:" : ""}${S.value}`;
if (a.isTypeFlagSet(S, _.TypeFlags.StringLiteral))
return `${a.isTypeFlagSet(S, _.TypeFlags.EnumLiteral) ? "enum:" : ""}string:${S.value}`;
if (a.isTypeFlagSet(S, _.TypeFlags.BigIntLiteral))
return bo(S.value);
if (h.isUniqueESSymbolType(S))
return S.escapedName;
if (D.isBooleanLiteralType(S, true))
return "true";
if (D.isBooleanLiteralType(S, false))
return "false";
}
function E_(S) {
var H;
if (((H = S.heritageClauses) === null || H === void 0 ? void 0 : H[0].token) === _.SyntaxKind.ExtendsKeyword)
return S.heritageClauses[0].types[0];
}
a.getBaseOfClassLikeExpression = E_;
} }), RV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/convert-comments.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(d, E, I, c) {
c === void 0 && (c = I);
var M = Object.getOwnPropertyDescriptor(E, I);
(!M || ("get" in M ? !E.__esModule : M.writable || M.configurable)) && (M = { enumerable: true, get: function() {
return E[I];
} }), Object.defineProperty(d, c, M);
} : function(d, E, I, c) {
c === void 0 && (c = I), d[c] = E[I];
}), v = a && a.__setModuleDefault || (Object.create ? function(d, E) {
Object.defineProperty(d, "default", { enumerable: true, value: E });
} : function(d, E) {
d.default = E;
}), h = a && a.__importStar || function(d) {
if (d && d.__esModule)
return d;
var E = {};
if (d != null)
for (var I in d)
I !== "default" && Object.prototype.hasOwnProperty.call(d, I) && _(E, d, I);
return v(E, d), E;
};
Object.defineProperty(a, "__esModule", { value: true }), a.convertComments = void 0;
var D = Q9(), P = h(vr()), y = E1(), m = x1();
function C(d, E) {
let I = [];
return (0, D.forEachComment)(d, (c, M) => {
let q = M.kind === P.SyntaxKind.SingleLineCommentTrivia ? m.AST_TOKEN_TYPES.Line : m.AST_TOKEN_TYPES.Block, W = [M.pos, M.end], K = (0, y.getLocFor)(W[0], W[1], d), ce = W[0] + 2, Ie = M.kind === P.SyntaxKind.SingleLineCommentTrivia ? W[1] - ce : W[1] - ce - 2;
I.push({ type: q, value: E.slice(ce, ce + Ie), range: W, loc: K });
}, d), I;
}
a.convertComments = C;
} }), Z9 = Oe({ "node_modules/eslint-visitor-keys/dist/eslint-visitor-keys.cjs"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true });
var _ = { AssignmentExpression: ["left", "right"], AssignmentPattern: ["left", "right"], ArrayExpression: ["elements"], ArrayPattern: ["elements"], ArrowFunctionExpression: ["params", "body"], AwaitExpression: ["argument"], BlockStatement: ["body"], BinaryExpression: ["left", "right"], BreakStatement: ["label"], CallExpression: ["callee", "arguments"], CatchClause: ["param", "body"], ChainExpression: ["expression"], ClassBody: ["body"], ClassDeclaration: ["id", "superClass", "body"], ClassExpression: ["id", "superClass", "body"], ConditionalExpression: ["test", "consequent", "alternate"], ContinueStatement: ["label"], DebuggerStatement: [], DoWhileStatement: ["body", "test"], EmptyStatement: [], ExportAllDeclaration: ["exported", "source"], ExportDefaultDeclaration: ["declaration"], ExportNamedDeclaration: ["declaration", "specifiers", "source"], ExportSpecifier: ["exported", "local"], ExpressionStatement: ["expression"], ExperimentalRestProperty: ["argument"], ExperimentalSpreadProperty: ["argument"], ForStatement: ["init", "test", "update", "body"], ForInStatement: ["left", "right", "body"], ForOfStatement: ["left", "right", "body"], FunctionDeclaration: ["id", "params", "body"], FunctionExpression: ["id", "params", "body"], Identifier: [], IfStatement: ["test", "consequent", "alternate"], ImportDeclaration: ["specifiers", "source"], ImportDefaultSpecifier: ["local"], ImportExpression: ["source"], ImportNamespaceSpecifier: ["local"], ImportSpecifier: ["imported", "local"], JSXAttribute: ["name", "value"], JSXClosingElement: ["name"], JSXElement: ["openingElement", "children", "closingElement"], JSXEmptyExpression: [], JSXExpressionContainer: ["expression"], JSXIdentifier: [], JSXMemberExpression: ["object", "property"], JSXNamespacedName: ["namespace", "name"], JSXOpeningElement: ["name", "attributes"], JSXSpreadAttribute: ["argument"], JSXText: [], JSXFragment: ["openingFragment", "children", "closingFragment"], JSXClosingFragment: [], JSXOpeningFragment: [], Literal: [], LabeledStatement: ["label", "body"], LogicalExpression: ["left", "right"], MemberExpression: ["object", "property"], MetaProperty: ["meta", "property"], MethodDefinition: ["key", "value"], NewExpression: ["callee", "arguments"], ObjectExpression: ["properties"], ObjectPattern: ["properties"], PrivateIdentifier: [], Program: ["body"], Property: ["key", "value"], PropertyDefinition: ["key", "value"], RestElement: ["argument"], ReturnStatement: ["argument"], SequenceExpression: ["expressions"], SpreadElement: ["argument"], StaticBlock: ["body"], Super: [], SwitchStatement: ["discriminant", "cases"], SwitchCase: ["test", "consequent"], TaggedTemplateExpression: ["tag", "quasi"], TemplateElement: [], TemplateLiteral: ["quasis", "expressions"], ThisExpression: [], ThrowStatement: ["argument"], TryStatement: ["block", "handler", "finalizer"], UnaryExpression: ["argument"], UpdateExpression: ["argument"], VariableDeclaration: ["declarations"], VariableDeclarator: ["id", "init"], WhileStatement: ["test", "body"], WithStatement: ["object", "body"], YieldExpression: ["argument"] }, v = Object.keys(_);
for (let m of v)
Object.freeze(_[m]);
Object.freeze(_);
var h = /* @__PURE__ */ new Set(["parent", "leadingComments", "trailingComments"]);
function D(m) {
return !h.has(m) && m[0] !== "_";
}
function P(m) {
return Object.keys(m).filter(D);
}
function y(m) {
let C = Object.assign({}, _);
for (let d of Object.keys(m))
if (Object.prototype.hasOwnProperty.call(C, d)) {
let E = new Set(m[d]);
for (let I of C[d])
E.add(I);
C[d] = Object.freeze(Array.from(E));
} else
C[d] = Object.freeze(Array.from(m[d]));
return Object.freeze(C);
}
a.KEYS = _, a.getKeys = P, a.unionWith = y;
} }), jV = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/@typescript-eslint/visitor-keys/dist/get-keys.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.getKeys = void 0;
var _ = Z9(), v = _.getKeys;
a.getKeys = v;
} }), JV = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/@typescript-eslint/visitor-keys/dist/visitor-keys.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(C, d, E, I) {
I === void 0 && (I = E);
var c = Object.getOwnPropertyDescriptor(d, E);
(!c || ("get" in c ? !d.__esModule : c.writable || c.configurable)) && (c = { enumerable: true, get: function() {
return d[E];
} }), Object.defineProperty(C, I, c);
} : function(C, d, E, I) {
I === void 0 && (I = E), C[I] = d[E];
}), v = a && a.__setModuleDefault || (Object.create ? function(C, d) {
Object.defineProperty(C, "default", { enumerable: true, value: d });
} : function(C, d) {
C.default = d;
}), h = a && a.__importStar || function(C) {
if (C && C.__esModule)
return C;
var d = {};
if (C != null)
for (var E in C)
E !== "default" && Object.prototype.hasOwnProperty.call(C, E) && _(d, C, E);
return v(d, C), d;
};
Object.defineProperty(a, "__esModule", { value: true }), a.visitorKeys = void 0;
var D = h(Z9()), P = (() => {
let C = ["typeParameters", "params", "returnType"], d = [...C, "body"], E = ["decorators", "key", "typeAnnotation"];
return { AnonymousFunction: d, Function: ["id", ...d], FunctionType: C, ClassDeclaration: ["decorators", "id", "typeParameters", "superClass", "superTypeParameters", "implements", "body"], AbstractPropertyDefinition: ["decorators", "key", "typeAnnotation"], PropertyDefinition: [...E, "value"], TypeAssertion: ["expression", "typeAnnotation"] };
})(), y = { AccessorProperty: P.PropertyDefinition, ArrayPattern: ["decorators", "elements", "typeAnnotation"], ArrowFunctionExpression: P.AnonymousFunction, AssignmentPattern: ["decorators", "left", "right", "typeAnnotation"], CallExpression: ["callee", "typeParameters", "arguments"], ClassDeclaration: P.ClassDeclaration, ClassExpression: P.ClassDeclaration, Decorator: ["expression"], ExportAllDeclaration: ["exported", "source", "assertions"], ExportNamedDeclaration: ["declaration", "specifiers", "source", "assertions"], FunctionDeclaration: P.Function, FunctionExpression: P.Function, Identifier: ["decorators", "typeAnnotation"], ImportAttribute: ["key", "value"], ImportDeclaration: ["specifiers", "source", "assertions"], ImportExpression: ["source", "attributes"], JSXClosingFragment: [], JSXOpeningElement: ["name", "typeParameters", "attributes"], JSXOpeningFragment: [], JSXSpreadChild: ["expression"], MethodDefinition: ["decorators", "key", "value", "typeParameters"], NewExpression: ["callee", "typeParameters", "arguments"], ObjectPattern: ["decorators", "properties", "typeAnnotation"], PropertyDefinition: P.PropertyDefinition, RestElement: ["decorators", "argument", "typeAnnotation"], StaticBlock: ["body"], TaggedTemplateExpression: ["tag", "typeParameters", "quasi"], TSAbstractAccessorProperty: P.AbstractPropertyDefinition, TSAbstractKeyword: [], TSAbstractMethodDefinition: ["key", "value"], TSAbstractPropertyDefinition: P.AbstractPropertyDefinition, TSAnyKeyword: [], TSArrayType: ["elementType"], TSAsExpression: P.TypeAssertion, TSAsyncKeyword: [], TSBigIntKeyword: [], TSBooleanKeyword: [], TSCallSignatureDeclaration: P.FunctionType, TSClassImplements: ["expression", "typeParameters"], TSConditionalType: ["checkType", "extendsType", "trueType", "falseType"], TSConstructorType: P.FunctionType, TSConstructSignatureDeclaration: P.FunctionType, TSDeclareFunction: P.Function, TSDeclareKeyword: [], TSEmptyBodyFunctionExpression: ["id", ...P.FunctionType], TSEnumDeclaration: ["id", "members"], TSEnumMember: ["id", "initializer"], TSExportAssignment: ["expression"], TSExportKeyword: [], TSExternalModuleReference: ["expression"], TSFunctionType: P.FunctionType, TSImportEqualsDeclaration: ["id", "moduleReference"], TSImportType: ["parameter", "qualifier", "typeParameters"], TSIndexedAccessType: ["indexType", "objectType"], TSIndexSignature: ["parameters", "typeAnnotation"], TSInferType: ["typeParameter"], TSInstantiationExpression: ["expression", "typeParameters"], TSInterfaceBody: ["body"], TSInterfaceDeclaration: ["id", "typeParameters", "extends", "body"], TSInterfaceHeritage: ["expression", "typeParameters"], TSIntersectionType: ["types"], TSIntrinsicKeyword: [], TSLiteralType: ["literal"], TSMappedType: ["nameType", "typeParameter", "typeAnnotation"], TSMethodSignature: ["typeParameters", "key", "params", "returnType"], TSModuleBlock: ["body"], TSModuleDeclaration: ["id", "body"], TSNamedTupleMember: ["label", "elementType"], TSNamespaceExportDeclaration: ["id"], TSNeverKeyword: [], TSNonNullExpression: ["expression"], TSNullKeyword: [], TSNumberKeyword: [], TSObjectKeyword: [], TSOptionalType: ["typeAnnotation"], TSParameterProperty: ["decorators", "parameter"], TSPrivateKeyword: [], TSPropertySignature: ["typeAnnotation", "key", "initializer"], TSProtectedKeyword: [], TSPublicKeyword: [], TSQualifiedName: ["left", "right"], TSReadonlyKeyword: [], TSRestType: ["typeAnnotation"], TSSatisfiesExpression: ["typeAnnotation", "expression"], TSStaticKeyword: [], TSStringKeyword: [], TSSymbolKeyword: [], TSTemplateLiteralType: ["quasis", "types"], TSThisType: [], TSTupleType: ["elementTypes"], TSTypeAliasDeclaration: ["id", "typeParameters", "typeAnnotation"], TSTypeAnnotation: ["typeAnnotation"], TSTypeAssertion: P.TypeAssertion, TSTypeLiteral: ["members"], TSTypeOperator: ["typeAnnotation"], TSTypeParameter: ["name", "constraint", "default"], TSTypeParameterDeclaration: ["params"], TSTypeParameterInstantiation: ["params"], TSTypePredicate: ["typeAnnotation", "parameterName"], TSTypeQuery: ["exprName", "typeParameters"], TSTypeReference: ["typeName", "typeParameters"], TSUndefinedKeyword: [], TSUnionType: ["types"], TSUnknownKeyword: [], TSVoidKeyword: [] }, m = D.unionWith(y);
a.visitorKeys = m;
} }), e5 = Oe({ "node_modules/@typescript-eslint/typescript-estree/node_modules/@typescript-eslint/visitor-keys/dist/index.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.visitorKeys = a.getKeys = void 0;
var _ = jV();
Object.defineProperty(a, "getKeys", { enumerable: true, get: function() {
return _.getKeys;
} });
var v = JV();
Object.defineProperty(a, "visitorKeys", { enumerable: true, get: function() {
return v.visitorKeys;
} });
} }), t5 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/simple-traverse.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.simpleTraverse = void 0;
var _ = e5();
function v(y) {
return y != null && typeof y == "object" && typeof y.type == "string";
}
function h(y, m) {
let C = y[m.type];
return C != null ? C : [];
}
var D = class {
constructor(y) {
let m = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
this.allVisitorKeys = _.visitorKeys, this.selectors = y, this.setParentPointers = m;
}
traverse(y, m) {
if (!v(y))
return;
this.setParentPointers && (y.parent = m), "enter" in this.selectors ? this.selectors.enter(y, m) : y.type in this.selectors && this.selectors[y.type](y, m);
let C = h(this.allVisitorKeys, y);
if (!(C.length < 1))
for (let d of C) {
let E = y[d];
if (Array.isArray(E))
for (let I of E)
this.traverse(I, y);
else
this.traverse(E, y);
}
}
};
function P(y, m) {
let C = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false;
new D(m, C).traverse(y, void 0);
}
a.simpleTraverse = P;
} }), FV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/ast-converter.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.astConverter = void 0;
var _ = G9(), v = RV(), h = E1(), D = t5();
function P(y, m, C) {
let { parseDiagnostics: d } = y;
if (d.length)
throw (0, _.convertError)(d[0]);
let E = new _.Converter(y, { errorOnUnknownASTType: m.errorOnUnknownASTType || false, shouldPreserveNodeMaps: C }), I = E.convertProgram();
(!m.range || !m.loc) && (0, D.simpleTraverse)(I, { enter: (M) => {
m.range || delete M.range, m.loc || delete M.loc;
} }), m.tokens && (I.tokens = (0, h.convertTokens)(y)), m.comment && (I.comments = (0, v.convertComments)(y, m.code));
let c = E.getASTMaps();
return { estree: I, astMaps: c };
}
a.astConverter = P;
} }), r5 = {};
m1(r5, { basename: () => o5, default: () => c5, delimiter: () => nT, dirname: () => s5, extname: () => _5, isAbsolute: () => mT, join: () => i5, normalize: () => dT, relative: () => a5, resolve: () => d1, sep: () => rT });
function n5(a, _) {
for (var v = 0, h = a.length - 1; h >= 0; h--) {
var D = a[h];
D === "." ? a.splice(h, 1) : D === ".." ? (a.splice(h, 1), v++) : v && (a.splice(h, 1), v--);
}
if (_)
for (; v--; v)
a.unshift("..");
return a;
}
function d1() {
for (var a = "", _ = false, v = arguments.length - 1; v >= -1 && !_; v--) {
var h = v >= 0 ? arguments[v] : "/";
if (typeof h != "string")
throw new TypeError("Arguments to path.resolve must be strings");
if (!h)
continue;
a = h + "/" + a, _ = h.charAt(0) === "/";
}
return a = n5(hT(a.split("/"), function(D) {
return !!D;
}), !_).join("/"), (_ ? "/" : "") + a || ".";
}
function dT(a) {
var _ = mT(a), v = l5(a, -1) === "/";
return a = n5(hT(a.split("/"), function(h) {
return !!h;
}), !_).join("/"), !a && !_ && (a = "."), a && v && (a += "/"), (_ ? "/" : "") + a;
}
function mT(a) {
return a.charAt(0) === "/";
}
function i5() {
var a = Array.prototype.slice.call(arguments, 0);
return dT(hT(a, function(_, v) {
if (typeof _ != "string")
throw new TypeError("Arguments to path.join must be strings");
return _;
}).join("/"));
}
function a5(a, _) {
a = d1(a).substr(1), _ = d1(_).substr(1);
function v(d) {
for (var E = 0; E < d.length && d[E] === ""; E++)
;
for (var I = d.length - 1; I >= 0 && d[I] === ""; I--)
;
return E > I ? [] : d.slice(E, I - E + 1);
}
for (var h = v(a.split("/")), D = v(_.split("/")), P = Math.min(h.length, D.length), y = P, m = 0; m < P; m++)
if (h[m] !== D[m]) {
y = m;
break;
}
for (var C = [], m = y; m < h.length; m++)
C.push("..");
return C = C.concat(D.slice(y)), C.join("/");
}
function s5(a) {
var _ = w1(a), v = _[0], h = _[1];
return !v && !h ? "." : (h && (h = h.substr(0, h.length - 1)), v + h);
}
function o5(a, _) {
var v = w1(a)[2];
return _ && v.substr(-1 * _.length) === _ && (v = v.substr(0, v.length - _.length)), v;
}
function _5(a) {
return w1(a)[3];
}
function hT(a, _) {
if (a.filter)
return a.filter(_);
for (var v = [], h = 0; h < a.length; h++)
_(a[h], h, a) && v.push(a[h]);
return v;
}
var x9, w1, rT, nT, c5, l5, BV = yp({ "node-modules-polyfills:path"() {
De(), x9 = /^(\/?|)([\s\S]*?)((?:\.{1,2}|[^\/]+?|)(\.[^.\/]*|))(?:[\/]*)$/, w1 = function(a) {
return x9.exec(a).slice(1);
}, rT = "/", nT = ":", c5 = { extname: _5, basename: o5, dirname: s5, sep: rT, delimiter: nT, relative: a5, join: i5, isAbsolute: mT, normalize: dT, resolve: d1 }, l5 = "ab".substr(-1) === "b" ? function(a, _, v) {
return a.substr(_, v);
} : function(a, _, v) {
return _ < 0 && (_ = a.length + _), a.substr(_, v);
};
} }), _o = Oe({ "node-modules-polyfills-commonjs:path"(a, _) {
De();
var v = (BV(), Li(r5));
if (v && v.default) {
_.exports = v.default;
for (let h in v)
_.exports[h] = v[h];
} else
v && (_.exports = v);
} }), d_ = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/create-program/shared.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(Ae, te, he, Pe) {
Pe === void 0 && (Pe = he);
var R = Object.getOwnPropertyDescriptor(te, he);
(!R || ("get" in R ? !te.__esModule : R.writable || R.configurable)) && (R = { enumerable: true, get: function() {
return te[he];
} }), Object.defineProperty(Ae, Pe, R);
} : function(Ae, te, he, Pe) {
Pe === void 0 && (Pe = he), Ae[Pe] = te[he];
}), v = a && a.__setModuleDefault || (Object.create ? function(Ae, te) {
Object.defineProperty(Ae, "default", { enumerable: true, value: te });
} : function(Ae, te) {
Ae.default = te;
}), h = a && a.__importStar || function(Ae) {
if (Ae && Ae.__esModule)
return Ae;
var te = {};
if (Ae != null)
for (var he in Ae)
he !== "default" && Object.prototype.hasOwnProperty.call(Ae, he) && _(te, Ae, he);
return v(te, Ae), te;
}, D = a && a.__importDefault || function(Ae) {
return Ae && Ae.__esModule ? Ae : { default: Ae };
};
Object.defineProperty(a, "__esModule", { value: true }), a.getModuleResolver = a.getAstFromProgram = a.getCanonicalFileName = a.ensureAbsolutePath = a.createHash = a.createDefaultCompilerOptionsFromExtra = a.canonicalDirname = a.CORE_COMPILER_OPTIONS = void 0;
var P = D(_o()), y = h(vr()), m = { noEmit: true, noUnusedLocals: true, noUnusedParameters: true };
a.CORE_COMPILER_OPTIONS = m;
var C = Object.assign(Object.assign({}, m), { allowNonTsExtensions: true, allowJs: true, checkJs: true });
function d(Ae) {
return Ae.debugLevel.has("typescript") ? Object.assign(Object.assign({}, C), { extendedDiagnostics: true }) : C;
}
a.createDefaultCompilerOptionsFromExtra = d;
var E = y.sys !== void 0 ? y.sys.useCaseSensitiveFileNames : true, I = E ? (Ae) => Ae : (Ae) => Ae.toLowerCase();
function c(Ae) {
let te = P.default.normalize(Ae);
return te.endsWith(P.default.sep) && (te = te.slice(0, -1)), I(te);
}
a.getCanonicalFileName = c;
function M(Ae, te) {
return P.default.isAbsolute(Ae) ? Ae : P.default.join(te || "/prettier-security-dirname-placeholder", Ae);
}
a.ensureAbsolutePath = M;
function q(Ae) {
return P.default.dirname(Ae);
}
a.canonicalDirname = q;
var W = [y.Extension.Dts, y.Extension.Dcts, y.Extension.Dmts];
function K(Ae) {
var te;
return Ae ? (te = W.find((he) => Ae.endsWith(he))) !== null && te !== void 0 ? te : P.default.extname(Ae) : null;
}
function ce(Ae, te) {
let he = Ae.getSourceFile(te.filePath), Pe = K(te.filePath), R = K(he == null ? void 0 : he.fileName);
if (Pe === R)
return he && { ast: he, program: Ae };
}
a.getAstFromProgram = ce;
function Ie(Ae) {
let te;
try {
throw new Error("Dynamic require is not supported");
} catch {
let Pe = ["Could not find the provided parserOptions.moduleResolver.", "Hint: use an absolute path if you are not in control over where the ESLint instance runs."];
throw new Error(Pe.join(`
`));
}
return te;
}
a.getModuleResolver = Ie;
function me(Ae) {
var te;
return !((te = y.sys) === null || te === void 0) && te.createHash ? y.sys.createHash(Ae) : Ae;
}
a.createHash = me;
} }), qV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/create-program/createDefaultProgram.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(I, c, M, q) {
q === void 0 && (q = M);
var W = Object.getOwnPropertyDescriptor(c, M);
(!W || ("get" in W ? !c.__esModule : W.writable || W.configurable)) && (W = { enumerable: true, get: function() {
return c[M];
} }), Object.defineProperty(I, q, W);
} : function(I, c, M, q) {
q === void 0 && (q = M), I[q] = c[M];
}), v = a && a.__setModuleDefault || (Object.create ? function(I, c) {
Object.defineProperty(I, "default", { enumerable: true, value: c });
} : function(I, c) {
I.default = c;
}), h = a && a.__importStar || function(I) {
if (I && I.__esModule)
return I;
var c = {};
if (I != null)
for (var M in I)
M !== "default" && Object.prototype.hasOwnProperty.call(I, M) && _(c, I, M);
return v(c, I), c;
}, D = a && a.__importDefault || function(I) {
return I && I.__esModule ? I : { default: I };
};
Object.defineProperty(a, "__esModule", { value: true }), a.createDefaultProgram = void 0;
var P = D(Ga()), y = D(_o()), m = h(vr()), C = d_(), d = (0, P.default)("typescript-eslint:typescript-estree:createDefaultProgram");
function E(I) {
var c;
if (d("Getting default program for: %s", I.filePath || "unnamed file"), ((c = I.projects) === null || c === void 0 ? void 0 : c.length) !== 1)
return;
let M = I.projects[0], q = m.getParsedCommandLineOfConfigFile(M, (0, C.createDefaultCompilerOptionsFromExtra)(I), Object.assign(Object.assign({}, m.sys), { onUnRecoverableConfigFileDiagnostic: () => {
} }));
if (!q)
return;
let W = m.createCompilerHost(q.options, true);
I.moduleResolver && (W.resolveModuleNames = (0, C.getModuleResolver)(I.moduleResolver).resolveModuleNames);
let K = W.readFile;
W.readFile = (me) => y.default.normalize(me) === y.default.normalize(I.filePath) ? I.code : K(me);
let ce = m.createProgram([I.filePath], q.options, W), Ie = ce.getSourceFile(I.filePath);
return Ie && { ast: Ie, program: ce };
}
a.createDefaultProgram = E;
} }), gT = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/create-program/getScriptKind.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(d, E, I, c) {
c === void 0 && (c = I);
var M = Object.getOwnPropertyDescriptor(E, I);
(!M || ("get" in M ? !E.__esModule : M.writable || M.configurable)) && (M = { enumerable: true, get: function() {
return E[I];
} }), Object.defineProperty(d, c, M);
} : function(d, E, I, c) {
c === void 0 && (c = I), d[c] = E[I];
}), v = a && a.__setModuleDefault || (Object.create ? function(d, E) {
Object.defineProperty(d, "default", { enumerable: true, value: E });
} : function(d, E) {
d.default = E;
}), h = a && a.__importStar || function(d) {
if (d && d.__esModule)
return d;
var E = {};
if (d != null)
for (var I in d)
I !== "default" && Object.prototype.hasOwnProperty.call(d, I) && _(E, d, I);
return v(E, d), E;
}, D = a && a.__importDefault || function(d) {
return d && d.__esModule ? d : { default: d };
};
Object.defineProperty(a, "__esModule", { value: true }), a.getLanguageVariant = a.getScriptKind = void 0;
var P = D(_o()), y = h(vr());
function m(d, E) {
switch (P.default.extname(d).toLowerCase()) {
case y.Extension.Js:
case y.Extension.Cjs:
case y.Extension.Mjs:
return y.ScriptKind.JS;
case y.Extension.Jsx:
return y.ScriptKind.JSX;
case y.Extension.Ts:
case y.Extension.Cts:
case y.Extension.Mts:
return y.ScriptKind.TS;
case y.Extension.Tsx:
return y.ScriptKind.TSX;
case y.Extension.Json:
return y.ScriptKind.JSON;
default:
return E ? y.ScriptKind.TSX : y.ScriptKind.TS;
}
}
a.getScriptKind = m;
function C(d) {
switch (d) {
case y.ScriptKind.TSX:
case y.ScriptKind.JSX:
case y.ScriptKind.JS:
case y.ScriptKind.JSON:
return y.LanguageVariant.JSX;
default:
return y.LanguageVariant.Standard;
}
}
a.getLanguageVariant = C;
} }), UV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/create-program/createIsolatedProgram.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(I, c, M, q) {
q === void 0 && (q = M);
var W = Object.getOwnPropertyDescriptor(c, M);
(!W || ("get" in W ? !c.__esModule : W.writable || W.configurable)) && (W = { enumerable: true, get: function() {
return c[M];
} }), Object.defineProperty(I, q, W);
} : function(I, c, M, q) {
q === void 0 && (q = M), I[q] = c[M];
}), v = a && a.__setModuleDefault || (Object.create ? function(I, c) {
Object.defineProperty(I, "default", { enumerable: true, value: c });
} : function(I, c) {
I.default = c;
}), h = a && a.__importStar || function(I) {
if (I && I.__esModule)
return I;
var c = {};
if (I != null)
for (var M in I)
M !== "default" && Object.prototype.hasOwnProperty.call(I, M) && _(c, I, M);
return v(c, I), c;
}, D = a && a.__importDefault || function(I) {
return I && I.__esModule ? I : { default: I };
};
Object.defineProperty(a, "__esModule", { value: true }), a.createIsolatedProgram = void 0;
var P = D(Ga()), y = h(vr()), m = gT(), C = d_(), d = (0, P.default)("typescript-eslint:typescript-estree:createIsolatedProgram");
function E(I) {
d("Getting isolated program in %s mode for: %s", I.jsx ? "TSX" : "TS", I.filePath);
let c = { fileExists() {
return true;
}, getCanonicalFileName() {
return I.filePath;
}, getCurrentDirectory() {
return "";
}, getDirectories() {
return [];
}, getDefaultLibFileName() {
return "lib.d.ts";
}, getNewLine() {
return `
`;
}, getSourceFile(W) {
return y.createSourceFile(W, I.code, y.ScriptTarget.Latest, true, (0, m.getScriptKind)(I.filePath, I.jsx));
}, readFile() {
}, useCaseSensitiveFileNames() {
return true;
}, writeFile() {
return null;
} }, M = y.createProgram([I.filePath], Object.assign({ noResolve: true, target: y.ScriptTarget.Latest, jsx: I.jsx ? y.JsxEmit.Preserve : void 0 }, (0, C.createDefaultCompilerOptionsFromExtra)(I)), c), q = M.getSourceFile(I.filePath);
if (!q)
throw new Error("Expected an ast to be returned for the single-file isolated program.");
return { ast: q, program: M };
}
a.createIsolatedProgram = E;
} }), zV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/create-program/describeFilePath.js"(a) {
"use strict";
De();
var _ = a && a.__importDefault || function(D) {
return D && D.__esModule ? D : { default: D };
};
Object.defineProperty(a, "__esModule", { value: true }), a.describeFilePath = void 0;
var v = _(_o());
function h(D, P) {
let y = v.default.relative(P, D);
return y && !y.startsWith("..") && !v.default.isAbsolute(y) ? `/${y}` : /^[(\w+:)\\/~]/.test(D) || /\.\.[/\\]\.\./.test(y) ? D : `/${y}`;
}
a.describeFilePath = h;
} }), u5 = {};
m1(u5, { default: () => p5 });
var p5, WV = yp({ "node-modules-polyfills:fs"() {
De(), p5 = {};
} }), yT = Oe({ "node-modules-polyfills-commonjs:fs"(a, _) {
De();
var v = (WV(), Li(u5));
if (v && v.default) {
_.exports = v.default;
for (let h in v)
_.exports[h] = v[h];
} else
v && (_.exports = v);
} }), f5 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/create-program/getWatchProgramsForProjects.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(Je, Xe, ee, je) {
je === void 0 && (je = ee);
var nt = Object.getOwnPropertyDescriptor(Xe, ee);
(!nt || ("get" in nt ? !Xe.__esModule : nt.writable || nt.configurable)) && (nt = { enumerable: true, get: function() {
return Xe[ee];
} }), Object.defineProperty(Je, je, nt);
} : function(Je, Xe, ee, je) {
je === void 0 && (je = ee), Je[je] = Xe[ee];
}), v = a && a.__setModuleDefault || (Object.create ? function(Je, Xe) {
Object.defineProperty(Je, "default", { enumerable: true, value: Xe });
} : function(Je, Xe) {
Je.default = Xe;
}), h = a && a.__importStar || function(Je) {
if (Je && Je.__esModule)
return Je;
var Xe = {};
if (Je != null)
for (var ee in Je)
ee !== "default" && Object.prototype.hasOwnProperty.call(Je, ee) && _(Xe, Je, ee);
return v(Xe, Je), Xe;
}, D = a && a.__importDefault || function(Je) {
return Je && Je.__esModule ? Je : { default: Je };
};
Object.defineProperty(a, "__esModule", { value: true }), a.getWatchProgramsForProjects = a.clearWatchCaches = void 0;
var P = D(Ga()), y = D(yT()), m = D(pT()), C = h(vr()), d = d_(), E = (0, P.default)("typescript-eslint:typescript-estree:createWatchProgram"), I = /* @__PURE__ */ new Map(), c = /* @__PURE__ */ new Map(), M = /* @__PURE__ */ new Map(), q = /* @__PURE__ */ new Map(), W = /* @__PURE__ */ new Map(), K = /* @__PURE__ */ new Map();
function ce() {
I.clear(), c.clear(), M.clear(), K.clear(), q.clear(), W.clear();
}
a.clearWatchCaches = ce;
function Ie(Je) {
return (Xe, ee) => {
let je = (0, d.getCanonicalFileName)(Xe), nt = (() => {
let Ze = Je.get(je);
return Ze || (Ze = /* @__PURE__ */ new Set(), Je.set(je, Ze)), Ze;
})();
return nt.add(ee), { close: () => {
nt.delete(ee);
} };
};
}
var me = { code: "", filePath: "" };
function Ae(Je) {
throw new Error(C.flattenDiagnosticMessageText(Je.messageText, C.sys.newLine));
}
function te(Je, Xe, ee) {
let je = ee.EXPERIMENTAL_useSourceOfProjectReferenceRedirect ? new Set(Xe.getSourceFiles().map((nt) => (0, d.getCanonicalFileName)(nt.fileName))) : new Set(Xe.getRootFileNames().map((nt) => (0, d.getCanonicalFileName)(nt)));
return q.set(Je, je), je;
}
function he(Je) {
let Xe = (0, d.getCanonicalFileName)(Je.filePath), ee = [];
me.code = Je.code, me.filePath = Xe;
let je = c.get(Xe), nt = (0, d.createHash)(Je.code);
K.get(Xe) !== nt && je && je.size > 0 && je.forEach((st) => st(Xe, C.FileWatcherEventKind.Changed));
let Ze = new Set(Je.projects);
for (let [st, tt] of I.entries()) {
if (!Ze.has(st))
continue;
let ct = q.get(st), ne = null;
if (ct || (ne = tt.getProgram().getProgram(), ct = te(st, ne, Je)), ct.has(Xe))
return E("Found existing program for file. %s", Xe), ne = ne != null ? ne : tt.getProgram().getProgram(), ne.getTypeChecker(), [ne];
}
E("File did not belong to any existing programs, moving to create/update. %s", Xe);
for (let st of Je.projects) {
let tt = I.get(st);
if (tt) {
let Fe = ke(tt, Xe, st);
if (!Fe)
continue;
if (Fe.getTypeChecker(), te(st, Fe, Je).has(Xe))
return E("Found updated program for file. %s", Xe), [Fe];
ee.push(Fe);
continue;
}
let ct = R(st, Je);
I.set(st, ct);
let ne = ct.getProgram().getProgram();
if (ne.getTypeChecker(), te(st, ne, Je).has(Xe))
return E("Found program for file. %s", Xe), [ne];
ee.push(ne);
}
return ee;
}
a.getWatchProgramsForProjects = he;
var Pe = m.default.satisfies(C.version, ">=3.9.0-beta", { includePrerelease: true });
function R(Je, Xe) {
E("Creating watch program for %s.", Je);
let ee = C.createWatchCompilerHost(Je, (0, d.createDefaultCompilerOptionsFromExtra)(Xe), C.sys, C.createAbstractBuilder, Ae, () => {
});
Xe.moduleResolver && (ee.resolveModuleNames = (0, d.getModuleResolver)(Xe.moduleResolver).resolveModuleNames);
let je = ee.readFile;
ee.readFile = (tt, ct) => {
let ne = (0, d.getCanonicalFileName)(tt), ge = ne === me.filePath ? me.code : je(ne, ct);
return ge !== void 0 && K.set(ne, (0, d.createHash)(ge)), ge;
}, ee.onUnRecoverableConfigFileDiagnostic = Ae, ee.afterProgramCreate = (tt) => {
let ct = tt.getConfigFileParsingDiagnostics().filter((ne) => ne.category === C.DiagnosticCategory.Error && ne.code !== 18003);
ct.length > 0 && Ae(ct[0]);
}, ee.watchFile = Ie(c), ee.watchDirectory = Ie(M);
let nt = ee.onCachedDirectoryStructureHostCreate;
ee.onCachedDirectoryStructureHostCreate = (tt) => {
let ct = tt.readDirectory;
tt.readDirectory = (ne, ge, Fe, at, Pt) => ct(ne, ge ? ge.concat(Xe.extraFileExtensions) : void 0, Fe, at, Pt), nt(tt);
}, ee.extraFileExtensions = Xe.extraFileExtensions.map((tt) => ({ extension: tt, isMixedContent: true, scriptKind: C.ScriptKind.Deferred })), ee.trace = E, ee.useSourceOfProjectReferenceRedirect = () => Xe.EXPERIMENTAL_useSourceOfProjectReferenceRedirect;
let Ze;
Pe ? (ee.setTimeout = void 0, ee.clearTimeout = void 0) : (E("Running without timeout fix"), ee.setTimeout = function(tt, ct) {
for (var ne = arguments.length, ge = new Array(ne > 2 ? ne - 2 : 0), Fe = 2; Fe < ne; Fe++)
ge[Fe - 2] = arguments[Fe];
return Ze = tt.bind(void 0, ...ge), Ze;
}, ee.clearTimeout = () => {
Ze = void 0;
});
let st = C.createWatchProgram(ee);
if (!Pe) {
let tt = st.getProgram;
st.getProgram = () => (Ze && Ze(), Ze = void 0, tt.call(st));
}
return st;
}
function pe(Je) {
let ee = y.default.statSync(Je).mtimeMs, je = W.get(Je);
return W.set(Je, ee), je === void 0 ? false : Math.abs(je - ee) > Number.EPSILON;
}
function ke(Je, Xe, ee) {
let je = Je.getProgram().getProgram();
if (cn.env.TSESTREE_NO_INVALIDATION === "true")
return je;
pe(ee) && (E("tsconfig has changed - triggering program update. %s", ee), c.get(ee).forEach((at) => at(ee, C.FileWatcherEventKind.Changed)), q.delete(ee));
let nt = je.getSourceFile(Xe);
if (nt)
return je;
E("File was not found in program - triggering folder update. %s", Xe);
let Ze = (0, d.canonicalDirname)(Xe), st = null, tt = Ze, ct = false;
for (; st !== tt; ) {
st = tt;
let at = M.get(st);
at && (at.forEach((Pt) => {
Ze !== st && Pt(Ze, C.FileWatcherEventKind.Changed), Pt(st, C.FileWatcherEventKind.Changed);
}), ct = true), tt = (0, d.canonicalDirname)(st);
}
if (!ct)
return E("No callback found for file, not part of this program. %s", Xe), null;
if (q.delete(ee), je = Je.getProgram().getProgram(), nt = je.getSourceFile(Xe), nt)
return je;
E("File was still not found in program after directory update - checking file deletions. %s", Xe);
let ge = je.getRootFileNames().find((at) => !y.default.existsSync(at));
if (!ge)
return null;
let Fe = c.get((0, d.getCanonicalFileName)(ge));
return Fe ? (E("Marking file as deleted. %s", ge), Fe.forEach((at) => at(ge, C.FileWatcherEventKind.Deleted)), q.delete(ee), je = Je.getProgram().getProgram(), nt = je.getSourceFile(Xe), nt ? je : (E("File was still not found in program after deletion check, assuming it is not part of this program. %s", Xe), null)) : (E("Could not find watch callbacks for root file. %s", ge), je);
}
} }), VV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/create-program/createProjectProgram.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(W, K, ce, Ie) {
Ie === void 0 && (Ie = ce);
var me = Object.getOwnPropertyDescriptor(K, ce);
(!me || ("get" in me ? !K.__esModule : me.writable || me.configurable)) && (me = { enumerable: true, get: function() {
return K[ce];
} }), Object.defineProperty(W, Ie, me);
} : function(W, K, ce, Ie) {
Ie === void 0 && (Ie = ce), W[Ie] = K[ce];
}), v = a && a.__setModuleDefault || (Object.create ? function(W, K) {
Object.defineProperty(W, "default", { enumerable: true, value: K });
} : function(W, K) {
W.default = K;
}), h = a && a.__importStar || function(W) {
if (W && W.__esModule)
return W;
var K = {};
if (W != null)
for (var ce in W)
ce !== "default" && Object.prototype.hasOwnProperty.call(W, ce) && _(K, W, ce);
return v(K, W), K;
}, D = a && a.__importDefault || function(W) {
return W && W.__esModule ? W : { default: W };
};
Object.defineProperty(a, "__esModule", { value: true }), a.createProjectProgram = void 0;
var P = D(Ga()), y = D(_o()), m = h(vr()), C = E1(), d = zV(), E = f5(), I = d_(), c = (0, P.default)("typescript-eslint:typescript-estree:createProjectProgram"), M = [m.Extension.Ts, m.Extension.Tsx, m.Extension.Js, m.Extension.Jsx, m.Extension.Mjs, m.Extension.Mts, m.Extension.Cjs, m.Extension.Cts];
function q(W) {
c("Creating project program for: %s", W.filePath);
let K = (0, E.getWatchProgramsForProjects)(W), ce = (0, C.firstDefined)(K, (ke) => (0, I.getAstFromProgram)(ke, W));
if (ce || W.createDefaultProgram)
return ce;
let Ie = (ke) => (0, d.describeFilePath)(ke, W.tsconfigRootDir), me = (0, d.describeFilePath)(W.filePath, W.tsconfigRootDir), Ae = W.projects.map(Ie), te = Ae.length === 1 ? Ae[0] : `
${Ae.map((ke) => `- ${ke}`).join(`
`)}`, he = [`ESLint was configured to run on \`${me}\` using \`parserOptions.project\`: ${te}`], Pe = false, R = W.extraFileExtensions || [];
R.forEach((ke) => {
ke.startsWith(".") || he.push(`Found unexpected extension \`${ke}\` specified with the \`parserOptions.extraFileExtensions\` option. Did you mean \`.${ke}\`?`), M.includes(ke) && he.push(`You unnecessarily included the extension \`${ke}\` with the \`parserOptions.extraFileExtensions\` option. This extension is already handled by the parser by default.`);
});
let pe = y.default.extname(W.filePath);
if (!M.includes(pe)) {
let ke = `The extension for the file (\`${pe}\`) is non-standard`;
R.length > 0 ? R.includes(pe) || (he.push(`${ke}. It should be added to your existing \`parserOptions.extraFileExtensions\`.`), Pe = true) : (he.push(`${ke}. You should add \`parserOptions.extraFileExtensions\` to your config.`), Pe = true);
}
if (!Pe) {
let [ke, Je] = W.projects.length === 1 ? ["that TSConfig does not", "that TSConfig"] : ["none of those TSConfigs", "one of those TSConfigs"];
he.push(`However, ${ke} include this file. Either:`, "- Change ESLint's list of included files to not include this file", `- Change ${Je} to include this file`, "- Create a new TSConfig that includes this file and include it in your parserOptions.project", "See the typescript-eslint docs for more info: https://typescript-eslint.io/linting/troubleshooting#i-get-errors-telling-me-eslint-was-configured-to-run--however-that-tsconfig-does-not--none-of-those-tsconfigs-include-this-file");
}
throw new Error(he.join(`
`));
}
a.createProjectProgram = q;
} }), HV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/create-program/createSourceFile.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(E, I, c, M) {
M === void 0 && (M = c);
var q = Object.getOwnPropertyDescriptor(I, c);
(!q || ("get" in q ? !I.__esModule : q.writable || q.configurable)) && (q = { enumerable: true, get: function() {
return I[c];
} }), Object.defineProperty(E, M, q);
} : function(E, I, c, M) {
M === void 0 && (M = c), E[M] = I[c];
}), v = a && a.__setModuleDefault || (Object.create ? function(E, I) {
Object.defineProperty(E, "default", { enumerable: true, value: I });
} : function(E, I) {
E.default = I;
}), h = a && a.__importStar || function(E) {
if (E && E.__esModule)
return E;
var I = {};
if (E != null)
for (var c in E)
c !== "default" && Object.prototype.hasOwnProperty.call(E, c) && _(I, E, c);
return v(I, E), I;
}, D = a && a.__importDefault || function(E) {
return E && E.__esModule ? E : { default: E };
};
Object.defineProperty(a, "__esModule", { value: true }), a.createSourceFile = void 0;
var P = D(Ga()), y = h(vr()), m = gT(), C = (0, P.default)("typescript-eslint:typescript-estree:createSourceFile");
function d(E) {
return C("Getting AST without type information in %s mode for: %s", E.jsx ? "TSX" : "TS", E.filePath), y.createSourceFile(E.filePath, E.code, y.ScriptTarget.Latest, true, (0, m.getScriptKind)(E.filePath, E.jsx));
}
a.createSourceFile = d;
} }), d5 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/create-program/useProvidedPrograms.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(q, W, K, ce) {
ce === void 0 && (ce = K);
var Ie = Object.getOwnPropertyDescriptor(W, K);
(!Ie || ("get" in Ie ? !W.__esModule : Ie.writable || Ie.configurable)) && (Ie = { enumerable: true, get: function() {
return W[K];
} }), Object.defineProperty(q, ce, Ie);
} : function(q, W, K, ce) {
ce === void 0 && (ce = K), q[ce] = W[K];
}), v = a && a.__setModuleDefault || (Object.create ? function(q, W) {
Object.defineProperty(q, "default", { enumerable: true, value: W });
} : function(q, W) {
q.default = W;
}), h = a && a.__importStar || function(q) {
if (q && q.__esModule)
return q;
var W = {};
if (q != null)
for (var K in q)
K !== "default" && Object.prototype.hasOwnProperty.call(q, K) && _(W, q, K);
return v(W, q), W;
}, D = a && a.__importDefault || function(q) {
return q && q.__esModule ? q : { default: q };
};
Object.defineProperty(a, "__esModule", { value: true }), a.createProgramFromConfigFile = a.useProvidedPrograms = void 0;
var P = D(Ga()), y = h(yT()), m = h(_o()), C = h(vr()), d = d_(), E = (0, P.default)("typescript-eslint:typescript-estree:useProvidedProgram");
function I(q, W) {
E("Retrieving ast for %s from provided program instance(s)", W.filePath);
let K;
for (let ce of q)
if (K = (0, d.getAstFromProgram)(ce, W), K)
break;
if (!K) {
let Ie = ['"parserOptions.programs" has been provided for @typescript-eslint/parser.', `The file was not found in any of the provided program instance(s): ${m.relative(W.tsconfigRootDir || "/prettier-security-dirname-placeholder", W.filePath)}`];
throw new Error(Ie.join(`
`));
}
return K.program.getTypeChecker(), K;
}
a.useProvidedPrograms = I;
function c(q, W) {
if (C.sys === void 0)
throw new Error("`createProgramFromConfigFile` is only supported in a Node-like environment.");
let ce = C.getParsedCommandLineOfConfigFile(q, d.CORE_COMPILER_OPTIONS, { onUnRecoverableConfigFileDiagnostic: (me) => {
throw new Error(M([me]));
}, fileExists: y.existsSync, getCurrentDirectory: () => W && m.resolve(W) || "/prettier-security-dirname-placeholder", readDirectory: C.sys.readDirectory, readFile: (me) => y.readFileSync(me, "utf-8"), useCaseSensitiveFileNames: C.sys.useCaseSensitiveFileNames });
if (ce.errors.length)
throw new Error(M(ce.errors));
let Ie = C.createCompilerHost(ce.options, true);
return C.createProgram(ce.fileNames, ce.options, Ie);
}
a.createProgramFromConfigFile = c;
function M(q) {
return C.formatDiagnostics(q, { getCanonicalFileName: (W) => W, getCurrentDirectory: cn.cwd, getNewLine: () => `
` });
}
} }), m5 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/parseSettings/ExpiringCache.js"(a) {
"use strict";
De();
var _ = a && a.__classPrivateFieldSet || function(m, C, d, E, I) {
if (E === "m")
throw new TypeError("Private method is not writable");
if (E === "a" && !I)
throw new TypeError("Private accessor was defined without a setter");
if (typeof C == "function" ? m !== C || !I : !C.has(m))
throw new TypeError("Cannot write private member to an object whose class did not declare it");
return E === "a" ? I.call(m, d) : I ? I.value = d : C.set(m, d), d;
}, v = a && a.__classPrivateFieldGet || function(m, C, d, E) {
if (d === "a" && !E)
throw new TypeError("Private accessor was defined without a getter");
if (typeof C == "function" ? m !== C || !E : !C.has(m))
throw new TypeError("Cannot read private member from an object whose class did not declare it");
return d === "m" ? E : d === "a" ? E.call(m) : E ? E.value : C.get(m);
}, h, D;
Object.defineProperty(a, "__esModule", { value: true }), a.ExpiringCache = a.DEFAULT_TSCONFIG_CACHE_DURATION_SECONDS = void 0, a.DEFAULT_TSCONFIG_CACHE_DURATION_SECONDS = 30;
var P = [0, 0], y = class {
constructor(m) {
h.set(this, void 0), D.set(this, /* @__PURE__ */ new Map()), _(this, h, m, "f");
}
set(m, C) {
return v(this, D, "f").set(m, { value: C, lastSeen: v(this, h, "f") === "Infinity" ? P : cn.hrtime() }), this;
}
get(m) {
let C = v(this, D, "f").get(m);
if ((C == null ? void 0 : C.value) != null) {
if (v(this, h, "f") === "Infinity" || cn.hrtime(C.lastSeen)[0] < v(this, h, "f"))
return C.value;
v(this, D, "f").delete(m);
}
}
clear() {
v(this, D, "f").clear();
}
};
a.ExpiringCache = y, h = /* @__PURE__ */ new WeakMap(), D = /* @__PURE__ */ new WeakMap();
} }), GV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/parseSettings/getProjectConfigFiles.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(E, I, c, M) {
M === void 0 && (M = c);
var q = Object.getOwnPropertyDescriptor(I, c);
(!q || ("get" in q ? !I.__esModule : q.writable || q.configurable)) && (q = { enumerable: true, get: function() {
return I[c];
} }), Object.defineProperty(E, M, q);
} : function(E, I, c, M) {
M === void 0 && (M = c), E[M] = I[c];
}), v = a && a.__setModuleDefault || (Object.create ? function(E, I) {
Object.defineProperty(E, "default", { enumerable: true, value: I });
} : function(E, I) {
E.default = I;
}), h = a && a.__importStar || function(E) {
if (E && E.__esModule)
return E;
var I = {};
if (E != null)
for (var c in E)
c !== "default" && Object.prototype.hasOwnProperty.call(E, c) && _(I, E, c);
return v(I, E), I;
}, D = a && a.__importDefault || function(E) {
return E && E.__esModule ? E : { default: E };
};
Object.defineProperty(a, "__esModule", { value: true }), a.getProjectConfigFiles = void 0;
var P = D(Ga()), y = h(yT()), m = h(_o()), C = (0, P.default)("typescript-eslint:typescript-estree:getProjectConfigFiles");
function d(E, I) {
var c;
if (I !== true)
return I === void 0 || Array.isArray(I) ? I : [I];
C("Looking for tsconfig.json at or above file: %s", E.filePath);
let M = m.dirname(E.filePath), q = [M];
do {
C("Checking tsconfig.json path: %s", M);
let W = m.join(M, "tsconfig.json"), K = (c = E.tsconfigMatchCache.get(M)) !== null && c !== void 0 ? c : y.existsSync(W) && W;
if (K) {
for (let ce of q)
E.tsconfigMatchCache.set(ce, K);
return [K];
}
M = m.dirname(M), q.push(M);
} while (M.length > 1 && M.length >= E.tsconfigRootDir.length);
throw new Error(`project was set to \`true\` but couldn't find any tsconfig.json relative to '${E.filePath}' within '${E.tsconfigRootDir}'.`);
}
a.getProjectConfigFiles = d;
} }), $V = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/parseSettings/inferSingleRun.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.inferSingleRun = void 0;
var _ = _o();
function v(h) {
return (h == null ? void 0 : h.project) == null || (h == null ? void 0 : h.programs) != null || cn.env.TSESTREE_SINGLE_RUN === "false" ? false : !!(cn.env.TSESTREE_SINGLE_RUN === "true" || h != null && h.allowAutomaticSingleRunInference && (cn.env.CI === "true" || cn.argv[1].endsWith((0, _.normalize)("node_modules/.bin/eslint"))));
}
a.inferSingleRun = v;
} }), KV = Oe({ "node_modules/is-extglob/index.js"(a, _) {
De(), _.exports = function(h) {
if (typeof h != "string" || h === "")
return false;
for (var D; D = /(\\).|([@?!+*]\(.*\))/g.exec(h); ) {
if (D[2])
return true;
h = h.slice(D.index + D[0].length);
}
return false;
};
} }), XV = Oe({ "node_modules/is-glob/index.js"(a, _) {
De();
var v = KV(), h = { "{": "}", "(": ")", "[": "]" }, D = function(y) {
if (y[0] === "!")
return true;
for (var m = 0, C = -2, d = -2, E = -2, I = -2, c = -2; m < y.length; ) {
if (y[m] === "*" || y[m + 1] === "?" && /[\].+)]/.test(y[m]) || d !== -1 && y[m] === "[" && y[m + 1] !== "]" && (d < m && (d = y.indexOf("]", m)), d > m && (c === -1 || c > d || (c = y.indexOf("\\", m), c === -1 || c > d))) || E !== -1 && y[m] === "{" && y[m + 1] !== "}" && (E = y.indexOf("}", m), E > m && (c = y.indexOf("\\", m), c === -1 || c > E)) || I !== -1 && y[m] === "(" && y[m + 1] === "?" && /[:!=]/.test(y[m + 2]) && y[m + 3] !== ")" && (I = y.indexOf(")", m), I > m && (c = y.indexOf("\\", m), c === -1 || c > I)) || C !== -1 && y[m] === "(" && y[m + 1] !== "|" && (C < m && (C = y.indexOf("|", m)), C !== -1 && y[C + 1] !== ")" && (I = y.indexOf(")", C), I > C && (c = y.indexOf("\\", C), c === -1 || c > I))))
return true;
if (y[m] === "\\") {
var M = y[m + 1];
m += 2;
var q = h[M];
if (q) {
var W = y.indexOf(q, m);
W !== -1 && (m = W + 1);
}
if (y[m] === "!")
return true;
} else
m++;
}
return false;
}, P = function(y) {
if (y[0] === "!")
return true;
for (var m = 0; m < y.length; ) {
if (/[*?{}()[\]]/.test(y[m]))
return true;
if (y[m] === "\\") {
var C = y[m + 1];
m += 2;
var d = h[C];
if (d) {
var E = y.indexOf(d, m);
E !== -1 && (m = E + 1);
}
if (y[m] === "!")
return true;
} else
m++;
}
return false;
};
_.exports = function(m, C) {
if (typeof m != "string" || m === "")
return false;
if (v(m))
return true;
var d = D;
return C && C.strict === false && (d = P), d(m);
};
} }), h5 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/parseSettings/resolveProjectList.js"(a) {
"use strict";
De();
var _ = a && a.__importDefault || function(M) {
return M && M.__esModule ? M : { default: M };
};
Object.defineProperty(a, "__esModule", { value: true }), a.clearGlobResolutionCache = a.resolveProjectList = a.clearGlobCache = void 0;
var v = _(Ga()), h = {}, D = _(XV()), P = d_(), y = m5(), m = (0, v.default)("typescript-eslint:typescript-estree:parser:parseSettings:resolveProjectList"), C = null;
function d() {
C == null || C.clear();
}
a.clearGlobCache = d;
function E(M) {
var q, W, K;
let ce = [];
if (typeof M.project == "string")
ce.push(M.project);
else if (Array.isArray(M.project))
for (let R of M.project)
typeof R == "string" && ce.push(R);
if (ce.length === 0)
return [];
let Ie = ((q = M.projectFolderIgnoreList) !== null && q !== void 0 ? q : ["**/node_modules/**"]).reduce((R, pe) => (typeof pe == "string" && R.push(pe), R), []).map((R) => R.startsWith("!") ? R : `!${R}`), me = I({ project: ce, projectFolderIgnoreList: Ie, tsconfigRootDir: M.tsconfigRootDir });
if (C == null)
C = new y.ExpiringCache(M.singleRun ? "Infinity" : (K = (W = M.cacheLifetime) === null || W === void 0 ? void 0 : W.glob) !== null && K !== void 0 ? K : y.DEFAULT_TSCONFIG_CACHE_DURATION_SECONDS);
else {
let R = C.get(me);
if (R)
return R;
}
let Ae = ce.filter((R) => !(0, D.default)(R)), te = ce.filter((R) => (0, D.default)(R)), he = new Set(Ae.concat(te.length === 0 ? [] : (0, h.sync)([...te, ...Ie], { cwd: M.tsconfigRootDir })).map((R) => (0, P.getCanonicalFileName)((0, P.ensureAbsolutePath)(R, M.tsconfigRootDir))));
m("parserOptions.project (excluding ignored) matched projects: %s", he);
let Pe = Array.from(he);
return C.set(me, Pe), Pe;
}
a.resolveProjectList = E;
function I(M) {
let { project: q, projectFolderIgnoreList: W, tsconfigRootDir: K } = M, ce = { tsconfigRootDir: K, project: q, projectFolderIgnoreList: [...W].sort() };
return (0, P.createHash)(JSON.stringify(ce));
}
function c() {
C == null || C.clear(), C = null;
}
a.clearGlobResolutionCache = c;
} }), YV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/parseSettings/warnAboutTSVersion.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(M, q, W, K) {
K === void 0 && (K = W);
var ce = Object.getOwnPropertyDescriptor(q, W);
(!ce || ("get" in ce ? !q.__esModule : ce.writable || ce.configurable)) && (ce = { enumerable: true, get: function() {
return q[W];
} }), Object.defineProperty(M, K, ce);
} : function(M, q, W, K) {
K === void 0 && (K = W), M[K] = q[W];
}), v = a && a.__setModuleDefault || (Object.create ? function(M, q) {
Object.defineProperty(M, "default", { enumerable: true, value: q });
} : function(M, q) {
M.default = q;
}), h = a && a.__importStar || function(M) {
if (M && M.__esModule)
return M;
var q = {};
if (M != null)
for (var W in M)
W !== "default" && Object.prototype.hasOwnProperty.call(M, W) && _(q, M, W);
return v(q, M), q;
}, D = a && a.__importDefault || function(M) {
return M && M.__esModule ? M : { default: M };
};
Object.defineProperty(a, "__esModule", { value: true }), a.warnAboutTSVersion = void 0;
var P = D(pT()), y = h(vr()), m = ">=3.3.1 <5.1.0", C = ["5.0.1-rc"], d = y.version, E = P.default.satisfies(d, [m].concat(C).join(" || ")), I = false;
function c(M) {
var q;
if (!E && !I) {
if (typeof cn > "u" ? false : (q = cn.stdout) === null || q === void 0 ? void 0 : q.isTTY) {
let K = "=============", ce = [K, "WARNING: You are currently running a version of TypeScript which is not officially supported by @typescript-eslint/typescript-estree.", "You may find that it works just fine, or you may not.", `SUPPORTED TYPESCRIPT VERSIONS: ${m}`, `YOUR TYPESCRIPT VERSION: ${d}`, "Please only submit bug reports when using the officially supported version.", K];
M.log(ce.join(`
`));
}
I = true;
}
}
a.warnAboutTSVersion = c;
} }), g5 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/parseSettings/createParseSettings.js"(a) {
"use strict";
De();
var _ = a && a.__importDefault || function(W) {
return W && W.__esModule ? W : { default: W };
};
Object.defineProperty(a, "__esModule", { value: true }), a.clearTSConfigMatchCache = a.createParseSettings = void 0;
var v = _(Ga()), h = d_(), D = m5(), P = GV(), y = $V(), m = h5(), C = YV(), d = (0, v.default)("typescript-eslint:typescript-estree:parser:parseSettings:createParseSettings"), E;
function I(W) {
let K = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
var ce, Ie, me;
let Ae = (0, y.inferSingleRun)(K), te = typeof K.tsconfigRootDir == "string" ? K.tsconfigRootDir : "/prettier-security-dirname-placeholder", he = { code: M(W), comment: K.comment === true, comments: [], createDefaultProgram: K.createDefaultProgram === true, debugLevel: K.debugLevel === true ? /* @__PURE__ */ new Set(["typescript-eslint"]) : Array.isArray(K.debugLevel) ? new Set(K.debugLevel) : /* @__PURE__ */ new Set(), errorOnTypeScriptSyntacticAndSemanticIssues: false, errorOnUnknownASTType: K.errorOnUnknownASTType === true, EXPERIMENTAL_useSourceOfProjectReferenceRedirect: K.EXPERIMENTAL_useSourceOfProjectReferenceRedirect === true, extraFileExtensions: Array.isArray(K.extraFileExtensions) && K.extraFileExtensions.every((Pe) => typeof Pe == "string") ? K.extraFileExtensions : [], filePath: (0, h.ensureAbsolutePath)(typeof K.filePath == "string" && K.filePath !== "" ? K.filePath : q(K.jsx), te), jsx: K.jsx === true, loc: K.loc === true, log: typeof K.loggerFn == "function" ? K.loggerFn : K.loggerFn === false ? () => {
} : console.log, moduleResolver: (ce = K.moduleResolver) !== null && ce !== void 0 ? ce : "", preserveNodeMaps: K.preserveNodeMaps !== false, programs: Array.isArray(K.programs) ? K.programs : null, projects: [], range: K.range === true, singleRun: Ae, tokens: K.tokens === true ? [] : null, tsconfigMatchCache: E != null ? E : E = new D.ExpiringCache(Ae ? "Infinity" : (me = (Ie = K.cacheLifetime) === null || Ie === void 0 ? void 0 : Ie.glob) !== null && me !== void 0 ? me : D.DEFAULT_TSCONFIG_CACHE_DURATION_SECONDS), tsconfigRootDir: te };
if (he.debugLevel.size > 0) {
let Pe = [];
he.debugLevel.has("typescript-eslint") && Pe.push("typescript-eslint:*"), (he.debugLevel.has("eslint") || v.default.enabled("eslint:*,-eslint:code-path")) && Pe.push("eslint:*,-eslint:code-path"), v.default.enable(Pe.join(","));
}
if (Array.isArray(K.programs)) {
if (!K.programs.length)
throw new Error("You have set parserOptions.programs to an empty array. This will cause all files to not be found in existing programs. Either provide one or more existing TypeScript Program instances in the array, or remove the parserOptions.programs setting.");
d("parserOptions.programs was provided, so parserOptions.project will be ignored.");
}
return he.programs || (he.projects = (0, m.resolveProjectList)({ cacheLifetime: K.cacheLifetime, project: (0, P.getProjectConfigFiles)(he, K.project), projectFolderIgnoreList: K.projectFolderIgnoreList, singleRun: he.singleRun, tsconfigRootDir: te })), (0, C.warnAboutTSVersion)(he), he;
}
a.createParseSettings = I;
function c() {
E == null || E.clear();
}
a.clearTSConfigMatchCache = c;
function M(W) {
return typeof W != "string" ? String(W) : W;
}
function q(W) {
return W ? "estree.tsx" : "estree.ts";
}
} }), QV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/semantic-or-syntactic-errors.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.getFirstSemanticOrSyntacticError = void 0;
var _ = vr();
function v(P, y) {
try {
let m = h(P.getSyntacticDiagnostics(y));
if (m.length)
return D(m[0]);
let C = h(P.getSemanticDiagnostics(y));
return C.length ? D(C[0]) : void 0;
} catch (m) {
console.warn(`Warning From TSC: "${m.message}`);
return;
}
}
a.getFirstSemanticOrSyntacticError = v;
function h(P) {
return P.filter((y) => {
switch (y.code) {
case 1013:
case 1014:
case 1044:
case 1045:
case 1048:
case 1049:
case 1070:
case 1071:
case 1085:
case 1090:
case 1096:
case 1097:
case 1098:
case 1099:
case 1117:
case 1121:
case 1123:
case 1141:
case 1162:
case 1164:
case 1172:
case 1173:
case 1175:
case 1176:
case 1190:
case 1196:
case 1200:
case 1206:
case 1211:
case 1242:
case 1246:
case 1255:
case 1308:
case 2364:
case 2369:
case 2452:
case 2462:
case 8017:
case 17012:
case 17013:
return true;
}
return false;
});
}
function D(P) {
return Object.assign(Object.assign({}, P), { message: (0, _.flattenDiagnosticMessageText)(P.messageText, _.sys.newLine) });
}
} }), y5 = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/parser.js"(a) {
"use strict";
De();
var _ = a && a.__importDefault || function(he) {
return he && he.__esModule ? he : { default: he };
};
Object.defineProperty(a, "__esModule", { value: true }), a.clearParseAndGenerateServicesCalls = a.clearProgramCache = a.parseWithNodeMaps = a.parseAndGenerateServices = a.parse = void 0;
var v = _(Ga()), h = FV(), D = G9(), P = qV(), y = UV(), m = VV(), C = HV(), d = d5(), E = g5(), I = QV(), c = (0, v.default)("typescript-eslint:typescript-estree:parser"), M = /* @__PURE__ */ new Map();
function q() {
M.clear();
}
a.clearProgramCache = q;
function W(he, Pe) {
return he.programs && (0, d.useProvidedPrograms)(he.programs, he) || Pe && (0, m.createProjectProgram)(he) || Pe && he.createDefaultProgram && (0, P.createDefaultProgram)(he) || (0, y.createIsolatedProgram)(he);
}
function K(he, Pe) {
let { ast: R } = ce(he, Pe, false);
return R;
}
a.parse = K;
function ce(he, Pe, R) {
let pe = (0, E.createParseSettings)(he, Pe);
if (Pe != null && Pe.errorOnTypeScriptSyntacticAndSemanticIssues)
throw new Error('"errorOnTypeScriptSyntacticAndSemanticIssues" is only supported for parseAndGenerateServices()');
let ke = (0, C.createSourceFile)(pe), { estree: Je, astMaps: Xe } = (0, h.astConverter)(ke, pe, R);
return { ast: Je, esTreeNodeToTSNodeMap: Xe.esTreeNodeToTSNodeMap, tsNodeToESTreeNodeMap: Xe.tsNodeToESTreeNodeMap };
}
function Ie(he, Pe) {
return ce(he, Pe, true);
}
a.parseWithNodeMaps = Ie;
var me = {};
function Ae() {
me = {};
}
a.clearParseAndGenerateServicesCalls = Ae;
function te(he, Pe) {
var R, pe;
let ke = (0, E.createParseSettings)(he, Pe);
Pe !== void 0 && typeof Pe.errorOnTypeScriptSyntacticAndSemanticIssues == "boolean" && Pe.errorOnTypeScriptSyntacticAndSemanticIssues && (ke.errorOnTypeScriptSyntacticAndSemanticIssues = true), ke.singleRun && !ke.programs && ((R = ke.projects) === null || R === void 0 ? void 0 : R.length) > 0 && (ke.programs = { *[Symbol.iterator]() {
for (let st of ke.projects) {
let tt = M.get(st);
if (tt)
yield tt;
else {
c("Detected single-run/CLI usage, creating Program once ahead of time for project: %s", st);
let ct = (0, d.createProgramFromConfigFile)(st);
M.set(st, ct), yield ct;
}
}
} });
let Je = ke.programs != null || ((pe = ke.projects) === null || pe === void 0 ? void 0 : pe.length) > 0;
ke.singleRun && Pe.filePath && (me[Pe.filePath] = (me[Pe.filePath] || 0) + 1);
let { ast: Xe, program: ee } = ke.singleRun && Pe.filePath && me[Pe.filePath] > 1 ? (0, y.createIsolatedProgram)(ke) : W(ke, Je), je = typeof ke.preserveNodeMaps == "boolean" ? ke.preserveNodeMaps : true, { estree: nt, astMaps: Ze } = (0, h.astConverter)(Xe, ke, je);
if (ee && ke.errorOnTypeScriptSyntacticAndSemanticIssues) {
let st = (0, I.getFirstSemanticOrSyntacticError)(ee, Xe);
if (st)
throw (0, D.convertError)(st);
}
return { ast: nt, services: { hasFullTypeInformation: Je, program: ee, esTreeNodeToTSNodeMap: Ze.esTreeNodeToTSNodeMap, tsNodeToESTreeNodeMap: Ze.tsNodeToESTreeNodeMap } };
}
a.parseAndGenerateServices = te;
} }), ZV = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/clear-caches.js"(a) {
"use strict";
De(), Object.defineProperty(a, "__esModule", { value: true }), a.clearProgramCache = a.clearCaches = void 0;
var _ = f5(), v = y5(), h = g5(), D = h5();
function P() {
(0, v.clearProgramCache)(), (0, _.clearWatchCaches)(), (0, h.clearTSConfigMatchCache)(), (0, D.clearGlobCache)();
}
a.clearCaches = P, a.clearProgramCache = P;
} }), eH = Oe({ "node_modules/@typescript-eslint/typescript-estree/package.json"(a, _) {
_.exports = { name: "@typescript-eslint/typescript-estree", version: "5.55.0", description: "A parser that converts TypeScript source code into an ESTree compatible form", main: "dist/index.js", types: "dist/index.d.ts", files: ["dist", "_ts3.4", "README.md", "LICENSE"], engines: { node: "^12.22.0 || ^14.17.0 || >=16.0.0" }, repository: { type: "git", url: "https://github.com/typescript-eslint/typescript-eslint.git", directory: "packages/typescript-estree" }, bugs: { url: "https://github.com/typescript-eslint/typescript-eslint/issues" }, license: "BSD-2-Clause", keywords: ["ast", "estree", "ecmascript", "javascript", "typescript", "parser", "syntax"], scripts: { build: "tsc -b tsconfig.build.json", postbuild: "downlevel-dts dist _ts3.4/dist", clean: "tsc -b tsconfig.build.json --clean", postclean: "rimraf dist && rimraf _ts3.4 && rimraf coverage", format: 'prettier --write "./**/*.{ts,mts,cts,tsx,js,mjs,cjs,jsx,json,md,css}" --ignore-path ../../.prettierignore', lint: "nx lint", test: "jest --coverage", typecheck: "tsc -p tsconfig.json --noEmit" }, dependencies: { "@typescript-eslint/types": "5.55.0", "@typescript-eslint/visitor-keys": "5.55.0", debug: "^4.3.4", globby: "^11.1.0", "is-glob": "^4.0.3", semver: "^7.3.7", tsutils: "^3.21.0" }, devDependencies: { "@babel/code-frame": "*", "@babel/parser": "*", "@types/babel__code-frame": "*", "@types/debug": "*", "@types/glob": "*", "@types/is-glob": "*", "@types/semver": "*", "@types/tmp": "*", glob: "*", "jest-specific-snapshot": "*", "make-dir": "*", tmp: "*", typescript: "*" }, peerDependenciesMeta: { typescript: { optional: true } }, funding: { type: "opencollective", url: "https://opencollective.com/typescript-eslint" }, typesVersions: { "<3.8": { "*": ["_ts3.4/*"] } }, gitHead: "877d73327fca3bdbe7e170e8b3a906d090a6de37" };
} }), tH = Oe({ "node_modules/@typescript-eslint/typescript-estree/dist/index.js"(a) {
"use strict";
De();
var _ = a && a.__createBinding || (Object.create ? function(C, d, E, I) {
I === void 0 && (I = E);
var c = Object.getOwnPropertyDescriptor(d, E);
(!c || ("get" in c ? !d.__esModule : c.writable || c.configurable)) && (c = { enumerable: true, get: function() {
return d[E];
} }), Object.defineProperty(C, I, c);
} : function(C, d, E, I) {
I === void 0 && (I = E), C[I] = d[E];
}), v = a && a.__exportStar || function(C, d) {
for (var E in C)
E !== "default" && !Object.prototype.hasOwnProperty.call(d, E) && _(d, C, E);
};
Object.defineProperty(a, "__esModule", { value: true }), a.version = a.visitorKeys = a.typescriptVersionIsAtLeast = a.createProgram = a.simpleTraverse = a.parseWithNodeMaps = a.parseAndGenerateServices = a.parse = void 0;
var h = y5();
Object.defineProperty(a, "parse", { enumerable: true, get: function() {
return h.parse;
} }), Object.defineProperty(a, "parseAndGenerateServices", { enumerable: true, get: function() {
return h.parseAndGenerateServices;
} }), Object.defineProperty(a, "parseWithNodeMaps", { enumerable: true, get: function() {
return h.parseWithNodeMaps;
} });
var D = t5();
Object.defineProperty(a, "simpleTraverse", { enumerable: true, get: function() {
return D.simpleTraverse;
} }), v(x1(), a);
var P = d5();
Object.defineProperty(a, "createProgram", { enumerable: true, get: function() {
return P.createProgramFromConfigFile;
} }), v(gT(), a);
var y = S1();
Object.defineProperty(a, "typescriptVersionIsAtLeast", { enumerable: true, get: function() {
return y.typescriptVersionIsAtLeast;
} }), v(fT(), a), v(ZV(), a);
var m = e5();
Object.defineProperty(a, "visitorKeys", { enumerable: true, get: function() {
return m.visitorKeys;
} }), a.version = eH().version;
} });
De();
var rH = w9(), nH = pW(), iH = SW(), aH = xW(), sH = PW(), { throwErrorForInvalidNodes: oH } = DW(), E9 = { loc: true, range: true, comment: true, jsx: true, tokens: true, loggerFn: false, project: [] };
function _H(a) {
let { message: _, lineNumber: v, column: h } = a;
return typeof v != "number" ? a : rH(_, { start: { line: v, column: h + 1 } });
}
function cH(a, _) {
let v = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, h = aH(a), D = lH(a), { parseWithNodeMaps: P } = tH(), { result: y, error: m } = nH(() => P(h, Object.assign(Object.assign({}, E9), {}, { jsx: D })), () => P(h, Object.assign(Object.assign({}, E9), {}, { jsx: !D })));
if (!y)
throw _H(m);
return v.originalText = a, oH(y, v), sH(y.ast, v);
}
function lH(a) {
return new RegExp(["(?:^[^\"'`]*)", "|", "(?:^[^/]{2}.*/>)"].join(""), "m").test(a);
}
v5.exports = { parsers: { typescript: iH(cH) } };
});
return uH();
});
}
});
// node_modules/prettier/parser-angular.js
var require_parser_angular = __commonJS({
"node_modules/prettier/parser-angular.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.angular = e();
}
})(function() {
"use strict";
var cr = (e, t) => () => (t || e((t = { exports: {} }).exports, t), t.exports);
var Br = cr((Mr, ar) => {
var ze = Object.defineProperty, ur = Object.getOwnPropertyDescriptor, He = Object.getOwnPropertyNames, lr = Object.prototype.hasOwnProperty, Y = (e, t) => function() {
return e && (t = (0, e[He(e)[0]])(e = 0)), t;
}, q = (e, t) => function() {
return t || (0, e[He(e)[0]])((t = { exports: {} }).exports, t), t.exports;
}, Xe = (e, t) => {
for (var r in t)
ze(e, r, { get: t[r], enumerable: true });
}, hr = (e, t, r, n2) => {
if (t && typeof t == "object" || typeof t == "function")
for (let s of He(t))
!lr.call(e, s) && s !== r && ze(e, s, { get: () => t[s], enumerable: !(n2 = ur(t, s)) || n2.enumerable });
return e;
}, be = (e) => hr(ze({}, "__esModule", { value: true }), e), L = Y({ ""() {
} }), pr = q({ "src/utils/is-non-empty-array.js"(e, t) {
"use strict";
L();
function r(n2) {
return Array.isArray(n2) && n2.length > 0;
}
t.exports = r;
} }), dr = q({ "src/language-js/loc.js"(e, t) {
"use strict";
L();
var r = pr();
function n2(l) {
var P, p;
let x = l.range ? l.range[0] : l.start, C = (P = (p = l.declaration) === null || p === void 0 ? void 0 : p.decorators) !== null && P !== void 0 ? P : l.decorators;
return r(C) ? Math.min(n2(C[0]), x) : x;
}
function s(l) {
return l.range ? l.range[1] : l.end;
}
function a(l, P) {
let p = n2(l);
return Number.isInteger(p) && p === n2(P);
}
function i(l, P) {
let p = s(l);
return Number.isInteger(p) && p === s(P);
}
function h(l, P) {
return a(l, P) && i(l, P);
}
t.exports = { locStart: n2, locEnd: s, hasSameLocStart: a, hasSameLoc: h };
} }), fr = q({ "node_modules/angular-estree-parser/node_modules/lines-and-columns/build/index.js"(e) {
"use strict";
L(), e.__esModule = true, e.LinesAndColumns = void 0;
var t = `
`, r = "\r", n2 = function() {
function s(a) {
this.string = a;
for (var i = [0], h = 0; h < a.length; )
switch (a[h]) {
case t:
h += t.length, i.push(h);
break;
case r:
h += r.length, a[h] === t && (h += t.length), i.push(h);
break;
default:
h++;
break;
}
this.offsets = i;
}
return s.prototype.locationForIndex = function(a) {
if (a < 0 || a > this.string.length)
return null;
for (var i = 0, h = this.offsets; h[i + 1] <= a; )
i++;
var l = a - h[i];
return { line: i, column: l };
}, s.prototype.indexForLocation = function(a) {
var i = a.line, h = a.column;
return i < 0 || i >= this.offsets.length || h < 0 || h > this.lengthOfLine(i) ? null : this.offsets[i] + h;
}, s.prototype.lengthOfLine = function(a) {
var i = this.offsets[a], h = a === this.offsets.length - 1 ? this.string.length : this.offsets[a + 1];
return h - i;
}, s;
}();
e.LinesAndColumns = n2, e.default = n2;
} }), gr = q({ "node_modules/angular-estree-parser/lib/context.js"(e) {
"use strict";
L(), Object.defineProperty(e, "__esModule", { value: true }), e.Context = void 0;
var t = fr(), r = class {
constructor(s) {
this.text = s, this.locator = new n2(this.text);
}
};
e.Context = r;
var n2 = class {
constructor(s) {
this._lineAndColumn = new t.default(s);
}
locationForIndex(s) {
let { line: a, column: i } = this._lineAndColumn.locationForIndex(s);
return { line: a + 1, column: i };
}
};
} }), Je = {};
Xe(Je, { AST: () => k, ASTWithName: () => W, ASTWithSource: () => G, AbsoluteSourceSpan: () => U, AstMemoryEfficientTransformer: () => Ct, AstTransformer: () => Pt, Binary: () => B, BindingPipe: () => fe, BoundElementProperty: () => It, Chain: () => oe, Conditional: () => ce, EmptyExpr: () => K, ExpressionBinding: () => Ze, FunctionCall: () => Pe, ImplicitReceiver: () => Oe, Interpolation: () => me, KeyedRead: () => he, KeyedWrite: () => de, LiteralArray: () => ge, LiteralMap: () => ve, LiteralPrimitive: () => $, MethodCall: () => ye, NonNullAssert: () => Se, ParseSpan: () => V, ParsedEvent: () => At, ParsedProperty: () => Et, ParsedPropertyType: () => se, ParsedVariable: () => _t, ParserError: () => ae, PrefixNot: () => xe, PropertyRead: () => ne, PropertyWrite: () => ue, Quote: () => Le, RecursiveAstVisitor: () => et, SafeKeyedRead: () => pe, SafeMethodCall: () => we, SafePropertyRead: () => le, ThisReceiver: () => Ye, Unary: () => F, VariableBinding: () => Re });
var ae, V, k, W, Le, K, Oe, Ye, oe, ce, ne, ue, le, he, pe, de, fe, $, ge, ve, me, B, F, xe, Se, ye, we, Pe, U, G, Re, Ze, et, Pt, Ct, Et, se, At, _t, It, tt = Y({ "node_modules/@angular/compiler/esm2015/src/expression_parser/ast.js"() {
L(), ae = class {
constructor(e, t, r, n2) {
this.input = t, this.errLocation = r, this.ctxLocation = n2, this.message = `Parser Error: ${e} ${r} [${t}] in ${n2}`;
}
}, V = class {
constructor(e, t) {
this.start = e, this.end = t;
}
toAbsolute(e) {
return new U(e + this.start, e + this.end);
}
}, k = class {
constructor(e, t) {
this.span = e, this.sourceSpan = t;
}
toString() {
return "AST";
}
}, W = class extends k {
constructor(e, t, r) {
super(e, t), this.nameSpan = r;
}
}, Le = class extends k {
constructor(e, t, r, n2, s) {
super(e, t), this.prefix = r, this.uninterpretedExpression = n2, this.location = s;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitQuote(this, t);
}
toString() {
return "Quote";
}
}, K = class extends k {
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
}
}, Oe = class extends k {
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitImplicitReceiver(this, t);
}
}, Ye = class extends Oe {
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
var r;
return (r = e.visitThisReceiver) === null || r === void 0 ? void 0 : r.call(e, this, t);
}
}, oe = class extends k {
constructor(e, t, r) {
super(e, t), this.expressions = r;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitChain(this, t);
}
}, ce = class extends k {
constructor(e, t, r, n2, s) {
super(e, t), this.condition = r, this.trueExp = n2, this.falseExp = s;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitConditional(this, t);
}
}, ne = class extends W {
constructor(e, t, r, n2, s) {
super(e, t, r), this.receiver = n2, this.name = s;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitPropertyRead(this, t);
}
}, ue = class extends W {
constructor(e, t, r, n2, s, a) {
super(e, t, r), this.receiver = n2, this.name = s, this.value = a;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitPropertyWrite(this, t);
}
}, le = class extends W {
constructor(e, t, r, n2, s) {
super(e, t, r), this.receiver = n2, this.name = s;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitSafePropertyRead(this, t);
}
}, he = class extends k {
constructor(e, t, r, n2) {
super(e, t), this.receiver = r, this.key = n2;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitKeyedRead(this, t);
}
}, pe = class extends k {
constructor(e, t, r, n2) {
super(e, t), this.receiver = r, this.key = n2;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitSafeKeyedRead(this, t);
}
}, de = class extends k {
constructor(e, t, r, n2, s) {
super(e, t), this.receiver = r, this.key = n2, this.value = s;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitKeyedWrite(this, t);
}
}, fe = class extends W {
constructor(e, t, r, n2, s, a) {
super(e, t, a), this.exp = r, this.name = n2, this.args = s;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitPipe(this, t);
}
}, $ = class extends k {
constructor(e, t, r) {
super(e, t), this.value = r;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitLiteralPrimitive(this, t);
}
}, ge = class extends k {
constructor(e, t, r) {
super(e, t), this.expressions = r;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitLiteralArray(this, t);
}
}, ve = class extends k {
constructor(e, t, r, n2) {
super(e, t), this.keys = r, this.values = n2;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitLiteralMap(this, t);
}
}, me = class extends k {
constructor(e, t, r, n2) {
super(e, t), this.strings = r, this.expressions = n2;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitInterpolation(this, t);
}
}, B = class extends k {
constructor(e, t, r, n2, s) {
super(e, t), this.operation = r, this.left = n2, this.right = s;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitBinary(this, t);
}
}, F = class extends B {
constructor(e, t, r, n2, s, a, i) {
super(e, t, s, a, i), this.operator = r, this.expr = n2;
}
static createMinus(e, t, r) {
return new F(e, t, "-", r, "-", new $(e, t, 0), r);
}
static createPlus(e, t, r) {
return new F(e, t, "+", r, "-", r, new $(e, t, 0));
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitUnary !== void 0 ? e.visitUnary(this, t) : e.visitBinary(this, t);
}
}, xe = class extends k {
constructor(e, t, r) {
super(e, t), this.expression = r;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitPrefixNot(this, t);
}
}, Se = class extends k {
constructor(e, t, r) {
super(e, t), this.expression = r;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitNonNullAssert(this, t);
}
}, ye = class extends W {
constructor(e, t, r, n2, s, a, i) {
super(e, t, r), this.receiver = n2, this.name = s, this.args = a, this.argumentSpan = i;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitMethodCall(this, t);
}
}, we = class extends W {
constructor(e, t, r, n2, s, a, i) {
super(e, t, r), this.receiver = n2, this.name = s, this.args = a, this.argumentSpan = i;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitSafeMethodCall(this, t);
}
}, Pe = class extends k {
constructor(e, t, r, n2) {
super(e, t), this.target = r, this.args = n2;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitFunctionCall(this, t);
}
}, U = class {
constructor(e, t) {
this.start = e, this.end = t;
}
}, G = class extends k {
constructor(e, t, r, n2, s) {
super(new V(0, t === null ? 0 : t.length), new U(n2, t === null ? n2 : n2 + t.length)), this.ast = e, this.source = t, this.location = r, this.errors = s;
}
visit(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
return e.visitASTWithSource ? e.visitASTWithSource(this, t) : this.ast.visit(e, t);
}
toString() {
return `${this.source} in ${this.location}`;
}
}, Re = class {
constructor(e, t, r) {
this.sourceSpan = e, this.key = t, this.value = r;
}
}, Ze = class {
constructor(e, t, r) {
this.sourceSpan = e, this.key = t, this.value = r;
}
}, et = class {
visit(e, t) {
e.visit(this, t);
}
visitUnary(e, t) {
this.visit(e.expr, t);
}
visitBinary(e, t) {
this.visit(e.left, t), this.visit(e.right, t);
}
visitChain(e, t) {
this.visitAll(e.expressions, t);
}
visitConditional(e, t) {
this.visit(e.condition, t), this.visit(e.trueExp, t), this.visit(e.falseExp, t);
}
visitPipe(e, t) {
this.visit(e.exp, t), this.visitAll(e.args, t);
}
visitFunctionCall(e, t) {
e.target && this.visit(e.target, t), this.visitAll(e.args, t);
}
visitImplicitReceiver(e, t) {
}
visitThisReceiver(e, t) {
}
visitInterpolation(e, t) {
this.visitAll(e.expressions, t);
}
visitKeyedRead(e, t) {
this.visit(e.receiver, t), this.visit(e.key, t);
}
visitKeyedWrite(e, t) {
this.visit(e.receiver, t), this.visit(e.key, t), this.visit(e.value, t);
}
visitLiteralArray(e, t) {
this.visitAll(e.expressions, t);
}
visitLiteralMap(e, t) {
this.visitAll(e.values, t);
}
visitLiteralPrimitive(e, t) {
}
visitMethodCall(e, t) {
this.visit(e.receiver, t), this.visitAll(e.args, t);
}
visitPrefixNot(e, t) {
this.visit(e.expression, t);
}
visitNonNullAssert(e, t) {
this.visit(e.expression, t);
}
visitPropertyRead(e, t) {
this.visit(e.receiver, t);
}
visitPropertyWrite(e, t) {
this.visit(e.receiver, t), this.visit(e.value, t);
}
visitSafePropertyRead(e, t) {
this.visit(e.receiver, t);
}
visitSafeMethodCall(e, t) {
this.visit(e.receiver, t), this.visitAll(e.args, t);
}
visitSafeKeyedRead(e, t) {
this.visit(e.receiver, t), this.visit(e.key, t);
}
visitQuote(e, t) {
}
visitAll(e, t) {
for (let r of e)
this.visit(r, t);
}
}, Pt = class {
visitImplicitReceiver(e, t) {
return e;
}
visitThisReceiver(e, t) {
return e;
}
visitInterpolation(e, t) {
return new me(e.span, e.sourceSpan, e.strings, this.visitAll(e.expressions));
}
visitLiteralPrimitive(e, t) {
return new $(e.span, e.sourceSpan, e.value);
}
visitPropertyRead(e, t) {
return new ne(e.span, e.sourceSpan, e.nameSpan, e.receiver.visit(this), e.name);
}
visitPropertyWrite(e, t) {
return new ue(e.span, e.sourceSpan, e.nameSpan, e.receiver.visit(this), e.name, e.value.visit(this));
}
visitSafePropertyRead(e, t) {
return new le(e.span, e.sourceSpan, e.nameSpan, e.receiver.visit(this), e.name);
}
visitMethodCall(e, t) {
return new ye(e.span, e.sourceSpan, e.nameSpan, e.receiver.visit(this), e.name, this.visitAll(e.args), e.argumentSpan);
}
visitSafeMethodCall(e, t) {
return new we(e.span, e.sourceSpan, e.nameSpan, e.receiver.visit(this), e.name, this.visitAll(e.args), e.argumentSpan);
}
visitFunctionCall(e, t) {
return new Pe(e.span, e.sourceSpan, e.target.visit(this), this.visitAll(e.args));
}
visitLiteralArray(e, t) {
return new ge(e.span, e.sourceSpan, this.visitAll(e.expressions));
}
visitLiteralMap(e, t) {
return new ve(e.span, e.sourceSpan, e.keys, this.visitAll(e.values));
}
visitUnary(e, t) {
switch (e.operator) {
case "+":
return F.createPlus(e.span, e.sourceSpan, e.expr.visit(this));
case "-":
return F.createMinus(e.span, e.sourceSpan, e.expr.visit(this));
default:
throw new Error(`Unknown unary operator ${e.operator}`);
}
}
visitBinary(e, t) {
return new B(e.span, e.sourceSpan, e.operation, e.left.visit(this), e.right.visit(this));
}
visitPrefixNot(e, t) {
return new xe(e.span, e.sourceSpan, e.expression.visit(this));
}
visitNonNullAssert(e, t) {
return new Se(e.span, e.sourceSpan, e.expression.visit(this));
}
visitConditional(e, t) {
return new ce(e.span, e.sourceSpan, e.condition.visit(this), e.trueExp.visit(this), e.falseExp.visit(this));
}
visitPipe(e, t) {
return new fe(e.span, e.sourceSpan, e.exp.visit(this), e.name, this.visitAll(e.args), e.nameSpan);
}
visitKeyedRead(e, t) {
return new he(e.span, e.sourceSpan, e.receiver.visit(this), e.key.visit(this));
}
visitKeyedWrite(e, t) {
return new de(e.span, e.sourceSpan, e.receiver.visit(this), e.key.visit(this), e.value.visit(this));
}
visitAll(e) {
let t = [];
for (let r = 0; r < e.length; ++r)
t[r] = e[r].visit(this);
return t;
}
visitChain(e, t) {
return new oe(e.span, e.sourceSpan, this.visitAll(e.expressions));
}
visitQuote(e, t) {
return new Le(e.span, e.sourceSpan, e.prefix, e.uninterpretedExpression, e.location);
}
visitSafeKeyedRead(e, t) {
return new pe(e.span, e.sourceSpan, e.receiver.visit(this), e.key.visit(this));
}
}, Ct = class {
visitImplicitReceiver(e, t) {
return e;
}
visitThisReceiver(e, t) {
return e;
}
visitInterpolation(e, t) {
let r = this.visitAll(e.expressions);
return r !== e.expressions ? new me(e.span, e.sourceSpan, e.strings, r) : e;
}
visitLiteralPrimitive(e, t) {
return e;
}
visitPropertyRead(e, t) {
let r = e.receiver.visit(this);
return r !== e.receiver ? new ne(e.span, e.sourceSpan, e.nameSpan, r, e.name) : e;
}
visitPropertyWrite(e, t) {
let r = e.receiver.visit(this), n2 = e.value.visit(this);
return r !== e.receiver || n2 !== e.value ? new ue(e.span, e.sourceSpan, e.nameSpan, r, e.name, n2) : e;
}
visitSafePropertyRead(e, t) {
let r = e.receiver.visit(this);
return r !== e.receiver ? new le(e.span, e.sourceSpan, e.nameSpan, r, e.name) : e;
}
visitMethodCall(e, t) {
let r = e.receiver.visit(this), n2 = this.visitAll(e.args);
return r !== e.receiver || n2 !== e.args ? new ye(e.span, e.sourceSpan, e.nameSpan, r, e.name, n2, e.argumentSpan) : e;
}
visitSafeMethodCall(e, t) {
let r = e.receiver.visit(this), n2 = this.visitAll(e.args);
return r !== e.receiver || n2 !== e.args ? new we(e.span, e.sourceSpan, e.nameSpan, r, e.name, n2, e.argumentSpan) : e;
}
visitFunctionCall(e, t) {
let r = e.target && e.target.visit(this), n2 = this.visitAll(e.args);
return r !== e.target || n2 !== e.args ? new Pe(e.span, e.sourceSpan, r, n2) : e;
}
visitLiteralArray(e, t) {
let r = this.visitAll(e.expressions);
return r !== e.expressions ? new ge(e.span, e.sourceSpan, r) : e;
}
visitLiteralMap(e, t) {
let r = this.visitAll(e.values);
return r !== e.values ? new ve(e.span, e.sourceSpan, e.keys, r) : e;
}
visitUnary(e, t) {
let r = e.expr.visit(this);
if (r !== e.expr)
switch (e.operator) {
case "+":
return F.createPlus(e.span, e.sourceSpan, r);
case "-":
return F.createMinus(e.span, e.sourceSpan, r);
default:
throw new Error(`Unknown unary operator ${e.operator}`);
}
return e;
}
visitBinary(e, t) {
let r = e.left.visit(this), n2 = e.right.visit(this);
return r !== e.left || n2 !== e.right ? new B(e.span, e.sourceSpan, e.operation, r, n2) : e;
}
visitPrefixNot(e, t) {
let r = e.expression.visit(this);
return r !== e.expression ? new xe(e.span, e.sourceSpan, r) : e;
}
visitNonNullAssert(e, t) {
let r = e.expression.visit(this);
return r !== e.expression ? new Se(e.span, e.sourceSpan, r) : e;
}
visitConditional(e, t) {
let r = e.condition.visit(this), n2 = e.trueExp.visit(this), s = e.falseExp.visit(this);
return r !== e.condition || n2 !== e.trueExp || s !== e.falseExp ? new ce(e.span, e.sourceSpan, r, n2, s) : e;
}
visitPipe(e, t) {
let r = e.exp.visit(this), n2 = this.visitAll(e.args);
return r !== e.exp || n2 !== e.args ? new fe(e.span, e.sourceSpan, r, e.name, n2, e.nameSpan) : e;
}
visitKeyedRead(e, t) {
let r = e.receiver.visit(this), n2 = e.key.visit(this);
return r !== e.receiver || n2 !== e.key ? new he(e.span, e.sourceSpan, r, n2) : e;
}
visitKeyedWrite(e, t) {
let r = e.receiver.visit(this), n2 = e.key.visit(this), s = e.value.visit(this);
return r !== e.receiver || n2 !== e.key || s !== e.value ? new de(e.span, e.sourceSpan, r, n2, s) : e;
}
visitAll(e) {
let t = [], r = false;
for (let n2 = 0; n2 < e.length; ++n2) {
let s = e[n2], a = s.visit(this);
t[n2] = a, r = r || a !== s;
}
return r ? t : e;
}
visitChain(e, t) {
let r = this.visitAll(e.expressions);
return r !== e.expressions ? new oe(e.span, e.sourceSpan, r) : e;
}
visitQuote(e, t) {
return e;
}
visitSafeKeyedRead(e, t) {
let r = e.receiver.visit(this), n2 = e.key.visit(this);
return r !== e.receiver || n2 !== e.key ? new pe(e.span, e.sourceSpan, r, n2) : e;
}
}, Et = class {
constructor(e, t, r, n2, s, a) {
this.name = e, this.expression = t, this.type = r, this.sourceSpan = n2, this.keySpan = s, this.valueSpan = a, this.isLiteral = this.type === se.LITERAL_ATTR, this.isAnimation = this.type === se.ANIMATION;
}
}, function(e) {
e[e.DEFAULT = 0] = "DEFAULT", e[e.LITERAL_ATTR = 1] = "LITERAL_ATTR", e[e.ANIMATION = 2] = "ANIMATION";
}(se || (se = {})), At = class {
constructor(e, t, r, n2, s, a, i) {
this.name = e, this.targetOrPhase = t, this.type = r, this.handler = n2, this.sourceSpan = s, this.handlerSpan = a, this.keySpan = i;
}
}, _t = class {
constructor(e, t, r, n2, s) {
this.name = e, this.value = t, this.sourceSpan = r, this.keySpan = n2, this.valueSpan = s;
}
}, It = class {
constructor(e, t, r, n2, s, a, i, h) {
this.name = e, this.type = t, this.securityContext = r, this.value = n2, this.unit = s, this.sourceSpan = a, this.keySpan = i, this.valueSpan = h;
}
};
} });
function vr(e) {
return e >= rt && e <= nt || e == dt;
}
function Q(e) {
return Mt <= e && e <= jt;
}
function mr(e) {
return e >= ht && e <= pt || e >= ut && e <= lt;
}
function mt(e) {
return e === at || e === st || e === Xt;
}
var Ce, rt, Ot, kt, Nt, bt, nt, Lt, st, Rt, it, Tt, je, at, Ee, z, $t, ot, ee, ct, H, Te, X, te, Bt, ie, Kt, Fe, Mt, jt, ut, Ft, lt, Ae, Ut, re, Wt, Be, ht, Gt, Vt, qt, Qt, Dt, zt, Ht, pt, $e, Ue, _e, dt, Xt, Jt = Y({ "node_modules/@angular/compiler/esm2015/src/chars.js"() {
L(), Ce = 0, rt = 9, Ot = 10, kt = 11, Nt = 12, bt = 13, nt = 32, Lt = 33, st = 34, Rt = 35, it = 36, Tt = 37, je = 38, at = 39, Ee = 40, z = 41, $t = 42, ot = 43, ee = 44, ct = 45, H = 46, Te = 47, X = 58, te = 59, Bt = 60, ie = 61, Kt = 62, Fe = 63, Mt = 48, jt = 57, ut = 65, Ft = 69, lt = 90, Ae = 91, Ut = 92, re = 93, Wt = 94, Be = 95, ht = 97, Gt = 101, Vt = 102, qt = 110, Qt = 114, Dt = 116, zt = 117, Ht = 118, pt = 122, $e = 123, Ue = 124, _e = 125, dt = 160, Xt = 96;
} }), Yt = {};
Xe(Yt, { EOF: () => Ie, Lexer: () => er, Token: () => M, TokenType: () => S, isIdentifier: () => Zt });
function xt(e, t, r) {
return new M(e, t, S.Character, r, String.fromCharCode(r));
}
function xr(e, t, r) {
return new M(e, t, S.Identifier, 0, r);
}
function Sr(e, t, r) {
return new M(e, t, S.PrivateIdentifier, 0, r);
}
function yr(e, t, r) {
return new M(e, t, S.Keyword, 0, r);
}
function Ke(e, t, r) {
return new M(e, t, S.Operator, 0, r);
}
function wr(e, t, r) {
return new M(e, t, S.String, 0, r);
}
function Pr(e, t, r) {
return new M(e, t, S.Number, r, "");
}
function Cr(e, t, r) {
return new M(e, t, S.Error, 0, r);
}
function We(e) {
return ht <= e && e <= pt || ut <= e && e <= lt || e == Be || e == it;
}
function Zt(e) {
if (e.length == 0)
return false;
let t = new Ve(e);
if (!We(t.peek))
return false;
for (t.advance(); t.peek !== Ce; ) {
if (!Ge(t.peek))
return false;
t.advance();
}
return true;
}
function Ge(e) {
return mr(e) || Q(e) || e == Be || e == it;
}
function Er(e) {
return e == Gt || e == Ft;
}
function Ar(e) {
return e == ct || e == ot;
}
function _r(e) {
switch (e) {
case qt:
return Ot;
case Vt:
return Nt;
case Qt:
return bt;
case Dt:
return rt;
case Ht:
return kt;
default:
return e;
}
}
function Ir(e) {
let t = parseInt(e);
if (isNaN(t))
throw new Error("Invalid integer literal when parsing " + e);
return t;
}
var S, St, er, M, Ie, Ve, tr = Y({ "node_modules/@angular/compiler/esm2015/src/expression_parser/lexer.js"() {
L(), Jt(), function(e) {
e[e.Character = 0] = "Character", e[e.Identifier = 1] = "Identifier", e[e.PrivateIdentifier = 2] = "PrivateIdentifier", e[e.Keyword = 3] = "Keyword", e[e.String = 4] = "String", e[e.Operator = 5] = "Operator", e[e.Number = 6] = "Number", e[e.Error = 7] = "Error";
}(S || (S = {})), St = ["var", "let", "as", "null", "undefined", "true", "false", "if", "else", "this"], er = class {
tokenize(e) {
let t = new Ve(e), r = [], n2 = t.scanToken();
for (; n2 != null; )
r.push(n2), n2 = t.scanToken();
return r;
}
}, M = class {
constructor(e, t, r, n2, s) {
this.index = e, this.end = t, this.type = r, this.numValue = n2, this.strValue = s;
}
isCharacter(e) {
return this.type == S.Character && this.numValue == e;
}
isNumber() {
return this.type == S.Number;
}
isString() {
return this.type == S.String;
}
isOperator(e) {
return this.type == S.Operator && this.strValue == e;
}
isIdentifier() {
return this.type == S.Identifier;
}
isPrivateIdentifier() {
return this.type == S.PrivateIdentifier;
}
isKeyword() {
return this.type == S.Keyword;
}
isKeywordLet() {
return this.type == S.Keyword && this.strValue == "let";
}
isKeywordAs() {
return this.type == S.Keyword && this.strValue == "as";
}
isKeywordNull() {
return this.type == S.Keyword && this.strValue == "null";
}
isKeywordUndefined() {
return this.type == S.Keyword && this.strValue == "undefined";
}
isKeywordTrue() {
return this.type == S.Keyword && this.strValue == "true";
}
isKeywordFalse() {
return this.type == S.Keyword && this.strValue == "false";
}
isKeywordThis() {
return this.type == S.Keyword && this.strValue == "this";
}
isError() {
return this.type == S.Error;
}
toNumber() {
return this.type == S.Number ? this.numValue : -1;
}
toString() {
switch (this.type) {
case S.Character:
case S.Identifier:
case S.Keyword:
case S.Operator:
case S.PrivateIdentifier:
case S.String:
case S.Error:
return this.strValue;
case S.Number:
return this.numValue.toString();
default:
return null;
}
}
}, Ie = new M(-1, -1, S.Character, 0, ""), Ve = class {
constructor(e) {
this.input = e, this.peek = 0, this.index = -1, this.length = e.length, this.advance();
}
advance() {
this.peek = ++this.index >= this.length ? Ce : this.input.charCodeAt(this.index);
}
scanToken() {
let e = this.input, t = this.length, r = this.peek, n2 = this.index;
for (; r <= nt; )
if (++n2 >= t) {
r = Ce;
break;
} else
r = e.charCodeAt(n2);
if (this.peek = r, this.index = n2, n2 >= t)
return null;
if (We(r))
return this.scanIdentifier();
if (Q(r))
return this.scanNumber(n2);
let s = n2;
switch (r) {
case H:
return this.advance(), Q(this.peek) ? this.scanNumber(s) : xt(s, this.index, H);
case Ee:
case z:
case $e:
case _e:
case Ae:
case re:
case ee:
case X:
case te:
return this.scanCharacter(s, r);
case at:
case st:
return this.scanString();
case Rt:
return this.scanPrivateIdentifier();
case ot:
case ct:
case $t:
case Te:
case Tt:
case Wt:
return this.scanOperator(s, String.fromCharCode(r));
case Fe:
return this.scanQuestion(s);
case Bt:
case Kt:
return this.scanComplexOperator(s, String.fromCharCode(r), ie, "=");
case Lt:
case ie:
return this.scanComplexOperator(s, String.fromCharCode(r), ie, "=", ie, "=");
case je:
return this.scanComplexOperator(s, "&", je, "&");
case Ue:
return this.scanComplexOperator(s, "|", Ue, "|");
case dt:
for (; vr(this.peek); )
this.advance();
return this.scanToken();
}
return this.advance(), this.error(`Unexpected character [${String.fromCharCode(r)}]`, 0);
}
scanCharacter(e, t) {
return this.advance(), xt(e, this.index, t);
}
scanOperator(e, t) {
return this.advance(), Ke(e, this.index, t);
}
scanComplexOperator(e, t, r, n2, s, a) {
this.advance();
let i = t;
return this.peek == r && (this.advance(), i += n2), s != null && this.peek == s && (this.advance(), i += a), Ke(e, this.index, i);
}
scanIdentifier() {
let e = this.index;
for (this.advance(); Ge(this.peek); )
this.advance();
let t = this.input.substring(e, this.index);
return St.indexOf(t) > -1 ? yr(e, this.index, t) : xr(e, this.index, t);
}
scanPrivateIdentifier() {
let e = this.index;
if (this.advance(), !We(this.peek))
return this.error("Invalid character [#]", -1);
for (; Ge(this.peek); )
this.advance();
let t = this.input.substring(e, this.index);
return Sr(e, this.index, t);
}
scanNumber(e) {
let t = this.index === e, r = false;
for (this.advance(); ; ) {
if (!Q(this.peek))
if (this.peek === Be) {
if (!Q(this.input.charCodeAt(this.index - 1)) || !Q(this.input.charCodeAt(this.index + 1)))
return this.error("Invalid numeric separator", 0);
r = true;
} else if (this.peek === H)
t = false;
else if (Er(this.peek)) {
if (this.advance(), Ar(this.peek) && this.advance(), !Q(this.peek))
return this.error("Invalid exponent", -1);
t = false;
} else
break;
this.advance();
}
let n2 = this.input.substring(e, this.index);
r && (n2 = n2.replace(/_/g, ""));
let s = t ? Ir(n2) : parseFloat(n2);
return Pr(e, this.index, s);
}
scanString() {
let e = this.index, t = this.peek;
this.advance();
let r = "", n2 = this.index, s = this.input;
for (; this.peek != t; )
if (this.peek == Ut) {
r += s.substring(n2, this.index), this.advance();
let i;
if (this.peek = this.peek, this.peek == zt) {
let h = s.substring(this.index + 1, this.index + 5);
if (/^[0-9a-f]+$/i.test(h))
i = parseInt(h, 16);
else
return this.error(`Invalid unicode escape [\\u${h}]`, 0);
for (let l = 0; l < 5; l++)
this.advance();
} else
i = _r(this.peek), this.advance();
r += String.fromCharCode(i), n2 = this.index;
} else {
if (this.peek == Ce)
return this.error("Unterminated quote", 0);
this.advance();
}
let a = s.substring(n2, this.index);
return this.advance(), wr(e, this.index, r + a);
}
scanQuestion(e) {
this.advance();
let t = "?";
return (this.peek === Fe || this.peek === H) && (t += this.peek === H ? "." : "?", this.advance()), Ke(e, this.index, t);
}
error(e, t) {
let r = this.index + t;
return Cr(r, this.index, `Lexer Error: ${e} at column ${r} in expression [${this.input}]`);
}
};
} });
function Or(e, t) {
if (t != null && !(Array.isArray(t) && t.length == 2))
throw new Error(`Expected '${e}' to be an array, [start, end].`);
if (t != null) {
let r = t[0], n2 = t[1];
rr.forEach((s) => {
if (s.test(r) || s.test(n2))
throw new Error(`['${r}', '${n2}'] contains unusable interpolation symbol.`);
});
}
}
var rr, kr = Y({ "node_modules/@angular/compiler/esm2015/src/assertions.js"() {
L(), rr = [/^\s*$/, /[<>]/, /^[{}]$/, /&(#|[a-z])/i, /^\/\//];
} }), Me, J, Nr = Y({ "node_modules/@angular/compiler/esm2015/src/ml_parser/interpolation_config.js"() {
L(), kr(), Me = class {
constructor(e, t) {
this.start = e, this.end = t;
}
static fromArray(e) {
return e ? (Or("interpolation", e), new Me(e[0], e[1])) : J;
}
}, J = new Me("{{", "}}");
} }), nr = {};
Xe(nr, { IvyParser: () => sr, Parser: () => De, SplitInterpolation: () => qe, TemplateBindingParseResult: () => Qe, _ParseAST: () => D });
var qe, Qe, De, sr, Z, D, yt, wt, br = Y({ "node_modules/@angular/compiler/esm2015/src/expression_parser/parser.js"() {
L(), Jt(), Nr(), tt(), tr(), qe = class {
constructor(e, t, r) {
this.strings = e, this.expressions = t, this.offsets = r;
}
}, Qe = class {
constructor(e, t, r) {
this.templateBindings = e, this.warnings = t, this.errors = r;
}
}, De = class {
constructor(e) {
this._lexer = e, this.errors = [], this.simpleExpressionChecker = yt;
}
parseAction(e, t, r) {
let n2 = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : J;
this._checkNoInterpolation(e, t, n2);
let s = this._stripComments(e), a = this._lexer.tokenize(this._stripComments(e)), i = new D(e, t, r, a, s.length, true, this.errors, e.length - s.length).parseChain();
return new G(i, e, t, r, this.errors);
}
parseBinding(e, t, r) {
let n2 = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : J, s = this._parseBindingAst(e, t, r, n2);
return new G(s, e, t, r, this.errors);
}
checkSimpleExpression(e) {
let t = new this.simpleExpressionChecker();
return e.visit(t), t.errors;
}
parseSimpleBinding(e, t, r) {
let n2 = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : J, s = this._parseBindingAst(e, t, r, n2), a = this.checkSimpleExpression(s);
return a.length > 0 && this._reportError(`Host binding expression cannot contain ${a.join(" ")}`, e, t), new G(s, e, t, r, this.errors);
}
_reportError(e, t, r, n2) {
this.errors.push(new ae(e, t, r, n2));
}
_parseBindingAst(e, t, r, n2) {
let s = this._parseQuote(e, t, r);
if (s != null)
return s;
this._checkNoInterpolation(e, t, n2);
let a = this._stripComments(e), i = this._lexer.tokenize(a);
return new D(e, t, r, i, a.length, false, this.errors, e.length - a.length).parseChain();
}
_parseQuote(e, t, r) {
if (e == null)
return null;
let n2 = e.indexOf(":");
if (n2 == -1)
return null;
let s = e.substring(0, n2).trim();
if (!Zt(s))
return null;
let a = e.substring(n2 + 1), i = new V(0, e.length);
return new Le(i, i.toAbsolute(r), s, a, t);
}
parseTemplateBindings(e, t, r, n2, s) {
let a = this._lexer.tokenize(t);
return new D(t, r, s, a, t.length, false, this.errors, 0).parseTemplateBindings({ source: e, span: new U(n2, n2 + e.length) });
}
parseInterpolation(e, t, r) {
let n2 = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : J, { strings: s, expressions: a, offsets: i } = this.splitInterpolation(e, t, n2);
if (a.length === 0)
return null;
let h = [];
for (let l = 0; l < a.length; ++l) {
let P = a[l].text, p = this._stripComments(P), x = this._lexer.tokenize(p), C = new D(e, t, r, x, p.length, false, this.errors, i[l] + (P.length - p.length)).parseChain();
h.push(C);
}
return this.createInterpolationAst(s.map((l) => l.text), h, e, t, r);
}
parseInterpolationExpression(e, t, r) {
let n2 = this._stripComments(e), s = this._lexer.tokenize(n2), a = new D(e, t, r, s, n2.length, false, this.errors, 0).parseChain(), i = ["", ""];
return this.createInterpolationAst(i, [a], e, t, r);
}
createInterpolationAst(e, t, r, n2, s) {
let a = new V(0, r.length), i = new me(a, a.toAbsolute(s), e, t);
return new G(i, r, n2, s, this.errors);
}
splitInterpolation(e, t) {
let r = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : J, n2 = [], s = [], a = [], i = 0, h = false, l = false, { start: P, end: p } = r;
for (; i < e.length; )
if (h) {
let x = i, C = x + P.length, b = this._getInterpolationEndIndex(e, p, C);
if (b === -1) {
h = false, l = true;
break;
}
let _ = b + p.length, R = e.substring(C, b);
R.trim().length === 0 && this._reportError("Blank expressions are not allowed in interpolated strings", e, `at column ${i} in`, t), s.push({ text: R, start: x, end: _ }), a.push(C), i = _, h = false;
} else {
let x = i;
i = e.indexOf(P, i), i === -1 && (i = e.length);
let C = e.substring(x, i);
n2.push({ text: C, start: x, end: i }), h = true;
}
if (!h)
if (l) {
let x = n2[n2.length - 1];
x.text += e.substring(i), x.end = e.length;
} else
n2.push({ text: e.substring(i), start: i, end: e.length });
return new qe(n2, s, a);
}
wrapLiteralPrimitive(e, t, r) {
let n2 = new V(0, e == null ? 0 : e.length);
return new G(new $(n2, n2.toAbsolute(r), e), e, t, r, this.errors);
}
_stripComments(e) {
let t = this._commentStart(e);
return t != null ? e.substring(0, t).trim() : e;
}
_commentStart(e) {
let t = null;
for (let r = 0; r < e.length - 1; r++) {
let n2 = e.charCodeAt(r), s = e.charCodeAt(r + 1);
if (n2 === Te && s == Te && t == null)
return r;
t === n2 ? t = null : t == null && mt(n2) && (t = n2);
}
return null;
}
_checkNoInterpolation(e, t, r) {
let { start: n2, end: s } = r, a = -1, i = -1;
for (let h of this._forEachUnquotedChar(e, 0))
if (a === -1)
e.startsWith(n2) && (a = h);
else if (i = this._getInterpolationEndIndex(e, s, h), i > -1)
break;
a > -1 && i > -1 && this._reportError(`Got interpolation (${n2}${s}) where expression was expected`, e, `at column ${a} in`, t);
}
_getInterpolationEndIndex(e, t, r) {
for (let n2 of this._forEachUnquotedChar(e, r)) {
if (e.startsWith(t, n2))
return n2;
if (e.startsWith("//", n2))
return e.indexOf(t, n2);
}
return -1;
}
*_forEachUnquotedChar(e, t) {
let r = null, n2 = 0;
for (let s = t; s < e.length; s++) {
let a = e[s];
mt(e.charCodeAt(s)) && (r === null || r === a) && n2 % 2 === 0 ? r = r === null ? a : null : r === null && (yield s), n2 = a === "\\" ? n2 + 1 : 0;
}
}
}, sr = class extends De {
constructor() {
super(...arguments), this.simpleExpressionChecker = wt;
}
}, function(e) {
e[e.None = 0] = "None", e[e.Writable = 1] = "Writable";
}(Z || (Z = {})), D = class {
constructor(e, t, r, n2, s, a, i, h) {
this.input = e, this.location = t, this.absoluteOffset = r, this.tokens = n2, this.inputLength = s, this.parseAction = a, this.errors = i, this.offset = h, this.rparensExpected = 0, this.rbracketsExpected = 0, this.rbracesExpected = 0, this.context = Z.None, this.sourceSpanCache = /* @__PURE__ */ new Map(), this.index = 0;
}
peek(e) {
let t = this.index + e;
return t < this.tokens.length ? this.tokens[t] : Ie;
}
get next() {
return this.peek(0);
}
get atEOF() {
return this.index >= this.tokens.length;
}
get inputIndex() {
return this.atEOF ? this.currentEndIndex : this.next.index + this.offset;
}
get currentEndIndex() {
return this.index > 0 ? this.peek(-1).end + this.offset : this.tokens.length === 0 ? this.inputLength + this.offset : this.next.index + this.offset;
}
get currentAbsoluteOffset() {
return this.absoluteOffset + this.inputIndex;
}
span(e, t) {
let r = this.currentEndIndex;
if (t !== void 0 && t > this.currentEndIndex && (r = t), e > r) {
let n2 = r;
r = e, e = n2;
}
return new V(e, r);
}
sourceSpan(e, t) {
let r = `${e}@${this.inputIndex}:${t}`;
return this.sourceSpanCache.has(r) || this.sourceSpanCache.set(r, this.span(e, t).toAbsolute(this.absoluteOffset)), this.sourceSpanCache.get(r);
}
advance() {
this.index++;
}
withContext(e, t) {
this.context |= e;
let r = t();
return this.context ^= e, r;
}
consumeOptionalCharacter(e) {
return this.next.isCharacter(e) ? (this.advance(), true) : false;
}
peekKeywordLet() {
return this.next.isKeywordLet();
}
peekKeywordAs() {
return this.next.isKeywordAs();
}
expectCharacter(e) {
this.consumeOptionalCharacter(e) || this.error(`Missing expected ${String.fromCharCode(e)}`);
}
consumeOptionalOperator(e) {
return this.next.isOperator(e) ? (this.advance(), true) : false;
}
expectOperator(e) {
this.consumeOptionalOperator(e) || this.error(`Missing expected operator ${e}`);
}
prettyPrintToken(e) {
return e === Ie ? "end of input" : `token ${e}`;
}
expectIdentifierOrKeyword() {
let e = this.next;
return !e.isIdentifier() && !e.isKeyword() ? (e.isPrivateIdentifier() ? this._reportErrorForPrivateIdentifier(e, "expected identifier or keyword") : this.error(`Unexpected ${this.prettyPrintToken(e)}, expected identifier or keyword`), null) : (this.advance(), e.toString());
}
expectIdentifierOrKeywordOrString() {
let e = this.next;
return !e.isIdentifier() && !e.isKeyword() && !e.isString() ? (e.isPrivateIdentifier() ? this._reportErrorForPrivateIdentifier(e, "expected identifier, keyword or string") : this.error(`Unexpected ${this.prettyPrintToken(e)}, expected identifier, keyword, or string`), "") : (this.advance(), e.toString());
}
parseChain() {
let e = [], t = this.inputIndex;
for (; this.index < this.tokens.length; ) {
let r = this.parsePipe();
if (e.push(r), this.consumeOptionalCharacter(te))
for (this.parseAction || this.error("Binding expression cannot contain chained expression"); this.consumeOptionalCharacter(te); )
;
else
this.index < this.tokens.length && this.error(`Unexpected token '${this.next}'`);
}
if (e.length == 0) {
let r = this.offset, n2 = this.offset + this.inputLength;
return new K(this.span(r, n2), this.sourceSpan(r, n2));
}
return e.length == 1 ? e[0] : new oe(this.span(t), this.sourceSpan(t), e);
}
parsePipe() {
let e = this.inputIndex, t = this.parseExpression();
if (this.consumeOptionalOperator("|")) {
this.parseAction && this.error("Cannot have a pipe in an action expression");
do {
let r = this.inputIndex, n2 = this.expectIdentifierOrKeyword(), s, a;
n2 !== null ? s = this.sourceSpan(r) : (n2 = "", a = this.next.index !== -1 ? this.next.index : this.inputLength + this.offset, s = new V(a, a).toAbsolute(this.absoluteOffset));
let i = [];
for (; this.consumeOptionalCharacter(X); )
i.push(this.parseExpression());
t = new fe(this.span(e), this.sourceSpan(e, a), t, n2, i, s);
} while (this.consumeOptionalOperator("|"));
}
return t;
}
parseExpression() {
return this.parseConditional();
}
parseConditional() {
let e = this.inputIndex, t = this.parseLogicalOr();
if (this.consumeOptionalOperator("?")) {
let r = this.parsePipe(), n2;
if (this.consumeOptionalCharacter(X))
n2 = this.parsePipe();
else {
let s = this.inputIndex, a = this.input.substring(e, s);
this.error(`Conditional expression ${a} requires all 3 expressions`), n2 = new K(this.span(e), this.sourceSpan(e));
}
return new ce(this.span(e), this.sourceSpan(e), t, r, n2);
} else
return t;
}
parseLogicalOr() {
let e = this.inputIndex, t = this.parseLogicalAnd();
for (; this.consumeOptionalOperator("||"); ) {
let r = this.parseLogicalAnd();
t = new B(this.span(e), this.sourceSpan(e), "||", t, r);
}
return t;
}
parseLogicalAnd() {
let e = this.inputIndex, t = this.parseNullishCoalescing();
for (; this.consumeOptionalOperator("&&"); ) {
let r = this.parseNullishCoalescing();
t = new B(this.span(e), this.sourceSpan(e), "&&", t, r);
}
return t;
}
parseNullishCoalescing() {
let e = this.inputIndex, t = this.parseEquality();
for (; this.consumeOptionalOperator("??"); ) {
let r = this.parseEquality();
t = new B(this.span(e), this.sourceSpan(e), "??", t, r);
}
return t;
}
parseEquality() {
let e = this.inputIndex, t = this.parseRelational();
for (; this.next.type == S.Operator; ) {
let r = this.next.strValue;
switch (r) {
case "==":
case "===":
case "!=":
case "!==":
this.advance();
let n2 = this.parseRelational();
t = new B(this.span(e), this.sourceSpan(e), r, t, n2);
continue;
}
break;
}
return t;
}
parseRelational() {
let e = this.inputIndex, t = this.parseAdditive();
for (; this.next.type == S.Operator; ) {
let r = this.next.strValue;
switch (r) {
case "<":
case ">":
case "<=":
case ">=":
this.advance();
let n2 = this.parseAdditive();
t = new B(this.span(e), this.sourceSpan(e), r, t, n2);
continue;
}
break;
}
return t;
}
parseAdditive() {
let e = this.inputIndex, t = this.parseMultiplicative();
for (; this.next.type == S.Operator; ) {
let r = this.next.strValue;
switch (r) {
case "+":
case "-":
this.advance();
let n2 = this.parseMultiplicative();
t = new B(this.span(e), this.sourceSpan(e), r, t, n2);
continue;
}
break;
}
return t;
}
parseMultiplicative() {
let e = this.inputIndex, t = this.parsePrefix();
for (; this.next.type == S.Operator; ) {
let r = this.next.strValue;
switch (r) {
case "*":
case "%":
case "/":
this.advance();
let n2 = this.parsePrefix();
t = new B(this.span(e), this.sourceSpan(e), r, t, n2);
continue;
}
break;
}
return t;
}
parsePrefix() {
if (this.next.type == S.Operator) {
let e = this.inputIndex, t = this.next.strValue, r;
switch (t) {
case "+":
return this.advance(), r = this.parsePrefix(), F.createPlus(this.span(e), this.sourceSpan(e), r);
case "-":
return this.advance(), r = this.parsePrefix(), F.createMinus(this.span(e), this.sourceSpan(e), r);
case "!":
return this.advance(), r = this.parsePrefix(), new xe(this.span(e), this.sourceSpan(e), r);
}
}
return this.parseCallChain();
}
parseCallChain() {
let e = this.inputIndex, t = this.parsePrimary();
for (; ; )
if (this.consumeOptionalCharacter(H))
t = this.parseAccessMemberOrMethodCall(t, e, false);
else if (this.consumeOptionalOperator("?."))
t = this.consumeOptionalCharacter(Ae) ? this.parseKeyedReadOrWrite(t, e, true) : this.parseAccessMemberOrMethodCall(t, e, true);
else if (this.consumeOptionalCharacter(Ae))
t = this.parseKeyedReadOrWrite(t, e, false);
else if (this.consumeOptionalCharacter(Ee)) {
this.rparensExpected++;
let r = this.parseCallArguments();
this.rparensExpected--, this.expectCharacter(z), t = new Pe(this.span(e), this.sourceSpan(e), t, r);
} else if (this.consumeOptionalOperator("!"))
t = new Se(this.span(e), this.sourceSpan(e), t);
else
return t;
}
parsePrimary() {
let e = this.inputIndex;
if (this.consumeOptionalCharacter(Ee)) {
this.rparensExpected++;
let t = this.parsePipe();
return this.rparensExpected--, this.expectCharacter(z), t;
} else {
if (this.next.isKeywordNull())
return this.advance(), new $(this.span(e), this.sourceSpan(e), null);
if (this.next.isKeywordUndefined())
return this.advance(), new $(this.span(e), this.sourceSpan(e), void 0);
if (this.next.isKeywordTrue())
return this.advance(), new $(this.span(e), this.sourceSpan(e), true);
if (this.next.isKeywordFalse())
return this.advance(), new $(this.span(e), this.sourceSpan(e), false);
if (this.next.isKeywordThis())
return this.advance(), new Ye(this.span(e), this.sourceSpan(e));
if (this.consumeOptionalCharacter(Ae)) {
this.rbracketsExpected++;
let t = this.parseExpressionList(re);
return this.rbracketsExpected--, this.expectCharacter(re), new ge(this.span(e), this.sourceSpan(e), t);
} else {
if (this.next.isCharacter($e))
return this.parseLiteralMap();
if (this.next.isIdentifier())
return this.parseAccessMemberOrMethodCall(new Oe(this.span(e), this.sourceSpan(e)), e, false);
if (this.next.isNumber()) {
let t = this.next.toNumber();
return this.advance(), new $(this.span(e), this.sourceSpan(e), t);
} else if (this.next.isString()) {
let t = this.next.toString();
return this.advance(), new $(this.span(e), this.sourceSpan(e), t);
} else
return this.next.isPrivateIdentifier() ? (this._reportErrorForPrivateIdentifier(this.next, null), new K(this.span(e), this.sourceSpan(e))) : this.index >= this.tokens.length ? (this.error(`Unexpected end of expression: ${this.input}`), new K(this.span(e), this.sourceSpan(e))) : (this.error(`Unexpected token ${this.next}`), new K(this.span(e), this.sourceSpan(e)));
}
}
}
parseExpressionList(e) {
let t = [];
do
if (!this.next.isCharacter(e))
t.push(this.parsePipe());
else
break;
while (this.consumeOptionalCharacter(ee));
return t;
}
parseLiteralMap() {
let e = [], t = [], r = this.inputIndex;
if (this.expectCharacter($e), !this.consumeOptionalCharacter(_e)) {
this.rbracesExpected++;
do {
let n2 = this.inputIndex, s = this.next.isString(), a = this.expectIdentifierOrKeywordOrString();
if (e.push({ key: a, quoted: s }), s)
this.expectCharacter(X), t.push(this.parsePipe());
else if (this.consumeOptionalCharacter(X))
t.push(this.parsePipe());
else {
let i = this.span(n2), h = this.sourceSpan(n2);
t.push(new ne(i, h, h, new Oe(i, h), a));
}
} while (this.consumeOptionalCharacter(ee));
this.rbracesExpected--, this.expectCharacter(_e);
}
return new ve(this.span(r), this.sourceSpan(r), e, t);
}
parseAccessMemberOrMethodCall(e, t, r) {
let n2 = this.inputIndex, s = this.withContext(Z.Writable, () => {
var i;
let h = (i = this.expectIdentifierOrKeyword()) !== null && i !== void 0 ? i : "";
return h.length === 0 && this.error("Expected identifier for property access", e.span.end), h;
}), a = this.sourceSpan(n2);
if (this.consumeOptionalCharacter(Ee)) {
let i = this.inputIndex;
this.rparensExpected++;
let h = this.parseCallArguments(), l = this.span(i, this.inputIndex).toAbsolute(this.absoluteOffset);
this.expectCharacter(z), this.rparensExpected--;
let P = this.span(t), p = this.sourceSpan(t);
return r ? new we(P, p, a, e, s, h, l) : new ye(P, p, a, e, s, h, l);
} else {
if (r)
return this.consumeOptionalOperator("=") ? (this.error("The '?.' operator cannot be used in the assignment"), new K(this.span(t), this.sourceSpan(t))) : new le(this.span(t), this.sourceSpan(t), a, e, s);
if (this.consumeOptionalOperator("=")) {
if (!this.parseAction)
return this.error("Bindings cannot contain assignments"), new K(this.span(t), this.sourceSpan(t));
let i = this.parseConditional();
return new ue(this.span(t), this.sourceSpan(t), a, e, s, i);
} else
return new ne(this.span(t), this.sourceSpan(t), a, e, s);
}
}
parseCallArguments() {
if (this.next.isCharacter(z))
return [];
let e = [];
do
e.push(this.parsePipe());
while (this.consumeOptionalCharacter(ee));
return e;
}
expectTemplateBindingKey() {
let e = "", t = false, r = this.currentAbsoluteOffset;
do
e += this.expectIdentifierOrKeywordOrString(), t = this.consumeOptionalOperator("-"), t && (e += "-");
while (t);
return { source: e, span: new U(r, r + e.length) };
}
parseTemplateBindings(e) {
let t = [];
for (t.push(...this.parseDirectiveKeywordBindings(e)); this.index < this.tokens.length; ) {
let r = this.parseLetBinding();
if (r)
t.push(r);
else {
let n2 = this.expectTemplateBindingKey(), s = this.parseAsBinding(n2);
s ? t.push(s) : (n2.source = e.source + n2.source.charAt(0).toUpperCase() + n2.source.substring(1), t.push(...this.parseDirectiveKeywordBindings(n2)));
}
this.consumeStatementTerminator();
}
return new Qe(t, [], this.errors);
}
parseKeyedReadOrWrite(e, t, r) {
return this.withContext(Z.Writable, () => {
this.rbracketsExpected++;
let n2 = this.parsePipe();
if (n2 instanceof K && this.error("Key access cannot be empty"), this.rbracketsExpected--, this.expectCharacter(re), this.consumeOptionalOperator("="))
if (r)
this.error("The '?.' operator cannot be used in the assignment");
else {
let s = this.parseConditional();
return new de(this.span(t), this.sourceSpan(t), e, n2, s);
}
else
return r ? new pe(this.span(t), this.sourceSpan(t), e, n2) : new he(this.span(t), this.sourceSpan(t), e, n2);
return new K(this.span(t), this.sourceSpan(t));
});
}
parseDirectiveKeywordBindings(e) {
let t = [];
this.consumeOptionalCharacter(X);
let r = this.getDirectiveBoundTarget(), n2 = this.currentAbsoluteOffset, s = this.parseAsBinding(e);
s || (this.consumeStatementTerminator(), n2 = this.currentAbsoluteOffset);
let a = new U(e.span.start, n2);
return t.push(new Ze(a, e, r)), s && t.push(s), t;
}
getDirectiveBoundTarget() {
if (this.next === Ie || this.peekKeywordAs() || this.peekKeywordLet())
return null;
let e = this.parsePipe(), { start: t, end: r } = e.span, n2 = this.input.substring(t, r);
return new G(e, n2, this.location, this.absoluteOffset + t, this.errors);
}
parseAsBinding(e) {
if (!this.peekKeywordAs())
return null;
this.advance();
let t = this.expectTemplateBindingKey();
this.consumeStatementTerminator();
let r = new U(e.span.start, this.currentAbsoluteOffset);
return new Re(r, t, e);
}
parseLetBinding() {
if (!this.peekKeywordLet())
return null;
let e = this.currentAbsoluteOffset;
this.advance();
let t = this.expectTemplateBindingKey(), r = null;
this.consumeOptionalOperator("=") && (r = this.expectTemplateBindingKey()), this.consumeStatementTerminator();
let n2 = new U(e, this.currentAbsoluteOffset);
return new Re(n2, t, r);
}
consumeStatementTerminator() {
this.consumeOptionalCharacter(te) || this.consumeOptionalCharacter(ee);
}
error(e) {
let t = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
this.errors.push(new ae(e, this.input, this.locationText(t), this.location)), this.skip();
}
locationText() {
let e = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : null;
return e == null && (e = this.index), e < this.tokens.length ? `at column ${this.tokens[e].index + 1} in` : "at the end of the expression";
}
_reportErrorForPrivateIdentifier(e, t) {
let r = `Private identifiers are not supported. Unexpected private identifier: ${e}`;
t !== null && (r += `, ${t}`), this.error(r);
}
skip() {
let e = this.next;
for (; this.index < this.tokens.length && !e.isCharacter(te) && !e.isOperator("|") && (this.rparensExpected <= 0 || !e.isCharacter(z)) && (this.rbracesExpected <= 0 || !e.isCharacter(_e)) && (this.rbracketsExpected <= 0 || !e.isCharacter(re)) && (!(this.context & Z.Writable) || !e.isOperator("=")); )
this.next.isError() && this.errors.push(new ae(this.next.toString(), this.input, this.locationText(), this.location)), this.advance(), e = this.next;
}
}, yt = class {
constructor() {
this.errors = [];
}
visitImplicitReceiver(e, t) {
}
visitThisReceiver(e, t) {
}
visitInterpolation(e, t) {
}
visitLiteralPrimitive(e, t) {
}
visitPropertyRead(e, t) {
}
visitPropertyWrite(e, t) {
}
visitSafePropertyRead(e, t) {
}
visitMethodCall(e, t) {
}
visitSafeMethodCall(e, t) {
}
visitFunctionCall(e, t) {
}
visitLiteralArray(e, t) {
this.visitAll(e.expressions, t);
}
visitLiteralMap(e, t) {
this.visitAll(e.values, t);
}
visitUnary(e, t) {
}
visitBinary(e, t) {
}
visitPrefixNot(e, t) {
}
visitNonNullAssert(e, t) {
}
visitConditional(e, t) {
}
visitPipe(e, t) {
this.errors.push("pipes");
}
visitKeyedRead(e, t) {
}
visitKeyedWrite(e, t) {
}
visitAll(e, t) {
return e.map((r) => r.visit(this, t));
}
visitChain(e, t) {
}
visitQuote(e, t) {
}
visitSafeKeyedRead(e, t) {
}
}, wt = class extends et {
constructor() {
super(...arguments), this.errors = [];
}
visitPipe() {
this.errors.push("pipes");
}
};
} }), ft = q({ "node_modules/angular-estree-parser/lib/utils.js"(e) {
"use strict";
L(), Object.defineProperty(e, "__esModule", { value: true }), e.getLast = e.toLowerCamelCase = e.findBackChar = e.findFrontChar = e.fitSpans = e.getNgType = e.parseNgInterpolation = e.parseNgTemplateBindings = e.parseNgAction = e.parseNgSimpleBinding = e.parseNgBinding = e.NG_PARSE_TEMPLATE_BINDINGS_FAKE_PREFIX = void 0;
var t = (tt(), be(Je)), r = (tr(), be(Yt)), n2 = (br(), be(nr)), s = "angular-estree-parser";
e.NG_PARSE_TEMPLATE_BINDINGS_FAKE_PREFIX = "NgEstreeParser";
var a = 0, i = [s, a];
function h() {
return new n2.Parser(new r.Lexer());
}
function l(o, d) {
let y = h(), { astInput: E, comments: A } = T(o, y), { ast: I, errors: j } = d(E, y);
return R(j), { ast: I, comments: A };
}
function P(o) {
return l(o, (d, y) => y.parseBinding(d, ...i));
}
e.parseNgBinding = P;
function p(o) {
return l(o, (d, y) => y.parseSimpleBinding(d, ...i));
}
e.parseNgSimpleBinding = p;
function x(o) {
return l(o, (d, y) => y.parseAction(d, ...i));
}
e.parseNgAction = x;
function C(o) {
let d = h(), { templateBindings: y, errors: E } = d.parseTemplateBindings(e.NG_PARSE_TEMPLATE_BINDINGS_FAKE_PREFIX, o, s, a, a);
return R(E), y;
}
e.parseNgTemplateBindings = C;
function b(o) {
let d = h(), { astInput: y, comments: E } = T(o, d), A = "{{", I = "}}", { ast: j, errors: or } = d.parseInterpolation(A + y + I, ...i);
R(or);
let gt = j.expressions[0], vt = /* @__PURE__ */ new Set();
return _(gt, (ke) => {
vt.has(ke) || (ke.start -= A.length, ke.end -= A.length, vt.add(ke));
}), { ast: gt, comments: E };
}
e.parseNgInterpolation = b;
function _(o, d) {
if (!(!o || typeof o != "object")) {
if (Array.isArray(o))
return o.forEach((y) => _(y, d));
for (let y of Object.keys(o)) {
let E = o[y];
y === "span" ? d(E) : _(E, d);
}
}
}
function R(o) {
if (o.length !== 0) {
let [{ message: d }] = o;
throw new SyntaxError(d.replace(/^Parser Error: | at column \d+ in [^]*$/g, ""));
}
}
function T(o, d) {
let y = d._commentStart(o);
return y === null ? { astInput: o, comments: [] } : { astInput: o.slice(0, y), comments: [{ type: "Comment", value: o.slice(y + 2), span: { start: y, end: o.length } }] };
}
function O(o) {
return t.Unary && o instanceof t.Unary ? "Unary" : o instanceof t.Binary ? "Binary" : o instanceof t.BindingPipe ? "BindingPipe" : o instanceof t.Chain ? "Chain" : o instanceof t.Conditional ? "Conditional" : o instanceof t.EmptyExpr ? "EmptyExpr" : o instanceof t.FunctionCall ? "FunctionCall" : o instanceof t.ImplicitReceiver ? "ImplicitReceiver" : o instanceof t.KeyedRead ? "KeyedRead" : o instanceof t.KeyedWrite ? "KeyedWrite" : o instanceof t.LiteralArray ? "LiteralArray" : o instanceof t.LiteralMap ? "LiteralMap" : o instanceof t.LiteralPrimitive ? "LiteralPrimitive" : o instanceof t.MethodCall ? "MethodCall" : o instanceof t.NonNullAssert ? "NonNullAssert" : o instanceof t.PrefixNot ? "PrefixNot" : o instanceof t.PropertyRead ? "PropertyRead" : o instanceof t.PropertyWrite ? "PropertyWrite" : o instanceof t.Quote ? "Quote" : o instanceof t.SafeMethodCall ? "SafeMethodCall" : o instanceof t.SafePropertyRead ? "SafePropertyRead" : o.type;
}
e.getNgType = O;
function N(o, d) {
let { start: y, end: E } = o, A = y, I = E;
for (; I !== A && /\s/.test(d[I - 1]); )
I--;
for (; A !== I && /\s/.test(d[A]); )
A++;
return { start: A, end: I };
}
function c(o, d) {
let { start: y, end: E } = o, A = y, I = E;
for (; I !== d.length && /\s/.test(d[I]); )
I++;
for (; A !== 0 && /\s/.test(d[A - 1]); )
A--;
return { start: A, end: I };
}
function g(o, d) {
return d[o.start - 1] === "(" && d[o.end] === ")" ? { start: o.start - 1, end: o.end + 1 } : o;
}
function u(o, d, y) {
let E = 0, A = { start: o.start, end: o.end };
for (; ; ) {
let I = c(A, d), j = g(I, d);
if (I.start === j.start && I.end === j.end)
break;
A.start = j.start, A.end = j.end, E++;
}
return { hasParens: (y ? E - 1 : E) !== 0, outerSpan: N(y ? { start: A.start + 1, end: A.end - 1 } : A, d), innerSpan: N(o, d) };
}
e.fitSpans = u;
function v(o, d, y) {
let E = d;
for (; !o.test(y[E]); )
if (--E < 0)
throw new Error(`Cannot find front char ${o} from index ${d} in ${JSON.stringify(y)}`);
return E;
}
e.findFrontChar = v;
function m(o, d, y) {
let E = d;
for (; !o.test(y[E]); )
if (++E >= y.length)
throw new Error(`Cannot find back char ${o} from index ${d} in ${JSON.stringify(y)}`);
return E;
}
e.findBackChar = m;
function f(o) {
return o.slice(0, 1).toLowerCase() + o.slice(1);
}
e.toLowerCamelCase = f;
function w(o) {
return o.length === 0 ? void 0 : o[o.length - 1];
}
e.getLast = w;
} }), ir = q({ "node_modules/angular-estree-parser/lib/transform.js"(e) {
"use strict";
L(), Object.defineProperty(e, "__esModule", { value: true }), e.transformSpan = e.transform = void 0;
var t = ft(), r = function(s, a) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false, h = t.getNgType(s);
switch (h) {
case "Unary": {
let { operator: c, expr: g } = s, u = l(g);
return p("UnaryExpression", { prefix: true, argument: u, operator: c }, s.span, { hasParentParens: i });
}
case "Binary": {
let { left: c, operation: g, right: u } = s, v = u.span.start === u.span.end, m = c.span.start === c.span.end;
if (v || m) {
let o = c.span.start === c.span.end ? l(u) : l(c);
return p("UnaryExpression", { prefix: true, argument: o, operator: v ? "+" : "-" }, { start: s.span.start, end: N(o) }, { hasParentParens: i });
}
let f = l(c), w = l(u);
return p(g === "&&" || g === "||" ? "LogicalExpression" : "BinaryExpression", { left: f, right: w, operator: g }, { start: O(f), end: N(w) }, { hasParentParens: i });
}
case "BindingPipe": {
let { exp: c, name: g, args: u } = s, v = l(c), m = b(/\S/, b(/\|/, N(v)) + 1), f = p("Identifier", { name: g }, { start: m, end: m + g.length }), w = u.map(l);
return p("NGPipeExpression", { left: v, right: f, arguments: w }, { start: O(v), end: N(w.length === 0 ? f : t.getLast(w)) }, { hasParentParens: i });
}
case "Chain": {
let { expressions: c } = s;
return p("NGChainedExpression", { expressions: c.map(l) }, s.span, { hasParentParens: i });
}
case "Comment": {
let { value: c } = s;
return p("CommentLine", { value: c }, s.span, { processSpan: false });
}
case "Conditional": {
let { condition: c, trueExp: g, falseExp: u } = s, v = l(c), m = l(g), f = l(u);
return p("ConditionalExpression", { test: v, consequent: m, alternate: f }, { start: O(v), end: N(f) }, { hasParentParens: i });
}
case "EmptyExpr":
return p("NGEmptyExpression", {}, s.span, { hasParentParens: i });
case "FunctionCall": {
let { target: c, args: g } = s, u = g.length === 1 ? [P(g[0])] : g.map(l), v = l(c);
return p("CallExpression", { callee: v, arguments: u }, { start: O(v), end: s.span.end }, { hasParentParens: i });
}
case "ImplicitReceiver":
return p("ThisExpression", {}, s.span, { hasParentParens: i });
case "KeyedRead": {
let { key: c } = s, g = Object.prototype.hasOwnProperty.call(s, "receiver") ? s.receiver : s.obj, u = l(c);
return x(g, u, { computed: true, optional: false }, { end: s.span.end, hasParentParens: i });
}
case "LiteralArray": {
let { expressions: c } = s;
return p("ArrayExpression", { elements: c.map(l) }, s.span, { hasParentParens: i });
}
case "LiteralMap": {
let { keys: c, values: g } = s, u = g.map((m) => l(m)), v = c.map((m, f) => {
let { key: w, quoted: o } = m, d = u[f], y = b(/\S/, f === 0 ? s.span.start + 1 : b(/,/, N(u[f - 1])) + 1), E = C(/\S/, C(/:/, O(d) - 1) - 1) + 1, A = { start: y, end: E }, I = o ? p("StringLiteral", { value: w }, A) : p("Identifier", { name: w }, A), j = I.end < I.start;
return p("ObjectProperty", { key: I, value: d, method: false, shorthand: j, computed: false }, { start: O(I), end: N(d) });
});
return p("ObjectExpression", { properties: v }, s.span, { hasParentParens: i });
}
case "LiteralPrimitive": {
let { value: c } = s;
switch (typeof c) {
case "boolean":
return p("BooleanLiteral", { value: c }, s.span, { hasParentParens: i });
case "number":
return p("NumericLiteral", { value: c }, s.span, { hasParentParens: i });
case "object":
return p("NullLiteral", {}, s.span, { hasParentParens: i });
case "string":
return p("StringLiteral", { value: c }, s.span, { hasParentParens: i });
case "undefined":
return p("Identifier", { name: "undefined" }, s.span, { hasParentParens: i });
default:
throw new Error(`Unexpected LiteralPrimitive value type ${typeof c}`);
}
}
case "MethodCall":
case "SafeMethodCall": {
let c = h === "SafeMethodCall", { receiver: g, name: u, args: v } = s, m = v.length === 1 ? [P(v[0])] : v.map(l), f = C(/\S/, C(/\(/, (m.length === 0 ? C(/\)/, s.span.end - 1) : O(m[0])) - 1) - 1) + 1, w = p("Identifier", { name: u }, { start: f - u.length, end: f }), o = x(g, w, { computed: false, optional: c }), d = R(o);
return p(c || d ? "OptionalCallExpression" : "CallExpression", { callee: o, arguments: m }, { start: O(o), end: s.span.end }, { hasParentParens: i });
}
case "NonNullAssert": {
let { expression: c } = s, g = l(c);
return p("TSNonNullExpression", { expression: g }, { start: O(g), end: s.span.end }, { hasParentParens: i });
}
case "PrefixNot": {
let { expression: c } = s, g = l(c);
return p("UnaryExpression", { prefix: true, operator: "!", argument: g }, { start: s.span.start, end: N(g) }, { hasParentParens: i });
}
case "PropertyRead":
case "SafePropertyRead": {
let c = h === "SafePropertyRead", { receiver: g, name: u } = s, v = C(/\S/, s.span.end - 1) + 1, m = p("Identifier", { name: u }, { start: v - u.length, end: v }, _(g) ? { hasParentParens: i } : {});
return x(g, m, { computed: false, optional: c }, { hasParentParens: i });
}
case "KeyedWrite": {
let { key: c, value: g } = s, u = Object.prototype.hasOwnProperty.call(s, "receiver") ? s.receiver : s.obj, v = l(c), m = l(g), f = x(u, v, { computed: true, optional: false }, { end: b(/\]/, N(v)) + 1 });
return p("AssignmentExpression", { left: f, operator: "=", right: m }, { start: O(f), end: N(m) }, { hasParentParens: i });
}
case "PropertyWrite": {
let { receiver: c, name: g, value: u } = s, v = l(u), m = C(/\S/, C(/=/, O(v) - 1) - 1) + 1, f = p("Identifier", { name: g }, { start: m - g.length, end: m }), w = x(c, f, { computed: false, optional: false });
return p("AssignmentExpression", { left: w, operator: "=", right: v }, { start: O(w), end: N(v) }, { hasParentParens: i });
}
case "Quote": {
let { prefix: c, uninterpretedExpression: g } = s;
return p("NGQuotedExpression", { prefix: c, value: g }, s.span, { hasParentParens: i });
}
default:
throw new Error(`Unexpected node ${h}`);
}
function l(c) {
return e.transform(c, a);
}
function P(c) {
return e.transform(c, a, true);
}
function p(c, g, u) {
let { processSpan: v = true, hasParentParens: m = false } = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : {}, f = Object.assign(Object.assign({ type: c }, n2(u, a, v, m)), g);
switch (c) {
case "Identifier": {
let w = f;
w.loc.identifierName = w.name;
break;
}
case "NumericLiteral": {
let w = f;
w.extra = Object.assign(Object.assign({}, w.extra), { raw: a.text.slice(w.start, w.end), rawValue: w.value });
break;
}
case "StringLiteral": {
let w = f;
w.extra = Object.assign(Object.assign({}, w.extra), { raw: a.text.slice(w.start, w.end), rawValue: w.value });
break;
}
}
return f;
}
function x(c, g, u) {
let { end: v = N(g), hasParentParens: m = false } = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : {};
if (_(c) || c.span.start === g.start)
return g;
let f = l(c), w = R(f);
return p(u.optional || w ? "OptionalMemberExpression" : "MemberExpression", Object.assign({ object: f, property: g, computed: u.computed }, u.optional ? { optional: true } : w ? { optional: false } : null), { start: O(f), end: v }, { hasParentParens: m });
}
function C(c, g) {
return t.findFrontChar(c, g, a.text);
}
function b(c, g) {
return t.findBackChar(c, g, a.text);
}
function _(c) {
return c.span.start >= c.span.end || /^\s+$/.test(a.text.slice(c.span.start, c.span.end));
}
function R(c) {
return (c.type === "OptionalCallExpression" || c.type === "OptionalMemberExpression") && !T(c);
}
function T(c) {
return c.extra && c.extra.parenthesized;
}
function O(c) {
return T(c) ? c.extra.parenStart : c.start;
}
function N(c) {
return T(c) ? c.extra.parenEnd : c.end;
}
};
e.transform = r;
function n2(s, a) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false, h = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : false;
if (!i) {
let { start: x, end: C } = s;
return { start: x, end: C, loc: { start: a.locator.locationForIndex(x), end: a.locator.locationForIndex(C) } };
}
let { outerSpan: l, innerSpan: P, hasParens: p } = t.fitSpans(s, a.text, h);
return Object.assign({ start: P.start, end: P.end, loc: { start: a.locator.locationForIndex(P.start), end: a.locator.locationForIndex(P.end) } }, p && { extra: { parenthesized: true, parenStart: l.start, parenEnd: l.end } });
}
e.transformSpan = n2;
} }), Lr = q({ "node_modules/angular-estree-parser/lib/transform-microsyntax.js"(e) {
"use strict";
L(), Object.defineProperty(e, "__esModule", { value: true }), e.transformTemplateBindings = void 0;
var t = (tt(), be(Je)), r = ir(), n2 = ft();
function s(a, i) {
a.forEach(N);
let [h] = a, { key: l } = h, P = i.text.slice(h.sourceSpan.start, h.sourceSpan.end).trim().length === 0 ? a.slice(1) : a, p = [], x = null;
for (let u = 0; u < P.length; u++) {
let v = P[u];
if (x && T(x) && O(v) && v.value && v.value.source === x.key.source) {
let m = _("NGMicrosyntaxKey", { name: v.key.source }, v.key.span), f = (d, y) => Object.assign(Object.assign({}, d), r.transformSpan({ start: d.start, end: y }, i)), w = (d) => Object.assign(Object.assign({}, f(d, m.end)), { alias: m }), o = p.pop();
if (o.type === "NGMicrosyntaxExpression")
p.push(w(o));
else if (o.type === "NGMicrosyntaxKeyedExpression") {
let d = w(o.expression);
p.push(f(Object.assign(Object.assign({}, o), { expression: d }), d.end));
} else
throw new Error(`Unexpected type ${o.type}`);
} else
p.push(C(v, u));
x = v;
}
return _("NGMicrosyntax", { body: p }, p.length === 0 ? a[0].sourceSpan : { start: p[0].start, end: p[p.length - 1].end });
function C(u, v) {
if (T(u)) {
let { key: m, value: f } = u;
return f ? v === 0 ? _("NGMicrosyntaxExpression", { expression: b(f.ast), alias: null }, f.sourceSpan) : _("NGMicrosyntaxKeyedExpression", { key: _("NGMicrosyntaxKey", { name: R(m.source) }, m.span), expression: _("NGMicrosyntaxExpression", { expression: b(f.ast), alias: null }, f.sourceSpan) }, { start: m.span.start, end: f.sourceSpan.end }) : _("NGMicrosyntaxKey", { name: R(m.source) }, m.span);
} else {
let { key: m, sourceSpan: f } = u;
if (/^let\s$/.test(i.text.slice(f.start, f.start + 4))) {
let { value: o } = u;
return _("NGMicrosyntaxLet", { key: _("NGMicrosyntaxKey", { name: m.source }, m.span), value: o ? _("NGMicrosyntaxKey", { name: o.source }, o.span) : null }, { start: f.start, end: o ? o.span.end : m.span.end });
} else {
let o = g(u);
return _("NGMicrosyntaxAs", { key: _("NGMicrosyntaxKey", { name: o.source }, o.span), alias: _("NGMicrosyntaxKey", { name: m.source }, m.span) }, { start: o.span.start, end: m.span.end });
}
}
}
function b(u) {
return r.transform(u, i);
}
function _(u, v, m) {
let f = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : true;
return Object.assign(Object.assign({ type: u }, r.transformSpan(m, i, f)), v);
}
function R(u) {
return n2.toLowerCamelCase(u.slice(l.source.length));
}
function T(u) {
return u instanceof t.ExpressionBinding;
}
function O(u) {
return u instanceof t.VariableBinding;
}
function N(u) {
c(u.key.span), O(u) && u.value && c(u.value.span);
}
function c(u) {
if (i.text[u.start] !== '"' && i.text[u.start] !== "'")
return;
let v = i.text[u.start], m = false;
for (let f = u.start + 1; f < i.text.length; f++)
switch (i.text[f]) {
case v:
if (!m) {
u.end = f + 1;
return;
}
default:
m = false;
break;
case "\\":
m = !m;
break;
}
}
function g(u) {
if (!u.value || u.value.source !== n2.NG_PARSE_TEMPLATE_BINDINGS_FAKE_PREFIX)
return u.value;
let v = n2.findBackChar(/\S/, u.sourceSpan.start, i.text);
return { source: "$implicit", span: { start: v, end: v } };
}
}
e.transformTemplateBindings = s;
} }), Rr = q({ "node_modules/angular-estree-parser/lib/index.js"(e) {
"use strict";
L(), Object.defineProperty(e, "__esModule", { value: true }), e.parseTemplateBindings = e.parseAction = e.parseInterpolation = e.parseSimpleBinding = e.parseBinding = void 0;
var t = gr(), r = ir(), n2 = Lr(), s = ft();
function a(x, C) {
let { ast: b, comments: _ } = C(x), R = new t.Context(x), T = (N) => r.transform(N, R), O = T(b);
return O.comments = _.map(T), O;
}
function i(x) {
return a(x, s.parseNgBinding);
}
e.parseBinding = i;
function h(x) {
return a(x, s.parseNgSimpleBinding);
}
e.parseSimpleBinding = h;
function l(x) {
return a(x, s.parseNgInterpolation);
}
e.parseInterpolation = l;
function P(x) {
return a(x, s.parseNgAction);
}
e.parseAction = P;
function p(x) {
return n2.transformTemplateBindings(s.parseNgTemplateBindings(x), new t.Context(x));
}
e.parseTemplateBindings = p;
} });
L();
var { locStart: Tr, locEnd: $r } = dr();
function Ne(e) {
return { astFormat: "estree", parse: (r, n2, s) => {
let a = Rr(), i = e(r, a);
return { type: "NGRoot", node: s.parser === "__ng_action" && i.type !== "NGChainedExpression" ? Object.assign(Object.assign({}, i), {}, { type: "NGChainedExpression", expressions: [i] }) : i };
}, locStart: Tr, locEnd: $r };
}
ar.exports = { parsers: { __ng_action: Ne((e, t) => t.parseAction(e)), __ng_binding: Ne((e, t) => t.parseBinding(e)), __ng_interpolation: Ne((e, t) => t.parseInterpolation(e)), __ng_directive: Ne((e, t) => t.parseTemplateBindings(e)) } };
});
return Br();
});
}
});
// node_modules/prettier/parser-espree.js
var require_parser_espree = __commonJS({
"node_modules/prettier/parser-espree.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.espree = e();
}
})(function() {
"use strict";
var C = (a, u) => () => (u || a((u = { exports: {} }).exports, u), u.exports);
var oe = C((tl, zr) => {
var Ye = function(a) {
return a && a.Math == Math && a;
};
zr.exports = Ye(typeof globalThis == "object" && globalThis) || Ye(typeof window == "object" && window) || Ye(typeof self == "object" && self) || Ye(typeof global == "object" && global) || function() {
return this;
}() || Function("return this")();
});
var me = C((rl, Gr) => {
Gr.exports = function(a) {
try {
return !!a();
} catch {
return true;
}
};
});
var xe = C((il, Hr) => {
var vn = me();
Hr.exports = !vn(function() {
return Object.defineProperty({}, 1, { get: function() {
return 7;
} })[1] != 7;
});
});
var bt = C((sl, Kr) => {
var gn = me();
Kr.exports = !gn(function() {
var a = function() {
}.bind();
return typeof a != "function" || a.hasOwnProperty("prototype");
});
});
var et = C((al, Xr) => {
var xn = bt(), Ze = Function.prototype.call;
Xr.exports = xn ? Ze.bind(Ze) : function() {
return Ze.apply(Ze, arguments);
};
});
var Yr = C(($r) => {
"use strict";
var Jr = {}.propertyIsEnumerable, Qr = Object.getOwnPropertyDescriptor, yn = Qr && !Jr.call({ 1: 2 }, 1);
$r.f = yn ? function(u) {
var o = Qr(this, u);
return !!o && o.enumerable;
} : Jr;
});
var _t = C((ul, Zr) => {
Zr.exports = function(a, u) {
return { enumerable: !(a & 1), configurable: !(a & 2), writable: !(a & 4), value: u };
};
});
var ye = C((ol, ri) => {
var ei = bt(), ti = Function.prototype, St = ti.call, An = ei && ti.bind.bind(St, St);
ri.exports = ei ? An : function(a) {
return function() {
return St.apply(a, arguments);
};
};
});
var ai = C((hl, si) => {
var ii = ye(), Cn = ii({}.toString), En = ii("".slice);
si.exports = function(a) {
return En(Cn(a), 8, -1);
};
});
var ui = C((ll, ni) => {
var bn = ye(), _n = me(), Sn = ai(), wt = Object, wn = bn("".split);
ni.exports = _n(function() {
return !wt("z").propertyIsEnumerable(0);
}) ? function(a) {
return Sn(a) == "String" ? wn(a, "") : wt(a);
} : wt;
});
var kt = C((cl, oi) => {
oi.exports = function(a) {
return a == null;
};
});
var Ft = C((pl, hi) => {
var kn = kt(), Fn = TypeError;
hi.exports = function(a) {
if (kn(a))
throw Fn("Can't call method on " + a);
return a;
};
});
var tt = C((fl, li) => {
var Bn = ui(), In = Ft();
li.exports = function(a) {
return Bn(In(a));
};
});
var It = C((dl, ci) => {
var Bt = typeof document == "object" && document.all, Tn = typeof Bt > "u" && Bt !== void 0;
ci.exports = { all: Bt, IS_HTMLDDA: Tn };
});
var le = C((ml, fi) => {
var pi = It(), Pn = pi.all;
fi.exports = pi.IS_HTMLDDA ? function(a) {
return typeof a == "function" || a === Pn;
} : function(a) {
return typeof a == "function";
};
});
var Pe = C((vl, vi) => {
var di = le(), mi = It(), Dn = mi.all;
vi.exports = mi.IS_HTMLDDA ? function(a) {
return typeof a == "object" ? a !== null : di(a) || a === Dn;
} : function(a) {
return typeof a == "object" ? a !== null : di(a);
};
});
var rt = C((gl, gi) => {
var Tt = oe(), Nn = le(), On = function(a) {
return Nn(a) ? a : void 0;
};
gi.exports = function(a, u) {
return arguments.length < 2 ? On(Tt[a]) : Tt[a] && Tt[a][u];
};
});
var yi = C((xl, xi) => {
var Ln = ye();
xi.exports = Ln({}.isPrototypeOf);
});
var Ci = C((yl, Ai) => {
var Vn = rt();
Ai.exports = Vn("navigator", "userAgent") || "";
});
var Fi = C((Al, ki) => {
var wi = oe(), Pt = Ci(), Ei = wi.process, bi = wi.Deno, _i = Ei && Ei.versions || bi && bi.version, Si = _i && _i.v8, ce, it;
Si && (ce = Si.split("."), it = ce[0] > 0 && ce[0] < 4 ? 1 : +(ce[0] + ce[1]));
!it && Pt && (ce = Pt.match(/Edge\/(\d+)/), (!ce || ce[1] >= 74) && (ce = Pt.match(/Chrome\/(\d+)/), ce && (it = +ce[1])));
ki.exports = it;
});
var Dt = C((Cl, Ii) => {
var Bi = Fi(), Rn = me();
Ii.exports = !!Object.getOwnPropertySymbols && !Rn(function() {
var a = Symbol();
return !String(a) || !(Object(a) instanceof Symbol) || !Symbol.sham && Bi && Bi < 41;
});
});
var Nt = C((El, Ti) => {
var jn = Dt();
Ti.exports = jn && !Symbol.sham && typeof Symbol.iterator == "symbol";
});
var Ot = C((bl, Pi) => {
var qn = rt(), Mn = le(), Un = yi(), Wn = Nt(), zn = Object;
Pi.exports = Wn ? function(a) {
return typeof a == "symbol";
} : function(a) {
var u = qn("Symbol");
return Mn(u) && Un(u.prototype, zn(a));
};
});
var Ni = C((_l, Di) => {
var Gn = String;
Di.exports = function(a) {
try {
return Gn(a);
} catch {
return "Object";
}
};
});
var Li = C((Sl, Oi) => {
var Hn = le(), Kn = Ni(), Xn = TypeError;
Oi.exports = function(a) {
if (Hn(a))
return a;
throw Xn(Kn(a) + " is not a function");
};
});
var Ri = C((wl, Vi) => {
var Jn = Li(), Qn = kt();
Vi.exports = function(a, u) {
var o = a[u];
return Qn(o) ? void 0 : Jn(o);
};
});
var qi = C((kl, ji) => {
var Lt = et(), Vt = le(), Rt = Pe(), $n = TypeError;
ji.exports = function(a, u) {
var o, l;
if (u === "string" && Vt(o = a.toString) && !Rt(l = Lt(o, a)) || Vt(o = a.valueOf) && !Rt(l = Lt(o, a)) || u !== "string" && Vt(o = a.toString) && !Rt(l = Lt(o, a)))
return l;
throw $n("Can't convert object to primitive value");
};
});
var Ui = C((Fl, Mi) => {
Mi.exports = false;
});
var st = C((Bl, zi) => {
var Wi = oe(), Yn = Object.defineProperty;
zi.exports = function(a, u) {
try {
Yn(Wi, a, { value: u, configurable: true, writable: true });
} catch {
Wi[a] = u;
}
return u;
};
});
var at = C((Il, Hi) => {
var Zn = oe(), eu = st(), Gi = "__core-js_shared__", tu = Zn[Gi] || eu(Gi, {});
Hi.exports = tu;
});
var jt = C((Tl, Xi) => {
var ru = Ui(), Ki = at();
(Xi.exports = function(a, u) {
return Ki[a] || (Ki[a] = u !== void 0 ? u : {});
})("versions", []).push({ version: "3.26.1", mode: ru ? "pure" : "global", copyright: "\xA9 2014-2022 Denis Pushkarev (zloirock.ru)", license: "https://github.com/zloirock/core-js/blob/v3.26.1/LICENSE", source: "https://github.com/zloirock/core-js" });
});
var Qi = C((Pl, Ji) => {
var iu = Ft(), su = Object;
Ji.exports = function(a) {
return su(iu(a));
};
});
var be = C((Dl, $i) => {
var au = ye(), nu = Qi(), uu = au({}.hasOwnProperty);
$i.exports = Object.hasOwn || function(u, o) {
return uu(nu(u), o);
};
});
var qt = C((Nl, Yi) => {
var ou = ye(), hu = 0, lu = Math.random(), cu = ou(1 .toString);
Yi.exports = function(a) {
return "Symbol(" + (a === void 0 ? "" : a) + ")_" + cu(++hu + lu, 36);
};
});
var ss = C((Ol, is) => {
var pu = oe(), fu = jt(), Zi = be(), du = qt(), es = Dt(), rs = Nt(), De = fu("wks"), we = pu.Symbol, ts = we && we.for, mu = rs ? we : we && we.withoutSetter || du;
is.exports = function(a) {
if (!Zi(De, a) || !(es || typeof De[a] == "string")) {
var u = "Symbol." + a;
es && Zi(we, a) ? De[a] = we[a] : rs && ts ? De[a] = ts(u) : De[a] = mu(u);
}
return De[a];
};
});
var os = C((Ll, us) => {
var vu = et(), as = Pe(), ns = Ot(), gu = Ri(), xu = qi(), yu = ss(), Au = TypeError, Cu = yu("toPrimitive");
us.exports = function(a, u) {
if (!as(a) || ns(a))
return a;
var o = gu(a, Cu), l;
if (o) {
if (u === void 0 && (u = "default"), l = vu(o, a, u), !as(l) || ns(l))
return l;
throw Au("Can't convert object to primitive value");
}
return u === void 0 && (u = "number"), xu(a, u);
};
});
var Mt = C((Vl, hs) => {
var Eu = os(), bu = Ot();
hs.exports = function(a) {
var u = Eu(a, "string");
return bu(u) ? u : u + "";
};
});
var ps = C((Rl, cs) => {
var _u = oe(), ls = Pe(), Ut = _u.document, Su = ls(Ut) && ls(Ut.createElement);
cs.exports = function(a) {
return Su ? Ut.createElement(a) : {};
};
});
var Wt = C((jl, fs2) => {
var wu = xe(), ku = me(), Fu = ps();
fs2.exports = !wu && !ku(function() {
return Object.defineProperty(Fu("div"), "a", { get: function() {
return 7;
} }).a != 7;
});
});
var zt = C((ms) => {
var Bu = xe(), Iu = et(), Tu = Yr(), Pu = _t(), Du = tt(), Nu = Mt(), Ou = be(), Lu = Wt(), ds = Object.getOwnPropertyDescriptor;
ms.f = Bu ? ds : function(u, o) {
if (u = Du(u), o = Nu(o), Lu)
try {
return ds(u, o);
} catch {
}
if (Ou(u, o))
return Pu(!Iu(Tu.f, u, o), u[o]);
};
});
var gs = C((Ml, vs) => {
var Vu = xe(), Ru = me();
vs.exports = Vu && Ru(function() {
return Object.defineProperty(function() {
}, "prototype", { value: 42, writable: false }).prototype != 42;
});
});
var nt = C((Ul, xs) => {
var ju = Pe(), qu = String, Mu = TypeError;
xs.exports = function(a) {
if (ju(a))
return a;
throw Mu(qu(a) + " is not an object");
};
});
var Me = C((As) => {
var Uu = xe(), Wu = Wt(), zu = gs(), ut = nt(), ys = Mt(), Gu = TypeError, Gt = Object.defineProperty, Hu = Object.getOwnPropertyDescriptor, Ht = "enumerable", Kt = "configurable", Xt = "writable";
As.f = Uu ? zu ? function(u, o, l) {
if (ut(u), o = ys(o), ut(l), typeof u == "function" && o === "prototype" && "value" in l && Xt in l && !l[Xt]) {
var v = Hu(u, o);
v && v[Xt] && (u[o] = l.value, l = { configurable: Kt in l ? l[Kt] : v[Kt], enumerable: Ht in l ? l[Ht] : v[Ht], writable: false });
}
return Gt(u, o, l);
} : Gt : function(u, o, l) {
if (ut(u), o = ys(o), ut(l), Wu)
try {
return Gt(u, o, l);
} catch {
}
if ("get" in l || "set" in l)
throw Gu("Accessors not supported");
return "value" in l && (u[o] = l.value), u;
};
});
var Jt = C((zl, Cs) => {
var Ku = xe(), Xu = Me(), Ju = _t();
Cs.exports = Ku ? function(a, u, o) {
return Xu.f(a, u, Ju(1, o));
} : function(a, u, o) {
return a[u] = o, a;
};
});
var _s = C((Gl, bs) => {
var Qt = xe(), Qu = be(), Es = Function.prototype, $u = Qt && Object.getOwnPropertyDescriptor, $t = Qu(Es, "name"), Yu = $t && function() {
}.name === "something", Zu = $t && (!Qt || Qt && $u(Es, "name").configurable);
bs.exports = { EXISTS: $t, PROPER: Yu, CONFIGURABLE: Zu };
});
var ws = C((Hl, Ss) => {
var eo = ye(), to = le(), Yt = at(), ro = eo(Function.toString);
to(Yt.inspectSource) || (Yt.inspectSource = function(a) {
return ro(a);
});
Ss.exports = Yt.inspectSource;
});
var Bs = C((Kl, Fs) => {
var io = oe(), so = le(), ks = io.WeakMap;
Fs.exports = so(ks) && /native code/.test(String(ks));
});
var Ps = C((Xl, Ts) => {
var ao = jt(), no = qt(), Is = ao("keys");
Ts.exports = function(a) {
return Is[a] || (Is[a] = no(a));
};
});
var Zt = C((Jl, Ds) => {
Ds.exports = {};
});
var Vs = C((Ql, Ls) => {
var uo = Bs(), Os = oe(), oo = Pe(), ho = Jt(), er = be(), tr = at(), lo = Ps(), co = Zt(), Ns = "Object already initialized", rr = Os.TypeError, po = Os.WeakMap, ot, Ue, ht, fo = function(a) {
return ht(a) ? Ue(a) : ot(a, {});
}, mo = function(a) {
return function(u) {
var o;
if (!oo(u) || (o = Ue(u)).type !== a)
throw rr("Incompatible receiver, " + a + " required");
return o;
};
};
uo || tr.state ? (pe = tr.state || (tr.state = new po()), pe.get = pe.get, pe.has = pe.has, pe.set = pe.set, ot = function(a, u) {
if (pe.has(a))
throw rr(Ns);
return u.facade = a, pe.set(a, u), u;
}, Ue = function(a) {
return pe.get(a) || {};
}, ht = function(a) {
return pe.has(a);
}) : (ke = lo("state"), co[ke] = true, ot = function(a, u) {
if (er(a, ke))
throw rr(Ns);
return u.facade = a, ho(a, ke, u), u;
}, Ue = function(a) {
return er(a, ke) ? a[ke] : {};
}, ht = function(a) {
return er(a, ke);
});
var pe, ke;
Ls.exports = { set: ot, get: Ue, has: ht, enforce: fo, getterFor: mo };
});
var sr = C(($l, js) => {
var vo = me(), go = le(), lt = be(), ir = xe(), xo = _s().CONFIGURABLE, yo = ws(), Rs = Vs(), Ao = Rs.enforce, Co = Rs.get, ct = Object.defineProperty, Eo = ir && !vo(function() {
return ct(function() {
}, "length", { value: 8 }).length !== 8;
}), bo = String(String).split("String"), _o = js.exports = function(a, u, o) {
String(u).slice(0, 7) === "Symbol(" && (u = "[" + String(u).replace(/^Symbol\(([^)]*)\)/, "$1") + "]"), o && o.getter && (u = "get " + u), o && o.setter && (u = "set " + u), (!lt(a, "name") || xo && a.name !== u) && (ir ? ct(a, "name", { value: u, configurable: true }) : a.name = u), Eo && o && lt(o, "arity") && a.length !== o.arity && ct(a, "length", { value: o.arity });
try {
o && lt(o, "constructor") && o.constructor ? ir && ct(a, "prototype", { writable: false }) : a.prototype && (a.prototype = void 0);
} catch {
}
var l = Ao(a);
return lt(l, "source") || (l.source = bo.join(typeof u == "string" ? u : "")), a;
};
Function.prototype.toString = _o(function() {
return go(this) && Co(this).source || yo(this);
}, "toString");
});
var Ms = C((Yl, qs) => {
var So = le(), wo = Me(), ko = sr(), Fo = st();
qs.exports = function(a, u, o, l) {
l || (l = {});
var v = l.enumerable, b = l.name !== void 0 ? l.name : u;
if (So(o) && ko(o, b, l), l.global)
v ? a[u] = o : Fo(u, o);
else {
try {
l.unsafe ? a[u] && (v = true) : delete a[u];
} catch {
}
v ? a[u] = o : wo.f(a, u, { value: o, enumerable: false, configurable: !l.nonConfigurable, writable: !l.nonWritable });
}
return a;
};
});
var Ws = C((Zl, Us) => {
var Bo = Math.ceil, Io = Math.floor;
Us.exports = Math.trunc || function(u) {
var o = +u;
return (o > 0 ? Io : Bo)(o);
};
});
var ar = C((ec, zs) => {
var To = Ws();
zs.exports = function(a) {
var u = +a;
return u !== u || u === 0 ? 0 : To(u);
};
});
var Hs = C((tc, Gs) => {
var Po = ar(), Do = Math.max, No = Math.min;
Gs.exports = function(a, u) {
var o = Po(a);
return o < 0 ? Do(o + u, 0) : No(o, u);
};
});
var Xs = C((rc, Ks) => {
var Oo = ar(), Lo = Math.min;
Ks.exports = function(a) {
return a > 0 ? Lo(Oo(a), 9007199254740991) : 0;
};
});
var Qs = C((ic, Js) => {
var Vo = Xs();
Js.exports = function(a) {
return Vo(a.length);
};
});
var Zs = C((sc, Ys) => {
var Ro = tt(), jo = Hs(), qo = Qs(), $s = function(a) {
return function(u, o, l) {
var v = Ro(u), b = qo(v), y = jo(l, b), I;
if (a && o != o) {
for (; b > y; )
if (I = v[y++], I != I)
return true;
} else
for (; b > y; y++)
if ((a || y in v) && v[y] === o)
return a || y || 0;
return !a && -1;
};
};
Ys.exports = { includes: $s(true), indexOf: $s(false) };
});
var ra = C((ac, ta) => {
var Mo = ye(), nr = be(), Uo = tt(), Wo = Zs().indexOf, zo = Zt(), ea = Mo([].push);
ta.exports = function(a, u) {
var o = Uo(a), l = 0, v = [], b;
for (b in o)
!nr(zo, b) && nr(o, b) && ea(v, b);
for (; u.length > l; )
nr(o, b = u[l++]) && (~Wo(v, b) || ea(v, b));
return v;
};
});
var sa = C((nc, ia) => {
ia.exports = ["constructor", "hasOwnProperty", "isPrototypeOf", "propertyIsEnumerable", "toLocaleString", "toString", "valueOf"];
});
var na = C((aa) => {
var Go = ra(), Ho = sa(), Ko = Ho.concat("length", "prototype");
aa.f = Object.getOwnPropertyNames || function(u) {
return Go(u, Ko);
};
});
var oa = C((ua) => {
ua.f = Object.getOwnPropertySymbols;
});
var la = C((hc, ha) => {
var Xo = rt(), Jo = ye(), Qo = na(), $o = oa(), Yo = nt(), Zo = Jo([].concat);
ha.exports = Xo("Reflect", "ownKeys") || function(u) {
var o = Qo.f(Yo(u)), l = $o.f;
return l ? Zo(o, l(u)) : o;
};
});
var fa = C((lc, pa) => {
var ca = be(), eh = la(), th = zt(), rh = Me();
pa.exports = function(a, u, o) {
for (var l = eh(u), v = rh.f, b = th.f, y = 0; y < l.length; y++) {
var I = l[y];
!ca(a, I) && !(o && ca(o, I)) && v(a, I, b(u, I));
}
};
});
var ma = C((cc, da) => {
var ih = me(), sh = le(), ah = /#|\.prototype\./, We = function(a, u) {
var o = uh[nh(a)];
return o == hh ? true : o == oh ? false : sh(u) ? ih(u) : !!u;
}, nh = We.normalize = function(a) {
return String(a).replace(ah, ".").toLowerCase();
}, uh = We.data = {}, oh = We.NATIVE = "N", hh = We.POLYFILL = "P";
da.exports = We;
});
var ga = C((pc, va) => {
var ur = oe(), lh = zt().f, ch = Jt(), ph = Ms(), fh = st(), dh = fa(), mh = ma();
va.exports = function(a, u) {
var o = a.target, l = a.global, v = a.stat, b, y, I, T, x, R;
if (l ? y = ur : v ? y = ur[o] || fh(o, {}) : y = (ur[o] || {}).prototype, y)
for (I in u) {
if (x = u[I], a.dontCallGetSet ? (R = lh(y, I), T = R && R.value) : T = y[I], b = mh(l ? I : o + (v ? "." : "#") + I, a.forced), !b && T !== void 0) {
if (typeof x == typeof T)
continue;
dh(x, T);
}
(a.sham || T && T.sham) && ch(x, "sham", true), ph(y, I, x, a);
}
};
});
var xa = C(() => {
var vh = ga(), or = oe();
vh({ global: true, forced: or.globalThis !== or }, { globalThis: or });
});
var ya = C(() => {
xa();
});
var Ea = C((gc, Ca) => {
var Aa = sr(), gh = Me();
Ca.exports = function(a, u, o) {
return o.get && Aa(o.get, u, { getter: true }), o.set && Aa(o.set, u, { setter: true }), gh.f(a, u, o);
};
});
var _a = C((xc, ba) => {
"use strict";
var xh = nt();
ba.exports = function() {
var a = xh(this), u = "";
return a.hasIndices && (u += "d"), a.global && (u += "g"), a.ignoreCase && (u += "i"), a.multiline && (u += "m"), a.dotAll && (u += "s"), a.unicode && (u += "u"), a.unicodeSets && (u += "v"), a.sticky && (u += "y"), u;
};
});
var ka = C(() => {
var yh = oe(), Ah = xe(), Ch = Ea(), Eh = _a(), bh = me(), Sa = yh.RegExp, wa = Sa.prototype, _h = Ah && bh(function() {
var a = true;
try {
Sa(".", "d");
} catch {
a = false;
}
var u = {}, o = "", l = a ? "dgimsy" : "gimsy", v = function(T, x) {
Object.defineProperty(u, T, { get: function() {
return o += x, true;
} });
}, b = { dotAll: "s", global: "g", ignoreCase: "i", multiline: "m", sticky: "y" };
a && (b.hasIndices = "d");
for (var y in b)
v(y, b[y]);
var I = Object.getOwnPropertyDescriptor(wa, "flags").get.call(u);
return I !== l || o !== l;
});
_h && Ch(wa, "flags", { configurable: true, get: Eh });
});
var Zh = C((Ec, Ka) => {
ya();
ka();
var pr = Object.defineProperty, Sh = Object.getOwnPropertyDescriptor, fr = Object.getOwnPropertyNames, wh = Object.prototype.hasOwnProperty, Fa = (a, u) => function() {
return a && (u = (0, a[fr(a)[0]])(a = 0)), u;
}, $ = (a, u) => function() {
return u || (0, a[fr(a)[0]])((u = { exports: {} }).exports, u), u.exports;
}, kh = (a, u) => {
for (var o in u)
pr(a, o, { get: u[o], enumerable: true });
}, Fh = (a, u, o, l) => {
if (u && typeof u == "object" || typeof u == "function")
for (let v of fr(u))
!wh.call(a, v) && v !== o && pr(a, v, { get: () => u[v], enumerable: !(l = Sh(u, v)) || l.enumerable });
return a;
}, Bh = (a) => Fh(pr({}, "__esModule", { value: true }), a), J = Fa({ ""() {
} }), dr = $({ "src/common/parser-create-error.js"(a, u) {
"use strict";
J();
function o(l, v) {
let b = new SyntaxError(l + " (" + v.start.line + ":" + v.start.column + ")");
return b.loc = v, b;
}
u.exports = o;
} }), Ba = $({ "src/utils/try-combinations.js"(a, u) {
"use strict";
J();
function o() {
let l;
for (var v = arguments.length, b = new Array(v), y = 0; y < v; y++)
b[y] = arguments[y];
for (let [I, T] of b.entries())
try {
return { result: T() };
} catch (x) {
I === 0 && (l = x);
}
return { error: l };
}
u.exports = o;
} }), Ia = {};
kh(Ia, { EOL: () => cr, arch: () => Ih, cpus: () => Va, default: () => Ua, endianness: () => Ta, freemem: () => Oa, getNetworkInterfaces: () => Ma, hostname: () => Pa, loadavg: () => Da, networkInterfaces: () => qa, platform: () => Th, release: () => ja, tmpDir: () => hr, tmpdir: () => lr, totalmem: () => La, type: () => Ra, uptime: () => Na });
function Ta() {
if (typeof pt > "u") {
var a = new ArrayBuffer(2), u = new Uint8Array(a), o = new Uint16Array(a);
if (u[0] = 1, u[1] = 2, o[0] === 258)
pt = "BE";
else if (o[0] === 513)
pt = "LE";
else
throw new Error("unable to figure out endianess");
}
return pt;
}
function Pa() {
return typeof globalThis.location < "u" ? globalThis.location.hostname : "";
}
function Da() {
return [];
}
function Na() {
return 0;
}
function Oa() {
return Number.MAX_VALUE;
}
function La() {
return Number.MAX_VALUE;
}
function Va() {
return [];
}
function Ra() {
return "Browser";
}
function ja() {
return typeof globalThis.navigator < "u" ? globalThis.navigator.appVersion : "";
}
function qa() {
}
function Ma() {
}
function Ih() {
return "javascript";
}
function Th() {
return "browser";
}
function hr() {
return "/tmp";
}
var pt, lr, cr, Ua, Ph = Fa({ "node-modules-polyfills:os"() {
J(), lr = hr, cr = `
`, Ua = { EOL: cr, tmpdir: lr, tmpDir: hr, networkInterfaces: qa, getNetworkInterfaces: Ma, release: ja, type: Ra, cpus: Va, totalmem: La, freemem: Oa, uptime: Na, loadavg: Da, hostname: Pa, endianness: Ta };
} }), Dh = $({ "node-modules-polyfills-commonjs:os"(a, u) {
J();
var o = (Ph(), Bh(Ia));
if (o && o.default) {
u.exports = o.default;
for (let l in o)
u.exports[l] = o[l];
} else
o && (u.exports = o);
} }), Nh = $({ "node_modules/detect-newline/index.js"(a, u) {
"use strict";
J();
var o = (l) => {
if (typeof l != "string")
throw new TypeError("Expected a string");
let v = l.match(/(?:\r?\n)/g) || [];
if (v.length === 0)
return;
let b = v.filter((I) => I === `\r
`).length, y = v.length - b;
return b > y ? `\r
` : `
`;
};
u.exports = o, u.exports.graceful = (l) => typeof l == "string" && o(l) || `
`;
} }), Oh = $({ "node_modules/jest-docblock/build/index.js"(a) {
"use strict";
J(), Object.defineProperty(a, "__esModule", { value: true }), a.extract = g, a.parse = G, a.parseWithComments = f, a.print = B, a.strip = w;
function u() {
let k = Dh();
return u = function() {
return k;
}, k;
}
function o() {
let k = l(Nh());
return o = function() {
return k;
}, k;
}
function l(k) {
return k && k.__esModule ? k : { default: k };
}
var v = /\*\/$/, b = /^\/\*\*?/, y = /^\s*(\/\*\*?(.|\r?\n)*?\*\/)/, I = /(^|\s+)\/\/([^\r\n]*)/g, T = /^(\r?\n)+/, x = /(?:^|\r?\n) *(@[^\r\n]*?) *\r?\n *(?![^@\r\n]*\/\/[^]*)([^@\r\n\s][^@\r\n]+?) *\r?\n/g, R = /(?:^|\r?\n) *@(\S+) *([^\r\n]*)/g, U = /(\r?\n|^) *\* ?/g, D = [];
function g(k) {
let X = k.match(y);
return X ? X[0].trimLeft() : "";
}
function w(k) {
let X = k.match(y);
return X && X[0] ? k.substring(X[0].length) : k;
}
function G(k) {
return f(k).pragmas;
}
function f(k) {
let X = (0, o().default)(k) || u().EOL;
k = k.replace(b, "").replace(v, "").replace(U, "$1");
let O = "";
for (; O !== k; )
O = k, k = k.replace(x, `${X}$1 $2${X}`);
k = k.replace(T, "").trimRight();
let i = /* @__PURE__ */ Object.create(null), S = k.replace(R, "").replace(T, "").trimRight(), F;
for (; F = R.exec(k); ) {
let j = F[2].replace(I, "");
typeof i[F[1]] == "string" || Array.isArray(i[F[1]]) ? i[F[1]] = D.concat(i[F[1]], j) : i[F[1]] = j;
}
return { comments: S, pragmas: i };
}
function B(k) {
let { comments: X = "", pragmas: O = {} } = k, i = (0, o().default)(X) || u().EOL, S = "/**", F = " *", j = " */", Z = Object.keys(O), ne = Z.map((ie) => V(ie, O[ie])).reduce((ie, Ne) => ie.concat(Ne), []).map((ie) => `${F} ${ie}${i}`).join("");
if (!X) {
if (Z.length === 0)
return "";
if (Z.length === 1 && !Array.isArray(O[Z[0]])) {
let ie = O[Z[0]];
return `${S} ${V(Z[0], ie)[0]}${j}`;
}
}
let ee = X.split(i).map((ie) => `${F} ${ie}`).join(i) + i;
return S + i + (X ? ee : "") + (X && Z.length ? F + i : "") + ne + j;
}
function V(k, X) {
return D.concat(X).map((O) => `@${k} ${O}`.trim());
}
} }), Lh = $({ "src/common/end-of-line.js"(a, u) {
"use strict";
J();
function o(y) {
let I = y.indexOf("\r");
return I >= 0 ? y.charAt(I + 1) === `
` ? "crlf" : "cr" : "lf";
}
function l(y) {
switch (y) {
case "cr":
return "\r";
case "crlf":
return `\r
`;
default:
return `
`;
}
}
function v(y, I) {
let T;
switch (I) {
case `
`:
T = /\n/g;
break;
case "\r":
T = /\r/g;
break;
case `\r
`:
T = /\r\n/g;
break;
default:
throw new Error(`Unexpected "eol" ${JSON.stringify(I)}.`);
}
let x = y.match(T);
return x ? x.length : 0;
}
function b(y) {
return y.replace(/\r\n?/g, `
`);
}
u.exports = { guessEndOfLine: o, convertEndOfLineToChars: l, countEndOfLineChars: v, normalizeEndOfLine: b };
} }), Vh = $({ "src/language-js/utils/get-shebang.js"(a, u) {
"use strict";
J();
function o(l) {
if (!l.startsWith("#!"))
return "";
let v = l.indexOf(`
`);
return v === -1 ? l : l.slice(0, v);
}
u.exports = o;
} }), Rh = $({ "src/language-js/pragma.js"(a, u) {
"use strict";
J();
var { parseWithComments: o, strip: l, extract: v, print: b } = Oh(), { normalizeEndOfLine: y } = Lh(), I = Vh();
function T(U) {
let D = I(U);
D && (U = U.slice(D.length + 1));
let g = v(U), { pragmas: w, comments: G } = o(g);
return { shebang: D, text: U, pragmas: w, comments: G };
}
function x(U) {
let D = Object.keys(T(U).pragmas);
return D.includes("prettier") || D.includes("format");
}
function R(U) {
let { shebang: D, text: g, pragmas: w, comments: G } = T(U), f = l(g), B = b({ pragmas: Object.assign({ format: "" }, w), comments: G.trimStart() });
return (D ? `${D}
` : "") + y(B) + (f.startsWith(`
`) ? `
` : `
`) + f;
}
u.exports = { hasPragma: x, insertPragma: R };
} }), jh = $({ "src/utils/is-non-empty-array.js"(a, u) {
"use strict";
J();
function o(l) {
return Array.isArray(l) && l.length > 0;
}
u.exports = o;
} }), Wa = $({ "src/language-js/loc.js"(a, u) {
"use strict";
J();
var o = jh();
function l(T) {
var x, R;
let U = T.range ? T.range[0] : T.start, D = (x = (R = T.declaration) === null || R === void 0 ? void 0 : R.decorators) !== null && x !== void 0 ? x : T.decorators;
return o(D) ? Math.min(l(D[0]), U) : U;
}
function v(T) {
return T.range ? T.range[1] : T.end;
}
function b(T, x) {
let R = l(T);
return Number.isInteger(R) && R === l(x);
}
function y(T, x) {
let R = v(T);
return Number.isInteger(R) && R === v(x);
}
function I(T, x) {
return b(T, x) && y(T, x);
}
u.exports = { locStart: l, locEnd: v, hasSameLocStart: b, hasSameLoc: I };
} }), za = $({ "src/language-js/parse/utils/create-parser.js"(a, u) {
"use strict";
J();
var { hasPragma: o } = Rh(), { locStart: l, locEnd: v } = Wa();
function b(y) {
return y = typeof y == "function" ? { parse: y } : y, Object.assign({ astFormat: "estree", hasPragma: o, locStart: l, locEnd: v }, y);
}
u.exports = b;
} }), qh = $({ "src/language-js/utils/is-ts-keyword-type.js"(a, u) {
"use strict";
J();
function o(l) {
let { type: v } = l;
return v.startsWith("TS") && v.endsWith("Keyword");
}
u.exports = o;
} }), Mh = $({ "src/language-js/utils/is-block-comment.js"(a, u) {
"use strict";
J();
var o = /* @__PURE__ */ new Set(["Block", "CommentBlock", "MultiLine"]), l = (v) => o.has(v == null ? void 0 : v.type);
u.exports = l;
} }), Uh = $({ "src/language-js/utils/is-type-cast-comment.js"(a, u) {
"use strict";
J();
var o = Mh();
function l(v) {
return o(v) && v.value[0] === "*" && /@(?:type|satisfies)\b/.test(v.value);
}
u.exports = l;
} }), Wh = $({ "src/utils/get-last.js"(a, u) {
"use strict";
J();
var o = (l) => l[l.length - 1];
u.exports = o;
} }), zh = $({ "src/language-js/parse/postprocess/visit-node.js"(a, u) {
"use strict";
J();
function o(l, v) {
if (Array.isArray(l)) {
for (let b = 0; b < l.length; b++)
l[b] = o(l[b], v);
return l;
}
if (l && typeof l == "object" && typeof l.type == "string") {
let b = Object.keys(l);
for (let y = 0; y < b.length; y++)
l[b[y]] = o(l[b[y]], v);
return v(l) || l;
}
return l;
}
u.exports = o;
} }), Gh = $({ "src/language-js/parse/postprocess/throw-syntax-error.js"(a, u) {
"use strict";
J();
var o = dr();
function l(v, b) {
let { start: y, end: I } = v.loc;
throw o(b, { start: { line: y.line, column: y.column + 1 }, end: { line: I.line, column: I.column + 1 } });
}
u.exports = l;
} }), Ga = $({ "src/language-js/parse/postprocess/index.js"(a, u) {
"use strict";
J();
var { locStart: o, locEnd: l } = Wa(), v = qh(), b = Uh(), y = Wh(), I = zh(), T = Gh();
function x(g, w) {
if (w.parser !== "typescript" && w.parser !== "flow" && w.parser !== "acorn" && w.parser !== "espree" && w.parser !== "meriyah") {
let f = /* @__PURE__ */ new Set();
g = I(g, (B) => {
B.leadingComments && B.leadingComments.some(b) && f.add(o(B));
}), g = I(g, (B) => {
if (B.type === "ParenthesizedExpression") {
let { expression: V } = B;
if (V.type === "TypeCastExpression")
return V.range = B.range, V;
let k = o(B);
if (!f.has(k))
return V.extra = Object.assign(Object.assign({}, V.extra), {}, { parenthesized: true }), V;
}
});
}
return g = I(g, (f) => {
switch (f.type) {
case "ChainExpression":
return R(f.expression);
case "LogicalExpression": {
if (U(f))
return D(f);
break;
}
case "VariableDeclaration": {
let B = y(f.declarations);
B && B.init && G(f, B);
break;
}
case "TSParenthesizedType":
return v(f.typeAnnotation) || f.typeAnnotation.type === "TSThisType" || (f.typeAnnotation.range = [o(f), l(f)]), f.typeAnnotation;
case "TSTypeParameter":
if (typeof f.name == "string") {
let B = o(f);
f.name = { type: "Identifier", name: f.name, range: [B, B + f.name.length] };
}
break;
case "ObjectExpression":
if (w.parser === "typescript") {
let B = f.properties.find((V) => V.type === "Property" && V.value.type === "TSEmptyBodyFunctionExpression");
B && T(B.value, "Unexpected token.");
}
break;
case "SequenceExpression": {
let B = y(f.expressions);
f.range = [o(f), Math.min(l(B), l(f))];
break;
}
case "TopicReference":
w.__isUsingHackPipeline = true;
break;
case "ExportAllDeclaration": {
let { exported: B } = f;
if (w.parser === "meriyah" && B && B.type === "Identifier") {
let V = w.originalText.slice(o(B), l(B));
(V.startsWith('"') || V.startsWith("'")) && (f.exported = Object.assign(Object.assign({}, f.exported), {}, { type: "Literal", value: f.exported.name, raw: V }));
}
break;
}
case "PropertyDefinition":
if (w.parser === "meriyah" && f.static && !f.computed && !f.key) {
let B = "static", V = o(f);
Object.assign(f, { static: false, key: { type: "Identifier", name: B, range: [V, V + B.length] } });
}
break;
}
}), g;
function G(f, B) {
w.originalText[l(B)] !== ";" && (f.range = [o(f), l(B)]);
}
}
function R(g) {
switch (g.type) {
case "CallExpression":
g.type = "OptionalCallExpression", g.callee = R(g.callee);
break;
case "MemberExpression":
g.type = "OptionalMemberExpression", g.object = R(g.object);
break;
case "TSNonNullExpression":
g.expression = R(g.expression);
break;
}
return g;
}
function U(g) {
return g.type === "LogicalExpression" && g.right.type === "LogicalExpression" && g.operator === g.right.operator;
}
function D(g) {
return U(g) ? D({ type: "LogicalExpression", operator: g.operator, left: D({ type: "LogicalExpression", operator: g.operator, left: g.left, right: g.right.left, range: [o(g.left), l(g.right.left)] }), right: g.right.right, range: [o(g), l(g)] }) : g;
}
u.exports = x;
} }), ft = $({ "node_modules/acorn/dist/acorn.js"(a, u) {
J(), function(o, l) {
typeof a == "object" && typeof u < "u" ? l(a) : typeof define == "function" && define.amd ? define(["exports"], l) : (o = typeof globalThis < "u" ? globalThis : o || self, l(o.acorn = {}));
}(a, function(o) {
"use strict";
var l = [509, 0, 227, 0, 150, 4, 294, 9, 1368, 2, 2, 1, 6, 3, 41, 2, 5, 0, 166, 1, 574, 3, 9, 9, 370, 1, 154, 10, 50, 3, 123, 2, 54, 14, 32, 10, 3, 1, 11, 3, 46, 10, 8, 0, 46, 9, 7, 2, 37, 13, 2, 9, 6, 1, 45, 0, 13, 2, 49, 13, 9, 3, 2, 11, 83, 11, 7, 0, 161, 11, 6, 9, 7, 3, 56, 1, 2, 6, 3, 1, 3, 2, 10, 0, 11, 1, 3, 6, 4, 4, 193, 17, 10, 9, 5, 0, 82, 19, 13, 9, 214, 6, 3, 8, 28, 1, 83, 16, 16, 9, 82, 12, 9, 9, 84, 14, 5, 9, 243, 14, 166, 9, 71, 5, 2, 1, 3, 3, 2, 0, 2, 1, 13, 9, 120, 6, 3, 6, 4, 0, 29, 9, 41, 6, 2, 3, 9, 0, 10, 10, 47, 15, 406, 7, 2, 7, 17, 9, 57, 21, 2, 13, 123, 5, 4, 0, 2, 1, 2, 6, 2, 0, 9, 9, 49, 4, 2, 1, 2, 4, 9, 9, 330, 3, 19306, 9, 87, 9, 39, 4, 60, 6, 26, 9, 1014, 0, 2, 54, 8, 3, 82, 0, 12, 1, 19628, 1, 4706, 45, 3, 22, 543, 4, 4, 5, 9, 7, 3, 6, 31, 3, 149, 2, 1418, 49, 513, 54, 5, 49, 9, 0, 15, 0, 23, 4, 2, 14, 1361, 6, 2, 16, 3, 6, 2, 1, 2, 4, 262, 6, 10, 9, 357, 0, 62, 13, 1495, 6, 110, 6, 6, 9, 4759, 9, 787719, 239], v = [0, 11, 2, 25, 2, 18, 2, 1, 2, 14, 3, 13, 35, 122, 70, 52, 268, 28, 4, 48, 48, 31, 14, 29, 6, 37, 11, 29, 3, 35, 5, 7, 2, 4, 43, 157, 19, 35, 5, 35, 5, 39, 9, 51, 13, 10, 2, 14, 2, 6, 2, 1, 2, 10, 2, 14, 2, 6, 2, 1, 68, 310, 10, 21, 11, 7, 25, 5, 2, 41, 2, 8, 70, 5, 3, 0, 2, 43, 2, 1, 4, 0, 3, 22, 11, 22, 10, 30, 66, 18, 2, 1, 11, 21, 11, 25, 71, 55, 7, 1, 65, 0, 16, 3, 2, 2, 2, 28, 43, 28, 4, 28, 36, 7, 2, 27, 28, 53, 11, 21, 11, 18, 14, 17, 111, 72, 56, 50, 14, 50, 14, 35, 349, 41, 7, 1, 79, 28, 11, 0, 9, 21, 43, 17, 47, 20, 28, 22, 13, 52, 58, 1, 3, 0, 14, 44, 33, 24, 27, 35, 30, 0, 3, 0, 9, 34, 4, 0, 13, 47, 15, 3, 22, 0, 2, 0, 36, 17, 2, 24, 85, 6, 2, 0, 2, 3, 2, 14, 2, 9, 8, 46, 39, 7, 3, 1, 3, 21, 2, 6, 2, 1, 2, 4, 4, 0, 19, 0, 13, 4, 159, 52, 19, 3, 21, 2, 31, 47, 21, 1, 2, 0, 185, 46, 42, 3, 37, 47, 21, 0, 60, 42, 14, 0, 72, 26, 38, 6, 186, 43, 117, 63, 32, 7, 3, 0, 3, 7, 2, 1, 2, 23, 16, 0, 2, 0, 95, 7, 3, 38, 17, 0, 2, 0, 29, 0, 11, 39, 8, 0, 22, 0, 12, 45, 20, 0, 19, 72, 264, 8, 2, 36, 18, 0, 50, 29, 113, 6, 2, 1, 2, 37, 22, 0, 26, 5, 2, 1, 2, 31, 15, 0, 328, 18, 190, 0, 80, 921, 103, 110, 18, 195, 2637, 96, 16, 1070, 4050, 582, 8634, 568, 8, 30, 18, 78, 18, 29, 19, 47, 17, 3, 32, 20, 6, 18, 689, 63, 129, 74, 6, 0, 67, 12, 65, 1, 2, 0, 29, 6135, 9, 1237, 43, 8, 8936, 3, 2, 6, 2, 1, 2, 290, 46, 2, 18, 3, 9, 395, 2309, 106, 6, 12, 4, 8, 8, 9, 5991, 84, 2, 70, 2, 1, 3, 0, 3, 1, 3, 3, 2, 11, 2, 0, 2, 6, 2, 64, 2, 3, 3, 7, 2, 6, 2, 27, 2, 3, 2, 4, 2, 0, 4, 6, 2, 339, 3, 24, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 7, 1845, 30, 482, 44, 11, 6, 17, 0, 322, 29, 19, 43, 1269, 6, 2, 3, 2, 1, 2, 14, 2, 196, 60, 67, 8, 0, 1205, 3, 2, 26, 2, 1, 2, 0, 3, 0, 2, 9, 2, 3, 2, 0, 2, 0, 7, 0, 5, 0, 2, 0, 2, 0, 2, 2, 2, 1, 2, 0, 3, 0, 2, 0, 2, 0, 2, 0, 2, 0, 2, 1, 2, 0, 3, 3, 2, 6, 2, 3, 2, 3, 2, 0, 2, 9, 2, 16, 6, 2, 2, 4, 2, 16, 4421, 42719, 33, 4152, 8, 221, 3, 5761, 15, 7472, 3104, 541, 1507, 4938], b = "\u200C\u200D\xB7\u0300-\u036F\u0387\u0483-\u0487\u0591-\u05BD\u05BF\u05C1\u05C2\u05C4\u05C5\u05C7\u0610-\u061A\u064B-\u0669\u0670\u06D6-\u06DC\u06DF-\u06E4\u06E7\u06E8\u06EA-\u06ED\u06F0-\u06F9\u0711\u0730-\u074A\u07A6-\u07B0\u07C0-\u07C9\u07EB-\u07F3\u07FD\u0816-\u0819\u081B-\u0823\u0825-\u0827\u0829-\u082D\u0859-\u085B\u0898-\u089F\u08CA-\u08E1\u08E3-\u0903\u093A-\u093C\u093E-\u094F\u0951-\u0957\u0962\u0963\u0966-\u096F\u0981-\u0983\u09BC\u09BE-\u09C4\u09C7\u09C8\u09CB-\u09CD\u09D7\u09E2\u09E3\u09E6-\u09EF\u09FE\u0A01-\u0A03\u0A3C\u0A3E-\u0A42\u0A47\u0A48\u0A4B-\u0A4D\u0A51\u0A66-\u0A71\u0A75\u0A81-\u0A83\u0ABC\u0ABE-\u0AC5\u0AC7-\u0AC9\u0ACB-\u0ACD\u0AE2\u0AE3\u0AE6-\u0AEF\u0AFA-\u0AFF\u0B01-\u0B03\u0B3C\u0B3E-\u0B44\u0B47\u0B48\u0B4B-\u0B4D\u0B55-\u0B57\u0B62\u0B63\u0B66-\u0B6F\u0B82\u0BBE-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCD\u0BD7\u0BE6-\u0BEF\u0C00-\u0C04\u0C3C\u0C3E-\u0C44\u0C46-\u0C48\u0C4A-\u0C4D\u0C55\u0C56\u0C62\u0C63\u0C66-\u0C6F\u0C81-\u0C83\u0CBC\u0CBE-\u0CC4\u0CC6-\u0CC8\u0CCA-\u0CCD\u0CD5\u0CD6\u0CE2\u0CE3\u0CE6-\u0CEF\u0D00-\u0D03\u0D3B\u0D3C\u0D3E-\u0D44\u0D46-\u0D48\u0D4A-\u0D4D\u0D57\u0D62\u0D63\u0D66-\u0D6F\u0D81-\u0D83\u0DCA\u0DCF-\u0DD4\u0DD6\u0DD8-\u0DDF\u0DE6-\u0DEF\u0DF2\u0DF3\u0E31\u0E34-\u0E3A\u0E47-\u0E4E\u0E50-\u0E59\u0EB1\u0EB4-\u0EBC\u0EC8-\u0ECD\u0ED0-\u0ED9\u0F18\u0F19\u0F20-\u0F29\u0F35\u0F37\u0F39\u0F3E\u0F3F\u0F71-\u0F84\u0F86\u0F87\u0F8D-\u0F97\u0F99-\u0FBC\u0FC6\u102B-\u103E\u1040-\u1049\u1056-\u1059\u105E-\u1060\u1062-\u1064\u1067-\u106D\u1071-\u1074\u1082-\u108D\u108F-\u109D\u135D-\u135F\u1369-\u1371\u1712-\u1715\u1732-\u1734\u1752\u1753\u1772\u1773\u17B4-\u17D3\u17DD\u17E0-\u17E9\u180B-\u180D\u180F-\u1819\u18A9\u1920-\u192B\u1930-\u193B\u1946-\u194F\u19D0-\u19DA\u1A17-\u1A1B\u1A55-\u1A5E\u1A60-\u1A7C\u1A7F-\u1A89\u1A90-\u1A99\u1AB0-\u1ABD\u1ABF-\u1ACE\u1B00-\u1B04\u1B34-\u1B44\u1B50-\u1B59\u1B6B-\u1B73\u1B80-\u1B82\u1BA1-\u1BAD\u1BB0-\u1BB9\u1BE6-\u1BF3\u1C24-\u1C37\u1C40-\u1C49\u1C50-\u1C59\u1CD0-\u1CD2\u1CD4-\u1CE8\u1CED\u1CF4\u1CF7-\u1CF9\u1DC0-\u1DFF\u203F\u2040\u2054\u20D0-\u20DC\u20E1\u20E5-\u20F0\u2CEF-\u2CF1\u2D7F\u2DE0-\u2DFF\u302A-\u302F\u3099\u309A\uA620-\uA629\uA66F\uA674-\uA67D\uA69E\uA69F\uA6F0\uA6F1\uA802\uA806\uA80B\uA823-\uA827\uA82C\uA880\uA881\uA8B4-\uA8C5\uA8D0-\uA8D9\uA8E0-\uA8F1\uA8FF-\uA909\uA926-\uA92D\uA947-\uA953\uA980-\uA983\uA9B3-\uA9C0\uA9D0-\uA9D9\uA9E5\uA9F0-\uA9F9\uAA29-\uAA36\uAA43\uAA4C\uAA4D\uAA50-\uAA59\uAA7B-\uAA7D\uAAB0\uAAB2-\uAAB4\uAAB7\uAAB8\uAABE\uAABF\uAAC1\uAAEB-\uAAEF\uAAF5\uAAF6\uABE3-\uABEA\uABEC\uABED\uABF0-\uABF9\uFB1E\uFE00-\uFE0F\uFE20-\uFE2F\uFE33\uFE34\uFE4D-\uFE4F\uFF10-\uFF19\uFF3F", y = "\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0370-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u048A-\u052F\u0531-\u0556\u0559\u0560-\u0588\u05D0-\u05EA\u05EF-\u05F2\u0620-\u064A\u066E\u066F\u0671-\u06D3\u06D5\u06E5\u06E6\u06EE\u06EF\u06FA-\u06FC\u06FF\u0710\u0712-\u072F\u074D-\u07A5\u07B1\u07CA-\u07EA\u07F4\u07F5\u07FA\u0800-\u0815\u081A\u0824\u0828\u0840-\u0858\u0860-\u086A\u0870-\u0887\u0889-\u088E\u08A0-\u08C9\u0904-\u0939\u093D\u0950\u0958-\u0961\u0971-\u0980\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD\u09CE\u09DC\u09DD\u09DF-\u09E1\u09F0\u09F1\u09FC\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A59-\u0A5C\u0A5E\u0A72-\u0A74\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABD\u0AD0\u0AE0\u0AE1\u0AF9\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3D\u0B5C\u0B5D\u0B5F-\u0B61\u0B71\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BD0\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C58-\u0C5A\u0C5D\u0C60\u0C61\u0C80\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CDD\u0CDE\u0CE0\u0CE1\u0CF1\u0CF2\u0D04-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D\u0D4E\u0D54-\u0D56\u0D5F-\u0D61\u0D7A-\u0D7F\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0E01-\u0E30\u0E32\u0E33\u0E40-\u0E46\u0E81\u0E82\u0E84\u0E86-\u0E8A\u0E8C-\u0EA3\u0EA5\u0EA7-\u0EB0\u0EB2\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6\u0EDC-\u0EDF\u0F00\u0F40-\u0F47\u0F49-\u0F6C\u0F88-\u0F8C\u1000-\u102A\u103F\u1050-\u1055\u105A-\u105D\u1061\u1065\u1066\u106E-\u1070\u1075-\u1081\u108E\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u1711\u171F-\u1731\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17D7\u17DC\u1820-\u1878\u1880-\u18A8\u18AA\u18B0-\u18F5\u1900-\u191E\u1950-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u1A00-\u1A16\u1A20-\u1A54\u1AA7\u1B05-\u1B33\u1B45-\u1B4C\u1B83-\u1BA0\u1BAE\u1BAF\u1BBA-\u1BE5\u1C00-\u1C23\u1C4D-\u1C4F\u1C5A-\u1C7D\u1C80-\u1C88\u1C90-\u1CBA\u1CBD-\u1CBF\u1CE9-\u1CEC\u1CEE-\u1CF3\u1CF5\u1CF6\u1CFA\u1D00-\u1DBF\u1E00-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2118-\u211D\u2124\u2126\u2128\u212A-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2CE4\u2CEB-\u2CEE\u2CF2\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u3005-\u3007\u3021-\u3029\u3031-\u3035\u3038-\u303C\u3041-\u3096\u309B-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312F\u3131-\u318E\u31A0-\u31BF\u31F0-\u31FF\u3400-\u4DBF\u4E00-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA61F\uA62A\uA62B\uA640-\uA66E\uA67F-\uA69D\uA6A0-\uA6EF\uA717-\uA71F\uA722-\uA788\uA78B-\uA7CA\uA7D0\uA7D1\uA7D3\uA7D5-\uA7D9\uA7F2-\uA801\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA840-\uA873\uA882-\uA8B3\uA8F2-\uA8F7\uA8FB\uA8FD\uA8FE\uA90A-\uA925\uA930-\uA946\uA960-\uA97C\uA984-\uA9B2\uA9CF\uA9E0-\uA9E4\uA9E6-\uA9EF\uA9FA-\uA9FE\uAA00-\uAA28\uAA40-\uAA42\uAA44-\uAA4B\uAA60-\uAA76\uAA7A\uAA7E-\uAAAF\uAAB1\uAAB5\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADD\uAAE0-\uAAEA\uAAF2-\uAAF4\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB69\uAB70-\uABE2\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE70-\uFE74\uFE76-\uFEFC\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC", I = { 3: "abstract boolean byte char class double enum export extends final float goto implements import int interface long native package private protected public short static super synchronized throws transient volatile", 5: "class enum extends super const export import", 6: "enum", strict: "implements interface let package private protected public static yield", strictBind: "eval arguments" }, T = "break case catch continue debugger default do else finally for function if return switch throw try var while with null true false instanceof typeof void delete new in this", x = { 5: T, "5module": T + " export import", 6: T + " const class extends export import super" }, R = /^in(stanceof)?$/, U = new RegExp("[" + y + "]"), D = new RegExp("[" + y + b + "]");
function g(e, t) {
for (var r = 65536, s = 0; s < t.length; s += 2) {
if (r += t[s], r > e)
return false;
if (r += t[s + 1], r >= e)
return true;
}
}
function w(e, t) {
return e < 65 ? e === 36 : e < 91 ? true : e < 97 ? e === 95 : e < 123 ? true : e <= 65535 ? e >= 170 && U.test(String.fromCharCode(e)) : t === false ? false : g(e, v);
}
function G(e, t) {
return e < 48 ? e === 36 : e < 58 ? true : e < 65 ? false : e < 91 ? true : e < 97 ? e === 95 : e < 123 ? true : e <= 65535 ? e >= 170 && D.test(String.fromCharCode(e)) : t === false ? false : g(e, v) || g(e, l);
}
var f = function(t, r) {
r === void 0 && (r = {}), this.label = t, this.keyword = r.keyword, this.beforeExpr = !!r.beforeExpr, this.startsExpr = !!r.startsExpr, this.isLoop = !!r.isLoop, this.isAssign = !!r.isAssign, this.prefix = !!r.prefix, this.postfix = !!r.postfix, this.binop = r.binop || null, this.updateContext = null;
};
function B(e, t) {
return new f(e, { beforeExpr: true, binop: t });
}
var V = { beforeExpr: true }, k = { startsExpr: true }, X = {};
function O(e, t) {
return t === void 0 && (t = {}), t.keyword = e, X[e] = new f(e, t);
}
var i = { num: new f("num", k), regexp: new f("regexp", k), string: new f("string", k), name: new f("name", k), privateId: new f("privateId", k), eof: new f("eof"), bracketL: new f("[", { beforeExpr: true, startsExpr: true }), bracketR: new f("]"), braceL: new f("{", { beforeExpr: true, startsExpr: true }), braceR: new f("}"), parenL: new f("(", { beforeExpr: true, startsExpr: true }), parenR: new f(")"), comma: new f(",", V), semi: new f(";", V), colon: new f(":", V), dot: new f("."), question: new f("?", V), questionDot: new f("?."), arrow: new f("=>", V), template: new f("template"), invalidTemplate: new f("invalidTemplate"), ellipsis: new f("...", V), backQuote: new f("`", k), dollarBraceL: new f("${", { beforeExpr: true, startsExpr: true }), eq: new f("=", { beforeExpr: true, isAssign: true }), assign: new f("_=", { beforeExpr: true, isAssign: true }), incDec: new f("++/--", { prefix: true, postfix: true, startsExpr: true }), prefix: new f("!/~", { beforeExpr: true, prefix: true, startsExpr: true }), logicalOR: B("||", 1), logicalAND: B("&&", 2), bitwiseOR: B("|", 3), bitwiseXOR: B("^", 4), bitwiseAND: B("&", 5), equality: B("==/!=/===/!==", 6), relational: B(">/<=/>=", 7), bitShift: B("<>>/>>>", 8), plusMin: new f("+/-", { beforeExpr: true, binop: 9, prefix: true, startsExpr: true }), modulo: B("%", 10), star: B("*", 10), slash: B("/", 10), starstar: new f("**", { beforeExpr: true }), coalesce: B("??", 1), _break: O("break"), _case: O("case", V), _catch: O("catch"), _continue: O("continue"), _debugger: O("debugger"), _default: O("default", V), _do: O("do", { isLoop: true, beforeExpr: true }), _else: O("else", V), _finally: O("finally"), _for: O("for", { isLoop: true }), _function: O("function", k), _if: O("if"), _return: O("return", V), _switch: O("switch"), _throw: O("throw", V), _try: O("try"), _var: O("var"), _const: O("const"), _while: O("while", { isLoop: true }), _with: O("with"), _new: O("new", { beforeExpr: true, startsExpr: true }), _this: O("this", k), _super: O("super", k), _class: O("class", k), _extends: O("extends", V), _export: O("export"), _import: O("import", k), _null: O("null", k), _true: O("true", k), _false: O("false", k), _in: O("in", { beforeExpr: true, binop: 7 }), _instanceof: O("instanceof", { beforeExpr: true, binop: 7 }), _typeof: O("typeof", { beforeExpr: true, prefix: true, startsExpr: true }), _void: O("void", { beforeExpr: true, prefix: true, startsExpr: true }), _delete: O("delete", { beforeExpr: true, prefix: true, startsExpr: true }) }, S = /\r\n?|\n|\u2028|\u2029/, F = new RegExp(S.source, "g");
function j(e) {
return e === 10 || e === 13 || e === 8232 || e === 8233;
}
function Z(e, t, r) {
r === void 0 && (r = e.length);
for (var s = t; s < r; s++) {
var n2 = e.charCodeAt(s);
if (j(n2))
return s < r - 1 && n2 === 13 && e.charCodeAt(s + 1) === 10 ? s + 2 : s + 1;
}
return -1;
}
var ne = /[\u1680\u2000-\u200a\u202f\u205f\u3000\ufeff]/, ee = /(?:\s|\/\/.*|\/\*[^]*?\*\/)*/g, ie = Object.prototype, Ne = ie.hasOwnProperty, p = ie.toString, P = Object.hasOwn || function(e, t) {
return Ne.call(e, t);
}, _ = Array.isArray || function(e) {
return p.call(e) === "[object Array]";
};
function d(e) {
return new RegExp("^(?:" + e.replace(/ /g, "|") + ")$");
}
function E(e) {
return e <= 65535 ? String.fromCharCode(e) : (e -= 65536, String.fromCharCode((e >> 10) + 55296, (e & 1023) + 56320));
}
var K = /(?:[\uD800-\uDBFF](?![\uDC00-\uDFFF])|(?:[^\uD800-\uDBFF]|^)[\uDC00-\uDFFF])/, H = function(t, r) {
this.line = t, this.column = r;
};
H.prototype.offset = function(t) {
return new H(this.line, this.column + t);
};
var te = function(t, r, s) {
this.start = r, this.end = s, t.sourceFile !== null && (this.source = t.sourceFile);
};
function ae(e, t) {
for (var r = 1, s = 0; ; ) {
var n2 = Z(e, s, t);
if (n2 < 0)
return new H(r, t - s);
++r, s = n2;
}
}
var fe = { ecmaVersion: null, sourceType: "script", onInsertedSemicolon: null, onTrailingComma: null, allowReserved: null, allowReturnOutsideFunction: false, allowImportExportEverywhere: false, allowAwaitOutsideFunction: null, allowSuperOutsideMethod: null, allowHashBang: false, locations: false, onToken: null, onComment: null, ranges: false, program: null, sourceFile: null, directSourceFile: null, preserveParens: false }, Ae = false;
function dt(e) {
var t = {};
for (var r in fe)
t[r] = e && P(e, r) ? e[r] : fe[r];
if (t.ecmaVersion === "latest" ? t.ecmaVersion = 1e8 : t.ecmaVersion == null ? (!Ae && typeof console == "object" && console.warn && (Ae = true, console.warn(`Since Acorn 8.0.0, options.ecmaVersion is required.
Defaulting to 2020, but this will stop working in the future.`)), t.ecmaVersion = 11) : t.ecmaVersion >= 2015 && (t.ecmaVersion -= 2009), t.allowReserved == null && (t.allowReserved = t.ecmaVersion < 5), e.allowHashBang == null && (t.allowHashBang = t.ecmaVersion >= 14), _(t.onToken)) {
var s = t.onToken;
t.onToken = function(n2) {
return s.push(n2);
};
}
return _(t.onComment) && (t.onComment = mt(t, t.onComment)), t;
}
function mt(e, t) {
return function(r, s, n2, h, c, m) {
var A = { type: r ? "Block" : "Line", value: s, start: n2, end: h };
e.locations && (A.loc = new te(this, c, m)), e.ranges && (A.range = [n2, h]), t.push(A);
};
}
var _e = 1, Ce = 2, Oe = 4, ze = 8, mr = 16, vr = 32, vt = 64, gr = 128, Le = 256, gt = _e | Ce | Le;
function xt(e, t) {
return Ce | (e ? Oe : 0) | (t ? ze : 0);
}
var Ge = 0, yt = 1, ve = 2, xr = 3, yr = 4, Ar = 5, Y = function(t, r, s) {
this.options = t = dt(t), this.sourceFile = t.sourceFile, this.keywords = d(x[t.ecmaVersion >= 6 ? 6 : t.sourceType === "module" ? "5module" : 5]);
var n2 = "";
t.allowReserved !== true && (n2 = I[t.ecmaVersion >= 6 ? 6 : t.ecmaVersion === 5 ? 5 : 3], t.sourceType === "module" && (n2 += " await")), this.reservedWords = d(n2);
var h = (n2 ? n2 + " " : "") + I.strict;
this.reservedWordsStrict = d(h), this.reservedWordsStrictBind = d(h + " " + I.strictBind), this.input = String(r), this.containsEsc = false, s ? (this.pos = s, this.lineStart = this.input.lastIndexOf(`
`, s - 1) + 1, this.curLine = this.input.slice(0, this.lineStart).split(S).length) : (this.pos = this.lineStart = 0, this.curLine = 1), this.type = i.eof, this.value = null, this.start = this.end = this.pos, this.startLoc = this.endLoc = this.curPosition(), this.lastTokEndLoc = this.lastTokStartLoc = null, this.lastTokStart = this.lastTokEnd = this.pos, this.context = this.initialContext(), this.exprAllowed = true, this.inModule = t.sourceType === "module", this.strict = this.inModule || this.strictDirective(this.pos), this.potentialArrowAt = -1, this.potentialArrowInForAwait = false, this.yieldPos = this.awaitPos = this.awaitIdentPos = 0, this.labels = [], this.undefinedExports = /* @__PURE__ */ Object.create(null), this.pos === 0 && t.allowHashBang && this.input.slice(0, 2) === "#!" && this.skipLineComment(2), this.scopeStack = [], this.enterScope(_e), this.regexpState = null, this.privateNameStack = [];
}, de = { inFunction: { configurable: true }, inGenerator: { configurable: true }, inAsync: { configurable: true }, canAwait: { configurable: true }, allowSuper: { configurable: true }, allowDirectSuper: { configurable: true }, treatFunctionsAsVar: { configurable: true }, allowNewDotTarget: { configurable: true }, inClassStaticBlock: { configurable: true } };
Y.prototype.parse = function() {
var t = this.options.program || this.startNode();
return this.nextToken(), this.parseTopLevel(t);
}, de.inFunction.get = function() {
return (this.currentVarScope().flags & Ce) > 0;
}, de.inGenerator.get = function() {
return (this.currentVarScope().flags & ze) > 0 && !this.currentVarScope().inClassFieldInit;
}, de.inAsync.get = function() {
return (this.currentVarScope().flags & Oe) > 0 && !this.currentVarScope().inClassFieldInit;
}, de.canAwait.get = function() {
for (var e = this.scopeStack.length - 1; e >= 0; e--) {
var t = this.scopeStack[e];
if (t.inClassFieldInit || t.flags & Le)
return false;
if (t.flags & Ce)
return (t.flags & Oe) > 0;
}
return this.inModule && this.options.ecmaVersion >= 13 || this.options.allowAwaitOutsideFunction;
}, de.allowSuper.get = function() {
var e = this.currentThisScope(), t = e.flags, r = e.inClassFieldInit;
return (t & vt) > 0 || r || this.options.allowSuperOutsideMethod;
}, de.allowDirectSuper.get = function() {
return (this.currentThisScope().flags & gr) > 0;
}, de.treatFunctionsAsVar.get = function() {
return this.treatFunctionsAsVarInScope(this.currentScope());
}, de.allowNewDotTarget.get = function() {
var e = this.currentThisScope(), t = e.flags, r = e.inClassFieldInit;
return (t & (Ce | Le)) > 0 || r;
}, de.inClassStaticBlock.get = function() {
return (this.currentVarScope().flags & Le) > 0;
}, Y.extend = function() {
for (var t = [], r = arguments.length; r--; )
t[r] = arguments[r];
for (var s = this, n2 = 0; n2 < t.length; n2++)
s = t[n2](s);
return s;
}, Y.parse = function(t, r) {
return new this(r, t).parse();
}, Y.parseExpressionAt = function(t, r, s) {
var n2 = new this(s, t, r);
return n2.nextToken(), n2.parseExpression();
}, Y.tokenizer = function(t, r) {
return new this(r, t);
}, Object.defineProperties(Y.prototype, de);
var se = Y.prototype, Xa = /^(?:'((?:\\.|[^'\\])*?)'|"((?:\\.|[^"\\])*?)")/;
se.strictDirective = function(e) {
if (this.options.ecmaVersion < 5)
return false;
for (; ; ) {
ee.lastIndex = e, e += ee.exec(this.input)[0].length;
var t = Xa.exec(this.input.slice(e));
if (!t)
return false;
if ((t[1] || t[2]) === "use strict") {
ee.lastIndex = e + t[0].length;
var r = ee.exec(this.input), s = r.index + r[0].length, n2 = this.input.charAt(s);
return n2 === ";" || n2 === "}" || S.test(r[0]) && !(/[(`.[+\-/*%<>=,?^&]/.test(n2) || n2 === "!" && this.input.charAt(s + 1) === "=");
}
e += t[0].length, ee.lastIndex = e, e += ee.exec(this.input)[0].length, this.input[e] === ";" && e++;
}
}, se.eat = function(e) {
return this.type === e ? (this.next(), true) : false;
}, se.isContextual = function(e) {
return this.type === i.name && this.value === e && !this.containsEsc;
}, se.eatContextual = function(e) {
return this.isContextual(e) ? (this.next(), true) : false;
}, se.expectContextual = function(e) {
this.eatContextual(e) || this.unexpected();
}, se.canInsertSemicolon = function() {
return this.type === i.eof || this.type === i.braceR || S.test(this.input.slice(this.lastTokEnd, this.start));
}, se.insertSemicolon = function() {
if (this.canInsertSemicolon())
return this.options.onInsertedSemicolon && this.options.onInsertedSemicolon(this.lastTokEnd, this.lastTokEndLoc), true;
}, se.semicolon = function() {
!this.eat(i.semi) && !this.insertSemicolon() && this.unexpected();
}, se.afterTrailingComma = function(e, t) {
if (this.type === e)
return this.options.onTrailingComma && this.options.onTrailingComma(this.lastTokStart, this.lastTokStartLoc), t || this.next(), true;
}, se.expect = function(e) {
this.eat(e) || this.unexpected();
}, se.unexpected = function(e) {
this.raise(e != null ? e : this.start, "Unexpected token");
};
var He = function() {
this.shorthandAssign = this.trailingComma = this.parenthesizedAssign = this.parenthesizedBind = this.doubleProto = -1;
};
se.checkPatternErrors = function(e, t) {
if (e) {
e.trailingComma > -1 && this.raiseRecoverable(e.trailingComma, "Comma is not permitted after the rest element");
var r = t ? e.parenthesizedAssign : e.parenthesizedBind;
r > -1 && this.raiseRecoverable(r, t ? "Assigning to rvalue" : "Parenthesized pattern");
}
}, se.checkExpressionErrors = function(e, t) {
if (!e)
return false;
var r = e.shorthandAssign, s = e.doubleProto;
if (!t)
return r >= 0 || s >= 0;
r >= 0 && this.raise(r, "Shorthand property assignments are valid only in destructuring patterns"), s >= 0 && this.raiseRecoverable(s, "Redefinition of __proto__ property");
}, se.checkYieldAwaitInDefaultParams = function() {
this.yieldPos && (!this.awaitPos || this.yieldPos < this.awaitPos) && this.raise(this.yieldPos, "Yield expression cannot be a default value"), this.awaitPos && this.raise(this.awaitPos, "Await expression cannot be a default value");
}, se.isSimpleAssignTarget = function(e) {
return e.type === "ParenthesizedExpression" ? this.isSimpleAssignTarget(e.expression) : e.type === "Identifier" || e.type === "MemberExpression";
};
var L = Y.prototype;
L.parseTopLevel = function(e) {
var t = /* @__PURE__ */ Object.create(null);
for (e.body || (e.body = []); this.type !== i.eof; ) {
var r = this.parseStatement(null, true, t);
e.body.push(r);
}
if (this.inModule)
for (var s = 0, n2 = Object.keys(this.undefinedExports); s < n2.length; s += 1) {
var h = n2[s];
this.raiseRecoverable(this.undefinedExports[h].start, "Export '" + h + "' is not defined");
}
return this.adaptDirectivePrologue(e.body), this.next(), e.sourceType = this.options.sourceType, this.finishNode(e, "Program");
};
var At = { kind: "loop" }, Ja = { kind: "switch" };
L.isLet = function(e) {
if (this.options.ecmaVersion < 6 || !this.isContextual("let"))
return false;
ee.lastIndex = this.pos;
var t = ee.exec(this.input), r = this.pos + t[0].length, s = this.input.charCodeAt(r);
if (s === 91 || s === 92 || s > 55295 && s < 56320)
return true;
if (e)
return false;
if (s === 123)
return true;
if (w(s, true)) {
for (var n2 = r + 1; G(s = this.input.charCodeAt(n2), true); )
++n2;
if (s === 92 || s > 55295 && s < 56320)
return true;
var h = this.input.slice(r, n2);
if (!R.test(h))
return true;
}
return false;
}, L.isAsyncFunction = function() {
if (this.options.ecmaVersion < 8 || !this.isContextual("async"))
return false;
ee.lastIndex = this.pos;
var e = ee.exec(this.input), t = this.pos + e[0].length, r;
return !S.test(this.input.slice(this.pos, t)) && this.input.slice(t, t + 8) === "function" && (t + 8 === this.input.length || !(G(r = this.input.charCodeAt(t + 8)) || r > 55295 && r < 56320));
}, L.parseStatement = function(e, t, r) {
var s = this.type, n2 = this.startNode(), h;
switch (this.isLet(e) && (s = i._var, h = "let"), s) {
case i._break:
case i._continue:
return this.parseBreakContinueStatement(n2, s.keyword);
case i._debugger:
return this.parseDebuggerStatement(n2);
case i._do:
return this.parseDoStatement(n2);
case i._for:
return this.parseForStatement(n2);
case i._function:
return e && (this.strict || e !== "if" && e !== "label") && this.options.ecmaVersion >= 6 && this.unexpected(), this.parseFunctionStatement(n2, false, !e);
case i._class:
return e && this.unexpected(), this.parseClass(n2, true);
case i._if:
return this.parseIfStatement(n2);
case i._return:
return this.parseReturnStatement(n2);
case i._switch:
return this.parseSwitchStatement(n2);
case i._throw:
return this.parseThrowStatement(n2);
case i._try:
return this.parseTryStatement(n2);
case i._const:
case i._var:
return h = h || this.value, e && h !== "var" && this.unexpected(), this.parseVarStatement(n2, h);
case i._while:
return this.parseWhileStatement(n2);
case i._with:
return this.parseWithStatement(n2);
case i.braceL:
return this.parseBlock(true, n2);
case i.semi:
return this.parseEmptyStatement(n2);
case i._export:
case i._import:
if (this.options.ecmaVersion > 10 && s === i._import) {
ee.lastIndex = this.pos;
var c = ee.exec(this.input), m = this.pos + c[0].length, A = this.input.charCodeAt(m);
if (A === 40 || A === 46)
return this.parseExpressionStatement(n2, this.parseExpression());
}
return this.options.allowImportExportEverywhere || (t || this.raise(this.start, "'import' and 'export' may only appear at the top level"), this.inModule || this.raise(this.start, "'import' and 'export' may appear only with 'sourceType: module'")), s === i._import ? this.parseImport(n2) : this.parseExport(n2, r);
default:
if (this.isAsyncFunction())
return e && this.unexpected(), this.next(), this.parseFunctionStatement(n2, true, !e);
var q = this.value, W = this.parseExpression();
return s === i.name && W.type === "Identifier" && this.eat(i.colon) ? this.parseLabeledStatement(n2, q, W, e) : this.parseExpressionStatement(n2, W);
}
}, L.parseBreakContinueStatement = function(e, t) {
var r = t === "break";
this.next(), this.eat(i.semi) || this.insertSemicolon() ? e.label = null : this.type !== i.name ? this.unexpected() : (e.label = this.parseIdent(), this.semicolon());
for (var s = 0; s < this.labels.length; ++s) {
var n2 = this.labels[s];
if ((e.label == null || n2.name === e.label.name) && (n2.kind != null && (r || n2.kind === "loop") || e.label && r))
break;
}
return s === this.labels.length && this.raise(e.start, "Unsyntactic " + t), this.finishNode(e, r ? "BreakStatement" : "ContinueStatement");
}, L.parseDebuggerStatement = function(e) {
return this.next(), this.semicolon(), this.finishNode(e, "DebuggerStatement");
}, L.parseDoStatement = function(e) {
return this.next(), this.labels.push(At), e.body = this.parseStatement("do"), this.labels.pop(), this.expect(i._while), e.test = this.parseParenExpression(), this.options.ecmaVersion >= 6 ? this.eat(i.semi) : this.semicolon(), this.finishNode(e, "DoWhileStatement");
}, L.parseForStatement = function(e) {
this.next();
var t = this.options.ecmaVersion >= 9 && this.canAwait && this.eatContextual("await") ? this.lastTokStart : -1;
if (this.labels.push(At), this.enterScope(0), this.expect(i.parenL), this.type === i.semi)
return t > -1 && this.unexpected(t), this.parseFor(e, null);
var r = this.isLet();
if (this.type === i._var || this.type === i._const || r) {
var s = this.startNode(), n2 = r ? "let" : this.value;
return this.next(), this.parseVar(s, true, n2), this.finishNode(s, "VariableDeclaration"), (this.type === i._in || this.options.ecmaVersion >= 6 && this.isContextual("of")) && s.declarations.length === 1 ? (this.options.ecmaVersion >= 9 && (this.type === i._in ? t > -1 && this.unexpected(t) : e.await = t > -1), this.parseForIn(e, s)) : (t > -1 && this.unexpected(t), this.parseFor(e, s));
}
var h = this.isContextual("let"), c = false, m = new He(), A = this.parseExpression(t > -1 ? "await" : true, m);
return this.type === i._in || (c = this.options.ecmaVersion >= 6 && this.isContextual("of")) ? (this.options.ecmaVersion >= 9 && (this.type === i._in ? t > -1 && this.unexpected(t) : e.await = t > -1), h && c && this.raise(A.start, "The left-hand side of a for-of loop may not start with 'let'."), this.toAssignable(A, false, m), this.checkLValPattern(A), this.parseForIn(e, A)) : (this.checkExpressionErrors(m, true), t > -1 && this.unexpected(t), this.parseFor(e, A));
}, L.parseFunctionStatement = function(e, t, r) {
return this.next(), this.parseFunction(e, Ve | (r ? 0 : Ct), false, t);
}, L.parseIfStatement = function(e) {
return this.next(), e.test = this.parseParenExpression(), e.consequent = this.parseStatement("if"), e.alternate = this.eat(i._else) ? this.parseStatement("if") : null, this.finishNode(e, "IfStatement");
}, L.parseReturnStatement = function(e) {
return !this.inFunction && !this.options.allowReturnOutsideFunction && this.raise(this.start, "'return' outside of function"), this.next(), this.eat(i.semi) || this.insertSemicolon() ? e.argument = null : (e.argument = this.parseExpression(), this.semicolon()), this.finishNode(e, "ReturnStatement");
}, L.parseSwitchStatement = function(e) {
this.next(), e.discriminant = this.parseParenExpression(), e.cases = [], this.expect(i.braceL), this.labels.push(Ja), this.enterScope(0);
for (var t, r = false; this.type !== i.braceR; )
if (this.type === i._case || this.type === i._default) {
var s = this.type === i._case;
t && this.finishNode(t, "SwitchCase"), e.cases.push(t = this.startNode()), t.consequent = [], this.next(), s ? t.test = this.parseExpression() : (r && this.raiseRecoverable(this.lastTokStart, "Multiple default clauses"), r = true, t.test = null), this.expect(i.colon);
} else
t || this.unexpected(), t.consequent.push(this.parseStatement(null));
return this.exitScope(), t && this.finishNode(t, "SwitchCase"), this.next(), this.labels.pop(), this.finishNode(e, "SwitchStatement");
}, L.parseThrowStatement = function(e) {
return this.next(), S.test(this.input.slice(this.lastTokEnd, this.start)) && this.raise(this.lastTokEnd, "Illegal newline after throw"), e.argument = this.parseExpression(), this.semicolon(), this.finishNode(e, "ThrowStatement");
};
var Qa = [];
L.parseTryStatement = function(e) {
if (this.next(), e.block = this.parseBlock(), e.handler = null, this.type === i._catch) {
var t = this.startNode();
if (this.next(), this.eat(i.parenL)) {
t.param = this.parseBindingAtom();
var r = t.param.type === "Identifier";
this.enterScope(r ? vr : 0), this.checkLValPattern(t.param, r ? yr : ve), this.expect(i.parenR);
} else
this.options.ecmaVersion < 10 && this.unexpected(), t.param = null, this.enterScope(0);
t.body = this.parseBlock(false), this.exitScope(), e.handler = this.finishNode(t, "CatchClause");
}
return e.finalizer = this.eat(i._finally) ? this.parseBlock() : null, !e.handler && !e.finalizer && this.raise(e.start, "Missing catch or finally clause"), this.finishNode(e, "TryStatement");
}, L.parseVarStatement = function(e, t) {
return this.next(), this.parseVar(e, false, t), this.semicolon(), this.finishNode(e, "VariableDeclaration");
}, L.parseWhileStatement = function(e) {
return this.next(), e.test = this.parseParenExpression(), this.labels.push(At), e.body = this.parseStatement("while"), this.labels.pop(), this.finishNode(e, "WhileStatement");
}, L.parseWithStatement = function(e) {
return this.strict && this.raise(this.start, "'with' in strict mode"), this.next(), e.object = this.parseParenExpression(), e.body = this.parseStatement("with"), this.finishNode(e, "WithStatement");
}, L.parseEmptyStatement = function(e) {
return this.next(), this.finishNode(e, "EmptyStatement");
}, L.parseLabeledStatement = function(e, t, r, s) {
for (var n2 = 0, h = this.labels; n2 < h.length; n2 += 1) {
var c = h[n2];
c.name === t && this.raise(r.start, "Label '" + t + "' is already declared");
}
for (var m = this.type.isLoop ? "loop" : this.type === i._switch ? "switch" : null, A = this.labels.length - 1; A >= 0; A--) {
var q = this.labels[A];
if (q.statementStart === e.start)
q.statementStart = this.start, q.kind = m;
else
break;
}
return this.labels.push({ name: t, kind: m, statementStart: this.start }), e.body = this.parseStatement(s ? s.indexOf("label") === -1 ? s + "label" : s : "label"), this.labels.pop(), e.label = r, this.finishNode(e, "LabeledStatement");
}, L.parseExpressionStatement = function(e, t) {
return e.expression = t, this.semicolon(), this.finishNode(e, "ExpressionStatement");
}, L.parseBlock = function(e, t, r) {
for (e === void 0 && (e = true), t === void 0 && (t = this.startNode()), t.body = [], this.expect(i.braceL), e && this.enterScope(0); this.type !== i.braceR; ) {
var s = this.parseStatement(null);
t.body.push(s);
}
return r && (this.strict = false), this.next(), e && this.exitScope(), this.finishNode(t, "BlockStatement");
}, L.parseFor = function(e, t) {
return e.init = t, this.expect(i.semi), e.test = this.type === i.semi ? null : this.parseExpression(), this.expect(i.semi), e.update = this.type === i.parenR ? null : this.parseExpression(), this.expect(i.parenR), e.body = this.parseStatement("for"), this.exitScope(), this.labels.pop(), this.finishNode(e, "ForStatement");
}, L.parseForIn = function(e, t) {
var r = this.type === i._in;
return this.next(), t.type === "VariableDeclaration" && t.declarations[0].init != null && (!r || this.options.ecmaVersion < 8 || this.strict || t.kind !== "var" || t.declarations[0].id.type !== "Identifier") && this.raise(t.start, (r ? "for-in" : "for-of") + " loop variable declaration may not have an initializer"), e.left = t, e.right = r ? this.parseExpression() : this.parseMaybeAssign(), this.expect(i.parenR), e.body = this.parseStatement("for"), this.exitScope(), this.labels.pop(), this.finishNode(e, r ? "ForInStatement" : "ForOfStatement");
}, L.parseVar = function(e, t, r) {
for (e.declarations = [], e.kind = r; ; ) {
var s = this.startNode();
if (this.parseVarId(s, r), this.eat(i.eq) ? s.init = this.parseMaybeAssign(t) : r === "const" && !(this.type === i._in || this.options.ecmaVersion >= 6 && this.isContextual("of")) ? this.unexpected() : s.id.type !== "Identifier" && !(t && (this.type === i._in || this.isContextual("of"))) ? this.raise(this.lastTokEnd, "Complex binding patterns require an initialization value") : s.init = null, e.declarations.push(this.finishNode(s, "VariableDeclarator")), !this.eat(i.comma))
break;
}
return e;
}, L.parseVarId = function(e, t) {
e.id = this.parseBindingAtom(), this.checkLValPattern(e.id, t === "var" ? yt : ve, false);
};
var Ve = 1, Ct = 2, Cr = 4;
L.parseFunction = function(e, t, r, s, n2) {
this.initFunction(e), (this.options.ecmaVersion >= 9 || this.options.ecmaVersion >= 6 && !s) && (this.type === i.star && t & Ct && this.unexpected(), e.generator = this.eat(i.star)), this.options.ecmaVersion >= 8 && (e.async = !!s), t & Ve && (e.id = t & Cr && this.type !== i.name ? null : this.parseIdent(), e.id && !(t & Ct) && this.checkLValSimple(e.id, this.strict || e.generator || e.async ? this.treatFunctionsAsVar ? yt : ve : xr));
var h = this.yieldPos, c = this.awaitPos, m = this.awaitIdentPos;
return this.yieldPos = 0, this.awaitPos = 0, this.awaitIdentPos = 0, this.enterScope(xt(e.async, e.generator)), t & Ve || (e.id = this.type === i.name ? this.parseIdent() : null), this.parseFunctionParams(e), this.parseFunctionBody(e, r, false, n2), this.yieldPos = h, this.awaitPos = c, this.awaitIdentPos = m, this.finishNode(e, t & Ve ? "FunctionDeclaration" : "FunctionExpression");
}, L.parseFunctionParams = function(e) {
this.expect(i.parenL), e.params = this.parseBindingList(i.parenR, false, this.options.ecmaVersion >= 8), this.checkYieldAwaitInDefaultParams();
}, L.parseClass = function(e, t) {
this.next();
var r = this.strict;
this.strict = true, this.parseClassId(e, t), this.parseClassSuper(e);
var s = this.enterClassBody(), n2 = this.startNode(), h = false;
for (n2.body = [], this.expect(i.braceL); this.type !== i.braceR; ) {
var c = this.parseClassElement(e.superClass !== null);
c && (n2.body.push(c), c.type === "MethodDefinition" && c.kind === "constructor" ? (h && this.raise(c.start, "Duplicate constructor in the same class"), h = true) : c.key && c.key.type === "PrivateIdentifier" && $a(s, c) && this.raiseRecoverable(c.key.start, "Identifier '#" + c.key.name + "' has already been declared"));
}
return this.strict = r, this.next(), e.body = this.finishNode(n2, "ClassBody"), this.exitClassBody(), this.finishNode(e, t ? "ClassDeclaration" : "ClassExpression");
}, L.parseClassElement = function(e) {
if (this.eat(i.semi))
return null;
var t = this.options.ecmaVersion, r = this.startNode(), s = "", n2 = false, h = false, c = "method", m = false;
if (this.eatContextual("static")) {
if (t >= 13 && this.eat(i.braceL))
return this.parseClassStaticBlock(r), r;
this.isClassElementNameStart() || this.type === i.star ? m = true : s = "static";
}
if (r.static = m, !s && t >= 8 && this.eatContextual("async") && ((this.isClassElementNameStart() || this.type === i.star) && !this.canInsertSemicolon() ? h = true : s = "async"), !s && (t >= 9 || !h) && this.eat(i.star) && (n2 = true), !s && !h && !n2) {
var A = this.value;
(this.eatContextual("get") || this.eatContextual("set")) && (this.isClassElementNameStart() ? c = A : s = A);
}
if (s ? (r.computed = false, r.key = this.startNodeAt(this.lastTokStart, this.lastTokStartLoc), r.key.name = s, this.finishNode(r.key, "Identifier")) : this.parseClassElementName(r), t < 13 || this.type === i.parenL || c !== "method" || n2 || h) {
var q = !r.static && Ke(r, "constructor"), W = q && e;
q && c !== "method" && this.raise(r.key.start, "Constructor can't have get/set modifier"), r.kind = q ? "constructor" : c, this.parseClassMethod(r, n2, h, W);
} else
this.parseClassField(r);
return r;
}, L.isClassElementNameStart = function() {
return this.type === i.name || this.type === i.privateId || this.type === i.num || this.type === i.string || this.type === i.bracketL || this.type.keyword;
}, L.parseClassElementName = function(e) {
this.type === i.privateId ? (this.value === "constructor" && this.raise(this.start, "Classes can't have an element named '#constructor'"), e.computed = false, e.key = this.parsePrivateIdent()) : this.parsePropertyName(e);
}, L.parseClassMethod = function(e, t, r, s) {
var n2 = e.key;
e.kind === "constructor" ? (t && this.raise(n2.start, "Constructor can't be a generator"), r && this.raise(n2.start, "Constructor can't be an async method")) : e.static && Ke(e, "prototype") && this.raise(n2.start, "Classes may not have a static property named prototype");
var h = e.value = this.parseMethod(t, r, s);
return e.kind === "get" && h.params.length !== 0 && this.raiseRecoverable(h.start, "getter should have no params"), e.kind === "set" && h.params.length !== 1 && this.raiseRecoverable(h.start, "setter should have exactly one param"), e.kind === "set" && h.params[0].type === "RestElement" && this.raiseRecoverable(h.params[0].start, "Setter cannot use rest params"), this.finishNode(e, "MethodDefinition");
}, L.parseClassField = function(e) {
if (Ke(e, "constructor") ? this.raise(e.key.start, "Classes can't have a field named 'constructor'") : e.static && Ke(e, "prototype") && this.raise(e.key.start, "Classes can't have a static field named 'prototype'"), this.eat(i.eq)) {
var t = this.currentThisScope(), r = t.inClassFieldInit;
t.inClassFieldInit = true, e.value = this.parseMaybeAssign(), t.inClassFieldInit = r;
} else
e.value = null;
return this.semicolon(), this.finishNode(e, "PropertyDefinition");
}, L.parseClassStaticBlock = function(e) {
e.body = [];
var t = this.labels;
for (this.labels = [], this.enterScope(Le | vt); this.type !== i.braceR; ) {
var r = this.parseStatement(null);
e.body.push(r);
}
return this.next(), this.exitScope(), this.labels = t, this.finishNode(e, "StaticBlock");
}, L.parseClassId = function(e, t) {
this.type === i.name ? (e.id = this.parseIdent(), t && this.checkLValSimple(e.id, ve, false)) : (t === true && this.unexpected(), e.id = null);
}, L.parseClassSuper = function(e) {
e.superClass = this.eat(i._extends) ? this.parseExprSubscripts(false) : null;
}, L.enterClassBody = function() {
var e = { declared: /* @__PURE__ */ Object.create(null), used: [] };
return this.privateNameStack.push(e), e.declared;
}, L.exitClassBody = function() {
for (var e = this.privateNameStack.pop(), t = e.declared, r = e.used, s = this.privateNameStack.length, n2 = s === 0 ? null : this.privateNameStack[s - 1], h = 0; h < r.length; ++h) {
var c = r[h];
P(t, c.name) || (n2 ? n2.used.push(c) : this.raiseRecoverable(c.start, "Private field '#" + c.name + "' must be declared in an enclosing class"));
}
};
function $a(e, t) {
var r = t.key.name, s = e[r], n2 = "true";
return t.type === "MethodDefinition" && (t.kind === "get" || t.kind === "set") && (n2 = (t.static ? "s" : "i") + t.kind), s === "iget" && n2 === "iset" || s === "iset" && n2 === "iget" || s === "sget" && n2 === "sset" || s === "sset" && n2 === "sget" ? (e[r] = "true", false) : s ? true : (e[r] = n2, false);
}
function Ke(e, t) {
var r = e.computed, s = e.key;
return !r && (s.type === "Identifier" && s.name === t || s.type === "Literal" && s.value === t);
}
L.parseExport = function(e, t) {
if (this.next(), this.eat(i.star))
return this.options.ecmaVersion >= 11 && (this.eatContextual("as") ? (e.exported = this.parseModuleExportName(), this.checkExport(t, e.exported, this.lastTokStart)) : e.exported = null), this.expectContextual("from"), this.type !== i.string && this.unexpected(), e.source = this.parseExprAtom(), this.semicolon(), this.finishNode(e, "ExportAllDeclaration");
if (this.eat(i._default)) {
this.checkExport(t, "default", this.lastTokStart);
var r;
if (this.type === i._function || (r = this.isAsyncFunction())) {
var s = this.startNode();
this.next(), r && this.next(), e.declaration = this.parseFunction(s, Ve | Cr, false, r);
} else if (this.type === i._class) {
var n2 = this.startNode();
e.declaration = this.parseClass(n2, "nullableID");
} else
e.declaration = this.parseMaybeAssign(), this.semicolon();
return this.finishNode(e, "ExportDefaultDeclaration");
}
if (this.shouldParseExportStatement())
e.declaration = this.parseStatement(null), e.declaration.type === "VariableDeclaration" ? this.checkVariableExport(t, e.declaration.declarations) : this.checkExport(t, e.declaration.id, e.declaration.id.start), e.specifiers = [], e.source = null;
else {
if (e.declaration = null, e.specifiers = this.parseExportSpecifiers(t), this.eatContextual("from"))
this.type !== i.string && this.unexpected(), e.source = this.parseExprAtom();
else {
for (var h = 0, c = e.specifiers; h < c.length; h += 1) {
var m = c[h];
this.checkUnreserved(m.local), this.checkLocalExport(m.local), m.local.type === "Literal" && this.raise(m.local.start, "A string literal cannot be used as an exported binding without `from`.");
}
e.source = null;
}
this.semicolon();
}
return this.finishNode(e, "ExportNamedDeclaration");
}, L.checkExport = function(e, t, r) {
e && (typeof t != "string" && (t = t.type === "Identifier" ? t.name : t.value), P(e, t) && this.raiseRecoverable(r, "Duplicate export '" + t + "'"), e[t] = true);
}, L.checkPatternExport = function(e, t) {
var r = t.type;
if (r === "Identifier")
this.checkExport(e, t, t.start);
else if (r === "ObjectPattern")
for (var s = 0, n2 = t.properties; s < n2.length; s += 1) {
var h = n2[s];
this.checkPatternExport(e, h);
}
else if (r === "ArrayPattern")
for (var c = 0, m = t.elements; c < m.length; c += 1) {
var A = m[c];
A && this.checkPatternExport(e, A);
}
else
r === "Property" ? this.checkPatternExport(e, t.value) : r === "AssignmentPattern" ? this.checkPatternExport(e, t.left) : r === "RestElement" ? this.checkPatternExport(e, t.argument) : r === "ParenthesizedExpression" && this.checkPatternExport(e, t.expression);
}, L.checkVariableExport = function(e, t) {
if (e)
for (var r = 0, s = t; r < s.length; r += 1) {
var n2 = s[r];
this.checkPatternExport(e, n2.id);
}
}, L.shouldParseExportStatement = function() {
return this.type.keyword === "var" || this.type.keyword === "const" || this.type.keyword === "class" || this.type.keyword === "function" || this.isLet() || this.isAsyncFunction();
}, L.parseExportSpecifiers = function(e) {
var t = [], r = true;
for (this.expect(i.braceL); !this.eat(i.braceR); ) {
if (r)
r = false;
else if (this.expect(i.comma), this.afterTrailingComma(i.braceR))
break;
var s = this.startNode();
s.local = this.parseModuleExportName(), s.exported = this.eatContextual("as") ? this.parseModuleExportName() : s.local, this.checkExport(e, s.exported, s.exported.start), t.push(this.finishNode(s, "ExportSpecifier"));
}
return t;
}, L.parseImport = function(e) {
return this.next(), this.type === i.string ? (e.specifiers = Qa, e.source = this.parseExprAtom()) : (e.specifiers = this.parseImportSpecifiers(), this.expectContextual("from"), e.source = this.type === i.string ? this.parseExprAtom() : this.unexpected()), this.semicolon(), this.finishNode(e, "ImportDeclaration");
}, L.parseImportSpecifiers = function() {
var e = [], t = true;
if (this.type === i.name) {
var r = this.startNode();
if (r.local = this.parseIdent(), this.checkLValSimple(r.local, ve), e.push(this.finishNode(r, "ImportDefaultSpecifier")), !this.eat(i.comma))
return e;
}
if (this.type === i.star) {
var s = this.startNode();
return this.next(), this.expectContextual("as"), s.local = this.parseIdent(), this.checkLValSimple(s.local, ve), e.push(this.finishNode(s, "ImportNamespaceSpecifier")), e;
}
for (this.expect(i.braceL); !this.eat(i.braceR); ) {
if (t)
t = false;
else if (this.expect(i.comma), this.afterTrailingComma(i.braceR))
break;
var n2 = this.startNode();
n2.imported = this.parseModuleExportName(), this.eatContextual("as") ? n2.local = this.parseIdent() : (this.checkUnreserved(n2.imported), n2.local = n2.imported), this.checkLValSimple(n2.local, ve), e.push(this.finishNode(n2, "ImportSpecifier"));
}
return e;
}, L.parseModuleExportName = function() {
if (this.options.ecmaVersion >= 13 && this.type === i.string) {
var e = this.parseLiteral(this.value);
return K.test(e.value) && this.raise(e.start, "An export name cannot include a lone surrogate."), e;
}
return this.parseIdent(true);
}, L.adaptDirectivePrologue = function(e) {
for (var t = 0; t < e.length && this.isDirectiveCandidate(e[t]); ++t)
e[t].directive = e[t].expression.raw.slice(1, -1);
}, L.isDirectiveCandidate = function(e) {
return this.options.ecmaVersion >= 5 && e.type === "ExpressionStatement" && e.expression.type === "Literal" && typeof e.expression.value == "string" && (this.input[e.start] === '"' || this.input[e.start] === "'");
};
var he = Y.prototype;
he.toAssignable = function(e, t, r) {
if (this.options.ecmaVersion >= 6 && e)
switch (e.type) {
case "Identifier":
this.inAsync && e.name === "await" && this.raise(e.start, "Cannot use 'await' as identifier inside an async function");
break;
case "ObjectPattern":
case "ArrayPattern":
case "AssignmentPattern":
case "RestElement":
break;
case "ObjectExpression":
e.type = "ObjectPattern", r && this.checkPatternErrors(r, true);
for (var s = 0, n2 = e.properties; s < n2.length; s += 1) {
var h = n2[s];
this.toAssignable(h, t), h.type === "RestElement" && (h.argument.type === "ArrayPattern" || h.argument.type === "ObjectPattern") && this.raise(h.argument.start, "Unexpected token");
}
break;
case "Property":
e.kind !== "init" && this.raise(e.key.start, "Object pattern can't contain getter or setter"), this.toAssignable(e.value, t);
break;
case "ArrayExpression":
e.type = "ArrayPattern", r && this.checkPatternErrors(r, true), this.toAssignableList(e.elements, t);
break;
case "SpreadElement":
e.type = "RestElement", this.toAssignable(e.argument, t), e.argument.type === "AssignmentPattern" && this.raise(e.argument.start, "Rest elements cannot have a default value");
break;
case "AssignmentExpression":
e.operator !== "=" && this.raise(e.left.end, "Only '=' operator can be used for specifying default value."), e.type = "AssignmentPattern", delete e.operator, this.toAssignable(e.left, t);
break;
case "ParenthesizedExpression":
this.toAssignable(e.expression, t, r);
break;
case "ChainExpression":
this.raiseRecoverable(e.start, "Optional chaining cannot appear in left-hand side");
break;
case "MemberExpression":
if (!t)
break;
default:
this.raise(e.start, "Assigning to rvalue");
}
else
r && this.checkPatternErrors(r, true);
return e;
}, he.toAssignableList = function(e, t) {
for (var r = e.length, s = 0; s < r; s++) {
var n2 = e[s];
n2 && this.toAssignable(n2, t);
}
if (r) {
var h = e[r - 1];
this.options.ecmaVersion === 6 && t && h && h.type === "RestElement" && h.argument.type !== "Identifier" && this.unexpected(h.argument.start);
}
return e;
}, he.parseSpread = function(e) {
var t = this.startNode();
return this.next(), t.argument = this.parseMaybeAssign(false, e), this.finishNode(t, "SpreadElement");
}, he.parseRestBinding = function() {
var e = this.startNode();
return this.next(), this.options.ecmaVersion === 6 && this.type !== i.name && this.unexpected(), e.argument = this.parseBindingAtom(), this.finishNode(e, "RestElement");
}, he.parseBindingAtom = function() {
if (this.options.ecmaVersion >= 6)
switch (this.type) {
case i.bracketL:
var e = this.startNode();
return this.next(), e.elements = this.parseBindingList(i.bracketR, true, true), this.finishNode(e, "ArrayPattern");
case i.braceL:
return this.parseObj(true);
}
return this.parseIdent();
}, he.parseBindingList = function(e, t, r) {
for (var s = [], n2 = true; !this.eat(e); )
if (n2 ? n2 = false : this.expect(i.comma), t && this.type === i.comma)
s.push(null);
else {
if (r && this.afterTrailingComma(e))
break;
if (this.type === i.ellipsis) {
var h = this.parseRestBinding();
this.parseBindingListItem(h), s.push(h), this.type === i.comma && this.raise(this.start, "Comma is not permitted after the rest element"), this.expect(e);
break;
} else {
var c = this.parseMaybeDefault(this.start, this.startLoc);
this.parseBindingListItem(c), s.push(c);
}
}
return s;
}, he.parseBindingListItem = function(e) {
return e;
}, he.parseMaybeDefault = function(e, t, r) {
if (r = r || this.parseBindingAtom(), this.options.ecmaVersion < 6 || !this.eat(i.eq))
return r;
var s = this.startNodeAt(e, t);
return s.left = r, s.right = this.parseMaybeAssign(), this.finishNode(s, "AssignmentPattern");
}, he.checkLValSimple = function(e, t, r) {
t === void 0 && (t = Ge);
var s = t !== Ge;
switch (e.type) {
case "Identifier":
this.strict && this.reservedWordsStrictBind.test(e.name) && this.raiseRecoverable(e.start, (s ? "Binding " : "Assigning to ") + e.name + " in strict mode"), s && (t === ve && e.name === "let" && this.raiseRecoverable(e.start, "let is disallowed as a lexically bound name"), r && (P(r, e.name) && this.raiseRecoverable(e.start, "Argument name clash"), r[e.name] = true), t !== Ar && this.declareName(e.name, t, e.start));
break;
case "ChainExpression":
this.raiseRecoverable(e.start, "Optional chaining cannot appear in left-hand side");
break;
case "MemberExpression":
s && this.raiseRecoverable(e.start, "Binding member expression");
break;
case "ParenthesizedExpression":
return s && this.raiseRecoverable(e.start, "Binding parenthesized expression"), this.checkLValSimple(e.expression, t, r);
default:
this.raise(e.start, (s ? "Binding" : "Assigning to") + " rvalue");
}
}, he.checkLValPattern = function(e, t, r) {
switch (t === void 0 && (t = Ge), e.type) {
case "ObjectPattern":
for (var s = 0, n2 = e.properties; s < n2.length; s += 1) {
var h = n2[s];
this.checkLValInnerPattern(h, t, r);
}
break;
case "ArrayPattern":
for (var c = 0, m = e.elements; c < m.length; c += 1) {
var A = m[c];
A && this.checkLValInnerPattern(A, t, r);
}
break;
default:
this.checkLValSimple(e, t, r);
}
}, he.checkLValInnerPattern = function(e, t, r) {
switch (t === void 0 && (t = Ge), e.type) {
case "Property":
this.checkLValInnerPattern(e.value, t, r);
break;
case "AssignmentPattern":
this.checkLValPattern(e.left, t, r);
break;
case "RestElement":
this.checkLValPattern(e.argument, t, r);
break;
default:
this.checkLValPattern(e, t, r);
}
};
var ue = function(t, r, s, n2, h) {
this.token = t, this.isExpr = !!r, this.preserveSpace = !!s, this.override = n2, this.generator = !!h;
}, Q = { b_stat: new ue("{", false), b_expr: new ue("{", true), b_tmpl: new ue("${", false), p_stat: new ue("(", false), p_expr: new ue("(", true), q_tmpl: new ue("`", true, true, function(e) {
return e.tryReadTemplateToken();
}), f_stat: new ue("function", false), f_expr: new ue("function", true), f_expr_gen: new ue("function", true, false, null, true), f_gen: new ue("function", false, false, null, true) }, Fe = Y.prototype;
Fe.initialContext = function() {
return [Q.b_stat];
}, Fe.curContext = function() {
return this.context[this.context.length - 1];
}, Fe.braceIsBlock = function(e) {
var t = this.curContext();
return t === Q.f_expr || t === Q.f_stat ? true : e === i.colon && (t === Q.b_stat || t === Q.b_expr) ? !t.isExpr : e === i._return || e === i.name && this.exprAllowed ? S.test(this.input.slice(this.lastTokEnd, this.start)) : e === i._else || e === i.semi || e === i.eof || e === i.parenR || e === i.arrow ? true : e === i.braceL ? t === Q.b_stat : e === i._var || e === i._const || e === i.name ? false : !this.exprAllowed;
}, Fe.inGeneratorContext = function() {
for (var e = this.context.length - 1; e >= 1; e--) {
var t = this.context[e];
if (t.token === "function")
return t.generator;
}
return false;
}, Fe.updateContext = function(e) {
var t, r = this.type;
r.keyword && e === i.dot ? this.exprAllowed = false : (t = r.updateContext) ? t.call(this, e) : this.exprAllowed = r.beforeExpr;
}, Fe.overrideContext = function(e) {
this.curContext() !== e && (this.context[this.context.length - 1] = e);
}, i.parenR.updateContext = i.braceR.updateContext = function() {
if (this.context.length === 1) {
this.exprAllowed = true;
return;
}
var e = this.context.pop();
e === Q.b_stat && this.curContext().token === "function" && (e = this.context.pop()), this.exprAllowed = !e.isExpr;
}, i.braceL.updateContext = function(e) {
this.context.push(this.braceIsBlock(e) ? Q.b_stat : Q.b_expr), this.exprAllowed = true;
}, i.dollarBraceL.updateContext = function() {
this.context.push(Q.b_tmpl), this.exprAllowed = true;
}, i.parenL.updateContext = function(e) {
var t = e === i._if || e === i._for || e === i._with || e === i._while;
this.context.push(t ? Q.p_stat : Q.p_expr), this.exprAllowed = true;
}, i.incDec.updateContext = function() {
}, i._function.updateContext = i._class.updateContext = function(e) {
e.beforeExpr && e !== i._else && !(e === i.semi && this.curContext() !== Q.p_stat) && !(e === i._return && S.test(this.input.slice(this.lastTokEnd, this.start))) && !((e === i.colon || e === i.braceL) && this.curContext() === Q.b_stat) ? this.context.push(Q.f_expr) : this.context.push(Q.f_stat), this.exprAllowed = false;
}, i.backQuote.updateContext = function() {
this.curContext() === Q.q_tmpl ? this.context.pop() : this.context.push(Q.q_tmpl), this.exprAllowed = false;
}, i.star.updateContext = function(e) {
if (e === i._function) {
var t = this.context.length - 1;
this.context[t] === Q.f_expr ? this.context[t] = Q.f_expr_gen : this.context[t] = Q.f_gen;
}
this.exprAllowed = true;
}, i.name.updateContext = function(e) {
var t = false;
this.options.ecmaVersion >= 6 && e !== i.dot && (this.value === "of" && !this.exprAllowed || this.value === "yield" && this.inGeneratorContext()) && (t = true), this.exprAllowed = t;
};
var M = Y.prototype;
M.checkPropClash = function(e, t, r) {
if (!(this.options.ecmaVersion >= 9 && e.type === "SpreadElement") && !(this.options.ecmaVersion >= 6 && (e.computed || e.method || e.shorthand))) {
var s = e.key, n2;
switch (s.type) {
case "Identifier":
n2 = s.name;
break;
case "Literal":
n2 = String(s.value);
break;
default:
return;
}
var h = e.kind;
if (this.options.ecmaVersion >= 6) {
n2 === "__proto__" && h === "init" && (t.proto && (r ? r.doubleProto < 0 && (r.doubleProto = s.start) : this.raiseRecoverable(s.start, "Redefinition of __proto__ property")), t.proto = true);
return;
}
n2 = "$" + n2;
var c = t[n2];
if (c) {
var m;
h === "init" ? m = this.strict && c.init || c.get || c.set : m = c.init || c[h], m && this.raiseRecoverable(s.start, "Redefinition of property");
} else
c = t[n2] = { init: false, get: false, set: false };
c[h] = true;
}
}, M.parseExpression = function(e, t) {
var r = this.start, s = this.startLoc, n2 = this.parseMaybeAssign(e, t);
if (this.type === i.comma) {
var h = this.startNodeAt(r, s);
for (h.expressions = [n2]; this.eat(i.comma); )
h.expressions.push(this.parseMaybeAssign(e, t));
return this.finishNode(h, "SequenceExpression");
}
return n2;
}, M.parseMaybeAssign = function(e, t, r) {
if (this.isContextual("yield")) {
if (this.inGenerator)
return this.parseYield(e);
this.exprAllowed = false;
}
var s = false, n2 = -1, h = -1, c = -1;
t ? (n2 = t.parenthesizedAssign, h = t.trailingComma, c = t.doubleProto, t.parenthesizedAssign = t.trailingComma = -1) : (t = new He(), s = true);
var m = this.start, A = this.startLoc;
(this.type === i.parenL || this.type === i.name) && (this.potentialArrowAt = this.start, this.potentialArrowInForAwait = e === "await");
var q = this.parseMaybeConditional(e, t);
if (r && (q = r.call(this, q, m, A)), this.type.isAssign) {
var W = this.startNodeAt(m, A);
return W.operator = this.value, this.type === i.eq && (q = this.toAssignable(q, false, t)), s || (t.parenthesizedAssign = t.trailingComma = t.doubleProto = -1), t.shorthandAssign >= q.start && (t.shorthandAssign = -1), this.type === i.eq ? this.checkLValPattern(q) : this.checkLValSimple(q), W.left = q, this.next(), W.right = this.parseMaybeAssign(e), c > -1 && (t.doubleProto = c), this.finishNode(W, "AssignmentExpression");
} else
s && this.checkExpressionErrors(t, true);
return n2 > -1 && (t.parenthesizedAssign = n2), h > -1 && (t.trailingComma = h), q;
}, M.parseMaybeConditional = function(e, t) {
var r = this.start, s = this.startLoc, n2 = this.parseExprOps(e, t);
if (this.checkExpressionErrors(t))
return n2;
if (this.eat(i.question)) {
var h = this.startNodeAt(r, s);
return h.test = n2, h.consequent = this.parseMaybeAssign(), this.expect(i.colon), h.alternate = this.parseMaybeAssign(e), this.finishNode(h, "ConditionalExpression");
}
return n2;
}, M.parseExprOps = function(e, t) {
var r = this.start, s = this.startLoc, n2 = this.parseMaybeUnary(t, false, false, e);
return this.checkExpressionErrors(t) || n2.start === r && n2.type === "ArrowFunctionExpression" ? n2 : this.parseExprOp(n2, r, s, -1, e);
}, M.parseExprOp = function(e, t, r, s, n2) {
var h = this.type.binop;
if (h != null && (!n2 || this.type !== i._in) && h > s) {
var c = this.type === i.logicalOR || this.type === i.logicalAND, m = this.type === i.coalesce;
m && (h = i.logicalAND.binop);
var A = this.value;
this.next();
var q = this.start, W = this.startLoc, re = this.parseExprOp(this.parseMaybeUnary(null, false, false, n2), q, W, h, n2), Se = this.buildBinary(t, r, e, re, A, c || m);
return (c && this.type === i.coalesce || m && (this.type === i.logicalOR || this.type === i.logicalAND)) && this.raiseRecoverable(this.start, "Logical expressions and coalesce expressions cannot be mixed. Wrap either by parentheses"), this.parseExprOp(Se, t, r, s, n2);
}
return e;
}, M.buildBinary = function(e, t, r, s, n2, h) {
s.type === "PrivateIdentifier" && this.raise(s.start, "Private identifier can only be left side of binary expression");
var c = this.startNodeAt(e, t);
return c.left = r, c.operator = n2, c.right = s, this.finishNode(c, h ? "LogicalExpression" : "BinaryExpression");
}, M.parseMaybeUnary = function(e, t, r, s) {
var n2 = this.start, h = this.startLoc, c;
if (this.isContextual("await") && this.canAwait)
c = this.parseAwait(s), t = true;
else if (this.type.prefix) {
var m = this.startNode(), A = this.type === i.incDec;
m.operator = this.value, m.prefix = true, this.next(), m.argument = this.parseMaybeUnary(null, true, A, s), this.checkExpressionErrors(e, true), A ? this.checkLValSimple(m.argument) : this.strict && m.operator === "delete" && m.argument.type === "Identifier" ? this.raiseRecoverable(m.start, "Deleting local variable in strict mode") : m.operator === "delete" && Er(m.argument) ? this.raiseRecoverable(m.start, "Private fields can not be deleted") : t = true, c = this.finishNode(m, A ? "UpdateExpression" : "UnaryExpression");
} else if (!t && this.type === i.privateId)
(s || this.privateNameStack.length === 0) && this.unexpected(), c = this.parsePrivateIdent(), this.type !== i._in && this.unexpected();
else {
if (c = this.parseExprSubscripts(e, s), this.checkExpressionErrors(e))
return c;
for (; this.type.postfix && !this.canInsertSemicolon(); ) {
var q = this.startNodeAt(n2, h);
q.operator = this.value, q.prefix = false, q.argument = c, this.checkLValSimple(c), this.next(), c = this.finishNode(q, "UpdateExpression");
}
}
if (!r && this.eat(i.starstar))
if (t)
this.unexpected(this.lastTokStart);
else
return this.buildBinary(n2, h, c, this.parseMaybeUnary(null, false, false, s), "**", false);
else
return c;
};
function Er(e) {
return e.type === "MemberExpression" && e.property.type === "PrivateIdentifier" || e.type === "ChainExpression" && Er(e.expression);
}
M.parseExprSubscripts = function(e, t) {
var r = this.start, s = this.startLoc, n2 = this.parseExprAtom(e, t);
if (n2.type === "ArrowFunctionExpression" && this.input.slice(this.lastTokStart, this.lastTokEnd) !== ")")
return n2;
var h = this.parseSubscripts(n2, r, s, false, t);
return e && h.type === "MemberExpression" && (e.parenthesizedAssign >= h.start && (e.parenthesizedAssign = -1), e.parenthesizedBind >= h.start && (e.parenthesizedBind = -1), e.trailingComma >= h.start && (e.trailingComma = -1)), h;
}, M.parseSubscripts = function(e, t, r, s, n2) {
for (var h = this.options.ecmaVersion >= 8 && e.type === "Identifier" && e.name === "async" && this.lastTokEnd === e.end && !this.canInsertSemicolon() && e.end - e.start === 5 && this.potentialArrowAt === e.start, c = false; ; ) {
var m = this.parseSubscript(e, t, r, s, h, c, n2);
if (m.optional && (c = true), m === e || m.type === "ArrowFunctionExpression") {
if (c) {
var A = this.startNodeAt(t, r);
A.expression = m, m = this.finishNode(A, "ChainExpression");
}
return m;
}
e = m;
}
}, M.parseSubscript = function(e, t, r, s, n2, h, c) {
var m = this.options.ecmaVersion >= 11, A = m && this.eat(i.questionDot);
s && A && this.raise(this.lastTokStart, "Optional chaining cannot appear in the callee of new expressions");
var q = this.eat(i.bracketL);
if (q || A && this.type !== i.parenL && this.type !== i.backQuote || this.eat(i.dot)) {
var W = this.startNodeAt(t, r);
W.object = e, q ? (W.property = this.parseExpression(), this.expect(i.bracketR)) : this.type === i.privateId && e.type !== "Super" ? W.property = this.parsePrivateIdent() : W.property = this.parseIdent(this.options.allowReserved !== "never"), W.computed = !!q, m && (W.optional = A), e = this.finishNode(W, "MemberExpression");
} else if (!s && this.eat(i.parenL)) {
var re = new He(), Se = this.yieldPos, qe = this.awaitPos, Be = this.awaitIdentPos;
this.yieldPos = 0, this.awaitPos = 0, this.awaitIdentPos = 0;
var $e = this.parseExprList(i.parenR, this.options.ecmaVersion >= 8, false, re);
if (n2 && !A && !this.canInsertSemicolon() && this.eat(i.arrow))
return this.checkPatternErrors(re, false), this.checkYieldAwaitInDefaultParams(), this.awaitIdentPos > 0 && this.raise(this.awaitIdentPos, "Cannot use 'await' as identifier inside an async function"), this.yieldPos = Se, this.awaitPos = qe, this.awaitIdentPos = Be, this.parseArrowExpression(this.startNodeAt(t, r), $e, true, c);
this.checkExpressionErrors(re, true), this.yieldPos = Se || this.yieldPos, this.awaitPos = qe || this.awaitPos, this.awaitIdentPos = Be || this.awaitIdentPos;
var Ie = this.startNodeAt(t, r);
Ie.callee = e, Ie.arguments = $e, m && (Ie.optional = A), e = this.finishNode(Ie, "CallExpression");
} else if (this.type === i.backQuote) {
(A || h) && this.raise(this.start, "Optional chaining cannot appear in the tag of tagged template expressions");
var Te = this.startNodeAt(t, r);
Te.tag = e, Te.quasi = this.parseTemplate({ isTagged: true }), e = this.finishNode(Te, "TaggedTemplateExpression");
}
return e;
}, M.parseExprAtom = function(e, t) {
this.type === i.slash && this.readRegexp();
var r, s = this.potentialArrowAt === this.start;
switch (this.type) {
case i._super:
return this.allowSuper || this.raise(this.start, "'super' keyword outside a method"), r = this.startNode(), this.next(), this.type === i.parenL && !this.allowDirectSuper && this.raise(r.start, "super() call outside constructor of a subclass"), this.type !== i.dot && this.type !== i.bracketL && this.type !== i.parenL && this.unexpected(), this.finishNode(r, "Super");
case i._this:
return r = this.startNode(), this.next(), this.finishNode(r, "ThisExpression");
case i.name:
var n2 = this.start, h = this.startLoc, c = this.containsEsc, m = this.parseIdent(false);
if (this.options.ecmaVersion >= 8 && !c && m.name === "async" && !this.canInsertSemicolon() && this.eat(i._function))
return this.overrideContext(Q.f_expr), this.parseFunction(this.startNodeAt(n2, h), 0, false, true, t);
if (s && !this.canInsertSemicolon()) {
if (this.eat(i.arrow))
return this.parseArrowExpression(this.startNodeAt(n2, h), [m], false, t);
if (this.options.ecmaVersion >= 8 && m.name === "async" && this.type === i.name && !c && (!this.potentialArrowInForAwait || this.value !== "of" || this.containsEsc))
return m = this.parseIdent(false), (this.canInsertSemicolon() || !this.eat(i.arrow)) && this.unexpected(), this.parseArrowExpression(this.startNodeAt(n2, h), [m], true, t);
}
return m;
case i.regexp:
var A = this.value;
return r = this.parseLiteral(A.value), r.regex = { pattern: A.pattern, flags: A.flags }, r;
case i.num:
case i.string:
return this.parseLiteral(this.value);
case i._null:
case i._true:
case i._false:
return r = this.startNode(), r.value = this.type === i._null ? null : this.type === i._true, r.raw = this.type.keyword, this.next(), this.finishNode(r, "Literal");
case i.parenL:
var q = this.start, W = this.parseParenAndDistinguishExpression(s, t);
return e && (e.parenthesizedAssign < 0 && !this.isSimpleAssignTarget(W) && (e.parenthesizedAssign = q), e.parenthesizedBind < 0 && (e.parenthesizedBind = q)), W;
case i.bracketL:
return r = this.startNode(), this.next(), r.elements = this.parseExprList(i.bracketR, true, true, e), this.finishNode(r, "ArrayExpression");
case i.braceL:
return this.overrideContext(Q.b_expr), this.parseObj(false, e);
case i._function:
return r = this.startNode(), this.next(), this.parseFunction(r, 0);
case i._class:
return this.parseClass(this.startNode(), false);
case i._new:
return this.parseNew();
case i.backQuote:
return this.parseTemplate();
case i._import:
return this.options.ecmaVersion >= 11 ? this.parseExprImport() : this.unexpected();
default:
this.unexpected();
}
}, M.parseExprImport = function() {
var e = this.startNode();
this.containsEsc && this.raiseRecoverable(this.start, "Escape sequence in keyword import");
var t = this.parseIdent(true);
switch (this.type) {
case i.parenL:
return this.parseDynamicImport(e);
case i.dot:
return e.meta = t, this.parseImportMeta(e);
default:
this.unexpected();
}
}, M.parseDynamicImport = function(e) {
if (this.next(), e.source = this.parseMaybeAssign(), !this.eat(i.parenR)) {
var t = this.start;
this.eat(i.comma) && this.eat(i.parenR) ? this.raiseRecoverable(t, "Trailing comma is not allowed in import()") : this.unexpected(t);
}
return this.finishNode(e, "ImportExpression");
}, M.parseImportMeta = function(e) {
this.next();
var t = this.containsEsc;
return e.property = this.parseIdent(true), e.property.name !== "meta" && this.raiseRecoverable(e.property.start, "The only valid meta property for import is 'import.meta'"), t && this.raiseRecoverable(e.start, "'import.meta' must not contain escaped characters"), this.options.sourceType !== "module" && !this.options.allowImportExportEverywhere && this.raiseRecoverable(e.start, "Cannot use 'import.meta' outside a module"), this.finishNode(e, "MetaProperty");
}, M.parseLiteral = function(e) {
var t = this.startNode();
return t.value = e, t.raw = this.input.slice(this.start, this.end), t.raw.charCodeAt(t.raw.length - 1) === 110 && (t.bigint = t.raw.slice(0, -1).replace(/_/g, "")), this.next(), this.finishNode(t, "Literal");
}, M.parseParenExpression = function() {
this.expect(i.parenL);
var e = this.parseExpression();
return this.expect(i.parenR), e;
}, M.parseParenAndDistinguishExpression = function(e, t) {
var r = this.start, s = this.startLoc, n2, h = this.options.ecmaVersion >= 8;
if (this.options.ecmaVersion >= 6) {
this.next();
var c = this.start, m = this.startLoc, A = [], q = true, W = false, re = new He(), Se = this.yieldPos, qe = this.awaitPos, Be;
for (this.yieldPos = 0, this.awaitPos = 0; this.type !== i.parenR; )
if (q ? q = false : this.expect(i.comma), h && this.afterTrailingComma(i.parenR, true)) {
W = true;
break;
} else if (this.type === i.ellipsis) {
Be = this.start, A.push(this.parseParenItem(this.parseRestBinding())), this.type === i.comma && this.raise(this.start, "Comma is not permitted after the rest element");
break;
} else
A.push(this.parseMaybeAssign(false, re, this.parseParenItem));
var $e = this.lastTokEnd, Ie = this.lastTokEndLoc;
if (this.expect(i.parenR), e && !this.canInsertSemicolon() && this.eat(i.arrow))
return this.checkPatternErrors(re, false), this.checkYieldAwaitInDefaultParams(), this.yieldPos = Se, this.awaitPos = qe, this.parseParenArrowList(r, s, A, t);
(!A.length || W) && this.unexpected(this.lastTokStart), Be && this.unexpected(Be), this.checkExpressionErrors(re, true), this.yieldPos = Se || this.yieldPos, this.awaitPos = qe || this.awaitPos, A.length > 1 ? (n2 = this.startNodeAt(c, m), n2.expressions = A, this.finishNodeAt(n2, "SequenceExpression", $e, Ie)) : n2 = A[0];
} else
n2 = this.parseParenExpression();
if (this.options.preserveParens) {
var Te = this.startNodeAt(r, s);
return Te.expression = n2, this.finishNode(Te, "ParenthesizedExpression");
} else
return n2;
}, M.parseParenItem = function(e) {
return e;
}, M.parseParenArrowList = function(e, t, r, s) {
return this.parseArrowExpression(this.startNodeAt(e, t), r, false, s);
};
var Ya = [];
M.parseNew = function() {
this.containsEsc && this.raiseRecoverable(this.start, "Escape sequence in keyword new");
var e = this.startNode(), t = this.parseIdent(true);
if (this.options.ecmaVersion >= 6 && this.eat(i.dot)) {
e.meta = t;
var r = this.containsEsc;
return e.property = this.parseIdent(true), e.property.name !== "target" && this.raiseRecoverable(e.property.start, "The only valid meta property for new is 'new.target'"), r && this.raiseRecoverable(e.start, "'new.target' must not contain escaped characters"), this.allowNewDotTarget || this.raiseRecoverable(e.start, "'new.target' can only be used in functions and class static block"), this.finishNode(e, "MetaProperty");
}
var s = this.start, n2 = this.startLoc, h = this.type === i._import;
return e.callee = this.parseSubscripts(this.parseExprAtom(), s, n2, true, false), h && e.callee.type === "ImportExpression" && this.raise(s, "Cannot use new with import()"), this.eat(i.parenL) ? e.arguments = this.parseExprList(i.parenR, this.options.ecmaVersion >= 8, false) : e.arguments = Ya, this.finishNode(e, "NewExpression");
}, M.parseTemplateElement = function(e) {
var t = e.isTagged, r = this.startNode();
return this.type === i.invalidTemplate ? (t || this.raiseRecoverable(this.start, "Bad escape sequence in untagged template literal"), r.value = { raw: this.value, cooked: null }) : r.value = { raw: this.input.slice(this.start, this.end).replace(/\r\n?/g, `
`), cooked: this.value }, this.next(), r.tail = this.type === i.backQuote, this.finishNode(r, "TemplateElement");
}, M.parseTemplate = function(e) {
e === void 0 && (e = {});
var t = e.isTagged;
t === void 0 && (t = false);
var r = this.startNode();
this.next(), r.expressions = [];
var s = this.parseTemplateElement({ isTagged: t });
for (r.quasis = [s]; !s.tail; )
this.type === i.eof && this.raise(this.pos, "Unterminated template literal"), this.expect(i.dollarBraceL), r.expressions.push(this.parseExpression()), this.expect(i.braceR), r.quasis.push(s = this.parseTemplateElement({ isTagged: t }));
return this.next(), this.finishNode(r, "TemplateLiteral");
}, M.isAsyncProp = function(e) {
return !e.computed && e.key.type === "Identifier" && e.key.name === "async" && (this.type === i.name || this.type === i.num || this.type === i.string || this.type === i.bracketL || this.type.keyword || this.options.ecmaVersion >= 9 && this.type === i.star) && !S.test(this.input.slice(this.lastTokEnd, this.start));
}, M.parseObj = function(e, t) {
var r = this.startNode(), s = true, n2 = {};
for (r.properties = [], this.next(); !this.eat(i.braceR); ) {
if (s)
s = false;
else if (this.expect(i.comma), this.options.ecmaVersion >= 5 && this.afterTrailingComma(i.braceR))
break;
var h = this.parseProperty(e, t);
e || this.checkPropClash(h, n2, t), r.properties.push(h);
}
return this.finishNode(r, e ? "ObjectPattern" : "ObjectExpression");
}, M.parseProperty = function(e, t) {
var r = this.startNode(), s, n2, h, c;
if (this.options.ecmaVersion >= 9 && this.eat(i.ellipsis))
return e ? (r.argument = this.parseIdent(false), this.type === i.comma && this.raise(this.start, "Comma is not permitted after the rest element"), this.finishNode(r, "RestElement")) : (r.argument = this.parseMaybeAssign(false, t), this.type === i.comma && t && t.trailingComma < 0 && (t.trailingComma = this.start), this.finishNode(r, "SpreadElement"));
this.options.ecmaVersion >= 6 && (r.method = false, r.shorthand = false, (e || t) && (h = this.start, c = this.startLoc), e || (s = this.eat(i.star)));
var m = this.containsEsc;
return this.parsePropertyName(r), !e && !m && this.options.ecmaVersion >= 8 && !s && this.isAsyncProp(r) ? (n2 = true, s = this.options.ecmaVersion >= 9 && this.eat(i.star), this.parsePropertyName(r, t)) : n2 = false, this.parsePropertyValue(r, e, s, n2, h, c, t, m), this.finishNode(r, "Property");
}, M.parsePropertyValue = function(e, t, r, s, n2, h, c, m) {
if ((r || s) && this.type === i.colon && this.unexpected(), this.eat(i.colon))
e.value = t ? this.parseMaybeDefault(this.start, this.startLoc) : this.parseMaybeAssign(false, c), e.kind = "init";
else if (this.options.ecmaVersion >= 6 && this.type === i.parenL)
t && this.unexpected(), e.kind = "init", e.method = true, e.value = this.parseMethod(r, s);
else if (!t && !m && this.options.ecmaVersion >= 5 && !e.computed && e.key.type === "Identifier" && (e.key.name === "get" || e.key.name === "set") && this.type !== i.comma && this.type !== i.braceR && this.type !== i.eq) {
(r || s) && this.unexpected(), e.kind = e.key.name, this.parsePropertyName(e), e.value = this.parseMethod(false);
var A = e.kind === "get" ? 0 : 1;
if (e.value.params.length !== A) {
var q = e.value.start;
e.kind === "get" ? this.raiseRecoverable(q, "getter should have no params") : this.raiseRecoverable(q, "setter should have exactly one param");
} else
e.kind === "set" && e.value.params[0].type === "RestElement" && this.raiseRecoverable(e.value.params[0].start, "Setter cannot use rest params");
} else
this.options.ecmaVersion >= 6 && !e.computed && e.key.type === "Identifier" ? ((r || s) && this.unexpected(), this.checkUnreserved(e.key), e.key.name === "await" && !this.awaitIdentPos && (this.awaitIdentPos = n2), e.kind = "init", t ? e.value = this.parseMaybeDefault(n2, h, this.copyNode(e.key)) : this.type === i.eq && c ? (c.shorthandAssign < 0 && (c.shorthandAssign = this.start), e.value = this.parseMaybeDefault(n2, h, this.copyNode(e.key))) : e.value = this.copyNode(e.key), e.shorthand = true) : this.unexpected();
}, M.parsePropertyName = function(e) {
if (this.options.ecmaVersion >= 6) {
if (this.eat(i.bracketL))
return e.computed = true, e.key = this.parseMaybeAssign(), this.expect(i.bracketR), e.key;
e.computed = false;
}
return e.key = this.type === i.num || this.type === i.string ? this.parseExprAtom() : this.parseIdent(this.options.allowReserved !== "never");
}, M.initFunction = function(e) {
e.id = null, this.options.ecmaVersion >= 6 && (e.generator = e.expression = false), this.options.ecmaVersion >= 8 && (e.async = false);
}, M.parseMethod = function(e, t, r) {
var s = this.startNode(), n2 = this.yieldPos, h = this.awaitPos, c = this.awaitIdentPos;
return this.initFunction(s), this.options.ecmaVersion >= 6 && (s.generator = e), this.options.ecmaVersion >= 8 && (s.async = !!t), this.yieldPos = 0, this.awaitPos = 0, this.awaitIdentPos = 0, this.enterScope(xt(t, s.generator) | vt | (r ? gr : 0)), this.expect(i.parenL), s.params = this.parseBindingList(i.parenR, false, this.options.ecmaVersion >= 8), this.checkYieldAwaitInDefaultParams(), this.parseFunctionBody(s, false, true, false), this.yieldPos = n2, this.awaitPos = h, this.awaitIdentPos = c, this.finishNode(s, "FunctionExpression");
}, M.parseArrowExpression = function(e, t, r, s) {
var n2 = this.yieldPos, h = this.awaitPos, c = this.awaitIdentPos;
return this.enterScope(xt(r, false) | mr), this.initFunction(e), this.options.ecmaVersion >= 8 && (e.async = !!r), this.yieldPos = 0, this.awaitPos = 0, this.awaitIdentPos = 0, e.params = this.toAssignableList(t, true), this.parseFunctionBody(e, true, false, s), this.yieldPos = n2, this.awaitPos = h, this.awaitIdentPos = c, this.finishNode(e, "ArrowFunctionExpression");
}, M.parseFunctionBody = function(e, t, r, s) {
var n2 = t && this.type !== i.braceL, h = this.strict, c = false;
if (n2)
e.body = this.parseMaybeAssign(s), e.expression = true, this.checkParams(e, false);
else {
var m = this.options.ecmaVersion >= 7 && !this.isSimpleParamList(e.params);
(!h || m) && (c = this.strictDirective(this.end), c && m && this.raiseRecoverable(e.start, "Illegal 'use strict' directive in function with non-simple parameter list"));
var A = this.labels;
this.labels = [], c && (this.strict = true), this.checkParams(e, !h && !c && !t && !r && this.isSimpleParamList(e.params)), this.strict && e.id && this.checkLValSimple(e.id, Ar), e.body = this.parseBlock(false, void 0, c && !h), e.expression = false, this.adaptDirectivePrologue(e.body.body), this.labels = A;
}
this.exitScope();
}, M.isSimpleParamList = function(e) {
for (var t = 0, r = e; t < r.length; t += 1) {
var s = r[t];
if (s.type !== "Identifier")
return false;
}
return true;
}, M.checkParams = function(e, t) {
for (var r = /* @__PURE__ */ Object.create(null), s = 0, n2 = e.params; s < n2.length; s += 1) {
var h = n2[s];
this.checkLValInnerPattern(h, yt, t ? null : r);
}
}, M.parseExprList = function(e, t, r, s) {
for (var n2 = [], h = true; !this.eat(e); ) {
if (h)
h = false;
else if (this.expect(i.comma), t && this.afterTrailingComma(e))
break;
var c = void 0;
r && this.type === i.comma ? c = null : this.type === i.ellipsis ? (c = this.parseSpread(s), s && this.type === i.comma && s.trailingComma < 0 && (s.trailingComma = this.start)) : c = this.parseMaybeAssign(false, s), n2.push(c);
}
return n2;
}, M.checkUnreserved = function(e) {
var t = e.start, r = e.end, s = e.name;
if (this.inGenerator && s === "yield" && this.raiseRecoverable(t, "Cannot use 'yield' as identifier inside a generator"), this.inAsync && s === "await" && this.raiseRecoverable(t, "Cannot use 'await' as identifier inside an async function"), this.currentThisScope().inClassFieldInit && s === "arguments" && this.raiseRecoverable(t, "Cannot use 'arguments' in class field initializer"), this.inClassStaticBlock && (s === "arguments" || s === "await") && this.raise(t, "Cannot use " + s + " in class static initialization block"), this.keywords.test(s) && this.raise(t, "Unexpected keyword '" + s + "'"), !(this.options.ecmaVersion < 6 && this.input.slice(t, r).indexOf("\\") !== -1)) {
var n2 = this.strict ? this.reservedWordsStrict : this.reservedWords;
n2.test(s) && (!this.inAsync && s === "await" && this.raiseRecoverable(t, "Cannot use keyword 'await' outside an async function"), this.raiseRecoverable(t, "The keyword '" + s + "' is reserved"));
}
}, M.parseIdent = function(e, t) {
var r = this.startNode();
return this.type === i.name ? r.name = this.value : this.type.keyword ? (r.name = this.type.keyword, (r.name === "class" || r.name === "function") && (this.lastTokEnd !== this.lastTokStart + 1 || this.input.charCodeAt(this.lastTokStart) !== 46) && this.context.pop()) : this.unexpected(), this.next(!!e), this.finishNode(r, "Identifier"), e || (this.checkUnreserved(r), r.name === "await" && !this.awaitIdentPos && (this.awaitIdentPos = r.start)), r;
}, M.parsePrivateIdent = function() {
var e = this.startNode();
return this.type === i.privateId ? e.name = this.value : this.unexpected(), this.next(), this.finishNode(e, "PrivateIdentifier"), this.privateNameStack.length === 0 ? this.raise(e.start, "Private field '#" + e.name + "' must be declared in an enclosing class") : this.privateNameStack[this.privateNameStack.length - 1].used.push(e), e;
}, M.parseYield = function(e) {
this.yieldPos || (this.yieldPos = this.start);
var t = this.startNode();
return this.next(), this.type === i.semi || this.canInsertSemicolon() || this.type !== i.star && !this.type.startsExpr ? (t.delegate = false, t.argument = null) : (t.delegate = this.eat(i.star), t.argument = this.parseMaybeAssign(e)), this.finishNode(t, "YieldExpression");
}, M.parseAwait = function(e) {
this.awaitPos || (this.awaitPos = this.start);
var t = this.startNode();
return this.next(), t.argument = this.parseMaybeUnary(null, true, false, e), this.finishNode(t, "AwaitExpression");
};
var Xe = Y.prototype;
Xe.raise = function(e, t) {
var r = ae(this.input, e);
t += " (" + r.line + ":" + r.column + ")";
var s = new SyntaxError(t);
throw s.pos = e, s.loc = r, s.raisedAt = this.pos, s;
}, Xe.raiseRecoverable = Xe.raise, Xe.curPosition = function() {
if (this.options.locations)
return new H(this.curLine, this.pos - this.lineStart);
};
var Ee = Y.prototype, Za = function(t) {
this.flags = t, this.var = [], this.lexical = [], this.functions = [], this.inClassFieldInit = false;
};
Ee.enterScope = function(e) {
this.scopeStack.push(new Za(e));
}, Ee.exitScope = function() {
this.scopeStack.pop();
}, Ee.treatFunctionsAsVarInScope = function(e) {
return e.flags & Ce || !this.inModule && e.flags & _e;
}, Ee.declareName = function(e, t, r) {
var s = false;
if (t === ve) {
var n2 = this.currentScope();
s = n2.lexical.indexOf(e) > -1 || n2.functions.indexOf(e) > -1 || n2.var.indexOf(e) > -1, n2.lexical.push(e), this.inModule && n2.flags & _e && delete this.undefinedExports[e];
} else if (t === yr) {
var h = this.currentScope();
h.lexical.push(e);
} else if (t === xr) {
var c = this.currentScope();
this.treatFunctionsAsVar ? s = c.lexical.indexOf(e) > -1 : s = c.lexical.indexOf(e) > -1 || c.var.indexOf(e) > -1, c.functions.push(e);
} else
for (var m = this.scopeStack.length - 1; m >= 0; --m) {
var A = this.scopeStack[m];
if (A.lexical.indexOf(e) > -1 && !(A.flags & vr && A.lexical[0] === e) || !this.treatFunctionsAsVarInScope(A) && A.functions.indexOf(e) > -1) {
s = true;
break;
}
if (A.var.push(e), this.inModule && A.flags & _e && delete this.undefinedExports[e], A.flags & gt)
break;
}
s && this.raiseRecoverable(r, "Identifier '" + e + "' has already been declared");
}, Ee.checkLocalExport = function(e) {
this.scopeStack[0].lexical.indexOf(e.name) === -1 && this.scopeStack[0].var.indexOf(e.name) === -1 && (this.undefinedExports[e.name] = e);
}, Ee.currentScope = function() {
return this.scopeStack[this.scopeStack.length - 1];
}, Ee.currentVarScope = function() {
for (var e = this.scopeStack.length - 1; ; e--) {
var t = this.scopeStack[e];
if (t.flags & gt)
return t;
}
}, Ee.currentThisScope = function() {
for (var e = this.scopeStack.length - 1; ; e--) {
var t = this.scopeStack[e];
if (t.flags & gt && !(t.flags & mr))
return t;
}
};
var Re = function(t, r, s) {
this.type = "", this.start = r, this.end = 0, t.options.locations && (this.loc = new te(t, s)), t.options.directSourceFile && (this.sourceFile = t.options.directSourceFile), t.options.ranges && (this.range = [r, 0]);
}, je = Y.prototype;
je.startNode = function() {
return new Re(this, this.start, this.startLoc);
}, je.startNodeAt = function(e, t) {
return new Re(this, e, t);
};
function br(e, t, r, s) {
return e.type = t, e.end = r, this.options.locations && (e.loc.end = s), this.options.ranges && (e.range[1] = r), e;
}
je.finishNode = function(e, t) {
return br.call(this, e, t, this.lastTokEnd, this.lastTokEndLoc);
}, je.finishNodeAt = function(e, t, r, s) {
return br.call(this, e, t, r, s);
}, je.copyNode = function(e) {
var t = new Re(this, e.start, this.startLoc);
for (var r in e)
t[r] = e[r];
return t;
};
var _r = "ASCII ASCII_Hex_Digit AHex Alphabetic Alpha Any Assigned Bidi_Control Bidi_C Bidi_Mirrored Bidi_M Case_Ignorable CI Cased Changes_When_Casefolded CWCF Changes_When_Casemapped CWCM Changes_When_Lowercased CWL Changes_When_NFKC_Casefolded CWKCF Changes_When_Titlecased CWT Changes_When_Uppercased CWU Dash Default_Ignorable_Code_Point DI Deprecated Dep Diacritic Dia Emoji Emoji_Component Emoji_Modifier Emoji_Modifier_Base Emoji_Presentation Extender Ext Grapheme_Base Gr_Base Grapheme_Extend Gr_Ext Hex_Digit Hex IDS_Binary_Operator IDSB IDS_Trinary_Operator IDST ID_Continue IDC ID_Start IDS Ideographic Ideo Join_Control Join_C Logical_Order_Exception LOE Lowercase Lower Math Noncharacter_Code_Point NChar Pattern_Syntax Pat_Syn Pattern_White_Space Pat_WS Quotation_Mark QMark Radical Regional_Indicator RI Sentence_Terminal STerm Soft_Dotted SD Terminal_Punctuation Term Unified_Ideograph UIdeo Uppercase Upper Variation_Selector VS White_Space space XID_Continue XIDC XID_Start XIDS", Sr = _r + " Extended_Pictographic", wr = Sr, kr = wr + " EBase EComp EMod EPres ExtPict", en = kr, tn = { 9: _r, 10: Sr, 11: wr, 12: kr, 13: en }, Fr = "Cased_Letter LC Close_Punctuation Pe Connector_Punctuation Pc Control Cc cntrl Currency_Symbol Sc Dash_Punctuation Pd Decimal_Number Nd digit Enclosing_Mark Me Final_Punctuation Pf Format Cf Initial_Punctuation Pi Letter L Letter_Number Nl Line_Separator Zl Lowercase_Letter Ll Mark M Combining_Mark Math_Symbol Sm Modifier_Letter Lm Modifier_Symbol Sk Nonspacing_Mark Mn Number N Open_Punctuation Ps Other C Other_Letter Lo Other_Number No Other_Punctuation Po Other_Symbol So Paragraph_Separator Zp Private_Use Co Punctuation P punct Separator Z Space_Separator Zs Spacing_Mark Mc Surrogate Cs Symbol S Titlecase_Letter Lt Unassigned Cn Uppercase_Letter Lu", Br = "Adlam Adlm Ahom Anatolian_Hieroglyphs Hluw Arabic Arab Armenian Armn Avestan Avst Balinese Bali Bamum Bamu Bassa_Vah Bass Batak Batk Bengali Beng Bhaiksuki Bhks Bopomofo Bopo Brahmi Brah Braille Brai Buginese Bugi Buhid Buhd Canadian_Aboriginal Cans Carian Cari Caucasian_Albanian Aghb Chakma Cakm Cham Cham Cherokee Cher Common Zyyy Coptic Copt Qaac Cuneiform Xsux Cypriot Cprt Cyrillic Cyrl Deseret Dsrt Devanagari Deva Duployan Dupl Egyptian_Hieroglyphs Egyp Elbasan Elba Ethiopic Ethi Georgian Geor Glagolitic Glag Gothic Goth Grantha Gran Greek Grek Gujarati Gujr Gurmukhi Guru Han Hani Hangul Hang Hanunoo Hano Hatran Hatr Hebrew Hebr Hiragana Hira Imperial_Aramaic Armi Inherited Zinh Qaai Inscriptional_Pahlavi Phli Inscriptional_Parthian Prti Javanese Java Kaithi Kthi Kannada Knda Katakana Kana Kayah_Li Kali Kharoshthi Khar Khmer Khmr Khojki Khoj Khudawadi Sind Lao Laoo Latin Latn Lepcha Lepc Limbu Limb Linear_A Lina Linear_B Linb Lisu Lisu Lycian Lyci Lydian Lydi Mahajani Mahj Malayalam Mlym Mandaic Mand Manichaean Mani Marchen Marc Masaram_Gondi Gonm Meetei_Mayek Mtei Mende_Kikakui Mend Meroitic_Cursive Merc Meroitic_Hieroglyphs Mero Miao Plrd Modi Mongolian Mong Mro Mroo Multani Mult Myanmar Mymr Nabataean Nbat New_Tai_Lue Talu Newa Newa Nko Nkoo Nushu Nshu Ogham Ogam Ol_Chiki Olck Old_Hungarian Hung Old_Italic Ital Old_North_Arabian Narb Old_Permic Perm Old_Persian Xpeo Old_South_Arabian Sarb Old_Turkic Orkh Oriya Orya Osage Osge Osmanya Osma Pahawh_Hmong Hmng Palmyrene Palm Pau_Cin_Hau Pauc Phags_Pa Phag Phoenician Phnx Psalter_Pahlavi Phlp Rejang Rjng Runic Runr Samaritan Samr Saurashtra Saur Sharada Shrd Shavian Shaw Siddham Sidd SignWriting Sgnw Sinhala Sinh Sora_Sompeng Sora Soyombo Soyo Sundanese Sund Syloti_Nagri Sylo Syriac Syrc Tagalog Tglg Tagbanwa Tagb Tai_Le Tale Tai_Tham Lana Tai_Viet Tavt Takri Takr Tamil Taml Tangut Tang Telugu Telu Thaana Thaa Thai Thai Tibetan Tibt Tifinagh Tfng Tirhuta Tirh Ugaritic Ugar Vai Vaii Warang_Citi Wara Yi Yiii Zanabazar_Square Zanb", Ir = Br + " Dogra Dogr Gunjala_Gondi Gong Hanifi_Rohingya Rohg Makasar Maka Medefaidrin Medf Old_Sogdian Sogo Sogdian Sogd", Tr = Ir + " Elymaic Elym Nandinagari Nand Nyiakeng_Puachue_Hmong Hmnp Wancho Wcho", Pr = Tr + " Chorasmian Chrs Diak Dives_Akuru Khitan_Small_Script Kits Yezi Yezidi", rn = Pr + " Cypro_Minoan Cpmn Old_Uyghur Ougr Tangsa Tnsa Toto Vithkuqi Vith", sn = { 9: Br, 10: Ir, 11: Tr, 12: Pr, 13: rn }, Dr = {};
function an(e) {
var t = Dr[e] = { binary: d(tn[e] + " " + Fr), nonBinary: { General_Category: d(Fr), Script: d(sn[e]) } };
t.nonBinary.Script_Extensions = t.nonBinary.Script, t.nonBinary.gc = t.nonBinary.General_Category, t.nonBinary.sc = t.nonBinary.Script, t.nonBinary.scx = t.nonBinary.Script_Extensions;
}
for (var Et = 0, Nr = [9, 10, 11, 12, 13]; Et < Nr.length; Et += 1) {
var nn = Nr[Et];
an(nn);
}
var N = Y.prototype, ge = function(t) {
this.parser = t, this.validFlags = "gim" + (t.options.ecmaVersion >= 6 ? "uy" : "") + (t.options.ecmaVersion >= 9 ? "s" : "") + (t.options.ecmaVersion >= 13 ? "d" : ""), this.unicodeProperties = Dr[t.options.ecmaVersion >= 13 ? 13 : t.options.ecmaVersion], this.source = "", this.flags = "", this.start = 0, this.switchU = false, this.switchN = false, this.pos = 0, this.lastIntValue = 0, this.lastStringValue = "", this.lastAssertionIsQuantifiable = false, this.numCapturingParens = 0, this.maxBackReference = 0, this.groupNames = [], this.backReferenceNames = [];
};
ge.prototype.reset = function(t, r, s) {
var n2 = s.indexOf("u") !== -1;
this.start = t | 0, this.source = r + "", this.flags = s, this.switchU = n2 && this.parser.options.ecmaVersion >= 6, this.switchN = n2 && this.parser.options.ecmaVersion >= 9;
}, ge.prototype.raise = function(t) {
this.parser.raiseRecoverable(this.start, "Invalid regular expression: /" + this.source + "/: " + t);
}, ge.prototype.at = function(t, r) {
r === void 0 && (r = false);
var s = this.source, n2 = s.length;
if (t >= n2)
return -1;
var h = s.charCodeAt(t);
if (!(r || this.switchU) || h <= 55295 || h >= 57344 || t + 1 >= n2)
return h;
var c = s.charCodeAt(t + 1);
return c >= 56320 && c <= 57343 ? (h << 10) + c - 56613888 : h;
}, ge.prototype.nextIndex = function(t, r) {
r === void 0 && (r = false);
var s = this.source, n2 = s.length;
if (t >= n2)
return n2;
var h = s.charCodeAt(t), c;
return !(r || this.switchU) || h <= 55295 || h >= 57344 || t + 1 >= n2 || (c = s.charCodeAt(t + 1)) < 56320 || c > 57343 ? t + 1 : t + 2;
}, ge.prototype.current = function(t) {
return t === void 0 && (t = false), this.at(this.pos, t);
}, ge.prototype.lookahead = function(t) {
return t === void 0 && (t = false), this.at(this.nextIndex(this.pos, t), t);
}, ge.prototype.advance = function(t) {
t === void 0 && (t = false), this.pos = this.nextIndex(this.pos, t);
}, ge.prototype.eat = function(t, r) {
return r === void 0 && (r = false), this.current(r) === t ? (this.advance(r), true) : false;
}, N.validateRegExpFlags = function(e) {
for (var t = e.validFlags, r = e.flags, s = 0; s < r.length; s++) {
var n2 = r.charAt(s);
t.indexOf(n2) === -1 && this.raise(e.start, "Invalid regular expression flag"), r.indexOf(n2, s + 1) > -1 && this.raise(e.start, "Duplicate regular expression flag");
}
}, N.validateRegExpPattern = function(e) {
this.regexp_pattern(e), !e.switchN && this.options.ecmaVersion >= 9 && e.groupNames.length > 0 && (e.switchN = true, this.regexp_pattern(e));
}, N.regexp_pattern = function(e) {
e.pos = 0, e.lastIntValue = 0, e.lastStringValue = "", e.lastAssertionIsQuantifiable = false, e.numCapturingParens = 0, e.maxBackReference = 0, e.groupNames.length = 0, e.backReferenceNames.length = 0, this.regexp_disjunction(e), e.pos !== e.source.length && (e.eat(41) && e.raise("Unmatched ')'"), (e.eat(93) || e.eat(125)) && e.raise("Lone quantifier brackets")), e.maxBackReference > e.numCapturingParens && e.raise("Invalid escape");
for (var t = 0, r = e.backReferenceNames; t < r.length; t += 1) {
var s = r[t];
e.groupNames.indexOf(s) === -1 && e.raise("Invalid named capture referenced");
}
}, N.regexp_disjunction = function(e) {
for (this.regexp_alternative(e); e.eat(124); )
this.regexp_alternative(e);
this.regexp_eatQuantifier(e, true) && e.raise("Nothing to repeat"), e.eat(123) && e.raise("Lone quantifier brackets");
}, N.regexp_alternative = function(e) {
for (; e.pos < e.source.length && this.regexp_eatTerm(e); )
;
}, N.regexp_eatTerm = function(e) {
return this.regexp_eatAssertion(e) ? (e.lastAssertionIsQuantifiable && this.regexp_eatQuantifier(e) && e.switchU && e.raise("Invalid quantifier"), true) : (e.switchU ? this.regexp_eatAtom(e) : this.regexp_eatExtendedAtom(e)) ? (this.regexp_eatQuantifier(e), true) : false;
}, N.regexp_eatAssertion = function(e) {
var t = e.pos;
if (e.lastAssertionIsQuantifiable = false, e.eat(94) || e.eat(36))
return true;
if (e.eat(92)) {
if (e.eat(66) || e.eat(98))
return true;
e.pos = t;
}
if (e.eat(40) && e.eat(63)) {
var r = false;
if (this.options.ecmaVersion >= 9 && (r = e.eat(60)), e.eat(61) || e.eat(33))
return this.regexp_disjunction(e), e.eat(41) || e.raise("Unterminated group"), e.lastAssertionIsQuantifiable = !r, true;
}
return e.pos = t, false;
}, N.regexp_eatQuantifier = function(e, t) {
return t === void 0 && (t = false), this.regexp_eatQuantifierPrefix(e, t) ? (e.eat(63), true) : false;
}, N.regexp_eatQuantifierPrefix = function(e, t) {
return e.eat(42) || e.eat(43) || e.eat(63) || this.regexp_eatBracedQuantifier(e, t);
}, N.regexp_eatBracedQuantifier = function(e, t) {
var r = e.pos;
if (e.eat(123)) {
var s = 0, n2 = -1;
if (this.regexp_eatDecimalDigits(e) && (s = e.lastIntValue, e.eat(44) && this.regexp_eatDecimalDigits(e) && (n2 = e.lastIntValue), e.eat(125)))
return n2 !== -1 && n2 < s && !t && e.raise("numbers out of order in {} quantifier"), true;
e.switchU && !t && e.raise("Incomplete quantifier"), e.pos = r;
}
return false;
}, N.regexp_eatAtom = function(e) {
return this.regexp_eatPatternCharacters(e) || e.eat(46) || this.regexp_eatReverseSolidusAtomEscape(e) || this.regexp_eatCharacterClass(e) || this.regexp_eatUncapturingGroup(e) || this.regexp_eatCapturingGroup(e);
}, N.regexp_eatReverseSolidusAtomEscape = function(e) {
var t = e.pos;
if (e.eat(92)) {
if (this.regexp_eatAtomEscape(e))
return true;
e.pos = t;
}
return false;
}, N.regexp_eatUncapturingGroup = function(e) {
var t = e.pos;
if (e.eat(40)) {
if (e.eat(63) && e.eat(58)) {
if (this.regexp_disjunction(e), e.eat(41))
return true;
e.raise("Unterminated group");
}
e.pos = t;
}
return false;
}, N.regexp_eatCapturingGroup = function(e) {
if (e.eat(40)) {
if (this.options.ecmaVersion >= 9 ? this.regexp_groupSpecifier(e) : e.current() === 63 && e.raise("Invalid group"), this.regexp_disjunction(e), e.eat(41))
return e.numCapturingParens += 1, true;
e.raise("Unterminated group");
}
return false;
}, N.regexp_eatExtendedAtom = function(e) {
return e.eat(46) || this.regexp_eatReverseSolidusAtomEscape(e) || this.regexp_eatCharacterClass(e) || this.regexp_eatUncapturingGroup(e) || this.regexp_eatCapturingGroup(e) || this.regexp_eatInvalidBracedQuantifier(e) || this.regexp_eatExtendedPatternCharacter(e);
}, N.regexp_eatInvalidBracedQuantifier = function(e) {
return this.regexp_eatBracedQuantifier(e, true) && e.raise("Nothing to repeat"), false;
}, N.regexp_eatSyntaxCharacter = function(e) {
var t = e.current();
return Or(t) ? (e.lastIntValue = t, e.advance(), true) : false;
};
function Or(e) {
return e === 36 || e >= 40 && e <= 43 || e === 46 || e === 63 || e >= 91 && e <= 94 || e >= 123 && e <= 125;
}
N.regexp_eatPatternCharacters = function(e) {
for (var t = e.pos, r = 0; (r = e.current()) !== -1 && !Or(r); )
e.advance();
return e.pos !== t;
}, N.regexp_eatExtendedPatternCharacter = function(e) {
var t = e.current();
return t !== -1 && t !== 36 && !(t >= 40 && t <= 43) && t !== 46 && t !== 63 && t !== 91 && t !== 94 && t !== 124 ? (e.advance(), true) : false;
}, N.regexp_groupSpecifier = function(e) {
if (e.eat(63)) {
if (this.regexp_eatGroupName(e)) {
e.groupNames.indexOf(e.lastStringValue) !== -1 && e.raise("Duplicate capture group name"), e.groupNames.push(e.lastStringValue);
return;
}
e.raise("Invalid group");
}
}, N.regexp_eatGroupName = function(e) {
if (e.lastStringValue = "", e.eat(60)) {
if (this.regexp_eatRegExpIdentifierName(e) && e.eat(62))
return true;
e.raise("Invalid capture group name");
}
return false;
}, N.regexp_eatRegExpIdentifierName = function(e) {
if (e.lastStringValue = "", this.regexp_eatRegExpIdentifierStart(e)) {
for (e.lastStringValue += E(e.lastIntValue); this.regexp_eatRegExpIdentifierPart(e); )
e.lastStringValue += E(e.lastIntValue);
return true;
}
return false;
}, N.regexp_eatRegExpIdentifierStart = function(e) {
var t = e.pos, r = this.options.ecmaVersion >= 11, s = e.current(r);
return e.advance(r), s === 92 && this.regexp_eatRegExpUnicodeEscapeSequence(e, r) && (s = e.lastIntValue), un(s) ? (e.lastIntValue = s, true) : (e.pos = t, false);
};
function un(e) {
return w(e, true) || e === 36 || e === 95;
}
N.regexp_eatRegExpIdentifierPart = function(e) {
var t = e.pos, r = this.options.ecmaVersion >= 11, s = e.current(r);
return e.advance(r), s === 92 && this.regexp_eatRegExpUnicodeEscapeSequence(e, r) && (s = e.lastIntValue), on(s) ? (e.lastIntValue = s, true) : (e.pos = t, false);
};
function on(e) {
return G(e, true) || e === 36 || e === 95 || e === 8204 || e === 8205;
}
N.regexp_eatAtomEscape = function(e) {
return this.regexp_eatBackReference(e) || this.regexp_eatCharacterClassEscape(e) || this.regexp_eatCharacterEscape(e) || e.switchN && this.regexp_eatKGroupName(e) ? true : (e.switchU && (e.current() === 99 && e.raise("Invalid unicode escape"), e.raise("Invalid escape")), false);
}, N.regexp_eatBackReference = function(e) {
var t = e.pos;
if (this.regexp_eatDecimalEscape(e)) {
var r = e.lastIntValue;
if (e.switchU)
return r > e.maxBackReference && (e.maxBackReference = r), true;
if (r <= e.numCapturingParens)
return true;
e.pos = t;
}
return false;
}, N.regexp_eatKGroupName = function(e) {
if (e.eat(107)) {
if (this.regexp_eatGroupName(e))
return e.backReferenceNames.push(e.lastStringValue), true;
e.raise("Invalid named reference");
}
return false;
}, N.regexp_eatCharacterEscape = function(e) {
return this.regexp_eatControlEscape(e) || this.regexp_eatCControlLetter(e) || this.regexp_eatZero(e) || this.regexp_eatHexEscapeSequence(e) || this.regexp_eatRegExpUnicodeEscapeSequence(e, false) || !e.switchU && this.regexp_eatLegacyOctalEscapeSequence(e) || this.regexp_eatIdentityEscape(e);
}, N.regexp_eatCControlLetter = function(e) {
var t = e.pos;
if (e.eat(99)) {
if (this.regexp_eatControlLetter(e))
return true;
e.pos = t;
}
return false;
}, N.regexp_eatZero = function(e) {
return e.current() === 48 && !Je(e.lookahead()) ? (e.lastIntValue = 0, e.advance(), true) : false;
}, N.regexp_eatControlEscape = function(e) {
var t = e.current();
return t === 116 ? (e.lastIntValue = 9, e.advance(), true) : t === 110 ? (e.lastIntValue = 10, e.advance(), true) : t === 118 ? (e.lastIntValue = 11, e.advance(), true) : t === 102 ? (e.lastIntValue = 12, e.advance(), true) : t === 114 ? (e.lastIntValue = 13, e.advance(), true) : false;
}, N.regexp_eatControlLetter = function(e) {
var t = e.current();
return Lr(t) ? (e.lastIntValue = t % 32, e.advance(), true) : false;
};
function Lr(e) {
return e >= 65 && e <= 90 || e >= 97 && e <= 122;
}
N.regexp_eatRegExpUnicodeEscapeSequence = function(e, t) {
t === void 0 && (t = false);
var r = e.pos, s = t || e.switchU;
if (e.eat(117)) {
if (this.regexp_eatFixedHexDigits(e, 4)) {
var n2 = e.lastIntValue;
if (s && n2 >= 55296 && n2 <= 56319) {
var h = e.pos;
if (e.eat(92) && e.eat(117) && this.regexp_eatFixedHexDigits(e, 4)) {
var c = e.lastIntValue;
if (c >= 56320 && c <= 57343)
return e.lastIntValue = (n2 - 55296) * 1024 + (c - 56320) + 65536, true;
}
e.pos = h, e.lastIntValue = n2;
}
return true;
}
if (s && e.eat(123) && this.regexp_eatHexDigits(e) && e.eat(125) && hn(e.lastIntValue))
return true;
s && e.raise("Invalid unicode escape"), e.pos = r;
}
return false;
};
function hn(e) {
return e >= 0 && e <= 1114111;
}
N.regexp_eatIdentityEscape = function(e) {
if (e.switchU)
return this.regexp_eatSyntaxCharacter(e) ? true : e.eat(47) ? (e.lastIntValue = 47, true) : false;
var t = e.current();
return t !== 99 && (!e.switchN || t !== 107) ? (e.lastIntValue = t, e.advance(), true) : false;
}, N.regexp_eatDecimalEscape = function(e) {
e.lastIntValue = 0;
var t = e.current();
if (t >= 49 && t <= 57) {
do
e.lastIntValue = 10 * e.lastIntValue + (t - 48), e.advance();
while ((t = e.current()) >= 48 && t <= 57);
return true;
}
return false;
}, N.regexp_eatCharacterClassEscape = function(e) {
var t = e.current();
if (ln(t))
return e.lastIntValue = -1, e.advance(), true;
if (e.switchU && this.options.ecmaVersion >= 9 && (t === 80 || t === 112)) {
if (e.lastIntValue = -1, e.advance(), e.eat(123) && this.regexp_eatUnicodePropertyValueExpression(e) && e.eat(125))
return true;
e.raise("Invalid property name");
}
return false;
};
function ln(e) {
return e === 100 || e === 68 || e === 115 || e === 83 || e === 119 || e === 87;
}
N.regexp_eatUnicodePropertyValueExpression = function(e) {
var t = e.pos;
if (this.regexp_eatUnicodePropertyName(e) && e.eat(61)) {
var r = e.lastStringValue;
if (this.regexp_eatUnicodePropertyValue(e)) {
var s = e.lastStringValue;
return this.regexp_validateUnicodePropertyNameAndValue(e, r, s), true;
}
}
if (e.pos = t, this.regexp_eatLoneUnicodePropertyNameOrValue(e)) {
var n2 = e.lastStringValue;
return this.regexp_validateUnicodePropertyNameOrValue(e, n2), true;
}
return false;
}, N.regexp_validateUnicodePropertyNameAndValue = function(e, t, r) {
P(e.unicodeProperties.nonBinary, t) || e.raise("Invalid property name"), e.unicodeProperties.nonBinary[t].test(r) || e.raise("Invalid property value");
}, N.regexp_validateUnicodePropertyNameOrValue = function(e, t) {
e.unicodeProperties.binary.test(t) || e.raise("Invalid property name");
}, N.regexp_eatUnicodePropertyName = function(e) {
var t = 0;
for (e.lastStringValue = ""; Vr(t = e.current()); )
e.lastStringValue += E(t), e.advance();
return e.lastStringValue !== "";
};
function Vr(e) {
return Lr(e) || e === 95;
}
N.regexp_eatUnicodePropertyValue = function(e) {
var t = 0;
for (e.lastStringValue = ""; cn(t = e.current()); )
e.lastStringValue += E(t), e.advance();
return e.lastStringValue !== "";
};
function cn(e) {
return Vr(e) || Je(e);
}
N.regexp_eatLoneUnicodePropertyNameOrValue = function(e) {
return this.regexp_eatUnicodePropertyValue(e);
}, N.regexp_eatCharacterClass = function(e) {
if (e.eat(91)) {
if (e.eat(94), this.regexp_classRanges(e), e.eat(93))
return true;
e.raise("Unterminated character class");
}
return false;
}, N.regexp_classRanges = function(e) {
for (; this.regexp_eatClassAtom(e); ) {
var t = e.lastIntValue;
if (e.eat(45) && this.regexp_eatClassAtom(e)) {
var r = e.lastIntValue;
e.switchU && (t === -1 || r === -1) && e.raise("Invalid character class"), t !== -1 && r !== -1 && t > r && e.raise("Range out of order in character class");
}
}
}, N.regexp_eatClassAtom = function(e) {
var t = e.pos;
if (e.eat(92)) {
if (this.regexp_eatClassEscape(e))
return true;
if (e.switchU) {
var r = e.current();
(r === 99 || qr(r)) && e.raise("Invalid class escape"), e.raise("Invalid escape");
}
e.pos = t;
}
var s = e.current();
return s !== 93 ? (e.lastIntValue = s, e.advance(), true) : false;
}, N.regexp_eatClassEscape = function(e) {
var t = e.pos;
if (e.eat(98))
return e.lastIntValue = 8, true;
if (e.switchU && e.eat(45))
return e.lastIntValue = 45, true;
if (!e.switchU && e.eat(99)) {
if (this.regexp_eatClassControlLetter(e))
return true;
e.pos = t;
}
return this.regexp_eatCharacterClassEscape(e) || this.regexp_eatCharacterEscape(e);
}, N.regexp_eatClassControlLetter = function(e) {
var t = e.current();
return Je(t) || t === 95 ? (e.lastIntValue = t % 32, e.advance(), true) : false;
}, N.regexp_eatHexEscapeSequence = function(e) {
var t = e.pos;
if (e.eat(120)) {
if (this.regexp_eatFixedHexDigits(e, 2))
return true;
e.switchU && e.raise("Invalid escape"), e.pos = t;
}
return false;
}, N.regexp_eatDecimalDigits = function(e) {
var t = e.pos, r = 0;
for (e.lastIntValue = 0; Je(r = e.current()); )
e.lastIntValue = 10 * e.lastIntValue + (r - 48), e.advance();
return e.pos !== t;
};
function Je(e) {
return e >= 48 && e <= 57;
}
N.regexp_eatHexDigits = function(e) {
var t = e.pos, r = 0;
for (e.lastIntValue = 0; Rr(r = e.current()); )
e.lastIntValue = 16 * e.lastIntValue + jr(r), e.advance();
return e.pos !== t;
};
function Rr(e) {
return e >= 48 && e <= 57 || e >= 65 && e <= 70 || e >= 97 && e <= 102;
}
function jr(e) {
return e >= 65 && e <= 70 ? 10 + (e - 65) : e >= 97 && e <= 102 ? 10 + (e - 97) : e - 48;
}
N.regexp_eatLegacyOctalEscapeSequence = function(e) {
if (this.regexp_eatOctalDigit(e)) {
var t = e.lastIntValue;
if (this.regexp_eatOctalDigit(e)) {
var r = e.lastIntValue;
t <= 3 && this.regexp_eatOctalDigit(e) ? e.lastIntValue = t * 64 + r * 8 + e.lastIntValue : e.lastIntValue = t * 8 + r;
} else
e.lastIntValue = t;
return true;
}
return false;
}, N.regexp_eatOctalDigit = function(e) {
var t = e.current();
return qr(t) ? (e.lastIntValue = t - 48, e.advance(), true) : (e.lastIntValue = 0, false);
};
function qr(e) {
return e >= 48 && e <= 55;
}
N.regexp_eatFixedHexDigits = function(e, t) {
var r = e.pos;
e.lastIntValue = 0;
for (var s = 0; s < t; ++s) {
var n2 = e.current();
if (!Rr(n2))
return e.pos = r, false;
e.lastIntValue = 16 * e.lastIntValue + jr(n2), e.advance();
}
return true;
};
var Qe = function(t) {
this.type = t.type, this.value = t.value, this.start = t.start, this.end = t.end, t.options.locations && (this.loc = new te(t, t.startLoc, t.endLoc)), t.options.ranges && (this.range = [t.start, t.end]);
}, z = Y.prototype;
z.next = function(e) {
!e && this.type.keyword && this.containsEsc && this.raiseRecoverable(this.start, "Escape sequence in keyword " + this.type.keyword), this.options.onToken && this.options.onToken(new Qe(this)), this.lastTokEnd = this.end, this.lastTokStart = this.start, this.lastTokEndLoc = this.endLoc, this.lastTokStartLoc = this.startLoc, this.nextToken();
}, z.getToken = function() {
return this.next(), new Qe(this);
}, typeof Symbol < "u" && (z[Symbol.iterator] = function() {
var e = this;
return { next: function() {
var t = e.getToken();
return { done: t.type === i.eof, value: t };
} };
}), z.nextToken = function() {
var e = this.curContext();
if ((!e || !e.preserveSpace) && this.skipSpace(), this.start = this.pos, this.options.locations && (this.startLoc = this.curPosition()), this.pos >= this.input.length)
return this.finishToken(i.eof);
if (e.override)
return e.override(this);
this.readToken(this.fullCharCodeAtPos());
}, z.readToken = function(e) {
return w(e, this.options.ecmaVersion >= 6) || e === 92 ? this.readWord() : this.getTokenFromCode(e);
}, z.fullCharCodeAtPos = function() {
var e = this.input.charCodeAt(this.pos);
if (e <= 55295 || e >= 56320)
return e;
var t = this.input.charCodeAt(this.pos + 1);
return t <= 56319 || t >= 57344 ? e : (e << 10) + t - 56613888;
}, z.skipBlockComment = function() {
var e = this.options.onComment && this.curPosition(), t = this.pos, r = this.input.indexOf("*/", this.pos += 2);
if (r === -1 && this.raise(this.pos - 2, "Unterminated comment"), this.pos = r + 2, this.options.locations)
for (var s = void 0, n2 = t; (s = Z(this.input, n2, this.pos)) > -1; )
++this.curLine, n2 = this.lineStart = s;
this.options.onComment && this.options.onComment(true, this.input.slice(t + 2, r), t, this.pos, e, this.curPosition());
}, z.skipLineComment = function(e) {
for (var t = this.pos, r = this.options.onComment && this.curPosition(), s = this.input.charCodeAt(this.pos += e); this.pos < this.input.length && !j(s); )
s = this.input.charCodeAt(++this.pos);
this.options.onComment && this.options.onComment(false, this.input.slice(t + e, this.pos), t, this.pos, r, this.curPosition());
}, z.skipSpace = function() {
e:
for (; this.pos < this.input.length; ) {
var e = this.input.charCodeAt(this.pos);
switch (e) {
case 32:
case 160:
++this.pos;
break;
case 13:
this.input.charCodeAt(this.pos + 1) === 10 && ++this.pos;
case 10:
case 8232:
case 8233:
++this.pos, this.options.locations && (++this.curLine, this.lineStart = this.pos);
break;
case 47:
switch (this.input.charCodeAt(this.pos + 1)) {
case 42:
this.skipBlockComment();
break;
case 47:
this.skipLineComment(2);
break;
default:
break e;
}
break;
default:
if (e > 8 && e < 14 || e >= 5760 && ne.test(String.fromCharCode(e)))
++this.pos;
else
break e;
}
}
}, z.finishToken = function(e, t) {
this.end = this.pos, this.options.locations && (this.endLoc = this.curPosition());
var r = this.type;
this.type = e, this.value = t, this.updateContext(r);
}, z.readToken_dot = function() {
var e = this.input.charCodeAt(this.pos + 1);
if (e >= 48 && e <= 57)
return this.readNumber(true);
var t = this.input.charCodeAt(this.pos + 2);
return this.options.ecmaVersion >= 6 && e === 46 && t === 46 ? (this.pos += 3, this.finishToken(i.ellipsis)) : (++this.pos, this.finishToken(i.dot));
}, z.readToken_slash = function() {
var e = this.input.charCodeAt(this.pos + 1);
return this.exprAllowed ? (++this.pos, this.readRegexp()) : e === 61 ? this.finishOp(i.assign, 2) : this.finishOp(i.slash, 1);
}, z.readToken_mult_modulo_exp = function(e) {
var t = this.input.charCodeAt(this.pos + 1), r = 1, s = e === 42 ? i.star : i.modulo;
return this.options.ecmaVersion >= 7 && e === 42 && t === 42 && (++r, s = i.starstar, t = this.input.charCodeAt(this.pos + 2)), t === 61 ? this.finishOp(i.assign, r + 1) : this.finishOp(s, r);
}, z.readToken_pipe_amp = function(e) {
var t = this.input.charCodeAt(this.pos + 1);
if (t === e) {
if (this.options.ecmaVersion >= 12) {
var r = this.input.charCodeAt(this.pos + 2);
if (r === 61)
return this.finishOp(i.assign, 3);
}
return this.finishOp(e === 124 ? i.logicalOR : i.logicalAND, 2);
}
return t === 61 ? this.finishOp(i.assign, 2) : this.finishOp(e === 124 ? i.bitwiseOR : i.bitwiseAND, 1);
}, z.readToken_caret = function() {
var e = this.input.charCodeAt(this.pos + 1);
return e === 61 ? this.finishOp(i.assign, 2) : this.finishOp(i.bitwiseXOR, 1);
}, z.readToken_plus_min = function(e) {
var t = this.input.charCodeAt(this.pos + 1);
return t === e ? t === 45 && !this.inModule && this.input.charCodeAt(this.pos + 2) === 62 && (this.lastTokEnd === 0 || S.test(this.input.slice(this.lastTokEnd, this.pos))) ? (this.skipLineComment(3), this.skipSpace(), this.nextToken()) : this.finishOp(i.incDec, 2) : t === 61 ? this.finishOp(i.assign, 2) : this.finishOp(i.plusMin, 1);
}, z.readToken_lt_gt = function(e) {
var t = this.input.charCodeAt(this.pos + 1), r = 1;
return t === e ? (r = e === 62 && this.input.charCodeAt(this.pos + 2) === 62 ? 3 : 2, this.input.charCodeAt(this.pos + r) === 61 ? this.finishOp(i.assign, r + 1) : this.finishOp(i.bitShift, r)) : t === 33 && e === 60 && !this.inModule && this.input.charCodeAt(this.pos + 2) === 45 && this.input.charCodeAt(this.pos + 3) === 45 ? (this.skipLineComment(4), this.skipSpace(), this.nextToken()) : (t === 61 && (r = 2), this.finishOp(i.relational, r));
}, z.readToken_eq_excl = function(e) {
var t = this.input.charCodeAt(this.pos + 1);
return t === 61 ? this.finishOp(i.equality, this.input.charCodeAt(this.pos + 2) === 61 ? 3 : 2) : e === 61 && t === 62 && this.options.ecmaVersion >= 6 ? (this.pos += 2, this.finishToken(i.arrow)) : this.finishOp(e === 61 ? i.eq : i.prefix, 1);
}, z.readToken_question = function() {
var e = this.options.ecmaVersion;
if (e >= 11) {
var t = this.input.charCodeAt(this.pos + 1);
if (t === 46) {
var r = this.input.charCodeAt(this.pos + 2);
if (r < 48 || r > 57)
return this.finishOp(i.questionDot, 2);
}
if (t === 63) {
if (e >= 12) {
var s = this.input.charCodeAt(this.pos + 2);
if (s === 61)
return this.finishOp(i.assign, 3);
}
return this.finishOp(i.coalesce, 2);
}
}
return this.finishOp(i.question, 1);
}, z.readToken_numberSign = function() {
var e = this.options.ecmaVersion, t = 35;
if (e >= 13 && (++this.pos, t = this.fullCharCodeAtPos(), w(t, true) || t === 92))
return this.finishToken(i.privateId, this.readWord1());
this.raise(this.pos, "Unexpected character '" + E(t) + "'");
}, z.getTokenFromCode = function(e) {
switch (e) {
case 46:
return this.readToken_dot();
case 40:
return ++this.pos, this.finishToken(i.parenL);
case 41:
return ++this.pos, this.finishToken(i.parenR);
case 59:
return ++this.pos, this.finishToken(i.semi);
case 44:
return ++this.pos, this.finishToken(i.comma);
case 91:
return ++this.pos, this.finishToken(i.bracketL);
case 93:
return ++this.pos, this.finishToken(i.bracketR);
case 123:
return ++this.pos, this.finishToken(i.braceL);
case 125:
return ++this.pos, this.finishToken(i.braceR);
case 58:
return ++this.pos, this.finishToken(i.colon);
case 96:
if (this.options.ecmaVersion < 6)
break;
return ++this.pos, this.finishToken(i.backQuote);
case 48:
var t = this.input.charCodeAt(this.pos + 1);
if (t === 120 || t === 88)
return this.readRadixNumber(16);
if (this.options.ecmaVersion >= 6) {
if (t === 111 || t === 79)
return this.readRadixNumber(8);
if (t === 98 || t === 66)
return this.readRadixNumber(2);
}
case 49:
case 50:
case 51:
case 52:
case 53:
case 54:
case 55:
case 56:
case 57:
return this.readNumber(false);
case 34:
case 39:
return this.readString(e);
case 47:
return this.readToken_slash();
case 37:
case 42:
return this.readToken_mult_modulo_exp(e);
case 124:
case 38:
return this.readToken_pipe_amp(e);
case 94:
return this.readToken_caret();
case 43:
case 45:
return this.readToken_plus_min(e);
case 60:
case 62:
return this.readToken_lt_gt(e);
case 61:
case 33:
return this.readToken_eq_excl(e);
case 63:
return this.readToken_question();
case 126:
return this.finishOp(i.prefix, 1);
case 35:
return this.readToken_numberSign();
}
this.raise(this.pos, "Unexpected character '" + E(e) + "'");
}, z.finishOp = function(e, t) {
var r = this.input.slice(this.pos, this.pos + t);
return this.pos += t, this.finishToken(e, r);
}, z.readRegexp = function() {
for (var e, t, r = this.pos; ; ) {
this.pos >= this.input.length && this.raise(r, "Unterminated regular expression");
var s = this.input.charAt(this.pos);
if (S.test(s) && this.raise(r, "Unterminated regular expression"), e)
e = false;
else {
if (s === "[")
t = true;
else if (s === "]" && t)
t = false;
else if (s === "/" && !t)
break;
e = s === "\\";
}
++this.pos;
}
var n2 = this.input.slice(r, this.pos);
++this.pos;
var h = this.pos, c = this.readWord1();
this.containsEsc && this.unexpected(h);
var m = this.regexpState || (this.regexpState = new ge(this));
m.reset(r, n2, c), this.validateRegExpFlags(m), this.validateRegExpPattern(m);
var A = null;
try {
A = new RegExp(n2, c);
} catch {
}
return this.finishToken(i.regexp, { pattern: n2, flags: c, value: A });
}, z.readInt = function(e, t, r) {
for (var s = this.options.ecmaVersion >= 12 && t === void 0, n2 = r && this.input.charCodeAt(this.pos) === 48, h = this.pos, c = 0, m = 0, A = 0, q = t == null ? 1 / 0 : t; A < q; ++A, ++this.pos) {
var W = this.input.charCodeAt(this.pos), re = void 0;
if (s && W === 95) {
n2 && this.raiseRecoverable(this.pos, "Numeric separator is not allowed in legacy octal numeric literals"), m === 95 && this.raiseRecoverable(this.pos, "Numeric separator must be exactly one underscore"), A === 0 && this.raiseRecoverable(this.pos, "Numeric separator is not allowed at the first of digits"), m = W;
continue;
}
if (W >= 97 ? re = W - 97 + 10 : W >= 65 ? re = W - 65 + 10 : W >= 48 && W <= 57 ? re = W - 48 : re = 1 / 0, re >= e)
break;
m = W, c = c * e + re;
}
return s && m === 95 && this.raiseRecoverable(this.pos - 1, "Numeric separator is not allowed at the last of digits"), this.pos === h || t != null && this.pos - h !== t ? null : c;
};
function pn(e, t) {
return t ? parseInt(e, 8) : parseFloat(e.replace(/_/g, ""));
}
function Mr(e) {
return typeof BigInt != "function" ? null : BigInt(e.replace(/_/g, ""));
}
z.readRadixNumber = function(e) {
var t = this.pos;
this.pos += 2;
var r = this.readInt(e);
return r == null && this.raise(this.start + 2, "Expected number in radix " + e), this.options.ecmaVersion >= 11 && this.input.charCodeAt(this.pos) === 110 ? (r = Mr(this.input.slice(t, this.pos)), ++this.pos) : w(this.fullCharCodeAtPos()) && this.raise(this.pos, "Identifier directly after number"), this.finishToken(i.num, r);
}, z.readNumber = function(e) {
var t = this.pos;
!e && this.readInt(10, void 0, true) === null && this.raise(t, "Invalid number");
var r = this.pos - t >= 2 && this.input.charCodeAt(t) === 48;
r && this.strict && this.raise(t, "Invalid number");
var s = this.input.charCodeAt(this.pos);
if (!r && !e && this.options.ecmaVersion >= 11 && s === 110) {
var n2 = Mr(this.input.slice(t, this.pos));
return ++this.pos, w(this.fullCharCodeAtPos()) && this.raise(this.pos, "Identifier directly after number"), this.finishToken(i.num, n2);
}
r && /[89]/.test(this.input.slice(t, this.pos)) && (r = false), s === 46 && !r && (++this.pos, this.readInt(10), s = this.input.charCodeAt(this.pos)), (s === 69 || s === 101) && !r && (s = this.input.charCodeAt(++this.pos), (s === 43 || s === 45) && ++this.pos, this.readInt(10) === null && this.raise(t, "Invalid number")), w(this.fullCharCodeAtPos()) && this.raise(this.pos, "Identifier directly after number");
var h = pn(this.input.slice(t, this.pos), r);
return this.finishToken(i.num, h);
}, z.readCodePoint = function() {
var e = this.input.charCodeAt(this.pos), t;
if (e === 123) {
this.options.ecmaVersion < 6 && this.unexpected();
var r = ++this.pos;
t = this.readHexChar(this.input.indexOf("}", this.pos) - this.pos), ++this.pos, t > 1114111 && this.invalidStringToken(r, "Code point out of bounds");
} else
t = this.readHexChar(4);
return t;
}, z.readString = function(e) {
for (var t = "", r = ++this.pos; ; ) {
this.pos >= this.input.length && this.raise(this.start, "Unterminated string constant");
var s = this.input.charCodeAt(this.pos);
if (s === e)
break;
s === 92 ? (t += this.input.slice(r, this.pos), t += this.readEscapedChar(false), r = this.pos) : s === 8232 || s === 8233 ? (this.options.ecmaVersion < 10 && this.raise(this.start, "Unterminated string constant"), ++this.pos, this.options.locations && (this.curLine++, this.lineStart = this.pos)) : (j(s) && this.raise(this.start, "Unterminated string constant"), ++this.pos);
}
return t += this.input.slice(r, this.pos++), this.finishToken(i.string, t);
};
var Ur = {};
z.tryReadTemplateToken = function() {
this.inTemplateElement = true;
try {
this.readTmplToken();
} catch (e) {
if (e === Ur)
this.readInvalidTemplateToken();
else
throw e;
}
this.inTemplateElement = false;
}, z.invalidStringToken = function(e, t) {
if (this.inTemplateElement && this.options.ecmaVersion >= 9)
throw Ur;
this.raise(e, t);
}, z.readTmplToken = function() {
for (var e = "", t = this.pos; ; ) {
this.pos >= this.input.length && this.raise(this.start, "Unterminated template");
var r = this.input.charCodeAt(this.pos);
if (r === 96 || r === 36 && this.input.charCodeAt(this.pos + 1) === 123)
return this.pos === this.start && (this.type === i.template || this.type === i.invalidTemplate) ? r === 36 ? (this.pos += 2, this.finishToken(i.dollarBraceL)) : (++this.pos, this.finishToken(i.backQuote)) : (e += this.input.slice(t, this.pos), this.finishToken(i.template, e));
if (r === 92)
e += this.input.slice(t, this.pos), e += this.readEscapedChar(true), t = this.pos;
else if (j(r)) {
switch (e += this.input.slice(t, this.pos), ++this.pos, r) {
case 13:
this.input.charCodeAt(this.pos) === 10 && ++this.pos;
case 10:
e += `
`;
break;
default:
e += String.fromCharCode(r);
break;
}
this.options.locations && (++this.curLine, this.lineStart = this.pos), t = this.pos;
} else
++this.pos;
}
}, z.readInvalidTemplateToken = function() {
for (; this.pos < this.input.length; this.pos++)
switch (this.input[this.pos]) {
case "\\":
++this.pos;
break;
case "$":
if (this.input[this.pos + 1] !== "{")
break;
case "`":
return this.finishToken(i.invalidTemplate, this.input.slice(this.start, this.pos));
}
this.raise(this.start, "Unterminated template");
}, z.readEscapedChar = function(e) {
var t = this.input.charCodeAt(++this.pos);
switch (++this.pos, t) {
case 110:
return `
`;
case 114:
return "\r";
case 120:
return String.fromCharCode(this.readHexChar(2));
case 117:
return E(this.readCodePoint());
case 116:
return " ";
case 98:
return "\b";
case 118:
return "\v";
case 102:
return "\f";
case 13:
this.input.charCodeAt(this.pos) === 10 && ++this.pos;
case 10:
return this.options.locations && (this.lineStart = this.pos, ++this.curLine), "";
case 56:
case 57:
if (this.strict && this.invalidStringToken(this.pos - 1, "Invalid escape sequence"), e) {
var r = this.pos - 1;
return this.invalidStringToken(r, "Invalid escape sequence in template string"), null;
}
default:
if (t >= 48 && t <= 55) {
var s = this.input.substr(this.pos - 1, 3).match(/^[0-7]+/)[0], n2 = parseInt(s, 8);
return n2 > 255 && (s = s.slice(0, -1), n2 = parseInt(s, 8)), this.pos += s.length - 1, t = this.input.charCodeAt(this.pos), (s !== "0" || t === 56 || t === 57) && (this.strict || e) && this.invalidStringToken(this.pos - 1 - s.length, e ? "Octal literal in template string" : "Octal literal in strict mode"), String.fromCharCode(n2);
}
return j(t) ? "" : String.fromCharCode(t);
}
}, z.readHexChar = function(e) {
var t = this.pos, r = this.readInt(16, e);
return r === null && this.invalidStringToken(t, "Bad character escape sequence"), r;
}, z.readWord1 = function() {
this.containsEsc = false;
for (var e = "", t = true, r = this.pos, s = this.options.ecmaVersion >= 6; this.pos < this.input.length; ) {
var n2 = this.fullCharCodeAtPos();
if (G(n2, s))
this.pos += n2 <= 65535 ? 1 : 2;
else if (n2 === 92) {
this.containsEsc = true, e += this.input.slice(r, this.pos);
var h = this.pos;
this.input.charCodeAt(++this.pos) !== 117 && this.invalidStringToken(this.pos, "Expecting Unicode escape sequence \\uXXXX"), ++this.pos;
var c = this.readCodePoint();
(t ? w : G)(c, s) || this.invalidStringToken(h, "Invalid Unicode escape"), e += E(c), r = this.pos;
} else
break;
t = false;
}
return e + this.input.slice(r, this.pos);
}, z.readWord = function() {
var e = this.readWord1(), t = i.name;
return this.keywords.test(e) && (t = X[e]), this.finishToken(t, e);
};
var Wr = "8.8.1";
Y.acorn = { Parser: Y, version: Wr, defaultOptions: fe, Position: H, SourceLocation: te, getLineInfo: ae, Node: Re, TokenType: f, tokTypes: i, keywordTypes: X, TokContext: ue, tokContexts: Q, isIdentifierChar: G, isIdentifierStart: w, Token: Qe, isNewLine: j, lineBreak: S, lineBreakG: F, nonASCIIwhitespace: ne };
function fn(e, t) {
return Y.parse(e, t);
}
function dn(e, t, r) {
return Y.parseExpressionAt(e, t, r);
}
function mn(e, t) {
return Y.tokenizer(e, t);
}
o.Node = Re, o.Parser = Y, o.Position = H, o.SourceLocation = te, o.TokContext = ue, o.Token = Qe, o.TokenType = f, o.defaultOptions = fe, o.getLineInfo = ae, o.isIdentifierChar = G, o.isIdentifierStart = w, o.isNewLine = j, o.keywordTypes = X, o.lineBreak = S, o.lineBreakG = F, o.nonASCIIwhitespace = ne, o.parse = fn, o.parseExpressionAt = dn, o.tokContexts = Q, o.tokTypes = i, o.tokenizer = mn, o.version = Wr, Object.defineProperty(o, "__esModule", { value: true });
});
} }), Hh = $({ "node_modules/acorn-jsx/xhtml.js"(a, u) {
J(), u.exports = { quot: '"', amp: "&", apos: "'", lt: "<", gt: ">", nbsp: "\xA0", iexcl: "\xA1", cent: "\xA2", pound: "\xA3", curren: "\xA4", yen: "\xA5", brvbar: "\xA6", sect: "\xA7", uml: "\xA8", copy: "\xA9", ordf: "\xAA", laquo: "\xAB", not: "\xAC", shy: "\xAD", reg: "\xAE", macr: "\xAF", deg: "\xB0", plusmn: "\xB1", sup2: "\xB2", sup3: "\xB3", acute: "\xB4", micro: "\xB5", para: "\xB6", middot: "\xB7", cedil: "\xB8", sup1: "\xB9", ordm: "\xBA", raquo: "\xBB", frac14: "\xBC", frac12: "\xBD", frac34: "\xBE", iquest: "\xBF", Agrave: "\xC0", Aacute: "\xC1", Acirc: "\xC2", Atilde: "\xC3", Auml: "\xC4", Aring: "\xC5", AElig: "\xC6", Ccedil: "\xC7", Egrave: "\xC8", Eacute: "\xC9", Ecirc: "\xCA", Euml: "\xCB", Igrave: "\xCC", Iacute: "\xCD", Icirc: "\xCE", Iuml: "\xCF", ETH: "\xD0", Ntilde: "\xD1", Ograve: "\xD2", Oacute: "\xD3", Ocirc: "\xD4", Otilde: "\xD5", Ouml: "\xD6", times: "\xD7", Oslash: "\xD8", Ugrave: "\xD9", Uacute: "\xDA", Ucirc: "\xDB", Uuml: "\xDC", Yacute: "\xDD", THORN: "\xDE", szlig: "\xDF", agrave: "\xE0", aacute: "\xE1", acirc: "\xE2", atilde: "\xE3", auml: "\xE4", aring: "\xE5", aelig: "\xE6", ccedil: "\xE7", egrave: "\xE8", eacute: "\xE9", ecirc: "\xEA", euml: "\xEB", igrave: "\xEC", iacute: "\xED", icirc: "\xEE", iuml: "\xEF", eth: "\xF0", ntilde: "\xF1", ograve: "\xF2", oacute: "\xF3", ocirc: "\xF4", otilde: "\xF5", ouml: "\xF6", divide: "\xF7", oslash: "\xF8", ugrave: "\xF9", uacute: "\xFA", ucirc: "\xFB", uuml: "\xFC", yacute: "\xFD", thorn: "\xFE", yuml: "\xFF", OElig: "\u0152", oelig: "\u0153", Scaron: "\u0160", scaron: "\u0161", Yuml: "\u0178", fnof: "\u0192", circ: "\u02C6", tilde: "\u02DC", Alpha: "\u0391", Beta: "\u0392", Gamma: "\u0393", Delta: "\u0394", Epsilon: "\u0395", Zeta: "\u0396", Eta: "\u0397", Theta: "\u0398", Iota: "\u0399", Kappa: "\u039A", Lambda: "\u039B", Mu: "\u039C", Nu: "\u039D", Xi: "\u039E", Omicron: "\u039F", Pi: "\u03A0", Rho: "\u03A1", Sigma: "\u03A3", Tau: "\u03A4", Upsilon: "\u03A5", Phi: "\u03A6", Chi: "\u03A7", Psi: "\u03A8", Omega: "\u03A9", alpha: "\u03B1", beta: "\u03B2", gamma: "\u03B3", delta: "\u03B4", epsilon: "\u03B5", zeta: "\u03B6", eta: "\u03B7", theta: "\u03B8", iota: "\u03B9", kappa: "\u03BA", lambda: "\u03BB", mu: "\u03BC", nu: "\u03BD", xi: "\u03BE", omicron: "\u03BF", pi: "\u03C0", rho: "\u03C1", sigmaf: "\u03C2", sigma: "\u03C3", tau: "\u03C4", upsilon: "\u03C5", phi: "\u03C6", chi: "\u03C7", psi: "\u03C8", omega: "\u03C9", thetasym: "\u03D1", upsih: "\u03D2", piv: "\u03D6", ensp: "\u2002", emsp: "\u2003", thinsp: "\u2009", zwnj: "\u200C", zwj: "\u200D", lrm: "\u200E", rlm: "\u200F", ndash: "\u2013", mdash: "\u2014", lsquo: "\u2018", rsquo: "\u2019", sbquo: "\u201A", ldquo: "\u201C", rdquo: "\u201D", bdquo: "\u201E", dagger: "\u2020", Dagger: "\u2021", bull: "\u2022", hellip: "\u2026", permil: "\u2030", prime: "\u2032", Prime: "\u2033", lsaquo: "\u2039", rsaquo: "\u203A", oline: "\u203E", frasl: "\u2044", euro: "\u20AC", image: "\u2111", weierp: "\u2118", real: "\u211C", trade: "\u2122", alefsym: "\u2135", larr: "\u2190", uarr: "\u2191", rarr: "\u2192", darr: "\u2193", harr: "\u2194", crarr: "\u21B5", lArr: "\u21D0", uArr: "\u21D1", rArr: "\u21D2", dArr: "\u21D3", hArr: "\u21D4", forall: "\u2200", part: "\u2202", exist: "\u2203", empty: "\u2205", nabla: "\u2207", isin: "\u2208", notin: "\u2209", ni: "\u220B", prod: "\u220F", sum: "\u2211", minus: "\u2212", lowast: "\u2217", radic: "\u221A", prop: "\u221D", infin: "\u221E", ang: "\u2220", and: "\u2227", or: "\u2228", cap: "\u2229", cup: "\u222A", int: "\u222B", there4: "\u2234", sim: "\u223C", cong: "\u2245", asymp: "\u2248", ne: "\u2260", equiv: "\u2261", le: "\u2264", ge: "\u2265", sub: "\u2282", sup: "\u2283", nsub: "\u2284", sube: "\u2286", supe: "\u2287", oplus: "\u2295", otimes: "\u2297", perp: "\u22A5", sdot: "\u22C5", lceil: "\u2308", rceil: "\u2309", lfloor: "\u230A", rfloor: "\u230B", lang: "\u2329", rang: "\u232A", loz: "\u25CA", spades: "\u2660", clubs: "\u2663", hearts: "\u2665", diams: "\u2666" };
} }), Ha = $({ "node_modules/acorn-jsx/index.js"(a, u) {
"use strict";
J();
var o = Hh(), l = /^[\da-fA-F]+$/, v = /^\d+$/, b = /* @__PURE__ */ new WeakMap();
function y(x) {
x = x.Parser.acorn || x;
let R = b.get(x);
if (!R) {
let U = x.tokTypes, D = x.TokContext, g = x.TokenType, w = new D("... ", true, true), B = { tc_oTag: w, tc_cTag: G, tc_expr: f }, V = { jsxName: new g("jsxName"), jsxText: new g("jsxText", { beforeExpr: true }), jsxTagStart: new g("jsxTagStart", { startsExpr: true }), jsxTagEnd: new g("jsxTagEnd") };
V.jsxTagStart.updateContext = function() {
this.context.push(f), this.context.push(w), this.exprAllowed = false;
}, V.jsxTagEnd.updateContext = function(k) {
let X = this.context.pop();
X === w && k === U.slash || X === G ? (this.context.pop(), this.exprAllowed = this.curContext() === f) : this.exprAllowed = true;
}, R = { tokContexts: B, tokTypes: V }, b.set(x, R);
}
return R;
}
function I(x) {
if (!x)
return x;
if (x.type === "JSXIdentifier")
return x.name;
if (x.type === "JSXNamespacedName")
return x.namespace.name + ":" + x.name.name;
if (x.type === "JSXMemberExpression")
return I(x.object) + "." + I(x.property);
}
u.exports = function(x) {
return x = x || {}, function(R) {
return T({ allowNamespaces: x.allowNamespaces !== false, allowNamespacedObjects: !!x.allowNamespacedObjects }, R);
};
}, Object.defineProperty(u.exports, "tokTypes", { get: function() {
return y(ft()).tokTypes;
}, configurable: true, enumerable: true });
function T(x, R) {
let U = R.acorn || ft(), D = y(U), g = U.tokTypes, w = D.tokTypes, G = U.tokContexts, f = D.tokContexts.tc_oTag, B = D.tokContexts.tc_cTag, V = D.tokContexts.tc_expr, k = U.isNewLine, X = U.isIdentifierStart, O = U.isIdentifierChar;
return class extends R {
static get acornJsx() {
return D;
}
jsx_readToken() {
let i = "", S = this.pos;
for (; ; ) {
this.pos >= this.input.length && this.raise(this.start, "Unterminated JSX contents");
let F = this.input.charCodeAt(this.pos);
switch (F) {
case 60:
case 123:
return this.pos === this.start ? F === 60 && this.exprAllowed ? (++this.pos, this.finishToken(w.jsxTagStart)) : this.getTokenFromCode(F) : (i += this.input.slice(S, this.pos), this.finishToken(w.jsxText, i));
case 38:
i += this.input.slice(S, this.pos), i += this.jsx_readEntity(), S = this.pos;
break;
case 62:
case 125:
this.raise(this.pos, "Unexpected token `" + this.input[this.pos] + "`. Did you mean `" + (F === 62 ? ">" : "}") + '` or `{"' + this.input[this.pos] + '"}`?');
default:
k(F) ? (i += this.input.slice(S, this.pos), i += this.jsx_readNewLine(true), S = this.pos) : ++this.pos;
}
}
}
jsx_readNewLine(i) {
let S = this.input.charCodeAt(this.pos), F;
return ++this.pos, S === 13 && this.input.charCodeAt(this.pos) === 10 ? (++this.pos, F = i ? `
` : `\r
`) : F = String.fromCharCode(S), this.options.locations && (++this.curLine, this.lineStart = this.pos), F;
}
jsx_readString(i) {
let S = "", F = ++this.pos;
for (; ; ) {
this.pos >= this.input.length && this.raise(this.start, "Unterminated string constant");
let j = this.input.charCodeAt(this.pos);
if (j === i)
break;
j === 38 ? (S += this.input.slice(F, this.pos), S += this.jsx_readEntity(), F = this.pos) : k(j) ? (S += this.input.slice(F, this.pos), S += this.jsx_readNewLine(false), F = this.pos) : ++this.pos;
}
return S += this.input.slice(F, this.pos++), this.finishToken(g.string, S);
}
jsx_readEntity() {
let i = "", S = 0, F, j = this.input[this.pos];
j !== "&" && this.raise(this.pos, "Entity must start with an ampersand");
let Z = ++this.pos;
for (; this.pos < this.input.length && S++ < 10; ) {
if (j = this.input[this.pos++], j === ";") {
i[0] === "#" ? i[1] === "x" ? (i = i.substr(2), l.test(i) && (F = String.fromCharCode(parseInt(i, 16)))) : (i = i.substr(1), v.test(i) && (F = String.fromCharCode(parseInt(i, 10)))) : F = o[i];
break;
}
i += j;
}
return F || (this.pos = Z, "&");
}
jsx_readWord() {
let i, S = this.pos;
do
i = this.input.charCodeAt(++this.pos);
while (O(i) || i === 45);
return this.finishToken(w.jsxName, this.input.slice(S, this.pos));
}
jsx_parseIdentifier() {
let i = this.startNode();
return this.type === w.jsxName ? i.name = this.value : this.type.keyword ? i.name = this.type.keyword : this.unexpected(), this.next(), this.finishNode(i, "JSXIdentifier");
}
jsx_parseNamespacedName() {
let i = this.start, S = this.startLoc, F = this.jsx_parseIdentifier();
if (!x.allowNamespaces || !this.eat(g.colon))
return F;
var j = this.startNodeAt(i, S);
return j.namespace = F, j.name = this.jsx_parseIdentifier(), this.finishNode(j, "JSXNamespacedName");
}
jsx_parseElementName() {
if (this.type === w.jsxTagEnd)
return "";
let i = this.start, S = this.startLoc, F = this.jsx_parseNamespacedName();
for (this.type === g.dot && F.type === "JSXNamespacedName" && !x.allowNamespacedObjects && this.unexpected(); this.eat(g.dot); ) {
let j = this.startNodeAt(i, S);
j.object = F, j.property = this.jsx_parseIdentifier(), F = this.finishNode(j, "JSXMemberExpression");
}
return F;
}
jsx_parseAttributeValue() {
switch (this.type) {
case g.braceL:
let i = this.jsx_parseExpressionContainer();
return i.expression.type === "JSXEmptyExpression" && this.raise(i.start, "JSX attributes must only be assigned a non-empty expression"), i;
case w.jsxTagStart:
case g.string:
return this.parseExprAtom();
default:
this.raise(this.start, "JSX value should be either an expression or a quoted JSX text");
}
}
jsx_parseEmptyExpression() {
let i = this.startNodeAt(this.lastTokEnd, this.lastTokEndLoc);
return this.finishNodeAt(i, "JSXEmptyExpression", this.start, this.startLoc);
}
jsx_parseExpressionContainer() {
let i = this.startNode();
return this.next(), i.expression = this.type === g.braceR ? this.jsx_parseEmptyExpression() : this.parseExpression(), this.expect(g.braceR), this.finishNode(i, "JSXExpressionContainer");
}
jsx_parseAttribute() {
let i = this.startNode();
return this.eat(g.braceL) ? (this.expect(g.ellipsis), i.argument = this.parseMaybeAssign(), this.expect(g.braceR), this.finishNode(i, "JSXSpreadAttribute")) : (i.name = this.jsx_parseNamespacedName(), i.value = this.eat(g.eq) ? this.jsx_parseAttributeValue() : null, this.finishNode(i, "JSXAttribute"));
}
jsx_parseOpeningElementAt(i, S) {
let F = this.startNodeAt(i, S);
F.attributes = [];
let j = this.jsx_parseElementName();
for (j && (F.name = j); this.type !== g.slash && this.type !== w.jsxTagEnd; )
F.attributes.push(this.jsx_parseAttribute());
return F.selfClosing = this.eat(g.slash), this.expect(w.jsxTagEnd), this.finishNode(F, j ? "JSXOpeningElement" : "JSXOpeningFragment");
}
jsx_parseClosingElementAt(i, S) {
let F = this.startNodeAt(i, S), j = this.jsx_parseElementName();
return j && (F.name = j), this.expect(w.jsxTagEnd), this.finishNode(F, j ? "JSXClosingElement" : "JSXClosingFragment");
}
jsx_parseElementAt(i, S) {
let F = this.startNodeAt(i, S), j = [], Z = this.jsx_parseOpeningElementAt(i, S), ne = null;
if (!Z.selfClosing) {
e:
for (; ; )
switch (this.type) {
case w.jsxTagStart:
if (i = this.start, S = this.startLoc, this.next(), this.eat(g.slash)) {
ne = this.jsx_parseClosingElementAt(i, S);
break e;
}
j.push(this.jsx_parseElementAt(i, S));
break;
case w.jsxText:
j.push(this.parseExprAtom());
break;
case g.braceL:
j.push(this.jsx_parseExpressionContainer());
break;
default:
this.unexpected();
}
I(ne.name) !== I(Z.name) && this.raise(ne.start, "Expected corresponding JSX closing tag for <" + I(Z.name) + ">");
}
let ee = Z.name ? "Element" : "Fragment";
return F["opening" + ee] = Z, F["closing" + ee] = ne, F.children = j, this.type === g.relational && this.value === "<" && this.raise(this.start, "Adjacent JSX elements must be wrapped in an enclosing tag"), this.finishNode(F, "JSX" + ee);
}
jsx_parseText() {
let i = this.parseLiteral(this.value);
return i.type = "JSXText", i;
}
jsx_parseElement() {
let i = this.start, S = this.startLoc;
return this.next(), this.jsx_parseElementAt(i, S);
}
parseExprAtom(i) {
return this.type === w.jsxText ? this.jsx_parseText() : this.type === w.jsxTagStart ? this.jsx_parseElement() : super.parseExprAtom(i);
}
readToken(i) {
let S = this.curContext();
if (S === V)
return this.jsx_readToken();
if (S === f || S === B) {
if (X(i))
return this.jsx_readWord();
if (i == 62)
return ++this.pos, this.finishToken(w.jsxTagEnd);
if ((i === 34 || i === 39) && S == f)
return this.jsx_readString(i);
}
return i === 60 && this.exprAllowed && this.input.charCodeAt(this.pos + 1) !== 33 ? (++this.pos, this.finishToken(w.jsxTagStart)) : super.readToken(i);
}
updateContext(i) {
if (this.type == g.braceL) {
var S = this.curContext();
S == f ? this.context.push(G.b_expr) : S == V ? this.context.push(G.b_tmpl) : super.updateContext(i), this.exprAllowed = true;
} else if (this.type === g.slash && i === w.jsxTagStart)
this.context.length -= 2, this.context.push(B), this.exprAllowed = false;
else
return super.updateContext(i);
}
};
}
} }), Kh = $({ "src/language-js/parse/acorn.js"(a, u) {
"use strict";
J();
var o = dr(), l = Ba(), v = za(), b = Ga(), y = { ecmaVersion: "latest", sourceType: "module", allowReserved: true, allowReturnOutsideFunction: true, allowImportExportEverywhere: true, allowAwaitOutsideFunction: true, allowSuperOutsideMethod: true, allowHashBang: true, locations: true, ranges: true };
function I(D) {
let { message: g, loc: w } = D;
if (!w)
return D;
let { line: G, column: f } = w;
return o(g.replace(/ \(\d+:\d+\)$/, ""), { start: { line: G, column: f + 1 } });
}
var T, x = () => {
if (!T) {
let { Parser: D } = ft(), g = Ha();
T = D.extend(g());
}
return T;
};
function R(D, g) {
let w = x(), G = [], f = [], B = w.parse(D, Object.assign(Object.assign({}, y), {}, { sourceType: g, onComment: G, onToken: f }));
return B.comments = G, B.tokens = f, B;
}
function U(D, g) {
let w = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, { result: G, error: f } = l(() => R(D, "module"), () => R(D, "script"));
if (!G)
throw I(f);
return w.originalText = D, b(G, w);
}
u.exports = v(U);
} }), Xh = $({ "src/language-js/parse/utils/replace-hashbang.js"(a, u) {
"use strict";
J();
function o(l) {
return l.charAt(0) === "#" && l.charAt(1) === "!" ? "//" + l.slice(2) : l;
}
u.exports = o;
} }), Jh = $({ "node_modules/espree/dist/espree.cjs"(a) {
"use strict";
J(), Object.defineProperty(a, "__esModule", { value: true });
var u = ft(), o = Ha(), l;
function v(p) {
return p && typeof p == "object" && "default" in p ? p : { default: p };
}
function b(p) {
if (p && p.__esModule)
return p;
var P = /* @__PURE__ */ Object.create(null);
return p && Object.keys(p).forEach(function(_) {
if (_ !== "default") {
var d = Object.getOwnPropertyDescriptor(p, _);
Object.defineProperty(P, _, d.get ? d : { enumerable: true, get: function() {
return p[_];
} });
}
}), P.default = p, Object.freeze(P);
}
var y = b(u), I = v(o), T = b(l), x = { Boolean: "Boolean", EOF: "", Identifier: "Identifier", PrivateIdentifier: "PrivateIdentifier", Keyword: "Keyword", Null: "Null", Numeric: "Numeric", Punctuator: "Punctuator", String: "String", RegularExpression: "RegularExpression", Template: "Template", JSXIdentifier: "JSXIdentifier", JSXText: "JSXText" };
function R(p, P) {
let _ = p[0], d = p[p.length - 1], E = { type: x.Template, value: P.slice(_.start, d.end) };
return _.loc && (E.loc = { start: _.loc.start, end: d.loc.end }), _.range && (E.start = _.range[0], E.end = d.range[1], E.range = [E.start, E.end]), E;
}
function U(p, P) {
this._acornTokTypes = p, this._tokens = [], this._curlyBrace = null, this._code = P;
}
U.prototype = { constructor: U, translate(p, P) {
let _ = p.type, d = this._acornTokTypes;
if (_ === d.name)
p.type = x.Identifier, p.value === "static" && (p.type = x.Keyword), P.ecmaVersion > 5 && (p.value === "yield" || p.value === "let") && (p.type = x.Keyword);
else if (_ === d.privateId)
p.type = x.PrivateIdentifier;
else if (_ === d.semi || _ === d.comma || _ === d.parenL || _ === d.parenR || _ === d.braceL || _ === d.braceR || _ === d.dot || _ === d.bracketL || _ === d.colon || _ === d.question || _ === d.bracketR || _ === d.ellipsis || _ === d.arrow || _ === d.jsxTagStart || _ === d.incDec || _ === d.starstar || _ === d.jsxTagEnd || _ === d.prefix || _ === d.questionDot || _.binop && !_.keyword || _.isAssign)
p.type = x.Punctuator, p.value = this._code.slice(p.start, p.end);
else if (_ === d.jsxName)
p.type = x.JSXIdentifier;
else if (_.label === "jsxText" || _ === d.jsxAttrValueToken)
p.type = x.JSXText;
else if (_.keyword)
_.keyword === "true" || _.keyword === "false" ? p.type = x.Boolean : _.keyword === "null" ? p.type = x.Null : p.type = x.Keyword;
else if (_ === d.num)
p.type = x.Numeric, p.value = this._code.slice(p.start, p.end);
else if (_ === d.string)
P.jsxAttrValueToken ? (P.jsxAttrValueToken = false, p.type = x.JSXText) : p.type = x.String, p.value = this._code.slice(p.start, p.end);
else if (_ === d.regexp) {
p.type = x.RegularExpression;
let E = p.value;
p.regex = { flags: E.flags, pattern: E.pattern }, p.value = `/${E.pattern}/${E.flags}`;
}
return p;
}, onToken(p, P) {
let _ = this, d = this._acornTokTypes, E = P.tokens, K = this._tokens;
function H() {
E.push(R(_._tokens, _._code)), _._tokens = [];
}
if (p.type === d.eof) {
this._curlyBrace && E.push(this.translate(this._curlyBrace, P));
return;
}
if (p.type === d.backQuote) {
this._curlyBrace && (E.push(this.translate(this._curlyBrace, P)), this._curlyBrace = null), K.push(p), K.length > 1 && H();
return;
}
if (p.type === d.dollarBraceL) {
K.push(p), H();
return;
}
if (p.type === d.braceR) {
this._curlyBrace && E.push(this.translate(this._curlyBrace, P)), this._curlyBrace = p;
return;
}
if (p.type === d.template || p.type === d.invalidTemplate) {
this._curlyBrace && (K.push(this._curlyBrace), this._curlyBrace = null), K.push(p);
return;
}
this._curlyBrace && (E.push(this.translate(this._curlyBrace, P)), this._curlyBrace = null), E.push(this.translate(p, P));
} };
var D = [3, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14];
function g() {
return D[D.length - 1];
}
function w() {
return [...D];
}
function G() {
let p = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : 5, P = p === "latest" ? g() : p;
if (typeof P != "number")
throw new Error(`ecmaVersion must be a number or "latest". Received value of type ${typeof p} instead.`);
if (P >= 2015 && (P -= 2009), !D.includes(P))
throw new Error("Invalid ecmaVersion.");
return P;
}
function f() {
let p = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : "script";
if (p === "script" || p === "module")
return p;
if (p === "commonjs")
return "script";
throw new Error("Invalid sourceType.");
}
function B(p) {
let P = G(p.ecmaVersion), _ = f(p.sourceType), d = p.range === true, E = p.loc === true;
if (P !== 3 && p.allowReserved)
throw new Error("`allowReserved` is only supported when ecmaVersion is 3");
if (typeof p.allowReserved < "u" && typeof p.allowReserved != "boolean")
throw new Error("`allowReserved`, when present, must be `true` or `false`");
let K = P === 3 ? p.allowReserved || "never" : false, H = p.ecmaFeatures || {}, te = p.sourceType === "commonjs" || Boolean(H.globalReturn);
if (_ === "module" && P < 6)
throw new Error("sourceType 'module' is not supported when ecmaVersion < 2015. Consider adding `{ ecmaVersion: 2015 }` to the parser options.");
return Object.assign({}, p, { ecmaVersion: P, sourceType: _, ranges: d, locations: E, allowReserved: K, allowReturnOutsideFunction: te });
}
var V = Symbol("espree's internal state"), k = Symbol("espree's esprimaFinishNode");
function X(p, P, _, d, E, K, H) {
let te;
p ? te = "Block" : H.slice(_, _ + 2) === "#!" ? te = "Hashbang" : te = "Line";
let ae = { type: te, value: P };
return typeof _ == "number" && (ae.start = _, ae.end = d, ae.range = [_, d]), typeof E == "object" && (ae.loc = { start: E, end: K }), ae;
}
var O = () => (p) => {
let P = Object.assign({}, p.acorn.tokTypes);
return p.acornJsx && Object.assign(P, p.acornJsx.tokTypes), class extends p {
constructor(d, E) {
(typeof d != "object" || d === null) && (d = {}), typeof E != "string" && !(E instanceof String) && (E = String(E));
let K = d.sourceType, H = B(d), te = H.ecmaFeatures || {}, ae = H.tokens === true ? new U(P, E) : null, fe = { originalSourceType: K || H.sourceType, tokens: ae ? [] : null, comments: H.comment === true ? [] : null, impliedStrict: te.impliedStrict === true && H.ecmaVersion >= 5, ecmaVersion: H.ecmaVersion, jsxAttrValueToken: false, lastToken: null, templateElements: [] };
super({ ecmaVersion: H.ecmaVersion, sourceType: H.sourceType, ranges: H.ranges, locations: H.locations, allowReserved: H.allowReserved, allowReturnOutsideFunction: H.allowReturnOutsideFunction, onToken: (Ae) => {
ae && ae.onToken(Ae, fe), Ae.type !== P.eof && (fe.lastToken = Ae);
}, onComment: (Ae, dt, mt, _e, Ce, Oe) => {
if (fe.comments) {
let ze = X(Ae, dt, mt, _e, Ce, Oe, E);
fe.comments.push(ze);
}
} }, E), this[V] = fe;
}
tokenize() {
do
this.next();
while (this.type !== P.eof);
this.next();
let d = this[V], E = d.tokens;
return d.comments && (E.comments = d.comments), E;
}
finishNode() {
let d = super.finishNode(...arguments);
return this[k](d);
}
finishNodeAt() {
let d = super.finishNodeAt(...arguments);
return this[k](d);
}
parse() {
let d = this[V], E = super.parse();
if (E.sourceType = d.originalSourceType, d.comments && (E.comments = d.comments), d.tokens && (E.tokens = d.tokens), E.body.length) {
let [K] = E.body;
E.range && (E.range[0] = K.range[0]), E.loc && (E.loc.start = K.loc.start), E.start = K.start;
}
return d.lastToken && (E.range && (E.range[1] = d.lastToken.range[1]), E.loc && (E.loc.end = d.lastToken.loc.end), E.end = d.lastToken.end), this[V].templateElements.forEach((K) => {
let te = K.tail ? 1 : 2;
K.start += -1, K.end += te, K.range && (K.range[0] += -1, K.range[1] += te), K.loc && (K.loc.start.column += -1, K.loc.end.column += te);
}), E;
}
parseTopLevel(d) {
return this[V].impliedStrict && (this.strict = true), super.parseTopLevel(d);
}
raise(d, E) {
let K = p.acorn.getLineInfo(this.input, d), H = new SyntaxError(E);
throw H.index = d, H.lineNumber = K.line, H.column = K.column + 1, H;
}
raiseRecoverable(d, E) {
this.raise(d, E);
}
unexpected(d) {
let E = "Unexpected token";
if (d != null) {
if (this.pos = d, this.options.locations)
for (; this.pos < this.lineStart; )
this.lineStart = this.input.lastIndexOf(`
`, this.lineStart - 2) + 1, --this.curLine;
this.nextToken();
}
this.end > this.start && (E += ` ${this.input.slice(this.start, this.end)}`), this.raise(this.start, E);
}
jsx_readString(d) {
let E = super.jsx_readString(d);
return this.type === P.string && (this[V].jsxAttrValueToken = true), E;
}
[k](d) {
return d.type === "TemplateElement" && this[V].templateElements.push(d), d.type.includes("Function") && !d.generator && (d.generator = false), d;
}
};
}, i = "9.4.1", S = { _regular: null, _jsx: null, get regular() {
return this._regular === null && (this._regular = y.Parser.extend(O())), this._regular;
}, get jsx() {
return this._jsx === null && (this._jsx = y.Parser.extend(I.default(), O())), this._jsx;
}, get(p) {
return Boolean(p && p.ecmaFeatures && p.ecmaFeatures.jsx) ? this.jsx : this.regular;
} };
function F(p, P) {
let _ = S.get(P);
return (!P || P.tokens !== true) && (P = Object.assign({}, P, { tokens: true })), new _(P, p).tokenize();
}
function j(p, P) {
let _ = S.get(P);
return new _(P, p).parse();
}
var Z = i, ne = function() {
return T.KEYS;
}(), ee = void 0, ie = g(), Ne = w();
a.Syntax = ee, a.VisitorKeys = ne, a.latestEcmaVersion = ie, a.parse = j, a.supportedEcmaVersions = Ne, a.tokenize = F, a.version = Z;
} }), Qh = $({ "src/language-js/parse/espree.js"(a, u) {
"use strict";
J();
var o = dr(), l = Ba(), v = za(), b = Xh(), y = Ga(), I = { ecmaVersion: "latest", range: true, loc: true, comment: true, tokens: true, sourceType: "module", ecmaFeatures: { jsx: true, globalReturn: true, impliedStrict: false } };
function T(R) {
let { message: U, lineNumber: D, column: g } = R;
return typeof D != "number" ? R : o(U, { start: { line: D, column: g } });
}
function x(R, U) {
let D = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, { parse: g } = Jh(), w = b(R), { result: G, error: f } = l(() => g(w, Object.assign(Object.assign({}, I), {}, { sourceType: "module" })), () => g(w, Object.assign(Object.assign({}, I), {}, { sourceType: "script" })));
if (!G)
throw T(f);
return D.originalText = R, y(G, D);
}
u.exports = v(x);
} });
J();
var $h = Kh(), Yh = Qh();
Ka.exports = { parsers: { acorn: $h, espree: Yh } };
});
return Zh();
});
}
});
// node_modules/prettier/parser-meriyah.js
var require_parser_meriyah = __commonJS({
"node_modules/prettier/parser-meriyah.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.meriyah = e();
}
})(function() {
"use strict";
var B = (a, g) => () => (g || a((g = { exports: {} }).exports, g), g.exports);
var k2 = B((X3, Fu) => {
var A1 = function(a) {
return a && a.Math == Math && a;
};
Fu.exports = A1(typeof globalThis == "object" && globalThis) || A1(typeof window == "object" && window) || A1(typeof self == "object" && self) || A1(typeof global == "object" && global) || function() {
return this;
}() || Function("return this")();
});
var D2 = B((z3, Lu) => {
Lu.exports = function(a) {
try {
return !!a();
} catch {
return true;
}
};
});
var S2 = B((W3, Ou) => {
var uo = D2();
Ou.exports = !uo(function() {
return Object.defineProperty({}, 1, { get: function() {
return 7;
} })[1] != 7;
});
});
var ue = B((K3, Tu) => {
var io = D2();
Tu.exports = !io(function() {
var a = function() {
}.bind();
return typeof a != "function" || a.hasOwnProperty("prototype");
});
});
var E1 = B((Y3, Iu) => {
var no = ue(), P1 = Function.prototype.call;
Iu.exports = no ? P1.bind(P1) : function() {
return P1.apply(P1, arguments);
};
});
var ju = B((Nu) => {
"use strict";
var Ru = {}.propertyIsEnumerable, Vu = Object.getOwnPropertyDescriptor, to = Vu && !Ru.call({ 1: 2 }, 1);
Nu.f = to ? function(g) {
var b = Vu(this, g);
return !!b && b.enumerable;
} : Ru;
});
var ie = B((Q3, _u) => {
_u.exports = function(a, g) {
return { enumerable: !(a & 1), configurable: !(a & 2), writable: !(a & 4), value: g };
};
});
var F2 = B((G3, Ju) => {
var Mu = ue(), Uu = Function.prototype, ne = Uu.call, oo = Mu && Uu.bind.bind(ne, ne);
Ju.exports = Mu ? oo : function(a) {
return function() {
return ne.apply(a, arguments);
};
};
});
var Xu = B((x3, Hu) => {
var $u = F2(), lo = $u({}.toString), fo = $u("".slice);
Hu.exports = function(a) {
return fo(lo(a), 8, -1);
};
});
var Wu = B((p3, zu) => {
var co = F2(), so = D2(), ao = Xu(), te = Object, go = co("".split);
zu.exports = so(function() {
return !te("z").propertyIsEnumerable(0);
}) ? function(a) {
return ao(a) == "String" ? go(a, "") : te(a);
} : te;
});
var oe = B((e6, Ku) => {
Ku.exports = function(a) {
return a == null;
};
});
var le = B((u6, Yu) => {
var ho = oe(), mo = TypeError;
Yu.exports = function(a) {
if (ho(a))
throw mo("Can't call method on " + a);
return a;
};
});
var C1 = B((i6, Zu) => {
var bo = Wu(), ko = le();
Zu.exports = function(a) {
return bo(ko(a));
};
});
var ce = B((n6, Qu) => {
var fe = typeof document == "object" && document.all, ro = typeof fe > "u" && fe !== void 0;
Qu.exports = { all: fe, IS_HTMLDDA: ro };
});
var A2 = B((t6, xu) => {
var Gu = ce(), vo = Gu.all;
xu.exports = Gu.IS_HTMLDDA ? function(a) {
return typeof a == "function" || a === vo;
} : function(a) {
return typeof a == "function";
};
});
var Z2 = B((o6, ui) => {
var pu = A2(), ei = ce(), yo = ei.all;
ui.exports = ei.IS_HTMLDDA ? function(a) {
return typeof a == "object" ? a !== null : pu(a) || a === yo;
} : function(a) {
return typeof a == "object" ? a !== null : pu(a);
};
});
var D1 = B((l6, ii) => {
var se = k2(), Ao = A2(), Po = function(a) {
return Ao(a) ? a : void 0;
};
ii.exports = function(a, g) {
return arguments.length < 2 ? Po(se[a]) : se[a] && se[a][g];
};
});
var ti = B((f6, ni) => {
var Eo = F2();
ni.exports = Eo({}.isPrototypeOf);
});
var li = B((c6, oi) => {
var Co = D1();
oi.exports = Co("navigator", "userAgent") || "";
});
var hi = B((s6, gi) => {
var di = k2(), ae = li(), fi = di.process, ci = di.Deno, si = fi && fi.versions || ci && ci.version, ai = si && si.v8, P2, w1;
ai && (P2 = ai.split("."), w1 = P2[0] > 0 && P2[0] < 4 ? 1 : +(P2[0] + P2[1]));
!w1 && ae && (P2 = ae.match(/Edge\/(\d+)/), (!P2 || P2[1] >= 74) && (P2 = ae.match(/Chrome\/(\d+)/), P2 && (w1 = +P2[1])));
gi.exports = w1;
});
var de = B((a6, bi) => {
var mi = hi(), Do = D2();
bi.exports = !!Object.getOwnPropertySymbols && !Do(function() {
var a = Symbol();
return !String(a) || !(Object(a) instanceof Symbol) || !Symbol.sham && mi && mi < 41;
});
});
var ge = B((d6, ki) => {
var wo = de();
ki.exports = wo && !Symbol.sham && typeof Symbol.iterator == "symbol";
});
var he = B((g6, ri) => {
var qo = D1(), Bo = A2(), So = ti(), Fo = ge(), Lo = Object;
ri.exports = Fo ? function(a) {
return typeof a == "symbol";
} : function(a) {
var g = qo("Symbol");
return Bo(g) && So(g.prototype, Lo(a));
};
});
var yi = B((h6, vi) => {
var Oo = String;
vi.exports = function(a) {
try {
return Oo(a);
} catch {
return "Object";
}
};
});
var Pi = B((m6, Ai) => {
var To = A2(), Io = yi(), Ro = TypeError;
Ai.exports = function(a) {
if (To(a))
return a;
throw Ro(Io(a) + " is not a function");
};
});
var Ci = B((b6, Ei) => {
var Vo = Pi(), No = oe();
Ei.exports = function(a, g) {
var b = a[g];
return No(b) ? void 0 : Vo(b);
};
});
var wi = B((k6, Di) => {
var me = E1(), be = A2(), ke = Z2(), jo = TypeError;
Di.exports = function(a, g) {
var b, f;
if (g === "string" && be(b = a.toString) && !ke(f = me(b, a)) || be(b = a.valueOf) && !ke(f = me(b, a)) || g !== "string" && be(b = a.toString) && !ke(f = me(b, a)))
return f;
throw jo("Can't convert object to primitive value");
};
});
var Bi = B((r6, qi) => {
qi.exports = false;
});
var q1 = B((v6, Fi) => {
var Si = k2(), _o = Object.defineProperty;
Fi.exports = function(a, g) {
try {
_o(Si, a, { value: g, configurable: true, writable: true });
} catch {
Si[a] = g;
}
return g;
};
});
var B1 = B((y6, Oi) => {
var Mo = k2(), Uo = q1(), Li = "__core-js_shared__", Jo = Mo[Li] || Uo(Li, {});
Oi.exports = Jo;
});
var re = B((A6, Ii) => {
var $o = Bi(), Ti = B1();
(Ii.exports = function(a, g) {
return Ti[a] || (Ti[a] = g !== void 0 ? g : {});
})("versions", []).push({ version: "3.26.1", mode: $o ? "pure" : "global", copyright: "\xA9 2014-2022 Denis Pushkarev (zloirock.ru)", license: "https://github.com/zloirock/core-js/blob/v3.26.1/LICENSE", source: "https://github.com/zloirock/core-js" });
});
var Vi = B((P6, Ri) => {
var Ho = le(), Xo = Object;
Ri.exports = function(a) {
return Xo(Ho(a));
};
});
var R2 = B((E6, Ni) => {
var zo = F2(), Wo = Vi(), Ko = zo({}.hasOwnProperty);
Ni.exports = Object.hasOwn || function(g, b) {
return Ko(Wo(g), b);
};
});
var ve = B((C6, ji) => {
var Yo = F2(), Zo = 0, Qo = Math.random(), Go = Yo(1 .toString);
ji.exports = function(a) {
return "Symbol(" + (a === void 0 ? "" : a) + ")_" + Go(++Zo + Qo, 36);
};
});
var Hi = B((D6, $i) => {
var xo = k2(), po = re(), _i = R2(), el = ve(), Mi = de(), Ji = ge(), Q2 = po("wks"), $2 = xo.Symbol, Ui = $2 && $2.for, ul = Ji ? $2 : $2 && $2.withoutSetter || el;
$i.exports = function(a) {
if (!_i(Q2, a) || !(Mi || typeof Q2[a] == "string")) {
var g = "Symbol." + a;
Mi && _i($2, a) ? Q2[a] = $2[a] : Ji && Ui ? Q2[a] = Ui(g) : Q2[a] = ul(g);
}
return Q2[a];
};
});
var Ki = B((w6, Wi) => {
var il = E1(), Xi = Z2(), zi = he(), nl = Ci(), tl = wi(), ol = Hi(), ll = TypeError, fl = ol("toPrimitive");
Wi.exports = function(a, g) {
if (!Xi(a) || zi(a))
return a;
var b = nl(a, fl), f;
if (b) {
if (g === void 0 && (g = "default"), f = il(b, a, g), !Xi(f) || zi(f))
return f;
throw ll("Can't convert object to primitive value");
}
return g === void 0 && (g = "number"), tl(a, g);
};
});
var ye = B((q6, Yi) => {
var cl = Ki(), sl = he();
Yi.exports = function(a) {
var g = cl(a, "string");
return sl(g) ? g : g + "";
};
});
var Gi = B((B6, Qi) => {
var al = k2(), Zi = Z2(), Ae = al.document, dl = Zi(Ae) && Zi(Ae.createElement);
Qi.exports = function(a) {
return dl ? Ae.createElement(a) : {};
};
});
var Pe = B((S6, xi) => {
var gl = S2(), hl = D2(), ml = Gi();
xi.exports = !gl && !hl(function() {
return Object.defineProperty(ml("div"), "a", { get: function() {
return 7;
} }).a != 7;
});
});
var Ee = B((en) => {
var bl = S2(), kl = E1(), rl = ju(), vl = ie(), yl = C1(), Al = ye(), Pl = R2(), El = Pe(), pi = Object.getOwnPropertyDescriptor;
en.f = bl ? pi : function(g, b) {
if (g = yl(g), b = Al(b), El)
try {
return pi(g, b);
} catch {
}
if (Pl(g, b))
return vl(!kl(rl.f, g, b), g[b]);
};
});
var nn = B((L6, un) => {
var Cl = S2(), Dl = D2();
un.exports = Cl && Dl(function() {
return Object.defineProperty(function() {
}, "prototype", { value: 42, writable: false }).prototype != 42;
});
});
var S1 = B((O6, tn) => {
var wl = Z2(), ql = String, Bl = TypeError;
tn.exports = function(a) {
if (wl(a))
return a;
throw Bl(ql(a) + " is not an object");
};
});
var u1 = B((ln) => {
var Sl = S2(), Fl = Pe(), Ll = nn(), F1 = S1(), on = ye(), Ol = TypeError, Ce = Object.defineProperty, Tl = Object.getOwnPropertyDescriptor, De = "enumerable", we = "configurable", qe = "writable";
ln.f = Sl ? Ll ? function(g, b, f) {
if (F1(g), b = on(b), F1(f), typeof g == "function" && b === "prototype" && "value" in f && qe in f && !f[qe]) {
var A = Tl(g, b);
A && A[qe] && (g[b] = f.value, f = { configurable: we in f ? f[we] : A[we], enumerable: De in f ? f[De] : A[De], writable: false });
}
return Ce(g, b, f);
} : Ce : function(g, b, f) {
if (F1(g), b = on(b), F1(f), Fl)
try {
return Ce(g, b, f);
} catch {
}
if ("get" in f || "set" in f)
throw Ol("Accessors not supported");
return "value" in f && (g[b] = f.value), g;
};
});
var Be = B((I6, fn) => {
var Il = S2(), Rl = u1(), Vl = ie();
fn.exports = Il ? function(a, g, b) {
return Rl.f(a, g, Vl(1, b));
} : function(a, g, b) {
return a[g] = b, a;
};
});
var an = B((R6, sn) => {
var Se = S2(), Nl = R2(), cn = Function.prototype, jl = Se && Object.getOwnPropertyDescriptor, Fe = Nl(cn, "name"), _l = Fe && function() {
}.name === "something", Ml = Fe && (!Se || Se && jl(cn, "name").configurable);
sn.exports = { EXISTS: Fe, PROPER: _l, CONFIGURABLE: Ml };
});
var gn = B((V6, dn) => {
var Ul = F2(), Jl = A2(), Le = B1(), $l = Ul(Function.toString);
Jl(Le.inspectSource) || (Le.inspectSource = function(a) {
return $l(a);
});
dn.exports = Le.inspectSource;
});
var bn = B((N6, mn) => {
var Hl = k2(), Xl = A2(), hn = Hl.WeakMap;
mn.exports = Xl(hn) && /native code/.test(String(hn));
});
var vn = B((j6, rn) => {
var zl = re(), Wl = ve(), kn = zl("keys");
rn.exports = function(a) {
return kn[a] || (kn[a] = Wl(a));
};
});
var Oe = B((_6, yn) => {
yn.exports = {};
});
var Cn = B((M6, En) => {
var Kl = bn(), Pn = k2(), Yl = Z2(), Zl = Be(), Te = R2(), Ie = B1(), Ql = vn(), Gl = Oe(), An = "Object already initialized", Re = Pn.TypeError, xl = Pn.WeakMap, L1, i1, O1, pl = function(a) {
return O1(a) ? i1(a) : L1(a, {});
}, e4 = function(a) {
return function(g) {
var b;
if (!Yl(g) || (b = i1(g)).type !== a)
throw Re("Incompatible receiver, " + a + " required");
return b;
};
};
Kl || Ie.state ? (E2 = Ie.state || (Ie.state = new xl()), E2.get = E2.get, E2.has = E2.has, E2.set = E2.set, L1 = function(a, g) {
if (E2.has(a))
throw Re(An);
return g.facade = a, E2.set(a, g), g;
}, i1 = function(a) {
return E2.get(a) || {};
}, O1 = function(a) {
return E2.has(a);
}) : (H2 = Ql("state"), Gl[H2] = true, L1 = function(a, g) {
if (Te(a, H2))
throw Re(An);
return g.facade = a, Zl(a, H2, g), g;
}, i1 = function(a) {
return Te(a, H2) ? a[H2] : {};
}, O1 = function(a) {
return Te(a, H2);
});
var E2, H2;
En.exports = { set: L1, get: i1, has: O1, enforce: pl, getterFor: e4 };
});
var Ne = B((U6, wn) => {
var u4 = D2(), i4 = A2(), T1 = R2(), Ve = S2(), n4 = an().CONFIGURABLE, t4 = gn(), Dn = Cn(), o4 = Dn.enforce, l4 = Dn.get, I1 = Object.defineProperty, f4 = Ve && !u4(function() {
return I1(function() {
}, "length", { value: 8 }).length !== 8;
}), c4 = String(String).split("String"), s4 = wn.exports = function(a, g, b) {
String(g).slice(0, 7) === "Symbol(" && (g = "[" + String(g).replace(/^Symbol\(([^)]*)\)/, "$1") + "]"), b && b.getter && (g = "get " + g), b && b.setter && (g = "set " + g), (!T1(a, "name") || n4 && a.name !== g) && (Ve ? I1(a, "name", { value: g, configurable: true }) : a.name = g), f4 && b && T1(b, "arity") && a.length !== b.arity && I1(a, "length", { value: b.arity });
try {
b && T1(b, "constructor") && b.constructor ? Ve && I1(a, "prototype", { writable: false }) : a.prototype && (a.prototype = void 0);
} catch {
}
var f = o4(a);
return T1(f, "source") || (f.source = c4.join(typeof g == "string" ? g : "")), a;
};
Function.prototype.toString = s4(function() {
return i4(this) && l4(this).source || t4(this);
}, "toString");
});
var Bn = B((J6, qn) => {
var a4 = A2(), d4 = u1(), g4 = Ne(), h4 = q1();
qn.exports = function(a, g, b, f) {
f || (f = {});
var A = f.enumerable, L = f.name !== void 0 ? f.name : g;
if (a4(b) && g4(b, L, f), f.global)
A ? a[g] = b : h4(g, b);
else {
try {
f.unsafe ? a[g] && (A = true) : delete a[g];
} catch {
}
A ? a[g] = b : d4.f(a, g, { value: b, enumerable: false, configurable: !f.nonConfigurable, writable: !f.nonWritable });
}
return a;
};
});
var Fn = B(($6, Sn) => {
var m4 = Math.ceil, b4 = Math.floor;
Sn.exports = Math.trunc || function(g) {
var b = +g;
return (b > 0 ? b4 : m4)(b);
};
});
var je = B((H6, Ln) => {
var k4 = Fn();
Ln.exports = function(a) {
var g = +a;
return g !== g || g === 0 ? 0 : k4(g);
};
});
var Tn = B((X6, On) => {
var r4 = je(), v4 = Math.max, y4 = Math.min;
On.exports = function(a, g) {
var b = r4(a);
return b < 0 ? v4(b + g, 0) : y4(b, g);
};
});
var Rn = B((z6, In) => {
var A4 = je(), P4 = Math.min;
In.exports = function(a) {
return a > 0 ? P4(A4(a), 9007199254740991) : 0;
};
});
var Nn = B((W6, Vn) => {
var E4 = Rn();
Vn.exports = function(a) {
return E4(a.length);
};
});
var Mn = B((K6, _n) => {
var C4 = C1(), D4 = Tn(), w4 = Nn(), jn = function(a) {
return function(g, b, f) {
var A = C4(g), L = w4(A), S = D4(f, L), V;
if (a && b != b) {
for (; L > S; )
if (V = A[S++], V != V)
return true;
} else
for (; L > S; S++)
if ((a || S in A) && A[S] === b)
return a || S || 0;
return !a && -1;
};
};
_n.exports = { includes: jn(true), indexOf: jn(false) };
});
var $n = B((Y6, Jn) => {
var q4 = F2(), _e = R2(), B4 = C1(), S4 = Mn().indexOf, F4 = Oe(), Un = q4([].push);
Jn.exports = function(a, g) {
var b = B4(a), f = 0, A = [], L;
for (L in b)
!_e(F4, L) && _e(b, L) && Un(A, L);
for (; g.length > f; )
_e(b, L = g[f++]) && (~S4(A, L) || Un(A, L));
return A;
};
});
var Xn = B((Z6, Hn) => {
Hn.exports = ["constructor", "hasOwnProperty", "isPrototypeOf", "propertyIsEnumerable", "toLocaleString", "toString", "valueOf"];
});
var Wn = B((zn) => {
var L4 = $n(), O4 = Xn(), T4 = O4.concat("length", "prototype");
zn.f = Object.getOwnPropertyNames || function(g) {
return L4(g, T4);
};
});
var Yn = B((Kn) => {
Kn.f = Object.getOwnPropertySymbols;
});
var Qn = B((x6, Zn) => {
var I4 = D1(), R4 = F2(), V4 = Wn(), N4 = Yn(), j4 = S1(), _4 = R4([].concat);
Zn.exports = I4("Reflect", "ownKeys") || function(g) {
var b = V4.f(j4(g)), f = N4.f;
return f ? _4(b, f(g)) : b;
};
});
var pn = B((p6, xn) => {
var Gn = R2(), M4 = Qn(), U4 = Ee(), J4 = u1();
xn.exports = function(a, g, b) {
for (var f = M4(g), A = J4.f, L = U4.f, S = 0; S < f.length; S++) {
var V = f[S];
!Gn(a, V) && !(b && Gn(b, V)) && A(a, V, L(g, V));
}
};
});
var u0 = B((ef, e0) => {
var $4 = D2(), H4 = A2(), X4 = /#|\.prototype\./, n1 = function(a, g) {
var b = W4[z4(a)];
return b == Y4 ? true : b == K4 ? false : H4(g) ? $4(g) : !!g;
}, z4 = n1.normalize = function(a) {
return String(a).replace(X4, ".").toLowerCase();
}, W4 = n1.data = {}, K4 = n1.NATIVE = "N", Y4 = n1.POLYFILL = "P";
e0.exports = n1;
});
var n0 = B((uf, i0) => {
var Me = k2(), Z4 = Ee().f, Q4 = Be(), G4 = Bn(), x4 = q1(), p4 = pn(), e3 = u0();
i0.exports = function(a, g) {
var b = a.target, f = a.global, A = a.stat, L, S, V, r, X, Y;
if (f ? S = Me : A ? S = Me[b] || x4(b, {}) : S = (Me[b] || {}).prototype, S)
for (V in g) {
if (X = g[V], a.dontCallGetSet ? (Y = Z4(S, V), r = Y && Y.value) : r = S[V], L = e3(f ? V : b + (A ? "." : "#") + V, a.forced), !L && r !== void 0) {
if (typeof X == typeof r)
continue;
p4(X, r);
}
(a.sham || r && r.sham) && Q4(X, "sham", true), G4(S, V, X, a);
}
};
});
var t0 = B(() => {
var u3 = n0(), Ue = k2();
u3({ global: true, forced: Ue.globalThis !== Ue }, { globalThis: Ue });
});
var o0 = B(() => {
t0();
});
var c0 = B((ff, f0) => {
var l0 = Ne(), i3 = u1();
f0.exports = function(a, g, b) {
return b.get && l0(b.get, g, { getter: true }), b.set && l0(b.set, g, { setter: true }), i3.f(a, g, b);
};
});
var a0 = B((cf, s0) => {
"use strict";
var n3 = S1();
s0.exports = function() {
var a = n3(this), g = "";
return a.hasIndices && (g += "d"), a.global && (g += "g"), a.ignoreCase && (g += "i"), a.multiline && (g += "m"), a.dotAll && (g += "s"), a.unicode && (g += "u"), a.unicodeSets && (g += "v"), a.sticky && (g += "y"), g;
};
});
var h0 = B(() => {
var t3 = k2(), o3 = S2(), l3 = c0(), f3 = a0(), c3 = D2(), d0 = t3.RegExp, g0 = d0.prototype, s3 = o3 && c3(function() {
var a = true;
try {
d0(".", "d");
} catch {
a = false;
}
var g = {}, b = "", f = a ? "dgimsy" : "gimsy", A = function(r, X) {
Object.defineProperty(g, r, { get: function() {
return b += X, true;
} });
}, L = { dotAll: "s", global: "g", ignoreCase: "i", multiline: "m", sticky: "y" };
a && (L.hasIndices = "d");
for (var S in L)
A(S, L[S]);
var V = Object.getOwnPropertyDescriptor(g0, "flags").get.call(g);
return V !== f || b !== f;
});
s3 && l3(g0, "flags", { configurable: true, get: f3 });
});
var $3 = B((df, O0) => {
o0();
h0();
var Xe = Object.defineProperty, a3 = Object.getOwnPropertyDescriptor, ze = Object.getOwnPropertyNames, d3 = Object.prototype.hasOwnProperty, b0 = (a, g) => function() {
return a && (g = (0, a[ze(a)[0]])(a = 0)), g;
}, t2 = (a, g) => function() {
return g || (0, a[ze(a)[0]])((g = { exports: {} }).exports, g), g.exports;
}, g3 = (a, g) => {
for (var b in g)
Xe(a, b, { get: g[b], enumerable: true });
}, h3 = (a, g, b, f) => {
if (g && typeof g == "object" || typeof g == "function")
for (let A of ze(g))
!d3.call(a, A) && A !== b && Xe(a, A, { get: () => g[A], enumerable: !(f = a3(g, A)) || f.enumerable });
return a;
}, m3 = (a) => h3(Xe({}, "__esModule", { value: true }), a), n2 = b0({ ""() {
} }), k0 = t2({ "src/common/parser-create-error.js"(a, g) {
"use strict";
n2();
function b(f, A) {
let L = new SyntaxError(f + " (" + A.start.line + ":" + A.start.column + ")");
return L.loc = A, L;
}
g.exports = b;
} }), b3 = t2({ "src/utils/try-combinations.js"(a, g) {
"use strict";
n2();
function b() {
let f;
for (var A = arguments.length, L = new Array(A), S = 0; S < A; S++)
L[S] = arguments[S];
for (let [V, r] of L.entries())
try {
return { result: r() };
} catch (X) {
V === 0 && (f = X);
}
return { error: f };
}
g.exports = b;
} }), r0 = {};
g3(r0, { EOL: () => He, arch: () => k3, cpus: () => D0, default: () => F0, endianness: () => v0, freemem: () => E0, getNetworkInterfaces: () => S0, hostname: () => y0, loadavg: () => A0, networkInterfaces: () => B0, platform: () => r3, release: () => q0, tmpDir: () => Je, tmpdir: () => $e, totalmem: () => C0, type: () => w0, uptime: () => P0 });
function v0() {
if (typeof R1 > "u") {
var a = new ArrayBuffer(2), g = new Uint8Array(a), b = new Uint16Array(a);
if (g[0] = 1, g[1] = 2, b[0] === 258)
R1 = "BE";
else if (b[0] === 513)
R1 = "LE";
else
throw new Error("unable to figure out endianess");
}
return R1;
}
function y0() {
return typeof globalThis.location < "u" ? globalThis.location.hostname : "";
}
function A0() {
return [];
}
function P0() {
return 0;
}
function E0() {
return Number.MAX_VALUE;
}
function C0() {
return Number.MAX_VALUE;
}
function D0() {
return [];
}
function w0() {
return "Browser";
}
function q0() {
return typeof globalThis.navigator < "u" ? globalThis.navigator.appVersion : "";
}
function B0() {
}
function S0() {
}
function k3() {
return "javascript";
}
function r3() {
return "browser";
}
function Je() {
return "/tmp";
}
var R1, $e, He, F0, v3 = b0({ "node-modules-polyfills:os"() {
n2(), $e = Je, He = `
`, F0 = { EOL: He, tmpdir: $e, tmpDir: Je, networkInterfaces: B0, getNetworkInterfaces: S0, release: q0, type: w0, cpus: D0, totalmem: C0, freemem: E0, uptime: P0, loadavg: A0, hostname: y0, endianness: v0 };
} }), y3 = t2({ "node-modules-polyfills-commonjs:os"(a, g) {
n2();
var b = (v3(), m3(r0));
if (b && b.default) {
g.exports = b.default;
for (let f in b)
g.exports[f] = b[f];
} else
b && (g.exports = b);
} }), A3 = t2({ "node_modules/detect-newline/index.js"(a, g) {
"use strict";
n2();
var b = (f) => {
if (typeof f != "string")
throw new TypeError("Expected a string");
let A = f.match(/(?:\r?\n)/g) || [];
if (A.length === 0)
return;
let L = A.filter((V) => V === `\r
`).length, S = A.length - L;
return L > S ? `\r
` : `
`;
};
g.exports = b, g.exports.graceful = (f) => typeof f == "string" && b(f) || `
`;
} }), P3 = t2({ "node_modules/jest-docblock/build/index.js"(a) {
"use strict";
n2(), Object.defineProperty(a, "__esModule", { value: true }), a.extract = T, a.parse = w2, a.parseWithComments = C, a.print = J, a.strip = z;
function g() {
let U = y3();
return g = function() {
return U;
}, U;
}
function b() {
let U = f(A3());
return b = function() {
return U;
}, U;
}
function f(U) {
return U && U.__esModule ? U : { default: U };
}
var A = /\*\/$/, L = /^\/\*\*?/, S = /^\s*(\/\*\*?(.|\r?\n)*?\*\/)/, V = /(^|\s+)\/\/([^\r\n]*)/g, r = /^(\r?\n)+/, X = /(?:^|\r?\n) *(@[^\r\n]*?) *\r?\n *(?![^@\r\n]*\/\/[^]*)([^@\r\n\s][^@\r\n]+?) *\r?\n/g, Y = /(?:^|\r?\n) *@(\S+) *([^\r\n]*)/g, G = /(\r?\n|^) *\* ?/g, u2 = [];
function T(U) {
let e2 = U.match(S);
return e2 ? e2[0].trimLeft() : "";
}
function z(U) {
let e2 = U.match(S);
return e2 && e2[0] ? U.substring(e2[0].length) : U;
}
function w2(U) {
return C(U).pragmas;
}
function C(U) {
let e2 = (0, b().default)(U) || g().EOL;
U = U.replace(L, "").replace(A, "").replace(G, "$1");
let g2 = "";
for (; g2 !== U; )
g2 = U, U = U.replace(X, `${e2}$1 $2${e2}`);
U = U.replace(r, "").trimRight();
let l2 = /* @__PURE__ */ Object.create(null), V2 = U.replace(Y, "").replace(r, "").trimRight(), f2;
for (; f2 = Y.exec(U); ) {
let N2 = f2[2].replace(V, "");
typeof l2[f2[1]] == "string" || Array.isArray(l2[f2[1]]) ? l2[f2[1]] = u2.concat(l2[f2[1]], N2) : l2[f2[1]] = N2;
}
return { comments: V2, pragmas: l2 };
}
function J(U) {
let { comments: e2 = "", pragmas: g2 = {} } = U, l2 = (0, b().default)(e2) || g().EOL, V2 = "/**", f2 = " *", N2 = " */", q2 = Object.keys(g2), V1 = q2.map((a2) => p(a2, g2[a2])).reduce((a2, t1) => a2.concat(t1), []).map((a2) => `${f2} ${a2}${l2}`).join("");
if (!e2) {
if (q2.length === 0)
return "";
if (q2.length === 1 && !Array.isArray(g2[q2[0]])) {
let a2 = g2[q2[0]];
return `${V2} ${p(q2[0], a2)[0]}${N2}`;
}
}
let N1 = e2.split(l2).map((a2) => `${f2} ${a2}`).join(l2) + l2;
return V2 + l2 + (e2 ? N1 : "") + (e2 && q2.length ? f2 + l2 : "") + V1 + N2;
}
function p(U, e2) {
return u2.concat(e2).map((g2) => `@${U} ${g2}`.trim());
}
} }), E3 = t2({ "src/common/end-of-line.js"(a, g) {
"use strict";
n2();
function b(S) {
let V = S.indexOf("\r");
return V >= 0 ? S.charAt(V + 1) === `
` ? "crlf" : "cr" : "lf";
}
function f(S) {
switch (S) {
case "cr":
return "\r";
case "crlf":
return `\r
`;
default:
return `
`;
}
}
function A(S, V) {
let r;
switch (V) {
case `
`:
r = /\n/g;
break;
case "\r":
r = /\r/g;
break;
case `\r
`:
r = /\r\n/g;
break;
default:
throw new Error(`Unexpected "eol" ${JSON.stringify(V)}.`);
}
let X = S.match(r);
return X ? X.length : 0;
}
function L(S) {
return S.replace(/\r\n?/g, `
`);
}
g.exports = { guessEndOfLine: b, convertEndOfLineToChars: f, countEndOfLineChars: A, normalizeEndOfLine: L };
} }), C3 = t2({ "src/language-js/utils/get-shebang.js"(a, g) {
"use strict";
n2();
function b(f) {
if (!f.startsWith("#!"))
return "";
let A = f.indexOf(`
`);
return A === -1 ? f : f.slice(0, A);
}
g.exports = b;
} }), D3 = t2({ "src/language-js/pragma.js"(a, g) {
"use strict";
n2();
var { parseWithComments: b, strip: f, extract: A, print: L } = P3(), { normalizeEndOfLine: S } = E3(), V = C3();
function r(G) {
let u2 = V(G);
u2 && (G = G.slice(u2.length + 1));
let T = A(G), { pragmas: z, comments: w2 } = b(T);
return { shebang: u2, text: G, pragmas: z, comments: w2 };
}
function X(G) {
let u2 = Object.keys(r(G).pragmas);
return u2.includes("prettier") || u2.includes("format");
}
function Y(G) {
let { shebang: u2, text: T, pragmas: z, comments: w2 } = r(G), C = f(T), J = L({ pragmas: Object.assign({ format: "" }, z), comments: w2.trimStart() });
return (u2 ? `${u2}
` : "") + S(J) + (C.startsWith(`
`) ? `
` : `
`) + C;
}
g.exports = { hasPragma: X, insertPragma: Y };
} }), w3 = t2({ "src/utils/is-non-empty-array.js"(a, g) {
"use strict";
n2();
function b(f) {
return Array.isArray(f) && f.length > 0;
}
g.exports = b;
} }), L0 = t2({ "src/language-js/loc.js"(a, g) {
"use strict";
n2();
var b = w3();
function f(r) {
var X, Y;
let G = r.range ? r.range[0] : r.start, u2 = (X = (Y = r.declaration) === null || Y === void 0 ? void 0 : Y.decorators) !== null && X !== void 0 ? X : r.decorators;
return b(u2) ? Math.min(f(u2[0]), G) : G;
}
function A(r) {
return r.range ? r.range[1] : r.end;
}
function L(r, X) {
let Y = f(r);
return Number.isInteger(Y) && Y === f(X);
}
function S(r, X) {
let Y = A(r);
return Number.isInteger(Y) && Y === A(X);
}
function V(r, X) {
return L(r, X) && S(r, X);
}
g.exports = { locStart: f, locEnd: A, hasSameLocStart: L, hasSameLoc: V };
} }), q3 = t2({ "src/language-js/parse/utils/create-parser.js"(a, g) {
"use strict";
n2();
var { hasPragma: b } = D3(), { locStart: f, locEnd: A } = L0();
function L(S) {
return S = typeof S == "function" ? { parse: S } : S, Object.assign({ astFormat: "estree", hasPragma: b, locStart: f, locEnd: A }, S);
}
g.exports = L;
} }), B3 = t2({ "src/language-js/utils/is-ts-keyword-type.js"(a, g) {
"use strict";
n2();
function b(f) {
let { type: A } = f;
return A.startsWith("TS") && A.endsWith("Keyword");
}
g.exports = b;
} }), S3 = t2({ "src/language-js/utils/is-block-comment.js"(a, g) {
"use strict";
n2();
var b = /* @__PURE__ */ new Set(["Block", "CommentBlock", "MultiLine"]), f = (A) => b.has(A == null ? void 0 : A.type);
g.exports = f;
} }), F3 = t2({ "src/language-js/utils/is-type-cast-comment.js"(a, g) {
"use strict";
n2();
var b = S3();
function f(A) {
return b(A) && A.value[0] === "*" && /@(?:type|satisfies)\b/.test(A.value);
}
g.exports = f;
} }), L3 = t2({ "src/utils/get-last.js"(a, g) {
"use strict";
n2();
var b = (f) => f[f.length - 1];
g.exports = b;
} }), O3 = t2({ "src/language-js/parse/postprocess/visit-node.js"(a, g) {
"use strict";
n2();
function b(f, A) {
if (Array.isArray(f)) {
for (let L = 0; L < f.length; L++)
f[L] = b(f[L], A);
return f;
}
if (f && typeof f == "object" && typeof f.type == "string") {
let L = Object.keys(f);
for (let S = 0; S < L.length; S++)
f[L[S]] = b(f[L[S]], A);
return A(f) || f;
}
return f;
}
g.exports = b;
} }), T3 = t2({ "src/language-js/parse/postprocess/throw-syntax-error.js"(a, g) {
"use strict";
n2();
var b = k0();
function f(A, L) {
let { start: S, end: V } = A.loc;
throw b(L, { start: { line: S.line, column: S.column + 1 }, end: { line: V.line, column: V.column + 1 } });
}
g.exports = f;
} }), I3 = t2({ "src/language-js/parse/postprocess/index.js"(a, g) {
"use strict";
n2();
var { locStart: b, locEnd: f } = L0(), A = B3(), L = F3(), S = L3(), V = O3(), r = T3();
function X(T, z) {
if (z.parser !== "typescript" && z.parser !== "flow" && z.parser !== "acorn" && z.parser !== "espree" && z.parser !== "meriyah") {
let C = /* @__PURE__ */ new Set();
T = V(T, (J) => {
J.leadingComments && J.leadingComments.some(L) && C.add(b(J));
}), T = V(T, (J) => {
if (J.type === "ParenthesizedExpression") {
let { expression: p } = J;
if (p.type === "TypeCastExpression")
return p.range = J.range, p;
let U = b(J);
if (!C.has(U))
return p.extra = Object.assign(Object.assign({}, p.extra), {}, { parenthesized: true }), p;
}
});
}
return T = V(T, (C) => {
switch (C.type) {
case "ChainExpression":
return Y(C.expression);
case "LogicalExpression": {
if (G(C))
return u2(C);
break;
}
case "VariableDeclaration": {
let J = S(C.declarations);
J && J.init && w2(C, J);
break;
}
case "TSParenthesizedType":
return A(C.typeAnnotation) || C.typeAnnotation.type === "TSThisType" || (C.typeAnnotation.range = [b(C), f(C)]), C.typeAnnotation;
case "TSTypeParameter":
if (typeof C.name == "string") {
let J = b(C);
C.name = { type: "Identifier", name: C.name, range: [J, J + C.name.length] };
}
break;
case "ObjectExpression":
if (z.parser === "typescript") {
let J = C.properties.find((p) => p.type === "Property" && p.value.type === "TSEmptyBodyFunctionExpression");
J && r(J.value, "Unexpected token.");
}
break;
case "SequenceExpression": {
let J = S(C.expressions);
C.range = [b(C), Math.min(f(J), f(C))];
break;
}
case "TopicReference":
z.__isUsingHackPipeline = true;
break;
case "ExportAllDeclaration": {
let { exported: J } = C;
if (z.parser === "meriyah" && J && J.type === "Identifier") {
let p = z.originalText.slice(b(J), f(J));
(p.startsWith('"') || p.startsWith("'")) && (C.exported = Object.assign(Object.assign({}, C.exported), {}, { type: "Literal", value: C.exported.name, raw: p }));
}
break;
}
case "PropertyDefinition":
if (z.parser === "meriyah" && C.static && !C.computed && !C.key) {
let J = "static", p = b(C);
Object.assign(C, { static: false, key: { type: "Identifier", name: J, range: [p, p + J.length] } });
}
break;
}
}), T;
function w2(C, J) {
z.originalText[f(J)] !== ";" && (C.range = [b(C), f(J)]);
}
}
function Y(T) {
switch (T.type) {
case "CallExpression":
T.type = "OptionalCallExpression", T.callee = Y(T.callee);
break;
case "MemberExpression":
T.type = "OptionalMemberExpression", T.object = Y(T.object);
break;
case "TSNonNullExpression":
T.expression = Y(T.expression);
break;
}
return T;
}
function G(T) {
return T.type === "LogicalExpression" && T.right.type === "LogicalExpression" && T.operator === T.right.operator;
}
function u2(T) {
return G(T) ? u2({ type: "LogicalExpression", operator: T.operator, left: u2({ type: "LogicalExpression", operator: T.operator, left: T.left, right: T.right.left, range: [b(T.left), f(T.right.left)] }), right: T.right.right, range: [b(T), f(T)] }) : T;
}
g.exports = X;
} }), R3 = t2({ "node_modules/meriyah/dist/meriyah.cjs"(a) {
"use strict";
n2(), Object.defineProperty(a, "__esModule", { value: true });
var g = { [0]: "Unexpected token", [28]: "Unexpected token: '%0'", [1]: "Octal escape sequences are not allowed in strict mode", [2]: "Octal escape sequences are not allowed in template strings", [3]: "Unexpected token `#`", [4]: "Illegal Unicode escape sequence", [5]: "Invalid code point %0", [6]: "Invalid hexadecimal escape sequence", [8]: "Octal literals are not allowed in strict mode", [7]: "Decimal integer literals with a leading zero are forbidden in strict mode", [9]: "Expected number in radix %0", [145]: "Invalid left-hand side assignment to a destructible right-hand side", [10]: "Non-number found after exponent indicator", [11]: "Invalid BigIntLiteral", [12]: "No identifiers allowed directly after numeric literal", [13]: "Escapes \\8 or \\9 are not syntactically valid escapes", [14]: "Unterminated string literal", [15]: "Unterminated template literal", [16]: "Multiline comment was not closed properly", [17]: "The identifier contained dynamic unicode escape that was not closed", [18]: "Illegal character '%0'", [19]: "Missing hexadecimal digits", [20]: "Invalid implicit octal", [21]: "Invalid line break in string literal", [22]: "Only unicode escapes are legal in identifier names", [23]: "Expected '%0'", [24]: "Invalid left-hand side in assignment", [25]: "Invalid left-hand side in async arrow", [26]: 'Calls to super must be in the "constructor" method of a class expression or class declaration that has a superclass', [27]: "Member access on super must be in a method", [29]: "Await expression not allowed in formal parameter", [30]: "Yield expression not allowed in formal parameter", [92]: "Unexpected token: 'escaped keyword'", [31]: "Unary expressions as the left operand of an exponentiation expression must be disambiguated with parentheses", [119]: "Async functions can only be declared at the top level or inside a block", [32]: "Unterminated regular expression", [33]: "Unexpected regular expression flag", [34]: "Duplicate regular expression flag '%0'", [35]: "%0 functions must have exactly %1 argument%2", [36]: "Setter function argument must not be a rest parameter", [37]: "%0 declaration must have a name in this context", [38]: "Function name may not contain any reserved words or be eval or arguments in strict mode", [39]: "The rest operator is missing an argument", [40]: "A getter cannot be a generator", [41]: "A computed property name must be followed by a colon or paren", [130]: "Object literal keys that are strings or numbers must be a method or have a colon", [43]: "Found `* async x(){}` but this should be `async * x(){}`", [42]: "Getters and setters can not be generators", [44]: "'%0' can not be generator method", [45]: "No line break is allowed after '=>'", [46]: "The left-hand side of the arrow can only be destructed through assignment", [47]: "The binding declaration is not destructible", [48]: "Async arrow can not be followed by new expression", [49]: "Classes may not have a static property named 'prototype'", [50]: "Class constructor may not be a %0", [51]: "Duplicate constructor method in class", [52]: "Invalid increment/decrement operand", [53]: "Invalid use of `new` keyword on an increment/decrement expression", [54]: "`=>` is an invalid assignment target", [55]: "Rest element may not have a trailing comma", [56]: "Missing initializer in %0 declaration", [57]: "'for-%0' loop head declarations can not have an initializer", [58]: "Invalid left-hand side in for-%0 loop: Must have a single binding", [59]: "Invalid shorthand property initializer", [60]: "Property name __proto__ appears more than once in object literal", [61]: "Let is disallowed as a lexically bound name", [62]: "Invalid use of '%0' inside new expression", [63]: "Illegal 'use strict' directive in function with non-simple parameter list", [64]: 'Identifier "let" disallowed as left-hand side expression in strict mode', [65]: "Illegal continue statement", [66]: "Illegal break statement", [67]: "Cannot have `let[...]` as a var name in strict mode", [68]: "Invalid destructuring assignment target", [69]: "Rest parameter may not have a default initializer", [70]: "The rest argument must the be last parameter", [71]: "Invalid rest argument", [73]: "In strict mode code, functions can only be declared at top level or inside a block", [74]: "In non-strict mode code, functions can only be declared at top level, inside a block, or as the body of an if statement", [75]: "Without web compatibility enabled functions can not be declared at top level, inside a block, or as the body of an if statement", [76]: "Class declaration can't appear in single-statement context", [77]: "Invalid left-hand side in for-%0", [78]: "Invalid assignment in for-%0", [79]: "for await (... of ...) is only valid in async functions and async generators", [80]: "The first token after the template expression should be a continuation of the template", [82]: "`let` declaration not allowed here and `let` cannot be a regular var name in strict mode", [81]: "`let \n [` is a restricted production at the start of a statement", [83]: "Catch clause requires exactly one parameter, not more (and no trailing comma)", [84]: "Catch clause parameter does not support default values", [85]: "Missing catch or finally after try", [86]: "More than one default clause in switch statement", [87]: "Illegal newline after throw", [88]: "Strict mode code may not include a with statement", [89]: "Illegal return statement", [90]: "The left hand side of the for-header binding declaration is not destructible", [91]: "new.target only allowed within functions", [93]: "'#' not followed by identifier", [99]: "Invalid keyword", [98]: "Can not use 'let' as a class name", [97]: "'A lexical declaration can't define a 'let' binding", [96]: "Can not use `let` as variable name in strict mode", [94]: "'%0' may not be used as an identifier in this context", [95]: "Await is only valid in async functions", [100]: "The %0 keyword can only be used with the module goal", [101]: "Unicode codepoint must not be greater than 0x10FFFF", [102]: "%0 source must be string", [103]: "Only a identifier can be used to indicate alias", [104]: "Only '*' or '{...}' can be imported after default", [105]: "Trailing decorator may be followed by method", [106]: "Decorators can't be used with a constructor", [108]: "HTML comments are only allowed with web compatibility (Annex B)", [109]: "The identifier 'let' must not be in expression position in strict mode", [110]: "Cannot assign to `eval` and `arguments` in strict mode", [111]: "The left-hand side of a for-of loop may not start with 'let'", [112]: "Block body arrows can not be immediately invoked without a group", [113]: "Block body arrows can not be immediately accessed without a group", [114]: "Unexpected strict mode reserved word", [115]: "Unexpected eval or arguments in strict mode", [116]: "Decorators must not be followed by a semicolon", [117]: "Calling delete on expression not allowed in strict mode", [118]: "Pattern can not have a tail", [120]: "Can not have a `yield` expression on the left side of a ternary", [121]: "An arrow function can not have a postfix update operator", [122]: "Invalid object literal key character after generator star", [123]: "Private fields can not be deleted", [125]: "Classes may not have a field called constructor", [124]: "Classes may not have a private element named constructor", [126]: "A class field initializer may not contain arguments", [127]: "Generators can only be declared at the top level or inside a block", [128]: "Async methods are a restricted production and cannot have a newline following it", [129]: "Unexpected character after object literal property name", [131]: "Invalid key token", [132]: "Label '%0' has already been declared", [133]: "continue statement must be nested within an iteration statement", [134]: "Undefined label '%0'", [135]: "Trailing comma is disallowed inside import(...) arguments", [136]: "import() requires exactly one argument", [137]: "Cannot use new with import(...)", [138]: "... is not allowed in import()", [139]: "Expected '=>'", [140]: "Duplicate binding '%0'", [141]: "Cannot export a duplicate name '%0'", [144]: "Duplicate %0 for-binding", [142]: "Exported binding '%0' needs to refer to a top-level declared variable", [143]: "Unexpected private field", [147]: "Numeric separators are not allowed at the end of numeric literals", [146]: "Only one underscore is allowed as numeric separator", [148]: "JSX value should be either an expression or a quoted JSX text", [149]: "Expected corresponding JSX closing tag for %0", [150]: "Adjacent JSX elements must be wrapped in an enclosing tag", [151]: "JSX attributes must only be assigned a non-empty 'expression'", [152]: "'%0' has already been declared", [153]: "'%0' shadowed a catch clause binding", [154]: "Dot property must be an identifier", [155]: "Encountered invalid input after spread/rest argument", [156]: "Catch without try", [157]: "Finally without try", [158]: "Expected corresponding closing tag for JSX fragment", [159]: "Coalescing and logical operators used together in the same expression must be disambiguated with parentheses", [160]: "Invalid tagged template on optional chain", [161]: "Invalid optional chain from super property", [162]: "Invalid optional chain from new expression", [163]: 'Cannot use "import.meta" outside a module', [164]: "Leading decorators must be attached to a class declaration" }, b = class extends SyntaxError {
constructor(e, u, i, n3) {
for (var t = arguments.length, o = new Array(t > 4 ? t - 4 : 0), l = 4; l < t; l++)
o[l - 4] = arguments[l];
let c = "[" + u + ":" + i + "]: " + g[n3].replace(/%(\d+)/g, (s, m) => o[m]);
super(`${c}`), this.index = e, this.line = u, this.column = i, this.description = c, this.loc = { line: u, column: i };
}
};
function f(e, u) {
for (var i = arguments.length, n3 = new Array(i > 2 ? i - 2 : 0), t = 2; t < i; t++)
n3[t - 2] = arguments[t];
throw new b(e.index, e.line, e.column, u, ...n3);
}
function A(e) {
throw new b(e.index, e.line, e.column, e.type, e.params);
}
function L(e, u, i, n3) {
for (var t = arguments.length, o = new Array(t > 4 ? t - 4 : 0), l = 4; l < t; l++)
o[l - 4] = arguments[l];
throw new b(e, u, i, n3, ...o);
}
function S(e, u, i, n3) {
throw new b(e, u, i, n3);
}
var V = ((e, u) => {
let i = new Uint32Array(104448), n3 = 0, t = 0;
for (; n3 < 3540; ) {
let o = e[n3++];
if (o < 0)
t -= o;
else {
let l = e[n3++];
o & 2 && (l = u[l]), o & 1 ? i.fill(l, t, t += e[n3++]) : i[t++] = l;
}
}
return i;
})([-1, 2, 24, 2, 25, 2, 5, -1, 0, 77595648, 3, 44, 2, 3, 0, 14, 2, 57, 2, 58, 3, 0, 3, 0, 3168796671, 0, 4294956992, 2, 1, 2, 0, 2, 59, 3, 0, 4, 0, 4294966523, 3, 0, 4, 2, 16, 2, 60, 2, 0, 0, 4294836735, 0, 3221225471, 0, 4294901942, 2, 61, 0, 134152192, 3, 0, 2, 0, 4294951935, 3, 0, 2, 0, 2683305983, 0, 2684354047, 2, 17, 2, 0, 0, 4294961151, 3, 0, 2, 2, 19, 2, 0, 0, 608174079, 2, 0, 2, 131, 2, 6, 2, 56, -1, 2, 37, 0, 4294443263, 2, 1, 3, 0, 3, 0, 4294901711, 2, 39, 0, 4089839103, 0, 2961209759, 0, 1342439375, 0, 4294543342, 0, 3547201023, 0, 1577204103, 0, 4194240, 0, 4294688750, 2, 2, 0, 80831, 0, 4261478351, 0, 4294549486, 2, 2, 0, 2967484831, 0, 196559, 0, 3594373100, 0, 3288319768, 0, 8469959, 2, 194, 2, 3, 0, 3825204735, 0, 123747807, 0, 65487, 0, 4294828015, 0, 4092591615, 0, 1080049119, 0, 458703, 2, 3, 2, 0, 0, 2163244511, 0, 4227923919, 0, 4236247022, 2, 66, 0, 4284449919, 0, 851904, 2, 4, 2, 11, 0, 67076095, -1, 2, 67, 0, 1073741743, 0, 4093591391, -1, 0, 50331649, 0, 3265266687, 2, 32, 0, 4294844415, 0, 4278190047, 2, 18, 2, 129, -1, 3, 0, 2, 2, 21, 2, 0, 2, 9, 2, 0, 2, 14, 2, 15, 3, 0, 10, 2, 69, 2, 0, 2, 70, 2, 71, 2, 72, 2, 0, 2, 73, 2, 0, 2, 10, 0, 261632, 2, 23, 3, 0, 2, 2, 12, 2, 4, 3, 0, 18, 2, 74, 2, 5, 3, 0, 2, 2, 75, 0, 2088959, 2, 27, 2, 8, 0, 909311, 3, 0, 2, 0, 814743551, 2, 41, 0, 67057664, 3, 0, 2, 2, 40, 2, 0, 2, 28, 2, 0, 2, 29, 2, 7, 0, 268374015, 2, 26, 2, 49, 2, 0, 2, 76, 0, 134153215, -1, 2, 6, 2, 0, 2, 7, 0, 2684354559, 0, 67044351, 0, 3221160064, 0, 1, -1, 3, 0, 2, 2, 42, 0, 1046528, 3, 0, 3, 2, 8, 2, 0, 2, 51, 0, 4294960127, 2, 9, 2, 38, 2, 10, 0, 4294377472, 2, 11, 3, 0, 7, 0, 4227858431, 3, 0, 8, 2, 12, 2, 0, 2, 78, 2, 9, 2, 0, 2, 79, 2, 80, 2, 81, -1, 2, 124, 0, 1048577, 2, 82, 2, 13, -1, 2, 13, 0, 131042, 2, 83, 2, 84, 2, 85, 2, 0, 2, 33, -83, 2, 0, 2, 53, 2, 7, 3, 0, 4, 0, 1046559, 2, 0, 2, 14, 2, 0, 0, 2147516671, 2, 20, 3, 86, 2, 2, 0, -16, 2, 87, 0, 524222462, 2, 4, 2, 0, 0, 4269801471, 2, 4, 2, 0, 2, 15, 2, 77, 2, 16, 3, 0, 2, 2, 47, 2, 0, -1, 2, 17, -16, 3, 0, 206, -2, 3, 0, 655, 2, 18, 3, 0, 36, 2, 68, -1, 2, 17, 2, 9, 3, 0, 8, 2, 89, 2, 121, 2, 0, 0, 3220242431, 3, 0, 3, 2, 19, 2, 90, 2, 91, 3, 0, 2, 2, 92, 2, 0, 2, 93, 2, 94, 2, 0, 0, 4351, 2, 0, 2, 8, 3, 0, 2, 0, 67043391, 0, 3909091327, 2, 0, 2, 22, 2, 8, 2, 18, 3, 0, 2, 0, 67076097, 2, 7, 2, 0, 2, 20, 0, 67059711, 0, 4236247039, 3, 0, 2, 0, 939524103, 0, 8191999, 2, 97, 2, 98, 2, 15, 2, 21, 3, 0, 3, 0, 67057663, 3, 0, 349, 2, 99, 2, 100, 2, 6, -264, 3, 0, 11, 2, 22, 3, 0, 2, 2, 31, -1, 0, 3774349439, 2, 101, 2, 102, 3, 0, 2, 2, 19, 2, 103, 3, 0, 10, 2, 9, 2, 17, 2, 0, 2, 45, 2, 0, 2, 30, 2, 104, 2, 23, 0, 1638399, 2, 172, 2, 105, 3, 0, 3, 2, 18, 2, 24, 2, 25, 2, 5, 2, 26, 2, 0, 2, 7, 2, 106, -1, 2, 107, 2, 108, 2, 109, -1, 3, 0, 3, 2, 11, -2, 2, 0, 2, 27, -3, 2, 150, -4, 2, 18, 2, 0, 2, 35, 0, 1, 2, 0, 2, 62, 2, 28, 2, 11, 2, 9, 2, 0, 2, 110, -1, 3, 0, 4, 2, 9, 2, 21, 2, 111, 2, 6, 2, 0, 2, 112, 2, 0, 2, 48, -4, 3, 0, 9, 2, 20, 2, 29, 2, 30, -4, 2, 113, 2, 114, 2, 29, 2, 20, 2, 7, -2, 2, 115, 2, 29, 2, 31, -2, 2, 0, 2, 116, -2, 0, 4277137519, 0, 2269118463, -1, 3, 18, 2, -1, 2, 32, 2, 36, 2, 0, 3, 29, 2, 2, 34, 2, 19, -3, 3, 0, 2, 2, 33, -1, 2, 0, 2, 34, 2, 0, 2, 34, 2, 0, 2, 46, -10, 2, 0, 0, 203775, -2, 2, 18, 2, 43, 2, 35, -2, 2, 17, 2, 117, 2, 20, 3, 0, 2, 2, 36, 0, 2147549120, 2, 0, 2, 11, 2, 17, 2, 135, 2, 0, 2, 37, 2, 52, 0, 5242879, 3, 0, 2, 0, 402644511, -1, 2, 120, 0, 1090519039, -2, 2, 122, 2, 38, 2, 0, 0, 67045375, 2, 39, 0, 4226678271, 0, 3766565279, 0, 2039759, -4, 3, 0, 2, 0, 3288270847, 0, 3, 3, 0, 2, 0, 67043519, -5, 2, 0, 0, 4282384383, 0, 1056964609, -1, 3, 0, 2, 0, 67043345, -1, 2, 0, 2, 40, 2, 41, -1, 2, 10, 2, 42, -6, 2, 0, 2, 11, -3, 3, 0, 2, 0, 2147484671, 2, 125, 0, 4190109695, 2, 50, -2, 2, 126, 0, 4244635647, 0, 27, 2, 0, 2, 7, 2, 43, 2, 0, 2, 63, -1, 2, 0, 2, 40, -8, 2, 54, 2, 44, 0, 67043329, 2, 127, 2, 45, 0, 8388351, -2, 2, 128, 0, 3028287487, 2, 46, 2, 130, 0, 33259519, 2, 41, -9, 2, 20, -5, 2, 64, -2, 3, 0, 28, 2, 31, -3, 3, 0, 3, 2, 47, 3, 0, 6, 2, 48, -85, 3, 0, 33, 2, 47, -126, 3, 0, 18, 2, 36, -269, 3, 0, 17, 2, 40, 2, 7, 2, 41, -2, 2, 17, 2, 49, 2, 0, 2, 20, 2, 50, 2, 132, 2, 23, -21, 3, 0, 2, -4, 3, 0, 2, 0, 4294936575, 2, 0, 0, 4294934783, -2, 0, 196635, 3, 0, 191, 2, 51, 3, 0, 38, 2, 29, -1, 2, 33, -279, 3, 0, 8, 2, 7, -1, 2, 133, 2, 52, 3, 0, 11, 2, 6, -72, 3, 0, 3, 2, 134, 0, 1677656575, -166, 0, 4161266656, 0, 4071, 0, 15360, -4, 0, 28, -13, 3, 0, 2, 2, 37, 2, 0, 2, 136, 2, 137, 2, 55, 2, 0, 2, 138, 2, 139, 2, 140, 3, 0, 10, 2, 141, 2, 142, 2, 15, 3, 37, 2, 3, 53, 2, 3, 54, 2, 0, 4294954999, 2, 0, -16, 2, 0, 2, 88, 2, 0, 0, 2105343, 0, 4160749584, 0, 65534, -42, 0, 4194303871, 0, 2011, -6, 2, 0, 0, 1073684479, 0, 17407, -11, 2, 0, 2, 31, -40, 3, 0, 6, 0, 8323103, -1, 3, 0, 2, 2, 42, -37, 2, 55, 2, 144, 2, 145, 2, 146, 2, 147, 2, 148, -105, 2, 24, -32, 3, 0, 1334, 2, 9, -1, 3, 0, 129, 2, 27, 3, 0, 6, 2, 9, 3, 0, 180, 2, 149, 3, 0, 233, 0, 1, -96, 3, 0, 16, 2, 9, -47, 3, 0, 154, 2, 56, -22381, 3, 0, 7, 2, 23, -6130, 3, 5, 2, -1, 0, 69207040, 3, 44, 2, 3, 0, 14, 2, 57, 2, 58, -3, 0, 3168731136, 0, 4294956864, 2, 1, 2, 0, 2, 59, 3, 0, 4, 0, 4294966275, 3, 0, 4, 2, 16, 2, 60, 2, 0, 2, 33, -1, 2, 17, 2, 61, -1, 2, 0, 2, 56, 0, 4294885376, 3, 0, 2, 0, 3145727, 0, 2617294944, 0, 4294770688, 2, 23, 2, 62, 3, 0, 2, 0, 131135, 2, 95, 0, 70256639, 0, 71303167, 0, 272, 2, 40, 2, 56, -1, 2, 37, 2, 30, -1, 2, 96, 2, 63, 0, 4278255616, 0, 4294836227, 0, 4294549473, 0, 600178175, 0, 2952806400, 0, 268632067, 0, 4294543328, 0, 57540095, 0, 1577058304, 0, 1835008, 0, 4294688736, 2, 65, 2, 64, 0, 33554435, 2, 123, 2, 65, 2, 151, 0, 131075, 0, 3594373096, 0, 67094296, 2, 64, -1, 0, 4294828e3, 0, 603979263, 2, 160, 0, 3, 0, 4294828001, 0, 602930687, 2, 183, 0, 393219, 0, 4294828016, 0, 671088639, 0, 2154840064, 0, 4227858435, 0, 4236247008, 2, 66, 2, 36, -1, 2, 4, 0, 917503, 2, 36, -1, 2, 67, 0, 537788335, 0, 4026531935, -1, 0, 1, -1, 2, 32, 2, 68, 0, 7936, -3, 2, 0, 0, 2147485695, 0, 1010761728, 0, 4292984930, 0, 16387, 2, 0, 2, 14, 2, 15, 3, 0, 10, 2, 69, 2, 0, 2, 70, 2, 71, 2, 72, 2, 0, 2, 73, 2, 0, 2, 11, -1, 2, 23, 3, 0, 2, 2, 12, 2, 4, 3, 0, 18, 2, 74, 2, 5, 3, 0, 2, 2, 75, 0, 253951, 3, 19, 2, 0, 122879, 2, 0, 2, 8, 0, 276824064, -2, 3, 0, 2, 2, 40, 2, 0, 0, 4294903295, 2, 0, 2, 29, 2, 7, -1, 2, 17, 2, 49, 2, 0, 2, 76, 2, 41, -1, 2, 20, 2, 0, 2, 27, -2, 0, 128, -2, 2, 77, 2, 8, 0, 4064, -1, 2, 119, 0, 4227907585, 2, 0, 2, 118, 2, 0, 2, 48, 2, 173, 2, 9, 2, 38, 2, 10, -1, 0, 74440192, 3, 0, 6, -2, 3, 0, 8, 2, 12, 2, 0, 2, 78, 2, 9, 2, 0, 2, 79, 2, 80, 2, 81, -3, 2, 82, 2, 13, -3, 2, 83, 2, 84, 2, 85, 2, 0, 2, 33, -83, 2, 0, 2, 53, 2, 7, 3, 0, 4, 0, 817183, 2, 0, 2, 14, 2, 0, 0, 33023, 2, 20, 3, 86, 2, -17, 2, 87, 0, 524157950, 2, 4, 2, 0, 2, 88, 2, 4, 2, 0, 2, 15, 2, 77, 2, 16, 3, 0, 2, 2, 47, 2, 0, -1, 2, 17, -16, 3, 0, 206, -2, 3, 0, 655, 2, 18, 3, 0, 36, 2, 68, -1, 2, 17, 2, 9, 3, 0, 8, 2, 89, 0, 3072, 2, 0, 0, 2147516415, 2, 9, 3, 0, 2, 2, 23, 2, 90, 2, 91, 3, 0, 2, 2, 92, 2, 0, 2, 93, 2, 94, 0, 4294965179, 0, 7, 2, 0, 2, 8, 2, 91, 2, 8, -1, 0, 1761345536, 2, 95, 0, 4294901823, 2, 36, 2, 18, 2, 96, 2, 34, 2, 166, 0, 2080440287, 2, 0, 2, 33, 2, 143, 0, 3296722943, 2, 0, 0, 1046675455, 0, 939524101, 0, 1837055, 2, 97, 2, 98, 2, 15, 2, 21, 3, 0, 3, 0, 7, 3, 0, 349, 2, 99, 2, 100, 2, 6, -264, 3, 0, 11, 2, 22, 3, 0, 2, 2, 31, -1, 0, 2700607615, 2, 101, 2, 102, 3, 0, 2, 2, 19, 2, 103, 3, 0, 10, 2, 9, 2, 17, 2, 0, 2, 45, 2, 0, 2, 30, 2, 104, -3, 2, 105, 3, 0, 3, 2, 18, -1, 3, 5, 2, 2, 26, 2, 0, 2, 7, 2, 106, -1, 2, 107, 2, 108, 2, 109, -1, 3, 0, 3, 2, 11, -2, 2, 0, 2, 27, -8, 2, 18, 2, 0, 2, 35, -1, 2, 0, 2, 62, 2, 28, 2, 29, 2, 9, 2, 0, 2, 110, -1, 3, 0, 4, 2, 9, 2, 17, 2, 111, 2, 6, 2, 0, 2, 112, 2, 0, 2, 48, -4, 3, 0, 9, 2, 20, 2, 29, 2, 30, -4, 2, 113, 2, 114, 2, 29, 2, 20, 2, 7, -2, 2, 115, 2, 29, 2, 31, -2, 2, 0, 2, 116, -2, 0, 4277075969, 2, 29, -1, 3, 18, 2, -1, 2, 32, 2, 117, 2, 0, 3, 29, 2, 2, 34, 2, 19, -3, 3, 0, 2, 2, 33, -1, 2, 0, 2, 34, 2, 0, 2, 34, 2, 0, 2, 48, -10, 2, 0, 0, 197631, -2, 2, 18, 2, 43, 2, 118, -2, 2, 17, 2, 117, 2, 20, 2, 119, 2, 51, -2, 2, 119, 2, 23, 2, 17, 2, 33, 2, 119, 2, 36, 0, 4294901904, 0, 4718591, 2, 119, 2, 34, 0, 335544350, -1, 2, 120, 2, 121, -2, 2, 122, 2, 38, 2, 7, -1, 2, 123, 2, 65, 0, 3758161920, 0, 3, -4, 2, 0, 2, 27, 0, 2147485568, 0, 3, 2, 0, 2, 23, 0, 176, -5, 2, 0, 2, 47, 2, 186, -1, 2, 0, 2, 23, 2, 197, -1, 2, 0, 0, 16779263, -2, 2, 11, -7, 2, 0, 2, 121, -3, 3, 0, 2, 2, 124, 2, 125, 0, 2147549183, 0, 2, -2, 2, 126, 2, 35, 0, 10, 0, 4294965249, 0, 67633151, 0, 4026597376, 2, 0, 0, 536871935, -1, 2, 0, 2, 40, -8, 2, 54, 2, 47, 0, 1, 2, 127, 2, 23, -3, 2, 128, 2, 35, 2, 129, 2, 130, 0, 16778239, -10, 2, 34, -5, 2, 64, -2, 3, 0, 28, 2, 31, -3, 3, 0, 3, 2, 47, 3, 0, 6, 2, 48, -85, 3, 0, 33, 2, 47, -126, 3, 0, 18, 2, 36, -269, 3, 0, 17, 2, 40, 2, 7, -3, 2, 17, 2, 131, 2, 0, 2, 23, 2, 48, 2, 132, 2, 23, -21, 3, 0, 2, -4, 3, 0, 2, 0, 67583, -1, 2, 103, -2, 0, 11, 3, 0, 191, 2, 51, 3, 0, 38, 2, 29, -1, 2, 33, -279, 3, 0, 8, 2, 7, -1, 2, 133, 2, 52, 3, 0, 11, 2, 6, -72, 3, 0, 3, 2, 134, 2, 135, -187, 3, 0, 2, 2, 37, 2, 0, 2, 136, 2, 137, 2, 55, 2, 0, 2, 138, 2, 139, 2, 140, 3, 0, 10, 2, 141, 2, 142, 2, 15, 3, 37, 2, 3, 53, 2, 3, 54, 2, 2, 143, -73, 2, 0, 0, 1065361407, 0, 16384, -11, 2, 0, 2, 121, -40, 3, 0, 6, 2, 117, -1, 3, 0, 2, 0, 2063, -37, 2, 55, 2, 144, 2, 145, 2, 146, 2, 147, 2, 148, -138, 3, 0, 1334, 2, 9, -1, 3, 0, 129, 2, 27, 3, 0, 6, 2, 9, 3, 0, 180, 2, 149, 3, 0, 233, 0, 1, -96, 3, 0, 16, 2, 9, -47, 3, 0, 154, 2, 56, -28517, 2, 0, 0, 1, -1, 2, 124, 2, 0, 0, 8193, -21, 2, 193, 0, 10255, 0, 4, -11, 2, 64, 2, 171, -1, 0, 71680, -1, 2, 161, 0, 4292900864, 0, 805306431, -5, 2, 150, -1, 2, 157, -1, 0, 6144, -2, 2, 127, -1, 2, 154, -1, 0, 2147532800, 2, 151, 2, 165, 2, 0, 2, 164, 0, 524032, 0, 4, -4, 2, 190, 0, 205128192, 0, 1333757536, 0, 2147483696, 0, 423953, 0, 747766272, 0, 2717763192, 0, 4286578751, 0, 278545, 2, 152, 0, 4294886464, 0, 33292336, 0, 417809, 2, 152, 0, 1327482464, 0, 4278190128, 0, 700594195, 0, 1006647527, 0, 4286497336, 0, 4160749631, 2, 153, 0, 469762560, 0, 4171219488, 0, 8323120, 2, 153, 0, 202375680, 0, 3214918176, 0, 4294508592, 2, 153, -1, 0, 983584, 0, 48, 0, 58720273, 0, 3489923072, 0, 10517376, 0, 4293066815, 0, 1, 0, 2013265920, 2, 177, 2, 0, 0, 2089, 0, 3221225552, 0, 201375904, 2, 0, -2, 0, 256, 0, 122880, 0, 16777216, 2, 150, 0, 4160757760, 2, 0, -6, 2, 167, -11, 0, 3263218176, -1, 0, 49664, 0, 2160197632, 0, 8388802, -1, 0, 12713984, -1, 2, 154, 2, 159, 2, 178, -2, 2, 162, -20, 0, 3758096385, -2, 2, 155, 0, 4292878336, 2, 90, 2, 169, 0, 4294057984, -2, 2, 163, 2, 156, 2, 175, -2, 2, 155, -1, 2, 182, -1, 2, 170, 2, 124, 0, 4026593280, 0, 14, 0, 4292919296, -1, 2, 158, 0, 939588608, -1, 0, 805306368, -1, 2, 124, 0, 1610612736, 2, 156, 2, 157, 2, 4, 2, 0, -2, 2, 158, 2, 159, -3, 0, 267386880, -1, 2, 160, 0, 7168, -1, 0, 65024, 2, 154, 2, 161, 2, 179, -7, 2, 168, -8, 2, 162, -1, 0, 1426112704, 2, 163, -1, 2, 164, 0, 271581216, 0, 2149777408, 2, 23, 2, 161, 2, 124, 0, 851967, 2, 180, -1, 2, 23, 2, 181, -4, 2, 158, -20, 2, 195, 2, 165, -56, 0, 3145728, 2, 185, -4, 2, 166, 2, 124, -4, 0, 32505856, -1, 2, 167, -1, 0, 2147385088, 2, 90, 1, 2155905152, 2, -3, 2, 103, 2, 0, 2, 168, -2, 2, 169, -6, 2, 170, 0, 4026597375, 0, 1, -1, 0, 1, -1, 2, 171, -3, 2, 117, 2, 64, -2, 2, 166, -2, 2, 176, 2, 124, -878, 2, 159, -36, 2, 172, -1, 2, 201, -10, 2, 188, -5, 2, 174, -6, 0, 4294965251, 2, 27, -1, 2, 173, -1, 2, 174, -2, 0, 4227874752, -3, 0, 2146435072, 2, 159, -2, 0, 1006649344, 2, 124, -1, 2, 90, 0, 201375744, -3, 0, 134217720, 2, 90, 0, 4286677377, 0, 32896, -1, 2, 158, -3, 2, 175, -349, 2, 176, 0, 1920, 2, 177, 3, 0, 264, -11, 2, 157, -2, 2, 178, 2, 0, 0, 520617856, 0, 2692743168, 0, 36, -3, 0, 524284, -11, 2, 23, -1, 2, 187, -1, 2, 184, 0, 3221291007, 2, 178, -1, 2, 202, 0, 2158720, -3, 2, 159, 0, 1, -4, 2, 124, 0, 3808625411, 0, 3489628288, 2, 200, 0, 1207959680, 0, 3221274624, 2, 0, -3, 2, 179, 0, 120, 0, 7340032, -2, 2, 180, 2, 4, 2, 23, 2, 163, 3, 0, 4, 2, 159, -1, 2, 181, 2, 177, -1, 0, 8176, 2, 182, 2, 179, 2, 183, -1, 0, 4290773232, 2, 0, -4, 2, 163, 2, 189, 0, 15728640, 2, 177, -1, 2, 161, -1, 0, 4294934512, 3, 0, 4, -9, 2, 90, 2, 170, 2, 184, 3, 0, 4, 0, 704, 0, 1849688064, 2, 185, -1, 2, 124, 0, 4294901887, 2, 0, 0, 130547712, 0, 1879048192, 2, 199, 3, 0, 2, -1, 2, 186, 2, 187, -1, 0, 17829776, 0, 2025848832, 0, 4261477888, -2, 2, 0, -1, 0, 4286580608, -1, 0, 29360128, 2, 192, 0, 16252928, 0, 3791388672, 2, 38, 3, 0, 2, -2, 2, 196, 2, 0, -1, 2, 103, -1, 0, 66584576, -1, 2, 191, 3, 0, 9, 2, 124, -1, 0, 4294755328, 3, 0, 2, -1, 2, 161, 2, 178, 3, 0, 2, 2, 23, 2, 188, 2, 90, -2, 0, 245760, 0, 2147418112, -1, 2, 150, 2, 203, 0, 4227923456, -1, 2, 164, 2, 161, 2, 90, -3, 0, 4292870145, 0, 262144, 2, 124, 3, 0, 2, 0, 1073758848, 2, 189, -1, 0, 4227921920, 2, 190, 0, 68289024, 0, 528402016, 0, 4292927536, 3, 0, 4, -2, 0, 268435456, 2, 91, -2, 2, 191, 3, 0, 5, -1, 2, 192, 2, 163, 2, 0, -2, 0, 4227923936, 2, 62, -1, 2, 155, 2, 95, 2, 0, 2, 154, 2, 158, 3, 0, 6, -1, 2, 177, 3, 0, 3, -2, 0, 2146959360, 0, 9440640, 0, 104857600, 0, 4227923840, 3, 0, 2, 0, 768, 2, 193, 2, 77, -2, 2, 161, -2, 2, 119, -1, 2, 155, 3, 0, 8, 0, 512, 0, 8388608, 2, 194, 2, 172, 2, 187, 0, 4286578944, 3, 0, 2, 0, 1152, 0, 1266679808, 2, 191, 0, 576, 0, 4261707776, 2, 95, 3, 0, 9, 2, 155, 3, 0, 5, 2, 16, -1, 0, 2147221504, -28, 2, 178, 3, 0, 3, -3, 0, 4292902912, -6, 2, 96, 3, 0, 85, -33, 0, 4294934528, 3, 0, 126, -18, 2, 195, 3, 0, 269, -17, 2, 155, 2, 124, 2, 198, 3, 0, 2, 2, 23, 0, 4290822144, -2, 0, 67174336, 0, 520093700, 2, 17, 3, 0, 21, -2, 2, 179, 3, 0, 3, -2, 0, 30720, -1, 0, 32512, 3, 0, 2, 0, 4294770656, -191, 2, 174, -38, 2, 170, 2, 0, 2, 196, 3, 0, 279, -8, 2, 124, 2, 0, 0, 4294508543, 0, 65295, -11, 2, 177, 3, 0, 72, -3, 0, 3758159872, 0, 201391616, 3, 0, 155, -7, 2, 170, -1, 0, 384, -1, 0, 133693440, -3, 2, 196, -2, 2, 26, 3, 0, 4, 2, 169, -2, 2, 90, 2, 155, 3, 0, 4, -2, 2, 164, -1, 2, 150, 0, 335552923, 2, 197, -1, 0, 538974272, 0, 2214592512, 0, 132e3, -10, 0, 192, -8, 0, 12288, -21, 0, 134213632, 0, 4294901761, 3, 0, 42, 0, 100663424, 0, 4294965284, 3, 0, 6, -1, 0, 3221282816, 2, 198, 3, 0, 11, -1, 2, 199, 3, 0, 40, -6, 0, 4286578784, 2, 0, -2, 0, 1006694400, 3, 0, 24, 2, 35, -1, 2, 94, 3, 0, 2, 0, 1, 2, 163, 3, 0, 6, 2, 197, 0, 4110942569, 0, 1432950139, 0, 2701658217, 0, 4026532864, 0, 4026532881, 2, 0, 2, 45, 3, 0, 8, -1, 2, 158, -2, 2, 169, 0, 98304, 0, 65537, 2, 170, -5, 0, 4294950912, 2, 0, 2, 118, 0, 65528, 2, 177, 0, 4294770176, 2, 26, 3, 0, 4, -30, 2, 174, 0, 3758153728, -3, 2, 169, -2, 2, 155, 2, 188, 2, 158, -1, 2, 191, -1, 2, 161, 0, 4294754304, 3, 0, 2, -3, 0, 33554432, -2, 2, 200, -3, 2, 169, 0, 4175478784, 2, 201, 0, 4286643712, 0, 4286644216, 2, 0, -4, 2, 202, -1, 2, 165, 0, 4227923967, 3, 0, 32, -1334, 2, 163, 2, 0, -129, 2, 94, -6, 2, 163, -180, 2, 203, -233, 2, 4, 3, 0, 96, -16, 2, 163, 3, 0, 47, -154, 2, 165, 3, 0, 22381, -7, 2, 17, 3, 0, 6128], [4294967295, 4294967291, 4092460543, 4294828031, 4294967294, 134217726, 268435455, 2147483647, 1048575, 1073741823, 3892314111, 134217727, 1061158911, 536805376, 4294910143, 4160749567, 4294901759, 4294901760, 536870911, 262143, 8388607, 4294902783, 4294918143, 65535, 67043328, 2281701374, 4294967232, 2097151, 4294903807, 4194303, 255, 67108863, 4294967039, 511, 524287, 131071, 127, 4292870143, 4294902271, 4294549487, 33554431, 1023, 67047423, 4294901888, 4286578687, 4294770687, 67043583, 32767, 15, 2047999, 67043343, 16777215, 4294902e3, 4294934527, 4294966783, 4294967279, 2047, 262083, 20511, 4290772991, 41943039, 493567, 4294959104, 603979775, 65536, 602799615, 805044223, 4294965206, 8191, 1031749119, 4294917631, 2134769663, 4286578493, 4282253311, 4294942719, 33540095, 4294905855, 4294967264, 2868854591, 1608515583, 265232348, 534519807, 2147614720, 1060109444, 4093640016, 17376, 2139062143, 224, 4169138175, 4294909951, 4286578688, 4294967292, 4294965759, 2044, 4292870144, 4294966272, 4294967280, 8289918, 4294934399, 4294901775, 4294965375, 1602223615, 4294967259, 4294443008, 268369920, 4292804608, 486341884, 4294963199, 3087007615, 1073692671, 4128527, 4279238655, 4294902015, 4294966591, 2445279231, 3670015, 3238002687, 31, 63, 4294967288, 4294705151, 4095, 3221208447, 4294549472, 2147483648, 4285526655, 4294966527, 4294705152, 4294966143, 64, 4294966719, 16383, 3774873592, 458752, 536807423, 67043839, 3758096383, 3959414372, 3755993023, 2080374783, 4294835295, 4294967103, 4160749565, 4087, 184024726, 2862017156, 1593309078, 268434431, 268434414, 4294901763, 536870912, 2952790016, 202506752, 139264, 402653184, 4261412864, 4227922944, 49152, 61440, 3758096384, 117440512, 65280, 3233808384, 3221225472, 2097152, 4294965248, 32768, 57152, 67108864, 4293918720, 4290772992, 25165824, 57344, 4227915776, 4278190080, 4227907584, 65520, 4026531840, 4227858432, 4160749568, 3758129152, 4294836224, 63488, 1073741824, 4294967040, 4194304, 251658240, 196608, 4294963200, 64512, 417808, 4227923712, 12582912, 50331648, 65472, 4294967168, 4294966784, 16, 4294917120, 2080374784, 4096, 65408, 524288, 65532]);
function r(e) {
return e.column++, e.currentChar = e.source.charCodeAt(++e.index);
}
function X(e, u) {
if ((u & 64512) !== 55296)
return 0;
let i = e.source.charCodeAt(e.index + 1);
return (i & 64512) !== 56320 ? 0 : (u = e.currentChar = 65536 + ((u & 1023) << 10) + (i & 1023), V[(u >>> 5) + 0] >>> u & 31 & 1 || f(e, 18, T(u)), e.index++, e.column++, 1);
}
function Y(e, u) {
e.currentChar = e.source.charCodeAt(++e.index), e.flags |= 1, u & 4 || (e.column = 0, e.line++);
}
function G(e) {
e.flags |= 1, e.currentChar = e.source.charCodeAt(++e.index), e.column = 0, e.line++;
}
function u2(e) {
return e === 160 || e === 65279 || e === 133 || e === 5760 || e >= 8192 && e <= 8203 || e === 8239 || e === 8287 || e === 12288 || e === 8201 || e === 65519;
}
function T(e) {
return e <= 65535 ? String.fromCharCode(e) : String.fromCharCode(e >>> 10) + String.fromCharCode(e & 1023);
}
function z(e) {
return e < 65 ? e - 48 : e - 65 + 10 & 15;
}
function w2(e) {
switch (e) {
case 134283266:
return "NumericLiteral";
case 134283267:
return "StringLiteral";
case 86021:
case 86022:
return "BooleanLiteral";
case 86023:
return "NullLiteral";
case 65540:
return "RegularExpression";
case 67174408:
case 67174409:
case 132:
return "TemplateLiteral";
default:
return (e & 143360) === 143360 ? "Identifier" : (e & 4096) === 4096 ? "Keyword" : "Punctuator";
}
}
var C = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1032, 0, 0, 2056, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 8192, 0, 3, 0, 0, 8192, 0, 0, 0, 256, 0, 33024, 0, 0, 242, 242, 114, 114, 114, 114, 114, 114, 594, 594, 0, 0, 16384, 0, 0, 0, 0, 67, 67, 67, 67, 67, 67, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 3, 0, 1, 0, 0, 4099, 0, 71, 71, 71, 71, 71, 71, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 7, 16384, 0, 0, 0, 0], J = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0], p = [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0];
function U(e) {
return e <= 127 ? J[e] : V[(e >>> 5) + 34816] >>> e & 31 & 1;
}
function e2(e) {
return e <= 127 ? p[e] : V[(e >>> 5) + 0] >>> e & 31 & 1 || e === 8204 || e === 8205;
}
var g2 = ["SingleLine", "MultiLine", "HTMLOpen", "HTMLClose", "HashbangComment"];
function l2(e) {
let u = e.source;
e.currentChar === 35 && u.charCodeAt(e.index + 1) === 33 && (r(e), r(e), f2(e, u, 0, 4, e.tokenPos, e.linePos, e.colPos));
}
function V2(e, u, i, n3, t, o, l, c) {
return n3 & 2048 && f(e, 0), f2(e, u, i, t, o, l, c);
}
function f2(e, u, i, n3, t, o, l) {
let { index: c } = e;
for (e.tokenPos = e.index, e.linePos = e.line, e.colPos = e.column; e.index < e.end; ) {
if (C[e.currentChar] & 8) {
let s = e.currentChar === 13;
G(e), s && e.index < e.end && e.currentChar === 10 && (e.currentChar = u.charCodeAt(++e.index));
break;
} else if ((e.currentChar ^ 8232) <= 1) {
G(e);
break;
}
r(e), e.tokenPos = e.index, e.linePos = e.line, e.colPos = e.column;
}
if (e.onComment) {
let s = { start: { line: o, column: l }, end: { line: e.linePos, column: e.colPos } };
e.onComment(g2[n3 & 255], u.slice(c, e.tokenPos), t, e.tokenPos, s);
}
return i | 1;
}
function N2(e, u, i) {
let { index: n3 } = e;
for (; e.index < e.end; )
if (e.currentChar < 43) {
let t = false;
for (; e.currentChar === 42; )
if (t || (i &= -5, t = true), r(e) === 47) {
if (r(e), e.onComment) {
let o = { start: { line: e.linePos, column: e.colPos }, end: { line: e.line, column: e.column } };
e.onComment(g2[1], u.slice(n3, e.index - 2), n3 - 2, e.index, o);
}
return e.tokenPos = e.index, e.linePos = e.line, e.colPos = e.column, i;
}
if (t)
continue;
C[e.currentChar] & 8 ? e.currentChar === 13 ? (i |= 5, G(e)) : (Y(e, i), i = i & -5 | 1) : r(e);
} else
(e.currentChar ^ 8232) <= 1 ? (i = i & -5 | 1, G(e)) : (i &= -5, r(e));
f(e, 16);
}
function q2(e, u) {
let i = e.index, n3 = 0;
e:
for (; ; ) {
let k = e.currentChar;
if (r(e), n3 & 1)
n3 &= -2;
else
switch (k) {
case 47:
if (n3)
break;
break e;
case 92:
n3 |= 1;
break;
case 91:
n3 |= 2;
break;
case 93:
n3 &= 1;
break;
case 13:
case 10:
case 8232:
case 8233:
f(e, 32);
}
if (e.index >= e.source.length)
return f(e, 32);
}
let t = e.index - 1, o = 0, l = e.currentChar, { index: c } = e;
for (; e2(l); ) {
switch (l) {
case 103:
o & 2 && f(e, 34, "g"), o |= 2;
break;
case 105:
o & 1 && f(e, 34, "i"), o |= 1;
break;
case 109:
o & 4 && f(e, 34, "m"), o |= 4;
break;
case 117:
o & 16 && f(e, 34, "g"), o |= 16;
break;
case 121:
o & 8 && f(e, 34, "y"), o |= 8;
break;
case 115:
o & 32 && f(e, 34, "s"), o |= 32;
break;
default:
f(e, 33);
}
l = r(e);
}
let s = e.source.slice(c, e.index), m = e.source.slice(i, t);
return e.tokenRegExp = { pattern: m, flags: s }, u & 512 && (e.tokenRaw = e.source.slice(e.tokenPos, e.index)), e.tokenValue = V1(e, m, s), 65540;
}
function V1(e, u, i) {
try {
return new RegExp(u, i);
} catch {
f(e, 32);
}
}
function N1(e, u, i) {
let { index: n3 } = e, t = "", o = r(e), l = e.index;
for (; !(C[o] & 8); ) {
if (o === i)
return t += e.source.slice(l, e.index), r(e), u & 512 && (e.tokenRaw = e.source.slice(n3, e.index)), e.tokenValue = t, 134283267;
if ((o & 8) === 8 && o === 92) {
if (t += e.source.slice(l, e.index), o = r(e), o < 127 || o === 8232 || o === 8233) {
let c = a2(e, u, o);
c >= 0 ? t += T(c) : t1(e, c, 0);
} else
t += T(o);
l = e.index + 1;
}
e.index >= e.end && f(e, 14), o = r(e);
}
f(e, 14);
}
function a2(e, u, i) {
switch (i) {
case 98:
return 8;
case 102:
return 12;
case 114:
return 13;
case 110:
return 10;
case 116:
return 9;
case 118:
return 11;
case 13:
if (e.index < e.end) {
let n3 = e.source.charCodeAt(e.index + 1);
n3 === 10 && (e.index = e.index + 1, e.currentChar = n3);
}
case 10:
case 8232:
case 8233:
return e.column = -1, e.line++, -1;
case 48:
case 49:
case 50:
case 51: {
let n3 = i - 48, t = e.index + 1, o = e.column + 1;
if (t < e.end) {
let l = e.source.charCodeAt(t);
if (C[l] & 32) {
if (u & 1024)
return -2;
if (e.currentChar = l, n3 = n3 << 3 | l - 48, t++, o++, t < e.end) {
let c = e.source.charCodeAt(t);
C[c] & 32 && (e.currentChar = c, n3 = n3 << 3 | c - 48, t++, o++);
}
e.flags |= 64, e.index = t - 1, e.column = o - 1;
} else if ((n3 !== 0 || C[l] & 512) && u & 1024)
return -2;
}
return n3;
}
case 52:
case 53:
case 54:
case 55: {
if (u & 1024)
return -2;
let n3 = i - 48, t = e.index + 1, o = e.column + 1;
if (t < e.end) {
let l = e.source.charCodeAt(t);
C[l] & 32 && (n3 = n3 << 3 | l - 48, e.currentChar = l, e.index = t, e.column = o);
}
return e.flags |= 64, n3;
}
case 120: {
let n3 = r(e);
if (!(C[n3] & 64))
return -4;
let t = z(n3), o = r(e);
if (!(C[o] & 64))
return -4;
let l = z(o);
return t << 4 | l;
}
case 117: {
let n3 = r(e);
if (e.currentChar === 123) {
let t = 0;
for (; C[r(e)] & 64; )
if (t = t << 4 | z(e.currentChar), t > 1114111)
return -5;
return e.currentChar < 1 || e.currentChar !== 125 ? -4 : t;
} else {
if (!(C[n3] & 64))
return -4;
let t = e.source.charCodeAt(e.index + 1);
if (!(C[t] & 64))
return -4;
let o = e.source.charCodeAt(e.index + 2);
if (!(C[o] & 64))
return -4;
let l = e.source.charCodeAt(e.index + 3);
return C[l] & 64 ? (e.index += 3, e.column += 3, e.currentChar = e.source.charCodeAt(e.index), z(n3) << 12 | z(t) << 8 | z(o) << 4 | z(l)) : -4;
}
}
case 56:
case 57:
if (!(u & 256))
return -3;
default:
return i;
}
}
function t1(e, u, i) {
switch (u) {
case -1:
return;
case -2:
f(e, i ? 2 : 1);
case -3:
f(e, 13);
case -4:
f(e, 6);
case -5:
f(e, 101);
}
}
function We(e, u) {
let { index: i } = e, n3 = 67174409, t = "", o = r(e);
for (; o !== 96; ) {
if (o === 36 && e.source.charCodeAt(e.index + 1) === 123) {
r(e), n3 = 67174408;
break;
} else if ((o & 8) === 8 && o === 92)
if (o = r(e), o > 126)
t += T(o);
else {
let l = a2(e, u | 1024, o);
if (l >= 0)
t += T(l);
else if (l !== -1 && u & 65536) {
t = void 0, o = T0(e, o), o < 0 && (n3 = 67174408);
break;
} else
t1(e, l, 1);
}
else
e.index < e.end && o === 13 && e.source.charCodeAt(e.index) === 10 && (t += T(o), e.currentChar = e.source.charCodeAt(++e.index)), ((o & 83) < 3 && o === 10 || (o ^ 8232) <= 1) && (e.column = -1, e.line++), t += T(o);
e.index >= e.end && f(e, 15), o = r(e);
}
return r(e), e.tokenValue = t, e.tokenRaw = e.source.slice(i + 1, e.index - (n3 === 67174409 ? 1 : 2)), n3;
}
function T0(e, u) {
for (; u !== 96; ) {
switch (u) {
case 36: {
let i = e.index + 1;
if (i < e.end && e.source.charCodeAt(i) === 123)
return e.index = i, e.column++, -u;
break;
}
case 10:
case 8232:
case 8233:
e.column = -1, e.line++;
}
e.index >= e.end && f(e, 15), u = r(e);
}
return u;
}
function I0(e, u) {
return e.index >= e.end && f(e, 0), e.index--, e.column--, We(e, u);
}
function Ke(e, u, i) {
let n3 = e.currentChar, t = 0, o = 9, l = i & 64 ? 0 : 1, c = 0, s = 0;
if (i & 64)
t = "." + o1(e, n3), n3 = e.currentChar, n3 === 110 && f(e, 11);
else {
if (n3 === 48)
if (n3 = r(e), (n3 | 32) === 120) {
for (i = 136, n3 = r(e); C[n3] & 4160; ) {
if (n3 === 95) {
s || f(e, 146), s = 0, n3 = r(e);
continue;
}
s = 1, t = t * 16 + z(n3), c++, n3 = r(e);
}
(c < 1 || !s) && f(e, c < 1 ? 19 : 147);
} else if ((n3 | 32) === 111) {
for (i = 132, n3 = r(e); C[n3] & 4128; ) {
if (n3 === 95) {
s || f(e, 146), s = 0, n3 = r(e);
continue;
}
s = 1, t = t * 8 + (n3 - 48), c++, n3 = r(e);
}
(c < 1 || !s) && f(e, c < 1 ? 0 : 147);
} else if ((n3 | 32) === 98) {
for (i = 130, n3 = r(e); C[n3] & 4224; ) {
if (n3 === 95) {
s || f(e, 146), s = 0, n3 = r(e);
continue;
}
s = 1, t = t * 2 + (n3 - 48), c++, n3 = r(e);
}
(c < 1 || !s) && f(e, c < 1 ? 0 : 147);
} else if (C[n3] & 32)
for (u & 1024 && f(e, 1), i = 1; C[n3] & 16; ) {
if (C[n3] & 512) {
i = 32, l = 0;
break;
}
t = t * 8 + (n3 - 48), n3 = r(e);
}
else
C[n3] & 512 ? (u & 1024 && f(e, 1), e.flags |= 64, i = 32) : n3 === 95 && f(e, 0);
if (i & 48) {
if (l) {
for (; o >= 0 && C[n3] & 4112; ) {
if (n3 === 95) {
n3 = r(e), (n3 === 95 || i & 32) && S(e.index, e.line, e.index + 1, 146), s = 1;
continue;
}
s = 0, t = 10 * t + (n3 - 48), n3 = r(e), --o;
}
if (s && S(e.index, e.line, e.index + 1, 147), o >= 0 && !U(n3) && n3 !== 46)
return e.tokenValue = t, u & 512 && (e.tokenRaw = e.source.slice(e.tokenPos, e.index)), 134283266;
}
t += o1(e, n3), n3 = e.currentChar, n3 === 46 && (r(e) === 95 && f(e, 0), i = 64, t += "." + o1(e, e.currentChar), n3 = e.currentChar);
}
}
let m = e.index, k = 0;
if (n3 === 110 && i & 128)
k = 1, n3 = r(e);
else if ((n3 | 32) === 101) {
n3 = r(e), C[n3] & 256 && (n3 = r(e));
let { index: h } = e;
(C[n3] & 16) < 1 && f(e, 10), t += e.source.substring(m, h) + o1(e, n3), n3 = e.currentChar;
}
return (e.index < e.end && C[n3] & 16 || U(n3)) && f(e, 12), k ? (e.tokenRaw = e.source.slice(e.tokenPos, e.index), e.tokenValue = BigInt(t), 134283389) : (e.tokenValue = i & 15 ? t : i & 32 ? parseFloat(e.source.substring(e.tokenPos, e.index)) : +t, u & 512 && (e.tokenRaw = e.source.slice(e.tokenPos, e.index)), 134283266);
}
function o1(e, u) {
let i = 0, n3 = e.index, t = "";
for (; C[u] & 4112; ) {
if (u === 95) {
let { index: o } = e;
u = r(e), u === 95 && S(e.index, e.line, e.index + 1, 146), i = 1, t += e.source.substring(n3, o), n3 = e.index;
continue;
}
i = 0, u = r(e);
}
return i && S(e.index, e.line, e.index + 1, 147), t + e.source.substring(n3, e.index);
}
var Z = ["end of source", "identifier", "number", "string", "regular expression", "false", "true", "null", "template continuation", "template tail", "=>", "(", "{", ".", "...", "}", ")", ";", ",", "[", "]", ":", "?", "'", '"', "", "/>", "++", "--", "=", "<<=", ">>=", ">>>=", "**=", "+=", "-=", "*=", "/=", "%=", "^=", "|=", "&=", "||=", "&&=", "??=", "typeof", "delete", "void", "!", "~", "+", "-", "in", "instanceof", "*", "%", "/", "**", "&&", "||", "===", "!==", "==", "!=", "<=", ">=", "<", ">", "<<", ">>", ">>>", "&", "|", "^", "var", "let", "const", "break", "case", "catch", "class", "continue", "debugger", "default", "do", "else", "export", "extends", "finally", "for", "function", "if", "import", "new", "return", "super", "switch", "this", "throw", "try", "while", "with", "implements", "interface", "package", "private", "protected", "public", "static", "yield", "as", "async", "await", "constructor", "get", "set", "from", "of", "enum", "eval", "arguments", "escaped keyword", "escaped future reserved keyword", "reserved if strict", "#", "BigIntLiteral", "??", "?.", "WhiteSpace", "Illegal", "LineTerminator", "PrivateField", "Template", "@", "target", "meta", "LineFeed", "Escaped", "JSXText"], Ye = Object.create(null, { this: { value: 86113 }, function: { value: 86106 }, if: { value: 20571 }, return: { value: 20574 }, var: { value: 86090 }, else: { value: 20565 }, for: { value: 20569 }, new: { value: 86109 }, in: { value: 8738868 }, typeof: { value: 16863277 }, while: { value: 20580 }, case: { value: 20558 }, break: { value: 20557 }, try: { value: 20579 }, catch: { value: 20559 }, delete: { value: 16863278 }, throw: { value: 86114 }, switch: { value: 86112 }, continue: { value: 20561 }, default: { value: 20563 }, instanceof: { value: 8476725 }, do: { value: 20564 }, void: { value: 16863279 }, finally: { value: 20568 }, async: { value: 209007 }, await: { value: 209008 }, class: { value: 86096 }, const: { value: 86092 }, constructor: { value: 12401 }, debugger: { value: 20562 }, export: { value: 20566 }, extends: { value: 20567 }, false: { value: 86021 }, from: { value: 12404 }, get: { value: 12402 }, implements: { value: 36966 }, import: { value: 86108 }, interface: { value: 36967 }, let: { value: 241739 }, null: { value: 86023 }, of: { value: 274549 }, package: { value: 36968 }, private: { value: 36969 }, protected: { value: 36970 }, public: { value: 36971 }, set: { value: 12403 }, static: { value: 36972 }, super: { value: 86111 }, true: { value: 86022 }, with: { value: 20581 }, yield: { value: 241773 }, enum: { value: 86134 }, eval: { value: 537079927 }, as: { value: 77934 }, arguments: { value: 537079928 }, target: { value: 143494 }, meta: { value: 143495 } });
function Ze(e, u, i) {
for (; p[r(e)]; )
;
return e.tokenValue = e.source.slice(e.tokenPos, e.index), e.currentChar !== 92 && e.currentChar < 126 ? Ye[e.tokenValue] || 208897 : j1(e, u, 0, i);
}
function R0(e, u) {
let i = Qe(e);
return e2(i) || f(e, 4), e.tokenValue = T(i), j1(e, u, 1, C[i] & 4);
}
function j1(e, u, i, n3) {
let t = e.index;
for (; e.index < e.end; )
if (e.currentChar === 92) {
e.tokenValue += e.source.slice(t, e.index), i = 1;
let l = Qe(e);
e2(l) || f(e, 4), n3 = n3 && C[l] & 4, e.tokenValue += T(l), t = e.index;
} else if (e2(e.currentChar) || X(e, e.currentChar))
r(e);
else
break;
e.index <= e.end && (e.tokenValue += e.source.slice(t, e.index));
let o = e.tokenValue.length;
if (n3 && o >= 2 && o <= 11) {
let l = Ye[e.tokenValue];
return l === void 0 ? 208897 : i ? u & 1024 ? l === 209008 && !(u & 4196352) ? l : l === 36972 || (l & 36864) === 36864 ? 122 : 121 : u & 1073741824 && !(u & 8192) && (l & 20480) === 20480 ? l : l === 241773 ? u & 1073741824 ? 143483 : u & 2097152 ? 121 : l : l === 209007 && u & 1073741824 ? 143483 : (l & 36864) === 36864 || l === 209008 && !(u & 4194304) ? l : 121 : l;
}
return 208897;
}
function V0(e) {
return U(r(e)) || f(e, 93), 131;
}
function Qe(e) {
return e.source.charCodeAt(e.index + 1) !== 117 && f(e, 4), e.currentChar = e.source.charCodeAt(e.index += 2), N0(e);
}
function N0(e) {
let u = 0, i = e.currentChar;
if (i === 123) {
let l = e.index - 2;
for (; C[r(e)] & 64; )
u = u << 4 | z(e.currentChar), u > 1114111 && S(l, e.line, e.index + 1, 101);
return e.currentChar !== 125 && S(l, e.line, e.index - 1, 6), r(e), u;
}
C[i] & 64 || f(e, 6);
let n3 = e.source.charCodeAt(e.index + 1);
C[n3] & 64 || f(e, 6);
let t = e.source.charCodeAt(e.index + 2);
C[t] & 64 || f(e, 6);
let o = e.source.charCodeAt(e.index + 3);
return C[o] & 64 || f(e, 6), u = z(i) << 12 | z(n3) << 8 | z(t) << 4 | z(o), e.currentChar = e.source.charCodeAt(e.index += 4), u;
}
var Ge = [129, 129, 129, 129, 129, 129, 129, 129, 129, 128, 136, 128, 128, 130, 129, 129, 129, 129, 129, 129, 129, 129, 129, 129, 129, 129, 129, 129, 129, 129, 129, 129, 128, 16842800, 134283267, 131, 208897, 8457015, 8455751, 134283267, 67174411, 16, 8457014, 25233970, 18, 25233971, 67108877, 8457016, 134283266, 134283266, 134283266, 134283266, 134283266, 134283266, 134283266, 134283266, 134283266, 134283266, 21, 1074790417, 8456258, 1077936157, 8456259, 22, 133, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 208897, 69271571, 137, 20, 8455497, 208897, 132, 4096, 4096, 4096, 4096, 4096, 4096, 4096, 208897, 4096, 208897, 208897, 4096, 208897, 4096, 208897, 4096, 208897, 4096, 4096, 4096, 208897, 4096, 4096, 208897, 4096, 4096, 2162700, 8455240, 1074790415, 16842801, 129];
function E(e, u) {
if (e.flags = (e.flags | 1) ^ 1, e.startPos = e.index, e.startColumn = e.column, e.startLine = e.line, e.token = xe(e, u, 0), e.onToken && e.token !== 1048576) {
let i = { start: { line: e.linePos, column: e.colPos }, end: { line: e.line, column: e.column } };
e.onToken(w2(e.token), e.tokenPos, e.index, i);
}
}
function xe(e, u, i) {
let n3 = e.index === 0, t = e.source, o = e.index, l = e.line, c = e.column;
for (; e.index < e.end; ) {
e.tokenPos = e.index, e.colPos = e.column, e.linePos = e.line;
let s = e.currentChar;
if (s <= 126) {
let m = Ge[s];
switch (m) {
case 67174411:
case 16:
case 2162700:
case 1074790415:
case 69271571:
case 20:
case 21:
case 1074790417:
case 18:
case 16842801:
case 133:
case 129:
return r(e), m;
case 208897:
return Ze(e, u, 0);
case 4096:
return Ze(e, u, 1);
case 134283266:
return Ke(e, u, 144);
case 134283267:
return N1(e, u, s);
case 132:
return We(e, u);
case 137:
return R0(e, u);
case 131:
return V0(e);
case 128:
r(e);
break;
case 130:
i |= 5, G(e);
break;
case 136:
Y(e, i), i = i & -5 | 1;
break;
case 8456258:
let k = r(e);
if (e.index < e.end) {
if (k === 60)
return e.index < e.end && r(e) === 61 ? (r(e), 4194334) : 8456516;
if (k === 61)
return r(e), 8456256;
if (k === 33) {
let d = e.index + 1;
if (d + 1 < e.end && t.charCodeAt(d) === 45 && t.charCodeAt(d + 1) == 45) {
e.column += 3, e.currentChar = t.charCodeAt(e.index += 3), i = V2(e, t, i, u, 2, e.tokenPos, e.linePos, e.colPos), o = e.tokenPos, l = e.linePos, c = e.colPos;
continue;
}
return 8456258;
}
if (k === 47) {
if ((u & 16) < 1)
return 8456258;
let d = e.index + 1;
if (d < e.end && (k = t.charCodeAt(d), k === 42 || k === 47))
break;
return r(e), 25;
}
}
return 8456258;
case 1077936157: {
r(e);
let d = e.currentChar;
return d === 61 ? r(e) === 61 ? (r(e), 8455996) : 8455998 : d === 62 ? (r(e), 10) : 1077936157;
}
case 16842800:
return r(e) !== 61 ? 16842800 : r(e) !== 61 ? 8455999 : (r(e), 8455997);
case 8457015:
return r(e) !== 61 ? 8457015 : (r(e), 4194342);
case 8457014: {
if (r(e), e.index >= e.end)
return 8457014;
let d = e.currentChar;
return d === 61 ? (r(e), 4194340) : d !== 42 ? 8457014 : r(e) !== 61 ? 8457273 : (r(e), 4194337);
}
case 8455497:
return r(e) !== 61 ? 8455497 : (r(e), 4194343);
case 25233970: {
r(e);
let d = e.currentChar;
return d === 43 ? (r(e), 33619995) : d === 61 ? (r(e), 4194338) : 25233970;
}
case 25233971: {
r(e);
let d = e.currentChar;
if (d === 45) {
if (r(e), (i & 1 || n3) && e.currentChar === 62) {
u & 256 || f(e, 108), r(e), i = V2(e, t, i, u, 3, o, l, c), o = e.tokenPos, l = e.linePos, c = e.colPos;
continue;
}
return 33619996;
}
return d === 61 ? (r(e), 4194339) : 25233971;
}
case 8457016: {
if (r(e), e.index < e.end) {
let d = e.currentChar;
if (d === 47) {
r(e), i = f2(e, t, i, 0, e.tokenPos, e.linePos, e.colPos), o = e.tokenPos, l = e.linePos, c = e.colPos;
continue;
}
if (d === 42) {
r(e), i = N2(e, t, i), o = e.tokenPos, l = e.linePos, c = e.colPos;
continue;
}
if (u & 32768)
return q2(e, u);
if (d === 61)
return r(e), 4259877;
}
return 8457016;
}
case 67108877:
let h = r(e);
if (h >= 48 && h <= 57)
return Ke(e, u, 80);
if (h === 46) {
let d = e.index + 1;
if (d < e.end && t.charCodeAt(d) === 46)
return e.column += 2, e.currentChar = t.charCodeAt(e.index += 2), 14;
}
return 67108877;
case 8455240: {
r(e);
let d = e.currentChar;
return d === 124 ? (r(e), e.currentChar === 61 ? (r(e), 4194346) : 8979003) : d === 61 ? (r(e), 4194344) : 8455240;
}
case 8456259: {
r(e);
let d = e.currentChar;
if (d === 61)
return r(e), 8456257;
if (d !== 62)
return 8456259;
if (r(e), e.index < e.end) {
let y = e.currentChar;
if (y === 62)
return r(e) === 61 ? (r(e), 4194336) : 8456518;
if (y === 61)
return r(e), 4194335;
}
return 8456517;
}
case 8455751: {
r(e);
let d = e.currentChar;
return d === 38 ? (r(e), e.currentChar === 61 ? (r(e), 4194347) : 8979258) : d === 61 ? (r(e), 4194345) : 8455751;
}
case 22: {
let d = r(e);
if (d === 63)
return r(e), e.currentChar === 61 ? (r(e), 4194348) : 276889982;
if (d === 46) {
let y = e.index + 1;
if (y < e.end && (d = t.charCodeAt(y), !(d >= 48 && d <= 57)))
return r(e), 67108991;
}
return 22;
}
}
} else {
if ((s ^ 8232) <= 1) {
i = i & -5 | 1, G(e);
continue;
}
if ((s & 64512) === 55296 || V[(s >>> 5) + 34816] >>> s & 31 & 1)
return (s & 64512) === 56320 && (s = (s & 1023) << 10 | s & 1023 | 65536, V[(s >>> 5) + 0] >>> s & 31 & 1 || f(e, 18, T(s)), e.index++, e.currentChar = s), e.column++, e.tokenValue = "", j1(e, u, 0, 0);
if (u2(s)) {
r(e);
continue;
}
f(e, 18, T(s));
}
}
return 1048576;
}
var j0 = { AElig: "\xC6", AMP: "&", Aacute: "\xC1", Abreve: "\u0102", Acirc: "\xC2", Acy: "\u0410", Afr: "\u{1D504}", Agrave: "\xC0", Alpha: "\u0391", Amacr: "\u0100", And: "\u2A53", Aogon: "\u0104", Aopf: "\u{1D538}", ApplyFunction: "\u2061", Aring: "\xC5", Ascr: "\u{1D49C}", Assign: "\u2254", Atilde: "\xC3", Auml: "\xC4", Backslash: "\u2216", Barv: "\u2AE7", Barwed: "\u2306", Bcy: "\u0411", Because: "\u2235", Bernoullis: "\u212C", Beta: "\u0392", Bfr: "\u{1D505}", Bopf: "\u{1D539}", Breve: "\u02D8", Bscr: "\u212C", Bumpeq: "\u224E", CHcy: "\u0427", COPY: "\xA9", Cacute: "\u0106", Cap: "\u22D2", CapitalDifferentialD: "\u2145", Cayleys: "\u212D", Ccaron: "\u010C", Ccedil: "\xC7", Ccirc: "\u0108", Cconint: "\u2230", Cdot: "\u010A", Cedilla: "\xB8", CenterDot: "\xB7", Cfr: "\u212D", Chi: "\u03A7", CircleDot: "\u2299", CircleMinus: "\u2296", CirclePlus: "\u2295", CircleTimes: "\u2297", ClockwiseContourIntegral: "\u2232", CloseCurlyDoubleQuote: "\u201D", CloseCurlyQuote: "\u2019", Colon: "\u2237", Colone: "\u2A74", Congruent: "\u2261", Conint: "\u222F", ContourIntegral: "\u222E", Copf: "\u2102", Coproduct: "\u2210", CounterClockwiseContourIntegral: "\u2233", Cross: "\u2A2F", Cscr: "\u{1D49E}", Cup: "\u22D3", CupCap: "\u224D", DD: "\u2145", DDotrahd: "\u2911", DJcy: "\u0402", DScy: "\u0405", DZcy: "\u040F", Dagger: "\u2021", Darr: "\u21A1", Dashv: "\u2AE4", Dcaron: "\u010E", Dcy: "\u0414", Del: "\u2207", Delta: "\u0394", Dfr: "\u{1D507}", DiacriticalAcute: "\xB4", DiacriticalDot: "\u02D9", DiacriticalDoubleAcute: "\u02DD", DiacriticalGrave: "`", DiacriticalTilde: "\u02DC", Diamond: "\u22C4", DifferentialD: "\u2146", Dopf: "\u{1D53B}", Dot: "\xA8", DotDot: "\u20DC", DotEqual: "\u2250", DoubleContourIntegral: "\u222F", DoubleDot: "\xA8", DoubleDownArrow: "\u21D3", DoubleLeftArrow: "\u21D0", DoubleLeftRightArrow: "\u21D4", DoubleLeftTee: "\u2AE4", DoubleLongLeftArrow: "\u27F8", DoubleLongLeftRightArrow: "\u27FA", DoubleLongRightArrow: "\u27F9", DoubleRightArrow: "\u21D2", DoubleRightTee: "\u22A8", DoubleUpArrow: "\u21D1", DoubleUpDownArrow: "\u21D5", DoubleVerticalBar: "\u2225", DownArrow: "\u2193", DownArrowBar: "\u2913", DownArrowUpArrow: "\u21F5", DownBreve: "\u0311", DownLeftRightVector: "\u2950", DownLeftTeeVector: "\u295E", DownLeftVector: "\u21BD", DownLeftVectorBar: "\u2956", DownRightTeeVector: "\u295F", DownRightVector: "\u21C1", DownRightVectorBar: "\u2957", DownTee: "\u22A4", DownTeeArrow: "\u21A7", Downarrow: "\u21D3", Dscr: "\u{1D49F}", Dstrok: "\u0110", ENG: "\u014A", ETH: "\xD0", Eacute: "\xC9", Ecaron: "\u011A", Ecirc: "\xCA", Ecy: "\u042D", Edot: "\u0116", Efr: "\u{1D508}", Egrave: "\xC8", Element: "\u2208", Emacr: "\u0112", EmptySmallSquare: "\u25FB", EmptyVerySmallSquare: "\u25AB", Eogon: "\u0118", Eopf: "\u{1D53C}", Epsilon: "\u0395", Equal: "\u2A75", EqualTilde: "\u2242", Equilibrium: "\u21CC", Escr: "\u2130", Esim: "\u2A73", Eta: "\u0397", Euml: "\xCB", Exists: "\u2203", ExponentialE: "\u2147", Fcy: "\u0424", Ffr: "\u{1D509}", FilledSmallSquare: "\u25FC", FilledVerySmallSquare: "\u25AA", Fopf: "\u{1D53D}", ForAll: "\u2200", Fouriertrf: "\u2131", Fscr: "\u2131", GJcy: "\u0403", GT: ">", Gamma: "\u0393", Gammad: "\u03DC", Gbreve: "\u011E", Gcedil: "\u0122", Gcirc: "\u011C", Gcy: "\u0413", Gdot: "\u0120", Gfr: "\u{1D50A}", Gg: "\u22D9", Gopf: "\u{1D53E}", GreaterEqual: "\u2265", GreaterEqualLess: "\u22DB", GreaterFullEqual: "\u2267", GreaterGreater: "\u2AA2", GreaterLess: "\u2277", GreaterSlantEqual: "\u2A7E", GreaterTilde: "\u2273", Gscr: "\u{1D4A2}", Gt: "\u226B", HARDcy: "\u042A", Hacek: "\u02C7", Hat: "^", Hcirc: "\u0124", Hfr: "\u210C", HilbertSpace: "\u210B", Hopf: "\u210D", HorizontalLine: "\u2500", Hscr: "\u210B", Hstrok: "\u0126", HumpDownHump: "\u224E", HumpEqual: "\u224F", IEcy: "\u0415", IJlig: "\u0132", IOcy: "\u0401", Iacute: "\xCD", Icirc: "\xCE", Icy: "\u0418", Idot: "\u0130", Ifr: "\u2111", Igrave: "\xCC", Im: "\u2111", Imacr: "\u012A", ImaginaryI: "\u2148", Implies: "\u21D2", Int: "\u222C", Integral: "\u222B", Intersection: "\u22C2", InvisibleComma: "\u2063", InvisibleTimes: "\u2062", Iogon: "\u012E", Iopf: "\u{1D540}", Iota: "\u0399", Iscr: "\u2110", Itilde: "\u0128", Iukcy: "\u0406", Iuml: "\xCF", Jcirc: "\u0134", Jcy: "\u0419", Jfr: "\u{1D50D}", Jopf: "\u{1D541}", Jscr: "\u{1D4A5}", Jsercy: "\u0408", Jukcy: "\u0404", KHcy: "\u0425", KJcy: "\u040C", Kappa: "\u039A", Kcedil: "\u0136", Kcy: "\u041A", Kfr: "\u{1D50E}", Kopf: "\u{1D542}", Kscr: "\u{1D4A6}", LJcy: "\u0409", LT: "<", Lacute: "\u0139", Lambda: "\u039B", Lang: "\u27EA", Laplacetrf: "\u2112", Larr: "\u219E", Lcaron: "\u013D", Lcedil: "\u013B", Lcy: "\u041B", LeftAngleBracket: "\u27E8", LeftArrow: "\u2190", LeftArrowBar: "\u21E4", LeftArrowRightArrow: "\u21C6", LeftCeiling: "\u2308", LeftDoubleBracket: "\u27E6", LeftDownTeeVector: "\u2961", LeftDownVector: "\u21C3", LeftDownVectorBar: "\u2959", LeftFloor: "\u230A", LeftRightArrow: "\u2194", LeftRightVector: "\u294E", LeftTee: "\u22A3", LeftTeeArrow: "\u21A4", LeftTeeVector: "\u295A", LeftTriangle: "\u22B2", LeftTriangleBar: "\u29CF", LeftTriangleEqual: "\u22B4", LeftUpDownVector: "\u2951", LeftUpTeeVector: "\u2960", LeftUpVector: "\u21BF", LeftUpVectorBar: "\u2958", LeftVector: "\u21BC", LeftVectorBar: "\u2952", Leftarrow: "\u21D0", Leftrightarrow: "\u21D4", LessEqualGreater: "\u22DA", LessFullEqual: "\u2266", LessGreater: "\u2276", LessLess: "\u2AA1", LessSlantEqual: "\u2A7D", LessTilde: "\u2272", Lfr: "\u{1D50F}", Ll: "\u22D8", Lleftarrow: "\u21DA", Lmidot: "\u013F", LongLeftArrow: "\u27F5", LongLeftRightArrow: "\u27F7", LongRightArrow: "\u27F6", Longleftarrow: "\u27F8", Longleftrightarrow: "\u27FA", Longrightarrow: "\u27F9", Lopf: "\u{1D543}", LowerLeftArrow: "\u2199", LowerRightArrow: "\u2198", Lscr: "\u2112", Lsh: "\u21B0", Lstrok: "\u0141", Lt: "\u226A", Map: "\u2905", Mcy: "\u041C", MediumSpace: "\u205F", Mellintrf: "\u2133", Mfr: "\u{1D510}", MinusPlus: "\u2213", Mopf: "\u{1D544}", Mscr: "\u2133", Mu: "\u039C", NJcy: "\u040A", Nacute: "\u0143", Ncaron: "\u0147", Ncedil: "\u0145", Ncy: "\u041D", NegativeMediumSpace: "\u200B", NegativeThickSpace: "\u200B", NegativeThinSpace: "\u200B", NegativeVeryThinSpace: "\u200B", NestedGreaterGreater: "\u226B", NestedLessLess: "\u226A", NewLine: `
`, Nfr: "\u{1D511}", NoBreak: "\u2060", NonBreakingSpace: "\xA0", Nopf: "\u2115", Not: "\u2AEC", NotCongruent: "\u2262", NotCupCap: "\u226D", NotDoubleVerticalBar: "\u2226", NotElement: "\u2209", NotEqual: "\u2260", NotEqualTilde: "\u2242\u0338", NotExists: "\u2204", NotGreater: "\u226F", NotGreaterEqual: "\u2271", NotGreaterFullEqual: "\u2267\u0338", NotGreaterGreater: "\u226B\u0338", NotGreaterLess: "\u2279", NotGreaterSlantEqual: "\u2A7E\u0338", NotGreaterTilde: "\u2275", NotHumpDownHump: "\u224E\u0338", NotHumpEqual: "\u224F\u0338", NotLeftTriangle: "\u22EA", NotLeftTriangleBar: "\u29CF\u0338", NotLeftTriangleEqual: "\u22EC", NotLess: "\u226E", NotLessEqual: "\u2270", NotLessGreater: "\u2278", NotLessLess: "\u226A\u0338", NotLessSlantEqual: "\u2A7D\u0338", NotLessTilde: "\u2274", NotNestedGreaterGreater: "\u2AA2\u0338", NotNestedLessLess: "\u2AA1\u0338", NotPrecedes: "\u2280", NotPrecedesEqual: "\u2AAF\u0338", NotPrecedesSlantEqual: "\u22E0", NotReverseElement: "\u220C", NotRightTriangle: "\u22EB", NotRightTriangleBar: "\u29D0\u0338", NotRightTriangleEqual: "\u22ED", NotSquareSubset: "\u228F\u0338", NotSquareSubsetEqual: "\u22E2", NotSquareSuperset: "\u2290\u0338", NotSquareSupersetEqual: "\u22E3", NotSubset: "\u2282\u20D2", NotSubsetEqual: "\u2288", NotSucceeds: "\u2281", NotSucceedsEqual: "\u2AB0\u0338", NotSucceedsSlantEqual: "\u22E1", NotSucceedsTilde: "\u227F\u0338", NotSuperset: "\u2283\u20D2", NotSupersetEqual: "\u2289", NotTilde: "\u2241", NotTildeEqual: "\u2244", NotTildeFullEqual: "\u2247", NotTildeTilde: "\u2249", NotVerticalBar: "\u2224", Nscr: "\u{1D4A9}", Ntilde: "\xD1", Nu: "\u039D", OElig: "\u0152", Oacute: "\xD3", Ocirc: "\xD4", Ocy: "\u041E", Odblac: "\u0150", Ofr: "\u{1D512}", Ograve: "\xD2", Omacr: "\u014C", Omega: "\u03A9", Omicron: "\u039F", Oopf: "\u{1D546}", OpenCurlyDoubleQuote: "\u201C", OpenCurlyQuote: "\u2018", Or: "\u2A54", Oscr: "\u{1D4AA}", Oslash: "\xD8", Otilde: "\xD5", Otimes: "\u2A37", Ouml: "\xD6", OverBar: "\u203E", OverBrace: "\u23DE", OverBracket: "\u23B4", OverParenthesis: "\u23DC", PartialD: "\u2202", Pcy: "\u041F", Pfr: "\u{1D513}", Phi: "\u03A6", Pi: "\u03A0", PlusMinus: "\xB1", Poincareplane: "\u210C", Popf: "\u2119", Pr: "\u2ABB", Precedes: "\u227A", PrecedesEqual: "\u2AAF", PrecedesSlantEqual: "\u227C", PrecedesTilde: "\u227E", Prime: "\u2033", Product: "\u220F", Proportion: "\u2237", Proportional: "\u221D", Pscr: "\u{1D4AB}", Psi: "\u03A8", QUOT: '"', Qfr: "\u{1D514}", Qopf: "\u211A", Qscr: "\u{1D4AC}", RBarr: "\u2910", REG: "\xAE", Racute: "\u0154", Rang: "\u27EB", Rarr: "\u21A0", Rarrtl: "\u2916", Rcaron: "\u0158", Rcedil: "\u0156", Rcy: "\u0420", Re: "\u211C", ReverseElement: "\u220B", ReverseEquilibrium: "\u21CB", ReverseUpEquilibrium: "\u296F", Rfr: "\u211C", Rho: "\u03A1", RightAngleBracket: "\u27E9", RightArrow: "\u2192", RightArrowBar: "\u21E5", RightArrowLeftArrow: "\u21C4", RightCeiling: "\u2309", RightDoubleBracket: "\u27E7", RightDownTeeVector: "\u295D", RightDownVector: "\u21C2", RightDownVectorBar: "\u2955", RightFloor: "\u230B", RightTee: "\u22A2", RightTeeArrow: "\u21A6", RightTeeVector: "\u295B", RightTriangle: "\u22B3", RightTriangleBar: "\u29D0", RightTriangleEqual: "\u22B5", RightUpDownVector: "\u294F", RightUpTeeVector: "\u295C", RightUpVector: "\u21BE", RightUpVectorBar: "\u2954", RightVector: "\u21C0", RightVectorBar: "\u2953", Rightarrow: "\u21D2", Ropf: "\u211D", RoundImplies: "\u2970", Rrightarrow: "\u21DB", Rscr: "\u211B", Rsh: "\u21B1", RuleDelayed: "\u29F4", SHCHcy: "\u0429", SHcy: "\u0428", SOFTcy: "\u042C", Sacute: "\u015A", Sc: "\u2ABC", Scaron: "\u0160", Scedil: "\u015E", Scirc: "\u015C", Scy: "\u0421", Sfr: "\u{1D516}", ShortDownArrow: "\u2193", ShortLeftArrow: "\u2190", ShortRightArrow: "\u2192", ShortUpArrow: "\u2191", Sigma: "\u03A3", SmallCircle: "\u2218", Sopf: "\u{1D54A}", Sqrt: "\u221A", Square: "\u25A1", SquareIntersection: "\u2293", SquareSubset: "\u228F", SquareSubsetEqual: "\u2291", SquareSuperset: "\u2290", SquareSupersetEqual: "\u2292", SquareUnion: "\u2294", Sscr: "\u{1D4AE}", Star: "\u22C6", Sub: "\u22D0", Subset: "\u22D0", SubsetEqual: "\u2286", Succeeds: "\u227B", SucceedsEqual: "\u2AB0", SucceedsSlantEqual: "\u227D", SucceedsTilde: "\u227F", SuchThat: "\u220B", Sum: "\u2211", Sup: "\u22D1", Superset: "\u2283", SupersetEqual: "\u2287", Supset: "\u22D1", THORN: "\xDE", TRADE: "\u2122", TSHcy: "\u040B", TScy: "\u0426", Tab: " ", Tau: "\u03A4", Tcaron: "\u0164", Tcedil: "\u0162", Tcy: "\u0422", Tfr: "\u{1D517}", Therefore: "\u2234", Theta: "\u0398", ThickSpace: "\u205F\u200A", ThinSpace: "\u2009", Tilde: "\u223C", TildeEqual: "\u2243", TildeFullEqual: "\u2245", TildeTilde: "\u2248", Topf: "\u{1D54B}", TripleDot: "\u20DB", Tscr: "\u{1D4AF}", Tstrok: "\u0166", Uacute: "\xDA", Uarr: "\u219F", Uarrocir: "\u2949", Ubrcy: "\u040E", Ubreve: "\u016C", Ucirc: "\xDB", Ucy: "\u0423", Udblac: "\u0170", Ufr: "\u{1D518}", Ugrave: "\xD9", Umacr: "\u016A", UnderBar: "_", UnderBrace: "\u23DF", UnderBracket: "\u23B5", UnderParenthesis: "\u23DD", Union: "\u22C3", UnionPlus: "\u228E", Uogon: "\u0172", Uopf: "\u{1D54C}", UpArrow: "\u2191", UpArrowBar: "\u2912", UpArrowDownArrow: "\u21C5", UpDownArrow: "\u2195", UpEquilibrium: "\u296E", UpTee: "\u22A5", UpTeeArrow: "\u21A5", Uparrow: "\u21D1", Updownarrow: "\u21D5", UpperLeftArrow: "\u2196", UpperRightArrow: "\u2197", Upsi: "\u03D2", Upsilon: "\u03A5", Uring: "\u016E", Uscr: "\u{1D4B0}", Utilde: "\u0168", Uuml: "\xDC", VDash: "\u22AB", Vbar: "\u2AEB", Vcy: "\u0412", Vdash: "\u22A9", Vdashl: "\u2AE6", Vee: "\u22C1", Verbar: "\u2016", Vert: "\u2016", VerticalBar: "\u2223", VerticalLine: "|", VerticalSeparator: "\u2758", VerticalTilde: "\u2240", VeryThinSpace: "\u200A", Vfr: "\u{1D519}", Vopf: "\u{1D54D}", Vscr: "\u{1D4B1}", Vvdash: "\u22AA", Wcirc: "\u0174", Wedge: "\u22C0", Wfr: "\u{1D51A}", Wopf: "\u{1D54E}", Wscr: "\u{1D4B2}", Xfr: "\u{1D51B}", Xi: "\u039E", Xopf: "\u{1D54F}", Xscr: "\u{1D4B3}", YAcy: "\u042F", YIcy: "\u0407", YUcy: "\u042E", Yacute: "\xDD", Ycirc: "\u0176", Ycy: "\u042B", Yfr: "\u{1D51C}", Yopf: "\u{1D550}", Yscr: "\u{1D4B4}", Yuml: "\u0178", ZHcy: "\u0416", Zacute: "\u0179", Zcaron: "\u017D", Zcy: "\u0417", Zdot: "\u017B", ZeroWidthSpace: "\u200B", Zeta: "\u0396", Zfr: "\u2128", Zopf: "\u2124", Zscr: "\u{1D4B5}", aacute: "\xE1", abreve: "\u0103", ac: "\u223E", acE: "\u223E\u0333", acd: "\u223F", acirc: "\xE2", acute: "\xB4", acy: "\u0430", aelig: "\xE6", af: "\u2061", afr: "\u{1D51E}", agrave: "\xE0", alefsym: "\u2135", aleph: "\u2135", alpha: "\u03B1", amacr: "\u0101", amalg: "\u2A3F", amp: "&", and: "\u2227", andand: "\u2A55", andd: "\u2A5C", andslope: "\u2A58", andv: "\u2A5A", ang: "\u2220", ange: "\u29A4", angle: "\u2220", angmsd: "\u2221", angmsdaa: "\u29A8", angmsdab: "\u29A9", angmsdac: "\u29AA", angmsdad: "\u29AB", angmsdae: "\u29AC", angmsdaf: "\u29AD", angmsdag: "\u29AE", angmsdah: "\u29AF", angrt: "\u221F", angrtvb: "\u22BE", angrtvbd: "\u299D", angsph: "\u2222", angst: "\xC5", angzarr: "\u237C", aogon: "\u0105", aopf: "\u{1D552}", ap: "\u2248", apE: "\u2A70", apacir: "\u2A6F", ape: "\u224A", apid: "\u224B", apos: "'", approx: "\u2248", approxeq: "\u224A", aring: "\xE5", ascr: "\u{1D4B6}", ast: "*", asymp: "\u2248", asympeq: "\u224D", atilde: "\xE3", auml: "\xE4", awconint: "\u2233", awint: "\u2A11", bNot: "\u2AED", backcong: "\u224C", backepsilon: "\u03F6", backprime: "\u2035", backsim: "\u223D", backsimeq: "\u22CD", barvee: "\u22BD", barwed: "\u2305", barwedge: "\u2305", bbrk: "\u23B5", bbrktbrk: "\u23B6", bcong: "\u224C", bcy: "\u0431", bdquo: "\u201E", becaus: "\u2235", because: "\u2235", bemptyv: "\u29B0", bepsi: "\u03F6", bernou: "\u212C", beta: "\u03B2", beth: "\u2136", between: "\u226C", bfr: "\u{1D51F}", bigcap: "\u22C2", bigcirc: "\u25EF", bigcup: "\u22C3", bigodot: "\u2A00", bigoplus: "\u2A01", bigotimes: "\u2A02", bigsqcup: "\u2A06", bigstar: "\u2605", bigtriangledown: "\u25BD", bigtriangleup: "\u25B3", biguplus: "\u2A04", bigvee: "\u22C1", bigwedge: "\u22C0", bkarow: "\u290D", blacklozenge: "\u29EB", blacksquare: "\u25AA", blacktriangle: "\u25B4", blacktriangledown: "\u25BE", blacktriangleleft: "\u25C2", blacktriangleright: "\u25B8", blank: "\u2423", blk12: "\u2592", blk14: "\u2591", blk34: "\u2593", block: "\u2588", bne: "=\u20E5", bnequiv: "\u2261\u20E5", bnot: "\u2310", bopf: "\u{1D553}", bot: "\u22A5", bottom: "\u22A5", bowtie: "\u22C8", boxDL: "\u2557", boxDR: "\u2554", boxDl: "\u2556", boxDr: "\u2553", boxH: "\u2550", boxHD: "\u2566", boxHU: "\u2569", boxHd: "\u2564", boxHu: "\u2567", boxUL: "\u255D", boxUR: "\u255A", boxUl: "\u255C", boxUr: "\u2559", boxV: "\u2551", boxVH: "\u256C", boxVL: "\u2563", boxVR: "\u2560", boxVh: "\u256B", boxVl: "\u2562", boxVr: "\u255F", boxbox: "\u29C9", boxdL: "\u2555", boxdR: "\u2552", boxdl: "\u2510", boxdr: "\u250C", boxh: "\u2500", boxhD: "\u2565", boxhU: "\u2568", boxhd: "\u252C", boxhu: "\u2534", boxminus: "\u229F", boxplus: "\u229E", boxtimes: "\u22A0", boxuL: "\u255B", boxuR: "\u2558", boxul: "\u2518", boxur: "\u2514", boxv: "\u2502", boxvH: "\u256A", boxvL: "\u2561", boxvR: "\u255E", boxvh: "\u253C", boxvl: "\u2524", boxvr: "\u251C", bprime: "\u2035", breve: "\u02D8", brvbar: "\xA6", bscr: "\u{1D4B7}", bsemi: "\u204F", bsim: "\u223D", bsime: "\u22CD", bsol: "\\", bsolb: "\u29C5", bsolhsub: "\u27C8", bull: "\u2022", bullet: "\u2022", bump: "\u224E", bumpE: "\u2AAE", bumpe: "\u224F", bumpeq: "\u224F", cacute: "\u0107", cap: "\u2229", capand: "\u2A44", capbrcup: "\u2A49", capcap: "\u2A4B", capcup: "\u2A47", capdot: "\u2A40", caps: "\u2229\uFE00", caret: "\u2041", caron: "\u02C7", ccaps: "\u2A4D", ccaron: "\u010D", ccedil: "\xE7", ccirc: "\u0109", ccups: "\u2A4C", ccupssm: "\u2A50", cdot: "\u010B", cedil: "\xB8", cemptyv: "\u29B2", cent: "\xA2", centerdot: "\xB7", cfr: "\u{1D520}", chcy: "\u0447", check: "\u2713", checkmark: "\u2713", chi: "\u03C7", cir: "\u25CB", cirE: "\u29C3", circ: "\u02C6", circeq: "\u2257", circlearrowleft: "\u21BA", circlearrowright: "\u21BB", circledR: "\xAE", circledS: "\u24C8", circledast: "\u229B", circledcirc: "\u229A", circleddash: "\u229D", cire: "\u2257", cirfnint: "\u2A10", cirmid: "\u2AEF", cirscir: "\u29C2", clubs: "\u2663", clubsuit: "\u2663", colon: ":", colone: "\u2254", coloneq: "\u2254", comma: ",", commat: "@", comp: "\u2201", compfn: "\u2218", complement: "\u2201", complexes: "\u2102", cong: "\u2245", congdot: "\u2A6D", conint: "\u222E", copf: "\u{1D554}", coprod: "\u2210", copy: "\xA9", copysr: "\u2117", crarr: "\u21B5", cross: "\u2717", cscr: "\u{1D4B8}", csub: "\u2ACF", csube: "\u2AD1", csup: "\u2AD0", csupe: "\u2AD2", ctdot: "\u22EF", cudarrl: "\u2938", cudarrr: "\u2935", cuepr: "\u22DE", cuesc: "\u22DF", cularr: "\u21B6", cularrp: "\u293D", cup: "\u222A", cupbrcap: "\u2A48", cupcap: "\u2A46", cupcup: "\u2A4A", cupdot: "\u228D", cupor: "\u2A45", cups: "\u222A\uFE00", curarr: "\u21B7", curarrm: "\u293C", curlyeqprec: "\u22DE", curlyeqsucc: "\u22DF", curlyvee: "\u22CE", curlywedge: "\u22CF", curren: "\xA4", curvearrowleft: "\u21B6", curvearrowright: "\u21B7", cuvee: "\u22CE", cuwed: "\u22CF", cwconint: "\u2232", cwint: "\u2231", cylcty: "\u232D", dArr: "\u21D3", dHar: "\u2965", dagger: "\u2020", daleth: "\u2138", darr: "\u2193", dash: "\u2010", dashv: "\u22A3", dbkarow: "\u290F", dblac: "\u02DD", dcaron: "\u010F", dcy: "\u0434", dd: "\u2146", ddagger: "\u2021", ddarr: "\u21CA", ddotseq: "\u2A77", deg: "\xB0", delta: "\u03B4", demptyv: "\u29B1", dfisht: "\u297F", dfr: "\u{1D521}", dharl: "\u21C3", dharr: "\u21C2", diam: "\u22C4", diamond: "\u22C4", diamondsuit: "\u2666", diams: "\u2666", die: "\xA8", digamma: "\u03DD", disin: "\u22F2", div: "\xF7", divide: "\xF7", divideontimes: "\u22C7", divonx: "\u22C7", djcy: "\u0452", dlcorn: "\u231E", dlcrop: "\u230D", dollar: "$", dopf: "\u{1D555}", dot: "\u02D9", doteq: "\u2250", doteqdot: "\u2251", dotminus: "\u2238", dotplus: "\u2214", dotsquare: "\u22A1", doublebarwedge: "\u2306", downarrow: "\u2193", downdownarrows: "\u21CA", downharpoonleft: "\u21C3", downharpoonright: "\u21C2", drbkarow: "\u2910", drcorn: "\u231F", drcrop: "\u230C", dscr: "\u{1D4B9}", dscy: "\u0455", dsol: "\u29F6", dstrok: "\u0111", dtdot: "\u22F1", dtri: "\u25BF", dtrif: "\u25BE", duarr: "\u21F5", duhar: "\u296F", dwangle: "\u29A6", dzcy: "\u045F", dzigrarr: "\u27FF", eDDot: "\u2A77", eDot: "\u2251", eacute: "\xE9", easter: "\u2A6E", ecaron: "\u011B", ecir: "\u2256", ecirc: "\xEA", ecolon: "\u2255", ecy: "\u044D", edot: "\u0117", ee: "\u2147", efDot: "\u2252", efr: "\u{1D522}", eg: "\u2A9A", egrave: "\xE8", egs: "\u2A96", egsdot: "\u2A98", el: "\u2A99", elinters: "\u23E7", ell: "\u2113", els: "\u2A95", elsdot: "\u2A97", emacr: "\u0113", empty: "\u2205", emptyset: "\u2205", emptyv: "\u2205", emsp13: "\u2004", emsp14: "\u2005", emsp: "\u2003", eng: "\u014B", ensp: "\u2002", eogon: "\u0119", eopf: "\u{1D556}", epar: "\u22D5", eparsl: "\u29E3", eplus: "\u2A71", epsi: "\u03B5", epsilon: "\u03B5", epsiv: "\u03F5", eqcirc: "\u2256", eqcolon: "\u2255", eqsim: "\u2242", eqslantgtr: "\u2A96", eqslantless: "\u2A95", equals: "=", equest: "\u225F", equiv: "\u2261", equivDD: "\u2A78", eqvparsl: "\u29E5", erDot: "\u2253", erarr: "\u2971", escr: "\u212F", esdot: "\u2250", esim: "\u2242", eta: "\u03B7", eth: "\xF0", euml: "\xEB", euro: "\u20AC", excl: "!", exist: "\u2203", expectation: "\u2130", exponentiale: "\u2147", fallingdotseq: "\u2252", fcy: "\u0444", female: "\u2640", ffilig: "\uFB03", fflig: "\uFB00", ffllig: "\uFB04", ffr: "\u{1D523}", filig: "\uFB01", fjlig: "fj", flat: "\u266D", fllig: "\uFB02", fltns: "\u25B1", fnof: "\u0192", fopf: "\u{1D557}", forall: "\u2200", fork: "\u22D4", forkv: "\u2AD9", fpartint: "\u2A0D", frac12: "\xBD", frac13: "\u2153", frac14: "\xBC", frac15: "\u2155", frac16: "\u2159", frac18: "\u215B", frac23: "\u2154", frac25: "\u2156", frac34: "\xBE", frac35: "\u2157", frac38: "\u215C", frac45: "\u2158", frac56: "\u215A", frac58: "\u215D", frac78: "\u215E", frasl: "\u2044", frown: "\u2322", fscr: "\u{1D4BB}", gE: "\u2267", gEl: "\u2A8C", gacute: "\u01F5", gamma: "\u03B3", gammad: "\u03DD", gap: "\u2A86", gbreve: "\u011F", gcirc: "\u011D", gcy: "\u0433", gdot: "\u0121", ge: "\u2265", gel: "\u22DB", geq: "\u2265", geqq: "\u2267", geqslant: "\u2A7E", ges: "\u2A7E", gescc: "\u2AA9", gesdot: "\u2A80", gesdoto: "\u2A82", gesdotol: "\u2A84", gesl: "\u22DB\uFE00", gesles: "\u2A94", gfr: "\u{1D524}", gg: "\u226B", ggg: "\u22D9", gimel: "\u2137", gjcy: "\u0453", gl: "\u2277", glE: "\u2A92", gla: "\u2AA5", glj: "\u2AA4", gnE: "\u2269", gnap: "\u2A8A", gnapprox: "\u2A8A", gne: "\u2A88", gneq: "\u2A88", gneqq: "\u2269", gnsim: "\u22E7", gopf: "\u{1D558}", grave: "`", gscr: "\u210A", gsim: "\u2273", gsime: "\u2A8E", gsiml: "\u2A90", gt: ">", gtcc: "\u2AA7", gtcir: "\u2A7A", gtdot: "\u22D7", gtlPar: "\u2995", gtquest: "\u2A7C", gtrapprox: "\u2A86", gtrarr: "\u2978", gtrdot: "\u22D7", gtreqless: "\u22DB", gtreqqless: "\u2A8C", gtrless: "\u2277", gtrsim: "\u2273", gvertneqq: "\u2269\uFE00", gvnE: "\u2269\uFE00", hArr: "\u21D4", hairsp: "\u200A", half: "\xBD", hamilt: "\u210B", hardcy: "\u044A", harr: "\u2194", harrcir: "\u2948", harrw: "\u21AD", hbar: "\u210F", hcirc: "\u0125", hearts: "\u2665", heartsuit: "\u2665", hellip: "\u2026", hercon: "\u22B9", hfr: "\u{1D525}", hksearow: "\u2925", hkswarow: "\u2926", hoarr: "\u21FF", homtht: "\u223B", hookleftarrow: "\u21A9", hookrightarrow: "\u21AA", hopf: "\u{1D559}", horbar: "\u2015", hscr: "\u{1D4BD}", hslash: "\u210F", hstrok: "\u0127", hybull: "\u2043", hyphen: "\u2010", iacute: "\xED", ic: "\u2063", icirc: "\xEE", icy: "\u0438", iecy: "\u0435", iexcl: "\xA1", iff: "\u21D4", ifr: "\u{1D526}", igrave: "\xEC", ii: "\u2148", iiiint: "\u2A0C", iiint: "\u222D", iinfin: "\u29DC", iiota: "\u2129", ijlig: "\u0133", imacr: "\u012B", image: "\u2111", imagline: "\u2110", imagpart: "\u2111", imath: "\u0131", imof: "\u22B7", imped: "\u01B5", in: "\u2208", incare: "\u2105", infin: "\u221E", infintie: "\u29DD", inodot: "\u0131", int: "\u222B", intcal: "\u22BA", integers: "\u2124", intercal: "\u22BA", intlarhk: "\u2A17", intprod: "\u2A3C", iocy: "\u0451", iogon: "\u012F", iopf: "\u{1D55A}", iota: "\u03B9", iprod: "\u2A3C", iquest: "\xBF", iscr: "\u{1D4BE}", isin: "\u2208", isinE: "\u22F9", isindot: "\u22F5", isins: "\u22F4", isinsv: "\u22F3", isinv: "\u2208", it: "\u2062", itilde: "\u0129", iukcy: "\u0456", iuml: "\xEF", jcirc: "\u0135", jcy: "\u0439", jfr: "\u{1D527}", jmath: "\u0237", jopf: "\u{1D55B}", jscr: "\u{1D4BF}", jsercy: "\u0458", jukcy: "\u0454", kappa: "\u03BA", kappav: "\u03F0", kcedil: "\u0137", kcy: "\u043A", kfr: "\u{1D528}", kgreen: "\u0138", khcy: "\u0445", kjcy: "\u045C", kopf: "\u{1D55C}", kscr: "\u{1D4C0}", lAarr: "\u21DA", lArr: "\u21D0", lAtail: "\u291B", lBarr: "\u290E", lE: "\u2266", lEg: "\u2A8B", lHar: "\u2962", lacute: "\u013A", laemptyv: "\u29B4", lagran: "\u2112", lambda: "\u03BB", lang: "\u27E8", langd: "\u2991", langle: "\u27E8", lap: "\u2A85", laquo: "\xAB", larr: "\u2190", larrb: "\u21E4", larrbfs: "\u291F", larrfs: "\u291D", larrhk: "\u21A9", larrlp: "\u21AB", larrpl: "\u2939", larrsim: "\u2973", larrtl: "\u21A2", lat: "\u2AAB", latail: "\u2919", late: "\u2AAD", lates: "\u2AAD\uFE00", lbarr: "\u290C", lbbrk: "\u2772", lbrace: "{", lbrack: "[", lbrke: "\u298B", lbrksld: "\u298F", lbrkslu: "\u298D", lcaron: "\u013E", lcedil: "\u013C", lceil: "\u2308", lcub: "{", lcy: "\u043B", ldca: "\u2936", ldquo: "\u201C", ldquor: "\u201E", ldrdhar: "\u2967", ldrushar: "\u294B", ldsh: "\u21B2", le: "\u2264", leftarrow: "\u2190", leftarrowtail: "\u21A2", leftharpoondown: "\u21BD", leftharpoonup: "\u21BC", leftleftarrows: "\u21C7", leftrightarrow: "\u2194", leftrightarrows: "\u21C6", leftrightharpoons: "\u21CB", leftrightsquigarrow: "\u21AD", leftthreetimes: "\u22CB", leg: "\u22DA", leq: "\u2264", leqq: "\u2266", leqslant: "\u2A7D", les: "\u2A7D", lescc: "\u2AA8", lesdot: "\u2A7F", lesdoto: "\u2A81", lesdotor: "\u2A83", lesg: "\u22DA\uFE00", lesges: "\u2A93", lessapprox: "\u2A85", lessdot: "\u22D6", lesseqgtr: "\u22DA", lesseqqgtr: "\u2A8B", lessgtr: "\u2276", lesssim: "\u2272", lfisht: "\u297C", lfloor: "\u230A", lfr: "\u{1D529}", lg: "\u2276", lgE: "\u2A91", lhard: "\u21BD", lharu: "\u21BC", lharul: "\u296A", lhblk: "\u2584", ljcy: "\u0459", ll: "\u226A", llarr: "\u21C7", llcorner: "\u231E", llhard: "\u296B", lltri: "\u25FA", lmidot: "\u0140", lmoust: "\u23B0", lmoustache: "\u23B0", lnE: "\u2268", lnap: "\u2A89", lnapprox: "\u2A89", lne: "\u2A87", lneq: "\u2A87", lneqq: "\u2268", lnsim: "\u22E6", loang: "\u27EC", loarr: "\u21FD", lobrk: "\u27E6", longleftarrow: "\u27F5", longleftrightarrow: "\u27F7", longmapsto: "\u27FC", longrightarrow: "\u27F6", looparrowleft: "\u21AB", looparrowright: "\u21AC", lopar: "\u2985", lopf: "\u{1D55D}", loplus: "\u2A2D", lotimes: "\u2A34", lowast: "\u2217", lowbar: "_", loz: "\u25CA", lozenge: "\u25CA", lozf: "\u29EB", lpar: "(", lparlt: "\u2993", lrarr: "\u21C6", lrcorner: "\u231F", lrhar: "\u21CB", lrhard: "\u296D", lrm: "\u200E", lrtri: "\u22BF", lsaquo: "\u2039", lscr: "\u{1D4C1}", lsh: "\u21B0", lsim: "\u2272", lsime: "\u2A8D", lsimg: "\u2A8F", lsqb: "[", lsquo: "\u2018", lsquor: "\u201A", lstrok: "\u0142", lt: "<", ltcc: "\u2AA6", ltcir: "\u2A79", ltdot: "\u22D6", lthree: "\u22CB", ltimes: "\u22C9", ltlarr: "\u2976", ltquest: "\u2A7B", ltrPar: "\u2996", ltri: "\u25C3", ltrie: "\u22B4", ltrif: "\u25C2", lurdshar: "\u294A", luruhar: "\u2966", lvertneqq: "\u2268\uFE00", lvnE: "\u2268\uFE00", mDDot: "\u223A", macr: "\xAF", male: "\u2642", malt: "\u2720", maltese: "\u2720", map: "\u21A6", mapsto: "\u21A6", mapstodown: "\u21A7", mapstoleft: "\u21A4", mapstoup: "\u21A5", marker: "\u25AE", mcomma: "\u2A29", mcy: "\u043C", mdash: "\u2014", measuredangle: "\u2221", mfr: "\u{1D52A}", mho: "\u2127", micro: "\xB5", mid: "\u2223", midast: "*", midcir: "\u2AF0", middot: "\xB7", minus: "\u2212", minusb: "\u229F", minusd: "\u2238", minusdu: "\u2A2A", mlcp: "\u2ADB", mldr: "\u2026", mnplus: "\u2213", models: "\u22A7", mopf: "\u{1D55E}", mp: "\u2213", mscr: "\u{1D4C2}", mstpos: "\u223E", mu: "\u03BC", multimap: "\u22B8", mumap: "\u22B8", nGg: "\u22D9\u0338", nGt: "\u226B\u20D2", nGtv: "\u226B\u0338", nLeftarrow: "\u21CD", nLeftrightarrow: "\u21CE", nLl: "\u22D8\u0338", nLt: "\u226A\u20D2", nLtv: "\u226A\u0338", nRightarrow: "\u21CF", nVDash: "\u22AF", nVdash: "\u22AE", nabla: "\u2207", nacute: "\u0144", nang: "\u2220\u20D2", nap: "\u2249", napE: "\u2A70\u0338", napid: "\u224B\u0338", napos: "\u0149", napprox: "\u2249", natur: "\u266E", natural: "\u266E", naturals: "\u2115", nbsp: "\xA0", nbump: "\u224E\u0338", nbumpe: "\u224F\u0338", ncap: "\u2A43", ncaron: "\u0148", ncedil: "\u0146", ncong: "\u2247", ncongdot: "\u2A6D\u0338", ncup: "\u2A42", ncy: "\u043D", ndash: "\u2013", ne: "\u2260", neArr: "\u21D7", nearhk: "\u2924", nearr: "\u2197", nearrow: "\u2197", nedot: "\u2250\u0338", nequiv: "\u2262", nesear: "\u2928", nesim: "\u2242\u0338", nexist: "\u2204", nexists: "\u2204", nfr: "\u{1D52B}", ngE: "\u2267\u0338", nge: "\u2271", ngeq: "\u2271", ngeqq: "\u2267\u0338", ngeqslant: "\u2A7E\u0338", nges: "\u2A7E\u0338", ngsim: "\u2275", ngt: "\u226F", ngtr: "\u226F", nhArr: "\u21CE", nharr: "\u21AE", nhpar: "\u2AF2", ni: "\u220B", nis: "\u22FC", nisd: "\u22FA", niv: "\u220B", njcy: "\u045A", nlArr: "\u21CD", nlE: "\u2266\u0338", nlarr: "\u219A", nldr: "\u2025", nle: "\u2270", nleftarrow: "\u219A", nleftrightarrow: "\u21AE", nleq: "\u2270", nleqq: "\u2266\u0338", nleqslant: "\u2A7D\u0338", nles: "\u2A7D\u0338", nless: "\u226E", nlsim: "\u2274", nlt: "\u226E", nltri: "\u22EA", nltrie: "\u22EC", nmid: "\u2224", nopf: "\u{1D55F}", not: "\xAC", notin: "\u2209", notinE: "\u22F9\u0338", notindot: "\u22F5\u0338", notinva: "\u2209", notinvb: "\u22F7", notinvc: "\u22F6", notni: "\u220C", notniva: "\u220C", notnivb: "\u22FE", notnivc: "\u22FD", npar: "\u2226", nparallel: "\u2226", nparsl: "\u2AFD\u20E5", npart: "\u2202\u0338", npolint: "\u2A14", npr: "\u2280", nprcue: "\u22E0", npre: "\u2AAF\u0338", nprec: "\u2280", npreceq: "\u2AAF\u0338", nrArr: "\u21CF", nrarr: "\u219B", nrarrc: "\u2933\u0338", nrarrw: "\u219D\u0338", nrightarrow: "\u219B", nrtri: "\u22EB", nrtrie: "\u22ED", nsc: "\u2281", nsccue: "\u22E1", nsce: "\u2AB0\u0338", nscr: "\u{1D4C3}", nshortmid: "\u2224", nshortparallel: "\u2226", nsim: "\u2241", nsime: "\u2244", nsimeq: "\u2244", nsmid: "\u2224", nspar: "\u2226", nsqsube: "\u22E2", nsqsupe: "\u22E3", nsub: "\u2284", nsubE: "\u2AC5\u0338", nsube: "\u2288", nsubset: "\u2282\u20D2", nsubseteq: "\u2288", nsubseteqq: "\u2AC5\u0338", nsucc: "\u2281", nsucceq: "\u2AB0\u0338", nsup: "\u2285", nsupE: "\u2AC6\u0338", nsupe: "\u2289", nsupset: "\u2283\u20D2", nsupseteq: "\u2289", nsupseteqq: "\u2AC6\u0338", ntgl: "\u2279", ntilde: "\xF1", ntlg: "\u2278", ntriangleleft: "\u22EA", ntrianglelefteq: "\u22EC", ntriangleright: "\u22EB", ntrianglerighteq: "\u22ED", nu: "\u03BD", num: "#", numero: "\u2116", numsp: "\u2007", nvDash: "\u22AD", nvHarr: "\u2904", nvap: "\u224D\u20D2", nvdash: "\u22AC", nvge: "\u2265\u20D2", nvgt: ">\u20D2", nvinfin: "\u29DE", nvlArr: "\u2902", nvle: "\u2264\u20D2", nvlt: "<\u20D2", nvltrie: "\u22B4\u20D2", nvrArr: "\u2903", nvrtrie: "\u22B5\u20D2", nvsim: "\u223C\u20D2", nwArr: "\u21D6", nwarhk: "\u2923", nwarr: "\u2196", nwarrow: "\u2196", nwnear: "\u2927", oS: "\u24C8", oacute: "\xF3", oast: "\u229B", ocir: "\u229A", ocirc: "\xF4", ocy: "\u043E", odash: "\u229D", odblac: "\u0151", odiv: "\u2A38", odot: "\u2299", odsold: "\u29BC", oelig: "\u0153", ofcir: "\u29BF", ofr: "\u{1D52C}", ogon: "\u02DB", ograve: "\xF2", ogt: "\u29C1", ohbar: "\u29B5", ohm: "\u03A9", oint: "\u222E", olarr: "\u21BA", olcir: "\u29BE", olcross: "\u29BB", oline: "\u203E", olt: "\u29C0", omacr: "\u014D", omega: "\u03C9", omicron: "\u03BF", omid: "\u29B6", ominus: "\u2296", oopf: "\u{1D560}", opar: "\u29B7", operp: "\u29B9", oplus: "\u2295", or: "\u2228", orarr: "\u21BB", ord: "\u2A5D", order: "\u2134", orderof: "\u2134", ordf: "\xAA", ordm: "\xBA", origof: "\u22B6", oror: "\u2A56", orslope: "\u2A57", orv: "\u2A5B", oscr: "\u2134", oslash: "\xF8", osol: "\u2298", otilde: "\xF5", otimes: "\u2297", otimesas: "\u2A36", ouml: "\xF6", ovbar: "\u233D", par: "\u2225", para: "\xB6", parallel: "\u2225", parsim: "\u2AF3", parsl: "\u2AFD", part: "\u2202", pcy: "\u043F", percnt: "%", period: ".", permil: "\u2030", perp: "\u22A5", pertenk: "\u2031", pfr: "\u{1D52D}", phi: "\u03C6", phiv: "\u03D5", phmmat: "\u2133", phone: "\u260E", pi: "\u03C0", pitchfork: "\u22D4", piv: "\u03D6", planck: "\u210F", planckh: "\u210E", plankv: "\u210F", plus: "+", plusacir: "\u2A23", plusb: "\u229E", pluscir: "\u2A22", plusdo: "\u2214", plusdu: "\u2A25", pluse: "\u2A72", plusmn: "\xB1", plussim: "\u2A26", plustwo: "\u2A27", pm: "\xB1", pointint: "\u2A15", popf: "\u{1D561}", pound: "\xA3", pr: "\u227A", prE: "\u2AB3", prap: "\u2AB7", prcue: "\u227C", pre: "\u2AAF", prec: "\u227A", precapprox: "\u2AB7", preccurlyeq: "\u227C", preceq: "\u2AAF", precnapprox: "\u2AB9", precneqq: "\u2AB5", precnsim: "\u22E8", precsim: "\u227E", prime: "\u2032", primes: "\u2119", prnE: "\u2AB5", prnap: "\u2AB9", prnsim: "\u22E8", prod: "\u220F", profalar: "\u232E", profline: "\u2312", profsurf: "\u2313", prop: "\u221D", propto: "\u221D", prsim: "\u227E", prurel: "\u22B0", pscr: "\u{1D4C5}", psi: "\u03C8", puncsp: "\u2008", qfr: "\u{1D52E}", qint: "\u2A0C", qopf: "\u{1D562}", qprime: "\u2057", qscr: "\u{1D4C6}", quaternions: "\u210D", quatint: "\u2A16", quest: "?", questeq: "\u225F", quot: '"', rAarr: "\u21DB", rArr: "\u21D2", rAtail: "\u291C", rBarr: "\u290F", rHar: "\u2964", race: "\u223D\u0331", racute: "\u0155", radic: "\u221A", raemptyv: "\u29B3", rang: "\u27E9", rangd: "\u2992", range: "\u29A5", rangle: "\u27E9", raquo: "\xBB", rarr: "\u2192", rarrap: "\u2975", rarrb: "\u21E5", rarrbfs: "\u2920", rarrc: "\u2933", rarrfs: "\u291E", rarrhk: "\u21AA", rarrlp: "\u21AC", rarrpl: "\u2945", rarrsim: "\u2974", rarrtl: "\u21A3", rarrw: "\u219D", ratail: "\u291A", ratio: "\u2236", rationals: "\u211A", rbarr: "\u290D", rbbrk: "\u2773", rbrace: "}", rbrack: "]", rbrke: "\u298C", rbrksld: "\u298E", rbrkslu: "\u2990", rcaron: "\u0159", rcedil: "\u0157", rceil: "\u2309", rcub: "}", rcy: "\u0440", rdca: "\u2937", rdldhar: "\u2969", rdquo: "\u201D", rdquor: "\u201D", rdsh: "\u21B3", real: "\u211C", realine: "\u211B", realpart: "\u211C", reals: "\u211D", rect: "\u25AD", reg: "\xAE", rfisht: "\u297D", rfloor: "\u230B", rfr: "\u{1D52F}", rhard: "\u21C1", rharu: "\u21C0", rharul: "\u296C", rho: "\u03C1", rhov: "\u03F1", rightarrow: "\u2192", rightarrowtail: "\u21A3", rightharpoondown: "\u21C1", rightharpoonup: "\u21C0", rightleftarrows: "\u21C4", rightleftharpoons: "\u21CC", rightrightarrows: "\u21C9", rightsquigarrow: "\u219D", rightthreetimes: "\u22CC", ring: "\u02DA", risingdotseq: "\u2253", rlarr: "\u21C4", rlhar: "\u21CC", rlm: "\u200F", rmoust: "\u23B1", rmoustache: "\u23B1", rnmid: "\u2AEE", roang: "\u27ED", roarr: "\u21FE", robrk: "\u27E7", ropar: "\u2986", ropf: "\u{1D563}", roplus: "\u2A2E", rotimes: "\u2A35", rpar: ")", rpargt: "\u2994", rppolint: "\u2A12", rrarr: "\u21C9", rsaquo: "\u203A", rscr: "\u{1D4C7}", rsh: "\u21B1", rsqb: "]", rsquo: "\u2019", rsquor: "\u2019", rthree: "\u22CC", rtimes: "\u22CA", rtri: "\u25B9", rtrie: "\u22B5", rtrif: "\u25B8", rtriltri: "\u29CE", ruluhar: "\u2968", rx: "\u211E", sacute: "\u015B", sbquo: "\u201A", sc: "\u227B", scE: "\u2AB4", scap: "\u2AB8", scaron: "\u0161", sccue: "\u227D", sce: "\u2AB0", scedil: "\u015F", scirc: "\u015D", scnE: "\u2AB6", scnap: "\u2ABA", scnsim: "\u22E9", scpolint: "\u2A13", scsim: "\u227F", scy: "\u0441", sdot: "\u22C5", sdotb: "\u22A1", sdote: "\u2A66", seArr: "\u21D8", searhk: "\u2925", searr: "\u2198", searrow: "\u2198", sect: "\xA7", semi: ";", seswar: "\u2929", setminus: "\u2216", setmn: "\u2216", sext: "\u2736", sfr: "\u{1D530}", sfrown: "\u2322", sharp: "\u266F", shchcy: "\u0449", shcy: "\u0448", shortmid: "\u2223", shortparallel: "\u2225", shy: "\xAD", sigma: "\u03C3", sigmaf: "\u03C2", sigmav: "\u03C2", sim: "\u223C", simdot: "\u2A6A", sime: "\u2243", simeq: "\u2243", simg: "\u2A9E", simgE: "\u2AA0", siml: "\u2A9D", simlE: "\u2A9F", simne: "\u2246", simplus: "\u2A24", simrarr: "\u2972", slarr: "\u2190", smallsetminus: "\u2216", smashp: "\u2A33", smeparsl: "\u29E4", smid: "\u2223", smile: "\u2323", smt: "\u2AAA", smte: "\u2AAC", smtes: "\u2AAC\uFE00", softcy: "\u044C", sol: "/", solb: "\u29C4", solbar: "\u233F", sopf: "\u{1D564}", spades: "\u2660", spadesuit: "\u2660", spar: "\u2225", sqcap: "\u2293", sqcaps: "\u2293\uFE00", sqcup: "\u2294", sqcups: "\u2294\uFE00", sqsub: "\u228F", sqsube: "\u2291", sqsubset: "\u228F", sqsubseteq: "\u2291", sqsup: "\u2290", sqsupe: "\u2292", sqsupset: "\u2290", sqsupseteq: "\u2292", squ: "\u25A1", square: "\u25A1", squarf: "\u25AA", squf: "\u25AA", srarr: "\u2192", sscr: "\u{1D4C8}", ssetmn: "\u2216", ssmile: "\u2323", sstarf: "\u22C6", star: "\u2606", starf: "\u2605", straightepsilon: "\u03F5", straightphi: "\u03D5", strns: "\xAF", sub: "\u2282", subE: "\u2AC5", subdot: "\u2ABD", sube: "\u2286", subedot: "\u2AC3", submult: "\u2AC1", subnE: "\u2ACB", subne: "\u228A", subplus: "\u2ABF", subrarr: "\u2979", subset: "\u2282", subseteq: "\u2286", subseteqq: "\u2AC5", subsetneq: "\u228A", subsetneqq: "\u2ACB", subsim: "\u2AC7", subsub: "\u2AD5", subsup: "\u2AD3", succ: "\u227B", succapprox: "\u2AB8", succcurlyeq: "\u227D", succeq: "\u2AB0", succnapprox: "\u2ABA", succneqq: "\u2AB6", succnsim: "\u22E9", succsim: "\u227F", sum: "\u2211", sung: "\u266A", sup1: "\xB9", sup2: "\xB2", sup3: "\xB3", sup: "\u2283", supE: "\u2AC6", supdot: "\u2ABE", supdsub: "\u2AD8", supe: "\u2287", supedot: "\u2AC4", suphsol: "\u27C9", suphsub: "\u2AD7", suplarr: "\u297B", supmult: "\u2AC2", supnE: "\u2ACC", supne: "\u228B", supplus: "\u2AC0", supset: "\u2283", supseteq: "\u2287", supseteqq: "\u2AC6", supsetneq: "\u228B", supsetneqq: "\u2ACC", supsim: "\u2AC8", supsub: "\u2AD4", supsup: "\u2AD6", swArr: "\u21D9", swarhk: "\u2926", swarr: "\u2199", swarrow: "\u2199", swnwar: "\u292A", szlig: "\xDF", target: "\u2316", tau: "\u03C4", tbrk: "\u23B4", tcaron: "\u0165", tcedil: "\u0163", tcy: "\u0442", tdot: "\u20DB", telrec: "\u2315", tfr: "\u{1D531}", there4: "\u2234", therefore: "\u2234", theta: "\u03B8", thetasym: "\u03D1", thetav: "\u03D1", thickapprox: "\u2248", thicksim: "\u223C", thinsp: "\u2009", thkap: "\u2248", thksim: "\u223C", thorn: "\xFE", tilde: "\u02DC", times: "\xD7", timesb: "\u22A0", timesbar: "\u2A31", timesd: "\u2A30", tint: "\u222D", toea: "\u2928", top: "\u22A4", topbot: "\u2336", topcir: "\u2AF1", topf: "\u{1D565}", topfork: "\u2ADA", tosa: "\u2929", tprime: "\u2034", trade: "\u2122", triangle: "\u25B5", triangledown: "\u25BF", triangleleft: "\u25C3", trianglelefteq: "\u22B4", triangleq: "\u225C", triangleright: "\u25B9", trianglerighteq: "\u22B5", tridot: "\u25EC", trie: "\u225C", triminus: "\u2A3A", triplus: "\u2A39", trisb: "\u29CD", tritime: "\u2A3B", trpezium: "\u23E2", tscr: "\u{1D4C9}", tscy: "\u0446", tshcy: "\u045B", tstrok: "\u0167", twixt: "\u226C", twoheadleftarrow: "\u219E", twoheadrightarrow: "\u21A0", uArr: "\u21D1", uHar: "\u2963", uacute: "\xFA", uarr: "\u2191", ubrcy: "\u045E", ubreve: "\u016D", ucirc: "\xFB", ucy: "\u0443", udarr: "\u21C5", udblac: "\u0171", udhar: "\u296E", ufisht: "\u297E", ufr: "\u{1D532}", ugrave: "\xF9", uharl: "\u21BF", uharr: "\u21BE", uhblk: "\u2580", ulcorn: "\u231C", ulcorner: "\u231C", ulcrop: "\u230F", ultri: "\u25F8", umacr: "\u016B", uml: "\xA8", uogon: "\u0173", uopf: "\u{1D566}", uparrow: "\u2191", updownarrow: "\u2195", upharpoonleft: "\u21BF", upharpoonright: "\u21BE", uplus: "\u228E", upsi: "\u03C5", upsih: "\u03D2", upsilon: "\u03C5", upuparrows: "\u21C8", urcorn: "\u231D", urcorner: "\u231D", urcrop: "\u230E", uring: "\u016F", urtri: "\u25F9", uscr: "\u{1D4CA}", utdot: "\u22F0", utilde: "\u0169", utri: "\u25B5", utrif: "\u25B4", uuarr: "\u21C8", uuml: "\xFC", uwangle: "\u29A7", vArr: "\u21D5", vBar: "\u2AE8", vBarv: "\u2AE9", vDash: "\u22A8", vangrt: "\u299C", varepsilon: "\u03F5", varkappa: "\u03F0", varnothing: "\u2205", varphi: "\u03D5", varpi: "\u03D6", varpropto: "\u221D", varr: "\u2195", varrho: "\u03F1", varsigma: "\u03C2", varsubsetneq: "\u228A\uFE00", varsubsetneqq: "\u2ACB\uFE00", varsupsetneq: "\u228B\uFE00", varsupsetneqq: "\u2ACC\uFE00", vartheta: "\u03D1", vartriangleleft: "\u22B2", vartriangleright: "\u22B3", vcy: "\u0432", vdash: "\u22A2", vee: "\u2228", veebar: "\u22BB", veeeq: "\u225A", vellip: "\u22EE", verbar: "|", vert: "|", vfr: "\u{1D533}", vltri: "\u22B2", vnsub: "\u2282\u20D2", vnsup: "\u2283\u20D2", vopf: "\u{1D567}", vprop: "\u221D", vrtri: "\u22B3", vscr: "\u{1D4CB}", vsubnE: "\u2ACB\uFE00", vsubne: "\u228A\uFE00", vsupnE: "\u2ACC\uFE00", vsupne: "\u228B\uFE00", vzigzag: "\u299A", wcirc: "\u0175", wedbar: "\u2A5F", wedge: "\u2227", wedgeq: "\u2259", weierp: "\u2118", wfr: "\u{1D534}", wopf: "\u{1D568}", wp: "\u2118", wr: "\u2240", wreath: "\u2240", wscr: "\u{1D4CC}", xcap: "\u22C2", xcirc: "\u25EF", xcup: "\u22C3", xdtri: "\u25BD", xfr: "\u{1D535}", xhArr: "\u27FA", xharr: "\u27F7", xi: "\u03BE", xlArr: "\u27F8", xlarr: "\u27F5", xmap: "\u27FC", xnis: "\u22FB", xodot: "\u2A00", xopf: "\u{1D569}", xoplus: "\u2A01", xotime: "\u2A02", xrArr: "\u27F9", xrarr: "\u27F6", xscr: "\u{1D4CD}", xsqcup: "\u2A06", xuplus: "\u2A04", xutri: "\u25B3", xvee: "\u22C1", xwedge: "\u22C0", yacute: "\xFD", yacy: "\u044F", ycirc: "\u0177", ycy: "\u044B", yen: "\xA5", yfr: "\u{1D536}", yicy: "\u0457", yopf: "\u{1D56A}", yscr: "\u{1D4CE}", yucy: "\u044E", yuml: "\xFF", zacute: "\u017A", zcaron: "\u017E", zcy: "\u0437", zdot: "\u017C", zeetrf: "\u2128", zeta: "\u03B6", zfr: "\u{1D537}", zhcy: "\u0436", zigrarr: "\u21DD", zopf: "\u{1D56B}", zscr: "\u{1D4CF}", zwj: "\u200D", zwnj: "\u200C" }, pe = { 0: 65533, 128: 8364, 130: 8218, 131: 402, 132: 8222, 133: 8230, 134: 8224, 135: 8225, 136: 710, 137: 8240, 138: 352, 139: 8249, 140: 338, 142: 381, 145: 8216, 146: 8217, 147: 8220, 148: 8221, 149: 8226, 150: 8211, 151: 8212, 152: 732, 153: 8482, 154: 353, 155: 8250, 156: 339, 158: 382, 159: 376 };
function _0(e) {
return e.replace(/&(?:[a-zA-Z]+|#[xX][\da-fA-F]+|#\d+);/g, (u) => {
if (u.charAt(1) === "#") {
let i = u.charAt(2), n3 = i === "X" || i === "x" ? parseInt(u.slice(3), 16) : parseInt(u.slice(2), 10);
return M0(n3);
}
return j0[u.slice(1, -1)] || u;
});
}
function M0(e) {
return e >= 55296 && e <= 57343 || e > 1114111 ? "\uFFFD" : (e in pe && (e = pe[e]), String.fromCodePoint(e));
}
function U0(e, u) {
return e.startPos = e.tokenPos = e.index, e.startColumn = e.colPos = e.column, e.startLine = e.linePos = e.line, e.token = C[e.currentChar] & 8192 ? J0(e, u) : xe(e, u, 0), e.token;
}
function J0(e, u) {
let i = e.currentChar, n3 = r(e), t = e.index;
for (; n3 !== i; )
e.index >= e.end && f(e, 14), n3 = r(e);
return n3 !== i && f(e, 14), e.tokenValue = e.source.slice(t, e.index), r(e), u & 512 && (e.tokenRaw = e.source.slice(e.tokenPos, e.index)), 134283267;
}
function j2(e, u) {
if (e.startPos = e.tokenPos = e.index, e.startColumn = e.colPos = e.column, e.startLine = e.linePos = e.line, e.index >= e.end)
return e.token = 1048576;
switch (Ge[e.source.charCodeAt(e.index)]) {
case 8456258: {
r(e), e.currentChar === 47 ? (r(e), e.token = 25) : e.token = 8456258;
break;
}
case 2162700: {
r(e), e.token = 2162700;
break;
}
default: {
let n3 = 0;
for (; e.index < e.end; ) {
let o = C[e.source.charCodeAt(e.index)];
if (o & 1024 ? (n3 |= 5, G(e)) : o & 2048 ? (Y(e, n3), n3 = n3 & -5 | 1) : r(e), C[e.currentChar] & 16384)
break;
}
let t = e.source.slice(e.tokenPos, e.index);
u & 512 && (e.tokenRaw = t), e.tokenValue = _0(t), e.token = 138;
}
}
return e.token;
}
function _1(e) {
if ((e.token & 143360) === 143360) {
let { index: u } = e, i = e.currentChar;
for (; C[i] & 32770; )
i = r(e);
e.tokenValue += e.source.slice(u, e.index);
}
return e.token = 208897, e.token;
}
function s2(e, u, i) {
!(e.flags & 1) && (e.token & 1048576) !== 1048576 && !i && f(e, 28, Z[e.token & 255]), M(e, u, 1074790417);
}
function eu(e, u, i, n3) {
return u - i < 13 && n3 === "use strict" && ((e.token & 1048576) === 1048576 || e.flags & 1) ? 1 : 0;
}
function M1(e, u, i) {
return e.token !== i ? 0 : (E(e, u), 1);
}
function M(e, u, i) {
return e.token !== i ? false : (E(e, u), true);
}
function q(e, u, i) {
e.token !== i && f(e, 23, Z[i & 255]), E(e, u);
}
function r2(e, u) {
switch (u.type) {
case "ArrayExpression":
u.type = "ArrayPattern";
let i = u.elements;
for (let t = 0, o = i.length; t < o; ++t) {
let l = i[t];
l && r2(e, l);
}
return;
case "ObjectExpression":
u.type = "ObjectPattern";
let n3 = u.properties;
for (let t = 0, o = n3.length; t < o; ++t)
r2(e, n3[t]);
return;
case "AssignmentExpression":
u.type = "AssignmentPattern", u.operator !== "=" && f(e, 68), delete u.operator, r2(e, u.left);
return;
case "Property":
r2(e, u.value);
return;
case "SpreadElement":
u.type = "RestElement", r2(e, u.argument);
}
}
function l1(e, u, i, n3, t) {
u & 1024 && ((n3 & 36864) === 36864 && f(e, 114), !t && (n3 & 537079808) === 537079808 && f(e, 115)), (n3 & 20480) === 20480 && f(e, 99), i & 24 && n3 === 241739 && f(e, 97), u & 4196352 && n3 === 209008 && f(e, 95), u & 2098176 && n3 === 241773 && f(e, 94, "yield");
}
function uu(e, u, i) {
u & 1024 && ((i & 36864) === 36864 && f(e, 114), (i & 537079808) === 537079808 && f(e, 115), i === 122 && f(e, 92), i === 121 && f(e, 92)), (i & 20480) === 20480 && f(e, 99), u & 4196352 && i === 209008 && f(e, 95), u & 2098176 && i === 241773 && f(e, 94, "yield");
}
function iu(e, u, i) {
return i === 209008 && (u & 4196352 && f(e, 95), e.destructible |= 128), i === 241773 && u & 2097152 && f(e, 94, "yield"), (i & 20480) === 20480 || (i & 36864) === 36864 || i == 122;
}
function $0(e) {
return e.property ? e.property.type === "PrivateIdentifier" : false;
}
function nu(e, u, i, n3) {
for (; u; ) {
if (u["$" + i])
return n3 && f(e, 133), 1;
n3 && u.loop && (n3 = 0), u = u.$;
}
return 0;
}
function H0(e, u, i) {
let n3 = u;
for (; n3; )
n3["$" + i] && f(e, 132, i), n3 = n3.$;
u["$" + i] = 1;
}
function v(e, u, i, n3, t, o) {
return u & 2 && (o.start = i, o.end = e.startPos, o.range = [i, e.startPos]), u & 4 && (o.loc = { start: { line: n3, column: t }, end: { line: e.startLine, column: e.startColumn } }, e.sourceFile && (o.loc.source = e.sourceFile)), o;
}
function f1(e) {
switch (e.type) {
case "JSXIdentifier":
return e.name;
case "JSXNamespacedName":
return e.namespace + ":" + e.name;
case "JSXMemberExpression":
return f1(e.object) + "." + f1(e.property);
}
}
function c1(e, u, i) {
let n3 = i2(_2(), 1024);
return L2(e, u, n3, i, 1, 0), n3;
}
function U1(e, u) {
let { index: i, line: n3, column: t } = e;
for (var o = arguments.length, l = new Array(o > 2 ? o - 2 : 0), c = 2; c < o; c++)
l[c - 2] = arguments[c];
return { type: u, params: l, index: i, line: n3, column: t };
}
function _2() {
return { parent: void 0, type: 2 };
}
function i2(e, u) {
return { parent: e, type: u, scopeError: void 0 };
}
function B2(e, u, i, n3, t, o) {
t & 4 ? tu(e, u, i, n3, t) : L2(e, u, i, n3, t, o), o & 64 && M2(e, n3);
}
function L2(e, u, i, n3, t, o) {
let l = i["#" + n3];
l && !(l & 2) && (t & 1 ? i.scopeError = U1(e, 140, n3) : u & 256 && l & 64 && o & 2 || f(e, 140, n3)), i.type & 128 && i.parent["#" + n3] && !(i.parent["#" + n3] & 2) && f(e, 140, n3), i.type & 1024 && l && !(l & 2) && t & 1 && (i.scopeError = U1(e, 140, n3)), i.type & 64 && i.parent["#" + n3] & 768 && f(e, 153, n3), i["#" + n3] = t;
}
function tu(e, u, i, n3, t) {
let o = i;
for (; o && !(o.type & 256); ) {
let l = o["#" + n3];
l & 248 && (u & 256 && !(u & 1024) && (t & 128 && l & 68 || l & 128 && t & 68) || f(e, 140, n3)), o === i && l & 1 && t & 1 && (o.scopeError = U1(e, 140, n3)), l & 768 && (!(l & 512) || !(u & 256) || u & 1024) && f(e, 140, n3), o["#" + n3] = t, o = o.parent;
}
}
function M2(e, u) {
e.exportedNames !== void 0 && u !== "" && (e.exportedNames["#" + u] && f(e, 141, u), e.exportedNames["#" + u] = 1);
}
function X0(e, u) {
e.exportedBindings !== void 0 && u !== "" && (e.exportedBindings["#" + u] = 1);
}
function z0(e, u) {
return function(i, n3, t, o, l) {
let c = { type: i, value: n3 };
e & 2 && (c.start = t, c.end = o, c.range = [t, o]), e & 4 && (c.loc = l), u.push(c);
};
}
function W0(e, u) {
return function(i, n3, t, o) {
let l = { token: i };
e & 2 && (l.start = n3, l.end = t, l.range = [n3, t]), e & 4 && (l.loc = o), u.push(l);
};
}
function J1(e, u) {
return e & 2098176 ? e & 2048 && u === 209008 || e & 2097152 && u === 241773 ? false : (u & 143360) === 143360 || (u & 12288) === 12288 : (u & 143360) === 143360 || (u & 12288) === 12288 || (u & 36864) === 36864;
}
function $1(e, u, i, n3) {
(i & 537079808) === 537079808 && (u & 1024 && f(e, 115), n3 && (e.flags |= 512)), J1(u, i) || f(e, 0);
}
function K0(e, u, i, n3) {
return { source: e, flags: 0, index: 0, line: 1, column: 0, startPos: 0, end: e.length, tokenPos: 0, startColumn: 0, colPos: 0, linePos: 1, startLine: 1, sourceFile: u, tokenValue: "", token: 1048576, tokenRaw: "", tokenRegExp: void 0, currentChar: e.charCodeAt(0), exportedNames: [], exportedBindings: [], assignable: 1, destructible: 0, onComment: i, onToken: n3, leadingDecorators: [] };
}
function H1(e, u, i) {
let n3 = "", t, o;
u != null && (u.module && (i |= 3072), u.next && (i |= 1), u.loc && (i |= 4), u.ranges && (i |= 2), u.uniqueKeyInPattern && (i |= -2147483648), u.lexical && (i |= 64), u.webcompat && (i |= 256), u.directives && (i |= 520), u.globalReturn && (i |= 32), u.raw && (i |= 512), u.preserveParens && (i |= 128), u.impliedStrict && (i |= 1024), u.jsx && (i |= 16), u.identifierPattern && (i |= 268435456), u.specDeviation && (i |= 536870912), u.source && (n3 = u.source), u.onComment != null && (t = Array.isArray(u.onComment) ? z0(i, u.onComment) : u.onComment), u.onToken != null && (o = Array.isArray(u.onToken) ? W0(i, u.onToken) : u.onToken));
let l = K0(e, n3, t, o);
i & 1 && l2(l);
let c = i & 64 ? _2() : void 0, s = [], m = "script";
if (i & 2048) {
if (m = "module", s = Z0(l, i | 8192, c), c)
for (let h in l.exportedBindings)
h[0] === "#" && !c[h] && f(l, 142, h.slice(1));
} else
s = Y0(l, i | 8192, c);
let k = { type: "Program", sourceType: m, body: s };
return i & 2 && (k.start = 0, k.end = e.length, k.range = [0, e.length]), i & 4 && (k.loc = { start: { line: 1, column: 0 }, end: { line: l.line, column: l.column } }, l.sourceFile && (k.loc.source = n3)), k;
}
function Y0(e, u, i) {
E(e, u | 32768 | 1073741824);
let n3 = [];
for (; e.token === 134283267; ) {
let { index: t, tokenPos: o, tokenValue: l, linePos: c, colPos: s, token: m } = e, k = c2(e, u);
eu(e, t, o, l) && (u |= 1024), n3.push(z1(e, u, k, m, o, c, s));
}
for (; e.token !== 1048576; )
n3.push(G2(e, u, i, 4, {}));
return n3;
}
function Z0(e, u, i) {
E(e, u | 32768);
let n3 = [];
if (u & 8)
for (; e.token === 134283267; ) {
let { tokenPos: t, linePos: o, colPos: l, token: c } = e;
n3.push(z1(e, u, c2(e, u), c, t, o, l));
}
for (; e.token !== 1048576; )
n3.push(Q0(e, u, i));
return n3;
}
function Q0(e, u, i) {
e.leadingDecorators = k1(e, u);
let n3;
switch (e.token) {
case 20566:
n3 = bt(e, u, i);
break;
case 86108:
n3 = ht(e, u, i);
break;
default:
n3 = G2(e, u, i, 4, {});
}
return e.leadingDecorators.length && f(e, 164), n3;
}
function G2(e, u, i, n3, t) {
let o = e.tokenPos, l = e.linePos, c = e.colPos;
switch (e.token) {
case 86106:
return I2(e, u, i, n3, 1, 0, 0, o, l, c);
case 133:
case 86096:
return x1(e, u, i, 0, o, l, c);
case 86092:
return W1(e, u, i, 16, 0, o, l, c);
case 241739:
return dt(e, u, i, n3, o, l, c);
case 20566:
f(e, 100, "export");
case 86108:
switch (E(e, u), e.token) {
case 67174411:
return hu(e, u, o, l, c);
case 67108877:
return gu(e, u, o, l, c);
default:
f(e, 100, "import");
}
case 209007:
return ou(e, u, i, n3, t, 1, o, l, c);
default:
return x2(e, u, i, n3, t, 1, o, l, c);
}
}
function x2(e, u, i, n3, t, o, l, c, s) {
switch (e.token) {
case 86090:
return fu(e, u, i, 0, l, c, s);
case 20574:
return x0(e, u, l, c, s);
case 20571:
return ut(e, u, i, t, l, c, s);
case 20569:
return gt(e, u, i, t, l, c, s);
case 20564:
return at(e, u, i, t, l, c, s);
case 20580:
return nt(e, u, i, t, l, c, s);
case 86112:
return it(e, u, i, t, l, c, s);
case 1074790417:
return p0(e, u, l, c, s);
case 2162700:
return s1(e, u, i && i2(i, 2), t, l, c, s);
case 86114:
return et(e, u, l, c, s);
case 20557:
return ot(e, u, t, l, c, s);
case 20561:
return tt(e, u, t, l, c, s);
case 20579:
return ct(e, u, i, t, l, c, s);
case 20581:
return lt(e, u, i, t, l, c, s);
case 20562:
return ft(e, u, l, c, s);
case 209007:
return ou(e, u, i, n3, t, 0, l, c, s);
case 20559:
f(e, 156);
case 20568:
f(e, 157);
case 86106:
f(e, u & 1024 ? 73 : (u & 256) < 1 ? 75 : 74);
case 86096:
f(e, 76);
default:
return G0(e, u, i, n3, t, o, l, c, s);
}
}
function G0(e, u, i, n3, t, o, l, c, s) {
let { tokenValue: m, token: k } = e, h;
switch (k) {
case 241739:
h = $(e, u, 0), u & 1024 && f(e, 82), e.token === 69271571 && f(e, 81);
break;
default:
h = d2(e, u, 2, 0, 1, 0, 0, 1, e.tokenPos, e.linePos, e.colPos);
}
return k & 143360 && e.token === 21 ? X1(e, u, i, n3, t, m, h, k, o, l, c, s) : (h = H(e, u, h, 0, 0, l, c, s), h = Q(e, u, 0, 0, l, c, s, h), e.token === 18 && (h = O2(e, u, 0, l, c, s, h)), X2(e, u, h, l, c, s));
}
function s1(e, u, i, n3, t, o, l) {
let c = [];
for (q(e, u | 32768, 2162700); e.token !== 1074790415; )
c.push(G2(e, u, i, 2, { $: n3 }));
return q(e, u | 32768, 1074790415), v(e, u, t, o, l, { type: "BlockStatement", body: c });
}
function x0(e, u, i, n3, t) {
(u & 32) < 1 && u & 8192 && f(e, 89), E(e, u | 32768);
let o = e.flags & 1 || e.token & 1048576 ? null : o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos);
return s2(e, u | 32768), v(e, u, i, n3, t, { type: "ReturnStatement", argument: o });
}
function X2(e, u, i, n3, t, o) {
return s2(e, u | 32768), v(e, u, n3, t, o, { type: "ExpressionStatement", expression: i });
}
function X1(e, u, i, n3, t, o, l, c, s, m, k, h) {
l1(e, u, 0, c, 1), H0(e, t, o), E(e, u | 32768);
let d = s && (u & 1024) < 1 && u & 256 && e.token === 86106 ? I2(e, u, i2(i, 2), n3, 0, 0, 0, e.tokenPos, e.linePos, e.colPos) : x2(e, u, i, n3, t, s, e.tokenPos, e.linePos, e.colPos);
return v(e, u, m, k, h, { type: "LabeledStatement", label: l, body: d });
}
function ou(e, u, i, n3, t, o, l, c, s) {
let { token: m, tokenValue: k } = e, h = $(e, u, 0);
if (e.token === 21)
return X1(e, u, i, n3, t, k, h, m, 1, l, c, s);
let d = e.flags & 1;
if (!d) {
if (e.token === 86106)
return o || f(e, 119), I2(e, u, i, n3, 1, 0, 1, l, c, s);
if ((e.token & 143360) === 143360)
return h = Pu(e, u, 1, l, c, s), e.token === 18 && (h = O2(e, u, 0, l, c, s, h)), X2(e, u, h, l, c, s);
}
return e.token === 67174411 ? h = G1(e, u, h, 1, 1, 0, d, l, c, s) : (e.token === 10 && ($1(e, u, m, 1), h = h1(e, u, e.tokenValue, h, 0, 1, 0, l, c, s)), e.assignable = 1), h = H(e, u, h, 0, 0, l, c, s), e.token === 18 && (h = O2(e, u, 0, l, c, s, h)), h = Q(e, u, 0, 0, l, c, s, h), e.assignable = 1, X2(e, u, h, l, c, s);
}
function z1(e, u, i, n3, t, o, l) {
return n3 !== 1074790417 && (e.assignable = 2, i = H(e, u, i, 0, 0, t, o, l), e.token !== 1074790417 && (i = Q(e, u, 0, 0, t, o, l, i), e.token === 18 && (i = O2(e, u, 0, t, o, l, i))), s2(e, u | 32768)), u & 8 && i.type === "Literal" && typeof i.value == "string" ? v(e, u, t, o, l, { type: "ExpressionStatement", expression: i, directive: i.raw.slice(1, -1) }) : v(e, u, t, o, l, { type: "ExpressionStatement", expression: i });
}
function p0(e, u, i, n3, t) {
return E(e, u | 32768), v(e, u, i, n3, t, { type: "EmptyStatement" });
}
function et(e, u, i, n3, t) {
E(e, u | 32768), e.flags & 1 && f(e, 87);
let o = o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos);
return s2(e, u | 32768), v(e, u, i, n3, t, { type: "ThrowStatement", argument: o });
}
function ut(e, u, i, n3, t, o, l) {
E(e, u), q(e, u | 32768, 67174411), e.assignable = 1;
let c = o2(e, u, 0, 1, e.tokenPos, e.line, e.colPos);
q(e, u | 32768, 16);
let s = lu(e, u, i, n3, e.tokenPos, e.linePos, e.colPos), m = null;
return e.token === 20565 && (E(e, u | 32768), m = lu(e, u, i, n3, e.tokenPos, e.linePos, e.colPos)), v(e, u, t, o, l, { type: "IfStatement", test: c, consequent: s, alternate: m });
}
function lu(e, u, i, n3, t, o, l) {
return u & 1024 || (u & 256) < 1 || e.token !== 86106 ? x2(e, u, i, 0, { $: n3 }, 0, e.tokenPos, e.linePos, e.colPos) : I2(e, u, i2(i, 2), 0, 0, 0, 0, t, o, l);
}
function it(e, u, i, n3, t, o, l) {
E(e, u), q(e, u | 32768, 67174411);
let c = o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos);
q(e, u, 16), q(e, u, 2162700);
let s = [], m = 0;
for (i && (i = i2(i, 8)); e.token !== 1074790415; ) {
let { tokenPos: k, linePos: h, colPos: d } = e, y = null, w = [];
for (M(e, u | 32768, 20558) ? y = o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos) : (q(e, u | 32768, 20563), m && f(e, 86), m = 1), q(e, u | 32768, 21); e.token !== 20558 && e.token !== 1074790415 && e.token !== 20563; )
w.push(G2(e, u | 4096, i, 2, { $: n3 }));
s.push(v(e, u, k, h, d, { type: "SwitchCase", test: y, consequent: w }));
}
return q(e, u | 32768, 1074790415), v(e, u, t, o, l, { type: "SwitchStatement", discriminant: c, cases: s });
}
function nt(e, u, i, n3, t, o, l) {
E(e, u), q(e, u | 32768, 67174411);
let c = o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos);
q(e, u | 32768, 16);
let s = p2(e, u, i, n3);
return v(e, u, t, o, l, { type: "WhileStatement", test: c, body: s });
}
function p2(e, u, i, n3) {
return x2(e, (u | 134217728) ^ 134217728 | 131072, i, 0, { loop: 1, $: n3 }, 0, e.tokenPos, e.linePos, e.colPos);
}
function tt(e, u, i, n3, t, o) {
(u & 131072) < 1 && f(e, 65), E(e, u);
let l = null;
if ((e.flags & 1) < 1 && e.token & 143360) {
let { tokenValue: c } = e;
l = $(e, u | 32768, 0), nu(e, i, c, 1) || f(e, 134, c);
}
return s2(e, u | 32768), v(e, u, n3, t, o, { type: "ContinueStatement", label: l });
}
function ot(e, u, i, n3, t, o) {
E(e, u | 32768);
let l = null;
if ((e.flags & 1) < 1 && e.token & 143360) {
let { tokenValue: c } = e;
l = $(e, u | 32768, 0), nu(e, i, c, 0) || f(e, 134, c);
} else
(u & 135168) < 1 && f(e, 66);
return s2(e, u | 32768), v(e, u, n3, t, o, { type: "BreakStatement", label: l });
}
function lt(e, u, i, n3, t, o, l) {
E(e, u), u & 1024 && f(e, 88), q(e, u | 32768, 67174411);
let c = o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos);
q(e, u | 32768, 16);
let s = x2(e, u, i, 2, n3, 0, e.tokenPos, e.linePos, e.colPos);
return v(e, u, t, o, l, { type: "WithStatement", object: c, body: s });
}
function ft(e, u, i, n3, t) {
return E(e, u | 32768), s2(e, u | 32768), v(e, u, i, n3, t, { type: "DebuggerStatement" });
}
function ct(e, u, i, n3, t, o, l) {
E(e, u | 32768);
let c = i ? i2(i, 32) : void 0, s = s1(e, u, c, { $: n3 }, e.tokenPos, e.linePos, e.colPos), { tokenPos: m, linePos: k, colPos: h } = e, d = M(e, u | 32768, 20559) ? st(e, u, i, n3, m, k, h) : null, y = null;
if (e.token === 20568) {
E(e, u | 32768);
let w = c ? i2(i, 4) : void 0;
y = s1(e, u, w, { $: n3 }, e.tokenPos, e.linePos, e.colPos);
}
return !d && !y && f(e, 85), v(e, u, t, o, l, { type: "TryStatement", block: s, handler: d, finalizer: y });
}
function st(e, u, i, n3, t, o, l) {
let c = null, s = i;
M(e, u, 67174411) && (i && (i = i2(i, 4)), c = Du(e, u, i, (e.token & 2097152) === 2097152 ? 256 : 512, 0, e.tokenPos, e.linePos, e.colPos), e.token === 18 ? f(e, 83) : e.token === 1077936157 && f(e, 84), q(e, u | 32768, 16), i && (s = i2(i, 64)));
let m = s1(e, u, s, { $: n3 }, e.tokenPos, e.linePos, e.colPos);
return v(e, u, t, o, l, { type: "CatchClause", param: c, body: m });
}
function at(e, u, i, n3, t, o, l) {
E(e, u | 32768);
let c = p2(e, u, i, n3);
q(e, u, 20580), q(e, u | 32768, 67174411);
let s = o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos);
return q(e, u | 32768, 16), M(e, u, 1074790417), v(e, u, t, o, l, { type: "DoWhileStatement", body: c, test: s });
}
function dt(e, u, i, n3, t, o, l) {
let { token: c, tokenValue: s } = e, m = $(e, u, 0);
if (e.token & 2240512) {
let k = z2(e, u, i, 8, 0);
return s2(e, u | 32768), v(e, u, t, o, l, { type: "VariableDeclaration", kind: "let", declarations: k });
}
if (e.assignable = 1, u & 1024 && f(e, 82), e.token === 21)
return X1(e, u, i, n3, {}, s, m, c, 0, t, o, l);
if (e.token === 10) {
let k;
u & 64 && (k = c1(e, u, s)), e.flags = (e.flags | 128) ^ 128, m = e1(e, u, k, [m], 0, t, o, l);
} else
m = H(e, u, m, 0, 0, t, o, l), m = Q(e, u, 0, 0, t, o, l, m);
return e.token === 18 && (m = O2(e, u, 0, t, o, l, m)), X2(e, u, m, t, o, l);
}
function W1(e, u, i, n3, t, o, l, c) {
E(e, u);
let s = z2(e, u, i, n3, t);
return s2(e, u | 32768), v(e, u, o, l, c, { type: "VariableDeclaration", kind: n3 & 8 ? "let" : "const", declarations: s });
}
function fu(e, u, i, n3, t, o, l) {
E(e, u);
let c = z2(e, u, i, 4, n3);
return s2(e, u | 32768), v(e, u, t, o, l, { type: "VariableDeclaration", kind: "var", declarations: c });
}
function z2(e, u, i, n3, t) {
let o = 1, l = [cu(e, u, i, n3, t)];
for (; M(e, u, 18); )
o++, l.push(cu(e, u, i, n3, t));
return o > 1 && t & 32 && e.token & 262144 && f(e, 58, Z[e.token & 255]), l;
}
function cu(e, u, i, n3, t) {
let { token: o, tokenPos: l, linePos: c, colPos: s } = e, m = null, k = Du(e, u, i, n3, t, l, c, s);
return e.token === 1077936157 ? (E(e, u | 32768), m = K(e, u, 1, 0, 0, e.tokenPos, e.linePos, e.colPos), (t & 32 || (o & 2097152) < 1) && (e.token === 274549 || e.token === 8738868 && (o & 2097152 || (n3 & 4) < 1 || u & 1024)) && L(l, e.line, e.index - 3, 57, e.token === 274549 ? "of" : "in")) : (n3 & 16 || (o & 2097152) > 0) && (e.token & 262144) !== 262144 && f(e, 56, n3 & 16 ? "const" : "destructuring"), v(e, u, l, c, s, { type: "VariableDeclarator", id: k, init: m });
}
function gt(e, u, i, n3, t, o, l) {
E(e, u);
let c = (u & 4194304) > 0 && M(e, u, 209008);
q(e, u | 32768, 67174411), i && (i = i2(i, 1));
let s = null, m = null, k = 0, h = null, d = e.token === 86090 || e.token === 241739 || e.token === 86092, y, { token: w, tokenPos: D, linePos: F, colPos: O } = e;
if (d ? w === 241739 ? (h = $(e, u, 0), e.token & 2240512 ? (e.token === 8738868 ? u & 1024 && f(e, 64) : h = v(e, u, D, F, O, { type: "VariableDeclaration", kind: "let", declarations: z2(e, u | 134217728, i, 8, 32) }), e.assignable = 1) : u & 1024 ? f(e, 64) : (d = false, e.assignable = 1, h = H(e, u, h, 0, 0, D, F, O), e.token === 274549 && f(e, 111))) : (E(e, u), h = v(e, u, D, F, O, w === 86090 ? { type: "VariableDeclaration", kind: "var", declarations: z2(e, u | 134217728, i, 4, 32) } : { type: "VariableDeclaration", kind: "const", declarations: z2(e, u | 134217728, i, 16, 32) }), e.assignable = 1) : w === 1074790417 ? c && f(e, 79) : (w & 2097152) === 2097152 ? (h = w === 2162700 ? b2(e, u, void 0, 1, 0, 0, 2, 32, D, F, O) : m2(e, u, void 0, 1, 0, 0, 2, 32, D, F, O), k = e.destructible, u & 256 && k & 64 && f(e, 60), e.assignable = k & 16 ? 2 : 1, h = H(e, u | 134217728, h, 0, 0, e.tokenPos, e.linePos, e.colPos)) : h = h2(e, u | 134217728, 1, 0, 1, D, F, O), (e.token & 262144) === 262144) {
if (e.token === 274549) {
e.assignable & 2 && f(e, 77, c ? "await" : "of"), r2(e, h), E(e, u | 32768), y = K(e, u, 1, 0, 0, e.tokenPos, e.linePos, e.colPos), q(e, u | 32768, 16);
let I = p2(e, u, i, n3);
return v(e, u, t, o, l, { type: "ForOfStatement", left: h, right: y, body: I, await: c });
}
e.assignable & 2 && f(e, 77, "in"), r2(e, h), E(e, u | 32768), c && f(e, 79), y = o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos), q(e, u | 32768, 16);
let x = p2(e, u, i, n3);
return v(e, u, t, o, l, { type: "ForInStatement", body: x, left: h, right: y });
}
c && f(e, 79), d || (k & 8 && e.token !== 1077936157 && f(e, 77, "loop"), h = Q(e, u | 134217728, 0, 0, D, F, O, h)), e.token === 18 && (h = O2(e, u, 0, e.tokenPos, e.linePos, e.colPos, h)), q(e, u | 32768, 1074790417), e.token !== 1074790417 && (s = o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos)), q(e, u | 32768, 1074790417), e.token !== 16 && (m = o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos)), q(e, u | 32768, 16);
let N = p2(e, u, i, n3);
return v(e, u, t, o, l, { type: "ForStatement", init: h, test: s, update: m, body: N });
}
function su(e, u, i) {
return J1(u, e.token) || f(e, 114), (e.token & 537079808) === 537079808 && f(e, 115), i && L2(e, u, i, e.tokenValue, 8, 0), $(e, u, 0);
}
function ht(e, u, i) {
let n3 = e.tokenPos, t = e.linePos, o = e.colPos;
E(e, u);
let l = null, { tokenPos: c, linePos: s, colPos: m } = e, k = [];
if (e.token === 134283267)
l = c2(e, u);
else {
if (e.token & 143360) {
let h = su(e, u, i);
if (k = [v(e, u, c, s, m, { type: "ImportDefaultSpecifier", local: h })], M(e, u, 18))
switch (e.token) {
case 8457014:
k.push(au(e, u, i));
break;
case 2162700:
du(e, u, i, k);
break;
default:
f(e, 104);
}
} else
switch (e.token) {
case 8457014:
k = [au(e, u, i)];
break;
case 2162700:
du(e, u, i, k);
break;
case 67174411:
return hu(e, u, n3, t, o);
case 67108877:
return gu(e, u, n3, t, o);
default:
f(e, 28, Z[e.token & 255]);
}
l = mt(e, u);
}
return s2(e, u | 32768), v(e, u, n3, t, o, { type: "ImportDeclaration", specifiers: k, source: l });
}
function au(e, u, i) {
let { tokenPos: n3, linePos: t, colPos: o } = e;
return E(e, u), q(e, u, 77934), (e.token & 134217728) === 134217728 && L(n3, e.line, e.index, 28, Z[e.token & 255]), v(e, u, n3, t, o, { type: "ImportNamespaceSpecifier", local: su(e, u, i) });
}
function mt(e, u) {
return M(e, u, 12404), e.token !== 134283267 && f(e, 102, "Import"), c2(e, u);
}
function du(e, u, i, n3) {
for (E(e, u); e.token & 143360; ) {
let { token: t, tokenValue: o, tokenPos: l, linePos: c, colPos: s } = e, m = $(e, u, 0), k;
M(e, u, 77934) ? ((e.token & 134217728) === 134217728 || e.token === 18 ? f(e, 103) : l1(e, u, 16, e.token, 0), o = e.tokenValue, k = $(e, u, 0)) : (l1(e, u, 16, t, 0), k = m), i && L2(e, u, i, o, 8, 0), n3.push(v(e, u, l, c, s, { type: "ImportSpecifier", local: k, imported: m })), e.token !== 1074790415 && q(e, u, 18);
}
return q(e, u, 1074790415), n3;
}
function gu(e, u, i, n3, t) {
let o = bu(e, u, v(e, u, i, n3, t, { type: "Identifier", name: "import" }), i, n3, t);
return o = H(e, u, o, 0, 0, i, n3, t), o = Q(e, u, 0, 0, i, n3, t, o), X2(e, u, o, i, n3, t);
}
function hu(e, u, i, n3, t) {
let o = ku(e, u, 0, i, n3, t);
return o = H(e, u, o, 0, 0, i, n3, t), X2(e, u, o, i, n3, t);
}
function bt(e, u, i) {
let n3 = e.tokenPos, t = e.linePos, o = e.colPos;
E(e, u | 32768);
let l = [], c = null, s = null, m;
if (M(e, u | 32768, 20563)) {
switch (e.token) {
case 86106: {
c = I2(e, u, i, 4, 1, 1, 0, e.tokenPos, e.linePos, e.colPos);
break;
}
case 133:
case 86096:
c = x1(e, u, i, 1, e.tokenPos, e.linePos, e.colPos);
break;
case 209007:
let { tokenPos: k, linePos: h, colPos: d } = e;
c = $(e, u, 0);
let { flags: y } = e;
(y & 1) < 1 && (e.token === 86106 ? c = I2(e, u, i, 4, 1, 1, 1, k, h, d) : e.token === 67174411 ? (c = G1(e, u, c, 1, 1, 0, y, k, h, d), c = H(e, u, c, 0, 0, k, h, d), c = Q(e, u, 0, 0, k, h, d, c)) : e.token & 143360 && (i && (i = c1(e, u, e.tokenValue)), c = $(e, u, 0), c = e1(e, u, i, [c], 1, k, h, d)));
break;
default:
c = K(e, u, 1, 0, 0, e.tokenPos, e.linePos, e.colPos), s2(e, u | 32768);
}
return i && M2(e, "default"), v(e, u, n3, t, o, { type: "ExportDefaultDeclaration", declaration: c });
}
switch (e.token) {
case 8457014: {
E(e, u);
let y = null;
return M(e, u, 77934) && (i && M2(e, e.tokenValue), y = $(e, u, 0)), q(e, u, 12404), e.token !== 134283267 && f(e, 102, "Export"), s = c2(e, u), s2(e, u | 32768), v(e, u, n3, t, o, { type: "ExportAllDeclaration", source: s, exported: y });
}
case 2162700: {
E(e, u);
let y = [], w = [];
for (; e.token & 143360; ) {
let { tokenPos: D, tokenValue: F, linePos: O, colPos: N } = e, x = $(e, u, 0), I;
e.token === 77934 ? (E(e, u), (e.token & 134217728) === 134217728 && f(e, 103), i && (y.push(e.tokenValue), w.push(F)), I = $(e, u, 0)) : (i && (y.push(e.tokenValue), w.push(e.tokenValue)), I = x), l.push(v(e, u, D, O, N, { type: "ExportSpecifier", local: x, exported: I })), e.token !== 1074790415 && q(e, u, 18);
}
if (q(e, u, 1074790415), M(e, u, 12404))
e.token !== 134283267 && f(e, 102, "Export"), s = c2(e, u);
else if (i) {
let D = 0, F = y.length;
for (; D < F; D++)
M2(e, y[D]);
for (D = 0, F = w.length; D < F; D++)
X0(e, w[D]);
}
s2(e, u | 32768);
break;
}
case 86096:
c = x1(e, u, i, 2, e.tokenPos, e.linePos, e.colPos);
break;
case 86106:
c = I2(e, u, i, 4, 1, 2, 0, e.tokenPos, e.linePos, e.colPos);
break;
case 241739:
c = W1(e, u, i, 8, 64, e.tokenPos, e.linePos, e.colPos);
break;
case 86092:
c = W1(e, u, i, 16, 64, e.tokenPos, e.linePos, e.colPos);
break;
case 86090:
c = fu(e, u, i, 64, e.tokenPos, e.linePos, e.colPos);
break;
case 209007:
let { tokenPos: k, linePos: h, colPos: d } = e;
if (E(e, u), (e.flags & 1) < 1 && e.token === 86106) {
c = I2(e, u, i, 4, 1, 2, 1, k, h, d), i && (m = c.id ? c.id.name : "", M2(e, m));
break;
}
default:
f(e, 28, Z[e.token & 255]);
}
return v(e, u, n3, t, o, { type: "ExportNamedDeclaration", declaration: c, specifiers: l, source: s });
}
function K(e, u, i, n3, t, o, l, c) {
let s = d2(e, u, 2, 0, i, n3, t, 1, o, l, c);
return s = H(e, u, s, t, 0, o, l, c), Q(e, u, t, 0, o, l, c, s);
}
function O2(e, u, i, n3, t, o, l) {
let c = [l];
for (; M(e, u | 32768, 18); )
c.push(K(e, u, 1, 0, i, e.tokenPos, e.linePos, e.colPos));
return v(e, u, n3, t, o, { type: "SequenceExpression", expressions: c });
}
function o2(e, u, i, n3, t, o, l) {
let c = K(e, u, n3, 0, i, t, o, l);
return e.token === 18 ? O2(e, u, i, t, o, l, c) : c;
}
function Q(e, u, i, n3, t, o, l, c) {
let { token: s } = e;
if ((s & 4194304) === 4194304) {
e.assignable & 2 && f(e, 24), (!n3 && s === 1077936157 && c.type === "ArrayExpression" || c.type === "ObjectExpression") && r2(e, c), E(e, u | 32768);
let m = K(e, u, 1, 1, i, e.tokenPos, e.linePos, e.colPos);
return e.assignable = 2, v(e, u, t, o, l, n3 ? { type: "AssignmentPattern", left: c, right: m } : { type: "AssignmentExpression", left: c, operator: Z[s & 255], right: m });
}
return (s & 8454144) === 8454144 && (c = T2(e, u, i, t, o, l, 4, s, c)), M(e, u | 32768, 22) && (c = U2(e, u, c, t, o, l)), c;
}
function a1(e, u, i, n3, t, o, l, c) {
let { token: s } = e;
E(e, u | 32768);
let m = K(e, u, 1, 1, i, e.tokenPos, e.linePos, e.colPos);
return c = v(e, u, t, o, l, n3 ? { type: "AssignmentPattern", left: c, right: m } : { type: "AssignmentExpression", left: c, operator: Z[s & 255], right: m }), e.assignable = 2, c;
}
function U2(e, u, i, n3, t, o) {
let l = K(e, (u | 134217728) ^ 134217728, 1, 0, 0, e.tokenPos, e.linePos, e.colPos);
q(e, u | 32768, 21), e.assignable = 1;
let c = K(e, u, 1, 0, 0, e.tokenPos, e.linePos, e.colPos);
return e.assignable = 2, v(e, u, n3, t, o, { type: "ConditionalExpression", test: i, consequent: l, alternate: c });
}
function T2(e, u, i, n3, t, o, l, c, s) {
let m = -((u & 134217728) > 0) & 8738868, k, h;
for (e.assignable = 2; e.token & 8454144 && (k = e.token, h = k & 3840, (k & 524288 && c & 268435456 || c & 524288 && k & 268435456) && f(e, 159), !(h + ((k === 8457273) << 8) - ((m === k) << 12) <= l)); )
E(e, u | 32768), s = v(e, u, n3, t, o, { type: k & 524288 || k & 268435456 ? "LogicalExpression" : "BinaryExpression", left: s, right: T2(e, u, i, e.tokenPos, e.linePos, e.colPos, h, k, h2(e, u, 0, i, 1, e.tokenPos, e.linePos, e.colPos)), operator: Z[k & 255] });
return e.token === 1077936157 && f(e, 24), s;
}
function kt(e, u, i, n3, t, o, l) {
i || f(e, 0);
let c = e.token;
E(e, u | 32768);
let s = h2(e, u, 0, l, 1, e.tokenPos, e.linePos, e.colPos);
return e.token === 8457273 && f(e, 31), u & 1024 && c === 16863278 && (s.type === "Identifier" ? f(e, 117) : $0(s) && f(e, 123)), e.assignable = 2, v(e, u, n3, t, o, { type: "UnaryExpression", operator: Z[c & 255], argument: s, prefix: true });
}
function rt(e, u, i, n3, t, o, l, c, s, m) {
let { token: k } = e, h = $(e, u, o), { flags: d } = e;
if ((d & 1) < 1) {
if (e.token === 86106)
return vu(e, u, 1, i, c, s, m);
if ((e.token & 143360) === 143360)
return n3 || f(e, 0), Pu(e, u, t, c, s, m);
}
return !l && e.token === 67174411 ? G1(e, u, h, t, 1, 0, d, c, s, m) : e.token === 10 ? ($1(e, u, k, 1), l && f(e, 48), h1(e, u, e.tokenValue, h, l, t, 0, c, s, m)) : h;
}
function vt(e, u, i, n3, t, o, l) {
if (i && (e.destructible |= 256), u & 2097152) {
E(e, u | 32768), u & 8388608 && f(e, 30), n3 || f(e, 24), e.token === 22 && f(e, 120);
let c = null, s = false;
return (e.flags & 1) < 1 && (s = M(e, u | 32768, 8457014), (e.token & 77824 || s) && (c = K(e, u, 1, 0, 0, e.tokenPos, e.linePos, e.colPos))), e.assignable = 2, v(e, u, t, o, l, { type: "YieldExpression", argument: c, delegate: s });
}
return u & 1024 && f(e, 94, "yield"), Q1(e, u, t, o, l);
}
function yt(e, u, i, n3, t, o, l) {
if (n3 && (e.destructible |= 128), u & 4194304 || u & 2048 && u & 8192) {
i && f(e, 0), u & 8388608 && L(e.index, e.line, e.index, 29), E(e, u | 32768);
let c = h2(e, u, 0, 0, 1, e.tokenPos, e.linePos, e.colPos);
return e.token === 8457273 && f(e, 31), e.assignable = 2, v(e, u, t, o, l, { type: "AwaitExpression", argument: c });
}
return u & 2048 && f(e, 95), Q1(e, u, t, o, l);
}
function d1(e, u, i, n3, t, o) {
let { tokenPos: l, linePos: c, colPos: s } = e;
q(e, u | 32768, 2162700);
let m = [], k = u;
if (e.token !== 1074790415) {
for (; e.token === 134283267; ) {
let { index: h, tokenPos: d, tokenValue: y, token: w } = e, D = c2(e, u);
eu(e, h, d, y) && (u |= 1024, e.flags & 128 && L(e.index, e.line, e.tokenPos, 63), e.flags & 64 && L(e.index, e.line, e.tokenPos, 8)), m.push(z1(e, u, D, w, d, e.linePos, e.colPos));
}
u & 1024 && (t && ((t & 537079808) === 537079808 && f(e, 115), (t & 36864) === 36864 && f(e, 38)), e.flags & 512 && f(e, 115), e.flags & 256 && f(e, 114)), u & 64 && i && o !== void 0 && (k & 1024) < 1 && (u & 8192) < 1 && A(o);
}
for (e.flags = (e.flags | 512 | 256 | 64) ^ 832, e.destructible = (e.destructible | 256) ^ 256; e.token !== 1074790415; )
m.push(G2(e, u, i, 4, {}));
return q(e, n3 & 24 ? u | 32768 : u, 1074790415), e.flags &= -193, e.token === 1077936157 && f(e, 24), v(e, u, l, c, s, { type: "BlockStatement", body: m });
}
function At(e, u, i, n3, t) {
switch (E(e, u), e.token) {
case 67108991:
f(e, 161);
case 67174411: {
(u & 524288) < 1 && f(e, 26), u & 16384 && f(e, 27), e.assignable = 2;
break;
}
case 69271571:
case 67108877: {
(u & 262144) < 1 && f(e, 27), u & 16384 && f(e, 27), e.assignable = 1;
break;
}
default:
f(e, 28, "super");
}
return v(e, u, i, n3, t, { type: "Super" });
}
function h2(e, u, i, n3, t, o, l, c) {
let s = d2(e, u, 2, 0, i, 0, n3, t, o, l, c);
return H(e, u, s, n3, 0, o, l, c);
}
function Pt(e, u, i, n3, t, o) {
e.assignable & 2 && f(e, 52);
let { token: l } = e;
return E(e, u), e.assignable = 2, v(e, u, n3, t, o, { type: "UpdateExpression", argument: i, operator: Z[l & 255], prefix: false });
}
function H(e, u, i, n3, t, o, l, c) {
if ((e.token & 33619968) === 33619968 && (e.flags & 1) < 1)
i = Pt(e, u, i, o, l, c);
else if ((e.token & 67108864) === 67108864) {
switch (u = (u | 134217728) ^ 134217728, e.token) {
case 67108877: {
E(e, (u | 1073741824 | 8192) ^ 8192), e.assignable = 1;
let s = mu(e, u);
i = v(e, u, o, l, c, { type: "MemberExpression", object: i, computed: false, property: s });
break;
}
case 69271571: {
let s = false;
(e.flags & 2048) === 2048 && (s = true, e.flags = (e.flags | 2048) ^ 2048), E(e, u | 32768);
let { tokenPos: m, linePos: k, colPos: h } = e, d = o2(e, u, n3, 1, m, k, h);
q(e, u, 20), e.assignable = 1, i = v(e, u, o, l, c, { type: "MemberExpression", object: i, computed: true, property: d }), s && (e.flags |= 2048);
break;
}
case 67174411: {
if ((e.flags & 1024) === 1024)
return e.flags = (e.flags | 1024) ^ 1024, i;
let s = false;
(e.flags & 2048) === 2048 && (s = true, e.flags = (e.flags | 2048) ^ 2048);
let m = Z1(e, u, n3);
e.assignable = 2, i = v(e, u, o, l, c, { type: "CallExpression", callee: i, arguments: m }), s && (e.flags |= 2048);
break;
}
case 67108991: {
E(e, (u | 1073741824 | 8192) ^ 8192), e.flags |= 2048, e.assignable = 2, i = Et(e, u, i, o, l, c);
break;
}
default:
(e.flags & 2048) === 2048 && f(e, 160), e.assignable = 2, i = v(e, u, o, l, c, { type: "TaggedTemplateExpression", tag: i, quasi: e.token === 67174408 ? Y1(e, u | 65536) : K1(e, u, e.tokenPos, e.linePos, e.colPos) });
}
i = H(e, u, i, 0, 1, o, l, c);
}
return t === 0 && (e.flags & 2048) === 2048 && (e.flags = (e.flags | 2048) ^ 2048, i = v(e, u, o, l, c, { type: "ChainExpression", expression: i })), i;
}
function Et(e, u, i, n3, t, o) {
let l = false, c;
if ((e.token === 69271571 || e.token === 67174411) && (e.flags & 2048) === 2048 && (l = true, e.flags = (e.flags | 2048) ^ 2048), e.token === 69271571) {
E(e, u | 32768);
let { tokenPos: s, linePos: m, colPos: k } = e, h = o2(e, u, 0, 1, s, m, k);
q(e, u, 20), e.assignable = 2, c = v(e, u, n3, t, o, { type: "MemberExpression", object: i, computed: true, optional: true, property: h });
} else if (e.token === 67174411) {
let s = Z1(e, u, 0);
e.assignable = 2, c = v(e, u, n3, t, o, { type: "CallExpression", callee: i, arguments: s, optional: true });
} else {
(e.token & 143360) < 1 && f(e, 154);
let s = $(e, u, 0);
e.assignable = 2, c = v(e, u, n3, t, o, { type: "MemberExpression", object: i, computed: false, optional: true, property: s });
}
return l && (e.flags |= 2048), c;
}
function mu(e, u) {
return (e.token & 143360) < 1 && e.token !== 131 && f(e, 154), u & 1 && e.token === 131 ? r1(e, u, e.tokenPos, e.linePos, e.colPos) : $(e, u, 0);
}
function Ct(e, u, i, n3, t, o, l) {
i && f(e, 53), n3 || f(e, 0);
let { token: c } = e;
E(e, u | 32768);
let s = h2(e, u, 0, 0, 1, e.tokenPos, e.linePos, e.colPos);
return e.assignable & 2 && f(e, 52), e.assignable = 2, v(e, u, t, o, l, { type: "UpdateExpression", argument: s, operator: Z[c & 255], prefix: true });
}
function d2(e, u, i, n3, t, o, l, c, s, m, k) {
if ((e.token & 143360) === 143360) {
switch (e.token) {
case 209008:
return yt(e, u, n3, l, s, m, k);
case 241773:
return vt(e, u, l, t, s, m, k);
case 209007:
return rt(e, u, l, c, t, o, n3, s, m, k);
}
let { token: h, tokenValue: d } = e, y = $(e, u | 65536, o);
return e.token === 10 ? (c || f(e, 0), $1(e, u, h, 1), h1(e, u, d, y, n3, t, 0, s, m, k)) : (u & 16384 && h === 537079928 && f(e, 126), h === 241739 && (u & 1024 && f(e, 109), i & 24 && f(e, 97)), e.assignable = u & 1024 && (h & 537079808) === 537079808 ? 2 : 1, y);
}
if ((e.token & 134217728) === 134217728)
return c2(e, u);
switch (e.token) {
case 33619995:
case 33619996:
return Ct(e, u, n3, c, s, m, k);
case 16863278:
case 16842800:
case 16842801:
case 25233970:
case 25233971:
case 16863277:
case 16863279:
return kt(e, u, c, s, m, k, l);
case 86106:
return vu(e, u, 0, l, s, m, k);
case 2162700:
return Ft(e, u, t ? 0 : 1, l, s, m, k);
case 69271571:
return St(e, u, t ? 0 : 1, l, s, m, k);
case 67174411:
return Ot(e, u, t, 1, 0, s, m, k);
case 86021:
case 86022:
case 86023:
return qt(e, u, s, m, k);
case 86113:
return Bt(e, u);
case 65540:
return Rt(e, u, s, m, k);
case 133:
case 86096:
return Vt(e, u, l, s, m, k);
case 86111:
return At(e, u, s, m, k);
case 67174409:
return K1(e, u, s, m, k);
case 67174408:
return Y1(e, u);
case 86109:
return Tt(e, u, l, s, m, k);
case 134283389:
return ru(e, u, s, m, k);
case 131:
return r1(e, u, s, m, k);
case 86108:
return Dt(e, u, n3, l, s, m, k);
case 8456258:
if (u & 16)
return ee(e, u, 1, s, m, k);
default:
if (J1(u, e.token))
return Q1(e, u, s, m, k);
f(e, 28, Z[e.token & 255]);
}
}
function Dt(e, u, i, n3, t, o, l) {
let c = $(e, u, 0);
return e.token === 67108877 ? bu(e, u, c, t, o, l) : (i && f(e, 137), c = ku(e, u, n3, t, o, l), e.assignable = 2, H(e, u, c, n3, 0, t, o, l));
}
function bu(e, u, i, n3, t, o) {
return u & 2048 || f(e, 163), E(e, u), e.token !== 143495 && e.tokenValue !== "meta" && f(e, 28, Z[e.token & 255]), e.assignable = 2, v(e, u, n3, t, o, { type: "MetaProperty", meta: i, property: $(e, u, 0) });
}
function ku(e, u, i, n3, t, o) {
q(e, u | 32768, 67174411), e.token === 14 && f(e, 138);
let l = K(e, u, 1, 0, i, e.tokenPos, e.linePos, e.colPos);
return q(e, u, 16), v(e, u, n3, t, o, { type: "ImportExpression", source: l });
}
function ru(e, u, i, n3, t) {
let { tokenRaw: o, tokenValue: l } = e;
return E(e, u), e.assignable = 2, v(e, u, i, n3, t, u & 512 ? { type: "Literal", value: l, bigint: o.slice(0, -1), raw: o } : { type: "Literal", value: l, bigint: o.slice(0, -1) });
}
function K1(e, u, i, n3, t) {
e.assignable = 2;
let { tokenValue: o, tokenRaw: l, tokenPos: c, linePos: s, colPos: m } = e;
q(e, u, 67174409);
let k = [g1(e, u, o, l, c, s, m, true)];
return v(e, u, i, n3, t, { type: "TemplateLiteral", expressions: [], quasis: k });
}
function Y1(e, u) {
u = (u | 134217728) ^ 134217728;
let { tokenValue: i, tokenRaw: n3, tokenPos: t, linePos: o, colPos: l } = e;
q(e, u | 32768, 67174408);
let c = [g1(e, u, i, n3, t, o, l, false)], s = [o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos)];
for (e.token !== 1074790415 && f(e, 80); (e.token = I0(e, u)) !== 67174409; ) {
let { tokenValue: m, tokenRaw: k, tokenPos: h, linePos: d, colPos: y } = e;
q(e, u | 32768, 67174408), c.push(g1(e, u, m, k, h, d, y, false)), s.push(o2(e, u, 0, 1, e.tokenPos, e.linePos, e.colPos)), e.token !== 1074790415 && f(e, 80);
}
{
let { tokenValue: m, tokenRaw: k, tokenPos: h, linePos: d, colPos: y } = e;
q(e, u, 67174409), c.push(g1(e, u, m, k, h, d, y, true));
}
return v(e, u, t, o, l, { type: "TemplateLiteral", expressions: s, quasis: c });
}
function g1(e, u, i, n3, t, o, l, c) {
let s = v(e, u, t, o, l, { type: "TemplateElement", value: { cooked: i, raw: n3 }, tail: c }), m = c ? 1 : 2;
return u & 2 && (s.start += 1, s.range[0] += 1, s.end -= m, s.range[1] -= m), u & 4 && (s.loc.start.column += 1, s.loc.end.column -= m), s;
}
function wt(e, u, i, n3, t) {
u = (u | 134217728) ^ 134217728, q(e, u | 32768, 14);
let o = K(e, u, 1, 0, 0, e.tokenPos, e.linePos, e.colPos);
return e.assignable = 1, v(e, u, i, n3, t, { type: "SpreadElement", argument: o });
}
function Z1(e, u, i) {
E(e, u | 32768);
let n3 = [];
if (e.token === 16)
return E(e, u), n3;
for (; e.token !== 16 && (e.token === 14 ? n3.push(wt(e, u, e.tokenPos, e.linePos, e.colPos)) : n3.push(K(e, u, 1, 0, i, e.tokenPos, e.linePos, e.colPos)), !(e.token !== 18 || (E(e, u | 32768), e.token === 16))); )
;
return q(e, u, 16), n3;
}
function $(e, u, i) {
let { tokenValue: n3, tokenPos: t, linePos: o, colPos: l } = e;
return E(e, u), v(e, u, t, o, l, u & 268435456 ? { type: "Identifier", name: n3, pattern: i === 1 } : { type: "Identifier", name: n3 });
}
function c2(e, u) {
let { tokenValue: i, tokenRaw: n3, tokenPos: t, linePos: o, colPos: l } = e;
return e.token === 134283389 ? ru(e, u, t, o, l) : (E(e, u), e.assignable = 2, v(e, u, t, o, l, u & 512 ? { type: "Literal", value: i, raw: n3 } : { type: "Literal", value: i }));
}
function qt(e, u, i, n3, t) {
let o = Z[e.token & 255], l = e.token === 86023 ? null : o === "true";
return E(e, u), e.assignable = 2, v(e, u, i, n3, t, u & 512 ? { type: "Literal", value: l, raw: o } : { type: "Literal", value: l });
}
function Bt(e, u) {
let { tokenPos: i, linePos: n3, colPos: t } = e;
return E(e, u), e.assignable = 2, v(e, u, i, n3, t, { type: "ThisExpression" });
}
function I2(e, u, i, n3, t, o, l, c, s, m) {
E(e, u | 32768);
let k = t ? M1(e, u, 8457014) : 0, h = null, d, y = i ? _2() : void 0;
if (e.token === 67174411)
(o & 1) < 1 && f(e, 37, "Function");
else {
let F = n3 & 4 && ((u & 8192) < 1 || (u & 2048) < 1) ? 4 : 64;
uu(e, u | (u & 3072) << 11, e.token), i && (F & 4 ? tu(e, u, i, e.tokenValue, F) : L2(e, u, i, e.tokenValue, F, n3), y = i2(y, 256), o && o & 2 && M2(e, e.tokenValue)), d = e.token, e.token & 143360 ? h = $(e, u, 0) : f(e, 28, Z[e.token & 255]);
}
u = (u | 32243712) ^ 32243712 | 67108864 | l * 2 + k << 21 | (k ? 0 : 1073741824), i && (y = i2(y, 512));
let w = Au(e, u | 8388608, y, 0, 1), D = d1(e, (u | 8192 | 4096 | 131072) ^ 143360, i ? i2(y, 128) : y, 8, d, i ? y.scopeError : void 0);
return v(e, u, c, s, m, { type: "FunctionDeclaration", id: h, params: w, body: D, async: l === 1, generator: k === 1 });
}
function vu(e, u, i, n3, t, o, l) {
E(e, u | 32768);
let c = M1(e, u, 8457014), s = i * 2 + c << 21, m = null, k, h = u & 64 ? _2() : void 0;
(e.token & 176128) > 0 && (uu(e, (u | 32243712) ^ 32243712 | s, e.token), h && (h = i2(h, 256)), k = e.token, m = $(e, u, 0)), u = (u | 32243712) ^ 32243712 | 67108864 | s | (c ? 0 : 1073741824), h && (h = i2(h, 512));
let d = Au(e, u | 8388608, h, n3, 1), y = d1(e, u & -134377473, h && i2(h, 128), 0, k, void 0);
return e.assignable = 2, v(e, u, t, o, l, { type: "FunctionExpression", id: m, params: d, body: y, async: i === 1, generator: c === 1 });
}
function St(e, u, i, n3, t, o, l) {
let c = m2(e, u, void 0, i, n3, 0, 2, 0, t, o, l);
return u & 256 && e.destructible & 64 && f(e, 60), e.destructible & 8 && f(e, 59), c;
}
function m2(e, u, i, n3, t, o, l, c, s, m, k) {
E(e, u | 32768);
let h = [], d = 0;
for (u = (u | 134217728) ^ 134217728; e.token !== 20; )
if (M(e, u | 32768, 18))
h.push(null);
else {
let w, { token: D, tokenPos: F, linePos: O, colPos: N, tokenValue: x } = e;
if (D & 143360)
if (w = d2(e, u, l, 0, 1, 0, t, 1, F, O, N), e.token === 1077936157) {
e.assignable & 2 && f(e, 24), E(e, u | 32768), i && B2(e, u, i, x, l, c);
let I = K(e, u, 1, 1, t, e.tokenPos, e.linePos, e.colPos);
w = v(e, u, F, O, N, o ? { type: "AssignmentPattern", left: w, right: I } : { type: "AssignmentExpression", operator: "=", left: w, right: I }), d |= e.destructible & 256 ? 256 : 0 | e.destructible & 128 ? 128 : 0;
} else
e.token === 18 || e.token === 20 ? (e.assignable & 2 ? d |= 16 : i && B2(e, u, i, x, l, c), d |= e.destructible & 256 ? 256 : 0 | e.destructible & 128 ? 128 : 0) : (d |= l & 1 ? 32 : (l & 2) < 1 ? 16 : 0, w = H(e, u, w, t, 0, F, O, N), e.token !== 18 && e.token !== 20 ? (e.token !== 1077936157 && (d |= 16), w = Q(e, u, t, o, F, O, N, w)) : e.token !== 1077936157 && (d |= e.assignable & 2 ? 16 : 32));
else
D & 2097152 ? (w = e.token === 2162700 ? b2(e, u, i, 0, t, o, l, c, F, O, N) : m2(e, u, i, 0, t, o, l, c, F, O, N), d |= e.destructible, e.assignable = e.destructible & 16 ? 2 : 1, e.token === 18 || e.token === 20 ? e.assignable & 2 && (d |= 16) : e.destructible & 8 ? f(e, 68) : (w = H(e, u, w, t, 0, F, O, N), d = e.assignable & 2 ? 16 : 0, e.token !== 18 && e.token !== 20 ? w = Q(e, u, t, o, F, O, N, w) : e.token !== 1077936157 && (d |= e.assignable & 2 ? 16 : 32))) : D === 14 ? (w = W2(e, u, i, 20, l, c, 0, t, o, F, O, N), d |= e.destructible, e.token !== 18 && e.token !== 20 && f(e, 28, Z[e.token & 255])) : (w = h2(e, u, 1, 0, 1, F, O, N), e.token !== 18 && e.token !== 20 ? (w = Q(e, u, t, o, F, O, N, w), (l & 3) < 1 && D === 67174411 && (d |= 16)) : e.assignable & 2 ? d |= 16 : D === 67174411 && (d |= e.assignable & 1 && l & 3 ? 32 : 16));
if (h.push(w), M(e, u | 32768, 18)) {
if (e.token === 20)
break;
} else
break;
}
q(e, u, 20);
let y = v(e, u, s, m, k, { type: o ? "ArrayPattern" : "ArrayExpression", elements: h });
return !n3 && e.token & 4194304 ? yu(e, u, d, t, o, s, m, k, y) : (e.destructible = d, y);
}
function yu(e, u, i, n3, t, o, l, c, s) {
e.token !== 1077936157 && f(e, 24), E(e, u | 32768), i & 16 && f(e, 24), t || r2(e, s);
let { tokenPos: m, linePos: k, colPos: h } = e, d = K(e, u, 1, 1, n3, m, k, h);
return e.destructible = (i | 64 | 8) ^ 72 | (e.destructible & 128 ? 128 : 0) | (e.destructible & 256 ? 256 : 0), v(e, u, o, l, c, t ? { type: "AssignmentPattern", left: s, right: d } : { type: "AssignmentExpression", left: s, operator: "=", right: d });
}
function W2(e, u, i, n3, t, o, l, c, s, m, k, h) {
E(e, u | 32768);
let d = null, y = 0, { token: w, tokenValue: D, tokenPos: F, linePos: O, colPos: N } = e;
if (w & 143360)
e.assignable = 1, d = d2(e, u, t, 0, 1, 0, c, 1, F, O, N), w = e.token, d = H(e, u, d, c, 0, F, O, N), e.token !== 18 && e.token !== n3 && (e.assignable & 2 && e.token === 1077936157 && f(e, 68), y |= 16, d = Q(e, u, c, s, F, O, N, d)), e.assignable & 2 ? y |= 16 : w === n3 || w === 18 ? i && B2(e, u, i, D, t, o) : y |= 32, y |= e.destructible & 128 ? 128 : 0;
else if (w === n3)
f(e, 39);
else if (w & 2097152)
d = e.token === 2162700 ? b2(e, u, i, 1, c, s, t, o, F, O, N) : m2(e, u, i, 1, c, s, t, o, F, O, N), w = e.token, w !== 1077936157 && w !== n3 && w !== 18 ? (e.destructible & 8 && f(e, 68), d = H(e, u, d, c, 0, F, O, N), y |= e.assignable & 2 ? 16 : 0, (e.token & 4194304) === 4194304 ? (e.token !== 1077936157 && (y |= 16), d = Q(e, u, c, s, F, O, N, d)) : ((e.token & 8454144) === 8454144 && (d = T2(e, u, 1, F, O, N, 4, w, d)), M(e, u | 32768, 22) && (d = U2(e, u, d, F, O, N)), y |= e.assignable & 2 ? 16 : 32)) : y |= n3 === 1074790415 && w !== 1077936157 ? 16 : e.destructible;
else {
y |= 32, d = h2(e, u, 1, c, 1, e.tokenPos, e.linePos, e.colPos);
let { token: x, tokenPos: I, linePos: W, colPos: P } = e;
return x === 1077936157 && x !== n3 && x !== 18 ? (e.assignable & 2 && f(e, 24), d = Q(e, u, c, s, I, W, P, d), y |= 16) : (x === 18 ? y |= 16 : x !== n3 && (d = Q(e, u, c, s, I, W, P, d)), y |= e.assignable & 1 ? 32 : 16), e.destructible = y, e.token !== n3 && e.token !== 18 && f(e, 155), v(e, u, m, k, h, { type: s ? "RestElement" : "SpreadElement", argument: d });
}
if (e.token !== n3)
if (t & 1 && (y |= l ? 16 : 32), M(e, u | 32768, 1077936157)) {
y & 16 && f(e, 24), r2(e, d);
let x = K(e, u, 1, 1, c, e.tokenPos, e.linePos, e.colPos);
d = v(e, u, F, O, N, s ? { type: "AssignmentPattern", left: d, right: x } : { type: "AssignmentExpression", left: d, operator: "=", right: x }), y = 16;
} else
y |= 16;
return e.destructible = y, v(e, u, m, k, h, { type: s ? "RestElement" : "SpreadElement", argument: d });
}
function v2(e, u, i, n3, t, o, l) {
let c = (i & 64) < 1 ? 31981568 : 14680064;
u = (u | c) ^ c | (i & 88) << 18 | 100925440;
let s = u & 64 ? i2(_2(), 512) : void 0, m = Lt(e, u | 8388608, s, i, 1, n3);
s && (s = i2(s, 128));
let k = d1(e, u & -134230017, s, 0, void 0, void 0);
return v(e, u, t, o, l, { type: "FunctionExpression", params: m, body: k, async: (i & 16) > 0, generator: (i & 8) > 0, id: null });
}
function Ft(e, u, i, n3, t, o, l) {
let c = b2(e, u, void 0, i, n3, 0, 2, 0, t, o, l);
return u & 256 && e.destructible & 64 && f(e, 60), e.destructible & 8 && f(e, 59), c;
}
function b2(e, u, i, n3, t, o, l, c, s, m, k) {
E(e, u);
let h = [], d = 0, y = 0;
for (u = (u | 134217728) ^ 134217728; e.token !== 1074790415; ) {
let { token: D, tokenValue: F, linePos: O, colPos: N, tokenPos: x } = e;
if (D === 14)
h.push(W2(e, u, i, 1074790415, l, c, 0, t, o, x, O, N));
else {
let I = 0, W = null, P, y2 = e.token;
if (e.token & 143360 || e.token === 121)
if (W = $(e, u, 0), e.token === 18 || e.token === 1074790415 || e.token === 1077936157)
if (I |= 4, u & 1024 && (D & 537079808) === 537079808 ? d |= 16 : l1(e, u, l, D, 0), i && B2(e, u, i, F, l, c), M(e, u | 32768, 1077936157)) {
d |= 8;
let R = K(e, u, 1, 1, t, e.tokenPos, e.linePos, e.colPos);
d |= e.destructible & 256 ? 256 : 0 | e.destructible & 128 ? 128 : 0, P = v(e, u, x, O, N, { type: "AssignmentPattern", left: u & -2147483648 ? Object.assign({}, W) : W, right: R });
} else
d |= (D === 209008 ? 128 : 0) | (D === 121 ? 16 : 0), P = u & -2147483648 ? Object.assign({}, W) : W;
else if (M(e, u | 32768, 21)) {
let { tokenPos: R, linePos: _, colPos: j } = e;
if (F === "__proto__" && y++, e.token & 143360) {
let J2 = e.token, Y2 = e.tokenValue;
d |= y2 === 121 ? 16 : 0, P = d2(e, u, l, 0, 1, 0, t, 1, R, _, j);
let { token: C2 } = e;
P = H(e, u, P, t, 0, R, _, j), e.token === 18 || e.token === 1074790415 ? C2 === 1077936157 || C2 === 1074790415 || C2 === 18 ? (d |= e.destructible & 128 ? 128 : 0, e.assignable & 2 ? d |= 16 : i && (J2 & 143360) === 143360 && B2(e, u, i, Y2, l, c)) : d |= e.assignable & 1 ? 32 : 16 : (e.token & 4194304) === 4194304 ? (e.assignable & 2 ? d |= 16 : C2 !== 1077936157 ? d |= 32 : i && B2(e, u, i, Y2, l, c), P = Q(e, u, t, o, R, _, j, P)) : (d |= 16, (e.token & 8454144) === 8454144 && (P = T2(e, u, 1, R, _, j, 4, C2, P)), M(e, u | 32768, 22) && (P = U2(e, u, P, R, _, j)));
} else
(e.token & 2097152) === 2097152 ? (P = e.token === 69271571 ? m2(e, u, i, 0, t, o, l, c, R, _, j) : b2(e, u, i, 0, t, o, l, c, R, _, j), d = e.destructible, e.assignable = d & 16 ? 2 : 1, e.token === 18 || e.token === 1074790415 ? e.assignable & 2 && (d |= 16) : e.destructible & 8 ? f(e, 68) : (P = H(e, u, P, t, 0, R, _, j), d = e.assignable & 2 ? 16 : 0, (e.token & 4194304) === 4194304 ? P = a1(e, u, t, o, R, _, j, P) : ((e.token & 8454144) === 8454144 && (P = T2(e, u, 1, R, _, j, 4, D, P)), M(e, u | 32768, 22) && (P = U2(e, u, P, R, _, j)), d |= e.assignable & 2 ? 16 : 32))) : (P = h2(e, u, 1, t, 1, R, _, j), d |= e.assignable & 1 ? 32 : 16, e.token === 18 || e.token === 1074790415 ? e.assignable & 2 && (d |= 16) : (P = H(e, u, P, t, 0, R, _, j), d = e.assignable & 2 ? 16 : 0, e.token !== 18 && D !== 1074790415 && (e.token !== 1077936157 && (d |= 16), P = Q(e, u, t, o, R, _, j, P))));
} else
e.token === 69271571 ? (d |= 16, D === 209007 && (I |= 16), I |= (D === 12402 ? 256 : D === 12403 ? 512 : 1) | 2, W = K2(e, u, t), d |= e.assignable, P = v2(e, u, I, t, e.tokenPos, e.linePos, e.colPos)) : e.token & 143360 ? (d |= 16, D === 121 && f(e, 92), D === 209007 && (e.flags & 1 && f(e, 128), I |= 16), W = $(e, u, 0), I |= D === 12402 ? 256 : D === 12403 ? 512 : 1, P = v2(e, u, I, t, e.tokenPos, e.linePos, e.colPos)) : e.token === 67174411 ? (d |= 16, I |= 1, P = v2(e, u, I, t, e.tokenPos, e.linePos, e.colPos)) : e.token === 8457014 ? (d |= 16, D === 12402 || D === 12403 ? f(e, 40) : D === 143483 && f(e, 92), E(e, u), I |= 9 | (D === 209007 ? 16 : 0), e.token & 143360 ? W = $(e, u, 0) : (e.token & 134217728) === 134217728 ? W = c2(e, u) : e.token === 69271571 ? (I |= 2, W = K2(e, u, t), d |= e.assignable) : f(e, 28, Z[e.token & 255]), P = v2(e, u, I, t, e.tokenPos, e.linePos, e.colPos)) : (e.token & 134217728) === 134217728 ? (D === 209007 && (I |= 16), I |= D === 12402 ? 256 : D === 12403 ? 512 : 1, d |= 16, W = c2(e, u), P = v2(e, u, I, t, e.tokenPos, e.linePos, e.colPos)) : f(e, 129);
else if ((e.token & 134217728) === 134217728)
if (W = c2(e, u), e.token === 21) {
q(e, u | 32768, 21);
let { tokenPos: R, linePos: _, colPos: j } = e;
if (F === "__proto__" && y++, e.token & 143360) {
P = d2(e, u, l, 0, 1, 0, t, 1, R, _, j);
let { token: J2, tokenValue: Y2 } = e;
P = H(e, u, P, t, 0, R, _, j), e.token === 18 || e.token === 1074790415 ? J2 === 1077936157 || J2 === 1074790415 || J2 === 18 ? e.assignable & 2 ? d |= 16 : i && B2(e, u, i, Y2, l, c) : d |= e.assignable & 1 ? 32 : 16 : e.token === 1077936157 ? (e.assignable & 2 && (d |= 16), P = Q(e, u, t, o, R, _, j, P)) : (d |= 16, P = Q(e, u, t, o, R, _, j, P));
} else
(e.token & 2097152) === 2097152 ? (P = e.token === 69271571 ? m2(e, u, i, 0, t, o, l, c, R, _, j) : b2(e, u, i, 0, t, o, l, c, R, _, j), d = e.destructible, e.assignable = d & 16 ? 2 : 1, e.token === 18 || e.token === 1074790415 ? e.assignable & 2 && (d |= 16) : (e.destructible & 8) !== 8 && (P = H(e, u, P, t, 0, R, _, j), d = e.assignable & 2 ? 16 : 0, (e.token & 4194304) === 4194304 ? P = a1(e, u, t, o, R, _, j, P) : ((e.token & 8454144) === 8454144 && (P = T2(e, u, 1, R, _, j, 4, D, P)), M(e, u | 32768, 22) && (P = U2(e, u, P, R, _, j)), d |= e.assignable & 2 ? 16 : 32))) : (P = h2(e, u, 1, 0, 1, R, _, j), d |= e.assignable & 1 ? 32 : 16, e.token === 18 || e.token === 1074790415 ? e.assignable & 2 && (d |= 16) : (P = H(e, u, P, t, 0, R, _, j), d = e.assignable & 1 ? 0 : 16, e.token !== 18 && e.token !== 1074790415 && (e.token !== 1077936157 && (d |= 16), P = Q(e, u, t, o, R, _, j, P))));
} else
e.token === 67174411 ? (I |= 1, P = v2(e, u, I, t, e.tokenPos, e.linePos, e.colPos), d = e.assignable | 16) : f(e, 130);
else if (e.token === 69271571)
if (W = K2(e, u, t), d |= e.destructible & 256 ? 256 : 0, I |= 2, e.token === 21) {
E(e, u | 32768);
let { tokenPos: R, linePos: _, colPos: j, tokenValue: J2, token: Y2 } = e;
if (e.token & 143360) {
P = d2(e, u, l, 0, 1, 0, t, 1, R, _, j);
let { token: C2 } = e;
P = H(e, u, P, t, 0, R, _, j), (e.token & 4194304) === 4194304 ? (d |= e.assignable & 2 ? 16 : C2 === 1077936157 ? 0 : 32, P = a1(e, u, t, o, R, _, j, P)) : e.token === 18 || e.token === 1074790415 ? C2 === 1077936157 || C2 === 1074790415 || C2 === 18 ? e.assignable & 2 ? d |= 16 : i && (Y2 & 143360) === 143360 && B2(e, u, i, J2, l, c) : d |= e.assignable & 1 ? 32 : 16 : (d |= 16, P = Q(e, u, t, o, R, _, j, P));
} else
(e.token & 2097152) === 2097152 ? (P = e.token === 69271571 ? m2(e, u, i, 0, t, o, l, c, R, _, j) : b2(e, u, i, 0, t, o, l, c, R, _, j), d = e.destructible, e.assignable = d & 16 ? 2 : 1, e.token === 18 || e.token === 1074790415 ? e.assignable & 2 && (d |= 16) : d & 8 ? f(e, 59) : (P = H(e, u, P, t, 0, R, _, j), d = e.assignable & 2 ? d | 16 : 0, (e.token & 4194304) === 4194304 ? (e.token !== 1077936157 && (d |= 16), P = a1(e, u, t, o, R, _, j, P)) : ((e.token & 8454144) === 8454144 && (P = T2(e, u, 1, R, _, j, 4, D, P)), M(e, u | 32768, 22) && (P = U2(e, u, P, R, _, j)), d |= e.assignable & 2 ? 16 : 32))) : (P = h2(e, u, 1, 0, 1, R, _, j), d |= e.assignable & 1 ? 32 : 16, e.token === 18 || e.token === 1074790415 ? e.assignable & 2 && (d |= 16) : (P = H(e, u, P, t, 0, R, _, j), d = e.assignable & 1 ? 0 : 16, e.token !== 18 && e.token !== 1074790415 && (e.token !== 1077936157 && (d |= 16), P = Q(e, u, t, o, R, _, j, P))));
} else
e.token === 67174411 ? (I |= 1, P = v2(e, u, I, t, e.tokenPos, O, N), d = 16) : f(e, 41);
else if (D === 8457014)
if (q(e, u | 32768, 8457014), I |= 8, e.token & 143360) {
let { token: R, line: _, index: j } = e;
W = $(e, u, 0), I |= 1, e.token === 67174411 ? (d |= 16, P = v2(e, u, I, t, e.tokenPos, e.linePos, e.colPos)) : L(j, _, j, R === 209007 ? 43 : R === 12402 || e.token === 12403 ? 42 : 44, Z[R & 255]);
} else
(e.token & 134217728) === 134217728 ? (d |= 16, W = c2(e, u), I |= 1, P = v2(e, u, I, t, x, O, N)) : e.token === 69271571 ? (d |= 16, I |= 3, W = K2(e, u, t), P = v2(e, u, I, t, e.tokenPos, e.linePos, e.colPos)) : f(e, 122);
else
f(e, 28, Z[D & 255]);
d |= e.destructible & 128 ? 128 : 0, e.destructible = d, h.push(v(e, u, x, O, N, { type: "Property", key: W, value: P, kind: I & 768 ? I & 512 ? "set" : "get" : "init", computed: (I & 2) > 0, method: (I & 1) > 0, shorthand: (I & 4) > 0 }));
}
if (d |= e.destructible, e.token !== 18)
break;
E(e, u);
}
q(e, u, 1074790415), y > 1 && (d |= 64);
let w = v(e, u, s, m, k, { type: o ? "ObjectPattern" : "ObjectExpression", properties: h });
return !n3 && e.token & 4194304 ? yu(e, u, d, t, o, s, m, k, w) : (e.destructible = d, w);
}
function Lt(e, u, i, n3, t, o) {
q(e, u, 67174411);
let l = [];
if (e.flags = (e.flags | 128) ^ 128, e.token === 16)
return n3 & 512 && f(e, 35, "Setter", "one", ""), E(e, u), l;
n3 & 256 && f(e, 35, "Getter", "no", "s"), n3 & 512 && e.token === 14 && f(e, 36), u = (u | 134217728) ^ 134217728;
let c = 0, s = 0;
for (; e.token !== 18; ) {
let m = null, { tokenPos: k, linePos: h, colPos: d } = e;
if (e.token & 143360 ? ((u & 1024) < 1 && ((e.token & 36864) === 36864 && (e.flags |= 256), (e.token & 537079808) === 537079808 && (e.flags |= 512)), m = p1(e, u, i, n3 | 1, 0, k, h, d)) : (e.token === 2162700 ? m = b2(e, u, i, 1, o, 1, t, 0, k, h, d) : e.token === 69271571 ? m = m2(e, u, i, 1, o, 1, t, 0, k, h, d) : e.token === 14 && (m = W2(e, u, i, 16, t, 0, 0, o, 1, k, h, d)), s = 1, e.destructible & 48 && f(e, 47)), e.token === 1077936157) {
E(e, u | 32768), s = 1;
let y = K(e, u, 1, 1, 0, e.tokenPos, e.linePos, e.colPos);
m = v(e, u, k, h, d, { type: "AssignmentPattern", left: m, right: y });
}
if (c++, l.push(m), !M(e, u, 18) || e.token === 16)
break;
}
return n3 & 512 && c !== 1 && f(e, 35, "Setter", "one", ""), i && i.scopeError !== void 0 && A(i.scopeError), s && (e.flags |= 128), q(e, u, 16), l;
}
function K2(e, u, i) {
E(e, u | 32768);
let n3 = K(e, (u | 134217728) ^ 134217728, 1, 0, i, e.tokenPos, e.linePos, e.colPos);
return q(e, u, 20), n3;
}
function Ot(e, u, i, n3, t, o, l, c) {
e.flags = (e.flags | 128) ^ 128;
let { tokenPos: s, linePos: m, colPos: k } = e;
E(e, u | 32768 | 1073741824);
let h = u & 64 ? i2(_2(), 1024) : void 0;
if (u = (u | 134217728) ^ 134217728, M(e, u, 16))
return m1(e, u, h, [], i, 0, o, l, c);
let d = 0;
e.destructible &= -385;
let y, w = [], D = 0, F = 0, { tokenPos: O, linePos: N, colPos: x } = e;
for (e.assignable = 1; e.token !== 16; ) {
let { token: I, tokenPos: W, linePos: P, colPos: y2 } = e;
if (I & 143360)
h && L2(e, u, h, e.tokenValue, 1, 0), y = d2(e, u, n3, 0, 1, 0, 1, 1, W, P, y2), e.token === 16 || e.token === 18 ? e.assignable & 2 ? (d |= 16, F = 1) : ((I & 537079808) === 537079808 || (I & 36864) === 36864) && (F = 1) : (e.token === 1077936157 ? F = 1 : d |= 16, y = H(e, u, y, 1, 0, W, P, y2), e.token !== 16 && e.token !== 18 && (y = Q(e, u, 1, 0, W, P, y2, y)));
else if ((I & 2097152) === 2097152)
y = I === 2162700 ? b2(e, u | 1073741824, h, 0, 1, 0, n3, t, W, P, y2) : m2(e, u | 1073741824, h, 0, 1, 0, n3, t, W, P, y2), d |= e.destructible, F = 1, e.assignable = 2, e.token !== 16 && e.token !== 18 && (d & 8 && f(e, 118), y = H(e, u, y, 0, 0, W, P, y2), d |= 16, e.token !== 16 && e.token !== 18 && (y = Q(e, u, 0, 0, W, P, y2, y)));
else if (I === 14) {
y = W2(e, u, h, 16, n3, t, 0, 1, 0, W, P, y2), e.destructible & 16 && f(e, 71), F = 1, D && (e.token === 16 || e.token === 18) && w.push(y), d |= 8;
break;
} else {
if (d |= 16, y = K(e, u, 1, 0, 1, W, P, y2), D && (e.token === 16 || e.token === 18) && w.push(y), e.token === 18 && (D || (D = 1, w = [y])), D) {
for (; M(e, u | 32768, 18); )
w.push(K(e, u, 1, 0, 1, e.tokenPos, e.linePos, e.colPos));
e.assignable = 2, y = v(e, u, O, N, x, { type: "SequenceExpression", expressions: w });
}
return q(e, u, 16), e.destructible = d, y;
}
if (D && (e.token === 16 || e.token === 18) && w.push(y), !M(e, u | 32768, 18))
break;
if (D || (D = 1, w = [y]), e.token === 16) {
d |= 8;
break;
}
}
return D && (e.assignable = 2, y = v(e, u, O, N, x, { type: "SequenceExpression", expressions: w })), q(e, u, 16), d & 16 && d & 8 && f(e, 145), d |= e.destructible & 256 ? 256 : 0 | e.destructible & 128 ? 128 : 0, e.token === 10 ? (d & 48 && f(e, 46), u & 4196352 && d & 128 && f(e, 29), u & 2098176 && d & 256 && f(e, 30), F && (e.flags |= 128), m1(e, u, h, D ? w : [y], i, 0, o, l, c)) : (d & 8 && f(e, 139), e.destructible = (e.destructible | 256) ^ 256 | d, u & 128 ? v(e, u, s, m, k, { type: "ParenthesizedExpression", expression: y }) : y);
}
function Q1(e, u, i, n3, t) {
let { tokenValue: o } = e, l = $(e, u, 0);
if (e.assignable = 1, e.token === 10) {
let c;
return u & 64 && (c = c1(e, u, o)), e.flags = (e.flags | 128) ^ 128, e1(e, u, c, [l], 0, i, n3, t);
}
return l;
}
function h1(e, u, i, n3, t, o, l, c, s, m) {
o || f(e, 54), t && f(e, 48), e.flags &= -129;
let k = u & 64 ? c1(e, u, i) : void 0;
return e1(e, u, k, [n3], l, c, s, m);
}
function m1(e, u, i, n3, t, o, l, c, s) {
t || f(e, 54);
for (let m = 0; m < n3.length; ++m)
r2(e, n3[m]);
return e1(e, u, i, n3, o, l, c, s);
}
function e1(e, u, i, n3, t, o, l, c) {
e.flags & 1 && f(e, 45), q(e, u | 32768, 10), u = (u | 15728640) ^ 15728640 | t << 22;
let s = e.token !== 2162700, m;
if (i && i.scopeError !== void 0 && A(i.scopeError), s)
m = K(e, u, 1, 0, 0, e.tokenPos, e.linePos, e.colPos);
else {
switch (i && (i = i2(i, 128)), m = d1(e, (u | 134221824 | 8192 | 16384) ^ 134246400, i, 16, void 0, void 0), e.token) {
case 69271571:
(e.flags & 1) < 1 && f(e, 112);
break;
case 67108877:
case 67174409:
case 22:
f(e, 113);
case 67174411:
(e.flags & 1) < 1 && f(e, 112), e.flags |= 1024;
break;
}
(e.token & 8454144) === 8454144 && (e.flags & 1) < 1 && f(e, 28, Z[e.token & 255]), (e.token & 33619968) === 33619968 && f(e, 121);
}
return e.assignable = 2, v(e, u, o, l, c, { type: "ArrowFunctionExpression", params: n3, body: m, async: t === 1, expression: s });
}
function Au(e, u, i, n3, t) {
q(e, u, 67174411), e.flags = (e.flags | 128) ^ 128;
let o = [];
if (M(e, u, 16))
return o;
u = (u | 134217728) ^ 134217728;
let l = 0;
for (; e.token !== 18; ) {
let c, { tokenPos: s, linePos: m, colPos: k } = e;
if (e.token & 143360 ? ((u & 1024) < 1 && ((e.token & 36864) === 36864 && (e.flags |= 256), (e.token & 537079808) === 537079808 && (e.flags |= 512)), c = p1(e, u, i, t | 1, 0, s, m, k)) : (e.token === 2162700 ? c = b2(e, u, i, 1, n3, 1, t, 0, s, m, k) : e.token === 69271571 ? c = m2(e, u, i, 1, n3, 1, t, 0, s, m, k) : e.token === 14 ? c = W2(e, u, i, 16, t, 0, 0, n3, 1, s, m, k) : f(e, 28, Z[e.token & 255]), l = 1, e.destructible & 48 && f(e, 47)), e.token === 1077936157) {
E(e, u | 32768), l = 1;
let h = K(e, u, 1, 1, n3, e.tokenPos, e.linePos, e.colPos);
c = v(e, u, s, m, k, { type: "AssignmentPattern", left: c, right: h });
}
if (o.push(c), !M(e, u, 18) || e.token === 16)
break;
}
return l && (e.flags |= 128), i && (l || u & 1024) && i.scopeError !== void 0 && A(i.scopeError), q(e, u, 16), o;
}
function b1(e, u, i, n3, t, o, l) {
let { token: c } = e;
if (c & 67108864) {
if (c === 67108877) {
E(e, u | 1073741824), e.assignable = 1;
let s = mu(e, u);
return b1(e, u, v(e, u, t, o, l, { type: "MemberExpression", object: i, computed: false, property: s }), 0, t, o, l);
} else if (c === 69271571) {
E(e, u | 32768);
let { tokenPos: s, linePos: m, colPos: k } = e, h = o2(e, u, n3, 1, s, m, k);
return q(e, u, 20), e.assignable = 1, b1(e, u, v(e, u, t, o, l, { type: "MemberExpression", object: i, computed: true, property: h }), 0, t, o, l);
} else if (c === 67174408 || c === 67174409)
return e.assignable = 2, b1(e, u, v(e, u, t, o, l, { type: "TaggedTemplateExpression", tag: i, quasi: e.token === 67174408 ? Y1(e, u | 65536) : K1(e, u, e.tokenPos, e.linePos, e.colPos) }), 0, t, o, l);
}
return i;
}
function Tt(e, u, i, n3, t, o) {
let l = $(e, u | 32768, 0), { tokenPos: c, linePos: s, colPos: m } = e;
if (M(e, u, 67108877)) {
if (u & 67108864 && e.token === 143494)
return e.assignable = 2, It(e, u, l, n3, t, o);
f(e, 91);
}
e.assignable = 2, (e.token & 16842752) === 16842752 && f(e, 62, Z[e.token & 255]);
let k = d2(e, u, 2, 1, 0, 0, i, 1, c, s, m);
u = (u | 134217728) ^ 134217728, e.token === 67108991 && f(e, 162);
let h = b1(e, u, k, i, c, s, m);
return e.assignable = 2, v(e, u, n3, t, o, { type: "NewExpression", callee: h, arguments: e.token === 67174411 ? Z1(e, u, i) : [] });
}
function It(e, u, i, n3, t, o) {
let l = $(e, u, 0);
return v(e, u, n3, t, o, { type: "MetaProperty", meta: i, property: l });
}
function Pu(e, u, i, n3, t, o) {
return e.token === 209008 && f(e, 29), u & 2098176 && e.token === 241773 && f(e, 30), (e.token & 537079808) === 537079808 && (e.flags |= 512), h1(e, u, e.tokenValue, $(e, u, 0), 0, i, 1, n3, t, o);
}
function G1(e, u, i, n3, t, o, l, c, s, m) {
E(e, u | 32768);
let k = u & 64 ? i2(_2(), 1024) : void 0;
if (u = (u | 134217728) ^ 134217728, M(e, u, 16))
return e.token === 10 ? (l & 1 && f(e, 45), m1(e, u, k, [], n3, 1, c, s, m)) : v(e, u, c, s, m, { type: "CallExpression", callee: i, arguments: [] });
let h = 0, d = null, y = 0;
e.destructible = (e.destructible | 256 | 128) ^ 384;
let w = [];
for (; e.token !== 16; ) {
let { token: D, tokenPos: F, linePos: O, colPos: N } = e;
if (D & 143360)
k && L2(e, u, k, e.tokenValue, t, 0), d = d2(e, u, t, 0, 1, 0, 1, 1, F, O, N), e.token === 16 || e.token === 18 ? e.assignable & 2 ? (h |= 16, y = 1) : (D & 537079808) === 537079808 ? e.flags |= 512 : (D & 36864) === 36864 && (e.flags |= 256) : (e.token === 1077936157 ? y = 1 : h |= 16, d = H(e, u, d, 1, 0, F, O, N), e.token !== 16 && e.token !== 18 && (d = Q(e, u, 1, 0, F, O, N, d)));
else if (D & 2097152)
d = D === 2162700 ? b2(e, u, k, 0, 1, 0, t, o, F, O, N) : m2(e, u, k, 0, 1, 0, t, o, F, O, N), h |= e.destructible, y = 1, e.token !== 16 && e.token !== 18 && (h & 8 && f(e, 118), d = H(e, u, d, 0, 0, F, O, N), h |= 16, (e.token & 8454144) === 8454144 && (d = T2(e, u, 1, c, s, m, 4, D, d)), M(e, u | 32768, 22) && (d = U2(e, u, d, c, s, m)));
else if (D === 14)
d = W2(e, u, k, 16, t, o, 1, 1, 0, F, O, N), h |= (e.token === 16 ? 0 : 16) | e.destructible, y = 1;
else {
for (d = K(e, u, 1, 0, 0, F, O, N), h = e.assignable, w.push(d); M(e, u | 32768, 18); )
w.push(K(e, u, 1, 0, 0, F, O, N));
return h |= e.assignable, q(e, u, 16), e.destructible = h | 16, e.assignable = 2, v(e, u, c, s, m, { type: "CallExpression", callee: i, arguments: w });
}
if (w.push(d), !M(e, u | 32768, 18))
break;
}
return q(e, u, 16), h |= e.destructible & 256 ? 256 : 0 | e.destructible & 128 ? 128 : 0, e.token === 10 ? (h & 48 && f(e, 25), (e.flags & 1 || l & 1) && f(e, 45), h & 128 && f(e, 29), u & 2098176 && h & 256 && f(e, 30), y && (e.flags |= 128), m1(e, u, k, w, n3, 1, c, s, m)) : (h & 8 && f(e, 59), e.assignable = 2, v(e, u, c, s, m, { type: "CallExpression", callee: i, arguments: w }));
}
function Rt(e, u, i, n3, t) {
let { tokenRaw: o, tokenRegExp: l, tokenValue: c } = e;
return E(e, u), e.assignable = 2, u & 512 ? v(e, u, i, n3, t, { type: "Literal", value: c, regex: l, raw: o }) : v(e, u, i, n3, t, { type: "Literal", value: c, regex: l });
}
function x1(e, u, i, n3, t, o, l) {
u = (u | 16777216 | 1024) ^ 16777216;
let c = k1(e, u);
c.length && (t = e.tokenPos, o = e.linePos, l = e.colPos), e.leadingDecorators.length && (e.leadingDecorators.push(...c), c = e.leadingDecorators, e.leadingDecorators = []), E(e, u);
let s = null, m = null, { tokenValue: k } = e;
e.token & 4096 && e.token !== 20567 ? (iu(e, u, e.token) && f(e, 114), (e.token & 537079808) === 537079808 && f(e, 115), i && (L2(e, u, i, k, 32, 0), n3 && n3 & 2 && M2(e, k)), s = $(e, u, 0)) : (n3 & 1) < 1 && f(e, 37, "Class");
let h = u;
M(e, u | 32768, 20567) ? (m = h2(e, u, 0, 0, 0, e.tokenPos, e.linePos, e.colPos), h |= 524288) : h = (h | 524288) ^ 524288;
let d = Eu(e, h, u, i, 2, 8, 0);
return v(e, u, t, o, l, u & 1 ? { type: "ClassDeclaration", id: s, superClass: m, decorators: c, body: d } : { type: "ClassDeclaration", id: s, superClass: m, body: d });
}
function Vt(e, u, i, n3, t, o) {
let l = null, c = null;
u = (u | 1024 | 16777216) ^ 16777216;
let s = k1(e, u);
s.length && (n3 = e.tokenPos, t = e.linePos, o = e.colPos), E(e, u), e.token & 4096 && e.token !== 20567 && (iu(e, u, e.token) && f(e, 114), (e.token & 537079808) === 537079808 && f(e, 115), l = $(e, u, 0));
let m = u;
M(e, u | 32768, 20567) ? (c = h2(e, u, 0, i, 0, e.tokenPos, e.linePos, e.colPos), m |= 524288) : m = (m | 524288) ^ 524288;
let k = Eu(e, m, u, void 0, 2, 0, i);
return e.assignable = 2, v(e, u, n3, t, o, u & 1 ? { type: "ClassExpression", id: l, superClass: c, decorators: s, body: k } : { type: "ClassExpression", id: l, superClass: c, body: k });
}
function k1(e, u) {
let i = [];
if (u & 1)
for (; e.token === 133; )
i.push(Nt(e, u, e.tokenPos, e.linePos, e.colPos));
return i;
}
function Nt(e, u, i, n3, t) {
E(e, u | 32768);
let o = d2(e, u, 2, 0, 1, 0, 0, 1, i, n3, t);
return o = H(e, u, o, 0, 0, i, n3, t), v(e, u, i, n3, t, { type: "Decorator", expression: o });
}
function Eu(e, u, i, n3, t, o, l) {
let { tokenPos: c, linePos: s, colPos: m } = e;
q(e, u | 32768, 2162700), u = (u | 134217728) ^ 134217728, e.flags = (e.flags | 32) ^ 32;
let k = [], h;
for (; e.token !== 1074790415; ) {
let d = 0;
if (h = k1(e, u), d = h.length, d > 0 && e.tokenValue === "constructor" && f(e, 106), e.token === 1074790415 && f(e, 105), M(e, u, 1074790417)) {
d > 0 && f(e, 116);
continue;
}
k.push(Cu(e, u, n3, i, t, h, 0, l, e.tokenPos, e.linePos, e.colPos));
}
return q(e, o & 8 ? u | 32768 : u, 1074790415), v(e, u, c, s, m, { type: "ClassBody", body: k });
}
function Cu(e, u, i, n3, t, o, l, c, s, m, k) {
let h = l ? 32 : 0, d = null, { token: y, tokenPos: w, linePos: D, colPos: F } = e;
if (y & 176128)
switch (d = $(e, u, 0), y) {
case 36972:
if (!l && e.token !== 67174411)
return Cu(e, u, i, n3, t, o, 1, c, s, m, k);
break;
case 209007:
if (e.token !== 67174411 && (e.flags & 1) < 1) {
if (u & 1 && (e.token & 1073741824) === 1073741824)
return v1(e, u, d, h, o, w, D, F);
h |= 16 | (M1(e, u, 8457014) ? 8 : 0);
}
break;
case 12402:
if (e.token !== 67174411) {
if (u & 1 && (e.token & 1073741824) === 1073741824)
return v1(e, u, d, h, o, w, D, F);
h |= 256;
}
break;
case 12403:
if (e.token !== 67174411) {
if (u & 1 && (e.token & 1073741824) === 1073741824)
return v1(e, u, d, h, o, w, D, F);
h |= 512;
}
break;
}
else
y === 69271571 ? (h |= 2, d = K2(e, n3, c)) : (y & 134217728) === 134217728 ? d = c2(e, u) : y === 8457014 ? (h |= 8, E(e, u)) : u & 1 && e.token === 131 ? (h |= 4096, d = r1(e, u | 16384, w, D, F)) : u & 1 && (e.token & 1073741824) === 1073741824 ? h |= 128 : y === 122 ? (d = $(e, u, 0), e.token !== 67174411 && f(e, 28, Z[e.token & 255])) : f(e, 28, Z[e.token & 255]);
if (h & 792 && (e.token & 143360 ? d = $(e, u, 0) : (e.token & 134217728) === 134217728 ? d = c2(e, u) : e.token === 69271571 ? (h |= 2, d = K2(e, u, 0)) : e.token === 122 ? d = $(e, u, 0) : u & 1 && e.token === 131 ? (h |= 4096, d = r1(e, u, w, D, F)) : f(e, 131)), (h & 2) < 1 && (e.tokenValue === "constructor" ? ((e.token & 1073741824) === 1073741824 ? f(e, 125) : (h & 32) < 1 && e.token === 67174411 && (h & 920 ? f(e, 50, "accessor") : (u & 524288) < 1 && (e.flags & 32 ? f(e, 51) : e.flags |= 32)), h |= 64) : (h & 4096) < 1 && h & 824 && e.tokenValue === "prototype" && f(e, 49)), u & 1 && e.token !== 67174411)
return v1(e, u, d, h, o, w, D, F);
let O = v2(e, u, h, c, e.tokenPos, e.linePos, e.colPos);
return v(e, u, s, m, k, u & 1 ? { type: "MethodDefinition", kind: (h & 32) < 1 && h & 64 ? "constructor" : h & 256 ? "get" : h & 512 ? "set" : "method", static: (h & 32) > 0, computed: (h & 2) > 0, key: d, decorators: o, value: O } : { type: "MethodDefinition", kind: (h & 32) < 1 && h & 64 ? "constructor" : h & 256 ? "get" : h & 512 ? "set" : "method", static: (h & 32) > 0, computed: (h & 2) > 0, key: d, value: O });
}
function r1(e, u, i, n3, t) {
E(e, u);
let { tokenValue: o } = e;
return o === "constructor" && f(e, 124), E(e, u), v(e, u, i, n3, t, { type: "PrivateIdentifier", name: o });
}
function v1(e, u, i, n3, t, o, l, c) {
let s = null;
if (n3 & 8 && f(e, 0), e.token === 1077936157) {
E(e, u | 32768);
let { tokenPos: m, linePos: k, colPos: h } = e;
e.token === 537079928 && f(e, 115), s = d2(e, u | 16384, 2, 0, 1, 0, 0, 1, m, k, h), (e.token & 1073741824) !== 1073741824 && (s = H(e, u | 16384, s, 0, 0, m, k, h), s = Q(e, u | 16384, 0, 0, m, k, h, s), e.token === 18 && (s = O2(e, u, 0, o, l, c, s)));
}
return v(e, u, o, l, c, { type: "PropertyDefinition", key: i, value: s, static: (n3 & 32) > 0, computed: (n3 & 2) > 0, decorators: t });
}
function Du(e, u, i, n3, t, o, l, c) {
if (e.token & 143360)
return p1(e, u, i, n3, t, o, l, c);
(e.token & 2097152) !== 2097152 && f(e, 28, Z[e.token & 255]);
let s = e.token === 69271571 ? m2(e, u, i, 1, 0, 1, n3, t, o, l, c) : b2(e, u, i, 1, 0, 1, n3, t, o, l, c);
return e.destructible & 16 && f(e, 47), e.destructible & 32 && f(e, 47), s;
}
function p1(e, u, i, n3, t, o, l, c) {
let { tokenValue: s, token: m } = e;
return u & 1024 && ((m & 537079808) === 537079808 ? f(e, 115) : (m & 36864) === 36864 && f(e, 114)), (m & 20480) === 20480 && f(e, 99), u & 2099200 && m === 241773 && f(e, 30), m === 241739 && n3 & 24 && f(e, 97), u & 4196352 && m === 209008 && f(e, 95), E(e, u), i && B2(e, u, i, s, n3, t), v(e, u, o, l, c, { type: "Identifier", name: s });
}
function ee(e, u, i, n3, t, o) {
if (E(e, u), e.token === 8456259)
return v(e, u, n3, t, o, { type: "JSXFragment", openingFragment: jt(e, u, n3, t, o), children: wu(e, u), closingFragment: Mt(e, u, i, e.tokenPos, e.linePos, e.colPos) });
let l = null, c = [], s = $t(e, u, i, n3, t, o);
if (!s.selfClosing) {
c = wu(e, u), l = _t(e, u, i, e.tokenPos, e.linePos, e.colPos);
let m = f1(l.name);
f1(s.name) !== m && f(e, 149, m);
}
return v(e, u, n3, t, o, { type: "JSXElement", children: c, openingElement: s, closingElement: l });
}
function jt(e, u, i, n3, t) {
return j2(e, u), v(e, u, i, n3, t, { type: "JSXOpeningFragment" });
}
function _t(e, u, i, n3, t, o) {
q(e, u, 25);
let l = qu(e, u, e.tokenPos, e.linePos, e.colPos);
return i ? q(e, u, 8456259) : e.token = j2(e, u), v(e, u, n3, t, o, { type: "JSXClosingElement", name: l });
}
function Mt(e, u, i, n3, t, o) {
return q(e, u, 25), q(e, u, 8456259), v(e, u, n3, t, o, { type: "JSXClosingFragment" });
}
function wu(e, u) {
let i = [];
for (; e.token !== 25; )
e.index = e.tokenPos = e.startPos, e.column = e.colPos = e.startColumn, e.line = e.linePos = e.startLine, j2(e, u), i.push(Ut(e, u, e.tokenPos, e.linePos, e.colPos));
return i;
}
function Ut(e, u, i, n3, t) {
if (e.token === 138)
return Jt(e, u, i, n3, t);
if (e.token === 2162700)
return Su(e, u, 0, 0, i, n3, t);
if (e.token === 8456258)
return ee(e, u, 0, i, n3, t);
f(e, 0);
}
function Jt(e, u, i, n3, t) {
j2(e, u);
let o = { type: "JSXText", value: e.tokenValue };
return u & 512 && (o.raw = e.tokenRaw), v(e, u, i, n3, t, o);
}
function $t(e, u, i, n3, t, o) {
(e.token & 143360) !== 143360 && (e.token & 4096) !== 4096 && f(e, 0);
let l = qu(e, u, e.tokenPos, e.linePos, e.colPos), c = Xt(e, u), s = e.token === 8457016;
return e.token === 8456259 ? j2(e, u) : (q(e, u, 8457016), i ? q(e, u, 8456259) : j2(e, u)), v(e, u, n3, t, o, { type: "JSXOpeningElement", name: l, attributes: c, selfClosing: s });
}
function qu(e, u, i, n3, t) {
_1(e);
let o = y1(e, u, i, n3, t);
if (e.token === 21)
return Bu(e, u, o, i, n3, t);
for (; M(e, u, 67108877); )
_1(e), o = Ht(e, u, o, i, n3, t);
return o;
}
function Ht(e, u, i, n3, t, o) {
let l = y1(e, u, e.tokenPos, e.linePos, e.colPos);
return v(e, u, n3, t, o, { type: "JSXMemberExpression", object: i, property: l });
}
function Xt(e, u) {
let i = [];
for (; e.token !== 8457016 && e.token !== 8456259 && e.token !== 1048576; )
i.push(Wt(e, u, e.tokenPos, e.linePos, e.colPos));
return i;
}
function zt(e, u, i, n3, t) {
E(e, u), q(e, u, 14);
let o = K(e, u, 1, 0, 0, e.tokenPos, e.linePos, e.colPos);
return q(e, u, 1074790415), v(e, u, i, n3, t, { type: "JSXSpreadAttribute", argument: o });
}
function Wt(e, u, i, n3, t) {
if (e.token === 2162700)
return zt(e, u, i, n3, t);
_1(e);
let o = null, l = y1(e, u, i, n3, t);
if (e.token === 21 && (l = Bu(e, u, l, i, n3, t)), e.token === 1077936157) {
let c = U0(e, u), { tokenPos: s, linePos: m, colPos: k } = e;
switch (c) {
case 134283267:
o = c2(e, u);
break;
case 8456258:
o = ee(e, u, 1, s, m, k);
break;
case 2162700:
o = Su(e, u, 1, 1, s, m, k);
break;
default:
f(e, 148);
}
}
return v(e, u, i, n3, t, { type: "JSXAttribute", value: o, name: l });
}
function Bu(e, u, i, n3, t, o) {
q(e, u, 21);
let l = y1(e, u, e.tokenPos, e.linePos, e.colPos);
return v(e, u, n3, t, o, { type: "JSXNamespacedName", namespace: i, name: l });
}
function Su(e, u, i, n3, t, o, l) {
E(e, u | 32768);
let { tokenPos: c, linePos: s, colPos: m } = e;
if (e.token === 14)
return Kt(e, u, c, s, m);
let k = null;
return e.token === 1074790415 ? (n3 && f(e, 151), k = Yt(e, u, e.startPos, e.startLine, e.startColumn)) : k = K(e, u, 1, 0, 0, c, s, m), i ? q(e, u, 1074790415) : j2(e, u), v(e, u, t, o, l, { type: "JSXExpressionContainer", expression: k });
}
function Kt(e, u, i, n3, t) {
q(e, u, 14);
let o = K(e, u, 1, 0, 0, e.tokenPos, e.linePos, e.colPos);
return q(e, u, 1074790415), v(e, u, i, n3, t, { type: "JSXSpreadChild", expression: o });
}
function Yt(e, u, i, n3, t) {
return e.startPos = e.tokenPos, e.startLine = e.linePos, e.startColumn = e.colPos, v(e, u, i, n3, t, { type: "JSXEmptyExpression" });
}
function y1(e, u, i, n3, t) {
let { tokenValue: o } = e;
return E(e, u), v(e, u, i, n3, t, { type: "JSXIdentifier", name: o });
}
var Zt = Object.freeze({ __proto__: null }), Qt = "4.2.1", Gt = Qt;
function xt(e, u) {
return H1(e, u, 0);
}
function pt(e, u) {
return H1(e, u, 3072);
}
function eo(e, u) {
return H1(e, u, 0);
}
a.ESTree = Zt, a.parse = eo, a.parseModule = pt, a.parseScript = xt, a.version = Gt;
} });
n2();
var V3 = k0(), N3 = b3(), j3 = q3(), _3 = I3(), M3 = { module: true, next: true, ranges: true, webcompat: true, loc: true, raw: true, directives: true, globalReturn: true, impliedStrict: false, preserveParens: false, lexical: false, identifierPattern: false, jsx: true, specDeviation: true, uniqueKeyInPattern: false };
function m0(a, g) {
let { parse: b } = R3(), f = [], A = [], L = b(a, Object.assign(Object.assign({}, M3), {}, { module: g, onComment: f, onToken: A }));
return L.comments = f, L.tokens = A, L;
}
function U3(a) {
let { message: g, line: b, column: f } = a, A = (g.match(/^\[(?\d+):(?\d+)]: (?.*)$/) || {}).groups;
return A && (g = A.message, typeof b != "number" && (b = Number(A.line), f = Number(A.column))), typeof b != "number" ? a : V3(g, { start: { line: b, column: f } });
}
function J3(a, g) {
let b = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, { result: f, error: A } = N3(() => m0(a, true), () => m0(a, false));
if (!f)
throw U3(A);
return b.originalText = a, _3(f, b);
}
O0.exports = { parsers: { meriyah: j3(J3) } };
});
return $3();
});
}
});
// node_modules/prettier/parser-postcss.js
var require_parser_postcss = __commonJS({
"node_modules/prettier/parser-postcss.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.postcss = e();
}
})(function() {
"use strict";
var U = (e, n2) => () => (n2 || e((n2 = { exports: {} }).exports, n2), n2.exports);
var pe = U((wp, Gt) => {
var er = function(e) {
return e && e.Math == Math && e;
};
Gt.exports = er(typeof globalThis == "object" && globalThis) || er(typeof window == "object" && window) || er(typeof self == "object" && self) || er(typeof global == "object" && global) || function() {
return this;
}() || Function("return this")();
});
var be = U((_p, Ht) => {
Ht.exports = function(e) {
try {
return !!e();
} catch {
return true;
}
};
});
var Oe = U((bp, Jt) => {
var _a = be();
Jt.exports = !_a(function() {
return Object.defineProperty({}, 1, { get: function() {
return 7;
} })[1] != 7;
});
});
var Tr = U((xp, Kt) => {
var ba = be();
Kt.exports = !ba(function() {
var e = function() {
}.bind();
return typeof e != "function" || e.hasOwnProperty("prototype");
});
});
var tr = U((Sp, Qt) => {
var xa = Tr(), rr = Function.prototype.call;
Qt.exports = xa ? rr.bind(rr) : function() {
return rr.apply(rr, arguments);
};
});
var en = U((Zt) => {
"use strict";
var Yt = {}.propertyIsEnumerable, Xt = Object.getOwnPropertyDescriptor, Sa = Xt && !Yt.call({ 1: 2 }, 1);
Zt.f = Sa ? function(n2) {
var i = Xt(this, n2);
return !!i && i.enumerable;
} : Yt;
});
var Er = U((Op, rn) => {
rn.exports = function(e, n2) {
return { enumerable: !(e & 1), configurable: !(e & 2), writable: !(e & 4), value: n2 };
};
});
var xe = U((Tp, sn) => {
var tn = Tr(), nn = Function.prototype, qr = nn.call, ka = tn && nn.bind.bind(qr, qr);
sn.exports = tn ? ka : function(e) {
return function() {
return qr.apply(e, arguments);
};
};
});
var un = U((Ep, an) => {
var on = xe(), Oa = on({}.toString), Ta = on("".slice);
an.exports = function(e) {
return Ta(Oa(e), 8, -1);
};
});
var ln = U((qp, cn) => {
var Ea = xe(), qa = be(), Aa = un(), Ar = Object, Pa = Ea("".split);
cn.exports = qa(function() {
return !Ar("z").propertyIsEnumerable(0);
}) ? function(e) {
return Aa(e) == "String" ? Pa(e, "") : Ar(e);
} : Ar;
});
var Pr = U((Ap, fn) => {
fn.exports = function(e) {
return e == null;
};
});
var Ir = U((Pp, pn) => {
var Ia = Pr(), Ra = TypeError;
pn.exports = function(e) {
if (Ia(e))
throw Ra("Can't call method on " + e);
return e;
};
});
var nr = U((Ip, hn) => {
var Ca = ln(), Na = Ir();
hn.exports = function(e) {
return Ca(Na(e));
};
});
var Cr = U((Rp, dn) => {
var Rr = typeof document == "object" && document.all, ja = typeof Rr > "u" && Rr !== void 0;
dn.exports = { all: Rr, IS_HTMLDDA: ja };
});
var he = U((Cp, mn) => {
var vn = Cr(), Ma = vn.all;
mn.exports = vn.IS_HTMLDDA ? function(e) {
return typeof e == "function" || e === Ma;
} : function(e) {
return typeof e == "function";
};
});
var Me = U((Np, wn) => {
var gn = he(), yn = Cr(), Da = yn.all;
wn.exports = yn.IS_HTMLDDA ? function(e) {
return typeof e == "object" ? e !== null : gn(e) || e === Da;
} : function(e) {
return typeof e == "object" ? e !== null : gn(e);
};
});
var ir = U((jp, _n) => {
var Nr = pe(), La = he(), za = function(e) {
return La(e) ? e : void 0;
};
_n.exports = function(e, n2) {
return arguments.length < 2 ? za(Nr[e]) : Nr[e] && Nr[e][n2];
};
});
var xn = U((Mp, bn) => {
var Ba = xe();
bn.exports = Ba({}.isPrototypeOf);
});
var kn = U((Dp, Sn) => {
var Fa = ir();
Sn.exports = Fa("navigator", "userAgent") || "";
});
var In = U((Lp, Pn) => {
var An = pe(), jr = kn(), On = An.process, Tn = An.Deno, En = On && On.versions || Tn && Tn.version, qn = En && En.v8, de, sr;
qn && (de = qn.split("."), sr = de[0] > 0 && de[0] < 4 ? 1 : +(de[0] + de[1]));
!sr && jr && (de = jr.match(/Edge\/(\d+)/), (!de || de[1] >= 74) && (de = jr.match(/Chrome\/(\d+)/), de && (sr = +de[1])));
Pn.exports = sr;
});
var Mr = U((zp, Cn) => {
var Rn = In(), Ua = be();
Cn.exports = !!Object.getOwnPropertySymbols && !Ua(function() {
var e = Symbol();
return !String(e) || !(Object(e) instanceof Symbol) || !Symbol.sham && Rn && Rn < 41;
});
});
var Dr = U((Bp, Nn) => {
var $a = Mr();
Nn.exports = $a && !Symbol.sham && typeof Symbol.iterator == "symbol";
});
var Lr = U((Fp, jn) => {
var Wa = ir(), Va = he(), Ga = xn(), Ha = Dr(), Ja = Object;
jn.exports = Ha ? function(e) {
return typeof e == "symbol";
} : function(e) {
var n2 = Wa("Symbol");
return Va(n2) && Ga(n2.prototype, Ja(e));
};
});
var Dn = U((Up, Mn) => {
var Ka = String;
Mn.exports = function(e) {
try {
return Ka(e);
} catch {
return "Object";
}
};
});
var zn = U(($p, Ln) => {
var Qa = he(), Ya = Dn(), Xa = TypeError;
Ln.exports = function(e) {
if (Qa(e))
return e;
throw Xa(Ya(e) + " is not a function");
};
});
var Fn = U((Wp, Bn) => {
var Za = zn(), eu = Pr();
Bn.exports = function(e, n2) {
var i = e[n2];
return eu(i) ? void 0 : Za(i);
};
});
var $n = U((Vp, Un) => {
var zr = tr(), Br = he(), Fr = Me(), ru = TypeError;
Un.exports = function(e, n2) {
var i, u;
if (n2 === "string" && Br(i = e.toString) && !Fr(u = zr(i, e)) || Br(i = e.valueOf) && !Fr(u = zr(i, e)) || n2 !== "string" && Br(i = e.toString) && !Fr(u = zr(i, e)))
return u;
throw ru("Can't convert object to primitive value");
};
});
var Vn = U((Gp, Wn) => {
Wn.exports = false;
});
var or = U((Hp, Hn) => {
var Gn = pe(), tu = Object.defineProperty;
Hn.exports = function(e, n2) {
try {
tu(Gn, e, { value: n2, configurable: true, writable: true });
} catch {
Gn[e] = n2;
}
return n2;
};
});
var ar = U((Jp, Kn) => {
var nu = pe(), iu = or(), Jn = "__core-js_shared__", su = nu[Jn] || iu(Jn, {});
Kn.exports = su;
});
var Ur = U((Kp, Yn) => {
var ou = Vn(), Qn = ar();
(Yn.exports = function(e, n2) {
return Qn[e] || (Qn[e] = n2 !== void 0 ? n2 : {});
})("versions", []).push({ version: "3.26.1", mode: ou ? "pure" : "global", copyright: "\xA9 2014-2022 Denis Pushkarev (zloirock.ru)", license: "https://github.com/zloirock/core-js/blob/v3.26.1/LICENSE", source: "https://github.com/zloirock/core-js" });
});
var Zn = U((Qp, Xn) => {
var au = Ir(), uu = Object;
Xn.exports = function(e) {
return uu(au(e));
};
});
var Te = U((Yp, ei) => {
var cu = xe(), lu = Zn(), fu = cu({}.hasOwnProperty);
ei.exports = Object.hasOwn || function(n2, i) {
return fu(lu(n2), i);
};
});
var $r = U((Xp, ri) => {
var pu = xe(), hu = 0, du = Math.random(), vu = pu(1 .toString);
ri.exports = function(e) {
return "Symbol(" + (e === void 0 ? "" : e) + ")_" + vu(++hu + du, 36);
};
});
var ai = U((Zp, oi) => {
var mu = pe(), gu = Ur(), ti = Te(), yu = $r(), ni = Mr(), si = Dr(), De = gu("wks"), Ee = mu.Symbol, ii = Ee && Ee.for, wu = si ? Ee : Ee && Ee.withoutSetter || yu;
oi.exports = function(e) {
if (!ti(De, e) || !(ni || typeof De[e] == "string")) {
var n2 = "Symbol." + e;
ni && ti(Ee, e) ? De[e] = Ee[e] : si && ii ? De[e] = ii(n2) : De[e] = wu(n2);
}
return De[e];
};
});
var fi = U((eh, li) => {
var _u = tr(), ui = Me(), ci = Lr(), bu = Fn(), xu = $n(), Su = ai(), ku = TypeError, Ou = Su("toPrimitive");
li.exports = function(e, n2) {
if (!ui(e) || ci(e))
return e;
var i = bu(e, Ou), u;
if (i) {
if (n2 === void 0 && (n2 = "default"), u = _u(i, e, n2), !ui(u) || ci(u))
return u;
throw ku("Can't convert object to primitive value");
}
return n2 === void 0 && (n2 = "number"), xu(e, n2);
};
});
var Wr = U((rh, pi) => {
var Tu = fi(), Eu = Lr();
pi.exports = function(e) {
var n2 = Tu(e, "string");
return Eu(n2) ? n2 : n2 + "";
};
});
var vi = U((th, di) => {
var qu = pe(), hi = Me(), Vr = qu.document, Au = hi(Vr) && hi(Vr.createElement);
di.exports = function(e) {
return Au ? Vr.createElement(e) : {};
};
});
var Gr = U((nh, mi) => {
var Pu = Oe(), Iu = be(), Ru = vi();
mi.exports = !Pu && !Iu(function() {
return Object.defineProperty(Ru("div"), "a", { get: function() {
return 7;
} }).a != 7;
});
});
var Hr = U((yi) => {
var Cu = Oe(), Nu = tr(), ju = en(), Mu = Er(), Du = nr(), Lu = Wr(), zu = Te(), Bu = Gr(), gi = Object.getOwnPropertyDescriptor;
yi.f = Cu ? gi : function(n2, i) {
if (n2 = Du(n2), i = Lu(i), Bu)
try {
return gi(n2, i);
} catch {
}
if (zu(n2, i))
return Mu(!Nu(ju.f, n2, i), n2[i]);
};
});
var _i = U((sh, wi) => {
var Fu = Oe(), Uu = be();
wi.exports = Fu && Uu(function() {
return Object.defineProperty(function() {
}, "prototype", { value: 42, writable: false }).prototype != 42;
});
});
var Jr = U((oh, bi) => {
var $u = Me(), Wu = String, Vu = TypeError;
bi.exports = function(e) {
if ($u(e))
return e;
throw Vu(Wu(e) + " is not an object");
};
});
var cr = U((Si) => {
var Gu = Oe(), Hu = Gr(), Ju = _i(), ur = Jr(), xi = Wr(), Ku = TypeError, Kr = Object.defineProperty, Qu = Object.getOwnPropertyDescriptor, Qr = "enumerable", Yr = "configurable", Xr = "writable";
Si.f = Gu ? Ju ? function(n2, i, u) {
if (ur(n2), i = xi(i), ur(u), typeof n2 == "function" && i === "prototype" && "value" in u && Xr in u && !u[Xr]) {
var o = Qu(n2, i);
o && o[Xr] && (n2[i] = u.value, u = { configurable: Yr in u ? u[Yr] : o[Yr], enumerable: Qr in u ? u[Qr] : o[Qr], writable: false });
}
return Kr(n2, i, u);
} : Kr : function(n2, i, u) {
if (ur(n2), i = xi(i), ur(u), Hu)
try {
return Kr(n2, i, u);
} catch {
}
if ("get" in u || "set" in u)
throw Ku("Accessors not supported");
return "value" in u && (n2[i] = u.value), n2;
};
});
var Zr = U((uh, ki) => {
var Yu = Oe(), Xu = cr(), Zu = Er();
ki.exports = Yu ? function(e, n2, i) {
return Xu.f(e, n2, Zu(1, i));
} : function(e, n2, i) {
return e[n2] = i, e;
};
});
var Ei = U((ch, Ti) => {
var et = Oe(), ec = Te(), Oi = Function.prototype, rc = et && Object.getOwnPropertyDescriptor, rt = ec(Oi, "name"), tc = rt && function() {
}.name === "something", nc = rt && (!et || et && rc(Oi, "name").configurable);
Ti.exports = { EXISTS: rt, PROPER: tc, CONFIGURABLE: nc };
});
var Ai = U((lh, qi) => {
var ic = xe(), sc = he(), tt = ar(), oc = ic(Function.toString);
sc(tt.inspectSource) || (tt.inspectSource = function(e) {
return oc(e);
});
qi.exports = tt.inspectSource;
});
var Ri = U((fh, Ii) => {
var ac = pe(), uc = he(), Pi = ac.WeakMap;
Ii.exports = uc(Pi) && /native code/.test(String(Pi));
});
var ji = U((ph, Ni) => {
var cc = Ur(), lc = $r(), Ci = cc("keys");
Ni.exports = function(e) {
return Ci[e] || (Ci[e] = lc(e));
};
});
var nt = U((hh, Mi) => {
Mi.exports = {};
});
var Bi = U((dh, zi) => {
var fc = Ri(), Li = pe(), pc = Me(), hc = Zr(), it = Te(), st = ar(), dc = ji(), vc = nt(), Di = "Object already initialized", ot = Li.TypeError, mc = Li.WeakMap, lr, Fe, fr, gc = function(e) {
return fr(e) ? Fe(e) : lr(e, {});
}, yc = function(e) {
return function(n2) {
var i;
if (!pc(n2) || (i = Fe(n2)).type !== e)
throw ot("Incompatible receiver, " + e + " required");
return i;
};
};
fc || st.state ? (ve = st.state || (st.state = new mc()), ve.get = ve.get, ve.has = ve.has, ve.set = ve.set, lr = function(e, n2) {
if (ve.has(e))
throw ot(Di);
return n2.facade = e, ve.set(e, n2), n2;
}, Fe = function(e) {
return ve.get(e) || {};
}, fr = function(e) {
return ve.has(e);
}) : (qe = dc("state"), vc[qe] = true, lr = function(e, n2) {
if (it(e, qe))
throw ot(Di);
return n2.facade = e, hc(e, qe, n2), n2;
}, Fe = function(e) {
return it(e, qe) ? e[qe] : {};
}, fr = function(e) {
return it(e, qe);
});
var ve, qe;
zi.exports = { set: lr, get: Fe, has: fr, enforce: gc, getterFor: yc };
});
var $i = U((vh, Ui) => {
var wc = be(), _c = he(), pr = Te(), at = Oe(), bc = Ei().CONFIGURABLE, xc = Ai(), Fi = Bi(), Sc = Fi.enforce, kc = Fi.get, hr = Object.defineProperty, Oc = at && !wc(function() {
return hr(function() {
}, "length", { value: 8 }).length !== 8;
}), Tc = String(String).split("String"), Ec = Ui.exports = function(e, n2, i) {
String(n2).slice(0, 7) === "Symbol(" && (n2 = "[" + String(n2).replace(/^Symbol\(([^)]*)\)/, "$1") + "]"), i && i.getter && (n2 = "get " + n2), i && i.setter && (n2 = "set " + n2), (!pr(e, "name") || bc && e.name !== n2) && (at ? hr(e, "name", { value: n2, configurable: true }) : e.name = n2), Oc && i && pr(i, "arity") && e.length !== i.arity && hr(e, "length", { value: i.arity });
try {
i && pr(i, "constructor") && i.constructor ? at && hr(e, "prototype", { writable: false }) : e.prototype && (e.prototype = void 0);
} catch {
}
var u = Sc(e);
return pr(u, "source") || (u.source = Tc.join(typeof n2 == "string" ? n2 : "")), e;
};
Function.prototype.toString = Ec(function() {
return _c(this) && kc(this).source || xc(this);
}, "toString");
});
var Vi = U((mh, Wi) => {
var qc = he(), Ac = cr(), Pc = $i(), Ic = or();
Wi.exports = function(e, n2, i, u) {
u || (u = {});
var o = u.enumerable, h = u.name !== void 0 ? u.name : n2;
if (qc(i) && Pc(i, h, u), u.global)
o ? e[n2] = i : Ic(n2, i);
else {
try {
u.unsafe ? e[n2] && (o = true) : delete e[n2];
} catch {
}
o ? e[n2] = i : Ac.f(e, n2, { value: i, enumerable: false, configurable: !u.nonConfigurable, writable: !u.nonWritable });
}
return e;
};
});
var Hi = U((gh, Gi) => {
var Rc = Math.ceil, Cc = Math.floor;
Gi.exports = Math.trunc || function(n2) {
var i = +n2;
return (i > 0 ? Cc : Rc)(i);
};
});
var ut = U((yh, Ji) => {
var Nc = Hi();
Ji.exports = function(e) {
var n2 = +e;
return n2 !== n2 || n2 === 0 ? 0 : Nc(n2);
};
});
var Qi = U((wh, Ki) => {
var jc = ut(), Mc = Math.max, Dc = Math.min;
Ki.exports = function(e, n2) {
var i = jc(e);
return i < 0 ? Mc(i + n2, 0) : Dc(i, n2);
};
});
var Xi = U((_h, Yi) => {
var Lc = ut(), zc = Math.min;
Yi.exports = function(e) {
return e > 0 ? zc(Lc(e), 9007199254740991) : 0;
};
});
var es = U((bh, Zi) => {
var Bc = Xi();
Zi.exports = function(e) {
return Bc(e.length);
};
});
var ns = U((xh, ts) => {
var Fc = nr(), Uc = Qi(), $c = es(), rs = function(e) {
return function(n2, i, u) {
var o = Fc(n2), h = $c(o), l = Uc(u, h), p;
if (e && i != i) {
for (; h > l; )
if (p = o[l++], p != p)
return true;
} else
for (; h > l; l++)
if ((e || l in o) && o[l] === i)
return e || l || 0;
return !e && -1;
};
};
ts.exports = { includes: rs(true), indexOf: rs(false) };
});
var os = U((Sh, ss) => {
var Wc = xe(), ct = Te(), Vc = nr(), Gc = ns().indexOf, Hc = nt(), is = Wc([].push);
ss.exports = function(e, n2) {
var i = Vc(e), u = 0, o = [], h;
for (h in i)
!ct(Hc, h) && ct(i, h) && is(o, h);
for (; n2.length > u; )
ct(i, h = n2[u++]) && (~Gc(o, h) || is(o, h));
return o;
};
});
var us = U((kh, as) => {
as.exports = ["constructor", "hasOwnProperty", "isPrototypeOf", "propertyIsEnumerable", "toLocaleString", "toString", "valueOf"];
});
var ls = U((cs) => {
var Jc = os(), Kc = us(), Qc = Kc.concat("length", "prototype");
cs.f = Object.getOwnPropertyNames || function(n2) {
return Jc(n2, Qc);
};
});
var ps = U((fs2) => {
fs2.f = Object.getOwnPropertySymbols;
});
var ds = U((Eh, hs) => {
var Yc = ir(), Xc = xe(), Zc = ls(), el = ps(), rl = Jr(), tl = Xc([].concat);
hs.exports = Yc("Reflect", "ownKeys") || function(n2) {
var i = Zc.f(rl(n2)), u = el.f;
return u ? tl(i, u(n2)) : i;
};
});
var gs = U((qh, ms) => {
var vs = Te(), nl = ds(), il = Hr(), sl = cr();
ms.exports = function(e, n2, i) {
for (var u = nl(n2), o = sl.f, h = il.f, l = 0; l < u.length; l++) {
var p = u[l];
!vs(e, p) && !(i && vs(i, p)) && o(e, p, h(n2, p));
}
};
});
var ws = U((Ah, ys) => {
var ol = be(), al = he(), ul = /#|\.prototype\./, Ue = function(e, n2) {
var i = ll[cl(e)];
return i == pl ? true : i == fl ? false : al(n2) ? ol(n2) : !!n2;
}, cl = Ue.normalize = function(e) {
return String(e).replace(ul, ".").toLowerCase();
}, ll = Ue.data = {}, fl = Ue.NATIVE = "N", pl = Ue.POLYFILL = "P";
ys.exports = Ue;
});
var bs = U((Ph, _s) => {
var lt = pe(), hl = Hr().f, dl = Zr(), vl = Vi(), ml = or(), gl = gs(), yl = ws();
_s.exports = function(e, n2) {
var i = e.target, u = e.global, o = e.stat, h, l, p, m, c, t;
if (u ? l = lt : o ? l = lt[i] || ml(i, {}) : l = (lt[i] || {}).prototype, l)
for (p in n2) {
if (c = n2[p], e.dontCallGetSet ? (t = hl(l, p), m = t && t.value) : m = l[p], h = yl(u ? p : i + (o ? "." : "#") + p, e.forced), !h && m !== void 0) {
if (typeof c == typeof m)
continue;
gl(c, m);
}
(e.sham || m && m.sham) && dl(c, "sham", true), vl(l, p, c, e);
}
};
});
var xs = U(() => {
var wl = bs(), ft = pe();
wl({ global: true, forced: ft.globalThis !== ft }, { globalThis: ft });
});
var Ss = U(() => {
xs();
});
var gp = U((Fh, wa) => {
Ss();
var Et = Object.defineProperty, _l = Object.getOwnPropertyDescriptor, qt = Object.getOwnPropertyNames, bl = Object.prototype.hasOwnProperty, Le = (e, n2) => function() {
return e && (n2 = (0, e[qt(e)[0]])(e = 0)), n2;
}, P = (e, n2) => function() {
return n2 || (0, e[qt(e)[0]])((n2 = { exports: {} }).exports, n2), n2.exports;
}, At = (e, n2) => {
for (var i in n2)
Et(e, i, { get: n2[i], enumerable: true });
}, xl = (e, n2, i, u) => {
if (n2 && typeof n2 == "object" || typeof n2 == "function")
for (let o of qt(n2))
!bl.call(e, o) && o !== i && Et(e, o, { get: () => n2[o], enumerable: !(u = _l(n2, o)) || u.enumerable });
return e;
}, Pt = (e) => xl(Et({}, "__esModule", { value: true }), e), A = Le({ ""() {
} }), Sl = P({ "src/common/parser-create-error.js"(e, n2) {
"use strict";
A();
function i(u, o) {
let h = new SyntaxError(u + " (" + o.start.line + ":" + o.start.column + ")");
return h.loc = o, h;
}
n2.exports = i;
} }), Us = P({ "src/utils/get-last.js"(e, n2) {
"use strict";
A();
var i = (u) => u[u.length - 1];
n2.exports = i;
} }), $s = P({ "src/utils/front-matter/parse.js"(e, n2) {
"use strict";
A();
var i = new RegExp("^(?-{3}|\\+{3})(?[^\\n]*)\\n(?:|(?.*?)\\n)(?\\k|\\.{3})[^\\S\\n]*(?:\\n|$)", "s");
function u(o) {
let h = o.match(i);
if (!h)
return { content: o };
let { startDelimiter: l, language: p, value: m = "", endDelimiter: c } = h.groups, t = p.trim() || "yaml";
if (l === "+++" && (t = "toml"), t !== "yaml" && l !== c)
return { content: o };
let [r] = h;
return { frontMatter: { type: "front-matter", lang: t, value: m, startDelimiter: l, endDelimiter: c, raw: r.replace(/\n$/, "") }, content: r.replace(/[^\n]/g, " ") + o.slice(r.length) };
}
n2.exports = u;
} }), Ws = {};
At(Ws, { EOL: () => bt, arch: () => kl, cpus: () => Ys, default: () => to, endianness: () => Vs, freemem: () => Ks, getNetworkInterfaces: () => ro, hostname: () => Gs, loadavg: () => Hs, networkInterfaces: () => eo, platform: () => Ol, release: () => Zs, tmpDir: () => wt, tmpdir: () => _t, totalmem: () => Qs, type: () => Xs, uptime: () => Js });
function Vs() {
if (typeof dr > "u") {
var e = new ArrayBuffer(2), n2 = new Uint8Array(e), i = new Uint16Array(e);
if (n2[0] = 1, n2[1] = 2, i[0] === 258)
dr = "BE";
else if (i[0] === 513)
dr = "LE";
else
throw new Error("unable to figure out endianess");
}
return dr;
}
function Gs() {
return typeof globalThis.location < "u" ? globalThis.location.hostname : "";
}
function Hs() {
return [];
}
function Js() {
return 0;
}
function Ks() {
return Number.MAX_VALUE;
}
function Qs() {
return Number.MAX_VALUE;
}
function Ys() {
return [];
}
function Xs() {
return "Browser";
}
function Zs() {
return typeof globalThis.navigator < "u" ? globalThis.navigator.appVersion : "";
}
function eo() {
}
function ro() {
}
function kl() {
return "javascript";
}
function Ol() {
return "browser";
}
function wt() {
return "/tmp";
}
var dr, _t, bt, to, Tl = Le({ "node-modules-polyfills:os"() {
A(), _t = wt, bt = `
`, to = { EOL: bt, tmpdir: _t, tmpDir: wt, networkInterfaces: eo, getNetworkInterfaces: ro, release: Zs, type: Xs, cpus: Ys, totalmem: Qs, freemem: Ks, uptime: Js, loadavg: Hs, hostname: Gs, endianness: Vs };
} }), El = P({ "node-modules-polyfills-commonjs:os"(e, n2) {
A();
var i = (Tl(), Pt(Ws));
if (i && i.default) {
n2.exports = i.default;
for (let u in i)
n2.exports[u] = i[u];
} else
i && (n2.exports = i);
} }), ql = P({ "node_modules/detect-newline/index.js"(e, n2) {
"use strict";
A();
var i = (u) => {
if (typeof u != "string")
throw new TypeError("Expected a string");
let o = u.match(/(?:\r?\n)/g) || [];
if (o.length === 0)
return;
let h = o.filter((p) => p === `\r
`).length, l = o.length - h;
return h > l ? `\r
` : `
`;
};
n2.exports = i, n2.exports.graceful = (u) => typeof u == "string" && i(u) || `
`;
} }), Al = P({ "node_modules/jest-docblock/build/index.js"(e) {
"use strict";
A(), Object.defineProperty(e, "__esModule", { value: true }), e.extract = s, e.parse = g, e.parseWithComments = v, e.print = y, e.strip = f;
function n2() {
let d = El();
return n2 = function() {
return d;
}, d;
}
function i() {
let d = u(ql());
return i = function() {
return d;
}, d;
}
function u(d) {
return d && d.__esModule ? d : { default: d };
}
var o = /\*\/$/, h = /^\/\*\*?/, l = /^\s*(\/\*\*?(.|\r?\n)*?\*\/)/, p = /(^|\s+)\/\/([^\r\n]*)/g, m = /^(\r?\n)+/, c = /(?:^|\r?\n) *(@[^\r\n]*?) *\r?\n *(?![^@\r\n]*\/\/[^]*)([^@\r\n\s][^@\r\n]+?) *\r?\n/g, t = /(?:^|\r?\n) *@(\S+) *([^\r\n]*)/g, r = /(\r?\n|^) *\* ?/g, a = [];
function s(d) {
let _ = d.match(l);
return _ ? _[0].trimLeft() : "";
}
function f(d) {
let _ = d.match(l);
return _ && _[0] ? d.substring(_[0].length) : d;
}
function g(d) {
return v(d).pragmas;
}
function v(d) {
let _ = (0, i().default)(d) || n2().EOL;
d = d.replace(h, "").replace(o, "").replace(r, "$1");
let k = "";
for (; k !== d; )
k = d, d = d.replace(c, `${_}$1 $2${_}`);
d = d.replace(m, "").trimRight();
let x = /* @__PURE__ */ Object.create(null), N = d.replace(t, "").replace(m, "").trimRight(), I;
for (; I = t.exec(d); ) {
let W = I[2].replace(p, "");
typeof x[I[1]] == "string" || Array.isArray(x[I[1]]) ? x[I[1]] = a.concat(x[I[1]], W) : x[I[1]] = W;
}
return { comments: N, pragmas: x };
}
function y(d) {
let { comments: _ = "", pragmas: k = {} } = d, x = (0, i().default)(_) || n2().EOL, N = "/**", I = " *", W = " */", $ = Object.keys(k), H = $.map((V) => w(V, k[V])).reduce((V, B) => V.concat(B), []).map((V) => `${I} ${V}${x}`).join("");
if (!_) {
if ($.length === 0)
return "";
if ($.length === 1 && !Array.isArray(k[$[0]])) {
let V = k[$[0]];
return `${N} ${w($[0], V)[0]}${W}`;
}
}
let D = _.split(x).map((V) => `${I} ${V}`).join(x) + x;
return N + x + (_ ? D : "") + (_ && $.length ? I + x : "") + H + W;
}
function w(d, _) {
return a.concat(_).map((k) => `@${d} ${k}`.trim());
}
} }), Pl = P({ "src/common/end-of-line.js"(e, n2) {
"use strict";
A();
function i(l) {
let p = l.indexOf("\r");
return p >= 0 ? l.charAt(p + 1) === `
` ? "crlf" : "cr" : "lf";
}
function u(l) {
switch (l) {
case "cr":
return "\r";
case "crlf":
return `\r
`;
default:
return `
`;
}
}
function o(l, p) {
let m;
switch (p) {
case `
`:
m = /\n/g;
break;
case "\r":
m = /\r/g;
break;
case `\r
`:
m = /\r\n/g;
break;
default:
throw new Error(`Unexpected "eol" ${JSON.stringify(p)}.`);
}
let c = l.match(m);
return c ? c.length : 0;
}
function h(l) {
return l.replace(/\r\n?/g, `
`);
}
n2.exports = { guessEndOfLine: i, convertEndOfLineToChars: u, countEndOfLineChars: o, normalizeEndOfLine: h };
} }), Il = P({ "src/language-js/utils/get-shebang.js"(e, n2) {
"use strict";
A();
function i(u) {
if (!u.startsWith("#!"))
return "";
let o = u.indexOf(`
`);
return o === -1 ? u : u.slice(0, o);
}
n2.exports = i;
} }), Rl = P({ "src/language-js/pragma.js"(e, n2) {
"use strict";
A();
var { parseWithComments: i, strip: u, extract: o, print: h } = Al(), { normalizeEndOfLine: l } = Pl(), p = Il();
function m(r) {
let a = p(r);
a && (r = r.slice(a.length + 1));
let s = o(r), { pragmas: f, comments: g } = i(s);
return { shebang: a, text: r, pragmas: f, comments: g };
}
function c(r) {
let a = Object.keys(m(r).pragmas);
return a.includes("prettier") || a.includes("format");
}
function t(r) {
let { shebang: a, text: s, pragmas: f, comments: g } = m(r), v = u(s), y = h({ pragmas: Object.assign({ format: "" }, f), comments: g.trimStart() });
return (a ? `${a}
` : "") + l(y) + (v.startsWith(`
`) ? `
` : `
`) + v;
}
n2.exports = { hasPragma: c, insertPragma: t };
} }), Cl = P({ "src/language-css/pragma.js"(e, n2) {
"use strict";
A();
var i = Rl(), u = $s();
function o(l) {
return i.hasPragma(u(l).content);
}
function h(l) {
let { frontMatter: p, content: m } = u(l);
return (p ? p.raw + `
` : "") + i.insertPragma(m);
}
n2.exports = { hasPragma: o, insertPragma: h };
} }), Nl = P({ "src/utils/text/skip.js"(e, n2) {
"use strict";
A();
function i(p) {
return (m, c, t) => {
let r = t && t.backwards;
if (c === false)
return false;
let { length: a } = m, s = c;
for (; s >= 0 && s < a; ) {
let f = m.charAt(s);
if (p instanceof RegExp) {
if (!p.test(f))
return s;
} else if (!p.includes(f))
return s;
r ? s-- : s++;
}
return s === -1 || s === a ? s : false;
};
}
var u = i(/\s/), o = i(" "), h = i(",; "), l = i(/[^\n\r]/);
n2.exports = { skipWhitespace: u, skipSpaces: o, skipToLineEnd: h, skipEverythingButNewLine: l };
} }), jl = P({ "src/utils/line-column-to-index.js"(e, n2) {
"use strict";
A(), n2.exports = function(i, u) {
let o = 0;
for (let h = 0; h < i.line - 1; ++h)
o = u.indexOf(`
`, o) + 1;
return o + i.column;
};
} }), no = P({ "src/language-css/loc.js"(e, n2) {
"use strict";
A();
var { skipEverythingButNewLine: i } = Nl(), u = Us(), o = jl();
function h(s, f) {
return typeof s.sourceIndex == "number" ? s.sourceIndex : s.source ? o(s.source.start, f) - 1 : null;
}
function l(s, f) {
if (s.type === "css-comment" && s.inline)
return i(f, s.source.startOffset);
let g = s.nodes && u(s.nodes);
return g && s.source && !s.source.end && (s = g), s.source && s.source.end ? o(s.source.end, f) : null;
}
function p(s, f) {
s.source && (s.source.startOffset = h(s, f), s.source.endOffset = l(s, f));
for (let g in s) {
let v = s[g];
g === "source" || !v || typeof v != "object" || (v.type === "value-root" || v.type === "value-unknown" ? m(v, c(s), v.text || v.value) : p(v, f));
}
}
function m(s, f, g) {
s.source && (s.source.startOffset = h(s, g) + f, s.source.endOffset = l(s, g) + f);
for (let v in s) {
let y = s[v];
v === "source" || !y || typeof y != "object" || m(y, f, g);
}
}
function c(s) {
let f = s.source.startOffset;
return typeof s.prop == "string" && (f += s.prop.length), s.type === "css-atrule" && typeof s.name == "string" && (f += 1 + s.name.length + s.raws.afterName.match(/^\s*:?\s*/)[0].length), s.type !== "css-atrule" && s.raws && typeof s.raws.between == "string" && (f += s.raws.between.length), f;
}
function t(s) {
let f = "initial", g = "initial", v, y = false, w = [];
for (let d = 0; d < s.length; d++) {
let _ = s[d];
switch (f) {
case "initial":
if (_ === "'") {
f = "single-quotes";
continue;
}
if (_ === '"') {
f = "double-quotes";
continue;
}
if ((_ === "u" || _ === "U") && s.slice(d, d + 4).toLowerCase() === "url(") {
f = "url", d += 3;
continue;
}
if (_ === "*" && s[d - 1] === "/") {
f = "comment-block";
continue;
}
if (_ === "/" && s[d - 1] === "/") {
f = "comment-inline", v = d - 1;
continue;
}
continue;
case "single-quotes":
if (_ === "'" && s[d - 1] !== "\\" && (f = g, g = "initial"), _ === `
` || _ === "\r")
return s;
continue;
case "double-quotes":
if (_ === '"' && s[d - 1] !== "\\" && (f = g, g = "initial"), _ === `
` || _ === "\r")
return s;
continue;
case "url":
if (_ === ")" && (f = "initial"), _ === `
` || _ === "\r")
return s;
if (_ === "'") {
f = "single-quotes", g = "url";
continue;
}
if (_ === '"') {
f = "double-quotes", g = "url";
continue;
}
continue;
case "comment-block":
_ === "/" && s[d - 1] === "*" && (f = "initial");
continue;
case "comment-inline":
(_ === '"' || _ === "'" || _ === "*") && (y = true), (_ === `
` || _ === "\r") && (y && w.push([v, d]), f = "initial", y = false);
continue;
}
}
for (let [d, _] of w)
s = s.slice(0, d) + s.slice(d, _).replace(/["'*]/g, " ") + s.slice(_);
return s;
}
function r(s) {
return s.source.startOffset;
}
function a(s) {
return s.source.endOffset;
}
n2.exports = { locStart: r, locEnd: a, calculateLoc: p, replaceQuotesInInlineComments: t };
} }), Ml = P({ "src/utils/is-non-empty-array.js"(e, n2) {
"use strict";
A();
function i(u) {
return Array.isArray(u) && u.length > 0;
}
n2.exports = i;
} }), Dl = P({ "src/language-css/utils/has-scss-interpolation.js"(e, n2) {
"use strict";
A();
var i = Ml();
function u(o) {
if (i(o)) {
for (let h = o.length - 1; h > 0; h--)
if (o[h].type === "word" && o[h].value === "{" && o[h - 1].type === "word" && o[h - 1].value.endsWith("#"))
return true;
}
return false;
}
n2.exports = u;
} }), Ll = P({ "src/language-css/utils/has-string-or-function.js"(e, n2) {
"use strict";
A();
function i(u) {
return u.some((o) => o.type === "string" || o.type === "func");
}
n2.exports = i;
} }), zl = P({ "src/language-css/utils/is-less-parser.js"(e, n2) {
"use strict";
A();
function i(u) {
return u.parser === "css" || u.parser === "less";
}
n2.exports = i;
} }), Bl = P({ "src/language-css/utils/is-scss.js"(e, n2) {
"use strict";
A();
function i(u, o) {
return u === "less" || u === "scss" ? u === "scss" : /(?:\w\s*:\s*[^:}]+|#){|@import[^\n]+(?:url|,)/.test(o);
}
n2.exports = i;
} }), Fl = P({ "src/language-css/utils/is-scss-nested-property-node.js"(e, n2) {
"use strict";
A();
function i(u) {
return u.selector ? u.selector.replace(/\/\*.*?\*\//, "").replace(/\/\/.*\n/, "").trim().endsWith(":") : false;
}
n2.exports = i;
} }), Ul = P({ "src/language-css/utils/is-scss-variable.js"(e, n2) {
"use strict";
A();
function i(u) {
return Boolean((u == null ? void 0 : u.type) === "word" && u.value.startsWith("$"));
}
n2.exports = i;
} }), $l = P({ "src/language-css/utils/stringify-node.js"(e, n2) {
"use strict";
A();
function i(u) {
var o, h, l;
if (u.groups) {
var p, m, c;
let y = ((p = u.open) === null || p === void 0 ? void 0 : p.value) || "", w = u.groups.map((_) => i(_)).join(((m = u.groups[0]) === null || m === void 0 ? void 0 : m.type) === "comma_group" ? "," : ""), d = ((c = u.close) === null || c === void 0 ? void 0 : c.value) || "";
return y + w + d;
}
let t = ((o = u.raws) === null || o === void 0 ? void 0 : o.before) || "", r = ((h = u.raws) === null || h === void 0 ? void 0 : h.quote) || "", a = u.type === "atword" ? "@" : "", s = u.value || "", f = u.unit || "", g = u.group ? i(u.group) : "", v = ((l = u.raws) === null || l === void 0 ? void 0 : l.after) || "";
return t + r + a + s + r + f + g + v;
}
n2.exports = i;
} }), Wl = P({ "src/language-css/utils/is-module-rule-name.js"(e, n2) {
"use strict";
A();
var i = /* @__PURE__ */ new Set(["import", "use", "forward"]);
function u(o) {
return i.has(o);
}
n2.exports = u;
} }), we = P({ "node_modules/postcss-values-parser/lib/node.js"(e, n2) {
"use strict";
A();
var i = function(u, o) {
let h = new u.constructor();
for (let l in u) {
if (!u.hasOwnProperty(l))
continue;
let p = u[l], m = typeof p;
l === "parent" && m === "object" ? o && (h[l] = o) : l === "source" ? h[l] = p : p instanceof Array ? h[l] = p.map((c) => i(c, h)) : l !== "before" && l !== "after" && l !== "between" && l !== "semicolon" && (m === "object" && p !== null && (p = i(p)), h[l] = p);
}
return h;
};
n2.exports = class {
constructor(o) {
o = o || {}, this.raws = { before: "", after: "" };
for (let h in o)
this[h] = o[h];
}
remove() {
return this.parent && this.parent.removeChild(this), this.parent = void 0, this;
}
toString() {
return [this.raws.before, String(this.value), this.raws.after].join("");
}
clone(o) {
o = o || {};
let h = i(this);
for (let l in o)
h[l] = o[l];
return h;
}
cloneBefore(o) {
o = o || {};
let h = this.clone(o);
return this.parent.insertBefore(this, h), h;
}
cloneAfter(o) {
o = o || {};
let h = this.clone(o);
return this.parent.insertAfter(this, h), h;
}
replaceWith() {
let o = Array.prototype.slice.call(arguments);
if (this.parent) {
for (let h of o)
this.parent.insertBefore(this, h);
this.remove();
}
return this;
}
moveTo(o) {
return this.cleanRaws(this.root() === o.root()), this.remove(), o.append(this), this;
}
moveBefore(o) {
return this.cleanRaws(this.root() === o.root()), this.remove(), o.parent.insertBefore(o, this), this;
}
moveAfter(o) {
return this.cleanRaws(this.root() === o.root()), this.remove(), o.parent.insertAfter(o, this), this;
}
next() {
let o = this.parent.index(this);
return this.parent.nodes[o + 1];
}
prev() {
let o = this.parent.index(this);
return this.parent.nodes[o - 1];
}
toJSON() {
let o = {};
for (let h in this) {
if (!this.hasOwnProperty(h) || h === "parent")
continue;
let l = this[h];
l instanceof Array ? o[h] = l.map((p) => typeof p == "object" && p.toJSON ? p.toJSON() : p) : typeof l == "object" && l.toJSON ? o[h] = l.toJSON() : o[h] = l;
}
return o;
}
root() {
let o = this;
for (; o.parent; )
o = o.parent;
return o;
}
cleanRaws(o) {
delete this.raws.before, delete this.raws.after, o || delete this.raws.between;
}
positionInside(o) {
let h = this.toString(), l = this.source.start.column, p = this.source.start.line;
for (let m = 0; m < o; m++)
h[m] === `
` ? (l = 1, p += 1) : l += 1;
return { line: p, column: l };
}
positionBy(o) {
let h = this.source.start;
if (Object(o).index)
h = this.positionInside(o.index);
else if (Object(o).word) {
let l = this.toString().indexOf(o.word);
l !== -1 && (h = this.positionInside(l));
}
return h;
}
};
} }), ae = P({ "node_modules/postcss-values-parser/lib/container.js"(e, n2) {
"use strict";
A();
var i = we(), u = class extends i {
constructor(o) {
super(o), this.nodes || (this.nodes = []);
}
push(o) {
return o.parent = this, this.nodes.push(o), this;
}
each(o) {
this.lastEach || (this.lastEach = 0), this.indexes || (this.indexes = {}), this.lastEach += 1;
let h = this.lastEach, l, p;
if (this.indexes[h] = 0, !!this.nodes) {
for (; this.indexes[h] < this.nodes.length && (l = this.indexes[h], p = o(this.nodes[l], l), p !== false); )
this.indexes[h] += 1;
return delete this.indexes[h], p;
}
}
walk(o) {
return this.each((h, l) => {
let p = o(h, l);
return p !== false && h.walk && (p = h.walk(o)), p;
});
}
walkType(o, h) {
if (!o || !h)
throw new Error("Parameters {type} and {callback} are required.");
let l = typeof o == "function";
return this.walk((p, m) => {
if (l && p instanceof o || !l && p.type === o)
return h.call(this, p, m);
});
}
append(o) {
return o.parent = this, this.nodes.push(o), this;
}
prepend(o) {
return o.parent = this, this.nodes.unshift(o), this;
}
cleanRaws(o) {
if (super.cleanRaws(o), this.nodes)
for (let h of this.nodes)
h.cleanRaws(o);
}
insertAfter(o, h) {
let l = this.index(o), p;
this.nodes.splice(l + 1, 0, h);
for (let m in this.indexes)
p = this.indexes[m], l <= p && (this.indexes[m] = p + this.nodes.length);
return this;
}
insertBefore(o, h) {
let l = this.index(o), p;
this.nodes.splice(l, 0, h);
for (let m in this.indexes)
p = this.indexes[m], l <= p && (this.indexes[m] = p + this.nodes.length);
return this;
}
removeChild(o) {
o = this.index(o), this.nodes[o].parent = void 0, this.nodes.splice(o, 1);
let h;
for (let l in this.indexes)
h = this.indexes[l], h >= o && (this.indexes[l] = h - 1);
return this;
}
removeAll() {
for (let o of this.nodes)
o.parent = void 0;
return this.nodes = [], this;
}
every(o) {
return this.nodes.every(o);
}
some(o) {
return this.nodes.some(o);
}
index(o) {
return typeof o == "number" ? o : this.nodes.indexOf(o);
}
get first() {
if (this.nodes)
return this.nodes[0];
}
get last() {
if (this.nodes)
return this.nodes[this.nodes.length - 1];
}
toString() {
let o = this.nodes.map(String).join("");
return this.value && (o = this.value + o), this.raws.before && (o = this.raws.before + o), this.raws.after && (o += this.raws.after), o;
}
};
u.registerWalker = (o) => {
let h = "walk" + o.name;
h.lastIndexOf("s") !== h.length - 1 && (h += "s"), !u.prototype[h] && (u.prototype[h] = function(l) {
return this.walkType(o, l);
});
}, n2.exports = u;
} }), Vl = P({ "node_modules/postcss-values-parser/lib/root.js"(e, n2) {
"use strict";
A();
var i = ae();
n2.exports = class extends i {
constructor(o) {
super(o), this.type = "root";
}
};
} }), io = P({ "node_modules/postcss-values-parser/lib/value.js"(e, n2) {
"use strict";
A();
var i = ae();
n2.exports = class extends i {
constructor(o) {
super(o), this.type = "value", this.unbalanced = 0;
}
};
} }), so = P({ "node_modules/postcss-values-parser/lib/atword.js"(e, n2) {
"use strict";
A();
var i = ae(), u = class extends i {
constructor(o) {
super(o), this.type = "atword";
}
toString() {
let o = this.quoted ? this.raws.quote : "";
return [this.raws.before, "@", String.prototype.toString.call(this.value), this.raws.after].join("");
}
};
i.registerWalker(u), n2.exports = u;
} }), oo = P({ "node_modules/postcss-values-parser/lib/colon.js"(e, n2) {
"use strict";
A();
var i = ae(), u = we(), o = class extends u {
constructor(h) {
super(h), this.type = "colon";
}
};
i.registerWalker(o), n2.exports = o;
} }), ao = P({ "node_modules/postcss-values-parser/lib/comma.js"(e, n2) {
"use strict";
A();
var i = ae(), u = we(), o = class extends u {
constructor(h) {
super(h), this.type = "comma";
}
};
i.registerWalker(o), n2.exports = o;
} }), uo = P({ "node_modules/postcss-values-parser/lib/comment.js"(e, n2) {
"use strict";
A();
var i = ae(), u = we(), o = class extends u {
constructor(h) {
super(h), this.type = "comment", this.inline = Object(h).inline || false;
}
toString() {
return [this.raws.before, this.inline ? "//" : "/*", String(this.value), this.inline ? "" : "*/", this.raws.after].join("");
}
};
i.registerWalker(o), n2.exports = o;
} }), co = P({ "node_modules/postcss-values-parser/lib/function.js"(e, n2) {
"use strict";
A();
var i = ae(), u = class extends i {
constructor(o) {
super(o), this.type = "func", this.unbalanced = -1;
}
};
i.registerWalker(u), n2.exports = u;
} }), lo = P({ "node_modules/postcss-values-parser/lib/number.js"(e, n2) {
"use strict";
A();
var i = ae(), u = we(), o = class extends u {
constructor(h) {
super(h), this.type = "number", this.unit = Object(h).unit || "";
}
toString() {
return [this.raws.before, String(this.value), this.unit, this.raws.after].join("");
}
};
i.registerWalker(o), n2.exports = o;
} }), fo = P({ "node_modules/postcss-values-parser/lib/operator.js"(e, n2) {
"use strict";
A();
var i = ae(), u = we(), o = class extends u {
constructor(h) {
super(h), this.type = "operator";
}
};
i.registerWalker(o), n2.exports = o;
} }), po = P({ "node_modules/postcss-values-parser/lib/paren.js"(e, n2) {
"use strict";
A();
var i = ae(), u = we(), o = class extends u {
constructor(h) {
super(h), this.type = "paren", this.parenType = "";
}
};
i.registerWalker(o), n2.exports = o;
} }), ho = P({ "node_modules/postcss-values-parser/lib/string.js"(e, n2) {
"use strict";
A();
var i = ae(), u = we(), o = class extends u {
constructor(h) {
super(h), this.type = "string";
}
toString() {
let h = this.quoted ? this.raws.quote : "";
return [this.raws.before, h, this.value + "", h, this.raws.after].join("");
}
};
i.registerWalker(o), n2.exports = o;
} }), vo = P({ "node_modules/postcss-values-parser/lib/word.js"(e, n2) {
"use strict";
A();
var i = ae(), u = we(), o = class extends u {
constructor(h) {
super(h), this.type = "word";
}
};
i.registerWalker(o), n2.exports = o;
} }), mo = P({ "node_modules/postcss-values-parser/lib/unicode-range.js"(e, n2) {
"use strict";
A();
var i = ae(), u = we(), o = class extends u {
constructor(h) {
super(h), this.type = "unicode-range";
}
};
i.registerWalker(o), n2.exports = o;
} });
function go() {
throw new Error("setTimeout has not been defined");
}
function yo() {
throw new Error("clearTimeout has not been defined");
}
function wo(e) {
if (Se === setTimeout)
return setTimeout(e, 0);
if ((Se === go || !Se) && setTimeout)
return Se = setTimeout, setTimeout(e, 0);
try {
return Se(e, 0);
} catch {
try {
return Se.call(null, e, 0);
} catch {
return Se.call(this, e, 0);
}
}
}
function Gl(e) {
if (ke === clearTimeout)
return clearTimeout(e);
if ((ke === yo || !ke) && clearTimeout)
return ke = clearTimeout, clearTimeout(e);
try {
return ke(e);
} catch {
try {
return ke.call(null, e);
} catch {
return ke.call(this, e);
}
}
}
function Hl() {
!Ne || !Ce || (Ne = false, Ce.length ? me = Ce.concat(me) : We = -1, me.length && _o());
}
function _o() {
if (!Ne) {
var e = wo(Hl);
Ne = true;
for (var n2 = me.length; n2; ) {
for (Ce = me, me = []; ++We < n2; )
Ce && Ce[We].run();
We = -1, n2 = me.length;
}
Ce = null, Ne = false, Gl(e);
}
}
function Jl(e) {
var n2 = new Array(arguments.length - 1);
if (arguments.length > 1)
for (var i = 1; i < arguments.length; i++)
n2[i - 1] = arguments[i];
me.push(new bo(e, n2)), me.length === 1 && !Ne && wo(_o);
}
function bo(e, n2) {
this.fun = e, this.array = n2;
}
function Ae() {
}
function Kl(e) {
throw new Error("process.binding is not supported");
}
function Ql() {
return "/";
}
function Yl(e) {
throw new Error("process.chdir is not supported");
}
function Xl() {
return 0;
}
function Zl(e) {
var n2 = xo.call(Ie) * 1e-3, i = Math.floor(n2), u = Math.floor(n2 % 1 * 1e9);
return e && (i = i - e[0], u = u - e[1], u < 0 && (i--, u += 1e9)), [i, u];
}
function ef() {
var e = new Date(), n2 = e - So;
return n2 / 1e3;
}
var Se, ke, me, Ne, Ce, We, ks, Os, Ts, Es, qs, As, Ps, Is, Rs, Cs, Ns, js, Ms, Ds, Ls, zs, Ie, xo, So, Bs, Ve, rf = Le({ "node-modules-polyfills:process"() {
A(), Se = go, ke = yo, typeof globalThis.setTimeout == "function" && (Se = setTimeout), typeof globalThis.clearTimeout == "function" && (ke = clearTimeout), me = [], Ne = false, We = -1, bo.prototype.run = function() {
this.fun.apply(null, this.array);
}, ks = "browser", Os = "browser", Ts = true, Es = {}, qs = [], As = "", Ps = {}, Is = {}, Rs = {}, Cs = Ae, Ns = Ae, js = Ae, Ms = Ae, Ds = Ae, Ls = Ae, zs = Ae, Ie = globalThis.performance || {}, xo = Ie.now || Ie.mozNow || Ie.msNow || Ie.oNow || Ie.webkitNow || function() {
return new Date().getTime();
}, So = new Date(), Bs = { nextTick: Jl, title: ks, browser: Ts, env: Es, argv: qs, version: As, versions: Ps, on: Cs, addListener: Ns, once: js, off: Ms, removeListener: Ds, removeAllListeners: Ls, emit: zs, binding: Kl, cwd: Ql, chdir: Yl, umask: Xl, hrtime: Zl, platform: Os, release: Is, config: Rs, uptime: ef }, Ve = Bs;
} }), pt, It, tf = Le({ "node_modules/rollup-plugin-node-polyfills/polyfills/inherits.js"() {
A(), typeof Object.create == "function" ? pt = function(n2, i) {
n2.super_ = i, n2.prototype = Object.create(i.prototype, { constructor: { value: n2, enumerable: false, writable: true, configurable: true } });
} : pt = function(n2, i) {
n2.super_ = i;
var u = function() {
};
u.prototype = i.prototype, n2.prototype = new u(), n2.prototype.constructor = n2;
}, It = pt;
} }), ko = {};
At(ko, { _extend: () => Mt, debuglog: () => Oo, default: () => No, deprecate: () => Rt, format: () => wr, inherits: () => It, inspect: () => ye, isArray: () => Ct, isBoolean: () => _r, isBuffer: () => Ao, isDate: () => gr, isError: () => He, isFunction: () => Je, isNull: () => Ke, isNullOrUndefined: () => To, isNumber: () => Nt, isObject: () => je, isPrimitive: () => qo, isRegExp: () => Ge, isString: () => Qe, isSymbol: () => Eo, isUndefined: () => ge, log: () => Po });
function wr(e) {
if (!Qe(e)) {
for (var n2 = [], i = 0; i < arguments.length; i++)
n2.push(ye(arguments[i]));
return n2.join(" ");
}
for (var i = 1, u = arguments, o = u.length, h = String(e).replace(Ro, function(p) {
if (p === "%%")
return "%";
if (i >= o)
return p;
switch (p) {
case "%s":
return String(u[i++]);
case "%d":
return Number(u[i++]);
case "%j":
try {
return JSON.stringify(u[i++]);
} catch {
return "[Circular]";
}
default:
return p;
}
}), l = u[i]; i < o; l = u[++i])
Ke(l) || !je(l) ? h += " " + l : h += " " + ye(l);
return h;
}
function Rt(e, n2) {
if (ge(globalThis.process))
return function() {
return Rt(e, n2).apply(this, arguments);
};
if (Ve.noDeprecation === true)
return e;
var i = false;
function u() {
if (!i) {
if (Ve.throwDeprecation)
throw new Error(n2);
Ve.traceDeprecation ? console.trace(n2) : console.error(n2), i = true;
}
return e.apply(this, arguments);
}
return u;
}
function Oo(e) {
if (ge(vt) && (vt = Ve.env.NODE_DEBUG || ""), e = e.toUpperCase(), !$e[e])
if (new RegExp("\\b" + e + "\\b", "i").test(vt)) {
var n2 = 0;
$e[e] = function() {
var i = wr.apply(null, arguments);
console.error("%s %d: %s", e, n2, i);
};
} else
$e[e] = function() {
};
return $e[e];
}
function ye(e, n2) {
var i = { seen: [], stylize: sf };
return arguments.length >= 3 && (i.depth = arguments[2]), arguments.length >= 4 && (i.colors = arguments[3]), _r(n2) ? i.showHidden = n2 : n2 && Mt(i, n2), ge(i.showHidden) && (i.showHidden = false), ge(i.depth) && (i.depth = 2), ge(i.colors) && (i.colors = false), ge(i.customInspect) && (i.customInspect = true), i.colors && (i.stylize = nf), mr(i, e, i.depth);
}
function nf(e, n2) {
var i = ye.styles[n2];
return i ? "\x1B[" + ye.colors[i][0] + "m" + e + "\x1B[" + ye.colors[i][1] + "m" : e;
}
function sf(e, n2) {
return e;
}
function of(e) {
var n2 = {};
return e.forEach(function(i, u) {
n2[i] = true;
}), n2;
}
function mr(e, n2, i) {
if (e.customInspect && n2 && Je(n2.inspect) && n2.inspect !== ye && !(n2.constructor && n2.constructor.prototype === n2)) {
var u = n2.inspect(i, e);
return Qe(u) || (u = mr(e, u, i)), u;
}
var o = af(e, n2);
if (o)
return o;
var h = Object.keys(n2), l = of(h);
if (e.showHidden && (h = Object.getOwnPropertyNames(n2)), He(n2) && (h.indexOf("message") >= 0 || h.indexOf("description") >= 0))
return ht(n2);
if (h.length === 0) {
if (Je(n2)) {
var p = n2.name ? ": " + n2.name : "";
return e.stylize("[Function" + p + "]", "special");
}
if (Ge(n2))
return e.stylize(RegExp.prototype.toString.call(n2), "regexp");
if (gr(n2))
return e.stylize(Date.prototype.toString.call(n2), "date");
if (He(n2))
return ht(n2);
}
var m = "", c = false, t = ["{", "}"];
if (Ct(n2) && (c = true, t = ["[", "]"]), Je(n2)) {
var r = n2.name ? ": " + n2.name : "";
m = " [Function" + r + "]";
}
if (Ge(n2) && (m = " " + RegExp.prototype.toString.call(n2)), gr(n2) && (m = " " + Date.prototype.toUTCString.call(n2)), He(n2) && (m = " " + ht(n2)), h.length === 0 && (!c || n2.length == 0))
return t[0] + m + t[1];
if (i < 0)
return Ge(n2) ? e.stylize(RegExp.prototype.toString.call(n2), "regexp") : e.stylize("[Object]", "special");
e.seen.push(n2);
var a;
return c ? a = uf(e, n2, i, l, h) : a = h.map(function(s) {
return xt(e, n2, i, l, s, c);
}), e.seen.pop(), cf(a, m, t);
}
function af(e, n2) {
if (ge(n2))
return e.stylize("undefined", "undefined");
if (Qe(n2)) {
var i = "'" + JSON.stringify(n2).replace(/^"|"$/g, "").replace(/'/g, "\\'").replace(/\\"/g, '"') + "'";
return e.stylize(i, "string");
}
if (Nt(n2))
return e.stylize("" + n2, "number");
if (_r(n2))
return e.stylize("" + n2, "boolean");
if (Ke(n2))
return e.stylize("null", "null");
}
function ht(e) {
return "[" + Error.prototype.toString.call(e) + "]";
}
function uf(e, n2, i, u, o) {
for (var h = [], l = 0, p = n2.length; l < p; ++l)
Io(n2, String(l)) ? h.push(xt(e, n2, i, u, String(l), true)) : h.push("");
return o.forEach(function(m) {
m.match(/^\d+$/) || h.push(xt(e, n2, i, u, m, true));
}), h;
}
function xt(e, n2, i, u, o, h) {
var l, p, m;
if (m = Object.getOwnPropertyDescriptor(n2, o) || { value: n2[o] }, m.get ? m.set ? p = e.stylize("[Getter/Setter]", "special") : p = e.stylize("[Getter]", "special") : m.set && (p = e.stylize("[Setter]", "special")), Io(u, o) || (l = "[" + o + "]"), p || (e.seen.indexOf(m.value) < 0 ? (Ke(i) ? p = mr(e, m.value, null) : p = mr(e, m.value, i - 1), p.indexOf(`
`) > -1 && (h ? p = p.split(`
`).map(function(c) {
return " " + c;
}).join(`
`).substr(2) : p = `
` + p.split(`
`).map(function(c) {
return " " + c;
}).join(`
`))) : p = e.stylize("[Circular]", "special")), ge(l)) {
if (h && o.match(/^\d+$/))
return p;
l = JSON.stringify("" + o), l.match(/^"([a-zA-Z_][a-zA-Z_0-9]*)"$/) ? (l = l.substr(1, l.length - 2), l = e.stylize(l, "name")) : (l = l.replace(/'/g, "\\'").replace(/\\"/g, '"').replace(/(^"|"$)/g, "'"), l = e.stylize(l, "string"));
}
return l + ": " + p;
}
function cf(e, n2, i) {
var u = 0, o = e.reduce(function(h, l) {
return u++, l.indexOf(`
`) >= 0 && u++, h + l.replace(/\u001b\[\d\d?m/g, "").length + 1;
}, 0);
return o > 60 ? i[0] + (n2 === "" ? "" : n2 + `
`) + " " + e.join(`,
`) + " " + i[1] : i[0] + n2 + " " + e.join(", ") + " " + i[1];
}
function Ct(e) {
return Array.isArray(e);
}
function _r(e) {
return typeof e == "boolean";
}
function Ke(e) {
return e === null;
}
function To(e) {
return e == null;
}
function Nt(e) {
return typeof e == "number";
}
function Qe(e) {
return typeof e == "string";
}
function Eo(e) {
return typeof e == "symbol";
}
function ge(e) {
return e === void 0;
}
function Ge(e) {
return je(e) && jt(e) === "[object RegExp]";
}
function je(e) {
return typeof e == "object" && e !== null;
}
function gr(e) {
return je(e) && jt(e) === "[object Date]";
}
function He(e) {
return je(e) && (jt(e) === "[object Error]" || e instanceof Error);
}
function Je(e) {
return typeof e == "function";
}
function qo(e) {
return e === null || typeof e == "boolean" || typeof e == "number" || typeof e == "string" || typeof e == "symbol" || typeof e > "u";
}
function Ao(e) {
return Buffer.isBuffer(e);
}
function jt(e) {
return Object.prototype.toString.call(e);
}
function dt(e) {
return e < 10 ? "0" + e.toString(10) : e.toString(10);
}
function lf() {
var e = new Date(), n2 = [dt(e.getHours()), dt(e.getMinutes()), dt(e.getSeconds())].join(":");
return [e.getDate(), Co[e.getMonth()], n2].join(" ");
}
function Po() {
console.log("%s - %s", lf(), wr.apply(null, arguments));
}
function Mt(e, n2) {
if (!n2 || !je(n2))
return e;
for (var i = Object.keys(n2), u = i.length; u--; )
e[i[u]] = n2[i[u]];
return e;
}
function Io(e, n2) {
return Object.prototype.hasOwnProperty.call(e, n2);
}
var Ro, $e, vt, Co, No, ff = Le({ "node-modules-polyfills:util"() {
A(), rf(), tf(), Ro = /%[sdj%]/g, $e = {}, ye.colors = { bold: [1, 22], italic: [3, 23], underline: [4, 24], inverse: [7, 27], white: [37, 39], grey: [90, 39], black: [30, 39], blue: [34, 39], cyan: [36, 39], green: [32, 39], magenta: [35, 39], red: [31, 39], yellow: [33, 39] }, ye.styles = { special: "cyan", number: "yellow", boolean: "yellow", undefined: "grey", null: "bold", string: "green", date: "magenta", regexp: "red" }, Co = ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"], No = { inherits: It, _extend: Mt, log: Po, isBuffer: Ao, isPrimitive: qo, isFunction: Je, isError: He, isDate: gr, isObject: je, isRegExp: Ge, isUndefined: ge, isSymbol: Eo, isString: Qe, isNumber: Nt, isNullOrUndefined: To, isNull: Ke, isBoolean: _r, isArray: Ct, inspect: ye, deprecate: Rt, format: wr, debuglog: Oo };
} }), pf = P({ "node-modules-polyfills-commonjs:util"(e, n2) {
A();
var i = (ff(), Pt(ko));
if (i && i.default) {
n2.exports = i.default;
for (let u in i)
n2.exports[u] = i[u];
} else
i && (n2.exports = i);
} }), hf = P({ "node_modules/postcss-values-parser/lib/errors/TokenizeError.js"(e, n2) {
"use strict";
A();
var i = class extends Error {
constructor(u) {
super(u), this.name = this.constructor.name, this.message = u || "An error ocurred while tokzenizing.", typeof Error.captureStackTrace == "function" ? Error.captureStackTrace(this, this.constructor) : this.stack = new Error(u).stack;
}
};
n2.exports = i;
} }), df = P({ "node_modules/postcss-values-parser/lib/tokenize.js"(e, n2) {
"use strict";
A();
var i = "{".charCodeAt(0), u = "}".charCodeAt(0), o = "(".charCodeAt(0), h = ")".charCodeAt(0), l = "'".charCodeAt(0), p = '"'.charCodeAt(0), m = "\\".charCodeAt(0), c = "/".charCodeAt(0), t = ".".charCodeAt(0), r = ",".charCodeAt(0), a = ":".charCodeAt(0), s = "*".charCodeAt(0), f = "-".charCodeAt(0), g = "+".charCodeAt(0), v = "#".charCodeAt(0), y = `
`.charCodeAt(0), w = " ".charCodeAt(0), d = "\f".charCodeAt(0), _ = " ".charCodeAt(0), k = "\r".charCodeAt(0), x = "@".charCodeAt(0), N = "e".charCodeAt(0), I = "E".charCodeAt(0), W = "0".charCodeAt(0), $ = "9".charCodeAt(0), H = "u".charCodeAt(0), D = "U".charCodeAt(0), V = /[ \n\t\r\{\(\)'"\\;,/]/g, B = /[ \n\t\r\(\)\{\}\*:;@!&'"\+\|~>,\[\]\\]|\/(?=\*)/g, O = /[ \n\t\r\(\)\{\}\*:;@!&'"\-\+\|~>,\[\]\\]|\//g, j = /^[a-z0-9]/i, C = /^[a-f0-9?\-]/i, R = pf(), X = hf();
n2.exports = function(Q, K) {
K = K || {};
let J = [], M = Q.valueOf(), Y = M.length, G = -1, E = 1, S = 0, b = 0, L = null, q, T, F, z, ee, te, ue, le, re, ne, oe, ie;
function ce(Ze) {
let _e = R.format("Unclosed %s at line: %d, column: %d, token: %d", Ze, E, S - G, S);
throw new X(_e);
}
function fe() {
let Ze = R.format("Syntax error at line: %d, column: %d, token: %d", E, S - G, S);
throw new X(Ze);
}
for (; S < Y; ) {
switch (q = M.charCodeAt(S), q === y && (G = S, E += 1), q) {
case y:
case w:
case _:
case k:
case d:
T = S;
do
T += 1, q = M.charCodeAt(T), q === y && (G = T, E += 1);
while (q === w || q === y || q === _ || q === k || q === d);
J.push(["space", M.slice(S, T), E, S - G, E, T - G, S]), S = T - 1;
break;
case a:
T = S + 1, J.push(["colon", M.slice(S, T), E, S - G, E, T - G, S]), S = T - 1;
break;
case r:
T = S + 1, J.push(["comma", M.slice(S, T), E, S - G, E, T - G, S]), S = T - 1;
break;
case i:
J.push(["{", "{", E, S - G, E, T - G, S]);
break;
case u:
J.push(["}", "}", E, S - G, E, T - G, S]);
break;
case o:
b++, L = !L && b === 1 && J.length > 0 && J[J.length - 1][0] === "word" && J[J.length - 1][1] === "url", J.push(["(", "(", E, S - G, E, T - G, S]);
break;
case h:
b--, L = L && b > 0, J.push([")", ")", E, S - G, E, T - G, S]);
break;
case l:
case p:
F = q === l ? "'" : '"', T = S;
do
for (ne = false, T = M.indexOf(F, T + 1), T === -1 && ce("quote", F), oe = T; M.charCodeAt(oe - 1) === m; )
oe -= 1, ne = !ne;
while (ne);
J.push(["string", M.slice(S, T + 1), E, S - G, E, T - G, S]), S = T;
break;
case x:
V.lastIndex = S + 1, V.test(M), V.lastIndex === 0 ? T = M.length - 1 : T = V.lastIndex - 2, J.push(["atword", M.slice(S, T + 1), E, S - G, E, T - G, S]), S = T;
break;
case m:
T = S, q = M.charCodeAt(T + 1), ue && q !== c && q !== w && q !== y && q !== _ && q !== k && q !== d && (T += 1), J.push(["word", M.slice(S, T + 1), E, S - G, E, T - G, S]), S = T;
break;
case g:
case f:
case s:
T = S + 1, ie = M.slice(S + 1, T + 1);
let Ze = M.slice(S - 1, S);
if (q === f && ie.charCodeAt(0) === f) {
T++, J.push(["word", M.slice(S, T), E, S - G, E, T - G, S]), S = T - 1;
break;
}
J.push(["operator", M.slice(S, T), E, S - G, E, T - G, S]), S = T - 1;
break;
default:
if (q === c && (M.charCodeAt(S + 1) === s || K.loose && !L && M.charCodeAt(S + 1) === c)) {
if (M.charCodeAt(S + 1) === s)
T = M.indexOf("*/", S + 2) + 1, T === 0 && ce("comment", "*/");
else {
let Be = M.indexOf(`
`, S + 2);
T = Be !== -1 ? Be - 1 : Y;
}
te = M.slice(S, T + 1), z = te.split(`
`), ee = z.length - 1, ee > 0 ? (le = E + ee, re = T - z[ee].length) : (le = E, re = G), J.push(["comment", te, E, S - G, le, T - re, S]), G = re, E = le, S = T;
} else if (q === v && !j.test(M.slice(S + 1, S + 2)))
T = S + 1, J.push(["#", M.slice(S, T), E, S - G, E, T - G, S]), S = T - 1;
else if ((q === H || q === D) && M.charCodeAt(S + 1) === g) {
T = S + 2;
do
T += 1, q = M.charCodeAt(T);
while (T < Y && C.test(M.slice(T, T + 1)));
J.push(["unicoderange", M.slice(S, T), E, S - G, E, T - G, S]), S = T - 1;
} else if (q === c)
T = S + 1, J.push(["operator", M.slice(S, T), E, S - G, E, T - G, S]), S = T - 1;
else {
let _e = B;
if (q >= W && q <= $ && (_e = O), _e.lastIndex = S + 1, _e.test(M), _e.lastIndex === 0 ? T = M.length - 1 : T = _e.lastIndex - 2, _e === O || q === t) {
let Be = M.charCodeAt(T), Wt = M.charCodeAt(T + 1), Vt = M.charCodeAt(T + 2);
(Be === N || Be === I) && (Wt === f || Wt === g) && Vt >= W && Vt <= $ && (O.lastIndex = T + 2, O.test(M), O.lastIndex === 0 ? T = M.length - 1 : T = O.lastIndex - 2);
}
J.push(["word", M.slice(S, T + 1), E, S - G, E, T - G, S]), S = T;
}
break;
}
S++;
}
return J;
};
} }), jo = P({ "node_modules/flatten/index.js"(e, n2) {
A(), n2.exports = function(u, o) {
if (o = typeof o == "number" ? o : 1 / 0, !o)
return Array.isArray(u) ? u.map(function(l) {
return l;
}) : u;
return h(u, 1);
function h(l, p) {
return l.reduce(function(m, c) {
return Array.isArray(c) && p < o ? m.concat(h(c, p + 1)) : m.concat(c);
}, []);
}
};
} }), Mo = P({ "node_modules/indexes-of/index.js"(e, n2) {
A(), n2.exports = function(i, u) {
for (var o = -1, h = []; (o = i.indexOf(u, o + 1)) !== -1; )
h.push(o);
return h;
};
} }), Do = P({ "node_modules/uniq/uniq.js"(e, n2) {
"use strict";
A();
function i(h, l) {
for (var p = 1, m = h.length, c = h[0], t = h[0], r = 1; r < m; ++r)
if (t = c, c = h[r], l(c, t)) {
if (r === p) {
p++;
continue;
}
h[p++] = c;
}
return h.length = p, h;
}
function u(h) {
for (var l = 1, p = h.length, m = h[0], c = h[0], t = 1; t < p; ++t, c = m)
if (c = m, m = h[t], m !== c) {
if (t === l) {
l++;
continue;
}
h[l++] = m;
}
return h.length = l, h;
}
function o(h, l, p) {
return h.length === 0 ? h : l ? (p || h.sort(l), i(h, l)) : (p || h.sort(), u(h));
}
n2.exports = o;
} }), vf = P({ "node_modules/postcss-values-parser/lib/errors/ParserError.js"(e, n2) {
"use strict";
A();
var i = class extends Error {
constructor(u) {
super(u), this.name = this.constructor.name, this.message = u || "An error ocurred while parsing.", typeof Error.captureStackTrace == "function" ? Error.captureStackTrace(this, this.constructor) : this.stack = new Error(u).stack;
}
};
n2.exports = i;
} }), mf = P({ "node_modules/postcss-values-parser/lib/parser.js"(e, n2) {
"use strict";
A();
var i = Vl(), u = io(), o = so(), h = oo(), l = ao(), p = uo(), m = co(), c = lo(), t = fo(), r = po(), a = ho(), s = vo(), f = mo(), g = df(), v = jo(), y = Mo(), w = Do(), d = vf();
function _(k) {
return k.sort((x, N) => x - N);
}
n2.exports = class {
constructor(x, N) {
let I = { loose: false };
this.cache = [], this.input = x, this.options = Object.assign({}, I, N), this.position = 0, this.unbalanced = 0, this.root = new i();
let W = new u();
this.root.append(W), this.current = W, this.tokens = g(x, this.options);
}
parse() {
return this.loop();
}
colon() {
let x = this.currToken;
this.newNode(new h({ value: x[1], source: { start: { line: x[2], column: x[3] }, end: { line: x[4], column: x[5] } }, sourceIndex: x[6] })), this.position++;
}
comma() {
let x = this.currToken;
this.newNode(new l({ value: x[1], source: { start: { line: x[2], column: x[3] }, end: { line: x[4], column: x[5] } }, sourceIndex: x[6] })), this.position++;
}
comment() {
let x = false, N = this.currToken[1].replace(/\/\*|\*\//g, ""), I;
this.options.loose && N.startsWith("//") && (N = N.substring(2), x = true), I = new p({ value: N, inline: x, source: { start: { line: this.currToken[2], column: this.currToken[3] }, end: { line: this.currToken[4], column: this.currToken[5] } }, sourceIndex: this.currToken[6] }), this.newNode(I), this.position++;
}
error(x, N) {
throw new d(x + ` at line: ${N[2]}, column ${N[3]}`);
}
loop() {
for (; this.position < this.tokens.length; )
this.parseTokens();
return !this.current.last && this.spaces ? this.current.raws.before += this.spaces : this.spaces && (this.current.last.raws.after += this.spaces), this.spaces = "", this.root;
}
operator() {
let x = this.currToken[1], N;
if (x === "+" || x === "-") {
if (this.options.loose || this.position > 0 && (this.current.type === "func" && this.current.value === "calc" ? this.prevToken[0] !== "space" && this.prevToken[0] !== "(" ? this.error("Syntax Error", this.currToken) : this.nextToken[0] !== "space" && this.nextToken[0] !== "word" ? this.error("Syntax Error", this.currToken) : this.nextToken[0] === "word" && this.current.last.type !== "operator" && this.current.last.value !== "(" && this.error("Syntax Error", this.currToken) : (this.nextToken[0] === "space" || this.nextToken[0] === "operator" || this.prevToken[0] === "operator") && this.error("Syntax Error", this.currToken)), this.options.loose) {
if ((!this.current.nodes.length || this.current.last && this.current.last.type === "operator") && this.nextToken[0] === "word")
return this.word();
} else if (this.nextToken[0] === "word")
return this.word();
}
return N = new t({ value: this.currToken[1], source: { start: { line: this.currToken[2], column: this.currToken[3] }, end: { line: this.currToken[2], column: this.currToken[3] } }, sourceIndex: this.currToken[4] }), this.position++, this.newNode(N);
}
parseTokens() {
switch (this.currToken[0]) {
case "space":
this.space();
break;
case "colon":
this.colon();
break;
case "comma":
this.comma();
break;
case "comment":
this.comment();
break;
case "(":
this.parenOpen();
break;
case ")":
this.parenClose();
break;
case "atword":
case "word":
this.word();
break;
case "operator":
this.operator();
break;
case "string":
this.string();
break;
case "unicoderange":
this.unicodeRange();
break;
default:
this.word();
break;
}
}
parenOpen() {
let x = 1, N = this.position + 1, I = this.currToken, W;
for (; N < this.tokens.length && x; ) {
let $ = this.tokens[N];
$[0] === "(" && x++, $[0] === ")" && x--, N++;
}
if (x && this.error("Expected closing parenthesis", I), W = this.current.last, W && W.type === "func" && W.unbalanced < 0 && (W.unbalanced = 0, this.current = W), this.current.unbalanced++, this.newNode(new r({ value: I[1], source: { start: { line: I[2], column: I[3] }, end: { line: I[4], column: I[5] } }, sourceIndex: I[6] })), this.position++, this.current.type === "func" && this.current.unbalanced && this.current.value === "url" && this.currToken[0] !== "string" && this.currToken[0] !== ")" && !this.options.loose) {
let $ = this.nextToken, H = this.currToken[1], D = { line: this.currToken[2], column: this.currToken[3] };
for (; $ && $[0] !== ")" && this.current.unbalanced; )
this.position++, H += this.currToken[1], $ = this.nextToken;
this.position !== this.tokens.length - 1 && (this.position++, this.newNode(new s({ value: H, source: { start: D, end: { line: this.currToken[4], column: this.currToken[5] } }, sourceIndex: this.currToken[6] })));
}
}
parenClose() {
let x = this.currToken;
this.newNode(new r({ value: x[1], source: { start: { line: x[2], column: x[3] }, end: { line: x[4], column: x[5] } }, sourceIndex: x[6] })), this.position++, !(this.position >= this.tokens.length - 1 && !this.current.unbalanced) && (this.current.unbalanced--, this.current.unbalanced < 0 && this.error("Expected opening parenthesis", x), !this.current.unbalanced && this.cache.length && (this.current = this.cache.pop()));
}
space() {
let x = this.currToken;
this.position === this.tokens.length - 1 || this.nextToken[0] === "," || this.nextToken[0] === ")" ? (this.current.last.raws.after += x[1], this.position++) : (this.spaces = x[1], this.position++);
}
unicodeRange() {
let x = this.currToken;
this.newNode(new f({ value: x[1], source: { start: { line: x[2], column: x[3] }, end: { line: x[4], column: x[5] } }, sourceIndex: x[6] })), this.position++;
}
splitWord() {
let x = this.nextToken, N = this.currToken[1], I = /^[\+\-]?((\d+(\.\d*)?)|(\.\d+))([eE][\+\-]?\d+)?/, W = /^(?!\#([a-z0-9]+))[\#\{\}]/gi, $, H;
if (!W.test(N))
for (; x && x[0] === "word"; ) {
this.position++;
let D = this.currToken[1];
N += D, x = this.nextToken;
}
$ = y(N, "@"), H = _(w(v([[0], $]))), H.forEach((D, V) => {
let B = H[V + 1] || N.length, O = N.slice(D, B), j;
if (~$.indexOf(D))
j = new o({ value: O.slice(1), source: { start: { line: this.currToken[2], column: this.currToken[3] + D }, end: { line: this.currToken[4], column: this.currToken[3] + (B - 1) } }, sourceIndex: this.currToken[6] + H[V] });
else if (I.test(this.currToken[1])) {
let C = O.replace(I, "");
j = new c({ value: O.replace(C, ""), source: { start: { line: this.currToken[2], column: this.currToken[3] + D }, end: { line: this.currToken[4], column: this.currToken[3] + (B - 1) } }, sourceIndex: this.currToken[6] + H[V], unit: C });
} else
j = new (x && x[0] === "(" ? m : s)({ value: O, source: { start: { line: this.currToken[2], column: this.currToken[3] + D }, end: { line: this.currToken[4], column: this.currToken[3] + (B - 1) } }, sourceIndex: this.currToken[6] + H[V] }), j.type === "word" ? (j.isHex = /^#(.+)/.test(O), j.isColor = /^#([0-9a-f]{3}|[0-9a-f]{4}|[0-9a-f]{6}|[0-9a-f]{8})$/i.test(O)) : this.cache.push(this.current);
this.newNode(j);
}), this.position++;
}
string() {
let x = this.currToken, N = this.currToken[1], I = /^(\"|\')/, W = I.test(N), $ = "", H;
W && ($ = N.match(I)[0], N = N.slice(1, N.length - 1)), H = new a({ value: N, source: { start: { line: x[2], column: x[3] }, end: { line: x[4], column: x[5] } }, sourceIndex: x[6], quoted: W }), H.raws.quote = $, this.newNode(H), this.position++;
}
word() {
return this.splitWord();
}
newNode(x) {
return this.spaces && (x.raws.before += this.spaces, this.spaces = ""), this.current.append(x);
}
get currToken() {
return this.tokens[this.position];
}
get nextToken() {
return this.tokens[this.position + 1];
}
get prevToken() {
return this.tokens[this.position - 1];
}
};
} }), gf = P({ "node_modules/postcss-values-parser/lib/index.js"(e, n2) {
"use strict";
A();
var i = mf(), u = so(), o = oo(), h = ao(), l = uo(), p = co(), m = lo(), c = fo(), t = po(), r = ho(), a = mo(), s = io(), f = vo(), g = function(v, y) {
return new i(v, y);
};
g.atword = function(v) {
return new u(v);
}, g.colon = function(v) {
return new o(Object.assign({ value: ":" }, v));
}, g.comma = function(v) {
return new h(Object.assign({ value: "," }, v));
}, g.comment = function(v) {
return new l(v);
}, g.func = function(v) {
return new p(v);
}, g.number = function(v) {
return new m(v);
}, g.operator = function(v) {
return new c(v);
}, g.paren = function(v) {
return new t(Object.assign({ value: "(" }, v));
}, g.string = function(v) {
return new r(Object.assign({ quote: "'" }, v));
}, g.value = function(v) {
return new s(v);
}, g.word = function(v) {
return new f(v);
}, g.unicodeRange = function(v) {
return new a(v);
}, n2.exports = g;
} }), ze = P({ "node_modules/postcss-selector-parser/dist/selectors/node.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = typeof Symbol == "function" && typeof Symbol.iterator == "symbol" ? function(l) {
return typeof l;
} : function(l) {
return l && typeof Symbol == "function" && l.constructor === Symbol && l !== Symbol.prototype ? "symbol" : typeof l;
};
function u(l, p) {
if (!(l instanceof p))
throw new TypeError("Cannot call a class as a function");
}
var o = function l(p, m) {
if ((typeof p > "u" ? "undefined" : i(p)) !== "object")
return p;
var c = new p.constructor();
for (var t in p)
if (p.hasOwnProperty(t)) {
var r = p[t], a = typeof r > "u" ? "undefined" : i(r);
t === "parent" && a === "object" ? m && (c[t] = m) : r instanceof Array ? c[t] = r.map(function(s) {
return l(s, c);
}) : c[t] = l(r, c);
}
return c;
}, h = function() {
function l() {
var p = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {};
u(this, l);
for (var m in p)
this[m] = p[m];
var c = p.spaces;
c = c === void 0 ? {} : c;
var t = c.before, r = t === void 0 ? "" : t, a = c.after, s = a === void 0 ? "" : a;
this.spaces = { before: r, after: s };
}
return l.prototype.remove = function() {
return this.parent && this.parent.removeChild(this), this.parent = void 0, this;
}, l.prototype.replaceWith = function() {
if (this.parent) {
for (var m in arguments)
this.parent.insertBefore(this, arguments[m]);
this.remove();
}
return this;
}, l.prototype.next = function() {
return this.parent.at(this.parent.index(this) + 1);
}, l.prototype.prev = function() {
return this.parent.at(this.parent.index(this) - 1);
}, l.prototype.clone = function() {
var m = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {}, c = o(this);
for (var t in m)
c[t] = m[t];
return c;
}, l.prototype.toString = function() {
return [this.spaces.before, String(this.value), this.spaces.after].join("");
}, l;
}();
e.default = h, n2.exports = e.default;
} }), se = P({ "node_modules/postcss-selector-parser/dist/selectors/types.js"(e) {
"use strict";
A(), e.__esModule = true;
var n2 = e.TAG = "tag", i = e.STRING = "string", u = e.SELECTOR = "selector", o = e.ROOT = "root", h = e.PSEUDO = "pseudo", l = e.NESTING = "nesting", p = e.ID = "id", m = e.COMMENT = "comment", c = e.COMBINATOR = "combinator", t = e.CLASS = "class", r = e.ATTRIBUTE = "attribute", a = e.UNIVERSAL = "universal";
} }), Dt = P({ "node_modules/postcss-selector-parser/dist/selectors/container.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = function() {
function s(f, g) {
for (var v = 0; v < g.length; v++) {
var y = g[v];
y.enumerable = y.enumerable || false, y.configurable = true, "value" in y && (y.writable = true), Object.defineProperty(f, y.key, y);
}
}
return function(f, g, v) {
return g && s(f.prototype, g), v && s(f, v), f;
};
}(), u = ze(), o = m(u), h = se(), l = p(h);
function p(s) {
if (s && s.__esModule)
return s;
var f = {};
if (s != null)
for (var g in s)
Object.prototype.hasOwnProperty.call(s, g) && (f[g] = s[g]);
return f.default = s, f;
}
function m(s) {
return s && s.__esModule ? s : { default: s };
}
function c(s, f) {
if (!(s instanceof f))
throw new TypeError("Cannot call a class as a function");
}
function t(s, f) {
if (!s)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return f && (typeof f == "object" || typeof f == "function") ? f : s;
}
function r(s, f) {
if (typeof f != "function" && f !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof f);
s.prototype = Object.create(f && f.prototype, { constructor: { value: s, enumerable: false, writable: true, configurable: true } }), f && (Object.setPrototypeOf ? Object.setPrototypeOf(s, f) : s.__proto__ = f);
}
var a = function(s) {
r(f, s);
function f(g) {
c(this, f);
var v = t(this, s.call(this, g));
return v.nodes || (v.nodes = []), v;
}
return f.prototype.append = function(v) {
return v.parent = this, this.nodes.push(v), this;
}, f.prototype.prepend = function(v) {
return v.parent = this, this.nodes.unshift(v), this;
}, f.prototype.at = function(v) {
return this.nodes[v];
}, f.prototype.index = function(v) {
return typeof v == "number" ? v : this.nodes.indexOf(v);
}, f.prototype.removeChild = function(v) {
v = this.index(v), this.at(v).parent = void 0, this.nodes.splice(v, 1);
var y = void 0;
for (var w in this.indexes)
y = this.indexes[w], y >= v && (this.indexes[w] = y - 1);
return this;
}, f.prototype.removeAll = function() {
for (var w = this.nodes, v = Array.isArray(w), y = 0, w = v ? w : w[Symbol.iterator](); ; ) {
var d;
if (v) {
if (y >= w.length)
break;
d = w[y++];
} else {
if (y = w.next(), y.done)
break;
d = y.value;
}
var _ = d;
_.parent = void 0;
}
return this.nodes = [], this;
}, f.prototype.empty = function() {
return this.removeAll();
}, f.prototype.insertAfter = function(v, y) {
var w = this.index(v);
this.nodes.splice(w + 1, 0, y);
var d = void 0;
for (var _ in this.indexes)
d = this.indexes[_], w <= d && (this.indexes[_] = d + this.nodes.length);
return this;
}, f.prototype.insertBefore = function(v, y) {
var w = this.index(v);
this.nodes.splice(w, 0, y);
var d = void 0;
for (var _ in this.indexes)
d = this.indexes[_], w <= d && (this.indexes[_] = d + this.nodes.length);
return this;
}, f.prototype.each = function(v) {
this.lastEach || (this.lastEach = 0), this.indexes || (this.indexes = {}), this.lastEach++;
var y = this.lastEach;
if (this.indexes[y] = 0, !!this.length) {
for (var w = void 0, d = void 0; this.indexes[y] < this.length && (w = this.indexes[y], d = v(this.at(w), w), d !== false); )
this.indexes[y] += 1;
if (delete this.indexes[y], d === false)
return false;
}
}, f.prototype.walk = function(v) {
return this.each(function(y, w) {
var d = v(y, w);
if (d !== false && y.length && (d = y.walk(v)), d === false)
return false;
});
}, f.prototype.walkAttributes = function(v) {
var y = this;
return this.walk(function(w) {
if (w.type === l.ATTRIBUTE)
return v.call(y, w);
});
}, f.prototype.walkClasses = function(v) {
var y = this;
return this.walk(function(w) {
if (w.type === l.CLASS)
return v.call(y, w);
});
}, f.prototype.walkCombinators = function(v) {
var y = this;
return this.walk(function(w) {
if (w.type === l.COMBINATOR)
return v.call(y, w);
});
}, f.prototype.walkComments = function(v) {
var y = this;
return this.walk(function(w) {
if (w.type === l.COMMENT)
return v.call(y, w);
});
}, f.prototype.walkIds = function(v) {
var y = this;
return this.walk(function(w) {
if (w.type === l.ID)
return v.call(y, w);
});
}, f.prototype.walkNesting = function(v) {
var y = this;
return this.walk(function(w) {
if (w.type === l.NESTING)
return v.call(y, w);
});
}, f.prototype.walkPseudos = function(v) {
var y = this;
return this.walk(function(w) {
if (w.type === l.PSEUDO)
return v.call(y, w);
});
}, f.prototype.walkTags = function(v) {
var y = this;
return this.walk(function(w) {
if (w.type === l.TAG)
return v.call(y, w);
});
}, f.prototype.walkUniversals = function(v) {
var y = this;
return this.walk(function(w) {
if (w.type === l.UNIVERSAL)
return v.call(y, w);
});
}, f.prototype.split = function(v) {
var y = this, w = [];
return this.reduce(function(d, _, k) {
var x = v.call(y, _);
return w.push(_), x ? (d.push(w), w = []) : k === y.length - 1 && d.push(w), d;
}, []);
}, f.prototype.map = function(v) {
return this.nodes.map(v);
}, f.prototype.reduce = function(v, y) {
return this.nodes.reduce(v, y);
}, f.prototype.every = function(v) {
return this.nodes.every(v);
}, f.prototype.some = function(v) {
return this.nodes.some(v);
}, f.prototype.filter = function(v) {
return this.nodes.filter(v);
}, f.prototype.sort = function(v) {
return this.nodes.sort(v);
}, f.prototype.toString = function() {
return this.map(String).join("");
}, i(f, [{ key: "first", get: function() {
return this.at(0);
} }, { key: "last", get: function() {
return this.at(this.length - 1);
} }, { key: "length", get: function() {
return this.nodes.length;
} }]), f;
}(o.default);
e.default = a, n2.exports = e.default;
} }), Lo = P({ "node_modules/postcss-selector-parser/dist/selectors/root.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = Dt(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.ROOT, s;
}
return r.prototype.toString = function() {
var s = this.reduce(function(f, g) {
var v = String(g);
return v ? f + v + "," : "";
}, "").slice(0, -1);
return this.trailingComma ? s + "," : s;
}, r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), zo = P({ "node_modules/postcss-selector-parser/dist/selectors/selector.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = Dt(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.SELECTOR, s;
}
return r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), Ye = P({ "node_modules/postcss-selector-parser/dist/selectors/namespace.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = function() {
function t(r, a) {
for (var s = 0; s < a.length; s++) {
var f = a[s];
f.enumerable = f.enumerable || false, f.configurable = true, "value" in f && (f.writable = true), Object.defineProperty(r, f.key, f);
}
}
return function(r, a, s) {
return a && t(r.prototype, a), s && t(r, s), r;
};
}(), u = ze(), o = h(u);
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r() {
return l(this, r), p(this, t.apply(this, arguments));
}
return r.prototype.toString = function() {
return [this.spaces.before, this.ns, String(this.value), this.spaces.after].join("");
}, i(r, [{ key: "ns", get: function() {
var s = this.namespace;
return s ? (typeof s == "string" ? s : "") + "|" : "";
} }]), r;
}(o.default);
e.default = c, n2.exports = e.default;
} }), Bo = P({ "node_modules/postcss-selector-parser/dist/selectors/className.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = Ye(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.CLASS, s;
}
return r.prototype.toString = function() {
return [this.spaces.before, this.ns, String("." + this.value), this.spaces.after].join("");
}, r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), Fo = P({ "node_modules/postcss-selector-parser/dist/selectors/comment.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = ze(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.COMMENT, s;
}
return r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), Uo = P({ "node_modules/postcss-selector-parser/dist/selectors/id.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = Ye(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.ID, s;
}
return r.prototype.toString = function() {
return [this.spaces.before, this.ns, String("#" + this.value), this.spaces.after].join("");
}, r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), $o = P({ "node_modules/postcss-selector-parser/dist/selectors/tag.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = Ye(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.TAG, s;
}
return r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), Wo = P({ "node_modules/postcss-selector-parser/dist/selectors/string.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = ze(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.STRING, s;
}
return r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), Vo = P({ "node_modules/postcss-selector-parser/dist/selectors/pseudo.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = Dt(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.PSEUDO, s;
}
return r.prototype.toString = function() {
var s = this.length ? "(" + this.map(String).join(",") + ")" : "";
return [this.spaces.before, String(this.value), s, this.spaces.after].join("");
}, r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), Go = P({ "node_modules/postcss-selector-parser/dist/selectors/attribute.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = Ye(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.ATTRIBUTE, s.raws = {}, s;
}
return r.prototype.toString = function() {
var s = [this.spaces.before, "[", this.ns, this.attribute];
return this.operator && s.push(this.operator), this.value && s.push(this.value), this.raws.insensitive ? s.push(this.raws.insensitive) : this.insensitive && s.push(" i"), s.push("]"), s.concat(this.spaces.after).join("");
}, r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), Ho = P({ "node_modules/postcss-selector-parser/dist/selectors/universal.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = Ye(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.UNIVERSAL, s.value = "*", s;
}
return r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), Jo = P({ "node_modules/postcss-selector-parser/dist/selectors/combinator.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = ze(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.COMBINATOR, s;
}
return r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), Ko = P({ "node_modules/postcss-selector-parser/dist/selectors/nesting.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = ze(), u = h(i), o = se();
function h(t) {
return t && t.__esModule ? t : { default: t };
}
function l(t, r) {
if (!(t instanceof r))
throw new TypeError("Cannot call a class as a function");
}
function p(t, r) {
if (!t)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return r && (typeof r == "object" || typeof r == "function") ? r : t;
}
function m(t, r) {
if (typeof r != "function" && r !== null)
throw new TypeError("Super expression must either be null or a function, not " + typeof r);
t.prototype = Object.create(r && r.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }), r && (Object.setPrototypeOf ? Object.setPrototypeOf(t, r) : t.__proto__ = r);
}
var c = function(t) {
m(r, t);
function r(a) {
l(this, r);
var s = p(this, t.call(this, a));
return s.type = o.NESTING, s.value = "&", s;
}
return r;
}(u.default);
e.default = c, n2.exports = e.default;
} }), yf = P({ "node_modules/postcss-selector-parser/dist/sortAscending.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = i;
function i(u) {
return u.sort(function(o, h) {
return o - h;
});
}
n2.exports = e.default;
} }), wf = P({ "node_modules/postcss-selector-parser/dist/tokenize.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = H;
var i = 39, u = 34, o = 92, h = 47, l = 10, p = 32, m = 12, c = 9, t = 13, r = 43, a = 62, s = 126, f = 124, g = 44, v = 40, y = 41, w = 91, d = 93, _ = 59, k = 42, x = 58, N = 38, I = 64, W = /[ \n\t\r\{\(\)'"\\;/]/g, $ = /[ \n\t\r\(\)\*:;@!&'"\+\|~>,\[\]\\]|\/(?=\*)/g;
function H(D) {
for (var V = [], B = D.css.valueOf(), O = void 0, j = void 0, C = void 0, R = void 0, X = void 0, Z = void 0, Q = void 0, K = void 0, J = void 0, M = void 0, Y = void 0, G = B.length, E = -1, S = 1, b = 0, L = function(T, F) {
if (D.safe)
B += F, j = B.length - 1;
else
throw D.error("Unclosed " + T, S, b - E, b);
}; b < G; ) {
switch (O = B.charCodeAt(b), O === l && (E = b, S += 1), O) {
case l:
case p:
case c:
case t:
case m:
j = b;
do
j += 1, O = B.charCodeAt(j), O === l && (E = j, S += 1);
while (O === p || O === l || O === c || O === t || O === m);
V.push(["space", B.slice(b, j), S, b - E, b]), b = j - 1;
break;
case r:
case a:
case s:
case f:
j = b;
do
j += 1, O = B.charCodeAt(j);
while (O === r || O === a || O === s || O === f);
V.push(["combinator", B.slice(b, j), S, b - E, b]), b = j - 1;
break;
case k:
V.push(["*", "*", S, b - E, b]);
break;
case N:
V.push(["&", "&", S, b - E, b]);
break;
case g:
V.push([",", ",", S, b - E, b]);
break;
case w:
V.push(["[", "[", S, b - E, b]);
break;
case d:
V.push(["]", "]", S, b - E, b]);
break;
case x:
V.push([":", ":", S, b - E, b]);
break;
case _:
V.push([";", ";", S, b - E, b]);
break;
case v:
V.push(["(", "(", S, b - E, b]);
break;
case y:
V.push([")", ")", S, b - E, b]);
break;
case i:
case u:
C = O === i ? "'" : '"', j = b;
do
for (M = false, j = B.indexOf(C, j + 1), j === -1 && L("quote", C), Y = j; B.charCodeAt(Y - 1) === o; )
Y -= 1, M = !M;
while (M);
V.push(["string", B.slice(b, j + 1), S, b - E, S, j - E, b]), b = j;
break;
case I:
W.lastIndex = b + 1, W.test(B), W.lastIndex === 0 ? j = B.length - 1 : j = W.lastIndex - 2, V.push(["at-word", B.slice(b, j + 1), S, b - E, S, j - E, b]), b = j;
break;
case o:
for (j = b, Q = true; B.charCodeAt(j + 1) === o; )
j += 1, Q = !Q;
O = B.charCodeAt(j + 1), Q && O !== h && O !== p && O !== l && O !== c && O !== t && O !== m && (j += 1), V.push(["word", B.slice(b, j + 1), S, b - E, S, j - E, b]), b = j;
break;
default:
O === h && B.charCodeAt(b + 1) === k ? (j = B.indexOf("*/", b + 2) + 1, j === 0 && L("comment", "*/"), Z = B.slice(b, j + 1), R = Z.split(`
`), X = R.length - 1, X > 0 ? (K = S + X, J = j - R[X].length) : (K = S, J = E), V.push(["comment", Z, S, b - E, K, j - J, b]), E = J, S = K, b = j) : ($.lastIndex = b + 1, $.test(B), $.lastIndex === 0 ? j = B.length - 1 : j = $.lastIndex - 2, V.push(["word", B.slice(b, j + 1), S, b - E, S, j - E, b]), b = j);
break;
}
b++;
}
return V;
}
n2.exports = e.default;
} }), _f = P({ "node_modules/postcss-selector-parser/dist/parser.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = function() {
function E(S, b) {
for (var L = 0; L < b.length; L++) {
var q = b[L];
q.enumerable = q.enumerable || false, q.configurable = true, "value" in q && (q.writable = true), Object.defineProperty(S, q.key, q);
}
}
return function(S, b, L) {
return b && E(S.prototype, b), L && E(S, L), S;
};
}(), u = jo(), o = M(u), h = Mo(), l = M(h), p = Do(), m = M(p), c = Lo(), t = M(c), r = zo(), a = M(r), s = Bo(), f = M(s), g = Fo(), v = M(g), y = Uo(), w = M(y), d = $o(), _ = M(d), k = Wo(), x = M(k), N = Vo(), I = M(N), W = Go(), $ = M(W), H = Ho(), D = M(H), V = Jo(), B = M(V), O = Ko(), j = M(O), C = yf(), R = M(C), X = wf(), Z = M(X), Q = se(), K = J(Q);
function J(E) {
if (E && E.__esModule)
return E;
var S = {};
if (E != null)
for (var b in E)
Object.prototype.hasOwnProperty.call(E, b) && (S[b] = E[b]);
return S.default = E, S;
}
function M(E) {
return E && E.__esModule ? E : { default: E };
}
function Y(E, S) {
if (!(E instanceof S))
throw new TypeError("Cannot call a class as a function");
}
var G = function() {
function E(S) {
Y(this, E), this.input = S, this.lossy = S.options.lossless === false, this.position = 0, this.root = new t.default();
var b = new a.default();
return this.root.append(b), this.current = b, this.lossy ? this.tokens = (0, Z.default)({ safe: S.safe, css: S.css.trim() }) : this.tokens = (0, Z.default)(S), this.loop();
}
return E.prototype.attribute = function() {
var b = "", L = void 0, q = this.currToken;
for (this.position++; this.position < this.tokens.length && this.currToken[0] !== "]"; )
b += this.tokens[this.position][1], this.position++;
this.position === this.tokens.length && !~b.indexOf("]") && this.error("Expected a closing square bracket.");
var T = b.split(/((?:[*~^$|]?=))([^]*)/), F = T[0].split(/(\|)/g), z = { operator: T[1], value: T[2], source: { start: { line: q[2], column: q[3] }, end: { line: this.currToken[2], column: this.currToken[3] } }, sourceIndex: q[4] };
if (F.length > 1 ? (F[0] === "" && (F[0] = true), z.attribute = this.parseValue(F[2]), z.namespace = this.parseNamespace(F[0])) : z.attribute = this.parseValue(T[0]), L = new $.default(z), T[2]) {
var ee = T[2].split(/(\s+i\s*?)$/), te = ee[0].trim();
L.value = this.lossy ? te : ee[0], ee[1] && (L.insensitive = true, this.lossy || (L.raws.insensitive = ee[1])), L.quoted = te[0] === "'" || te[0] === '"', L.raws.unquoted = L.quoted ? te.slice(1, -1) : te;
}
this.newNode(L), this.position++;
}, E.prototype.combinator = function() {
if (this.currToken[1] === "|")
return this.namespace();
for (var b = new B.default({ value: "", source: { start: { line: this.currToken[2], column: this.currToken[3] }, end: { line: this.currToken[2], column: this.currToken[3] } }, sourceIndex: this.currToken[4] }); this.position < this.tokens.length && this.currToken && (this.currToken[0] === "space" || this.currToken[0] === "combinator"); )
this.nextToken && this.nextToken[0] === "combinator" ? (b.spaces.before = this.parseSpace(this.currToken[1]), b.source.start.line = this.nextToken[2], b.source.start.column = this.nextToken[3], b.source.end.column = this.nextToken[3], b.source.end.line = this.nextToken[2], b.sourceIndex = this.nextToken[4]) : this.prevToken && this.prevToken[0] === "combinator" ? b.spaces.after = this.parseSpace(this.currToken[1]) : this.currToken[0] === "combinator" ? b.value = this.currToken[1] : this.currToken[0] === "space" && (b.value = this.parseSpace(this.currToken[1], " ")), this.position++;
return this.newNode(b);
}, E.prototype.comma = function() {
if (this.position === this.tokens.length - 1) {
this.root.trailingComma = true, this.position++;
return;
}
var b = new a.default();
this.current.parent.append(b), this.current = b, this.position++;
}, E.prototype.comment = function() {
var b = new v.default({ value: this.currToken[1], source: { start: { line: this.currToken[2], column: this.currToken[3] }, end: { line: this.currToken[4], column: this.currToken[5] } }, sourceIndex: this.currToken[6] });
this.newNode(b), this.position++;
}, E.prototype.error = function(b) {
throw new this.input.error(b);
}, E.prototype.missingBackslash = function() {
return this.error("Expected a backslash preceding the semicolon.");
}, E.prototype.missingParenthesis = function() {
return this.error("Expected opening parenthesis.");
}, E.prototype.missingSquareBracket = function() {
return this.error("Expected opening square bracket.");
}, E.prototype.namespace = function() {
var b = this.prevToken && this.prevToken[1] || true;
if (this.nextToken[0] === "word")
return this.position++, this.word(b);
if (this.nextToken[0] === "*")
return this.position++, this.universal(b);
}, E.prototype.nesting = function() {
this.newNode(new j.default({ value: this.currToken[1], source: { start: { line: this.currToken[2], column: this.currToken[3] }, end: { line: this.currToken[2], column: this.currToken[3] } }, sourceIndex: this.currToken[4] })), this.position++;
}, E.prototype.parentheses = function() {
var b = this.current.last;
if (b && b.type === K.PSEUDO) {
var L = new a.default(), q = this.current;
b.append(L), this.current = L;
var T = 1;
for (this.position++; this.position < this.tokens.length && T; )
this.currToken[0] === "(" && T++, this.currToken[0] === ")" && T--, T ? this.parse() : (L.parent.source.end.line = this.currToken[2], L.parent.source.end.column = this.currToken[3], this.position++);
T && this.error("Expected closing parenthesis."), this.current = q;
} else {
var F = 1;
for (this.position++, b.value += "("; this.position < this.tokens.length && F; )
this.currToken[0] === "(" && F++, this.currToken[0] === ")" && F--, b.value += this.parseParenthesisToken(this.currToken), this.position++;
F && this.error("Expected closing parenthesis.");
}
}, E.prototype.pseudo = function() {
for (var b = this, L = "", q = this.currToken; this.currToken && this.currToken[0] === ":"; )
L += this.currToken[1], this.position++;
if (!this.currToken)
return this.error("Expected pseudo-class or pseudo-element");
if (this.currToken[0] === "word") {
var T = void 0;
this.splitWord(false, function(F, z) {
L += F, T = new I.default({ value: L, source: { start: { line: q[2], column: q[3] }, end: { line: b.currToken[4], column: b.currToken[5] } }, sourceIndex: q[4] }), b.newNode(T), z > 1 && b.nextToken && b.nextToken[0] === "(" && b.error("Misplaced parenthesis.");
});
} else
this.error('Unexpected "' + this.currToken[0] + '" found.');
}, E.prototype.space = function() {
var b = this.currToken;
this.position === 0 || this.prevToken[0] === "," || this.prevToken[0] === "(" ? (this.spaces = this.parseSpace(b[1]), this.position++) : this.position === this.tokens.length - 1 || this.nextToken[0] === "," || this.nextToken[0] === ")" ? (this.current.last.spaces.after = this.parseSpace(b[1]), this.position++) : this.combinator();
}, E.prototype.string = function() {
var b = this.currToken;
this.newNode(new x.default({ value: this.currToken[1], source: { start: { line: b[2], column: b[3] }, end: { line: b[4], column: b[5] } }, sourceIndex: b[6] })), this.position++;
}, E.prototype.universal = function(b) {
var L = this.nextToken;
if (L && L[1] === "|")
return this.position++, this.namespace();
this.newNode(new D.default({ value: this.currToken[1], source: { start: { line: this.currToken[2], column: this.currToken[3] }, end: { line: this.currToken[2], column: this.currToken[3] } }, sourceIndex: this.currToken[4] }), b), this.position++;
}, E.prototype.splitWord = function(b, L) {
for (var q = this, T = this.nextToken, F = this.currToken[1]; T && T[0] === "word"; ) {
this.position++;
var z = this.currToken[1];
if (F += z, z.lastIndexOf("\\") === z.length - 1) {
var ee = this.nextToken;
ee && ee[0] === "space" && (F += this.parseSpace(ee[1], " "), this.position++);
}
T = this.nextToken;
}
var te = (0, l.default)(F, "."), ue = (0, l.default)(F, "#"), le = (0, l.default)(F, "#{");
le.length && (ue = ue.filter(function(ne) {
return !~le.indexOf(ne);
}));
var re = (0, R.default)((0, m.default)((0, o.default)([[0], te, ue])));
re.forEach(function(ne, oe) {
var ie = re[oe + 1] || F.length, ce = F.slice(ne, ie);
if (oe === 0 && L)
return L.call(q, ce, re.length);
var fe = void 0;
~te.indexOf(ne) ? fe = new f.default({ value: ce.slice(1), source: { start: { line: q.currToken[2], column: q.currToken[3] + ne }, end: { line: q.currToken[4], column: q.currToken[3] + (ie - 1) } }, sourceIndex: q.currToken[6] + re[oe] }) : ~ue.indexOf(ne) ? fe = new w.default({ value: ce.slice(1), source: { start: { line: q.currToken[2], column: q.currToken[3] + ne }, end: { line: q.currToken[4], column: q.currToken[3] + (ie - 1) } }, sourceIndex: q.currToken[6] + re[oe] }) : fe = new _.default({ value: ce, source: { start: { line: q.currToken[2], column: q.currToken[3] + ne }, end: { line: q.currToken[4], column: q.currToken[3] + (ie - 1) } }, sourceIndex: q.currToken[6] + re[oe] }), q.newNode(fe, b);
}), this.position++;
}, E.prototype.word = function(b) {
var L = this.nextToken;
return L && L[1] === "|" ? (this.position++, this.namespace()) : this.splitWord(b);
}, E.prototype.loop = function() {
for (; this.position < this.tokens.length; )
this.parse(true);
return this.root;
}, E.prototype.parse = function(b) {
switch (this.currToken[0]) {
case "space":
this.space();
break;
case "comment":
this.comment();
break;
case "(":
this.parentheses();
break;
case ")":
b && this.missingParenthesis();
break;
case "[":
this.attribute();
break;
case "]":
this.missingSquareBracket();
break;
case "at-word":
case "word":
this.word();
break;
case ":":
this.pseudo();
break;
case ";":
this.missingBackslash();
break;
case ",":
this.comma();
break;
case "*":
this.universal();
break;
case "&":
this.nesting();
break;
case "combinator":
this.combinator();
break;
case "string":
this.string();
break;
}
}, E.prototype.parseNamespace = function(b) {
if (this.lossy && typeof b == "string") {
var L = b.trim();
return L.length ? L : true;
}
return b;
}, E.prototype.parseSpace = function(b, L) {
return this.lossy ? L || "" : b;
}, E.prototype.parseValue = function(b) {
return this.lossy && b && typeof b == "string" ? b.trim() : b;
}, E.prototype.parseParenthesisToken = function(b) {
return this.lossy ? b[0] === "space" ? this.parseSpace(b[1], " ") : this.parseValue(b[1]) : b[1];
}, E.prototype.newNode = function(b, L) {
return L && (b.namespace = this.parseNamespace(L)), this.spaces && (b.spaces.before = this.spaces, this.spaces = ""), this.current.append(b);
}, i(E, [{ key: "currToken", get: function() {
return this.tokens[this.position];
} }, { key: "nextToken", get: function() {
return this.tokens[this.position + 1];
} }, { key: "prevToken", get: function() {
return this.tokens[this.position - 1];
} }]), E;
}();
e.default = G, n2.exports = e.default;
} }), bf = P({ "node_modules/postcss-selector-parser/dist/processor.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = function() {
function m(c, t) {
for (var r = 0; r < t.length; r++) {
var a = t[r];
a.enumerable = a.enumerable || false, a.configurable = true, "value" in a && (a.writable = true), Object.defineProperty(c, a.key, a);
}
}
return function(c, t, r) {
return t && m(c.prototype, t), r && m(c, r), c;
};
}(), u = _f(), o = h(u);
function h(m) {
return m && m.__esModule ? m : { default: m };
}
function l(m, c) {
if (!(m instanceof c))
throw new TypeError("Cannot call a class as a function");
}
var p = function() {
function m(c) {
return l(this, m), this.func = c || function() {
}, this;
}
return m.prototype.process = function(t) {
var r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {}, a = new o.default({ css: t, error: function(f) {
throw new Error(f);
}, options: r });
return this.res = a, this.func(a), this;
}, i(m, [{ key: "result", get: function() {
return String(this.res);
} }]), m;
}();
e.default = p, n2.exports = e.default;
} }), xf = P({ "node_modules/postcss-selector-parser/dist/index.js"(e, n2) {
"use strict";
A(), e.__esModule = true;
var i = bf(), u = O(i), o = Go(), h = O(o), l = Bo(), p = O(l), m = Jo(), c = O(m), t = Fo(), r = O(t), a = Uo(), s = O(a), f = Ko(), g = O(f), v = Vo(), y = O(v), w = Lo(), d = O(w), _ = zo(), k = O(_), x = Wo(), N = O(x), I = $o(), W = O(I), $ = Ho(), H = O($), D = se(), V = B(D);
function B(C) {
if (C && C.__esModule)
return C;
var R = {};
if (C != null)
for (var X in C)
Object.prototype.hasOwnProperty.call(C, X) && (R[X] = C[X]);
return R.default = C, R;
}
function O(C) {
return C && C.__esModule ? C : { default: C };
}
var j = function(R) {
return new u.default(R);
};
j.attribute = function(C) {
return new h.default(C);
}, j.className = function(C) {
return new p.default(C);
}, j.combinator = function(C) {
return new c.default(C);
}, j.comment = function(C) {
return new r.default(C);
}, j.id = function(C) {
return new s.default(C);
}, j.nesting = function(C) {
return new g.default(C);
}, j.pseudo = function(C) {
return new y.default(C);
}, j.root = function(C) {
return new d.default(C);
}, j.selector = function(C) {
return new k.default(C);
}, j.string = function(C) {
return new N.default(C);
}, j.tag = function(C) {
return new W.default(C);
}, j.universal = function(C) {
return new H.default(C);
}, Object.keys(V).forEach(function(C) {
C !== "__esModule" && (j[C] = V[C]);
}), e.default = j, n2.exports = e.default;
} }), Qo = P({ "node_modules/postcss-media-query-parser/dist/nodes/Node.js"(e) {
"use strict";
A(), Object.defineProperty(e, "__esModule", { value: true });
function n2(i) {
this.after = i.after, this.before = i.before, this.type = i.type, this.value = i.value, this.sourceIndex = i.sourceIndex;
}
e.default = n2;
} }), Yo = P({ "node_modules/postcss-media-query-parser/dist/nodes/Container.js"(e) {
"use strict";
A(), Object.defineProperty(e, "__esModule", { value: true });
var n2 = Qo(), i = u(n2);
function u(h) {
return h && h.__esModule ? h : { default: h };
}
function o(h) {
var l = this;
this.constructor(h), this.nodes = h.nodes, this.after === void 0 && (this.after = this.nodes.length > 0 ? this.nodes[this.nodes.length - 1].after : ""), this.before === void 0 && (this.before = this.nodes.length > 0 ? this.nodes[0].before : ""), this.sourceIndex === void 0 && (this.sourceIndex = this.before.length), this.nodes.forEach(function(p) {
p.parent = l;
});
}
o.prototype = Object.create(i.default.prototype), o.constructor = i.default, o.prototype.walk = function(l, p) {
for (var m = typeof l == "string" || l instanceof RegExp, c = m ? p : l, t = typeof l == "string" ? new RegExp(l) : l, r = 0; r < this.nodes.length; r++) {
var a = this.nodes[r], s = m ? t.test(a.type) : true;
if (s && c && c(a, r, this.nodes) === false || a.nodes && a.walk(l, p) === false)
return false;
}
return true;
}, o.prototype.each = function() {
for (var l = arguments.length <= 0 || arguments[0] === void 0 ? function() {
} : arguments[0], p = 0; p < this.nodes.length; p++) {
var m = this.nodes[p];
if (l(m, p, this.nodes) === false)
return false;
}
return true;
}, e.default = o;
} }), Sf = P({ "node_modules/postcss-media-query-parser/dist/parsers.js"(e) {
"use strict";
A(), Object.defineProperty(e, "__esModule", { value: true }), e.parseMediaFeature = l, e.parseMediaQuery = p, e.parseMediaList = m;
var n2 = Qo(), i = h(n2), u = Yo(), o = h(u);
function h(c) {
return c && c.__esModule ? c : { default: c };
}
function l(c) {
var t = arguments.length <= 1 || arguments[1] === void 0 ? 0 : arguments[1], r = [{ mode: "normal", character: null }], a = [], s = 0, f = "", g = null, v = null, y = t, w = c;
c[0] === "(" && c[c.length - 1] === ")" && (w = c.substring(1, c.length - 1), y++);
for (var d = 0; d < w.length; d++) {
var _ = w[d];
if ((_ === "'" || _ === '"') && (r[s].isCalculationEnabled === true ? (r.push({ mode: "string", isCalculationEnabled: false, character: _ }), s++) : r[s].mode === "string" && r[s].character === _ && w[d - 1] !== "\\" && (r.pop(), s--)), _ === "{" ? (r.push({ mode: "interpolation", isCalculationEnabled: true }), s++) : _ === "}" && (r.pop(), s--), r[s].mode === "normal" && _ === ":") {
var k = w.substring(d + 1);
v = { type: "value", before: /^(\s*)/.exec(k)[1], after: /(\s*)$/.exec(k)[1], value: k.trim() }, v.sourceIndex = v.before.length + d + 1 + y, g = { type: "colon", sourceIndex: d + y, after: v.before, value: ":" };
break;
}
f += _;
}
return f = { type: "media-feature", before: /^(\s*)/.exec(f)[1], after: /(\s*)$/.exec(f)[1], value: f.trim() }, f.sourceIndex = f.before.length + y, a.push(f), g !== null && (g.before = f.after, a.push(g)), v !== null && a.push(v), a;
}
function p(c) {
var t = arguments.length <= 1 || arguments[1] === void 0 ? 0 : arguments[1], r = [], a = 0, s = false, f = void 0;
function g() {
return { before: "", after: "", value: "" };
}
f = g();
for (var v = 0; v < c.length; v++) {
var y = c[v];
s ? (f.value += y, (y === "{" || y === "(") && a++, (y === ")" || y === "}") && a--) : y.search(/\s/) !== -1 ? f.before += y : (y === "(" && (f.type = "media-feature-expression", a++), f.value = y, f.sourceIndex = t + v, s = true), s && a === 0 && (y === ")" || v === c.length - 1 || c[v + 1].search(/\s/) !== -1) && (["not", "only", "and"].indexOf(f.value) !== -1 && (f.type = "keyword"), f.type === "media-feature-expression" && (f.nodes = l(f.value, f.sourceIndex)), r.push(Array.isArray(f.nodes) ? new o.default(f) : new i.default(f)), f = g(), s = false);
}
for (var w = 0; w < r.length; w++)
if (f = r[w], w > 0 && (r[w - 1].after = f.before), f.type === void 0) {
if (w > 0) {
if (r[w - 1].type === "media-feature-expression") {
f.type = "keyword";
continue;
}
if (r[w - 1].value === "not" || r[w - 1].value === "only") {
f.type = "media-type";
continue;
}
if (r[w - 1].value === "and") {
f.type = "media-feature-expression";
continue;
}
r[w - 1].type === "media-type" && (r[w + 1] ? f.type = r[w + 1].type === "media-feature-expression" ? "keyword" : "media-feature-expression" : f.type = "media-feature-expression");
}
if (w === 0) {
if (!r[w + 1]) {
f.type = "media-type";
continue;
}
if (r[w + 1] && (r[w + 1].type === "media-feature-expression" || r[w + 1].type === "keyword")) {
f.type = "media-type";
continue;
}
if (r[w + 2]) {
if (r[w + 2].type === "media-feature-expression") {
f.type = "media-type", r[w + 1].type = "keyword";
continue;
}
if (r[w + 2].type === "keyword") {
f.type = "keyword", r[w + 1].type = "media-type";
continue;
}
}
if (r[w + 3] && r[w + 3].type === "media-feature-expression") {
f.type = "keyword", r[w + 1].type = "media-type", r[w + 2].type = "keyword";
continue;
}
}
}
return r;
}
function m(c) {
var t = [], r = 0, a = 0, s = /^(\s*)url\s*\(/.exec(c);
if (s !== null) {
for (var f = s[0].length, g = 1; g > 0; ) {
var v = c[f];
v === "(" && g++, v === ")" && g--, f++;
}
t.unshift(new i.default({ type: "url", value: c.substring(0, f).trim(), sourceIndex: s[1].length, before: s[1], after: /^(\s*)/.exec(c.substring(f))[1] })), r = f;
}
for (var y = r; y < c.length; y++) {
var w = c[y];
if (w === "(" && a++, w === ")" && a--, a === 0 && w === ",") {
var d = c.substring(r, y), _ = /^(\s*)/.exec(d)[1];
t.push(new o.default({ type: "media-query", value: d.trim(), sourceIndex: r + _.length, nodes: p(d, r), before: _, after: /(\s*)$/.exec(d)[1] })), r = y + 1;
}
}
var k = c.substring(r), x = /^(\s*)/.exec(k)[1];
return t.push(new o.default({ type: "media-query", value: k.trim(), sourceIndex: r + x.length, nodes: p(k, r), before: x, after: /(\s*)$/.exec(k)[1] })), t;
}
} }), kf = P({ "node_modules/postcss-media-query-parser/dist/index.js"(e) {
"use strict";
A(), Object.defineProperty(e, "__esModule", { value: true }), e.default = h;
var n2 = Yo(), i = o(n2), u = Sf();
function o(l) {
return l && l.__esModule ? l : { default: l };
}
function h(l) {
return new i.default({ nodes: (0, u.parseMediaList)(l), type: "media-query-list", value: l.trim() });
}
} }), Xo = {};
At(Xo, { basename: () => na, default: () => sa, delimiter: () => kt, dirname: () => ta, extname: () => ia, isAbsolute: () => zt, join: () => ea, normalize: () => Lt, relative: () => ra, resolve: () => yr, sep: () => St });
function Zo(e, n2) {
for (var i = 0, u = e.length - 1; u >= 0; u--) {
var o = e[u];
o === "." ? e.splice(u, 1) : o === ".." ? (e.splice(u, 1), i++) : i && (e.splice(u, 1), i--);
}
if (n2)
for (; i--; i)
e.unshift("..");
return e;
}
function yr() {
for (var e = "", n2 = false, i = arguments.length - 1; i >= -1 && !n2; i--) {
var u = i >= 0 ? arguments[i] : "/";
if (typeof u != "string")
throw new TypeError("Arguments to path.resolve must be strings");
if (!u)
continue;
e = u + "/" + e, n2 = u.charAt(0) === "/";
}
return e = Zo(Bt(e.split("/"), function(o) {
return !!o;
}), !n2).join("/"), (n2 ? "/" : "") + e || ".";
}
function Lt(e) {
var n2 = zt(e), i = oa(e, -1) === "/";
return e = Zo(Bt(e.split("/"), function(u) {
return !!u;
}), !n2).join("/"), !e && !n2 && (e = "."), e && i && (e += "/"), (n2 ? "/" : "") + e;
}
function zt(e) {
return e.charAt(0) === "/";
}
function ea() {
var e = Array.prototype.slice.call(arguments, 0);
return Lt(Bt(e, function(n2, i) {
if (typeof n2 != "string")
throw new TypeError("Arguments to path.join must be strings");
return n2;
}).join("/"));
}
function ra(e, n2) {
e = yr(e).substr(1), n2 = yr(n2).substr(1);
function i(c) {
for (var t = 0; t < c.length && c[t] === ""; t++)
;
for (var r = c.length - 1; r >= 0 && c[r] === ""; r--)
;
return t > r ? [] : c.slice(t, r - t + 1);
}
for (var u = i(e.split("/")), o = i(n2.split("/")), h = Math.min(u.length, o.length), l = h, p = 0; p < h; p++)
if (u[p] !== o[p]) {
l = p;
break;
}
for (var m = [], p = l; p < u.length; p++)
m.push("..");
return m = m.concat(o.slice(l)), m.join("/");
}
function ta(e) {
var n2 = br(e), i = n2[0], u = n2[1];
return !i && !u ? "." : (u && (u = u.substr(0, u.length - 1)), i + u);
}
function na(e, n2) {
var i = br(e)[2];
return n2 && i.substr(-1 * n2.length) === n2 && (i = i.substr(0, i.length - n2.length)), i;
}
function ia(e) {
return br(e)[3];
}
function Bt(e, n2) {
if (e.filter)
return e.filter(n2);
for (var i = [], u = 0; u < e.length; u++)
n2(e[u], u, e) && i.push(e[u]);
return i;
}
var Fs, br, St, kt, sa, oa, Of = Le({ "node-modules-polyfills:path"() {
A(), Fs = /^(\/?|)([\s\S]*?)((?:\.{1,2}|[^\/]+?|)(\.[^.\/]*|))(?:[\/]*)$/, br = function(e) {
return Fs.exec(e).slice(1);
}, St = "/", kt = ":", sa = { extname: ia, basename: na, dirname: ta, sep: St, delimiter: kt, relative: ra, join: ea, isAbsolute: zt, normalize: Lt, resolve: yr }, oa = "ab".substr(-1) === "b" ? function(e, n2, i) {
return e.substr(n2, i);
} : function(e, n2, i) {
return n2 < 0 && (n2 = e.length + n2), e.substr(n2, i);
};
} }), Tf = P({ "node-modules-polyfills-commonjs:path"(e, n2) {
A();
var i = (Of(), Pt(Xo));
if (i && i.default) {
n2.exports = i.default;
for (let u in i)
n2.exports[u] = i[u];
} else
i && (n2.exports = i);
} }), Ef = P({ "node_modules/picocolors/picocolors.browser.js"(e, n2) {
A();
var i = String, u = function() {
return { isColorSupported: false, reset: i, bold: i, dim: i, italic: i, underline: i, inverse: i, hidden: i, strikethrough: i, black: i, red: i, green: i, yellow: i, blue: i, magenta: i, cyan: i, white: i, gray: i, bgBlack: i, bgRed: i, bgGreen: i, bgYellow: i, bgBlue: i, bgMagenta: i, bgCyan: i, bgWhite: i };
};
n2.exports = u(), n2.exports.createColors = u;
} }), qf = P({ "(disabled):node_modules/postcss/lib/terminal-highlight"() {
A();
} }), aa = P({ "node_modules/postcss/lib/css-syntax-error.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = o(Ef()), u = o(qf());
function o(g) {
return g && g.__esModule ? g : { default: g };
}
function h(g) {
if (g === void 0)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return g;
}
function l(g, v) {
g.prototype = Object.create(v.prototype), g.prototype.constructor = g, g.__proto__ = v;
}
function p(g) {
var v = typeof Map == "function" ? /* @__PURE__ */ new Map() : void 0;
return p = function(w) {
if (w === null || !t(w))
return w;
if (typeof w != "function")
throw new TypeError("Super expression must either be null or a function");
if (typeof v < "u") {
if (v.has(w))
return v.get(w);
v.set(w, d);
}
function d() {
return m(w, arguments, a(this).constructor);
}
return d.prototype = Object.create(w.prototype, { constructor: { value: d, enumerable: false, writable: true, configurable: true } }), r(d, w);
}, p(g);
}
function m(g, v, y) {
return c() ? m = Reflect.construct : m = function(d, _, k) {
var x = [null];
x.push.apply(x, _);
var N = Function.bind.apply(d, x), I = new N();
return k && r(I, k.prototype), I;
}, m.apply(null, arguments);
}
function c() {
if (typeof Reflect > "u" || !Reflect.construct || Reflect.construct.sham)
return false;
if (typeof Proxy == "function")
return true;
try {
return Date.prototype.toString.call(Reflect.construct(Date, [], function() {
})), true;
} catch {
return false;
}
}
function t(g) {
return Function.toString.call(g).indexOf("[native code]") !== -1;
}
function r(g, v) {
return r = Object.setPrototypeOf || function(w, d) {
return w.__proto__ = d, w;
}, r(g, v);
}
function a(g) {
return a = Object.setPrototypeOf ? Object.getPrototypeOf : function(y) {
return y.__proto__ || Object.getPrototypeOf(y);
}, a(g);
}
var s = function(g) {
l(v, g);
function v(w, d, _, k, x, N) {
var I;
return I = g.call(this, w) || this, I.name = "CssSyntaxError", I.reason = w, x && (I.file = x), k && (I.source = k), N && (I.plugin = N), typeof d < "u" && typeof _ < "u" && (I.line = d, I.column = _), I.setMessage(), Error.captureStackTrace && Error.captureStackTrace(h(I), v), I;
}
var y = v.prototype;
return y.setMessage = function() {
this.message = this.plugin ? this.plugin + ": " : "", this.message += this.file ? this.file : "", typeof this.line < "u" && (this.message += ":" + this.line + ":" + this.column), this.message += ": " + this.reason;
}, y.showSourceCode = function(d) {
var _ = this;
if (!this.source)
return "";
var k = this.source;
u.default && (typeof d > "u" && (d = i.default.isColorSupported), d && (k = (0, u.default)(k)));
var x = k.split(/\r?\n/), N = Math.max(this.line - 3, 0), I = Math.min(this.line + 2, x.length), W = String(I).length;
function $(D) {
return d && i.default.red ? i.default.red(i.default.bold(D)) : D;
}
function H(D) {
return d && i.default.gray ? i.default.gray(D) : D;
}
return x.slice(N, I).map(function(D, V) {
var B = N + 1 + V, O = " " + (" " + B).slice(-W) + " | ";
if (B === _.line) {
var j = H(O.replace(/\d/g, " ")) + D.slice(0, _.column - 1).replace(/[^\t]/g, " ");
return $(">") + H(O) + D + `
` + j + $("^");
}
return " " + H(O) + D;
}).join(`
`);
}, y.toString = function() {
var d = this.showSourceCode();
return d && (d = `
` + d + `
`), this.name + ": " + this.message + d;
}, v;
}(p(Error)), f = s;
e.default = f, n2.exports = e.default;
} }), Af = P({ "node_modules/postcss/lib/previous-map.js"(e, n2) {
A(), n2.exports = class {
};
} }), xr = P({ "node_modules/postcss/lib/input.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = h(Tf()), u = h(aa()), o = h(Af());
function h(r) {
return r && r.__esModule ? r : { default: r };
}
function l(r, a) {
for (var s = 0; s < a.length; s++) {
var f = a[s];
f.enumerable = f.enumerable || false, f.configurable = true, "value" in f && (f.writable = true), Object.defineProperty(r, f.key, f);
}
}
function p(r, a, s) {
return a && l(r.prototype, a), s && l(r, s), r;
}
var m = 0, c = function() {
function r(s, f) {
if (f === void 0 && (f = {}), s === null || typeof s > "u" || typeof s == "object" && !s.toString)
throw new Error("PostCSS received " + s + " instead of CSS string");
this.css = s.toString(), this.css[0] === "\uFEFF" || this.css[0] === "\uFFFE" ? (this.hasBOM = true, this.css = this.css.slice(1)) : this.hasBOM = false, f.from && (/^\w+:\/\//.test(f.from) || i.default.isAbsolute(f.from) ? this.file = f.from : this.file = i.default.resolve(f.from));
var g = new o.default(this.css, f);
if (g.text) {
this.map = g;
var v = g.consumer().file;
!this.file && v && (this.file = this.mapResolve(v));
}
this.file || (m += 1, this.id = ""), this.map && (this.map.file = this.from);
}
var a = r.prototype;
return a.error = function(f, g, v, y) {
y === void 0 && (y = {});
var w, d = this.origin(g, v);
return d ? w = new u.default(f, d.line, d.column, d.source, d.file, y.plugin) : w = new u.default(f, g, v, this.css, this.file, y.plugin), w.input = { line: g, column: v, source: this.css }, this.file && (w.input.file = this.file), w;
}, a.origin = function(f, g) {
if (!this.map)
return false;
var v = this.map.consumer(), y = v.originalPositionFor({ line: f, column: g });
if (!y.source)
return false;
var w = { file: this.mapResolve(y.source), line: y.line, column: y.column }, d = v.sourceContentFor(y.source);
return d && (w.source = d), w;
}, a.mapResolve = function(f) {
return /^\w+:\/\//.test(f) ? f : i.default.resolve(this.map.consumer().sourceRoot || ".", f);
}, p(r, [{ key: "from", get: function() {
return this.file || this.id;
} }]), r;
}(), t = c;
e.default = t, n2.exports = e.default;
} }), Sr = P({ "node_modules/postcss/lib/stringifier.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = { colon: ": ", indent: " ", beforeDecl: `
`, beforeRule: `
`, beforeOpen: " ", beforeClose: `
`, beforeComment: `
`, after: `
`, emptyBody: "", commentLeft: " ", commentRight: " ", semicolon: false };
function u(l) {
return l[0].toUpperCase() + l.slice(1);
}
var o = function() {
function l(m) {
this.builder = m;
}
var p = l.prototype;
return p.stringify = function(c, t) {
this[c.type](c, t);
}, p.root = function(c) {
this.body(c), c.raws.after && this.builder(c.raws.after);
}, p.comment = function(c) {
var t = this.raw(c, "left", "commentLeft"), r = this.raw(c, "right", "commentRight");
this.builder("/*" + t + c.text + r + "*/", c);
}, p.decl = function(c, t) {
var r = this.raw(c, "between", "colon"), a = c.prop + r + this.rawValue(c, "value");
c.important && (a += c.raws.important || " !important"), t && (a += ";"), this.builder(a, c);
}, p.rule = function(c) {
this.block(c, this.rawValue(c, "selector")), c.raws.ownSemicolon && this.builder(c.raws.ownSemicolon, c, "end");
}, p.atrule = function(c, t) {
var r = "@" + c.name, a = c.params ? this.rawValue(c, "params") : "";
if (typeof c.raws.afterName < "u" ? r += c.raws.afterName : a && (r += " "), c.nodes)
this.block(c, r + a);
else {
var s = (c.raws.between || "") + (t ? ";" : "");
this.builder(r + a + s, c);
}
}, p.body = function(c) {
for (var t = c.nodes.length - 1; t > 0 && c.nodes[t].type === "comment"; )
t -= 1;
for (var r = this.raw(c, "semicolon"), a = 0; a < c.nodes.length; a++) {
var s = c.nodes[a], f = this.raw(s, "before");
f && this.builder(f), this.stringify(s, t !== a || r);
}
}, p.block = function(c, t) {
var r = this.raw(c, "between", "beforeOpen");
this.builder(t + r + "{", c, "start");
var a;
c.nodes && c.nodes.length ? (this.body(c), a = this.raw(c, "after")) : a = this.raw(c, "after", "emptyBody"), a && this.builder(a), this.builder("}", c, "end");
}, p.raw = function(c, t, r) {
var a;
if (r || (r = t), t && (a = c.raws[t], typeof a < "u"))
return a;
var s = c.parent;
if (r === "before" && (!s || s.type === "root" && s.first === c))
return "";
if (!s)
return i[r];
var f = c.root();
if (f.rawCache || (f.rawCache = {}), typeof f.rawCache[r] < "u")
return f.rawCache[r];
if (r === "before" || r === "after")
return this.beforeAfter(c, r);
var g = "raw" + u(r);
return this[g] ? a = this[g](f, c) : f.walk(function(v) {
if (a = v.raws[t], typeof a < "u")
return false;
}), typeof a > "u" && (a = i[r]), f.rawCache[r] = a, a;
}, p.rawSemicolon = function(c) {
var t;
return c.walk(function(r) {
if (r.nodes && r.nodes.length && r.last.type === "decl" && (t = r.raws.semicolon, typeof t < "u"))
return false;
}), t;
}, p.rawEmptyBody = function(c) {
var t;
return c.walk(function(r) {
if (r.nodes && r.nodes.length === 0 && (t = r.raws.after, typeof t < "u"))
return false;
}), t;
}, p.rawIndent = function(c) {
if (c.raws.indent)
return c.raws.indent;
var t;
return c.walk(function(r) {
var a = r.parent;
if (a && a !== c && a.parent && a.parent === c && typeof r.raws.before < "u") {
var s = r.raws.before.split(`
`);
return t = s[s.length - 1], t = t.replace(/[^\s]/g, ""), false;
}
}), t;
}, p.rawBeforeComment = function(c, t) {
var r;
return c.walkComments(function(a) {
if (typeof a.raws.before < "u")
return r = a.raws.before, r.indexOf(`
`) !== -1 && (r = r.replace(/[^\n]+$/, "")), false;
}), typeof r > "u" ? r = this.raw(t, null, "beforeDecl") : r && (r = r.replace(/[^\s]/g, "")), r;
}, p.rawBeforeDecl = function(c, t) {
var r;
return c.walkDecls(function(a) {
if (typeof a.raws.before < "u")
return r = a.raws.before, r.indexOf(`
`) !== -1 && (r = r.replace(/[^\n]+$/, "")), false;
}), typeof r > "u" ? r = this.raw(t, null, "beforeRule") : r && (r = r.replace(/[^\s]/g, "")), r;
}, p.rawBeforeRule = function(c) {
var t;
return c.walk(function(r) {
if (r.nodes && (r.parent !== c || c.first !== r) && typeof r.raws.before < "u")
return t = r.raws.before, t.indexOf(`
`) !== -1 && (t = t.replace(/[^\n]+$/, "")), false;
}), t && (t = t.replace(/[^\s]/g, "")), t;
}, p.rawBeforeClose = function(c) {
var t;
return c.walk(function(r) {
if (r.nodes && r.nodes.length > 0 && typeof r.raws.after < "u")
return t = r.raws.after, t.indexOf(`
`) !== -1 && (t = t.replace(/[^\n]+$/, "")), false;
}), t && (t = t.replace(/[^\s]/g, "")), t;
}, p.rawBeforeOpen = function(c) {
var t;
return c.walk(function(r) {
if (r.type !== "decl" && (t = r.raws.between, typeof t < "u"))
return false;
}), t;
}, p.rawColon = function(c) {
var t;
return c.walkDecls(function(r) {
if (typeof r.raws.between < "u")
return t = r.raws.between.replace(/[^\s:]/g, ""), false;
}), t;
}, p.beforeAfter = function(c, t) {
var r;
c.type === "decl" ? r = this.raw(c, null, "beforeDecl") : c.type === "comment" ? r = this.raw(c, null, "beforeComment") : t === "before" ? r = this.raw(c, null, "beforeRule") : r = this.raw(c, null, "beforeClose");
for (var a = c.parent, s = 0; a && a.type !== "root"; )
s += 1, a = a.parent;
if (r.indexOf(`
`) !== -1) {
var f = this.raw(c, null, "indent");
if (f.length)
for (var g = 0; g < s; g++)
r += f;
}
return r;
}, p.rawValue = function(c, t) {
var r = c[t], a = c.raws[t];
return a && a.value === r ? a.raw : r;
}, l;
}(), h = o;
e.default = h, n2.exports = e.default;
} }), ua = P({ "node_modules/postcss/lib/stringify.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = u(Sr());
function u(l) {
return l && l.__esModule ? l : { default: l };
}
function o(l, p) {
var m = new i.default(p);
m.stringify(l);
}
var h = o;
e.default = h, n2.exports = e.default;
} }), Ft = P({ "node_modules/postcss/lib/node.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = h(aa()), u = h(Sr()), o = h(ua());
function h(c) {
return c && c.__esModule ? c : { default: c };
}
function l(c, t) {
var r = new c.constructor();
for (var a in c)
if (c.hasOwnProperty(a)) {
var s = c[a], f = typeof s;
a === "parent" && f === "object" ? t && (r[a] = t) : a === "source" ? r[a] = s : s instanceof Array ? r[a] = s.map(function(g) {
return l(g, r);
}) : (f === "object" && s !== null && (s = l(s)), r[a] = s);
}
return r;
}
var p = function() {
function c(r) {
r === void 0 && (r = {}), this.raws = {};
for (var a in r)
this[a] = r[a];
}
var t = c.prototype;
return t.error = function(a, s) {
if (s === void 0 && (s = {}), this.source) {
var f = this.positionBy(s);
return this.source.input.error(a, f.line, f.column, s);
}
return new i.default(a);
}, t.warn = function(a, s, f) {
var g = { node: this };
for (var v in f)
g[v] = f[v];
return a.warn(s, g);
}, t.remove = function() {
return this.parent && this.parent.removeChild(this), this.parent = void 0, this;
}, t.toString = function(a) {
a === void 0 && (a = o.default), a.stringify && (a = a.stringify);
var s = "";
return a(this, function(f) {
s += f;
}), s;
}, t.clone = function(a) {
a === void 0 && (a = {});
var s = l(this);
for (var f in a)
s[f] = a[f];
return s;
}, t.cloneBefore = function(a) {
a === void 0 && (a = {});
var s = this.clone(a);
return this.parent.insertBefore(this, s), s;
}, t.cloneAfter = function(a) {
a === void 0 && (a = {});
var s = this.clone(a);
return this.parent.insertAfter(this, s), s;
}, t.replaceWith = function() {
if (this.parent) {
for (var a = arguments.length, s = new Array(a), f = 0; f < a; f++)
s[f] = arguments[f];
for (var g = 0, v = s; g < v.length; g++) {
var y = v[g];
this.parent.insertBefore(this, y);
}
this.remove();
}
return this;
}, t.next = function() {
if (this.parent) {
var a = this.parent.index(this);
return this.parent.nodes[a + 1];
}
}, t.prev = function() {
if (this.parent) {
var a = this.parent.index(this);
return this.parent.nodes[a - 1];
}
}, t.before = function(a) {
return this.parent.insertBefore(this, a), this;
}, t.after = function(a) {
return this.parent.insertAfter(this, a), this;
}, t.toJSON = function() {
var a = {};
for (var s in this)
if (this.hasOwnProperty(s) && s !== "parent") {
var f = this[s];
f instanceof Array ? a[s] = f.map(function(g) {
return typeof g == "object" && g.toJSON ? g.toJSON() : g;
}) : typeof f == "object" && f.toJSON ? a[s] = f.toJSON() : a[s] = f;
}
return a;
}, t.raw = function(a, s) {
var f = new u.default();
return f.raw(this, a, s);
}, t.root = function() {
for (var a = this; a.parent; )
a = a.parent;
return a;
}, t.cleanRaws = function(a) {
delete this.raws.before, delete this.raws.after, a || delete this.raws.between;
}, t.positionInside = function(a) {
for (var s = this.toString(), f = this.source.start.column, g = this.source.start.line, v = 0; v < a; v++)
s[v] === `
` ? (f = 1, g += 1) : f += 1;
return { line: g, column: f };
}, t.positionBy = function(a) {
var s = this.source.start;
if (a.index)
s = this.positionInside(a.index);
else if (a.word) {
var f = this.toString().indexOf(a.word);
f !== -1 && (s = this.positionInside(f));
}
return s;
}, c;
}(), m = p;
e.default = m, n2.exports = e.default;
} }), kr = P({ "node_modules/postcss/lib/comment.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = u(Ft());
function u(p) {
return p && p.__esModule ? p : { default: p };
}
function o(p, m) {
p.prototype = Object.create(m.prototype), p.prototype.constructor = p, p.__proto__ = m;
}
var h = function(p) {
o(m, p);
function m(c) {
var t;
return t = p.call(this, c) || this, t.type = "comment", t;
}
return m;
}(i.default), l = h;
e.default = l, n2.exports = e.default;
} }), ca = P({ "node_modules/postcss/lib/declaration.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = u(Ft());
function u(p) {
return p && p.__esModule ? p : { default: p };
}
function o(p, m) {
p.prototype = Object.create(m.prototype), p.prototype.constructor = p, p.__proto__ = m;
}
var h = function(p) {
o(m, p);
function m(c) {
var t;
return t = p.call(this, c) || this, t.type = "decl", t;
}
return m;
}(i.default), l = h;
e.default = l, n2.exports = e.default;
} }), Ut = P({ "node_modules/postcss/lib/tokenize.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = W;
var i = "'".charCodeAt(0), u = '"'.charCodeAt(0), o = "\\".charCodeAt(0), h = "/".charCodeAt(0), l = `
`.charCodeAt(0), p = " ".charCodeAt(0), m = "\f".charCodeAt(0), c = " ".charCodeAt(0), t = "\r".charCodeAt(0), r = "[".charCodeAt(0), a = "]".charCodeAt(0), s = "(".charCodeAt(0), f = ")".charCodeAt(0), g = "{".charCodeAt(0), v = "}".charCodeAt(0), y = ";".charCodeAt(0), w = "*".charCodeAt(0), d = ":".charCodeAt(0), _ = "@".charCodeAt(0), k = /[ \n\t\r\f{}()'"\\;/[\]#]/g, x = /[ \n\t\r\f(){}:;@!'"\\\][#]|\/(?=\*)/g, N = /.[\\/("'\n]/, I = /[a-f0-9]/i;
function W($, H) {
H === void 0 && (H = {});
var D = $.css.valueOf(), V = H.ignoreErrors, B, O, j, C, R, X, Z, Q, K, J, M, Y, G, E, S = D.length, b = -1, L = 1, q = 0, T = [], F = [];
function z() {
return q;
}
function ee(re) {
throw $.error("Unclosed " + re, L, q - b);
}
function te() {
return F.length === 0 && q >= S;
}
function ue(re) {
if (F.length)
return F.pop();
if (!(q >= S)) {
var ne = re ? re.ignoreUnclosed : false;
switch (B = D.charCodeAt(q), (B === l || B === m || B === t && D.charCodeAt(q + 1) !== l) && (b = q, L += 1), B) {
case l:
case p:
case c:
case t:
case m:
O = q;
do
O += 1, B = D.charCodeAt(O), B === l && (b = O, L += 1);
while (B === p || B === l || B === c || B === t || B === m);
E = ["space", D.slice(q, O)], q = O - 1;
break;
case r:
case a:
case g:
case v:
case d:
case y:
case f:
var oe = String.fromCharCode(B);
E = [oe, oe, L, q - b];
break;
case s:
if (Y = T.length ? T.pop()[1] : "", G = D.charCodeAt(q + 1), Y === "url" && G !== i && G !== u && G !== p && G !== l && G !== c && G !== m && G !== t) {
O = q;
do {
if (J = false, O = D.indexOf(")", O + 1), O === -1)
if (V || ne) {
O = q;
break;
} else
ee("bracket");
for (M = O; D.charCodeAt(M - 1) === o; )
M -= 1, J = !J;
} while (J);
E = ["brackets", D.slice(q, O + 1), L, q - b, L, O - b], q = O;
} else
O = D.indexOf(")", q + 1), X = D.slice(q, O + 1), O === -1 || N.test(X) ? E = ["(", "(", L, q - b] : (E = ["brackets", X, L, q - b, L, O - b], q = O);
break;
case i:
case u:
j = B === i ? "'" : '"', O = q;
do {
if (J = false, O = D.indexOf(j, O + 1), O === -1)
if (V || ne) {
O = q + 1;
break;
} else
ee("string");
for (M = O; D.charCodeAt(M - 1) === o; )
M -= 1, J = !J;
} while (J);
X = D.slice(q, O + 1), C = X.split(`
`), R = C.length - 1, R > 0 ? (Q = L + R, K = O - C[R].length) : (Q = L, K = b), E = ["string", D.slice(q, O + 1), L, q - b, Q, O - K], b = K, L = Q, q = O;
break;
case _:
k.lastIndex = q + 1, k.test(D), k.lastIndex === 0 ? O = D.length - 1 : O = k.lastIndex - 2, E = ["at-word", D.slice(q, O + 1), L, q - b, L, O - b], q = O;
break;
case o:
for (O = q, Z = true; D.charCodeAt(O + 1) === o; )
O += 1, Z = !Z;
if (B = D.charCodeAt(O + 1), Z && B !== h && B !== p && B !== l && B !== c && B !== t && B !== m && (O += 1, I.test(D.charAt(O)))) {
for (; I.test(D.charAt(O + 1)); )
O += 1;
D.charCodeAt(O + 1) === p && (O += 1);
}
E = ["word", D.slice(q, O + 1), L, q - b, L, O - b], q = O;
break;
default:
B === h && D.charCodeAt(q + 1) === w ? (O = D.indexOf("*/", q + 2) + 1, O === 0 && (V || ne ? O = D.length : ee("comment")), X = D.slice(q, O + 1), C = X.split(`
`), R = C.length - 1, R > 0 ? (Q = L + R, K = O - C[R].length) : (Q = L, K = b), E = ["comment", X, L, q - b, Q, O - K], b = K, L = Q, q = O) : (x.lastIndex = q + 1, x.test(D), x.lastIndex === 0 ? O = D.length - 1 : O = x.lastIndex - 2, E = ["word", D.slice(q, O + 1), L, q - b, L, O - b], T.push(E), q = O);
break;
}
return q++, E;
}
}
function le(re) {
F.push(re);
}
return { back: le, nextToken: ue, endOfFile: te, position: z };
}
n2.exports = e.default;
} }), la = P({ "node_modules/postcss/lib/parse.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = o($t()), u = o(xr());
function o(p) {
return p && p.__esModule ? p : { default: p };
}
function h(p, m) {
var c = new u.default(p, m), t = new i.default(c);
try {
t.parse();
} catch (r) {
throw r;
}
return t.root;
}
var l = h;
e.default = l, n2.exports = e.default;
} }), Pf = P({ "node_modules/postcss/lib/list.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = { split: function(h, l, p) {
for (var m = [], c = "", t = false, r = 0, a = false, s = false, f = 0; f < h.length; f++) {
var g = h[f];
a ? s ? s = false : g === "\\" ? s = true : g === a && (a = false) : g === '"' || g === "'" ? a = g : g === "(" ? r += 1 : g === ")" ? r > 0 && (r -= 1) : r === 0 && l.indexOf(g) !== -1 && (t = true), t ? (c !== "" && m.push(c.trim()), c = "", t = false) : c += g;
}
return (p || c !== "") && m.push(c.trim()), m;
}, space: function(h) {
var l = [" ", `
`, " "];
return i.split(h, l);
}, comma: function(h) {
return i.split(h, [","], true);
} }, u = i;
e.default = u, n2.exports = e.default;
} }), fa = P({ "node_modules/postcss/lib/rule.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = o(Or()), u = o(Pf());
function o(t) {
return t && t.__esModule ? t : { default: t };
}
function h(t, r) {
for (var a = 0; a < r.length; a++) {
var s = r[a];
s.enumerable = s.enumerable || false, s.configurable = true, "value" in s && (s.writable = true), Object.defineProperty(t, s.key, s);
}
}
function l(t, r, a) {
return r && h(t.prototype, r), a && h(t, a), t;
}
function p(t, r) {
t.prototype = Object.create(r.prototype), t.prototype.constructor = t, t.__proto__ = r;
}
var m = function(t) {
p(r, t);
function r(a) {
var s;
return s = t.call(this, a) || this, s.type = "rule", s.nodes || (s.nodes = []), s;
}
return l(r, [{ key: "selectors", get: function() {
return u.default.comma(this.selector);
}, set: function(s) {
var f = this.selector ? this.selector.match(/,\s*/) : null, g = f ? f[0] : "," + this.raw("between", "beforeOpen");
this.selector = s.join(g);
} }]), r;
}(i.default), c = m;
e.default = c, n2.exports = e.default;
} }), Or = P({ "node_modules/postcss/lib/container.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = h(ca()), u = h(kr()), o = h(Ft());
function h(g) {
return g && g.__esModule ? g : { default: g };
}
function l(g, v) {
var y;
if (typeof Symbol > "u" || g[Symbol.iterator] == null) {
if (Array.isArray(g) || (y = p(g)) || v && g && typeof g.length == "number") {
y && (g = y);
var w = 0;
return function() {
return w >= g.length ? { done: true } : { done: false, value: g[w++] };
};
}
throw new TypeError(`Invalid attempt to iterate non-iterable instance.
In order to be iterable, non-array objects must have a [Symbol.iterator]() method.`);
}
return y = g[Symbol.iterator](), y.next.bind(y);
}
function p(g, v) {
if (g) {
if (typeof g == "string")
return m(g, v);
var y = Object.prototype.toString.call(g).slice(8, -1);
if (y === "Object" && g.constructor && (y = g.constructor.name), y === "Map" || y === "Set")
return Array.from(g);
if (y === "Arguments" || /^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(y))
return m(g, v);
}
}
function m(g, v) {
(v == null || v > g.length) && (v = g.length);
for (var y = 0, w = new Array(v); y < v; y++)
w[y] = g[y];
return w;
}
function c(g, v) {
for (var y = 0; y < v.length; y++) {
var w = v[y];
w.enumerable = w.enumerable || false, w.configurable = true, "value" in w && (w.writable = true), Object.defineProperty(g, w.key, w);
}
}
function t(g, v, y) {
return v && c(g.prototype, v), y && c(g, y), g;
}
function r(g, v) {
g.prototype = Object.create(v.prototype), g.prototype.constructor = g, g.__proto__ = v;
}
function a(g) {
return g.map(function(v) {
return v.nodes && (v.nodes = a(v.nodes)), delete v.source, v;
});
}
var s = function(g) {
r(v, g);
function v() {
return g.apply(this, arguments) || this;
}
var y = v.prototype;
return y.push = function(d) {
return d.parent = this, this.nodes.push(d), this;
}, y.each = function(d) {
this.lastEach || (this.lastEach = 0), this.indexes || (this.indexes = {}), this.lastEach += 1;
var _ = this.lastEach;
if (this.indexes[_] = 0, !!this.nodes) {
for (var k, x; this.indexes[_] < this.nodes.length && (k = this.indexes[_], x = d(this.nodes[k], k), x !== false); )
this.indexes[_] += 1;
return delete this.indexes[_], x;
}
}, y.walk = function(d) {
return this.each(function(_, k) {
var x;
try {
x = d(_, k);
} catch (I) {
if (I.postcssNode = _, I.stack && _.source && /\n\s{4}at /.test(I.stack)) {
var N = _.source;
I.stack = I.stack.replace(/\n\s{4}at /, "$&" + N.input.from + ":" + N.start.line + ":" + N.start.column + "$&");
}
throw I;
}
return x !== false && _.walk && (x = _.walk(d)), x;
});
}, y.walkDecls = function(d, _) {
return _ ? d instanceof RegExp ? this.walk(function(k, x) {
if (k.type === "decl" && d.test(k.prop))
return _(k, x);
}) : this.walk(function(k, x) {
if (k.type === "decl" && k.prop === d)
return _(k, x);
}) : (_ = d, this.walk(function(k, x) {
if (k.type === "decl")
return _(k, x);
}));
}, y.walkRules = function(d, _) {
return _ ? d instanceof RegExp ? this.walk(function(k, x) {
if (k.type === "rule" && d.test(k.selector))
return _(k, x);
}) : this.walk(function(k, x) {
if (k.type === "rule" && k.selector === d)
return _(k, x);
}) : (_ = d, this.walk(function(k, x) {
if (k.type === "rule")
return _(k, x);
}));
}, y.walkAtRules = function(d, _) {
return _ ? d instanceof RegExp ? this.walk(function(k, x) {
if (k.type === "atrule" && d.test(k.name))
return _(k, x);
}) : this.walk(function(k, x) {
if (k.type === "atrule" && k.name === d)
return _(k, x);
}) : (_ = d, this.walk(function(k, x) {
if (k.type === "atrule")
return _(k, x);
}));
}, y.walkComments = function(d) {
return this.walk(function(_, k) {
if (_.type === "comment")
return d(_, k);
});
}, y.append = function() {
for (var d = arguments.length, _ = new Array(d), k = 0; k < d; k++)
_[k] = arguments[k];
for (var x = 0, N = _; x < N.length; x++)
for (var I = N[x], W = this.normalize(I, this.last), $ = l(W), H; !(H = $()).done; ) {
var D = H.value;
this.nodes.push(D);
}
return this;
}, y.prepend = function() {
for (var d = arguments.length, _ = new Array(d), k = 0; k < d; k++)
_[k] = arguments[k];
_ = _.reverse();
for (var x = l(_), N; !(N = x()).done; ) {
for (var I = N.value, W = this.normalize(I, this.first, "prepend").reverse(), $ = l(W), H; !(H = $()).done; ) {
var D = H.value;
this.nodes.unshift(D);
}
for (var V in this.indexes)
this.indexes[V] = this.indexes[V] + W.length;
}
return this;
}, y.cleanRaws = function(d) {
if (g.prototype.cleanRaws.call(this, d), this.nodes)
for (var _ = l(this.nodes), k; !(k = _()).done; ) {
var x = k.value;
x.cleanRaws(d);
}
}, y.insertBefore = function(d, _) {
d = this.index(d);
for (var k = d === 0 ? "prepend" : false, x = this.normalize(_, this.nodes[d], k).reverse(), N = l(x), I; !(I = N()).done; ) {
var W = I.value;
this.nodes.splice(d, 0, W);
}
var $;
for (var H in this.indexes)
$ = this.indexes[H], d <= $ && (this.indexes[H] = $ + x.length);
return this;
}, y.insertAfter = function(d, _) {
d = this.index(d);
for (var k = this.normalize(_, this.nodes[d]).reverse(), x = l(k), N; !(N = x()).done; ) {
var I = N.value;
this.nodes.splice(d + 1, 0, I);
}
var W;
for (var $ in this.indexes)
W = this.indexes[$], d < W && (this.indexes[$] = W + k.length);
return this;
}, y.removeChild = function(d) {
d = this.index(d), this.nodes[d].parent = void 0, this.nodes.splice(d, 1);
var _;
for (var k in this.indexes)
_ = this.indexes[k], _ >= d && (this.indexes[k] = _ - 1);
return this;
}, y.removeAll = function() {
for (var d = l(this.nodes), _; !(_ = d()).done; ) {
var k = _.value;
k.parent = void 0;
}
return this.nodes = [], this;
}, y.replaceValues = function(d, _, k) {
return k || (k = _, _ = {}), this.walkDecls(function(x) {
_.props && _.props.indexOf(x.prop) === -1 || _.fast && x.value.indexOf(_.fast) === -1 || (x.value = x.value.replace(d, k));
}), this;
}, y.every = function(d) {
return this.nodes.every(d);
}, y.some = function(d) {
return this.nodes.some(d);
}, y.index = function(d) {
return typeof d == "number" ? d : this.nodes.indexOf(d);
}, y.normalize = function(d, _) {
var k = this;
if (typeof d == "string") {
var x = la();
d = a(x(d).nodes);
} else if (Array.isArray(d)) {
d = d.slice(0);
for (var N = l(d), I; !(I = N()).done; ) {
var W = I.value;
W.parent && W.parent.removeChild(W, "ignore");
}
} else if (d.type === "root") {
d = d.nodes.slice(0);
for (var $ = l(d), H; !(H = $()).done; ) {
var D = H.value;
D.parent && D.parent.removeChild(D, "ignore");
}
} else if (d.type)
d = [d];
else if (d.prop) {
if (typeof d.value > "u")
throw new Error("Value field is missed in node creation");
typeof d.value != "string" && (d.value = String(d.value)), d = [new i.default(d)];
} else if (d.selector) {
var V = fa();
d = [new V(d)];
} else if (d.name) {
var B = pa();
d = [new B(d)];
} else if (d.text)
d = [new u.default(d)];
else
throw new Error("Unknown node type in node creation");
var O = d.map(function(j) {
return j.parent && j.parent.removeChild(j), typeof j.raws.before > "u" && _ && typeof _.raws.before < "u" && (j.raws.before = _.raws.before.replace(/[^\s]/g, "")), j.parent = k, j;
});
return O;
}, t(v, [{ key: "first", get: function() {
if (this.nodes)
return this.nodes[0];
} }, { key: "last", get: function() {
if (this.nodes)
return this.nodes[this.nodes.length - 1];
} }]), v;
}(o.default), f = s;
e.default = f, n2.exports = e.default;
} }), pa = P({ "node_modules/postcss/lib/at-rule.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = u(Or());
function u(p) {
return p && p.__esModule ? p : { default: p };
}
function o(p, m) {
p.prototype = Object.create(m.prototype), p.prototype.constructor = p, p.__proto__ = m;
}
var h = function(p) {
o(m, p);
function m(t) {
var r;
return r = p.call(this, t) || this, r.type = "atrule", r;
}
var c = m.prototype;
return c.append = function() {
var r;
this.nodes || (this.nodes = []);
for (var a = arguments.length, s = new Array(a), f = 0; f < a; f++)
s[f] = arguments[f];
return (r = p.prototype.append).call.apply(r, [this].concat(s));
}, c.prepend = function() {
var r;
this.nodes || (this.nodes = []);
for (var a = arguments.length, s = new Array(a), f = 0; f < a; f++)
s[f] = arguments[f];
return (r = p.prototype.prepend).call.apply(r, [this].concat(s));
}, m;
}(i.default), l = h;
e.default = l, n2.exports = e.default;
} }), If = P({ "node_modules/postcss/lib/map-generator.js"(e, n2) {
A(), n2.exports = class {
generate() {
}
};
} }), Rf = P({ "node_modules/postcss/lib/warn-once.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = u;
var i = {};
function u(o) {
i[o] || (i[o] = true, typeof console < "u" && console.warn && console.warn(o));
}
n2.exports = e.default;
} }), Cf = P({ "node_modules/postcss/lib/warning.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = function() {
function o(l, p) {
if (p === void 0 && (p = {}), this.type = "warning", this.text = l, p.node && p.node.source) {
var m = p.node.positionBy(p);
this.line = m.line, this.column = m.column;
}
for (var c in p)
this[c] = p[c];
}
var h = o.prototype;
return h.toString = function() {
return this.node ? this.node.error(this.text, { plugin: this.plugin, index: this.index, word: this.word }).message : this.plugin ? this.plugin + ": " + this.text : this.text;
}, o;
}(), u = i;
e.default = u, n2.exports = e.default;
} }), Nf = P({ "node_modules/postcss/lib/result.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = u(Cf());
function u(m) {
return m && m.__esModule ? m : { default: m };
}
function o(m, c) {
for (var t = 0; t < c.length; t++) {
var r = c[t];
r.enumerable = r.enumerable || false, r.configurable = true, "value" in r && (r.writable = true), Object.defineProperty(m, r.key, r);
}
}
function h(m, c, t) {
return c && o(m.prototype, c), t && o(m, t), m;
}
var l = function() {
function m(t, r, a) {
this.processor = t, this.messages = [], this.root = r, this.opts = a, this.css = void 0, this.map = void 0;
}
var c = m.prototype;
return c.toString = function() {
return this.css;
}, c.warn = function(r, a) {
a === void 0 && (a = {}), a.plugin || this.lastPlugin && this.lastPlugin.postcssPlugin && (a.plugin = this.lastPlugin.postcssPlugin);
var s = new i.default(r, a);
return this.messages.push(s), s;
}, c.warnings = function() {
return this.messages.filter(function(r) {
return r.type === "warning";
});
}, h(m, [{ key: "content", get: function() {
return this.css;
} }]), m;
}(), p = l;
e.default = p, n2.exports = e.default;
} }), ha = P({ "node_modules/postcss/lib/lazy-result.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = p(If()), u = p(ua()), o = p(Rf()), h = p(Nf()), l = p(la());
function p(v) {
return v && v.__esModule ? v : { default: v };
}
function m(v, y) {
var w;
if (typeof Symbol > "u" || v[Symbol.iterator] == null) {
if (Array.isArray(v) || (w = c(v)) || y && v && typeof v.length == "number") {
w && (v = w);
var d = 0;
return function() {
return d >= v.length ? { done: true } : { done: false, value: v[d++] };
};
}
throw new TypeError(`Invalid attempt to iterate non-iterable instance.
In order to be iterable, non-array objects must have a [Symbol.iterator]() method.`);
}
return w = v[Symbol.iterator](), w.next.bind(w);
}
function c(v, y) {
if (v) {
if (typeof v == "string")
return t(v, y);
var w = Object.prototype.toString.call(v).slice(8, -1);
if (w === "Object" && v.constructor && (w = v.constructor.name), w === "Map" || w === "Set")
return Array.from(v);
if (w === "Arguments" || /^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(w))
return t(v, y);
}
}
function t(v, y) {
(y == null || y > v.length) && (y = v.length);
for (var w = 0, d = new Array(y); w < y; w++)
d[w] = v[w];
return d;
}
function r(v, y) {
for (var w = 0; w < y.length; w++) {
var d = y[w];
d.enumerable = d.enumerable || false, d.configurable = true, "value" in d && (d.writable = true), Object.defineProperty(v, d.key, d);
}
}
function a(v, y, w) {
return y && r(v.prototype, y), w && r(v, w), v;
}
function s(v) {
return typeof v == "object" && typeof v.then == "function";
}
var f = function() {
function v(w, d, _) {
this.stringified = false, this.processed = false;
var k;
if (typeof d == "object" && d !== null && d.type === "root")
k = d;
else if (d instanceof v || d instanceof h.default)
k = d.root, d.map && (typeof _.map > "u" && (_.map = {}), _.map.inline || (_.map.inline = false), _.map.prev = d.map);
else {
var x = l.default;
_.syntax && (x = _.syntax.parse), _.parser && (x = _.parser), x.parse && (x = x.parse);
try {
k = x(d, _);
} catch (N) {
this.error = N;
}
}
this.result = new h.default(w, k, _);
}
var y = v.prototype;
return y.warnings = function() {
return this.sync().warnings();
}, y.toString = function() {
return this.css;
}, y.then = function(d, _) {
return this.async().then(d, _);
}, y.catch = function(d) {
return this.async().catch(d);
}, y.finally = function(d) {
return this.async().then(d, d);
}, y.handleError = function(d, _) {
try {
if (this.error = d, d.name === "CssSyntaxError" && !d.plugin)
d.plugin = _.postcssPlugin, d.setMessage();
else if (_.postcssVersion && false)
var k, x, N, I, W;
} catch ($) {
console && console.error && console.error($);
}
}, y.asyncTick = function(d, _) {
var k = this;
if (this.plugin >= this.processor.plugins.length)
return this.processed = true, d();
try {
var x = this.processor.plugins[this.plugin], N = this.run(x);
this.plugin += 1, s(N) ? N.then(function() {
k.asyncTick(d, _);
}).catch(function(I) {
k.handleError(I, x), k.processed = true, _(I);
}) : this.asyncTick(d, _);
} catch (I) {
this.processed = true, _(I);
}
}, y.async = function() {
var d = this;
return this.processed ? new Promise(function(_, k) {
d.error ? k(d.error) : _(d.stringify());
}) : this.processing ? this.processing : (this.processing = new Promise(function(_, k) {
if (d.error)
return k(d.error);
d.plugin = 0, d.asyncTick(_, k);
}).then(function() {
return d.processed = true, d.stringify();
}), this.processing);
}, y.sync = function() {
if (this.processed)
return this.result;
if (this.processed = true, this.processing)
throw new Error("Use process(css).then(cb) to work with async plugins");
if (this.error)
throw this.error;
for (var d = m(this.result.processor.plugins), _; !(_ = d()).done; ) {
var k = _.value, x = this.run(k);
if (s(x))
throw new Error("Use process(css).then(cb) to work with async plugins");
}
return this.result;
}, y.run = function(d) {
this.result.lastPlugin = d;
try {
return d(this.result.root, this.result);
} catch (_) {
throw this.handleError(_, d), _;
}
}, y.stringify = function() {
if (this.stringified)
return this.result;
this.stringified = true, this.sync();
var d = this.result.opts, _ = u.default;
d.syntax && (_ = d.syntax.stringify), d.stringifier && (_ = d.stringifier), _.stringify && (_ = _.stringify);
var k = new i.default(_, this.result.root, this.result.opts), x = k.generate();
return this.result.css = x[0], this.result.map = x[1], this.result;
}, a(v, [{ key: "processor", get: function() {
return this.result.processor;
} }, { key: "opts", get: function() {
return this.result.opts;
} }, { key: "css", get: function() {
return this.stringify().css;
} }, { key: "content", get: function() {
return this.stringify().content;
} }, { key: "map", get: function() {
return this.stringify().map;
} }, { key: "root", get: function() {
return this.sync().root;
} }, { key: "messages", get: function() {
return this.sync().messages;
} }]), v;
}(), g = f;
e.default = g, n2.exports = e.default;
} }), jf = P({ "node_modules/postcss/lib/processor.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = u(ha());
function u(c) {
return c && c.__esModule ? c : { default: c };
}
function o(c, t) {
var r;
if (typeof Symbol > "u" || c[Symbol.iterator] == null) {
if (Array.isArray(c) || (r = h(c)) || t && c && typeof c.length == "number") {
r && (c = r);
var a = 0;
return function() {
return a >= c.length ? { done: true } : { done: false, value: c[a++] };
};
}
throw new TypeError(`Invalid attempt to iterate non-iterable instance.
In order to be iterable, non-array objects must have a [Symbol.iterator]() method.`);
}
return r = c[Symbol.iterator](), r.next.bind(r);
}
function h(c, t) {
if (c) {
if (typeof c == "string")
return l(c, t);
var r = Object.prototype.toString.call(c).slice(8, -1);
if (r === "Object" && c.constructor && (r = c.constructor.name), r === "Map" || r === "Set")
return Array.from(c);
if (r === "Arguments" || /^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(r))
return l(c, t);
}
}
function l(c, t) {
(t == null || t > c.length) && (t = c.length);
for (var r = 0, a = new Array(t); r < t; r++)
a[r] = c[r];
return a;
}
var p = function() {
function c(r) {
r === void 0 && (r = []), this.version = "7.0.39", this.plugins = this.normalize(r);
}
var t = c.prototype;
return t.use = function(a) {
return this.plugins = this.plugins.concat(this.normalize([a])), this;
}, t.process = function(r) {
function a(s) {
return r.apply(this, arguments);
}
return a.toString = function() {
return r.toString();
}, a;
}(function(r, a) {
return a === void 0 && (a = {}), this.plugins.length === 0 && (a.parser, a.stringifier), new i.default(this, r, a);
}), t.normalize = function(a) {
for (var s = [], f = o(a), g; !(g = f()).done; ) {
var v = g.value;
if (v.postcss === true) {
var y = v();
throw new Error("PostCSS plugin " + y.postcssPlugin + ` requires PostCSS 8.
Migration guide for end-users:
https://github.com/postcss/postcss/wiki/PostCSS-8-for-end-users`);
}
if (v.postcss && (v = v.postcss), typeof v == "object" && Array.isArray(v.plugins))
s = s.concat(v.plugins);
else if (typeof v == "function")
s.push(v);
else if (!(typeof v == "object" && (v.parse || v.stringify)))
throw typeof v == "object" && v.postcssPlugin ? new Error("PostCSS plugin " + v.postcssPlugin + ` requires PostCSS 8.
Migration guide for end-users:
https://github.com/postcss/postcss/wiki/PostCSS-8-for-end-users`) : new Error(v + " is not a PostCSS plugin");
}
return s;
}, c;
}(), m = p;
e.default = m, n2.exports = e.default;
} }), Mf = P({ "node_modules/postcss/lib/root.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = u(Or());
function u(t) {
return t && t.__esModule ? t : { default: t };
}
function o(t, r) {
var a;
if (typeof Symbol > "u" || t[Symbol.iterator] == null) {
if (Array.isArray(t) || (a = h(t)) || r && t && typeof t.length == "number") {
a && (t = a);
var s = 0;
return function() {
return s >= t.length ? { done: true } : { done: false, value: t[s++] };
};
}
throw new TypeError(`Invalid attempt to iterate non-iterable instance.
In order to be iterable, non-array objects must have a [Symbol.iterator]() method.`);
}
return a = t[Symbol.iterator](), a.next.bind(a);
}
function h(t, r) {
if (t) {
if (typeof t == "string")
return l(t, r);
var a = Object.prototype.toString.call(t).slice(8, -1);
if (a === "Object" && t.constructor && (a = t.constructor.name), a === "Map" || a === "Set")
return Array.from(t);
if (a === "Arguments" || /^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(a))
return l(t, r);
}
}
function l(t, r) {
(r == null || r > t.length) && (r = t.length);
for (var a = 0, s = new Array(r); a < r; a++)
s[a] = t[a];
return s;
}
function p(t, r) {
t.prototype = Object.create(r.prototype), t.prototype.constructor = t, t.__proto__ = r;
}
var m = function(t) {
p(r, t);
function r(s) {
var f;
return f = t.call(this, s) || this, f.type = "root", f.nodes || (f.nodes = []), f;
}
var a = r.prototype;
return a.removeChild = function(f, g) {
var v = this.index(f);
return !g && v === 0 && this.nodes.length > 1 && (this.nodes[1].raws.before = this.nodes[v].raws.before), t.prototype.removeChild.call(this, f);
}, a.normalize = function(f, g, v) {
var y = t.prototype.normalize.call(this, f);
if (g) {
if (v === "prepend")
this.nodes.length > 1 ? g.raws.before = this.nodes[1].raws.before : delete g.raws.before;
else if (this.first !== g)
for (var w = o(y), d; !(d = w()).done; ) {
var _ = d.value;
_.raws.before = g.raws.before;
}
}
return y;
}, a.toResult = function(f) {
f === void 0 && (f = {});
var g = ha(), v = jf(), y = new g(new v(), this, f);
return y.stringify();
}, r;
}(i.default), c = m;
e.default = c, n2.exports = e.default;
} }), $t = P({ "node_modules/postcss/lib/parser.js"(e, n2) {
"use strict";
A(), e.__esModule = true, e.default = void 0;
var i = m(ca()), u = m(Ut()), o = m(kr()), h = m(pa()), l = m(Mf()), p = m(fa());
function m(t) {
return t && t.__esModule ? t : { default: t };
}
var c = function() {
function t(a) {
this.input = a, this.root = new l.default(), this.current = this.root, this.spaces = "", this.semicolon = false, this.createTokenizer(), this.root.source = { input: a, start: { line: 1, column: 1 } };
}
var r = t.prototype;
return r.createTokenizer = function() {
this.tokenizer = (0, u.default)(this.input);
}, r.parse = function() {
for (var s; !this.tokenizer.endOfFile(); )
switch (s = this.tokenizer.nextToken(), s[0]) {
case "space":
this.spaces += s[1];
break;
case ";":
this.freeSemicolon(s);
break;
case "}":
this.end(s);
break;
case "comment":
this.comment(s);
break;
case "at-word":
this.atrule(s);
break;
case "{":
this.emptyRule(s);
break;
default:
this.other(s);
break;
}
this.endFile();
}, r.comment = function(s) {
var f = new o.default();
this.init(f, s[2], s[3]), f.source.end = { line: s[4], column: s[5] };
var g = s[1].slice(2, -2);
if (/^\s*$/.test(g))
f.text = "", f.raws.left = g, f.raws.right = "";
else {
var v = g.match(/^(\s*)([^]*[^\s])(\s*)$/);
f.text = v[2], f.raws.left = v[1], f.raws.right = v[3];
}
}, r.emptyRule = function(s) {
var f = new p.default();
this.init(f, s[2], s[3]), f.selector = "", f.raws.between = "", this.current = f;
}, r.other = function(s) {
for (var f = false, g = null, v = false, y = null, w = [], d = [], _ = s; _; ) {
if (g = _[0], d.push(_), g === "(" || g === "[")
y || (y = _), w.push(g === "(" ? ")" : "]");
else if (w.length === 0)
if (g === ";")
if (v) {
this.decl(d);
return;
} else
break;
else if (g === "{") {
this.rule(d);
return;
} else if (g === "}") {
this.tokenizer.back(d.pop()), f = true;
break;
} else
g === ":" && (v = true);
else
g === w[w.length - 1] && (w.pop(), w.length === 0 && (y = null));
_ = this.tokenizer.nextToken();
}
if (this.tokenizer.endOfFile() && (f = true), w.length > 0 && this.unclosedBracket(y), f && v) {
for (; d.length && (_ = d[d.length - 1][0], !(_ !== "space" && _ !== "comment")); )
this.tokenizer.back(d.pop());
this.decl(d);
} else
this.unknownWord(d);
}, r.rule = function(s) {
s.pop();
var f = new p.default();
this.init(f, s[0][2], s[0][3]), f.raws.between = this.spacesAndCommentsFromEnd(s), this.raw(f, "selector", s), this.current = f;
}, r.decl = function(s) {
var f = new i.default();
this.init(f);
var g = s[s.length - 1];
for (g[0] === ";" && (this.semicolon = true, s.pop()), g[4] ? f.source.end = { line: g[4], column: g[5] } : f.source.end = { line: g[2], column: g[3] }; s[0][0] !== "word"; )
s.length === 1 && this.unknownWord(s), f.raws.before += s.shift()[1];
for (f.source.start = { line: s[0][2], column: s[0][3] }, f.prop = ""; s.length; ) {
var v = s[0][0];
if (v === ":" || v === "space" || v === "comment")
break;
f.prop += s.shift()[1];
}
f.raws.between = "";
for (var y; s.length; )
if (y = s.shift(), y[0] === ":") {
f.raws.between += y[1];
break;
} else
y[0] === "word" && /\w/.test(y[1]) && this.unknownWord([y]), f.raws.between += y[1];
(f.prop[0] === "_" || f.prop[0] === "*") && (f.raws.before += f.prop[0], f.prop = f.prop.slice(1)), f.raws.between += this.spacesAndCommentsFromStart(s), this.precheckMissedSemicolon(s);
for (var w = s.length - 1; w > 0; w--) {
if (y = s[w], y[1].toLowerCase() === "!important") {
f.important = true;
var d = this.stringFrom(s, w);
d = this.spacesFromEnd(s) + d, d !== " !important" && (f.raws.important = d);
break;
} else if (y[1].toLowerCase() === "important") {
for (var _ = s.slice(0), k = "", x = w; x > 0; x--) {
var N = _[x][0];
if (k.trim().indexOf("!") === 0 && N !== "space")
break;
k = _.pop()[1] + k;
}
k.trim().indexOf("!") === 0 && (f.important = true, f.raws.important = k, s = _);
}
if (y[0] !== "space" && y[0] !== "comment")
break;
}
this.raw(f, "value", s), f.value.indexOf(":") !== -1 && this.checkMissedSemicolon(s);
}, r.atrule = function(s) {
var f = new h.default();
f.name = s[1].slice(1), f.name === "" && this.unnamedAtrule(f, s), this.init(f, s[2], s[3]);
for (var g, v, y = false, w = false, d = []; !this.tokenizer.endOfFile(); ) {
if (s = this.tokenizer.nextToken(), s[0] === ";") {
f.source.end = { line: s[2], column: s[3] }, this.semicolon = true;
break;
} else if (s[0] === "{") {
w = true;
break;
} else if (s[0] === "}") {
if (d.length > 0) {
for (v = d.length - 1, g = d[v]; g && g[0] === "space"; )
g = d[--v];
g && (f.source.end = { line: g[4], column: g[5] });
}
this.end(s);
break;
} else
d.push(s);
if (this.tokenizer.endOfFile()) {
y = true;
break;
}
}
f.raws.between = this.spacesAndCommentsFromEnd(d), d.length ? (f.raws.afterName = this.spacesAndCommentsFromStart(d), this.raw(f, "params", d), y && (s = d[d.length - 1], f.source.end = { line: s[4], column: s[5] }, this.spaces = f.raws.between, f.raws.between = "")) : (f.raws.afterName = "", f.params = ""), w && (f.nodes = [], this.current = f);
}, r.end = function(s) {
this.current.nodes && this.current.nodes.length && (this.current.raws.semicolon = this.semicolon), this.semicolon = false, this.current.raws.after = (this.current.raws.after || "") + this.spaces, this.spaces = "", this.current.parent ? (this.current.source.end = { line: s[2], column: s[3] }, this.current = this.current.parent) : this.unexpectedClose(s);
}, r.endFile = function() {
this.current.parent && this.unclosedBlock(), this.current.nodes && this.current.nodes.length && (this.current.raws.semicolon = this.semicolon), this.current.raws.after = (this.current.raws.after || "") + this.spaces;
}, r.freeSemicolon = function(s) {
if (this.spaces += s[1], this.current.nodes) {
var f = this.current.nodes[this.current.nodes.length - 1];
f && f.type === "rule" && !f.raws.ownSemicolon && (f.raws.ownSemicolon = this.spaces, this.spaces = "");
}
}, r.init = function(s, f, g) {
this.current.push(s), s.source = { start: { line: f, column: g }, input: this.input }, s.raws.before = this.spaces, this.spaces = "", s.type !== "comment" && (this.semicolon = false);
}, r.raw = function(s, f, g) {
for (var v, y, w = g.length, d = "", _ = true, k, x, N = /^([.|#])?([\w])+/i, I = 0; I < w; I += 1) {
if (v = g[I], y = v[0], y === "comment" && s.type === "rule") {
x = g[I - 1], k = g[I + 1], x[0] !== "space" && k[0] !== "space" && N.test(x[1]) && N.test(k[1]) ? d += v[1] : _ = false;
continue;
}
y === "comment" || y === "space" && I === w - 1 ? _ = false : d += v[1];
}
if (!_) {
var W = g.reduce(function($, H) {
return $ + H[1];
}, "");
s.raws[f] = { value: d, raw: W };
}
s[f] = d;
}, r.spacesAndCommentsFromEnd = function(s) {
for (var f, g = ""; s.length && (f = s[s.length - 1][0], !(f !== "space" && f !== "comment")); )
g = s.pop()[1] + g;
return g;
}, r.spacesAndCommentsFromStart = function(s) {
for (var f, g = ""; s.length && (f = s[0][0], !(f !== "space" && f !== "comment")); )
g += s.shift()[1];
return g;
}, r.spacesFromEnd = function(s) {
for (var f, g = ""; s.length && (f = s[s.length - 1][0], f === "space"); )
g = s.pop()[1] + g;
return g;
}, r.stringFrom = function(s, f) {
for (var g = "", v = f; v < s.length; v++)
g += s[v][1];
return s.splice(f, s.length - f), g;
}, r.colon = function(s) {
for (var f = 0, g, v, y, w = 0; w < s.length; w++) {
if (g = s[w], v = g[0], v === "(" && (f += 1), v === ")" && (f -= 1), f === 0 && v === ":")
if (!y)
this.doubleColon(g);
else {
if (y[0] === "word" && y[1] === "progid")
continue;
return w;
}
y = g;
}
return false;
}, r.unclosedBracket = function(s) {
throw this.input.error("Unclosed bracket", s[2], s[3]);
}, r.unknownWord = function(s) {
throw this.input.error("Unknown word", s[0][2], s[0][3]);
}, r.unexpectedClose = function(s) {
throw this.input.error("Unexpected }", s[2], s[3]);
}, r.unclosedBlock = function() {
var s = this.current.source.start;
throw this.input.error("Unclosed block", s.line, s.column);
}, r.doubleColon = function(s) {
throw this.input.error("Double colon", s[2], s[3]);
}, r.unnamedAtrule = function(s, f) {
throw this.input.error("At-rule without name", f[2], f[3]);
}, r.precheckMissedSemicolon = function() {
}, r.checkMissedSemicolon = function(s) {
var f = this.colon(s);
if (f !== false) {
for (var g = 0, v, y = f - 1; y >= 0 && (v = s[y], !(v[0] !== "space" && (g += 1, g === 2))); y--)
;
throw this.input.error("Missed semicolon", v[2], v[3]);
}
}, t;
}();
e.default = c, n2.exports = e.default;
} }), Df = P({ "node_modules/postcss-less/lib/nodes/inline-comment.js"(e, n2) {
A();
var i = Ut(), u = xr();
n2.exports = { isInlineComment(o) {
if (o[0] === "word" && o[1].slice(0, 2) === "//") {
let h = o, l = [], p;
for (; o; ) {
if (/\r?\n/.test(o[1])) {
if (/['"].*\r?\n/.test(o[1])) {
l.push(o[1].substring(0, o[1].indexOf(`
`)));
let c = o[1].substring(o[1].indexOf(`
`));
c += this.input.css.valueOf().substring(this.tokenizer.position()), this.input = new u(c), this.tokenizer = i(this.input);
} else
this.tokenizer.back(o);
break;
}
l.push(o[1]), p = o, o = this.tokenizer.nextToken({ ignoreUnclosed: true });
}
let m = ["comment", l.join(""), h[2], h[3], p[2], p[3]];
return this.inlineComment(m), true;
} else if (o[1] === "/") {
let h = this.tokenizer.nextToken({ ignoreUnclosed: true });
if (h[0] === "comment" && /^\/\*/.test(h[1]))
return h[0] = "word", h[1] = h[1].slice(1), o[1] = "//", this.tokenizer.back(h), n2.exports.isInlineComment.bind(this)(o);
}
return false;
} };
} }), Lf = P({ "node_modules/postcss-less/lib/nodes/interpolation.js"(e, n2) {
A(), n2.exports = { interpolation(i) {
let u = i, o = [i], h = ["word", "{", "}"];
if (i = this.tokenizer.nextToken(), u[1].length > 1 || i[0] !== "{")
return this.tokenizer.back(i), false;
for (; i && h.includes(i[0]); )
o.push(i), i = this.tokenizer.nextToken();
let l = o.map((r) => r[1]);
[u] = o;
let p = o.pop(), m = [u[2], u[3]], c = [p[4] || p[2], p[5] || p[3]], t = ["word", l.join("")].concat(m, c);
return this.tokenizer.back(i), this.tokenizer.back(t), true;
} };
} }), zf = P({ "node_modules/postcss-less/lib/nodes/mixin.js"(e, n2) {
A();
var i = /^#[0-9a-fA-F]{6}$|^#[0-9a-fA-F]{3}$/, u = /\.[0-9]/, o = (h) => {
let [, l] = h, [p] = l;
return (p === "." || p === "#") && i.test(l) === false && u.test(l) === false;
};
n2.exports = { isMixinToken: o };
} }), Bf = P({ "node_modules/postcss-less/lib/nodes/import.js"(e, n2) {
A();
var i = Ut(), u = /^url\((.+)\)/;
n2.exports = (o) => {
let { name: h, params: l = "" } = o;
if (h === "import" && l.length) {
o.import = true;
let p = i({ css: l });
for (o.filename = l.replace(u, "$1"); !p.endOfFile(); ) {
let [m, c] = p.nextToken();
if (m === "word" && c === "url")
return;
if (m === "brackets") {
o.options = c, o.filename = l.replace(c, "").trim();
break;
}
}
}
};
} }), Ff = P({ "node_modules/postcss-less/lib/nodes/variable.js"(e, n2) {
A();
var i = /:$/, u = /^:(\s+)?/;
n2.exports = (o) => {
let { name: h, params: l = "" } = o;
if (o.name.slice(-1) === ":") {
if (i.test(h)) {
let [p] = h.match(i);
o.name = h.replace(p, ""), o.raws.afterName = p + (o.raws.afterName || ""), o.variable = true, o.value = o.params;
}
if (u.test(l)) {
let [p] = l.match(u);
o.value = l.replace(p, ""), o.raws.afterName = (o.raws.afterName || "") + p, o.variable = true;
}
}
};
} }), Uf = P({ "node_modules/postcss-less/lib/LessParser.js"(e, n2) {
A();
var i = kr(), u = $t(), { isInlineComment: o } = Df(), { interpolation: h } = Lf(), { isMixinToken: l } = zf(), p = Bf(), m = Ff(), c = /(!\s*important)$/i;
n2.exports = class extends u {
constructor() {
super(...arguments), this.lastNode = null;
}
atrule(r) {
h.bind(this)(r) || (super.atrule(r), p(this.lastNode), m(this.lastNode));
}
decl() {
super.decl(...arguments), /extend\(.+\)/i.test(this.lastNode.value) && (this.lastNode.extend = true);
}
each(r) {
r[0][1] = ` ${r[0][1]}`;
let a = r.findIndex((y) => y[0] === "("), s = r.reverse().find((y) => y[0] === ")"), f = r.reverse().indexOf(s), v = r.splice(a, f).map((y) => y[1]).join("");
for (let y of r.reverse())
this.tokenizer.back(y);
this.atrule(this.tokenizer.nextToken()), this.lastNode.function = true, this.lastNode.params = v;
}
init(r, a, s) {
super.init(r, a, s), this.lastNode = r;
}
inlineComment(r) {
let a = new i(), s = r[1].slice(2);
if (this.init(a, r[2], r[3]), a.source.end = { line: r[4], column: r[5] }, a.inline = true, a.raws.begin = "//", /^\s*$/.test(s))
a.text = "", a.raws.left = s, a.raws.right = "";
else {
let f = s.match(/^(\s*)([^]*[^\s])(\s*)$/);
[, a.raws.left, a.text, a.raws.right] = f;
}
}
mixin(r) {
let [a] = r, s = a[1].slice(0, 1), f = r.findIndex((d) => d[0] === "brackets"), g = r.findIndex((d) => d[0] === "("), v = "";
if ((f < 0 || f > 3) && g > 0) {
let d = r.reduce((V, B, O) => B[0] === ")" ? O : V), k = r.slice(g, d + g).map((V) => V[1]).join(""), [x] = r.slice(g), N = [x[2], x[3]], [I] = r.slice(d, d + 1), W = [I[2], I[3]], $ = ["brackets", k].concat(N, W), H = r.slice(0, g), D = r.slice(d + 1);
r = H, r.push($), r = r.concat(D);
}
let y = [];
for (let d of r)
if ((d[1] === "!" || y.length) && y.push(d), d[1] === "important")
break;
if (y.length) {
let [d] = y, _ = r.indexOf(d), k = y[y.length - 1], x = [d[2], d[3]], N = [k[4], k[5]], W = ["word", y.map(($) => $[1]).join("")].concat(x, N);
r.splice(_, y.length, W);
}
let w = r.findIndex((d) => c.test(d[1]));
w > 0 && ([, v] = r[w], r.splice(w, 1));
for (let d of r.reverse())
this.tokenizer.back(d);
this.atrule(this.tokenizer.nextToken()), this.lastNode.mixin = true, this.lastNode.raws.identifier = s, v && (this.lastNode.important = true, this.lastNode.raws.important = v);
}
other(r) {
o.bind(this)(r) || super.other(r);
}
rule(r) {
let a = r[r.length - 1], s = r[r.length - 2];
if (s[0] === "at-word" && a[0] === "{" && (this.tokenizer.back(a), h.bind(this)(s))) {
let g = this.tokenizer.nextToken();
r = r.slice(0, r.length - 2).concat([g]);
for (let v of r.reverse())
this.tokenizer.back(v);
return;
}
super.rule(r), /:extend\(.+\)/i.test(this.lastNode.selector) && (this.lastNode.extend = true);
}
unknownWord(r) {
let [a] = r;
if (r[0][1] === "each" && r[1][0] === "(") {
this.each(r);
return;
}
if (l(a)) {
this.mixin(r);
return;
}
super.unknownWord(r);
}
};
} }), $f = P({ "node_modules/postcss-less/lib/LessStringifier.js"(e, n2) {
A();
var i = Sr();
n2.exports = class extends i {
atrule(o, h) {
if (!o.mixin && !o.variable && !o.function) {
super.atrule(o, h);
return;
}
let p = `${o.function ? "" : o.raws.identifier || "@"}${o.name}`, m = o.params ? this.rawValue(o, "params") : "", c = o.raws.important || "";
if (o.variable && (m = o.value), typeof o.raws.afterName < "u" ? p += o.raws.afterName : m && (p += " "), o.nodes)
this.block(o, p + m + c);
else {
let t = (o.raws.between || "") + c + (h ? ";" : "");
this.builder(p + m + t, o);
}
}
comment(o) {
if (o.inline) {
let h = this.raw(o, "left", "commentLeft"), l = this.raw(o, "right", "commentRight");
this.builder(`//${h}${o.text}${l}`, o);
} else
super.comment(o);
}
};
} }), Wf = P({ "node_modules/postcss-less/lib/index.js"(e, n2) {
A();
var i = xr(), u = Uf(), o = $f();
n2.exports = { parse(h, l) {
let p = new i(h, l), m = new u(p);
return m.parse(), m.root;
}, stringify(h, l) {
new o(l).stringify(h);
}, nodeToString(h) {
let l = "";
return n2.exports.stringify(h, (p) => {
l += p;
}), l;
} };
} }), Vf = P({ "node_modules/postcss-scss/lib/scss-stringifier.js"(e, n2) {
"use strict";
A();
function i(h, l) {
h.prototype = Object.create(l.prototype), h.prototype.constructor = h, h.__proto__ = l;
}
var u = Sr(), o = function(h) {
i(l, h);
function l() {
return h.apply(this, arguments) || this;
}
var p = l.prototype;
return p.comment = function(c) {
var t = this.raw(c, "left", "commentLeft"), r = this.raw(c, "right", "commentRight");
if (c.raws.inline) {
var a = c.raws.text || c.text;
this.builder("//" + t + a + r, c);
} else
this.builder("/*" + t + c.text + r + "*/", c);
}, p.decl = function(c, t) {
if (!c.isNested)
h.prototype.decl.call(this, c, t);
else {
var r = this.raw(c, "between", "colon"), a = c.prop + r + this.rawValue(c, "value");
c.important && (a += c.raws.important || " !important"), this.builder(a + "{", c, "start");
var s;
c.nodes && c.nodes.length ? (this.body(c), s = this.raw(c, "after")) : s = this.raw(c, "after", "emptyBody"), s && this.builder(s), this.builder("}", c, "end");
}
}, p.rawValue = function(c, t) {
var r = c[t], a = c.raws[t];
return a && a.value === r ? a.scss ? a.scss : a.raw : r;
}, l;
}(u);
n2.exports = o;
} }), Gf = P({ "node_modules/postcss-scss/lib/scss-stringify.js"(e, n2) {
"use strict";
A();
var i = Vf();
n2.exports = function(o, h) {
var l = new i(h);
l.stringify(o);
};
} }), Hf = P({ "node_modules/postcss-scss/lib/nested-declaration.js"(e, n2) {
"use strict";
A();
function i(h, l) {
h.prototype = Object.create(l.prototype), h.prototype.constructor = h, h.__proto__ = l;
}
var u = Or(), o = function(h) {
i(l, h);
function l(p) {
var m;
return m = h.call(this, p) || this, m.type = "decl", m.isNested = true, m.nodes || (m.nodes = []), m;
}
return l;
}(u);
n2.exports = o;
} }), Jf = P({ "node_modules/postcss-scss/lib/scss-tokenize.js"(e, n2) {
"use strict";
A();
var i = "'".charCodeAt(0), u = '"'.charCodeAt(0), o = "\\".charCodeAt(0), h = "/".charCodeAt(0), l = `
`.charCodeAt(0), p = " ".charCodeAt(0), m = "\f".charCodeAt(0), c = " ".charCodeAt(0), t = "\r".charCodeAt(0), r = "[".charCodeAt(0), a = "]".charCodeAt(0), s = "(".charCodeAt(0), f = ")".charCodeAt(0), g = "{".charCodeAt(0), v = "}".charCodeAt(0), y = ";".charCodeAt(0), w = "*".charCodeAt(0), d = ":".charCodeAt(0), _ = "@".charCodeAt(0), k = ",".charCodeAt(0), x = "#".charCodeAt(0), N = /[ \n\t\r\f{}()'"\\;/[\]#]/g, I = /[ \n\t\r\f(){}:;@!'"\\\][#]|\/(?=\*)/g, W = /.[\\/("'\n]/, $ = /[a-f0-9]/i, H = /[\r\f\n]/g;
n2.exports = function(V, B) {
B === void 0 && (B = {});
var O = V.css.valueOf(), j = B.ignoreErrors, C, R, X, Z, Q, K, J, M, Y, G, E, S, b, L, q = O.length, T = -1, F = 1, z = 0, ee = [], te = [];
function ue(ie) {
throw V.error("Unclosed " + ie, F, z - T);
}
function le() {
return te.length === 0 && z >= q;
}
function re() {
for (var ie = 1, ce = false, fe = false; ie > 0; )
R += 1, O.length <= R && ue("interpolation"), C = O.charCodeAt(R), S = O.charCodeAt(R + 1), ce ? !fe && C === ce ? (ce = false, fe = false) : C === o ? fe = !G : fe && (fe = false) : C === i || C === u ? ce = C : C === v ? ie -= 1 : C === x && S === g && (ie += 1);
}
function ne() {
if (te.length)
return te.pop();
if (!(z >= q)) {
switch (C = O.charCodeAt(z), (C === l || C === m || C === t && O.charCodeAt(z + 1) !== l) && (T = z, F += 1), C) {
case l:
case p:
case c:
case t:
case m:
R = z;
do
R += 1, C = O.charCodeAt(R), C === l && (T = R, F += 1);
while (C === p || C === l || C === c || C === t || C === m);
b = ["space", O.slice(z, R)], z = R - 1;
break;
case r:
b = ["[", "[", F, z - T];
break;
case a:
b = ["]", "]", F, z - T];
break;
case g:
b = ["{", "{", F, z - T];
break;
case v:
b = ["}", "}", F, z - T];
break;
case k:
b = ["word", ",", F, z - T, F, z - T + 1];
break;
case d:
b = [":", ":", F, z - T];
break;
case y:
b = [";", ";", F, z - T];
break;
case s:
if (E = ee.length ? ee.pop()[1] : "", S = O.charCodeAt(z + 1), E === "url" && S !== i && S !== u) {
for (L = 1, G = false, R = z + 1; R <= O.length - 1; ) {
if (S = O.charCodeAt(R), S === o)
G = !G;
else if (S === s)
L += 1;
else if (S === f && (L -= 1, L === 0))
break;
R += 1;
}
K = O.slice(z, R + 1), Z = K.split(`
`), Q = Z.length - 1, Q > 0 ? (M = F + Q, Y = R - Z[Q].length) : (M = F, Y = T), b = ["brackets", K, F, z - T, M, R - Y], T = Y, F = M, z = R;
} else
R = O.indexOf(")", z + 1), K = O.slice(z, R + 1), R === -1 || W.test(K) ? b = ["(", "(", F, z - T] : (b = ["brackets", K, F, z - T, F, R - T], z = R);
break;
case f:
b = [")", ")", F, z - T];
break;
case i:
case u:
for (X = C, R = z, G = false; R < q && (R++, R === q && ue("string"), C = O.charCodeAt(R), S = O.charCodeAt(R + 1), !(!G && C === X)); )
C === o ? G = !G : G ? G = false : C === x && S === g && re();
K = O.slice(z, R + 1), Z = K.split(`
`), Q = Z.length - 1, Q > 0 ? (M = F + Q, Y = R - Z[Q].length) : (M = F, Y = T), b = ["string", O.slice(z, R + 1), F, z - T, M, R - Y], T = Y, F = M, z = R;
break;
case _:
N.lastIndex = z + 1, N.test(O), N.lastIndex === 0 ? R = O.length - 1 : R = N.lastIndex - 2, b = ["at-word", O.slice(z, R + 1), F, z - T, F, R - T], z = R;
break;
case o:
for (R = z, J = true; O.charCodeAt(R + 1) === o; )
R += 1, J = !J;
if (C = O.charCodeAt(R + 1), J && C !== h && C !== p && C !== l && C !== c && C !== t && C !== m && (R += 1, $.test(O.charAt(R)))) {
for (; $.test(O.charAt(R + 1)); )
R += 1;
O.charCodeAt(R + 1) === p && (R += 1);
}
b = ["word", O.slice(z, R + 1), F, z - T, F, R - T], z = R;
break;
default:
S = O.charCodeAt(z + 1), C === x && S === g ? (R = z, re(), K = O.slice(z, R + 1), Z = K.split(`
`), Q = Z.length - 1, Q > 0 ? (M = F + Q, Y = R - Z[Q].length) : (M = F, Y = T), b = ["word", K, F, z - T, M, R - Y], T = Y, F = M, z = R) : C === h && S === w ? (R = O.indexOf("*/", z + 2) + 1, R === 0 && (j ? R = O.length : ue("comment")), K = O.slice(z, R + 1), Z = K.split(`
`), Q = Z.length - 1, Q > 0 ? (M = F + Q, Y = R - Z[Q].length) : (M = F, Y = T), b = ["comment", K, F, z - T, M, R - Y], T = Y, F = M, z = R) : C === h && S === h ? (H.lastIndex = z + 1, H.test(O), H.lastIndex === 0 ? R = O.length - 1 : R = H.lastIndex - 2, K = O.slice(z, R + 1), b = ["comment", K, F, z - T, F, R - T, "inline"], z = R) : (I.lastIndex = z + 1, I.test(O), I.lastIndex === 0 ? R = O.length - 1 : R = I.lastIndex - 2, b = ["word", O.slice(z, R + 1), F, z - T, F, R - T], ee.push(b), z = R);
break;
}
return z++, b;
}
}
function oe(ie) {
te.push(ie);
}
return { back: oe, nextToken: ne, endOfFile: le };
};
} }), Kf = P({ "node_modules/postcss-scss/lib/scss-parser.js"(e, n2) {
"use strict";
A();
function i(m, c) {
m.prototype = Object.create(c.prototype), m.prototype.constructor = m, m.__proto__ = c;
}
var u = kr(), o = $t(), h = Hf(), l = Jf(), p = function(m) {
i(c, m);
function c() {
return m.apply(this, arguments) || this;
}
var t = c.prototype;
return t.createTokenizer = function() {
this.tokenizer = l(this.input);
}, t.rule = function(a) {
for (var s = false, f = 0, g = "", w = a, v = Array.isArray(w), y = 0, w = v ? w : w[Symbol.iterator](); ; ) {
var d;
if (v) {
if (y >= w.length)
break;
d = w[y++];
} else {
if (y = w.next(), y.done)
break;
d = y.value;
}
var _ = d;
if (s)
_[0] !== "comment" && _[0] !== "{" && (g += _[1]);
else {
if (_[0] === "space" && _[1].indexOf(`
`) !== -1)
break;
_[0] === "(" ? f += 1 : _[0] === ")" ? f -= 1 : f === 0 && _[0] === ":" && (s = true);
}
}
if (!s || g.trim() === "" || /^[a-zA-Z-:#]/.test(g))
m.prototype.rule.call(this, a);
else {
a.pop();
var k = new h();
this.init(k);
var x = a[a.length - 1];
for (x[4] ? k.source.end = { line: x[4], column: x[5] } : k.source.end = { line: x[2], column: x[3] }; a[0][0] !== "word"; )
k.raws.before += a.shift()[1];
for (k.source.start = { line: a[0][2], column: a[0][3] }, k.prop = ""; a.length; ) {
var N = a[0][0];
if (N === ":" || N === "space" || N === "comment")
break;
k.prop += a.shift()[1];
}
k.raws.between = "";
for (var I; a.length; )
if (I = a.shift(), I[0] === ":") {
k.raws.between += I[1];
break;
} else
k.raws.between += I[1];
(k.prop[0] === "_" || k.prop[0] === "*") && (k.raws.before += k.prop[0], k.prop = k.prop.slice(1)), k.raws.between += this.spacesAndCommentsFromStart(a), this.precheckMissedSemicolon(a);
for (var W = a.length - 1; W > 0; W--) {
if (I = a[W], I[1] === "!important") {
k.important = true;
var $ = this.stringFrom(a, W);
$ = this.spacesFromEnd(a) + $, $ !== " !important" && (k.raws.important = $);
break;
} else if (I[1] === "important") {
for (var H = a.slice(0), D = "", V = W; V > 0; V--) {
var B = H[V][0];
if (D.trim().indexOf("!") === 0 && B !== "space")
break;
D = H.pop()[1] + D;
}
D.trim().indexOf("!") === 0 && (k.important = true, k.raws.important = D, a = H);
}
if (I[0] !== "space" && I[0] !== "comment")
break;
}
this.raw(k, "value", a), k.value.indexOf(":") !== -1 && this.checkMissedSemicolon(a), this.current = k;
}
}, t.comment = function(a) {
if (a[6] === "inline") {
var s = new u();
this.init(s, a[2], a[3]), s.raws.inline = true, s.source.end = { line: a[4], column: a[5] };
var f = a[1].slice(2);
if (/^\s*$/.test(f))
s.text = "", s.raws.left = f, s.raws.right = "";
else {
var g = f.match(/^(\s*)([^]*[^\s])(\s*)$/), v = g[2].replace(/(\*\/|\/\*)/g, "*//*");
s.text = v, s.raws.left = g[1], s.raws.right = g[3], s.raws.text = g[2];
}
} else
m.prototype.comment.call(this, a);
}, t.raw = function(a, s, f) {
if (m.prototype.raw.call(this, a, s, f), a.raws[s]) {
var g = a.raws[s].raw;
a.raws[s].raw = f.reduce(function(v, y) {
if (y[0] === "comment" && y[6] === "inline") {
var w = y[1].slice(2).replace(/(\*\/|\/\*)/g, "*//*");
return v + "/*" + w + "*/";
} else
return v + y[1];
}, ""), g !== a.raws[s].raw && (a.raws[s].scss = g);
}
}, c;
}(o);
n2.exports = p;
} }), Qf = P({ "node_modules/postcss-scss/lib/scss-parse.js"(e, n2) {
"use strict";
A();
var i = xr(), u = Kf();
n2.exports = function(h, l) {
var p = new i(h, l), m = new u(p);
return m.parse(), m.root;
};
} }), Yf = P({ "node_modules/postcss-scss/lib/scss-syntax.js"(e, n2) {
"use strict";
A();
var i = Gf(), u = Qf();
n2.exports = { parse: u, stringify: i };
} });
A();
var Xf = Sl(), mt = Us(), Zf = $s(), { hasPragma: ep } = Cl(), { locStart: rp, locEnd: tp } = no(), { calculateLoc: np, replaceQuotesInInlineComments: ip } = no(), sp = Dl(), op = Ll(), gt = zl(), da = Bl(), ap = Fl(), up = Ul(), cp = $l(), lp = Wl(), fp = (e) => {
for (; e.parent; )
e = e.parent;
return e;
};
function pp(e, n2) {
let { nodes: i } = e, u = { open: null, close: null, groups: [], type: "paren_group" }, o = [u], h = u, l = { groups: [], type: "comma_group" }, p = [l];
for (let m = 0; m < i.length; ++m) {
let c = i[m];
if (da(n2.parser, c.value) && c.type === "number" && c.unit === ".." && mt(c.value) === "." && (c.value = c.value.slice(0, -1), c.unit = "..."), c.type === "func" && c.value === "selector" && (c.group.groups = [Re(fp(e).text.slice(c.group.open.sourceIndex + 1, c.group.close.sourceIndex))]), c.type === "func" && c.value === "url") {
let t = c.group && c.group.groups || [], r = [];
for (let a = 0; a < t.length; a++) {
let s = t[a];
s.type === "comma_group" ? r = [...r, ...s.groups] : r.push(s);
}
if (sp(r) || !op(r) && !up(r[0])) {
let a = cp({ groups: c.group.groups });
c.group.groups = [a.trim()];
}
}
if (c.type === "paren" && c.value === "(")
u = { open: c, close: null, groups: [], type: "paren_group" }, o.push(u), l = { groups: [], type: "comma_group" }, p.push(l);
else if (c.type === "paren" && c.value === ")") {
if (l.groups.length > 0 && u.groups.push(l), u.close = c, p.length === 1)
throw new Error("Unbalanced parenthesis");
p.pop(), l = mt(p), l.groups.push(u), o.pop(), u = mt(o);
} else
c.type === "comma" ? (u.groups.push(l), l = { groups: [], type: "comma_group" }, p[p.length - 1] = l) : l.groups.push(c);
}
return l.groups.length > 0 && u.groups.push(l), h;
}
function vr(e) {
return e.type === "paren_group" && !e.open && !e.close && e.groups.length === 1 || e.type === "comma_group" && e.groups.length === 1 ? vr(e.groups[0]) : e.type === "paren_group" || e.type === "comma_group" ? Object.assign(Object.assign({}, e), {}, { groups: e.groups.map(vr) }) : e;
}
function Xe(e, n2, i) {
if (e && typeof e == "object") {
delete e.parent;
for (let u in e)
Xe(e[u], n2, i), u === "type" && typeof e[u] == "string" && !e[u].startsWith(n2) && (!i || !i.test(e[u])) && (e[u] = n2 + e[u]);
}
return e;
}
function va(e) {
if (e && typeof e == "object") {
delete e.parent;
for (let n2 in e)
va(e[n2]);
!Array.isArray(e) && e.value && !e.type && (e.type = "unknown");
}
return e;
}
function ma(e, n2) {
if (e && typeof e == "object") {
for (let i in e)
i !== "parent" && (ma(e[i], n2), i === "nodes" && (e.group = vr(pp(e, n2)), delete e[i]));
delete e.parent;
}
return e;
}
function Pe(e, n2) {
let i = gf(), u = null;
try {
u = i(e, { loose: true }).parse();
} catch {
return { type: "value-unknown", value: e };
}
u.text = e;
let o = ma(u, n2);
return Xe(o, "value-", /^selector-/);
}
function Re(e) {
if (/\/\/|\/\*/.test(e))
return { type: "selector-unknown", value: e.trim() };
let n2 = xf(), i = null;
try {
n2((u) => {
i = u;
}).process(e);
} catch {
return { type: "selector-unknown", value: e };
}
return Xe(i, "selector-");
}
function hp(e) {
let n2 = kf().default, i = null;
try {
i = n2(e);
} catch {
return { type: "selector-unknown", value: e };
}
return Xe(va(i), "media-");
}
var dp = /(\s*)(!default).*$/, vp = /(\s*)(!global).*$/;
function ga(e, n2) {
if (e && typeof e == "object") {
delete e.parent;
for (let m in e)
ga(e[m], n2);
if (!e.type)
return e;
e.raws || (e.raws = {});
let h = "";
if (typeof e.selector == "string") {
var i;
h = e.raws.selector ? (i = e.raws.selector.scss) !== null && i !== void 0 ? i : e.raws.selector.raw : e.selector, e.raws.between && e.raws.between.trim().length > 0 && (h += e.raws.between), e.raws.selector = h;
}
let l = "";
if (typeof e.value == "string") {
var u;
l = e.raws.value ? (u = e.raws.value.scss) !== null && u !== void 0 ? u : e.raws.value.raw : e.value, l = l.trim(), e.raws.value = l;
}
let p = "";
if (typeof e.params == "string") {
var o;
p = e.raws.params ? (o = e.raws.params.scss) !== null && o !== void 0 ? o : e.raws.params.raw : e.params, e.raws.afterName && e.raws.afterName.trim().length > 0 && (p = e.raws.afterName + p), e.raws.between && e.raws.between.trim().length > 0 && (p = p + e.raws.between), p = p.trim(), e.raws.params = p;
}
if (h.trim().length > 0)
return h.startsWith("@") && h.endsWith(":") ? e : e.mixin ? (e.selector = Pe(h, n2), e) : (ap(e) && (e.isSCSSNesterProperty = true), e.selector = Re(h), e);
if (l.length > 0) {
let m = l.match(dp);
m && (l = l.slice(0, m.index), e.scssDefault = true, m[0].trim() !== "!default" && (e.raws.scssDefault = m[0]));
let c = l.match(vp);
if (c && (l = l.slice(0, c.index), e.scssGlobal = true, c[0].trim() !== "!global" && (e.raws.scssGlobal = c[0])), l.startsWith("progid:"))
return { type: "value-unknown", value: l };
e.value = Pe(l, n2);
}
if (gt(n2) && e.type === "css-decl" && l.startsWith("extend(") && (e.extend || (e.extend = e.raws.between === ":"), e.extend && !e.selector && (delete e.value, e.selector = Re(l.slice(7, -1)))), e.type === "css-atrule") {
if (gt(n2)) {
if (e.mixin) {
let m = e.raws.identifier + e.name + e.raws.afterName + e.raws.params;
return e.selector = Re(m), delete e.params, e;
}
if (e.function)
return e;
}
if (n2.parser === "css" && e.name === "custom-selector") {
let m = e.params.match(/:--\S+\s+/)[0].trim();
return e.customSelector = m, e.selector = Re(e.params.slice(m.length).trim()), delete e.params, e;
}
if (gt(n2)) {
if (e.name.includes(":") && !e.params) {
e.variable = true;
let m = e.name.split(":");
e.name = m[0], e.value = Pe(m.slice(1).join(":"), n2);
}
if (!["page", "nest", "keyframes"].includes(e.name) && e.params && e.params[0] === ":") {
e.variable = true;
let m = e.params.slice(1);
m && (e.value = Pe(m, n2)), e.raws.afterName += ":";
}
if (e.variable)
return delete e.params, e.value || delete e.value, e;
}
}
if (e.type === "css-atrule" && p.length > 0) {
let { name: m } = e, c = e.name.toLowerCase();
return m === "warn" || m === "error" ? (e.params = { type: "media-unknown", value: p }, e) : m === "extend" || m === "nest" ? (e.selector = Re(p), delete e.params, e) : m === "at-root" ? (/^\(\s*(?:without|with)\s*:.+\)$/s.test(p) ? e.params = Pe(p, n2) : (e.selector = Re(p), delete e.params), e) : lp(c) ? (e.import = true, delete e.filename, e.params = Pe(p, n2), e) : ["namespace", "supports", "if", "else", "for", "each", "while", "debug", "mixin", "include", "function", "return", "define-mixin", "add-mixin"].includes(m) ? (p = p.replace(/(\$\S+?)(\s+)?\.{3}/, "$1...$2"), p = p.replace(/^(?!if)(\S+)(\s+)\(/, "$1($2"), e.value = Pe(p, n2), delete e.params, e) : ["media", "custom-media"].includes(c) ? p.includes("#{") ? { type: "media-unknown", value: p } : (e.params = hp(p), e) : (e.params = p, e);
}
}
return e;
}
function ya(e, n2, i) {
let u = Zf(n2), { frontMatter: o } = u;
n2 = u.content;
let h;
try {
h = e(n2);
} catch (l) {
let { name: p, reason: m, line: c, column: t } = l;
throw typeof c != "number" ? l : Xf(`${p}: ${m}`, { start: { line: c, column: t } });
}
return h = ga(Xe(h, "css-"), i), np(h, n2), o && (o.source = { startOffset: 0, endOffset: o.raw.length }, h.nodes.unshift(o)), h;
}
function mp(e, n2) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, o = da(i.parser, e) ? [Tt, Ot] : [Ot, Tt], h;
for (let l of o)
try {
return l(e, n2, i);
} catch (p) {
h = h || p;
}
if (h)
throw h;
}
function Ot(e, n2) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, u = Wf();
return ya((o) => u.parse(ip(o)), e, i);
}
function Tt(e, n2) {
let i = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, { parse: u } = Yf();
return ya(u, e, i);
}
var yt = { astFormat: "postcss", hasPragma: ep, locStart: rp, locEnd: tp };
wa.exports = { parsers: { css: Object.assign(Object.assign({}, yt), {}, { parse: mp }), less: Object.assign(Object.assign({}, yt), {}, { parse: Ot }), scss: Object.assign(Object.assign({}, yt), {}, { parse: Tt }) } };
});
return gp();
});
}
});
// node_modules/prettier/parser-glimmer.js
var require_parser_glimmer = __commonJS({
"node_modules/prettier/parser-glimmer.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.glimmer = e();
}
})(function() {
"use strict";
var it = (t, f) => () => (f || t((f = { exports: {} }).exports, f), f.exports);
var Zt = it((nr, $e) => {
var xe = Object.getOwnPropertyNames, st = (t, f) => function() {
return t && (f = (0, t[xe(t)[0]])(t = 0)), f;
}, I = (t, f) => function() {
return f || (0, t[xe(t)[0]])((f = { exports: {} }).exports, f), f.exports;
}, F = st({ ""() {
} }), at = I({ "node_modules/lines-and-columns/build/index.cjs"(t) {
"use strict";
F(), t.__esModule = true, t.LinesAndColumns = void 0;
var f = `
`, h = "\r", d = function() {
function c(o) {
this.length = o.length;
for (var e = [0], r = 0; r < o.length; )
switch (o[r]) {
case f:
r += f.length, e.push(r);
break;
case h:
r += h.length, o[r] === f && (r += f.length), e.push(r);
break;
default:
r++;
break;
}
this.offsets = e;
}
return c.prototype.locationForIndex = function(o) {
if (o < 0 || o > this.length)
return null;
for (var e = 0, r = this.offsets; r[e + 1] <= o; )
e++;
var a = o - r[e];
return { line: e, column: a };
}, c.prototype.indexForLocation = function(o) {
var e = o.line, r = o.column;
return e < 0 || e >= this.offsets.length || r < 0 || r > this.lengthOfLine(e) ? null : this.offsets[e] + r;
}, c.prototype.lengthOfLine = function(o) {
var e = this.offsets[o], r = o === this.offsets.length - 1 ? this.length : this.offsets[o + 1];
return r - e;
}, c;
}();
t.LinesAndColumns = d;
} }), ut = I({ "src/common/parser-create-error.js"(t, f) {
"use strict";
F();
function h(d, c) {
let o = new SyntaxError(d + " (" + c.start.line + ":" + c.start.column + ")");
return o.loc = c, o;
}
f.exports = h;
} }), ot = I({ "src/language-handlebars/loc.js"(t, f) {
"use strict";
F();
function h(c) {
return c.loc.start.offset;
}
function d(c) {
return c.loc.end.offset;
}
f.exports = { locStart: h, locEnd: d };
} }), fe = I({ "node_modules/@glimmer/env/dist/commonjs/es5/index.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true });
var f = t.DEBUG = false, h = t.CI = false;
} }), lt = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/array-utils.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.emptyArray = h, t.isEmptyArray = o, t.EMPTY_NUMBER_ARRAY = t.EMPTY_STRING_ARRAY = t.EMPTY_ARRAY = void 0;
var f = Object.freeze([]);
t.EMPTY_ARRAY = f;
function h() {
return f;
}
var d = h();
t.EMPTY_STRING_ARRAY = d;
var c = h();
t.EMPTY_NUMBER_ARRAY = c;
function o(e) {
return e === f;
}
} }), Pe = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/assert.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.debugAssert = h, t.prodAssert = d, t.deprecate = c, t.default = void 0;
var f = X();
function h(e, r) {
if (!e)
throw new Error(r || "assertion failure");
}
function d() {
}
function c(e) {
f.LOCAL_LOGGER.warn(`DEPRECATION: ${e}`);
}
var o = h;
t.default = o;
} }), ct = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/collections.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.dict = f, t.isDict = h, t.isObject = d, t.StackImpl = void 0;
function f() {
return /* @__PURE__ */ Object.create(null);
}
function h(o) {
return o != null;
}
function d(o) {
return typeof o == "function" || typeof o == "object" && o !== null;
}
var c = class {
constructor() {
let o = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : [];
this.current = null, this.stack = o;
}
get size() {
return this.stack.length;
}
push(o) {
this.current = o, this.stack.push(o);
}
pop() {
let o = this.stack.pop(), e = this.stack.length;
return this.current = e === 0 ? null : this.stack[e - 1], o === void 0 ? null : o;
}
nth(o) {
let e = this.stack.length;
return e < o ? null : this.stack[e - o];
}
isEmpty() {
return this.stack.length === 0;
}
toArray() {
return this.stack;
}
};
t.StackImpl = c;
} }), ht = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/dom.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.clearElement = f;
function f(h) {
let d = h.firstChild;
for (; d; ) {
let c = d.nextSibling;
h.removeChild(d), d = c;
}
}
} }), dt = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/is-serialization-first-node.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.isSerializationFirstNode = h, t.SERIALIZATION_FIRST_NODE_STRING = void 0;
var f = "%+b:0%";
t.SERIALIZATION_FIRST_NODE_STRING = f;
function h(d) {
return d.nodeValue === f;
}
} }), pt = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/object-utils.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.fillNulls = h, t.values = d, t.assign = void 0;
var f = Object.assign;
t.assign = f;
function h(c) {
let o = new Array(c);
for (let e = 0; e < c; e++)
o[e] = null;
return o;
}
function d(c) {
let o = [];
for (let e in c)
o.push(c[e]);
return o;
}
} }), je = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/intern.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = f;
function f(h) {
let d = {};
d[h] = 1;
for (let c in d)
if (c === h)
return c;
return h;
}
} }), me = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/platform-utils.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.keys = o, t.unwrap = e, t.expect = r, t.unreachable = a, t.exhausted = p, t.enumerableSymbol = s, t.symbol = t.tuple = t.HAS_NATIVE_SYMBOL = t.HAS_NATIVE_PROXY = void 0;
var f = h(je());
function h(i) {
return i && i.__esModule ? i : { default: i };
}
var d = typeof Proxy == "function";
t.HAS_NATIVE_PROXY = d;
var c = function() {
return typeof Symbol != "function" ? false : typeof Symbol() == "symbol";
}();
t.HAS_NATIVE_SYMBOL = c;
function o(i) {
return Object.keys(i);
}
function e(i) {
if (i == null)
throw new Error("Expected value to be present");
return i;
}
function r(i, l) {
if (i == null)
throw new Error(l);
return i;
}
function a() {
let i = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : "unreachable";
return new Error(i);
}
function p(i) {
throw new Error(`Exhausted ${i}`);
}
var n2 = function() {
for (var i = arguments.length, l = new Array(i), b = 0; b < i; b++)
l[b] = arguments[b];
return l;
};
t.tuple = n2;
function s(i) {
return (0, f.default)(`__${i}${Math.floor(Math.random() * Date.now())}__`);
}
var u = c ? Symbol : s;
t.symbol = u;
} }), ft = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/string.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.strip = f;
function f(h) {
let d = "";
for (var c = arguments.length, o = new Array(c > 1 ? c - 1 : 0), e = 1; e < c; e++)
o[e - 1] = arguments[e];
for (let n2 = 0; n2 < h.length; n2++) {
let s = h[n2], u = o[n2] !== void 0 ? String(o[n2]) : "";
d += `${s}${u}`;
}
let r = d.split(`
`);
for (; r.length && r[0].match(/^\s*$/); )
r.shift();
for (; r.length && r[r.length - 1].match(/^\s*$/); )
r.pop();
let a = 1 / 0;
for (let n2 of r) {
let s = n2.match(/^\s*/)[0].length;
a = Math.min(a, s);
}
let p = [];
for (let n2 of r)
p.push(n2.slice(a));
return p.join(`
`);
}
} }), mt = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/immediate.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.isHandle = h, t.isNonPrimitiveHandle = d, t.constants = c, t.isSmallInt = o, t.encodeNegative = e, t.decodeNegative = r, t.encodePositive = a, t.decodePositive = p, t.encodeHandle = n2, t.decodeHandle = s, t.encodeImmediate = u, t.decodeImmediate = i;
var f = Pe();
function h(l) {
return l >= 0;
}
function d(l) {
return l > 3;
}
function c() {
for (var l = arguments.length, b = new Array(l), P = 0; P < l; P++)
b[P] = arguments[P];
return [false, true, null, void 0, ...b];
}
function o(l) {
return l % 1 === 0 && l <= 536870911 && l >= -536870912;
}
function e(l) {
return l & -536870913;
}
function r(l) {
return l | 536870912;
}
function a(l) {
return ~l;
}
function p(l) {
return ~l;
}
function n2(l) {
return l;
}
function s(l) {
return l;
}
function u(l) {
return l |= 0, l < 0 ? e(l) : a(l);
}
function i(l) {
return l |= 0, l > -536870913 ? p(l) : r(l);
}
[1, 2, 3].forEach((l) => l), [1, -1].forEach((l) => i(u(l)));
} }), gt = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/template.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.unwrapHandle = f, t.unwrapTemplate = h, t.extractHandle = d, t.isOkHandle = c, t.isErrHandle = o;
function f(e) {
if (typeof e == "number")
return e;
{
let r = e.errors[0];
throw new Error(`Compile Error: ${r.problem} @ ${r.span.start}..${r.span.end}`);
}
}
function h(e) {
if (e.result === "error")
throw new Error(`Compile Error: ${e.problem} @ ${e.span.start}..${e.span.end}`);
return e;
}
function d(e) {
return typeof e == "number" ? e : e.handle;
}
function c(e) {
return typeof e == "number";
}
function o(e) {
return typeof e == "number";
}
} }), bt = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/weak-set.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = void 0;
var f = typeof WeakSet == "function" ? WeakSet : class {
constructor() {
this._map = /* @__PURE__ */ new WeakMap();
}
add(d) {
return this._map.set(d, true), this;
}
delete(d) {
return this._map.delete(d);
}
has(d) {
return this._map.has(d);
}
};
t.default = f;
} }), vt = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/simple-cast.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.castToSimple = h, t.castToBrowser = d, t.checkNode = r;
var f = me();
function h(p) {
return o(p) || e(p), p;
}
function d(p, n2) {
if (p == null)
return null;
if (typeof document === void 0)
throw new Error("Attempted to cast to a browser node in a non-browser context");
if (o(p))
return p;
if (p.ownerDocument !== document)
throw new Error("Attempted to cast to a browser node with a node that was not created from this document");
return r(p, n2);
}
function c(p, n2) {
return new Error(`cannot cast a ${p} into ${n2}`);
}
function o(p) {
return p.nodeType === 9;
}
function e(p) {
return p.nodeType === 1;
}
function r(p, n2) {
let s = false;
if (p !== null)
if (typeof n2 == "string")
s = a(p, n2);
else if (Array.isArray(n2))
s = n2.some((u) => a(p, u));
else
throw (0, f.unreachable)();
if (s)
return p;
throw c(`SimpleElement(${p})`, n2);
}
function a(p, n2) {
switch (n2) {
case "NODE":
return true;
case "HTML":
return p instanceof HTMLElement;
case "SVG":
return p instanceof SVGElement;
case "ELEMENT":
return p instanceof Element;
default:
if (n2.toUpperCase() === n2)
throw new Error("BUG: this code is missing handling for a generic node type");
return p instanceof Element && p.tagName.toLowerCase() === n2;
}
}
} }), yt = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/present.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.isPresent = f, t.ifPresent = h, t.toPresentOption = d, t.assertPresent = c, t.mapPresent = o;
function f(e) {
return e.length > 0;
}
function h(e, r, a) {
return f(e) ? r(e) : a();
}
function d(e) {
return f(e) ? e : null;
}
function c(e) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : "unexpected empty list";
if (!f(e))
throw new Error(r);
}
function o(e, r) {
if (e === null)
return null;
let a = [];
for (let p of e)
a.push(r(p));
return a;
}
} }), At = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/untouchable-this.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = d;
var f = fe(), h = me();
function d(c) {
let o = null;
if (f.DEBUG && h.HAS_NATIVE_PROXY) {
let e = (r) => {
throw new Error(`You accessed \`this.${String(r)}\` from a function passed to the ${c}, but the function itself was not bound to a valid \`this\` context. Consider updating to use a bound function (for instance, use an arrow function, \`() => {}\`).`);
};
o = new Proxy({}, { get(r, a) {
e(a);
}, set(r, a) {
return e(a), false;
}, has(r, a) {
return e(a), false;
} });
}
return o;
}
} }), Et = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/debug-to-string.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = void 0;
var f = fe(), h;
if (f.DEBUG) {
let c = (r) => {
let a = r.name;
if (a === void 0) {
let p = Function.prototype.toString.call(r).match(/function (\w+)\s*\(/);
a = p && p[1] || "";
}
return a.replace(/^bound /, "");
}, o = (r) => {
let a, p;
return r.constructor && typeof r.constructor == "function" && (p = c(r.constructor)), "toString" in r && r.toString !== Object.prototype.toString && r.toString !== Function.prototype.toString && (a = r.toString()), a && a.match(/<.*:ember\d+>/) && p && p[0] !== "_" && p.length > 2 && p !== "Class" ? a.replace(/<.*:/, `<${p}:`) : a || p;
}, e = (r) => String(r);
h = (r) => typeof r == "function" ? c(r) || "(unknown function)" : typeof r == "object" && r !== null ? o(r) || "(unknown object)" : e(r);
}
var d = h;
t.default = d;
} }), _t = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/lib/debug-steps.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.logStep = t.verifySteps = t.endTestSteps = t.beginTestSteps = void 0;
var f = d(Pe()), h = me();
function d(a) {
return a && a.__esModule ? a : { default: a };
}
var c;
t.beginTestSteps = c;
var o;
t.endTestSteps = o;
var e;
t.verifySteps = e;
var r;
t.logStep = r;
} }), X = I({ "node_modules/@glimmer/util/dist/commonjs/es2017/index.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true });
var f = { LOCAL_LOGGER: true, LOGGER: true, assertNever: true, assert: true, deprecate: true, dict: true, isDict: true, isObject: true, Stack: true, isSerializationFirstNode: true, SERIALIZATION_FIRST_NODE_STRING: true, assign: true, fillNulls: true, values: true, _WeakSet: true, castToSimple: true, castToBrowser: true, checkNode: true, intern: true, buildUntouchableThis: true, debugToString: true, beginTestSteps: true, endTestSteps: true, logStep: true, verifySteps: true };
t.assertNever = x, Object.defineProperty(t, "assert", { enumerable: true, get: function() {
return d.default;
} }), Object.defineProperty(t, "deprecate", { enumerable: true, get: function() {
return d.deprecate;
} }), Object.defineProperty(t, "dict", { enumerable: true, get: function() {
return c.dict;
} }), Object.defineProperty(t, "isDict", { enumerable: true, get: function() {
return c.isDict;
} }), Object.defineProperty(t, "isObject", { enumerable: true, get: function() {
return c.isObject;
} }), Object.defineProperty(t, "Stack", { enumerable: true, get: function() {
return c.StackImpl;
} }), Object.defineProperty(t, "isSerializationFirstNode", { enumerable: true, get: function() {
return e.isSerializationFirstNode;
} }), Object.defineProperty(t, "SERIALIZATION_FIRST_NODE_STRING", { enumerable: true, get: function() {
return e.SERIALIZATION_FIRST_NODE_STRING;
} }), Object.defineProperty(t, "assign", { enumerable: true, get: function() {
return r.assign;
} }), Object.defineProperty(t, "fillNulls", { enumerable: true, get: function() {
return r.fillNulls;
} }), Object.defineProperty(t, "values", { enumerable: true, get: function() {
return r.values;
} }), Object.defineProperty(t, "_WeakSet", { enumerable: true, get: function() {
return u.default;
} }), Object.defineProperty(t, "castToSimple", { enumerable: true, get: function() {
return i.castToSimple;
} }), Object.defineProperty(t, "castToBrowser", { enumerable: true, get: function() {
return i.castToBrowser;
} }), Object.defineProperty(t, "checkNode", { enumerable: true, get: function() {
return i.checkNode;
} }), Object.defineProperty(t, "intern", { enumerable: true, get: function() {
return b.default;
} }), Object.defineProperty(t, "buildUntouchableThis", { enumerable: true, get: function() {
return P.default;
} }), Object.defineProperty(t, "debugToString", { enumerable: true, get: function() {
return E.default;
} }), Object.defineProperty(t, "beginTestSteps", { enumerable: true, get: function() {
return v.beginTestSteps;
} }), Object.defineProperty(t, "endTestSteps", { enumerable: true, get: function() {
return v.endTestSteps;
} }), Object.defineProperty(t, "logStep", { enumerable: true, get: function() {
return v.logStep;
} }), Object.defineProperty(t, "verifySteps", { enumerable: true, get: function() {
return v.verifySteps;
} }), t.LOGGER = t.LOCAL_LOGGER = void 0;
var h = lt();
Object.keys(h).forEach(function(w) {
w === "default" || w === "__esModule" || Object.prototype.hasOwnProperty.call(f, w) || Object.defineProperty(t, w, { enumerable: true, get: function() {
return h[w];
} });
});
var d = g(Pe()), c = ct(), o = ht();
Object.keys(o).forEach(function(w) {
w === "default" || w === "__esModule" || Object.prototype.hasOwnProperty.call(f, w) || Object.defineProperty(t, w, { enumerable: true, get: function() {
return o[w];
} });
});
var e = dt(), r = pt(), a = me();
Object.keys(a).forEach(function(w) {
w === "default" || w === "__esModule" || Object.prototype.hasOwnProperty.call(f, w) || Object.defineProperty(t, w, { enumerable: true, get: function() {
return a[w];
} });
});
var p = ft();
Object.keys(p).forEach(function(w) {
w === "default" || w === "__esModule" || Object.prototype.hasOwnProperty.call(f, w) || Object.defineProperty(t, w, { enumerable: true, get: function() {
return p[w];
} });
});
var n2 = mt();
Object.keys(n2).forEach(function(w) {
w === "default" || w === "__esModule" || Object.prototype.hasOwnProperty.call(f, w) || Object.defineProperty(t, w, { enumerable: true, get: function() {
return n2[w];
} });
});
var s = gt();
Object.keys(s).forEach(function(w) {
w === "default" || w === "__esModule" || Object.prototype.hasOwnProperty.call(f, w) || Object.defineProperty(t, w, { enumerable: true, get: function() {
return s[w];
} });
});
var u = _(bt()), i = vt(), l = yt();
Object.keys(l).forEach(function(w) {
w === "default" || w === "__esModule" || Object.prototype.hasOwnProperty.call(f, w) || Object.defineProperty(t, w, { enumerable: true, get: function() {
return l[w];
} });
});
var b = _(je()), P = _(At()), E = _(Et()), v = _t();
function _(w) {
return w && w.__esModule ? w : { default: w };
}
function y() {
if (typeof WeakMap != "function")
return null;
var w = /* @__PURE__ */ new WeakMap();
return y = function() {
return w;
}, w;
}
function g(w) {
if (w && w.__esModule)
return w;
if (w === null || typeof w != "object" && typeof w != "function")
return { default: w };
var H = y();
if (H && H.has(w))
return H.get(w);
var m = {}, C = Object.defineProperty && Object.getOwnPropertyDescriptor;
for (var S in w)
if (Object.prototype.hasOwnProperty.call(w, S)) {
var R = C ? Object.getOwnPropertyDescriptor(w, S) : null;
R && (R.get || R.set) ? Object.defineProperty(m, S, R) : m[S] = w[S];
}
return m.default = w, H && H.set(w, m), m;
}
var L = console;
t.LOCAL_LOGGER = L;
var j = console;
t.LOGGER = j;
function x(w) {
let H = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : "unexpected unreachable branch";
throw j.log("unreachable", w), j.log(`${H} :: ${JSON.stringify(w)} (${w})`), new Error("code reached unreachable");
}
} }), ge = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/source/location.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.isLocatedWithPositionsArray = a, t.isLocatedWithPositions = p, t.BROKEN_LOCATION = t.NON_EXISTENT_LOCATION = t.TEMPORARY_LOCATION = t.SYNTHETIC = t.SYNTHETIC_LOCATION = t.UNKNOWN_POSITION = void 0;
var f = X(), h = Object.freeze({ line: 1, column: 0 });
t.UNKNOWN_POSITION = h;
var d = Object.freeze({ source: "(synthetic)", start: h, end: h });
t.SYNTHETIC_LOCATION = d;
var c = d;
t.SYNTHETIC = c;
var o = Object.freeze({ source: "(temporary)", start: h, end: h });
t.TEMPORARY_LOCATION = o;
var e = Object.freeze({ source: "(nonexistent)", start: h, end: h });
t.NON_EXISTENT_LOCATION = e;
var r = Object.freeze({ source: "(broken)", start: h, end: h });
t.BROKEN_LOCATION = r;
function a(n2) {
return (0, f.isPresent)(n2) && n2.every(p);
}
function p(n2) {
return n2.loc !== void 0;
}
} }), le = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/source/slice.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.SourceSlice = void 0;
var f = ue(), h = class {
constructor(d) {
this.loc = d.loc, this.chars = d.chars;
}
static synthetic(d) {
let c = f.SourceSpan.synthetic(d);
return new h({ loc: c, chars: d });
}
static load(d, c) {
return new h({ loc: f.SourceSpan.load(d, c[1]), chars: c[0] });
}
getString() {
return this.chars;
}
serialize() {
return [this.chars, this.loc.serialize()];
}
};
t.SourceSlice = h;
} }), Me = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/source/loc/match.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.match = e, t.IsInvisible = t.MatchAny = void 0;
var f = X(), h = "MATCH_ANY";
t.MatchAny = h;
var d = "IS_INVISIBLE";
t.IsInvisible = d;
var c = class {
constructor(p) {
this._whens = p;
}
first(p) {
for (let n2 of this._whens) {
let s = n2.match(p);
if ((0, f.isPresent)(s))
return s[0];
}
return null;
}
}, o = class {
constructor() {
this._map = /* @__PURE__ */ new Map();
}
get(p, n2) {
let s = this._map.get(p);
return s || (s = n2(), this._map.set(p, s), s);
}
add(p, n2) {
this._map.set(p, n2);
}
match(p) {
let n2 = a(p), s = [], u = this._map.get(n2), i = this._map.get(h);
return u && s.push(u), i && s.push(i), s;
}
};
function e(p) {
return p(new r()).check();
}
var r = class {
constructor() {
this._whens = new o();
}
check() {
return (p, n2) => this.matchFor(p.kind, n2.kind)(p, n2);
}
matchFor(p, n2) {
let s = this._whens.match(p);
return new c(s).first(n2);
}
when(p, n2, s) {
return this._whens.get(p, () => new o()).add(n2, s), this;
}
};
function a(p) {
switch (p) {
case "Broken":
case "InternalsSynthetic":
case "NonExistent":
return d;
default:
return p;
}
}
} }), He = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/source/loc/offset.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.InvisiblePosition = t.HbsPosition = t.CharPosition = t.SourceOffset = t.BROKEN = void 0;
var f = ge(), h = Me(), d = Ve(), c = "BROKEN";
t.BROKEN = c;
var o = class {
constructor(n2) {
this.data = n2;
}
static forHbsPos(n2, s) {
return new r(n2, s, null).wrap();
}
static broken() {
let n2 = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : f.UNKNOWN_POSITION;
return new a("Broken", n2).wrap();
}
get offset() {
let n2 = this.data.toCharPos();
return n2 === null ? null : n2.offset;
}
eql(n2) {
return p(this.data, n2.data);
}
until(n2) {
return (0, d.span)(this.data, n2.data);
}
move(n2) {
let s = this.data.toCharPos();
if (s === null)
return o.broken();
{
let u = s.offset + n2;
return s.source.check(u) ? new e(s.source, u).wrap() : o.broken();
}
}
collapsed() {
return (0, d.span)(this.data, this.data);
}
toJSON() {
return this.data.toJSON();
}
};
t.SourceOffset = o;
var e = class {
constructor(n2, s) {
this.source = n2, this.charPos = s, this.kind = "CharPosition", this._locPos = null;
}
toCharPos() {
return this;
}
toJSON() {
let n2 = this.toHbsPos();
return n2 === null ? f.UNKNOWN_POSITION : n2.toJSON();
}
wrap() {
return new o(this);
}
get offset() {
return this.charPos;
}
toHbsPos() {
let n2 = this._locPos;
if (n2 === null) {
let s = this.source.hbsPosFor(this.charPos);
s === null ? this._locPos = n2 = c : this._locPos = n2 = new r(this.source, s, this.charPos);
}
return n2 === c ? null : n2;
}
};
t.CharPosition = e;
var r = class {
constructor(n2, s) {
let u = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : null;
this.source = n2, this.hbsPos = s, this.kind = "HbsPosition", this._charPos = u === null ? null : new e(n2, u);
}
toCharPos() {
let n2 = this._charPos;
if (n2 === null) {
let s = this.source.charPosFor(this.hbsPos);
s === null ? this._charPos = n2 = c : this._charPos = n2 = new e(this.source, s);
}
return n2 === c ? null : n2;
}
toJSON() {
return this.hbsPos;
}
wrap() {
return new o(this);
}
toHbsPos() {
return this;
}
};
t.HbsPosition = r;
var a = class {
constructor(n2, s) {
this.kind = n2, this.pos = s;
}
toCharPos() {
return null;
}
toJSON() {
return this.pos;
}
wrap() {
return new o(this);
}
get offset() {
return null;
}
};
t.InvisiblePosition = a;
var p = (0, h.match)((n2) => n2.when("HbsPosition", "HbsPosition", (s, u) => {
let { hbsPos: i } = s, { hbsPos: l } = u;
return i.column === l.column && i.line === l.line;
}).when("CharPosition", "CharPosition", (s, u) => {
let { charPos: i } = s, { charPos: l } = u;
return i === l;
}).when("CharPosition", "HbsPosition", (s, u) => {
let { offset: i } = s;
var l;
return i === ((l = u.toCharPos()) === null || l === void 0 ? void 0 : l.offset);
}).when("HbsPosition", "CharPosition", (s, u) => {
let { offset: i } = u;
var l;
return ((l = s.toCharPos()) === null || l === void 0 ? void 0 : l.offset) === i;
}).when(h.MatchAny, h.MatchAny, () => false));
} }), Ve = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/source/loc/span.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.span = t.HbsSpan = t.SourceSpan = void 0;
var f = fe(), h = X(), d = ge(), c = le(), o = Me(), e = He(), r = class {
constructor(u) {
this.data = u, this.isInvisible = u.kind !== "CharPosition" && u.kind !== "HbsPosition";
}
static get NON_EXISTENT() {
return new n2("NonExistent", d.NON_EXISTENT_LOCATION).wrap();
}
static load(u, i) {
if (typeof i == "number")
return r.forCharPositions(u, i, i);
if (typeof i == "string")
return r.synthetic(i);
if (Array.isArray(i))
return r.forCharPositions(u, i[0], i[1]);
if (i === "NonExistent")
return r.NON_EXISTENT;
if (i === "Broken")
return r.broken(d.BROKEN_LOCATION);
(0, h.assertNever)(i);
}
static forHbsLoc(u, i) {
let l = new e.HbsPosition(u, i.start), b = new e.HbsPosition(u, i.end);
return new p(u, { start: l, end: b }, i).wrap();
}
static forCharPositions(u, i, l) {
let b = new e.CharPosition(u, i), P = new e.CharPosition(u, l);
return new a(u, { start: b, end: P }).wrap();
}
static synthetic(u) {
return new n2("InternalsSynthetic", d.NON_EXISTENT_LOCATION, u).wrap();
}
static broken() {
let u = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : d.BROKEN_LOCATION;
return new n2("Broken", u).wrap();
}
getStart() {
return this.data.getStart().wrap();
}
getEnd() {
return this.data.getEnd().wrap();
}
get loc() {
let u = this.data.toHbsSpan();
return u === null ? d.BROKEN_LOCATION : u.toHbsLoc();
}
get module() {
return this.data.getModule();
}
get startPosition() {
return this.loc.start;
}
get endPosition() {
return this.loc.end;
}
toJSON() {
return this.loc;
}
withStart(u) {
return s(u.data, this.data.getEnd());
}
withEnd(u) {
return s(this.data.getStart(), u.data);
}
asString() {
return this.data.asString();
}
toSlice(u) {
let i = this.data.asString();
return f.DEBUG && u !== void 0 && i !== u && console.warn(`unexpectedly found ${JSON.stringify(i)} when slicing source, but expected ${JSON.stringify(u)}`), new c.SourceSlice({ loc: this, chars: u || i });
}
get start() {
return this.loc.start;
}
set start(u) {
this.data.locDidUpdate({ start: u });
}
get end() {
return this.loc.end;
}
set end(u) {
this.data.locDidUpdate({ end: u });
}
get source() {
return this.module;
}
collapse(u) {
switch (u) {
case "start":
return this.getStart().collapsed();
case "end":
return this.getEnd().collapsed();
}
}
extend(u) {
return s(this.data.getStart(), u.data.getEnd());
}
serialize() {
return this.data.serialize();
}
slice(u) {
let { skipStart: i = 0, skipEnd: l = 0 } = u;
return s(this.getStart().move(i).data, this.getEnd().move(-l).data);
}
sliceStartChars(u) {
let { skipStart: i = 0, chars: l } = u;
return s(this.getStart().move(i).data, this.getStart().move(i + l).data);
}
sliceEndChars(u) {
let { skipEnd: i = 0, chars: l } = u;
return s(this.getEnd().move(i - l).data, this.getStart().move(-i).data);
}
};
t.SourceSpan = r;
var a = class {
constructor(u, i) {
this.source = u, this.charPositions = i, this.kind = "CharPosition", this._locPosSpan = null;
}
wrap() {
return new r(this);
}
asString() {
return this.source.slice(this.charPositions.start.charPos, this.charPositions.end.charPos);
}
getModule() {
return this.source.module;
}
getStart() {
return this.charPositions.start;
}
getEnd() {
return this.charPositions.end;
}
locDidUpdate() {
}
toHbsSpan() {
let u = this._locPosSpan;
if (u === null) {
let i = this.charPositions.start.toHbsPos(), l = this.charPositions.end.toHbsPos();
i === null || l === null ? u = this._locPosSpan = e.BROKEN : u = this._locPosSpan = new p(this.source, { start: i, end: l });
}
return u === e.BROKEN ? null : u;
}
serialize() {
let { start: { charPos: u }, end: { charPos: i } } = this.charPositions;
return u === i ? u : [u, i];
}
toCharPosSpan() {
return this;
}
}, p = class {
constructor(u, i) {
let l = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : null;
this.source = u, this.hbsPositions = i, this.kind = "HbsPosition", this._charPosSpan = null, this._providedHbsLoc = l;
}
serialize() {
let u = this.toCharPosSpan();
return u === null ? "Broken" : u.wrap().serialize();
}
wrap() {
return new r(this);
}
updateProvided(u, i) {
this._providedHbsLoc && (this._providedHbsLoc[i] = u), this._charPosSpan = null, this._providedHbsLoc = { start: u, end: u };
}
locDidUpdate(u) {
let { start: i, end: l } = u;
i !== void 0 && (this.updateProvided(i, "start"), this.hbsPositions.start = new e.HbsPosition(this.source, i, null)), l !== void 0 && (this.updateProvided(l, "end"), this.hbsPositions.end = new e.HbsPosition(this.source, l, null));
}
asString() {
let u = this.toCharPosSpan();
return u === null ? "" : u.asString();
}
getModule() {
return this.source.module;
}
getStart() {
return this.hbsPositions.start;
}
getEnd() {
return this.hbsPositions.end;
}
toHbsLoc() {
return { start: this.hbsPositions.start.hbsPos, end: this.hbsPositions.end.hbsPos };
}
toHbsSpan() {
return this;
}
toCharPosSpan() {
let u = this._charPosSpan;
if (u === null) {
let i = this.hbsPositions.start.toCharPos(), l = this.hbsPositions.end.toCharPos();
if (i && l)
u = this._charPosSpan = new a(this.source, { start: i, end: l });
else
return u = this._charPosSpan = e.BROKEN, null;
}
return u === e.BROKEN ? null : u;
}
};
t.HbsSpan = p;
var n2 = class {
constructor(u, i) {
let l = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : null;
this.kind = u, this.loc = i, this.string = l;
}
serialize() {
switch (this.kind) {
case "Broken":
case "NonExistent":
return this.kind;
case "InternalsSynthetic":
return this.string || "";
}
}
wrap() {
return new r(this);
}
asString() {
return this.string || "";
}
locDidUpdate(u) {
let { start: i, end: l } = u;
i !== void 0 && (this.loc.start = i), l !== void 0 && (this.loc.end = l);
}
getModule() {
return "an unknown module";
}
getStart() {
return new e.InvisiblePosition(this.kind, this.loc.start);
}
getEnd() {
return new e.InvisiblePosition(this.kind, this.loc.end);
}
toCharPosSpan() {
return this;
}
toHbsSpan() {
return null;
}
toHbsLoc() {
return d.BROKEN_LOCATION;
}
}, s = (0, o.match)((u) => u.when("HbsPosition", "HbsPosition", (i, l) => new p(i.source, { start: i, end: l }).wrap()).when("CharPosition", "CharPosition", (i, l) => new a(i.source, { start: i, end: l }).wrap()).when("CharPosition", "HbsPosition", (i, l) => {
let b = l.toCharPos();
return b === null ? new n2("Broken", d.BROKEN_LOCATION).wrap() : s(i, b);
}).when("HbsPosition", "CharPosition", (i, l) => {
let b = i.toCharPos();
return b === null ? new n2("Broken", d.BROKEN_LOCATION).wrap() : s(b, l);
}).when(o.IsInvisible, o.MatchAny, (i) => new n2(i.kind, d.BROKEN_LOCATION).wrap()).when(o.MatchAny, o.IsInvisible, (i, l) => new n2(l.kind, d.BROKEN_LOCATION).wrap()));
t.span = s;
} }), ue = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/source/span.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), Object.defineProperty(t, "SourceSpan", { enumerable: true, get: function() {
return f.SourceSpan;
} }), Object.defineProperty(t, "SourceOffset", { enumerable: true, get: function() {
return h.SourceOffset;
} });
var f = Ve(), h = He();
} }), De = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/source/source.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.Source = void 0;
var f = fe(), h = X(), d = ue(), c = class {
constructor(o) {
let e = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : "an unknown module";
this.source = o, this.module = e;
}
check(o) {
return o >= 0 && o <= this.source.length;
}
slice(o, e) {
return this.source.slice(o, e);
}
offsetFor(o, e) {
return d.SourceOffset.forHbsPos(this, { line: o, column: e });
}
spanFor(o) {
let { start: e, end: r } = o;
return d.SourceSpan.forHbsLoc(this, { start: { line: e.line, column: e.column }, end: { line: r.line, column: r.column } });
}
hbsPosFor(o) {
let e = 0, r = 0;
if (o > this.source.length)
return null;
for (; ; ) {
let a = this.source.indexOf(`
`, r);
if (o <= a || a === -1)
return { line: e + 1, column: o - r };
e += 1, r = a + 1;
}
}
charPosFor(o) {
let { line: e, column: r } = o, p = this.source.length, n2 = 0, s = 0;
for (; ; ) {
if (s >= p)
return p;
let u = this.source.indexOf(`
`, s);
if (u === -1 && (u = this.source.length), n2 === e - 1) {
if (s + r > u)
return u;
if (f.DEBUG) {
let i = this.hbsPosFor(s + r);
}
return s + r;
} else {
if (u === -1)
return 0;
n2 += 1, s = u + 1;
}
}
}
};
t.Source = c;
} }), we = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v1/legacy-interop.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.PathExpressionImplV1 = void 0;
var f = h(ke());
function h(c) {
return c && c.__esModule ? c : { default: c };
}
var d = class {
constructor(c, o, e, r) {
this.original = c, this.loc = r, this.type = "PathExpression", this.this = false, this.data = false, this._head = void 0;
let a = e.slice();
o.type === "ThisHead" ? this.this = true : o.type === "AtHead" ? (this.data = true, a.unshift(o.name.slice(1))) : a.unshift(o.name), this.parts = a;
}
get head() {
if (this._head)
return this._head;
let c;
this.this ? c = "this" : this.data ? c = `@${this.parts[0]}` : c = this.parts[0];
let o = this.loc.collapse("start").sliceStartChars({ chars: c.length }).loc;
return this._head = f.default.head(c, o);
}
get tail() {
return this.this ? this.parts : this.parts.slice(1);
}
};
t.PathExpressionImplV1 = d;
} }), ke = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v1/public-builders.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = void 0;
var f = X(), h = ge(), d = De(), c = ue(), o = we(), e;
function r() {
return e || (e = new d.Source("", "(synthetic)")), e;
}
function a(T, N, k, B, O, q) {
return typeof T == "string" && (T = m(T)), { type: "MustacheStatement", path: T, params: N || [], hash: k || S([]), escaped: !B, trusting: !!B, loc: U(O || null), strip: q || { open: false, close: false } };
}
function p(T, N, k, B, O, q, z, A, Q) {
let D, $;
return B.type === "Template" ? D = (0, f.assign)({}, B, { type: "Block" }) : D = B, O != null && O.type === "Template" ? $ = (0, f.assign)({}, O, { type: "Block" }) : $ = O, { type: "BlockStatement", path: m(T), params: N || [], hash: k || S([]), program: D || null, inverse: $ || null, loc: U(q || null), openStrip: z || { open: false, close: false }, inverseStrip: A || { open: false, close: false }, closeStrip: Q || { open: false, close: false } };
}
function n2(T, N, k, B) {
return { type: "ElementModifierStatement", path: m(T), params: N || [], hash: k || S([]), loc: U(B || null) };
}
function s(T, N, k, B, O) {
return { type: "PartialStatement", name: T, params: N || [], hash: k || S([]), indent: B || "", strip: { open: false, close: false }, loc: U(O || null) };
}
function u(T, N) {
return { type: "CommentStatement", value: T, loc: U(N || null) };
}
function i(T, N) {
return { type: "MustacheCommentStatement", value: T, loc: U(N || null) };
}
function l(T, N) {
if (!(0, f.isPresent)(T))
throw new Error("b.concat requires at least one part");
return { type: "ConcatStatement", parts: T || [], loc: U(N || null) };
}
function b(T) {
let N = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {}, { attrs: k, blockParams: B, modifiers: O, comments: q, children: z, loc: A } = N, Q, D = false;
return typeof T == "object" ? (D = T.selfClosing, Q = T.name) : T.slice(-1) === "/" ? (Q = T.slice(0, -1), D = true) : Q = T, { type: "ElementNode", tag: Q, selfClosing: D, attributes: k || [], blockParams: B || [], modifiers: O || [], comments: q || [], children: z || [], loc: U(A || null) };
}
function P(T, N, k) {
return { type: "AttrNode", name: T, value: N, loc: U(k || null) };
}
function E(T, N) {
return { type: "TextNode", chars: T || "", loc: U(N || null) };
}
function v(T, N, k, B) {
return { type: "SubExpression", path: m(T), params: N || [], hash: k || S([]), loc: U(B || null) };
}
function _(T) {
switch (T.type) {
case "AtHead":
return { original: T.name, parts: [T.name] };
case "ThisHead":
return { original: "this", parts: [] };
case "VarHead":
return { original: T.name, parts: [T.name] };
}
}
function y(T, N) {
let [k, ...B] = T.split("."), O;
return k === "this" ? O = { type: "ThisHead", loc: U(N || null) } : k[0] === "@" ? O = { type: "AtHead", name: k, loc: U(N || null) } : O = { type: "VarHead", name: k, loc: U(N || null) }, { head: O, tail: B };
}
function g(T) {
return { type: "ThisHead", loc: U(T || null) };
}
function L(T, N) {
return { type: "AtHead", name: T, loc: U(N || null) };
}
function j(T, N) {
return { type: "VarHead", name: T, loc: U(N || null) };
}
function x(T, N) {
return T[0] === "@" ? L(T, N) : T === "this" ? g(N) : j(T, N);
}
function w(T, N) {
return { type: "NamedBlockName", name: T, loc: U(N || null) };
}
function H(T, N, k) {
let { original: B, parts: O } = _(T), q = [...O, ...N], z = [...B, ...q].join(".");
return new o.PathExpressionImplV1(z, T, N, U(k || null));
}
function m(T, N) {
if (typeof T != "string") {
if ("type" in T)
return T;
{
let { head: O, tail: q } = y(T.head, c.SourceSpan.broken()), { original: z } = _(O);
return new o.PathExpressionImplV1([z, ...q].join("."), O, q, U(N || null));
}
}
let { head: k, tail: B } = y(T, c.SourceSpan.broken());
return new o.PathExpressionImplV1(T, k, B, U(N || null));
}
function C(T, N, k) {
return { type: T, value: N, original: N, loc: U(k || null) };
}
function S(T, N) {
return { type: "Hash", pairs: T || [], loc: U(N || null) };
}
function R(T, N, k) {
return { type: "HashPair", key: T, value: N, loc: U(k || null) };
}
function M(T, N, k) {
return { type: "Template", body: T || [], blockParams: N || [], loc: U(k || null) };
}
function V(T, N) {
let k = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false, B = arguments.length > 3 ? arguments[3] : void 0;
return { type: "Block", body: T || [], blockParams: N || [], chained: k, loc: U(B || null) };
}
function G(T, N, k) {
return { type: "Template", body: T || [], blockParams: N || [], loc: U(k || null) };
}
function K(T, N) {
return { line: T, column: N };
}
function U() {
for (var T = arguments.length, N = new Array(T), k = 0; k < T; k++)
N[k] = arguments[k];
if (N.length === 1) {
let B = N[0];
return B && typeof B == "object" ? c.SourceSpan.forHbsLoc(r(), B) : c.SourceSpan.forHbsLoc(r(), h.SYNTHETIC_LOCATION);
} else {
let [B, O, q, z, A] = N, Q = A ? new d.Source("", A) : r();
return c.SourceSpan.forHbsLoc(Q, { start: { line: B, column: O }, end: { line: q, column: z } });
}
}
var Z = { mustache: a, block: p, partial: s, comment: u, mustacheComment: i, element: b, elementModifier: n2, attr: P, text: E, sexpr: v, concat: l, hash: S, pair: R, literal: C, program: M, blockItself: V, template: G, loc: U, pos: K, path: m, fullPath: H, head: x, at: L, var: j, this: g, blockName: w, string: W("StringLiteral"), boolean: W("BooleanLiteral"), number: W("NumberLiteral"), undefined() {
return C("UndefinedLiteral", void 0);
}, null() {
return C("NullLiteral", null);
} };
t.default = Z;
function W(T) {
return function(N, k) {
return C(T, N, k);
};
}
} }), St = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v1/nodes-v1.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true });
} }), Ct = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v1/api.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true });
var f = St();
Object.keys(f).forEach(function(h) {
h === "default" || h === "__esModule" || Object.defineProperty(t, h, { enumerable: true, get: function() {
return f[h];
} });
});
} }), Pt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/objects/resolution.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.loadResolution = o, t.ARGUMENT_RESOLUTION = t.LooseModeResolution = t.STRICT_RESOLUTION = t.StrictResolution = void 0;
var f = class {
constructor() {
this.isAngleBracket = false;
}
resolution() {
return 31;
}
serialize() {
return "Strict";
}
};
t.StrictResolution = f;
var h = new f();
t.STRICT_RESOLUTION = h;
var d = class {
constructor(e) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
this.ambiguity = e, this.isAngleBracket = r;
}
static namespaced(e) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
return new d({ namespaces: [e], fallback: false }, r);
}
static fallback() {
return new d({ namespaces: [], fallback: true });
}
static append(e) {
let { invoke: r } = e;
return new d({ namespaces: ["Component", "Helper"], fallback: !r });
}
static trustingAppend(e) {
let { invoke: r } = e;
return new d({ namespaces: ["Helper"], fallback: !r });
}
static attr() {
return new d({ namespaces: ["Helper"], fallback: true });
}
resolution() {
if (this.ambiguity.namespaces.length === 0)
return 31;
if (this.ambiguity.namespaces.length === 1) {
if (this.ambiguity.fallback)
return 36;
switch (this.ambiguity.namespaces[0]) {
case "Helper":
return 37;
case "Modifier":
return 38;
case "Component":
return 39;
}
} else
return this.ambiguity.fallback ? 34 : 35;
}
serialize() {
return this.ambiguity.namespaces.length === 0 ? "Loose" : this.ambiguity.namespaces.length === 1 ? this.ambiguity.fallback ? ["ambiguous", "Attr"] : ["ns", this.ambiguity.namespaces[0]] : this.ambiguity.fallback ? ["ambiguous", "Append"] : ["ambiguous", "Invoke"];
}
};
t.LooseModeResolution = d;
var c = d.fallback();
t.ARGUMENT_RESOLUTION = c;
function o(e) {
if (typeof e == "string")
switch (e) {
case "Loose":
return d.fallback();
case "Strict":
return h;
}
switch (e[0]) {
case "ambiguous":
switch (e[1]) {
case "Append":
return d.append({ invoke: false });
case "Attr":
return d.attr();
case "Invoke":
return d.append({ invoke: true });
}
case "ns":
return d.namespaced(e[1]);
}
}
} }), ne = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/objects/node.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.node = h;
var f = X();
function h(d) {
if (d !== void 0) {
let c = d;
return { fields() {
return class {
constructor(o) {
this.type = c, (0, f.assign)(this, o);
}
};
} };
} else
return { fields() {
return class {
constructor(c) {
(0, f.assign)(this, c);
}
};
} };
}
} }), be = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/objects/args.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.NamedArgument = t.NamedArguments = t.PositionalArguments = t.Args = void 0;
var f = ne(), h = class extends (0, f.node)().fields() {
static empty(e) {
return new h({ loc: e, positional: d.empty(e), named: c.empty(e) });
}
static named(e) {
return new h({ loc: e.loc, positional: d.empty(e.loc.collapse("end")), named: e });
}
nth(e) {
return this.positional.nth(e);
}
get(e) {
return this.named.get(e);
}
isEmpty() {
return this.positional.isEmpty() && this.named.isEmpty();
}
};
t.Args = h;
var d = class extends (0, f.node)().fields() {
static empty(e) {
return new d({ loc: e, exprs: [] });
}
get size() {
return this.exprs.length;
}
nth(e) {
return this.exprs[e] || null;
}
isEmpty() {
return this.exprs.length === 0;
}
};
t.PositionalArguments = d;
var c = class extends (0, f.node)().fields() {
static empty(e) {
return new c({ loc: e, entries: [] });
}
get size() {
return this.entries.length;
}
get(e) {
let r = this.entries.filter((a) => a.name.chars === e)[0];
return r ? r.value : null;
}
isEmpty() {
return this.entries.length === 0;
}
};
t.NamedArguments = c;
var o = class {
constructor(e) {
this.loc = e.name.loc.extend(e.value.loc), this.name = e.name, this.value = e.value;
}
};
t.NamedArgument = o;
} }), Dt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/objects/attr-block.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.ElementModifier = t.ComponentArg = t.SplatAttr = t.HtmlAttr = void 0;
var f = be(), h = ne(), d = class extends (0, h.node)("HtmlAttr").fields() {
};
t.HtmlAttr = d;
var c = class extends (0, h.node)("SplatAttr").fields() {
};
t.SplatAttr = c;
var o = class extends (0, h.node)().fields() {
toNamedArgument() {
return new f.NamedArgument({ name: this.name, value: this.value });
}
};
t.ComponentArg = o;
var e = class extends (0, h.node)("ElementModifier").fields() {
};
t.ElementModifier = e;
} }), wt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/objects/base.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true });
} }), ce = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/source/span-list.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.loc = d, t.hasSpan = c, t.maybeLoc = o, t.SpanList = void 0;
var f = ue(), h = class {
constructor() {
let e = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : [];
this._span = e;
}
static range(e) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : f.SourceSpan.NON_EXISTENT;
return new h(e.map(d)).getRangeOffset(r);
}
add(e) {
this._span.push(e);
}
getRangeOffset(e) {
if (this._span.length === 0)
return e;
{
let r = this._span[0], a = this._span[this._span.length - 1];
return r.extend(a);
}
}
};
t.SpanList = h;
function d(e) {
if (Array.isArray(e)) {
let r = e[0], a = e[e.length - 1];
return d(r).extend(d(a));
} else
return e instanceof f.SourceSpan ? e : e.loc;
}
function c(e) {
return !(Array.isArray(e) && e.length === 0);
}
function o(e, r) {
return c(e) ? d(e) : r;
}
} }), kt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/objects/content.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.SimpleElement = t.InvokeComponent = t.InvokeBlock = t.AppendContent = t.HtmlComment = t.HtmlText = t.GlimmerComment = void 0;
var f = ce(), h = be(), d = ne(), c = class extends (0, d.node)("GlimmerComment").fields() {
};
t.GlimmerComment = c;
var o = class extends (0, d.node)("HtmlText").fields() {
};
t.HtmlText = o;
var e = class extends (0, d.node)("HtmlComment").fields() {
};
t.HtmlComment = e;
var r = class extends (0, d.node)("AppendContent").fields() {
get callee() {
return this.value.type === "Call" ? this.value.callee : this.value;
}
get args() {
return this.value.type === "Call" ? this.value.args : h.Args.empty(this.value.loc.collapse("end"));
}
};
t.AppendContent = r;
var a = class extends (0, d.node)("InvokeBlock").fields() {
};
t.InvokeBlock = a;
var p = class extends (0, d.node)("InvokeComponent").fields() {
get args() {
let s = this.componentArgs.map((u) => u.toNamedArgument());
return h.Args.named(new h.NamedArguments({ loc: f.SpanList.range(s, this.callee.loc.collapse("end")), entries: s }));
}
};
t.InvokeComponent = p;
var n2 = class extends (0, d.node)("SimpleElement").fields() {
get args() {
let s = this.componentArgs.map((u) => u.toNamedArgument());
return h.Args.named(new h.NamedArguments({ loc: f.SpanList.range(s, this.tag.loc.collapse("end")), entries: s }));
}
};
t.SimpleElement = n2;
} }), Tt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/objects/expr.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.isLiteral = c, t.InterpolateExpression = t.DeprecatedCallExpression = t.CallExpression = t.PathExpression = t.LiteralExpression = void 0;
var f = le(), h = ne(), d = class extends (0, h.node)("Literal").fields() {
toSlice() {
return new f.SourceSlice({ loc: this.loc, chars: this.value });
}
};
t.LiteralExpression = d;
function c(p, n2) {
return p.type === "Literal" ? n2 === void 0 ? true : n2 === "null" ? p.value === null : typeof p.value === n2 : false;
}
var o = class extends (0, h.node)("Path").fields() {
};
t.PathExpression = o;
var e = class extends (0, h.node)("Call").fields() {
};
t.CallExpression = e;
var r = class extends (0, h.node)("DeprecatedCall").fields() {
};
t.DeprecatedCallExpression = r;
var a = class extends (0, h.node)("Interpolate").fields() {
};
t.InterpolateExpression = a;
} }), Bt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/objects/refs.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.FreeVarReference = t.LocalVarReference = t.ArgReference = t.ThisReference = void 0;
var f = ne(), h = class extends (0, f.node)("This").fields() {
};
t.ThisReference = h;
var d = class extends (0, f.node)("Arg").fields() {
};
t.ArgReference = d;
var c = class extends (0, f.node)("Local").fields() {
};
t.LocalVarReference = c;
var o = class extends (0, f.node)("Free").fields() {
};
t.FreeVarReference = o;
} }), Ot = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/objects/internal-node.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.NamedBlock = t.NamedBlocks = t.Block = t.Template = void 0;
var f = ce(), h = be(), d = ne(), c = class extends (0, d.node)().fields() {
};
t.Template = c;
var o = class extends (0, d.node)().fields() {
};
t.Block = o;
var e = class extends (0, d.node)().fields() {
get(a) {
return this.blocks.filter((p) => p.name.chars === a)[0] || null;
}
};
t.NamedBlocks = e;
var r = class extends (0, d.node)().fields() {
get args() {
let a = this.componentArgs.map((p) => p.toNamedArgument());
return h.Args.named(new h.NamedArguments({ loc: f.SpanList.range(a, this.name.loc.collapse("end")), entries: a }));
}
};
t.NamedBlock = r;
} }), ve = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/api.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true });
var f = Pt();
Object.keys(f).forEach(function(n2) {
n2 === "default" || n2 === "__esModule" || Object.defineProperty(t, n2, { enumerable: true, get: function() {
return f[n2];
} });
});
var h = ne();
Object.keys(h).forEach(function(n2) {
n2 === "default" || n2 === "__esModule" || Object.defineProperty(t, n2, { enumerable: true, get: function() {
return h[n2];
} });
});
var d = be();
Object.keys(d).forEach(function(n2) {
n2 === "default" || n2 === "__esModule" || Object.defineProperty(t, n2, { enumerable: true, get: function() {
return d[n2];
} });
});
var c = Dt();
Object.keys(c).forEach(function(n2) {
n2 === "default" || n2 === "__esModule" || Object.defineProperty(t, n2, { enumerable: true, get: function() {
return c[n2];
} });
});
var o = wt();
Object.keys(o).forEach(function(n2) {
n2 === "default" || n2 === "__esModule" || Object.defineProperty(t, n2, { enumerable: true, get: function() {
return o[n2];
} });
});
var e = kt();
Object.keys(e).forEach(function(n2) {
n2 === "default" || n2 === "__esModule" || Object.defineProperty(t, n2, { enumerable: true, get: function() {
return e[n2];
} });
});
var r = Tt();
Object.keys(r).forEach(function(n2) {
n2 === "default" || n2 === "__esModule" || Object.defineProperty(t, n2, { enumerable: true, get: function() {
return r[n2];
} });
});
var a = Bt();
Object.keys(a).forEach(function(n2) {
n2 === "default" || n2 === "__esModule" || Object.defineProperty(t, n2, { enumerable: true, get: function() {
return a[n2];
} });
});
var p = Ot();
Object.keys(p).forEach(function(n2) {
n2 === "default" || n2 === "__esModule" || Object.defineProperty(t, n2, { enumerable: true, get: function() {
return p[n2];
} });
});
} }), Ue = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/generation/util.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.escapeAttrValue = r, t.escapeText = a, t.sortByLoc = p;
var f = /[\xA0"&]/, h = new RegExp(f.source, "g"), d = /[\xA0&<>]/, c = new RegExp(d.source, "g");
function o(n2) {
switch (n2.charCodeAt(0)) {
case 160:
return " ";
case 34:
return """;
case 38:
return "&";
default:
return n2;
}
}
function e(n2) {
switch (n2.charCodeAt(0)) {
case 160:
return " ";
case 38:
return "&";
case 60:
return "<";
case 62:
return ">";
default:
return n2;
}
}
function r(n2) {
return f.test(n2) ? n2.replace(h, o) : n2;
}
function a(n2) {
return d.test(n2) ? n2.replace(c, e) : n2;
}
function p(n2, s) {
return n2.loc.isInvisible || s.loc.isInvisible ? 0 : n2.loc.startPosition.line < s.loc.startPosition.line || n2.loc.startPosition.line === s.loc.startPosition.line && n2.loc.startPosition.column < s.loc.startPosition.column ? -1 : n2.loc.startPosition.line === s.loc.startPosition.line && n2.loc.startPosition.column === s.loc.startPosition.column ? 0 : 1;
}
} }), Te = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/generation/printer.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = t.voidMap = void 0;
var f = Ue(), h = /* @__PURE__ */ Object.create(null);
t.voidMap = h;
var d = "area base br col command embed hr img input keygen link meta param source track wbr";
d.split(" ").forEach((e) => {
h[e] = true;
});
var c = /\S/, o = class {
constructor(e) {
this.buffer = "", this.options = e;
}
handledByOverride(e) {
let r = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : false;
if (this.options.override !== void 0) {
let a = this.options.override(e, this.options);
if (typeof a == "string")
return r && a !== "" && c.test(a[0]) && (a = ` ${a}`), this.buffer += a, true;
}
return false;
}
Node(e) {
switch (e.type) {
case "MustacheStatement":
case "BlockStatement":
case "PartialStatement":
case "MustacheCommentStatement":
case "CommentStatement":
case "TextNode":
case "ElementNode":
case "AttrNode":
case "Block":
case "Template":
return this.TopLevelStatement(e);
case "StringLiteral":
case "BooleanLiteral":
case "NumberLiteral":
case "UndefinedLiteral":
case "NullLiteral":
case "PathExpression":
case "SubExpression":
return this.Expression(e);
case "Program":
return this.Block(e);
case "ConcatStatement":
return this.ConcatStatement(e);
case "Hash":
return this.Hash(e);
case "HashPair":
return this.HashPair(e);
case "ElementModifierStatement":
return this.ElementModifierStatement(e);
}
}
Expression(e) {
switch (e.type) {
case "StringLiteral":
case "BooleanLiteral":
case "NumberLiteral":
case "UndefinedLiteral":
case "NullLiteral":
return this.Literal(e);
case "PathExpression":
return this.PathExpression(e);
case "SubExpression":
return this.SubExpression(e);
}
}
Literal(e) {
switch (e.type) {
case "StringLiteral":
return this.StringLiteral(e);
case "BooleanLiteral":
return this.BooleanLiteral(e);
case "NumberLiteral":
return this.NumberLiteral(e);
case "UndefinedLiteral":
return this.UndefinedLiteral(e);
case "NullLiteral":
return this.NullLiteral(e);
}
}
TopLevelStatement(e) {
switch (e.type) {
case "MustacheStatement":
return this.MustacheStatement(e);
case "BlockStatement":
return this.BlockStatement(e);
case "PartialStatement":
return this.PartialStatement(e);
case "MustacheCommentStatement":
return this.MustacheCommentStatement(e);
case "CommentStatement":
return this.CommentStatement(e);
case "TextNode":
return this.TextNode(e);
case "ElementNode":
return this.ElementNode(e);
case "Block":
case "Template":
return this.Block(e);
case "AttrNode":
return this.AttrNode(e);
}
}
Block(e) {
if (e.chained) {
let r = e.body[0];
r.chained = true;
}
this.handledByOverride(e) || this.TopLevelStatements(e.body);
}
TopLevelStatements(e) {
e.forEach((r) => this.TopLevelStatement(r));
}
ElementNode(e) {
this.handledByOverride(e) || (this.OpenElementNode(e), this.TopLevelStatements(e.children), this.CloseElementNode(e));
}
OpenElementNode(e) {
this.buffer += `<${e.tag}`;
let r = [...e.attributes, ...e.modifiers, ...e.comments].sort(f.sortByLoc);
for (let a of r)
switch (this.buffer += " ", a.type) {
case "AttrNode":
this.AttrNode(a);
break;
case "ElementModifierStatement":
this.ElementModifierStatement(a);
break;
case "MustacheCommentStatement":
this.MustacheCommentStatement(a);
break;
}
e.blockParams.length && this.BlockParams(e.blockParams), e.selfClosing && (this.buffer += " /"), this.buffer += ">";
}
CloseElementNode(e) {
e.selfClosing || h[e.tag.toLowerCase()] || (this.buffer += `${e.tag}>`);
}
AttrNode(e) {
if (this.handledByOverride(e))
return;
let { name: r, value: a } = e;
this.buffer += r, (a.type !== "TextNode" || a.chars.length > 0) && (this.buffer += "=", this.AttrNodeValue(a));
}
AttrNodeValue(e) {
e.type === "TextNode" ? (this.buffer += '"', this.TextNode(e, true), this.buffer += '"') : this.Node(e);
}
TextNode(e, r) {
this.handledByOverride(e) || (this.options.entityEncoding === "raw" ? this.buffer += e.chars : r ? this.buffer += (0, f.escapeAttrValue)(e.chars) : this.buffer += (0, f.escapeText)(e.chars));
}
MustacheStatement(e) {
this.handledByOverride(e) || (this.buffer += e.escaped ? "{{" : "{{{", e.strip.open && (this.buffer += "~"), this.Expression(e.path), this.Params(e.params), this.Hash(e.hash), e.strip.close && (this.buffer += "~"), this.buffer += e.escaped ? "}}" : "}}}");
}
BlockStatement(e) {
this.handledByOverride(e) || (e.chained ? (this.buffer += e.inverseStrip.open ? "{{~" : "{{", this.buffer += "else ") : this.buffer += e.openStrip.open ? "{{~#" : "{{#", this.Expression(e.path), this.Params(e.params), this.Hash(e.hash), e.program.blockParams.length && this.BlockParams(e.program.blockParams), e.chained ? this.buffer += e.inverseStrip.close ? "~}}" : "}}" : this.buffer += e.openStrip.close ? "~}}" : "}}", this.Block(e.program), e.inverse && (e.inverse.chained || (this.buffer += e.inverseStrip.open ? "{{~" : "{{", this.buffer += "else", this.buffer += e.inverseStrip.close ? "~}}" : "}}"), this.Block(e.inverse)), e.chained || (this.buffer += e.closeStrip.open ? "{{~/" : "{{/", this.Expression(e.path), this.buffer += e.closeStrip.close ? "~}}" : "}}"));
}
BlockParams(e) {
this.buffer += ` as |${e.join(" ")}|`;
}
PartialStatement(e) {
this.handledByOverride(e) || (this.buffer += "{{>", this.Expression(e.name), this.Params(e.params), this.Hash(e.hash), this.buffer += "}}");
}
ConcatStatement(e) {
this.handledByOverride(e) || (this.buffer += '"', e.parts.forEach((r) => {
r.type === "TextNode" ? this.TextNode(r, true) : this.Node(r);
}), this.buffer += '"');
}
MustacheCommentStatement(e) {
this.handledByOverride(e) || (this.buffer += `{{!--${e.value}--}}`);
}
ElementModifierStatement(e) {
this.handledByOverride(e) || (this.buffer += "{{", this.Expression(e.path), this.Params(e.params), this.Hash(e.hash), this.buffer += "}}");
}
CommentStatement(e) {
this.handledByOverride(e) || (this.buffer += ``);
}
PathExpression(e) {
this.handledByOverride(e) || (this.buffer += e.original);
}
SubExpression(e) {
this.handledByOverride(e) || (this.buffer += "(", this.Expression(e.path), this.Params(e.params), this.Hash(e.hash), this.buffer += ")");
}
Params(e) {
e.length && e.forEach((r) => {
this.buffer += " ", this.Expression(r);
});
}
Hash(e) {
this.handledByOverride(e, true) || e.pairs.forEach((r) => {
this.buffer += " ", this.HashPair(r);
});
}
HashPair(e) {
this.handledByOverride(e) || (this.buffer += e.key, this.buffer += "=", this.Node(e.value));
}
StringLiteral(e) {
this.handledByOverride(e) || (this.buffer += JSON.stringify(e.value));
}
BooleanLiteral(e) {
this.handledByOverride(e) || (this.buffer += e.value);
}
NumberLiteral(e) {
this.handledByOverride(e) || (this.buffer += e.value);
}
UndefinedLiteral(e) {
this.handledByOverride(e) || (this.buffer += "undefined");
}
NullLiteral(e) {
this.handledByOverride(e) || (this.buffer += "null");
}
print(e) {
let { options: r } = this;
if (r.override) {
let a = r.override(e, r);
if (a !== void 0)
return a;
}
return this.buffer = "", this.Node(e), this.buffer;
}
};
t.default = o;
} }), Be = I({ "node_modules/@handlebars/parser/dist/cjs/exception.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true });
var f = ["description", "fileName", "lineNumber", "endLineNumber", "message", "name", "number", "stack"];
function h(d, c) {
var o = c && c.loc, e, r, a, p;
o && (e = o.start.line, r = o.end.line, a = o.start.column, p = o.end.column, d += " - " + e + ":" + a);
for (var n2 = Error.prototype.constructor.call(this, d), s = 0; s < f.length; s++)
this[f[s]] = n2[f[s]];
Error.captureStackTrace && Error.captureStackTrace(this, h);
try {
o && (this.lineNumber = e, this.endLineNumber = r, Object.defineProperty ? (Object.defineProperty(this, "column", { value: a, enumerable: true }), Object.defineProperty(this, "endColumn", { value: p, enumerable: true })) : (this.column = a, this.endColumn = p));
} catch {
}
}
h.prototype = new Error(), t.default = h;
} }), Oe = I({ "node_modules/@handlebars/parser/dist/cjs/visitor.js"(t) {
"use strict";
F();
var f = t && t.__importDefault || function(r) {
return r && r.__esModule ? r : { default: r };
};
Object.defineProperty(t, "__esModule", { value: true });
var h = f(Be());
function d() {
this.parents = [];
}
d.prototype = { constructor: d, mutating: false, acceptKey: function(r, a) {
var p = this.accept(r[a]);
if (this.mutating) {
if (p && !d.prototype[p.type])
throw new h.default('Unexpected node type "' + p.type + '" found when accepting ' + a + " on " + r.type);
r[a] = p;
}
}, acceptRequired: function(r, a) {
if (this.acceptKey(r, a), !r[a])
throw new h.default(r.type + " requires " + a);
}, acceptArray: function(r) {
for (var a = 0, p = r.length; a < p; a++)
this.acceptKey(r, a), r[a] || (r.splice(a, 1), a--, p--);
}, accept: function(r) {
if (r) {
if (!this[r.type])
throw new h.default("Unknown type: " + r.type, r);
this.current && this.parents.unshift(this.current), this.current = r;
var a = this[r.type](r);
if (this.current = this.parents.shift(), !this.mutating || a)
return a;
if (a !== false)
return r;
}
}, Program: function(r) {
this.acceptArray(r.body);
}, MustacheStatement: c, Decorator: c, BlockStatement: o, DecoratorBlock: o, PartialStatement: e, PartialBlockStatement: function(r) {
e.call(this, r), this.acceptKey(r, "program");
}, ContentStatement: function() {
}, CommentStatement: function() {
}, SubExpression: c, PathExpression: function() {
}, StringLiteral: function() {
}, NumberLiteral: function() {
}, BooleanLiteral: function() {
}, UndefinedLiteral: function() {
}, NullLiteral: function() {
}, Hash: function(r) {
this.acceptArray(r.pairs);
}, HashPair: function(r) {
this.acceptRequired(r, "value");
} };
function c(r) {
this.acceptRequired(r, "path"), this.acceptArray(r.params), this.acceptKey(r, "hash");
}
function o(r) {
c.call(this, r), this.acceptKey(r, "program"), this.acceptKey(r, "inverse");
}
function e(r) {
this.acceptRequired(r, "name"), this.acceptArray(r.params), this.acceptKey(r, "hash");
}
t.default = d;
} }), ze = I({ "node_modules/@handlebars/parser/dist/cjs/whitespace-control.js"(t) {
"use strict";
F();
var f = t && t.__importDefault || function(a) {
return a && a.__esModule ? a : { default: a };
};
Object.defineProperty(t, "__esModule", { value: true });
var h = f(Oe());
function d(a) {
a === void 0 && (a = {}), this.options = a;
}
d.prototype = new h.default(), d.prototype.Program = function(a) {
var p = !this.options.ignoreStandalone, n2 = !this.isRootSeen;
this.isRootSeen = true;
for (var s = a.body, u = 0, i = s.length; u < i; u++) {
var l = s[u], b = this.accept(l);
if (b) {
var P = c(s, u, n2), E = o(s, u, n2), v = b.openStandalone && P, _ = b.closeStandalone && E, y = b.inlineStandalone && P && E;
b.close && e(s, u, true), b.open && r(s, u, true), p && y && (e(s, u), r(s, u) && l.type === "PartialStatement" && (l.indent = /([ \t]+$)/.exec(s[u - 1].original)[1])), p && v && (e((l.program || l.inverse).body), r(s, u)), p && _ && (e(s, u), r((l.inverse || l.program).body));
}
}
return a;
}, d.prototype.BlockStatement = d.prototype.DecoratorBlock = d.prototype.PartialBlockStatement = function(a) {
this.accept(a.program), this.accept(a.inverse);
var p = a.program || a.inverse, n2 = a.program && a.inverse, s = n2, u = n2;
if (n2 && n2.chained)
for (s = n2.body[0].program; u.chained; )
u = u.body[u.body.length - 1].program;
var i = { open: a.openStrip.open, close: a.closeStrip.close, openStandalone: o(p.body), closeStandalone: c((s || p).body) };
if (a.openStrip.close && e(p.body, null, true), n2) {
var l = a.inverseStrip;
l.open && r(p.body, null, true), l.close && e(s.body, null, true), a.closeStrip.open && r(u.body, null, true), !this.options.ignoreStandalone && c(p.body) && o(s.body) && (r(p.body), e(s.body));
} else
a.closeStrip.open && r(p.body, null, true);
return i;
}, d.prototype.Decorator = d.prototype.MustacheStatement = function(a) {
return a.strip;
}, d.prototype.PartialStatement = d.prototype.CommentStatement = function(a) {
var p = a.strip || {};
return { inlineStandalone: true, open: p.open, close: p.close };
};
function c(a, p, n2) {
p === void 0 && (p = a.length);
var s = a[p - 1], u = a[p - 2];
if (!s)
return n2;
if (s.type === "ContentStatement")
return (u || !n2 ? /\r?\n\s*?$/ : /(^|\r?\n)\s*?$/).test(s.original);
}
function o(a, p, n2) {
p === void 0 && (p = -1);
var s = a[p + 1], u = a[p + 2];
if (!s)
return n2;
if (s.type === "ContentStatement")
return (u || !n2 ? /^\s*?\r?\n/ : /^\s*?(\r?\n|$)/).test(s.original);
}
function e(a, p, n2) {
var s = a[p == null ? 0 : p + 1];
if (!(!s || s.type !== "ContentStatement" || !n2 && s.rightStripped)) {
var u = s.value;
s.value = s.value.replace(n2 ? /^\s+/ : /^[ \t]*\r?\n?/, ""), s.rightStripped = s.value !== u;
}
}
function r(a, p, n2) {
var s = a[p == null ? a.length - 1 : p - 1];
if (!(!s || s.type !== "ContentStatement" || !n2 && s.leftStripped)) {
var u = s.value;
return s.value = s.value.replace(n2 ? /\s+$/ : /[ \t]+$/, ""), s.leftStripped = s.value !== u, s.leftStripped;
}
}
t.default = d;
} }), Ge = I({ "node_modules/@handlebars/parser/dist/cjs/parser.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true });
var f = function() {
var h = function(N, k, B, O) {
for (B = B || {}, O = N.length; O--; B[N[O]] = k)
;
return B;
}, d = [2, 44], c = [1, 20], o = [5, 14, 15, 19, 29, 34, 39, 44, 47, 48, 52, 56, 60], e = [1, 35], r = [1, 38], a = [1, 30], p = [1, 31], n2 = [1, 32], s = [1, 33], u = [1, 34], i = [1, 37], l = [14, 15, 19, 29, 34, 39, 44, 47, 48, 52, 56, 60], b = [14, 15, 19, 29, 34, 44, 47, 48, 52, 56, 60], P = [15, 18], E = [14, 15, 19, 29, 34, 47, 48, 52, 56, 60], v = [33, 64, 71, 79, 80, 81, 82, 83, 84], _ = [23, 33, 55, 64, 67, 71, 74, 79, 80, 81, 82, 83, 84], y = [1, 51], g = [23, 33, 55, 64, 67, 71, 74, 79, 80, 81, 82, 83, 84, 86], L = [2, 43], j = [55, 64, 71, 79, 80, 81, 82, 83, 84], x = [1, 58], w = [1, 59], H = [1, 66], m = [33, 64, 71, 74, 79, 80, 81, 82, 83, 84], C = [23, 64, 71, 79, 80, 81, 82, 83, 84], S = [1, 76], R = [64, 67, 71, 79, 80, 81, 82, 83, 84], M = [33, 74], V = [23, 33, 55, 67, 71, 74], G = [1, 106], K = [1, 118], U = [71, 76], Z = { trace: function() {
}, yy: {}, symbols_: { error: 2, root: 3, program: 4, EOF: 5, program_repetition0: 6, statement: 7, mustache: 8, block: 9, rawBlock: 10, partial: 11, partialBlock: 12, content: 13, COMMENT: 14, CONTENT: 15, openRawBlock: 16, rawBlock_repetition0: 17, END_RAW_BLOCK: 18, OPEN_RAW_BLOCK: 19, helperName: 20, openRawBlock_repetition0: 21, openRawBlock_option0: 22, CLOSE_RAW_BLOCK: 23, openBlock: 24, block_option0: 25, closeBlock: 26, openInverse: 27, block_option1: 28, OPEN_BLOCK: 29, openBlock_repetition0: 30, openBlock_option0: 31, openBlock_option1: 32, CLOSE: 33, OPEN_INVERSE: 34, openInverse_repetition0: 35, openInverse_option0: 36, openInverse_option1: 37, openInverseChain: 38, OPEN_INVERSE_CHAIN: 39, openInverseChain_repetition0: 40, openInverseChain_option0: 41, openInverseChain_option1: 42, inverseAndProgram: 43, INVERSE: 44, inverseChain: 45, inverseChain_option0: 46, OPEN_ENDBLOCK: 47, OPEN: 48, expr: 49, mustache_repetition0: 50, mustache_option0: 51, OPEN_UNESCAPED: 52, mustache_repetition1: 53, mustache_option1: 54, CLOSE_UNESCAPED: 55, OPEN_PARTIAL: 56, partial_repetition0: 57, partial_option0: 58, openPartialBlock: 59, OPEN_PARTIAL_BLOCK: 60, openPartialBlock_repetition0: 61, openPartialBlock_option0: 62, sexpr: 63, OPEN_SEXPR: 64, sexpr_repetition0: 65, sexpr_option0: 66, CLOSE_SEXPR: 67, hash: 68, hash_repetition_plus0: 69, hashSegment: 70, ID: 71, EQUALS: 72, blockParams: 73, OPEN_BLOCK_PARAMS: 74, blockParams_repetition_plus0: 75, CLOSE_BLOCK_PARAMS: 76, path: 77, dataName: 78, STRING: 79, NUMBER: 80, BOOLEAN: 81, UNDEFINED: 82, NULL: 83, DATA: 84, pathSegments: 85, SEP: 86, $accept: 0, $end: 1 }, terminals_: { 2: "error", 5: "EOF", 14: "COMMENT", 15: "CONTENT", 18: "END_RAW_BLOCK", 19: "OPEN_RAW_BLOCK", 23: "CLOSE_RAW_BLOCK", 29: "OPEN_BLOCK", 33: "CLOSE", 34: "OPEN_INVERSE", 39: "OPEN_INVERSE_CHAIN", 44: "INVERSE", 47: "OPEN_ENDBLOCK", 48: "OPEN", 52: "OPEN_UNESCAPED", 55: "CLOSE_UNESCAPED", 56: "OPEN_PARTIAL", 60: "OPEN_PARTIAL_BLOCK", 64: "OPEN_SEXPR", 67: "CLOSE_SEXPR", 71: "ID", 72: "EQUALS", 74: "OPEN_BLOCK_PARAMS", 76: "CLOSE_BLOCK_PARAMS", 79: "STRING", 80: "NUMBER", 81: "BOOLEAN", 82: "UNDEFINED", 83: "NULL", 84: "DATA", 86: "SEP" }, productions_: [0, [3, 2], [4, 1], [7, 1], [7, 1], [7, 1], [7, 1], [7, 1], [7, 1], [7, 1], [13, 1], [10, 3], [16, 5], [9, 4], [9, 4], [24, 6], [27, 6], [38, 6], [43, 2], [45, 3], [45, 1], [26, 3], [8, 5], [8, 5], [11, 5], [12, 3], [59, 5], [49, 1], [49, 1], [63, 5], [68, 1], [70, 3], [73, 3], [20, 1], [20, 1], [20, 1], [20, 1], [20, 1], [20, 1], [20, 1], [78, 2], [77, 1], [85, 3], [85, 1], [6, 0], [6, 2], [17, 0], [17, 2], [21, 0], [21, 2], [22, 0], [22, 1], [25, 0], [25, 1], [28, 0], [28, 1], [30, 0], [30, 2], [31, 0], [31, 1], [32, 0], [32, 1], [35, 0], [35, 2], [36, 0], [36, 1], [37, 0], [37, 1], [40, 0], [40, 2], [41, 0], [41, 1], [42, 0], [42, 1], [46, 0], [46, 1], [50, 0], [50, 2], [51, 0], [51, 1], [53, 0], [53, 2], [54, 0], [54, 1], [57, 0], [57, 2], [58, 0], [58, 1], [61, 0], [61, 2], [62, 0], [62, 1], [65, 0], [65, 2], [66, 0], [66, 1], [69, 1], [69, 2], [75, 1], [75, 2]], performAction: function(k, B, O, q, z, A, Q) {
var D = A.length - 1;
switch (z) {
case 1:
return A[D - 1];
case 2:
this.$ = q.prepareProgram(A[D]);
break;
case 3:
case 4:
case 5:
case 6:
case 7:
case 8:
case 20:
case 27:
case 28:
case 33:
case 34:
this.$ = A[D];
break;
case 9:
this.$ = { type: "CommentStatement", value: q.stripComment(A[D]), strip: q.stripFlags(A[D], A[D]), loc: q.locInfo(this._$) };
break;
case 10:
this.$ = { type: "ContentStatement", original: A[D], value: A[D], loc: q.locInfo(this._$) };
break;
case 11:
this.$ = q.prepareRawBlock(A[D - 2], A[D - 1], A[D], this._$);
break;
case 12:
this.$ = { path: A[D - 3], params: A[D - 2], hash: A[D - 1] };
break;
case 13:
this.$ = q.prepareBlock(A[D - 3], A[D - 2], A[D - 1], A[D], false, this._$);
break;
case 14:
this.$ = q.prepareBlock(A[D - 3], A[D - 2], A[D - 1], A[D], true, this._$);
break;
case 15:
this.$ = { open: A[D - 5], path: A[D - 4], params: A[D - 3], hash: A[D - 2], blockParams: A[D - 1], strip: q.stripFlags(A[D - 5], A[D]) };
break;
case 16:
case 17:
this.$ = { path: A[D - 4], params: A[D - 3], hash: A[D - 2], blockParams: A[D - 1], strip: q.stripFlags(A[D - 5], A[D]) };
break;
case 18:
this.$ = { strip: q.stripFlags(A[D - 1], A[D - 1]), program: A[D] };
break;
case 19:
var $ = q.prepareBlock(A[D - 2], A[D - 1], A[D], A[D], false, this._$), oe = q.prepareProgram([$], A[D - 1].loc);
oe.chained = true, this.$ = { strip: A[D - 2].strip, program: oe, chain: true };
break;
case 21:
this.$ = { path: A[D - 1], strip: q.stripFlags(A[D - 2], A[D]) };
break;
case 22:
case 23:
this.$ = q.prepareMustache(A[D - 3], A[D - 2], A[D - 1], A[D - 4], q.stripFlags(A[D - 4], A[D]), this._$);
break;
case 24:
this.$ = { type: "PartialStatement", name: A[D - 3], params: A[D - 2], hash: A[D - 1], indent: "", strip: q.stripFlags(A[D - 4], A[D]), loc: q.locInfo(this._$) };
break;
case 25:
this.$ = q.preparePartialBlock(A[D - 2], A[D - 1], A[D], this._$);
break;
case 26:
this.$ = { path: A[D - 3], params: A[D - 2], hash: A[D - 1], strip: q.stripFlags(A[D - 4], A[D]) };
break;
case 29:
this.$ = { type: "SubExpression", path: A[D - 3], params: A[D - 2], hash: A[D - 1], loc: q.locInfo(this._$) };
break;
case 30:
this.$ = { type: "Hash", pairs: A[D], loc: q.locInfo(this._$) };
break;
case 31:
this.$ = { type: "HashPair", key: q.id(A[D - 2]), value: A[D], loc: q.locInfo(this._$) };
break;
case 32:
this.$ = q.id(A[D - 1]);
break;
case 35:
this.$ = { type: "StringLiteral", value: A[D], original: A[D], loc: q.locInfo(this._$) };
break;
case 36:
this.$ = { type: "NumberLiteral", value: Number(A[D]), original: Number(A[D]), loc: q.locInfo(this._$) };
break;
case 37:
this.$ = { type: "BooleanLiteral", value: A[D] === "true", original: A[D] === "true", loc: q.locInfo(this._$) };
break;
case 38:
this.$ = { type: "UndefinedLiteral", original: void 0, value: void 0, loc: q.locInfo(this._$) };
break;
case 39:
this.$ = { type: "NullLiteral", original: null, value: null, loc: q.locInfo(this._$) };
break;
case 40:
this.$ = q.preparePath(true, A[D], this._$);
break;
case 41:
this.$ = q.preparePath(false, A[D], this._$);
break;
case 42:
A[D - 2].push({ part: q.id(A[D]), original: A[D], separator: A[D - 1] }), this.$ = A[D - 2];
break;
case 43:
this.$ = [{ part: q.id(A[D]), original: A[D] }];
break;
case 44:
case 46:
case 48:
case 56:
case 62:
case 68:
case 76:
case 80:
case 84:
case 88:
case 92:
this.$ = [];
break;
case 45:
case 47:
case 49:
case 57:
case 63:
case 69:
case 77:
case 81:
case 85:
case 89:
case 93:
case 97:
case 99:
A[D - 1].push(A[D]);
break;
case 96:
case 98:
this.$ = [A[D]];
break;
}
}, table: [h([5, 14, 15, 19, 29, 34, 48, 52, 56, 60], d, { 3: 1, 4: 2, 6: 3 }), { 1: [3] }, { 5: [1, 4] }, h([5, 39, 44, 47], [2, 2], { 7: 5, 8: 6, 9: 7, 10: 8, 11: 9, 12: 10, 13: 11, 24: 15, 27: 16, 16: 17, 59: 19, 14: [1, 12], 15: c, 19: [1, 23], 29: [1, 21], 34: [1, 22], 48: [1, 13], 52: [1, 14], 56: [1, 18], 60: [1, 24] }), { 1: [2, 1] }, h(o, [2, 45]), h(o, [2, 3]), h(o, [2, 4]), h(o, [2, 5]), h(o, [2, 6]), h(o, [2, 7]), h(o, [2, 8]), h(o, [2, 9]), { 20: 26, 49: 25, 63: 27, 64: e, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, { 20: 26, 49: 39, 63: 27, 64: e, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, h(l, d, { 6: 3, 4: 40 }), h(b, d, { 6: 3, 4: 41 }), h(P, [2, 46], { 17: 42 }), { 20: 26, 49: 43, 63: 27, 64: e, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, h(E, d, { 6: 3, 4: 44 }), h([5, 14, 15, 18, 19, 29, 34, 39, 44, 47, 48, 52, 56, 60], [2, 10]), { 20: 45, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, { 20: 46, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, { 20: 47, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, { 20: 26, 49: 48, 63: 27, 64: e, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, h(v, [2, 76], { 50: 49 }), h(_, [2, 27]), h(_, [2, 28]), h(_, [2, 33]), h(_, [2, 34]), h(_, [2, 35]), h(_, [2, 36]), h(_, [2, 37]), h(_, [2, 38]), h(_, [2, 39]), { 20: 26, 49: 50, 63: 27, 64: e, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, h(_, [2, 41], { 86: y }), { 71: r, 85: 52 }, h(g, L), h(j, [2, 80], { 53: 53 }), { 25: 54, 38: 56, 39: x, 43: 57, 44: w, 45: 55, 47: [2, 52] }, { 28: 60, 43: 61, 44: w, 47: [2, 54] }, { 13: 63, 15: c, 18: [1, 62] }, h(v, [2, 84], { 57: 64 }), { 26: 65, 47: H }, h(m, [2, 56], { 30: 67 }), h(m, [2, 62], { 35: 68 }), h(C, [2, 48], { 21: 69 }), h(v, [2, 88], { 61: 70 }), { 20: 26, 33: [2, 78], 49: 72, 51: 71, 63: 27, 64: e, 68: 73, 69: 74, 70: 75, 71: S, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, h(R, [2, 92], { 65: 77 }), { 71: [1, 78] }, h(_, [2, 40], { 86: y }), { 20: 26, 49: 80, 54: 79, 55: [2, 82], 63: 27, 64: e, 68: 81, 69: 74, 70: 75, 71: S, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, { 26: 82, 47: H }, { 47: [2, 53] }, h(l, d, { 6: 3, 4: 83 }), { 47: [2, 20] }, { 20: 84, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, h(E, d, { 6: 3, 4: 85 }), { 26: 86, 47: H }, { 47: [2, 55] }, h(o, [2, 11]), h(P, [2, 47]), { 20: 26, 33: [2, 86], 49: 88, 58: 87, 63: 27, 64: e, 68: 89, 69: 74, 70: 75, 71: S, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, h(o, [2, 25]), { 20: 90, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, h(M, [2, 58], { 20: 26, 63: 27, 77: 28, 78: 29, 85: 36, 69: 74, 70: 75, 31: 91, 49: 92, 68: 93, 64: e, 71: S, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i }), h(M, [2, 64], { 20: 26, 63: 27, 77: 28, 78: 29, 85: 36, 69: 74, 70: 75, 36: 94, 49: 95, 68: 96, 64: e, 71: S, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i }), { 20: 26, 22: 97, 23: [2, 50], 49: 98, 63: 27, 64: e, 68: 99, 69: 74, 70: 75, 71: S, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, { 20: 26, 33: [2, 90], 49: 101, 62: 100, 63: 27, 64: e, 68: 102, 69: 74, 70: 75, 71: S, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, { 33: [1, 103] }, h(v, [2, 77]), { 33: [2, 79] }, h([23, 33, 55, 67, 74], [2, 30], { 70: 104, 71: [1, 105] }), h(V, [2, 96]), h(g, L, { 72: G }), { 20: 26, 49: 108, 63: 27, 64: e, 66: 107, 67: [2, 94], 68: 109, 69: 74, 70: 75, 71: S, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, h(g, [2, 42]), { 55: [1, 110] }, h(j, [2, 81]), { 55: [2, 83] }, h(o, [2, 13]), { 38: 56, 39: x, 43: 57, 44: w, 45: 112, 46: 111, 47: [2, 74] }, h(m, [2, 68], { 40: 113 }), { 47: [2, 18] }, h(o, [2, 14]), { 33: [1, 114] }, h(v, [2, 85]), { 33: [2, 87] }, { 33: [1, 115] }, { 32: 116, 33: [2, 60], 73: 117, 74: K }, h(m, [2, 57]), h(M, [2, 59]), { 33: [2, 66], 37: 119, 73: 120, 74: K }, h(m, [2, 63]), h(M, [2, 65]), { 23: [1, 121] }, h(C, [2, 49]), { 23: [2, 51] }, { 33: [1, 122] }, h(v, [2, 89]), { 33: [2, 91] }, h(o, [2, 22]), h(V, [2, 97]), { 72: G }, { 20: 26, 49: 123, 63: 27, 64: e, 71: r, 77: 28, 78: 29, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i, 85: 36 }, { 67: [1, 124] }, h(R, [2, 93]), { 67: [2, 95] }, h(o, [2, 23]), { 47: [2, 19] }, { 47: [2, 75] }, h(M, [2, 70], { 20: 26, 63: 27, 77: 28, 78: 29, 85: 36, 69: 74, 70: 75, 41: 125, 49: 126, 68: 127, 64: e, 71: S, 79: a, 80: p, 81: n2, 82: s, 83: u, 84: i }), h(o, [2, 24]), h(o, [2, 21]), { 33: [1, 128] }, { 33: [2, 61] }, { 71: [1, 130], 75: 129 }, { 33: [1, 131] }, { 33: [2, 67] }, h(P, [2, 12]), h(E, [2, 26]), h(V, [2, 31]), h(_, [2, 29]), { 33: [2, 72], 42: 132, 73: 133, 74: K }, h(m, [2, 69]), h(M, [2, 71]), h(l, [2, 15]), { 71: [1, 135], 76: [1, 134] }, h(U, [2, 98]), h(b, [2, 16]), { 33: [1, 136] }, { 33: [2, 73] }, { 33: [2, 32] }, h(U, [2, 99]), h(l, [2, 17])], defaultActions: { 4: [2, 1], 55: [2, 53], 57: [2, 20], 61: [2, 55], 73: [2, 79], 81: [2, 83], 85: [2, 18], 89: [2, 87], 99: [2, 51], 102: [2, 91], 109: [2, 95], 111: [2, 19], 112: [2, 75], 117: [2, 61], 120: [2, 67], 133: [2, 73], 134: [2, 32] }, parseError: function(k, B) {
if (B.recoverable)
this.trace(k);
else {
var O = new Error(k);
throw O.hash = B, O;
}
}, parse: function(k) {
var B = this, O = [0], q = [], z = [null], A = [], Q = this.table, D = "", $ = 0, oe = 0, Ie = 0, et = 2, Re = 1, tt = A.slice.call(arguments, 1), Y = Object.create(this.lexer), ie = { yy: {} };
for (var Ae in this.yy)
Object.prototype.hasOwnProperty.call(this.yy, Ae) && (ie.yy[Ae] = this.yy[Ae]);
Y.setInput(k, ie.yy), ie.yy.lexer = Y, ie.yy.parser = this, typeof Y.yylloc > "u" && (Y.yylloc = {});
var Ee = Y.yylloc;
A.push(Ee);
var rt = Y.options && Y.options.ranges;
typeof ie.yy.parseError == "function" ? this.parseError = ie.yy.parseError : this.parseError = Object.getPrototypeOf(this).parseError;
function $t(te) {
O.length = O.length - 2 * te, z.length = z.length - te, A.length = A.length - te;
}
e:
var nt = function() {
var te;
return te = Y.lex() || Re, typeof te != "number" && (te = B.symbols_[te] || te), te;
};
for (var J, _e, se, ee, er, Se, ae = {}, de, re, qe, pe; ; ) {
if (se = O[O.length - 1], this.defaultActions[se] ? ee = this.defaultActions[se] : ((J === null || typeof J > "u") && (J = nt()), ee = Q[se] && Q[se][J]), typeof ee > "u" || !ee.length || !ee[0]) {
var Ce = "";
pe = [];
for (de in Q[se])
this.terminals_[de] && de > et && pe.push("'" + this.terminals_[de] + "'");
Y.showPosition ? Ce = "Parse error on line " + ($ + 1) + `:
` + Y.showPosition() + `
Expecting ` + pe.join(", ") + ", got '" + (this.terminals_[J] || J) + "'" : Ce = "Parse error on line " + ($ + 1) + ": Unexpected " + (J == Re ? "end of input" : "'" + (this.terminals_[J] || J) + "'"), this.parseError(Ce, { text: Y.match, token: this.terminals_[J] || J, line: Y.yylineno, loc: Ee, expected: pe });
}
if (ee[0] instanceof Array && ee.length > 1)
throw new Error("Parse Error: multiple actions possible at state: " + se + ", token: " + J);
switch (ee[0]) {
case 1:
O.push(J), z.push(Y.yytext), A.push(Y.yylloc), O.push(ee[1]), J = null, _e ? (J = _e, _e = null) : (oe = Y.yyleng, D = Y.yytext, $ = Y.yylineno, Ee = Y.yylloc, Ie > 0 && Ie--);
break;
case 2:
if (re = this.productions_[ee[1]][1], ae.$ = z[z.length - re], ae._$ = { first_line: A[A.length - (re || 1)].first_line, last_line: A[A.length - 1].last_line, first_column: A[A.length - (re || 1)].first_column, last_column: A[A.length - 1].last_column }, rt && (ae._$.range = [A[A.length - (re || 1)].range[0], A[A.length - 1].range[1]]), Se = this.performAction.apply(ae, [D, oe, $, ie.yy, ee[1], z, A].concat(tt)), typeof Se < "u")
return Se;
re && (O = O.slice(0, -1 * re * 2), z = z.slice(0, -1 * re), A = A.slice(0, -1 * re)), O.push(this.productions_[ee[1]][0]), z.push(ae.$), A.push(ae._$), qe = Q[O[O.length - 2]][O[O.length - 1]], O.push(qe);
break;
case 3:
return true;
}
}
return true;
} }, W = function() {
var N = { EOF: 1, parseError: function(B, O) {
if (this.yy.parser)
this.yy.parser.parseError(B, O);
else
throw new Error(B);
}, setInput: function(k, B) {
return this.yy = B || this.yy || {}, this._input = k, this._more = this._backtrack = this.done = false, this.yylineno = this.yyleng = 0, this.yytext = this.matched = this.match = "", this.conditionStack = ["INITIAL"], this.yylloc = { first_line: 1, first_column: 0, last_line: 1, last_column: 0 }, this.options.ranges && (this.yylloc.range = [0, 0]), this.offset = 0, this;
}, input: function() {
var k = this._input[0];
this.yytext += k, this.yyleng++, this.offset++, this.match += k, this.matched += k;
var B = k.match(/(?:\r\n?|\n).*/g);
return B ? (this.yylineno++, this.yylloc.last_line++) : this.yylloc.last_column++, this.options.ranges && this.yylloc.range[1]++, this._input = this._input.slice(1), k;
}, unput: function(k) {
var B = k.length, O = k.split(/(?:\r\n?|\n)/g);
this._input = k + this._input, this.yytext = this.yytext.substr(0, this.yytext.length - B), this.offset -= B;
var q = this.match.split(/(?:\r\n?|\n)/g);
this.match = this.match.substr(0, this.match.length - 1), this.matched = this.matched.substr(0, this.matched.length - 1), O.length - 1 && (this.yylineno -= O.length - 1);
var z = this.yylloc.range;
return this.yylloc = { first_line: this.yylloc.first_line, last_line: this.yylineno + 1, first_column: this.yylloc.first_column, last_column: O ? (O.length === q.length ? this.yylloc.first_column : 0) + q[q.length - O.length].length - O[0].length : this.yylloc.first_column - B }, this.options.ranges && (this.yylloc.range = [z[0], z[0] + this.yyleng - B]), this.yyleng = this.yytext.length, this;
}, more: function() {
return this._more = true, this;
}, reject: function() {
if (this.options.backtrack_lexer)
this._backtrack = true;
else
return this.parseError("Lexical error on line " + (this.yylineno + 1) + `. You can only invoke reject() in the lexer when the lexer is of the backtracking persuasion (options.backtrack_lexer = true).
` + this.showPosition(), { text: "", token: null, line: this.yylineno });
return this;
}, less: function(k) {
this.unput(this.match.slice(k));
}, pastInput: function() {
var k = this.matched.substr(0, this.matched.length - this.match.length);
return (k.length > 20 ? "..." : "") + k.substr(-20).replace(/\n/g, "");
}, upcomingInput: function() {
var k = this.match;
return k.length < 20 && (k += this._input.substr(0, 20 - k.length)), (k.substr(0, 20) + (k.length > 20 ? "..." : "")).replace(/\n/g, "");
}, showPosition: function() {
var k = this.pastInput(), B = new Array(k.length + 1).join("-");
return k + this.upcomingInput() + `
` + B + "^";
}, test_match: function(k, B) {
var O, q, z;
if (this.options.backtrack_lexer && (z = { yylineno: this.yylineno, yylloc: { first_line: this.yylloc.first_line, last_line: this.last_line, first_column: this.yylloc.first_column, last_column: this.yylloc.last_column }, yytext: this.yytext, match: this.match, matches: this.matches, matched: this.matched, yyleng: this.yyleng, offset: this.offset, _more: this._more, _input: this._input, yy: this.yy, conditionStack: this.conditionStack.slice(0), done: this.done }, this.options.ranges && (z.yylloc.range = this.yylloc.range.slice(0))), q = k[0].match(/(?:\r\n?|\n).*/g), q && (this.yylineno += q.length), this.yylloc = { first_line: this.yylloc.last_line, last_line: this.yylineno + 1, first_column: this.yylloc.last_column, last_column: q ? q[q.length - 1].length - q[q.length - 1].match(/\r?\n?/)[0].length : this.yylloc.last_column + k[0].length }, this.yytext += k[0], this.match += k[0], this.matches = k, this.yyleng = this.yytext.length, this.options.ranges && (this.yylloc.range = [this.offset, this.offset += this.yyleng]), this._more = false, this._backtrack = false, this._input = this._input.slice(k[0].length), this.matched += k[0], O = this.performAction.call(this, this.yy, this, B, this.conditionStack[this.conditionStack.length - 1]), this.done && this._input && (this.done = false), O)
return O;
if (this._backtrack) {
for (var A in z)
this[A] = z[A];
return false;
}
return false;
}, next: function() {
if (this.done)
return this.EOF;
this._input || (this.done = true);
var k, B, O, q;
this._more || (this.yytext = "", this.match = "");
for (var z = this._currentRules(), A = 0; A < z.length; A++)
if (O = this._input.match(this.rules[z[A]]), O && (!B || O[0].length > B[0].length)) {
if (B = O, q = A, this.options.backtrack_lexer) {
if (k = this.test_match(O, z[A]), k !== false)
return k;
if (this._backtrack) {
B = false;
continue;
} else
return false;
} else if (!this.options.flex)
break;
}
return B ? (k = this.test_match(B, z[q]), k !== false ? k : false) : this._input === "" ? this.EOF : this.parseError("Lexical error on line " + (this.yylineno + 1) + `. Unrecognized text.
` + this.showPosition(), { text: "", token: null, line: this.yylineno });
}, lex: function() {
var B = this.next();
return B || this.lex();
}, begin: function(B) {
this.conditionStack.push(B);
}, popState: function() {
var B = this.conditionStack.length - 1;
return B > 0 ? this.conditionStack.pop() : this.conditionStack[0];
}, _currentRules: function() {
return this.conditionStack.length && this.conditionStack[this.conditionStack.length - 1] ? this.conditions[this.conditionStack[this.conditionStack.length - 1]].rules : this.conditions.INITIAL.rules;
}, topState: function(B) {
return B = this.conditionStack.length - 1 - Math.abs(B || 0), B >= 0 ? this.conditionStack[B] : "INITIAL";
}, pushState: function(B) {
this.begin(B);
}, stateStackSize: function() {
return this.conditionStack.length;
}, options: {}, performAction: function(B, O, q, z) {
function A(D, $) {
return O.yytext = O.yytext.substring(D, O.yyleng - $ + D);
}
var Q = z;
switch (q) {
case 0:
if (O.yytext.slice(-2) === "\\\\" ? (A(0, 1), this.begin("mu")) : O.yytext.slice(-1) === "\\" ? (A(0, 1), this.begin("emu")) : this.begin("mu"), O.yytext)
return 15;
break;
case 1:
return 15;
case 2:
return this.popState(), 15;
break;
case 3:
return this.begin("raw"), 15;
break;
case 4:
return this.popState(), this.conditionStack[this.conditionStack.length - 1] === "raw" ? 15 : (A(5, 9), 18);
case 5:
return 15;
case 6:
return this.popState(), 14;
break;
case 7:
return 64;
case 8:
return 67;
case 9:
return 19;
case 10:
return this.popState(), this.begin("raw"), 23;
break;
case 11:
return 56;
case 12:
return 60;
case 13:
return 29;
case 14:
return 47;
case 15:
return this.popState(), 44;
break;
case 16:
return this.popState(), 44;
break;
case 17:
return 34;
case 18:
return 39;
case 19:
return 52;
case 20:
return 48;
case 21:
this.unput(O.yytext), this.popState(), this.begin("com");
break;
case 22:
return this.popState(), 14;
break;
case 23:
return 48;
case 24:
return 72;
case 25:
return 71;
case 26:
return 71;
case 27:
return 86;
case 28:
break;
case 29:
return this.popState(), 55;
break;
case 30:
return this.popState(), 33;
break;
case 31:
return O.yytext = A(1, 2).replace(/\\"/g, '"'), 79;
break;
case 32:
return O.yytext = A(1, 2).replace(/\\'/g, "'"), 79;
break;
case 33:
return 84;
case 34:
return 81;
case 35:
return 81;
case 36:
return 82;
case 37:
return 83;
case 38:
return 80;
case 39:
return 74;
case 40:
return 76;
case 41:
return 71;
case 42:
return O.yytext = O.yytext.replace(/\\([\\\]])/g, "$1"), 71;
break;
case 43:
return "INVALID";
case 44:
return 5;
}
}, rules: [/^(?:[^\x00]*?(?=(\{\{)))/, /^(?:[^\x00]+)/, /^(?:[^\x00]{2,}?(?=(\{\{|\\\{\{|\\\\\{\{|$)))/, /^(?:\{\{\{\{(?=[^/]))/, /^(?:\{\{\{\{\/[^\s!"#%-,\.\/;->@\[-\^`\{-~]+(?=[=}\s\/.])\}\}\}\})/, /^(?:[^\x00]+?(?=(\{\{\{\{)))/, /^(?:[\s\S]*?--(~)?\}\})/, /^(?:\()/, /^(?:\))/, /^(?:\{\{\{\{)/, /^(?:\}\}\}\})/, /^(?:\{\{(~)?>)/, /^(?:\{\{(~)?#>)/, /^(?:\{\{(~)?#\*?)/, /^(?:\{\{(~)?\/)/, /^(?:\{\{(~)?\^\s*(~)?\}\})/, /^(?:\{\{(~)?\s*else\s*(~)?\}\})/, /^(?:\{\{(~)?\^)/, /^(?:\{\{(~)?\s*else\b)/, /^(?:\{\{(~)?\{)/, /^(?:\{\{(~)?&)/, /^(?:\{\{(~)?!--)/, /^(?:\{\{(~)?![\s\S]*?\}\})/, /^(?:\{\{(~)?\*?)/, /^(?:=)/, /^(?:\.\.)/, /^(?:\.(?=([=~}\s\/.)|])))/, /^(?:[\/.])/, /^(?:\s+)/, /^(?:\}(~)?\}\})/, /^(?:(~)?\}\})/, /^(?:"(\\["]|[^"])*")/, /^(?:'(\\[']|[^'])*')/, /^(?:@)/, /^(?:true(?=([~}\s)])))/, /^(?:false(?=([~}\s)])))/, /^(?:undefined(?=([~}\s)])))/, /^(?:null(?=([~}\s)])))/, /^(?:-?[0-9]+(?:\.[0-9]+)?(?=([~}\s)])))/, /^(?:as\s+\|)/, /^(?:\|)/, /^(?:([^\s!"#%-,\.\/;->@\[-\^`\{-~]+(?=([=~}\s\/.)|]))))/, /^(?:\[(\\\]|[^\]])*\])/, /^(?:.)/, /^(?:$)/], conditions: { mu: { rules: [7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44], inclusive: false }, emu: { rules: [2], inclusive: false }, com: { rules: [6], inclusive: false }, raw: { rules: [3, 4, 5], inclusive: false }, INITIAL: { rules: [0, 1, 44], inclusive: true } } };
return N;
}();
Z.lexer = W;
function T() {
this.yy = {};
}
return T.prototype = Z, Z.Parser = T, new T();
}();
t.default = f;
} }), Nt = I({ "node_modules/@handlebars/parser/dist/cjs/printer.js"(t) {
"use strict";
F();
var f = t && t.__importDefault || function(o) {
return o && o.__esModule ? o : { default: o };
};
Object.defineProperty(t, "__esModule", { value: true }), t.PrintVisitor = t.print = void 0;
var h = f(Oe());
function d(o) {
return new c().accept(o);
}
t.print = d;
function c() {
this.padding = 0;
}
t.PrintVisitor = c, c.prototype = new h.default(), c.prototype.pad = function(o) {
for (var e = "", r = 0, a = this.padding; r < a; r++)
e += " ";
return e += o + `
`, e;
}, c.prototype.Program = function(o) {
var e = "", r = o.body, a, p;
if (o.blockParams) {
var n2 = "BLOCK PARAMS: [";
for (a = 0, p = o.blockParams.length; a < p; a++)
n2 += " " + o.blockParams[a];
n2 += " ]", e += this.pad(n2);
}
for (a = 0, p = r.length; a < p; a++)
e += this.accept(r[a]);
return this.padding--, e;
}, c.prototype.MustacheStatement = function(o) {
return this.pad("{{ " + this.SubExpression(o) + " }}");
}, c.prototype.Decorator = function(o) {
return this.pad("{{ DIRECTIVE " + this.SubExpression(o) + " }}");
}, c.prototype.BlockStatement = c.prototype.DecoratorBlock = function(o) {
var e = "";
return e += this.pad((o.type === "DecoratorBlock" ? "DIRECTIVE " : "") + "BLOCK:"), this.padding++, e += this.pad(this.SubExpression(o)), o.program && (e += this.pad("PROGRAM:"), this.padding++, e += this.accept(o.program), this.padding--), o.inverse && (o.program && this.padding++, e += this.pad("{{^}}"), this.padding++, e += this.accept(o.inverse), this.padding--, o.program && this.padding--), this.padding--, e;
}, c.prototype.PartialStatement = function(o) {
var e = "PARTIAL:" + o.name.original;
return o.params[0] && (e += " " + this.accept(o.params[0])), o.hash && (e += " " + this.accept(o.hash)), this.pad("{{> " + e + " }}");
}, c.prototype.PartialBlockStatement = function(o) {
var e = "PARTIAL BLOCK:" + o.name.original;
return o.params[0] && (e += " " + this.accept(o.params[0])), o.hash && (e += " " + this.accept(o.hash)), e += " " + this.pad("PROGRAM:"), this.padding++, e += this.accept(o.program), this.padding--, this.pad("{{> " + e + " }}");
}, c.prototype.ContentStatement = function(o) {
return this.pad("CONTENT[ '" + o.value + "' ]");
}, c.prototype.CommentStatement = function(o) {
return this.pad("{{! '" + o.value + "' }}");
}, c.prototype.SubExpression = function(o) {
for (var e = o.params, r = [], a, p = 0, n2 = e.length; p < n2; p++)
r.push(this.accept(e[p]));
return e = "[" + r.join(", ") + "]", a = o.hash ? " " + this.accept(o.hash) : "", this.accept(o.path) + " " + e + a;
}, c.prototype.PathExpression = function(o) {
var e = o.parts.join("/");
return (o.data ? "@" : "") + "PATH:" + e;
}, c.prototype.StringLiteral = function(o) {
return '"' + o.value + '"';
}, c.prototype.NumberLiteral = function(o) {
return "NUMBER{" + o.value + "}";
}, c.prototype.BooleanLiteral = function(o) {
return "BOOLEAN{" + o.value + "}";
}, c.prototype.UndefinedLiteral = function() {
return "UNDEFINED";
}, c.prototype.NullLiteral = function() {
return "NULL";
}, c.prototype.Hash = function(o) {
for (var e = o.pairs, r = [], a = 0, p = e.length; a < p; a++)
r.push(this.accept(e[a]));
return "HASH{" + r.join(", ") + "}";
}, c.prototype.HashPair = function(o) {
return o.key + "=" + this.accept(o.value);
};
} }), Lt = I({ "node_modules/@handlebars/parser/dist/cjs/helpers.js"(t) {
"use strict";
F();
var f = t && t.__importDefault || function(l) {
return l && l.__esModule ? l : { default: l };
};
Object.defineProperty(t, "__esModule", { value: true }), t.preparePartialBlock = t.prepareProgram = t.prepareBlock = t.prepareRawBlock = t.prepareMustache = t.preparePath = t.stripComment = t.stripFlags = t.id = t.SourceLocation = void 0;
var h = f(Be());
function d(l, b) {
if (b = b.path ? b.path.original : b, l.path.original !== b) {
var P = { loc: l.path.loc };
throw new h.default(l.path.original + " doesn't match " + b, P);
}
}
function c(l, b) {
this.source = l, this.start = { line: b.first_line, column: b.first_column }, this.end = { line: b.last_line, column: b.last_column };
}
t.SourceLocation = c;
function o(l) {
return /^\[.*\]$/.test(l) ? l.substring(1, l.length - 1) : l;
}
t.id = o;
function e(l, b) {
return { open: l.charAt(2) === "~", close: b.charAt(b.length - 3) === "~" };
}
t.stripFlags = e;
function r(l) {
return l.replace(/^\{\{~?!-?-?/, "").replace(/-?-?~?\}\}$/, "");
}
t.stripComment = r;
function a(l, b, P) {
P = this.locInfo(P);
for (var E = l ? "@" : "", v = [], _ = 0, y = 0, g = b.length; y < g; y++) {
var L = b[y].part, j = b[y].original !== L;
if (E += (b[y].separator || "") + L, !j && (L === ".." || L === "." || L === "this")) {
if (v.length > 0)
throw new h.default("Invalid path: " + E, { loc: P });
L === ".." && _++;
} else
v.push(L);
}
return { type: "PathExpression", data: l, depth: _, parts: v, original: E, loc: P };
}
t.preparePath = a;
function p(l, b, P, E, v, _) {
var y = E.charAt(3) || E.charAt(2), g = y !== "{" && y !== "&", L = /\*/.test(E);
return { type: L ? "Decorator" : "MustacheStatement", path: l, params: b, hash: P, escaped: g, strip: v, loc: this.locInfo(_) };
}
t.prepareMustache = p;
function n2(l, b, P, E) {
d(l, P), E = this.locInfo(E);
var v = { type: "Program", body: b, strip: {}, loc: E };
return { type: "BlockStatement", path: l.path, params: l.params, hash: l.hash, program: v, openStrip: {}, inverseStrip: {}, closeStrip: {}, loc: E };
}
t.prepareRawBlock = n2;
function s(l, b, P, E, v, _) {
E && E.path && d(l, E);
var y = /\*/.test(l.open);
b.blockParams = l.blockParams;
var g, L;
if (P) {
if (y)
throw new h.default("Unexpected inverse block on decorator", P);
P.chain && (P.program.body[0].closeStrip = E.strip), L = P.strip, g = P.program;
}
return v && (v = g, g = b, b = v), { type: y ? "DecoratorBlock" : "BlockStatement", path: l.path, params: l.params, hash: l.hash, program: b, inverse: g, openStrip: l.strip, inverseStrip: L, closeStrip: E && E.strip, loc: this.locInfo(_) };
}
t.prepareBlock = s;
function u(l, b) {
if (!b && l.length) {
var P = l[0].loc, E = l[l.length - 1].loc;
P && E && (b = { source: P.source, start: { line: P.start.line, column: P.start.column }, end: { line: E.end.line, column: E.end.column } });
}
return { type: "Program", body: l, strip: {}, loc: b };
}
t.prepareProgram = u;
function i(l, b, P, E) {
return d(l, P), { type: "PartialBlockStatement", name: l.path, params: l.params, hash: l.hash, program: b, openStrip: l.strip, closeStrip: P && P.strip, loc: this.locInfo(E) };
}
t.preparePartialBlock = i;
} }), Ft = I({ "node_modules/@handlebars/parser/dist/cjs/parse.js"(t) {
"use strict";
F();
var f = t && t.__createBinding || (Object.create ? function(u, i, l, b) {
b === void 0 && (b = l), Object.defineProperty(u, b, { enumerable: true, get: function() {
return i[l];
} });
} : function(u, i, l, b) {
b === void 0 && (b = l), u[b] = i[l];
}), h = t && t.__setModuleDefault || (Object.create ? function(u, i) {
Object.defineProperty(u, "default", { enumerable: true, value: i });
} : function(u, i) {
u.default = i;
}), d = t && t.__importStar || function(u) {
if (u && u.__esModule)
return u;
var i = {};
if (u != null)
for (var l in u)
l !== "default" && Object.prototype.hasOwnProperty.call(u, l) && f(i, u, l);
return h(i, u), i;
}, c = t && t.__importDefault || function(u) {
return u && u.__esModule ? u : { default: u };
};
Object.defineProperty(t, "__esModule", { value: true }), t.parse = t.parseWithoutProcessing = void 0;
var o = c(Ge()), e = c(ze()), r = d(Lt()), a = {};
for (p in r)
Object.prototype.hasOwnProperty.call(r, p) && (a[p] = r[p]);
var p;
function n2(u, i) {
if (u.type === "Program")
return u;
o.default.yy = a, o.default.yy.locInfo = function(b) {
return new r.SourceLocation(i && i.srcName, b);
};
var l = o.default.parse(u);
return l;
}
t.parseWithoutProcessing = n2;
function s(u, i) {
var l = n2(u, i), b = new e.default(i);
return b.accept(l);
}
t.parse = s;
} }), It = I({ "node_modules/@handlebars/parser/dist/cjs/index.js"(t) {
"use strict";
F();
var f = t && t.__importDefault || function(a) {
return a && a.__esModule ? a : { default: a };
};
Object.defineProperty(t, "__esModule", { value: true }), t.parseWithoutProcessing = t.parse = t.PrintVisitor = t.print = t.Exception = t.parser = t.WhitespaceControl = t.Visitor = void 0;
var h = Oe();
Object.defineProperty(t, "Visitor", { enumerable: true, get: function() {
return f(h).default;
} });
var d = ze();
Object.defineProperty(t, "WhitespaceControl", { enumerable: true, get: function() {
return f(d).default;
} });
var c = Ge();
Object.defineProperty(t, "parser", { enumerable: true, get: function() {
return f(c).default;
} });
var o = Be();
Object.defineProperty(t, "Exception", { enumerable: true, get: function() {
return f(o).default;
} });
var e = Nt();
Object.defineProperty(t, "print", { enumerable: true, get: function() {
return e.print;
} }), Object.defineProperty(t, "PrintVisitor", { enumerable: true, get: function() {
return e.PrintVisitor;
} });
var r = Ft();
Object.defineProperty(t, "parse", { enumerable: true, get: function() {
return r.parse;
} }), Object.defineProperty(t, "parseWithoutProcessing", { enumerable: true, get: function() {
return r.parseWithoutProcessing;
} });
} }), Ke = I({ "node_modules/simple-html-tokenizer/dist/simple-html-tokenizer.js"(t, f) {
F(), function(h, d) {
typeof t == "object" && typeof f < "u" ? d(t) : typeof define == "function" && define.amd ? define(["exports"], d) : d(h.HTML5Tokenizer = {});
}(t, function(h) {
"use strict";
var d = { Aacute: "\xC1", aacute: "\xE1", Abreve: "\u0102", abreve: "\u0103", ac: "\u223E", acd: "\u223F", acE: "\u223E\u0333", Acirc: "\xC2", acirc: "\xE2", acute: "\xB4", Acy: "\u0410", acy: "\u0430", AElig: "\xC6", aelig: "\xE6", af: "\u2061", Afr: "\u{1D504}", afr: "\u{1D51E}", Agrave: "\xC0", agrave: "\xE0", alefsym: "\u2135", aleph: "\u2135", Alpha: "\u0391", alpha: "\u03B1", Amacr: "\u0100", amacr: "\u0101", amalg: "\u2A3F", amp: "&", AMP: "&", andand: "\u2A55", And: "\u2A53", and: "\u2227", andd: "\u2A5C", andslope: "\u2A58", andv: "\u2A5A", ang: "\u2220", ange: "\u29A4", angle: "\u2220", angmsdaa: "\u29A8", angmsdab: "\u29A9", angmsdac: "\u29AA", angmsdad: "\u29AB", angmsdae: "\u29AC", angmsdaf: "\u29AD", angmsdag: "\u29AE", angmsdah: "\u29AF", angmsd: "\u2221", angrt: "\u221F", angrtvb: "\u22BE", angrtvbd: "\u299D", angsph: "\u2222", angst: "\xC5", angzarr: "\u237C", Aogon: "\u0104", aogon: "\u0105", Aopf: "\u{1D538}", aopf: "\u{1D552}", apacir: "\u2A6F", ap: "\u2248", apE: "\u2A70", ape: "\u224A", apid: "\u224B", apos: "'", ApplyFunction: "\u2061", approx: "\u2248", approxeq: "\u224A", Aring: "\xC5", aring: "\xE5", Ascr: "\u{1D49C}", ascr: "\u{1D4B6}", Assign: "\u2254", ast: "*", asymp: "\u2248", asympeq: "\u224D", Atilde: "\xC3", atilde: "\xE3", Auml: "\xC4", auml: "\xE4", awconint: "\u2233", awint: "\u2A11", backcong: "\u224C", backepsilon: "\u03F6", backprime: "\u2035", backsim: "\u223D", backsimeq: "\u22CD", Backslash: "\u2216", Barv: "\u2AE7", barvee: "\u22BD", barwed: "\u2305", Barwed: "\u2306", barwedge: "\u2305", bbrk: "\u23B5", bbrktbrk: "\u23B6", bcong: "\u224C", Bcy: "\u0411", bcy: "\u0431", bdquo: "\u201E", becaus: "\u2235", because: "\u2235", Because: "\u2235", bemptyv: "\u29B0", bepsi: "\u03F6", bernou: "\u212C", Bernoullis: "\u212C", Beta: "\u0392", beta: "\u03B2", beth: "\u2136", between: "\u226C", Bfr: "\u{1D505}", bfr: "\u{1D51F}", bigcap: "\u22C2", bigcirc: "\u25EF", bigcup: "\u22C3", bigodot: "\u2A00", bigoplus: "\u2A01", bigotimes: "\u2A02", bigsqcup: "\u2A06", bigstar: "\u2605", bigtriangledown: "\u25BD", bigtriangleup: "\u25B3", biguplus: "\u2A04", bigvee: "\u22C1", bigwedge: "\u22C0", bkarow: "\u290D", blacklozenge: "\u29EB", blacksquare: "\u25AA", blacktriangle: "\u25B4", blacktriangledown: "\u25BE", blacktriangleleft: "\u25C2", blacktriangleright: "\u25B8", blank: "\u2423", blk12: "\u2592", blk14: "\u2591", blk34: "\u2593", block: "\u2588", bne: "=\u20E5", bnequiv: "\u2261\u20E5", bNot: "\u2AED", bnot: "\u2310", Bopf: "\u{1D539}", bopf: "\u{1D553}", bot: "\u22A5", bottom: "\u22A5", bowtie: "\u22C8", boxbox: "\u29C9", boxdl: "\u2510", boxdL: "\u2555", boxDl: "\u2556", boxDL: "\u2557", boxdr: "\u250C", boxdR: "\u2552", boxDr: "\u2553", boxDR: "\u2554", boxh: "\u2500", boxH: "\u2550", boxhd: "\u252C", boxHd: "\u2564", boxhD: "\u2565", boxHD: "\u2566", boxhu: "\u2534", boxHu: "\u2567", boxhU: "\u2568", boxHU: "\u2569", boxminus: "\u229F", boxplus: "\u229E", boxtimes: "\u22A0", boxul: "\u2518", boxuL: "\u255B", boxUl: "\u255C", boxUL: "\u255D", boxur: "\u2514", boxuR: "\u2558", boxUr: "\u2559", boxUR: "\u255A", boxv: "\u2502", boxV: "\u2551", boxvh: "\u253C", boxvH: "\u256A", boxVh: "\u256B", boxVH: "\u256C", boxvl: "\u2524", boxvL: "\u2561", boxVl: "\u2562", boxVL: "\u2563", boxvr: "\u251C", boxvR: "\u255E", boxVr: "\u255F", boxVR: "\u2560", bprime: "\u2035", breve: "\u02D8", Breve: "\u02D8", brvbar: "\xA6", bscr: "\u{1D4B7}", Bscr: "\u212C", bsemi: "\u204F", bsim: "\u223D", bsime: "\u22CD", bsolb: "\u29C5", bsol: "\\", bsolhsub: "\u27C8", bull: "\u2022", bullet: "\u2022", bump: "\u224E", bumpE: "\u2AAE", bumpe: "\u224F", Bumpeq: "\u224E", bumpeq: "\u224F", Cacute: "\u0106", cacute: "\u0107", capand: "\u2A44", capbrcup: "\u2A49", capcap: "\u2A4B", cap: "\u2229", Cap: "\u22D2", capcup: "\u2A47", capdot: "\u2A40", CapitalDifferentialD: "\u2145", caps: "\u2229\uFE00", caret: "\u2041", caron: "\u02C7", Cayleys: "\u212D", ccaps: "\u2A4D", Ccaron: "\u010C", ccaron: "\u010D", Ccedil: "\xC7", ccedil: "\xE7", Ccirc: "\u0108", ccirc: "\u0109", Cconint: "\u2230", ccups: "\u2A4C", ccupssm: "\u2A50", Cdot: "\u010A", cdot: "\u010B", cedil: "\xB8", Cedilla: "\xB8", cemptyv: "\u29B2", cent: "\xA2", centerdot: "\xB7", CenterDot: "\xB7", cfr: "\u{1D520}", Cfr: "\u212D", CHcy: "\u0427", chcy: "\u0447", check: "\u2713", checkmark: "\u2713", Chi: "\u03A7", chi: "\u03C7", circ: "\u02C6", circeq: "\u2257", circlearrowleft: "\u21BA", circlearrowright: "\u21BB", circledast: "\u229B", circledcirc: "\u229A", circleddash: "\u229D", CircleDot: "\u2299", circledR: "\xAE", circledS: "\u24C8", CircleMinus: "\u2296", CirclePlus: "\u2295", CircleTimes: "\u2297", cir: "\u25CB", cirE: "\u29C3", cire: "\u2257", cirfnint: "\u2A10", cirmid: "\u2AEF", cirscir: "\u29C2", ClockwiseContourIntegral: "\u2232", CloseCurlyDoubleQuote: "\u201D", CloseCurlyQuote: "\u2019", clubs: "\u2663", clubsuit: "\u2663", colon: ":", Colon: "\u2237", Colone: "\u2A74", colone: "\u2254", coloneq: "\u2254", comma: ",", commat: "@", comp: "\u2201", compfn: "\u2218", complement: "\u2201", complexes: "\u2102", cong: "\u2245", congdot: "\u2A6D", Congruent: "\u2261", conint: "\u222E", Conint: "\u222F", ContourIntegral: "\u222E", copf: "\u{1D554}", Copf: "\u2102", coprod: "\u2210", Coproduct: "\u2210", copy: "\xA9", COPY: "\xA9", copysr: "\u2117", CounterClockwiseContourIntegral: "\u2233", crarr: "\u21B5", cross: "\u2717", Cross: "\u2A2F", Cscr: "\u{1D49E}", cscr: "\u{1D4B8}", csub: "\u2ACF", csube: "\u2AD1", csup: "\u2AD0", csupe: "\u2AD2", ctdot: "\u22EF", cudarrl: "\u2938", cudarrr: "\u2935", cuepr: "\u22DE", cuesc: "\u22DF", cularr: "\u21B6", cularrp: "\u293D", cupbrcap: "\u2A48", cupcap: "\u2A46", CupCap: "\u224D", cup: "\u222A", Cup: "\u22D3", cupcup: "\u2A4A", cupdot: "\u228D", cupor: "\u2A45", cups: "\u222A\uFE00", curarr: "\u21B7", curarrm: "\u293C", curlyeqprec: "\u22DE", curlyeqsucc: "\u22DF", curlyvee: "\u22CE", curlywedge: "\u22CF", curren: "\xA4", curvearrowleft: "\u21B6", curvearrowright: "\u21B7", cuvee: "\u22CE", cuwed: "\u22CF", cwconint: "\u2232", cwint: "\u2231", cylcty: "\u232D", dagger: "\u2020", Dagger: "\u2021", daleth: "\u2138", darr: "\u2193", Darr: "\u21A1", dArr: "\u21D3", dash: "\u2010", Dashv: "\u2AE4", dashv: "\u22A3", dbkarow: "\u290F", dblac: "\u02DD", Dcaron: "\u010E", dcaron: "\u010F", Dcy: "\u0414", dcy: "\u0434", ddagger: "\u2021", ddarr: "\u21CA", DD: "\u2145", dd: "\u2146", DDotrahd: "\u2911", ddotseq: "\u2A77", deg: "\xB0", Del: "\u2207", Delta: "\u0394", delta: "\u03B4", demptyv: "\u29B1", dfisht: "\u297F", Dfr: "\u{1D507}", dfr: "\u{1D521}", dHar: "\u2965", dharl: "\u21C3", dharr: "\u21C2", DiacriticalAcute: "\xB4", DiacriticalDot: "\u02D9", DiacriticalDoubleAcute: "\u02DD", DiacriticalGrave: "`", DiacriticalTilde: "\u02DC", diam: "\u22C4", diamond: "\u22C4", Diamond: "\u22C4", diamondsuit: "\u2666", diams: "\u2666", die: "\xA8", DifferentialD: "\u2146", digamma: "\u03DD", disin: "\u22F2", div: "\xF7", divide: "\xF7", divideontimes: "\u22C7", divonx: "\u22C7", DJcy: "\u0402", djcy: "\u0452", dlcorn: "\u231E", dlcrop: "\u230D", dollar: "$", Dopf: "\u{1D53B}", dopf: "\u{1D555}", Dot: "\xA8", dot: "\u02D9", DotDot: "\u20DC", doteq: "\u2250", doteqdot: "\u2251", DotEqual: "\u2250", dotminus: "\u2238", dotplus: "\u2214", dotsquare: "\u22A1", doublebarwedge: "\u2306", DoubleContourIntegral: "\u222F", DoubleDot: "\xA8", DoubleDownArrow: "\u21D3", DoubleLeftArrow: "\u21D0", DoubleLeftRightArrow: "\u21D4", DoubleLeftTee: "\u2AE4", DoubleLongLeftArrow: "\u27F8", DoubleLongLeftRightArrow: "\u27FA", DoubleLongRightArrow: "\u27F9", DoubleRightArrow: "\u21D2", DoubleRightTee: "\u22A8", DoubleUpArrow: "\u21D1", DoubleUpDownArrow: "\u21D5", DoubleVerticalBar: "\u2225", DownArrowBar: "\u2913", downarrow: "\u2193", DownArrow: "\u2193", Downarrow: "\u21D3", DownArrowUpArrow: "\u21F5", DownBreve: "\u0311", downdownarrows: "\u21CA", downharpoonleft: "\u21C3", downharpoonright: "\u21C2", DownLeftRightVector: "\u2950", DownLeftTeeVector: "\u295E", DownLeftVectorBar: "\u2956", DownLeftVector: "\u21BD", DownRightTeeVector: "\u295F", DownRightVectorBar: "\u2957", DownRightVector: "\u21C1", DownTeeArrow: "\u21A7", DownTee: "\u22A4", drbkarow: "\u2910", drcorn: "\u231F", drcrop: "\u230C", Dscr: "\u{1D49F}", dscr: "\u{1D4B9}", DScy: "\u0405", dscy: "\u0455", dsol: "\u29F6", Dstrok: "\u0110", dstrok: "\u0111", dtdot: "\u22F1", dtri: "\u25BF", dtrif: "\u25BE", duarr: "\u21F5", duhar: "\u296F", dwangle: "\u29A6", DZcy: "\u040F", dzcy: "\u045F", dzigrarr: "\u27FF", Eacute: "\xC9", eacute: "\xE9", easter: "\u2A6E", Ecaron: "\u011A", ecaron: "\u011B", Ecirc: "\xCA", ecirc: "\xEA", ecir: "\u2256", ecolon: "\u2255", Ecy: "\u042D", ecy: "\u044D", eDDot: "\u2A77", Edot: "\u0116", edot: "\u0117", eDot: "\u2251", ee: "\u2147", efDot: "\u2252", Efr: "\u{1D508}", efr: "\u{1D522}", eg: "\u2A9A", Egrave: "\xC8", egrave: "\xE8", egs: "\u2A96", egsdot: "\u2A98", el: "\u2A99", Element: "\u2208", elinters: "\u23E7", ell: "\u2113", els: "\u2A95", elsdot: "\u2A97", Emacr: "\u0112", emacr: "\u0113", empty: "\u2205", emptyset: "\u2205", EmptySmallSquare: "\u25FB", emptyv: "\u2205", EmptyVerySmallSquare: "\u25AB", emsp13: "\u2004", emsp14: "\u2005", emsp: "\u2003", ENG: "\u014A", eng: "\u014B", ensp: "\u2002", Eogon: "\u0118", eogon: "\u0119", Eopf: "\u{1D53C}", eopf: "\u{1D556}", epar: "\u22D5", eparsl: "\u29E3", eplus: "\u2A71", epsi: "\u03B5", Epsilon: "\u0395", epsilon: "\u03B5", epsiv: "\u03F5", eqcirc: "\u2256", eqcolon: "\u2255", eqsim: "\u2242", eqslantgtr: "\u2A96", eqslantless: "\u2A95", Equal: "\u2A75", equals: "=", EqualTilde: "\u2242", equest: "\u225F", Equilibrium: "\u21CC", equiv: "\u2261", equivDD: "\u2A78", eqvparsl: "\u29E5", erarr: "\u2971", erDot: "\u2253", escr: "\u212F", Escr: "\u2130", esdot: "\u2250", Esim: "\u2A73", esim: "\u2242", Eta: "\u0397", eta: "\u03B7", ETH: "\xD0", eth: "\xF0", Euml: "\xCB", euml: "\xEB", euro: "\u20AC", excl: "!", exist: "\u2203", Exists: "\u2203", expectation: "\u2130", exponentiale: "\u2147", ExponentialE: "\u2147", fallingdotseq: "\u2252", Fcy: "\u0424", fcy: "\u0444", female: "\u2640", ffilig: "\uFB03", fflig: "\uFB00", ffllig: "\uFB04", Ffr: "\u{1D509}", ffr: "\u{1D523}", filig: "\uFB01", FilledSmallSquare: "\u25FC", FilledVerySmallSquare: "\u25AA", fjlig: "fj", flat: "\u266D", fllig: "\uFB02", fltns: "\u25B1", fnof: "\u0192", Fopf: "\u{1D53D}", fopf: "\u{1D557}", forall: "\u2200", ForAll: "\u2200", fork: "\u22D4", forkv: "\u2AD9", Fouriertrf: "\u2131", fpartint: "\u2A0D", frac12: "\xBD", frac13: "\u2153", frac14: "\xBC", frac15: "\u2155", frac16: "\u2159", frac18: "\u215B", frac23: "\u2154", frac25: "\u2156", frac34: "\xBE", frac35: "\u2157", frac38: "\u215C", frac45: "\u2158", frac56: "\u215A", frac58: "\u215D", frac78: "\u215E", frasl: "\u2044", frown: "\u2322", fscr: "\u{1D4BB}", Fscr: "\u2131", gacute: "\u01F5", Gamma: "\u0393", gamma: "\u03B3", Gammad: "\u03DC", gammad: "\u03DD", gap: "\u2A86", Gbreve: "\u011E", gbreve: "\u011F", Gcedil: "\u0122", Gcirc: "\u011C", gcirc: "\u011D", Gcy: "\u0413", gcy: "\u0433", Gdot: "\u0120", gdot: "\u0121", ge: "\u2265", gE: "\u2267", gEl: "\u2A8C", gel: "\u22DB", geq: "\u2265", geqq: "\u2267", geqslant: "\u2A7E", gescc: "\u2AA9", ges: "\u2A7E", gesdot: "\u2A80", gesdoto: "\u2A82", gesdotol: "\u2A84", gesl: "\u22DB\uFE00", gesles: "\u2A94", Gfr: "\u{1D50A}", gfr: "\u{1D524}", gg: "\u226B", Gg: "\u22D9", ggg: "\u22D9", gimel: "\u2137", GJcy: "\u0403", gjcy: "\u0453", gla: "\u2AA5", gl: "\u2277", glE: "\u2A92", glj: "\u2AA4", gnap: "\u2A8A", gnapprox: "\u2A8A", gne: "\u2A88", gnE: "\u2269", gneq: "\u2A88", gneqq: "\u2269", gnsim: "\u22E7", Gopf: "\u{1D53E}", gopf: "\u{1D558}", grave: "`", GreaterEqual: "\u2265", GreaterEqualLess: "\u22DB", GreaterFullEqual: "\u2267", GreaterGreater: "\u2AA2", GreaterLess: "\u2277", GreaterSlantEqual: "\u2A7E", GreaterTilde: "\u2273", Gscr: "\u{1D4A2}", gscr: "\u210A", gsim: "\u2273", gsime: "\u2A8E", gsiml: "\u2A90", gtcc: "\u2AA7", gtcir: "\u2A7A", gt: ">", GT: ">", Gt: "\u226B", gtdot: "\u22D7", gtlPar: "\u2995", gtquest: "\u2A7C", gtrapprox: "\u2A86", gtrarr: "\u2978", gtrdot: "\u22D7", gtreqless: "\u22DB", gtreqqless: "\u2A8C", gtrless: "\u2277", gtrsim: "\u2273", gvertneqq: "\u2269\uFE00", gvnE: "\u2269\uFE00", Hacek: "\u02C7", hairsp: "\u200A", half: "\xBD", hamilt: "\u210B", HARDcy: "\u042A", hardcy: "\u044A", harrcir: "\u2948", harr: "\u2194", hArr: "\u21D4", harrw: "\u21AD", Hat: "^", hbar: "\u210F", Hcirc: "\u0124", hcirc: "\u0125", hearts: "\u2665", heartsuit: "\u2665", hellip: "\u2026", hercon: "\u22B9", hfr: "\u{1D525}", Hfr: "\u210C", HilbertSpace: "\u210B", hksearow: "\u2925", hkswarow: "\u2926", hoarr: "\u21FF", homtht: "\u223B", hookleftarrow: "\u21A9", hookrightarrow: "\u21AA", hopf: "\u{1D559}", Hopf: "\u210D", horbar: "\u2015", HorizontalLine: "\u2500", hscr: "\u{1D4BD}", Hscr: "\u210B", hslash: "\u210F", Hstrok: "\u0126", hstrok: "\u0127", HumpDownHump: "\u224E", HumpEqual: "\u224F", hybull: "\u2043", hyphen: "\u2010", Iacute: "\xCD", iacute: "\xED", ic: "\u2063", Icirc: "\xCE", icirc: "\xEE", Icy: "\u0418", icy: "\u0438", Idot: "\u0130", IEcy: "\u0415", iecy: "\u0435", iexcl: "\xA1", iff: "\u21D4", ifr: "\u{1D526}", Ifr: "\u2111", Igrave: "\xCC", igrave: "\xEC", ii: "\u2148", iiiint: "\u2A0C", iiint: "\u222D", iinfin: "\u29DC", iiota: "\u2129", IJlig: "\u0132", ijlig: "\u0133", Imacr: "\u012A", imacr: "\u012B", image: "\u2111", ImaginaryI: "\u2148", imagline: "\u2110", imagpart: "\u2111", imath: "\u0131", Im: "\u2111", imof: "\u22B7", imped: "\u01B5", Implies: "\u21D2", incare: "\u2105", in: "\u2208", infin: "\u221E", infintie: "\u29DD", inodot: "\u0131", intcal: "\u22BA", int: "\u222B", Int: "\u222C", integers: "\u2124", Integral: "\u222B", intercal: "\u22BA", Intersection: "\u22C2", intlarhk: "\u2A17", intprod: "\u2A3C", InvisibleComma: "\u2063", InvisibleTimes: "\u2062", IOcy: "\u0401", iocy: "\u0451", Iogon: "\u012E", iogon: "\u012F", Iopf: "\u{1D540}", iopf: "\u{1D55A}", Iota: "\u0399", iota: "\u03B9", iprod: "\u2A3C", iquest: "\xBF", iscr: "\u{1D4BE}", Iscr: "\u2110", isin: "\u2208", isindot: "\u22F5", isinE: "\u22F9", isins: "\u22F4", isinsv: "\u22F3", isinv: "\u2208", it: "\u2062", Itilde: "\u0128", itilde: "\u0129", Iukcy: "\u0406", iukcy: "\u0456", Iuml: "\xCF", iuml: "\xEF", Jcirc: "\u0134", jcirc: "\u0135", Jcy: "\u0419", jcy: "\u0439", Jfr: "\u{1D50D}", jfr: "\u{1D527}", jmath: "\u0237", Jopf: "\u{1D541}", jopf: "\u{1D55B}", Jscr: "\u{1D4A5}", jscr: "\u{1D4BF}", Jsercy: "\u0408", jsercy: "\u0458", Jukcy: "\u0404", jukcy: "\u0454", Kappa: "\u039A", kappa: "\u03BA", kappav: "\u03F0", Kcedil: "\u0136", kcedil: "\u0137", Kcy: "\u041A", kcy: "\u043A", Kfr: "\u{1D50E}", kfr: "\u{1D528}", kgreen: "\u0138", KHcy: "\u0425", khcy: "\u0445", KJcy: "\u040C", kjcy: "\u045C", Kopf: "\u{1D542}", kopf: "\u{1D55C}", Kscr: "\u{1D4A6}", kscr: "\u{1D4C0}", lAarr: "\u21DA", Lacute: "\u0139", lacute: "\u013A", laemptyv: "\u29B4", lagran: "\u2112", Lambda: "\u039B", lambda: "\u03BB", lang: "\u27E8", Lang: "\u27EA", langd: "\u2991", langle: "\u27E8", lap: "\u2A85", Laplacetrf: "\u2112", laquo: "\xAB", larrb: "\u21E4", larrbfs: "\u291F", larr: "\u2190", Larr: "\u219E", lArr: "\u21D0", larrfs: "\u291D", larrhk: "\u21A9", larrlp: "\u21AB", larrpl: "\u2939", larrsim: "\u2973", larrtl: "\u21A2", latail: "\u2919", lAtail: "\u291B", lat: "\u2AAB", late: "\u2AAD", lates: "\u2AAD\uFE00", lbarr: "\u290C", lBarr: "\u290E", lbbrk: "\u2772", lbrace: "{", lbrack: "[", lbrke: "\u298B", lbrksld: "\u298F", lbrkslu: "\u298D", Lcaron: "\u013D", lcaron: "\u013E", Lcedil: "\u013B", lcedil: "\u013C", lceil: "\u2308", lcub: "{", Lcy: "\u041B", lcy: "\u043B", ldca: "\u2936", ldquo: "\u201C", ldquor: "\u201E", ldrdhar: "\u2967", ldrushar: "\u294B", ldsh: "\u21B2", le: "\u2264", lE: "\u2266", LeftAngleBracket: "\u27E8", LeftArrowBar: "\u21E4", leftarrow: "\u2190", LeftArrow: "\u2190", Leftarrow: "\u21D0", LeftArrowRightArrow: "\u21C6", leftarrowtail: "\u21A2", LeftCeiling: "\u2308", LeftDoubleBracket: "\u27E6", LeftDownTeeVector: "\u2961", LeftDownVectorBar: "\u2959", LeftDownVector: "\u21C3", LeftFloor: "\u230A", leftharpoondown: "\u21BD", leftharpoonup: "\u21BC", leftleftarrows: "\u21C7", leftrightarrow: "\u2194", LeftRightArrow: "\u2194", Leftrightarrow: "\u21D4", leftrightarrows: "\u21C6", leftrightharpoons: "\u21CB", leftrightsquigarrow: "\u21AD", LeftRightVector: "\u294E", LeftTeeArrow: "\u21A4", LeftTee: "\u22A3", LeftTeeVector: "\u295A", leftthreetimes: "\u22CB", LeftTriangleBar: "\u29CF", LeftTriangle: "\u22B2", LeftTriangleEqual: "\u22B4", LeftUpDownVector: "\u2951", LeftUpTeeVector: "\u2960", LeftUpVectorBar: "\u2958", LeftUpVector: "\u21BF", LeftVectorBar: "\u2952", LeftVector: "\u21BC", lEg: "\u2A8B", leg: "\u22DA", leq: "\u2264", leqq: "\u2266", leqslant: "\u2A7D", lescc: "\u2AA8", les: "\u2A7D", lesdot: "\u2A7F", lesdoto: "\u2A81", lesdotor: "\u2A83", lesg: "\u22DA\uFE00", lesges: "\u2A93", lessapprox: "\u2A85", lessdot: "\u22D6", lesseqgtr: "\u22DA", lesseqqgtr: "\u2A8B", LessEqualGreater: "\u22DA", LessFullEqual: "\u2266", LessGreater: "\u2276", lessgtr: "\u2276", LessLess: "\u2AA1", lesssim: "\u2272", LessSlantEqual: "\u2A7D", LessTilde: "\u2272", lfisht: "\u297C", lfloor: "\u230A", Lfr: "\u{1D50F}", lfr: "\u{1D529}", lg: "\u2276", lgE: "\u2A91", lHar: "\u2962", lhard: "\u21BD", lharu: "\u21BC", lharul: "\u296A", lhblk: "\u2584", LJcy: "\u0409", ljcy: "\u0459", llarr: "\u21C7", ll: "\u226A", Ll: "\u22D8", llcorner: "\u231E", Lleftarrow: "\u21DA", llhard: "\u296B", lltri: "\u25FA", Lmidot: "\u013F", lmidot: "\u0140", lmoustache: "\u23B0", lmoust: "\u23B0", lnap: "\u2A89", lnapprox: "\u2A89", lne: "\u2A87", lnE: "\u2268", lneq: "\u2A87", lneqq: "\u2268", lnsim: "\u22E6", loang: "\u27EC", loarr: "\u21FD", lobrk: "\u27E6", longleftarrow: "\u27F5", LongLeftArrow: "\u27F5", Longleftarrow: "\u27F8", longleftrightarrow: "\u27F7", LongLeftRightArrow: "\u27F7", Longleftrightarrow: "\u27FA", longmapsto: "\u27FC", longrightarrow: "\u27F6", LongRightArrow: "\u27F6", Longrightarrow: "\u27F9", looparrowleft: "\u21AB", looparrowright: "\u21AC", lopar: "\u2985", Lopf: "\u{1D543}", lopf: "\u{1D55D}", loplus: "\u2A2D", lotimes: "\u2A34", lowast: "\u2217", lowbar: "_", LowerLeftArrow: "\u2199", LowerRightArrow: "\u2198", loz: "\u25CA", lozenge: "\u25CA", lozf: "\u29EB", lpar: "(", lparlt: "\u2993", lrarr: "\u21C6", lrcorner: "\u231F", lrhar: "\u21CB", lrhard: "\u296D", lrm: "\u200E", lrtri: "\u22BF", lsaquo: "\u2039", lscr: "\u{1D4C1}", Lscr: "\u2112", lsh: "\u21B0", Lsh: "\u21B0", lsim: "\u2272", lsime: "\u2A8D", lsimg: "\u2A8F", lsqb: "[", lsquo: "\u2018", lsquor: "\u201A", Lstrok: "\u0141", lstrok: "\u0142", ltcc: "\u2AA6", ltcir: "\u2A79", lt: "<", LT: "<", Lt: "\u226A", ltdot: "\u22D6", lthree: "\u22CB", ltimes: "\u22C9", ltlarr: "\u2976", ltquest: "\u2A7B", ltri: "\u25C3", ltrie: "\u22B4", ltrif: "\u25C2", ltrPar: "\u2996", lurdshar: "\u294A", luruhar: "\u2966", lvertneqq: "\u2268\uFE00", lvnE: "\u2268\uFE00", macr: "\xAF", male: "\u2642", malt: "\u2720", maltese: "\u2720", Map: "\u2905", map: "\u21A6", mapsto: "\u21A6", mapstodown: "\u21A7", mapstoleft: "\u21A4", mapstoup: "\u21A5", marker: "\u25AE", mcomma: "\u2A29", Mcy: "\u041C", mcy: "\u043C", mdash: "\u2014", mDDot: "\u223A", measuredangle: "\u2221", MediumSpace: "\u205F", Mellintrf: "\u2133", Mfr: "\u{1D510}", mfr: "\u{1D52A}", mho: "\u2127", micro: "\xB5", midast: "*", midcir: "\u2AF0", mid: "\u2223", middot: "\xB7", minusb: "\u229F", minus: "\u2212", minusd: "\u2238", minusdu: "\u2A2A", MinusPlus: "\u2213", mlcp: "\u2ADB", mldr: "\u2026", mnplus: "\u2213", models: "\u22A7", Mopf: "\u{1D544}", mopf: "\u{1D55E}", mp: "\u2213", mscr: "\u{1D4C2}", Mscr: "\u2133", mstpos: "\u223E", Mu: "\u039C", mu: "\u03BC", multimap: "\u22B8", mumap: "\u22B8", nabla: "\u2207", Nacute: "\u0143", nacute: "\u0144", nang: "\u2220\u20D2", nap: "\u2249", napE: "\u2A70\u0338", napid: "\u224B\u0338", napos: "\u0149", napprox: "\u2249", natural: "\u266E", naturals: "\u2115", natur: "\u266E", nbsp: "\xA0", nbump: "\u224E\u0338", nbumpe: "\u224F\u0338", ncap: "\u2A43", Ncaron: "\u0147", ncaron: "\u0148", Ncedil: "\u0145", ncedil: "\u0146", ncong: "\u2247", ncongdot: "\u2A6D\u0338", ncup: "\u2A42", Ncy: "\u041D", ncy: "\u043D", ndash: "\u2013", nearhk: "\u2924", nearr: "\u2197", neArr: "\u21D7", nearrow: "\u2197", ne: "\u2260", nedot: "\u2250\u0338", NegativeMediumSpace: "\u200B", NegativeThickSpace: "\u200B", NegativeThinSpace: "\u200B", NegativeVeryThinSpace: "\u200B", nequiv: "\u2262", nesear: "\u2928", nesim: "\u2242\u0338", NestedGreaterGreater: "\u226B", NestedLessLess: "\u226A", NewLine: `
`, nexist: "\u2204", nexists: "\u2204", Nfr: "\u{1D511}", nfr: "\u{1D52B}", ngE: "\u2267\u0338", nge: "\u2271", ngeq: "\u2271", ngeqq: "\u2267\u0338", ngeqslant: "\u2A7E\u0338", nges: "\u2A7E\u0338", nGg: "\u22D9\u0338", ngsim: "\u2275", nGt: "\u226B\u20D2", ngt: "\u226F", ngtr: "\u226F", nGtv: "\u226B\u0338", nharr: "\u21AE", nhArr: "\u21CE", nhpar: "\u2AF2", ni: "\u220B", nis: "\u22FC", nisd: "\u22FA", niv: "\u220B", NJcy: "\u040A", njcy: "\u045A", nlarr: "\u219A", nlArr: "\u21CD", nldr: "\u2025", nlE: "\u2266\u0338", nle: "\u2270", nleftarrow: "\u219A", nLeftarrow: "\u21CD", nleftrightarrow: "\u21AE", nLeftrightarrow: "\u21CE", nleq: "\u2270", nleqq: "\u2266\u0338", nleqslant: "\u2A7D\u0338", nles: "\u2A7D\u0338", nless: "\u226E", nLl: "\u22D8\u0338", nlsim: "\u2274", nLt: "\u226A\u20D2", nlt: "\u226E", nltri: "\u22EA", nltrie: "\u22EC", nLtv: "\u226A\u0338", nmid: "\u2224", NoBreak: "\u2060", NonBreakingSpace: "\xA0", nopf: "\u{1D55F}", Nopf: "\u2115", Not: "\u2AEC", not: "\xAC", NotCongruent: "\u2262", NotCupCap: "\u226D", NotDoubleVerticalBar: "\u2226", NotElement: "\u2209", NotEqual: "\u2260", NotEqualTilde: "\u2242\u0338", NotExists: "\u2204", NotGreater: "\u226F", NotGreaterEqual: "\u2271", NotGreaterFullEqual: "\u2267\u0338", NotGreaterGreater: "\u226B\u0338", NotGreaterLess: "\u2279", NotGreaterSlantEqual: "\u2A7E\u0338", NotGreaterTilde: "\u2275", NotHumpDownHump: "\u224E\u0338", NotHumpEqual: "\u224F\u0338", notin: "\u2209", notindot: "\u22F5\u0338", notinE: "\u22F9\u0338", notinva: "\u2209", notinvb: "\u22F7", notinvc: "\u22F6", NotLeftTriangleBar: "\u29CF\u0338", NotLeftTriangle: "\u22EA", NotLeftTriangleEqual: "\u22EC", NotLess: "\u226E", NotLessEqual: "\u2270", NotLessGreater: "\u2278", NotLessLess: "\u226A\u0338", NotLessSlantEqual: "\u2A7D\u0338", NotLessTilde: "\u2274", NotNestedGreaterGreater: "\u2AA2\u0338", NotNestedLessLess: "\u2AA1\u0338", notni: "\u220C", notniva: "\u220C", notnivb: "\u22FE", notnivc: "\u22FD", NotPrecedes: "\u2280", NotPrecedesEqual: "\u2AAF\u0338", NotPrecedesSlantEqual: "\u22E0", NotReverseElement: "\u220C", NotRightTriangleBar: "\u29D0\u0338", NotRightTriangle: "\u22EB", NotRightTriangleEqual: "\u22ED", NotSquareSubset: "\u228F\u0338", NotSquareSubsetEqual: "\u22E2", NotSquareSuperset: "\u2290\u0338", NotSquareSupersetEqual: "\u22E3", NotSubset: "\u2282\u20D2", NotSubsetEqual: "\u2288", NotSucceeds: "\u2281", NotSucceedsEqual: "\u2AB0\u0338", NotSucceedsSlantEqual: "\u22E1", NotSucceedsTilde: "\u227F\u0338", NotSuperset: "\u2283\u20D2", NotSupersetEqual: "\u2289", NotTilde: "\u2241", NotTildeEqual: "\u2244", NotTildeFullEqual: "\u2247", NotTildeTilde: "\u2249", NotVerticalBar: "\u2224", nparallel: "\u2226", npar: "\u2226", nparsl: "\u2AFD\u20E5", npart: "\u2202\u0338", npolint: "\u2A14", npr: "\u2280", nprcue: "\u22E0", nprec: "\u2280", npreceq: "\u2AAF\u0338", npre: "\u2AAF\u0338", nrarrc: "\u2933\u0338", nrarr: "\u219B", nrArr: "\u21CF", nrarrw: "\u219D\u0338", nrightarrow: "\u219B", nRightarrow: "\u21CF", nrtri: "\u22EB", nrtrie: "\u22ED", nsc: "\u2281", nsccue: "\u22E1", nsce: "\u2AB0\u0338", Nscr: "\u{1D4A9}", nscr: "\u{1D4C3}", nshortmid: "\u2224", nshortparallel: "\u2226", nsim: "\u2241", nsime: "\u2244", nsimeq: "\u2244", nsmid: "\u2224", nspar: "\u2226", nsqsube: "\u22E2", nsqsupe: "\u22E3", nsub: "\u2284", nsubE: "\u2AC5\u0338", nsube: "\u2288", nsubset: "\u2282\u20D2", nsubseteq: "\u2288", nsubseteqq: "\u2AC5\u0338", nsucc: "\u2281", nsucceq: "\u2AB0\u0338", nsup: "\u2285", nsupE: "\u2AC6\u0338", nsupe: "\u2289", nsupset: "\u2283\u20D2", nsupseteq: "\u2289", nsupseteqq: "\u2AC6\u0338", ntgl: "\u2279", Ntilde: "\xD1", ntilde: "\xF1", ntlg: "\u2278", ntriangleleft: "\u22EA", ntrianglelefteq: "\u22EC", ntriangleright: "\u22EB", ntrianglerighteq: "\u22ED", Nu: "\u039D", nu: "\u03BD", num: "#", numero: "\u2116", numsp: "\u2007", nvap: "\u224D\u20D2", nvdash: "\u22AC", nvDash: "\u22AD", nVdash: "\u22AE", nVDash: "\u22AF", nvge: "\u2265\u20D2", nvgt: ">\u20D2", nvHarr: "\u2904", nvinfin: "\u29DE", nvlArr: "\u2902", nvle: "\u2264\u20D2", nvlt: "<\u20D2", nvltrie: "\u22B4\u20D2", nvrArr: "\u2903", nvrtrie: "\u22B5\u20D2", nvsim: "\u223C\u20D2", nwarhk: "\u2923", nwarr: "\u2196", nwArr: "\u21D6", nwarrow: "\u2196", nwnear: "\u2927", Oacute: "\xD3", oacute: "\xF3", oast: "\u229B", Ocirc: "\xD4", ocirc: "\xF4", ocir: "\u229A", Ocy: "\u041E", ocy: "\u043E", odash: "\u229D", Odblac: "\u0150", odblac: "\u0151", odiv: "\u2A38", odot: "\u2299", odsold: "\u29BC", OElig: "\u0152", oelig: "\u0153", ofcir: "\u29BF", Ofr: "\u{1D512}", ofr: "\u{1D52C}", ogon: "\u02DB", Ograve: "\xD2", ograve: "\xF2", ogt: "\u29C1", ohbar: "\u29B5", ohm: "\u03A9", oint: "\u222E", olarr: "\u21BA", olcir: "\u29BE", olcross: "\u29BB", oline: "\u203E", olt: "\u29C0", Omacr: "\u014C", omacr: "\u014D", Omega: "\u03A9", omega: "\u03C9", Omicron: "\u039F", omicron: "\u03BF", omid: "\u29B6", ominus: "\u2296", Oopf: "\u{1D546}", oopf: "\u{1D560}", opar: "\u29B7", OpenCurlyDoubleQuote: "\u201C", OpenCurlyQuote: "\u2018", operp: "\u29B9", oplus: "\u2295", orarr: "\u21BB", Or: "\u2A54", or: "\u2228", ord: "\u2A5D", order: "\u2134", orderof: "\u2134", ordf: "\xAA", ordm: "\xBA", origof: "\u22B6", oror: "\u2A56", orslope: "\u2A57", orv: "\u2A5B", oS: "\u24C8", Oscr: "\u{1D4AA}", oscr: "\u2134", Oslash: "\xD8", oslash: "\xF8", osol: "\u2298", Otilde: "\xD5", otilde: "\xF5", otimesas: "\u2A36", Otimes: "\u2A37", otimes: "\u2297", Ouml: "\xD6", ouml: "\xF6", ovbar: "\u233D", OverBar: "\u203E", OverBrace: "\u23DE", OverBracket: "\u23B4", OverParenthesis: "\u23DC", para: "\xB6", parallel: "\u2225", par: "\u2225", parsim: "\u2AF3", parsl: "\u2AFD", part: "\u2202", PartialD: "\u2202", Pcy: "\u041F", pcy: "\u043F", percnt: "%", period: ".", permil: "\u2030", perp: "\u22A5", pertenk: "\u2031", Pfr: "\u{1D513}", pfr: "\u{1D52D}", Phi: "\u03A6", phi: "\u03C6", phiv: "\u03D5", phmmat: "\u2133", phone: "\u260E", Pi: "\u03A0", pi: "\u03C0", pitchfork: "\u22D4", piv: "\u03D6", planck: "\u210F", planckh: "\u210E", plankv: "\u210F", plusacir: "\u2A23", plusb: "\u229E", pluscir: "\u2A22", plus: "+", plusdo: "\u2214", plusdu: "\u2A25", pluse: "\u2A72", PlusMinus: "\xB1", plusmn: "\xB1", plussim: "\u2A26", plustwo: "\u2A27", pm: "\xB1", Poincareplane: "\u210C", pointint: "\u2A15", popf: "\u{1D561}", Popf: "\u2119", pound: "\xA3", prap: "\u2AB7", Pr: "\u2ABB", pr: "\u227A", prcue: "\u227C", precapprox: "\u2AB7", prec: "\u227A", preccurlyeq: "\u227C", Precedes: "\u227A", PrecedesEqual: "\u2AAF", PrecedesSlantEqual: "\u227C", PrecedesTilde: "\u227E", preceq: "\u2AAF", precnapprox: "\u2AB9", precneqq: "\u2AB5", precnsim: "\u22E8", pre: "\u2AAF", prE: "\u2AB3", precsim: "\u227E", prime: "\u2032", Prime: "\u2033", primes: "\u2119", prnap: "\u2AB9", prnE: "\u2AB5", prnsim: "\u22E8", prod: "\u220F", Product: "\u220F", profalar: "\u232E", profline: "\u2312", profsurf: "\u2313", prop: "\u221D", Proportional: "\u221D", Proportion: "\u2237", propto: "\u221D", prsim: "\u227E", prurel: "\u22B0", Pscr: "\u{1D4AB}", pscr: "\u{1D4C5}", Psi: "\u03A8", psi: "\u03C8", puncsp: "\u2008", Qfr: "\u{1D514}", qfr: "\u{1D52E}", qint: "\u2A0C", qopf: "\u{1D562}", Qopf: "\u211A", qprime: "\u2057", Qscr: "\u{1D4AC}", qscr: "\u{1D4C6}", quaternions: "\u210D", quatint: "\u2A16", quest: "?", questeq: "\u225F", quot: '"', QUOT: '"', rAarr: "\u21DB", race: "\u223D\u0331", Racute: "\u0154", racute: "\u0155", radic: "\u221A", raemptyv: "\u29B3", rang: "\u27E9", Rang: "\u27EB", rangd: "\u2992", range: "\u29A5", rangle: "\u27E9", raquo: "\xBB", rarrap: "\u2975", rarrb: "\u21E5", rarrbfs: "\u2920", rarrc: "\u2933", rarr: "\u2192", Rarr: "\u21A0", rArr: "\u21D2", rarrfs: "\u291E", rarrhk: "\u21AA", rarrlp: "\u21AC", rarrpl: "\u2945", rarrsim: "\u2974", Rarrtl: "\u2916", rarrtl: "\u21A3", rarrw: "\u219D", ratail: "\u291A", rAtail: "\u291C", ratio: "\u2236", rationals: "\u211A", rbarr: "\u290D", rBarr: "\u290F", RBarr: "\u2910", rbbrk: "\u2773", rbrace: "}", rbrack: "]", rbrke: "\u298C", rbrksld: "\u298E", rbrkslu: "\u2990", Rcaron: "\u0158", rcaron: "\u0159", Rcedil: "\u0156", rcedil: "\u0157", rceil: "\u2309", rcub: "}", Rcy: "\u0420", rcy: "\u0440", rdca: "\u2937", rdldhar: "\u2969", rdquo: "\u201D", rdquor: "\u201D", rdsh: "\u21B3", real: "\u211C", realine: "\u211B", realpart: "\u211C", reals: "\u211D", Re: "\u211C", rect: "\u25AD", reg: "\xAE", REG: "\xAE", ReverseElement: "\u220B", ReverseEquilibrium: "\u21CB", ReverseUpEquilibrium: "\u296F", rfisht: "\u297D", rfloor: "\u230B", rfr: "\u{1D52F}", Rfr: "\u211C", rHar: "\u2964", rhard: "\u21C1", rharu: "\u21C0", rharul: "\u296C", Rho: "\u03A1", rho: "\u03C1", rhov: "\u03F1", RightAngleBracket: "\u27E9", RightArrowBar: "\u21E5", rightarrow: "\u2192", RightArrow: "\u2192", Rightarrow: "\u21D2", RightArrowLeftArrow: "\u21C4", rightarrowtail: "\u21A3", RightCeiling: "\u2309", RightDoubleBracket: "\u27E7", RightDownTeeVector: "\u295D", RightDownVectorBar: "\u2955", RightDownVector: "\u21C2", RightFloor: "\u230B", rightharpoondown: "\u21C1", rightharpoonup: "\u21C0", rightleftarrows: "\u21C4", rightleftharpoons: "\u21CC", rightrightarrows: "\u21C9", rightsquigarrow: "\u219D", RightTeeArrow: "\u21A6", RightTee: "\u22A2", RightTeeVector: "\u295B", rightthreetimes: "\u22CC", RightTriangleBar: "\u29D0", RightTriangle: "\u22B3", RightTriangleEqual: "\u22B5", RightUpDownVector: "\u294F", RightUpTeeVector: "\u295C", RightUpVectorBar: "\u2954", RightUpVector: "\u21BE", RightVectorBar: "\u2953", RightVector: "\u21C0", ring: "\u02DA", risingdotseq: "\u2253", rlarr: "\u21C4", rlhar: "\u21CC", rlm: "\u200F", rmoustache: "\u23B1", rmoust: "\u23B1", rnmid: "\u2AEE", roang: "\u27ED", roarr: "\u21FE", robrk: "\u27E7", ropar: "\u2986", ropf: "\u{1D563}", Ropf: "\u211D", roplus: "\u2A2E", rotimes: "\u2A35", RoundImplies: "\u2970", rpar: ")", rpargt: "\u2994", rppolint: "\u2A12", rrarr: "\u21C9", Rrightarrow: "\u21DB", rsaquo: "\u203A", rscr: "\u{1D4C7}", Rscr: "\u211B", rsh: "\u21B1", Rsh: "\u21B1", rsqb: "]", rsquo: "\u2019", rsquor: "\u2019", rthree: "\u22CC", rtimes: "\u22CA", rtri: "\u25B9", rtrie: "\u22B5", rtrif: "\u25B8", rtriltri: "\u29CE", RuleDelayed: "\u29F4", ruluhar: "\u2968", rx: "\u211E", Sacute: "\u015A", sacute: "\u015B", sbquo: "\u201A", scap: "\u2AB8", Scaron: "\u0160", scaron: "\u0161", Sc: "\u2ABC", sc: "\u227B", sccue: "\u227D", sce: "\u2AB0", scE: "\u2AB4", Scedil: "\u015E", scedil: "\u015F", Scirc: "\u015C", scirc: "\u015D", scnap: "\u2ABA", scnE: "\u2AB6", scnsim: "\u22E9", scpolint: "\u2A13", scsim: "\u227F", Scy: "\u0421", scy: "\u0441", sdotb: "\u22A1", sdot: "\u22C5", sdote: "\u2A66", searhk: "\u2925", searr: "\u2198", seArr: "\u21D8", searrow: "\u2198", sect: "\xA7", semi: ";", seswar: "\u2929", setminus: "\u2216", setmn: "\u2216", sext: "\u2736", Sfr: "\u{1D516}", sfr: "\u{1D530}", sfrown: "\u2322", sharp: "\u266F", SHCHcy: "\u0429", shchcy: "\u0449", SHcy: "\u0428", shcy: "\u0448", ShortDownArrow: "\u2193", ShortLeftArrow: "\u2190", shortmid: "\u2223", shortparallel: "\u2225", ShortRightArrow: "\u2192", ShortUpArrow: "\u2191", shy: "\xAD", Sigma: "\u03A3", sigma: "\u03C3", sigmaf: "\u03C2", sigmav: "\u03C2", sim: "\u223C", simdot: "\u2A6A", sime: "\u2243", simeq: "\u2243", simg: "\u2A9E", simgE: "\u2AA0", siml: "\u2A9D", simlE: "\u2A9F", simne: "\u2246", simplus: "\u2A24", simrarr: "\u2972", slarr: "\u2190", SmallCircle: "\u2218", smallsetminus: "\u2216", smashp: "\u2A33", smeparsl: "\u29E4", smid: "\u2223", smile: "\u2323", smt: "\u2AAA", smte: "\u2AAC", smtes: "\u2AAC\uFE00", SOFTcy: "\u042C", softcy: "\u044C", solbar: "\u233F", solb: "\u29C4", sol: "/", Sopf: "\u{1D54A}", sopf: "\u{1D564}", spades: "\u2660", spadesuit: "\u2660", spar: "\u2225", sqcap: "\u2293", sqcaps: "\u2293\uFE00", sqcup: "\u2294", sqcups: "\u2294\uFE00", Sqrt: "\u221A", sqsub: "\u228F", sqsube: "\u2291", sqsubset: "\u228F", sqsubseteq: "\u2291", sqsup: "\u2290", sqsupe: "\u2292", sqsupset: "\u2290", sqsupseteq: "\u2292", square: "\u25A1", Square: "\u25A1", SquareIntersection: "\u2293", SquareSubset: "\u228F", SquareSubsetEqual: "\u2291", SquareSuperset: "\u2290", SquareSupersetEqual: "\u2292", SquareUnion: "\u2294", squarf: "\u25AA", squ: "\u25A1", squf: "\u25AA", srarr: "\u2192", Sscr: "\u{1D4AE}", sscr: "\u{1D4C8}", ssetmn: "\u2216", ssmile: "\u2323", sstarf: "\u22C6", Star: "\u22C6", star: "\u2606", starf: "\u2605", straightepsilon: "\u03F5", straightphi: "\u03D5", strns: "\xAF", sub: "\u2282", Sub: "\u22D0", subdot: "\u2ABD", subE: "\u2AC5", sube: "\u2286", subedot: "\u2AC3", submult: "\u2AC1", subnE: "\u2ACB", subne: "\u228A", subplus: "\u2ABF", subrarr: "\u2979", subset: "\u2282", Subset: "\u22D0", subseteq: "\u2286", subseteqq: "\u2AC5", SubsetEqual: "\u2286", subsetneq: "\u228A", subsetneqq: "\u2ACB", subsim: "\u2AC7", subsub: "\u2AD5", subsup: "\u2AD3", succapprox: "\u2AB8", succ: "\u227B", succcurlyeq: "\u227D", Succeeds: "\u227B", SucceedsEqual: "\u2AB0", SucceedsSlantEqual: "\u227D", SucceedsTilde: "\u227F", succeq: "\u2AB0", succnapprox: "\u2ABA", succneqq: "\u2AB6", succnsim: "\u22E9", succsim: "\u227F", SuchThat: "\u220B", sum: "\u2211", Sum: "\u2211", sung: "\u266A", sup1: "\xB9", sup2: "\xB2", sup3: "\xB3", sup: "\u2283", Sup: "\u22D1", supdot: "\u2ABE", supdsub: "\u2AD8", supE: "\u2AC6", supe: "\u2287", supedot: "\u2AC4", Superset: "\u2283", SupersetEqual: "\u2287", suphsol: "\u27C9", suphsub: "\u2AD7", suplarr: "\u297B", supmult: "\u2AC2", supnE: "\u2ACC", supne: "\u228B", supplus: "\u2AC0", supset: "\u2283", Supset: "\u22D1", supseteq: "\u2287", supseteqq: "\u2AC6", supsetneq: "\u228B", supsetneqq: "\u2ACC", supsim: "\u2AC8", supsub: "\u2AD4", supsup: "\u2AD6", swarhk: "\u2926", swarr: "\u2199", swArr: "\u21D9", swarrow: "\u2199", swnwar: "\u292A", szlig: "\xDF", Tab: " ", target: "\u2316", Tau: "\u03A4", tau: "\u03C4", tbrk: "\u23B4", Tcaron: "\u0164", tcaron: "\u0165", Tcedil: "\u0162", tcedil: "\u0163", Tcy: "\u0422", tcy: "\u0442", tdot: "\u20DB", telrec: "\u2315", Tfr: "\u{1D517}", tfr: "\u{1D531}", there4: "\u2234", therefore: "\u2234", Therefore: "\u2234", Theta: "\u0398", theta: "\u03B8", thetasym: "\u03D1", thetav: "\u03D1", thickapprox: "\u2248", thicksim: "\u223C", ThickSpace: "\u205F\u200A", ThinSpace: "\u2009", thinsp: "\u2009", thkap: "\u2248", thksim: "\u223C", THORN: "\xDE", thorn: "\xFE", tilde: "\u02DC", Tilde: "\u223C", TildeEqual: "\u2243", TildeFullEqual: "\u2245", TildeTilde: "\u2248", timesbar: "\u2A31", timesb: "\u22A0", times: "\xD7", timesd: "\u2A30", tint: "\u222D", toea: "\u2928", topbot: "\u2336", topcir: "\u2AF1", top: "\u22A4", Topf: "\u{1D54B}", topf: "\u{1D565}", topfork: "\u2ADA", tosa: "\u2929", tprime: "\u2034", trade: "\u2122", TRADE: "\u2122", triangle: "\u25B5", triangledown: "\u25BF", triangleleft: "\u25C3", trianglelefteq: "\u22B4", triangleq: "\u225C", triangleright: "\u25B9", trianglerighteq: "\u22B5", tridot: "\u25EC", trie: "\u225C", triminus: "\u2A3A", TripleDot: "\u20DB", triplus: "\u2A39", trisb: "\u29CD", tritime: "\u2A3B", trpezium: "\u23E2", Tscr: "\u{1D4AF}", tscr: "\u{1D4C9}", TScy: "\u0426", tscy: "\u0446", TSHcy: "\u040B", tshcy: "\u045B", Tstrok: "\u0166", tstrok: "\u0167", twixt: "\u226C", twoheadleftarrow: "\u219E", twoheadrightarrow: "\u21A0", Uacute: "\xDA", uacute: "\xFA", uarr: "\u2191", Uarr: "\u219F", uArr: "\u21D1", Uarrocir: "\u2949", Ubrcy: "\u040E", ubrcy: "\u045E", Ubreve: "\u016C", ubreve: "\u016D", Ucirc: "\xDB", ucirc: "\xFB", Ucy: "\u0423", ucy: "\u0443", udarr: "\u21C5", Udblac: "\u0170", udblac: "\u0171", udhar: "\u296E", ufisht: "\u297E", Ufr: "\u{1D518}", ufr: "\u{1D532}", Ugrave: "\xD9", ugrave: "\xF9", uHar: "\u2963", uharl: "\u21BF", uharr: "\u21BE", uhblk: "\u2580", ulcorn: "\u231C", ulcorner: "\u231C", ulcrop: "\u230F", ultri: "\u25F8", Umacr: "\u016A", umacr: "\u016B", uml: "\xA8", UnderBar: "_", UnderBrace: "\u23DF", UnderBracket: "\u23B5", UnderParenthesis: "\u23DD", Union: "\u22C3", UnionPlus: "\u228E", Uogon: "\u0172", uogon: "\u0173", Uopf: "\u{1D54C}", uopf: "\u{1D566}", UpArrowBar: "\u2912", uparrow: "\u2191", UpArrow: "\u2191", Uparrow: "\u21D1", UpArrowDownArrow: "\u21C5", updownarrow: "\u2195", UpDownArrow: "\u2195", Updownarrow: "\u21D5", UpEquilibrium: "\u296E", upharpoonleft: "\u21BF", upharpoonright: "\u21BE", uplus: "\u228E", UpperLeftArrow: "\u2196", UpperRightArrow: "\u2197", upsi: "\u03C5", Upsi: "\u03D2", upsih: "\u03D2", Upsilon: "\u03A5", upsilon: "\u03C5", UpTeeArrow: "\u21A5", UpTee: "\u22A5", upuparrows: "\u21C8", urcorn: "\u231D", urcorner: "\u231D", urcrop: "\u230E", Uring: "\u016E", uring: "\u016F", urtri: "\u25F9", Uscr: "\u{1D4B0}", uscr: "\u{1D4CA}", utdot: "\u22F0", Utilde: "\u0168", utilde: "\u0169", utri: "\u25B5", utrif: "\u25B4", uuarr: "\u21C8", Uuml: "\xDC", uuml: "\xFC", uwangle: "\u29A7", vangrt: "\u299C", varepsilon: "\u03F5", varkappa: "\u03F0", varnothing: "\u2205", varphi: "\u03D5", varpi: "\u03D6", varpropto: "\u221D", varr: "\u2195", vArr: "\u21D5", varrho: "\u03F1", varsigma: "\u03C2", varsubsetneq: "\u228A\uFE00", varsubsetneqq: "\u2ACB\uFE00", varsupsetneq: "\u228B\uFE00", varsupsetneqq: "\u2ACC\uFE00", vartheta: "\u03D1", vartriangleleft: "\u22B2", vartriangleright: "\u22B3", vBar: "\u2AE8", Vbar: "\u2AEB", vBarv: "\u2AE9", Vcy: "\u0412", vcy: "\u0432", vdash: "\u22A2", vDash: "\u22A8", Vdash: "\u22A9", VDash: "\u22AB", Vdashl: "\u2AE6", veebar: "\u22BB", vee: "\u2228", Vee: "\u22C1", veeeq: "\u225A", vellip: "\u22EE", verbar: "|", Verbar: "\u2016", vert: "|", Vert: "\u2016", VerticalBar: "\u2223", VerticalLine: "|", VerticalSeparator: "\u2758", VerticalTilde: "\u2240", VeryThinSpace: "\u200A", Vfr: "\u{1D519}", vfr: "\u{1D533}", vltri: "\u22B2", vnsub: "\u2282\u20D2", vnsup: "\u2283\u20D2", Vopf: "\u{1D54D}", vopf: "\u{1D567}", vprop: "\u221D", vrtri: "\u22B3", Vscr: "\u{1D4B1}", vscr: "\u{1D4CB}", vsubnE: "\u2ACB\uFE00", vsubne: "\u228A\uFE00", vsupnE: "\u2ACC\uFE00", vsupne: "\u228B\uFE00", Vvdash: "\u22AA", vzigzag: "\u299A", Wcirc: "\u0174", wcirc: "\u0175", wedbar: "\u2A5F", wedge: "\u2227", Wedge: "\u22C0", wedgeq: "\u2259", weierp: "\u2118", Wfr: "\u{1D51A}", wfr: "\u{1D534}", Wopf: "\u{1D54E}", wopf: "\u{1D568}", wp: "\u2118", wr: "\u2240", wreath: "\u2240", Wscr: "\u{1D4B2}", wscr: "\u{1D4CC}", xcap: "\u22C2", xcirc: "\u25EF", xcup: "\u22C3", xdtri: "\u25BD", Xfr: "\u{1D51B}", xfr: "\u{1D535}", xharr: "\u27F7", xhArr: "\u27FA", Xi: "\u039E", xi: "\u03BE", xlarr: "\u27F5", xlArr: "\u27F8", xmap: "\u27FC", xnis: "\u22FB", xodot: "\u2A00", Xopf: "\u{1D54F}", xopf: "\u{1D569}", xoplus: "\u2A01", xotime: "\u2A02", xrarr: "\u27F6", xrArr: "\u27F9", Xscr: "\u{1D4B3}", xscr: "\u{1D4CD}", xsqcup: "\u2A06", xuplus: "\u2A04", xutri: "\u25B3", xvee: "\u22C1", xwedge: "\u22C0", Yacute: "\xDD", yacute: "\xFD", YAcy: "\u042F", yacy: "\u044F", Ycirc: "\u0176", ycirc: "\u0177", Ycy: "\u042B", ycy: "\u044B", yen: "\xA5", Yfr: "\u{1D51C}", yfr: "\u{1D536}", YIcy: "\u0407", yicy: "\u0457", Yopf: "\u{1D550}", yopf: "\u{1D56A}", Yscr: "\u{1D4B4}", yscr: "\u{1D4CE}", YUcy: "\u042E", yucy: "\u044E", yuml: "\xFF", Yuml: "\u0178", Zacute: "\u0179", zacute: "\u017A", Zcaron: "\u017D", zcaron: "\u017E", Zcy: "\u0417", zcy: "\u0437", Zdot: "\u017B", zdot: "\u017C", zeetrf: "\u2128", ZeroWidthSpace: "\u200B", Zeta: "\u0396", zeta: "\u03B6", zfr: "\u{1D537}", Zfr: "\u2128", ZHcy: "\u0416", zhcy: "\u0436", zigrarr: "\u21DD", zopf: "\u{1D56B}", Zopf: "\u2124", Zscr: "\u{1D4B5}", zscr: "\u{1D4CF}", zwj: "\u200D", zwnj: "\u200C" }, c = /^#[xX]([A-Fa-f0-9]+)$/, o = /^#([0-9]+)$/, e = /^([A-Za-z0-9]+)$/, r = function() {
function E(v) {
this.named = v;
}
return E.prototype.parse = function(v) {
if (v) {
var _ = v.match(c);
if (_)
return String.fromCharCode(parseInt(_[1], 16));
if (_ = v.match(o), _)
return String.fromCharCode(parseInt(_[1], 10));
if (_ = v.match(e), _)
return this.named[_[1]];
}
}, E;
}(), a = /[\t\n\f ]/, p = /[A-Za-z]/, n2 = /\r\n?/g;
function s(E) {
return a.test(E);
}
function u(E) {
return p.test(E);
}
function i(E) {
return E.replace(n2, `
`);
}
var l = function() {
function E(v, _, y) {
y === void 0 && (y = "precompile"), this.delegate = v, this.entityParser = _, this.mode = y, this.state = "beforeData", this.line = -1, this.column = -1, this.input = "", this.index = -1, this.tagNameBuffer = "", this.states = { beforeData: function() {
var g = this.peek();
if (g === "<" && !this.isIgnoredEndTag())
this.transitionTo("tagOpen"), this.markTagStart(), this.consume();
else {
if (this.mode === "precompile" && g === `
`) {
var L = this.tagNameBuffer.toLowerCase();
(L === "pre" || L === "textarea") && this.consume();
}
this.transitionTo("data"), this.delegate.beginData();
}
}, data: function() {
var g = this.peek(), L = this.tagNameBuffer;
g === "<" && !this.isIgnoredEndTag() ? (this.delegate.finishData(), this.transitionTo("tagOpen"), this.markTagStart(), this.consume()) : g === "&" && L !== "script" && L !== "style" ? (this.consume(), this.delegate.appendToData(this.consumeCharRef() || "&")) : (this.consume(), this.delegate.appendToData(g));
}, tagOpen: function() {
var g = this.consume();
g === "!" ? this.transitionTo("markupDeclarationOpen") : g === "/" ? this.transitionTo("endTagOpen") : (g === "@" || g === ":" || u(g)) && (this.transitionTo("tagName"), this.tagNameBuffer = "", this.delegate.beginStartTag(), this.appendToTagName(g));
}, markupDeclarationOpen: function() {
var g = this.consume();
if (g === "-" && this.peek() === "-")
this.consume(), this.transitionTo("commentStart"), this.delegate.beginComment();
else {
var L = g.toUpperCase() + this.input.substring(this.index, this.index + 6).toUpperCase();
L === "DOCTYPE" && (this.consume(), this.consume(), this.consume(), this.consume(), this.consume(), this.consume(), this.transitionTo("doctype"), this.delegate.beginDoctype && this.delegate.beginDoctype());
}
}, doctype: function() {
var g = this.consume();
s(g) && this.transitionTo("beforeDoctypeName");
}, beforeDoctypeName: function() {
var g = this.consume();
s(g) || (this.transitionTo("doctypeName"), this.delegate.appendToDoctypeName && this.delegate.appendToDoctypeName(g.toLowerCase()));
}, doctypeName: function() {
var g = this.consume();
s(g) ? this.transitionTo("afterDoctypeName") : g === ">" ? (this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData")) : this.delegate.appendToDoctypeName && this.delegate.appendToDoctypeName(g.toLowerCase());
}, afterDoctypeName: function() {
var g = this.consume();
if (!s(g))
if (g === ">")
this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData");
else {
var L = g.toUpperCase() + this.input.substring(this.index, this.index + 5).toUpperCase(), j = L.toUpperCase() === "PUBLIC", x = L.toUpperCase() === "SYSTEM";
(j || x) && (this.consume(), this.consume(), this.consume(), this.consume(), this.consume(), this.consume()), j ? this.transitionTo("afterDoctypePublicKeyword") : x && this.transitionTo("afterDoctypeSystemKeyword");
}
}, afterDoctypePublicKeyword: function() {
var g = this.peek();
s(g) ? (this.transitionTo("beforeDoctypePublicIdentifier"), this.consume()) : g === '"' ? (this.transitionTo("doctypePublicIdentifierDoubleQuoted"), this.consume()) : g === "'" ? (this.transitionTo("doctypePublicIdentifierSingleQuoted"), this.consume()) : g === ">" && (this.consume(), this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData"));
}, doctypePublicIdentifierDoubleQuoted: function() {
var g = this.consume();
g === '"' ? this.transitionTo("afterDoctypePublicIdentifier") : g === ">" ? (this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData")) : this.delegate.appendToDoctypePublicIdentifier && this.delegate.appendToDoctypePublicIdentifier(g);
}, doctypePublicIdentifierSingleQuoted: function() {
var g = this.consume();
g === "'" ? this.transitionTo("afterDoctypePublicIdentifier") : g === ">" ? (this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData")) : this.delegate.appendToDoctypePublicIdentifier && this.delegate.appendToDoctypePublicIdentifier(g);
}, afterDoctypePublicIdentifier: function() {
var g = this.consume();
s(g) ? this.transitionTo("betweenDoctypePublicAndSystemIdentifiers") : g === ">" ? (this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData")) : g === '"' ? this.transitionTo("doctypeSystemIdentifierDoubleQuoted") : g === "'" && this.transitionTo("doctypeSystemIdentifierSingleQuoted");
}, betweenDoctypePublicAndSystemIdentifiers: function() {
var g = this.consume();
s(g) || (g === ">" ? (this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData")) : g === '"' ? this.transitionTo("doctypeSystemIdentifierDoubleQuoted") : g === "'" && this.transitionTo("doctypeSystemIdentifierSingleQuoted"));
}, doctypeSystemIdentifierDoubleQuoted: function() {
var g = this.consume();
g === '"' ? this.transitionTo("afterDoctypeSystemIdentifier") : g === ">" ? (this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData")) : this.delegate.appendToDoctypeSystemIdentifier && this.delegate.appendToDoctypeSystemIdentifier(g);
}, doctypeSystemIdentifierSingleQuoted: function() {
var g = this.consume();
g === "'" ? this.transitionTo("afterDoctypeSystemIdentifier") : g === ">" ? (this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData")) : this.delegate.appendToDoctypeSystemIdentifier && this.delegate.appendToDoctypeSystemIdentifier(g);
}, afterDoctypeSystemIdentifier: function() {
var g = this.consume();
s(g) || g === ">" && (this.delegate.endDoctype && this.delegate.endDoctype(), this.transitionTo("beforeData"));
}, commentStart: function() {
var g = this.consume();
g === "-" ? this.transitionTo("commentStartDash") : g === ">" ? (this.delegate.finishComment(), this.transitionTo("beforeData")) : (this.delegate.appendToCommentData(g), this.transitionTo("comment"));
}, commentStartDash: function() {
var g = this.consume();
g === "-" ? this.transitionTo("commentEnd") : g === ">" ? (this.delegate.finishComment(), this.transitionTo("beforeData")) : (this.delegate.appendToCommentData("-"), this.transitionTo("comment"));
}, comment: function() {
var g = this.consume();
g === "-" ? this.transitionTo("commentEndDash") : this.delegate.appendToCommentData(g);
}, commentEndDash: function() {
var g = this.consume();
g === "-" ? this.transitionTo("commentEnd") : (this.delegate.appendToCommentData("-" + g), this.transitionTo("comment"));
}, commentEnd: function() {
var g = this.consume();
g === ">" ? (this.delegate.finishComment(), this.transitionTo("beforeData")) : (this.delegate.appendToCommentData("--" + g), this.transitionTo("comment"));
}, tagName: function() {
var g = this.consume();
s(g) ? this.transitionTo("beforeAttributeName") : g === "/" ? this.transitionTo("selfClosingStartTag") : g === ">" ? (this.delegate.finishTag(), this.transitionTo("beforeData")) : this.appendToTagName(g);
}, endTagName: function() {
var g = this.consume();
s(g) ? (this.transitionTo("beforeAttributeName"), this.tagNameBuffer = "") : g === "/" ? (this.transitionTo("selfClosingStartTag"), this.tagNameBuffer = "") : g === ">" ? (this.delegate.finishTag(), this.transitionTo("beforeData"), this.tagNameBuffer = "") : this.appendToTagName(g);
}, beforeAttributeName: function() {
var g = this.peek();
if (s(g)) {
this.consume();
return;
} else
g === "/" ? (this.transitionTo("selfClosingStartTag"), this.consume()) : g === ">" ? (this.consume(), this.delegate.finishTag(), this.transitionTo("beforeData")) : g === "=" ? (this.delegate.reportSyntaxError("attribute name cannot start with equals sign"), this.transitionTo("attributeName"), this.delegate.beginAttribute(), this.consume(), this.delegate.appendToAttributeName(g)) : (this.transitionTo("attributeName"), this.delegate.beginAttribute());
}, attributeName: function() {
var g = this.peek();
s(g) ? (this.transitionTo("afterAttributeName"), this.consume()) : g === "/" ? (this.delegate.beginAttributeValue(false), this.delegate.finishAttributeValue(), this.consume(), this.transitionTo("selfClosingStartTag")) : g === "=" ? (this.transitionTo("beforeAttributeValue"), this.consume()) : g === ">" ? (this.delegate.beginAttributeValue(false), this.delegate.finishAttributeValue(), this.consume(), this.delegate.finishTag(), this.transitionTo("beforeData")) : g === '"' || g === "'" || g === "<" ? (this.delegate.reportSyntaxError(g + " is not a valid character within attribute names"), this.consume(), this.delegate.appendToAttributeName(g)) : (this.consume(), this.delegate.appendToAttributeName(g));
}, afterAttributeName: function() {
var g = this.peek();
if (s(g)) {
this.consume();
return;
} else
g === "/" ? (this.delegate.beginAttributeValue(false), this.delegate.finishAttributeValue(), this.consume(), this.transitionTo("selfClosingStartTag")) : g === "=" ? (this.consume(), this.transitionTo("beforeAttributeValue")) : g === ">" ? (this.delegate.beginAttributeValue(false), this.delegate.finishAttributeValue(), this.consume(), this.delegate.finishTag(), this.transitionTo("beforeData")) : (this.delegate.beginAttributeValue(false), this.delegate.finishAttributeValue(), this.transitionTo("attributeName"), this.delegate.beginAttribute(), this.consume(), this.delegate.appendToAttributeName(g));
}, beforeAttributeValue: function() {
var g = this.peek();
s(g) ? this.consume() : g === '"' ? (this.transitionTo("attributeValueDoubleQuoted"), this.delegate.beginAttributeValue(true), this.consume()) : g === "'" ? (this.transitionTo("attributeValueSingleQuoted"), this.delegate.beginAttributeValue(true), this.consume()) : g === ">" ? (this.delegate.beginAttributeValue(false), this.delegate.finishAttributeValue(), this.consume(), this.delegate.finishTag(), this.transitionTo("beforeData")) : (this.transitionTo("attributeValueUnquoted"), this.delegate.beginAttributeValue(false), this.consume(), this.delegate.appendToAttributeValue(g));
}, attributeValueDoubleQuoted: function() {
var g = this.consume();
g === '"' ? (this.delegate.finishAttributeValue(), this.transitionTo("afterAttributeValueQuoted")) : g === "&" ? this.delegate.appendToAttributeValue(this.consumeCharRef() || "&") : this.delegate.appendToAttributeValue(g);
}, attributeValueSingleQuoted: function() {
var g = this.consume();
g === "'" ? (this.delegate.finishAttributeValue(), this.transitionTo("afterAttributeValueQuoted")) : g === "&" ? this.delegate.appendToAttributeValue(this.consumeCharRef() || "&") : this.delegate.appendToAttributeValue(g);
}, attributeValueUnquoted: function() {
var g = this.peek();
s(g) ? (this.delegate.finishAttributeValue(), this.consume(), this.transitionTo("beforeAttributeName")) : g === "/" ? (this.delegate.finishAttributeValue(), this.consume(), this.transitionTo("selfClosingStartTag")) : g === "&" ? (this.consume(), this.delegate.appendToAttributeValue(this.consumeCharRef() || "&")) : g === ">" ? (this.delegate.finishAttributeValue(), this.consume(), this.delegate.finishTag(), this.transitionTo("beforeData")) : (this.consume(), this.delegate.appendToAttributeValue(g));
}, afterAttributeValueQuoted: function() {
var g = this.peek();
s(g) ? (this.consume(), this.transitionTo("beforeAttributeName")) : g === "/" ? (this.consume(), this.transitionTo("selfClosingStartTag")) : g === ">" ? (this.consume(), this.delegate.finishTag(), this.transitionTo("beforeData")) : this.transitionTo("beforeAttributeName");
}, selfClosingStartTag: function() {
var g = this.peek();
g === ">" ? (this.consume(), this.delegate.markTagAsSelfClosing(), this.delegate.finishTag(), this.transitionTo("beforeData")) : this.transitionTo("beforeAttributeName");
}, endTagOpen: function() {
var g = this.consume();
(g === "@" || g === ":" || u(g)) && (this.transitionTo("endTagName"), this.tagNameBuffer = "", this.delegate.beginEndTag(), this.appendToTagName(g));
} }, this.reset();
}
return E.prototype.reset = function() {
this.transitionTo("beforeData"), this.input = "", this.tagNameBuffer = "", this.index = 0, this.line = 1, this.column = 0, this.delegate.reset();
}, E.prototype.transitionTo = function(v) {
this.state = v;
}, E.prototype.tokenize = function(v) {
this.reset(), this.tokenizePart(v), this.tokenizeEOF();
}, E.prototype.tokenizePart = function(v) {
for (this.input += i(v); this.index < this.input.length; ) {
var _ = this.states[this.state];
if (_ !== void 0)
_.call(this);
else
throw new Error("unhandled state " + this.state);
}
}, E.prototype.tokenizeEOF = function() {
this.flushData();
}, E.prototype.flushData = function() {
this.state === "data" && (this.delegate.finishData(), this.transitionTo("beforeData"));
}, E.prototype.peek = function() {
return this.input.charAt(this.index);
}, E.prototype.consume = function() {
var v = this.peek();
return this.index++, v === `
` ? (this.line++, this.column = 0) : this.column++, v;
}, E.prototype.consumeCharRef = function() {
var v = this.input.indexOf(";", this.index);
if (v !== -1) {
var _ = this.input.slice(this.index, v), y = this.entityParser.parse(_);
if (y) {
for (var g = _.length; g; )
this.consume(), g--;
return this.consume(), y;
}
}
}, E.prototype.markTagStart = function() {
this.delegate.tagOpen();
}, E.prototype.appendToTagName = function(v) {
this.tagNameBuffer += v, this.delegate.appendToTagName(v);
}, E.prototype.isIgnoredEndTag = function() {
var v = this.tagNameBuffer;
return v === "title" && this.input.substring(this.index, this.index + 8) !== "" || v === "style" && this.input.substring(this.index, this.index + 8) !== "" || v === "script" && this.input.substring(this.index, this.index + 9) !== "<\/script>";
}, E;
}(), b = function() {
function E(v, _) {
_ === void 0 && (_ = {}), this.options = _, this.token = null, this.startLine = 1, this.startColumn = 0, this.tokens = [], this.tokenizer = new l(this, v, _.mode), this._currentAttribute = void 0;
}
return E.prototype.tokenize = function(v) {
return this.tokens = [], this.tokenizer.tokenize(v), this.tokens;
}, E.prototype.tokenizePart = function(v) {
return this.tokens = [], this.tokenizer.tokenizePart(v), this.tokens;
}, E.prototype.tokenizeEOF = function() {
return this.tokens = [], this.tokenizer.tokenizeEOF(), this.tokens[0];
}, E.prototype.reset = function() {
this.token = null, this.startLine = 1, this.startColumn = 0;
}, E.prototype.current = function() {
var v = this.token;
if (v === null)
throw new Error("token was unexpectedly null");
if (arguments.length === 0)
return v;
for (var _ = 0; _ < arguments.length; _++)
if (v.type === arguments[_])
return v;
throw new Error("token type was unexpectedly " + v.type);
}, E.prototype.push = function(v) {
this.token = v, this.tokens.push(v);
}, E.prototype.currentAttribute = function() {
return this._currentAttribute;
}, E.prototype.addLocInfo = function() {
this.options.loc && (this.current().loc = { start: { line: this.startLine, column: this.startColumn }, end: { line: this.tokenizer.line, column: this.tokenizer.column } }), this.startLine = this.tokenizer.line, this.startColumn = this.tokenizer.column;
}, E.prototype.beginDoctype = function() {
this.push({ type: "Doctype", name: "" });
}, E.prototype.appendToDoctypeName = function(v) {
this.current("Doctype").name += v;
}, E.prototype.appendToDoctypePublicIdentifier = function(v) {
var _ = this.current("Doctype");
_.publicIdentifier === void 0 ? _.publicIdentifier = v : _.publicIdentifier += v;
}, E.prototype.appendToDoctypeSystemIdentifier = function(v) {
var _ = this.current("Doctype");
_.systemIdentifier === void 0 ? _.systemIdentifier = v : _.systemIdentifier += v;
}, E.prototype.endDoctype = function() {
this.addLocInfo();
}, E.prototype.beginData = function() {
this.push({ type: "Chars", chars: "" });
}, E.prototype.appendToData = function(v) {
this.current("Chars").chars += v;
}, E.prototype.finishData = function() {
this.addLocInfo();
}, E.prototype.beginComment = function() {
this.push({ type: "Comment", chars: "" });
}, E.prototype.appendToCommentData = function(v) {
this.current("Comment").chars += v;
}, E.prototype.finishComment = function() {
this.addLocInfo();
}, E.prototype.tagOpen = function() {
}, E.prototype.beginStartTag = function() {
this.push({ type: "StartTag", tagName: "", attributes: [], selfClosing: false });
}, E.prototype.beginEndTag = function() {
this.push({ type: "EndTag", tagName: "" });
}, E.prototype.finishTag = function() {
this.addLocInfo();
}, E.prototype.markTagAsSelfClosing = function() {
this.current("StartTag").selfClosing = true;
}, E.prototype.appendToTagName = function(v) {
this.current("StartTag", "EndTag").tagName += v;
}, E.prototype.beginAttribute = function() {
this._currentAttribute = ["", "", false];
}, E.prototype.appendToAttributeName = function(v) {
this.currentAttribute()[0] += v;
}, E.prototype.beginAttributeValue = function(v) {
this.currentAttribute()[2] = v;
}, E.prototype.appendToAttributeValue = function(v) {
this.currentAttribute()[1] += v;
}, E.prototype.finishAttributeValue = function() {
this.current("StartTag").attributes.push(this._currentAttribute);
}, E.prototype.reportSyntaxError = function(v) {
this.current().syntaxError = v;
}, E;
}();
function P(E, v) {
var _ = new b(new r(d), v);
return _.tokenize(E);
}
h.HTML5NamedCharRefs = d, h.EntityParser = r, h.EventedTokenizer = l, h.Tokenizer = b, h.tokenize = P, Object.defineProperty(h, "__esModule", { value: true });
});
} }), We = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/generation/print.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = d;
var f = h(Te());
function h(c) {
return c && c.__esModule ? c : { default: c };
}
function d(c) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : { entityEncoding: "transformed" };
return c ? new f.default(o).print(c) : "";
}
} }), he = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/syntax-error.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.generateSyntaxError = f;
function f(h, d) {
let { module: c, loc: o } = d, { line: e, column: r } = o.start, a = d.asString(), p = a ? `
|
| ${a.split(`
`).join(`
| `)}
|
` : "", n2 = new Error(`${h}: ${p}(error occurred in '${c}' @ line ${e} : column ${r})`);
return n2.name = "SyntaxError", n2.location = d, n2.code = a, n2;
}
} }), Rt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v1/visitor-keys.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = void 0;
var f = X(), h = { Program: (0, f.tuple)("body"), Template: (0, f.tuple)("body"), Block: (0, f.tuple)("body"), MustacheStatement: (0, f.tuple)("path", "params", "hash"), BlockStatement: (0, f.tuple)("path", "params", "hash", "program", "inverse"), ElementModifierStatement: (0, f.tuple)("path", "params", "hash"), PartialStatement: (0, f.tuple)("name", "params", "hash"), CommentStatement: (0, f.tuple)(), MustacheCommentStatement: (0, f.tuple)(), ElementNode: (0, f.tuple)("attributes", "modifiers", "children", "comments"), AttrNode: (0, f.tuple)("value"), TextNode: (0, f.tuple)(), ConcatStatement: (0, f.tuple)("parts"), SubExpression: (0, f.tuple)("path", "params", "hash"), PathExpression: (0, f.tuple)(), PathHead: (0, f.tuple)(), StringLiteral: (0, f.tuple)(), BooleanLiteral: (0, f.tuple)(), NumberLiteral: (0, f.tuple)(), NullLiteral: (0, f.tuple)(), UndefinedLiteral: (0, f.tuple)(), Hash: (0, f.tuple)("pairs"), HashPair: (0, f.tuple)("value"), NamedBlock: (0, f.tuple)("attributes", "modifiers", "children", "comments"), SimpleElement: (0, f.tuple)("attributes", "modifiers", "children", "comments"), Component: (0, f.tuple)("head", "attributes", "modifiers", "children", "comments") }, d = h;
t.default = d;
} }), Ye = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/traversal/errors.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.cannotRemoveNode = d, t.cannotReplaceNode = c, t.cannotReplaceOrRemoveInKeyHandlerYet = o, t.default = void 0;
var f = function() {
e.prototype = Object.create(Error.prototype), e.prototype.constructor = e;
function e(r, a, p, n2) {
let s = Error.call(this, r);
this.key = n2, this.message = r, this.node = a, this.parent = p, this.stack = s.stack;
}
return e;
}(), h = f;
t.default = h;
function d(e, r, a) {
return new f("Cannot remove a node unless it is part of an array", e, r, a);
}
function c(e, r, a) {
return new f("Cannot replace a node with multiple nodes unless it is part of an array", e, r, a);
}
function o(e, r) {
return new f("Replacing and removing in key handlers is not yet supported.", e, null, r);
}
} }), Qe = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/traversal/path.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = void 0;
var f = class {
constructor(d) {
let c = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null, o = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : null;
this.node = d, this.parent = c, this.parentKey = o;
}
get parentNode() {
return this.parent ? this.parent.node : null;
}
parents() {
return { [Symbol.iterator]: () => new h(this) };
}
};
t.default = f;
var h = class {
constructor(d) {
this.path = d;
}
next() {
return this.path.parent ? (this.path = this.path.parent, { done: false, value: this.path }) : { done: true, value: null };
}
};
} }), Ne = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/traversal/traverse.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = E;
var f = X(), h = o(Rt()), d = Ye(), c = o(Qe());
function o(v) {
return v && v.__esModule ? v : { default: v };
}
function e(v) {
return typeof v == "function" ? v : v.enter;
}
function r(v) {
if (typeof v != "function")
return v.exit;
}
function a(v, _) {
let y = typeof v != "function" ? v.keys : void 0;
if (y === void 0)
return;
let g = y[_];
return g !== void 0 ? g : y.All;
}
function p(v, _) {
if ((_ === "Template" || _ === "Block") && v.Program)
return v.Program;
let y = v[_];
return y !== void 0 ? y : v.All;
}
function n2(v, _) {
let { node: y, parent: g, parentKey: L } = _, j = p(v, y.type), x, w;
j !== void 0 && (x = e(j), w = r(j));
let H;
if (x !== void 0 && (H = x(y, _)), H != null)
if (JSON.stringify(y) === JSON.stringify(H))
H = void 0;
else {
if (Array.isArray(H))
return l(v, H, g, L), H;
{
let m = new c.default(H, g, L);
return n2(v, m) || H;
}
}
if (H === void 0) {
let m = h.default[y.type];
for (let C = 0; C < m.length; C++) {
let S = m[C];
i(v, j, _, S);
}
w !== void 0 && (H = w(y, _));
}
return H;
}
function s(v, _) {
return v[_];
}
function u(v, _, y) {
v[_] = y;
}
function i(v, _, y, g) {
let { node: L } = y, j = s(L, g);
if (!j)
return;
let x, w;
if (_ !== void 0) {
let H = a(_, g);
H !== void 0 && (x = e(H), w = r(H));
}
if (x !== void 0 && x(L, g) !== void 0)
throw (0, d.cannotReplaceOrRemoveInKeyHandlerYet)(L, g);
if (Array.isArray(j))
l(v, j, y, g);
else {
let H = new c.default(j, y, g), m = n2(v, H);
m !== void 0 && b(L, g, j, m);
}
if (w !== void 0 && w(L, g) !== void 0)
throw (0, d.cannotReplaceOrRemoveInKeyHandlerYet)(L, g);
}
function l(v, _, y, g) {
for (let L = 0; L < _.length; L++) {
let j = _[L], x = new c.default(j, y, g), w = n2(v, x);
w !== void 0 && (L += P(_, L, w) - 1);
}
}
function b(v, _, y, g) {
if (g === null)
throw (0, d.cannotRemoveNode)(y, v, _);
if (Array.isArray(g))
if (g.length === 1)
u(v, _, g[0]);
else
throw g.length === 0 ? (0, d.cannotRemoveNode)(y, v, _) : (0, d.cannotReplaceNode)(y, v, _);
else
u(v, _, g);
}
function P(v, _, y) {
return y === null ? (v.splice(_, 1), 0) : Array.isArray(y) ? (v.splice(_, 1, ...y), y.length) : (v.splice(_, 1, y), 1);
}
function E(v, _) {
let y = new c.default(v);
n2(_, y);
}
} }), Je = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/traversal/walker.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = void 0;
var f = class {
constructor(d) {
this.order = d, this.stack = [];
}
visit(d, c) {
d && (this.stack.push(d), this.order === "post" ? (this.children(d, c), c(d, this)) : (c(d, this), this.children(d, c)), this.stack.pop());
}
children(d, c) {
switch (d.type) {
case "Block":
case "Template":
return h.Program(this, d, c);
case "ElementNode":
return h.ElementNode(this, d, c);
case "BlockStatement":
return h.BlockStatement(this, d, c);
default:
return;
}
}
};
t.default = f;
var h = { Program(d, c, o) {
for (let e = 0; e < c.body.length; e++)
d.visit(c.body[e], o);
}, Template(d, c, o) {
for (let e = 0; e < c.body.length; e++)
d.visit(c.body[e], o);
}, Block(d, c, o) {
for (let e = 0; e < c.body.length; e++)
d.visit(c.body[e], o);
}, ElementNode(d, c, o) {
for (let e = 0; e < c.children.length; e++)
d.visit(c.children[e], o);
}, BlockStatement(d, c, o) {
d.visit(c.program, o), d.visit(c.inverse || null, o);
} };
} }), ye = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/utils.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.parseElementBlockParams = d, t.childrenFor = o, t.appendChild = e, t.isHBSLiteral = r, t.printLiteral = a, t.isUpperCase = p, t.isLowerCase = n2;
var f = he(), h = /[!"#%-,\.\/;->@\[-\^`\{-~]/;
function d(s) {
let u = c(s);
u && (s.blockParams = u);
}
function c(s) {
let u = s.attributes.length, i = [];
for (let b = 0; b < u; b++)
i.push(s.attributes[b].name);
let l = i.indexOf("as");
if (l === -1 && i.length > 0 && i[i.length - 1].charAt(0) === "|")
throw (0, f.generateSyntaxError)("Block parameters must be preceded by the `as` keyword, detected block parameters without `as`", s.loc);
if (l !== -1 && u > l && i[l + 1].charAt(0) === "|") {
let b = i.slice(l).join(" ");
if (b.charAt(b.length - 1) !== "|" || b.match(/\|/g).length !== 2)
throw (0, f.generateSyntaxError)("Invalid block parameters syntax, '" + b + "'", s.loc);
let P = [];
for (let E = l + 1; E < u; E++) {
let v = i[E].replace(/\|/g, "");
if (v !== "") {
if (h.test(v))
throw (0, f.generateSyntaxError)("Invalid identifier for block parameters, '" + v + "'", s.loc);
P.push(v);
}
}
if (P.length === 0)
throw (0, f.generateSyntaxError)("Cannot use zero block parameters", s.loc);
return s.attributes = s.attributes.slice(0, l), P;
}
return null;
}
function o(s) {
switch (s.type) {
case "Block":
case "Template":
return s.body;
case "ElementNode":
return s.children;
}
}
function e(s, u) {
o(s).push(u);
}
function r(s) {
return s.type === "StringLiteral" || s.type === "BooleanLiteral" || s.type === "NumberLiteral" || s.type === "NullLiteral" || s.type === "UndefinedLiteral";
}
function a(s) {
return s.type === "UndefinedLiteral" ? "undefined" : JSON.stringify(s.value);
}
function p(s) {
return s[0] === s[0].toUpperCase() && s[0] !== s[0].toLowerCase();
}
function n2(s) {
return s[0] === s[0].toLowerCase() && s[0] !== s[0].toUpperCase();
}
} }), Le = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v1/parser-builders.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.default = void 0;
var f = X(), h = we(), d = { close: false, open: false }, c = class {
pos(r, a) {
return { line: r, column: a };
}
blockItself(r) {
let { body: a, blockParams: p, chained: n2 = false, loc: s } = r;
return { type: "Block", body: a || [], blockParams: p || [], chained: n2, loc: s };
}
template(r) {
let { body: a, blockParams: p, loc: n2 } = r;
return { type: "Template", body: a || [], blockParams: p || [], loc: n2 };
}
mustache(r) {
let { path: a, params: p, hash: n2, trusting: s, loc: u, strip: i = d } = r;
return { type: "MustacheStatement", path: a, params: p, hash: n2, escaped: !s, trusting: s, loc: u, strip: i || { open: false, close: false } };
}
block(r) {
let { path: a, params: p, hash: n2, defaultBlock: s, elseBlock: u = null, loc: i, openStrip: l = d, inverseStrip: b = d, closeStrip: P = d } = r;
return { type: "BlockStatement", path: a, params: p, hash: n2, program: s, inverse: u, loc: i, openStrip: l, inverseStrip: b, closeStrip: P };
}
comment(r, a) {
return { type: "CommentStatement", value: r, loc: a };
}
mustacheComment(r, a) {
return { type: "MustacheCommentStatement", value: r, loc: a };
}
concat(r, a) {
return { type: "ConcatStatement", parts: r, loc: a };
}
element(r) {
let { tag: a, selfClosing: p, attrs: n2, blockParams: s, modifiers: u, comments: i, children: l, loc: b } = r;
return { type: "ElementNode", tag: a, selfClosing: p, attributes: n2 || [], blockParams: s || [], modifiers: u || [], comments: i || [], children: l || [], loc: b };
}
elementModifier(r) {
let { path: a, params: p, hash: n2, loc: s } = r;
return { type: "ElementModifierStatement", path: a, params: p, hash: n2, loc: s };
}
attr(r) {
let { name: a, value: p, loc: n2 } = r;
return { type: "AttrNode", name: a, value: p, loc: n2 };
}
text(r) {
let { chars: a, loc: p } = r;
return { type: "TextNode", chars: a, loc: p };
}
sexpr(r) {
let { path: a, params: p, hash: n2, loc: s } = r;
return { type: "SubExpression", path: a, params: p, hash: n2, loc: s };
}
path(r) {
let { head: a, tail: p, loc: n2 } = r, { original: s } = o(a), u = [...s, ...p].join(".");
return new h.PathExpressionImplV1(u, a, p, n2);
}
head(r, a) {
return r[0] === "@" ? this.atName(r, a) : r === "this" ? this.this(a) : this.var(r, a);
}
this(r) {
return { type: "ThisHead", loc: r };
}
atName(r, a) {
return { type: "AtHead", name: r, loc: a };
}
var(r, a) {
return { type: "VarHead", name: r, loc: a };
}
hash(r, a) {
return { type: "Hash", pairs: r || [], loc: a };
}
pair(r) {
let { key: a, value: p, loc: n2 } = r;
return { type: "HashPair", key: a, value: p, loc: n2 };
}
literal(r) {
let { type: a, value: p, loc: n2 } = r;
return { type: a, value: p, original: p, loc: n2 };
}
undefined() {
return this.literal({ type: "UndefinedLiteral", value: void 0 });
}
null() {
return this.literal({ type: "NullLiteral", value: null });
}
string(r, a) {
return this.literal({ type: "StringLiteral", value: r, loc: a });
}
boolean(r, a) {
return this.literal({ type: "BooleanLiteral", value: r, loc: a });
}
number(r, a) {
return this.literal({ type: "NumberLiteral", value: r, loc: a });
}
};
function o(r) {
switch (r.type) {
case "AtHead":
return { original: r.name, parts: [r.name] };
case "ThisHead":
return { original: "this", parts: [] };
case "VarHead":
return { original: r.name, parts: [r.name] };
}
}
var e = new c();
t.default = e;
} }), qt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/parser.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.Parser = void 0;
var f = X(), h = Ke(), d = class {
constructor(c) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : new h.EntityParser(h.HTML5NamedCharRefs), e = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : "precompile";
this.elementStack = [], this.currentAttribute = null, this.currentNode = null, this.source = c, this.lines = c.source.split(/(?:\r\n?|\n)/g), this.tokenizer = new h.EventedTokenizer(this, o, e);
}
offset() {
let { line: c, column: o } = this.tokenizer;
return this.source.offsetFor(c, o);
}
pos(c) {
let { line: o, column: e } = c;
return this.source.offsetFor(o, e);
}
finish(c) {
return (0, f.assign)({}, c, { loc: c.loc.until(this.offset()) });
}
get currentAttr() {
return this.currentAttribute;
}
get currentTag() {
return this.currentNode;
}
get currentStartTag() {
return this.currentNode;
}
get currentEndTag() {
return this.currentNode;
}
get currentComment() {
return this.currentNode;
}
get currentData() {
return this.currentNode;
}
acceptTemplate(c) {
return this[c.type](c);
}
acceptNode(c) {
return this[c.type](c);
}
currentElement() {
return this.elementStack[this.elementStack.length - 1];
}
sourceForNode(c, o) {
let e = c.loc.start.line - 1, r = e - 1, a = c.loc.start.column, p = [], n2, s, u;
for (o ? (s = o.loc.end.line - 1, u = o.loc.end.column) : (s = c.loc.end.line - 1, u = c.loc.end.column); r < s; )
r++, n2 = this.lines[r], r === e ? e === s ? p.push(n2.slice(a, u)) : p.push(n2.slice(a)) : r === s ? p.push(n2.slice(0, u)) : p.push(n2);
return p.join(`
`);
}
};
t.Parser = d;
} }), xt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/parser/handlebars-node-visitors.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.HandlebarsNodeVisitors = void 0;
var f = qt(), h = ge(), d = he(), c = ye(), o = we(), e = r(Le());
function r(i) {
return i && i.__esModule ? i : { default: i };
}
var a = class extends f.Parser {
get isTopLevel() {
return this.elementStack.length === 0;
}
Program(i) {
let l = [], b;
this.isTopLevel ? b = e.default.template({ body: l, blockParams: i.blockParams, loc: this.source.spanFor(i.loc) }) : b = e.default.blockItself({ body: l, blockParams: i.blockParams, chained: i.chained, loc: this.source.spanFor(i.loc) });
let P, E = i.body.length;
if (this.elementStack.push(b), E === 0)
return this.elementStack.pop();
for (P = 0; P < E; P++)
this.acceptNode(i.body[P]);
let v = this.elementStack.pop();
if (v !== b) {
let _ = v;
throw (0, d.generateSyntaxError)(`Unclosed element \`${_.tag}\``, _.loc);
}
return b;
}
BlockStatement(i) {
if (this.tokenizer.state === "comment") {
this.appendToCommentData(this.sourceForNode(i));
return;
}
if (this.tokenizer.state !== "data" && this.tokenizer.state !== "beforeData")
throw (0, d.generateSyntaxError)("A block may only be used inside an HTML element or another block.", this.source.spanFor(i.loc));
let { path: l, params: b, hash: P } = s(this, i);
i.program.loc || (i.program.loc = h.NON_EXISTENT_LOCATION), i.inverse && !i.inverse.loc && (i.inverse.loc = h.NON_EXISTENT_LOCATION);
let E = this.Program(i.program), v = i.inverse ? this.Program(i.inverse) : null, _ = e.default.block({ path: l, params: b, hash: P, defaultBlock: E, elseBlock: v, loc: this.source.spanFor(i.loc), openStrip: i.openStrip, inverseStrip: i.inverseStrip, closeStrip: i.closeStrip }), y = this.currentElement();
(0, c.appendChild)(y, _);
}
MustacheStatement(i) {
let { tokenizer: l } = this;
if (l.state === "comment") {
this.appendToCommentData(this.sourceForNode(i));
return;
}
let b, { escaped: P, loc: E, strip: v } = i;
if ((0, c.isHBSLiteral)(i.path))
b = e.default.mustache({ path: this.acceptNode(i.path), params: [], hash: e.default.hash([], this.source.spanFor(i.path.loc).collapse("end")), trusting: !P, loc: this.source.spanFor(E), strip: v });
else {
let { path: _, params: y, hash: g } = s(this, i);
b = e.default.mustache({ path: _, params: y, hash: g, trusting: !P, loc: this.source.spanFor(E), strip: v });
}
switch (l.state) {
case "tagOpen":
case "tagName":
throw (0, d.generateSyntaxError)("Cannot use mustaches in an elements tagname", b.loc);
case "beforeAttributeName":
u(this.currentStartTag, b);
break;
case "attributeName":
case "afterAttributeName":
this.beginAttributeValue(false), this.finishAttributeValue(), u(this.currentStartTag, b), l.transitionTo("beforeAttributeName");
break;
case "afterAttributeValueQuoted":
u(this.currentStartTag, b), l.transitionTo("beforeAttributeName");
break;
case "beforeAttributeValue":
this.beginAttributeValue(false), this.appendDynamicAttributeValuePart(b), l.transitionTo("attributeValueUnquoted");
break;
case "attributeValueDoubleQuoted":
case "attributeValueSingleQuoted":
case "attributeValueUnquoted":
this.appendDynamicAttributeValuePart(b);
break;
default:
(0, c.appendChild)(this.currentElement(), b);
}
return b;
}
appendDynamicAttributeValuePart(i) {
this.finalizeTextPart();
let l = this.currentAttr;
l.isDynamic = true, l.parts.push(i);
}
finalizeTextPart() {
let l = this.currentAttr.currentPart;
l !== null && (this.currentAttr.parts.push(l), this.startTextPart());
}
startTextPart() {
this.currentAttr.currentPart = null;
}
ContentStatement(i) {
n2(this.tokenizer, i), this.tokenizer.tokenizePart(i.value), this.tokenizer.flushData();
}
CommentStatement(i) {
let { tokenizer: l } = this;
if (l.state === "comment")
return this.appendToCommentData(this.sourceForNode(i)), null;
let { value: b, loc: P } = i, E = e.default.mustacheComment(b, this.source.spanFor(P));
switch (l.state) {
case "beforeAttributeName":
case "afterAttributeName":
this.currentStartTag.comments.push(E);
break;
case "beforeData":
case "data":
(0, c.appendChild)(this.currentElement(), E);
break;
default:
throw (0, d.generateSyntaxError)(`Using a Handlebars comment when in the \`${l.state}\` state is not supported`, this.source.spanFor(i.loc));
}
return E;
}
PartialStatement(i) {
throw (0, d.generateSyntaxError)("Handlebars partials are not supported", this.source.spanFor(i.loc));
}
PartialBlockStatement(i) {
throw (0, d.generateSyntaxError)("Handlebars partial blocks are not supported", this.source.spanFor(i.loc));
}
Decorator(i) {
throw (0, d.generateSyntaxError)("Handlebars decorators are not supported", this.source.spanFor(i.loc));
}
DecoratorBlock(i) {
throw (0, d.generateSyntaxError)("Handlebars decorator blocks are not supported", this.source.spanFor(i.loc));
}
SubExpression(i) {
let { path: l, params: b, hash: P } = s(this, i);
return e.default.sexpr({ path: l, params: b, hash: P, loc: this.source.spanFor(i.loc) });
}
PathExpression(i) {
let { original: l } = i, b;
if (l.indexOf("/") !== -1) {
if (l.slice(0, 2) === "./")
throw (0, d.generateSyntaxError)('Using "./" is not supported in Glimmer and unnecessary', this.source.spanFor(i.loc));
if (l.slice(0, 3) === "../")
throw (0, d.generateSyntaxError)('Changing context using "../" is not supported in Glimmer', this.source.spanFor(i.loc));
if (l.indexOf(".") !== -1)
throw (0, d.generateSyntaxError)("Mixing '.' and '/' in paths is not supported in Glimmer; use only '.' to separate property paths", this.source.spanFor(i.loc));
b = [i.parts.join("/")];
} else {
if (l === ".")
throw (0, d.generateSyntaxError)("'.' is not a supported path in Glimmer; check for a path with a trailing '.'", this.source.spanFor(i.loc));
b = i.parts;
}
let P = false;
l.match(/^this(\..+)?$/) && (P = true);
let E;
if (P)
E = { type: "ThisHead", loc: { start: i.loc.start, end: { line: i.loc.start.line, column: i.loc.start.column + 4 } } };
else if (i.data) {
let v = b.shift();
if (v === void 0)
throw (0, d.generateSyntaxError)("Attempted to parse a path expression, but it was not valid. Paths beginning with @ must start with a-z.", this.source.spanFor(i.loc));
E = { type: "AtHead", name: `@${v}`, loc: { start: i.loc.start, end: { line: i.loc.start.line, column: i.loc.start.column + v.length + 1 } } };
} else {
let v = b.shift();
if (v === void 0)
throw (0, d.generateSyntaxError)("Attempted to parse a path expression, but it was not valid. Paths must start with a-z or A-Z.", this.source.spanFor(i.loc));
E = { type: "VarHead", name: v, loc: { start: i.loc.start, end: { line: i.loc.start.line, column: i.loc.start.column + v.length } } };
}
return new o.PathExpressionImplV1(i.original, E, b, this.source.spanFor(i.loc));
}
Hash(i) {
let l = [];
for (let b = 0; b < i.pairs.length; b++) {
let P = i.pairs[b];
l.push(e.default.pair({ key: P.key, value: this.acceptNode(P.value), loc: this.source.spanFor(P.loc) }));
}
return e.default.hash(l, this.source.spanFor(i.loc));
}
StringLiteral(i) {
return e.default.literal({ type: "StringLiteral", value: i.value, loc: i.loc });
}
BooleanLiteral(i) {
return e.default.literal({ type: "BooleanLiteral", value: i.value, loc: i.loc });
}
NumberLiteral(i) {
return e.default.literal({ type: "NumberLiteral", value: i.value, loc: i.loc });
}
UndefinedLiteral(i) {
return e.default.literal({ type: "UndefinedLiteral", value: void 0, loc: i.loc });
}
NullLiteral(i) {
return e.default.literal({ type: "NullLiteral", value: null, loc: i.loc });
}
};
t.HandlebarsNodeVisitors = a;
function p(i, l) {
if (l === "")
return { lines: i.split(`
`).length - 1, columns: 0 };
let P = i.split(l)[0].split(/\n/), E = P.length - 1;
return { lines: E, columns: P[E].length };
}
function n2(i, l) {
let b = l.loc.start.line, P = l.loc.start.column, E = p(l.original, l.value);
b = b + E.lines, E.lines ? P = E.columns : P = P + E.columns, i.line = b, i.column = P;
}
function s(i, l) {
if (l.path.type.endsWith("Literal")) {
let _ = l.path, y = "";
throw _.type === "BooleanLiteral" ? y = _.original.toString() : _.type === "StringLiteral" ? y = `"${_.original}"` : _.type === "NullLiteral" ? y = "null" : _.type === "NumberLiteral" ? y = _.value.toString() : y = "undefined", (0, d.generateSyntaxError)(`${_.type} "${_.type === "StringLiteral" ? _.original : y}" cannot be called as a sub-expression, replace (${y}) with ${y}`, i.source.spanFor(_.loc));
}
let b = l.path.type === "PathExpression" ? i.PathExpression(l.path) : i.SubExpression(l.path), P = l.params ? l.params.map((_) => i.acceptNode(_)) : [], E = P.length > 0 ? P[P.length - 1].loc : b.loc, v = l.hash ? i.Hash(l.hash) : { type: "Hash", pairs: [], loc: i.source.spanFor(E).collapse("end") };
return { path: b, params: P, hash: v };
}
function u(i, l) {
let { path: b, params: P, hash: E, loc: v } = l;
if ((0, c.isHBSLiteral)(b)) {
let y = `{{${(0, c.printLiteral)(b)}}}`, g = `<${i.name} ... ${y} ...`;
throw (0, d.generateSyntaxError)(`In ${g}, ${y} is not a valid modifier`, l.loc);
}
let _ = e.default.elementModifier({ path: b, params: P, hash: E, loc: v });
i.modifiers.push(_);
}
} }), Fe = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/parser/tokenizer-event-handlers.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.preprocess = _, t.TokenizerEventHandlers = void 0;
var f = X(), h = It(), d = Ke(), c = b(We()), o = Te(), e = De(), r = ue(), a = he(), p = b(Ne()), n2 = b(Je()), s = ye(), u = b(Le()), i = b(ke()), l = xt();
function b(y) {
return y && y.__esModule ? y : { default: y };
}
var P = class extends l.HandlebarsNodeVisitors {
constructor() {
super(...arguments), this.tagOpenLine = 0, this.tagOpenColumn = 0;
}
reset() {
this.currentNode = null;
}
beginComment() {
this.currentNode = u.default.comment("", this.source.offsetFor(this.tagOpenLine, this.tagOpenColumn));
}
appendToCommentData(y) {
this.currentComment.value += y;
}
finishComment() {
(0, s.appendChild)(this.currentElement(), this.finish(this.currentComment));
}
beginData() {
this.currentNode = u.default.text({ chars: "", loc: this.offset().collapsed() });
}
appendToData(y) {
this.currentData.chars += y;
}
finishData() {
this.currentData.loc = this.currentData.loc.withEnd(this.offset()), (0, s.appendChild)(this.currentElement(), this.currentData);
}
tagOpen() {
this.tagOpenLine = this.tokenizer.line, this.tagOpenColumn = this.tokenizer.column;
}
beginStartTag() {
this.currentNode = { type: "StartTag", name: "", attributes: [], modifiers: [], comments: [], selfClosing: false, loc: this.source.offsetFor(this.tagOpenLine, this.tagOpenColumn) };
}
beginEndTag() {
this.currentNode = { type: "EndTag", name: "", attributes: [], modifiers: [], comments: [], selfClosing: false, loc: this.source.offsetFor(this.tagOpenLine, this.tagOpenColumn) };
}
finishTag() {
let y = this.finish(this.currentTag);
if (y.type === "StartTag") {
if (this.finishStartTag(), y.name === ":")
throw (0, a.generateSyntaxError)("Invalid named block named detected, you may have created a named block without a name, or you may have began your name with a number. Named blocks must have names that are at least one character long, and begin with a lower case letter", this.source.spanFor({ start: this.currentTag.loc.toJSON(), end: this.offset().toJSON() }));
(o.voidMap[y.name] || y.selfClosing) && this.finishEndTag(true);
} else
y.type === "EndTag" && this.finishEndTag(false);
}
finishStartTag() {
let { name: y, attributes: g, modifiers: L, comments: j, selfClosing: x, loc: w } = this.finish(this.currentStartTag), H = u.default.element({ tag: y, selfClosing: x, attrs: g, modifiers: L, comments: j, children: [], blockParams: [], loc: w });
this.elementStack.push(H);
}
finishEndTag(y) {
let g = this.finish(this.currentTag), L = this.elementStack.pop(), j = this.currentElement();
this.validateEndTag(g, L, y), L.loc = L.loc.withEnd(this.offset()), (0, s.parseElementBlockParams)(L), (0, s.appendChild)(j, L);
}
markTagAsSelfClosing() {
this.currentTag.selfClosing = true;
}
appendToTagName(y) {
this.currentTag.name += y;
}
beginAttribute() {
let y = this.offset();
this.currentAttribute = { name: "", parts: [], currentPart: null, isQuoted: false, isDynamic: false, start: y, valueSpan: y.collapsed() };
}
appendToAttributeName(y) {
this.currentAttr.name += y;
}
beginAttributeValue(y) {
this.currentAttr.isQuoted = y, this.startTextPart(), this.currentAttr.valueSpan = this.offset().collapsed();
}
appendToAttributeValue(y) {
let g = this.currentAttr.parts, L = g[g.length - 1], j = this.currentAttr.currentPart;
if (j)
j.chars += y, j.loc = j.loc.withEnd(this.offset());
else {
let x = this.offset();
y === `
` ? x = L ? L.loc.getEnd() : this.currentAttr.valueSpan.getStart() : x = x.move(-1), this.currentAttr.currentPart = u.default.text({ chars: y, loc: x.collapsed() });
}
}
finishAttributeValue() {
this.finalizeTextPart();
let y = this.currentTag, g = this.offset();
if (y.type === "EndTag")
throw (0, a.generateSyntaxError)("Invalid end tag: closing tag must not have attributes", this.source.spanFor({ start: y.loc.toJSON(), end: g.toJSON() }));
let { name: L, parts: j, start: x, isQuoted: w, isDynamic: H, valueSpan: m } = this.currentAttr, C = this.assembleAttributeValue(j, w, H, x.until(g));
C.loc = m.withEnd(g);
let S = u.default.attr({ name: L, value: C, loc: x.until(g) });
this.currentStartTag.attributes.push(S);
}
reportSyntaxError(y) {
throw (0, a.generateSyntaxError)(y, this.offset().collapsed());
}
assembleConcatenatedValue(y) {
for (let j = 0; j < y.length; j++) {
let x = y[j];
if (x.type !== "MustacheStatement" && x.type !== "TextNode")
throw (0, a.generateSyntaxError)("Unsupported node in quoted attribute value: " + x.type, x.loc);
}
(0, f.assertPresent)(y, "the concatenation parts of an element should not be empty");
let g = y[0], L = y[y.length - 1];
return u.default.concat(y, this.source.spanFor(g.loc).extend(this.source.spanFor(L.loc)));
}
validateEndTag(y, g, L) {
let j;
if (o.voidMap[y.name] && !L ? j = `<${y.name}> elements do not need end tags. You should remove it` : g.tag === void 0 ? j = `Closing tag ${y.name}> without an open tag` : g.tag !== y.name && (j = `Closing tag ${y.name}> did not match last open tag <${g.tag}> (on line ${g.loc.startPosition.line})`), j)
throw (0, a.generateSyntaxError)(j, y.loc);
}
assembleAttributeValue(y, g, L, j) {
if (L) {
if (g)
return this.assembleConcatenatedValue(y);
if (y.length === 1 || y.length === 2 && y[1].type === "TextNode" && y[1].chars === "/")
return y[0];
throw (0, a.generateSyntaxError)("An unquoted attribute value must be a string or a mustache, preceded by whitespace or a '=' character, and followed by whitespace, a '>' character, or '/>'", j);
} else
return y.length > 0 ? y[0] : u.default.text({ chars: "", loc: j });
}
};
t.TokenizerEventHandlers = P;
var E = { parse: _, builders: i.default, print: c.default, traverse: p.default, Walker: n2.default }, v = class extends d.EntityParser {
constructor() {
super({});
}
parse() {
}
};
function _(y) {
let g = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
var L, j, x;
let w = g.mode || "precompile", H, m;
typeof y == "string" ? (H = new e.Source(y, (L = g.meta) === null || L === void 0 ? void 0 : L.moduleName), w === "codemod" ? m = (0, h.parseWithoutProcessing)(y, g.parseOptions) : m = (0, h.parse)(y, g.parseOptions)) : y instanceof e.Source ? (H = y, w === "codemod" ? m = (0, h.parseWithoutProcessing)(y.source, g.parseOptions) : m = (0, h.parse)(y.source, g.parseOptions)) : (H = new e.Source("", (j = g.meta) === null || j === void 0 ? void 0 : j.moduleName), m = y);
let C;
w === "codemod" && (C = new v());
let S = r.SourceSpan.forCharPositions(H, 0, H.source.length);
m.loc = { source: "(program)", start: S.startPosition, end: S.endPosition };
let R = new P(H, C, w).acceptTemplate(m);
if (g.strictMode && (R.blockParams = (x = g.locals) !== null && x !== void 0 ? x : []), g && g.plugins && g.plugins.ast)
for (let M = 0, V = g.plugins.ast.length; M < V; M++) {
let G = g.plugins.ast[M], K = (0, f.assign)({}, g, { syntax: E }, { plugins: void 0 }), U = G(K);
(0, p.default)(R, U.visitor);
}
return R;
}
} }), Xe = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/symbol-table.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.BlockSymbolTable = t.ProgramSymbolTable = t.SymbolTable = void 0;
var f = X(), h = ye(), d = class {
static top(e, r) {
return new c(e, r);
}
child(e) {
let r = e.map((a) => this.allocate(a));
return new o(this, e, r);
}
};
t.SymbolTable = d;
var c = class extends d {
constructor(e, r) {
super(), this.templateLocals = e, this.customizeComponentName = r, this.symbols = [], this.upvars = [], this.size = 1, this.named = (0, f.dict)(), this.blocks = (0, f.dict)(), this.usedTemplateLocals = [], this._hasEval = false;
}
getUsedTemplateLocals() {
return this.usedTemplateLocals;
}
setHasEval() {
this._hasEval = true;
}
get hasEval() {
return this._hasEval;
}
has(e) {
return this.templateLocals.indexOf(e) !== -1;
}
get(e) {
let r = this.usedTemplateLocals.indexOf(e);
return r !== -1 ? [r, true] : (r = this.usedTemplateLocals.length, this.usedTemplateLocals.push(e), [r, true]);
}
getLocalsMap() {
return (0, f.dict)();
}
getEvalInfo() {
let e = this.getLocalsMap();
return Object.keys(e).map((r) => e[r]);
}
allocateFree(e, r) {
r.resolution() === 39 && r.isAngleBracket && (0, h.isUpperCase)(e) && (e = this.customizeComponentName(e));
let a = this.upvars.indexOf(e);
return a !== -1 || (a = this.upvars.length, this.upvars.push(e)), a;
}
allocateNamed(e) {
let r = this.named[e];
return r || (r = this.named[e] = this.allocate(e)), r;
}
allocateBlock(e) {
e === "inverse" && (e = "else");
let r = this.blocks[e];
return r || (r = this.blocks[e] = this.allocate(`&${e}`)), r;
}
allocate(e) {
return this.symbols.push(e), this.size++;
}
};
t.ProgramSymbolTable = c;
var o = class extends d {
constructor(e, r, a) {
super(), this.parent = e, this.symbols = r, this.slots = a;
}
get locals() {
return this.symbols;
}
has(e) {
return this.symbols.indexOf(e) !== -1 || this.parent.has(e);
}
get(e) {
let r = this.symbols.indexOf(e);
return r === -1 ? this.parent.get(e) : [this.slots[r], false];
}
getLocalsMap() {
let e = this.parent.getLocalsMap();
return this.symbols.forEach((r) => e[r] = this.get(r)[0]), e;
}
getEvalInfo() {
let e = this.getLocalsMap();
return Object.keys(e).map((r) => e[r]);
}
setHasEval() {
this.parent.setHasEval();
}
allocateFree(e, r) {
return this.parent.allocateFree(e, r);
}
allocateNamed(e) {
return this.parent.allocateNamed(e);
}
allocateBlock(e) {
return this.parent.allocateBlock(e);
}
allocate(e) {
return this.parent.allocate(e);
}
};
t.BlockSymbolTable = o;
} }), jt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/builders.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.BuildElement = t.Builder = void 0;
var f = X(), h = le(), d = ce(), c = e(ve());
function o() {
if (typeof WeakMap != "function")
return null;
var n2 = /* @__PURE__ */ new WeakMap();
return o = function() {
return n2;
}, n2;
}
function e(n2) {
if (n2 && n2.__esModule)
return n2;
if (n2 === null || typeof n2 != "object" && typeof n2 != "function")
return { default: n2 };
var s = o();
if (s && s.has(n2))
return s.get(n2);
var u = {}, i = Object.defineProperty && Object.getOwnPropertyDescriptor;
for (var l in n2)
if (Object.prototype.hasOwnProperty.call(n2, l)) {
var b = i ? Object.getOwnPropertyDescriptor(n2, l) : null;
b && (b.get || b.set) ? Object.defineProperty(u, l, b) : u[l] = n2[l];
}
return u.default = n2, s && s.set(n2, u), u;
}
var r = function(n2, s) {
var u = {};
for (var i in n2)
Object.prototype.hasOwnProperty.call(n2, i) && s.indexOf(i) < 0 && (u[i] = n2[i]);
if (n2 != null && typeof Object.getOwnPropertySymbols == "function")
for (var l = 0, i = Object.getOwnPropertySymbols(n2); l < i.length; l++)
s.indexOf(i[l]) < 0 && Object.prototype.propertyIsEnumerable.call(n2, i[l]) && (u[i[l]] = n2[i[l]]);
return u;
}, a = class {
template(n2, s, u) {
return new c.Template({ table: n2, body: s, loc: u });
}
block(n2, s, u) {
return new c.Block({ scope: n2, body: s, loc: u });
}
namedBlock(n2, s, u) {
return new c.NamedBlock({ name: n2, block: s, attrs: [], componentArgs: [], modifiers: [], loc: u });
}
simpleNamedBlock(n2, s, u) {
return new p({ selfClosing: false, attrs: [], componentArgs: [], modifiers: [], comments: [] }).named(n2, s, u);
}
slice(n2, s) {
return new h.SourceSlice({ loc: s, chars: n2 });
}
args(n2, s, u) {
return new c.Args({ loc: u, positional: n2, named: s });
}
positional(n2, s) {
return new c.PositionalArguments({ loc: s, exprs: n2 });
}
namedArgument(n2, s) {
return new c.NamedArgument({ name: n2, value: s });
}
named(n2, s) {
return new c.NamedArguments({ loc: s, entries: n2 });
}
attr(n2, s) {
let { name: u, value: i, trusting: l } = n2;
return new c.HtmlAttr({ loc: s, name: u, value: i, trusting: l });
}
splatAttr(n2, s) {
return new c.SplatAttr({ symbol: n2, loc: s });
}
arg(n2, s) {
let { name: u, value: i, trusting: l } = n2;
return new c.ComponentArg({ name: u, value: i, trusting: l, loc: s });
}
path(n2, s, u) {
return new c.PathExpression({ loc: u, ref: n2, tail: s });
}
self(n2) {
return new c.ThisReference({ loc: n2 });
}
at(n2, s, u) {
return new c.ArgReference({ loc: u, name: new h.SourceSlice({ loc: u, chars: n2 }), symbol: s });
}
freeVar(n2) {
let { name: s, context: u, symbol: i, loc: l } = n2;
return new c.FreeVarReference({ name: s, resolution: u, symbol: i, loc: l });
}
localVar(n2, s, u, i) {
return new c.LocalVarReference({ loc: i, name: n2, isTemplateLocal: u, symbol: s });
}
sexp(n2, s) {
return new c.CallExpression({ loc: s, callee: n2.callee, args: n2.args });
}
deprecatedCall(n2, s, u) {
return new c.DeprecatedCallExpression({ loc: u, arg: n2, callee: s });
}
interpolate(n2, s) {
return (0, f.assertPresent)(n2), new c.InterpolateExpression({ loc: s, parts: n2 });
}
literal(n2, s) {
return new c.LiteralExpression({ loc: s, value: n2 });
}
append(n2, s) {
let { table: u, trusting: i, value: l } = n2;
return new c.AppendContent({ table: u, trusting: i, value: l, loc: s });
}
modifier(n2, s) {
let { callee: u, args: i } = n2;
return new c.ElementModifier({ loc: s, callee: u, args: i });
}
namedBlocks(n2, s) {
return new c.NamedBlocks({ loc: s, blocks: n2 });
}
blockStatement(n2, s) {
var { symbols: u, program: i, inverse: l = null } = n2, b = r(n2, ["symbols", "program", "inverse"]);
let P = i.loc, E = [this.namedBlock(h.SourceSlice.synthetic("default"), i, i.loc)];
return l && (P = P.extend(l.loc), E.push(this.namedBlock(h.SourceSlice.synthetic("else"), l, l.loc))), new c.InvokeBlock({ loc: s, blocks: this.namedBlocks(E, P), callee: b.callee, args: b.args });
}
element(n2) {
return new p(n2);
}
};
t.Builder = a;
var p = class {
constructor(n2) {
this.base = n2, this.builder = new a();
}
simple(n2, s, u) {
return new c.SimpleElement((0, f.assign)({ tag: n2, body: s, componentArgs: [], loc: u }, this.base));
}
named(n2, s, u) {
return new c.NamedBlock((0, f.assign)({ name: n2, block: s, componentArgs: [], loc: u }, this.base));
}
selfClosingComponent(n2, s) {
return new c.InvokeComponent((0, f.assign)({ loc: s, callee: n2, blocks: new c.NamedBlocks({ blocks: [], loc: s.sliceEndChars({ skipEnd: 1, chars: 1 }) }) }, this.base));
}
componentWithDefaultBlock(n2, s, u, i) {
let l = this.builder.block(u, s, i), b = this.builder.namedBlock(h.SourceSlice.synthetic("default"), l, i);
return new c.InvokeComponent((0, f.assign)({ loc: i, callee: n2, blocks: this.builder.namedBlocks([b], b.loc) }, this.base));
}
componentWithNamedBlocks(n2, s, u) {
return new c.InvokeComponent((0, f.assign)({ loc: u, callee: n2, blocks: this.builder.namedBlocks(s, d.SpanList.range(s)) }, this.base));
}
};
t.BuildElement = p;
} }), Mt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/loose-resolution.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.SexpSyntaxContext = c, t.ModifierSyntaxContext = o, t.BlockSyntaxContext = e, t.ComponentSyntaxContext = r, t.AttrValueSyntaxContext = a, t.AppendSyntaxContext = p;
var f = d(ve());
function h() {
if (typeof WeakMap != "function")
return null;
var i = /* @__PURE__ */ new WeakMap();
return h = function() {
return i;
}, i;
}
function d(i) {
if (i && i.__esModule)
return i;
if (i === null || typeof i != "object" && typeof i != "function")
return { default: i };
var l = h();
if (l && l.has(i))
return l.get(i);
var b = {}, P = Object.defineProperty && Object.getOwnPropertyDescriptor;
for (var E in i)
if (Object.prototype.hasOwnProperty.call(i, E)) {
var v = P ? Object.getOwnPropertyDescriptor(i, E) : null;
v && (v.get || v.set) ? Object.defineProperty(b, E, v) : b[E] = i[E];
}
return b.default = i, l && l.set(i, b), b;
}
function c(i) {
return n2(i) ? f.LooseModeResolution.namespaced("Helper") : null;
}
function o(i) {
return n2(i) ? f.LooseModeResolution.namespaced("Modifier") : null;
}
function e(i) {
return n2(i) ? f.LooseModeResolution.namespaced("Component") : f.LooseModeResolution.fallback();
}
function r(i) {
return s(i) ? f.LooseModeResolution.namespaced("Component", true) : null;
}
function a(i) {
let l = n2(i), b = u(i);
return l ? b ? f.LooseModeResolution.namespaced("Helper") : f.LooseModeResolution.attr() : b ? f.STRICT_RESOLUTION : f.LooseModeResolution.fallback();
}
function p(i) {
let l = n2(i), b = u(i), P = i.trusting;
return l ? P ? f.LooseModeResolution.trustingAppend({ invoke: b }) : f.LooseModeResolution.append({ invoke: b }) : f.LooseModeResolution.fallback();
}
function n2(i) {
let l = i.path;
return s(l);
}
function s(i) {
return i.type === "PathExpression" && i.head.type === "VarHead" ? i.tail.length === 0 : false;
}
function u(i) {
return i.params.length > 0 || i.hash.pairs.length > 0;
}
} }), Ht = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/v2-a/normalize.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.normalize = P, t.BlockContext = void 0;
var f = X(), h = b(Te()), d = Fe(), c = le(), o = ce(), e = Xe(), r = he(), a = ye(), p = b(Le()), n2 = l(ve()), s = jt(), u = Mt();
function i() {
if (typeof WeakMap != "function")
return null;
var m = /* @__PURE__ */ new WeakMap();
return i = function() {
return m;
}, m;
}
function l(m) {
if (m && m.__esModule)
return m;
if (m === null || typeof m != "object" && typeof m != "function")
return { default: m };
var C = i();
if (C && C.has(m))
return C.get(m);
var S = {}, R = Object.defineProperty && Object.getOwnPropertyDescriptor;
for (var M in m)
if (Object.prototype.hasOwnProperty.call(m, M)) {
var V = R ? Object.getOwnPropertyDescriptor(m, M) : null;
V && (V.get || V.set) ? Object.defineProperty(S, M, V) : S[M] = m[M];
}
return S.default = m, C && C.set(m, S), S;
}
function b(m) {
return m && m.__esModule ? m : { default: m };
}
function P(m) {
let C = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
var S;
let R = (0, d.preprocess)(m, C), M = (0, f.assign)({ strictMode: false, locals: [] }, C), V = e.SymbolTable.top(M.locals, (S = C.customizeComponentName) !== null && S !== void 0 ? S : (W) => W), G = new E(m, M, V), K = new _(G), U = new L(G.loc(R.loc), R.body.map((W) => K.normalize(W)), G).assertTemplate(V), Z = V.getUsedTemplateLocals();
return [U, Z];
}
var E = class {
constructor(m, C, S) {
this.source = m, this.options = C, this.table = S, this.builder = new s.Builder();
}
get strict() {
return this.options.strictMode || false;
}
loc(m) {
return this.source.spanFor(m);
}
resolutionFor(m, C) {
if (this.strict)
return { resolution: n2.STRICT_RESOLUTION };
if (this.isFreeVar(m)) {
let S = C(m);
return S === null ? { resolution: "error", path: w(m), head: H(m) } : { resolution: S };
} else
return { resolution: n2.STRICT_RESOLUTION };
}
isFreeVar(m) {
return m.type === "PathExpression" ? m.head.type !== "VarHead" ? false : !this.table.has(m.head.name) : m.path.type === "PathExpression" ? this.isFreeVar(m.path) : false;
}
hasBinding(m) {
return this.table.has(m);
}
child(m) {
return new E(this.source, this.options, this.table.child(m));
}
customizeComponentName(m) {
return this.options.customizeComponentName ? this.options.customizeComponentName(m) : m;
}
};
t.BlockContext = E;
var v = class {
constructor(m) {
this.block = m;
}
normalize(m, C) {
switch (m.type) {
case "NullLiteral":
case "BooleanLiteral":
case "NumberLiteral":
case "StringLiteral":
case "UndefinedLiteral":
return this.block.builder.literal(m.value, this.block.loc(m.loc));
case "PathExpression":
return this.path(m, C);
case "SubExpression": {
let S = this.block.resolutionFor(m, u.SexpSyntaxContext);
if (S.resolution === "error")
throw (0, r.generateSyntaxError)(`You attempted to invoke a path (\`${S.path}\`) but ${S.head} was not in scope`, m.loc);
return this.block.builder.sexp(this.callParts(m, S.resolution), this.block.loc(m.loc));
}
}
}
path(m, C) {
let S = this.block.loc(m.head.loc), R = [], M = S;
for (let V of m.tail)
M = M.sliceStartChars({ chars: V.length, skipStart: 1 }), R.push(new c.SourceSlice({ loc: M, chars: V }));
return this.block.builder.path(this.ref(m.head, C), R, this.block.loc(m.loc));
}
callParts(m, C) {
let { path: S, params: R, hash: M } = m, V = this.normalize(S, C), G = R.map((N) => this.normalize(N, n2.ARGUMENT_RESOLUTION)), K = o.SpanList.range(G, V.loc.collapse("end")), U = this.block.loc(M.loc), Z = o.SpanList.range([K, U]), W = this.block.builder.positional(R.map((N) => this.normalize(N, n2.ARGUMENT_RESOLUTION)), K), T = this.block.builder.named(M.pairs.map((N) => this.namedArgument(N)), this.block.loc(M.loc));
return { callee: V, args: this.block.builder.args(W, T, Z) };
}
namedArgument(m) {
let S = this.block.loc(m.loc).sliceStartChars({ chars: m.key.length });
return this.block.builder.namedArgument(new c.SourceSlice({ chars: m.key, loc: S }), this.normalize(m.value, n2.ARGUMENT_RESOLUTION));
}
ref(m, C) {
let { block: S } = this, { builder: R, table: M } = S, V = S.loc(m.loc);
switch (m.type) {
case "ThisHead":
return R.self(V);
case "AtHead": {
let G = M.allocateNamed(m.name);
return R.at(m.name, G, V);
}
case "VarHead":
if (S.hasBinding(m.name)) {
let [G, K] = M.get(m.name);
return S.builder.localVar(m.name, G, K, V);
} else {
let G = S.strict ? n2.STRICT_RESOLUTION : C, K = S.table.allocateFree(m.name, G);
return S.builder.freeVar({ name: m.name, context: G, symbol: K, loc: V });
}
}
}
}, _ = class {
constructor(m) {
this.block = m;
}
normalize(m) {
switch (m.type) {
case "PartialStatement":
throw new Error("Handlebars partial syntax ({{> ...}}) is not allowed in Glimmer");
case "BlockStatement":
return this.BlockStatement(m);
case "ElementNode":
return new y(this.block).ElementNode(m);
case "MustacheStatement":
return this.MustacheStatement(m);
case "MustacheCommentStatement":
return this.MustacheCommentStatement(m);
case "CommentStatement": {
let C = this.block.loc(m.loc);
return new n2.HtmlComment({ loc: C, text: C.slice({ skipStart: 4, skipEnd: 3 }).toSlice(m.value) });
}
case "TextNode":
return new n2.HtmlText({ loc: this.block.loc(m.loc), chars: m.chars });
}
}
MustacheCommentStatement(m) {
let C = this.block.loc(m.loc), S;
return C.asString().slice(0, 5) === "{{!--" ? S = C.slice({ skipStart: 5, skipEnd: 4 }) : S = C.slice({ skipStart: 3, skipEnd: 2 }), new n2.GlimmerComment({ loc: C, text: S.toSlice(m.value) });
}
MustacheStatement(m) {
let { escaped: C } = m, S = this.block.loc(m.loc), R = this.expr.callParts({ path: m.path, params: m.params, hash: m.hash }, (0, u.AppendSyntaxContext)(m)), M = R.args.isEmpty() ? R.callee : this.block.builder.sexp(R, S);
return this.block.builder.append({ table: this.block.table, trusting: !C, value: M }, S);
}
BlockStatement(m) {
let { program: C, inverse: S } = m, R = this.block.loc(m.loc), M = this.block.resolutionFor(m, u.BlockSyntaxContext);
if (M.resolution === "error")
throw (0, r.generateSyntaxError)(`You attempted to invoke a path (\`{{#${M.path}}}\`) but ${M.head} was not in scope`, R);
let V = this.expr.callParts(m, M.resolution);
return this.block.builder.blockStatement((0, f.assign)({ symbols: this.block.table, program: this.Block(C), inverse: S ? this.Block(S) : null }, V), R);
}
Block(m) {
let { body: C, loc: S, blockParams: R } = m, M = this.block.child(R), V = new _(M);
return new j(this.block.loc(S), C.map((G) => V.normalize(G)), this.block).assertBlock(M.table);
}
get expr() {
return new v(this.block);
}
}, y = class {
constructor(m) {
this.ctx = m;
}
ElementNode(m) {
let { tag: C, selfClosing: S, comments: R } = m, M = this.ctx.loc(m.loc), [V, ...G] = C.split("."), K = this.classifyTag(V, G, m.loc), U = m.attributes.filter((A) => A.name[0] !== "@").map((A) => this.attr(A)), Z = m.attributes.filter((A) => A.name[0] === "@").map((A) => this.arg(A)), W = m.modifiers.map((A) => this.modifier(A)), T = this.ctx.child(m.blockParams), N = new _(T), k = m.children.map((A) => N.normalize(A)), B = this.ctx.builder.element({ selfClosing: S, attrs: U, componentArgs: Z, modifiers: W, comments: R.map((A) => new _(this.ctx).MustacheCommentStatement(A)) }), O = new x(B, M, k, this.ctx), z = this.ctx.loc(m.loc).sliceStartChars({ chars: C.length, skipStart: 1 });
if (K === "ElementHead")
return C[0] === ":" ? O.assertNamedBlock(z.slice({ skipStart: 1 }).toSlice(C.slice(1)), T.table) : O.assertElement(z.toSlice(C), m.blockParams.length > 0);
if (m.selfClosing)
return B.selfClosingComponent(K, M);
{
let A = O.assertComponent(C, T.table, m.blockParams.length > 0);
return B.componentWithNamedBlocks(K, A, M);
}
}
modifier(m) {
let C = this.ctx.resolutionFor(m, u.ModifierSyntaxContext);
if (C.resolution === "error")
throw (0, r.generateSyntaxError)(`You attempted to invoke a path (\`{{#${C.path}}}\`) as a modifier, but ${C.head} was not in scope. Try adding \`this\` to the beginning of the path`, m.loc);
let S = this.expr.callParts(m, C.resolution);
return this.ctx.builder.modifier(S, this.ctx.loc(m.loc));
}
mustacheAttr(m) {
let C = this.ctx.builder.sexp(this.expr.callParts(m, (0, u.AttrValueSyntaxContext)(m)), this.ctx.loc(m.loc));
return C.args.isEmpty() ? C.callee : C;
}
attrPart(m) {
switch (m.type) {
case "MustacheStatement":
return { expr: this.mustacheAttr(m), trusting: !m.escaped };
case "TextNode":
return { expr: this.ctx.builder.literal(m.chars, this.ctx.loc(m.loc)), trusting: true };
}
}
attrValue(m) {
switch (m.type) {
case "ConcatStatement": {
let C = m.parts.map((S) => this.attrPart(S).expr);
return { expr: this.ctx.builder.interpolate(C, this.ctx.loc(m.loc)), trusting: false };
}
default:
return this.attrPart(m);
}
}
attr(m) {
if (m.name === "...attributes")
return this.ctx.builder.splatAttr(this.ctx.table.allocateBlock("attrs"), this.ctx.loc(m.loc));
let C = this.ctx.loc(m.loc), S = C.sliceStartChars({ chars: m.name.length }).toSlice(m.name), R = this.attrValue(m.value);
return this.ctx.builder.attr({ name: S, value: R.expr, trusting: R.trusting }, C);
}
maybeDeprecatedCall(m, C) {
if (this.ctx.strict || C.type !== "MustacheStatement")
return null;
let { path: S } = C;
if (S.type !== "PathExpression" || S.head.type !== "VarHead")
return null;
let { name: R } = S.head;
if (R === "has-block" || R === "has-block-params" || this.ctx.hasBinding(R) || S.tail.length !== 0 || C.params.length !== 0 || C.hash.pairs.length !== 0)
return null;
let M = n2.LooseModeResolution.attr(), V = this.ctx.builder.freeVar({ name: R, context: M, symbol: this.ctx.table.allocateFree(R, M), loc: S.loc });
return { expr: this.ctx.builder.deprecatedCall(m, V, C.loc), trusting: false };
}
arg(m) {
let C = this.ctx.loc(m.loc), S = C.sliceStartChars({ chars: m.name.length }).toSlice(m.name), R = this.maybeDeprecatedCall(S, m.value) || this.attrValue(m.value);
return this.ctx.builder.arg({ name: S, value: R.expr, trusting: R.trusting }, C);
}
classifyTag(m, C, S) {
let R = (0, a.isUpperCase)(m), M = m[0] === "@" || m === "this" || this.ctx.hasBinding(m);
if (this.ctx.strict && !M) {
if (R)
throw (0, r.generateSyntaxError)(`Attempted to invoke a component that was not in scope in a strict mode template, \`<${m}>\`. If you wanted to create an element with that name, convert it to lowercase - \`<${m.toLowerCase()}>\``, S);
return "ElementHead";
}
let V = M || R, G = S.sliceStartChars({ skipStart: 1, chars: m.length }), K = C.reduce((W, T) => W + 1 + T.length, 0), U = G.getEnd().move(K), Z = G.withEnd(U);
if (V) {
let W = p.default.path({ head: p.default.head(m, G), tail: C, loc: Z }), T = this.ctx.resolutionFor(W, u.ComponentSyntaxContext);
if (T.resolution === "error")
throw (0, r.generateSyntaxError)(`You attempted to invoke a path (\`<${T.path}>\`) but ${T.head} was not in scope`, S);
return new v(this.ctx).normalize(W, T.resolution);
}
if (C.length > 0)
throw (0, r.generateSyntaxError)(`You used ${m}.${C.join(".")} as a tag name, but ${m} is not in scope`, S);
return "ElementHead";
}
get expr() {
return new v(this.ctx);
}
}, g = class {
constructor(m, C, S) {
this.loc = m, this.children = C, this.block = S, this.namedBlocks = C.filter((R) => R instanceof n2.NamedBlock), this.hasSemanticContent = Boolean(C.filter((R) => {
if (R instanceof n2.NamedBlock)
return false;
switch (R.type) {
case "GlimmerComment":
case "HtmlComment":
return false;
case "HtmlText":
return !/^\s*$/.exec(R.chars);
default:
return true;
}
}).length), this.nonBlockChildren = C.filter((R) => !(R instanceof n2.NamedBlock));
}
}, L = class extends g {
assertTemplate(m) {
if ((0, f.isPresent)(this.namedBlocks))
throw (0, r.generateSyntaxError)("Unexpected named block at the top-level of a template", this.loc);
return this.block.builder.template(m, this.nonBlockChildren, this.block.loc(this.loc));
}
}, j = class extends g {
assertBlock(m) {
if ((0, f.isPresent)(this.namedBlocks))
throw (0, r.generateSyntaxError)("Unexpected named block nested in a normal block", this.loc);
return this.block.builder.block(m, this.nonBlockChildren, this.loc);
}
}, x = class extends g {
constructor(m, C, S, R) {
super(C, S, R), this.el = m;
}
assertNamedBlock(m, C) {
if (this.el.base.selfClosing)
throw (0, r.generateSyntaxError)(`<:${m.chars}/> is not a valid named block: named blocks cannot be self-closing`, this.loc);
if ((0, f.isPresent)(this.namedBlocks))
throw (0, r.generateSyntaxError)(`Unexpected named block inside <:${m.chars}> named block: named blocks cannot contain nested named blocks`, this.loc);
if (!(0, a.isLowerCase)(m.chars))
throw (0, r.generateSyntaxError)(`<:${m.chars}> is not a valid named block, and named blocks must begin with a lowercase letter`, this.loc);
if (this.el.base.attrs.length > 0 || this.el.base.componentArgs.length > 0 || this.el.base.modifiers.length > 0)
throw (0, r.generateSyntaxError)(`named block <:${m.chars}> cannot have attributes, arguments, or modifiers`, this.loc);
let S = o.SpanList.range(this.nonBlockChildren, this.loc);
return this.block.builder.namedBlock(m, this.block.builder.block(C, this.nonBlockChildren, S), this.loc);
}
assertElement(m, C) {
if (C)
throw (0, r.generateSyntaxError)(`Unexpected block params in <${m}>: simple elements cannot have block params`, this.loc);
if ((0, f.isPresent)(this.namedBlocks)) {
let S = this.namedBlocks.map((R) => R.name);
if (S.length === 1)
throw (0, r.generateSyntaxError)(`Unexpected named block <:foo> inside <${m.chars}> HTML element`, this.loc);
{
let R = S.map((M) => `<:${M.chars}>`).join(", ");
throw (0, r.generateSyntaxError)(`Unexpected named blocks inside <${m.chars}> HTML element (${R})`, this.loc);
}
}
return this.el.simple(m, this.nonBlockChildren, this.loc);
}
assertComponent(m, C, S) {
if ((0, f.isPresent)(this.namedBlocks) && this.hasSemanticContent)
throw (0, r.generateSyntaxError)(`Unexpected content inside <${m}> component invocation: when using named blocks, the tag cannot contain other content`, this.loc);
if ((0, f.isPresent)(this.namedBlocks)) {
if (S)
throw (0, r.generateSyntaxError)(`Unexpected block params list on <${m}> component invocation: when passing named blocks, the invocation tag cannot take block params`, this.loc);
let R = /* @__PURE__ */ new Set();
for (let M of this.namedBlocks) {
let V = M.name.chars;
if (R.has(V))
throw (0, r.generateSyntaxError)(`Component had two named blocks with the same name, \`<:${V}>\`. Only one block with a given name may be passed`, this.loc);
if (V === "inverse" && R.has("else") || V === "else" && R.has("inverse"))
throw (0, r.generateSyntaxError)("Component has both <:else> and <:inverse> block. <:inverse> is an alias for <:else>", this.loc);
R.add(V);
}
return this.namedBlocks;
} else
return [this.block.builder.namedBlock(c.SourceSlice.synthetic("default"), this.block.builder.block(C, this.nonBlockChildren, this.loc), this.loc)];
}
};
function w(m) {
return m.type !== "PathExpression" && m.path.type === "PathExpression" ? w(m.path) : new h.default({ entityEncoding: "raw" }).print(m);
}
function H(m) {
if (m.type === "PathExpression")
switch (m.head.type) {
case "AtHead":
case "VarHead":
return m.head.name;
case "ThisHead":
return "this";
}
else
return m.path.type === "PathExpression" ? H(m.path) : new h.default({ entityEncoding: "raw" }).print(m);
}
} }), Ze = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/keywords.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.isKeyword = f, t.KEYWORDS_TYPES = void 0;
function f(d) {
return d in h;
}
var h = { component: ["Call", "Append", "Block"], debugger: ["Append"], "each-in": ["Block"], each: ["Block"], "has-block-params": ["Call", "Append"], "has-block": ["Call", "Append"], helper: ["Call", "Append"], if: ["Call", "Append", "Block"], "in-element": ["Block"], let: ["Block"], "link-to": ["Append", "Block"], log: ["Call", "Append"], modifier: ["Call"], mount: ["Append"], mut: ["Call", "Append"], outlet: ["Append"], "query-params": ["Call"], readonly: ["Call", "Append"], unbound: ["Call", "Append"], unless: ["Call", "Append", "Block"], with: ["Block"], yield: ["Append"] };
t.KEYWORDS_TYPES = h;
} }), Vt = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/lib/get-template-locals.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), t.getTemplateLocals = r;
var f = Ze(), h = Fe(), d = c(Ne());
function c(a) {
return a && a.__esModule ? a : { default: a };
}
function o(a, p, n2) {
if (a.type === "PathExpression") {
if (a.head.type === "AtHead" || a.head.type === "ThisHead")
return;
let s = a.head.name;
if (p.indexOf(s) === -1)
return s;
} else if (a.type === "ElementNode") {
let { tag: s } = a, u = s.charAt(0);
return u === ":" || u === "@" || !n2.includeHtmlElements && s.indexOf(".") === -1 && s.toLowerCase() === s || s.substr(0, 5) === "this." || p.indexOf(s) !== -1 ? void 0 : s;
}
}
function e(a, p, n2, s) {
let u = o(p, n2, s);
(Array.isArray(u) ? u : [u]).forEach((i) => {
i !== void 0 && i[0] !== "@" && a.add(i.split(".")[0]);
});
}
function r(a) {
let p = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : { includeHtmlElements: false, includeKeywords: false }, n2 = (0, h.preprocess)(a), s = /* @__PURE__ */ new Set(), u = [];
(0, d.default)(n2, { Block: { enter(l) {
let { blockParams: b } = l;
b.forEach((P) => {
u.push(P);
});
}, exit(l) {
let { blockParams: b } = l;
b.forEach(() => {
u.pop();
});
} }, ElementNode: { enter(l) {
l.blockParams.forEach((b) => {
u.push(b);
}), e(s, l, u, p);
}, exit(l) {
let { blockParams: b } = l;
b.forEach(() => {
u.pop();
});
} }, PathExpression(l) {
e(s, l, u, p);
} });
let i = [];
return s.forEach((l) => i.push(l)), p != null && p.includeKeywords || (i = i.filter((l) => !(0, f.isKeyword)(l))), i;
}
} }), Ut = I({ "node_modules/@glimmer/syntax/dist/commonjs/es2017/index.js"(t) {
"use strict";
F(), Object.defineProperty(t, "__esModule", { value: true }), Object.defineProperty(t, "Source", { enumerable: true, get: function() {
return f.Source;
} }), Object.defineProperty(t, "builders", { enumerable: true, get: function() {
return h.default;
} }), Object.defineProperty(t, "normalize", { enumerable: true, get: function() {
return o.normalize;
} }), Object.defineProperty(t, "SymbolTable", { enumerable: true, get: function() {
return e.SymbolTable;
} }), Object.defineProperty(t, "BlockSymbolTable", { enumerable: true, get: function() {
return e.BlockSymbolTable;
} }), Object.defineProperty(t, "ProgramSymbolTable", { enumerable: true, get: function() {
return e.ProgramSymbolTable;
} }), Object.defineProperty(t, "generateSyntaxError", { enumerable: true, get: function() {
return r.generateSyntaxError;
} }), Object.defineProperty(t, "preprocess", { enumerable: true, get: function() {
return a.preprocess;
} }), Object.defineProperty(t, "print", { enumerable: true, get: function() {
return p.default;
} }), Object.defineProperty(t, "sortByLoc", { enumerable: true, get: function() {
return n2.sortByLoc;
} }), Object.defineProperty(t, "Walker", { enumerable: true, get: function() {
return s.default;
} }), Object.defineProperty(t, "Path", { enumerable: true, get: function() {
return s.default;
} }), Object.defineProperty(t, "traverse", { enumerable: true, get: function() {
return u.default;
} }), Object.defineProperty(t, "cannotRemoveNode", { enumerable: true, get: function() {
return i.cannotRemoveNode;
} }), Object.defineProperty(t, "cannotReplaceNode", { enumerable: true, get: function() {
return i.cannotReplaceNode;
} }), Object.defineProperty(t, "WalkerPath", { enumerable: true, get: function() {
return l.default;
} }), Object.defineProperty(t, "isKeyword", { enumerable: true, get: function() {
return b.isKeyword;
} }), Object.defineProperty(t, "KEYWORDS_TYPES", { enumerable: true, get: function() {
return b.KEYWORDS_TYPES;
} }), Object.defineProperty(t, "getTemplateLocals", { enumerable: true, get: function() {
return P.getTemplateLocals;
} }), Object.defineProperty(t, "SourceSlice", { enumerable: true, get: function() {
return E.SourceSlice;
} }), Object.defineProperty(t, "SourceSpan", { enumerable: true, get: function() {
return v.SourceSpan;
} }), Object.defineProperty(t, "SpanList", { enumerable: true, get: function() {
return _.SpanList;
} }), Object.defineProperty(t, "maybeLoc", { enumerable: true, get: function() {
return _.maybeLoc;
} }), Object.defineProperty(t, "loc", { enumerable: true, get: function() {
return _.loc;
} }), Object.defineProperty(t, "hasSpan", { enumerable: true, get: function() {
return _.hasSpan;
} }), Object.defineProperty(t, "node", { enumerable: true, get: function() {
return y.node;
} }), t.ASTv2 = t.AST = t.ASTv1 = void 0;
var f = De(), h = j(ke()), d = L(Ct());
t.ASTv1 = d, t.AST = d;
var c = L(ve());
t.ASTv2 = c;
var o = Ht(), e = Xe(), r = he(), a = Fe(), p = j(We()), n2 = Ue(), s = j(Je()), u = j(Ne()), i = Ye(), l = j(Qe()), b = Ze(), P = Vt(), E = le(), v = ue(), _ = ce(), y = ne();
function g() {
if (typeof WeakMap != "function")
return null;
var x = /* @__PURE__ */ new WeakMap();
return g = function() {
return x;
}, x;
}
function L(x) {
if (x && x.__esModule)
return x;
if (x === null || typeof x != "object" && typeof x != "function")
return { default: x };
var w = g();
if (w && w.has(x))
return w.get(x);
var H = {}, m = Object.defineProperty && Object.getOwnPropertyDescriptor;
for (var C in x)
if (Object.prototype.hasOwnProperty.call(x, C)) {
var S = m ? Object.getOwnPropertyDescriptor(x, C) : null;
S && (S.get || S.set) ? Object.defineProperty(H, C, S) : H[C] = x[C];
}
return H.default = x, w && w.set(x, H), H;
}
function j(x) {
return x && x.__esModule ? x : { default: x };
}
} });
F();
var { LinesAndColumns: zt } = at(), Gt = ut(), { locStart: Kt, locEnd: Wt } = ot();
function Yt() {
return { name: "addBackslash", visitor: { All(t) {
var f;
let h = (f = t.children) !== null && f !== void 0 ? f : t.body;
if (h)
for (let d = 0; d < h.length - 1; d++)
h[d].type === "TextNode" && h[d + 1].type === "MustacheStatement" && (h[d].chars = h[d].chars.replace(/\\$/, "\\\\"));
} } };
}
function Qt(t) {
let f = new zt(t), h = (d) => {
let { line: c, column: o } = d;
return f.indexForLocation({ line: c - 1, column: o });
};
return () => ({ name: "addOffset", visitor: { All(d) {
let { start: c, end: o } = d.loc;
c.offset = h(c), o.offset = h(o);
} } });
}
function Jt(t) {
let { preprocess: f } = Ut(), h;
try {
h = f(t, { mode: "codemod", plugins: { ast: [Yt, Qt(t)] } });
} catch (d) {
let c = Xt(d);
throw c ? Gt(d.message, c) : d;
}
return h;
}
function Xt(t) {
let { location: f, hash: h } = t;
if (f) {
let { start: d, end: c } = f;
return typeof c.line != "number" ? { start: d } : f;
}
if (h) {
let { loc: { last_line: d, last_column: c } } = h;
return { start: { line: d, column: c + 1 } };
}
}
$e.exports = { parsers: { glimmer: { parse: Jt, astFormat: "glimmer", locStart: Kt, locEnd: Wt } } };
});
return Zt();
});
}
});
// node_modules/prettier/parser-graphql.js
var require_parser_graphql = __commonJS({
"node_modules/prettier/parser-graphql.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.graphql = e();
}
})(function() {
"use strict";
var oe = (a, d) => () => (d || a((d = { exports: {} }).exports, d), d.exports);
var be = oe((Ce, ae) => {
var H = Object.getOwnPropertyNames, se = (a, d) => function() {
return a && (d = (0, a[H(a)[0]])(a = 0)), d;
}, L = (a, d) => function() {
return d || (0, a[H(a)[0]])((d = { exports: {} }).exports, d), d.exports;
}, K = se({ ""() {
} }), ce = L({ "src/common/parser-create-error.js"(a, d) {
"use strict";
K();
function i(c, r) {
let _ = new SyntaxError(c + " (" + r.start.line + ":" + r.start.column + ")");
return _.loc = r, _;
}
d.exports = i;
} }), ue = L({ "src/utils/try-combinations.js"(a, d) {
"use strict";
K();
function i() {
let c;
for (var r = arguments.length, _ = new Array(r), E = 0; E < r; E++)
_[E] = arguments[E];
for (let [k, O] of _.entries())
try {
return { result: O() };
} catch (A) {
k === 0 && (c = A);
}
return { error: c };
}
d.exports = i;
} }), le = L({ "src/language-graphql/pragma.js"(a, d) {
"use strict";
K();
function i(r) {
return /^\s*#[^\S\n]*@(?:format|prettier)\s*(?:\n|$)/.test(r);
}
function c(r) {
return `# @format
` + r;
}
d.exports = { hasPragma: i, insertPragma: c };
} }), pe = L({ "src/language-graphql/loc.js"(a, d) {
"use strict";
K();
function i(r) {
return typeof r.start == "number" ? r.start : r.loc && r.loc.start;
}
function c(r) {
return typeof r.end == "number" ? r.end : r.loc && r.loc.end;
}
d.exports = { locStart: i, locEnd: c };
} }), fe = L({ "node_modules/graphql/jsutils/isObjectLike.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.default = i;
function d(c) {
return typeof Symbol == "function" && typeof Symbol.iterator == "symbol" ? d = function(_) {
return typeof _;
} : d = function(_) {
return _ && typeof Symbol == "function" && _.constructor === Symbol && _ !== Symbol.prototype ? "symbol" : typeof _;
}, d(c);
}
function i(c) {
return d(c) == "object" && c !== null;
}
} }), z = L({ "node_modules/graphql/polyfills/symbols.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.SYMBOL_TO_STRING_TAG = a.SYMBOL_ASYNC_ITERATOR = a.SYMBOL_ITERATOR = void 0;
var d = typeof Symbol == "function" && Symbol.iterator != null ? Symbol.iterator : "@@iterator";
a.SYMBOL_ITERATOR = d;
var i = typeof Symbol == "function" && Symbol.asyncIterator != null ? Symbol.asyncIterator : "@@asyncIterator";
a.SYMBOL_ASYNC_ITERATOR = i;
var c = typeof Symbol == "function" && Symbol.toStringTag != null ? Symbol.toStringTag : "@@toStringTag";
a.SYMBOL_TO_STRING_TAG = c;
} }), $ = L({ "node_modules/graphql/language/location.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.getLocation = d;
function d(i, c) {
for (var r = /\r\n|[\n\r]/g, _ = 1, E = c + 1, k; (k = r.exec(i.body)) && k.index < c; )
_ += 1, E = c + 1 - (k.index + k[0].length);
return { line: _, column: E };
}
} }), de = L({ "node_modules/graphql/language/printLocation.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.printLocation = i, a.printSourceLocation = c;
var d = $();
function i(k) {
return c(k.source, (0, d.getLocation)(k.source, k.start));
}
function c(k, O) {
var A = k.locationOffset.column - 1, N = _(A) + k.body, g = O.line - 1, D = k.locationOffset.line - 1, v = O.line + D, I = O.line === 1 ? A : 0, s = O.column + I, p = "".concat(k.name, ":").concat(v, ":").concat(s, `
`), e = N.split(/\r\n|[\n\r]/g), n2 = e[g];
if (n2.length > 120) {
for (var t = Math.floor(s / 80), u = s % 80, y = [], f = 0; f < n2.length; f += 80)
y.push(n2.slice(f, f + 80));
return p + r([["".concat(v), y[0]]].concat(y.slice(1, t + 1).map(function(m) {
return ["", m];
}), [[" ", _(u - 1) + "^"], ["", y[t + 1]]]));
}
return p + r([["".concat(v - 1), e[g - 1]], ["".concat(v), n2], ["", _(s - 1) + "^"], ["".concat(v + 1), e[g + 1]]]);
}
function r(k) {
var O = k.filter(function(N) {
var g = N[0], D = N[1];
return D !== void 0;
}), A = Math.max.apply(Math, O.map(function(N) {
var g = N[0];
return g.length;
}));
return O.map(function(N) {
var g = N[0], D = N[1];
return E(A, g) + (D ? " | " + D : " |");
}).join(`
`);
}
function _(k) {
return Array(k + 1).join(" ");
}
function E(k, O) {
return _(k - O.length) + O;
}
} }), W = L({ "node_modules/graphql/error/GraphQLError.js"(a) {
"use strict";
K();
function d(f) {
return typeof Symbol == "function" && typeof Symbol.iterator == "symbol" ? d = function(o) {
return typeof o;
} : d = function(o) {
return o && typeof Symbol == "function" && o.constructor === Symbol && o !== Symbol.prototype ? "symbol" : typeof o;
}, d(f);
}
Object.defineProperty(a, "__esModule", { value: true }), a.printError = y, a.GraphQLError = void 0;
var i = E(fe()), c = z(), r = $(), _ = de();
function E(f) {
return f && f.__esModule ? f : { default: f };
}
function k(f, m) {
if (!(f instanceof m))
throw new TypeError("Cannot call a class as a function");
}
function O(f, m) {
for (var o = 0; o < m.length; o++) {
var h = m[o];
h.enumerable = h.enumerable || false, h.configurable = true, "value" in h && (h.writable = true), Object.defineProperty(f, h.key, h);
}
}
function A(f, m, o) {
return m && O(f.prototype, m), o && O(f, o), f;
}
function N(f, m) {
if (typeof m != "function" && m !== null)
throw new TypeError("Super expression must either be null or a function");
f.prototype = Object.create(m && m.prototype, { constructor: { value: f, writable: true, configurable: true } }), m && n2(f, m);
}
function g(f) {
var m = p();
return function() {
var h = t(f), l;
if (m) {
var T = t(this).constructor;
l = Reflect.construct(h, arguments, T);
} else
l = h.apply(this, arguments);
return D(this, l);
};
}
function D(f, m) {
return m && (d(m) === "object" || typeof m == "function") ? m : v(f);
}
function v(f) {
if (f === void 0)
throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
return f;
}
function I(f) {
var m = typeof Map == "function" ? /* @__PURE__ */ new Map() : void 0;
return I = function(h) {
if (h === null || !e(h))
return h;
if (typeof h != "function")
throw new TypeError("Super expression must either be null or a function");
if (typeof m < "u") {
if (m.has(h))
return m.get(h);
m.set(h, l);
}
function l() {
return s(h, arguments, t(this).constructor);
}
return l.prototype = Object.create(h.prototype, { constructor: { value: l, enumerable: false, writable: true, configurable: true } }), n2(l, h);
}, I(f);
}
function s(f, m, o) {
return p() ? s = Reflect.construct : s = function(l, T, S) {
var x = [null];
x.push.apply(x, T);
var b = Function.bind.apply(l, x), M = new b();
return S && n2(M, S.prototype), M;
}, s.apply(null, arguments);
}
function p() {
if (typeof Reflect > "u" || !Reflect.construct || Reflect.construct.sham)
return false;
if (typeof Proxy == "function")
return true;
try {
return Date.prototype.toString.call(Reflect.construct(Date, [], function() {
})), true;
} catch {
return false;
}
}
function e(f) {
return Function.toString.call(f).indexOf("[native code]") !== -1;
}
function n2(f, m) {
return n2 = Object.setPrototypeOf || function(h, l) {
return h.__proto__ = l, h;
}, n2(f, m);
}
function t(f) {
return t = Object.setPrototypeOf ? Object.getPrototypeOf : function(o) {
return o.__proto__ || Object.getPrototypeOf(o);
}, t(f);
}
var u = function(f) {
N(o, f);
var m = g(o);
function o(h, l, T, S, x, b, M) {
var U, V, q, G, C;
k(this, o), C = m.call(this, h);
var R = Array.isArray(l) ? l.length !== 0 ? l : void 0 : l ? [l] : void 0, Y = T;
if (!Y && R) {
var J;
Y = (J = R[0].loc) === null || J === void 0 ? void 0 : J.source;
}
var F = S;
!F && R && (F = R.reduce(function(w, P) {
return P.loc && w.push(P.loc.start), w;
}, [])), F && F.length === 0 && (F = void 0);
var B;
S && T ? B = S.map(function(w) {
return (0, r.getLocation)(T, w);
}) : R && (B = R.reduce(function(w, P) {
return P.loc && w.push((0, r.getLocation)(P.loc.source, P.loc.start)), w;
}, []));
var j = M;
if (j == null && b != null) {
var Q = b.extensions;
(0, i.default)(Q) && (j = Q);
}
return Object.defineProperties(v(C), { name: { value: "GraphQLError" }, message: { value: h, enumerable: true, writable: true }, locations: { value: (U = B) !== null && U !== void 0 ? U : void 0, enumerable: B != null }, path: { value: x != null ? x : void 0, enumerable: x != null }, nodes: { value: R != null ? R : void 0 }, source: { value: (V = Y) !== null && V !== void 0 ? V : void 0 }, positions: { value: (q = F) !== null && q !== void 0 ? q : void 0 }, originalError: { value: b }, extensions: { value: (G = j) !== null && G !== void 0 ? G : void 0, enumerable: j != null } }), b != null && b.stack ? (Object.defineProperty(v(C), "stack", { value: b.stack, writable: true, configurable: true }), D(C)) : (Error.captureStackTrace ? Error.captureStackTrace(v(C), o) : Object.defineProperty(v(C), "stack", { value: Error().stack, writable: true, configurable: true }), C);
}
return A(o, [{ key: "toString", value: function() {
return y(this);
} }, { key: c.SYMBOL_TO_STRING_TAG, get: function() {
return "Object";
} }]), o;
}(I(Error));
a.GraphQLError = u;
function y(f) {
var m = f.message;
if (f.nodes)
for (var o = 0, h = f.nodes; o < h.length; o++) {
var l = h[o];
l.loc && (m += `
` + (0, _.printLocation)(l.loc));
}
else if (f.source && f.locations)
for (var T = 0, S = f.locations; T < S.length; T++) {
var x = S[T];
m += `
` + (0, _.printSourceLocation)(f.source, x);
}
return m;
}
} }), Z = L({ "node_modules/graphql/error/syntaxError.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.syntaxError = i;
var d = W();
function i(c, r, _) {
return new d.GraphQLError("Syntax Error: ".concat(_), void 0, c, [r]);
}
} }), he = L({ "node_modules/graphql/language/kinds.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.Kind = void 0;
var d = Object.freeze({ NAME: "Name", DOCUMENT: "Document", OPERATION_DEFINITION: "OperationDefinition", VARIABLE_DEFINITION: "VariableDefinition", SELECTION_SET: "SelectionSet", FIELD: "Field", ARGUMENT: "Argument", FRAGMENT_SPREAD: "FragmentSpread", INLINE_FRAGMENT: "InlineFragment", FRAGMENT_DEFINITION: "FragmentDefinition", VARIABLE: "Variable", INT: "IntValue", FLOAT: "FloatValue", STRING: "StringValue", BOOLEAN: "BooleanValue", NULL: "NullValue", ENUM: "EnumValue", LIST: "ListValue", OBJECT: "ObjectValue", OBJECT_FIELD: "ObjectField", DIRECTIVE: "Directive", NAMED_TYPE: "NamedType", LIST_TYPE: "ListType", NON_NULL_TYPE: "NonNullType", SCHEMA_DEFINITION: "SchemaDefinition", OPERATION_TYPE_DEFINITION: "OperationTypeDefinition", SCALAR_TYPE_DEFINITION: "ScalarTypeDefinition", OBJECT_TYPE_DEFINITION: "ObjectTypeDefinition", FIELD_DEFINITION: "FieldDefinition", INPUT_VALUE_DEFINITION: "InputValueDefinition", INTERFACE_TYPE_DEFINITION: "InterfaceTypeDefinition", UNION_TYPE_DEFINITION: "UnionTypeDefinition", ENUM_TYPE_DEFINITION: "EnumTypeDefinition", ENUM_VALUE_DEFINITION: "EnumValueDefinition", INPUT_OBJECT_TYPE_DEFINITION: "InputObjectTypeDefinition", DIRECTIVE_DEFINITION: "DirectiveDefinition", SCHEMA_EXTENSION: "SchemaExtension", SCALAR_TYPE_EXTENSION: "ScalarTypeExtension", OBJECT_TYPE_EXTENSION: "ObjectTypeExtension", INTERFACE_TYPE_EXTENSION: "InterfaceTypeExtension", UNION_TYPE_EXTENSION: "UnionTypeExtension", ENUM_TYPE_EXTENSION: "EnumTypeExtension", INPUT_OBJECT_TYPE_EXTENSION: "InputObjectTypeExtension" });
a.Kind = d;
} }), ve = L({ "node_modules/graphql/jsutils/invariant.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.default = d;
function d(i, c) {
var r = Boolean(i);
if (!r)
throw new Error(c != null ? c : "Unexpected invariant triggered.");
}
} }), ee = L({ "node_modules/graphql/jsutils/nodejsCustomInspectSymbol.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.default = void 0;
var d = typeof Symbol == "function" && typeof Symbol.for == "function" ? Symbol.for("nodejs.util.inspect.custom") : void 0, i = d;
a.default = i;
} }), Te = L({ "node_modules/graphql/jsutils/defineInspect.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.default = r;
var d = c(ve()), i = c(ee());
function c(_) {
return _ && _.__esModule ? _ : { default: _ };
}
function r(_) {
var E = _.prototype.toJSON;
typeof E == "function" || (0, d.default)(0), _.prototype.inspect = E, i.default && (_.prototype[i.default] = E);
}
} }), te = L({ "node_modules/graphql/language/ast.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.isNode = _, a.Token = a.Location = void 0;
var d = i(Te());
function i(E) {
return E && E.__esModule ? E : { default: E };
}
var c = function() {
function E(O, A, N) {
this.start = O.start, this.end = A.end, this.startToken = O, this.endToken = A, this.source = N;
}
var k = E.prototype;
return k.toJSON = function() {
return { start: this.start, end: this.end };
}, E;
}();
a.Location = c, (0, d.default)(c);
var r = function() {
function E(O, A, N, g, D, v, I) {
this.kind = O, this.start = A, this.end = N, this.line = g, this.column = D, this.value = I, this.prev = v, this.next = null;
}
var k = E.prototype;
return k.toJSON = function() {
return { kind: this.kind, value: this.value, line: this.line, column: this.column };
}, E;
}();
a.Token = r, (0, d.default)(r);
function _(E) {
return E != null && typeof E.kind == "string";
}
} }), ne = L({ "node_modules/graphql/language/tokenKind.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.TokenKind = void 0;
var d = Object.freeze({ SOF: "", EOF: "", BANG: "!", DOLLAR: "$", AMP: "&", PAREN_L: "(", PAREN_R: ")", SPREAD: "...", COLON: ":", EQUALS: "=", AT: "@", BRACKET_L: "[", BRACKET_R: "]", BRACE_L: "{", PIPE: "|", BRACE_R: "}", NAME: "Name", INT: "Int", FLOAT: "Float", STRING: "String", BLOCK_STRING: "BlockString", COMMENT: "Comment" });
a.TokenKind = d;
} }), re = L({ "node_modules/graphql/jsutils/inspect.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.default = E;
var d = i(ee());
function i(v) {
return v && v.__esModule ? v : { default: v };
}
function c(v) {
return typeof Symbol == "function" && typeof Symbol.iterator == "symbol" ? c = function(s) {
return typeof s;
} : c = function(s) {
return s && typeof Symbol == "function" && s.constructor === Symbol && s !== Symbol.prototype ? "symbol" : typeof s;
}, c(v);
}
var r = 10, _ = 2;
function E(v) {
return k(v, []);
}
function k(v, I) {
switch (c(v)) {
case "string":
return JSON.stringify(v);
case "function":
return v.name ? "[function ".concat(v.name, "]") : "[function]";
case "object":
return v === null ? "null" : O(v, I);
default:
return String(v);
}
}
function O(v, I) {
if (I.indexOf(v) !== -1)
return "[Circular]";
var s = [].concat(I, [v]), p = g(v);
if (p !== void 0) {
var e = p.call(v);
if (e !== v)
return typeof e == "string" ? e : k(e, s);
} else if (Array.isArray(v))
return N(v, s);
return A(v, s);
}
function A(v, I) {
var s = Object.keys(v);
if (s.length === 0)
return "{}";
if (I.length > _)
return "[" + D(v) + "]";
var p = s.map(function(e) {
var n2 = k(v[e], I);
return e + ": " + n2;
});
return "{ " + p.join(", ") + " }";
}
function N(v, I) {
if (v.length === 0)
return "[]";
if (I.length > _)
return "[Array]";
for (var s = Math.min(r, v.length), p = v.length - s, e = [], n2 = 0; n2 < s; ++n2)
e.push(k(v[n2], I));
return p === 1 ? e.push("... 1 more item") : p > 1 && e.push("... ".concat(p, " more items")), "[" + e.join(", ") + "]";
}
function g(v) {
var I = v[String(d.default)];
if (typeof I == "function")
return I;
if (typeof v.inspect == "function")
return v.inspect;
}
function D(v) {
var I = Object.prototype.toString.call(v).replace(/^\[object /, "").replace(/]$/, "");
if (I === "Object" && typeof v.constructor == "function") {
var s = v.constructor.name;
if (typeof s == "string" && s !== "")
return s;
}
return I;
}
} }), _e = L({ "node_modules/graphql/jsutils/devAssert.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.default = d;
function d(i, c) {
var r = Boolean(i);
if (!r)
throw new Error(c);
}
} }), Ee = L({ "node_modules/graphql/jsutils/instanceOf.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.default = void 0;
var d = i(re());
function i(r) {
return r && r.__esModule ? r : { default: r };
}
var c = function(_, E) {
return _ instanceof E;
};
a.default = c;
} }), me = L({ "node_modules/graphql/language/source.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.isSource = A, a.Source = void 0;
var d = z(), i = _(re()), c = _(_e()), r = _(Ee());
function _(N) {
return N && N.__esModule ? N : { default: N };
}
function E(N, g) {
for (var D = 0; D < g.length; D++) {
var v = g[D];
v.enumerable = v.enumerable || false, v.configurable = true, "value" in v && (v.writable = true), Object.defineProperty(N, v.key, v);
}
}
function k(N, g, D) {
return g && E(N.prototype, g), D && E(N, D), N;
}
var O = function() {
function N(g) {
var D = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : "GraphQL request", v = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : { line: 1, column: 1 };
typeof g == "string" || (0, c.default)(0, "Body must be a string. Received: ".concat((0, i.default)(g), ".")), this.body = g, this.name = D, this.locationOffset = v, this.locationOffset.line > 0 || (0, c.default)(0, "line in locationOffset is 1-indexed and must be positive."), this.locationOffset.column > 0 || (0, c.default)(0, "column in locationOffset is 1-indexed and must be positive.");
}
return k(N, [{ key: d.SYMBOL_TO_STRING_TAG, get: function() {
return "Source";
} }]), N;
}();
a.Source = O;
function A(N) {
return (0, r.default)(N, O);
}
} }), ye = L({ "node_modules/graphql/language/directiveLocation.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.DirectiveLocation = void 0;
var d = Object.freeze({ QUERY: "QUERY", MUTATION: "MUTATION", SUBSCRIPTION: "SUBSCRIPTION", FIELD: "FIELD", FRAGMENT_DEFINITION: "FRAGMENT_DEFINITION", FRAGMENT_SPREAD: "FRAGMENT_SPREAD", INLINE_FRAGMENT: "INLINE_FRAGMENT", VARIABLE_DEFINITION: "VARIABLE_DEFINITION", SCHEMA: "SCHEMA", SCALAR: "SCALAR", OBJECT: "OBJECT", FIELD_DEFINITION: "FIELD_DEFINITION", ARGUMENT_DEFINITION: "ARGUMENT_DEFINITION", INTERFACE: "INTERFACE", UNION: "UNION", ENUM: "ENUM", ENUM_VALUE: "ENUM_VALUE", INPUT_OBJECT: "INPUT_OBJECT", INPUT_FIELD_DEFINITION: "INPUT_FIELD_DEFINITION" });
a.DirectiveLocation = d;
} }), ke = L({ "node_modules/graphql/language/blockString.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.dedentBlockStringValue = d, a.getBlockStringIndentation = c, a.printBlockString = r;
function d(_) {
var E = _.split(/\r\n|[\n\r]/g), k = c(_);
if (k !== 0)
for (var O = 1; O < E.length; O++)
E[O] = E[O].slice(k);
for (var A = 0; A < E.length && i(E[A]); )
++A;
for (var N = E.length; N > A && i(E[N - 1]); )
--N;
return E.slice(A, N).join(`
`);
}
function i(_) {
for (var E = 0; E < _.length; ++E)
if (_[E] !== " " && _[E] !== " ")
return false;
return true;
}
function c(_) {
for (var E, k = true, O = true, A = 0, N = null, g = 0; g < _.length; ++g)
switch (_.charCodeAt(g)) {
case 13:
_.charCodeAt(g + 1) === 10 && ++g;
case 10:
k = false, O = true, A = 0;
break;
case 9:
case 32:
++A;
break;
default:
O && !k && (N === null || A < N) && (N = A), O = false;
}
return (E = N) !== null && E !== void 0 ? E : 0;
}
function r(_) {
var E = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : "", k = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : false, O = _.indexOf(`
`) === -1, A = _[0] === " " || _[0] === " ", N = _[_.length - 1] === '"', g = _[_.length - 1] === "\\", D = !O || N || g || k, v = "";
return D && !(O && A) && (v += `
` + E), v += E ? _.replace(/\n/g, `
` + E) : _, D && (v += `
`), '"""' + v.replace(/"""/g, '\\"""') + '"""';
}
} }), Ne = L({ "node_modules/graphql/language/lexer.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.isPunctuatorTokenKind = E, a.Lexer = void 0;
var d = Z(), i = te(), c = ne(), r = ke(), _ = function() {
function t(y) {
var f = new i.Token(c.TokenKind.SOF, 0, 0, 0, 0, null);
this.source = y, this.lastToken = f, this.token = f, this.line = 1, this.lineStart = 0;
}
var u = t.prototype;
return u.advance = function() {
this.lastToken = this.token;
var f = this.token = this.lookahead();
return f;
}, u.lookahead = function() {
var f = this.token;
if (f.kind !== c.TokenKind.EOF)
do {
var m;
f = (m = f.next) !== null && m !== void 0 ? m : f.next = O(this, f);
} while (f.kind === c.TokenKind.COMMENT);
return f;
}, t;
}();
a.Lexer = _;
function E(t) {
return t === c.TokenKind.BANG || t === c.TokenKind.DOLLAR || t === c.TokenKind.AMP || t === c.TokenKind.PAREN_L || t === c.TokenKind.PAREN_R || t === c.TokenKind.SPREAD || t === c.TokenKind.COLON || t === c.TokenKind.EQUALS || t === c.TokenKind.AT || t === c.TokenKind.BRACKET_L || t === c.TokenKind.BRACKET_R || t === c.TokenKind.BRACE_L || t === c.TokenKind.PIPE || t === c.TokenKind.BRACE_R;
}
function k(t) {
return isNaN(t) ? c.TokenKind.EOF : t < 127 ? JSON.stringify(String.fromCharCode(t)) : '"\\u'.concat(("00" + t.toString(16).toUpperCase()).slice(-4), '"');
}
function O(t, u) {
for (var y = t.source, f = y.body, m = f.length, o = u.end; o < m; ) {
var h = f.charCodeAt(o), l = t.line, T = 1 + o - t.lineStart;
switch (h) {
case 65279:
case 9:
case 32:
case 44:
++o;
continue;
case 10:
++o, ++t.line, t.lineStart = o;
continue;
case 13:
f.charCodeAt(o + 1) === 10 ? o += 2 : ++o, ++t.line, t.lineStart = o;
continue;
case 33:
return new i.Token(c.TokenKind.BANG, o, o + 1, l, T, u);
case 35:
return N(y, o, l, T, u);
case 36:
return new i.Token(c.TokenKind.DOLLAR, o, o + 1, l, T, u);
case 38:
return new i.Token(c.TokenKind.AMP, o, o + 1, l, T, u);
case 40:
return new i.Token(c.TokenKind.PAREN_L, o, o + 1, l, T, u);
case 41:
return new i.Token(c.TokenKind.PAREN_R, o, o + 1, l, T, u);
case 46:
if (f.charCodeAt(o + 1) === 46 && f.charCodeAt(o + 2) === 46)
return new i.Token(c.TokenKind.SPREAD, o, o + 3, l, T, u);
break;
case 58:
return new i.Token(c.TokenKind.COLON, o, o + 1, l, T, u);
case 61:
return new i.Token(c.TokenKind.EQUALS, o, o + 1, l, T, u);
case 64:
return new i.Token(c.TokenKind.AT, o, o + 1, l, T, u);
case 91:
return new i.Token(c.TokenKind.BRACKET_L, o, o + 1, l, T, u);
case 93:
return new i.Token(c.TokenKind.BRACKET_R, o, o + 1, l, T, u);
case 123:
return new i.Token(c.TokenKind.BRACE_L, o, o + 1, l, T, u);
case 124:
return new i.Token(c.TokenKind.PIPE, o, o + 1, l, T, u);
case 125:
return new i.Token(c.TokenKind.BRACE_R, o, o + 1, l, T, u);
case 34:
return f.charCodeAt(o + 1) === 34 && f.charCodeAt(o + 2) === 34 ? I(y, o, l, T, u, t) : v(y, o, l, T, u);
case 45:
case 48:
case 49:
case 50:
case 51:
case 52:
case 53:
case 54:
case 55:
case 56:
case 57:
return g(y, o, h, l, T, u);
case 65:
case 66:
case 67:
case 68:
case 69:
case 70:
case 71:
case 72:
case 73:
case 74:
case 75:
case 76:
case 77:
case 78:
case 79:
case 80:
case 81:
case 82:
case 83:
case 84:
case 85:
case 86:
case 87:
case 88:
case 89:
case 90:
case 95:
case 97:
case 98:
case 99:
case 100:
case 101:
case 102:
case 103:
case 104:
case 105:
case 106:
case 107:
case 108:
case 109:
case 110:
case 111:
case 112:
case 113:
case 114:
case 115:
case 116:
case 117:
case 118:
case 119:
case 120:
case 121:
case 122:
return e(y, o, l, T, u);
}
throw (0, d.syntaxError)(y, o, A(h));
}
var S = t.line, x = 1 + o - t.lineStart;
return new i.Token(c.TokenKind.EOF, m, m, S, x, u);
}
function A(t) {
return t < 32 && t !== 9 && t !== 10 && t !== 13 ? "Cannot contain the invalid character ".concat(k(t), ".") : t === 39 ? `Unexpected single quote character ('), did you mean to use a double quote (")?` : "Cannot parse the unexpected character ".concat(k(t), ".");
}
function N(t, u, y, f, m) {
var o = t.body, h, l = u;
do
h = o.charCodeAt(++l);
while (!isNaN(h) && (h > 31 || h === 9));
return new i.Token(c.TokenKind.COMMENT, u, l, y, f, m, o.slice(u + 1, l));
}
function g(t, u, y, f, m, o) {
var h = t.body, l = y, T = u, S = false;
if (l === 45 && (l = h.charCodeAt(++T)), l === 48) {
if (l = h.charCodeAt(++T), l >= 48 && l <= 57)
throw (0, d.syntaxError)(t, T, "Invalid number, unexpected digit after 0: ".concat(k(l), "."));
} else
T = D(t, T, l), l = h.charCodeAt(T);
if (l === 46 && (S = true, l = h.charCodeAt(++T), T = D(t, T, l), l = h.charCodeAt(T)), (l === 69 || l === 101) && (S = true, l = h.charCodeAt(++T), (l === 43 || l === 45) && (l = h.charCodeAt(++T)), T = D(t, T, l), l = h.charCodeAt(T)), l === 46 || n2(l))
throw (0, d.syntaxError)(t, T, "Invalid number, expected digit but got: ".concat(k(l), "."));
return new i.Token(S ? c.TokenKind.FLOAT : c.TokenKind.INT, u, T, f, m, o, h.slice(u, T));
}
function D(t, u, y) {
var f = t.body, m = u, o = y;
if (o >= 48 && o <= 57) {
do
o = f.charCodeAt(++m);
while (o >= 48 && o <= 57);
return m;
}
throw (0, d.syntaxError)(t, m, "Invalid number, expected digit but got: ".concat(k(o), "."));
}
function v(t, u, y, f, m) {
for (var o = t.body, h = u + 1, l = h, T = 0, S = ""; h < o.length && !isNaN(T = o.charCodeAt(h)) && T !== 10 && T !== 13; ) {
if (T === 34)
return S += o.slice(l, h), new i.Token(c.TokenKind.STRING, u, h + 1, y, f, m, S);
if (T < 32 && T !== 9)
throw (0, d.syntaxError)(t, h, "Invalid character within String: ".concat(k(T), "."));
if (++h, T === 92) {
switch (S += o.slice(l, h - 1), T = o.charCodeAt(h), T) {
case 34:
S += '"';
break;
case 47:
S += "/";
break;
case 92:
S += "\\";
break;
case 98:
S += "\b";
break;
case 102:
S += "\f";
break;
case 110:
S += `
`;
break;
case 114:
S += "\r";
break;
case 116:
S += " ";
break;
case 117: {
var x = s(o.charCodeAt(h + 1), o.charCodeAt(h + 2), o.charCodeAt(h + 3), o.charCodeAt(h + 4));
if (x < 0) {
var b = o.slice(h + 1, h + 5);
throw (0, d.syntaxError)(t, h, "Invalid character escape sequence: \\u".concat(b, "."));
}
S += String.fromCharCode(x), h += 4;
break;
}
default:
throw (0, d.syntaxError)(t, h, "Invalid character escape sequence: \\".concat(String.fromCharCode(T), "."));
}
++h, l = h;
}
}
throw (0, d.syntaxError)(t, h, "Unterminated string.");
}
function I(t, u, y, f, m, o) {
for (var h = t.body, l = u + 3, T = l, S = 0, x = ""; l < h.length && !isNaN(S = h.charCodeAt(l)); ) {
if (S === 34 && h.charCodeAt(l + 1) === 34 && h.charCodeAt(l + 2) === 34)
return x += h.slice(T, l), new i.Token(c.TokenKind.BLOCK_STRING, u, l + 3, y, f, m, (0, r.dedentBlockStringValue)(x));
if (S < 32 && S !== 9 && S !== 10 && S !== 13)
throw (0, d.syntaxError)(t, l, "Invalid character within String: ".concat(k(S), "."));
S === 10 ? (++l, ++o.line, o.lineStart = l) : S === 13 ? (h.charCodeAt(l + 1) === 10 ? l += 2 : ++l, ++o.line, o.lineStart = l) : S === 92 && h.charCodeAt(l + 1) === 34 && h.charCodeAt(l + 2) === 34 && h.charCodeAt(l + 3) === 34 ? (x += h.slice(T, l) + '"""', l += 4, T = l) : ++l;
}
throw (0, d.syntaxError)(t, l, "Unterminated string.");
}
function s(t, u, y, f) {
return p(t) << 12 | p(u) << 8 | p(y) << 4 | p(f);
}
function p(t) {
return t >= 48 && t <= 57 ? t - 48 : t >= 65 && t <= 70 ? t - 55 : t >= 97 && t <= 102 ? t - 87 : -1;
}
function e(t, u, y, f, m) {
for (var o = t.body, h = o.length, l = u + 1, T = 0; l !== h && !isNaN(T = o.charCodeAt(l)) && (T === 95 || T >= 48 && T <= 57 || T >= 65 && T <= 90 || T >= 97 && T <= 122); )
++l;
return new i.Token(c.TokenKind.NAME, u, l, y, f, m, o.slice(u, l));
}
function n2(t) {
return t === 95 || t >= 65 && t <= 90 || t >= 97 && t <= 122;
}
} }), Oe = L({ "node_modules/graphql/language/parser.js"(a) {
"use strict";
K(), Object.defineProperty(a, "__esModule", { value: true }), a.parse = O, a.parseValue = A, a.parseType = N, a.Parser = void 0;
var d = Z(), i = he(), c = te(), r = ne(), _ = me(), E = ye(), k = Ne();
function O(I, s) {
var p = new g(I, s);
return p.parseDocument();
}
function A(I, s) {
var p = new g(I, s);
p.expectToken(r.TokenKind.SOF);
var e = p.parseValueLiteral(false);
return p.expectToken(r.TokenKind.EOF), e;
}
function N(I, s) {
var p = new g(I, s);
p.expectToken(r.TokenKind.SOF);
var e = p.parseTypeReference();
return p.expectToken(r.TokenKind.EOF), e;
}
var g = function() {
function I(p, e) {
var n2 = (0, _.isSource)(p) ? p : new _.Source(p);
this._lexer = new k.Lexer(n2), this._options = e;
}
var s = I.prototype;
return s.parseName = function() {
var e = this.expectToken(r.TokenKind.NAME);
return { kind: i.Kind.NAME, value: e.value, loc: this.loc(e) };
}, s.parseDocument = function() {
var e = this._lexer.token;
return { kind: i.Kind.DOCUMENT, definitions: this.many(r.TokenKind.SOF, this.parseDefinition, r.TokenKind.EOF), loc: this.loc(e) };
}, s.parseDefinition = function() {
if (this.peek(r.TokenKind.NAME))
switch (this._lexer.token.value) {
case "query":
case "mutation":
case "subscription":
return this.parseOperationDefinition();
case "fragment":
return this.parseFragmentDefinition();
case "schema":
case "scalar":
case "type":
case "interface":
case "union":
case "enum":
case "input":
case "directive":
return this.parseTypeSystemDefinition();
case "extend":
return this.parseTypeSystemExtension();
}
else {
if (this.peek(r.TokenKind.BRACE_L))
return this.parseOperationDefinition();
if (this.peekDescription())
return this.parseTypeSystemDefinition();
}
throw this.unexpected();
}, s.parseOperationDefinition = function() {
var e = this._lexer.token;
if (this.peek(r.TokenKind.BRACE_L))
return { kind: i.Kind.OPERATION_DEFINITION, operation: "query", name: void 0, variableDefinitions: [], directives: [], selectionSet: this.parseSelectionSet(), loc: this.loc(e) };
var n2 = this.parseOperationType(), t;
return this.peek(r.TokenKind.NAME) && (t = this.parseName()), { kind: i.Kind.OPERATION_DEFINITION, operation: n2, name: t, variableDefinitions: this.parseVariableDefinitions(), directives: this.parseDirectives(false), selectionSet: this.parseSelectionSet(), loc: this.loc(e) };
}, s.parseOperationType = function() {
var e = this.expectToken(r.TokenKind.NAME);
switch (e.value) {
case "query":
return "query";
case "mutation":
return "mutation";
case "subscription":
return "subscription";
}
throw this.unexpected(e);
}, s.parseVariableDefinitions = function() {
return this.optionalMany(r.TokenKind.PAREN_L, this.parseVariableDefinition, r.TokenKind.PAREN_R);
}, s.parseVariableDefinition = function() {
var e = this._lexer.token;
return { kind: i.Kind.VARIABLE_DEFINITION, variable: this.parseVariable(), type: (this.expectToken(r.TokenKind.COLON), this.parseTypeReference()), defaultValue: this.expectOptionalToken(r.TokenKind.EQUALS) ? this.parseValueLiteral(true) : void 0, directives: this.parseDirectives(true), loc: this.loc(e) };
}, s.parseVariable = function() {
var e = this._lexer.token;
return this.expectToken(r.TokenKind.DOLLAR), { kind: i.Kind.VARIABLE, name: this.parseName(), loc: this.loc(e) };
}, s.parseSelectionSet = function() {
var e = this._lexer.token;
return { kind: i.Kind.SELECTION_SET, selections: this.many(r.TokenKind.BRACE_L, this.parseSelection, r.TokenKind.BRACE_R), loc: this.loc(e) };
}, s.parseSelection = function() {
return this.peek(r.TokenKind.SPREAD) ? this.parseFragment() : this.parseField();
}, s.parseField = function() {
var e = this._lexer.token, n2 = this.parseName(), t, u;
return this.expectOptionalToken(r.TokenKind.COLON) ? (t = n2, u = this.parseName()) : u = n2, { kind: i.Kind.FIELD, alias: t, name: u, arguments: this.parseArguments(false), directives: this.parseDirectives(false), selectionSet: this.peek(r.TokenKind.BRACE_L) ? this.parseSelectionSet() : void 0, loc: this.loc(e) };
}, s.parseArguments = function(e) {
var n2 = e ? this.parseConstArgument : this.parseArgument;
return this.optionalMany(r.TokenKind.PAREN_L, n2, r.TokenKind.PAREN_R);
}, s.parseArgument = function() {
var e = this._lexer.token, n2 = this.parseName();
return this.expectToken(r.TokenKind.COLON), { kind: i.Kind.ARGUMENT, name: n2, value: this.parseValueLiteral(false), loc: this.loc(e) };
}, s.parseConstArgument = function() {
var e = this._lexer.token;
return { kind: i.Kind.ARGUMENT, name: this.parseName(), value: (this.expectToken(r.TokenKind.COLON), this.parseValueLiteral(true)), loc: this.loc(e) };
}, s.parseFragment = function() {
var e = this._lexer.token;
this.expectToken(r.TokenKind.SPREAD);
var n2 = this.expectOptionalKeyword("on");
return !n2 && this.peek(r.TokenKind.NAME) ? { kind: i.Kind.FRAGMENT_SPREAD, name: this.parseFragmentName(), directives: this.parseDirectives(false), loc: this.loc(e) } : { kind: i.Kind.INLINE_FRAGMENT, typeCondition: n2 ? this.parseNamedType() : void 0, directives: this.parseDirectives(false), selectionSet: this.parseSelectionSet(), loc: this.loc(e) };
}, s.parseFragmentDefinition = function() {
var e, n2 = this._lexer.token;
return this.expectKeyword("fragment"), ((e = this._options) === null || e === void 0 ? void 0 : e.experimentalFragmentVariables) === true ? { kind: i.Kind.FRAGMENT_DEFINITION, name: this.parseFragmentName(), variableDefinitions: this.parseVariableDefinitions(), typeCondition: (this.expectKeyword("on"), this.parseNamedType()), directives: this.parseDirectives(false), selectionSet: this.parseSelectionSet(), loc: this.loc(n2) } : { kind: i.Kind.FRAGMENT_DEFINITION, name: this.parseFragmentName(), typeCondition: (this.expectKeyword("on"), this.parseNamedType()), directives: this.parseDirectives(false), selectionSet: this.parseSelectionSet(), loc: this.loc(n2) };
}, s.parseFragmentName = function() {
if (this._lexer.token.value === "on")
throw this.unexpected();
return this.parseName();
}, s.parseValueLiteral = function(e) {
var n2 = this._lexer.token;
switch (n2.kind) {
case r.TokenKind.BRACKET_L:
return this.parseList(e);
case r.TokenKind.BRACE_L:
return this.parseObject(e);
case r.TokenKind.INT:
return this._lexer.advance(), { kind: i.Kind.INT, value: n2.value, loc: this.loc(n2) };
case r.TokenKind.FLOAT:
return this._lexer.advance(), { kind: i.Kind.FLOAT, value: n2.value, loc: this.loc(n2) };
case r.TokenKind.STRING:
case r.TokenKind.BLOCK_STRING:
return this.parseStringLiteral();
case r.TokenKind.NAME:
switch (this._lexer.advance(), n2.value) {
case "true":
return { kind: i.Kind.BOOLEAN, value: true, loc: this.loc(n2) };
case "false":
return { kind: i.Kind.BOOLEAN, value: false, loc: this.loc(n2) };
case "null":
return { kind: i.Kind.NULL, loc: this.loc(n2) };
default:
return { kind: i.Kind.ENUM, value: n2.value, loc: this.loc(n2) };
}
case r.TokenKind.DOLLAR:
if (!e)
return this.parseVariable();
break;
}
throw this.unexpected();
}, s.parseStringLiteral = function() {
var e = this._lexer.token;
return this._lexer.advance(), { kind: i.Kind.STRING, value: e.value, block: e.kind === r.TokenKind.BLOCK_STRING, loc: this.loc(e) };
}, s.parseList = function(e) {
var n2 = this, t = this._lexer.token, u = function() {
return n2.parseValueLiteral(e);
};
return { kind: i.Kind.LIST, values: this.any(r.TokenKind.BRACKET_L, u, r.TokenKind.BRACKET_R), loc: this.loc(t) };
}, s.parseObject = function(e) {
var n2 = this, t = this._lexer.token, u = function() {
return n2.parseObjectField(e);
};
return { kind: i.Kind.OBJECT, fields: this.any(r.TokenKind.BRACE_L, u, r.TokenKind.BRACE_R), loc: this.loc(t) };
}, s.parseObjectField = function(e) {
var n2 = this._lexer.token, t = this.parseName();
return this.expectToken(r.TokenKind.COLON), { kind: i.Kind.OBJECT_FIELD, name: t, value: this.parseValueLiteral(e), loc: this.loc(n2) };
}, s.parseDirectives = function(e) {
for (var n2 = []; this.peek(r.TokenKind.AT); )
n2.push(this.parseDirective(e));
return n2;
}, s.parseDirective = function(e) {
var n2 = this._lexer.token;
return this.expectToken(r.TokenKind.AT), { kind: i.Kind.DIRECTIVE, name: this.parseName(), arguments: this.parseArguments(e), loc: this.loc(n2) };
}, s.parseTypeReference = function() {
var e = this._lexer.token, n2;
return this.expectOptionalToken(r.TokenKind.BRACKET_L) ? (n2 = this.parseTypeReference(), this.expectToken(r.TokenKind.BRACKET_R), n2 = { kind: i.Kind.LIST_TYPE, type: n2, loc: this.loc(e) }) : n2 = this.parseNamedType(), this.expectOptionalToken(r.TokenKind.BANG) ? { kind: i.Kind.NON_NULL_TYPE, type: n2, loc: this.loc(e) } : n2;
}, s.parseNamedType = function() {
var e = this._lexer.token;
return { kind: i.Kind.NAMED_TYPE, name: this.parseName(), loc: this.loc(e) };
}, s.parseTypeSystemDefinition = function() {
var e = this.peekDescription() ? this._lexer.lookahead() : this._lexer.token;
if (e.kind === r.TokenKind.NAME)
switch (e.value) {
case "schema":
return this.parseSchemaDefinition();
case "scalar":
return this.parseScalarTypeDefinition();
case "type":
return this.parseObjectTypeDefinition();
case "interface":
return this.parseInterfaceTypeDefinition();
case "union":
return this.parseUnionTypeDefinition();
case "enum":
return this.parseEnumTypeDefinition();
case "input":
return this.parseInputObjectTypeDefinition();
case "directive":
return this.parseDirectiveDefinition();
}
throw this.unexpected(e);
}, s.peekDescription = function() {
return this.peek(r.TokenKind.STRING) || this.peek(r.TokenKind.BLOCK_STRING);
}, s.parseDescription = function() {
if (this.peekDescription())
return this.parseStringLiteral();
}, s.parseSchemaDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription();
this.expectKeyword("schema");
var t = this.parseDirectives(true), u = this.many(r.TokenKind.BRACE_L, this.parseOperationTypeDefinition, r.TokenKind.BRACE_R);
return { kind: i.Kind.SCHEMA_DEFINITION, description: n2, directives: t, operationTypes: u, loc: this.loc(e) };
}, s.parseOperationTypeDefinition = function() {
var e = this._lexer.token, n2 = this.parseOperationType();
this.expectToken(r.TokenKind.COLON);
var t = this.parseNamedType();
return { kind: i.Kind.OPERATION_TYPE_DEFINITION, operation: n2, type: t, loc: this.loc(e) };
}, s.parseScalarTypeDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription();
this.expectKeyword("scalar");
var t = this.parseName(), u = this.parseDirectives(true);
return { kind: i.Kind.SCALAR_TYPE_DEFINITION, description: n2, name: t, directives: u, loc: this.loc(e) };
}, s.parseObjectTypeDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription();
this.expectKeyword("type");
var t = this.parseName(), u = this.parseImplementsInterfaces(), y = this.parseDirectives(true), f = this.parseFieldsDefinition();
return { kind: i.Kind.OBJECT_TYPE_DEFINITION, description: n2, name: t, interfaces: u, directives: y, fields: f, loc: this.loc(e) };
}, s.parseImplementsInterfaces = function() {
var e;
if (!this.expectOptionalKeyword("implements"))
return [];
if (((e = this._options) === null || e === void 0 ? void 0 : e.allowLegacySDLImplementsInterfaces) === true) {
var n2 = [];
this.expectOptionalToken(r.TokenKind.AMP);
do
n2.push(this.parseNamedType());
while (this.expectOptionalToken(r.TokenKind.AMP) || this.peek(r.TokenKind.NAME));
return n2;
}
return this.delimitedMany(r.TokenKind.AMP, this.parseNamedType);
}, s.parseFieldsDefinition = function() {
var e;
return ((e = this._options) === null || e === void 0 ? void 0 : e.allowLegacySDLEmptyFields) === true && this.peek(r.TokenKind.BRACE_L) && this._lexer.lookahead().kind === r.TokenKind.BRACE_R ? (this._lexer.advance(), this._lexer.advance(), []) : this.optionalMany(r.TokenKind.BRACE_L, this.parseFieldDefinition, r.TokenKind.BRACE_R);
}, s.parseFieldDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription(), t = this.parseName(), u = this.parseArgumentDefs();
this.expectToken(r.TokenKind.COLON);
var y = this.parseTypeReference(), f = this.parseDirectives(true);
return { kind: i.Kind.FIELD_DEFINITION, description: n2, name: t, arguments: u, type: y, directives: f, loc: this.loc(e) };
}, s.parseArgumentDefs = function() {
return this.optionalMany(r.TokenKind.PAREN_L, this.parseInputValueDef, r.TokenKind.PAREN_R);
}, s.parseInputValueDef = function() {
var e = this._lexer.token, n2 = this.parseDescription(), t = this.parseName();
this.expectToken(r.TokenKind.COLON);
var u = this.parseTypeReference(), y;
this.expectOptionalToken(r.TokenKind.EQUALS) && (y = this.parseValueLiteral(true));
var f = this.parseDirectives(true);
return { kind: i.Kind.INPUT_VALUE_DEFINITION, description: n2, name: t, type: u, defaultValue: y, directives: f, loc: this.loc(e) };
}, s.parseInterfaceTypeDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription();
this.expectKeyword("interface");
var t = this.parseName(), u = this.parseImplementsInterfaces(), y = this.parseDirectives(true), f = this.parseFieldsDefinition();
return { kind: i.Kind.INTERFACE_TYPE_DEFINITION, description: n2, name: t, interfaces: u, directives: y, fields: f, loc: this.loc(e) };
}, s.parseUnionTypeDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription();
this.expectKeyword("union");
var t = this.parseName(), u = this.parseDirectives(true), y = this.parseUnionMemberTypes();
return { kind: i.Kind.UNION_TYPE_DEFINITION, description: n2, name: t, directives: u, types: y, loc: this.loc(e) };
}, s.parseUnionMemberTypes = function() {
return this.expectOptionalToken(r.TokenKind.EQUALS) ? this.delimitedMany(r.TokenKind.PIPE, this.parseNamedType) : [];
}, s.parseEnumTypeDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription();
this.expectKeyword("enum");
var t = this.parseName(), u = this.parseDirectives(true), y = this.parseEnumValuesDefinition();
return { kind: i.Kind.ENUM_TYPE_DEFINITION, description: n2, name: t, directives: u, values: y, loc: this.loc(e) };
}, s.parseEnumValuesDefinition = function() {
return this.optionalMany(r.TokenKind.BRACE_L, this.parseEnumValueDefinition, r.TokenKind.BRACE_R);
}, s.parseEnumValueDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription(), t = this.parseName(), u = this.parseDirectives(true);
return { kind: i.Kind.ENUM_VALUE_DEFINITION, description: n2, name: t, directives: u, loc: this.loc(e) };
}, s.parseInputObjectTypeDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription();
this.expectKeyword("input");
var t = this.parseName(), u = this.parseDirectives(true), y = this.parseInputFieldsDefinition();
return { kind: i.Kind.INPUT_OBJECT_TYPE_DEFINITION, description: n2, name: t, directives: u, fields: y, loc: this.loc(e) };
}, s.parseInputFieldsDefinition = function() {
return this.optionalMany(r.TokenKind.BRACE_L, this.parseInputValueDef, r.TokenKind.BRACE_R);
}, s.parseTypeSystemExtension = function() {
var e = this._lexer.lookahead();
if (e.kind === r.TokenKind.NAME)
switch (e.value) {
case "schema":
return this.parseSchemaExtension();
case "scalar":
return this.parseScalarTypeExtension();
case "type":
return this.parseObjectTypeExtension();
case "interface":
return this.parseInterfaceTypeExtension();
case "union":
return this.parseUnionTypeExtension();
case "enum":
return this.parseEnumTypeExtension();
case "input":
return this.parseInputObjectTypeExtension();
}
throw this.unexpected(e);
}, s.parseSchemaExtension = function() {
var e = this._lexer.token;
this.expectKeyword("extend"), this.expectKeyword("schema");
var n2 = this.parseDirectives(true), t = this.optionalMany(r.TokenKind.BRACE_L, this.parseOperationTypeDefinition, r.TokenKind.BRACE_R);
if (n2.length === 0 && t.length === 0)
throw this.unexpected();
return { kind: i.Kind.SCHEMA_EXTENSION, directives: n2, operationTypes: t, loc: this.loc(e) };
}, s.parseScalarTypeExtension = function() {
var e = this._lexer.token;
this.expectKeyword("extend"), this.expectKeyword("scalar");
var n2 = this.parseName(), t = this.parseDirectives(true);
if (t.length === 0)
throw this.unexpected();
return { kind: i.Kind.SCALAR_TYPE_EXTENSION, name: n2, directives: t, loc: this.loc(e) };
}, s.parseObjectTypeExtension = function() {
var e = this._lexer.token;
this.expectKeyword("extend"), this.expectKeyword("type");
var n2 = this.parseName(), t = this.parseImplementsInterfaces(), u = this.parseDirectives(true), y = this.parseFieldsDefinition();
if (t.length === 0 && u.length === 0 && y.length === 0)
throw this.unexpected();
return { kind: i.Kind.OBJECT_TYPE_EXTENSION, name: n2, interfaces: t, directives: u, fields: y, loc: this.loc(e) };
}, s.parseInterfaceTypeExtension = function() {
var e = this._lexer.token;
this.expectKeyword("extend"), this.expectKeyword("interface");
var n2 = this.parseName(), t = this.parseImplementsInterfaces(), u = this.parseDirectives(true), y = this.parseFieldsDefinition();
if (t.length === 0 && u.length === 0 && y.length === 0)
throw this.unexpected();
return { kind: i.Kind.INTERFACE_TYPE_EXTENSION, name: n2, interfaces: t, directives: u, fields: y, loc: this.loc(e) };
}, s.parseUnionTypeExtension = function() {
var e = this._lexer.token;
this.expectKeyword("extend"), this.expectKeyword("union");
var n2 = this.parseName(), t = this.parseDirectives(true), u = this.parseUnionMemberTypes();
if (t.length === 0 && u.length === 0)
throw this.unexpected();
return { kind: i.Kind.UNION_TYPE_EXTENSION, name: n2, directives: t, types: u, loc: this.loc(e) };
}, s.parseEnumTypeExtension = function() {
var e = this._lexer.token;
this.expectKeyword("extend"), this.expectKeyword("enum");
var n2 = this.parseName(), t = this.parseDirectives(true), u = this.parseEnumValuesDefinition();
if (t.length === 0 && u.length === 0)
throw this.unexpected();
return { kind: i.Kind.ENUM_TYPE_EXTENSION, name: n2, directives: t, values: u, loc: this.loc(e) };
}, s.parseInputObjectTypeExtension = function() {
var e = this._lexer.token;
this.expectKeyword("extend"), this.expectKeyword("input");
var n2 = this.parseName(), t = this.parseDirectives(true), u = this.parseInputFieldsDefinition();
if (t.length === 0 && u.length === 0)
throw this.unexpected();
return { kind: i.Kind.INPUT_OBJECT_TYPE_EXTENSION, name: n2, directives: t, fields: u, loc: this.loc(e) };
}, s.parseDirectiveDefinition = function() {
var e = this._lexer.token, n2 = this.parseDescription();
this.expectKeyword("directive"), this.expectToken(r.TokenKind.AT);
var t = this.parseName(), u = this.parseArgumentDefs(), y = this.expectOptionalKeyword("repeatable");
this.expectKeyword("on");
var f = this.parseDirectiveLocations();
return { kind: i.Kind.DIRECTIVE_DEFINITION, description: n2, name: t, arguments: u, repeatable: y, locations: f, loc: this.loc(e) };
}, s.parseDirectiveLocations = function() {
return this.delimitedMany(r.TokenKind.PIPE, this.parseDirectiveLocation);
}, s.parseDirectiveLocation = function() {
var e = this._lexer.token, n2 = this.parseName();
if (E.DirectiveLocation[n2.value] !== void 0)
return n2;
throw this.unexpected(e);
}, s.loc = function(e) {
var n2;
if (((n2 = this._options) === null || n2 === void 0 ? void 0 : n2.noLocation) !== true)
return new c.Location(e, this._lexer.lastToken, this._lexer.source);
}, s.peek = function(e) {
return this._lexer.token.kind === e;
}, s.expectToken = function(e) {
var n2 = this._lexer.token;
if (n2.kind === e)
return this._lexer.advance(), n2;
throw (0, d.syntaxError)(this._lexer.source, n2.start, "Expected ".concat(v(e), ", found ").concat(D(n2), "."));
}, s.expectOptionalToken = function(e) {
var n2 = this._lexer.token;
if (n2.kind === e)
return this._lexer.advance(), n2;
}, s.expectKeyword = function(e) {
var n2 = this._lexer.token;
if (n2.kind === r.TokenKind.NAME && n2.value === e)
this._lexer.advance();
else
throw (0, d.syntaxError)(this._lexer.source, n2.start, 'Expected "'.concat(e, '", found ').concat(D(n2), "."));
}, s.expectOptionalKeyword = function(e) {
var n2 = this._lexer.token;
return n2.kind === r.TokenKind.NAME && n2.value === e ? (this._lexer.advance(), true) : false;
}, s.unexpected = function(e) {
var n2 = e != null ? e : this._lexer.token;
return (0, d.syntaxError)(this._lexer.source, n2.start, "Unexpected ".concat(D(n2), "."));
}, s.any = function(e, n2, t) {
this.expectToken(e);
for (var u = []; !this.expectOptionalToken(t); )
u.push(n2.call(this));
return u;
}, s.optionalMany = function(e, n2, t) {
if (this.expectOptionalToken(e)) {
var u = [];
do
u.push(n2.call(this));
while (!this.expectOptionalToken(t));
return u;
}
return [];
}, s.many = function(e, n2, t) {
this.expectToken(e);
var u = [];
do
u.push(n2.call(this));
while (!this.expectOptionalToken(t));
return u;
}, s.delimitedMany = function(e, n2) {
this.expectOptionalToken(e);
var t = [];
do
t.push(n2.call(this));
while (this.expectOptionalToken(e));
return t;
}, I;
}();
a.Parser = g;
function D(I) {
var s = I.value;
return v(I.kind) + (s != null ? ' "'.concat(s, '"') : "");
}
function v(I) {
return (0, k.isPunctuatorTokenKind)(I) ? '"'.concat(I, '"') : I;
}
} });
K();
var Ie = ce(), ge = ue(), { hasPragma: Se } = le(), { locStart: Ae, locEnd: De } = pe();
function Ke(a) {
let d = [], { startToken: i } = a.loc, { next: c } = i;
for (; c.kind !== ""; )
c.kind === "Comment" && (Object.assign(c, { column: c.column - 1 }), d.push(c)), c = c.next;
return d;
}
function ie(a) {
if (a && typeof a == "object") {
delete a.startToken, delete a.endToken, delete a.prev, delete a.next;
for (let d in a)
ie(a[d]);
}
return a;
}
var X = { allowLegacySDLImplementsInterfaces: false, experimentalFragmentVariables: true };
function Le(a) {
let { GraphQLError: d } = W();
if (a instanceof d) {
let { message: i, locations: [c] } = a;
return Ie(i, { start: c });
}
return a;
}
function xe(a) {
let { parse: d } = Oe(), { result: i, error: c } = ge(() => d(a, Object.assign({}, X)), () => d(a, Object.assign(Object.assign({}, X), {}, { allowLegacySDLImplementsInterfaces: true })));
if (!i)
throw Le(c);
return i.comments = Ke(i), ie(i), i;
}
ae.exports = { parsers: { graphql: { parse: xe, astFormat: "graphql", hasPragma: Se, locStart: Ae, locEnd: De } } };
});
return be();
});
}
});
// node_modules/prettier/parser-markdown.js
var require_parser_markdown = __commonJS({
"node_modules/prettier/parser-markdown.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.markdown = e();
}
})(function() {
"use strict";
var $ = (e, r) => () => (r || e((r = { exports: {} }).exports, r), r.exports);
var Fe = $((nf, yu) => {
var tr = function(e) {
return e && e.Math == Math && e;
};
yu.exports = tr(typeof globalThis == "object" && globalThis) || tr(typeof window == "object" && window) || tr(typeof self == "object" && self) || tr(typeof global == "object" && global) || function() {
return this;
}() || Function("return this")();
});
var Ae = $((af, wu) => {
wu.exports = function(e) {
try {
return !!e();
} catch {
return true;
}
};
});
var Be = $((of, Bu) => {
var fa = Ae();
Bu.exports = !fa(function() {
return Object.defineProperty({}, 1, { get: function() {
return 7;
} })[1] != 7;
});
});
var nr = $((sf, ku) => {
var pa = Ae();
ku.exports = !pa(function() {
var e = function() {
}.bind();
return typeof e != "function" || e.hasOwnProperty("prototype");
});
});
var Oe = $((cf, qu) => {
var da = nr(), ir = Function.prototype.call;
qu.exports = da ? ir.bind(ir) : function() {
return ir.apply(ir, arguments);
};
});
var Su = $((Iu) => {
"use strict";
var _u = {}.propertyIsEnumerable, Ou = Object.getOwnPropertyDescriptor, ha = Ou && !_u.call({ 1: 2 }, 1);
Iu.f = ha ? function(r) {
var u = Ou(this, r);
return !!u && u.enumerable;
} : _u;
});
var ar = $((Df, Tu) => {
Tu.exports = function(e, r) {
return { enumerable: !(e & 1), configurable: !(e & 2), writable: !(e & 4), value: r };
};
});
var ve = $((ff, Ru) => {
var Nu = nr(), Lu = Function.prototype, wr = Lu.call, va = Nu && Lu.bind.bind(wr, wr);
Ru.exports = Nu ? va : function(e) {
return function() {
return wr.apply(e, arguments);
};
};
});
var Ve = $((pf, Pu) => {
var ju = ve(), ma = ju({}.toString), Ea = ju("".slice);
Pu.exports = function(e) {
return Ea(ma(e), 8, -1);
};
});
var zu = $((df, Mu) => {
var Ca = ve(), ga = Ae(), Fa = Ve(), Br = Object, Aa = Ca("".split);
Mu.exports = ga(function() {
return !Br("z").propertyIsEnumerable(0);
}) ? function(e) {
return Fa(e) == "String" ? Aa(e, "") : Br(e);
} : Br;
});
var or = $((hf, $u) => {
$u.exports = function(e) {
return e == null;
};
});
var kr = $((vf, Uu) => {
var xa = or(), ba = TypeError;
Uu.exports = function(e) {
if (xa(e))
throw ba("Can't call method on " + e);
return e;
};
});
var sr = $((mf, Gu) => {
var ya = zu(), wa = kr();
Gu.exports = function(e) {
return ya(wa(e));
};
});
var _r = $((Ef, Vu) => {
var qr = typeof document == "object" && document.all, Ba = typeof qr > "u" && qr !== void 0;
Vu.exports = { all: qr, IS_HTMLDDA: Ba };
});
var de = $((Cf, Xu) => {
var Hu = _r(), ka = Hu.all;
Xu.exports = Hu.IS_HTMLDDA ? function(e) {
return typeof e == "function" || e === ka;
} : function(e) {
return typeof e == "function";
};
});
var Ie = $((gf, Yu) => {
var Wu = de(), Ku = _r(), qa = Ku.all;
Yu.exports = Ku.IS_HTMLDDA ? function(e) {
return typeof e == "object" ? e !== null : Wu(e) || e === qa;
} : function(e) {
return typeof e == "object" ? e !== null : Wu(e);
};
});
var He = $((Ff, Ju) => {
var Or = Fe(), _a = de(), Oa = function(e) {
return _a(e) ? e : void 0;
};
Ju.exports = function(e, r) {
return arguments.length < 2 ? Oa(Or[e]) : Or[e] && Or[e][r];
};
});
var Ir = $((Af, Zu) => {
var Ia = ve();
Zu.exports = Ia({}.isPrototypeOf);
});
var et = $((xf, Qu) => {
var Sa = He();
Qu.exports = Sa("navigator", "userAgent") || "";
});
var ot = $((bf, at) => {
var it = Fe(), Sr = et(), rt = it.process, ut = it.Deno, tt = rt && rt.versions || ut && ut.version, nt = tt && tt.v8, me, cr;
nt && (me = nt.split("."), cr = me[0] > 0 && me[0] < 4 ? 1 : +(me[0] + me[1]));
!cr && Sr && (me = Sr.match(/Edge\/(\d+)/), (!me || me[1] >= 74) && (me = Sr.match(/Chrome\/(\d+)/), me && (cr = +me[1])));
at.exports = cr;
});
var Tr = $((yf, ct) => {
var st = ot(), Ta = Ae();
ct.exports = !!Object.getOwnPropertySymbols && !Ta(function() {
var e = Symbol();
return !String(e) || !(Object(e) instanceof Symbol) || !Symbol.sham && st && st < 41;
});
});
var Nr = $((wf, lt) => {
var Na = Tr();
lt.exports = Na && !Symbol.sham && typeof Symbol.iterator == "symbol";
});
var Lr = $((Bf, Dt) => {
var La = He(), Ra = de(), ja = Ir(), Pa = Nr(), Ma = Object;
Dt.exports = Pa ? function(e) {
return typeof e == "symbol";
} : function(e) {
var r = La("Symbol");
return Ra(r) && ja(r.prototype, Ma(e));
};
});
var lr = $((kf, ft) => {
var za = String;
ft.exports = function(e) {
try {
return za(e);
} catch {
return "Object";
}
};
});
var Xe = $((qf, pt) => {
var $a = de(), Ua = lr(), Ga = TypeError;
pt.exports = function(e) {
if ($a(e))
return e;
throw Ga(Ua(e) + " is not a function");
};
});
var Dr = $((_f, dt) => {
var Va = Xe(), Ha = or();
dt.exports = function(e, r) {
var u = e[r];
return Ha(u) ? void 0 : Va(u);
};
});
var vt = $((Of, ht) => {
var Rr = Oe(), jr = de(), Pr = Ie(), Xa = TypeError;
ht.exports = function(e, r) {
var u, t;
if (r === "string" && jr(u = e.toString) && !Pr(t = Rr(u, e)) || jr(u = e.valueOf) && !Pr(t = Rr(u, e)) || r !== "string" && jr(u = e.toString) && !Pr(t = Rr(u, e)))
return t;
throw Xa("Can't convert object to primitive value");
};
});
var Et = $((If, mt) => {
mt.exports = false;
});
var fr = $((Sf, gt) => {
var Ct = Fe(), Wa = Object.defineProperty;
gt.exports = function(e, r) {
try {
Wa(Ct, e, { value: r, configurable: true, writable: true });
} catch {
Ct[e] = r;
}
return r;
};
});
var pr = $((Tf, At) => {
var Ka = Fe(), Ya = fr(), Ft = "__core-js_shared__", Ja = Ka[Ft] || Ya(Ft, {});
At.exports = Ja;
});
var Mr = $((Nf, bt) => {
var Za = Et(), xt = pr();
(bt.exports = function(e, r) {
return xt[e] || (xt[e] = r !== void 0 ? r : {});
})("versions", []).push({ version: "3.26.1", mode: Za ? "pure" : "global", copyright: "\xA9 2014-2022 Denis Pushkarev (zloirock.ru)", license: "https://github.com/zloirock/core-js/blob/v3.26.1/LICENSE", source: "https://github.com/zloirock/core-js" });
});
var zr = $((Lf, yt) => {
var Qa = kr(), eo = Object;
yt.exports = function(e) {
return eo(Qa(e));
};
});
var ke = $((Rf, wt) => {
var ro = ve(), uo = zr(), to = ro({}.hasOwnProperty);
wt.exports = Object.hasOwn || function(r, u) {
return to(uo(r), u);
};
});
var $r = $((jf, Bt) => {
var no = ve(), io = 0, ao = Math.random(), oo = no(1 .toString);
Bt.exports = function(e) {
return "Symbol(" + (e === void 0 ? "" : e) + ")_" + oo(++io + ao, 36);
};
});
var Te = $((Pf, It) => {
var so = Fe(), co = Mr(), kt = ke(), lo = $r(), qt = Tr(), Ot = Nr(), Le = co("wks"), Se = so.Symbol, _t = Se && Se.for, Do = Ot ? Se : Se && Se.withoutSetter || lo;
It.exports = function(e) {
if (!kt(Le, e) || !(qt || typeof Le[e] == "string")) {
var r = "Symbol." + e;
qt && kt(Se, e) ? Le[e] = Se[e] : Ot && _t ? Le[e] = _t(r) : Le[e] = Do(r);
}
return Le[e];
};
});
var Lt = $((Mf, Nt) => {
var fo = Oe(), St = Ie(), Tt = Lr(), po = Dr(), ho = vt(), vo = Te(), mo = TypeError, Eo = vo("toPrimitive");
Nt.exports = function(e, r) {
if (!St(e) || Tt(e))
return e;
var u = po(e, Eo), t;
if (u) {
if (r === void 0 && (r = "default"), t = fo(u, e, r), !St(t) || Tt(t))
return t;
throw mo("Can't convert object to primitive value");
}
return r === void 0 && (r = "number"), ho(e, r);
};
});
var dr = $((zf, Rt) => {
var Co = Lt(), go = Lr();
Rt.exports = function(e) {
var r = Co(e, "string");
return go(r) ? r : r + "";
};
});
var Mt = $(($f, Pt) => {
var Fo = Fe(), jt = Ie(), Ur = Fo.document, Ao = jt(Ur) && jt(Ur.createElement);
Pt.exports = function(e) {
return Ao ? Ur.createElement(e) : {};
};
});
var Gr = $((Uf, zt) => {
var xo = Be(), bo = Ae(), yo = Mt();
zt.exports = !xo && !bo(function() {
return Object.defineProperty(yo("div"), "a", { get: function() {
return 7;
} }).a != 7;
});
});
var Vr = $((Ut) => {
var wo = Be(), Bo = Oe(), ko = Su(), qo = ar(), _o = sr(), Oo = dr(), Io = ke(), So = Gr(), $t = Object.getOwnPropertyDescriptor;
Ut.f = wo ? $t : function(r, u) {
if (r = _o(r), u = Oo(u), So)
try {
return $t(r, u);
} catch {
}
if (Io(r, u))
return qo(!Bo(ko.f, r, u), r[u]);
};
});
var Vt = $((Vf, Gt) => {
var To = Be(), No = Ae();
Gt.exports = To && No(function() {
return Object.defineProperty(function() {
}, "prototype", { value: 42, writable: false }).prototype != 42;
});
});
var Re = $((Hf, Ht) => {
var Lo = Ie(), Ro = String, jo = TypeError;
Ht.exports = function(e) {
if (Lo(e))
return e;
throw jo(Ro(e) + " is not an object");
};
});
var We = $((Wt) => {
var Po = Be(), Mo = Gr(), zo = Vt(), hr = Re(), Xt = dr(), $o = TypeError, Hr = Object.defineProperty, Uo = Object.getOwnPropertyDescriptor, Xr = "enumerable", Wr = "configurable", Kr = "writable";
Wt.f = Po ? zo ? function(r, u, t) {
if (hr(r), u = Xt(u), hr(t), typeof r == "function" && u === "prototype" && "value" in t && Kr in t && !t[Kr]) {
var a = Uo(r, u);
a && a[Kr] && (r[u] = t.value, t = { configurable: Wr in t ? t[Wr] : a[Wr], enumerable: Xr in t ? t[Xr] : a[Xr], writable: false });
}
return Hr(r, u, t);
} : Hr : function(r, u, t) {
if (hr(r), u = Xt(u), hr(t), Mo)
try {
return Hr(r, u, t);
} catch {
}
if ("get" in t || "set" in t)
throw $o("Accessors not supported");
return "value" in t && (r[u] = t.value), r;
};
});
var Yr = $((Wf, Kt) => {
var Go = Be(), Vo = We(), Ho = ar();
Kt.exports = Go ? function(e, r, u) {
return Vo.f(e, r, Ho(1, u));
} : function(e, r, u) {
return e[r] = u, e;
};
});
var Zt = $((Kf, Jt) => {
var Jr = Be(), Xo = ke(), Yt = Function.prototype, Wo = Jr && Object.getOwnPropertyDescriptor, Zr = Xo(Yt, "name"), Ko = Zr && function() {
}.name === "something", Yo = Zr && (!Jr || Jr && Wo(Yt, "name").configurable);
Jt.exports = { EXISTS: Zr, PROPER: Ko, CONFIGURABLE: Yo };
});
var eu = $((Yf, Qt) => {
var Jo = ve(), Zo = de(), Qr = pr(), Qo = Jo(Function.toString);
Zo(Qr.inspectSource) || (Qr.inspectSource = function(e) {
return Qo(e);
});
Qt.exports = Qr.inspectSource;
});
var un = $((Jf, rn) => {
var es = Fe(), rs = de(), en = es.WeakMap;
rn.exports = rs(en) && /native code/.test(String(en));
});
var an = $((Zf, nn) => {
var us = Mr(), ts = $r(), tn = us("keys");
nn.exports = function(e) {
return tn[e] || (tn[e] = ts(e));
};
});
var ru = $((Qf, on) => {
on.exports = {};
});
var Dn = $((ep, ln) => {
var ns = un(), cn = Fe(), is = Ie(), as = Yr(), uu = ke(), tu = pr(), os = an(), ss = ru(), sn = "Object already initialized", nu = cn.TypeError, cs = cn.WeakMap, vr, Ke, mr, ls = function(e) {
return mr(e) ? Ke(e) : vr(e, {});
}, Ds = function(e) {
return function(r) {
var u;
if (!is(r) || (u = Ke(r)).type !== e)
throw nu("Incompatible receiver, " + e + " required");
return u;
};
};
ns || tu.state ? (Ee = tu.state || (tu.state = new cs()), Ee.get = Ee.get, Ee.has = Ee.has, Ee.set = Ee.set, vr = function(e, r) {
if (Ee.has(e))
throw nu(sn);
return r.facade = e, Ee.set(e, r), r;
}, Ke = function(e) {
return Ee.get(e) || {};
}, mr = function(e) {
return Ee.has(e);
}) : (Ne = os("state"), ss[Ne] = true, vr = function(e, r) {
if (uu(e, Ne))
throw nu(sn);
return r.facade = e, as(e, Ne, r), r;
}, Ke = function(e) {
return uu(e, Ne) ? e[Ne] : {};
}, mr = function(e) {
return uu(e, Ne);
});
var Ee, Ne;
ln.exports = { set: vr, get: Ke, has: mr, enforce: ls, getterFor: Ds };
});
var dn = $((rp, pn) => {
var fs2 = Ae(), ps = de(), Er = ke(), iu = Be(), ds = Zt().CONFIGURABLE, hs = eu(), fn = Dn(), vs = fn.enforce, ms = fn.get, Cr = Object.defineProperty, Es = iu && !fs2(function() {
return Cr(function() {
}, "length", { value: 8 }).length !== 8;
}), Cs = String(String).split("String"), gs = pn.exports = function(e, r, u) {
String(r).slice(0, 7) === "Symbol(" && (r = "[" + String(r).replace(/^Symbol\(([^)]*)\)/, "$1") + "]"), u && u.getter && (r = "get " + r), u && u.setter && (r = "set " + r), (!Er(e, "name") || ds && e.name !== r) && (iu ? Cr(e, "name", { value: r, configurable: true }) : e.name = r), Es && u && Er(u, "arity") && e.length !== u.arity && Cr(e, "length", { value: u.arity });
try {
u && Er(u, "constructor") && u.constructor ? iu && Cr(e, "prototype", { writable: false }) : e.prototype && (e.prototype = void 0);
} catch {
}
var t = vs(e);
return Er(t, "source") || (t.source = Cs.join(typeof r == "string" ? r : "")), e;
};
Function.prototype.toString = gs(function() {
return ps(this) && ms(this).source || hs(this);
}, "toString");
});
var vn = $((up, hn) => {
var Fs = de(), As = We(), xs = dn(), bs = fr();
hn.exports = function(e, r, u, t) {
t || (t = {});
var a = t.enumerable, n2 = t.name !== void 0 ? t.name : r;
if (Fs(u) && xs(u, n2, t), t.global)
a ? e[r] = u : bs(r, u);
else {
try {
t.unsafe ? e[r] && (a = true) : delete e[r];
} catch {
}
a ? e[r] = u : As.f(e, r, { value: u, enumerable: false, configurable: !t.nonConfigurable, writable: !t.nonWritable });
}
return e;
};
});
var En = $((tp, mn) => {
var ys = Math.ceil, ws = Math.floor;
mn.exports = Math.trunc || function(r) {
var u = +r;
return (u > 0 ? ws : ys)(u);
};
});
var au = $((np, Cn) => {
var Bs = En();
Cn.exports = function(e) {
var r = +e;
return r !== r || r === 0 ? 0 : Bs(r);
};
});
var Fn = $((ip, gn) => {
var ks = au(), qs = Math.max, _s = Math.min;
gn.exports = function(e, r) {
var u = ks(e);
return u < 0 ? qs(u + r, 0) : _s(u, r);
};
});
var xn = $((ap, An) => {
var Os = au(), Is = Math.min;
An.exports = function(e) {
return e > 0 ? Is(Os(e), 9007199254740991) : 0;
};
});
var Ye = $((op, bn) => {
var Ss = xn();
bn.exports = function(e) {
return Ss(e.length);
};
});
var Bn = $((sp, wn) => {
var Ts = sr(), Ns = Fn(), Ls = Ye(), yn = function(e) {
return function(r, u, t) {
var a = Ts(r), n2 = Ls(a), s = Ns(t, n2), c;
if (e && u != u) {
for (; n2 > s; )
if (c = a[s++], c != c)
return true;
} else
for (; n2 > s; s++)
if ((e || s in a) && a[s] === u)
return e || s || 0;
return !e && -1;
};
};
wn.exports = { includes: yn(true), indexOf: yn(false) };
});
var _n = $((cp, qn) => {
var Rs = ve(), ou = ke(), js = sr(), Ps = Bn().indexOf, Ms = ru(), kn = Rs([].push);
qn.exports = function(e, r) {
var u = js(e), t = 0, a = [], n2;
for (n2 in u)
!ou(Ms, n2) && ou(u, n2) && kn(a, n2);
for (; r.length > t; )
ou(u, n2 = r[t++]) && (~Ps(a, n2) || kn(a, n2));
return a;
};
});
var In = $((lp, On) => {
On.exports = ["constructor", "hasOwnProperty", "isPrototypeOf", "propertyIsEnumerable", "toLocaleString", "toString", "valueOf"];
});
var Tn = $((Sn) => {
var zs = _n(), $s = In(), Us = $s.concat("length", "prototype");
Sn.f = Object.getOwnPropertyNames || function(r) {
return zs(r, Us);
};
});
var Ln = $((Nn) => {
Nn.f = Object.getOwnPropertySymbols;
});
var jn = $((pp, Rn) => {
var Gs = He(), Vs = ve(), Hs = Tn(), Xs = Ln(), Ws = Re(), Ks = Vs([].concat);
Rn.exports = Gs("Reflect", "ownKeys") || function(r) {
var u = Hs.f(Ws(r)), t = Xs.f;
return t ? Ks(u, t(r)) : u;
};
});
var zn = $((dp, Mn) => {
var Pn = ke(), Ys = jn(), Js = Vr(), Zs = We();
Mn.exports = function(e, r, u) {
for (var t = Ys(r), a = Zs.f, n2 = Js.f, s = 0; s < t.length; s++) {
var c = t[s];
!Pn(e, c) && !(u && Pn(u, c)) && a(e, c, n2(r, c));
}
};
});
var Un = $((hp, $n) => {
var Qs = Ae(), ec = de(), rc = /#|\.prototype\./, Je = function(e, r) {
var u = tc[uc(e)];
return u == ic ? true : u == nc ? false : ec(r) ? Qs(r) : !!r;
}, uc = Je.normalize = function(e) {
return String(e).replace(rc, ".").toLowerCase();
}, tc = Je.data = {}, nc = Je.NATIVE = "N", ic = Je.POLYFILL = "P";
$n.exports = Je;
});
var cu = $((vp, Gn) => {
var su = Fe(), ac = Vr().f, oc = Yr(), sc = vn(), cc = fr(), lc = zn(), Dc = Un();
Gn.exports = function(e, r) {
var u = e.target, t = e.global, a = e.stat, n2, s, c, i, D, o;
if (t ? s = su : a ? s = su[u] || cc(u, {}) : s = (su[u] || {}).prototype, s)
for (c in r) {
if (D = r[c], e.dontCallGetSet ? (o = ac(s, c), i = o && o.value) : i = s[c], n2 = Dc(t ? c : u + (a ? "." : "#") + c, e.forced), !n2 && i !== void 0) {
if (typeof D == typeof i)
continue;
lc(D, i);
}
(e.sham || i && i.sham) && oc(D, "sham", true), sc(s, c, D, e);
}
};
});
var lu = $((mp, Vn) => {
var fc = Ve();
Vn.exports = Array.isArray || function(r) {
return fc(r) == "Array";
};
});
var Xn = $((Ep, Hn) => {
var pc = TypeError, dc = 9007199254740991;
Hn.exports = function(e) {
if (e > dc)
throw pc("Maximum allowed index exceeded");
return e;
};
});
var Kn = $((Cp, Wn) => {
var hc = Ve(), vc = ve();
Wn.exports = function(e) {
if (hc(e) === "Function")
return vc(e);
};
});
var Du = $((gp, Jn) => {
var Yn = Kn(), mc = Xe(), Ec = nr(), Cc = Yn(Yn.bind);
Jn.exports = function(e, r) {
return mc(e), r === void 0 ? e : Ec ? Cc(e, r) : function() {
return e.apply(r, arguments);
};
};
});
var ei = $((Fp, Qn) => {
"use strict";
var gc = lu(), Fc = Ye(), Ac = Xn(), xc = Du(), Zn = function(e, r, u, t, a, n2, s, c) {
for (var i = a, D = 0, o = s ? xc(s, c) : false, l, d; D < t; )
D in u && (l = o ? o(u[D], D, r) : u[D], n2 > 0 && gc(l) ? (d = Fc(l), i = Zn(e, r, l, d, i, n2 - 1) - 1) : (Ac(i + 1), e[i] = l), i++), D++;
return i;
};
Qn.exports = Zn;
});
var ti = $((Ap, ui) => {
var bc = Te(), yc = bc("toStringTag"), ri = {};
ri[yc] = "z";
ui.exports = String(ri) === "[object z]";
});
var fu = $((xp, ni) => {
var wc = ti(), Bc = de(), gr = Ve(), kc = Te(), qc = kc("toStringTag"), _c = Object, Oc = gr(function() {
return arguments;
}()) == "Arguments", Ic = function(e, r) {
try {
return e[r];
} catch {
}
};
ni.exports = wc ? gr : function(e) {
var r, u, t;
return e === void 0 ? "Undefined" : e === null ? "Null" : typeof (u = Ic(r = _c(e), qc)) == "string" ? u : Oc ? gr(r) : (t = gr(r)) == "Object" && Bc(r.callee) ? "Arguments" : t;
};
});
var li = $((bp, ci) => {
var Sc = ve(), Tc = Ae(), ii = de(), Nc = fu(), Lc = He(), Rc = eu(), ai = function() {
}, jc = [], oi = Lc("Reflect", "construct"), pu = /^\s*(?:class|function)\b/, Pc = Sc(pu.exec), Mc = !pu.exec(ai), Ze = function(r) {
if (!ii(r))
return false;
try {
return oi(ai, jc, r), true;
} catch {
return false;
}
}, si = function(r) {
if (!ii(r))
return false;
switch (Nc(r)) {
case "AsyncFunction":
case "GeneratorFunction":
case "AsyncGeneratorFunction":
return false;
}
try {
return Mc || !!Pc(pu, Rc(r));
} catch {
return true;
}
};
si.sham = true;
ci.exports = !oi || Tc(function() {
var e;
return Ze(Ze.call) || !Ze(Object) || !Ze(function() {
e = true;
}) || e;
}) ? si : Ze;
});
var di = $((yp, pi) => {
var Di = lu(), zc = li(), $c = Ie(), Uc = Te(), Gc = Uc("species"), fi = Array;
pi.exports = function(e) {
var r;
return Di(e) && (r = e.constructor, zc(r) && (r === fi || Di(r.prototype)) ? r = void 0 : $c(r) && (r = r[Gc], r === null && (r = void 0))), r === void 0 ? fi : r;
};
});
var vi = $((wp, hi) => {
var Vc = di();
hi.exports = function(e, r) {
return new (Vc(e))(r === 0 ? 0 : r);
};
});
var mi = $(() => {
"use strict";
var Hc = cu(), Xc = ei(), Wc = Xe(), Kc = zr(), Yc = Ye(), Jc = vi();
Hc({ target: "Array", proto: true }, { flatMap: function(r) {
var u = Kc(this), t = Yc(u), a;
return Wc(r), a = Jc(u, 0), a.length = Xc(a, u, u, t, 0, 1, r, arguments.length > 1 ? arguments[1] : void 0), a;
} });
});
var du = $((qp, Ei) => {
Ei.exports = {};
});
var gi = $((_p, Ci) => {
var Zc = Te(), Qc = du(), el = Zc("iterator"), rl = Array.prototype;
Ci.exports = function(e) {
return e !== void 0 && (Qc.Array === e || rl[el] === e);
};
});
var hu = $((Op, Ai) => {
var ul = fu(), Fi = Dr(), tl = or(), nl = du(), il = Te(), al = il("iterator");
Ai.exports = function(e) {
if (!tl(e))
return Fi(e, al) || Fi(e, "@@iterator") || nl[ul(e)];
};
});
var bi = $((Ip, xi) => {
var ol = Oe(), sl = Xe(), cl = Re(), ll = lr(), Dl = hu(), fl = TypeError;
xi.exports = function(e, r) {
var u = arguments.length < 2 ? Dl(e) : r;
if (sl(u))
return cl(ol(u, e));
throw fl(ll(e) + " is not iterable");
};
});
var Bi = $((Sp, wi) => {
var pl = Oe(), yi = Re(), dl = Dr();
wi.exports = function(e, r, u) {
var t, a;
yi(e);
try {
if (t = dl(e, "return"), !t) {
if (r === "throw")
throw u;
return u;
}
t = pl(t, e);
} catch (n2) {
a = true, t = n2;
}
if (r === "throw")
throw u;
if (a)
throw t;
return yi(t), u;
};
});
var Ii = $((Tp, Oi) => {
var hl = Du(), vl = Oe(), ml = Re(), El = lr(), Cl = gi(), gl = Ye(), ki = Ir(), Fl = bi(), Al = hu(), qi = Bi(), xl = TypeError, Fr = function(e, r) {
this.stopped = e, this.result = r;
}, _i = Fr.prototype;
Oi.exports = function(e, r, u) {
var t = u && u.that, a = !!(u && u.AS_ENTRIES), n2 = !!(u && u.IS_RECORD), s = !!(u && u.IS_ITERATOR), c = !!(u && u.INTERRUPTED), i = hl(r, t), D, o, l, d, p, g, F, E = function(f) {
return D && qi(D, "normal", f), new Fr(true, f);
}, b = function(f) {
return a ? (ml(f), c ? i(f[0], f[1], E) : i(f[0], f[1])) : c ? i(f, E) : i(f);
};
if (n2)
D = e.iterator;
else if (s)
D = e;
else {
if (o = Al(e), !o)
throw xl(El(e) + " is not iterable");
if (Cl(o)) {
for (l = 0, d = gl(e); d > l; l++)
if (p = b(e[l]), p && ki(_i, p))
return p;
return new Fr(false);
}
D = Fl(e, o);
}
for (g = n2 ? e.next : D.next; !(F = vl(g, D)).done; ) {
try {
p = b(F.value);
} catch (f) {
qi(D, "throw", f);
}
if (typeof p == "object" && p && ki(_i, p))
return p;
}
return new Fr(false);
};
});
var Ti = $((Np, Si) => {
"use strict";
var bl = dr(), yl = We(), wl = ar();
Si.exports = function(e, r, u) {
var t = bl(r);
t in e ? yl.f(e, t, wl(0, u)) : e[t] = u;
};
});
var Ni = $(() => {
var Bl = cu(), kl = Ii(), ql = Ti();
Bl({ target: "Object", stat: true }, { fromEntries: function(r) {
var u = {};
return kl(r, function(t, a) {
ql(u, t, a);
}, { AS_ENTRIES: true }), u;
} });
});
var uf = $((jp, la) => {
var _l = ["cliName", "cliCategory", "cliDescription"];
function Ol(e, r) {
if (e == null)
return {};
var u = Il(e, r), t, a;
if (Object.getOwnPropertySymbols) {
var n3 = Object.getOwnPropertySymbols(e);
for (a = 0; a < n3.length; a++)
t = n3[a], !(r.indexOf(t) >= 0) && Object.prototype.propertyIsEnumerable.call(e, t) && (u[t] = e[t]);
}
return u;
}
function Il(e, r) {
if (e == null)
return {};
var u = {}, t = Object.keys(e), a, n3;
for (n3 = 0; n3 < t.length; n3++)
a = t[n3], !(r.indexOf(a) >= 0) && (u[a] = e[a]);
return u;
}
mi();
Ni();
var Sl = Object.create, Ar = Object.defineProperty, Tl = Object.getOwnPropertyDescriptor, vu = Object.getOwnPropertyNames, Nl = Object.getPrototypeOf, Ll = Object.prototype.hasOwnProperty, je = (e, r) => function() {
return e && (r = (0, e[vu(e)[0]])(e = 0)), r;
}, S = (e, r) => function() {
return r || (0, e[vu(e)[0]])((r = { exports: {} }).exports, r), r.exports;
}, Pi = (e, r) => {
for (var u in r)
Ar(e, u, { get: r[u], enumerable: true });
}, Mi = (e, r, u, t) => {
if (r && typeof r == "object" || typeof r == "function")
for (let a of vu(r))
!Ll.call(e, a) && a !== u && Ar(e, a, { get: () => r[a], enumerable: !(t = Tl(r, a)) || t.enumerable });
return e;
}, Rl = (e, r, u) => (u = e != null ? Sl(Nl(e)) : {}, Mi(r || !e || !e.__esModule ? Ar(u, "default", { value: e, enumerable: true }) : u, e)), zi = (e) => Mi(Ar({}, "__esModule", { value: true }), e), Qe, I = je({ ""() {
Qe = { env: {}, argv: [] };
} }), Pe = S({ "node_modules/xtend/immutable.js"(e, r) {
I(), r.exports = t;
var u = Object.prototype.hasOwnProperty;
function t() {
for (var a = {}, n3 = 0; n3 < arguments.length; n3++) {
var s = arguments[n3];
for (var c in s)
u.call(s, c) && (a[c] = s[c]);
}
return a;
}
} }), jl = S({ "node_modules/inherits/inherits_browser.js"(e, r) {
I(), typeof Object.create == "function" ? r.exports = function(t, a) {
a && (t.super_ = a, t.prototype = Object.create(a.prototype, { constructor: { value: t, enumerable: false, writable: true, configurable: true } }));
} : r.exports = function(t, a) {
if (a) {
t.super_ = a;
var n3 = function() {
};
n3.prototype = a.prototype, t.prototype = new n3(), t.prototype.constructor = t;
}
};
} }), Pl = S({ "node_modules/unherit/index.js"(e, r) {
"use strict";
I();
var u = Pe(), t = jl();
r.exports = a;
function a(n3) {
var s, c, i;
t(o, n3), t(D, o), s = o.prototype;
for (c in s)
i = s[c], i && typeof i == "object" && (s[c] = "concat" in i ? i.concat() : u(i));
return o;
function D(l) {
return n3.apply(this, l);
}
function o() {
return this instanceof o ? n3.apply(this, arguments) : new D(arguments);
}
}
} }), Ml = S({ "node_modules/state-toggle/index.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a, n3) {
return s;
function s() {
var c = n3 || this, i = c[t];
return c[t] = !a, D;
function D() {
c[t] = i;
}
}
}
} }), zl = S({ "node_modules/vfile-location/index.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t) {
for (var a = String(t), n3 = [], s = /\r?\n|\r/g; s.exec(a); )
n3.push(s.lastIndex);
return n3.push(a.length + 1), { toPoint: c, toPosition: c, toOffset: i };
function c(D) {
var o = -1;
if (D > -1 && D < n3[n3.length - 1]) {
for (; ++o < n3.length; )
if (n3[o] > D)
return { line: o + 1, column: D - (n3[o - 1] || 0) + 1, offset: D };
}
return {};
}
function i(D) {
var o = D && D.line, l = D && D.column, d;
return !isNaN(o) && !isNaN(l) && o - 1 in n3 && (d = (n3[o - 2] || 0) + l - 1 || 0), d > -1 && d < n3[n3.length - 1] ? d : -1;
}
}
} }), $l = S({ "node_modules/remark-parse/lib/unescape.js"(e, r) {
"use strict";
I(), r.exports = t;
var u = "\\";
function t(a, n3) {
return s;
function s(c) {
for (var i = 0, D = c.indexOf(u), o = a[n3], l = [], d; D !== -1; )
l.push(c.slice(i, D)), i = D + 1, d = c.charAt(i), (!d || o.indexOf(d) === -1) && l.push(u), D = c.indexOf(u, i + 1);
return l.push(c.slice(i)), l.join("");
}
}
} }), Ul = S({ "node_modules/character-entities-legacy/index.json"(e, r) {
r.exports = { AElig: "\xC6", AMP: "&", Aacute: "\xC1", Acirc: "\xC2", Agrave: "\xC0", Aring: "\xC5", Atilde: "\xC3", Auml: "\xC4", COPY: "\xA9", Ccedil: "\xC7", ETH: "\xD0", Eacute: "\xC9", Ecirc: "\xCA", Egrave: "\xC8", Euml: "\xCB", GT: ">", Iacute: "\xCD", Icirc: "\xCE", Igrave: "\xCC", Iuml: "\xCF", LT: "<", Ntilde: "\xD1", Oacute: "\xD3", Ocirc: "\xD4", Ograve: "\xD2", Oslash: "\xD8", Otilde: "\xD5", Ouml: "\xD6", QUOT: '"', REG: "\xAE", THORN: "\xDE", Uacute: "\xDA", Ucirc: "\xDB", Ugrave: "\xD9", Uuml: "\xDC", Yacute: "\xDD", aacute: "\xE1", acirc: "\xE2", acute: "\xB4", aelig: "\xE6", agrave: "\xE0", amp: "&", aring: "\xE5", atilde: "\xE3", auml: "\xE4", brvbar: "\xA6", ccedil: "\xE7", cedil: "\xB8", cent: "\xA2", copy: "\xA9", curren: "\xA4", deg: "\xB0", divide: "\xF7", eacute: "\xE9", ecirc: "\xEA", egrave: "\xE8", eth: "\xF0", euml: "\xEB", frac12: "\xBD", frac14: "\xBC", frac34: "\xBE", gt: ">", iacute: "\xED", icirc: "\xEE", iexcl: "\xA1", igrave: "\xEC", iquest: "\xBF", iuml: "\xEF", laquo: "\xAB", lt: "<", macr: "\xAF", micro: "\xB5", middot: "\xB7", nbsp: "\xA0", not: "\xAC", ntilde: "\xF1", oacute: "\xF3", ocirc: "\xF4", ograve: "\xF2", ordf: "\xAA", ordm: "\xBA", oslash: "\xF8", otilde: "\xF5", ouml: "\xF6", para: "\xB6", plusmn: "\xB1", pound: "\xA3", quot: '"', raquo: "\xBB", reg: "\xAE", sect: "\xA7", shy: "\xAD", sup1: "\xB9", sup2: "\xB2", sup3: "\xB3", szlig: "\xDF", thorn: "\xFE", times: "\xD7", uacute: "\xFA", ucirc: "\xFB", ugrave: "\xF9", uml: "\xA8", uuml: "\xFC", yacute: "\xFD", yen: "\xA5", yuml: "\xFF" };
} }), Gl = S({ "node_modules/character-reference-invalid/index.json"(e, r) {
r.exports = { 0: "\uFFFD", 128: "\u20AC", 130: "\u201A", 131: "\u0192", 132: "\u201E", 133: "\u2026", 134: "\u2020", 135: "\u2021", 136: "\u02C6", 137: "\u2030", 138: "\u0160", 139: "\u2039", 140: "\u0152", 142: "\u017D", 145: "\u2018", 146: "\u2019", 147: "\u201C", 148: "\u201D", 149: "\u2022", 150: "\u2013", 151: "\u2014", 152: "\u02DC", 153: "\u2122", 154: "\u0161", 155: "\u203A", 156: "\u0153", 158: "\u017E", 159: "\u0178" };
} }), Me = S({ "node_modules/is-decimal/index.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t) {
var a = typeof t == "string" ? t.charCodeAt(0) : t;
return a >= 48 && a <= 57;
}
} }), Vl = S({ "node_modules/is-hexadecimal/index.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t) {
var a = typeof t == "string" ? t.charCodeAt(0) : t;
return a >= 97 && a <= 102 || a >= 65 && a <= 70 || a >= 48 && a <= 57;
}
} }), er = S({ "node_modules/is-alphabetical/index.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t) {
var a = typeof t == "string" ? t.charCodeAt(0) : t;
return a >= 97 && a <= 122 || a >= 65 && a <= 90;
}
} }), Hl = S({ "node_modules/is-alphanumerical/index.js"(e, r) {
"use strict";
I();
var u = er(), t = Me();
r.exports = a;
function a(n3) {
return u(n3) || t(n3);
}
} }), Xl = S({ "node_modules/character-entities/index.json"(e, r) {
r.exports = { AEli: "\xC6", AElig: "\xC6", AM: "&", AMP: "&", Aacut: "\xC1", Aacute: "\xC1", Abreve: "\u0102", Acir: "\xC2", Acirc: "\xC2", Acy: "\u0410", Afr: "\u{1D504}", Agrav: "\xC0", Agrave: "\xC0", Alpha: "\u0391", Amacr: "\u0100", And: "\u2A53", Aogon: "\u0104", Aopf: "\u{1D538}", ApplyFunction: "\u2061", Arin: "\xC5", Aring: "\xC5", Ascr: "\u{1D49C}", Assign: "\u2254", Atild: "\xC3", Atilde: "\xC3", Aum: "\xC4", Auml: "\xC4", Backslash: "\u2216", Barv: "\u2AE7", Barwed: "\u2306", Bcy: "\u0411", Because: "\u2235", Bernoullis: "\u212C", Beta: "\u0392", Bfr: "\u{1D505}", Bopf: "\u{1D539}", Breve: "\u02D8", Bscr: "\u212C", Bumpeq: "\u224E", CHcy: "\u0427", COP: "\xA9", COPY: "\xA9", Cacute: "\u0106", Cap: "\u22D2", CapitalDifferentialD: "\u2145", Cayleys: "\u212D", Ccaron: "\u010C", Ccedi: "\xC7", Ccedil: "\xC7", Ccirc: "\u0108", Cconint: "\u2230", Cdot: "\u010A", Cedilla: "\xB8", CenterDot: "\xB7", Cfr: "\u212D", Chi: "\u03A7", CircleDot: "\u2299", CircleMinus: "\u2296", CirclePlus: "\u2295", CircleTimes: "\u2297", ClockwiseContourIntegral: "\u2232", CloseCurlyDoubleQuote: "\u201D", CloseCurlyQuote: "\u2019", Colon: "\u2237", Colone: "\u2A74", Congruent: "\u2261", Conint: "\u222F", ContourIntegral: "\u222E", Copf: "\u2102", Coproduct: "\u2210", CounterClockwiseContourIntegral: "\u2233", Cross: "\u2A2F", Cscr: "\u{1D49E}", Cup: "\u22D3", CupCap: "\u224D", DD: "\u2145", DDotrahd: "\u2911", DJcy: "\u0402", DScy: "\u0405", DZcy: "\u040F", Dagger: "\u2021", Darr: "\u21A1", Dashv: "\u2AE4", Dcaron: "\u010E", Dcy: "\u0414", Del: "\u2207", Delta: "\u0394", Dfr: "\u{1D507}", DiacriticalAcute: "\xB4", DiacriticalDot: "\u02D9", DiacriticalDoubleAcute: "\u02DD", DiacriticalGrave: "`", DiacriticalTilde: "\u02DC", Diamond: "\u22C4", DifferentialD: "\u2146", Dopf: "\u{1D53B}", Dot: "\xA8", DotDot: "\u20DC", DotEqual: "\u2250", DoubleContourIntegral: "\u222F", DoubleDot: "\xA8", DoubleDownArrow: "\u21D3", DoubleLeftArrow: "\u21D0", DoubleLeftRightArrow: "\u21D4", DoubleLeftTee: "\u2AE4", DoubleLongLeftArrow: "\u27F8", DoubleLongLeftRightArrow: "\u27FA", DoubleLongRightArrow: "\u27F9", DoubleRightArrow: "\u21D2", DoubleRightTee: "\u22A8", DoubleUpArrow: "\u21D1", DoubleUpDownArrow: "\u21D5", DoubleVerticalBar: "\u2225", DownArrow: "\u2193", DownArrowBar: "\u2913", DownArrowUpArrow: "\u21F5", DownBreve: "\u0311", DownLeftRightVector: "\u2950", DownLeftTeeVector: "\u295E", DownLeftVector: "\u21BD", DownLeftVectorBar: "\u2956", DownRightTeeVector: "\u295F", DownRightVector: "\u21C1", DownRightVectorBar: "\u2957", DownTee: "\u22A4", DownTeeArrow: "\u21A7", Downarrow: "\u21D3", Dscr: "\u{1D49F}", Dstrok: "\u0110", ENG: "\u014A", ET: "\xD0", ETH: "\xD0", Eacut: "\xC9", Eacute: "\xC9", Ecaron: "\u011A", Ecir: "\xCA", Ecirc: "\xCA", Ecy: "\u042D", Edot: "\u0116", Efr: "\u{1D508}", Egrav: "\xC8", Egrave: "\xC8", Element: "\u2208", Emacr: "\u0112", EmptySmallSquare: "\u25FB", EmptyVerySmallSquare: "\u25AB", Eogon: "\u0118", Eopf: "\u{1D53C}", Epsilon: "\u0395", Equal: "\u2A75", EqualTilde: "\u2242", Equilibrium: "\u21CC", Escr: "\u2130", Esim: "\u2A73", Eta: "\u0397", Eum: "\xCB", Euml: "\xCB", Exists: "\u2203", ExponentialE: "\u2147", Fcy: "\u0424", Ffr: "\u{1D509}", FilledSmallSquare: "\u25FC", FilledVerySmallSquare: "\u25AA", Fopf: "\u{1D53D}", ForAll: "\u2200", Fouriertrf: "\u2131", Fscr: "\u2131", GJcy: "\u0403", G: ">", GT: ">", Gamma: "\u0393", Gammad: "\u03DC", Gbreve: "\u011E", Gcedil: "\u0122", Gcirc: "\u011C", Gcy: "\u0413", Gdot: "\u0120", Gfr: "\u{1D50A}", Gg: "\u22D9", Gopf: "\u{1D53E}", GreaterEqual: "\u2265", GreaterEqualLess: "\u22DB", GreaterFullEqual: "\u2267", GreaterGreater: "\u2AA2", GreaterLess: "\u2277", GreaterSlantEqual: "\u2A7E", GreaterTilde: "\u2273", Gscr: "\u{1D4A2}", Gt: "\u226B", HARDcy: "\u042A", Hacek: "\u02C7", Hat: "^", Hcirc: "\u0124", Hfr: "\u210C", HilbertSpace: "\u210B", Hopf: "\u210D", HorizontalLine: "\u2500", Hscr: "\u210B", Hstrok: "\u0126", HumpDownHump: "\u224E", HumpEqual: "\u224F", IEcy: "\u0415", IJlig: "\u0132", IOcy: "\u0401", Iacut: "\xCD", Iacute: "\xCD", Icir: "\xCE", Icirc: "\xCE", Icy: "\u0418", Idot: "\u0130", Ifr: "\u2111", Igrav: "\xCC", Igrave: "\xCC", Im: "\u2111", Imacr: "\u012A", ImaginaryI: "\u2148", Implies: "\u21D2", Int: "\u222C", Integral: "\u222B", Intersection: "\u22C2", InvisibleComma: "\u2063", InvisibleTimes: "\u2062", Iogon: "\u012E", Iopf: "\u{1D540}", Iota: "\u0399", Iscr: "\u2110", Itilde: "\u0128", Iukcy: "\u0406", Ium: "\xCF", Iuml: "\xCF", Jcirc: "\u0134", Jcy: "\u0419", Jfr: "\u{1D50D}", Jopf: "\u{1D541}", Jscr: "\u{1D4A5}", Jsercy: "\u0408", Jukcy: "\u0404", KHcy: "\u0425", KJcy: "\u040C", Kappa: "\u039A", Kcedil: "\u0136", Kcy: "\u041A", Kfr: "\u{1D50E}", Kopf: "\u{1D542}", Kscr: "\u{1D4A6}", LJcy: "\u0409", L: "<", LT: "<", Lacute: "\u0139", Lambda: "\u039B", Lang: "\u27EA", Laplacetrf: "\u2112", Larr: "\u219E", Lcaron: "\u013D", Lcedil: "\u013B", Lcy: "\u041B", LeftAngleBracket: "\u27E8", LeftArrow: "\u2190", LeftArrowBar: "\u21E4", LeftArrowRightArrow: "\u21C6", LeftCeiling: "\u2308", LeftDoubleBracket: "\u27E6", LeftDownTeeVector: "\u2961", LeftDownVector: "\u21C3", LeftDownVectorBar: "\u2959", LeftFloor: "\u230A", LeftRightArrow: "\u2194", LeftRightVector: "\u294E", LeftTee: "\u22A3", LeftTeeArrow: "\u21A4", LeftTeeVector: "\u295A", LeftTriangle: "\u22B2", LeftTriangleBar: "\u29CF", LeftTriangleEqual: "\u22B4", LeftUpDownVector: "\u2951", LeftUpTeeVector: "\u2960", LeftUpVector: "\u21BF", LeftUpVectorBar: "\u2958", LeftVector: "\u21BC", LeftVectorBar: "\u2952", Leftarrow: "\u21D0", Leftrightarrow: "\u21D4", LessEqualGreater: "\u22DA", LessFullEqual: "\u2266", LessGreater: "\u2276", LessLess: "\u2AA1", LessSlantEqual: "\u2A7D", LessTilde: "\u2272", Lfr: "\u{1D50F}", Ll: "\u22D8", Lleftarrow: "\u21DA", Lmidot: "\u013F", LongLeftArrow: "\u27F5", LongLeftRightArrow: "\u27F7", LongRightArrow: "\u27F6", Longleftarrow: "\u27F8", Longleftrightarrow: "\u27FA", Longrightarrow: "\u27F9", Lopf: "\u{1D543}", LowerLeftArrow: "\u2199", LowerRightArrow: "\u2198", Lscr: "\u2112", Lsh: "\u21B0", Lstrok: "\u0141", Lt: "\u226A", Map: "\u2905", Mcy: "\u041C", MediumSpace: "\u205F", Mellintrf: "\u2133", Mfr: "\u{1D510}", MinusPlus: "\u2213", Mopf: "\u{1D544}", Mscr: "\u2133", Mu: "\u039C", NJcy: "\u040A", Nacute: "\u0143", Ncaron: "\u0147", Ncedil: "\u0145", Ncy: "\u041D", NegativeMediumSpace: "\u200B", NegativeThickSpace: "\u200B", NegativeThinSpace: "\u200B", NegativeVeryThinSpace: "\u200B", NestedGreaterGreater: "\u226B", NestedLessLess: "\u226A", NewLine: `
`, Nfr: "\u{1D511}", NoBreak: "\u2060", NonBreakingSpace: "\xA0", Nopf: "\u2115", Not: "\u2AEC", NotCongruent: "\u2262", NotCupCap: "\u226D", NotDoubleVerticalBar: "\u2226", NotElement: "\u2209", NotEqual: "\u2260", NotEqualTilde: "\u2242\u0338", NotExists: "\u2204", NotGreater: "\u226F", NotGreaterEqual: "\u2271", NotGreaterFullEqual: "\u2267\u0338", NotGreaterGreater: "\u226B\u0338", NotGreaterLess: "\u2279", NotGreaterSlantEqual: "\u2A7E\u0338", NotGreaterTilde: "\u2275", NotHumpDownHump: "\u224E\u0338", NotHumpEqual: "\u224F\u0338", NotLeftTriangle: "\u22EA", NotLeftTriangleBar: "\u29CF\u0338", NotLeftTriangleEqual: "\u22EC", NotLess: "\u226E", NotLessEqual: "\u2270", NotLessGreater: "\u2278", NotLessLess: "\u226A\u0338", NotLessSlantEqual: "\u2A7D\u0338", NotLessTilde: "\u2274", NotNestedGreaterGreater: "\u2AA2\u0338", NotNestedLessLess: "\u2AA1\u0338", NotPrecedes: "\u2280", NotPrecedesEqual: "\u2AAF\u0338", NotPrecedesSlantEqual: "\u22E0", NotReverseElement: "\u220C", NotRightTriangle: "\u22EB", NotRightTriangleBar: "\u29D0\u0338", NotRightTriangleEqual: "\u22ED", NotSquareSubset: "\u228F\u0338", NotSquareSubsetEqual: "\u22E2", NotSquareSuperset: "\u2290\u0338", NotSquareSupersetEqual: "\u22E3", NotSubset: "\u2282\u20D2", NotSubsetEqual: "\u2288", NotSucceeds: "\u2281", NotSucceedsEqual: "\u2AB0\u0338", NotSucceedsSlantEqual: "\u22E1", NotSucceedsTilde: "\u227F\u0338", NotSuperset: "\u2283\u20D2", NotSupersetEqual: "\u2289", NotTilde: "\u2241", NotTildeEqual: "\u2244", NotTildeFullEqual: "\u2247", NotTildeTilde: "\u2249", NotVerticalBar: "\u2224", Nscr: "\u{1D4A9}", Ntild: "\xD1", Ntilde: "\xD1", Nu: "\u039D", OElig: "\u0152", Oacut: "\xD3", Oacute: "\xD3", Ocir: "\xD4", Ocirc: "\xD4", Ocy: "\u041E", Odblac: "\u0150", Ofr: "\u{1D512}", Ograv: "\xD2", Ograve: "\xD2", Omacr: "\u014C", Omega: "\u03A9", Omicron: "\u039F", Oopf: "\u{1D546}", OpenCurlyDoubleQuote: "\u201C", OpenCurlyQuote: "\u2018", Or: "\u2A54", Oscr: "\u{1D4AA}", Oslas: "\xD8", Oslash: "\xD8", Otild: "\xD5", Otilde: "\xD5", Otimes: "\u2A37", Oum: "\xD6", Ouml: "\xD6", OverBar: "\u203E", OverBrace: "\u23DE", OverBracket: "\u23B4", OverParenthesis: "\u23DC", PartialD: "\u2202", Pcy: "\u041F", Pfr: "\u{1D513}", Phi: "\u03A6", Pi: "\u03A0", PlusMinus: "\xB1", Poincareplane: "\u210C", Popf: "\u2119", Pr: "\u2ABB", Precedes: "\u227A", PrecedesEqual: "\u2AAF", PrecedesSlantEqual: "\u227C", PrecedesTilde: "\u227E", Prime: "\u2033", Product: "\u220F", Proportion: "\u2237", Proportional: "\u221D", Pscr: "\u{1D4AB}", Psi: "\u03A8", QUO: '"', QUOT: '"', Qfr: "\u{1D514}", Qopf: "\u211A", Qscr: "\u{1D4AC}", RBarr: "\u2910", RE: "\xAE", REG: "\xAE", Racute: "\u0154", Rang: "\u27EB", Rarr: "\u21A0", Rarrtl: "\u2916", Rcaron: "\u0158", Rcedil: "\u0156", Rcy: "\u0420", Re: "\u211C", ReverseElement: "\u220B", ReverseEquilibrium: "\u21CB", ReverseUpEquilibrium: "\u296F", Rfr: "\u211C", Rho: "\u03A1", RightAngleBracket: "\u27E9", RightArrow: "\u2192", RightArrowBar: "\u21E5", RightArrowLeftArrow: "\u21C4", RightCeiling: "\u2309", RightDoubleBracket: "\u27E7", RightDownTeeVector: "\u295D", RightDownVector: "\u21C2", RightDownVectorBar: "\u2955", RightFloor: "\u230B", RightTee: "\u22A2", RightTeeArrow: "\u21A6", RightTeeVector: "\u295B", RightTriangle: "\u22B3", RightTriangleBar: "\u29D0", RightTriangleEqual: "\u22B5", RightUpDownVector: "\u294F", RightUpTeeVector: "\u295C", RightUpVector: "\u21BE", RightUpVectorBar: "\u2954", RightVector: "\u21C0", RightVectorBar: "\u2953", Rightarrow: "\u21D2", Ropf: "\u211D", RoundImplies: "\u2970", Rrightarrow: "\u21DB", Rscr: "\u211B", Rsh: "\u21B1", RuleDelayed: "\u29F4", SHCHcy: "\u0429", SHcy: "\u0428", SOFTcy: "\u042C", Sacute: "\u015A", Sc: "\u2ABC", Scaron: "\u0160", Scedil: "\u015E", Scirc: "\u015C", Scy: "\u0421", Sfr: "\u{1D516}", ShortDownArrow: "\u2193", ShortLeftArrow: "\u2190", ShortRightArrow: "\u2192", ShortUpArrow: "\u2191", Sigma: "\u03A3", SmallCircle: "\u2218", Sopf: "\u{1D54A}", Sqrt: "\u221A", Square: "\u25A1", SquareIntersection: "\u2293", SquareSubset: "\u228F", SquareSubsetEqual: "\u2291", SquareSuperset: "\u2290", SquareSupersetEqual: "\u2292", SquareUnion: "\u2294", Sscr: "\u{1D4AE}", Star: "\u22C6", Sub: "\u22D0", Subset: "\u22D0", SubsetEqual: "\u2286", Succeeds: "\u227B", SucceedsEqual: "\u2AB0", SucceedsSlantEqual: "\u227D", SucceedsTilde: "\u227F", SuchThat: "\u220B", Sum: "\u2211", Sup: "\u22D1", Superset: "\u2283", SupersetEqual: "\u2287", Supset: "\u22D1", THOR: "\xDE", THORN: "\xDE", TRADE: "\u2122", TSHcy: "\u040B", TScy: "\u0426", Tab: " ", Tau: "\u03A4", Tcaron: "\u0164", Tcedil: "\u0162", Tcy: "\u0422", Tfr: "\u{1D517}", Therefore: "\u2234", Theta: "\u0398", ThickSpace: "\u205F\u200A", ThinSpace: "\u2009", Tilde: "\u223C", TildeEqual: "\u2243", TildeFullEqual: "\u2245", TildeTilde: "\u2248", Topf: "\u{1D54B}", TripleDot: "\u20DB", Tscr: "\u{1D4AF}", Tstrok: "\u0166", Uacut: "\xDA", Uacute: "\xDA", Uarr: "\u219F", Uarrocir: "\u2949", Ubrcy: "\u040E", Ubreve: "\u016C", Ucir: "\xDB", Ucirc: "\xDB", Ucy: "\u0423", Udblac: "\u0170", Ufr: "\u{1D518}", Ugrav: "\xD9", Ugrave: "\xD9", Umacr: "\u016A", UnderBar: "_", UnderBrace: "\u23DF", UnderBracket: "\u23B5", UnderParenthesis: "\u23DD", Union: "\u22C3", UnionPlus: "\u228E", Uogon: "\u0172", Uopf: "\u{1D54C}", UpArrow: "\u2191", UpArrowBar: "\u2912", UpArrowDownArrow: "\u21C5", UpDownArrow: "\u2195", UpEquilibrium: "\u296E", UpTee: "\u22A5", UpTeeArrow: "\u21A5", Uparrow: "\u21D1", Updownarrow: "\u21D5", UpperLeftArrow: "\u2196", UpperRightArrow: "\u2197", Upsi: "\u03D2", Upsilon: "\u03A5", Uring: "\u016E", Uscr: "\u{1D4B0}", Utilde: "\u0168", Uum: "\xDC", Uuml: "\xDC", VDash: "\u22AB", Vbar: "\u2AEB", Vcy: "\u0412", Vdash: "\u22A9", Vdashl: "\u2AE6", Vee: "\u22C1", Verbar: "\u2016", Vert: "\u2016", VerticalBar: "\u2223", VerticalLine: "|", VerticalSeparator: "\u2758", VerticalTilde: "\u2240", VeryThinSpace: "\u200A", Vfr: "\u{1D519}", Vopf: "\u{1D54D}", Vscr: "\u{1D4B1}", Vvdash: "\u22AA", Wcirc: "\u0174", Wedge: "\u22C0", Wfr: "\u{1D51A}", Wopf: "\u{1D54E}", Wscr: "\u{1D4B2}", Xfr: "\u{1D51B}", Xi: "\u039E", Xopf: "\u{1D54F}", Xscr: "\u{1D4B3}", YAcy: "\u042F", YIcy: "\u0407", YUcy: "\u042E", Yacut: "\xDD", Yacute: "\xDD", Ycirc: "\u0176", Ycy: "\u042B", Yfr: "\u{1D51C}", Yopf: "\u{1D550}", Yscr: "\u{1D4B4}", Yuml: "\u0178", ZHcy: "\u0416", Zacute: "\u0179", Zcaron: "\u017D", Zcy: "\u0417", Zdot: "\u017B", ZeroWidthSpace: "\u200B", Zeta: "\u0396", Zfr: "\u2128", Zopf: "\u2124", Zscr: "\u{1D4B5}", aacut: "\xE1", aacute: "\xE1", abreve: "\u0103", ac: "\u223E", acE: "\u223E\u0333", acd: "\u223F", acir: "\xE2", acirc: "\xE2", acut: "\xB4", acute: "\xB4", acy: "\u0430", aeli: "\xE6", aelig: "\xE6", af: "\u2061", afr: "\u{1D51E}", agrav: "\xE0", agrave: "\xE0", alefsym: "\u2135", aleph: "\u2135", alpha: "\u03B1", amacr: "\u0101", amalg: "\u2A3F", am: "&", amp: "&", and: "\u2227", andand: "\u2A55", andd: "\u2A5C", andslope: "\u2A58", andv: "\u2A5A", ang: "\u2220", ange: "\u29A4", angle: "\u2220", angmsd: "\u2221", angmsdaa: "\u29A8", angmsdab: "\u29A9", angmsdac: "\u29AA", angmsdad: "\u29AB", angmsdae: "\u29AC", angmsdaf: "\u29AD", angmsdag: "\u29AE", angmsdah: "\u29AF", angrt: "\u221F", angrtvb: "\u22BE", angrtvbd: "\u299D", angsph: "\u2222", angst: "\xC5", angzarr: "\u237C", aogon: "\u0105", aopf: "\u{1D552}", ap: "\u2248", apE: "\u2A70", apacir: "\u2A6F", ape: "\u224A", apid: "\u224B", apos: "'", approx: "\u2248", approxeq: "\u224A", arin: "\xE5", aring: "\xE5", ascr: "\u{1D4B6}", ast: "*", asymp: "\u2248", asympeq: "\u224D", atild: "\xE3", atilde: "\xE3", aum: "\xE4", auml: "\xE4", awconint: "\u2233", awint: "\u2A11", bNot: "\u2AED", backcong: "\u224C", backepsilon: "\u03F6", backprime: "\u2035", backsim: "\u223D", backsimeq: "\u22CD", barvee: "\u22BD", barwed: "\u2305", barwedge: "\u2305", bbrk: "\u23B5", bbrktbrk: "\u23B6", bcong: "\u224C", bcy: "\u0431", bdquo: "\u201E", becaus: "\u2235", because: "\u2235", bemptyv: "\u29B0", bepsi: "\u03F6", bernou: "\u212C", beta: "\u03B2", beth: "\u2136", between: "\u226C", bfr: "\u{1D51F}", bigcap: "\u22C2", bigcirc: "\u25EF", bigcup: "\u22C3", bigodot: "\u2A00", bigoplus: "\u2A01", bigotimes: "\u2A02", bigsqcup: "\u2A06", bigstar: "\u2605", bigtriangledown: "\u25BD", bigtriangleup: "\u25B3", biguplus: "\u2A04", bigvee: "\u22C1", bigwedge: "\u22C0", bkarow: "\u290D", blacklozenge: "\u29EB", blacksquare: "\u25AA", blacktriangle: "\u25B4", blacktriangledown: "\u25BE", blacktriangleleft: "\u25C2", blacktriangleright: "\u25B8", blank: "\u2423", blk12: "\u2592", blk14: "\u2591", blk34: "\u2593", block: "\u2588", bne: "=\u20E5", bnequiv: "\u2261\u20E5", bnot: "\u2310", bopf: "\u{1D553}", bot: "\u22A5", bottom: "\u22A5", bowtie: "\u22C8", boxDL: "\u2557", boxDR: "\u2554", boxDl: "\u2556", boxDr: "\u2553", boxH: "\u2550", boxHD: "\u2566", boxHU: "\u2569", boxHd: "\u2564", boxHu: "\u2567", boxUL: "\u255D", boxUR: "\u255A", boxUl: "\u255C", boxUr: "\u2559", boxV: "\u2551", boxVH: "\u256C", boxVL: "\u2563", boxVR: "\u2560", boxVh: "\u256B", boxVl: "\u2562", boxVr: "\u255F", boxbox: "\u29C9", boxdL: "\u2555", boxdR: "\u2552", boxdl: "\u2510", boxdr: "\u250C", boxh: "\u2500", boxhD: "\u2565", boxhU: "\u2568", boxhd: "\u252C", boxhu: "\u2534", boxminus: "\u229F", boxplus: "\u229E", boxtimes: "\u22A0", boxuL: "\u255B", boxuR: "\u2558", boxul: "\u2518", boxur: "\u2514", boxv: "\u2502", boxvH: "\u256A", boxvL: "\u2561", boxvR: "\u255E", boxvh: "\u253C", boxvl: "\u2524", boxvr: "\u251C", bprime: "\u2035", breve: "\u02D8", brvba: "\xA6", brvbar: "\xA6", bscr: "\u{1D4B7}", bsemi: "\u204F", bsim: "\u223D", bsime: "\u22CD", bsol: "\\", bsolb: "\u29C5", bsolhsub: "\u27C8", bull: "\u2022", bullet: "\u2022", bump: "\u224E", bumpE: "\u2AAE", bumpe: "\u224F", bumpeq: "\u224F", cacute: "\u0107", cap: "\u2229", capand: "\u2A44", capbrcup: "\u2A49", capcap: "\u2A4B", capcup: "\u2A47", capdot: "\u2A40", caps: "\u2229\uFE00", caret: "\u2041", caron: "\u02C7", ccaps: "\u2A4D", ccaron: "\u010D", ccedi: "\xE7", ccedil: "\xE7", ccirc: "\u0109", ccups: "\u2A4C", ccupssm: "\u2A50", cdot: "\u010B", cedi: "\xB8", cedil: "\xB8", cemptyv: "\u29B2", cen: "\xA2", cent: "\xA2", centerdot: "\xB7", cfr: "\u{1D520}", chcy: "\u0447", check: "\u2713", checkmark: "\u2713", chi: "\u03C7", cir: "\u25CB", cirE: "\u29C3", circ: "\u02C6", circeq: "\u2257", circlearrowleft: "\u21BA", circlearrowright: "\u21BB", circledR: "\xAE", circledS: "\u24C8", circledast: "\u229B", circledcirc: "\u229A", circleddash: "\u229D", cire: "\u2257", cirfnint: "\u2A10", cirmid: "\u2AEF", cirscir: "\u29C2", clubs: "\u2663", clubsuit: "\u2663", colon: ":", colone: "\u2254", coloneq: "\u2254", comma: ",", commat: "@", comp: "\u2201", compfn: "\u2218", complement: "\u2201", complexes: "\u2102", cong: "\u2245", congdot: "\u2A6D", conint: "\u222E", copf: "\u{1D554}", coprod: "\u2210", cop: "\xA9", copy: "\xA9", copysr: "\u2117", crarr: "\u21B5", cross: "\u2717", cscr: "\u{1D4B8}", csub: "\u2ACF", csube: "\u2AD1", csup: "\u2AD0", csupe: "\u2AD2", ctdot: "\u22EF", cudarrl: "\u2938", cudarrr: "\u2935", cuepr: "\u22DE", cuesc: "\u22DF", cularr: "\u21B6", cularrp: "\u293D", cup: "\u222A", cupbrcap: "\u2A48", cupcap: "\u2A46", cupcup: "\u2A4A", cupdot: "\u228D", cupor: "\u2A45", cups: "\u222A\uFE00", curarr: "\u21B7", curarrm: "\u293C", curlyeqprec: "\u22DE", curlyeqsucc: "\u22DF", curlyvee: "\u22CE", curlywedge: "\u22CF", curre: "\xA4", curren: "\xA4", curvearrowleft: "\u21B6", curvearrowright: "\u21B7", cuvee: "\u22CE", cuwed: "\u22CF", cwconint: "\u2232", cwint: "\u2231", cylcty: "\u232D", dArr: "\u21D3", dHar: "\u2965", dagger: "\u2020", daleth: "\u2138", darr: "\u2193", dash: "\u2010", dashv: "\u22A3", dbkarow: "\u290F", dblac: "\u02DD", dcaron: "\u010F", dcy: "\u0434", dd: "\u2146", ddagger: "\u2021", ddarr: "\u21CA", ddotseq: "\u2A77", de: "\xB0", deg: "\xB0", delta: "\u03B4", demptyv: "\u29B1", dfisht: "\u297F", dfr: "\u{1D521}", dharl: "\u21C3", dharr: "\u21C2", diam: "\u22C4", diamond: "\u22C4", diamondsuit: "\u2666", diams: "\u2666", die: "\xA8", digamma: "\u03DD", disin: "\u22F2", div: "\xF7", divid: "\xF7", divide: "\xF7", divideontimes: "\u22C7", divonx: "\u22C7", djcy: "\u0452", dlcorn: "\u231E", dlcrop: "\u230D", dollar: "$", dopf: "\u{1D555}", dot: "\u02D9", doteq: "\u2250", doteqdot: "\u2251", dotminus: "\u2238", dotplus: "\u2214", dotsquare: "\u22A1", doublebarwedge: "\u2306", downarrow: "\u2193", downdownarrows: "\u21CA", downharpoonleft: "\u21C3", downharpoonright: "\u21C2", drbkarow: "\u2910", drcorn: "\u231F", drcrop: "\u230C", dscr: "\u{1D4B9}", dscy: "\u0455", dsol: "\u29F6", dstrok: "\u0111", dtdot: "\u22F1", dtri: "\u25BF", dtrif: "\u25BE", duarr: "\u21F5", duhar: "\u296F", dwangle: "\u29A6", dzcy: "\u045F", dzigrarr: "\u27FF", eDDot: "\u2A77", eDot: "\u2251", eacut: "\xE9", eacute: "\xE9", easter: "\u2A6E", ecaron: "\u011B", ecir: "\xEA", ecirc: "\xEA", ecolon: "\u2255", ecy: "\u044D", edot: "\u0117", ee: "\u2147", efDot: "\u2252", efr: "\u{1D522}", eg: "\u2A9A", egrav: "\xE8", egrave: "\xE8", egs: "\u2A96", egsdot: "\u2A98", el: "\u2A99", elinters: "\u23E7", ell: "\u2113", els: "\u2A95", elsdot: "\u2A97", emacr: "\u0113", empty: "\u2205", emptyset: "\u2205", emptyv: "\u2205", emsp13: "\u2004", emsp14: "\u2005", emsp: "\u2003", eng: "\u014B", ensp: "\u2002", eogon: "\u0119", eopf: "\u{1D556}", epar: "\u22D5", eparsl: "\u29E3", eplus: "\u2A71", epsi: "\u03B5", epsilon: "\u03B5", epsiv: "\u03F5", eqcirc: "\u2256", eqcolon: "\u2255", eqsim: "\u2242", eqslantgtr: "\u2A96", eqslantless: "\u2A95", equals: "=", equest: "\u225F", equiv: "\u2261", equivDD: "\u2A78", eqvparsl: "\u29E5", erDot: "\u2253", erarr: "\u2971", escr: "\u212F", esdot: "\u2250", esim: "\u2242", eta: "\u03B7", et: "\xF0", eth: "\xF0", eum: "\xEB", euml: "\xEB", euro: "\u20AC", excl: "!", exist: "\u2203", expectation: "\u2130", exponentiale: "\u2147", fallingdotseq: "\u2252", fcy: "\u0444", female: "\u2640", ffilig: "\uFB03", fflig: "\uFB00", ffllig: "\uFB04", ffr: "\u{1D523}", filig: "\uFB01", fjlig: "fj", flat: "\u266D", fllig: "\uFB02", fltns: "\u25B1", fnof: "\u0192", fopf: "\u{1D557}", forall: "\u2200", fork: "\u22D4", forkv: "\u2AD9", fpartint: "\u2A0D", frac1: "\xBC", frac12: "\xBD", frac13: "\u2153", frac14: "\xBC", frac15: "\u2155", frac16: "\u2159", frac18: "\u215B", frac23: "\u2154", frac25: "\u2156", frac3: "\xBE", frac34: "\xBE", frac35: "\u2157", frac38: "\u215C", frac45: "\u2158", frac56: "\u215A", frac58: "\u215D", frac78: "\u215E", frasl: "\u2044", frown: "\u2322", fscr: "\u{1D4BB}", gE: "\u2267", gEl: "\u2A8C", gacute: "\u01F5", gamma: "\u03B3", gammad: "\u03DD", gap: "\u2A86", gbreve: "\u011F", gcirc: "\u011D", gcy: "\u0433", gdot: "\u0121", ge: "\u2265", gel: "\u22DB", geq: "\u2265", geqq: "\u2267", geqslant: "\u2A7E", ges: "\u2A7E", gescc: "\u2AA9", gesdot: "\u2A80", gesdoto: "\u2A82", gesdotol: "\u2A84", gesl: "\u22DB\uFE00", gesles: "\u2A94", gfr: "\u{1D524}", gg: "\u226B", ggg: "\u22D9", gimel: "\u2137", gjcy: "\u0453", gl: "\u2277", glE: "\u2A92", gla: "\u2AA5", glj: "\u2AA4", gnE: "\u2269", gnap: "\u2A8A", gnapprox: "\u2A8A", gne: "\u2A88", gneq: "\u2A88", gneqq: "\u2269", gnsim: "\u22E7", gopf: "\u{1D558}", grave: "`", gscr: "\u210A", gsim: "\u2273", gsime: "\u2A8E", gsiml: "\u2A90", g: ">", gt: ">", gtcc: "\u2AA7", gtcir: "\u2A7A", gtdot: "\u22D7", gtlPar: "\u2995", gtquest: "\u2A7C", gtrapprox: "\u2A86", gtrarr: "\u2978", gtrdot: "\u22D7", gtreqless: "\u22DB", gtreqqless: "\u2A8C", gtrless: "\u2277", gtrsim: "\u2273", gvertneqq: "\u2269\uFE00", gvnE: "\u2269\uFE00", hArr: "\u21D4", hairsp: "\u200A", half: "\xBD", hamilt: "\u210B", hardcy: "\u044A", harr: "\u2194", harrcir: "\u2948", harrw: "\u21AD", hbar: "\u210F", hcirc: "\u0125", hearts: "\u2665", heartsuit: "\u2665", hellip: "\u2026", hercon: "\u22B9", hfr: "\u{1D525}", hksearow: "\u2925", hkswarow: "\u2926", hoarr: "\u21FF", homtht: "\u223B", hookleftarrow: "\u21A9", hookrightarrow: "\u21AA", hopf: "\u{1D559}", horbar: "\u2015", hscr: "\u{1D4BD}", hslash: "\u210F", hstrok: "\u0127", hybull: "\u2043", hyphen: "\u2010", iacut: "\xED", iacute: "\xED", ic: "\u2063", icir: "\xEE", icirc: "\xEE", icy: "\u0438", iecy: "\u0435", iexc: "\xA1", iexcl: "\xA1", iff: "\u21D4", ifr: "\u{1D526}", igrav: "\xEC", igrave: "\xEC", ii: "\u2148", iiiint: "\u2A0C", iiint: "\u222D", iinfin: "\u29DC", iiota: "\u2129", ijlig: "\u0133", imacr: "\u012B", image: "\u2111", imagline: "\u2110", imagpart: "\u2111", imath: "\u0131", imof: "\u22B7", imped: "\u01B5", in: "\u2208", incare: "\u2105", infin: "\u221E", infintie: "\u29DD", inodot: "\u0131", int: "\u222B", intcal: "\u22BA", integers: "\u2124", intercal: "\u22BA", intlarhk: "\u2A17", intprod: "\u2A3C", iocy: "\u0451", iogon: "\u012F", iopf: "\u{1D55A}", iota: "\u03B9", iprod: "\u2A3C", iques: "\xBF", iquest: "\xBF", iscr: "\u{1D4BE}", isin: "\u2208", isinE: "\u22F9", isindot: "\u22F5", isins: "\u22F4", isinsv: "\u22F3", isinv: "\u2208", it: "\u2062", itilde: "\u0129", iukcy: "\u0456", ium: "\xEF", iuml: "\xEF", jcirc: "\u0135", jcy: "\u0439", jfr: "\u{1D527}", jmath: "\u0237", jopf: "\u{1D55B}", jscr: "\u{1D4BF}", jsercy: "\u0458", jukcy: "\u0454", kappa: "\u03BA", kappav: "\u03F0", kcedil: "\u0137", kcy: "\u043A", kfr: "\u{1D528}", kgreen: "\u0138", khcy: "\u0445", kjcy: "\u045C", kopf: "\u{1D55C}", kscr: "\u{1D4C0}", lAarr: "\u21DA", lArr: "\u21D0", lAtail: "\u291B", lBarr: "\u290E", lE: "\u2266", lEg: "\u2A8B", lHar: "\u2962", lacute: "\u013A", laemptyv: "\u29B4", lagran: "\u2112", lambda: "\u03BB", lang: "\u27E8", langd: "\u2991", langle: "\u27E8", lap: "\u2A85", laqu: "\xAB", laquo: "\xAB", larr: "\u2190", larrb: "\u21E4", larrbfs: "\u291F", larrfs: "\u291D", larrhk: "\u21A9", larrlp: "\u21AB", larrpl: "\u2939", larrsim: "\u2973", larrtl: "\u21A2", lat: "\u2AAB", latail: "\u2919", late: "\u2AAD", lates: "\u2AAD\uFE00", lbarr: "\u290C", lbbrk: "\u2772", lbrace: "{", lbrack: "[", lbrke: "\u298B", lbrksld: "\u298F", lbrkslu: "\u298D", lcaron: "\u013E", lcedil: "\u013C", lceil: "\u2308", lcub: "{", lcy: "\u043B", ldca: "\u2936", ldquo: "\u201C", ldquor: "\u201E", ldrdhar: "\u2967", ldrushar: "\u294B", ldsh: "\u21B2", le: "\u2264", leftarrow: "\u2190", leftarrowtail: "\u21A2", leftharpoondown: "\u21BD", leftharpoonup: "\u21BC", leftleftarrows: "\u21C7", leftrightarrow: "\u2194", leftrightarrows: "\u21C6", leftrightharpoons: "\u21CB", leftrightsquigarrow: "\u21AD", leftthreetimes: "\u22CB", leg: "\u22DA", leq: "\u2264", leqq: "\u2266", leqslant: "\u2A7D", les: "\u2A7D", lescc: "\u2AA8", lesdot: "\u2A7F", lesdoto: "\u2A81", lesdotor: "\u2A83", lesg: "\u22DA\uFE00", lesges: "\u2A93", lessapprox: "\u2A85", lessdot: "\u22D6", lesseqgtr: "\u22DA", lesseqqgtr: "\u2A8B", lessgtr: "\u2276", lesssim: "\u2272", lfisht: "\u297C", lfloor: "\u230A", lfr: "\u{1D529}", lg: "\u2276", lgE: "\u2A91", lhard: "\u21BD", lharu: "\u21BC", lharul: "\u296A", lhblk: "\u2584", ljcy: "\u0459", ll: "\u226A", llarr: "\u21C7", llcorner: "\u231E", llhard: "\u296B", lltri: "\u25FA", lmidot: "\u0140", lmoust: "\u23B0", lmoustache: "\u23B0", lnE: "\u2268", lnap: "\u2A89", lnapprox: "\u2A89", lne: "\u2A87", lneq: "\u2A87", lneqq: "\u2268", lnsim: "\u22E6", loang: "\u27EC", loarr: "\u21FD", lobrk: "\u27E6", longleftarrow: "\u27F5", longleftrightarrow: "\u27F7", longmapsto: "\u27FC", longrightarrow: "\u27F6", looparrowleft: "\u21AB", looparrowright: "\u21AC", lopar: "\u2985", lopf: "\u{1D55D}", loplus: "\u2A2D", lotimes: "\u2A34", lowast: "\u2217", lowbar: "_", loz: "\u25CA", lozenge: "\u25CA", lozf: "\u29EB", lpar: "(", lparlt: "\u2993", lrarr: "\u21C6", lrcorner: "\u231F", lrhar: "\u21CB", lrhard: "\u296D", lrm: "\u200E", lrtri: "\u22BF", lsaquo: "\u2039", lscr: "\u{1D4C1}", lsh: "\u21B0", lsim: "\u2272", lsime: "\u2A8D", lsimg: "\u2A8F", lsqb: "[", lsquo: "\u2018", lsquor: "\u201A", lstrok: "\u0142", l: "<", lt: "<", ltcc: "\u2AA6", ltcir: "\u2A79", ltdot: "\u22D6", lthree: "\u22CB", ltimes: "\u22C9", ltlarr: "\u2976", ltquest: "\u2A7B", ltrPar: "\u2996", ltri: "\u25C3", ltrie: "\u22B4", ltrif: "\u25C2", lurdshar: "\u294A", luruhar: "\u2966", lvertneqq: "\u2268\uFE00", lvnE: "\u2268\uFE00", mDDot: "\u223A", mac: "\xAF", macr: "\xAF", male: "\u2642", malt: "\u2720", maltese: "\u2720", map: "\u21A6", mapsto: "\u21A6", mapstodown: "\u21A7", mapstoleft: "\u21A4", mapstoup: "\u21A5", marker: "\u25AE", mcomma: "\u2A29", mcy: "\u043C", mdash: "\u2014", measuredangle: "\u2221", mfr: "\u{1D52A}", mho: "\u2127", micr: "\xB5", micro: "\xB5", mid: "\u2223", midast: "*", midcir: "\u2AF0", middo: "\xB7", middot: "\xB7", minus: "\u2212", minusb: "\u229F", minusd: "\u2238", minusdu: "\u2A2A", mlcp: "\u2ADB", mldr: "\u2026", mnplus: "\u2213", models: "\u22A7", mopf: "\u{1D55E}", mp: "\u2213", mscr: "\u{1D4C2}", mstpos: "\u223E", mu: "\u03BC", multimap: "\u22B8", mumap: "\u22B8", nGg: "\u22D9\u0338", nGt: "\u226B\u20D2", nGtv: "\u226B\u0338", nLeftarrow: "\u21CD", nLeftrightarrow: "\u21CE", nLl: "\u22D8\u0338", nLt: "\u226A\u20D2", nLtv: "\u226A\u0338", nRightarrow: "\u21CF", nVDash: "\u22AF", nVdash: "\u22AE", nabla: "\u2207", nacute: "\u0144", nang: "\u2220\u20D2", nap: "\u2249", napE: "\u2A70\u0338", napid: "\u224B\u0338", napos: "\u0149", napprox: "\u2249", natur: "\u266E", natural: "\u266E", naturals: "\u2115", nbs: "\xA0", nbsp: "\xA0", nbump: "\u224E\u0338", nbumpe: "\u224F\u0338", ncap: "\u2A43", ncaron: "\u0148", ncedil: "\u0146", ncong: "\u2247", ncongdot: "\u2A6D\u0338", ncup: "\u2A42", ncy: "\u043D", ndash: "\u2013", ne: "\u2260", neArr: "\u21D7", nearhk: "\u2924", nearr: "\u2197", nearrow: "\u2197", nedot: "\u2250\u0338", nequiv: "\u2262", nesear: "\u2928", nesim: "\u2242\u0338", nexist: "\u2204", nexists: "\u2204", nfr: "\u{1D52B}", ngE: "\u2267\u0338", nge: "\u2271", ngeq: "\u2271", ngeqq: "\u2267\u0338", ngeqslant: "\u2A7E\u0338", nges: "\u2A7E\u0338", ngsim: "\u2275", ngt: "\u226F", ngtr: "\u226F", nhArr: "\u21CE", nharr: "\u21AE", nhpar: "\u2AF2", ni: "\u220B", nis: "\u22FC", nisd: "\u22FA", niv: "\u220B", njcy: "\u045A", nlArr: "\u21CD", nlE: "\u2266\u0338", nlarr: "\u219A", nldr: "\u2025", nle: "\u2270", nleftarrow: "\u219A", nleftrightarrow: "\u21AE", nleq: "\u2270", nleqq: "\u2266\u0338", nleqslant: "\u2A7D\u0338", nles: "\u2A7D\u0338", nless: "\u226E", nlsim: "\u2274", nlt: "\u226E", nltri: "\u22EA", nltrie: "\u22EC", nmid: "\u2224", nopf: "\u{1D55F}", no: "\xAC", not: "\xAC", notin: "\u2209", notinE: "\u22F9\u0338", notindot: "\u22F5\u0338", notinva: "\u2209", notinvb: "\u22F7", notinvc: "\u22F6", notni: "\u220C", notniva: "\u220C", notnivb: "\u22FE", notnivc: "\u22FD", npar: "\u2226", nparallel: "\u2226", nparsl: "\u2AFD\u20E5", npart: "\u2202\u0338", npolint: "\u2A14", npr: "\u2280", nprcue: "\u22E0", npre: "\u2AAF\u0338", nprec: "\u2280", npreceq: "\u2AAF\u0338", nrArr: "\u21CF", nrarr: "\u219B", nrarrc: "\u2933\u0338", nrarrw: "\u219D\u0338", nrightarrow: "\u219B", nrtri: "\u22EB", nrtrie: "\u22ED", nsc: "\u2281", nsccue: "\u22E1", nsce: "\u2AB0\u0338", nscr: "\u{1D4C3}", nshortmid: "\u2224", nshortparallel: "\u2226", nsim: "\u2241", nsime: "\u2244", nsimeq: "\u2244", nsmid: "\u2224", nspar: "\u2226", nsqsube: "\u22E2", nsqsupe: "\u22E3", nsub: "\u2284", nsubE: "\u2AC5\u0338", nsube: "\u2288", nsubset: "\u2282\u20D2", nsubseteq: "\u2288", nsubseteqq: "\u2AC5\u0338", nsucc: "\u2281", nsucceq: "\u2AB0\u0338", nsup: "\u2285", nsupE: "\u2AC6\u0338", nsupe: "\u2289", nsupset: "\u2283\u20D2", nsupseteq: "\u2289", nsupseteqq: "\u2AC6\u0338", ntgl: "\u2279", ntild: "\xF1", ntilde: "\xF1", ntlg: "\u2278", ntriangleleft: "\u22EA", ntrianglelefteq: "\u22EC", ntriangleright: "\u22EB", ntrianglerighteq: "\u22ED", nu: "\u03BD", num: "#", numero: "\u2116", numsp: "\u2007", nvDash: "\u22AD", nvHarr: "\u2904", nvap: "\u224D\u20D2", nvdash: "\u22AC", nvge: "\u2265\u20D2", nvgt: ">\u20D2", nvinfin: "\u29DE", nvlArr: "\u2902", nvle: "\u2264\u20D2", nvlt: "<\u20D2", nvltrie: "\u22B4\u20D2", nvrArr: "\u2903", nvrtrie: "\u22B5\u20D2", nvsim: "\u223C\u20D2", nwArr: "\u21D6", nwarhk: "\u2923", nwarr: "\u2196", nwarrow: "\u2196", nwnear: "\u2927", oS: "\u24C8", oacut: "\xF3", oacute: "\xF3", oast: "\u229B", ocir: "\xF4", ocirc: "\xF4", ocy: "\u043E", odash: "\u229D", odblac: "\u0151", odiv: "\u2A38", odot: "\u2299", odsold: "\u29BC", oelig: "\u0153", ofcir: "\u29BF", ofr: "\u{1D52C}", ogon: "\u02DB", ograv: "\xF2", ograve: "\xF2", ogt: "\u29C1", ohbar: "\u29B5", ohm: "\u03A9", oint: "\u222E", olarr: "\u21BA", olcir: "\u29BE", olcross: "\u29BB", oline: "\u203E", olt: "\u29C0", omacr: "\u014D", omega: "\u03C9", omicron: "\u03BF", omid: "\u29B6", ominus: "\u2296", oopf: "\u{1D560}", opar: "\u29B7", operp: "\u29B9", oplus: "\u2295", or: "\u2228", orarr: "\u21BB", ord: "\xBA", order: "\u2134", orderof: "\u2134", ordf: "\xAA", ordm: "\xBA", origof: "\u22B6", oror: "\u2A56", orslope: "\u2A57", orv: "\u2A5B", oscr: "\u2134", oslas: "\xF8", oslash: "\xF8", osol: "\u2298", otild: "\xF5", otilde: "\xF5", otimes: "\u2297", otimesas: "\u2A36", oum: "\xF6", ouml: "\xF6", ovbar: "\u233D", par: "\xB6", para: "\xB6", parallel: "\u2225", parsim: "\u2AF3", parsl: "\u2AFD", part: "\u2202", pcy: "\u043F", percnt: "%", period: ".", permil: "\u2030", perp: "\u22A5", pertenk: "\u2031", pfr: "\u{1D52D}", phi: "\u03C6", phiv: "\u03D5", phmmat: "\u2133", phone: "\u260E", pi: "\u03C0", pitchfork: "\u22D4", piv: "\u03D6", planck: "\u210F", planckh: "\u210E", plankv: "\u210F", plus: "+", plusacir: "\u2A23", plusb: "\u229E", pluscir: "\u2A22", plusdo: "\u2214", plusdu: "\u2A25", pluse: "\u2A72", plusm: "\xB1", plusmn: "\xB1", plussim: "\u2A26", plustwo: "\u2A27", pm: "\xB1", pointint: "\u2A15", popf: "\u{1D561}", poun: "\xA3", pound: "\xA3", pr: "\u227A", prE: "\u2AB3", prap: "\u2AB7", prcue: "\u227C", pre: "\u2AAF", prec: "\u227A", precapprox: "\u2AB7", preccurlyeq: "\u227C", preceq: "\u2AAF", precnapprox: "\u2AB9", precneqq: "\u2AB5", precnsim: "\u22E8", precsim: "\u227E", prime: "\u2032", primes: "\u2119", prnE: "\u2AB5", prnap: "\u2AB9", prnsim: "\u22E8", prod: "\u220F", profalar: "\u232E", profline: "\u2312", profsurf: "\u2313", prop: "\u221D", propto: "\u221D", prsim: "\u227E", prurel: "\u22B0", pscr: "\u{1D4C5}", psi: "\u03C8", puncsp: "\u2008", qfr: "\u{1D52E}", qint: "\u2A0C", qopf: "\u{1D562}", qprime: "\u2057", qscr: "\u{1D4C6}", quaternions: "\u210D", quatint: "\u2A16", quest: "?", questeq: "\u225F", quo: '"', quot: '"', rAarr: "\u21DB", rArr: "\u21D2", rAtail: "\u291C", rBarr: "\u290F", rHar: "\u2964", race: "\u223D\u0331", racute: "\u0155", radic: "\u221A", raemptyv: "\u29B3", rang: "\u27E9", rangd: "\u2992", range: "\u29A5", rangle: "\u27E9", raqu: "\xBB", raquo: "\xBB", rarr: "\u2192", rarrap: "\u2975", rarrb: "\u21E5", rarrbfs: "\u2920", rarrc: "\u2933", rarrfs: "\u291E", rarrhk: "\u21AA", rarrlp: "\u21AC", rarrpl: "\u2945", rarrsim: "\u2974", rarrtl: "\u21A3", rarrw: "\u219D", ratail: "\u291A", ratio: "\u2236", rationals: "\u211A", rbarr: "\u290D", rbbrk: "\u2773", rbrace: "}", rbrack: "]", rbrke: "\u298C", rbrksld: "\u298E", rbrkslu: "\u2990", rcaron: "\u0159", rcedil: "\u0157", rceil: "\u2309", rcub: "}", rcy: "\u0440", rdca: "\u2937", rdldhar: "\u2969", rdquo: "\u201D", rdquor: "\u201D", rdsh: "\u21B3", real: "\u211C", realine: "\u211B", realpart: "\u211C", reals: "\u211D", rect: "\u25AD", re: "\xAE", reg: "\xAE", rfisht: "\u297D", rfloor: "\u230B", rfr: "\u{1D52F}", rhard: "\u21C1", rharu: "\u21C0", rharul: "\u296C", rho: "\u03C1", rhov: "\u03F1", rightarrow: "\u2192", rightarrowtail: "\u21A3", rightharpoondown: "\u21C1", rightharpoonup: "\u21C0", rightleftarrows: "\u21C4", rightleftharpoons: "\u21CC", rightrightarrows: "\u21C9", rightsquigarrow: "\u219D", rightthreetimes: "\u22CC", ring: "\u02DA", risingdotseq: "\u2253", rlarr: "\u21C4", rlhar: "\u21CC", rlm: "\u200F", rmoust: "\u23B1", rmoustache: "\u23B1", rnmid: "\u2AEE", roang: "\u27ED", roarr: "\u21FE", robrk: "\u27E7", ropar: "\u2986", ropf: "\u{1D563}", roplus: "\u2A2E", rotimes: "\u2A35", rpar: ")", rpargt: "\u2994", rppolint: "\u2A12", rrarr: "\u21C9", rsaquo: "\u203A", rscr: "\u{1D4C7}", rsh: "\u21B1", rsqb: "]", rsquo: "\u2019", rsquor: "\u2019", rthree: "\u22CC", rtimes: "\u22CA", rtri: "\u25B9", rtrie: "\u22B5", rtrif: "\u25B8", rtriltri: "\u29CE", ruluhar: "\u2968", rx: "\u211E", sacute: "\u015B", sbquo: "\u201A", sc: "\u227B", scE: "\u2AB4", scap: "\u2AB8", scaron: "\u0161", sccue: "\u227D", sce: "\u2AB0", scedil: "\u015F", scirc: "\u015D", scnE: "\u2AB6", scnap: "\u2ABA", scnsim: "\u22E9", scpolint: "\u2A13", scsim: "\u227F", scy: "\u0441", sdot: "\u22C5", sdotb: "\u22A1", sdote: "\u2A66", seArr: "\u21D8", searhk: "\u2925", searr: "\u2198", searrow: "\u2198", sec: "\xA7", sect: "\xA7", semi: ";", seswar: "\u2929", setminus: "\u2216", setmn: "\u2216", sext: "\u2736", sfr: "\u{1D530}", sfrown: "\u2322", sharp: "\u266F", shchcy: "\u0449", shcy: "\u0448", shortmid: "\u2223", shortparallel: "\u2225", sh: "\xAD", shy: "\xAD", sigma: "\u03C3", sigmaf: "\u03C2", sigmav: "\u03C2", sim: "\u223C", simdot: "\u2A6A", sime: "\u2243", simeq: "\u2243", simg: "\u2A9E", simgE: "\u2AA0", siml: "\u2A9D", simlE: "\u2A9F", simne: "\u2246", simplus: "\u2A24", simrarr: "\u2972", slarr: "\u2190", smallsetminus: "\u2216", smashp: "\u2A33", smeparsl: "\u29E4", smid: "\u2223", smile: "\u2323", smt: "\u2AAA", smte: "\u2AAC", smtes: "\u2AAC\uFE00", softcy: "\u044C", sol: "/", solb: "\u29C4", solbar: "\u233F", sopf: "\u{1D564}", spades: "\u2660", spadesuit: "\u2660", spar: "\u2225", sqcap: "\u2293", sqcaps: "\u2293\uFE00", sqcup: "\u2294", sqcups: "\u2294\uFE00", sqsub: "\u228F", sqsube: "\u2291", sqsubset: "\u228F", sqsubseteq: "\u2291", sqsup: "\u2290", sqsupe: "\u2292", sqsupset: "\u2290", sqsupseteq: "\u2292", squ: "\u25A1", square: "\u25A1", squarf: "\u25AA", squf: "\u25AA", srarr: "\u2192", sscr: "\u{1D4C8}", ssetmn: "\u2216", ssmile: "\u2323", sstarf: "\u22C6", star: "\u2606", starf: "\u2605", straightepsilon: "\u03F5", straightphi: "\u03D5", strns: "\xAF", sub: "\u2282", subE: "\u2AC5", subdot: "\u2ABD", sube: "\u2286", subedot: "\u2AC3", submult: "\u2AC1", subnE: "\u2ACB", subne: "\u228A", subplus: "\u2ABF", subrarr: "\u2979", subset: "\u2282", subseteq: "\u2286", subseteqq: "\u2AC5", subsetneq: "\u228A", subsetneqq: "\u2ACB", subsim: "\u2AC7", subsub: "\u2AD5", subsup: "\u2AD3", succ: "\u227B", succapprox: "\u2AB8", succcurlyeq: "\u227D", succeq: "\u2AB0", succnapprox: "\u2ABA", succneqq: "\u2AB6", succnsim: "\u22E9", succsim: "\u227F", sum: "\u2211", sung: "\u266A", sup: "\u2283", sup1: "\xB9", sup2: "\xB2", sup3: "\xB3", supE: "\u2AC6", supdot: "\u2ABE", supdsub: "\u2AD8", supe: "\u2287", supedot: "\u2AC4", suphsol: "\u27C9", suphsub: "\u2AD7", suplarr: "\u297B", supmult: "\u2AC2", supnE: "\u2ACC", supne: "\u228B", supplus: "\u2AC0", supset: "\u2283", supseteq: "\u2287", supseteqq: "\u2AC6", supsetneq: "\u228B", supsetneqq: "\u2ACC", supsim: "\u2AC8", supsub: "\u2AD4", supsup: "\u2AD6", swArr: "\u21D9", swarhk: "\u2926", swarr: "\u2199", swarrow: "\u2199", swnwar: "\u292A", szli: "\xDF", szlig: "\xDF", target: "\u2316", tau: "\u03C4", tbrk: "\u23B4", tcaron: "\u0165", tcedil: "\u0163", tcy: "\u0442", tdot: "\u20DB", telrec: "\u2315", tfr: "\u{1D531}", there4: "\u2234", therefore: "\u2234", theta: "\u03B8", thetasym: "\u03D1", thetav: "\u03D1", thickapprox: "\u2248", thicksim: "\u223C", thinsp: "\u2009", thkap: "\u2248", thksim: "\u223C", thor: "\xFE", thorn: "\xFE", tilde: "\u02DC", time: "\xD7", times: "\xD7", timesb: "\u22A0", timesbar: "\u2A31", timesd: "\u2A30", tint: "\u222D", toea: "\u2928", top: "\u22A4", topbot: "\u2336", topcir: "\u2AF1", topf: "\u{1D565}", topfork: "\u2ADA", tosa: "\u2929", tprime: "\u2034", trade: "\u2122", triangle: "\u25B5", triangledown: "\u25BF", triangleleft: "\u25C3", trianglelefteq: "\u22B4", triangleq: "\u225C", triangleright: "\u25B9", trianglerighteq: "\u22B5", tridot: "\u25EC", trie: "\u225C", triminus: "\u2A3A", triplus: "\u2A39", trisb: "\u29CD", tritime: "\u2A3B", trpezium: "\u23E2", tscr: "\u{1D4C9}", tscy: "\u0446", tshcy: "\u045B", tstrok: "\u0167", twixt: "\u226C", twoheadleftarrow: "\u219E", twoheadrightarrow: "\u21A0", uArr: "\u21D1", uHar: "\u2963", uacut: "\xFA", uacute: "\xFA", uarr: "\u2191", ubrcy: "\u045E", ubreve: "\u016D", ucir: "\xFB", ucirc: "\xFB", ucy: "\u0443", udarr: "\u21C5", udblac: "\u0171", udhar: "\u296E", ufisht: "\u297E", ufr: "\u{1D532}", ugrav: "\xF9", ugrave: "\xF9", uharl: "\u21BF", uharr: "\u21BE", uhblk: "\u2580", ulcorn: "\u231C", ulcorner: "\u231C", ulcrop: "\u230F", ultri: "\u25F8", umacr: "\u016B", um: "\xA8", uml: "\xA8", uogon: "\u0173", uopf: "\u{1D566}", uparrow: "\u2191", updownarrow: "\u2195", upharpoonleft: "\u21BF", upharpoonright: "\u21BE", uplus: "\u228E", upsi: "\u03C5", upsih: "\u03D2", upsilon: "\u03C5", upuparrows: "\u21C8", urcorn: "\u231D", urcorner: "\u231D", urcrop: "\u230E", uring: "\u016F", urtri: "\u25F9", uscr: "\u{1D4CA}", utdot: "\u22F0", utilde: "\u0169", utri: "\u25B5", utrif: "\u25B4", uuarr: "\u21C8", uum: "\xFC", uuml: "\xFC", uwangle: "\u29A7", vArr: "\u21D5", vBar: "\u2AE8", vBarv: "\u2AE9", vDash: "\u22A8", vangrt: "\u299C", varepsilon: "\u03F5", varkappa: "\u03F0", varnothing: "\u2205", varphi: "\u03D5", varpi: "\u03D6", varpropto: "\u221D", varr: "\u2195", varrho: "\u03F1", varsigma: "\u03C2", varsubsetneq: "\u228A\uFE00", varsubsetneqq: "\u2ACB\uFE00", varsupsetneq: "\u228B\uFE00", varsupsetneqq: "\u2ACC\uFE00", vartheta: "\u03D1", vartriangleleft: "\u22B2", vartriangleright: "\u22B3", vcy: "\u0432", vdash: "\u22A2", vee: "\u2228", veebar: "\u22BB", veeeq: "\u225A", vellip: "\u22EE", verbar: "|", vert: "|", vfr: "\u{1D533}", vltri: "\u22B2", vnsub: "\u2282\u20D2", vnsup: "\u2283\u20D2", vopf: "\u{1D567}", vprop: "\u221D", vrtri: "\u22B3", vscr: "\u{1D4CB}", vsubnE: "\u2ACB\uFE00", vsubne: "\u228A\uFE00", vsupnE: "\u2ACC\uFE00", vsupne: "\u228B\uFE00", vzigzag: "\u299A", wcirc: "\u0175", wedbar: "\u2A5F", wedge: "\u2227", wedgeq: "\u2259", weierp: "\u2118", wfr: "\u{1D534}", wopf: "\u{1D568}", wp: "\u2118", wr: "\u2240", wreath: "\u2240", wscr: "\u{1D4CC}", xcap: "\u22C2", xcirc: "\u25EF", xcup: "\u22C3", xdtri: "\u25BD", xfr: "\u{1D535}", xhArr: "\u27FA", xharr: "\u27F7", xi: "\u03BE", xlArr: "\u27F8", xlarr: "\u27F5", xmap: "\u27FC", xnis: "\u22FB", xodot: "\u2A00", xopf: "\u{1D569}", xoplus: "\u2A01", xotime: "\u2A02", xrArr: "\u27F9", xrarr: "\u27F6", xscr: "\u{1D4CD}", xsqcup: "\u2A06", xuplus: "\u2A04", xutri: "\u25B3", xvee: "\u22C1", xwedge: "\u22C0", yacut: "\xFD", yacute: "\xFD", yacy: "\u044F", ycirc: "\u0177", ycy: "\u044B", ye: "\xA5", yen: "\xA5", yfr: "\u{1D536}", yicy: "\u0457", yopf: "\u{1D56A}", yscr: "\u{1D4CE}", yucy: "\u044E", yum: "\xFF", yuml: "\xFF", zacute: "\u017A", zcaron: "\u017E", zcy: "\u0437", zdot: "\u017C", zeetrf: "\u2128", zeta: "\u03B6", zfr: "\u{1D537}", zhcy: "\u0436", zigrarr: "\u21DD", zopf: "\u{1D56B}", zscr: "\u{1D4CF}", zwj: "\u200D", zwnj: "\u200C" };
} }), Wl = S({ "node_modules/parse-entities/decode-entity.js"(e, r) {
"use strict";
I();
var u = Xl();
r.exports = a;
var t = {}.hasOwnProperty;
function a(n3) {
return t.call(u, n3) ? u[n3] : false;
}
} }), xr = S({ "node_modules/parse-entities/index.js"(e, r) {
"use strict";
I();
var u = Ul(), t = Gl(), a = Me(), n3 = Vl(), s = Hl(), c = Wl();
r.exports = J;
var i = {}.hasOwnProperty, D = String.fromCharCode, o = Function.prototype, l = { warning: null, reference: null, text: null, warningContext: null, referenceContext: null, textContext: null, position: {}, additional: null, attribute: false, nonTerminated: true }, d = 9, p = 10, g = 12, F = 32, E = 38, b = 59, f = 60, x = 61, v = 35, h = 88, m = 120, C = 65533, w = "named", q = "hexadecimal", L = "decimal", B = {};
B[q] = 16, B[L] = 10;
var O = {};
O[w] = s, O[L] = a, O[q] = n3;
var T = 1, P = 2, A = 3, j = 4, H = 5, G = 6, X = 7, R = {};
R[T] = "Named character references must be terminated by a semicolon", R[P] = "Numeric character references must be terminated by a semicolon", R[A] = "Named character references cannot be empty", R[j] = "Numeric character references cannot be empty", R[H] = "Named character references must be known", R[G] = "Numeric character references cannot be disallowed", R[X] = "Numeric character references cannot be outside the permissible Unicode range";
function J(k, y) {
var _ = {}, N, V;
y || (y = {});
for (V in l)
N = y[V], _[V] = N == null ? l[V] : N;
return (_.position.indent || _.position.start) && (_.indent = _.position.indent || [], _.position = _.position.start), z(k, _);
}
function z(k, y) {
var _ = y.additional, N = y.nonTerminated, V = y.text, W = y.reference, K = y.warning, ee = y.textContext, Y = y.referenceContext, ue = y.warningContext, le = y.position, ce = y.indent || [], te = k.length, Z = 0, Q = -1, De = le.column || 1, ye = le.line || 1, fe = "", he = [], ae, pe, ne, re, we, oe, ie, Ce, rr, br, qe, $e, _e, xe, Fu, Ue, ur, ge, se;
for (typeof _ == "string" && (_ = _.charCodeAt(0)), Ue = Ge(), Ce = K ? Da : o, Z--, te++; ++Z < te; )
if (we === p && (De = ce[Q] || 1), we = k.charCodeAt(Z), we === E) {
if (ie = k.charCodeAt(Z + 1), ie === d || ie === p || ie === g || ie === F || ie === E || ie === f || ie !== ie || _ && ie === _) {
fe += D(we), De++;
continue;
}
for (_e = Z + 1, $e = _e, se = _e, ie === v ? (se = ++$e, ie = k.charCodeAt(se), ie === h || ie === m ? (xe = q, se = ++$e) : xe = L) : xe = w, ae = "", qe = "", re = "", Fu = O[xe], se--; ++se < te && (ie = k.charCodeAt(se), !!Fu(ie)); )
re += D(ie), xe === w && i.call(u, re) && (ae = re, qe = u[re]);
ne = k.charCodeAt(se) === b, ne && (se++, pe = xe === w ? c(re) : false, pe && (ae = re, qe = pe)), ge = 1 + se - _e, !ne && !N || (re ? xe === w ? (ne && !qe ? Ce(H, 1) : (ae !== re && (se = $e + ae.length, ge = 1 + se - $e, ne = false), ne || (rr = ae ? T : A, y.attribute ? (ie = k.charCodeAt(se), ie === x ? (Ce(rr, ge), qe = null) : s(ie) ? qe = null : Ce(rr, ge)) : Ce(rr, ge))), oe = qe) : (ne || Ce(P, ge), oe = parseInt(re, B[xe]), M(oe) ? (Ce(X, ge), oe = D(C)) : oe in t ? (Ce(G, ge), oe = t[oe]) : (br = "", U(oe) && Ce(G, ge), oe > 65535 && (oe -= 65536, br += D(oe >>> 10 | 55296), oe = 56320 | oe & 1023), oe = br + D(oe))) : xe !== w && Ce(j, ge)), oe ? (Au(), Ue = Ge(), Z = se - 1, De += se - _e + 1, he.push(oe), ur = Ge(), ur.offset++, W && W.call(Y, oe, { start: Ue, end: ur }, k.slice(_e - 1, se)), Ue = ur) : (re = k.slice(_e - 1, se), fe += re, De += re.length, Z = se - 1);
} else
we === 10 && (ye++, Q++, De = 0), we === we ? (fe += D(we), De++) : Au();
return he.join("");
function Ge() {
return { line: ye, column: De, offset: Z + (le.offset || 0) };
}
function Da(xu, bu) {
var yr = Ge();
yr.column += bu, yr.offset += bu, K.call(ue, R[xu], yr, xu);
}
function Au() {
fe && (he.push(fe), V && V.call(ee, fe, { start: Ue, end: Ge() }), fe = "");
}
}
function M(k) {
return k >= 55296 && k <= 57343 || k > 1114111;
}
function U(k) {
return k >= 1 && k <= 8 || k === 11 || k >= 13 && k <= 31 || k >= 127 && k <= 159 || k >= 64976 && k <= 65007 || (k & 65535) === 65535 || (k & 65535) === 65534;
}
} }), Kl = S({ "node_modules/remark-parse/lib/decode.js"(e, r) {
"use strict";
I();
var u = Pe(), t = xr();
r.exports = a;
function a(n3) {
return c.raw = i, c;
function s(o) {
for (var l = n3.offset, d = o.line, p = []; ++d && d in l; )
p.push((l[d] || 0) + 1);
return { start: o, indent: p };
}
function c(o, l, d) {
t(o, { position: s(l), warning: D, text: d, reference: d, textContext: n3, referenceContext: n3 });
}
function i(o, l, d) {
return t(o, u(d, { position: s(l), warning: D }));
}
function D(o, l, d) {
d !== 3 && n3.file.message(o, l);
}
}
} }), Yl = S({ "node_modules/remark-parse/lib/tokenizer.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(s) {
return c;
function c(i, D) {
var o = this, l = o.offset, d = [], p = o[s + "Methods"], g = o[s + "Tokenizers"], F = D.line, E = D.column, b, f, x, v, h, m;
if (!i)
return d;
for (P.now = q, P.file = o.file, C(""); i; ) {
for (b = -1, f = p.length, h = false; ++b < f && (v = p[b], x = g[v], !(x && (!x.onlyAtStart || o.atStart) && (!x.notInList || !o.inList) && (!x.notInBlock || !o.inBlock) && (!x.notInLink || !o.inLink) && (m = i.length, x.apply(o, [P, i]), h = m !== i.length, h))); )
;
h || o.file.fail(new Error("Infinite loop"), P.now());
}
return o.eof = q(), d;
function C(A) {
for (var j = -1, H = A.indexOf(`
`); H !== -1; )
F++, j = H, H = A.indexOf(`
`, H + 1);
j === -1 ? E += A.length : E = A.length - j, F in l && (j !== -1 ? E += l[F] : E <= l[F] && (E = l[F] + 1));
}
function w() {
var A = [], j = F + 1;
return function() {
for (var H = F + 1; j < H; )
A.push((l[j] || 0) + 1), j++;
return A;
};
}
function q() {
var A = { line: F, column: E };
return A.offset = o.toOffset(A), A;
}
function L(A) {
this.start = A, this.end = q();
}
function B(A) {
i.slice(0, A.length) !== A && o.file.fail(new Error("Incorrectly eaten value: please report this warning on https://git.io/vg5Ft"), q());
}
function O() {
var A = q();
return j;
function j(H, G) {
var X = H.position, R = X ? X.start : A, J = [], z = X && X.end.line, M = A.line;
if (H.position = new L(R), X && G && X.indent) {
if (J = X.indent, z < M) {
for (; ++z < M; )
J.push((l[z] || 0) + 1);
J.push(A.column);
}
G = J.concat(G);
}
return H.position.indent = G || [], H;
}
}
function T(A, j) {
var H = j ? j.children : d, G = H[H.length - 1], X;
return G && A.type === G.type && (A.type === "text" || A.type === "blockquote") && t(G) && t(A) && (X = A.type === "text" ? a : n3, A = X.call(o, G, A)), A !== G && H.push(A), o.atStart && d.length !== 0 && o.exitStart(), A;
}
function P(A) {
var j = w(), H = O(), G = q();
return B(A), X.reset = R, R.test = J, X.test = J, i = i.slice(A.length), C(A), j = j(), X;
function X(z, M) {
return H(T(H(z), M), j);
}
function R() {
var z = X.apply(null, arguments);
return F = G.line, E = G.column, i = A + i, z;
}
function J() {
var z = H({});
return F = G.line, E = G.column, i = A + i, z.position;
}
}
}
}
function t(s) {
var c, i;
return s.type !== "text" || !s.position ? true : (c = s.position.start, i = s.position.end, c.line !== i.line || i.column - c.column === s.value.length);
}
function a(s, c) {
return s.value += c.value, s;
}
function n3(s, c) {
return this.options.commonmark || this.options.gfm ? c : (s.children = s.children.concat(c.children), s);
}
} }), Jl = S({ "node_modules/markdown-escapes/index.js"(e, r) {
"use strict";
I(), r.exports = n3;
var u = ["\\", "`", "*", "{", "}", "[", "]", "(", ")", "#", "+", "-", ".", "!", "_", ">"], t = u.concat(["~", "|"]), a = t.concat([`
`, '"', "$", "%", "&", "'", ",", "/", ":", ";", "<", "=", "?", "@", "^"]);
n3.default = u, n3.gfm = t, n3.commonmark = a;
function n3(s) {
var c = s || {};
return c.commonmark ? a : c.gfm ? t : u;
}
} }), Zl = S({ "node_modules/remark-parse/lib/block-elements.js"(e, r) {
"use strict";
I(), r.exports = ["address", "article", "aside", "base", "basefont", "blockquote", "body", "caption", "center", "col", "colgroup", "dd", "details", "dialog", "dir", "div", "dl", "dt", "fieldset", "figcaption", "figure", "footer", "form", "frame", "frameset", "h1", "h2", "h3", "h4", "h5", "h6", "head", "header", "hgroup", "hr", "html", "iframe", "legend", "li", "link", "main", "menu", "menuitem", "meta", "nav", "noframes", "ol", "optgroup", "option", "p", "param", "pre", "section", "source", "title", "summary", "table", "tbody", "td", "tfoot", "th", "thead", "title", "tr", "track", "ul"];
} }), $i = S({ "node_modules/remark-parse/lib/defaults.js"(e, r) {
"use strict";
I(), r.exports = { position: true, gfm: true, commonmark: false, pedantic: false, blocks: Zl() };
} }), Ql = S({ "node_modules/remark-parse/lib/set-options.js"(e, r) {
"use strict";
I();
var u = Pe(), t = Jl(), a = $i();
r.exports = n3;
function n3(s) {
var c = this, i = c.options, D, o;
if (s == null)
s = {};
else if (typeof s == "object")
s = u(s);
else
throw new Error("Invalid value `" + s + "` for setting `options`");
for (D in a) {
if (o = s[D], o == null && (o = i[D]), D !== "blocks" && typeof o != "boolean" || D === "blocks" && typeof o != "object")
throw new Error("Invalid value `" + o + "` for setting `options." + D + "`");
s[D] = o;
}
return c.options = s, c.escape = t(s), c;
}
} }), eD = S({ "node_modules/unist-util-is/convert.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(c) {
if (c == null)
return s;
if (typeof c == "string")
return n3(c);
if (typeof c == "object")
return "length" in c ? a(c) : t(c);
if (typeof c == "function")
return c;
throw new Error("Expected function, string, or object as test");
}
function t(c) {
return i;
function i(D) {
var o;
for (o in c)
if (D[o] !== c[o])
return false;
return true;
}
}
function a(c) {
for (var i = [], D = -1; ++D < c.length; )
i[D] = u(c[D]);
return o;
function o() {
for (var l = -1; ++l < i.length; )
if (i[l].apply(this, arguments))
return true;
return false;
}
}
function n3(c) {
return i;
function i(D) {
return Boolean(D && D.type === c);
}
}
function s() {
return true;
}
} }), rD = S({ "node_modules/unist-util-visit-parents/color.browser.js"(e, r) {
I(), r.exports = u;
function u(t) {
return t;
}
} }), uD = S({ "node_modules/unist-util-visit-parents/index.js"(e, r) {
"use strict";
I(), r.exports = c;
var u = eD(), t = rD(), a = true, n3 = "skip", s = false;
c.CONTINUE = a, c.SKIP = n3, c.EXIT = s;
function c(D, o, l, d) {
var p, g;
typeof o == "function" && typeof l != "function" && (d = l, l = o, o = null), g = u(o), p = d ? -1 : 1, F(D, null, [])();
function F(E, b, f) {
var x = typeof E == "object" && E !== null ? E : {}, v;
return typeof x.type == "string" && (v = typeof x.tagName == "string" ? x.tagName : typeof x.name == "string" ? x.name : void 0, h.displayName = "node (" + t(x.type + (v ? "<" + v + ">" : "")) + ")"), h;
function h() {
var m = f.concat(E), C = [], w, q;
if ((!o || g(E, b, f[f.length - 1] || null)) && (C = i(l(E, f)), C[0] === s))
return C;
if (E.children && C[0] !== n3)
for (q = (d ? E.children.length : -1) + p; q > -1 && q < E.children.length; ) {
if (w = F(E.children[q], q, m)(), w[0] === s)
return w;
q = typeof w[1] == "number" ? w[1] : q + p;
}
return C;
}
}
}
function i(D) {
return D !== null && typeof D == "object" && "length" in D ? D : typeof D == "number" ? [a, D] : [D];
}
} }), tD = S({ "node_modules/unist-util-visit/index.js"(e, r) {
"use strict";
I(), r.exports = s;
var u = uD(), t = u.CONTINUE, a = u.SKIP, n3 = u.EXIT;
s.CONTINUE = t, s.SKIP = a, s.EXIT = n3;
function s(c, i, D, o) {
typeof i == "function" && typeof D != "function" && (o = D, D = i, i = null), u(c, i, l, o);
function l(d, p) {
var g = p[p.length - 1], F = g ? g.children.indexOf(d) : null;
return D(d, F, g);
}
}
} }), nD = S({ "node_modules/unist-util-remove-position/index.js"(e, r) {
"use strict";
I();
var u = tD();
r.exports = t;
function t(s, c) {
return u(s, c ? a : n3), s;
}
function a(s) {
delete s.position;
}
function n3(s) {
s.position = void 0;
}
} }), iD = S({ "node_modules/remark-parse/lib/parse.js"(e, r) {
"use strict";
I();
var u = Pe(), t = nD();
r.exports = s;
var a = `
`, n3 = /\r\n|\r/g;
function s() {
var c = this, i = String(c.file), D = { line: 1, column: 1, offset: 0 }, o = u(D), l;
return i = i.replace(n3, a), i.charCodeAt(0) === 65279 && (i = i.slice(1), o.column++, o.offset++), l = { type: "root", children: c.tokenizeBlock(i, o), position: { start: D, end: c.eof || u(D) } }, c.options.position || t(l, true), l;
}
} }), aD = S({ "node_modules/remark-parse/lib/tokenize/blank-line.js"(e, r) {
"use strict";
I();
var u = /^[ \t]*(\n|$)/;
r.exports = t;
function t(a, n3, s) {
for (var c, i = "", D = 0, o = n3.length; D < o && (c = u.exec(n3.slice(D)), c != null); )
D += c[0].length, i += c[0];
if (i !== "") {
if (s)
return true;
a(i);
}
}
} }), mu = S({ "node_modules/repeat-string/index.js"(e, r) {
"use strict";
I();
var u = "", t;
r.exports = a;
function a(n3, s) {
if (typeof n3 != "string")
throw new TypeError("expected a string");
if (s === 1)
return n3;
if (s === 2)
return n3 + n3;
var c = n3.length * s;
if (t !== n3 || typeof t > "u")
t = n3, u = "";
else if (u.length >= c)
return u.substr(0, c);
for (; c > u.length && s > 1; )
s & 1 && (u += n3), s >>= 1, n3 += n3;
return u += n3, u = u.substr(0, c), u;
}
} }), Ui = S({ "node_modules/trim-trailing-lines/index.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t) {
return String(t).replace(/\n+$/, "");
}
} }), oD = S({ "node_modules/remark-parse/lib/tokenize/code-indented.js"(e, r) {
"use strict";
I();
var u = mu(), t = Ui();
r.exports = D;
var a = `
`, n3 = " ", s = " ", c = 4, i = u(s, c);
function D(o, l, d) {
for (var p = -1, g = l.length, F = "", E = "", b = "", f = "", x, v, h; ++p < g; )
if (x = l.charAt(p), h)
if (h = false, F += b, E += f, b = "", f = "", x === a)
b = x, f = x;
else
for (F += x, E += x; ++p < g; ) {
if (x = l.charAt(p), !x || x === a) {
f = x, b = x;
break;
}
F += x, E += x;
}
else if (x === s && l.charAt(p + 1) === x && l.charAt(p + 2) === x && l.charAt(p + 3) === x)
b += i, p += 3, h = true;
else if (x === n3)
b += x, h = true;
else {
for (v = ""; x === n3 || x === s; )
v += x, x = l.charAt(++p);
if (x !== a)
break;
b += v + x, f += x;
}
if (E)
return d ? true : o(F)({ type: "code", lang: null, meta: null, value: t(E) });
}
} }), sD = S({ "node_modules/remark-parse/lib/tokenize/code-fenced.js"(e, r) {
"use strict";
I(), r.exports = D;
var u = `
`, t = " ", a = " ", n3 = "~", s = "`", c = 3, i = 4;
function D(o, l, d) {
var p = this, g = p.options.gfm, F = l.length + 1, E = 0, b = "", f, x, v, h, m, C, w, q, L, B, O, T, P;
if (g) {
for (; E < F && (v = l.charAt(E), !(v !== a && v !== t)); )
b += v, E++;
if (T = E, v = l.charAt(E), !(v !== n3 && v !== s)) {
for (E++, x = v, f = 1, b += v; E < F && (v = l.charAt(E), v === x); )
b += v, f++, E++;
if (!(f < c)) {
for (; E < F && (v = l.charAt(E), !(v !== a && v !== t)); )
b += v, E++;
for (h = "", w = ""; E < F && (v = l.charAt(E), !(v === u || x === s && v === x)); )
v === a || v === t ? w += v : (h += w + v, w = ""), E++;
if (v = l.charAt(E), !(v && v !== u)) {
if (d)
return true;
P = o.now(), P.column += b.length, P.offset += b.length, b += h, h = p.decode.raw(p.unescape(h), P), w && (b += w), w = "", B = "", O = "", q = "", L = "";
for (var A = true; E < F; ) {
if (v = l.charAt(E), q += B, L += O, B = "", O = "", v !== u) {
q += v, O += v, E++;
continue;
}
for (A ? (b += v, A = false) : (B += v, O += v), w = "", E++; E < F && (v = l.charAt(E), v === a); )
w += v, E++;
if (B += w, O += w.slice(T), !(w.length >= i)) {
for (w = ""; E < F && (v = l.charAt(E), v === x); )
w += v, E++;
if (B += w, O += w, !(w.length < f)) {
for (w = ""; E < F && (v = l.charAt(E), !(v !== a && v !== t)); )
B += v, O += v, E++;
if (!v || v === u)
break;
}
}
}
for (b += q + B, E = -1, F = h.length; ++E < F; )
if (v = h.charAt(E), v === a || v === t)
m || (m = h.slice(0, E));
else if (m) {
C = h.slice(E);
break;
}
return o(b)({ type: "code", lang: m || h || null, meta: C || null, value: L });
}
}
}
}
}
} }), ze = S({ "node_modules/trim/index.js"(e, r) {
I(), e = r.exports = u;
function u(t) {
return t.replace(/^\s*|\s*$/g, "");
}
e.left = function(t) {
return t.replace(/^\s*/, "");
}, e.right = function(t) {
return t.replace(/\s*$/, "");
};
} }), Eu = S({ "node_modules/remark-parse/lib/util/interrupt.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a, n3, s) {
for (var c = t.length, i = -1, D, o; ++i < c; )
if (D = t[i], o = D[1] || {}, !(o.pedantic !== void 0 && o.pedantic !== n3.options.pedantic) && !(o.commonmark !== void 0 && o.commonmark !== n3.options.commonmark) && a[D[0]].apply(n3, s))
return true;
return false;
}
} }), cD = S({ "node_modules/remark-parse/lib/tokenize/blockquote.js"(e, r) {
"use strict";
I();
var u = ze(), t = Eu();
r.exports = i;
var a = `
`, n3 = " ", s = " ", c = ">";
function i(D, o, l) {
for (var d = this, p = d.offset, g = d.blockTokenizers, F = d.interruptBlockquote, E = D.now(), b = E.line, f = o.length, x = [], v = [], h = [], m, C = 0, w, q, L, B, O, T, P, A; C < f && (w = o.charAt(C), !(w !== s && w !== n3)); )
C++;
if (o.charAt(C) === c) {
if (l)
return true;
for (C = 0; C < f; ) {
for (L = o.indexOf(a, C), T = C, P = false, L === -1 && (L = f); C < f && (w = o.charAt(C), !(w !== s && w !== n3)); )
C++;
if (o.charAt(C) === c ? (C++, P = true, o.charAt(C) === s && C++) : C = T, B = o.slice(C, L), !P && !u(B)) {
C = T;
break;
}
if (!P && (q = o.slice(C), t(F, g, d, [D, q, true])))
break;
O = T === C ? B : o.slice(T, L), h.push(C - T), x.push(O), v.push(B), C = L + 1;
}
for (C = -1, f = h.length, m = D(x.join(a)); ++C < f; )
p[b] = (p[b] || 0) + h[C], b++;
return A = d.enterBlock(), v = d.tokenizeBlock(v.join(a), E), A(), m({ type: "blockquote", children: v });
}
}
} }), lD = S({ "node_modules/remark-parse/lib/tokenize/heading-atx.js"(e, r) {
"use strict";
I(), r.exports = c;
var u = `
`, t = " ", a = " ", n3 = "#", s = 6;
function c(i, D, o) {
for (var l = this, d = l.options.pedantic, p = D.length + 1, g = -1, F = i.now(), E = "", b = "", f, x, v; ++g < p; ) {
if (f = D.charAt(g), f !== a && f !== t) {
g--;
break;
}
E += f;
}
for (v = 0; ++g <= p; ) {
if (f = D.charAt(g), f !== n3) {
g--;
break;
}
E += f, v++;
}
if (!(v > s) && !(!v || !d && D.charAt(g + 1) === n3)) {
for (p = D.length + 1, x = ""; ++g < p; ) {
if (f = D.charAt(g), f !== a && f !== t) {
g--;
break;
}
x += f;
}
if (!(!d && x.length === 0 && f && f !== u)) {
if (o)
return true;
for (E += x, x = "", b = ""; ++g < p && (f = D.charAt(g), !(!f || f === u)); ) {
if (f !== a && f !== t && f !== n3) {
b += x + f, x = "";
continue;
}
for (; f === a || f === t; )
x += f, f = D.charAt(++g);
if (!d && b && !x && f === n3) {
b += f;
continue;
}
for (; f === n3; )
x += f, f = D.charAt(++g);
for (; f === a || f === t; )
x += f, f = D.charAt(++g);
g--;
}
return F.column += E.length, F.offset += E.length, E += b + x, i(E)({ type: "heading", depth: v, children: l.tokenizeInline(b, F) });
}
}
}
} }), DD = S({ "node_modules/remark-parse/lib/tokenize/thematic-break.js"(e, r) {
"use strict";
I(), r.exports = D;
var u = " ", t = `
`, a = " ", n3 = "*", s = "-", c = "_", i = 3;
function D(o, l, d) {
for (var p = -1, g = l.length + 1, F = "", E, b, f, x; ++p < g && (E = l.charAt(p), !(E !== u && E !== a)); )
F += E;
if (!(E !== n3 && E !== s && E !== c))
for (b = E, F += E, f = 1, x = ""; ++p < g; )
if (E = l.charAt(p), E === b)
f++, F += x + b, x = "";
else if (E === a)
x += E;
else
return f >= i && (!E || E === t) ? (F += x, d ? true : o(F)({ type: "thematicBreak" })) : void 0;
}
} }), Gi = S({ "node_modules/remark-parse/lib/util/get-indentation.js"(e, r) {
"use strict";
I(), r.exports = s;
var u = " ", t = " ", a = 1, n3 = 4;
function s(c) {
for (var i = 0, D = 0, o = c.charAt(i), l = {}, d, p = 0; o === u || o === t; ) {
for (d = o === u ? n3 : a, D += d, d > 1 && (D = Math.floor(D / d) * d); p < D; )
l[++p] = i;
o = c.charAt(++i);
}
return { indent: D, stops: l };
}
} }), fD = S({ "node_modules/remark-parse/lib/util/remove-indentation.js"(e, r) {
"use strict";
I();
var u = ze(), t = mu(), a = Gi();
r.exports = i;
var n3 = `
`, s = " ", c = "!";
function i(D, o) {
var l = D.split(n3), d = l.length + 1, p = 1 / 0, g = [], F, E, b;
for (l.unshift(t(s, o) + c); d--; )
if (E = a(l[d]), g[d] = E.stops, u(l[d]).length !== 0)
if (E.indent)
E.indent > 0 && E.indent < p && (p = E.indent);
else {
p = 1 / 0;
break;
}
if (p !== 1 / 0)
for (d = l.length; d--; ) {
for (b = g[d], F = p; F && !(F in b); )
F--;
l[d] = l[d].slice(b[F] + 1);
}
return l.shift(), l.join(n3);
}
} }), pD = S({ "node_modules/remark-parse/lib/tokenize/list.js"(e, r) {
"use strict";
I();
var u = ze(), t = mu(), a = Me(), n3 = Gi(), s = fD(), c = Eu();
r.exports = w;
var i = "*", D = "_", o = "+", l = "-", d = ".", p = " ", g = `
`, F = " ", E = ")", b = "x", f = 4, x = /\n\n(?!\s*$)/, v = /^\[([ X\tx])][ \t]/, h = /^([ \t]*)([*+-]|\d+[.)])( {1,4}(?! )| |\t|$|(?=\n))([^\n]*)/, m = /^([ \t]*)([*+-]|\d+[.)])([ \t]+)/, C = /^( {1,4}|\t)?/gm;
function w(O, T, P) {
for (var A = this, j = A.options.commonmark, H = A.options.pedantic, G = A.blockTokenizers, X = A.interruptList, R = 0, J = T.length, z = null, M, U, k, y, _, N, V, W, K, ee, Y, ue, le, ce, te, Z, Q, De, ye, fe = false, he, ae, pe, ne; R < J && (y = T.charAt(R), !(y !== F && y !== p)); )
R++;
if (y = T.charAt(R), y === i || y === o || y === l)
_ = y, k = false;
else {
for (k = true, U = ""; R < J && (y = T.charAt(R), !!a(y)); )
U += y, R++;
if (y = T.charAt(R), !U || !(y === d || j && y === E) || P && U !== "1")
return;
z = parseInt(U, 10), _ = y;
}
if (y = T.charAt(++R), !(y !== p && y !== F && (H || y !== g && y !== ""))) {
if (P)
return true;
for (R = 0, ce = [], te = [], Z = []; R < J; ) {
for (N = T.indexOf(g, R), V = R, W = false, ne = false, N === -1 && (N = J), M = 0; R < J; ) {
if (y = T.charAt(R), y === F)
M += f - M % f;
else if (y === p)
M++;
else
break;
R++;
}
if (Q && M >= Q.indent && (ne = true), y = T.charAt(R), K = null, !ne) {
if (y === i || y === o || y === l)
K = y, R++, M++;
else {
for (U = ""; R < J && (y = T.charAt(R), !!a(y)); )
U += y, R++;
y = T.charAt(R), R++, U && (y === d || j && y === E) && (K = y, M += U.length + 1);
}
if (K)
if (y = T.charAt(R), y === F)
M += f - M % f, R++;
else if (y === p) {
for (pe = R + f; R < pe && T.charAt(R) === p; )
R++, M++;
R === pe && T.charAt(R) === p && (R -= f - 1, M -= f - 1);
} else
y !== g && y !== "" && (K = null);
}
if (K) {
if (!H && _ !== K)
break;
W = true;
} else
!j && !ne && T.charAt(V) === p ? ne = true : j && Q && (ne = M >= Q.indent || M > f), W = false, R = V;
if (Y = T.slice(V, N), ee = V === R ? Y : T.slice(R, N), (K === i || K === D || K === l) && G.thematicBreak.call(A, O, Y, true))
break;
if (ue = le, le = !W && !u(ee).length, ne && Q)
Q.value = Q.value.concat(Z, Y), te = te.concat(Z, Y), Z = [];
else if (W)
Z.length !== 0 && (fe = true, Q.value.push(""), Q.trail = Z.concat()), Q = { value: [Y], indent: M, trail: [] }, ce.push(Q), te = te.concat(Z, Y), Z = [];
else if (le) {
if (ue && !j)
break;
Z.push(Y);
} else {
if (ue || c(X, G, A, [O, Y, true]))
break;
Q.value = Q.value.concat(Z, Y), te = te.concat(Z, Y), Z = [];
}
R = N + 1;
}
for (he = O(te.join(g)).reset({ type: "list", ordered: k, start: z, spread: fe, children: [] }), De = A.enterList(), ye = A.enterBlock(), R = -1, J = ce.length; ++R < J; )
Q = ce[R].value.join(g), ae = O.now(), O(Q)(q(A, Q, ae), he), Q = ce[R].trail.join(g), R !== J - 1 && (Q += g), O(Q);
return De(), ye(), he;
}
}
function q(O, T, P) {
var A = O.offset, j = O.options.pedantic ? L : B, H = null, G, X;
return T = j.apply(null, arguments), O.options.gfm && (G = T.match(v), G && (X = G[0].length, H = G[1].toLowerCase() === b, A[P.line] += X, T = T.slice(X))), { type: "listItem", spread: x.test(T), checked: H, children: O.tokenizeBlock(T, P) };
}
function L(O, T, P) {
var A = O.offset, j = P.line;
return T = T.replace(m, H), j = P.line, T.replace(C, H);
function H(G) {
return A[j] = (A[j] || 0) + G.length, j++, "";
}
}
function B(O, T, P) {
var A = O.offset, j = P.line, H, G, X, R, J, z, M;
for (T = T.replace(h, U), R = T.split(g), J = s(T, n3(H).indent).split(g), J[0] = X, A[j] = (A[j] || 0) + G.length, j++, z = 0, M = R.length; ++z < M; )
A[j] = (A[j] || 0) + R[z].length - J[z].length, j++;
return J.join(g);
function U(k, y, _, N, V) {
return G = y + _ + N, X = V, Number(_) < 10 && G.length % 2 === 1 && (_ = p + _), H = y + t(p, _.length) + N, H + X;
}
}
} }), dD = S({ "node_modules/remark-parse/lib/tokenize/heading-setext.js"(e, r) {
"use strict";
I(), r.exports = o;
var u = `
`, t = " ", a = " ", n3 = "=", s = "-", c = 3, i = 1, D = 2;
function o(l, d, p) {
for (var g = this, F = l.now(), E = d.length, b = -1, f = "", x, v, h, m, C; ++b < E; ) {
if (h = d.charAt(b), h !== a || b >= c) {
b--;
break;
}
f += h;
}
for (x = "", v = ""; ++b < E; ) {
if (h = d.charAt(b), h === u) {
b--;
break;
}
h === a || h === t ? v += h : (x += v + h, v = "");
}
if (F.column += f.length, F.offset += f.length, f += x + v, h = d.charAt(++b), m = d.charAt(++b), !(h !== u || m !== n3 && m !== s)) {
for (f += h, v = m, C = m === n3 ? i : D; ++b < E; ) {
if (h = d.charAt(b), h !== m) {
if (h !== u)
return;
b--;
break;
}
v += h;
}
return p ? true : l(f + v)({ type: "heading", depth: C, children: g.tokenizeInline(x, F) });
}
}
} }), Vi = S({ "node_modules/remark-parse/lib/util/html.js"(e) {
"use strict";
I();
var r = "[a-zA-Z_:][a-zA-Z0-9:._-]*", u = "[^\"'=<>`\\u0000-\\u0020]+", t = "'[^']*'", a = '"[^"]*"', n3 = "(?:" + u + "|" + t + "|" + a + ")", s = "(?:\\s+" + r + "(?:\\s*=\\s*" + n3 + ")?)", c = "<[A-Za-z][A-Za-z0-9\\-]*" + s + "*\\s*\\/?>", i = "<\\/[A-Za-z][A-Za-z0-9\\-]*\\s*>", D = "|", o = "<[?].*?[?]>", l = "]*>", d = "";
e.openCloseTag = new RegExp("^(?:" + c + "|" + i + ")"), e.tag = new RegExp("^(?:" + c + "|" + i + "|" + D + "|" + o + "|" + l + "|" + d + ")");
} }), hD = S({ "node_modules/remark-parse/lib/tokenize/html-block.js"(e, r) {
"use strict";
I();
var u = Vi().openCloseTag;
r.exports = x;
var t = " ", a = " ", n3 = `
`, s = "<", c = /^<(script|pre|style)(?=(\s|>|$))/i, i = /<\/(script|pre|style)>/i, D = /^/, l = /^<\?/, d = /\?>/, p = /^/, F = /^/, b = /^$/, f = new RegExp(u.source + "\\s*$");
function x(v, h, m) {
for (var C = this, w = C.options.blocks.join("|"), q = new RegExp("^?(" + w + ")(?=(\\s|/?>|$))", "i"), L = h.length, B = 0, O, T, P, A, j, H, G, X = [[c, i, true], [D, o, true], [l, d, true], [p, g, true], [F, E, true], [q, b, true], [f, b, false]]; B < L && (A = h.charAt(B), !(A !== t && A !== a)); )
B++;
if (h.charAt(B) === s) {
for (O = h.indexOf(n3, B + 1), O = O === -1 ? L : O, T = h.slice(B, O), P = -1, j = X.length; ++P < j; )
if (X[P][0].test(T)) {
H = X[P];
break;
}
if (H) {
if (m)
return H[2];
if (B = O, !H[1].test(T))
for (; B < L; ) {
if (O = h.indexOf(n3, B + 1), O = O === -1 ? L : O, T = h.slice(B + 1, O), H[1].test(T)) {
T && (B = O);
break;
}
B = O;
}
return G = h.slice(0, B), v(G)({ type: "html", value: G });
}
}
}
} }), be = S({ "node_modules/is-whitespace-character/index.js"(e, r) {
"use strict";
I(), r.exports = a;
var u = String.fromCharCode, t = /\s/;
function a(n3) {
return t.test(typeof n3 == "number" ? u(n3) : n3.charAt(0));
}
} }), vD = S({ "node_modules/collapse-white-space/index.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t) {
return String(t).replace(/\s+/g, " ");
}
} }), Hi = S({ "node_modules/remark-parse/lib/util/normalize.js"(e, r) {
"use strict";
I();
var u = vD();
r.exports = t;
function t(a) {
return u(a).toLowerCase();
}
} }), mD = S({ "node_modules/remark-parse/lib/tokenize/definition.js"(e, r) {
"use strict";
I();
var u = be(), t = Hi();
r.exports = b;
var a = '"', n3 = "'", s = "\\", c = `
`, i = " ", D = " ", o = "[", l = "]", d = "(", p = ")", g = ":", F = "<", E = ">";
function b(v, h, m) {
for (var C = this, w = C.options.commonmark, q = 0, L = h.length, B = "", O, T, P, A, j, H, G, X; q < L && (A = h.charAt(q), !(A !== D && A !== i)); )
B += A, q++;
if (A = h.charAt(q), A === o) {
for (q++, B += A, P = ""; q < L && (A = h.charAt(q), A !== l); )
A === s && (P += A, q++, A = h.charAt(q)), P += A, q++;
if (!(!P || h.charAt(q) !== l || h.charAt(q + 1) !== g)) {
for (H = P, B += P + l + g, q = B.length, P = ""; q < L && (A = h.charAt(q), !(A !== i && A !== D && A !== c)); )
B += A, q++;
if (A = h.charAt(q), P = "", O = B, A === F) {
for (q++; q < L && (A = h.charAt(q), !!f(A)); )
P += A, q++;
if (A = h.charAt(q), A === f.delimiter)
B += F + P + A, q++;
else {
if (w)
return;
q -= P.length + 1, P = "";
}
}
if (!P) {
for (; q < L && (A = h.charAt(q), !!x(A)); )
P += A, q++;
B += P;
}
if (P) {
for (G = P, P = ""; q < L && (A = h.charAt(q), !(A !== i && A !== D && A !== c)); )
P += A, q++;
if (A = h.charAt(q), j = null, A === a ? j = a : A === n3 ? j = n3 : A === d && (j = p), !j)
P = "", q = B.length;
else if (P) {
for (B += P + A, q = B.length, P = ""; q < L && (A = h.charAt(q), A !== j); ) {
if (A === c) {
if (q++, A = h.charAt(q), A === c || A === j)
return;
P += c;
}
P += A, q++;
}
if (A = h.charAt(q), A !== j)
return;
T = B, B += P + A, q++, X = P, P = "";
} else
return;
for (; q < L && (A = h.charAt(q), !(A !== i && A !== D)); )
B += A, q++;
if (A = h.charAt(q), !A || A === c)
return m ? true : (O = v(O).test().end, G = C.decode.raw(C.unescape(G), O, { nonTerminated: false }), X && (T = v(T).test().end, X = C.decode.raw(C.unescape(X), T)), v(B)({ type: "definition", identifier: t(H), label: H, title: X || null, url: G }));
}
}
}
}
function f(v) {
return v !== E && v !== o && v !== l;
}
f.delimiter = E;
function x(v) {
return v !== o && v !== l && !u(v);
}
} }), ED = S({ "node_modules/remark-parse/lib/tokenize/table.js"(e, r) {
"use strict";
I();
var u = be();
r.exports = F;
var t = " ", a = `
`, n3 = " ", s = "-", c = ":", i = "\\", D = "|", o = 1, l = 2, d = "left", p = "center", g = "right";
function F(E, b, f) {
var x = this, v, h, m, C, w, q, L, B, O, T, P, A, j, H, G, X, R, J, z, M, U, k;
if (x.options.gfm) {
for (v = 0, X = 0, q = b.length + 1, L = []; v < q; ) {
if (M = b.indexOf(a, v), U = b.indexOf(D, v + 1), M === -1 && (M = b.length), U === -1 || U > M) {
if (X < l)
return;
break;
}
L.push(b.slice(v, M)), X++, v = M + 1;
}
for (C = L.join(a), h = L.splice(1, 1)[0] || [], v = 0, q = h.length, X--, m = false, P = []; v < q; ) {
if (O = h.charAt(v), O === D) {
if (T = null, m === false) {
if (k === false)
return;
} else
P.push(m), m = false;
k = false;
} else if (O === s)
T = true, m = m || null;
else if (O === c)
m === d ? m = p : T && m === null ? m = g : m = d;
else if (!u(O))
return;
v++;
}
if (m !== false && P.push(m), !(P.length < o)) {
if (f)
return true;
for (G = -1, J = [], z = E(C).reset({ type: "table", align: P, children: J }); ++G < X; ) {
for (R = L[G], w = { type: "tableRow", children: [] }, G && E(a), E(R).reset(w, z), q = R.length + 1, v = 0, B = "", A = "", j = true; v < q; ) {
if (O = R.charAt(v), O === t || O === n3) {
A ? B += O : E(O), v++;
continue;
}
O === "" || O === D ? j ? E(O) : ((A || O) && !j && (C = A, B.length > 1 && (O ? (C += B.slice(0, -1), B = B.charAt(B.length - 1)) : (C += B, B = "")), H = E.now(), E(C)({ type: "tableCell", children: x.tokenizeInline(A, H) }, w)), E(B + O), B = "", A = "") : (B && (A += B, B = ""), A += O, O === i && v !== q - 2 && (A += R.charAt(v + 1), v++)), j = false, v++;
}
G || E(a + h);
}
return z;
}
}
}
} }), CD = S({ "node_modules/remark-parse/lib/tokenize/paragraph.js"(e, r) {
"use strict";
I();
var u = ze(), t = Ui(), a = Eu();
r.exports = D;
var n3 = " ", s = `
`, c = " ", i = 4;
function D(o, l, d) {
for (var p = this, g = p.options, F = g.commonmark, E = p.blockTokenizers, b = p.interruptParagraph, f = l.indexOf(s), x = l.length, v, h, m, C, w; f < x; ) {
if (f === -1) {
f = x;
break;
}
if (l.charAt(f + 1) === s)
break;
if (F) {
for (C = 0, v = f + 1; v < x; ) {
if (m = l.charAt(v), m === n3) {
C = i;
break;
} else if (m === c)
C++;
else
break;
v++;
}
if (C >= i && m !== s) {
f = l.indexOf(s, f + 1);
continue;
}
}
if (h = l.slice(f + 1), a(b, E, p, [o, h, true]))
break;
if (v = f, f = l.indexOf(s, f + 1), f !== -1 && u(l.slice(v, f)) === "") {
f = v;
break;
}
}
return h = l.slice(0, f), d ? true : (w = o.now(), h = t(h), o(h)({ type: "paragraph", children: p.tokenizeInline(h, w) }));
}
} }), gD = S({ "node_modules/remark-parse/lib/locate/escape.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a) {
return t.indexOf("\\", a);
}
} }), FD = S({ "node_modules/remark-parse/lib/tokenize/escape.js"(e, r) {
"use strict";
I();
var u = gD();
r.exports = n3, n3.locator = u;
var t = `
`, a = "\\";
function n3(s, c, i) {
var D = this, o, l;
if (c.charAt(0) === a && (o = c.charAt(1), D.escape.indexOf(o) !== -1))
return i ? true : (o === t ? l = { type: "break" } : l = { type: "text", value: o }, s(a + o)(l));
}
} }), Xi = S({ "node_modules/remark-parse/lib/locate/tag.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a) {
return t.indexOf("<", a);
}
} }), AD = S({ "node_modules/remark-parse/lib/tokenize/auto-link.js"(e, r) {
"use strict";
I();
var u = be(), t = xr(), a = Xi();
r.exports = l, l.locator = a, l.notInLink = true;
var n3 = "<", s = ">", c = "@", i = "/", D = "mailto:", o = D.length;
function l(d, p, g) {
var F = this, E = "", b = p.length, f = 0, x = "", v = false, h = "", m, C, w, q, L;
if (p.charAt(0) === n3) {
for (f++, E = n3; f < b && (m = p.charAt(f), !(u(m) || m === s || m === c || m === ":" && p.charAt(f + 1) === i)); )
x += m, f++;
if (x) {
if (h += x, x = "", m = p.charAt(f), h += m, f++, m === c)
v = true;
else {
if (m !== ":" || p.charAt(f + 1) !== i)
return;
h += i, f++;
}
for (; f < b && (m = p.charAt(f), !(u(m) || m === s)); )
x += m, f++;
if (m = p.charAt(f), !(!x || m !== s))
return g ? true : (h += x, w = h, E += h + m, C = d.now(), C.column++, C.offset++, v && (h.slice(0, o).toLowerCase() === D ? (w = w.slice(o), C.column += o, C.offset += o) : h = D + h), q = F.inlineTokenizers, F.inlineTokenizers = { text: q.text }, L = F.enterLink(), w = F.tokenizeInline(w, C), F.inlineTokenizers = q, L(), d(E)({ type: "link", title: null, url: t(h, { nonTerminated: false }), children: w }));
}
}
}
} }), xD = S({ "node_modules/ccount/index.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a) {
var n3 = String(t), s = 0, c;
if (typeof a != "string")
throw new Error("Expected character");
for (c = n3.indexOf(a); c !== -1; )
s++, c = n3.indexOf(a, c + a.length);
return s;
}
} }), bD = S({ "node_modules/remark-parse/lib/locate/url.js"(e, r) {
"use strict";
I(), r.exports = t;
var u = ["www.", "http://", "https://"];
function t(a, n3) {
var s = -1, c, i, D;
if (!this.options.gfm)
return s;
for (i = u.length, c = -1; ++c < i; )
D = a.indexOf(u[c], n3), D !== -1 && (s === -1 || D < s) && (s = D);
return s;
}
} }), yD = S({ "node_modules/remark-parse/lib/tokenize/url.js"(e, r) {
"use strict";
I();
var u = xD(), t = xr(), a = Me(), n3 = er(), s = be(), c = bD();
r.exports = C, C.locator = c, C.notInLink = true;
var i = 33, D = 38, o = 41, l = 42, d = 44, p = 45, g = 46, F = 58, E = 59, b = 63, f = 60, x = 95, v = 126, h = "(", m = ")";
function C(w, q, L) {
var B = this, O = B.options.gfm, T = B.inlineTokenizers, P = q.length, A = -1, j = false, H, G, X, R, J, z, M, U, k, y, _, N, V, W;
if (O) {
if (q.slice(0, 4) === "www.")
j = true, R = 4;
else if (q.slice(0, 7).toLowerCase() === "http://")
R = 7;
else if (q.slice(0, 8).toLowerCase() === "https://")
R = 8;
else
return;
for (A = R - 1, X = R, H = []; R < P; ) {
if (M = q.charCodeAt(R), M === g) {
if (A === R - 1)
break;
H.push(R), A = R, R++;
continue;
}
if (a(M) || n3(M) || M === p || M === x) {
R++;
continue;
}
break;
}
if (M === g && (H.pop(), R--), H[0] !== void 0 && (G = H.length < 2 ? X : H[H.length - 2] + 1, q.slice(G, R).indexOf("_") === -1)) {
if (L)
return true;
for (U = R, J = R; R < P && (M = q.charCodeAt(R), !(s(M) || M === f)); )
R++, M === i || M === l || M === d || M === g || M === F || M === b || M === x || M === v || (U = R);
if (R = U, q.charCodeAt(R - 1) === o)
for (z = q.slice(J, R), k = u(z, h), y = u(z, m); y > k; )
R = J + z.lastIndexOf(m), z = q.slice(J, R), y--;
if (q.charCodeAt(R - 1) === E && (R--, n3(q.charCodeAt(R - 1)))) {
for (U = R - 2; n3(q.charCodeAt(U)); )
U--;
q.charCodeAt(U) === D && (R = U);
}
return _ = q.slice(0, R), V = t(_, { nonTerminated: false }), j && (V = "http://" + V), W = B.enterLink(), B.inlineTokenizers = { text: T.text }, N = B.tokenizeInline(_, w.now()), B.inlineTokenizers = T, W(), w(_)({ type: "link", title: null, url: V, children: N });
}
}
}
} }), wD = S({ "node_modules/remark-parse/lib/locate/email.js"(e, r) {
"use strict";
I();
var u = Me(), t = er(), a = 43, n3 = 45, s = 46, c = 95;
r.exports = i;
function i(o, l) {
var d = this, p, g;
if (!this.options.gfm || (p = o.indexOf("@", l), p === -1))
return -1;
if (g = p, g === l || !D(o.charCodeAt(g - 1)))
return i.call(d, o, p + 1);
for (; g > l && D(o.charCodeAt(g - 1)); )
g--;
return g;
}
function D(o) {
return u(o) || t(o) || o === a || o === n3 || o === s || o === c;
}
} }), BD = S({ "node_modules/remark-parse/lib/tokenize/email.js"(e, r) {
"use strict";
I();
var u = xr(), t = Me(), a = er(), n3 = wD();
r.exports = l, l.locator = n3, l.notInLink = true;
var s = 43, c = 45, i = 46, D = 64, o = 95;
function l(d, p, g) {
var F = this, E = F.options.gfm, b = F.inlineTokenizers, f = 0, x = p.length, v = -1, h, m, C, w;
if (E) {
for (h = p.charCodeAt(f); t(h) || a(h) || h === s || h === c || h === i || h === o; )
h = p.charCodeAt(++f);
if (f !== 0 && h === D) {
for (f++; f < x; ) {
if (h = p.charCodeAt(f), t(h) || a(h) || h === c || h === i || h === o) {
f++, v === -1 && h === i && (v = f);
continue;
}
break;
}
if (!(v === -1 || v === f || h === c || h === o))
return h === i && f--, m = p.slice(0, f), g ? true : (w = F.enterLink(), F.inlineTokenizers = { text: b.text }, C = F.tokenizeInline(m, d.now()), F.inlineTokenizers = b, w(), d(m)({ type: "link", title: null, url: "mailto:" + u(m, { nonTerminated: false }), children: C }));
}
}
}
} }), kD = S({ "node_modules/remark-parse/lib/tokenize/html-inline.js"(e, r) {
"use strict";
I();
var u = er(), t = Xi(), a = Vi().tag;
r.exports = l, l.locator = t;
var n3 = "<", s = "?", c = "!", i = "/", D = /^/i;
function l(d, p, g) {
var F = this, E = p.length, b, f;
if (!(p.charAt(0) !== n3 || E < 3) && (b = p.charAt(1), !(!u(b) && b !== s && b !== c && b !== i) && (f = p.match(a), !!f)))
return g ? true : (f = f[0], !F.inLink && D.test(f) ? F.inLink = true : F.inLink && o.test(f) && (F.inLink = false), d(f)({ type: "html", value: f }));
}
} }), Wi = S({ "node_modules/remark-parse/lib/locate/link.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a) {
var n3 = t.indexOf("[", a), s = t.indexOf("![", a);
return s === -1 || n3 < s ? n3 : s;
}
} }), qD = S({ "node_modules/remark-parse/lib/tokenize/link.js"(e, r) {
"use strict";
I();
var u = be(), t = Wi();
r.exports = E, E.locator = t;
var a = `
`, n3 = "!", s = '"', c = "'", i = "(", D = ")", o = "<", l = ">", d = "[", p = "\\", g = "]", F = "`";
function E(b, f, x) {
var v = this, h = "", m = 0, C = f.charAt(0), w = v.options.pedantic, q = v.options.commonmark, L = v.options.gfm, B, O, T, P, A, j, H, G, X, R, J, z, M, U, k, y, _, N;
if (C === n3 && (G = true, h = C, C = f.charAt(++m)), C === d && !(!G && v.inLink)) {
for (h += C, U = "", m++, J = f.length, y = b.now(), M = 0, y.column += m, y.offset += m; m < J; ) {
if (C = f.charAt(m), j = C, C === F) {
for (O = 1; f.charAt(m + 1) === F; )
j += C, m++, O++;
T ? O >= T && (T = 0) : T = O;
} else if (C === p)
m++, j += f.charAt(m);
else if ((!T || L) && C === d)
M++;
else if ((!T || L) && C === g)
if (M)
M--;
else {
if (f.charAt(m + 1) !== i)
return;
j += i, B = true, m++;
break;
}
U += j, j = "", m++;
}
if (B) {
for (X = U, h += U + j, m++; m < J && (C = f.charAt(m), !!u(C)); )
h += C, m++;
if (C = f.charAt(m), U = "", P = h, C === o) {
for (m++, P += o; m < J && (C = f.charAt(m), C !== l); ) {
if (q && C === a)
return;
U += C, m++;
}
if (f.charAt(m) !== l)
return;
h += o + U + l, k = U, m++;
} else {
for (C = null, j = ""; m < J && (C = f.charAt(m), !(j && (C === s || C === c || q && C === i))); ) {
if (u(C)) {
if (!w)
break;
j += C;
} else {
if (C === i)
M++;
else if (C === D) {
if (M === 0)
break;
M--;
}
U += j, j = "", C === p && (U += p, C = f.charAt(++m)), U += C;
}
m++;
}
h += U, k = U, m = h.length;
}
for (U = ""; m < J && (C = f.charAt(m), !!u(C)); )
U += C, m++;
if (C = f.charAt(m), h += U, U && (C === s || C === c || q && C === i))
if (m++, h += C, U = "", R = C === i ? D : C, A = h, q) {
for (; m < J && (C = f.charAt(m), C !== R); )
C === p && (U += p, C = f.charAt(++m)), m++, U += C;
if (C = f.charAt(m), C !== R)
return;
for (z = U, h += U + C, m++; m < J && (C = f.charAt(m), !!u(C)); )
h += C, m++;
} else
for (j = ""; m < J; ) {
if (C = f.charAt(m), C === R)
H && (U += R + j, j = ""), H = true;
else if (!H)
U += C;
else if (C === D) {
h += U + R + j, z = U;
break;
} else
u(C) ? j += C : (U += R + j + C, j = "", H = false);
m++;
}
if (f.charAt(m) === D)
return x ? true : (h += D, k = v.decode.raw(v.unescape(k), b(P).test().end, { nonTerminated: false }), z && (A = b(A).test().end, z = v.decode.raw(v.unescape(z), A)), N = { type: G ? "image" : "link", title: z || null, url: k }, G ? N.alt = v.decode.raw(v.unescape(X), y) || null : (_ = v.enterLink(), N.children = v.tokenizeInline(X, y), _()), b(h)(N));
}
}
}
} }), _D = S({ "node_modules/remark-parse/lib/tokenize/reference.js"(e, r) {
"use strict";
I();
var u = be(), t = Wi(), a = Hi();
r.exports = g, g.locator = t;
var n3 = "link", s = "image", c = "shortcut", i = "collapsed", D = "full", o = "!", l = "[", d = "\\", p = "]";
function g(F, E, b) {
var f = this, x = f.options.commonmark, v = E.charAt(0), h = 0, m = E.length, C = "", w = "", q = n3, L = c, B, O, T, P, A, j, H, G;
if (v === o && (q = s, w = v, v = E.charAt(++h)), v === l) {
for (h++, w += v, j = "", G = 0; h < m; ) {
if (v = E.charAt(h), v === l)
H = true, G++;
else if (v === p) {
if (!G)
break;
G--;
}
v === d && (j += d, v = E.charAt(++h)), j += v, h++;
}
if (C = j, B = j, v = E.charAt(h), v === p) {
if (h++, C += v, j = "", !x)
for (; h < m && (v = E.charAt(h), !!u(v)); )
j += v, h++;
if (v = E.charAt(h), v === l) {
for (O = "", j += v, h++; h < m && (v = E.charAt(h), !(v === l || v === p)); )
v === d && (O += d, v = E.charAt(++h)), O += v, h++;
v = E.charAt(h), v === p ? (L = O ? D : i, j += O + v, h++) : O = "", C += j, j = "";
} else {
if (!B)
return;
O = B;
}
if (!(L !== D && H))
return C = w + C, q === n3 && f.inLink ? null : b ? true : (T = F.now(), T.column += w.length, T.offset += w.length, O = L === D ? O : B, P = { type: q + "Reference", identifier: a(O), label: O, referenceType: L }, q === n3 ? (A = f.enterLink(), P.children = f.tokenizeInline(B, T), A()) : P.alt = f.decode.raw(f.unescape(B), T) || null, F(C)(P));
}
}
}
} }), OD = S({ "node_modules/remark-parse/lib/locate/strong.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a) {
var n3 = t.indexOf("**", a), s = t.indexOf("__", a);
return s === -1 ? n3 : n3 === -1 || s < n3 ? s : n3;
}
} }), ID = S({ "node_modules/remark-parse/lib/tokenize/strong.js"(e, r) {
"use strict";
I();
var u = ze(), t = be(), a = OD();
r.exports = i, i.locator = a;
var n3 = "\\", s = "*", c = "_";
function i(D, o, l) {
var d = this, p = 0, g = o.charAt(p), F, E, b, f, x, v, h;
if (!(g !== s && g !== c || o.charAt(++p) !== g) && (E = d.options.pedantic, b = g, x = b + b, v = o.length, p++, f = "", g = "", !(E && t(o.charAt(p)))))
for (; p < v; ) {
if (h = g, g = o.charAt(p), g === b && o.charAt(p + 1) === b && (!E || !t(h)) && (g = o.charAt(p + 2), g !== b))
return u(f) ? l ? true : (F = D.now(), F.column += 2, F.offset += 2, D(x + f + x)({ type: "strong", children: d.tokenizeInline(f, F) })) : void 0;
!E && g === n3 && (f += g, g = o.charAt(++p)), f += g, p++;
}
}
} }), SD = S({ "node_modules/is-word-character/index.js"(e, r) {
"use strict";
I(), r.exports = a;
var u = String.fromCharCode, t = /\w/;
function a(n3) {
return t.test(typeof n3 == "number" ? u(n3) : n3.charAt(0));
}
} }), TD = S({ "node_modules/remark-parse/lib/locate/emphasis.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a) {
var n3 = t.indexOf("*", a), s = t.indexOf("_", a);
return s === -1 ? n3 : n3 === -1 || s < n3 ? s : n3;
}
} }), ND = S({ "node_modules/remark-parse/lib/tokenize/emphasis.js"(e, r) {
"use strict";
I();
var u = ze(), t = SD(), a = be(), n3 = TD();
r.exports = D, D.locator = n3;
var s = "*", c = "_", i = "\\";
function D(o, l, d) {
var p = this, g = 0, F = l.charAt(g), E, b, f, x, v, h, m;
if (!(F !== s && F !== c) && (b = p.options.pedantic, v = F, f = F, h = l.length, g++, x = "", F = "", !(b && a(l.charAt(g)))))
for (; g < h; ) {
if (m = F, F = l.charAt(g), F === f && (!b || !a(m))) {
if (F = l.charAt(++g), F !== f) {
if (!u(x) || m === f)
return;
if (!b && f === c && t(F)) {
x += f;
continue;
}
return d ? true : (E = o.now(), E.column++, E.offset++, o(v + x + f)({ type: "emphasis", children: p.tokenizeInline(x, E) }));
}
x += f;
}
!b && F === i && (x += F, F = l.charAt(++g)), x += F, g++;
}
}
} }), LD = S({ "node_modules/remark-parse/lib/locate/delete.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a) {
return t.indexOf("~~", a);
}
} }), RD = S({ "node_modules/remark-parse/lib/tokenize/delete.js"(e, r) {
"use strict";
I();
var u = be(), t = LD();
r.exports = s, s.locator = t;
var a = "~", n3 = "~~";
function s(c, i, D) {
var o = this, l = "", d = "", p = "", g = "", F, E, b;
if (!(!o.options.gfm || i.charAt(0) !== a || i.charAt(1) !== a || u(i.charAt(2))))
for (F = 1, E = i.length, b = c.now(), b.column += 2, b.offset += 2; ++F < E; ) {
if (l = i.charAt(F), l === a && d === a && (!p || !u(p)))
return D ? true : c(n3 + g + n3)({ type: "delete", children: o.tokenizeInline(g, b) });
g += d, p = d, d = l;
}
}
} }), jD = S({ "node_modules/remark-parse/lib/locate/code-inline.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a) {
return t.indexOf("`", a);
}
} }), PD = S({ "node_modules/remark-parse/lib/tokenize/code-inline.js"(e, r) {
"use strict";
I();
var u = jD();
r.exports = s, s.locator = u;
var t = 10, a = 32, n3 = 96;
function s(c, i, D) {
for (var o = i.length, l = 0, d, p, g, F, E, b; l < o && i.charCodeAt(l) === n3; )
l++;
if (!(l === 0 || l === o)) {
for (d = l, E = i.charCodeAt(l); l < o; ) {
if (F = E, E = i.charCodeAt(l + 1), F === n3) {
if (p === void 0 && (p = l), g = l + 1, E !== n3 && g - p === d) {
b = true;
break;
}
} else
p !== void 0 && (p = void 0, g = void 0);
l++;
}
if (b) {
if (D)
return true;
if (l = d, o = p, F = i.charCodeAt(l), E = i.charCodeAt(o - 1), b = false, o - l > 2 && (F === a || F === t) && (E === a || E === t)) {
for (l++, o--; l < o; ) {
if (F = i.charCodeAt(l), F !== a && F !== t) {
b = true;
break;
}
l++;
}
b === true && (d++, p--);
}
return c(i.slice(0, g))({ type: "inlineCode", value: i.slice(d, p) });
}
}
}
} }), MD = S({ "node_modules/remark-parse/lib/locate/break.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a) {
for (var n3 = t.indexOf(`
`, a); n3 > a && t.charAt(n3 - 1) === " "; )
n3--;
return n3;
}
} }), zD = S({ "node_modules/remark-parse/lib/tokenize/break.js"(e, r) {
"use strict";
I();
var u = MD();
r.exports = s, s.locator = u;
var t = " ", a = `
`, n3 = 2;
function s(c, i, D) {
for (var o = i.length, l = -1, d = "", p; ++l < o; ) {
if (p = i.charAt(l), p === a)
return l < n3 ? void 0 : D ? true : (d += p, c(d)({ type: "break" }));
if (p !== t)
return;
d += p;
}
}
} }), $D = S({ "node_modules/remark-parse/lib/tokenize/text.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t, a, n3) {
var s = this, c, i, D, o, l, d, p, g, F, E;
if (n3)
return true;
for (c = s.inlineMethods, o = c.length, i = s.inlineTokenizers, D = -1, F = a.length; ++D < o; )
g = c[D], !(g === "text" || !i[g]) && (p = i[g].locator, p || t.file.fail("Missing locator: `" + g + "`"), d = p.call(s, a, 1), d !== -1 && d < F && (F = d));
l = a.slice(0, F), E = t.now(), s.decode(l, E, b);
function b(f, x, v) {
t(v || f)({ type: "text", value: f });
}
}
} }), UD = S({ "node_modules/remark-parse/lib/parser.js"(e, r) {
"use strict";
I();
var u = Pe(), t = Ml(), a = zl(), n3 = $l(), s = Kl(), c = Yl();
r.exports = i;
function i(l, d) {
this.file = d, this.offset = {}, this.options = u(this.options), this.setOptions({}), this.inList = false, this.inBlock = false, this.inLink = false, this.atStart = true, this.toOffset = a(d).toOffset, this.unescape = n3(this, "escape"), this.decode = s(this);
}
var D = i.prototype;
D.setOptions = Ql(), D.parse = iD(), D.options = $i(), D.exitStart = t("atStart", true), D.enterList = t("inList", false), D.enterLink = t("inLink", false), D.enterBlock = t("inBlock", false), D.interruptParagraph = [["thematicBreak"], ["list"], ["atxHeading"], ["fencedCode"], ["blockquote"], ["html"], ["setextHeading", { commonmark: false }], ["definition", { commonmark: false }]], D.interruptList = [["atxHeading", { pedantic: false }], ["fencedCode", { pedantic: false }], ["thematicBreak", { pedantic: false }], ["definition", { commonmark: false }]], D.interruptBlockquote = [["indentedCode", { commonmark: true }], ["fencedCode", { commonmark: true }], ["atxHeading", { commonmark: true }], ["setextHeading", { commonmark: true }], ["thematicBreak", { commonmark: true }], ["html", { commonmark: true }], ["list", { commonmark: true }], ["definition", { commonmark: false }]], D.blockTokenizers = { blankLine: aD(), indentedCode: oD(), fencedCode: sD(), blockquote: cD(), atxHeading: lD(), thematicBreak: DD(), list: pD(), setextHeading: dD(), html: hD(), definition: mD(), table: ED(), paragraph: CD() }, D.inlineTokenizers = { escape: FD(), autoLink: AD(), url: yD(), email: BD(), html: kD(), link: qD(), reference: _D(), strong: ID(), emphasis: ND(), deletion: RD(), code: PD(), break: zD(), text: $D() }, D.blockMethods = o(D.blockTokenizers), D.inlineMethods = o(D.inlineTokenizers), D.tokenizeBlock = c("block"), D.tokenizeInline = c("inline"), D.tokenizeFactory = c;
function o(l) {
var d = [], p;
for (p in l)
d.push(p);
return d;
}
} }), GD = S({ "node_modules/remark-parse/index.js"(e, r) {
"use strict";
I();
var u = Pl(), t = Pe(), a = UD();
r.exports = n3, n3.Parser = a;
function n3(s) {
var c = this.data("settings"), i = u(a);
i.prototype.options = t(i.prototype.options, c, s), this.Parser = i;
}
} }), VD = S({ "node_modules/bail/index.js"(e, r) {
"use strict";
I(), r.exports = u;
function u(t) {
if (t)
throw t;
}
} }), Ki = S({ "node_modules/is-buffer/index.js"(e, r) {
I(), r.exports = function(t) {
return t != null && t.constructor != null && typeof t.constructor.isBuffer == "function" && t.constructor.isBuffer(t);
};
} }), HD = S({ "node_modules/extend/index.js"(e, r) {
"use strict";
I();
var u = Object.prototype.hasOwnProperty, t = Object.prototype.toString, a = Object.defineProperty, n3 = Object.getOwnPropertyDescriptor, s = function(l) {
return typeof Array.isArray == "function" ? Array.isArray(l) : t.call(l) === "[object Array]";
}, c = function(l) {
if (!l || t.call(l) !== "[object Object]")
return false;
var d = u.call(l, "constructor"), p = l.constructor && l.constructor.prototype && u.call(l.constructor.prototype, "isPrototypeOf");
if (l.constructor && !d && !p)
return false;
var g;
for (g in l)
;
return typeof g > "u" || u.call(l, g);
}, i = function(l, d) {
a && d.name === "__proto__" ? a(l, d.name, { enumerable: true, configurable: true, value: d.newValue, writable: true }) : l[d.name] = d.newValue;
}, D = function(l, d) {
if (d === "__proto__")
if (u.call(l, d)) {
if (n3)
return n3(l, d).value;
} else
return;
return l[d];
};
r.exports = function o() {
var l, d, p, g, F, E, b = arguments[0], f = 1, x = arguments.length, v = false;
for (typeof b == "boolean" && (v = b, b = arguments[1] || {}, f = 2), (b == null || typeof b != "object" && typeof b != "function") && (b = {}); f < x; ++f)
if (l = arguments[f], l != null)
for (d in l)
p = D(b, d), g = D(l, d), b !== g && (v && g && (c(g) || (F = s(g))) ? (F ? (F = false, E = p && s(p) ? p : []) : E = p && c(p) ? p : {}, i(b, { name: d, newValue: o(v, E, g) })) : typeof g < "u" && i(b, { name: d, newValue: g }));
return b;
};
} }), XD = S({ "node_modules/is-plain-obj/index.js"(e, r) {
"use strict";
I(), r.exports = (u) => {
if (Object.prototype.toString.call(u) !== "[object Object]")
return false;
let t = Object.getPrototypeOf(u);
return t === null || t === Object.prototype;
};
} }), WD = S({ "node_modules/trough/wrap.js"(e, r) {
"use strict";
I();
var u = [].slice;
r.exports = t;
function t(a, n3) {
var s;
return c;
function c() {
var o = u.call(arguments, 0), l = a.length > o.length, d;
l && o.push(i);
try {
d = a.apply(null, o);
} catch (p) {
if (l && s)
throw p;
return i(p);
}
l || (d && typeof d.then == "function" ? d.then(D, i) : d instanceof Error ? i(d) : D(d));
}
function i() {
s || (s = true, n3.apply(null, arguments));
}
function D(o) {
i(null, o);
}
}
} }), KD = S({ "node_modules/trough/index.js"(e, r) {
"use strict";
I();
var u = WD();
r.exports = a, a.wrap = u;
var t = [].slice;
function a() {
var n3 = [], s = {};
return s.run = c, s.use = i, s;
function c() {
var D = -1, o = t.call(arguments, 0, -1), l = arguments[arguments.length - 1];
if (typeof l != "function")
throw new Error("Expected function as last argument, not " + l);
d.apply(null, [null].concat(o));
function d(p) {
var g = n3[++D], F = t.call(arguments, 0), E = F.slice(1), b = o.length, f = -1;
if (p) {
l(p);
return;
}
for (; ++f < b; )
(E[f] === null || E[f] === void 0) && (E[f] = o[f]);
o = E, g ? u(g, d).apply(null, o) : l.apply(null, [null].concat(o));
}
}
function i(D) {
if (typeof D != "function")
throw new Error("Expected `fn` to be a function, not " + D);
return n3.push(D), s;
}
}
} }), YD = S({ "node_modules/unist-util-stringify-position/index.js"(e, r) {
"use strict";
I();
var u = {}.hasOwnProperty;
r.exports = t;
function t(c) {
return !c || typeof c != "object" ? "" : u.call(c, "position") || u.call(c, "type") ? n3(c.position) : u.call(c, "start") || u.call(c, "end") ? n3(c) : u.call(c, "line") || u.call(c, "column") ? a(c) : "";
}
function a(c) {
return (!c || typeof c != "object") && (c = {}), s(c.line) + ":" + s(c.column);
}
function n3(c) {
return (!c || typeof c != "object") && (c = {}), a(c.start) + "-" + a(c.end);
}
function s(c) {
return c && typeof c == "number" ? c : 1;
}
} }), JD = S({ "node_modules/vfile-message/index.js"(e, r) {
"use strict";
I();
var u = YD();
r.exports = n3;
function t() {
}
t.prototype = Error.prototype, n3.prototype = new t();
var a = n3.prototype;
a.file = "", a.name = "", a.reason = "", a.message = "", a.stack = "", a.fatal = null, a.column = null, a.line = null;
function n3(c, i, D) {
var o, l, d;
typeof i == "string" && (D = i, i = null), o = s(D), l = u(i) || "1:1", d = { start: { line: null, column: null }, end: { line: null, column: null } }, i && i.position && (i = i.position), i && (i.start ? (d = i, i = i.start) : d.start = i), c.stack && (this.stack = c.stack, c = c.message), this.message = c, this.name = l, this.reason = c, this.line = i ? i.line : null, this.column = i ? i.column : null, this.location = d, this.source = o[0], this.ruleId = o[1];
}
function s(c) {
var i = [null, null], D;
return typeof c == "string" && (D = c.indexOf(":"), D === -1 ? i[1] = c : (i[0] = c.slice(0, D), i[1] = c.slice(D + 1))), i;
}
} }), ZD = S({ "node_modules/vfile/lib/minpath.browser.js"(e) {
"use strict";
I(), e.basename = r, e.dirname = u, e.extname = t, e.join = a, e.sep = "/";
function r(i, D) {
var o = 0, l = -1, d, p, g, F;
if (D !== void 0 && typeof D != "string")
throw new TypeError('"ext" argument must be a string');
if (c(i), d = i.length, D === void 0 || !D.length || D.length > i.length) {
for (; d--; )
if (i.charCodeAt(d) === 47) {
if (g) {
o = d + 1;
break;
}
} else
l < 0 && (g = true, l = d + 1);
return l < 0 ? "" : i.slice(o, l);
}
if (D === i)
return "";
for (p = -1, F = D.length - 1; d--; )
if (i.charCodeAt(d) === 47) {
if (g) {
o = d + 1;
break;
}
} else
p < 0 && (g = true, p = d + 1), F > -1 && (i.charCodeAt(d) === D.charCodeAt(F--) ? F < 0 && (l = d) : (F = -1, l = p));
return o === l ? l = p : l < 0 && (l = i.length), i.slice(o, l);
}
function u(i) {
var D, o, l;
if (c(i), !i.length)
return ".";
for (D = -1, l = i.length; --l; )
if (i.charCodeAt(l) === 47) {
if (o) {
D = l;
break;
}
} else
o || (o = true);
return D < 0 ? i.charCodeAt(0) === 47 ? "/" : "." : D === 1 && i.charCodeAt(0) === 47 ? "//" : i.slice(0, D);
}
function t(i) {
var D = -1, o = 0, l = -1, d = 0, p, g, F;
for (c(i), F = i.length; F--; ) {
if (g = i.charCodeAt(F), g === 47) {
if (p) {
o = F + 1;
break;
}
continue;
}
l < 0 && (p = true, l = F + 1), g === 46 ? D < 0 ? D = F : d !== 1 && (d = 1) : D > -1 && (d = -1);
}
return D < 0 || l < 0 || d === 0 || d === 1 && D === l - 1 && D === o + 1 ? "" : i.slice(D, l);
}
function a() {
for (var i = -1, D; ++i < arguments.length; )
c(arguments[i]), arguments[i] && (D = D === void 0 ? arguments[i] : D + "/" + arguments[i]);
return D === void 0 ? "." : n3(D);
}
function n3(i) {
var D, o;
return c(i), D = i.charCodeAt(0) === 47, o = s(i, !D), !o.length && !D && (o = "."), o.length && i.charCodeAt(i.length - 1) === 47 && (o += "/"), D ? "/" + o : o;
}
function s(i, D) {
for (var o = "", l = 0, d = -1, p = 0, g = -1, F, E; ++g <= i.length; ) {
if (g < i.length)
F = i.charCodeAt(g);
else {
if (F === 47)
break;
F = 47;
}
if (F === 47) {
if (!(d === g - 1 || p === 1))
if (d !== g - 1 && p === 2) {
if (o.length < 2 || l !== 2 || o.charCodeAt(o.length - 1) !== 46 || o.charCodeAt(o.length - 2) !== 46) {
if (o.length > 2) {
if (E = o.lastIndexOf("/"), E !== o.length - 1) {
E < 0 ? (o = "", l = 0) : (o = o.slice(0, E), l = o.length - 1 - o.lastIndexOf("/")), d = g, p = 0;
continue;
}
} else if (o.length) {
o = "", l = 0, d = g, p = 0;
continue;
}
}
D && (o = o.length ? o + "/.." : "..", l = 2);
} else
o.length ? o += "/" + i.slice(d + 1, g) : o = i.slice(d + 1, g), l = g - d - 1;
d = g, p = 0;
} else
F === 46 && p > -1 ? p++ : p = -1;
}
return o;
}
function c(i) {
if (typeof i != "string")
throw new TypeError("Path must be a string. Received " + JSON.stringify(i));
}
} }), QD = S({ "node_modules/vfile/lib/minproc.browser.js"(e) {
"use strict";
I(), e.cwd = r;
function r() {
return "/";
}
} }), e2 = S({ "node_modules/vfile/lib/core.js"(e, r) {
"use strict";
I();
var u = ZD(), t = QD(), a = Ki();
r.exports = c;
var n3 = {}.hasOwnProperty, s = ["history", "path", "basename", "stem", "extname", "dirname"];
c.prototype.toString = f, Object.defineProperty(c.prototype, "path", { get: i, set: D }), Object.defineProperty(c.prototype, "dirname", { get: o, set: l }), Object.defineProperty(c.prototype, "basename", { get: d, set: p }), Object.defineProperty(c.prototype, "extname", { get: g, set: F }), Object.defineProperty(c.prototype, "stem", { get: E, set: b });
function c(m) {
var C, w;
if (!m)
m = {};
else if (typeof m == "string" || a(m))
m = { contents: m };
else if ("message" in m && "messages" in m)
return m;
if (!(this instanceof c))
return new c(m);
for (this.data = {}, this.messages = [], this.history = [], this.cwd = t.cwd(), w = -1; ++w < s.length; )
C = s[w], n3.call(m, C) && (this[C] = m[C]);
for (C in m)
s.indexOf(C) < 0 && (this[C] = m[C]);
}
function i() {
return this.history[this.history.length - 1];
}
function D(m) {
v(m, "path"), this.path !== m && this.history.push(m);
}
function o() {
return typeof this.path == "string" ? u.dirname(this.path) : void 0;
}
function l(m) {
h(this.path, "dirname"), this.path = u.join(m || "", this.basename);
}
function d() {
return typeof this.path == "string" ? u.basename(this.path) : void 0;
}
function p(m) {
v(m, "basename"), x(m, "basename"), this.path = u.join(this.dirname || "", m);
}
function g() {
return typeof this.path == "string" ? u.extname(this.path) : void 0;
}
function F(m) {
if (x(m, "extname"), h(this.path, "extname"), m) {
if (m.charCodeAt(0) !== 46)
throw new Error("`extname` must start with `.`");
if (m.indexOf(".", 1) > -1)
throw new Error("`extname` cannot contain multiple dots");
}
this.path = u.join(this.dirname, this.stem + (m || ""));
}
function E() {
return typeof this.path == "string" ? u.basename(this.path, this.extname) : void 0;
}
function b(m) {
v(m, "stem"), x(m, "stem"), this.path = u.join(this.dirname || "", m + (this.extname || ""));
}
function f(m) {
return (this.contents || "").toString(m);
}
function x(m, C) {
if (m && m.indexOf(u.sep) > -1)
throw new Error("`" + C + "` cannot be a path: did not expect `" + u.sep + "`");
}
function v(m, C) {
if (!m)
throw new Error("`" + C + "` cannot be empty");
}
function h(m, C) {
if (!m)
throw new Error("Setting `" + C + "` requires `path` to be set too");
}
} }), r2 = S({ "node_modules/vfile/lib/index.js"(e, r) {
"use strict";
I();
var u = JD(), t = e2();
r.exports = t, t.prototype.message = a, t.prototype.info = s, t.prototype.fail = n3;
function a(c, i, D) {
var o = new u(c, i, D);
return this.path && (o.name = this.path + ":" + o.name, o.file = this.path), o.fatal = false, this.messages.push(o), o;
}
function n3() {
var c = this.message.apply(this, arguments);
throw c.fatal = true, c;
}
function s() {
var c = this.message.apply(this, arguments);
return c.fatal = null, c;
}
} }), u2 = S({ "node_modules/vfile/index.js"(e, r) {
"use strict";
I(), r.exports = r2();
} }), t2 = S({ "node_modules/unified/index.js"(e, r) {
"use strict";
I();
var u = VD(), t = Ki(), a = HD(), n3 = XD(), s = KD(), c = u2();
r.exports = g().freeze();
var i = [].slice, D = {}.hasOwnProperty, o = s().use(l).use(d).use(p);
function l(m, C) {
C.tree = m.parse(C.file);
}
function d(m, C, w) {
m.run(C.tree, C.file, q);
function q(L, B, O) {
L ? w(L) : (C.tree = B, C.file = O, w());
}
}
function p(m, C) {
var w = m.stringify(C.tree, C.file);
w == null || (typeof w == "string" || t(w) ? C.file.contents = w : C.file.result = w);
}
function g() {
var m = [], C = s(), w = {}, q = -1, L;
return B.data = T, B.freeze = O, B.attachers = m, B.use = P, B.parse = j, B.stringify = X, B.run = H, B.runSync = G, B.process = R, B.processSync = J, B;
function B() {
for (var z = g(), M = -1; ++M < m.length; )
z.use.apply(null, m[M]);
return z.data(a(true, {}, w)), z;
}
function O() {
var z, M;
if (L)
return B;
for (; ++q < m.length; )
z = m[q], z[1] !== false && (z[1] === true && (z[1] = void 0), M = z[0].apply(B, z.slice(1)), typeof M == "function" && C.use(M));
return L = true, q = 1 / 0, B;
}
function T(z, M) {
return typeof z == "string" ? arguments.length === 2 ? (x("data", L), w[z] = M, B) : D.call(w, z) && w[z] || null : z ? (x("data", L), w = z, B) : w;
}
function P(z) {
var M;
if (x("use", L), z != null)
if (typeof z == "function")
_.apply(null, arguments);
else if (typeof z == "object")
"length" in z ? y(z) : U(z);
else
throw new Error("Expected usable value, not `" + z + "`");
return M && (w.settings = a(w.settings || {}, M)), B;
function U(N) {
y(N.plugins), N.settings && (M = a(M || {}, N.settings));
}
function k(N) {
if (typeof N == "function")
_(N);
else if (typeof N == "object")
"length" in N ? _.apply(null, N) : U(N);
else
throw new Error("Expected usable value, not `" + N + "`");
}
function y(N) {
var V = -1;
if (N != null)
if (typeof N == "object" && "length" in N)
for (; ++V < N.length; )
k(N[V]);
else
throw new Error("Expected a list of plugins, not `" + N + "`");
}
function _(N, V) {
var W = A(N);
W ? (n3(W[1]) && n3(V) && (V = a(true, W[1], V)), W[1] = V) : m.push(i.call(arguments));
}
}
function A(z) {
for (var M = -1; ++M < m.length; )
if (m[M][0] === z)
return m[M];
}
function j(z) {
var M = c(z), U;
return O(), U = B.Parser, b("parse", U), F(U, "parse") ? new U(String(M), M).parse() : U(String(M), M);
}
function H(z, M, U) {
if (v(z), O(), !U && typeof M == "function" && (U = M, M = null), !U)
return new Promise(k);
k(null, U);
function k(y, _) {
C.run(z, c(M), N);
function N(V, W, K) {
W = W || z, V ? _(V) : y ? y(W) : U(null, W, K);
}
}
}
function G(z, M) {
var U, k;
return H(z, M, y), h("runSync", "run", k), U;
function y(_, N) {
k = true, U = N, u(_);
}
}
function X(z, M) {
var U = c(M), k;
return O(), k = B.Compiler, f("stringify", k), v(z), F(k, "compile") ? new k(z, U).compile() : k(z, U);
}
function R(z, M) {
if (O(), b("process", B.Parser), f("process", B.Compiler), !M)
return new Promise(U);
U(null, M);
function U(k, y) {
var _ = c(z);
o.run(B, { file: _ }, N);
function N(V) {
V ? y(V) : k ? k(_) : M(null, _);
}
}
}
function J(z) {
var M, U;
return O(), b("processSync", B.Parser), f("processSync", B.Compiler), M = c(z), R(M, k), h("processSync", "process", U), M;
function k(y) {
U = true, u(y);
}
}
}
function F(m, C) {
return typeof m == "function" && m.prototype && (E(m.prototype) || C in m.prototype);
}
function E(m) {
var C;
for (C in m)
return true;
return false;
}
function b(m, C) {
if (typeof C != "function")
throw new Error("Cannot `" + m + "` without `Parser`");
}
function f(m, C) {
if (typeof C != "function")
throw new Error("Cannot `" + m + "` without `Compiler`");
}
function x(m, C) {
if (C)
throw new Error("Cannot invoke `" + m + "` on a frozen processor.\nCreate a new processor first, by invoking it: use `processor()` instead of `processor`.");
}
function v(m) {
if (!m || typeof m.type != "string")
throw new Error("Expected node, got `" + m + "`");
}
function h(m, C, w) {
if (!w)
throw new Error("`" + m + "` finished async. Use `" + C + "` instead");
}
} }), Yi = S({ "node_modules/remark-math/util.js"(e) {
I(), e.isRemarkParser = r, e.isRemarkCompiler = u;
function r(t) {
return Boolean(t && t.prototype && t.prototype.blockTokenizers);
}
function u(t) {
return Boolean(t && t.prototype && t.prototype.visitors);
}
} }), n2 = S({ "node_modules/remark-math/inline.js"(e, r) {
I();
var u = Yi();
r.exports = l;
var t = 9, a = 32, n3 = 36, s = 48, c = 57, i = 92, D = ["math", "math-inline"], o = "math-display";
function l(g) {
let F = this.Parser, E = this.Compiler;
u.isRemarkParser(F) && d(F, g), u.isRemarkCompiler(E) && p(E, g);
}
function d(g, F) {
let E = g.prototype, b = E.inlineMethods;
x.locator = f, E.inlineTokenizers.math = x, b.splice(b.indexOf("text"), 0, "math");
function f(v, h) {
return v.indexOf("$", h);
}
function x(v, h, m) {
let C = h.length, w = false, q = false, L = 0, B, O, T, P, A, j, H;
if (h.charCodeAt(L) === i && (q = true, L++), h.charCodeAt(L) === n3) {
if (L++, q)
return m ? true : v(h.slice(0, L))({ type: "text", value: "$" });
if (h.charCodeAt(L) === n3 && (w = true, L++), T = h.charCodeAt(L), !(T === a || T === t)) {
for (P = L; L < C; ) {
if (O = T, T = h.charCodeAt(L + 1), O === n3) {
if (B = h.charCodeAt(L - 1), B !== a && B !== t && (T !== T || T < s || T > c) && (!w || T === n3)) {
A = L - 1, L++, w && L++, j = L;
break;
}
} else
O === i && (L++, T = h.charCodeAt(L + 1));
L++;
}
if (j !== void 0)
return m ? true : (H = h.slice(P, A + 1), v(h.slice(0, j))({ type: "inlineMath", value: H, data: { hName: "span", hProperties: { className: D.concat(w && F.inlineMathDouble ? [o] : []) }, hChildren: [{ type: "text", value: H }] } }));
}
}
}
}
function p(g) {
let F = g.prototype;
F.visitors.inlineMath = E;
function E(b) {
let f = "$";
return (b.data && b.data.hProperties && b.data.hProperties.className || []).includes(o) && (f = "$$"), f + b.value + f;
}
}
} }), i2 = S({ "node_modules/remark-math/block.js"(e, r) {
I();
var u = Yi();
r.exports = o;
var t = 10, a = 32, n3 = 36, s = `
`, c = "$", i = 2, D = ["math", "math-display"];
function o() {
let p = this.Parser, g = this.Compiler;
u.isRemarkParser(p) && l(p), u.isRemarkCompiler(g) && d(g);
}
function l(p) {
let g = p.prototype, F = g.blockMethods, E = g.interruptParagraph, b = g.interruptList, f = g.interruptBlockquote;
g.blockTokenizers.math = x, F.splice(F.indexOf("fencedCode") + 1, 0, "math"), E.splice(E.indexOf("fencedCode") + 1, 0, ["math"]), b.splice(b.indexOf("fencedCode") + 1, 0, ["math"]), f.splice(f.indexOf("fencedCode") + 1, 0, ["math"]);
function x(v, h, m) {
var C = h.length, w = 0;
let q, L, B, O, T, P, A, j, H, G, X;
for (; w < C && h.charCodeAt(w) === a; )
w++;
for (T = w; w < C && h.charCodeAt(w) === n3; )
w++;
if (P = w - T, !(P < i)) {
for (; w < C && h.charCodeAt(w) === a; )
w++;
for (A = w; w < C; ) {
if (q = h.charCodeAt(w), q === n3)
return;
if (q === t)
break;
w++;
}
if (h.charCodeAt(w) === t) {
if (m)
return true;
for (L = [], A !== w && L.push(h.slice(A, w)), w++, B = h.indexOf(s, w + 1), B = B === -1 ? C : B; w < C; ) {
for (j = false, G = w, X = B, O = B, H = 0; O > G && h.charCodeAt(O - 1) === a; )
O--;
for (; O > G && h.charCodeAt(O - 1) === n3; )
H++, O--;
for (P <= H && h.indexOf(c, G) === O && (j = true, X = O); G <= X && G - w < T && h.charCodeAt(G) === a; )
G++;
if (j)
for (; X > G && h.charCodeAt(X - 1) === a; )
X--;
if ((!j || G !== X) && L.push(h.slice(G, X)), j)
break;
w = B + 1, B = h.indexOf(s, w + 1), B = B === -1 ? C : B;
}
return L = L.join(`
`), v(h.slice(0, B))({ type: "math", value: L, data: { hName: "div", hProperties: { className: D.concat() }, hChildren: [{ type: "text", value: L }] } });
}
}
}
}
function d(p) {
let g = p.prototype;
g.visitors.math = F;
function F(E) {
return `$$
` + E.value + `
$$`;
}
}
} }), a2 = S({ "node_modules/remark-math/index.js"(e, r) {
I();
var u = n2(), t = i2();
r.exports = a;
function a(n3) {
var s = n3 || {};
t.call(this, s), u.call(this, s);
}
} }), o2 = S({ "node_modules/remark-footnotes/index.js"(e, r) {
"use strict";
I(), r.exports = g;
var u = 9, t = 10, a = 32, n3 = 33, s = 58, c = 91, i = 92, D = 93, o = 94, l = 96, d = 4, p = 1024;
function g(h) {
var m = this.Parser, C = this.Compiler;
F(m) && b(m, h), E(C) && f(C);
}
function F(h) {
return Boolean(h && h.prototype && h.prototype.blockTokenizers);
}
function E(h) {
return Boolean(h && h.prototype && h.prototype.visitors);
}
function b(h, m) {
for (var C = m || {}, w = h.prototype, q = w.blockTokenizers, L = w.inlineTokenizers, B = w.blockMethods, O = w.inlineMethods, T = q.definition, P = L.reference, A = [], j = -1, H = B.length, G; ++j < H; )
G = B[j], !(G === "newline" || G === "indentedCode" || G === "paragraph" || G === "footnoteDefinition") && A.push([G]);
A.push(["footnoteDefinition"]), C.inlineNotes && (x(O, "reference", "inlineNote"), L.inlineNote = J), x(B, "definition", "footnoteDefinition"), x(O, "reference", "footnoteCall"), q.definition = M, q.footnoteDefinition = X, L.footnoteCall = R, L.reference = z, w.interruptFootnoteDefinition = A, z.locator = P.locator, R.locator = U, J.locator = k;
function X(y, _, N) {
for (var V = this, W = V.interruptFootnoteDefinition, K = V.offset, ee = _.length + 1, Y = 0, ue = [], le, ce, te, Z, Q, De, ye, fe, he, ae, pe, ne, re; Y < ee && (Z = _.charCodeAt(Y), !(Z !== u && Z !== a)); )
Y++;
if (_.charCodeAt(Y++) === c && _.charCodeAt(Y++) === o) {
for (ce = Y; Y < ee; ) {
if (Z = _.charCodeAt(Y), Z !== Z || Z === t || Z === u || Z === a)
return;
if (Z === D) {
te = Y, Y++;
break;
}
Y++;
}
if (!(te === void 0 || ce === te || _.charCodeAt(Y++) !== s)) {
if (N)
return true;
for (le = _.slice(ce, te), Q = y.now(), he = 0, ae = 0, pe = Y, ne = []; Y < ee; ) {
if (Z = _.charCodeAt(Y), Z !== Z || Z === t)
re = { start: he, contentStart: pe || Y, contentEnd: Y, end: Y }, ne.push(re), Z === t && (he = Y + 1, ae = 0, pe = void 0, re.end = he);
else if (ae !== void 0)
if (Z === a || Z === u)
ae += Z === a ? 1 : d - ae % d, ae > d && (ae = void 0, pe = Y);
else {
if (ae < d && re && (re.contentStart === re.contentEnd || v(W, q, V, [y, _.slice(Y, p), true])))
break;
ae = void 0, pe = Y;
}
Y++;
}
for (Y = -1, ee = ne.length; ee > 0 && (re = ne[ee - 1], re.contentStart === re.contentEnd); )
ee--;
for (De = y(_.slice(0, re.contentEnd)); ++Y < ee; )
re = ne[Y], K[Q.line + Y] = (K[Q.line + Y] || 0) + (re.contentStart - re.start), ue.push(_.slice(re.contentStart, re.end));
return ye = V.enterBlock(), fe = V.tokenizeBlock(ue.join(""), Q), ye(), De({ type: "footnoteDefinition", identifier: le.toLowerCase(), label: le, children: fe });
}
}
}
function R(y, _, N) {
var V = _.length + 1, W = 0, K, ee, Y, ue;
if (_.charCodeAt(W++) === c && _.charCodeAt(W++) === o) {
for (ee = W; W < V; ) {
if (ue = _.charCodeAt(W), ue !== ue || ue === t || ue === u || ue === a)
return;
if (ue === D) {
Y = W, W++;
break;
}
W++;
}
if (!(Y === void 0 || ee === Y))
return N ? true : (K = _.slice(ee, Y), y(_.slice(0, W))({ type: "footnoteReference", identifier: K.toLowerCase(), label: K }));
}
}
function J(y, _, N) {
var V = this, W = _.length + 1, K = 0, ee = 0, Y, ue, le, ce, te, Z, Q;
if (_.charCodeAt(K++) === o && _.charCodeAt(K++) === c) {
for (le = K; K < W; ) {
if (ue = _.charCodeAt(K), ue !== ue)
return;
if (Z === void 0)
if (ue === i)
K += 2;
else if (ue === c)
ee++, K++;
else if (ue === D)
if (ee === 0) {
ce = K, K++;
break;
} else
ee--, K++;
else if (ue === l) {
for (te = K, Z = 1; _.charCodeAt(te + Z) === l; )
Z++;
K += Z;
} else
K++;
else if (ue === l) {
for (te = K, Q = 1; _.charCodeAt(te + Q) === l; )
Q++;
K += Q, Z === Q && (Z = void 0), Q = void 0;
} else
K++;
}
if (ce !== void 0)
return N ? true : (Y = y.now(), Y.column += 2, Y.offset += 2, y(_.slice(0, K))({ type: "footnote", children: V.tokenizeInline(_.slice(le, ce), Y) }));
}
}
function z(y, _, N) {
var V = 0;
if (_.charCodeAt(V) === n3 && V++, _.charCodeAt(V) === c && _.charCodeAt(V + 1) !== o)
return P.call(this, y, _, N);
}
function M(y, _, N) {
for (var V = 0, W = _.charCodeAt(V); W === a || W === u; )
W = _.charCodeAt(++V);
if (W === c && _.charCodeAt(V + 1) !== o)
return T.call(this, y, _, N);
}
function U(y, _) {
return y.indexOf("[", _);
}
function k(y, _) {
return y.indexOf("^[", _);
}
}
function f(h) {
var m = h.prototype.visitors, C = " ";
m.footnote = w, m.footnoteReference = q, m.footnoteDefinition = L;
function w(B) {
return "^[" + this.all(B).join("") + "]";
}
function q(B) {
return "[^" + (B.label || B.identifier) + "]";
}
function L(B) {
for (var O = this.all(B).join(`
`).split(`
`), T = 0, P = O.length, A; ++T < P; )
A = O[T], A !== "" && (O[T] = C + A);
return "[^" + (B.label || B.identifier) + "]: " + O.join(`
`);
}
}
function x(h, m, C) {
h.splice(h.indexOf(m), 0, C);
}
function v(h, m, C, w) {
for (var q = h.length, L = -1; ++L < q; )
if (m[h[L][0]].apply(C, w))
return true;
return false;
}
} }), Ji = S({ "src/utils/front-matter/parse.js"(e, r) {
"use strict";
I();
var u = new RegExp("^(?-{3}|\\+{3})(?[^\\n]*)\\n(?:|(?.*?)\\n)(?\\k|\\.{3})[^\\S\\n]*(?:\\n|$)", "s");
function t(a) {
let n3 = a.match(u);
if (!n3)
return { content: a };
let { startDelimiter: s, language: c, value: i = "", endDelimiter: D } = n3.groups, o = c.trim() || "yaml";
if (s === "+++" && (o = "toml"), o !== "yaml" && s !== D)
return { content: a };
let [l] = n3;
return { frontMatter: { type: "front-matter", lang: o, value: i, startDelimiter: s, endDelimiter: D, raw: l.replace(/\n$/, "") }, content: l.replace(/[^\n]/g, " ") + a.slice(l.length) };
}
r.exports = t;
} }), s2 = S({ "src/language-markdown/pragma.js"(e, r) {
"use strict";
I();
var u = Ji(), t = ["format", "prettier"];
function a(n3) {
let s = `@(${t.join("|")})`, c = new RegExp([``, `{\\s*\\/\\*\\s*${s}\\s*\\*\\/\\s*}`, ``].join("|"), "m"), i = n3.match(c);
return (i == null ? void 0 : i.index) === 0;
}
r.exports = { startWithPragma: a, hasPragma: (n3) => a(u(n3).content.trimStart()), insertPragma: (n3) => {
let s = u(n3), c = ``;
return s.frontMatter ? `${s.frontMatter.raw}
${c}
${s.content}` : `${c}
${s.content}`;
} };
} }), Zi = S({ "src/language-markdown/loc.js"(e, r) {
"use strict";
I();
function u(a) {
return a.position.start.offset;
}
function t(a) {
return a.position.end.offset;
}
r.exports = { locStart: u, locEnd: t };
} }), Qi = S({ "src/language-markdown/mdx.js"(e, r) {
"use strict";
I();
var u = /^import\s/, t = /^export\s/, a = "[a-z][a-z0-9]*(\\.[a-z][a-z0-9]*)*|", n3 = /|/, s = /^{\s*\/\*(.*)\*\/\s*}/, c = `
`, i = (p) => u.test(p), D = (p) => t.test(p), o = (p, g) => {
let F = g.indexOf(c), E = g.slice(0, F);
if (D(E) || i(E))
return p(E)({ type: D(E) ? "export" : "import", value: E });
}, l = (p, g) => {
let F = s.exec(g);
if (F)
return p(F[0])({ type: "esComment", value: F[1].trim() });
};
o.locator = (p) => D(p) || i(p) ? -1 : 1, l.locator = (p, g) => p.indexOf("{", g);
function d() {
let { Parser: p } = this, { blockTokenizers: g, blockMethods: F, inlineTokenizers: E, inlineMethods: b } = p.prototype;
g.esSyntax = o, E.esComment = l, F.splice(F.indexOf("paragraph"), 0, "esSyntax"), b.splice(b.indexOf("text"), 0, "esComment");
}
r.exports = { esSyntax: d, BLOCKS_REGEX: a, COMMENT_REGEX: n3 };
} }), ea = {};
Pi(ea, { default: () => c2 });
function c2(e) {
if (typeof e != "string")
throw new TypeError("Expected a string");
return e.replace(/[|\\{}()[\]^$+*?.]/g, "\\$&").replace(/-/g, "\\x2d");
}
var l2 = je({ "node_modules/escape-string-regexp/index.js"() {
I();
} }), D2 = S({ "src/utils/get-last.js"(e, r) {
"use strict";
I();
var u = (t) => t[t.length - 1];
r.exports = u;
} }), ra = S({ "node_modules/semver/internal/debug.js"(e, r) {
I();
var u = typeof Qe == "object" && Qe.env && Qe.env.NODE_DEBUG && /\bsemver\b/i.test(Qe.env.NODE_DEBUG) ? function() {
for (var t = arguments.length, a = new Array(t), n3 = 0; n3 < t; n3++)
a[n3] = arguments[n3];
return console.error("SEMVER", ...a);
} : () => {
};
r.exports = u;
} }), ua = S({ "node_modules/semver/internal/constants.js"(e, r) {
I();
var u = "2.0.0", t = 256, a = Number.MAX_SAFE_INTEGER || 9007199254740991, n3 = 16;
r.exports = { SEMVER_SPEC_VERSION: u, MAX_LENGTH: t, MAX_SAFE_INTEGER: a, MAX_SAFE_COMPONENT_LENGTH: n3 };
} }), f2 = S({ "node_modules/semver/internal/re.js"(e, r) {
I();
var { MAX_SAFE_COMPONENT_LENGTH: u } = ua(), t = ra();
e = r.exports = {};
var a = e.re = [], n3 = e.src = [], s = e.t = {}, c = 0, i = (D, o, l) => {
let d = c++;
t(D, d, o), s[D] = d, n3[d] = o, a[d] = new RegExp(o, l ? "g" : void 0);
};
i("NUMERICIDENTIFIER", "0|[1-9]\\d*"), i("NUMERICIDENTIFIERLOOSE", "[0-9]+"), i("NONNUMERICIDENTIFIER", "\\d*[a-zA-Z-][a-zA-Z0-9-]*"), i("MAINVERSION", `(${n3[s.NUMERICIDENTIFIER]})\\.(${n3[s.NUMERICIDENTIFIER]})\\.(${n3[s.NUMERICIDENTIFIER]})`), i("MAINVERSIONLOOSE", `(${n3[s.NUMERICIDENTIFIERLOOSE]})\\.(${n3[s.NUMERICIDENTIFIERLOOSE]})\\.(${n3[s.NUMERICIDENTIFIERLOOSE]})`), i("PRERELEASEIDENTIFIER", `(?:${n3[s.NUMERICIDENTIFIER]}|${n3[s.NONNUMERICIDENTIFIER]})`), i("PRERELEASEIDENTIFIERLOOSE", `(?:${n3[s.NUMERICIDENTIFIERLOOSE]}|${n3[s.NONNUMERICIDENTIFIER]})`), i("PRERELEASE", `(?:-(${n3[s.PRERELEASEIDENTIFIER]}(?:\\.${n3[s.PRERELEASEIDENTIFIER]})*))`), i("PRERELEASELOOSE", `(?:-?(${n3[s.PRERELEASEIDENTIFIERLOOSE]}(?:\\.${n3[s.PRERELEASEIDENTIFIERLOOSE]})*))`), i("BUILDIDENTIFIER", "[0-9A-Za-z-]+"), i("BUILD", `(?:\\+(${n3[s.BUILDIDENTIFIER]}(?:\\.${n3[s.BUILDIDENTIFIER]})*))`), i("FULLPLAIN", `v?${n3[s.MAINVERSION]}${n3[s.PRERELEASE]}?${n3[s.BUILD]}?`), i("FULL", `^${n3[s.FULLPLAIN]}$`), i("LOOSEPLAIN", `[v=\\s]*${n3[s.MAINVERSIONLOOSE]}${n3[s.PRERELEASELOOSE]}?${n3[s.BUILD]}?`), i("LOOSE", `^${n3[s.LOOSEPLAIN]}$`), i("GTLT", "((?:<|>)?=?)"), i("XRANGEIDENTIFIERLOOSE", `${n3[s.NUMERICIDENTIFIERLOOSE]}|x|X|\\*`), i("XRANGEIDENTIFIER", `${n3[s.NUMERICIDENTIFIER]}|x|X|\\*`), i("XRANGEPLAIN", `[v=\\s]*(${n3[s.XRANGEIDENTIFIER]})(?:\\.(${n3[s.XRANGEIDENTIFIER]})(?:\\.(${n3[s.XRANGEIDENTIFIER]})(?:${n3[s.PRERELEASE]})?${n3[s.BUILD]}?)?)?`), i("XRANGEPLAINLOOSE", `[v=\\s]*(${n3[s.XRANGEIDENTIFIERLOOSE]})(?:\\.(${n3[s.XRANGEIDENTIFIERLOOSE]})(?:\\.(${n3[s.XRANGEIDENTIFIERLOOSE]})(?:${n3[s.PRERELEASELOOSE]})?${n3[s.BUILD]}?)?)?`), i("XRANGE", `^${n3[s.GTLT]}\\s*${n3[s.XRANGEPLAIN]}$`), i("XRANGELOOSE", `^${n3[s.GTLT]}\\s*${n3[s.XRANGEPLAINLOOSE]}$`), i("COERCE", `(^|[^\\d])(\\d{1,${u}})(?:\\.(\\d{1,${u}}))?(?:\\.(\\d{1,${u}}))?(?:$|[^\\d])`), i("COERCERTL", n3[s.COERCE], true), i("LONETILDE", "(?:~>?)"), i("TILDETRIM", `(\\s*)${n3[s.LONETILDE]}\\s+`, true), e.tildeTrimReplace = "$1~", i("TILDE", `^${n3[s.LONETILDE]}${n3[s.XRANGEPLAIN]}$`), i("TILDELOOSE", `^${n3[s.LONETILDE]}${n3[s.XRANGEPLAINLOOSE]}$`), i("LONECARET", "(?:\\^)"), i("CARETTRIM", `(\\s*)${n3[s.LONECARET]}\\s+`, true), e.caretTrimReplace = "$1^", i("CARET", `^${n3[s.LONECARET]}${n3[s.XRANGEPLAIN]}$`), i("CARETLOOSE", `^${n3[s.LONECARET]}${n3[s.XRANGEPLAINLOOSE]}$`), i("COMPARATORLOOSE", `^${n3[s.GTLT]}\\s*(${n3[s.LOOSEPLAIN]})$|^$`), i("COMPARATOR", `^${n3[s.GTLT]}\\s*(${n3[s.FULLPLAIN]})$|^$`), i("COMPARATORTRIM", `(\\s*)${n3[s.GTLT]}\\s*(${n3[s.LOOSEPLAIN]}|${n3[s.XRANGEPLAIN]})`, true), e.comparatorTrimReplace = "$1$2$3", i("HYPHENRANGE", `^\\s*(${n3[s.XRANGEPLAIN]})\\s+-\\s+(${n3[s.XRANGEPLAIN]})\\s*$`), i("HYPHENRANGELOOSE", `^\\s*(${n3[s.XRANGEPLAINLOOSE]})\\s+-\\s+(${n3[s.XRANGEPLAINLOOSE]})\\s*$`), i("STAR", "(<|>)?=?\\s*\\*"), i("GTE0", "^\\s*>=\\s*0\\.0\\.0\\s*$"), i("GTE0PRE", "^\\s*>=\\s*0\\.0\\.0-0\\s*$");
} }), p2 = S({ "node_modules/semver/internal/parse-options.js"(e, r) {
I();
var u = ["includePrerelease", "loose", "rtl"], t = (a) => a ? typeof a != "object" ? { loose: true } : u.filter((n3) => a[n3]).reduce((n3, s) => (n3[s] = true, n3), {}) : {};
r.exports = t;
} }), d2 = S({ "node_modules/semver/internal/identifiers.js"(e, r) {
I();
var u = /^[0-9]+$/, t = (n3, s) => {
let c = u.test(n3), i = u.test(s);
return c && i && (n3 = +n3, s = +s), n3 === s ? 0 : c && !i ? -1 : i && !c ? 1 : n3 < s ? -1 : 1;
}, a = (n3, s) => t(s, n3);
r.exports = { compareIdentifiers: t, rcompareIdentifiers: a };
} }), h2 = S({ "node_modules/semver/classes/semver.js"(e, r) {
I();
var u = ra(), { MAX_LENGTH: t, MAX_SAFE_INTEGER: a } = ua(), { re: n3, t: s } = f2(), c = p2(), { compareIdentifiers: i } = d2(), D = class {
constructor(o, l) {
if (l = c(l), o instanceof D) {
if (o.loose === !!l.loose && o.includePrerelease === !!l.includePrerelease)
return o;
o = o.version;
} else if (typeof o != "string")
throw new TypeError(`Invalid Version: ${o}`);
if (o.length > t)
throw new TypeError(`version is longer than ${t} characters`);
u("SemVer", o, l), this.options = l, this.loose = !!l.loose, this.includePrerelease = !!l.includePrerelease;
let d = o.trim().match(l.loose ? n3[s.LOOSE] : n3[s.FULL]);
if (!d)
throw new TypeError(`Invalid Version: ${o}`);
if (this.raw = o, this.major = +d[1], this.minor = +d[2], this.patch = +d[3], this.major > a || this.major < 0)
throw new TypeError("Invalid major version");
if (this.minor > a || this.minor < 0)
throw new TypeError("Invalid minor version");
if (this.patch > a || this.patch < 0)
throw new TypeError("Invalid patch version");
d[4] ? this.prerelease = d[4].split(".").map((p) => {
if (/^[0-9]+$/.test(p)) {
let g = +p;
if (g >= 0 && g < a)
return g;
}
return p;
}) : this.prerelease = [], this.build = d[5] ? d[5].split(".") : [], this.format();
}
format() {
return this.version = `${this.major}.${this.minor}.${this.patch}`, this.prerelease.length && (this.version += `-${this.prerelease.join(".")}`), this.version;
}
toString() {
return this.version;
}
compare(o) {
if (u("SemVer.compare", this.version, this.options, o), !(o instanceof D)) {
if (typeof o == "string" && o === this.version)
return 0;
o = new D(o, this.options);
}
return o.version === this.version ? 0 : this.compareMain(o) || this.comparePre(o);
}
compareMain(o) {
return o instanceof D || (o = new D(o, this.options)), i(this.major, o.major) || i(this.minor, o.minor) || i(this.patch, o.patch);
}
comparePre(o) {
if (o instanceof D || (o = new D(o, this.options)), this.prerelease.length && !o.prerelease.length)
return -1;
if (!this.prerelease.length && o.prerelease.length)
return 1;
if (!this.prerelease.length && !o.prerelease.length)
return 0;
let l = 0;
do {
let d = this.prerelease[l], p = o.prerelease[l];
if (u("prerelease compare", l, d, p), d === void 0 && p === void 0)
return 0;
if (p === void 0)
return 1;
if (d === void 0)
return -1;
if (d === p)
continue;
return i(d, p);
} while (++l);
}
compareBuild(o) {
o instanceof D || (o = new D(o, this.options));
let l = 0;
do {
let d = this.build[l], p = o.build[l];
if (u("prerelease compare", l, d, p), d === void 0 && p === void 0)
return 0;
if (p === void 0)
return 1;
if (d === void 0)
return -1;
if (d === p)
continue;
return i(d, p);
} while (++l);
}
inc(o, l) {
switch (o) {
case "premajor":
this.prerelease.length = 0, this.patch = 0, this.minor = 0, this.major++, this.inc("pre", l);
break;
case "preminor":
this.prerelease.length = 0, this.patch = 0, this.minor++, this.inc("pre", l);
break;
case "prepatch":
this.prerelease.length = 0, this.inc("patch", l), this.inc("pre", l);
break;
case "prerelease":
this.prerelease.length === 0 && this.inc("patch", l), this.inc("pre", l);
break;
case "major":
(this.minor !== 0 || this.patch !== 0 || this.prerelease.length === 0) && this.major++, this.minor = 0, this.patch = 0, this.prerelease = [];
break;
case "minor":
(this.patch !== 0 || this.prerelease.length === 0) && this.minor++, this.patch = 0, this.prerelease = [];
break;
case "patch":
this.prerelease.length === 0 && this.patch++, this.prerelease = [];
break;
case "pre":
if (this.prerelease.length === 0)
this.prerelease = [0];
else {
let d = this.prerelease.length;
for (; --d >= 0; )
typeof this.prerelease[d] == "number" && (this.prerelease[d]++, d = -2);
d === -1 && this.prerelease.push(0);
}
l && (i(this.prerelease[0], l) === 0 ? isNaN(this.prerelease[1]) && (this.prerelease = [l, 0]) : this.prerelease = [l, 0]);
break;
default:
throw new Error(`invalid increment argument: ${o}`);
}
return this.format(), this.raw = this.version, this;
}
};
r.exports = D;
} }), Cu = S({ "node_modules/semver/functions/compare.js"(e, r) {
I();
var u = h2(), t = (a, n3, s) => new u(a, s).compare(new u(n3, s));
r.exports = t;
} }), v2 = S({ "node_modules/semver/functions/lt.js"(e, r) {
I();
var u = Cu(), t = (a, n3, s) => u(a, n3, s) < 0;
r.exports = t;
} }), m2 = S({ "node_modules/semver/functions/gte.js"(e, r) {
I();
var u = Cu(), t = (a, n3, s) => u(a, n3, s) >= 0;
r.exports = t;
} }), E2 = S({ "src/utils/arrayify.js"(e, r) {
"use strict";
I(), r.exports = (u, t) => Object.entries(u).map((a) => {
let [n3, s] = a;
return Object.assign({ [t]: n3 }, s);
});
} }), C2 = S({ "package.json"(e, r) {
r.exports = { version: "2.8.8" };
} }), g2 = S({ "node_modules/outdent/lib/index.js"(e, r) {
"use strict";
I(), Object.defineProperty(e, "__esModule", { value: true }), e.outdent = void 0;
function u() {
for (var f = [], x = 0; x < arguments.length; x++)
f[x] = arguments[x];
}
function t() {
return typeof WeakMap < "u" ? /* @__PURE__ */ new WeakMap() : a();
}
function a() {
return { add: u, delete: u, get: u, set: u, has: function(f) {
return false;
} };
}
var n3 = Object.prototype.hasOwnProperty, s = function(f, x) {
return n3.call(f, x);
};
function c(f, x) {
for (var v in x)
s(x, v) && (f[v] = x[v]);
return f;
}
var i = /^[ \t]*(?:\r\n|\r|\n)/, D = /(?:\r\n|\r|\n)[ \t]*$/, o = /^(?:[\r\n]|$)/, l = /(?:\r\n|\r|\n)([ \t]*)(?:[^ \t\r\n]|$)/, d = /^[ \t]*[\r\n][ \t\r\n]*$/;
function p(f, x, v) {
var h = 0, m = f[0].match(l);
m && (h = m[1].length);
var C = "(\\r\\n|\\r|\\n).{0," + h + "}", w = new RegExp(C, "g");
x && (f = f.slice(1));
var q = v.newline, L = v.trimLeadingNewline, B = v.trimTrailingNewline, O = typeof q == "string", T = f.length, P = f.map(function(A, j) {
return A = A.replace(w, "$1"), j === 0 && L && (A = A.replace(i, "")), j === T - 1 && B && (A = A.replace(D, "")), O && (A = A.replace(/\r\n|\n|\r/g, function(H) {
return q;
})), A;
});
return P;
}
function g(f, x) {
for (var v = "", h = 0, m = f.length; h < m; h++)
v += f[h], h < m - 1 && (v += x[h]);
return v;
}
function F(f) {
return s(f, "raw") && s(f, "length");
}
function E(f) {
var x = t(), v = t();
function h(C) {
for (var w = [], q = 1; q < arguments.length; q++)
w[q - 1] = arguments[q];
if (F(C)) {
var L = C, B = (w[0] === h || w[0] === b) && d.test(L[0]) && o.test(L[1]), O = B ? v : x, T = O.get(L);
if (T || (T = p(L, B, f), O.set(L, T)), w.length === 0)
return T[0];
var P = g(T, B ? w.slice(1) : w);
return P;
} else
return E(c(c({}, f), C || {}));
}
var m = c(h, { string: function(C) {
return p([C], false, f)[0];
} });
return m;
}
var b = E({ trimLeadingNewline: true, trimTrailingNewline: true });
if (e.outdent = b, e.default = b, typeof r < "u")
try {
r.exports = b, Object.defineProperty(b, "__esModule", { value: true }), b.default = b, b.outdent = b;
} catch {
}
} }), F2 = S({ "src/main/core-options.js"(e, r) {
"use strict";
I();
var { outdent: u } = g2(), t = "Config", a = "Editor", n3 = "Format", s = "Other", c = "Output", i = "Global", D = "Special", o = { cursorOffset: { since: "1.4.0", category: D, type: "int", default: -1, range: { start: -1, end: Number.POSITIVE_INFINITY, step: 1 }, description: u`
Print (to stderr) where a cursor at the given position would move to after formatting.
This option cannot be used with --range-start and --range-end.
`, cliCategory: a }, endOfLine: { since: "1.15.0", category: i, type: "choice", default: [{ since: "1.15.0", value: "auto" }, { since: "2.0.0", value: "lf" }], description: "Which end of line characters to apply.", choices: [{ value: "lf", description: "Line Feed only (\\n), common on Linux and macOS as well as inside git repos" }, { value: "crlf", description: "Carriage Return + Line Feed characters (\\r\\n), common on Windows" }, { value: "cr", description: "Carriage Return character only (\\r), used very rarely" }, { value: "auto", description: u`
Maintain existing
(mixed values within one file are normalised by looking at what's used after the first line)
` }] }, filepath: { since: "1.4.0", category: D, type: "path", description: "Specify the input filepath. This will be used to do parser inference.", cliName: "stdin-filepath", cliCategory: s, cliDescription: "Path to the file to pretend that stdin comes from." }, insertPragma: { since: "1.8.0", category: D, type: "boolean", default: false, description: "Insert @format pragma into file's first docblock comment.", cliCategory: s }, parser: { since: "0.0.10", category: i, type: "choice", default: [{ since: "0.0.10", value: "babylon" }, { since: "1.13.0", value: void 0 }], description: "Which parser to use.", exception: (l) => typeof l == "string" || typeof l == "function", choices: [{ value: "flow", description: "Flow" }, { value: "babel", since: "1.16.0", description: "JavaScript" }, { value: "babel-flow", since: "1.16.0", description: "Flow" }, { value: "babel-ts", since: "2.0.0", description: "TypeScript" }, { value: "typescript", since: "1.4.0", description: "TypeScript" }, { value: "acorn", since: "2.6.0", description: "JavaScript" }, { value: "espree", since: "2.2.0", description: "JavaScript" }, { value: "meriyah", since: "2.2.0", description: "JavaScript" }, { value: "css", since: "1.7.1", description: "CSS" }, { value: "less", since: "1.7.1", description: "Less" }, { value: "scss", since: "1.7.1", description: "SCSS" }, { value: "json", since: "1.5.0", description: "JSON" }, { value: "json5", since: "1.13.0", description: "JSON5" }, { value: "json-stringify", since: "1.13.0", description: "JSON.stringify" }, { value: "graphql", since: "1.5.0", description: "GraphQL" }, { value: "markdown", since: "1.8.0", description: "Markdown" }, { value: "mdx", since: "1.15.0", description: "MDX" }, { value: "vue", since: "1.10.0", description: "Vue" }, { value: "yaml", since: "1.14.0", description: "YAML" }, { value: "glimmer", since: "2.3.0", description: "Ember / Handlebars" }, { value: "html", since: "1.15.0", description: "HTML" }, { value: "angular", since: "1.15.0", description: "Angular" }, { value: "lwc", since: "1.17.0", description: "Lightning Web Components" }] }, plugins: { since: "1.10.0", type: "path", array: true, default: [{ value: [] }], category: i, description: "Add a plugin. Multiple plugins can be passed as separate `--plugin`s.", exception: (l) => typeof l == "string" || typeof l == "object", cliName: "plugin", cliCategory: t }, pluginSearchDirs: { since: "1.13.0", type: "path", array: true, default: [{ value: [] }], category: i, description: u`
Custom directory that contains prettier plugins in node_modules subdirectory.
Overrides default behavior when plugins are searched relatively to the location of Prettier.
Multiple values are accepted.
`, exception: (l) => typeof l == "string" || typeof l == "object", cliName: "plugin-search-dir", cliCategory: t }, printWidth: { since: "0.0.0", category: i, type: "int", default: 80, description: "The line length where Prettier will try wrap.", range: { start: 0, end: Number.POSITIVE_INFINITY, step: 1 } }, rangeEnd: { since: "1.4.0", category: D, type: "int", default: Number.POSITIVE_INFINITY, range: { start: 0, end: Number.POSITIVE_INFINITY, step: 1 }, description: u`
Format code ending at a given character offset (exclusive).
The range will extend forwards to the end of the selected statement.
This option cannot be used with --cursor-offset.
`, cliCategory: a }, rangeStart: { since: "1.4.0", category: D, type: "int", default: 0, range: { start: 0, end: Number.POSITIVE_INFINITY, step: 1 }, description: u`
Format code starting at a given character offset.
The range will extend backwards to the start of the first line containing the selected statement.
This option cannot be used with --cursor-offset.
`, cliCategory: a }, requirePragma: { since: "1.7.0", category: D, type: "boolean", default: false, description: u`
Require either '@prettier' or '@format' to be present in the file's first docblock comment
in order for it to be formatted.
`, cliCategory: s }, tabWidth: { type: "int", category: i, default: 2, description: "Number of spaces per indentation level.", range: { start: 0, end: Number.POSITIVE_INFINITY, step: 1 } }, useTabs: { since: "1.0.0", category: i, type: "boolean", default: false, description: "Indent with tabs instead of spaces." }, embeddedLanguageFormatting: { since: "2.1.0", category: i, type: "choice", default: [{ since: "2.1.0", value: "auto" }], description: "Control how Prettier formats quoted code embedded in the file.", choices: [{ value: "auto", description: "Format embedded code if Prettier can automatically identify it." }, { value: "off", description: "Never automatically format embedded code." }] } };
r.exports = { CATEGORY_CONFIG: t, CATEGORY_EDITOR: a, CATEGORY_FORMAT: n3, CATEGORY_OTHER: s, CATEGORY_OUTPUT: c, CATEGORY_GLOBAL: i, CATEGORY_SPECIAL: D, options: o };
} }), A2 = S({ "src/main/support.js"(e, r) {
"use strict";
I();
var u = { compare: Cu(), lt: v2(), gte: m2() }, t = E2(), a = C2().version, n3 = F2().options;
function s() {
let { plugins: i = [], showUnreleased: D = false, showDeprecated: o = false, showInternal: l = false } = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {}, d = a.split("-", 1)[0], p = i.flatMap((f) => f.languages || []).filter(F), g = t(Object.assign({}, ...i.map((f) => {
let { options: x } = f;
return x;
}), n3), "name").filter((f) => F(f) && E(f)).sort((f, x) => f.name === x.name ? 0 : f.name < x.name ? -1 : 1).map(b).map((f) => {
f = Object.assign({}, f), Array.isArray(f.default) && (f.default = f.default.length === 1 ? f.default[0].value : f.default.filter(F).sort((v, h) => u.compare(h.since, v.since))[0].value), Array.isArray(f.choices) && (f.choices = f.choices.filter((v) => F(v) && E(v)), f.name === "parser" && c(f, p, i));
let x = Object.fromEntries(i.filter((v) => v.defaultOptions && v.defaultOptions[f.name] !== void 0).map((v) => [v.name, v.defaultOptions[f.name]]));
return Object.assign(Object.assign({}, f), {}, { pluginDefaults: x });
});
return { languages: p, options: g };
function F(f) {
return D || !("since" in f) || f.since && u.gte(d, f.since);
}
function E(f) {
return o || !("deprecated" in f) || f.deprecated && u.lt(d, f.deprecated);
}
function b(f) {
if (l)
return f;
let { cliName: x, cliCategory: v, cliDescription: h } = f;
return Ol(f, _l);
}
}
function c(i, D, o) {
let l = new Set(i.choices.map((d) => d.value));
for (let d of D)
if (d.parsers) {
for (let p of d.parsers)
if (!l.has(p)) {
l.add(p);
let g = o.find((E) => E.parsers && E.parsers[p]), F = d.name;
g && g.name && (F += ` (plugin: ${g.name})`), i.choices.push({ value: p, description: F });
}
}
}
r.exports = { getSupportInfo: s };
} }), x2 = S({ "src/utils/is-non-empty-array.js"(e, r) {
"use strict";
I();
function u(t) {
return Array.isArray(t) && t.length > 0;
}
r.exports = u;
} });
function b2() {
let { onlyFirst: e = false } = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {}, r = ["[\\u001B\\u009B][[\\]()#;?]*(?:(?:(?:(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]+)*|[a-zA-Z\\d]+(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]*)*)?\\u0007)", "(?:(?:\\d{1,4}(?:;\\d{0,4})*)?[\\dA-PR-TZcf-ntqry=><~]))"].join("|");
return new RegExp(r, e ? void 0 : "g");
}
var y2 = je({ "node_modules/strip-ansi/node_modules/ansi-regex/index.js"() {
I();
} });
function w2(e) {
if (typeof e != "string")
throw new TypeError(`Expected a \`string\`, got \`${typeof e}\``);
return e.replace(b2(), "");
}
var B2 = je({ "node_modules/strip-ansi/index.js"() {
I(), y2();
} });
function k2(e) {
return Number.isInteger(e) ? e >= 4352 && (e <= 4447 || e === 9001 || e === 9002 || 11904 <= e && e <= 12871 && e !== 12351 || 12880 <= e && e <= 19903 || 19968 <= e && e <= 42182 || 43360 <= e && e <= 43388 || 44032 <= e && e <= 55203 || 63744 <= e && e <= 64255 || 65040 <= e && e <= 65049 || 65072 <= e && e <= 65131 || 65281 <= e && e <= 65376 || 65504 <= e && e <= 65510 || 110592 <= e && e <= 110593 || 127488 <= e && e <= 127569 || 131072 <= e && e <= 262141) : false;
}
var q2 = je({ "node_modules/is-fullwidth-code-point/index.js"() {
I();
} }), _2 = S({ "node_modules/emoji-regex/index.js"(e, r) {
"use strict";
I(), r.exports = function() {
return /\uD83C\uDFF4\uDB40\uDC67\uDB40\uDC62(?:\uDB40\uDC77\uDB40\uDC6C\uDB40\uDC73|\uDB40\uDC73\uDB40\uDC63\uDB40\uDC74|\uDB40\uDC65\uDB40\uDC6E\uDB40\uDC67)\uDB40\uDC7F|(?:\uD83E\uDDD1\uD83C\uDFFF\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFF\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB-\uDFFE])|(?:\uD83E\uDDD1\uD83C\uDFFE\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFE\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB-\uDFFD\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFD\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFD\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB\uDFFC\uDFFE\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFC\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFC\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB\uDFFD-\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFB\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFB\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFC-\uDFFF])|\uD83D\uDC68(?:\uD83C\uDFFB(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF]))|\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFC-\uDFFF])|[\u2695\u2696\u2708]\uFE0F|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))?|(?:\uD83C[\uDFFC-\uDFFF])\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF]))|\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83D\uDC68|(?:\uD83D[\uDC68\uDC69])\u200D(?:\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67]))|\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFE])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFD\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB\uDFFC\uDFFE\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB\uDFFD-\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])\uFE0F|\u200D(?:(?:\uD83D[\uDC68\uDC69])\u200D(?:\uD83D[\uDC66\uDC67])|\uD83D[\uDC66\uDC67])|\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC)?|(?:\uD83D\uDC69(?:\uD83C\uDFFB\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69])|(?:\uD83C[\uDFFC-\uDFFF])\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69]))|\uD83E\uDDD1(?:\uD83C[\uDFFB-\uDFFF])\u200D\uD83E\uDD1D\u200D\uD83E\uDDD1)(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC69\u200D\uD83D\uDC69\u200D(?:\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67]))|\uD83D\uDC69(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFB\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))|\uD83E\uDDD1(?:\u200D(?:\uD83E\uDD1D\u200D\uD83E\uDDD1|\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFB\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))|\uD83D\uDC69\u200D\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC69\u200D\uD83D\uDC69\u200D(?:\uD83D[\uDC66\uDC67])|\uD83D\uDC69\u200D\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|(?:\uD83D\uDC41\uFE0F\u200D\uD83D\uDDE8|\uD83E\uDDD1(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\uD83C\uDFFB\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])|\uD83D\uDC69(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\uD83C\uDFFB\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])|\uD83D\uDE36\u200D\uD83C\uDF2B|\uD83C\uDFF3\uFE0F\u200D\u26A7|\uD83D\uDC3B\u200D\u2744|(?:(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC6F|\uD83E[\uDD3C\uDDDE\uDDDF])\u200D[\u2640\u2642]|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])\u200D[\u2640\u2642]|\uD83C\uDFF4\u200D\u2620|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])\u200D[\u2640\u2642]|[\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u2328\u23CF\u23ED-\u23EF\u23F1\u23F2\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB\u25FC\u2600-\u2604\u260E\u2611\u2618\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u265F\u2660\u2663\u2665\u2666\u2668\u267B\u267E\u2692\u2694-\u2697\u2699\u269B\u269C\u26A0\u26A7\u26B0\u26B1\u26C8\u26CF\u26D1\u26D3\u26E9\u26F0\u26F1\u26F4\u26F7\u26F8\u2702\u2708\u2709\u270F\u2712\u2714\u2716\u271D\u2721\u2733\u2734\u2744\u2747\u2763\u27A1\u2934\u2935\u2B05-\u2B07\u3030\u303D\u3297\u3299]|\uD83C[\uDD70\uDD71\uDD7E\uDD7F\uDE02\uDE37\uDF21\uDF24-\uDF2C\uDF36\uDF7D\uDF96\uDF97\uDF99-\uDF9B\uDF9E\uDF9F\uDFCD\uDFCE\uDFD4-\uDFDF\uDFF5\uDFF7]|\uD83D[\uDC3F\uDCFD\uDD49\uDD4A\uDD6F\uDD70\uDD73\uDD76-\uDD79\uDD87\uDD8A-\uDD8D\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA\uDECB\uDECD-\uDECF\uDEE0-\uDEE5\uDEE9\uDEF0\uDEF3])\uFE0F|\uD83C\uDFF3\uFE0F\u200D\uD83C\uDF08|\uD83D\uDC69\u200D\uD83D\uDC67|\uD83D\uDC69\u200D\uD83D\uDC66|\uD83D\uDE35\u200D\uD83D\uDCAB|\uD83D\uDE2E\u200D\uD83D\uDCA8|\uD83D\uDC15\u200D\uD83E\uDDBA|\uD83E\uDDD1(?:\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC|\uD83C\uDFFB)?|\uD83D\uDC69(?:\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC|\uD83C\uDFFB)?|\uD83C\uDDFD\uD83C\uDDF0|\uD83C\uDDF6\uD83C\uDDE6|\uD83C\uDDF4\uD83C\uDDF2|\uD83D\uDC08\u200D\u2B1B|\u2764\uFE0F\u200D(?:\uD83D\uDD25|\uD83E\uDE79)|\uD83D\uDC41\uFE0F|\uD83C\uDFF3\uFE0F|\uD83C\uDDFF(?:\uD83C[\uDDE6\uDDF2\uDDFC])|\uD83C\uDDFE(?:\uD83C[\uDDEA\uDDF9])|\uD83C\uDDFC(?:\uD83C[\uDDEB\uDDF8])|\uD83C\uDDFB(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDEE\uDDF3\uDDFA])|\uD83C\uDDFA(?:\uD83C[\uDDE6\uDDEC\uDDF2\uDDF3\uDDF8\uDDFE\uDDFF])|\uD83C\uDDF9(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDED\uDDEF-\uDDF4\uDDF7\uDDF9\uDDFB\uDDFC\uDDFF])|\uD83C\uDDF8(?:\uD83C[\uDDE6-\uDDEA\uDDEC-\uDDF4\uDDF7-\uDDF9\uDDFB\uDDFD-\uDDFF])|\uD83C\uDDF7(?:\uD83C[\uDDEA\uDDF4\uDDF8\uDDFA\uDDFC])|\uD83C\uDDF5(?:\uD83C[\uDDE6\uDDEA-\uDDED\uDDF0-\uDDF3\uDDF7-\uDDF9\uDDFC\uDDFE])|\uD83C\uDDF3(?:\uD83C[\uDDE6\uDDE8\uDDEA-\uDDEC\uDDEE\uDDF1\uDDF4\uDDF5\uDDF7\uDDFA\uDDFF])|\uD83C\uDDF2(?:\uD83C[\uDDE6\uDDE8-\uDDED\uDDF0-\uDDFF])|\uD83C\uDDF1(?:\uD83C[\uDDE6-\uDDE8\uDDEE\uDDF0\uDDF7-\uDDFB\uDDFE])|\uD83C\uDDF0(?:\uD83C[\uDDEA\uDDEC-\uDDEE\uDDF2\uDDF3\uDDF5\uDDF7\uDDFC\uDDFE\uDDFF])|\uD83C\uDDEF(?:\uD83C[\uDDEA\uDDF2\uDDF4\uDDF5])|\uD83C\uDDEE(?:\uD83C[\uDDE8-\uDDEA\uDDF1-\uDDF4\uDDF6-\uDDF9])|\uD83C\uDDED(?:\uD83C[\uDDF0\uDDF2\uDDF3\uDDF7\uDDF9\uDDFA])|\uD83C\uDDEC(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEE\uDDF1-\uDDF3\uDDF5-\uDDFA\uDDFC\uDDFE])|\uD83C\uDDEB(?:\uD83C[\uDDEE-\uDDF0\uDDF2\uDDF4\uDDF7])|\uD83C\uDDEA(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDED\uDDF7-\uDDFA])|\uD83C\uDDE9(?:\uD83C[\uDDEA\uDDEC\uDDEF\uDDF0\uDDF2\uDDF4\uDDFF])|\uD83C\uDDE8(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDEE\uDDF0-\uDDF5\uDDF7\uDDFA-\uDDFF])|\uD83C\uDDE7(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEF\uDDF1-\uDDF4\uDDF6-\uDDF9\uDDFB\uDDFC\uDDFE\uDDFF])|\uD83C\uDDE6(?:\uD83C[\uDDE8-\uDDEC\uDDEE\uDDF1\uDDF2\uDDF4\uDDF6-\uDDFA\uDDFC\uDDFD\uDDFF])|[#\*0-9]\uFE0F\u20E3|\u2764\uFE0F|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])|\uD83C\uDFF4|(?:[\u270A\u270B]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC6B-\uDC6D\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDC8F\uDC91\uDCAA\uDD7A\uDD95\uDD96\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD34\uDD36\uDD77\uDDB5\uDDB6\uDDBB\uDDD2\uDDD3\uDDD5])(?:\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u270C\u270D]|\uD83D[\uDD74\uDD90])(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])|[\u270A\u270B]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC08\uDC15\uDC3B\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC6B-\uDC6D\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDC8F\uDC91\uDCAA\uDD7A\uDD95\uDD96\uDE2E\uDE35\uDE36\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD34\uDD36\uDD77\uDDB5\uDDB6\uDDBB\uDDD2\uDDD3\uDDD5]|\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD]|\uD83D\uDC6F|\uD83E[\uDD3C\uDDDE\uDDDF]|[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF84\uDF86-\uDF93\uDFA0-\uDFC1\uDFC5\uDFC6\uDFC8\uDFC9\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC07\uDC09-\uDC14\uDC16-\uDC3A\uDC3C-\uDC3E\uDC40\uDC44\uDC45\uDC51-\uDC65\uDC6A\uDC79-\uDC7B\uDC7D-\uDC80\uDC84\uDC88-\uDC8E\uDC90\uDC92-\uDCA9\uDCAB-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDDA4\uDDFB-\uDE2D\uDE2F-\uDE34\uDE37-\uDE44\uDE48-\uDE4A\uDE80-\uDEA2\uDEA4-\uDEB3\uDEB7-\uDEBF\uDEC1-\uDEC5\uDED0-\uDED2\uDED5-\uDED7\uDEEB\uDEEC\uDEF4-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0D\uDD0E\uDD10-\uDD17\uDD1D\uDD20-\uDD25\uDD27-\uDD2F\uDD3A\uDD3F-\uDD45\uDD47-\uDD76\uDD78\uDD7A-\uDDB4\uDDB7\uDDBA\uDDBC-\uDDCB\uDDD0\uDDE0-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6]|(?:[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u270A\u270B\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF93\uDFA0-\uDFCA\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF4\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC3E\uDC40\uDC42-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDD7A\uDD95\uDD96\uDDA4\uDDFB-\uDE4F\uDE80-\uDEC5\uDECC\uDED0-\uDED2\uDED5-\uDED7\uDEEB\uDEEC\uDEF4-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0C-\uDD3A\uDD3C-\uDD45\uDD47-\uDD78\uDD7A-\uDDCB\uDDCD-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6])|(?:[#\*0-9\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u231A\u231B\u2328\u23CF\u23E9-\u23F3\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB-\u25FE\u2600-\u2604\u260E\u2611\u2614\u2615\u2618\u261D\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u2648-\u2653\u265F\u2660\u2663\u2665\u2666\u2668\u267B\u267E\u267F\u2692-\u2697\u2699\u269B\u269C\u26A0\u26A1\u26A7\u26AA\u26AB\u26B0\u26B1\u26BD\u26BE\u26C4\u26C5\u26C8\u26CE\u26CF\u26D1\u26D3\u26D4\u26E9\u26EA\u26F0-\u26F5\u26F7-\u26FA\u26FD\u2702\u2705\u2708-\u270D\u270F\u2712\u2714\u2716\u271D\u2721\u2728\u2733\u2734\u2744\u2747\u274C\u274E\u2753-\u2755\u2757\u2763\u2764\u2795-\u2797\u27A1\u27B0\u27BF\u2934\u2935\u2B05-\u2B07\u2B1B\u2B1C\u2B50\u2B55\u3030\u303D\u3297\u3299]|\uD83C[\uDC04\uDCCF\uDD70\uDD71\uDD7E\uDD7F\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE02\uDE1A\uDE2F\uDE32-\uDE3A\uDE50\uDE51\uDF00-\uDF21\uDF24-\uDF93\uDF96\uDF97\uDF99-\uDF9B\uDF9E-\uDFF0\uDFF3-\uDFF5\uDFF7-\uDFFF]|\uD83D[\uDC00-\uDCFD\uDCFF-\uDD3D\uDD49-\uDD4E\uDD50-\uDD67\uDD6F\uDD70\uDD73-\uDD7A\uDD87\uDD8A-\uDD8D\uDD90\uDD95\uDD96\uDDA4\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA-\uDE4F\uDE80-\uDEC5\uDECB-\uDED2\uDED5-\uDED7\uDEE0-\uDEE5\uDEE9\uDEEB\uDEEC\uDEF0\uDEF3-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0C-\uDD3A\uDD3C-\uDD45\uDD47-\uDD78\uDD7A-\uDDCB\uDDCD-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6])\uFE0F|(?:[\u261D\u26F9\u270A-\u270D]|\uD83C[\uDF85\uDFC2-\uDFC4\uDFC7\uDFCA-\uDFCC]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66-\uDC78\uDC7C\uDC81-\uDC83\uDC85-\uDC87\uDC8F\uDC91\uDCAA\uDD74\uDD75\uDD7A\uDD90\uDD95\uDD96\uDE45-\uDE47\uDE4B-\uDE4F\uDEA3\uDEB4-\uDEB6\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1F\uDD26\uDD30-\uDD39\uDD3C-\uDD3E\uDD77\uDDB5\uDDB6\uDDB8\uDDB9\uDDBB\uDDCD-\uDDCF\uDDD1-\uDDDD])/g;
};
} }), ta = {};
Pi(ta, { default: () => O2 });
function O2(e) {
if (typeof e != "string" || e.length === 0 || (e = w2(e), e.length === 0))
return 0;
e = e.replace((0, na.default)(), " ");
let r = 0;
for (let u = 0; u < e.length; u++) {
let t = e.codePointAt(u);
t <= 31 || t >= 127 && t <= 159 || t >= 768 && t <= 879 || (t > 65535 && u++, r += k2(t) ? 2 : 1);
}
return r;
}
var na, I2 = je({ "node_modules/string-width/index.js"() {
I(), B2(), q2(), na = Rl(_2());
} }), S2 = S({ "src/utils/get-string-width.js"(e, r) {
"use strict";
I();
var u = (I2(), zi(ta)).default, t = /[^\x20-\x7F]/;
function a(n3) {
return n3 ? t.test(n3) ? u(n3) : n3.length : 0;
}
r.exports = a;
} }), gu = S({ "src/utils/text/skip.js"(e, r) {
"use strict";
I();
function u(c) {
return (i, D, o) => {
let l = o && o.backwards;
if (D === false)
return false;
let { length: d } = i, p = D;
for (; p >= 0 && p < d; ) {
let g = i.charAt(p);
if (c instanceof RegExp) {
if (!c.test(g))
return p;
} else if (!c.includes(g))
return p;
l ? p-- : p++;
}
return p === -1 || p === d ? p : false;
};
}
var t = u(/\s/), a = u(" "), n3 = u(",; "), s = u(/[^\n\r]/);
r.exports = { skipWhitespace: t, skipSpaces: a, skipToLineEnd: n3, skipEverythingButNewLine: s };
} }), ia = S({ "src/utils/text/skip-inline-comment.js"(e, r) {
"use strict";
I();
function u(t, a) {
if (a === false)
return false;
if (t.charAt(a) === "/" && t.charAt(a + 1) === "*") {
for (let n3 = a + 2; n3 < t.length; ++n3)
if (t.charAt(n3) === "*" && t.charAt(n3 + 1) === "/")
return n3 + 2;
}
return a;
}
r.exports = u;
} }), aa = S({ "src/utils/text/skip-trailing-comment.js"(e, r) {
"use strict";
I();
var { skipEverythingButNewLine: u } = gu();
function t(a, n3) {
return n3 === false ? false : a.charAt(n3) === "/" && a.charAt(n3 + 1) === "/" ? u(a, n3) : n3;
}
r.exports = t;
} }), oa = S({ "src/utils/text/skip-newline.js"(e, r) {
"use strict";
I();
function u(t, a, n3) {
let s = n3 && n3.backwards;
if (a === false)
return false;
let c = t.charAt(a);
if (s) {
if (t.charAt(a - 1) === "\r" && c === `
`)
return a - 2;
if (c === `
` || c === "\r" || c === "\u2028" || c === "\u2029")
return a - 1;
} else {
if (c === "\r" && t.charAt(a + 1) === `
`)
return a + 2;
if (c === `
` || c === "\r" || c === "\u2028" || c === "\u2029")
return a + 1;
}
return a;
}
r.exports = u;
} }), T2 = S({ "src/utils/text/get-next-non-space-non-comment-character-index-with-start-index.js"(e, r) {
"use strict";
I();
var u = ia(), t = oa(), a = aa(), { skipSpaces: n3 } = gu();
function s(c, i) {
let D = null, o = i;
for (; o !== D; )
D = o, o = n3(c, o), o = u(c, o), o = a(c, o), o = t(c, o);
return o;
}
r.exports = s;
} }), N2 = S({ "src/common/util.js"(e, r) {
"use strict";
I();
var { default: u } = (l2(), zi(ea)), t = D2(), { getSupportInfo: a } = A2(), n3 = x2(), s = S2(), { skipWhitespace: c, skipSpaces: i, skipToLineEnd: D, skipEverythingButNewLine: o } = gu(), l = ia(), d = aa(), p = oa(), g = T2(), F = (k) => k[k.length - 2];
function E(k) {
return (y, _, N) => {
let V = N && N.backwards;
if (_ === false)
return false;
let { length: W } = y, K = _;
for (; K >= 0 && K < W; ) {
let ee = y.charAt(K);
if (k instanceof RegExp) {
if (!k.test(ee))
return K;
} else if (!k.includes(ee))
return K;
V ? K-- : K++;
}
return K === -1 || K === W ? K : false;
};
}
function b(k, y) {
let _ = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, N = i(k, _.backwards ? y - 1 : y, _), V = p(k, N, _);
return N !== V;
}
function f(k, y, _) {
for (let N = y; N < _; ++N)
if (k.charAt(N) === `
`)
return true;
return false;
}
function x(k, y, _) {
let N = _(y) - 1;
N = i(k, N, { backwards: true }), N = p(k, N, { backwards: true }), N = i(k, N, { backwards: true });
let V = p(k, N, { backwards: true });
return N !== V;
}
function v(k, y) {
let _ = null, N = y;
for (; N !== _; )
_ = N, N = D(k, N), N = l(k, N), N = i(k, N);
return N = d(k, N), N = p(k, N), N !== false && b(k, N);
}
function h(k, y, _) {
return v(k, _(y));
}
function m(k, y, _) {
return g(k, _(y));
}
function C(k, y, _) {
return k.charAt(m(k, y, _));
}
function w(k, y) {
let _ = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {};
return i(k, _.backwards ? y - 1 : y, _) !== y;
}
function q(k, y) {
let _ = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : 0, N = 0;
for (let V = _; V < k.length; ++V)
k[V] === " " ? N = N + y - N % y : N++;
return N;
}
function L(k, y) {
let _ = k.lastIndexOf(`
`);
return _ === -1 ? 0 : q(k.slice(_ + 1).match(/^[\t ]*/)[0], y);
}
function B(k, y) {
let _ = { quote: '"', regex: /"/g, escaped: """ }, N = { quote: "'", regex: /'/g, escaped: "'" }, V = y === "'" ? N : _, W = V === N ? _ : N, K = V;
if (k.includes(V.quote) || k.includes(W.quote)) {
let ee = (k.match(V.regex) || []).length, Y = (k.match(W.regex) || []).length;
K = ee > Y ? W : V;
}
return K;
}
function O(k, y) {
let _ = k.slice(1, -1), N = y.parser === "json" || y.parser === "json5" && y.quoteProps === "preserve" && !y.singleQuote ? '"' : y.__isInHtmlAttribute ? "'" : B(_, y.singleQuote ? "'" : '"').quote;
return T(_, N, !(y.parser === "css" || y.parser === "less" || y.parser === "scss" || y.__embeddedInHtml));
}
function T(k, y, _) {
let N = y === '"' ? "'" : '"', V = /\\(.)|(["'])/gs, W = k.replace(V, (K, ee, Y) => ee === N ? ee : Y === y ? "\\" + Y : Y || (_ && /^[^\n\r"'0-7\\bfnrt-vx\u2028\u2029]$/.test(ee) ? ee : "\\" + ee));
return y + W + y;
}
function P(k) {
return k.toLowerCase().replace(/^([+-]?[\d.]+e)(?:\+|(-))?0*(\d)/, "$1$2$3").replace(/^([+-]?[\d.]+)e[+-]?0+$/, "$1").replace(/^([+-])?\./, "$10.").replace(/(\.\d+?)0+(?=e|$)/, "$1").replace(/\.(?=e|$)/, "");
}
function A(k, y) {
let _ = k.match(new RegExp(`(${u(y)})+`, "g"));
return _ === null ? 0 : _.reduce((N, V) => Math.max(N, V.length / y.length), 0);
}
function j(k, y) {
let _ = k.match(new RegExp(`(${u(y)})+`, "g"));
if (_ === null)
return 0;
let N = /* @__PURE__ */ new Map(), V = 0;
for (let W of _) {
let K = W.length / y.length;
N.set(K, true), K > V && (V = K);
}
for (let W = 1; W < V; W++)
if (!N.get(W))
return W;
return V + 1;
}
function H(k, y) {
(k.comments || (k.comments = [])).push(y), y.printed = false, y.nodeDescription = U(k);
}
function G(k, y) {
y.leading = true, y.trailing = false, H(k, y);
}
function X(k, y, _) {
y.leading = false, y.trailing = false, _ && (y.marker = _), H(k, y);
}
function R(k, y) {
y.leading = false, y.trailing = true, H(k, y);
}
function J(k, y) {
let { languages: _ } = a({ plugins: y.plugins }), N = _.find((V) => {
let { name: W } = V;
return W.toLowerCase() === k;
}) || _.find((V) => {
let { aliases: W } = V;
return Array.isArray(W) && W.includes(k);
}) || _.find((V) => {
let { extensions: W } = V;
return Array.isArray(W) && W.includes(`.${k}`);
});
return N && N.parsers[0];
}
function z(k) {
return k && k.type === "front-matter";
}
function M(k) {
let y = /* @__PURE__ */ new WeakMap();
return function(_) {
return y.has(_) || y.set(_, Symbol(k)), y.get(_);
};
}
function U(k) {
let y = k.type || k.kind || "(unknown type)", _ = String(k.name || k.id && (typeof k.id == "object" ? k.id.name : k.id) || k.key && (typeof k.key == "object" ? k.key.name : k.key) || k.value && (typeof k.value == "object" ? "" : String(k.value)) || k.operator || "");
return _.length > 20 && (_ = _.slice(0, 19) + "\u2026"), y + (_ ? " " + _ : "");
}
r.exports = { inferParserByLanguage: J, getStringWidth: s, getMaxContinuousCount: A, getMinNotPresentContinuousCount: j, getPenultimate: F, getLast: t, getNextNonSpaceNonCommentCharacterIndexWithStartIndex: g, getNextNonSpaceNonCommentCharacterIndex: m, getNextNonSpaceNonCommentCharacter: C, skip: E, skipWhitespace: c, skipSpaces: i, skipToLineEnd: D, skipEverythingButNewLine: o, skipInlineComment: l, skipTrailingComment: d, skipNewline: p, isNextLineEmptyAfterIndex: v, isNextLineEmpty: h, isPreviousLineEmpty: x, hasNewline: b, hasNewlineInRange: f, hasSpaces: w, getAlignmentSize: q, getIndentSize: L, getPreferredQuote: B, printString: O, printNumber: P, makeString: T, addLeadingComment: G, addDanglingComment: X, addTrailingComment: R, isFrontMatterNode: z, isNonEmptyArray: n3, createGroupIdMapper: M };
} }), L2 = S({ "src/language-markdown/constants.evaluate.js"(e, r) {
r.exports = { cjkPattern: "(?:[\\u02ea-\\u02eb\\u1100-\\u11ff\\u2e80-\\u2e99\\u2e9b-\\u2ef3\\u2f00-\\u2fd5\\u2ff0-\\u303f\\u3041-\\u3096\\u3099-\\u309f\\u30a1-\\u30fa\\u30fc-\\u30ff\\u3105-\\u312f\\u3131-\\u318e\\u3190-\\u3191\\u3196-\\u31ba\\u31c0-\\u31e3\\u31f0-\\u321e\\u322a-\\u3247\\u3260-\\u327e\\u328a-\\u32b0\\u32c0-\\u32cb\\u32d0-\\u3370\\u337b-\\u337f\\u33e0-\\u33fe\\u3400-\\u4db5\\u4e00-\\u9fef\\ua960-\\ua97c\\uac00-\\ud7a3\\ud7b0-\\ud7c6\\ud7cb-\\ud7fb\\uf900-\\ufa6d\\ufa70-\\ufad9\\ufe10-\\ufe1f\\ufe30-\\ufe6f\\uff00-\\uffef]|[\\ud840-\\ud868\\ud86a-\\ud86c\\ud86f-\\ud872\\ud874-\\ud879][\\udc00-\\udfff]|\\ud82c[\\udc00-\\udd1e\\udd50-\\udd52\\udd64-\\udd67]|\\ud83c[\\ude00\\ude50-\\ude51]|\\ud869[\\udc00-\\uded6\\udf00-\\udfff]|\\ud86d[\\udc00-\\udf34\\udf40-\\udfff]|\\ud86e[\\udc00-\\udc1d\\udc20-\\udfff]|\\ud873[\\udc00-\\udea1\\udeb0-\\udfff]|\\ud87a[\\udc00-\\udfe0]|\\ud87e[\\udc00-\\ude1d])(?:[\\ufe00-\\ufe0f]|\\udb40[\\udd00-\\uddef])?", kPattern: "[\\u1100-\\u11ff\\u3001-\\u3003\\u3008-\\u3011\\u3013-\\u301f\\u302e-\\u3030\\u3037\\u30fb\\u3131-\\u318e\\u3200-\\u321e\\u3260-\\u327e\\ua960-\\ua97c\\uac00-\\ud7a3\\ud7b0-\\ud7c6\\ud7cb-\\ud7fb\\ufe45-\\ufe46\\uff61-\\uff65\\uffa0-\\uffbe\\uffc2-\\uffc7\\uffca-\\uffcf\\uffd2-\\uffd7\\uffda-\\uffdc]", punctuationPattern: "[\\u0021-\\u002f\\u003a-\\u0040\\u005b-\\u0060\\u007b-\\u007e\\u00a1\\u00a7\\u00ab\\u00b6-\\u00b7\\u00bb\\u00bf\\u037e\\u0387\\u055a-\\u055f\\u0589-\\u058a\\u05be\\u05c0\\u05c3\\u05c6\\u05f3-\\u05f4\\u0609-\\u060a\\u060c-\\u060d\\u061b\\u061e-\\u061f\\u066a-\\u066d\\u06d4\\u0700-\\u070d\\u07f7-\\u07f9\\u0830-\\u083e\\u085e\\u0964-\\u0965\\u0970\\u09fd\\u0a76\\u0af0\\u0c77\\u0c84\\u0df4\\u0e4f\\u0e5a-\\u0e5b\\u0f04-\\u0f12\\u0f14\\u0f3a-\\u0f3d\\u0f85\\u0fd0-\\u0fd4\\u0fd9-\\u0fda\\u104a-\\u104f\\u10fb\\u1360-\\u1368\\u1400\\u166e\\u169b-\\u169c\\u16eb-\\u16ed\\u1735-\\u1736\\u17d4-\\u17d6\\u17d8-\\u17da\\u1800-\\u180a\\u1944-\\u1945\\u1a1e-\\u1a1f\\u1aa0-\\u1aa6\\u1aa8-\\u1aad\\u1b5a-\\u1b60\\u1bfc-\\u1bff\\u1c3b-\\u1c3f\\u1c7e-\\u1c7f\\u1cc0-\\u1cc7\\u1cd3\\u2010-\\u2027\\u2030-\\u2043\\u2045-\\u2051\\u2053-\\u205e\\u207d-\\u207e\\u208d-\\u208e\\u2308-\\u230b\\u2329-\\u232a\\u2768-\\u2775\\u27c5-\\u27c6\\u27e6-\\u27ef\\u2983-\\u2998\\u29d8-\\u29db\\u29fc-\\u29fd\\u2cf9-\\u2cfc\\u2cfe-\\u2cff\\u2d70\\u2e00-\\u2e2e\\u2e30-\\u2e4f\\u3001-\\u3003\\u3008-\\u3011\\u3014-\\u301f\\u3030\\u303d\\u30a0\\u30fb\\ua4fe-\\ua4ff\\ua60d-\\ua60f\\ua673\\ua67e\\ua6f2-\\ua6f7\\ua874-\\ua877\\ua8ce-\\ua8cf\\ua8f8-\\ua8fa\\ua8fc\\ua92e-\\ua92f\\ua95f\\ua9c1-\\ua9cd\\ua9de-\\ua9df\\uaa5c-\\uaa5f\\uaade-\\uaadf\\uaaf0-\\uaaf1\\uabeb\\ufd3e-\\ufd3f\\ufe10-\\ufe19\\ufe30-\\ufe52\\ufe54-\\ufe61\\ufe63\\ufe68\\ufe6a-\\ufe6b\\uff01-\\uff03\\uff05-\\uff0a\\uff0c-\\uff0f\\uff1a-\\uff1b\\uff1f-\\uff20\\uff3b-\\uff3d\\uff3f\\uff5b\\uff5d\\uff5f-\\uff65]|\\ud800[\\udd00-\\udd02\\udf9f\\udfd0]|\\ud801[\\udd6f]|\\ud802[\\udc57\\udd1f\\udd3f\\ude50-\\ude58\\ude7f\\udef0-\\udef6\\udf39-\\udf3f\\udf99-\\udf9c]|\\ud803[\\udf55-\\udf59]|\\ud804[\\udc47-\\udc4d\\udcbb-\\udcbc\\udcbe-\\udcc1\\udd40-\\udd43\\udd74-\\udd75\\uddc5-\\uddc8\\uddcd\\udddb\\udddd-\\udddf\\ude38-\\ude3d\\udea9]|\\ud805[\\udc4b-\\udc4f\\udc5b\\udc5d\\udcc6\\uddc1-\\uddd7\\ude41-\\ude43\\ude60-\\ude6c\\udf3c-\\udf3e]|\\ud806[\\udc3b\\udde2\\ude3f-\\ude46\\ude9a-\\ude9c\\ude9e-\\udea2]|\\ud807[\\udc41-\\udc45\\udc70-\\udc71\\udef7-\\udef8\\udfff]|\\ud809[\\udc70-\\udc74]|\\ud81a[\\ude6e-\\ude6f\\udef5\\udf37-\\udf3b\\udf44]|\\ud81b[\\ude97-\\ude9a\\udfe2]|\\ud82f[\\udc9f]|\\ud836[\\ude87-\\ude8b]|\\ud83a[\\udd5e-\\udd5f]" };
} }), R2 = S({ "src/language-markdown/utils.js"(e, r) {
"use strict";
I();
var { getLast: u } = N2(), { locStart: t, locEnd: a } = Zi(), { cjkPattern: n3, kPattern: s, punctuationPattern: c } = L2(), i = ["liquidNode", "inlineCode", "emphasis", "esComment", "strong", "delete", "wikiLink", "link", "linkReference", "image", "imageReference", "footnote", "footnoteReference", "sentence", "whitespace", "word", "break", "inlineMath"], D = [...i, "tableCell", "paragraph", "heading"], o = new RegExp(s), l = new RegExp(c);
function d(f, x) {
let v = "non-cjk", h = "cj-letter", m = "k-letter", C = "cjk-punctuation", w = [], q = (x.proseWrap === "preserve" ? f : f.replace(new RegExp(`(${n3})
(${n3})`, "g"), "$1$2")).split(/([\t\n ]+)/);
for (let [B, O] of q.entries()) {
if (B % 2 === 1) {
w.push({ type: "whitespace", value: /\n/.test(O) ? `
` : " " });
continue;
}
if ((B === 0 || B === q.length - 1) && O === "")
continue;
let T = O.split(new RegExp(`(${n3})`));
for (let [P, A] of T.entries())
if (!((P === 0 || P === T.length - 1) && A === "")) {
if (P % 2 === 0) {
A !== "" && L({ type: "word", value: A, kind: v, hasLeadingPunctuation: l.test(A[0]), hasTrailingPunctuation: l.test(u(A)) });
continue;
}
L(l.test(A) ? { type: "word", value: A, kind: C, hasLeadingPunctuation: true, hasTrailingPunctuation: true } : { type: "word", value: A, kind: o.test(A) ? m : h, hasLeadingPunctuation: false, hasTrailingPunctuation: false });
}
}
return w;
function L(B) {
let O = u(w);
O && O.type === "word" && (O.kind === v && B.kind === h && !O.hasTrailingPunctuation || O.kind === h && B.kind === v && !B.hasLeadingPunctuation ? w.push({ type: "whitespace", value: " " }) : !T(v, C) && ![O.value, B.value].some((P) => /\u3000/.test(P)) && w.push({ type: "whitespace", value: "" })), w.push(B);
function T(P, A) {
return O.kind === P && B.kind === A || O.kind === A && B.kind === P;
}
}
}
function p(f, x) {
let [, v, h, m] = x.slice(f.position.start.offset, f.position.end.offset).match(/^\s*(\d+)(\.|\))(\s*)/);
return { numberText: v, marker: h, leadingSpaces: m };
}
function g(f, x) {
if (!f.ordered || f.children.length < 2)
return false;
let v = Number(p(f.children[0], x.originalText).numberText), h = Number(p(f.children[1], x.originalText).numberText);
if (v === 0 && f.children.length > 2) {
let m = Number(p(f.children[2], x.originalText).numberText);
return h === 1 && m === 1;
}
return h === 1;
}
function F(f, x) {
let { value: v } = f;
return f.position.end.offset === x.length && v.endsWith(`
`) && x.endsWith(`
`) ? v.slice(0, -1) : v;
}
function E(f, x) {
return function v(h, m, C) {
let w = Object.assign({}, x(h, m, C));
return w.children && (w.children = w.children.map((q, L) => v(q, L, [w, ...C]))), w;
}(f, null, []);
}
function b(f) {
if ((f == null ? void 0 : f.type) !== "link" || f.children.length !== 1)
return false;
let [x] = f.children;
return t(f) === t(x) && a(f) === a(x);
}
r.exports = { mapAst: E, splitText: d, punctuationPattern: c, getFencedCodeBlockValue: F, getOrderedListItemInfo: p, hasGitDiffFriendlyOrderedList: g, INLINE_NODE_TYPES: i, INLINE_NODE_WRAPPER_TYPES: D, isAutolink: b };
} }), j2 = S({ "src/language-markdown/unified-plugins/html-to-jsx.js"(e, r) {
"use strict";
I();
var u = Qi(), { mapAst: t, INLINE_NODE_WRAPPER_TYPES: a } = R2();
function n3() {
return (s) => t(s, (c, i, D) => {
let [o] = D;
return c.type !== "html" || u.COMMENT_REGEX.test(c.value) || a.includes(o.type) ? c : Object.assign(Object.assign({}, c), {}, { type: "jsx" });
});
}
r.exports = n3;
} }), P2 = S({ "src/language-markdown/unified-plugins/front-matter.js"(e, r) {
"use strict";
I();
var u = Ji();
function t() {
let a = this.Parser.prototype;
a.blockMethods = ["frontMatter", ...a.blockMethods], a.blockTokenizers.frontMatter = n3;
function n3(s, c) {
let i = u(c);
if (i.frontMatter)
return s(i.frontMatter.raw)(i.frontMatter);
}
n3.onlyAtStart = true;
}
r.exports = t;
} }), M2 = S({ "src/language-markdown/unified-plugins/liquid.js"(e, r) {
"use strict";
I();
function u() {
let t = this.Parser.prototype, a = t.inlineMethods;
a.splice(a.indexOf("text"), 0, "liquid"), t.inlineTokenizers.liquid = n3;
function n3(s, c) {
let i = c.match(/^({%.*?%}|{{.*?}})/s);
if (i)
return s(i[0])({ type: "liquidNode", value: i[0] });
}
n3.locator = function(s, c) {
return s.indexOf("{", c);
};
}
r.exports = u;
} }), z2 = S({ "src/language-markdown/unified-plugins/wiki-link.js"(e, r) {
"use strict";
I();
function u() {
let t = "wikiLink", a = /^\[\[(?.+?)]]/s, n3 = this.Parser.prototype, s = n3.inlineMethods;
s.splice(s.indexOf("link"), 0, t), n3.inlineTokenizers.wikiLink = c;
function c(i, D) {
let o = a.exec(D);
if (o) {
let l = o.groups.linkContents.trim();
return i(o[0])({ type: t, value: l });
}
}
c.locator = function(i, D) {
return i.indexOf("[", D);
};
}
r.exports = u;
} }), $2 = S({ "src/language-markdown/unified-plugins/loose-items.js"(e, r) {
"use strict";
I();
function u() {
let t = this.Parser.prototype, a = t.blockTokenizers.list;
function n3(s, c, i) {
return c.type === "listItem" && (c.loose = c.spread || s.charAt(s.length - 1) === `
`, c.loose && (i.loose = true)), c;
}
t.blockTokenizers.list = function(c, i, D) {
function o(l) {
let d = c(l);
function p(g, F) {
return d(n3(l, g, F), F);
}
return p.reset = function(g, F) {
return d.reset(n3(l, g, F), F);
}, p;
}
return o.now = c.now, a.call(this, o, i, D);
};
}
r.exports = u;
} });
I();
var U2 = GD(), G2 = t2(), V2 = a2(), H2 = o2(), X2 = s2(), { locStart: W2, locEnd: K2 } = Zi(), Li = Qi(), Y2 = j2(), J2 = P2(), Z2 = M2(), Q2 = z2(), ef = $2();
function sa(e) {
let { isMDX: r } = e;
return (u) => {
let t = G2().use(U2, Object.assign({ commonmark: true }, r && { blocks: [Li.BLOCKS_REGEX] })).use(H2).use(J2).use(V2).use(r ? Li.esSyntax : Ri).use(Z2).use(r ? Y2 : Ri).use(Q2).use(ef);
return t.runSync(t.parse(u));
};
}
function Ri(e) {
return e;
}
var ca = { astFormat: "mdast", hasPragma: X2.hasPragma, locStart: W2, locEnd: K2 }, ji = Object.assign(Object.assign({}, ca), {}, { parse: sa({ isMDX: false }) }), rf = Object.assign(Object.assign({}, ca), {}, { parse: sa({ isMDX: true }) });
la.exports = { parsers: { remark: ji, markdown: ji, mdx: rf } };
});
return uf();
});
}
});
// node_modules/prettier/parser-html.js
var require_parser_html = __commonJS({
"node_modules/prettier/parser-html.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.html = e();
}
})(function() {
"use strict";
var S = (e, r) => () => (r || e((r = { exports: {} }).exports, r), r.exports);
var ee = S((cc, Kr) => {
var Ne = function(e) {
return e && e.Math == Math && e;
};
Kr.exports = Ne(typeof globalThis == "object" && globalThis) || Ne(typeof window == "object" && window) || Ne(typeof self == "object" && self) || Ne(typeof global == "object" && global) || function() {
return this;
}() || Function("return this")();
});
var se = S((hc, Jr) => {
Jr.exports = function(e) {
try {
return !!e();
} catch {
return true;
}
};
});
var ae = S((pc, Zr) => {
var qs = se();
Zr.exports = !qs(function() {
return Object.defineProperty({}, 1, { get: function() {
return 7;
} })[1] != 7;
});
});
var Oe = S((fc, eu) => {
var Is = se();
eu.exports = !Is(function() {
var e = function() {
}.bind();
return typeof e != "function" || e.hasOwnProperty("prototype");
});
});
var De = S((dc, ru) => {
var Rs = Oe(), qe = Function.prototype.call;
ru.exports = Rs ? qe.bind(qe) : function() {
return qe.apply(qe, arguments);
};
});
var su = S((nu) => {
"use strict";
var uu = {}.propertyIsEnumerable, tu = Object.getOwnPropertyDescriptor, xs = tu && !uu.call({ 1: 2 }, 1);
nu.f = xs ? function(r) {
var u = tu(this, r);
return !!u && u.enumerable;
} : uu;
});
var Ie = S((Cc, iu) => {
iu.exports = function(e, r) {
return { enumerable: !(e & 1), configurable: !(e & 2), writable: !(e & 4), value: r };
};
});
var re = S((mc, Du) => {
var au = Oe(), ou = Function.prototype, er = ou.call, Ps = au && ou.bind.bind(er, er);
Du.exports = au ? Ps : function(e) {
return function() {
return er.apply(e, arguments);
};
};
});
var me = S((gc, cu) => {
var lu = re(), ks = lu({}.toString), Ls = lu("".slice);
cu.exports = function(e) {
return Ls(ks(e), 8, -1);
};
});
var pu = S((Fc, hu) => {
var $s = re(), Ms = se(), js = me(), rr = Object, Us = $s("".split);
hu.exports = Ms(function() {
return !rr("z").propertyIsEnumerable(0);
}) ? function(e) {
return js(e) == "String" ? Us(e, "") : rr(e);
} : rr;
});
var Re = S((Ac, fu) => {
fu.exports = function(e) {
return e == null;
};
});
var ur = S((vc, du) => {
var Gs = Re(), Vs = TypeError;
du.exports = function(e) {
if (Gs(e))
throw Vs("Can't call method on " + e);
return e;
};
});
var xe = S((_c, Eu) => {
var Xs = pu(), Hs = ur();
Eu.exports = function(e) {
return Xs(Hs(e));
};
});
var nr = S((Sc, Cu) => {
var tr = typeof document == "object" && document.all, zs = typeof tr > "u" && tr !== void 0;
Cu.exports = { all: tr, IS_HTMLDDA: zs };
});
var Y = S((yc, gu) => {
var mu = nr(), Ws = mu.all;
gu.exports = mu.IS_HTMLDDA ? function(e) {
return typeof e == "function" || e === Ws;
} : function(e) {
return typeof e == "function";
};
});
var le = S((Tc, vu) => {
var Fu = Y(), Au = nr(), Ys = Au.all;
vu.exports = Au.IS_HTMLDDA ? function(e) {
return typeof e == "object" ? e !== null : Fu(e) || e === Ys;
} : function(e) {
return typeof e == "object" ? e !== null : Fu(e);
};
});
var ge = S((Bc, _u) => {
var sr = ee(), Qs = Y(), Ks = function(e) {
return Qs(e) ? e : void 0;
};
_u.exports = function(e, r) {
return arguments.length < 2 ? Ks(sr[e]) : sr[e] && sr[e][r];
};
});
var ir = S((bc, Su) => {
var Js = re();
Su.exports = Js({}.isPrototypeOf);
});
var Tu = S((wc, yu) => {
var Zs = ge();
yu.exports = Zs("navigator", "userAgent") || "";
});
var Iu = S((Nc, qu) => {
var Ou = ee(), ar = Tu(), Bu = Ou.process, bu = Ou.Deno, wu = Bu && Bu.versions || bu && bu.version, Nu = wu && wu.v8, ue, Pe;
Nu && (ue = Nu.split("."), Pe = ue[0] > 0 && ue[0] < 4 ? 1 : +(ue[0] + ue[1]));
!Pe && ar && (ue = ar.match(/Edge\/(\d+)/), (!ue || ue[1] >= 74) && (ue = ar.match(/Chrome\/(\d+)/), ue && (Pe = +ue[1])));
qu.exports = Pe;
});
var or = S((Oc, xu) => {
var Ru = Iu(), ei = se();
xu.exports = !!Object.getOwnPropertySymbols && !ei(function() {
var e = Symbol();
return !String(e) || !(Object(e) instanceof Symbol) || !Symbol.sham && Ru && Ru < 41;
});
});
var Dr = S((qc, Pu) => {
var ri = or();
Pu.exports = ri && !Symbol.sham && typeof Symbol.iterator == "symbol";
});
var lr = S((Ic, ku) => {
var ui = ge(), ti = Y(), ni = ir(), si = Dr(), ii = Object;
ku.exports = si ? function(e) {
return typeof e == "symbol";
} : function(e) {
var r = ui("Symbol");
return ti(r) && ni(r.prototype, ii(e));
};
});
var ke = S((Rc, Lu) => {
var ai = String;
Lu.exports = function(e) {
try {
return ai(e);
} catch {
return "Object";
}
};
});
var Fe = S((xc, $u) => {
var oi = Y(), Di = ke(), li = TypeError;
$u.exports = function(e) {
if (oi(e))
return e;
throw li(Di(e) + " is not a function");
};
});
var Le = S((Pc, Mu) => {
var ci = Fe(), hi = Re();
Mu.exports = function(e, r) {
var u = e[r];
return hi(u) ? void 0 : ci(u);
};
});
var Uu = S((kc, ju) => {
var cr = De(), hr = Y(), pr = le(), pi = TypeError;
ju.exports = function(e, r) {
var u, n2;
if (r === "string" && hr(u = e.toString) && !pr(n2 = cr(u, e)) || hr(u = e.valueOf) && !pr(n2 = cr(u, e)) || r !== "string" && hr(u = e.toString) && !pr(n2 = cr(u, e)))
return n2;
throw pi("Can't convert object to primitive value");
};
});
var Vu = S((Lc, Gu) => {
Gu.exports = false;
});
var $e = S(($c, Hu) => {
var Xu = ee(), fi = Object.defineProperty;
Hu.exports = function(e, r) {
try {
fi(Xu, e, { value: r, configurable: true, writable: true });
} catch {
Xu[e] = r;
}
return r;
};
});
var Me = S((Mc, Wu) => {
var di = ee(), Ei = $e(), zu = "__core-js_shared__", Ci = di[zu] || Ei(zu, {});
Wu.exports = Ci;
});
var fr = S((jc, Qu) => {
var mi = Vu(), Yu = Me();
(Qu.exports = function(e, r) {
return Yu[e] || (Yu[e] = r !== void 0 ? r : {});
})("versions", []).push({ version: "3.26.1", mode: mi ? "pure" : "global", copyright: "\xA9 2014-2022 Denis Pushkarev (zloirock.ru)", license: "https://github.com/zloirock/core-js/blob/v3.26.1/LICENSE", source: "https://github.com/zloirock/core-js" });
});
var dr = S((Uc, Ku) => {
var gi = ur(), Fi = Object;
Ku.exports = function(e) {
return Fi(gi(e));
};
});
var oe = S((Gc, Ju) => {
var Ai = re(), vi = dr(), _i = Ai({}.hasOwnProperty);
Ju.exports = Object.hasOwn || function(r, u) {
return _i(vi(r), u);
};
});
var Er = S((Vc, Zu) => {
var Si = re(), yi = 0, Ti = Math.random(), Bi = Si(1 .toString);
Zu.exports = function(e) {
return "Symbol(" + (e === void 0 ? "" : e) + ")_" + Bi(++yi + Ti, 36);
};
});
var he = S((Xc, nt) => {
var bi = ee(), wi = fr(), et = oe(), Ni = Er(), rt = or(), tt = Dr(), fe = wi("wks"), ce = bi.Symbol, ut = ce && ce.for, Oi = tt ? ce : ce && ce.withoutSetter || Ni;
nt.exports = function(e) {
if (!et(fe, e) || !(rt || typeof fe[e] == "string")) {
var r = "Symbol." + e;
rt && et(ce, e) ? fe[e] = ce[e] : tt && ut ? fe[e] = ut(r) : fe[e] = Oi(r);
}
return fe[e];
};
});
var ot = S((Hc, at) => {
var qi = De(), st = le(), it = lr(), Ii = Le(), Ri = Uu(), xi = he(), Pi = TypeError, ki = xi("toPrimitive");
at.exports = function(e, r) {
if (!st(e) || it(e))
return e;
var u = Ii(e, ki), n2;
if (u) {
if (r === void 0 && (r = "default"), n2 = qi(u, e, r), !st(n2) || it(n2))
return n2;
throw Pi("Can't convert object to primitive value");
}
return r === void 0 && (r = "number"), Ri(e, r);
};
});
var je = S((zc, Dt) => {
var Li = ot(), $i = lr();
Dt.exports = function(e) {
var r = Li(e, "string");
return $i(r) ? r : r + "";
};
});
var ht = S((Wc, ct) => {
var Mi = ee(), lt = le(), Cr = Mi.document, ji = lt(Cr) && lt(Cr.createElement);
ct.exports = function(e) {
return ji ? Cr.createElement(e) : {};
};
});
var mr = S((Yc, pt) => {
var Ui = ae(), Gi = se(), Vi = ht();
pt.exports = !Ui && !Gi(function() {
return Object.defineProperty(Vi("div"), "a", { get: function() {
return 7;
} }).a != 7;
});
});
var gr = S((dt) => {
var Xi = ae(), Hi = De(), zi = su(), Wi = Ie(), Yi = xe(), Qi = je(), Ki = oe(), Ji = mr(), ft = Object.getOwnPropertyDescriptor;
dt.f = Xi ? ft : function(r, u) {
if (r = Yi(r), u = Qi(u), Ji)
try {
return ft(r, u);
} catch {
}
if (Ki(r, u))
return Wi(!Hi(zi.f, r, u), r[u]);
};
});
var Ct = S((Kc, Et) => {
var Zi = ae(), ea = se();
Et.exports = Zi && ea(function() {
return Object.defineProperty(function() {
}, "prototype", { value: 42, writable: false }).prototype != 42;
});
});
var de = S((Jc, mt) => {
var ra = le(), ua = String, ta = TypeError;
mt.exports = function(e) {
if (ra(e))
return e;
throw ta(ua(e) + " is not an object");
};
});
var Ae = S((Ft) => {
var na = ae(), sa = mr(), ia = Ct(), Ue = de(), gt = je(), aa = TypeError, Fr = Object.defineProperty, oa = Object.getOwnPropertyDescriptor, Ar = "enumerable", vr = "configurable", _r = "writable";
Ft.f = na ? ia ? function(r, u, n2) {
if (Ue(r), u = gt(u), Ue(n2), typeof r == "function" && u === "prototype" && "value" in n2 && _r in n2 && !n2[_r]) {
var D = oa(r, u);
D && D[_r] && (r[u] = n2.value, n2 = { configurable: vr in n2 ? n2[vr] : D[vr], enumerable: Ar in n2 ? n2[Ar] : D[Ar], writable: false });
}
return Fr(r, u, n2);
} : Fr : function(r, u, n2) {
if (Ue(r), u = gt(u), Ue(n2), sa)
try {
return Fr(r, u, n2);
} catch {
}
if ("get" in n2 || "set" in n2)
throw aa("Accessors not supported");
return "value" in n2 && (r[u] = n2.value), r;
};
});
var Sr = S((e2, At) => {
var Da = ae(), la = Ae(), ca = Ie();
At.exports = Da ? function(e, r, u) {
return la.f(e, r, ca(1, u));
} : function(e, r, u) {
return e[r] = u, e;
};
});
var St = S((r2, _t) => {
var yr = ae(), ha = oe(), vt = Function.prototype, pa = yr && Object.getOwnPropertyDescriptor, Tr = ha(vt, "name"), fa = Tr && function() {
}.name === "something", da = Tr && (!yr || yr && pa(vt, "name").configurable);
_t.exports = { EXISTS: Tr, PROPER: fa, CONFIGURABLE: da };
});
var br = S((u2, yt) => {
var Ea = re(), Ca = Y(), Br = Me(), ma = Ea(Function.toString);
Ca(Br.inspectSource) || (Br.inspectSource = function(e) {
return ma(e);
});
yt.exports = Br.inspectSource;
});
var bt = S((t2, Bt) => {
var ga = ee(), Fa = Y(), Tt = ga.WeakMap;
Bt.exports = Fa(Tt) && /native code/.test(String(Tt));
});
var Ot = S((n2, Nt) => {
var Aa = fr(), va = Er(), wt = Aa("keys");
Nt.exports = function(e) {
return wt[e] || (wt[e] = va(e));
};
});
var wr = S((s2, qt) => {
qt.exports = {};
});
var Pt = S((i2, xt) => {
var _a = bt(), Rt = ee(), Sa = le(), ya = Sr(), Nr = oe(), Or = Me(), Ta = Ot(), Ba = wr(), It = "Object already initialized", qr = Rt.TypeError, ba = Rt.WeakMap, Ge, ve, Ve, wa = function(e) {
return Ve(e) ? ve(e) : Ge(e, {});
}, Na = function(e) {
return function(r) {
var u;
if (!Sa(r) || (u = ve(r)).type !== e)
throw qr("Incompatible receiver, " + e + " required");
return u;
};
};
_a || Or.state ? (te = Or.state || (Or.state = new ba()), te.get = te.get, te.has = te.has, te.set = te.set, Ge = function(e, r) {
if (te.has(e))
throw qr(It);
return r.facade = e, te.set(e, r), r;
}, ve = function(e) {
return te.get(e) || {};
}, Ve = function(e) {
return te.has(e);
}) : (pe = Ta("state"), Ba[pe] = true, Ge = function(e, r) {
if (Nr(e, pe))
throw qr(It);
return r.facade = e, ya(e, pe, r), r;
}, ve = function(e) {
return Nr(e, pe) ? e[pe] : {};
}, Ve = function(e) {
return Nr(e, pe);
});
var te, pe;
xt.exports = { set: Ge, get: ve, has: Ve, enforce: wa, getterFor: Na };
});
var $t = S((a2, Lt) => {
var Oa = se(), qa = Y(), Xe = oe(), Ir = ae(), Ia = St().CONFIGURABLE, Ra = br(), kt = Pt(), xa = kt.enforce, Pa = kt.get, He = Object.defineProperty, ka = Ir && !Oa(function() {
return He(function() {
}, "length", { value: 8 }).length !== 8;
}), La = String(String).split("String"), $a = Lt.exports = function(e, r, u) {
String(r).slice(0, 7) === "Symbol(" && (r = "[" + String(r).replace(/^Symbol\(([^)]*)\)/, "$1") + "]"), u && u.getter && (r = "get " + r), u && u.setter && (r = "set " + r), (!Xe(e, "name") || Ia && e.name !== r) && (Ir ? He(e, "name", { value: r, configurable: true }) : e.name = r), ka && u && Xe(u, "arity") && e.length !== u.arity && He(e, "length", { value: u.arity });
try {
u && Xe(u, "constructor") && u.constructor ? Ir && He(e, "prototype", { writable: false }) : e.prototype && (e.prototype = void 0);
} catch {
}
var n2 = xa(e);
return Xe(n2, "source") || (n2.source = La.join(typeof r == "string" ? r : "")), e;
};
Function.prototype.toString = $a(function() {
return qa(this) && Pa(this).source || Ra(this);
}, "toString");
});
var jt = S((o2, Mt) => {
var Ma = Y(), ja = Ae(), Ua = $t(), Ga = $e();
Mt.exports = function(e, r, u, n2) {
n2 || (n2 = {});
var D = n2.enumerable, s = n2.name !== void 0 ? n2.name : r;
if (Ma(u) && Ua(u, s, n2), n2.global)
D ? e[r] = u : Ga(r, u);
else {
try {
n2.unsafe ? e[r] && (D = true) : delete e[r];
} catch {
}
D ? e[r] = u : ja.f(e, r, { value: u, enumerable: false, configurable: !n2.nonConfigurable, writable: !n2.nonWritable });
}
return e;
};
});
var Gt = S((D2, Ut) => {
var Va = Math.ceil, Xa = Math.floor;
Ut.exports = Math.trunc || function(r) {
var u = +r;
return (u > 0 ? Xa : Va)(u);
};
});
var Rr = S((l2, Vt) => {
var Ha = Gt();
Vt.exports = function(e) {
var r = +e;
return r !== r || r === 0 ? 0 : Ha(r);
};
});
var Ht = S((c2, Xt) => {
var za = Rr(), Wa = Math.max, Ya = Math.min;
Xt.exports = function(e, r) {
var u = za(e);
return u < 0 ? Wa(u + r, 0) : Ya(u, r);
};
});
var Wt = S((h2, zt) => {
var Qa = Rr(), Ka = Math.min;
zt.exports = function(e) {
return e > 0 ? Ka(Qa(e), 9007199254740991) : 0;
};
});
var _e = S((p2, Yt) => {
var Ja = Wt();
Yt.exports = function(e) {
return Ja(e.length);
};
});
var Jt = S((f2, Kt) => {
var Za = xe(), eo = Ht(), ro = _e(), Qt = function(e) {
return function(r, u, n2) {
var D = Za(r), s = ro(D), i = eo(n2, s), f;
if (e && u != u) {
for (; s > i; )
if (f = D[i++], f != f)
return true;
} else
for (; s > i; i++)
if ((e || i in D) && D[i] === u)
return e || i || 0;
return !e && -1;
};
};
Kt.exports = { includes: Qt(true), indexOf: Qt(false) };
});
var rn = S((d2, en) => {
var uo = re(), xr = oe(), to = xe(), no = Jt().indexOf, so = wr(), Zt = uo([].push);
en.exports = function(e, r) {
var u = to(e), n2 = 0, D = [], s;
for (s in u)
!xr(so, s) && xr(u, s) && Zt(D, s);
for (; r.length > n2; )
xr(u, s = r[n2++]) && (~no(D, s) || Zt(D, s));
return D;
};
});
var tn = S((E2, un) => {
un.exports = ["constructor", "hasOwnProperty", "isPrototypeOf", "propertyIsEnumerable", "toLocaleString", "toString", "valueOf"];
});
var sn = S((nn) => {
var io = rn(), ao = tn(), oo = ao.concat("length", "prototype");
nn.f = Object.getOwnPropertyNames || function(r) {
return io(r, oo);
};
});
var on = S((an) => {
an.f = Object.getOwnPropertySymbols;
});
var ln = S((g2, Dn) => {
var Do = ge(), lo = re(), co = sn(), ho = on(), po = de(), fo = lo([].concat);
Dn.exports = Do("Reflect", "ownKeys") || function(r) {
var u = co.f(po(r)), n2 = ho.f;
return n2 ? fo(u, n2(r)) : u;
};
});
var pn = S((F2, hn) => {
var cn = oe(), Eo = ln(), Co = gr(), mo = Ae();
hn.exports = function(e, r, u) {
for (var n2 = Eo(r), D = mo.f, s = Co.f, i = 0; i < n2.length; i++) {
var f = n2[i];
!cn(e, f) && !(u && cn(u, f)) && D(e, f, s(r, f));
}
};
});
var dn = S((A2, fn) => {
var go = se(), Fo = Y(), Ao = /#|\.prototype\./, Se = function(e, r) {
var u = _o[vo(e)];
return u == yo ? true : u == So ? false : Fo(r) ? go(r) : !!r;
}, vo = Se.normalize = function(e) {
return String(e).replace(Ao, ".").toLowerCase();
}, _o = Se.data = {}, So = Se.NATIVE = "N", yo = Se.POLYFILL = "P";
fn.exports = Se;
});
var ze = S((v2, En) => {
var Pr = ee(), To = gr().f, Bo = Sr(), bo = jt(), wo = $e(), No = pn(), Oo = dn();
En.exports = function(e, r) {
var u = e.target, n2 = e.global, D = e.stat, s, i, f, c, F, a;
if (n2 ? i = Pr : D ? i = Pr[u] || wo(u, {}) : i = (Pr[u] || {}).prototype, i)
for (f in r) {
if (F = r[f], e.dontCallGetSet ? (a = To(i, f), c = a && a.value) : c = i[f], s = Oo(n2 ? f : u + (D ? "." : "#") + f, e.forced), !s && c !== void 0) {
if (typeof F == typeof c)
continue;
No(F, c);
}
(e.sham || c && c.sham) && Bo(F, "sham", true), bo(i, f, F, e);
}
};
});
var Cn = S(() => {
var qo = ze(), kr = ee();
qo({ global: true, forced: kr.globalThis !== kr }, { globalThis: kr });
});
var mn = S(() => {
Cn();
});
var Lr = S((B2, gn) => {
var Io = me();
gn.exports = Array.isArray || function(r) {
return Io(r) == "Array";
};
});
var An = S((b2, Fn) => {
var Ro = TypeError, xo = 9007199254740991;
Fn.exports = function(e) {
if (e > xo)
throw Ro("Maximum allowed index exceeded");
return e;
};
});
var _n = S((w2, vn) => {
var Po = me(), ko = re();
vn.exports = function(e) {
if (Po(e) === "Function")
return ko(e);
};
});
var $r = S((N2, yn) => {
var Sn = _n(), Lo = Fe(), $o = Oe(), Mo = Sn(Sn.bind);
yn.exports = function(e, r) {
return Lo(e), r === void 0 ? e : $o ? Mo(e, r) : function() {
return e.apply(r, arguments);
};
};
});
var bn = S((O2, Bn) => {
"use strict";
var jo = Lr(), Uo = _e(), Go = An(), Vo = $r(), Tn = function(e, r, u, n2, D, s, i, f) {
for (var c = D, F = 0, a = i ? Vo(i, f) : false, l, h; F < n2; )
F in u && (l = a ? a(u[F], F, r) : u[F], s > 0 && jo(l) ? (h = Uo(l), c = Tn(e, r, l, h, c, s - 1) - 1) : (Go(c + 1), e[c] = l), c++), F++;
return c;
};
Bn.exports = Tn;
});
var On = S((q2, Nn) => {
var Xo = he(), Ho = Xo("toStringTag"), wn = {};
wn[Ho] = "z";
Nn.exports = String(wn) === "[object z]";
});
var Mr = S((I2, qn) => {
var zo = On(), Wo = Y(), We = me(), Yo = he(), Qo = Yo("toStringTag"), Ko = Object, Jo = We(function() {
return arguments;
}()) == "Arguments", Zo = function(e, r) {
try {
return e[r];
} catch {
}
};
qn.exports = zo ? We : function(e) {
var r, u, n2;
return e === void 0 ? "Undefined" : e === null ? "Null" : typeof (u = Zo(r = Ko(e), Qo)) == "string" ? u : Jo ? We(r) : (n2 = We(r)) == "Object" && Wo(r.callee) ? "Arguments" : n2;
};
});
var Ln = S((R2, kn) => {
var eD = re(), rD = se(), In = Y(), uD = Mr(), tD = ge(), nD = br(), Rn = function() {
}, sD = [], xn = tD("Reflect", "construct"), jr = /^\s*(?:class|function)\b/, iD = eD(jr.exec), aD = !jr.exec(Rn), ye = function(r) {
if (!In(r))
return false;
try {
return xn(Rn, sD, r), true;
} catch {
return false;
}
}, Pn = function(r) {
if (!In(r))
return false;
switch (uD(r)) {
case "AsyncFunction":
case "GeneratorFunction":
case "AsyncGeneratorFunction":
return false;
}
try {
return aD || !!iD(jr, nD(r));
} catch {
return true;
}
};
Pn.sham = true;
kn.exports = !xn || rD(function() {
var e;
return ye(ye.call) || !ye(Object) || !ye(function() {
e = true;
}) || e;
}) ? Pn : ye;
});
var Un = S((x2, jn) => {
var $n = Lr(), oD = Ln(), DD = le(), lD = he(), cD = lD("species"), Mn = Array;
jn.exports = function(e) {
var r;
return $n(e) && (r = e.constructor, oD(r) && (r === Mn || $n(r.prototype)) ? r = void 0 : DD(r) && (r = r[cD], r === null && (r = void 0))), r === void 0 ? Mn : r;
};
});
var Vn = S((P2, Gn) => {
var hD = Un();
Gn.exports = function(e, r) {
return new (hD(e))(r === 0 ? 0 : r);
};
});
var Xn = S(() => {
"use strict";
var pD = ze(), fD = bn(), dD = Fe(), ED = dr(), CD = _e(), mD = Vn();
pD({ target: "Array", proto: true }, { flatMap: function(r) {
var u = ED(this), n2 = CD(u), D;
return dD(r), D = mD(u, 0), D.length = fD(D, u, u, n2, 0, 1, r, arguments.length > 1 ? arguments[1] : void 0), D;
} });
});
var Ur = S(($2, Hn) => {
Hn.exports = {};
});
var Wn = S((M2, zn) => {
var gD = he(), FD = Ur(), AD = gD("iterator"), vD = Array.prototype;
zn.exports = function(e) {
return e !== void 0 && (FD.Array === e || vD[AD] === e);
};
});
var Gr = S((j2, Qn) => {
var _D = Mr(), Yn = Le(), SD = Re(), yD = Ur(), TD = he(), BD = TD("iterator");
Qn.exports = function(e) {
if (!SD(e))
return Yn(e, BD) || Yn(e, "@@iterator") || yD[_D(e)];
};
});
var Jn = S((U2, Kn) => {
var bD = De(), wD = Fe(), ND = de(), OD = ke(), qD = Gr(), ID = TypeError;
Kn.exports = function(e, r) {
var u = arguments.length < 2 ? qD(e) : r;
if (wD(u))
return ND(bD(u, e));
throw ID(OD(e) + " is not iterable");
};
});
var rs = S((G2, es) => {
var RD = De(), Zn = de(), xD = Le();
es.exports = function(e, r, u) {
var n2, D;
Zn(e);
try {
if (n2 = xD(e, "return"), !n2) {
if (r === "throw")
throw u;
return u;
}
n2 = RD(n2, e);
} catch (s) {
D = true, n2 = s;
}
if (r === "throw")
throw u;
if (D)
throw n2;
return Zn(n2), u;
};
});
var is = S((V2, ss) => {
var PD = $r(), kD = De(), LD = de(), $D = ke(), MD = Wn(), jD = _e(), us = ir(), UD = Jn(), GD = Gr(), ts = rs(), VD = TypeError, Ye = function(e, r) {
this.stopped = e, this.result = r;
}, ns = Ye.prototype;
ss.exports = function(e, r, u) {
var n2 = u && u.that, D = !!(u && u.AS_ENTRIES), s = !!(u && u.IS_RECORD), i = !!(u && u.IS_ITERATOR), f = !!(u && u.INTERRUPTED), c = PD(r, n2), F, a, l, h, C, d, m, T = function(g) {
return F && ts(F, "normal", g), new Ye(true, g);
}, w = function(g) {
return D ? (LD(g), f ? c(g[0], g[1], T) : c(g[0], g[1])) : f ? c(g, T) : c(g);
};
if (s)
F = e.iterator;
else if (i)
F = e;
else {
if (a = GD(e), !a)
throw VD($D(e) + " is not iterable");
if (MD(a)) {
for (l = 0, h = jD(e); h > l; l++)
if (C = w(e[l]), C && us(ns, C))
return C;
return new Ye(false);
}
F = UD(e, a);
}
for (d = s ? e.next : F.next; !(m = kD(d, F)).done; ) {
try {
C = w(m.value);
} catch (g) {
ts(F, "throw", g);
}
if (typeof C == "object" && C && us(ns, C))
return C;
}
return new Ye(false);
};
});
var os = S((X2, as) => {
"use strict";
var XD = je(), HD = Ae(), zD = Ie();
as.exports = function(e, r, u) {
var n2 = XD(r);
n2 in e ? HD.f(e, n2, zD(0, u)) : e[n2] = u;
};
});
var Ds = S(() => {
var WD = ze(), YD = is(), QD = os();
WD({ target: "Object", stat: true }, { fromEntries: function(r) {
var u = {};
return YD(r, function(n2, D) {
QD(u, n2, D);
}, { AS_ENTRIES: true }), u;
} });
});
var Dc = S((W2, Os) => {
var KD = ["cliName", "cliCategory", "cliDescription"];
function JD(e, r) {
if (e == null)
return {};
var u = ZD(e, r), n2, D;
if (Object.getOwnPropertySymbols) {
var s = Object.getOwnPropertySymbols(e);
for (D = 0; D < s.length; D++)
n2 = s[D], !(r.indexOf(n2) >= 0) && Object.prototype.propertyIsEnumerable.call(e, n2) && (u[n2] = e[n2]);
}
return u;
}
function ZD(e, r) {
if (e == null)
return {};
var u = {}, n2 = Object.keys(e), D, s;
for (s = 0; s < n2.length; s++)
D = n2[s], !(r.indexOf(D) >= 0) && (u[D] = e[D]);
return u;
}
mn();
Xn();
Ds();
var el = Object.create, Je = Object.defineProperty, rl = Object.getOwnPropertyDescriptor, Xr = Object.getOwnPropertyNames, ul = Object.getPrototypeOf, tl = Object.prototype.hasOwnProperty, Ee = (e, r) => function() {
return e && (r = (0, e[Xr(e)[0]])(e = 0)), r;
}, I = (e, r) => function() {
return r || (0, e[Xr(e)[0]])((r = { exports: {} }).exports, r), r.exports;
}, ps = (e, r) => {
for (var u in r)
Je(e, u, { get: r[u], enumerable: true });
}, fs2 = (e, r, u, n2) => {
if (r && typeof r == "object" || typeof r == "function")
for (let D of Xr(r))
!tl.call(e, D) && D !== u && Je(e, D, { get: () => r[D], enumerable: !(n2 = rl(r, D)) || n2.enumerable });
return e;
}, nl = (e, r, u) => (u = e != null ? el(ul(e)) : {}, fs2(r || !e || !e.__esModule ? Je(u, "default", { value: e, enumerable: true }) : u, e)), ds = (e) => fs2(Je({}, "__esModule", { value: true }), e), Te, q = Ee({ ""() {
Te = { env: {}, argv: [] };
} }), Es = I({ "node_modules/angular-html-parser/lib/compiler/src/chars.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true }), e.$EOF = 0, e.$BSPACE = 8, e.$TAB = 9, e.$LF = 10, e.$VTAB = 11, e.$FF = 12, e.$CR = 13, e.$SPACE = 32, e.$BANG = 33, e.$DQ = 34, e.$HASH = 35, e.$$ = 36, e.$PERCENT = 37, e.$AMPERSAND = 38, e.$SQ = 39, e.$LPAREN = 40, e.$RPAREN = 41, e.$STAR = 42, e.$PLUS = 43, e.$COMMA = 44, e.$MINUS = 45, e.$PERIOD = 46, e.$SLASH = 47, e.$COLON = 58, e.$SEMICOLON = 59, e.$LT = 60, e.$EQ = 61, e.$GT = 62, e.$QUESTION = 63, e.$0 = 48, e.$7 = 55, e.$9 = 57, e.$A = 65, e.$E = 69, e.$F = 70, e.$X = 88, e.$Z = 90, e.$LBRACKET = 91, e.$BACKSLASH = 92, e.$RBRACKET = 93, e.$CARET = 94, e.$_ = 95, e.$a = 97, e.$b = 98, e.$e = 101, e.$f = 102, e.$n = 110, e.$r = 114, e.$t = 116, e.$u = 117, e.$v = 118, e.$x = 120, e.$z = 122, e.$LBRACE = 123, e.$BAR = 124, e.$RBRACE = 125, e.$NBSP = 160, e.$PIPE = 124, e.$TILDA = 126, e.$AT = 64, e.$BT = 96;
function r(f) {
return f >= e.$TAB && f <= e.$SPACE || f == e.$NBSP;
}
e.isWhitespace = r;
function u(f) {
return e.$0 <= f && f <= e.$9;
}
e.isDigit = u;
function n2(f) {
return f >= e.$a && f <= e.$z || f >= e.$A && f <= e.$Z;
}
e.isAsciiLetter = n2;
function D(f) {
return f >= e.$a && f <= e.$f || f >= e.$A && f <= e.$F || u(f);
}
e.isAsciiHexDigit = D;
function s(f) {
return f === e.$LF || f === e.$CR;
}
e.isNewLine = s;
function i(f) {
return e.$0 <= f && f <= e.$7;
}
e.isOctalDigit = i;
} }), sl = I({ "node_modules/angular-html-parser/lib/compiler/src/aot/static_symbol.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = class {
constructor(n2, D, s) {
this.filePath = n2, this.name = D, this.members = s;
}
assertNoMembers() {
if (this.members.length)
throw new Error(`Illegal state: symbol without members expected, but got ${JSON.stringify(this)}.`);
}
};
e.StaticSymbol = r;
var u = class {
constructor() {
this.cache = /* @__PURE__ */ new Map();
}
get(n2, D, s) {
s = s || [];
let i = s.length ? `.${s.join(".")}` : "", f = `"${n2}".${D}${i}`, c = this.cache.get(f);
return c || (c = new r(n2, D, s), this.cache.set(f, c)), c;
}
};
e.StaticSymbolCache = u;
} }), il = I({ "node_modules/angular-html-parser/lib/compiler/src/util.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = /-+([a-z0-9])/g;
function u(o) {
return o.replace(r, function() {
for (var E = arguments.length, p = new Array(E), A = 0; A < E; A++)
p[A] = arguments[A];
return p[1].toUpperCase();
});
}
e.dashCaseToCamelCase = u;
function n2(o, E) {
return s(o, ":", E);
}
e.splitAtColon = n2;
function D(o, E) {
return s(o, ".", E);
}
e.splitAtPeriod = D;
function s(o, E, p) {
let A = o.indexOf(E);
return A == -1 ? p : [o.slice(0, A).trim(), o.slice(A + 1).trim()];
}
function i(o, E, p) {
return Array.isArray(o) ? E.visitArray(o, p) : g(o) ? E.visitStringMap(o, p) : o == null || typeof o == "string" || typeof o == "number" || typeof o == "boolean" ? E.visitPrimitive(o, p) : E.visitOther(o, p);
}
e.visitValue = i;
function f(o) {
return o != null;
}
e.isDefined = f;
function c(o) {
return o === void 0 ? null : o;
}
e.noUndefined = c;
var F = class {
visitArray(o, E) {
return o.map((p) => i(p, this, E));
}
visitStringMap(o, E) {
let p = {};
return Object.keys(o).forEach((A) => {
p[A] = i(o[A], this, E);
}), p;
}
visitPrimitive(o, E) {
return o;
}
visitOther(o, E) {
return o;
}
};
e.ValueTransformer = F, e.SyncAsync = { assertSync: (o) => {
if (_(o))
throw new Error("Illegal state: value cannot be a promise");
return o;
}, then: (o, E) => _(o) ? o.then(E) : E(o), all: (o) => o.some(_) ? Promise.all(o) : o };
function a(o) {
throw new Error(`Internal Error: ${o}`);
}
e.error = a;
function l(o, E) {
let p = Error(o);
return p[h] = true, E && (p[C] = E), p;
}
e.syntaxError = l;
var h = "ngSyntaxError", C = "ngParseErrors";
function d(o) {
return o[h];
}
e.isSyntaxError = d;
function m(o) {
return o[C] || [];
}
e.getParseErrors = m;
function T(o) {
return o.replace(/([.*+?^=!:${}()|[\]\/\\])/g, "\\$1");
}
e.escapeRegExp = T;
var w = Object.getPrototypeOf({});
function g(o) {
return typeof o == "object" && o !== null && Object.getPrototypeOf(o) === w;
}
function N(o) {
let E = "";
for (let p = 0; p < o.length; p++) {
let A = o.charCodeAt(p);
if (A >= 55296 && A <= 56319 && o.length > p + 1) {
let P = o.charCodeAt(p + 1);
P >= 56320 && P <= 57343 && (p++, A = (A - 55296 << 10) + P - 56320 + 65536);
}
A <= 127 ? E += String.fromCharCode(A) : A <= 2047 ? E += String.fromCharCode(A >> 6 & 31 | 192, A & 63 | 128) : A <= 65535 ? E += String.fromCharCode(A >> 12 | 224, A >> 6 & 63 | 128, A & 63 | 128) : A <= 2097151 && (E += String.fromCharCode(A >> 18 & 7 | 240, A >> 12 & 63 | 128, A >> 6 & 63 | 128, A & 63 | 128));
}
return E;
}
e.utf8Encode = N;
function R(o) {
if (typeof o == "string")
return o;
if (o instanceof Array)
return "[" + o.map(R).join(", ") + "]";
if (o == null)
return "" + o;
if (o.overriddenName)
return `${o.overriddenName}`;
if (o.name)
return `${o.name}`;
if (!o.toString)
return "object";
let E = o.toString();
if (E == null)
return "" + E;
let p = E.indexOf(`
`);
return p === -1 ? E : E.substring(0, p);
}
e.stringify = R;
function j(o) {
return typeof o == "function" && o.hasOwnProperty("__forward_ref__") ? o() : o;
}
e.resolveForwardRef = j;
function _(o) {
return !!o && typeof o.then == "function";
}
e.isPromise = _;
var O = class {
constructor(o) {
this.full = o;
let E = o.split(".");
this.major = E[0], this.minor = E[1], this.patch = E.slice(2).join(".");
}
};
e.Version = O;
var x = typeof window < "u" && window, k = typeof self < "u" && typeof WorkerGlobalScope < "u" && self instanceof WorkerGlobalScope && self, $ = typeof globalThis < "u" && globalThis, t = $ || x || k;
e.global = t;
} }), al = I({ "node_modules/angular-html-parser/lib/compiler/src/compile_metadata.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = sl(), u = il(), n2 = /^(?:(?:\[([^\]]+)\])|(?:\(([^\)]+)\)))|(\@[-\w]+)$/;
function D(p) {
return p.replace(/\W/g, "_");
}
e.sanitizeIdentifier = D;
var s = 0;
function i(p) {
if (!p || !p.reference)
return null;
let A = p.reference;
if (A instanceof r.StaticSymbol)
return A.name;
if (A.__anonymousType)
return A.__anonymousType;
let P = u.stringify(A);
return P.indexOf("(") >= 0 ? (P = `anonymous_${s++}`, A.__anonymousType = P) : P = D(P), P;
}
e.identifierName = i;
function f(p) {
let A = p.reference;
return A instanceof r.StaticSymbol ? A.filePath : `./${u.stringify(A)}`;
}
e.identifierModuleUrl = f;
function c(p, A) {
return `View_${i({ reference: p })}_${A}`;
}
e.viewClassName = c;
function F(p) {
return `RenderType_${i({ reference: p })}`;
}
e.rendererTypeName = F;
function a(p) {
return `HostView_${i({ reference: p })}`;
}
e.hostViewClassName = a;
function l(p) {
return `${i({ reference: p })}NgFactory`;
}
e.componentFactoryName = l;
var h;
(function(p) {
p[p.Pipe = 0] = "Pipe", p[p.Directive = 1] = "Directive", p[p.NgModule = 2] = "NgModule", p[p.Injectable = 3] = "Injectable";
})(h = e.CompileSummaryKind || (e.CompileSummaryKind = {}));
function C(p) {
return p.value != null ? D(p.value) : i(p.identifier);
}
e.tokenName = C;
function d(p) {
return p.identifier != null ? p.identifier.reference : p.value;
}
e.tokenReference = d;
var m = class {
constructor() {
let { moduleUrl: p, styles: A, styleUrls: P } = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {};
this.moduleUrl = p || null, this.styles = _(A), this.styleUrls = _(P);
}
};
e.CompileStylesheetMetadata = m;
var T = class {
constructor(p) {
let { encapsulation: A, template: P, templateUrl: M, htmlAst: z, styles: V, styleUrls: X, externalStylesheets: H, animations: Q, ngContentSelectors: K, interpolation: J, isInline: v, preserveWhitespaces: y } = p;
if (this.encapsulation = A, this.template = P, this.templateUrl = M, this.htmlAst = z, this.styles = _(V), this.styleUrls = _(X), this.externalStylesheets = _(H), this.animations = Q ? x(Q) : [], this.ngContentSelectors = K || [], J && J.length != 2)
throw new Error("'interpolation' should have a start and an end symbol.");
this.interpolation = J, this.isInline = v, this.preserveWhitespaces = y;
}
toSummary() {
return { ngContentSelectors: this.ngContentSelectors, encapsulation: this.encapsulation, styles: this.styles, animations: this.animations };
}
};
e.CompileTemplateMetadata = T;
var w = class {
static create(p) {
let { isHost: A, type: P, isComponent: M, selector: z, exportAs: V, changeDetection: X, inputs: H, outputs: Q, host: K, providers: J, viewProviders: v, queries: y, guards: B, viewQueries: b, entryComponents: L, template: U, componentViewType: G, rendererType: W, componentFactory: ne } = p, be = {}, we = {}, Wr = {};
K != null && Object.keys(K).forEach((Z) => {
let ie = K[Z], Ce = Z.match(n2);
Ce === null ? Wr[Z] = ie : Ce[1] != null ? we[Ce[1]] = ie : Ce[2] != null && (be[Ce[2]] = ie);
});
let Yr = {};
H != null && H.forEach((Z) => {
let ie = u.splitAtColon(Z, [Z, Z]);
Yr[ie[0]] = ie[1];
});
let Qr = {};
return Q != null && Q.forEach((Z) => {
let ie = u.splitAtColon(Z, [Z, Z]);
Qr[ie[0]] = ie[1];
}), new w({ isHost: A, type: P, isComponent: !!M, selector: z, exportAs: V, changeDetection: X, inputs: Yr, outputs: Qr, hostListeners: be, hostProperties: we, hostAttributes: Wr, providers: J, viewProviders: v, queries: y, guards: B, viewQueries: b, entryComponents: L, template: U, componentViewType: G, rendererType: W, componentFactory: ne });
}
constructor(p) {
let { isHost: A, type: P, isComponent: M, selector: z, exportAs: V, changeDetection: X, inputs: H, outputs: Q, hostListeners: K, hostProperties: J, hostAttributes: v, providers: y, viewProviders: B, queries: b, guards: L, viewQueries: U, entryComponents: G, template: W, componentViewType: ne, rendererType: be, componentFactory: we } = p;
this.isHost = !!A, this.type = P, this.isComponent = M, this.selector = z, this.exportAs = V, this.changeDetection = X, this.inputs = H, this.outputs = Q, this.hostListeners = K, this.hostProperties = J, this.hostAttributes = v, this.providers = _(y), this.viewProviders = _(B), this.queries = _(b), this.guards = L, this.viewQueries = _(U), this.entryComponents = _(G), this.template = W, this.componentViewType = ne, this.rendererType = be, this.componentFactory = we;
}
toSummary() {
return { summaryKind: h.Directive, type: this.type, isComponent: this.isComponent, selector: this.selector, exportAs: this.exportAs, inputs: this.inputs, outputs: this.outputs, hostListeners: this.hostListeners, hostProperties: this.hostProperties, hostAttributes: this.hostAttributes, providers: this.providers, viewProviders: this.viewProviders, queries: this.queries, guards: this.guards, viewQueries: this.viewQueries, entryComponents: this.entryComponents, changeDetection: this.changeDetection, template: this.template && this.template.toSummary(), componentViewType: this.componentViewType, rendererType: this.rendererType, componentFactory: this.componentFactory };
}
};
e.CompileDirectiveMetadata = w;
var g = class {
constructor(p) {
let { type: A, name: P, pure: M } = p;
this.type = A, this.name = P, this.pure = !!M;
}
toSummary() {
return { summaryKind: h.Pipe, type: this.type, name: this.name, pure: this.pure };
}
};
e.CompilePipeMetadata = g;
var N = class {
};
e.CompileShallowModuleMetadata = N;
var R = class {
constructor(p) {
let { type: A, providers: P, declaredDirectives: M, exportedDirectives: z, declaredPipes: V, exportedPipes: X, entryComponents: H, bootstrapComponents: Q, importedModules: K, exportedModules: J, schemas: v, transitiveModule: y, id: B } = p;
this.type = A || null, this.declaredDirectives = _(M), this.exportedDirectives = _(z), this.declaredPipes = _(V), this.exportedPipes = _(X), this.providers = _(P), this.entryComponents = _(H), this.bootstrapComponents = _(Q), this.importedModules = _(K), this.exportedModules = _(J), this.schemas = _(v), this.id = B || null, this.transitiveModule = y || null;
}
toSummary() {
let p = this.transitiveModule;
return { summaryKind: h.NgModule, type: this.type, entryComponents: p.entryComponents, providers: p.providers, modules: p.modules, exportedDirectives: p.exportedDirectives, exportedPipes: p.exportedPipes };
}
};
e.CompileNgModuleMetadata = R;
var j = class {
constructor() {
this.directivesSet = /* @__PURE__ */ new Set(), this.directives = [], this.exportedDirectivesSet = /* @__PURE__ */ new Set(), this.exportedDirectives = [], this.pipesSet = /* @__PURE__ */ new Set(), this.pipes = [], this.exportedPipesSet = /* @__PURE__ */ new Set(), this.exportedPipes = [], this.modulesSet = /* @__PURE__ */ new Set(), this.modules = [], this.entryComponentsSet = /* @__PURE__ */ new Set(), this.entryComponents = [], this.providers = [];
}
addProvider(p, A) {
this.providers.push({ provider: p, module: A });
}
addDirective(p) {
this.directivesSet.has(p.reference) || (this.directivesSet.add(p.reference), this.directives.push(p));
}
addExportedDirective(p) {
this.exportedDirectivesSet.has(p.reference) || (this.exportedDirectivesSet.add(p.reference), this.exportedDirectives.push(p));
}
addPipe(p) {
this.pipesSet.has(p.reference) || (this.pipesSet.add(p.reference), this.pipes.push(p));
}
addExportedPipe(p) {
this.exportedPipesSet.has(p.reference) || (this.exportedPipesSet.add(p.reference), this.exportedPipes.push(p));
}
addModule(p) {
this.modulesSet.has(p.reference) || (this.modulesSet.add(p.reference), this.modules.push(p));
}
addEntryComponent(p) {
this.entryComponentsSet.has(p.componentType) || (this.entryComponentsSet.add(p.componentType), this.entryComponents.push(p));
}
};
e.TransitiveCompileNgModuleMetadata = j;
function _(p) {
return p || [];
}
var O = class {
constructor(p, A) {
let { useClass: P, useValue: M, useExisting: z, useFactory: V, deps: X, multi: H } = A;
this.token = p, this.useClass = P || null, this.useValue = M, this.useExisting = z, this.useFactory = V || null, this.dependencies = X || null, this.multi = !!H;
}
};
e.ProviderMeta = O;
function x(p) {
return p.reduce((A, P) => {
let M = Array.isArray(P) ? x(P) : P;
return A.concat(M);
}, []);
}
e.flatten = x;
function k(p) {
return p.replace(/(\w+:\/\/[\w:-]+)?(\/+)?/, "ng:///");
}
function $(p, A, P) {
let M;
return P.isInline ? A.type.reference instanceof r.StaticSymbol ? M = `${A.type.reference.filePath}.${A.type.reference.name}.html` : M = `${i(p)}/${i(A.type)}.html` : M = P.templateUrl, A.type.reference instanceof r.StaticSymbol ? M : k(M);
}
e.templateSourceUrl = $;
function t(p, A) {
let P = p.moduleUrl.split(/\/\\/g), M = P[P.length - 1];
return k(`css/${A}${M}.ngstyle.js`);
}
e.sharedStylesheetJitUrl = t;
function o(p) {
return k(`${i(p.type)}/module.ngfactory.js`);
}
e.ngModuleJitUrl = o;
function E(p, A) {
return k(`${i(p)}/${i(A.type)}.ngfactory.js`);
}
e.templateJitUrl = E;
} }), Be = I({ "node_modules/angular-html-parser/lib/compiler/src/parse_util.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = Es(), u = al(), n2 = class {
constructor(a, l, h, C) {
this.file = a, this.offset = l, this.line = h, this.col = C;
}
toString() {
return this.offset != null ? `${this.file.url}@${this.line}:${this.col}` : this.file.url;
}
moveBy(a) {
let l = this.file.content, h = l.length, C = this.offset, d = this.line, m = this.col;
for (; C > 0 && a < 0; )
if (C--, a++, l.charCodeAt(C) == r.$LF) {
d--;
let w = l.substr(0, C - 1).lastIndexOf(String.fromCharCode(r.$LF));
m = w > 0 ? C - w : C;
} else
m--;
for (; C < h && a > 0; ) {
let T = l.charCodeAt(C);
C++, a--, T == r.$LF ? (d++, m = 0) : m++;
}
return new n2(this.file, C, d, m);
}
getContext(a, l) {
let h = this.file.content, C = this.offset;
if (C != null) {
C > h.length - 1 && (C = h.length - 1);
let d = C, m = 0, T = 0;
for (; m < a && C > 0 && (C--, m++, !(h[C] == `
` && ++T == l)); )
;
for (m = 0, T = 0; m < a && d < h.length - 1 && (d++, m++, !(h[d] == `
` && ++T == l)); )
;
return { before: h.substring(C, this.offset), after: h.substring(this.offset, d + 1) };
}
return null;
}
};
e.ParseLocation = n2;
var D = class {
constructor(a, l) {
this.content = a, this.url = l;
}
};
e.ParseSourceFile = D;
var s = class {
constructor(a, l) {
let h = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : null;
this.start = a, this.end = l, this.details = h;
}
toString() {
return this.start.file.content.substring(this.start.offset, this.end.offset);
}
};
e.ParseSourceSpan = s, e.EMPTY_PARSE_LOCATION = new n2(new D("", ""), 0, 0, 0), e.EMPTY_SOURCE_SPAN = new s(e.EMPTY_PARSE_LOCATION, e.EMPTY_PARSE_LOCATION);
var i;
(function(a) {
a[a.WARNING = 0] = "WARNING", a[a.ERROR = 1] = "ERROR";
})(i = e.ParseErrorLevel || (e.ParseErrorLevel = {}));
var f = class {
constructor(a, l) {
let h = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : i.ERROR;
this.span = a, this.msg = l, this.level = h;
}
contextualMessage() {
let a = this.span.start.getContext(100, 3);
return a ? `${this.msg} ("${a.before}[${i[this.level]} ->]${a.after}")` : this.msg;
}
toString() {
let a = this.span.details ? `, ${this.span.details}` : "";
return `${this.contextualMessage()}: ${this.span.start}${a}`;
}
};
e.ParseError = f;
function c(a, l) {
let h = u.identifierModuleUrl(l), C = h != null ? `in ${a} ${u.identifierName(l)} in ${h}` : `in ${a} ${u.identifierName(l)}`, d = new D("", C);
return new s(new n2(d, -1, -1, -1), new n2(d, -1, -1, -1));
}
e.typeSourceSpan = c;
function F(a, l, h) {
let C = `in ${a} ${l} in ${h}`, d = new D("", C);
return new s(new n2(d, -1, -1, -1), new n2(d, -1, -1, -1));
}
e.r3JitTypeSourceSpan = F;
} }), ol = I({ "src/utils/front-matter/parse.js"(e, r) {
"use strict";
q();
var u = new RegExp("^(?-{3}|\\+{3})(?[^\\n]*)\\n(?:|(?.*?)\\n)(?\\k|\\.{3})[^\\S\\n]*(?:\\n|$)", "s");
function n2(D) {
let s = D.match(u);
if (!s)
return { content: D };
let { startDelimiter: i, language: f, value: c = "", endDelimiter: F } = s.groups, a = f.trim() || "yaml";
if (i === "+++" && (a = "toml"), a !== "yaml" && i !== F)
return { content: D };
let [l] = s;
return { frontMatter: { type: "front-matter", lang: a, value: c, startDelimiter: i, endDelimiter: F, raw: l.replace(/\n$/, "") }, content: l.replace(/[^\n]/g, " ") + D.slice(l.length) };
}
r.exports = n2;
} }), Cs = I({ "src/utils/get-last.js"(e, r) {
"use strict";
q();
var u = (n2) => n2[n2.length - 1];
r.exports = u;
} }), Dl = I({ "src/common/parser-create-error.js"(e, r) {
"use strict";
q();
function u(n2, D) {
let s = new SyntaxError(n2 + " (" + D.start.line + ":" + D.start.column + ")");
return s.loc = D, s;
}
r.exports = u;
} }), ms = {};
ps(ms, { default: () => ll });
function ll(e) {
if (typeof e != "string")
throw new TypeError("Expected a string");
return e.replace(/[|\\{}()[\]^$+*?.]/g, "\\$&").replace(/-/g, "\\x2d");
}
var cl = Ee({ "node_modules/escape-string-regexp/index.js"() {
q();
} }), gs = I({ "node_modules/semver/internal/debug.js"(e, r) {
q();
var u = typeof Te == "object" && Te.env && Te.env.NODE_DEBUG && /\bsemver\b/i.test(Te.env.NODE_DEBUG) ? function() {
for (var n2 = arguments.length, D = new Array(n2), s = 0; s < n2; s++)
D[s] = arguments[s];
return console.error("SEMVER", ...D);
} : () => {
};
r.exports = u;
} }), Fs = I({ "node_modules/semver/internal/constants.js"(e, r) {
q();
var u = "2.0.0", n2 = 256, D = Number.MAX_SAFE_INTEGER || 9007199254740991, s = 16;
r.exports = { SEMVER_SPEC_VERSION: u, MAX_LENGTH: n2, MAX_SAFE_INTEGER: D, MAX_SAFE_COMPONENT_LENGTH: s };
} }), hl = I({ "node_modules/semver/internal/re.js"(e, r) {
q();
var { MAX_SAFE_COMPONENT_LENGTH: u } = Fs(), n2 = gs();
e = r.exports = {};
var D = e.re = [], s = e.src = [], i = e.t = {}, f = 0, c = (F, a, l) => {
let h = f++;
n2(F, h, a), i[F] = h, s[h] = a, D[h] = new RegExp(a, l ? "g" : void 0);
};
c("NUMERICIDENTIFIER", "0|[1-9]\\d*"), c("NUMERICIDENTIFIERLOOSE", "[0-9]+"), c("NONNUMERICIDENTIFIER", "\\d*[a-zA-Z-][a-zA-Z0-9-]*"), c("MAINVERSION", `(${s[i.NUMERICIDENTIFIER]})\\.(${s[i.NUMERICIDENTIFIER]})\\.(${s[i.NUMERICIDENTIFIER]})`), c("MAINVERSIONLOOSE", `(${s[i.NUMERICIDENTIFIERLOOSE]})\\.(${s[i.NUMERICIDENTIFIERLOOSE]})\\.(${s[i.NUMERICIDENTIFIERLOOSE]})`), c("PRERELEASEIDENTIFIER", `(?:${s[i.NUMERICIDENTIFIER]}|${s[i.NONNUMERICIDENTIFIER]})`), c("PRERELEASEIDENTIFIERLOOSE", `(?:${s[i.NUMERICIDENTIFIERLOOSE]}|${s[i.NONNUMERICIDENTIFIER]})`), c("PRERELEASE", `(?:-(${s[i.PRERELEASEIDENTIFIER]}(?:\\.${s[i.PRERELEASEIDENTIFIER]})*))`), c("PRERELEASELOOSE", `(?:-?(${s[i.PRERELEASEIDENTIFIERLOOSE]}(?:\\.${s[i.PRERELEASEIDENTIFIERLOOSE]})*))`), c("BUILDIDENTIFIER", "[0-9A-Za-z-]+"), c("BUILD", `(?:\\+(${s[i.BUILDIDENTIFIER]}(?:\\.${s[i.BUILDIDENTIFIER]})*))`), c("FULLPLAIN", `v?${s[i.MAINVERSION]}${s[i.PRERELEASE]}?${s[i.BUILD]}?`), c("FULL", `^${s[i.FULLPLAIN]}$`), c("LOOSEPLAIN", `[v=\\s]*${s[i.MAINVERSIONLOOSE]}${s[i.PRERELEASELOOSE]}?${s[i.BUILD]}?`), c("LOOSE", `^${s[i.LOOSEPLAIN]}$`), c("GTLT", "((?:<|>)?=?)"), c("XRANGEIDENTIFIERLOOSE", `${s[i.NUMERICIDENTIFIERLOOSE]}|x|X|\\*`), c("XRANGEIDENTIFIER", `${s[i.NUMERICIDENTIFIER]}|x|X|\\*`), c("XRANGEPLAIN", `[v=\\s]*(${s[i.XRANGEIDENTIFIER]})(?:\\.(${s[i.XRANGEIDENTIFIER]})(?:\\.(${s[i.XRANGEIDENTIFIER]})(?:${s[i.PRERELEASE]})?${s[i.BUILD]}?)?)?`), c("XRANGEPLAINLOOSE", `[v=\\s]*(${s[i.XRANGEIDENTIFIERLOOSE]})(?:\\.(${s[i.XRANGEIDENTIFIERLOOSE]})(?:\\.(${s[i.XRANGEIDENTIFIERLOOSE]})(?:${s[i.PRERELEASELOOSE]})?${s[i.BUILD]}?)?)?`), c("XRANGE", `^${s[i.GTLT]}\\s*${s[i.XRANGEPLAIN]}$`), c("XRANGELOOSE", `^${s[i.GTLT]}\\s*${s[i.XRANGEPLAINLOOSE]}$`), c("COERCE", `(^|[^\\d])(\\d{1,${u}})(?:\\.(\\d{1,${u}}))?(?:\\.(\\d{1,${u}}))?(?:$|[^\\d])`), c("COERCERTL", s[i.COERCE], true), c("LONETILDE", "(?:~>?)"), c("TILDETRIM", `(\\s*)${s[i.LONETILDE]}\\s+`, true), e.tildeTrimReplace = "$1~", c("TILDE", `^${s[i.LONETILDE]}${s[i.XRANGEPLAIN]}$`), c("TILDELOOSE", `^${s[i.LONETILDE]}${s[i.XRANGEPLAINLOOSE]}$`), c("LONECARET", "(?:\\^)"), c("CARETTRIM", `(\\s*)${s[i.LONECARET]}\\s+`, true), e.caretTrimReplace = "$1^", c("CARET", `^${s[i.LONECARET]}${s[i.XRANGEPLAIN]}$`), c("CARETLOOSE", `^${s[i.LONECARET]}${s[i.XRANGEPLAINLOOSE]}$`), c("COMPARATORLOOSE", `^${s[i.GTLT]}\\s*(${s[i.LOOSEPLAIN]})$|^$`), c("COMPARATOR", `^${s[i.GTLT]}\\s*(${s[i.FULLPLAIN]})$|^$`), c("COMPARATORTRIM", `(\\s*)${s[i.GTLT]}\\s*(${s[i.LOOSEPLAIN]}|${s[i.XRANGEPLAIN]})`, true), e.comparatorTrimReplace = "$1$2$3", c("HYPHENRANGE", `^\\s*(${s[i.XRANGEPLAIN]})\\s+-\\s+(${s[i.XRANGEPLAIN]})\\s*$`), c("HYPHENRANGELOOSE", `^\\s*(${s[i.XRANGEPLAINLOOSE]})\\s+-\\s+(${s[i.XRANGEPLAINLOOSE]})\\s*$`), c("STAR", "(<|>)?=?\\s*\\*"), c("GTE0", "^\\s*>=\\s*0\\.0\\.0\\s*$"), c("GTE0PRE", "^\\s*>=\\s*0\\.0\\.0-0\\s*$");
} }), pl = I({ "node_modules/semver/internal/parse-options.js"(e, r) {
q();
var u = ["includePrerelease", "loose", "rtl"], n2 = (D) => D ? typeof D != "object" ? { loose: true } : u.filter((s) => D[s]).reduce((s, i) => (s[i] = true, s), {}) : {};
r.exports = n2;
} }), fl = I({ "node_modules/semver/internal/identifiers.js"(e, r) {
q();
var u = /^[0-9]+$/, n2 = (s, i) => {
let f = u.test(s), c = u.test(i);
return f && c && (s = +s, i = +i), s === i ? 0 : f && !c ? -1 : c && !f ? 1 : s < i ? -1 : 1;
}, D = (s, i) => n2(i, s);
r.exports = { compareIdentifiers: n2, rcompareIdentifiers: D };
} }), dl = I({ "node_modules/semver/classes/semver.js"(e, r) {
q();
var u = gs(), { MAX_LENGTH: n2, MAX_SAFE_INTEGER: D } = Fs(), { re: s, t: i } = hl(), f = pl(), { compareIdentifiers: c } = fl(), F = class {
constructor(a, l) {
if (l = f(l), a instanceof F) {
if (a.loose === !!l.loose && a.includePrerelease === !!l.includePrerelease)
return a;
a = a.version;
} else if (typeof a != "string")
throw new TypeError(`Invalid Version: ${a}`);
if (a.length > n2)
throw new TypeError(`version is longer than ${n2} characters`);
u("SemVer", a, l), this.options = l, this.loose = !!l.loose, this.includePrerelease = !!l.includePrerelease;
let h = a.trim().match(l.loose ? s[i.LOOSE] : s[i.FULL]);
if (!h)
throw new TypeError(`Invalid Version: ${a}`);
if (this.raw = a, this.major = +h[1], this.minor = +h[2], this.patch = +h[3], this.major > D || this.major < 0)
throw new TypeError("Invalid major version");
if (this.minor > D || this.minor < 0)
throw new TypeError("Invalid minor version");
if (this.patch > D || this.patch < 0)
throw new TypeError("Invalid patch version");
h[4] ? this.prerelease = h[4].split(".").map((C) => {
if (/^[0-9]+$/.test(C)) {
let d = +C;
if (d >= 0 && d < D)
return d;
}
return C;
}) : this.prerelease = [], this.build = h[5] ? h[5].split(".") : [], this.format();
}
format() {
return this.version = `${this.major}.${this.minor}.${this.patch}`, this.prerelease.length && (this.version += `-${this.prerelease.join(".")}`), this.version;
}
toString() {
return this.version;
}
compare(a) {
if (u("SemVer.compare", this.version, this.options, a), !(a instanceof F)) {
if (typeof a == "string" && a === this.version)
return 0;
a = new F(a, this.options);
}
return a.version === this.version ? 0 : this.compareMain(a) || this.comparePre(a);
}
compareMain(a) {
return a instanceof F || (a = new F(a, this.options)), c(this.major, a.major) || c(this.minor, a.minor) || c(this.patch, a.patch);
}
comparePre(a) {
if (a instanceof F || (a = new F(a, this.options)), this.prerelease.length && !a.prerelease.length)
return -1;
if (!this.prerelease.length && a.prerelease.length)
return 1;
if (!this.prerelease.length && !a.prerelease.length)
return 0;
let l = 0;
do {
let h = this.prerelease[l], C = a.prerelease[l];
if (u("prerelease compare", l, h, C), h === void 0 && C === void 0)
return 0;
if (C === void 0)
return 1;
if (h === void 0)
return -1;
if (h === C)
continue;
return c(h, C);
} while (++l);
}
compareBuild(a) {
a instanceof F || (a = new F(a, this.options));
let l = 0;
do {
let h = this.build[l], C = a.build[l];
if (u("prerelease compare", l, h, C), h === void 0 && C === void 0)
return 0;
if (C === void 0)
return 1;
if (h === void 0)
return -1;
if (h === C)
continue;
return c(h, C);
} while (++l);
}
inc(a, l) {
switch (a) {
case "premajor":
this.prerelease.length = 0, this.patch = 0, this.minor = 0, this.major++, this.inc("pre", l);
break;
case "preminor":
this.prerelease.length = 0, this.patch = 0, this.minor++, this.inc("pre", l);
break;
case "prepatch":
this.prerelease.length = 0, this.inc("patch", l), this.inc("pre", l);
break;
case "prerelease":
this.prerelease.length === 0 && this.inc("patch", l), this.inc("pre", l);
break;
case "major":
(this.minor !== 0 || this.patch !== 0 || this.prerelease.length === 0) && this.major++, this.minor = 0, this.patch = 0, this.prerelease = [];
break;
case "minor":
(this.patch !== 0 || this.prerelease.length === 0) && this.minor++, this.patch = 0, this.prerelease = [];
break;
case "patch":
this.prerelease.length === 0 && this.patch++, this.prerelease = [];
break;
case "pre":
if (this.prerelease.length === 0)
this.prerelease = [0];
else {
let h = this.prerelease.length;
for (; --h >= 0; )
typeof this.prerelease[h] == "number" && (this.prerelease[h]++, h = -2);
h === -1 && this.prerelease.push(0);
}
l && (c(this.prerelease[0], l) === 0 ? isNaN(this.prerelease[1]) && (this.prerelease = [l, 0]) : this.prerelease = [l, 0]);
break;
default:
throw new Error(`invalid increment argument: ${a}`);
}
return this.format(), this.raw = this.version, this;
}
};
r.exports = F;
} }), Hr = I({ "node_modules/semver/functions/compare.js"(e, r) {
q();
var u = dl(), n2 = (D, s, i) => new u(D, i).compare(new u(s, i));
r.exports = n2;
} }), El = I({ "node_modules/semver/functions/lt.js"(e, r) {
q();
var u = Hr(), n2 = (D, s, i) => u(D, s, i) < 0;
r.exports = n2;
} }), Cl = I({ "node_modules/semver/functions/gte.js"(e, r) {
q();
var u = Hr(), n2 = (D, s, i) => u(D, s, i) >= 0;
r.exports = n2;
} }), ml = I({ "src/utils/arrayify.js"(e, r) {
"use strict";
q(), r.exports = (u, n2) => Object.entries(u).map((D) => {
let [s, i] = D;
return Object.assign({ [n2]: s }, i);
});
} }), gl = I({ "package.json"(e, r) {
r.exports = { version: "2.8.8" };
} }), Fl = I({ "node_modules/outdent/lib/index.js"(e, r) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true }), e.outdent = void 0;
function u() {
for (var g = [], N = 0; N < arguments.length; N++)
g[N] = arguments[N];
}
function n2() {
return typeof WeakMap < "u" ? /* @__PURE__ */ new WeakMap() : D();
}
function D() {
return { add: u, delete: u, get: u, set: u, has: function(g) {
return false;
} };
}
var s = Object.prototype.hasOwnProperty, i = function(g, N) {
return s.call(g, N);
};
function f(g, N) {
for (var R in N)
i(N, R) && (g[R] = N[R]);
return g;
}
var c = /^[ \t]*(?:\r\n|\r|\n)/, F = /(?:\r\n|\r|\n)[ \t]*$/, a = /^(?:[\r\n]|$)/, l = /(?:\r\n|\r|\n)([ \t]*)(?:[^ \t\r\n]|$)/, h = /^[ \t]*[\r\n][ \t\r\n]*$/;
function C(g, N, R) {
var j = 0, _ = g[0].match(l);
_ && (j = _[1].length);
var O = "(\\r\\n|\\r|\\n).{0," + j + "}", x = new RegExp(O, "g");
N && (g = g.slice(1));
var k = R.newline, $ = R.trimLeadingNewline, t = R.trimTrailingNewline, o = typeof k == "string", E = g.length, p = g.map(function(A, P) {
return A = A.replace(x, "$1"), P === 0 && $ && (A = A.replace(c, "")), P === E - 1 && t && (A = A.replace(F, "")), o && (A = A.replace(/\r\n|\n|\r/g, function(M) {
return k;
})), A;
});
return p;
}
function d(g, N) {
for (var R = "", j = 0, _ = g.length; j < _; j++)
R += g[j], j < _ - 1 && (R += N[j]);
return R;
}
function m(g) {
return i(g, "raw") && i(g, "length");
}
function T(g) {
var N = n2(), R = n2();
function j(O) {
for (var x = [], k = 1; k < arguments.length; k++)
x[k - 1] = arguments[k];
if (m(O)) {
var $ = O, t = (x[0] === j || x[0] === w) && h.test($[0]) && a.test($[1]), o = t ? R : N, E = o.get($);
if (E || (E = C($, t, g), o.set($, E)), x.length === 0)
return E[0];
var p = d(E, t ? x.slice(1) : x);
return p;
} else
return T(f(f({}, g), O || {}));
}
var _ = f(j, { string: function(O) {
return C([O], false, g)[0];
} });
return _;
}
var w = T({ trimLeadingNewline: true, trimTrailingNewline: true });
if (e.outdent = w, e.default = w, typeof r < "u")
try {
r.exports = w, Object.defineProperty(w, "__esModule", { value: true }), w.default = w, w.outdent = w;
} catch {
}
} }), Al = I({ "src/main/core-options.js"(e, r) {
"use strict";
q();
var { outdent: u } = Fl(), n2 = "Config", D = "Editor", s = "Format", i = "Other", f = "Output", c = "Global", F = "Special", a = { cursorOffset: { since: "1.4.0", category: F, type: "int", default: -1, range: { start: -1, end: Number.POSITIVE_INFINITY, step: 1 }, description: u`
Print (to stderr) where a cursor at the given position would move to after formatting.
This option cannot be used with --range-start and --range-end.
`, cliCategory: D }, endOfLine: { since: "1.15.0", category: c, type: "choice", default: [{ since: "1.15.0", value: "auto" }, { since: "2.0.0", value: "lf" }], description: "Which end of line characters to apply.", choices: [{ value: "lf", description: "Line Feed only (\\n), common on Linux and macOS as well as inside git repos" }, { value: "crlf", description: "Carriage Return + Line Feed characters (\\r\\n), common on Windows" }, { value: "cr", description: "Carriage Return character only (\\r), used very rarely" }, { value: "auto", description: u`
Maintain existing
(mixed values within one file are normalised by looking at what's used after the first line)
` }] }, filepath: { since: "1.4.0", category: F, type: "path", description: "Specify the input filepath. This will be used to do parser inference.", cliName: "stdin-filepath", cliCategory: i, cliDescription: "Path to the file to pretend that stdin comes from." }, insertPragma: { since: "1.8.0", category: F, type: "boolean", default: false, description: "Insert @format pragma into file's first docblock comment.", cliCategory: i }, parser: { since: "0.0.10", category: c, type: "choice", default: [{ since: "0.0.10", value: "babylon" }, { since: "1.13.0", value: void 0 }], description: "Which parser to use.", exception: (l) => typeof l == "string" || typeof l == "function", choices: [{ value: "flow", description: "Flow" }, { value: "babel", since: "1.16.0", description: "JavaScript" }, { value: "babel-flow", since: "1.16.0", description: "Flow" }, { value: "babel-ts", since: "2.0.0", description: "TypeScript" }, { value: "typescript", since: "1.4.0", description: "TypeScript" }, { value: "acorn", since: "2.6.0", description: "JavaScript" }, { value: "espree", since: "2.2.0", description: "JavaScript" }, { value: "meriyah", since: "2.2.0", description: "JavaScript" }, { value: "css", since: "1.7.1", description: "CSS" }, { value: "less", since: "1.7.1", description: "Less" }, { value: "scss", since: "1.7.1", description: "SCSS" }, { value: "json", since: "1.5.0", description: "JSON" }, { value: "json5", since: "1.13.0", description: "JSON5" }, { value: "json-stringify", since: "1.13.0", description: "JSON.stringify" }, { value: "graphql", since: "1.5.0", description: "GraphQL" }, { value: "markdown", since: "1.8.0", description: "Markdown" }, { value: "mdx", since: "1.15.0", description: "MDX" }, { value: "vue", since: "1.10.0", description: "Vue" }, { value: "yaml", since: "1.14.0", description: "YAML" }, { value: "glimmer", since: "2.3.0", description: "Ember / Handlebars" }, { value: "html", since: "1.15.0", description: "HTML" }, { value: "angular", since: "1.15.0", description: "Angular" }, { value: "lwc", since: "1.17.0", description: "Lightning Web Components" }] }, plugins: { since: "1.10.0", type: "path", array: true, default: [{ value: [] }], category: c, description: "Add a plugin. Multiple plugins can be passed as separate `--plugin`s.", exception: (l) => typeof l == "string" || typeof l == "object", cliName: "plugin", cliCategory: n2 }, pluginSearchDirs: { since: "1.13.0", type: "path", array: true, default: [{ value: [] }], category: c, description: u`
Custom directory that contains prettier plugins in node_modules subdirectory.
Overrides default behavior when plugins are searched relatively to the location of Prettier.
Multiple values are accepted.
`, exception: (l) => typeof l == "string" || typeof l == "object", cliName: "plugin-search-dir", cliCategory: n2 }, printWidth: { since: "0.0.0", category: c, type: "int", default: 80, description: "The line length where Prettier will try wrap.", range: { start: 0, end: Number.POSITIVE_INFINITY, step: 1 } }, rangeEnd: { since: "1.4.0", category: F, type: "int", default: Number.POSITIVE_INFINITY, range: { start: 0, end: Number.POSITIVE_INFINITY, step: 1 }, description: u`
Format code ending at a given character offset (exclusive).
The range will extend forwards to the end of the selected statement.
This option cannot be used with --cursor-offset.
`, cliCategory: D }, rangeStart: { since: "1.4.0", category: F, type: "int", default: 0, range: { start: 0, end: Number.POSITIVE_INFINITY, step: 1 }, description: u`
Format code starting at a given character offset.
The range will extend backwards to the start of the first line containing the selected statement.
This option cannot be used with --cursor-offset.
`, cliCategory: D }, requirePragma: { since: "1.7.0", category: F, type: "boolean", default: false, description: u`
Require either '@prettier' or '@format' to be present in the file's first docblock comment
in order for it to be formatted.
`, cliCategory: i }, tabWidth: { type: "int", category: c, default: 2, description: "Number of spaces per indentation level.", range: { start: 0, end: Number.POSITIVE_INFINITY, step: 1 } }, useTabs: { since: "1.0.0", category: c, type: "boolean", default: false, description: "Indent with tabs instead of spaces." }, embeddedLanguageFormatting: { since: "2.1.0", category: c, type: "choice", default: [{ since: "2.1.0", value: "auto" }], description: "Control how Prettier formats quoted code embedded in the file.", choices: [{ value: "auto", description: "Format embedded code if Prettier can automatically identify it." }, { value: "off", description: "Never automatically format embedded code." }] } };
r.exports = { CATEGORY_CONFIG: n2, CATEGORY_EDITOR: D, CATEGORY_FORMAT: s, CATEGORY_OTHER: i, CATEGORY_OUTPUT: f, CATEGORY_GLOBAL: c, CATEGORY_SPECIAL: F, options: a };
} }), vl = I({ "src/main/support.js"(e, r) {
"use strict";
q();
var u = { compare: Hr(), lt: El(), gte: Cl() }, n2 = ml(), D = gl().version, s = Al().options;
function i() {
let { plugins: c = [], showUnreleased: F = false, showDeprecated: a = false, showInternal: l = false } = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {}, h = D.split("-", 1)[0], C = c.flatMap((g) => g.languages || []).filter(m), d = n2(Object.assign({}, ...c.map((g) => {
let { options: N } = g;
return N;
}), s), "name").filter((g) => m(g) && T(g)).sort((g, N) => g.name === N.name ? 0 : g.name < N.name ? -1 : 1).map(w).map((g) => {
g = Object.assign({}, g), Array.isArray(g.default) && (g.default = g.default.length === 1 ? g.default[0].value : g.default.filter(m).sort((R, j) => u.compare(j.since, R.since))[0].value), Array.isArray(g.choices) && (g.choices = g.choices.filter((R) => m(R) && T(R)), g.name === "parser" && f(g, C, c));
let N = Object.fromEntries(c.filter((R) => R.defaultOptions && R.defaultOptions[g.name] !== void 0).map((R) => [R.name, R.defaultOptions[g.name]]));
return Object.assign(Object.assign({}, g), {}, { pluginDefaults: N });
});
return { languages: C, options: d };
function m(g) {
return F || !("since" in g) || g.since && u.gte(h, g.since);
}
function T(g) {
return a || !("deprecated" in g) || g.deprecated && u.lt(h, g.deprecated);
}
function w(g) {
if (l)
return g;
let { cliName: N, cliCategory: R, cliDescription: j } = g;
return JD(g, KD);
}
}
function f(c, F, a) {
let l = new Set(c.choices.map((h) => h.value));
for (let h of F)
if (h.parsers) {
for (let C of h.parsers)
if (!l.has(C)) {
l.add(C);
let d = a.find((T) => T.parsers && T.parsers[C]), m = h.name;
d && d.name && (m += ` (plugin: ${d.name})`), c.choices.push({ value: C, description: m });
}
}
}
r.exports = { getSupportInfo: i };
} }), _l = I({ "src/utils/is-non-empty-array.js"(e, r) {
"use strict";
q();
function u(n2) {
return Array.isArray(n2) && n2.length > 0;
}
r.exports = u;
} });
function Sl() {
let { onlyFirst: e = false } = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {}, r = ["[\\u001B\\u009B][[\\]()#;?]*(?:(?:(?:(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]+)*|[a-zA-Z\\d]+(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]*)*)?\\u0007)", "(?:(?:\\d{1,4}(?:;\\d{0,4})*)?[\\dA-PR-TZcf-ntqry=><~]))"].join("|");
return new RegExp(r, e ? void 0 : "g");
}
var yl = Ee({ "node_modules/strip-ansi/node_modules/ansi-regex/index.js"() {
q();
} });
function Tl(e) {
if (typeof e != "string")
throw new TypeError(`Expected a \`string\`, got \`${typeof e}\``);
return e.replace(Sl(), "");
}
var Bl = Ee({ "node_modules/strip-ansi/index.js"() {
q(), yl();
} });
function bl(e) {
return Number.isInteger(e) ? e >= 4352 && (e <= 4447 || e === 9001 || e === 9002 || 11904 <= e && e <= 12871 && e !== 12351 || 12880 <= e && e <= 19903 || 19968 <= e && e <= 42182 || 43360 <= e && e <= 43388 || 44032 <= e && e <= 55203 || 63744 <= e && e <= 64255 || 65040 <= e && e <= 65049 || 65072 <= e && e <= 65131 || 65281 <= e && e <= 65376 || 65504 <= e && e <= 65510 || 110592 <= e && e <= 110593 || 127488 <= e && e <= 127569 || 131072 <= e && e <= 262141) : false;
}
var wl = Ee({ "node_modules/is-fullwidth-code-point/index.js"() {
q();
} }), Nl = I({ "node_modules/emoji-regex/index.js"(e, r) {
"use strict";
q(), r.exports = function() {
return /\uD83C\uDFF4\uDB40\uDC67\uDB40\uDC62(?:\uDB40\uDC77\uDB40\uDC6C\uDB40\uDC73|\uDB40\uDC73\uDB40\uDC63\uDB40\uDC74|\uDB40\uDC65\uDB40\uDC6E\uDB40\uDC67)\uDB40\uDC7F|(?:\uD83E\uDDD1\uD83C\uDFFF\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFF\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB-\uDFFE])|(?:\uD83E\uDDD1\uD83C\uDFFE\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFE\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB-\uDFFD\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFD\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFD\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB\uDFFC\uDFFE\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFC\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFC\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB\uDFFD-\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFB\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFB\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFC-\uDFFF])|\uD83D\uDC68(?:\uD83C\uDFFB(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF]))|\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFC-\uDFFF])|[\u2695\u2696\u2708]\uFE0F|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))?|(?:\uD83C[\uDFFC-\uDFFF])\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF]))|\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83D\uDC68|(?:\uD83D[\uDC68\uDC69])\u200D(?:\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67]))|\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFE])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFD\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB\uDFFC\uDFFE\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB\uDFFD-\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])\uFE0F|\u200D(?:(?:\uD83D[\uDC68\uDC69])\u200D(?:\uD83D[\uDC66\uDC67])|\uD83D[\uDC66\uDC67])|\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC)?|(?:\uD83D\uDC69(?:\uD83C\uDFFB\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69])|(?:\uD83C[\uDFFC-\uDFFF])\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69]))|\uD83E\uDDD1(?:\uD83C[\uDFFB-\uDFFF])\u200D\uD83E\uDD1D\u200D\uD83E\uDDD1)(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC69\u200D\uD83D\uDC69\u200D(?:\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67]))|\uD83D\uDC69(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFB\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))|\uD83E\uDDD1(?:\u200D(?:\uD83E\uDD1D\u200D\uD83E\uDDD1|\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFB\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))|\uD83D\uDC69\u200D\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC69\u200D\uD83D\uDC69\u200D(?:\uD83D[\uDC66\uDC67])|\uD83D\uDC69\u200D\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|(?:\uD83D\uDC41\uFE0F\u200D\uD83D\uDDE8|\uD83E\uDDD1(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\uD83C\uDFFB\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])|\uD83D\uDC69(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\uD83C\uDFFB\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])|\uD83D\uDE36\u200D\uD83C\uDF2B|\uD83C\uDFF3\uFE0F\u200D\u26A7|\uD83D\uDC3B\u200D\u2744|(?:(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC6F|\uD83E[\uDD3C\uDDDE\uDDDF])\u200D[\u2640\u2642]|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])\u200D[\u2640\u2642]|\uD83C\uDFF4\u200D\u2620|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])\u200D[\u2640\u2642]|[\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u2328\u23CF\u23ED-\u23EF\u23F1\u23F2\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB\u25FC\u2600-\u2604\u260E\u2611\u2618\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u265F\u2660\u2663\u2665\u2666\u2668\u267B\u267E\u2692\u2694-\u2697\u2699\u269B\u269C\u26A0\u26A7\u26B0\u26B1\u26C8\u26CF\u26D1\u26D3\u26E9\u26F0\u26F1\u26F4\u26F7\u26F8\u2702\u2708\u2709\u270F\u2712\u2714\u2716\u271D\u2721\u2733\u2734\u2744\u2747\u2763\u27A1\u2934\u2935\u2B05-\u2B07\u3030\u303D\u3297\u3299]|\uD83C[\uDD70\uDD71\uDD7E\uDD7F\uDE02\uDE37\uDF21\uDF24-\uDF2C\uDF36\uDF7D\uDF96\uDF97\uDF99-\uDF9B\uDF9E\uDF9F\uDFCD\uDFCE\uDFD4-\uDFDF\uDFF5\uDFF7]|\uD83D[\uDC3F\uDCFD\uDD49\uDD4A\uDD6F\uDD70\uDD73\uDD76-\uDD79\uDD87\uDD8A-\uDD8D\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA\uDECB\uDECD-\uDECF\uDEE0-\uDEE5\uDEE9\uDEF0\uDEF3])\uFE0F|\uD83C\uDFF3\uFE0F\u200D\uD83C\uDF08|\uD83D\uDC69\u200D\uD83D\uDC67|\uD83D\uDC69\u200D\uD83D\uDC66|\uD83D\uDE35\u200D\uD83D\uDCAB|\uD83D\uDE2E\u200D\uD83D\uDCA8|\uD83D\uDC15\u200D\uD83E\uDDBA|\uD83E\uDDD1(?:\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC|\uD83C\uDFFB)?|\uD83D\uDC69(?:\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC|\uD83C\uDFFB)?|\uD83C\uDDFD\uD83C\uDDF0|\uD83C\uDDF6\uD83C\uDDE6|\uD83C\uDDF4\uD83C\uDDF2|\uD83D\uDC08\u200D\u2B1B|\u2764\uFE0F\u200D(?:\uD83D\uDD25|\uD83E\uDE79)|\uD83D\uDC41\uFE0F|\uD83C\uDFF3\uFE0F|\uD83C\uDDFF(?:\uD83C[\uDDE6\uDDF2\uDDFC])|\uD83C\uDDFE(?:\uD83C[\uDDEA\uDDF9])|\uD83C\uDDFC(?:\uD83C[\uDDEB\uDDF8])|\uD83C\uDDFB(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDEE\uDDF3\uDDFA])|\uD83C\uDDFA(?:\uD83C[\uDDE6\uDDEC\uDDF2\uDDF3\uDDF8\uDDFE\uDDFF])|\uD83C\uDDF9(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDED\uDDEF-\uDDF4\uDDF7\uDDF9\uDDFB\uDDFC\uDDFF])|\uD83C\uDDF8(?:\uD83C[\uDDE6-\uDDEA\uDDEC-\uDDF4\uDDF7-\uDDF9\uDDFB\uDDFD-\uDDFF])|\uD83C\uDDF7(?:\uD83C[\uDDEA\uDDF4\uDDF8\uDDFA\uDDFC])|\uD83C\uDDF5(?:\uD83C[\uDDE6\uDDEA-\uDDED\uDDF0-\uDDF3\uDDF7-\uDDF9\uDDFC\uDDFE])|\uD83C\uDDF3(?:\uD83C[\uDDE6\uDDE8\uDDEA-\uDDEC\uDDEE\uDDF1\uDDF4\uDDF5\uDDF7\uDDFA\uDDFF])|\uD83C\uDDF2(?:\uD83C[\uDDE6\uDDE8-\uDDED\uDDF0-\uDDFF])|\uD83C\uDDF1(?:\uD83C[\uDDE6-\uDDE8\uDDEE\uDDF0\uDDF7-\uDDFB\uDDFE])|\uD83C\uDDF0(?:\uD83C[\uDDEA\uDDEC-\uDDEE\uDDF2\uDDF3\uDDF5\uDDF7\uDDFC\uDDFE\uDDFF])|\uD83C\uDDEF(?:\uD83C[\uDDEA\uDDF2\uDDF4\uDDF5])|\uD83C\uDDEE(?:\uD83C[\uDDE8-\uDDEA\uDDF1-\uDDF4\uDDF6-\uDDF9])|\uD83C\uDDED(?:\uD83C[\uDDF0\uDDF2\uDDF3\uDDF7\uDDF9\uDDFA])|\uD83C\uDDEC(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEE\uDDF1-\uDDF3\uDDF5-\uDDFA\uDDFC\uDDFE])|\uD83C\uDDEB(?:\uD83C[\uDDEE-\uDDF0\uDDF2\uDDF4\uDDF7])|\uD83C\uDDEA(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDED\uDDF7-\uDDFA])|\uD83C\uDDE9(?:\uD83C[\uDDEA\uDDEC\uDDEF\uDDF0\uDDF2\uDDF4\uDDFF])|\uD83C\uDDE8(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDEE\uDDF0-\uDDF5\uDDF7\uDDFA-\uDDFF])|\uD83C\uDDE7(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEF\uDDF1-\uDDF4\uDDF6-\uDDF9\uDDFB\uDDFC\uDDFE\uDDFF])|\uD83C\uDDE6(?:\uD83C[\uDDE8-\uDDEC\uDDEE\uDDF1\uDDF2\uDDF4\uDDF6-\uDDFA\uDDFC\uDDFD\uDDFF])|[#\*0-9]\uFE0F\u20E3|\u2764\uFE0F|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])|\uD83C\uDFF4|(?:[\u270A\u270B]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC6B-\uDC6D\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDC8F\uDC91\uDCAA\uDD7A\uDD95\uDD96\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD34\uDD36\uDD77\uDDB5\uDDB6\uDDBB\uDDD2\uDDD3\uDDD5])(?:\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u270C\u270D]|\uD83D[\uDD74\uDD90])(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])|[\u270A\u270B]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC08\uDC15\uDC3B\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC6B-\uDC6D\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDC8F\uDC91\uDCAA\uDD7A\uDD95\uDD96\uDE2E\uDE35\uDE36\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD34\uDD36\uDD77\uDDB5\uDDB6\uDDBB\uDDD2\uDDD3\uDDD5]|\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD]|\uD83D\uDC6F|\uD83E[\uDD3C\uDDDE\uDDDF]|[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF84\uDF86-\uDF93\uDFA0-\uDFC1\uDFC5\uDFC6\uDFC8\uDFC9\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC07\uDC09-\uDC14\uDC16-\uDC3A\uDC3C-\uDC3E\uDC40\uDC44\uDC45\uDC51-\uDC65\uDC6A\uDC79-\uDC7B\uDC7D-\uDC80\uDC84\uDC88-\uDC8E\uDC90\uDC92-\uDCA9\uDCAB-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDDA4\uDDFB-\uDE2D\uDE2F-\uDE34\uDE37-\uDE44\uDE48-\uDE4A\uDE80-\uDEA2\uDEA4-\uDEB3\uDEB7-\uDEBF\uDEC1-\uDEC5\uDED0-\uDED2\uDED5-\uDED7\uDEEB\uDEEC\uDEF4-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0D\uDD0E\uDD10-\uDD17\uDD1D\uDD20-\uDD25\uDD27-\uDD2F\uDD3A\uDD3F-\uDD45\uDD47-\uDD76\uDD78\uDD7A-\uDDB4\uDDB7\uDDBA\uDDBC-\uDDCB\uDDD0\uDDE0-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6]|(?:[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u270A\u270B\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF93\uDFA0-\uDFCA\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF4\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC3E\uDC40\uDC42-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDD7A\uDD95\uDD96\uDDA4\uDDFB-\uDE4F\uDE80-\uDEC5\uDECC\uDED0-\uDED2\uDED5-\uDED7\uDEEB\uDEEC\uDEF4-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0C-\uDD3A\uDD3C-\uDD45\uDD47-\uDD78\uDD7A-\uDDCB\uDDCD-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6])|(?:[#\*0-9\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u231A\u231B\u2328\u23CF\u23E9-\u23F3\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB-\u25FE\u2600-\u2604\u260E\u2611\u2614\u2615\u2618\u261D\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u2648-\u2653\u265F\u2660\u2663\u2665\u2666\u2668\u267B\u267E\u267F\u2692-\u2697\u2699\u269B\u269C\u26A0\u26A1\u26A7\u26AA\u26AB\u26B0\u26B1\u26BD\u26BE\u26C4\u26C5\u26C8\u26CE\u26CF\u26D1\u26D3\u26D4\u26E9\u26EA\u26F0-\u26F5\u26F7-\u26FA\u26FD\u2702\u2705\u2708-\u270D\u270F\u2712\u2714\u2716\u271D\u2721\u2728\u2733\u2734\u2744\u2747\u274C\u274E\u2753-\u2755\u2757\u2763\u2764\u2795-\u2797\u27A1\u27B0\u27BF\u2934\u2935\u2B05-\u2B07\u2B1B\u2B1C\u2B50\u2B55\u3030\u303D\u3297\u3299]|\uD83C[\uDC04\uDCCF\uDD70\uDD71\uDD7E\uDD7F\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE02\uDE1A\uDE2F\uDE32-\uDE3A\uDE50\uDE51\uDF00-\uDF21\uDF24-\uDF93\uDF96\uDF97\uDF99-\uDF9B\uDF9E-\uDFF0\uDFF3-\uDFF5\uDFF7-\uDFFF]|\uD83D[\uDC00-\uDCFD\uDCFF-\uDD3D\uDD49-\uDD4E\uDD50-\uDD67\uDD6F\uDD70\uDD73-\uDD7A\uDD87\uDD8A-\uDD8D\uDD90\uDD95\uDD96\uDDA4\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA-\uDE4F\uDE80-\uDEC5\uDECB-\uDED2\uDED5-\uDED7\uDEE0-\uDEE5\uDEE9\uDEEB\uDEEC\uDEF0\uDEF3-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0C-\uDD3A\uDD3C-\uDD45\uDD47-\uDD78\uDD7A-\uDDCB\uDDCD-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6])\uFE0F|(?:[\u261D\u26F9\u270A-\u270D]|\uD83C[\uDF85\uDFC2-\uDFC4\uDFC7\uDFCA-\uDFCC]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66-\uDC78\uDC7C\uDC81-\uDC83\uDC85-\uDC87\uDC8F\uDC91\uDCAA\uDD74\uDD75\uDD7A\uDD90\uDD95\uDD96\uDE45-\uDE47\uDE4B-\uDE4F\uDEA3\uDEB4-\uDEB6\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1F\uDD26\uDD30-\uDD39\uDD3C-\uDD3E\uDD77\uDDB5\uDDB6\uDDB8\uDDB9\uDDBB\uDDCD-\uDDCF\uDDD1-\uDDDD])/g;
};
} }), As = {};
ps(As, { default: () => Ol });
function Ol(e) {
if (typeof e != "string" || e.length === 0 || (e = Tl(e), e.length === 0))
return 0;
e = e.replace((0, vs.default)(), " ");
let r = 0;
for (let u = 0; u < e.length; u++) {
let n2 = e.codePointAt(u);
n2 <= 31 || n2 >= 127 && n2 <= 159 || n2 >= 768 && n2 <= 879 || (n2 > 65535 && u++, r += bl(n2) ? 2 : 1);
}
return r;
}
var vs, ql = Ee({ "node_modules/string-width/index.js"() {
q(), Bl(), wl(), vs = nl(Nl());
} }), Il = I({ "src/utils/get-string-width.js"(e, r) {
"use strict";
q();
var u = (ql(), ds(As)).default, n2 = /[^\x20-\x7F]/;
function D(s) {
return s ? n2.test(s) ? u(s) : s.length : 0;
}
r.exports = D;
} }), zr = I({ "src/utils/text/skip.js"(e, r) {
"use strict";
q();
function u(f) {
return (c, F, a) => {
let l = a && a.backwards;
if (F === false)
return false;
let { length: h } = c, C = F;
for (; C >= 0 && C < h; ) {
let d = c.charAt(C);
if (f instanceof RegExp) {
if (!f.test(d))
return C;
} else if (!f.includes(d))
return C;
l ? C-- : C++;
}
return C === -1 || C === h ? C : false;
};
}
var n2 = u(/\s/), D = u(" "), s = u(",; "), i = u(/[^\n\r]/);
r.exports = { skipWhitespace: n2, skipSpaces: D, skipToLineEnd: s, skipEverythingButNewLine: i };
} }), _s = I({ "src/utils/text/skip-inline-comment.js"(e, r) {
"use strict";
q();
function u(n2, D) {
if (D === false)
return false;
if (n2.charAt(D) === "/" && n2.charAt(D + 1) === "*") {
for (let s = D + 2; s < n2.length; ++s)
if (n2.charAt(s) === "*" && n2.charAt(s + 1) === "/")
return s + 2;
}
return D;
}
r.exports = u;
} }), Ss = I({ "src/utils/text/skip-trailing-comment.js"(e, r) {
"use strict";
q();
var { skipEverythingButNewLine: u } = zr();
function n2(D, s) {
return s === false ? false : D.charAt(s) === "/" && D.charAt(s + 1) === "/" ? u(D, s) : s;
}
r.exports = n2;
} }), ys = I({ "src/utils/text/skip-newline.js"(e, r) {
"use strict";
q();
function u(n2, D, s) {
let i = s && s.backwards;
if (D === false)
return false;
let f = n2.charAt(D);
if (i) {
if (n2.charAt(D - 1) === "\r" && f === `
`)
return D - 2;
if (f === `
` || f === "\r" || f === "\u2028" || f === "\u2029")
return D - 1;
} else {
if (f === "\r" && n2.charAt(D + 1) === `
`)
return D + 2;
if (f === `
` || f === "\r" || f === "\u2028" || f === "\u2029")
return D + 1;
}
return D;
}
r.exports = u;
} }), Rl = I({ "src/utils/text/get-next-non-space-non-comment-character-index-with-start-index.js"(e, r) {
"use strict";
q();
var u = _s(), n2 = ys(), D = Ss(), { skipSpaces: s } = zr();
function i(f, c) {
let F = null, a = c;
for (; a !== F; )
F = a, a = s(f, a), a = u(f, a), a = D(f, a), a = n2(f, a);
return a;
}
r.exports = i;
} }), xl = I({ "src/common/util.js"(e, r) {
"use strict";
q();
var { default: u } = (cl(), ds(ms)), n2 = Cs(), { getSupportInfo: D } = vl(), s = _l(), i = Il(), { skipWhitespace: f, skipSpaces: c, skipToLineEnd: F, skipEverythingButNewLine: a } = zr(), l = _s(), h = Ss(), C = ys(), d = Rl(), m = (v) => v[v.length - 2];
function T(v) {
return (y, B, b) => {
let L = b && b.backwards;
if (B === false)
return false;
let { length: U } = y, G = B;
for (; G >= 0 && G < U; ) {
let W = y.charAt(G);
if (v instanceof RegExp) {
if (!v.test(W))
return G;
} else if (!v.includes(W))
return G;
L ? G-- : G++;
}
return G === -1 || G === U ? G : false;
};
}
function w(v, y) {
let B = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {}, b = c(v, B.backwards ? y - 1 : y, B), L = C(v, b, B);
return b !== L;
}
function g(v, y, B) {
for (let b = y; b < B; ++b)
if (v.charAt(b) === `
`)
return true;
return false;
}
function N(v, y, B) {
let b = B(y) - 1;
b = c(v, b, { backwards: true }), b = C(v, b, { backwards: true }), b = c(v, b, { backwards: true });
let L = C(v, b, { backwards: true });
return b !== L;
}
function R(v, y) {
let B = null, b = y;
for (; b !== B; )
B = b, b = F(v, b), b = l(v, b), b = c(v, b);
return b = h(v, b), b = C(v, b), b !== false && w(v, b);
}
function j(v, y, B) {
return R(v, B(y));
}
function _(v, y, B) {
return d(v, B(y));
}
function O(v, y, B) {
return v.charAt(_(v, y, B));
}
function x(v, y) {
let B = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {};
return c(v, B.backwards ? y - 1 : y, B) !== y;
}
function k(v, y) {
let B = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : 0, b = 0;
for (let L = B; L < v.length; ++L)
v[L] === " " ? b = b + y - b % y : b++;
return b;
}
function $(v, y) {
let B = v.lastIndexOf(`
`);
return B === -1 ? 0 : k(v.slice(B + 1).match(/^[\t ]*/)[0], y);
}
function t(v, y) {
let B = { quote: '"', regex: /"/g, escaped: """ }, b = { quote: "'", regex: /'/g, escaped: "'" }, L = y === "'" ? b : B, U = L === b ? B : b, G = L;
if (v.includes(L.quote) || v.includes(U.quote)) {
let W = (v.match(L.regex) || []).length, ne = (v.match(U.regex) || []).length;
G = W > ne ? U : L;
}
return G;
}
function o(v, y) {
let B = v.slice(1, -1), b = y.parser === "json" || y.parser === "json5" && y.quoteProps === "preserve" && !y.singleQuote ? '"' : y.__isInHtmlAttribute ? "'" : t(B, y.singleQuote ? "'" : '"').quote;
return E(B, b, !(y.parser === "css" || y.parser === "less" || y.parser === "scss" || y.__embeddedInHtml));
}
function E(v, y, B) {
let b = y === '"' ? "'" : '"', L = /\\(.)|(["'])/gs, U = v.replace(L, (G, W, ne) => W === b ? W : ne === y ? "\\" + ne : ne || (B && /^[^\n\r"'0-7\\bfnrt-vx\u2028\u2029]$/.test(W) ? W : "\\" + W));
return y + U + y;
}
function p(v) {
return v.toLowerCase().replace(/^([+-]?[\d.]+e)(?:\+|(-))?0*(\d)/, "$1$2$3").replace(/^([+-]?[\d.]+)e[+-]?0+$/, "$1").replace(/^([+-])?\./, "$10.").replace(/(\.\d+?)0+(?=e|$)/, "$1").replace(/\.(?=e|$)/, "");
}
function A(v, y) {
let B = v.match(new RegExp(`(${u(y)})+`, "g"));
return B === null ? 0 : B.reduce((b, L) => Math.max(b, L.length / y.length), 0);
}
function P(v, y) {
let B = v.match(new RegExp(`(${u(y)})+`, "g"));
if (B === null)
return 0;
let b = /* @__PURE__ */ new Map(), L = 0;
for (let U of B) {
let G = U.length / y.length;
b.set(G, true), G > L && (L = G);
}
for (let U = 1; U < L; U++)
if (!b.get(U))
return U;
return L + 1;
}
function M(v, y) {
(v.comments || (v.comments = [])).push(y), y.printed = false, y.nodeDescription = J(v);
}
function z(v, y) {
y.leading = true, y.trailing = false, M(v, y);
}
function V(v, y, B) {
y.leading = false, y.trailing = false, B && (y.marker = B), M(v, y);
}
function X(v, y) {
y.leading = false, y.trailing = true, M(v, y);
}
function H(v, y) {
let { languages: B } = D({ plugins: y.plugins }), b = B.find((L) => {
let { name: U } = L;
return U.toLowerCase() === v;
}) || B.find((L) => {
let { aliases: U } = L;
return Array.isArray(U) && U.includes(v);
}) || B.find((L) => {
let { extensions: U } = L;
return Array.isArray(U) && U.includes(`.${v}`);
});
return b && b.parsers[0];
}
function Q(v) {
return v && v.type === "front-matter";
}
function K(v) {
let y = /* @__PURE__ */ new WeakMap();
return function(B) {
return y.has(B) || y.set(B, Symbol(v)), y.get(B);
};
}
function J(v) {
let y = v.type || v.kind || "(unknown type)", B = String(v.name || v.id && (typeof v.id == "object" ? v.id.name : v.id) || v.key && (typeof v.key == "object" ? v.key.name : v.key) || v.value && (typeof v.value == "object" ? "" : String(v.value)) || v.operator || "");
return B.length > 20 && (B = B.slice(0, 19) + "\u2026"), y + (B ? " " + B : "");
}
r.exports = { inferParserByLanguage: H, getStringWidth: i, getMaxContinuousCount: A, getMinNotPresentContinuousCount: P, getPenultimate: m, getLast: n2, getNextNonSpaceNonCommentCharacterIndexWithStartIndex: d, getNextNonSpaceNonCommentCharacterIndex: _, getNextNonSpaceNonCommentCharacter: O, skip: T, skipWhitespace: f, skipSpaces: c, skipToLineEnd: F, skipEverythingButNewLine: a, skipInlineComment: l, skipTrailingComment: h, skipNewline: C, isNextLineEmptyAfterIndex: R, isNextLineEmpty: j, isPreviousLineEmpty: N, hasNewline: w, hasNewlineInRange: g, hasSpaces: x, getAlignmentSize: k, getIndentSize: $, getPreferredQuote: t, printString: o, printNumber: p, makeString: E, addLeadingComment: z, addDanglingComment: V, addTrailingComment: X, isFrontMatterNode: Q, isNonEmptyArray: s, createGroupIdMapper: K };
} }), Pl = I({ "vendors/html-tag-names.json"(e, r) {
r.exports = { htmlTagNames: ["a", "abbr", "acronym", "address", "applet", "area", "article", "aside", "audio", "b", "base", "basefont", "bdi", "bdo", "bgsound", "big", "blink", "blockquote", "body", "br", "button", "canvas", "caption", "center", "cite", "code", "col", "colgroup", "command", "content", "data", "datalist", "dd", "del", "details", "dfn", "dialog", "dir", "div", "dl", "dt", "element", "em", "embed", "fieldset", "figcaption", "figure", "font", "footer", "form", "frame", "frameset", "h1", "h2", "h3", "h4", "h5", "h6", "head", "header", "hgroup", "hr", "html", "i", "iframe", "image", "img", "input", "ins", "isindex", "kbd", "keygen", "label", "legend", "li", "link", "listing", "main", "map", "mark", "marquee", "math", "menu", "menuitem", "meta", "meter", "multicol", "nav", "nextid", "nobr", "noembed", "noframes", "noscript", "object", "ol", "optgroup", "option", "output", "p", "param", "picture", "plaintext", "pre", "progress", "q", "rb", "rbc", "rp", "rt", "rtc", "ruby", "s", "samp", "script", "section", "select", "shadow", "slot", "small", "source", "spacer", "span", "strike", "strong", "style", "sub", "summary", "sup", "svg", "table", "tbody", "td", "template", "textarea", "tfoot", "th", "thead", "time", "title", "tr", "track", "tt", "u", "ul", "var", "video", "wbr", "xmp"] };
} }), Ts = I({ "src/language-html/utils/array-to-map.js"(e, r) {
"use strict";
q();
function u(n2) {
let D = /* @__PURE__ */ Object.create(null);
for (let s of n2)
D[s] = true;
return D;
}
r.exports = u;
} }), kl = I({ "src/language-html/utils/html-tag-names.js"(e, r) {
"use strict";
q();
var { htmlTagNames: u } = Pl(), n2 = Ts(), D = n2(u);
r.exports = D;
} }), Ll = I({ "vendors/html-element-attributes.json"(e, r) {
r.exports = { htmlElementAttributes: { "*": ["accesskey", "autocapitalize", "autofocus", "class", "contenteditable", "dir", "draggable", "enterkeyhint", "hidden", "id", "inputmode", "is", "itemid", "itemprop", "itemref", "itemscope", "itemtype", "lang", "nonce", "slot", "spellcheck", "style", "tabindex", "title", "translate"], a: ["charset", "coords", "download", "href", "hreflang", "name", "ping", "referrerpolicy", "rel", "rev", "shape", "target", "type"], applet: ["align", "alt", "archive", "code", "codebase", "height", "hspace", "name", "object", "vspace", "width"], area: ["alt", "coords", "download", "href", "hreflang", "nohref", "ping", "referrerpolicy", "rel", "shape", "target", "type"], audio: ["autoplay", "controls", "crossorigin", "loop", "muted", "preload", "src"], base: ["href", "target"], basefont: ["color", "face", "size"], blockquote: ["cite"], body: ["alink", "background", "bgcolor", "link", "text", "vlink"], br: ["clear"], button: ["disabled", "form", "formaction", "formenctype", "formmethod", "formnovalidate", "formtarget", "name", "type", "value"], canvas: ["height", "width"], caption: ["align"], col: ["align", "char", "charoff", "span", "valign", "width"], colgroup: ["align", "char", "charoff", "span", "valign", "width"], data: ["value"], del: ["cite", "datetime"], details: ["open"], dialog: ["open"], dir: ["compact"], div: ["align"], dl: ["compact"], embed: ["height", "src", "type", "width"], fieldset: ["disabled", "form", "name"], font: ["color", "face", "size"], form: ["accept", "accept-charset", "action", "autocomplete", "enctype", "method", "name", "novalidate", "target"], frame: ["frameborder", "longdesc", "marginheight", "marginwidth", "name", "noresize", "scrolling", "src"], frameset: ["cols", "rows"], h1: ["align"], h2: ["align"], h3: ["align"], h4: ["align"], h5: ["align"], h6: ["align"], head: ["profile"], hr: ["align", "noshade", "size", "width"], html: ["manifest", "version"], iframe: ["align", "allow", "allowfullscreen", "allowpaymentrequest", "allowusermedia", "frameborder", "height", "loading", "longdesc", "marginheight", "marginwidth", "name", "referrerpolicy", "sandbox", "scrolling", "src", "srcdoc", "width"], img: ["align", "alt", "border", "crossorigin", "decoding", "height", "hspace", "ismap", "loading", "longdesc", "name", "referrerpolicy", "sizes", "src", "srcset", "usemap", "vspace", "width"], input: ["accept", "align", "alt", "autocomplete", "checked", "dirname", "disabled", "form", "formaction", "formenctype", "formmethod", "formnovalidate", "formtarget", "height", "ismap", "list", "max", "maxlength", "min", "minlength", "multiple", "name", "pattern", "placeholder", "readonly", "required", "size", "src", "step", "type", "usemap", "value", "width"], ins: ["cite", "datetime"], isindex: ["prompt"], label: ["for", "form"], legend: ["align"], li: ["type", "value"], link: ["as", "charset", "color", "crossorigin", "disabled", "href", "hreflang", "imagesizes", "imagesrcset", "integrity", "media", "referrerpolicy", "rel", "rev", "sizes", "target", "type"], map: ["name"], menu: ["compact"], meta: ["charset", "content", "http-equiv", "media", "name", "scheme"], meter: ["high", "low", "max", "min", "optimum", "value"], object: ["align", "archive", "border", "classid", "codebase", "codetype", "data", "declare", "form", "height", "hspace", "name", "standby", "type", "typemustmatch", "usemap", "vspace", "width"], ol: ["compact", "reversed", "start", "type"], optgroup: ["disabled", "label"], option: ["disabled", "label", "selected", "value"], output: ["for", "form", "name"], p: ["align"], param: ["name", "type", "value", "valuetype"], pre: ["width"], progress: ["max", "value"], q: ["cite"], script: ["async", "charset", "crossorigin", "defer", "integrity", "language", "nomodule", "referrerpolicy", "src", "type"], select: ["autocomplete", "disabled", "form", "multiple", "name", "required", "size"], slot: ["name"], source: ["height", "media", "sizes", "src", "srcset", "type", "width"], style: ["media", "type"], table: ["align", "bgcolor", "border", "cellpadding", "cellspacing", "frame", "rules", "summary", "width"], tbody: ["align", "char", "charoff", "valign"], td: ["abbr", "align", "axis", "bgcolor", "char", "charoff", "colspan", "headers", "height", "nowrap", "rowspan", "scope", "valign", "width"], textarea: ["autocomplete", "cols", "dirname", "disabled", "form", "maxlength", "minlength", "name", "placeholder", "readonly", "required", "rows", "wrap"], tfoot: ["align", "char", "charoff", "valign"], th: ["abbr", "align", "axis", "bgcolor", "char", "charoff", "colspan", "headers", "height", "nowrap", "rowspan", "scope", "valign", "width"], thead: ["align", "char", "charoff", "valign"], time: ["datetime"], tr: ["align", "bgcolor", "char", "charoff", "valign"], track: ["default", "kind", "label", "src", "srclang"], ul: ["compact", "type"], video: ["autoplay", "controls", "crossorigin", "height", "loop", "muted", "playsinline", "poster", "preload", "src", "width"] } };
} }), $l = I({ "src/language-html/utils/map-object.js"(e, r) {
"use strict";
q();
function u(n2, D) {
let s = /* @__PURE__ */ Object.create(null);
for (let [i, f] of Object.entries(n2))
s[i] = D(f, i);
return s;
}
r.exports = u;
} }), Ml = I({ "src/language-html/utils/html-elements-attributes.js"(e, r) {
"use strict";
q();
var { htmlElementAttributes: u } = Ll(), n2 = $l(), D = Ts(), s = n2(u, D);
r.exports = s;
} }), jl = I({ "src/language-html/utils/is-unknown-namespace.js"(e, r) {
"use strict";
q();
function u(n2) {
return n2.type === "element" && !n2.hasExplicitNamespace && !["html", "svg"].includes(n2.namespace);
}
r.exports = u;
} }), Ul = I({ "src/language-html/pragma.js"(e, r) {
"use strict";
q();
function u(D) {
return /^\s*/.test(D);
}
function n2(D) {
return `
` + D.replace(/^\s*\n/, "");
}
r.exports = { hasPragma: u, insertPragma: n2 };
} }), Gl = I({ "src/language-html/ast.js"(e, r) {
"use strict";
q();
var u = { attrs: true, children: true }, n2 = /* @__PURE__ */ new Set(["parent"]), D = class {
constructor() {
let i = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {};
for (let f of /* @__PURE__ */ new Set([...n2, ...Object.keys(i)]))
this.setProperty(f, i[f]);
}
setProperty(i, f) {
if (this[i] !== f) {
if (i in u && (f = f.map((c) => this.createChild(c))), !n2.has(i)) {
this[i] = f;
return;
}
Object.defineProperty(this, i, { value: f, enumerable: false, configurable: true });
}
}
map(i) {
let f;
for (let c in u) {
let F = this[c];
if (F) {
let a = s(F, (l) => l.map(i));
f !== F && (f || (f = new D({ parent: this.parent })), f.setProperty(c, a));
}
}
if (f)
for (let c in this)
c in u || (f[c] = this[c]);
return i(f || this);
}
walk(i) {
for (let f in u) {
let c = this[f];
if (c)
for (let F = 0; F < c.length; F++)
c[F].walk(i);
}
i(this);
}
createChild(i) {
let f = i instanceof D ? i.clone() : new D(i);
return f.setProperty("parent", this), f;
}
insertChildBefore(i, f) {
this.children.splice(this.children.indexOf(i), 0, this.createChild(f));
}
removeChild(i) {
this.children.splice(this.children.indexOf(i), 1);
}
replaceChild(i, f) {
this.children[this.children.indexOf(i)] = this.createChild(f);
}
clone() {
return new D(this);
}
get firstChild() {
var i;
return (i = this.children) === null || i === void 0 ? void 0 : i[0];
}
get lastChild() {
var i;
return (i = this.children) === null || i === void 0 ? void 0 : i[this.children.length - 1];
}
get prev() {
var i;
return (i = this.parent) === null || i === void 0 ? void 0 : i.children[this.parent.children.indexOf(this) - 1];
}
get next() {
var i;
return (i = this.parent) === null || i === void 0 ? void 0 : i.children[this.parent.children.indexOf(this) + 1];
}
get rawName() {
return this.hasExplicitNamespace ? this.fullName : this.name;
}
get fullName() {
return this.namespace ? this.namespace + ":" + this.name : this.name;
}
get attrMap() {
return Object.fromEntries(this.attrs.map((i) => [i.fullName, i.value]));
}
};
function s(i, f) {
let c = i.map(f);
return c.some((F, a) => F !== i[a]) ? c : i;
}
r.exports = { Node: D };
} }), Vl = I({ "src/language-html/conditional-comment.js"(e, r) {
"use strict";
q();
var { ParseSourceSpan: u } = Be(), n2 = [{ regex: /^(\[if([^\]]*)]>)(.*?) {
try {
return [true, F(C, m).children];
} catch {
return [false, [{ type: "text", value: C, sourceSpan: new u(m, T) }]];
}
})();
return { type: "ieConditionalComment", complete: w, children: g, condition: h.trim().replace(/\s+/g, " "), sourceSpan: c.sourceSpan, startSourceSpan: new u(c.sourceSpan.start, m), endSourceSpan: new u(T, c.sourceSpan.end) };
}
function i(c, F, a) {
let [, l] = a;
return { type: "ieConditionalStartComment", condition: l.trim().replace(/\s+/g, " "), sourceSpan: c.sourceSpan };
}
function f(c) {
return { type: "ieConditionalEndComment", sourceSpan: c.sourceSpan };
}
r.exports = { parseIeConditionalComment: D };
} }), Xl = I({ "src/language-html/loc.js"(e, r) {
"use strict";
q();
function u(D) {
return D.sourceSpan.start.offset;
}
function n2(D) {
return D.sourceSpan.end.offset;
}
r.exports = { locStart: u, locEnd: n2 };
} }), Ze = I({ "node_modules/angular-html-parser/lib/compiler/src/ml_parser/tags.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r;
(function(c) {
c[c.RAW_TEXT = 0] = "RAW_TEXT", c[c.ESCAPABLE_RAW_TEXT = 1] = "ESCAPABLE_RAW_TEXT", c[c.PARSABLE_DATA = 2] = "PARSABLE_DATA";
})(r = e.TagContentType || (e.TagContentType = {}));
function u(c) {
if (c[0] != ":")
return [null, c];
let F = c.indexOf(":", 1);
if (F == -1)
throw new Error(`Unsupported format "${c}" expecting ":namespace:name"`);
return [c.slice(1, F), c.slice(F + 1)];
}
e.splitNsName = u;
function n2(c) {
return u(c)[1] === "ng-container";
}
e.isNgContainer = n2;
function D(c) {
return u(c)[1] === "ng-content";
}
e.isNgContent = D;
function s(c) {
return u(c)[1] === "ng-template";
}
e.isNgTemplate = s;
function i(c) {
return c === null ? null : u(c)[0];
}
e.getNsPrefix = i;
function f(c, F) {
return c ? `:${c}:${F}` : F;
}
e.mergeNsAndName = f, e.NAMED_ENTITIES = { Aacute: "\xC1", aacute: "\xE1", Abreve: "\u0102", abreve: "\u0103", ac: "\u223E", acd: "\u223F", acE: "\u223E\u0333", Acirc: "\xC2", acirc: "\xE2", acute: "\xB4", Acy: "\u0410", acy: "\u0430", AElig: "\xC6", aelig: "\xE6", af: "\u2061", Afr: "\u{1D504}", afr: "\u{1D51E}", Agrave: "\xC0", agrave: "\xE0", alefsym: "\u2135", aleph: "\u2135", Alpha: "\u0391", alpha: "\u03B1", Amacr: "\u0100", amacr: "\u0101", amalg: "\u2A3F", AMP: "&", amp: "&", And: "\u2A53", and: "\u2227", andand: "\u2A55", andd: "\u2A5C", andslope: "\u2A58", andv: "\u2A5A", ang: "\u2220", ange: "\u29A4", angle: "\u2220", angmsd: "\u2221", angmsdaa: "\u29A8", angmsdab: "\u29A9", angmsdac: "\u29AA", angmsdad: "\u29AB", angmsdae: "\u29AC", angmsdaf: "\u29AD", angmsdag: "\u29AE", angmsdah: "\u29AF", angrt: "\u221F", angrtvb: "\u22BE", angrtvbd: "\u299D", angsph: "\u2222", angst: "\xC5", angzarr: "\u237C", Aogon: "\u0104", aogon: "\u0105", Aopf: "\u{1D538}", aopf: "\u{1D552}", ap: "\u2248", apacir: "\u2A6F", apE: "\u2A70", ape: "\u224A", apid: "\u224B", apos: "'", ApplyFunction: "\u2061", approx: "\u2248", approxeq: "\u224A", Aring: "\xC5", aring: "\xE5", Ascr: "\u{1D49C}", ascr: "\u{1D4B6}", Assign: "\u2254", ast: "*", asymp: "\u2248", asympeq: "\u224D", Atilde: "\xC3", atilde: "\xE3", Auml: "\xC4", auml: "\xE4", awconint: "\u2233", awint: "\u2A11", backcong: "\u224C", backepsilon: "\u03F6", backprime: "\u2035", backsim: "\u223D", backsimeq: "\u22CD", Backslash: "\u2216", Barv: "\u2AE7", barvee: "\u22BD", Barwed: "\u2306", barwed: "\u2305", barwedge: "\u2305", bbrk: "\u23B5", bbrktbrk: "\u23B6", bcong: "\u224C", Bcy: "\u0411", bcy: "\u0431", bdquo: "\u201E", becaus: "\u2235", Because: "\u2235", because: "\u2235", bemptyv: "\u29B0", bepsi: "\u03F6", bernou: "\u212C", Bernoullis: "\u212C", Beta: "\u0392", beta: "\u03B2", beth: "\u2136", between: "\u226C", Bfr: "\u{1D505}", bfr: "\u{1D51F}", bigcap: "\u22C2", bigcirc: "\u25EF", bigcup: "\u22C3", bigodot: "\u2A00", bigoplus: "\u2A01", bigotimes: "\u2A02", bigsqcup: "\u2A06", bigstar: "\u2605", bigtriangledown: "\u25BD", bigtriangleup: "\u25B3", biguplus: "\u2A04", bigvee: "\u22C1", bigwedge: "\u22C0", bkarow: "\u290D", blacklozenge: "\u29EB", blacksquare: "\u25AA", blacktriangle: "\u25B4", blacktriangledown: "\u25BE", blacktriangleleft: "\u25C2", blacktriangleright: "\u25B8", blank: "\u2423", blk12: "\u2592", blk14: "\u2591", blk34: "\u2593", block: "\u2588", bne: "=\u20E5", bnequiv: "\u2261\u20E5", bNot: "\u2AED", bnot: "\u2310", Bopf: "\u{1D539}", bopf: "\u{1D553}", bot: "\u22A5", bottom: "\u22A5", bowtie: "\u22C8", boxbox: "\u29C9", boxDL: "\u2557", boxDl: "\u2556", boxdL: "\u2555", boxdl: "\u2510", boxDR: "\u2554", boxDr: "\u2553", boxdR: "\u2552", boxdr: "\u250C", boxH: "\u2550", boxh: "\u2500", boxHD: "\u2566", boxHd: "\u2564", boxhD: "\u2565", boxhd: "\u252C", boxHU: "\u2569", boxHu: "\u2567", boxhU: "\u2568", boxhu: "\u2534", boxminus: "\u229F", boxplus: "\u229E", boxtimes: "\u22A0", boxUL: "\u255D", boxUl: "\u255C", boxuL: "\u255B", boxul: "\u2518", boxUR: "\u255A", boxUr: "\u2559", boxuR: "\u2558", boxur: "\u2514", boxV: "\u2551", boxv: "\u2502", boxVH: "\u256C", boxVh: "\u256B", boxvH: "\u256A", boxvh: "\u253C", boxVL: "\u2563", boxVl: "\u2562", boxvL: "\u2561", boxvl: "\u2524", boxVR: "\u2560", boxVr: "\u255F", boxvR: "\u255E", boxvr: "\u251C", bprime: "\u2035", Breve: "\u02D8", breve: "\u02D8", brvbar: "\xA6", Bscr: "\u212C", bscr: "\u{1D4B7}", bsemi: "\u204F", bsim: "\u223D", bsime: "\u22CD", bsol: "\\", bsolb: "\u29C5", bsolhsub: "\u27C8", bull: "\u2022", bullet: "\u2022", bump: "\u224E", bumpE: "\u2AAE", bumpe: "\u224F", Bumpeq: "\u224E", bumpeq: "\u224F", Cacute: "\u0106", cacute: "\u0107", Cap: "\u22D2", cap: "\u2229", capand: "\u2A44", capbrcup: "\u2A49", capcap: "\u2A4B", capcup: "\u2A47", capdot: "\u2A40", CapitalDifferentialD: "\u2145", caps: "\u2229\uFE00", caret: "\u2041", caron: "\u02C7", Cayleys: "\u212D", ccaps: "\u2A4D", Ccaron: "\u010C", ccaron: "\u010D", Ccedil: "\xC7", ccedil: "\xE7", Ccirc: "\u0108", ccirc: "\u0109", Cconint: "\u2230", ccups: "\u2A4C", ccupssm: "\u2A50", Cdot: "\u010A", cdot: "\u010B", cedil: "\xB8", Cedilla: "\xB8", cemptyv: "\u29B2", cent: "\xA2", CenterDot: "\xB7", centerdot: "\xB7", Cfr: "\u212D", cfr: "\u{1D520}", CHcy: "\u0427", chcy: "\u0447", check: "\u2713", checkmark: "\u2713", Chi: "\u03A7", chi: "\u03C7", cir: "\u25CB", circ: "\u02C6", circeq: "\u2257", circlearrowleft: "\u21BA", circlearrowright: "\u21BB", circledast: "\u229B", circledcirc: "\u229A", circleddash: "\u229D", CircleDot: "\u2299", circledR: "\xAE", circledS: "\u24C8", CircleMinus: "\u2296", CirclePlus: "\u2295", CircleTimes: "\u2297", cirE: "\u29C3", cire: "\u2257", cirfnint: "\u2A10", cirmid: "\u2AEF", cirscir: "\u29C2", ClockwiseContourIntegral: "\u2232", CloseCurlyDoubleQuote: "\u201D", CloseCurlyQuote: "\u2019", clubs: "\u2663", clubsuit: "\u2663", Colon: "\u2237", colon: ":", Colone: "\u2A74", colone: "\u2254", coloneq: "\u2254", comma: ",", commat: "@", comp: "\u2201", compfn: "\u2218", complement: "\u2201", complexes: "\u2102", cong: "\u2245", congdot: "\u2A6D", Congruent: "\u2261", Conint: "\u222F", conint: "\u222E", ContourIntegral: "\u222E", Copf: "\u2102", copf: "\u{1D554}", coprod: "\u2210", Coproduct: "\u2210", COPY: "\xA9", copy: "\xA9", copysr: "\u2117", CounterClockwiseContourIntegral: "\u2233", crarr: "\u21B5", Cross: "\u2A2F", cross: "\u2717", Cscr: "\u{1D49E}", cscr: "\u{1D4B8}", csub: "\u2ACF", csube: "\u2AD1", csup: "\u2AD0", csupe: "\u2AD2", ctdot: "\u22EF", cudarrl: "\u2938", cudarrr: "\u2935", cuepr: "\u22DE", cuesc: "\u22DF", cularr: "\u21B6", cularrp: "\u293D", Cup: "\u22D3", cup: "\u222A", cupbrcap: "\u2A48", CupCap: "\u224D", cupcap: "\u2A46", cupcup: "\u2A4A", cupdot: "\u228D", cupor: "\u2A45", cups: "\u222A\uFE00", curarr: "\u21B7", curarrm: "\u293C", curlyeqprec: "\u22DE", curlyeqsucc: "\u22DF", curlyvee: "\u22CE", curlywedge: "\u22CF", curren: "\xA4", curvearrowleft: "\u21B6", curvearrowright: "\u21B7", cuvee: "\u22CE", cuwed: "\u22CF", cwconint: "\u2232", cwint: "\u2231", cylcty: "\u232D", Dagger: "\u2021", dagger: "\u2020", daleth: "\u2138", Darr: "\u21A1", dArr: "\u21D3", darr: "\u2193", dash: "\u2010", Dashv: "\u2AE4", dashv: "\u22A3", dbkarow: "\u290F", dblac: "\u02DD", Dcaron: "\u010E", dcaron: "\u010F", Dcy: "\u0414", dcy: "\u0434", DD: "\u2145", dd: "\u2146", ddagger: "\u2021", ddarr: "\u21CA", DDotrahd: "\u2911", ddotseq: "\u2A77", deg: "\xB0", Del: "\u2207", Delta: "\u0394", delta: "\u03B4", demptyv: "\u29B1", dfisht: "\u297F", Dfr: "\u{1D507}", dfr: "\u{1D521}", dHar: "\u2965", dharl: "\u21C3", dharr: "\u21C2", DiacriticalAcute: "\xB4", DiacriticalDot: "\u02D9", DiacriticalDoubleAcute: "\u02DD", DiacriticalGrave: "`", DiacriticalTilde: "\u02DC", diam: "\u22C4", Diamond: "\u22C4", diamond: "\u22C4", diamondsuit: "\u2666", diams: "\u2666", die: "\xA8", DifferentialD: "\u2146", digamma: "\u03DD", disin: "\u22F2", div: "\xF7", divide: "\xF7", divideontimes: "\u22C7", divonx: "\u22C7", DJcy: "\u0402", djcy: "\u0452", dlcorn: "\u231E", dlcrop: "\u230D", dollar: "$", Dopf: "\u{1D53B}", dopf: "\u{1D555}", Dot: "\xA8", dot: "\u02D9", DotDot: "\u20DC", doteq: "\u2250", doteqdot: "\u2251", DotEqual: "\u2250", dotminus: "\u2238", dotplus: "\u2214", dotsquare: "\u22A1", doublebarwedge: "\u2306", DoubleContourIntegral: "\u222F", DoubleDot: "\xA8", DoubleDownArrow: "\u21D3", DoubleLeftArrow: "\u21D0", DoubleLeftRightArrow: "\u21D4", DoubleLeftTee: "\u2AE4", DoubleLongLeftArrow: "\u27F8", DoubleLongLeftRightArrow: "\u27FA", DoubleLongRightArrow: "\u27F9", DoubleRightArrow: "\u21D2", DoubleRightTee: "\u22A8", DoubleUpArrow: "\u21D1", DoubleUpDownArrow: "\u21D5", DoubleVerticalBar: "\u2225", DownArrow: "\u2193", Downarrow: "\u21D3", downarrow: "\u2193", DownArrowBar: "\u2913", DownArrowUpArrow: "\u21F5", DownBreve: "\u0311", downdownarrows: "\u21CA", downharpoonleft: "\u21C3", downharpoonright: "\u21C2", DownLeftRightVector: "\u2950", DownLeftTeeVector: "\u295E", DownLeftVector: "\u21BD", DownLeftVectorBar: "\u2956", DownRightTeeVector: "\u295F", DownRightVector: "\u21C1", DownRightVectorBar: "\u2957", DownTee: "\u22A4", DownTeeArrow: "\u21A7", drbkarow: "\u2910", drcorn: "\u231F", drcrop: "\u230C", Dscr: "\u{1D49F}", dscr: "\u{1D4B9}", DScy: "\u0405", dscy: "\u0455", dsol: "\u29F6", Dstrok: "\u0110", dstrok: "\u0111", dtdot: "\u22F1", dtri: "\u25BF", dtrif: "\u25BE", duarr: "\u21F5", duhar: "\u296F", dwangle: "\u29A6", DZcy: "\u040F", dzcy: "\u045F", dzigrarr: "\u27FF", Eacute: "\xC9", eacute: "\xE9", easter: "\u2A6E", Ecaron: "\u011A", ecaron: "\u011B", ecir: "\u2256", Ecirc: "\xCA", ecirc: "\xEA", ecolon: "\u2255", Ecy: "\u042D", ecy: "\u044D", eDDot: "\u2A77", Edot: "\u0116", eDot: "\u2251", edot: "\u0117", ee: "\u2147", efDot: "\u2252", Efr: "\u{1D508}", efr: "\u{1D522}", eg: "\u2A9A", Egrave: "\xC8", egrave: "\xE8", egs: "\u2A96", egsdot: "\u2A98", el: "\u2A99", Element: "\u2208", elinters: "\u23E7", ell: "\u2113", els: "\u2A95", elsdot: "\u2A97", Emacr: "\u0112", emacr: "\u0113", empty: "\u2205", emptyset: "\u2205", EmptySmallSquare: "\u25FB", emptyv: "\u2205", EmptyVerySmallSquare: "\u25AB", emsp: "\u2003", emsp13: "\u2004", emsp14: "\u2005", ENG: "\u014A", eng: "\u014B", ensp: "\u2002", Eogon: "\u0118", eogon: "\u0119", Eopf: "\u{1D53C}", eopf: "\u{1D556}", epar: "\u22D5", eparsl: "\u29E3", eplus: "\u2A71", epsi: "\u03B5", Epsilon: "\u0395", epsilon: "\u03B5", epsiv: "\u03F5", eqcirc: "\u2256", eqcolon: "\u2255", eqsim: "\u2242", eqslantgtr: "\u2A96", eqslantless: "\u2A95", Equal: "\u2A75", equals: "=", EqualTilde: "\u2242", equest: "\u225F", Equilibrium: "\u21CC", equiv: "\u2261", equivDD: "\u2A78", eqvparsl: "\u29E5", erarr: "\u2971", erDot: "\u2253", Escr: "\u2130", escr: "\u212F", esdot: "\u2250", Esim: "\u2A73", esim: "\u2242", Eta: "\u0397", eta: "\u03B7", ETH: "\xD0", eth: "\xF0", Euml: "\xCB", euml: "\xEB", euro: "\u20AC", excl: "!", exist: "\u2203", Exists: "\u2203", expectation: "\u2130", ExponentialE: "\u2147", exponentiale: "\u2147", fallingdotseq: "\u2252", Fcy: "\u0424", fcy: "\u0444", female: "\u2640", ffilig: "\uFB03", fflig: "\uFB00", ffllig: "\uFB04", Ffr: "\u{1D509}", ffr: "\u{1D523}", filig: "\uFB01", FilledSmallSquare: "\u25FC", FilledVerySmallSquare: "\u25AA", fjlig: "fj", flat: "\u266D", fllig: "\uFB02", fltns: "\u25B1", fnof: "\u0192", Fopf: "\u{1D53D}", fopf: "\u{1D557}", ForAll: "\u2200", forall: "\u2200", fork: "\u22D4", forkv: "\u2AD9", Fouriertrf: "\u2131", fpartint: "\u2A0D", frac12: "\xBD", frac13: "\u2153", frac14: "\xBC", frac15: "\u2155", frac16: "\u2159", frac18: "\u215B", frac23: "\u2154", frac25: "\u2156", frac34: "\xBE", frac35: "\u2157", frac38: "\u215C", frac45: "\u2158", frac56: "\u215A", frac58: "\u215D", frac78: "\u215E", frasl: "\u2044", frown: "\u2322", Fscr: "\u2131", fscr: "\u{1D4BB}", gacute: "\u01F5", Gamma: "\u0393", gamma: "\u03B3", Gammad: "\u03DC", gammad: "\u03DD", gap: "\u2A86", Gbreve: "\u011E", gbreve: "\u011F", Gcedil: "\u0122", Gcirc: "\u011C", gcirc: "\u011D", Gcy: "\u0413", gcy: "\u0433", Gdot: "\u0120", gdot: "\u0121", gE: "\u2267", ge: "\u2265", gEl: "\u2A8C", gel: "\u22DB", geq: "\u2265", geqq: "\u2267", geqslant: "\u2A7E", ges: "\u2A7E", gescc: "\u2AA9", gesdot: "\u2A80", gesdoto: "\u2A82", gesdotol: "\u2A84", gesl: "\u22DB\uFE00", gesles: "\u2A94", Gfr: "\u{1D50A}", gfr: "\u{1D524}", Gg: "\u22D9", gg: "\u226B", ggg: "\u22D9", gimel: "\u2137", GJcy: "\u0403", gjcy: "\u0453", gl: "\u2277", gla: "\u2AA5", glE: "\u2A92", glj: "\u2AA4", gnap: "\u2A8A", gnapprox: "\u2A8A", gnE: "\u2269", gne: "\u2A88", gneq: "\u2A88", gneqq: "\u2269", gnsim: "\u22E7", Gopf: "\u{1D53E}", gopf: "\u{1D558}", grave: "`", GreaterEqual: "\u2265", GreaterEqualLess: "\u22DB", GreaterFullEqual: "\u2267", GreaterGreater: "\u2AA2", GreaterLess: "\u2277", GreaterSlantEqual: "\u2A7E", GreaterTilde: "\u2273", Gscr: "\u{1D4A2}", gscr: "\u210A", gsim: "\u2273", gsime: "\u2A8E", gsiml: "\u2A90", GT: ">", Gt: "\u226B", gt: ">", gtcc: "\u2AA7", gtcir: "\u2A7A", gtdot: "\u22D7", gtlPar: "\u2995", gtquest: "\u2A7C", gtrapprox: "\u2A86", gtrarr: "\u2978", gtrdot: "\u22D7", gtreqless: "\u22DB", gtreqqless: "\u2A8C", gtrless: "\u2277", gtrsim: "\u2273", gvertneqq: "\u2269\uFE00", gvnE: "\u2269\uFE00", Hacek: "\u02C7", hairsp: "\u200A", half: "\xBD", hamilt: "\u210B", HARDcy: "\u042A", hardcy: "\u044A", hArr: "\u21D4", harr: "\u2194", harrcir: "\u2948", harrw: "\u21AD", Hat: "^", hbar: "\u210F", Hcirc: "\u0124", hcirc: "\u0125", hearts: "\u2665", heartsuit: "\u2665", hellip: "\u2026", hercon: "\u22B9", Hfr: "\u210C", hfr: "\u{1D525}", HilbertSpace: "\u210B", hksearow: "\u2925", hkswarow: "\u2926", hoarr: "\u21FF", homtht: "\u223B", hookleftarrow: "\u21A9", hookrightarrow: "\u21AA", Hopf: "\u210D", hopf: "\u{1D559}", horbar: "\u2015", HorizontalLine: "\u2500", Hscr: "\u210B", hscr: "\u{1D4BD}", hslash: "\u210F", Hstrok: "\u0126", hstrok: "\u0127", HumpDownHump: "\u224E", HumpEqual: "\u224F", hybull: "\u2043", hyphen: "\u2010", Iacute: "\xCD", iacute: "\xED", ic: "\u2063", Icirc: "\xCE", icirc: "\xEE", Icy: "\u0418", icy: "\u0438", Idot: "\u0130", IEcy: "\u0415", iecy: "\u0435", iexcl: "\xA1", iff: "\u21D4", Ifr: "\u2111", ifr: "\u{1D526}", Igrave: "\xCC", igrave: "\xEC", ii: "\u2148", iiiint: "\u2A0C", iiint: "\u222D", iinfin: "\u29DC", iiota: "\u2129", IJlig: "\u0132", ijlig: "\u0133", Im: "\u2111", Imacr: "\u012A", imacr: "\u012B", image: "\u2111", ImaginaryI: "\u2148", imagline: "\u2110", imagpart: "\u2111", imath: "\u0131", imof: "\u22B7", imped: "\u01B5", Implies: "\u21D2", in: "\u2208", incare: "\u2105", infin: "\u221E", infintie: "\u29DD", inodot: "\u0131", Int: "\u222C", int: "\u222B", intcal: "\u22BA", integers: "\u2124", Integral: "\u222B", intercal: "\u22BA", Intersection: "\u22C2", intlarhk: "\u2A17", intprod: "\u2A3C", InvisibleComma: "\u2063", InvisibleTimes: "\u2062", IOcy: "\u0401", iocy: "\u0451", Iogon: "\u012E", iogon: "\u012F", Iopf: "\u{1D540}", iopf: "\u{1D55A}", Iota: "\u0399", iota: "\u03B9", iprod: "\u2A3C", iquest: "\xBF", Iscr: "\u2110", iscr: "\u{1D4BE}", isin: "\u2208", isindot: "\u22F5", isinE: "\u22F9", isins: "\u22F4", isinsv: "\u22F3", isinv: "\u2208", it: "\u2062", Itilde: "\u0128", itilde: "\u0129", Iukcy: "\u0406", iukcy: "\u0456", Iuml: "\xCF", iuml: "\xEF", Jcirc: "\u0134", jcirc: "\u0135", Jcy: "\u0419", jcy: "\u0439", Jfr: "\u{1D50D}", jfr: "\u{1D527}", jmath: "\u0237", Jopf: "\u{1D541}", jopf: "\u{1D55B}", Jscr: "\u{1D4A5}", jscr: "\u{1D4BF}", Jsercy: "\u0408", jsercy: "\u0458", Jukcy: "\u0404", jukcy: "\u0454", Kappa: "\u039A", kappa: "\u03BA", kappav: "\u03F0", Kcedil: "\u0136", kcedil: "\u0137", Kcy: "\u041A", kcy: "\u043A", Kfr: "\u{1D50E}", kfr: "\u{1D528}", kgreen: "\u0138", KHcy: "\u0425", khcy: "\u0445", KJcy: "\u040C", kjcy: "\u045C", Kopf: "\u{1D542}", kopf: "\u{1D55C}", Kscr: "\u{1D4A6}", kscr: "\u{1D4C0}", lAarr: "\u21DA", Lacute: "\u0139", lacute: "\u013A", laemptyv: "\u29B4", lagran: "\u2112", Lambda: "\u039B", lambda: "\u03BB", Lang: "\u27EA", lang: "\u27E8", langd: "\u2991", langle: "\u27E8", lap: "\u2A85", Laplacetrf: "\u2112", laquo: "\xAB", Larr: "\u219E", lArr: "\u21D0", larr: "\u2190", larrb: "\u21E4", larrbfs: "\u291F", larrfs: "\u291D", larrhk: "\u21A9", larrlp: "\u21AB", larrpl: "\u2939", larrsim: "\u2973", larrtl: "\u21A2", lat: "\u2AAB", lAtail: "\u291B", latail: "\u2919", late: "\u2AAD", lates: "\u2AAD\uFE00", lBarr: "\u290E", lbarr: "\u290C", lbbrk: "\u2772", lbrace: "{", lbrack: "[", lbrke: "\u298B", lbrksld: "\u298F", lbrkslu: "\u298D", Lcaron: "\u013D", lcaron: "\u013E", Lcedil: "\u013B", lcedil: "\u013C", lceil: "\u2308", lcub: "{", Lcy: "\u041B", lcy: "\u043B", ldca: "\u2936", ldquo: "\u201C", ldquor: "\u201E", ldrdhar: "\u2967", ldrushar: "\u294B", ldsh: "\u21B2", lE: "\u2266", le: "\u2264", LeftAngleBracket: "\u27E8", LeftArrow: "\u2190", Leftarrow: "\u21D0", leftarrow: "\u2190", LeftArrowBar: "\u21E4", LeftArrowRightArrow: "\u21C6", leftarrowtail: "\u21A2", LeftCeiling: "\u2308", LeftDoubleBracket: "\u27E6", LeftDownTeeVector: "\u2961", LeftDownVector: "\u21C3", LeftDownVectorBar: "\u2959", LeftFloor: "\u230A", leftharpoondown: "\u21BD", leftharpoonup: "\u21BC", leftleftarrows: "\u21C7", LeftRightArrow: "\u2194", Leftrightarrow: "\u21D4", leftrightarrow: "\u2194", leftrightarrows: "\u21C6", leftrightharpoons: "\u21CB", leftrightsquigarrow: "\u21AD", LeftRightVector: "\u294E", LeftTee: "\u22A3", LeftTeeArrow: "\u21A4", LeftTeeVector: "\u295A", leftthreetimes: "\u22CB", LeftTriangle: "\u22B2", LeftTriangleBar: "\u29CF", LeftTriangleEqual: "\u22B4", LeftUpDownVector: "\u2951", LeftUpTeeVector: "\u2960", LeftUpVector: "\u21BF", LeftUpVectorBar: "\u2958", LeftVector: "\u21BC", LeftVectorBar: "\u2952", lEg: "\u2A8B", leg: "\u22DA", leq: "\u2264", leqq: "\u2266", leqslant: "\u2A7D", les: "\u2A7D", lescc: "\u2AA8", lesdot: "\u2A7F", lesdoto: "\u2A81", lesdotor: "\u2A83", lesg: "\u22DA\uFE00", lesges: "\u2A93", lessapprox: "\u2A85", lessdot: "\u22D6", lesseqgtr: "\u22DA", lesseqqgtr: "\u2A8B", LessEqualGreater: "\u22DA", LessFullEqual: "\u2266", LessGreater: "\u2276", lessgtr: "\u2276", LessLess: "\u2AA1", lesssim: "\u2272", LessSlantEqual: "\u2A7D", LessTilde: "\u2272", lfisht: "\u297C", lfloor: "\u230A", Lfr: "\u{1D50F}", lfr: "\u{1D529}", lg: "\u2276", lgE: "\u2A91", lHar: "\u2962", lhard: "\u21BD", lharu: "\u21BC", lharul: "\u296A", lhblk: "\u2584", LJcy: "\u0409", ljcy: "\u0459", Ll: "\u22D8", ll: "\u226A", llarr: "\u21C7", llcorner: "\u231E", Lleftarrow: "\u21DA", llhard: "\u296B", lltri: "\u25FA", Lmidot: "\u013F", lmidot: "\u0140", lmoust: "\u23B0", lmoustache: "\u23B0", lnap: "\u2A89", lnapprox: "\u2A89", lnE: "\u2268", lne: "\u2A87", lneq: "\u2A87", lneqq: "\u2268", lnsim: "\u22E6", loang: "\u27EC", loarr: "\u21FD", lobrk: "\u27E6", LongLeftArrow: "\u27F5", Longleftarrow: "\u27F8", longleftarrow: "\u27F5", LongLeftRightArrow: "\u27F7", Longleftrightarrow: "\u27FA", longleftrightarrow: "\u27F7", longmapsto: "\u27FC", LongRightArrow: "\u27F6", Longrightarrow: "\u27F9", longrightarrow: "\u27F6", looparrowleft: "\u21AB", looparrowright: "\u21AC", lopar: "\u2985", Lopf: "\u{1D543}", lopf: "\u{1D55D}", loplus: "\u2A2D", lotimes: "\u2A34", lowast: "\u2217", lowbar: "_", LowerLeftArrow: "\u2199", LowerRightArrow: "\u2198", loz: "\u25CA", lozenge: "\u25CA", lozf: "\u29EB", lpar: "(", lparlt: "\u2993", lrarr: "\u21C6", lrcorner: "\u231F", lrhar: "\u21CB", lrhard: "\u296D", lrm: "\u200E", lrtri: "\u22BF", lsaquo: "\u2039", Lscr: "\u2112", lscr: "\u{1D4C1}", Lsh: "\u21B0", lsh: "\u21B0", lsim: "\u2272", lsime: "\u2A8D", lsimg: "\u2A8F", lsqb: "[", lsquo: "\u2018", lsquor: "\u201A", Lstrok: "\u0141", lstrok: "\u0142", LT: "<", Lt: "\u226A", lt: "<", ltcc: "\u2AA6", ltcir: "\u2A79", ltdot: "\u22D6", lthree: "\u22CB", ltimes: "\u22C9", ltlarr: "\u2976", ltquest: "\u2A7B", ltri: "\u25C3", ltrie: "\u22B4", ltrif: "\u25C2", ltrPar: "\u2996", lurdshar: "\u294A", luruhar: "\u2966", lvertneqq: "\u2268\uFE00", lvnE: "\u2268\uFE00", macr: "\xAF", male: "\u2642", malt: "\u2720", maltese: "\u2720", Map: "\u2905", map: "\u21A6", mapsto: "\u21A6", mapstodown: "\u21A7", mapstoleft: "\u21A4", mapstoup: "\u21A5", marker: "\u25AE", mcomma: "\u2A29", Mcy: "\u041C", mcy: "\u043C", mdash: "\u2014", mDDot: "\u223A", measuredangle: "\u2221", MediumSpace: "\u205F", Mellintrf: "\u2133", Mfr: "\u{1D510}", mfr: "\u{1D52A}", mho: "\u2127", micro: "\xB5", mid: "\u2223", midast: "*", midcir: "\u2AF0", middot: "\xB7", minus: "\u2212", minusb: "\u229F", minusd: "\u2238", minusdu: "\u2A2A", MinusPlus: "\u2213", mlcp: "\u2ADB", mldr: "\u2026", mnplus: "\u2213", models: "\u22A7", Mopf: "\u{1D544}", mopf: "\u{1D55E}", mp: "\u2213", Mscr: "\u2133", mscr: "\u{1D4C2}", mstpos: "\u223E", Mu: "\u039C", mu: "\u03BC", multimap: "\u22B8", mumap: "\u22B8", nabla: "\u2207", Nacute: "\u0143", nacute: "\u0144", nang: "\u2220\u20D2", nap: "\u2249", napE: "\u2A70\u0338", napid: "\u224B\u0338", napos: "\u0149", napprox: "\u2249", natur: "\u266E", natural: "\u266E", naturals: "\u2115", nbsp: "\xA0", nbump: "\u224E\u0338", nbumpe: "\u224F\u0338", ncap: "\u2A43", Ncaron: "\u0147", ncaron: "\u0148", Ncedil: "\u0145", ncedil: "\u0146", ncong: "\u2247", ncongdot: "\u2A6D\u0338", ncup: "\u2A42", Ncy: "\u041D", ncy: "\u043D", ndash: "\u2013", ne: "\u2260", nearhk: "\u2924", neArr: "\u21D7", nearr: "\u2197", nearrow: "\u2197", nedot: "\u2250\u0338", NegativeMediumSpace: "\u200B", NegativeThickSpace: "\u200B", NegativeThinSpace: "\u200B", NegativeVeryThinSpace: "\u200B", nequiv: "\u2262", nesear: "\u2928", nesim: "\u2242\u0338", NestedGreaterGreater: "\u226B", NestedLessLess: "\u226A", NewLine: `
`, nexist: "\u2204", nexists: "\u2204", Nfr: "\u{1D511}", nfr: "\u{1D52B}", ngE: "\u2267\u0338", nge: "\u2271", ngeq: "\u2271", ngeqq: "\u2267\u0338", ngeqslant: "\u2A7E\u0338", nges: "\u2A7E\u0338", nGg: "\u22D9\u0338", ngsim: "\u2275", nGt: "\u226B\u20D2", ngt: "\u226F", ngtr: "\u226F", nGtv: "\u226B\u0338", nhArr: "\u21CE", nharr: "\u21AE", nhpar: "\u2AF2", ni: "\u220B", nis: "\u22FC", nisd: "\u22FA", niv: "\u220B", NJcy: "\u040A", njcy: "\u045A", nlArr: "\u21CD", nlarr: "\u219A", nldr: "\u2025", nlE: "\u2266\u0338", nle: "\u2270", nLeftarrow: "\u21CD", nleftarrow: "\u219A", nLeftrightarrow: "\u21CE", nleftrightarrow: "\u21AE", nleq: "\u2270", nleqq: "\u2266\u0338", nleqslant: "\u2A7D\u0338", nles: "\u2A7D\u0338", nless: "\u226E", nLl: "\u22D8\u0338", nlsim: "\u2274", nLt: "\u226A\u20D2", nlt: "\u226E", nltri: "\u22EA", nltrie: "\u22EC", nLtv: "\u226A\u0338", nmid: "\u2224", NoBreak: "\u2060", NonBreakingSpace: "\xA0", Nopf: "\u2115", nopf: "\u{1D55F}", Not: "\u2AEC", not: "\xAC", NotCongruent: "\u2262", NotCupCap: "\u226D", NotDoubleVerticalBar: "\u2226", NotElement: "\u2209", NotEqual: "\u2260", NotEqualTilde: "\u2242\u0338", NotExists: "\u2204", NotGreater: "\u226F", NotGreaterEqual: "\u2271", NotGreaterFullEqual: "\u2267\u0338", NotGreaterGreater: "\u226B\u0338", NotGreaterLess: "\u2279", NotGreaterSlantEqual: "\u2A7E\u0338", NotGreaterTilde: "\u2275", NotHumpDownHump: "\u224E\u0338", NotHumpEqual: "\u224F\u0338", notin: "\u2209", notindot: "\u22F5\u0338", notinE: "\u22F9\u0338", notinva: "\u2209", notinvb: "\u22F7", notinvc: "\u22F6", NotLeftTriangle: "\u22EA", NotLeftTriangleBar: "\u29CF\u0338", NotLeftTriangleEqual: "\u22EC", NotLess: "\u226E", NotLessEqual: "\u2270", NotLessGreater: "\u2278", NotLessLess: "\u226A\u0338", NotLessSlantEqual: "\u2A7D\u0338", NotLessTilde: "\u2274", NotNestedGreaterGreater: "\u2AA2\u0338", NotNestedLessLess: "\u2AA1\u0338", notni: "\u220C", notniva: "\u220C", notnivb: "\u22FE", notnivc: "\u22FD", NotPrecedes: "\u2280", NotPrecedesEqual: "\u2AAF\u0338", NotPrecedesSlantEqual: "\u22E0", NotReverseElement: "\u220C", NotRightTriangle: "\u22EB", NotRightTriangleBar: "\u29D0\u0338", NotRightTriangleEqual: "\u22ED", NotSquareSubset: "\u228F\u0338", NotSquareSubsetEqual: "\u22E2", NotSquareSuperset: "\u2290\u0338", NotSquareSupersetEqual: "\u22E3", NotSubset: "\u2282\u20D2", NotSubsetEqual: "\u2288", NotSucceeds: "\u2281", NotSucceedsEqual: "\u2AB0\u0338", NotSucceedsSlantEqual: "\u22E1", NotSucceedsTilde: "\u227F\u0338", NotSuperset: "\u2283\u20D2", NotSupersetEqual: "\u2289", NotTilde: "\u2241", NotTildeEqual: "\u2244", NotTildeFullEqual: "\u2247", NotTildeTilde: "\u2249", NotVerticalBar: "\u2224", npar: "\u2226", nparallel: "\u2226", nparsl: "\u2AFD\u20E5", npart: "\u2202\u0338", npolint: "\u2A14", npr: "\u2280", nprcue: "\u22E0", npre: "\u2AAF\u0338", nprec: "\u2280", npreceq: "\u2AAF\u0338", nrArr: "\u21CF", nrarr: "\u219B", nrarrc: "\u2933\u0338", nrarrw: "\u219D\u0338", nRightarrow: "\u21CF", nrightarrow: "\u219B", nrtri: "\u22EB", nrtrie: "\u22ED", nsc: "\u2281", nsccue: "\u22E1", nsce: "\u2AB0\u0338", Nscr: "\u{1D4A9}", nscr: "\u{1D4C3}", nshortmid: "\u2224", nshortparallel: "\u2226", nsim: "\u2241", nsime: "\u2244", nsimeq: "\u2244", nsmid: "\u2224", nspar: "\u2226", nsqsube: "\u22E2", nsqsupe: "\u22E3", nsub: "\u2284", nsubE: "\u2AC5\u0338", nsube: "\u2288", nsubset: "\u2282\u20D2", nsubseteq: "\u2288", nsubseteqq: "\u2AC5\u0338", nsucc: "\u2281", nsucceq: "\u2AB0\u0338", nsup: "\u2285", nsupE: "\u2AC6\u0338", nsupe: "\u2289", nsupset: "\u2283\u20D2", nsupseteq: "\u2289", nsupseteqq: "\u2AC6\u0338", ntgl: "\u2279", Ntilde: "\xD1", ntilde: "\xF1", ntlg: "\u2278", ntriangleleft: "\u22EA", ntrianglelefteq: "\u22EC", ntriangleright: "\u22EB", ntrianglerighteq: "\u22ED", Nu: "\u039D", nu: "\u03BD", num: "#", numero: "\u2116", numsp: "\u2007", nvap: "\u224D\u20D2", nVDash: "\u22AF", nVdash: "\u22AE", nvDash: "\u22AD", nvdash: "\u22AC", nvge: "\u2265\u20D2", nvgt: ">\u20D2", nvHarr: "\u2904", nvinfin: "\u29DE", nvlArr: "\u2902", nvle: "\u2264\u20D2", nvlt: "<\u20D2", nvltrie: "\u22B4\u20D2", nvrArr: "\u2903", nvrtrie: "\u22B5\u20D2", nvsim: "\u223C\u20D2", nwarhk: "\u2923", nwArr: "\u21D6", nwarr: "\u2196", nwarrow: "\u2196", nwnear: "\u2927", Oacute: "\xD3", oacute: "\xF3", oast: "\u229B", ocir: "\u229A", Ocirc: "\xD4", ocirc: "\xF4", Ocy: "\u041E", ocy: "\u043E", odash: "\u229D", Odblac: "\u0150", odblac: "\u0151", odiv: "\u2A38", odot: "\u2299", odsold: "\u29BC", OElig: "\u0152", oelig: "\u0153", ofcir: "\u29BF", Ofr: "\u{1D512}", ofr: "\u{1D52C}", ogon: "\u02DB", Ograve: "\xD2", ograve: "\xF2", ogt: "\u29C1", ohbar: "\u29B5", ohm: "\u03A9", oint: "\u222E", olarr: "\u21BA", olcir: "\u29BE", olcross: "\u29BB", oline: "\u203E", olt: "\u29C0", Omacr: "\u014C", omacr: "\u014D", Omega: "\u03A9", omega: "\u03C9", Omicron: "\u039F", omicron: "\u03BF", omid: "\u29B6", ominus: "\u2296", Oopf: "\u{1D546}", oopf: "\u{1D560}", opar: "\u29B7", OpenCurlyDoubleQuote: "\u201C", OpenCurlyQuote: "\u2018", operp: "\u29B9", oplus: "\u2295", Or: "\u2A54", or: "\u2228", orarr: "\u21BB", ord: "\u2A5D", order: "\u2134", orderof: "\u2134", ordf: "\xAA", ordm: "\xBA", origof: "\u22B6", oror: "\u2A56", orslope: "\u2A57", orv: "\u2A5B", oS: "\u24C8", Oscr: "\u{1D4AA}", oscr: "\u2134", Oslash: "\xD8", oslash: "\xF8", osol: "\u2298", Otilde: "\xD5", otilde: "\xF5", Otimes: "\u2A37", otimes: "\u2297", otimesas: "\u2A36", Ouml: "\xD6", ouml: "\xF6", ovbar: "\u233D", OverBar: "\u203E", OverBrace: "\u23DE", OverBracket: "\u23B4", OverParenthesis: "\u23DC", par: "\u2225", para: "\xB6", parallel: "\u2225", parsim: "\u2AF3", parsl: "\u2AFD", part: "\u2202", PartialD: "\u2202", Pcy: "\u041F", pcy: "\u043F", percnt: "%", period: ".", permil: "\u2030", perp: "\u22A5", pertenk: "\u2031", Pfr: "\u{1D513}", pfr: "\u{1D52D}", Phi: "\u03A6", phi: "\u03C6", phiv: "\u03D5", phmmat: "\u2133", phone: "\u260E", Pi: "\u03A0", pi: "\u03C0", pitchfork: "\u22D4", piv: "\u03D6", planck: "\u210F", planckh: "\u210E", plankv: "\u210F", plus: "+", plusacir: "\u2A23", plusb: "\u229E", pluscir: "\u2A22", plusdo: "\u2214", plusdu: "\u2A25", pluse: "\u2A72", PlusMinus: "\xB1", plusmn: "\xB1", plussim: "\u2A26", plustwo: "\u2A27", pm: "\xB1", Poincareplane: "\u210C", pointint: "\u2A15", Popf: "\u2119", popf: "\u{1D561}", pound: "\xA3", Pr: "\u2ABB", pr: "\u227A", prap: "\u2AB7", prcue: "\u227C", prE: "\u2AB3", pre: "\u2AAF", prec: "\u227A", precapprox: "\u2AB7", preccurlyeq: "\u227C", Precedes: "\u227A", PrecedesEqual: "\u2AAF", PrecedesSlantEqual: "\u227C", PrecedesTilde: "\u227E", preceq: "\u2AAF", precnapprox: "\u2AB9", precneqq: "\u2AB5", precnsim: "\u22E8", precsim: "\u227E", Prime: "\u2033", prime: "\u2032", primes: "\u2119", prnap: "\u2AB9", prnE: "\u2AB5", prnsim: "\u22E8", prod: "\u220F", Product: "\u220F", profalar: "\u232E", profline: "\u2312", profsurf: "\u2313", prop: "\u221D", Proportion: "\u2237", Proportional: "\u221D", propto: "\u221D", prsim: "\u227E", prurel: "\u22B0", Pscr: "\u{1D4AB}", pscr: "\u{1D4C5}", Psi: "\u03A8", psi: "\u03C8", puncsp: "\u2008", Qfr: "\u{1D514}", qfr: "\u{1D52E}", qint: "\u2A0C", Qopf: "\u211A", qopf: "\u{1D562}", qprime: "\u2057", Qscr: "\u{1D4AC}", qscr: "\u{1D4C6}", quaternions: "\u210D", quatint: "\u2A16", quest: "?", questeq: "\u225F", QUOT: '"', quot: '"', rAarr: "\u21DB", race: "\u223D\u0331", Racute: "\u0154", racute: "\u0155", radic: "\u221A", raemptyv: "\u29B3", Rang: "\u27EB", rang: "\u27E9", rangd: "\u2992", range: "\u29A5", rangle: "\u27E9", raquo: "\xBB", Rarr: "\u21A0", rArr: "\u21D2", rarr: "\u2192", rarrap: "\u2975", rarrb: "\u21E5", rarrbfs: "\u2920", rarrc: "\u2933", rarrfs: "\u291E", rarrhk: "\u21AA", rarrlp: "\u21AC", rarrpl: "\u2945", rarrsim: "\u2974", Rarrtl: "\u2916", rarrtl: "\u21A3", rarrw: "\u219D", rAtail: "\u291C", ratail: "\u291A", ratio: "\u2236", rationals: "\u211A", RBarr: "\u2910", rBarr: "\u290F", rbarr: "\u290D", rbbrk: "\u2773", rbrace: "}", rbrack: "]", rbrke: "\u298C", rbrksld: "\u298E", rbrkslu: "\u2990", Rcaron: "\u0158", rcaron: "\u0159", Rcedil: "\u0156", rcedil: "\u0157", rceil: "\u2309", rcub: "}", Rcy: "\u0420", rcy: "\u0440", rdca: "\u2937", rdldhar: "\u2969", rdquo: "\u201D", rdquor: "\u201D", rdsh: "\u21B3", Re: "\u211C", real: "\u211C", realine: "\u211B", realpart: "\u211C", reals: "\u211D", rect: "\u25AD", REG: "\xAE", reg: "\xAE", ReverseElement: "\u220B", ReverseEquilibrium: "\u21CB", ReverseUpEquilibrium: "\u296F", rfisht: "\u297D", rfloor: "\u230B", Rfr: "\u211C", rfr: "\u{1D52F}", rHar: "\u2964", rhard: "\u21C1", rharu: "\u21C0", rharul: "\u296C", Rho: "\u03A1", rho: "\u03C1", rhov: "\u03F1", RightAngleBracket: "\u27E9", RightArrow: "\u2192", Rightarrow: "\u21D2", rightarrow: "\u2192", RightArrowBar: "\u21E5", RightArrowLeftArrow: "\u21C4", rightarrowtail: "\u21A3", RightCeiling: "\u2309", RightDoubleBracket: "\u27E7", RightDownTeeVector: "\u295D", RightDownVector: "\u21C2", RightDownVectorBar: "\u2955", RightFloor: "\u230B", rightharpoondown: "\u21C1", rightharpoonup: "\u21C0", rightleftarrows: "\u21C4", rightleftharpoons: "\u21CC", rightrightarrows: "\u21C9", rightsquigarrow: "\u219D", RightTee: "\u22A2", RightTeeArrow: "\u21A6", RightTeeVector: "\u295B", rightthreetimes: "\u22CC", RightTriangle: "\u22B3", RightTriangleBar: "\u29D0", RightTriangleEqual: "\u22B5", RightUpDownVector: "\u294F", RightUpTeeVector: "\u295C", RightUpVector: "\u21BE", RightUpVectorBar: "\u2954", RightVector: "\u21C0", RightVectorBar: "\u2953", ring: "\u02DA", risingdotseq: "\u2253", rlarr: "\u21C4", rlhar: "\u21CC", rlm: "\u200F", rmoust: "\u23B1", rmoustache: "\u23B1", rnmid: "\u2AEE", roang: "\u27ED", roarr: "\u21FE", robrk: "\u27E7", ropar: "\u2986", Ropf: "\u211D", ropf: "\u{1D563}", roplus: "\u2A2E", rotimes: "\u2A35", RoundImplies: "\u2970", rpar: ")", rpargt: "\u2994", rppolint: "\u2A12", rrarr: "\u21C9", Rrightarrow: "\u21DB", rsaquo: "\u203A", Rscr: "\u211B", rscr: "\u{1D4C7}", Rsh: "\u21B1", rsh: "\u21B1", rsqb: "]", rsquo: "\u2019", rsquor: "\u2019", rthree: "\u22CC", rtimes: "\u22CA", rtri: "\u25B9", rtrie: "\u22B5", rtrif: "\u25B8", rtriltri: "\u29CE", RuleDelayed: "\u29F4", ruluhar: "\u2968", rx: "\u211E", Sacute: "\u015A", sacute: "\u015B", sbquo: "\u201A", Sc: "\u2ABC", sc: "\u227B", scap: "\u2AB8", Scaron: "\u0160", scaron: "\u0161", sccue: "\u227D", scE: "\u2AB4", sce: "\u2AB0", Scedil: "\u015E", scedil: "\u015F", Scirc: "\u015C", scirc: "\u015D", scnap: "\u2ABA", scnE: "\u2AB6", scnsim: "\u22E9", scpolint: "\u2A13", scsim: "\u227F", Scy: "\u0421", scy: "\u0441", sdot: "\u22C5", sdotb: "\u22A1", sdote: "\u2A66", searhk: "\u2925", seArr: "\u21D8", searr: "\u2198", searrow: "\u2198", sect: "\xA7", semi: ";", seswar: "\u2929", setminus: "\u2216", setmn: "\u2216", sext: "\u2736", Sfr: "\u{1D516}", sfr: "\u{1D530}", sfrown: "\u2322", sharp: "\u266F", SHCHcy: "\u0429", shchcy: "\u0449", SHcy: "\u0428", shcy: "\u0448", ShortDownArrow: "\u2193", ShortLeftArrow: "\u2190", shortmid: "\u2223", shortparallel: "\u2225", ShortRightArrow: "\u2192", ShortUpArrow: "\u2191", shy: "\xAD", Sigma: "\u03A3", sigma: "\u03C3", sigmaf: "\u03C2", sigmav: "\u03C2", sim: "\u223C", simdot: "\u2A6A", sime: "\u2243", simeq: "\u2243", simg: "\u2A9E", simgE: "\u2AA0", siml: "\u2A9D", simlE: "\u2A9F", simne: "\u2246", simplus: "\u2A24", simrarr: "\u2972", slarr: "\u2190", SmallCircle: "\u2218", smallsetminus: "\u2216", smashp: "\u2A33", smeparsl: "\u29E4", smid: "\u2223", smile: "\u2323", smt: "\u2AAA", smte: "\u2AAC", smtes: "\u2AAC\uFE00", SOFTcy: "\u042C", softcy: "\u044C", sol: "/", solb: "\u29C4", solbar: "\u233F", Sopf: "\u{1D54A}", sopf: "\u{1D564}", spades: "\u2660", spadesuit: "\u2660", spar: "\u2225", sqcap: "\u2293", sqcaps: "\u2293\uFE00", sqcup: "\u2294", sqcups: "\u2294\uFE00", Sqrt: "\u221A", sqsub: "\u228F", sqsube: "\u2291", sqsubset: "\u228F", sqsubseteq: "\u2291", sqsup: "\u2290", sqsupe: "\u2292", sqsupset: "\u2290", sqsupseteq: "\u2292", squ: "\u25A1", Square: "\u25A1", square: "\u25A1", SquareIntersection: "\u2293", SquareSubset: "\u228F", SquareSubsetEqual: "\u2291", SquareSuperset: "\u2290", SquareSupersetEqual: "\u2292", SquareUnion: "\u2294", squarf: "\u25AA", squf: "\u25AA", srarr: "\u2192", Sscr: "\u{1D4AE}", sscr: "\u{1D4C8}", ssetmn: "\u2216", ssmile: "\u2323", sstarf: "\u22C6", Star: "\u22C6", star: "\u2606", starf: "\u2605", straightepsilon: "\u03F5", straightphi: "\u03D5", strns: "\xAF", Sub: "\u22D0", sub: "\u2282", subdot: "\u2ABD", subE: "\u2AC5", sube: "\u2286", subedot: "\u2AC3", submult: "\u2AC1", subnE: "\u2ACB", subne: "\u228A", subplus: "\u2ABF", subrarr: "\u2979", Subset: "\u22D0", subset: "\u2282", subseteq: "\u2286", subseteqq: "\u2AC5", SubsetEqual: "\u2286", subsetneq: "\u228A", subsetneqq: "\u2ACB", subsim: "\u2AC7", subsub: "\u2AD5", subsup: "\u2AD3", succ: "\u227B", succapprox: "\u2AB8", succcurlyeq: "\u227D", Succeeds: "\u227B", SucceedsEqual: "\u2AB0", SucceedsSlantEqual: "\u227D", SucceedsTilde: "\u227F", succeq: "\u2AB0", succnapprox: "\u2ABA", succneqq: "\u2AB6", succnsim: "\u22E9", succsim: "\u227F", SuchThat: "\u220B", Sum: "\u2211", sum: "\u2211", sung: "\u266A", Sup: "\u22D1", sup: "\u2283", sup1: "\xB9", sup2: "\xB2", sup3: "\xB3", supdot: "\u2ABE", supdsub: "\u2AD8", supE: "\u2AC6", supe: "\u2287", supedot: "\u2AC4", Superset: "\u2283", SupersetEqual: "\u2287", suphsol: "\u27C9", suphsub: "\u2AD7", suplarr: "\u297B", supmult: "\u2AC2", supnE: "\u2ACC", supne: "\u228B", supplus: "\u2AC0", Supset: "\u22D1", supset: "\u2283", supseteq: "\u2287", supseteqq: "\u2AC6", supsetneq: "\u228B", supsetneqq: "\u2ACC", supsim: "\u2AC8", supsub: "\u2AD4", supsup: "\u2AD6", swarhk: "\u2926", swArr: "\u21D9", swarr: "\u2199", swarrow: "\u2199", swnwar: "\u292A", szlig: "\xDF", Tab: " ", target: "\u2316", Tau: "\u03A4", tau: "\u03C4", tbrk: "\u23B4", Tcaron: "\u0164", tcaron: "\u0165", Tcedil: "\u0162", tcedil: "\u0163", Tcy: "\u0422", tcy: "\u0442", tdot: "\u20DB", telrec: "\u2315", Tfr: "\u{1D517}", tfr: "\u{1D531}", there4: "\u2234", Therefore: "\u2234", therefore: "\u2234", Theta: "\u0398", theta: "\u03B8", thetasym: "\u03D1", thetav: "\u03D1", thickapprox: "\u2248", thicksim: "\u223C", ThickSpace: "\u205F\u200A", thinsp: "\u2009", ThinSpace: "\u2009", thkap: "\u2248", thksim: "\u223C", THORN: "\xDE", thorn: "\xFE", Tilde: "\u223C", tilde: "\u02DC", TildeEqual: "\u2243", TildeFullEqual: "\u2245", TildeTilde: "\u2248", times: "\xD7", timesb: "\u22A0", timesbar: "\u2A31", timesd: "\u2A30", tint: "\u222D", toea: "\u2928", top: "\u22A4", topbot: "\u2336", topcir: "\u2AF1", Topf: "\u{1D54B}", topf: "\u{1D565}", topfork: "\u2ADA", tosa: "\u2929", tprime: "\u2034", TRADE: "\u2122", trade: "\u2122", triangle: "\u25B5", triangledown: "\u25BF", triangleleft: "\u25C3", trianglelefteq: "\u22B4", triangleq: "\u225C", triangleright: "\u25B9", trianglerighteq: "\u22B5", tridot: "\u25EC", trie: "\u225C", triminus: "\u2A3A", TripleDot: "\u20DB", triplus: "\u2A39", trisb: "\u29CD", tritime: "\u2A3B", trpezium: "\u23E2", Tscr: "\u{1D4AF}", tscr: "\u{1D4C9}", TScy: "\u0426", tscy: "\u0446", TSHcy: "\u040B", tshcy: "\u045B", Tstrok: "\u0166", tstrok: "\u0167", twixt: "\u226C", twoheadleftarrow: "\u219E", twoheadrightarrow: "\u21A0", Uacute: "\xDA", uacute: "\xFA", Uarr: "\u219F", uArr: "\u21D1", uarr: "\u2191", Uarrocir: "\u2949", Ubrcy: "\u040E", ubrcy: "\u045E", Ubreve: "\u016C", ubreve: "\u016D", Ucirc: "\xDB", ucirc: "\xFB", Ucy: "\u0423", ucy: "\u0443", udarr: "\u21C5", Udblac: "\u0170", udblac: "\u0171", udhar: "\u296E", ufisht: "\u297E", Ufr: "\u{1D518}", ufr: "\u{1D532}", Ugrave: "\xD9", ugrave: "\xF9", uHar: "\u2963", uharl: "\u21BF", uharr: "\u21BE", uhblk: "\u2580", ulcorn: "\u231C", ulcorner: "\u231C", ulcrop: "\u230F", ultri: "\u25F8", Umacr: "\u016A", umacr: "\u016B", uml: "\xA8", UnderBar: "_", UnderBrace: "\u23DF", UnderBracket: "\u23B5", UnderParenthesis: "\u23DD", Union: "\u22C3", UnionPlus: "\u228E", Uogon: "\u0172", uogon: "\u0173", Uopf: "\u{1D54C}", uopf: "\u{1D566}", UpArrow: "\u2191", Uparrow: "\u21D1", uparrow: "\u2191", UpArrowBar: "\u2912", UpArrowDownArrow: "\u21C5", UpDownArrow: "\u2195", Updownarrow: "\u21D5", updownarrow: "\u2195", UpEquilibrium: "\u296E", upharpoonleft: "\u21BF", upharpoonright: "\u21BE", uplus: "\u228E", UpperLeftArrow: "\u2196", UpperRightArrow: "\u2197", Upsi: "\u03D2", upsi: "\u03C5", upsih: "\u03D2", Upsilon: "\u03A5", upsilon: "\u03C5", UpTee: "\u22A5", UpTeeArrow: "\u21A5", upuparrows: "\u21C8", urcorn: "\u231D", urcorner: "\u231D", urcrop: "\u230E", Uring: "\u016E", uring: "\u016F", urtri: "\u25F9", Uscr: "\u{1D4B0}", uscr: "\u{1D4CA}", utdot: "\u22F0", Utilde: "\u0168", utilde: "\u0169", utri: "\u25B5", utrif: "\u25B4", uuarr: "\u21C8", Uuml: "\xDC", uuml: "\xFC", uwangle: "\u29A7", vangrt: "\u299C", varepsilon: "\u03F5", varkappa: "\u03F0", varnothing: "\u2205", varphi: "\u03D5", varpi: "\u03D6", varpropto: "\u221D", vArr: "\u21D5", varr: "\u2195", varrho: "\u03F1", varsigma: "\u03C2", varsubsetneq: "\u228A\uFE00", varsubsetneqq: "\u2ACB\uFE00", varsupsetneq: "\u228B\uFE00", varsupsetneqq: "\u2ACC\uFE00", vartheta: "\u03D1", vartriangleleft: "\u22B2", vartriangleright: "\u22B3", Vbar: "\u2AEB", vBar: "\u2AE8", vBarv: "\u2AE9", Vcy: "\u0412", vcy: "\u0432", VDash: "\u22AB", Vdash: "\u22A9", vDash: "\u22A8", vdash: "\u22A2", Vdashl: "\u2AE6", Vee: "\u22C1", vee: "\u2228", veebar: "\u22BB", veeeq: "\u225A", vellip: "\u22EE", Verbar: "\u2016", verbar: "|", Vert: "\u2016", vert: "|", VerticalBar: "\u2223", VerticalLine: "|", VerticalSeparator: "\u2758", VerticalTilde: "\u2240", VeryThinSpace: "\u200A", Vfr: "\u{1D519}", vfr: "\u{1D533}", vltri: "\u22B2", vnsub: "\u2282\u20D2", vnsup: "\u2283\u20D2", Vopf: "\u{1D54D}", vopf: "\u{1D567}", vprop: "\u221D", vrtri: "\u22B3", Vscr: "\u{1D4B1}", vscr: "\u{1D4CB}", vsubnE: "\u2ACB\uFE00", vsubne: "\u228A\uFE00", vsupnE: "\u2ACC\uFE00", vsupne: "\u228B\uFE00", Vvdash: "\u22AA", vzigzag: "\u299A", Wcirc: "\u0174", wcirc: "\u0175", wedbar: "\u2A5F", Wedge: "\u22C0", wedge: "\u2227", wedgeq: "\u2259", weierp: "\u2118", Wfr: "\u{1D51A}", wfr: "\u{1D534}", Wopf: "\u{1D54E}", wopf: "\u{1D568}", wp: "\u2118", wr: "\u2240", wreath: "\u2240", Wscr: "\u{1D4B2}", wscr: "\u{1D4CC}", xcap: "\u22C2", xcirc: "\u25EF", xcup: "\u22C3", xdtri: "\u25BD", Xfr: "\u{1D51B}", xfr: "\u{1D535}", xhArr: "\u27FA", xharr: "\u27F7", Xi: "\u039E", xi: "\u03BE", xlArr: "\u27F8", xlarr: "\u27F5", xmap: "\u27FC", xnis: "\u22FB", xodot: "\u2A00", Xopf: "\u{1D54F}", xopf: "\u{1D569}", xoplus: "\u2A01", xotime: "\u2A02", xrArr: "\u27F9", xrarr: "\u27F6", Xscr: "\u{1D4B3}", xscr: "\u{1D4CD}", xsqcup: "\u2A06", xuplus: "\u2A04", xutri: "\u25B3", xvee: "\u22C1", xwedge: "\u22C0", Yacute: "\xDD", yacute: "\xFD", YAcy: "\u042F", yacy: "\u044F", Ycirc: "\u0176", ycirc: "\u0177", Ycy: "\u042B", ycy: "\u044B", yen: "\xA5", Yfr: "\u{1D51C}", yfr: "\u{1D536}", YIcy: "\u0407", yicy: "\u0457", Yopf: "\u{1D550}", yopf: "\u{1D56A}", Yscr: "\u{1D4B4}", yscr: "\u{1D4CE}", YUcy: "\u042E", yucy: "\u044E", Yuml: "\u0178", yuml: "\xFF", Zacute: "\u0179", zacute: "\u017A", Zcaron: "\u017D", zcaron: "\u017E", Zcy: "\u0417", zcy: "\u0437", Zdot: "\u017B", zdot: "\u017C", zeetrf: "\u2128", ZeroWidthSpace: "\u200B", Zeta: "\u0396", zeta: "\u03B6", Zfr: "\u2128", zfr: "\u{1D537}", ZHcy: "\u0416", zhcy: "\u0436", zigrarr: "\u21DD", Zopf: "\u2124", zopf: "\u{1D56B}", Zscr: "\u{1D4B5}", zscr: "\u{1D4CF}", zwj: "\u200D", zwnj: "\u200C" }, e.NGSP_UNICODE = "\uE500", e.NAMED_ENTITIES.ngsp = e.NGSP_UNICODE;
} }), Bs = I({ "node_modules/angular-html-parser/lib/compiler/src/ml_parser/html_tags.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = Ze(), u = class {
constructor() {
let { closedByChildren: i, implicitNamespacePrefix: f, contentType: c = r.TagContentType.PARSABLE_DATA, closedByParent: F = false, isVoid: a = false, ignoreFirstLf: l = false } = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {};
this.closedByChildren = {}, this.closedByParent = false, this.canSelfClose = false, i && i.length > 0 && i.forEach((h) => this.closedByChildren[h] = true), this.isVoid = a, this.closedByParent = F || a, this.implicitNamespacePrefix = f || null, this.contentType = c, this.ignoreFirstLf = l;
}
isClosedByChild(i) {
return this.isVoid || i.toLowerCase() in this.closedByChildren;
}
};
e.HtmlTagDefinition = u;
var n2, D;
function s(i) {
return D || (n2 = new u(), D = { base: new u({ isVoid: true }), meta: new u({ isVoid: true }), area: new u({ isVoid: true }), embed: new u({ isVoid: true }), link: new u({ isVoid: true }), img: new u({ isVoid: true }), input: new u({ isVoid: true }), param: new u({ isVoid: true }), hr: new u({ isVoid: true }), br: new u({ isVoid: true }), source: new u({ isVoid: true }), track: new u({ isVoid: true }), wbr: new u({ isVoid: true }), p: new u({ closedByChildren: ["address", "article", "aside", "blockquote", "div", "dl", "fieldset", "footer", "form", "h1", "h2", "h3", "h4", "h5", "h6", "header", "hgroup", "hr", "main", "nav", "ol", "p", "pre", "section", "table", "ul"], closedByParent: true }), thead: new u({ closedByChildren: ["tbody", "tfoot"] }), tbody: new u({ closedByChildren: ["tbody", "tfoot"], closedByParent: true }), tfoot: new u({ closedByChildren: ["tbody"], closedByParent: true }), tr: new u({ closedByChildren: ["tr"], closedByParent: true }), td: new u({ closedByChildren: ["td", "th"], closedByParent: true }), th: new u({ closedByChildren: ["td", "th"], closedByParent: true }), col: new u({ isVoid: true }), svg: new u({ implicitNamespacePrefix: "svg" }), math: new u({ implicitNamespacePrefix: "math" }), li: new u({ closedByChildren: ["li"], closedByParent: true }), dt: new u({ closedByChildren: ["dt", "dd"] }), dd: new u({ closedByChildren: ["dt", "dd"], closedByParent: true }), rb: new u({ closedByChildren: ["rb", "rt", "rtc", "rp"], closedByParent: true }), rt: new u({ closedByChildren: ["rb", "rt", "rtc", "rp"], closedByParent: true }), rtc: new u({ closedByChildren: ["rb", "rtc", "rp"], closedByParent: true }), rp: new u({ closedByChildren: ["rb", "rt", "rtc", "rp"], closedByParent: true }), optgroup: new u({ closedByChildren: ["optgroup"], closedByParent: true }), option: new u({ closedByChildren: ["option", "optgroup"], closedByParent: true }), pre: new u({ ignoreFirstLf: true }), listing: new u({ ignoreFirstLf: true }), style: new u({ contentType: r.TagContentType.RAW_TEXT }), script: new u({ contentType: r.TagContentType.RAW_TEXT }), title: new u({ contentType: r.TagContentType.ESCAPABLE_RAW_TEXT }), textarea: new u({ contentType: r.TagContentType.ESCAPABLE_RAW_TEXT, ignoreFirstLf: true }) }), D[i] || n2;
}
e.getHtmlTagDefinition = s;
} }), Hl = I({ "node_modules/angular-html-parser/lib/compiler/src/ast_path.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = class {
constructor(u) {
let n2 = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : -1;
this.path = u, this.position = n2;
}
get empty() {
return !this.path || !this.path.length;
}
get head() {
return this.path[0];
}
get tail() {
return this.path[this.path.length - 1];
}
parentOf(u) {
return u && this.path[this.path.indexOf(u) - 1];
}
childOf(u) {
return this.path[this.path.indexOf(u) + 1];
}
first(u) {
for (let n2 = this.path.length - 1; n2 >= 0; n2--) {
let D = this.path[n2];
if (D instanceof u)
return D;
}
}
push(u) {
this.path.push(u);
}
pop() {
return this.path.pop();
}
};
e.AstPath = r;
} }), bs = I({ "node_modules/angular-html-parser/lib/compiler/src/ml_parser/ast.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = Hl(), u = class {
constructor(d, m, T) {
this.value = d, this.sourceSpan = m, this.i18n = T, this.type = "text";
}
visit(d, m) {
return d.visitText(this, m);
}
};
e.Text = u;
var n2 = class {
constructor(d, m) {
this.value = d, this.sourceSpan = m, this.type = "cdata";
}
visit(d, m) {
return d.visitCdata(this, m);
}
};
e.CDATA = n2;
var D = class {
constructor(d, m, T, w, g, N) {
this.switchValue = d, this.type = m, this.cases = T, this.sourceSpan = w, this.switchValueSourceSpan = g, this.i18n = N;
}
visit(d, m) {
return d.visitExpansion(this, m);
}
};
e.Expansion = D;
var s = class {
constructor(d, m, T, w, g) {
this.value = d, this.expression = m, this.sourceSpan = T, this.valueSourceSpan = w, this.expSourceSpan = g;
}
visit(d, m) {
return d.visitExpansionCase(this, m);
}
};
e.ExpansionCase = s;
var i = class {
constructor(d, m, T) {
let w = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : null, g = arguments.length > 4 && arguments[4] !== void 0 ? arguments[4] : null, N = arguments.length > 5 && arguments[5] !== void 0 ? arguments[5] : null;
this.name = d, this.value = m, this.sourceSpan = T, this.valueSpan = w, this.nameSpan = g, this.i18n = N, this.type = "attribute";
}
visit(d, m) {
return d.visitAttribute(this, m);
}
};
e.Attribute = i;
var f = class {
constructor(d, m, T, w) {
let g = arguments.length > 4 && arguments[4] !== void 0 ? arguments[4] : null, N = arguments.length > 5 && arguments[5] !== void 0 ? arguments[5] : null, R = arguments.length > 6 && arguments[6] !== void 0 ? arguments[6] : null, j = arguments.length > 7 && arguments[7] !== void 0 ? arguments[7] : null;
this.name = d, this.attrs = m, this.children = T, this.sourceSpan = w, this.startSourceSpan = g, this.endSourceSpan = N, this.nameSpan = R, this.i18n = j, this.type = "element";
}
visit(d, m) {
return d.visitElement(this, m);
}
};
e.Element = f;
var c = class {
constructor(d, m) {
this.value = d, this.sourceSpan = m, this.type = "comment";
}
visit(d, m) {
return d.visitComment(this, m);
}
};
e.Comment = c;
var F = class {
constructor(d, m) {
this.value = d, this.sourceSpan = m, this.type = "docType";
}
visit(d, m) {
return d.visitDocType(this, m);
}
};
e.DocType = F;
function a(d, m) {
let T = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : null, w = [], g = d.visit ? (N) => d.visit(N, T) || N.visit(d, T) : (N) => N.visit(d, T);
return m.forEach((N) => {
let R = g(N);
R && w.push(R);
}), w;
}
e.visitAll = a;
var l = class {
constructor() {
}
visitElement(d, m) {
this.visitChildren(m, (T) => {
T(d.attrs), T(d.children);
});
}
visitAttribute(d, m) {
}
visitText(d, m) {
}
visitCdata(d, m) {
}
visitComment(d, m) {
}
visitDocType(d, m) {
}
visitExpansion(d, m) {
return this.visitChildren(m, (T) => {
T(d.cases);
});
}
visitExpansionCase(d, m) {
}
visitChildren(d, m) {
let T = [], w = this;
function g(N) {
N && T.push(a(w, N, d));
}
return m(g), Array.prototype.concat.apply([], T);
}
};
e.RecursiveVisitor = l;
function h(d) {
let m = d.sourceSpan.start.offset, T = d.sourceSpan.end.offset;
return d instanceof f && (d.endSourceSpan ? T = d.endSourceSpan.end.offset : d.children && d.children.length && (T = h(d.children[d.children.length - 1]).end)), { start: m, end: T };
}
function C(d, m) {
let T = [], w = new class extends l {
visit(g, N) {
let R = h(g);
if (R.start <= m && m < R.end)
T.push(g);
else
return true;
}
}();
return a(w, d), new r.AstPath(T, m);
}
e.findNode = C;
} }), zl = I({ "node_modules/angular-html-parser/lib/compiler/src/assertions.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
function r(D, s) {
if (s != null) {
if (!Array.isArray(s))
throw new Error(`Expected '${D}' to be an array of strings.`);
for (let i = 0; i < s.length; i += 1)
if (typeof s[i] != "string")
throw new Error(`Expected '${D}' to be an array of strings.`);
}
}
e.assertArrayOfStrings = r;
var u = [/^\s*$/, /[<>]/, /^[{}]$/, /&(#|[a-z])/i, /^\/\//];
function n2(D, s) {
if (s != null && !(Array.isArray(s) && s.length == 2))
throw new Error(`Expected '${D}' to be an array, [start, end].`);
if (s != null) {
let i = s[0], f = s[1];
u.forEach((c) => {
if (c.test(i) || c.test(f))
throw new Error(`['${i}', '${f}'] contains unusable interpolation symbol.`);
});
}
}
e.assertInterpolationSymbols = n2;
} }), Wl = I({ "node_modules/angular-html-parser/lib/compiler/src/ml_parser/interpolation_config.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = zl(), u = class {
constructor(n2, D) {
this.start = n2, this.end = D;
}
static fromArray(n2) {
return n2 ? (r.assertInterpolationSymbols("interpolation", n2), new u(n2[0], n2[1])) : e.DEFAULT_INTERPOLATION_CONFIG;
}
};
e.InterpolationConfig = u, e.DEFAULT_INTERPOLATION_CONFIG = new u("{{", "}}");
} }), Yl = I({ "node_modules/angular-html-parser/lib/compiler/src/ml_parser/lexer.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = Es(), u = Be(), n2 = Wl(), D = Ze(), s;
(function(t) {
t[t.TAG_OPEN_START = 0] = "TAG_OPEN_START", t[t.TAG_OPEN_END = 1] = "TAG_OPEN_END", t[t.TAG_OPEN_END_VOID = 2] = "TAG_OPEN_END_VOID", t[t.TAG_CLOSE = 3] = "TAG_CLOSE", t[t.TEXT = 4] = "TEXT", t[t.ESCAPABLE_RAW_TEXT = 5] = "ESCAPABLE_RAW_TEXT", t[t.RAW_TEXT = 6] = "RAW_TEXT", t[t.COMMENT_START = 7] = "COMMENT_START", t[t.COMMENT_END = 8] = "COMMENT_END", t[t.CDATA_START = 9] = "CDATA_START", t[t.CDATA_END = 10] = "CDATA_END", t[t.ATTR_NAME = 11] = "ATTR_NAME", t[t.ATTR_QUOTE = 12] = "ATTR_QUOTE", t[t.ATTR_VALUE = 13] = "ATTR_VALUE", t[t.DOC_TYPE_START = 14] = "DOC_TYPE_START", t[t.DOC_TYPE_END = 15] = "DOC_TYPE_END", t[t.EXPANSION_FORM_START = 16] = "EXPANSION_FORM_START", t[t.EXPANSION_CASE_VALUE = 17] = "EXPANSION_CASE_VALUE", t[t.EXPANSION_CASE_EXP_START = 18] = "EXPANSION_CASE_EXP_START", t[t.EXPANSION_CASE_EXP_END = 19] = "EXPANSION_CASE_EXP_END", t[t.EXPANSION_FORM_END = 20] = "EXPANSION_FORM_END", t[t.EOF = 21] = "EOF";
})(s = e.TokenType || (e.TokenType = {}));
var i = class {
constructor(t, o, E) {
this.type = t, this.parts = o, this.sourceSpan = E;
}
};
e.Token = i;
var f = class extends u.ParseError {
constructor(t, o, E) {
super(E, t), this.tokenType = o;
}
};
e.TokenError = f;
var c = class {
constructor(t, o) {
this.tokens = t, this.errors = o;
}
};
e.TokenizeResult = c;
function F(t, o, E) {
let p = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : {};
return new d(new u.ParseSourceFile(t, o), E, p).tokenize();
}
e.tokenize = F;
var a = /\r\n?/g;
function l(t) {
return `Unexpected character "${t === r.$EOF ? "EOF" : String.fromCharCode(t)}"`;
}
function h(t) {
return `Unknown entity "${t}" - use the ";" or ";" syntax`;
}
var C = class {
constructor(t) {
this.error = t;
}
}, d = class {
constructor(t, o, E) {
this._getTagContentType = o, this._currentTokenStart = null, this._currentTokenType = null, this._expansionCaseStack = [], this._inInterpolation = false, this._fullNameStack = [], this.tokens = [], this.errors = [], this._tokenizeIcu = E.tokenizeExpansionForms || false, this._interpolationConfig = E.interpolationConfig || n2.DEFAULT_INTERPOLATION_CONFIG, this._leadingTriviaCodePoints = E.leadingTriviaChars && E.leadingTriviaChars.map((A) => A.codePointAt(0) || 0), this._canSelfClose = E.canSelfClose || false, this._allowHtmComponentClosingTags = E.allowHtmComponentClosingTags || false;
let p = E.range || { endPos: t.content.length, startPos: 0, startLine: 0, startCol: 0 };
this._cursor = E.escapedString ? new k(t, p) : new x(t, p);
try {
this._cursor.init();
} catch (A) {
this.handleError(A);
}
}
_processCarriageReturns(t) {
return t.replace(a, `
`);
}
tokenize() {
for (; this._cursor.peek() !== r.$EOF; ) {
let t = this._cursor.clone();
try {
if (this._attemptCharCode(r.$LT))
if (this._attemptCharCode(r.$BANG))
this._attemptStr("[CDATA[") ? this._consumeCdata(t) : this._attemptStr("--") ? this._consumeComment(t) : this._attemptStrCaseInsensitive("doctype") ? this._consumeDocType(t) : this._consumeBogusComment(t);
else if (this._attemptCharCode(r.$SLASH))
this._consumeTagClose(t);
else {
let o = this._cursor.clone();
this._attemptCharCode(r.$QUESTION) ? (this._cursor = o, this._consumeBogusComment(t)) : this._consumeTagOpen(t);
}
else
this._tokenizeIcu && this._tokenizeExpansionForm() || this._consumeText();
} catch (o) {
this.handleError(o);
}
}
return this._beginToken(s.EOF), this._endToken([]), new c(O(this.tokens), this.errors);
}
_tokenizeExpansionForm() {
if (this.isExpansionFormStart())
return this._consumeExpansionFormStart(), true;
if (R(this._cursor.peek()) && this._isInExpansionForm())
return this._consumeExpansionCaseStart(), true;
if (this._cursor.peek() === r.$RBRACE) {
if (this._isInExpansionCase())
return this._consumeExpansionCaseEnd(), true;
if (this._isInExpansionForm())
return this._consumeExpansionFormEnd(), true;
}
return false;
}
_beginToken(t) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : this._cursor.clone();
this._currentTokenStart = o, this._currentTokenType = t;
}
_endToken(t) {
let o = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : this._cursor.clone();
if (this._currentTokenStart === null)
throw new f("Programming error - attempted to end a token when there was no start to the token", this._currentTokenType, this._cursor.getSpan(o));
if (this._currentTokenType === null)
throw new f("Programming error - attempted to end a token which has no token type", null, this._cursor.getSpan(this._currentTokenStart));
let E = new i(this._currentTokenType, t, this._cursor.getSpan(this._currentTokenStart, this._leadingTriviaCodePoints));
return this.tokens.push(E), this._currentTokenStart = null, this._currentTokenType = null, E;
}
_createError(t, o) {
this._isInExpansionForm() && (t += ` (Do you have an unescaped "{" in your template? Use "{{ '{' }}") to escape it.)`);
let E = new f(t, this._currentTokenType, o);
return this._currentTokenStart = null, this._currentTokenType = null, new C(E);
}
handleError(t) {
if (t instanceof $ && (t = this._createError(t.msg, this._cursor.getSpan(t.cursor))), t instanceof C)
this.errors.push(t.error);
else
throw t;
}
_attemptCharCode(t) {
return this._cursor.peek() === t ? (this._cursor.advance(), true) : false;
}
_attemptCharCodeCaseInsensitive(t) {
return j(this._cursor.peek(), t) ? (this._cursor.advance(), true) : false;
}
_requireCharCode(t) {
let o = this._cursor.clone();
if (!this._attemptCharCode(t))
throw this._createError(l(this._cursor.peek()), this._cursor.getSpan(o));
}
_attemptStr(t) {
let o = t.length;
if (this._cursor.charsLeft() < o)
return false;
let E = this._cursor.clone();
for (let p = 0; p < o; p++)
if (!this._attemptCharCode(t.charCodeAt(p)))
return this._cursor = E, false;
return true;
}
_attemptStrCaseInsensitive(t) {
for (let o = 0; o < t.length; o++)
if (!this._attemptCharCodeCaseInsensitive(t.charCodeAt(o)))
return false;
return true;
}
_requireStr(t) {
let o = this._cursor.clone();
if (!this._attemptStr(t))
throw this._createError(l(this._cursor.peek()), this._cursor.getSpan(o));
}
_requireStrCaseInsensitive(t) {
let o = this._cursor.clone();
if (!this._attemptStrCaseInsensitive(t))
throw this._createError(l(this._cursor.peek()), this._cursor.getSpan(o));
}
_attemptCharCodeUntilFn(t) {
for (; !t(this._cursor.peek()); )
this._cursor.advance();
}
_requireCharCodeUntilFn(t, o) {
let E = this._cursor.clone();
if (this._attemptCharCodeUntilFn(t), this._cursor.clone().diff(E) < o)
throw this._createError(l(this._cursor.peek()), this._cursor.getSpan(E));
}
_attemptUntilChar(t) {
for (; this._cursor.peek() !== t; )
this._cursor.advance();
}
_readChar(t) {
if (t && this._cursor.peek() === r.$AMPERSAND)
return this._decodeEntity();
{
let o = String.fromCodePoint(this._cursor.peek());
return this._cursor.advance(), o;
}
}
_decodeEntity() {
let t = this._cursor.clone();
if (this._cursor.advance(), this._attemptCharCode(r.$HASH)) {
let o = this._attemptCharCode(r.$x) || this._attemptCharCode(r.$X), E = this._cursor.clone();
if (this._attemptCharCodeUntilFn(g), this._cursor.peek() != r.$SEMICOLON)
throw this._createError(l(this._cursor.peek()), this._cursor.getSpan());
let p = this._cursor.getChars(E);
this._cursor.advance();
try {
let A = parseInt(p, o ? 16 : 10);
return String.fromCharCode(A);
} catch {
throw this._createError(h(this._cursor.getChars(t)), this._cursor.getSpan());
}
} else {
let o = this._cursor.clone();
if (this._attemptCharCodeUntilFn(N), this._cursor.peek() != r.$SEMICOLON)
return this._cursor = o, "&";
let E = this._cursor.getChars(o);
this._cursor.advance();
let p = D.NAMED_ENTITIES[E];
if (!p)
throw this._createError(h(E), this._cursor.getSpan(t));
return p;
}
}
_consumeRawText(t, o) {
this._beginToken(t ? s.ESCAPABLE_RAW_TEXT : s.RAW_TEXT);
let E = [];
for (; ; ) {
let p = this._cursor.clone(), A = o();
if (this._cursor = p, A)
break;
E.push(this._readChar(t));
}
return this._endToken([this._processCarriageReturns(E.join(""))]);
}
_consumeComment(t) {
this._beginToken(s.COMMENT_START, t), this._endToken([]), this._consumeRawText(false, () => this._attemptStr("-->")), this._beginToken(s.COMMENT_END), this._requireStr("-->"), this._endToken([]);
}
_consumeBogusComment(t) {
this._beginToken(s.COMMENT_START, t), this._endToken([]), this._consumeRawText(false, () => this._cursor.peek() === r.$GT), this._beginToken(s.COMMENT_END), this._cursor.advance(), this._endToken([]);
}
_consumeCdata(t) {
this._beginToken(s.CDATA_START, t), this._endToken([]), this._consumeRawText(false, () => this._attemptStr("]]>")), this._beginToken(s.CDATA_END), this._requireStr("]]>"), this._endToken([]);
}
_consumeDocType(t) {
this._beginToken(s.DOC_TYPE_START, t), this._endToken([]), this._consumeRawText(false, () => this._cursor.peek() === r.$GT), this._beginToken(s.DOC_TYPE_END), this._cursor.advance(), this._endToken([]);
}
_consumePrefixAndName() {
let t = this._cursor.clone(), o = "";
for (; this._cursor.peek() !== r.$COLON && !w(this._cursor.peek()); )
this._cursor.advance();
let E;
this._cursor.peek() === r.$COLON ? (o = this._cursor.getChars(t), this._cursor.advance(), E = this._cursor.clone()) : E = t, this._requireCharCodeUntilFn(T, o === "" ? 0 : 1);
let p = this._cursor.getChars(E);
return [o, p];
}
_consumeTagOpen(t) {
let o, E, p, A = this.tokens.length, P = this._cursor.clone(), M = [];
try {
if (!r.isAsciiLetter(this._cursor.peek()))
throw this._createError(l(this._cursor.peek()), this._cursor.getSpan(t));
for (p = this._consumeTagOpenStart(t), E = p.parts[0], o = p.parts[1], this._attemptCharCodeUntilFn(m); this._cursor.peek() !== r.$SLASH && this._cursor.peek() !== r.$GT; ) {
let [V, X] = this._consumeAttributeName();
if (this._attemptCharCodeUntilFn(m), this._attemptCharCode(r.$EQ)) {
this._attemptCharCodeUntilFn(m);
let H = this._consumeAttributeValue();
M.push({ prefix: V, name: X, value: H });
} else
M.push({ prefix: V, name: X });
this._attemptCharCodeUntilFn(m);
}
this._consumeTagOpenEnd();
} catch (V) {
if (V instanceof C) {
this._cursor = P, p && (this.tokens.length = A), this._beginToken(s.TEXT, t), this._endToken(["<"]);
return;
}
throw V;
}
if (this._canSelfClose && this.tokens[this.tokens.length - 1].type === s.TAG_OPEN_END_VOID)
return;
let z = this._getTagContentType(o, E, this._fullNameStack.length > 0, M);
this._handleFullNameStackForTagOpen(E, o), z === D.TagContentType.RAW_TEXT ? this._consumeRawTextWithTagClose(E, o, false) : z === D.TagContentType.ESCAPABLE_RAW_TEXT && this._consumeRawTextWithTagClose(E, o, true);
}
_consumeRawTextWithTagClose(t, o, E) {
let p = this._consumeRawText(E, () => !this._attemptCharCode(r.$LT) || !this._attemptCharCode(r.$SLASH) || (this._attemptCharCodeUntilFn(m), !this._attemptStrCaseInsensitive(t ? `${t}:${o}` : o)) ? false : (this._attemptCharCodeUntilFn(m), this._attemptCharCode(r.$GT)));
this._beginToken(s.TAG_CLOSE), this._requireCharCodeUntilFn((A) => A === r.$GT, 3), this._cursor.advance(), this._endToken([t, o]), this._handleFullNameStackForTagClose(t, o);
}
_consumeTagOpenStart(t) {
this._beginToken(s.TAG_OPEN_START, t);
let o = this._consumePrefixAndName();
return this._endToken(o);
}
_consumeAttributeName() {
let t = this._cursor.peek();
if (t === r.$SQ || t === r.$DQ)
throw this._createError(l(t), this._cursor.getSpan());
this._beginToken(s.ATTR_NAME);
let o = this._consumePrefixAndName();
return this._endToken(o), o;
}
_consumeAttributeValue() {
let t;
if (this._cursor.peek() === r.$SQ || this._cursor.peek() === r.$DQ) {
this._beginToken(s.ATTR_QUOTE);
let o = this._cursor.peek();
this._cursor.advance(), this._endToken([String.fromCodePoint(o)]), this._beginToken(s.ATTR_VALUE);
let E = [];
for (; this._cursor.peek() !== o; )
E.push(this._readChar(true));
t = this._processCarriageReturns(E.join("")), this._endToken([t]), this._beginToken(s.ATTR_QUOTE), this._cursor.advance(), this._endToken([String.fromCodePoint(o)]);
} else {
this._beginToken(s.ATTR_VALUE);
let o = this._cursor.clone();
this._requireCharCodeUntilFn(T, 1), t = this._processCarriageReturns(this._cursor.getChars(o)), this._endToken([t]);
}
return t;
}
_consumeTagOpenEnd() {
let t = this._attemptCharCode(r.$SLASH) ? s.TAG_OPEN_END_VOID : s.TAG_OPEN_END;
this._beginToken(t), this._requireCharCode(r.$GT), this._endToken([]);
}
_consumeTagClose(t) {
if (this._beginToken(s.TAG_CLOSE, t), this._attemptCharCodeUntilFn(m), this._allowHtmComponentClosingTags && this._attemptCharCode(r.$SLASH))
this._attemptCharCodeUntilFn(m), this._requireCharCode(r.$GT), this._endToken([]);
else {
let [o, E] = this._consumePrefixAndName();
this._attemptCharCodeUntilFn(m), this._requireCharCode(r.$GT), this._endToken([o, E]), this._handleFullNameStackForTagClose(o, E);
}
}
_consumeExpansionFormStart() {
this._beginToken(s.EXPANSION_FORM_START), this._requireCharCode(r.$LBRACE), this._endToken([]), this._expansionCaseStack.push(s.EXPANSION_FORM_START), this._beginToken(s.RAW_TEXT);
let t = this._readUntil(r.$COMMA);
this._endToken([t]), this._requireCharCode(r.$COMMA), this._attemptCharCodeUntilFn(m), this._beginToken(s.RAW_TEXT);
let o = this._readUntil(r.$COMMA);
this._endToken([o]), this._requireCharCode(r.$COMMA), this._attemptCharCodeUntilFn(m);
}
_consumeExpansionCaseStart() {
this._beginToken(s.EXPANSION_CASE_VALUE);
let t = this._readUntil(r.$LBRACE).trim();
this._endToken([t]), this._attemptCharCodeUntilFn(m), this._beginToken(s.EXPANSION_CASE_EXP_START), this._requireCharCode(r.$LBRACE), this._endToken([]), this._attemptCharCodeUntilFn(m), this._expansionCaseStack.push(s.EXPANSION_CASE_EXP_START);
}
_consumeExpansionCaseEnd() {
this._beginToken(s.EXPANSION_CASE_EXP_END), this._requireCharCode(r.$RBRACE), this._endToken([]), this._attemptCharCodeUntilFn(m), this._expansionCaseStack.pop();
}
_consumeExpansionFormEnd() {
this._beginToken(s.EXPANSION_FORM_END), this._requireCharCode(r.$RBRACE), this._endToken([]), this._expansionCaseStack.pop();
}
_consumeText() {
let t = this._cursor.clone();
this._beginToken(s.TEXT, t);
let o = [];
do
this._interpolationConfig && this._attemptStr(this._interpolationConfig.start) ? (o.push(this._interpolationConfig.start), this._inInterpolation = true) : this._interpolationConfig && this._inInterpolation && this._attemptStr(this._interpolationConfig.end) ? (o.push(this._interpolationConfig.end), this._inInterpolation = false) : o.push(this._readChar(true));
while (!this._isTextEnd());
this._endToken([this._processCarriageReturns(o.join(""))]);
}
_isTextEnd() {
return !!(this._cursor.peek() === r.$LT || this._cursor.peek() === r.$EOF || this._tokenizeIcu && !this._inInterpolation && (this.isExpansionFormStart() || this._cursor.peek() === r.$RBRACE && this._isInExpansionCase()));
}
_readUntil(t) {
let o = this._cursor.clone();
return this._attemptUntilChar(t), this._cursor.getChars(o);
}
_isInExpansionCase() {
return this._expansionCaseStack.length > 0 && this._expansionCaseStack[this._expansionCaseStack.length - 1] === s.EXPANSION_CASE_EXP_START;
}
_isInExpansionForm() {
return this._expansionCaseStack.length > 0 && this._expansionCaseStack[this._expansionCaseStack.length - 1] === s.EXPANSION_FORM_START;
}
isExpansionFormStart() {
if (this._cursor.peek() !== r.$LBRACE)
return false;
if (this._interpolationConfig) {
let t = this._cursor.clone(), o = this._attemptStr(this._interpolationConfig.start);
return this._cursor = t, !o;
}
return true;
}
_handleFullNameStackForTagOpen(t, o) {
let E = D.mergeNsAndName(t, o);
(this._fullNameStack.length === 0 || this._fullNameStack[this._fullNameStack.length - 1] === E) && this._fullNameStack.push(E);
}
_handleFullNameStackForTagClose(t, o) {
let E = D.mergeNsAndName(t, o);
this._fullNameStack.length !== 0 && this._fullNameStack[this._fullNameStack.length - 1] === E && this._fullNameStack.pop();
}
};
function m(t) {
return !r.isWhitespace(t) || t === r.$EOF;
}
function T(t) {
return r.isWhitespace(t) || t === r.$GT || t === r.$SLASH || t === r.$SQ || t === r.$DQ || t === r.$EQ;
}
function w(t) {
return (t < r.$a || r.$z < t) && (t < r.$A || r.$Z < t) && (t < r.$0 || t > r.$9);
}
function g(t) {
return t == r.$SEMICOLON || t == r.$EOF || !r.isAsciiHexDigit(t);
}
function N(t) {
return t == r.$SEMICOLON || t == r.$EOF || !r.isAsciiLetter(t);
}
function R(t) {
return t === r.$EQ || r.isAsciiLetter(t) || r.isDigit(t);
}
function j(t, o) {
return _(t) == _(o);
}
function _(t) {
return t >= r.$a && t <= r.$z ? t - r.$a + r.$A : t;
}
function O(t) {
let o = [], E;
for (let p = 0; p < t.length; p++) {
let A = t[p];
E && E.type == s.TEXT && A.type == s.TEXT ? (E.parts[0] += A.parts[0], E.sourceSpan.end = A.sourceSpan.end) : (E = A, o.push(E));
}
return o;
}
var x = class {
constructor(t, o) {
if (t instanceof x)
this.file = t.file, this.input = t.input, this.end = t.end, this.state = Object.assign({}, t.state);
else {
if (!o)
throw new Error("Programming error: the range argument must be provided with a file argument.");
this.file = t, this.input = t.content, this.end = o.endPos, this.state = { peek: -1, offset: o.startPos, line: o.startLine, column: o.startCol };
}
}
clone() {
return new x(this);
}
peek() {
return this.state.peek;
}
charsLeft() {
return this.end - this.state.offset;
}
diff(t) {
return this.state.offset - t.state.offset;
}
advance() {
this.advanceState(this.state);
}
init() {
this.updatePeek(this.state);
}
getSpan(t, o) {
if (t = t || this, o)
for (t = t.clone(); this.diff(t) > 0 && o.indexOf(t.peek()) !== -1; )
t.advance();
return new u.ParseSourceSpan(new u.ParseLocation(t.file, t.state.offset, t.state.line, t.state.column), new u.ParseLocation(this.file, this.state.offset, this.state.line, this.state.column));
}
getChars(t) {
return this.input.substring(t.state.offset, this.state.offset);
}
charAt(t) {
return this.input.charCodeAt(t);
}
advanceState(t) {
if (t.offset >= this.end)
throw this.state = t, new $('Unexpected character "EOF"', this);
let o = this.charAt(t.offset);
o === r.$LF ? (t.line++, t.column = 0) : r.isNewLine(o) || t.column++, t.offset++, this.updatePeek(t);
}
updatePeek(t) {
t.peek = t.offset >= this.end ? r.$EOF : this.charAt(t.offset);
}
}, k = class extends x {
constructor(t, o) {
t instanceof k ? (super(t), this.internalState = Object.assign({}, t.internalState)) : (super(t, o), this.internalState = this.state);
}
advance() {
this.state = this.internalState, super.advance(), this.processEscapeSequence();
}
init() {
super.init(), this.processEscapeSequence();
}
clone() {
return new k(this);
}
getChars(t) {
let o = t.clone(), E = "";
for (; o.internalState.offset < this.internalState.offset; )
E += String.fromCodePoint(o.peek()), o.advance();
return E;
}
processEscapeSequence() {
let t = () => this.internalState.peek;
if (t() === r.$BACKSLASH)
if (this.internalState = Object.assign({}, this.state), this.advanceState(this.internalState), t() === r.$n)
this.state.peek = r.$LF;
else if (t() === r.$r)
this.state.peek = r.$CR;
else if (t() === r.$v)
this.state.peek = r.$VTAB;
else if (t() === r.$t)
this.state.peek = r.$TAB;
else if (t() === r.$b)
this.state.peek = r.$BSPACE;
else if (t() === r.$f)
this.state.peek = r.$FF;
else if (t() === r.$u)
if (this.advanceState(this.internalState), t() === r.$LBRACE) {
this.advanceState(this.internalState);
let o = this.clone(), E = 0;
for (; t() !== r.$RBRACE; )
this.advanceState(this.internalState), E++;
this.state.peek = this.decodeHexDigits(o, E);
} else {
let o = this.clone();
this.advanceState(this.internalState), this.advanceState(this.internalState), this.advanceState(this.internalState), this.state.peek = this.decodeHexDigits(o, 4);
}
else if (t() === r.$x) {
this.advanceState(this.internalState);
let o = this.clone();
this.advanceState(this.internalState), this.state.peek = this.decodeHexDigits(o, 2);
} else if (r.isOctalDigit(t())) {
let o = "", E = 0, p = this.clone();
for (; r.isOctalDigit(t()) && E < 3; )
p = this.clone(), o += String.fromCodePoint(t()), this.advanceState(this.internalState), E++;
this.state.peek = parseInt(o, 8), this.internalState = p.internalState;
} else
r.isNewLine(this.internalState.peek) ? (this.advanceState(this.internalState), this.state = this.internalState) : this.state.peek = this.internalState.peek;
}
decodeHexDigits(t, o) {
let E = this.input.substr(t.internalState.offset, o), p = parseInt(E, 16);
if (isNaN(p))
throw t.state = t.internalState, new $("Invalid hexadecimal escape sequence", t);
return p;
}
}, $ = class {
constructor(t, o) {
this.msg = t, this.cursor = o;
}
};
e.CursorError = $;
} }), ls = I({ "node_modules/angular-html-parser/lib/compiler/src/ml_parser/parser.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = Be(), u = bs(), n2 = Yl(), D = Ze(), s = class extends r.ParseError {
constructor(a, l, h) {
super(l, h), this.elementName = a;
}
static create(a, l, h) {
return new s(a, l, h);
}
};
e.TreeError = s;
var i = class {
constructor(a, l) {
this.rootNodes = a, this.errors = l;
}
};
e.ParseTreeResult = i;
var f = class {
constructor(a) {
this.getTagDefinition = a;
}
parse(a, l, h) {
let C = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : false, d = arguments.length > 4 ? arguments[4] : void 0, m = (x) => function(k) {
for (var $ = arguments.length, t = new Array($ > 1 ? $ - 1 : 0), o = 1; o < $; o++)
t[o - 1] = arguments[o];
return x(k.toLowerCase(), ...t);
}, T = C ? this.getTagDefinition : m(this.getTagDefinition), w = (x) => T(x).contentType, g = C ? d : m(d), N = d ? (x, k, $, t) => {
let o = g(x, k, $, t);
return o !== void 0 ? o : w(x);
} : w, R = n2.tokenize(a, l, N, h), j = h && h.canSelfClose || false, _ = h && h.allowHtmComponentClosingTags || false, O = new c(R.tokens, T, j, _, C).build();
return new i(O.rootNodes, R.errors.concat(O.errors));
}
};
e.Parser = f;
var c = class {
constructor(a, l, h, C, d) {
this.tokens = a, this.getTagDefinition = l, this.canSelfClose = h, this.allowHtmComponentClosingTags = C, this.isTagNameCaseSensitive = d, this._index = -1, this._rootNodes = [], this._errors = [], this._elementStack = [], this._advance();
}
build() {
for (; this._peek.type !== n2.TokenType.EOF; )
this._peek.type === n2.TokenType.TAG_OPEN_START ? this._consumeStartTag(this._advance()) : this._peek.type === n2.TokenType.TAG_CLOSE ? (this._closeVoidElement(), this._consumeEndTag(this._advance())) : this._peek.type === n2.TokenType.CDATA_START ? (this._closeVoidElement(), this._consumeCdata(this._advance())) : this._peek.type === n2.TokenType.COMMENT_START ? (this._closeVoidElement(), this._consumeComment(this._advance())) : this._peek.type === n2.TokenType.TEXT || this._peek.type === n2.TokenType.RAW_TEXT || this._peek.type === n2.TokenType.ESCAPABLE_RAW_TEXT ? (this._closeVoidElement(), this._consumeText(this._advance())) : this._peek.type === n2.TokenType.EXPANSION_FORM_START ? this._consumeExpansion(this._advance()) : this._peek.type === n2.TokenType.DOC_TYPE_START ? this._consumeDocType(this._advance()) : this._advance();
return new i(this._rootNodes, this._errors);
}
_advance() {
let a = this._peek;
return this._index < this.tokens.length - 1 && this._index++, this._peek = this.tokens[this._index], a;
}
_advanceIf(a) {
return this._peek.type === a ? this._advance() : null;
}
_consumeCdata(a) {
let l = this._advance(), h = this._getText(l), C = this._advanceIf(n2.TokenType.CDATA_END);
this._addToParent(new u.CDATA(h, new r.ParseSourceSpan(a.sourceSpan.start, (C || l).sourceSpan.end)));
}
_consumeComment(a) {
let l = this._advanceIf(n2.TokenType.RAW_TEXT), h = this._advanceIf(n2.TokenType.COMMENT_END), C = l != null ? l.parts[0].trim() : null, d = new r.ParseSourceSpan(a.sourceSpan.start, (h || l || a).sourceSpan.end);
this._addToParent(new u.Comment(C, d));
}
_consumeDocType(a) {
let l = this._advanceIf(n2.TokenType.RAW_TEXT), h = this._advanceIf(n2.TokenType.DOC_TYPE_END), C = l != null ? l.parts[0].trim() : null, d = new r.ParseSourceSpan(a.sourceSpan.start, (h || l || a).sourceSpan.end);
this._addToParent(new u.DocType(C, d));
}
_consumeExpansion(a) {
let l = this._advance(), h = this._advance(), C = [];
for (; this._peek.type === n2.TokenType.EXPANSION_CASE_VALUE; ) {
let m = this._parseExpansionCase();
if (!m)
return;
C.push(m);
}
if (this._peek.type !== n2.TokenType.EXPANSION_FORM_END) {
this._errors.push(s.create(null, this._peek.sourceSpan, "Invalid ICU message. Missing '}'."));
return;
}
let d = new r.ParseSourceSpan(a.sourceSpan.start, this._peek.sourceSpan.end);
this._addToParent(new u.Expansion(l.parts[0], h.parts[0], C, d, l.sourceSpan)), this._advance();
}
_parseExpansionCase() {
let a = this._advance();
if (this._peek.type !== n2.TokenType.EXPANSION_CASE_EXP_START)
return this._errors.push(s.create(null, this._peek.sourceSpan, "Invalid ICU message. Missing '{'.")), null;
let l = this._advance(), h = this._collectExpansionExpTokens(l);
if (!h)
return null;
let C = this._advance();
h.push(new n2.Token(n2.TokenType.EOF, [], C.sourceSpan));
let d = new c(h, this.getTagDefinition, this.canSelfClose, this.allowHtmComponentClosingTags, this.isTagNameCaseSensitive).build();
if (d.errors.length > 0)
return this._errors = this._errors.concat(d.errors), null;
let m = new r.ParseSourceSpan(a.sourceSpan.start, C.sourceSpan.end), T = new r.ParseSourceSpan(l.sourceSpan.start, C.sourceSpan.end);
return new u.ExpansionCase(a.parts[0], d.rootNodes, m, a.sourceSpan, T);
}
_collectExpansionExpTokens(a) {
let l = [], h = [n2.TokenType.EXPANSION_CASE_EXP_START];
for (; ; ) {
if ((this._peek.type === n2.TokenType.EXPANSION_FORM_START || this._peek.type === n2.TokenType.EXPANSION_CASE_EXP_START) && h.push(this._peek.type), this._peek.type === n2.TokenType.EXPANSION_CASE_EXP_END)
if (F(h, n2.TokenType.EXPANSION_CASE_EXP_START)) {
if (h.pop(), h.length == 0)
return l;
} else
return this._errors.push(s.create(null, a.sourceSpan, "Invalid ICU message. Missing '}'.")), null;
if (this._peek.type === n2.TokenType.EXPANSION_FORM_END)
if (F(h, n2.TokenType.EXPANSION_FORM_START))
h.pop();
else
return this._errors.push(s.create(null, a.sourceSpan, "Invalid ICU message. Missing '}'.")), null;
if (this._peek.type === n2.TokenType.EOF)
return this._errors.push(s.create(null, a.sourceSpan, "Invalid ICU message. Missing '}'.")), null;
l.push(this._advance());
}
}
_getText(a) {
let l = a.parts[0];
if (l.length > 0 && l[0] == `
`) {
let h = this._getParentElement();
h != null && h.children.length == 0 && this.getTagDefinition(h.name).ignoreFirstLf && (l = l.substring(1));
}
return l;
}
_consumeText(a) {
let l = this._getText(a);
l.length > 0 && this._addToParent(new u.Text(l, a.sourceSpan));
}
_closeVoidElement() {
let a = this._getParentElement();
a && this.getTagDefinition(a.name).isVoid && this._elementStack.pop();
}
_consumeStartTag(a) {
let l = a.parts[0], h = a.parts[1], C = [];
for (; this._peek.type === n2.TokenType.ATTR_NAME; )
C.push(this._consumeAttr(this._advance()));
let d = this._getElementFullName(l, h, this._getParentElement()), m = false;
if (this._peek.type === n2.TokenType.TAG_OPEN_END_VOID) {
this._advance(), m = true;
let R = this.getTagDefinition(d);
this.canSelfClose || R.canSelfClose || D.getNsPrefix(d) !== null || R.isVoid || this._errors.push(s.create(d, a.sourceSpan, `Only void and foreign elements can be self closed "${a.parts[1]}"`));
} else
this._peek.type === n2.TokenType.TAG_OPEN_END && (this._advance(), m = false);
let T = this._peek.sourceSpan.start, w = new r.ParseSourceSpan(a.sourceSpan.start, T), g = new r.ParseSourceSpan(a.sourceSpan.start.moveBy(1), a.sourceSpan.end), N = new u.Element(d, C, [], w, w, void 0, g);
this._pushElement(N), m && (this._popElement(d), N.endSourceSpan = w);
}
_pushElement(a) {
let l = this._getParentElement();
l && this.getTagDefinition(l.name).isClosedByChild(a.name) && this._elementStack.pop(), this._addToParent(a), this._elementStack.push(a);
}
_consumeEndTag(a) {
let l = this.allowHtmComponentClosingTags && a.parts.length === 0 ? null : this._getElementFullName(a.parts[0], a.parts[1], this._getParentElement());
if (this._getParentElement() && (this._getParentElement().endSourceSpan = a.sourceSpan), l && this.getTagDefinition(l).isVoid)
this._errors.push(s.create(l, a.sourceSpan, `Void elements do not have end tags "${a.parts[1]}"`));
else if (!this._popElement(l)) {
let h = `Unexpected closing tag "${l}". It may happen when the tag has already been closed by another tag. For more info see https://www.w3.org/TR/html5/syntax.html#closing-elements-that-have-implied-end-tags`;
this._errors.push(s.create(l, a.sourceSpan, h));
}
}
_popElement(a) {
for (let l = this._elementStack.length - 1; l >= 0; l--) {
let h = this._elementStack[l];
if (!a || (D.getNsPrefix(h.name) ? h.name == a : h.name.toLowerCase() == a.toLowerCase()))
return this._elementStack.splice(l, this._elementStack.length - l), true;
if (!this.getTagDefinition(h.name).closedByParent)
return false;
}
return false;
}
_consumeAttr(a) {
let l = D.mergeNsAndName(a.parts[0], a.parts[1]), h = a.sourceSpan.end, C = "", d, m;
if (this._peek.type === n2.TokenType.ATTR_QUOTE && (m = this._advance().sourceSpan.start), this._peek.type === n2.TokenType.ATTR_VALUE) {
let T = this._advance();
C = T.parts[0], h = T.sourceSpan.end, d = T.sourceSpan;
}
return this._peek.type === n2.TokenType.ATTR_QUOTE && (h = this._advance().sourceSpan.end, d = new r.ParseSourceSpan(m, h)), new u.Attribute(l, C, new r.ParseSourceSpan(a.sourceSpan.start, h), d, a.sourceSpan);
}
_getParentElement() {
return this._elementStack.length > 0 ? this._elementStack[this._elementStack.length - 1] : null;
}
_getParentElementSkippingContainers() {
let a = null;
for (let l = this._elementStack.length - 1; l >= 0; l--) {
if (!D.isNgContainer(this._elementStack[l].name))
return { parent: this._elementStack[l], container: a };
a = this._elementStack[l];
}
return { parent: null, container: a };
}
_addToParent(a) {
let l = this._getParentElement();
l != null ? l.children.push(a) : this._rootNodes.push(a);
}
_insertBeforeContainer(a, l, h) {
if (!l)
this._addToParent(h), this._elementStack.push(h);
else {
if (a) {
let C = a.children.indexOf(l);
a.children[C] = h;
} else
this._rootNodes.push(h);
h.children.push(l), this._elementStack.splice(this._elementStack.indexOf(l), 0, h);
}
}
_getElementFullName(a, l, h) {
return a === "" && (a = this.getTagDefinition(l).implicitNamespacePrefix || "", a === "" && h != null && (a = D.getNsPrefix(h.name))), D.mergeNsAndName(a, l);
}
};
function F(a, l) {
return a.length > 0 && a[a.length - 1] === l;
}
} }), Ql = I({ "node_modules/angular-html-parser/lib/compiler/src/ml_parser/html_parser.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = Bs(), u = ls(), n2 = ls();
e.ParseTreeResult = n2.ParseTreeResult, e.TreeError = n2.TreeError;
var D = class extends u.Parser {
constructor() {
super(r.getHtmlTagDefinition);
}
parse(s, i, f) {
let c = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : false, F = arguments.length > 4 ? arguments[4] : void 0;
return super.parse(s, i, f, c, F);
}
};
e.HtmlParser = D;
} }), ws = I({ "node_modules/angular-html-parser/lib/angular-html-parser/src/index.js"(e) {
"use strict";
q(), Object.defineProperty(e, "__esModule", { value: true });
var r = Ql(), u = Ze();
e.TagContentType = u.TagContentType;
var n2 = null, D = () => (n2 || (n2 = new r.HtmlParser()), n2);
function s(i) {
let f = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {}, { canSelfClose: c = false, allowHtmComponentClosingTags: F = false, isTagNameCaseSensitive: a = false, getTagContentType: l } = f;
return D().parse(i, "angular-html-parser", { tokenizeExpansionForms: false, interpolationConfig: void 0, canSelfClose: c, allowHtmComponentClosingTags: F }, a, l);
}
e.parse = s;
} });
q();
var { ParseSourceSpan: Qe, ParseLocation: cs, ParseSourceFile: Kl } = Be(), Jl = ol(), Zl = Cs(), ec = Dl(), { inferParserByLanguage: rc } = xl(), uc = kl(), Vr = Ml(), hs = jl(), { hasPragma: tc } = Ul(), { Node: nc } = Gl(), { parseIeConditionalComment: sc } = Vl(), { locStart: ic, locEnd: ac } = Xl();
function oc(e, r, u) {
let { canSelfClose: n2, normalizeTagName: D, normalizeAttributeName: s, allowHtmComponentClosingTags: i, isTagNameCaseSensitive: f, getTagContentType: c } = r, F = ws(), { RecursiveVisitor: a, visitAll: l } = bs(), { ParseSourceSpan: h } = Be(), { getHtmlTagDefinition: C } = Bs(), { rootNodes: d, errors: m } = F.parse(e, { canSelfClose: n2, allowHtmComponentClosingTags: i, isTagNameCaseSensitive: f, getTagContentType: c });
if (u.parser === "vue")
if (d.some((O) => O.type === "docType" && O.value === "html" || O.type === "element" && O.name.toLowerCase() === "html")) {
n2 = true, D = true, s = true, i = true, f = false;
let O = F.parse(e, { canSelfClose: n2, allowHtmComponentClosingTags: i, isTagNameCaseSensitive: f });
d = O.rootNodes, m = O.errors;
} else {
let O = (x) => {
if (!x || x.type !== "element" || x.name !== "template")
return false;
let k = x.attrs.find((t) => t.name === "lang"), $ = k && k.value;
return !$ || rc($, u) === "html";
};
if (d.some(O)) {
let x, k = () => F.parse(e, { canSelfClose: n2, allowHtmComponentClosingTags: i, isTagNameCaseSensitive: f }), $ = () => x || (x = k()), t = (o) => $().rootNodes.find((E) => {
let { startSourceSpan: p } = E;
return p && p.start.offset === o.startSourceSpan.start.offset;
});
for (let o = 0; o < d.length; o++) {
let E = d[o], { endSourceSpan: p, startSourceSpan: A } = E;
if (p === null)
m = $().errors, d[o] = t(E) || E;
else if (O(E)) {
let M = $(), z = A.end.offset, V = p.start.offset;
for (let X of M.errors) {
let { offset: H } = X.span.start;
if (z < H && H < V) {
m = [X];
break;
}
}
d[o] = t(E) || E;
}
}
}
}
if (m.length > 0) {
let { msg: _, span: { start: O, end: x } } = m[0];
throw ec(_, { start: { line: O.line + 1, column: O.col + 1 }, end: { line: x.line + 1, column: x.col + 1 } });
}
let T = (_) => {
let O = _.name.startsWith(":") ? _.name.slice(1).split(":")[0] : null, x = _.nameSpan.toString(), k = O !== null && x.startsWith(`${O}:`), $ = k ? x.slice(O.length + 1) : x;
_.name = $, _.namespace = O, _.hasExplicitNamespace = k;
}, w = (_) => {
switch (_.type) {
case "element":
T(_);
for (let O of _.attrs)
T(O), O.valueSpan ? (O.value = O.valueSpan.toString(), /["']/.test(O.value[0]) && (O.value = O.value.slice(1, -1))) : O.value = null;
break;
case "comment":
_.value = _.sourceSpan.toString().slice(4, -3);
break;
case "text":
_.value = _.sourceSpan.toString();
break;
}
}, g = (_, O) => {
let x = _.toLowerCase();
return O(x) ? x : _;
}, N = (_) => {
if (_.type === "element" && (D && (!_.namespace || _.namespace === _.tagDefinition.implicitNamespacePrefix || hs(_)) && (_.name = g(_.name, (O) => O in uc)), s)) {
let O = Vr[_.name] || /* @__PURE__ */ Object.create(null);
for (let x of _.attrs)
x.namespace || (x.name = g(x.name, (k) => _.name in Vr && (k in Vr["*"] || k in O)));
}
}, R = (_) => {
_.sourceSpan && _.endSourceSpan && (_.sourceSpan = new h(_.sourceSpan.start, _.endSourceSpan.end));
}, j = (_) => {
if (_.type === "element") {
let O = C(f ? _.name : _.name.toLowerCase());
!_.namespace || _.namespace === O.implicitNamespacePrefix || hs(_) ? _.tagDefinition = O : _.tagDefinition = C("");
}
};
return l(new class extends a {
visit(_) {
w(_), j(_), N(_), R(_);
}
}(), d), d;
}
function Ns(e, r, u) {
let n2 = arguments.length > 3 && arguments[3] !== void 0 ? arguments[3] : true, { frontMatter: D, content: s } = n2 ? Jl(e) : { frontMatter: null, content: e }, i = new Kl(e, r.filepath), f = new cs(i, 0, 0, 0), c = f.moveBy(e.length), F = { type: "root", sourceSpan: new Qe(f, c), children: oc(s, u, r) };
if (D) {
let h = new cs(i, 0, 0, 0), C = h.moveBy(D.raw.length);
D.sourceSpan = new Qe(h, C), F.children.unshift(D);
}
let a = new nc(F), l = (h, C) => {
let { offset: d } = C, m = e.slice(0, d).replace(/[^\n\r]/g, " "), w = Ns(m + h, r, u, false);
w.sourceSpan = new Qe(C, Zl(w.children).sourceSpan.end);
let g = w.children[0];
return g.length === d ? w.children.shift() : (g.sourceSpan = new Qe(g.sourceSpan.start.moveBy(d), g.sourceSpan.end), g.value = g.value.slice(d)), w;
};
return a.walk((h) => {
if (h.type === "comment") {
let C = sc(h, l);
C && h.parent.replaceChild(h, C);
}
}), a;
}
function Ke() {
let { name: e, canSelfClose: r = false, normalizeTagName: u = false, normalizeAttributeName: n2 = false, allowHtmComponentClosingTags: D = false, isTagNameCaseSensitive: s = false, getTagContentType: i } = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {};
return { parse: (f, c, F) => Ns(f, Object.assign({ parser: e }, F), { canSelfClose: r, normalizeTagName: u, normalizeAttributeName: n2, allowHtmComponentClosingTags: D, isTagNameCaseSensitive: s, getTagContentType: i }), hasPragma: tc, astFormat: "html", locStart: ic, locEnd: ac };
}
Os.exports = { parsers: { html: Ke({ name: "html", canSelfClose: true, normalizeTagName: true, normalizeAttributeName: true, allowHtmComponentClosingTags: true }), angular: Ke({ name: "angular", canSelfClose: true }), vue: Ke({ name: "vue", canSelfClose: true, isTagNameCaseSensitive: true, getTagContentType: (e, r, u, n2) => {
if (e.toLowerCase() !== "html" && !u && (e !== "template" || n2.some((D) => {
let { name: s, value: i } = D;
return s === "lang" && i !== "html" && i !== "" && i !== void 0;
})))
return ws().TagContentType.RAW_TEXT;
} }), lwc: Ke({ name: "lwc" }) } };
});
return Dc();
});
}
});
// node_modules/prettier/parser-yaml.js
var require_parser_yaml = __commonJS({
"node_modules/prettier/parser-yaml.js"(exports, module2) {
(function(e) {
if (typeof exports == "object" && typeof module2 == "object")
module2.exports = e();
else if (typeof define == "function" && define.amd)
define(e);
else {
var i = typeof globalThis < "u" ? globalThis : typeof global < "u" ? global : typeof self < "u" ? self : this || {};
i.prettierPlugins = i.prettierPlugins || {}, i.prettierPlugins.yaml = e();
}
})(function() {
"use strict";
var yt = (n2, e) => () => (e || n2((e = { exports: {} }).exports, e), e.exports);
var ln = yt((un, at) => {
var Ye = Object.defineProperty, bt = Object.getOwnPropertyDescriptor, De = Object.getOwnPropertyNames, wt = Object.prototype.hasOwnProperty, Ke = (n2, e) => function() {
return n2 && (e = (0, n2[De(n2)[0]])(n2 = 0)), e;
}, D = (n2, e) => function() {
return e || (0, n2[De(n2)[0]])((e = { exports: {} }).exports, e), e.exports;
}, St = (n2, e) => {
for (var r in e)
Ye(n2, r, { get: e[r], enumerable: true });
}, Et = (n2, e, r, c) => {
if (e && typeof e == "object" || typeof e == "function")
for (let h of De(e))
!wt.call(n2, h) && h !== r && Ye(n2, h, { get: () => e[h], enumerable: !(c = bt(e, h)) || c.enumerable });
return n2;
}, se = (n2) => Et(Ye({}, "__esModule", { value: true }), n2), Te, Y = Ke({ ""() {
Te = { env: {}, argv: [] };
} }), Mt = D({ "src/common/parser-create-error.js"(n2, e) {
"use strict";
Y();
function r(c, h) {
let d = new SyntaxError(c + " (" + h.start.line + ":" + h.start.column + ")");
return d.loc = h, d;
}
e.exports = r;
} }), Ot = D({ "src/language-yaml/pragma.js"(n2, e) {
"use strict";
Y();
function r(d) {
return /^\s*@(?:prettier|format)\s*$/.test(d);
}
function c(d) {
return /^\s*#[^\S\n]*@(?:prettier|format)\s*?(?:\n|$)/.test(d);
}
function h(d) {
return `# @format
${d}`;
}
e.exports = { isPragma: r, hasPragma: c, insertPragma: h };
} }), Lt = D({ "src/language-yaml/loc.js"(n2, e) {
"use strict";
Y();
function r(h) {
return h.position.start.offset;
}
function c(h) {
return h.position.end.offset;
}
e.exports = { locStart: r, locEnd: c };
} }), te = {};
St(te, { __assign: () => qe, __asyncDelegator: () => Yt, __asyncGenerator: () => jt, __asyncValues: () => Dt, __await: () => Ce, __awaiter: () => Pt, __classPrivateFieldGet: () => Qt, __classPrivateFieldSet: () => Ut, __createBinding: () => Rt, __decorate: () => Tt, __exportStar: () => qt, __extends: () => At, __generator: () => It, __importDefault: () => Vt, __importStar: () => Wt, __makeTemplateObject: () => Ft, __metadata: () => kt, __param: () => Ct, __read: () => Je, __rest: () => Nt, __spread: () => $t, __spreadArrays: () => Bt, __values: () => je });
function At(n2, e) {
Re(n2, e);
function r() {
this.constructor = n2;
}
n2.prototype = e === null ? Object.create(e) : (r.prototype = e.prototype, new r());
}
function Nt(n2, e) {
var r = {};
for (var c in n2)
Object.prototype.hasOwnProperty.call(n2, c) && e.indexOf(c) < 0 && (r[c] = n2[c]);
if (n2 != null && typeof Object.getOwnPropertySymbols == "function")
for (var h = 0, c = Object.getOwnPropertySymbols(n2); h < c.length; h++)
e.indexOf(c[h]) < 0 && Object.prototype.propertyIsEnumerable.call(n2, c[h]) && (r[c[h]] = n2[c[h]]);
return r;
}
function Tt(n2, e, r, c) {
var h = arguments.length, d = h < 3 ? e : c === null ? c = Object.getOwnPropertyDescriptor(e, r) : c, y;
if (typeof Reflect == "object" && typeof Reflect.decorate == "function")
d = Reflect.decorate(n2, e, r, c);
else
for (var E = n2.length - 1; E >= 0; E--)
(y = n2[E]) && (d = (h < 3 ? y(d) : h > 3 ? y(e, r, d) : y(e, r)) || d);
return h > 3 && d && Object.defineProperty(e, r, d), d;
}
function Ct(n2, e) {
return function(r, c) {
e(r, c, n2);
};
}
function kt(n2, e) {
if (typeof Reflect == "object" && typeof Reflect.metadata == "function")
return Reflect.metadata(n2, e);
}
function Pt(n2, e, r, c) {
function h(d) {
return d instanceof r ? d : new r(function(y) {
y(d);
});
}
return new (r || (r = Promise))(function(d, y) {
function E(M) {
try {
S(c.next(M));
} catch (T) {
y(T);
}
}
function I(M) {
try {
S(c.throw(M));
} catch (T) {
y(T);
}
}
function S(M) {
M.done ? d(M.value) : h(M.value).then(E, I);
}
S((c = c.apply(n2, e || [])).next());
});
}
function It(n2, e) {
var r = { label: 0, sent: function() {
if (d[0] & 1)
throw d[1];
return d[1];
}, trys: [], ops: [] }, c, h, d, y;
return y = { next: E(0), throw: E(1), return: E(2) }, typeof Symbol == "function" && (y[Symbol.iterator] = function() {
return this;
}), y;
function E(S) {
return function(M) {
return I([S, M]);
};
}
function I(S) {
if (c)
throw new TypeError("Generator is already executing.");
for (; r; )
try {
if (c = 1, h && (d = S[0] & 2 ? h.return : S[0] ? h.throw || ((d = h.return) && d.call(h), 0) : h.next) && !(d = d.call(h, S[1])).done)
return d;
switch (h = 0, d && (S = [S[0] & 2, d.value]), S[0]) {
case 0:
case 1:
d = S;
break;
case 4:
return r.label++, { value: S[1], done: false };
case 5:
r.label++, h = S[1], S = [0];
continue;
case 7:
S = r.ops.pop(), r.trys.pop();
continue;
default:
if (d = r.trys, !(d = d.length > 0 && d[d.length - 1]) && (S[0] === 6 || S[0] === 2)) {
r = 0;
continue;
}
if (S[0] === 3 && (!d || S[1] > d[0] && S[1] < d[3])) {
r.label = S[1];
break;
}
if (S[0] === 6 && r.label < d[1]) {
r.label = d[1], d = S;
break;
}
if (d && r.label < d[2]) {
r.label = d[2], r.ops.push(S);
break;
}
d[2] && r.ops.pop(), r.trys.pop();
continue;
}
S = e.call(n2, r);
} catch (M) {
S = [6, M], h = 0;
} finally {
c = d = 0;
}
if (S[0] & 5)
throw S[1];
return { value: S[0] ? S[1] : void 0, done: true };
}
}
function Rt(n2, e, r, c) {
c === void 0 && (c = r), n2[c] = e[r];
}
function qt(n2, e) {
for (var r in n2)
r !== "default" && !e.hasOwnProperty(r) && (e[r] = n2[r]);
}
function je(n2) {
var e = typeof Symbol == "function" && Symbol.iterator, r = e && n2[e], c = 0;
if (r)
return r.call(n2);
if (n2 && typeof n2.length == "number")
return { next: function() {
return n2 && c >= n2.length && (n2 = void 0), { value: n2 && n2[c++], done: !n2 };
} };
throw new TypeError(e ? "Object is not iterable." : "Symbol.iterator is not defined.");
}
function Je(n2, e) {
var r = typeof Symbol == "function" && n2[Symbol.iterator];
if (!r)
return n2;
var c = r.call(n2), h, d = [], y;
try {
for (; (e === void 0 || e-- > 0) && !(h = c.next()).done; )
d.push(h.value);
} catch (E) {
y = { error: E };
} finally {
try {
h && !h.done && (r = c.return) && r.call(c);
} finally {
if (y)
throw y.error;
}
}
return d;
}
function $t() {
for (var n2 = [], e = 0; e < arguments.length; e++)
n2 = n2.concat(Je(arguments[e]));
return n2;
}
function Bt() {
for (var n2 = 0, e = 0, r = arguments.length; e < r; e++)
n2 += arguments[e].length;
for (var c = Array(n2), h = 0, e = 0; e < r; e++)
for (var d = arguments[e], y = 0, E = d.length; y < E; y++, h++)
c[h] = d[y];
return c;
}
function Ce(n2) {
return this instanceof Ce ? (this.v = n2, this) : new Ce(n2);
}
function jt(n2, e, r) {
if (!Symbol.asyncIterator)
throw new TypeError("Symbol.asyncIterator is not defined.");
var c = r.apply(n2, e || []), h, d = [];
return h = {}, y("next"), y("throw"), y("return"), h[Symbol.asyncIterator] = function() {
return this;
}, h;
function y(P) {
c[P] && (h[P] = function(C) {
return new Promise(function(q, R) {
d.push([P, C, q, R]) > 1 || E(P, C);
});
});
}
function E(P, C) {
try {
I(c[P](C));
} catch (q) {
T(d[0][3], q);
}
}
function I(P) {
P.value instanceof Ce ? Promise.resolve(P.value.v).then(S, M) : T(d[0][2], P);
}
function S(P) {
E("next", P);
}
function M(P) {
E("throw", P);
}
function T(P, C) {
P(C), d.shift(), d.length && E(d[0][0], d[0][1]);
}
}
function Yt(n2) {
var e, r;
return e = {}, c("next"), c("throw", function(h) {
throw h;
}), c("return"), e[Symbol.iterator] = function() {
return this;
}, e;
function c(h, d) {
e[h] = n2[h] ? function(y) {
return (r = !r) ? { value: Ce(n2[h](y)), done: h === "return" } : d ? d(y) : y;
} : d;
}
}
function Dt(n2) {
if (!Symbol.asyncIterator)
throw new TypeError("Symbol.asyncIterator is not defined.");
var e = n2[Symbol.asyncIterator], r;
return e ? e.call(n2) : (n2 = typeof je == "function" ? je(n2) : n2[Symbol.iterator](), r = {}, c("next"), c("throw"), c("return"), r[Symbol.asyncIterator] = function() {
return this;
}, r);
function c(d) {
r[d] = n2[d] && function(y) {
return new Promise(function(E, I) {
y = n2[d](y), h(E, I, y.done, y.value);
});
};
}
function h(d, y, E, I) {
Promise.resolve(I).then(function(S) {
d({ value: S, done: E });
}, y);
}
}
function Ft(n2, e) {
return Object.defineProperty ? Object.defineProperty(n2, "raw", { value: e }) : n2.raw = e, n2;
}
function Wt(n2) {
if (n2 && n2.__esModule)
return n2;
var e = {};
if (n2 != null)
for (var r in n2)
Object.hasOwnProperty.call(n2, r) && (e[r] = n2[r]);
return e.default = n2, e;
}
function Vt(n2) {
return n2 && n2.__esModule ? n2 : { default: n2 };
}
function Qt(n2, e) {
if (!e.has(n2))
throw new TypeError("attempted to get private field on non-instance");
return e.get(n2);
}
function Ut(n2, e, r) {
if (!e.has(n2))
throw new TypeError("attempted to set private field on non-instance");
return e.set(n2, r), r;
}
var Re, qe, ie = Ke({ "node_modules/tslib/tslib.es6.js"() {
Y(), Re = function(n2, e) {
return Re = Object.setPrototypeOf || { __proto__: [] } instanceof Array && function(r, c) {
r.__proto__ = c;
} || function(r, c) {
for (var h in c)
c.hasOwnProperty(h) && (r[h] = c[h]);
}, Re(n2, e);
}, qe = function() {
return qe = Object.assign || function(e) {
for (var r, c = 1, h = arguments.length; c < h; c++) {
r = arguments[c];
for (var d in r)
Object.prototype.hasOwnProperty.call(r, d) && (e[d] = r[d]);
}
return e;
}, qe.apply(this, arguments);
};
} }), Kt = D({ "node_modules/yaml-unist-parser/node_modules/lines-and-columns/build/index.js"(n2) {
"use strict";
Y(), n2.__esModule = true, n2.LinesAndColumns = void 0;
var e = `
`, r = "\r", c = function() {
function h(d) {
this.string = d;
for (var y = [0], E = 0; E < d.length; )
switch (d[E]) {
case e:
E += e.length, y.push(E);
break;
case r:
E += r.length, d[E] === e && (E += e.length), y.push(E);
break;
default:
E++;
break;
}
this.offsets = y;
}
return h.prototype.locationForIndex = function(d) {
if (d < 0 || d > this.string.length)
return null;
for (var y = 0, E = this.offsets; E[y + 1] <= d; )
y++;
var I = d - E[y];
return { line: y, column: I };
}, h.prototype.indexForLocation = function(d) {
var y = d.line, E = d.column;
return y < 0 || y >= this.offsets.length || E < 0 || E > this.lengthOfLine(y) ? null : this.offsets[y] + E;
}, h.prototype.lengthOfLine = function(d) {
var y = this.offsets[d], E = d === this.offsets.length - 1 ? this.string.length : this.offsets[d + 1];
return E - y;
}, h;
}();
n2.LinesAndColumns = c, n2.default = c;
} }), Jt = D({ "node_modules/yaml-unist-parser/lib/utils/define-parents.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c) {
c === void 0 && (c = null), "children" in r && r.children.forEach(function(h) {
return e(h, r);
}), "anchor" in r && r.anchor && e(r.anchor, r), "tag" in r && r.tag && e(r.tag, r), "leadingComments" in r && r.leadingComments.forEach(function(h) {
return e(h, r);
}), "middleComments" in r && r.middleComments.forEach(function(h) {
return e(h, r);
}), "indicatorComment" in r && r.indicatorComment && e(r.indicatorComment, r), "trailingComment" in r && r.trailingComment && e(r.trailingComment, r), "endComments" in r && r.endComments.forEach(function(h) {
return e(h, r);
}), Object.defineProperty(r, "_parent", { value: c, enumerable: false });
}
n2.defineParents = e;
} }), Fe = D({ "node_modules/yaml-unist-parser/lib/utils/get-point-text.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r) {
return r.line + ":" + r.column;
}
n2.getPointText = e;
} }), xt = D({ "node_modules/yaml-unist-parser/lib/attach.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Jt(), r = Fe();
function c(S) {
e.defineParents(S);
var M = h(S), T = S.children.slice();
S.comments.sort(function(P, C) {
return P.position.start.offset - C.position.end.offset;
}).filter(function(P) {
return !P._parent;
}).forEach(function(P) {
for (; T.length > 1 && P.position.start.line > T[0].position.end.line; )
T.shift();
y(P, M, T[0]);
});
}
n2.attachComments = c;
function h(S) {
for (var M = Array.from(new Array(S.position.end.line), function() {
return {};
}), T = 0, P = S.comments; T < P.length; T++) {
var C = P[T];
M[C.position.start.line - 1].comment = C;
}
return d(M, S), M;
}
function d(S, M) {
if (M.position.start.offset !== M.position.end.offset) {
if ("leadingComments" in M) {
var T = M.position.start, P = S[T.line - 1].leadingAttachableNode;
(!P || T.column < P.position.start.column) && (S[T.line - 1].leadingAttachableNode = M);
}
if ("trailingComment" in M && M.position.end.column > 1 && M.type !== "document" && M.type !== "documentHead") {
var C = M.position.end, q = S[C.line - 1].trailingAttachableNode;
(!q || C.column >= q.position.end.column) && (S[C.line - 1].trailingAttachableNode = M);
}
if (M.type !== "root" && M.type !== "document" && M.type !== "documentHead" && M.type !== "documentBody")
for (var R = M.position, T = R.start, C = R.end, B = [C.line].concat(T.line === C.line ? [] : T.line), U = 0, f = B; U < f.length; U++) {
var i = f[U], t = S[i - 1].trailingNode;
(!t || C.column >= t.position.end.column) && (S[i - 1].trailingNode = M);
}
"children" in M && M.children.forEach(function(s) {
d(S, s);
});
}
}
function y(S, M, T) {
var P = S.position.start.line, C = M[P - 1].trailingAttachableNode;
if (C) {
if (C.trailingComment)
throw new Error("Unexpected multiple trailing comment at " + r.getPointText(S.position.start));
e.defineParents(S, C), C.trailingComment = S;
return;
}
for (var q = P; q >= T.position.start.line; q--) {
var R = M[q - 1].trailingNode, B = void 0;
if (R)
B = R;
else if (q !== P && M[q - 1].comment)
B = M[q - 1].comment._parent;
else
continue;
if ((B.type === "sequence" || B.type === "mapping") && (B = B.children[0]), B.type === "mappingItem") {
var U = B.children, f = U[0], i = U[1];
B = I(f) ? f : i;
}
for (; ; ) {
if (E(B, S)) {
e.defineParents(S, B), B.endComments.push(S);
return;
}
if (!B._parent)
break;
B = B._parent;
}
break;
}
for (var q = P + 1; q <= T.position.end.line; q++) {
var t = M[q - 1].leadingAttachableNode;
if (t) {
e.defineParents(S, t), t.leadingComments.push(S);
return;
}
}
var s = T.children[1];
e.defineParents(S, s), s.endComments.push(S);
}
function E(S, M) {
if (S.position.start.offset < M.position.start.offset && S.position.end.offset > M.position.end.offset)
switch (S.type) {
case "flowMapping":
case "flowSequence":
return S.children.length === 0 || M.position.start.line > S.children[S.children.length - 1].position.end.line;
}
if (M.position.end.offset < S.position.end.offset)
return false;
switch (S.type) {
case "sequenceItem":
return M.position.start.column > S.position.start.column;
case "mappingKey":
case "mappingValue":
return M.position.start.column > S._parent.position.start.column && (S.children.length === 0 || S.children.length === 1 && S.children[0].type !== "blockFolded" && S.children[0].type !== "blockLiteral") && (S.type === "mappingValue" || I(S));
default:
return false;
}
}
function I(S) {
return S.position.start !== S.position.end && (S.children.length === 0 || S.position.start.offset !== S.children[0].position.start.offset);
}
} }), me = D({ "node_modules/yaml-unist-parser/lib/factories/node.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c) {
return { type: r, position: c };
}
n2.createNode = e;
} }), Ht = D({ "node_modules/yaml-unist-parser/lib/factories/root.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = me();
function c(h, d, y) {
return e.__assign(e.__assign({}, r.createNode("root", h)), { children: d, comments: y });
}
n2.createRoot = c;
} }), Gt = D({ "node_modules/yaml-unist-parser/lib/preprocess.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r) {
switch (r.type) {
case "DOCUMENT":
for (var c = r.contents.length - 1; c >= 0; c--)
r.contents[c].type === "BLANK_LINE" ? r.contents.splice(c, 1) : e(r.contents[c]);
for (var c = r.directives.length - 1; c >= 0; c--)
r.directives[c].type === "BLANK_LINE" && r.directives.splice(c, 1);
break;
case "FLOW_MAP":
case "FLOW_SEQ":
case "MAP":
case "SEQ":
for (var c = r.items.length - 1; c >= 0; c--) {
var h = r.items[c];
"char" in h || (h.type === "BLANK_LINE" ? r.items.splice(c, 1) : e(h));
}
break;
case "MAP_KEY":
case "MAP_VALUE":
case "SEQ_ITEM":
r.node && e(r.node);
break;
case "ALIAS":
case "BLANK_LINE":
case "BLOCK_FOLDED":
case "BLOCK_LITERAL":
case "COMMENT":
case "DIRECTIVE":
case "PLAIN":
case "QUOTE_DOUBLE":
case "QUOTE_SINGLE":
break;
default:
throw new Error("Unexpected node type " + JSON.stringify(r.type));
}
}
n2.removeCstBlankLine = e;
} }), Oe = D({ "node_modules/yaml-unist-parser/lib/factories/leading-comment-attachable.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e() {
return { leadingComments: [] };
}
n2.createLeadingCommentAttachable = e;
} }), $e = D({ "node_modules/yaml-unist-parser/lib/factories/trailing-comment-attachable.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r) {
return r === void 0 && (r = null), { trailingComment: r };
}
n2.createTrailingCommentAttachable = e;
} }), Se = D({ "node_modules/yaml-unist-parser/lib/factories/comment-attachable.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Oe(), c = $e();
function h() {
return e.__assign(e.__assign({}, r.createLeadingCommentAttachable()), c.createTrailingCommentAttachable());
}
n2.createCommentAttachable = h;
} }), zt = D({ "node_modules/yaml-unist-parser/lib/factories/alias.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Se(), c = me();
function h(d, y, E) {
return e.__assign(e.__assign(e.__assign(e.__assign({}, c.createNode("alias", d)), r.createCommentAttachable()), y), { value: E });
}
n2.createAlias = h;
} }), Zt = D({ "node_modules/yaml-unist-parser/lib/transforms/alias.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = zt();
function r(c, h) {
var d = c.cstNode;
return e.createAlias(h.transformRange({ origStart: d.valueRange.origStart - 1, origEnd: d.valueRange.origEnd }), h.transformContent(c), d.rawValue);
}
n2.transformAlias = r;
} }), Xt = D({ "node_modules/yaml-unist-parser/lib/factories/block-folded.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te));
function r(c) {
return e.__assign(e.__assign({}, c), { type: "blockFolded" });
}
n2.createBlockFolded = r;
} }), er = D({ "node_modules/yaml-unist-parser/lib/factories/block-value.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Oe(), c = me();
function h(d, y, E, I, S, M) {
return e.__assign(e.__assign(e.__assign(e.__assign({}, c.createNode("blockValue", d)), r.createLeadingCommentAttachable()), y), { chomping: E, indent: I, value: S, indicatorComment: M });
}
n2.createBlockValue = h;
} }), xe = D({ "node_modules/yaml-unist-parser/lib/constants.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e;
(function(r) {
r.Tag = "!", r.Anchor = "&", r.Comment = "#";
})(e = n2.PropLeadingCharacter || (n2.PropLeadingCharacter = {}));
} }), tr = D({ "node_modules/yaml-unist-parser/lib/factories/anchor.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = me();
function c(h, d) {
return e.__assign(e.__assign({}, r.createNode("anchor", h)), { value: d });
}
n2.createAnchor = c;
} }), We = D({ "node_modules/yaml-unist-parser/lib/factories/comment.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = me();
function c(h, d) {
return e.__assign(e.__assign({}, r.createNode("comment", h)), { value: d });
}
n2.createComment = c;
} }), rr = D({ "node_modules/yaml-unist-parser/lib/factories/content.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c, h) {
return { anchor: c, tag: r, middleComments: h };
}
n2.createContent = e;
} }), nr = D({ "node_modules/yaml-unist-parser/lib/factories/tag.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = me();
function c(h, d) {
return e.__assign(e.__assign({}, r.createNode("tag", h)), { value: d });
}
n2.createTag = c;
} }), He = D({ "node_modules/yaml-unist-parser/lib/transforms/content.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = xe(), r = tr(), c = We(), h = rr(), d = nr();
function y(E, I, S) {
S === void 0 && (S = function() {
return false;
});
for (var M = E.cstNode, T = [], P = null, C = null, q = null, R = 0, B = M.props; R < B.length; R++) {
var U = B[R], f = I.text[U.origStart];
switch (f) {
case e.PropLeadingCharacter.Tag:
P = P || U, C = d.createTag(I.transformRange(U), E.tag);
break;
case e.PropLeadingCharacter.Anchor:
P = P || U, q = r.createAnchor(I.transformRange(U), M.anchor);
break;
case e.PropLeadingCharacter.Comment: {
var i = c.createComment(I.transformRange(U), I.text.slice(U.origStart + 1, U.origEnd));
I.comments.push(i), !S(i) && P && P.origEnd <= U.origStart && U.origEnd <= M.valueRange.origStart && T.push(i);
break;
}
default:
throw new Error("Unexpected leading character " + JSON.stringify(f));
}
}
return h.createContent(C, q, T);
}
n2.transformContent = y;
} }), Ge = D({ "node_modules/yaml-unist-parser/lib/transforms/block-value.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = er(), r = Fe(), c = He(), h;
(function(y) {
y.CLIP = "clip", y.STRIP = "strip", y.KEEP = "keep";
})(h || (h = {}));
function d(y, E) {
var I = y.cstNode, S = 1, M = I.chomping === "CLIP" ? 0 : 1, T = I.header.origEnd - I.header.origStart, P = T - S - M !== 0, C = E.transformRange({ origStart: I.header.origStart, origEnd: I.valueRange.origEnd }), q = null, R = c.transformContent(y, E, function(B) {
var U = C.start.offset < B.position.start.offset && B.position.end.offset < C.end.offset;
if (!U)
return false;
if (q)
throw new Error("Unexpected multiple indicator comments at " + r.getPointText(B.position.start));
return q = B, true;
});
return e.createBlockValue(C, R, h[I.chomping], P ? I.blockIndent : null, I.strValue, q);
}
n2.transformAstBlockValue = d;
} }), sr = D({ "node_modules/yaml-unist-parser/lib/transforms/block-folded.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Xt(), r = Ge();
function c(h, d) {
return e.createBlockFolded(r.transformAstBlockValue(h, d));
}
n2.transformBlockFolded = c;
} }), ir = D({ "node_modules/yaml-unist-parser/lib/factories/block-literal.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te));
function r(c) {
return e.__assign(e.__assign({}, c), { type: "blockLiteral" });
}
n2.createBlockLiteral = r;
} }), ar = D({ "node_modules/yaml-unist-parser/lib/transforms/block-literal.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = ir(), r = Ge();
function c(h, d) {
return e.createBlockLiteral(r.transformAstBlockValue(h, d));
}
n2.transformBlockLiteral = c;
} }), or = D({ "node_modules/yaml-unist-parser/lib/transforms/comment.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = We();
function r(c, h) {
return e.createComment(h.transformRange(c.range), c.comment);
}
n2.transformComment = r;
} }), lr = D({ "node_modules/yaml-unist-parser/lib/factories/directive.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Se(), c = me();
function h(d, y, E) {
return e.__assign(e.__assign(e.__assign({}, c.createNode("directive", d)), r.createCommentAttachable()), { name: y, parameters: E });
}
n2.createDirective = h;
} }), Ve = D({ "node_modules/yaml-unist-parser/lib/utils/extract-prop-comments.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = xe(), r = We();
function c(h, d) {
for (var y = 0, E = h.props; y < E.length; y++) {
var I = E[y], S = d.text[I.origStart];
switch (S) {
case e.PropLeadingCharacter.Comment:
d.comments.push(r.createComment(d.transformRange(I), d.text.slice(I.origStart + 1, I.origEnd)));
break;
default:
throw new Error("Unexpected leading character " + JSON.stringify(S));
}
}
}
n2.extractPropComments = c;
} }), cr = D({ "node_modules/yaml-unist-parser/lib/transforms/directive.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = lr(), r = Ve();
function c(h, d) {
return r.extractPropComments(h, d), e.createDirective(d.transformRange(h.range), h.name, h.parameters);
}
n2.transformDirective = c;
} }), ur = D({ "node_modules/yaml-unist-parser/lib/factories/document.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = me(), c = $e();
function h(d, y, E, I) {
return e.__assign(e.__assign(e.__assign({}, r.createNode("document", d)), c.createTrailingCommentAttachable(I)), { children: [y, E] });
}
n2.createDocument = h;
} }), Le = D({ "node_modules/yaml-unist-parser/lib/factories/position.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(c, h) {
return { start: c, end: h };
}
n2.createPosition = e;
function r(c) {
return { start: c, end: c };
}
n2.createEmptyPosition = r;
} }), Ee = D({ "node_modules/yaml-unist-parser/lib/factories/end-comment-attachable.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r) {
return r === void 0 && (r = []), { endComments: r };
}
n2.createEndCommentAttachable = e;
} }), fr = D({ "node_modules/yaml-unist-parser/lib/factories/document-body.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Ee(), c = me();
function h(d, y, E) {
return e.__assign(e.__assign(e.__assign({}, c.createNode("documentBody", d)), r.createEndCommentAttachable(E)), { children: y ? [y] : [] });
}
n2.createDocumentBody = h;
} }), Ae = D({ "node_modules/yaml-unist-parser/lib/utils/get-last.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r) {
return r[r.length - 1];
}
n2.getLast = e;
} }), ze = D({ "node_modules/yaml-unist-parser/lib/utils/get-match-index.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c) {
var h = r.match(c);
return h ? h.index : -1;
}
n2.getMatchIndex = e;
} }), mr = D({ "node_modules/yaml-unist-parser/lib/transforms/document-body.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = fr(), c = Ae(), h = ze(), d = Fe();
function y(S, M, T) {
var P, C = S.cstNode, q = E(C, M, T), R = q.comments, B = q.endComments, U = q.documentTrailingComment, f = q.documentHeadTrailingComment, i = M.transformNode(S.contents), t = I(C, i, M), s = t.position, a = t.documentEndPoint;
return (P = M.comments).push.apply(P, e.__spreadArrays(R, B)), { documentBody: r.createDocumentBody(s, i, B), documentEndPoint: a, documentTrailingComment: U, documentHeadTrailingComment: f };
}
n2.transformDocumentBody = y;
function E(S, M, T) {
for (var P = [], C = [], q = [], R = [], B = false, U = S.contents.length - 1; U >= 0; U--) {
var f = S.contents[U];
if (f.type === "COMMENT") {
var i = M.transformNode(f);
T && T.line === i.position.start.line ? R.unshift(i) : B ? P.unshift(i) : i.position.start.offset >= S.valueRange.origEnd ? q.unshift(i) : P.unshift(i);
} else
B = true;
}
if (q.length > 1)
throw new Error("Unexpected multiple document trailing comments at " + d.getPointText(q[1].position.start));
if (R.length > 1)
throw new Error("Unexpected multiple documentHead trailing comments at " + d.getPointText(R[1].position.start));
return { comments: P, endComments: C, documentTrailingComment: c.getLast(q) || null, documentHeadTrailingComment: c.getLast(R) || null };
}
function I(S, M, T) {
var P = h.getMatchIndex(T.text.slice(S.valueRange.origEnd), /^\.\.\./), C = P === -1 ? S.valueRange.origEnd : Math.max(0, S.valueRange.origEnd - 1);
T.text[C - 1] === "\r" && C--;
var q = T.transformRange({ origStart: M !== null ? M.position.start.offset : C, origEnd: C }), R = P === -1 ? q.end : T.transformOffset(S.valueRange.origEnd + 3);
return { position: q, documentEndPoint: R };
}
} }), dr = D({ "node_modules/yaml-unist-parser/lib/factories/document-head.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Ee(), c = me(), h = $e();
function d(y, E, I, S) {
return e.__assign(e.__assign(e.__assign(e.__assign({}, c.createNode("documentHead", y)), r.createEndCommentAttachable(I)), h.createTrailingCommentAttachable(S)), { children: E });
}
n2.createDocumentHead = d;
} }), hr = D({ "node_modules/yaml-unist-parser/lib/transforms/document-head.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = dr(), c = ze();
function h(E, I) {
var S, M = E.cstNode, T = d(M, I), P = T.directives, C = T.comments, q = T.endComments, R = y(M, P, I), B = R.position, U = R.endMarkerPoint;
(S = I.comments).push.apply(S, e.__spreadArrays(C, q));
var f = function(i) {
return i && I.comments.push(i), r.createDocumentHead(B, P, q, i);
};
return { createDocumentHeadWithTrailingComment: f, documentHeadEndMarkerPoint: U };
}
n2.transformDocumentHead = h;
function d(E, I) {
for (var S = [], M = [], T = [], P = false, C = E.directives.length - 1; C >= 0; C--) {
var q = I.transformNode(E.directives[C]);
q.type === "comment" ? P ? M.unshift(q) : T.unshift(q) : (P = true, S.unshift(q));
}
return { directives: S, comments: M, endComments: T };
}
function y(E, I, S) {
var M = c.getMatchIndex(S.text.slice(0, E.valueRange.origStart), /---\s*$/);
M > 0 && !/[\r\n]/.test(S.text[M - 1]) && (M = -1);
var T = M === -1 ? { origStart: E.valueRange.origStart, origEnd: E.valueRange.origStart } : { origStart: M, origEnd: M + 3 };
return I.length !== 0 && (T.origStart = I[0].position.start.offset), { position: S.transformRange(T), endMarkerPoint: M === -1 ? null : S.transformOffset(M) };
}
} }), gr = D({ "node_modules/yaml-unist-parser/lib/transforms/document.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = ur(), r = Le(), c = mr(), h = hr();
function d(y, E) {
var I = h.transformDocumentHead(y, E), S = I.createDocumentHeadWithTrailingComment, M = I.documentHeadEndMarkerPoint, T = c.transformDocumentBody(y, E, M), P = T.documentBody, C = T.documentEndPoint, q = T.documentTrailingComment, R = T.documentHeadTrailingComment, B = S(R);
return q && E.comments.push(q), e.createDocument(r.createPosition(B.position.start, C), B, P, q);
}
n2.transformDocument = d;
} }), Ze = D({ "node_modules/yaml-unist-parser/lib/factories/flow-collection.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Se(), c = Ee(), h = me();
function d(y, E, I) {
return e.__assign(e.__assign(e.__assign(e.__assign(e.__assign({}, h.createNode("flowCollection", y)), r.createCommentAttachable()), c.createEndCommentAttachable()), E), { children: I });
}
n2.createFlowCollection = d;
} }), pr = D({ "node_modules/yaml-unist-parser/lib/factories/flow-mapping.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Ze();
function c(h, d, y) {
return e.__assign(e.__assign({}, r.createFlowCollection(h, d, y)), { type: "flowMapping" });
}
n2.createFlowMapping = c;
} }), Xe = D({ "node_modules/yaml-unist-parser/lib/factories/flow-mapping-item.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Oe(), c = me();
function h(d, y, E) {
return e.__assign(e.__assign(e.__assign({}, c.createNode("flowMappingItem", d)), r.createLeadingCommentAttachable()), { children: [y, E] });
}
n2.createFlowMappingItem = h;
} }), Be = D({ "node_modules/yaml-unist-parser/lib/utils/extract-comments.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c) {
for (var h = [], d = 0, y = r; d < y.length; d++) {
var E = y[d];
E && "type" in E && E.type === "COMMENT" ? c.comments.push(c.transformNode(E)) : h.push(E);
}
return h;
}
n2.extractComments = e;
} }), et = D({ "node_modules/yaml-unist-parser/lib/utils/get-flow-map-item-additional-ranges.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r) {
var c = ["?", ":"].map(function(y) {
var E = r.find(function(I) {
return "char" in I && I.char === y;
});
return E ? { origStart: E.origOffset, origEnd: E.origOffset + 1 } : null;
}), h = c[0], d = c[1];
return { additionalKeyRange: h, additionalValueRange: d };
}
n2.getFlowMapItemAdditionalRanges = e;
} }), tt = D({ "node_modules/yaml-unist-parser/lib/utils/create-slicer.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c) {
var h = c;
return function(d) {
return r.slice(h, h = d);
};
}
n2.createSlicer = e;
} }), rt = D({ "node_modules/yaml-unist-parser/lib/utils/group-cst-flow-collection-items.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = tt();
function r(c) {
for (var h = [], d = e.createSlicer(c, 1), y = false, E = 1; E < c.length - 1; E++) {
var I = c[E];
if ("char" in I && I.char === ",") {
h.push(d(E)), d(E + 1), y = false;
continue;
}
y = true;
}
return y && h.push(d(c.length - 1)), h;
}
n2.groupCstFlowCollectionItems = r;
} }), _r = D({ "node_modules/yaml-unist-parser/lib/factories/mapping-key.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Ee(), c = me(), h = $e();
function d(y, E) {
return e.__assign(e.__assign(e.__assign(e.__assign({}, c.createNode("mappingKey", y)), h.createTrailingCommentAttachable()), r.createEndCommentAttachable()), { children: E ? [E] : [] });
}
n2.createMappingKey = d;
} }), vr = D({ "node_modules/yaml-unist-parser/lib/factories/mapping-value.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Se(), c = Ee(), h = me();
function d(y, E) {
return e.__assign(e.__assign(e.__assign(e.__assign({}, h.createNode("mappingValue", y)), r.createCommentAttachable()), c.createEndCommentAttachable()), { children: E ? [E] : [] });
}
n2.createMappingValue = d;
} }), Qe = D({ "node_modules/yaml-unist-parser/lib/transforms/pair.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = _r(), r = vr(), c = Le();
function h(d, y, E, I, S) {
var M = y.transformNode(d.key), T = y.transformNode(d.value), P = M || I ? e.createMappingKey(y.transformRange({ origStart: I ? I.origStart : M.position.start.offset, origEnd: M ? M.position.end.offset : I.origStart + 1 }), M) : null, C = T || S ? r.createMappingValue(y.transformRange({ origStart: S ? S.origStart : T.position.start.offset, origEnd: T ? T.position.end.offset : S.origStart + 1 }), T) : null;
return E(c.createPosition(P ? P.position.start : C.position.start, C ? C.position.end : P.position.end), P || e.createMappingKey(c.createEmptyPosition(C.position.start), null), C || r.createMappingValue(c.createEmptyPosition(P.position.end), null));
}
n2.transformAstPair = h;
} }), yr = D({ "node_modules/yaml-unist-parser/lib/transforms/flow-map.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = pr(), r = Xe(), c = Be(), h = et(), d = Ae(), y = rt(), E = Qe();
function I(S, M) {
var T = c.extractComments(S.cstNode.items, M), P = y.groupCstFlowCollectionItems(T), C = S.items.map(function(B, U) {
var f = P[U], i = h.getFlowMapItemAdditionalRanges(f), t = i.additionalKeyRange, s = i.additionalValueRange;
return E.transformAstPair(B, M, r.createFlowMappingItem, t, s);
}), q = T[0], R = d.getLast(T);
return e.createFlowMapping(M.transformRange({ origStart: q.origOffset, origEnd: R.origOffset + 1 }), M.transformContent(S), C);
}
n2.transformFlowMap = I;
} }), br = D({ "node_modules/yaml-unist-parser/lib/factories/flow-sequence.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Ze();
function c(h, d, y) {
return e.__assign(e.__assign({}, r.createFlowCollection(h, d, y)), { type: "flowSequence" });
}
n2.createFlowSequence = c;
} }), wr = D({ "node_modules/yaml-unist-parser/lib/factories/flow-sequence-item.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = me();
function c(h, d) {
return e.__assign(e.__assign({}, r.createNode("flowSequenceItem", h)), { children: [d] });
}
n2.createFlowSequenceItem = c;
} }), Sr = D({ "node_modules/yaml-unist-parser/lib/transforms/flow-seq.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Xe(), r = br(), c = wr(), h = Le(), d = Be(), y = et(), E = Ae(), I = rt(), S = Qe();
function M(T, P) {
var C = d.extractComments(T.cstNode.items, P), q = I.groupCstFlowCollectionItems(C), R = T.items.map(function(f, i) {
if (f.type !== "PAIR") {
var t = P.transformNode(f);
return c.createFlowSequenceItem(h.createPosition(t.position.start, t.position.end), t);
} else {
var s = q[i], a = y.getFlowMapItemAdditionalRanges(s), m = a.additionalKeyRange, g = a.additionalValueRange;
return S.transformAstPair(f, P, e.createFlowMappingItem, m, g);
}
}), B = C[0], U = E.getLast(C);
return r.createFlowSequence(P.transformRange({ origStart: B.origOffset, origEnd: U.origOffset + 1 }), P.transformContent(T), R);
}
n2.transformFlowSeq = M;
} }), Er = D({ "node_modules/yaml-unist-parser/lib/factories/mapping.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Oe(), c = me();
function h(d, y, E) {
return e.__assign(e.__assign(e.__assign(e.__assign({}, c.createNode("mapping", d)), r.createLeadingCommentAttachable()), y), { children: E });
}
n2.createMapping = h;
} }), Mr = D({ "node_modules/yaml-unist-parser/lib/factories/mapping-item.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Oe(), c = me();
function h(d, y, E) {
return e.__assign(e.__assign(e.__assign({}, c.createNode("mappingItem", d)), r.createLeadingCommentAttachable()), { children: [y, E] });
}
n2.createMappingItem = h;
} }), Or = D({ "node_modules/yaml-unist-parser/lib/transforms/map.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Er(), r = Mr(), c = Le(), h = tt(), d = Be(), y = Ve(), E = Ae(), I = Qe();
function S(T, P) {
var C = T.cstNode;
C.items.filter(function(U) {
return U.type === "MAP_KEY" || U.type === "MAP_VALUE";
}).forEach(function(U) {
return y.extractPropComments(U, P);
});
var q = d.extractComments(C.items, P), R = M(q), B = T.items.map(function(U, f) {
var i = R[f], t = i[0].type === "MAP_VALUE" ? [null, i[0].range] : [i[0].range, i.length === 1 ? null : i[1].range], s = t[0], a = t[1];
return I.transformAstPair(U, P, r.createMappingItem, s, a);
});
return e.createMapping(c.createPosition(B[0].position.start, E.getLast(B).position.end), P.transformContent(T), B);
}
n2.transformMap = S;
function M(T) {
for (var P = [], C = h.createSlicer(T, 0), q = false, R = 0; R < T.length; R++) {
var B = T[R];
if (B.type === "MAP_VALUE") {
P.push(C(R + 1)), q = false;
continue;
}
q && P.push(C(R)), q = true;
}
return q && P.push(C(1 / 0)), P;
}
} }), Lr = D({ "node_modules/yaml-unist-parser/lib/factories/plain.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Se(), c = me();
function h(d, y, E) {
return e.__assign(e.__assign(e.__assign(e.__assign({}, c.createNode("plain", d)), r.createCommentAttachable()), y), { value: E });
}
n2.createPlain = h;
} }), Ar = D({ "node_modules/yaml-unist-parser/lib/utils/find-last-char-index.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c, h) {
for (var d = c; d >= 0; d--)
if (h.test(r[d]))
return d;
return -1;
}
n2.findLastCharIndex = e;
} }), Nr = D({ "node_modules/yaml-unist-parser/lib/transforms/plain.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Lr(), r = Ar();
function c(h, d) {
var y = h.cstNode;
return e.createPlain(d.transformRange({ origStart: y.valueRange.origStart, origEnd: r.findLastCharIndex(d.text, y.valueRange.origEnd - 1, /\S/) + 1 }), d.transformContent(h), y.strValue);
}
n2.transformPlain = c;
} }), Tr = D({ "node_modules/yaml-unist-parser/lib/factories/quote-double.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te));
function r(c) {
return e.__assign(e.__assign({}, c), { type: "quoteDouble" });
}
n2.createQuoteDouble = r;
} }), Cr = D({ "node_modules/yaml-unist-parser/lib/factories/quote-value.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Se(), c = me();
function h(d, y, E) {
return e.__assign(e.__assign(e.__assign(e.__assign({}, c.createNode("quoteValue", d)), y), r.createCommentAttachable()), { value: E });
}
n2.createQuoteValue = h;
} }), nt = D({ "node_modules/yaml-unist-parser/lib/transforms/quote-value.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Cr();
function r(c, h) {
var d = c.cstNode;
return e.createQuoteValue(h.transformRange(d.valueRange), h.transformContent(c), d.strValue);
}
n2.transformAstQuoteValue = r;
} }), kr = D({ "node_modules/yaml-unist-parser/lib/transforms/quote-double.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Tr(), r = nt();
function c(h, d) {
return e.createQuoteDouble(r.transformAstQuoteValue(h, d));
}
n2.transformQuoteDouble = c;
} }), Pr = D({ "node_modules/yaml-unist-parser/lib/factories/quote-single.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te));
function r(c) {
return e.__assign(e.__assign({}, c), { type: "quoteSingle" });
}
n2.createQuoteSingle = r;
} }), Ir = D({ "node_modules/yaml-unist-parser/lib/transforms/quote-single.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Pr(), r = nt();
function c(h, d) {
return e.createQuoteSingle(r.transformAstQuoteValue(h, d));
}
n2.transformQuoteSingle = c;
} }), Rr = D({ "node_modules/yaml-unist-parser/lib/factories/sequence.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Ee(), c = Oe(), h = me();
function d(y, E, I) {
return e.__assign(e.__assign(e.__assign(e.__assign(e.__assign({}, h.createNode("sequence", y)), c.createLeadingCommentAttachable()), r.createEndCommentAttachable()), E), { children: I });
}
n2.createSequence = d;
} }), qr = D({ "node_modules/yaml-unist-parser/lib/factories/sequence-item.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te)), r = Se(), c = Ee(), h = me();
function d(y, E) {
return e.__assign(e.__assign(e.__assign(e.__assign({}, h.createNode("sequenceItem", y)), r.createCommentAttachable()), c.createEndCommentAttachable()), { children: E ? [E] : [] });
}
n2.createSequenceItem = d;
} }), $r = D({ "node_modules/yaml-unist-parser/lib/transforms/seq.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Le(), r = Rr(), c = qr(), h = Be(), d = Ve(), y = Ae();
function E(I, S) {
var M = h.extractComments(I.cstNode.items, S), T = M.map(function(P, C) {
d.extractPropComments(P, S);
var q = S.transformNode(I.items[C]);
return c.createSequenceItem(e.createPosition(S.transformOffset(P.valueRange.origStart), q === null ? S.transformOffset(P.valueRange.origStart + 1) : q.position.end), q);
});
return r.createSequence(e.createPosition(T[0].position.start, y.getLast(T).position.end), S.transformContent(I), T);
}
n2.transformSeq = E;
} }), Br = D({ "node_modules/yaml-unist-parser/lib/transform.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Zt(), r = sr(), c = ar(), h = or(), d = cr(), y = gr(), E = yr(), I = Sr(), S = Or(), M = Nr(), T = kr(), P = Ir(), C = $r();
function q(R, B) {
if (R === null || R.type === void 0 && R.value === null)
return null;
switch (R.type) {
case "ALIAS":
return e.transformAlias(R, B);
case "BLOCK_FOLDED":
return r.transformBlockFolded(R, B);
case "BLOCK_LITERAL":
return c.transformBlockLiteral(R, B);
case "COMMENT":
return h.transformComment(R, B);
case "DIRECTIVE":
return d.transformDirective(R, B);
case "DOCUMENT":
return y.transformDocument(R, B);
case "FLOW_MAP":
return E.transformFlowMap(R, B);
case "FLOW_SEQ":
return I.transformFlowSeq(R, B);
case "MAP":
return S.transformMap(R, B);
case "PLAIN":
return M.transformPlain(R, B);
case "QUOTE_DOUBLE":
return T.transformQuoteDouble(R, B);
case "QUOTE_SINGLE":
return P.transformQuoteSingle(R, B);
case "SEQ":
return C.transformSeq(R, B);
default:
throw new Error("Unexpected node type " + R.type);
}
}
n2.transformNode = q;
} }), jr = D({ "node_modules/yaml-unist-parser/lib/factories/error.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c, h) {
var d = new SyntaxError(r);
return d.name = "YAMLSyntaxError", d.source = c, d.position = h, d;
}
n2.createError = e;
} }), Yr = D({ "node_modules/yaml-unist-parser/lib/transforms/error.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = jr();
function r(c, h) {
var d = c.source.range || c.source.valueRange;
return e.createError(c.message, h.text, h.transformRange(d));
}
n2.transformError = r;
} }), Dr = D({ "node_modules/yaml-unist-parser/lib/factories/point.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c, h) {
return { offset: r, line: c, column: h };
}
n2.createPoint = e;
} }), Fr = D({ "node_modules/yaml-unist-parser/lib/transforms/offset.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Dr();
function r(c, h) {
c < 0 ? c = 0 : c > h.text.length && (c = h.text.length);
var d = h.locator.locationForIndex(c);
return e.createPoint(c, d.line + 1, d.column + 1);
}
n2.transformOffset = r;
} }), Wr = D({ "node_modules/yaml-unist-parser/lib/transforms/range.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Le();
function r(c, h) {
return e.createPosition(h.transformOffset(c.origStart), h.transformOffset(c.origEnd));
}
n2.transformRange = r;
} }), Vr = D({ "node_modules/yaml-unist-parser/lib/utils/add-orig-range.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = true;
function r(y) {
if (!y.setOrigRanges()) {
var E = function(I) {
if (h(I))
return I.origStart = I.start, I.origEnd = I.end, e;
if (d(I))
return I.origOffset = I.offset, e;
};
y.forEach(function(I) {
return c(I, E);
});
}
}
n2.addOrigRange = r;
function c(y, E) {
if (!(!y || typeof y != "object") && E(y) !== e)
for (var I = 0, S = Object.keys(y); I < S.length; I++) {
var M = S[I];
if (!(M === "context" || M === "error")) {
var T = y[M];
Array.isArray(T) ? T.forEach(function(P) {
return c(P, E);
}) : c(T, E);
}
}
}
function h(y) {
return typeof y.start == "number";
}
function d(y) {
return typeof y.offset == "number";
}
} }), Qr = D({ "node_modules/yaml-unist-parser/lib/utils/remove-fake-nodes.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r) {
if ("children" in r) {
if (r.children.length === 1) {
var c = r.children[0];
if (c.type === "plain" && c.tag === null && c.anchor === null && c.value === "")
return r.children.splice(0, 1), r;
}
r.children.forEach(e);
}
return r;
}
n2.removeFakeNodes = e;
} }), Ur = D({ "node_modules/yaml-unist-parser/lib/utils/create-updater.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
function e(r, c, h, d) {
var y = c(r);
return function(E) {
d(y, E) && h(r, y = E);
};
}
n2.createUpdater = e;
} }), Kr = D({ "node_modules/yaml-unist-parser/lib/utils/update-positions.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Ur(), r = Ae();
function c(M) {
if (!(M === null || !("children" in M))) {
var T = M.children;
if (T.forEach(c), M.type === "document") {
var P = M.children, C = P[0], q = P[1];
C.position.start.offset === C.position.end.offset ? C.position.start = C.position.end = q.position.start : q.position.start.offset === q.position.end.offset && (q.position.start = q.position.end = C.position.end);
}
var R = e.createUpdater(M.position, h, d, I), B = e.createUpdater(M.position, y, E, S);
"endComments" in M && M.endComments.length !== 0 && (R(M.endComments[0].position.start), B(r.getLast(M.endComments).position.end));
var U = T.filter(function(t) {
return t !== null;
});
if (U.length !== 0) {
var f = U[0], i = r.getLast(U);
R(f.position.start), B(i.position.end), "leadingComments" in f && f.leadingComments.length !== 0 && R(f.leadingComments[0].position.start), "tag" in f && f.tag && R(f.tag.position.start), "anchor" in f && f.anchor && R(f.anchor.position.start), "trailingComment" in i && i.trailingComment && B(i.trailingComment.position.end);
}
}
}
n2.updatePositions = c;
function h(M) {
return M.start;
}
function d(M, T) {
M.start = T;
}
function y(M) {
return M.end;
}
function E(M, T) {
M.end = T;
}
function I(M, T) {
return T.offset < M.offset;
}
function S(M, T) {
return T.offset > M.offset;
}
} }), Me = D({ "node_modules/yaml/dist/PlainValue-ec8e588e.js"(n2) {
"use strict";
Y();
var e = { ANCHOR: "&", COMMENT: "#", TAG: "!", DIRECTIVES_END: "-", DOCUMENT_END: "." }, r = { ALIAS: "ALIAS", BLANK_LINE: "BLANK_LINE", BLOCK_FOLDED: "BLOCK_FOLDED", BLOCK_LITERAL: "BLOCK_LITERAL", COMMENT: "COMMENT", DIRECTIVE: "DIRECTIVE", DOCUMENT: "DOCUMENT", FLOW_MAP: "FLOW_MAP", FLOW_SEQ: "FLOW_SEQ", MAP: "MAP", MAP_KEY: "MAP_KEY", MAP_VALUE: "MAP_VALUE", PLAIN: "PLAIN", QUOTE_DOUBLE: "QUOTE_DOUBLE", QUOTE_SINGLE: "QUOTE_SINGLE", SEQ: "SEQ", SEQ_ITEM: "SEQ_ITEM" }, c = "tag:yaml.org,2002:", h = { MAP: "tag:yaml.org,2002:map", SEQ: "tag:yaml.org,2002:seq", STR: "tag:yaml.org,2002:str" };
function d(i) {
let t = [0], s = i.indexOf(`
`);
for (; s !== -1; )
s += 1, t.push(s), s = i.indexOf(`
`, s);
return t;
}
function y(i) {
let t, s;
return typeof i == "string" ? (t = d(i), s = i) : (Array.isArray(i) && (i = i[0]), i && i.context && (i.lineStarts || (i.lineStarts = d(i.context.src)), t = i.lineStarts, s = i.context.src)), { lineStarts: t, src: s };
}
function E(i, t) {
if (typeof i != "number" || i < 0)
return null;
let { lineStarts: s, src: a } = y(t);
if (!s || !a || i > a.length)
return null;
for (let g = 0; g < s.length; ++g) {
let u = s[g];
if (i < u)
return { line: g, col: i - s[g - 1] + 1 };
if (i === u)
return { line: g + 1, col: 1 };
}
let m = s.length;
return { line: m, col: i - s[m - 1] + 1 };
}
function I(i, t) {
let { lineStarts: s, src: a } = y(t);
if (!s || !(i >= 1) || i > s.length)
return null;
let m = s[i - 1], g = s[i];
for (; g && g > m && a[g - 1] === `
`; )
--g;
return a.slice(m, g);
}
function S(i, t) {
let { start: s, end: a } = i, m = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : 80, g = I(s.line, t);
if (!g)
return null;
let { col: u } = s;
if (g.length > m)
if (u <= m - 10)
g = g.substr(0, m - 1) + "\u2026";
else {
let K = Math.round(m / 2);
g.length > u + K && (g = g.substr(0, u + K - 1) + "\u2026"), u -= g.length - m, g = "\u2026" + g.substr(1 - m);
}
let p = 1, L = "";
a && (a.line === s.line && u + (a.col - s.col) <= m + 1 ? p = a.col - s.col : (p = Math.min(g.length + 1, m) - u, L = "\u2026"));
let k = u > 1 ? " ".repeat(u - 1) : "", $ = "^".repeat(p);
return `${g}
${k}${$}${L}`;
}
var M = class {
static copy(i) {
return new M(i.start, i.end);
}
constructor(i, t) {
this.start = i, this.end = t || i;
}
isEmpty() {
return typeof this.start != "number" || !this.end || this.end <= this.start;
}
setOrigRange(i, t) {
let { start: s, end: a } = this;
if (i.length === 0 || a <= i[0])
return this.origStart = s, this.origEnd = a, t;
let m = t;
for (; m < i.length && !(i[m] > s); )
++m;
this.origStart = s + m;
let g = m;
for (; m < i.length && !(i[m] >= a); )
++m;
return this.origEnd = a + m, g;
}
}, T = class {
static addStringTerminator(i, t, s) {
if (s[s.length - 1] === `
`)
return s;
let a = T.endOfWhiteSpace(i, t);
return a >= i.length || i[a] === `
` ? s + `
` : s;
}
static atDocumentBoundary(i, t, s) {
let a = i[t];
if (!a)
return true;
let m = i[t - 1];
if (m && m !== `
`)
return false;
if (s) {
if (a !== s)
return false;
} else if (a !== e.DIRECTIVES_END && a !== e.DOCUMENT_END)
return false;
let g = i[t + 1], u = i[t + 2];
if (g !== a || u !== a)
return false;
let p = i[t + 3];
return !p || p === `
` || p === " " || p === " ";
}
static endOfIdentifier(i, t) {
let s = i[t], a = s === "<", m = a ? [`
`, " ", " ", ">"] : [`
`, " ", " ", "[", "]", "{", "}", ","];
for (; s && m.indexOf(s) === -1; )
s = i[t += 1];
return a && s === ">" && (t += 1), t;
}
static endOfIndent(i, t) {
let s = i[t];
for (; s === " "; )
s = i[t += 1];
return t;
}
static endOfLine(i, t) {
let s = i[t];
for (; s && s !== `
`; )
s = i[t += 1];
return t;
}
static endOfWhiteSpace(i, t) {
let s = i[t];
for (; s === " " || s === " "; )
s = i[t += 1];
return t;
}
static startOfLine(i, t) {
let s = i[t - 1];
if (s === `
`)
return t;
for (; s && s !== `
`; )
s = i[t -= 1];
return t + 1;
}
static endOfBlockIndent(i, t, s) {
let a = T.endOfIndent(i, s);
if (a > s + t)
return a;
{
let m = T.endOfWhiteSpace(i, a), g = i[m];
if (!g || g === `
`)
return m;
}
return null;
}
static atBlank(i, t, s) {
let a = i[t];
return a === `
` || a === " " || a === " " || s && !a;
}
static nextNodeIsIndented(i, t, s) {
return !i || t < 0 ? false : t > 0 ? true : s && i === "-";
}
static normalizeOffset(i, t) {
let s = i[t];
return s ? s !== `
` && i[t - 1] === `
` ? t - 1 : T.endOfWhiteSpace(i, t) : t;
}
static foldNewline(i, t, s) {
let a = 0, m = false, g = "", u = i[t + 1];
for (; u === " " || u === " " || u === `
`; ) {
switch (u) {
case `
`:
a = 0, t += 1, g += `
`;
break;
case " ":
a <= s && (m = true), t = T.endOfWhiteSpace(i, t + 2) - 1;
break;
case " ":
a += 1, t += 1;
break;
}
u = i[t + 1];
}
return g || (g = " "), u && a <= s && (m = true), { fold: g, offset: t, error: m };
}
constructor(i, t, s) {
Object.defineProperty(this, "context", { value: s || null, writable: true }), this.error = null, this.range = null, this.valueRange = null, this.props = t || [], this.type = i, this.value = null;
}
getPropValue(i, t, s) {
if (!this.context)
return null;
let { src: a } = this.context, m = this.props[i];
return m && a[m.start] === t ? a.slice(m.start + (s ? 1 : 0), m.end) : null;
}
get anchor() {
for (let i = 0; i < this.props.length; ++i) {
let t = this.getPropValue(i, e.ANCHOR, true);
if (t != null)
return t;
}
return null;
}
get comment() {
let i = [];
for (let t = 0; t < this.props.length; ++t) {
let s = this.getPropValue(t, e.COMMENT, true);
s != null && i.push(s);
}
return i.length > 0 ? i.join(`
`) : null;
}
commentHasRequiredWhitespace(i) {
let { src: t } = this.context;
if (this.header && i === this.header.end || !this.valueRange)
return false;
let { end: s } = this.valueRange;
return i !== s || T.atBlank(t, s - 1);
}
get hasComment() {
if (this.context) {
let { src: i } = this.context;
for (let t = 0; t < this.props.length; ++t)
if (i[this.props[t].start] === e.COMMENT)
return true;
}
return false;
}
get hasProps() {
if (this.context) {
let { src: i } = this.context;
for (let t = 0; t < this.props.length; ++t)
if (i[this.props[t].start] !== e.COMMENT)
return true;
}
return false;
}
get includesTrailingLines() {
return false;
}
get jsonLike() {
return [r.FLOW_MAP, r.FLOW_SEQ, r.QUOTE_DOUBLE, r.QUOTE_SINGLE].indexOf(this.type) !== -1;
}
get rangeAsLinePos() {
if (!this.range || !this.context)
return;
let i = E(this.range.start, this.context.root);
if (!i)
return;
let t = E(this.range.end, this.context.root);
return { start: i, end: t };
}
get rawValue() {
if (!this.valueRange || !this.context)
return null;
let { start: i, end: t } = this.valueRange;
return this.context.src.slice(i, t);
}
get tag() {
for (let i = 0; i < this.props.length; ++i) {
let t = this.getPropValue(i, e.TAG, false);
if (t != null) {
if (t[1] === "<")
return { verbatim: t.slice(2, -1) };
{
let [s, a, m] = t.match(/^(.*!)([^!]*)$/);
return { handle: a, suffix: m };
}
}
}
return null;
}
get valueRangeContainsNewline() {
if (!this.valueRange || !this.context)
return false;
let { start: i, end: t } = this.valueRange, { src: s } = this.context;
for (let a = i; a < t; ++a)
if (s[a] === `
`)
return true;
return false;
}
parseComment(i) {
let { src: t } = this.context;
if (t[i] === e.COMMENT) {
let s = T.endOfLine(t, i + 1), a = new M(i, s);
return this.props.push(a), s;
}
return i;
}
setOrigRanges(i, t) {
return this.range && (t = this.range.setOrigRange(i, t)), this.valueRange && this.valueRange.setOrigRange(i, t), this.props.forEach((s) => s.setOrigRange(i, t)), t;
}
toString() {
let { context: { src: i }, range: t, value: s } = this;
if (s != null)
return s;
let a = i.slice(t.start, t.end);
return T.addStringTerminator(i, t.end, a);
}
}, P = class extends Error {
constructor(i, t, s) {
if (!s || !(t instanceof T))
throw new Error(`Invalid arguments for new ${i}`);
super(), this.name = i, this.message = s, this.source = t;
}
makePretty() {
if (!this.source)
return;
this.nodeType = this.source.type;
let i = this.source.context && this.source.context.root;
if (typeof this.offset == "number") {
this.range = new M(this.offset, this.offset + 1);
let t = i && E(this.offset, i);
if (t) {
let s = { line: t.line, col: t.col + 1 };
this.linePos = { start: t, end: s };
}
delete this.offset;
} else
this.range = this.source.range, this.linePos = this.source.rangeAsLinePos;
if (this.linePos) {
let { line: t, col: s } = this.linePos.start;
this.message += ` at line ${t}, column ${s}`;
let a = i && S(this.linePos, i);
a && (this.message += `:
${a}
`);
}
delete this.source;
}
}, C = class extends P {
constructor(i, t) {
super("YAMLReferenceError", i, t);
}
}, q = class extends P {
constructor(i, t) {
super("YAMLSemanticError", i, t);
}
}, R = class extends P {
constructor(i, t) {
super("YAMLSyntaxError", i, t);
}
}, B = class extends P {
constructor(i, t) {
super("YAMLWarning", i, t);
}
};
function U(i, t, s) {
return t in i ? Object.defineProperty(i, t, { value: s, enumerable: true, configurable: true, writable: true }) : i[t] = s, i;
}
var f = class extends T {
static endOfLine(i, t, s) {
let a = i[t], m = t;
for (; a && a !== `
` && !(s && (a === "[" || a === "]" || a === "{" || a === "}" || a === ",")); ) {
let g = i[m + 1];
if (a === ":" && (!g || g === `
` || g === " " || g === " " || s && g === ",") || (a === " " || a === " ") && g === "#")
break;
m += 1, a = g;
}
return m;
}
get strValue() {
if (!this.valueRange || !this.context)
return null;
let { start: i, end: t } = this.valueRange, { src: s } = this.context, a = s[t - 1];
for (; i < t && (a === `
` || a === " " || a === " "); )
a = s[--t - 1];
let m = "";
for (let u = i; u < t; ++u) {
let p = s[u];
if (p === `
`) {
let { fold: L, offset: k } = T.foldNewline(s, u, -1);
m += L, u = k;
} else if (p === " " || p === " ") {
let L = u, k = s[u + 1];
for (; u < t && (k === " " || k === " "); )
u += 1, k = s[u + 1];
k !== `
` && (m += u > L ? s.slice(L, u + 1) : p);
} else
m += p;
}
let g = s[i];
switch (g) {
case " ": {
let u = "Plain value cannot start with a tab character";
return { errors: [new q(this, u)], str: m };
}
case "@":
case "`": {
let u = `Plain value cannot start with reserved character ${g}`;
return { errors: [new q(this, u)], str: m };
}
default:
return m;
}
}
parseBlockValue(i) {
let { indent: t, inFlow: s, src: a } = this.context, m = i, g = i;
for (let u = a[m]; u === `
` && !T.atDocumentBoundary(a, m + 1); u = a[m]) {
let p = T.endOfBlockIndent(a, t, m + 1);
if (p === null || a[p] === "#")
break;
a[p] === `
` ? m = p : (g = f.endOfLine(a, p, s), m = g);
}
return this.valueRange.isEmpty() && (this.valueRange.start = i), this.valueRange.end = g, g;
}
parse(i, t) {
this.context = i;
let { inFlow: s, src: a } = i, m = t, g = a[m];
return g && g !== "#" && g !== `
` && (m = f.endOfLine(a, t, s)), this.valueRange = new M(t, m), m = T.endOfWhiteSpace(a, m), m = this.parseComment(m), (!this.hasComment || this.valueRange.isEmpty()) && (m = this.parseBlockValue(m)), m;
}
};
n2.Char = e, n2.Node = T, n2.PlainValue = f, n2.Range = M, n2.Type = r, n2.YAMLError = P, n2.YAMLReferenceError = C, n2.YAMLSemanticError = q, n2.YAMLSyntaxError = R, n2.YAMLWarning = B, n2._defineProperty = U, n2.defaultTagPrefix = c, n2.defaultTags = h;
} }), Jr = D({ "node_modules/yaml/dist/parse-cst.js"(n2) {
"use strict";
Y();
var e = Me(), r = class extends e.Node {
constructor() {
super(e.Type.BLANK_LINE);
}
get includesTrailingLines() {
return true;
}
parse(f, i) {
return this.context = f, this.range = new e.Range(i, i + 1), i + 1;
}
}, c = class extends e.Node {
constructor(f, i) {
super(f, i), this.node = null;
}
get includesTrailingLines() {
return !!this.node && this.node.includesTrailingLines;
}
parse(f, i) {
this.context = f;
let { parseNode: t, src: s } = f, { atLineStart: a, lineStart: m } = f;
!a && this.type === e.Type.SEQ_ITEM && (this.error = new e.YAMLSemanticError(this, "Sequence items must not have preceding content on the same line"));
let g = a ? i - m : f.indent, u = e.Node.endOfWhiteSpace(s, i + 1), p = s[u], L = p === "#", k = [], $ = null;
for (; p === `
` || p === "#"; ) {
if (p === "#") {
let V = e.Node.endOfLine(s, u + 1);
k.push(new e.Range(u, V)), u = V;
} else {
a = true, m = u + 1;
let V = e.Node.endOfWhiteSpace(s, m);
s[V] === `
` && k.length === 0 && ($ = new r(), m = $.parse({ src: s }, m)), u = e.Node.endOfIndent(s, m);
}
p = s[u];
}
if (e.Node.nextNodeIsIndented(p, u - (m + g), this.type !== e.Type.SEQ_ITEM) ? this.node = t({ atLineStart: a, inCollection: false, indent: g, lineStart: m, parent: this }, u) : p && m > i + 1 && (u = m - 1), this.node) {
if ($) {
let V = f.parent.items || f.parent.contents;
V && V.push($);
}
k.length && Array.prototype.push.apply(this.props, k), u = this.node.range.end;
} else if (L) {
let V = k[0];
this.props.push(V), u = V.end;
} else
u = e.Node.endOfLine(s, i + 1);
let K = this.node ? this.node.valueRange.end : u;
return this.valueRange = new e.Range(i, K), u;
}
setOrigRanges(f, i) {
return i = super.setOrigRanges(f, i), this.node ? this.node.setOrigRanges(f, i) : i;
}
toString() {
let { context: { src: f }, node: i, range: t, value: s } = this;
if (s != null)
return s;
let a = i ? f.slice(t.start, i.range.start) + String(i) : f.slice(t.start, t.end);
return e.Node.addStringTerminator(f, t.end, a);
}
}, h = class extends e.Node {
constructor() {
super(e.Type.COMMENT);
}
parse(f, i) {
this.context = f;
let t = this.parseComment(i);
return this.range = new e.Range(i, t), t;
}
};
function d(f) {
let i = f;
for (; i instanceof c; )
i = i.node;
if (!(i instanceof y))
return null;
let t = i.items.length, s = -1;
for (let g = t - 1; g >= 0; --g) {
let u = i.items[g];
if (u.type === e.Type.COMMENT) {
let { indent: p, lineStart: L } = u.context;
if (p > 0 && u.range.start >= L + p)
break;
s = g;
} else if (u.type === e.Type.BLANK_LINE)
s = g;
else
break;
}
if (s === -1)
return null;
let a = i.items.splice(s, t - s), m = a[0].range.start;
for (; i.range.end = m, i.valueRange && i.valueRange.end > m && (i.valueRange.end = m), i !== f; )
i = i.context.parent;
return a;
}
var y = class extends e.Node {
static nextContentHasIndent(f, i, t) {
let s = e.Node.endOfLine(f, i) + 1;
i = e.Node.endOfWhiteSpace(f, s);
let a = f[i];
return a ? i >= s + t ? true : a !== "#" && a !== `
` ? false : y.nextContentHasIndent(f, i, t) : false;
}
constructor(f) {
super(f.type === e.Type.SEQ_ITEM ? e.Type.SEQ : e.Type.MAP);
for (let t = f.props.length - 1; t >= 0; --t)
if (f.props[t].start < f.context.lineStart) {
this.props = f.props.slice(0, t + 1), f.props = f.props.slice(t + 1);
let s = f.props[0] || f.valueRange;
f.range.start = s.start;
break;
}
this.items = [f];
let i = d(f);
i && Array.prototype.push.apply(this.items, i);
}
get includesTrailingLines() {
return this.items.length > 0;
}
parse(f, i) {
this.context = f;
let { parseNode: t, src: s } = f, a = e.Node.startOfLine(s, i), m = this.items[0];
m.context.parent = this, this.valueRange = e.Range.copy(m.valueRange);
let g = m.range.start - m.context.lineStart, u = i;
u = e.Node.normalizeOffset(s, u);
let p = s[u], L = e.Node.endOfWhiteSpace(s, a) === u, k = false;
for (; p; ) {
for (; p === `
` || p === "#"; ) {
if (L && p === `
` && !k) {
let V = new r();
if (u = V.parse({ src: s }, u), this.valueRange.end = u, u >= s.length) {
p = null;
break;
}
this.items.push(V), u -= 1;
} else if (p === "#") {
if (u < a + g && !y.nextContentHasIndent(s, u, g))
return u;
let V = new h();
if (u = V.parse({ indent: g, lineStart: a, src: s }, u), this.items.push(V), this.valueRange.end = u, u >= s.length) {
p = null;
break;
}
}
if (a = u + 1, u = e.Node.endOfIndent(s, a), e.Node.atBlank(s, u)) {
let V = e.Node.endOfWhiteSpace(s, u), z = s[V];
(!z || z === `
` || z === "#") && (u = V);
}
p = s[u], L = true;
}
if (!p)
break;
if (u !== a + g && (L || p !== ":")) {
if (u < a + g) {
a > i && (u = a);
break;
} else if (!this.error) {
let V = "All collection items must start at the same column";
this.error = new e.YAMLSyntaxError(this, V);
}
}
if (m.type === e.Type.SEQ_ITEM) {
if (p !== "-") {
a > i && (u = a);
break;
}
} else if (p === "-" && !this.error) {
let V = s[u + 1];
if (!V || V === `
` || V === " " || V === " ") {
let z = "A collection cannot be both a mapping and a sequence";
this.error = new e.YAMLSyntaxError(this, z);
}
}
let $ = t({ atLineStart: L, inCollection: true, indent: g, lineStart: a, parent: this }, u);
if (!$)
return u;
if (this.items.push($), this.valueRange.end = $.valueRange.end, u = e.Node.normalizeOffset(s, $.range.end), p = s[u], L = false, k = $.includesTrailingLines, p) {
let V = u - 1, z = s[V];
for (; z === " " || z === " "; )
z = s[--V];
z === `
` && (a = V + 1, L = true);
}
let K = d($);
K && Array.prototype.push.apply(this.items, K);
}
return u;
}
setOrigRanges(f, i) {
return i = super.setOrigRanges(f, i), this.items.forEach((t) => {
i = t.setOrigRanges(f, i);
}), i;
}
toString() {
let { context: { src: f }, items: i, range: t, value: s } = this;
if (s != null)
return s;
let a = f.slice(t.start, i[0].range.start) + String(i[0]);
for (let m = 1; m < i.length; ++m) {
let g = i[m], { atLineStart: u, indent: p } = g.context;
if (u)
for (let L = 0; L < p; ++L)
a += " ";
a += String(g);
}
return e.Node.addStringTerminator(f, t.end, a);
}
}, E = class extends e.Node {
constructor() {
super(e.Type.DIRECTIVE), this.name = null;
}
get parameters() {
let f = this.rawValue;
return f ? f.trim().split(/[ \t]+/) : [];
}
parseName(f) {
let { src: i } = this.context, t = f, s = i[t];
for (; s && s !== `
` && s !== " " && s !== " "; )
s = i[t += 1];
return this.name = i.slice(f, t), t;
}
parseParameters(f) {
let { src: i } = this.context, t = f, s = i[t];
for (; s && s !== `
` && s !== "#"; )
s = i[t += 1];
return this.valueRange = new e.Range(f, t), t;
}
parse(f, i) {
this.context = f;
let t = this.parseName(i + 1);
return t = this.parseParameters(t), t = this.parseComment(t), this.range = new e.Range(i, t), t;
}
}, I = class extends e.Node {
static startCommentOrEndBlankLine(f, i) {
let t = e.Node.endOfWhiteSpace(f, i), s = f[t];
return s === "#" || s === `
` ? t : i;
}
constructor() {
super(e.Type.DOCUMENT), this.directives = null, this.contents = null, this.directivesEndMarker = null, this.documentEndMarker = null;
}
parseDirectives(f) {
let { src: i } = this.context;
this.directives = [];
let t = true, s = false, a = f;
for (; !e.Node.atDocumentBoundary(i, a, e.Char.DIRECTIVES_END); )
switch (a = I.startCommentOrEndBlankLine(i, a), i[a]) {
case `
`:
if (t) {
let m = new r();
a = m.parse({ src: i }, a), a < i.length && this.directives.push(m);
} else
a += 1, t = true;
break;
case "#":
{
let m = new h();
a = m.parse({ src: i }, a), this.directives.push(m), t = false;
}
break;
case "%":
{
let m = new E();
a = m.parse({ parent: this, src: i }, a), this.directives.push(m), s = true, t = false;
}
break;
default:
return s ? this.error = new e.YAMLSemanticError(this, "Missing directives-end indicator line") : this.directives.length > 0 && (this.contents = this.directives, this.directives = []), a;
}
return i[a] ? (this.directivesEndMarker = new e.Range(a, a + 3), a + 3) : (s ? this.error = new e.YAMLSemanticError(this, "Missing directives-end indicator line") : this.directives.length > 0 && (this.contents = this.directives, this.directives = []), a);
}
parseContents(f) {
let { parseNode: i, src: t } = this.context;
this.contents || (this.contents = []);
let s = f;
for (; t[s - 1] === "-"; )
s -= 1;
let a = e.Node.endOfWhiteSpace(t, f), m = s === f;
for (this.valueRange = new e.Range(a); !e.Node.atDocumentBoundary(t, a, e.Char.DOCUMENT_END); ) {
switch (t[a]) {
case `
`:
if (m) {
let g = new r();
a = g.parse({ src: t }, a), a < t.length && this.contents.push(g);
} else
a += 1, m = true;
s = a;
break;
case "#":
{
let g = new h();
a = g.parse({ src: t }, a), this.contents.push(g), m = false;
}
break;
default: {
let g = e.Node.endOfIndent(t, a), p = i({ atLineStart: m, indent: -1, inFlow: false, inCollection: false, lineStart: s, parent: this }, g);
if (!p)
return this.valueRange.end = g;
this.contents.push(p), a = p.range.end, m = false;
let L = d(p);
L && Array.prototype.push.apply(this.contents, L);
}
}
a = I.startCommentOrEndBlankLine(t, a);
}
if (this.valueRange.end = a, t[a] && (this.documentEndMarker = new e.Range(a, a + 3), a += 3, t[a])) {
if (a = e.Node.endOfWhiteSpace(t, a), t[a] === "#") {
let g = new h();
a = g.parse({ src: t }, a), this.contents.push(g);
}
switch (t[a]) {
case `
`:
a += 1;
break;
case void 0:
break;
default:
this.error = new e.YAMLSyntaxError(this, "Document end marker line cannot have a non-comment suffix");
}
}
return a;
}
parse(f, i) {
f.root = this, this.context = f;
let { src: t } = f, s = t.charCodeAt(i) === 65279 ? i + 1 : i;
return s = this.parseDirectives(s), s = this.parseContents(s), s;
}
setOrigRanges(f, i) {
return i = super.setOrigRanges(f, i), this.directives.forEach((t) => {
i = t.setOrigRanges(f, i);
}), this.directivesEndMarker && (i = this.directivesEndMarker.setOrigRange(f, i)), this.contents.forEach((t) => {
i = t.setOrigRanges(f, i);
}), this.documentEndMarker && (i = this.documentEndMarker.setOrigRange(f, i)), i;
}
toString() {
let { contents: f, directives: i, value: t } = this;
if (t != null)
return t;
let s = i.join("");
return f.length > 0 && ((i.length > 0 || f[0].type === e.Type.COMMENT) && (s += `---
`), s += f.join("")), s[s.length - 1] !== `
` && (s += `
`), s;
}
}, S = class extends e.Node {
parse(f, i) {
this.context = f;
let { src: t } = f, s = e.Node.endOfIdentifier(t, i + 1);
return this.valueRange = new e.Range(i + 1, s), s = e.Node.endOfWhiteSpace(t, s), s = this.parseComment(s), s;
}
}, M = { CLIP: "CLIP", KEEP: "KEEP", STRIP: "STRIP" }, T = class extends e.Node {
constructor(f, i) {
super(f, i), this.blockIndent = null, this.chomping = M.CLIP, this.header = null;
}
get includesTrailingLines() {
return this.chomping === M.KEEP;
}
get strValue() {
if (!this.valueRange || !this.context)
return null;
let { start: f, end: i } = this.valueRange, { indent: t, src: s } = this.context;
if (this.valueRange.isEmpty())
return "";
let a = null, m = s[i - 1];
for (; m === `
` || m === " " || m === " "; ) {
if (i -= 1, i <= f) {
if (this.chomping === M.KEEP)
break;
return "";
}
m === `
` && (a = i), m = s[i - 1];
}
let g = i + 1;
a && (this.chomping === M.KEEP ? (g = a, i = this.valueRange.end) : i = a);
let u = t + this.blockIndent, p = this.type === e.Type.BLOCK_FOLDED, L = true, k = "", $ = "", K = false;
for (let V = f; V < i; ++V) {
for (let ae = 0; ae < u && s[V] === " "; ++ae)
V += 1;
let z = s[V];
if (z === `
`)
$ === `
` ? k += `
` : $ = `
`;
else {
let ae = e.Node.endOfLine(s, V), ue = s.slice(V, ae);
V = ae, p && (z === " " || z === " ") && V < g ? ($ === " " ? $ = `
` : !K && !L && $ === `
` && ($ = `
`), k += $ + ue, $ = ae < i && s[ae] || "", K = true) : (k += $ + ue, $ = p && V < g ? " " : `
`, K = false), L && ue !== "" && (L = false);
}
}
return this.chomping === M.STRIP ? k : k + `
`;
}
parseBlockHeader(f) {
let { src: i } = this.context, t = f + 1, s = "";
for (; ; ) {
let a = i[t];
switch (a) {
case "-":
this.chomping = M.STRIP;
break;
case "+":
this.chomping = M.KEEP;
break;
case "0":
case "1":
case "2":
case "3":
case "4":
case "5":
case "6":
case "7":
case "8":
case "9":
s += a;
break;
default:
return this.blockIndent = Number(s) || null, this.header = new e.Range(f, t), t;
}
t += 1;
}
}
parseBlockValue(f) {
let { indent: i, src: t } = this.context, s = !!this.blockIndent, a = f, m = f, g = 1;
for (let u = t[a]; u === `
` && (a += 1, !e.Node.atDocumentBoundary(t, a)); u = t[a]) {
let p = e.Node.endOfBlockIndent(t, i, a);
if (p === null)
break;
let L = t[p], k = p - (a + i);
if (this.blockIndent) {
if (L && L !== `
` && k < this.blockIndent) {
if (t[p] === "#")
break;
if (!this.error) {
let K = `Block scalars must not be less indented than their ${s ? "explicit indentation indicator" : "first line"}`;
this.error = new e.YAMLSemanticError(this, K);
}
}
} else if (t[p] !== `
`) {
if (k < g) {
let $ = "Block scalars with more-indented leading empty lines must use an explicit indentation indicator";
this.error = new e.YAMLSemanticError(this, $);
}
this.blockIndent = k;
} else
k > g && (g = k);
t[p] === `
` ? a = p : a = m = e.Node.endOfLine(t, p);
}
return this.chomping !== M.KEEP && (a = t[m] ? m + 1 : m), this.valueRange = new e.Range(f + 1, a), a;
}
parse(f, i) {
this.context = f;
let { src: t } = f, s = this.parseBlockHeader(i);
return s = e.Node.endOfWhiteSpace(t, s), s = this.parseComment(s), s = this.parseBlockValue(s), s;
}
setOrigRanges(f, i) {
return i = super.setOrigRanges(f, i), this.header ? this.header.setOrigRange(f, i) : i;
}
}, P = class extends e.Node {
constructor(f, i) {
super(f, i), this.items = null;
}
prevNodeIsJsonLike() {
let f = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : this.items.length, i = this.items[f - 1];
return !!i && (i.jsonLike || i.type === e.Type.COMMENT && this.prevNodeIsJsonLike(f - 1));
}
parse(f, i) {
this.context = f;
let { parseNode: t, src: s } = f, { indent: a, lineStart: m } = f, g = s[i];
this.items = [{ char: g, offset: i }];
let u = e.Node.endOfWhiteSpace(s, i + 1);
for (g = s[u]; g && g !== "]" && g !== "}"; ) {
switch (g) {
case `
`:
{
m = u + 1;
let p = e.Node.endOfWhiteSpace(s, m);
if (s[p] === `
`) {
let L = new r();
m = L.parse({ src: s }, m), this.items.push(L);
}
if (u = e.Node.endOfIndent(s, m), u <= m + a && (g = s[u], u < m + a || g !== "]" && g !== "}")) {
let L = "Insufficient indentation in flow collection";
this.error = new e.YAMLSemanticError(this, L);
}
}
break;
case ",":
this.items.push({ char: g, offset: u }), u += 1;
break;
case "#":
{
let p = new h();
u = p.parse({ src: s }, u), this.items.push(p);
}
break;
case "?":
case ":": {
let p = s[u + 1];
if (p === `
` || p === " " || p === " " || p === "," || g === ":" && this.prevNodeIsJsonLike()) {
this.items.push({ char: g, offset: u }), u += 1;
break;
}
}
default: {
let p = t({ atLineStart: false, inCollection: false, inFlow: true, indent: -1, lineStart: m, parent: this }, u);
if (!p)
return this.valueRange = new e.Range(i, u), u;
this.items.push(p), u = e.Node.normalizeOffset(s, p.range.end);
}
}
u = e.Node.endOfWhiteSpace(s, u), g = s[u];
}
return this.valueRange = new e.Range(i, u + 1), g && (this.items.push({ char: g, offset: u }), u = e.Node.endOfWhiteSpace(s, u + 1), u = this.parseComment(u)), u;
}
setOrigRanges(f, i) {
return i = super.setOrigRanges(f, i), this.items.forEach((t) => {
if (t instanceof e.Node)
i = t.setOrigRanges(f, i);
else if (f.length === 0)
t.origOffset = t.offset;
else {
let s = i;
for (; s < f.length && !(f[s] > t.offset); )
++s;
t.origOffset = t.offset + s, i = s;
}
}), i;
}
toString() {
let { context: { src: f }, items: i, range: t, value: s } = this;
if (s != null)
return s;
let a = i.filter((u) => u instanceof e.Node), m = "", g = t.start;
return a.forEach((u) => {
let p = f.slice(g, u.range.start);
g = u.range.end, m += p + String(u), m[m.length - 1] === `
` && f[g - 1] !== `
` && f[g] === `
` && (g += 1);
}), m += f.slice(g, t.end), e.Node.addStringTerminator(f, t.end, m);
}
}, C = class extends e.Node {
static endOfQuote(f, i) {
let t = f[i];
for (; t && t !== '"'; )
i += t === "\\" ? 2 : 1, t = f[i];
return i + 1;
}
get strValue() {
if (!this.valueRange || !this.context)
return null;
let f = [], { start: i, end: t } = this.valueRange, { indent: s, src: a } = this.context;
a[t - 1] !== '"' && f.push(new e.YAMLSyntaxError(this, 'Missing closing "quote'));
let m = "";
for (let g = i + 1; g < t - 1; ++g) {
let u = a[g];
if (u === `
`) {
e.Node.atDocumentBoundary(a, g + 1) && f.push(new e.YAMLSemanticError(this, "Document boundary indicators are not allowed within string values"));
let { fold: p, offset: L, error: k } = e.Node.foldNewline(a, g, s);
m += p, g = L, k && f.push(new e.YAMLSemanticError(this, "Multi-line double-quoted string needs to be sufficiently indented"));
} else if (u === "\\")
switch (g += 1, a[g]) {
case "0":
m += "\0";
break;
case "a":
m += "\x07";
break;
case "b":
m += "\b";
break;
case "e":
m += "\x1B";
break;
case "f":
m += "\f";
break;
case "n":
m += `
`;
break;
case "r":
m += "\r";
break;
case "t":
m += " ";
break;
case "v":
m += "\v";
break;
case "N":
m += "\x85";
break;
case "_":
m += "\xA0";
break;
case "L":
m += "\u2028";
break;
case "P":
m += "\u2029";
break;
case " ":
m += " ";
break;
case '"':
m += '"';
break;
case "/":
m += "/";
break;
case "\\":
m += "\\";
break;
case " ":
m += " ";
break;
case "x":
m += this.parseCharCode(g + 1, 2, f), g += 2;
break;
case "u":
m += this.parseCharCode(g + 1, 4, f), g += 4;
break;
case "U":
m += this.parseCharCode(g + 1, 8, f), g += 8;
break;
case `
`:
for (; a[g + 1] === " " || a[g + 1] === " "; )
g += 1;
break;
default:
f.push(new e.YAMLSyntaxError(this, `Invalid escape sequence ${a.substr(g - 1, 2)}`)), m += "\\" + a[g];
}
else if (u === " " || u === " ") {
let p = g, L = a[g + 1];
for (; L === " " || L === " "; )
g += 1, L = a[g + 1];
L !== `
` && (m += g > p ? a.slice(p, g + 1) : u);
} else
m += u;
}
return f.length > 0 ? { errors: f, str: m } : m;
}
parseCharCode(f, i, t) {
let { src: s } = this.context, a = s.substr(f, i), g = a.length === i && /^[0-9a-fA-F]+$/.test(a) ? parseInt(a, 16) : NaN;
return isNaN(g) ? (t.push(new e.YAMLSyntaxError(this, `Invalid escape sequence ${s.substr(f - 2, i + 2)}`)), s.substr(f - 2, i + 2)) : String.fromCodePoint(g);
}
parse(f, i) {
this.context = f;
let { src: t } = f, s = C.endOfQuote(t, i + 1);
return this.valueRange = new e.Range(i, s), s = e.Node.endOfWhiteSpace(t, s), s = this.parseComment(s), s;
}
}, q = class extends e.Node {
static endOfQuote(f, i) {
let t = f[i];
for (; t; )
if (t === "'") {
if (f[i + 1] !== "'")
break;
t = f[i += 2];
} else
t = f[i += 1];
return i + 1;
}
get strValue() {
if (!this.valueRange || !this.context)
return null;
let f = [], { start: i, end: t } = this.valueRange, { indent: s, src: a } = this.context;
a[t - 1] !== "'" && f.push(new e.YAMLSyntaxError(this, "Missing closing 'quote"));
let m = "";
for (let g = i + 1; g < t - 1; ++g) {
let u = a[g];
if (u === `
`) {
e.Node.atDocumentBoundary(a, g + 1) && f.push(new e.YAMLSemanticError(this, "Document boundary indicators are not allowed within string values"));
let { fold: p, offset: L, error: k } = e.Node.foldNewline(a, g, s);
m += p, g = L, k && f.push(new e.YAMLSemanticError(this, "Multi-line single-quoted string needs to be sufficiently indented"));
} else if (u === "'")
m += u, g += 1, a[g] !== "'" && f.push(new e.YAMLSyntaxError(this, "Unescaped single quote? This should not happen."));
else if (u === " " || u === " ") {
let p = g, L = a[g + 1];
for (; L === " " || L === " "; )
g += 1, L = a[g + 1];
L !== `
` && (m += g > p ? a.slice(p, g + 1) : u);
} else
m += u;
}
return f.length > 0 ? { errors: f, str: m } : m;
}
parse(f, i) {
this.context = f;
let { src: t } = f, s = q.endOfQuote(t, i + 1);
return this.valueRange = new e.Range(i, s), s = e.Node.endOfWhiteSpace(t, s), s = this.parseComment(s), s;
}
};
function R(f, i) {
switch (f) {
case e.Type.ALIAS:
return new S(f, i);
case e.Type.BLOCK_FOLDED:
case e.Type.BLOCK_LITERAL:
return new T(f, i);
case e.Type.FLOW_MAP:
case e.Type.FLOW_SEQ:
return new P(f, i);
case e.Type.MAP_KEY:
case e.Type.MAP_VALUE:
case e.Type.SEQ_ITEM:
return new c(f, i);
case e.Type.COMMENT:
case e.Type.PLAIN:
return new e.PlainValue(f, i);
case e.Type.QUOTE_DOUBLE:
return new C(f, i);
case e.Type.QUOTE_SINGLE:
return new q(f, i);
default:
return null;
}
}
var B = class {
static parseType(f, i, t) {
switch (f[i]) {
case "*":
return e.Type.ALIAS;
case ">":
return e.Type.BLOCK_FOLDED;
case "|":
return e.Type.BLOCK_LITERAL;
case "{":
return e.Type.FLOW_MAP;
case "[":
return e.Type.FLOW_SEQ;
case "?":
return !t && e.Node.atBlank(f, i + 1, true) ? e.Type.MAP_KEY : e.Type.PLAIN;
case ":":
return !t && e.Node.atBlank(f, i + 1, true) ? e.Type.MAP_VALUE : e.Type.PLAIN;
case "-":
return !t && e.Node.atBlank(f, i + 1, true) ? e.Type.SEQ_ITEM : e.Type.PLAIN;
case '"':
return e.Type.QUOTE_DOUBLE;
case "'":
return e.Type.QUOTE_SINGLE;
default:
return e.Type.PLAIN;
}
}
constructor() {
let f = arguments.length > 0 && arguments[0] !== void 0 ? arguments[0] : {}, { atLineStart: i, inCollection: t, inFlow: s, indent: a, lineStart: m, parent: g } = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : {};
e._defineProperty(this, "parseNode", (u, p) => {
if (e.Node.atDocumentBoundary(this.src, p))
return null;
let L = new B(this, u), { props: k, type: $, valueStart: K } = L.parseProps(p), V = R($, k), z = V.parse(L, K);
if (V.range = new e.Range(p, z), z <= p && (V.error = new Error("Node#parse consumed no characters"), V.error.parseEnd = z, V.error.source = V, V.range.end = p + 1), L.nodeStartsCollection(V)) {
!V.error && !L.atLineStart && L.parent.type === e.Type.DOCUMENT && (V.error = new e.YAMLSyntaxError(V, "Block collection must not have preceding content here (e.g. directives-end indicator)"));
let ae = new y(V);
return z = ae.parse(new B(L), z), ae.range = new e.Range(p, z), ae;
}
return V;
}), this.atLineStart = i != null ? i : f.atLineStart || false, this.inCollection = t != null ? t : f.inCollection || false, this.inFlow = s != null ? s : f.inFlow || false, this.indent = a != null ? a : f.indent, this.lineStart = m != null ? m : f.lineStart, this.parent = g != null ? g : f.parent || {}, this.root = f.root, this.src = f.src;
}
nodeStartsCollection(f) {
let { inCollection: i, inFlow: t, src: s } = this;
if (i || t)
return false;
if (f instanceof c)
return true;
let a = f.range.end;
return s[a] === `
` || s[a - 1] === `
` ? false : (a = e.Node.endOfWhiteSpace(s, a), s[a] === ":");
}
parseProps(f) {
let { inFlow: i, parent: t, src: s } = this, a = [], m = false;
f = this.atLineStart ? e.Node.endOfIndent(s, f) : e.Node.endOfWhiteSpace(s, f);
let g = s[f];
for (; g === e.Char.ANCHOR || g === e.Char.COMMENT || g === e.Char.TAG || g === `
`; ) {
if (g === `
`) {
let p = f, L;
do
L = p + 1, p = e.Node.endOfIndent(s, L);
while (s[p] === `
`);
let k = p - (L + this.indent), $ = t.type === e.Type.SEQ_ITEM && t.context.atLineStart;
if (s[p] !== "#" && !e.Node.nextNodeIsIndented(s[p], k, !$))
break;
this.atLineStart = true, this.lineStart = L, m = false, f = p;
} else if (g === e.Char.COMMENT) {
let p = e.Node.endOfLine(s, f + 1);
a.push(new e.Range(f, p)), f = p;
} else {
let p = e.Node.endOfIdentifier(s, f + 1);
g === e.Char.TAG && s[p] === "," && /^[a-zA-Z0-9-]+\.[a-zA-Z0-9-]+,\d\d\d\d(-\d\d){0,2}\/\S/.test(s.slice(f + 1, p + 13)) && (p = e.Node.endOfIdentifier(s, p + 5)), a.push(new e.Range(f, p)), m = true, f = e.Node.endOfWhiteSpace(s, p);
}
g = s[f];
}
m && g === ":" && e.Node.atBlank(s, f + 1, true) && (f -= 1);
let u = B.parseType(s, f, i);
return { props: a, type: u, valueStart: f };
}
};
function U(f) {
let i = [];
f.indexOf("\r") !== -1 && (f = f.replace(/\r\n?/g, (a, m) => (a.length > 1 && i.push(m), `
`)));
let t = [], s = 0;
do {
let a = new I(), m = new B({ src: f });
s = a.parse(m, s), t.push(a);
} while (s < f.length);
return t.setOrigRanges = () => {
if (i.length === 0)
return false;
for (let m = 1; m < i.length; ++m)
i[m] -= m;
let a = 0;
for (let m = 0; m < t.length; ++m)
a = t[m].setOrigRanges(i, a);
return i.splice(0, i.length), true;
}, t.toString = () => t.join(`...
`), t;
}
n2.parse = U;
} }), ke = D({ "node_modules/yaml/dist/resolveSeq-d03cb037.js"(n2) {
"use strict";
Y();
var e = Me();
function r(o, l, _) {
return _ ? `#${_.replace(/[\s\S]^/gm, `$&${l}#`)}
${l}${o}` : o;
}
function c(o, l, _) {
return _ ? _.indexOf(`
`) === -1 ? `${o} #${_}` : `${o}
` + _.replace(/^/gm, `${l || ""}#`) : o;
}
var h = class {
};
function d(o, l, _) {
if (Array.isArray(o))
return o.map((v, b) => d(v, String(b), _));
if (o && typeof o.toJSON == "function") {
let v = _ && _.anchors && _.anchors.get(o);
v && (_.onCreate = (w) => {
v.res = w, delete _.onCreate;
});
let b = o.toJSON(l, _);
return v && _.onCreate && _.onCreate(b), b;
}
return (!_ || !_.keep) && typeof o == "bigint" ? Number(o) : o;
}
var y = class extends h {
constructor(o) {
super(), this.value = o;
}
toJSON(o, l) {
return l && l.keep ? this.value : d(this.value, o, l);
}
toString() {
return String(this.value);
}
};
function E(o, l, _) {
let v = _;
for (let b = l.length - 1; b >= 0; --b) {
let w = l[b];
if (Number.isInteger(w) && w >= 0) {
let A = [];
A[w] = v, v = A;
} else {
let A = {};
Object.defineProperty(A, w, { value: v, writable: true, enumerable: true, configurable: true }), v = A;
}
}
return o.createNode(v, false);
}
var I = (o) => o == null || typeof o == "object" && o[Symbol.iterator]().next().done, S = class extends h {
constructor(o) {
super(), e._defineProperty(this, "items", []), this.schema = o;
}
addIn(o, l) {
if (I(o))
this.add(l);
else {
let [_, ...v] = o, b = this.get(_, true);
if (b instanceof S)
b.addIn(v, l);
else if (b === void 0 && this.schema)
this.set(_, E(this.schema, v, l));
else
throw new Error(`Expected YAML collection at ${_}. Remaining path: ${v}`);
}
}
deleteIn(o) {
let [l, ..._] = o;
if (_.length === 0)
return this.delete(l);
let v = this.get(l, true);
if (v instanceof S)
return v.deleteIn(_);
throw new Error(`Expected YAML collection at ${l}. Remaining path: ${_}`);
}
getIn(o, l) {
let [_, ...v] = o, b = this.get(_, true);
return v.length === 0 ? !l && b instanceof y ? b.value : b : b instanceof S ? b.getIn(v, l) : void 0;
}
hasAllNullValues() {
return this.items.every((o) => {
if (!o || o.type !== "PAIR")
return false;
let l = o.value;
return l == null || l instanceof y && l.value == null && !l.commentBefore && !l.comment && !l.tag;
});
}
hasIn(o) {
let [l, ..._] = o;
if (_.length === 0)
return this.has(l);
let v = this.get(l, true);
return v instanceof S ? v.hasIn(_) : false;
}
setIn(o, l) {
let [_, ...v] = o;
if (v.length === 0)
this.set(_, l);
else {
let b = this.get(_, true);
if (b instanceof S)
b.setIn(v, l);
else if (b === void 0 && this.schema)
this.set(_, E(this.schema, v, l));
else
throw new Error(`Expected YAML collection at ${_}. Remaining path: ${v}`);
}
}
toJSON() {
return null;
}
toString(o, l, _, v) {
let { blockItem: b, flowChars: w, isMap: A, itemIndent: N } = l, { indent: j, indentStep: F, stringify: Q } = o, H = this.type === e.Type.FLOW_MAP || this.type === e.Type.FLOW_SEQ || o.inFlow;
H && (N += F);
let oe = A && this.hasAllNullValues();
o = Object.assign({}, o, { allNullValues: oe, indent: N, inFlow: H, type: null });
let le = false, Z = false, ee = this.items.reduce((de, ne, he) => {
let ce;
ne && (!le && ne.spaceBefore && de.push({ type: "comment", str: "" }), ne.commentBefore && ne.commentBefore.match(/^.*$/gm).forEach((Ie) => {
de.push({ type: "comment", str: `#${Ie}` });
}), ne.comment && (ce = ne.comment), H && (!le && ne.spaceBefore || ne.commentBefore || ne.comment || ne.key && (ne.key.commentBefore || ne.key.comment) || ne.value && (ne.value.commentBefore || ne.value.comment)) && (Z = true)), le = false;
let fe = Q(ne, o, () => ce = null, () => le = true);
return H && !Z && fe.includes(`
`) && (Z = true), H && he < this.items.length - 1 && (fe += ","), fe = c(fe, N, ce), le && (ce || H) && (le = false), de.push({ type: "item", str: fe }), de;
}, []), X;
if (ee.length === 0)
X = w.start + w.end;
else if (H) {
let { start: de, end: ne } = w, he = ee.map((ce) => ce.str);
if (Z || he.reduce((ce, fe) => ce + fe.length + 2, 2) > S.maxFlowStringSingleLineLength) {
X = de;
for (let ce of he)
X += ce ? `
${F}${j}${ce}` : `
`;
X += `
${j}${ne}`;
} else
X = `${de} ${he.join(" ")} ${ne}`;
} else {
let de = ee.map(b);
X = de.shift();
for (let ne of de)
X += ne ? `
${j}${ne}` : `
`;
}
return this.comment ? (X += `
` + this.comment.replace(/^/gm, `${j}#`), _ && _()) : le && v && v(), X;
}
};
e._defineProperty(S, "maxFlowStringSingleLineLength", 60);
function M(o) {
let l = o instanceof y ? o.value : o;
return l && typeof l == "string" && (l = Number(l)), Number.isInteger(l) && l >= 0 ? l : null;
}
var T = class extends S {
add(o) {
this.items.push(o);
}
delete(o) {
let l = M(o);
return typeof l != "number" ? false : this.items.splice(l, 1).length > 0;
}
get(o, l) {
let _ = M(o);
if (typeof _ != "number")
return;
let v = this.items[_];
return !l && v instanceof y ? v.value : v;
}
has(o) {
let l = M(o);
return typeof l == "number" && l < this.items.length;
}
set(o, l) {
let _ = M(o);
if (typeof _ != "number")
throw new Error(`Expected a valid index, not ${o}.`);
this.items[_] = l;
}
toJSON(o, l) {
let _ = [];
l && l.onCreate && l.onCreate(_);
let v = 0;
for (let b of this.items)
_.push(d(b, String(v++), l));
return _;
}
toString(o, l, _) {
return o ? super.toString(o, { blockItem: (v) => v.type === "comment" ? v.str : `- ${v.str}`, flowChars: { start: "[", end: "]" }, isMap: false, itemIndent: (o.indent || "") + " " }, l, _) : JSON.stringify(this);
}
}, P = (o, l, _) => l === null ? "" : typeof l != "object" ? String(l) : o instanceof h && _ && _.doc ? o.toString({ anchors: /* @__PURE__ */ Object.create(null), doc: _.doc, indent: "", indentStep: _.indentStep, inFlow: true, inStringifyKey: true, stringify: _.stringify }) : JSON.stringify(l), C = class extends h {
constructor(o) {
let l = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : null;
super(), this.key = o, this.value = l, this.type = C.Type.PAIR;
}
get commentBefore() {
return this.key instanceof h ? this.key.commentBefore : void 0;
}
set commentBefore(o) {
if (this.key == null && (this.key = new y(null)), this.key instanceof h)
this.key.commentBefore = o;
else {
let l = "Pair.commentBefore is an alias for Pair.key.commentBefore. To set it, the key must be a Node.";
throw new Error(l);
}
}
addToJSMap(o, l) {
let _ = d(this.key, "", o);
if (l instanceof Map) {
let v = d(this.value, _, o);
l.set(_, v);
} else if (l instanceof Set)
l.add(_);
else {
let v = P(this.key, _, o), b = d(this.value, v, o);
v in l ? Object.defineProperty(l, v, { value: b, writable: true, enumerable: true, configurable: true }) : l[v] = b;
}
return l;
}
toJSON(o, l) {
let _ = l && l.mapAsMap ? /* @__PURE__ */ new Map() : {};
return this.addToJSMap(l, _);
}
toString(o, l, _) {
if (!o || !o.doc)
return JSON.stringify(this);
let { indent: v, indentSeq: b, simpleKeys: w } = o.doc.options, { key: A, value: N } = this, j = A instanceof h && A.comment;
if (w) {
if (j)
throw new Error("With simple keys, key nodes cannot have comments");
if (A instanceof S) {
let ce = "With simple keys, collection cannot be used as a key value";
throw new Error(ce);
}
}
let F = !w && (!A || j || (A instanceof h ? A instanceof S || A.type === e.Type.BLOCK_FOLDED || A.type === e.Type.BLOCK_LITERAL : typeof A == "object")), { doc: Q, indent: H, indentStep: oe, stringify: le } = o;
o = Object.assign({}, o, { implicitKey: !F, indent: H + oe });
let Z = false, ee = le(A, o, () => j = null, () => Z = true);
if (ee = c(ee, o.indent, j), !F && ee.length > 1024) {
if (w)
throw new Error("With simple keys, single line scalar must not span more than 1024 characters");
F = true;
}
if (o.allNullValues && !w)
return this.comment ? (ee = c(ee, o.indent, this.comment), l && l()) : Z && !j && _ && _(), o.inFlow && !F ? ee : `? ${ee}`;
ee = F ? `? ${ee}
${H}:` : `${ee}:`, this.comment && (ee = c(ee, o.indent, this.comment), l && l());
let X = "", de = null;
if (N instanceof h) {
if (N.spaceBefore && (X = `
`), N.commentBefore) {
let ce = N.commentBefore.replace(/^/gm, `${o.indent}#`);
X += `
${ce}`;
}
de = N.comment;
} else
N && typeof N == "object" && (N = Q.schema.createNode(N, true));
o.implicitKey = false, !F && !this.comment && N instanceof y && (o.indentAtStart = ee.length + 1), Z = false, !b && v >= 2 && !o.inFlow && !F && N instanceof T && N.type !== e.Type.FLOW_SEQ && !N.tag && !Q.anchors.getName(N) && (o.indent = o.indent.substr(2));
let ne = le(N, o, () => de = null, () => Z = true), he = " ";
return X || this.comment ? he = `${X}
${o.indent}` : !F && N instanceof S ? (!(ne[0] === "[" || ne[0] === "{") || ne.includes(`
`)) && (he = `
${o.indent}`) : ne[0] === `
` && (he = ""), Z && !de && _ && _(), c(ee + he + ne, o.indent, de);
}
};
e._defineProperty(C, "Type", { PAIR: "PAIR", MERGE_PAIR: "MERGE_PAIR" });
var q = (o, l) => {
if (o instanceof R) {
let _ = l.get(o.source);
return _.count * _.aliasCount;
} else if (o instanceof S) {
let _ = 0;
for (let v of o.items) {
let b = q(v, l);
b > _ && (_ = b);
}
return _;
} else if (o instanceof C) {
let _ = q(o.key, l), v = q(o.value, l);
return Math.max(_, v);
}
return 1;
}, R = class extends h {
static stringify(o, l) {
let { range: _, source: v } = o, { anchors: b, doc: w, implicitKey: A, inStringifyKey: N } = l, j = Object.keys(b).find((Q) => b[Q] === v);
if (!j && N && (j = w.anchors.getName(v) || w.anchors.newName()), j)
return `*${j}${A ? " " : ""}`;
let F = w.anchors.getName(v) ? "Alias node must be after source node" : "Source node not found for alias node";
throw new Error(`${F} [${_}]`);
}
constructor(o) {
super(), this.source = o, this.type = e.Type.ALIAS;
}
set tag(o) {
throw new Error("Alias nodes cannot have tags");
}
toJSON(o, l) {
if (!l)
return d(this.source, o, l);
let { anchors: _, maxAliasCount: v } = l, b = _.get(this.source);
if (!b || b.res === void 0) {
let w = "This should not happen: Alias anchor was not resolved?";
throw this.cstNode ? new e.YAMLReferenceError(this.cstNode, w) : new ReferenceError(w);
}
if (v >= 0 && (b.count += 1, b.aliasCount === 0 && (b.aliasCount = q(this.source, _)), b.count * b.aliasCount > v)) {
let w = "Excessive alias count indicates a resource exhaustion attack";
throw this.cstNode ? new e.YAMLReferenceError(this.cstNode, w) : new ReferenceError(w);
}
return b.res;
}
toString(o) {
return R.stringify(this, o);
}
};
e._defineProperty(R, "default", true);
function B(o, l) {
let _ = l instanceof y ? l.value : l;
for (let v of o)
if (v instanceof C && (v.key === l || v.key === _ || v.key && v.key.value === _))
return v;
}
var U = class extends S {
add(o, l) {
o ? o instanceof C || (o = new C(o.key || o, o.value)) : o = new C(o);
let _ = B(this.items, o.key), v = this.schema && this.schema.sortMapEntries;
if (_)
if (l)
_.value = o.value;
else
throw new Error(`Key ${o.key} already set`);
else if (v) {
let b = this.items.findIndex((w) => v(o, w) < 0);
b === -1 ? this.items.push(o) : this.items.splice(b, 0, o);
} else
this.items.push(o);
}
delete(o) {
let l = B(this.items, o);
return l ? this.items.splice(this.items.indexOf(l), 1).length > 0 : false;
}
get(o, l) {
let _ = B(this.items, o), v = _ && _.value;
return !l && v instanceof y ? v.value : v;
}
has(o) {
return !!B(this.items, o);
}
set(o, l) {
this.add(new C(o, l), true);
}
toJSON(o, l, _) {
let v = _ ? new _() : l && l.mapAsMap ? /* @__PURE__ */ new Map() : {};
l && l.onCreate && l.onCreate(v);
for (let b of this.items)
b.addToJSMap(l, v);
return v;
}
toString(o, l, _) {
if (!o)
return JSON.stringify(this);
for (let v of this.items)
if (!(v instanceof C))
throw new Error(`Map items must all be pairs; found ${JSON.stringify(v)} instead`);
return super.toString(o, { blockItem: (v) => v.str, flowChars: { start: "{", end: "}" }, isMap: true, itemIndent: o.indent || "" }, l, _);
}
}, f = "<<", i = class extends C {
constructor(o) {
if (o instanceof C) {
let l = o.value;
l instanceof T || (l = new T(), l.items.push(o.value), l.range = o.value.range), super(o.key, l), this.range = o.range;
} else
super(new y(f), new T());
this.type = C.Type.MERGE_PAIR;
}
addToJSMap(o, l) {
for (let { source: _ } of this.value.items) {
if (!(_ instanceof U))
throw new Error("Merge sources must be maps");
let v = _.toJSON(null, o, Map);
for (let [b, w] of v)
l instanceof Map ? l.has(b) || l.set(b, w) : l instanceof Set ? l.add(b) : Object.prototype.hasOwnProperty.call(l, b) || Object.defineProperty(l, b, { value: w, writable: true, enumerable: true, configurable: true });
}
return l;
}
toString(o, l) {
let _ = this.value;
if (_.items.length > 1)
return super.toString(o, l);
this.value = _.items[0];
let v = super.toString(o, l);
return this.value = _, v;
}
}, t = { defaultType: e.Type.BLOCK_LITERAL, lineWidth: 76 }, s = { trueStr: "true", falseStr: "false" }, a = { asBigInt: false }, m = { nullStr: "null" }, g = { defaultType: e.Type.PLAIN, doubleQuoted: { jsonEncoding: false, minMultiLineLength: 40 }, fold: { lineWidth: 80, minContentWidth: 20 } };
function u(o, l, _) {
for (let { format: v, test: b, resolve: w } of l)
if (b) {
let A = o.match(b);
if (A) {
let N = w.apply(null, A);
return N instanceof y || (N = new y(N)), v && (N.format = v), N;
}
}
return _ && (o = _(o)), new y(o);
}
var p = "flow", L = "block", k = "quoted", $ = (o, l) => {
let _ = o[l + 1];
for (; _ === " " || _ === " "; ) {
do
_ = o[l += 1];
while (_ && _ !== `
`);
_ = o[l + 1];
}
return l;
};
function K(o, l, _, v) {
let { indentAtStart: b, lineWidth: w = 80, minContentWidth: A = 20, onFold: N, onOverflow: j } = v;
if (!w || w < 0)
return o;
let F = Math.max(1 + A, 1 + w - l.length);
if (o.length <= F)
return o;
let Q = [], H = {}, oe = w - l.length;
typeof b == "number" && (b > w - Math.max(2, A) ? Q.push(0) : oe = w - b);
let le, Z, ee = false, X = -1, de = -1, ne = -1;
_ === L && (X = $(o, X), X !== -1 && (oe = X + F));
for (let ce; ce = o[X += 1]; ) {
if (_ === k && ce === "\\") {
switch (de = X, o[X + 1]) {
case "x":
X += 3;
break;
case "u":
X += 5;
break;
case "U":
X += 9;
break;
default:
X += 1;
}
ne = X;
}
if (ce === `
`)
_ === L && (X = $(o, X)), oe = X + F, le = void 0;
else {
if (ce === " " && Z && Z !== " " && Z !== `
` && Z !== " ") {
let fe = o[X + 1];
fe && fe !== " " && fe !== `
` && fe !== " " && (le = X);
}
if (X >= oe)
if (le)
Q.push(le), oe = le + F, le = void 0;
else if (_ === k) {
for (; Z === " " || Z === " "; )
Z = ce, ce = o[X += 1], ee = true;
let fe = X > ne + 1 ? X - 2 : de - 1;
if (H[fe])
return o;
Q.push(fe), H[fe] = true, oe = fe + F, le = void 0;
} else
ee = true;
}
Z = ce;
}
if (ee && j && j(), Q.length === 0)
return o;
N && N();
let he = o.slice(0, Q[0]);
for (let ce = 0; ce < Q.length; ++ce) {
let fe = Q[ce], Ie = Q[ce + 1] || o.length;
fe === 0 ? he = `
${l}${o.slice(0, Ie)}` : (_ === k && H[fe] && (he += `${o[fe]}\\`), he += `
${l}${o.slice(fe + 1, Ie)}`);
}
return he;
}
var V = (o) => {
let { indentAtStart: l } = o;
return l ? Object.assign({ indentAtStart: l }, g.fold) : g.fold;
}, z = (o) => /^(%|---|\.\.\.)/m.test(o);
function ae(o, l, _) {
if (!l || l < 0)
return false;
let v = l - _, b = o.length;
if (b <= v)
return false;
for (let w = 0, A = 0; w < b; ++w)
if (o[w] === `
`) {
if (w - A > v)
return true;
if (A = w + 1, b - A <= v)
return false;
}
return true;
}
function ue(o, l) {
let { implicitKey: _ } = l, { jsonEncoding: v, minMultiLineLength: b } = g.doubleQuoted, w = JSON.stringify(o);
if (v)
return w;
let A = l.indent || (z(o) ? " " : ""), N = "", j = 0;
for (let F = 0, Q = w[F]; Q; Q = w[++F])
if (Q === " " && w[F + 1] === "\\" && w[F + 2] === "n" && (N += w.slice(j, F) + "\\ ", F += 1, j = F, Q = "\\"), Q === "\\")
switch (w[F + 1]) {
case "u":
{
N += w.slice(j, F);
let H = w.substr(F + 2, 4);
switch (H) {
case "0000":
N += "\\0";
break;
case "0007":
N += "\\a";
break;
case "000b":
N += "\\v";
break;
case "001b":
N += "\\e";
break;
case "0085":
N += "\\N";
break;
case "00a0":
N += "\\_";
break;
case "2028":
N += "\\L";
break;
case "2029":
N += "\\P";
break;
default:
H.substr(0, 2) === "00" ? N += "\\x" + H.substr(2) : N += w.substr(F, 6);
}
F += 5, j = F + 1;
}
break;
case "n":
if (_ || w[F + 2] === '"' || w.length < b)
F += 1;
else {
for (N += w.slice(j, F) + `
`; w[F + 2] === "\\" && w[F + 3] === "n" && w[F + 4] !== '"'; )
N += `
`, F += 2;
N += A, w[F + 2] === " " && (N += "\\"), F += 1, j = F + 1;
}
break;
default:
F += 1;
}
return N = j ? N + w.slice(j) : w, _ ? N : K(N, A, k, V(l));
}
function pe(o, l) {
if (l.implicitKey) {
if (/\n/.test(o))
return ue(o, l);
} else if (/[ \t]\n|\n[ \t]/.test(o))
return ue(o, l);
let _ = l.indent || (z(o) ? " " : ""), v = "'" + o.replace(/'/g, "''").replace(/\n+/g, `$&
${_}`) + "'";
return l.implicitKey ? v : K(v, _, p, V(l));
}
function ge(o, l, _, v) {
let { comment: b, type: w, value: A } = o;
if (/\n[\t ]+$/.test(A) || /^\s*$/.test(A))
return ue(A, l);
let N = l.indent || (l.forceBlockIndent || z(A) ? " " : ""), j = N ? "2" : "1", F = w === e.Type.BLOCK_FOLDED ? false : w === e.Type.BLOCK_LITERAL ? true : !ae(A, g.fold.lineWidth, N.length), Q = F ? "|" : ">";
if (!A)
return Q + `
`;
let H = "", oe = "";
if (A = A.replace(/[\n\t ]*$/, (Z) => {
let ee = Z.indexOf(`
`);
return ee === -1 ? Q += "-" : (A === Z || ee !== Z.length - 1) && (Q += "+", v && v()), oe = Z.replace(/\n$/, ""), "";
}).replace(/^[\n ]*/, (Z) => {
Z.indexOf(" ") !== -1 && (Q += j);
let ee = Z.match(/ +$/);
return ee ? (H = Z.slice(0, -ee[0].length), ee[0]) : (H = Z, "");
}), oe && (oe = oe.replace(/\n+(?!\n|$)/g, `$&${N}`)), H && (H = H.replace(/\n+/g, `$&${N}`)), b && (Q += " #" + b.replace(/ ?[\r\n]+/g, " "), _ && _()), !A)
return `${Q}${j}
${N}${oe}`;
if (F)
return A = A.replace(/\n+/g, `$&${N}`), `${Q}
${N}${H}${A}${oe}`;
A = A.replace(/\n+/g, `
$&`).replace(/(?:^|\n)([\t ].*)(?:([\n\t ]*)\n(?![\n\t ]))?/g, "$1$2").replace(/\n+/g, `$&${N}`);
let le = K(`${H}${A}${oe}`, N, L, g.fold);
return `${Q}
${N}${le}`;
}
function O(o, l, _, v) {
let { comment: b, type: w, value: A } = o, { actualString: N, implicitKey: j, indent: F, inFlow: Q } = l;
if (j && /[\n[\]{},]/.test(A) || Q && /[[\]{},]/.test(A))
return ue(A, l);
if (!A || /^[\n\t ,[\]{}#&*!|>'"%@`]|^[?-]$|^[?-][ \t]|[\n:][ \t]|[ \t]\n|[\n\t ]#|[\n\t :]$/.test(A))
return j || Q || A.indexOf(`
`) === -1 ? A.indexOf('"') !== -1 && A.indexOf("'") === -1 ? pe(A, l) : ue(A, l) : ge(o, l, _, v);
if (!j && !Q && w !== e.Type.PLAIN && A.indexOf(`
`) !== -1)
return ge(o, l, _, v);
if (F === "" && z(A))
return l.forceBlockIndent = true, ge(o, l, _, v);
let H = A.replace(/\n+/g, `$&
${F}`);
if (N) {
let { tags: le } = l.doc.schema;
if (typeof u(H, le, le.scalarFallback).value != "string")
return ue(A, l);
}
let oe = j ? H : K(H, F, p, V(l));
return b && !Q && (oe.indexOf(`
`) !== -1 || b.indexOf(`
`) !== -1) ? (_ && _(), r(oe, F, b)) : oe;
}
function W(o, l, _, v) {
let { defaultType: b } = g, { implicitKey: w, inFlow: A } = l, { type: N, value: j } = o;
typeof j != "string" && (j = String(j), o = Object.assign({}, o, { value: j }));
let F = (H) => {
switch (H) {
case e.Type.BLOCK_FOLDED:
case e.Type.BLOCK_LITERAL:
return ge(o, l, _, v);
case e.Type.QUOTE_DOUBLE:
return ue(j, l);
case e.Type.QUOTE_SINGLE:
return pe(j, l);
case e.Type.PLAIN:
return O(o, l, _, v);
default:
return null;
}
};
(N !== e.Type.QUOTE_DOUBLE && /[\x00-\x08\x0b-\x1f\x7f-\x9f]/.test(j) || (w || A) && (N === e.Type.BLOCK_FOLDED || N === e.Type.BLOCK_LITERAL)) && (N = e.Type.QUOTE_DOUBLE);
let Q = F(N);
if (Q === null && (Q = F(b), Q === null))
throw new Error(`Unsupported default string type ${b}`);
return Q;
}
function J(o) {
let { format: l, minFractionDigits: _, tag: v, value: b } = o;
if (typeof b == "bigint")
return String(b);
if (!isFinite(b))
return isNaN(b) ? ".nan" : b < 0 ? "-.inf" : ".inf";
let w = JSON.stringify(b);
if (!l && _ && (!v || v === "tag:yaml.org,2002:float") && /^\d/.test(w)) {
let A = w.indexOf(".");
A < 0 && (A = w.length, w += ".");
let N = _ - (w.length - A - 1);
for (; N-- > 0; )
w += "0";
}
return w;
}
function x(o, l) {
let _, v;
switch (l.type) {
case e.Type.FLOW_MAP:
_ = "}", v = "flow map";
break;
case e.Type.FLOW_SEQ:
_ = "]", v = "flow sequence";
break;
default:
o.push(new e.YAMLSemanticError(l, "Not a flow collection!?"));
return;
}
let b;
for (let w = l.items.length - 1; w >= 0; --w) {
let A = l.items[w];
if (!A || A.type !== e.Type.COMMENT) {
b = A;
break;
}
}
if (b && b.char !== _) {
let w = `Expected ${v} to end with ${_}`, A;
typeof b.offset == "number" ? (A = new e.YAMLSemanticError(l, w), A.offset = b.offset + 1) : (A = new e.YAMLSemanticError(b, w), b.range && b.range.end && (A.offset = b.range.end - b.range.start)), o.push(A);
}
}
function G(o, l) {
let _ = l.context.src[l.range.start - 1];
if (_ !== `
` && _ !== " " && _ !== " ") {
let v = "Comments must be separated from other tokens by white space characters";
o.push(new e.YAMLSemanticError(l, v));
}
}
function re(o, l) {
let _ = String(l), v = _.substr(0, 8) + "..." + _.substr(-8);
return new e.YAMLSemanticError(o, `The "${v}" key is too long`);
}
function _e(o, l) {
for (let { afterKey: _, before: v, comment: b } of l) {
let w = o.items[v];
w ? (_ && w.value && (w = w.value), b === void 0 ? (_ || !w.commentBefore) && (w.spaceBefore = true) : w.commentBefore ? w.commentBefore += `
` + b : w.commentBefore = b) : b !== void 0 && (o.comment ? o.comment += `
` + b : o.comment = b);
}
}
function ye(o, l) {
let _ = l.strValue;
return _ ? typeof _ == "string" ? _ : (_.errors.forEach((v) => {
v.source || (v.source = l), o.errors.push(v);
}), _.str) : "";
}
function be(o, l) {
let { handle: _, suffix: v } = l.tag, b = o.tagPrefixes.find((w) => w.handle === _);
if (!b) {
let w = o.getDefaults().tagPrefixes;
if (w && (b = w.find((A) => A.handle === _)), !b)
throw new e.YAMLSemanticError(l, `The ${_} tag handle is non-default and was not declared.`);
}
if (!v)
throw new e.YAMLSemanticError(l, `The ${_} tag has no suffix.`);
if (_ === "!" && (o.version || o.options.version) === "1.0") {
if (v[0] === "^")
return o.warnings.push(new e.YAMLWarning(l, "YAML 1.0 ^ tag expansion is not supported")), v;
if (/[:/]/.test(v)) {
let w = v.match(/^([a-z0-9-]+)\/(.*)/i);
return w ? `tag:${w[1]}.yaml.org,2002:${w[2]}` : `tag:${v}`;
}
}
return b.prefix + decodeURIComponent(v);
}
function ve(o, l) {
let { tag: _, type: v } = l, b = false;
if (_) {
let { handle: w, suffix: A, verbatim: N } = _;
if (N) {
if (N !== "!" && N !== "!!")
return N;
let j = `Verbatim tags aren't resolved, so ${N} is invalid.`;
o.errors.push(new e.YAMLSemanticError(l, j));
} else if (w === "!" && !A)
b = true;
else
try {
return be(o, l);
} catch (j) {
o.errors.push(j);
}
}
switch (v) {
case e.Type.BLOCK_FOLDED:
case e.Type.BLOCK_LITERAL:
case e.Type.QUOTE_DOUBLE:
case e.Type.QUOTE_SINGLE:
return e.defaultTags.STR;
case e.Type.FLOW_MAP:
case e.Type.MAP:
return e.defaultTags.MAP;
case e.Type.FLOW_SEQ:
case e.Type.SEQ:
return e.defaultTags.SEQ;
case e.Type.PLAIN:
return b ? e.defaultTags.STR : null;
default:
return null;
}
}
function Ne(o, l, _) {
let { tags: v } = o.schema, b = [];
for (let A of v)
if (A.tag === _)
if (A.test)
b.push(A);
else {
let N = A.resolve(o, l);
return N instanceof S ? N : new y(N);
}
let w = ye(o, l);
return typeof w == "string" && b.length > 0 ? u(w, b, v.scalarFallback) : null;
}
function Pe(o) {
let { type: l } = o;
switch (l) {
case e.Type.FLOW_MAP:
case e.Type.MAP:
return e.defaultTags.MAP;
case e.Type.FLOW_SEQ:
case e.Type.SEQ:
return e.defaultTags.SEQ;
default:
return e.defaultTags.STR;
}
}
function ot(o, l, _) {
try {
let v = Ne(o, l, _);
if (v)
return _ && l.tag && (v.tag = _), v;
} catch (v) {
return v.source || (v.source = l), o.errors.push(v), null;
}
try {
let v = Pe(l);
if (!v)
throw new Error(`The tag ${_} is unavailable`);
let b = `The tag ${_} is unavailable, falling back to ${v}`;
o.warnings.push(new e.YAMLWarning(l, b));
let w = Ne(o, l, v);
return w.tag = _, w;
} catch (v) {
let b = new e.YAMLReferenceError(l, v.message);
return b.stack = v.stack, o.errors.push(b), null;
}
}
var lt = (o) => {
if (!o)
return false;
let { type: l } = o;
return l === e.Type.MAP_KEY || l === e.Type.MAP_VALUE || l === e.Type.SEQ_ITEM;
};
function ct(o, l) {
let _ = { before: [], after: [] }, v = false, b = false, w = lt(l.context.parent) ? l.context.parent.props.concat(l.props) : l.props;
for (let { start: A, end: N } of w)
switch (l.context.src[A]) {
case e.Char.COMMENT: {
if (!l.commentHasRequiredWhitespace(A)) {
let H = "Comments must be separated from other tokens by white space characters";
o.push(new e.YAMLSemanticError(l, H));
}
let { header: j, valueRange: F } = l;
(F && (A > F.start || j && A > j.start) ? _.after : _.before).push(l.context.src.slice(A + 1, N));
break;
}
case e.Char.ANCHOR:
if (v) {
let j = "A node can have at most one anchor";
o.push(new e.YAMLSemanticError(l, j));
}
v = true;
break;
case e.Char.TAG:
if (b) {
let j = "A node can have at most one tag";
o.push(new e.YAMLSemanticError(l, j));
}
b = true;
break;
}
return { comments: _, hasAnchor: v, hasTag: b };
}
function ut(o, l) {
let { anchors: _, errors: v, schema: b } = o;
if (l.type === e.Type.ALIAS) {
let A = l.rawValue, N = _.getNode(A);
if (!N) {
let F = `Aliased anchor not found: ${A}`;
return v.push(new e.YAMLReferenceError(l, F)), null;
}
let j = new R(N);
return _._cstAliases.push(j), j;
}
let w = ve(o, l);
if (w)
return ot(o, l, w);
if (l.type !== e.Type.PLAIN) {
let A = `Failed to resolve ${l.type} node here`;
return v.push(new e.YAMLSyntaxError(l, A)), null;
}
try {
let A = ye(o, l);
return u(A, b.tags, b.tags.scalarFallback);
} catch (A) {
return A.source || (A.source = l), v.push(A), null;
}
}
function we(o, l) {
if (!l)
return null;
l.error && o.errors.push(l.error);
let { comments: _, hasAnchor: v, hasTag: b } = ct(o.errors, l);
if (v) {
let { anchors: A } = o, N = l.anchor, j = A.getNode(N);
j && (A.map[A.newName(N)] = j), A.map[N] = l;
}
if (l.type === e.Type.ALIAS && (v || b)) {
let A = "An alias node must not specify any properties";
o.errors.push(new e.YAMLSemanticError(l, A));
}
let w = ut(o, l);
if (w) {
w.range = [l.range.start, l.range.end], o.options.keepCstNodes && (w.cstNode = l), o.options.keepNodeTypes && (w.type = l.type);
let A = _.before.join(`
`);
A && (w.commentBefore = w.commentBefore ? `${w.commentBefore}
${A}` : A);
let N = _.after.join(`
`);
N && (w.comment = w.comment ? `${w.comment}
${N}` : N);
}
return l.resolved = w;
}
function ft(o, l) {
if (l.type !== e.Type.MAP && l.type !== e.Type.FLOW_MAP) {
let A = `A ${l.type} node cannot be resolved as a mapping`;
return o.errors.push(new e.YAMLSyntaxError(l, A)), null;
}
let { comments: _, items: v } = l.type === e.Type.FLOW_MAP ? gt(o, l) : ht(o, l), b = new U();
b.items = v, _e(b, _);
let w = false;
for (let A = 0; A < v.length; ++A) {
let { key: N } = v[A];
if (N instanceof S && (w = true), o.schema.merge && N && N.value === f) {
v[A] = new i(v[A]);
let j = v[A].value.items, F = null;
j.some((Q) => {
if (Q instanceof R) {
let { type: H } = Q.source;
return H === e.Type.MAP || H === e.Type.FLOW_MAP ? false : F = "Merge nodes aliases can only point to maps";
}
return F = "Merge nodes can only have Alias nodes as values";
}), F && o.errors.push(new e.YAMLSemanticError(l, F));
} else
for (let j = A + 1; j < v.length; ++j) {
let { key: F } = v[j];
if (N === F || N && F && Object.prototype.hasOwnProperty.call(N, "value") && N.value === F.value) {
let Q = `Map keys must be unique; "${N}" is repeated`;
o.errors.push(new e.YAMLSemanticError(l, Q));
break;
}
}
}
if (w && !o.options.mapAsMap) {
let A = "Keys with collection values will be stringified as YAML due to JS Object restrictions. Use mapAsMap: true to avoid this.";
o.warnings.push(new e.YAMLWarning(l, A));
}
return l.resolved = b, b;
}
var mt = (o) => {
let { context: { lineStart: l, node: _, src: v }, props: b } = o;
if (b.length === 0)
return false;
let { start: w } = b[0];
if (_ && w > _.valueRange.start || v[w] !== e.Char.COMMENT)
return false;
for (let A = l; A < w; ++A)
if (v[A] === `
`)
return false;
return true;
};
function dt(o, l) {
if (!mt(o))
return;
let _ = o.getPropValue(0, e.Char.COMMENT, true), v = false, b = l.value.commentBefore;
if (b && b.startsWith(_))
l.value.commentBefore = b.substr(_.length + 1), v = true;
else {
let w = l.value.comment;
!o.node && w && w.startsWith(_) && (l.value.comment = w.substr(_.length + 1), v = true);
}
v && (l.comment = _);
}
function ht(o, l) {
let _ = [], v = [], b, w = null;
for (let A = 0; A < l.items.length; ++A) {
let N = l.items[A];
switch (N.type) {
case e.Type.BLANK_LINE:
_.push({ afterKey: !!b, before: v.length });
break;
case e.Type.COMMENT:
_.push({ afterKey: !!b, before: v.length, comment: N.comment });
break;
case e.Type.MAP_KEY:
b !== void 0 && v.push(new C(b)), N.error && o.errors.push(N.error), b = we(o, N.node), w = null;
break;
case e.Type.MAP_VALUE:
{
if (b === void 0 && (b = null), N.error && o.errors.push(N.error), !N.context.atLineStart && N.node && N.node.type === e.Type.MAP && !N.node.context.atLineStart) {
let Q = "Nested mappings are not allowed in compact mappings";
o.errors.push(new e.YAMLSemanticError(N.node, Q));
}
let j = N.node;
if (!j && N.props.length > 0) {
j = new e.PlainValue(e.Type.PLAIN, []), j.context = { parent: N, src: N.context.src };
let Q = N.range.start + 1;
if (j.range = { start: Q, end: Q }, j.valueRange = { start: Q, end: Q }, typeof N.range.origStart == "number") {
let H = N.range.origStart + 1;
j.range.origStart = j.range.origEnd = H, j.valueRange.origStart = j.valueRange.origEnd = H;
}
}
let F = new C(b, we(o, j));
dt(N, F), v.push(F), b && typeof w == "number" && N.range.start > w + 1024 && o.errors.push(re(l, b)), b = void 0, w = null;
}
break;
default:
b !== void 0 && v.push(new C(b)), b = we(o, N), w = N.range.start, N.error && o.errors.push(N.error);
e:
for (let j = A + 1; ; ++j) {
let F = l.items[j];
switch (F && F.type) {
case e.Type.BLANK_LINE:
case e.Type.COMMENT:
continue e;
case e.Type.MAP_VALUE:
break e;
default: {
let Q = "Implicit map keys need to be followed by map values";
o.errors.push(new e.YAMLSemanticError(N, Q));
break e;
}
}
}
if (N.valueRangeContainsNewline) {
let j = "Implicit map keys need to be on a single line";
o.errors.push(new e.YAMLSemanticError(N, j));
}
}
}
return b !== void 0 && v.push(new C(b)), { comments: _, items: v };
}
function gt(o, l) {
let _ = [], v = [], b, w = false, A = "{";
for (let N = 0; N < l.items.length; ++N) {
let j = l.items[N];
if (typeof j.char == "string") {
let { char: F, offset: Q } = j;
if (F === "?" && b === void 0 && !w) {
w = true, A = ":";
continue;
}
if (F === ":") {
if (b === void 0 && (b = null), A === ":") {
A = ",";
continue;
}
} else if (w && (b === void 0 && F !== "," && (b = null), w = false), b !== void 0 && (v.push(new C(b)), b = void 0, F === ",")) {
A = ":";
continue;
}
if (F === "}") {
if (N === l.items.length - 1)
continue;
} else if (F === A) {
A = ":";
continue;
}
let H = `Flow map contains an unexpected ${F}`, oe = new e.YAMLSyntaxError(l, H);
oe.offset = Q, o.errors.push(oe);
} else
j.type === e.Type.BLANK_LINE ? _.push({ afterKey: !!b, before: v.length }) : j.type === e.Type.COMMENT ? (G(o.errors, j), _.push({ afterKey: !!b, before: v.length, comment: j.comment })) : b === void 0 ? (A === "," && o.errors.push(new e.YAMLSemanticError(j, "Separator , missing in flow map")), b = we(o, j)) : (A !== "," && o.errors.push(new e.YAMLSemanticError(j, "Indicator : missing in flow map entry")), v.push(new C(b, we(o, j))), b = void 0, w = false);
}
return x(o.errors, l), b !== void 0 && v.push(new C(b)), { comments: _, items: v };
}
function pt(o, l) {
if (l.type !== e.Type.SEQ && l.type !== e.Type.FLOW_SEQ) {
let w = `A ${l.type} node cannot be resolved as a sequence`;
return o.errors.push(new e.YAMLSyntaxError(l, w)), null;
}
let { comments: _, items: v } = l.type === e.Type.FLOW_SEQ ? vt(o, l) : _t(o, l), b = new T();
if (b.items = v, _e(b, _), !o.options.mapAsMap && v.some((w) => w instanceof C && w.key instanceof S)) {
let w = "Keys with collection values will be stringified as YAML due to JS Object restrictions. Use mapAsMap: true to avoid this.";
o.warnings.push(new e.YAMLWarning(l, w));
}
return l.resolved = b, b;
}
function _t(o, l) {
let _ = [], v = [];
for (let b = 0; b < l.items.length; ++b) {
let w = l.items[b];
switch (w.type) {
case e.Type.BLANK_LINE:
_.push({ before: v.length });
break;
case e.Type.COMMENT:
_.push({ comment: w.comment, before: v.length });
break;
case e.Type.SEQ_ITEM:
if (w.error && o.errors.push(w.error), v.push(we(o, w.node)), w.hasProps) {
let A = "Sequence items cannot have tags or anchors before the - indicator";
o.errors.push(new e.YAMLSemanticError(w, A));
}
break;
default:
w.error && o.errors.push(w.error), o.errors.push(new e.YAMLSyntaxError(w, `Unexpected ${w.type} node in sequence`));
}
}
return { comments: _, items: v };
}
function vt(o, l) {
let _ = [], v = [], b = false, w, A = null, N = "[", j = null;
for (let F = 0; F < l.items.length; ++F) {
let Q = l.items[F];
if (typeof Q.char == "string") {
let { char: H, offset: oe } = Q;
if (H !== ":" && (b || w !== void 0) && (b && w === void 0 && (w = N ? v.pop() : null), v.push(new C(w)), b = false, w = void 0, A = null), H === N)
N = null;
else if (!N && H === "?")
b = true;
else if (N !== "[" && H === ":" && w === void 0) {
if (N === ",") {
if (w = v.pop(), w instanceof C) {
let le = "Chaining flow sequence pairs is invalid", Z = new e.YAMLSemanticError(l, le);
Z.offset = oe, o.errors.push(Z);
}
if (!b && typeof A == "number") {
let le = Q.range ? Q.range.start : Q.offset;
le > A + 1024 && o.errors.push(re(l, w));
let { src: Z } = j.context;
for (let ee = A; ee < le; ++ee)
if (Z[ee] === `
`) {
let X = "Implicit keys of flow sequence pairs need to be on a single line";
o.errors.push(new e.YAMLSemanticError(j, X));
break;
}
}
} else
w = null;
A = null, b = false, N = null;
} else if (N === "[" || H !== "]" || F < l.items.length - 1) {
let le = `Flow sequence contains an unexpected ${H}`, Z = new e.YAMLSyntaxError(l, le);
Z.offset = oe, o.errors.push(Z);
}
} else if (Q.type === e.Type.BLANK_LINE)
_.push({ before: v.length });
else if (Q.type === e.Type.COMMENT)
G(o.errors, Q), _.push({ comment: Q.comment, before: v.length });
else {
if (N) {
let oe = `Expected a ${N} in flow sequence`;
o.errors.push(new e.YAMLSemanticError(Q, oe));
}
let H = we(o, Q);
w === void 0 ? (v.push(H), j = Q) : (v.push(new C(w, H)), w = void 0), A = Q.range.start, N = ",";
}
}
return x(o.errors, l), w !== void 0 && v.push(new C(w)), { comments: _, items: v };
}
n2.Alias = R, n2.Collection = S, n2.Merge = i, n2.Node = h, n2.Pair = C, n2.Scalar = y, n2.YAMLMap = U, n2.YAMLSeq = T, n2.addComment = c, n2.binaryOptions = t, n2.boolOptions = s, n2.findPair = B, n2.intOptions = a, n2.isEmptyPath = I, n2.nullOptions = m, n2.resolveMap = ft, n2.resolveNode = we, n2.resolveSeq = pt, n2.resolveString = ye, n2.strOptions = g, n2.stringifyNumber = J, n2.stringifyString = W, n2.toJSON = d;
} }), st = D({ "node_modules/yaml/dist/warnings-1000a372.js"(n2) {
"use strict";
Y();
var e = Me(), r = ke(), c = { identify: (u) => u instanceof Uint8Array, default: false, tag: "tag:yaml.org,2002:binary", resolve: (u, p) => {
let L = r.resolveString(u, p);
if (typeof Buffer == "function")
return Buffer.from(L, "base64");
if (typeof atob == "function") {
let k = atob(L.replace(/[\n\r]/g, "")), $ = new Uint8Array(k.length);
for (let K = 0; K < k.length; ++K)
$[K] = k.charCodeAt(K);
return $;
} else {
let k = "This environment does not support reading binary tags; either Buffer or atob is required";
return u.errors.push(new e.YAMLReferenceError(p, k)), null;
}
}, options: r.binaryOptions, stringify: (u, p, L, k) => {
let { comment: $, type: K, value: V } = u, z;
if (typeof Buffer == "function")
z = V instanceof Buffer ? V.toString("base64") : Buffer.from(V.buffer).toString("base64");
else if (typeof btoa == "function") {
let ae = "";
for (let ue = 0; ue < V.length; ++ue)
ae += String.fromCharCode(V[ue]);
z = btoa(ae);
} else
throw new Error("This environment does not support writing binary tags; either Buffer or btoa is required");
if (K || (K = r.binaryOptions.defaultType), K === e.Type.QUOTE_DOUBLE)
V = z;
else {
let { lineWidth: ae } = r.binaryOptions, ue = Math.ceil(z.length / ae), pe = new Array(ue);
for (let ge = 0, O = 0; ge < ue; ++ge, O += ae)
pe[ge] = z.substr(O, ae);
V = pe.join(K === e.Type.BLOCK_LITERAL ? `
` : " ");
}
return r.stringifyString({ comment: $, type: K, value: V }, p, L, k);
} };
function h(u, p) {
let L = r.resolveSeq(u, p);
for (let k = 0; k < L.items.length; ++k) {
let $ = L.items[k];
if (!($ instanceof r.Pair)) {
if ($ instanceof r.YAMLMap) {
if ($.items.length > 1) {
let V = "Each pair must have its own sequence indicator";
throw new e.YAMLSemanticError(p, V);
}
let K = $.items[0] || new r.Pair();
$.commentBefore && (K.commentBefore = K.commentBefore ? `${$.commentBefore}
${K.commentBefore}` : $.commentBefore), $.comment && (K.comment = K.comment ? `${$.comment}
${K.comment}` : $.comment), $ = K;
}
L.items[k] = $ instanceof r.Pair ? $ : new r.Pair($);
}
}
return L;
}
function d(u, p, L) {
let k = new r.YAMLSeq(u);
k.tag = "tag:yaml.org,2002:pairs";
for (let $ of p) {
let K, V;
if (Array.isArray($))
if ($.length === 2)
K = $[0], V = $[1];
else
throw new TypeError(`Expected [key, value] tuple: ${$}`);
else if ($ && $ instanceof Object) {
let ae = Object.keys($);
if (ae.length === 1)
K = ae[0], V = $[K];
else
throw new TypeError(`Expected { key: value } tuple: ${$}`);
} else
K = $;
let z = u.createPair(K, V, L);
k.items.push(z);
}
return k;
}
var y = { default: false, tag: "tag:yaml.org,2002:pairs", resolve: h, createNode: d }, E = class extends r.YAMLSeq {
constructor() {
super(), e._defineProperty(this, "add", r.YAMLMap.prototype.add.bind(this)), e._defineProperty(this, "delete", r.YAMLMap.prototype.delete.bind(this)), e._defineProperty(this, "get", r.YAMLMap.prototype.get.bind(this)), e._defineProperty(this, "has", r.YAMLMap.prototype.has.bind(this)), e._defineProperty(this, "set", r.YAMLMap.prototype.set.bind(this)), this.tag = E.tag;
}
toJSON(u, p) {
let L = /* @__PURE__ */ new Map();
p && p.onCreate && p.onCreate(L);
for (let k of this.items) {
let $, K;
if (k instanceof r.Pair ? ($ = r.toJSON(k.key, "", p), K = r.toJSON(k.value, $, p)) : $ = r.toJSON(k, "", p), L.has($))
throw new Error("Ordered maps must not include duplicate keys");
L.set($, K);
}
return L;
}
};
e._defineProperty(E, "tag", "tag:yaml.org,2002:omap");
function I(u, p) {
let L = h(u, p), k = [];
for (let { key: $ } of L.items)
if ($ instanceof r.Scalar)
if (k.includes($.value)) {
let K = "Ordered maps must not include duplicate keys";
throw new e.YAMLSemanticError(p, K);
} else
k.push($.value);
return Object.assign(new E(), L);
}
function S(u, p, L) {
let k = d(u, p, L), $ = new E();
return $.items = k.items, $;
}
var M = { identify: (u) => u instanceof Map, nodeClass: E, default: false, tag: "tag:yaml.org,2002:omap", resolve: I, createNode: S }, T = class extends r.YAMLMap {
constructor() {
super(), this.tag = T.tag;
}
add(u) {
let p = u instanceof r.Pair ? u : new r.Pair(u);
r.findPair(this.items, p.key) || this.items.push(p);
}
get(u, p) {
let L = r.findPair(this.items, u);
return !p && L instanceof r.Pair ? L.key instanceof r.Scalar ? L.key.value : L.key : L;
}
set(u, p) {
if (typeof p != "boolean")
throw new Error(`Expected boolean value for set(key, value) in a YAML set, not ${typeof p}`);
let L = r.findPair(this.items, u);
L && !p ? this.items.splice(this.items.indexOf(L), 1) : !L && p && this.items.push(new r.Pair(u));
}
toJSON(u, p) {
return super.toJSON(u, p, Set);
}
toString(u, p, L) {
if (!u)
return JSON.stringify(this);
if (this.hasAllNullValues())
return super.toString(u, p, L);
throw new Error("Set items must all have null values");
}
};
e._defineProperty(T, "tag", "tag:yaml.org,2002:set");
function P(u, p) {
let L = r.resolveMap(u, p);
if (!L.hasAllNullValues())
throw new e.YAMLSemanticError(p, "Set items must all have null values");
return Object.assign(new T(), L);
}
function C(u, p, L) {
let k = new T();
for (let $ of p)
k.items.push(u.createPair($, null, L));
return k;
}
var q = { identify: (u) => u instanceof Set, nodeClass: T, default: false, tag: "tag:yaml.org,2002:set", resolve: P, createNode: C }, R = (u, p) => {
let L = p.split(":").reduce((k, $) => k * 60 + Number($), 0);
return u === "-" ? -L : L;
}, B = (u) => {
let { value: p } = u;
if (isNaN(p) || !isFinite(p))
return r.stringifyNumber(p);
let L = "";
p < 0 && (L = "-", p = Math.abs(p));
let k = [p % 60];
return p < 60 ? k.unshift(0) : (p = Math.round((p - k[0]) / 60), k.unshift(p % 60), p >= 60 && (p = Math.round((p - k[0]) / 60), k.unshift(p))), L + k.map(($) => $ < 10 ? "0" + String($) : String($)).join(":").replace(/000000\d*$/, "");
}, U = { identify: (u) => typeof u == "number", default: true, tag: "tag:yaml.org,2002:int", format: "TIME", test: /^([-+]?)([0-9][0-9_]*(?::[0-5]?[0-9])+)$/, resolve: (u, p, L) => R(p, L.replace(/_/g, "")), stringify: B }, f = { identify: (u) => typeof u == "number", default: true, tag: "tag:yaml.org,2002:float", format: "TIME", test: /^([-+]?)([0-9][0-9_]*(?::[0-5]?[0-9])+\.[0-9_]*)$/, resolve: (u, p, L) => R(p, L.replace(/_/g, "")), stringify: B }, i = { identify: (u) => u instanceof Date, default: true, tag: "tag:yaml.org,2002:timestamp", test: RegExp("^(?:([0-9]{4})-([0-9]{1,2})-([0-9]{1,2})(?:(?:t|T|[ \\t]+)([0-9]{1,2}):([0-9]{1,2}):([0-9]{1,2}(\\.[0-9]+)?)(?:[ \\t]*(Z|[-+][012]?[0-9](?::[0-9]{2})?))?)?)$"), resolve: (u, p, L, k, $, K, V, z, ae) => {
z && (z = (z + "00").substr(1, 3));
let ue = Date.UTC(p, L - 1, k, $ || 0, K || 0, V || 0, z || 0);
if (ae && ae !== "Z") {
let pe = R(ae[0], ae.slice(1));
Math.abs(pe) < 30 && (pe *= 60), ue -= 6e4 * pe;
}
return new Date(ue);
}, stringify: (u) => {
let { value: p } = u;
return p.toISOString().replace(/((T00:00)?:00)?\.000Z$/, "");
} };
function t(u) {
let p = typeof Te < "u" && Te.env || {};
return u ? typeof YAML_SILENCE_DEPRECATION_WARNINGS < "u" ? !YAML_SILENCE_DEPRECATION_WARNINGS : !p.YAML_SILENCE_DEPRECATION_WARNINGS : typeof YAML_SILENCE_WARNINGS < "u" ? !YAML_SILENCE_WARNINGS : !p.YAML_SILENCE_WARNINGS;
}
function s(u, p) {
if (t(false)) {
let L = typeof Te < "u" && Te.emitWarning;
L ? L(u, p) : console.warn(p ? `${p}: ${u}` : u);
}
}
function a(u) {
if (t(true)) {
let p = u.replace(/.*yaml[/\\]/i, "").replace(/\.js$/, "").replace(/\\/g, "/");
s(`The endpoint 'yaml/${p}' will be removed in a future release.`, "DeprecationWarning");
}
}
var m = {};
function g(u, p) {
if (!m[u] && t(true)) {
m[u] = true;
let L = `The option '${u}' will be removed in a future release`;
L += p ? `, use '${p}' instead.` : ".", s(L, "DeprecationWarning");
}
}
n2.binary = c, n2.floatTime = f, n2.intTime = U, n2.omap = M, n2.pairs = y, n2.set = q, n2.timestamp = i, n2.warn = s, n2.warnFileDeprecation = a, n2.warnOptionDeprecation = g;
} }), it = D({ "node_modules/yaml/dist/Schema-88e323a7.js"(n2) {
"use strict";
Y();
var e = Me(), r = ke(), c = st();
function h(O, W, J) {
let x = new r.YAMLMap(O);
if (W instanceof Map)
for (let [G, re] of W)
x.items.push(O.createPair(G, re, J));
else if (W && typeof W == "object")
for (let G of Object.keys(W))
x.items.push(O.createPair(G, W[G], J));
return typeof O.sortMapEntries == "function" && x.items.sort(O.sortMapEntries), x;
}
var d = { createNode: h, default: true, nodeClass: r.YAMLMap, tag: "tag:yaml.org,2002:map", resolve: r.resolveMap };
function y(O, W, J) {
let x = new r.YAMLSeq(O);
if (W && W[Symbol.iterator])
for (let G of W) {
let re = O.createNode(G, J.wrapScalars, null, J);
x.items.push(re);
}
return x;
}
var E = { createNode: y, default: true, nodeClass: r.YAMLSeq, tag: "tag:yaml.org,2002:seq", resolve: r.resolveSeq }, I = { identify: (O) => typeof O == "string", default: true, tag: "tag:yaml.org,2002:str", resolve: r.resolveString, stringify(O, W, J, x) {
return W = Object.assign({ actualString: true }, W), r.stringifyString(O, W, J, x);
}, options: r.strOptions }, S = [d, E, I], M = (O) => typeof O == "bigint" || Number.isInteger(O), T = (O, W, J) => r.intOptions.asBigInt ? BigInt(O) : parseInt(W, J);
function P(O, W, J) {
let { value: x } = O;
return M(x) && x >= 0 ? J + x.toString(W) : r.stringifyNumber(O);
}
var C = { identify: (O) => O == null, createNode: (O, W, J) => J.wrapScalars ? new r.Scalar(null) : null, default: true, tag: "tag:yaml.org,2002:null", test: /^(?:~|[Nn]ull|NULL)?$/, resolve: () => null, options: r.nullOptions, stringify: () => r.nullOptions.nullStr }, q = { identify: (O) => typeof O == "boolean", default: true, tag: "tag:yaml.org,2002:bool", test: /^(?:[Tt]rue|TRUE|[Ff]alse|FALSE)$/, resolve: (O) => O[0] === "t" || O[0] === "T", options: r.boolOptions, stringify: (O) => {
let { value: W } = O;
return W ? r.boolOptions.trueStr : r.boolOptions.falseStr;
} }, R = { identify: (O) => M(O) && O >= 0, default: true, tag: "tag:yaml.org,2002:int", format: "OCT", test: /^0o([0-7]+)$/, resolve: (O, W) => T(O, W, 8), options: r.intOptions, stringify: (O) => P(O, 8, "0o") }, B = { identify: M, default: true, tag: "tag:yaml.org,2002:int", test: /^[-+]?[0-9]+$/, resolve: (O) => T(O, O, 10), options: r.intOptions, stringify: r.stringifyNumber }, U = { identify: (O) => M(O) && O >= 0, default: true, tag: "tag:yaml.org,2002:int", format: "HEX", test: /^0x([0-9a-fA-F]+)$/, resolve: (O, W) => T(O, W, 16), options: r.intOptions, stringify: (O) => P(O, 16, "0x") }, f = { identify: (O) => typeof O == "number", default: true, tag: "tag:yaml.org,2002:float", test: /^(?:[-+]?\.inf|(\.nan))$/i, resolve: (O, W) => W ? NaN : O[0] === "-" ? Number.NEGATIVE_INFINITY : Number.POSITIVE_INFINITY, stringify: r.stringifyNumber }, i = { identify: (O) => typeof O == "number", default: true, tag: "tag:yaml.org,2002:float", format: "EXP", test: /^[-+]?(?:\.[0-9]+|[0-9]+(?:\.[0-9]*)?)[eE][-+]?[0-9]+$/, resolve: (O) => parseFloat(O), stringify: (O) => {
let { value: W } = O;
return Number(W).toExponential();
} }, t = { identify: (O) => typeof O == "number", default: true, tag: "tag:yaml.org,2002:float", test: /^[-+]?(?:\.([0-9]+)|[0-9]+\.([0-9]*))$/, resolve(O, W, J) {
let x = W || J, G = new r.Scalar(parseFloat(O));
return x && x[x.length - 1] === "0" && (G.minFractionDigits = x.length), G;
}, stringify: r.stringifyNumber }, s = S.concat([C, q, R, B, U, f, i, t]), a = (O) => typeof O == "bigint" || Number.isInteger(O), m = (O) => {
let { value: W } = O;
return JSON.stringify(W);
}, g = [d, E, { identify: (O) => typeof O == "string", default: true, tag: "tag:yaml.org,2002:str", resolve: r.resolveString, stringify: m }, { identify: (O) => O == null, createNode: (O, W, J) => J.wrapScalars ? new r.Scalar(null) : null, default: true, tag: "tag:yaml.org,2002:null", test: /^null$/, resolve: () => null, stringify: m }, { identify: (O) => typeof O == "boolean", default: true, tag: "tag:yaml.org,2002:bool", test: /^true|false$/, resolve: (O) => O === "true", stringify: m }, { identify: a, default: true, tag: "tag:yaml.org,2002:int", test: /^-?(?:0|[1-9][0-9]*)$/, resolve: (O) => r.intOptions.asBigInt ? BigInt(O) : parseInt(O, 10), stringify: (O) => {
let { value: W } = O;
return a(W) ? W.toString() : JSON.stringify(W);
} }, { identify: (O) => typeof O == "number", default: true, tag: "tag:yaml.org,2002:float", test: /^-?(?:0|[1-9][0-9]*)(?:\.[0-9]*)?(?:[eE][-+]?[0-9]+)?$/, resolve: (O) => parseFloat(O), stringify: m }];
g.scalarFallback = (O) => {
throw new SyntaxError(`Unresolved plain scalar ${JSON.stringify(O)}`);
};
var u = (O) => {
let { value: W } = O;
return W ? r.boolOptions.trueStr : r.boolOptions.falseStr;
}, p = (O) => typeof O == "bigint" || Number.isInteger(O);
function L(O, W, J) {
let x = W.replace(/_/g, "");
if (r.intOptions.asBigInt) {
switch (J) {
case 2:
x = `0b${x}`;
break;
case 8:
x = `0o${x}`;
break;
case 16:
x = `0x${x}`;
break;
}
let re = BigInt(x);
return O === "-" ? BigInt(-1) * re : re;
}
let G = parseInt(x, J);
return O === "-" ? -1 * G : G;
}
function k(O, W, J) {
let { value: x } = O;
if (p(x)) {
let G = x.toString(W);
return x < 0 ? "-" + J + G.substr(1) : J + G;
}
return r.stringifyNumber(O);
}
var $ = S.concat([{ identify: (O) => O == null, createNode: (O, W, J) => J.wrapScalars ? new r.Scalar(null) : null, default: true, tag: "tag:yaml.org,2002:null", test: /^(?:~|[Nn]ull|NULL)?$/, resolve: () => null, options: r.nullOptions, stringify: () => r.nullOptions.nullStr }, { identify: (O) => typeof O == "boolean", default: true, tag: "tag:yaml.org,2002:bool", test: /^(?:Y|y|[Yy]es|YES|[Tt]rue|TRUE|[Oo]n|ON)$/, resolve: () => true, options: r.boolOptions, stringify: u }, { identify: (O) => typeof O == "boolean", default: true, tag: "tag:yaml.org,2002:bool", test: /^(?:N|n|[Nn]o|NO|[Ff]alse|FALSE|[Oo]ff|OFF)$/i, resolve: () => false, options: r.boolOptions, stringify: u }, { identify: p, default: true, tag: "tag:yaml.org,2002:int", format: "BIN", test: /^([-+]?)0b([0-1_]+)$/, resolve: (O, W, J) => L(W, J, 2), stringify: (O) => k(O, 2, "0b") }, { identify: p, default: true, tag: "tag:yaml.org,2002:int", format: "OCT", test: /^([-+]?)0([0-7_]+)$/, resolve: (O, W, J) => L(W, J, 8), stringify: (O) => k(O, 8, "0") }, { identify: p, default: true, tag: "tag:yaml.org,2002:int", test: /^([-+]?)([0-9][0-9_]*)$/, resolve: (O, W, J) => L(W, J, 10), stringify: r.stringifyNumber }, { identify: p, default: true, tag: "tag:yaml.org,2002:int", format: "HEX", test: /^([-+]?)0x([0-9a-fA-F_]+)$/, resolve: (O, W, J) => L(W, J, 16), stringify: (O) => k(O, 16, "0x") }, { identify: (O) => typeof O == "number", default: true, tag: "tag:yaml.org,2002:float", test: /^(?:[-+]?\.inf|(\.nan))$/i, resolve: (O, W) => W ? NaN : O[0] === "-" ? Number.NEGATIVE_INFINITY : Number.POSITIVE_INFINITY, stringify: r.stringifyNumber }, { identify: (O) => typeof O == "number", default: true, tag: "tag:yaml.org,2002:float", format: "EXP", test: /^[-+]?([0-9][0-9_]*)?(\.[0-9_]*)?[eE][-+]?[0-9]+$/, resolve: (O) => parseFloat(O.replace(/_/g, "")), stringify: (O) => {
let { value: W } = O;
return Number(W).toExponential();
} }, { identify: (O) => typeof O == "number", default: true, tag: "tag:yaml.org,2002:float", test: /^[-+]?(?:[0-9][0-9_]*)?\.([0-9_]*)$/, resolve(O, W) {
let J = new r.Scalar(parseFloat(O.replace(/_/g, "")));
if (W) {
let x = W.replace(/_/g, "");
x[x.length - 1] === "0" && (J.minFractionDigits = x.length);
}
return J;
}, stringify: r.stringifyNumber }], c.binary, c.omap, c.pairs, c.set, c.intTime, c.floatTime, c.timestamp), K = { core: s, failsafe: S, json: g, yaml11: $ }, V = { binary: c.binary, bool: q, float: t, floatExp: i, floatNaN: f, floatTime: c.floatTime, int: B, intHex: U, intOct: R, intTime: c.intTime, map: d, null: C, omap: c.omap, pairs: c.pairs, seq: E, set: c.set, timestamp: c.timestamp };
function z(O, W, J) {
if (W) {
let x = J.filter((re) => re.tag === W), G = x.find((re) => !re.format) || x[0];
if (!G)
throw new Error(`Tag ${W} not found`);
return G;
}
return J.find((x) => (x.identify && x.identify(O) || x.class && O instanceof x.class) && !x.format);
}
function ae(O, W, J) {
if (O instanceof r.Node)
return O;
let { defaultPrefix: x, onTagObj: G, prevObjects: re, schema: _e, wrapScalars: ye } = J;
W && W.startsWith("!!") && (W = x + W.slice(2));
let be = z(O, W, _e.tags);
if (!be) {
if (typeof O.toJSON == "function" && (O = O.toJSON()), !O || typeof O != "object")
return ye ? new r.Scalar(O) : O;
be = O instanceof Map ? d : O[Symbol.iterator] ? E : d;
}
G && (G(be), delete J.onTagObj);
let ve = { value: void 0, node: void 0 };
if (O && typeof O == "object" && re) {
let Ne = re.get(O);
if (Ne) {
let Pe = new r.Alias(Ne);
return J.aliasNodes.push(Pe), Pe;
}
ve.value = O, re.set(O, ve);
}
return ve.node = be.createNode ? be.createNode(J.schema, O, J) : ye ? new r.Scalar(O) : O, W && ve.node instanceof r.Node && (ve.node.tag = W), ve.node;
}
function ue(O, W, J, x) {
let G = O[x.replace(/\W/g, "")];
if (!G) {
let re = Object.keys(O).map((_e) => JSON.stringify(_e)).join(", ");
throw new Error(`Unknown schema "${x}"; use one of ${re}`);
}
if (Array.isArray(J))
for (let re of J)
G = G.concat(re);
else
typeof J == "function" && (G = J(G.slice()));
for (let re = 0; re < G.length; ++re) {
let _e = G[re];
if (typeof _e == "string") {
let ye = W[_e];
if (!ye) {
let be = Object.keys(W).map((ve) => JSON.stringify(ve)).join(", ");
throw new Error(`Unknown custom tag "${_e}"; use one of ${be}`);
}
G[re] = ye;
}
}
return G;
}
var pe = (O, W) => O.key < W.key ? -1 : O.key > W.key ? 1 : 0, ge = class {
constructor(O) {
let { customTags: W, merge: J, schema: x, sortMapEntries: G, tags: re } = O;
this.merge = !!J, this.name = x, this.sortMapEntries = G === true ? pe : G || null, !W && re && c.warnOptionDeprecation("tags", "customTags"), this.tags = ue(K, V, W || re, x);
}
createNode(O, W, J, x) {
let G = { defaultPrefix: ge.defaultPrefix, schema: this, wrapScalars: W }, re = x ? Object.assign(x, G) : G;
return ae(O, J, re);
}
createPair(O, W, J) {
J || (J = { wrapScalars: true });
let x = this.createNode(O, J.wrapScalars, null, J), G = this.createNode(W, J.wrapScalars, null, J);
return new r.Pair(x, G);
}
};
e._defineProperty(ge, "defaultPrefix", e.defaultTagPrefix), e._defineProperty(ge, "defaultTags", e.defaultTags), n2.Schema = ge;
} }), xr = D({ "node_modules/yaml/dist/Document-9b4560a1.js"(n2) {
"use strict";
Y();
var e = Me(), r = ke(), c = it(), h = { anchorPrefix: "a", customTags: null, indent: 2, indentSeq: true, keepCstNodes: false, keepNodeTypes: true, keepBlobsInJSON: true, mapAsMap: false, maxAliasCount: 100, prettyErrors: false, simpleKeys: false, version: "1.2" }, d = { get binary() {
return r.binaryOptions;
}, set binary(t) {
Object.assign(r.binaryOptions, t);
}, get bool() {
return r.boolOptions;
}, set bool(t) {
Object.assign(r.boolOptions, t);
}, get int() {
return r.intOptions;
}, set int(t) {
Object.assign(r.intOptions, t);
}, get null() {
return r.nullOptions;
}, set null(t) {
Object.assign(r.nullOptions, t);
}, get str() {
return r.strOptions;
}, set str(t) {
Object.assign(r.strOptions, t);
} }, y = { "1.0": { schema: "yaml-1.1", merge: true, tagPrefixes: [{ handle: "!", prefix: e.defaultTagPrefix }, { handle: "!!", prefix: "tag:private.yaml.org,2002:" }] }, 1.1: { schema: "yaml-1.1", merge: true, tagPrefixes: [{ handle: "!", prefix: "!" }, { handle: "!!", prefix: e.defaultTagPrefix }] }, 1.2: { schema: "core", merge: false, tagPrefixes: [{ handle: "!", prefix: "!" }, { handle: "!!", prefix: e.defaultTagPrefix }] } };
function E(t, s) {
if ((t.version || t.options.version) === "1.0") {
let g = s.match(/^tag:private\.yaml\.org,2002:([^:/]+)$/);
if (g)
return "!" + g[1];
let u = s.match(/^tag:([a-zA-Z0-9-]+)\.yaml\.org,2002:(.*)/);
return u ? `!${u[1]}/${u[2]}` : `!${s.replace(/^tag:/, "")}`;
}
let a = t.tagPrefixes.find((g) => s.indexOf(g.prefix) === 0);
if (!a) {
let g = t.getDefaults().tagPrefixes;
a = g && g.find((u) => s.indexOf(u.prefix) === 0);
}
if (!a)
return s[0] === "!" ? s : `!<${s}>`;
let m = s.substr(a.prefix.length).replace(/[!,[\]{}]/g, (g) => ({ "!": "%21", ",": "%2C", "[": "%5B", "]": "%5D", "{": "%7B", "}": "%7D" })[g]);
return a.handle + m;
}
function I(t, s) {
if (s instanceof r.Alias)
return r.Alias;
if (s.tag) {
let g = t.filter((u) => u.tag === s.tag);
if (g.length > 0)
return g.find((u) => u.format === s.format) || g[0];
}
let a, m;
if (s instanceof r.Scalar) {
m = s.value;
let g = t.filter((u) => u.identify && u.identify(m) || u.class && m instanceof u.class);
a = g.find((u) => u.format === s.format) || g.find((u) => !u.format);
} else
m = s, a = t.find((g) => g.nodeClass && m instanceof g.nodeClass);
if (!a) {
let g = m && m.constructor ? m.constructor.name : typeof m;
throw new Error(`Tag not resolved for ${g} value`);
}
return a;
}
function S(t, s, a) {
let { anchors: m, doc: g } = a, u = [], p = g.anchors.getName(t);
return p && (m[p] = t, u.push(`&${p}`)), t.tag ? u.push(E(g, t.tag)) : s.default || u.push(E(g, s.tag)), u.join(" ");
}
function M(t, s, a, m) {
let { anchors: g, schema: u } = s.doc, p;
if (!(t instanceof r.Node)) {
let $ = { aliasNodes: [], onTagObj: (K) => p = K, prevObjects: /* @__PURE__ */ new Map() };
t = u.createNode(t, true, null, $);
for (let K of $.aliasNodes) {
K.source = K.source.node;
let V = g.getName(K.source);
V || (V = g.newName(), g.map[V] = K.source);
}
}
if (t instanceof r.Pair)
return t.toString(s, a, m);
p || (p = I(u.tags, t));
let L = S(t, p, s);
L.length > 0 && (s.indentAtStart = (s.indentAtStart || 0) + L.length + 1);
let k = typeof p.stringify == "function" ? p.stringify(t, s, a, m) : t instanceof r.Scalar ? r.stringifyString(t, s, a, m) : t.toString(s, a, m);
return L ? t instanceof r.Scalar || k[0] === "{" || k[0] === "[" ? `${L} ${k}` : `${L}
${s.indent}${k}` : k;
}
var T = class {
static validAnchorNode(t) {
return t instanceof r.Scalar || t instanceof r.YAMLSeq || t instanceof r.YAMLMap;
}
constructor(t) {
e._defineProperty(this, "map", /* @__PURE__ */ Object.create(null)), this.prefix = t;
}
createAlias(t, s) {
return this.setAnchor(t, s), new r.Alias(t);
}
createMergePair() {
let t = new r.Merge();
for (var s = arguments.length, a = new Array(s), m = 0; m < s; m++)
a[m] = arguments[m];
return t.value.items = a.map((g) => {
if (g instanceof r.Alias) {
if (g.source instanceof r.YAMLMap)
return g;
} else if (g instanceof r.YAMLMap)
return this.createAlias(g);
throw new Error("Merge sources must be Map nodes or their Aliases");
}), t;
}
getName(t) {
let { map: s } = this;
return Object.keys(s).find((a) => s[a] === t);
}
getNames() {
return Object.keys(this.map);
}
getNode(t) {
return this.map[t];
}
newName(t) {
t || (t = this.prefix);
let s = Object.keys(this.map);
for (let a = 1; ; ++a) {
let m = `${t}${a}`;
if (!s.includes(m))
return m;
}
}
resolveNodes() {
let { map: t, _cstAliases: s } = this;
Object.keys(t).forEach((a) => {
t[a] = t[a].resolved;
}), s.forEach((a) => {
a.source = a.source.resolved;
}), delete this._cstAliases;
}
setAnchor(t, s) {
if (t != null && !T.validAnchorNode(t))
throw new Error("Anchors may only be set for Scalar, Seq and Map nodes");
if (s && /[\x00-\x19\s,[\]{}]/.test(s))
throw new Error("Anchor names must not contain whitespace or control characters");
let { map: a } = this, m = t && Object.keys(a).find((g) => a[g] === t);
if (m)
if (s)
m !== s && (delete a[m], a[s] = t);
else
return m;
else {
if (!s) {
if (!t)
return null;
s = this.newName();
}
a[s] = t;
}
return s;
}
}, P = (t, s) => {
if (t && typeof t == "object") {
let { tag: a } = t;
t instanceof r.Collection ? (a && (s[a] = true), t.items.forEach((m) => P(m, s))) : t instanceof r.Pair ? (P(t.key, s), P(t.value, s)) : t instanceof r.Scalar && a && (s[a] = true);
}
return s;
}, C = (t) => Object.keys(P(t, {}));
function q(t, s) {
let a = { before: [], after: [] }, m, g = false;
for (let u of s)
if (u.valueRange) {
if (m !== void 0) {
let L = "Document contains trailing content not separated by a ... or --- line";
t.errors.push(new e.YAMLSyntaxError(u, L));
break;
}
let p = r.resolveNode(t, u);
g && (p.spaceBefore = true, g = false), m = p;
} else
u.comment !== null ? (m === void 0 ? a.before : a.after).push(u.comment) : u.type === e.Type.BLANK_LINE && (g = true, m === void 0 && a.before.length > 0 && !t.commentBefore && (t.commentBefore = a.before.join(`
`), a.before = []));
if (t.contents = m || null, !m)
t.comment = a.before.concat(a.after).join(`
`) || null;
else {
let u = a.before.join(`
`);
if (u) {
let p = m instanceof r.Collection && m.items[0] ? m.items[0] : m;
p.commentBefore = p.commentBefore ? `${u}
${p.commentBefore}` : u;
}
t.comment = a.after.join(`
`) || null;
}
}
function R(t, s) {
let { tagPrefixes: a } = t, [m, g] = s.parameters;
if (!m || !g) {
let u = "Insufficient parameters given for %TAG directive";
throw new e.YAMLSemanticError(s, u);
}
if (a.some((u) => u.handle === m)) {
let u = "The %TAG directive must only be given at most once per handle in the same document.";
throw new e.YAMLSemanticError(s, u);
}
return { handle: m, prefix: g };
}
function B(t, s) {
let [a] = s.parameters;
if (s.name === "YAML:1.0" && (a = "1.0"), !a) {
let m = "Insufficient parameters given for %YAML directive";
throw new e.YAMLSemanticError(s, m);
}
if (!y[a]) {
let g = `Document will be parsed as YAML ${t.version || t.options.version} rather than YAML ${a}`;
t.warnings.push(new e.YAMLWarning(s, g));
}
return a;
}
function U(t, s, a) {
let m = [], g = false;
for (let u of s) {
let { comment: p, name: L } = u;
switch (L) {
case "TAG":
try {
t.tagPrefixes.push(R(t, u));
} catch (k) {
t.errors.push(k);
}
g = true;
break;
case "YAML":
case "YAML:1.0":
if (t.version) {
let k = "The %YAML directive must only be given at most once per document.";
t.errors.push(new e.YAMLSemanticError(u, k));
}
try {
t.version = B(t, u);
} catch (k) {
t.errors.push(k);
}
g = true;
break;
default:
if (L) {
let k = `YAML only supports %TAG and %YAML directives, and not %${L}`;
t.warnings.push(new e.YAMLWarning(u, k));
}
}
p && m.push(p);
}
if (a && !g && (t.version || a.version || t.options.version) === "1.1") {
let u = (p) => {
let { handle: L, prefix: k } = p;
return { handle: L, prefix: k };
};
t.tagPrefixes = a.tagPrefixes.map(u), t.version = a.version;
}
t.commentBefore = m.join(`
`) || null;
}
function f(t) {
if (t instanceof r.Collection)
return true;
throw new Error("Expected a YAML collection as document contents");
}
var i = class {
constructor(t) {
this.anchors = new T(t.anchorPrefix), this.commentBefore = null, this.comment = null, this.contents = null, this.directivesEndMarker = null, this.errors = [], this.options = t, this.schema = null, this.tagPrefixes = [], this.version = null, this.warnings = [];
}
add(t) {
return f(this.contents), this.contents.add(t);
}
addIn(t, s) {
f(this.contents), this.contents.addIn(t, s);
}
delete(t) {
return f(this.contents), this.contents.delete(t);
}
deleteIn(t) {
return r.isEmptyPath(t) ? this.contents == null ? false : (this.contents = null, true) : (f(this.contents), this.contents.deleteIn(t));
}
getDefaults() {
return i.defaults[this.version] || i.defaults[this.options.version] || {};
}
get(t, s) {
return this.contents instanceof r.Collection ? this.contents.get(t, s) : void 0;
}
getIn(t, s) {
return r.isEmptyPath(t) ? !s && this.contents instanceof r.Scalar ? this.contents.value : this.contents : this.contents instanceof r.Collection ? this.contents.getIn(t, s) : void 0;
}
has(t) {
return this.contents instanceof r.Collection ? this.contents.has(t) : false;
}
hasIn(t) {
return r.isEmptyPath(t) ? this.contents !== void 0 : this.contents instanceof r.Collection ? this.contents.hasIn(t) : false;
}
set(t, s) {
f(this.contents), this.contents.set(t, s);
}
setIn(t, s) {
r.isEmptyPath(t) ? this.contents = s : (f(this.contents), this.contents.setIn(t, s));
}
setSchema(t, s) {
if (!t && !s && this.schema)
return;
typeof t == "number" && (t = t.toFixed(1)), t === "1.0" || t === "1.1" || t === "1.2" ? (this.version ? this.version = t : this.options.version = t, delete this.options.schema) : t && typeof t == "string" && (this.options.schema = t), Array.isArray(s) && (this.options.customTags = s);
let a = Object.assign({}, this.getDefaults(), this.options);
this.schema = new c.Schema(a);
}
parse(t, s) {
this.options.keepCstNodes && (this.cstNode = t), this.options.keepNodeTypes && (this.type = "DOCUMENT");
let { directives: a = [], contents: m = [], directivesEndMarker: g, error: u, valueRange: p } = t;
if (u && (u.source || (u.source = this), this.errors.push(u)), U(this, a, s), g && (this.directivesEndMarker = true), this.range = p ? [p.start, p.end] : null, this.setSchema(), this.anchors._cstAliases = [], q(this, m), this.anchors.resolveNodes(), this.options.prettyErrors) {
for (let L of this.errors)
L instanceof e.YAMLError && L.makePretty();
for (let L of this.warnings)
L instanceof e.YAMLError && L.makePretty();
}
return this;
}
listNonDefaultTags() {
return C(this.contents).filter((t) => t.indexOf(c.Schema.defaultPrefix) !== 0);
}
setTagPrefix(t, s) {
if (t[0] !== "!" || t[t.length - 1] !== "!")
throw new Error("Handle must start and end with !");
if (s) {
let a = this.tagPrefixes.find((m) => m.handle === t);
a ? a.prefix = s : this.tagPrefixes.push({ handle: t, prefix: s });
} else
this.tagPrefixes = this.tagPrefixes.filter((a) => a.handle !== t);
}
toJSON(t, s) {
let { keepBlobsInJSON: a, mapAsMap: m, maxAliasCount: g } = this.options, u = a && (typeof t != "string" || !(this.contents instanceof r.Scalar)), p = { doc: this, indentStep: " ", keep: u, mapAsMap: u && !!m, maxAliasCount: g, stringify: M }, L = Object.keys(this.anchors.map);
L.length > 0 && (p.anchors = new Map(L.map(($) => [this.anchors.map[$], { alias: [], aliasCount: 0, count: 1 }])));
let k = r.toJSON(this.contents, t, p);
if (typeof s == "function" && p.anchors)
for (let { count: $, res: K } of p.anchors.values())
s(K, $);
return k;
}
toString() {
if (this.errors.length > 0)
throw new Error("Document with errors cannot be stringified");
let t = this.options.indent;
if (!Number.isInteger(t) || t <= 0) {
let L = JSON.stringify(t);
throw new Error(`"indent" option must be a positive integer, not ${L}`);
}
this.setSchema();
let s = [], a = false;
if (this.version) {
let L = "%YAML 1.2";
this.schema.name === "yaml-1.1" && (this.version === "1.0" ? L = "%YAML:1.0" : this.version === "1.1" && (L = "%YAML 1.1")), s.push(L), a = true;
}
let m = this.listNonDefaultTags();
this.tagPrefixes.forEach((L) => {
let { handle: k, prefix: $ } = L;
m.some((K) => K.indexOf($) === 0) && (s.push(`%TAG ${k} ${$}`), a = true);
}), (a || this.directivesEndMarker) && s.push("---"), this.commentBefore && ((a || !this.directivesEndMarker) && s.unshift(""), s.unshift(this.commentBefore.replace(/^/gm, "#")));
let g = { anchors: /* @__PURE__ */ Object.create(null), doc: this, indent: "", indentStep: " ".repeat(t), stringify: M }, u = false, p = null;
if (this.contents) {
this.contents instanceof r.Node && (this.contents.spaceBefore && (a || this.directivesEndMarker) && s.push(""), this.contents.commentBefore && s.push(this.contents.commentBefore.replace(/^/gm, "#")), g.forceBlockIndent = !!this.comment, p = this.contents.comment);
let L = p ? null : () => u = true, k = M(this.contents, g, () => p = null, L);
s.push(r.addComment(k, "", p));
} else
this.contents !== void 0 && s.push(M(this.contents, g));
return this.comment && ((!u || p) && s[s.length - 1] !== "" && s.push(""), s.push(this.comment.replace(/^/gm, "#"))), s.join(`
`) + `
`;
}
};
e._defineProperty(i, "defaults", y), n2.Document = i, n2.defaultOptions = h, n2.scalarOptions = d;
} }), Hr = D({ "node_modules/yaml/dist/index.js"(n2) {
"use strict";
Y();
var e = Jr(), r = xr(), c = it(), h = Me(), d = st();
ke();
function y(C) {
let q = arguments.length > 1 && arguments[1] !== void 0 ? arguments[1] : true, R = arguments.length > 2 ? arguments[2] : void 0;
R === void 0 && typeof q == "string" && (R = q, q = true);
let B = Object.assign({}, r.Document.defaults[r.defaultOptions.version], r.defaultOptions);
return new c.Schema(B).createNode(C, q, R);
}
var E = class extends r.Document {
constructor(C) {
super(Object.assign({}, r.defaultOptions, C));
}
};
function I(C, q) {
let R = [], B;
for (let U of e.parse(C)) {
let f = new E(q);
f.parse(U, B), R.push(f), B = f;
}
return R;
}
function S(C, q) {
let R = e.parse(C), B = new E(q).parse(R[0]);
if (R.length > 1) {
let U = "Source contains multiple documents; please use YAML.parseAllDocuments()";
B.errors.unshift(new h.YAMLSemanticError(R[1], U));
}
return B;
}
function M(C, q) {
let R = S(C, q);
if (R.warnings.forEach((B) => d.warn(B)), R.errors.length > 0)
throw R.errors[0];
return R.toJSON();
}
function T(C, q) {
let R = new E(q);
return R.contents = C, String(R);
}
var P = { createNode: y, defaultOptions: r.defaultOptions, Document: E, parse: M, parseAllDocuments: I, parseCST: e.parse, parseDocument: S, scalarOptions: r.scalarOptions, stringify: T };
n2.YAML = P;
} }), Ue = D({ "node_modules/yaml/index.js"(n2, e) {
Y(), e.exports = Hr().YAML;
} }), Gr = D({ "node_modules/yaml/dist/util.js"(n2) {
"use strict";
Y();
var e = ke(), r = Me();
n2.findPair = e.findPair, n2.parseMap = e.resolveMap, n2.parseSeq = e.resolveSeq, n2.stringifyNumber = e.stringifyNumber, n2.stringifyString = e.stringifyString, n2.toJSON = e.toJSON, n2.Type = r.Type, n2.YAMLError = r.YAMLError, n2.YAMLReferenceError = r.YAMLReferenceError, n2.YAMLSemanticError = r.YAMLSemanticError, n2.YAMLSyntaxError = r.YAMLSyntaxError, n2.YAMLWarning = r.YAMLWarning;
} }), zr = D({ "node_modules/yaml/util.js"(n2) {
Y();
var e = Gr();
n2.findPair = e.findPair, n2.toJSON = e.toJSON, n2.parseMap = e.parseMap, n2.parseSeq = e.parseSeq, n2.stringifyNumber = e.stringifyNumber, n2.stringifyString = e.stringifyString, n2.Type = e.Type, n2.YAMLError = e.YAMLError, n2.YAMLReferenceError = e.YAMLReferenceError, n2.YAMLSemanticError = e.YAMLSemanticError, n2.YAMLSyntaxError = e.YAMLSyntaxError, n2.YAMLWarning = e.YAMLWarning;
} }), Zr = D({ "node_modules/yaml-unist-parser/lib/yaml.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Ue();
n2.Document = e.Document;
var r = Ue();
n2.parseCST = r.parseCST;
var c = zr();
n2.YAMLError = c.YAMLError, n2.YAMLSyntaxError = c.YAMLSyntaxError, n2.YAMLSemanticError = c.YAMLSemanticError;
} }), Xr = D({ "node_modules/yaml-unist-parser/lib/parse.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = Kt(), r = xt(), c = Ht(), h = Gt(), d = Br(), y = He(), E = Yr(), I = Fr(), S = Wr(), M = Vr(), T = Qr(), P = Kr(), C = Zr();
function q(R) {
var B = C.parseCST(R);
M.addOrigRange(B);
for (var U = B.map(function(k) {
return new C.Document({ merge: false, keepCstNodes: true }).parse(k);
}), f = new e.default(R), i = [], t = { text: R, locator: f, comments: i, transformOffset: function(k) {
return I.transformOffset(k, t);
}, transformRange: function(k) {
return S.transformRange(k, t);
}, transformNode: function(k) {
return d.transformNode(k, t);
}, transformContent: function(k) {
return y.transformContent(k, t);
} }, s = 0, a = U; s < a.length; s++)
for (var m = a[s], g = 0, u = m.errors; g < u.length; g++) {
var p = u[g];
if (!(p instanceof C.YAMLSemanticError && p.message === 'Map keys must be unique; "<<" is repeated'))
throw E.transformError(p, t);
}
U.forEach(function(k) {
return h.removeCstBlankLine(k.cstNode);
});
var L = c.createRoot(t.transformRange({ origStart: 0, origEnd: t.text.length }), U.map(t.transformNode), i);
return r.attachComments(L), P.updatePositions(L), T.removeFakeNodes(L), L;
}
n2.parse = q;
} }), en = D({ "node_modules/yaml-unist-parser/lib/index.js"(n2) {
"use strict";
Y(), n2.__esModule = true;
var e = (ie(), se(te));
e.__exportStar(Xr(), n2);
} });
Y();
var tn = Mt(), { hasPragma: rn } = Ot(), { locStart: nn, locEnd: sn } = Lt();
function an(n2) {
let { parse: e } = en();
try {
let r = e(n2);
return delete r.comments, r;
} catch (r) {
throw r != null && r.position ? tn(r.message, r.position) : r;
}
}
var on = { astFormat: "yaml", parse: an, hasPragma: rn, locStart: nn, locEnd: sn };
at.exports = { parsers: { yaml: on } };
});
return ln();
});
}
});
// node_modules/prettier/index.js
var require_prettier = __commonJS({
"node_modules/prettier/index.js"(exports, module2) {
"use strict";
var __getOwnPropNames2 = Object.getOwnPropertyNames;
var __commonJS2 = (cb, mod) => function __require() {
return mod || (0, cb[__getOwnPropNames2(cb)[0]])((mod = { exports: {} }).exports, mod), mod.exports;
};
var require_global = __commonJS2({
"node_modules/core-js/internals/global.js"(exports2, module22) {
var check = function(it) {
return it && it.Math == Math && it;
};
module22.exports = check(typeof globalThis == "object" && globalThis) || check(typeof window == "object" && window) || check(typeof self == "object" && self) || check(typeof global == "object" && global) || function() {
return this;
}() || Function("return this")();
}
});
var require_fails = __commonJS2({
"node_modules/core-js/internals/fails.js"(exports2, module22) {
module22.exports = function(exec) {
try {
return !!exec();
} catch (error) {
return true;
}
};
}
});
var require_descriptors = __commonJS2({
"node_modules/core-js/internals/descriptors.js"(exports2, module22) {
var fails = require_fails();
module22.exports = !fails(function() {
return Object.defineProperty({}, 1, { get: function() {
return 7;
} })[1] != 7;
});
}
});
var require_function_bind_native = __commonJS2({
"node_modules/core-js/internals/function-bind-native.js"(exports2, module22) {
var fails = require_fails();
module22.exports = !fails(function() {
var test = function() {
}.bind();
return typeof test != "function" || test.hasOwnProperty("prototype");
});
}
});
var require_function_call = __commonJS2({
"node_modules/core-js/internals/function-call.js"(exports2, module22) {
var NATIVE_BIND = require_function_bind_native();
var call = Function.prototype.call;
module22.exports = NATIVE_BIND ? call.bind(call) : function() {
return call.apply(call, arguments);
};
}
});
var require_object_property_is_enumerable = __commonJS2({
"node_modules/core-js/internals/object-property-is-enumerable.js"(exports2) {
"use strict";
var $propertyIsEnumerable = {}.propertyIsEnumerable;
var getOwnPropertyDescriptor = Object.getOwnPropertyDescriptor;
var NASHORN_BUG = getOwnPropertyDescriptor && !$propertyIsEnumerable.call({ 1: 2 }, 1);
exports2.f = NASHORN_BUG ? function propertyIsEnumerable(V) {
var descriptor = getOwnPropertyDescriptor(this, V);
return !!descriptor && descriptor.enumerable;
} : $propertyIsEnumerable;
}
});
var require_create_property_descriptor = __commonJS2({
"node_modules/core-js/internals/create-property-descriptor.js"(exports2, module22) {
module22.exports = function(bitmap, value) {
return {
enumerable: !(bitmap & 1),
configurable: !(bitmap & 2),
writable: !(bitmap & 4),
value
};
};
}
});
var require_function_uncurry_this = __commonJS2({
"node_modules/core-js/internals/function-uncurry-this.js"(exports2, module22) {
var NATIVE_BIND = require_function_bind_native();
var FunctionPrototype = Function.prototype;
var call = FunctionPrototype.call;
var uncurryThisWithBind = NATIVE_BIND && FunctionPrototype.bind.bind(call, call);
module22.exports = NATIVE_BIND ? uncurryThisWithBind : function(fn) {
return function() {
return call.apply(fn, arguments);
};
};
}
});
var require_classof_raw = __commonJS2({
"node_modules/core-js/internals/classof-raw.js"(exports2, module22) {
var uncurryThis = require_function_uncurry_this();
var toString2 = uncurryThis({}.toString);
var stringSlice = uncurryThis("".slice);
module22.exports = function(it) {
return stringSlice(toString2(it), 8, -1);
};
}
});
var require_indexed_object = __commonJS2({
"node_modules/core-js/internals/indexed-object.js"(exports2, module22) {
var uncurryThis = require_function_uncurry_this();
var fails = require_fails();
var classof = require_classof_raw();
var $Object = Object;
var split = uncurryThis("".split);
module22.exports = fails(function() {
return !$Object("z").propertyIsEnumerable(0);
}) ? function(it) {
return classof(it) == "String" ? split(it, "") : $Object(it);
} : $Object;
}
});
var require_is_null_or_undefined = __commonJS2({
"node_modules/core-js/internals/is-null-or-undefined.js"(exports2, module22) {
module22.exports = function(it) {
return it === null || it === void 0;
};
}
});
var require_require_object_coercible = __commonJS2({
"node_modules/core-js/internals/require-object-coercible.js"(exports2, module22) {
var isNullOrUndefined = require_is_null_or_undefined();
var $TypeError = TypeError;
module22.exports = function(it) {
if (isNullOrUndefined(it))
throw $TypeError("Can't call method on " + it);
return it;
};
}
});
var require_to_indexed_object = __commonJS2({
"node_modules/core-js/internals/to-indexed-object.js"(exports2, module22) {
var IndexedObject = require_indexed_object();
var requireObjectCoercible = require_require_object_coercible();
module22.exports = function(it) {
return IndexedObject(requireObjectCoercible(it));
};
}
});
var require_document_all = __commonJS2({
"node_modules/core-js/internals/document-all.js"(exports2, module22) {
var documentAll = typeof document == "object" && document.all;
var IS_HTMLDDA = typeof documentAll == "undefined" && documentAll !== void 0;
module22.exports = {
all: documentAll,
IS_HTMLDDA
};
}
});
var require_is_callable = __commonJS2({
"node_modules/core-js/internals/is-callable.js"(exports2, module22) {
var $documentAll = require_document_all();
var documentAll = $documentAll.all;
module22.exports = $documentAll.IS_HTMLDDA ? function(argument) {
return typeof argument == "function" || argument === documentAll;
} : function(argument) {
return typeof argument == "function";
};
}
});
var require_is_object = __commonJS2({
"node_modules/core-js/internals/is-object.js"(exports2, module22) {
var isCallable = require_is_callable();
var $documentAll = require_document_all();
var documentAll = $documentAll.all;
module22.exports = $documentAll.IS_HTMLDDA ? function(it) {
return typeof it == "object" ? it !== null : isCallable(it) || it === documentAll;
} : function(it) {
return typeof it == "object" ? it !== null : isCallable(it);
};
}
});
var require_get_built_in = __commonJS2({
"node_modules/core-js/internals/get-built-in.js"(exports2, module22) {
var global2 = require_global();
var isCallable = require_is_callable();
var aFunction = function(argument) {
return isCallable(argument) ? argument : void 0;
};
module22.exports = function(namespace, method) {
return arguments.length < 2 ? aFunction(global2[namespace]) : global2[namespace] && global2[namespace][method];
};
}
});
var require_object_is_prototype_of = __commonJS2({
"node_modules/core-js/internals/object-is-prototype-of.js"(exports2, module22) {
var uncurryThis = require_function_uncurry_this();
module22.exports = uncurryThis({}.isPrototypeOf);
}
});
var require_engine_user_agent = __commonJS2({
"node_modules/core-js/internals/engine-user-agent.js"(exports2, module22) {
var getBuiltIn = require_get_built_in();
module22.exports = getBuiltIn("navigator", "userAgent") || "";
}
});
var require_engine_v8_version = __commonJS2({
"node_modules/core-js/internals/engine-v8-version.js"(exports2, module22) {
var global2 = require_global();
var userAgent = require_engine_user_agent();
var process2 = global2.process;
var Deno = global2.Deno;
var versions = process2 && process2.versions || Deno && Deno.version;
var v8 = versions && versions.v8;
var match;
var version2;
if (v8) {
match = v8.split(".");
version2 = match[0] > 0 && match[0] < 4 ? 1 : +(match[0] + match[1]);
}
if (!version2 && userAgent) {
match = userAgent.match(/Edge\/(\d+)/);
if (!match || match[1] >= 74) {
match = userAgent.match(/Chrome\/(\d+)/);
if (match)
version2 = +match[1];
}
}
module22.exports = version2;
}
});
var require_symbol_constructor_detection = __commonJS2({
"node_modules/core-js/internals/symbol-constructor-detection.js"(exports2, module22) {
var V8_VERSION = require_engine_v8_version();
var fails = require_fails();
module22.exports = !!Object.getOwnPropertySymbols && !fails(function() {
var symbol = Symbol();
return !String(symbol) || !(Object(symbol) instanceof Symbol) || !Symbol.sham && V8_VERSION && V8_VERSION < 41;
});
}
});
var require_use_symbol_as_uid = __commonJS2({
"node_modules/core-js/internals/use-symbol-as-uid.js"(exports2, module22) {
var NATIVE_SYMBOL = require_symbol_constructor_detection();
module22.exports = NATIVE_SYMBOL && !Symbol.sham && typeof Symbol.iterator == "symbol";
}
});
var require_is_symbol = __commonJS2({
"node_modules/core-js/internals/is-symbol.js"(exports2, module22) {
var getBuiltIn = require_get_built_in();
var isCallable = require_is_callable();
var isPrototypeOf = require_object_is_prototype_of();
var USE_SYMBOL_AS_UID = require_use_symbol_as_uid();
var $Object = Object;
module22.exports = USE_SYMBOL_AS_UID ? function(it) {
return typeof it == "symbol";
} : function(it) {
var $Symbol = getBuiltIn("Symbol");
return isCallable($Symbol) && isPrototypeOf($Symbol.prototype, $Object(it));
};
}
});
var require_try_to_string = __commonJS2({
"node_modules/core-js/internals/try-to-string.js"(exports2, module22) {
var $String = String;
module22.exports = function(argument) {
try {
return $String(argument);
} catch (error) {
return "Object";
}
};
}
});
var require_a_callable = __commonJS2({
"node_modules/core-js/internals/a-callable.js"(exports2, module22) {
var isCallable = require_is_callable();
var tryToString = require_try_to_string();
var $TypeError = TypeError;
module22.exports = function(argument) {
if (isCallable(argument))
return argument;
throw $TypeError(tryToString(argument) + " is not a function");
};
}
});
var require_get_method = __commonJS2({
"node_modules/core-js/internals/get-method.js"(exports2, module22) {
var aCallable = require_a_callable();
var isNullOrUndefined = require_is_null_or_undefined();
module22.exports = function(V, P) {
var func = V[P];
return isNullOrUndefined(func) ? void 0 : aCallable(func);
};
}
});
var require_ordinary_to_primitive = __commonJS2({
"node_modules/core-js/internals/ordinary-to-primitive.js"(exports2, module22) {
var call = require_function_call();
var isCallable = require_is_callable();
var isObject2 = require_is_object();
var $TypeError = TypeError;
module22.exports = function(input, pref) {
var fn, val;
if (pref === "string" && isCallable(fn = input.toString) && !isObject2(val = call(fn, input)))
return val;
if (isCallable(fn = input.valueOf) && !isObject2(val = call(fn, input)))
return val;
if (pref !== "string" && isCallable(fn = input.toString) && !isObject2(val = call(fn, input)))
return val;
throw $TypeError("Can't convert object to primitive value");
};
}
});
var require_is_pure = __commonJS2({
"node_modules/core-js/internals/is-pure.js"(exports2, module22) {
module22.exports = false;
}
});
var require_define_global_property = __commonJS2({
"node_modules/core-js/internals/define-global-property.js"(exports2, module22) {
var global2 = require_global();
var defineProperty = Object.defineProperty;
module22.exports = function(key, value) {
try {
defineProperty(global2, key, { value, configurable: true, writable: true });
} catch (error) {
global2[key] = value;
}
return value;
};
}
});
var require_shared_store = __commonJS2({
"node_modules/core-js/internals/shared-store.js"(exports2, module22) {
var global2 = require_global();
var defineGlobalProperty = require_define_global_property();
var SHARED = "__core-js_shared__";
var store = global2[SHARED] || defineGlobalProperty(SHARED, {});
module22.exports = store;
}
});
var require_shared = __commonJS2({
"node_modules/core-js/internals/shared.js"(exports2, module22) {
var IS_PURE = require_is_pure();
var store = require_shared_store();
(module22.exports = function(key, value) {
return store[key] || (store[key] = value !== void 0 ? value : {});
})("versions", []).push({
version: "3.26.1",
mode: IS_PURE ? "pure" : "global",
copyright: "\xA9 2014-2022 Denis Pushkarev (zloirock.ru)",
license: "https://github.com/zloirock/core-js/blob/v3.26.1/LICENSE",
source: "https://github.com/zloirock/core-js"
});
}
});
var require_to_object = __commonJS2({
"node_modules/core-js/internals/to-object.js"(exports2, module22) {
var requireObjectCoercible = require_require_object_coercible();
var $Object = Object;
module22.exports = function(argument) {
return $Object(requireObjectCoercible(argument));
};
}
});
var require_has_own_property = __commonJS2({
"node_modules/core-js/internals/has-own-property.js"(exports2, module22) {
var uncurryThis = require_function_uncurry_this();
var toObject = require_to_object();
var hasOwnProperty = uncurryThis({}.hasOwnProperty);
module22.exports = Object.hasOwn || function hasOwn(it, key) {
return hasOwnProperty(toObject(it), key);
};
}
});
var require_uid = __commonJS2({
"node_modules/core-js/internals/uid.js"(exports2, module22) {
var uncurryThis = require_function_uncurry_this();
var id = 0;
var postfix = Math.random();
var toString2 = uncurryThis(1 .toString);
module22.exports = function(key) {
return "Symbol(" + (key === void 0 ? "" : key) + ")_" + toString2(++id + postfix, 36);
};
}
});
var require_well_known_symbol = __commonJS2({
"node_modules/core-js/internals/well-known-symbol.js"(exports2, module22) {
var global2 = require_global();
var shared = require_shared();
var hasOwn = require_has_own_property();
var uid = require_uid();
var NATIVE_SYMBOL = require_symbol_constructor_detection();
var USE_SYMBOL_AS_UID = require_use_symbol_as_uid();
var WellKnownSymbolsStore = shared("wks");
var Symbol2 = global2.Symbol;
var symbolFor = Symbol2 && Symbol2["for"];
var createWellKnownSymbol = USE_SYMBOL_AS_UID ? Symbol2 : Symbol2 && Symbol2.withoutSetter || uid;
module22.exports = function(name) {
if (!hasOwn(WellKnownSymbolsStore, name) || !(NATIVE_SYMBOL || typeof WellKnownSymbolsStore[name] == "string")) {
var description = "Symbol." + name;
if (NATIVE_SYMBOL && hasOwn(Symbol2, name)) {
WellKnownSymbolsStore[name] = Symbol2[name];
} else if (USE_SYMBOL_AS_UID && symbolFor) {
WellKnownSymbolsStore[name] = symbolFor(description);
} else {
WellKnownSymbolsStore[name] = createWellKnownSymbol(description);
}
}
return WellKnownSymbolsStore[name];
};
}
});
var require_to_primitive = __commonJS2({
"node_modules/core-js/internals/to-primitive.js"(exports2, module22) {
var call = require_function_call();
var isObject2 = require_is_object();
var isSymbol = require_is_symbol();
var getMethod = require_get_method();
var ordinaryToPrimitive = require_ordinary_to_primitive();
var wellKnownSymbol = require_well_known_symbol();
var $TypeError = TypeError;
var TO_PRIMITIVE = wellKnownSymbol("toPrimitive");
module22.exports = function(input, pref) {
if (!isObject2(input) || isSymbol(input))
return input;
var exoticToPrim = getMethod(input, TO_PRIMITIVE);
var result;
if (exoticToPrim) {
if (pref === void 0)
pref = "default";
result = call(exoticToPrim, input, pref);
if (!isObject2(result) || isSymbol(result))
return result;
throw $TypeError("Can't convert object to primitive value");
}
if (pref === void 0)
pref = "number";
return ordinaryToPrimitive(input, pref);
};
}
});
var require_to_property_key = __commonJS2({
"node_modules/core-js/internals/to-property-key.js"(exports2, module22) {
var toPrimitive = require_to_primitive();
var isSymbol = require_is_symbol();
module22.exports = function(argument) {
var key = toPrimitive(argument, "string");
return isSymbol(key) ? key : key + "";
};
}
});
var require_document_create_element = __commonJS2({
"node_modules/core-js/internals/document-create-element.js"(exports2, module22) {
var global2 = require_global();
var isObject2 = require_is_object();
var document2 = global2.document;
var EXISTS = isObject2(document2) && isObject2(document2.createElement);
module22.exports = function(it) {
return EXISTS ? document2.createElement(it) : {};
};
}
});
var require_ie8_dom_define = __commonJS2({
"node_modules/core-js/internals/ie8-dom-define.js"(exports2, module22) {
var DESCRIPTORS = require_descriptors();
var fails = require_fails();
var createElement = require_document_create_element();
module22.exports = !DESCRIPTORS && !fails(function() {
return Object.defineProperty(createElement("div"), "a", {
get: function() {
return 7;
}
}).a != 7;
});
}
});
var require_object_get_own_property_descriptor = __commonJS2({
"node_modules/core-js/internals/object-get-own-property-descriptor.js"(exports2) {
var DESCRIPTORS = require_descriptors();
var call = require_function_call();
var propertyIsEnumerableModule = require_object_property_is_enumerable();
var createPropertyDescriptor = require_create_property_descriptor();
var toIndexedObject = require_to_indexed_object();
var toPropertyKey = require_to_property_key();
var hasOwn = require_has_own_property();
var IE8_DOM_DEFINE = require_ie8_dom_define();
var $getOwnPropertyDescriptor = Object.getOwnPropertyDescriptor;
exports2.f = DESCRIPTORS ? $getOwnPropertyDescriptor : function getOwnPropertyDescriptor(O, P) {
O = toIndexedObject(O);
P = toPropertyKey(P);
if (IE8_DOM_DEFINE)
try {
return $getOwnPropertyDescriptor(O, P);
} catch (error) {
}
if (hasOwn(O, P))
return createPropertyDescriptor(!call(propertyIsEnumerableModule.f, O, P), O[P]);
};
}
});
var require_v8_prototype_define_bug = __commonJS2({
"node_modules/core-js/internals/v8-prototype-define-bug.js"(exports2, module22) {
var DESCRIPTORS = require_descriptors();
var fails = require_fails();
module22.exports = DESCRIPTORS && fails(function() {
return Object.defineProperty(function() {
}, "prototype", {
value: 42,
writable: false
}).prototype != 42;
});
}
});
var require_an_object = __commonJS2({
"node_modules/core-js/internals/an-object.js"(exports2, module22) {
var isObject2 = require_is_object();
var $String = String;
var $TypeError = TypeError;
module22.exports = function(argument) {
if (isObject2(argument))
return argument;
throw $TypeError($String(argument) + " is not an object");
};
}
});
var require_object_define_property = __commonJS2({
"node_modules/core-js/internals/object-define-property.js"(exports2) {
var DESCRIPTORS = require_descriptors();
var IE8_DOM_DEFINE = require_ie8_dom_define();
var V8_PROTOTYPE_DEFINE_BUG = require_v8_prototype_define_bug();
var anObject = require_an_object();
var toPropertyKey = require_to_property_key();
var $TypeError = TypeError;
var $defineProperty = Object.defineProperty;
var $getOwnPropertyDescriptor = Object.getOwnPropertyDescriptor;
var ENUMERABLE = "enumerable";
var CONFIGURABLE = "configurable";
var WRITABLE = "writable";
exports2.f = DESCRIPTORS ? V8_PROTOTYPE_DEFINE_BUG ? function defineProperty(O, P, Attributes) {
anObject(O);
P = toPropertyKey(P);
anObject(Attributes);
if (typeof O === "function" && P === "prototype" && "value" in Attributes && WRITABLE in Attributes && !Attributes[WRITABLE]) {
var current = $getOwnPropertyDescriptor(O, P);
if (current && current[WRITABLE]) {
O[P] = Attributes.value;
Attributes = {
configurable: CONFIGURABLE in Attributes ? Attributes[CONFIGURABLE] : current[CONFIGURABLE],
enumerable: ENUMERABLE in Attributes ? Attributes[ENUMERABLE] : current[ENUMERABLE],
writable: false
};
}
}
return $defineProperty(O, P, Attributes);
} : $defineProperty : function defineProperty(O, P, Attributes) {
anObject(O);
P = toPropertyKey(P);
anObject(Attributes);
if (IE8_DOM_DEFINE)
try {
return $defineProperty(O, P, Attributes);
} catch (error) {
}
if ("get" in Attributes || "set" in Attributes)
throw $TypeError("Accessors not supported");
if ("value" in Attributes)
O[P] = Attributes.value;
return O;
};
}
});
var require_create_non_enumerable_property = __commonJS2({
"node_modules/core-js/internals/create-non-enumerable-property.js"(exports2, module22) {
var DESCRIPTORS = require_descriptors();
var definePropertyModule = require_object_define_property();
var createPropertyDescriptor = require_create_property_descriptor();
module22.exports = DESCRIPTORS ? function(object, key, value) {
return definePropertyModule.f(object, key, createPropertyDescriptor(1, value));
} : function(object, key, value) {
object[key] = value;
return object;
};
}
});
var require_function_name = __commonJS2({
"node_modules/core-js/internals/function-name.js"(exports2, module22) {
var DESCRIPTORS = require_descriptors();
var hasOwn = require_has_own_property();
var FunctionPrototype = Function.prototype;
var getDescriptor = DESCRIPTORS && Object.getOwnPropertyDescriptor;
var EXISTS = hasOwn(FunctionPrototype, "name");
var PROPER = EXISTS && function something() {
}.name === "something";
var CONFIGURABLE = EXISTS && (!DESCRIPTORS || DESCRIPTORS && getDescriptor(FunctionPrototype, "name").configurable);
module22.exports = {
EXISTS,
PROPER,
CONFIGURABLE
};
}
});
var require_inspect_source = __commonJS2({
"node_modules/core-js/internals/inspect-source.js"(exports2, module22) {
var uncurryThis = require_function_uncurry_this();
var isCallable = require_is_callable();
var store = require_shared_store();
var functionToString = uncurryThis(Function.toString);
if (!isCallable(store.inspectSource)) {
store.inspectSource = function(it) {
return functionToString(it);
};
}
module22.exports = store.inspectSource;
}
});
var require_weak_map_basic_detection = __commonJS2({
"node_modules/core-js/internals/weak-map-basic-detection.js"(exports2, module22) {
var global2 = require_global();
var isCallable = require_is_callable();
var WeakMap2 = global2.WeakMap;
module22.exports = isCallable(WeakMap2) && /native code/.test(String(WeakMap2));
}
});
var require_shared_key = __commonJS2({
"node_modules/core-js/internals/shared-key.js"(exports2, module22) {
var shared = require_shared();
var uid = require_uid();
var keys = shared("keys");
module22.exports = function(key) {
return keys[key] || (keys[key] = uid(key));
};
}
});
var require_hidden_keys = __commonJS2({
"node_modules/core-js/internals/hidden-keys.js"(exports2, module22) {
module22.exports = {};
}
});
var require_internal_state = __commonJS2({
"node_modules/core-js/internals/internal-state.js"(exports2, module22) {
var NATIVE_WEAK_MAP = require_weak_map_basic_detection();
var global2 = require_global();
var isObject2 = require_is_object();
var createNonEnumerableProperty = require_create_non_enumerable_property();
var hasOwn = require_has_own_property();
var shared = require_shared_store();
var sharedKey = require_shared_key();
var hiddenKeys = require_hidden_keys();
var OBJECT_ALREADY_INITIALIZED = "Object already initialized";
var TypeError2 = global2.TypeError;
var WeakMap2 = global2.WeakMap;
var set;
var get;
var has;
var enforce = function(it) {
return has(it) ? get(it) : set(it, {});
};
var getterFor = function(TYPE) {
return function(it) {
var state;
if (!isObject2(it) || (state = get(it)).type !== TYPE) {
throw TypeError2("Incompatible receiver, " + TYPE + " required");
}
return state;
};
};
if (NATIVE_WEAK_MAP || shared.state) {
store = shared.state || (shared.state = new WeakMap2());
store.get = store.get;
store.has = store.has;
store.set = store.set;
set = function(it, metadata) {
if (store.has(it))
throw TypeError2(OBJECT_ALREADY_INITIALIZED);
metadata.facade = it;
store.set(it, metadata);
return metadata;
};
get = function(it) {
return store.get(it) || {};
};
has = function(it) {
return store.has(it);
};
} else {
STATE = sharedKey("state");
hiddenKeys[STATE] = true;
set = function(it, metadata) {
if (hasOwn(it, STATE))
throw TypeError2(OBJECT_ALREADY_INITIALIZED);
metadata.facade = it;
createNonEnumerableProperty(it, STATE, metadata);
return metadata;
};
get = function(it) {
return hasOwn(it, STATE) ? it[STATE] : {};
};
has = function(it) {
return hasOwn(it, STATE);
};
}
var store;
var STATE;
module22.exports = {
set,
get,
has,
enforce,
getterFor
};
}
});
var require_make_built_in = __commonJS2({
"node_modules/core-js/internals/make-built-in.js"(exports2, module22) {
var fails = require_fails();
var isCallable = require_is_callable();
var hasOwn = require_has_own_property();
var DESCRIPTORS = require_descriptors();
var CONFIGURABLE_FUNCTION_NAME = require_function_name().CONFIGURABLE;
var inspectSource = require_inspect_source();
var InternalStateModule = require_internal_state();
var enforceInternalState = InternalStateModule.enforce;
var getInternalState = InternalStateModule.get;
var defineProperty = Object.defineProperty;
var CONFIGURABLE_LENGTH = DESCRIPTORS && !fails(function() {
return defineProperty(function() {
}, "length", { value: 8 }).length !== 8;
});
var TEMPLATE = String(String).split("String");
var makeBuiltIn = module22.exports = function(value, name, options) {
if (String(name).slice(0, 7) === "Symbol(") {
name = "[" + String(name).replace(/^Symbol\(([^)]*)\)/, "$1") + "]";
}
if (options && options.getter)
name = "get " + name;
if (options && options.setter)
name = "set " + name;
if (!hasOwn(value, "name") || CONFIGURABLE_FUNCTION_NAME && value.name !== name) {
if (DESCRIPTORS)
defineProperty(value, "name", { value: name, configurable: true });
else
value.name = name;
}
if (CONFIGURABLE_LENGTH && options && hasOwn(options, "arity") && value.length !== options.arity) {
defineProperty(value, "length", { value: options.arity });
}
try {
if (options && hasOwn(options, "constructor") && options.constructor) {
if (DESCRIPTORS)
defineProperty(value, "prototype", { writable: false });
} else if (value.prototype)
value.prototype = void 0;
} catch (error) {
}
var state = enforceInternalState(value);
if (!hasOwn(state, "source")) {
state.source = TEMPLATE.join(typeof name == "string" ? name : "");
}
return value;
};
Function.prototype.toString = makeBuiltIn(function toString2() {
return isCallable(this) && getInternalState(this).source || inspectSource(this);
}, "toString");
}
});
var require_define_built_in = __commonJS2({
"node_modules/core-js/internals/define-built-in.js"(exports2, module22) {
var isCallable = require_is_callable();
var definePropertyModule = require_object_define_property();
var makeBuiltIn = require_make_built_in();
var defineGlobalProperty = require_define_global_property();
module22.exports = function(O, key, value, options) {
if (!options)
options = {};
var simple = options.enumerable;
var name = options.name !== void 0 ? options.name : key;
if (isCallable(value))
makeBuiltIn(value, name, options);
if (options.global) {
if (simple)
O[key] = value;
else
defineGlobalProperty(key, value);
} else {
try {
if (!options.unsafe)
delete O[key];
else if (O[key])
simple = true;
} catch (error) {
}
if (simple)
O[key] = value;
else
definePropertyModule.f(O, key, {
value,
enumerable: false,
configurable: !options.nonConfigurable,
writable: !options.nonWritable
});
}
return O;
};
}
});
var require_math_trunc = __commonJS2({
"node_modules/core-js/internals/math-trunc.js"(exports2, module22) {
var ceil = Math.ceil;
var floor = Math.floor;
module22.exports = Math.trunc || function trunc(x) {
var n2 = +x;
return (n2 > 0 ? floor : ceil)(n2);
};
}
});
var require_to_integer_or_infinity = __commonJS2({
"node_modules/core-js/internals/to-integer-or-infinity.js"(exports2, module22) {
var trunc = require_math_trunc();
module22.exports = function(argument) {
var number = +argument;
return number !== number || number === 0 ? 0 : trunc(number);
};
}
});
var require_to_absolute_index = __commonJS2({
"node_modules/core-js/internals/to-absolute-index.js"(exports2, module22) {
var toIntegerOrInfinity = require_to_integer_or_infinity();
var max = Math.max;
var min = Math.min;
module22.exports = function(index, length) {
var integer = toIntegerOrInfinity(index);
return integer < 0 ? max(integer + length, 0) : min(integer, length);
};
}
});
var require_to_length = __commonJS2({
"node_modules/core-js/internals/to-length.js"(exports2, module22) {
var toIntegerOrInfinity = require_to_integer_or_infinity();
var min = Math.min;
module22.exports = function(argument) {
return argument > 0 ? min(toIntegerOrInfinity(argument), 9007199254740991) : 0;
};
}
});
var require_length_of_array_like = __commonJS2({
"node_modules/core-js/internals/length-of-array-like.js"(exports2, module22) {
var toLength = require_to_length();
module22.exports = function(obj) {
return toLength(obj.length);
};
}
});
var require_array_includes = __commonJS2({
"node_modules/core-js/internals/array-includes.js"(exports2, module22) {
var toIndexedObject = require_to_indexed_object();
var toAbsoluteIndex = require_to_absolute_index();
var lengthOfArrayLike = require_length_of_array_like();
var createMethod = function(IS_INCLUDES) {
return function($this, el, fromIndex) {
var O = toIndexedObject($this);
var length = lengthOfArrayLike(O);
var index = toAbsoluteIndex(fromIndex, length);
var value;
if (IS_INCLUDES && el != el)
while (length > index) {
value = O[index++];
if (value != value)
return true;
}
else
for (; length > index; index++) {
if ((IS_INCLUDES || index in O) && O[index] === el)
return IS_INCLUDES || index || 0;
}
return !IS_INCLUDES && -1;
};
};
module22.exports = {
includes: createMethod(true),
indexOf: createMethod(false)
};
}
});
var require_object_keys_internal = __commonJS2({
"node_modules/core-js/internals/object-keys-internal.js"(exports2, module22) {
var uncurryThis = require_function_uncurry_this();
var hasOwn = require_has_own_property();
var toIndexedObject = require_to_indexed_object();
var indexOf = require_array_includes().indexOf;
var hiddenKeys = require_hidden_keys();
var push = uncurryThis([].push);
module22.exports = function(object, names) {
var O = toIndexedObject(object);
var i = 0;
var result = [];
var key;
for (key in O)
!hasOwn(hiddenKeys, key) && hasOwn(O, key) && push(result, key);
while (names.length > i)
if (hasOwn(O, key = names[i++])) {
~indexOf(result, key) || push(result, key);
}
return result;
};
}
});
var require_enum_bug_keys = __commonJS2({
"node_modules/core-js/internals/enum-bug-keys.js"(exports2, module22) {
module22.exports = [
"constructor",
"hasOwnProperty",
"isPrototypeOf",
"propertyIsEnumerable",
"toLocaleString",
"toString",
"valueOf"
];
}
});
var require_object_get_own_property_names = __commonJS2({
"node_modules/core-js/internals/object-get-own-property-names.js"(exports2) {
var internalObjectKeys = require_object_keys_internal();
var enumBugKeys = require_enum_bug_keys();
var hiddenKeys = enumBugKeys.concat("length", "prototype");
exports2.f = Object.getOwnPropertyNames || function getOwnPropertyNames(O) {
return internalObjectKeys(O, hiddenKeys);
};
}
});
var require_object_get_own_property_symbols = __commonJS2({
"node_modules/core-js/internals/object-get-own-property-symbols.js"(exports2) {
exports2.f = Object.getOwnPropertySymbols;
}
});
var require_own_keys = __commonJS2({
"node_modules/core-js/internals/own-keys.js"(exports2, module22) {
var getBuiltIn = require_get_built_in();
var uncurryThis = require_function_uncurry_this();
var getOwnPropertyNamesModule = require_object_get_own_property_names();
var getOwnPropertySymbolsModule = require_object_get_own_property_symbols();
var anObject = require_an_object();
var concat = uncurryThis([].concat);
module22.exports = getBuiltIn("Reflect", "ownKeys") || function ownKeys(it) {
var keys = getOwnPropertyNamesModule.f(anObject(it));
var getOwnPropertySymbols = getOwnPropertySymbolsModule.f;
return getOwnPropertySymbols ? concat(keys, getOwnPropertySymbols(it)) : keys;
};
}
});
var require_copy_constructor_properties = __commonJS2({
"node_modules/core-js/internals/copy-constructor-properties.js"(exports2, module22) {
var hasOwn = require_has_own_property();
var ownKeys = require_own_keys();
var getOwnPropertyDescriptorModule = require_object_get_own_property_descriptor();
var definePropertyModule = require_object_define_property();
module22.exports = function(target, source, exceptions) {
var keys = ownKeys(source);
var defineProperty = definePropertyModule.f;
var getOwnPropertyDescriptor = getOwnPropertyDescriptorModule.f;
for (var i = 0; i < keys.length; i++) {
var key = keys[i];
if (!hasOwn(target, key) && !(exceptions && hasOwn(exceptions, key))) {
defineProperty(target, key, getOwnPropertyDescriptor(source, key));
}
}
};
}
});
var require_is_forced = __commonJS2({
"node_modules/core-js/internals/is-forced.js"(exports2, module22) {
var fails = require_fails();
var isCallable = require_is_callable();
var replacement = /#|\.prototype\./;
var isForced = function(feature, detection) {
var value = data[normalize(feature)];
return value == POLYFILL ? true : value == NATIVE ? false : isCallable(detection) ? fails(detection) : !!detection;
};
var normalize = isForced.normalize = function(string) {
return String(string).replace(replacement, ".").toLowerCase();
};
var data = isForced.data = {};
var NATIVE = isForced.NATIVE = "N";
var POLYFILL = isForced.POLYFILL = "P";
module22.exports = isForced;
}
});
var require_export = __commonJS2({
"node_modules/core-js/internals/export.js"(exports2, module22) {
var global2 = require_global();
var getOwnPropertyDescriptor = require_object_get_own_property_descriptor().f;
var createNonEnumerableProperty = require_create_non_enumerable_property();
var defineBuiltIn = require_define_built_in();
var defineGlobalProperty = require_define_global_property();
var copyConstructorProperties = require_copy_constructor_properties();
var isForced = require_is_forced();
module22.exports = function(options, source) {
var TARGET = options.target;
var GLOBAL = options.global;
var STATIC = options.stat;
var FORCED, target, key, targetProperty, sourceProperty, descriptor;
if (GLOBAL) {
target = global2;
} else if (STATIC) {
target = global2[TARGET] || defineGlobalProperty(TARGET, {});
} else {
target = (global2[TARGET] || {}).prototype;
}
if (target)
for (key in source) {
sourceProperty = source[key];
if (options.dontCallGetSet) {
descriptor = getOwnPropertyDescriptor(target, key);
targetProperty = descriptor && descriptor.value;
} else
targetProperty = target[key];
FORCED = isForced(GLOBAL ? key : TARGET + (STATIC ? "." : "#") + key, options.forced);
if (!FORCED && targetProperty !== void 0) {
if (typeof sourceProperty == typeof targetProperty)
continue;
copyConstructorProperties(sourceProperty, targetProperty);
}
if (options.sham || targetProperty && targetProperty.sham) {
createNonEnumerableProperty(sourceProperty, "sham", true);
}
defineBuiltIn(target, key, sourceProperty, options);
}
};
}
});
var require_is_array = __commonJS2({
"node_modules/core-js/internals/is-array.js"(exports2, module22) {
var classof = require_classof_raw();
module22.exports = Array.isArray || function isArray(argument) {
return classof(argument) == "Array";
};
}
});
var require_does_not_exceed_safe_integer = __commonJS2({
"node_modules/core-js/internals/does-not-exceed-safe-integer.js"(exports2, module22) {
var $TypeError = TypeError;
var MAX_SAFE_INTEGER = 9007199254740991;
module22.exports = function(it) {
if (it > MAX_SAFE_INTEGER)
throw $TypeError("Maximum allowed index exceeded");
return it;
};
}
});
var require_function_uncurry_this_clause = __commonJS2({
"node_modules/core-js/internals/function-uncurry-this-clause.js"(exports2, module22) {
var classofRaw = require_classof_raw();
var uncurryThis = require_function_uncurry_this();
module22.exports = function(fn) {
if (classofRaw(fn) === "Function")
return uncurryThis(fn);
};
}
});
var require_function_bind_context = __commonJS2({
"node_modules/core-js/internals/function-bind-context.js"(exports2, module22) {
var uncurryThis = require_function_uncurry_this_clause();
var aCallable = require_a_callable();
var NATIVE_BIND = require_function_bind_native();
var bind = uncurryThis(uncurryThis.bind);
module22.exports = function(fn, that) {
aCallable(fn);
return that === void 0 ? fn : NATIVE_BIND ? bind(fn, that) : function() {
return fn.apply(that, arguments);
};
};
}
});
var require_flatten_into_array = __commonJS2({
"node_modules/core-js/internals/flatten-into-array.js"(exports2, module22) {
"use strict";
var isArray = require_is_array();
var lengthOfArrayLike = require_length_of_array_like();
var doesNotExceedSafeInteger = require_does_not_exceed_safe_integer();
var bind = require_function_bind_context();
var flattenIntoArray = function(target, original, source, sourceLen, start, depth, mapper, thisArg) {
var targetIndex = start;
var sourceIndex = 0;
var mapFn = mapper ? bind(mapper, thisArg) : false;
var element, elementLen;
while (sourceIndex < sourceLen) {
if (sourceIndex in source) {
element = mapFn ? mapFn(source[sourceIndex], sourceIndex, original) : source[sourceIndex];
if (depth > 0 && isArray(element)) {
elementLen = lengthOfArrayLike(element);
targetIndex = flattenIntoArray(target, original, element, elementLen, targetIndex, depth - 1) - 1;
} else {
doesNotExceedSafeInteger(targetIndex + 1);
target[targetIndex] = element;
}
targetIndex++;
}
sourceIndex++;
}
return targetIndex;
};
module22.exports = flattenIntoArray;
}
});
var require_to_string_tag_support = __commonJS2({
"node_modules/core-js/internals/to-string-tag-support.js"(exports2, module22) {
var wellKnownSymbol = require_well_known_symbol();
var TO_STRING_TAG = wellKnownSymbol("toStringTag");
var test = {};
test[TO_STRING_TAG] = "z";
module22.exports = String(test) === "[object z]";
}
});
var require_classof = __commonJS2({
"node_modules/core-js/internals/classof.js"(exports2, module22) {
var TO_STRING_TAG_SUPPORT = require_to_string_tag_support();
var isCallable = require_is_callable();
var classofRaw = require_classof_raw();
var wellKnownSymbol = require_well_known_symbol();
var TO_STRING_TAG = wellKnownSymbol("toStringTag");
var $Object = Object;
var CORRECT_ARGUMENTS = classofRaw(function() {
return arguments;
}()) == "Arguments";
var tryGet = function(it, key) {
try {
return it[key];
} catch (error) {
}
};
module22.exports = TO_STRING_TAG_SUPPORT ? classofRaw : function(it) {
var O, tag, result;
return it === void 0 ? "Undefined" : it === null ? "Null" : typeof (tag = tryGet(O = $Object(it), TO_STRING_TAG)) == "string" ? tag : CORRECT_ARGUMENTS ? classofRaw(O) : (result = classofRaw(O)) == "Object" && isCallable(O.callee) ? "Arguments" : result;
};
}
});
var require_is_constructor = __commonJS2({
"node_modules/core-js/internals/is-constructor.js"(exports2, module22) {
var uncurryThis = require_function_uncurry_this();
var fails = require_fails();
var isCallable = require_is_callable();
var classof = require_classof();
var getBuiltIn = require_get_built_in();
var inspectSource = require_inspect_source();
var noop = function() {
};
var empty = [];
var construct = getBuiltIn("Reflect", "construct");
var constructorRegExp = /^\s*(?:class|function)\b/;
var exec = uncurryThis(constructorRegExp.exec);
var INCORRECT_TO_STRING = !constructorRegExp.exec(noop);
var isConstructorModern = function isConstructor(argument) {
if (!isCallable(argument))
return false;
try {
construct(noop, empty, argument);
return true;
} catch (error) {
return false;
}
};
var isConstructorLegacy = function isConstructor(argument) {
if (!isCallable(argument))
return false;
switch (classof(argument)) {
case "AsyncFunction":
case "GeneratorFunction":
case "AsyncGeneratorFunction":
return false;
}
try {
return INCORRECT_TO_STRING || !!exec(constructorRegExp, inspectSource(argument));
} catch (error) {
return true;
}
};
isConstructorLegacy.sham = true;
module22.exports = !construct || fails(function() {
var called;
return isConstructorModern(isConstructorModern.call) || !isConstructorModern(Object) || !isConstructorModern(function() {
called = true;
}) || called;
}) ? isConstructorLegacy : isConstructorModern;
}
});
var require_array_species_constructor = __commonJS2({
"node_modules/core-js/internals/array-species-constructor.js"(exports2, module22) {
var isArray = require_is_array();
var isConstructor = require_is_constructor();
var isObject2 = require_is_object();
var wellKnownSymbol = require_well_known_symbol();
var SPECIES = wellKnownSymbol("species");
var $Array = Array;
module22.exports = function(originalArray) {
var C;
if (isArray(originalArray)) {
C = originalArray.constructor;
if (isConstructor(C) && (C === $Array || isArray(C.prototype)))
C = void 0;
else if (isObject2(C)) {
C = C[SPECIES];
if (C === null)
C = void 0;
}
}
return C === void 0 ? $Array : C;
};
}
});
var require_array_species_create = __commonJS2({
"node_modules/core-js/internals/array-species-create.js"(exports2, module22) {
var arraySpeciesConstructor = require_array_species_constructor();
module22.exports = function(originalArray, length) {
return new (arraySpeciesConstructor(originalArray))(length === 0 ? 0 : length);
};
}
});
var require_es_array_flat_map = __commonJS2({
"node_modules/core-js/modules/es.array.flat-map.js"() {
"use strict";
var $ = require_export();
var flattenIntoArray = require_flatten_into_array();
var aCallable = require_a_callable();
var toObject = require_to_object();
var lengthOfArrayLike = require_length_of_array_like();
var arraySpeciesCreate = require_array_species_create();
$({ target: "Array", proto: true }, {
flatMap: function flatMap(callbackfn) {
var O = toObject(this);
var sourceLen = lengthOfArrayLike(O);
var A;
aCallable(callbackfn);
A = arraySpeciesCreate(O, 0);
A.length = flattenIntoArray(A, O, O, sourceLen, 0, 1, callbackfn, arguments.length > 1 ? arguments[1] : void 0);
return A;
}
});
}
});
var require_iterators = __commonJS2({
"node_modules/core-js/internals/iterators.js"(exports2, module22) {
module22.exports = {};
}
});
var require_is_array_iterator_method = __commonJS2({
"node_modules/core-js/internals/is-array-iterator-method.js"(exports2, module22) {
var wellKnownSymbol = require_well_known_symbol();
var Iterators = require_iterators();
var ITERATOR = wellKnownSymbol("iterator");
var ArrayPrototype = Array.prototype;
module22.exports = function(it) {
return it !== void 0 && (Iterators.Array === it || ArrayPrototype[ITERATOR] === it);
};
}
});
var require_get_iterator_method = __commonJS2({
"node_modules/core-js/internals/get-iterator-method.js"(exports2, module22) {
var classof = require_classof();
var getMethod = require_get_method();
var isNullOrUndefined = require_is_null_or_undefined();
var Iterators = require_iterators();
var wellKnownSymbol = require_well_known_symbol();
var ITERATOR = wellKnownSymbol("iterator");
module22.exports = function(it) {
if (!isNullOrUndefined(it))
return getMethod(it, ITERATOR) || getMethod(it, "@@iterator") || Iterators[classof(it)];
};
}
});
var require_get_iterator = __commonJS2({
"node_modules/core-js/internals/get-iterator.js"(exports2, module22) {
var call = require_function_call();
var aCallable = require_a_callable();
var anObject = require_an_object();
var tryToString = require_try_to_string();
var getIteratorMethod = require_get_iterator_method();
var $TypeError = TypeError;
module22.exports = function(argument, usingIterator) {
var iteratorMethod = arguments.length < 2 ? getIteratorMethod(argument) : usingIterator;
if (aCallable(iteratorMethod))
return anObject(call(iteratorMethod, argument));
throw $TypeError(tryToString(argument) + " is not iterable");
};
}
});
var require_iterator_close = __commonJS2({
"node_modules/core-js/internals/iterator-close.js"(exports2, module22) {
var call = require_function_call();
var anObject = require_an_object();
var getMethod = require_get_method();
module22.exports = function(iterator, kind, value) {
var innerResult, innerError;
anObject(iterator);
try {
innerResult = getMethod(iterator, "return");
if (!innerResult) {
if (kind === "throw")
throw value;
return value;
}
innerResult = call(innerResult, iterator);
} catch (error) {
innerError = true;
innerResult = error;
}
if (kind === "throw")
throw value;
if (innerError)
throw innerResult;
anObject(innerResult);
return value;
};
}
});
var require_iterate = __commonJS2({
"node_modules/core-js/internals/iterate.js"(exports2, module22) {
var bind = require_function_bind_context();
var call = require_function_call();
var anObject = require_an_object();
var tryToString = require_try_to_string();
var isArrayIteratorMethod = require_is_array_iterator_method();
var lengthOfArrayLike = require_length_of_array_like();
var isPrototypeOf = require_object_is_prototype_of();
var getIterator = require_get_iterator();
var getIteratorMethod = require_get_iterator_method();
var iteratorClose = require_iterator_close();
var $TypeError = TypeError;
var Result = function(stopped, result) {
this.stopped = stopped;
this.result = result;
};
var ResultPrototype = Result.prototype;
module22.exports = function(iterable, unboundFunction, options) {
var that = options && options.that;
var AS_ENTRIES = !!(options && options.AS_ENTRIES);
var IS_RECORD = !!(options && options.IS_RECORD);
var IS_ITERATOR = !!(options && options.IS_ITERATOR);
var INTERRUPTED = !!(options && options.INTERRUPTED);
var fn = bind(unboundFunction, that);
var iterator, iterFn, index, length, result, next, step;
var stop = function(condition) {
if (iterator)
iteratorClose(iterator, "normal", condition);
return new Result(true, condition);
};
var callFn = function(value) {
if (AS_ENTRIES) {
anObject(value);
return INTERRUPTED ? fn(value[0], value[1], stop) : fn(value[0], value[1]);
}
return INTERRUPTED ? fn(value, stop) : fn(value);
};
if (IS_RECORD) {
iterator = iterable.iterator;
} else if (IS_ITERATOR) {
iterator = iterable;
} else {
iterFn = getIteratorMethod(iterable);
if (!iterFn)
throw $TypeError(tryToString(iterable) + " is not iterable");
if (isArrayIteratorMethod(iterFn)) {
for (index = 0, length = lengthOfArrayLike(iterable); length > index; index++) {
result = callFn(iterable[index]);
if (result && isPrototypeOf(ResultPrototype, result))
return result;
}
return new Result(false);
}
iterator = getIterator(iterable, iterFn);
}
next = IS_RECORD ? iterable.next : iterator.next;
while (!(step = call(next, iterator)).done) {
try {
result = callFn(step.value);
} catch (error) {
iteratorClose(iterator, "throw", error);
}
if (typeof result == "object" && result && isPrototypeOf(ResultPrototype, result))
return result;
}
return new Result(false);
};
}
});
var require_create_property = __commonJS2({
"node_modules/core-js/internals/create-property.js"(exports2, module22) {
"use strict";
var toPropertyKey = require_to_property_key();
var definePropertyModule = require_object_define_property();
var createPropertyDescriptor = require_create_property_descriptor();
module22.exports = function(object, key, value) {
var propertyKey = toPropertyKey(key);
if (propertyKey in object)
definePropertyModule.f(object, propertyKey, createPropertyDescriptor(0, value));
else
object[propertyKey] = value;
};
}
});
var require_es_object_from_entries = __commonJS2({
"node_modules/core-js/modules/es.object.from-entries.js"() {
var $ = require_export();
var iterate = require_iterate();
var createProperty = require_create_property();
$({ target: "Object", stat: true }, {
fromEntries: function fromEntries(iterable) {
var obj = {};
iterate(iterable, function(k, v) {
createProperty(obj, k, v);
}, { AS_ENTRIES: true });
return obj;
}
});
}
});
var require_define_built_in_accessor = __commonJS2({
"node_modules/core-js/internals/define-built-in-accessor.js"(exports2, module22) {
var makeBuiltIn = require_make_built_in();
var defineProperty = require_object_define_property();
module22.exports = function(target, name, descriptor) {
if (descriptor.get)
makeBuiltIn(descriptor.get, name, { getter: true });
if (descriptor.set)
makeBuiltIn(descriptor.set, name, { setter: true });
return defineProperty.f(target, name, descriptor);
};
}
});
var require_regexp_flags = __commonJS2({
"node_modules/core-js/internals/regexp-flags.js"(exports2, module22) {
"use strict";
var anObject = require_an_object();
module22.exports = function() {
var that = anObject(this);
var result = "";
if (that.hasIndices)
result += "d";
if (that.global)
result += "g";
if (that.ignoreCase)
result += "i";
if (that.multiline)
result += "m";
if (that.dotAll)
result += "s";
if (that.unicode)
result += "u";
if (that.unicodeSets)
result += "v";
if (that.sticky)
result += "y";
return result;
};
}
});
var require_es_regexp_flags = __commonJS2({
"node_modules/core-js/modules/es.regexp.flags.js"() {
var global2 = require_global();
var DESCRIPTORS = require_descriptors();
var defineBuiltInAccessor = require_define_built_in_accessor();
var regExpFlags = require_regexp_flags();
var fails = require_fails();
var RegExp2 = global2.RegExp;
var RegExpPrototype = RegExp2.prototype;
var FORCED = DESCRIPTORS && fails(function() {
var INDICES_SUPPORT = true;
try {
RegExp2(".", "d");
} catch (error) {
INDICES_SUPPORT = false;
}
var O = {};
var calls = "";
var expected = INDICES_SUPPORT ? "dgimsy" : "gimsy";
var addGetter = function(key2, chr) {
Object.defineProperty(O, key2, { get: function() {
calls += chr;
return true;
} });
};
var pairs = {
dotAll: "s",
global: "g",
ignoreCase: "i",
multiline: "m",
sticky: "y"
};
if (INDICES_SUPPORT)
pairs.hasIndices = "d";
for (var key in pairs)
addGetter(key, pairs[key]);
var result = Object.getOwnPropertyDescriptor(RegExpPrototype, "flags").get.call(O);
return result !== expected || calls !== expected;
});
if (FORCED)
defineBuiltInAccessor(RegExpPrototype, "flags", {
configurable: true,
get: regExpFlags
});
}
});
var require_es_array_flat = __commonJS2({
"node_modules/core-js/modules/es.array.flat.js"() {
"use strict";
var $ = require_export();
var flattenIntoArray = require_flatten_into_array();
var toObject = require_to_object();
var lengthOfArrayLike = require_length_of_array_like();
var toIntegerOrInfinity = require_to_integer_or_infinity();
var arraySpeciesCreate = require_array_species_create();
$({ target: "Array", proto: true }, {
flat: function flat() {
var depthArg = arguments.length ? arguments[0] : void 0;
var O = toObject(this);
var sourceLen = lengthOfArrayLike(O);
var A = arraySpeciesCreate(O, 0);
A.length = flattenIntoArray(A, O, O, sourceLen, 0, depthArg === void 0 ? 1 : toIntegerOrInfinity(depthArg));
return A;
}
});
}
});
var _excluded = ["cliName", "cliCategory", "cliDescription"];
var _excluded2 = ["_"];
var _excluded3 = ["overrides"];
var _excluded4 = ["languageId"];
function _objectWithoutProperties(source, excluded) {
if (source == null)
return {};
var target = _objectWithoutPropertiesLoose(source, excluded);
var key, i;
if (Object.getOwnPropertySymbols) {
var sourceSymbolKeys = Object.getOwnPropertySymbols(source);
for (i = 0; i < sourceSymbolKeys.length; i++) {
key = sourceSymbolKeys[i];
if (excluded.indexOf(key) >= 0)
continue;
if (!Object.prototype.propertyIsEnumerable.call(source, key))
continue;
target[key] = source[key];
}
}
return target;
}
function _objectWithoutPropertiesLoose(source, excluded) {
if (source == null)
return {};
var target = {};
var sourceKeys = Object.keys(source);
var key, i;
for (i = 0; i < sourceKeys.length; i++) {
key = sourceKeys[i];
if (excluded.indexOf(key) >= 0)
continue;
target[key] = source[key];
}
return target;
}
require_es_array_flat_map();
require_es_object_from_entries();
require_es_regexp_flags();
require_es_array_flat();
var __create2 = Object.create;
var __defProp2 = Object.defineProperty;
var __getOwnPropDesc2 = Object.getOwnPropertyDescriptor;
var __getOwnPropNames22 = Object.getOwnPropertyNames;
var __getProtoOf2 = Object.getPrototypeOf;
var __hasOwnProp2 = Object.prototype.hasOwnProperty;
var __esm = (fn, res) => function __init() {
return fn && (res = (0, fn[__getOwnPropNames22(fn)[0]])(fn = 0)), res;
};
var __commonJS22 = (cb, mod) => function __require() {
return mod || (0, cb[__getOwnPropNames22(cb)[0]])((mod = {
exports: {}
}).exports, mod), mod.exports;
};
var __export = (target, all) => {
for (var name in all)
__defProp2(target, name, {
get: all[name],
enumerable: true
});
};
var __copyProps2 = (to, from, except, desc) => {
if (from && typeof from === "object" || typeof from === "function") {
for (let key of __getOwnPropNames22(from))
if (!__hasOwnProp2.call(to, key) && key !== except)
__defProp2(to, key, {
get: () => from[key],
enumerable: !(desc = __getOwnPropDesc2(from, key)) || desc.enumerable
});
}
return to;
};
var __toESM2 = (mod, isNodeMode, target) => (target = mod != null ? __create2(__getProtoOf2(mod)) : {}, __copyProps2(isNodeMode || !mod || !mod.__esModule ? __defProp2(target, "default", {
value: mod,
enumerable: true
}) : target, mod));
var __toCommonJS = (mod) => __copyProps2(__defProp2({}, "__esModule", {
value: true
}), mod);
var require_base = __commonJS22({
"node_modules/diff/lib/diff/base.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2["default"] = Diff;
function Diff() {
}
Diff.prototype = {
diff: function diff(oldString, newString) {
var options = arguments.length > 2 && arguments[2] !== void 0 ? arguments[2] : {};
var callback = options.callback;
if (typeof options === "function") {
callback = options;
options = {};
}
this.options = options;
var self2 = this;
function done(value) {
if (callback) {
setTimeout(function() {
callback(void 0, value);
}, 0);
return true;
} else {
return value;
}
}
oldString = this.castInput(oldString);
newString = this.castInput(newString);
oldString = this.removeEmpty(this.tokenize(oldString));
newString = this.removeEmpty(this.tokenize(newString));
var newLen = newString.length, oldLen = oldString.length;
var editLength = 1;
var maxEditLength = newLen + oldLen;
var bestPath = [{
newPos: -1,
components: []
}];
var oldPos = this.extractCommon(bestPath[0], newString, oldString, 0);
if (bestPath[0].newPos + 1 >= newLen && oldPos + 1 >= oldLen) {
return done([{
value: this.join(newString),
count: newString.length
}]);
}
function execEditLength() {
for (var diagonalPath = -1 * editLength; diagonalPath <= editLength; diagonalPath += 2) {
var basePath = void 0;
var addPath = bestPath[diagonalPath - 1], removePath = bestPath[diagonalPath + 1], _oldPos = (removePath ? removePath.newPos : 0) - diagonalPath;
if (addPath) {
bestPath[diagonalPath - 1] = void 0;
}
var canAdd = addPath && addPath.newPos + 1 < newLen, canRemove = removePath && 0 <= _oldPos && _oldPos < oldLen;
if (!canAdd && !canRemove) {
bestPath[diagonalPath] = void 0;
continue;
}
if (!canAdd || canRemove && addPath.newPos < removePath.newPos) {
basePath = clonePath(removePath);
self2.pushComponent(basePath.components, void 0, true);
} else {
basePath = addPath;
basePath.newPos++;
self2.pushComponent(basePath.components, true, void 0);
}
_oldPos = self2.extractCommon(basePath, newString, oldString, diagonalPath);
if (basePath.newPos + 1 >= newLen && _oldPos + 1 >= oldLen) {
return done(buildValues(self2, basePath.components, newString, oldString, self2.useLongestToken));
} else {
bestPath[diagonalPath] = basePath;
}
}
editLength++;
}
if (callback) {
(function exec() {
setTimeout(function() {
if (editLength > maxEditLength) {
return callback();
}
if (!execEditLength()) {
exec();
}
}, 0);
})();
} else {
while (editLength <= maxEditLength) {
var ret = execEditLength();
if (ret) {
return ret;
}
}
}
},
pushComponent: function pushComponent(components, added, removed) {
var last = components[components.length - 1];
if (last && last.added === added && last.removed === removed) {
components[components.length - 1] = {
count: last.count + 1,
added,
removed
};
} else {
components.push({
count: 1,
added,
removed
});
}
},
extractCommon: function extractCommon(basePath, newString, oldString, diagonalPath) {
var newLen = newString.length, oldLen = oldString.length, newPos = basePath.newPos, oldPos = newPos - diagonalPath, commonCount = 0;
while (newPos + 1 < newLen && oldPos + 1 < oldLen && this.equals(newString[newPos + 1], oldString[oldPos + 1])) {
newPos++;
oldPos++;
commonCount++;
}
if (commonCount) {
basePath.components.push({
count: commonCount
});
}
basePath.newPos = newPos;
return oldPos;
},
equals: function equals(left, right) {
if (this.options.comparator) {
return this.options.comparator(left, right);
} else {
return left === right || this.options.ignoreCase && left.toLowerCase() === right.toLowerCase();
}
},
removeEmpty: function removeEmpty(array) {
var ret = [];
for (var i = 0; i < array.length; i++) {
if (array[i]) {
ret.push(array[i]);
}
}
return ret;
},
castInput: function castInput(value) {
return value;
},
tokenize: function tokenize(value) {
return value.split("");
},
join: function join(chars) {
return chars.join("");
}
};
function buildValues(diff, components, newString, oldString, useLongestToken) {
var componentPos = 0, componentLen = components.length, newPos = 0, oldPos = 0;
for (; componentPos < componentLen; componentPos++) {
var component = components[componentPos];
if (!component.removed) {
if (!component.added && useLongestToken) {
var value = newString.slice(newPos, newPos + component.count);
value = value.map(function(value2, i) {
var oldValue = oldString[oldPos + i];
return oldValue.length > value2.length ? oldValue : value2;
});
component.value = diff.join(value);
} else {
component.value = diff.join(newString.slice(newPos, newPos + component.count));
}
newPos += component.count;
if (!component.added) {
oldPos += component.count;
}
} else {
component.value = diff.join(oldString.slice(oldPos, oldPos + component.count));
oldPos += component.count;
if (componentPos && components[componentPos - 1].added) {
var tmp = components[componentPos - 1];
components[componentPos - 1] = components[componentPos];
components[componentPos] = tmp;
}
}
}
var lastComponent = components[componentLen - 1];
if (componentLen > 1 && typeof lastComponent.value === "string" && (lastComponent.added || lastComponent.removed) && diff.equals("", lastComponent.value)) {
components[componentLen - 2].value += lastComponent.value;
components.pop();
}
return components;
}
function clonePath(path) {
return {
newPos: path.newPos,
components: path.components.slice(0)
};
}
}
});
var require_array = __commonJS22({
"node_modules/diff/lib/diff/array.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.diffArrays = diffArrays;
exports2.arrayDiff = void 0;
var _base = _interopRequireDefault(require_base());
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : {
"default": obj
};
}
var arrayDiff = new _base["default"]();
exports2.arrayDiff = arrayDiff;
arrayDiff.tokenize = function(value) {
return value.slice();
};
arrayDiff.join = arrayDiff.removeEmpty = function(value) {
return value;
};
function diffArrays(oldArr, newArr, callback) {
return arrayDiff.diff(oldArr, newArr, callback);
}
}
});
var escape_string_regexp_exports = {};
__export(escape_string_regexp_exports, {
default: () => escapeStringRegexp
});
function escapeStringRegexp(string) {
if (typeof string !== "string") {
throw new TypeError("Expected a string");
}
return string.replace(/[|\\{}()[\]^$+*?.]/g, "\\$&").replace(/-/g, "\\x2d");
}
var init_escape_string_regexp = __esm({
"node_modules/escape-string-regexp/index.js"() {
}
});
var require_get_last = __commonJS22({
"src/utils/get-last.js"(exports2, module22) {
"use strict";
var getLast = (arr) => arr[arr.length - 1];
module22.exports = getLast;
}
});
var require_debug = __commonJS22({
"node_modules/semver/internal/debug.js"(exports2, module22) {
var debug = typeof process === "object" && process.env && process.env.NODE_DEBUG && /\bsemver\b/i.test(process.env.NODE_DEBUG) ? (...args) => console.error("SEMVER", ...args) : () => {
};
module22.exports = debug;
}
});
var require_constants = __commonJS22({
"node_modules/semver/internal/constants.js"(exports2, module22) {
var SEMVER_SPEC_VERSION = "2.0.0";
var MAX_LENGTH = 256;
var MAX_SAFE_INTEGER = Number.MAX_SAFE_INTEGER || 9007199254740991;
var MAX_SAFE_COMPONENT_LENGTH = 16;
module22.exports = {
SEMVER_SPEC_VERSION,
MAX_LENGTH,
MAX_SAFE_INTEGER,
MAX_SAFE_COMPONENT_LENGTH
};
}
});
var require_re = __commonJS22({
"node_modules/semver/internal/re.js"(exports2, module22) {
var {
MAX_SAFE_COMPONENT_LENGTH
} = require_constants();
var debug = require_debug();
exports2 = module22.exports = {};
var re = exports2.re = [];
var src = exports2.src = [];
var t = exports2.t = {};
var R = 0;
var createToken = (name, value, isGlobal) => {
const index = R++;
debug(name, index, value);
t[name] = index;
src[index] = value;
re[index] = new RegExp(value, isGlobal ? "g" : void 0);
};
createToken("NUMERICIDENTIFIER", "0|[1-9]\\d*");
createToken("NUMERICIDENTIFIERLOOSE", "[0-9]+");
createToken("NONNUMERICIDENTIFIER", "\\d*[a-zA-Z-][a-zA-Z0-9-]*");
createToken("MAINVERSION", `(${src[t.NUMERICIDENTIFIER]})\\.(${src[t.NUMERICIDENTIFIER]})\\.(${src[t.NUMERICIDENTIFIER]})`);
createToken("MAINVERSIONLOOSE", `(${src[t.NUMERICIDENTIFIERLOOSE]})\\.(${src[t.NUMERICIDENTIFIERLOOSE]})\\.(${src[t.NUMERICIDENTIFIERLOOSE]})`);
createToken("PRERELEASEIDENTIFIER", `(?:${src[t.NUMERICIDENTIFIER]}|${src[t.NONNUMERICIDENTIFIER]})`);
createToken("PRERELEASEIDENTIFIERLOOSE", `(?:${src[t.NUMERICIDENTIFIERLOOSE]}|${src[t.NONNUMERICIDENTIFIER]})`);
createToken("PRERELEASE", `(?:-(${src[t.PRERELEASEIDENTIFIER]}(?:\\.${src[t.PRERELEASEIDENTIFIER]})*))`);
createToken("PRERELEASELOOSE", `(?:-?(${src[t.PRERELEASEIDENTIFIERLOOSE]}(?:\\.${src[t.PRERELEASEIDENTIFIERLOOSE]})*))`);
createToken("BUILDIDENTIFIER", "[0-9A-Za-z-]+");
createToken("BUILD", `(?:\\+(${src[t.BUILDIDENTIFIER]}(?:\\.${src[t.BUILDIDENTIFIER]})*))`);
createToken("FULLPLAIN", `v?${src[t.MAINVERSION]}${src[t.PRERELEASE]}?${src[t.BUILD]}?`);
createToken("FULL", `^${src[t.FULLPLAIN]}$`);
createToken("LOOSEPLAIN", `[v=\\s]*${src[t.MAINVERSIONLOOSE]}${src[t.PRERELEASELOOSE]}?${src[t.BUILD]}?`);
createToken("LOOSE", `^${src[t.LOOSEPLAIN]}$`);
createToken("GTLT", "((?:<|>)?=?)");
createToken("XRANGEIDENTIFIERLOOSE", `${src[t.NUMERICIDENTIFIERLOOSE]}|x|X|\\*`);
createToken("XRANGEIDENTIFIER", `${src[t.NUMERICIDENTIFIER]}|x|X|\\*`);
createToken("XRANGEPLAIN", `[v=\\s]*(${src[t.XRANGEIDENTIFIER]})(?:\\.(${src[t.XRANGEIDENTIFIER]})(?:\\.(${src[t.XRANGEIDENTIFIER]})(?:${src[t.PRERELEASE]})?${src[t.BUILD]}?)?)?`);
createToken("XRANGEPLAINLOOSE", `[v=\\s]*(${src[t.XRANGEIDENTIFIERLOOSE]})(?:\\.(${src[t.XRANGEIDENTIFIERLOOSE]})(?:\\.(${src[t.XRANGEIDENTIFIERLOOSE]})(?:${src[t.PRERELEASELOOSE]})?${src[t.BUILD]}?)?)?`);
createToken("XRANGE", `^${src[t.GTLT]}\\s*${src[t.XRANGEPLAIN]}$`);
createToken("XRANGELOOSE", `^${src[t.GTLT]}\\s*${src[t.XRANGEPLAINLOOSE]}$`);
createToken("COERCE", `${"(^|[^\\d])(\\d{1,"}${MAX_SAFE_COMPONENT_LENGTH}})(?:\\.(\\d{1,${MAX_SAFE_COMPONENT_LENGTH}}))?(?:\\.(\\d{1,${MAX_SAFE_COMPONENT_LENGTH}}))?(?:$|[^\\d])`);
createToken("COERCERTL", src[t.COERCE], true);
createToken("LONETILDE", "(?:~>?)");
createToken("TILDETRIM", `(\\s*)${src[t.LONETILDE]}\\s+`, true);
exports2.tildeTrimReplace = "$1~";
createToken("TILDE", `^${src[t.LONETILDE]}${src[t.XRANGEPLAIN]}$`);
createToken("TILDELOOSE", `^${src[t.LONETILDE]}${src[t.XRANGEPLAINLOOSE]}$`);
createToken("LONECARET", "(?:\\^)");
createToken("CARETTRIM", `(\\s*)${src[t.LONECARET]}\\s+`, true);
exports2.caretTrimReplace = "$1^";
createToken("CARET", `^${src[t.LONECARET]}${src[t.XRANGEPLAIN]}$`);
createToken("CARETLOOSE", `^${src[t.LONECARET]}${src[t.XRANGEPLAINLOOSE]}$`);
createToken("COMPARATORLOOSE", `^${src[t.GTLT]}\\s*(${src[t.LOOSEPLAIN]})$|^$`);
createToken("COMPARATOR", `^${src[t.GTLT]}\\s*(${src[t.FULLPLAIN]})$|^$`);
createToken("COMPARATORTRIM", `(\\s*)${src[t.GTLT]}\\s*(${src[t.LOOSEPLAIN]}|${src[t.XRANGEPLAIN]})`, true);
exports2.comparatorTrimReplace = "$1$2$3";
createToken("HYPHENRANGE", `^\\s*(${src[t.XRANGEPLAIN]})\\s+-\\s+(${src[t.XRANGEPLAIN]})\\s*$`);
createToken("HYPHENRANGELOOSE", `^\\s*(${src[t.XRANGEPLAINLOOSE]})\\s+-\\s+(${src[t.XRANGEPLAINLOOSE]})\\s*$`);
createToken("STAR", "(<|>)?=?\\s*\\*");
createToken("GTE0", "^\\s*>=\\s*0\\.0\\.0\\s*$");
createToken("GTE0PRE", "^\\s*>=\\s*0\\.0\\.0-0\\s*$");
}
});
var require_parse_options = __commonJS22({
"node_modules/semver/internal/parse-options.js"(exports2, module22) {
var opts = ["includePrerelease", "loose", "rtl"];
var parseOptions = (options) => !options ? {} : typeof options !== "object" ? {
loose: true
} : opts.filter((k) => options[k]).reduce((o, k) => {
o[k] = true;
return o;
}, {});
module22.exports = parseOptions;
}
});
var require_identifiers = __commonJS22({
"node_modules/semver/internal/identifiers.js"(exports2, module22) {
var numeric = /^[0-9]+$/;
var compareIdentifiers = (a, b) => {
const anum = numeric.test(a);
const bnum = numeric.test(b);
if (anum && bnum) {
a = +a;
b = +b;
}
return a === b ? 0 : anum && !bnum ? -1 : bnum && !anum ? 1 : a < b ? -1 : 1;
};
var rcompareIdentifiers = (a, b) => compareIdentifiers(b, a);
module22.exports = {
compareIdentifiers,
rcompareIdentifiers
};
}
});
var require_semver = __commonJS22({
"node_modules/semver/classes/semver.js"(exports2, module22) {
var debug = require_debug();
var {
MAX_LENGTH,
MAX_SAFE_INTEGER
} = require_constants();
var {
re,
t
} = require_re();
var parseOptions = require_parse_options();
var {
compareIdentifiers
} = require_identifiers();
var SemVer = class {
constructor(version2, options) {
options = parseOptions(options);
if (version2 instanceof SemVer) {
if (version2.loose === !!options.loose && version2.includePrerelease === !!options.includePrerelease) {
return version2;
} else {
version2 = version2.version;
}
} else if (typeof version2 !== "string") {
throw new TypeError(`Invalid Version: ${version2}`);
}
if (version2.length > MAX_LENGTH) {
throw new TypeError(`version is longer than ${MAX_LENGTH} characters`);
}
debug("SemVer", version2, options);
this.options = options;
this.loose = !!options.loose;
this.includePrerelease = !!options.includePrerelease;
const m = version2.trim().match(options.loose ? re[t.LOOSE] : re[t.FULL]);
if (!m) {
throw new TypeError(`Invalid Version: ${version2}`);
}
this.raw = version2;
this.major = +m[1];
this.minor = +m[2];
this.patch = +m[3];
if (this.major > MAX_SAFE_INTEGER || this.major < 0) {
throw new TypeError("Invalid major version");
}
if (this.minor > MAX_SAFE_INTEGER || this.minor < 0) {
throw new TypeError("Invalid minor version");
}
if (this.patch > MAX_SAFE_INTEGER || this.patch < 0) {
throw new TypeError("Invalid patch version");
}
if (!m[4]) {
this.prerelease = [];
} else {
this.prerelease = m[4].split(".").map((id) => {
if (/^[0-9]+$/.test(id)) {
const num = +id;
if (num >= 0 && num < MAX_SAFE_INTEGER) {
return num;
}
}
return id;
});
}
this.build = m[5] ? m[5].split(".") : [];
this.format();
}
format() {
this.version = `${this.major}.${this.minor}.${this.patch}`;
if (this.prerelease.length) {
this.version += `-${this.prerelease.join(".")}`;
}
return this.version;
}
toString() {
return this.version;
}
compare(other) {
debug("SemVer.compare", this.version, this.options, other);
if (!(other instanceof SemVer)) {
if (typeof other === "string" && other === this.version) {
return 0;
}
other = new SemVer(other, this.options);
}
if (other.version === this.version) {
return 0;
}
return this.compareMain(other) || this.comparePre(other);
}
compareMain(other) {
if (!(other instanceof SemVer)) {
other = new SemVer(other, this.options);
}
return compareIdentifiers(this.major, other.major) || compareIdentifiers(this.minor, other.minor) || compareIdentifiers(this.patch, other.patch);
}
comparePre(other) {
if (!(other instanceof SemVer)) {
other = new SemVer(other, this.options);
}
if (this.prerelease.length && !other.prerelease.length) {
return -1;
} else if (!this.prerelease.length && other.prerelease.length) {
return 1;
} else if (!this.prerelease.length && !other.prerelease.length) {
return 0;
}
let i = 0;
do {
const a = this.prerelease[i];
const b = other.prerelease[i];
debug("prerelease compare", i, a, b);
if (a === void 0 && b === void 0) {
return 0;
} else if (b === void 0) {
return 1;
} else if (a === void 0) {
return -1;
} else if (a === b) {
continue;
} else {
return compareIdentifiers(a, b);
}
} while (++i);
}
compareBuild(other) {
if (!(other instanceof SemVer)) {
other = new SemVer(other, this.options);
}
let i = 0;
do {
const a = this.build[i];
const b = other.build[i];
debug("prerelease compare", i, a, b);
if (a === void 0 && b === void 0) {
return 0;
} else if (b === void 0) {
return 1;
} else if (a === void 0) {
return -1;
} else if (a === b) {
continue;
} else {
return compareIdentifiers(a, b);
}
} while (++i);
}
inc(release, identifier) {
switch (release) {
case "premajor":
this.prerelease.length = 0;
this.patch = 0;
this.minor = 0;
this.major++;
this.inc("pre", identifier);
break;
case "preminor":
this.prerelease.length = 0;
this.patch = 0;
this.minor++;
this.inc("pre", identifier);
break;
case "prepatch":
this.prerelease.length = 0;
this.inc("patch", identifier);
this.inc("pre", identifier);
break;
case "prerelease":
if (this.prerelease.length === 0) {
this.inc("patch", identifier);
}
this.inc("pre", identifier);
break;
case "major":
if (this.minor !== 0 || this.patch !== 0 || this.prerelease.length === 0) {
this.major++;
}
this.minor = 0;
this.patch = 0;
this.prerelease = [];
break;
case "minor":
if (this.patch !== 0 || this.prerelease.length === 0) {
this.minor++;
}
this.patch = 0;
this.prerelease = [];
break;
case "patch":
if (this.prerelease.length === 0) {
this.patch++;
}
this.prerelease = [];
break;
case "pre":
if (this.prerelease.length === 0) {
this.prerelease = [0];
} else {
let i = this.prerelease.length;
while (--i >= 0) {
if (typeof this.prerelease[i] === "number") {
this.prerelease[i]++;
i = -2;
}
}
if (i === -1) {
this.prerelease.push(0);
}
}
if (identifier) {
if (compareIdentifiers(this.prerelease[0], identifier) === 0) {
if (isNaN(this.prerelease[1])) {
this.prerelease = [identifier, 0];
}
} else {
this.prerelease = [identifier, 0];
}
}
break;
default:
throw new Error(`invalid increment argument: ${release}`);
}
this.format();
this.raw = this.version;
return this;
}
};
module22.exports = SemVer;
}
});
var require_compare = __commonJS22({
"node_modules/semver/functions/compare.js"(exports2, module22) {
var SemVer = require_semver();
var compare = (a, b, loose) => new SemVer(a, loose).compare(new SemVer(b, loose));
module22.exports = compare;
}
});
var require_lt = __commonJS22({
"node_modules/semver/functions/lt.js"(exports2, module22) {
var compare = require_compare();
var lt = (a, b, loose) => compare(a, b, loose) < 0;
module22.exports = lt;
}
});
var require_gte = __commonJS22({
"node_modules/semver/functions/gte.js"(exports2, module22) {
var compare = require_compare();
var gte = (a, b, loose) => compare(a, b, loose) >= 0;
module22.exports = gte;
}
});
var require_arrayify = __commonJS22({
"src/utils/arrayify.js"(exports2, module22) {
"use strict";
module22.exports = (object, keyName) => Object.entries(object).map(([key, value]) => Object.assign({
[keyName]: key
}, value));
}
});
var require_lib8 = __commonJS22({
"node_modules/outdent/lib/index.js"(exports2, module22) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.outdent = void 0;
function noop() {
var args = [];
for (var _i = 0; _i < arguments.length; _i++) {
args[_i] = arguments[_i];
}
}
function createWeakMap() {
if (typeof WeakMap !== "undefined") {
return /* @__PURE__ */ new WeakMap();
} else {
return fakeSetOrMap();
}
}
function fakeSetOrMap() {
return {
add: noop,
delete: noop,
get: noop,
set: noop,
has: function(k) {
return false;
}
};
}
var hop = Object.prototype.hasOwnProperty;
var has = function(obj, prop) {
return hop.call(obj, prop);
};
function extend(target, source) {
for (var prop in source) {
if (has(source, prop)) {
target[prop] = source[prop];
}
}
return target;
}
var reLeadingNewline = /^[ \t]*(?:\r\n|\r|\n)/;
var reTrailingNewline = /(?:\r\n|\r|\n)[ \t]*$/;
var reStartsWithNewlineOrIsEmpty = /^(?:[\r\n]|$)/;
var reDetectIndentation = /(?:\r\n|\r|\n)([ \t]*)(?:[^ \t\r\n]|$)/;
var reOnlyWhitespaceWithAtLeastOneNewline = /^[ \t]*[\r\n][ \t\r\n]*$/;
function _outdentArray(strings, firstInterpolatedValueSetsIndentationLevel, options) {
var indentationLevel = 0;
var match = strings[0].match(reDetectIndentation);
if (match) {
indentationLevel = match[1].length;
}
var reSource = "(\\r\\n|\\r|\\n).{0," + indentationLevel + "}";
var reMatchIndent = new RegExp(reSource, "g");
if (firstInterpolatedValueSetsIndentationLevel) {
strings = strings.slice(1);
}
var newline = options.newline, trimLeadingNewline = options.trimLeadingNewline, trimTrailingNewline = options.trimTrailingNewline;
var normalizeNewlines = typeof newline === "string";
var l = strings.length;
var outdentedStrings = strings.map(function(v, i) {
v = v.replace(reMatchIndent, "$1");
if (i === 0 && trimLeadingNewline) {
v = v.replace(reLeadingNewline, "");
}
if (i === l - 1 && trimTrailingNewline) {
v = v.replace(reTrailingNewline, "");
}
if (normalizeNewlines) {
v = v.replace(/\r\n|\n|\r/g, function(_) {
return newline;
});
}
return v;
});
return outdentedStrings;
}
function concatStringsAndValues(strings, values) {
var ret = "";
for (var i = 0, l = strings.length; i < l; i++) {
ret += strings[i];
if (i < l - 1) {
ret += values[i];
}
}
return ret;
}
function isTemplateStringsArray(v) {
return has(v, "raw") && has(v, "length");
}
function createInstance(options) {
var arrayAutoIndentCache = createWeakMap();
var arrayFirstInterpSetsIndentCache = createWeakMap();
function outdent(stringsOrOptions) {
var values = [];
for (var _i = 1; _i < arguments.length; _i++) {
values[_i - 1] = arguments[_i];
}
if (isTemplateStringsArray(stringsOrOptions)) {
var strings = stringsOrOptions;
var firstInterpolatedValueSetsIndentationLevel = (values[0] === outdent || values[0] === defaultOutdent) && reOnlyWhitespaceWithAtLeastOneNewline.test(strings[0]) && reStartsWithNewlineOrIsEmpty.test(strings[1]);
var cache = firstInterpolatedValueSetsIndentationLevel ? arrayFirstInterpSetsIndentCache : arrayAutoIndentCache;
var renderedArray = cache.get(strings);
if (!renderedArray) {
renderedArray = _outdentArray(strings, firstInterpolatedValueSetsIndentationLevel, options);
cache.set(strings, renderedArray);
}
if (values.length === 0) {
return renderedArray[0];
}
var rendered = concatStringsAndValues(renderedArray, firstInterpolatedValueSetsIndentationLevel ? values.slice(1) : values);
return rendered;
} else {
return createInstance(extend(extend({}, options), stringsOrOptions || {}));
}
}
var fullOutdent = extend(outdent, {
string: function(str) {
return _outdentArray([str], false, options)[0];
}
});
return fullOutdent;
}
var defaultOutdent = createInstance({
trimLeadingNewline: true,
trimTrailingNewline: true
});
exports2.outdent = defaultOutdent;
exports2.default = defaultOutdent;
if (typeof module22 !== "undefined") {
try {
module22.exports = defaultOutdent;
Object.defineProperty(defaultOutdent, "__esModule", {
value: true
});
defaultOutdent.default = defaultOutdent;
defaultOutdent.outdent = defaultOutdent;
} catch (e) {
}
}
}
});
var require_core_options = __commonJS22({
"src/main/core-options.js"(exports2, module22) {
"use strict";
var {
outdent
} = require_lib8();
var CATEGORY_CONFIG = "Config";
var CATEGORY_EDITOR = "Editor";
var CATEGORY_FORMAT = "Format";
var CATEGORY_OTHER = "Other";
var CATEGORY_OUTPUT = "Output";
var CATEGORY_GLOBAL = "Global";
var CATEGORY_SPECIAL = "Special";
var options = {
cursorOffset: {
since: "1.4.0",
category: CATEGORY_SPECIAL,
type: "int",
default: -1,
range: {
start: -1,
end: Number.POSITIVE_INFINITY,
step: 1
},
description: outdent`
Print (to stderr) where a cursor at the given position would move to after formatting.
This option cannot be used with --range-start and --range-end.
`,
cliCategory: CATEGORY_EDITOR
},
endOfLine: {
since: "1.15.0",
category: CATEGORY_GLOBAL,
type: "choice",
default: [{
since: "1.15.0",
value: "auto"
}, {
since: "2.0.0",
value: "lf"
}],
description: "Which end of line characters to apply.",
choices: [{
value: "lf",
description: "Line Feed only (\\n), common on Linux and macOS as well as inside git repos"
}, {
value: "crlf",
description: "Carriage Return + Line Feed characters (\\r\\n), common on Windows"
}, {
value: "cr",
description: "Carriage Return character only (\\r), used very rarely"
}, {
value: "auto",
description: outdent`
Maintain existing
(mixed values within one file are normalised by looking at what's used after the first line)
`
}]
},
filepath: {
since: "1.4.0",
category: CATEGORY_SPECIAL,
type: "path",
description: "Specify the input filepath. This will be used to do parser inference.",
cliName: "stdin-filepath",
cliCategory: CATEGORY_OTHER,
cliDescription: "Path to the file to pretend that stdin comes from."
},
insertPragma: {
since: "1.8.0",
category: CATEGORY_SPECIAL,
type: "boolean",
default: false,
description: "Insert @format pragma into file's first docblock comment.",
cliCategory: CATEGORY_OTHER
},
parser: {
since: "0.0.10",
category: CATEGORY_GLOBAL,
type: "choice",
default: [{
since: "0.0.10",
value: "babylon"
}, {
since: "1.13.0",
value: void 0
}],
description: "Which parser to use.",
exception: (value) => typeof value === "string" || typeof value === "function",
choices: [{
value: "flow",
description: "Flow"
}, {
value: "babel",
since: "1.16.0",
description: "JavaScript"
}, {
value: "babel-flow",
since: "1.16.0",
description: "Flow"
}, {
value: "babel-ts",
since: "2.0.0",
description: "TypeScript"
}, {
value: "typescript",
since: "1.4.0",
description: "TypeScript"
}, {
value: "acorn",
since: "2.6.0",
description: "JavaScript"
}, {
value: "espree",
since: "2.2.0",
description: "JavaScript"
}, {
value: "meriyah",
since: "2.2.0",
description: "JavaScript"
}, {
value: "css",
since: "1.7.1",
description: "CSS"
}, {
value: "less",
since: "1.7.1",
description: "Less"
}, {
value: "scss",
since: "1.7.1",
description: "SCSS"
}, {
value: "json",
since: "1.5.0",
description: "JSON"
}, {
value: "json5",
since: "1.13.0",
description: "JSON5"
}, {
value: "json-stringify",
since: "1.13.0",
description: "JSON.stringify"
}, {
value: "graphql",
since: "1.5.0",
description: "GraphQL"
}, {
value: "markdown",
since: "1.8.0",
description: "Markdown"
}, {
value: "mdx",
since: "1.15.0",
description: "MDX"
}, {
value: "vue",
since: "1.10.0",
description: "Vue"
}, {
value: "yaml",
since: "1.14.0",
description: "YAML"
}, {
value: "glimmer",
since: "2.3.0",
description: "Ember / Handlebars"
}, {
value: "html",
since: "1.15.0",
description: "HTML"
}, {
value: "angular",
since: "1.15.0",
description: "Angular"
}, {
value: "lwc",
since: "1.17.0",
description: "Lightning Web Components"
}]
},
plugins: {
since: "1.10.0",
type: "path",
array: true,
default: [{
value: []
}],
category: CATEGORY_GLOBAL,
description: "Add a plugin. Multiple plugins can be passed as separate `--plugin`s.",
exception: (value) => typeof value === "string" || typeof value === "object",
cliName: "plugin",
cliCategory: CATEGORY_CONFIG
},
pluginSearchDirs: {
since: "1.13.0",
type: "path",
array: true,
default: [{
value: []
}],
category: CATEGORY_GLOBAL,
description: outdent`
Custom directory that contains prettier plugins in node_modules subdirectory.
Overrides default behavior when plugins are searched relatively to the location of Prettier.
Multiple values are accepted.
`,
exception: (value) => typeof value === "string" || typeof value === "object",
cliName: "plugin-search-dir",
cliCategory: CATEGORY_CONFIG
},
printWidth: {
since: "0.0.0",
category: CATEGORY_GLOBAL,
type: "int",
default: 80,
description: "The line length where Prettier will try wrap.",
range: {
start: 0,
end: Number.POSITIVE_INFINITY,
step: 1
}
},
rangeEnd: {
since: "1.4.0",
category: CATEGORY_SPECIAL,
type: "int",
default: Number.POSITIVE_INFINITY,
range: {
start: 0,
end: Number.POSITIVE_INFINITY,
step: 1
},
description: outdent`
Format code ending at a given character offset (exclusive).
The range will extend forwards to the end of the selected statement.
This option cannot be used with --cursor-offset.
`,
cliCategory: CATEGORY_EDITOR
},
rangeStart: {
since: "1.4.0",
category: CATEGORY_SPECIAL,
type: "int",
default: 0,
range: {
start: 0,
end: Number.POSITIVE_INFINITY,
step: 1
},
description: outdent`
Format code starting at a given character offset.
The range will extend backwards to the start of the first line containing the selected statement.
This option cannot be used with --cursor-offset.
`,
cliCategory: CATEGORY_EDITOR
},
requirePragma: {
since: "1.7.0",
category: CATEGORY_SPECIAL,
type: "boolean",
default: false,
description: outdent`
Require either '@prettier' or '@format' to be present in the file's first docblock comment
in order for it to be formatted.
`,
cliCategory: CATEGORY_OTHER
},
tabWidth: {
type: "int",
category: CATEGORY_GLOBAL,
default: 2,
description: "Number of spaces per indentation level.",
range: {
start: 0,
end: Number.POSITIVE_INFINITY,
step: 1
}
},
useTabs: {
since: "1.0.0",
category: CATEGORY_GLOBAL,
type: "boolean",
default: false,
description: "Indent with tabs instead of spaces."
},
embeddedLanguageFormatting: {
since: "2.1.0",
category: CATEGORY_GLOBAL,
type: "choice",
default: [{
since: "2.1.0",
value: "auto"
}],
description: "Control how Prettier formats quoted code embedded in the file.",
choices: [{
value: "auto",
description: "Format embedded code if Prettier can automatically identify it."
}, {
value: "off",
description: "Never automatically format embedded code."
}]
}
};
module22.exports = {
CATEGORY_CONFIG,
CATEGORY_EDITOR,
CATEGORY_FORMAT,
CATEGORY_OTHER,
CATEGORY_OUTPUT,
CATEGORY_GLOBAL,
CATEGORY_SPECIAL,
options
};
}
});
var require_support = __commonJS22({
"src/main/support.js"(exports2, module22) {
"use strict";
var semver = {
compare: require_compare(),
lt: require_lt(),
gte: require_gte()
};
var arrayify = require_arrayify();
var currentVersion = require_package().version;
var coreOptions = require_core_options().options;
function getSupportInfo2({
plugins: plugins2 = [],
showUnreleased = false,
showDeprecated = false,
showInternal = false
} = {}) {
const version2 = currentVersion.split("-", 1)[0];
const languages = plugins2.flatMap((plugin) => plugin.languages || []).filter(filterSince);
const options = arrayify(Object.assign({}, ...plugins2.map(({
options: options2
}) => options2), coreOptions), "name").filter((option) => filterSince(option) && filterDeprecated(option)).sort((a, b) => a.name === b.name ? 0 : a.name < b.name ? -1 : 1).map(mapInternal).map((option) => {
option = Object.assign({}, option);
if (Array.isArray(option.default)) {
option.default = option.default.length === 1 ? option.default[0].value : option.default.filter(filterSince).sort((info1, info2) => semver.compare(info2.since, info1.since))[0].value;
}
if (Array.isArray(option.choices)) {
option.choices = option.choices.filter((option2) => filterSince(option2) && filterDeprecated(option2));
if (option.name === "parser") {
collectParsersFromLanguages(option, languages, plugins2);
}
}
const pluginDefaults = Object.fromEntries(plugins2.filter((plugin) => plugin.defaultOptions && plugin.defaultOptions[option.name] !== void 0).map((plugin) => [plugin.name, plugin.defaultOptions[option.name]]));
return Object.assign(Object.assign({}, option), {}, {
pluginDefaults
});
});
return {
languages,
options
};
function filterSince(object) {
return showUnreleased || !("since" in object) || object.since && semver.gte(version2, object.since);
}
function filterDeprecated(object) {
return showDeprecated || !("deprecated" in object) || object.deprecated && semver.lt(version2, object.deprecated);
}
function mapInternal(object) {
if (showInternal) {
return object;
}
const {
cliName,
cliCategory,
cliDescription
} = object, newObject = _objectWithoutProperties(object, _excluded);
return newObject;
}
}
function collectParsersFromLanguages(option, languages, plugins2) {
const existingValues = new Set(option.choices.map((choice) => choice.value));
for (const language of languages) {
if (language.parsers) {
for (const value of language.parsers) {
if (!existingValues.has(value)) {
existingValues.add(value);
const plugin = plugins2.find((plugin2) => plugin2.parsers && plugin2.parsers[value]);
let description = language.name;
if (plugin && plugin.name) {
description += ` (plugin: ${plugin.name})`;
}
option.choices.push({
value,
description
});
}
}
}
}
}
module22.exports = {
getSupportInfo: getSupportInfo2
};
}
});
var require_is_non_empty_array = __commonJS22({
"src/utils/is-non-empty-array.js"(exports2, module22) {
"use strict";
function isNonEmptyArray(object) {
return Array.isArray(object) && object.length > 0;
}
module22.exports = isNonEmptyArray;
}
});
function ansiRegex({
onlyFirst = false
} = {}) {
const pattern = ["[\\u001B\\u009B][[\\]()#;?]*(?:(?:(?:(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]+)*|[a-zA-Z\\d]+(?:;[-a-zA-Z\\d\\/#&.:=?%@~_]*)*)?\\u0007)", "(?:(?:\\d{1,4}(?:;\\d{0,4})*)?[\\dA-PR-TZcf-ntqry=><~]))"].join("|");
return new RegExp(pattern, onlyFirst ? void 0 : "g");
}
var init_ansi_regex = __esm({
"node_modules/strip-ansi/node_modules/ansi-regex/index.js"() {
}
});
function stripAnsi(string) {
if (typeof string !== "string") {
throw new TypeError(`Expected a \`string\`, got \`${typeof string}\``);
}
return string.replace(ansiRegex(), "");
}
var init_strip_ansi = __esm({
"node_modules/strip-ansi/index.js"() {
init_ansi_regex();
}
});
function isFullwidthCodePoint(codePoint) {
if (!Number.isInteger(codePoint)) {
return false;
}
return codePoint >= 4352 && (codePoint <= 4447 || codePoint === 9001 || codePoint === 9002 || 11904 <= codePoint && codePoint <= 12871 && codePoint !== 12351 || 12880 <= codePoint && codePoint <= 19903 || 19968 <= codePoint && codePoint <= 42182 || 43360 <= codePoint && codePoint <= 43388 || 44032 <= codePoint && codePoint <= 55203 || 63744 <= codePoint && codePoint <= 64255 || 65040 <= codePoint && codePoint <= 65049 || 65072 <= codePoint && codePoint <= 65131 || 65281 <= codePoint && codePoint <= 65376 || 65504 <= codePoint && codePoint <= 65510 || 110592 <= codePoint && codePoint <= 110593 || 127488 <= codePoint && codePoint <= 127569 || 131072 <= codePoint && codePoint <= 262141);
}
var init_is_fullwidth_code_point = __esm({
"node_modules/is-fullwidth-code-point/index.js"() {
}
});
var require_emoji_regex = __commonJS22({
"node_modules/emoji-regex/index.js"(exports2, module22) {
"use strict";
module22.exports = function() {
return /\uD83C\uDFF4\uDB40\uDC67\uDB40\uDC62(?:\uDB40\uDC77\uDB40\uDC6C\uDB40\uDC73|\uDB40\uDC73\uDB40\uDC63\uDB40\uDC74|\uDB40\uDC65\uDB40\uDC6E\uDB40\uDC67)\uDB40\uDC7F|(?:\uD83E\uDDD1\uD83C\uDFFF\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFF\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB-\uDFFE])|(?:\uD83E\uDDD1\uD83C\uDFFE\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFE\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB-\uDFFD\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFD\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFD\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB\uDFFC\uDFFE\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFC\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFC\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFB\uDFFD-\uDFFF])|(?:\uD83E\uDDD1\uD83C\uDFFB\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83E\uDDD1|\uD83D\uDC69\uD83C\uDFFB\u200D\uD83E\uDD1D\u200D(?:\uD83D[\uDC68\uDC69]))(?:\uD83C[\uDFFC-\uDFFF])|\uD83D\uDC68(?:\uD83C\uDFFB(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF]))|\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFC-\uDFFF])|[\u2695\u2696\u2708]\uFE0F|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))?|(?:\uD83C[\uDFFC-\uDFFF])\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFF]))|\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D)?\uD83D\uDC68|(?:\uD83D[\uDC68\uDC69])\u200D(?:\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67]))|\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFE])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB-\uDFFD\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB\uDFFC\uDFFE\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83E\uDD1D\u200D\uD83D\uDC68(?:\uD83C[\uDFFB\uDFFD-\uDFFF])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])\uFE0F|\u200D(?:(?:\uD83D[\uDC68\uDC69])\u200D(?:\uD83D[\uDC66\uDC67])|\uD83D[\uDC66\uDC67])|\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC)?|(?:\uD83D\uDC69(?:\uD83C\uDFFB\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69])|(?:\uD83C[\uDFFC-\uDFFF])\u200D\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69]))|\uD83E\uDDD1(?:\uD83C[\uDFFB-\uDFFF])\u200D\uD83E\uDD1D\u200D\uD83E\uDDD1)(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC69\u200D\uD83D\uDC69\u200D(?:\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67]))|\uD83D\uDC69(?:\u200D(?:\u2764\uFE0F\u200D(?:\uD83D\uDC8B\u200D(?:\uD83D[\uDC68\uDC69])|\uD83D[\uDC68\uDC69])|\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFB\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))|\uD83E\uDDD1(?:\u200D(?:\uD83E\uDD1D\u200D\uD83E\uDDD1|\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFF\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFE\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFD\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFC\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD])|\uD83C\uDFFB\u200D(?:\uD83C[\uDF3E\uDF73\uDF7C\uDF84\uDF93\uDFA4\uDFA8\uDFEB\uDFED]|\uD83D[\uDCBB\uDCBC\uDD27\uDD2C\uDE80\uDE92]|\uD83E[\uDDAF-\uDDB3\uDDBC\uDDBD]))|\uD83D\uDC69\u200D\uD83D\uDC66\u200D\uD83D\uDC66|\uD83D\uDC69\u200D\uD83D\uDC69\u200D(?:\uD83D[\uDC66\uDC67])|\uD83D\uDC69\u200D\uD83D\uDC67\u200D(?:\uD83D[\uDC66\uDC67])|(?:\uD83D\uDC41\uFE0F\u200D\uD83D\uDDE8|\uD83E\uDDD1(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\uD83C\uDFFB\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])|\uD83D\uDC69(?:\uD83C\uDFFF\u200D[\u2695\u2696\u2708]|\uD83C\uDFFE\u200D[\u2695\u2696\u2708]|\uD83C\uDFFD\u200D[\u2695\u2696\u2708]|\uD83C\uDFFC\u200D[\u2695\u2696\u2708]|\uD83C\uDFFB\u200D[\u2695\u2696\u2708]|\u200D[\u2695\u2696\u2708])|\uD83D\uDE36\u200D\uD83C\uDF2B|\uD83C\uDFF3\uFE0F\u200D\u26A7|\uD83D\uDC3B\u200D\u2744|(?:(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|\uD83D\uDC6F|\uD83E[\uDD3C\uDDDE\uDDDF])\u200D[\u2640\u2642]|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])\u200D[\u2640\u2642]|\uD83C\uDFF4\u200D\u2620|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])\u200D[\u2640\u2642]|[\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u2328\u23CF\u23ED-\u23EF\u23F1\u23F2\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB\u25FC\u2600-\u2604\u260E\u2611\u2618\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u265F\u2660\u2663\u2665\u2666\u2668\u267B\u267E\u2692\u2694-\u2697\u2699\u269B\u269C\u26A0\u26A7\u26B0\u26B1\u26C8\u26CF\u26D1\u26D3\u26E9\u26F0\u26F1\u26F4\u26F7\u26F8\u2702\u2708\u2709\u270F\u2712\u2714\u2716\u271D\u2721\u2733\u2734\u2744\u2747\u2763\u27A1\u2934\u2935\u2B05-\u2B07\u3030\u303D\u3297\u3299]|\uD83C[\uDD70\uDD71\uDD7E\uDD7F\uDE02\uDE37\uDF21\uDF24-\uDF2C\uDF36\uDF7D\uDF96\uDF97\uDF99-\uDF9B\uDF9E\uDF9F\uDFCD\uDFCE\uDFD4-\uDFDF\uDFF5\uDFF7]|\uD83D[\uDC3F\uDCFD\uDD49\uDD4A\uDD6F\uDD70\uDD73\uDD76-\uDD79\uDD87\uDD8A-\uDD8D\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA\uDECB\uDECD-\uDECF\uDEE0-\uDEE5\uDEE9\uDEF0\uDEF3])\uFE0F|\uD83C\uDFF3\uFE0F\u200D\uD83C\uDF08|\uD83D\uDC69\u200D\uD83D\uDC67|\uD83D\uDC69\u200D\uD83D\uDC66|\uD83D\uDE35\u200D\uD83D\uDCAB|\uD83D\uDE2E\u200D\uD83D\uDCA8|\uD83D\uDC15\u200D\uD83E\uDDBA|\uD83E\uDDD1(?:\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC|\uD83C\uDFFB)?|\uD83D\uDC69(?:\uD83C\uDFFF|\uD83C\uDFFE|\uD83C\uDFFD|\uD83C\uDFFC|\uD83C\uDFFB)?|\uD83C\uDDFD\uD83C\uDDF0|\uD83C\uDDF6\uD83C\uDDE6|\uD83C\uDDF4\uD83C\uDDF2|\uD83D\uDC08\u200D\u2B1B|\u2764\uFE0F\u200D(?:\uD83D\uDD25|\uD83E\uDE79)|\uD83D\uDC41\uFE0F|\uD83C\uDFF3\uFE0F|\uD83C\uDDFF(?:\uD83C[\uDDE6\uDDF2\uDDFC])|\uD83C\uDDFE(?:\uD83C[\uDDEA\uDDF9])|\uD83C\uDDFC(?:\uD83C[\uDDEB\uDDF8])|\uD83C\uDDFB(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDEE\uDDF3\uDDFA])|\uD83C\uDDFA(?:\uD83C[\uDDE6\uDDEC\uDDF2\uDDF3\uDDF8\uDDFE\uDDFF])|\uD83C\uDDF9(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDED\uDDEF-\uDDF4\uDDF7\uDDF9\uDDFB\uDDFC\uDDFF])|\uD83C\uDDF8(?:\uD83C[\uDDE6-\uDDEA\uDDEC-\uDDF4\uDDF7-\uDDF9\uDDFB\uDDFD-\uDDFF])|\uD83C\uDDF7(?:\uD83C[\uDDEA\uDDF4\uDDF8\uDDFA\uDDFC])|\uD83C\uDDF5(?:\uD83C[\uDDE6\uDDEA-\uDDED\uDDF0-\uDDF3\uDDF7-\uDDF9\uDDFC\uDDFE])|\uD83C\uDDF3(?:\uD83C[\uDDE6\uDDE8\uDDEA-\uDDEC\uDDEE\uDDF1\uDDF4\uDDF5\uDDF7\uDDFA\uDDFF])|\uD83C\uDDF2(?:\uD83C[\uDDE6\uDDE8-\uDDED\uDDF0-\uDDFF])|\uD83C\uDDF1(?:\uD83C[\uDDE6-\uDDE8\uDDEE\uDDF0\uDDF7-\uDDFB\uDDFE])|\uD83C\uDDF0(?:\uD83C[\uDDEA\uDDEC-\uDDEE\uDDF2\uDDF3\uDDF5\uDDF7\uDDFC\uDDFE\uDDFF])|\uD83C\uDDEF(?:\uD83C[\uDDEA\uDDF2\uDDF4\uDDF5])|\uD83C\uDDEE(?:\uD83C[\uDDE8-\uDDEA\uDDF1-\uDDF4\uDDF6-\uDDF9])|\uD83C\uDDED(?:\uD83C[\uDDF0\uDDF2\uDDF3\uDDF7\uDDF9\uDDFA])|\uD83C\uDDEC(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEE\uDDF1-\uDDF3\uDDF5-\uDDFA\uDDFC\uDDFE])|\uD83C\uDDEB(?:\uD83C[\uDDEE-\uDDF0\uDDF2\uDDF4\uDDF7])|\uD83C\uDDEA(?:\uD83C[\uDDE6\uDDE8\uDDEA\uDDEC\uDDED\uDDF7-\uDDFA])|\uD83C\uDDE9(?:\uD83C[\uDDEA\uDDEC\uDDEF\uDDF0\uDDF2\uDDF4\uDDFF])|\uD83C\uDDE8(?:\uD83C[\uDDE6\uDDE8\uDDE9\uDDEB-\uDDEE\uDDF0-\uDDF5\uDDF7\uDDFA-\uDDFF])|\uD83C\uDDE7(?:\uD83C[\uDDE6\uDDE7\uDDE9-\uDDEF\uDDF1-\uDDF4\uDDF6-\uDDF9\uDDFB\uDDFC\uDDFE\uDDFF])|\uD83C\uDDE6(?:\uD83C[\uDDE8-\uDDEC\uDDEE\uDDF1\uDDF2\uDDF4\uDDF6-\uDDFA\uDDFC\uDDFD\uDDFF])|[#\*0-9]\uFE0F\u20E3|\u2764\uFE0F|(?:\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD])(?:\uD83C[\uDFFB-\uDFFF])|(?:\u26F9|\uD83C[\uDFCB\uDFCC]|\uD83D\uDD75)(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])|\uD83C\uDFF4|(?:[\u270A\u270B]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC6B-\uDC6D\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDC8F\uDC91\uDCAA\uDD7A\uDD95\uDD96\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD34\uDD36\uDD77\uDDB5\uDDB6\uDDBB\uDDD2\uDDD3\uDDD5])(?:\uD83C[\uDFFB-\uDFFF])|(?:[\u261D\u270C\u270D]|\uD83D[\uDD74\uDD90])(?:\uFE0F|\uD83C[\uDFFB-\uDFFF])|[\u270A\u270B]|\uD83C[\uDF85\uDFC2\uDFC7]|\uD83D[\uDC08\uDC15\uDC3B\uDC42\uDC43\uDC46-\uDC50\uDC66\uDC67\uDC6B-\uDC6D\uDC72\uDC74-\uDC76\uDC78\uDC7C\uDC83\uDC85\uDC8F\uDC91\uDCAA\uDD7A\uDD95\uDD96\uDE2E\uDE35\uDE36\uDE4C\uDE4F\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1C\uDD1E\uDD1F\uDD30-\uDD34\uDD36\uDD77\uDDB5\uDDB6\uDDBB\uDDD2\uDDD3\uDDD5]|\uD83C[\uDFC3\uDFC4\uDFCA]|\uD83D[\uDC6E\uDC70\uDC71\uDC73\uDC77\uDC81\uDC82\uDC86\uDC87\uDE45-\uDE47\uDE4B\uDE4D\uDE4E\uDEA3\uDEB4-\uDEB6]|\uD83E[\uDD26\uDD35\uDD37-\uDD39\uDD3D\uDD3E\uDDB8\uDDB9\uDDCD-\uDDCF\uDDD4\uDDD6-\uDDDD]|\uD83D\uDC6F|\uD83E[\uDD3C\uDDDE\uDDDF]|[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF84\uDF86-\uDF93\uDFA0-\uDFC1\uDFC5\uDFC6\uDFC8\uDFC9\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC07\uDC09-\uDC14\uDC16-\uDC3A\uDC3C-\uDC3E\uDC40\uDC44\uDC45\uDC51-\uDC65\uDC6A\uDC79-\uDC7B\uDC7D-\uDC80\uDC84\uDC88-\uDC8E\uDC90\uDC92-\uDCA9\uDCAB-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDDA4\uDDFB-\uDE2D\uDE2F-\uDE34\uDE37-\uDE44\uDE48-\uDE4A\uDE80-\uDEA2\uDEA4-\uDEB3\uDEB7-\uDEBF\uDEC1-\uDEC5\uDED0-\uDED2\uDED5-\uDED7\uDEEB\uDEEC\uDEF4-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0D\uDD0E\uDD10-\uDD17\uDD1D\uDD20-\uDD25\uDD27-\uDD2F\uDD3A\uDD3F-\uDD45\uDD47-\uDD76\uDD78\uDD7A-\uDDB4\uDDB7\uDDBA\uDDBC-\uDDCB\uDDD0\uDDE0-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6]|(?:[\u231A\u231B\u23E9-\u23EC\u23F0\u23F3\u25FD\u25FE\u2614\u2615\u2648-\u2653\u267F\u2693\u26A1\u26AA\u26AB\u26BD\u26BE\u26C4\u26C5\u26CE\u26D4\u26EA\u26F2\u26F3\u26F5\u26FA\u26FD\u2705\u270A\u270B\u2728\u274C\u274E\u2753-\u2755\u2757\u2795-\u2797\u27B0\u27BF\u2B1B\u2B1C\u2B50\u2B55]|\uD83C[\uDC04\uDCCF\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE1A\uDE2F\uDE32-\uDE36\uDE38-\uDE3A\uDE50\uDE51\uDF00-\uDF20\uDF2D-\uDF35\uDF37-\uDF7C\uDF7E-\uDF93\uDFA0-\uDFCA\uDFCF-\uDFD3\uDFE0-\uDFF0\uDFF4\uDFF8-\uDFFF]|\uD83D[\uDC00-\uDC3E\uDC40\uDC42-\uDCFC\uDCFF-\uDD3D\uDD4B-\uDD4E\uDD50-\uDD67\uDD7A\uDD95\uDD96\uDDA4\uDDFB-\uDE4F\uDE80-\uDEC5\uDECC\uDED0-\uDED2\uDED5-\uDED7\uDEEB\uDEEC\uDEF4-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0C-\uDD3A\uDD3C-\uDD45\uDD47-\uDD78\uDD7A-\uDDCB\uDDCD-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6])|(?:[#\*0-9\xA9\xAE\u203C\u2049\u2122\u2139\u2194-\u2199\u21A9\u21AA\u231A\u231B\u2328\u23CF\u23E9-\u23F3\u23F8-\u23FA\u24C2\u25AA\u25AB\u25B6\u25C0\u25FB-\u25FE\u2600-\u2604\u260E\u2611\u2614\u2615\u2618\u261D\u2620\u2622\u2623\u2626\u262A\u262E\u262F\u2638-\u263A\u2640\u2642\u2648-\u2653\u265F\u2660\u2663\u2665\u2666\u2668\u267B\u267E\u267F\u2692-\u2697\u2699\u269B\u269C\u26A0\u26A1\u26A7\u26AA\u26AB\u26B0\u26B1\u26BD\u26BE\u26C4\u26C5\u26C8\u26CE\u26CF\u26D1\u26D3\u26D4\u26E9\u26EA\u26F0-\u26F5\u26F7-\u26FA\u26FD\u2702\u2705\u2708-\u270D\u270F\u2712\u2714\u2716\u271D\u2721\u2728\u2733\u2734\u2744\u2747\u274C\u274E\u2753-\u2755\u2757\u2763\u2764\u2795-\u2797\u27A1\u27B0\u27BF\u2934\u2935\u2B05-\u2B07\u2B1B\u2B1C\u2B50\u2B55\u3030\u303D\u3297\u3299]|\uD83C[\uDC04\uDCCF\uDD70\uDD71\uDD7E\uDD7F\uDD8E\uDD91-\uDD9A\uDDE6-\uDDFF\uDE01\uDE02\uDE1A\uDE2F\uDE32-\uDE3A\uDE50\uDE51\uDF00-\uDF21\uDF24-\uDF93\uDF96\uDF97\uDF99-\uDF9B\uDF9E-\uDFF0\uDFF3-\uDFF5\uDFF7-\uDFFF]|\uD83D[\uDC00-\uDCFD\uDCFF-\uDD3D\uDD49-\uDD4E\uDD50-\uDD67\uDD6F\uDD70\uDD73-\uDD7A\uDD87\uDD8A-\uDD8D\uDD90\uDD95\uDD96\uDDA4\uDDA5\uDDA8\uDDB1\uDDB2\uDDBC\uDDC2-\uDDC4\uDDD1-\uDDD3\uDDDC-\uDDDE\uDDE1\uDDE3\uDDE8\uDDEF\uDDF3\uDDFA-\uDE4F\uDE80-\uDEC5\uDECB-\uDED2\uDED5-\uDED7\uDEE0-\uDEE5\uDEE9\uDEEB\uDEEC\uDEF0\uDEF3-\uDEFC\uDFE0-\uDFEB]|\uD83E[\uDD0C-\uDD3A\uDD3C-\uDD45\uDD47-\uDD78\uDD7A-\uDDCB\uDDCD-\uDDFF\uDE70-\uDE74\uDE78-\uDE7A\uDE80-\uDE86\uDE90-\uDEA8\uDEB0-\uDEB6\uDEC0-\uDEC2\uDED0-\uDED6])\uFE0F|(?:[\u261D\u26F9\u270A-\u270D]|\uD83C[\uDF85\uDFC2-\uDFC4\uDFC7\uDFCA-\uDFCC]|\uD83D[\uDC42\uDC43\uDC46-\uDC50\uDC66-\uDC78\uDC7C\uDC81-\uDC83\uDC85-\uDC87\uDC8F\uDC91\uDCAA\uDD74\uDD75\uDD7A\uDD90\uDD95\uDD96\uDE45-\uDE47\uDE4B-\uDE4F\uDEA3\uDEB4-\uDEB6\uDEC0\uDECC]|\uD83E[\uDD0C\uDD0F\uDD18-\uDD1F\uDD26\uDD30-\uDD39\uDD3C-\uDD3E\uDD77\uDDB5\uDDB6\uDDB8\uDDB9\uDDBB\uDDCD-\uDDCF\uDDD1-\uDDDD])/g;
};
}
});
var string_width_exports = {};
__export(string_width_exports, {
default: () => stringWidth
});
function stringWidth(string) {
if (typeof string !== "string" || string.length === 0) {
return 0;
}
string = stripAnsi(string);
if (string.length === 0) {
return 0;
}
string = string.replace((0, import_emoji_regex.default)(), " ");
let width = 0;
for (let index = 0; index < string.length; index++) {
const codePoint = string.codePointAt(index);
if (codePoint <= 31 || codePoint >= 127 && codePoint <= 159) {
continue;
}
if (codePoint >= 768 && codePoint <= 879) {
continue;
}
if (codePoint > 65535) {
index++;
}
width += isFullwidthCodePoint(codePoint) ? 2 : 1;
}
return width;
}
var import_emoji_regex;
var init_string_width = __esm({
"node_modules/string-width/index.js"() {
init_strip_ansi();
init_is_fullwidth_code_point();
import_emoji_regex = __toESM2(require_emoji_regex());
}
});
var require_get_string_width = __commonJS22({
"src/utils/get-string-width.js"(exports2, module22) {
"use strict";
var stringWidth2 = (init_string_width(), __toCommonJS(string_width_exports)).default;
var notAsciiRegex = /[^\x20-\x7F]/;
function getStringWidth(text) {
if (!text) {
return 0;
}
if (!notAsciiRegex.test(text)) {
return text.length;
}
return stringWidth2(text);
}
module22.exports = getStringWidth;
}
});
var require_skip = __commonJS22({
"src/utils/text/skip.js"(exports2, module22) {
"use strict";
function skip(chars) {
return (text, index, opts) => {
const backwards = opts && opts.backwards;
if (index === false) {
return false;
}
const {
length
} = text;
let cursor = index;
while (cursor >= 0 && cursor < length) {
const c = text.charAt(cursor);
if (chars instanceof RegExp) {
if (!chars.test(c)) {
return cursor;
}
} else if (!chars.includes(c)) {
return cursor;
}
backwards ? cursor-- : cursor++;
}
if (cursor === -1 || cursor === length) {
return cursor;
}
return false;
};
}
var skipWhitespace = skip(/\s/);
var skipSpaces = skip(" ");
var skipToLineEnd = skip(",; ");
var skipEverythingButNewLine = skip(/[^\n\r]/);
module22.exports = {
skipWhitespace,
skipSpaces,
skipToLineEnd,
skipEverythingButNewLine
};
}
});
var require_skip_inline_comment = __commonJS22({
"src/utils/text/skip-inline-comment.js"(exports2, module22) {
"use strict";
function skipInlineComment(text, index) {
if (index === false) {
return false;
}
if (text.charAt(index) === "/" && text.charAt(index + 1) === "*") {
for (let i = index + 2; i < text.length; ++i) {
if (text.charAt(i) === "*" && text.charAt(i + 1) === "/") {
return i + 2;
}
}
}
return index;
}
module22.exports = skipInlineComment;
}
});
var require_skip_trailing_comment = __commonJS22({
"src/utils/text/skip-trailing-comment.js"(exports2, module22) {
"use strict";
var {
skipEverythingButNewLine
} = require_skip();
function skipTrailingComment(text, index) {
if (index === false) {
return false;
}
if (text.charAt(index) === "/" && text.charAt(index + 1) === "/") {
return skipEverythingButNewLine(text, index);
}
return index;
}
module22.exports = skipTrailingComment;
}
});
var require_skip_newline = __commonJS22({
"src/utils/text/skip-newline.js"(exports2, module22) {
"use strict";
function skipNewline(text, index, opts) {
const backwards = opts && opts.backwards;
if (index === false) {
return false;
}
const atIndex = text.charAt(index);
if (backwards) {
if (text.charAt(index - 1) === "\r" && atIndex === "\n") {
return index - 2;
}
if (atIndex === "\n" || atIndex === "\r" || atIndex === "\u2028" || atIndex === "\u2029") {
return index - 1;
}
} else {
if (atIndex === "\r" && text.charAt(index + 1) === "\n") {
return index + 2;
}
if (atIndex === "\n" || atIndex === "\r" || atIndex === "\u2028" || atIndex === "\u2029") {
return index + 1;
}
}
return index;
}
module22.exports = skipNewline;
}
});
var require_get_next_non_space_non_comment_character_index_with_start_index = __commonJS22({
"src/utils/text/get-next-non-space-non-comment-character-index-with-start-index.js"(exports2, module22) {
"use strict";
var skipInlineComment = require_skip_inline_comment();
var skipNewline = require_skip_newline();
var skipTrailingComment = require_skip_trailing_comment();
var {
skipSpaces
} = require_skip();
function getNextNonSpaceNonCommentCharacterIndexWithStartIndex(text, idx) {
let oldIdx = null;
let nextIdx = idx;
while (nextIdx !== oldIdx) {
oldIdx = nextIdx;
nextIdx = skipSpaces(text, nextIdx);
nextIdx = skipInlineComment(text, nextIdx);
nextIdx = skipTrailingComment(text, nextIdx);
nextIdx = skipNewline(text, nextIdx);
}
return nextIdx;
}
module22.exports = getNextNonSpaceNonCommentCharacterIndexWithStartIndex;
}
});
var require_util = __commonJS22({
"src/common/util.js"(exports2, module22) {
"use strict";
var {
default: escapeStringRegexp2
} = (init_escape_string_regexp(), __toCommonJS(escape_string_regexp_exports));
var getLast = require_get_last();
var {
getSupportInfo: getSupportInfo2
} = require_support();
var isNonEmptyArray = require_is_non_empty_array();
var getStringWidth = require_get_string_width();
var {
skipWhitespace,
skipSpaces,
skipToLineEnd,
skipEverythingButNewLine
} = require_skip();
var skipInlineComment = require_skip_inline_comment();
var skipTrailingComment = require_skip_trailing_comment();
var skipNewline = require_skip_newline();
var getNextNonSpaceNonCommentCharacterIndexWithStartIndex = require_get_next_non_space_non_comment_character_index_with_start_index();
var getPenultimate = (arr) => arr[arr.length - 2];
function skip(chars) {
return (text, index, opts) => {
const backwards = opts && opts.backwards;
if (index === false) {
return false;
}
const {
length
} = text;
let cursor = index;
while (cursor >= 0 && cursor < length) {
const c = text.charAt(cursor);
if (chars instanceof RegExp) {
if (!chars.test(c)) {
return cursor;
}
} else if (!chars.includes(c)) {
return cursor;
}
backwards ? cursor-- : cursor++;
}
if (cursor === -1 || cursor === length) {
return cursor;
}
return false;
};
}
function hasNewline(text, index, opts = {}) {
const idx = skipSpaces(text, opts.backwards ? index - 1 : index, opts);
const idx2 = skipNewline(text, idx, opts);
return idx !== idx2;
}
function hasNewlineInRange(text, start, end) {
for (let i = start; i < end; ++i) {
if (text.charAt(i) === "\n") {
return true;
}
}
return false;
}
function isPreviousLineEmpty(text, node, locStart) {
let idx = locStart(node) - 1;
idx = skipSpaces(text, idx, {
backwards: true
});
idx = skipNewline(text, idx, {
backwards: true
});
idx = skipSpaces(text, idx, {
backwards: true
});
const idx2 = skipNewline(text, idx, {
backwards: true
});
return idx !== idx2;
}
function isNextLineEmptyAfterIndex(text, index) {
let oldIdx = null;
let idx = index;
while (idx !== oldIdx) {
oldIdx = idx;
idx = skipToLineEnd(text, idx);
idx = skipInlineComment(text, idx);
idx = skipSpaces(text, idx);
}
idx = skipTrailingComment(text, idx);
idx = skipNewline(text, idx);
return idx !== false && hasNewline(text, idx);
}
function isNextLineEmpty(text, node, locEnd) {
return isNextLineEmptyAfterIndex(text, locEnd(node));
}
function getNextNonSpaceNonCommentCharacterIndex(text, node, locEnd) {
return getNextNonSpaceNonCommentCharacterIndexWithStartIndex(text, locEnd(node));
}
function getNextNonSpaceNonCommentCharacter(text, node, locEnd) {
return text.charAt(getNextNonSpaceNonCommentCharacterIndex(text, node, locEnd));
}
function hasSpaces(text, index, opts = {}) {
const idx = skipSpaces(text, opts.backwards ? index - 1 : index, opts);
return idx !== index;
}
function getAlignmentSize(value, tabWidth, startIndex = 0) {
let size = 0;
for (let i = startIndex; i < value.length; ++i) {
if (value[i] === " ") {
size = size + tabWidth - size % tabWidth;
} else {
size++;
}
}
return size;
}
function getIndentSize(value, tabWidth) {
const lastNewlineIndex = value.lastIndexOf("\n");
if (lastNewlineIndex === -1) {
return 0;
}
return getAlignmentSize(value.slice(lastNewlineIndex + 1).match(/^[\t ]*/)[0], tabWidth);
}
function getPreferredQuote(rawContent, preferredQuote) {
const double = {
quote: '"',
regex: /"/g,
escaped: """
};
const single = {
quote: "'",
regex: /'/g,
escaped: "'"
};
const preferred = preferredQuote === "'" ? single : double;
const alternate = preferred === single ? double : single;
let result = preferred;
if (rawContent.includes(preferred.quote) || rawContent.includes(alternate.quote)) {
const numPreferredQuotes = (rawContent.match(preferred.regex) || []).length;
const numAlternateQuotes = (rawContent.match(alternate.regex) || []).length;
result = numPreferredQuotes > numAlternateQuotes ? alternate : preferred;
}
return result;
}
function printString(raw, options) {
const rawContent = raw.slice(1, -1);
const enclosingQuote = options.parser === "json" || options.parser === "json5" && options.quoteProps === "preserve" && !options.singleQuote ? '"' : options.__isInHtmlAttribute ? "'" : getPreferredQuote(rawContent, options.singleQuote ? "'" : '"').quote;
return makeString(rawContent, enclosingQuote, !(options.parser === "css" || options.parser === "less" || options.parser === "scss" || options.__embeddedInHtml));
}
function makeString(rawContent, enclosingQuote, unescapeUnnecessaryEscapes) {
const otherQuote = enclosingQuote === '"' ? "'" : '"';
const regex = /\\(.)|(["'])/gs;
const newContent = rawContent.replace(regex, (match, escaped, quote2) => {
if (escaped === otherQuote) {
return escaped;
}
if (quote2 === enclosingQuote) {
return "\\" + quote2;
}
if (quote2) {
return quote2;
}
return unescapeUnnecessaryEscapes && /^[^\n\r"'0-7\\bfnrt-vx\u2028\u2029]$/.test(escaped) ? escaped : "\\" + escaped;
});
return enclosingQuote + newContent + enclosingQuote;
}
function printNumber(rawNumber) {
return rawNumber.toLowerCase().replace(/^([+-]?[\d.]+e)(?:\+|(-))?0*(\d)/, "$1$2$3").replace(/^([+-]?[\d.]+)e[+-]?0+$/, "$1").replace(/^([+-])?\./, "$10.").replace(/(\.\d+?)0+(?=e|$)/, "$1").replace(/\.(?=e|$)/, "");
}
function getMaxContinuousCount(str, target) {
const results = str.match(new RegExp(`(${escapeStringRegexp2(target)})+`, "g"));
if (results === null) {
return 0;
}
return results.reduce((maxCount, result) => Math.max(maxCount, result.length / target.length), 0);
}
function getMinNotPresentContinuousCount(str, target) {
const matches = str.match(new RegExp(`(${escapeStringRegexp2(target)})+`, "g"));
if (matches === null) {
return 0;
}
const countPresent = /* @__PURE__ */ new Map();
let max = 0;
for (const match of matches) {
const count = match.length / target.length;
countPresent.set(count, true);
if (count > max) {
max = count;
}
}
for (let i = 1; i < max; i++) {
if (!countPresent.get(i)) {
return i;
}
}
return max + 1;
}
function addCommentHelper(node, comment) {
const comments = node.comments || (node.comments = []);
comments.push(comment);
comment.printed = false;
comment.nodeDescription = describeNodeForDebugging(node);
}
function addLeadingComment(node, comment) {
comment.leading = true;
comment.trailing = false;
addCommentHelper(node, comment);
}
function addDanglingComment(node, comment, marker) {
comment.leading = false;
comment.trailing = false;
if (marker) {
comment.marker = marker;
}
addCommentHelper(node, comment);
}
function addTrailingComment(node, comment) {
comment.leading = false;
comment.trailing = true;
addCommentHelper(node, comment);
}
function inferParserByLanguage(language, options) {
const {
languages
} = getSupportInfo2({
plugins: options.plugins
});
const matched = languages.find(({
name
}) => name.toLowerCase() === language) || languages.find(({
aliases
}) => Array.isArray(aliases) && aliases.includes(language)) || languages.find(({
extensions
}) => Array.isArray(extensions) && extensions.includes(`.${language}`));
return matched && matched.parsers[0];
}
function isFrontMatterNode(node) {
return node && node.type === "front-matter";
}
function createGroupIdMapper(description) {
const groupIds = /* @__PURE__ */ new WeakMap();
return function(node) {
if (!groupIds.has(node)) {
groupIds.set(node, Symbol(description));
}
return groupIds.get(node);
};
}
function describeNodeForDebugging(node) {
const nodeType = node.type || node.kind || "(unknown type)";
let nodeName = String(node.name || node.id && (typeof node.id === "object" ? node.id.name : node.id) || node.key && (typeof node.key === "object" ? node.key.name : node.key) || node.value && (typeof node.value === "object" ? "" : String(node.value)) || node.operator || "");
if (nodeName.length > 20) {
nodeName = nodeName.slice(0, 19) + "\u2026";
}
return nodeType + (nodeName ? " " + nodeName : "");
}
module22.exports = {
inferParserByLanguage,
getStringWidth,
getMaxContinuousCount,
getMinNotPresentContinuousCount,
getPenultimate,
getLast,
getNextNonSpaceNonCommentCharacterIndexWithStartIndex,
getNextNonSpaceNonCommentCharacterIndex,
getNextNonSpaceNonCommentCharacter,
skip,
skipWhitespace,
skipSpaces,
skipToLineEnd,
skipEverythingButNewLine,
skipInlineComment,
skipTrailingComment,
skipNewline,
isNextLineEmptyAfterIndex,
isNextLineEmpty,
isPreviousLineEmpty,
hasNewline,
hasNewlineInRange,
hasSpaces,
getAlignmentSize,
getIndentSize,
getPreferredQuote,
printString,
printNumber,
makeString,
addLeadingComment,
addDanglingComment,
addTrailingComment,
isFrontMatterNode,
isNonEmptyArray,
createGroupIdMapper
};
}
});
var require_end_of_line = __commonJS22({
"src/common/end-of-line.js"(exports2, module22) {
"use strict";
function guessEndOfLine(text) {
const index = text.indexOf("\r");
if (index >= 0) {
return text.charAt(index + 1) === "\n" ? "crlf" : "cr";
}
return "lf";
}
function convertEndOfLineToChars(value) {
switch (value) {
case "cr":
return "\r";
case "crlf":
return "\r\n";
default:
return "\n";
}
}
function countEndOfLineChars(text, eol) {
let regex;
switch (eol) {
case "\n":
regex = /\n/g;
break;
case "\r":
regex = /\r/g;
break;
case "\r\n":
regex = /\r\n/g;
break;
default:
throw new Error(`Unexpected "eol" ${JSON.stringify(eol)}.`);
}
const endOfLines = text.match(regex);
return endOfLines ? endOfLines.length : 0;
}
function normalizeEndOfLine(text) {
return text.replace(/\r\n?/g, "\n");
}
module22.exports = {
guessEndOfLine,
convertEndOfLineToChars,
countEndOfLineChars,
normalizeEndOfLine
};
}
});
var require_errors = __commonJS22({
"src/common/errors.js"(exports2, module22) {
"use strict";
var ConfigError = class extends Error {
};
var DebugError = class extends Error {
};
var UndefinedParserError = class extends Error {
};
var ArgExpansionBailout = class extends Error {
};
module22.exports = {
ConfigError,
DebugError,
UndefinedParserError,
ArgExpansionBailout
};
}
});
var tslib_es6_exports = {};
__export(tslib_es6_exports, {
__assign: () => __assign,
__asyncDelegator: () => __asyncDelegator,
__asyncGenerator: () => __asyncGenerator,
__asyncValues: () => __asyncValues,
__await: () => __await,
__awaiter: () => __awaiter,
__classPrivateFieldGet: () => __classPrivateFieldGet,
__classPrivateFieldSet: () => __classPrivateFieldSet,
__createBinding: () => __createBinding,
__decorate: () => __decorate,
__exportStar: () => __exportStar,
__extends: () => __extends,
__generator: () => __generator,
__importDefault: () => __importDefault,
__importStar: () => __importStar,
__makeTemplateObject: () => __makeTemplateObject,
__metadata: () => __metadata,
__param: () => __param,
__read: () => __read,
__rest: () => __rest,
__spread: () => __spread,
__spreadArrays: () => __spreadArrays,
__values: () => __values
});
function __extends(d, b) {
extendStatics(d, b);
function __() {
this.constructor = d;
}
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
}
function __rest(s, e) {
var t = {};
for (var p in s)
if (Object.prototype.hasOwnProperty.call(s, p) && e.indexOf(p) < 0)
t[p] = s[p];
if (s != null && typeof Object.getOwnPropertySymbols === "function")
for (var i = 0, p = Object.getOwnPropertySymbols(s); i < p.length; i++) {
if (e.indexOf(p[i]) < 0 && Object.prototype.propertyIsEnumerable.call(s, p[i]))
t[p[i]] = s[p[i]];
}
return t;
}
function __decorate(decorators, target, key, desc) {
var c = arguments.length, r = c < 3 ? target : desc === null ? desc = Object.getOwnPropertyDescriptor(target, key) : desc, d;
if (typeof Reflect === "object" && typeof Reflect.decorate === "function")
r = Reflect.decorate(decorators, target, key, desc);
else
for (var i = decorators.length - 1; i >= 0; i--)
if (d = decorators[i])
r = (c < 3 ? d(r) : c > 3 ? d(target, key, r) : d(target, key)) || r;
return c > 3 && r && Object.defineProperty(target, key, r), r;
}
function __param(paramIndex, decorator) {
return function(target, key) {
decorator(target, key, paramIndex);
};
}
function __metadata(metadataKey, metadataValue) {
if (typeof Reflect === "object" && typeof Reflect.metadata === "function")
return Reflect.metadata(metadataKey, metadataValue);
}
function __awaiter(thisArg, _arguments, P, generator) {
function adopt(value) {
return value instanceof P ? value : new P(function(resolve) {
resolve(value);
});
}
return new (P || (P = Promise))(function(resolve, reject) {
function fulfilled(value) {
try {
step(generator.next(value));
} catch (e) {
reject(e);
}
}
function rejected(value) {
try {
step(generator["throw"](value));
} catch (e) {
reject(e);
}
}
function step(result) {
result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected);
}
step((generator = generator.apply(thisArg, _arguments || [])).next());
});
}
function __generator(thisArg, body) {
var _ = {
label: 0,
sent: function() {
if (t[0] & 1)
throw t[1];
return t[1];
},
trys: [],
ops: []
}, f, y, t, g;
return g = {
next: verb(0),
"throw": verb(1),
"return": verb(2)
}, typeof Symbol === "function" && (g[Symbol.iterator] = function() {
return this;
}), g;
function verb(n2) {
return function(v) {
return step([n2, v]);
};
}
function step(op) {
if (f)
throw new TypeError("Generator is already executing.");
while (_)
try {
if (f = 1, y && (t = op[0] & 2 ? y["return"] : op[0] ? y["throw"] || ((t = y["return"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done)
return t;
if (y = 0, t)
op = [op[0] & 2, t.value];
switch (op[0]) {
case 0:
case 1:
t = op;
break;
case 4:
_.label++;
return {
value: op[1],
done: false
};
case 5:
_.label++;
y = op[1];
op = [0];
continue;
case 7:
op = _.ops.pop();
_.trys.pop();
continue;
default:
if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) {
_ = 0;
continue;
}
if (op[0] === 3 && (!t || op[1] > t[0] && op[1] < t[3])) {
_.label = op[1];
break;
}
if (op[0] === 6 && _.label < t[1]) {
_.label = t[1];
t = op;
break;
}
if (t && _.label < t[2]) {
_.label = t[2];
_.ops.push(op);
break;
}
if (t[2])
_.ops.pop();
_.trys.pop();
continue;
}
op = body.call(thisArg, _);
} catch (e) {
op = [6, e];
y = 0;
} finally {
f = t = 0;
}
if (op[0] & 5)
throw op[1];
return {
value: op[0] ? op[1] : void 0,
done: true
};
}
}
function __createBinding(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
o[k2] = m[k];
}
function __exportStar(m, exports2) {
for (var p in m)
if (p !== "default" && !exports2.hasOwnProperty(p))
exports2[p] = m[p];
}
function __values(o) {
var s = typeof Symbol === "function" && Symbol.iterator, m = s && o[s], i = 0;
if (m)
return m.call(o);
if (o && typeof o.length === "number")
return {
next: function() {
if (o && i >= o.length)
o = void 0;
return {
value: o && o[i++],
done: !o
};
}
};
throw new TypeError(s ? "Object is not iterable." : "Symbol.iterator is not defined.");
}
function __read(o, n2) {
var m = typeof Symbol === "function" && o[Symbol.iterator];
if (!m)
return o;
var i = m.call(o), r, ar = [], e;
try {
while ((n2 === void 0 || n2-- > 0) && !(r = i.next()).done)
ar.push(r.value);
} catch (error) {
e = {
error
};
} finally {
try {
if (r && !r.done && (m = i["return"]))
m.call(i);
} finally {
if (e)
throw e.error;
}
}
return ar;
}
function __spread() {
for (var ar = [], i = 0; i < arguments.length; i++)
ar = ar.concat(__read(arguments[i]));
return ar;
}
function __spreadArrays() {
for (var s = 0, i = 0, il = arguments.length; i < il; i++)
s += arguments[i].length;
for (var r = Array(s), k = 0, i = 0; i < il; i++)
for (var a = arguments[i], j = 0, jl = a.length; j < jl; j++, k++)
r[k] = a[j];
return r;
}
function __await(v) {
return this instanceof __await ? (this.v = v, this) : new __await(v);
}
function __asyncGenerator(thisArg, _arguments, generator) {
if (!Symbol.asyncIterator)
throw new TypeError("Symbol.asyncIterator is not defined.");
var g = generator.apply(thisArg, _arguments || []), i, q = [];
return i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function() {
return this;
}, i;
function verb(n2) {
if (g[n2])
i[n2] = function(v) {
return new Promise(function(a, b) {
q.push([n2, v, a, b]) > 1 || resume(n2, v);
});
};
}
function resume(n2, v) {
try {
step(g[n2](v));
} catch (e) {
settle(q[0][3], e);
}
}
function step(r) {
r.value instanceof __await ? Promise.resolve(r.value.v).then(fulfill, reject) : settle(q[0][2], r);
}
function fulfill(value) {
resume("next", value);
}
function reject(value) {
resume("throw", value);
}
function settle(f, v) {
if (f(v), q.shift(), q.length)
resume(q[0][0], q[0][1]);
}
}
function __asyncDelegator(o) {
var i, p;
return i = {}, verb("next"), verb("throw", function(e) {
throw e;
}), verb("return"), i[Symbol.iterator] = function() {
return this;
}, i;
function verb(n2, f) {
i[n2] = o[n2] ? function(v) {
return (p = !p) ? {
value: __await(o[n2](v)),
done: n2 === "return"
} : f ? f(v) : v;
} : f;
}
}
function __asyncValues(o) {
if (!Symbol.asyncIterator)
throw new TypeError("Symbol.asyncIterator is not defined.");
var m = o[Symbol.asyncIterator], i;
return m ? m.call(o) : (o = typeof __values === "function" ? __values(o) : o[Symbol.iterator](), i = {}, verb("next"), verb("throw"), verb("return"), i[Symbol.asyncIterator] = function() {
return this;
}, i);
function verb(n2) {
i[n2] = o[n2] && function(v) {
return new Promise(function(resolve, reject) {
v = o[n2](v), settle(resolve, reject, v.done, v.value);
});
};
}
function settle(resolve, reject, d, v) {
Promise.resolve(v).then(function(v2) {
resolve({
value: v2,
done: d
});
}, reject);
}
}
function __makeTemplateObject(cooked, raw) {
if (Object.defineProperty) {
Object.defineProperty(cooked, "raw", {
value: raw
});
} else {
cooked.raw = raw;
}
return cooked;
}
function __importStar(mod) {
if (mod && mod.__esModule)
return mod;
var result = {};
if (mod != null) {
for (var k in mod)
if (Object.hasOwnProperty.call(mod, k))
result[k] = mod[k];
}
result.default = mod;
return result;
}
function __importDefault(mod) {
return mod && mod.__esModule ? mod : {
default: mod
};
}
function __classPrivateFieldGet(receiver, privateMap) {
if (!privateMap.has(receiver)) {
throw new TypeError("attempted to get private field on non-instance");
}
return privateMap.get(receiver);
}
function __classPrivateFieldSet(receiver, privateMap, value) {
if (!privateMap.has(receiver)) {
throw new TypeError("attempted to set private field on non-instance");
}
privateMap.set(receiver, value);
return value;
}
var extendStatics;
var __assign;
var init_tslib_es6 = __esm({
"node_modules/tslib/tslib.es6.js"() {
extendStatics = function(d, b) {
extendStatics = Object.setPrototypeOf || {
__proto__: []
} instanceof Array && function(d2, b2) {
d2.__proto__ = b2;
} || function(d2, b2) {
for (var p in b2)
if (b2.hasOwnProperty(p))
d2[p] = b2[p];
};
return extendStatics(d, b);
};
__assign = function() {
__assign = Object.assign || function __assign2(t) {
for (var s, i = 1, n2 = arguments.length; i < n2; i++) {
s = arguments[i];
for (var p in s)
if (Object.prototype.hasOwnProperty.call(s, p))
t[p] = s[p];
}
return t;
};
return __assign.apply(this, arguments);
};
}
});
var require_api = __commonJS22({
"node_modules/vnopts/lib/descriptors/api.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.apiDescriptor = {
key: (key) => /^[$_a-zA-Z][$_a-zA-Z0-9]*$/.test(key) ? key : JSON.stringify(key),
value(value) {
if (value === null || typeof value !== "object") {
return JSON.stringify(value);
}
if (Array.isArray(value)) {
return `[${value.map((subValue) => exports2.apiDescriptor.value(subValue)).join(", ")}]`;
}
const keys = Object.keys(value);
return keys.length === 0 ? "{}" : `{ ${keys.map((key) => `${exports2.apiDescriptor.key(key)}: ${exports2.apiDescriptor.value(value[key])}`).join(", ")} }`;
},
pair: ({
key,
value
}) => exports2.apiDescriptor.value({
[key]: value
})
};
}
});
var require_descriptors2 = __commonJS22({
"node_modules/vnopts/lib/descriptors/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var tslib_1 = (init_tslib_es6(), __toCommonJS(tslib_es6_exports));
tslib_1.__exportStar(require_api(), exports2);
}
});
var require_escape_string_regexp = __commonJS22({
"node_modules/vnopts/node_modules/escape-string-regexp/index.js"(exports2, module22) {
"use strict";
var matchOperatorsRe = /[|\\{}()[\]^$+*?.]/g;
module22.exports = function(str) {
if (typeof str !== "string") {
throw new TypeError("Expected a string");
}
return str.replace(matchOperatorsRe, "\\$&");
};
}
});
var require_color_name = __commonJS22({
"node_modules/color-name/index.js"(exports2, module22) {
"use strict";
module22.exports = {
"aliceblue": [240, 248, 255],
"antiquewhite": [250, 235, 215],
"aqua": [0, 255, 255],
"aquamarine": [127, 255, 212],
"azure": [240, 255, 255],
"beige": [245, 245, 220],
"bisque": [255, 228, 196],
"black": [0, 0, 0],
"blanchedalmond": [255, 235, 205],
"blue": [0, 0, 255],
"blueviolet": [138, 43, 226],
"brown": [165, 42, 42],
"burlywood": [222, 184, 135],
"cadetblue": [95, 158, 160],
"chartreuse": [127, 255, 0],
"chocolate": [210, 105, 30],
"coral": [255, 127, 80],
"cornflowerblue": [100, 149, 237],
"cornsilk": [255, 248, 220],
"crimson": [220, 20, 60],
"cyan": [0, 255, 255],
"darkblue": [0, 0, 139],
"darkcyan": [0, 139, 139],
"darkgoldenrod": [184, 134, 11],
"darkgray": [169, 169, 169],
"darkgreen": [0, 100, 0],
"darkgrey": [169, 169, 169],
"darkkhaki": [189, 183, 107],
"darkmagenta": [139, 0, 139],
"darkolivegreen": [85, 107, 47],
"darkorange": [255, 140, 0],
"darkorchid": [153, 50, 204],
"darkred": [139, 0, 0],
"darksalmon": [233, 150, 122],
"darkseagreen": [143, 188, 143],
"darkslateblue": [72, 61, 139],
"darkslategray": [47, 79, 79],
"darkslategrey": [47, 79, 79],
"darkturquoise": [0, 206, 209],
"darkviolet": [148, 0, 211],
"deeppink": [255, 20, 147],
"deepskyblue": [0, 191, 255],
"dimgray": [105, 105, 105],
"dimgrey": [105, 105, 105],
"dodgerblue": [30, 144, 255],
"firebrick": [178, 34, 34],
"floralwhite": [255, 250, 240],
"forestgreen": [34, 139, 34],
"fuchsia": [255, 0, 255],
"gainsboro": [220, 220, 220],
"ghostwhite": [248, 248, 255],
"gold": [255, 215, 0],
"goldenrod": [218, 165, 32],
"gray": [128, 128, 128],
"green": [0, 128, 0],
"greenyellow": [173, 255, 47],
"grey": [128, 128, 128],
"honeydew": [240, 255, 240],
"hotpink": [255, 105, 180],
"indianred": [205, 92, 92],
"indigo": [75, 0, 130],
"ivory": [255, 255, 240],
"khaki": [240, 230, 140],
"lavender": [230, 230, 250],
"lavenderblush": [255, 240, 245],
"lawngreen": [124, 252, 0],
"lemonchiffon": [255, 250, 205],
"lightblue": [173, 216, 230],
"lightcoral": [240, 128, 128],
"lightcyan": [224, 255, 255],
"lightgoldenrodyellow": [250, 250, 210],
"lightgray": [211, 211, 211],
"lightgreen": [144, 238, 144],
"lightgrey": [211, 211, 211],
"lightpink": [255, 182, 193],
"lightsalmon": [255, 160, 122],
"lightseagreen": [32, 178, 170],
"lightskyblue": [135, 206, 250],
"lightslategray": [119, 136, 153],
"lightslategrey": [119, 136, 153],
"lightsteelblue": [176, 196, 222],
"lightyellow": [255, 255, 224],
"lime": [0, 255, 0],
"limegreen": [50, 205, 50],
"linen": [250, 240, 230],
"magenta": [255, 0, 255],
"maroon": [128, 0, 0],
"mediumaquamarine": [102, 205, 170],
"mediumblue": [0, 0, 205],
"mediumorchid": [186, 85, 211],
"mediumpurple": [147, 112, 219],
"mediumseagreen": [60, 179, 113],
"mediumslateblue": [123, 104, 238],
"mediumspringgreen": [0, 250, 154],
"mediumturquoise": [72, 209, 204],
"mediumvioletred": [199, 21, 133],
"midnightblue": [25, 25, 112],
"mintcream": [245, 255, 250],
"mistyrose": [255, 228, 225],
"moccasin": [255, 228, 181],
"navajowhite": [255, 222, 173],
"navy": [0, 0, 128],
"oldlace": [253, 245, 230],
"olive": [128, 128, 0],
"olivedrab": [107, 142, 35],
"orange": [255, 165, 0],
"orangered": [255, 69, 0],
"orchid": [218, 112, 214],
"palegoldenrod": [238, 232, 170],
"palegreen": [152, 251, 152],
"paleturquoise": [175, 238, 238],
"palevioletred": [219, 112, 147],
"papayawhip": [255, 239, 213],
"peachpuff": [255, 218, 185],
"peru": [205, 133, 63],
"pink": [255, 192, 203],
"plum": [221, 160, 221],
"powderblue": [176, 224, 230],
"purple": [128, 0, 128],
"rebeccapurple": [102, 51, 153],
"red": [255, 0, 0],
"rosybrown": [188, 143, 143],
"royalblue": [65, 105, 225],
"saddlebrown": [139, 69, 19],
"salmon": [250, 128, 114],
"sandybrown": [244, 164, 96],
"seagreen": [46, 139, 87],
"seashell": [255, 245, 238],
"sienna": [160, 82, 45],
"silver": [192, 192, 192],
"skyblue": [135, 206, 235],
"slateblue": [106, 90, 205],
"slategray": [112, 128, 144],
"slategrey": [112, 128, 144],
"snow": [255, 250, 250],
"springgreen": [0, 255, 127],
"steelblue": [70, 130, 180],
"tan": [210, 180, 140],
"teal": [0, 128, 128],
"thistle": [216, 191, 216],
"tomato": [255, 99, 71],
"turquoise": [64, 224, 208],
"violet": [238, 130, 238],
"wheat": [245, 222, 179],
"white": [255, 255, 255],
"whitesmoke": [245, 245, 245],
"yellow": [255, 255, 0],
"yellowgreen": [154, 205, 50]
};
}
});
var require_conversions = __commonJS22({
"node_modules/color-convert/conversions.js"(exports2, module22) {
var cssKeywords = require_color_name();
var reverseKeywords = {};
for (key in cssKeywords) {
if (cssKeywords.hasOwnProperty(key)) {
reverseKeywords[cssKeywords[key]] = key;
}
}
var key;
var convert = module22.exports = {
rgb: {
channels: 3,
labels: "rgb"
},
hsl: {
channels: 3,
labels: "hsl"
},
hsv: {
channels: 3,
labels: "hsv"
},
hwb: {
channels: 3,
labels: "hwb"
},
cmyk: {
channels: 4,
labels: "cmyk"
},
xyz: {
channels: 3,
labels: "xyz"
},
lab: {
channels: 3,
labels: "lab"
},
lch: {
channels: 3,
labels: "lch"
},
hex: {
channels: 1,
labels: ["hex"]
},
keyword: {
channels: 1,
labels: ["keyword"]
},
ansi16: {
channels: 1,
labels: ["ansi16"]
},
ansi256: {
channels: 1,
labels: ["ansi256"]
},
hcg: {
channels: 3,
labels: ["h", "c", "g"]
},
apple: {
channels: 3,
labels: ["r16", "g16", "b16"]
},
gray: {
channels: 1,
labels: ["gray"]
}
};
for (model in convert) {
if (convert.hasOwnProperty(model)) {
if (!("channels" in convert[model])) {
throw new Error("missing channels property: " + model);
}
if (!("labels" in convert[model])) {
throw new Error("missing channel labels property: " + model);
}
if (convert[model].labels.length !== convert[model].channels) {
throw new Error("channel and label counts mismatch: " + model);
}
channels = convert[model].channels;
labels = convert[model].labels;
delete convert[model].channels;
delete convert[model].labels;
Object.defineProperty(convert[model], "channels", {
value: channels
});
Object.defineProperty(convert[model], "labels", {
value: labels
});
}
}
var channels;
var labels;
var model;
convert.rgb.hsl = function(rgb) {
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
var min = Math.min(r, g, b);
var max = Math.max(r, g, b);
var delta = max - min;
var h;
var s;
var l;
if (max === min) {
h = 0;
} else if (r === max) {
h = (g - b) / delta;
} else if (g === max) {
h = 2 + (b - r) / delta;
} else if (b === max) {
h = 4 + (r - g) / delta;
}
h = Math.min(h * 60, 360);
if (h < 0) {
h += 360;
}
l = (min + max) / 2;
if (max === min) {
s = 0;
} else if (l <= 0.5) {
s = delta / (max + min);
} else {
s = delta / (2 - max - min);
}
return [h, s * 100, l * 100];
};
convert.rgb.hsv = function(rgb) {
var rdif;
var gdif;
var bdif;
var h;
var s;
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
var v = Math.max(r, g, b);
var diff = v - Math.min(r, g, b);
var diffc = function(c) {
return (v - c) / 6 / diff + 1 / 2;
};
if (diff === 0) {
h = s = 0;
} else {
s = diff / v;
rdif = diffc(r);
gdif = diffc(g);
bdif = diffc(b);
if (r === v) {
h = bdif - gdif;
} else if (g === v) {
h = 1 / 3 + rdif - bdif;
} else if (b === v) {
h = 2 / 3 + gdif - rdif;
}
if (h < 0) {
h += 1;
} else if (h > 1) {
h -= 1;
}
}
return [h * 360, s * 100, v * 100];
};
convert.rgb.hwb = function(rgb) {
var r = rgb[0];
var g = rgb[1];
var b = rgb[2];
var h = convert.rgb.hsl(rgb)[0];
var w = 1 / 255 * Math.min(r, Math.min(g, b));
b = 1 - 1 / 255 * Math.max(r, Math.max(g, b));
return [h, w * 100, b * 100];
};
convert.rgb.cmyk = function(rgb) {
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
var c;
var m;
var y;
var k;
k = Math.min(1 - r, 1 - g, 1 - b);
c = (1 - r - k) / (1 - k) || 0;
m = (1 - g - k) / (1 - k) || 0;
y = (1 - b - k) / (1 - k) || 0;
return [c * 100, m * 100, y * 100, k * 100];
};
function comparativeDistance(x, y) {
return Math.pow(x[0] - y[0], 2) + Math.pow(x[1] - y[1], 2) + Math.pow(x[2] - y[2], 2);
}
convert.rgb.keyword = function(rgb) {
var reversed = reverseKeywords[rgb];
if (reversed) {
return reversed;
}
var currentClosestDistance = Infinity;
var currentClosestKeyword;
for (var keyword in cssKeywords) {
if (cssKeywords.hasOwnProperty(keyword)) {
var value = cssKeywords[keyword];
var distance = comparativeDistance(rgb, value);
if (distance < currentClosestDistance) {
currentClosestDistance = distance;
currentClosestKeyword = keyword;
}
}
}
return currentClosestKeyword;
};
convert.keyword.rgb = function(keyword) {
return cssKeywords[keyword];
};
convert.rgb.xyz = function(rgb) {
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
r = r > 0.04045 ? Math.pow((r + 0.055) / 1.055, 2.4) : r / 12.92;
g = g > 0.04045 ? Math.pow((g + 0.055) / 1.055, 2.4) : g / 12.92;
b = b > 0.04045 ? Math.pow((b + 0.055) / 1.055, 2.4) : b / 12.92;
var x = r * 0.4124 + g * 0.3576 + b * 0.1805;
var y = r * 0.2126 + g * 0.7152 + b * 0.0722;
var z = r * 0.0193 + g * 0.1192 + b * 0.9505;
return [x * 100, y * 100, z * 100];
};
convert.rgb.lab = function(rgb) {
var xyz = convert.rgb.xyz(rgb);
var x = xyz[0];
var y = xyz[1];
var z = xyz[2];
var l;
var a;
var b;
x /= 95.047;
y /= 100;
z /= 108.883;
x = x > 8856e-6 ? Math.pow(x, 1 / 3) : 7.787 * x + 16 / 116;
y = y > 8856e-6 ? Math.pow(y, 1 / 3) : 7.787 * y + 16 / 116;
z = z > 8856e-6 ? Math.pow(z, 1 / 3) : 7.787 * z + 16 / 116;
l = 116 * y - 16;
a = 500 * (x - y);
b = 200 * (y - z);
return [l, a, b];
};
convert.hsl.rgb = function(hsl) {
var h = hsl[0] / 360;
var s = hsl[1] / 100;
var l = hsl[2] / 100;
var t1;
var t2;
var t3;
var rgb;
var val;
if (s === 0) {
val = l * 255;
return [val, val, val];
}
if (l < 0.5) {
t2 = l * (1 + s);
} else {
t2 = l + s - l * s;
}
t1 = 2 * l - t2;
rgb = [0, 0, 0];
for (var i = 0; i < 3; i++) {
t3 = h + 1 / 3 * -(i - 1);
if (t3 < 0) {
t3++;
}
if (t3 > 1) {
t3--;
}
if (6 * t3 < 1) {
val = t1 + (t2 - t1) * 6 * t3;
} else if (2 * t3 < 1) {
val = t2;
} else if (3 * t3 < 2) {
val = t1 + (t2 - t1) * (2 / 3 - t3) * 6;
} else {
val = t1;
}
rgb[i] = val * 255;
}
return rgb;
};
convert.hsl.hsv = function(hsl) {
var h = hsl[0];
var s = hsl[1] / 100;
var l = hsl[2] / 100;
var smin = s;
var lmin = Math.max(l, 0.01);
var sv;
var v;
l *= 2;
s *= l <= 1 ? l : 2 - l;
smin *= lmin <= 1 ? lmin : 2 - lmin;
v = (l + s) / 2;
sv = l === 0 ? 2 * smin / (lmin + smin) : 2 * s / (l + s);
return [h, sv * 100, v * 100];
};
convert.hsv.rgb = function(hsv) {
var h = hsv[0] / 60;
var s = hsv[1] / 100;
var v = hsv[2] / 100;
var hi = Math.floor(h) % 6;
var f = h - Math.floor(h);
var p = 255 * v * (1 - s);
var q = 255 * v * (1 - s * f);
var t = 255 * v * (1 - s * (1 - f));
v *= 255;
switch (hi) {
case 0:
return [v, t, p];
case 1:
return [q, v, p];
case 2:
return [p, v, t];
case 3:
return [p, q, v];
case 4:
return [t, p, v];
case 5:
return [v, p, q];
}
};
convert.hsv.hsl = function(hsv) {
var h = hsv[0];
var s = hsv[1] / 100;
var v = hsv[2] / 100;
var vmin = Math.max(v, 0.01);
var lmin;
var sl;
var l;
l = (2 - s) * v;
lmin = (2 - s) * vmin;
sl = s * vmin;
sl /= lmin <= 1 ? lmin : 2 - lmin;
sl = sl || 0;
l /= 2;
return [h, sl * 100, l * 100];
};
convert.hwb.rgb = function(hwb) {
var h = hwb[0] / 360;
var wh = hwb[1] / 100;
var bl = hwb[2] / 100;
var ratio = wh + bl;
var i;
var v;
var f;
var n2;
if (ratio > 1) {
wh /= ratio;
bl /= ratio;
}
i = Math.floor(6 * h);
v = 1 - bl;
f = 6 * h - i;
if ((i & 1) !== 0) {
f = 1 - f;
}
n2 = wh + f * (v - wh);
var r;
var g;
var b;
switch (i) {
default:
case 6:
case 0:
r = v;
g = n2;
b = wh;
break;
case 1:
r = n2;
g = v;
b = wh;
break;
case 2:
r = wh;
g = v;
b = n2;
break;
case 3:
r = wh;
g = n2;
b = v;
break;
case 4:
r = n2;
g = wh;
b = v;
break;
case 5:
r = v;
g = wh;
b = n2;
break;
}
return [r * 255, g * 255, b * 255];
};
convert.cmyk.rgb = function(cmyk) {
var c = cmyk[0] / 100;
var m = cmyk[1] / 100;
var y = cmyk[2] / 100;
var k = cmyk[3] / 100;
var r;
var g;
var b;
r = 1 - Math.min(1, c * (1 - k) + k);
g = 1 - Math.min(1, m * (1 - k) + k);
b = 1 - Math.min(1, y * (1 - k) + k);
return [r * 255, g * 255, b * 255];
};
convert.xyz.rgb = function(xyz) {
var x = xyz[0] / 100;
var y = xyz[1] / 100;
var z = xyz[2] / 100;
var r;
var g;
var b;
r = x * 3.2406 + y * -1.5372 + z * -0.4986;
g = x * -0.9689 + y * 1.8758 + z * 0.0415;
b = x * 0.0557 + y * -0.204 + z * 1.057;
r = r > 31308e-7 ? 1.055 * Math.pow(r, 1 / 2.4) - 0.055 : r * 12.92;
g = g > 31308e-7 ? 1.055 * Math.pow(g, 1 / 2.4) - 0.055 : g * 12.92;
b = b > 31308e-7 ? 1.055 * Math.pow(b, 1 / 2.4) - 0.055 : b * 12.92;
r = Math.min(Math.max(0, r), 1);
g = Math.min(Math.max(0, g), 1);
b = Math.min(Math.max(0, b), 1);
return [r * 255, g * 255, b * 255];
};
convert.xyz.lab = function(xyz) {
var x = xyz[0];
var y = xyz[1];
var z = xyz[2];
var l;
var a;
var b;
x /= 95.047;
y /= 100;
z /= 108.883;
x = x > 8856e-6 ? Math.pow(x, 1 / 3) : 7.787 * x + 16 / 116;
y = y > 8856e-6 ? Math.pow(y, 1 / 3) : 7.787 * y + 16 / 116;
z = z > 8856e-6 ? Math.pow(z, 1 / 3) : 7.787 * z + 16 / 116;
l = 116 * y - 16;
a = 500 * (x - y);
b = 200 * (y - z);
return [l, a, b];
};
convert.lab.xyz = function(lab) {
var l = lab[0];
var a = lab[1];
var b = lab[2];
var x;
var y;
var z;
y = (l + 16) / 116;
x = a / 500 + y;
z = y - b / 200;
var y2 = Math.pow(y, 3);
var x2 = Math.pow(x, 3);
var z2 = Math.pow(z, 3);
y = y2 > 8856e-6 ? y2 : (y - 16 / 116) / 7.787;
x = x2 > 8856e-6 ? x2 : (x - 16 / 116) / 7.787;
z = z2 > 8856e-6 ? z2 : (z - 16 / 116) / 7.787;
x *= 95.047;
y *= 100;
z *= 108.883;
return [x, y, z];
};
convert.lab.lch = function(lab) {
var l = lab[0];
var a = lab[1];
var b = lab[2];
var hr;
var h;
var c;
hr = Math.atan2(b, a);
h = hr * 360 / 2 / Math.PI;
if (h < 0) {
h += 360;
}
c = Math.sqrt(a * a + b * b);
return [l, c, h];
};
convert.lch.lab = function(lch) {
var l = lch[0];
var c = lch[1];
var h = lch[2];
var a;
var b;
var hr;
hr = h / 360 * 2 * Math.PI;
a = c * Math.cos(hr);
b = c * Math.sin(hr);
return [l, a, b];
};
convert.rgb.ansi16 = function(args) {
var r = args[0];
var g = args[1];
var b = args[2];
var value = 1 in arguments ? arguments[1] : convert.rgb.hsv(args)[2];
value = Math.round(value / 50);
if (value === 0) {
return 30;
}
var ansi = 30 + (Math.round(b / 255) << 2 | Math.round(g / 255) << 1 | Math.round(r / 255));
if (value === 2) {
ansi += 60;
}
return ansi;
};
convert.hsv.ansi16 = function(args) {
return convert.rgb.ansi16(convert.hsv.rgb(args), args[2]);
};
convert.rgb.ansi256 = function(args) {
var r = args[0];
var g = args[1];
var b = args[2];
if (r === g && g === b) {
if (r < 8) {
return 16;
}
if (r > 248) {
return 231;
}
return Math.round((r - 8) / 247 * 24) + 232;
}
var ansi = 16 + 36 * Math.round(r / 255 * 5) + 6 * Math.round(g / 255 * 5) + Math.round(b / 255 * 5);
return ansi;
};
convert.ansi16.rgb = function(args) {
var color = args % 10;
if (color === 0 || color === 7) {
if (args > 50) {
color += 3.5;
}
color = color / 10.5 * 255;
return [color, color, color];
}
var mult = (~~(args > 50) + 1) * 0.5;
var r = (color & 1) * mult * 255;
var g = (color >> 1 & 1) * mult * 255;
var b = (color >> 2 & 1) * mult * 255;
return [r, g, b];
};
convert.ansi256.rgb = function(args) {
if (args >= 232) {
var c = (args - 232) * 10 + 8;
return [c, c, c];
}
args -= 16;
var rem;
var r = Math.floor(args / 36) / 5 * 255;
var g = Math.floor((rem = args % 36) / 6) / 5 * 255;
var b = rem % 6 / 5 * 255;
return [r, g, b];
};
convert.rgb.hex = function(args) {
var integer = ((Math.round(args[0]) & 255) << 16) + ((Math.round(args[1]) & 255) << 8) + (Math.round(args[2]) & 255);
var string = integer.toString(16).toUpperCase();
return "000000".substring(string.length) + string;
};
convert.hex.rgb = function(args) {
var match = args.toString(16).match(/[a-f0-9]{6}|[a-f0-9]{3}/i);
if (!match) {
return [0, 0, 0];
}
var colorString = match[0];
if (match[0].length === 3) {
colorString = colorString.split("").map(function(char2) {
return char2 + char2;
}).join("");
}
var integer = parseInt(colorString, 16);
var r = integer >> 16 & 255;
var g = integer >> 8 & 255;
var b = integer & 255;
return [r, g, b];
};
convert.rgb.hcg = function(rgb) {
var r = rgb[0] / 255;
var g = rgb[1] / 255;
var b = rgb[2] / 255;
var max = Math.max(Math.max(r, g), b);
var min = Math.min(Math.min(r, g), b);
var chroma = max - min;
var grayscale;
var hue;
if (chroma < 1) {
grayscale = min / (1 - chroma);
} else {
grayscale = 0;
}
if (chroma <= 0) {
hue = 0;
} else if (max === r) {
hue = (g - b) / chroma % 6;
} else if (max === g) {
hue = 2 + (b - r) / chroma;
} else {
hue = 4 + (r - g) / chroma + 4;
}
hue /= 6;
hue %= 1;
return [hue * 360, chroma * 100, grayscale * 100];
};
convert.hsl.hcg = function(hsl) {
var s = hsl[1] / 100;
var l = hsl[2] / 100;
var c = 1;
var f = 0;
if (l < 0.5) {
c = 2 * s * l;
} else {
c = 2 * s * (1 - l);
}
if (c < 1) {
f = (l - 0.5 * c) / (1 - c);
}
return [hsl[0], c * 100, f * 100];
};
convert.hsv.hcg = function(hsv) {
var s = hsv[1] / 100;
var v = hsv[2] / 100;
var c = s * v;
var f = 0;
if (c < 1) {
f = (v - c) / (1 - c);
}
return [hsv[0], c * 100, f * 100];
};
convert.hcg.rgb = function(hcg) {
var h = hcg[0] / 360;
var c = hcg[1] / 100;
var g = hcg[2] / 100;
if (c === 0) {
return [g * 255, g * 255, g * 255];
}
var pure = [0, 0, 0];
var hi = h % 1 * 6;
var v = hi % 1;
var w = 1 - v;
var mg = 0;
switch (Math.floor(hi)) {
case 0:
pure[0] = 1;
pure[1] = v;
pure[2] = 0;
break;
case 1:
pure[0] = w;
pure[1] = 1;
pure[2] = 0;
break;
case 2:
pure[0] = 0;
pure[1] = 1;
pure[2] = v;
break;
case 3:
pure[0] = 0;
pure[1] = w;
pure[2] = 1;
break;
case 4:
pure[0] = v;
pure[1] = 0;
pure[2] = 1;
break;
default:
pure[0] = 1;
pure[1] = 0;
pure[2] = w;
}
mg = (1 - c) * g;
return [(c * pure[0] + mg) * 255, (c * pure[1] + mg) * 255, (c * pure[2] + mg) * 255];
};
convert.hcg.hsv = function(hcg) {
var c = hcg[1] / 100;
var g = hcg[2] / 100;
var v = c + g * (1 - c);
var f = 0;
if (v > 0) {
f = c / v;
}
return [hcg[0], f * 100, v * 100];
};
convert.hcg.hsl = function(hcg) {
var c = hcg[1] / 100;
var g = hcg[2] / 100;
var l = g * (1 - c) + 0.5 * c;
var s = 0;
if (l > 0 && l < 0.5) {
s = c / (2 * l);
} else if (l >= 0.5 && l < 1) {
s = c / (2 * (1 - l));
}
return [hcg[0], s * 100, l * 100];
};
convert.hcg.hwb = function(hcg) {
var c = hcg[1] / 100;
var g = hcg[2] / 100;
var v = c + g * (1 - c);
return [hcg[0], (v - c) * 100, (1 - v) * 100];
};
convert.hwb.hcg = function(hwb) {
var w = hwb[1] / 100;
var b = hwb[2] / 100;
var v = 1 - b;
var c = v - w;
var g = 0;
if (c < 1) {
g = (v - c) / (1 - c);
}
return [hwb[0], c * 100, g * 100];
};
convert.apple.rgb = function(apple) {
return [apple[0] / 65535 * 255, apple[1] / 65535 * 255, apple[2] / 65535 * 255];
};
convert.rgb.apple = function(rgb) {
return [rgb[0] / 255 * 65535, rgb[1] / 255 * 65535, rgb[2] / 255 * 65535];
};
convert.gray.rgb = function(args) {
return [args[0] / 100 * 255, args[0] / 100 * 255, args[0] / 100 * 255];
};
convert.gray.hsl = convert.gray.hsv = function(args) {
return [0, 0, args[0]];
};
convert.gray.hwb = function(gray) {
return [0, 100, gray[0]];
};
convert.gray.cmyk = function(gray) {
return [0, 0, 0, gray[0]];
};
convert.gray.lab = function(gray) {
return [gray[0], 0, 0];
};
convert.gray.hex = function(gray) {
var val = Math.round(gray[0] / 100 * 255) & 255;
var integer = (val << 16) + (val << 8) + val;
var string = integer.toString(16).toUpperCase();
return "000000".substring(string.length) + string;
};
convert.rgb.gray = function(rgb) {
var val = (rgb[0] + rgb[1] + rgb[2]) / 3;
return [val / 255 * 100];
};
}
});
var require_route = __commonJS22({
"node_modules/color-convert/route.js"(exports2, module22) {
var conversions = require_conversions();
function buildGraph() {
var graph = {};
var models = Object.keys(conversions);
for (var len = models.length, i = 0; i < len; i++) {
graph[models[i]] = {
distance: -1,
parent: null
};
}
return graph;
}
function deriveBFS(fromModel) {
var graph = buildGraph();
var queue = [fromModel];
graph[fromModel].distance = 0;
while (queue.length) {
var current = queue.pop();
var adjacents = Object.keys(conversions[current]);
for (var len = adjacents.length, i = 0; i < len; i++) {
var adjacent = adjacents[i];
var node = graph[adjacent];
if (node.distance === -1) {
node.distance = graph[current].distance + 1;
node.parent = current;
queue.unshift(adjacent);
}
}
}
return graph;
}
function link(from, to) {
return function(args) {
return to(from(args));
};
}
function wrapConversion(toModel, graph) {
var path = [graph[toModel].parent, toModel];
var fn = conversions[graph[toModel].parent][toModel];
var cur = graph[toModel].parent;
while (graph[cur].parent) {
path.unshift(graph[cur].parent);
fn = link(conversions[graph[cur].parent][cur], fn);
cur = graph[cur].parent;
}
fn.conversion = path;
return fn;
}
module22.exports = function(fromModel) {
var graph = deriveBFS(fromModel);
var conversion = {};
var models = Object.keys(graph);
for (var len = models.length, i = 0; i < len; i++) {
var toModel = models[i];
var node = graph[toModel];
if (node.parent === null) {
continue;
}
conversion[toModel] = wrapConversion(toModel, graph);
}
return conversion;
};
}
});
var require_color_convert = __commonJS22({
"node_modules/color-convert/index.js"(exports2, module22) {
var conversions = require_conversions();
var route = require_route();
var convert = {};
var models = Object.keys(conversions);
function wrapRaw(fn) {
var wrappedFn = function(args) {
if (args === void 0 || args === null) {
return args;
}
if (arguments.length > 1) {
args = Array.prototype.slice.call(arguments);
}
return fn(args);
};
if ("conversion" in fn) {
wrappedFn.conversion = fn.conversion;
}
return wrappedFn;
}
function wrapRounded(fn) {
var wrappedFn = function(args) {
if (args === void 0 || args === null) {
return args;
}
if (arguments.length > 1) {
args = Array.prototype.slice.call(arguments);
}
var result = fn(args);
if (typeof result === "object") {
for (var len = result.length, i = 0; i < len; i++) {
result[i] = Math.round(result[i]);
}
}
return result;
};
if ("conversion" in fn) {
wrappedFn.conversion = fn.conversion;
}
return wrappedFn;
}
models.forEach(function(fromModel) {
convert[fromModel] = {};
Object.defineProperty(convert[fromModel], "channels", {
value: conversions[fromModel].channels
});
Object.defineProperty(convert[fromModel], "labels", {
value: conversions[fromModel].labels
});
var routes = route(fromModel);
var routeModels = Object.keys(routes);
routeModels.forEach(function(toModel) {
var fn = routes[toModel];
convert[fromModel][toModel] = wrapRounded(fn);
convert[fromModel][toModel].raw = wrapRaw(fn);
});
});
module22.exports = convert;
}
});
var require_ansi_styles = __commonJS22({
"node_modules/ansi-styles/index.js"(exports2, module22) {
"use strict";
var colorConvert = require_color_convert();
var wrapAnsi16 = (fn, offset) => function() {
const code = fn.apply(colorConvert, arguments);
return `\x1B[${code + offset}m`;
};
var wrapAnsi256 = (fn, offset) => function() {
const code = fn.apply(colorConvert, arguments);
return `\x1B[${38 + offset};5;${code}m`;
};
var wrapAnsi16m = (fn, offset) => function() {
const rgb = fn.apply(colorConvert, arguments);
return `\x1B[${38 + offset};2;${rgb[0]};${rgb[1]};${rgb[2]}m`;
};
function assembleStyles() {
const codes = /* @__PURE__ */ new Map();
const styles = {
modifier: {
reset: [0, 0],
bold: [1, 22],
dim: [2, 22],
italic: [3, 23],
underline: [4, 24],
inverse: [7, 27],
hidden: [8, 28],
strikethrough: [9, 29]
},
color: {
black: [30, 39],
red: [31, 39],
green: [32, 39],
yellow: [33, 39],
blue: [34, 39],
magenta: [35, 39],
cyan: [36, 39],
white: [37, 39],
gray: [90, 39],
redBright: [91, 39],
greenBright: [92, 39],
yellowBright: [93, 39],
blueBright: [94, 39],
magentaBright: [95, 39],
cyanBright: [96, 39],
whiteBright: [97, 39]
},
bgColor: {
bgBlack: [40, 49],
bgRed: [41, 49],
bgGreen: [42, 49],
bgYellow: [43, 49],
bgBlue: [44, 49],
bgMagenta: [45, 49],
bgCyan: [46, 49],
bgWhite: [47, 49],
bgBlackBright: [100, 49],
bgRedBright: [101, 49],
bgGreenBright: [102, 49],
bgYellowBright: [103, 49],
bgBlueBright: [104, 49],
bgMagentaBright: [105, 49],
bgCyanBright: [106, 49],
bgWhiteBright: [107, 49]
}
};
styles.color.grey = styles.color.gray;
for (const groupName of Object.keys(styles)) {
const group = styles[groupName];
for (const styleName of Object.keys(group)) {
const style = group[styleName];
styles[styleName] = {
open: `\x1B[${style[0]}m`,
close: `\x1B[${style[1]}m`
};
group[styleName] = styles[styleName];
codes.set(style[0], style[1]);
}
Object.defineProperty(styles, groupName, {
value: group,
enumerable: false
});
Object.defineProperty(styles, "codes", {
value: codes,
enumerable: false
});
}
const ansi2ansi = (n2) => n2;
const rgb2rgb = (r, g, b) => [r, g, b];
styles.color.close = "\x1B[39m";
styles.bgColor.close = "\x1B[49m";
styles.color.ansi = {
ansi: wrapAnsi16(ansi2ansi, 0)
};
styles.color.ansi256 = {
ansi256: wrapAnsi256(ansi2ansi, 0)
};
styles.color.ansi16m = {
rgb: wrapAnsi16m(rgb2rgb, 0)
};
styles.bgColor.ansi = {
ansi: wrapAnsi16(ansi2ansi, 10)
};
styles.bgColor.ansi256 = {
ansi256: wrapAnsi256(ansi2ansi, 10)
};
styles.bgColor.ansi16m = {
rgb: wrapAnsi16m(rgb2rgb, 10)
};
for (let key of Object.keys(colorConvert)) {
if (typeof colorConvert[key] !== "object") {
continue;
}
const suite = colorConvert[key];
if (key === "ansi16") {
key = "ansi";
}
if ("ansi16" in suite) {
styles.color.ansi[key] = wrapAnsi16(suite.ansi16, 0);
styles.bgColor.ansi[key] = wrapAnsi16(suite.ansi16, 10);
}
if ("ansi256" in suite) {
styles.color.ansi256[key] = wrapAnsi256(suite.ansi256, 0);
styles.bgColor.ansi256[key] = wrapAnsi256(suite.ansi256, 10);
}
if ("rgb" in suite) {
styles.color.ansi16m[key] = wrapAnsi16m(suite.rgb, 0);
styles.bgColor.ansi16m[key] = wrapAnsi16m(suite.rgb, 10);
}
}
return styles;
}
Object.defineProperty(module22, "exports", {
enumerable: true,
get: assembleStyles
});
}
});
var require_has_flag = __commonJS22({
"node_modules/vnopts/node_modules/has-flag/index.js"(exports2, module22) {
"use strict";
module22.exports = (flag, argv) => {
argv = argv || process.argv;
const prefix = flag.startsWith("-") ? "" : flag.length === 1 ? "-" : "--";
const pos = argv.indexOf(prefix + flag);
const terminatorPos = argv.indexOf("--");
return pos !== -1 && (terminatorPos === -1 ? true : pos < terminatorPos);
};
}
});
var require_supports_color = __commonJS22({
"node_modules/vnopts/node_modules/supports-color/index.js"(exports2, module22) {
"use strict";
var os = require("os");
var hasFlag = require_has_flag();
var env = process.env;
var forceColor;
if (hasFlag("no-color") || hasFlag("no-colors") || hasFlag("color=false")) {
forceColor = false;
} else if (hasFlag("color") || hasFlag("colors") || hasFlag("color=true") || hasFlag("color=always")) {
forceColor = true;
}
if ("FORCE_COLOR" in env) {
forceColor = env.FORCE_COLOR.length === 0 || parseInt(env.FORCE_COLOR, 10) !== 0;
}
function translateLevel(level) {
if (level === 0) {
return false;
}
return {
level,
hasBasic: true,
has256: level >= 2,
has16m: level >= 3
};
}
function supportsColor(stream) {
if (forceColor === false) {
return 0;
}
if (hasFlag("color=16m") || hasFlag("color=full") || hasFlag("color=truecolor")) {
return 3;
}
if (hasFlag("color=256")) {
return 2;
}
if (stream && !stream.isTTY && forceColor !== true) {
return 0;
}
const min = forceColor ? 1 : 0;
if (process.platform === "win32") {
const osRelease = os.release().split(".");
if (Number(process.versions.node.split(".")[0]) >= 8 && Number(osRelease[0]) >= 10 && Number(osRelease[2]) >= 10586) {
return Number(osRelease[2]) >= 14931 ? 3 : 2;
}
return 1;
}
if ("CI" in env) {
if (["TRAVIS", "CIRCLECI", "APPVEYOR", "GITLAB_CI"].some((sign) => sign in env) || env.CI_NAME === "codeship") {
return 1;
}
return min;
}
if ("TEAMCITY_VERSION" in env) {
return /^(9\.(0*[1-9]\d*)\.|\d{2,}\.)/.test(env.TEAMCITY_VERSION) ? 1 : 0;
}
if (env.COLORTERM === "truecolor") {
return 3;
}
if ("TERM_PROGRAM" in env) {
const version2 = parseInt((env.TERM_PROGRAM_VERSION || "").split(".")[0], 10);
switch (env.TERM_PROGRAM) {
case "iTerm.app":
return version2 >= 3 ? 3 : 2;
case "Apple_Terminal":
return 2;
}
}
if (/-256(color)?$/i.test(env.TERM)) {
return 2;
}
if (/^screen|^xterm|^vt100|^vt220|^rxvt|color|ansi|cygwin|linux/i.test(env.TERM)) {
return 1;
}
if ("COLORTERM" in env) {
return 1;
}
if (env.TERM === "dumb") {
return min;
}
return min;
}
function getSupportLevel(stream) {
const level = supportsColor(stream);
return translateLevel(level);
}
module22.exports = {
supportsColor: getSupportLevel,
stdout: getSupportLevel(process.stdout),
stderr: getSupportLevel(process.stderr)
};
}
});
var require_templates = __commonJS22({
"node_modules/vnopts/node_modules/chalk/templates.js"(exports2, module22) {
"use strict";
var TEMPLATE_REGEX = /(?:\\(u[a-f\d]{4}|x[a-f\d]{2}|.))|(?:\{(~)?(\w+(?:\([^)]*\))?(?:\.\w+(?:\([^)]*\))?)*)(?:[ \t]|(?=\r?\n)))|(\})|((?:.|[\r\n\f])+?)/gi;
var STYLE_REGEX = /(?:^|\.)(\w+)(?:\(([^)]*)\))?/g;
var STRING_REGEX = /^(['"])((?:\\.|(?!\1)[^\\])*)\1$/;
var ESCAPE_REGEX = /\\(u[a-f\d]{4}|x[a-f\d]{2}|.)|([^\\])/gi;
var ESCAPES = /* @__PURE__ */ new Map([["n", "\n"], ["r", "\r"], ["t", " "], ["b", "\b"], ["f", "\f"], ["v", "\v"], ["0", "\0"], ["\\", "\\"], ["e", "\x1B"], ["a", "\x07"]]);
function unescape2(c) {
if (c[0] === "u" && c.length === 5 || c[0] === "x" && c.length === 3) {
return String.fromCharCode(parseInt(c.slice(1), 16));
}
return ESCAPES.get(c) || c;
}
function parseArguments(name, args) {
const results = [];
const chunks = args.trim().split(/\s*,\s*/g);
let matches;
for (const chunk of chunks) {
if (!isNaN(chunk)) {
results.push(Number(chunk));
} else if (matches = chunk.match(STRING_REGEX)) {
results.push(matches[2].replace(ESCAPE_REGEX, (m, escape, chr) => escape ? unescape2(escape) : chr));
} else {
throw new Error(`Invalid Chalk template style argument: ${chunk} (in style '${name}')`);
}
}
return results;
}
function parseStyle(style) {
STYLE_REGEX.lastIndex = 0;
const results = [];
let matches;
while ((matches = STYLE_REGEX.exec(style)) !== null) {
const name = matches[1];
if (matches[2]) {
const args = parseArguments(name, matches[2]);
results.push([name].concat(args));
} else {
results.push([name]);
}
}
return results;
}
function buildStyle(chalk, styles) {
const enabled = {};
for (const layer of styles) {
for (const style of layer.styles) {
enabled[style[0]] = layer.inverse ? null : style.slice(1);
}
}
let current = chalk;
for (const styleName of Object.keys(enabled)) {
if (Array.isArray(enabled[styleName])) {
if (!(styleName in current)) {
throw new Error(`Unknown Chalk style: ${styleName}`);
}
if (enabled[styleName].length > 0) {
current = current[styleName].apply(current, enabled[styleName]);
} else {
current = current[styleName];
}
}
}
return current;
}
module22.exports = (chalk, tmp) => {
const styles = [];
const chunks = [];
let chunk = [];
tmp.replace(TEMPLATE_REGEX, (m, escapeChar, inverse, style, close, chr) => {
if (escapeChar) {
chunk.push(unescape2(escapeChar));
} else if (style) {
const str = chunk.join("");
chunk = [];
chunks.push(styles.length === 0 ? str : buildStyle(chalk, styles)(str));
styles.push({
inverse,
styles: parseStyle(style)
});
} else if (close) {
if (styles.length === 0) {
throw new Error("Found extraneous } in Chalk template literal");
}
chunks.push(buildStyle(chalk, styles)(chunk.join("")));
chunk = [];
styles.pop();
} else {
chunk.push(chr);
}
});
chunks.push(chunk.join(""));
if (styles.length > 0) {
const errMsg = `Chalk template literal is missing ${styles.length} closing bracket${styles.length === 1 ? "" : "s"} (\`}\`)`;
throw new Error(errMsg);
}
return chunks.join("");
};
}
});
var require_chalk = __commonJS22({
"node_modules/vnopts/node_modules/chalk/index.js"(exports2, module22) {
"use strict";
var escapeStringRegexp2 = require_escape_string_regexp();
var ansiStyles = require_ansi_styles();
var stdoutColor = require_supports_color().stdout;
var template = require_templates();
var isSimpleWindowsTerm = process.platform === "win32" && !(process.env.TERM || "").toLowerCase().startsWith("xterm");
var levelMapping = ["ansi", "ansi", "ansi256", "ansi16m"];
var skipModels = /* @__PURE__ */ new Set(["gray"]);
var styles = /* @__PURE__ */ Object.create(null);
function applyOptions(obj, options) {
options = options || {};
const scLevel = stdoutColor ? stdoutColor.level : 0;
obj.level = options.level === void 0 ? scLevel : options.level;
obj.enabled = "enabled" in options ? options.enabled : obj.level > 0;
}
function Chalk(options) {
if (!this || !(this instanceof Chalk) || this.template) {
const chalk = {};
applyOptions(chalk, options);
chalk.template = function() {
const args = [].slice.call(arguments);
return chalkTag.apply(null, [chalk.template].concat(args));
};
Object.setPrototypeOf(chalk, Chalk.prototype);
Object.setPrototypeOf(chalk.template, chalk);
chalk.template.constructor = Chalk;
return chalk.template;
}
applyOptions(this, options);
}
if (isSimpleWindowsTerm) {
ansiStyles.blue.open = "\x1B[94m";
}
for (const key of Object.keys(ansiStyles)) {
ansiStyles[key].closeRe = new RegExp(escapeStringRegexp2(ansiStyles[key].close), "g");
styles[key] = {
get() {
const codes = ansiStyles[key];
return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, key);
}
};
}
styles.visible = {
get() {
return build.call(this, this._styles || [], true, "visible");
}
};
ansiStyles.color.closeRe = new RegExp(escapeStringRegexp2(ansiStyles.color.close), "g");
for (const model of Object.keys(ansiStyles.color.ansi)) {
if (skipModels.has(model)) {
continue;
}
styles[model] = {
get() {
const level = this.level;
return function() {
const open = ansiStyles.color[levelMapping[level]][model].apply(null, arguments);
const codes = {
open,
close: ansiStyles.color.close,
closeRe: ansiStyles.color.closeRe
};
return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, model);
};
}
};
}
ansiStyles.bgColor.closeRe = new RegExp(escapeStringRegexp2(ansiStyles.bgColor.close), "g");
for (const model of Object.keys(ansiStyles.bgColor.ansi)) {
if (skipModels.has(model)) {
continue;
}
const bgModel = "bg" + model[0].toUpperCase() + model.slice(1);
styles[bgModel] = {
get() {
const level = this.level;
return function() {
const open = ansiStyles.bgColor[levelMapping[level]][model].apply(null, arguments);
const codes = {
open,
close: ansiStyles.bgColor.close,
closeRe: ansiStyles.bgColor.closeRe
};
return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, model);
};
}
};
}
var proto = Object.defineProperties(() => {
}, styles);
function build(_styles, _empty, key) {
const builder = function() {
return applyStyle.apply(builder, arguments);
};
builder._styles = _styles;
builder._empty = _empty;
const self2 = this;
Object.defineProperty(builder, "level", {
enumerable: true,
get() {
return self2.level;
},
set(level) {
self2.level = level;
}
});
Object.defineProperty(builder, "enabled", {
enumerable: true,
get() {
return self2.enabled;
},
set(enabled) {
self2.enabled = enabled;
}
});
builder.hasGrey = this.hasGrey || key === "gray" || key === "grey";
builder.__proto__ = proto;
return builder;
}
function applyStyle() {
const args = arguments;
const argsLen = args.length;
let str = String(arguments[0]);
if (argsLen === 0) {
return "";
}
if (argsLen > 1) {
for (let a = 1; a < argsLen; a++) {
str += " " + args[a];
}
}
if (!this.enabled || this.level <= 0 || !str) {
return this._empty ? "" : str;
}
const originalDim = ansiStyles.dim.open;
if (isSimpleWindowsTerm && this.hasGrey) {
ansiStyles.dim.open = "";
}
for (const code of this._styles.slice().reverse()) {
str = code.open + str.replace(code.closeRe, code.open) + code.close;
str = str.replace(/\r?\n/g, `${code.close}$&${code.open}`);
}
ansiStyles.dim.open = originalDim;
return str;
}
function chalkTag(chalk, strings) {
if (!Array.isArray(strings)) {
return [].slice.call(arguments, 1).join(" ");
}
const args = [].slice.call(arguments, 2);
const parts = [strings.raw[0]];
for (let i = 1; i < strings.length; i++) {
parts.push(String(args[i - 1]).replace(/[{}\\]/g, "\\$&"));
parts.push(String(strings.raw[i]));
}
return template(chalk, parts.join(""));
}
Object.defineProperties(Chalk.prototype, styles);
module22.exports = Chalk();
module22.exports.supportsColor = stdoutColor;
module22.exports.default = module22.exports;
}
});
var require_common = __commonJS22({
"node_modules/vnopts/lib/handlers/deprecated/common.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var chalk_1 = require_chalk();
exports2.commonDeprecatedHandler = (keyOrPair, redirectTo, {
descriptor
}) => {
const messages = [`${chalk_1.default.yellow(typeof keyOrPair === "string" ? descriptor.key(keyOrPair) : descriptor.pair(keyOrPair))} is deprecated`];
if (redirectTo) {
messages.push(`we now treat it as ${chalk_1.default.blue(typeof redirectTo === "string" ? descriptor.key(redirectTo) : descriptor.pair(redirectTo))}`);
}
return messages.join("; ") + ".";
};
}
});
var require_deprecated = __commonJS22({
"node_modules/vnopts/lib/handlers/deprecated/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var tslib_1 = (init_tslib_es6(), __toCommonJS(tslib_es6_exports));
tslib_1.__exportStar(require_common(), exports2);
}
});
var require_common2 = __commonJS22({
"node_modules/vnopts/lib/handlers/invalid/common.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var chalk_1 = require_chalk();
exports2.commonInvalidHandler = (key, value, utils) => [`Invalid ${chalk_1.default.red(utils.descriptor.key(key))} value.`, `Expected ${chalk_1.default.blue(utils.schemas[key].expected(utils))},`, `but received ${chalk_1.default.red(utils.descriptor.value(value))}.`].join(" ");
}
});
var require_invalid = __commonJS22({
"node_modules/vnopts/lib/handlers/invalid/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var tslib_1 = (init_tslib_es6(), __toCommonJS(tslib_es6_exports));
tslib_1.__exportStar(require_common2(), exports2);
}
});
var require_leven = __commonJS22({
"node_modules/vnopts/node_modules/leven/index.js"(exports2, module22) {
"use strict";
var arr = [];
var charCodeCache = [];
module22.exports = function(a, b) {
if (a === b) {
return 0;
}
var swap = a;
if (a.length > b.length) {
a = b;
b = swap;
}
var aLen = a.length;
var bLen = b.length;
if (aLen === 0) {
return bLen;
}
if (bLen === 0) {
return aLen;
}
while (aLen > 0 && a.charCodeAt(~-aLen) === b.charCodeAt(~-bLen)) {
aLen--;
bLen--;
}
if (aLen === 0) {
return bLen;
}
var start = 0;
while (start < aLen && a.charCodeAt(start) === b.charCodeAt(start)) {
start++;
}
aLen -= start;
bLen -= start;
if (aLen === 0) {
return bLen;
}
var bCharCode;
var ret;
var tmp;
var tmp2;
var i = 0;
var j = 0;
while (i < aLen) {
charCodeCache[start + i] = a.charCodeAt(start + i);
arr[i] = ++i;
}
while (j < bLen) {
bCharCode = b.charCodeAt(start + j);
tmp = j++;
ret = j;
for (i = 0; i < aLen; i++) {
tmp2 = bCharCode === charCodeCache[start + i] ? tmp : tmp + 1;
tmp = arr[i];
ret = arr[i] = tmp > ret ? tmp2 > ret ? ret + 1 : tmp2 : tmp2 > tmp ? tmp + 1 : tmp2;
}
}
return ret;
};
}
});
var require_leven2 = __commonJS22({
"node_modules/vnopts/lib/handlers/unknown/leven.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var chalk_1 = require_chalk();
var leven = require_leven();
exports2.levenUnknownHandler = (key, value, {
descriptor,
logger,
schemas
}) => {
const messages = [`Ignored unknown option ${chalk_1.default.yellow(descriptor.pair({
key,
value
}))}.`];
const suggestion = Object.keys(schemas).sort().find((knownKey) => leven(key, knownKey) < 3);
if (suggestion) {
messages.push(`Did you mean ${chalk_1.default.blue(descriptor.key(suggestion))}?`);
}
logger.warn(messages.join(" "));
};
}
});
var require_unknown = __commonJS22({
"node_modules/vnopts/lib/handlers/unknown/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var tslib_1 = (init_tslib_es6(), __toCommonJS(tslib_es6_exports));
tslib_1.__exportStar(require_leven2(), exports2);
}
});
var require_handlers = __commonJS22({
"node_modules/vnopts/lib/handlers/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var tslib_1 = (init_tslib_es6(), __toCommonJS(tslib_es6_exports));
tslib_1.__exportStar(require_deprecated(), exports2);
tslib_1.__exportStar(require_invalid(), exports2);
tslib_1.__exportStar(require_unknown(), exports2);
}
});
var require_schema = __commonJS22({
"node_modules/vnopts/lib/schema.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var HANDLER_KEYS = ["default", "expected", "validate", "deprecated", "forward", "redirect", "overlap", "preprocess", "postprocess"];
function createSchema(SchemaConstructor, parameters) {
const schema = new SchemaConstructor(parameters);
const subSchema = Object.create(schema);
for (const handlerKey of HANDLER_KEYS) {
if (handlerKey in parameters) {
subSchema[handlerKey] = normalizeHandler(parameters[handlerKey], schema, Schema.prototype[handlerKey].length);
}
}
return subSchema;
}
exports2.createSchema = createSchema;
var Schema = class {
constructor(parameters) {
this.name = parameters.name;
}
static create(parameters) {
return createSchema(this, parameters);
}
default(_utils) {
return void 0;
}
expected(_utils) {
return "nothing";
}
validate(_value, _utils) {
return false;
}
deprecated(_value, _utils) {
return false;
}
forward(_value, _utils) {
return void 0;
}
redirect(_value, _utils) {
return void 0;
}
overlap(currentValue, _newValue, _utils) {
return currentValue;
}
preprocess(value, _utils) {
return value;
}
postprocess(value, _utils) {
return value;
}
};
exports2.Schema = Schema;
function normalizeHandler(handler, superSchema, handlerArgumentsLength) {
return typeof handler === "function" ? (...args) => handler(...args.slice(0, handlerArgumentsLength - 1), superSchema, ...args.slice(handlerArgumentsLength - 1)) : () => handler;
}
}
});
var require_alias = __commonJS22({
"node_modules/vnopts/lib/schemas/alias.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var schema_1 = require_schema();
var AliasSchema = class extends schema_1.Schema {
constructor(parameters) {
super(parameters);
this._sourceName = parameters.sourceName;
}
expected(utils) {
return utils.schemas[this._sourceName].expected(utils);
}
validate(value, utils) {
return utils.schemas[this._sourceName].validate(value, utils);
}
redirect(_value, _utils) {
return this._sourceName;
}
};
exports2.AliasSchema = AliasSchema;
}
});
var require_any = __commonJS22({
"node_modules/vnopts/lib/schemas/any.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var schema_1 = require_schema();
var AnySchema = class extends schema_1.Schema {
expected() {
return "anything";
}
validate() {
return true;
}
};
exports2.AnySchema = AnySchema;
}
});
var require_array2 = __commonJS22({
"node_modules/vnopts/lib/schemas/array.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var tslib_1 = (init_tslib_es6(), __toCommonJS(tslib_es6_exports));
var schema_1 = require_schema();
var ArraySchema = class extends schema_1.Schema {
constructor(_a) {
var {
valueSchema,
name = valueSchema.name
} = _a, handlers = tslib_1.__rest(_a, ["valueSchema", "name"]);
super(Object.assign({}, handlers, {
name
}));
this._valueSchema = valueSchema;
}
expected(utils) {
return `an array of ${this._valueSchema.expected(utils)}`;
}
validate(value, utils) {
if (!Array.isArray(value)) {
return false;
}
const invalidValues = [];
for (const subValue of value) {
const subValidateResult = utils.normalizeValidateResult(this._valueSchema.validate(subValue, utils), subValue);
if (subValidateResult !== true) {
invalidValues.push(subValidateResult.value);
}
}
return invalidValues.length === 0 ? true : {
value: invalidValues
};
}
deprecated(value, utils) {
const deprecatedResult = [];
for (const subValue of value) {
const subDeprecatedResult = utils.normalizeDeprecatedResult(this._valueSchema.deprecated(subValue, utils), subValue);
if (subDeprecatedResult !== false) {
deprecatedResult.push(...subDeprecatedResult.map(({
value: deprecatedValue
}) => ({
value: [deprecatedValue]
})));
}
}
return deprecatedResult;
}
forward(value, utils) {
const forwardResult = [];
for (const subValue of value) {
const subForwardResult = utils.normalizeForwardResult(this._valueSchema.forward(subValue, utils), subValue);
forwardResult.push(...subForwardResult.map(wrapTransferResult));
}
return forwardResult;
}
redirect(value, utils) {
const remain = [];
const redirect = [];
for (const subValue of value) {
const subRedirectResult = utils.normalizeRedirectResult(this._valueSchema.redirect(subValue, utils), subValue);
if ("remain" in subRedirectResult) {
remain.push(subRedirectResult.remain);
}
redirect.push(...subRedirectResult.redirect.map(wrapTransferResult));
}
return remain.length === 0 ? {
redirect
} : {
redirect,
remain
};
}
overlap(currentValue, newValue) {
return currentValue.concat(newValue);
}
};
exports2.ArraySchema = ArraySchema;
function wrapTransferResult({
from,
to
}) {
return {
from: [from],
to
};
}
}
});
var require_boolean = __commonJS22({
"node_modules/vnopts/lib/schemas/boolean.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var schema_1 = require_schema();
var BooleanSchema = class extends schema_1.Schema {
expected() {
return "true or false";
}
validate(value) {
return typeof value === "boolean";
}
};
exports2.BooleanSchema = BooleanSchema;
}
});
var require_utils = __commonJS22({
"node_modules/vnopts/lib/utils.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
function recordFromArray(array, mainKey) {
const record = /* @__PURE__ */ Object.create(null);
for (const value of array) {
const key = value[mainKey];
if (record[key]) {
throw new Error(`Duplicate ${mainKey} ${JSON.stringify(key)}`);
}
record[key] = value;
}
return record;
}
exports2.recordFromArray = recordFromArray;
function mapFromArray(array, mainKey) {
const map = /* @__PURE__ */ new Map();
for (const value of array) {
const key = value[mainKey];
if (map.has(key)) {
throw new Error(`Duplicate ${mainKey} ${JSON.stringify(key)}`);
}
map.set(key, value);
}
return map;
}
exports2.mapFromArray = mapFromArray;
function createAutoChecklist() {
const map = /* @__PURE__ */ Object.create(null);
return (id) => {
const idString = JSON.stringify(id);
if (map[idString]) {
return true;
}
map[idString] = true;
return false;
};
}
exports2.createAutoChecklist = createAutoChecklist;
function partition(array, predicate) {
const trueArray = [];
const falseArray = [];
for (const value of array) {
if (predicate(value)) {
trueArray.push(value);
} else {
falseArray.push(value);
}
}
return [trueArray, falseArray];
}
exports2.partition = partition;
function isInt(value) {
return value === Math.floor(value);
}
exports2.isInt = isInt;
function comparePrimitive(a, b) {
if (a === b) {
return 0;
}
const typeofA = typeof a;
const typeofB = typeof b;
const orders = ["undefined", "object", "boolean", "number", "string"];
if (typeofA !== typeofB) {
return orders.indexOf(typeofA) - orders.indexOf(typeofB);
}
if (typeofA !== "string") {
return Number(a) - Number(b);
}
return a.localeCompare(b);
}
exports2.comparePrimitive = comparePrimitive;
function normalizeDefaultResult(result) {
return result === void 0 ? {} : result;
}
exports2.normalizeDefaultResult = normalizeDefaultResult;
function normalizeValidateResult(result, value) {
return result === true ? true : result === false ? {
value
} : result;
}
exports2.normalizeValidateResult = normalizeValidateResult;
function normalizeDeprecatedResult(result, value, doNotNormalizeTrue = false) {
return result === false ? false : result === true ? doNotNormalizeTrue ? true : [{
value
}] : "value" in result ? [result] : result.length === 0 ? false : result;
}
exports2.normalizeDeprecatedResult = normalizeDeprecatedResult;
function normalizeTransferResult(result, value) {
return typeof result === "string" || "key" in result ? {
from: value,
to: result
} : "from" in result ? {
from: result.from,
to: result.to
} : {
from: value,
to: result.to
};
}
exports2.normalizeTransferResult = normalizeTransferResult;
function normalizeForwardResult(result, value) {
return result === void 0 ? [] : Array.isArray(result) ? result.map((transferResult) => normalizeTransferResult(transferResult, value)) : [normalizeTransferResult(result, value)];
}
exports2.normalizeForwardResult = normalizeForwardResult;
function normalizeRedirectResult(result, value) {
const redirect = normalizeForwardResult(typeof result === "object" && "redirect" in result ? result.redirect : result, value);
return redirect.length === 0 ? {
remain: value,
redirect
} : typeof result === "object" && "remain" in result ? {
remain: result.remain,
redirect
} : {
redirect
};
}
exports2.normalizeRedirectResult = normalizeRedirectResult;
}
});
var require_choice = __commonJS22({
"node_modules/vnopts/lib/schemas/choice.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var schema_1 = require_schema();
var utils_1 = require_utils();
var ChoiceSchema = class extends schema_1.Schema {
constructor(parameters) {
super(parameters);
this._choices = utils_1.mapFromArray(parameters.choices.map((choice) => choice && typeof choice === "object" ? choice : {
value: choice
}), "value");
}
expected({
descriptor
}) {
const choiceValues = Array.from(this._choices.keys()).map((value) => this._choices.get(value)).filter((choiceInfo) => !choiceInfo.deprecated).map((choiceInfo) => choiceInfo.value).sort(utils_1.comparePrimitive).map(descriptor.value);
const head = choiceValues.slice(0, -2);
const tail = choiceValues.slice(-2);
return head.concat(tail.join(" or ")).join(", ");
}
validate(value) {
return this._choices.has(value);
}
deprecated(value) {
const choiceInfo = this._choices.get(value);
return choiceInfo && choiceInfo.deprecated ? {
value
} : false;
}
forward(value) {
const choiceInfo = this._choices.get(value);
return choiceInfo ? choiceInfo.forward : void 0;
}
redirect(value) {
const choiceInfo = this._choices.get(value);
return choiceInfo ? choiceInfo.redirect : void 0;
}
};
exports2.ChoiceSchema = ChoiceSchema;
}
});
var require_number = __commonJS22({
"node_modules/vnopts/lib/schemas/number.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var schema_1 = require_schema();
var NumberSchema = class extends schema_1.Schema {
expected() {
return "a number";
}
validate(value, _utils) {
return typeof value === "number";
}
};
exports2.NumberSchema = NumberSchema;
}
});
var require_integer = __commonJS22({
"node_modules/vnopts/lib/schemas/integer.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var utils_1 = require_utils();
var number_1 = require_number();
var IntegerSchema = class extends number_1.NumberSchema {
expected() {
return "an integer";
}
validate(value, utils) {
return utils.normalizeValidateResult(super.validate(value, utils), value) === true && utils_1.isInt(value);
}
};
exports2.IntegerSchema = IntegerSchema;
}
});
var require_string = __commonJS22({
"node_modules/vnopts/lib/schemas/string.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var schema_1 = require_schema();
var StringSchema = class extends schema_1.Schema {
expected() {
return "a string";
}
validate(value) {
return typeof value === "string";
}
};
exports2.StringSchema = StringSchema;
}
});
var require_schemas = __commonJS22({
"node_modules/vnopts/lib/schemas/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var tslib_1 = (init_tslib_es6(), __toCommonJS(tslib_es6_exports));
tslib_1.__exportStar(require_alias(), exports2);
tslib_1.__exportStar(require_any(), exports2);
tslib_1.__exportStar(require_array2(), exports2);
tslib_1.__exportStar(require_boolean(), exports2);
tslib_1.__exportStar(require_choice(), exports2);
tslib_1.__exportStar(require_integer(), exports2);
tslib_1.__exportStar(require_number(), exports2);
tslib_1.__exportStar(require_string(), exports2);
}
});
var require_defaults = __commonJS22({
"node_modules/vnopts/lib/defaults.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var api_1 = require_api();
var common_1 = require_common();
var invalid_1 = require_invalid();
var leven_1 = require_leven2();
exports2.defaultDescriptor = api_1.apiDescriptor;
exports2.defaultUnknownHandler = leven_1.levenUnknownHandler;
exports2.defaultInvalidHandler = invalid_1.commonInvalidHandler;
exports2.defaultDeprecatedHandler = common_1.commonDeprecatedHandler;
}
});
var require_normalize = __commonJS22({
"node_modules/vnopts/lib/normalize.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var defaults_1 = require_defaults();
var utils_1 = require_utils();
exports2.normalize = (options, schemas, opts) => new Normalizer(schemas, opts).normalize(options);
var Normalizer = class {
constructor(schemas, opts) {
const {
logger = console,
descriptor = defaults_1.defaultDescriptor,
unknown = defaults_1.defaultUnknownHandler,
invalid = defaults_1.defaultInvalidHandler,
deprecated = defaults_1.defaultDeprecatedHandler
} = opts || {};
this._utils = {
descriptor,
logger: logger || {
warn: () => {
}
},
schemas: utils_1.recordFromArray(schemas, "name"),
normalizeDefaultResult: utils_1.normalizeDefaultResult,
normalizeDeprecatedResult: utils_1.normalizeDeprecatedResult,
normalizeForwardResult: utils_1.normalizeForwardResult,
normalizeRedirectResult: utils_1.normalizeRedirectResult,
normalizeValidateResult: utils_1.normalizeValidateResult
};
this._unknownHandler = unknown;
this._invalidHandler = invalid;
this._deprecatedHandler = deprecated;
this.cleanHistory();
}
cleanHistory() {
this._hasDeprecationWarned = utils_1.createAutoChecklist();
}
normalize(options) {
const normalized = {};
const restOptionsArray = [options];
const applyNormalization = () => {
while (restOptionsArray.length !== 0) {
const currentOptions = restOptionsArray.shift();
const transferredOptionsArray = this._applyNormalization(currentOptions, normalized);
restOptionsArray.push(...transferredOptionsArray);
}
};
applyNormalization();
for (const key of Object.keys(this._utils.schemas)) {
const schema = this._utils.schemas[key];
if (!(key in normalized)) {
const defaultResult = utils_1.normalizeDefaultResult(schema.default(this._utils));
if ("value" in defaultResult) {
restOptionsArray.push({
[key]: defaultResult.value
});
}
}
}
applyNormalization();
for (const key of Object.keys(this._utils.schemas)) {
const schema = this._utils.schemas[key];
if (key in normalized) {
normalized[key] = schema.postprocess(normalized[key], this._utils);
}
}
return normalized;
}
_applyNormalization(options, normalized) {
const transferredOptionsArray = [];
const [knownOptionNames, unknownOptionNames] = utils_1.partition(Object.keys(options), (key) => key in this._utils.schemas);
for (const key of knownOptionNames) {
const schema = this._utils.schemas[key];
const value = schema.preprocess(options[key], this._utils);
const validateResult = utils_1.normalizeValidateResult(schema.validate(value, this._utils), value);
if (validateResult !== true) {
const {
value: invalidValue
} = validateResult;
const errorMessageOrError = this._invalidHandler(key, invalidValue, this._utils);
throw typeof errorMessageOrError === "string" ? new Error(errorMessageOrError) : errorMessageOrError;
}
const appendTransferredOptions = ({
from,
to
}) => {
transferredOptionsArray.push(typeof to === "string" ? {
[to]: from
} : {
[to.key]: to.value
});
};
const warnDeprecated = ({
value: currentValue,
redirectTo
}) => {
const deprecatedResult = utils_1.normalizeDeprecatedResult(schema.deprecated(currentValue, this._utils), value, true);
if (deprecatedResult === false) {
return;
}
if (deprecatedResult === true) {
if (!this._hasDeprecationWarned(key)) {
this._utils.logger.warn(this._deprecatedHandler(key, redirectTo, this._utils));
}
} else {
for (const {
value: deprecatedValue
} of deprecatedResult) {
const pair = {
key,
value: deprecatedValue
};
if (!this._hasDeprecationWarned(pair)) {
const redirectToPair = typeof redirectTo === "string" ? {
key: redirectTo,
value: deprecatedValue
} : redirectTo;
this._utils.logger.warn(this._deprecatedHandler(pair, redirectToPair, this._utils));
}
}
}
};
const forwardResult = utils_1.normalizeForwardResult(schema.forward(value, this._utils), value);
forwardResult.forEach(appendTransferredOptions);
const redirectResult = utils_1.normalizeRedirectResult(schema.redirect(value, this._utils), value);
redirectResult.redirect.forEach(appendTransferredOptions);
if ("remain" in redirectResult) {
const remainingValue = redirectResult.remain;
normalized[key] = key in normalized ? schema.overlap(normalized[key], remainingValue, this._utils) : remainingValue;
warnDeprecated({
value: remainingValue
});
}
for (const {
from,
to
} of redirectResult.redirect) {
warnDeprecated({
value: from,
redirectTo: to
});
}
}
for (const key of unknownOptionNames) {
const value = options[key];
const unknownResult = this._unknownHandler(key, value, this._utils);
if (unknownResult) {
for (const unknownKey of Object.keys(unknownResult)) {
const unknownOption = {
[unknownKey]: unknownResult[unknownKey]
};
if (unknownKey in this._utils.schemas) {
transferredOptionsArray.push(unknownOption);
} else {
Object.assign(normalized, unknownOption);
}
}
}
}
return transferredOptionsArray;
}
};
exports2.Normalizer = Normalizer;
}
});
var require_lib22 = __commonJS22({
"node_modules/vnopts/lib/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var tslib_1 = (init_tslib_es6(), __toCommonJS(tslib_es6_exports));
tslib_1.__exportStar(require_descriptors2(), exports2);
tslib_1.__exportStar(require_handlers(), exports2);
tslib_1.__exportStar(require_schemas(), exports2);
tslib_1.__exportStar(require_normalize(), exports2);
tslib_1.__exportStar(require_schema(), exports2);
}
});
var require_options_normalizer = __commonJS22({
"src/main/options-normalizer.js"(exports2, module22) {
"use strict";
var vnopts = require_lib22();
var getLast = require_get_last();
var cliDescriptor = {
key: (key) => key.length === 1 ? `-${key}` : `--${key}`,
value: (value) => vnopts.apiDescriptor.value(value),
pair: ({
key,
value
}) => value === false ? `--no-${key}` : value === true ? cliDescriptor.key(key) : value === "" ? `${cliDescriptor.key(key)} without an argument` : `${cliDescriptor.key(key)}=${value}`
};
var getFlagSchema = ({
colorsModule,
levenshteinDistance
}) => class FlagSchema extends vnopts.ChoiceSchema {
constructor({
name,
flags
}) {
super({
name,
choices: flags
});
this._flags = [...flags].sort();
}
preprocess(value, utils) {
if (typeof value === "string" && value.length > 0 && !this._flags.includes(value)) {
const suggestion = this._flags.find((flag) => levenshteinDistance(flag, value) < 3);
if (suggestion) {
utils.logger.warn([`Unknown flag ${colorsModule.yellow(utils.descriptor.value(value))},`, `did you mean ${colorsModule.blue(utils.descriptor.value(suggestion))}?`].join(" "));
return suggestion;
}
}
return value;
}
expected() {
return "a flag";
}
};
var hasDeprecationWarned;
function normalizeOptions(options, optionInfos, {
logger = false,
isCLI = false,
passThrough = false,
colorsModule = null,
levenshteinDistance = null
} = {}) {
const unknown = !passThrough ? (key, value, options2) => {
const _options2$schemas = options2.schemas, {
_
} = _options2$schemas, schemas2 = _objectWithoutProperties(_options2$schemas, _excluded2);
return vnopts.levenUnknownHandler(key, value, Object.assign(Object.assign({}, options2), {}, {
schemas: schemas2
}));
} : Array.isArray(passThrough) ? (key, value) => !passThrough.includes(key) ? void 0 : {
[key]: value
} : (key, value) => ({
[key]: value
});
const descriptor = isCLI ? cliDescriptor : vnopts.apiDescriptor;
const schemas = optionInfosToSchemas(optionInfos, {
isCLI,
colorsModule,
levenshteinDistance
});
const normalizer = new vnopts.Normalizer(schemas, {
logger,
unknown,
descriptor
});
const shouldSuppressDuplicateDeprecationWarnings = logger !== false;
if (shouldSuppressDuplicateDeprecationWarnings && hasDeprecationWarned) {
normalizer._hasDeprecationWarned = hasDeprecationWarned;
}
const normalized = normalizer.normalize(options);
if (shouldSuppressDuplicateDeprecationWarnings) {
hasDeprecationWarned = normalizer._hasDeprecationWarned;
}
if (isCLI && normalized["plugin-search"] === false) {
normalized["plugin-search-dir"] = false;
}
return normalized;
}
function optionInfosToSchemas(optionInfos, {
isCLI,
colorsModule,
levenshteinDistance
}) {
const schemas = [];
if (isCLI) {
schemas.push(vnopts.AnySchema.create({
name: "_"
}));
}
for (const optionInfo of optionInfos) {
schemas.push(optionInfoToSchema(optionInfo, {
isCLI,
optionInfos,
colorsModule,
levenshteinDistance
}));
if (optionInfo.alias && isCLI) {
schemas.push(vnopts.AliasSchema.create({
name: optionInfo.alias,
sourceName: optionInfo.name
}));
}
}
return schemas;
}
function optionInfoToSchema(optionInfo, {
isCLI,
optionInfos,
colorsModule,
levenshteinDistance
}) {
const {
name
} = optionInfo;
if (name === "plugin-search-dir" || name === "pluginSearchDirs") {
return vnopts.AnySchema.create({
name,
preprocess(value) {
if (value === false) {
return value;
}
value = Array.isArray(value) ? value : [value];
return value;
},
validate(value) {
if (value === false) {
return true;
}
return value.every((dir) => typeof dir === "string");
},
expected() {
return "false or paths to plugin search dir";
}
});
}
const parameters = {
name
};
let SchemaConstructor;
const handlers = {};
switch (optionInfo.type) {
case "int":
SchemaConstructor = vnopts.IntegerSchema;
if (isCLI) {
parameters.preprocess = Number;
}
break;
case "string":
SchemaConstructor = vnopts.StringSchema;
break;
case "choice":
SchemaConstructor = vnopts.ChoiceSchema;
parameters.choices = optionInfo.choices.map((choiceInfo) => typeof choiceInfo === "object" && choiceInfo.redirect ? Object.assign(Object.assign({}, choiceInfo), {}, {
redirect: {
to: {
key: optionInfo.name,
value: choiceInfo.redirect
}
}
}) : choiceInfo);
break;
case "boolean":
SchemaConstructor = vnopts.BooleanSchema;
break;
case "flag":
SchemaConstructor = getFlagSchema({
colorsModule,
levenshteinDistance
});
parameters.flags = optionInfos.flatMap((optionInfo2) => [optionInfo2.alias, optionInfo2.description && optionInfo2.name, optionInfo2.oppositeDescription && `no-${optionInfo2.name}`].filter(Boolean));
break;
case "path":
SchemaConstructor = vnopts.StringSchema;
break;
default:
throw new Error(`Unexpected type ${optionInfo.type}`);
}
if (optionInfo.exception) {
parameters.validate = (value, schema, utils) => optionInfo.exception(value) || schema.validate(value, utils);
} else {
parameters.validate = (value, schema, utils) => value === void 0 || schema.validate(value, utils);
}
if (optionInfo.redirect) {
handlers.redirect = (value) => !value ? void 0 : {
to: {
key: optionInfo.redirect.option,
value: optionInfo.redirect.value
}
};
}
if (optionInfo.deprecated) {
handlers.deprecated = true;
}
if (isCLI && !optionInfo.array) {
const originalPreprocess = parameters.preprocess || ((x) => x);
parameters.preprocess = (value, schema, utils) => schema.preprocess(originalPreprocess(Array.isArray(value) ? getLast(value) : value), utils);
}
return optionInfo.array ? vnopts.ArraySchema.create(Object.assign(Object.assign(Object.assign({}, isCLI ? {
preprocess: (v) => Array.isArray(v) ? v : [v]
} : {}), handlers), {}, {
valueSchema: SchemaConstructor.create(parameters)
})) : SchemaConstructor.create(Object.assign(Object.assign({}, parameters), handlers));
}
function normalizeApiOptions(options, optionInfos, opts) {
return normalizeOptions(options, optionInfos, opts);
}
function normalizeCliOptions(options, optionInfos, opts) {
if (false) {
if (!opts.colorsModule) {
throw new Error("'colorsModule' option is required.");
}
if (!opts.levenshteinDistance) {
throw new Error("'levenshteinDistance' option is required.");
}
}
return normalizeOptions(options, optionInfos, Object.assign({
isCLI: true
}, opts));
}
module22.exports = {
normalizeApiOptions,
normalizeCliOptions
};
}
});
var require_loc = __commonJS22({
"src/language-js/loc.js"(exports2, module22) {
"use strict";
var isNonEmptyArray = require_is_non_empty_array();
function locStart(node) {
var _node$declaration$dec, _node$declaration;
const start = node.range ? node.range[0] : node.start;
const decorators = (_node$declaration$dec = (_node$declaration = node.declaration) === null || _node$declaration === void 0 ? void 0 : _node$declaration.decorators) !== null && _node$declaration$dec !== void 0 ? _node$declaration$dec : node.decorators;
if (isNonEmptyArray(decorators)) {
return Math.min(locStart(decorators[0]), start);
}
return start;
}
function locEnd(node) {
return node.range ? node.range[1] : node.end;
}
function hasSameLocStart(nodeA, nodeB) {
const nodeAStart = locStart(nodeA);
return Number.isInteger(nodeAStart) && nodeAStart === locStart(nodeB);
}
function hasSameLocEnd(nodeA, nodeB) {
const nodeAEnd = locEnd(nodeA);
return Number.isInteger(nodeAEnd) && nodeAEnd === locEnd(nodeB);
}
function hasSameLoc(nodeA, nodeB) {
return hasSameLocStart(nodeA, nodeB) && hasSameLocEnd(nodeA, nodeB);
}
module22.exports = {
locStart,
locEnd,
hasSameLocStart,
hasSameLoc
};
}
});
var require_load_parser = __commonJS22({
"src/main/load-parser.js"(exports2, module22) {
"use strict";
var path = require("path");
var {
ConfigError
} = require_errors();
var {
locStart,
locEnd
} = require_loc();
function requireParser(parser) {
try {
return {
parse: require(path.resolve(process.cwd(), parser)),
astFormat: "estree",
locStart,
locEnd
};
} catch {
throw new ConfigError(`Couldn't resolve parser "${parser}"`);
}
}
module22.exports = requireParser;
}
});
var require_js_tokens = __commonJS22({
"node_modules/js-tokens/index.js"(exports2) {
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.default = /((['"])(?:(?!\2|\\).|\\(?:\r\n|[\s\S]))*(\2)?|`(?:[^`\\$]|\\[\s\S]|\$(?!\{)|\$\{(?:[^{}]|\{[^}]*\}?)*\}?)*(`)?)|(\/\/.*)|(\/\*(?:[^*]|\*(?!\/))*(\*\/)?)|(\/(?!\*)(?:\[(?:(?![\]\\]).|\\.)*\]|(?![\/\]\\]).|\\.)+\/(?:(?!\s*(?:\b|[\u0080-\uFFFF$\\'"~({]|[+\-!](?!=)|\.?\d))|[gmiyus]{1,6}\b(?![\u0080-\uFFFF$\\]|\s*(?:[+\-*%&|^<>!=?({]|\/(?![\/*])))))|(0[xX][\da-fA-F]+|0[oO][0-7]+|0[bB][01]+|(?:\d*\.\d+|\d+\.?)(?:[eE][+-]?\d+)?)|((?!\d)(?:(?!\s)[$\w\u0080-\uFFFF]|\\u[\da-fA-F]{4}|\\u\{[\da-fA-F]+\})+)|(--|\+\+|&&|\|\||=>|\.{3}|(?:[+\-\/%&|^]|\*{1,2}|<{1,2}|>{1,3}|!=?|={1,2})=?|[?~.,:;[\](){}])|(\s+)|(^$|[\s\S])/g;
exports2.matchToToken = function(match) {
var token = {
type: "invalid",
value: match[0],
closed: void 0
};
if (match[1])
token.type = "string", token.closed = !!(match[3] || match[4]);
else if (match[5])
token.type = "comment";
else if (match[6])
token.type = "comment", token.closed = !!match[7];
else if (match[8])
token.type = "regex";
else if (match[9])
token.type = "number";
else if (match[10])
token.type = "name";
else if (match[11])
token.type = "punctuator";
else if (match[12])
token.type = "whitespace";
return token;
};
}
});
var require_identifier = __commonJS22({
"node_modules/@babel/helper-validator-identifier/lib/identifier.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.isIdentifierChar = isIdentifierChar;
exports2.isIdentifierName = isIdentifierName;
exports2.isIdentifierStart = isIdentifierStart;
var nonASCIIidentifierStartChars = "\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0370-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u048A-\u052F\u0531-\u0556\u0559\u0560-\u0588\u05D0-\u05EA\u05EF-\u05F2\u0620-\u064A\u066E\u066F\u0671-\u06D3\u06D5\u06E5\u06E6\u06EE\u06EF\u06FA-\u06FC\u06FF\u0710\u0712-\u072F\u074D-\u07A5\u07B1\u07CA-\u07EA\u07F4\u07F5\u07FA\u0800-\u0815\u081A\u0824\u0828\u0840-\u0858\u0860-\u086A\u0870-\u0887\u0889-\u088E\u08A0-\u08C9\u0904-\u0939\u093D\u0950\u0958-\u0961\u0971-\u0980\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD\u09CE\u09DC\u09DD\u09DF-\u09E1\u09F0\u09F1\u09FC\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A59-\u0A5C\u0A5E\u0A72-\u0A74\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABD\u0AD0\u0AE0\u0AE1\u0AF9\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3D\u0B5C\u0B5D\u0B5F-\u0B61\u0B71\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BD0\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C58-\u0C5A\u0C5D\u0C60\u0C61\u0C80\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CDD\u0CDE\u0CE0\u0CE1\u0CF1\u0CF2\u0D04-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D\u0D4E\u0D54-\u0D56\u0D5F-\u0D61\u0D7A-\u0D7F\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0E01-\u0E30\u0E32\u0E33\u0E40-\u0E46\u0E81\u0E82\u0E84\u0E86-\u0E8A\u0E8C-\u0EA3\u0EA5\u0EA7-\u0EB0\u0EB2\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6\u0EDC-\u0EDF\u0F00\u0F40-\u0F47\u0F49-\u0F6C\u0F88-\u0F8C\u1000-\u102A\u103F\u1050-\u1055\u105A-\u105D\u1061\u1065\u1066\u106E-\u1070\u1075-\u1081\u108E\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u1711\u171F-\u1731\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17D7\u17DC\u1820-\u1878\u1880-\u18A8\u18AA\u18B0-\u18F5\u1900-\u191E\u1950-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u1A00-\u1A16\u1A20-\u1A54\u1AA7\u1B05-\u1B33\u1B45-\u1B4C\u1B83-\u1BA0\u1BAE\u1BAF\u1BBA-\u1BE5\u1C00-\u1C23\u1C4D-\u1C4F\u1C5A-\u1C7D\u1C80-\u1C88\u1C90-\u1CBA\u1CBD-\u1CBF\u1CE9-\u1CEC\u1CEE-\u1CF3\u1CF5\u1CF6\u1CFA\u1D00-\u1DBF\u1E00-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2118-\u211D\u2124\u2126\u2128\u212A-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2CE4\u2CEB-\u2CEE\u2CF2\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u3005-\u3007\u3021-\u3029\u3031-\u3035\u3038-\u303C\u3041-\u3096\u309B-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312F\u3131-\u318E\u31A0-\u31BF\u31F0-\u31FF\u3400-\u4DBF\u4E00-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA61F\uA62A\uA62B\uA640-\uA66E\uA67F-\uA69D\uA6A0-\uA6EF\uA717-\uA71F\uA722-\uA788\uA78B-\uA7CA\uA7D0\uA7D1\uA7D3\uA7D5-\uA7D9\uA7F2-\uA801\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA840-\uA873\uA882-\uA8B3\uA8F2-\uA8F7\uA8FB\uA8FD\uA8FE\uA90A-\uA925\uA930-\uA946\uA960-\uA97C\uA984-\uA9B2\uA9CF\uA9E0-\uA9E4\uA9E6-\uA9EF\uA9FA-\uA9FE\uAA00-\uAA28\uAA40-\uAA42\uAA44-\uAA4B\uAA60-\uAA76\uAA7A\uAA7E-\uAAAF\uAAB1\uAAB5\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADD\uAAE0-\uAAEA\uAAF2-\uAAF4\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB69\uAB70-\uABE2\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE70-\uFE74\uFE76-\uFEFC\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC";
var nonASCIIidentifierChars = "\u200C\u200D\xB7\u0300-\u036F\u0387\u0483-\u0487\u0591-\u05BD\u05BF\u05C1\u05C2\u05C4\u05C5\u05C7\u0610-\u061A\u064B-\u0669\u0670\u06D6-\u06DC\u06DF-\u06E4\u06E7\u06E8\u06EA-\u06ED\u06F0-\u06F9\u0711\u0730-\u074A\u07A6-\u07B0\u07C0-\u07C9\u07EB-\u07F3\u07FD\u0816-\u0819\u081B-\u0823\u0825-\u0827\u0829-\u082D\u0859-\u085B\u0898-\u089F\u08CA-\u08E1\u08E3-\u0903\u093A-\u093C\u093E-\u094F\u0951-\u0957\u0962\u0963\u0966-\u096F\u0981-\u0983\u09BC\u09BE-\u09C4\u09C7\u09C8\u09CB-\u09CD\u09D7\u09E2\u09E3\u09E6-\u09EF\u09FE\u0A01-\u0A03\u0A3C\u0A3E-\u0A42\u0A47\u0A48\u0A4B-\u0A4D\u0A51\u0A66-\u0A71\u0A75\u0A81-\u0A83\u0ABC\u0ABE-\u0AC5\u0AC7-\u0AC9\u0ACB-\u0ACD\u0AE2\u0AE3\u0AE6-\u0AEF\u0AFA-\u0AFF\u0B01-\u0B03\u0B3C\u0B3E-\u0B44\u0B47\u0B48\u0B4B-\u0B4D\u0B55-\u0B57\u0B62\u0B63\u0B66-\u0B6F\u0B82\u0BBE-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCD\u0BD7\u0BE6-\u0BEF\u0C00-\u0C04\u0C3C\u0C3E-\u0C44\u0C46-\u0C48\u0C4A-\u0C4D\u0C55\u0C56\u0C62\u0C63\u0C66-\u0C6F\u0C81-\u0C83\u0CBC\u0CBE-\u0CC4\u0CC6-\u0CC8\u0CCA-\u0CCD\u0CD5\u0CD6\u0CE2\u0CE3\u0CE6-\u0CEF\u0CF3\u0D00-\u0D03\u0D3B\u0D3C\u0D3E-\u0D44\u0D46-\u0D48\u0D4A-\u0D4D\u0D57\u0D62\u0D63\u0D66-\u0D6F\u0D81-\u0D83\u0DCA\u0DCF-\u0DD4\u0DD6\u0DD8-\u0DDF\u0DE6-\u0DEF\u0DF2\u0DF3\u0E31\u0E34-\u0E3A\u0E47-\u0E4E\u0E50-\u0E59\u0EB1\u0EB4-\u0EBC\u0EC8-\u0ECE\u0ED0-\u0ED9\u0F18\u0F19\u0F20-\u0F29\u0F35\u0F37\u0F39\u0F3E\u0F3F\u0F71-\u0F84\u0F86\u0F87\u0F8D-\u0F97\u0F99-\u0FBC\u0FC6\u102B-\u103E\u1040-\u1049\u1056-\u1059\u105E-\u1060\u1062-\u1064\u1067-\u106D\u1071-\u1074\u1082-\u108D\u108F-\u109D\u135D-\u135F\u1369-\u1371\u1712-\u1715\u1732-\u1734\u1752\u1753\u1772\u1773\u17B4-\u17D3\u17DD\u17E0-\u17E9\u180B-\u180D\u180F-\u1819\u18A9\u1920-\u192B\u1930-\u193B\u1946-\u194F\u19D0-\u19DA\u1A17-\u1A1B\u1A55-\u1A5E\u1A60-\u1A7C\u1A7F-\u1A89\u1A90-\u1A99\u1AB0-\u1ABD\u1ABF-\u1ACE\u1B00-\u1B04\u1B34-\u1B44\u1B50-\u1B59\u1B6B-\u1B73\u1B80-\u1B82\u1BA1-\u1BAD\u1BB0-\u1BB9\u1BE6-\u1BF3\u1C24-\u1C37\u1C40-\u1C49\u1C50-\u1C59\u1CD0-\u1CD2\u1CD4-\u1CE8\u1CED\u1CF4\u1CF7-\u1CF9\u1DC0-\u1DFF\u203F\u2040\u2054\u20D0-\u20DC\u20E1\u20E5-\u20F0\u2CEF-\u2CF1\u2D7F\u2DE0-\u2DFF\u302A-\u302F\u3099\u309A\uA620-\uA629\uA66F\uA674-\uA67D\uA69E\uA69F\uA6F0\uA6F1\uA802\uA806\uA80B\uA823-\uA827\uA82C\uA880\uA881\uA8B4-\uA8C5\uA8D0-\uA8D9\uA8E0-\uA8F1\uA8FF-\uA909\uA926-\uA92D\uA947-\uA953\uA980-\uA983\uA9B3-\uA9C0\uA9D0-\uA9D9\uA9E5\uA9F0-\uA9F9\uAA29-\uAA36\uAA43\uAA4C\uAA4D\uAA50-\uAA59\uAA7B-\uAA7D\uAAB0\uAAB2-\uAAB4\uAAB7\uAAB8\uAABE\uAABF\uAAC1\uAAEB-\uAAEF\uAAF5\uAAF6\uABE3-\uABEA\uABEC\uABED\uABF0-\uABF9\uFB1E\uFE00-\uFE0F\uFE20-\uFE2F\uFE33\uFE34\uFE4D-\uFE4F\uFF10-\uFF19\uFF3F";
var nonASCIIidentifierStart = new RegExp("[" + nonASCIIidentifierStartChars + "]");
var nonASCIIidentifier = new RegExp("[" + nonASCIIidentifierStartChars + nonASCIIidentifierChars + "]");
nonASCIIidentifierStartChars = nonASCIIidentifierChars = null;
var astralIdentifierStartCodes = [0, 11, 2, 25, 2, 18, 2, 1, 2, 14, 3, 13, 35, 122, 70, 52, 268, 28, 4, 48, 48, 31, 14, 29, 6, 37, 11, 29, 3, 35, 5, 7, 2, 4, 43, 157, 19, 35, 5, 35, 5, 39, 9, 51, 13, 10, 2, 14, 2, 6, 2, 1, 2, 10, 2, 14, 2, 6, 2, 1, 68, 310, 10, 21, 11, 7, 25, 5, 2, 41, 2, 8, 70, 5, 3, 0, 2, 43, 2, 1, 4, 0, 3, 22, 11, 22, 10, 30, 66, 18, 2, 1, 11, 21, 11, 25, 71, 55, 7, 1, 65, 0, 16, 3, 2, 2, 2, 28, 43, 28, 4, 28, 36, 7, 2, 27, 28, 53, 11, 21, 11, 18, 14, 17, 111, 72, 56, 50, 14, 50, 14, 35, 349, 41, 7, 1, 79, 28, 11, 0, 9, 21, 43, 17, 47, 20, 28, 22, 13, 52, 58, 1, 3, 0, 14, 44, 33, 24, 27, 35, 30, 0, 3, 0, 9, 34, 4, 0, 13, 47, 15, 3, 22, 0, 2, 0, 36, 17, 2, 24, 20, 1, 64, 6, 2, 0, 2, 3, 2, 14, 2, 9, 8, 46, 39, 7, 3, 1, 3, 21, 2, 6, 2, 1, 2, 4, 4, 0, 19, 0, 13, 4, 159, 52, 19, 3, 21, 2, 31, 47, 21, 1, 2, 0, 185, 46, 42, 3, 37, 47, 21, 0, 60, 42, 14, 0, 72, 26, 38, 6, 186, 43, 117, 63, 32, 7, 3, 0, 3, 7, 2, 1, 2, 23, 16, 0, 2, 0, 95, 7, 3, 38, 17, 0, 2, 0, 29, 0, 11, 39, 8, 0, 22, 0, 12, 45, 20, 0, 19, 72, 264, 8, 2, 36, 18, 0, 50, 29, 113, 6, 2, 1, 2, 37, 22, 0, 26, 5, 2, 1, 2, 31, 15, 0, 328, 18, 16, 0, 2, 12, 2, 33, 125, 0, 80, 921, 103, 110, 18, 195, 2637, 96, 16, 1071, 18, 5, 4026, 582, 8634, 568, 8, 30, 18, 78, 18, 29, 19, 47, 17, 3, 32, 20, 6, 18, 689, 63, 129, 74, 6, 0, 67, 12, 65, 1, 2, 0, 29, 6135, 9, 1237, 43, 8, 8936, 3, 2, 6, 2, 1, 2, 290, 16, 0, 30, 2, 3, 0, 15, 3, 9, 395, 2309, 106, 6, 12, 4, 8, 8, 9, 5991, 84, 2, 70, 2, 1, 3, 0, 3, 1, 3, 3, 2, 11, 2, 0, 2, 6, 2, 64, 2, 3, 3, 7, 2, 6, 2, 27, 2, 3, 2, 4, 2, 0, 4, 6, 2, 339, 3, 24, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 30, 2, 24, 2, 7, 1845, 30, 7, 5, 262, 61, 147, 44, 11, 6, 17, 0, 322, 29, 19, 43, 485, 27, 757, 6, 2, 3, 2, 1, 2, 14, 2, 196, 60, 67, 8, 0, 1205, 3, 2, 26, 2, 1, 2, 0, 3, 0, 2, 9, 2, 3, 2, 0, 2, 0, 7, 0, 5, 0, 2, 0, 2, 0, 2, 2, 2, 1, 2, 0, 3, 0, 2, 0, 2, 0, 2, 0, 2, 0, 2, 1, 2, 0, 3, 3, 2, 6, 2, 3, 2, 3, 2, 0, 2, 9, 2, 16, 6, 2, 2, 4, 2, 16, 4421, 42719, 33, 4153, 7, 221, 3, 5761, 15, 7472, 3104, 541, 1507, 4938, 6, 4191];
var astralIdentifierCodes = [509, 0, 227, 0, 150, 4, 294, 9, 1368, 2, 2, 1, 6, 3, 41, 2, 5, 0, 166, 1, 574, 3, 9, 9, 370, 1, 81, 2, 71, 10, 50, 3, 123, 2, 54, 14, 32, 10, 3, 1, 11, 3, 46, 10, 8, 0, 46, 9, 7, 2, 37, 13, 2, 9, 6, 1, 45, 0, 13, 2, 49, 13, 9, 3, 2, 11, 83, 11, 7, 0, 3, 0, 158, 11, 6, 9, 7, 3, 56, 1, 2, 6, 3, 1, 3, 2, 10, 0, 11, 1, 3, 6, 4, 4, 193, 17, 10, 9, 5, 0, 82, 19, 13, 9, 214, 6, 3, 8, 28, 1, 83, 16, 16, 9, 82, 12, 9, 9, 84, 14, 5, 9, 243, 14, 166, 9, 71, 5, 2, 1, 3, 3, 2, 0, 2, 1, 13, 9, 120, 6, 3, 6, 4, 0, 29, 9, 41, 6, 2, 3, 9, 0, 10, 10, 47, 15, 406, 7, 2, 7, 17, 9, 57, 21, 2, 13, 123, 5, 4, 0, 2, 1, 2, 6, 2, 0, 9, 9, 49, 4, 2, 1, 2, 4, 9, 9, 330, 3, 10, 1, 2, 0, 49, 6, 4, 4, 14, 9, 5351, 0, 7, 14, 13835, 9, 87, 9, 39, 4, 60, 6, 26, 9, 1014, 0, 2, 54, 8, 3, 82, 0, 12, 1, 19628, 1, 4706, 45, 3, 22, 543, 4, 4, 5, 9, 7, 3, 6, 31, 3, 149, 2, 1418, 49, 513, 54, 5, 49, 9, 0, 15, 0, 23, 4, 2, 14, 1361, 6, 2, 16, 3, 6, 2, 1, 2, 4, 101, 0, 161, 6, 10, 9, 357, 0, 62, 13, 499, 13, 983, 6, 110, 6, 6, 9, 4759, 9, 787719, 239];
function isInAstralSet(code, set) {
let pos = 65536;
for (let i = 0, length = set.length; i < length; i += 2) {
pos += set[i];
if (pos > code)
return false;
pos += set[i + 1];
if (pos >= code)
return true;
}
return false;
}
function isIdentifierStart(code) {
if (code < 65)
return code === 36;
if (code <= 90)
return true;
if (code < 97)
return code === 95;
if (code <= 122)
return true;
if (code <= 65535) {
return code >= 170 && nonASCIIidentifierStart.test(String.fromCharCode(code));
}
return isInAstralSet(code, astralIdentifierStartCodes);
}
function isIdentifierChar(code) {
if (code < 48)
return code === 36;
if (code < 58)
return true;
if (code < 65)
return false;
if (code <= 90)
return true;
if (code < 97)
return code === 95;
if (code <= 122)
return true;
if (code <= 65535) {
return code >= 170 && nonASCIIidentifier.test(String.fromCharCode(code));
}
return isInAstralSet(code, astralIdentifierStartCodes) || isInAstralSet(code, astralIdentifierCodes);
}
function isIdentifierName(name) {
let isFirst = true;
for (let i = 0; i < name.length; i++) {
let cp = name.charCodeAt(i);
if ((cp & 64512) === 55296 && i + 1 < name.length) {
const trail = name.charCodeAt(++i);
if ((trail & 64512) === 56320) {
cp = 65536 + ((cp & 1023) << 10) + (trail & 1023);
}
}
if (isFirst) {
isFirst = false;
if (!isIdentifierStart(cp)) {
return false;
}
} else if (!isIdentifierChar(cp)) {
return false;
}
}
return !isFirst;
}
}
});
var require_keyword = __commonJS22({
"node_modules/@babel/helper-validator-identifier/lib/keyword.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.isKeyword = isKeyword;
exports2.isReservedWord = isReservedWord;
exports2.isStrictBindOnlyReservedWord = isStrictBindOnlyReservedWord;
exports2.isStrictBindReservedWord = isStrictBindReservedWord;
exports2.isStrictReservedWord = isStrictReservedWord;
var reservedWords = {
keyword: ["break", "case", "catch", "continue", "debugger", "default", "do", "else", "finally", "for", "function", "if", "return", "switch", "throw", "try", "var", "const", "while", "with", "new", "this", "super", "class", "extends", "export", "import", "null", "true", "false", "in", "instanceof", "typeof", "void", "delete"],
strict: ["implements", "interface", "let", "package", "private", "protected", "public", "static", "yield"],
strictBind: ["eval", "arguments"]
};
var keywords = new Set(reservedWords.keyword);
var reservedWordsStrictSet = new Set(reservedWords.strict);
var reservedWordsStrictBindSet = new Set(reservedWords.strictBind);
function isReservedWord(word, inModule) {
return inModule && word === "await" || word === "enum";
}
function isStrictReservedWord(word, inModule) {
return isReservedWord(word, inModule) || reservedWordsStrictSet.has(word);
}
function isStrictBindOnlyReservedWord(word) {
return reservedWordsStrictBindSet.has(word);
}
function isStrictBindReservedWord(word, inModule) {
return isStrictReservedWord(word, inModule) || isStrictBindOnlyReservedWord(word);
}
function isKeyword(word) {
return keywords.has(word);
}
}
});
var require_lib32 = __commonJS22({
"node_modules/@babel/helper-validator-identifier/lib/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
Object.defineProperty(exports2, "isIdentifierChar", {
enumerable: true,
get: function() {
return _identifier.isIdentifierChar;
}
});
Object.defineProperty(exports2, "isIdentifierName", {
enumerable: true,
get: function() {
return _identifier.isIdentifierName;
}
});
Object.defineProperty(exports2, "isIdentifierStart", {
enumerable: true,
get: function() {
return _identifier.isIdentifierStart;
}
});
Object.defineProperty(exports2, "isKeyword", {
enumerable: true,
get: function() {
return _keyword.isKeyword;
}
});
Object.defineProperty(exports2, "isReservedWord", {
enumerable: true,
get: function() {
return _keyword.isReservedWord;
}
});
Object.defineProperty(exports2, "isStrictBindOnlyReservedWord", {
enumerable: true,
get: function() {
return _keyword.isStrictBindOnlyReservedWord;
}
});
Object.defineProperty(exports2, "isStrictBindReservedWord", {
enumerable: true,
get: function() {
return _keyword.isStrictBindReservedWord;
}
});
Object.defineProperty(exports2, "isStrictReservedWord", {
enumerable: true,
get: function() {
return _keyword.isStrictReservedWord;
}
});
var _identifier = require_identifier();
var _keyword = require_keyword();
}
});
var require_escape_string_regexp2 = __commonJS22({
"node_modules/@babel/highlight/node_modules/escape-string-regexp/index.js"(exports2, module22) {
"use strict";
var matchOperatorsRe = /[|\\{}()[\]^$+*?.]/g;
module22.exports = function(str) {
if (typeof str !== "string") {
throw new TypeError("Expected a string");
}
return str.replace(matchOperatorsRe, "\\$&");
};
}
});
var require_has_flag2 = __commonJS22({
"node_modules/@babel/highlight/node_modules/has-flag/index.js"(exports2, module22) {
"use strict";
module22.exports = (flag, argv) => {
argv = argv || process.argv;
const prefix = flag.startsWith("-") ? "" : flag.length === 1 ? "-" : "--";
const pos = argv.indexOf(prefix + flag);
const terminatorPos = argv.indexOf("--");
return pos !== -1 && (terminatorPos === -1 ? true : pos < terminatorPos);
};
}
});
var require_supports_color2 = __commonJS22({
"node_modules/@babel/highlight/node_modules/supports-color/index.js"(exports2, module22) {
"use strict";
var os = require("os");
var hasFlag = require_has_flag2();
var env = process.env;
var forceColor;
if (hasFlag("no-color") || hasFlag("no-colors") || hasFlag("color=false")) {
forceColor = false;
} else if (hasFlag("color") || hasFlag("colors") || hasFlag("color=true") || hasFlag("color=always")) {
forceColor = true;
}
if ("FORCE_COLOR" in env) {
forceColor = env.FORCE_COLOR.length === 0 || parseInt(env.FORCE_COLOR, 10) !== 0;
}
function translateLevel(level) {
if (level === 0) {
return false;
}
return {
level,
hasBasic: true,
has256: level >= 2,
has16m: level >= 3
};
}
function supportsColor(stream) {
if (forceColor === false) {
return 0;
}
if (hasFlag("color=16m") || hasFlag("color=full") || hasFlag("color=truecolor")) {
return 3;
}
if (hasFlag("color=256")) {
return 2;
}
if (stream && !stream.isTTY && forceColor !== true) {
return 0;
}
const min = forceColor ? 1 : 0;
if (process.platform === "win32") {
const osRelease = os.release().split(".");
if (Number(process.versions.node.split(".")[0]) >= 8 && Number(osRelease[0]) >= 10 && Number(osRelease[2]) >= 10586) {
return Number(osRelease[2]) >= 14931 ? 3 : 2;
}
return 1;
}
if ("CI" in env) {
if (["TRAVIS", "CIRCLECI", "APPVEYOR", "GITLAB_CI"].some((sign) => sign in env) || env.CI_NAME === "codeship") {
return 1;
}
return min;
}
if ("TEAMCITY_VERSION" in env) {
return /^(9\.(0*[1-9]\d*)\.|\d{2,}\.)/.test(env.TEAMCITY_VERSION) ? 1 : 0;
}
if (env.COLORTERM === "truecolor") {
return 3;
}
if ("TERM_PROGRAM" in env) {
const version2 = parseInt((env.TERM_PROGRAM_VERSION || "").split(".")[0], 10);
switch (env.TERM_PROGRAM) {
case "iTerm.app":
return version2 >= 3 ? 3 : 2;
case "Apple_Terminal":
return 2;
}
}
if (/-256(color)?$/i.test(env.TERM)) {
return 2;
}
if (/^screen|^xterm|^vt100|^vt220|^rxvt|color|ansi|cygwin|linux/i.test(env.TERM)) {
return 1;
}
if ("COLORTERM" in env) {
return 1;
}
if (env.TERM === "dumb") {
return min;
}
return min;
}
function getSupportLevel(stream) {
const level = supportsColor(stream);
return translateLevel(level);
}
module22.exports = {
supportsColor: getSupportLevel,
stdout: getSupportLevel(process.stdout),
stderr: getSupportLevel(process.stderr)
};
}
});
var require_templates2 = __commonJS22({
"node_modules/@babel/highlight/node_modules/chalk/templates.js"(exports2, module22) {
"use strict";
var TEMPLATE_REGEX = /(?:\\(u[a-f\d]{4}|x[a-f\d]{2}|.))|(?:\{(~)?(\w+(?:\([^)]*\))?(?:\.\w+(?:\([^)]*\))?)*)(?:[ \t]|(?=\r?\n)))|(\})|((?:.|[\r\n\f])+?)/gi;
var STYLE_REGEX = /(?:^|\.)(\w+)(?:\(([^)]*)\))?/g;
var STRING_REGEX = /^(['"])((?:\\.|(?!\1)[^\\])*)\1$/;
var ESCAPE_REGEX = /\\(u[a-f\d]{4}|x[a-f\d]{2}|.)|([^\\])/gi;
var ESCAPES = /* @__PURE__ */ new Map([["n", "\n"], ["r", "\r"], ["t", " "], ["b", "\b"], ["f", "\f"], ["v", "\v"], ["0", "\0"], ["\\", "\\"], ["e", "\x1B"], ["a", "\x07"]]);
function unescape2(c) {
if (c[0] === "u" && c.length === 5 || c[0] === "x" && c.length === 3) {
return String.fromCharCode(parseInt(c.slice(1), 16));
}
return ESCAPES.get(c) || c;
}
function parseArguments(name, args) {
const results = [];
const chunks = args.trim().split(/\s*,\s*/g);
let matches;
for (const chunk of chunks) {
if (!isNaN(chunk)) {
results.push(Number(chunk));
} else if (matches = chunk.match(STRING_REGEX)) {
results.push(matches[2].replace(ESCAPE_REGEX, (m, escape, chr) => escape ? unescape2(escape) : chr));
} else {
throw new Error(`Invalid Chalk template style argument: ${chunk} (in style '${name}')`);
}
}
return results;
}
function parseStyle(style) {
STYLE_REGEX.lastIndex = 0;
const results = [];
let matches;
while ((matches = STYLE_REGEX.exec(style)) !== null) {
const name = matches[1];
if (matches[2]) {
const args = parseArguments(name, matches[2]);
results.push([name].concat(args));
} else {
results.push([name]);
}
}
return results;
}
function buildStyle(chalk, styles) {
const enabled = {};
for (const layer of styles) {
for (const style of layer.styles) {
enabled[style[0]] = layer.inverse ? null : style.slice(1);
}
}
let current = chalk;
for (const styleName of Object.keys(enabled)) {
if (Array.isArray(enabled[styleName])) {
if (!(styleName in current)) {
throw new Error(`Unknown Chalk style: ${styleName}`);
}
if (enabled[styleName].length > 0) {
current = current[styleName].apply(current, enabled[styleName]);
} else {
current = current[styleName];
}
}
}
return current;
}
module22.exports = (chalk, tmp) => {
const styles = [];
const chunks = [];
let chunk = [];
tmp.replace(TEMPLATE_REGEX, (m, escapeChar, inverse, style, close, chr) => {
if (escapeChar) {
chunk.push(unescape2(escapeChar));
} else if (style) {
const str = chunk.join("");
chunk = [];
chunks.push(styles.length === 0 ? str : buildStyle(chalk, styles)(str));
styles.push({
inverse,
styles: parseStyle(style)
});
} else if (close) {
if (styles.length === 0) {
throw new Error("Found extraneous } in Chalk template literal");
}
chunks.push(buildStyle(chalk, styles)(chunk.join("")));
chunk = [];
styles.pop();
} else {
chunk.push(chr);
}
});
chunks.push(chunk.join(""));
if (styles.length > 0) {
const errMsg = `Chalk template literal is missing ${styles.length} closing bracket${styles.length === 1 ? "" : "s"} (\`}\`)`;
throw new Error(errMsg);
}
return chunks.join("");
};
}
});
var require_chalk2 = __commonJS22({
"node_modules/@babel/highlight/node_modules/chalk/index.js"(exports2, module22) {
"use strict";
var escapeStringRegexp2 = require_escape_string_regexp2();
var ansiStyles = require_ansi_styles();
var stdoutColor = require_supports_color2().stdout;
var template = require_templates2();
var isSimpleWindowsTerm = process.platform === "win32" && !(process.env.TERM || "").toLowerCase().startsWith("xterm");
var levelMapping = ["ansi", "ansi", "ansi256", "ansi16m"];
var skipModels = /* @__PURE__ */ new Set(["gray"]);
var styles = /* @__PURE__ */ Object.create(null);
function applyOptions(obj, options) {
options = options || {};
const scLevel = stdoutColor ? stdoutColor.level : 0;
obj.level = options.level === void 0 ? scLevel : options.level;
obj.enabled = "enabled" in options ? options.enabled : obj.level > 0;
}
function Chalk(options) {
if (!this || !(this instanceof Chalk) || this.template) {
const chalk = {};
applyOptions(chalk, options);
chalk.template = function() {
const args = [].slice.call(arguments);
return chalkTag.apply(null, [chalk.template].concat(args));
};
Object.setPrototypeOf(chalk, Chalk.prototype);
Object.setPrototypeOf(chalk.template, chalk);
chalk.template.constructor = Chalk;
return chalk.template;
}
applyOptions(this, options);
}
if (isSimpleWindowsTerm) {
ansiStyles.blue.open = "\x1B[94m";
}
for (const key of Object.keys(ansiStyles)) {
ansiStyles[key].closeRe = new RegExp(escapeStringRegexp2(ansiStyles[key].close), "g");
styles[key] = {
get() {
const codes = ansiStyles[key];
return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, key);
}
};
}
styles.visible = {
get() {
return build.call(this, this._styles || [], true, "visible");
}
};
ansiStyles.color.closeRe = new RegExp(escapeStringRegexp2(ansiStyles.color.close), "g");
for (const model of Object.keys(ansiStyles.color.ansi)) {
if (skipModels.has(model)) {
continue;
}
styles[model] = {
get() {
const level = this.level;
return function() {
const open = ansiStyles.color[levelMapping[level]][model].apply(null, arguments);
const codes = {
open,
close: ansiStyles.color.close,
closeRe: ansiStyles.color.closeRe
};
return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, model);
};
}
};
}
ansiStyles.bgColor.closeRe = new RegExp(escapeStringRegexp2(ansiStyles.bgColor.close), "g");
for (const model of Object.keys(ansiStyles.bgColor.ansi)) {
if (skipModels.has(model)) {
continue;
}
const bgModel = "bg" + model[0].toUpperCase() + model.slice(1);
styles[bgModel] = {
get() {
const level = this.level;
return function() {
const open = ansiStyles.bgColor[levelMapping[level]][model].apply(null, arguments);
const codes = {
open,
close: ansiStyles.bgColor.close,
closeRe: ansiStyles.bgColor.closeRe
};
return build.call(this, this._styles ? this._styles.concat(codes) : [codes], this._empty, model);
};
}
};
}
var proto = Object.defineProperties(() => {
}, styles);
function build(_styles, _empty, key) {
const builder = function() {
return applyStyle.apply(builder, arguments);
};
builder._styles = _styles;
builder._empty = _empty;
const self2 = this;
Object.defineProperty(builder, "level", {
enumerable: true,
get() {
return self2.level;
},
set(level) {
self2.level = level;
}
});
Object.defineProperty(builder, "enabled", {
enumerable: true,
get() {
return self2.enabled;
},
set(enabled) {
self2.enabled = enabled;
}
});
builder.hasGrey = this.hasGrey || key === "gray" || key === "grey";
builder.__proto__ = proto;
return builder;
}
function applyStyle() {
const args = arguments;
const argsLen = args.length;
let str = String(arguments[0]);
if (argsLen === 0) {
return "";
}
if (argsLen > 1) {
for (let a = 1; a < argsLen; a++) {
str += " " + args[a];
}
}
if (!this.enabled || this.level <= 0 || !str) {
return this._empty ? "" : str;
}
const originalDim = ansiStyles.dim.open;
if (isSimpleWindowsTerm && this.hasGrey) {
ansiStyles.dim.open = "";
}
for (const code of this._styles.slice().reverse()) {
str = code.open + str.replace(code.closeRe, code.open) + code.close;
str = str.replace(/\r?\n/g, `${code.close}$&${code.open}`);
}
ansiStyles.dim.open = originalDim;
return str;
}
function chalkTag(chalk, strings) {
if (!Array.isArray(strings)) {
return [].slice.call(arguments, 1).join(" ");
}
const args = [].slice.call(arguments, 2);
const parts = [strings.raw[0]];
for (let i = 1; i < strings.length; i++) {
parts.push(String(args[i - 1]).replace(/[{}\\]/g, "\\$&"));
parts.push(String(strings.raw[i]));
}
return template(chalk, parts.join(""));
}
Object.defineProperties(Chalk.prototype, styles);
module22.exports = Chalk();
module22.exports.supportsColor = stdoutColor;
module22.exports.default = module22.exports;
}
});
var require_lib42 = __commonJS22({
"node_modules/@babel/highlight/lib/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.default = highlight;
exports2.getChalk = getChalk;
exports2.shouldHighlight = shouldHighlight;
var _jsTokens = require_js_tokens();
var _helperValidatorIdentifier = require_lib32();
var _chalk = require_chalk2();
var sometimesKeywords = /* @__PURE__ */ new Set(["as", "async", "from", "get", "of", "set"]);
function getDefs(chalk) {
return {
keyword: chalk.cyan,
capitalized: chalk.yellow,
jsxIdentifier: chalk.yellow,
punctuator: chalk.yellow,
number: chalk.magenta,
string: chalk.green,
regex: chalk.magenta,
comment: chalk.grey,
invalid: chalk.white.bgRed.bold
};
}
var NEWLINE = /\r\n|[\n\r\u2028\u2029]/;
var BRACKET = /^[()[\]{}]$/;
var tokenize;
{
const JSX_TAG = /^[a-z][\w-]*$/i;
const getTokenType = function(token, offset, text) {
if (token.type === "name") {
if ((0, _helperValidatorIdentifier.isKeyword)(token.value) || (0, _helperValidatorIdentifier.isStrictReservedWord)(token.value, true) || sometimesKeywords.has(token.value)) {
return "keyword";
}
if (JSX_TAG.test(token.value) && (text[offset - 1] === "<" || text.slice(offset - 2, offset) == "")) {
return "jsxIdentifier";
}
if (token.value[0] !== token.value[0].toLowerCase()) {
return "capitalized";
}
}
if (token.type === "punctuator" && BRACKET.test(token.value)) {
return "bracket";
}
if (token.type === "invalid" && (token.value === "@" || token.value === "#")) {
return "punctuator";
}
return token.type;
};
tokenize = function* (text) {
let match;
while (match = _jsTokens.default.exec(text)) {
const token = _jsTokens.matchToToken(match);
yield {
type: getTokenType(token, match.index, text),
value: token.value
};
}
};
}
function highlightTokens(defs, text) {
let highlighted = "";
for (const {
type,
value
} of tokenize(text)) {
const colorize = defs[type];
if (colorize) {
highlighted += value.split(NEWLINE).map((str) => colorize(str)).join("\n");
} else {
highlighted += value;
}
}
return highlighted;
}
function shouldHighlight(options) {
return !!_chalk.supportsColor || options.forceColor;
}
function getChalk(options) {
return options.forceColor ? new _chalk.constructor({
enabled: true,
level: 1
}) : _chalk;
}
function highlight(code, options = {}) {
if (code !== "" && shouldHighlight(options)) {
const chalk = getChalk(options);
const defs = getDefs(chalk);
return highlightTokens(defs, code);
} else {
return code;
}
}
}
});
var require_lib52 = __commonJS22({
"node_modules/@babel/code-frame/lib/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.codeFrameColumns = codeFrameColumns;
exports2.default = _default;
var _highlight = require_lib42();
var deprecationWarningShown = false;
function getDefs(chalk) {
return {
gutter: chalk.grey,
marker: chalk.red.bold,
message: chalk.red.bold
};
}
var NEWLINE = /\r\n|[\n\r\u2028\u2029]/;
function getMarkerLines(loc, source, opts) {
const startLoc = Object.assign({
column: 0,
line: -1
}, loc.start);
const endLoc = Object.assign({}, startLoc, loc.end);
const {
linesAbove = 2,
linesBelow = 3
} = opts || {};
const startLine = startLoc.line;
const startColumn = startLoc.column;
const endLine = endLoc.line;
const endColumn = endLoc.column;
let start = Math.max(startLine - (linesAbove + 1), 0);
let end = Math.min(source.length, endLine + linesBelow);
if (startLine === -1) {
start = 0;
}
if (endLine === -1) {
end = source.length;
}
const lineDiff = endLine - startLine;
const markerLines = {};
if (lineDiff) {
for (let i = 0; i <= lineDiff; i++) {
const lineNumber = i + startLine;
if (!startColumn) {
markerLines[lineNumber] = true;
} else if (i === 0) {
const sourceLength = source[lineNumber - 1].length;
markerLines[lineNumber] = [startColumn, sourceLength - startColumn + 1];
} else if (i === lineDiff) {
markerLines[lineNumber] = [0, endColumn];
} else {
const sourceLength = source[lineNumber - i].length;
markerLines[lineNumber] = [0, sourceLength];
}
}
} else {
if (startColumn === endColumn) {
if (startColumn) {
markerLines[startLine] = [startColumn, 0];
} else {
markerLines[startLine] = true;
}
} else {
markerLines[startLine] = [startColumn, endColumn - startColumn];
}
}
return {
start,
end,
markerLines
};
}
function codeFrameColumns(rawLines, loc, opts = {}) {
const highlighted = (opts.highlightCode || opts.forceColor) && (0, _highlight.shouldHighlight)(opts);
const chalk = (0, _highlight.getChalk)(opts);
const defs = getDefs(chalk);
const maybeHighlight = (chalkFn, string) => {
return highlighted ? chalkFn(string) : string;
};
const lines = rawLines.split(NEWLINE);
const {
start,
end,
markerLines
} = getMarkerLines(loc, lines, opts);
const hasColumns = loc.start && typeof loc.start.column === "number";
const numberMaxWidth = String(end).length;
const highlightedLines = highlighted ? (0, _highlight.default)(rawLines, opts) : rawLines;
let frame = highlightedLines.split(NEWLINE, end).slice(start, end).map((line, index) => {
const number = start + 1 + index;
const paddedNumber = ` ${number}`.slice(-numberMaxWidth);
const gutter = ` ${paddedNumber} |`;
const hasMarker = markerLines[number];
const lastMarkerLine = !markerLines[number + 1];
if (hasMarker) {
let markerLine = "";
if (Array.isArray(hasMarker)) {
const markerSpacing = line.slice(0, Math.max(hasMarker[0] - 1, 0)).replace(/[^\t]/g, " ");
const numberOfMarkers = hasMarker[1] || 1;
markerLine = ["\n ", maybeHighlight(defs.gutter, gutter.replace(/\d/g, " ")), " ", markerSpacing, maybeHighlight(defs.marker, "^").repeat(numberOfMarkers)].join("");
if (lastMarkerLine && opts.message) {
markerLine += " " + maybeHighlight(defs.message, opts.message);
}
}
return [maybeHighlight(defs.marker, ">"), maybeHighlight(defs.gutter, gutter), line.length > 0 ? ` ${line}` : "", markerLine].join("");
} else {
return ` ${maybeHighlight(defs.gutter, gutter)}${line.length > 0 ? ` ${line}` : ""}`;
}
}).join("\n");
if (opts.message && !hasColumns) {
frame = `${" ".repeat(numberMaxWidth + 1)}${opts.message}
${frame}`;
}
if (highlighted) {
return chalk.reset(frame);
} else {
return frame;
}
}
function _default(rawLines, lineNumber, colNumber, opts = {}) {
if (!deprecationWarningShown) {
deprecationWarningShown = true;
const message = "Passing lineNumber and colNumber is deprecated to @babel/code-frame. Please use `codeFrameColumns`.";
if (process.emitWarning) {
process.emitWarning(message, "DeprecationWarning");
} else {
const deprecationError = new Error(message);
deprecationError.name = "DeprecationWarning";
console.warn(new Error(message));
}
}
colNumber = Math.max(colNumber, 0);
const location = {
start: {
column: colNumber,
line: lineNumber
}
};
return codeFrameColumns(rawLines, location, opts);
}
}
});
var require_parser = __commonJS22({
"src/main/parser.js"(exports2, module22) {
"use strict";
var {
ConfigError
} = require_errors();
var jsLoc = require_loc();
var loadParser = require_load_parser();
var {
locStart,
locEnd
} = jsLoc;
var ownNames = Object.getOwnPropertyNames;
var ownDescriptor = Object.getOwnPropertyDescriptor;
function getParsers(options) {
const parsers = {};
for (const plugin of options.plugins) {
if (!plugin.parsers) {
continue;
}
for (const name of ownNames(plugin.parsers)) {
Object.defineProperty(parsers, name, ownDescriptor(plugin.parsers, name));
}
}
return parsers;
}
function resolveParser(opts, parsers = getParsers(opts)) {
if (typeof opts.parser === "function") {
return {
parse: opts.parser,
astFormat: "estree",
locStart,
locEnd
};
}
if (typeof opts.parser === "string") {
if (Object.prototype.hasOwnProperty.call(parsers, opts.parser)) {
return parsers[opts.parser];
}
if (false) {
throw new ConfigError(`Couldn't resolve parser "${opts.parser}". Parsers must be explicitly added to the standalone bundle.`);
}
return loadParser(opts.parser);
}
}
function parse2(text, opts) {
const parsers = getParsers(opts);
const parsersForCustomParserApi = Object.defineProperties({}, Object.fromEntries(Object.keys(parsers).map((parserName) => [parserName, {
enumerable: true,
get() {
return parsers[parserName].parse;
}
}])));
const parser = resolveParser(opts, parsers);
try {
if (parser.preprocess) {
text = parser.preprocess(text, opts);
}
return {
text,
ast: parser.parse(text, parsersForCustomParserApi, opts)
};
} catch (error) {
const {
loc
} = error;
if (loc) {
const {
codeFrameColumns
} = require_lib52();
error.codeFrame = codeFrameColumns(text, loc, {
highlightCode: true
});
error.message += "\n" + error.codeFrame;
throw error;
}
throw error;
}
}
module22.exports = {
parse: parse2,
resolveParser
};
}
});
var require_readlines = __commonJS22({
"node_modules/n-readlines/readlines.js"(exports2, module22) {
"use strict";
var fs2 = require("fs");
var LineByLine = class {
constructor(file, options) {
options = options || {};
if (!options.readChunk)
options.readChunk = 1024;
if (!options.newLineCharacter) {
options.newLineCharacter = 10;
} else {
options.newLineCharacter = options.newLineCharacter.charCodeAt(0);
}
if (typeof file === "number") {
this.fd = file;
} else {
this.fd = fs2.openSync(file, "r");
}
this.options = options;
this.newLineCharacter = options.newLineCharacter;
this.reset();
}
_searchInBuffer(buffer, hexNeedle) {
let found = -1;
for (let i = 0; i <= buffer.length; i++) {
let b_byte = buffer[i];
if (b_byte === hexNeedle) {
found = i;
break;
}
}
return found;
}
reset() {
this.eofReached = false;
this.linesCache = [];
this.fdPosition = 0;
}
close() {
fs2.closeSync(this.fd);
this.fd = null;
}
_extractLines(buffer) {
let line;
const lines = [];
let bufferPosition = 0;
let lastNewLineBufferPosition = 0;
while (true) {
let bufferPositionValue = buffer[bufferPosition++];
if (bufferPositionValue === this.newLineCharacter) {
line = buffer.slice(lastNewLineBufferPosition, bufferPosition);
lines.push(line);
lastNewLineBufferPosition = bufferPosition;
} else if (bufferPositionValue === void 0) {
break;
}
}
let leftovers = buffer.slice(lastNewLineBufferPosition, bufferPosition);
if (leftovers.length) {
lines.push(leftovers);
}
return lines;
}
_readChunk(lineLeftovers) {
let totalBytesRead = 0;
let bytesRead;
const buffers = [];
do {
const readBuffer = new Buffer(this.options.readChunk);
bytesRead = fs2.readSync(this.fd, readBuffer, 0, this.options.readChunk, this.fdPosition);
totalBytesRead = totalBytesRead + bytesRead;
this.fdPosition = this.fdPosition + bytesRead;
buffers.push(readBuffer);
} while (bytesRead && this._searchInBuffer(buffers[buffers.length - 1], this.options.newLineCharacter) === -1);
let bufferData = Buffer.concat(buffers);
if (bytesRead < this.options.readChunk) {
this.eofReached = true;
bufferData = bufferData.slice(0, totalBytesRead);
}
if (totalBytesRead) {
this.linesCache = this._extractLines(bufferData);
if (lineLeftovers) {
this.linesCache[0] = Buffer.concat([lineLeftovers, this.linesCache[0]]);
}
}
return totalBytesRead;
}
next() {
if (!this.fd)
return false;
let line = false;
if (this.eofReached && this.linesCache.length === 0) {
return line;
}
let bytesRead;
if (!this.linesCache.length) {
bytesRead = this._readChunk();
}
if (this.linesCache.length) {
line = this.linesCache.shift();
const lastLineCharacter = line[line.length - 1];
if (lastLineCharacter !== this.newLineCharacter) {
bytesRead = this._readChunk(line);
if (bytesRead) {
line = this.linesCache.shift();
}
}
}
if (this.eofReached && this.linesCache.length === 0) {
this.close();
}
if (line && line[line.length - 1] === this.newLineCharacter) {
line = line.slice(0, line.length - 1);
}
return line;
}
};
module22.exports = LineByLine;
}
});
var require_get_interpreter = __commonJS22({
"src/utils/get-interpreter.js"(exports2, module22) {
"use strict";
var fs2 = require("fs");
var readlines = require_readlines();
function getInterpreter(filepath) {
if (typeof filepath !== "string") {
return "";
}
let fd;
try {
fd = fs2.openSync(filepath, "r");
} catch {
return "";
}
try {
const liner = new readlines(fd);
const firstLine = liner.next().toString("utf8");
const m1 = firstLine.match(/^#!\/(?:usr\/)?bin\/env\s+(\S+)/);
if (m1) {
return m1[1];
}
const m2 = firstLine.match(/^#!\/(?:usr\/(?:local\/)?)?bin\/(\S+)/);
if (m2) {
return m2[1];
}
return "";
} catch {
return "";
} finally {
try {
fs2.closeSync(fd);
} catch {
}
}
}
module22.exports = getInterpreter;
}
});
var require_options = __commonJS22({
"src/main/options.js"(exports2, module22) {
"use strict";
var path = require("path");
var {
UndefinedParserError
} = require_errors();
var {
getSupportInfo: getSupportInfo2
} = require_support();
var normalizer = require_options_normalizer();
var {
resolveParser
} = require_parser();
var hiddenDefaults = {
astFormat: "estree",
printer: {},
originalText: void 0,
locStart: null,
locEnd: null
};
function normalize(options, opts = {}) {
const rawOptions = Object.assign({}, options);
const supportOptions = getSupportInfo2({
plugins: options.plugins,
showUnreleased: true,
showDeprecated: true
}).options;
const defaults = Object.assign(Object.assign({}, hiddenDefaults), Object.fromEntries(supportOptions.filter((optionInfo) => optionInfo.default !== void 0).map((option) => [option.name, option.default])));
if (!rawOptions.parser) {
if (!rawOptions.filepath) {
const logger = opts.logger || console;
logger.warn("No parser and no filepath given, using 'babel' the parser now but this will throw an error in the future. Please specify a parser or a filepath so one can be inferred.");
rawOptions.parser = "babel";
} else {
rawOptions.parser = inferParser(rawOptions.filepath, rawOptions.plugins);
if (!rawOptions.parser) {
throw new UndefinedParserError(`No parser could be inferred for file: ${rawOptions.filepath}`);
}
}
}
const parser = resolveParser(normalizer.normalizeApiOptions(rawOptions, [supportOptions.find((x) => x.name === "parser")], {
passThrough: true,
logger: false
}));
rawOptions.astFormat = parser.astFormat;
rawOptions.locEnd = parser.locEnd;
rawOptions.locStart = parser.locStart;
const plugin = getPlugin(rawOptions);
rawOptions.printer = plugin.printers[rawOptions.astFormat];
const pluginDefaults = Object.fromEntries(supportOptions.filter((optionInfo) => optionInfo.pluginDefaults && optionInfo.pluginDefaults[plugin.name] !== void 0).map((optionInfo) => [optionInfo.name, optionInfo.pluginDefaults[plugin.name]]));
const mixedDefaults = Object.assign(Object.assign({}, defaults), pluginDefaults);
for (const [k, value] of Object.entries(mixedDefaults)) {
if (rawOptions[k] === null || rawOptions[k] === void 0) {
rawOptions[k] = value;
}
}
if (rawOptions.parser === "json") {
rawOptions.trailingComma = "none";
}
return normalizer.normalizeApiOptions(rawOptions, supportOptions, Object.assign({
passThrough: Object.keys(hiddenDefaults)
}, opts));
}
function getPlugin(options) {
const {
astFormat
} = options;
if (!astFormat) {
throw new Error("getPlugin() requires astFormat to be set");
}
const printerPlugin = options.plugins.find((plugin) => plugin.printers && plugin.printers[astFormat]);
if (!printerPlugin) {
throw new Error(`Couldn't find plugin for AST format "${astFormat}"`);
}
return printerPlugin;
}
function inferParser(filepath, plugins2) {
const filename = path.basename(filepath).toLowerCase();
const languages = getSupportInfo2({
plugins: plugins2
}).languages.filter((language2) => language2.since !== null);
let language = languages.find((language2) => language2.extensions && language2.extensions.some((extension) => filename.endsWith(extension)) || language2.filenames && language2.filenames.some((name) => name.toLowerCase() === filename));
if (!language && !filename.includes(".")) {
const getInterpreter = require_get_interpreter();
const interpreter = getInterpreter(filepath);
language = languages.find((language2) => language2.interpreters && language2.interpreters.includes(interpreter));
}
return language && language.parsers[0];
}
module22.exports = {
normalize,
hiddenDefaults,
inferParser
};
}
});
var require_massage_ast = __commonJS22({
"src/main/massage-ast.js"(exports2, module22) {
"use strict";
function massageAST(ast, options, parent) {
if (Array.isArray(ast)) {
return ast.map((e) => massageAST(e, options, parent)).filter(Boolean);
}
if (!ast || typeof ast !== "object") {
return ast;
}
const cleanFunction = options.printer.massageAstNode;
let ignoredProperties;
if (cleanFunction && cleanFunction.ignoredProperties) {
ignoredProperties = cleanFunction.ignoredProperties;
} else {
ignoredProperties = /* @__PURE__ */ new Set();
}
const newObj = {};
for (const [key, value] of Object.entries(ast)) {
if (!ignoredProperties.has(key) && typeof value !== "function") {
newObj[key] = massageAST(value, options, ast);
}
}
if (cleanFunction) {
const result = cleanFunction(ast, newObj, parent);
if (result === null) {
return;
}
if (result) {
return result;
}
}
return newObj;
}
module22.exports = massageAST;
}
});
var require_comments = __commonJS22({
"src/main/comments.js"(exports2, module22) {
"use strict";
var assert = require("assert");
var {
builders: {
line,
hardline,
breakParent,
indent,
lineSuffix,
join,
cursor
}
} = require_doc();
var {
hasNewline,
skipNewline,
skipSpaces,
isPreviousLineEmpty,
addLeadingComment,
addDanglingComment,
addTrailingComment
} = require_util();
var childNodesCache = /* @__PURE__ */ new WeakMap();
function getSortedChildNodes(node, options, resultArray) {
if (!node) {
return;
}
const {
printer,
locStart,
locEnd
} = options;
if (resultArray) {
if (printer.canAttachComment && printer.canAttachComment(node)) {
let i;
for (i = resultArray.length - 1; i >= 0; --i) {
if (locStart(resultArray[i]) <= locStart(node) && locEnd(resultArray[i]) <= locEnd(node)) {
break;
}
}
resultArray.splice(i + 1, 0, node);
return;
}
} else if (childNodesCache.has(node)) {
return childNodesCache.get(node);
}
const childNodes = printer.getCommentChildNodes && printer.getCommentChildNodes(node, options) || typeof node === "object" && Object.entries(node).filter(([key]) => key !== "enclosingNode" && key !== "precedingNode" && key !== "followingNode" && key !== "tokens" && key !== "comments" && key !== "parent").map(([, value]) => value);
if (!childNodes) {
return;
}
if (!resultArray) {
resultArray = [];
childNodesCache.set(node, resultArray);
}
for (const childNode of childNodes) {
getSortedChildNodes(childNode, options, resultArray);
}
return resultArray;
}
function decorateComment(node, comment, options, enclosingNode) {
const {
locStart,
locEnd
} = options;
const commentStart = locStart(comment);
const commentEnd = locEnd(comment);
const childNodes = getSortedChildNodes(node, options);
let precedingNode;
let followingNode;
let left = 0;
let right = childNodes.length;
while (left < right) {
const middle = left + right >> 1;
const child = childNodes[middle];
const start = locStart(child);
const end = locEnd(child);
if (start <= commentStart && commentEnd <= end) {
return decorateComment(child, comment, options, child);
}
if (end <= commentStart) {
precedingNode = child;
left = middle + 1;
continue;
}
if (commentEnd <= start) {
followingNode = child;
right = middle;
continue;
}
throw new Error("Comment location overlaps with node location");
}
if (enclosingNode && enclosingNode.type === "TemplateLiteral") {
const {
quasis
} = enclosingNode;
const commentIndex = findExpressionIndexForComment(quasis, comment, options);
if (precedingNode && findExpressionIndexForComment(quasis, precedingNode, options) !== commentIndex) {
precedingNode = null;
}
if (followingNode && findExpressionIndexForComment(quasis, followingNode, options) !== commentIndex) {
followingNode = null;
}
}
return {
enclosingNode,
precedingNode,
followingNode
};
}
var returnFalse = () => false;
function attach(comments, ast, text, options) {
if (!Array.isArray(comments)) {
return;
}
const tiesToBreak = [];
const {
locStart,
locEnd,
printer: {
handleComments = {}
}
} = options;
const {
avoidAstMutation,
ownLine: handleOwnLineComment = returnFalse,
endOfLine: handleEndOfLineComment = returnFalse,
remaining: handleRemainingComment = returnFalse
} = handleComments;
const decoratedComments = comments.map((comment, index) => Object.assign(Object.assign({}, decorateComment(ast, comment, options)), {}, {
comment,
text,
options,
ast,
isLastComment: comments.length - 1 === index
}));
for (const [index, context] of decoratedComments.entries()) {
const {
comment,
precedingNode,
enclosingNode,
followingNode,
text: text2,
options: options2,
ast: ast2,
isLastComment
} = context;
if (options2.parser === "json" || options2.parser === "json5" || options2.parser === "__js_expression" || options2.parser === "__vue_expression" || options2.parser === "__vue_ts_expression") {
if (locStart(comment) - locStart(ast2) <= 0) {
addLeadingComment(ast2, comment);
continue;
}
if (locEnd(comment) - locEnd(ast2) >= 0) {
addTrailingComment(ast2, comment);
continue;
}
}
let args;
if (avoidAstMutation) {
args = [context];
} else {
comment.enclosingNode = enclosingNode;
comment.precedingNode = precedingNode;
comment.followingNode = followingNode;
args = [comment, text2, options2, ast2, isLastComment];
}
if (isOwnLineComment(text2, options2, decoratedComments, index)) {
comment.placement = "ownLine";
if (handleOwnLineComment(...args)) {
} else if (followingNode) {
addLeadingComment(followingNode, comment);
} else if (precedingNode) {
addTrailingComment(precedingNode, comment);
} else if (enclosingNode) {
addDanglingComment(enclosingNode, comment);
} else {
addDanglingComment(ast2, comment);
}
} else if (isEndOfLineComment(text2, options2, decoratedComments, index)) {
comment.placement = "endOfLine";
if (handleEndOfLineComment(...args)) {
} else if (precedingNode) {
addTrailingComment(precedingNode, comment);
} else if (followingNode) {
addLeadingComment(followingNode, comment);
} else if (enclosingNode) {
addDanglingComment(enclosingNode, comment);
} else {
addDanglingComment(ast2, comment);
}
} else {
comment.placement = "remaining";
if (handleRemainingComment(...args)) {
} else if (precedingNode && followingNode) {
const tieCount = tiesToBreak.length;
if (tieCount > 0) {
const lastTie = tiesToBreak[tieCount - 1];
if (lastTie.followingNode !== followingNode) {
breakTies(tiesToBreak, text2, options2);
}
}
tiesToBreak.push(context);
} else if (precedingNode) {
addTrailingComment(precedingNode, comment);
} else if (followingNode) {
addLeadingComment(followingNode, comment);
} else if (enclosingNode) {
addDanglingComment(enclosingNode, comment);
} else {
addDanglingComment(ast2, comment);
}
}
}
breakTies(tiesToBreak, text, options);
if (!avoidAstMutation) {
for (const comment of comments) {
delete comment.precedingNode;
delete comment.enclosingNode;
delete comment.followingNode;
}
}
}
var isAllEmptyAndNoLineBreak = (text) => !/[\S\n\u2028\u2029]/.test(text);
function isOwnLineComment(text, options, decoratedComments, commentIndex) {
const {
comment,
precedingNode
} = decoratedComments[commentIndex];
const {
locStart,
locEnd
} = options;
let start = locStart(comment);
if (precedingNode) {
for (let index = commentIndex - 1; index >= 0; index--) {
const {
comment: comment2,
precedingNode: currentCommentPrecedingNode
} = decoratedComments[index];
if (currentCommentPrecedingNode !== precedingNode || !isAllEmptyAndNoLineBreak(text.slice(locEnd(comment2), start))) {
break;
}
start = locStart(comment2);
}
}
return hasNewline(text, start, {
backwards: true
});
}
function isEndOfLineComment(text, options, decoratedComments, commentIndex) {
const {
comment,
followingNode
} = decoratedComments[commentIndex];
const {
locStart,
locEnd
} = options;
let end = locEnd(comment);
if (followingNode) {
for (let index = commentIndex + 1; index < decoratedComments.length; index++) {
const {
comment: comment2,
followingNode: currentCommentFollowingNode
} = decoratedComments[index];
if (currentCommentFollowingNode !== followingNode || !isAllEmptyAndNoLineBreak(text.slice(end, locStart(comment2)))) {
break;
}
end = locEnd(comment2);
}
}
return hasNewline(text, end);
}
function breakTies(tiesToBreak, text, options) {
const tieCount = tiesToBreak.length;
if (tieCount === 0) {
return;
}
const {
precedingNode,
followingNode,
enclosingNode
} = tiesToBreak[0];
const gapRegExp = options.printer.getGapRegex && options.printer.getGapRegex(enclosingNode) || /^[\s(]*$/;
let gapEndPos = options.locStart(followingNode);
let indexOfFirstLeadingComment;
for (indexOfFirstLeadingComment = tieCount; indexOfFirstLeadingComment > 0; --indexOfFirstLeadingComment) {
const {
comment,
precedingNode: currentCommentPrecedingNode,
followingNode: currentCommentFollowingNode
} = tiesToBreak[indexOfFirstLeadingComment - 1];
assert.strictEqual(currentCommentPrecedingNode, precedingNode);
assert.strictEqual(currentCommentFollowingNode, followingNode);
const gap = text.slice(options.locEnd(comment), gapEndPos);
if (gapRegExp.test(gap)) {
gapEndPos = options.locStart(comment);
} else {
break;
}
}
for (const [i, {
comment
}] of tiesToBreak.entries()) {
if (i < indexOfFirstLeadingComment) {
addTrailingComment(precedingNode, comment);
} else {
addLeadingComment(followingNode, comment);
}
}
for (const node of [precedingNode, followingNode]) {
if (node.comments && node.comments.length > 1) {
node.comments.sort((a, b) => options.locStart(a) - options.locStart(b));
}
}
tiesToBreak.length = 0;
}
function printComment(path, options) {
const comment = path.getValue();
comment.printed = true;
return options.printer.printComment(path, options);
}
function findExpressionIndexForComment(quasis, comment, options) {
const startPos = options.locStart(comment) - 1;
for (let i = 1; i < quasis.length; ++i) {
if (startPos < options.locStart(quasis[i])) {
return i - 1;
}
}
return 0;
}
function printLeadingComment(path, options) {
const comment = path.getValue();
const parts = [printComment(path, options)];
const {
printer,
originalText,
locStart,
locEnd
} = options;
const isBlock = printer.isBlockComment && printer.isBlockComment(comment);
if (isBlock) {
const lineBreak = hasNewline(originalText, locEnd(comment)) ? hasNewline(originalText, locStart(comment), {
backwards: true
}) ? hardline : line : " ";
parts.push(lineBreak);
} else {
parts.push(hardline);
}
const index = skipNewline(originalText, skipSpaces(originalText, locEnd(comment)));
if (index !== false && hasNewline(originalText, index)) {
parts.push(hardline);
}
return parts;
}
function printTrailingComment(path, options) {
const comment = path.getValue();
const printed = printComment(path, options);
const {
printer,
originalText,
locStart
} = options;
const isBlock = printer.isBlockComment && printer.isBlockComment(comment);
if (hasNewline(originalText, locStart(comment), {
backwards: true
})) {
const isLineBeforeEmpty = isPreviousLineEmpty(originalText, comment, locStart);
return lineSuffix([hardline, isLineBeforeEmpty ? hardline : "", printed]);
}
let parts = [" ", printed];
if (!isBlock) {
parts = [lineSuffix(parts), breakParent];
}
return parts;
}
function printDanglingComments(path, options, sameIndent, filter) {
const parts = [];
const node = path.getValue();
if (!node || !node.comments) {
return "";
}
path.each(() => {
const comment = path.getValue();
if (!comment.leading && !comment.trailing && (!filter || filter(comment))) {
parts.push(printComment(path, options));
}
}, "comments");
if (parts.length === 0) {
return "";
}
if (sameIndent) {
return join(hardline, parts);
}
return indent([hardline, join(hardline, parts)]);
}
function printCommentsSeparately(path, options, ignored) {
const value = path.getValue();
if (!value) {
return {};
}
let comments = value.comments || [];
if (ignored) {
comments = comments.filter((comment) => !ignored.has(comment));
}
const isCursorNode = value === options.cursorNode;
if (comments.length === 0) {
const maybeCursor = isCursorNode ? cursor : "";
return {
leading: maybeCursor,
trailing: maybeCursor
};
}
const leadingParts = [];
const trailingParts = [];
path.each(() => {
const comment = path.getValue();
if (ignored && ignored.has(comment)) {
return;
}
const {
leading,
trailing
} = comment;
if (leading) {
leadingParts.push(printLeadingComment(path, options));
} else if (trailing) {
trailingParts.push(printTrailingComment(path, options));
}
}, "comments");
if (isCursorNode) {
leadingParts.unshift(cursor);
trailingParts.push(cursor);
}
return {
leading: leadingParts,
trailing: trailingParts
};
}
function printComments(path, doc2, options, ignored) {
const {
leading,
trailing
} = printCommentsSeparately(path, options, ignored);
if (!leading && !trailing) {
return doc2;
}
return [leading, doc2, trailing];
}
function ensureAllCommentsPrinted(astComments) {
if (!astComments) {
return;
}
for (const comment of astComments) {
if (!comment.printed) {
throw new Error('Comment "' + comment.value.trim() + '" was not printed. Please report this error!');
}
delete comment.printed;
}
}
module22.exports = {
attach,
printComments,
printCommentsSeparately,
printDanglingComments,
getSortedChildNodes,
ensureAllCommentsPrinted
};
}
});
var require_ast_path = __commonJS22({
"src/common/ast-path.js"(exports2, module22) {
"use strict";
var getLast = require_get_last();
function getNodeHelper(path, count) {
const stackIndex = getNodeStackIndexHelper(path.stack, count);
return stackIndex === -1 ? null : path.stack[stackIndex];
}
function getNodeStackIndexHelper(stack, count) {
for (let i = stack.length - 1; i >= 0; i -= 2) {
const value = stack[i];
if (value && !Array.isArray(value) && --count < 0) {
return i;
}
}
return -1;
}
var AstPath = class {
constructor(value) {
this.stack = [value];
}
getName() {
const {
stack
} = this;
const {
length
} = stack;
if (length > 1) {
return stack[length - 2];
}
return null;
}
getValue() {
return getLast(this.stack);
}
getNode(count = 0) {
return getNodeHelper(this, count);
}
getParentNode(count = 0) {
return getNodeHelper(this, count + 1);
}
call(callback, ...names) {
const {
stack
} = this;
const {
length
} = stack;
let value = getLast(stack);
for (const name of names) {
value = value[name];
stack.push(name, value);
}
const result = callback(this);
stack.length = length;
return result;
}
callParent(callback, count = 0) {
const stackIndex = getNodeStackIndexHelper(this.stack, count + 1);
const parentValues = this.stack.splice(stackIndex + 1);
const result = callback(this);
this.stack.push(...parentValues);
return result;
}
each(callback, ...names) {
const {
stack
} = this;
const {
length
} = stack;
let value = getLast(stack);
for (const name of names) {
value = value[name];
stack.push(name, value);
}
for (let i = 0; i < value.length; ++i) {
stack.push(i, value[i]);
callback(this, i, value);
stack.length -= 2;
}
stack.length = length;
}
map(callback, ...names) {
const result = [];
this.each((path, index, value) => {
result[index] = callback(path, index, value);
}, ...names);
return result;
}
try(callback) {
const {
stack
} = this;
const stackBackup = [...stack];
try {
return callback();
} finally {
stack.length = 0;
stack.push(...stackBackup);
}
}
match(...predicates) {
let stackPointer = this.stack.length - 1;
let name = null;
let node = this.stack[stackPointer--];
for (const predicate of predicates) {
if (node === void 0) {
return false;
}
let number = null;
if (typeof name === "number") {
number = name;
name = this.stack[stackPointer--];
node = this.stack[stackPointer--];
}
if (predicate && !predicate(node, name, number)) {
return false;
}
name = this.stack[stackPointer--];
node = this.stack[stackPointer--];
}
return true;
}
findAncestor(predicate) {
let stackPointer = this.stack.length - 1;
let name = null;
let node = this.stack[stackPointer--];
while (node) {
let number = null;
if (typeof name === "number") {
number = name;
name = this.stack[stackPointer--];
node = this.stack[stackPointer--];
}
if (name !== null && predicate(node, name, number)) {
return node;
}
name = this.stack[stackPointer--];
node = this.stack[stackPointer--];
}
}
};
module22.exports = AstPath;
}
});
var require_multiparser = __commonJS22({
"src/main/multiparser.js"(exports2, module22) {
"use strict";
var {
utils: {
stripTrailingHardline
}
} = require_doc();
var {
normalize
} = require_options();
var comments = require_comments();
function printSubtree(path, print, options, printAstToDoc) {
if (options.printer.embed && options.embeddedLanguageFormatting === "auto") {
return options.printer.embed(path, print, (text, partialNextOptions, textToDocOptions) => textToDoc(text, partialNextOptions, options, printAstToDoc, textToDocOptions), options);
}
}
function textToDoc(text, partialNextOptions, parentOptions, printAstToDoc, {
stripTrailingHardline: shouldStripTrailingHardline = false
} = {}) {
const nextOptions = normalize(Object.assign(Object.assign(Object.assign({}, parentOptions), partialNextOptions), {}, {
parentParser: parentOptions.parser,
originalText: text
}), {
passThrough: true
});
const result = require_parser().parse(text, nextOptions);
const {
ast
} = result;
text = result.text;
const astComments = ast.comments;
delete ast.comments;
comments.attach(astComments, ast, text, nextOptions);
nextOptions[Symbol.for("comments")] = astComments || [];
nextOptions[Symbol.for("tokens")] = ast.tokens || [];
const doc2 = printAstToDoc(ast, nextOptions);
comments.ensureAllCommentsPrinted(astComments);
if (shouldStripTrailingHardline) {
if (typeof doc2 === "string") {
return doc2.replace(/(?:\r?\n)*$/, "");
}
return stripTrailingHardline(doc2);
}
return doc2;
}
module22.exports = {
printSubtree
};
}
});
var require_ast_to_doc = __commonJS22({
"src/main/ast-to-doc.js"(exports2, module22) {
"use strict";
var AstPath = require_ast_path();
var {
builders: {
hardline,
addAlignmentToDoc
},
utils: {
propagateBreaks
}
} = require_doc();
var {
printComments
} = require_comments();
var multiparser = require_multiparser();
function printAstToDoc(ast, options, alignmentSize = 0) {
const {
printer
} = options;
if (printer.preprocess) {
ast = printer.preprocess(ast, options);
}
const cache = /* @__PURE__ */ new Map();
const path = new AstPath(ast);
let doc2 = mainPrint();
if (alignmentSize > 0) {
doc2 = addAlignmentToDoc([hardline, doc2], alignmentSize, options.tabWidth);
}
propagateBreaks(doc2);
return doc2;
function mainPrint(selector, args) {
if (selector === void 0 || selector === path) {
return mainPrintInternal(args);
}
if (Array.isArray(selector)) {
return path.call(() => mainPrintInternal(args), ...selector);
}
return path.call(() => mainPrintInternal(args), selector);
}
function mainPrintInternal(args) {
const value = path.getValue();
const shouldCache = value && typeof value === "object" && args === void 0;
if (shouldCache && cache.has(value)) {
return cache.get(value);
}
const doc3 = callPluginPrintFunction(path, options, mainPrint, args);
if (shouldCache) {
cache.set(value, doc3);
}
return doc3;
}
}
function printPrettierIgnoredNode(node, options) {
const {
originalText,
[Symbol.for("comments")]: comments,
locStart,
locEnd
} = options;
const start = locStart(node);
const end = locEnd(node);
const printedComments = /* @__PURE__ */ new Set();
for (const comment of comments) {
if (locStart(comment) >= start && locEnd(comment) <= end) {
comment.printed = true;
printedComments.add(comment);
}
}
return {
doc: originalText.slice(start, end),
printedComments
};
}
function callPluginPrintFunction(path, options, printPath, args) {
const node = path.getValue();
const {
printer
} = options;
let doc2;
let printedComments;
if (printer.hasPrettierIgnore && printer.hasPrettierIgnore(path)) {
({
doc: doc2,
printedComments
} = printPrettierIgnoredNode(node, options));
} else {
if (node) {
try {
doc2 = multiparser.printSubtree(path, printPath, options, printAstToDoc);
} catch (error) {
if (process.env.PRETTIER_DEBUG) {
throw error;
}
}
}
if (!doc2) {
doc2 = printer.print(path, options, printPath, args);
}
}
if (!printer.willPrintOwnComments || !printer.willPrintOwnComments(path, options)) {
doc2 = printComments(path, doc2, options, printedComments);
}
return doc2;
}
module22.exports = printAstToDoc;
}
});
var require_range_util = __commonJS22({
"src/main/range-util.js"(exports2, module22) {
"use strict";
var assert = require("assert");
var comments = require_comments();
var isJsonParser = ({
parser
}) => parser === "json" || parser === "json5" || parser === "json-stringify";
function findCommonAncestor(startNodeAndParents, endNodeAndParents) {
const startNodeAndAncestors = [startNodeAndParents.node, ...startNodeAndParents.parentNodes];
const endNodeAndAncestors = /* @__PURE__ */ new Set([endNodeAndParents.node, ...endNodeAndParents.parentNodes]);
return startNodeAndAncestors.find((node) => jsonSourceElements.has(node.type) && endNodeAndAncestors.has(node));
}
function dropRootParents(parents) {
let lastParentIndex = parents.length - 1;
for (; ; ) {
const parent = parents[lastParentIndex];
if (parent && (parent.type === "Program" || parent.type === "File")) {
lastParentIndex--;
} else {
break;
}
}
return parents.slice(0, lastParentIndex + 1);
}
function findSiblingAncestors(startNodeAndParents, endNodeAndParents, {
locStart,
locEnd
}) {
let resultStartNode = startNodeAndParents.node;
let resultEndNode = endNodeAndParents.node;
if (resultStartNode === resultEndNode) {
return {
startNode: resultStartNode,
endNode: resultEndNode
};
}
const startNodeStart = locStart(startNodeAndParents.node);
for (const endParent of dropRootParents(endNodeAndParents.parentNodes)) {
if (locStart(endParent) >= startNodeStart) {
resultEndNode = endParent;
} else {
break;
}
}
const endNodeEnd = locEnd(endNodeAndParents.node);
for (const startParent of dropRootParents(startNodeAndParents.parentNodes)) {
if (locEnd(startParent) <= endNodeEnd) {
resultStartNode = startParent;
} else {
break;
}
if (resultStartNode === resultEndNode) {
break;
}
}
return {
startNode: resultStartNode,
endNode: resultEndNode
};
}
function findNodeAtOffset(node, offset, options, predicate, parentNodes = [], type) {
const {
locStart,
locEnd
} = options;
const start = locStart(node);
const end = locEnd(node);
if (offset > end || offset < start || type === "rangeEnd" && offset === start || type === "rangeStart" && offset === end) {
return;
}
for (const childNode of comments.getSortedChildNodes(node, options)) {
const childResult = findNodeAtOffset(childNode, offset, options, predicate, [node, ...parentNodes], type);
if (childResult) {
return childResult;
}
}
if (!predicate || predicate(node, parentNodes[0])) {
return {
node,
parentNodes
};
}
}
function isJsSourceElement(type, parentType) {
return parentType !== "DeclareExportDeclaration" && type !== "TypeParameterDeclaration" && (type === "Directive" || type === "TypeAlias" || type === "TSExportAssignment" || type.startsWith("Declare") || type.startsWith("TSDeclare") || type.endsWith("Statement") || type.endsWith("Declaration"));
}
var jsonSourceElements = /* @__PURE__ */ new Set(["ObjectExpression", "ArrayExpression", "StringLiteral", "NumericLiteral", "BooleanLiteral", "NullLiteral", "UnaryExpression", "TemplateLiteral"]);
var graphqlSourceElements = /* @__PURE__ */ new Set(["OperationDefinition", "FragmentDefinition", "VariableDefinition", "TypeExtensionDefinition", "ObjectTypeDefinition", "FieldDefinition", "DirectiveDefinition", "EnumTypeDefinition", "EnumValueDefinition", "InputValueDefinition", "InputObjectTypeDefinition", "SchemaDefinition", "OperationTypeDefinition", "InterfaceTypeDefinition", "UnionTypeDefinition", "ScalarTypeDefinition"]);
function isSourceElement(opts, node, parentNode) {
if (!node) {
return false;
}
switch (opts.parser) {
case "flow":
case "babel":
case "babel-flow":
case "babel-ts":
case "typescript":
case "acorn":
case "espree":
case "meriyah":
case "__babel_estree":
return isJsSourceElement(node.type, parentNode && parentNode.type);
case "json":
case "json5":
case "json-stringify":
return jsonSourceElements.has(node.type);
case "graphql":
return graphqlSourceElements.has(node.kind);
case "vue":
return node.tag !== "root";
}
return false;
}
function calculateRange(text, opts, ast) {
let {
rangeStart: start,
rangeEnd: end,
locStart,
locEnd
} = opts;
assert.ok(end > start);
const firstNonWhitespaceCharacterIndex = text.slice(start, end).search(/\S/);
const isAllWhitespace = firstNonWhitespaceCharacterIndex === -1;
if (!isAllWhitespace) {
start += firstNonWhitespaceCharacterIndex;
for (; end > start; --end) {
if (/\S/.test(text[end - 1])) {
break;
}
}
}
const startNodeAndParents = findNodeAtOffset(ast, start, opts, (node, parentNode) => isSourceElement(opts, node, parentNode), [], "rangeStart");
const endNodeAndParents = isAllWhitespace ? startNodeAndParents : findNodeAtOffset(ast, end, opts, (node) => isSourceElement(opts, node), [], "rangeEnd");
if (!startNodeAndParents || !endNodeAndParents) {
return {
rangeStart: 0,
rangeEnd: 0
};
}
let startNode;
let endNode;
if (isJsonParser(opts)) {
const commonAncestor = findCommonAncestor(startNodeAndParents, endNodeAndParents);
startNode = commonAncestor;
endNode = commonAncestor;
} else {
({
startNode,
endNode
} = findSiblingAncestors(startNodeAndParents, endNodeAndParents, opts));
}
return {
rangeStart: Math.min(locStart(startNode), locStart(endNode)),
rangeEnd: Math.max(locEnd(startNode), locEnd(endNode))
};
}
module22.exports = {
calculateRange,
findNodeAtOffset
};
}
});
var require_core = __commonJS22({
"src/main/core.js"(exports2, module22) {
"use strict";
var {
diffArrays
} = require_array();
var {
printer: {
printDocToString
},
debug: {
printDocToDebug
}
} = require_doc();
var {
getAlignmentSize
} = require_util();
var {
guessEndOfLine,
convertEndOfLineToChars,
countEndOfLineChars,
normalizeEndOfLine
} = require_end_of_line();
var normalizeOptions = require_options().normalize;
var massageAST = require_massage_ast();
var comments = require_comments();
var parser = require_parser();
var printAstToDoc = require_ast_to_doc();
var rangeUtil = require_range_util();
var BOM = "\uFEFF";
var CURSOR = Symbol("cursor");
function attachComments(text, ast, opts) {
const astComments = ast.comments;
if (astComments) {
delete ast.comments;
comments.attach(astComments, ast, text, opts);
}
opts[Symbol.for("comments")] = astComments || [];
opts[Symbol.for("tokens")] = ast.tokens || [];
opts.originalText = text;
return astComments;
}
function coreFormat(originalText, opts, addAlignmentSize = 0) {
if (!originalText || originalText.trim().length === 0) {
return {
formatted: "",
cursorOffset: -1,
comments: []
};
}
const {
ast,
text
} = parser.parse(originalText, opts);
if (opts.cursorOffset >= 0) {
const nodeResult = rangeUtil.findNodeAtOffset(ast, opts.cursorOffset, opts);
if (nodeResult && nodeResult.node) {
opts.cursorNode = nodeResult.node;
}
}
const astComments = attachComments(text, ast, opts);
const doc2 = printAstToDoc(ast, opts, addAlignmentSize);
const result = printDocToString(doc2, opts);
comments.ensureAllCommentsPrinted(astComments);
if (addAlignmentSize > 0) {
const trimmed = result.formatted.trim();
if (result.cursorNodeStart !== void 0) {
result.cursorNodeStart -= result.formatted.indexOf(trimmed);
}
result.formatted = trimmed + convertEndOfLineToChars(opts.endOfLine);
}
if (opts.cursorOffset >= 0) {
let oldCursorNodeStart;
let oldCursorNodeText;
let cursorOffsetRelativeToOldCursorNode;
let newCursorNodeStart;
let newCursorNodeText;
if (opts.cursorNode && result.cursorNodeText) {
oldCursorNodeStart = opts.locStart(opts.cursorNode);
oldCursorNodeText = text.slice(oldCursorNodeStart, opts.locEnd(opts.cursorNode));
cursorOffsetRelativeToOldCursorNode = opts.cursorOffset - oldCursorNodeStart;
newCursorNodeStart = result.cursorNodeStart;
newCursorNodeText = result.cursorNodeText;
} else {
oldCursorNodeStart = 0;
oldCursorNodeText = text;
cursorOffsetRelativeToOldCursorNode = opts.cursorOffset;
newCursorNodeStart = 0;
newCursorNodeText = result.formatted;
}
if (oldCursorNodeText === newCursorNodeText) {
return {
formatted: result.formatted,
cursorOffset: newCursorNodeStart + cursorOffsetRelativeToOldCursorNode,
comments: astComments
};
}
const oldCursorNodeCharArray = [...oldCursorNodeText];
oldCursorNodeCharArray.splice(cursorOffsetRelativeToOldCursorNode, 0, CURSOR);
const newCursorNodeCharArray = [...newCursorNodeText];
const cursorNodeDiff = diffArrays(oldCursorNodeCharArray, newCursorNodeCharArray);
let cursorOffset = newCursorNodeStart;
for (const entry of cursorNodeDiff) {
if (entry.removed) {
if (entry.value.includes(CURSOR)) {
break;
}
} else {
cursorOffset += entry.count;
}
}
return {
formatted: result.formatted,
cursorOffset,
comments: astComments
};
}
return {
formatted: result.formatted,
cursorOffset: -1,
comments: astComments
};
}
function formatRange(originalText, opts) {
const {
ast,
text
} = parser.parse(originalText, opts);
const {
rangeStart,
rangeEnd
} = rangeUtil.calculateRange(text, opts, ast);
const rangeString = text.slice(rangeStart, rangeEnd);
const rangeStart2 = Math.min(rangeStart, text.lastIndexOf("\n", rangeStart) + 1);
const indentString = text.slice(rangeStart2, rangeStart).match(/^\s*/)[0];
const alignmentSize = getAlignmentSize(indentString, opts.tabWidth);
const rangeResult = coreFormat(rangeString, Object.assign(Object.assign({}, opts), {}, {
rangeStart: 0,
rangeEnd: Number.POSITIVE_INFINITY,
cursorOffset: opts.cursorOffset > rangeStart && opts.cursorOffset <= rangeEnd ? opts.cursorOffset - rangeStart : -1,
endOfLine: "lf"
}), alignmentSize);
const rangeTrimmed = rangeResult.formatted.trimEnd();
let {
cursorOffset
} = opts;
if (cursorOffset > rangeEnd) {
cursorOffset += rangeTrimmed.length - rangeString.length;
} else if (rangeResult.cursorOffset >= 0) {
cursorOffset = rangeResult.cursorOffset + rangeStart;
}
let formatted = text.slice(0, rangeStart) + rangeTrimmed + text.slice(rangeEnd);
if (opts.endOfLine !== "lf") {
const eol = convertEndOfLineToChars(opts.endOfLine);
if (cursorOffset >= 0 && eol === "\r\n") {
cursorOffset += countEndOfLineChars(formatted.slice(0, cursorOffset), "\n");
}
formatted = formatted.replace(/\n/g, eol);
}
return {
formatted,
cursorOffset,
comments: rangeResult.comments
};
}
function ensureIndexInText(text, index, defaultValue) {
if (typeof index !== "number" || Number.isNaN(index) || index < 0 || index > text.length) {
return defaultValue;
}
return index;
}
function normalizeIndexes(text, options) {
let {
cursorOffset,
rangeStart,
rangeEnd
} = options;
cursorOffset = ensureIndexInText(text, cursorOffset, -1);
rangeStart = ensureIndexInText(text, rangeStart, 0);
rangeEnd = ensureIndexInText(text, rangeEnd, text.length);
return Object.assign(Object.assign({}, options), {}, {
cursorOffset,
rangeStart,
rangeEnd
});
}
function normalizeInputAndOptions(text, options) {
let {
cursorOffset,
rangeStart,
rangeEnd,
endOfLine
} = normalizeIndexes(text, options);
const hasBOM = text.charAt(0) === BOM;
if (hasBOM) {
text = text.slice(1);
cursorOffset--;
rangeStart--;
rangeEnd--;
}
if (endOfLine === "auto") {
endOfLine = guessEndOfLine(text);
}
if (text.includes("\r")) {
const countCrlfBefore = (index) => countEndOfLineChars(text.slice(0, Math.max(index, 0)), "\r\n");
cursorOffset -= countCrlfBefore(cursorOffset);
rangeStart -= countCrlfBefore(rangeStart);
rangeEnd -= countCrlfBefore(rangeEnd);
text = normalizeEndOfLine(text);
}
return {
hasBOM,
text,
options: normalizeIndexes(text, Object.assign(Object.assign({}, options), {}, {
cursorOffset,
rangeStart,
rangeEnd,
endOfLine
}))
};
}
function hasPragma(text, options) {
const selectedParser = parser.resolveParser(options);
return !selectedParser.hasPragma || selectedParser.hasPragma(text);
}
function formatWithCursor2(originalText, originalOptions) {
let {
hasBOM,
text,
options
} = normalizeInputAndOptions(originalText, normalizeOptions(originalOptions));
if (options.rangeStart >= options.rangeEnd && text !== "" || options.requirePragma && !hasPragma(text, options)) {
return {
formatted: originalText,
cursorOffset: originalOptions.cursorOffset,
comments: []
};
}
let result;
if (options.rangeStart > 0 || options.rangeEnd < text.length) {
result = formatRange(text, options);
} else {
if (!options.requirePragma && options.insertPragma && options.printer.insertPragma && !hasPragma(text, options)) {
text = options.printer.insertPragma(text);
}
result = coreFormat(text, options);
}
if (hasBOM) {
result.formatted = BOM + result.formatted;
if (result.cursorOffset >= 0) {
result.cursorOffset++;
}
}
return result;
}
module22.exports = {
formatWithCursor: formatWithCursor2,
parse(originalText, originalOptions, massage) {
const {
text,
options
} = normalizeInputAndOptions(originalText, normalizeOptions(originalOptions));
const parsed = parser.parse(text, options);
if (massage) {
parsed.ast = massageAST(parsed.ast, options);
}
return parsed;
},
formatAST(ast, options) {
options = normalizeOptions(options);
const doc2 = printAstToDoc(ast, options);
return printDocToString(doc2, options);
},
formatDoc(doc2, options) {
return formatWithCursor2(printDocToDebug(doc2), Object.assign(Object.assign({}, options), {}, {
parser: "__js_expression"
})).formatted;
},
printToDoc(originalText, options) {
options = normalizeOptions(options);
const {
ast,
text
} = parser.parse(originalText, options);
attachComments(text, ast, options);
return printAstToDoc(ast, options);
},
printDocToString(doc2, options) {
return printDocToString(doc2, normalizeOptions(options));
}
};
}
});
var require_utils2 = __commonJS22({
"node_modules/braces/lib/utils.js"(exports2) {
"use strict";
exports2.isInteger = (num) => {
if (typeof num === "number") {
return Number.isInteger(num);
}
if (typeof num === "string" && num.trim() !== "") {
return Number.isInteger(Number(num));
}
return false;
};
exports2.find = (node, type) => node.nodes.find((node2) => node2.type === type);
exports2.exceedsLimit = (min, max, step = 1, limit) => {
if (limit === false)
return false;
if (!exports2.isInteger(min) || !exports2.isInteger(max))
return false;
return (Number(max) - Number(min)) / Number(step) >= limit;
};
exports2.escapeNode = (block, n2 = 0, type) => {
let node = block.nodes[n2];
if (!node)
return;
if (type && node.type === type || node.type === "open" || node.type === "close") {
if (node.escaped !== true) {
node.value = "\\" + node.value;
node.escaped = true;
}
}
};
exports2.encloseBrace = (node) => {
if (node.type !== "brace")
return false;
if (node.commas >> 0 + node.ranges >> 0 === 0) {
node.invalid = true;
return true;
}
return false;
};
exports2.isInvalidBrace = (block) => {
if (block.type !== "brace")
return false;
if (block.invalid === true || block.dollar)
return true;
if (block.commas >> 0 + block.ranges >> 0 === 0) {
block.invalid = true;
return true;
}
if (block.open !== true || block.close !== true) {
block.invalid = true;
return true;
}
return false;
};
exports2.isOpenOrClose = (node) => {
if (node.type === "open" || node.type === "close") {
return true;
}
return node.open === true || node.close === true;
};
exports2.reduce = (nodes) => nodes.reduce((acc, node) => {
if (node.type === "text")
acc.push(node.value);
if (node.type === "range")
node.type = "text";
return acc;
}, []);
exports2.flatten = (...args) => {
const result = [];
const flat = (arr) => {
for (let i = 0; i < arr.length; i++) {
let ele = arr[i];
Array.isArray(ele) ? flat(ele, result) : ele !== void 0 && result.push(ele);
}
return result;
};
flat(args);
return result;
};
}
});
var require_stringify3 = __commonJS22({
"node_modules/braces/lib/stringify.js"(exports2, module22) {
"use strict";
var utils = require_utils2();
module22.exports = (ast, options = {}) => {
let stringify = (node, parent = {}) => {
let invalidBlock = options.escapeInvalid && utils.isInvalidBrace(parent);
let invalidNode = node.invalid === true && options.escapeInvalid === true;
let output = "";
if (node.value) {
if ((invalidBlock || invalidNode) && utils.isOpenOrClose(node)) {
return "\\" + node.value;
}
return node.value;
}
if (node.value) {
return node.value;
}
if (node.nodes) {
for (let child of node.nodes) {
output += stringify(child);
}
}
return output;
};
return stringify(ast);
};
}
});
var require_is_number = __commonJS22({
"node_modules/is-number/index.js"(exports2, module22) {
"use strict";
module22.exports = function(num) {
if (typeof num === "number") {
return num - num === 0;
}
if (typeof num === "string" && num.trim() !== "") {
return Number.isFinite ? Number.isFinite(+num) : isFinite(+num);
}
return false;
};
}
});
var require_to_regex_range = __commonJS22({
"node_modules/to-regex-range/index.js"(exports2, module22) {
"use strict";
var isNumber = require_is_number();
var toRegexRange = (min, max, options) => {
if (isNumber(min) === false) {
throw new TypeError("toRegexRange: expected the first argument to be a number");
}
if (max === void 0 || min === max) {
return String(min);
}
if (isNumber(max) === false) {
throw new TypeError("toRegexRange: expected the second argument to be a number.");
}
let opts = Object.assign({
relaxZeros: true
}, options);
if (typeof opts.strictZeros === "boolean") {
opts.relaxZeros = opts.strictZeros === false;
}
let relax = String(opts.relaxZeros);
let shorthand = String(opts.shorthand);
let capture = String(opts.capture);
let wrap = String(opts.wrap);
let cacheKey = min + ":" + max + "=" + relax + shorthand + capture + wrap;
if (toRegexRange.cache.hasOwnProperty(cacheKey)) {
return toRegexRange.cache[cacheKey].result;
}
let a = Math.min(min, max);
let b = Math.max(min, max);
if (Math.abs(a - b) === 1) {
let result = min + "|" + max;
if (opts.capture) {
return `(${result})`;
}
if (opts.wrap === false) {
return result;
}
return `(?:${result})`;
}
let isPadded = hasPadding(min) || hasPadding(max);
let state = {
min,
max,
a,
b
};
let positives = [];
let negatives = [];
if (isPadded) {
state.isPadded = isPadded;
state.maxLen = String(state.max).length;
}
if (a < 0) {
let newMin = b < 0 ? Math.abs(b) : 1;
negatives = splitToPatterns(newMin, Math.abs(a), state, opts);
a = state.a = 0;
}
if (b >= 0) {
positives = splitToPatterns(a, b, state, opts);
}
state.negatives = negatives;
state.positives = positives;
state.result = collatePatterns(negatives, positives, opts);
if (opts.capture === true) {
state.result = `(${state.result})`;
} else if (opts.wrap !== false && positives.length + negatives.length > 1) {
state.result = `(?:${state.result})`;
}
toRegexRange.cache[cacheKey] = state;
return state.result;
};
function collatePatterns(neg, pos, options) {
let onlyNegative = filterPatterns(neg, pos, "-", false, options) || [];
let onlyPositive = filterPatterns(pos, neg, "", false, options) || [];
let intersected = filterPatterns(neg, pos, "-?", true, options) || [];
let subpatterns = onlyNegative.concat(intersected).concat(onlyPositive);
return subpatterns.join("|");
}
function splitToRanges(min, max) {
let nines = 1;
let zeros = 1;
let stop = countNines(min, nines);
let stops = /* @__PURE__ */ new Set([max]);
while (min <= stop && stop <= max) {
stops.add(stop);
nines += 1;
stop = countNines(min, nines);
}
stop = countZeros(max + 1, zeros) - 1;
while (min < stop && stop <= max) {
stops.add(stop);
zeros += 1;
stop = countZeros(max + 1, zeros) - 1;
}
stops = [...stops];
stops.sort(compare);
return stops;
}
function rangeToPattern(start, stop, options) {
if (start === stop) {
return {
pattern: start,
count: [],
digits: 0
};
}
let zipped = zip(start, stop);
let digits = zipped.length;
let pattern = "";
let count = 0;
for (let i = 0; i < digits; i++) {
let [startDigit, stopDigit] = zipped[i];
if (startDigit === stopDigit) {
pattern += startDigit;
} else if (startDigit !== "0" || stopDigit !== "9") {
pattern += toCharacterClass(startDigit, stopDigit, options);
} else {
count++;
}
}
if (count) {
pattern += options.shorthand === true ? "\\d" : "[0-9]";
}
return {
pattern,
count: [count],
digits
};
}
function splitToPatterns(min, max, tok, options) {
let ranges = splitToRanges(min, max);
let tokens = [];
let start = min;
let prev;
for (let i = 0; i < ranges.length; i++) {
let max2 = ranges[i];
let obj = rangeToPattern(String(start), String(max2), options);
let zeros = "";
if (!tok.isPadded && prev && prev.pattern === obj.pattern) {
if (prev.count.length > 1) {
prev.count.pop();
}
prev.count.push(obj.count[0]);
prev.string = prev.pattern + toQuantifier(prev.count);
start = max2 + 1;
continue;
}
if (tok.isPadded) {
zeros = padZeros(max2, tok, options);
}
obj.string = zeros + obj.pattern + toQuantifier(obj.count);
tokens.push(obj);
start = max2 + 1;
prev = obj;
}
return tokens;
}
function filterPatterns(arr, comparison, prefix, intersection, options) {
let result = [];
for (let ele of arr) {
let {
string
} = ele;
if (!intersection && !contains(comparison, "string", string)) {
result.push(prefix + string);
}
if (intersection && contains(comparison, "string", string)) {
result.push(prefix + string);
}
}
return result;
}
function zip(a, b) {
let arr = [];
for (let i = 0; i < a.length; i++)
arr.push([a[i], b[i]]);
return arr;
}
function compare(a, b) {
return a > b ? 1 : b > a ? -1 : 0;
}
function contains(arr, key, val) {
return arr.some((ele) => ele[key] === val);
}
function countNines(min, len) {
return Number(String(min).slice(0, -len) + "9".repeat(len));
}
function countZeros(integer, zeros) {
return integer - integer % Math.pow(10, zeros);
}
function toQuantifier(digits) {
let [start = 0, stop = ""] = digits;
if (stop || start > 1) {
return `{${start + (stop ? "," + stop : "")}}`;
}
return "";
}
function toCharacterClass(a, b, options) {
return `[${a}${b - a === 1 ? "" : "-"}${b}]`;
}
function hasPadding(str) {
return /^-?(0+)\d/.test(str);
}
function padZeros(value, tok, options) {
if (!tok.isPadded) {
return value;
}
let diff = Math.abs(tok.maxLen - String(value).length);
let relax = options.relaxZeros !== false;
switch (diff) {
case 0:
return "";
case 1:
return relax ? "0?" : "0";
case 2:
return relax ? "0{0,2}" : "00";
default: {
return relax ? `0{0,${diff}}` : `0{${diff}}`;
}
}
}
toRegexRange.cache = {};
toRegexRange.clearCache = () => toRegexRange.cache = {};
module22.exports = toRegexRange;
}
});
var require_fill_range = __commonJS22({
"node_modules/fill-range/index.js"(exports2, module22) {
"use strict";
var util = require("util");
var toRegexRange = require_to_regex_range();
var isObject2 = (val) => val !== null && typeof val === "object" && !Array.isArray(val);
var transform2 = (toNumber) => {
return (value) => toNumber === true ? Number(value) : String(value);
};
var isValidValue = (value) => {
return typeof value === "number" || typeof value === "string" && value !== "";
};
var isNumber = (num) => Number.isInteger(+num);
var zeros = (input) => {
let value = `${input}`;
let index = -1;
if (value[0] === "-")
value = value.slice(1);
if (value === "0")
return false;
while (value[++index] === "0")
;
return index > 0;
};
var stringify = (start, end, options) => {
if (typeof start === "string" || typeof end === "string") {
return true;
}
return options.stringify === true;
};
var pad = (input, maxLength, toNumber) => {
if (maxLength > 0) {
let dash = input[0] === "-" ? "-" : "";
if (dash)
input = input.slice(1);
input = dash + input.padStart(dash ? maxLength - 1 : maxLength, "0");
}
if (toNumber === false) {
return String(input);
}
return input;
};
var toMaxLen = (input, maxLength) => {
let negative = input[0] === "-" ? "-" : "";
if (negative) {
input = input.slice(1);
maxLength--;
}
while (input.length < maxLength)
input = "0" + input;
return negative ? "-" + input : input;
};
var toSequence = (parts, options) => {
parts.negatives.sort((a, b) => a < b ? -1 : a > b ? 1 : 0);
parts.positives.sort((a, b) => a < b ? -1 : a > b ? 1 : 0);
let prefix = options.capture ? "" : "?:";
let positives = "";
let negatives = "";
let result;
if (parts.positives.length) {
positives = parts.positives.join("|");
}
if (parts.negatives.length) {
negatives = `-(${prefix}${parts.negatives.join("|")})`;
}
if (positives && negatives) {
result = `${positives}|${negatives}`;
} else {
result = positives || negatives;
}
if (options.wrap) {
return `(${prefix}${result})`;
}
return result;
};
var toRange = (a, b, isNumbers, options) => {
if (isNumbers) {
return toRegexRange(a, b, Object.assign({
wrap: false
}, options));
}
let start = String.fromCharCode(a);
if (a === b)
return start;
let stop = String.fromCharCode(b);
return `[${start}-${stop}]`;
};
var toRegex = (start, end, options) => {
if (Array.isArray(start)) {
let wrap = options.wrap === true;
let prefix = options.capture ? "" : "?:";
return wrap ? `(${prefix}${start.join("|")})` : start.join("|");
}
return toRegexRange(start, end, options);
};
var rangeError = (...args) => {
return new RangeError("Invalid range arguments: " + util.inspect(...args));
};
var invalidRange = (start, end, options) => {
if (options.strictRanges === true)
throw rangeError([start, end]);
return [];
};
var invalidStep = (step, options) => {
if (options.strictRanges === true) {
throw new TypeError(`Expected step "${step}" to be a number`);
}
return [];
};
var fillNumbers = (start, end, step = 1, options = {}) => {
let a = Number(start);
let b = Number(end);
if (!Number.isInteger(a) || !Number.isInteger(b)) {
if (options.strictRanges === true)
throw rangeError([start, end]);
return [];
}
if (a === 0)
a = 0;
if (b === 0)
b = 0;
let descending = a > b;
let startString = String(start);
let endString = String(end);
let stepString = String(step);
step = Math.max(Math.abs(step), 1);
let padded = zeros(startString) || zeros(endString) || zeros(stepString);
let maxLen = padded ? Math.max(startString.length, endString.length, stepString.length) : 0;
let toNumber = padded === false && stringify(start, end, options) === false;
let format2 = options.transform || transform2(toNumber);
if (options.toRegex && step === 1) {
return toRange(toMaxLen(start, maxLen), toMaxLen(end, maxLen), true, options);
}
let parts = {
negatives: [],
positives: []
};
let push = (num) => parts[num < 0 ? "negatives" : "positives"].push(Math.abs(num));
let range = [];
let index = 0;
while (descending ? a >= b : a <= b) {
if (options.toRegex === true && step > 1) {
push(a);
} else {
range.push(pad(format2(a, index), maxLen, toNumber));
}
a = descending ? a - step : a + step;
index++;
}
if (options.toRegex === true) {
return step > 1 ? toSequence(parts, options) : toRegex(range, null, Object.assign({
wrap: false
}, options));
}
return range;
};
var fillLetters = (start, end, step = 1, options = {}) => {
if (!isNumber(start) && start.length > 1 || !isNumber(end) && end.length > 1) {
return invalidRange(start, end, options);
}
let format2 = options.transform || ((val) => String.fromCharCode(val));
let a = `${start}`.charCodeAt(0);
let b = `${end}`.charCodeAt(0);
let descending = a > b;
let min = Math.min(a, b);
let max = Math.max(a, b);
if (options.toRegex && step === 1) {
return toRange(min, max, false, options);
}
let range = [];
let index = 0;
while (descending ? a >= b : a <= b) {
range.push(format2(a, index));
a = descending ? a - step : a + step;
index++;
}
if (options.toRegex === true) {
return toRegex(range, null, {
wrap: false,
options
});
}
return range;
};
var fill = (start, end, step, options = {}) => {
if (end == null && isValidValue(start)) {
return [start];
}
if (!isValidValue(start) || !isValidValue(end)) {
return invalidRange(start, end, options);
}
if (typeof step === "function") {
return fill(start, end, 1, {
transform: step
});
}
if (isObject2(step)) {
return fill(start, end, 0, step);
}
let opts = Object.assign({}, options);
if (opts.capture === true)
opts.wrap = true;
step = step || opts.step || 1;
if (!isNumber(step)) {
if (step != null && !isObject2(step))
return invalidStep(step, opts);
return fill(start, end, 1, step);
}
if (isNumber(start) && isNumber(end)) {
return fillNumbers(start, end, step, opts);
}
return fillLetters(start, end, Math.max(Math.abs(step), 1), opts);
};
module22.exports = fill;
}
});
var require_compile3 = __commonJS22({
"node_modules/braces/lib/compile.js"(exports2, module22) {
"use strict";
var fill = require_fill_range();
var utils = require_utils2();
var compile = (ast, options = {}) => {
let walk = (node, parent = {}) => {
let invalidBlock = utils.isInvalidBrace(parent);
let invalidNode = node.invalid === true && options.escapeInvalid === true;
let invalid = invalidBlock === true || invalidNode === true;
let prefix = options.escapeInvalid === true ? "\\" : "";
let output = "";
if (node.isOpen === true) {
return prefix + node.value;
}
if (node.isClose === true) {
return prefix + node.value;
}
if (node.type === "open") {
return invalid ? prefix + node.value : "(";
}
if (node.type === "close") {
return invalid ? prefix + node.value : ")";
}
if (node.type === "comma") {
return node.prev.type === "comma" ? "" : invalid ? node.value : "|";
}
if (node.value) {
return node.value;
}
if (node.nodes && node.ranges > 0) {
let args = utils.reduce(node.nodes);
let range = fill(...args, Object.assign(Object.assign({}, options), {}, {
wrap: false,
toRegex: true
}));
if (range.length !== 0) {
return args.length > 1 && range.length > 1 ? `(${range})` : range;
}
}
if (node.nodes) {
for (let child of node.nodes) {
output += walk(child, node);
}
}
return output;
};
return walk(ast);
};
module22.exports = compile;
}
});
var require_expand = __commonJS22({
"node_modules/braces/lib/expand.js"(exports2, module22) {
"use strict";
var fill = require_fill_range();
var stringify = require_stringify3();
var utils = require_utils2();
var append = (queue = "", stash = "", enclose = false) => {
let result = [];
queue = [].concat(queue);
stash = [].concat(stash);
if (!stash.length)
return queue;
if (!queue.length) {
return enclose ? utils.flatten(stash).map((ele) => `{${ele}}`) : stash;
}
for (let item of queue) {
if (Array.isArray(item)) {
for (let value of item) {
result.push(append(value, stash, enclose));
}
} else {
for (let ele of stash) {
if (enclose === true && typeof ele === "string")
ele = `{${ele}}`;
result.push(Array.isArray(ele) ? append(item, ele, enclose) : item + ele);
}
}
}
return utils.flatten(result);
};
var expand = (ast, options = {}) => {
let rangeLimit = options.rangeLimit === void 0 ? 1e3 : options.rangeLimit;
let walk = (node, parent = {}) => {
node.queue = [];
let p = parent;
let q = parent.queue;
while (p.type !== "brace" && p.type !== "root" && p.parent) {
p = p.parent;
q = p.queue;
}
if (node.invalid || node.dollar) {
q.push(append(q.pop(), stringify(node, options)));
return;
}
if (node.type === "brace" && node.invalid !== true && node.nodes.length === 2) {
q.push(append(q.pop(), ["{}"]));
return;
}
if (node.nodes && node.ranges > 0) {
let args = utils.reduce(node.nodes);
if (utils.exceedsLimit(...args, options.step, rangeLimit)) {
throw new RangeError("expanded array length exceeds range limit. Use options.rangeLimit to increase or disable the limit.");
}
let range = fill(...args, options);
if (range.length === 0) {
range = stringify(node, options);
}
q.push(append(q.pop(), range));
node.nodes = [];
return;
}
let enclose = utils.encloseBrace(node);
let queue = node.queue;
let block = node;
while (block.type !== "brace" && block.type !== "root" && block.parent) {
block = block.parent;
queue = block.queue;
}
for (let i = 0; i < node.nodes.length; i++) {
let child = node.nodes[i];
if (child.type === "comma" && node.type === "brace") {
if (i === 1)
queue.push("");
queue.push("");
continue;
}
if (child.type === "close") {
q.push(append(q.pop(), queue, enclose));
continue;
}
if (child.value && child.type !== "open") {
queue.push(append(queue.pop(), child.value));
continue;
}
if (child.nodes) {
walk(child, node);
}
}
return queue;
};
return utils.flatten(walk(ast));
};
module22.exports = expand;
}
});
var require_constants2 = __commonJS22({
"node_modules/braces/lib/constants.js"(exports2, module22) {
"use strict";
module22.exports = {
MAX_LENGTH: 1024 * 64,
CHAR_0: "0",
CHAR_9: "9",
CHAR_UPPERCASE_A: "A",
CHAR_LOWERCASE_A: "a",
CHAR_UPPERCASE_Z: "Z",
CHAR_LOWERCASE_Z: "z",
CHAR_LEFT_PARENTHESES: "(",
CHAR_RIGHT_PARENTHESES: ")",
CHAR_ASTERISK: "*",
CHAR_AMPERSAND: "&",
CHAR_AT: "@",
CHAR_BACKSLASH: "\\",
CHAR_BACKTICK: "`",
CHAR_CARRIAGE_RETURN: "\r",
CHAR_CIRCUMFLEX_ACCENT: "^",
CHAR_COLON: ":",
CHAR_COMMA: ",",
CHAR_DOLLAR: "$",
CHAR_DOT: ".",
CHAR_DOUBLE_QUOTE: '"',
CHAR_EQUAL: "=",
CHAR_EXCLAMATION_MARK: "!",
CHAR_FORM_FEED: "\f",
CHAR_FORWARD_SLASH: "/",
CHAR_HASH: "#",
CHAR_HYPHEN_MINUS: "-",
CHAR_LEFT_ANGLE_BRACKET: "<",
CHAR_LEFT_CURLY_BRACE: "{",
CHAR_LEFT_SQUARE_BRACKET: "[",
CHAR_LINE_FEED: "\n",
CHAR_NO_BREAK_SPACE: "\xA0",
CHAR_PERCENT: "%",
CHAR_PLUS: "+",
CHAR_QUESTION_MARK: "?",
CHAR_RIGHT_ANGLE_BRACKET: ">",
CHAR_RIGHT_CURLY_BRACE: "}",
CHAR_RIGHT_SQUARE_BRACKET: "]",
CHAR_SEMICOLON: ";",
CHAR_SINGLE_QUOTE: "'",
CHAR_SPACE: " ",
CHAR_TAB: " ",
CHAR_UNDERSCORE: "_",
CHAR_VERTICAL_LINE: "|",
CHAR_ZERO_WIDTH_NOBREAK_SPACE: "\uFEFF"
};
}
});
var require_parse4 = __commonJS22({
"node_modules/braces/lib/parse.js"(exports2, module22) {
"use strict";
var stringify = require_stringify3();
var {
MAX_LENGTH,
CHAR_BACKSLASH,
CHAR_BACKTICK,
CHAR_COMMA,
CHAR_DOT,
CHAR_LEFT_PARENTHESES,
CHAR_RIGHT_PARENTHESES,
CHAR_LEFT_CURLY_BRACE,
CHAR_RIGHT_CURLY_BRACE,
CHAR_LEFT_SQUARE_BRACKET,
CHAR_RIGHT_SQUARE_BRACKET,
CHAR_DOUBLE_QUOTE,
CHAR_SINGLE_QUOTE,
CHAR_NO_BREAK_SPACE,
CHAR_ZERO_WIDTH_NOBREAK_SPACE
} = require_constants2();
var parse2 = (input, options = {}) => {
if (typeof input !== "string") {
throw new TypeError("Expected a string");
}
let opts = options || {};
let max = typeof opts.maxLength === "number" ? Math.min(MAX_LENGTH, opts.maxLength) : MAX_LENGTH;
if (input.length > max) {
throw new SyntaxError(`Input length (${input.length}), exceeds max characters (${max})`);
}
let ast = {
type: "root",
input,
nodes: []
};
let stack = [ast];
let block = ast;
let prev = ast;
let brackets = 0;
let length = input.length;
let index = 0;
let depth = 0;
let value;
let memo = {};
const advance = () => input[index++];
const push = (node) => {
if (node.type === "text" && prev.type === "dot") {
prev.type = "text";
}
if (prev && prev.type === "text" && node.type === "text") {
prev.value += node.value;
return;
}
block.nodes.push(node);
node.parent = block;
node.prev = prev;
prev = node;
return node;
};
push({
type: "bos"
});
while (index < length) {
block = stack[stack.length - 1];
value = advance();
if (value === CHAR_ZERO_WIDTH_NOBREAK_SPACE || value === CHAR_NO_BREAK_SPACE) {
continue;
}
if (value === CHAR_BACKSLASH) {
push({
type: "text",
value: (options.keepEscaping ? value : "") + advance()
});
continue;
}
if (value === CHAR_RIGHT_SQUARE_BRACKET) {
push({
type: "text",
value: "\\" + value
});
continue;
}
if (value === CHAR_LEFT_SQUARE_BRACKET) {
brackets++;
let closed = true;
let next;
while (index < length && (next = advance())) {
value += next;
if (next === CHAR_LEFT_SQUARE_BRACKET) {
brackets++;
continue;
}
if (next === CHAR_BACKSLASH) {
value += advance();
continue;
}
if (next === CHAR_RIGHT_SQUARE_BRACKET) {
brackets--;
if (brackets === 0) {
break;
}
}
}
push({
type: "text",
value
});
continue;
}
if (value === CHAR_LEFT_PARENTHESES) {
block = push({
type: "paren",
nodes: []
});
stack.push(block);
push({
type: "text",
value
});
continue;
}
if (value === CHAR_RIGHT_PARENTHESES) {
if (block.type !== "paren") {
push({
type: "text",
value
});
continue;
}
block = stack.pop();
push({
type: "text",
value
});
block = stack[stack.length - 1];
continue;
}
if (value === CHAR_DOUBLE_QUOTE || value === CHAR_SINGLE_QUOTE || value === CHAR_BACKTICK) {
let open = value;
let next;
if (options.keepQuotes !== true) {
value = "";
}
while (index < length && (next = advance())) {
if (next === CHAR_BACKSLASH) {
value += next + advance();
continue;
}
if (next === open) {
if (options.keepQuotes === true)
value += next;
break;
}
value += next;
}
push({
type: "text",
value
});
continue;
}
if (value === CHAR_LEFT_CURLY_BRACE) {
depth++;
let dollar = prev.value && prev.value.slice(-1) === "$" || block.dollar === true;
let brace = {
type: "brace",
open: true,
close: false,
dollar,
depth,
commas: 0,
ranges: 0,
nodes: []
};
block = push(brace);
stack.push(block);
push({
type: "open",
value
});
continue;
}
if (value === CHAR_RIGHT_CURLY_BRACE) {
if (block.type !== "brace") {
push({
type: "text",
value
});
continue;
}
let type = "close";
block = stack.pop();
block.close = true;
push({
type,
value
});
depth--;
block = stack[stack.length - 1];
continue;
}
if (value === CHAR_COMMA && depth > 0) {
if (block.ranges > 0) {
block.ranges = 0;
let open = block.nodes.shift();
block.nodes = [open, {
type: "text",
value: stringify(block)
}];
}
push({
type: "comma",
value
});
block.commas++;
continue;
}
if (value === CHAR_DOT && depth > 0 && block.commas === 0) {
let siblings = block.nodes;
if (depth === 0 || siblings.length === 0) {
push({
type: "text",
value
});
continue;
}
if (prev.type === "dot") {
block.range = [];
prev.value += value;
prev.type = "range";
if (block.nodes.length !== 3 && block.nodes.length !== 5) {
block.invalid = true;
block.ranges = 0;
prev.type = "text";
continue;
}
block.ranges++;
block.args = [];
continue;
}
if (prev.type === "range") {
siblings.pop();
let before = siblings[siblings.length - 1];
before.value += prev.value + value;
prev = before;
block.ranges--;
continue;
}
push({
type: "dot",
value
});
continue;
}
push({
type: "text",
value
});
}
do {
block = stack.pop();
if (block.type !== "root") {
block.nodes.forEach((node) => {
if (!node.nodes) {
if (node.type === "open")
node.isOpen = true;
if (node.type === "close")
node.isClose = true;
if (!node.nodes)
node.type = "text";
node.invalid = true;
}
});
let parent = stack[stack.length - 1];
let index2 = parent.nodes.indexOf(block);
parent.nodes.splice(index2, 1, ...block.nodes);
}
} while (stack.length > 0);
push({
type: "eos"
});
return ast;
};
module22.exports = parse2;
}
});
var require_braces = __commonJS22({
"node_modules/braces/index.js"(exports2, module22) {
"use strict";
var stringify = require_stringify3();
var compile = require_compile3();
var expand = require_expand();
var parse2 = require_parse4();
var braces = (input, options = {}) => {
let output = [];
if (Array.isArray(input)) {
for (let pattern of input) {
let result = braces.create(pattern, options);
if (Array.isArray(result)) {
output.push(...result);
} else {
output.push(result);
}
}
} else {
output = [].concat(braces.create(input, options));
}
if (options && options.expand === true && options.nodupes === true) {
output = [...new Set(output)];
}
return output;
};
braces.parse = (input, options = {}) => parse2(input, options);
braces.stringify = (input, options = {}) => {
if (typeof input === "string") {
return stringify(braces.parse(input, options), options);
}
return stringify(input, options);
};
braces.compile = (input, options = {}) => {
if (typeof input === "string") {
input = braces.parse(input, options);
}
return compile(input, options);
};
braces.expand = (input, options = {}) => {
if (typeof input === "string") {
input = braces.parse(input, options);
}
let result = expand(input, options);
if (options.noempty === true) {
result = result.filter(Boolean);
}
if (options.nodupes === true) {
result = [...new Set(result)];
}
return result;
};
braces.create = (input, options = {}) => {
if (input === "" || input.length < 3) {
return [input];
}
return options.expand !== true ? braces.compile(input, options) : braces.expand(input, options);
};
module22.exports = braces;
}
});
var require_constants3 = __commonJS22({
"node_modules/picomatch/lib/constants.js"(exports2, module22) {
"use strict";
var path = require("path");
var WIN_SLASH = "\\\\/";
var WIN_NO_SLASH = `[^${WIN_SLASH}]`;
var DOT_LITERAL = "\\.";
var PLUS_LITERAL = "\\+";
var QMARK_LITERAL = "\\?";
var SLASH_LITERAL = "\\/";
var ONE_CHAR = "(?=.)";
var QMARK = "[^/]";
var END_ANCHOR = `(?:${SLASH_LITERAL}|$)`;
var START_ANCHOR = `(?:^|${SLASH_LITERAL})`;
var DOTS_SLASH = `${DOT_LITERAL}{1,2}${END_ANCHOR}`;
var NO_DOT = `(?!${DOT_LITERAL})`;
var NO_DOTS = `(?!${START_ANCHOR}${DOTS_SLASH})`;
var NO_DOT_SLASH = `(?!${DOT_LITERAL}{0,1}${END_ANCHOR})`;
var NO_DOTS_SLASH = `(?!${DOTS_SLASH})`;
var QMARK_NO_DOT = `[^.${SLASH_LITERAL}]`;
var STAR = `${QMARK}*?`;
var POSIX_CHARS = {
DOT_LITERAL,
PLUS_LITERAL,
QMARK_LITERAL,
SLASH_LITERAL,
ONE_CHAR,
QMARK,
END_ANCHOR,
DOTS_SLASH,
NO_DOT,
NO_DOTS,
NO_DOT_SLASH,
NO_DOTS_SLASH,
QMARK_NO_DOT,
STAR,
START_ANCHOR
};
var WINDOWS_CHARS = Object.assign(Object.assign({}, POSIX_CHARS), {}, {
SLASH_LITERAL: `[${WIN_SLASH}]`,
QMARK: WIN_NO_SLASH,
STAR: `${WIN_NO_SLASH}*?`,
DOTS_SLASH: `${DOT_LITERAL}{1,2}(?:[${WIN_SLASH}]|$)`,
NO_DOT: `(?!${DOT_LITERAL})`,
NO_DOTS: `(?!(?:^|[${WIN_SLASH}])${DOT_LITERAL}{1,2}(?:[${WIN_SLASH}]|$))`,
NO_DOT_SLASH: `(?!${DOT_LITERAL}{0,1}(?:[${WIN_SLASH}]|$))`,
NO_DOTS_SLASH: `(?!${DOT_LITERAL}{1,2}(?:[${WIN_SLASH}]|$))`,
QMARK_NO_DOT: `[^.${WIN_SLASH}]`,
START_ANCHOR: `(?:^|[${WIN_SLASH}])`,
END_ANCHOR: `(?:[${WIN_SLASH}]|$)`
});
var POSIX_REGEX_SOURCE = {
alnum: "a-zA-Z0-9",
alpha: "a-zA-Z",
ascii: "\\x00-\\x7F",
blank: " \\t",
cntrl: "\\x00-\\x1F\\x7F",
digit: "0-9",
graph: "\\x21-\\x7E",
lower: "a-z",
print: "\\x20-\\x7E ",
punct: "\\-!\"#$%&'()\\*+,./:;<=>?@[\\]^_`{|}~",
space: " \\t\\r\\n\\v\\f",
upper: "A-Z",
word: "A-Za-z0-9_",
xdigit: "A-Fa-f0-9"
};
module22.exports = {
MAX_LENGTH: 1024 * 64,
POSIX_REGEX_SOURCE,
REGEX_BACKSLASH: /\\(?![*+?^${}(|)[\]])/g,
REGEX_NON_SPECIAL_CHARS: /^[^@![\].,$*+?^{}()|\\/]+/,
REGEX_SPECIAL_CHARS: /[-*+?.^${}(|)[\]]/,
REGEX_SPECIAL_CHARS_BACKREF: /(\\?)((\W)(\3*))/g,
REGEX_SPECIAL_CHARS_GLOBAL: /([-*+?.^${}(|)[\]])/g,
REGEX_REMOVE_BACKSLASH: /(?:\[.*?[^\\]\]|\\(?=.))/g,
REPLACEMENTS: {
"***": "*",
"**/**": "**",
"**/**/**": "**"
},
CHAR_0: 48,
CHAR_9: 57,
CHAR_UPPERCASE_A: 65,
CHAR_LOWERCASE_A: 97,
CHAR_UPPERCASE_Z: 90,
CHAR_LOWERCASE_Z: 122,
CHAR_LEFT_PARENTHESES: 40,
CHAR_RIGHT_PARENTHESES: 41,
CHAR_ASTERISK: 42,
CHAR_AMPERSAND: 38,
CHAR_AT: 64,
CHAR_BACKWARD_SLASH: 92,
CHAR_CARRIAGE_RETURN: 13,
CHAR_CIRCUMFLEX_ACCENT: 94,
CHAR_COLON: 58,
CHAR_COMMA: 44,
CHAR_DOT: 46,
CHAR_DOUBLE_QUOTE: 34,
CHAR_EQUAL: 61,
CHAR_EXCLAMATION_MARK: 33,
CHAR_FORM_FEED: 12,
CHAR_FORWARD_SLASH: 47,
CHAR_GRAVE_ACCENT: 96,
CHAR_HASH: 35,
CHAR_HYPHEN_MINUS: 45,
CHAR_LEFT_ANGLE_BRACKET: 60,
CHAR_LEFT_CURLY_BRACE: 123,
CHAR_LEFT_SQUARE_BRACKET: 91,
CHAR_LINE_FEED: 10,
CHAR_NO_BREAK_SPACE: 160,
CHAR_PERCENT: 37,
CHAR_PLUS: 43,
CHAR_QUESTION_MARK: 63,
CHAR_RIGHT_ANGLE_BRACKET: 62,
CHAR_RIGHT_CURLY_BRACE: 125,
CHAR_RIGHT_SQUARE_BRACKET: 93,
CHAR_SEMICOLON: 59,
CHAR_SINGLE_QUOTE: 39,
CHAR_SPACE: 32,
CHAR_TAB: 9,
CHAR_UNDERSCORE: 95,
CHAR_VERTICAL_LINE: 124,
CHAR_ZERO_WIDTH_NOBREAK_SPACE: 65279,
SEP: path.sep,
extglobChars(chars) {
return {
"!": {
type: "negate",
open: "(?:(?!(?:",
close: `))${chars.STAR})`
},
"?": {
type: "qmark",
open: "(?:",
close: ")?"
},
"+": {
type: "plus",
open: "(?:",
close: ")+"
},
"*": {
type: "star",
open: "(?:",
close: ")*"
},
"@": {
type: "at",
open: "(?:",
close: ")"
}
};
},
globChars(win32) {
return win32 === true ? WINDOWS_CHARS : POSIX_CHARS;
}
};
}
});
var require_utils3 = __commonJS22({
"node_modules/picomatch/lib/utils.js"(exports2) {
"use strict";
var path = require("path");
var win32 = process.platform === "win32";
var {
REGEX_BACKSLASH,
REGEX_REMOVE_BACKSLASH,
REGEX_SPECIAL_CHARS,
REGEX_SPECIAL_CHARS_GLOBAL
} = require_constants3();
exports2.isObject = (val) => val !== null && typeof val === "object" && !Array.isArray(val);
exports2.hasRegexChars = (str) => REGEX_SPECIAL_CHARS.test(str);
exports2.isRegexChar = (str) => str.length === 1 && exports2.hasRegexChars(str);
exports2.escapeRegex = (str) => str.replace(REGEX_SPECIAL_CHARS_GLOBAL, "\\$1");
exports2.toPosixSlashes = (str) => str.replace(REGEX_BACKSLASH, "/");
exports2.removeBackslashes = (str) => {
return str.replace(REGEX_REMOVE_BACKSLASH, (match) => {
return match === "\\" ? "" : match;
});
};
exports2.supportsLookbehinds = () => {
const segs = process.version.slice(1).split(".").map(Number);
if (segs.length === 3 && segs[0] >= 9 || segs[0] === 8 && segs[1] >= 10) {
return true;
}
return false;
};
exports2.isWindows = (options) => {
if (options && typeof options.windows === "boolean") {
return options.windows;
}
return win32 === true || path.sep === "\\";
};
exports2.escapeLast = (input, char2, lastIdx) => {
const idx = input.lastIndexOf(char2, lastIdx);
if (idx === -1)
return input;
if (input[idx - 1] === "\\")
return exports2.escapeLast(input, char2, idx - 1);
return `${input.slice(0, idx)}\\${input.slice(idx)}`;
};
exports2.removePrefix = (input, state = {}) => {
let output = input;
if (output.startsWith("./")) {
output = output.slice(2);
state.prefix = "./";
}
return output;
};
exports2.wrapOutput = (input, state = {}, options = {}) => {
const prepend = options.contains ? "" : "^";
const append = options.contains ? "" : "$";
let output = `${prepend}(?:${input})${append}`;
if (state.negated === true) {
output = `(?:^(?!${output}).*$)`;
}
return output;
};
}
});
var require_scan = __commonJS22({
"node_modules/picomatch/lib/scan.js"(exports2, module22) {
"use strict";
var utils = require_utils3();
var {
CHAR_ASTERISK,
CHAR_AT,
CHAR_BACKWARD_SLASH,
CHAR_COMMA,
CHAR_DOT,
CHAR_EXCLAMATION_MARK,
CHAR_FORWARD_SLASH,
CHAR_LEFT_CURLY_BRACE,
CHAR_LEFT_PARENTHESES,
CHAR_LEFT_SQUARE_BRACKET,
CHAR_PLUS,
CHAR_QUESTION_MARK,
CHAR_RIGHT_CURLY_BRACE,
CHAR_RIGHT_PARENTHESES,
CHAR_RIGHT_SQUARE_BRACKET
} = require_constants3();
var isPathSeparator = (code) => {
return code === CHAR_FORWARD_SLASH || code === CHAR_BACKWARD_SLASH;
};
var depth = (token) => {
if (token.isPrefix !== true) {
token.depth = token.isGlobstar ? Infinity : 1;
}
};
var scan = (input, options) => {
const opts = options || {};
const length = input.length - 1;
const scanToEnd = opts.parts === true || opts.scanToEnd === true;
const slashes = [];
const tokens = [];
const parts = [];
let str = input;
let index = -1;
let start = 0;
let lastIndex = 0;
let isBrace = false;
let isBracket = false;
let isGlob = false;
let isExtglob = false;
let isGlobstar = false;
let braceEscaped = false;
let backslashes = false;
let negated = false;
let negatedExtglob = false;
let finished = false;
let braces = 0;
let prev;
let code;
let token = {
value: "",
depth: 0,
isGlob: false
};
const eos = () => index >= length;
const peek = () => str.charCodeAt(index + 1);
const advance = () => {
prev = code;
return str.charCodeAt(++index);
};
while (index < length) {
code = advance();
let next;
if (code === CHAR_BACKWARD_SLASH) {
backslashes = token.backslashes = true;
code = advance();
if (code === CHAR_LEFT_CURLY_BRACE) {
braceEscaped = true;
}
continue;
}
if (braceEscaped === true || code === CHAR_LEFT_CURLY_BRACE) {
braces++;
while (eos() !== true && (code = advance())) {
if (code === CHAR_BACKWARD_SLASH) {
backslashes = token.backslashes = true;
advance();
continue;
}
if (code === CHAR_LEFT_CURLY_BRACE) {
braces++;
continue;
}
if (braceEscaped !== true && code === CHAR_DOT && (code = advance()) === CHAR_DOT) {
isBrace = token.isBrace = true;
isGlob = token.isGlob = true;
finished = true;
if (scanToEnd === true) {
continue;
}
break;
}
if (braceEscaped !== true && code === CHAR_COMMA) {
isBrace = token.isBrace = true;
isGlob = token.isGlob = true;
finished = true;
if (scanToEnd === true) {
continue;
}
break;
}
if (code === CHAR_RIGHT_CURLY_BRACE) {
braces--;
if (braces === 0) {
braceEscaped = false;
isBrace = token.isBrace = true;
finished = true;
break;
}
}
}
if (scanToEnd === true) {
continue;
}
break;
}
if (code === CHAR_FORWARD_SLASH) {
slashes.push(index);
tokens.push(token);
token = {
value: "",
depth: 0,
isGlob: false
};
if (finished === true)
continue;
if (prev === CHAR_DOT && index === start + 1) {
start += 2;
continue;
}
lastIndex = index + 1;
continue;
}
if (opts.noext !== true) {
const isExtglobChar = code === CHAR_PLUS || code === CHAR_AT || code === CHAR_ASTERISK || code === CHAR_QUESTION_MARK || code === CHAR_EXCLAMATION_MARK;
if (isExtglobChar === true && peek() === CHAR_LEFT_PARENTHESES) {
isGlob = token.isGlob = true;
isExtglob = token.isExtglob = true;
finished = true;
if (code === CHAR_EXCLAMATION_MARK && index === start) {
negatedExtglob = true;
}
if (scanToEnd === true) {
while (eos() !== true && (code = advance())) {
if (code === CHAR_BACKWARD_SLASH) {
backslashes = token.backslashes = true;
code = advance();
continue;
}
if (code === CHAR_RIGHT_PARENTHESES) {
isGlob = token.isGlob = true;
finished = true;
break;
}
}
continue;
}
break;
}
}
if (code === CHAR_ASTERISK) {
if (prev === CHAR_ASTERISK)
isGlobstar = token.isGlobstar = true;
isGlob = token.isGlob = true;
finished = true;
if (scanToEnd === true) {
continue;
}
break;
}
if (code === CHAR_QUESTION_MARK) {
isGlob = token.isGlob = true;
finished = true;
if (scanToEnd === true) {
continue;
}
break;
}
if (code === CHAR_LEFT_SQUARE_BRACKET) {
while (eos() !== true && (next = advance())) {
if (next === CHAR_BACKWARD_SLASH) {
backslashes = token.backslashes = true;
advance();
continue;
}
if (next === CHAR_RIGHT_SQUARE_BRACKET) {
isBracket = token.isBracket = true;
isGlob = token.isGlob = true;
finished = true;
break;
}
}
if (scanToEnd === true) {
continue;
}
break;
}
if (opts.nonegate !== true && code === CHAR_EXCLAMATION_MARK && index === start) {
negated = token.negated = true;
start++;
continue;
}
if (opts.noparen !== true && code === CHAR_LEFT_PARENTHESES) {
isGlob = token.isGlob = true;
if (scanToEnd === true) {
while (eos() !== true && (code = advance())) {
if (code === CHAR_LEFT_PARENTHESES) {
backslashes = token.backslashes = true;
code = advance();
continue;
}
if (code === CHAR_RIGHT_PARENTHESES) {
finished = true;
break;
}
}
continue;
}
break;
}
if (isGlob === true) {
finished = true;
if (scanToEnd === true) {
continue;
}
break;
}
}
if (opts.noext === true) {
isExtglob = false;
isGlob = false;
}
let base = str;
let prefix = "";
let glob = "";
if (start > 0) {
prefix = str.slice(0, start);
str = str.slice(start);
lastIndex -= start;
}
if (base && isGlob === true && lastIndex > 0) {
base = str.slice(0, lastIndex);
glob = str.slice(lastIndex);
} else if (isGlob === true) {
base = "";
glob = str;
} else {
base = str;
}
if (base && base !== "" && base !== "/" && base !== str) {
if (isPathSeparator(base.charCodeAt(base.length - 1))) {
base = base.slice(0, -1);
}
}
if (opts.unescape === true) {
if (glob)
glob = utils.removeBackslashes(glob);
if (base && backslashes === true) {
base = utils.removeBackslashes(base);
}
}
const state = {
prefix,
input,
start,
base,
glob,
isBrace,
isBracket,
isGlob,
isExtglob,
isGlobstar,
negated,
negatedExtglob
};
if (opts.tokens === true) {
state.maxDepth = 0;
if (!isPathSeparator(code)) {
tokens.push(token);
}
state.tokens = tokens;
}
if (opts.parts === true || opts.tokens === true) {
let prevIndex;
for (let idx = 0; idx < slashes.length; idx++) {
const n2 = prevIndex ? prevIndex + 1 : start;
const i = slashes[idx];
const value = input.slice(n2, i);
if (opts.tokens) {
if (idx === 0 && start !== 0) {
tokens[idx].isPrefix = true;
tokens[idx].value = prefix;
} else {
tokens[idx].value = value;
}
depth(tokens[idx]);
state.maxDepth += tokens[idx].depth;
}
if (idx !== 0 || value !== "") {
parts.push(value);
}
prevIndex = i;
}
if (prevIndex && prevIndex + 1 < input.length) {
const value = input.slice(prevIndex + 1);
parts.push(value);
if (opts.tokens) {
tokens[tokens.length - 1].value = value;
depth(tokens[tokens.length - 1]);
state.maxDepth += tokens[tokens.length - 1].depth;
}
}
state.slashes = slashes;
state.parts = parts;
}
return state;
};
module22.exports = scan;
}
});
var require_parse22 = __commonJS22({
"node_modules/picomatch/lib/parse.js"(exports2, module22) {
"use strict";
var constants = require_constants3();
var utils = require_utils3();
var {
MAX_LENGTH,
POSIX_REGEX_SOURCE,
REGEX_NON_SPECIAL_CHARS,
REGEX_SPECIAL_CHARS_BACKREF,
REPLACEMENTS
} = constants;
var expandRange = (args, options) => {
if (typeof options.expandRange === "function") {
return options.expandRange(...args, options);
}
args.sort();
const value = `[${args.join("-")}]`;
try {
new RegExp(value);
} catch (ex) {
return args.map((v) => utils.escapeRegex(v)).join("..");
}
return value;
};
var syntaxError = (type, char2) => {
return `Missing ${type}: "${char2}" - use "\\\\${char2}" to match literal characters`;
};
var parse2 = (input, options) => {
if (typeof input !== "string") {
throw new TypeError("Expected a string");
}
input = REPLACEMENTS[input] || input;
const opts = Object.assign({}, options);
const max = typeof opts.maxLength === "number" ? Math.min(MAX_LENGTH, opts.maxLength) : MAX_LENGTH;
let len = input.length;
if (len > max) {
throw new SyntaxError(`Input length: ${len}, exceeds maximum allowed length: ${max}`);
}
const bos = {
type: "bos",
value: "",
output: opts.prepend || ""
};
const tokens = [bos];
const capture = opts.capture ? "" : "?:";
const win32 = utils.isWindows(options);
const PLATFORM_CHARS = constants.globChars(win32);
const EXTGLOB_CHARS = constants.extglobChars(PLATFORM_CHARS);
const {
DOT_LITERAL,
PLUS_LITERAL,
SLASH_LITERAL,
ONE_CHAR,
DOTS_SLASH,
NO_DOT,
NO_DOT_SLASH,
NO_DOTS_SLASH,
QMARK,
QMARK_NO_DOT,
STAR,
START_ANCHOR
} = PLATFORM_CHARS;
const globstar = (opts2) => {
return `(${capture}(?:(?!${START_ANCHOR}${opts2.dot ? DOTS_SLASH : DOT_LITERAL}).)*?)`;
};
const nodot = opts.dot ? "" : NO_DOT;
const qmarkNoDot = opts.dot ? QMARK : QMARK_NO_DOT;
let star = opts.bash === true ? globstar(opts) : STAR;
if (opts.capture) {
star = `(${star})`;
}
if (typeof opts.noext === "boolean") {
opts.noextglob = opts.noext;
}
const state = {
input,
index: -1,
start: 0,
dot: opts.dot === true,
consumed: "",
output: "",
prefix: "",
backtrack: false,
negated: false,
brackets: 0,
braces: 0,
parens: 0,
quotes: 0,
globstar: false,
tokens
};
input = utils.removePrefix(input, state);
len = input.length;
const extglobs = [];
const braces = [];
const stack = [];
let prev = bos;
let value;
const eos = () => state.index === len - 1;
const peek = state.peek = (n2 = 1) => input[state.index + n2];
const advance = state.advance = () => input[++state.index] || "";
const remaining = () => input.slice(state.index + 1);
const consume = (value2 = "", num = 0) => {
state.consumed += value2;
state.index += num;
};
const append = (token) => {
state.output += token.output != null ? token.output : token.value;
consume(token.value);
};
const negate = () => {
let count = 1;
while (peek() === "!" && (peek(2) !== "(" || peek(3) === "?")) {
advance();
state.start++;
count++;
}
if (count % 2 === 0) {
return false;
}
state.negated = true;
state.start++;
return true;
};
const increment = (type) => {
state[type]++;
stack.push(type);
};
const decrement = (type) => {
state[type]--;
stack.pop();
};
const push = (tok) => {
if (prev.type === "globstar") {
const isBrace = state.braces > 0 && (tok.type === "comma" || tok.type === "brace");
const isExtglob = tok.extglob === true || extglobs.length && (tok.type === "pipe" || tok.type === "paren");
if (tok.type !== "slash" && tok.type !== "paren" && !isBrace && !isExtglob) {
state.output = state.output.slice(0, -prev.output.length);
prev.type = "star";
prev.value = "*";
prev.output = star;
state.output += prev.output;
}
}
if (extglobs.length && tok.type !== "paren") {
extglobs[extglobs.length - 1].inner += tok.value;
}
if (tok.value || tok.output)
append(tok);
if (prev && prev.type === "text" && tok.type === "text") {
prev.value += tok.value;
prev.output = (prev.output || "") + tok.value;
return;
}
tok.prev = prev;
tokens.push(tok);
prev = tok;
};
const extglobOpen = (type, value2) => {
const token = Object.assign(Object.assign({}, EXTGLOB_CHARS[value2]), {}, {
conditions: 1,
inner: ""
});
token.prev = prev;
token.parens = state.parens;
token.output = state.output;
const output = (opts.capture ? "(" : "") + token.open;
increment("parens");
push({
type,
value: value2,
output: state.output ? "" : ONE_CHAR
});
push({
type: "paren",
extglob: true,
value: advance(),
output
});
extglobs.push(token);
};
const extglobClose = (token) => {
let output = token.close + (opts.capture ? ")" : "");
let rest;
if (token.type === "negate") {
let extglobStar = star;
if (token.inner && token.inner.length > 1 && token.inner.includes("/")) {
extglobStar = globstar(opts);
}
if (extglobStar !== star || eos() || /^\)+$/.test(remaining())) {
output = token.close = `)$))${extglobStar}`;
}
if (token.inner.includes("*") && (rest = remaining()) && /^\.[^\\/.]+$/.test(rest)) {
const expression = parse2(rest, Object.assign(Object.assign({}, options), {}, {
fastpaths: false
})).output;
output = token.close = `)${expression})${extglobStar})`;
}
if (token.prev.type === "bos") {
state.negatedExtglob = true;
}
}
push({
type: "paren",
extglob: true,
value,
output
});
decrement("parens");
};
if (opts.fastpaths !== false && !/(^[*!]|[/()[\]{}"])/.test(input)) {
let backslashes = false;
let output = input.replace(REGEX_SPECIAL_CHARS_BACKREF, (m, esc, chars, first, rest, index) => {
if (first === "\\") {
backslashes = true;
return m;
}
if (first === "?") {
if (esc) {
return esc + first + (rest ? QMARK.repeat(rest.length) : "");
}
if (index === 0) {
return qmarkNoDot + (rest ? QMARK.repeat(rest.length) : "");
}
return QMARK.repeat(chars.length);
}
if (first === ".") {
return DOT_LITERAL.repeat(chars.length);
}
if (first === "*") {
if (esc) {
return esc + first + (rest ? star : "");
}
return star;
}
return esc ? m : `\\${m}`;
});
if (backslashes === true) {
if (opts.unescape === true) {
output = output.replace(/\\/g, "");
} else {
output = output.replace(/\\+/g, (m) => {
return m.length % 2 === 0 ? "\\\\" : m ? "\\" : "";
});
}
}
if (output === input && opts.contains === true) {
state.output = input;
return state;
}
state.output = utils.wrapOutput(output, state, options);
return state;
}
while (!eos()) {
value = advance();
if (value === "\0") {
continue;
}
if (value === "\\") {
const next = peek();
if (next === "/" && opts.bash !== true) {
continue;
}
if (next === "." || next === ";") {
continue;
}
if (!next) {
value += "\\";
push({
type: "text",
value
});
continue;
}
const match = /^\\+/.exec(remaining());
let slashes = 0;
if (match && match[0].length > 2) {
slashes = match[0].length;
state.index += slashes;
if (slashes % 2 !== 0) {
value += "\\";
}
}
if (opts.unescape === true) {
value = advance();
} else {
value += advance();
}
if (state.brackets === 0) {
push({
type: "text",
value
});
continue;
}
}
if (state.brackets > 0 && (value !== "]" || prev.value === "[" || prev.value === "[^")) {
if (opts.posix !== false && value === ":") {
const inner = prev.value.slice(1);
if (inner.includes("[")) {
prev.posix = true;
if (inner.includes(":")) {
const idx = prev.value.lastIndexOf("[");
const pre = prev.value.slice(0, idx);
const rest2 = prev.value.slice(idx + 2);
const posix = POSIX_REGEX_SOURCE[rest2];
if (posix) {
prev.value = pre + posix;
state.backtrack = true;
advance();
if (!bos.output && tokens.indexOf(prev) === 1) {
bos.output = ONE_CHAR;
}
continue;
}
}
}
}
if (value === "[" && peek() !== ":" || value === "-" && peek() === "]") {
value = `\\${value}`;
}
if (value === "]" && (prev.value === "[" || prev.value === "[^")) {
value = `\\${value}`;
}
if (opts.posix === true && value === "!" && prev.value === "[") {
value = "^";
}
prev.value += value;
append({
value
});
continue;
}
if (state.quotes === 1 && value !== '"') {
value = utils.escapeRegex(value);
prev.value += value;
append({
value
});
continue;
}
if (value === '"') {
state.quotes = state.quotes === 1 ? 0 : 1;
if (opts.keepQuotes === true) {
push({
type: "text",
value
});
}
continue;
}
if (value === "(") {
increment("parens");
push({
type: "paren",
value
});
continue;
}
if (value === ")") {
if (state.parens === 0 && opts.strictBrackets === true) {
throw new SyntaxError(syntaxError("opening", "("));
}
const extglob = extglobs[extglobs.length - 1];
if (extglob && state.parens === extglob.parens + 1) {
extglobClose(extglobs.pop());
continue;
}
push({
type: "paren",
value,
output: state.parens ? ")" : "\\)"
});
decrement("parens");
continue;
}
if (value === "[") {
if (opts.nobracket === true || !remaining().includes("]")) {
if (opts.nobracket !== true && opts.strictBrackets === true) {
throw new SyntaxError(syntaxError("closing", "]"));
}
value = `\\${value}`;
} else {
increment("brackets");
}
push({
type: "bracket",
value
});
continue;
}
if (value === "]") {
if (opts.nobracket === true || prev && prev.type === "bracket" && prev.value.length === 1) {
push({
type: "text",
value,
output: `\\${value}`
});
continue;
}
if (state.brackets === 0) {
if (opts.strictBrackets === true) {
throw new SyntaxError(syntaxError("opening", "["));
}
push({
type: "text",
value,
output: `\\${value}`
});
continue;
}
decrement("brackets");
const prevValue = prev.value.slice(1);
if (prev.posix !== true && prevValue[0] === "^" && !prevValue.includes("/")) {
value = `/${value}`;
}
prev.value += value;
append({
value
});
if (opts.literalBrackets === false || utils.hasRegexChars(prevValue)) {
continue;
}
const escaped = utils.escapeRegex(prev.value);
state.output = state.output.slice(0, -prev.value.length);
if (opts.literalBrackets === true) {
state.output += escaped;
prev.value = escaped;
continue;
}
prev.value = `(${capture}${escaped}|${prev.value})`;
state.output += prev.value;
continue;
}
if (value === "{" && opts.nobrace !== true) {
increment("braces");
const open = {
type: "brace",
value,
output: "(",
outputIndex: state.output.length,
tokensIndex: state.tokens.length
};
braces.push(open);
push(open);
continue;
}
if (value === "}") {
const brace = braces[braces.length - 1];
if (opts.nobrace === true || !brace) {
push({
type: "text",
value,
output: value
});
continue;
}
let output = ")";
if (brace.dots === true) {
const arr = tokens.slice();
const range = [];
for (let i = arr.length - 1; i >= 0; i--) {
tokens.pop();
if (arr[i].type === "brace") {
break;
}
if (arr[i].type !== "dots") {
range.unshift(arr[i].value);
}
}
output = expandRange(range, opts);
state.backtrack = true;
}
if (brace.comma !== true && brace.dots !== true) {
const out = state.output.slice(0, brace.outputIndex);
const toks = state.tokens.slice(brace.tokensIndex);
brace.value = brace.output = "\\{";
value = output = "\\}";
state.output = out;
for (const t of toks) {
state.output += t.output || t.value;
}
}
push({
type: "brace",
value,
output
});
decrement("braces");
braces.pop();
continue;
}
if (value === "|") {
if (extglobs.length > 0) {
extglobs[extglobs.length - 1].conditions++;
}
push({
type: "text",
value
});
continue;
}
if (value === ",") {
let output = value;
const brace = braces[braces.length - 1];
if (brace && stack[stack.length - 1] === "braces") {
brace.comma = true;
output = "|";
}
push({
type: "comma",
value,
output
});
continue;
}
if (value === "/") {
if (prev.type === "dot" && state.index === state.start + 1) {
state.start = state.index + 1;
state.consumed = "";
state.output = "";
tokens.pop();
prev = bos;
continue;
}
push({
type: "slash",
value,
output: SLASH_LITERAL
});
continue;
}
if (value === ".") {
if (state.braces > 0 && prev.type === "dot") {
if (prev.value === ".")
prev.output = DOT_LITERAL;
const brace = braces[braces.length - 1];
prev.type = "dots";
prev.output += value;
prev.value += value;
brace.dots = true;
continue;
}
if (state.braces + state.parens === 0 && prev.type !== "bos" && prev.type !== "slash") {
push({
type: "text",
value,
output: DOT_LITERAL
});
continue;
}
push({
type: "dot",
value,
output: DOT_LITERAL
});
continue;
}
if (value === "?") {
const isGroup = prev && prev.value === "(";
if (!isGroup && opts.noextglob !== true && peek() === "(" && peek(2) !== "?") {
extglobOpen("qmark", value);
continue;
}
if (prev && prev.type === "paren") {
const next = peek();
let output = value;
if (next === "<" && !utils.supportsLookbehinds()) {
throw new Error("Node.js v10 or higher is required for regex lookbehinds");
}
if (prev.value === "(" && !/[!=<:]/.test(next) || next === "<" && !/<([!=]|\w+>)/.test(remaining())) {
output = `\\${value}`;
}
push({
type: "text",
value,
output
});
continue;
}
if (opts.dot !== true && (prev.type === "slash" || prev.type === "bos")) {
push({
type: "qmark",
value,
output: QMARK_NO_DOT
});
continue;
}
push({
type: "qmark",
value,
output: QMARK
});
continue;
}
if (value === "!") {
if (opts.noextglob !== true && peek() === "(") {
if (peek(2) !== "?" || !/[!=<:]/.test(peek(3))) {
extglobOpen("negate", value);
continue;
}
}
if (opts.nonegate !== true && state.index === 0) {
negate();
continue;
}
}
if (value === "+") {
if (opts.noextglob !== true && peek() === "(" && peek(2) !== "?") {
extglobOpen("plus", value);
continue;
}
if (prev && prev.value === "(" || opts.regex === false) {
push({
type: "plus",
value,
output: PLUS_LITERAL
});
continue;
}
if (prev && (prev.type === "bracket" || prev.type === "paren" || prev.type === "brace") || state.parens > 0) {
push({
type: "plus",
value
});
continue;
}
push({
type: "plus",
value: PLUS_LITERAL
});
continue;
}
if (value === "@") {
if (opts.noextglob !== true && peek() === "(" && peek(2) !== "?") {
push({
type: "at",
extglob: true,
value,
output: ""
});
continue;
}
push({
type: "text",
value
});
continue;
}
if (value !== "*") {
if (value === "$" || value === "^") {
value = `\\${value}`;
}
const match = REGEX_NON_SPECIAL_CHARS.exec(remaining());
if (match) {
value += match[0];
state.index += match[0].length;
}
push({
type: "text",
value
});
continue;
}
if (prev && (prev.type === "globstar" || prev.star === true)) {
prev.type = "star";
prev.star = true;
prev.value += value;
prev.output = star;
state.backtrack = true;
state.globstar = true;
consume(value);
continue;
}
let rest = remaining();
if (opts.noextglob !== true && /^\([^?]/.test(rest)) {
extglobOpen("star", value);
continue;
}
if (prev.type === "star") {
if (opts.noglobstar === true) {
consume(value);
continue;
}
const prior = prev.prev;
const before = prior.prev;
const isStart = prior.type === "slash" || prior.type === "bos";
const afterStar = before && (before.type === "star" || before.type === "globstar");
if (opts.bash === true && (!isStart || rest[0] && rest[0] !== "/")) {
push({
type: "star",
value,
output: ""
});
continue;
}
const isBrace = state.braces > 0 && (prior.type === "comma" || prior.type === "brace");
const isExtglob = extglobs.length && (prior.type === "pipe" || prior.type === "paren");
if (!isStart && prior.type !== "paren" && !isBrace && !isExtglob) {
push({
type: "star",
value,
output: ""
});
continue;
}
while (rest.slice(0, 3) === "/**") {
const after = input[state.index + 4];
if (after && after !== "/") {
break;
}
rest = rest.slice(3);
consume("/**", 3);
}
if (prior.type === "bos" && eos()) {
prev.type = "globstar";
prev.value += value;
prev.output = globstar(opts);
state.output = prev.output;
state.globstar = true;
consume(value);
continue;
}
if (prior.type === "slash" && prior.prev.type !== "bos" && !afterStar && eos()) {
state.output = state.output.slice(0, -(prior.output + prev.output).length);
prior.output = `(?:${prior.output}`;
prev.type = "globstar";
prev.output = globstar(opts) + (opts.strictSlashes ? ")" : "|$)");
prev.value += value;
state.globstar = true;
state.output += prior.output + prev.output;
consume(value);
continue;
}
if (prior.type === "slash" && prior.prev.type !== "bos" && rest[0] === "/") {
const end = rest[1] !== void 0 ? "|$" : "";
state.output = state.output.slice(0, -(prior.output + prev.output).length);
prior.output = `(?:${prior.output}`;
prev.type = "globstar";
prev.output = `${globstar(opts)}${SLASH_LITERAL}|${SLASH_LITERAL}${end})`;
prev.value += value;
state.output += prior.output + prev.output;
state.globstar = true;
consume(value + advance());
push({
type: "slash",
value: "/",
output: ""
});
continue;
}
if (prior.type === "bos" && rest[0] === "/") {
prev.type = "globstar";
prev.value += value;
prev.output = `(?:^|${SLASH_LITERAL}|${globstar(opts)}${SLASH_LITERAL})`;
state.output = prev.output;
state.globstar = true;
consume(value + advance());
push({
type: "slash",
value: "/",
output: ""
});
continue;
}
state.output = state.output.slice(0, -prev.output.length);
prev.type = "globstar";
prev.output = globstar(opts);
prev.value += value;
state.output += prev.output;
state.globstar = true;
consume(value);
continue;
}
const token = {
type: "star",
value,
output: star
};
if (opts.bash === true) {
token.output = ".*?";
if (prev.type === "bos" || prev.type === "slash") {
token.output = nodot + token.output;
}
push(token);
continue;
}
if (prev && (prev.type === "bracket" || prev.type === "paren") && opts.regex === true) {
token.output = value;
push(token);
continue;
}
if (state.index === state.start || prev.type === "slash" || prev.type === "dot") {
if (prev.type === "dot") {
state.output += NO_DOT_SLASH;
prev.output += NO_DOT_SLASH;
} else if (opts.dot === true) {
state.output += NO_DOTS_SLASH;
prev.output += NO_DOTS_SLASH;
} else {
state.output += nodot;
prev.output += nodot;
}
if (peek() !== "*") {
state.output += ONE_CHAR;
prev.output += ONE_CHAR;
}
}
push(token);
}
while (state.brackets > 0) {
if (opts.strictBrackets === true)
throw new SyntaxError(syntaxError("closing", "]"));
state.output = utils.escapeLast(state.output, "[");
decrement("brackets");
}
while (state.parens > 0) {
if (opts.strictBrackets === true)
throw new SyntaxError(syntaxError("closing", ")"));
state.output = utils.escapeLast(state.output, "(");
decrement("parens");
}
while (state.braces > 0) {
if (opts.strictBrackets === true)
throw new SyntaxError(syntaxError("closing", "}"));
state.output = utils.escapeLast(state.output, "{");
decrement("braces");
}
if (opts.strictSlashes !== true && (prev.type === "star" || prev.type === "bracket")) {
push({
type: "maybe_slash",
value: "",
output: `${SLASH_LITERAL}?`
});
}
if (state.backtrack === true) {
state.output = "";
for (const token of state.tokens) {
state.output += token.output != null ? token.output : token.value;
if (token.suffix) {
state.output += token.suffix;
}
}
}
return state;
};
parse2.fastpaths = (input, options) => {
const opts = Object.assign({}, options);
const max = typeof opts.maxLength === "number" ? Math.min(MAX_LENGTH, opts.maxLength) : MAX_LENGTH;
const len = input.length;
if (len > max) {
throw new SyntaxError(`Input length: ${len}, exceeds maximum allowed length: ${max}`);
}
input = REPLACEMENTS[input] || input;
const win32 = utils.isWindows(options);
const {
DOT_LITERAL,
SLASH_LITERAL,
ONE_CHAR,
DOTS_SLASH,
NO_DOT,
NO_DOTS,
NO_DOTS_SLASH,
STAR,
START_ANCHOR
} = constants.globChars(win32);
const nodot = opts.dot ? NO_DOTS : NO_DOT;
const slashDot = opts.dot ? NO_DOTS_SLASH : NO_DOT;
const capture = opts.capture ? "" : "?:";
const state = {
negated: false,
prefix: ""
};
let star = opts.bash === true ? ".*?" : STAR;
if (opts.capture) {
star = `(${star})`;
}
const globstar = (opts2) => {
if (opts2.noglobstar === true)
return star;
return `(${capture}(?:(?!${START_ANCHOR}${opts2.dot ? DOTS_SLASH : DOT_LITERAL}).)*?)`;
};
const create = (str) => {
switch (str) {
case "*":
return `${nodot}${ONE_CHAR}${star}`;
case ".*":
return `${DOT_LITERAL}${ONE_CHAR}${star}`;
case "*.*":
return `${nodot}${star}${DOT_LITERAL}${ONE_CHAR}${star}`;
case "*/*":
return `${nodot}${star}${SLASH_LITERAL}${ONE_CHAR}${slashDot}${star}`;
case "**":
return nodot + globstar(opts);
case "**/*":
return `(?:${nodot}${globstar(opts)}${SLASH_LITERAL})?${slashDot}${ONE_CHAR}${star}`;
case "**/*.*":
return `(?:${nodot}${globstar(opts)}${SLASH_LITERAL})?${slashDot}${star}${DOT_LITERAL}${ONE_CHAR}${star}`;
case "**/.*":
return `(?:${nodot}${globstar(opts)}${SLASH_LITERAL})?${DOT_LITERAL}${ONE_CHAR}${star}`;
default: {
const match = /^(.*?)\.(\w+)$/.exec(str);
if (!match)
return;
const source2 = create(match[1]);
if (!source2)
return;
return source2 + DOT_LITERAL + match[2];
}
}
};
const output = utils.removePrefix(input, state);
let source = create(output);
if (source && opts.strictSlashes !== true) {
source += `${SLASH_LITERAL}?`;
}
return source;
};
module22.exports = parse2;
}
});
var require_picomatch = __commonJS22({
"node_modules/picomatch/lib/picomatch.js"(exports2, module22) {
"use strict";
var path = require("path");
var scan = require_scan();
var parse2 = require_parse22();
var utils = require_utils3();
var constants = require_constants3();
var isObject2 = (val) => val && typeof val === "object" && !Array.isArray(val);
var picomatch = (glob, options, returnState = false) => {
if (Array.isArray(glob)) {
const fns = glob.map((input) => picomatch(input, options, returnState));
const arrayMatcher = (str) => {
for (const isMatch of fns) {
const state2 = isMatch(str);
if (state2)
return state2;
}
return false;
};
return arrayMatcher;
}
const isState = isObject2(glob) && glob.tokens && glob.input;
if (glob === "" || typeof glob !== "string" && !isState) {
throw new TypeError("Expected pattern to be a non-empty string");
}
const opts = options || {};
const posix = utils.isWindows(options);
const regex = isState ? picomatch.compileRe(glob, options) : picomatch.makeRe(glob, options, false, true);
const state = regex.state;
delete regex.state;
let isIgnored = () => false;
if (opts.ignore) {
const ignoreOpts = Object.assign(Object.assign({}, options), {}, {
ignore: null,
onMatch: null,
onResult: null
});
isIgnored = picomatch(opts.ignore, ignoreOpts, returnState);
}
const matcher = (input, returnObject = false) => {
const {
isMatch,
match,
output
} = picomatch.test(input, regex, options, {
glob,
posix
});
const result = {
glob,
state,
regex,
posix,
input,
output,
match,
isMatch
};
if (typeof opts.onResult === "function") {
opts.onResult(result);
}
if (isMatch === false) {
result.isMatch = false;
return returnObject ? result : false;
}
if (isIgnored(input)) {
if (typeof opts.onIgnore === "function") {
opts.onIgnore(result);
}
result.isMatch = false;
return returnObject ? result : false;
}
if (typeof opts.onMatch === "function") {
opts.onMatch(result);
}
return returnObject ? result : true;
};
if (returnState) {
matcher.state = state;
}
return matcher;
};
picomatch.test = (input, regex, options, {
glob,
posix
} = {}) => {
if (typeof input !== "string") {
throw new TypeError("Expected input to be a string");
}
if (input === "") {
return {
isMatch: false,
output: ""
};
}
const opts = options || {};
const format2 = opts.format || (posix ? utils.toPosixSlashes : null);
let match = input === glob;
let output = match && format2 ? format2(input) : input;
if (match === false) {
output = format2 ? format2(input) : input;
match = output === glob;
}
if (match === false || opts.capture === true) {
if (opts.matchBase === true || opts.basename === true) {
match = picomatch.matchBase(input, regex, options, posix);
} else {
match = regex.exec(output);
}
}
return {
isMatch: Boolean(match),
match,
output
};
};
picomatch.matchBase = (input, glob, options, posix = utils.isWindows(options)) => {
const regex = glob instanceof RegExp ? glob : picomatch.makeRe(glob, options);
return regex.test(path.basename(input));
};
picomatch.isMatch = (str, patterns, options) => picomatch(patterns, options)(str);
picomatch.parse = (pattern, options) => {
if (Array.isArray(pattern))
return pattern.map((p) => picomatch.parse(p, options));
return parse2(pattern, Object.assign(Object.assign({}, options), {}, {
fastpaths: false
}));
};
picomatch.scan = (input, options) => scan(input, options);
picomatch.compileRe = (state, options, returnOutput = false, returnState = false) => {
if (returnOutput === true) {
return state.output;
}
const opts = options || {};
const prepend = opts.contains ? "" : "^";
const append = opts.contains ? "" : "$";
let source = `${prepend}(?:${state.output})${append}`;
if (state && state.negated === true) {
source = `^(?!${source}).*$`;
}
const regex = picomatch.toRegex(source, options);
if (returnState === true) {
regex.state = state;
}
return regex;
};
picomatch.makeRe = (input, options = {}, returnOutput = false, returnState = false) => {
if (!input || typeof input !== "string") {
throw new TypeError("Expected a non-empty string");
}
let parsed = {
negated: false,
fastpaths: true
};
if (options.fastpaths !== false && (input[0] === "." || input[0] === "*")) {
parsed.output = parse2.fastpaths(input, options);
}
if (!parsed.output) {
parsed = parse2(input, options);
}
return picomatch.compileRe(parsed, options, returnOutput, returnState);
};
picomatch.toRegex = (source, options) => {
try {
const opts = options || {};
return new RegExp(source, opts.flags || (opts.nocase ? "i" : ""));
} catch (err) {
if (options && options.debug === true)
throw err;
return /$^/;
}
};
picomatch.constants = constants;
module22.exports = picomatch;
}
});
var require_picomatch2 = __commonJS22({
"node_modules/picomatch/index.js"(exports2, module22) {
"use strict";
module22.exports = require_picomatch();
}
});
var require_micromatch = __commonJS22({
"node_modules/micromatch/index.js"(exports2, module22) {
"use strict";
var util = require("util");
var braces = require_braces();
var picomatch = require_picomatch2();
var utils = require_utils3();
var isEmptyString = (val) => val === "" || val === "./";
var micromatch = (list, patterns, options) => {
patterns = [].concat(patterns);
list = [].concat(list);
let omit = /* @__PURE__ */ new Set();
let keep = /* @__PURE__ */ new Set();
let items = /* @__PURE__ */ new Set();
let negatives = 0;
let onResult = (state) => {
items.add(state.output);
if (options && options.onResult) {
options.onResult(state);
}
};
for (let i = 0; i < patterns.length; i++) {
let isMatch = picomatch(String(patterns[i]), Object.assign(Object.assign({}, options), {}, {
onResult
}), true);
let negated = isMatch.state.negated || isMatch.state.negatedExtglob;
if (negated)
negatives++;
for (let item of list) {
let matched = isMatch(item, true);
let match = negated ? !matched.isMatch : matched.isMatch;
if (!match)
continue;
if (negated) {
omit.add(matched.output);
} else {
omit.delete(matched.output);
keep.add(matched.output);
}
}
}
let result = negatives === patterns.length ? [...items] : [...keep];
let matches = result.filter((item) => !omit.has(item));
if (options && matches.length === 0) {
if (options.failglob === true) {
throw new Error(`No matches found for "${patterns.join(", ")}"`);
}
if (options.nonull === true || options.nullglob === true) {
return options.unescape ? patterns.map((p) => p.replace(/\\/g, "")) : patterns;
}
}
return matches;
};
micromatch.match = micromatch;
micromatch.matcher = (pattern, options) => picomatch(pattern, options);
micromatch.isMatch = (str, patterns, options) => picomatch(patterns, options)(str);
micromatch.any = micromatch.isMatch;
micromatch.not = (list, patterns, options = {}) => {
patterns = [].concat(patterns).map(String);
let result = /* @__PURE__ */ new Set();
let items = [];
let onResult = (state) => {
if (options.onResult)
options.onResult(state);
items.push(state.output);
};
let matches = new Set(micromatch(list, patterns, Object.assign(Object.assign({}, options), {}, {
onResult
})));
for (let item of items) {
if (!matches.has(item)) {
result.add(item);
}
}
return [...result];
};
micromatch.contains = (str, pattern, options) => {
if (typeof str !== "string") {
throw new TypeError(`Expected a string: "${util.inspect(str)}"`);
}
if (Array.isArray(pattern)) {
return pattern.some((p) => micromatch.contains(str, p, options));
}
if (typeof pattern === "string") {
if (isEmptyString(str) || isEmptyString(pattern)) {
return false;
}
if (str.includes(pattern) || str.startsWith("./") && str.slice(2).includes(pattern)) {
return true;
}
}
return micromatch.isMatch(str, pattern, Object.assign(Object.assign({}, options), {}, {
contains: true
}));
};
micromatch.matchKeys = (obj, patterns, options) => {
if (!utils.isObject(obj)) {
throw new TypeError("Expected the first argument to be an object");
}
let keys = micromatch(Object.keys(obj), patterns, options);
let res = {};
for (let key of keys)
res[key] = obj[key];
return res;
};
micromatch.some = (list, patterns, options) => {
let items = [].concat(list);
for (let pattern of [].concat(patterns)) {
let isMatch = picomatch(String(pattern), options);
if (items.some((item) => isMatch(item))) {
return true;
}
}
return false;
};
micromatch.every = (list, patterns, options) => {
let items = [].concat(list);
for (let pattern of [].concat(patterns)) {
let isMatch = picomatch(String(pattern), options);
if (!items.every((item) => isMatch(item))) {
return false;
}
}
return true;
};
micromatch.all = (str, patterns, options) => {
if (typeof str !== "string") {
throw new TypeError(`Expected a string: "${util.inspect(str)}"`);
}
return [].concat(patterns).every((p) => picomatch(p, options)(str));
};
micromatch.capture = (glob, input, options) => {
let posix = utils.isWindows(options);
let regex = picomatch.makeRe(String(glob), Object.assign(Object.assign({}, options), {}, {
capture: true
}));
let match = regex.exec(posix ? utils.toPosixSlashes(input) : input);
if (match) {
return match.slice(1).map((v) => v === void 0 ? "" : v);
}
};
micromatch.makeRe = (...args) => picomatch.makeRe(...args);
micromatch.scan = (...args) => picomatch.scan(...args);
micromatch.parse = (patterns, options) => {
let res = [];
for (let pattern of [].concat(patterns || [])) {
for (let str of braces(String(pattern), options)) {
res.push(picomatch.parse(str, options));
}
}
return res;
};
micromatch.braces = (pattern, options) => {
if (typeof pattern !== "string")
throw new TypeError("Expected a string");
if (options && options.nobrace === true || !/\{.*\}/.test(pattern)) {
return [pattern];
}
return braces(pattern, options);
};
micromatch.braceExpand = (pattern, options) => {
if (typeof pattern !== "string")
throw new TypeError("Expected a string");
return micromatch.braces(pattern, Object.assign(Object.assign({}, options), {}, {
expand: true
}));
};
module22.exports = micromatch;
}
});
var require_parser2 = __commonJS22({
"node_modules/@iarna/toml/lib/parser.js"(exports2, module22) {
"use strict";
var ParserEND = 1114112;
var ParserError = class extends Error {
constructor(msg, filename, linenumber) {
super("[ParserError] " + msg, filename, linenumber);
this.name = "ParserError";
this.code = "ParserError";
if (Error.captureStackTrace)
Error.captureStackTrace(this, ParserError);
}
};
var State = class {
constructor(parser) {
this.parser = parser;
this.buf = "";
this.returned = null;
this.result = null;
this.resultTable = null;
this.resultArr = null;
}
};
var Parser = class {
constructor() {
this.pos = 0;
this.col = 0;
this.line = 0;
this.obj = {};
this.ctx = this.obj;
this.stack = [];
this._buf = "";
this.char = null;
this.ii = 0;
this.state = new State(this.parseStart);
}
parse(str) {
if (str.length === 0 || str.length == null)
return;
this._buf = String(str);
this.ii = -1;
this.char = -1;
let getNext;
while (getNext === false || this.nextChar()) {
getNext = this.runOne();
}
this._buf = null;
}
nextChar() {
if (this.char === 10) {
++this.line;
this.col = -1;
}
++this.ii;
this.char = this._buf.codePointAt(this.ii);
++this.pos;
++this.col;
return this.haveBuffer();
}
haveBuffer() {
return this.ii < this._buf.length;
}
runOne() {
return this.state.parser.call(this, this.state.returned);
}
finish() {
this.char = ParserEND;
let last;
do {
last = this.state.parser;
this.runOne();
} while (this.state.parser !== last);
this.ctx = null;
this.state = null;
this._buf = null;
return this.obj;
}
next(fn) {
if (typeof fn !== "function")
throw new ParserError("Tried to set state to non-existent state: " + JSON.stringify(fn));
this.state.parser = fn;
}
goto(fn) {
this.next(fn);
return this.runOne();
}
call(fn, returnWith) {
if (returnWith)
this.next(returnWith);
this.stack.push(this.state);
this.state = new State(fn);
}
callNow(fn, returnWith) {
this.call(fn, returnWith);
return this.runOne();
}
return(value) {
if (this.stack.length === 0)
throw this.error(new ParserError("Stack underflow"));
if (value === void 0)
value = this.state.buf;
this.state = this.stack.pop();
this.state.returned = value;
}
returnNow(value) {
this.return(value);
return this.runOne();
}
consume() {
if (this.char === ParserEND)
throw this.error(new ParserError("Unexpected end-of-buffer"));
this.state.buf += this._buf[this.ii];
}
error(err) {
err.line = this.line;
err.col = this.col;
err.pos = this.pos;
return err;
}
parseStart() {
throw new ParserError("Must declare a parseStart method");
}
};
Parser.END = ParserEND;
Parser.Error = ParserError;
module22.exports = Parser;
}
});
var require_create_datetime = __commonJS22({
"node_modules/@iarna/toml/lib/create-datetime.js"(exports2, module22) {
"use strict";
module22.exports = (value) => {
const date = new Date(value);
if (isNaN(date)) {
throw new TypeError("Invalid Datetime");
} else {
return date;
}
};
}
});
var require_format_num = __commonJS22({
"node_modules/@iarna/toml/lib/format-num.js"(exports2, module22) {
"use strict";
module22.exports = (d, num) => {
num = String(num);
while (num.length < d)
num = "0" + num;
return num;
};
}
});
var require_create_datetime_float = __commonJS22({
"node_modules/@iarna/toml/lib/create-datetime-float.js"(exports2, module22) {
"use strict";
var f = require_format_num();
var FloatingDateTime = class extends Date {
constructor(value) {
super(value + "Z");
this.isFloating = true;
}
toISOString() {
const date = `${this.getUTCFullYear()}-${f(2, this.getUTCMonth() + 1)}-${f(2, this.getUTCDate())}`;
const time = `${f(2, this.getUTCHours())}:${f(2, this.getUTCMinutes())}:${f(2, this.getUTCSeconds())}.${f(3, this.getUTCMilliseconds())}`;
return `${date}T${time}`;
}
};
module22.exports = (value) => {
const date = new FloatingDateTime(value);
if (isNaN(date)) {
throw new TypeError("Invalid Datetime");
} else {
return date;
}
};
}
});
var require_create_date = __commonJS22({
"node_modules/@iarna/toml/lib/create-date.js"(exports2, module22) {
"use strict";
var f = require_format_num();
var DateTime = global.Date;
var Date2 = class extends DateTime {
constructor(value) {
super(value);
this.isDate = true;
}
toISOString() {
return `${this.getUTCFullYear()}-${f(2, this.getUTCMonth() + 1)}-${f(2, this.getUTCDate())}`;
}
};
module22.exports = (value) => {
const date = new Date2(value);
if (isNaN(date)) {
throw new TypeError("Invalid Datetime");
} else {
return date;
}
};
}
});
var require_create_time = __commonJS22({
"node_modules/@iarna/toml/lib/create-time.js"(exports2, module22) {
"use strict";
var f = require_format_num();
var Time = class extends Date {
constructor(value) {
super(`0000-01-01T${value}Z`);
this.isTime = true;
}
toISOString() {
return `${f(2, this.getUTCHours())}:${f(2, this.getUTCMinutes())}:${f(2, this.getUTCSeconds())}.${f(3, this.getUTCMilliseconds())}`;
}
};
module22.exports = (value) => {
const date = new Time(value);
if (isNaN(date)) {
throw new TypeError("Invalid Datetime");
} else {
return date;
}
};
}
});
var require_toml_parser = __commonJS22({
"node_modules/@iarna/toml/lib/toml-parser.js"(exports2, module22) {
"use strict";
module22.exports = makeParserClass(require_parser2());
module22.exports.makeParserClass = makeParserClass;
var TomlError = class extends Error {
constructor(msg) {
super(msg);
this.name = "TomlError";
if (Error.captureStackTrace)
Error.captureStackTrace(this, TomlError);
this.fromTOML = true;
this.wrapped = null;
}
};
TomlError.wrap = (err) => {
const terr = new TomlError(err.message);
terr.code = err.code;
terr.wrapped = err;
return terr;
};
module22.exports.TomlError = TomlError;
var createDateTime = require_create_datetime();
var createDateTimeFloat = require_create_datetime_float();
var createDate = require_create_date();
var createTime = require_create_time();
var CTRL_I = 9;
var CTRL_J = 10;
var CTRL_M = 13;
var CTRL_CHAR_BOUNDARY = 31;
var CHAR_SP = 32;
var CHAR_QUOT = 34;
var CHAR_NUM = 35;
var CHAR_APOS = 39;
var CHAR_PLUS = 43;
var CHAR_COMMA = 44;
var CHAR_HYPHEN = 45;
var CHAR_PERIOD = 46;
var CHAR_0 = 48;
var CHAR_1 = 49;
var CHAR_7 = 55;
var CHAR_9 = 57;
var CHAR_COLON = 58;
var CHAR_EQUALS = 61;
var CHAR_A = 65;
var CHAR_E = 69;
var CHAR_F = 70;
var CHAR_T = 84;
var CHAR_U = 85;
var CHAR_Z = 90;
var CHAR_LOWBAR = 95;
var CHAR_a = 97;
var CHAR_b = 98;
var CHAR_e = 101;
var CHAR_f = 102;
var CHAR_i = 105;
var CHAR_l = 108;
var CHAR_n = 110;
var CHAR_o = 111;
var CHAR_r = 114;
var CHAR_s = 115;
var CHAR_t = 116;
var CHAR_u = 117;
var CHAR_x = 120;
var CHAR_z = 122;
var CHAR_LCUB = 123;
var CHAR_RCUB = 125;
var CHAR_LSQB = 91;
var CHAR_BSOL = 92;
var CHAR_RSQB = 93;
var CHAR_DEL = 127;
var SURROGATE_FIRST = 55296;
var SURROGATE_LAST = 57343;
var escapes = {
[CHAR_b]: "\b",
[CHAR_t]: " ",
[CHAR_n]: "\n",
[CHAR_f]: "\f",
[CHAR_r]: "\r",
[CHAR_QUOT]: '"',
[CHAR_BSOL]: "\\"
};
function isDigit(cp) {
return cp >= CHAR_0 && cp <= CHAR_9;
}
function isHexit(cp) {
return cp >= CHAR_A && cp <= CHAR_F || cp >= CHAR_a && cp <= CHAR_f || cp >= CHAR_0 && cp <= CHAR_9;
}
function isBit(cp) {
return cp === CHAR_1 || cp === CHAR_0;
}
function isOctit(cp) {
return cp >= CHAR_0 && cp <= CHAR_7;
}
function isAlphaNumQuoteHyphen(cp) {
return cp >= CHAR_A && cp <= CHAR_Z || cp >= CHAR_a && cp <= CHAR_z || cp >= CHAR_0 && cp <= CHAR_9 || cp === CHAR_APOS || cp === CHAR_QUOT || cp === CHAR_LOWBAR || cp === CHAR_HYPHEN;
}
function isAlphaNumHyphen(cp) {
return cp >= CHAR_A && cp <= CHAR_Z || cp >= CHAR_a && cp <= CHAR_z || cp >= CHAR_0 && cp <= CHAR_9 || cp === CHAR_LOWBAR || cp === CHAR_HYPHEN;
}
var _type = Symbol("type");
var _declared = Symbol("declared");
var hasOwnProperty = Object.prototype.hasOwnProperty;
var defineProperty = Object.defineProperty;
var descriptor = {
configurable: true,
enumerable: true,
writable: true,
value: void 0
};
function hasKey(obj, key) {
if (hasOwnProperty.call(obj, key))
return true;
if (key === "__proto__")
defineProperty(obj, "__proto__", descriptor);
return false;
}
var INLINE_TABLE = Symbol("inline-table");
function InlineTable() {
return Object.defineProperties({}, {
[_type]: {
value: INLINE_TABLE
}
});
}
function isInlineTable(obj) {
if (obj === null || typeof obj !== "object")
return false;
return obj[_type] === INLINE_TABLE;
}
var TABLE = Symbol("table");
function Table() {
return Object.defineProperties({}, {
[_type]: {
value: TABLE
},
[_declared]: {
value: false,
writable: true
}
});
}
function isTable(obj) {
if (obj === null || typeof obj !== "object")
return false;
return obj[_type] === TABLE;
}
var _contentType = Symbol("content-type");
var INLINE_LIST = Symbol("inline-list");
function InlineList(type) {
return Object.defineProperties([], {
[_type]: {
value: INLINE_LIST
},
[_contentType]: {
value: type
}
});
}
function isInlineList(obj) {
if (obj === null || typeof obj !== "object")
return false;
return obj[_type] === INLINE_LIST;
}
var LIST = Symbol("list");
function List() {
return Object.defineProperties([], {
[_type]: {
value: LIST
}
});
}
function isList(obj) {
if (obj === null || typeof obj !== "object")
return false;
return obj[_type] === LIST;
}
var _custom;
try {
const utilInspect = require("util").inspect;
_custom = utilInspect.custom;
} catch (_) {
}
var _inspect = _custom || "inspect";
var BoxedBigInt = class {
constructor(value) {
try {
this.value = global.BigInt.asIntN(64, value);
} catch (_) {
this.value = null;
}
Object.defineProperty(this, _type, {
value: INTEGER
});
}
isNaN() {
return this.value === null;
}
toString() {
return String(this.value);
}
[_inspect]() {
return `[BigInt: ${this.toString()}]}`;
}
valueOf() {
return this.value;
}
};
var INTEGER = Symbol("integer");
function Integer(value) {
let num = Number(value);
if (Object.is(num, -0))
num = 0;
if (global.BigInt && !Number.isSafeInteger(num)) {
return new BoxedBigInt(value);
} else {
return Object.defineProperties(new Number(num), {
isNaN: {
value: function() {
return isNaN(this);
}
},
[_type]: {
value: INTEGER
},
[_inspect]: {
value: () => `[Integer: ${value}]`
}
});
}
}
function isInteger(obj) {
if (obj === null || typeof obj !== "object")
return false;
return obj[_type] === INTEGER;
}
var FLOAT = Symbol("float");
function Float(value) {
return Object.defineProperties(new Number(value), {
[_type]: {
value: FLOAT
},
[_inspect]: {
value: () => `[Float: ${value}]`
}
});
}
function isFloat(obj) {
if (obj === null || typeof obj !== "object")
return false;
return obj[_type] === FLOAT;
}
function tomlType(value) {
const type = typeof value;
if (type === "object") {
if (value === null)
return "null";
if (value instanceof Date)
return "datetime";
if (_type in value) {
switch (value[_type]) {
case INLINE_TABLE:
return "inline-table";
case INLINE_LIST:
return "inline-list";
case TABLE:
return "table";
case LIST:
return "list";
case FLOAT:
return "float";
case INTEGER:
return "integer";
}
}
}
return type;
}
function makeParserClass(Parser) {
class TOMLParser extends Parser {
constructor() {
super();
this.ctx = this.obj = Table();
}
atEndOfWord() {
return this.char === CHAR_NUM || this.char === CTRL_I || this.char === CHAR_SP || this.atEndOfLine();
}
atEndOfLine() {
return this.char === Parser.END || this.char === CTRL_J || this.char === CTRL_M;
}
parseStart() {
if (this.char === Parser.END) {
return null;
} else if (this.char === CHAR_LSQB) {
return this.call(this.parseTableOrList);
} else if (this.char === CHAR_NUM) {
return this.call(this.parseComment);
} else if (this.char === CTRL_J || this.char === CHAR_SP || this.char === CTRL_I || this.char === CTRL_M) {
return null;
} else if (isAlphaNumQuoteHyphen(this.char)) {
return this.callNow(this.parseAssignStatement);
} else {
throw this.error(new TomlError(`Unknown character "${this.char}"`));
}
}
parseWhitespaceToEOL() {
if (this.char === CHAR_SP || this.char === CTRL_I || this.char === CTRL_M) {
return null;
} else if (this.char === CHAR_NUM) {
return this.goto(this.parseComment);
} else if (this.char === Parser.END || this.char === CTRL_J) {
return this.return();
} else {
throw this.error(new TomlError("Unexpected character, expected only whitespace or comments till end of line"));
}
}
parseAssignStatement() {
return this.callNow(this.parseAssign, this.recordAssignStatement);
}
recordAssignStatement(kv) {
let target = this.ctx;
let finalKey = kv.key.pop();
for (let kw of kv.key) {
if (hasKey(target, kw) && (!isTable(target[kw]) || target[kw][_declared])) {
throw this.error(new TomlError("Can't redefine existing key"));
}
target = target[kw] = target[kw] || Table();
}
if (hasKey(target, finalKey)) {
throw this.error(new TomlError("Can't redefine existing key"));
}
if (isInteger(kv.value) || isFloat(kv.value)) {
target[finalKey] = kv.value.valueOf();
} else {
target[finalKey] = kv.value;
}
return this.goto(this.parseWhitespaceToEOL);
}
parseAssign() {
return this.callNow(this.parseKeyword, this.recordAssignKeyword);
}
recordAssignKeyword(key) {
if (this.state.resultTable) {
this.state.resultTable.push(key);
} else {
this.state.resultTable = [key];
}
return this.goto(this.parseAssignKeywordPreDot);
}
parseAssignKeywordPreDot() {
if (this.char === CHAR_PERIOD) {
return this.next(this.parseAssignKeywordPostDot);
} else if (this.char !== CHAR_SP && this.char !== CTRL_I) {
return this.goto(this.parseAssignEqual);
}
}
parseAssignKeywordPostDot() {
if (this.char !== CHAR_SP && this.char !== CTRL_I) {
return this.callNow(this.parseKeyword, this.recordAssignKeyword);
}
}
parseAssignEqual() {
if (this.char === CHAR_EQUALS) {
return this.next(this.parseAssignPreValue);
} else {
throw this.error(new TomlError('Invalid character, expected "="'));
}
}
parseAssignPreValue() {
if (this.char === CHAR_SP || this.char === CTRL_I) {
return null;
} else {
return this.callNow(this.parseValue, this.recordAssignValue);
}
}
recordAssignValue(value) {
return this.returnNow({
key: this.state.resultTable,
value
});
}
parseComment() {
do {
if (this.char === Parser.END || this.char === CTRL_J) {
return this.return();
}
} while (this.nextChar());
}
parseTableOrList() {
if (this.char === CHAR_LSQB) {
this.next(this.parseList);
} else {
return this.goto(this.parseTable);
}
}
parseTable() {
this.ctx = this.obj;
return this.goto(this.parseTableNext);
}
parseTableNext() {
if (this.char === CHAR_SP || this.char === CTRL_I) {
return null;
} else {
return this.callNow(this.parseKeyword, this.parseTableMore);
}
}
parseTableMore(keyword) {
if (this.char === CHAR_SP || this.char === CTRL_I) {
return null;
} else if (this.char === CHAR_RSQB) {
if (hasKey(this.ctx, keyword) && (!isTable(this.ctx[keyword]) || this.ctx[keyword][_declared])) {
throw this.error(new TomlError("Can't redefine existing key"));
} else {
this.ctx = this.ctx[keyword] = this.ctx[keyword] || Table();
this.ctx[_declared] = true;
}
return this.next(this.parseWhitespaceToEOL);
} else if (this.char === CHAR_PERIOD) {
if (!hasKey(this.ctx, keyword)) {
this.ctx = this.ctx[keyword] = Table();
} else if (isTable(this.ctx[keyword])) {
this.ctx = this.ctx[keyword];
} else if (isList(this.ctx[keyword])) {
this.ctx = this.ctx[keyword][this.ctx[keyword].length - 1];
} else {
throw this.error(new TomlError("Can't redefine existing key"));
}
return this.next(this.parseTableNext);
} else {
throw this.error(new TomlError("Unexpected character, expected whitespace, . or ]"));
}
}
parseList() {
this.ctx = this.obj;
return this.goto(this.parseListNext);
}
parseListNext() {
if (this.char === CHAR_SP || this.char === CTRL_I) {
return null;
} else {
return this.callNow(this.parseKeyword, this.parseListMore);
}
}
parseListMore(keyword) {
if (this.char === CHAR_SP || this.char === CTRL_I) {
return null;
} else if (this.char === CHAR_RSQB) {
if (!hasKey(this.ctx, keyword)) {
this.ctx[keyword] = List();
}
if (isInlineList(this.ctx[keyword])) {
throw this.error(new TomlError("Can't extend an inline array"));
} else if (isList(this.ctx[keyword])) {
const next = Table();
this.ctx[keyword].push(next);
this.ctx = next;
} else {
throw this.error(new TomlError("Can't redefine an existing key"));
}
return this.next(this.parseListEnd);
} else if (this.char === CHAR_PERIOD) {
if (!hasKey(this.ctx, keyword)) {
this.ctx = this.ctx[keyword] = Table();
} else if (isInlineList(this.ctx[keyword])) {
throw this.error(new TomlError("Can't extend an inline array"));
} else if (isInlineTable(this.ctx[keyword])) {
throw this.error(new TomlError("Can't extend an inline table"));
} else if (isList(this.ctx[keyword])) {
this.ctx = this.ctx[keyword][this.ctx[keyword].length - 1];
} else if (isTable(this.ctx[keyword])) {
this.ctx = this.ctx[keyword];
} else {
throw this.error(new TomlError("Can't redefine an existing key"));
}
return this.next(this.parseListNext);
} else {
throw this.error(new TomlError("Unexpected character, expected whitespace, . or ]"));
}
}
parseListEnd(keyword) {
if (this.char === CHAR_RSQB) {
return this.next(this.parseWhitespaceToEOL);
} else {
throw this.error(new TomlError("Unexpected character, expected whitespace, . or ]"));
}
}
parseValue() {
if (this.char === Parser.END) {
throw this.error(new TomlError("Key without value"));
} else if (this.char === CHAR_QUOT) {
return this.next(this.parseDoubleString);
}
if (this.char === CHAR_APOS) {
return this.next(this.parseSingleString);
} else if (this.char === CHAR_HYPHEN || this.char === CHAR_PLUS) {
return this.goto(this.parseNumberSign);
} else if (this.char === CHAR_i) {
return this.next(this.parseInf);
} else if (this.char === CHAR_n) {
return this.next(this.parseNan);
} else if (isDigit(this.char)) {
return this.goto(this.parseNumberOrDateTime);
} else if (this.char === CHAR_t || this.char === CHAR_f) {
return this.goto(this.parseBoolean);
} else if (this.char === CHAR_LSQB) {
return this.call(this.parseInlineList, this.recordValue);
} else if (this.char === CHAR_LCUB) {
return this.call(this.parseInlineTable, this.recordValue);
} else {
throw this.error(new TomlError("Unexpected character, expecting string, number, datetime, boolean, inline array or inline table"));
}
}
recordValue(value) {
return this.returnNow(value);
}
parseInf() {
if (this.char === CHAR_n) {
return this.next(this.parseInf2);
} else {
throw this.error(new TomlError('Unexpected character, expected "inf", "+inf" or "-inf"'));
}
}
parseInf2() {
if (this.char === CHAR_f) {
if (this.state.buf === "-") {
return this.return(-Infinity);
} else {
return this.return(Infinity);
}
} else {
throw this.error(new TomlError('Unexpected character, expected "inf", "+inf" or "-inf"'));
}
}
parseNan() {
if (this.char === CHAR_a) {
return this.next(this.parseNan2);
} else {
throw this.error(new TomlError('Unexpected character, expected "nan"'));
}
}
parseNan2() {
if (this.char === CHAR_n) {
return this.return(NaN);
} else {
throw this.error(new TomlError('Unexpected character, expected "nan"'));
}
}
parseKeyword() {
if (this.char === CHAR_QUOT) {
return this.next(this.parseBasicString);
} else if (this.char === CHAR_APOS) {
return this.next(this.parseLiteralString);
} else {
return this.goto(this.parseBareKey);
}
}
parseBareKey() {
do {
if (this.char === Parser.END) {
throw this.error(new TomlError("Key ended without value"));
} else if (isAlphaNumHyphen(this.char)) {
this.consume();
} else if (this.state.buf.length === 0) {
throw this.error(new TomlError("Empty bare keys are not allowed"));
} else {
return this.returnNow();
}
} while (this.nextChar());
}
parseSingleString() {
if (this.char === CHAR_APOS) {
return this.next(this.parseLiteralMultiStringMaybe);
} else {
return this.goto(this.parseLiteralString);
}
}
parseLiteralString() {
do {
if (this.char === CHAR_APOS) {
return this.return();
} else if (this.atEndOfLine()) {
throw this.error(new TomlError("Unterminated string"));
} else if (this.char === CHAR_DEL || this.char <= CTRL_CHAR_BOUNDARY && this.char !== CTRL_I) {
throw this.errorControlCharInString();
} else {
this.consume();
}
} while (this.nextChar());
}
parseLiteralMultiStringMaybe() {
if (this.char === CHAR_APOS) {
return this.next(this.parseLiteralMultiString);
} else {
return this.returnNow();
}
}
parseLiteralMultiString() {
if (this.char === CTRL_M) {
return null;
} else if (this.char === CTRL_J) {
return this.next(this.parseLiteralMultiStringContent);
} else {
return this.goto(this.parseLiteralMultiStringContent);
}
}
parseLiteralMultiStringContent() {
do {
if (this.char === CHAR_APOS) {
return this.next(this.parseLiteralMultiEnd);
} else if (this.char === Parser.END) {
throw this.error(new TomlError("Unterminated multi-line string"));
} else if (this.char === CHAR_DEL || this.char <= CTRL_CHAR_BOUNDARY && this.char !== CTRL_I && this.char !== CTRL_J && this.char !== CTRL_M) {
throw this.errorControlCharInString();
} else {
this.consume();
}
} while (this.nextChar());
}
parseLiteralMultiEnd() {
if (this.char === CHAR_APOS) {
return this.next(this.parseLiteralMultiEnd2);
} else {
this.state.buf += "'";
return this.goto(this.parseLiteralMultiStringContent);
}
}
parseLiteralMultiEnd2() {
if (this.char === CHAR_APOS) {
return this.return();
} else {
this.state.buf += "''";
return this.goto(this.parseLiteralMultiStringContent);
}
}
parseDoubleString() {
if (this.char === CHAR_QUOT) {
return this.next(this.parseMultiStringMaybe);
} else {
return this.goto(this.parseBasicString);
}
}
parseBasicString() {
do {
if (this.char === CHAR_BSOL) {
return this.call(this.parseEscape, this.recordEscapeReplacement);
} else if (this.char === CHAR_QUOT) {
return this.return();
} else if (this.atEndOfLine()) {
throw this.error(new TomlError("Unterminated string"));
} else if (this.char === CHAR_DEL || this.char <= CTRL_CHAR_BOUNDARY && this.char !== CTRL_I) {
throw this.errorControlCharInString();
} else {
this.consume();
}
} while (this.nextChar());
}
recordEscapeReplacement(replacement) {
this.state.buf += replacement;
return this.goto(this.parseBasicString);
}
parseMultiStringMaybe() {
if (this.char === CHAR_QUOT) {
return this.next(this.parseMultiString);
} else {
return this.returnNow();
}
}
parseMultiString() {
if (this.char === CTRL_M) {
return null;
} else if (this.char === CTRL_J) {
return this.next(this.parseMultiStringContent);
} else {
return this.goto(this.parseMultiStringContent);
}
}
parseMultiStringContent() {
do {
if (this.char === CHAR_BSOL) {
return this.call(this.parseMultiEscape, this.recordMultiEscapeReplacement);
} else if (this.char === CHAR_QUOT) {
return this.next(this.parseMultiEnd);
} else if (this.char === Parser.END) {
throw this.error(new TomlError("Unterminated multi-line string"));
} else if (this.char === CHAR_DEL || this.char <= CTRL_CHAR_BOUNDARY && this.char !== CTRL_I && this.char !== CTRL_J && this.char !== CTRL_M) {
throw this.errorControlCharInString();
} else {
this.consume();
}
} while (this.nextChar());
}
errorControlCharInString() {
let displayCode = "\\u00";
if (this.char < 16) {
displayCode += "0";
}
displayCode += this.char.toString(16);
return this.error(new TomlError(`Control characters (codes < 0x1f and 0x7f) are not allowed in strings, use ${displayCode} instead`));
}
recordMultiEscapeReplacement(replacement) {
this.state.buf += replacement;
return this.goto(this.parseMultiStringContent);
}
parseMultiEnd() {
if (this.char === CHAR_QUOT) {
return this.next(this.parseMultiEnd2);
} else {
this.state.buf += '"';
return this.goto(this.parseMultiStringContent);
}
}
parseMultiEnd2() {
if (this.char === CHAR_QUOT) {
return this.return();
} else {
this.state.buf += '""';
return this.goto(this.parseMultiStringContent);
}
}
parseMultiEscape() {
if (this.char === CTRL_M || this.char === CTRL_J) {
return this.next(this.parseMultiTrim);
} else if (this.char === CHAR_SP || this.char === CTRL_I) {
return this.next(this.parsePreMultiTrim);
} else {
return this.goto(this.parseEscape);
}
}
parsePreMultiTrim() {
if (this.char === CHAR_SP || this.char === CTRL_I) {
return null;
} else if (this.char === CTRL_M || this.char === CTRL_J) {
return this.next(this.parseMultiTrim);
} else {
throw this.error(new TomlError("Can't escape whitespace"));
}
}
parseMultiTrim() {
if (this.char === CTRL_J || this.char === CHAR_SP || this.char === CTRL_I || this.char === CTRL_M) {
return null;
} else {
return this.returnNow();
}
}
parseEscape() {
if (this.char in escapes) {
return this.return(escapes[this.char]);
} else if (this.char === CHAR_u) {
return this.call(this.parseSmallUnicode, this.parseUnicodeReturn);
} else if (this.char === CHAR_U) {
return this.call(this.parseLargeUnicode, this.parseUnicodeReturn);
} else {
throw this.error(new TomlError("Unknown escape character: " + this.char));
}
}
parseUnicodeReturn(char2) {
try {
const codePoint = parseInt(char2, 16);
if (codePoint >= SURROGATE_FIRST && codePoint <= SURROGATE_LAST) {
throw this.error(new TomlError("Invalid unicode, character in range 0xD800 - 0xDFFF is reserved"));
}
return this.returnNow(String.fromCodePoint(codePoint));
} catch (err) {
throw this.error(TomlError.wrap(err));
}
}
parseSmallUnicode() {
if (!isHexit(this.char)) {
throw this.error(new TomlError("Invalid character in unicode sequence, expected hex"));
} else {
this.consume();
if (this.state.buf.length >= 4)
return this.return();
}
}
parseLargeUnicode() {
if (!isHexit(this.char)) {
throw this.error(new TomlError("Invalid character in unicode sequence, expected hex"));
} else {
this.consume();
if (this.state.buf.length >= 8)
return this.return();
}
}
parseNumberSign() {
this.consume();
return this.next(this.parseMaybeSignedInfOrNan);
}
parseMaybeSignedInfOrNan() {
if (this.char === CHAR_i) {
return this.next(this.parseInf);
} else if (this.char === CHAR_n) {
return this.next(this.parseNan);
} else {
return this.callNow(this.parseNoUnder, this.parseNumberIntegerStart);
}
}
parseNumberIntegerStart() {
if (this.char === CHAR_0) {
this.consume();
return this.next(this.parseNumberIntegerExponentOrDecimal);
} else {
return this.goto(this.parseNumberInteger);
}
}
parseNumberIntegerExponentOrDecimal() {
if (this.char === CHAR_PERIOD) {
this.consume();
return this.call(this.parseNoUnder, this.parseNumberFloat);
} else if (this.char === CHAR_E || this.char === CHAR_e) {
this.consume();
return this.next(this.parseNumberExponentSign);
} else {
return this.returnNow(Integer(this.state.buf));
}
}
parseNumberInteger() {
if (isDigit(this.char)) {
this.consume();
} else if (this.char === CHAR_LOWBAR) {
return this.call(this.parseNoUnder);
} else if (this.char === CHAR_E || this.char === CHAR_e) {
this.consume();
return this.next(this.parseNumberExponentSign);
} else if (this.char === CHAR_PERIOD) {
this.consume();
return this.call(this.parseNoUnder, this.parseNumberFloat);
} else {
const result = Integer(this.state.buf);
if (result.isNaN()) {
throw this.error(new TomlError("Invalid number"));
} else {
return this.returnNow(result);
}
}
}
parseNoUnder() {
if (this.char === CHAR_LOWBAR || this.char === CHAR_PERIOD || this.char === CHAR_E || this.char === CHAR_e) {
throw this.error(new TomlError("Unexpected character, expected digit"));
} else if (this.atEndOfWord()) {
throw this.error(new TomlError("Incomplete number"));
}
return this.returnNow();
}
parseNoUnderHexOctBinLiteral() {
if (this.char === CHAR_LOWBAR || this.char === CHAR_PERIOD) {
throw this.error(new TomlError("Unexpected character, expected digit"));
} else if (this.atEndOfWord()) {
throw this.error(new TomlError("Incomplete number"));
}
return this.returnNow();
}
parseNumberFloat() {
if (this.char === CHAR_LOWBAR) {
return this.call(this.parseNoUnder, this.parseNumberFloat);
} else if (isDigit(this.char)) {
this.consume();
} else if (this.char === CHAR_E || this.char === CHAR_e) {
this.consume();
return this.next(this.parseNumberExponentSign);
} else {
return this.returnNow(Float(this.state.buf));
}
}
parseNumberExponentSign() {
if (isDigit(this.char)) {
return this.goto(this.parseNumberExponent);
} else if (this.char === CHAR_HYPHEN || this.char === CHAR_PLUS) {
this.consume();
this.call(this.parseNoUnder, this.parseNumberExponent);
} else {
throw this.error(new TomlError("Unexpected character, expected -, + or digit"));
}
}
parseNumberExponent() {
if (isDigit(this.char)) {
this.consume();
} else if (this.char === CHAR_LOWBAR) {
return this.call(this.parseNoUnder);
} else {
return this.returnNow(Float(this.state.buf));
}
}
parseNumberOrDateTime() {
if (this.char === CHAR_0) {
this.consume();
return this.next(this.parseNumberBaseOrDateTime);
} else {
return this.goto(this.parseNumberOrDateTimeOnly);
}
}
parseNumberOrDateTimeOnly() {
if (this.char === CHAR_LOWBAR) {
return this.call(this.parseNoUnder, this.parseNumberInteger);
} else if (isDigit(this.char)) {
this.consume();
if (this.state.buf.length > 4)
this.next(this.parseNumberInteger);
} else if (this.char === CHAR_E || this.char === CHAR_e) {
this.consume();
return this.next(this.parseNumberExponentSign);
} else if (this.char === CHAR_PERIOD) {
this.consume();
return this.call(this.parseNoUnder, this.parseNumberFloat);
} else if (this.char === CHAR_HYPHEN) {
return this.goto(this.parseDateTime);
} else if (this.char === CHAR_COLON) {
return this.goto(this.parseOnlyTimeHour);
} else {
return this.returnNow(Integer(this.state.buf));
}
}
parseDateTimeOnly() {
if (this.state.buf.length < 4) {
if (isDigit(this.char)) {
return this.consume();
} else if (this.char === CHAR_COLON) {
return this.goto(this.parseOnlyTimeHour);
} else {
throw this.error(new TomlError("Expected digit while parsing year part of a date"));
}
} else {
if (this.char === CHAR_HYPHEN) {
return this.goto(this.parseDateTime);
} else {
throw this.error(new TomlError("Expected hyphen (-) while parsing year part of date"));
}
}
}
parseNumberBaseOrDateTime() {
if (this.char === CHAR_b) {
this.consume();
return this.call(this.parseNoUnderHexOctBinLiteral, this.parseIntegerBin);
} else if (this.char === CHAR_o) {
this.consume();
return this.call(this.parseNoUnderHexOctBinLiteral, this.parseIntegerOct);
} else if (this.char === CHAR_x) {
this.consume();
return this.call(this.parseNoUnderHexOctBinLiteral, this.parseIntegerHex);
} else if (this.char === CHAR_PERIOD) {
return this.goto(this.parseNumberInteger);
} else if (isDigit(this.char)) {
return this.goto(this.parseDateTimeOnly);
} else {
return this.returnNow(Integer(this.state.buf));
}
}
parseIntegerHex() {
if (isHexit(this.char)) {
this.consume();
} else if (this.char === CHAR_LOWBAR) {
return this.call(this.parseNoUnderHexOctBinLiteral);
} else {
const result = Integer(this.state.buf);
if (result.isNaN()) {
throw this.error(new TomlError("Invalid number"));
} else {
return this.returnNow(result);
}
}
}
parseIntegerOct() {
if (isOctit(this.char)) {
this.consume();
} else if (this.char === CHAR_LOWBAR) {
return this.call(this.parseNoUnderHexOctBinLiteral);
} else {
const result = Integer(this.state.buf);
if (result.isNaN()) {
throw this.error(new TomlError("Invalid number"));
} else {
return this.returnNow(result);
}
}
}
parseIntegerBin() {
if (isBit(this.char)) {
this.consume();
} else if (this.char === CHAR_LOWBAR) {
return this.call(this.parseNoUnderHexOctBinLiteral);
} else {
const result = Integer(this.state.buf);
if (result.isNaN()) {
throw this.error(new TomlError("Invalid number"));
} else {
return this.returnNow(result);
}
}
}
parseDateTime() {
if (this.state.buf.length < 4) {
throw this.error(new TomlError("Years less than 1000 must be zero padded to four characters"));
}
this.state.result = this.state.buf;
this.state.buf = "";
return this.next(this.parseDateMonth);
}
parseDateMonth() {
if (this.char === CHAR_HYPHEN) {
if (this.state.buf.length < 2) {
throw this.error(new TomlError("Months less than 10 must be zero padded to two characters"));
}
this.state.result += "-" + this.state.buf;
this.state.buf = "";
return this.next(this.parseDateDay);
} else if (isDigit(this.char)) {
this.consume();
} else {
throw this.error(new TomlError("Incomplete datetime"));
}
}
parseDateDay() {
if (this.char === CHAR_T || this.char === CHAR_SP) {
if (this.state.buf.length < 2) {
throw this.error(new TomlError("Days less than 10 must be zero padded to two characters"));
}
this.state.result += "-" + this.state.buf;
this.state.buf = "";
return this.next(this.parseStartTimeHour);
} else if (this.atEndOfWord()) {
return this.returnNow(createDate(this.state.result + "-" + this.state.buf));
} else if (isDigit(this.char)) {
this.consume();
} else {
throw this.error(new TomlError("Incomplete datetime"));
}
}
parseStartTimeHour() {
if (this.atEndOfWord()) {
return this.returnNow(createDate(this.state.result));
} else {
return this.goto(this.parseTimeHour);
}
}
parseTimeHour() {
if (this.char === CHAR_COLON) {
if (this.state.buf.length < 2) {
throw this.error(new TomlError("Hours less than 10 must be zero padded to two characters"));
}
this.state.result += "T" + this.state.buf;
this.state.buf = "";
return this.next(this.parseTimeMin);
} else if (isDigit(this.char)) {
this.consume();
} else {
throw this.error(new TomlError("Incomplete datetime"));
}
}
parseTimeMin() {
if (this.state.buf.length < 2 && isDigit(this.char)) {
this.consume();
} else if (this.state.buf.length === 2 && this.char === CHAR_COLON) {
this.state.result += ":" + this.state.buf;
this.state.buf = "";
return this.next(this.parseTimeSec);
} else {
throw this.error(new TomlError("Incomplete datetime"));
}
}
parseTimeSec() {
if (isDigit(this.char)) {
this.consume();
if (this.state.buf.length === 2) {
this.state.result += ":" + this.state.buf;
this.state.buf = "";
return this.next(this.parseTimeZoneOrFraction);
}
} else {
throw this.error(new TomlError("Incomplete datetime"));
}
}
parseOnlyTimeHour() {
if (this.char === CHAR_COLON) {
if (this.state.buf.length < 2) {
throw this.error(new TomlError("Hours less than 10 must be zero padded to two characters"));
}
this.state.result = this.state.buf;
this.state.buf = "";
return this.next(this.parseOnlyTimeMin);
} else {
throw this.error(new TomlError("Incomplete time"));
}
}
parseOnlyTimeMin() {
if (this.state.buf.length < 2 && isDigit(this.char)) {
this.consume();
} else if (this.state.buf.length === 2 && this.char === CHAR_COLON) {
this.state.result += ":" + this.state.buf;
this.state.buf = "";
return this.next(this.parseOnlyTimeSec);
} else {
throw this.error(new TomlError("Incomplete time"));
}
}
parseOnlyTimeSec() {
if (isDigit(this.char)) {
this.consume();
if (this.state.buf.length === 2) {
return this.next(this.parseOnlyTimeFractionMaybe);
}
} else {
throw this.error(new TomlError("Incomplete time"));
}
}
parseOnlyTimeFractionMaybe() {
this.state.result += ":" + this.state.buf;
if (this.char === CHAR_PERIOD) {
this.state.buf = "";
this.next(this.parseOnlyTimeFraction);
} else {
return this.return(createTime(this.state.result));
}
}
parseOnlyTimeFraction() {
if (isDigit(this.char)) {
this.consume();
} else if (this.atEndOfWord()) {
if (this.state.buf.length === 0)
throw this.error(new TomlError("Expected digit in milliseconds"));
return this.returnNow(createTime(this.state.result + "." + this.state.buf));
} else {
throw this.error(new TomlError("Unexpected character in datetime, expected period (.), minus (-), plus (+) or Z"));
}
}
parseTimeZoneOrFraction() {
if (this.char === CHAR_PERIOD) {
this.consume();
this.next(this.parseDateTimeFraction);
} else if (this.char === CHAR_HYPHEN || this.char === CHAR_PLUS) {
this.consume();
this.next(this.parseTimeZoneHour);
} else if (this.char === CHAR_Z) {
this.consume();
return this.return(createDateTime(this.state.result + this.state.buf));
} else if (this.atEndOfWord()) {
return this.returnNow(createDateTimeFloat(this.state.result + this.state.buf));
} else {
throw this.error(new TomlError("Unexpected character in datetime, expected period (.), minus (-), plus (+) or Z"));
}
}
parseDateTimeFraction() {
if (isDigit(this.char)) {
this.consume();
} else if (this.state.buf.length === 1) {
throw this.error(new TomlError("Expected digit in milliseconds"));
} else if (this.char === CHAR_HYPHEN || this.char === CHAR_PLUS) {
this.consume();
this.next(this.parseTimeZoneHour);
} else if (this.char === CHAR_Z) {
this.consume();
return this.return(createDateTime(this.state.result + this.state.buf));
} else if (this.atEndOfWord()) {
return this.returnNow(createDateTimeFloat(this.state.result + this.state.buf));
} else {
throw this.error(new TomlError("Unexpected character in datetime, expected period (.), minus (-), plus (+) or Z"));
}
}
parseTimeZoneHour() {
if (isDigit(this.char)) {
this.consume();
if (/\d\d$/.test(this.state.buf))
return this.next(this.parseTimeZoneSep);
} else {
throw this.error(new TomlError("Unexpected character in datetime, expected digit"));
}
}
parseTimeZoneSep() {
if (this.char === CHAR_COLON) {
this.consume();
this.next(this.parseTimeZoneMin);
} else {
throw this.error(new TomlError("Unexpected character in datetime, expected colon"));
}
}
parseTimeZoneMin() {
if (isDigit(this.char)) {
this.consume();
if (/\d\d$/.test(this.state.buf))
return this.return(createDateTime(this.state.result + this.state.buf));
} else {
throw this.error(new TomlError("Unexpected character in datetime, expected digit"));
}
}
parseBoolean() {
if (this.char === CHAR_t) {
this.consume();
return this.next(this.parseTrue_r);
} else if (this.char === CHAR_f) {
this.consume();
return this.next(this.parseFalse_a);
}
}
parseTrue_r() {
if (this.char === CHAR_r) {
this.consume();
return this.next(this.parseTrue_u);
} else {
throw this.error(new TomlError("Invalid boolean, expected true or false"));
}
}
parseTrue_u() {
if (this.char === CHAR_u) {
this.consume();
return this.next(this.parseTrue_e);
} else {
throw this.error(new TomlError("Invalid boolean, expected true or false"));
}
}
parseTrue_e() {
if (this.char === CHAR_e) {
return this.return(true);
} else {
throw this.error(new TomlError("Invalid boolean, expected true or false"));
}
}
parseFalse_a() {
if (this.char === CHAR_a) {
this.consume();
return this.next(this.parseFalse_l);
} else {
throw this.error(new TomlError("Invalid boolean, expected true or false"));
}
}
parseFalse_l() {
if (this.char === CHAR_l) {
this.consume();
return this.next(this.parseFalse_s);
} else {
throw this.error(new TomlError("Invalid boolean, expected true or false"));
}
}
parseFalse_s() {
if (this.char === CHAR_s) {
this.consume();
return this.next(this.parseFalse_e);
} else {
throw this.error(new TomlError("Invalid boolean, expected true or false"));
}
}
parseFalse_e() {
if (this.char === CHAR_e) {
return this.return(false);
} else {
throw this.error(new TomlError("Invalid boolean, expected true or false"));
}
}
parseInlineList() {
if (this.char === CHAR_SP || this.char === CTRL_I || this.char === CTRL_M || this.char === CTRL_J) {
return null;
} else if (this.char === Parser.END) {
throw this.error(new TomlError("Unterminated inline array"));
} else if (this.char === CHAR_NUM) {
return this.call(this.parseComment);
} else if (this.char === CHAR_RSQB) {
return this.return(this.state.resultArr || InlineList());
} else {
return this.callNow(this.parseValue, this.recordInlineListValue);
}
}
recordInlineListValue(value) {
if (this.state.resultArr) {
const listType = this.state.resultArr[_contentType];
const valueType = tomlType(value);
if (listType !== valueType) {
throw this.error(new TomlError(`Inline lists must be a single type, not a mix of ${listType} and ${valueType}`));
}
} else {
this.state.resultArr = InlineList(tomlType(value));
}
if (isFloat(value) || isInteger(value)) {
this.state.resultArr.push(value.valueOf());
} else {
this.state.resultArr.push(value);
}
return this.goto(this.parseInlineListNext);
}
parseInlineListNext() {
if (this.char === CHAR_SP || this.char === CTRL_I || this.char === CTRL_M || this.char === CTRL_J) {
return null;
} else if (this.char === CHAR_NUM) {
return this.call(this.parseComment);
} else if (this.char === CHAR_COMMA) {
return this.next(this.parseInlineList);
} else if (this.char === CHAR_RSQB) {
return this.goto(this.parseInlineList);
} else {
throw this.error(new TomlError("Invalid character, expected whitespace, comma (,) or close bracket (])"));
}
}
parseInlineTable() {
if (this.char === CHAR_SP || this.char === CTRL_I) {
return null;
} else if (this.char === Parser.END || this.char === CHAR_NUM || this.char === CTRL_J || this.char === CTRL_M) {
throw this.error(new TomlError("Unterminated inline array"));
} else if (this.char === CHAR_RCUB) {
return this.return(this.state.resultTable || InlineTable());
} else {
if (!this.state.resultTable)
this.state.resultTable = InlineTable();
return this.callNow(this.parseAssign, this.recordInlineTableValue);
}
}
recordInlineTableValue(kv) {
let target = this.state.resultTable;
let finalKey = kv.key.pop();
for (let kw of kv.key) {
if (hasKey(target, kw) && (!isTable(target[kw]) || target[kw][_declared])) {
throw this.error(new TomlError("Can't redefine existing key"));
}
target = target[kw] = target[kw] || Table();
}
if (hasKey(target, finalKey)) {
throw this.error(new TomlError("Can't redefine existing key"));
}
if (isInteger(kv.value) || isFloat(kv.value)) {
target[finalKey] = kv.value.valueOf();
} else {
target[finalKey] = kv.value;
}
return this.goto(this.parseInlineTableNext);
}
parseInlineTableNext() {
if (this.char === CHAR_SP || this.char === CTRL_I) {
return null;
} else if (this.char === Parser.END || this.char === CHAR_NUM || this.char === CTRL_J || this.char === CTRL_M) {
throw this.error(new TomlError("Unterminated inline array"));
} else if (this.char === CHAR_COMMA) {
return this.next(this.parseInlineTable);
} else if (this.char === CHAR_RCUB) {
return this.goto(this.parseInlineTable);
} else {
throw this.error(new TomlError("Invalid character, expected whitespace, comma (,) or close bracket (])"));
}
}
}
return TOMLParser;
}
}
});
var require_parse_pretty_error = __commonJS22({
"node_modules/@iarna/toml/parse-pretty-error.js"(exports2, module22) {
"use strict";
module22.exports = prettyError;
function prettyError(err, buf) {
if (err.pos == null || err.line == null)
return err;
let msg = err.message;
msg += ` at row ${err.line + 1}, col ${err.col + 1}, pos ${err.pos}:
`;
if (buf && buf.split) {
const lines = buf.split(/\n/);
const lineNumWidth = String(Math.min(lines.length, err.line + 3)).length;
let linePadding = " ";
while (linePadding.length < lineNumWidth)
linePadding += " ";
for (let ii = Math.max(0, err.line - 1); ii < Math.min(lines.length, err.line + 2); ++ii) {
let lineNum = String(ii + 1);
if (lineNum.length < lineNumWidth)
lineNum = " " + lineNum;
if (err.line === ii) {
msg += lineNum + "> " + lines[ii] + "\n";
msg += linePadding + " ";
for (let hh = 0; hh < err.col; ++hh) {
msg += " ";
}
msg += "^\n";
} else {
msg += lineNum + ": " + lines[ii] + "\n";
}
}
}
err.message = msg + "\n";
return err;
}
}
});
var require_parse_string = __commonJS22({
"node_modules/@iarna/toml/parse-string.js"(exports2, module22) {
"use strict";
module22.exports = parseString;
var TOMLParser = require_toml_parser();
var prettyError = require_parse_pretty_error();
function parseString(str) {
if (global.Buffer && global.Buffer.isBuffer(str)) {
str = str.toString("utf8");
}
const parser = new TOMLParser();
try {
parser.parse(str);
return parser.finish();
} catch (err) {
throw prettyError(err, str);
}
}
}
});
var require_load_toml = __commonJS22({
"src/utils/load-toml.js"(exports2, module22) {
"use strict";
var parse2 = require_parse_string();
module22.exports = function(filePath2, content) {
try {
return parse2(content);
} catch (error) {
error.message = `TOML Error in ${filePath2}:
${error.message}`;
throw error;
}
};
}
});
var require_unicode = __commonJS22({
"node_modules/json5/lib/unicode.js"(exports2, module22) {
module22.exports.Space_Separator = /[\u1680\u2000-\u200A\u202F\u205F\u3000]/;
module22.exports.ID_Start = /[\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0370-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u048A-\u052F\u0531-\u0556\u0559\u0561-\u0587\u05D0-\u05EA\u05F0-\u05F2\u0620-\u064A\u066E\u066F\u0671-\u06D3\u06D5\u06E5\u06E6\u06EE\u06EF\u06FA-\u06FC\u06FF\u0710\u0712-\u072F\u074D-\u07A5\u07B1\u07CA-\u07EA\u07F4\u07F5\u07FA\u0800-\u0815\u081A\u0824\u0828\u0840-\u0858\u0860-\u086A\u08A0-\u08B4\u08B6-\u08BD\u0904-\u0939\u093D\u0950\u0958-\u0961\u0971-\u0980\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD\u09CE\u09DC\u09DD\u09DF-\u09E1\u09F0\u09F1\u09FC\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A59-\u0A5C\u0A5E\u0A72-\u0A74\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABD\u0AD0\u0AE0\u0AE1\u0AF9\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3D\u0B5C\u0B5D\u0B5F-\u0B61\u0B71\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BD0\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C58-\u0C5A\u0C60\u0C61\u0C80\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CDE\u0CE0\u0CE1\u0CF1\u0CF2\u0D05-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D\u0D4E\u0D54-\u0D56\u0D5F-\u0D61\u0D7A-\u0D7F\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0E01-\u0E30\u0E32\u0E33\u0E40-\u0E46\u0E81\u0E82\u0E84\u0E87\u0E88\u0E8A\u0E8D\u0E94-\u0E97\u0E99-\u0E9F\u0EA1-\u0EA3\u0EA5\u0EA7\u0EAA\u0EAB\u0EAD-\u0EB0\u0EB2\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6\u0EDC-\u0EDF\u0F00\u0F40-\u0F47\u0F49-\u0F6C\u0F88-\u0F8C\u1000-\u102A\u103F\u1050-\u1055\u105A-\u105D\u1061\u1065\u1066\u106E-\u1070\u1075-\u1081\u108E\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u170C\u170E-\u1711\u1720-\u1731\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17D7\u17DC\u1820-\u1877\u1880-\u1884\u1887-\u18A8\u18AA\u18B0-\u18F5\u1900-\u191E\u1950-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u1A00-\u1A16\u1A20-\u1A54\u1AA7\u1B05-\u1B33\u1B45-\u1B4B\u1B83-\u1BA0\u1BAE\u1BAF\u1BBA-\u1BE5\u1C00-\u1C23\u1C4D-\u1C4F\u1C5A-\u1C7D\u1C80-\u1C88\u1CE9-\u1CEC\u1CEE-\u1CF1\u1CF5\u1CF6\u1D00-\u1DBF\u1E00-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2119-\u211D\u2124\u2126\u2128\u212A-\u212D\u212F-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2C2E\u2C30-\u2C5E\u2C60-\u2CE4\u2CEB-\u2CEE\u2CF2\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u2E2F\u3005-\u3007\u3021-\u3029\u3031-\u3035\u3038-\u303C\u3041-\u3096\u309D-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312E\u3131-\u318E\u31A0-\u31BA\u31F0-\u31FF\u3400-\u4DB5\u4E00-\u9FEA\uA000-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA61F\uA62A\uA62B\uA640-\uA66E\uA67F-\uA69D\uA6A0-\uA6EF\uA717-\uA71F\uA722-\uA788\uA78B-\uA7AE\uA7B0-\uA7B7\uA7F7-\uA801\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA840-\uA873\uA882-\uA8B3\uA8F2-\uA8F7\uA8FB\uA8FD\uA90A-\uA925\uA930-\uA946\uA960-\uA97C\uA984-\uA9B2\uA9CF\uA9E0-\uA9E4\uA9E6-\uA9EF\uA9FA-\uA9FE\uAA00-\uAA28\uAA40-\uAA42\uAA44-\uAA4B\uAA60-\uAA76\uAA7A\uAA7E-\uAAAF\uAAB1\uAAB5\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADD\uAAE0-\uAAEA\uAAF2-\uAAF4\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB65\uAB70-\uABE2\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE70-\uFE74\uFE76-\uFEFC\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC]|\uD800[\uDC00-\uDC0B\uDC0D-\uDC26\uDC28-\uDC3A\uDC3C\uDC3D\uDC3F-\uDC4D\uDC50-\uDC5D\uDC80-\uDCFA\uDD40-\uDD74\uDE80-\uDE9C\uDEA0-\uDED0\uDF00-\uDF1F\uDF2D-\uDF4A\uDF50-\uDF75\uDF80-\uDF9D\uDFA0-\uDFC3\uDFC8-\uDFCF\uDFD1-\uDFD5]|\uD801[\uDC00-\uDC9D\uDCB0-\uDCD3\uDCD8-\uDCFB\uDD00-\uDD27\uDD30-\uDD63\uDE00-\uDF36\uDF40-\uDF55\uDF60-\uDF67]|\uD802[\uDC00-\uDC05\uDC08\uDC0A-\uDC35\uDC37\uDC38\uDC3C\uDC3F-\uDC55\uDC60-\uDC76\uDC80-\uDC9E\uDCE0-\uDCF2\uDCF4\uDCF5\uDD00-\uDD15\uDD20-\uDD39\uDD80-\uDDB7\uDDBE\uDDBF\uDE00\uDE10-\uDE13\uDE15-\uDE17\uDE19-\uDE33\uDE60-\uDE7C\uDE80-\uDE9C\uDEC0-\uDEC7\uDEC9-\uDEE4\uDF00-\uDF35\uDF40-\uDF55\uDF60-\uDF72\uDF80-\uDF91]|\uD803[\uDC00-\uDC48\uDC80-\uDCB2\uDCC0-\uDCF2]|\uD804[\uDC03-\uDC37\uDC83-\uDCAF\uDCD0-\uDCE8\uDD03-\uDD26\uDD50-\uDD72\uDD76\uDD83-\uDDB2\uDDC1-\uDDC4\uDDDA\uDDDC\uDE00-\uDE11\uDE13-\uDE2B\uDE80-\uDE86\uDE88\uDE8A-\uDE8D\uDE8F-\uDE9D\uDE9F-\uDEA8\uDEB0-\uDEDE\uDF05-\uDF0C\uDF0F\uDF10\uDF13-\uDF28\uDF2A-\uDF30\uDF32\uDF33\uDF35-\uDF39\uDF3D\uDF50\uDF5D-\uDF61]|\uD805[\uDC00-\uDC34\uDC47-\uDC4A\uDC80-\uDCAF\uDCC4\uDCC5\uDCC7\uDD80-\uDDAE\uDDD8-\uDDDB\uDE00-\uDE2F\uDE44\uDE80-\uDEAA\uDF00-\uDF19]|\uD806[\uDCA0-\uDCDF\uDCFF\uDE00\uDE0B-\uDE32\uDE3A\uDE50\uDE5C-\uDE83\uDE86-\uDE89\uDEC0-\uDEF8]|\uD807[\uDC00-\uDC08\uDC0A-\uDC2E\uDC40\uDC72-\uDC8F\uDD00-\uDD06\uDD08\uDD09\uDD0B-\uDD30\uDD46]|\uD808[\uDC00-\uDF99]|\uD809[\uDC00-\uDC6E\uDC80-\uDD43]|[\uD80C\uD81C-\uD820\uD840-\uD868\uD86A-\uD86C\uD86F-\uD872\uD874-\uD879][\uDC00-\uDFFF]|\uD80D[\uDC00-\uDC2E]|\uD811[\uDC00-\uDE46]|\uD81A[\uDC00-\uDE38\uDE40-\uDE5E\uDED0-\uDEED\uDF00-\uDF2F\uDF40-\uDF43\uDF63-\uDF77\uDF7D-\uDF8F]|\uD81B[\uDF00-\uDF44\uDF50\uDF93-\uDF9F\uDFE0\uDFE1]|\uD821[\uDC00-\uDFEC]|\uD822[\uDC00-\uDEF2]|\uD82C[\uDC00-\uDD1E\uDD70-\uDEFB]|\uD82F[\uDC00-\uDC6A\uDC70-\uDC7C\uDC80-\uDC88\uDC90-\uDC99]|\uD835[\uDC00-\uDC54\uDC56-\uDC9C\uDC9E\uDC9F\uDCA2\uDCA5\uDCA6\uDCA9-\uDCAC\uDCAE-\uDCB9\uDCBB\uDCBD-\uDCC3\uDCC5-\uDD05\uDD07-\uDD0A\uDD0D-\uDD14\uDD16-\uDD1C\uDD1E-\uDD39\uDD3B-\uDD3E\uDD40-\uDD44\uDD46\uDD4A-\uDD50\uDD52-\uDEA5\uDEA8-\uDEC0\uDEC2-\uDEDA\uDEDC-\uDEFA\uDEFC-\uDF14\uDF16-\uDF34\uDF36-\uDF4E\uDF50-\uDF6E\uDF70-\uDF88\uDF8A-\uDFA8\uDFAA-\uDFC2\uDFC4-\uDFCB]|\uD83A[\uDC00-\uDCC4\uDD00-\uDD43]|\uD83B[\uDE00-\uDE03\uDE05-\uDE1F\uDE21\uDE22\uDE24\uDE27\uDE29-\uDE32\uDE34-\uDE37\uDE39\uDE3B\uDE42\uDE47\uDE49\uDE4B\uDE4D-\uDE4F\uDE51\uDE52\uDE54\uDE57\uDE59\uDE5B\uDE5D\uDE5F\uDE61\uDE62\uDE64\uDE67-\uDE6A\uDE6C-\uDE72\uDE74-\uDE77\uDE79-\uDE7C\uDE7E\uDE80-\uDE89\uDE8B-\uDE9B\uDEA1-\uDEA3\uDEA5-\uDEA9\uDEAB-\uDEBB]|\uD869[\uDC00-\uDED6\uDF00-\uDFFF]|\uD86D[\uDC00-\uDF34\uDF40-\uDFFF]|\uD86E[\uDC00-\uDC1D\uDC20-\uDFFF]|\uD873[\uDC00-\uDEA1\uDEB0-\uDFFF]|\uD87A[\uDC00-\uDFE0]|\uD87E[\uDC00-\uDE1D]/;
module22.exports.ID_Continue = /[\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0300-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u0483-\u0487\u048A-\u052F\u0531-\u0556\u0559\u0561-\u0587\u0591-\u05BD\u05BF\u05C1\u05C2\u05C4\u05C5\u05C7\u05D0-\u05EA\u05F0-\u05F2\u0610-\u061A\u0620-\u0669\u066E-\u06D3\u06D5-\u06DC\u06DF-\u06E8\u06EA-\u06FC\u06FF\u0710-\u074A\u074D-\u07B1\u07C0-\u07F5\u07FA\u0800-\u082D\u0840-\u085B\u0860-\u086A\u08A0-\u08B4\u08B6-\u08BD\u08D4-\u08E1\u08E3-\u0963\u0966-\u096F\u0971-\u0983\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BC-\u09C4\u09C7\u09C8\u09CB-\u09CE\u09D7\u09DC\u09DD\u09DF-\u09E3\u09E6-\u09F1\u09FC\u0A01-\u0A03\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A3C\u0A3E-\u0A42\u0A47\u0A48\u0A4B-\u0A4D\u0A51\u0A59-\u0A5C\u0A5E\u0A66-\u0A75\u0A81-\u0A83\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABC-\u0AC5\u0AC7-\u0AC9\u0ACB-\u0ACD\u0AD0\u0AE0-\u0AE3\u0AE6-\u0AEF\u0AF9-\u0AFF\u0B01-\u0B03\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3C-\u0B44\u0B47\u0B48\u0B4B-\u0B4D\u0B56\u0B57\u0B5C\u0B5D\u0B5F-\u0B63\u0B66-\u0B6F\u0B71\u0B82\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BBE-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCD\u0BD0\u0BD7\u0BE6-\u0BEF\u0C00-\u0C03\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D-\u0C44\u0C46-\u0C48\u0C4A-\u0C4D\u0C55\u0C56\u0C58-\u0C5A\u0C60-\u0C63\u0C66-\u0C6F\u0C80-\u0C83\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBC-\u0CC4\u0CC6-\u0CC8\u0CCA-\u0CCD\u0CD5\u0CD6\u0CDE\u0CE0-\u0CE3\u0CE6-\u0CEF\u0CF1\u0CF2\u0D00-\u0D03\u0D05-\u0D0C\u0D0E-\u0D10\u0D12-\u0D44\u0D46-\u0D48\u0D4A-\u0D4E\u0D54-\u0D57\u0D5F-\u0D63\u0D66-\u0D6F\u0D7A-\u0D7F\u0D82\u0D83\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0DCA\u0DCF-\u0DD4\u0DD6\u0DD8-\u0DDF\u0DE6-\u0DEF\u0DF2\u0DF3\u0E01-\u0E3A\u0E40-\u0E4E\u0E50-\u0E59\u0E81\u0E82\u0E84\u0E87\u0E88\u0E8A\u0E8D\u0E94-\u0E97\u0E99-\u0E9F\u0EA1-\u0EA3\u0EA5\u0EA7\u0EAA\u0EAB\u0EAD-\u0EB9\u0EBB-\u0EBD\u0EC0-\u0EC4\u0EC6\u0EC8-\u0ECD\u0ED0-\u0ED9\u0EDC-\u0EDF\u0F00\u0F18\u0F19\u0F20-\u0F29\u0F35\u0F37\u0F39\u0F3E-\u0F47\u0F49-\u0F6C\u0F71-\u0F84\u0F86-\u0F97\u0F99-\u0FBC\u0FC6\u1000-\u1049\u1050-\u109D\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u135D-\u135F\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u170C\u170E-\u1714\u1720-\u1734\u1740-\u1753\u1760-\u176C\u176E-\u1770\u1772\u1773\u1780-\u17D3\u17D7\u17DC\u17DD\u17E0-\u17E9\u180B-\u180D\u1810-\u1819\u1820-\u1877\u1880-\u18AA\u18B0-\u18F5\u1900-\u191E\u1920-\u192B\u1930-\u193B\u1946-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u19D0-\u19D9\u1A00-\u1A1B\u1A20-\u1A5E\u1A60-\u1A7C\u1A7F-\u1A89\u1A90-\u1A99\u1AA7\u1AB0-\u1ABD\u1B00-\u1B4B\u1B50-\u1B59\u1B6B-\u1B73\u1B80-\u1BF3\u1C00-\u1C37\u1C40-\u1C49\u1C4D-\u1C7D\u1C80-\u1C88\u1CD0-\u1CD2\u1CD4-\u1CF9\u1D00-\u1DF9\u1DFB-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u203F\u2040\u2054\u2071\u207F\u2090-\u209C\u20D0-\u20DC\u20E1\u20E5-\u20F0\u2102\u2107\u210A-\u2113\u2115\u2119-\u211D\u2124\u2126\u2128\u212A-\u212D\u212F-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2C2E\u2C30-\u2C5E\u2C60-\u2CE4\u2CEB-\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D7F-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u2DE0-\u2DFF\u2E2F\u3005-\u3007\u3021-\u302F\u3031-\u3035\u3038-\u303C\u3041-\u3096\u3099\u309A\u309D-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312E\u3131-\u318E\u31A0-\u31BA\u31F0-\u31FF\u3400-\u4DB5\u4E00-\u9FEA\uA000-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA62B\uA640-\uA66F\uA674-\uA67D\uA67F-\uA6F1\uA717-\uA71F\uA722-\uA788\uA78B-\uA7AE\uA7B0-\uA7B7\uA7F7-\uA827\uA840-\uA873\uA880-\uA8C5\uA8D0-\uA8D9\uA8E0-\uA8F7\uA8FB\uA8FD\uA900-\uA92D\uA930-\uA953\uA960-\uA97C\uA980-\uA9C0\uA9CF-\uA9D9\uA9E0-\uA9FE\uAA00-\uAA36\uAA40-\uAA4D\uAA50-\uAA59\uAA60-\uAA76\uAA7A-\uAAC2\uAADB-\uAADD\uAAE0-\uAAEF\uAAF2-\uAAF6\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB65\uAB70-\uABEA\uABEC\uABED\uABF0-\uABF9\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE00-\uFE0F\uFE20-\uFE2F\uFE33\uFE34\uFE4D-\uFE4F\uFE70-\uFE74\uFE76-\uFEFC\uFF10-\uFF19\uFF21-\uFF3A\uFF3F\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC]|\uD800[\uDC00-\uDC0B\uDC0D-\uDC26\uDC28-\uDC3A\uDC3C\uDC3D\uDC3F-\uDC4D\uDC50-\uDC5D\uDC80-\uDCFA\uDD40-\uDD74\uDDFD\uDE80-\uDE9C\uDEA0-\uDED0\uDEE0\uDF00-\uDF1F\uDF2D-\uDF4A\uDF50-\uDF7A\uDF80-\uDF9D\uDFA0-\uDFC3\uDFC8-\uDFCF\uDFD1-\uDFD5]|\uD801[\uDC00-\uDC9D\uDCA0-\uDCA9\uDCB0-\uDCD3\uDCD8-\uDCFB\uDD00-\uDD27\uDD30-\uDD63\uDE00-\uDF36\uDF40-\uDF55\uDF60-\uDF67]|\uD802[\uDC00-\uDC05\uDC08\uDC0A-\uDC35\uDC37\uDC38\uDC3C\uDC3F-\uDC55\uDC60-\uDC76\uDC80-\uDC9E\uDCE0-\uDCF2\uDCF4\uDCF5\uDD00-\uDD15\uDD20-\uDD39\uDD80-\uDDB7\uDDBE\uDDBF\uDE00-\uDE03\uDE05\uDE06\uDE0C-\uDE13\uDE15-\uDE17\uDE19-\uDE33\uDE38-\uDE3A\uDE3F\uDE60-\uDE7C\uDE80-\uDE9C\uDEC0-\uDEC7\uDEC9-\uDEE6\uDF00-\uDF35\uDF40-\uDF55\uDF60-\uDF72\uDF80-\uDF91]|\uD803[\uDC00-\uDC48\uDC80-\uDCB2\uDCC0-\uDCF2]|\uD804[\uDC00-\uDC46\uDC66-\uDC6F\uDC7F-\uDCBA\uDCD0-\uDCE8\uDCF0-\uDCF9\uDD00-\uDD34\uDD36-\uDD3F\uDD50-\uDD73\uDD76\uDD80-\uDDC4\uDDCA-\uDDCC\uDDD0-\uDDDA\uDDDC\uDE00-\uDE11\uDE13-\uDE37\uDE3E\uDE80-\uDE86\uDE88\uDE8A-\uDE8D\uDE8F-\uDE9D\uDE9F-\uDEA8\uDEB0-\uDEEA\uDEF0-\uDEF9\uDF00-\uDF03\uDF05-\uDF0C\uDF0F\uDF10\uDF13-\uDF28\uDF2A-\uDF30\uDF32\uDF33\uDF35-\uDF39\uDF3C-\uDF44\uDF47\uDF48\uDF4B-\uDF4D\uDF50\uDF57\uDF5D-\uDF63\uDF66-\uDF6C\uDF70-\uDF74]|\uD805[\uDC00-\uDC4A\uDC50-\uDC59\uDC80-\uDCC5\uDCC7\uDCD0-\uDCD9\uDD80-\uDDB5\uDDB8-\uDDC0\uDDD8-\uDDDD\uDE00-\uDE40\uDE44\uDE50-\uDE59\uDE80-\uDEB7\uDEC0-\uDEC9\uDF00-\uDF19\uDF1D-\uDF2B\uDF30-\uDF39]|\uD806[\uDCA0-\uDCE9\uDCFF\uDE00-\uDE3E\uDE47\uDE50-\uDE83\uDE86-\uDE99\uDEC0-\uDEF8]|\uD807[\uDC00-\uDC08\uDC0A-\uDC36\uDC38-\uDC40\uDC50-\uDC59\uDC72-\uDC8F\uDC92-\uDCA7\uDCA9-\uDCB6\uDD00-\uDD06\uDD08\uDD09\uDD0B-\uDD36\uDD3A\uDD3C\uDD3D\uDD3F-\uDD47\uDD50-\uDD59]|\uD808[\uDC00-\uDF99]|\uD809[\uDC00-\uDC6E\uDC80-\uDD43]|[\uD80C\uD81C-\uD820\uD840-\uD868\uD86A-\uD86C\uD86F-\uD872\uD874-\uD879][\uDC00-\uDFFF]|\uD80D[\uDC00-\uDC2E]|\uD811[\uDC00-\uDE46]|\uD81A[\uDC00-\uDE38\uDE40-\uDE5E\uDE60-\uDE69\uDED0-\uDEED\uDEF0-\uDEF4\uDF00-\uDF36\uDF40-\uDF43\uDF50-\uDF59\uDF63-\uDF77\uDF7D-\uDF8F]|\uD81B[\uDF00-\uDF44\uDF50-\uDF7E\uDF8F-\uDF9F\uDFE0\uDFE1]|\uD821[\uDC00-\uDFEC]|\uD822[\uDC00-\uDEF2]|\uD82C[\uDC00-\uDD1E\uDD70-\uDEFB]|\uD82F[\uDC00-\uDC6A\uDC70-\uDC7C\uDC80-\uDC88\uDC90-\uDC99\uDC9D\uDC9E]|\uD834[\uDD65-\uDD69\uDD6D-\uDD72\uDD7B-\uDD82\uDD85-\uDD8B\uDDAA-\uDDAD\uDE42-\uDE44]|\uD835[\uDC00-\uDC54\uDC56-\uDC9C\uDC9E\uDC9F\uDCA2\uDCA5\uDCA6\uDCA9-\uDCAC\uDCAE-\uDCB9\uDCBB\uDCBD-\uDCC3\uDCC5-\uDD05\uDD07-\uDD0A\uDD0D-\uDD14\uDD16-\uDD1C\uDD1E-\uDD39\uDD3B-\uDD3E\uDD40-\uDD44\uDD46\uDD4A-\uDD50\uDD52-\uDEA5\uDEA8-\uDEC0\uDEC2-\uDEDA\uDEDC-\uDEFA\uDEFC-\uDF14\uDF16-\uDF34\uDF36-\uDF4E\uDF50-\uDF6E\uDF70-\uDF88\uDF8A-\uDFA8\uDFAA-\uDFC2\uDFC4-\uDFCB\uDFCE-\uDFFF]|\uD836[\uDE00-\uDE36\uDE3B-\uDE6C\uDE75\uDE84\uDE9B-\uDE9F\uDEA1-\uDEAF]|\uD838[\uDC00-\uDC06\uDC08-\uDC18\uDC1B-\uDC21\uDC23\uDC24\uDC26-\uDC2A]|\uD83A[\uDC00-\uDCC4\uDCD0-\uDCD6\uDD00-\uDD4A\uDD50-\uDD59]|\uD83B[\uDE00-\uDE03\uDE05-\uDE1F\uDE21\uDE22\uDE24\uDE27\uDE29-\uDE32\uDE34-\uDE37\uDE39\uDE3B\uDE42\uDE47\uDE49\uDE4B\uDE4D-\uDE4F\uDE51\uDE52\uDE54\uDE57\uDE59\uDE5B\uDE5D\uDE5F\uDE61\uDE62\uDE64\uDE67-\uDE6A\uDE6C-\uDE72\uDE74-\uDE77\uDE79-\uDE7C\uDE7E\uDE80-\uDE89\uDE8B-\uDE9B\uDEA1-\uDEA3\uDEA5-\uDEA9\uDEAB-\uDEBB]|\uD869[\uDC00-\uDED6\uDF00-\uDFFF]|\uD86D[\uDC00-\uDF34\uDF40-\uDFFF]|\uD86E[\uDC00-\uDC1D\uDC20-\uDFFF]|\uD873[\uDC00-\uDEA1\uDEB0-\uDFFF]|\uD87A[\uDC00-\uDFE0]|\uD87E[\uDC00-\uDE1D]|\uDB40[\uDD00-\uDDEF]/;
}
});
var require_util2 = __commonJS22({
"node_modules/json5/lib/util.js"(exports2, module22) {
var unicode = require_unicode();
module22.exports = {
isSpaceSeparator(c) {
return typeof c === "string" && unicode.Space_Separator.test(c);
},
isIdStartChar(c) {
return typeof c === "string" && (c >= "a" && c <= "z" || c >= "A" && c <= "Z" || c === "$" || c === "_" || unicode.ID_Start.test(c));
},
isIdContinueChar(c) {
return typeof c === "string" && (c >= "a" && c <= "z" || c >= "A" && c <= "Z" || c >= "0" && c <= "9" || c === "$" || c === "_" || c === "\u200C" || c === "\u200D" || unicode.ID_Continue.test(c));
},
isDigit(c) {
return typeof c === "string" && /[0-9]/.test(c);
},
isHexDigit(c) {
return typeof c === "string" && /[0-9A-Fa-f]/.test(c);
}
};
}
});
var require_parse32 = __commonJS22({
"node_modules/json5/lib/parse.js"(exports2, module22) {
var util = require_util2();
var source;
var parseState;
var stack;
var pos;
var line;
var column;
var token;
var key;
var root;
module22.exports = function parse2(text, reviver) {
source = String(text);
parseState = "start";
stack = [];
pos = 0;
line = 1;
column = 0;
token = void 0;
key = void 0;
root = void 0;
do {
token = lex();
parseStates[parseState]();
} while (token.type !== "eof");
if (typeof reviver === "function") {
return internalize({
"": root
}, "", reviver);
}
return root;
};
function internalize(holder, name, reviver) {
const value = holder[name];
if (value != null && typeof value === "object") {
if (Array.isArray(value)) {
for (let i = 0; i < value.length; i++) {
const key2 = String(i);
const replacement = internalize(value, key2, reviver);
if (replacement === void 0) {
delete value[key2];
} else {
Object.defineProperty(value, key2, {
value: replacement,
writable: true,
enumerable: true,
configurable: true
});
}
}
} else {
for (const key2 in value) {
const replacement = internalize(value, key2, reviver);
if (replacement === void 0) {
delete value[key2];
} else {
Object.defineProperty(value, key2, {
value: replacement,
writable: true,
enumerable: true,
configurable: true
});
}
}
}
}
return reviver.call(holder, name, value);
}
var lexState;
var buffer;
var doubleQuote;
var sign;
var c;
function lex() {
lexState = "default";
buffer = "";
doubleQuote = false;
sign = 1;
for (; ; ) {
c = peek();
const token2 = lexStates[lexState]();
if (token2) {
return token2;
}
}
}
function peek() {
if (source[pos]) {
return String.fromCodePoint(source.codePointAt(pos));
}
}
function read() {
const c2 = peek();
if (c2 === "\n") {
line++;
column = 0;
} else if (c2) {
column += c2.length;
} else {
column++;
}
if (c2) {
pos += c2.length;
}
return c2;
}
var lexStates = {
default() {
switch (c) {
case " ":
case "\v":
case "\f":
case " ":
case "\xA0":
case "\uFEFF":
case "\n":
case "\r":
case "\u2028":
case "\u2029":
read();
return;
case "/":
read();
lexState = "comment";
return;
case void 0:
read();
return newToken("eof");
}
if (util.isSpaceSeparator(c)) {
read();
return;
}
return lexStates[parseState]();
},
comment() {
switch (c) {
case "*":
read();
lexState = "multiLineComment";
return;
case "/":
read();
lexState = "singleLineComment";
return;
}
throw invalidChar(read());
},
multiLineComment() {
switch (c) {
case "*":
read();
lexState = "multiLineCommentAsterisk";
return;
case void 0:
throw invalidChar(read());
}
read();
},
multiLineCommentAsterisk() {
switch (c) {
case "*":
read();
return;
case "/":
read();
lexState = "default";
return;
case void 0:
throw invalidChar(read());
}
read();
lexState = "multiLineComment";
},
singleLineComment() {
switch (c) {
case "\n":
case "\r":
case "\u2028":
case "\u2029":
read();
lexState = "default";
return;
case void 0:
read();
return newToken("eof");
}
read();
},
value() {
switch (c) {
case "{":
case "[":
return newToken("punctuator", read());
case "n":
read();
literal("ull");
return newToken("null", null);
case "t":
read();
literal("rue");
return newToken("boolean", true);
case "f":
read();
literal("alse");
return newToken("boolean", false);
case "-":
case "+":
if (read() === "-") {
sign = -1;
}
lexState = "sign";
return;
case ".":
buffer = read();
lexState = "decimalPointLeading";
return;
case "0":
buffer = read();
lexState = "zero";
return;
case "1":
case "2":
case "3":
case "4":
case "5":
case "6":
case "7":
case "8":
case "9":
buffer = read();
lexState = "decimalInteger";
return;
case "I":
read();
literal("nfinity");
return newToken("numeric", Infinity);
case "N":
read();
literal("aN");
return newToken("numeric", NaN);
case '"':
case "'":
doubleQuote = read() === '"';
buffer = "";
lexState = "string";
return;
}
throw invalidChar(read());
},
identifierNameStartEscape() {
if (c !== "u") {
throw invalidChar(read());
}
read();
const u = unicodeEscape();
switch (u) {
case "$":
case "_":
break;
default:
if (!util.isIdStartChar(u)) {
throw invalidIdentifier();
}
break;
}
buffer += u;
lexState = "identifierName";
},
identifierName() {
switch (c) {
case "$":
case "_":
case "\u200C":
case "\u200D":
buffer += read();
return;
case "\\":
read();
lexState = "identifierNameEscape";
return;
}
if (util.isIdContinueChar(c)) {
buffer += read();
return;
}
return newToken("identifier", buffer);
},
identifierNameEscape() {
if (c !== "u") {
throw invalidChar(read());
}
read();
const u = unicodeEscape();
switch (u) {
case "$":
case "_":
case "\u200C":
case "\u200D":
break;
default:
if (!util.isIdContinueChar(u)) {
throw invalidIdentifier();
}
break;
}
buffer += u;
lexState = "identifierName";
},
sign() {
switch (c) {
case ".":
buffer = read();
lexState = "decimalPointLeading";
return;
case "0":
buffer = read();
lexState = "zero";
return;
case "1":
case "2":
case "3":
case "4":
case "5":
case "6":
case "7":
case "8":
case "9":
buffer = read();
lexState = "decimalInteger";
return;
case "I":
read();
literal("nfinity");
return newToken("numeric", sign * Infinity);
case "N":
read();
literal("aN");
return newToken("numeric", NaN);
}
throw invalidChar(read());
},
zero() {
switch (c) {
case ".":
buffer += read();
lexState = "decimalPoint";
return;
case "e":
case "E":
buffer += read();
lexState = "decimalExponent";
return;
case "x":
case "X":
buffer += read();
lexState = "hexadecimal";
return;
}
return newToken("numeric", sign * 0);
},
decimalInteger() {
switch (c) {
case ".":
buffer += read();
lexState = "decimalPoint";
return;
case "e":
case "E":
buffer += read();
lexState = "decimalExponent";
return;
}
if (util.isDigit(c)) {
buffer += read();
return;
}
return newToken("numeric", sign * Number(buffer));
},
decimalPointLeading() {
if (util.isDigit(c)) {
buffer += read();
lexState = "decimalFraction";
return;
}
throw invalidChar(read());
},
decimalPoint() {
switch (c) {
case "e":
case "E":
buffer += read();
lexState = "decimalExponent";
return;
}
if (util.isDigit(c)) {
buffer += read();
lexState = "decimalFraction";
return;
}
return newToken("numeric", sign * Number(buffer));
},
decimalFraction() {
switch (c) {
case "e":
case "E":
buffer += read();
lexState = "decimalExponent";
return;
}
if (util.isDigit(c)) {
buffer += read();
return;
}
return newToken("numeric", sign * Number(buffer));
},
decimalExponent() {
switch (c) {
case "+":
case "-":
buffer += read();
lexState = "decimalExponentSign";
return;
}
if (util.isDigit(c)) {
buffer += read();
lexState = "decimalExponentInteger";
return;
}
throw invalidChar(read());
},
decimalExponentSign() {
if (util.isDigit(c)) {
buffer += read();
lexState = "decimalExponentInteger";
return;
}
throw invalidChar(read());
},
decimalExponentInteger() {
if (util.isDigit(c)) {
buffer += read();
return;
}
return newToken("numeric", sign * Number(buffer));
},
hexadecimal() {
if (util.isHexDigit(c)) {
buffer += read();
lexState = "hexadecimalInteger";
return;
}
throw invalidChar(read());
},
hexadecimalInteger() {
if (util.isHexDigit(c)) {
buffer += read();
return;
}
return newToken("numeric", sign * Number(buffer));
},
string() {
switch (c) {
case "\\":
read();
buffer += escape();
return;
case '"':
if (doubleQuote) {
read();
return newToken("string", buffer);
}
buffer += read();
return;
case "'":
if (!doubleQuote) {
read();
return newToken("string", buffer);
}
buffer += read();
return;
case "\n":
case "\r":
throw invalidChar(read());
case "\u2028":
case "\u2029":
separatorChar(c);
break;
case void 0:
throw invalidChar(read());
}
buffer += read();
},
start() {
switch (c) {
case "{":
case "[":
return newToken("punctuator", read());
}
lexState = "value";
},
beforePropertyName() {
switch (c) {
case "$":
case "_":
buffer = read();
lexState = "identifierName";
return;
case "\\":
read();
lexState = "identifierNameStartEscape";
return;
case "}":
return newToken("punctuator", read());
case '"':
case "'":
doubleQuote = read() === '"';
lexState = "string";
return;
}
if (util.isIdStartChar(c)) {
buffer += read();
lexState = "identifierName";
return;
}
throw invalidChar(read());
},
afterPropertyName() {
if (c === ":") {
return newToken("punctuator", read());
}
throw invalidChar(read());
},
beforePropertyValue() {
lexState = "value";
},
afterPropertyValue() {
switch (c) {
case ",":
case "}":
return newToken("punctuator", read());
}
throw invalidChar(read());
},
beforeArrayValue() {
if (c === "]") {
return newToken("punctuator", read());
}
lexState = "value";
},
afterArrayValue() {
switch (c) {
case ",":
case "]":
return newToken("punctuator", read());
}
throw invalidChar(read());
},
end() {
throw invalidChar(read());
}
};
function newToken(type, value) {
return {
type,
value,
line,
column
};
}
function literal(s) {
for (const c2 of s) {
const p = peek();
if (p !== c2) {
throw invalidChar(read());
}
read();
}
}
function escape() {
const c2 = peek();
switch (c2) {
case "b":
read();
return "\b";
case "f":
read();
return "\f";
case "n":
read();
return "\n";
case "r":
read();
return "\r";
case "t":
read();
return " ";
case "v":
read();
return "\v";
case "0":
read();
if (util.isDigit(peek())) {
throw invalidChar(read());
}
return "\0";
case "x":
read();
return hexEscape();
case "u":
read();
return unicodeEscape();
case "\n":
case "\u2028":
case "\u2029":
read();
return "";
case "\r":
read();
if (peek() === "\n") {
read();
}
return "";
case "1":
case "2":
case "3":
case "4":
case "5":
case "6":
case "7":
case "8":
case "9":
throw invalidChar(read());
case void 0:
throw invalidChar(read());
}
return read();
}
function hexEscape() {
let buffer2 = "";
let c2 = peek();
if (!util.isHexDigit(c2)) {
throw invalidChar(read());
}
buffer2 += read();
c2 = peek();
if (!util.isHexDigit(c2)) {
throw invalidChar(read());
}
buffer2 += read();
return String.fromCodePoint(parseInt(buffer2, 16));
}
function unicodeEscape() {
let buffer2 = "";
let count = 4;
while (count-- > 0) {
const c2 = peek();
if (!util.isHexDigit(c2)) {
throw invalidChar(read());
}
buffer2 += read();
}
return String.fromCodePoint(parseInt(buffer2, 16));
}
var parseStates = {
start() {
if (token.type === "eof") {
throw invalidEOF();
}
push();
},
beforePropertyName() {
switch (token.type) {
case "identifier":
case "string":
key = token.value;
parseState = "afterPropertyName";
return;
case "punctuator":
pop();
return;
case "eof":
throw invalidEOF();
}
},
afterPropertyName() {
if (token.type === "eof") {
throw invalidEOF();
}
parseState = "beforePropertyValue";
},
beforePropertyValue() {
if (token.type === "eof") {
throw invalidEOF();
}
push();
},
beforeArrayValue() {
if (token.type === "eof") {
throw invalidEOF();
}
if (token.type === "punctuator" && token.value === "]") {
pop();
return;
}
push();
},
afterPropertyValue() {
if (token.type === "eof") {
throw invalidEOF();
}
switch (token.value) {
case ",":
parseState = "beforePropertyName";
return;
case "}":
pop();
}
},
afterArrayValue() {
if (token.type === "eof") {
throw invalidEOF();
}
switch (token.value) {
case ",":
parseState = "beforeArrayValue";
return;
case "]":
pop();
}
},
end() {
}
};
function push() {
let value;
switch (token.type) {
case "punctuator":
switch (token.value) {
case "{":
value = {};
break;
case "[":
value = [];
break;
}
break;
case "null":
case "boolean":
case "numeric":
case "string":
value = token.value;
break;
}
if (root === void 0) {
root = value;
} else {
const parent = stack[stack.length - 1];
if (Array.isArray(parent)) {
parent.push(value);
} else {
Object.defineProperty(parent, key, {
value,
writable: true,
enumerable: true,
configurable: true
});
}
}
if (value !== null && typeof value === "object") {
stack.push(value);
if (Array.isArray(value)) {
parseState = "beforeArrayValue";
} else {
parseState = "beforePropertyName";
}
} else {
const current = stack[stack.length - 1];
if (current == null) {
parseState = "end";
} else if (Array.isArray(current)) {
parseState = "afterArrayValue";
} else {
parseState = "afterPropertyValue";
}
}
}
function pop() {
stack.pop();
const current = stack[stack.length - 1];
if (current == null) {
parseState = "end";
} else if (Array.isArray(current)) {
parseState = "afterArrayValue";
} else {
parseState = "afterPropertyValue";
}
}
function invalidChar(c2) {
if (c2 === void 0) {
return syntaxError(`JSON5: invalid end of input at ${line}:${column}`);
}
return syntaxError(`JSON5: invalid character '${formatChar(c2)}' at ${line}:${column}`);
}
function invalidEOF() {
return syntaxError(`JSON5: invalid end of input at ${line}:${column}`);
}
function invalidIdentifier() {
column -= 5;
return syntaxError(`JSON5: invalid identifier character at ${line}:${column}`);
}
function separatorChar(c2) {
console.warn(`JSON5: '${formatChar(c2)}' in strings is not valid ECMAScript; consider escaping`);
}
function formatChar(c2) {
const replacements = {
"'": "\\'",
'"': '\\"',
"\\": "\\\\",
"\b": "\\b",
"\f": "\\f",
"\n": "\\n",
"\r": "\\r",
" ": "\\t",
"\v": "\\v",
"\0": "\\0",
"\u2028": "\\u2028",
"\u2029": "\\u2029"
};
if (replacements[c2]) {
return replacements[c2];
}
if (c2 < " ") {
const hexString = c2.charCodeAt(0).toString(16);
return "\\x" + ("00" + hexString).substring(hexString.length);
}
return c2;
}
function syntaxError(message) {
const err = new SyntaxError(message);
err.lineNumber = line;
err.columnNumber = column;
return err;
}
}
});
var require_stringify22 = __commonJS22({
"node_modules/json5/lib/stringify.js"(exports2, module22) {
var util = require_util2();
module22.exports = function stringify(value, replacer, space) {
const stack = [];
let indent = "";
let propertyList;
let replacerFunc;
let gap = "";
let quote2;
if (replacer != null && typeof replacer === "object" && !Array.isArray(replacer)) {
space = replacer.space;
quote2 = replacer.quote;
replacer = replacer.replacer;
}
if (typeof replacer === "function") {
replacerFunc = replacer;
} else if (Array.isArray(replacer)) {
propertyList = [];
for (const v of replacer) {
let item;
if (typeof v === "string") {
item = v;
} else if (typeof v === "number" || v instanceof String || v instanceof Number) {
item = String(v);
}
if (item !== void 0 && propertyList.indexOf(item) < 0) {
propertyList.push(item);
}
}
}
if (space instanceof Number) {
space = Number(space);
} else if (space instanceof String) {
space = String(space);
}
if (typeof space === "number") {
if (space > 0) {
space = Math.min(10, Math.floor(space));
gap = " ".substr(0, space);
}
} else if (typeof space === "string") {
gap = space.substr(0, 10);
}
return serializeProperty("", {
"": value
});
function serializeProperty(key, holder) {
let value2 = holder[key];
if (value2 != null) {
if (typeof value2.toJSON5 === "function") {
value2 = value2.toJSON5(key);
} else if (typeof value2.toJSON === "function") {
value2 = value2.toJSON(key);
}
}
if (replacerFunc) {
value2 = replacerFunc.call(holder, key, value2);
}
if (value2 instanceof Number) {
value2 = Number(value2);
} else if (value2 instanceof String) {
value2 = String(value2);
} else if (value2 instanceof Boolean) {
value2 = value2.valueOf();
}
switch (value2) {
case null:
return "null";
case true:
return "true";
case false:
return "false";
}
if (typeof value2 === "string") {
return quoteString(value2, false);
}
if (typeof value2 === "number") {
return String(value2);
}
if (typeof value2 === "object") {
return Array.isArray(value2) ? serializeArray(value2) : serializeObject(value2);
}
return void 0;
}
function quoteString(value2) {
const quotes = {
"'": 0.1,
'"': 0.2
};
const replacements = {
"'": "\\'",
'"': '\\"',
"\\": "\\\\",
"\b": "\\b",
"\f": "\\f",
"\n": "\\n",
"\r": "\\r",
" ": "\\t",
"\v": "\\v",
"\0": "\\0",
"\u2028": "\\u2028",
"\u2029": "\\u2029"
};
let product = "";
for (let i = 0; i < value2.length; i++) {
const c = value2[i];
switch (c) {
case "'":
case '"':
quotes[c]++;
product += c;
continue;
case "\0":
if (util.isDigit(value2[i + 1])) {
product += "\\x00";
continue;
}
}
if (replacements[c]) {
product += replacements[c];
continue;
}
if (c < " ") {
let hexString = c.charCodeAt(0).toString(16);
product += "\\x" + ("00" + hexString).substring(hexString.length);
continue;
}
product += c;
}
const quoteChar = quote2 || Object.keys(quotes).reduce((a, b) => quotes[a] < quotes[b] ? a : b);
product = product.replace(new RegExp(quoteChar, "g"), replacements[quoteChar]);
return quoteChar + product + quoteChar;
}
function serializeObject(value2) {
if (stack.indexOf(value2) >= 0) {
throw TypeError("Converting circular structure to JSON5");
}
stack.push(value2);
let stepback = indent;
indent = indent + gap;
let keys = propertyList || Object.keys(value2);
let partial = [];
for (const key of keys) {
const propertyString = serializeProperty(key, value2);
if (propertyString !== void 0) {
let member = serializeKey(key) + ":";
if (gap !== "") {
member += " ";
}
member += propertyString;
partial.push(member);
}
}
let final;
if (partial.length === 0) {
final = "{}";
} else {
let properties;
if (gap === "") {
properties = partial.join(",");
final = "{" + properties + "}";
} else {
let separator = ",\n" + indent;
properties = partial.join(separator);
final = "{\n" + indent + properties + ",\n" + stepback + "}";
}
}
stack.pop();
indent = stepback;
return final;
}
function serializeKey(key) {
if (key.length === 0) {
return quoteString(key, true);
}
const firstChar = String.fromCodePoint(key.codePointAt(0));
if (!util.isIdStartChar(firstChar)) {
return quoteString(key, true);
}
for (let i = firstChar.length; i < key.length; i++) {
if (!util.isIdContinueChar(String.fromCodePoint(key.codePointAt(i)))) {
return quoteString(key, true);
}
}
return key;
}
function serializeArray(value2) {
if (stack.indexOf(value2) >= 0) {
throw TypeError("Converting circular structure to JSON5");
}
stack.push(value2);
let stepback = indent;
indent = indent + gap;
let partial = [];
for (let i = 0; i < value2.length; i++) {
const propertyString = serializeProperty(String(i), value2);
partial.push(propertyString !== void 0 ? propertyString : "null");
}
let final;
if (partial.length === 0) {
final = "[]";
} else {
if (gap === "") {
let properties = partial.join(",");
final = "[" + properties + "]";
} else {
let separator = ",\n" + indent;
let properties = partial.join(separator);
final = "[\n" + indent + properties + ",\n" + stepback + "]";
}
}
stack.pop();
indent = stepback;
return final;
}
};
}
});
var require_lib62 = __commonJS22({
"node_modules/json5/lib/index.js"(exports2, module22) {
var parse2 = require_parse32();
var stringify = require_stringify22();
var JSON5 = {
parse: parse2,
stringify
};
module22.exports = JSON5;
}
});
var require_load_json5 = __commonJS22({
"src/utils/load-json5.js"(exports2, module22) {
"use strict";
var {
parse: parse2
} = require_lib62();
module22.exports = function(filePath2, content) {
try {
return parse2(content);
} catch (error) {
error.message = `JSON5 Error in ${filePath2}:
${error.message}`;
throw error;
}
};
}
});
var require_partition = __commonJS22({
"src/utils/partition.js"(exports2, module22) {
"use strict";
function partition(array, predicate) {
const result = [[], []];
for (const value of array) {
result[predicate(value) ? 0 : 1].push(value);
}
return result;
}
module22.exports = partition;
}
});
var require_homedir = __commonJS22({
"node_modules/resolve/lib/homedir.js"(exports2, module22) {
"use strict";
var os = require("os");
module22.exports = os.homedir || function homedir() {
var home = process.env.HOME;
var user = process.env.LOGNAME || process.env.USER || process.env.LNAME || process.env.USERNAME;
if (process.platform === "win32") {
return process.env.USERPROFILE || process.env.HOMEDRIVE + process.env.HOMEPATH || home || null;
}
if (process.platform === "darwin") {
return home || (user ? "/Users/" + user : null);
}
if (process.platform === "linux") {
return home || (process.getuid() === 0 ? "/root" : user ? "/home/" + user : null);
}
return home || null;
};
}
});
var require_caller = __commonJS22({
"node_modules/resolve/lib/caller.js"(exports2, module22) {
module22.exports = function() {
var origPrepareStackTrace = Error.prepareStackTrace;
Error.prepareStackTrace = function(_, stack2) {
return stack2;
};
var stack = new Error().stack;
Error.prepareStackTrace = origPrepareStackTrace;
return stack[2].getFileName();
};
}
});
var require_path_parse = __commonJS22({
"node_modules/path-parse/index.js"(exports2, module22) {
"use strict";
var isWindows = process.platform === "win32";
var splitWindowsRe = /^(((?:[a-zA-Z]:|[\\\/]{2}[^\\\/]+[\\\/]+[^\\\/]+)?[\\\/]?)(?:[^\\\/]*[\\\/])*)((\.{1,2}|[^\\\/]+?|)(\.[^.\/\\]*|))[\\\/]*$/;
var win32 = {};
function win32SplitPath(filename) {
return splitWindowsRe.exec(filename).slice(1);
}
win32.parse = function(pathString) {
if (typeof pathString !== "string") {
throw new TypeError("Parameter 'pathString' must be a string, not " + typeof pathString);
}
var allParts = win32SplitPath(pathString);
if (!allParts || allParts.length !== 5) {
throw new TypeError("Invalid path '" + pathString + "'");
}
return {
root: allParts[1],
dir: allParts[0] === allParts[1] ? allParts[0] : allParts[0].slice(0, -1),
base: allParts[2],
ext: allParts[4],
name: allParts[3]
};
};
var splitPathRe = /^((\/?)(?:[^\/]*\/)*)((\.{1,2}|[^\/]+?|)(\.[^.\/]*|))[\/]*$/;
var posix = {};
function posixSplitPath(filename) {
return splitPathRe.exec(filename).slice(1);
}
posix.parse = function(pathString) {
if (typeof pathString !== "string") {
throw new TypeError("Parameter 'pathString' must be a string, not " + typeof pathString);
}
var allParts = posixSplitPath(pathString);
if (!allParts || allParts.length !== 5) {
throw new TypeError("Invalid path '" + pathString + "'");
}
return {
root: allParts[1],
dir: allParts[0].slice(0, -1),
base: allParts[2],
ext: allParts[4],
name: allParts[3]
};
};
if (isWindows)
module22.exports = win32.parse;
else
module22.exports = posix.parse;
module22.exports.posix = posix.parse;
module22.exports.win32 = win32.parse;
}
});
var require_node_modules_paths = __commonJS22({
"node_modules/resolve/lib/node-modules-paths.js"(exports2, module22) {
var path = require("path");
var parse2 = path.parse || require_path_parse();
var getNodeModulesDirs = function getNodeModulesDirs2(absoluteStart, modules) {
var prefix = "/";
if (/^([A-Za-z]:)/.test(absoluteStart)) {
prefix = "";
} else if (/^\\\\/.test(absoluteStart)) {
prefix = "\\\\";
}
var paths = [absoluteStart];
var parsed = parse2(absoluteStart);
while (parsed.dir !== paths[paths.length - 1]) {
paths.push(parsed.dir);
parsed = parse2(parsed.dir);
}
return paths.reduce(function(dirs, aPath) {
return dirs.concat(modules.map(function(moduleDir) {
return path.resolve(prefix, aPath, moduleDir);
}));
}, []);
};
module22.exports = function nodeModulesPaths(start, opts, request) {
var modules = opts && opts.moduleDirectory ? [].concat(opts.moduleDirectory) : ["node_modules"];
if (opts && typeof opts.paths === "function") {
return opts.paths(request, start, function() {
return getNodeModulesDirs(start, modules);
}, opts);
}
var dirs = getNodeModulesDirs(start, modules);
return opts && opts.paths ? dirs.concat(opts.paths) : dirs;
};
}
});
var require_normalize_options = __commonJS22({
"node_modules/resolve/lib/normalize-options.js"(exports2, module22) {
module22.exports = function(x, opts) {
return opts || {};
};
}
});
var require_implementation = __commonJS22({
"node_modules/function-bind/implementation.js"(exports2, module22) {
"use strict";
var ERROR_MESSAGE = "Function.prototype.bind called on incompatible ";
var slice = Array.prototype.slice;
var toStr = Object.prototype.toString;
var funcType = "[object Function]";
module22.exports = function bind(that) {
var target = this;
if (typeof target !== "function" || toStr.call(target) !== funcType) {
throw new TypeError(ERROR_MESSAGE + target);
}
var args = slice.call(arguments, 1);
var bound;
var binder = function() {
if (this instanceof bound) {
var result = target.apply(this, args.concat(slice.call(arguments)));
if (Object(result) === result) {
return result;
}
return this;
} else {
return target.apply(that, args.concat(slice.call(arguments)));
}
};
var boundLength = Math.max(0, target.length - args.length);
var boundArgs = [];
for (var i = 0; i < boundLength; i++) {
boundArgs.push("$" + i);
}
bound = Function("binder", "return function (" + boundArgs.join(",") + "){ return binder.apply(this,arguments); }")(binder);
if (target.prototype) {
var Empty = function Empty2() {
};
Empty.prototype = target.prototype;
bound.prototype = new Empty();
Empty.prototype = null;
}
return bound;
};
}
});
var require_function_bind = __commonJS22({
"node_modules/function-bind/index.js"(exports2, module22) {
"use strict";
var implementation = require_implementation();
module22.exports = Function.prototype.bind || implementation;
}
});
var require_src = __commonJS22({
"node_modules/has/src/index.js"(exports2, module22) {
"use strict";
var bind = require_function_bind();
module22.exports = bind.call(Function.call, Object.prototype.hasOwnProperty);
}
});
var require_core2 = __commonJS22({
"node_modules/is-core-module/core.json"(exports2, module22) {
module22.exports = {
assert: true,
"node:assert": [">= 14.18 && < 15", ">= 16"],
"assert/strict": ">= 15",
"node:assert/strict": ">= 16",
async_hooks: ">= 8",
"node:async_hooks": [">= 14.18 && < 15", ">= 16"],
buffer_ieee754: ">= 0.5 && < 0.9.7",
buffer: true,
"node:buffer": [">= 14.18 && < 15", ">= 16"],
child_process: true,
"node:child_process": [">= 14.18 && < 15", ">= 16"],
cluster: ">= 0.5",
"node:cluster": [">= 14.18 && < 15", ">= 16"],
console: true,
"node:console": [">= 14.18 && < 15", ">= 16"],
constants: true,
"node:constants": [">= 14.18 && < 15", ">= 16"],
crypto: true,
"node:crypto": [">= 14.18 && < 15", ">= 16"],
_debug_agent: ">= 1 && < 8",
_debugger: "< 8",
dgram: true,
"node:dgram": [">= 14.18 && < 15", ">= 16"],
diagnostics_channel: [">= 14.17 && < 15", ">= 15.1"],
"node:diagnostics_channel": [">= 14.18 && < 15", ">= 16"],
dns: true,
"node:dns": [">= 14.18 && < 15", ">= 16"],
"dns/promises": ">= 15",
"node:dns/promises": ">= 16",
domain: ">= 0.7.12",
"node:domain": [">= 14.18 && < 15", ">= 16"],
events: true,
"node:events": [">= 14.18 && < 15", ">= 16"],
freelist: "< 6",
fs: true,
"node:fs": [">= 14.18 && < 15", ">= 16"],
"fs/promises": [">= 10 && < 10.1", ">= 14"],
"node:fs/promises": [">= 14.18 && < 15", ">= 16"],
_http_agent: ">= 0.11.1",
"node:_http_agent": [">= 14.18 && < 15", ">= 16"],
_http_client: ">= 0.11.1",
"node:_http_client": [">= 14.18 && < 15", ">= 16"],
_http_common: ">= 0.11.1",
"node:_http_common": [">= 14.18 && < 15", ">= 16"],
_http_incoming: ">= 0.11.1",
"node:_http_incoming": [">= 14.18 && < 15", ">= 16"],
_http_outgoing: ">= 0.11.1",
"node:_http_outgoing": [">= 14.18 && < 15", ">= 16"],
_http_server: ">= 0.11.1",
"node:_http_server": [">= 14.18 && < 15", ">= 16"],
http: true,
"node:http": [">= 14.18 && < 15", ">= 16"],
http2: ">= 8.8",
"node:http2": [">= 14.18 && < 15", ">= 16"],
https: true,
"node:https": [">= 14.18 && < 15", ">= 16"],
inspector: ">= 8",
"node:inspector": [">= 14.18 && < 15", ">= 16"],
"inspector/promises": [">= 19"],
"node:inspector/promises": [">= 19"],
_linklist: "< 8",
module: true,
"node:module": [">= 14.18 && < 15", ">= 16"],
net: true,
"node:net": [">= 14.18 && < 15", ">= 16"],
"node-inspect/lib/_inspect": ">= 7.6 && < 12",
"node-inspect/lib/internal/inspect_client": ">= 7.6 && < 12",
"node-inspect/lib/internal/inspect_repl": ">= 7.6 && < 12",
os: true,
"node:os": [">= 14.18 && < 15", ">= 16"],
path: true,
"node:path": [">= 14.18 && < 15", ">= 16"],
"path/posix": ">= 15.3",
"node:path/posix": ">= 16",
"path/win32": ">= 15.3",
"node:path/win32": ">= 16",
perf_hooks: ">= 8.5",
"node:perf_hooks": [">= 14.18 && < 15", ">= 16"],
process: ">= 1",
"node:process": [">= 14.18 && < 15", ">= 16"],
punycode: ">= 0.5",
"node:punycode": [">= 14.18 && < 15", ">= 16"],
querystring: true,
"node:querystring": [">= 14.18 && < 15", ">= 16"],
readline: true,
"node:readline": [">= 14.18 && < 15", ">= 16"],
"readline/promises": ">= 17",
"node:readline/promises": ">= 17",
repl: true,
"node:repl": [">= 14.18 && < 15", ">= 16"],
smalloc: ">= 0.11.5 && < 3",
_stream_duplex: ">= 0.9.4",
"node:_stream_duplex": [">= 14.18 && < 15", ">= 16"],
_stream_transform: ">= 0.9.4",
"node:_stream_transform": [">= 14.18 && < 15", ">= 16"],
_stream_wrap: ">= 1.4.1",
"node:_stream_wrap": [">= 14.18 && < 15", ">= 16"],
_stream_passthrough: ">= 0.9.4",
"node:_stream_passthrough": [">= 14.18 && < 15", ">= 16"],
_stream_readable: ">= 0.9.4",
"node:_stream_readable": [">= 14.18 && < 15", ">= 16"],
_stream_writable: ">= 0.9.4",
"node:_stream_writable": [">= 14.18 && < 15", ">= 16"],
stream: true,
"node:stream": [">= 14.18 && < 15", ">= 16"],
"stream/consumers": ">= 16.7",
"node:stream/consumers": ">= 16.7",
"stream/promises": ">= 15",
"node:stream/promises": ">= 16",
"stream/web": ">= 16.5",
"node:stream/web": ">= 16.5",
string_decoder: true,
"node:string_decoder": [">= 14.18 && < 15", ">= 16"],
sys: [">= 0.4 && < 0.7", ">= 0.8"],
"node:sys": [">= 14.18 && < 15", ">= 16"],
"node:test": [">= 16.17 && < 17", ">= 18"],
timers: true,
"node:timers": [">= 14.18 && < 15", ">= 16"],
"timers/promises": ">= 15",
"node:timers/promises": ">= 16",
_tls_common: ">= 0.11.13",
"node:_tls_common": [">= 14.18 && < 15", ">= 16"],
_tls_legacy: ">= 0.11.3 && < 10",
_tls_wrap: ">= 0.11.3",
"node:_tls_wrap": [">= 14.18 && < 15", ">= 16"],
tls: true,
"node:tls": [">= 14.18 && < 15", ">= 16"],
trace_events: ">= 10",
"node:trace_events": [">= 14.18 && < 15", ">= 16"],
tty: true,
"node:tty": [">= 14.18 && < 15", ">= 16"],
url: true,
"node:url": [">= 14.18 && < 15", ">= 16"],
util: true,
"node:util": [">= 14.18 && < 15", ">= 16"],
"util/types": ">= 15.3",
"node:util/types": ">= 16",
"v8/tools/arguments": ">= 10 && < 12",
"v8/tools/codemap": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/consarray": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/csvparser": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/logreader": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/profile_view": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/splaytree": [">= 4.4 && < 5", ">= 5.2 && < 12"],
v8: ">= 1",
"node:v8": [">= 14.18 && < 15", ">= 16"],
vm: true,
"node:vm": [">= 14.18 && < 15", ">= 16"],
wasi: ">= 13.4 && < 13.5",
worker_threads: ">= 11.7",
"node:worker_threads": [">= 14.18 && < 15", ">= 16"],
zlib: ">= 0.5",
"node:zlib": [">= 14.18 && < 15", ">= 16"]
};
}
});
var require_is_core_module = __commonJS22({
"node_modules/is-core-module/index.js"(exports2, module22) {
"use strict";
var has = require_src();
function specifierIncluded(current, specifier) {
var nodeParts = current.split(".");
var parts = specifier.split(" ");
var op = parts.length > 1 ? parts[0] : "=";
var versionParts = (parts.length > 1 ? parts[1] : parts[0]).split(".");
for (var i = 0; i < 3; ++i) {
var cur = parseInt(nodeParts[i] || 0, 10);
var ver = parseInt(versionParts[i] || 0, 10);
if (cur === ver) {
continue;
}
if (op === "<") {
return cur < ver;
}
if (op === ">=") {
return cur >= ver;
}
return false;
}
return op === ">=";
}
function matchesRange(current, range) {
var specifiers = range.split(/ ?&& ?/);
if (specifiers.length === 0) {
return false;
}
for (var i = 0; i < specifiers.length; ++i) {
if (!specifierIncluded(current, specifiers[i])) {
return false;
}
}
return true;
}
function versionIncluded(nodeVersion, specifierValue) {
if (typeof specifierValue === "boolean") {
return specifierValue;
}
var current = typeof nodeVersion === "undefined" ? process.versions && process.versions.node : nodeVersion;
if (typeof current !== "string") {
throw new TypeError(typeof nodeVersion === "undefined" ? "Unable to determine current node version" : "If provided, a valid node version is required");
}
if (specifierValue && typeof specifierValue === "object") {
for (var i = 0; i < specifierValue.length; ++i) {
if (matchesRange(current, specifierValue[i])) {
return true;
}
}
return false;
}
return matchesRange(current, specifierValue);
}
var data = require_core2();
module22.exports = function isCore(x, nodeVersion) {
return has(data, x) && versionIncluded(nodeVersion, data[x]);
};
}
});
var require_async = __commonJS22({
"node_modules/resolve/lib/async.js"(exports2, module22) {
var fs2 = require("fs");
var getHomedir = require_homedir();
var path = require("path");
var caller = require_caller();
var nodeModulesPaths = require_node_modules_paths();
var normalizeOptions = require_normalize_options();
var isCore = require_is_core_module();
var realpathFS = process.platform !== "win32" && fs2.realpath && typeof fs2.realpath.native === "function" ? fs2.realpath.native : fs2.realpath;
var homedir = getHomedir();
var defaultPaths = function() {
return [path.join(homedir, ".node_modules"), path.join(homedir, ".node_libraries")];
};
var defaultIsFile = function isFile(file, cb) {
fs2.stat(file, function(err, stat2) {
if (!err) {
return cb(null, stat2.isFile() || stat2.isFIFO());
}
if (err.code === "ENOENT" || err.code === "ENOTDIR")
return cb(null, false);
return cb(err);
});
};
var defaultIsDir = function isDirectory(dir, cb) {
fs2.stat(dir, function(err, stat2) {
if (!err) {
return cb(null, stat2.isDirectory());
}
if (err.code === "ENOENT" || err.code === "ENOTDIR")
return cb(null, false);
return cb(err);
});
};
var defaultRealpath = function realpath(x, cb) {
realpathFS(x, function(realpathErr, realPath) {
if (realpathErr && realpathErr.code !== "ENOENT")
cb(realpathErr);
else
cb(null, realpathErr ? x : realPath);
});
};
var maybeRealpath = function maybeRealpath2(realpath, x, opts, cb) {
if (opts && opts.preserveSymlinks === false) {
realpath(x, cb);
} else {
cb(null, x);
}
};
var defaultReadPackage = function defaultReadPackage2(readFile, pkgfile, cb) {
readFile(pkgfile, function(readFileErr, body) {
if (readFileErr)
cb(readFileErr);
else {
try {
var pkg = JSON.parse(body);
cb(null, pkg);
} catch (jsonErr) {
cb(null);
}
}
});
};
var getPackageCandidates = function getPackageCandidates2(x, start, opts) {
var dirs = nodeModulesPaths(start, opts, x);
for (var i = 0; i < dirs.length; i++) {
dirs[i] = path.join(dirs[i], x);
}
return dirs;
};
module22.exports = function resolve(x, options, callback) {
var cb = callback;
var opts = options;
if (typeof options === "function") {
cb = opts;
opts = {};
}
if (typeof x !== "string") {
var err = new TypeError("Path must be a string.");
return process.nextTick(function() {
cb(err);
});
}
opts = normalizeOptions(x, opts);
var isFile = opts.isFile || defaultIsFile;
var isDirectory = opts.isDirectory || defaultIsDir;
var readFile = opts.readFile || fs2.readFile;
var realpath = opts.realpath || defaultRealpath;
var readPackage = opts.readPackage || defaultReadPackage;
if (opts.readFile && opts.readPackage) {
var conflictErr = new TypeError("`readFile` and `readPackage` are mutually exclusive.");
return process.nextTick(function() {
cb(conflictErr);
});
}
var packageIterator = opts.packageIterator;
var extensions = opts.extensions || [".js"];
var includeCoreModules = opts.includeCoreModules !== false;
var basedir = opts.basedir || path.dirname(caller());
var parent = opts.filename || basedir;
opts.paths = opts.paths || defaultPaths();
var absoluteStart = path.resolve(basedir);
maybeRealpath(realpath, absoluteStart, opts, function(err2, realStart) {
if (err2)
cb(err2);
else
init(realStart);
});
var res;
function init(basedir2) {
if (/^(?:\.\.?(?:\/|$)|\/|([A-Za-z]:)?[/\\])/.test(x)) {
res = path.resolve(basedir2, x);
if (x === "." || x === ".." || x.slice(-1) === "/")
res += "/";
if (/\/$/.test(x) && res === basedir2) {
loadAsDirectory(res, opts.package, onfile);
} else
loadAsFile(res, opts.package, onfile);
} else if (includeCoreModules && isCore(x)) {
return cb(null, x);
} else
loadNodeModules(x, basedir2, function(err2, n2, pkg) {
if (err2)
cb(err2);
else if (n2) {
return maybeRealpath(realpath, n2, opts, function(err3, realN) {
if (err3) {
cb(err3);
} else {
cb(null, realN, pkg);
}
});
} else {
var moduleError = new Error("Cannot find module '" + x + "' from '" + parent + "'");
moduleError.code = "MODULE_NOT_FOUND";
cb(moduleError);
}
});
}
function onfile(err2, m, pkg) {
if (err2)
cb(err2);
else if (m)
cb(null, m, pkg);
else
loadAsDirectory(res, function(err3, d, pkg2) {
if (err3)
cb(err3);
else if (d) {
maybeRealpath(realpath, d, opts, function(err4, realD) {
if (err4) {
cb(err4);
} else {
cb(null, realD, pkg2);
}
});
} else {
var moduleError = new Error("Cannot find module '" + x + "' from '" + parent + "'");
moduleError.code = "MODULE_NOT_FOUND";
cb(moduleError);
}
});
}
function loadAsFile(x2, thePackage, callback2) {
var loadAsFilePackage = thePackage;
var cb2 = callback2;
if (typeof loadAsFilePackage === "function") {
cb2 = loadAsFilePackage;
loadAsFilePackage = void 0;
}
var exts = [""].concat(extensions);
load(exts, x2, loadAsFilePackage);
function load(exts2, x3, loadPackage) {
if (exts2.length === 0)
return cb2(null, void 0, loadPackage);
var file = x3 + exts2[0];
var pkg = loadPackage;
if (pkg)
onpkg(null, pkg);
else
loadpkg(path.dirname(file), onpkg);
function onpkg(err2, pkg_, dir) {
pkg = pkg_;
if (err2)
return cb2(err2);
if (dir && pkg && opts.pathFilter) {
var rfile = path.relative(dir, file);
var rel = rfile.slice(0, rfile.length - exts2[0].length);
var r = opts.pathFilter(pkg, x3, rel);
if (r)
return load([""].concat(extensions.slice()), path.resolve(dir, r), pkg);
}
isFile(file, onex);
}
function onex(err2, ex) {
if (err2)
return cb2(err2);
if (ex)
return cb2(null, file, pkg);
load(exts2.slice(1), x3, pkg);
}
}
}
function loadpkg(dir, cb2) {
if (dir === "" || dir === "/")
return cb2(null);
if (process.platform === "win32" && /^\w:[/\\]*$/.test(dir)) {
return cb2(null);
}
if (/[/\\]node_modules[/\\]*$/.test(dir))
return cb2(null);
maybeRealpath(realpath, dir, opts, function(unwrapErr, pkgdir) {
if (unwrapErr)
return loadpkg(path.dirname(dir), cb2);
var pkgfile = path.join(pkgdir, "package.json");
isFile(pkgfile, function(err2, ex) {
if (!ex)
return loadpkg(path.dirname(dir), cb2);
readPackage(readFile, pkgfile, function(err3, pkgParam) {
if (err3)
cb2(err3);
var pkg = pkgParam;
if (pkg && opts.packageFilter) {
pkg = opts.packageFilter(pkg, pkgfile);
}
cb2(null, pkg, dir);
});
});
});
}
function loadAsDirectory(x2, loadAsDirectoryPackage, callback2) {
var cb2 = callback2;
var fpkg = loadAsDirectoryPackage;
if (typeof fpkg === "function") {
cb2 = fpkg;
fpkg = opts.package;
}
maybeRealpath(realpath, x2, opts, function(unwrapErr, pkgdir) {
if (unwrapErr)
return cb2(unwrapErr);
var pkgfile = path.join(pkgdir, "package.json");
isFile(pkgfile, function(err2, ex) {
if (err2)
return cb2(err2);
if (!ex)
return loadAsFile(path.join(x2, "index"), fpkg, cb2);
readPackage(readFile, pkgfile, function(err3, pkgParam) {
if (err3)
return cb2(err3);
var pkg = pkgParam;
if (pkg && opts.packageFilter) {
pkg = opts.packageFilter(pkg, pkgfile);
}
if (pkg && pkg.main) {
if (typeof pkg.main !== "string") {
var mainError = new TypeError("package \u201C" + pkg.name + "\u201D `main` must be a string");
mainError.code = "INVALID_PACKAGE_MAIN";
return cb2(mainError);
}
if (pkg.main === "." || pkg.main === "./") {
pkg.main = "index";
}
loadAsFile(path.resolve(x2, pkg.main), pkg, function(err4, m, pkg2) {
if (err4)
return cb2(err4);
if (m)
return cb2(null, m, pkg2);
if (!pkg2)
return loadAsFile(path.join(x2, "index"), pkg2, cb2);
var dir = path.resolve(x2, pkg2.main);
loadAsDirectory(dir, pkg2, function(err5, n2, pkg3) {
if (err5)
return cb2(err5);
if (n2)
return cb2(null, n2, pkg3);
loadAsFile(path.join(x2, "index"), pkg3, cb2);
});
});
return;
}
loadAsFile(path.join(x2, "/index"), pkg, cb2);
});
});
});
}
function processDirs(cb2, dirs) {
if (dirs.length === 0)
return cb2(null, void 0);
var dir = dirs[0];
isDirectory(path.dirname(dir), isdir);
function isdir(err2, isdir2) {
if (err2)
return cb2(err2);
if (!isdir2)
return processDirs(cb2, dirs.slice(1));
loadAsFile(dir, opts.package, onfile2);
}
function onfile2(err2, m, pkg) {
if (err2)
return cb2(err2);
if (m)
return cb2(null, m, pkg);
loadAsDirectory(dir, opts.package, ondir);
}
function ondir(err2, n2, pkg) {
if (err2)
return cb2(err2);
if (n2)
return cb2(null, n2, pkg);
processDirs(cb2, dirs.slice(1));
}
}
function loadNodeModules(x2, start, cb2) {
var thunk = function() {
return getPackageCandidates(x2, start, opts);
};
processDirs(cb2, packageIterator ? packageIterator(x2, start, thunk, opts) : thunk());
}
};
}
});
var require_core3 = __commonJS22({
"node_modules/resolve/lib/core.json"(exports2, module22) {
module22.exports = {
assert: true,
"node:assert": [">= 14.18 && < 15", ">= 16"],
"assert/strict": ">= 15",
"node:assert/strict": ">= 16",
async_hooks: ">= 8",
"node:async_hooks": [">= 14.18 && < 15", ">= 16"],
buffer_ieee754: ">= 0.5 && < 0.9.7",
buffer: true,
"node:buffer": [">= 14.18 && < 15", ">= 16"],
child_process: true,
"node:child_process": [">= 14.18 && < 15", ">= 16"],
cluster: ">= 0.5",
"node:cluster": [">= 14.18 && < 15", ">= 16"],
console: true,
"node:console": [">= 14.18 && < 15", ">= 16"],
constants: true,
"node:constants": [">= 14.18 && < 15", ">= 16"],
crypto: true,
"node:crypto": [">= 14.18 && < 15", ">= 16"],
_debug_agent: ">= 1 && < 8",
_debugger: "< 8",
dgram: true,
"node:dgram": [">= 14.18 && < 15", ">= 16"],
diagnostics_channel: [">= 14.17 && < 15", ">= 15.1"],
"node:diagnostics_channel": [">= 14.18 && < 15", ">= 16"],
dns: true,
"node:dns": [">= 14.18 && < 15", ">= 16"],
"dns/promises": ">= 15",
"node:dns/promises": ">= 16",
domain: ">= 0.7.12",
"node:domain": [">= 14.18 && < 15", ">= 16"],
events: true,
"node:events": [">= 14.18 && < 15", ">= 16"],
freelist: "< 6",
fs: true,
"node:fs": [">= 14.18 && < 15", ">= 16"],
"fs/promises": [">= 10 && < 10.1", ">= 14"],
"node:fs/promises": [">= 14.18 && < 15", ">= 16"],
_http_agent: ">= 0.11.1",
"node:_http_agent": [">= 14.18 && < 15", ">= 16"],
_http_client: ">= 0.11.1",
"node:_http_client": [">= 14.18 && < 15", ">= 16"],
_http_common: ">= 0.11.1",
"node:_http_common": [">= 14.18 && < 15", ">= 16"],
_http_incoming: ">= 0.11.1",
"node:_http_incoming": [">= 14.18 && < 15", ">= 16"],
_http_outgoing: ">= 0.11.1",
"node:_http_outgoing": [">= 14.18 && < 15", ">= 16"],
_http_server: ">= 0.11.1",
"node:_http_server": [">= 14.18 && < 15", ">= 16"],
http: true,
"node:http": [">= 14.18 && < 15", ">= 16"],
http2: ">= 8.8",
"node:http2": [">= 14.18 && < 15", ">= 16"],
https: true,
"node:https": [">= 14.18 && < 15", ">= 16"],
inspector: ">= 8",
"node:inspector": [">= 14.18 && < 15", ">= 16"],
_linklist: "< 8",
module: true,
"node:module": [">= 14.18 && < 15", ">= 16"],
net: true,
"node:net": [">= 14.18 && < 15", ">= 16"],
"node-inspect/lib/_inspect": ">= 7.6 && < 12",
"node-inspect/lib/internal/inspect_client": ">= 7.6 && < 12",
"node-inspect/lib/internal/inspect_repl": ">= 7.6 && < 12",
os: true,
"node:os": [">= 14.18 && < 15", ">= 16"],
path: true,
"node:path": [">= 14.18 && < 15", ">= 16"],
"path/posix": ">= 15.3",
"node:path/posix": ">= 16",
"path/win32": ">= 15.3",
"node:path/win32": ">= 16",
perf_hooks: ">= 8.5",
"node:perf_hooks": [">= 14.18 && < 15", ">= 16"],
process: ">= 1",
"node:process": [">= 14.18 && < 15", ">= 16"],
punycode: ">= 0.5",
"node:punycode": [">= 14.18 && < 15", ">= 16"],
querystring: true,
"node:querystring": [">= 14.18 && < 15", ">= 16"],
readline: true,
"node:readline": [">= 14.18 && < 15", ">= 16"],
"readline/promises": ">= 17",
"node:readline/promises": ">= 17",
repl: true,
"node:repl": [">= 14.18 && < 15", ">= 16"],
smalloc: ">= 0.11.5 && < 3",
_stream_duplex: ">= 0.9.4",
"node:_stream_duplex": [">= 14.18 && < 15", ">= 16"],
_stream_transform: ">= 0.9.4",
"node:_stream_transform": [">= 14.18 && < 15", ">= 16"],
_stream_wrap: ">= 1.4.1",
"node:_stream_wrap": [">= 14.18 && < 15", ">= 16"],
_stream_passthrough: ">= 0.9.4",
"node:_stream_passthrough": [">= 14.18 && < 15", ">= 16"],
_stream_readable: ">= 0.9.4",
"node:_stream_readable": [">= 14.18 && < 15", ">= 16"],
_stream_writable: ">= 0.9.4",
"node:_stream_writable": [">= 14.18 && < 15", ">= 16"],
stream: true,
"node:stream": [">= 14.18 && < 15", ">= 16"],
"stream/consumers": ">= 16.7",
"node:stream/consumers": ">= 16.7",
"stream/promises": ">= 15",
"node:stream/promises": ">= 16",
"stream/web": ">= 16.5",
"node:stream/web": ">= 16.5",
string_decoder: true,
"node:string_decoder": [">= 14.18 && < 15", ">= 16"],
sys: [">= 0.4 && < 0.7", ">= 0.8"],
"node:sys": [">= 14.18 && < 15", ">= 16"],
"node:test": ">= 18",
timers: true,
"node:timers": [">= 14.18 && < 15", ">= 16"],
"timers/promises": ">= 15",
"node:timers/promises": ">= 16",
_tls_common: ">= 0.11.13",
"node:_tls_common": [">= 14.18 && < 15", ">= 16"],
_tls_legacy: ">= 0.11.3 && < 10",
_tls_wrap: ">= 0.11.3",
"node:_tls_wrap": [">= 14.18 && < 15", ">= 16"],
tls: true,
"node:tls": [">= 14.18 && < 15", ">= 16"],
trace_events: ">= 10",
"node:trace_events": [">= 14.18 && < 15", ">= 16"],
tty: true,
"node:tty": [">= 14.18 && < 15", ">= 16"],
url: true,
"node:url": [">= 14.18 && < 15", ">= 16"],
util: true,
"node:util": [">= 14.18 && < 15", ">= 16"],
"util/types": ">= 15.3",
"node:util/types": ">= 16",
"v8/tools/arguments": ">= 10 && < 12",
"v8/tools/codemap": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/consarray": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/csvparser": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/logreader": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/profile_view": [">= 4.4 && < 5", ">= 5.2 && < 12"],
"v8/tools/splaytree": [">= 4.4 && < 5", ">= 5.2 && < 12"],
v8: ">= 1",
"node:v8": [">= 14.18 && < 15", ">= 16"],
vm: true,
"node:vm": [">= 14.18 && < 15", ">= 16"],
wasi: ">= 13.4 && < 13.5",
worker_threads: ">= 11.7",
"node:worker_threads": [">= 14.18 && < 15", ">= 16"],
zlib: ">= 0.5",
"node:zlib": [">= 14.18 && < 15", ">= 16"]
};
}
});
var require_core4 = __commonJS22({
"node_modules/resolve/lib/core.js"(exports2, module22) {
var current = process.versions && process.versions.node && process.versions.node.split(".") || [];
function specifierIncluded(specifier) {
var parts = specifier.split(" ");
var op = parts.length > 1 ? parts[0] : "=";
var versionParts = (parts.length > 1 ? parts[1] : parts[0]).split(".");
for (var i = 0; i < 3; ++i) {
var cur = parseInt(current[i] || 0, 10);
var ver = parseInt(versionParts[i] || 0, 10);
if (cur === ver) {
continue;
}
if (op === "<") {
return cur < ver;
} else if (op === ">=") {
return cur >= ver;
}
return false;
}
return op === ">=";
}
function matchesRange(range) {
var specifiers = range.split(/ ?&& ?/);
if (specifiers.length === 0) {
return false;
}
for (var i = 0; i < specifiers.length; ++i) {
if (!specifierIncluded(specifiers[i])) {
return false;
}
}
return true;
}
function versionIncluded(specifierValue) {
if (typeof specifierValue === "boolean") {
return specifierValue;
}
if (specifierValue && typeof specifierValue === "object") {
for (var i = 0; i < specifierValue.length; ++i) {
if (matchesRange(specifierValue[i])) {
return true;
}
}
return false;
}
return matchesRange(specifierValue);
}
var data = require_core3();
var core2 = {};
for (mod in data) {
if (Object.prototype.hasOwnProperty.call(data, mod)) {
core2[mod] = versionIncluded(data[mod]);
}
}
var mod;
module22.exports = core2;
}
});
var require_is_core = __commonJS22({
"node_modules/resolve/lib/is-core.js"(exports2, module22) {
var isCoreModule = require_is_core_module();
module22.exports = function isCore(x) {
return isCoreModule(x);
};
}
});
var require_sync = __commonJS22({
"node_modules/resolve/lib/sync.js"(exports2, module22) {
var isCore = require_is_core_module();
var fs2 = require("fs");
var path = require("path");
var getHomedir = require_homedir();
var caller = require_caller();
var nodeModulesPaths = require_node_modules_paths();
var normalizeOptions = require_normalize_options();
var realpathFS = process.platform !== "win32" && fs2.realpathSync && typeof fs2.realpathSync.native === "function" ? fs2.realpathSync.native : fs2.realpathSync;
var homedir = getHomedir();
var defaultPaths = function() {
return [path.join(homedir, ".node_modules"), path.join(homedir, ".node_libraries")];
};
var defaultIsFile = function isFile(file) {
try {
var stat2 = fs2.statSync(file, {
throwIfNoEntry: false
});
} catch (e) {
if (e && (e.code === "ENOENT" || e.code === "ENOTDIR"))
return false;
throw e;
}
return !!stat2 && (stat2.isFile() || stat2.isFIFO());
};
var defaultIsDir = function isDirectory(dir) {
try {
var stat2 = fs2.statSync(dir, {
throwIfNoEntry: false
});
} catch (e) {
if (e && (e.code === "ENOENT" || e.code === "ENOTDIR"))
return false;
throw e;
}
return !!stat2 && stat2.isDirectory();
};
var defaultRealpathSync = function realpathSync(x) {
try {
return realpathFS(x);
} catch (realpathErr) {
if (realpathErr.code !== "ENOENT") {
throw realpathErr;
}
}
return x;
};
var maybeRealpathSync = function maybeRealpathSync2(realpathSync, x, opts) {
if (opts && opts.preserveSymlinks === false) {
return realpathSync(x);
}
return x;
};
var defaultReadPackageSync = function defaultReadPackageSync2(readFileSync2, pkgfile) {
var body = readFileSync2(pkgfile);
try {
var pkg = JSON.parse(body);
return pkg;
} catch (jsonErr) {
}
};
var getPackageCandidates = function getPackageCandidates2(x, start, opts) {
var dirs = nodeModulesPaths(start, opts, x);
for (var i = 0; i < dirs.length; i++) {
dirs[i] = path.join(dirs[i], x);
}
return dirs;
};
module22.exports = function resolveSync(x, options) {
if (typeof x !== "string") {
throw new TypeError("Path must be a string.");
}
var opts = normalizeOptions(x, options);
var isFile = opts.isFile || defaultIsFile;
var readFileSync2 = opts.readFileSync || fs2.readFileSync;
var isDirectory = opts.isDirectory || defaultIsDir;
var realpathSync = opts.realpathSync || defaultRealpathSync;
var readPackageSync = opts.readPackageSync || defaultReadPackageSync;
if (opts.readFileSync && opts.readPackageSync) {
throw new TypeError("`readFileSync` and `readPackageSync` are mutually exclusive.");
}
var packageIterator = opts.packageIterator;
var extensions = opts.extensions || [".js"];
var includeCoreModules = opts.includeCoreModules !== false;
var basedir = opts.basedir || path.dirname(caller());
var parent = opts.filename || basedir;
opts.paths = opts.paths || defaultPaths();
var absoluteStart = maybeRealpathSync(realpathSync, path.resolve(basedir), opts);
if (/^(?:\.\.?(?:\/|$)|\/|([A-Za-z]:)?[/\\])/.test(x)) {
var res = path.resolve(absoluteStart, x);
if (x === "." || x === ".." || x.slice(-1) === "/")
res += "/";
var m = loadAsFileSync(res) || loadAsDirectorySync(res);
if (m)
return maybeRealpathSync(realpathSync, m, opts);
} else if (includeCoreModules && isCore(x)) {
return x;
} else {
var n2 = loadNodeModulesSync(x, absoluteStart);
if (n2)
return maybeRealpathSync(realpathSync, n2, opts);
}
var err = new Error("Cannot find module '" + x + "' from '" + parent + "'");
err.code = "MODULE_NOT_FOUND";
throw err;
function loadAsFileSync(x2) {
var pkg = loadpkg(path.dirname(x2));
if (pkg && pkg.dir && pkg.pkg && opts.pathFilter) {
var rfile = path.relative(pkg.dir, x2);
var r = opts.pathFilter(pkg.pkg, x2, rfile);
if (r) {
x2 = path.resolve(pkg.dir, r);
}
}
if (isFile(x2)) {
return x2;
}
for (var i = 0; i < extensions.length; i++) {
var file = x2 + extensions[i];
if (isFile(file)) {
return file;
}
}
}
function loadpkg(dir) {
if (dir === "" || dir === "/")
return;
if (process.platform === "win32" && /^\w:[/\\]*$/.test(dir)) {
return;
}
if (/[/\\]node_modules[/\\]*$/.test(dir))
return;
var pkgfile = path.join(maybeRealpathSync(realpathSync, dir, opts), "package.json");
if (!isFile(pkgfile)) {
return loadpkg(path.dirname(dir));
}
var pkg = readPackageSync(readFileSync2, pkgfile);
if (pkg && opts.packageFilter) {
pkg = opts.packageFilter(pkg, dir);
}
return {
pkg,
dir
};
}
function loadAsDirectorySync(x2) {
var pkgfile = path.join(maybeRealpathSync(realpathSync, x2, opts), "/package.json");
if (isFile(pkgfile)) {
try {
var pkg = readPackageSync(readFileSync2, pkgfile);
} catch (e) {
}
if (pkg && opts.packageFilter) {
pkg = opts.packageFilter(pkg, x2);
}
if (pkg && pkg.main) {
if (typeof pkg.main !== "string") {
var mainError = new TypeError("package \u201C" + pkg.name + "\u201D `main` must be a string");
mainError.code = "INVALID_PACKAGE_MAIN";
throw mainError;
}
if (pkg.main === "." || pkg.main === "./") {
pkg.main = "index";
}
try {
var m2 = loadAsFileSync(path.resolve(x2, pkg.main));
if (m2)
return m2;
var n22 = loadAsDirectorySync(path.resolve(x2, pkg.main));
if (n22)
return n22;
} catch (e) {
}
}
}
return loadAsFileSync(path.join(x2, "/index"));
}
function loadNodeModulesSync(x2, start) {
var thunk = function() {
return getPackageCandidates(x2, start, opts);
};
var dirs = packageIterator ? packageIterator(x2, start, thunk, opts) : thunk();
for (var i = 0; i < dirs.length; i++) {
var dir = dirs[i];
if (isDirectory(path.dirname(dir))) {
var m2 = loadAsFileSync(dir);
if (m2)
return m2;
var n22 = loadAsDirectorySync(dir);
if (n22)
return n22;
}
}
}
};
}
});
var require_resolve = __commonJS22({
"node_modules/resolve/index.js"(exports2, module22) {
var async = require_async();
async.core = require_core4();
async.isCore = require_is_core();
async.sync = require_sync();
module22.exports = async;
}
});
var require_resolve2 = __commonJS22({
"src/common/resolve.js"(exports2, module22) {
"use strict";
var {
resolve
} = require;
if (resolve.length === 1 || process.env.PRETTIER_FALLBACK_RESOLVE) {
resolve = (id, options) => {
let basedir;
if (options && options.paths && options.paths.length === 1) {
basedir = options.paths[0];
}
return require_resolve().sync(id, {
basedir
});
};
}
module22.exports = resolve;
}
});
function mimicFunction(to, from, {
ignoreNonConfigurable = false
} = {}) {
const {
name
} = to;
for (const property of Reflect.ownKeys(from)) {
copyProperty(to, from, property, ignoreNonConfigurable);
}
changePrototype(to, from);
changeToString(to, from, name);
return to;
}
var copyProperty;
var canCopyProperty;
var changePrototype;
var wrappedToString;
var toStringDescriptor;
var toStringName;
var changeToString;
var init_mimic_fn = __esm({
"node_modules/mimic-fn/index.js"() {
copyProperty = (to, from, property, ignoreNonConfigurable) => {
if (property === "length" || property === "prototype") {
return;
}
if (property === "arguments" || property === "caller") {
return;
}
const toDescriptor = Object.getOwnPropertyDescriptor(to, property);
const fromDescriptor = Object.getOwnPropertyDescriptor(from, property);
if (!canCopyProperty(toDescriptor, fromDescriptor) && ignoreNonConfigurable) {
return;
}
Object.defineProperty(to, property, fromDescriptor);
};
canCopyProperty = function(toDescriptor, fromDescriptor) {
return toDescriptor === void 0 || toDescriptor.configurable || toDescriptor.writable === fromDescriptor.writable && toDescriptor.enumerable === fromDescriptor.enumerable && toDescriptor.configurable === fromDescriptor.configurable && (toDescriptor.writable || toDescriptor.value === fromDescriptor.value);
};
changePrototype = (to, from) => {
const fromPrototype = Object.getPrototypeOf(from);
if (fromPrototype === Object.getPrototypeOf(to)) {
return;
}
Object.setPrototypeOf(to, fromPrototype);
};
wrappedToString = (withName, fromBody) => `/* Wrapped ${withName}*/
${fromBody}`;
toStringDescriptor = Object.getOwnPropertyDescriptor(Function.prototype, "toString");
toStringName = Object.getOwnPropertyDescriptor(Function.prototype.toString, "name");
changeToString = (to, from, name) => {
const withName = name === "" ? "" : `with ${name.trim()}() `;
const newToString = wrappedToString.bind(null, withName, from.toString());
Object.defineProperty(newToString, "name", toStringName);
Object.defineProperty(to, "toString", Object.assign(Object.assign({}, toStringDescriptor), {}, {
value: newToString
}));
};
}
});
var require_p_defer = __commonJS22({
"node_modules/p-defer/index.js"(exports2, module22) {
"use strict";
module22.exports = () => {
const ret = {};
ret.promise = new Promise((resolve, reject) => {
ret.resolve = resolve;
ret.reject = reject;
});
return ret;
};
}
});
var require_dist2 = __commonJS22({
"node_modules/map-age-cleaner/dist/index.js"(exports2, module22) {
"use strict";
var __awaiter2 = exports2 && exports2.__awaiter || function(thisArg, _arguments, P, generator) {
return new (P || (P = Promise))(function(resolve, reject) {
function fulfilled(value) {
try {
step(generator.next(value));
} catch (e) {
reject(e);
}
}
function rejected(value) {
try {
step(generator["throw"](value));
} catch (e) {
reject(e);
}
}
function step(result) {
result.done ? resolve(result.value) : new P(function(resolve2) {
resolve2(result.value);
}).then(fulfilled, rejected);
}
step((generator = generator.apply(thisArg, _arguments || [])).next());
});
};
var __importDefault2 = exports2 && exports2.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : {
"default": mod
};
};
Object.defineProperty(exports2, "__esModule", {
value: true
});
var p_defer_1 = __importDefault2(require_p_defer());
function mapAgeCleaner2(map, property = "maxAge") {
let processingKey;
let processingTimer;
let processingDeferred;
const cleanup = () => __awaiter2(this, void 0, void 0, function* () {
if (processingKey !== void 0) {
return;
}
const setupTimer = (item) => __awaiter2(this, void 0, void 0, function* () {
processingDeferred = p_defer_1.default();
const delay = item[1][property] - Date.now();
if (delay <= 0) {
map.delete(item[0]);
processingDeferred.resolve();
return;
}
processingKey = item[0];
processingTimer = setTimeout(() => {
map.delete(item[0]);
if (processingDeferred) {
processingDeferred.resolve();
}
}, delay);
if (typeof processingTimer.unref === "function") {
processingTimer.unref();
}
return processingDeferred.promise;
});
try {
for (const entry of map) {
yield setupTimer(entry);
}
} catch (_a) {
}
processingKey = void 0;
});
const reset = () => {
processingKey = void 0;
if (processingTimer !== void 0) {
clearTimeout(processingTimer);
processingTimer = void 0;
}
if (processingDeferred !== void 0) {
processingDeferred.reject(void 0);
processingDeferred = void 0;
}
};
const originalSet = map.set.bind(map);
map.set = (key, value) => {
if (map.has(key)) {
map.delete(key);
}
const result = originalSet(key, value);
if (processingKey && processingKey === key) {
reset();
}
cleanup();
return result;
};
cleanup();
return map;
}
exports2.default = mapAgeCleaner2;
module22.exports = mapAgeCleaner2;
module22.exports.default = mapAgeCleaner2;
}
});
var dist_exports = {};
__export(dist_exports, {
default: () => mem,
memClear: () => memClear,
memDecorator: () => memDecorator
});
function mem(fn, {
cacheKey,
cache = /* @__PURE__ */ new Map(),
maxAge
} = {}) {
if (typeof maxAge === "number") {
(0, import_map_age_cleaner.default)(cache);
}
const memoized = function(...arguments_) {
const key = cacheKey ? cacheKey(arguments_) : arguments_[0];
const cacheItem = cache.get(key);
if (cacheItem) {
return cacheItem.data;
}
const result = fn.apply(this, arguments_);
cache.set(key, {
data: result,
maxAge: maxAge ? Date.now() + maxAge : Number.POSITIVE_INFINITY
});
return result;
};
mimicFunction(memoized, fn, {
ignoreNonConfigurable: true
});
cacheStore.set(memoized, cache);
return memoized;
}
function memDecorator(options = {}) {
const instanceMap = /* @__PURE__ */ new WeakMap();
return (target, propertyKey, descriptor) => {
const input = target[propertyKey];
if (typeof input !== "function") {
throw new TypeError("The decorated value must be a function");
}
delete descriptor.value;
delete descriptor.writable;
descriptor.get = function() {
if (!instanceMap.has(this)) {
const value = mem(input, options);
instanceMap.set(this, value);
return value;
}
return instanceMap.get(this);
};
};
}
function memClear(fn) {
const cache = cacheStore.get(fn);
if (!cache) {
throw new TypeError("Can't clear a function that was not memoized!");
}
if (typeof cache.clear !== "function") {
throw new TypeError("The cache Map can't be cleared!");
}
cache.clear();
}
var import_map_age_cleaner;
var cacheStore;
var init_dist = __esm({
"node_modules/mem/dist/index.js"() {
init_mimic_fn();
import_map_age_cleaner = __toESM2(require_dist2());
cacheStore = /* @__PURE__ */ new WeakMap();
}
});
var require_pseudomap = __commonJS22({
"node_modules/pseudomap/pseudomap.js"(exports2, module22) {
var hasOwnProperty = Object.prototype.hasOwnProperty;
module22.exports = PseudoMap;
function PseudoMap(set2) {
if (!(this instanceof PseudoMap))
throw new TypeError("Constructor PseudoMap requires 'new'");
this.clear();
if (set2) {
if (set2 instanceof PseudoMap || typeof Map === "function" && set2 instanceof Map)
set2.forEach(function(value, key) {
this.set(key, value);
}, this);
else if (Array.isArray(set2))
set2.forEach(function(kv) {
this.set(kv[0], kv[1]);
}, this);
else
throw new TypeError("invalid argument");
}
}
PseudoMap.prototype.forEach = function(fn, thisp) {
thisp = thisp || this;
Object.keys(this._data).forEach(function(k) {
if (k !== "size")
fn.call(thisp, this._data[k].value, this._data[k].key);
}, this);
};
PseudoMap.prototype.has = function(k) {
return !!find(this._data, k);
};
PseudoMap.prototype.get = function(k) {
var res = find(this._data, k);
return res && res.value;
};
PseudoMap.prototype.set = function(k, v) {
set(this._data, k, v);
};
PseudoMap.prototype.delete = function(k) {
var res = find(this._data, k);
if (res) {
delete this._data[res._index];
this._data.size--;
}
};
PseudoMap.prototype.clear = function() {
var data = /* @__PURE__ */ Object.create(null);
data.size = 0;
Object.defineProperty(this, "_data", {
value: data,
enumerable: false,
configurable: true,
writable: false
});
};
Object.defineProperty(PseudoMap.prototype, "size", {
get: function() {
return this._data.size;
},
set: function(n2) {
},
enumerable: true,
configurable: true
});
PseudoMap.prototype.values = PseudoMap.prototype.keys = PseudoMap.prototype.entries = function() {
throw new Error("iterators are not implemented in this version");
};
function same(a, b) {
return a === b || a !== a && b !== b;
}
function Entry(k, v, i) {
this.key = k;
this.value = v;
this._index = i;
}
function find(data, k) {
for (var i = 0, s = "_" + k, key = s; hasOwnProperty.call(data, key); key = s + i++) {
if (same(data[key].key, k))
return data[key];
}
}
function set(data, k, v) {
for (var i = 0, s = "_" + k, key = s; hasOwnProperty.call(data, key); key = s + i++) {
if (same(data[key].key, k)) {
data[key].value = v;
return;
}
}
data.size++;
data[key] = new Entry(k, v, key);
}
}
});
var require_map = __commonJS22({
"node_modules/pseudomap/map.js"(exports2, module22) {
if (process.env.npm_package_name === "pseudomap" && process.env.npm_lifecycle_script === "test")
process.env.TEST_PSEUDOMAP = "true";
if (typeof Map === "function" && !process.env.TEST_PSEUDOMAP) {
module22.exports = Map;
} else {
module22.exports = require_pseudomap();
}
}
});
var require_yallist = __commonJS22({
"node_modules/editorconfig/node_modules/yallist/yallist.js"(exports2, module22) {
module22.exports = Yallist;
Yallist.Node = Node;
Yallist.create = Yallist;
function Yallist(list) {
var self2 = this;
if (!(self2 instanceof Yallist)) {
self2 = new Yallist();
}
self2.tail = null;
self2.head = null;
self2.length = 0;
if (list && typeof list.forEach === "function") {
list.forEach(function(item) {
self2.push(item);
});
} else if (arguments.length > 0) {
for (var i = 0, l = arguments.length; i < l; i++) {
self2.push(arguments[i]);
}
}
return self2;
}
Yallist.prototype.removeNode = function(node) {
if (node.list !== this) {
throw new Error("removing node which does not belong to this list");
}
var next = node.next;
var prev = node.prev;
if (next) {
next.prev = prev;
}
if (prev) {
prev.next = next;
}
if (node === this.head) {
this.head = next;
}
if (node === this.tail) {
this.tail = prev;
}
node.list.length--;
node.next = null;
node.prev = null;
node.list = null;
};
Yallist.prototype.unshiftNode = function(node) {
if (node === this.head) {
return;
}
if (node.list) {
node.list.removeNode(node);
}
var head = this.head;
node.list = this;
node.next = head;
if (head) {
head.prev = node;
}
this.head = node;
if (!this.tail) {
this.tail = node;
}
this.length++;
};
Yallist.prototype.pushNode = function(node) {
if (node === this.tail) {
return;
}
if (node.list) {
node.list.removeNode(node);
}
var tail = this.tail;
node.list = this;
node.prev = tail;
if (tail) {
tail.next = node;
}
this.tail = node;
if (!this.head) {
this.head = node;
}
this.length++;
};
Yallist.prototype.push = function() {
for (var i = 0, l = arguments.length; i < l; i++) {
push(this, arguments[i]);
}
return this.length;
};
Yallist.prototype.unshift = function() {
for (var i = 0, l = arguments.length; i < l; i++) {
unshift(this, arguments[i]);
}
return this.length;
};
Yallist.prototype.pop = function() {
if (!this.tail) {
return void 0;
}
var res = this.tail.value;
this.tail = this.tail.prev;
if (this.tail) {
this.tail.next = null;
} else {
this.head = null;
}
this.length--;
return res;
};
Yallist.prototype.shift = function() {
if (!this.head) {
return void 0;
}
var res = this.head.value;
this.head = this.head.next;
if (this.head) {
this.head.prev = null;
} else {
this.tail = null;
}
this.length--;
return res;
};
Yallist.prototype.forEach = function(fn, thisp) {
thisp = thisp || this;
for (var walker = this.head, i = 0; walker !== null; i++) {
fn.call(thisp, walker.value, i, this);
walker = walker.next;
}
};
Yallist.prototype.forEachReverse = function(fn, thisp) {
thisp = thisp || this;
for (var walker = this.tail, i = this.length - 1; walker !== null; i--) {
fn.call(thisp, walker.value, i, this);
walker = walker.prev;
}
};
Yallist.prototype.get = function(n2) {
for (var i = 0, walker = this.head; walker !== null && i < n2; i++) {
walker = walker.next;
}
if (i === n2 && walker !== null) {
return walker.value;
}
};
Yallist.prototype.getReverse = function(n2) {
for (var i = 0, walker = this.tail; walker !== null && i < n2; i++) {
walker = walker.prev;
}
if (i === n2 && walker !== null) {
return walker.value;
}
};
Yallist.prototype.map = function(fn, thisp) {
thisp = thisp || this;
var res = new Yallist();
for (var walker = this.head; walker !== null; ) {
res.push(fn.call(thisp, walker.value, this));
walker = walker.next;
}
return res;
};
Yallist.prototype.mapReverse = function(fn, thisp) {
thisp = thisp || this;
var res = new Yallist();
for (var walker = this.tail; walker !== null; ) {
res.push(fn.call(thisp, walker.value, this));
walker = walker.prev;
}
return res;
};
Yallist.prototype.reduce = function(fn, initial) {
var acc;
var walker = this.head;
if (arguments.length > 1) {
acc = initial;
} else if (this.head) {
walker = this.head.next;
acc = this.head.value;
} else {
throw new TypeError("Reduce of empty list with no initial value");
}
for (var i = 0; walker !== null; i++) {
acc = fn(acc, walker.value, i);
walker = walker.next;
}
return acc;
};
Yallist.prototype.reduceReverse = function(fn, initial) {
var acc;
var walker = this.tail;
if (arguments.length > 1) {
acc = initial;
} else if (this.tail) {
walker = this.tail.prev;
acc = this.tail.value;
} else {
throw new TypeError("Reduce of empty list with no initial value");
}
for (var i = this.length - 1; walker !== null; i--) {
acc = fn(acc, walker.value, i);
walker = walker.prev;
}
return acc;
};
Yallist.prototype.toArray = function() {
var arr = new Array(this.length);
for (var i = 0, walker = this.head; walker !== null; i++) {
arr[i] = walker.value;
walker = walker.next;
}
return arr;
};
Yallist.prototype.toArrayReverse = function() {
var arr = new Array(this.length);
for (var i = 0, walker = this.tail; walker !== null; i++) {
arr[i] = walker.value;
walker = walker.prev;
}
return arr;
};
Yallist.prototype.slice = function(from, to) {
to = to || this.length;
if (to < 0) {
to += this.length;
}
from = from || 0;
if (from < 0) {
from += this.length;
}
var ret = new Yallist();
if (to < from || to < 0) {
return ret;
}
if (from < 0) {
from = 0;
}
if (to > this.length) {
to = this.length;
}
for (var i = 0, walker = this.head; walker !== null && i < from; i++) {
walker = walker.next;
}
for (; walker !== null && i < to; i++, walker = walker.next) {
ret.push(walker.value);
}
return ret;
};
Yallist.prototype.sliceReverse = function(from, to) {
to = to || this.length;
if (to < 0) {
to += this.length;
}
from = from || 0;
if (from < 0) {
from += this.length;
}
var ret = new Yallist();
if (to < from || to < 0) {
return ret;
}
if (from < 0) {
from = 0;
}
if (to > this.length) {
to = this.length;
}
for (var i = this.length, walker = this.tail; walker !== null && i > to; i--) {
walker = walker.prev;
}
for (; walker !== null && i > from; i--, walker = walker.prev) {
ret.push(walker.value);
}
return ret;
};
Yallist.prototype.reverse = function() {
var head = this.head;
var tail = this.tail;
for (var walker = head; walker !== null; walker = walker.prev) {
var p = walker.prev;
walker.prev = walker.next;
walker.next = p;
}
this.head = tail;
this.tail = head;
return this;
};
function push(self2, item) {
self2.tail = new Node(item, self2.tail, null, self2);
if (!self2.head) {
self2.head = self2.tail;
}
self2.length++;
}
function unshift(self2, item) {
self2.head = new Node(item, null, self2.head, self2);
if (!self2.tail) {
self2.tail = self2.head;
}
self2.length++;
}
function Node(value, prev, next, list) {
if (!(this instanceof Node)) {
return new Node(value, prev, next, list);
}
this.list = list;
this.value = value;
if (prev) {
prev.next = this;
this.prev = prev;
} else {
this.prev = null;
}
if (next) {
next.prev = this;
this.next = next;
} else {
this.next = null;
}
}
}
});
var require_lru_cache = __commonJS22({
"node_modules/editorconfig/node_modules/lru-cache/index.js"(exports2, module22) {
"use strict";
module22.exports = LRUCache;
var Map2 = require_map();
var util = require("util");
var Yallist = require_yallist();
var hasSymbol = typeof Symbol === "function" && process.env._nodeLRUCacheForceNoSymbol !== "1";
var makeSymbol;
if (hasSymbol) {
makeSymbol = function(key) {
return Symbol(key);
};
} else {
makeSymbol = function(key) {
return "_" + key;
};
}
var MAX = makeSymbol("max");
var LENGTH = makeSymbol("length");
var LENGTH_CALCULATOR = makeSymbol("lengthCalculator");
var ALLOW_STALE = makeSymbol("allowStale");
var MAX_AGE = makeSymbol("maxAge");
var DISPOSE = makeSymbol("dispose");
var NO_DISPOSE_ON_SET = makeSymbol("noDisposeOnSet");
var LRU_LIST = makeSymbol("lruList");
var CACHE = makeSymbol("cache");
function naiveLength() {
return 1;
}
function LRUCache(options) {
if (!(this instanceof LRUCache)) {
return new LRUCache(options);
}
if (typeof options === "number") {
options = {
max: options
};
}
if (!options) {
options = {};
}
var max = this[MAX] = options.max;
if (!max || !(typeof max === "number") || max <= 0) {
this[MAX] = Infinity;
}
var lc = options.length || naiveLength;
if (typeof lc !== "function") {
lc = naiveLength;
}
this[LENGTH_CALCULATOR] = lc;
this[ALLOW_STALE] = options.stale || false;
this[MAX_AGE] = options.maxAge || 0;
this[DISPOSE] = options.dispose;
this[NO_DISPOSE_ON_SET] = options.noDisposeOnSet || false;
this.reset();
}
Object.defineProperty(LRUCache.prototype, "max", {
set: function(mL) {
if (!mL || !(typeof mL === "number") || mL <= 0) {
mL = Infinity;
}
this[MAX] = mL;
trim(this);
},
get: function() {
return this[MAX];
},
enumerable: true
});
Object.defineProperty(LRUCache.prototype, "allowStale", {
set: function(allowStale) {
this[ALLOW_STALE] = !!allowStale;
},
get: function() {
return this[ALLOW_STALE];
},
enumerable: true
});
Object.defineProperty(LRUCache.prototype, "maxAge", {
set: function(mA) {
if (!mA || !(typeof mA === "number") || mA < 0) {
mA = 0;
}
this[MAX_AGE] = mA;
trim(this);
},
get: function() {
return this[MAX_AGE];
},
enumerable: true
});
Object.defineProperty(LRUCache.prototype, "lengthCalculator", {
set: function(lC) {
if (typeof lC !== "function") {
lC = naiveLength;
}
if (lC !== this[LENGTH_CALCULATOR]) {
this[LENGTH_CALCULATOR] = lC;
this[LENGTH] = 0;
this[LRU_LIST].forEach(function(hit) {
hit.length = this[LENGTH_CALCULATOR](hit.value, hit.key);
this[LENGTH] += hit.length;
}, this);
}
trim(this);
},
get: function() {
return this[LENGTH_CALCULATOR];
},
enumerable: true
});
Object.defineProperty(LRUCache.prototype, "length", {
get: function() {
return this[LENGTH];
},
enumerable: true
});
Object.defineProperty(LRUCache.prototype, "itemCount", {
get: function() {
return this[LRU_LIST].length;
},
enumerable: true
});
LRUCache.prototype.rforEach = function(fn, thisp) {
thisp = thisp || this;
for (var walker = this[LRU_LIST].tail; walker !== null; ) {
var prev = walker.prev;
forEachStep(this, fn, walker, thisp);
walker = prev;
}
};
function forEachStep(self2, fn, node, thisp) {
var hit = node.value;
if (isStale(self2, hit)) {
del(self2, node);
if (!self2[ALLOW_STALE]) {
hit = void 0;
}
}
if (hit) {
fn.call(thisp, hit.value, hit.key, self2);
}
}
LRUCache.prototype.forEach = function(fn, thisp) {
thisp = thisp || this;
for (var walker = this[LRU_LIST].head; walker !== null; ) {
var next = walker.next;
forEachStep(this, fn, walker, thisp);
walker = next;
}
};
LRUCache.prototype.keys = function() {
return this[LRU_LIST].toArray().map(function(k) {
return k.key;
}, this);
};
LRUCache.prototype.values = function() {
return this[LRU_LIST].toArray().map(function(k) {
return k.value;
}, this);
};
LRUCache.prototype.reset = function() {
if (this[DISPOSE] && this[LRU_LIST] && this[LRU_LIST].length) {
this[LRU_LIST].forEach(function(hit) {
this[DISPOSE](hit.key, hit.value);
}, this);
}
this[CACHE] = new Map2();
this[LRU_LIST] = new Yallist();
this[LENGTH] = 0;
};
LRUCache.prototype.dump = function() {
return this[LRU_LIST].map(function(hit) {
if (!isStale(this, hit)) {
return {
k: hit.key,
v: hit.value,
e: hit.now + (hit.maxAge || 0)
};
}
}, this).toArray().filter(function(h) {
return h;
});
};
LRUCache.prototype.dumpLru = function() {
return this[LRU_LIST];
};
LRUCache.prototype.inspect = function(n2, opts) {
var str = "LRUCache {";
var extras = false;
var as = this[ALLOW_STALE];
if (as) {
str += "\n allowStale: true";
extras = true;
}
var max = this[MAX];
if (max && max !== Infinity) {
if (extras) {
str += ",";
}
str += "\n max: " + util.inspect(max, opts);
extras = true;
}
var maxAge = this[MAX_AGE];
if (maxAge) {
if (extras) {
str += ",";
}
str += "\n maxAge: " + util.inspect(maxAge, opts);
extras = true;
}
var lc = this[LENGTH_CALCULATOR];
if (lc && lc !== naiveLength) {
if (extras) {
str += ",";
}
str += "\n length: " + util.inspect(this[LENGTH], opts);
extras = true;
}
var didFirst = false;
this[LRU_LIST].forEach(function(item) {
if (didFirst) {
str += ",\n ";
} else {
if (extras) {
str += ",\n";
}
didFirst = true;
str += "\n ";
}
var key = util.inspect(item.key).split("\n").join("\n ");
var val = {
value: item.value
};
if (item.maxAge !== maxAge) {
val.maxAge = item.maxAge;
}
if (lc !== naiveLength) {
val.length = item.length;
}
if (isStale(this, item)) {
val.stale = true;
}
val = util.inspect(val, opts).split("\n").join("\n ");
str += key + " => " + val;
});
if (didFirst || extras) {
str += "\n";
}
str += "}";
return str;
};
LRUCache.prototype.set = function(key, value, maxAge) {
maxAge = maxAge || this[MAX_AGE];
var now = maxAge ? Date.now() : 0;
var len = this[LENGTH_CALCULATOR](value, key);
if (this[CACHE].has(key)) {
if (len > this[MAX]) {
del(this, this[CACHE].get(key));
return false;
}
var node = this[CACHE].get(key);
var item = node.value;
if (this[DISPOSE]) {
if (!this[NO_DISPOSE_ON_SET]) {
this[DISPOSE](key, item.value);
}
}
item.now = now;
item.maxAge = maxAge;
item.value = value;
this[LENGTH] += len - item.length;
item.length = len;
this.get(key);
trim(this);
return true;
}
var hit = new Entry(key, value, len, now, maxAge);
if (hit.length > this[MAX]) {
if (this[DISPOSE]) {
this[DISPOSE](key, value);
}
return false;
}
this[LENGTH] += hit.length;
this[LRU_LIST].unshift(hit);
this[CACHE].set(key, this[LRU_LIST].head);
trim(this);
return true;
};
LRUCache.prototype.has = function(key) {
if (!this[CACHE].has(key))
return false;
var hit = this[CACHE].get(key).value;
if (isStale(this, hit)) {
return false;
}
return true;
};
LRUCache.prototype.get = function(key) {
return get(this, key, true);
};
LRUCache.prototype.peek = function(key) {
return get(this, key, false);
};
LRUCache.prototype.pop = function() {
var node = this[LRU_LIST].tail;
if (!node)
return null;
del(this, node);
return node.value;
};
LRUCache.prototype.del = function(key) {
del(this, this[CACHE].get(key));
};
LRUCache.prototype.load = function(arr) {
this.reset();
var now = Date.now();
for (var l = arr.length - 1; l >= 0; l--) {
var hit = arr[l];
var expiresAt = hit.e || 0;
if (expiresAt === 0) {
this.set(hit.k, hit.v);
} else {
var maxAge = expiresAt - now;
if (maxAge > 0) {
this.set(hit.k, hit.v, maxAge);
}
}
}
};
LRUCache.prototype.prune = function() {
var self2 = this;
this[CACHE].forEach(function(value, key) {
get(self2, key, false);
});
};
function get(self2, key, doUse) {
var node = self2[CACHE].get(key);
if (node) {
var hit = node.value;
if (isStale(self2, hit)) {
del(self2, node);
if (!self2[ALLOW_STALE])
hit = void 0;
} else {
if (doUse) {
self2[LRU_LIST].unshiftNode(node);
}
}
if (hit)
hit = hit.value;
}
return hit;
}
function isStale(self2, hit) {
if (!hit || !hit.maxAge && !self2[MAX_AGE]) {
return false;
}
var stale = false;
var diff = Date.now() - hit.now;
if (hit.maxAge) {
stale = diff > hit.maxAge;
} else {
stale = self2[MAX_AGE] && diff > self2[MAX_AGE];
}
return stale;
}
function trim(self2) {
if (self2[LENGTH] > self2[MAX]) {
for (var walker = self2[LRU_LIST].tail; self2[LENGTH] > self2[MAX] && walker !== null; ) {
var prev = walker.prev;
del(self2, walker);
walker = prev;
}
}
}
function del(self2, node) {
if (node) {
var hit = node.value;
if (self2[DISPOSE]) {
self2[DISPOSE](hit.key, hit.value);
}
self2[LENGTH] -= hit.length;
self2[CACHE].delete(hit.key);
self2[LRU_LIST].removeNode(node);
}
}
function Entry(key, value, length, now, maxAge) {
this.key = key;
this.value = value;
this.length = length;
this.now = now;
this.maxAge = maxAge || 0;
}
}
});
var require_sigmund = __commonJS22({
"node_modules/sigmund/sigmund.js"(exports2, module22) {
module22.exports = sigmund;
function sigmund(subject, maxSessions) {
maxSessions = maxSessions || 10;
var notes = [];
var analysis = "";
var RE = RegExp;
function psychoAnalyze(subject2, session) {
if (session > maxSessions)
return;
if (typeof subject2 === "function" || typeof subject2 === "undefined") {
return;
}
if (typeof subject2 !== "object" || !subject2 || subject2 instanceof RE) {
analysis += subject2;
return;
}
if (notes.indexOf(subject2) !== -1 || session === maxSessions)
return;
notes.push(subject2);
analysis += "{";
Object.keys(subject2).forEach(function(issue, _, __) {
if (issue.charAt(0) === "_")
return;
var to = typeof subject2[issue];
if (to === "function" || to === "undefined")
return;
analysis += issue;
psychoAnalyze(subject2[issue], session + 1);
});
}
psychoAnalyze(subject, 0);
return analysis;
}
}
});
var require_fnmatch = __commonJS22({
"node_modules/editorconfig/src/lib/fnmatch.js"(exports2, module22) {
var platform = typeof process === "object" ? process.platform : "win32";
if (module22)
module22.exports = minimatch;
else
exports2.minimatch = minimatch;
minimatch.Minimatch = Minimatch;
var LRU = require_lru_cache();
var cache = minimatch.cache = new LRU({
max: 100
});
var GLOBSTAR = minimatch.GLOBSTAR = Minimatch.GLOBSTAR = {};
var sigmund = require_sigmund();
var path = require("path");
var qmark = "[^/]";
var star = qmark + "*?";
var twoStarDot = "(?:(?!(?:\\/|^)(?:\\.{1,2})($|\\/)).)*?";
var twoStarNoDot = "(?:(?!(?:\\/|^)\\.).)*?";
var reSpecials = charSet("().*{}+?[]^$\\!");
function charSet(s) {
return s.split("").reduce(function(set, c) {
set[c] = true;
return set;
}, {});
}
var slashSplit = /\/+/;
minimatch.monkeyPatch = monkeyPatch;
function monkeyPatch() {
var desc = Object.getOwnPropertyDescriptor(String.prototype, "match");
var orig = desc.value;
desc.value = function(p) {
if (p instanceof Minimatch)
return p.match(this);
return orig.call(this, p);
};
Object.defineProperty(String.prototype, desc);
}
minimatch.filter = filter;
function filter(pattern, options) {
options = options || {};
return function(p, i, list) {
return minimatch(p, pattern, options);
};
}
function ext(a, b) {
a = a || {};
b = b || {};
var t = {};
Object.keys(b).forEach(function(k) {
t[k] = b[k];
});
Object.keys(a).forEach(function(k) {
t[k] = a[k];
});
return t;
}
minimatch.defaults = function(def) {
if (!def || !Object.keys(def).length)
return minimatch;
var orig = minimatch;
var m = function minimatch2(p, pattern, options) {
return orig.minimatch(p, pattern, ext(def, options));
};
m.Minimatch = function Minimatch2(pattern, options) {
return new orig.Minimatch(pattern, ext(def, options));
};
return m;
};
Minimatch.defaults = function(def) {
if (!def || !Object.keys(def).length)
return Minimatch;
return minimatch.defaults(def).Minimatch;
};
function minimatch(p, pattern, options) {
if (typeof pattern !== "string") {
throw new TypeError("glob pattern string required");
}
if (!options)
options = {};
if (!options.nocomment && pattern.charAt(0) === "#") {
return false;
}
if (pattern.trim() === "")
return p === "";
return new Minimatch(pattern, options).match(p);
}
function Minimatch(pattern, options) {
if (!(this instanceof Minimatch)) {
return new Minimatch(pattern, options, cache);
}
if (typeof pattern !== "string") {
throw new TypeError("glob pattern string required");
}
if (!options)
options = {};
if (platform === "win32") {
pattern = pattern.split("\\").join("/");
}
var cacheKey = pattern + "\n" + sigmund(options);
var cached = minimatch.cache.get(cacheKey);
if (cached)
return cached;
minimatch.cache.set(cacheKey, this);
this.options = options;
this.set = [];
this.pattern = pattern;
this.regexp = null;
this.negate = false;
this.comment = false;
this.empty = false;
this.make();
}
Minimatch.prototype.make = make;
function make() {
if (this._made)
return;
var pattern = this.pattern;
var options = this.options;
if (!options.nocomment && pattern.charAt(0) === "#") {
this.comment = true;
return;
}
if (!pattern) {
this.empty = true;
return;
}
this.parseNegate();
var set = this.globSet = this.braceExpand();
if (options.debug)
console.error(this.pattern, set);
set = this.globParts = set.map(function(s) {
return s.split(slashSplit);
});
if (options.debug)
console.error(this.pattern, set);
set = set.map(function(s, si, set2) {
return s.map(this.parse, this);
}, this);
if (options.debug)
console.error(this.pattern, set);
set = set.filter(function(s) {
return -1 === s.indexOf(false);
});
if (options.debug)
console.error(this.pattern, set);
this.set = set;
}
Minimatch.prototype.parseNegate = parseNegate;
function parseNegate() {
var pattern = this.pattern, negate = false, options = this.options, negateOffset = 0;
if (options.nonegate)
return;
for (var i = 0, l = pattern.length; i < l && pattern.charAt(i) === "!"; i++) {
negate = !negate;
negateOffset++;
}
if (negateOffset)
this.pattern = pattern.substr(negateOffset);
this.negate = negate;
}
minimatch.braceExpand = function(pattern, options) {
return new Minimatch(pattern, options).braceExpand();
};
Minimatch.prototype.braceExpand = braceExpand;
function braceExpand(pattern, options) {
options = options || this.options;
pattern = typeof pattern === "undefined" ? this.pattern : pattern;
if (typeof pattern === "undefined") {
throw new Error("undefined pattern");
}
if (options.nobrace || !pattern.match(/\{.*\}/)) {
return [pattern];
}
var escaping = false;
if (pattern.charAt(0) !== "{") {
var prefix = null;
for (var i = 0, l = pattern.length; i < l; i++) {
var c = pattern.charAt(i);
if (c === "\\") {
escaping = !escaping;
} else if (c === "{" && !escaping) {
prefix = pattern.substr(0, i);
break;
}
}
if (prefix === null) {
return [pattern];
}
var tail = braceExpand(pattern.substr(i), options);
return tail.map(function(t) {
return prefix + t;
});
}
var numset = pattern.match(/^\{(-?[0-9]+)\.\.(-?[0-9]+)\}/);
if (numset) {
var suf = braceExpand(pattern.substr(numset[0].length), options), start = +numset[1], end = +numset[2], inc = start > end ? -1 : 1, set = [];
for (var i = start; i != end + inc; i += inc) {
for (var ii = 0, ll = suf.length; ii < ll; ii++) {
set.push(i + suf[ii]);
}
}
return set;
}
var i = 1, depth = 1, set = [], member = "", sawEnd = false, escaping = false;
function addMember() {
set.push(member);
member = "";
}
FOR:
for (i = 1, l = pattern.length; i < l; i++) {
var c = pattern.charAt(i);
if (escaping) {
escaping = false;
member += "\\" + c;
} else {
switch (c) {
case "\\":
escaping = true;
continue;
case "{":
depth++;
member += "{";
continue;
case "}":
depth--;
if (depth === 0) {
addMember();
i++;
break FOR;
} else {
member += c;
continue;
}
case ",":
if (depth === 1) {
addMember();
} else {
member += c;
}
continue;
default:
member += c;
continue;
}
}
}
if (depth !== 0) {
return braceExpand("\\" + pattern, options);
}
var suf = braceExpand(pattern.substr(i), options);
var addBraces = set.length === 1;
set = set.map(function(p) {
return braceExpand(p, options);
});
set = set.reduce(function(l2, r) {
return l2.concat(r);
});
if (addBraces) {
set = set.map(function(s) {
return "{" + s + "}";
});
}
var ret = [];
for (var i = 0, l = set.length; i < l; i++) {
for (var ii = 0, ll = suf.length; ii < ll; ii++) {
ret.push(set[i] + suf[ii]);
}
}
return ret;
}
Minimatch.prototype.parse = parse2;
var SUBPARSE = {};
function parse2(pattern, isSub) {
var options = this.options;
if (!options.noglobstar && pattern === "**")
return GLOBSTAR;
if (pattern === "")
return "";
var re = "", hasMagic = !!options.nocase, escaping = false, patternListStack = [], plType, stateChar, inClass = false, reClassStart = -1, classStart = -1, patternStart = pattern.charAt(0) === "." ? "" : options.dot ? "(?!(?:^|\\/)\\.{1,2}(?:$|\\/))" : "(?!\\.)";
function clearStateChar() {
if (stateChar) {
switch (stateChar) {
case "*":
re += star;
hasMagic = true;
break;
case "?":
re += qmark;
hasMagic = true;
break;
default:
re += "\\" + stateChar;
break;
}
stateChar = false;
}
}
for (var i = 0, len = pattern.length, c; i < len && (c = pattern.charAt(i)); i++) {
if (options.debug) {
console.error("%s %s %s %j", pattern, i, re, c);
}
if (escaping && reSpecials[c]) {
re += "\\" + c;
escaping = false;
continue;
}
SWITCH:
switch (c) {
case "/":
return false;
case "\\":
clearStateChar();
escaping = true;
continue;
case "?":
case "*":
case "+":
case "@":
case "!":
if (options.debug) {
console.error("%s %s %s %j <-- stateChar", pattern, i, re, c);
}
if (inClass) {
if (c === "!" && i === classStart + 1)
c = "^";
re += c;
continue;
}
clearStateChar();
stateChar = c;
if (options.noext)
clearStateChar();
continue;
case "(":
if (inClass) {
re += "(";
continue;
}
if (!stateChar) {
re += "\\(";
continue;
}
plType = stateChar;
patternListStack.push({
type: plType,
start: i - 1,
reStart: re.length
});
re += stateChar === "!" ? "(?:(?!" : "(?:";
stateChar = false;
continue;
case ")":
if (inClass || !patternListStack.length) {
re += "\\)";
continue;
}
hasMagic = true;
re += ")";
plType = patternListStack.pop().type;
switch (plType) {
case "!":
re += "[^/]*?)";
break;
case "?":
case "+":
case "*":
re += plType;
case "@":
break;
}
continue;
case "|":
if (inClass || !patternListStack.length || escaping) {
re += "\\|";
escaping = false;
continue;
}
re += "|";
continue;
case "[":
clearStateChar();
if (inClass) {
re += "\\" + c;
continue;
}
inClass = true;
classStart = i;
reClassStart = re.length;
re += c;
continue;
case "]":
if (i === classStart + 1 || !inClass) {
re += "\\" + c;
escaping = false;
continue;
}
hasMagic = true;
inClass = false;
re += c;
continue;
default:
clearStateChar();
if (escaping) {
escaping = false;
} else if (reSpecials[c] && !(c === "^" && inClass)) {
re += "\\";
}
re += c;
}
}
if (inClass) {
var cs = pattern.substr(classStart + 1), sp = this.parse(cs, SUBPARSE);
re = re.substr(0, reClassStart) + "\\[" + sp[0];
hasMagic = hasMagic || sp[1];
}
var pl;
while (pl = patternListStack.pop()) {
var tail = re.slice(pl.reStart + 3);
tail = tail.replace(/((?:\\{2})*)(\\?)\|/g, function(_, $1, $2) {
if (!$2) {
$2 = "\\";
}
return $1 + $1 + $2 + "|";
});
var t = pl.type === "*" ? star : pl.type === "?" ? qmark : "\\" + pl.type;
hasMagic = true;
re = re.slice(0, pl.reStart) + t + "\\(" + tail;
}
clearStateChar();
if (escaping) {
re += "\\\\";
}
var addPatternStart = false;
switch (re.charAt(0)) {
case ".":
case "[":
case "(":
addPatternStart = true;
}
if (re !== "" && hasMagic)
re = "(?=.)" + re;
if (addPatternStart)
re = patternStart + re;
if (isSub === SUBPARSE) {
return [re, hasMagic];
}
if (!hasMagic) {
return globUnescape(pattern);
}
var flags = options.nocase ? "i" : "", regExp = new RegExp("^" + re + "$", flags);
regExp._glob = pattern;
regExp._src = re;
return regExp;
}
minimatch.makeRe = function(pattern, options) {
return new Minimatch(pattern, options || {}).makeRe();
};
Minimatch.prototype.makeRe = makeRe;
function makeRe() {
if (this.regexp || this.regexp === false)
return this.regexp;
var set = this.set;
if (!set.length)
return this.regexp = false;
var options = this.options;
var twoStar = options.noglobstar ? star : options.dot ? twoStarDot : twoStarNoDot, flags = options.nocase ? "i" : "";
var re = set.map(function(pattern) {
return pattern.map(function(p) {
return p === GLOBSTAR ? twoStar : typeof p === "string" ? regExpEscape(p) : p._src;
}).join("\\/");
}).join("|");
re = "^(?:" + re + ")$";
if (this.negate)
re = "^(?!" + re + ").*$";
try {
return this.regexp = new RegExp(re, flags);
} catch (ex) {
return this.regexp = false;
}
}
minimatch.match = function(list, pattern, options) {
var mm = new Minimatch(pattern, options);
list = list.filter(function(f) {
return mm.match(f);
});
if (options.nonull && !list.length) {
list.push(pattern);
}
return list;
};
Minimatch.prototype.match = match;
function match(f, partial) {
if (this.comment)
return false;
if (this.empty)
return f === "";
if (f === "/" && partial)
return true;
var options = this.options;
if (platform === "win32") {
f = f.split("\\").join("/");
}
f = f.split(slashSplit);
if (options.debug) {
console.error(this.pattern, "split", f);
}
var set = this.set;
for (var i = 0, l = set.length; i < l; i++) {
var pattern = set[i];
var hit = this.matchOne(f, pattern, partial);
if (hit) {
if (options.flipNegate)
return true;
return !this.negate;
}
}
if (options.flipNegate)
return false;
return this.negate;
}
Minimatch.prototype.matchOne = function(file, pattern, partial) {
var options = this.options;
if (options.debug) {
console.error("matchOne", {
"this": this,
file,
pattern
});
}
if (options.matchBase && pattern.length === 1) {
file = path.basename(file.join("/")).split("/");
}
if (options.debug) {
console.error("matchOne", file.length, pattern.length);
}
for (var fi = 0, pi = 0, fl = file.length, pl = pattern.length; fi < fl && pi < pl; fi++, pi++) {
if (options.debug) {
console.error("matchOne loop");
}
var p = pattern[pi], f = file[fi];
if (options.debug) {
console.error(pattern, p, f);
}
if (p === false)
return false;
if (p === GLOBSTAR) {
if (options.debug)
console.error("GLOBSTAR", [pattern, p, f]);
var fr = fi, pr = pi + 1;
if (pr === pl) {
if (options.debug)
console.error("** at the end");
for (; fi < fl; fi++) {
if (file[fi] === "." || file[fi] === ".." || !options.dot && file[fi].charAt(0) === ".")
return false;
}
return true;
}
WHILE:
while (fr < fl) {
var swallowee = file[fr];
if (options.debug) {
console.error("\nglobstar while", file, fr, pattern, pr, swallowee);
}
if (this.matchOne(file.slice(fr), pattern.slice(pr), partial)) {
if (options.debug)
console.error("globstar found match!", fr, fl, swallowee);
return true;
} else {
if (swallowee === "." || swallowee === ".." || !options.dot && swallowee.charAt(0) === ".") {
if (options.debug)
console.error("dot detected!", file, fr, pattern, pr);
break WHILE;
}
if (options.debug)
console.error("globstar swallow a segment, and continue");
fr++;
}
}
if (partial) {
if (fr === fl)
return true;
}
return false;
}
var hit;
if (typeof p === "string") {
if (options.nocase) {
hit = f.toLowerCase() === p.toLowerCase();
} else {
hit = f === p;
}
if (options.debug) {
console.error("string match", p, f, hit);
}
} else {
hit = f.match(p);
if (options.debug) {
console.error("pattern match", p, f, hit);
}
}
if (!hit)
return false;
}
if (fi === fl && pi === pl) {
return true;
} else if (fi === fl) {
return partial;
} else if (pi === pl) {
var emptyFileEnd = fi === fl - 1 && file[fi] === "";
return emptyFileEnd;
}
throw new Error("wtf?");
};
function globUnescape(s) {
return s.replace(/\\(.)/g, "$1");
}
function regExpEscape(s) {
return s.replace(/[-[\]{}()*+?.,\\^$|#\s]/g, "\\$&");
}
}
});
var require_ini = __commonJS22({
"node_modules/editorconfig/src/lib/ini.js"(exports2) {
"use strict";
var __awaiter2 = exports2 && exports2.__awaiter || function(thisArg, _arguments, P, generator) {
return new (P || (P = Promise))(function(resolve, reject) {
function fulfilled(value) {
try {
step(generator.next(value));
} catch (e) {
reject(e);
}
}
function rejected(value) {
try {
step(generator["throw"](value));
} catch (e) {
reject(e);
}
}
function step(result) {
result.done ? resolve(result.value) : new P(function(resolve2) {
resolve2(result.value);
}).then(fulfilled, rejected);
}
step((generator = generator.apply(thisArg, _arguments || [])).next());
});
};
var __generator2 = exports2 && exports2.__generator || function(thisArg, body) {
var _ = {
label: 0,
sent: function() {
if (t[0] & 1)
throw t[1];
return t[1];
},
trys: [],
ops: []
}, f, y, t, g;
return g = {
next: verb(0),
"throw": verb(1),
"return": verb(2)
}, typeof Symbol === "function" && (g[Symbol.iterator] = function() {
return this;
}), g;
function verb(n2) {
return function(v) {
return step([n2, v]);
};
}
function step(op) {
if (f)
throw new TypeError("Generator is already executing.");
while (_)
try {
if (f = 1, y && (t = op[0] & 2 ? y["return"] : op[0] ? y["throw"] || ((t = y["return"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done)
return t;
if (y = 0, t)
op = [op[0] & 2, t.value];
switch (op[0]) {
case 0:
case 1:
t = op;
break;
case 4:
_.label++;
return {
value: op[1],
done: false
};
case 5:
_.label++;
y = op[1];
op = [0];
continue;
case 7:
op = _.ops.pop();
_.trys.pop();
continue;
default:
if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) {
_ = 0;
continue;
}
if (op[0] === 3 && (!t || op[1] > t[0] && op[1] < t[3])) {
_.label = op[1];
break;
}
if (op[0] === 6 && _.label < t[1]) {
_.label = t[1];
t = op;
break;
}
if (t && _.label < t[2]) {
_.label = t[2];
_.ops.push(op);
break;
}
if (t[2])
_.ops.pop();
_.trys.pop();
continue;
}
op = body.call(thisArg, _);
} catch (e) {
op = [6, e];
y = 0;
} finally {
f = t = 0;
}
if (op[0] & 5)
throw op[1];
return {
value: op[0] ? op[1] : void 0,
done: true
};
}
};
var __importStar2 = exports2 && exports2.__importStar || function(mod) {
if (mod && mod.__esModule)
return mod;
var result = {};
if (mod != null) {
for (var k in mod)
if (Object.hasOwnProperty.call(mod, k))
result[k] = mod[k];
}
result["default"] = mod;
return result;
};
Object.defineProperty(exports2, "__esModule", {
value: true
});
var fs2 = __importStar2(require("fs"));
var regex = {
section: /^\s*\[(([^#;]|\\#|\\;)+)\]\s*([#;].*)?$/,
param: /^\s*([\w\.\-\_]+)\s*[=:]\s*(.*?)\s*([#;].*)?$/,
comment: /^\s*[#;].*$/
};
function parse2(file) {
return __awaiter2(this, void 0, void 0, function() {
return __generator2(this, function(_a) {
return [2, new Promise(function(resolve, reject) {
fs2.readFile(file, "utf8", function(err, data) {
if (err) {
reject(err);
return;
}
resolve(parseString(data));
});
})];
});
});
}
exports2.parse = parse2;
function parseSync(file) {
return parseString(fs2.readFileSync(file, "utf8"));
}
exports2.parseSync = parseSync;
function parseString(data) {
var sectionBody = {};
var sectionName = null;
var value = [[sectionName, sectionBody]];
var lines = data.split(/\r\n|\r|\n/);
lines.forEach(function(line) {
var match;
if (regex.comment.test(line)) {
return;
}
if (regex.param.test(line)) {
match = line.match(regex.param);
sectionBody[match[1]] = match[2];
} else if (regex.section.test(line)) {
match = line.match(regex.section);
sectionName = match[1];
sectionBody = {};
value.push([sectionName, sectionBody]);
}
});
return value;
}
exports2.parseString = parseString;
}
});
var require_package2 = __commonJS22({
"node_modules/editorconfig/package.json"(exports2, module22) {
module22.exports = {
name: "editorconfig",
version: "0.15.3",
description: "EditorConfig File Locator and Interpreter for Node.js",
keywords: ["editorconfig", "core"],
main: "src/index.js",
contributors: ["Hong Xu (topbug.net)", "Jed Mao (https://github.com/jedmao/)", "Trey Hunner (http://treyhunner.com)"],
directories: {
bin: "./bin",
lib: "./lib"
},
scripts: {
clean: "rimraf dist",
prebuild: "npm run clean",
build: "tsc",
pretest: "npm run lint && npm run build && npm run copy && cmake .",
test: "ctest .",
"pretest:ci": "npm run pretest",
"test:ci": "ctest -VV --output-on-failure .",
lint: "npm run eclint && npm run tslint",
eclint: 'eclint check --indent_size ignore "src/**"',
tslint: "tslint --project tsconfig.json --exclude package.json",
copy: "cpy .npmignore LICENSE README.md CHANGELOG.md dist && cpy bin/* dist/bin && cpy src/lib/fnmatch*.* dist/src/lib",
prepub: "npm run lint && npm run build && npm run copy",
pub: "npm publish ./dist"
},
repository: {
type: "git",
url: "git://github.com/editorconfig/editorconfig-core-js.git"
},
bugs: "https://github.com/editorconfig/editorconfig-core-js/issues",
author: "EditorConfig Team",
license: "MIT",
dependencies: {
commander: "^2.19.0",
"lru-cache": "^4.1.5",
semver: "^5.6.0",
sigmund: "^1.0.1"
},
devDependencies: {
"@types/mocha": "^5.2.6",
"@types/node": "^10.12.29",
"@types/semver": "^5.5.0",
"cpy-cli": "^2.0.0",
eclint: "^2.8.1",
mocha: "^5.2.0",
rimraf: "^2.6.3",
should: "^13.2.3",
tslint: "^5.13.1",
typescript: "^3.3.3333"
}
};
}
});
var require_src2 = __commonJS22({
"node_modules/editorconfig/src/index.js"(exports2) {
"use strict";
var __awaiter2 = exports2 && exports2.__awaiter || function(thisArg, _arguments, P, generator) {
return new (P || (P = Promise))(function(resolve, reject) {
function fulfilled(value) {
try {
step(generator.next(value));
} catch (e) {
reject(e);
}
}
function rejected(value) {
try {
step(generator["throw"](value));
} catch (e) {
reject(e);
}
}
function step(result) {
result.done ? resolve(result.value) : new P(function(resolve2) {
resolve2(result.value);
}).then(fulfilled, rejected);
}
step((generator = generator.apply(thisArg, _arguments || [])).next());
});
};
var __generator2 = exports2 && exports2.__generator || function(thisArg, body) {
var _ = {
label: 0,
sent: function() {
if (t[0] & 1)
throw t[1];
return t[1];
},
trys: [],
ops: []
}, f, y, t, g;
return g = {
next: verb(0),
"throw": verb(1),
"return": verb(2)
}, typeof Symbol === "function" && (g[Symbol.iterator] = function() {
return this;
}), g;
function verb(n2) {
return function(v) {
return step([n2, v]);
};
}
function step(op) {
if (f)
throw new TypeError("Generator is already executing.");
while (_)
try {
if (f = 1, y && (t = op[0] & 2 ? y["return"] : op[0] ? y["throw"] || ((t = y["return"]) && t.call(y), 0) : y.next) && !(t = t.call(y, op[1])).done)
return t;
if (y = 0, t)
op = [op[0] & 2, t.value];
switch (op[0]) {
case 0:
case 1:
t = op;
break;
case 4:
_.label++;
return {
value: op[1],
done: false
};
case 5:
_.label++;
y = op[1];
op = [0];
continue;
case 7:
op = _.ops.pop();
_.trys.pop();
continue;
default:
if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) {
_ = 0;
continue;
}
if (op[0] === 3 && (!t || op[1] > t[0] && op[1] < t[3])) {
_.label = op[1];
break;
}
if (op[0] === 6 && _.label < t[1]) {
_.label = t[1];
t = op;
break;
}
if (t && _.label < t[2]) {
_.label = t[2];
_.ops.push(op);
break;
}
if (t[2])
_.ops.pop();
_.trys.pop();
continue;
}
op = body.call(thisArg, _);
} catch (e) {
op = [6, e];
y = 0;
} finally {
f = t = 0;
}
if (op[0] & 5)
throw op[1];
return {
value: op[0] ? op[1] : void 0,
done: true
};
}
};
var __importStar2 = exports2 && exports2.__importStar || function(mod) {
if (mod && mod.__esModule)
return mod;
var result = {};
if (mod != null) {
for (var k in mod)
if (Object.hasOwnProperty.call(mod, k))
result[k] = mod[k];
}
result["default"] = mod;
return result;
};
var __importDefault2 = exports2 && exports2.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : {
"default": mod
};
};
Object.defineProperty(exports2, "__esModule", {
value: true
});
var fs2 = __importStar2(require("fs"));
var path = __importStar2(require("path"));
var semver = {
gte: require_gte()
};
var fnmatch_1 = __importDefault2(require_fnmatch());
var ini_1 = require_ini();
exports2.parseString = ini_1.parseString;
var package_json_1 = __importDefault2(require_package2());
var knownProps = {
end_of_line: true,
indent_style: true,
indent_size: true,
insert_final_newline: true,
trim_trailing_whitespace: true,
charset: true
};
function fnmatch(filepath, glob) {
var matchOptions = {
matchBase: true,
dot: true,
noext: true
};
glob = glob.replace(/\*\*/g, "{*,**/**/**}");
return fnmatch_1.default(filepath, glob, matchOptions);
}
function getConfigFileNames(filepath, options) {
var paths = [];
do {
filepath = path.dirname(filepath);
paths.push(path.join(filepath, options.config));
} while (filepath !== options.root);
return paths;
}
function processMatches(matches, version2) {
if ("indent_style" in matches && matches.indent_style === "tab" && !("indent_size" in matches) && semver.gte(version2, "0.10.0")) {
matches.indent_size = "tab";
}
if ("indent_size" in matches && !("tab_width" in matches) && matches.indent_size !== "tab") {
matches.tab_width = matches.indent_size;
}
if ("indent_size" in matches && "tab_width" in matches && matches.indent_size === "tab") {
matches.indent_size = matches.tab_width;
}
return matches;
}
function processOptions(options, filepath) {
if (options === void 0) {
options = {};
}
return {
config: options.config || ".editorconfig",
version: options.version || package_json_1.default.version,
root: path.resolve(options.root || path.parse(filepath).root)
};
}
function buildFullGlob(pathPrefix, glob) {
switch (glob.indexOf("/")) {
case -1:
glob = "**/" + glob;
break;
case 0:
glob = glob.substring(1);
break;
default:
break;
}
return path.join(pathPrefix, glob);
}
function extendProps(props, options) {
if (props === void 0) {
props = {};
}
if (options === void 0) {
options = {};
}
for (var key in options) {
if (options.hasOwnProperty(key)) {
var value = options[key];
var key2 = key.toLowerCase();
var value2 = value;
if (knownProps[key2]) {
value2 = value.toLowerCase();
}
try {
value2 = JSON.parse(value);
} catch (e) {
}
if (typeof value === "undefined" || value === null) {
value2 = String(value);
}
props[key2] = value2;
}
}
return props;
}
function parseFromConfigs(configs, filepath, options) {
return processMatches(configs.reverse().reduce(function(matches, file) {
var pathPrefix = path.dirname(file.name);
file.contents.forEach(function(section) {
var glob = section[0];
var options2 = section[1];
if (!glob) {
return;
}
var fullGlob = buildFullGlob(pathPrefix, glob);
if (!fnmatch(filepath, fullGlob)) {
return;
}
matches = extendProps(matches, options2);
});
return matches;
}, {}), options.version);
}
function getConfigsForFiles(files) {
var configs = [];
for (var i in files) {
if (files.hasOwnProperty(i)) {
var file = files[i];
var contents = ini_1.parseString(file.contents);
configs.push({
name: file.name,
contents
});
if ((contents[0][1].root || "").toLowerCase() === "true") {
break;
}
}
}
return configs;
}
function readConfigFiles(filepaths) {
return __awaiter2(this, void 0, void 0, function() {
return __generator2(this, function(_a) {
return [2, Promise.all(filepaths.map(function(name) {
return new Promise(function(resolve) {
fs2.readFile(name, "utf8", function(err, data) {
resolve({
name,
contents: err ? "" : data
});
});
});
}))];
});
});
}
function readConfigFilesSync(filepaths) {
var files = [];
var file;
filepaths.forEach(function(filepath) {
try {
file = fs2.readFileSync(filepath, "utf8");
} catch (e) {
file = "";
}
files.push({
name: filepath,
contents: file
});
});
return files;
}
function opts(filepath, options) {
if (options === void 0) {
options = {};
}
var resolvedFilePath = path.resolve(filepath);
return [resolvedFilePath, processOptions(options, resolvedFilePath)];
}
function parseFromFiles(filepath, files, options) {
if (options === void 0) {
options = {};
}
return __awaiter2(this, void 0, void 0, function() {
var _a, resolvedFilePath, processedOptions;
return __generator2(this, function(_b) {
_a = opts(filepath, options), resolvedFilePath = _a[0], processedOptions = _a[1];
return [2, files.then(getConfigsForFiles).then(function(configs) {
return parseFromConfigs(configs, resolvedFilePath, processedOptions);
})];
});
});
}
exports2.parseFromFiles = parseFromFiles;
function parseFromFilesSync(filepath, files, options) {
if (options === void 0) {
options = {};
}
var _a = opts(filepath, options), resolvedFilePath = _a[0], processedOptions = _a[1];
return parseFromConfigs(getConfigsForFiles(files), resolvedFilePath, processedOptions);
}
exports2.parseFromFilesSync = parseFromFilesSync;
function parse2(_filepath, _options) {
if (_options === void 0) {
_options = {};
}
return __awaiter2(this, void 0, void 0, function() {
var _a, resolvedFilePath, processedOptions, filepaths;
return __generator2(this, function(_b) {
_a = opts(_filepath, _options), resolvedFilePath = _a[0], processedOptions = _a[1];
filepaths = getConfigFileNames(resolvedFilePath, processedOptions);
return [2, readConfigFiles(filepaths).then(getConfigsForFiles).then(function(configs) {
return parseFromConfigs(configs, resolvedFilePath, processedOptions);
})];
});
});
}
exports2.parse = parse2;
function parseSync(_filepath, _options) {
if (_options === void 0) {
_options = {};
}
var _a = opts(_filepath, _options), resolvedFilePath = _a[0], processedOptions = _a[1];
var filepaths = getConfigFileNames(resolvedFilePath, processedOptions);
var files = readConfigFilesSync(filepaths);
return parseFromConfigs(getConfigsForFiles(files), resolvedFilePath, processedOptions);
}
exports2.parseSync = parseSync;
}
});
var require_editorconfig_to_prettier = __commonJS22({
"node_modules/editorconfig-to-prettier/index.js"(exports2, module22) {
module22.exports = editorConfigToPrettier;
function removeUnset(editorConfig) {
const result = {};
const keys = Object.keys(editorConfig);
for (let i = 0; i < keys.length; i++) {
const key = keys[i];
if (editorConfig[key] === "unset") {
continue;
}
result[key] = editorConfig[key];
}
return result;
}
function editorConfigToPrettier(editorConfig) {
if (!editorConfig) {
return null;
}
editorConfig = removeUnset(editorConfig);
if (Object.keys(editorConfig).length === 0) {
return null;
}
const result = {};
if (editorConfig.indent_style) {
result.useTabs = editorConfig.indent_style === "tab";
}
if (editorConfig.indent_size === "tab") {
result.useTabs = true;
}
if (result.useTabs && editorConfig.tab_width) {
result.tabWidth = editorConfig.tab_width;
} else if (editorConfig.indent_style === "space" && editorConfig.indent_size && editorConfig.indent_size !== "tab") {
result.tabWidth = editorConfig.indent_size;
} else if (editorConfig.tab_width !== void 0) {
result.tabWidth = editorConfig.tab_width;
}
if (editorConfig.max_line_length) {
if (editorConfig.max_line_length === "off") {
result.printWidth = Number.POSITIVE_INFINITY;
} else {
result.printWidth = editorConfig.max_line_length;
}
}
if (editorConfig.quote_type === "single") {
result.singleQuote = true;
} else if (editorConfig.quote_type === "double") {
result.singleQuote = false;
}
if (["cr", "crlf", "lf"].indexOf(editorConfig.end_of_line) !== -1) {
result.endOfLine = editorConfig.end_of_line;
}
if (editorConfig.insert_final_newline === false || editorConfig.insert_final_newline === true) {
result.insertFinalNewline = editorConfig.insert_final_newline;
}
return result;
}
}
});
var require_find_project_root = __commonJS22({
"src/config/find-project-root.js"(exports2, module22) {
"use strict";
var fs2 = require("fs");
var path = require("path");
var MARKERS = [".git", ".hg"];
var markerExists = (directory) => MARKERS.some((mark) => fs2.existsSync(path.join(directory, mark)));
function findProjectRoot(directory) {
while (!markerExists(directory)) {
const parentDirectory = path.resolve(directory, "..");
if (parentDirectory === directory) {
break;
}
directory = parentDirectory;
}
return directory;
}
module22.exports = findProjectRoot;
}
});
var require_resolve_config_editorconfig = __commonJS22({
"src/config/resolve-config-editorconfig.js"(exports2, module22) {
"use strict";
var path = require("path");
var editorconfig = require_src2();
var editorConfigToPrettier = require_editorconfig_to_prettier();
var {
default: mem2,
memClear: memClear2
} = (init_dist(), __toCommonJS(dist_exports));
var findProjectRoot = require_find_project_root();
var jsonStringifyMem = (fn) => mem2(fn, {
cacheKey: JSON.stringify
});
var maybeParse = (filePath2, parse2) => filePath2 && parse2(filePath2, {
root: findProjectRoot(path.dirname(path.resolve(filePath2)))
});
var editorconfigAsyncNoCache = async (filePath2) => editorConfigToPrettier(await maybeParse(filePath2, editorconfig.parse));
var editorconfigAsyncWithCache = jsonStringifyMem(editorconfigAsyncNoCache);
var editorconfigSyncNoCache = (filePath2) => editorConfigToPrettier(maybeParse(filePath2, editorconfig.parseSync));
var editorconfigSyncWithCache = jsonStringifyMem(editorconfigSyncNoCache);
function getLoadFunction(opts) {
if (!opts.editorconfig) {
return () => null;
}
if (opts.sync) {
return opts.cache ? editorconfigSyncWithCache : editorconfigSyncNoCache;
}
return opts.cache ? editorconfigAsyncWithCache : editorconfigAsyncNoCache;
}
function clearCache() {
memClear2(editorconfigSyncWithCache);
memClear2(editorconfigAsyncWithCache);
}
module22.exports = {
getLoadFunction,
clearCache
};
}
});
var require_resolve_config = __commonJS22({
"src/config/resolve-config.js"(exports2, module22) {
"use strict";
var path = require("path");
var micromatch = require_micromatch();
var thirdParty = require_third_party();
var loadToml = require_load_toml();
var loadJson5 = require_load_json5();
var partition = require_partition();
var resolve = require_resolve2();
var {
default: mem2,
memClear: memClear2
} = (init_dist(), __toCommonJS(dist_exports));
var resolveEditorConfig = require_resolve_config_editorconfig();
var getExplorerMemoized = mem2((opts) => {
const cosmiconfig = thirdParty["cosmiconfig" + (opts.sync ? "Sync" : "")];
const explorer = cosmiconfig("prettier", {
cache: opts.cache,
transform: (result) => {
if (result && result.config) {
if (typeof result.config === "string") {
const dir = path.dirname(result.filepath);
const modulePath = resolve(result.config, {
paths: [dir]
});
result.config = require(modulePath);
}
if (typeof result.config !== "object") {
throw new TypeError(`Config is only allowed to be an object, but received ${typeof result.config} in "${result.filepath}"`);
}
delete result.config.$schema;
}
return result;
},
searchPlaces: ["package.json", ".prettierrc", ".prettierrc.json", ".prettierrc.yaml", ".prettierrc.yml", ".prettierrc.json5", ".prettierrc.js", ".prettierrc.cjs", "prettier.config.js", "prettier.config.cjs", ".prettierrc.toml"],
loaders: {
".toml": loadToml,
".json5": loadJson5
}
});
return explorer;
}, {
cacheKey: JSON.stringify
});
function getExplorer(opts) {
opts = Object.assign({
sync: false,
cache: false
}, opts);
return getExplorerMemoized(opts);
}
function _resolveConfig(filePath2, opts, sync) {
opts = Object.assign({
useCache: true
}, opts);
const loadOpts = {
cache: Boolean(opts.useCache),
sync: Boolean(sync),
editorconfig: Boolean(opts.editorconfig)
};
const {
load,
search
} = getExplorer(loadOpts);
const loadEditorConfig = resolveEditorConfig.getLoadFunction(loadOpts);
const arr = [opts.config ? load(opts.config) : search(filePath2), loadEditorConfig(filePath2)];
const unwrapAndMerge = ([result, editorConfigured]) => {
const merged = Object.assign(Object.assign({}, editorConfigured), mergeOverrides(result, filePath2));
for (const optionName of ["plugins", "pluginSearchDirs"]) {
if (Array.isArray(merged[optionName])) {
merged[optionName] = merged[optionName].map((value) => typeof value === "string" && value.startsWith(".") ? path.resolve(path.dirname(result.filepath), value) : value);
}
}
if (!result && !editorConfigured) {
return null;
}
delete merged.insertFinalNewline;
return merged;
};
if (loadOpts.sync) {
return unwrapAndMerge(arr);
}
return Promise.all(arr).then(unwrapAndMerge);
}
var resolveConfig = (filePath2, opts) => _resolveConfig(filePath2, opts, false);
resolveConfig.sync = (filePath2, opts) => _resolveConfig(filePath2, opts, true);
function clearCache() {
memClear2(getExplorerMemoized);
resolveEditorConfig.clearCache();
}
async function resolveConfigFile(filePath2) {
const {
search
} = getExplorer({
sync: false
});
const result = await search(filePath2);
return result ? result.filepath : null;
}
resolveConfigFile.sync = (filePath2) => {
const {
search
} = getExplorer({
sync: true
});
const result = search(filePath2);
return result ? result.filepath : null;
};
function mergeOverrides(configResult, filePath2) {
const {
config: config2,
filepath: configPath
} = configResult || {};
const _ref = config2 || {}, {
overrides
} = _ref, options = _objectWithoutProperties(_ref, _excluded3);
if (filePath2 && overrides) {
const relativeFilePath = path.relative(path.dirname(configPath), filePath2);
for (const override of overrides) {
if (pathMatchesGlobs(relativeFilePath, override.files, override.excludeFiles)) {
Object.assign(options, override.options);
}
}
}
return options;
}
function pathMatchesGlobs(filePath2, patterns, excludedPatterns) {
const patternList = Array.isArray(patterns) ? patterns : [patterns];
const [withSlashes, withoutSlashes] = partition(patternList, (pattern) => pattern.includes("/"));
return micromatch.isMatch(filePath2, withoutSlashes, {
ignore: excludedPatterns,
basename: true,
dot: true
}) || micromatch.isMatch(filePath2, withSlashes, {
ignore: excludedPatterns,
basename: false,
dot: true
});
}
module22.exports = {
resolveConfig,
resolveConfigFile,
clearCache
};
}
});
var require_ignore = __commonJS22({
"node_modules/ignore/index.js"(exports2, module22) {
function makeArray(subject) {
return Array.isArray(subject) ? subject : [subject];
}
var EMPTY = "";
var SPACE = " ";
var ESCAPE = "\\";
var REGEX_TEST_BLANK_LINE = /^\s+$/;
var REGEX_REPLACE_LEADING_EXCAPED_EXCLAMATION = /^\\!/;
var REGEX_REPLACE_LEADING_EXCAPED_HASH = /^\\#/;
var REGEX_SPLITALL_CRLF = /\r?\n/g;
var REGEX_TEST_INVALID_PATH = /^\.*\/|^\.+$/;
var SLASH = "/";
var KEY_IGNORE = typeof Symbol !== "undefined" ? Symbol.for("node-ignore") : "node-ignore";
var define2 = (object, key, value) => Object.defineProperty(object, key, {
value
});
var REGEX_REGEXP_RANGE = /([0-z])-([0-z])/g;
var RETURN_FALSE = () => false;
var sanitizeRange = (range) => range.replace(REGEX_REGEXP_RANGE, (match, from, to) => from.charCodeAt(0) <= to.charCodeAt(0) ? match : EMPTY);
var cleanRangeBackSlash = (slashes) => {
const {
length
} = slashes;
return slashes.slice(0, length - length % 2);
};
var REPLACERS = [[/\\?\s+$/, (match) => match.indexOf("\\") === 0 ? SPACE : EMPTY], [/\\\s/g, () => SPACE], [/[\\$.|*+(){^]/g, (match) => `\\${match}`], [/(?!\\)\?/g, () => "[^/]"], [/^\//, () => "^"], [/\//g, () => "\\/"], [/^\^*\\\*\\\*\\\//, () => "^(?:.*\\/)?"], [/^(?=[^^])/, function startingReplacer() {
return !/\/(?!$)/.test(this) ? "(?:^|\\/)" : "^";
}], [/\\\/\\\*\\\*(?=\\\/|$)/g, (_, index, str) => index + 6 < str.length ? "(?:\\/[^\\/]+)*" : "\\/.+"], [/(^|[^\\]+)\\\*(?=.+)/g, (_, p1) => `${p1}[^\\/]*`], [/\\\\\\(?=[$.|*+(){^])/g, () => ESCAPE], [/\\\\/g, () => ESCAPE], [/(\\)?\[([^\]/]*?)(\\*)($|\])/g, (match, leadEscape, range, endEscape, close) => leadEscape === ESCAPE ? `\\[${range}${cleanRangeBackSlash(endEscape)}${close}` : close === "]" ? endEscape.length % 2 === 0 ? `[${sanitizeRange(range)}${endEscape}]` : "[]" : "[]"], [/(?:[^*])$/, (match) => /\/$/.test(match) ? `${match}$` : `${match}(?=$|\\/$)`], [/(\^|\\\/)?\\\*$/, (_, p1) => {
const prefix = p1 ? `${p1}[^/]+` : "[^/]*";
return `${prefix}(?=$|\\/$)`;
}]];
var regexCache = /* @__PURE__ */ Object.create(null);
var makeRegex = (pattern, ignoreCase) => {
let source = regexCache[pattern];
if (!source) {
source = REPLACERS.reduce((prev, current) => prev.replace(current[0], current[1].bind(pattern)), pattern);
regexCache[pattern] = source;
}
return ignoreCase ? new RegExp(source, "i") : new RegExp(source);
};
var isString = (subject) => typeof subject === "string";
var checkPattern = (pattern) => pattern && isString(pattern) && !REGEX_TEST_BLANK_LINE.test(pattern) && pattern.indexOf("#") !== 0;
var splitPattern = (pattern) => pattern.split(REGEX_SPLITALL_CRLF);
var IgnoreRule = class {
constructor(origin, pattern, negative, regex) {
this.origin = origin;
this.pattern = pattern;
this.negative = negative;
this.regex = regex;
}
};
var createRule = (pattern, ignoreCase) => {
const origin = pattern;
let negative = false;
if (pattern.indexOf("!") === 0) {
negative = true;
pattern = pattern.substr(1);
}
pattern = pattern.replace(REGEX_REPLACE_LEADING_EXCAPED_EXCLAMATION, "!").replace(REGEX_REPLACE_LEADING_EXCAPED_HASH, "#");
const regex = makeRegex(pattern, ignoreCase);
return new IgnoreRule(origin, pattern, negative, regex);
};
var throwError = (message, Ctor) => {
throw new Ctor(message);
};
var checkPath = (path, originalPath, doThrow) => {
if (!isString(path)) {
return doThrow(`path must be a string, but got \`${originalPath}\``, TypeError);
}
if (!path) {
return doThrow(`path must not be empty`, TypeError);
}
if (checkPath.isNotRelative(path)) {
const r = "`path.relative()`d";
return doThrow(`path should be a ${r} string, but got "${originalPath}"`, RangeError);
}
return true;
};
var isNotRelative = (path) => REGEX_TEST_INVALID_PATH.test(path);
checkPath.isNotRelative = isNotRelative;
checkPath.convert = (p) => p;
var Ignore = class {
constructor({
ignorecase = true,
ignoreCase = ignorecase,
allowRelativePaths = false
} = {}) {
define2(this, KEY_IGNORE, true);
this._rules = [];
this._ignoreCase = ignoreCase;
this._allowRelativePaths = allowRelativePaths;
this._initCache();
}
_initCache() {
this._ignoreCache = /* @__PURE__ */ Object.create(null);
this._testCache = /* @__PURE__ */ Object.create(null);
}
_addPattern(pattern) {
if (pattern && pattern[KEY_IGNORE]) {
this._rules = this._rules.concat(pattern._rules);
this._added = true;
return;
}
if (checkPattern(pattern)) {
const rule = createRule(pattern, this._ignoreCase);
this._added = true;
this._rules.push(rule);
}
}
add(pattern) {
this._added = false;
makeArray(isString(pattern) ? splitPattern(pattern) : pattern).forEach(this._addPattern, this);
if (this._added) {
this._initCache();
}
return this;
}
addPattern(pattern) {
return this.add(pattern);
}
_testOne(path, checkUnignored) {
let ignored = false;
let unignored = false;
this._rules.forEach((rule) => {
const {
negative
} = rule;
if (unignored === negative && ignored !== unignored || negative && !ignored && !unignored && !checkUnignored) {
return;
}
const matched = rule.regex.test(path);
if (matched) {
ignored = !negative;
unignored = negative;
}
});
return {
ignored,
unignored
};
}
_test(originalPath, cache, checkUnignored, slices) {
const path = originalPath && checkPath.convert(originalPath);
checkPath(path, originalPath, this._allowRelativePaths ? RETURN_FALSE : throwError);
return this._t(path, cache, checkUnignored, slices);
}
_t(path, cache, checkUnignored, slices) {
if (path in cache) {
return cache[path];
}
if (!slices) {
slices = path.split(SLASH);
}
slices.pop();
if (!slices.length) {
return cache[path] = this._testOne(path, checkUnignored);
}
const parent = this._t(slices.join(SLASH) + SLASH, cache, checkUnignored, slices);
return cache[path] = parent.ignored ? parent : this._testOne(path, checkUnignored);
}
ignores(path) {
return this._test(path, this._ignoreCache, false).ignored;
}
createFilter() {
return (path) => !this.ignores(path);
}
filter(paths) {
return makeArray(paths).filter(this.createFilter());
}
test(path) {
return this._test(path, this._testCache, true);
}
};
var factory = (options) => new Ignore(options);
var isPathValid = (path) => checkPath(path && checkPath.convert(path), path, RETURN_FALSE);
factory.isPathValid = isPathValid;
factory.default = factory;
module22.exports = factory;
if (typeof process !== "undefined" && (process.env && process.env.IGNORE_TEST_WIN32 || process.platform === "win32")) {
const makePosix = (str) => /^\\\\\?\\/.test(str) || /["<>|\u0000-\u001F]+/u.test(str) ? str : str.replace(/\\/g, "/");
checkPath.convert = makePosix;
const REGIX_IS_WINDOWS_PATH_ABSOLUTE = /^[a-z]:\//i;
checkPath.isNotRelative = (path) => REGIX_IS_WINDOWS_PATH_ABSOLUTE.test(path) || isNotRelative(path);
}
}
});
var require_get_file_content_or_null = __commonJS22({
"src/utils/get-file-content-or-null.js"(exports2, module22) {
"use strict";
var fs2 = require("fs");
var fsAsync = fs2.promises;
async function getFileContentOrNull(filename) {
try {
return await fsAsync.readFile(filename, "utf8");
} catch (error) {
return handleError(filename, error);
}
}
getFileContentOrNull.sync = function(filename) {
try {
return fs2.readFileSync(filename, "utf8");
} catch (error) {
return handleError(filename, error);
}
};
function handleError(filename, error) {
if (error && error.code === "ENOENT") {
return null;
}
throw new Error(`Unable to read ${filename}: ${error.message}`);
}
module22.exports = getFileContentOrNull;
}
});
var require_create_ignorer = __commonJS22({
"src/common/create-ignorer.js"(exports2, module22) {
"use strict";
var path = require("path");
var ignore = require_ignore().default;
var getFileContentOrNull = require_get_file_content_or_null();
async function createIgnorer(ignorePath, withNodeModules) {
const ignoreContent = ignorePath ? await getFileContentOrNull(path.resolve(ignorePath)) : null;
return _createIgnorer(ignoreContent, withNodeModules);
}
createIgnorer.sync = function(ignorePath, withNodeModules) {
const ignoreContent = !ignorePath ? null : getFileContentOrNull.sync(path.resolve(ignorePath));
return _createIgnorer(ignoreContent, withNodeModules);
};
function _createIgnorer(ignoreContent, withNodeModules) {
const ignorer = ignore({
allowRelativePaths: true
}).add(ignoreContent || "");
if (!withNodeModules) {
ignorer.add("node_modules");
}
return ignorer;
}
module22.exports = createIgnorer;
}
});
var require_get_file_info = __commonJS22({
"src/common/get-file-info.js"(exports2, module22) {
"use strict";
var path = require("path");
var options = require_options();
var config2 = require_resolve_config();
var createIgnorer = require_create_ignorer();
async function getFileInfo2(filePath2, opts) {
if (typeof filePath2 !== "string") {
throw new TypeError(`expect \`filePath\` to be a string, got \`${typeof filePath2}\``);
}
const ignorer = await createIgnorer(opts.ignorePath, opts.withNodeModules);
return _getFileInfo({
ignorer,
filePath: filePath2,
plugins: opts.plugins,
resolveConfig: opts.resolveConfig,
ignorePath: opts.ignorePath,
sync: false
});
}
getFileInfo2.sync = function(filePath2, opts) {
if (typeof filePath2 !== "string") {
throw new TypeError(`expect \`filePath\` to be a string, got \`${typeof filePath2}\``);
}
const ignorer = createIgnorer.sync(opts.ignorePath, opts.withNodeModules);
return _getFileInfo({
ignorer,
filePath: filePath2,
plugins: opts.plugins,
resolveConfig: opts.resolveConfig,
ignorePath: opts.ignorePath,
sync: true
});
};
function getFileParser(resolvedConfig, filePath2, plugins2) {
if (resolvedConfig && resolvedConfig.parser) {
return resolvedConfig.parser;
}
const inferredParser = options.inferParser(filePath2, plugins2);
if (inferredParser) {
return inferredParser;
}
return null;
}
function _getFileInfo({
ignorer,
filePath: filePath2,
plugins: plugins2,
resolveConfig = false,
ignorePath,
sync = false
}) {
const normalizedFilePath = normalizeFilePath(filePath2, ignorePath);
const fileInfo = {
ignored: ignorer.ignores(normalizedFilePath),
inferredParser: null
};
if (fileInfo.ignored) {
return fileInfo;
}
let resolvedConfig;
if (resolveConfig) {
if (sync) {
resolvedConfig = config2.resolveConfig.sync(filePath2);
} else {
return config2.resolveConfig(filePath2).then((resolvedConfig2) => {
fileInfo.inferredParser = getFileParser(resolvedConfig2, filePath2, plugins2);
return fileInfo;
});
}
}
fileInfo.inferredParser = getFileParser(resolvedConfig, filePath2, plugins2);
return fileInfo;
}
function normalizeFilePath(filePath2, ignorePath) {
return ignorePath ? path.relative(path.dirname(ignorePath), filePath2) : filePath2;
}
module22.exports = getFileInfo2;
}
});
var require_util_shared = __commonJS22({
"src/common/util-shared.js"(exports2, module22) {
"use strict";
var {
getMaxContinuousCount,
getStringWidth,
getAlignmentSize,
getIndentSize,
skip,
skipWhitespace,
skipSpaces,
skipNewline,
skipToLineEnd,
skipEverythingButNewLine,
skipInlineComment,
skipTrailingComment,
hasNewline,
hasNewlineInRange,
hasSpaces,
isNextLineEmpty,
isNextLineEmptyAfterIndex,
isPreviousLineEmpty,
getNextNonSpaceNonCommentCharacterIndex,
makeString,
addLeadingComment,
addDanglingComment,
addTrailingComment
} = require_util();
module22.exports = {
getMaxContinuousCount,
getStringWidth,
getAlignmentSize,
getIndentSize,
skip,
skipWhitespace,
skipSpaces,
skipNewline,
skipToLineEnd,
skipEverythingButNewLine,
skipInlineComment,
skipTrailingComment,
hasNewline,
hasNewlineInRange,
hasSpaces,
isNextLineEmpty,
isNextLineEmptyAfterIndex,
isPreviousLineEmpty,
getNextNonSpaceNonCommentCharacterIndex,
makeString,
addLeadingComment,
addDanglingComment,
addTrailingComment
};
}
});
var require_array3 = __commonJS22({
"node_modules/fast-glob/out/utils/array.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.splitWhen = exports2.flatten = void 0;
function flatten(items) {
return items.reduce((collection, item) => [].concat(collection, item), []);
}
exports2.flatten = flatten;
function splitWhen(items, predicate) {
const result = [[]];
let groupIndex = 0;
for (const item of items) {
if (predicate(item)) {
groupIndex++;
result[groupIndex] = [];
} else {
result[groupIndex].push(item);
}
}
return result;
}
exports2.splitWhen = splitWhen;
}
});
var require_errno = __commonJS22({
"node_modules/fast-glob/out/utils/errno.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.isEnoentCodeError = void 0;
function isEnoentCodeError(error) {
return error.code === "ENOENT";
}
exports2.isEnoentCodeError = isEnoentCodeError;
}
});
var require_fs = __commonJS22({
"node_modules/fast-glob/out/utils/fs.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.createDirentFromStats = void 0;
var DirentFromStats = class {
constructor(name, stats) {
this.name = name;
this.isBlockDevice = stats.isBlockDevice.bind(stats);
this.isCharacterDevice = stats.isCharacterDevice.bind(stats);
this.isDirectory = stats.isDirectory.bind(stats);
this.isFIFO = stats.isFIFO.bind(stats);
this.isFile = stats.isFile.bind(stats);
this.isSocket = stats.isSocket.bind(stats);
this.isSymbolicLink = stats.isSymbolicLink.bind(stats);
}
};
function createDirentFromStats(name, stats) {
return new DirentFromStats(name, stats);
}
exports2.createDirentFromStats = createDirentFromStats;
}
});
var require_path = __commonJS22({
"node_modules/fast-glob/out/utils/path.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.removeLeadingDotSegment = exports2.escape = exports2.makeAbsolute = exports2.unixify = void 0;
var path = require("path");
var LEADING_DOT_SEGMENT_CHARACTERS_COUNT = 2;
var UNESCAPED_GLOB_SYMBOLS_RE = /(\\?)([()*?[\]{|}]|^!|[!+@](?=\())/g;
function unixify(filepath) {
return filepath.replace(/\\/g, "/");
}
exports2.unixify = unixify;
function makeAbsolute(cwd, filepath) {
return path.resolve(cwd, filepath);
}
exports2.makeAbsolute = makeAbsolute;
function escape(pattern) {
return pattern.replace(UNESCAPED_GLOB_SYMBOLS_RE, "\\$2");
}
exports2.escape = escape;
function removeLeadingDotSegment(entry) {
if (entry.charAt(0) === ".") {
const secondCharactery = entry.charAt(1);
if (secondCharactery === "/" || secondCharactery === "\\") {
return entry.slice(LEADING_DOT_SEGMENT_CHARACTERS_COUNT);
}
}
return entry;
}
exports2.removeLeadingDotSegment = removeLeadingDotSegment;
}
});
var require_is_extglob = __commonJS22({
"node_modules/is-extglob/index.js"(exports2, module22) {
module22.exports = function isExtglob(str) {
if (typeof str !== "string" || str === "") {
return false;
}
var match;
while (match = /(\\).|([@?!+*]\(.*\))/g.exec(str)) {
if (match[2])
return true;
str = str.slice(match.index + match[0].length);
}
return false;
};
}
});
var require_is_glob = __commonJS22({
"node_modules/is-glob/index.js"(exports2, module22) {
var isExtglob = require_is_extglob();
var chars = {
"{": "}",
"(": ")",
"[": "]"
};
var strictCheck = function(str) {
if (str[0] === "!") {
return true;
}
var index = 0;
var pipeIndex = -2;
var closeSquareIndex = -2;
var closeCurlyIndex = -2;
var closeParenIndex = -2;
var backSlashIndex = -2;
while (index < str.length) {
if (str[index] === "*") {
return true;
}
if (str[index + 1] === "?" && /[\].+)]/.test(str[index])) {
return true;
}
if (closeSquareIndex !== -1 && str[index] === "[" && str[index + 1] !== "]") {
if (closeSquareIndex < index) {
closeSquareIndex = str.indexOf("]", index);
}
if (closeSquareIndex > index) {
if (backSlashIndex === -1 || backSlashIndex > closeSquareIndex) {
return true;
}
backSlashIndex = str.indexOf("\\", index);
if (backSlashIndex === -1 || backSlashIndex > closeSquareIndex) {
return true;
}
}
}
if (closeCurlyIndex !== -1 && str[index] === "{" && str[index + 1] !== "}") {
closeCurlyIndex = str.indexOf("}", index);
if (closeCurlyIndex > index) {
backSlashIndex = str.indexOf("\\", index);
if (backSlashIndex === -1 || backSlashIndex > closeCurlyIndex) {
return true;
}
}
}
if (closeParenIndex !== -1 && str[index] === "(" && str[index + 1] === "?" && /[:!=]/.test(str[index + 2]) && str[index + 3] !== ")") {
closeParenIndex = str.indexOf(")", index);
if (closeParenIndex > index) {
backSlashIndex = str.indexOf("\\", index);
if (backSlashIndex === -1 || backSlashIndex > closeParenIndex) {
return true;
}
}
}
if (pipeIndex !== -1 && str[index] === "(" && str[index + 1] !== "|") {
if (pipeIndex < index) {
pipeIndex = str.indexOf("|", index);
}
if (pipeIndex !== -1 && str[pipeIndex + 1] !== ")") {
closeParenIndex = str.indexOf(")", pipeIndex);
if (closeParenIndex > pipeIndex) {
backSlashIndex = str.indexOf("\\", pipeIndex);
if (backSlashIndex === -1 || backSlashIndex > closeParenIndex) {
return true;
}
}
}
}
if (str[index] === "\\") {
var open = str[index + 1];
index += 2;
var close = chars[open];
if (close) {
var n2 = str.indexOf(close, index);
if (n2 !== -1) {
index = n2 + 1;
}
}
if (str[index] === "!") {
return true;
}
} else {
index++;
}
}
return false;
};
var relaxedCheck = function(str) {
if (str[0] === "!") {
return true;
}
var index = 0;
while (index < str.length) {
if (/[*?{}()[\]]/.test(str[index])) {
return true;
}
if (str[index] === "\\") {
var open = str[index + 1];
index += 2;
var close = chars[open];
if (close) {
var n2 = str.indexOf(close, index);
if (n2 !== -1) {
index = n2 + 1;
}
}
if (str[index] === "!") {
return true;
}
} else {
index++;
}
}
return false;
};
module22.exports = function isGlob(str, options) {
if (typeof str !== "string" || str === "") {
return false;
}
if (isExtglob(str)) {
return true;
}
var check = strictCheck;
if (options && options.strict === false) {
check = relaxedCheck;
}
return check(str);
};
}
});
var require_glob_parent = __commonJS22({
"node_modules/glob-parent/index.js"(exports2, module22) {
"use strict";
var isGlob = require_is_glob();
var pathPosixDirname = require("path").posix.dirname;
var isWin32 = require("os").platform() === "win32";
var slash = "/";
var backslash = /\\/g;
var enclosure = /[\{\[].*[\}\]]$/;
var globby = /(^|[^\\])([\{\[]|\([^\)]+$)/;
var escaped = /\\([\!\*\?\|\[\]\(\)\{\}])/g;
module22.exports = function globParent(str, opts) {
var options = Object.assign({
flipBackslashes: true
}, opts);
if (options.flipBackslashes && isWin32 && str.indexOf(slash) < 0) {
str = str.replace(backslash, slash);
}
if (enclosure.test(str)) {
str += slash;
}
str += "a";
do {
str = pathPosixDirname(str);
} while (isGlob(str) || globby.test(str));
return str.replace(escaped, "$1");
};
}
});
var require_pattern = __commonJS22({
"node_modules/fast-glob/out/utils/pattern.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.matchAny = exports2.convertPatternsToRe = exports2.makeRe = exports2.getPatternParts = exports2.expandBraceExpansion = exports2.expandPatternsWithBraceExpansion = exports2.isAffectDepthOfReadingPattern = exports2.endsWithSlashGlobStar = exports2.hasGlobStar = exports2.getBaseDirectory = exports2.isPatternRelatedToParentDirectory = exports2.getPatternsOutsideCurrentDirectory = exports2.getPatternsInsideCurrentDirectory = exports2.getPositivePatterns = exports2.getNegativePatterns = exports2.isPositivePattern = exports2.isNegativePattern = exports2.convertToNegativePattern = exports2.convertToPositivePattern = exports2.isDynamicPattern = exports2.isStaticPattern = void 0;
var path = require("path");
var globParent = require_glob_parent();
var micromatch = require_micromatch();
var GLOBSTAR = "**";
var ESCAPE_SYMBOL = "\\";
var COMMON_GLOB_SYMBOLS_RE = /[*?]|^!/;
var REGEX_CHARACTER_CLASS_SYMBOLS_RE = /\[[^[]*]/;
var REGEX_GROUP_SYMBOLS_RE = /(?:^|[^!*+?@])\([^(]*\|[^|]*\)/;
var GLOB_EXTENSION_SYMBOLS_RE = /[!*+?@]\([^(]*\)/;
var BRACE_EXPANSION_SEPARATORS_RE = /,|\.\./;
function isStaticPattern(pattern, options = {}) {
return !isDynamicPattern(pattern, options);
}
exports2.isStaticPattern = isStaticPattern;
function isDynamicPattern(pattern, options = {}) {
if (pattern === "") {
return false;
}
if (options.caseSensitiveMatch === false || pattern.includes(ESCAPE_SYMBOL)) {
return true;
}
if (COMMON_GLOB_SYMBOLS_RE.test(pattern) || REGEX_CHARACTER_CLASS_SYMBOLS_RE.test(pattern) || REGEX_GROUP_SYMBOLS_RE.test(pattern)) {
return true;
}
if (options.extglob !== false && GLOB_EXTENSION_SYMBOLS_RE.test(pattern)) {
return true;
}
if (options.braceExpansion !== false && hasBraceExpansion(pattern)) {
return true;
}
return false;
}
exports2.isDynamicPattern = isDynamicPattern;
function hasBraceExpansion(pattern) {
const openingBraceIndex = pattern.indexOf("{");
if (openingBraceIndex === -1) {
return false;
}
const closingBraceIndex = pattern.indexOf("}", openingBraceIndex + 1);
if (closingBraceIndex === -1) {
return false;
}
const braceContent = pattern.slice(openingBraceIndex, closingBraceIndex);
return BRACE_EXPANSION_SEPARATORS_RE.test(braceContent);
}
function convertToPositivePattern(pattern) {
return isNegativePattern(pattern) ? pattern.slice(1) : pattern;
}
exports2.convertToPositivePattern = convertToPositivePattern;
function convertToNegativePattern(pattern) {
return "!" + pattern;
}
exports2.convertToNegativePattern = convertToNegativePattern;
function isNegativePattern(pattern) {
return pattern.startsWith("!") && pattern[1] !== "(";
}
exports2.isNegativePattern = isNegativePattern;
function isPositivePattern(pattern) {
return !isNegativePattern(pattern);
}
exports2.isPositivePattern = isPositivePattern;
function getNegativePatterns(patterns) {
return patterns.filter(isNegativePattern);
}
exports2.getNegativePatterns = getNegativePatterns;
function getPositivePatterns(patterns) {
return patterns.filter(isPositivePattern);
}
exports2.getPositivePatterns = getPositivePatterns;
function getPatternsInsideCurrentDirectory(patterns) {
return patterns.filter((pattern) => !isPatternRelatedToParentDirectory(pattern));
}
exports2.getPatternsInsideCurrentDirectory = getPatternsInsideCurrentDirectory;
function getPatternsOutsideCurrentDirectory(patterns) {
return patterns.filter(isPatternRelatedToParentDirectory);
}
exports2.getPatternsOutsideCurrentDirectory = getPatternsOutsideCurrentDirectory;
function isPatternRelatedToParentDirectory(pattern) {
return pattern.startsWith("..") || pattern.startsWith("./..");
}
exports2.isPatternRelatedToParentDirectory = isPatternRelatedToParentDirectory;
function getBaseDirectory(pattern) {
return globParent(pattern, {
flipBackslashes: false
});
}
exports2.getBaseDirectory = getBaseDirectory;
function hasGlobStar(pattern) {
return pattern.includes(GLOBSTAR);
}
exports2.hasGlobStar = hasGlobStar;
function endsWithSlashGlobStar(pattern) {
return pattern.endsWith("/" + GLOBSTAR);
}
exports2.endsWithSlashGlobStar = endsWithSlashGlobStar;
function isAffectDepthOfReadingPattern(pattern) {
const basename = path.basename(pattern);
return endsWithSlashGlobStar(pattern) || isStaticPattern(basename);
}
exports2.isAffectDepthOfReadingPattern = isAffectDepthOfReadingPattern;
function expandPatternsWithBraceExpansion(patterns) {
return patterns.reduce((collection, pattern) => {
return collection.concat(expandBraceExpansion(pattern));
}, []);
}
exports2.expandPatternsWithBraceExpansion = expandPatternsWithBraceExpansion;
function expandBraceExpansion(pattern) {
return micromatch.braces(pattern, {
expand: true,
nodupes: true
});
}
exports2.expandBraceExpansion = expandBraceExpansion;
function getPatternParts(pattern, options) {
let {
parts
} = micromatch.scan(pattern, Object.assign(Object.assign({}, options), {
parts: true
}));
if (parts.length === 0) {
parts = [pattern];
}
if (parts[0].startsWith("/")) {
parts[0] = parts[0].slice(1);
parts.unshift("");
}
return parts;
}
exports2.getPatternParts = getPatternParts;
function makeRe(pattern, options) {
return micromatch.makeRe(pattern, options);
}
exports2.makeRe = makeRe;
function convertPatternsToRe(patterns, options) {
return patterns.map((pattern) => makeRe(pattern, options));
}
exports2.convertPatternsToRe = convertPatternsToRe;
function matchAny(entry, patternsRe) {
return patternsRe.some((patternRe) => patternRe.test(entry));
}
exports2.matchAny = matchAny;
}
});
var require_merge2 = __commonJS22({
"node_modules/merge2/index.js"(exports2, module22) {
"use strict";
var Stream = require("stream");
var PassThrough = Stream.PassThrough;
var slice = Array.prototype.slice;
module22.exports = merge2;
function merge2() {
const streamsQueue = [];
const args = slice.call(arguments);
let merging = false;
let options = args[args.length - 1];
if (options && !Array.isArray(options) && options.pipe == null) {
args.pop();
} else {
options = {};
}
const doEnd = options.end !== false;
const doPipeError = options.pipeError === true;
if (options.objectMode == null) {
options.objectMode = true;
}
if (options.highWaterMark == null) {
options.highWaterMark = 64 * 1024;
}
const mergedStream = PassThrough(options);
function addStream() {
for (let i = 0, len = arguments.length; i < len; i++) {
streamsQueue.push(pauseStreams(arguments[i], options));
}
mergeStream();
return this;
}
function mergeStream() {
if (merging) {
return;
}
merging = true;
let streams = streamsQueue.shift();
if (!streams) {
process.nextTick(endStream);
return;
}
if (!Array.isArray(streams)) {
streams = [streams];
}
let pipesCount = streams.length + 1;
function next() {
if (--pipesCount > 0) {
return;
}
merging = false;
mergeStream();
}
function pipe(stream) {
function onend() {
stream.removeListener("merge2UnpipeEnd", onend);
stream.removeListener("end", onend);
if (doPipeError) {
stream.removeListener("error", onerror);
}
next();
}
function onerror(err) {
mergedStream.emit("error", err);
}
if (stream._readableState.endEmitted) {
return next();
}
stream.on("merge2UnpipeEnd", onend);
stream.on("end", onend);
if (doPipeError) {
stream.on("error", onerror);
}
stream.pipe(mergedStream, {
end: false
});
stream.resume();
}
for (let i = 0; i < streams.length; i++) {
pipe(streams[i]);
}
next();
}
function endStream() {
merging = false;
mergedStream.emit("queueDrain");
if (doEnd) {
mergedStream.end();
}
}
mergedStream.setMaxListeners(0);
mergedStream.add = addStream;
mergedStream.on("unpipe", function(stream) {
stream.emit("merge2UnpipeEnd");
});
if (args.length) {
addStream.apply(null, args);
}
return mergedStream;
}
function pauseStreams(streams, options) {
if (!Array.isArray(streams)) {
if (!streams._readableState && streams.pipe) {
streams = streams.pipe(PassThrough(options));
}
if (!streams._readableState || !streams.pause || !streams.pipe) {
throw new Error("Only readable stream can be merged.");
}
streams.pause();
} else {
for (let i = 0, len = streams.length; i < len; i++) {
streams[i] = pauseStreams(streams[i], options);
}
}
return streams;
}
}
});
var require_stream = __commonJS22({
"node_modules/fast-glob/out/utils/stream.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.merge = void 0;
var merge2 = require_merge2();
function merge(streams) {
const mergedStream = merge2(streams);
streams.forEach((stream) => {
stream.once("error", (error) => mergedStream.emit("error", error));
});
mergedStream.once("close", () => propagateCloseEventToSources(streams));
mergedStream.once("end", () => propagateCloseEventToSources(streams));
return mergedStream;
}
exports2.merge = merge;
function propagateCloseEventToSources(streams) {
streams.forEach((stream) => stream.emit("close"));
}
}
});
var require_string2 = __commonJS22({
"node_modules/fast-glob/out/utils/string.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.isEmpty = exports2.isString = void 0;
function isString(input) {
return typeof input === "string";
}
exports2.isString = isString;
function isEmpty(input) {
return input === "";
}
exports2.isEmpty = isEmpty;
}
});
var require_utils4 = __commonJS22({
"node_modules/fast-glob/out/utils/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.string = exports2.stream = exports2.pattern = exports2.path = exports2.fs = exports2.errno = exports2.array = void 0;
var array = require_array3();
exports2.array = array;
var errno = require_errno();
exports2.errno = errno;
var fs2 = require_fs();
exports2.fs = fs2;
var path = require_path();
exports2.path = path;
var pattern = require_pattern();
exports2.pattern = pattern;
var stream = require_stream();
exports2.stream = stream;
var string = require_string2();
exports2.string = string;
}
});
var require_tasks = __commonJS22({
"node_modules/fast-glob/out/managers/tasks.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.convertPatternGroupToTask = exports2.convertPatternGroupsToTasks = exports2.groupPatternsByBaseDirectory = exports2.getNegativePatternsAsPositive = exports2.getPositivePatterns = exports2.convertPatternsToTasks = exports2.generate = void 0;
var utils = require_utils4();
function generate(patterns, settings) {
const positivePatterns = getPositivePatterns(patterns);
const negativePatterns = getNegativePatternsAsPositive(patterns, settings.ignore);
const staticPatterns = positivePatterns.filter((pattern) => utils.pattern.isStaticPattern(pattern, settings));
const dynamicPatterns = positivePatterns.filter((pattern) => utils.pattern.isDynamicPattern(pattern, settings));
const staticTasks = convertPatternsToTasks(staticPatterns, negativePatterns, false);
const dynamicTasks = convertPatternsToTasks(dynamicPatterns, negativePatterns, true);
return staticTasks.concat(dynamicTasks);
}
exports2.generate = generate;
function convertPatternsToTasks(positive, negative, dynamic) {
const tasks = [];
const patternsOutsideCurrentDirectory = utils.pattern.getPatternsOutsideCurrentDirectory(positive);
const patternsInsideCurrentDirectory = utils.pattern.getPatternsInsideCurrentDirectory(positive);
const outsideCurrentDirectoryGroup = groupPatternsByBaseDirectory(patternsOutsideCurrentDirectory);
const insideCurrentDirectoryGroup = groupPatternsByBaseDirectory(patternsInsideCurrentDirectory);
tasks.push(...convertPatternGroupsToTasks(outsideCurrentDirectoryGroup, negative, dynamic));
if ("." in insideCurrentDirectoryGroup) {
tasks.push(convertPatternGroupToTask(".", patternsInsideCurrentDirectory, negative, dynamic));
} else {
tasks.push(...convertPatternGroupsToTasks(insideCurrentDirectoryGroup, negative, dynamic));
}
return tasks;
}
exports2.convertPatternsToTasks = convertPatternsToTasks;
function getPositivePatterns(patterns) {
return utils.pattern.getPositivePatterns(patterns);
}
exports2.getPositivePatterns = getPositivePatterns;
function getNegativePatternsAsPositive(patterns, ignore) {
const negative = utils.pattern.getNegativePatterns(patterns).concat(ignore);
const positive = negative.map(utils.pattern.convertToPositivePattern);
return positive;
}
exports2.getNegativePatternsAsPositive = getNegativePatternsAsPositive;
function groupPatternsByBaseDirectory(patterns) {
const group = {};
return patterns.reduce((collection, pattern) => {
const base = utils.pattern.getBaseDirectory(pattern);
if (base in collection) {
collection[base].push(pattern);
} else {
collection[base] = [pattern];
}
return collection;
}, group);
}
exports2.groupPatternsByBaseDirectory = groupPatternsByBaseDirectory;
function convertPatternGroupsToTasks(positive, negative, dynamic) {
return Object.keys(positive).map((base) => {
return convertPatternGroupToTask(base, positive[base], negative, dynamic);
});
}
exports2.convertPatternGroupsToTasks = convertPatternGroupsToTasks;
function convertPatternGroupToTask(base, positive, negative, dynamic) {
return {
dynamic,
positive,
negative,
base,
patterns: [].concat(positive, negative.map(utils.pattern.convertToNegativePattern))
};
}
exports2.convertPatternGroupToTask = convertPatternGroupToTask;
}
});
var require_patterns = __commonJS22({
"node_modules/fast-glob/out/managers/patterns.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.removeDuplicateSlashes = exports2.transform = void 0;
var DOUBLE_SLASH_RE = /(?!^)\/{2,}/g;
function transform2(patterns) {
return patterns.map((pattern) => removeDuplicateSlashes(pattern));
}
exports2.transform = transform2;
function removeDuplicateSlashes(pattern) {
return pattern.replace(DOUBLE_SLASH_RE, "/");
}
exports2.removeDuplicateSlashes = removeDuplicateSlashes;
}
});
var require_async2 = __commonJS22({
"node_modules/@nodelib/fs.stat/out/providers/async.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.read = void 0;
function read(path, settings, callback) {
settings.fs.lstat(path, (lstatError, lstat) => {
if (lstatError !== null) {
callFailureCallback(callback, lstatError);
return;
}
if (!lstat.isSymbolicLink() || !settings.followSymbolicLink) {
callSuccessCallback(callback, lstat);
return;
}
settings.fs.stat(path, (statError, stat2) => {
if (statError !== null) {
if (settings.throwErrorOnBrokenSymbolicLink) {
callFailureCallback(callback, statError);
return;
}
callSuccessCallback(callback, lstat);
return;
}
if (settings.markSymbolicLink) {
stat2.isSymbolicLink = () => true;
}
callSuccessCallback(callback, stat2);
});
});
}
exports2.read = read;
function callFailureCallback(callback, error) {
callback(error);
}
function callSuccessCallback(callback, result) {
callback(null, result);
}
}
});
var require_sync2 = __commonJS22({
"node_modules/@nodelib/fs.stat/out/providers/sync.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.read = void 0;
function read(path, settings) {
const lstat = settings.fs.lstatSync(path);
if (!lstat.isSymbolicLink() || !settings.followSymbolicLink) {
return lstat;
}
try {
const stat2 = settings.fs.statSync(path);
if (settings.markSymbolicLink) {
stat2.isSymbolicLink = () => true;
}
return stat2;
} catch (error) {
if (!settings.throwErrorOnBrokenSymbolicLink) {
return lstat;
}
throw error;
}
}
exports2.read = read;
}
});
var require_fs2 = __commonJS22({
"node_modules/@nodelib/fs.stat/out/adapters/fs.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.createFileSystemAdapter = exports2.FILE_SYSTEM_ADAPTER = void 0;
var fs2 = require("fs");
exports2.FILE_SYSTEM_ADAPTER = {
lstat: fs2.lstat,
stat: fs2.stat,
lstatSync: fs2.lstatSync,
statSync: fs2.statSync
};
function createFileSystemAdapter(fsMethods) {
if (fsMethods === void 0) {
return exports2.FILE_SYSTEM_ADAPTER;
}
return Object.assign(Object.assign({}, exports2.FILE_SYSTEM_ADAPTER), fsMethods);
}
exports2.createFileSystemAdapter = createFileSystemAdapter;
}
});
var require_settings = __commonJS22({
"node_modules/@nodelib/fs.stat/out/settings.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var fs2 = require_fs2();
var Settings = class {
constructor(_options = {}) {
this._options = _options;
this.followSymbolicLink = this._getValue(this._options.followSymbolicLink, true);
this.fs = fs2.createFileSystemAdapter(this._options.fs);
this.markSymbolicLink = this._getValue(this._options.markSymbolicLink, false);
this.throwErrorOnBrokenSymbolicLink = this._getValue(this._options.throwErrorOnBrokenSymbolicLink, true);
}
_getValue(option, value) {
return option !== null && option !== void 0 ? option : value;
}
};
exports2.default = Settings;
}
});
var require_out = __commonJS22({
"node_modules/@nodelib/fs.stat/out/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.statSync = exports2.stat = exports2.Settings = void 0;
var async = require_async2();
var sync = require_sync2();
var settings_1 = require_settings();
exports2.Settings = settings_1.default;
function stat2(path, optionsOrSettingsOrCallback, callback) {
if (typeof optionsOrSettingsOrCallback === "function") {
async.read(path, getSettings(), optionsOrSettingsOrCallback);
return;
}
async.read(path, getSettings(optionsOrSettingsOrCallback), callback);
}
exports2.stat = stat2;
function statSync(path, optionsOrSettings) {
const settings = getSettings(optionsOrSettings);
return sync.read(path, settings);
}
exports2.statSync = statSync;
function getSettings(settingsOrOptions = {}) {
if (settingsOrOptions instanceof settings_1.default) {
return settingsOrOptions;
}
return new settings_1.default(settingsOrOptions);
}
}
});
var require_queue_microtask = __commonJS22({
"node_modules/queue-microtask/index.js"(exports2, module22) {
var promise;
module22.exports = typeof queueMicrotask === "function" ? queueMicrotask.bind(typeof window !== "undefined" ? window : global) : (cb) => (promise || (promise = Promise.resolve())).then(cb).catch((err) => setTimeout(() => {
throw err;
}, 0));
}
});
var require_run_parallel = __commonJS22({
"node_modules/run-parallel/index.js"(exports2, module22) {
module22.exports = runParallel;
var queueMicrotask2 = require_queue_microtask();
function runParallel(tasks, cb) {
let results, pending, keys;
let isSync = true;
if (Array.isArray(tasks)) {
results = [];
pending = tasks.length;
} else {
keys = Object.keys(tasks);
results = {};
pending = keys.length;
}
function done(err) {
function end() {
if (cb)
cb(err, results);
cb = null;
}
if (isSync)
queueMicrotask2(end);
else
end();
}
function each(i, err, result) {
results[i] = result;
if (--pending === 0 || err) {
done(err);
}
}
if (!pending) {
done(null);
} else if (keys) {
keys.forEach(function(key) {
tasks[key](function(err, result) {
each(key, err, result);
});
});
} else {
tasks.forEach(function(task, i) {
task(function(err, result) {
each(i, err, result);
});
});
}
isSync = false;
}
}
});
var require_constants4 = __commonJS22({
"node_modules/@nodelib/fs.scandir/out/constants.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.IS_SUPPORT_READDIR_WITH_FILE_TYPES = void 0;
var NODE_PROCESS_VERSION_PARTS = process.versions.node.split(".");
if (NODE_PROCESS_VERSION_PARTS[0] === void 0 || NODE_PROCESS_VERSION_PARTS[1] === void 0) {
throw new Error(`Unexpected behavior. The 'process.versions.node' variable has invalid value: ${process.versions.node}`);
}
var MAJOR_VERSION = Number.parseInt(NODE_PROCESS_VERSION_PARTS[0], 10);
var MINOR_VERSION = Number.parseInt(NODE_PROCESS_VERSION_PARTS[1], 10);
var SUPPORTED_MAJOR_VERSION = 10;
var SUPPORTED_MINOR_VERSION = 10;
var IS_MATCHED_BY_MAJOR = MAJOR_VERSION > SUPPORTED_MAJOR_VERSION;
var IS_MATCHED_BY_MAJOR_AND_MINOR = MAJOR_VERSION === SUPPORTED_MAJOR_VERSION && MINOR_VERSION >= SUPPORTED_MINOR_VERSION;
exports2.IS_SUPPORT_READDIR_WITH_FILE_TYPES = IS_MATCHED_BY_MAJOR || IS_MATCHED_BY_MAJOR_AND_MINOR;
}
});
var require_fs3 = __commonJS22({
"node_modules/@nodelib/fs.scandir/out/utils/fs.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.createDirentFromStats = void 0;
var DirentFromStats = class {
constructor(name, stats) {
this.name = name;
this.isBlockDevice = stats.isBlockDevice.bind(stats);
this.isCharacterDevice = stats.isCharacterDevice.bind(stats);
this.isDirectory = stats.isDirectory.bind(stats);
this.isFIFO = stats.isFIFO.bind(stats);
this.isFile = stats.isFile.bind(stats);
this.isSocket = stats.isSocket.bind(stats);
this.isSymbolicLink = stats.isSymbolicLink.bind(stats);
}
};
function createDirentFromStats(name, stats) {
return new DirentFromStats(name, stats);
}
exports2.createDirentFromStats = createDirentFromStats;
}
});
var require_utils5 = __commonJS22({
"node_modules/@nodelib/fs.scandir/out/utils/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.fs = void 0;
var fs2 = require_fs3();
exports2.fs = fs2;
}
});
var require_common3 = __commonJS22({
"node_modules/@nodelib/fs.scandir/out/providers/common.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.joinPathSegments = void 0;
function joinPathSegments(a, b, separator) {
if (a.endsWith(separator)) {
return a + b;
}
return a + separator + b;
}
exports2.joinPathSegments = joinPathSegments;
}
});
var require_async3 = __commonJS22({
"node_modules/@nodelib/fs.scandir/out/providers/async.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.readdir = exports2.readdirWithFileTypes = exports2.read = void 0;
var fsStat = require_out();
var rpl = require_run_parallel();
var constants_1 = require_constants4();
var utils = require_utils5();
var common = require_common3();
function read(directory, settings, callback) {
if (!settings.stats && constants_1.IS_SUPPORT_READDIR_WITH_FILE_TYPES) {
readdirWithFileTypes(directory, settings, callback);
return;
}
readdir(directory, settings, callback);
}
exports2.read = read;
function readdirWithFileTypes(directory, settings, callback) {
settings.fs.readdir(directory, {
withFileTypes: true
}, (readdirError, dirents) => {
if (readdirError !== null) {
callFailureCallback(callback, readdirError);
return;
}
const entries = dirents.map((dirent) => ({
dirent,
name: dirent.name,
path: common.joinPathSegments(directory, dirent.name, settings.pathSegmentSeparator)
}));
if (!settings.followSymbolicLinks) {
callSuccessCallback(callback, entries);
return;
}
const tasks = entries.map((entry) => makeRplTaskEntry(entry, settings));
rpl(tasks, (rplError, rplEntries) => {
if (rplError !== null) {
callFailureCallback(callback, rplError);
return;
}
callSuccessCallback(callback, rplEntries);
});
});
}
exports2.readdirWithFileTypes = readdirWithFileTypes;
function makeRplTaskEntry(entry, settings) {
return (done) => {
if (!entry.dirent.isSymbolicLink()) {
done(null, entry);
return;
}
settings.fs.stat(entry.path, (statError, stats) => {
if (statError !== null) {
if (settings.throwErrorOnBrokenSymbolicLink) {
done(statError);
return;
}
done(null, entry);
return;
}
entry.dirent = utils.fs.createDirentFromStats(entry.name, stats);
done(null, entry);
});
};
}
function readdir(directory, settings, callback) {
settings.fs.readdir(directory, (readdirError, names) => {
if (readdirError !== null) {
callFailureCallback(callback, readdirError);
return;
}
const tasks = names.map((name) => {
const path = common.joinPathSegments(directory, name, settings.pathSegmentSeparator);
return (done) => {
fsStat.stat(path, settings.fsStatSettings, (error, stats) => {
if (error !== null) {
done(error);
return;
}
const entry = {
name,
path,
dirent: utils.fs.createDirentFromStats(name, stats)
};
if (settings.stats) {
entry.stats = stats;
}
done(null, entry);
});
};
});
rpl(tasks, (rplError, entries) => {
if (rplError !== null) {
callFailureCallback(callback, rplError);
return;
}
callSuccessCallback(callback, entries);
});
});
}
exports2.readdir = readdir;
function callFailureCallback(callback, error) {
callback(error);
}
function callSuccessCallback(callback, result) {
callback(null, result);
}
}
});
var require_sync3 = __commonJS22({
"node_modules/@nodelib/fs.scandir/out/providers/sync.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.readdir = exports2.readdirWithFileTypes = exports2.read = void 0;
var fsStat = require_out();
var constants_1 = require_constants4();
var utils = require_utils5();
var common = require_common3();
function read(directory, settings) {
if (!settings.stats && constants_1.IS_SUPPORT_READDIR_WITH_FILE_TYPES) {
return readdirWithFileTypes(directory, settings);
}
return readdir(directory, settings);
}
exports2.read = read;
function readdirWithFileTypes(directory, settings) {
const dirents = settings.fs.readdirSync(directory, {
withFileTypes: true
});
return dirents.map((dirent) => {
const entry = {
dirent,
name: dirent.name,
path: common.joinPathSegments(directory, dirent.name, settings.pathSegmentSeparator)
};
if (entry.dirent.isSymbolicLink() && settings.followSymbolicLinks) {
try {
const stats = settings.fs.statSync(entry.path);
entry.dirent = utils.fs.createDirentFromStats(entry.name, stats);
} catch (error) {
if (settings.throwErrorOnBrokenSymbolicLink) {
throw error;
}
}
}
return entry;
});
}
exports2.readdirWithFileTypes = readdirWithFileTypes;
function readdir(directory, settings) {
const names = settings.fs.readdirSync(directory);
return names.map((name) => {
const entryPath = common.joinPathSegments(directory, name, settings.pathSegmentSeparator);
const stats = fsStat.statSync(entryPath, settings.fsStatSettings);
const entry = {
name,
path: entryPath,
dirent: utils.fs.createDirentFromStats(name, stats)
};
if (settings.stats) {
entry.stats = stats;
}
return entry;
});
}
exports2.readdir = readdir;
}
});
var require_fs4 = __commonJS22({
"node_modules/@nodelib/fs.scandir/out/adapters/fs.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.createFileSystemAdapter = exports2.FILE_SYSTEM_ADAPTER = void 0;
var fs2 = require("fs");
exports2.FILE_SYSTEM_ADAPTER = {
lstat: fs2.lstat,
stat: fs2.stat,
lstatSync: fs2.lstatSync,
statSync: fs2.statSync,
readdir: fs2.readdir,
readdirSync: fs2.readdirSync
};
function createFileSystemAdapter(fsMethods) {
if (fsMethods === void 0) {
return exports2.FILE_SYSTEM_ADAPTER;
}
return Object.assign(Object.assign({}, exports2.FILE_SYSTEM_ADAPTER), fsMethods);
}
exports2.createFileSystemAdapter = createFileSystemAdapter;
}
});
var require_settings2 = __commonJS22({
"node_modules/@nodelib/fs.scandir/out/settings.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var path = require("path");
var fsStat = require_out();
var fs2 = require_fs4();
var Settings = class {
constructor(_options = {}) {
this._options = _options;
this.followSymbolicLinks = this._getValue(this._options.followSymbolicLinks, false);
this.fs = fs2.createFileSystemAdapter(this._options.fs);
this.pathSegmentSeparator = this._getValue(this._options.pathSegmentSeparator, path.sep);
this.stats = this._getValue(this._options.stats, false);
this.throwErrorOnBrokenSymbolicLink = this._getValue(this._options.throwErrorOnBrokenSymbolicLink, true);
this.fsStatSettings = new fsStat.Settings({
followSymbolicLink: this.followSymbolicLinks,
fs: this.fs,
throwErrorOnBrokenSymbolicLink: this.throwErrorOnBrokenSymbolicLink
});
}
_getValue(option, value) {
return option !== null && option !== void 0 ? option : value;
}
};
exports2.default = Settings;
}
});
var require_out2 = __commonJS22({
"node_modules/@nodelib/fs.scandir/out/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.Settings = exports2.scandirSync = exports2.scandir = void 0;
var async = require_async3();
var sync = require_sync3();
var settings_1 = require_settings2();
exports2.Settings = settings_1.default;
function scandir(path, optionsOrSettingsOrCallback, callback) {
if (typeof optionsOrSettingsOrCallback === "function") {
async.read(path, getSettings(), optionsOrSettingsOrCallback);
return;
}
async.read(path, getSettings(optionsOrSettingsOrCallback), callback);
}
exports2.scandir = scandir;
function scandirSync(path, optionsOrSettings) {
const settings = getSettings(optionsOrSettings);
return sync.read(path, settings);
}
exports2.scandirSync = scandirSync;
function getSettings(settingsOrOptions = {}) {
if (settingsOrOptions instanceof settings_1.default) {
return settingsOrOptions;
}
return new settings_1.default(settingsOrOptions);
}
}
});
var require_reusify = __commonJS22({
"node_modules/reusify/reusify.js"(exports2, module22) {
"use strict";
function reusify(Constructor) {
var head = new Constructor();
var tail = head;
function get() {
var current = head;
if (current.next) {
head = current.next;
} else {
head = new Constructor();
tail = head;
}
current.next = null;
return current;
}
function release(obj) {
tail.next = obj;
tail = obj;
}
return {
get,
release
};
}
module22.exports = reusify;
}
});
var require_queue = __commonJS22({
"node_modules/fastq/queue.js"(exports2, module22) {
"use strict";
var reusify = require_reusify();
function fastqueue(context, worker, concurrency) {
if (typeof context === "function") {
concurrency = worker;
worker = context;
context = null;
}
if (concurrency < 1) {
throw new Error("fastqueue concurrency must be greater than 1");
}
var cache = reusify(Task);
var queueHead = null;
var queueTail = null;
var _running = 0;
var errorHandler = null;
var self2 = {
push,
drain: noop,
saturated: noop,
pause,
paused: false,
concurrency,
running,
resume,
idle,
length,
getQueue,
unshift,
empty: noop,
kill,
killAndDrain,
error
};
return self2;
function running() {
return _running;
}
function pause() {
self2.paused = true;
}
function length() {
var current = queueHead;
var counter = 0;
while (current) {
current = current.next;
counter++;
}
return counter;
}
function getQueue() {
var current = queueHead;
var tasks = [];
while (current) {
tasks.push(current.value);
current = current.next;
}
return tasks;
}
function resume() {
if (!self2.paused)
return;
self2.paused = false;
for (var i = 0; i < self2.concurrency; i++) {
_running++;
release();
}
}
function idle() {
return _running === 0 && self2.length() === 0;
}
function push(value, done) {
var current = cache.get();
current.context = context;
current.release = release;
current.value = value;
current.callback = done || noop;
current.errorHandler = errorHandler;
if (_running === self2.concurrency || self2.paused) {
if (queueTail) {
queueTail.next = current;
queueTail = current;
} else {
queueHead = current;
queueTail = current;
self2.saturated();
}
} else {
_running++;
worker.call(context, current.value, current.worked);
}
}
function unshift(value, done) {
var current = cache.get();
current.context = context;
current.release = release;
current.value = value;
current.callback = done || noop;
if (_running === self2.concurrency || self2.paused) {
if (queueHead) {
current.next = queueHead;
queueHead = current;
} else {
queueHead = current;
queueTail = current;
self2.saturated();
}
} else {
_running++;
worker.call(context, current.value, current.worked);
}
}
function release(holder) {
if (holder) {
cache.release(holder);
}
var next = queueHead;
if (next) {
if (!self2.paused) {
if (queueTail === queueHead) {
queueTail = null;
}
queueHead = next.next;
next.next = null;
worker.call(context, next.value, next.worked);
if (queueTail === null) {
self2.empty();
}
} else {
_running--;
}
} else if (--_running === 0) {
self2.drain();
}
}
function kill() {
queueHead = null;
queueTail = null;
self2.drain = noop;
}
function killAndDrain() {
queueHead = null;
queueTail = null;
self2.drain();
self2.drain = noop;
}
function error(handler) {
errorHandler = handler;
}
}
function noop() {
}
function Task() {
this.value = null;
this.callback = noop;
this.next = null;
this.release = noop;
this.context = null;
this.errorHandler = null;
var self2 = this;
this.worked = function worked(err, result) {
var callback = self2.callback;
var errorHandler = self2.errorHandler;
var val = self2.value;
self2.value = null;
self2.callback = noop;
if (self2.errorHandler) {
errorHandler(err, val);
}
callback.call(self2.context, err, result);
self2.release(self2);
};
}
function queueAsPromised(context, worker, concurrency) {
if (typeof context === "function") {
concurrency = worker;
worker = context;
context = null;
}
function asyncWrapper(arg, cb) {
worker.call(this, arg).then(function(res) {
cb(null, res);
}, cb);
}
var queue = fastqueue(context, asyncWrapper, concurrency);
var pushCb = queue.push;
var unshiftCb = queue.unshift;
queue.push = push;
queue.unshift = unshift;
queue.drained = drained;
return queue;
function push(value) {
var p = new Promise(function(resolve, reject) {
pushCb(value, function(err, result) {
if (err) {
reject(err);
return;
}
resolve(result);
});
});
p.catch(noop);
return p;
}
function unshift(value) {
var p = new Promise(function(resolve, reject) {
unshiftCb(value, function(err, result) {
if (err) {
reject(err);
return;
}
resolve(result);
});
});
p.catch(noop);
return p;
}
function drained() {
var previousDrain = queue.drain;
var p = new Promise(function(resolve) {
queue.drain = function() {
previousDrain();
resolve();
};
});
return p;
}
}
module22.exports = fastqueue;
module22.exports.promise = queueAsPromised;
}
});
var require_common4 = __commonJS22({
"node_modules/@nodelib/fs.walk/out/readers/common.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.joinPathSegments = exports2.replacePathSegmentSeparator = exports2.isAppliedFilter = exports2.isFatalError = void 0;
function isFatalError(settings, error) {
if (settings.errorFilter === null) {
return true;
}
return !settings.errorFilter(error);
}
exports2.isFatalError = isFatalError;
function isAppliedFilter(filter, value) {
return filter === null || filter(value);
}
exports2.isAppliedFilter = isAppliedFilter;
function replacePathSegmentSeparator(filepath, separator) {
return filepath.split(/[/\\]/).join(separator);
}
exports2.replacePathSegmentSeparator = replacePathSegmentSeparator;
function joinPathSegments(a, b, separator) {
if (a === "") {
return b;
}
if (a.endsWith(separator)) {
return a + b;
}
return a + separator + b;
}
exports2.joinPathSegments = joinPathSegments;
}
});
var require_reader = __commonJS22({
"node_modules/@nodelib/fs.walk/out/readers/reader.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var common = require_common4();
var Reader = class {
constructor(_root, _settings) {
this._root = _root;
this._settings = _settings;
this._root = common.replacePathSegmentSeparator(_root, _settings.pathSegmentSeparator);
}
};
exports2.default = Reader;
}
});
var require_async4 = __commonJS22({
"node_modules/@nodelib/fs.walk/out/readers/async.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var events_1 = require("events");
var fsScandir = require_out2();
var fastq = require_queue();
var common = require_common4();
var reader_1 = require_reader();
var AsyncReader = class extends reader_1.default {
constructor(_root, _settings) {
super(_root, _settings);
this._settings = _settings;
this._scandir = fsScandir.scandir;
this._emitter = new events_1.EventEmitter();
this._queue = fastq(this._worker.bind(this), this._settings.concurrency);
this._isFatalError = false;
this._isDestroyed = false;
this._queue.drain = () => {
if (!this._isFatalError) {
this._emitter.emit("end");
}
};
}
read() {
this._isFatalError = false;
this._isDestroyed = false;
setImmediate(() => {
this._pushToQueue(this._root, this._settings.basePath);
});
return this._emitter;
}
get isDestroyed() {
return this._isDestroyed;
}
destroy() {
if (this._isDestroyed) {
throw new Error("The reader is already destroyed");
}
this._isDestroyed = true;
this._queue.killAndDrain();
}
onEntry(callback) {
this._emitter.on("entry", callback);
}
onError(callback) {
this._emitter.once("error", callback);
}
onEnd(callback) {
this._emitter.once("end", callback);
}
_pushToQueue(directory, base) {
const queueItem = {
directory,
base
};
this._queue.push(queueItem, (error) => {
if (error !== null) {
this._handleError(error);
}
});
}
_worker(item, done) {
this._scandir(item.directory, this._settings.fsScandirSettings, (error, entries) => {
if (error !== null) {
done(error, void 0);
return;
}
for (const entry of entries) {
this._handleEntry(entry, item.base);
}
done(null, void 0);
});
}
_handleError(error) {
if (this._isDestroyed || !common.isFatalError(this._settings, error)) {
return;
}
this._isFatalError = true;
this._isDestroyed = true;
this._emitter.emit("error", error);
}
_handleEntry(entry, base) {
if (this._isDestroyed || this._isFatalError) {
return;
}
const fullpath = entry.path;
if (base !== void 0) {
entry.path = common.joinPathSegments(base, entry.name, this._settings.pathSegmentSeparator);
}
if (common.isAppliedFilter(this._settings.entryFilter, entry)) {
this._emitEntry(entry);
}
if (entry.dirent.isDirectory() && common.isAppliedFilter(this._settings.deepFilter, entry)) {
this._pushToQueue(fullpath, base === void 0 ? void 0 : entry.path);
}
}
_emitEntry(entry) {
this._emitter.emit("entry", entry);
}
};
exports2.default = AsyncReader;
}
});
var require_async5 = __commonJS22({
"node_modules/@nodelib/fs.walk/out/providers/async.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var async_1 = require_async4();
var AsyncProvider = class {
constructor(_root, _settings) {
this._root = _root;
this._settings = _settings;
this._reader = new async_1.default(this._root, this._settings);
this._storage = [];
}
read(callback) {
this._reader.onError((error) => {
callFailureCallback(callback, error);
});
this._reader.onEntry((entry) => {
this._storage.push(entry);
});
this._reader.onEnd(() => {
callSuccessCallback(callback, this._storage);
});
this._reader.read();
}
};
exports2.default = AsyncProvider;
function callFailureCallback(callback, error) {
callback(error);
}
function callSuccessCallback(callback, entries) {
callback(null, entries);
}
}
});
var require_stream2 = __commonJS22({
"node_modules/@nodelib/fs.walk/out/providers/stream.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var stream_1 = require("stream");
var async_1 = require_async4();
var StreamProvider = class {
constructor(_root, _settings) {
this._root = _root;
this._settings = _settings;
this._reader = new async_1.default(this._root, this._settings);
this._stream = new stream_1.Readable({
objectMode: true,
read: () => {
},
destroy: () => {
if (!this._reader.isDestroyed) {
this._reader.destroy();
}
}
});
}
read() {
this._reader.onError((error) => {
this._stream.emit("error", error);
});
this._reader.onEntry((entry) => {
this._stream.push(entry);
});
this._reader.onEnd(() => {
this._stream.push(null);
});
this._reader.read();
return this._stream;
}
};
exports2.default = StreamProvider;
}
});
var require_sync4 = __commonJS22({
"node_modules/@nodelib/fs.walk/out/readers/sync.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var fsScandir = require_out2();
var common = require_common4();
var reader_1 = require_reader();
var SyncReader = class extends reader_1.default {
constructor() {
super(...arguments);
this._scandir = fsScandir.scandirSync;
this._storage = [];
this._queue = /* @__PURE__ */ new Set();
}
read() {
this._pushToQueue(this._root, this._settings.basePath);
this._handleQueue();
return this._storage;
}
_pushToQueue(directory, base) {
this._queue.add({
directory,
base
});
}
_handleQueue() {
for (const item of this._queue.values()) {
this._handleDirectory(item.directory, item.base);
}
}
_handleDirectory(directory, base) {
try {
const entries = this._scandir(directory, this._settings.fsScandirSettings);
for (const entry of entries) {
this._handleEntry(entry, base);
}
} catch (error) {
this._handleError(error);
}
}
_handleError(error) {
if (!common.isFatalError(this._settings, error)) {
return;
}
throw error;
}
_handleEntry(entry, base) {
const fullpath = entry.path;
if (base !== void 0) {
entry.path = common.joinPathSegments(base, entry.name, this._settings.pathSegmentSeparator);
}
if (common.isAppliedFilter(this._settings.entryFilter, entry)) {
this._pushToStorage(entry);
}
if (entry.dirent.isDirectory() && common.isAppliedFilter(this._settings.deepFilter, entry)) {
this._pushToQueue(fullpath, base === void 0 ? void 0 : entry.path);
}
}
_pushToStorage(entry) {
this._storage.push(entry);
}
};
exports2.default = SyncReader;
}
});
var require_sync5 = __commonJS22({
"node_modules/@nodelib/fs.walk/out/providers/sync.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var sync_1 = require_sync4();
var SyncProvider = class {
constructor(_root, _settings) {
this._root = _root;
this._settings = _settings;
this._reader = new sync_1.default(this._root, this._settings);
}
read() {
return this._reader.read();
}
};
exports2.default = SyncProvider;
}
});
var require_settings3 = __commonJS22({
"node_modules/@nodelib/fs.walk/out/settings.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var path = require("path");
var fsScandir = require_out2();
var Settings = class {
constructor(_options = {}) {
this._options = _options;
this.basePath = this._getValue(this._options.basePath, void 0);
this.concurrency = this._getValue(this._options.concurrency, Number.POSITIVE_INFINITY);
this.deepFilter = this._getValue(this._options.deepFilter, null);
this.entryFilter = this._getValue(this._options.entryFilter, null);
this.errorFilter = this._getValue(this._options.errorFilter, null);
this.pathSegmentSeparator = this._getValue(this._options.pathSegmentSeparator, path.sep);
this.fsScandirSettings = new fsScandir.Settings({
followSymbolicLinks: this._options.followSymbolicLinks,
fs: this._options.fs,
pathSegmentSeparator: this._options.pathSegmentSeparator,
stats: this._options.stats,
throwErrorOnBrokenSymbolicLink: this._options.throwErrorOnBrokenSymbolicLink
});
}
_getValue(option, value) {
return option !== null && option !== void 0 ? option : value;
}
};
exports2.default = Settings;
}
});
var require_out3 = __commonJS22({
"node_modules/@nodelib/fs.walk/out/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.Settings = exports2.walkStream = exports2.walkSync = exports2.walk = void 0;
var async_1 = require_async5();
var stream_1 = require_stream2();
var sync_1 = require_sync5();
var settings_1 = require_settings3();
exports2.Settings = settings_1.default;
function walk(directory, optionsOrSettingsOrCallback, callback) {
if (typeof optionsOrSettingsOrCallback === "function") {
new async_1.default(directory, getSettings()).read(optionsOrSettingsOrCallback);
return;
}
new async_1.default(directory, getSettings(optionsOrSettingsOrCallback)).read(callback);
}
exports2.walk = walk;
function walkSync(directory, optionsOrSettings) {
const settings = getSettings(optionsOrSettings);
const provider = new sync_1.default(directory, settings);
return provider.read();
}
exports2.walkSync = walkSync;
function walkStream(directory, optionsOrSettings) {
const settings = getSettings(optionsOrSettings);
const provider = new stream_1.default(directory, settings);
return provider.read();
}
exports2.walkStream = walkStream;
function getSettings(settingsOrOptions = {}) {
if (settingsOrOptions instanceof settings_1.default) {
return settingsOrOptions;
}
return new settings_1.default(settingsOrOptions);
}
}
});
var require_reader2 = __commonJS22({
"node_modules/fast-glob/out/readers/reader.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var path = require("path");
var fsStat = require_out();
var utils = require_utils4();
var Reader = class {
constructor(_settings) {
this._settings = _settings;
this._fsStatSettings = new fsStat.Settings({
followSymbolicLink: this._settings.followSymbolicLinks,
fs: this._settings.fs,
throwErrorOnBrokenSymbolicLink: this._settings.followSymbolicLinks
});
}
_getFullEntryPath(filepath) {
return path.resolve(this._settings.cwd, filepath);
}
_makeEntry(stats, pattern) {
const entry = {
name: pattern,
path: pattern,
dirent: utils.fs.createDirentFromStats(pattern, stats)
};
if (this._settings.stats) {
entry.stats = stats;
}
return entry;
}
_isFatalError(error) {
return !utils.errno.isEnoentCodeError(error) && !this._settings.suppressErrors;
}
};
exports2.default = Reader;
}
});
var require_stream3 = __commonJS22({
"node_modules/fast-glob/out/readers/stream.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var stream_1 = require("stream");
var fsStat = require_out();
var fsWalk = require_out3();
var reader_1 = require_reader2();
var ReaderStream = class extends reader_1.default {
constructor() {
super(...arguments);
this._walkStream = fsWalk.walkStream;
this._stat = fsStat.stat;
}
dynamic(root, options) {
return this._walkStream(root, options);
}
static(patterns, options) {
const filepaths = patterns.map(this._getFullEntryPath, this);
const stream = new stream_1.PassThrough({
objectMode: true
});
stream._write = (index, _enc, done) => {
return this._getEntry(filepaths[index], patterns[index], options).then((entry) => {
if (entry !== null && options.entryFilter(entry)) {
stream.push(entry);
}
if (index === filepaths.length - 1) {
stream.end();
}
done();
}).catch(done);
};
for (let i = 0; i < filepaths.length; i++) {
stream.write(i);
}
return stream;
}
_getEntry(filepath, pattern, options) {
return this._getStat(filepath).then((stats) => this._makeEntry(stats, pattern)).catch((error) => {
if (options.errorFilter(error)) {
return null;
}
throw error;
});
}
_getStat(filepath) {
return new Promise((resolve, reject) => {
this._stat(filepath, this._fsStatSettings, (error, stats) => {
return error === null ? resolve(stats) : reject(error);
});
});
}
};
exports2.default = ReaderStream;
}
});
var require_async6 = __commonJS22({
"node_modules/fast-glob/out/readers/async.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var fsWalk = require_out3();
var reader_1 = require_reader2();
var stream_1 = require_stream3();
var ReaderAsync = class extends reader_1.default {
constructor() {
super(...arguments);
this._walkAsync = fsWalk.walk;
this._readerStream = new stream_1.default(this._settings);
}
dynamic(root, options) {
return new Promise((resolve, reject) => {
this._walkAsync(root, options, (error, entries) => {
if (error === null) {
resolve(entries);
} else {
reject(error);
}
});
});
}
async static(patterns, options) {
const entries = [];
const stream = this._readerStream.static(patterns, options);
return new Promise((resolve, reject) => {
stream.once("error", reject);
stream.on("data", (entry) => entries.push(entry));
stream.once("end", () => resolve(entries));
});
}
};
exports2.default = ReaderAsync;
}
});
var require_matcher2 = __commonJS22({
"node_modules/fast-glob/out/providers/matchers/matcher.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var utils = require_utils4();
var Matcher = class {
constructor(_patterns, _settings, _micromatchOptions) {
this._patterns = _patterns;
this._settings = _settings;
this._micromatchOptions = _micromatchOptions;
this._storage = [];
this._fillStorage();
}
_fillStorage() {
const patterns = utils.pattern.expandPatternsWithBraceExpansion(this._patterns);
for (const pattern of patterns) {
const segments = this._getPatternSegments(pattern);
const sections = this._splitSegmentsIntoSections(segments);
this._storage.push({
complete: sections.length <= 1,
pattern,
segments,
sections
});
}
}
_getPatternSegments(pattern) {
const parts = utils.pattern.getPatternParts(pattern, this._micromatchOptions);
return parts.map((part) => {
const dynamic = utils.pattern.isDynamicPattern(part, this._settings);
if (!dynamic) {
return {
dynamic: false,
pattern: part
};
}
return {
dynamic: true,
pattern: part,
patternRe: utils.pattern.makeRe(part, this._micromatchOptions)
};
});
}
_splitSegmentsIntoSections(segments) {
return utils.array.splitWhen(segments, (segment) => segment.dynamic && utils.pattern.hasGlobStar(segment.pattern));
}
};
exports2.default = Matcher;
}
});
var require_partial = __commonJS22({
"node_modules/fast-glob/out/providers/matchers/partial.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var matcher_1 = require_matcher2();
var PartialMatcher = class extends matcher_1.default {
match(filepath) {
const parts = filepath.split("/");
const levels = parts.length;
const patterns = this._storage.filter((info) => !info.complete || info.segments.length > levels);
for (const pattern of patterns) {
const section = pattern.sections[0];
if (!pattern.complete && levels > section.length) {
return true;
}
const match = parts.every((part, index) => {
const segment = pattern.segments[index];
if (segment.dynamic && segment.patternRe.test(part)) {
return true;
}
if (!segment.dynamic && segment.pattern === part) {
return true;
}
return false;
});
if (match) {
return true;
}
}
return false;
}
};
exports2.default = PartialMatcher;
}
});
var require_deep = __commonJS22({
"node_modules/fast-glob/out/providers/filters/deep.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var utils = require_utils4();
var partial_1 = require_partial();
var DeepFilter = class {
constructor(_settings, _micromatchOptions) {
this._settings = _settings;
this._micromatchOptions = _micromatchOptions;
}
getFilter(basePath, positive, negative) {
const matcher = this._getMatcher(positive);
const negativeRe = this._getNegativePatternsRe(negative);
return (entry) => this._filter(basePath, entry, matcher, negativeRe);
}
_getMatcher(patterns) {
return new partial_1.default(patterns, this._settings, this._micromatchOptions);
}
_getNegativePatternsRe(patterns) {
const affectDepthOfReadingPatterns = patterns.filter(utils.pattern.isAffectDepthOfReadingPattern);
return utils.pattern.convertPatternsToRe(affectDepthOfReadingPatterns, this._micromatchOptions);
}
_filter(basePath, entry, matcher, negativeRe) {
if (this._isSkippedByDeep(basePath, entry.path)) {
return false;
}
if (this._isSkippedSymbolicLink(entry)) {
return false;
}
const filepath = utils.path.removeLeadingDotSegment(entry.path);
if (this._isSkippedByPositivePatterns(filepath, matcher)) {
return false;
}
return this._isSkippedByNegativePatterns(filepath, negativeRe);
}
_isSkippedByDeep(basePath, entryPath) {
if (this._settings.deep === Infinity) {
return false;
}
return this._getEntryLevel(basePath, entryPath) >= this._settings.deep;
}
_getEntryLevel(basePath, entryPath) {
const entryPathDepth = entryPath.split("/").length;
if (basePath === "") {
return entryPathDepth;
}
const basePathDepth = basePath.split("/").length;
return entryPathDepth - basePathDepth;
}
_isSkippedSymbolicLink(entry) {
return !this._settings.followSymbolicLinks && entry.dirent.isSymbolicLink();
}
_isSkippedByPositivePatterns(entryPath, matcher) {
return !this._settings.baseNameMatch && !matcher.match(entryPath);
}
_isSkippedByNegativePatterns(entryPath, patternsRe) {
return !utils.pattern.matchAny(entryPath, patternsRe);
}
};
exports2.default = DeepFilter;
}
});
var require_entry = __commonJS22({
"node_modules/fast-glob/out/providers/filters/entry.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var utils = require_utils4();
var EntryFilter = class {
constructor(_settings, _micromatchOptions) {
this._settings = _settings;
this._micromatchOptions = _micromatchOptions;
this.index = /* @__PURE__ */ new Map();
}
getFilter(positive, negative) {
const positiveRe = utils.pattern.convertPatternsToRe(positive, this._micromatchOptions);
const negativeRe = utils.pattern.convertPatternsToRe(negative, this._micromatchOptions);
return (entry) => this._filter(entry, positiveRe, negativeRe);
}
_filter(entry, positiveRe, negativeRe) {
if (this._settings.unique && this._isDuplicateEntry(entry)) {
return false;
}
if (this._onlyFileFilter(entry) || this._onlyDirectoryFilter(entry)) {
return false;
}
if (this._isSkippedByAbsoluteNegativePatterns(entry.path, negativeRe)) {
return false;
}
const filepath = this._settings.baseNameMatch ? entry.name : entry.path;
const isDirectory = entry.dirent.isDirectory();
const isMatched = this._isMatchToPatterns(filepath, positiveRe, isDirectory) && !this._isMatchToPatterns(entry.path, negativeRe, isDirectory);
if (this._settings.unique && isMatched) {
this._createIndexRecord(entry);
}
return isMatched;
}
_isDuplicateEntry(entry) {
return this.index.has(entry.path);
}
_createIndexRecord(entry) {
this.index.set(entry.path, void 0);
}
_onlyFileFilter(entry) {
return this._settings.onlyFiles && !entry.dirent.isFile();
}
_onlyDirectoryFilter(entry) {
return this._settings.onlyDirectories && !entry.dirent.isDirectory();
}
_isSkippedByAbsoluteNegativePatterns(entryPath, patternsRe) {
if (!this._settings.absolute) {
return false;
}
const fullpath = utils.path.makeAbsolute(this._settings.cwd, entryPath);
return utils.pattern.matchAny(fullpath, patternsRe);
}
_isMatchToPatterns(entryPath, patternsRe, isDirectory) {
const filepath = utils.path.removeLeadingDotSegment(entryPath);
const isMatched = utils.pattern.matchAny(filepath, patternsRe);
if (!isMatched && isDirectory) {
return utils.pattern.matchAny(filepath + "/", patternsRe);
}
return isMatched;
}
};
exports2.default = EntryFilter;
}
});
var require_error = __commonJS22({
"node_modules/fast-glob/out/providers/filters/error.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var utils = require_utils4();
var ErrorFilter = class {
constructor(_settings) {
this._settings = _settings;
}
getFilter() {
return (error) => this._isNonFatalError(error);
}
_isNonFatalError(error) {
return utils.errno.isEnoentCodeError(error) || this._settings.suppressErrors;
}
};
exports2.default = ErrorFilter;
}
});
var require_entry2 = __commonJS22({
"node_modules/fast-glob/out/providers/transformers/entry.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var utils = require_utils4();
var EntryTransformer = class {
constructor(_settings) {
this._settings = _settings;
}
getTransformer() {
return (entry) => this._transform(entry);
}
_transform(entry) {
let filepath = entry.path;
if (this._settings.absolute) {
filepath = utils.path.makeAbsolute(this._settings.cwd, filepath);
filepath = utils.path.unixify(filepath);
}
if (this._settings.markDirectories && entry.dirent.isDirectory()) {
filepath += "/";
}
if (!this._settings.objectMode) {
return filepath;
}
return Object.assign(Object.assign({}, entry), {
path: filepath
});
}
};
exports2.default = EntryTransformer;
}
});
var require_provider = __commonJS22({
"node_modules/fast-glob/out/providers/provider.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var path = require("path");
var deep_1 = require_deep();
var entry_1 = require_entry();
var error_1 = require_error();
var entry_2 = require_entry2();
var Provider = class {
constructor(_settings) {
this._settings = _settings;
this.errorFilter = new error_1.default(this._settings);
this.entryFilter = new entry_1.default(this._settings, this._getMicromatchOptions());
this.deepFilter = new deep_1.default(this._settings, this._getMicromatchOptions());
this.entryTransformer = new entry_2.default(this._settings);
}
_getRootDirectory(task) {
return path.resolve(this._settings.cwd, task.base);
}
_getReaderOptions(task) {
const basePath = task.base === "." ? "" : task.base;
return {
basePath,
pathSegmentSeparator: "/",
concurrency: this._settings.concurrency,
deepFilter: this.deepFilter.getFilter(basePath, task.positive, task.negative),
entryFilter: this.entryFilter.getFilter(task.positive, task.negative),
errorFilter: this.errorFilter.getFilter(),
followSymbolicLinks: this._settings.followSymbolicLinks,
fs: this._settings.fs,
stats: this._settings.stats,
throwErrorOnBrokenSymbolicLink: this._settings.throwErrorOnBrokenSymbolicLink,
transform: this.entryTransformer.getTransformer()
};
}
_getMicromatchOptions() {
return {
dot: this._settings.dot,
matchBase: this._settings.baseNameMatch,
nobrace: !this._settings.braceExpansion,
nocase: !this._settings.caseSensitiveMatch,
noext: !this._settings.extglob,
noglobstar: !this._settings.globstar,
posix: true,
strictSlashes: false
};
}
};
exports2.default = Provider;
}
});
var require_async7 = __commonJS22({
"node_modules/fast-glob/out/providers/async.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var async_1 = require_async6();
var provider_1 = require_provider();
var ProviderAsync = class extends provider_1.default {
constructor() {
super(...arguments);
this._reader = new async_1.default(this._settings);
}
async read(task) {
const root = this._getRootDirectory(task);
const options = this._getReaderOptions(task);
const entries = await this.api(root, task, options);
return entries.map((entry) => options.transform(entry));
}
api(root, task, options) {
if (task.dynamic) {
return this._reader.dynamic(root, options);
}
return this._reader.static(task.patterns, options);
}
};
exports2.default = ProviderAsync;
}
});
var require_stream4 = __commonJS22({
"node_modules/fast-glob/out/providers/stream.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var stream_1 = require("stream");
var stream_2 = require_stream3();
var provider_1 = require_provider();
var ProviderStream = class extends provider_1.default {
constructor() {
super(...arguments);
this._reader = new stream_2.default(this._settings);
}
read(task) {
const root = this._getRootDirectory(task);
const options = this._getReaderOptions(task);
const source = this.api(root, task, options);
const destination = new stream_1.Readable({
objectMode: true,
read: () => {
}
});
source.once("error", (error) => destination.emit("error", error)).on("data", (entry) => destination.emit("data", options.transform(entry))).once("end", () => destination.emit("end"));
destination.once("close", () => source.destroy());
return destination;
}
api(root, task, options) {
if (task.dynamic) {
return this._reader.dynamic(root, options);
}
return this._reader.static(task.patterns, options);
}
};
exports2.default = ProviderStream;
}
});
var require_sync6 = __commonJS22({
"node_modules/fast-glob/out/readers/sync.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var fsStat = require_out();
var fsWalk = require_out3();
var reader_1 = require_reader2();
var ReaderSync = class extends reader_1.default {
constructor() {
super(...arguments);
this._walkSync = fsWalk.walkSync;
this._statSync = fsStat.statSync;
}
dynamic(root, options) {
return this._walkSync(root, options);
}
static(patterns, options) {
const entries = [];
for (const pattern of patterns) {
const filepath = this._getFullEntryPath(pattern);
const entry = this._getEntry(filepath, pattern, options);
if (entry === null || !options.entryFilter(entry)) {
continue;
}
entries.push(entry);
}
return entries;
}
_getEntry(filepath, pattern, options) {
try {
const stats = this._getStat(filepath);
return this._makeEntry(stats, pattern);
} catch (error) {
if (options.errorFilter(error)) {
return null;
}
throw error;
}
}
_getStat(filepath) {
return this._statSync(filepath, this._fsStatSettings);
}
};
exports2.default = ReaderSync;
}
});
var require_sync7 = __commonJS22({
"node_modules/fast-glob/out/providers/sync.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var sync_1 = require_sync6();
var provider_1 = require_provider();
var ProviderSync = class extends provider_1.default {
constructor() {
super(...arguments);
this._reader = new sync_1.default(this._settings);
}
read(task) {
const root = this._getRootDirectory(task);
const options = this._getReaderOptions(task);
const entries = this.api(root, task, options);
return entries.map(options.transform);
}
api(root, task, options) {
if (task.dynamic) {
return this._reader.dynamic(root, options);
}
return this._reader.static(task.patterns, options);
}
};
exports2.default = ProviderSync;
}
});
var require_settings4 = __commonJS22({
"node_modules/fast-glob/out/settings.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.DEFAULT_FILE_SYSTEM_ADAPTER = void 0;
var fs2 = require("fs");
var os = require("os");
var CPU_COUNT = Math.max(os.cpus().length, 1);
exports2.DEFAULT_FILE_SYSTEM_ADAPTER = {
lstat: fs2.lstat,
lstatSync: fs2.lstatSync,
stat: fs2.stat,
statSync: fs2.statSync,
readdir: fs2.readdir,
readdirSync: fs2.readdirSync
};
var Settings = class {
constructor(_options = {}) {
this._options = _options;
this.absolute = this._getValue(this._options.absolute, false);
this.baseNameMatch = this._getValue(this._options.baseNameMatch, false);
this.braceExpansion = this._getValue(this._options.braceExpansion, true);
this.caseSensitiveMatch = this._getValue(this._options.caseSensitiveMatch, true);
this.concurrency = this._getValue(this._options.concurrency, CPU_COUNT);
this.cwd = this._getValue(this._options.cwd, process.cwd());
this.deep = this._getValue(this._options.deep, Infinity);
this.dot = this._getValue(this._options.dot, false);
this.extglob = this._getValue(this._options.extglob, true);
this.followSymbolicLinks = this._getValue(this._options.followSymbolicLinks, true);
this.fs = this._getFileSystemMethods(this._options.fs);
this.globstar = this._getValue(this._options.globstar, true);
this.ignore = this._getValue(this._options.ignore, []);
this.markDirectories = this._getValue(this._options.markDirectories, false);
this.objectMode = this._getValue(this._options.objectMode, false);
this.onlyDirectories = this._getValue(this._options.onlyDirectories, false);
this.onlyFiles = this._getValue(this._options.onlyFiles, true);
this.stats = this._getValue(this._options.stats, false);
this.suppressErrors = this._getValue(this._options.suppressErrors, false);
this.throwErrorOnBrokenSymbolicLink = this._getValue(this._options.throwErrorOnBrokenSymbolicLink, false);
this.unique = this._getValue(this._options.unique, true);
if (this.onlyDirectories) {
this.onlyFiles = false;
}
if (this.stats) {
this.objectMode = true;
}
}
_getValue(option, value) {
return option === void 0 ? value : option;
}
_getFileSystemMethods(methods = {}) {
return Object.assign(Object.assign({}, exports2.DEFAULT_FILE_SYSTEM_ADAPTER), methods);
}
};
exports2.default = Settings;
}
});
var require_out4 = __commonJS22({
"node_modules/fast-glob/out/index.js"(exports2, module22) {
"use strict";
var taskManager = require_tasks();
var patternManager = require_patterns();
var async_1 = require_async7();
var stream_1 = require_stream4();
var sync_1 = require_sync7();
var settings_1 = require_settings4();
var utils = require_utils4();
async function FastGlob(source, options) {
assertPatternsInput(source);
const works = getWorks(source, async_1.default, options);
const result = await Promise.all(works);
return utils.array.flatten(result);
}
(function(FastGlob2) {
function sync(source, options) {
assertPatternsInput(source);
const works = getWorks(source, sync_1.default, options);
return utils.array.flatten(works);
}
FastGlob2.sync = sync;
function stream(source, options) {
assertPatternsInput(source);
const works = getWorks(source, stream_1.default, options);
return utils.stream.merge(works);
}
FastGlob2.stream = stream;
function generateTasks(source, options) {
assertPatternsInput(source);
const patterns = patternManager.transform([].concat(source));
const settings = new settings_1.default(options);
return taskManager.generate(patterns, settings);
}
FastGlob2.generateTasks = generateTasks;
function isDynamicPattern(source, options) {
assertPatternsInput(source);
const settings = new settings_1.default(options);
return utils.pattern.isDynamicPattern(source, settings);
}
FastGlob2.isDynamicPattern = isDynamicPattern;
function escapePath(source) {
assertPatternsInput(source);
return utils.path.escape(source);
}
FastGlob2.escapePath = escapePath;
})(FastGlob || (FastGlob = {}));
function getWorks(source, _Provider, options) {
const patterns = patternManager.transform([].concat(source));
const settings = new settings_1.default(options);
const tasks = taskManager.generate(patterns, settings);
const provider = new _Provider(settings);
return tasks.map(provider.read, provider);
}
function assertPatternsInput(input) {
const source = [].concat(input);
const isValidSource = source.every((item) => utils.string.isString(item) && !utils.string.isEmpty(item));
if (!isValidSource) {
throw new TypeError("Patterns must be a string (non empty) or an array of strings");
}
}
module22.exports = FastGlob;
}
});
var require_uniq_by_key = __commonJS22({
"src/utils/uniq-by-key.js"(exports2, module22) {
"use strict";
function uniqByKey(array, key) {
const result = [];
const seen = /* @__PURE__ */ new Set();
for (const element of array) {
const value = element[key];
if (!seen.has(value)) {
seen.add(value);
result.push(element);
}
}
return result;
}
module22.exports = uniqByKey;
}
});
var require_create_language = __commonJS22({
"src/utils/create-language.js"(exports2, module22) {
"use strict";
module22.exports = function(linguistData, override) {
const {
languageId
} = linguistData, rest = _objectWithoutProperties(linguistData, _excluded4);
return Object.assign(Object.assign({
linguistLanguageId: languageId
}, rest), override(linguistData));
};
}
});
var require_ast = __commonJS22({
"node_modules/esutils/lib/ast.js"(exports2, module22) {
(function() {
"use strict";
function isExpression(node) {
if (node == null) {
return false;
}
switch (node.type) {
case "ArrayExpression":
case "AssignmentExpression":
case "BinaryExpression":
case "CallExpression":
case "ConditionalExpression":
case "FunctionExpression":
case "Identifier":
case "Literal":
case "LogicalExpression":
case "MemberExpression":
case "NewExpression":
case "ObjectExpression":
case "SequenceExpression":
case "ThisExpression":
case "UnaryExpression":
case "UpdateExpression":
return true;
}
return false;
}
function isIterationStatement(node) {
if (node == null) {
return false;
}
switch (node.type) {
case "DoWhileStatement":
case "ForInStatement":
case "ForStatement":
case "WhileStatement":
return true;
}
return false;
}
function isStatement(node) {
if (node == null) {
return false;
}
switch (node.type) {
case "BlockStatement":
case "BreakStatement":
case "ContinueStatement":
case "DebuggerStatement":
case "DoWhileStatement":
case "EmptyStatement":
case "ExpressionStatement":
case "ForInStatement":
case "ForStatement":
case "IfStatement":
case "LabeledStatement":
case "ReturnStatement":
case "SwitchStatement":
case "ThrowStatement":
case "TryStatement":
case "VariableDeclaration":
case "WhileStatement":
case "WithStatement":
return true;
}
return false;
}
function isSourceElement(node) {
return isStatement(node) || node != null && node.type === "FunctionDeclaration";
}
function trailingStatement(node) {
switch (node.type) {
case "IfStatement":
if (node.alternate != null) {
return node.alternate;
}
return node.consequent;
case "LabeledStatement":
case "ForStatement":
case "ForInStatement":
case "WhileStatement":
case "WithStatement":
return node.body;
}
return null;
}
function isProblematicIfStatement(node) {
var current;
if (node.type !== "IfStatement") {
return false;
}
if (node.alternate == null) {
return false;
}
current = node.consequent;
do {
if (current.type === "IfStatement") {
if (current.alternate == null) {
return true;
}
}
current = trailingStatement(current);
} while (current);
return false;
}
module22.exports = {
isExpression,
isStatement,
isIterationStatement,
isSourceElement,
isProblematicIfStatement,
trailingStatement
};
})();
}
});
var require_code = __commonJS22({
"node_modules/esutils/lib/code.js"(exports2, module22) {
(function() {
"use strict";
var ES6Regex, ES5Regex, NON_ASCII_WHITESPACES, IDENTIFIER_START, IDENTIFIER_PART, ch;
ES5Regex = {
NonAsciiIdentifierStart: /[\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0370-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u048A-\u052F\u0531-\u0556\u0559\u0561-\u0587\u05D0-\u05EA\u05F0-\u05F2\u0620-\u064A\u066E\u066F\u0671-\u06D3\u06D5\u06E5\u06E6\u06EE\u06EF\u06FA-\u06FC\u06FF\u0710\u0712-\u072F\u074D-\u07A5\u07B1\u07CA-\u07EA\u07F4\u07F5\u07FA\u0800-\u0815\u081A\u0824\u0828\u0840-\u0858\u08A0-\u08B4\u08B6-\u08BD\u0904-\u0939\u093D\u0950\u0958-\u0961\u0971-\u0980\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD\u09CE\u09DC\u09DD\u09DF-\u09E1\u09F0\u09F1\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A59-\u0A5C\u0A5E\u0A72-\u0A74\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABD\u0AD0\u0AE0\u0AE1\u0AF9\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3D\u0B5C\u0B5D\u0B5F-\u0B61\u0B71\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BD0\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C58-\u0C5A\u0C60\u0C61\u0C80\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CDE\u0CE0\u0CE1\u0CF1\u0CF2\u0D05-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D\u0D4E\u0D54-\u0D56\u0D5F-\u0D61\u0D7A-\u0D7F\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0E01-\u0E30\u0E32\u0E33\u0E40-\u0E46\u0E81\u0E82\u0E84\u0E87\u0E88\u0E8A\u0E8D\u0E94-\u0E97\u0E99-\u0E9F\u0EA1-\u0EA3\u0EA5\u0EA7\u0EAA\u0EAB\u0EAD-\u0EB0\u0EB2\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6\u0EDC-\u0EDF\u0F00\u0F40-\u0F47\u0F49-\u0F6C\u0F88-\u0F8C\u1000-\u102A\u103F\u1050-\u1055\u105A-\u105D\u1061\u1065\u1066\u106E-\u1070\u1075-\u1081\u108E\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u170C\u170E-\u1711\u1720-\u1731\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17D7\u17DC\u1820-\u1877\u1880-\u1884\u1887-\u18A8\u18AA\u18B0-\u18F5\u1900-\u191E\u1950-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u1A00-\u1A16\u1A20-\u1A54\u1AA7\u1B05-\u1B33\u1B45-\u1B4B\u1B83-\u1BA0\u1BAE\u1BAF\u1BBA-\u1BE5\u1C00-\u1C23\u1C4D-\u1C4F\u1C5A-\u1C7D\u1C80-\u1C88\u1CE9-\u1CEC\u1CEE-\u1CF1\u1CF5\u1CF6\u1D00-\u1DBF\u1E00-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2119-\u211D\u2124\u2126\u2128\u212A-\u212D\u212F-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2C2E\u2C30-\u2C5E\u2C60-\u2CE4\u2CEB-\u2CEE\u2CF2\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u2E2F\u3005-\u3007\u3021-\u3029\u3031-\u3035\u3038-\u303C\u3041-\u3096\u309D-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312D\u3131-\u318E\u31A0-\u31BA\u31F0-\u31FF\u3400-\u4DB5\u4E00-\u9FD5\uA000-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA61F\uA62A\uA62B\uA640-\uA66E\uA67F-\uA69D\uA6A0-\uA6EF\uA717-\uA71F\uA722-\uA788\uA78B-\uA7AE\uA7B0-\uA7B7\uA7F7-\uA801\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA840-\uA873\uA882-\uA8B3\uA8F2-\uA8F7\uA8FB\uA8FD\uA90A-\uA925\uA930-\uA946\uA960-\uA97C\uA984-\uA9B2\uA9CF\uA9E0-\uA9E4\uA9E6-\uA9EF\uA9FA-\uA9FE\uAA00-\uAA28\uAA40-\uAA42\uAA44-\uAA4B\uAA60-\uAA76\uAA7A\uAA7E-\uAAAF\uAAB1\uAAB5\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADD\uAAE0-\uAAEA\uAAF2-\uAAF4\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB65\uAB70-\uABE2\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE70-\uFE74\uFE76-\uFEFC\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC]/,
NonAsciiIdentifierPart: /[\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0300-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u0483-\u0487\u048A-\u052F\u0531-\u0556\u0559\u0561-\u0587\u0591-\u05BD\u05BF\u05C1\u05C2\u05C4\u05C5\u05C7\u05D0-\u05EA\u05F0-\u05F2\u0610-\u061A\u0620-\u0669\u066E-\u06D3\u06D5-\u06DC\u06DF-\u06E8\u06EA-\u06FC\u06FF\u0710-\u074A\u074D-\u07B1\u07C0-\u07F5\u07FA\u0800-\u082D\u0840-\u085B\u08A0-\u08B4\u08B6-\u08BD\u08D4-\u08E1\u08E3-\u0963\u0966-\u096F\u0971-\u0983\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BC-\u09C4\u09C7\u09C8\u09CB-\u09CE\u09D7\u09DC\u09DD\u09DF-\u09E3\u09E6-\u09F1\u0A01-\u0A03\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A3C\u0A3E-\u0A42\u0A47\u0A48\u0A4B-\u0A4D\u0A51\u0A59-\u0A5C\u0A5E\u0A66-\u0A75\u0A81-\u0A83\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABC-\u0AC5\u0AC7-\u0AC9\u0ACB-\u0ACD\u0AD0\u0AE0-\u0AE3\u0AE6-\u0AEF\u0AF9\u0B01-\u0B03\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3C-\u0B44\u0B47\u0B48\u0B4B-\u0B4D\u0B56\u0B57\u0B5C\u0B5D\u0B5F-\u0B63\u0B66-\u0B6F\u0B71\u0B82\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BBE-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCD\u0BD0\u0BD7\u0BE6-\u0BEF\u0C00-\u0C03\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D-\u0C44\u0C46-\u0C48\u0C4A-\u0C4D\u0C55\u0C56\u0C58-\u0C5A\u0C60-\u0C63\u0C66-\u0C6F\u0C80-\u0C83\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBC-\u0CC4\u0CC6-\u0CC8\u0CCA-\u0CCD\u0CD5\u0CD6\u0CDE\u0CE0-\u0CE3\u0CE6-\u0CEF\u0CF1\u0CF2\u0D01-\u0D03\u0D05-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D-\u0D44\u0D46-\u0D48\u0D4A-\u0D4E\u0D54-\u0D57\u0D5F-\u0D63\u0D66-\u0D6F\u0D7A-\u0D7F\u0D82\u0D83\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0DCA\u0DCF-\u0DD4\u0DD6\u0DD8-\u0DDF\u0DE6-\u0DEF\u0DF2\u0DF3\u0E01-\u0E3A\u0E40-\u0E4E\u0E50-\u0E59\u0E81\u0E82\u0E84\u0E87\u0E88\u0E8A\u0E8D\u0E94-\u0E97\u0E99-\u0E9F\u0EA1-\u0EA3\u0EA5\u0EA7\u0EAA\u0EAB\u0EAD-\u0EB9\u0EBB-\u0EBD\u0EC0-\u0EC4\u0EC6\u0EC8-\u0ECD\u0ED0-\u0ED9\u0EDC-\u0EDF\u0F00\u0F18\u0F19\u0F20-\u0F29\u0F35\u0F37\u0F39\u0F3E-\u0F47\u0F49-\u0F6C\u0F71-\u0F84\u0F86-\u0F97\u0F99-\u0FBC\u0FC6\u1000-\u1049\u1050-\u109D\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u135D-\u135F\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u170C\u170E-\u1714\u1720-\u1734\u1740-\u1753\u1760-\u176C\u176E-\u1770\u1772\u1773\u1780-\u17D3\u17D7\u17DC\u17DD\u17E0-\u17E9\u180B-\u180D\u1810-\u1819\u1820-\u1877\u1880-\u18AA\u18B0-\u18F5\u1900-\u191E\u1920-\u192B\u1930-\u193B\u1946-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u19D0-\u19D9\u1A00-\u1A1B\u1A20-\u1A5E\u1A60-\u1A7C\u1A7F-\u1A89\u1A90-\u1A99\u1AA7\u1AB0-\u1ABD\u1B00-\u1B4B\u1B50-\u1B59\u1B6B-\u1B73\u1B80-\u1BF3\u1C00-\u1C37\u1C40-\u1C49\u1C4D-\u1C7D\u1C80-\u1C88\u1CD0-\u1CD2\u1CD4-\u1CF6\u1CF8\u1CF9\u1D00-\u1DF5\u1DFB-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u200C\u200D\u203F\u2040\u2054\u2071\u207F\u2090-\u209C\u20D0-\u20DC\u20E1\u20E5-\u20F0\u2102\u2107\u210A-\u2113\u2115\u2119-\u211D\u2124\u2126\u2128\u212A-\u212D\u212F-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2C2E\u2C30-\u2C5E\u2C60-\u2CE4\u2CEB-\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D7F-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u2DE0-\u2DFF\u2E2F\u3005-\u3007\u3021-\u302F\u3031-\u3035\u3038-\u303C\u3041-\u3096\u3099\u309A\u309D-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312D\u3131-\u318E\u31A0-\u31BA\u31F0-\u31FF\u3400-\u4DB5\u4E00-\u9FD5\uA000-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA62B\uA640-\uA66F\uA674-\uA67D\uA67F-\uA6F1\uA717-\uA71F\uA722-\uA788\uA78B-\uA7AE\uA7B0-\uA7B7\uA7F7-\uA827\uA840-\uA873\uA880-\uA8C5\uA8D0-\uA8D9\uA8E0-\uA8F7\uA8FB\uA8FD\uA900-\uA92D\uA930-\uA953\uA960-\uA97C\uA980-\uA9C0\uA9CF-\uA9D9\uA9E0-\uA9FE\uAA00-\uAA36\uAA40-\uAA4D\uAA50-\uAA59\uAA60-\uAA76\uAA7A-\uAAC2\uAADB-\uAADD\uAAE0-\uAAEF\uAAF2-\uAAF6\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB65\uAB70-\uABEA\uABEC\uABED\uABF0-\uABF9\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE00-\uFE0F\uFE20-\uFE2F\uFE33\uFE34\uFE4D-\uFE4F\uFE70-\uFE74\uFE76-\uFEFC\uFF10-\uFF19\uFF21-\uFF3A\uFF3F\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC]/
};
ES6Regex = {
NonAsciiIdentifierStart: /[\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0370-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u048A-\u052F\u0531-\u0556\u0559\u0561-\u0587\u05D0-\u05EA\u05F0-\u05F2\u0620-\u064A\u066E\u066F\u0671-\u06D3\u06D5\u06E5\u06E6\u06EE\u06EF\u06FA-\u06FC\u06FF\u0710\u0712-\u072F\u074D-\u07A5\u07B1\u07CA-\u07EA\u07F4\u07F5\u07FA\u0800-\u0815\u081A\u0824\u0828\u0840-\u0858\u08A0-\u08B4\u08B6-\u08BD\u0904-\u0939\u093D\u0950\u0958-\u0961\u0971-\u0980\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD\u09CE\u09DC\u09DD\u09DF-\u09E1\u09F0\u09F1\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A59-\u0A5C\u0A5E\u0A72-\u0A74\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABD\u0AD0\u0AE0\u0AE1\u0AF9\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3D\u0B5C\u0B5D\u0B5F-\u0B61\u0B71\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BD0\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C58-\u0C5A\u0C60\u0C61\u0C80\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CDE\u0CE0\u0CE1\u0CF1\u0CF2\u0D05-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D\u0D4E\u0D54-\u0D56\u0D5F-\u0D61\u0D7A-\u0D7F\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0E01-\u0E30\u0E32\u0E33\u0E40-\u0E46\u0E81\u0E82\u0E84\u0E87\u0E88\u0E8A\u0E8D\u0E94-\u0E97\u0E99-\u0E9F\u0EA1-\u0EA3\u0EA5\u0EA7\u0EAA\u0EAB\u0EAD-\u0EB0\u0EB2\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6\u0EDC-\u0EDF\u0F00\u0F40-\u0F47\u0F49-\u0F6C\u0F88-\u0F8C\u1000-\u102A\u103F\u1050-\u1055\u105A-\u105D\u1061\u1065\u1066\u106E-\u1070\u1075-\u1081\u108E\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u170C\u170E-\u1711\u1720-\u1731\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17D7\u17DC\u1820-\u1877\u1880-\u18A8\u18AA\u18B0-\u18F5\u1900-\u191E\u1950-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u1A00-\u1A16\u1A20-\u1A54\u1AA7\u1B05-\u1B33\u1B45-\u1B4B\u1B83-\u1BA0\u1BAE\u1BAF\u1BBA-\u1BE5\u1C00-\u1C23\u1C4D-\u1C4F\u1C5A-\u1C7D\u1C80-\u1C88\u1CE9-\u1CEC\u1CEE-\u1CF1\u1CF5\u1CF6\u1D00-\u1DBF\u1E00-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2118-\u211D\u2124\u2126\u2128\u212A-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2C2E\u2C30-\u2C5E\u2C60-\u2CE4\u2CEB-\u2CEE\u2CF2\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u3005-\u3007\u3021-\u3029\u3031-\u3035\u3038-\u303C\u3041-\u3096\u309B-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312D\u3131-\u318E\u31A0-\u31BA\u31F0-\u31FF\u3400-\u4DB5\u4E00-\u9FD5\uA000-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA61F\uA62A\uA62B\uA640-\uA66E\uA67F-\uA69D\uA6A0-\uA6EF\uA717-\uA71F\uA722-\uA788\uA78B-\uA7AE\uA7B0-\uA7B7\uA7F7-\uA801\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA840-\uA873\uA882-\uA8B3\uA8F2-\uA8F7\uA8FB\uA8FD\uA90A-\uA925\uA930-\uA946\uA960-\uA97C\uA984-\uA9B2\uA9CF\uA9E0-\uA9E4\uA9E6-\uA9EF\uA9FA-\uA9FE\uAA00-\uAA28\uAA40-\uAA42\uAA44-\uAA4B\uAA60-\uAA76\uAA7A\uAA7E-\uAAAF\uAAB1\uAAB5\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADD\uAAE0-\uAAEA\uAAF2-\uAAF4\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB65\uAB70-\uABE2\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE70-\uFE74\uFE76-\uFEFC\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC]|\uD800[\uDC00-\uDC0B\uDC0D-\uDC26\uDC28-\uDC3A\uDC3C\uDC3D\uDC3F-\uDC4D\uDC50-\uDC5D\uDC80-\uDCFA\uDD40-\uDD74\uDE80-\uDE9C\uDEA0-\uDED0\uDF00-\uDF1F\uDF30-\uDF4A\uDF50-\uDF75\uDF80-\uDF9D\uDFA0-\uDFC3\uDFC8-\uDFCF\uDFD1-\uDFD5]|\uD801[\uDC00-\uDC9D\uDCB0-\uDCD3\uDCD8-\uDCFB\uDD00-\uDD27\uDD30-\uDD63\uDE00-\uDF36\uDF40-\uDF55\uDF60-\uDF67]|\uD802[\uDC00-\uDC05\uDC08\uDC0A-\uDC35\uDC37\uDC38\uDC3C\uDC3F-\uDC55\uDC60-\uDC76\uDC80-\uDC9E\uDCE0-\uDCF2\uDCF4\uDCF5\uDD00-\uDD15\uDD20-\uDD39\uDD80-\uDDB7\uDDBE\uDDBF\uDE00\uDE10-\uDE13\uDE15-\uDE17\uDE19-\uDE33\uDE60-\uDE7C\uDE80-\uDE9C\uDEC0-\uDEC7\uDEC9-\uDEE4\uDF00-\uDF35\uDF40-\uDF55\uDF60-\uDF72\uDF80-\uDF91]|\uD803[\uDC00-\uDC48\uDC80-\uDCB2\uDCC0-\uDCF2]|\uD804[\uDC03-\uDC37\uDC83-\uDCAF\uDCD0-\uDCE8\uDD03-\uDD26\uDD50-\uDD72\uDD76\uDD83-\uDDB2\uDDC1-\uDDC4\uDDDA\uDDDC\uDE00-\uDE11\uDE13-\uDE2B\uDE80-\uDE86\uDE88\uDE8A-\uDE8D\uDE8F-\uDE9D\uDE9F-\uDEA8\uDEB0-\uDEDE\uDF05-\uDF0C\uDF0F\uDF10\uDF13-\uDF28\uDF2A-\uDF30\uDF32\uDF33\uDF35-\uDF39\uDF3D\uDF50\uDF5D-\uDF61]|\uD805[\uDC00-\uDC34\uDC47-\uDC4A\uDC80-\uDCAF\uDCC4\uDCC5\uDCC7\uDD80-\uDDAE\uDDD8-\uDDDB\uDE00-\uDE2F\uDE44\uDE80-\uDEAA\uDF00-\uDF19]|\uD806[\uDCA0-\uDCDF\uDCFF\uDEC0-\uDEF8]|\uD807[\uDC00-\uDC08\uDC0A-\uDC2E\uDC40\uDC72-\uDC8F]|\uD808[\uDC00-\uDF99]|\uD809[\uDC00-\uDC6E\uDC80-\uDD43]|[\uD80C\uD81C-\uD820\uD840-\uD868\uD86A-\uD86C\uD86F-\uD872][\uDC00-\uDFFF]|\uD80D[\uDC00-\uDC2E]|\uD811[\uDC00-\uDE46]|\uD81A[\uDC00-\uDE38\uDE40-\uDE5E\uDED0-\uDEED\uDF00-\uDF2F\uDF40-\uDF43\uDF63-\uDF77\uDF7D-\uDF8F]|\uD81B[\uDF00-\uDF44\uDF50\uDF93-\uDF9F\uDFE0]|\uD821[\uDC00-\uDFEC]|\uD822[\uDC00-\uDEF2]|\uD82C[\uDC00\uDC01]|\uD82F[\uDC00-\uDC6A\uDC70-\uDC7C\uDC80-\uDC88\uDC90-\uDC99]|\uD835[\uDC00-\uDC54\uDC56-\uDC9C\uDC9E\uDC9F\uDCA2\uDCA5\uDCA6\uDCA9-\uDCAC\uDCAE-\uDCB9\uDCBB\uDCBD-\uDCC3\uDCC5-\uDD05\uDD07-\uDD0A\uDD0D-\uDD14\uDD16-\uDD1C\uDD1E-\uDD39\uDD3B-\uDD3E\uDD40-\uDD44\uDD46\uDD4A-\uDD50\uDD52-\uDEA5\uDEA8-\uDEC0\uDEC2-\uDEDA\uDEDC-\uDEFA\uDEFC-\uDF14\uDF16-\uDF34\uDF36-\uDF4E\uDF50-\uDF6E\uDF70-\uDF88\uDF8A-\uDFA8\uDFAA-\uDFC2\uDFC4-\uDFCB]|\uD83A[\uDC00-\uDCC4\uDD00-\uDD43]|\uD83B[\uDE00-\uDE03\uDE05-\uDE1F\uDE21\uDE22\uDE24\uDE27\uDE29-\uDE32\uDE34-\uDE37\uDE39\uDE3B\uDE42\uDE47\uDE49\uDE4B\uDE4D-\uDE4F\uDE51\uDE52\uDE54\uDE57\uDE59\uDE5B\uDE5D\uDE5F\uDE61\uDE62\uDE64\uDE67-\uDE6A\uDE6C-\uDE72\uDE74-\uDE77\uDE79-\uDE7C\uDE7E\uDE80-\uDE89\uDE8B-\uDE9B\uDEA1-\uDEA3\uDEA5-\uDEA9\uDEAB-\uDEBB]|\uD869[\uDC00-\uDED6\uDF00-\uDFFF]|\uD86D[\uDC00-\uDF34\uDF40-\uDFFF]|\uD86E[\uDC00-\uDC1D\uDC20-\uDFFF]|\uD873[\uDC00-\uDEA1]|\uD87E[\uDC00-\uDE1D]/,
NonAsciiIdentifierPart: /[\xAA\xB5\xB7\xBA\xC0-\xD6\xD8-\xF6\xF8-\u02C1\u02C6-\u02D1\u02E0-\u02E4\u02EC\u02EE\u0300-\u0374\u0376\u0377\u037A-\u037D\u037F\u0386-\u038A\u038C\u038E-\u03A1\u03A3-\u03F5\u03F7-\u0481\u0483-\u0487\u048A-\u052F\u0531-\u0556\u0559\u0561-\u0587\u0591-\u05BD\u05BF\u05C1\u05C2\u05C4\u05C5\u05C7\u05D0-\u05EA\u05F0-\u05F2\u0610-\u061A\u0620-\u0669\u066E-\u06D3\u06D5-\u06DC\u06DF-\u06E8\u06EA-\u06FC\u06FF\u0710-\u074A\u074D-\u07B1\u07C0-\u07F5\u07FA\u0800-\u082D\u0840-\u085B\u08A0-\u08B4\u08B6-\u08BD\u08D4-\u08E1\u08E3-\u0963\u0966-\u096F\u0971-\u0983\u0985-\u098C\u098F\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BC-\u09C4\u09C7\u09C8\u09CB-\u09CE\u09D7\u09DC\u09DD\u09DF-\u09E3\u09E6-\u09F1\u0A01-\u0A03\u0A05-\u0A0A\u0A0F\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A3C\u0A3E-\u0A42\u0A47\u0A48\u0A4B-\u0A4D\u0A51\u0A59-\u0A5C\u0A5E\u0A66-\u0A75\u0A81-\u0A83\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2\u0AB3\u0AB5-\u0AB9\u0ABC-\u0AC5\u0AC7-\u0AC9\u0ACB-\u0ACD\u0AD0\u0AE0-\u0AE3\u0AE6-\u0AEF\u0AF9\u0B01-\u0B03\u0B05-\u0B0C\u0B0F\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32\u0B33\u0B35-\u0B39\u0B3C-\u0B44\u0B47\u0B48\u0B4B-\u0B4D\u0B56\u0B57\u0B5C\u0B5D\u0B5F-\u0B63\u0B66-\u0B6F\u0B71\u0B82\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9\u0BBE-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCD\u0BD0\u0BD7\u0BE6-\u0BEF\u0C00-\u0C03\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C39\u0C3D-\u0C44\u0C46-\u0C48\u0C4A-\u0C4D\u0C55\u0C56\u0C58-\u0C5A\u0C60-\u0C63\u0C66-\u0C6F\u0C80-\u0C83\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBC-\u0CC4\u0CC6-\u0CC8\u0CCA-\u0CCD\u0CD5\u0CD6\u0CDE\u0CE0-\u0CE3\u0CE6-\u0CEF\u0CF1\u0CF2\u0D01-\u0D03\u0D05-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D-\u0D44\u0D46-\u0D48\u0D4A-\u0D4E\u0D54-\u0D57\u0D5F-\u0D63\u0D66-\u0D6F\u0D7A-\u0D7F\u0D82\u0D83\u0D85-\u0D96\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0DCA\u0DCF-\u0DD4\u0DD6\u0DD8-\u0DDF\u0DE6-\u0DEF\u0DF2\u0DF3\u0E01-\u0E3A\u0E40-\u0E4E\u0E50-\u0E59\u0E81\u0E82\u0E84\u0E87\u0E88\u0E8A\u0E8D\u0E94-\u0E97\u0E99-\u0E9F\u0EA1-\u0EA3\u0EA5\u0EA7\u0EAA\u0EAB\u0EAD-\u0EB9\u0EBB-\u0EBD\u0EC0-\u0EC4\u0EC6\u0EC8-\u0ECD\u0ED0-\u0ED9\u0EDC-\u0EDF\u0F00\u0F18\u0F19\u0F20-\u0F29\u0F35\u0F37\u0F39\u0F3E-\u0F47\u0F49-\u0F6C\u0F71-\u0F84\u0F86-\u0F97\u0F99-\u0FBC\u0FC6\u1000-\u1049\u1050-\u109D\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FC-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u135D-\u135F\u1369-\u1371\u1380-\u138F\u13A0-\u13F5\u13F8-\u13FD\u1401-\u166C\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EE-\u16F8\u1700-\u170C\u170E-\u1714\u1720-\u1734\u1740-\u1753\u1760-\u176C\u176E-\u1770\u1772\u1773\u1780-\u17D3\u17D7\u17DC\u17DD\u17E0-\u17E9\u180B-\u180D\u1810-\u1819\u1820-\u1877\u1880-\u18AA\u18B0-\u18F5\u1900-\u191E\u1920-\u192B\u1930-\u193B\u1946-\u196D\u1970-\u1974\u1980-\u19AB\u19B0-\u19C9\u19D0-\u19DA\u1A00-\u1A1B\u1A20-\u1A5E\u1A60-\u1A7C\u1A7F-\u1A89\u1A90-\u1A99\u1AA7\u1AB0-\u1ABD\u1B00-\u1B4B\u1B50-\u1B59\u1B6B-\u1B73\u1B80-\u1BF3\u1C00-\u1C37\u1C40-\u1C49\u1C4D-\u1C7D\u1C80-\u1C88\u1CD0-\u1CD2\u1CD4-\u1CF6\u1CF8\u1CF9\u1D00-\u1DF5\u1DFB-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u200C\u200D\u203F\u2040\u2054\u2071\u207F\u2090-\u209C\u20D0-\u20DC\u20E1\u20E5-\u20F0\u2102\u2107\u210A-\u2113\u2115\u2118-\u211D\u2124\u2126\u2128\u212A-\u2139\u213C-\u213F\u2145-\u2149\u214E\u2160-\u2188\u2C00-\u2C2E\u2C30-\u2C5E\u2C60-\u2CE4\u2CEB-\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F\u2D7F-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u2DE0-\u2DFF\u3005-\u3007\u3021-\u302F\u3031-\u3035\u3038-\u303C\u3041-\u3096\u3099-\u309F\u30A1-\u30FA\u30FC-\u30FF\u3105-\u312D\u3131-\u318E\u31A0-\u31BA\u31F0-\u31FF\u3400-\u4DB5\u4E00-\u9FD5\uA000-\uA48C\uA4D0-\uA4FD\uA500-\uA60C\uA610-\uA62B\uA640-\uA66F\uA674-\uA67D\uA67F-\uA6F1\uA717-\uA71F\uA722-\uA788\uA78B-\uA7AE\uA7B0-\uA7B7\uA7F7-\uA827\uA840-\uA873\uA880-\uA8C5\uA8D0-\uA8D9\uA8E0-\uA8F7\uA8FB\uA8FD\uA900-\uA92D\uA930-\uA953\uA960-\uA97C\uA980-\uA9C0\uA9CF-\uA9D9\uA9E0-\uA9FE\uAA00-\uAA36\uAA40-\uAA4D\uAA50-\uAA59\uAA60-\uAA76\uAA7A-\uAAC2\uAADB-\uAADD\uAAE0-\uAAEF\uAAF2-\uAAF6\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E\uAB30-\uAB5A\uAB5C-\uAB65\uAB70-\uABEA\uABEC\uABED\uABF0-\uABF9\uAC00-\uD7A3\uD7B0-\uD7C6\uD7CB-\uD7FB\uF900-\uFA6D\uFA70-\uFAD9\uFB00-\uFB06\uFB13-\uFB17\uFB1D-\uFB28\uFB2A-\uFB36\uFB38-\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46-\uFBB1\uFBD3-\uFD3D\uFD50-\uFD8F\uFD92-\uFDC7\uFDF0-\uFDFB\uFE00-\uFE0F\uFE20-\uFE2F\uFE33\uFE34\uFE4D-\uFE4F\uFE70-\uFE74\uFE76-\uFEFC\uFF10-\uFF19\uFF21-\uFF3A\uFF3F\uFF41-\uFF5A\uFF66-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF\uFFD2-\uFFD7\uFFDA-\uFFDC]|\uD800[\uDC00-\uDC0B\uDC0D-\uDC26\uDC28-\uDC3A\uDC3C\uDC3D\uDC3F-\uDC4D\uDC50-\uDC5D\uDC80-\uDCFA\uDD40-\uDD74\uDDFD\uDE80-\uDE9C\uDEA0-\uDED0\uDEE0\uDF00-\uDF1F\uDF30-\uDF4A\uDF50-\uDF7A\uDF80-\uDF9D\uDFA0-\uDFC3\uDFC8-\uDFCF\uDFD1-\uDFD5]|\uD801[\uDC00-\uDC9D\uDCA0-\uDCA9\uDCB0-\uDCD3\uDCD8-\uDCFB\uDD00-\uDD27\uDD30-\uDD63\uDE00-\uDF36\uDF40-\uDF55\uDF60-\uDF67]|\uD802[\uDC00-\uDC05\uDC08\uDC0A-\uDC35\uDC37\uDC38\uDC3C\uDC3F-\uDC55\uDC60-\uDC76\uDC80-\uDC9E\uDCE0-\uDCF2\uDCF4\uDCF5\uDD00-\uDD15\uDD20-\uDD39\uDD80-\uDDB7\uDDBE\uDDBF\uDE00-\uDE03\uDE05\uDE06\uDE0C-\uDE13\uDE15-\uDE17\uDE19-\uDE33\uDE38-\uDE3A\uDE3F\uDE60-\uDE7C\uDE80-\uDE9C\uDEC0-\uDEC7\uDEC9-\uDEE6\uDF00-\uDF35\uDF40-\uDF55\uDF60-\uDF72\uDF80-\uDF91]|\uD803[\uDC00-\uDC48\uDC80-\uDCB2\uDCC0-\uDCF2]|\uD804[\uDC00-\uDC46\uDC66-\uDC6F\uDC7F-\uDCBA\uDCD0-\uDCE8\uDCF0-\uDCF9\uDD00-\uDD34\uDD36-\uDD3F\uDD50-\uDD73\uDD76\uDD80-\uDDC4\uDDCA-\uDDCC\uDDD0-\uDDDA\uDDDC\uDE00-\uDE11\uDE13-\uDE37\uDE3E\uDE80-\uDE86\uDE88\uDE8A-\uDE8D\uDE8F-\uDE9D\uDE9F-\uDEA8\uDEB0-\uDEEA\uDEF0-\uDEF9\uDF00-\uDF03\uDF05-\uDF0C\uDF0F\uDF10\uDF13-\uDF28\uDF2A-\uDF30\uDF32\uDF33\uDF35-\uDF39\uDF3C-\uDF44\uDF47\uDF48\uDF4B-\uDF4D\uDF50\uDF57\uDF5D-\uDF63\uDF66-\uDF6C\uDF70-\uDF74]|\uD805[\uDC00-\uDC4A\uDC50-\uDC59\uDC80-\uDCC5\uDCC7\uDCD0-\uDCD9\uDD80-\uDDB5\uDDB8-\uDDC0\uDDD8-\uDDDD\uDE00-\uDE40\uDE44\uDE50-\uDE59\uDE80-\uDEB7\uDEC0-\uDEC9\uDF00-\uDF19\uDF1D-\uDF2B\uDF30-\uDF39]|\uD806[\uDCA0-\uDCE9\uDCFF\uDEC0-\uDEF8]|\uD807[\uDC00-\uDC08\uDC0A-\uDC36\uDC38-\uDC40\uDC50-\uDC59\uDC72-\uDC8F\uDC92-\uDCA7\uDCA9-\uDCB6]|\uD808[\uDC00-\uDF99]|\uD809[\uDC00-\uDC6E\uDC80-\uDD43]|[\uD80C\uD81C-\uD820\uD840-\uD868\uD86A-\uD86C\uD86F-\uD872][\uDC00-\uDFFF]|\uD80D[\uDC00-\uDC2E]|\uD811[\uDC00-\uDE46]|\uD81A[\uDC00-\uDE38\uDE40-\uDE5E\uDE60-\uDE69\uDED0-\uDEED\uDEF0-\uDEF4\uDF00-\uDF36\uDF40-\uDF43\uDF50-\uDF59\uDF63-\uDF77\uDF7D-\uDF8F]|\uD81B[\uDF00-\uDF44\uDF50-\uDF7E\uDF8F-\uDF9F\uDFE0]|\uD821[\uDC00-\uDFEC]|\uD822[\uDC00-\uDEF2]|\uD82C[\uDC00\uDC01]|\uD82F[\uDC00-\uDC6A\uDC70-\uDC7C\uDC80-\uDC88\uDC90-\uDC99\uDC9D\uDC9E]|\uD834[\uDD65-\uDD69\uDD6D-\uDD72\uDD7B-\uDD82\uDD85-\uDD8B\uDDAA-\uDDAD\uDE42-\uDE44]|\uD835[\uDC00-\uDC54\uDC56-\uDC9C\uDC9E\uDC9F\uDCA2\uDCA5\uDCA6\uDCA9-\uDCAC\uDCAE-\uDCB9\uDCBB\uDCBD-\uDCC3\uDCC5-\uDD05\uDD07-\uDD0A\uDD0D-\uDD14\uDD16-\uDD1C\uDD1E-\uDD39\uDD3B-\uDD3E\uDD40-\uDD44\uDD46\uDD4A-\uDD50\uDD52-\uDEA5\uDEA8-\uDEC0\uDEC2-\uDEDA\uDEDC-\uDEFA\uDEFC-\uDF14\uDF16-\uDF34\uDF36-\uDF4E\uDF50-\uDF6E\uDF70-\uDF88\uDF8A-\uDFA8\uDFAA-\uDFC2\uDFC4-\uDFCB\uDFCE-\uDFFF]|\uD836[\uDE00-\uDE36\uDE3B-\uDE6C\uDE75\uDE84\uDE9B-\uDE9F\uDEA1-\uDEAF]|\uD838[\uDC00-\uDC06\uDC08-\uDC18\uDC1B-\uDC21\uDC23\uDC24\uDC26-\uDC2A]|\uD83A[\uDC00-\uDCC4\uDCD0-\uDCD6\uDD00-\uDD4A\uDD50-\uDD59]|\uD83B[\uDE00-\uDE03\uDE05-\uDE1F\uDE21\uDE22\uDE24\uDE27\uDE29-\uDE32\uDE34-\uDE37\uDE39\uDE3B\uDE42\uDE47\uDE49\uDE4B\uDE4D-\uDE4F\uDE51\uDE52\uDE54\uDE57\uDE59\uDE5B\uDE5D\uDE5F\uDE61\uDE62\uDE64\uDE67-\uDE6A\uDE6C-\uDE72\uDE74-\uDE77\uDE79-\uDE7C\uDE7E\uDE80-\uDE89\uDE8B-\uDE9B\uDEA1-\uDEA3\uDEA5-\uDEA9\uDEAB-\uDEBB]|\uD869[\uDC00-\uDED6\uDF00-\uDFFF]|\uD86D[\uDC00-\uDF34\uDF40-\uDFFF]|\uD86E[\uDC00-\uDC1D\uDC20-\uDFFF]|\uD873[\uDC00-\uDEA1]|\uD87E[\uDC00-\uDE1D]|\uDB40[\uDD00-\uDDEF]/
};
function isDecimalDigit(ch2) {
return 48 <= ch2 && ch2 <= 57;
}
function isHexDigit(ch2) {
return 48 <= ch2 && ch2 <= 57 || 97 <= ch2 && ch2 <= 102 || 65 <= ch2 && ch2 <= 70;
}
function isOctalDigit(ch2) {
return ch2 >= 48 && ch2 <= 55;
}
NON_ASCII_WHITESPACES = [5760, 8192, 8193, 8194, 8195, 8196, 8197, 8198, 8199, 8200, 8201, 8202, 8239, 8287, 12288, 65279];
function isWhiteSpace(ch2) {
return ch2 === 32 || ch2 === 9 || ch2 === 11 || ch2 === 12 || ch2 === 160 || ch2 >= 5760 && NON_ASCII_WHITESPACES.indexOf(ch2) >= 0;
}
function isLineTerminator(ch2) {
return ch2 === 10 || ch2 === 13 || ch2 === 8232 || ch2 === 8233;
}
function fromCodePoint(cp) {
if (cp <= 65535) {
return String.fromCharCode(cp);
}
var cu1 = String.fromCharCode(Math.floor((cp - 65536) / 1024) + 55296);
var cu2 = String.fromCharCode((cp - 65536) % 1024 + 56320);
return cu1 + cu2;
}
IDENTIFIER_START = new Array(128);
for (ch = 0; ch < 128; ++ch) {
IDENTIFIER_START[ch] = ch >= 97 && ch <= 122 || ch >= 65 && ch <= 90 || ch === 36 || ch === 95;
}
IDENTIFIER_PART = new Array(128);
for (ch = 0; ch < 128; ++ch) {
IDENTIFIER_PART[ch] = ch >= 97 && ch <= 122 || ch >= 65 && ch <= 90 || ch >= 48 && ch <= 57 || ch === 36 || ch === 95;
}
function isIdentifierStartES5(ch2) {
return ch2 < 128 ? IDENTIFIER_START[ch2] : ES5Regex.NonAsciiIdentifierStart.test(fromCodePoint(ch2));
}
function isIdentifierPartES5(ch2) {
return ch2 < 128 ? IDENTIFIER_PART[ch2] : ES5Regex.NonAsciiIdentifierPart.test(fromCodePoint(ch2));
}
function isIdentifierStartES6(ch2) {
return ch2 < 128 ? IDENTIFIER_START[ch2] : ES6Regex.NonAsciiIdentifierStart.test(fromCodePoint(ch2));
}
function isIdentifierPartES6(ch2) {
return ch2 < 128 ? IDENTIFIER_PART[ch2] : ES6Regex.NonAsciiIdentifierPart.test(fromCodePoint(ch2));
}
module22.exports = {
isDecimalDigit,
isHexDigit,
isOctalDigit,
isWhiteSpace,
isLineTerminator,
isIdentifierStartES5,
isIdentifierPartES5,
isIdentifierStartES6,
isIdentifierPartES6
};
})();
}
});
var require_keyword2 = __commonJS22({
"node_modules/esutils/lib/keyword.js"(exports2, module22) {
(function() {
"use strict";
var code = require_code();
function isStrictModeReservedWordES6(id) {
switch (id) {
case "implements":
case "interface":
case "package":
case "private":
case "protected":
case "public":
case "static":
case "let":
return true;
default:
return false;
}
}
function isKeywordES5(id, strict) {
if (!strict && id === "yield") {
return false;
}
return isKeywordES6(id, strict);
}
function isKeywordES6(id, strict) {
if (strict && isStrictModeReservedWordES6(id)) {
return true;
}
switch (id.length) {
case 2:
return id === "if" || id === "in" || id === "do";
case 3:
return id === "var" || id === "for" || id === "new" || id === "try";
case 4:
return id === "this" || id === "else" || id === "case" || id === "void" || id === "with" || id === "enum";
case 5:
return id === "while" || id === "break" || id === "catch" || id === "throw" || id === "const" || id === "yield" || id === "class" || id === "super";
case 6:
return id === "return" || id === "typeof" || id === "delete" || id === "switch" || id === "export" || id === "import";
case 7:
return id === "default" || id === "finally" || id === "extends";
case 8:
return id === "function" || id === "continue" || id === "debugger";
case 10:
return id === "instanceof";
default:
return false;
}
}
function isReservedWordES5(id, strict) {
return id === "null" || id === "true" || id === "false" || isKeywordES5(id, strict);
}
function isReservedWordES6(id, strict) {
return id === "null" || id === "true" || id === "false" || isKeywordES6(id, strict);
}
function isRestrictedWord(id) {
return id === "eval" || id === "arguments";
}
function isIdentifierNameES5(id) {
var i, iz, ch;
if (id.length === 0) {
return false;
}
ch = id.charCodeAt(0);
if (!code.isIdentifierStartES5(ch)) {
return false;
}
for (i = 1, iz = id.length; i < iz; ++i) {
ch = id.charCodeAt(i);
if (!code.isIdentifierPartES5(ch)) {
return false;
}
}
return true;
}
function decodeUtf16(lead, trail) {
return (lead - 55296) * 1024 + (trail - 56320) + 65536;
}
function isIdentifierNameES6(id) {
var i, iz, ch, lowCh, check;
if (id.length === 0) {
return false;
}
check = code.isIdentifierStartES6;
for (i = 0, iz = id.length; i < iz; ++i) {
ch = id.charCodeAt(i);
if (55296 <= ch && ch <= 56319) {
++i;
if (i >= iz) {
return false;
}
lowCh = id.charCodeAt(i);
if (!(56320 <= lowCh && lowCh <= 57343)) {
return false;
}
ch = decodeUtf16(ch, lowCh);
}
if (!check(ch)) {
return false;
}
check = code.isIdentifierPartES6;
}
return true;
}
function isIdentifierES5(id, strict) {
return isIdentifierNameES5(id) && !isReservedWordES5(id, strict);
}
function isIdentifierES6(id, strict) {
return isIdentifierNameES6(id) && !isReservedWordES6(id, strict);
}
module22.exports = {
isKeywordES5,
isKeywordES6,
isReservedWordES5,
isReservedWordES6,
isRestrictedWord,
isIdentifierNameES5,
isIdentifierNameES6,
isIdentifierES5,
isIdentifierES6
};
})();
}
});
var require_utils6 = __commonJS22({
"node_modules/esutils/lib/utils.js"(exports2) {
(function() {
"use strict";
exports2.ast = require_ast();
exports2.code = require_code();
exports2.keyword = require_keyword2();
})();
}
});
var require_is_block_comment = __commonJS22({
"src/language-js/utils/is-block-comment.js"(exports2, module22) {
"use strict";
var BLOCK_COMMENT_TYPES = /* @__PURE__ */ new Set(["Block", "CommentBlock", "MultiLine"]);
var isBlockComment = (comment) => BLOCK_COMMENT_TYPES.has(comment === null || comment === void 0 ? void 0 : comment.type);
module22.exports = isBlockComment;
}
});
var require_is_node_matches = __commonJS22({
"src/language-js/utils/is-node-matches.js"(exports2, module22) {
"use strict";
function isNodeMatchesNameOrPath(node, nameOrPath) {
const names = nameOrPath.split(".");
for (let index = names.length - 1; index >= 0; index--) {
const name = names[index];
if (index === 0) {
return node.type === "Identifier" && node.name === name;
}
if (node.type !== "MemberExpression" || node.optional || node.computed || node.property.type !== "Identifier" || node.property.name !== name) {
return false;
}
node = node.object;
}
}
function isNodeMatches(node, nameOrPaths) {
return nameOrPaths.some((nameOrPath) => isNodeMatchesNameOrPath(node, nameOrPath));
}
module22.exports = isNodeMatches;
}
});
var require_utils7 = __commonJS22({
"src/language-js/utils/index.js"(exports2, module22) {
"use strict";
var isIdentifierName = require_utils6().keyword.isIdentifierNameES5;
var {
getLast,
hasNewline,
skipWhitespace,
isNonEmptyArray,
isNextLineEmptyAfterIndex,
getStringWidth
} = require_util();
var {
locStart,
locEnd,
hasSameLocStart
} = require_loc();
var isBlockComment = require_is_block_comment();
var isNodeMatches = require_is_node_matches();
var NON_LINE_TERMINATING_WHITE_SPACE = "(?:(?=.)\\s)";
var FLOW_SHORTHAND_ANNOTATION = new RegExp(`^${NON_LINE_TERMINATING_WHITE_SPACE}*:`);
var FLOW_ANNOTATION = new RegExp(`^${NON_LINE_TERMINATING_WHITE_SPACE}*::`);
function hasFlowShorthandAnnotationComment(node) {
var _node$extra, _node$trailingComment;
return ((_node$extra = node.extra) === null || _node$extra === void 0 ? void 0 : _node$extra.parenthesized) && isBlockComment((_node$trailingComment = node.trailingComments) === null || _node$trailingComment === void 0 ? void 0 : _node$trailingComment[0]) && FLOW_SHORTHAND_ANNOTATION.test(node.trailingComments[0].value);
}
function hasFlowAnnotationComment(comments) {
const firstComment = comments === null || comments === void 0 ? void 0 : comments[0];
return isBlockComment(firstComment) && FLOW_ANNOTATION.test(firstComment.value);
}
function hasNode(node, fn) {
if (!node || typeof node !== "object") {
return false;
}
if (Array.isArray(node)) {
return node.some((value) => hasNode(value, fn));
}
const result = fn(node);
return typeof result === "boolean" ? result : Object.values(node).some((value) => hasNode(value, fn));
}
function hasNakedLeftSide(node) {
return node.type === "AssignmentExpression" || node.type === "BinaryExpression" || node.type === "LogicalExpression" || node.type === "NGPipeExpression" || node.type === "ConditionalExpression" || isCallExpression(node) || isMemberExpression(node) || node.type === "SequenceExpression" || node.type === "TaggedTemplateExpression" || node.type === "BindExpression" || node.type === "UpdateExpression" && !node.prefix || isTSTypeExpression(node) || node.type === "TSNonNullExpression";
}
function getLeftSide(node) {
var _ref2, _ref3, _ref4, _ref5, _ref6, _node$left;
if (node.expressions) {
return node.expressions[0];
}
return (_ref2 = (_ref3 = (_ref4 = (_ref5 = (_ref6 = (_node$left = node.left) !== null && _node$left !== void 0 ? _node$left : node.test) !== null && _ref6 !== void 0 ? _ref6 : node.callee) !== null && _ref5 !== void 0 ? _ref5 : node.object) !== null && _ref4 !== void 0 ? _ref4 : node.tag) !== null && _ref3 !== void 0 ? _ref3 : node.argument) !== null && _ref2 !== void 0 ? _ref2 : node.expression;
}
function getLeftSidePathName(path, node) {
if (node.expressions) {
return ["expressions", 0];
}
if (node.left) {
return ["left"];
}
if (node.test) {
return ["test"];
}
if (node.object) {
return ["object"];
}
if (node.callee) {
return ["callee"];
}
if (node.tag) {
return ["tag"];
}
if (node.argument) {
return ["argument"];
}
if (node.expression) {
return ["expression"];
}
throw new Error("Unexpected node has no left side.");
}
function createTypeCheckFunction(types) {
types = new Set(types);
return (node) => types.has(node === null || node === void 0 ? void 0 : node.type);
}
var isLineComment = createTypeCheckFunction(["Line", "CommentLine", "SingleLine", "HashbangComment", "HTMLOpen", "HTMLClose"]);
var isExportDeclaration = createTypeCheckFunction(["ExportDefaultDeclaration", "ExportDefaultSpecifier", "DeclareExportDeclaration", "ExportNamedDeclaration", "ExportAllDeclaration"]);
function getParentExportDeclaration(path) {
const parentNode = path.getParentNode();
if (path.getName() === "declaration" && isExportDeclaration(parentNode)) {
return parentNode;
}
return null;
}
var isLiteral = createTypeCheckFunction(["BooleanLiteral", "DirectiveLiteral", "Literal", "NullLiteral", "NumericLiteral", "BigIntLiteral", "DecimalLiteral", "RegExpLiteral", "StringLiteral", "TemplateLiteral", "TSTypeLiteral", "JSXText"]);
function isNumericLiteral(node) {
return node.type === "NumericLiteral" || node.type === "Literal" && typeof node.value === "number";
}
function isSignedNumericLiteral(node) {
return node.type === "UnaryExpression" && (node.operator === "+" || node.operator === "-") && isNumericLiteral(node.argument);
}
function isStringLiteral(node) {
return node.type === "StringLiteral" || node.type === "Literal" && typeof node.value === "string";
}
var isObjectType = createTypeCheckFunction(["ObjectTypeAnnotation", "TSTypeLiteral", "TSMappedType"]);
var isFunctionOrArrowExpression = createTypeCheckFunction(["FunctionExpression", "ArrowFunctionExpression"]);
function isFunctionOrArrowExpressionWithBody(node) {
return node.type === "FunctionExpression" || node.type === "ArrowFunctionExpression" && node.body.type === "BlockStatement";
}
function isAngularTestWrapper(node) {
return isCallExpression(node) && node.callee.type === "Identifier" && ["async", "inject", "fakeAsync", "waitForAsync"].includes(node.callee.name);
}
var isJsxNode = createTypeCheckFunction(["JSXElement", "JSXFragment"]);
function isTheOnlyJsxElementInMarkdown(options, path) {
if (options.parentParser !== "markdown" && options.parentParser !== "mdx") {
return false;
}
const node = path.getNode();
if (!node.expression || !isJsxNode(node.expression)) {
return false;
}
const parent = path.getParentNode();
return parent.type === "Program" && parent.body.length === 1;
}
function isGetterOrSetter(node) {
return node.kind === "get" || node.kind === "set";
}
function isFunctionNotation(node) {
return isGetterOrSetter(node) || hasSameLocStart(node, node.value);
}
function isObjectTypePropertyAFunction(node) {
return (node.type === "ObjectTypeProperty" || node.type === "ObjectTypeInternalSlot") && node.value.type === "FunctionTypeAnnotation" && !node.static && !isFunctionNotation(node);
}
function isTypeAnnotationAFunction(node) {
return (node.type === "TypeAnnotation" || node.type === "TSTypeAnnotation") && node.typeAnnotation.type === "FunctionTypeAnnotation" && !node.static && !hasSameLocStart(node, node.typeAnnotation);
}
var isBinaryish = createTypeCheckFunction(["BinaryExpression", "LogicalExpression", "NGPipeExpression"]);
function isMemberish(node) {
return isMemberExpression(node) || node.type === "BindExpression" && Boolean(node.object);
}
var simpleTypeAnnotations = /* @__PURE__ */ new Set(["AnyTypeAnnotation", "TSAnyKeyword", "NullLiteralTypeAnnotation", "TSNullKeyword", "ThisTypeAnnotation", "TSThisType", "NumberTypeAnnotation", "TSNumberKeyword", "VoidTypeAnnotation", "TSVoidKeyword", "BooleanTypeAnnotation", "TSBooleanKeyword", "BigIntTypeAnnotation", "TSBigIntKeyword", "SymbolTypeAnnotation", "TSSymbolKeyword", "StringTypeAnnotation", "TSStringKeyword", "BooleanLiteralTypeAnnotation", "StringLiteralTypeAnnotation", "BigIntLiteralTypeAnnotation", "NumberLiteralTypeAnnotation", "TSLiteralType", "TSTemplateLiteralType", "EmptyTypeAnnotation", "MixedTypeAnnotation", "TSNeverKeyword", "TSObjectKeyword", "TSUndefinedKeyword", "TSUnknownKeyword"]);
function isSimpleType(node) {
if (!node) {
return false;
}
if ((node.type === "GenericTypeAnnotation" || node.type === "TSTypeReference") && !node.typeParameters) {
return true;
}
if (simpleTypeAnnotations.has(node.type)) {
return true;
}
return false;
}
function isUnitTestSetUp(node) {
const unitTestSetUpRe = /^(?:before|after)(?:Each|All)$/;
return node.callee.type === "Identifier" && unitTestSetUpRe.test(node.callee.name) && node.arguments.length === 1;
}
var testCallCalleePatterns = ["it", "it.only", "it.skip", "describe", "describe.only", "describe.skip", "test", "test.only", "test.skip", "test.step", "test.describe", "test.describe.only", "test.describe.parallel", "test.describe.parallel.only", "test.describe.serial", "test.describe.serial.only", "skip", "xit", "xdescribe", "xtest", "fit", "fdescribe", "ftest"];
function isTestCallCallee(node) {
return isNodeMatches(node, testCallCalleePatterns);
}
function isTestCall(node, parent) {
if (node.type !== "CallExpression") {
return false;
}
if (node.arguments.length === 1) {
if (isAngularTestWrapper(node) && parent && isTestCall(parent)) {
return isFunctionOrArrowExpression(node.arguments[0]);
}
if (isUnitTestSetUp(node)) {
return isAngularTestWrapper(node.arguments[0]);
}
} else if (node.arguments.length === 2 || node.arguments.length === 3) {
if ((node.arguments[0].type === "TemplateLiteral" || isStringLiteral(node.arguments[0])) && isTestCallCallee(node.callee)) {
if (node.arguments[2] && !isNumericLiteral(node.arguments[2])) {
return false;
}
return (node.arguments.length === 2 ? isFunctionOrArrowExpression(node.arguments[1]) : isFunctionOrArrowExpressionWithBody(node.arguments[1]) && getFunctionParameters(node.arguments[1]).length <= 1) || isAngularTestWrapper(node.arguments[1]);
}
}
return false;
}
var isCallExpression = createTypeCheckFunction(["CallExpression", "OptionalCallExpression"]);
var isMemberExpression = createTypeCheckFunction(["MemberExpression", "OptionalMemberExpression"]);
function isSimpleTemplateLiteral(node) {
let expressionsKey = "expressions";
if (node.type === "TSTemplateLiteralType") {
expressionsKey = "types";
}
const expressions = node[expressionsKey];
if (expressions.length === 0) {
return false;
}
return expressions.every((expr) => {
if (hasComment(expr)) {
return false;
}
if (expr.type === "Identifier" || expr.type === "ThisExpression") {
return true;
}
if (isMemberExpression(expr)) {
let head = expr;
while (isMemberExpression(head)) {
if (head.property.type !== "Identifier" && head.property.type !== "Literal" && head.property.type !== "StringLiteral" && head.property.type !== "NumericLiteral") {
return false;
}
head = head.object;
if (hasComment(head)) {
return false;
}
}
if (head.type === "Identifier" || head.type === "ThisExpression") {
return true;
}
return false;
}
return false;
});
}
function getTypeScriptMappedTypeModifier(tokenNode, keyword) {
if (tokenNode === "+" || tokenNode === "-") {
return tokenNode + keyword;
}
return keyword;
}
function isFlowAnnotationComment(text, typeAnnotation) {
const start = locStart(typeAnnotation);
const end = skipWhitespace(text, locEnd(typeAnnotation));
return end !== false && text.slice(start, start + 2) === "/*" && text.slice(end, end + 2) === "*/";
}
function hasLeadingOwnLineComment(text, node) {
if (isJsxNode(node)) {
return hasNodeIgnoreComment(node);
}
return hasComment(node, CommentCheckFlags.Leading, (comment) => hasNewline(text, locEnd(comment)));
}
function isStringPropSafeToUnquote(node, options) {
return options.parser !== "json" && isStringLiteral(node.key) && rawText(node.key).slice(1, -1) === node.key.value && (isIdentifierName(node.key.value) && !(options.parser === "babel-ts" && node.type === "ClassProperty" || options.parser === "typescript" && node.type === "PropertyDefinition") || isSimpleNumber(node.key.value) && String(Number(node.key.value)) === node.key.value && (options.parser === "babel" || options.parser === "acorn" || options.parser === "espree" || options.parser === "meriyah" || options.parser === "__babel_estree"));
}
function isSimpleNumber(numberString) {
return /^(?:\d+|\d+\.\d+)$/.test(numberString);
}
function isJestEachTemplateLiteral(node, parentNode) {
const jestEachTriggerRegex = /^[fx]?(?:describe|it|test)$/;
return parentNode.type === "TaggedTemplateExpression" && parentNode.quasi === node && parentNode.tag.type === "MemberExpression" && parentNode.tag.property.type === "Identifier" && parentNode.tag.property.name === "each" && (parentNode.tag.object.type === "Identifier" && jestEachTriggerRegex.test(parentNode.tag.object.name) || parentNode.tag.object.type === "MemberExpression" && parentNode.tag.object.property.type === "Identifier" && (parentNode.tag.object.property.name === "only" || parentNode.tag.object.property.name === "skip") && parentNode.tag.object.object.type === "Identifier" && jestEachTriggerRegex.test(parentNode.tag.object.object.name));
}
function templateLiteralHasNewLines(template) {
return template.quasis.some((quasi) => quasi.value.raw.includes("\n"));
}
function isTemplateOnItsOwnLine(node, text) {
return (node.type === "TemplateLiteral" && templateLiteralHasNewLines(node) || node.type === "TaggedTemplateExpression" && templateLiteralHasNewLines(node.quasi)) && !hasNewline(text, locStart(node), {
backwards: true
});
}
function needsHardlineAfterDanglingComment(node) {
if (!hasComment(node)) {
return false;
}
const lastDanglingComment = getLast(getComments(node, CommentCheckFlags.Dangling));
return lastDanglingComment && !isBlockComment(lastDanglingComment);
}
function isFunctionCompositionArgs(args) {
if (args.length <= 1) {
return false;
}
let count = 0;
for (const arg of args) {
if (isFunctionOrArrowExpression(arg)) {
count += 1;
if (count > 1) {
return true;
}
} else if (isCallExpression(arg)) {
for (const childArg of arg.arguments) {
if (isFunctionOrArrowExpression(childArg)) {
return true;
}
}
}
}
return false;
}
function isLongCurriedCallExpression(path) {
const node = path.getValue();
const parent = path.getParentNode();
return isCallExpression(node) && isCallExpression(parent) && parent.callee === node && node.arguments.length > parent.arguments.length && parent.arguments.length > 0;
}
function isSimpleCallArgument(node, depth) {
if (depth >= 2) {
return false;
}
const isChildSimple = (child) => isSimpleCallArgument(child, depth + 1);
const regexpPattern = node.type === "Literal" && "regex" in node && node.regex.pattern || node.type === "RegExpLiteral" && node.pattern;
if (regexpPattern && getStringWidth(regexpPattern) > 5) {
return false;
}
if (node.type === "Literal" || node.type === "BigIntLiteral" || node.type === "DecimalLiteral" || node.type === "BooleanLiteral" || node.type === "NullLiteral" || node.type === "NumericLiteral" || node.type === "RegExpLiteral" || node.type === "StringLiteral" || node.type === "Identifier" || node.type === "ThisExpression" || node.type === "Super" || node.type === "PrivateName" || node.type === "PrivateIdentifier" || node.type === "ArgumentPlaceholder" || node.type === "Import") {
return true;
}
if (node.type === "TemplateLiteral") {
return node.quasis.every((element) => !element.value.raw.includes("\n")) && node.expressions.every(isChildSimple);
}
if (node.type === "ObjectExpression") {
return node.properties.every((p) => !p.computed && (p.shorthand || p.value && isChildSimple(p.value)));
}
if (node.type === "ArrayExpression") {
return node.elements.every((x) => x === null || isChildSimple(x));
}
if (isCallLikeExpression(node)) {
return (node.type === "ImportExpression" || isSimpleCallArgument(node.callee, depth)) && getCallArguments(node).every(isChildSimple);
}
if (isMemberExpression(node)) {
return isSimpleCallArgument(node.object, depth) && isSimpleCallArgument(node.property, depth);
}
const targetUnaryExpressionOperators = {
"!": true,
"-": true,
"+": true,
"~": true
};
if (node.type === "UnaryExpression" && targetUnaryExpressionOperators[node.operator]) {
return isSimpleCallArgument(node.argument, depth);
}
const targetUpdateExpressionOperators = {
"++": true,
"--": true
};
if (node.type === "UpdateExpression" && targetUpdateExpressionOperators[node.operator]) {
return isSimpleCallArgument(node.argument, depth);
}
if (node.type === "TSNonNullExpression") {
return isSimpleCallArgument(node.expression, depth);
}
return false;
}
function rawText(node) {
var _node$extra$raw, _node$extra2;
return (_node$extra$raw = (_node$extra2 = node.extra) === null || _node$extra2 === void 0 ? void 0 : _node$extra2.raw) !== null && _node$extra$raw !== void 0 ? _node$extra$raw : node.raw;
}
function identity(x) {
return x;
}
function isTSXFile(options) {
return options.filepath && /\.tsx$/i.test(options.filepath);
}
function shouldPrintComma(options, level = "es5") {
return options.trailingComma === "es5" && level === "es5" || options.trailingComma === "all" && (level === "all" || level === "es5");
}
function startsWithNoLookaheadToken(node, predicate) {
switch (node.type) {
case "BinaryExpression":
case "LogicalExpression":
case "AssignmentExpression":
case "NGPipeExpression":
return startsWithNoLookaheadToken(node.left, predicate);
case "MemberExpression":
case "OptionalMemberExpression":
return startsWithNoLookaheadToken(node.object, predicate);
case "TaggedTemplateExpression":
if (node.tag.type === "FunctionExpression") {
return false;
}
return startsWithNoLookaheadToken(node.tag, predicate);
case "CallExpression":
case "OptionalCallExpression":
if (node.callee.type === "FunctionExpression") {
return false;
}
return startsWithNoLookaheadToken(node.callee, predicate);
case "ConditionalExpression":
return startsWithNoLookaheadToken(node.test, predicate);
case "UpdateExpression":
return !node.prefix && startsWithNoLookaheadToken(node.argument, predicate);
case "BindExpression":
return node.object && startsWithNoLookaheadToken(node.object, predicate);
case "SequenceExpression":
return startsWithNoLookaheadToken(node.expressions[0], predicate);
case "TSSatisfiesExpression":
case "TSAsExpression":
case "TSNonNullExpression":
return startsWithNoLookaheadToken(node.expression, predicate);
default:
return predicate(node);
}
}
var equalityOperators = {
"==": true,
"!=": true,
"===": true,
"!==": true
};
var multiplicativeOperators = {
"*": true,
"/": true,
"%": true
};
var bitshiftOperators = {
">>": true,
">>>": true,
"<<": true
};
function shouldFlatten(parentOp, nodeOp) {
if (getPrecedence(nodeOp) !== getPrecedence(parentOp)) {
return false;
}
if (parentOp === "**") {
return false;
}
if (equalityOperators[parentOp] && equalityOperators[nodeOp]) {
return false;
}
if (nodeOp === "%" && multiplicativeOperators[parentOp] || parentOp === "%" && multiplicativeOperators[nodeOp]) {
return false;
}
if (nodeOp !== parentOp && multiplicativeOperators[nodeOp] && multiplicativeOperators[parentOp]) {
return false;
}
if (bitshiftOperators[parentOp] && bitshiftOperators[nodeOp]) {
return false;
}
return true;
}
var PRECEDENCE = new Map([["|>"], ["??"], ["||"], ["&&"], ["|"], ["^"], ["&"], ["==", "===", "!=", "!=="], ["<", ">", "<=", ">=", "in", "instanceof"], [">>", "<<", ">>>"], ["+", "-"], ["*", "/", "%"], ["**"]].flatMap((operators, index) => operators.map((operator) => [operator, index])));
function getPrecedence(operator) {
return PRECEDENCE.get(operator);
}
function isBitwiseOperator(operator) {
return Boolean(bitshiftOperators[operator]) || operator === "|" || operator === "^" || operator === "&";
}
function hasRestParameter(node) {
var _getLast;
if (node.rest) {
return true;
}
const parameters = getFunctionParameters(node);
return ((_getLast = getLast(parameters)) === null || _getLast === void 0 ? void 0 : _getLast.type) === "RestElement";
}
var functionParametersCache = /* @__PURE__ */ new WeakMap();
function getFunctionParameters(node) {
if (functionParametersCache.has(node)) {
return functionParametersCache.get(node);
}
const parameters = [];
if (node.this) {
parameters.push(node.this);
}
if (Array.isArray(node.parameters)) {
parameters.push(...node.parameters);
} else if (Array.isArray(node.params)) {
parameters.push(...node.params);
}
if (node.rest) {
parameters.push(node.rest);
}
functionParametersCache.set(node, parameters);
return parameters;
}
function iterateFunctionParametersPath(path, iteratee) {
const node = path.getValue();
let index = 0;
const callback = (childPath) => iteratee(childPath, index++);
if (node.this) {
path.call(callback, "this");
}
if (Array.isArray(node.parameters)) {
path.each(callback, "parameters");
} else if (Array.isArray(node.params)) {
path.each(callback, "params");
}
if (node.rest) {
path.call(callback, "rest");
}
}
var callArgumentsCache = /* @__PURE__ */ new WeakMap();
function getCallArguments(node) {
if (callArgumentsCache.has(node)) {
return callArgumentsCache.get(node);
}
let args = node.arguments;
if (node.type === "ImportExpression") {
args = [node.source];
if (node.attributes) {
args.push(node.attributes);
}
}
callArgumentsCache.set(node, args);
return args;
}
function iterateCallArgumentsPath(path, iteratee) {
const node = path.getValue();
if (node.type === "ImportExpression") {
path.call((sourcePath) => iteratee(sourcePath, 0), "source");
if (node.attributes) {
path.call((sourcePath) => iteratee(sourcePath, 1), "attributes");
}
} else {
path.each(iteratee, "arguments");
}
}
function isPrettierIgnoreComment(comment) {
return comment.value.trim() === "prettier-ignore" && !comment.unignore;
}
function hasNodeIgnoreComment(node) {
return node && (node.prettierIgnore || hasComment(node, CommentCheckFlags.PrettierIgnore));
}
function hasIgnoreComment(path) {
const node = path.getValue();
return hasNodeIgnoreComment(node);
}
var CommentCheckFlags = {
Leading: 1 << 1,
Trailing: 1 << 2,
Dangling: 1 << 3,
Block: 1 << 4,
Line: 1 << 5,
PrettierIgnore: 1 << 6,
First: 1 << 7,
Last: 1 << 8
};
var getCommentTestFunction = (flags, fn) => {
if (typeof flags === "function") {
fn = flags;
flags = 0;
}
if (flags || fn) {
return (comment, index, comments) => !(flags & CommentCheckFlags.Leading && !comment.leading || flags & CommentCheckFlags.Trailing && !comment.trailing || flags & CommentCheckFlags.Dangling && (comment.leading || comment.trailing) || flags & CommentCheckFlags.Block && !isBlockComment(comment) || flags & CommentCheckFlags.Line && !isLineComment(comment) || flags & CommentCheckFlags.First && index !== 0 || flags & CommentCheckFlags.Last && index !== comments.length - 1 || flags & CommentCheckFlags.PrettierIgnore && !isPrettierIgnoreComment(comment) || fn && !fn(comment));
}
};
function hasComment(node, flags, fn) {
if (!isNonEmptyArray(node === null || node === void 0 ? void 0 : node.comments)) {
return false;
}
const test = getCommentTestFunction(flags, fn);
return test ? node.comments.some(test) : true;
}
function getComments(node, flags, fn) {
if (!Array.isArray(node === null || node === void 0 ? void 0 : node.comments)) {
return [];
}
const test = getCommentTestFunction(flags, fn);
return test ? node.comments.filter(test) : node.comments;
}
var isNextLineEmpty = (node, {
originalText
}) => isNextLineEmptyAfterIndex(originalText, locEnd(node));
function isCallLikeExpression(node) {
return isCallExpression(node) || node.type === "NewExpression" || node.type === "ImportExpression";
}
function isObjectProperty(node) {
return node && (node.type === "ObjectProperty" || node.type === "Property" && !node.method && node.kind === "init");
}
function isEnabledHackPipeline(options) {
return Boolean(options.__isUsingHackPipeline);
}
var markerForIfWithoutBlockAndSameLineComment = Symbol("ifWithoutBlockAndSameLineComment");
function isTSTypeExpression(node) {
return node.type === "TSAsExpression" || node.type === "TSSatisfiesExpression";
}
module22.exports = {
getFunctionParameters,
iterateFunctionParametersPath,
getCallArguments,
iterateCallArgumentsPath,
hasRestParameter,
getLeftSide,
getLeftSidePathName,
getParentExportDeclaration,
getTypeScriptMappedTypeModifier,
hasFlowAnnotationComment,
hasFlowShorthandAnnotationComment,
hasLeadingOwnLineComment,
hasNakedLeftSide,
hasNode,
hasIgnoreComment,
hasNodeIgnoreComment,
identity,
isBinaryish,
isCallLikeExpression,
isEnabledHackPipeline,
isLineComment,
isPrettierIgnoreComment,
isCallExpression,
isMemberExpression,
isExportDeclaration,
isFlowAnnotationComment,
isFunctionCompositionArgs,
isFunctionNotation,
isFunctionOrArrowExpression,
isGetterOrSetter,
isJestEachTemplateLiteral,
isJsxNode,
isLiteral,
isLongCurriedCallExpression,
isSimpleCallArgument,
isMemberish,
isNumericLiteral,
isSignedNumericLiteral,
isObjectProperty,
isObjectType,
isObjectTypePropertyAFunction,
isSimpleType,
isSimpleNumber,
isSimpleTemplateLiteral,
isStringLiteral,
isStringPropSafeToUnquote,
isTemplateOnItsOwnLine,
isTestCall,
isTheOnlyJsxElementInMarkdown,
isTSXFile,
isTypeAnnotationAFunction,
isNextLineEmpty,
needsHardlineAfterDanglingComment,
rawText,
shouldPrintComma,
isBitwiseOperator,
shouldFlatten,
startsWithNoLookaheadToken,
getPrecedence,
hasComment,
getComments,
CommentCheckFlags,
markerForIfWithoutBlockAndSameLineComment,
isTSTypeExpression
};
}
});
var require_template_literal = __commonJS22({
"src/language-js/print/template-literal.js"(exports2, module22) {
"use strict";
var getLast = require_get_last();
var {
getStringWidth,
getIndentSize
} = require_util();
var {
builders: {
join,
hardline,
softline,
group,
indent,
align,
lineSuffixBoundary,
addAlignmentToDoc
},
printer: {
printDocToString
},
utils: {
mapDoc
}
} = require_doc();
var {
isBinaryish,
isJestEachTemplateLiteral,
isSimpleTemplateLiteral,
hasComment,
isMemberExpression,
isTSTypeExpression
} = require_utils7();
function printTemplateLiteral(path, print, options) {
const node = path.getValue();
const isTemplateLiteral = node.type === "TemplateLiteral";
if (isTemplateLiteral && isJestEachTemplateLiteral(node, path.getParentNode())) {
const printed = printJestEachTemplateLiteral(path, options, print);
if (printed) {
return printed;
}
}
let expressionsKey = "expressions";
if (node.type === "TSTemplateLiteralType") {
expressionsKey = "types";
}
const parts = [];
let expressions = path.map(print, expressionsKey);
const isSimple = isSimpleTemplateLiteral(node);
if (isSimple) {
expressions = expressions.map((doc2) => printDocToString(doc2, Object.assign(Object.assign({}, options), {}, {
printWidth: Number.POSITIVE_INFINITY
})).formatted);
}
parts.push(lineSuffixBoundary, "`");
path.each((childPath) => {
const i = childPath.getName();
parts.push(print());
if (i < expressions.length) {
const {
tabWidth
} = options;
const quasi = childPath.getValue();
const indentSize = getIndentSize(quasi.value.raw, tabWidth);
let printed = expressions[i];
if (!isSimple) {
const expression = node[expressionsKey][i];
if (hasComment(expression) || isMemberExpression(expression) || expression.type === "ConditionalExpression" || expression.type === "SequenceExpression" || isTSTypeExpression(expression) || isBinaryish(expression)) {
printed = [indent([softline, printed]), softline];
}
}
const aligned = indentSize === 0 && quasi.value.raw.endsWith("\n") ? align(Number.NEGATIVE_INFINITY, printed) : addAlignmentToDoc(printed, indentSize, tabWidth);
parts.push(group(["${", aligned, lineSuffixBoundary, "}"]));
}
}, "quasis");
parts.push("`");
return parts;
}
function printJestEachTemplateLiteral(path, options, print) {
const node = path.getNode();
const headerNames = node.quasis[0].value.raw.trim().split(/\s*\|\s*/);
if (headerNames.length > 1 || headerNames.some((headerName) => headerName.length > 0)) {
options.__inJestEach = true;
const expressions = path.map(print, "expressions");
options.__inJestEach = false;
const parts = [];
const stringifiedExpressions = expressions.map((doc2) => "${" + printDocToString(doc2, Object.assign(Object.assign({}, options), {}, {
printWidth: Number.POSITIVE_INFINITY,
endOfLine: "lf"
})).formatted + "}");
const tableBody = [{
hasLineBreak: false,
cells: []
}];
for (let i = 1; i < node.quasis.length; i++) {
const row = getLast(tableBody);
const correspondingExpression = stringifiedExpressions[i - 1];
row.cells.push(correspondingExpression);
if (correspondingExpression.includes("\n")) {
row.hasLineBreak = true;
}
if (node.quasis[i].value.raw.includes("\n")) {
tableBody.push({
hasLineBreak: false,
cells: []
});
}
}
const maxColumnCount = Math.max(headerNames.length, ...tableBody.map((row) => row.cells.length));
const maxColumnWidths = Array.from({
length: maxColumnCount
}).fill(0);
const table = [{
cells: headerNames
}, ...tableBody.filter((row) => row.cells.length > 0)];
for (const {
cells
} of table.filter((row) => !row.hasLineBreak)) {
for (const [index, cell] of cells.entries()) {
maxColumnWidths[index] = Math.max(maxColumnWidths[index], getStringWidth(cell));
}
}
parts.push(lineSuffixBoundary, "`", indent([hardline, join(hardline, table.map((row) => join(" | ", row.cells.map((cell, index) => row.hasLineBreak ? cell : cell + " ".repeat(maxColumnWidths[index] - getStringWidth(cell))))))]), hardline, "`");
return parts;
}
}
function printTemplateExpression(path, print) {
const node = path.getValue();
let printed = print();
if (hasComment(node)) {
printed = group([indent([softline, printed]), softline]);
}
return ["${", printed, lineSuffixBoundary, "}"];
}
function printTemplateExpressions(path, print) {
return path.map((path2) => printTemplateExpression(path2, print), "expressions");
}
function escapeTemplateCharacters(doc2, raw) {
return mapDoc(doc2, (currentDoc) => {
if (typeof currentDoc === "string") {
return raw ? currentDoc.replace(/(\\*)`/g, "$1$1\\`") : uncookTemplateElementValue(currentDoc);
}
return currentDoc;
});
}
function uncookTemplateElementValue(cookedValue) {
return cookedValue.replace(/([\\`]|\${)/g, "\\$1");
}
module22.exports = {
printTemplateLiteral,
printTemplateExpressions,
escapeTemplateCharacters,
uncookTemplateElementValue
};
}
});
var require_markdown = __commonJS22({
"src/language-js/embed/markdown.js"(exports2, module22) {
"use strict";
var {
builders: {
indent,
softline,
literalline,
dedentToRoot
}
} = require_doc();
var {
escapeTemplateCharacters
} = require_template_literal();
function format2(path, print, textToDoc) {
const node = path.getValue();
let text = node.quasis[0].value.raw.replace(/((?:\\\\)*)\\`/g, (_, backslashes) => "\\".repeat(backslashes.length / 2) + "`");
const indentation = getIndentation(text);
const hasIndent = indentation !== "";
if (hasIndent) {
text = text.replace(new RegExp(`^${indentation}`, "gm"), "");
}
const doc2 = escapeTemplateCharacters(textToDoc(text, {
parser: "markdown",
__inJsTemplate: true
}, {
stripTrailingHardline: true
}), true);
return ["`", hasIndent ? indent([softline, doc2]) : [literalline, dedentToRoot(doc2)], softline, "`"];
}
function getIndentation(str) {
const firstMatchedIndent = str.match(/^([^\S\n]*)\S/m);
return firstMatchedIndent === null ? "" : firstMatchedIndent[1];
}
module22.exports = format2;
}
});
var require_css = __commonJS22({
"src/language-js/embed/css.js"(exports2, module22) {
"use strict";
var {
isNonEmptyArray
} = require_util();
var {
builders: {
indent,
hardline,
softline
},
utils: {
mapDoc,
replaceEndOfLine,
cleanDoc
}
} = require_doc();
var {
printTemplateExpressions
} = require_template_literal();
function format2(path, print, textToDoc) {
const node = path.getValue();
const rawQuasis = node.quasis.map((q) => q.value.raw);
let placeholderID = 0;
const text = rawQuasis.reduce((prevVal, currVal, idx) => idx === 0 ? currVal : prevVal + "@prettier-placeholder-" + placeholderID++ + "-id" + currVal, "");
const doc2 = textToDoc(text, {
parser: "scss"
}, {
stripTrailingHardline: true
});
const expressionDocs = printTemplateExpressions(path, print);
return transformCssDoc(doc2, node, expressionDocs);
}
function transformCssDoc(quasisDoc, parentNode, expressionDocs) {
const isEmpty = parentNode.quasis.length === 1 && !parentNode.quasis[0].value.raw.trim();
if (isEmpty) {
return "``";
}
const newDoc = replacePlaceholders(quasisDoc, expressionDocs);
if (!newDoc) {
throw new Error("Couldn't insert all the expressions");
}
return ["`", indent([hardline, newDoc]), softline, "`"];
}
function replacePlaceholders(quasisDoc, expressionDocs) {
if (!isNonEmptyArray(expressionDocs)) {
return quasisDoc;
}
let replaceCounter = 0;
const newDoc = mapDoc(cleanDoc(quasisDoc), (doc2) => {
if (typeof doc2 !== "string" || !doc2.includes("@prettier-placeholder")) {
return doc2;
}
return doc2.split(/@prettier-placeholder-(\d+)-id/).map((component, idx) => {
if (idx % 2 === 0) {
return replaceEndOfLine(component);
}
replaceCounter++;
return expressionDocs[component];
});
});
return expressionDocs.length === replaceCounter ? newDoc : null;
}
module22.exports = format2;
}
});
var require_graphql = __commonJS22({
"src/language-js/embed/graphql.js"(exports2, module22) {
"use strict";
var {
builders: {
indent,
join,
hardline
}
} = require_doc();
var {
escapeTemplateCharacters,
printTemplateExpressions
} = require_template_literal();
function format2(path, print, textToDoc) {
const node = path.getValue();
const numQuasis = node.quasis.length;
if (numQuasis === 1 && node.quasis[0].value.raw.trim() === "") {
return "``";
}
const expressionDocs = printTemplateExpressions(path, print);
const parts = [];
for (let i = 0; i < numQuasis; i++) {
const templateElement = node.quasis[i];
const isFirst = i === 0;
const isLast = i === numQuasis - 1;
const text = templateElement.value.cooked;
const lines = text.split("\n");
const numLines = lines.length;
const expressionDoc = expressionDocs[i];
const startsWithBlankLine = numLines > 2 && lines[0].trim() === "" && lines[1].trim() === "";
const endsWithBlankLine = numLines > 2 && lines[numLines - 1].trim() === "" && lines[numLines - 2].trim() === "";
const commentsAndWhitespaceOnly = lines.every((line) => /^\s*(?:#[^\n\r]*)?$/.test(line));
if (!isLast && /#[^\n\r]*$/.test(lines[numLines - 1])) {
return null;
}
let doc2 = null;
if (commentsAndWhitespaceOnly) {
doc2 = printGraphqlComments(lines);
} else {
doc2 = textToDoc(text, {
parser: "graphql"
}, {
stripTrailingHardline: true
});
}
if (doc2) {
doc2 = escapeTemplateCharacters(doc2, false);
if (!isFirst && startsWithBlankLine) {
parts.push("");
}
parts.push(doc2);
if (!isLast && endsWithBlankLine) {
parts.push("");
}
} else if (!isFirst && !isLast && startsWithBlankLine) {
parts.push("");
}
if (expressionDoc) {
parts.push(expressionDoc);
}
}
return ["`", indent([hardline, join(hardline, parts)]), hardline, "`"];
}
function printGraphqlComments(lines) {
const parts = [];
let seenComment = false;
const array = lines.map((textLine) => textLine.trim());
for (const [i, textLine] of array.entries()) {
if (textLine === "") {
continue;
}
if (array[i - 1] === "" && seenComment) {
parts.push([hardline, textLine]);
} else {
parts.push(textLine);
}
seenComment = true;
}
return parts.length === 0 ? null : join(hardline, parts);
}
module22.exports = format2;
}
});
var require_html2 = __commonJS22({
"src/language-js/embed/html.js"(exports2, module22) {
"use strict";
var {
builders: {
indent,
line,
hardline,
group
},
utils: {
mapDoc
}
} = require_doc();
var {
printTemplateExpressions,
uncookTemplateElementValue
} = require_template_literal();
var htmlTemplateLiteralCounter = 0;
function format2(path, print, textToDoc, options, {
parser
}) {
const node = path.getValue();
const counter = htmlTemplateLiteralCounter;
htmlTemplateLiteralCounter = htmlTemplateLiteralCounter + 1 >>> 0;
const composePlaceholder = (index) => `PRETTIER_HTML_PLACEHOLDER_${index}_${counter}_IN_JS`;
const text = node.quasis.map((quasi, index, quasis) => index === quasis.length - 1 ? quasi.value.cooked : quasi.value.cooked + composePlaceholder(index)).join("");
const expressionDocs = printTemplateExpressions(path, print);
if (expressionDocs.length === 0 && text.trim().length === 0) {
return "``";
}
const placeholderRegex = new RegExp(composePlaceholder("(\\d+)"), "g");
let topLevelCount = 0;
const doc2 = textToDoc(text, {
parser,
__onHtmlRoot(root) {
topLevelCount = root.children.length;
}
}, {
stripTrailingHardline: true
});
const contentDoc = mapDoc(doc2, (doc3) => {
if (typeof doc3 !== "string") {
return doc3;
}
const parts = [];
const components = doc3.split(placeholderRegex);
for (let i = 0; i < components.length; i++) {
let component = components[i];
if (i % 2 === 0) {
if (component) {
component = uncookTemplateElementValue(component);
if (options.__embeddedInHtml) {
component = component.replace(/<\/(script)\b/gi, "<\\/$1");
}
parts.push(component);
}
continue;
}
const placeholderIndex = Number(component);
parts.push(expressionDocs[placeholderIndex]);
}
return parts;
});
const leadingWhitespace = /^\s/.test(text) ? " " : "";
const trailingWhitespace = /\s$/.test(text) ? " " : "";
const linebreak = options.htmlWhitespaceSensitivity === "ignore" ? hardline : leadingWhitespace && trailingWhitespace ? line : null;
if (linebreak) {
return group(["`", indent([linebreak, group(contentDoc)]), linebreak, "`"]);
}
return group(["`", leadingWhitespace, topLevelCount > 1 ? indent(group(contentDoc)) : group(contentDoc), trailingWhitespace, "`"]);
}
module22.exports = format2;
}
});
var require_embed = __commonJS22({
"src/language-js/embed.js"(exports2, module22) {
"use strict";
var {
hasComment,
CommentCheckFlags,
isObjectProperty
} = require_utils7();
var formatMarkdown = require_markdown();
var formatCss = require_css();
var formatGraphql = require_graphql();
var formatHtml = require_html2();
function getLanguage(path) {
if (isStyledJsx(path) || isStyledComponents(path) || isCssProp(path) || isAngularComponentStyles(path)) {
return "css";
}
if (isGraphQL(path)) {
return "graphql";
}
if (isHtml(path)) {
return "html";
}
if (isAngularComponentTemplate(path)) {
return "angular";
}
if (isMarkdown(path)) {
return "markdown";
}
}
function embed(path, print, textToDoc, options) {
const node = path.getValue();
if (node.type !== "TemplateLiteral" || hasInvalidCookedValue(node)) {
return;
}
const language = getLanguage(path);
if (!language) {
return;
}
if (language === "markdown") {
return formatMarkdown(path, print, textToDoc);
}
if (language === "css") {
return formatCss(path, print, textToDoc);
}
if (language === "graphql") {
return formatGraphql(path, print, textToDoc);
}
if (language === "html" || language === "angular") {
return formatHtml(path, print, textToDoc, options, {
parser: language
});
}
}
function isMarkdown(path) {
const node = path.getValue();
const parent = path.getParentNode();
return parent && parent.type === "TaggedTemplateExpression" && node.quasis.length === 1 && parent.tag.type === "Identifier" && (parent.tag.name === "md" || parent.tag.name === "markdown");
}
function isStyledJsx(path) {
const node = path.getValue();
const parent = path.getParentNode();
const parentParent = path.getParentNode(1);
return parentParent && node.quasis && parent.type === "JSXExpressionContainer" && parentParent.type === "JSXElement" && parentParent.openingElement.name.name === "style" && parentParent.openingElement.attributes.some((attribute) => attribute.name.name === "jsx") || parent && parent.type === "TaggedTemplateExpression" && parent.tag.type === "Identifier" && parent.tag.name === "css" || parent && parent.type === "TaggedTemplateExpression" && parent.tag.type === "MemberExpression" && parent.tag.object.name === "css" && (parent.tag.property.name === "global" || parent.tag.property.name === "resolve");
}
function isAngularComponentStyles(path) {
return path.match((node) => node.type === "TemplateLiteral", (node, name) => node.type === "ArrayExpression" && name === "elements", (node, name) => isObjectProperty(node) && node.key.type === "Identifier" && node.key.name === "styles" && name === "value", ...angularComponentObjectExpressionPredicates);
}
function isAngularComponentTemplate(path) {
return path.match((node) => node.type === "TemplateLiteral", (node, name) => isObjectProperty(node) && node.key.type === "Identifier" && node.key.name === "template" && name === "value", ...angularComponentObjectExpressionPredicates);
}
var angularComponentObjectExpressionPredicates = [(node, name) => node.type === "ObjectExpression" && name === "properties", (node, name) => node.type === "CallExpression" && node.callee.type === "Identifier" && node.callee.name === "Component" && name === "arguments", (node, name) => node.type === "Decorator" && name === "expression"];
function isStyledComponents(path) {
const parent = path.getParentNode();
if (!parent || parent.type !== "TaggedTemplateExpression") {
return false;
}
const tag = parent.tag.type === "ParenthesizedExpression" ? parent.tag.expression : parent.tag;
switch (tag.type) {
case "MemberExpression":
return isStyledIdentifier(tag.object) || isStyledExtend(tag);
case "CallExpression":
return isStyledIdentifier(tag.callee) || tag.callee.type === "MemberExpression" && (tag.callee.object.type === "MemberExpression" && (isStyledIdentifier(tag.callee.object.object) || isStyledExtend(tag.callee.object)) || tag.callee.object.type === "CallExpression" && isStyledIdentifier(tag.callee.object.callee));
case "Identifier":
return tag.name === "css";
default:
return false;
}
}
function isCssProp(path) {
const parent = path.getParentNode();
const parentParent = path.getParentNode(1);
return parentParent && parent.type === "JSXExpressionContainer" && parentParent.type === "JSXAttribute" && parentParent.name.type === "JSXIdentifier" && parentParent.name.name === "css";
}
function isStyledIdentifier(node) {
return node.type === "Identifier" && node.name === "styled";
}
function isStyledExtend(node) {
return /^[A-Z]/.test(node.object.name) && node.property.name === "extend";
}
function isGraphQL(path) {
const node = path.getValue();
const parent = path.getParentNode();
return hasLanguageComment(node, "GraphQL") || parent && (parent.type === "TaggedTemplateExpression" && (parent.tag.type === "MemberExpression" && parent.tag.object.name === "graphql" && parent.tag.property.name === "experimental" || parent.tag.type === "Identifier" && (parent.tag.name === "gql" || parent.tag.name === "graphql")) || parent.type === "CallExpression" && parent.callee.type === "Identifier" && parent.callee.name === "graphql");
}
function hasLanguageComment(node, languageName) {
return hasComment(node, CommentCheckFlags.Block | CommentCheckFlags.Leading, ({
value
}) => value === ` ${languageName} `);
}
function isHtml(path) {
return hasLanguageComment(path.getValue(), "HTML") || path.match((node) => node.type === "TemplateLiteral", (node, name) => node.type === "TaggedTemplateExpression" && node.tag.type === "Identifier" && node.tag.name === "html" && name === "quasi");
}
function hasInvalidCookedValue({
quasis
}) {
return quasis.some(({
value: {
cooked
}
}) => cooked === null);
}
module22.exports = embed;
}
});
var require_clean = __commonJS22({
"src/language-js/clean.js"(exports2, module22) {
"use strict";
var isBlockComment = require_is_block_comment();
var ignoredProperties = /* @__PURE__ */ new Set(["range", "raw", "comments", "leadingComments", "trailingComments", "innerComments", "extra", "start", "end", "loc", "flags", "errors", "tokens"]);
var removeTemplateElementsValue = (node) => {
for (const templateElement of node.quasis) {
delete templateElement.value;
}
};
function clean(ast, newObj, parent) {
if (ast.type === "Program") {
delete newObj.sourceType;
}
if (ast.type === "BigIntLiteral" || ast.type === "BigIntLiteralTypeAnnotation") {
if (newObj.value) {
newObj.value = newObj.value.toLowerCase();
}
}
if (ast.type === "BigIntLiteral" || ast.type === "Literal") {
if (newObj.bigint) {
newObj.bigint = newObj.bigint.toLowerCase();
}
}
if (ast.type === "DecimalLiteral") {
newObj.value = Number(newObj.value);
}
if (ast.type === "Literal" && newObj.decimal) {
newObj.decimal = Number(newObj.decimal);
}
if (ast.type === "EmptyStatement") {
return null;
}
if (ast.type === "JSXText") {
return null;
}
if (ast.type === "JSXExpressionContainer" && (ast.expression.type === "Literal" || ast.expression.type === "StringLiteral") && ast.expression.value === " ") {
return null;
}
if ((ast.type === "Property" || ast.type === "ObjectProperty" || ast.type === "MethodDefinition" || ast.type === "ClassProperty" || ast.type === "ClassMethod" || ast.type === "PropertyDefinition" || ast.type === "TSDeclareMethod" || ast.type === "TSPropertySignature" || ast.type === "ObjectTypeProperty") && typeof ast.key === "object" && ast.key && (ast.key.type === "Literal" || ast.key.type === "NumericLiteral" || ast.key.type === "StringLiteral" || ast.key.type === "Identifier")) {
delete newObj.key;
}
if (ast.type === "JSXElement" && ast.openingElement.name.name === "style" && ast.openingElement.attributes.some((attr) => attr.name.name === "jsx")) {
for (const {
type,
expression: expression2
} of newObj.children) {
if (type === "JSXExpressionContainer" && expression2.type === "TemplateLiteral") {
removeTemplateElementsValue(expression2);
}
}
}
if (ast.type === "JSXAttribute" && ast.name.name === "css" && ast.value.type === "JSXExpressionContainer" && ast.value.expression.type === "TemplateLiteral") {
removeTemplateElementsValue(newObj.value.expression);
}
if (ast.type === "JSXAttribute" && ast.value && ast.value.type === "Literal" && /["']|"|'/.test(ast.value.value)) {
newObj.value.value = newObj.value.value.replace(/["']|"|'/g, '"');
}
const expression = ast.expression || ast.callee;
if (ast.type === "Decorator" && expression.type === "CallExpression" && expression.callee.name === "Component" && expression.arguments.length === 1) {
const astProps = ast.expression.arguments[0].properties;
for (const [index, prop] of newObj.expression.arguments[0].properties.entries()) {
switch (astProps[index].key.name) {
case "styles":
if (prop.value.type === "ArrayExpression") {
removeTemplateElementsValue(prop.value.elements[0]);
}
break;
case "template":
if (prop.value.type === "TemplateLiteral") {
removeTemplateElementsValue(prop.value);
}
break;
}
}
}
if (ast.type === "TaggedTemplateExpression" && (ast.tag.type === "MemberExpression" || ast.tag.type === "Identifier" && (ast.tag.name === "gql" || ast.tag.name === "graphql" || ast.tag.name === "css" || ast.tag.name === "md" || ast.tag.name === "markdown" || ast.tag.name === "html") || ast.tag.type === "CallExpression")) {
removeTemplateElementsValue(newObj.quasi);
}
if (ast.type === "TemplateLiteral") {
var _ast$leadingComments;
const hasLanguageComment = (_ast$leadingComments = ast.leadingComments) === null || _ast$leadingComments === void 0 ? void 0 : _ast$leadingComments.some((comment) => isBlockComment(comment) && ["GraphQL", "HTML"].some((languageName) => comment.value === ` ${languageName} `));
if (hasLanguageComment || parent.type === "CallExpression" && parent.callee.name === "graphql" || !ast.leadingComments) {
removeTemplateElementsValue(newObj);
}
}
if (ast.type === "InterpreterDirective") {
newObj.value = newObj.value.trimEnd();
}
if ((ast.type === "TSIntersectionType" || ast.type === "TSUnionType") && ast.types.length === 1) {
return newObj.types[0];
}
}
clean.ignoredProperties = ignoredProperties;
module22.exports = clean;
}
});
var require_detect_newline = __commonJS22({
"node_modules/detect-newline/index.js"(exports2, module22) {
"use strict";
var detectNewline = (string) => {
if (typeof string !== "string") {
throw new TypeError("Expected a string");
}
const newlines = string.match(/(?:\r?\n)/g) || [];
if (newlines.length === 0) {
return;
}
const crlf = newlines.filter((newline) => newline === "\r\n").length;
const lf = newlines.length - crlf;
return crlf > lf ? "\r\n" : "\n";
};
module22.exports = detectNewline;
module22.exports.graceful = (string) => typeof string === "string" && detectNewline(string) || "\n";
}
});
var require_build = __commonJS22({
"node_modules/jest-docblock/build/index.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.extract = extract;
exports2.parse = parse2;
exports2.parseWithComments = parseWithComments;
exports2.print = print;
exports2.strip = strip;
function _os() {
const data = require("os");
_os = function() {
return data;
};
return data;
}
function _detectNewline() {
const data = _interopRequireDefault(require_detect_newline());
_detectNewline = function() {
return data;
};
return data;
}
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : {
default: obj
};
}
var commentEndRe = /\*\/$/;
var commentStartRe = /^\/\*\*?/;
var docblockRe = /^\s*(\/\*\*?(.|\r?\n)*?\*\/)/;
var lineCommentRe = /(^|\s+)\/\/([^\r\n]*)/g;
var ltrimNewlineRe = /^(\r?\n)+/;
var multilineRe = /(?:^|\r?\n) *(@[^\r\n]*?) *\r?\n *(?![^@\r\n]*\/\/[^]*)([^@\r\n\s][^@\r\n]+?) *\r?\n/g;
var propertyRe = /(?:^|\r?\n) *@(\S+) *([^\r\n]*)/g;
var stringStartRe = /(\r?\n|^) *\* ?/g;
var STRING_ARRAY = [];
function extract(contents) {
const match = contents.match(docblockRe);
return match ? match[0].trimLeft() : "";
}
function strip(contents) {
const match = contents.match(docblockRe);
return match && match[0] ? contents.substring(match[0].length) : contents;
}
function parse2(docblock) {
return parseWithComments(docblock).pragmas;
}
function parseWithComments(docblock) {
const line = (0, _detectNewline().default)(docblock) || _os().EOL;
docblock = docblock.replace(commentStartRe, "").replace(commentEndRe, "").replace(stringStartRe, "$1");
let prev = "";
while (prev !== docblock) {
prev = docblock;
docblock = docblock.replace(multilineRe, `${line}$1 $2${line}`);
}
docblock = docblock.replace(ltrimNewlineRe, "").trimRight();
const result = /* @__PURE__ */ Object.create(null);
const comments = docblock.replace(propertyRe, "").replace(ltrimNewlineRe, "").trimRight();
let match;
while (match = propertyRe.exec(docblock)) {
const nextPragma = match[2].replace(lineCommentRe, "");
if (typeof result[match[1]] === "string" || Array.isArray(result[match[1]])) {
result[match[1]] = STRING_ARRAY.concat(result[match[1]], nextPragma);
} else {
result[match[1]] = nextPragma;
}
}
return {
comments,
pragmas: result
};
}
function print({
comments = "",
pragmas = {}
}) {
const line = (0, _detectNewline().default)(comments) || _os().EOL;
const head = "/**";
const start = " *";
const tail = " */";
const keys = Object.keys(pragmas);
const printedObject = keys.map((key) => printKeyValues(key, pragmas[key])).reduce((arr, next) => arr.concat(next), []).map((keyValue) => `${start} ${keyValue}${line}`).join("");
if (!comments) {
if (keys.length === 0) {
return "";
}
if (keys.length === 1 && !Array.isArray(pragmas[keys[0]])) {
const value = pragmas[keys[0]];
return `${head} ${printKeyValues(keys[0], value)[0]}${tail}`;
}
}
const printedComments = comments.split(line).map((textLine) => `${start} ${textLine}`).join(line) + line;
return head + line + (comments ? printedComments : "") + (comments && keys.length ? start + line : "") + printedObject + tail;
}
function printKeyValues(key, valueOrArray) {
return STRING_ARRAY.concat(valueOrArray).map((value) => `@${key} ${value}`.trim());
}
}
});
var require_get_shebang = __commonJS22({
"src/language-js/utils/get-shebang.js"(exports2, module22) {
"use strict";
function getShebang(text) {
if (!text.startsWith("#!")) {
return "";
}
const index = text.indexOf("\n");
if (index === -1) {
return text;
}
return text.slice(0, index);
}
module22.exports = getShebang;
}
});
var require_pragma = __commonJS22({
"src/language-js/pragma.js"(exports2, module22) {
"use strict";
var {
parseWithComments,
strip,
extract,
print
} = require_build();
var {
normalizeEndOfLine
} = require_end_of_line();
var getShebang = require_get_shebang();
function parseDocBlock(text) {
const shebang = getShebang(text);
if (shebang) {
text = text.slice(shebang.length + 1);
}
const docBlock = extract(text);
const {
pragmas,
comments
} = parseWithComments(docBlock);
return {
shebang,
text,
pragmas,
comments
};
}
function hasPragma(text) {
const pragmas = Object.keys(parseDocBlock(text).pragmas);
return pragmas.includes("prettier") || pragmas.includes("format");
}
function insertPragma(originalText) {
const {
shebang,
text,
pragmas,
comments
} = parseDocBlock(originalText);
const strippedText = strip(text);
const docBlock = print({
pragmas: Object.assign({
format: ""
}, pragmas),
comments: comments.trimStart()
});
return (shebang ? `${shebang}
` : "") + normalizeEndOfLine(docBlock) + (strippedText.startsWith("\n") ? "\n" : "\n\n") + strippedText;
}
module22.exports = {
hasPragma,
insertPragma
};
}
});
var require_is_type_cast_comment = __commonJS22({
"src/language-js/utils/is-type-cast-comment.js"(exports2, module22) {
"use strict";
var isBlockComment = require_is_block_comment();
function isTypeCastComment(comment) {
return isBlockComment(comment) && comment.value[0] === "*" && /@(?:type|satisfies)\b/.test(comment.value);
}
module22.exports = isTypeCastComment;
}
});
var require_comments2 = __commonJS22({
"src/language-js/comments.js"(exports2, module22) {
"use strict";
var {
getLast,
hasNewline,
getNextNonSpaceNonCommentCharacterIndexWithStartIndex,
getNextNonSpaceNonCommentCharacter,
hasNewlineInRange,
addLeadingComment,
addTrailingComment,
addDanglingComment,
getNextNonSpaceNonCommentCharacterIndex,
isNonEmptyArray
} = require_util();
var {
getFunctionParameters,
isPrettierIgnoreComment,
isJsxNode,
hasFlowShorthandAnnotationComment,
hasFlowAnnotationComment,
hasIgnoreComment,
isCallLikeExpression,
getCallArguments,
isCallExpression,
isMemberExpression,
isObjectProperty,
isLineComment,
getComments,
CommentCheckFlags,
markerForIfWithoutBlockAndSameLineComment
} = require_utils7();
var {
locStart,
locEnd
} = require_loc();
var isBlockComment = require_is_block_comment();
var isTypeCastComment = require_is_type_cast_comment();
function handleOwnLineComment(context) {
return [handleIgnoreComments, handleLastFunctionArgComments, handleMemberExpressionComments, handleIfStatementComments, handleWhileComments, handleTryStatementComments, handleClassComments, handleForComments, handleUnionTypeComments, handleOnlyComments, handleModuleSpecifiersComments, handleAssignmentPatternComments, handleMethodNameComments, handleLabeledStatementComments, handleBreakAndContinueStatementComments].some((fn) => fn(context));
}
function handleEndOfLineComment(context) {
return [handleClosureTypeCastComments, handleLastFunctionArgComments, handleConditionalExpressionComments, handleModuleSpecifiersComments, handleIfStatementComments, handleWhileComments, handleTryStatementComments, handleClassComments, handleLabeledStatementComments, handleCallExpressionComments, handlePropertyComments, handleOnlyComments, handleVariableDeclaratorComments, handleBreakAndContinueStatementComments, handleSwitchDefaultCaseComments].some((fn) => fn(context));
}
function handleRemainingComment(context) {
return [handleIgnoreComments, handleIfStatementComments, handleWhileComments, handleObjectPropertyAssignment, handleCommentInEmptyParens, handleMethodNameComments, handleOnlyComments, handleCommentAfterArrowParams, handleFunctionNameComments, handleTSMappedTypeComments, handleBreakAndContinueStatementComments, handleTSFunctionTrailingComments].some((fn) => fn(context));
}
function addBlockStatementFirstComment(node, comment) {
const firstNonEmptyNode = (node.body || node.properties).find(({
type
}) => type !== "EmptyStatement");
if (firstNonEmptyNode) {
addLeadingComment(firstNonEmptyNode, comment);
} else {
addDanglingComment(node, comment);
}
}
function addBlockOrNotComment(node, comment) {
if (node.type === "BlockStatement") {
addBlockStatementFirstComment(node, comment);
} else {
addLeadingComment(node, comment);
}
}
function handleClosureTypeCastComments({
comment,
followingNode
}) {
if (followingNode && isTypeCastComment(comment)) {
addLeadingComment(followingNode, comment);
return true;
}
return false;
}
function handleIfStatementComments({
comment,
precedingNode,
enclosingNode,
followingNode,
text
}) {
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) !== "IfStatement" || !followingNode) {
return false;
}
const nextCharacter = getNextNonSpaceNonCommentCharacter(text, comment, locEnd);
if (nextCharacter === ")") {
addTrailingComment(precedingNode, comment);
return true;
}
if (precedingNode === enclosingNode.consequent && followingNode === enclosingNode.alternate) {
if (precedingNode.type === "BlockStatement") {
addTrailingComment(precedingNode, comment);
} else {
const isSingleLineComment = comment.type === "SingleLine" || comment.loc.start.line === comment.loc.end.line;
const isSameLineComment = comment.loc.start.line === precedingNode.loc.start.line;
if (isSingleLineComment && isSameLineComment) {
addDanglingComment(precedingNode, comment, markerForIfWithoutBlockAndSameLineComment);
} else {
addDanglingComment(enclosingNode, comment);
}
}
return true;
}
if (followingNode.type === "BlockStatement") {
addBlockStatementFirstComment(followingNode, comment);
return true;
}
if (followingNode.type === "IfStatement") {
addBlockOrNotComment(followingNode.consequent, comment);
return true;
}
if (enclosingNode.consequent === followingNode) {
addLeadingComment(followingNode, comment);
return true;
}
return false;
}
function handleWhileComments({
comment,
precedingNode,
enclosingNode,
followingNode,
text
}) {
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) !== "WhileStatement" || !followingNode) {
return false;
}
const nextCharacter = getNextNonSpaceNonCommentCharacter(text, comment, locEnd);
if (nextCharacter === ")") {
addTrailingComment(precedingNode, comment);
return true;
}
if (followingNode.type === "BlockStatement") {
addBlockStatementFirstComment(followingNode, comment);
return true;
}
if (enclosingNode.body === followingNode) {
addLeadingComment(followingNode, comment);
return true;
}
return false;
}
function handleTryStatementComments({
comment,
precedingNode,
enclosingNode,
followingNode
}) {
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) !== "TryStatement" && (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) !== "CatchClause" || !followingNode) {
return false;
}
if (enclosingNode.type === "CatchClause" && precedingNode) {
addTrailingComment(precedingNode, comment);
return true;
}
if (followingNode.type === "BlockStatement") {
addBlockStatementFirstComment(followingNode, comment);
return true;
}
if (followingNode.type === "TryStatement") {
addBlockOrNotComment(followingNode.finalizer, comment);
return true;
}
if (followingNode.type === "CatchClause") {
addBlockOrNotComment(followingNode.body, comment);
return true;
}
return false;
}
function handleMemberExpressionComments({
comment,
enclosingNode,
followingNode
}) {
if (isMemberExpression(enclosingNode) && (followingNode === null || followingNode === void 0 ? void 0 : followingNode.type) === "Identifier") {
addLeadingComment(enclosingNode, comment);
return true;
}
return false;
}
function handleConditionalExpressionComments({
comment,
precedingNode,
enclosingNode,
followingNode,
text
}) {
const isSameLineAsPrecedingNode = precedingNode && !hasNewlineInRange(text, locEnd(precedingNode), locStart(comment));
if ((!precedingNode || !isSameLineAsPrecedingNode) && ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ConditionalExpression" || (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "TSConditionalType") && followingNode) {
addLeadingComment(followingNode, comment);
return true;
}
return false;
}
function handleObjectPropertyAssignment({
comment,
precedingNode,
enclosingNode
}) {
if (isObjectProperty(enclosingNode) && enclosingNode.shorthand && enclosingNode.key === precedingNode && enclosingNode.value.type === "AssignmentPattern") {
addTrailingComment(enclosingNode.value.left, comment);
return true;
}
return false;
}
var classLikeNodeTypes = /* @__PURE__ */ new Set(["ClassDeclaration", "ClassExpression", "DeclareClass", "DeclareInterface", "InterfaceDeclaration", "TSInterfaceDeclaration"]);
function handleClassComments({
comment,
precedingNode,
enclosingNode,
followingNode
}) {
if (classLikeNodeTypes.has(enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type)) {
if (isNonEmptyArray(enclosingNode.decorators) && !(followingNode && followingNode.type === "Decorator")) {
addTrailingComment(getLast(enclosingNode.decorators), comment);
return true;
}
if (enclosingNode.body && followingNode === enclosingNode.body) {
addBlockStatementFirstComment(enclosingNode.body, comment);
return true;
}
if (followingNode) {
if (enclosingNode.superClass && followingNode === enclosingNode.superClass && precedingNode && (precedingNode === enclosingNode.id || precedingNode === enclosingNode.typeParameters)) {
addTrailingComment(precedingNode, comment);
return true;
}
for (const prop of ["implements", "extends", "mixins"]) {
if (enclosingNode[prop] && followingNode === enclosingNode[prop][0]) {
if (precedingNode && (precedingNode === enclosingNode.id || precedingNode === enclosingNode.typeParameters || precedingNode === enclosingNode.superClass)) {
addTrailingComment(precedingNode, comment);
} else {
addDanglingComment(enclosingNode, comment, prop);
}
return true;
}
}
}
}
return false;
}
var propertyLikeNodeTypes = /* @__PURE__ */ new Set(["ClassMethod", "ClassProperty", "PropertyDefinition", "TSAbstractPropertyDefinition", "TSAbstractMethodDefinition", "TSDeclareMethod", "MethodDefinition", "ClassAccessorProperty", "AccessorProperty", "TSAbstractAccessorProperty"]);
function handleMethodNameComments({
comment,
precedingNode,
enclosingNode,
text
}) {
if (enclosingNode && precedingNode && getNextNonSpaceNonCommentCharacter(text, comment, locEnd) === "(" && (enclosingNode.type === "Property" || enclosingNode.type === "TSDeclareMethod" || enclosingNode.type === "TSAbstractMethodDefinition") && precedingNode.type === "Identifier" && enclosingNode.key === precedingNode && getNextNonSpaceNonCommentCharacter(text, precedingNode, locEnd) !== ":") {
addTrailingComment(precedingNode, comment);
return true;
}
if ((precedingNode === null || precedingNode === void 0 ? void 0 : precedingNode.type) === "Decorator" && propertyLikeNodeTypes.has(enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type)) {
addTrailingComment(precedingNode, comment);
return true;
}
return false;
}
var functionLikeNodeTypes = /* @__PURE__ */ new Set(["FunctionDeclaration", "FunctionExpression", "ClassMethod", "MethodDefinition", "ObjectMethod"]);
function handleFunctionNameComments({
comment,
precedingNode,
enclosingNode,
text
}) {
if (getNextNonSpaceNonCommentCharacter(text, comment, locEnd) !== "(") {
return false;
}
if (precedingNode && functionLikeNodeTypes.has(enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type)) {
addTrailingComment(precedingNode, comment);
return true;
}
return false;
}
function handleCommentAfterArrowParams({
comment,
enclosingNode,
text
}) {
if (!((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ArrowFunctionExpression")) {
return false;
}
const index = getNextNonSpaceNonCommentCharacterIndex(text, comment, locEnd);
if (index !== false && text.slice(index, index + 2) === "=>") {
addDanglingComment(enclosingNode, comment);
return true;
}
return false;
}
function handleCommentInEmptyParens({
comment,
enclosingNode,
text
}) {
if (getNextNonSpaceNonCommentCharacter(text, comment, locEnd) !== ")") {
return false;
}
if (enclosingNode && (isRealFunctionLikeNode(enclosingNode) && getFunctionParameters(enclosingNode).length === 0 || isCallLikeExpression(enclosingNode) && getCallArguments(enclosingNode).length === 0)) {
addDanglingComment(enclosingNode, comment);
return true;
}
if (((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "MethodDefinition" || (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "TSAbstractMethodDefinition") && getFunctionParameters(enclosingNode.value).length === 0) {
addDanglingComment(enclosingNode.value, comment);
return true;
}
return false;
}
function handleLastFunctionArgComments({
comment,
precedingNode,
enclosingNode,
followingNode,
text
}) {
if ((precedingNode === null || precedingNode === void 0 ? void 0 : precedingNode.type) === "FunctionTypeParam" && (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "FunctionTypeAnnotation" && (followingNode === null || followingNode === void 0 ? void 0 : followingNode.type) !== "FunctionTypeParam") {
addTrailingComment(precedingNode, comment);
return true;
}
if (((precedingNode === null || precedingNode === void 0 ? void 0 : precedingNode.type) === "Identifier" || (precedingNode === null || precedingNode === void 0 ? void 0 : precedingNode.type) === "AssignmentPattern") && enclosingNode && isRealFunctionLikeNode(enclosingNode) && getNextNonSpaceNonCommentCharacter(text, comment, locEnd) === ")") {
addTrailingComment(precedingNode, comment);
return true;
}
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "FunctionDeclaration" && (followingNode === null || followingNode === void 0 ? void 0 : followingNode.type) === "BlockStatement") {
const functionParamRightParenIndex = (() => {
const parameters = getFunctionParameters(enclosingNode);
if (parameters.length > 0) {
return getNextNonSpaceNonCommentCharacterIndexWithStartIndex(text, locEnd(getLast(parameters)));
}
const functionParamLeftParenIndex = getNextNonSpaceNonCommentCharacterIndexWithStartIndex(text, locEnd(enclosingNode.id));
return functionParamLeftParenIndex !== false && getNextNonSpaceNonCommentCharacterIndexWithStartIndex(text, functionParamLeftParenIndex + 1);
})();
if (locStart(comment) > functionParamRightParenIndex) {
addBlockStatementFirstComment(followingNode, comment);
return true;
}
}
return false;
}
function handleLabeledStatementComments({
comment,
enclosingNode
}) {
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "LabeledStatement") {
addLeadingComment(enclosingNode, comment);
return true;
}
return false;
}
function handleBreakAndContinueStatementComments({
comment,
enclosingNode
}) {
if (((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ContinueStatement" || (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "BreakStatement") && !enclosingNode.label) {
addTrailingComment(enclosingNode, comment);
return true;
}
return false;
}
function handleCallExpressionComments({
comment,
precedingNode,
enclosingNode
}) {
if (isCallExpression(enclosingNode) && precedingNode && enclosingNode.callee === precedingNode && enclosingNode.arguments.length > 0) {
addLeadingComment(enclosingNode.arguments[0], comment);
return true;
}
return false;
}
function handleUnionTypeComments({
comment,
precedingNode,
enclosingNode,
followingNode
}) {
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "UnionTypeAnnotation" || (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "TSUnionType") {
if (isPrettierIgnoreComment(comment)) {
followingNode.prettierIgnore = true;
comment.unignore = true;
}
if (precedingNode) {
addTrailingComment(precedingNode, comment);
return true;
}
return false;
}
if (((followingNode === null || followingNode === void 0 ? void 0 : followingNode.type) === "UnionTypeAnnotation" || (followingNode === null || followingNode === void 0 ? void 0 : followingNode.type) === "TSUnionType") && isPrettierIgnoreComment(comment)) {
followingNode.types[0].prettierIgnore = true;
comment.unignore = true;
}
return false;
}
function handlePropertyComments({
comment,
enclosingNode
}) {
if (isObjectProperty(enclosingNode)) {
addLeadingComment(enclosingNode, comment);
return true;
}
return false;
}
function handleOnlyComments({
comment,
enclosingNode,
followingNode,
ast,
isLastComment
}) {
if (ast && ast.body && ast.body.length === 0) {
if (isLastComment) {
addDanglingComment(ast, comment);
} else {
addLeadingComment(ast, comment);
}
return true;
}
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "Program" && (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.body.length) === 0 && !isNonEmptyArray(enclosingNode.directives)) {
if (isLastComment) {
addDanglingComment(enclosingNode, comment);
} else {
addLeadingComment(enclosingNode, comment);
}
return true;
}
if ((followingNode === null || followingNode === void 0 ? void 0 : followingNode.type) === "Program" && (followingNode === null || followingNode === void 0 ? void 0 : followingNode.body.length) === 0 && (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ModuleExpression") {
addDanglingComment(followingNode, comment);
return true;
}
return false;
}
function handleForComments({
comment,
enclosingNode
}) {
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ForInStatement" || (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ForOfStatement") {
addLeadingComment(enclosingNode, comment);
return true;
}
return false;
}
function handleModuleSpecifiersComments({
comment,
precedingNode,
enclosingNode,
text
}) {
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ImportSpecifier" || (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ExportSpecifier") {
addLeadingComment(enclosingNode, comment);
return true;
}
const isImportDeclaration = (precedingNode === null || precedingNode === void 0 ? void 0 : precedingNode.type) === "ImportSpecifier" && (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ImportDeclaration";
const isExportDeclaration = (precedingNode === null || precedingNode === void 0 ? void 0 : precedingNode.type) === "ExportSpecifier" && (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "ExportNamedDeclaration";
if ((isImportDeclaration || isExportDeclaration) && hasNewline(text, locEnd(comment))) {
addTrailingComment(precedingNode, comment);
return true;
}
return false;
}
function handleAssignmentPatternComments({
comment,
enclosingNode
}) {
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "AssignmentPattern") {
addLeadingComment(enclosingNode, comment);
return true;
}
return false;
}
var assignmentLikeNodeTypes = /* @__PURE__ */ new Set(["VariableDeclarator", "AssignmentExpression", "TypeAlias", "TSTypeAliasDeclaration"]);
var complexExprNodeTypes = /* @__PURE__ */ new Set(["ObjectExpression", "ArrayExpression", "TemplateLiteral", "TaggedTemplateExpression", "ObjectTypeAnnotation", "TSTypeLiteral"]);
function handleVariableDeclaratorComments({
comment,
enclosingNode,
followingNode
}) {
if (assignmentLikeNodeTypes.has(enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) && followingNode && (complexExprNodeTypes.has(followingNode.type) || isBlockComment(comment))) {
addLeadingComment(followingNode, comment);
return true;
}
return false;
}
function handleTSFunctionTrailingComments({
comment,
enclosingNode,
followingNode,
text
}) {
if (!followingNode && ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "TSMethodSignature" || (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "TSDeclareFunction" || (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "TSAbstractMethodDefinition") && getNextNonSpaceNonCommentCharacter(text, comment, locEnd) === ";") {
addTrailingComment(enclosingNode, comment);
return true;
}
return false;
}
function handleIgnoreComments({
comment,
enclosingNode,
followingNode
}) {
if (isPrettierIgnoreComment(comment) && (enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) === "TSMappedType" && (followingNode === null || followingNode === void 0 ? void 0 : followingNode.type) === "TSTypeParameter" && followingNode.constraint) {
enclosingNode.prettierIgnore = true;
comment.unignore = true;
return true;
}
}
function handleTSMappedTypeComments({
comment,
precedingNode,
enclosingNode,
followingNode
}) {
if ((enclosingNode === null || enclosingNode === void 0 ? void 0 : enclosingNode.type) !== "TSMappedType") {
return false;
}
if ((followingNode === null || followingNode === void 0 ? void 0 : followingNode.type) === "TSTypeParameter" && followingNode.name) {
addLeadingComment(followingNode.name, comment);
return true;
}
if ((precedingNode === null || precedingNode === void 0 ? void 0 : precedingNode.type) === "TSTypeParameter" && precedingNode.constraint) {
addTrailingComment(precedingNode.constraint, comment);
return true;
}
return false;
}
function handleSwitchDefaultCaseComments({
comment,
enclosingNode,
followingNode
}) {
if (!enclosingNode || enclosingNode.type !== "SwitchCase" || enclosingNode.test || !followingNode || followingNode !== enclosingNode.consequent[0]) {
return false;
}
if (followingNode.type === "BlockStatement" && isLineComment(comment)) {
addBlockStatementFirstComment(followingNode, comment);
} else {
addDanglingComment(enclosingNode, comment);
}
return true;
}
function isRealFunctionLikeNode(node) {
return node.type === "ArrowFunctionExpression" || node.type === "FunctionExpression" || node.type === "FunctionDeclaration" || node.type === "ObjectMethod" || node.type === "ClassMethod" || node.type === "TSDeclareFunction" || node.type === "TSCallSignatureDeclaration" || node.type === "TSConstructSignatureDeclaration" || node.type === "TSMethodSignature" || node.type === "TSConstructorType" || node.type === "TSFunctionType" || node.type === "TSDeclareMethod";
}
function getCommentChildNodes(node, options) {
if ((options.parser === "typescript" || options.parser === "flow" || options.parser === "acorn" || options.parser === "espree" || options.parser === "meriyah" || options.parser === "__babel_estree") && node.type === "MethodDefinition" && node.value && node.value.type === "FunctionExpression" && getFunctionParameters(node.value).length === 0 && !node.value.returnType && !isNonEmptyArray(node.value.typeParameters) && node.value.body) {
return [...node.decorators || [], node.key, node.value.body];
}
}
function willPrintOwnComments(path) {
const node = path.getValue();
const parent = path.getParentNode();
const hasFlowAnnotations = (node2) => hasFlowAnnotationComment(getComments(node2, CommentCheckFlags.Leading)) || hasFlowAnnotationComment(getComments(node2, CommentCheckFlags.Trailing));
return (node && (isJsxNode(node) || hasFlowShorthandAnnotationComment(node) || isCallExpression(parent) && hasFlowAnnotations(node)) || parent && (parent.type === "JSXSpreadAttribute" || parent.type === "JSXSpreadChild" || parent.type === "UnionTypeAnnotation" || parent.type === "TSUnionType" || (parent.type === "ClassDeclaration" || parent.type === "ClassExpression") && parent.superClass === node)) && (!hasIgnoreComment(path) || parent.type === "UnionTypeAnnotation" || parent.type === "TSUnionType");
}
module22.exports = {
handleOwnLineComment,
handleEndOfLineComment,
handleRemainingComment,
getCommentChildNodes,
willPrintOwnComments
};
}
});
var require_needs_parens = __commonJS22({
"src/language-js/needs-parens.js"(exports2, module22) {
"use strict";
var getLast = require_get_last();
var isNonEmptyArray = require_is_non_empty_array();
var {
getFunctionParameters,
getLeftSidePathName,
hasFlowShorthandAnnotationComment,
hasNakedLeftSide,
hasNode,
isBitwiseOperator,
startsWithNoLookaheadToken,
shouldFlatten,
getPrecedence,
isCallExpression,
isMemberExpression,
isObjectProperty,
isTSTypeExpression
} = require_utils7();
function needsParens(path, options) {
const parent = path.getParentNode();
if (!parent) {
return false;
}
const name = path.getName();
const node = path.getNode();
if (options.__isInHtmlInterpolation && !options.bracketSpacing && endsWithRightBracket(node) && isFollowedByRightBracket(path)) {
return true;
}
if (isStatement(node)) {
return false;
}
if (options.parser !== "flow" && hasFlowShorthandAnnotationComment(path.getValue())) {
return true;
}
if (node.type === "Identifier") {
if (node.extra && node.extra.parenthesized && /^PRETTIER_HTML_PLACEHOLDER_\d+_\d+_IN_JS$/.test(node.name)) {
return true;
}
if (name === "left" && (node.name === "async" && !parent.await || node.name === "let") && parent.type === "ForOfStatement") {
return true;
}
if (node.name === "let") {
var _path$findAncestor;
const expression = (_path$findAncestor = path.findAncestor((node2) => node2.type === "ForOfStatement")) === null || _path$findAncestor === void 0 ? void 0 : _path$findAncestor.left;
if (expression && startsWithNoLookaheadToken(expression, (leftmostNode) => leftmostNode === node)) {
return true;
}
}
if (name === "object" && node.name === "let" && parent.type === "MemberExpression" && parent.computed && !parent.optional) {
const statement = path.findAncestor((node2) => node2.type === "ExpressionStatement" || node2.type === "ForStatement" || node2.type === "ForInStatement");
const expression = !statement ? void 0 : statement.type === "ExpressionStatement" ? statement.expression : statement.type === "ForStatement" ? statement.init : statement.left;
if (expression && startsWithNoLookaheadToken(expression, (leftmostNode) => leftmostNode === node)) {
return true;
}
}
return false;
}
if (node.type === "ObjectExpression" || node.type === "FunctionExpression" || node.type === "ClassExpression" || node.type === "DoExpression") {
var _path$findAncestor2;
const expression = (_path$findAncestor2 = path.findAncestor((node2) => node2.type === "ExpressionStatement")) === null || _path$findAncestor2 === void 0 ? void 0 : _path$findAncestor2.expression;
if (expression && startsWithNoLookaheadToken(expression, (leftmostNode) => leftmostNode === node)) {
return true;
}
}
switch (parent.type) {
case "ParenthesizedExpression":
return false;
case "ClassDeclaration":
case "ClassExpression": {
if (name === "superClass" && (node.type === "ArrowFunctionExpression" || node.type === "AssignmentExpression" || node.type === "AwaitExpression" || node.type === "BinaryExpression" || node.type === "ConditionalExpression" || node.type === "LogicalExpression" || node.type === "NewExpression" || node.type === "ObjectExpression" || node.type === "SequenceExpression" || node.type === "TaggedTemplateExpression" || node.type === "UnaryExpression" || node.type === "UpdateExpression" || node.type === "YieldExpression" || node.type === "TSNonNullExpression")) {
return true;
}
break;
}
case "ExportDefaultDeclaration": {
return shouldWrapFunctionForExportDefault(path, options) || node.type === "SequenceExpression";
}
case "Decorator": {
if (name === "expression") {
if (isMemberExpression(node) && node.computed) {
return true;
}
let hasCallExpression = false;
let hasMemberExpression = false;
let current = node;
while (current) {
switch (current.type) {
case "MemberExpression":
hasMemberExpression = true;
current = current.object;
break;
case "CallExpression":
if (hasMemberExpression || hasCallExpression) {
return options.parser !== "typescript";
}
hasCallExpression = true;
current = current.callee;
break;
case "Identifier":
return false;
case "TaggedTemplateExpression":
return options.parser !== "typescript";
default:
return true;
}
}
return true;
}
break;
}
case "ArrowFunctionExpression": {
if (name === "body" && node.type !== "SequenceExpression" && startsWithNoLookaheadToken(node, (node2) => node2.type === "ObjectExpression")) {
return true;
}
break;
}
}
switch (node.type) {
case "UpdateExpression":
if (parent.type === "UnaryExpression") {
return node.prefix && (node.operator === "++" && parent.operator === "+" || node.operator === "--" && parent.operator === "-");
}
case "UnaryExpression":
switch (parent.type) {
case "UnaryExpression":
return node.operator === parent.operator && (node.operator === "+" || node.operator === "-");
case "BindExpression":
return true;
case "MemberExpression":
case "OptionalMemberExpression":
return name === "object";
case "TaggedTemplateExpression":
return true;
case "NewExpression":
case "CallExpression":
case "OptionalCallExpression":
return name === "callee";
case "BinaryExpression":
return name === "left" && parent.operator === "**";
case "TSNonNullExpression":
return true;
default:
return false;
}
case "BinaryExpression": {
if (parent.type === "UpdateExpression") {
return true;
}
if (node.operator === "in" && isPathInForStatementInitializer(path)) {
return true;
}
if (node.operator === "|>" && node.extra && node.extra.parenthesized) {
const grandParent = path.getParentNode(1);
if (grandParent.type === "BinaryExpression" && grandParent.operator === "|>") {
return true;
}
}
}
case "TSTypeAssertion":
case "TSAsExpression":
case "TSSatisfiesExpression":
case "LogicalExpression":
switch (parent.type) {
case "TSSatisfiesExpression":
case "TSAsExpression":
return !isTSTypeExpression(node);
case "ConditionalExpression":
return isTSTypeExpression(node);
case "CallExpression":
case "NewExpression":
case "OptionalCallExpression":
return name === "callee";
case "ClassExpression":
case "ClassDeclaration":
return name === "superClass";
case "TSTypeAssertion":
case "TaggedTemplateExpression":
case "UnaryExpression":
case "JSXSpreadAttribute":
case "SpreadElement":
case "SpreadProperty":
case "BindExpression":
case "AwaitExpression":
case "TSNonNullExpression":
case "UpdateExpression":
return true;
case "MemberExpression":
case "OptionalMemberExpression":
return name === "object";
case "AssignmentExpression":
case "AssignmentPattern":
return name === "left" && (node.type === "TSTypeAssertion" || isTSTypeExpression(node));
case "LogicalExpression":
if (node.type === "LogicalExpression") {
return parent.operator !== node.operator;
}
case "BinaryExpression": {
const {
operator,
type
} = node;
if (!operator && type !== "TSTypeAssertion") {
return true;
}
const precedence = getPrecedence(operator);
const parentOperator = parent.operator;
const parentPrecedence = getPrecedence(parentOperator);
if (parentPrecedence > precedence) {
return true;
}
if (name === "right" && parentPrecedence === precedence) {
return true;
}
if (parentPrecedence === precedence && !shouldFlatten(parentOperator, operator)) {
return true;
}
if (parentPrecedence < precedence && operator === "%") {
return parentOperator === "+" || parentOperator === "-";
}
if (isBitwiseOperator(parentOperator)) {
return true;
}
return false;
}
default:
return false;
}
case "SequenceExpression":
switch (parent.type) {
case "ReturnStatement":
return false;
case "ForStatement":
return false;
case "ExpressionStatement":
return name !== "expression";
case "ArrowFunctionExpression":
return name !== "body";
default:
return true;
}
case "YieldExpression":
if (parent.type === "UnaryExpression" || parent.type === "AwaitExpression" || isTSTypeExpression(parent) || parent.type === "TSNonNullExpression") {
return true;
}
case "AwaitExpression":
switch (parent.type) {
case "TaggedTemplateExpression":
case "UnaryExpression":
case "LogicalExpression":
case "SpreadElement":
case "SpreadProperty":
case "TSAsExpression":
case "TSSatisfiesExpression":
case "TSNonNullExpression":
case "BindExpression":
return true;
case "MemberExpression":
case "OptionalMemberExpression":
return name === "object";
case "NewExpression":
case "CallExpression":
case "OptionalCallExpression":
return name === "callee";
case "ConditionalExpression":
return name === "test";
case "BinaryExpression": {
if (!node.argument && parent.operator === "|>") {
return false;
}
return true;
}
default:
return false;
}
case "TSConditionalType":
case "TSFunctionType":
case "TSConstructorType":
if (name === "extendsType" && parent.type === "TSConditionalType") {
if (node.type === "TSConditionalType") {
return true;
}
let {
typeAnnotation
} = node.returnType || node.typeAnnotation;
if (typeAnnotation.type === "TSTypePredicate" && typeAnnotation.typeAnnotation) {
typeAnnotation = typeAnnotation.typeAnnotation.typeAnnotation;
}
if (typeAnnotation.type === "TSInferType" && typeAnnotation.typeParameter.constraint) {
return true;
}
}
if (name === "checkType" && parent.type === "TSConditionalType") {
return true;
}
case "TSUnionType":
case "TSIntersectionType":
if ((parent.type === "TSUnionType" || parent.type === "TSIntersectionType") && parent.types.length > 1 && (!node.types || node.types.length > 1)) {
return true;
}
case "TSInferType":
if (node.type === "TSInferType" && parent.type === "TSRestType") {
return false;
}
case "TSTypeOperator":
return parent.type === "TSArrayType" || parent.type === "TSOptionalType" || parent.type === "TSRestType" || name === "objectType" && parent.type === "TSIndexedAccessType" || parent.type === "TSTypeOperator" || parent.type === "TSTypeAnnotation" && path.getParentNode(1).type.startsWith("TSJSDoc");
case "TSTypeQuery":
return name === "objectType" && parent.type === "TSIndexedAccessType" || name === "elementType" && parent.type === "TSArrayType";
case "TypeofTypeAnnotation":
return name === "objectType" && (parent.type === "IndexedAccessType" || parent.type === "OptionalIndexedAccessType") || name === "elementType" && parent.type === "ArrayTypeAnnotation";
case "ArrayTypeAnnotation":
return parent.type === "NullableTypeAnnotation";
case "IntersectionTypeAnnotation":
case "UnionTypeAnnotation":
return parent.type === "ArrayTypeAnnotation" || parent.type === "NullableTypeAnnotation" || parent.type === "IntersectionTypeAnnotation" || parent.type === "UnionTypeAnnotation" || name === "objectType" && (parent.type === "IndexedAccessType" || parent.type === "OptionalIndexedAccessType");
case "NullableTypeAnnotation":
return parent.type === "ArrayTypeAnnotation" || name === "objectType" && (parent.type === "IndexedAccessType" || parent.type === "OptionalIndexedAccessType");
case "FunctionTypeAnnotation": {
const ancestor = parent.type === "NullableTypeAnnotation" ? path.getParentNode(1) : parent;
return ancestor.type === "UnionTypeAnnotation" || ancestor.type === "IntersectionTypeAnnotation" || ancestor.type === "ArrayTypeAnnotation" || name === "objectType" && (ancestor.type === "IndexedAccessType" || ancestor.type === "OptionalIndexedAccessType") || ancestor.type === "NullableTypeAnnotation" || parent.type === "FunctionTypeParam" && parent.name === null && getFunctionParameters(node).some((param) => param.typeAnnotation && param.typeAnnotation.type === "NullableTypeAnnotation");
}
case "OptionalIndexedAccessType":
return name === "objectType" && parent.type === "IndexedAccessType";
case "StringLiteral":
case "NumericLiteral":
case "Literal":
if (typeof node.value === "string" && parent.type === "ExpressionStatement" && !parent.directive) {
const grandParent = path.getParentNode(1);
return grandParent.type === "Program" || grandParent.type === "BlockStatement";
}
return name === "object" && parent.type === "MemberExpression" && typeof node.value === "number";
case "AssignmentExpression": {
const grandParent = path.getParentNode(1);
if (name === "body" && parent.type === "ArrowFunctionExpression") {
return true;
}
if (name === "key" && (parent.type === "ClassProperty" || parent.type === "PropertyDefinition") && parent.computed) {
return false;
}
if ((name === "init" || name === "update") && parent.type === "ForStatement") {
return false;
}
if (parent.type === "ExpressionStatement") {
return node.left.type === "ObjectPattern";
}
if (name === "key" && parent.type === "TSPropertySignature") {
return false;
}
if (parent.type === "AssignmentExpression") {
return false;
}
if (parent.type === "SequenceExpression" && grandParent && grandParent.type === "ForStatement" && (grandParent.init === parent || grandParent.update === parent)) {
return false;
}
if (name === "value" && parent.type === "Property" && grandParent && grandParent.type === "ObjectPattern" && grandParent.properties.includes(parent)) {
return false;
}
if (parent.type === "NGChainedExpression") {
return false;
}
return true;
}
case "ConditionalExpression":
switch (parent.type) {
case "TaggedTemplateExpression":
case "UnaryExpression":
case "SpreadElement":
case "SpreadProperty":
case "BinaryExpression":
case "LogicalExpression":
case "NGPipeExpression":
case "ExportDefaultDeclaration":
case "AwaitExpression":
case "JSXSpreadAttribute":
case "TSTypeAssertion":
case "TypeCastExpression":
case "TSAsExpression":
case "TSSatisfiesExpression":
case "TSNonNullExpression":
return true;
case "NewExpression":
case "CallExpression":
case "OptionalCallExpression":
return name === "callee";
case "ConditionalExpression":
return name === "test";
case "MemberExpression":
case "OptionalMemberExpression":
return name === "object";
default:
return false;
}
case "FunctionExpression":
switch (parent.type) {
case "NewExpression":
case "CallExpression":
case "OptionalCallExpression":
return name === "callee";
case "TaggedTemplateExpression":
return true;
default:
return false;
}
case "ArrowFunctionExpression":
switch (parent.type) {
case "BinaryExpression":
return parent.operator !== "|>" || node.extra && node.extra.parenthesized;
case "NewExpression":
case "CallExpression":
case "OptionalCallExpression":
return name === "callee";
case "MemberExpression":
case "OptionalMemberExpression":
return name === "object";
case "TSAsExpression":
case "TSSatisfiesExpression":
case "TSNonNullExpression":
case "BindExpression":
case "TaggedTemplateExpression":
case "UnaryExpression":
case "LogicalExpression":
case "AwaitExpression":
case "TSTypeAssertion":
return true;
case "ConditionalExpression":
return name === "test";
default:
return false;
}
case "ClassExpression":
if (isNonEmptyArray(node.decorators)) {
return true;
}
switch (parent.type) {
case "NewExpression":
return name === "callee";
default:
return false;
}
case "OptionalMemberExpression":
case "OptionalCallExpression": {
const parentParent = path.getParentNode(1);
if (name === "object" && parent.type === "MemberExpression" || name === "callee" && (parent.type === "CallExpression" || parent.type === "NewExpression") || parent.type === "TSNonNullExpression" && parentParent.type === "MemberExpression" && parentParent.object === parent) {
return true;
}
}
case "CallExpression":
case "MemberExpression":
case "TaggedTemplateExpression":
case "TSNonNullExpression":
if (name === "callee" && (parent.type === "BindExpression" || parent.type === "NewExpression")) {
let object = node;
while (object) {
switch (object.type) {
case "CallExpression":
case "OptionalCallExpression":
return true;
case "MemberExpression":
case "OptionalMemberExpression":
case "BindExpression":
object = object.object;
break;
case "TaggedTemplateExpression":
object = object.tag;
break;
case "TSNonNullExpression":
object = object.expression;
break;
default:
return false;
}
}
}
return false;
case "BindExpression":
return name === "callee" && (parent.type === "BindExpression" || parent.type === "NewExpression") || name === "object" && isMemberExpression(parent);
case "NGPipeExpression":
if (parent.type === "NGRoot" || parent.type === "NGMicrosyntaxExpression" || parent.type === "ObjectProperty" && !(node.extra && node.extra.parenthesized) || parent.type === "ArrayExpression" || isCallExpression(parent) && parent.arguments[name] === node || name === "right" && parent.type === "NGPipeExpression" || name === "property" && parent.type === "MemberExpression" || parent.type === "AssignmentExpression") {
return false;
}
return true;
case "JSXFragment":
case "JSXElement":
return name === "callee" || name === "left" && parent.type === "BinaryExpression" && parent.operator === "<" || parent.type !== "ArrayExpression" && parent.type !== "ArrowFunctionExpression" && parent.type !== "AssignmentExpression" && parent.type !== "AssignmentPattern" && parent.type !== "BinaryExpression" && parent.type !== "NewExpression" && parent.type !== "ConditionalExpression" && parent.type !== "ExpressionStatement" && parent.type !== "JsExpressionRoot" && parent.type !== "JSXAttribute" && parent.type !== "JSXElement" && parent.type !== "JSXExpressionContainer" && parent.type !== "JSXFragment" && parent.type !== "LogicalExpression" && !isCallExpression(parent) && !isObjectProperty(parent) && parent.type !== "ReturnStatement" && parent.type !== "ThrowStatement" && parent.type !== "TypeCastExpression" && parent.type !== "VariableDeclarator" && parent.type !== "YieldExpression";
case "TypeAnnotation":
return name === "returnType" && parent.type === "ArrowFunctionExpression" && includesFunctionTypeInObjectType(node);
}
return false;
}
function isStatement(node) {
return node.type === "BlockStatement" || node.type === "BreakStatement" || node.type === "ClassBody" || node.type === "ClassDeclaration" || node.type === "ClassMethod" || node.type === "ClassProperty" || node.type === "PropertyDefinition" || node.type === "ClassPrivateProperty" || node.type === "ContinueStatement" || node.type === "DebuggerStatement" || node.type === "DeclareClass" || node.type === "DeclareExportAllDeclaration" || node.type === "DeclareExportDeclaration" || node.type === "DeclareFunction" || node.type === "DeclareInterface" || node.type === "DeclareModule" || node.type === "DeclareModuleExports" || node.type === "DeclareVariable" || node.type === "DoWhileStatement" || node.type === "EnumDeclaration" || node.type === "ExportAllDeclaration" || node.type === "ExportDefaultDeclaration" || node.type === "ExportNamedDeclaration" || node.type === "ExpressionStatement" || node.type === "ForInStatement" || node.type === "ForOfStatement" || node.type === "ForStatement" || node.type === "FunctionDeclaration" || node.type === "IfStatement" || node.type === "ImportDeclaration" || node.type === "InterfaceDeclaration" || node.type === "LabeledStatement" || node.type === "MethodDefinition" || node.type === "ReturnStatement" || node.type === "SwitchStatement" || node.type === "ThrowStatement" || node.type === "TryStatement" || node.type === "TSDeclareFunction" || node.type === "TSEnumDeclaration" || node.type === "TSImportEqualsDeclaration" || node.type === "TSInterfaceDeclaration" || node.type === "TSModuleDeclaration" || node.type === "TSNamespaceExportDeclaration" || node.type === "TypeAlias" || node.type === "VariableDeclaration" || node.type === "WhileStatement" || node.type === "WithStatement";
}
function isPathInForStatementInitializer(path) {
let i = 0;
let node = path.getValue();
while (node) {
const parent = path.getParentNode(i++);
if (parent && parent.type === "ForStatement" && parent.init === node) {
return true;
}
node = parent;
}
return false;
}
function includesFunctionTypeInObjectType(node) {
return hasNode(node, (n1) => n1.type === "ObjectTypeAnnotation" && hasNode(n1, (n2) => n2.type === "FunctionTypeAnnotation" || void 0) || void 0);
}
function endsWithRightBracket(node) {
switch (node.type) {
case "ObjectExpression":
return true;
default:
return false;
}
}
function isFollowedByRightBracket(path) {
const node = path.getValue();
const parent = path.getParentNode();
const name = path.getName();
switch (parent.type) {
case "NGPipeExpression":
if (typeof name === "number" && parent.arguments[name] === node && parent.arguments.length - 1 === name) {
return path.callParent(isFollowedByRightBracket);
}
break;
case "ObjectProperty":
if (name === "value") {
const parentParent = path.getParentNode(1);
return getLast(parentParent.properties) === parent;
}
break;
case "BinaryExpression":
case "LogicalExpression":
if (name === "right") {
return path.callParent(isFollowedByRightBracket);
}
break;
case "ConditionalExpression":
if (name === "alternate") {
return path.callParent(isFollowedByRightBracket);
}
break;
case "UnaryExpression":
if (parent.prefix) {
return path.callParent(isFollowedByRightBracket);
}
break;
}
return false;
}
function shouldWrapFunctionForExportDefault(path, options) {
const node = path.getValue();
const parent = path.getParentNode();
if (node.type === "FunctionExpression" || node.type === "ClassExpression") {
return parent.type === "ExportDefaultDeclaration" || !needsParens(path, options);
}
if (!hasNakedLeftSide(node) || parent.type !== "ExportDefaultDeclaration" && needsParens(path, options)) {
return false;
}
return path.call((childPath) => shouldWrapFunctionForExportDefault(childPath, options), ...getLeftSidePathName(path, node));
}
module22.exports = needsParens;
}
});
var require_print_preprocess = __commonJS22({
"src/language-js/print-preprocess.js"(exports2, module22) {
"use strict";
function preprocess(ast, options) {
switch (options.parser) {
case "json":
case "json5":
case "json-stringify":
case "__js_expression":
case "__vue_expression":
case "__vue_ts_expression":
return Object.assign(Object.assign({}, ast), {}, {
type: options.parser.startsWith("__") ? "JsExpressionRoot" : "JsonRoot",
node: ast,
comments: [],
rootMarker: options.rootMarker
});
default:
return ast;
}
}
module22.exports = preprocess;
}
});
var require_html_binding = __commonJS22({
"src/language-js/print/html-binding.js"(exports2, module22) {
"use strict";
var {
builders: {
join,
line,
group,
softline,
indent
}
} = require_doc();
function printHtmlBinding(path, options, print) {
const node = path.getValue();
if (options.__onHtmlBindingRoot && path.getName() === null) {
options.__onHtmlBindingRoot(node, options);
}
if (node.type !== "File") {
return;
}
if (options.__isVueForBindingLeft) {
return path.call((functionDeclarationPath) => {
const printed = join([",", line], functionDeclarationPath.map(print, "params"));
const {
params
} = functionDeclarationPath.getValue();
if (params.length === 1) {
return printed;
}
return ["(", indent([softline, group(printed)]), softline, ")"];
}, "program", "body", 0);
}
if (options.__isVueBindings) {
return path.call((functionDeclarationPath) => join([",", line], functionDeclarationPath.map(print, "params")), "program", "body", 0);
}
}
function isVueEventBindingExpression(node) {
switch (node.type) {
case "MemberExpression":
switch (node.property.type) {
case "Identifier":
case "NumericLiteral":
case "StringLiteral":
return isVueEventBindingExpression(node.object);
}
return false;
case "Identifier":
return true;
default:
return false;
}
}
module22.exports = {
isVueEventBindingExpression,
printHtmlBinding
};
}
});
var require_binaryish = __commonJS22({
"src/language-js/print/binaryish.js"(exports2, module22) {
"use strict";
var {
printComments
} = require_comments();
var {
getLast
} = require_util();
var {
builders: {
join,
line,
softline,
group,
indent,
align,
indentIfBreak
},
utils: {
cleanDoc,
getDocParts,
isConcat
}
} = require_doc();
var {
hasLeadingOwnLineComment,
isBinaryish,
isJsxNode,
shouldFlatten,
hasComment,
CommentCheckFlags,
isCallExpression,
isMemberExpression,
isObjectProperty,
isEnabledHackPipeline
} = require_utils7();
var uid = 0;
function printBinaryishExpression(path, options, print) {
const node = path.getValue();
const parent = path.getParentNode();
const parentParent = path.getParentNode(1);
const isInsideParenthesis = node !== parent.body && (parent.type === "IfStatement" || parent.type === "WhileStatement" || parent.type === "SwitchStatement" || parent.type === "DoWhileStatement");
const isHackPipeline = isEnabledHackPipeline(options) && node.operator === "|>";
const parts = printBinaryishExpressions(path, print, options, false, isInsideParenthesis);
if (isInsideParenthesis) {
return parts;
}
if (isHackPipeline) {
return group(parts);
}
if (isCallExpression(parent) && parent.callee === node || parent.type === "UnaryExpression" || isMemberExpression(parent) && !parent.computed) {
return group([indent([softline, ...parts]), softline]);
}
const shouldNotIndent = parent.type === "ReturnStatement" || parent.type === "ThrowStatement" || parent.type === "JSXExpressionContainer" && parentParent.type === "JSXAttribute" || node.operator !== "|" && parent.type === "JsExpressionRoot" || node.type !== "NGPipeExpression" && (parent.type === "NGRoot" && options.parser === "__ng_binding" || parent.type === "NGMicrosyntaxExpression" && parentParent.type === "NGMicrosyntax" && parentParent.body.length === 1) || node === parent.body && parent.type === "ArrowFunctionExpression" || node !== parent.body && parent.type === "ForStatement" || parent.type === "ConditionalExpression" && parentParent.type !== "ReturnStatement" && parentParent.type !== "ThrowStatement" && !isCallExpression(parentParent) || parent.type === "TemplateLiteral";
const shouldIndentIfInlining = parent.type === "AssignmentExpression" || parent.type === "VariableDeclarator" || parent.type === "ClassProperty" || parent.type === "PropertyDefinition" || parent.type === "TSAbstractPropertyDefinition" || parent.type === "ClassPrivateProperty" || isObjectProperty(parent);
const samePrecedenceSubExpression = isBinaryish(node.left) && shouldFlatten(node.operator, node.left.operator);
if (shouldNotIndent || shouldInlineLogicalExpression(node) && !samePrecedenceSubExpression || !shouldInlineLogicalExpression(node) && shouldIndentIfInlining) {
return group(parts);
}
if (parts.length === 0) {
return "";
}
const hasJsx = isJsxNode(node.right);
const firstGroupIndex = parts.findIndex((part) => typeof part !== "string" && !Array.isArray(part) && part.type === "group");
const headParts = parts.slice(0, firstGroupIndex === -1 ? 1 : firstGroupIndex + 1);
const rest = parts.slice(headParts.length, hasJsx ? -1 : void 0);
const groupId = Symbol("logicalChain-" + ++uid);
const chain = group([...headParts, indent(rest)], {
id: groupId
});
if (!hasJsx) {
return chain;
}
const jsxPart = getLast(parts);
return group([chain, indentIfBreak(jsxPart, {
groupId
})]);
}
function printBinaryishExpressions(path, print, options, isNested, isInsideParenthesis) {
const node = path.getValue();
if (!isBinaryish(node)) {
return [group(print())];
}
let parts = [];
if (shouldFlatten(node.operator, node.left.operator)) {
parts = path.call((left) => printBinaryishExpressions(left, print, options, true, isInsideParenthesis), "left");
} else {
parts.push(group(print("left")));
}
const shouldInline = shouldInlineLogicalExpression(node);
const lineBeforeOperator = (node.operator === "|>" || node.type === "NGPipeExpression" || node.operator === "|" && options.parser === "__vue_expression") && !hasLeadingOwnLineComment(options.originalText, node.right);
const operator = node.type === "NGPipeExpression" ? "|" : node.operator;
const rightSuffix = node.type === "NGPipeExpression" && node.arguments.length > 0 ? group(indent([line, ": ", join([line, ": "], path.map(print, "arguments").map((arg) => align(2, group(arg))))])) : "";
let right;
if (shouldInline) {
right = [operator, " ", print("right"), rightSuffix];
} else {
const isHackPipeline = isEnabledHackPipeline(options) && operator === "|>";
const rightContent = isHackPipeline ? path.call((left) => printBinaryishExpressions(left, print, options, true, isInsideParenthesis), "right") : print("right");
right = [lineBeforeOperator ? line : "", operator, lineBeforeOperator ? " " : line, rightContent, rightSuffix];
}
const parent = path.getParentNode();
const shouldBreak = hasComment(node.left, CommentCheckFlags.Trailing | CommentCheckFlags.Line);
const shouldGroup = shouldBreak || !(isInsideParenthesis && node.type === "LogicalExpression") && parent.type !== node.type && node.left.type !== node.type && node.right.type !== node.type;
parts.push(lineBeforeOperator ? "" : " ", shouldGroup ? group(right, {
shouldBreak
}) : right);
if (isNested && hasComment(node)) {
const printed = cleanDoc(printComments(path, parts, options));
if (isConcat(printed) || printed.type === "fill") {
return getDocParts(printed);
}
return [printed];
}
return parts;
}
function shouldInlineLogicalExpression(node) {
if (node.type !== "LogicalExpression") {
return false;
}
if (node.right.type === "ObjectExpression" && node.right.properties.length > 0) {
return true;
}
if (node.right.type === "ArrayExpression" && node.right.elements.length > 0) {
return true;
}
if (isJsxNode(node.right)) {
return true;
}
return false;
}
module22.exports = {
printBinaryishExpression,
shouldInlineLogicalExpression
};
}
});
var require_angular = __commonJS22({
"src/language-js/print/angular.js"(exports2, module22) {
"use strict";
var {
builders: {
join,
line,
group
}
} = require_doc();
var {
hasNode,
hasComment,
getComments
} = require_utils7();
var {
printBinaryishExpression
} = require_binaryish();
function printAngular(path, options, print) {
const node = path.getValue();
if (!node.type.startsWith("NG")) {
return;
}
switch (node.type) {
case "NGRoot":
return [print("node"), !hasComment(node.node) ? "" : " //" + getComments(node.node)[0].value.trimEnd()];
case "NGPipeExpression":
return printBinaryishExpression(path, options, print);
case "NGChainedExpression":
return group(join([";", line], path.map((childPath) => hasNgSideEffect(childPath) ? print() : ["(", print(), ")"], "expressions")));
case "NGEmptyExpression":
return "";
case "NGQuotedExpression":
return [node.prefix, ": ", node.value.trim()];
case "NGMicrosyntax":
return path.map((childPath, index) => [index === 0 ? "" : isNgForOf(childPath.getValue(), index, node) ? " " : [";", line], print()], "body");
case "NGMicrosyntaxKey":
return /^[$_a-z][\w$]*(?:-[$_a-z][\w$])*$/i.test(node.name) ? node.name : JSON.stringify(node.name);
case "NGMicrosyntaxExpression":
return [print("expression"), node.alias === null ? "" : [" as ", print("alias")]];
case "NGMicrosyntaxKeyedExpression": {
const index = path.getName();
const parentNode = path.getParentNode();
const shouldNotPrintColon = isNgForOf(node, index, parentNode) || (index === 1 && (node.key.name === "then" || node.key.name === "else") || index === 2 && node.key.name === "else" && parentNode.body[index - 1].type === "NGMicrosyntaxKeyedExpression" && parentNode.body[index - 1].key.name === "then") && parentNode.body[0].type === "NGMicrosyntaxExpression";
return [print("key"), shouldNotPrintColon ? " " : ": ", print("expression")];
}
case "NGMicrosyntaxLet":
return ["let ", print("key"), node.value === null ? "" : [" = ", print("value")]];
case "NGMicrosyntaxAs":
return [print("key"), " as ", print("alias")];
default:
throw new Error(`Unknown Angular node type: ${JSON.stringify(node.type)}.`);
}
}
function isNgForOf(node, index, parentNode) {
return node.type === "NGMicrosyntaxKeyedExpression" && node.key.name === "of" && index === 1 && parentNode.body[0].type === "NGMicrosyntaxLet" && parentNode.body[0].value === null;
}
function hasNgSideEffect(path) {
return hasNode(path.getValue(), (node) => {
switch (node.type) {
case void 0:
return false;
case "CallExpression":
case "OptionalCallExpression":
case "AssignmentExpression":
return true;
}
});
}
module22.exports = {
printAngular
};
}
});
var require_jsx = __commonJS22({
"src/language-js/print/jsx.js"(exports2, module22) {
"use strict";
var {
printComments,
printDanglingComments,
printCommentsSeparately
} = require_comments();
var {
builders: {
line,
hardline,
softline,
group,
indent,
conditionalGroup,
fill,
ifBreak,
lineSuffixBoundary,
join
},
utils: {
willBreak
}
} = require_doc();
var {
getLast,
getPreferredQuote
} = require_util();
var {
isJsxNode,
rawText,
isCallExpression,
isStringLiteral,
isBinaryish,
hasComment,
CommentCheckFlags,
hasNodeIgnoreComment
} = require_utils7();
var pathNeedsParens = require_needs_parens();
var {
willPrintOwnComments
} = require_comments2();
var isEmptyStringOrAnyLine = (doc2) => doc2 === "" || doc2 === line || doc2 === hardline || doc2 === softline;
function printJsxElementInternal(path, options, print) {
const node = path.getValue();
if (node.type === "JSXElement" && isEmptyJsxElement(node)) {
return [print("openingElement"), print("closingElement")];
}
const openingLines = node.type === "JSXElement" ? print("openingElement") : print("openingFragment");
const closingLines = node.type === "JSXElement" ? print("closingElement") : print("closingFragment");
if (node.children.length === 1 && node.children[0].type === "JSXExpressionContainer" && (node.children[0].expression.type === "TemplateLiteral" || node.children[0].expression.type === "TaggedTemplateExpression")) {
return [openingLines, ...path.map(print, "children"), closingLines];
}
node.children = node.children.map((child) => {
if (isJsxWhitespaceExpression(child)) {
return {
type: "JSXText",
value: " ",
raw: " "
};
}
return child;
});
const containsTag = node.children.some(isJsxNode);
const containsMultipleExpressions = node.children.filter((child) => child.type === "JSXExpressionContainer").length > 1;
const containsMultipleAttributes = node.type === "JSXElement" && node.openingElement.attributes.length > 1;
let forcedBreak = willBreak(openingLines) || containsTag || containsMultipleAttributes || containsMultipleExpressions;
const isMdxBlock = path.getParentNode().rootMarker === "mdx";
const rawJsxWhitespace = options.singleQuote ? "{' '}" : '{" "}';
const jsxWhitespace = isMdxBlock ? " " : ifBreak([rawJsxWhitespace, softline], " ");
const isFacebookTranslationTag = node.openingElement && node.openingElement.name && node.openingElement.name.name === "fbt";
const children = printJsxChildren(path, options, print, jsxWhitespace, isFacebookTranslationTag);
const containsText = node.children.some((child) => isMeaningfulJsxText(child));
for (let i = children.length - 2; i >= 0; i--) {
const isPairOfEmptyStrings = children[i] === "" && children[i + 1] === "";
const isPairOfHardlines = children[i] === hardline && children[i + 1] === "" && children[i + 2] === hardline;
const isLineFollowedByJsxWhitespace = (children[i] === softline || children[i] === hardline) && children[i + 1] === "" && children[i + 2] === jsxWhitespace;
const isJsxWhitespaceFollowedByLine = children[i] === jsxWhitespace && children[i + 1] === "" && (children[i + 2] === softline || children[i + 2] === hardline);
const isDoubleJsxWhitespace = children[i] === jsxWhitespace && children[i + 1] === "" && children[i + 2] === jsxWhitespace;
const isPairOfHardOrSoftLines = children[i] === softline && children[i + 1] === "" && children[i + 2] === hardline || children[i] === hardline && children[i + 1] === "" && children[i + 2] === softline;
if (isPairOfHardlines && containsText || isPairOfEmptyStrings || isLineFollowedByJsxWhitespace || isDoubleJsxWhitespace || isPairOfHardOrSoftLines) {
children.splice(i, 2);
} else if (isJsxWhitespaceFollowedByLine) {
children.splice(i + 1, 2);
}
}
while (children.length > 0 && isEmptyStringOrAnyLine(getLast(children))) {
children.pop();
}
while (children.length > 1 && isEmptyStringOrAnyLine(children[0]) && isEmptyStringOrAnyLine(children[1])) {
children.shift();
children.shift();
}
const multilineChildren = [];
for (const [i, child] of children.entries()) {
if (child === jsxWhitespace) {
if (i === 1 && children[i - 1] === "") {
if (children.length === 2) {
multilineChildren.push(rawJsxWhitespace);
continue;
}
multilineChildren.push([rawJsxWhitespace, hardline]);
continue;
} else if (i === children.length - 1) {
multilineChildren.push(rawJsxWhitespace);
continue;
} else if (children[i - 1] === "" && children[i - 2] === hardline) {
multilineChildren.push(rawJsxWhitespace);
continue;
}
}
multilineChildren.push(child);
if (willBreak(child)) {
forcedBreak = true;
}
}
const content = containsText ? fill(multilineChildren) : group(multilineChildren, {
shouldBreak: true
});
if (isMdxBlock) {
return content;
}
const multiLineElem = group([openingLines, indent([hardline, content]), hardline, closingLines]);
if (forcedBreak) {
return multiLineElem;
}
return conditionalGroup([group([openingLines, ...children, closingLines]), multiLineElem]);
}
function printJsxChildren(path, options, print, jsxWhitespace, isFacebookTranslationTag) {
const parts = [];
path.each((childPath, i, children) => {
const child = childPath.getValue();
if (child.type === "JSXText") {
const text = rawText(child);
if (isMeaningfulJsxText(child)) {
const words = text.split(matchJsxWhitespaceRegex);
if (words[0] === "") {
parts.push("");
words.shift();
if (/\n/.test(words[0])) {
const next = children[i + 1];
parts.push(separatorWithWhitespace(isFacebookTranslationTag, words[1], child, next));
} else {
parts.push(jsxWhitespace);
}
words.shift();
}
let endWhitespace;
if (getLast(words) === "") {
words.pop();
endWhitespace = words.pop();
}
if (words.length === 0) {
return;
}
for (const [i2, word] of words.entries()) {
if (i2 % 2 === 1) {
parts.push(line);
} else {
parts.push(word);
}
}
if (endWhitespace !== void 0) {
if (/\n/.test(endWhitespace)) {
const next = children[i + 1];
parts.push(separatorWithWhitespace(isFacebookTranslationTag, getLast(parts), child, next));
} else {
parts.push(jsxWhitespace);
}
} else {
const next = children[i + 1];
parts.push(separatorNoWhitespace(isFacebookTranslationTag, getLast(parts), child, next));
}
} else if (/\n/.test(text)) {
if (text.match(/\n/g).length > 1) {
parts.push("", hardline);
}
} else {
parts.push("", jsxWhitespace);
}
} else {
const printedChild = print();
parts.push(printedChild);
const next = children[i + 1];
const directlyFollowedByMeaningfulText = next && isMeaningfulJsxText(next);
if (directlyFollowedByMeaningfulText) {
const firstWord = trimJsxWhitespace(rawText(next)).split(matchJsxWhitespaceRegex)[0];
parts.push(separatorNoWhitespace(isFacebookTranslationTag, firstWord, child, next));
} else {
parts.push(hardline);
}
}
}, "children");
return parts;
}
function separatorNoWhitespace(isFacebookTranslationTag, child, childNode, nextNode) {
if (isFacebookTranslationTag) {
return "";
}
if (childNode.type === "JSXElement" && !childNode.closingElement || nextNode && nextNode.type === "JSXElement" && !nextNode.closingElement) {
return child.length === 1 ? softline : hardline;
}
return softline;
}
function separatorWithWhitespace(isFacebookTranslationTag, child, childNode, nextNode) {
if (isFacebookTranslationTag) {
return hardline;
}
if (child.length === 1) {
return childNode.type === "JSXElement" && !childNode.closingElement || nextNode && nextNode.type === "JSXElement" && !nextNode.closingElement ? hardline : softline;
}
return hardline;
}
function maybeWrapJsxElementInParens(path, elem, options) {
const parent = path.getParentNode();
if (!parent) {
return elem;
}
const NO_WRAP_PARENTS = {
ArrayExpression: true,
JSXAttribute: true,
JSXElement: true,
JSXExpressionContainer: true,
JSXFragment: true,
ExpressionStatement: true,
CallExpression: true,
OptionalCallExpression: true,
ConditionalExpression: true,
JsExpressionRoot: true
};
if (NO_WRAP_PARENTS[parent.type]) {
return elem;
}
const shouldBreak = path.match(void 0, (node) => node.type === "ArrowFunctionExpression", isCallExpression, (node) => node.type === "JSXExpressionContainer");
const needsParens = pathNeedsParens(path, options);
return group([needsParens ? "" : ifBreak("("), indent([softline, elem]), softline, needsParens ? "" : ifBreak(")")], {
shouldBreak
});
}
function printJsxAttribute(path, options, print) {
const node = path.getValue();
const parts = [];
parts.push(print("name"));
if (node.value) {
let res;
if (isStringLiteral(node.value)) {
const raw = rawText(node.value);
let final = raw.slice(1, -1).replace(/'/g, "'").replace(/"/g, '"');
const {
escaped,
quote: quote2,
regex
} = getPreferredQuote(final, options.jsxSingleQuote ? "'" : '"');
final = final.replace(regex, escaped);
const {
leading,
trailing
} = path.call(() => printCommentsSeparately(path, options), "value");
res = [leading, quote2, final, quote2, trailing];
} else {
res = print("value");
}
parts.push("=", res);
}
return parts;
}
function printJsxExpressionContainer(path, options, print) {
const node = path.getValue();
const shouldInline = (node2, parent) => node2.type === "JSXEmptyExpression" || !hasComment(node2) && (node2.type === "ArrayExpression" || node2.type === "ObjectExpression" || node2.type === "ArrowFunctionExpression" || node2.type === "AwaitExpression" && (shouldInline(node2.argument, node2) || node2.argument.type === "JSXElement") || isCallExpression(node2) || node2.type === "FunctionExpression" || node2.type === "TemplateLiteral" || node2.type === "TaggedTemplateExpression" || node2.type === "DoExpression" || isJsxNode(parent) && (node2.type === "ConditionalExpression" || isBinaryish(node2)));
if (shouldInline(node.expression, path.getParentNode(0))) {
return group(["{", print("expression"), lineSuffixBoundary, "}"]);
}
return group(["{", indent([softline, print("expression")]), softline, lineSuffixBoundary, "}"]);
}
function printJsxOpeningElement(path, options, print) {
const node = path.getValue();
const nameHasComments = node.name && hasComment(node.name) || node.typeParameters && hasComment(node.typeParameters);
if (node.selfClosing && node.attributes.length === 0 && !nameHasComments) {
return ["<", print("name"), print("typeParameters"), " />"];
}
if (node.attributes && node.attributes.length === 1 && node.attributes[0].value && isStringLiteral(node.attributes[0].value) && !node.attributes[0].value.value.includes("\n") && !nameHasComments && !hasComment(node.attributes[0])) {
return group(["<", print("name"), print("typeParameters"), " ", ...path.map(print, "attributes"), node.selfClosing ? " />" : ">"]);
}
const shouldBreak = node.attributes && node.attributes.some((attr) => attr.value && isStringLiteral(attr.value) && attr.value.value.includes("\n"));
const attributeLine = options.singleAttributePerLine && node.attributes.length > 1 ? hardline : line;
return group(["<", print("name"), print("typeParameters"), indent(path.map(() => [attributeLine, print()], "attributes")), ...printEndOfOpeningTag(node, options, nameHasComments)], {
shouldBreak
});
}
function printEndOfOpeningTag(node, options, nameHasComments) {
if (node.selfClosing) {
return [line, "/>"];
}
const bracketSameLine = shouldPrintBracketSameLine(node, options, nameHasComments);
if (bracketSameLine) {
return [">"];
}
return [softline, ">"];
}
function shouldPrintBracketSameLine(node, options, nameHasComments) {
const lastAttrHasTrailingComments = node.attributes.length > 0 && hasComment(getLast(node.attributes), CommentCheckFlags.Trailing);
return node.attributes.length === 0 && !nameHasComments || (options.bracketSameLine || options.jsxBracketSameLine) && (!nameHasComments || node.attributes.length > 0) && !lastAttrHasTrailingComments;
}
function printJsxClosingElement(path, options, print) {
const node = path.getValue();
const parts = [];
parts.push("");
const printed = print("name");
if (hasComment(node.name, CommentCheckFlags.Leading | CommentCheckFlags.Line)) {
parts.push(indent([hardline, printed]), hardline);
} else if (hasComment(node.name, CommentCheckFlags.Leading | CommentCheckFlags.Block)) {
parts.push(" ", printed);
} else {
parts.push(printed);
}
parts.push(">");
return parts;
}
function printJsxOpeningClosingFragment(path, options) {
const node = path.getValue();
const nodeHasComment = hasComment(node);
const hasOwnLineComment = hasComment(node, CommentCheckFlags.Line);
const isOpeningFragment = node.type === "JSXOpeningFragment";
return [isOpeningFragment ? "<" : "", indent([hasOwnLineComment ? hardline : nodeHasComment && !isOpeningFragment ? " " : "", printDanglingComments(path, options, true)]), hasOwnLineComment ? hardline : "", ">"];
}
function printJsxElement(path, options, print) {
const elem = printComments(path, printJsxElementInternal(path, options, print), options);
return maybeWrapJsxElementInParens(path, elem, options);
}
function printJsxEmptyExpression(path, options) {
const node = path.getValue();
const requiresHardline = hasComment(node, CommentCheckFlags.Line);
return [printDanglingComments(path, options, !requiresHardline), requiresHardline ? hardline : ""];
}
function printJsxSpreadAttribute(path, options, print) {
const node = path.getValue();
return ["{", path.call((p) => {
const printed = ["...", print()];
const node2 = p.getValue();
if (!hasComment(node2) || !willPrintOwnComments(p)) {
return printed;
}
return [indent([softline, printComments(p, printed, options)]), softline];
}, node.type === "JSXSpreadAttribute" ? "argument" : "expression"), "}"];
}
function printJsx(path, options, print) {
const node = path.getValue();
if (!node.type.startsWith("JSX")) {
return;
}
switch (node.type) {
case "JSXAttribute":
return printJsxAttribute(path, options, print);
case "JSXIdentifier":
return String(node.name);
case "JSXNamespacedName":
return join(":", [print("namespace"), print("name")]);
case "JSXMemberExpression":
return join(".", [print("object"), print("property")]);
case "JSXSpreadAttribute":
return printJsxSpreadAttribute(path, options, print);
case "JSXSpreadChild": {
const printJsxSpreadChild = printJsxSpreadAttribute;
return printJsxSpreadChild(path, options, print);
}
case "JSXExpressionContainer":
return printJsxExpressionContainer(path, options, print);
case "JSXFragment":
case "JSXElement":
return printJsxElement(path, options, print);
case "JSXOpeningElement":
return printJsxOpeningElement(path, options, print);
case "JSXClosingElement":
return printJsxClosingElement(path, options, print);
case "JSXOpeningFragment":
case "JSXClosingFragment":
return printJsxOpeningClosingFragment(path, options);
case "JSXEmptyExpression":
return printJsxEmptyExpression(path, options);
case "JSXText":
throw new Error("JSXText should be handled by JSXElement");
default:
throw new Error(`Unknown JSX node type: ${JSON.stringify(node.type)}.`);
}
}
var jsxWhitespaceChars = " \n\r ";
var matchJsxWhitespaceRegex = new RegExp("([" + jsxWhitespaceChars + "]+)");
var containsNonJsxWhitespaceRegex = new RegExp("[^" + jsxWhitespaceChars + "]");
var trimJsxWhitespace = (text) => text.replace(new RegExp("(?:^" + matchJsxWhitespaceRegex.source + "|" + matchJsxWhitespaceRegex.source + "$)"), "");
function isEmptyJsxElement(node) {
if (node.children.length === 0) {
return true;
}
if (node.children.length > 1) {
return false;
}
const child = node.children[0];
return child.type === "JSXText" && !isMeaningfulJsxText(child);
}
function isMeaningfulJsxText(node) {
return node.type === "JSXText" && (containsNonJsxWhitespaceRegex.test(rawText(node)) || !/\n/.test(rawText(node)));
}
function isJsxWhitespaceExpression(node) {
return node.type === "JSXExpressionContainer" && isStringLiteral(node.expression) && node.expression.value === " " && !hasComment(node.expression);
}
function hasJsxIgnoreComment(path) {
const node = path.getValue();
const parent = path.getParentNode();
if (!parent || !node || !isJsxNode(node) || !isJsxNode(parent)) {
return false;
}
const index = parent.children.indexOf(node);
let prevSibling = null;
for (let i = index; i > 0; i--) {
const candidate = parent.children[i - 1];
if (candidate.type === "JSXText" && !isMeaningfulJsxText(candidate)) {
continue;
}
prevSibling = candidate;
break;
}
return prevSibling && prevSibling.type === "JSXExpressionContainer" && prevSibling.expression.type === "JSXEmptyExpression" && hasNodeIgnoreComment(prevSibling.expression);
}
module22.exports = {
hasJsxIgnoreComment,
printJsx
};
}
});
var require_doc_builders = __commonJS22({
"src/document/doc-builders.js"(exports2, module22) {
"use strict";
function concat(parts) {
if (false) {
for (const part of parts) {
assertDoc(part);
}
}
return {
type: "concat",
parts
};
}
function indent(contents) {
if (false) {
assertDoc(contents);
}
return {
type: "indent",
contents
};
}
function align(widthOrString, contents) {
if (false) {
assertDoc(contents);
}
return {
type: "align",
contents,
n: widthOrString
};
}
function group(contents, opts = {}) {
if (false) {
assertDoc(contents);
}
return {
type: "group",
id: opts.id,
contents,
break: Boolean(opts.shouldBreak),
expandedStates: opts.expandedStates
};
}
function dedentToRoot(contents) {
return align(Number.NEGATIVE_INFINITY, contents);
}
function markAsRoot(contents) {
return align({
type: "root"
}, contents);
}
function dedent(contents) {
return align(-1, contents);
}
function conditionalGroup(states, opts) {
return group(states[0], Object.assign(Object.assign({}, opts), {}, {
expandedStates: states
}));
}
function fill(parts) {
if (false) {
for (const part of parts) {
assertDoc(part);
}
}
return {
type: "fill",
parts
};
}
function ifBreak(breakContents, flatContents, opts = {}) {
if (false) {
if (breakContents) {
assertDoc(breakContents);
}
if (flatContents) {
assertDoc(flatContents);
}
}
return {
type: "if-break",
breakContents,
flatContents,
groupId: opts.groupId
};
}
function indentIfBreak(contents, opts) {
return {
type: "indent-if-break",
contents,
groupId: opts.groupId,
negate: opts.negate
};
}
function lineSuffix(contents) {
if (false) {
assertDoc(contents);
}
return {
type: "line-suffix",
contents
};
}
var lineSuffixBoundary = {
type: "line-suffix-boundary"
};
var breakParent = {
type: "break-parent"
};
var trim = {
type: "trim"
};
var hardlineWithoutBreakParent = {
type: "line",
hard: true
};
var literallineWithoutBreakParent = {
type: "line",
hard: true,
literal: true
};
var line = {
type: "line"
};
var softline = {
type: "line",
soft: true
};
var hardline = concat([hardlineWithoutBreakParent, breakParent]);
var literalline = concat([literallineWithoutBreakParent, breakParent]);
var cursor = {
type: "cursor",
placeholder: Symbol("cursor")
};
function join(sep, arr) {
const res = [];
for (let i = 0; i < arr.length; i++) {
if (i !== 0) {
res.push(sep);
}
res.push(arr[i]);
}
return concat(res);
}
function addAlignmentToDoc(doc2, size, tabWidth) {
let aligned = doc2;
if (size > 0) {
for (let i = 0; i < Math.floor(size / tabWidth); ++i) {
aligned = indent(aligned);
}
aligned = align(size % tabWidth, aligned);
aligned = align(Number.NEGATIVE_INFINITY, aligned);
}
return aligned;
}
function label(label2, contents) {
return {
type: "label",
label: label2,
contents
};
}
module22.exports = {
concat,
join,
line,
softline,
hardline,
literalline,
group,
conditionalGroup,
fill,
lineSuffix,
lineSuffixBoundary,
cursor,
breakParent,
ifBreak,
trim,
indent,
indentIfBreak,
align,
addAlignmentToDoc,
markAsRoot,
dedentToRoot,
dedent,
hardlineWithoutBreakParent,
literallineWithoutBreakParent,
label
};
}
});
var require_doc_utils = __commonJS22({
"src/document/doc-utils.js"(exports2, module22) {
"use strict";
var getLast = require_get_last();
var {
literalline,
join
} = require_doc_builders();
var isConcat = (doc2) => Array.isArray(doc2) || doc2 && doc2.type === "concat";
var getDocParts = (doc2) => {
if (Array.isArray(doc2)) {
return doc2;
}
if (doc2.type !== "concat" && doc2.type !== "fill") {
throw new Error("Expect doc type to be `concat` or `fill`.");
}
return doc2.parts;
};
var traverseDocOnExitStackMarker = {};
function traverseDoc(doc2, onEnter, onExit, shouldTraverseConditionalGroups) {
const docsStack = [doc2];
while (docsStack.length > 0) {
const doc3 = docsStack.pop();
if (doc3 === traverseDocOnExitStackMarker) {
onExit(docsStack.pop());
continue;
}
if (onExit) {
docsStack.push(doc3, traverseDocOnExitStackMarker);
}
if (!onEnter || onEnter(doc3) !== false) {
if (isConcat(doc3) || doc3.type === "fill") {
const parts = getDocParts(doc3);
for (let ic = parts.length, i = ic - 1; i >= 0; --i) {
docsStack.push(parts[i]);
}
} else if (doc3.type === "if-break") {
if (doc3.flatContents) {
docsStack.push(doc3.flatContents);
}
if (doc3.breakContents) {
docsStack.push(doc3.breakContents);
}
} else if (doc3.type === "group" && doc3.expandedStates) {
if (shouldTraverseConditionalGroups) {
for (let ic = doc3.expandedStates.length, i = ic - 1; i >= 0; --i) {
docsStack.push(doc3.expandedStates[i]);
}
} else {
docsStack.push(doc3.contents);
}
} else if (doc3.contents) {
docsStack.push(doc3.contents);
}
}
}
}
function mapDoc(doc2, cb) {
const mapped = /* @__PURE__ */ new Map();
return rec(doc2);
function rec(doc3) {
if (mapped.has(doc3)) {
return mapped.get(doc3);
}
const result = process2(doc3);
mapped.set(doc3, result);
return result;
}
function process2(doc3) {
if (Array.isArray(doc3)) {
return cb(doc3.map(rec));
}
if (doc3.type === "concat" || doc3.type === "fill") {
const parts = doc3.parts.map(rec);
return cb(Object.assign(Object.assign({}, doc3), {}, {
parts
}));
}
if (doc3.type === "if-break") {
const breakContents = doc3.breakContents && rec(doc3.breakContents);
const flatContents = doc3.flatContents && rec(doc3.flatContents);
return cb(Object.assign(Object.assign({}, doc3), {}, {
breakContents,
flatContents
}));
}
if (doc3.type === "group" && doc3.expandedStates) {
const expandedStates = doc3.expandedStates.map(rec);
const contents = expandedStates[0];
return cb(Object.assign(Object.assign({}, doc3), {}, {
contents,
expandedStates
}));
}
if (doc3.contents) {
const contents = rec(doc3.contents);
return cb(Object.assign(Object.assign({}, doc3), {}, {
contents
}));
}
return cb(doc3);
}
}
function findInDoc(doc2, fn, defaultValue) {
let result = defaultValue;
let hasStopped = false;
function findInDocOnEnterFn(doc3) {
const maybeResult = fn(doc3);
if (maybeResult !== void 0) {
hasStopped = true;
result = maybeResult;
}
if (hasStopped) {
return false;
}
}
traverseDoc(doc2, findInDocOnEnterFn);
return result;
}
function willBreakFn(doc2) {
if (doc2.type === "group" && doc2.break) {
return true;
}
if (doc2.type === "line" && doc2.hard) {
return true;
}
if (doc2.type === "break-parent") {
return true;
}
}
function willBreak(doc2) {
return findInDoc(doc2, willBreakFn, false);
}
function breakParentGroup(groupStack) {
if (groupStack.length > 0) {
const parentGroup = getLast(groupStack);
if (!parentGroup.expandedStates && !parentGroup.break) {
parentGroup.break = "propagated";
}
}
return null;
}
function propagateBreaks(doc2) {
const alreadyVisitedSet = /* @__PURE__ */ new Set();
const groupStack = [];
function propagateBreaksOnEnterFn(doc3) {
if (doc3.type === "break-parent") {
breakParentGroup(groupStack);
}
if (doc3.type === "group") {
groupStack.push(doc3);
if (alreadyVisitedSet.has(doc3)) {
return false;
}
alreadyVisitedSet.add(doc3);
}
}
function propagateBreaksOnExitFn(doc3) {
if (doc3.type === "group") {
const group = groupStack.pop();
if (group.break) {
breakParentGroup(groupStack);
}
}
}
traverseDoc(doc2, propagateBreaksOnEnterFn, propagateBreaksOnExitFn, true);
}
function removeLinesFn(doc2) {
if (doc2.type === "line" && !doc2.hard) {
return doc2.soft ? "" : " ";
}
if (doc2.type === "if-break") {
return doc2.flatContents || "";
}
return doc2;
}
function removeLines(doc2) {
return mapDoc(doc2, removeLinesFn);
}
var isHardline = (doc2, nextDoc) => doc2 && doc2.type === "line" && doc2.hard && nextDoc && nextDoc.type === "break-parent";
function stripDocTrailingHardlineFromDoc(doc2) {
if (!doc2) {
return doc2;
}
if (isConcat(doc2) || doc2.type === "fill") {
const parts = getDocParts(doc2);
while (parts.length > 1 && isHardline(...parts.slice(-2))) {
parts.length -= 2;
}
if (parts.length > 0) {
const lastPart = stripDocTrailingHardlineFromDoc(getLast(parts));
parts[parts.length - 1] = lastPart;
}
return Array.isArray(doc2) ? parts : Object.assign(Object.assign({}, doc2), {}, {
parts
});
}
switch (doc2.type) {
case "align":
case "indent":
case "indent-if-break":
case "group":
case "line-suffix":
case "label": {
const contents = stripDocTrailingHardlineFromDoc(doc2.contents);
return Object.assign(Object.assign({}, doc2), {}, {
contents
});
}
case "if-break": {
const breakContents = stripDocTrailingHardlineFromDoc(doc2.breakContents);
const flatContents = stripDocTrailingHardlineFromDoc(doc2.flatContents);
return Object.assign(Object.assign({}, doc2), {}, {
breakContents,
flatContents
});
}
}
return doc2;
}
function stripTrailingHardline(doc2) {
return stripDocTrailingHardlineFromDoc(cleanDoc(doc2));
}
function cleanDocFn(doc2) {
switch (doc2.type) {
case "fill":
if (doc2.parts.every((part) => part === "")) {
return "";
}
break;
case "group":
if (!doc2.contents && !doc2.id && !doc2.break && !doc2.expandedStates) {
return "";
}
if (doc2.contents.type === "group" && doc2.contents.id === doc2.id && doc2.contents.break === doc2.break && doc2.contents.expandedStates === doc2.expandedStates) {
return doc2.contents;
}
break;
case "align":
case "indent":
case "indent-if-break":
case "line-suffix":
if (!doc2.contents) {
return "";
}
break;
case "if-break":
if (!doc2.flatContents && !doc2.breakContents) {
return "";
}
break;
}
if (!isConcat(doc2)) {
return doc2;
}
const parts = [];
for (const part of getDocParts(doc2)) {
if (!part) {
continue;
}
const [currentPart, ...restParts] = isConcat(part) ? getDocParts(part) : [part];
if (typeof currentPart === "string" && typeof getLast(parts) === "string") {
parts[parts.length - 1] += currentPart;
} else {
parts.push(currentPart);
}
parts.push(...restParts);
}
if (parts.length === 0) {
return "";
}
if (parts.length === 1) {
return parts[0];
}
return Array.isArray(doc2) ? parts : Object.assign(Object.assign({}, doc2), {}, {
parts
});
}
function cleanDoc(doc2) {
return mapDoc(doc2, (currentDoc) => cleanDocFn(currentDoc));
}
function normalizeParts(parts) {
const newParts = [];
const restParts = parts.filter(Boolean);
while (restParts.length > 0) {
const part = restParts.shift();
if (!part) {
continue;
}
if (isConcat(part)) {
restParts.unshift(...getDocParts(part));
continue;
}
if (newParts.length > 0 && typeof getLast(newParts) === "string" && typeof part === "string") {
newParts[newParts.length - 1] += part;
continue;
}
newParts.push(part);
}
return newParts;
}
function normalizeDoc(doc2) {
return mapDoc(doc2, (currentDoc) => {
if (Array.isArray(currentDoc)) {
return normalizeParts(currentDoc);
}
if (!currentDoc.parts) {
return currentDoc;
}
return Object.assign(Object.assign({}, currentDoc), {}, {
parts: normalizeParts(currentDoc.parts)
});
});
}
function replaceEndOfLine(doc2) {
return mapDoc(doc2, (currentDoc) => typeof currentDoc === "string" && currentDoc.includes("\n") ? replaceTextEndOfLine(currentDoc) : currentDoc);
}
function replaceTextEndOfLine(text, replacement = literalline) {
return join(replacement, text.split("\n")).parts;
}
function canBreakFn(doc2) {
if (doc2.type === "line") {
return true;
}
}
function canBreak(doc2) {
return findInDoc(doc2, canBreakFn, false);
}
module22.exports = {
isConcat,
getDocParts,
willBreak,
traverseDoc,
findInDoc,
mapDoc,
propagateBreaks,
removeLines,
stripTrailingHardline,
normalizeParts,
normalizeDoc,
cleanDoc,
replaceTextEndOfLine,
replaceEndOfLine,
canBreak
};
}
});
var require_misc = __commonJS22({
"src/language-js/print/misc.js"(exports2, module22) {
"use strict";
var {
isNonEmptyArray
} = require_util();
var {
builders: {
indent,
join,
line
}
} = require_doc();
var {
isFlowAnnotationComment
} = require_utils7();
function printOptionalToken(path) {
const node = path.getValue();
if (!node.optional || node.type === "Identifier" && node === path.getParentNode().key) {
return "";
}
if (node.type === "OptionalCallExpression" || node.type === "OptionalMemberExpression" && node.computed) {
return "?.";
}
return "?";
}
function printDefiniteToken(path) {
return path.getValue().definite || path.match(void 0, (node, name) => name === "id" && node.type === "VariableDeclarator" && node.definite) ? "!" : "";
}
function printFunctionTypeParameters(path, options, print) {
const fun = path.getValue();
if (fun.typeArguments) {
return print("typeArguments");
}
if (fun.typeParameters) {
return print("typeParameters");
}
return "";
}
function printTypeAnnotation(path, options, print) {
const node = path.getValue();
if (!node.typeAnnotation) {
return "";
}
const parentNode = path.getParentNode();
const isFunctionDeclarationIdentifier = parentNode.type === "DeclareFunction" && parentNode.id === node;
if (isFlowAnnotationComment(options.originalText, node.typeAnnotation)) {
return [" /*: ", print("typeAnnotation"), " */"];
}
return [isFunctionDeclarationIdentifier ? "" : ": ", print("typeAnnotation")];
}
function printBindExpressionCallee(path, options, print) {
return ["::", print("callee")];
}
function printTypeScriptModifiers(path, options, print) {
const node = path.getValue();
if (!isNonEmptyArray(node.modifiers)) {
return "";
}
return [join(" ", path.map(print, "modifiers")), " "];
}
function adjustClause(node, clause, forceSpace) {
if (node.type === "EmptyStatement") {
return ";";
}
if (node.type === "BlockStatement" || forceSpace) {
return [" ", clause];
}
return indent([line, clause]);
}
function printRestSpread(path, options, print) {
return ["...", print("argument"), printTypeAnnotation(path, options, print)];
}
function printDirective(rawText, options) {
const rawContent = rawText.slice(1, -1);
if (rawContent.includes('"') || rawContent.includes("'")) {
return rawText;
}
const enclosingQuote = options.singleQuote ? "'" : '"';
return enclosingQuote + rawContent + enclosingQuote;
}
module22.exports = {
printOptionalToken,
printDefiniteToken,
printFunctionTypeParameters,
printBindExpressionCallee,
printTypeScriptModifiers,
printTypeAnnotation,
printRestSpread,
adjustClause,
printDirective
};
}
});
var require_array4 = __commonJS22({
"src/language-js/print/array.js"(exports2, module22) {
"use strict";
var {
printDanglingComments
} = require_comments();
var {
builders: {
line,
softline,
hardline,
group,
indent,
ifBreak,
fill
}
} = require_doc();
var {
getLast,
hasNewline
} = require_util();
var {
shouldPrintComma,
hasComment,
CommentCheckFlags,
isNextLineEmpty,
isNumericLiteral,
isSignedNumericLiteral
} = require_utils7();
var {
locStart
} = require_loc();
var {
printOptionalToken,
printTypeAnnotation
} = require_misc();
function printArray(path, options, print) {
const node = path.getValue();
const parts = [];
const openBracket = node.type === "TupleExpression" ? "#[" : "[";
const closeBracket = "]";
if (node.elements.length === 0) {
if (!hasComment(node, CommentCheckFlags.Dangling)) {
parts.push(openBracket, closeBracket);
} else {
parts.push(group([openBracket, printDanglingComments(path, options), softline, closeBracket]));
}
} else {
const lastElem = getLast(node.elements);
const canHaveTrailingComma = !(lastElem && lastElem.type === "RestElement");
const needsForcedTrailingComma = lastElem === null;
const groupId = Symbol("array");
const shouldBreak = !options.__inJestEach && node.elements.length > 1 && node.elements.every((element, i, elements) => {
const elementType = element && element.type;
if (elementType !== "ArrayExpression" && elementType !== "ObjectExpression") {
return false;
}
const nextElement = elements[i + 1];
if (nextElement && elementType !== nextElement.type) {
return false;
}
const itemsKey = elementType === "ArrayExpression" ? "elements" : "properties";
return element[itemsKey] && element[itemsKey].length > 1;
});
const shouldUseConciseFormatting = isConciselyPrintedArray(node, options);
const trailingComma = !canHaveTrailingComma ? "" : needsForcedTrailingComma ? "," : !shouldPrintComma(options) ? "" : shouldUseConciseFormatting ? ifBreak(",", "", {
groupId
}) : ifBreak(",");
parts.push(group([openBracket, indent([softline, shouldUseConciseFormatting ? printArrayItemsConcisely(path, options, print, trailingComma) : [printArrayItems(path, options, "elements", print), trailingComma], printDanglingComments(path, options, true)]), softline, closeBracket], {
shouldBreak,
id: groupId
}));
}
parts.push(printOptionalToken(path), printTypeAnnotation(path, options, print));
return parts;
}
function isConciselyPrintedArray(node, options) {
return node.elements.length > 1 && node.elements.every((element) => element && (isNumericLiteral(element) || isSignedNumericLiteral(element) && !hasComment(element.argument)) && !hasComment(element, CommentCheckFlags.Trailing | CommentCheckFlags.Line, (comment) => !hasNewline(options.originalText, locStart(comment), {
backwards: true
})));
}
function printArrayItems(path, options, printPath, print) {
const printedElements = [];
let separatorParts = [];
path.each((childPath) => {
printedElements.push(separatorParts, group(print()));
separatorParts = [",", line];
if (childPath.getValue() && isNextLineEmpty(childPath.getValue(), options)) {
separatorParts.push(softline);
}
}, printPath);
return printedElements;
}
function printArrayItemsConcisely(path, options, print, trailingComma) {
const parts = [];
path.each((childPath, i, elements) => {
const isLast = i === elements.length - 1;
parts.push([print(), isLast ? trailingComma : ","]);
if (!isLast) {
parts.push(isNextLineEmpty(childPath.getValue(), options) ? [hardline, hardline] : hasComment(elements[i + 1], CommentCheckFlags.Leading | CommentCheckFlags.Line) ? hardline : line);
}
}, "elements");
return fill(parts);
}
module22.exports = {
printArray,
printArrayItems,
isConciselyPrintedArray
};
}
});
var require_call_arguments = __commonJS22({
"src/language-js/print/call-arguments.js"(exports2, module22) {
"use strict";
var {
printDanglingComments
} = require_comments();
var {
getLast,
getPenultimate
} = require_util();
var {
getFunctionParameters,
hasComment,
CommentCheckFlags,
isFunctionCompositionArgs,
isJsxNode,
isLongCurriedCallExpression,
shouldPrintComma,
getCallArguments,
iterateCallArgumentsPath,
isNextLineEmpty,
isCallExpression,
isStringLiteral,
isObjectProperty,
isTSTypeExpression
} = require_utils7();
var {
builders: {
line,
hardline,
softline,
group,
indent,
conditionalGroup,
ifBreak,
breakParent
},
utils: {
willBreak
}
} = require_doc();
var {
ArgExpansionBailout
} = require_errors();
var {
isConciselyPrintedArray
} = require_array4();
function printCallArguments(path, options, print) {
const node = path.getValue();
const isDynamicImport = node.type === "ImportExpression";
const args = getCallArguments(node);
if (args.length === 0) {
return ["(", printDanglingComments(path, options, true), ")"];
}
if (isReactHookCallWithDepsArray(args)) {
return ["(", print(["arguments", 0]), ", ", print(["arguments", 1]), ")"];
}
let anyArgEmptyLine = false;
let hasEmptyLineFollowingFirstArg = false;
const lastArgIndex = args.length - 1;
const printedArguments = [];
iterateCallArgumentsPath(path, (argPath, index) => {
const arg = argPath.getNode();
const parts = [print()];
if (index === lastArgIndex) {
} else if (isNextLineEmpty(arg, options)) {
if (index === 0) {
hasEmptyLineFollowingFirstArg = true;
}
anyArgEmptyLine = true;
parts.push(",", hardline, hardline);
} else {
parts.push(",", line);
}
printedArguments.push(parts);
});
const maybeTrailingComma = !(isDynamicImport || node.callee && node.callee.type === "Import") && shouldPrintComma(options, "all") ? "," : "";
function allArgsBrokenOut() {
return group(["(", indent([line, ...printedArguments]), maybeTrailingComma, line, ")"], {
shouldBreak: true
});
}
if (anyArgEmptyLine || path.getParentNode().type !== "Decorator" && isFunctionCompositionArgs(args)) {
return allArgsBrokenOut();
}
const shouldGroupFirst = shouldGroupFirstArg(args);
const shouldGroupLast = shouldGroupLastArg(args, options);
if (shouldGroupFirst || shouldGroupLast) {
if (shouldGroupFirst ? printedArguments.slice(1).some(willBreak) : printedArguments.slice(0, -1).some(willBreak)) {
return allArgsBrokenOut();
}
let printedExpanded = [];
try {
path.try(() => {
iterateCallArgumentsPath(path, (argPath, i) => {
if (shouldGroupFirst && i === 0) {
printedExpanded = [[print([], {
expandFirstArg: true
}), printedArguments.length > 1 ? "," : "", hasEmptyLineFollowingFirstArg ? hardline : line, hasEmptyLineFollowingFirstArg ? hardline : ""], ...printedArguments.slice(1)];
}
if (shouldGroupLast && i === lastArgIndex) {
printedExpanded = [...printedArguments.slice(0, -1), print([], {
expandLastArg: true
})];
}
});
});
} catch (caught) {
if (caught instanceof ArgExpansionBailout) {
return allArgsBrokenOut();
}
throw caught;
}
return [printedArguments.some(willBreak) ? breakParent : "", conditionalGroup([["(", ...printedExpanded, ")"], shouldGroupFirst ? ["(", group(printedExpanded[0], {
shouldBreak: true
}), ...printedExpanded.slice(1), ")"] : ["(", ...printedArguments.slice(0, -1), group(getLast(printedExpanded), {
shouldBreak: true
}), ")"], allArgsBrokenOut()])];
}
const contents = ["(", indent([softline, ...printedArguments]), ifBreak(maybeTrailingComma), softline, ")"];
if (isLongCurriedCallExpression(path)) {
return contents;
}
return group(contents, {
shouldBreak: printedArguments.some(willBreak) || anyArgEmptyLine
});
}
function couldGroupArg(arg, arrowChainRecursion = false) {
return arg.type === "ObjectExpression" && (arg.properties.length > 0 || hasComment(arg)) || arg.type === "ArrayExpression" && (arg.elements.length > 0 || hasComment(arg)) || arg.type === "TSTypeAssertion" && couldGroupArg(arg.expression) || isTSTypeExpression(arg) && couldGroupArg(arg.expression) || arg.type === "FunctionExpression" || arg.type === "ArrowFunctionExpression" && (!arg.returnType || !arg.returnType.typeAnnotation || arg.returnType.typeAnnotation.type !== "TSTypeReference" || isNonEmptyBlockStatement(arg.body)) && (arg.body.type === "BlockStatement" || arg.body.type === "ArrowFunctionExpression" && couldGroupArg(arg.body, true) || arg.body.type === "ObjectExpression" || arg.body.type === "ArrayExpression" || !arrowChainRecursion && (isCallExpression(arg.body) || arg.body.type === "ConditionalExpression") || isJsxNode(arg.body)) || arg.type === "DoExpression" || arg.type === "ModuleExpression";
}
function shouldGroupLastArg(args, options) {
const lastArg = getLast(args);
const penultimateArg = getPenultimate(args);
return !hasComment(lastArg, CommentCheckFlags.Leading) && !hasComment(lastArg, CommentCheckFlags.Trailing) && couldGroupArg(lastArg) && (!penultimateArg || penultimateArg.type !== lastArg.type) && (args.length !== 2 || penultimateArg.type !== "ArrowFunctionExpression" || lastArg.type !== "ArrayExpression") && !(args.length > 1 && lastArg.type === "ArrayExpression" && isConciselyPrintedArray(lastArg, options));
}
function shouldGroupFirstArg(args) {
if (args.length !== 2) {
return false;
}
const [firstArg, secondArg] = args;
if (firstArg.type === "ModuleExpression" && isTypeModuleObjectExpression(secondArg)) {
return true;
}
return !hasComment(firstArg) && (firstArg.type === "FunctionExpression" || firstArg.type === "ArrowFunctionExpression" && firstArg.body.type === "BlockStatement") && secondArg.type !== "FunctionExpression" && secondArg.type !== "ArrowFunctionExpression" && secondArg.type !== "ConditionalExpression" && !couldGroupArg(secondArg);
}
function isReactHookCallWithDepsArray(args) {
return args.length === 2 && args[0].type === "ArrowFunctionExpression" && getFunctionParameters(args[0]).length === 0 && args[0].body.type === "BlockStatement" && args[1].type === "ArrayExpression" && !args.some((arg) => hasComment(arg));
}
function isNonEmptyBlockStatement(node) {
return node.type === "BlockStatement" && (node.body.some((node2) => node2.type !== "EmptyStatement") || hasComment(node, CommentCheckFlags.Dangling));
}
function isTypeModuleObjectExpression(node) {
return node.type === "ObjectExpression" && node.properties.length === 1 && isObjectProperty(node.properties[0]) && node.properties[0].key.type === "Identifier" && node.properties[0].key.name === "type" && isStringLiteral(node.properties[0].value) && node.properties[0].value.value === "module";
}
module22.exports = printCallArguments;
}
});
var require_member = __commonJS22({
"src/language-js/print/member.js"(exports2, module22) {
"use strict";
var {
builders: {
softline,
group,
indent,
label
}
} = require_doc();
var {
isNumericLiteral,
isMemberExpression,
isCallExpression
} = require_utils7();
var {
printOptionalToken
} = require_misc();
function printMemberExpression(path, options, print) {
const node = path.getValue();
const parent = path.getParentNode();
let firstNonMemberParent;
let i = 0;
do {
firstNonMemberParent = path.getParentNode(i);
i++;
} while (firstNonMemberParent && (isMemberExpression(firstNonMemberParent) || firstNonMemberParent.type === "TSNonNullExpression"));
const objectDoc = print("object");
const lookupDoc = printMemberLookup(path, options, print);
const shouldInline = firstNonMemberParent && (firstNonMemberParent.type === "NewExpression" || firstNonMemberParent.type === "BindExpression" || firstNonMemberParent.type === "AssignmentExpression" && firstNonMemberParent.left.type !== "Identifier") || node.computed || node.object.type === "Identifier" && node.property.type === "Identifier" && !isMemberExpression(parent) || (parent.type === "AssignmentExpression" || parent.type === "VariableDeclarator") && (isCallExpression(node.object) && node.object.arguments.length > 0 || node.object.type === "TSNonNullExpression" && isCallExpression(node.object.expression) && node.object.expression.arguments.length > 0 || objectDoc.label === "member-chain");
return label(objectDoc.label === "member-chain" ? "member-chain" : "member", [objectDoc, shouldInline ? lookupDoc : group(indent([softline, lookupDoc]))]);
}
function printMemberLookup(path, options, print) {
const property = print("property");
const node = path.getValue();
const optional = printOptionalToken(path);
if (!node.computed) {
return [optional, ".", property];
}
if (!node.property || isNumericLiteral(node.property)) {
return [optional, "[", property, "]"];
}
return group([optional, "[", indent([softline, property]), softline, "]"]);
}
module22.exports = {
printMemberExpression,
printMemberLookup
};
}
});
var require_member_chain = __commonJS22({
"src/language-js/print/member-chain.js"(exports2, module22) {
"use strict";
var {
printComments
} = require_comments();
var {
getLast,
isNextLineEmptyAfterIndex,
getNextNonSpaceNonCommentCharacterIndex
} = require_util();
var pathNeedsParens = require_needs_parens();
var {
isCallExpression,
isMemberExpression,
isFunctionOrArrowExpression,
isLongCurriedCallExpression,
isMemberish,
isNumericLiteral,
isSimpleCallArgument,
hasComment,
CommentCheckFlags,
isNextLineEmpty
} = require_utils7();
var {
locEnd
} = require_loc();
var {
builders: {
join,
hardline,
group,
indent,
conditionalGroup,
breakParent,
label
},
utils: {
willBreak
}
} = require_doc();
var printCallArguments = require_call_arguments();
var {
printMemberLookup
} = require_member();
var {
printOptionalToken,
printFunctionTypeParameters,
printBindExpressionCallee
} = require_misc();
function printMemberChain(path, options, print) {
const parent = path.getParentNode();
const isExpressionStatement = !parent || parent.type === "ExpressionStatement";
const printedNodes = [];
function shouldInsertEmptyLineAfter(node2) {
const {
originalText
} = options;
const nextCharIndex = getNextNonSpaceNonCommentCharacterIndex(originalText, node2, locEnd);
const nextChar = originalText.charAt(nextCharIndex);
if (nextChar === ")") {
return nextCharIndex !== false && isNextLineEmptyAfterIndex(originalText, nextCharIndex + 1);
}
return isNextLineEmpty(node2, options);
}
function rec(path2) {
const node2 = path2.getValue();
if (isCallExpression(node2) && (isMemberish(node2.callee) || isCallExpression(node2.callee))) {
printedNodes.unshift({
node: node2,
printed: [printComments(path2, [printOptionalToken(path2), printFunctionTypeParameters(path2, options, print), printCallArguments(path2, options, print)], options), shouldInsertEmptyLineAfter(node2) ? hardline : ""]
});
path2.call((callee) => rec(callee), "callee");
} else if (isMemberish(node2)) {
printedNodes.unshift({
node: node2,
needsParens: pathNeedsParens(path2, options),
printed: printComments(path2, isMemberExpression(node2) ? printMemberLookup(path2, options, print) : printBindExpressionCallee(path2, options, print), options)
});
path2.call((object) => rec(object), "object");
} else if (node2.type === "TSNonNullExpression") {
printedNodes.unshift({
node: node2,
printed: printComments(path2, "!", options)
});
path2.call((expression) => rec(expression), "expression");
} else {
printedNodes.unshift({
node: node2,
printed: print()
});
}
}
const node = path.getValue();
printedNodes.unshift({
node,
printed: [printOptionalToken(path), printFunctionTypeParameters(path, options, print), printCallArguments(path, options, print)]
});
if (node.callee) {
path.call((callee) => rec(callee), "callee");
}
const groups = [];
let currentGroup = [printedNodes[0]];
let i = 1;
for (; i < printedNodes.length; ++i) {
if (printedNodes[i].node.type === "TSNonNullExpression" || isCallExpression(printedNodes[i].node) || isMemberExpression(printedNodes[i].node) && printedNodes[i].node.computed && isNumericLiteral(printedNodes[i].node.property)) {
currentGroup.push(printedNodes[i]);
} else {
break;
}
}
if (!isCallExpression(printedNodes[0].node)) {
for (; i + 1 < printedNodes.length; ++i) {
if (isMemberish(printedNodes[i].node) && isMemberish(printedNodes[i + 1].node)) {
currentGroup.push(printedNodes[i]);
} else {
break;
}
}
}
groups.push(currentGroup);
currentGroup = [];
let hasSeenCallExpression = false;
for (; i < printedNodes.length; ++i) {
if (hasSeenCallExpression && isMemberish(printedNodes[i].node)) {
if (printedNodes[i].node.computed && isNumericLiteral(printedNodes[i].node.property)) {
currentGroup.push(printedNodes[i]);
continue;
}
groups.push(currentGroup);
currentGroup = [];
hasSeenCallExpression = false;
}
if (isCallExpression(printedNodes[i].node) || printedNodes[i].node.type === "ImportExpression") {
hasSeenCallExpression = true;
}
currentGroup.push(printedNodes[i]);
if (hasComment(printedNodes[i].node, CommentCheckFlags.Trailing)) {
groups.push(currentGroup);
currentGroup = [];
hasSeenCallExpression = false;
}
}
if (currentGroup.length > 0) {
groups.push(currentGroup);
}
function isFactory(name) {
return /^[A-Z]|^[$_]+$/.test(name);
}
function isShort(name) {
return name.length <= options.tabWidth;
}
function shouldNotWrap(groups2) {
const hasComputed = groups2[1].length > 0 && groups2[1][0].node.computed;
if (groups2[0].length === 1) {
const firstNode = groups2[0][0].node;
return firstNode.type === "ThisExpression" || firstNode.type === "Identifier" && (isFactory(firstNode.name) || isExpressionStatement && isShort(firstNode.name) || hasComputed);
}
const lastNode = getLast(groups2[0]).node;
return isMemberExpression(lastNode) && lastNode.property.type === "Identifier" && (isFactory(lastNode.property.name) || hasComputed);
}
const shouldMerge = groups.length >= 2 && !hasComment(groups[1][0].node) && shouldNotWrap(groups);
function printGroup(printedGroup) {
const printed = printedGroup.map((tuple) => tuple.printed);
if (printedGroup.length > 0 && getLast(printedGroup).needsParens) {
return ["(", ...printed, ")"];
}
return printed;
}
function printIndentedGroup(groups2) {
if (groups2.length === 0) {
return "";
}
return indent(group([hardline, join(hardline, groups2.map(printGroup))]));
}
const printedGroups = groups.map(printGroup);
const oneLine = printedGroups;
const cutoff = shouldMerge ? 3 : 2;
const flatGroups = groups.flat();
const nodeHasComment = flatGroups.slice(1, -1).some((node2) => hasComment(node2.node, CommentCheckFlags.Leading)) || flatGroups.slice(0, -1).some((node2) => hasComment(node2.node, CommentCheckFlags.Trailing)) || groups[cutoff] && hasComment(groups[cutoff][0].node, CommentCheckFlags.Leading);
if (groups.length <= cutoff && !nodeHasComment) {
if (isLongCurriedCallExpression(path)) {
return oneLine;
}
return group(oneLine);
}
const lastNodeBeforeIndent = getLast(groups[shouldMerge ? 1 : 0]).node;
const shouldHaveEmptyLineBeforeIndent = !isCallExpression(lastNodeBeforeIndent) && shouldInsertEmptyLineAfter(lastNodeBeforeIndent);
const expanded = [printGroup(groups[0]), shouldMerge ? groups.slice(1, 2).map(printGroup) : "", shouldHaveEmptyLineBeforeIndent ? hardline : "", printIndentedGroup(groups.slice(shouldMerge ? 2 : 1))];
const callExpressions = printedNodes.map(({
node: node2
}) => node2).filter(isCallExpression);
function lastGroupWillBreakAndOtherCallsHaveFunctionArguments() {
const lastGroupNode = getLast(getLast(groups)).node;
const lastGroupDoc = getLast(printedGroups);
return isCallExpression(lastGroupNode) && willBreak(lastGroupDoc) && callExpressions.slice(0, -1).some((node2) => node2.arguments.some(isFunctionOrArrowExpression));
}
let result;
if (nodeHasComment || callExpressions.length > 2 && callExpressions.some((expr) => !expr.arguments.every((arg) => isSimpleCallArgument(arg, 0))) || printedGroups.slice(0, -1).some(willBreak) || lastGroupWillBreakAndOtherCallsHaveFunctionArguments()) {
result = group(expanded);
} else {
result = [willBreak(oneLine) || shouldHaveEmptyLineBeforeIndent ? breakParent : "", conditionalGroup([oneLine, expanded])];
}
return label("member-chain", result);
}
module22.exports = printMemberChain;
}
});
var require_call_expression = __commonJS22({
"src/language-js/print/call-expression.js"(exports2, module22) {
"use strict";
var {
builders: {
join,
group
}
} = require_doc();
var pathNeedsParens = require_needs_parens();
var {
getCallArguments,
hasFlowAnnotationComment,
isCallExpression,
isMemberish,
isStringLiteral,
isTemplateOnItsOwnLine,
isTestCall,
iterateCallArgumentsPath
} = require_utils7();
var printMemberChain = require_member_chain();
var printCallArguments = require_call_arguments();
var {
printOptionalToken,
printFunctionTypeParameters
} = require_misc();
function printCallExpression(path, options, print) {
const node = path.getValue();
const parentNode = path.getParentNode();
const isNew = node.type === "NewExpression";
const isDynamicImport = node.type === "ImportExpression";
const optional = printOptionalToken(path);
const args = getCallArguments(node);
if (args.length > 0 && (!isDynamicImport && !isNew && isCommonsJsOrAmdCall(node, parentNode) || args.length === 1 && isTemplateOnItsOwnLine(args[0], options.originalText) || !isNew && isTestCall(node, parentNode))) {
const printed = [];
iterateCallArgumentsPath(path, () => {
printed.push(print());
});
return [isNew ? "new " : "", print("callee"), optional, printFunctionTypeParameters(path, options, print), "(", join(", ", printed), ")"];
}
const isIdentifierWithFlowAnnotation = (options.parser === "babel" || options.parser === "babel-flow") && node.callee && node.callee.type === "Identifier" && hasFlowAnnotationComment(node.callee.trailingComments);
if (isIdentifierWithFlowAnnotation) {
node.callee.trailingComments[0].printed = true;
}
if (!isDynamicImport && !isNew && isMemberish(node.callee) && !path.call((path2) => pathNeedsParens(path2, options), "callee")) {
return printMemberChain(path, options, print);
}
const contents = [isNew ? "new " : "", isDynamicImport ? "import" : print("callee"), optional, isIdentifierWithFlowAnnotation ? `/*:: ${node.callee.trailingComments[0].value.slice(2).trim()} */` : "", printFunctionTypeParameters(path, options, print), printCallArguments(path, options, print)];
if (isDynamicImport || isCallExpression(node.callee)) {
return group(contents);
}
return contents;
}
function isCommonsJsOrAmdCall(node, parentNode) {
if (node.callee.type !== "Identifier") {
return false;
}
if (node.callee.name === "require") {
return true;
}
if (node.callee.name === "define") {
const args = getCallArguments(node);
return parentNode.type === "ExpressionStatement" && (args.length === 1 || args.length === 2 && args[0].type === "ArrayExpression" || args.length === 3 && isStringLiteral(args[0]) && args[1].type === "ArrayExpression");
}
return false;
}
module22.exports = {
printCallExpression
};
}
});
var require_assignment = __commonJS22({
"src/language-js/print/assignment.js"(exports2, module22) {
"use strict";
var {
isNonEmptyArray,
getStringWidth
} = require_util();
var {
builders: {
line,
group,
indent,
indentIfBreak,
lineSuffixBoundary
},
utils: {
cleanDoc,
willBreak,
canBreak
}
} = require_doc();
var {
hasLeadingOwnLineComment,
isBinaryish,
isStringLiteral,
isLiteral,
isNumericLiteral,
isCallExpression,
isMemberExpression,
getCallArguments,
rawText,
hasComment,
isSignedNumericLiteral,
isObjectProperty
} = require_utils7();
var {
shouldInlineLogicalExpression
} = require_binaryish();
var {
printCallExpression
} = require_call_expression();
function printAssignment(path, options, print, leftDoc, operator, rightPropertyName) {
const layout = chooseLayout(path, options, print, leftDoc, rightPropertyName);
const rightDoc = print(rightPropertyName, {
assignmentLayout: layout
});
switch (layout) {
case "break-after-operator":
return group([group(leftDoc), operator, group(indent([line, rightDoc]))]);
case "never-break-after-operator":
return group([group(leftDoc), operator, " ", rightDoc]);
case "fluid": {
const groupId = Symbol("assignment");
return group([group(leftDoc), operator, group(indent(line), {
id: groupId
}), lineSuffixBoundary, indentIfBreak(rightDoc, {
groupId
})]);
}
case "break-lhs":
return group([leftDoc, operator, " ", group(rightDoc)]);
case "chain":
return [group(leftDoc), operator, line, rightDoc];
case "chain-tail":
return [group(leftDoc), operator, indent([line, rightDoc])];
case "chain-tail-arrow-chain":
return [group(leftDoc), operator, rightDoc];
case "only-left":
return leftDoc;
}
}
function printAssignmentExpression(path, options, print) {
const node = path.getValue();
return printAssignment(path, options, print, print("left"), [" ", node.operator], "right");
}
function printVariableDeclarator(path, options, print) {
return printAssignment(path, options, print, print("id"), " =", "init");
}
function chooseLayout(path, options, print, leftDoc, rightPropertyName) {
const node = path.getValue();
const rightNode = node[rightPropertyName];
if (!rightNode) {
return "only-left";
}
const isTail = !isAssignment(rightNode);
const shouldUseChainFormatting = path.match(isAssignment, isAssignmentOrVariableDeclarator, (node2) => !isTail || node2.type !== "ExpressionStatement" && node2.type !== "VariableDeclaration");
if (shouldUseChainFormatting) {
return !isTail ? "chain" : rightNode.type === "ArrowFunctionExpression" && rightNode.body.type === "ArrowFunctionExpression" ? "chain-tail-arrow-chain" : "chain-tail";
}
const isHeadOfLongChain = !isTail && isAssignment(rightNode.right);
if (isHeadOfLongChain || hasLeadingOwnLineComment(options.originalText, rightNode)) {
return "break-after-operator";
}
if (rightNode.type === "CallExpression" && rightNode.callee.name === "require" || options.parser === "json5" || options.parser === "json") {
return "never-break-after-operator";
}
if (isComplexDestructuring(node) || isComplexTypeAliasParams(node) || hasComplexTypeAnnotation(node) || isArrowFunctionVariableDeclarator(node) && canBreak(leftDoc)) {
return "break-lhs";
}
const hasShortKey = isObjectPropertyWithShortKey(node, leftDoc, options);
if (path.call(() => shouldBreakAfterOperator(path, options, print, hasShortKey), rightPropertyName)) {
return "break-after-operator";
}
if (hasShortKey || rightNode.type === "TemplateLiteral" || rightNode.type === "TaggedTemplateExpression" || rightNode.type === "BooleanLiteral" || isNumericLiteral(rightNode) || rightNode.type === "ClassExpression") {
return "never-break-after-operator";
}
return "fluid";
}
function shouldBreakAfterOperator(path, options, print, hasShortKey) {
const rightNode = path.getValue();
if (isBinaryish(rightNode) && !shouldInlineLogicalExpression(rightNode)) {
return true;
}
switch (rightNode.type) {
case "StringLiteralTypeAnnotation":
case "SequenceExpression":
return true;
case "ConditionalExpression": {
const {
test
} = rightNode;
return isBinaryish(test) && !shouldInlineLogicalExpression(test);
}
case "ClassExpression":
return isNonEmptyArray(rightNode.decorators);
}
if (hasShortKey) {
return false;
}
let node = rightNode;
const propertiesForPath = [];
for (; ; ) {
if (node.type === "UnaryExpression") {
node = node.argument;
propertiesForPath.push("argument");
} else if (node.type === "TSNonNullExpression") {
node = node.expression;
propertiesForPath.push("expression");
} else {
break;
}
}
if (isStringLiteral(node) || path.call(() => isPoorlyBreakableMemberOrCallChain(path, options, print), ...propertiesForPath)) {
return true;
}
return false;
}
function isComplexDestructuring(node) {
if (isAssignmentOrVariableDeclarator(node)) {
const leftNode = node.left || node.id;
return leftNode.type === "ObjectPattern" && leftNode.properties.length > 2 && leftNode.properties.some((property) => isObjectProperty(property) && (!property.shorthand || property.value && property.value.type === "AssignmentPattern"));
}
return false;
}
function isAssignment(node) {
return node.type === "AssignmentExpression";
}
function isAssignmentOrVariableDeclarator(node) {
return isAssignment(node) || node.type === "VariableDeclarator";
}
function isComplexTypeAliasParams(node) {
const typeParams = getTypeParametersFromTypeAlias(node);
if (isNonEmptyArray(typeParams)) {
const constraintPropertyName = node.type === "TSTypeAliasDeclaration" ? "constraint" : "bound";
if (typeParams.length > 1 && typeParams.some((param) => param[constraintPropertyName] || param.default)) {
return true;
}
}
return false;
}
function getTypeParametersFromTypeAlias(node) {
if (isTypeAlias(node) && node.typeParameters && node.typeParameters.params) {
return node.typeParameters.params;
}
return null;
}
function isTypeAlias(node) {
return node.type === "TSTypeAliasDeclaration" || node.type === "TypeAlias";
}
function hasComplexTypeAnnotation(node) {
if (node.type !== "VariableDeclarator") {
return false;
}
const {
typeAnnotation
} = node.id;
if (!typeAnnotation || !typeAnnotation.typeAnnotation) {
return false;
}
const typeParams = getTypeParametersFromTypeReference(typeAnnotation.typeAnnotation);
return isNonEmptyArray(typeParams) && typeParams.length > 1 && typeParams.some((param) => isNonEmptyArray(getTypeParametersFromTypeReference(param)) || param.type === "TSConditionalType");
}
function isArrowFunctionVariableDeclarator(node) {
return node.type === "VariableDeclarator" && node.init && node.init.type === "ArrowFunctionExpression";
}
function getTypeParametersFromTypeReference(node) {
if (isTypeReference(node) && node.typeParameters && node.typeParameters.params) {
return node.typeParameters.params;
}
return null;
}
function isTypeReference(node) {
return node.type === "TSTypeReference" || node.type === "GenericTypeAnnotation";
}
function isPoorlyBreakableMemberOrCallChain(path, options, print, deep = false) {
const node = path.getValue();
const goDeeper = () => isPoorlyBreakableMemberOrCallChain(path, options, print, true);
if (node.type === "TSNonNullExpression") {
return path.call(goDeeper, "expression");
}
if (isCallExpression(node)) {
const doc2 = printCallExpression(path, options, print);
if (doc2.label === "member-chain") {
return false;
}
const args = getCallArguments(node);
const isPoorlyBreakableCall = args.length === 0 || args.length === 1 && isLoneShortArgument(args[0], options);
if (!isPoorlyBreakableCall) {
return false;
}
if (isCallExpressionWithComplexTypeArguments(node, print)) {
return false;
}
return path.call(goDeeper, "callee");
}
if (isMemberExpression(node)) {
return path.call(goDeeper, "object");
}
return deep && (node.type === "Identifier" || node.type === "ThisExpression");
}
var LONE_SHORT_ARGUMENT_THRESHOLD_RATE = 0.25;
function isLoneShortArgument(node, {
printWidth
}) {
if (hasComment(node)) {
return false;
}
const threshold = printWidth * LONE_SHORT_ARGUMENT_THRESHOLD_RATE;
if (node.type === "ThisExpression" || node.type === "Identifier" && node.name.length <= threshold || isSignedNumericLiteral(node) && !hasComment(node.argument)) {
return true;
}
const regexpPattern = node.type === "Literal" && "regex" in node && node.regex.pattern || node.type === "RegExpLiteral" && node.pattern;
if (regexpPattern) {
return regexpPattern.length <= threshold;
}
if (isStringLiteral(node)) {
return rawText(node).length <= threshold;
}
if (node.type === "TemplateLiteral") {
return node.expressions.length === 0 && node.quasis[0].value.raw.length <= threshold && !node.quasis[0].value.raw.includes("\n");
}
return isLiteral(node);
}
function isObjectPropertyWithShortKey(node, keyDoc, options) {
if (!isObjectProperty(node)) {
return false;
}
keyDoc = cleanDoc(keyDoc);
const MIN_OVERLAP_FOR_BREAK = 3;
return typeof keyDoc === "string" && getStringWidth(keyDoc) < options.tabWidth + MIN_OVERLAP_FOR_BREAK;
}
function isCallExpressionWithComplexTypeArguments(node, print) {
const typeArgs = getTypeArgumentsFromCallExpression(node);
if (isNonEmptyArray(typeArgs)) {
if (typeArgs.length > 1) {
return true;
}
if (typeArgs.length === 1) {
const firstArg = typeArgs[0];
if (firstArg.type === "TSUnionType" || firstArg.type === "UnionTypeAnnotation" || firstArg.type === "TSIntersectionType" || firstArg.type === "IntersectionTypeAnnotation" || firstArg.type === "TSTypeLiteral" || firstArg.type === "ObjectTypeAnnotation") {
return true;
}
}
const typeArgsKeyName = node.typeParameters ? "typeParameters" : "typeArguments";
if (willBreak(print(typeArgsKeyName))) {
return true;
}
}
return false;
}
function getTypeArgumentsFromCallExpression(node) {
return node.typeParameters && node.typeParameters.params || node.typeArguments && node.typeArguments.params;
}
module22.exports = {
printVariableDeclarator,
printAssignmentExpression,
printAssignment,
isArrowFunctionVariableDeclarator
};
}
});
var require_function_parameters = __commonJS22({
"src/language-js/print/function-parameters.js"(exports2, module22) {
"use strict";
var {
getNextNonSpaceNonCommentCharacter
} = require_util();
var {
printDanglingComments
} = require_comments();
var {
builders: {
line,
hardline,
softline,
group,
indent,
ifBreak
},
utils: {
removeLines,
willBreak
}
} = require_doc();
var {
getFunctionParameters,
iterateFunctionParametersPath,
isSimpleType,
isTestCall,
isTypeAnnotationAFunction,
isObjectType,
isObjectTypePropertyAFunction,
hasRestParameter,
shouldPrintComma,
hasComment,
isNextLineEmpty
} = require_utils7();
var {
locEnd
} = require_loc();
var {
ArgExpansionBailout
} = require_errors();
var {
printFunctionTypeParameters
} = require_misc();
function printFunctionParameters(path, print, options, expandArg, printTypeParams) {
const functionNode = path.getValue();
const parameters = getFunctionParameters(functionNode);
const typeParams = printTypeParams ? printFunctionTypeParameters(path, options, print) : "";
if (parameters.length === 0) {
return [typeParams, "(", printDanglingComments(path, options, true, (comment) => getNextNonSpaceNonCommentCharacter(options.originalText, comment, locEnd) === ")"), ")"];
}
const parent = path.getParentNode();
const isParametersInTestCall = isTestCall(parent);
const shouldHugParameters = shouldHugFunctionParameters(functionNode);
const printed = [];
iterateFunctionParametersPath(path, (parameterPath, index) => {
const isLastParameter = index === parameters.length - 1;
if (isLastParameter && functionNode.rest) {
printed.push("...");
}
printed.push(print());
if (isLastParameter) {
return;
}
printed.push(",");
if (isParametersInTestCall || shouldHugParameters) {
printed.push(" ");
} else if (isNextLineEmpty(parameters[index], options)) {
printed.push(hardline, hardline);
} else {
printed.push(line);
}
});
if (expandArg) {
if (willBreak(typeParams) || willBreak(printed)) {
throw new ArgExpansionBailout();
}
return group([removeLines(typeParams), "(", removeLines(printed), ")"]);
}
const hasNotParameterDecorator = parameters.every((node) => !node.decorators);
if (shouldHugParameters && hasNotParameterDecorator) {
return [typeParams, "(", ...printed, ")"];
}
if (isParametersInTestCall) {
return [typeParams, "(", ...printed, ")"];
}
const isFlowShorthandWithOneArg = (isObjectTypePropertyAFunction(parent) || isTypeAnnotationAFunction(parent) || parent.type === "TypeAlias" || parent.type === "UnionTypeAnnotation" || parent.type === "TSUnionType" || parent.type === "IntersectionTypeAnnotation" || parent.type === "FunctionTypeAnnotation" && parent.returnType === functionNode) && parameters.length === 1 && parameters[0].name === null && functionNode.this !== parameters[0] && parameters[0].typeAnnotation && functionNode.typeParameters === null && isSimpleType(parameters[0].typeAnnotation) && !functionNode.rest;
if (isFlowShorthandWithOneArg) {
if (options.arrowParens === "always") {
return ["(", ...printed, ")"];
}
return printed;
}
return [typeParams, "(", indent([softline, ...printed]), ifBreak(!hasRestParameter(functionNode) && shouldPrintComma(options, "all") ? "," : ""), softline, ")"];
}
function shouldHugFunctionParameters(node) {
if (!node) {
return false;
}
const parameters = getFunctionParameters(node);
if (parameters.length !== 1) {
return false;
}
const [parameter] = parameters;
return !hasComment(parameter) && (parameter.type === "ObjectPattern" || parameter.type === "ArrayPattern" || parameter.type === "Identifier" && parameter.typeAnnotation && (parameter.typeAnnotation.type === "TypeAnnotation" || parameter.typeAnnotation.type === "TSTypeAnnotation") && isObjectType(parameter.typeAnnotation.typeAnnotation) || parameter.type === "FunctionTypeParam" && isObjectType(parameter.typeAnnotation) || parameter.type === "AssignmentPattern" && (parameter.left.type === "ObjectPattern" || parameter.left.type === "ArrayPattern") && (parameter.right.type === "Identifier" || parameter.right.type === "ObjectExpression" && parameter.right.properties.length === 0 || parameter.right.type === "ArrayExpression" && parameter.right.elements.length === 0));
}
function getReturnTypeNode(functionNode) {
let returnTypeNode;
if (functionNode.returnType) {
returnTypeNode = functionNode.returnType;
if (returnTypeNode.typeAnnotation) {
returnTypeNode = returnTypeNode.typeAnnotation;
}
} else if (functionNode.typeAnnotation) {
returnTypeNode = functionNode.typeAnnotation;
}
return returnTypeNode;
}
function shouldGroupFunctionParameters(functionNode, returnTypeDoc) {
const returnTypeNode = getReturnTypeNode(functionNode);
if (!returnTypeNode) {
return false;
}
const typeParameters = functionNode.typeParameters && functionNode.typeParameters.params;
if (typeParameters) {
if (typeParameters.length > 1) {
return false;
}
if (typeParameters.length === 1) {
const typeParameter = typeParameters[0];
if (typeParameter.constraint || typeParameter.default) {
return false;
}
}
}
return getFunctionParameters(functionNode).length === 1 && (isObjectType(returnTypeNode) || willBreak(returnTypeDoc));
}
module22.exports = {
printFunctionParameters,
shouldHugFunctionParameters,
shouldGroupFunctionParameters
};
}
});
var require_type_annotation = __commonJS22({
"src/language-js/print/type-annotation.js"(exports2, module22) {
"use strict";
var {
printComments,
printDanglingComments
} = require_comments();
var {
isNonEmptyArray
} = require_util();
var {
builders: {
group,
join,
line,
softline,
indent,
align,
ifBreak
}
} = require_doc();
var pathNeedsParens = require_needs_parens();
var {
locStart
} = require_loc();
var {
isSimpleType,
isObjectType,
hasLeadingOwnLineComment,
isObjectTypePropertyAFunction,
shouldPrintComma
} = require_utils7();
var {
printAssignment
} = require_assignment();
var {
printFunctionParameters,
shouldGroupFunctionParameters
} = require_function_parameters();
var {
printArrayItems
} = require_array4();
function shouldHugType(node) {
if (isSimpleType(node) || isObjectType(node)) {
return true;
}
if (node.type === "UnionTypeAnnotation" || node.type === "TSUnionType") {
const voidCount = node.types.filter((node2) => node2.type === "VoidTypeAnnotation" || node2.type === "TSVoidKeyword" || node2.type === "NullLiteralTypeAnnotation" || node2.type === "TSNullKeyword").length;
const hasObject = node.types.some((node2) => node2.type === "ObjectTypeAnnotation" || node2.type === "TSTypeLiteral" || node2.type === "GenericTypeAnnotation" || node2.type === "TSTypeReference");
if (node.types.length - 1 === voidCount && hasObject) {
return true;
}
}
return false;
}
function printOpaqueType(path, options, print) {
const semi = options.semi ? ";" : "";
const node = path.getValue();
const parts = [];
parts.push("opaque type ", print("id"), print("typeParameters"));
if (node.supertype) {
parts.push(": ", print("supertype"));
}
if (node.impltype) {
parts.push(" = ", print("impltype"));
}
parts.push(semi);
return parts;
}
function printTypeAlias(path, options, print) {
const semi = options.semi ? ";" : "";
const node = path.getValue();
const parts = [];
if (node.declare) {
parts.push("declare ");
}
parts.push("type ", print("id"), print("typeParameters"));
const rightPropertyName = node.type === "TSTypeAliasDeclaration" ? "typeAnnotation" : "right";
return [printAssignment(path, options, print, parts, " =", rightPropertyName), semi];
}
function printIntersectionType(path, options, print) {
const node = path.getValue();
const types = path.map(print, "types");
const result = [];
let wasIndented = false;
for (let i = 0; i < types.length; ++i) {
if (i === 0) {
result.push(types[i]);
} else if (isObjectType(node.types[i - 1]) && isObjectType(node.types[i])) {
result.push([" & ", wasIndented ? indent(types[i]) : types[i]]);
} else if (!isObjectType(node.types[i - 1]) && !isObjectType(node.types[i])) {
result.push(indent([" &", line, types[i]]));
} else {
if (i > 1) {
wasIndented = true;
}
result.push(" & ", i > 1 ? indent(types[i]) : types[i]);
}
}
return group(result);
}
function printUnionType(path, options, print) {
const node = path.getValue();
const parent = path.getParentNode();
const shouldIndent = parent.type !== "TypeParameterInstantiation" && parent.type !== "TSTypeParameterInstantiation" && parent.type !== "GenericTypeAnnotation" && parent.type !== "TSTypeReference" && parent.type !== "TSTypeAssertion" && parent.type !== "TupleTypeAnnotation" && parent.type !== "TSTupleType" && !(parent.type === "FunctionTypeParam" && !parent.name && path.getParentNode(1).this !== parent) && !((parent.type === "TypeAlias" || parent.type === "VariableDeclarator" || parent.type === "TSTypeAliasDeclaration") && hasLeadingOwnLineComment(options.originalText, node));
const shouldHug = shouldHugType(node);
const printed = path.map((typePath) => {
let printedType = print();
if (!shouldHug) {
printedType = align(2, printedType);
}
return printComments(typePath, printedType, options);
}, "types");
if (shouldHug) {
return join(" | ", printed);
}
const shouldAddStartLine = shouldIndent && !hasLeadingOwnLineComment(options.originalText, node);
const code = [ifBreak([shouldAddStartLine ? line : "", "| "]), join([line, "| "], printed)];
if (pathNeedsParens(path, options)) {
return group([indent(code), softline]);
}
if (parent.type === "TupleTypeAnnotation" && parent.types.length > 1 || parent.type === "TSTupleType" && parent.elementTypes.length > 1) {
return group([indent([ifBreak(["(", softline]), code]), softline, ifBreak(")")]);
}
return group(shouldIndent ? indent(code) : code);
}
function printFunctionType(path, options, print) {
const node = path.getValue();
const parts = [];
const parent = path.getParentNode(0);
const parentParent = path.getParentNode(1);
const parentParentParent = path.getParentNode(2);
let isArrowFunctionTypeAnnotation = node.type === "TSFunctionType" || !((parent.type === "ObjectTypeProperty" || parent.type === "ObjectTypeInternalSlot") && !parent.variance && !parent.optional && locStart(parent) === locStart(node) || parent.type === "ObjectTypeCallProperty" || parentParentParent && parentParentParent.type === "DeclareFunction");
let needsColon = isArrowFunctionTypeAnnotation && (parent.type === "TypeAnnotation" || parent.type === "TSTypeAnnotation");
const needsParens = needsColon && isArrowFunctionTypeAnnotation && (parent.type === "TypeAnnotation" || parent.type === "TSTypeAnnotation") && parentParent.type === "ArrowFunctionExpression";
if (isObjectTypePropertyAFunction(parent)) {
isArrowFunctionTypeAnnotation = true;
needsColon = true;
}
if (needsParens) {
parts.push("(");
}
const parametersDoc = printFunctionParameters(path, print, options, false, true);
const returnTypeDoc = node.returnType || node.predicate || node.typeAnnotation ? [isArrowFunctionTypeAnnotation ? " => " : ": ", print("returnType"), print("predicate"), print("typeAnnotation")] : "";
const shouldGroupParameters = shouldGroupFunctionParameters(node, returnTypeDoc);
parts.push(shouldGroupParameters ? group(parametersDoc) : parametersDoc);
if (returnTypeDoc) {
parts.push(returnTypeDoc);
}
if (needsParens) {
parts.push(")");
}
return group(parts);
}
function printTupleType(path, options, print) {
const node = path.getValue();
const typesField = node.type === "TSTupleType" ? "elementTypes" : "types";
const types = node[typesField];
const isNonEmptyTuple = isNonEmptyArray(types);
const bracketsDelimiterLine = isNonEmptyTuple ? softline : "";
return group(["[", indent([bracketsDelimiterLine, printArrayItems(path, options, typesField, print)]), ifBreak(isNonEmptyTuple && shouldPrintComma(options, "all") ? "," : ""), printDanglingComments(path, options, true), bracketsDelimiterLine, "]"]);
}
function printIndexedAccessType(path, options, print) {
const node = path.getValue();
const leftDelimiter = node.type === "OptionalIndexedAccessType" && node.optional ? "?.[" : "[";
return [print("objectType"), leftDelimiter, print("indexType"), "]"];
}
function printJSDocType(path, print, token) {
const node = path.getValue();
return [node.postfix ? "" : token, print("typeAnnotation"), node.postfix ? token : ""];
}
module22.exports = {
printOpaqueType,
printTypeAlias,
printIntersectionType,
printUnionType,
printFunctionType,
printTupleType,
printIndexedAccessType,
shouldHugType,
printJSDocType
};
}
});
var require_type_parameters = __commonJS22({
"src/language-js/print/type-parameters.js"(exports2, module22) {
"use strict";
var {
printDanglingComments
} = require_comments();
var {
builders: {
join,
line,
hardline,
softline,
group,
indent,
ifBreak
}
} = require_doc();
var {
isTestCall,
hasComment,
CommentCheckFlags,
isTSXFile,
shouldPrintComma,
getFunctionParameters,
isObjectType,
getTypeScriptMappedTypeModifier
} = require_utils7();
var {
createGroupIdMapper
} = require_util();
var {
shouldHugType
} = require_type_annotation();
var {
isArrowFunctionVariableDeclarator
} = require_assignment();
var getTypeParametersGroupId = createGroupIdMapper("typeParameters");
function printTypeParameters(path, options, print, paramsKey) {
const node = path.getValue();
if (!node[paramsKey]) {
return "";
}
if (!Array.isArray(node[paramsKey])) {
return print(paramsKey);
}
const grandparent = path.getNode(2);
const isParameterInTestCall = grandparent && isTestCall(grandparent);
const isArrowFunctionVariable = path.match((node2) => !(node2[paramsKey].length === 1 && isObjectType(node2[paramsKey][0])), void 0, (node2, name) => name === "typeAnnotation", (node2) => node2.type === "Identifier", isArrowFunctionVariableDeclarator);
const shouldInline = node[paramsKey].length === 0 || !isArrowFunctionVariable && (isParameterInTestCall || node[paramsKey].length === 1 && (node[paramsKey][0].type === "NullableTypeAnnotation" || shouldHugType(node[paramsKey][0])));
if (shouldInline) {
return ["<", join(", ", path.map(print, paramsKey)), printDanglingCommentsForInline(path, options), ">"];
}
const trailingComma = node.type === "TSTypeParameterInstantiation" ? "" : getFunctionParameters(node).length === 1 && isTSXFile(options) && !node[paramsKey][0].constraint && path.getParentNode().type === "ArrowFunctionExpression" ? "," : shouldPrintComma(options, "all") ? ifBreak(",") : "";
return group(["<", indent([softline, join([",", line], path.map(print, paramsKey))]), trailingComma, softline, ">"], {
id: getTypeParametersGroupId(node)
});
}
function printDanglingCommentsForInline(path, options) {
const node = path.getValue();
if (!hasComment(node, CommentCheckFlags.Dangling)) {
return "";
}
const hasOnlyBlockComments = !hasComment(node, CommentCheckFlags.Line);
const printed = printDanglingComments(path, options, hasOnlyBlockComments);
if (hasOnlyBlockComments) {
return printed;
}
return [printed, hardline];
}
function printTypeParameter(path, options, print) {
const node = path.getValue();
const parts = [node.type === "TSTypeParameter" && node.const ? "const " : ""];
const parent = path.getParentNode();
if (parent.type === "TSMappedType") {
if (parent.readonly) {
parts.push(getTypeScriptMappedTypeModifier(parent.readonly, "readonly"), " ");
}
parts.push("[", print("name"));
if (node.constraint) {
parts.push(" in ", print("constraint"));
}
if (parent.nameType) {
parts.push(" as ", path.callParent(() => print("nameType")));
}
parts.push("]");
return parts;
}
if (node.variance) {
parts.push(print("variance"));
}
if (node.in) {
parts.push("in ");
}
if (node.out) {
parts.push("out ");
}
parts.push(print("name"));
if (node.bound) {
parts.push(": ", print("bound"));
}
if (node.constraint) {
parts.push(" extends ", print("constraint"));
}
if (node.default) {
parts.push(" = ", print("default"));
}
return parts;
}
module22.exports = {
printTypeParameter,
printTypeParameters,
getTypeParametersGroupId
};
}
});
var require_property = __commonJS22({
"src/language-js/print/property.js"(exports2, module22) {
"use strict";
var {
printComments
} = require_comments();
var {
printString,
printNumber
} = require_util();
var {
isNumericLiteral,
isSimpleNumber,
isStringLiteral,
isStringPropSafeToUnquote,
rawText
} = require_utils7();
var {
printAssignment
} = require_assignment();
var needsQuoteProps = /* @__PURE__ */ new WeakMap();
function printPropertyKey(path, options, print) {
const node = path.getNode();
if (node.computed) {
return ["[", print("key"), "]"];
}
const parent = path.getParentNode();
const {
key
} = node;
if (options.quoteProps === "consistent" && !needsQuoteProps.has(parent)) {
const objectHasStringProp = (parent.properties || parent.body || parent.members).some((prop) => !prop.computed && prop.key && isStringLiteral(prop.key) && !isStringPropSafeToUnquote(prop, options));
needsQuoteProps.set(parent, objectHasStringProp);
}
if ((key.type === "Identifier" || isNumericLiteral(key) && isSimpleNumber(printNumber(rawText(key))) && String(key.value) === printNumber(rawText(key)) && !(options.parser === "typescript" || options.parser === "babel-ts")) && (options.parser === "json" || options.quoteProps === "consistent" && needsQuoteProps.get(parent))) {
const prop = printString(JSON.stringify(key.type === "Identifier" ? key.name : key.value.toString()), options);
return path.call((keyPath) => printComments(keyPath, prop, options), "key");
}
if (isStringPropSafeToUnquote(node, options) && (options.quoteProps === "as-needed" || options.quoteProps === "consistent" && !needsQuoteProps.get(parent))) {
return path.call((keyPath) => printComments(keyPath, /^\d/.test(key.value) ? printNumber(key.value) : key.value, options), "key");
}
return print("key");
}
function printProperty(path, options, print) {
const node = path.getValue();
if (node.shorthand) {
return print("value");
}
return printAssignment(path, options, print, printPropertyKey(path, options, print), ":", "value");
}
module22.exports = {
printProperty,
printPropertyKey
};
}
});
var require_function = __commonJS22({
"src/language-js/print/function.js"(exports2, module22) {
"use strict";
var assert = require("assert");
var {
printDanglingComments,
printCommentsSeparately
} = require_comments();
var getLast = require_get_last();
var {
getNextNonSpaceNonCommentCharacterIndex
} = require_util();
var {
builders: {
line,
softline,
group,
indent,
ifBreak,
hardline,
join,
indentIfBreak
},
utils: {
removeLines,
willBreak
}
} = require_doc();
var {
ArgExpansionBailout
} = require_errors();
var {
getFunctionParameters,
hasLeadingOwnLineComment,
isFlowAnnotationComment,
isJsxNode,
isTemplateOnItsOwnLine,
shouldPrintComma,
startsWithNoLookaheadToken,
isBinaryish,
isLineComment,
hasComment,
getComments,
CommentCheckFlags,
isCallLikeExpression,
isCallExpression,
getCallArguments,
hasNakedLeftSide,
getLeftSide
} = require_utils7();
var {
locEnd
} = require_loc();
var {
printFunctionParameters,
shouldGroupFunctionParameters
} = require_function_parameters();
var {
printPropertyKey
} = require_property();
var {
printFunctionTypeParameters
} = require_misc();
function printFunction(path, print, options, args) {
const node = path.getValue();
let expandArg = false;
if ((node.type === "FunctionDeclaration" || node.type === "FunctionExpression") && args && args.expandLastArg) {
const parent = path.getParentNode();
if (isCallExpression(parent) && getCallArguments(parent).length > 1) {
expandArg = true;
}
}
const parts = [];
if (node.type === "TSDeclareFunction" && node.declare) {
parts.push("declare ");
}
if (node.async) {
parts.push("async ");
}
if (node.generator) {
parts.push("function* ");
} else {
parts.push("function ");
}
if (node.id) {
parts.push(print("id"));
}
const parametersDoc = printFunctionParameters(path, print, options, expandArg);
const returnTypeDoc = printReturnType(path, print, options);
const shouldGroupParameters = shouldGroupFunctionParameters(node, returnTypeDoc);
parts.push(printFunctionTypeParameters(path, options, print), group([shouldGroupParameters ? group(parametersDoc) : parametersDoc, returnTypeDoc]), node.body ? " " : "", print("body"));
if (options.semi && (node.declare || !node.body)) {
parts.push(";");
}
return parts;
}
function printMethod(path, options, print) {
const node = path.getNode();
const {
kind
} = node;
const value = node.value || node;
const parts = [];
if (!kind || kind === "init" || kind === "method" || kind === "constructor") {
if (value.async) {
parts.push("async ");
}
} else {
assert.ok(kind === "get" || kind === "set");
parts.push(kind, " ");
}
if (value.generator) {
parts.push("*");
}
parts.push(printPropertyKey(path, options, print), node.optional || node.key.optional ? "?" : "");
if (node === value) {
parts.push(printMethodInternal(path, options, print));
} else if (value.type === "FunctionExpression") {
parts.push(path.call((path2) => printMethodInternal(path2, options, print), "value"));
} else {
parts.push(print("value"));
}
return parts;
}
function printMethodInternal(path, options, print) {
const node = path.getNode();
const parametersDoc = printFunctionParameters(path, print, options);
const returnTypeDoc = printReturnType(path, print, options);
const shouldGroupParameters = shouldGroupFunctionParameters(node, returnTypeDoc);
const parts = [printFunctionTypeParameters(path, options, print), group([shouldGroupParameters ? group(parametersDoc) : parametersDoc, returnTypeDoc])];
if (node.body) {
parts.push(" ", print("body"));
} else {
parts.push(options.semi ? ";" : "");
}
return parts;
}
function printArrowFunctionSignature(path, options, print, args) {
const node = path.getValue();
const parts = [];
if (node.async) {
parts.push("async ");
}
if (shouldPrintParamsWithoutParens(path, options)) {
parts.push(print(["params", 0]));
} else {
const expandArg = args && (args.expandLastArg || args.expandFirstArg);
let returnTypeDoc = printReturnType(path, print, options);
if (expandArg) {
if (willBreak(returnTypeDoc)) {
throw new ArgExpansionBailout();
}
returnTypeDoc = group(removeLines(returnTypeDoc));
}
parts.push(group([printFunctionParameters(path, print, options, expandArg, true), returnTypeDoc]));
}
const dangling = printDanglingComments(path, options, true, (comment) => {
const nextCharacter = getNextNonSpaceNonCommentCharacterIndex(options.originalText, comment, locEnd);
return nextCharacter !== false && options.originalText.slice(nextCharacter, nextCharacter + 2) === "=>";
});
if (dangling) {
parts.push(" ", dangling);
}
return parts;
}
function printArrowChain(path, args, signatures, shouldBreak, bodyDoc, tailNode) {
const name = path.getName();
const parent = path.getParentNode();
const isCallee = isCallLikeExpression(parent) && name === "callee";
const isAssignmentRhs = Boolean(args && args.assignmentLayout);
const shouldPutBodyOnSeparateLine = tailNode.body.type !== "BlockStatement" && tailNode.body.type !== "ObjectExpression" && tailNode.body.type !== "SequenceExpression";
const shouldBreakBeforeChain = isCallee && shouldPutBodyOnSeparateLine || args && args.assignmentLayout === "chain-tail-arrow-chain";
const groupId = Symbol("arrow-chain");
if (tailNode.body.type === "SequenceExpression") {
bodyDoc = group(["(", indent([softline, bodyDoc]), softline, ")"]);
}
return group([group(indent([isCallee || isAssignmentRhs ? softline : "", group(join([" =>", line], signatures), {
shouldBreak
})]), {
id: groupId,
shouldBreak: shouldBreakBeforeChain
}), " =>", indentIfBreak(shouldPutBodyOnSeparateLine ? indent([line, bodyDoc]) : [" ", bodyDoc], {
groupId
}), isCallee ? ifBreak(softline, "", {
groupId
}) : ""]);
}
function printArrowFunction(path, options, print, args) {
let node = path.getValue();
const signatures = [];
const body = [];
let chainShouldBreak = false;
(function rec() {
const doc2 = printArrowFunctionSignature(path, options, print, args);
if (signatures.length === 0) {
signatures.push(doc2);
} else {
const {
leading,
trailing
} = printCommentsSeparately(path, options);
signatures.push([leading, doc2]);
body.unshift(trailing);
}
chainShouldBreak = chainShouldBreak || node.returnType && getFunctionParameters(node).length > 0 || node.typeParameters || getFunctionParameters(node).some((param) => param.type !== "Identifier");
if (node.body.type !== "ArrowFunctionExpression" || args && args.expandLastArg) {
body.unshift(print("body", args));
} else {
node = node.body;
path.call(rec, "body");
}
})();
if (signatures.length > 1) {
return printArrowChain(path, args, signatures, chainShouldBreak, body, node);
}
const parts = signatures;
parts.push(" =>");
if (!hasLeadingOwnLineComment(options.originalText, node.body) && (node.body.type === "ArrayExpression" || node.body.type === "ObjectExpression" || node.body.type === "BlockStatement" || isJsxNode(node.body) || isTemplateOnItsOwnLine(node.body, options.originalText) || node.body.type === "ArrowFunctionExpression" || node.body.type === "DoExpression")) {
return group([...parts, " ", body]);
}
if (node.body.type === "SequenceExpression") {
return group([...parts, group([" (", indent([softline, body]), softline, ")"])]);
}
const shouldAddSoftLine = (args && args.expandLastArg || path.getParentNode().type === "JSXExpressionContainer") && !hasComment(node);
const printTrailingComma = args && args.expandLastArg && shouldPrintComma(options, "all");
const shouldAddParens = node.body.type === "ConditionalExpression" && !startsWithNoLookaheadToken(node.body, (node2) => node2.type === "ObjectExpression");
return group([...parts, group([indent([line, shouldAddParens ? ifBreak("", "(") : "", body, shouldAddParens ? ifBreak("", ")") : ""]), shouldAddSoftLine ? [ifBreak(printTrailingComma ? "," : ""), softline] : ""])]);
}
function canPrintParamsWithoutParens(node) {
const parameters = getFunctionParameters(node);
return parameters.length === 1 && !node.typeParameters && !hasComment(node, CommentCheckFlags.Dangling) && parameters[0].type === "Identifier" && !parameters[0].typeAnnotation && !hasComment(parameters[0]) && !parameters[0].optional && !node.predicate && !node.returnType;
}
function shouldPrintParamsWithoutParens(path, options) {
if (options.arrowParens === "always") {
return false;
}
if (options.arrowParens === "avoid") {
const node = path.getValue();
return canPrintParamsWithoutParens(node);
}
return false;
}
function printReturnType(path, print, options) {
const node = path.getValue();
const returnType = print("returnType");
if (node.returnType && isFlowAnnotationComment(options.originalText, node.returnType)) {
return [" /*: ", returnType, " */"];
}
const parts = [returnType];
if (node.returnType && node.returnType.typeAnnotation) {
parts.unshift(": ");
}
if (node.predicate) {
parts.push(node.returnType ? " " : ": ", print("predicate"));
}
return parts;
}
function printReturnOrThrowArgument(path, options, print) {
const node = path.getValue();
const semi = options.semi ? ";" : "";
const parts = [];
if (node.argument) {
if (returnArgumentHasLeadingComment(options, node.argument)) {
parts.push([" (", indent([hardline, print("argument")]), hardline, ")"]);
} else if (isBinaryish(node.argument) || node.argument.type === "SequenceExpression") {
parts.push(group([ifBreak(" (", " "), indent([softline, print("argument")]), softline, ifBreak(")")]));
} else {
parts.push(" ", print("argument"));
}
}
const comments = getComments(node);
const lastComment = getLast(comments);
const isLastCommentLine = lastComment && isLineComment(lastComment);
if (isLastCommentLine) {
parts.push(semi);
}
if (hasComment(node, CommentCheckFlags.Dangling)) {
parts.push(" ", printDanglingComments(path, options, true));
}
if (!isLastCommentLine) {
parts.push(semi);
}
return parts;
}
function printReturnStatement(path, options, print) {
return ["return", printReturnOrThrowArgument(path, options, print)];
}
function printThrowStatement(path, options, print) {
return ["throw", printReturnOrThrowArgument(path, options, print)];
}
function returnArgumentHasLeadingComment(options, argument) {
if (hasLeadingOwnLineComment(options.originalText, argument)) {
return true;
}
if (hasNakedLeftSide(argument)) {
let leftMost = argument;
let newLeftMost;
while (newLeftMost = getLeftSide(leftMost)) {
leftMost = newLeftMost;
if (hasLeadingOwnLineComment(options.originalText, leftMost)) {
return true;
}
}
}
return false;
}
module22.exports = {
printFunction,
printArrowFunction,
printMethod,
printReturnStatement,
printThrowStatement,
printMethodInternal,
shouldPrintParamsWithoutParens
};
}
});
var require_decorators = __commonJS22({
"src/language-js/print/decorators.js"(exports2, module22) {
"use strict";
var {
isNonEmptyArray,
hasNewline
} = require_util();
var {
builders: {
line,
hardline,
join,
breakParent,
group
}
} = require_doc();
var {
locStart,
locEnd
} = require_loc();
var {
getParentExportDeclaration
} = require_utils7();
function printClassMemberDecorators(path, options, print) {
const node = path.getValue();
return group([join(line, path.map(print, "decorators")), hasNewlineBetweenOrAfterDecorators(node, options) ? hardline : line]);
}
function printDecoratorsBeforeExport(path, options, print) {
return [join(hardline, path.map(print, "declaration", "decorators")), hardline];
}
function printDecorators(path, options, print) {
const node = path.getValue();
const {
decorators
} = node;
if (!isNonEmptyArray(decorators) || hasDecoratorsBeforeExport(path.getParentNode())) {
return;
}
const shouldBreak = node.type === "ClassExpression" || node.type === "ClassDeclaration" || hasNewlineBetweenOrAfterDecorators(node, options);
return [getParentExportDeclaration(path) ? hardline : shouldBreak ? breakParent : "", join(line, path.map(print, "decorators")), line];
}
function hasNewlineBetweenOrAfterDecorators(node, options) {
return node.decorators.some((decorator) => hasNewline(options.originalText, locEnd(decorator)));
}
function hasDecoratorsBeforeExport(node) {
if (node.type !== "ExportDefaultDeclaration" && node.type !== "ExportNamedDeclaration" && node.type !== "DeclareExportDeclaration") {
return false;
}
const decorators = node.declaration && node.declaration.decorators;
return isNonEmptyArray(decorators) && locStart(node) === locStart(decorators[0]);
}
module22.exports = {
printDecorators,
printClassMemberDecorators,
printDecoratorsBeforeExport,
hasDecoratorsBeforeExport
};
}
});
var require_class = __commonJS22({
"src/language-js/print/class.js"(exports2, module22) {
"use strict";
var {
isNonEmptyArray,
createGroupIdMapper
} = require_util();
var {
printComments,
printDanglingComments
} = require_comments();
var {
builders: {
join,
line,
hardline,
softline,
group,
indent,
ifBreak
}
} = require_doc();
var {
hasComment,
CommentCheckFlags
} = require_utils7();
var {
getTypeParametersGroupId
} = require_type_parameters();
var {
printMethod
} = require_function();
var {
printOptionalToken,
printTypeAnnotation,
printDefiniteToken
} = require_misc();
var {
printPropertyKey
} = require_property();
var {
printAssignment
} = require_assignment();
var {
printClassMemberDecorators
} = require_decorators();
function printClass(path, options, print) {
const node = path.getValue();
const parts = [];
if (node.declare) {
parts.push("declare ");
}
if (node.abstract) {
parts.push("abstract ");
}
parts.push("class");
const groupMode = node.id && hasComment(node.id, CommentCheckFlags.Trailing) || node.typeParameters && hasComment(node.typeParameters, CommentCheckFlags.Trailing) || node.superClass && hasComment(node.superClass) || isNonEmptyArray(node.extends) || isNonEmptyArray(node.mixins) || isNonEmptyArray(node.implements);
const partsGroup = [];
const extendsParts = [];
if (node.id) {
partsGroup.push(" ", print("id"));
}
partsGroup.push(print("typeParameters"));
if (node.superClass) {
const printed = [printSuperClass(path, options, print), print("superTypeParameters")];
const printedWithComments = path.call((superClass) => ["extends ", printComments(superClass, printed, options)], "superClass");
if (groupMode) {
extendsParts.push(line, group(printedWithComments));
} else {
extendsParts.push(" ", printedWithComments);
}
} else {
extendsParts.push(printList(path, options, print, "extends"));
}
extendsParts.push(printList(path, options, print, "mixins"), printList(path, options, print, "implements"));
if (groupMode) {
let printedPartsGroup;
if (shouldIndentOnlyHeritageClauses(node)) {
printedPartsGroup = [...partsGroup, indent(extendsParts)];
} else {
printedPartsGroup = indent([...partsGroup, extendsParts]);
}
parts.push(group(printedPartsGroup, {
id: getHeritageGroupId(node)
}));
} else {
parts.push(...partsGroup, ...extendsParts);
}
parts.push(" ", print("body"));
return parts;
}
var getHeritageGroupId = createGroupIdMapper("heritageGroup");
function printHardlineAfterHeritage(node) {
return ifBreak(hardline, "", {
groupId: getHeritageGroupId(node)
});
}
function hasMultipleHeritage(node) {
return ["superClass", "extends", "mixins", "implements"].filter((key) => Boolean(node[key])).length > 1;
}
function shouldIndentOnlyHeritageClauses(node) {
return node.typeParameters && !hasComment(node.typeParameters, CommentCheckFlags.Trailing | CommentCheckFlags.Line) && !hasMultipleHeritage(node);
}
function printList(path, options, print, listName) {
const node = path.getValue();
if (!isNonEmptyArray(node[listName])) {
return "";
}
const printedLeadingComments = printDanglingComments(path, options, true, ({
marker
}) => marker === listName);
return [shouldIndentOnlyHeritageClauses(node) ? ifBreak(" ", line, {
groupId: getTypeParametersGroupId(node.typeParameters)
}) : line, printedLeadingComments, printedLeadingComments && hardline, listName, group(indent([line, join([",", line], path.map(print, listName))]))];
}
function printSuperClass(path, options, print) {
const printed = print("superClass");
const parent = path.getParentNode();
if (parent.type === "AssignmentExpression") {
return group(ifBreak(["(", indent([softline, printed]), softline, ")"], printed));
}
return printed;
}
function printClassMethod(path, options, print) {
const node = path.getValue();
const parts = [];
if (isNonEmptyArray(node.decorators)) {
parts.push(printClassMemberDecorators(path, options, print));
}
if (node.accessibility) {
parts.push(node.accessibility + " ");
}
if (node.readonly) {
parts.push("readonly ");
}
if (node.declare) {
parts.push("declare ");
}
if (node.static) {
parts.push("static ");
}
if (node.type === "TSAbstractMethodDefinition" || node.abstract) {
parts.push("abstract ");
}
if (node.override) {
parts.push("override ");
}
parts.push(printMethod(path, options, print));
return parts;
}
function printClassProperty(path, options, print) {
const node = path.getValue();
const parts = [];
const semi = options.semi ? ";" : "";
if (isNonEmptyArray(node.decorators)) {
parts.push(printClassMemberDecorators(path, options, print));
}
if (node.accessibility) {
parts.push(node.accessibility + " ");
}
if (node.declare) {
parts.push("declare ");
}
if (node.static) {
parts.push("static ");
}
if (node.type === "TSAbstractPropertyDefinition" || node.type === "TSAbstractAccessorProperty" || node.abstract) {
parts.push("abstract ");
}
if (node.override) {
parts.push("override ");
}
if (node.readonly) {
parts.push("readonly ");
}
if (node.variance) {
parts.push(print("variance"));
}
if (node.type === "ClassAccessorProperty" || node.type === "AccessorProperty" || node.type === "TSAbstractAccessorProperty") {
parts.push("accessor ");
}
parts.push(printPropertyKey(path, options, print), printOptionalToken(path), printDefiniteToken(path), printTypeAnnotation(path, options, print));
return [printAssignment(path, options, print, parts, " =", "value"), semi];
}
module22.exports = {
printClass,
printClassMethod,
printClassProperty,
printHardlineAfterHeritage
};
}
});
var require_interface = __commonJS22({
"src/language-js/print/interface.js"(exports2, module22) {
"use strict";
var {
isNonEmptyArray
} = require_util();
var {
builders: {
join,
line,
group,
indent,
ifBreak
}
} = require_doc();
var {
hasComment,
identity,
CommentCheckFlags
} = require_utils7();
var {
getTypeParametersGroupId
} = require_type_parameters();
var {
printTypeScriptModifiers
} = require_misc();
function printInterface(path, options, print) {
const node = path.getValue();
const parts = [];
if (node.declare) {
parts.push("declare ");
}
if (node.type === "TSInterfaceDeclaration") {
parts.push(node.abstract ? "abstract " : "", printTypeScriptModifiers(path, options, print));
}
parts.push("interface");
const partsGroup = [];
const extendsParts = [];
if (node.type !== "InterfaceTypeAnnotation") {
partsGroup.push(" ", print("id"), print("typeParameters"));
}
const shouldIndentOnlyHeritageClauses = node.typeParameters && !hasComment(node.typeParameters, CommentCheckFlags.Trailing | CommentCheckFlags.Line);
if (isNonEmptyArray(node.extends)) {
extendsParts.push(shouldIndentOnlyHeritageClauses ? ifBreak(" ", line, {
groupId: getTypeParametersGroupId(node.typeParameters)
}) : line, "extends ", (node.extends.length === 1 ? identity : indent)(join([",", line], path.map(print, "extends"))));
}
if (node.id && hasComment(node.id, CommentCheckFlags.Trailing) || isNonEmptyArray(node.extends)) {
if (shouldIndentOnlyHeritageClauses) {
parts.push(group([...partsGroup, indent(extendsParts)]));
} else {
parts.push(group(indent([...partsGroup, ...extendsParts])));
}
} else {
parts.push(...partsGroup, ...extendsParts);
}
parts.push(" ", print("body"));
return group(parts);
}
module22.exports = {
printInterface
};
}
});
var require_module = __commonJS22({
"src/language-js/print/module.js"(exports2, module22) {
"use strict";
var {
isNonEmptyArray
} = require_util();
var {
builders: {
softline,
group,
indent,
join,
line,
ifBreak,
hardline
}
} = require_doc();
var {
printDanglingComments
} = require_comments();
var {
hasComment,
CommentCheckFlags,
shouldPrintComma,
needsHardlineAfterDanglingComment,
isStringLiteral,
rawText
} = require_utils7();
var {
locStart,
hasSameLoc
} = require_loc();
var {
hasDecoratorsBeforeExport,
printDecoratorsBeforeExport
} = require_decorators();
function printImportDeclaration(path, options, print) {
const node = path.getValue();
const semi = options.semi ? ";" : "";
const parts = [];
const {
importKind
} = node;
parts.push("import");
if (importKind && importKind !== "value") {
parts.push(" ", importKind);
}
parts.push(printModuleSpecifiers(path, options, print), printModuleSource(path, options, print), printImportAssertions(path, options, print), semi);
return parts;
}
function printExportDeclaration(path, options, print) {
const node = path.getValue();
const parts = [];
if (hasDecoratorsBeforeExport(node)) {
parts.push(printDecoratorsBeforeExport(path, options, print));
}
const {
type,
exportKind,
declaration
} = node;
parts.push("export");
const isDefaultExport = node.default || type === "ExportDefaultDeclaration";
if (isDefaultExport) {
parts.push(" default");
}
if (hasComment(node, CommentCheckFlags.Dangling)) {
parts.push(" ", printDanglingComments(path, options, true));
if (needsHardlineAfterDanglingComment(node)) {
parts.push(hardline);
}
}
if (declaration) {
parts.push(" ", print("declaration"));
} else {
parts.push(exportKind === "type" ? " type" : "", printModuleSpecifiers(path, options, print), printModuleSource(path, options, print), printImportAssertions(path, options, print));
}
if (shouldExportDeclarationPrintSemi(node, options)) {
parts.push(";");
}
return parts;
}
function printExportAllDeclaration(path, options, print) {
const node = path.getValue();
const semi = options.semi ? ";" : "";
const parts = [];
const {
exportKind,
exported
} = node;
parts.push("export");
if (exportKind === "type") {
parts.push(" type");
}
parts.push(" *");
if (exported) {
parts.push(" as ", print("exported"));
}
parts.push(printModuleSource(path, options, print), printImportAssertions(path, options, print), semi);
return parts;
}
function shouldExportDeclarationPrintSemi(node, options) {
if (!options.semi) {
return false;
}
const {
type,
declaration
} = node;
const isDefaultExport = node.default || type === "ExportDefaultDeclaration";
if (!declaration) {
return true;
}
const {
type: declarationType
} = declaration;
if (isDefaultExport && declarationType !== "ClassDeclaration" && declarationType !== "FunctionDeclaration" && declarationType !== "TSInterfaceDeclaration" && declarationType !== "DeclareClass" && declarationType !== "DeclareFunction" && declarationType !== "TSDeclareFunction" && declarationType !== "EnumDeclaration") {
return true;
}
return false;
}
function printModuleSource(path, options, print) {
const node = path.getValue();
if (!node.source) {
return "";
}
const parts = [];
if (!shouldNotPrintSpecifiers(node, options)) {
parts.push(" from");
}
parts.push(" ", print("source"));
return parts;
}
function printModuleSpecifiers(path, options, print) {
const node = path.getValue();
if (shouldNotPrintSpecifiers(node, options)) {
return "";
}
const parts = [" "];
if (isNonEmptyArray(node.specifiers)) {
const standaloneSpecifiers = [];
const groupedSpecifiers = [];
path.each(() => {
const specifierType = path.getValue().type;
if (specifierType === "ExportNamespaceSpecifier" || specifierType === "ExportDefaultSpecifier" || specifierType === "ImportNamespaceSpecifier" || specifierType === "ImportDefaultSpecifier") {
standaloneSpecifiers.push(print());
} else if (specifierType === "ExportSpecifier" || specifierType === "ImportSpecifier") {
groupedSpecifiers.push(print());
} else {
throw new Error(`Unknown specifier type ${JSON.stringify(specifierType)}`);
}
}, "specifiers");
parts.push(join(", ", standaloneSpecifiers));
if (groupedSpecifiers.length > 0) {
if (standaloneSpecifiers.length > 0) {
parts.push(", ");
}
const canBreak = groupedSpecifiers.length > 1 || standaloneSpecifiers.length > 0 || node.specifiers.some((node2) => hasComment(node2));
if (canBreak) {
parts.push(group(["{", indent([options.bracketSpacing ? line : softline, join([",", line], groupedSpecifiers)]), ifBreak(shouldPrintComma(options) ? "," : ""), options.bracketSpacing ? line : softline, "}"]));
} else {
parts.push(["{", options.bracketSpacing ? " " : "", ...groupedSpecifiers, options.bracketSpacing ? " " : "", "}"]);
}
}
} else {
parts.push("{}");
}
return parts;
}
function shouldNotPrintSpecifiers(node, options) {
const {
type,
importKind,
source,
specifiers
} = node;
if (type !== "ImportDeclaration" || isNonEmptyArray(specifiers) || importKind === "type") {
return false;
}
return !/{\s*}/.test(options.originalText.slice(locStart(node), locStart(source)));
}
function printImportAssertions(path, options, print) {
const node = path.getNode();
if (isNonEmptyArray(node.assertions)) {
return [" assert {", options.bracketSpacing ? " " : "", join(", ", path.map(print, "assertions")), options.bracketSpacing ? " " : "", "}"];
}
return "";
}
function printModuleSpecifier(path, options, print) {
const node = path.getNode();
const {
type
} = node;
const parts = [];
const kind = type === "ImportSpecifier" ? node.importKind : node.exportKind;
if (kind && kind !== "value") {
parts.push(kind, " ");
}
const isImport = type.startsWith("Import");
const leftSideProperty = isImport ? "imported" : "local";
const rightSideProperty = isImport ? "local" : "exported";
const leftSideNode = node[leftSideProperty];
const rightSideNode = node[rightSideProperty];
let left = "";
let right = "";
if (type === "ExportNamespaceSpecifier" || type === "ImportNamespaceSpecifier") {
left = "*";
} else if (leftSideNode) {
left = print(leftSideProperty);
}
if (rightSideNode && !isShorthandSpecifier(node)) {
right = print(rightSideProperty);
}
parts.push(left, left && right ? " as " : "", right);
return parts;
}
function isShorthandSpecifier(specifier) {
if (specifier.type !== "ImportSpecifier" && specifier.type !== "ExportSpecifier") {
return false;
}
const {
local,
[specifier.type === "ImportSpecifier" ? "imported" : "exported"]: importedOrExported
} = specifier;
if (local.type !== importedOrExported.type || !hasSameLoc(local, importedOrExported)) {
return false;
}
if (isStringLiteral(local)) {
return local.value === importedOrExported.value && rawText(local) === rawText(importedOrExported);
}
switch (local.type) {
case "Identifier":
return local.name === importedOrExported.name;
default:
return false;
}
}
module22.exports = {
printImportDeclaration,
printExportDeclaration,
printExportAllDeclaration,
printModuleSpecifier
};
}
});
var require_object = __commonJS22({
"src/language-js/print/object.js"(exports2, module22) {
"use strict";
var {
printDanglingComments
} = require_comments();
var {
builders: {
line,
softline,
group,
indent,
ifBreak,
hardline
}
} = require_doc();
var {
getLast,
hasNewlineInRange,
hasNewline,
isNonEmptyArray
} = require_util();
var {
shouldPrintComma,
hasComment,
getComments,
CommentCheckFlags,
isNextLineEmpty
} = require_utils7();
var {
locStart,
locEnd
} = require_loc();
var {
printOptionalToken,
printTypeAnnotation
} = require_misc();
var {
shouldHugFunctionParameters
} = require_function_parameters();
var {
shouldHugType
} = require_type_annotation();
var {
printHardlineAfterHeritage
} = require_class();
function printObject(path, options, print) {
const semi = options.semi ? ";" : "";
const node = path.getValue();
let propertiesField;
if (node.type === "TSTypeLiteral") {
propertiesField = "members";
} else if (node.type === "TSInterfaceBody") {
propertiesField = "body";
} else {
propertiesField = "properties";
}
const isTypeAnnotation = node.type === "ObjectTypeAnnotation";
const fields = [propertiesField];
if (isTypeAnnotation) {
fields.push("indexers", "callProperties", "internalSlots");
}
const firstProperty = fields.map((field) => node[field][0]).sort((a, b) => locStart(a) - locStart(b))[0];
const parent = path.getParentNode(0);
const isFlowInterfaceLikeBody = isTypeAnnotation && parent && (parent.type === "InterfaceDeclaration" || parent.type === "DeclareInterface" || parent.type === "DeclareClass") && path.getName() === "body";
const shouldBreak = node.type === "TSInterfaceBody" || isFlowInterfaceLikeBody || node.type === "ObjectPattern" && parent.type !== "FunctionDeclaration" && parent.type !== "FunctionExpression" && parent.type !== "ArrowFunctionExpression" && parent.type !== "ObjectMethod" && parent.type !== "ClassMethod" && parent.type !== "ClassPrivateMethod" && parent.type !== "AssignmentPattern" && parent.type !== "CatchClause" && node.properties.some((property) => property.value && (property.value.type === "ObjectPattern" || property.value.type === "ArrayPattern")) || node.type !== "ObjectPattern" && firstProperty && hasNewlineInRange(options.originalText, locStart(node), locStart(firstProperty));
const separator = isFlowInterfaceLikeBody ? ";" : node.type === "TSInterfaceBody" || node.type === "TSTypeLiteral" ? ifBreak(semi, ";") : ",";
const leftBrace = node.type === "RecordExpression" ? "#{" : node.exact ? "{|" : "{";
const rightBrace = node.exact ? "|}" : "}";
const propsAndLoc = [];
for (const field of fields) {
path.each((childPath) => {
const node2 = childPath.getValue();
propsAndLoc.push({
node: node2,
printed: print(),
loc: locStart(node2)
});
}, field);
}
if (fields.length > 1) {
propsAndLoc.sort((a, b) => a.loc - b.loc);
}
let separatorParts = [];
const props = propsAndLoc.map((prop) => {
const result = [...separatorParts, group(prop.printed)];
separatorParts = [separator, line];
if ((prop.node.type === "TSPropertySignature" || prop.node.type === "TSMethodSignature" || prop.node.type === "TSConstructSignatureDeclaration") && hasComment(prop.node, CommentCheckFlags.PrettierIgnore)) {
separatorParts.shift();
}
if (isNextLineEmpty(prop.node, options)) {
separatorParts.push(hardline);
}
return result;
});
if (node.inexact) {
let printed;
if (hasComment(node, CommentCheckFlags.Dangling)) {
const hasLineComments = hasComment(node, CommentCheckFlags.Line);
const printedDanglingComments = printDanglingComments(path, options, true);
printed = [printedDanglingComments, hasLineComments || hasNewline(options.originalText, locEnd(getLast(getComments(node)))) ? hardline : line, "..."];
} else {
printed = ["..."];
}
props.push([...separatorParts, ...printed]);
}
const lastElem = getLast(node[propertiesField]);
const canHaveTrailingSeparator = !(node.inexact || lastElem && lastElem.type === "RestElement" || lastElem && (lastElem.type === "TSPropertySignature" || lastElem.type === "TSCallSignatureDeclaration" || lastElem.type === "TSMethodSignature" || lastElem.type === "TSConstructSignatureDeclaration") && hasComment(lastElem, CommentCheckFlags.PrettierIgnore));
let content;
if (props.length === 0) {
if (!hasComment(node, CommentCheckFlags.Dangling)) {
return [leftBrace, rightBrace, printTypeAnnotation(path, options, print)];
}
content = group([leftBrace, printDanglingComments(path, options), softline, rightBrace, printOptionalToken(path), printTypeAnnotation(path, options, print)]);
} else {
content = [isFlowInterfaceLikeBody && isNonEmptyArray(node.properties) ? printHardlineAfterHeritage(parent) : "", leftBrace, indent([options.bracketSpacing ? line : softline, ...props]), ifBreak(canHaveTrailingSeparator && (separator !== "," || shouldPrintComma(options)) ? separator : ""), options.bracketSpacing ? line : softline, rightBrace, printOptionalToken(path), printTypeAnnotation(path, options, print)];
}
if (path.match((node2) => node2.type === "ObjectPattern" && !node2.decorators, (node2, name, number) => shouldHugFunctionParameters(node2) && (name === "params" || name === "parameters" || name === "this" || name === "rest") && number === 0) || path.match(shouldHugType, (node2, name) => name === "typeAnnotation", (node2, name) => name === "typeAnnotation", (node2, name, number) => shouldHugFunctionParameters(node2) && (name === "params" || name === "parameters" || name === "this" || name === "rest") && number === 0) || !shouldBreak && path.match((node2) => node2.type === "ObjectPattern", (node2) => node2.type === "AssignmentExpression" || node2.type === "VariableDeclarator")) {
return content;
}
return group(content, {
shouldBreak
});
}
module22.exports = {
printObject
};
}
});
var require_flow = __commonJS22({
"src/language-js/print/flow.js"(exports2, module22) {
"use strict";
var assert = require("assert");
var {
printDanglingComments
} = require_comments();
var {
printString,
printNumber
} = require_util();
var {
builders: {
hardline,
softline,
group,
indent
}
} = require_doc();
var {
getParentExportDeclaration,
isFunctionNotation,
isGetterOrSetter,
rawText,
shouldPrintComma
} = require_utils7();
var {
locStart,
locEnd
} = require_loc();
var {
replaceTextEndOfLine
} = require_doc_utils();
var {
printClass
} = require_class();
var {
printOpaqueType,
printTypeAlias,
printIntersectionType,
printUnionType,
printFunctionType,
printTupleType,
printIndexedAccessType
} = require_type_annotation();
var {
printInterface
} = require_interface();
var {
printTypeParameter,
printTypeParameters
} = require_type_parameters();
var {
printExportDeclaration,
printExportAllDeclaration
} = require_module();
var {
printArrayItems
} = require_array4();
var {
printObject
} = require_object();
var {
printPropertyKey
} = require_property();
var {
printOptionalToken,
printTypeAnnotation,
printRestSpread
} = require_misc();
function printFlow(path, options, print) {
const node = path.getValue();
const semi = options.semi ? ";" : "";
const parts = [];
switch (node.type) {
case "DeclareClass":
return printFlowDeclaration(path, printClass(path, options, print));
case "DeclareFunction":
return printFlowDeclaration(path, ["function ", print("id"), node.predicate ? " " : "", print("predicate"), semi]);
case "DeclareModule":
return printFlowDeclaration(path, ["module ", print("id"), " ", print("body")]);
case "DeclareModuleExports":
return printFlowDeclaration(path, ["module.exports", ": ", print("typeAnnotation"), semi]);
case "DeclareVariable":
return printFlowDeclaration(path, ["var ", print("id"), semi]);
case "DeclareOpaqueType":
return printFlowDeclaration(path, printOpaqueType(path, options, print));
case "DeclareInterface":
return printFlowDeclaration(path, printInterface(path, options, print));
case "DeclareTypeAlias":
return printFlowDeclaration(path, printTypeAlias(path, options, print));
case "DeclareExportDeclaration":
return printFlowDeclaration(path, printExportDeclaration(path, options, print));
case "DeclareExportAllDeclaration":
return printFlowDeclaration(path, printExportAllDeclaration(path, options, print));
case "OpaqueType":
return printOpaqueType(path, options, print);
case "TypeAlias":
return printTypeAlias(path, options, print);
case "IntersectionTypeAnnotation":
return printIntersectionType(path, options, print);
case "UnionTypeAnnotation":
return printUnionType(path, options, print);
case "FunctionTypeAnnotation":
return printFunctionType(path, options, print);
case "TupleTypeAnnotation":
return printTupleType(path, options, print);
case "GenericTypeAnnotation":
return [print("id"), printTypeParameters(path, options, print, "typeParameters")];
case "IndexedAccessType":
case "OptionalIndexedAccessType":
return printIndexedAccessType(path, options, print);
case "TypeAnnotation":
return print("typeAnnotation");
case "TypeParameter":
return printTypeParameter(path, options, print);
case "TypeofTypeAnnotation":
return ["typeof ", print("argument")];
case "ExistsTypeAnnotation":
return "*";
case "EmptyTypeAnnotation":
return "empty";
case "MixedTypeAnnotation":
return "mixed";
case "ArrayTypeAnnotation":
return [print("elementType"), "[]"];
case "BooleanLiteralTypeAnnotation":
return String(node.value);
case "EnumDeclaration":
return ["enum ", print("id"), " ", print("body")];
case "EnumBooleanBody":
case "EnumNumberBody":
case "EnumStringBody":
case "EnumSymbolBody": {
if (node.type === "EnumSymbolBody" || node.explicitType) {
let type = null;
switch (node.type) {
case "EnumBooleanBody":
type = "boolean";
break;
case "EnumNumberBody":
type = "number";
break;
case "EnumStringBody":
type = "string";
break;
case "EnumSymbolBody":
type = "symbol";
break;
}
parts.push("of ", type, " ");
}
if (node.members.length === 0 && !node.hasUnknownMembers) {
parts.push(group(["{", printDanglingComments(path, options), softline, "}"]));
} else {
const members = node.members.length > 0 ? [hardline, printArrayItems(path, options, "members", print), node.hasUnknownMembers || shouldPrintComma(options) ? "," : ""] : [];
parts.push(group(["{", indent([...members, ...node.hasUnknownMembers ? [hardline, "..."] : []]), printDanglingComments(path, options, true), hardline, "}"]));
}
return parts;
}
case "EnumBooleanMember":
case "EnumNumberMember":
case "EnumStringMember":
return [print("id"), " = ", typeof node.init === "object" ? print("init") : String(node.init)];
case "EnumDefaultedMember":
return print("id");
case "FunctionTypeParam": {
const name = node.name ? print("name") : path.getParentNode().this === node ? "this" : "";
return [name, printOptionalToken(path), name ? ": " : "", print("typeAnnotation")];
}
case "InterfaceDeclaration":
case "InterfaceTypeAnnotation":
return printInterface(path, options, print);
case "ClassImplements":
case "InterfaceExtends":
return [print("id"), print("typeParameters")];
case "NullableTypeAnnotation":
return ["?", print("typeAnnotation")];
case "Variance": {
const {
kind
} = node;
assert.ok(kind === "plus" || kind === "minus");
return kind === "plus" ? "+" : "-";
}
case "ObjectTypeCallProperty":
if (node.static) {
parts.push("static ");
}
parts.push(print("value"));
return parts;
case "ObjectTypeIndexer": {
return [node.static ? "static " : "", node.variance ? print("variance") : "", "[", print("id"), node.id ? ": " : "", print("key"), "]: ", print("value")];
}
case "ObjectTypeProperty": {
let modifier = "";
if (node.proto) {
modifier = "proto ";
} else if (node.static) {
modifier = "static ";
}
return [modifier, isGetterOrSetter(node) ? node.kind + " " : "", node.variance ? print("variance") : "", printPropertyKey(path, options, print), printOptionalToken(path), isFunctionNotation(node) ? "" : ": ", print("value")];
}
case "ObjectTypeAnnotation":
return printObject(path, options, print);
case "ObjectTypeInternalSlot":
return [node.static ? "static " : "", "[[", print("id"), "]]", printOptionalToken(path), node.method ? "" : ": ", print("value")];
case "ObjectTypeSpreadProperty":
return printRestSpread(path, options, print);
case "QualifiedTypeofIdentifier":
case "QualifiedTypeIdentifier":
return [print("qualification"), ".", print("id")];
case "StringLiteralTypeAnnotation":
return replaceTextEndOfLine(printString(rawText(node), options));
case "NumberLiteralTypeAnnotation":
assert.strictEqual(typeof node.value, "number");
case "BigIntLiteralTypeAnnotation":
if (node.extra) {
return printNumber(node.extra.raw);
}
return printNumber(node.raw);
case "TypeCastExpression": {
return ["(", print("expression"), printTypeAnnotation(path, options, print), ")"];
}
case "TypeParameterDeclaration":
case "TypeParameterInstantiation": {
const printed = printTypeParameters(path, options, print, "params");
if (options.parser === "flow") {
const start = locStart(node);
const end = locEnd(node);
const commentStartIndex = options.originalText.lastIndexOf("/*", start);
const commentEndIndex = options.originalText.indexOf("*/", end);
if (commentStartIndex !== -1 && commentEndIndex !== -1) {
const comment = options.originalText.slice(commentStartIndex + 2, commentEndIndex).trim();
if (comment.startsWith("::") && !comment.includes("/*") && !comment.includes("*/")) {
return ["/*:: ", printed, " */"];
}
}
}
return printed;
}
case "InferredPredicate":
return "%checks";
case "DeclaredPredicate":
return ["%checks(", print("value"), ")"];
case "AnyTypeAnnotation":
return "any";
case "BooleanTypeAnnotation":
return "boolean";
case "BigIntTypeAnnotation":
return "bigint";
case "NullLiteralTypeAnnotation":
return "null";
case "NumberTypeAnnotation":
return "number";
case "SymbolTypeAnnotation":
return "symbol";
case "StringTypeAnnotation":
return "string";
case "VoidTypeAnnotation":
return "void";
case "ThisTypeAnnotation":
return "this";
case "Node":
case "Printable":
case "SourceLocation":
case "Position":
case "Statement":
case "Function":
case "Pattern":
case "Expression":
case "Declaration":
case "Specifier":
case "NamedSpecifier":
case "Comment":
case "MemberTypeAnnotation":
case "Type":
throw new Error("unprintable type: " + JSON.stringify(node.type));
}
}
function printFlowDeclaration(path, printed) {
const parentExportDecl = getParentExportDeclaration(path);
if (parentExportDecl) {
assert.strictEqual(parentExportDecl.type, "DeclareExportDeclaration");
return printed;
}
return ["declare ", printed];
}
module22.exports = {
printFlow
};
}
});
var require_is_ts_keyword_type = __commonJS22({
"src/language-js/utils/is-ts-keyword-type.js"(exports2, module22) {
"use strict";
function isTsKeywordType({
type
}) {
return type.startsWith("TS") && type.endsWith("Keyword");
}
module22.exports = isTsKeywordType;
}
});
var require_ternary = __commonJS22({
"src/language-js/print/ternary.js"(exports2, module22) {
"use strict";
var {
hasNewlineInRange
} = require_util();
var {
isJsxNode,
getComments,
isCallExpression,
isMemberExpression,
isTSTypeExpression
} = require_utils7();
var {
locStart,
locEnd
} = require_loc();
var isBlockComment = require_is_block_comment();
var {
builders: {
line,
softline,
group,
indent,
align,
ifBreak,
dedent,
breakParent
}
} = require_doc();
function conditionalExpressionChainContainsJsx(node) {
const conditionalExpressions = [node];
for (let index = 0; index < conditionalExpressions.length; index++) {
const conditionalExpression = conditionalExpressions[index];
for (const property of ["test", "consequent", "alternate"]) {
const node2 = conditionalExpression[property];
if (isJsxNode(node2)) {
return true;
}
if (node2.type === "ConditionalExpression") {
conditionalExpressions.push(node2);
}
}
}
return false;
}
function printTernaryTest(path, options, print) {
const node = path.getValue();
const isConditionalExpression = node.type === "ConditionalExpression";
const alternateNodePropertyName = isConditionalExpression ? "alternate" : "falseType";
const parent = path.getParentNode();
const printed = isConditionalExpression ? print("test") : [print("checkType"), " ", "extends", " ", print("extendsType")];
if (parent.type === node.type && parent[alternateNodePropertyName] === node) {
return align(2, printed);
}
return printed;
}
var ancestorNameMap = /* @__PURE__ */ new Map([["AssignmentExpression", "right"], ["VariableDeclarator", "init"], ["ReturnStatement", "argument"], ["ThrowStatement", "argument"], ["UnaryExpression", "argument"], ["YieldExpression", "argument"]]);
function shouldExtraIndentForConditionalExpression(path) {
const node = path.getValue();
if (node.type !== "ConditionalExpression") {
return false;
}
let parent;
let child = node;
for (let ancestorCount = 0; !parent; ancestorCount++) {
const node2 = path.getParentNode(ancestorCount);
if (isCallExpression(node2) && node2.callee === child || isMemberExpression(node2) && node2.object === child || node2.type === "TSNonNullExpression" && node2.expression === child) {
child = node2;
continue;
}
if (node2.type === "NewExpression" && node2.callee === child || isTSTypeExpression(node2) && node2.expression === child) {
parent = path.getParentNode(ancestorCount + 1);
child = node2;
} else {
parent = node2;
}
}
if (child === node) {
return false;
}
return parent[ancestorNameMap.get(parent.type)] === child;
}
function printTernary(path, options, print) {
const node = path.getValue();
const isConditionalExpression = node.type === "ConditionalExpression";
const consequentNodePropertyName = isConditionalExpression ? "consequent" : "trueType";
const alternateNodePropertyName = isConditionalExpression ? "alternate" : "falseType";
const testNodePropertyNames = isConditionalExpression ? ["test"] : ["checkType", "extendsType"];
const consequentNode = node[consequentNodePropertyName];
const alternateNode = node[alternateNodePropertyName];
const parts = [];
let jsxMode = false;
const parent = path.getParentNode();
const isParentTest = parent.type === node.type && testNodePropertyNames.some((prop) => parent[prop] === node);
let forceNoIndent = parent.type === node.type && !isParentTest;
let currentParent;
let previousParent;
let i = 0;
do {
previousParent = currentParent || node;
currentParent = path.getParentNode(i);
i++;
} while (currentParent && currentParent.type === node.type && testNodePropertyNames.every((prop) => currentParent[prop] !== previousParent));
const firstNonConditionalParent = currentParent || parent;
const lastConditionalParent = previousParent;
if (isConditionalExpression && (isJsxNode(node[testNodePropertyNames[0]]) || isJsxNode(consequentNode) || isJsxNode(alternateNode) || conditionalExpressionChainContainsJsx(lastConditionalParent))) {
jsxMode = true;
forceNoIndent = true;
const wrap = (doc2) => [ifBreak("("), indent([softline, doc2]), softline, ifBreak(")")];
const isNil = (node2) => node2.type === "NullLiteral" || node2.type === "Literal" && node2.value === null || node2.type === "Identifier" && node2.name === "undefined";
parts.push(" ? ", isNil(consequentNode) ? print(consequentNodePropertyName) : wrap(print(consequentNodePropertyName)), " : ", alternateNode.type === node.type || isNil(alternateNode) ? print(alternateNodePropertyName) : wrap(print(alternateNodePropertyName)));
} else {
const part = [line, "? ", consequentNode.type === node.type ? ifBreak("", "(") : "", align(2, print(consequentNodePropertyName)), consequentNode.type === node.type ? ifBreak("", ")") : "", line, ": ", alternateNode.type === node.type ? print(alternateNodePropertyName) : align(2, print(alternateNodePropertyName))];
parts.push(parent.type !== node.type || parent[alternateNodePropertyName] === node || isParentTest ? part : options.useTabs ? dedent(indent(part)) : align(Math.max(0, options.tabWidth - 2), part));
}
const comments = [...testNodePropertyNames.map((propertyName) => getComments(node[propertyName])), getComments(consequentNode), getComments(alternateNode)].flat();
const shouldBreak = comments.some((comment) => isBlockComment(comment) && hasNewlineInRange(options.originalText, locStart(comment), locEnd(comment)));
const maybeGroup = (doc2) => parent === firstNonConditionalParent ? group(doc2, {
shouldBreak
}) : shouldBreak ? [doc2, breakParent] : doc2;
const breakClosingParen = !jsxMode && (isMemberExpression(parent) || parent.type === "NGPipeExpression" && parent.left === node) && !parent.computed;
const shouldExtraIndent = shouldExtraIndentForConditionalExpression(path);
const result = maybeGroup([printTernaryTest(path, options, print), forceNoIndent ? parts : indent(parts), isConditionalExpression && breakClosingParen && !shouldExtraIndent ? softline : ""]);
return isParentTest || shouldExtraIndent ? group([indent([softline, result]), softline]) : result;
}
module22.exports = {
printTernary
};
}
});
var require_statement = __commonJS22({
"src/language-js/print/statement.js"(exports2, module22) {
"use strict";
var {
builders: {
hardline
}
} = require_doc();
var pathNeedsParens = require_needs_parens();
var {
getLeftSidePathName,
hasNakedLeftSide,
isJsxNode,
isTheOnlyJsxElementInMarkdown,
hasComment,
CommentCheckFlags,
isNextLineEmpty
} = require_utils7();
var {
shouldPrintParamsWithoutParens
} = require_function();
function printStatementSequence(path, options, print, property) {
const node = path.getValue();
const parts = [];
const isClassBody = node.type === "ClassBody";
const lastStatement = getLastStatement(node[property]);
path.each((path2, index, statements) => {
const node2 = path2.getValue();
if (node2.type === "EmptyStatement") {
return;
}
const printed = print();
if (!options.semi && !isClassBody && !isTheOnlyJsxElementInMarkdown(options, path2) && statementNeedsASIProtection(path2, options)) {
if (hasComment(node2, CommentCheckFlags.Leading)) {
parts.push(print([], {
needsSemi: true
}));
} else {
parts.push(";", printed);
}
} else {
parts.push(printed);
}
if (!options.semi && isClassBody && isClassProperty(node2) && shouldPrintSemicolonAfterClassProperty(node2, statements[index + 1])) {
parts.push(";");
}
if (node2 !== lastStatement) {
parts.push(hardline);
if (isNextLineEmpty(node2, options)) {
parts.push(hardline);
}
}
}, property);
return parts;
}
function getLastStatement(statements) {
for (let i = statements.length - 1; i >= 0; i--) {
const statement = statements[i];
if (statement.type !== "EmptyStatement") {
return statement;
}
}
}
function statementNeedsASIProtection(path, options) {
const node = path.getNode();
if (node.type !== "ExpressionStatement") {
return false;
}
return path.call((childPath) => expressionNeedsASIProtection(childPath, options), "expression");
}
function expressionNeedsASIProtection(path, options) {
const node = path.getValue();
switch (node.type) {
case "ParenthesizedExpression":
case "TypeCastExpression":
case "ArrayExpression":
case "ArrayPattern":
case "TemplateLiteral":
case "TemplateElement":
case "RegExpLiteral":
return true;
case "ArrowFunctionExpression": {
if (!shouldPrintParamsWithoutParens(path, options)) {
return true;
}
break;
}
case "UnaryExpression": {
const {
prefix,
operator
} = node;
if (prefix && (operator === "+" || operator === "-")) {
return true;
}
break;
}
case "BindExpression": {
if (!node.object) {
return true;
}
break;
}
case "Literal": {
if (node.regex) {
return true;
}
break;
}
default: {
if (isJsxNode(node)) {
return true;
}
}
}
if (pathNeedsParens(path, options)) {
return true;
}
if (!hasNakedLeftSide(node)) {
return false;
}
return path.call((childPath) => expressionNeedsASIProtection(childPath, options), ...getLeftSidePathName(path, node));
}
function printBody(path, options, print) {
return printStatementSequence(path, options, print, "body");
}
function printSwitchCaseConsequent(path, options, print) {
return printStatementSequence(path, options, print, "consequent");
}
var isClassProperty = ({
type
}) => type === "ClassProperty" || type === "PropertyDefinition" || type === "ClassPrivateProperty" || type === "ClassAccessorProperty" || type === "AccessorProperty" || type === "TSAbstractPropertyDefinition" || type === "TSAbstractAccessorProperty";
function shouldPrintSemicolonAfterClassProperty(node, nextNode) {
const {
type,
name
} = node.key;
if (!node.computed && type === "Identifier" && (name === "static" || name === "get" || name === "set" || name === "accessor") && !node.value && !node.typeAnnotation) {
return true;
}
if (!nextNode) {
return false;
}
if (nextNode.static || nextNode.accessibility) {
return false;
}
if (!nextNode.computed) {
const name2 = nextNode.key && nextNode.key.name;
if (name2 === "in" || name2 === "instanceof") {
return true;
}
}
if (isClassProperty(nextNode) && nextNode.variance && !nextNode.static && !nextNode.declare) {
return true;
}
switch (nextNode.type) {
case "ClassProperty":
case "PropertyDefinition":
case "TSAbstractPropertyDefinition":
return nextNode.computed;
case "MethodDefinition":
case "TSAbstractMethodDefinition":
case "ClassMethod":
case "ClassPrivateMethod": {
const isAsync = nextNode.value ? nextNode.value.async : nextNode.async;
if (isAsync || nextNode.kind === "get" || nextNode.kind === "set") {
return false;
}
const isGenerator = nextNode.value ? nextNode.value.generator : nextNode.generator;
if (nextNode.computed || isGenerator) {
return true;
}
return false;
}
case "TSIndexSignature":
return true;
}
return false;
}
module22.exports = {
printBody,
printSwitchCaseConsequent
};
}
});
var require_block = __commonJS22({
"src/language-js/print/block.js"(exports2, module22) {
"use strict";
var {
printDanglingComments
} = require_comments();
var {
isNonEmptyArray
} = require_util();
var {
builders: {
hardline,
indent
}
} = require_doc();
var {
hasComment,
CommentCheckFlags,
isNextLineEmpty
} = require_utils7();
var {
printHardlineAfterHeritage
} = require_class();
var {
printBody
} = require_statement();
function printBlock(path, options, print) {
const node = path.getValue();
const parts = [];
if (node.type === "StaticBlock") {
parts.push("static ");
}
if (node.type === "ClassBody" && isNonEmptyArray(node.body)) {
const parent = path.getParentNode();
parts.push(printHardlineAfterHeritage(parent));
}
parts.push("{");
const printed = printBlockBody(path, options, print);
if (printed) {
parts.push(indent([hardline, printed]), hardline);
} else {
const parent = path.getParentNode();
const parentParent = path.getParentNode(1);
if (!(parent.type === "ArrowFunctionExpression" || parent.type === "FunctionExpression" || parent.type === "FunctionDeclaration" || parent.type === "ObjectMethod" || parent.type === "ClassMethod" || parent.type === "ClassPrivateMethod" || parent.type === "ForStatement" || parent.type === "WhileStatement" || parent.type === "DoWhileStatement" || parent.type === "DoExpression" || parent.type === "CatchClause" && !parentParent.finalizer || parent.type === "TSModuleDeclaration" || parent.type === "TSDeclareFunction" || node.type === "StaticBlock" || node.type === "ClassBody")) {
parts.push(hardline);
}
}
parts.push("}");
return parts;
}
function printBlockBody(path, options, print) {
const node = path.getValue();
const nodeHasDirectives = isNonEmptyArray(node.directives);
const nodeHasBody = node.body.some((node2) => node2.type !== "EmptyStatement");
const nodeHasComment = hasComment(node, CommentCheckFlags.Dangling);
if (!nodeHasDirectives && !nodeHasBody && !nodeHasComment) {
return "";
}
const parts = [];
if (nodeHasDirectives) {
path.each((childPath, index, directives) => {
parts.push(print());
if (index < directives.length - 1 || nodeHasBody || nodeHasComment) {
parts.push(hardline);
if (isNextLineEmpty(childPath.getValue(), options)) {
parts.push(hardline);
}
}
}, "directives");
}
if (nodeHasBody) {
parts.push(printBody(path, options, print));
}
if (nodeHasComment) {
parts.push(printDanglingComments(path, options, true));
}
if (node.type === "Program") {
const parent = path.getParentNode();
if (!parent || parent.type !== "ModuleExpression") {
parts.push(hardline);
}
}
return parts;
}
module22.exports = {
printBlock,
printBlockBody
};
}
});
var require_typescript = __commonJS22({
"src/language-js/print/typescript.js"(exports2, module22) {
"use strict";
var {
printDanglingComments
} = require_comments();
var {
hasNewlineInRange
} = require_util();
var {
builders: {
join,
line,
hardline,
softline,
group,
indent,
conditionalGroup,
ifBreak
}
} = require_doc();
var {
isStringLiteral,
getTypeScriptMappedTypeModifier,
shouldPrintComma,
isCallExpression,
isMemberExpression
} = require_utils7();
var isTsKeywordType = require_is_ts_keyword_type();
var {
locStart,
locEnd
} = require_loc();
var {
printOptionalToken,
printTypeScriptModifiers
} = require_misc();
var {
printTernary
} = require_ternary();
var {
printFunctionParameters,
shouldGroupFunctionParameters
} = require_function_parameters();
var {
printTemplateLiteral
} = require_template_literal();
var {
printArrayItems
} = require_array4();
var {
printObject
} = require_object();
var {
printClassProperty,
printClassMethod
} = require_class();
var {
printTypeParameter,
printTypeParameters
} = require_type_parameters();
var {
printPropertyKey
} = require_property();
var {
printFunction,
printMethodInternal
} = require_function();
var {
printInterface
} = require_interface();
var {
printBlock
} = require_block();
var {
printTypeAlias,
printIntersectionType,
printUnionType,
printFunctionType,
printTupleType,
printIndexedAccessType,
printJSDocType
} = require_type_annotation();
function printTypescript(path, options, print) {
const node = path.getValue();
if (!node.type.startsWith("TS")) {
return;
}
if (isTsKeywordType(node)) {
return node.type.slice(2, -7).toLowerCase();
}
const semi = options.semi ? ";" : "";
const parts = [];
switch (node.type) {
case "TSThisType":
return "this";
case "TSTypeAssertion": {
const shouldBreakAfterCast = !(node.expression.type === "ArrayExpression" || node.expression.type === "ObjectExpression");
const castGroup = group(["<", indent([softline, print("typeAnnotation")]), softline, ">"]);
const exprContents = [ifBreak("("), indent([softline, print("expression")]), softline, ifBreak(")")];
if (shouldBreakAfterCast) {
return conditionalGroup([[castGroup, print("expression")], [castGroup, group(exprContents, {
shouldBreak: true
})], [castGroup, print("expression")]]);
}
return group([castGroup, print("expression")]);
}
case "TSDeclareFunction":
return printFunction(path, print, options);
case "TSExportAssignment":
return ["export = ", print("expression"), semi];
case "TSModuleBlock":
return printBlock(path, options, print);
case "TSInterfaceBody":
case "TSTypeLiteral":
return printObject(path, options, print);
case "TSTypeAliasDeclaration":
return printTypeAlias(path, options, print);
case "TSQualifiedName":
return join(".", [print("left"), print("right")]);
case "TSAbstractMethodDefinition":
case "TSDeclareMethod":
return printClassMethod(path, options, print);
case "TSAbstractAccessorProperty":
case "TSAbstractPropertyDefinition":
return printClassProperty(path, options, print);
case "TSInterfaceHeritage":
case "TSExpressionWithTypeArguments":
parts.push(print("expression"));
if (node.typeParameters) {
parts.push(print("typeParameters"));
}
return parts;
case "TSTemplateLiteralType":
return printTemplateLiteral(path, print, options);
case "TSNamedTupleMember":
return [print("label"), node.optional ? "?" : "", ": ", print("elementType")];
case "TSRestType":
return ["...", print("typeAnnotation")];
case "TSOptionalType":
return [print("typeAnnotation"), "?"];
case "TSInterfaceDeclaration":
return printInterface(path, options, print);
case "TSClassImplements":
return [print("expression"), print("typeParameters")];
case "TSTypeParameterDeclaration":
case "TSTypeParameterInstantiation":
return printTypeParameters(path, options, print, "params");
case "TSTypeParameter":
return printTypeParameter(path, options, print);
case "TSSatisfiesExpression":
case "TSAsExpression": {
const operator = node.type === "TSAsExpression" ? "as" : "satisfies";
parts.push(print("expression"), ` ${operator} `, print("typeAnnotation"));
const parent = path.getParentNode();
if (isCallExpression(parent) && parent.callee === node || isMemberExpression(parent) && parent.object === node) {
return group([indent([softline, ...parts]), softline]);
}
return parts;
}
case "TSArrayType":
return [print("elementType"), "[]"];
case "TSPropertySignature": {
if (node.readonly) {
parts.push("readonly ");
}
parts.push(printPropertyKey(path, options, print), printOptionalToken(path));
if (node.typeAnnotation) {
parts.push(": ", print("typeAnnotation"));
}
if (node.initializer) {
parts.push(" = ", print("initializer"));
}
return parts;
}
case "TSParameterProperty":
if (node.accessibility) {
parts.push(node.accessibility + " ");
}
if (node.export) {
parts.push("export ");
}
if (node.static) {
parts.push("static ");
}
if (node.override) {
parts.push("override ");
}
if (node.readonly) {
parts.push("readonly ");
}
parts.push(print("parameter"));
return parts;
case "TSTypeQuery":
return ["typeof ", print("exprName"), print("typeParameters")];
case "TSIndexSignature": {
const parent = path.getParentNode();
const trailingComma = node.parameters.length > 1 ? ifBreak(shouldPrintComma(options) ? "," : "") : "";
const parametersGroup = group([indent([softline, join([", ", softline], path.map(print, "parameters"))]), trailingComma, softline]);
return [node.export ? "export " : "", node.accessibility ? [node.accessibility, " "] : "", node.static ? "static " : "", node.readonly ? "readonly " : "", node.declare ? "declare " : "", "[", node.parameters ? parametersGroup : "", node.typeAnnotation ? "]: " : "]", node.typeAnnotation ? print("typeAnnotation") : "", parent.type === "ClassBody" ? semi : ""];
}
case "TSTypePredicate":
return [node.asserts ? "asserts " : "", print("parameterName"), node.typeAnnotation ? [" is ", print("typeAnnotation")] : ""];
case "TSNonNullExpression":
return [print("expression"), "!"];
case "TSImportType":
return [!node.isTypeOf ? "" : "typeof ", "import(", print(node.parameter ? "parameter" : "argument"), ")", !node.qualifier ? "" : [".", print("qualifier")], printTypeParameters(path, options, print, "typeParameters")];
case "TSLiteralType":
return print("literal");
case "TSIndexedAccessType":
return printIndexedAccessType(path, options, print);
case "TSConstructSignatureDeclaration":
case "TSCallSignatureDeclaration":
case "TSConstructorType": {
if (node.type === "TSConstructorType" && node.abstract) {
parts.push("abstract ");
}
if (node.type !== "TSCallSignatureDeclaration") {
parts.push("new ");
}
parts.push(group(printFunctionParameters(path, print, options, false, true)));
if (node.returnType || node.typeAnnotation) {
const isType = node.type === "TSConstructorType";
parts.push(isType ? " => " : ": ", print("returnType"), print("typeAnnotation"));
}
return parts;
}
case "TSTypeOperator":
return [node.operator, " ", print("typeAnnotation")];
case "TSMappedType": {
const shouldBreak = hasNewlineInRange(options.originalText, locStart(node), locEnd(node));
return group(["{", indent([options.bracketSpacing ? line : softline, print("typeParameter"), node.optional ? getTypeScriptMappedTypeModifier(node.optional, "?") : "", node.typeAnnotation ? ": " : "", print("typeAnnotation"), ifBreak(semi)]), printDanglingComments(path, options, true), options.bracketSpacing ? line : softline, "}"], {
shouldBreak
});
}
case "TSMethodSignature": {
const kind = node.kind && node.kind !== "method" ? `${node.kind} ` : "";
parts.push(node.accessibility ? [node.accessibility, " "] : "", kind, node.export ? "export " : "", node.static ? "static " : "", node.readonly ? "readonly " : "", node.abstract ? "abstract " : "", node.declare ? "declare " : "", node.computed ? "[" : "", print("key"), node.computed ? "]" : "", printOptionalToken(path));
const parametersDoc = printFunctionParameters(path, print, options, false, true);
const returnTypePropertyName = node.returnType ? "returnType" : "typeAnnotation";
const returnTypeNode = node[returnTypePropertyName];
const returnTypeDoc = returnTypeNode ? print(returnTypePropertyName) : "";
const shouldGroupParameters = shouldGroupFunctionParameters(node, returnTypeDoc);
parts.push(shouldGroupParameters ? group(parametersDoc) : parametersDoc);
if (returnTypeNode) {
parts.push(": ", group(returnTypeDoc));
}
return group(parts);
}
case "TSNamespaceExportDeclaration":
parts.push("export as namespace ", print("id"));
if (options.semi) {
parts.push(";");
}
return group(parts);
case "TSEnumDeclaration":
if (node.declare) {
parts.push("declare ");
}
if (node.modifiers) {
parts.push(printTypeScriptModifiers(path, options, print));
}
if (node.const) {
parts.push("const ");
}
parts.push("enum ", print("id"), " ");
if (node.members.length === 0) {
parts.push(group(["{", printDanglingComments(path, options), softline, "}"]));
} else {
parts.push(group(["{", indent([hardline, printArrayItems(path, options, "members", print), shouldPrintComma(options, "es5") ? "," : ""]), printDanglingComments(path, options, true), hardline, "}"]));
}
return parts;
case "TSEnumMember":
if (node.computed) {
parts.push("[", print("id"), "]");
} else {
parts.push(print("id"));
}
if (node.initializer) {
parts.push(" = ", print("initializer"));
}
return parts;
case "TSImportEqualsDeclaration":
if (node.isExport) {
parts.push("export ");
}
parts.push("import ");
if (node.importKind && node.importKind !== "value") {
parts.push(node.importKind, " ");
}
parts.push(print("id"), " = ", print("moduleReference"));
if (options.semi) {
parts.push(";");
}
return group(parts);
case "TSExternalModuleReference":
return ["require(", print("expression"), ")"];
case "TSModuleDeclaration": {
const parent = path.getParentNode();
const isExternalModule = isStringLiteral(node.id);
const parentIsDeclaration = parent.type === "TSModuleDeclaration";
const bodyIsDeclaration = node.body && node.body.type === "TSModuleDeclaration";
if (parentIsDeclaration) {
parts.push(".");
} else {
if (node.declare) {
parts.push("declare ");
}
parts.push(printTypeScriptModifiers(path, options, print));
const textBetweenNodeAndItsId = options.originalText.slice(locStart(node), locStart(node.id));
const isGlobalDeclaration2 = node.id.type === "Identifier" && node.id.name === "global" && !/namespace|module/.test(textBetweenNodeAndItsId);
if (!isGlobalDeclaration2) {
parts.push(isExternalModule || /(?:^|\s)module(?:\s|$)/.test(textBetweenNodeAndItsId) ? "module " : "namespace ");
}
}
parts.push(print("id"));
if (bodyIsDeclaration) {
parts.push(print("body"));
} else if (node.body) {
parts.push(" ", group(print("body")));
} else {
parts.push(semi);
}
return parts;
}
case "TSConditionalType":
return printTernary(path, options, print);
case "TSInferType":
return ["infer", " ", print("typeParameter")];
case "TSIntersectionType":
return printIntersectionType(path, options, print);
case "TSUnionType":
return printUnionType(path, options, print);
case "TSFunctionType":
return printFunctionType(path, options, print);
case "TSTupleType":
return printTupleType(path, options, print);
case "TSTypeReference":
return [print("typeName"), printTypeParameters(path, options, print, "typeParameters")];
case "TSTypeAnnotation":
return print("typeAnnotation");
case "TSEmptyBodyFunctionExpression":
return printMethodInternal(path, options, print);
case "TSJSDocAllType":
return "*";
case "TSJSDocUnknownType":
return "?";
case "TSJSDocNullableType":
return printJSDocType(path, print, "?");
case "TSJSDocNonNullableType":
return printJSDocType(path, print, "!");
case "TSInstantiationExpression":
return [print("expression"), print("typeParameters")];
default:
throw new Error(`Unknown TypeScript node type: ${JSON.stringify(node.type)}.`);
}
}
module22.exports = {
printTypescript
};
}
});
var require_comment2 = __commonJS22({
"src/language-js/print/comment.js"(exports2, module22) {
"use strict";
var {
hasNewline
} = require_util();
var {
builders: {
join,
hardline
},
utils: {
replaceTextEndOfLine
}
} = require_doc();
var {
isLineComment
} = require_utils7();
var {
locStart,
locEnd
} = require_loc();
var isBlockComment = require_is_block_comment();
function printComment(commentPath, options) {
const comment = commentPath.getValue();
if (isLineComment(comment)) {
return options.originalText.slice(locStart(comment), locEnd(comment)).trimEnd();
}
if (isBlockComment(comment)) {
if (isIndentableBlockComment(comment)) {
const printed = printIndentableBlockComment(comment);
if (comment.trailing && !hasNewline(options.originalText, locStart(comment), {
backwards: true
})) {
return [hardline, printed];
}
return printed;
}
const commentEnd = locEnd(comment);
const isInsideFlowComment = options.originalText.slice(commentEnd - 3, commentEnd) === "*-/";
return ["/*", replaceTextEndOfLine(comment.value), isInsideFlowComment ? "*-/" : "*/"];
}
throw new Error("Not a comment: " + JSON.stringify(comment));
}
function isIndentableBlockComment(comment) {
const lines = `*${comment.value}*`.split("\n");
return lines.length > 1 && lines.every((line) => line.trim()[0] === "*");
}
function printIndentableBlockComment(comment) {
const lines = comment.value.split("\n");
return ["/*", join(hardline, lines.map((line, index) => index === 0 ? line.trimEnd() : " " + (index < lines.length - 1 ? line.trim() : line.trimStart()))), "*/"];
}
module22.exports = {
printComment
};
}
});
var require_literal = __commonJS22({
"src/language-js/print/literal.js"(exports2, module22) {
"use strict";
var {
printString,
printNumber
} = require_util();
var {
replaceTextEndOfLine
} = require_doc_utils();
var {
printDirective
} = require_misc();
function printLiteral(path, options) {
const node = path.getNode();
switch (node.type) {
case "RegExpLiteral":
return printRegex(node);
case "BigIntLiteral":
return printBigInt(node.bigint || node.extra.raw);
case "NumericLiteral":
return printNumber(node.extra.raw);
case "StringLiteral":
return replaceTextEndOfLine(printString(node.extra.raw, options));
case "NullLiteral":
return "null";
case "BooleanLiteral":
return String(node.value);
case "DecimalLiteral":
return printNumber(node.value) + "m";
case "Literal": {
if (node.regex) {
return printRegex(node.regex);
}
if (node.bigint) {
return printBigInt(node.raw);
}
if (node.decimal) {
return printNumber(node.decimal) + "m";
}
const {
value
} = node;
if (typeof value === "number") {
return printNumber(node.raw);
}
if (typeof value === "string") {
return isDirective(path) ? printDirective(node.raw, options) : replaceTextEndOfLine(printString(node.raw, options));
}
return String(value);
}
}
}
function isDirective(path) {
if (path.getName() !== "expression") {
return;
}
const parent = path.getParentNode();
return parent.type === "ExpressionStatement" && parent.directive;
}
function printBigInt(raw) {
return raw.toLowerCase();
}
function printRegex({
pattern,
flags
}) {
flags = [...flags].sort().join("");
return `/${pattern}/${flags}`;
}
module22.exports = {
printLiteral
};
}
});
var require_printer_estree = __commonJS22({
"src/language-js/printer-estree.js"(exports2, module22) {
"use strict";
var {
printDanglingComments
} = require_comments();
var {
hasNewline
} = require_util();
var {
builders: {
join,
line,
hardline,
softline,
group,
indent
},
utils: {
replaceTextEndOfLine
}
} = require_doc();
var embed = require_embed();
var clean = require_clean();
var {
insertPragma
} = require_pragma();
var handleComments = require_comments2();
var pathNeedsParens = require_needs_parens();
var preprocess = require_print_preprocess();
var {
hasFlowShorthandAnnotationComment,
hasComment,
CommentCheckFlags,
isTheOnlyJsxElementInMarkdown,
isLineComment,
isNextLineEmpty,
needsHardlineAfterDanglingComment,
hasIgnoreComment,
isCallExpression,
isMemberExpression,
markerForIfWithoutBlockAndSameLineComment
} = require_utils7();
var {
locStart,
locEnd
} = require_loc();
var isBlockComment = require_is_block_comment();
var {
printHtmlBinding,
isVueEventBindingExpression
} = require_html_binding();
var {
printAngular
} = require_angular();
var {
printJsx,
hasJsxIgnoreComment
} = require_jsx();
var {
printFlow
} = require_flow();
var {
printTypescript
} = require_typescript();
var {
printOptionalToken,
printBindExpressionCallee,
printTypeAnnotation,
adjustClause,
printRestSpread,
printDefiniteToken,
printDirective
} = require_misc();
var {
printImportDeclaration,
printExportDeclaration,
printExportAllDeclaration,
printModuleSpecifier
} = require_module();
var {
printTernary
} = require_ternary();
var {
printTemplateLiteral
} = require_template_literal();
var {
printArray
} = require_array4();
var {
printObject
} = require_object();
var {
printClass,
printClassMethod,
printClassProperty
} = require_class();
var {
printProperty
} = require_property();
var {
printFunction,
printArrowFunction,
printMethod,
printReturnStatement,
printThrowStatement
} = require_function();
var {
printCallExpression
} = require_call_expression();
var {
printVariableDeclarator,
printAssignmentExpression
} = require_assignment();
var {
printBinaryishExpression
} = require_binaryish();
var {
printSwitchCaseConsequent
} = require_statement();
var {
printMemberExpression
} = require_member();
var {
printBlock,
printBlockBody
} = require_block();
var {
printComment
} = require_comment2();
var {
printLiteral
} = require_literal();
var {
printDecorators
} = require_decorators();
function genericPrint(path, options, print, args) {
const printed = printPathNoParens(path, options, print, args);
if (!printed) {
return "";
}
const node = path.getValue();
const {
type
} = node;
if (type === "ClassMethod" || type === "ClassPrivateMethod" || type === "ClassProperty" || type === "ClassAccessorProperty" || type === "AccessorProperty" || type === "TSAbstractAccessorProperty" || type === "PropertyDefinition" || type === "TSAbstractPropertyDefinition" || type === "ClassPrivateProperty" || type === "MethodDefinition" || type === "TSAbstractMethodDefinition" || type === "TSDeclareMethod") {
return printed;
}
let parts = [printed];
const printedDecorators = printDecorators(path, options, print);
const isClassExpressionWithDecorators = node.type === "ClassExpression" && printedDecorators;
if (printedDecorators) {
parts = [...printedDecorators, printed];
if (!isClassExpressionWithDecorators) {
return group(parts);
}
}
const needsParens = pathNeedsParens(path, options);
if (!needsParens) {
if (args && args.needsSemi) {
parts.unshift(";");
}
if (parts.length === 1 && parts[0] === printed) {
return printed;
}
return parts;
}
if (isClassExpressionWithDecorators) {
parts = [indent([line, ...parts])];
}
parts.unshift("(");
if (args && args.needsSemi) {
parts.unshift(";");
}
if (hasFlowShorthandAnnotationComment(node)) {
const [comment] = node.trailingComments;
parts.push(" /*", comment.value.trimStart(), "*/");
comment.printed = true;
}
if (isClassExpressionWithDecorators) {
parts.push(line);
}
parts.push(")");
return parts;
}
function printPathNoParens(path, options, print, args) {
const node = path.getValue();
const semi = options.semi ? ";" : "";
if (!node) {
return "";
}
if (typeof node === "string") {
return node;
}
for (const printer of [printLiteral, printHtmlBinding, printAngular, printJsx, printFlow, printTypescript]) {
const printed = printer(path, options, print);
if (typeof printed !== "undefined") {
return printed;
}
}
let parts = [];
switch (node.type) {
case "JsExpressionRoot":
return print("node");
case "JsonRoot":
return [print("node"), hardline];
case "File":
if (node.program && node.program.interpreter) {
parts.push(print(["program", "interpreter"]));
}
parts.push(print("program"));
return parts;
case "Program":
return printBlockBody(path, options, print);
case "EmptyStatement":
return "";
case "ExpressionStatement": {
if (options.parser === "__vue_event_binding" || options.parser === "__vue_ts_event_binding") {
const parent = path.getParentNode();
if (parent.type === "Program" && parent.body.length === 1 && parent.body[0] === node) {
return [print("expression"), isVueEventBindingExpression(node.expression) ? ";" : ""];
}
}
const danglingComment = printDanglingComments(path, options, true, ({
marker
}) => marker === markerForIfWithoutBlockAndSameLineComment);
return [print("expression"), isTheOnlyJsxElementInMarkdown(options, path) ? "" : semi, danglingComment ? [" ", danglingComment] : ""];
}
case "ParenthesizedExpression": {
const shouldHug = !hasComment(node.expression) && (node.expression.type === "ObjectExpression" || node.expression.type === "ArrayExpression");
if (shouldHug) {
return ["(", print("expression"), ")"];
}
return group(["(", indent([softline, print("expression")]), softline, ")"]);
}
case "AssignmentExpression":
return printAssignmentExpression(path, options, print);
case "VariableDeclarator":
return printVariableDeclarator(path, options, print);
case "BinaryExpression":
case "LogicalExpression":
return printBinaryishExpression(path, options, print);
case "AssignmentPattern":
return [print("left"), " = ", print("right")];
case "OptionalMemberExpression":
case "MemberExpression": {
return printMemberExpression(path, options, print);
}
case "MetaProperty":
return [print("meta"), ".", print("property")];
case "BindExpression":
if (node.object) {
parts.push(print("object"));
}
parts.push(group(indent([softline, printBindExpressionCallee(path, options, print)])));
return parts;
case "Identifier": {
return [node.name, printOptionalToken(path), printDefiniteToken(path), printTypeAnnotation(path, options, print)];
}
case "V8IntrinsicIdentifier":
return ["%", node.name];
case "SpreadElement":
case "SpreadElementPattern":
case "SpreadProperty":
case "SpreadPropertyPattern":
case "RestElement":
return printRestSpread(path, options, print);
case "FunctionDeclaration":
case "FunctionExpression":
return printFunction(path, print, options, args);
case "ArrowFunctionExpression":
return printArrowFunction(path, options, print, args);
case "YieldExpression":
parts.push("yield");
if (node.delegate) {
parts.push("*");
}
if (node.argument) {
parts.push(" ", print("argument"));
}
return parts;
case "AwaitExpression": {
parts.push("await");
if (node.argument) {
parts.push(" ", print("argument"));
const parent = path.getParentNode();
if (isCallExpression(parent) && parent.callee === node || isMemberExpression(parent) && parent.object === node) {
parts = [indent([softline, ...parts]), softline];
const parentAwaitOrBlock = path.findAncestor((node2) => node2.type === "AwaitExpression" || node2.type === "BlockStatement");
if (!parentAwaitOrBlock || parentAwaitOrBlock.type !== "AwaitExpression") {
return group(parts);
}
}
}
return parts;
}
case "ExportDefaultDeclaration":
case "ExportNamedDeclaration":
return printExportDeclaration(path, options, print);
case "ExportAllDeclaration":
return printExportAllDeclaration(path, options, print);
case "ImportDeclaration":
return printImportDeclaration(path, options, print);
case "ImportSpecifier":
case "ExportSpecifier":
case "ImportNamespaceSpecifier":
case "ExportNamespaceSpecifier":
case "ImportDefaultSpecifier":
case "ExportDefaultSpecifier":
return printModuleSpecifier(path, options, print);
case "ImportAttribute":
return [print("key"), ": ", print("value")];
case "Import":
return "import";
case "BlockStatement":
case "StaticBlock":
case "ClassBody":
return printBlock(path, options, print);
case "ThrowStatement":
return printThrowStatement(path, options, print);
case "ReturnStatement":
return printReturnStatement(path, options, print);
case "NewExpression":
case "ImportExpression":
case "OptionalCallExpression":
case "CallExpression":
return printCallExpression(path, options, print);
case "ObjectExpression":
case "ObjectPattern":
case "RecordExpression":
return printObject(path, options, print);
case "ObjectProperty":
case "Property":
if (node.method || node.kind === "get" || node.kind === "set") {
return printMethod(path, options, print);
}
return printProperty(path, options, print);
case "ObjectMethod":
return printMethod(path, options, print);
case "Decorator":
return ["@", print("expression")];
case "ArrayExpression":
case "ArrayPattern":
case "TupleExpression":
return printArray(path, options, print);
case "SequenceExpression": {
const parent = path.getParentNode(0);
if (parent.type === "ExpressionStatement" || parent.type === "ForStatement") {
const parts2 = [];
path.each((expressionPath, index) => {
if (index === 0) {
parts2.push(print());
} else {
parts2.push(",", indent([line, print()]));
}
}, "expressions");
return group(parts2);
}
return group(join([",", line], path.map(print, "expressions")));
}
case "ThisExpression":
return "this";
case "Super":
return "super";
case "Directive":
return [print("value"), semi];
case "DirectiveLiteral":
return printDirective(node.extra.raw, options);
case "UnaryExpression":
parts.push(node.operator);
if (/[a-z]$/.test(node.operator)) {
parts.push(" ");
}
if (hasComment(node.argument)) {
parts.push(group(["(", indent([softline, print("argument")]), softline, ")"]));
} else {
parts.push(print("argument"));
}
return parts;
case "UpdateExpression":
parts.push(print("argument"), node.operator);
if (node.prefix) {
parts.reverse();
}
return parts;
case "ConditionalExpression":
return printTernary(path, options, print);
case "VariableDeclaration": {
const printed = path.map(print, "declarations");
const parentNode = path.getParentNode();
const isParentForLoop = parentNode.type === "ForStatement" || parentNode.type === "ForInStatement" || parentNode.type === "ForOfStatement";
const hasValue = node.declarations.some((decl) => decl.init);
let firstVariable;
if (printed.length === 1 && !hasComment(node.declarations[0])) {
firstVariable = printed[0];
} else if (printed.length > 0) {
firstVariable = indent(printed[0]);
}
parts = [node.declare ? "declare " : "", node.kind, firstVariable ? [" ", firstVariable] : "", indent(printed.slice(1).map((p) => [",", hasValue && !isParentForLoop ? hardline : line, p]))];
if (!(isParentForLoop && parentNode.body !== node)) {
parts.push(semi);
}
return group(parts);
}
case "WithStatement":
return group(["with (", print("object"), ")", adjustClause(node.body, print("body"))]);
case "IfStatement": {
const con = adjustClause(node.consequent, print("consequent"));
const opening = group(["if (", group([indent([softline, print("test")]), softline]), ")", con]);
parts.push(opening);
if (node.alternate) {
const commentOnOwnLine = hasComment(node.consequent, CommentCheckFlags.Trailing | CommentCheckFlags.Line) || needsHardlineAfterDanglingComment(node);
const elseOnSameLine = node.consequent.type === "BlockStatement" && !commentOnOwnLine;
parts.push(elseOnSameLine ? " " : hardline);
if (hasComment(node, CommentCheckFlags.Dangling)) {
parts.push(printDanglingComments(path, options, true), commentOnOwnLine ? hardline : " ");
}
parts.push("else", group(adjustClause(node.alternate, print("alternate"), node.alternate.type === "IfStatement")));
}
return parts;
}
case "ForStatement": {
const body = adjustClause(node.body, print("body"));
const dangling = printDanglingComments(path, options, true);
const printedComments = dangling ? [dangling, softline] : "";
if (!node.init && !node.test && !node.update) {
return [printedComments, group(["for (;;)", body])];
}
return [printedComments, group(["for (", group([indent([softline, print("init"), ";", line, print("test"), ";", line, print("update")]), softline]), ")", body])];
}
case "WhileStatement":
return group(["while (", group([indent([softline, print("test")]), softline]), ")", adjustClause(node.body, print("body"))]);
case "ForInStatement":
return group(["for (", print("left"), " in ", print("right"), ")", adjustClause(node.body, print("body"))]);
case "ForOfStatement":
return group(["for", node.await ? " await" : "", " (", print("left"), " of ", print("right"), ")", adjustClause(node.body, print("body"))]);
case "DoWhileStatement": {
const clause = adjustClause(node.body, print("body"));
const doBody = group(["do", clause]);
parts = [doBody];
if (node.body.type === "BlockStatement") {
parts.push(" ");
} else {
parts.push(hardline);
}
parts.push("while (", group([indent([softline, print("test")]), softline]), ")", semi);
return parts;
}
case "DoExpression":
return [node.async ? "async " : "", "do ", print("body")];
case "BreakStatement":
parts.push("break");
if (node.label) {
parts.push(" ", print("label"));
}
parts.push(semi);
return parts;
case "ContinueStatement":
parts.push("continue");
if (node.label) {
parts.push(" ", print("label"));
}
parts.push(semi);
return parts;
case "LabeledStatement":
if (node.body.type === "EmptyStatement") {
return [print("label"), ":;"];
}
return [print("label"), ": ", print("body")];
case "TryStatement":
return ["try ", print("block"), node.handler ? [" ", print("handler")] : "", node.finalizer ? [" finally ", print("finalizer")] : ""];
case "CatchClause":
if (node.param) {
const parameterHasComments = hasComment(node.param, (comment) => !isBlockComment(comment) || comment.leading && hasNewline(options.originalText, locEnd(comment)) || comment.trailing && hasNewline(options.originalText, locStart(comment), {
backwards: true
}));
const param = print("param");
return ["catch ", parameterHasComments ? ["(", indent([softline, param]), softline, ") "] : ["(", param, ") "], print("body")];
}
return ["catch ", print("body")];
case "SwitchStatement":
return [group(["switch (", indent([softline, print("discriminant")]), softline, ")"]), " {", node.cases.length > 0 ? indent([hardline, join(hardline, path.map((casePath, index, cases) => {
const caseNode = casePath.getValue();
return [print(), index !== cases.length - 1 && isNextLineEmpty(caseNode, options) ? hardline : ""];
}, "cases"))]) : "", hardline, "}"];
case "SwitchCase": {
if (node.test) {
parts.push("case ", print("test"), ":");
} else {
parts.push("default:");
}
if (hasComment(node, CommentCheckFlags.Dangling)) {
parts.push(" ", printDanglingComments(path, options, true));
}
const consequent = node.consequent.filter((node2) => node2.type !== "EmptyStatement");
if (consequent.length > 0) {
const cons = printSwitchCaseConsequent(path, options, print);
parts.push(consequent.length === 1 && consequent[0].type === "BlockStatement" ? [" ", cons] : indent([hardline, cons]));
}
return parts;
}
case "DebuggerStatement":
return ["debugger", semi];
case "ClassDeclaration":
case "ClassExpression":
return printClass(path, options, print);
case "ClassMethod":
case "ClassPrivateMethod":
case "MethodDefinition":
return printClassMethod(path, options, print);
case "ClassProperty":
case "PropertyDefinition":
case "ClassPrivateProperty":
case "ClassAccessorProperty":
case "AccessorProperty":
return printClassProperty(path, options, print);
case "TemplateElement":
return replaceTextEndOfLine(node.value.raw);
case "TemplateLiteral":
return printTemplateLiteral(path, print, options);
case "TaggedTemplateExpression":
return [print("tag"), print("typeParameters"), print("quasi")];
case "PrivateIdentifier":
return ["#", print("name")];
case "PrivateName":
return ["#", print("id")];
case "InterpreterDirective":
parts.push("#!", node.value, hardline);
if (isNextLineEmpty(node, options)) {
parts.push(hardline);
}
return parts;
case "TopicReference":
return "%";
case "ArgumentPlaceholder":
return "?";
case "ModuleExpression": {
parts.push("module {");
const printed = print("body");
if (printed) {
parts.push(indent([hardline, printed]), hardline);
}
parts.push("}");
return parts;
}
default:
throw new Error("unknown type: " + JSON.stringify(node.type));
}
}
function canAttachComment(node) {
return node.type && !isBlockComment(node) && !isLineComment(node) && node.type !== "EmptyStatement" && node.type !== "TemplateElement" && node.type !== "Import" && node.type !== "TSEmptyBodyFunctionExpression";
}
module22.exports = {
preprocess,
print: genericPrint,
embed,
insertPragma,
massageAstNode: clean,
hasPrettierIgnore(path) {
return hasIgnoreComment(path) || hasJsxIgnoreComment(path);
},
willPrintOwnComments: handleComments.willPrintOwnComments,
canAttachComment,
printComment,
isBlockComment,
handleComments: {
avoidAstMutation: true,
ownLine: handleComments.handleOwnLineComment,
endOfLine: handleComments.handleEndOfLineComment,
remaining: handleComments.handleRemainingComment
},
getCommentChildNodes: handleComments.getCommentChildNodes
};
}
});
var require_printer_estree_json = __commonJS22({
"src/language-js/printer-estree-json.js"(exports2, module22) {
"use strict";
var {
builders: {
hardline,
indent,
join
}
} = require_doc();
var preprocess = require_print_preprocess();
function genericPrint(path, options, print) {
const node = path.getValue();
switch (node.type) {
case "JsonRoot":
return [print("node"), hardline];
case "ArrayExpression": {
if (node.elements.length === 0) {
return "[]";
}
const printed = path.map(() => path.getValue() === null ? "null" : print(), "elements");
return ["[", indent([hardline, join([",", hardline], printed)]), hardline, "]"];
}
case "ObjectExpression":
return node.properties.length === 0 ? "{}" : ["{", indent([hardline, join([",", hardline], path.map(print, "properties"))]), hardline, "}"];
case "ObjectProperty":
return [print("key"), ": ", print("value")];
case "UnaryExpression":
return [node.operator === "+" ? "" : node.operator, print("argument")];
case "NullLiteral":
return "null";
case "BooleanLiteral":
return node.value ? "true" : "false";
case "StringLiteral":
return JSON.stringify(node.value);
case "NumericLiteral":
return isObjectKey(path) ? JSON.stringify(String(node.value)) : JSON.stringify(node.value);
case "Identifier":
return isObjectKey(path) ? JSON.stringify(node.name) : node.name;
case "TemplateLiteral":
return print(["quasis", 0]);
case "TemplateElement":
return JSON.stringify(node.value.cooked);
default:
throw new Error("unknown type: " + JSON.stringify(node.type));
}
}
function isObjectKey(path) {
return path.getName() === "key" && path.getParentNode().type === "ObjectProperty";
}
var ignoredProperties = /* @__PURE__ */ new Set(["start", "end", "extra", "loc", "comments", "leadingComments", "trailingComments", "innerComments", "errors", "range", "tokens"]);
function clean(node, newNode) {
const {
type
} = node;
if (type === "ObjectProperty") {
const {
key
} = node;
if (key.type === "Identifier") {
newNode.key = {
type: "StringLiteral",
value: key.name
};
} else if (key.type === "NumericLiteral") {
newNode.key = {
type: "StringLiteral",
value: String(key.value)
};
}
return;
}
if (type === "UnaryExpression" && node.operator === "+") {
return newNode.argument;
}
if (type === "ArrayExpression") {
for (const [index, element] of node.elements.entries()) {
if (element === null) {
newNode.elements.splice(index, 0, {
type: "NullLiteral"
});
}
}
return;
}
if (type === "TemplateLiteral") {
return {
type: "StringLiteral",
value: node.quasis[0].value.cooked
};
}
}
clean.ignoredProperties = ignoredProperties;
module22.exports = {
preprocess,
print: genericPrint,
massageAstNode: clean
};
}
});
var require_common_options = __commonJS22({
"src/common/common-options.js"(exports2, module22) {
"use strict";
var CATEGORY_COMMON = "Common";
module22.exports = {
bracketSpacing: {
since: "0.0.0",
category: CATEGORY_COMMON,
type: "boolean",
default: true,
description: "Print spaces between brackets.",
oppositeDescription: "Do not print spaces between brackets."
},
singleQuote: {
since: "0.0.0",
category: CATEGORY_COMMON,
type: "boolean",
default: false,
description: "Use single quotes instead of double quotes."
},
proseWrap: {
since: "1.8.2",
category: CATEGORY_COMMON,
type: "choice",
default: [{
since: "1.8.2",
value: true
}, {
since: "1.9.0",
value: "preserve"
}],
description: "How to wrap prose.",
choices: [{
since: "1.9.0",
value: "always",
description: "Wrap prose if it exceeds the print width."
}, {
since: "1.9.0",
value: "never",
description: "Do not wrap prose."
}, {
since: "1.9.0",
value: "preserve",
description: "Wrap prose as-is."
}]
},
bracketSameLine: {
since: "2.4.0",
category: CATEGORY_COMMON,
type: "boolean",
default: false,
description: "Put > of opening tags on the last line instead of on a new line."
},
singleAttributePerLine: {
since: "2.6.0",
category: CATEGORY_COMMON,
type: "boolean",
default: false,
description: "Enforce single attribute per line in HTML, Vue and JSX."
}
};
}
});
var require_options2 = __commonJS22({
"src/language-js/options.js"(exports2, module22) {
"use strict";
var commonOptions = require_common_options();
var CATEGORY_JAVASCRIPT = "JavaScript";
module22.exports = {
arrowParens: {
since: "1.9.0",
category: CATEGORY_JAVASCRIPT,
type: "choice",
default: [{
since: "1.9.0",
value: "avoid"
}, {
since: "2.0.0",
value: "always"
}],
description: "Include parentheses around a sole arrow function parameter.",
choices: [{
value: "always",
description: "Always include parens. Example: `(x) => x`"
}, {
value: "avoid",
description: "Omit parens when possible. Example: `x => x`"
}]
},
bracketSameLine: commonOptions.bracketSameLine,
bracketSpacing: commonOptions.bracketSpacing,
jsxBracketSameLine: {
since: "0.17.0",
category: CATEGORY_JAVASCRIPT,
type: "boolean",
description: "Put > on the last line instead of at a new line.",
deprecated: "2.4.0"
},
semi: {
since: "1.0.0",
category: CATEGORY_JAVASCRIPT,
type: "boolean",
default: true,
description: "Print semicolons.",
oppositeDescription: "Do not print semicolons, except at the beginning of lines which may need them."
},
singleQuote: commonOptions.singleQuote,
jsxSingleQuote: {
since: "1.15.0",
category: CATEGORY_JAVASCRIPT,
type: "boolean",
default: false,
description: "Use single quotes in JSX."
},
quoteProps: {
since: "1.17.0",
category: CATEGORY_JAVASCRIPT,
type: "choice",
default: "as-needed",
description: "Change when properties in objects are quoted.",
choices: [{
value: "as-needed",
description: "Only add quotes around object properties where required."
}, {
value: "consistent",
description: "If at least one property in an object requires quotes, quote all properties."
}, {
value: "preserve",
description: "Respect the input use of quotes in object properties."
}]
},
trailingComma: {
since: "0.0.0",
category: CATEGORY_JAVASCRIPT,
type: "choice",
default: [{
since: "0.0.0",
value: false
}, {
since: "0.19.0",
value: "none"
}, {
since: "2.0.0",
value: "es5"
}],
description: "Print trailing commas wherever possible when multi-line.",
choices: [{
value: "es5",
description: "Trailing commas where valid in ES5 (objects, arrays, etc.)"
}, {
value: "none",
description: "No trailing commas."
}, {
value: "all",
description: "Trailing commas wherever possible (including function arguments)."
}]
},
singleAttributePerLine: commonOptions.singleAttributePerLine
};
}
});
var require_parsers = __commonJS22({
"src/language-js/parse/parsers.js"(exports2, module22) {
"use strict";
module22.exports = {
get babel() {
return require_parser_babel().parsers.babel;
},
get "babel-flow"() {
return require_parser_babel().parsers["babel-flow"];
},
get "babel-ts"() {
return require_parser_babel().parsers["babel-ts"];
},
get json() {
return require_parser_babel().parsers.json;
},
get json5() {
return require_parser_babel().parsers.json5;
},
get "json-stringify"() {
return require_parser_babel().parsers["json-stringify"];
},
get __js_expression() {
return require_parser_babel().parsers.__js_expression;
},
get __vue_expression() {
return require_parser_babel().parsers.__vue_expression;
},
get __vue_ts_expression() {
return require_parser_babel().parsers.__vue_ts_expression;
},
get __vue_event_binding() {
return require_parser_babel().parsers.__vue_event_binding;
},
get __vue_ts_event_binding() {
return require_parser_babel().parsers.__vue_ts_event_binding;
},
get flow() {
return require_parser_flow().parsers.flow;
},
get typescript() {
return require_parser_typescript().parsers.typescript;
},
get __ng_action() {
return require_parser_angular().parsers.__ng_action;
},
get __ng_binding() {
return require_parser_angular().parsers.__ng_binding;
},
get __ng_interpolation() {
return require_parser_angular().parsers.__ng_interpolation;
},
get __ng_directive() {
return require_parser_angular().parsers.__ng_directive;
},
get acorn() {
return require_parser_espree().parsers.acorn;
},
get espree() {
return require_parser_espree().parsers.espree;
},
get meriyah() {
return require_parser_meriyah().parsers.meriyah;
},
get __babel_estree() {
return require_parser_babel().parsers.__babel_estree;
}
};
}
});
var require_JavaScript = __commonJS22({
"node_modules/linguist-languages/data/JavaScript.json"(exports2, module22) {
module22.exports = {
name: "JavaScript",
type: "programming",
tmScope: "source.js",
aceMode: "javascript",
codemirrorMode: "javascript",
codemirrorMimeType: "text/javascript",
color: "#f1e05a",
aliases: ["js", "node"],
extensions: [".js", "._js", ".bones", ".cjs", ".es", ".es6", ".frag", ".gs", ".jake", ".javascript", ".jsb", ".jscad", ".jsfl", ".jslib", ".jsm", ".jspre", ".jss", ".jsx", ".mjs", ".njs", ".pac", ".sjs", ".ssjs", ".xsjs", ".xsjslib"],
filenames: ["Jakefile"],
interpreters: ["chakra", "d8", "gjs", "js", "node", "nodejs", "qjs", "rhino", "v8", "v8-shell"],
languageId: 183
};
}
});
var require_TypeScript = __commonJS22({
"node_modules/linguist-languages/data/TypeScript.json"(exports2, module22) {
module22.exports = {
name: "TypeScript",
type: "programming",
color: "#3178c6",
aliases: ["ts"],
interpreters: ["deno", "ts-node"],
extensions: [".ts", ".cts", ".mts"],
tmScope: "source.ts",
aceMode: "typescript",
codemirrorMode: "javascript",
codemirrorMimeType: "application/typescript",
languageId: 378
};
}
});
var require_TSX = __commonJS22({
"node_modules/linguist-languages/data/TSX.json"(exports2, module22) {
module22.exports = {
name: "TSX",
type: "programming",
color: "#3178c6",
group: "TypeScript",
extensions: [".tsx"],
tmScope: "source.tsx",
aceMode: "javascript",
codemirrorMode: "jsx",
codemirrorMimeType: "text/jsx",
languageId: 94901924
};
}
});
var require_JSON = __commonJS22({
"node_modules/linguist-languages/data/JSON.json"(exports2, module22) {
module22.exports = {
name: "JSON",
type: "data",
color: "#292929",
tmScope: "source.json",
aceMode: "json",
codemirrorMode: "javascript",
codemirrorMimeType: "application/json",
aliases: ["geojson", "jsonl", "topojson"],
extensions: [".json", ".4DForm", ".4DProject", ".avsc", ".geojson", ".gltf", ".har", ".ice", ".JSON-tmLanguage", ".jsonl", ".mcmeta", ".tfstate", ".tfstate.backup", ".topojson", ".webapp", ".webmanifest", ".yy", ".yyp"],
filenames: [".arcconfig", ".auto-changelog", ".c8rc", ".htmlhintrc", ".imgbotconfig", ".nycrc", ".tern-config", ".tern-project", ".watchmanconfig", "Pipfile.lock", "composer.lock", "mcmod.info"],
languageId: 174
};
}
});
var require_JSON_with_Comments = __commonJS22({
"node_modules/linguist-languages/data/JSON with Comments.json"(exports2, module22) {
module22.exports = {
name: "JSON with Comments",
type: "data",
color: "#292929",
group: "JSON",
tmScope: "source.js",
aceMode: "javascript",
codemirrorMode: "javascript",
codemirrorMimeType: "text/javascript",
aliases: ["jsonc"],
extensions: [".jsonc", ".code-snippets", ".sublime-build", ".sublime-commands", ".sublime-completions", ".sublime-keymap", ".sublime-macro", ".sublime-menu", ".sublime-mousemap", ".sublime-project", ".sublime-settings", ".sublime-theme", ".sublime-workspace", ".sublime_metrics", ".sublime_session"],
filenames: [".babelrc", ".devcontainer.json", ".eslintrc.json", ".jscsrc", ".jshintrc", ".jslintrc", "api-extractor.json", "devcontainer.json", "jsconfig.json", "language-configuration.json", "tsconfig.json", "tslint.json"],
languageId: 423
};
}
});
var require_JSON5 = __commonJS22({
"node_modules/linguist-languages/data/JSON5.json"(exports2, module22) {
module22.exports = {
name: "JSON5",
type: "data",
color: "#267CB9",
extensions: [".json5"],
tmScope: "source.js",
aceMode: "javascript",
codemirrorMode: "javascript",
codemirrorMimeType: "application/json",
languageId: 175
};
}
});
var require_language_js = __commonJS22({
"src/language-js/index.js"(exports2, module22) {
"use strict";
var createLanguage = require_create_language();
var estreePrinter = require_printer_estree();
var estreeJsonPrinter = require_printer_estree_json();
var options = require_options2();
var parsers = require_parsers();
var languages = [createLanguage(require_JavaScript(), (data) => ({
since: "0.0.0",
parsers: ["babel", "acorn", "espree", "meriyah", "babel-flow", "babel-ts", "flow", "typescript"],
vscodeLanguageIds: ["javascript", "mongo"],
interpreters: [...data.interpreters, "zx"],
extensions: [...data.extensions.filter((extension) => extension !== ".jsx"), ".wxs"]
})), createLanguage(require_JavaScript(), () => ({
name: "Flow",
since: "0.0.0",
parsers: ["flow", "babel-flow"],
vscodeLanguageIds: ["javascript"],
aliases: [],
filenames: [],
extensions: [".js.flow"]
})), createLanguage(require_JavaScript(), () => ({
name: "JSX",
since: "0.0.0",
parsers: ["babel", "babel-flow", "babel-ts", "flow", "typescript", "espree", "meriyah"],
vscodeLanguageIds: ["javascriptreact"],
aliases: void 0,
filenames: void 0,
extensions: [".jsx"],
group: "JavaScript",
interpreters: void 0,
tmScope: "source.js.jsx",
aceMode: "javascript",
codemirrorMode: "jsx",
codemirrorMimeType: "text/jsx",
color: void 0
})), createLanguage(require_TypeScript(), () => ({
since: "1.4.0",
parsers: ["typescript", "babel-ts"],
vscodeLanguageIds: ["typescript"]
})), createLanguage(require_TSX(), () => ({
since: "1.4.0",
parsers: ["typescript", "babel-ts"],
vscodeLanguageIds: ["typescriptreact"]
})), createLanguage(require_JSON(), () => ({
name: "JSON.stringify",
since: "1.13.0",
parsers: ["json-stringify"],
vscodeLanguageIds: ["json"],
extensions: [".importmap"],
filenames: ["package.json", "package-lock.json", "composer.json"]
})), createLanguage(require_JSON(), (data) => ({
since: "1.5.0",
parsers: ["json"],
vscodeLanguageIds: ["json"],
extensions: data.extensions.filter((extension) => extension !== ".jsonl")
})), createLanguage(require_JSON_with_Comments(), (data) => ({
since: "1.5.0",
parsers: ["json"],
vscodeLanguageIds: ["jsonc"],
filenames: [...data.filenames, ".eslintrc", ".swcrc"]
})), createLanguage(require_JSON5(), () => ({
since: "1.13.0",
parsers: ["json5"],
vscodeLanguageIds: ["json5"]
}))];
var printers = {
estree: estreePrinter,
"estree-json": estreeJsonPrinter
};
module22.exports = {
languages,
options,
printers,
parsers
};
}
});
var require_clean2 = __commonJS22({
"src/language-css/clean.js"(exports2, module22) {
"use strict";
var {
isFrontMatterNode
} = require_util();
var getLast = require_get_last();
var ignoredProperties = /* @__PURE__ */ new Set(["raw", "raws", "sourceIndex", "source", "before", "after", "trailingComma"]);
function clean(ast, newObj, parent) {
if (isFrontMatterNode(ast) && ast.lang === "yaml") {
delete newObj.value;
}
if (ast.type === "css-comment" && parent.type === "css-root" && parent.nodes.length > 0) {
if (parent.nodes[0] === ast || isFrontMatterNode(parent.nodes[0]) && parent.nodes[1] === ast) {
delete newObj.text;
if (/^\*\s*@(?:format|prettier)\s*$/.test(ast.text)) {
return null;
}
}
if (parent.type === "css-root" && getLast(parent.nodes) === ast) {
return null;
}
}
if (ast.type === "value-root") {
delete newObj.text;
}
if (ast.type === "media-query" || ast.type === "media-query-list" || ast.type === "media-feature-expression") {
delete newObj.value;
}
if (ast.type === "css-rule") {
delete newObj.params;
}
if (ast.type === "selector-combinator") {
newObj.value = newObj.value.replace(/\s+/g, " ");
}
if (ast.type === "media-feature") {
newObj.value = newObj.value.replace(/ /g, "");
}
if (ast.type === "value-word" && (ast.isColor && ast.isHex || ["initial", "inherit", "unset", "revert"].includes(newObj.value.replace().toLowerCase())) || ast.type === "media-feature" || ast.type === "selector-root-invalid" || ast.type === "selector-pseudo") {
newObj.value = newObj.value.toLowerCase();
}
if (ast.type === "css-decl") {
newObj.prop = newObj.prop.toLowerCase();
}
if (ast.type === "css-atrule" || ast.type === "css-import") {
newObj.name = newObj.name.toLowerCase();
}
if (ast.type === "value-number") {
newObj.unit = newObj.unit.toLowerCase();
}
if ((ast.type === "media-feature" || ast.type === "media-keyword" || ast.type === "media-type" || ast.type === "media-unknown" || ast.type === "media-url" || ast.type === "media-value" || ast.type === "selector-attribute" || ast.type === "selector-string" || ast.type === "selector-class" || ast.type === "selector-combinator" || ast.type === "value-string") && newObj.value) {
newObj.value = cleanCSSStrings(newObj.value);
}
if (ast.type === "selector-attribute") {
newObj.attribute = newObj.attribute.trim();
if (newObj.namespace) {
if (typeof newObj.namespace === "string") {
newObj.namespace = newObj.namespace.trim();
if (newObj.namespace.length === 0) {
newObj.namespace = true;
}
}
}
if (newObj.value) {
newObj.value = newObj.value.trim().replace(/^["']|["']$/g, "");
delete newObj.quoted;
}
}
if ((ast.type === "media-value" || ast.type === "media-type" || ast.type === "value-number" || ast.type === "selector-root-invalid" || ast.type === "selector-class" || ast.type === "selector-combinator" || ast.type === "selector-tag") && newObj.value) {
newObj.value = newObj.value.replace(/([\d+.Ee-]+)([A-Za-z]*)/g, (match, numStr, unit) => {
const num = Number(numStr);
return Number.isNaN(num) ? match : num + unit.toLowerCase();
});
}
if (ast.type === "selector-tag") {
const lowercasedValue = ast.value.toLowerCase();
if (["from", "to"].includes(lowercasedValue)) {
newObj.value = lowercasedValue;
}
}
if (ast.type === "css-atrule" && ast.name.toLowerCase() === "supports") {
delete newObj.value;
}
if (ast.type === "selector-unknown") {
delete newObj.value;
}
if (ast.type === "value-comma_group") {
const index = ast.groups.findIndex((node) => node.type === "value-number" && node.unit === "...");
if (index !== -1) {
newObj.groups[index].unit = "";
newObj.groups.splice(index + 1, 0, {
type: "value-word",
value: "...",
isColor: false,
isHex: false
});
}
}
if (ast.type === "value-comma_group" && ast.groups.some((node) => node.type === "value-atword" && node.value.endsWith("[") || node.type === "value-word" && node.value.startsWith("]"))) {
return {
type: "value-atword",
value: ast.groups.map((node) => node.value).join(""),
group: {
open: null,
close: null,
groups: [],
type: "value-paren_group"
}
};
}
}
clean.ignoredProperties = ignoredProperties;
function cleanCSSStrings(value) {
return value.replace(/'/g, '"').replace(/\\([^\dA-Fa-f])/g, "$1");
}
module22.exports = clean;
}
});
var require_print = __commonJS22({
"src/utils/front-matter/print.js"(exports2, module22) {
"use strict";
var {
builders: {
hardline,
markAsRoot
}
} = require_doc();
function print(node, textToDoc) {
if (node.lang === "yaml") {
const value = node.value.trim();
const doc2 = value ? textToDoc(value, {
parser: "yaml"
}, {
stripTrailingHardline: true
}) : "";
return markAsRoot([node.startDelimiter, hardline, doc2, doc2 ? hardline : "", node.endDelimiter]);
}
}
module22.exports = print;
}
});
var require_embed2 = __commonJS22({
"src/language-css/embed.js"(exports2, module22) {
"use strict";
var {
builders: {
hardline
}
} = require_doc();
var printFrontMatter = require_print();
function embed(path, print, textToDoc) {
const node = path.getValue();
if (node.type === "front-matter") {
const doc2 = printFrontMatter(node, textToDoc);
return doc2 ? [doc2, hardline] : "";
}
}
module22.exports = embed;
}
});
var require_parse42 = __commonJS22({
"src/utils/front-matter/parse.js"(exports2, module22) {
"use strict";
var frontMatterRegex = new RegExp("^(?-{3}|\\+{3})(?[^\\n]*)\\n(?:|(?.*?)\\n)(?\\k|\\.{3})[^\\S\\n]*(?:\\n|$)", "s");
function parse2(text) {
const match = text.match(frontMatterRegex);
if (!match) {
return {
content: text
};
}
const {
startDelimiter,
language,
value = "",
endDelimiter
} = match.groups;
let lang = language.trim() || "yaml";
if (startDelimiter === "+++") {
lang = "toml";
}
if (lang !== "yaml" && startDelimiter !== endDelimiter) {
return {
content: text
};
}
const [raw] = match;
const frontMatter = {
type: "front-matter",
lang,
value,
startDelimiter,
endDelimiter,
raw: raw.replace(/\n$/, "")
};
return {
frontMatter,
content: raw.replace(/[^\n]/g, " ") + text.slice(raw.length)
};
}
module22.exports = parse2;
}
});
var require_pragma2 = __commonJS22({
"src/language-css/pragma.js"(exports2, module22) {
"use strict";
var jsPragma = require_pragma();
var parseFrontMatter = require_parse42();
function hasPragma(text) {
return jsPragma.hasPragma(parseFrontMatter(text).content);
}
function insertPragma(text) {
const {
frontMatter,
content
} = parseFrontMatter(text);
return (frontMatter ? frontMatter.raw + "\n\n" : "") + jsPragma.insertPragma(content);
}
module22.exports = {
hasPragma,
insertPragma
};
}
});
var require_utils8 = __commonJS22({
"src/language-css/utils/index.js"(exports2, module22) {
"use strict";
var colorAdjusterFunctions = /* @__PURE__ */ new Set(["red", "green", "blue", "alpha", "a", "rgb", "hue", "h", "saturation", "s", "lightness", "l", "whiteness", "w", "blackness", "b", "tint", "shade", "blend", "blenda", "contrast", "hsl", "hsla", "hwb", "hwba"]);
function getAncestorCounter(path, typeOrTypes) {
const types = Array.isArray(typeOrTypes) ? typeOrTypes : [typeOrTypes];
let counter = -1;
let ancestorNode;
while (ancestorNode = path.getParentNode(++counter)) {
if (types.includes(ancestorNode.type)) {
return counter;
}
}
return -1;
}
function getAncestorNode(path, typeOrTypes) {
const counter = getAncestorCounter(path, typeOrTypes);
return counter === -1 ? null : path.getParentNode(counter);
}
function getPropOfDeclNode(path) {
var _declAncestorNode$pro;
const declAncestorNode = getAncestorNode(path, "css-decl");
return declAncestorNode === null || declAncestorNode === void 0 ? void 0 : (_declAncestorNode$pro = declAncestorNode.prop) === null || _declAncestorNode$pro === void 0 ? void 0 : _declAncestorNode$pro.toLowerCase();
}
var wideKeywords = /* @__PURE__ */ new Set(["initial", "inherit", "unset", "revert"]);
function isWideKeywords(value) {
return wideKeywords.has(value.toLowerCase());
}
function isKeyframeAtRuleKeywords(path, value) {
const atRuleAncestorNode = getAncestorNode(path, "css-atrule");
return (atRuleAncestorNode === null || atRuleAncestorNode === void 0 ? void 0 : atRuleAncestorNode.name) && atRuleAncestorNode.name.toLowerCase().endsWith("keyframes") && ["from", "to"].includes(value.toLowerCase());
}
function maybeToLowerCase(value) {
return value.includes("$") || value.includes("@") || value.includes("#") || value.startsWith("%") || value.startsWith("--") || value.startsWith(":--") || value.includes("(") && value.includes(")") ? value : value.toLowerCase();
}
function insideValueFunctionNode(path, functionName) {
var _funcAncestorNode$val;
const funcAncestorNode = getAncestorNode(path, "value-func");
return (funcAncestorNode === null || funcAncestorNode === void 0 ? void 0 : (_funcAncestorNode$val = funcAncestorNode.value) === null || _funcAncestorNode$val === void 0 ? void 0 : _funcAncestorNode$val.toLowerCase()) === functionName;
}
function insideICSSRuleNode(path) {
var _ruleAncestorNode$raw;
const ruleAncestorNode = getAncestorNode(path, "css-rule");
const selector = ruleAncestorNode === null || ruleAncestorNode === void 0 ? void 0 : (_ruleAncestorNode$raw = ruleAncestorNode.raws) === null || _ruleAncestorNode$raw === void 0 ? void 0 : _ruleAncestorNode$raw.selector;
return selector && (selector.startsWith(":import") || selector.startsWith(":export"));
}
function insideAtRuleNode(path, atRuleNameOrAtRuleNames) {
const atRuleNames = Array.isArray(atRuleNameOrAtRuleNames) ? atRuleNameOrAtRuleNames : [atRuleNameOrAtRuleNames];
const atRuleAncestorNode = getAncestorNode(path, "css-atrule");
return atRuleAncestorNode && atRuleNames.includes(atRuleAncestorNode.name.toLowerCase());
}
function insideURLFunctionInImportAtRuleNode(path) {
const node = path.getValue();
const atRuleAncestorNode = getAncestorNode(path, "css-atrule");
return (atRuleAncestorNode === null || atRuleAncestorNode === void 0 ? void 0 : atRuleAncestorNode.name) === "import" && node.groups[0].value === "url" && node.groups.length === 2;
}
function isURLFunctionNode(node) {
return node.type === "value-func" && node.value.toLowerCase() === "url";
}
function isLastNode(path, node) {
var _path$getParentNode;
const nodes = (_path$getParentNode = path.getParentNode()) === null || _path$getParentNode === void 0 ? void 0 : _path$getParentNode.nodes;
return nodes && nodes.indexOf(node) === nodes.length - 1;
}
function isDetachedRulesetDeclarationNode(node) {
const {
selector
} = node;
if (!selector) {
return false;
}
return typeof selector === "string" && /^@.+:.*$/.test(selector) || selector.value && /^@.+:.*$/.test(selector.value);
}
function isForKeywordNode(node) {
return node.type === "value-word" && ["from", "through", "end"].includes(node.value);
}
function isIfElseKeywordNode(node) {
return node.type === "value-word" && ["and", "or", "not"].includes(node.value);
}
function isEachKeywordNode(node) {
return node.type === "value-word" && node.value === "in";
}
function isMultiplicationNode(node) {
return node.type === "value-operator" && node.value === "*";
}
function isDivisionNode(node) {
return node.type === "value-operator" && node.value === "/";
}
function isAdditionNode(node) {
return node.type === "value-operator" && node.value === "+";
}
function isSubtractionNode(node) {
return node.type === "value-operator" && node.value === "-";
}
function isModuloNode(node) {
return node.type === "value-operator" && node.value === "%";
}
function isMathOperatorNode(node) {
return isMultiplicationNode(node) || isDivisionNode(node) || isAdditionNode(node) || isSubtractionNode(node) || isModuloNode(node);
}
function isEqualityOperatorNode(node) {
return node.type === "value-word" && ["==", "!="].includes(node.value);
}
function isRelationalOperatorNode(node) {
return node.type === "value-word" && ["<", ">", "<=", ">="].includes(node.value);
}
function isSCSSControlDirectiveNode(node) {
return node.type === "css-atrule" && ["if", "else", "for", "each", "while"].includes(node.name);
}
function isDetachedRulesetCallNode(node) {
var _node$raws;
return ((_node$raws = node.raws) === null || _node$raws === void 0 ? void 0 : _node$raws.params) && /^\(\s*\)$/.test(node.raws.params);
}
function isTemplatePlaceholderNode(node) {
return node.name.startsWith("prettier-placeholder");
}
function isTemplatePropNode(node) {
return node.prop.startsWith("@prettier-placeholder");
}
function isPostcssSimpleVarNode(currentNode, nextNode) {
return currentNode.value === "$$" && currentNode.type === "value-func" && (nextNode === null || nextNode === void 0 ? void 0 : nextNode.type) === "value-word" && !nextNode.raws.before;
}
function hasComposesNode(node) {
var _node$value, _node$value$group;
return ((_node$value = node.value) === null || _node$value === void 0 ? void 0 : _node$value.type) === "value-root" && ((_node$value$group = node.value.group) === null || _node$value$group === void 0 ? void 0 : _node$value$group.type) === "value-value" && node.prop.toLowerCase() === "composes";
}
function hasParensAroundNode(node) {
var _node$value2, _node$value2$group, _node$value2$group$gr;
return ((_node$value2 = node.value) === null || _node$value2 === void 0 ? void 0 : (_node$value2$group = _node$value2.group) === null || _node$value2$group === void 0 ? void 0 : (_node$value2$group$gr = _node$value2$group.group) === null || _node$value2$group$gr === void 0 ? void 0 : _node$value2$group$gr.type) === "value-paren_group" && node.value.group.group.open !== null && node.value.group.group.close !== null;
}
function hasEmptyRawBefore(node) {
var _node$raws2;
return ((_node$raws2 = node.raws) === null || _node$raws2 === void 0 ? void 0 : _node$raws2.before) === "";
}
function isKeyValuePairNode(node) {
var _node$groups, _node$groups$;
return node.type === "value-comma_group" && ((_node$groups = node.groups) === null || _node$groups === void 0 ? void 0 : (_node$groups$ = _node$groups[1]) === null || _node$groups$ === void 0 ? void 0 : _node$groups$.type) === "value-colon";
}
function isKeyValuePairInParenGroupNode(node) {
var _node$groups2;
return node.type === "value-paren_group" && ((_node$groups2 = node.groups) === null || _node$groups2 === void 0 ? void 0 : _node$groups2[0]) && isKeyValuePairNode(node.groups[0]);
}
function isSCSSMapItemNode(path) {
var _declNode$prop;
const node = path.getValue();
if (node.groups.length === 0) {
return false;
}
const parentParentNode = path.getParentNode(1);
if (!isKeyValuePairInParenGroupNode(node) && !(parentParentNode && isKeyValuePairInParenGroupNode(parentParentNode))) {
return false;
}
const declNode = getAncestorNode(path, "css-decl");
if (declNode !== null && declNode !== void 0 && (_declNode$prop = declNode.prop) !== null && _declNode$prop !== void 0 && _declNode$prop.startsWith("$")) {
return true;
}
if (isKeyValuePairInParenGroupNode(parentParentNode)) {
return true;
}
if (parentParentNode.type === "value-func") {
return true;
}
return false;
}
function isInlineValueCommentNode(node) {
return node.type === "value-comment" && node.inline;
}
function isHashNode(node) {
return node.type === "value-word" && node.value === "#";
}
function isLeftCurlyBraceNode(node) {
return node.type === "value-word" && node.value === "{";
}
function isRightCurlyBraceNode(node) {
return node.type === "value-word" && node.value === "}";
}
function isWordNode(node) {
return ["value-word", "value-atword"].includes(node.type);
}
function isColonNode(node) {
return (node === null || node === void 0 ? void 0 : node.type) === "value-colon";
}
function isKeyInValuePairNode(node, parentNode) {
if (!isKeyValuePairNode(parentNode)) {
return false;
}
const {
groups
} = parentNode;
const index = groups.indexOf(node);
if (index === -1) {
return false;
}
return isColonNode(groups[index + 1]);
}
function isMediaAndSupportsKeywords(node) {
return node.value && ["not", "and", "or"].includes(node.value.toLowerCase());
}
function isColorAdjusterFuncNode(node) {
if (node.type !== "value-func") {
return false;
}
return colorAdjusterFunctions.has(node.value.toLowerCase());
}
function lastLineHasInlineComment(text) {
return /\/\//.test(text.split(/[\n\r]/).pop());
}
function isAtWordPlaceholderNode(node) {
return (node === null || node === void 0 ? void 0 : node.type) === "value-atword" && node.value.startsWith("prettier-placeholder-");
}
function isConfigurationNode(node, parentNode) {
var _node$open, _node$close;
if (((_node$open = node.open) === null || _node$open === void 0 ? void 0 : _node$open.value) !== "(" || ((_node$close = node.close) === null || _node$close === void 0 ? void 0 : _node$close.value) !== ")" || node.groups.some((group) => group.type !== "value-comma_group")) {
return false;
}
if (parentNode.type === "value-comma_group") {
const prevIdx = parentNode.groups.indexOf(node) - 1;
const maybeWithNode = parentNode.groups[prevIdx];
if ((maybeWithNode === null || maybeWithNode === void 0 ? void 0 : maybeWithNode.type) === "value-word" && maybeWithNode.value === "with") {
return true;
}
}
return false;
}
function isParenGroupNode(node) {
var _node$open2, _node$close2;
return node.type === "value-paren_group" && ((_node$open2 = node.open) === null || _node$open2 === void 0 ? void 0 : _node$open2.value) === "(" && ((_node$close2 = node.close) === null || _node$close2 === void 0 ? void 0 : _node$close2.value) === ")";
}
module22.exports = {
getAncestorCounter,
getAncestorNode,
getPropOfDeclNode,
maybeToLowerCase,
insideValueFunctionNode,
insideICSSRuleNode,
insideAtRuleNode,
insideURLFunctionInImportAtRuleNode,
isKeyframeAtRuleKeywords,
isWideKeywords,
isLastNode,
isSCSSControlDirectiveNode,
isDetachedRulesetDeclarationNode,
isRelationalOperatorNode,
isEqualityOperatorNode,
isMultiplicationNode,
isDivisionNode,
isAdditionNode,
isSubtractionNode,
isModuloNode,
isMathOperatorNode,
isEachKeywordNode,
isForKeywordNode,
isURLFunctionNode,
isIfElseKeywordNode,
hasComposesNode,
hasParensAroundNode,
hasEmptyRawBefore,
isDetachedRulesetCallNode,
isTemplatePlaceholderNode,
isTemplatePropNode,
isPostcssSimpleVarNode,
isKeyValuePairNode,
isKeyValuePairInParenGroupNode,
isKeyInValuePairNode,
isSCSSMapItemNode,
isInlineValueCommentNode,
isHashNode,
isLeftCurlyBraceNode,
isRightCurlyBraceNode,
isWordNode,
isColonNode,
isMediaAndSupportsKeywords,
isColorAdjusterFuncNode,
lastLineHasInlineComment,
isAtWordPlaceholderNode,
isConfigurationNode,
isParenGroupNode
};
}
});
var require_line_column_to_index = __commonJS22({
"src/utils/line-column-to-index.js"(exports2, module22) {
"use strict";
module22.exports = function(lineColumn, text) {
let index = 0;
for (let i = 0; i < lineColumn.line - 1; ++i) {
index = text.indexOf("\n", index) + 1;
}
return index + lineColumn.column;
};
}
});
var require_loc2 = __commonJS22({
"src/language-css/loc.js"(exports2, module22) {
"use strict";
var {
skipEverythingButNewLine
} = require_skip();
var getLast = require_get_last();
var lineColumnToIndex = require_line_column_to_index();
function calculateLocStart(node, text) {
if (typeof node.sourceIndex === "number") {
return node.sourceIndex;
}
return node.source ? lineColumnToIndex(node.source.start, text) - 1 : null;
}
function calculateLocEnd(node, text) {
if (node.type === "css-comment" && node.inline) {
return skipEverythingButNewLine(text, node.source.startOffset);
}
const endNode = node.nodes && getLast(node.nodes);
if (endNode && node.source && !node.source.end) {
node = endNode;
}
if (node.source && node.source.end) {
return lineColumnToIndex(node.source.end, text);
}
return null;
}
function calculateLoc(node, text) {
if (node.source) {
node.source.startOffset = calculateLocStart(node, text);
node.source.endOffset = calculateLocEnd(node, text);
}
for (const key in node) {
const child = node[key];
if (key === "source" || !child || typeof child !== "object") {
continue;
}
if (child.type === "value-root" || child.type === "value-unknown") {
calculateValueNodeLoc(child, getValueRootOffset(node), child.text || child.value);
} else {
calculateLoc(child, text);
}
}
}
function calculateValueNodeLoc(node, rootOffset, text) {
if (node.source) {
node.source.startOffset = calculateLocStart(node, text) + rootOffset;
node.source.endOffset = calculateLocEnd(node, text) + rootOffset;
}
for (const key in node) {
const child = node[key];
if (key === "source" || !child || typeof child !== "object") {
continue;
}
calculateValueNodeLoc(child, rootOffset, text);
}
}
function getValueRootOffset(node) {
let result = node.source.startOffset;
if (typeof node.prop === "string") {
result += node.prop.length;
}
if (node.type === "css-atrule" && typeof node.name === "string") {
result += 1 + node.name.length + node.raws.afterName.match(/^\s*:?\s*/)[0].length;
}
if (node.type !== "css-atrule" && node.raws && typeof node.raws.between === "string") {
result += node.raws.between.length;
}
return result;
}
function replaceQuotesInInlineComments(text) {
let state = "initial";
let stateToReturnFromQuotes = "initial";
let inlineCommentStartIndex;
let inlineCommentContainsQuotes = false;
const inlineCommentsToReplace = [];
for (let i = 0; i < text.length; i++) {
const c = text[i];
switch (state) {
case "initial":
if (c === "'") {
state = "single-quotes";
continue;
}
if (c === '"') {
state = "double-quotes";
continue;
}
if ((c === "u" || c === "U") && text.slice(i, i + 4).toLowerCase() === "url(") {
state = "url";
i += 3;
continue;
}
if (c === "*" && text[i - 1] === "/") {
state = "comment-block";
continue;
}
if (c === "/" && text[i - 1] === "/") {
state = "comment-inline";
inlineCommentStartIndex = i - 1;
continue;
}
continue;
case "single-quotes":
if (c === "'" && text[i - 1] !== "\\") {
state = stateToReturnFromQuotes;
stateToReturnFromQuotes = "initial";
}
if (c === "\n" || c === "\r") {
return text;
}
continue;
case "double-quotes":
if (c === '"' && text[i - 1] !== "\\") {
state = stateToReturnFromQuotes;
stateToReturnFromQuotes = "initial";
}
if (c === "\n" || c === "\r") {
return text;
}
continue;
case "url":
if (c === ")") {
state = "initial";
}
if (c === "\n" || c === "\r") {
return text;
}
if (c === "'") {
state = "single-quotes";
stateToReturnFromQuotes = "url";
continue;
}
if (c === '"') {
state = "double-quotes";
stateToReturnFromQuotes = "url";
continue;
}
continue;
case "comment-block":
if (c === "/" && text[i - 1] === "*") {
state = "initial";
}
continue;
case "comment-inline":
if (c === '"' || c === "'" || c === "*") {
inlineCommentContainsQuotes = true;
}
if (c === "\n" || c === "\r") {
if (inlineCommentContainsQuotes) {
inlineCommentsToReplace.push([inlineCommentStartIndex, i]);
}
state = "initial";
inlineCommentContainsQuotes = false;
}
continue;
}
}
for (const [start, end] of inlineCommentsToReplace) {
text = text.slice(0, start) + text.slice(start, end).replace(/["'*]/g, " ") + text.slice(end);
}
return text;
}
function locStart(node) {
return node.source.startOffset;
}
function locEnd(node) {
return node.source.endOffset;
}
module22.exports = {
locStart,
locEnd,
calculateLoc,
replaceQuotesInInlineComments
};
}
});
var require_is_less_parser = __commonJS22({
"src/language-css/utils/is-less-parser.js"(exports2, module22) {
"use strict";
function isLessParser(options) {
return options.parser === "css" || options.parser === "less";
}
module22.exports = isLessParser;
}
});
var require_is_scss = __commonJS22({
"src/language-css/utils/is-scss.js"(exports2, module22) {
"use strict";
function isSCSS(parser, text) {
const hasExplicitParserChoice = parser === "less" || parser === "scss";
const IS_POSSIBLY_SCSS = /(?:\w\s*:\s*[^:}]+|#){|@import[^\n]+(?:url|,)/;
return hasExplicitParserChoice ? parser === "scss" : IS_POSSIBLY_SCSS.test(text);
}
module22.exports = isSCSS;
}
});
var require_css_units_evaluate = __commonJS22({
"src/language-css/utils/css-units.evaluate.js"(exports2, module22) {
module22.exports = {
em: "em",
rem: "rem",
ex: "ex",
rex: "rex",
cap: "cap",
rcap: "rcap",
ch: "ch",
rch: "rch",
ic: "ic",
ric: "ric",
lh: "lh",
rlh: "rlh",
vw: "vw",
svw: "svw",
lvw: "lvw",
dvw: "dvw",
vh: "vh",
svh: "svh",
lvh: "lvh",
dvh: "dvh",
vi: "vi",
svi: "svi",
lvi: "lvi",
dvi: "dvi",
vb: "vb",
svb: "svb",
lvb: "lvb",
dvb: "dvb",
vmin: "vmin",
svmin: "svmin",
lvmin: "lvmin",
dvmin: "dvmin",
vmax: "vmax",
svmax: "svmax",
lvmax: "lvmax",
dvmax: "dvmax",
cm: "cm",
mm: "mm",
q: "Q",
in: "in",
pt: "pt",
pc: "pc",
px: "px",
deg: "deg",
grad: "grad",
rad: "rad",
turn: "turn",
s: "s",
ms: "ms",
hz: "Hz",
khz: "kHz",
dpi: "dpi",
dpcm: "dpcm",
dppx: "dppx",
x: "x"
};
}
});
var require_print_unit = __commonJS22({
"src/language-css/utils/print-unit.js"(exports2, module22) {
"use strict";
var CSS_UNITS = require_css_units_evaluate();
function printUnit(unit) {
const lowercased = unit.toLowerCase();
return Object.prototype.hasOwnProperty.call(CSS_UNITS, lowercased) ? CSS_UNITS[lowercased] : unit;
}
module22.exports = printUnit;
}
});
var require_printer_postcss = __commonJS22({
"src/language-css/printer-postcss.js"(exports2, module22) {
"use strict";
var getLast = require_get_last();
var {
printNumber,
printString,
hasNewline,
isFrontMatterNode,
isNextLineEmpty,
isNonEmptyArray
} = require_util();
var {
builders: {
join,
line,
hardline,
softline,
group,
fill,
indent,
dedent,
ifBreak,
breakParent
},
utils: {
removeLines,
getDocParts
}
} = require_doc();
var clean = require_clean2();
var embed = require_embed2();
var {
insertPragma
} = require_pragma2();
var {
getAncestorNode,
getPropOfDeclNode,
maybeToLowerCase,
insideValueFunctionNode,
insideICSSRuleNode,
insideAtRuleNode,
insideURLFunctionInImportAtRuleNode,
isKeyframeAtRuleKeywords,
isWideKeywords,
isLastNode,
isSCSSControlDirectiveNode,
isDetachedRulesetDeclarationNode,
isRelationalOperatorNode,
isEqualityOperatorNode,
isMultiplicationNode,
isDivisionNode,
isAdditionNode,
isSubtractionNode,
isMathOperatorNode,
isEachKeywordNode,
isForKeywordNode,
isURLFunctionNode,
isIfElseKeywordNode,
hasComposesNode,
hasParensAroundNode,
hasEmptyRawBefore,
isKeyValuePairNode,
isKeyInValuePairNode,
isDetachedRulesetCallNode,
isTemplatePlaceholderNode,
isTemplatePropNode,
isPostcssSimpleVarNode,
isSCSSMapItemNode,
isInlineValueCommentNode,
isHashNode,
isLeftCurlyBraceNode,
isRightCurlyBraceNode,
isWordNode,
isColonNode,
isMediaAndSupportsKeywords,
isColorAdjusterFuncNode,
lastLineHasInlineComment,
isAtWordPlaceholderNode,
isConfigurationNode,
isParenGroupNode
} = require_utils8();
var {
locStart,
locEnd
} = require_loc2();
var isLessParser = require_is_less_parser();
var isSCSS = require_is_scss();
var printUnit = require_print_unit();
function shouldPrintComma(options) {
return options.trailingComma === "es5" || options.trailingComma === "all";
}
function genericPrint(path, options, print) {
const node = path.getValue();
if (!node) {
return "";
}
if (typeof node === "string") {
return node;
}
switch (node.type) {
case "front-matter":
return [node.raw, hardline];
case "css-root": {
const nodes = printNodeSequence(path, options, print);
let after = node.raws.after.trim();
if (after.startsWith(";")) {
after = after.slice(1).trim();
}
return [nodes, after ? ` ${after}` : "", getDocParts(nodes).length > 0 ? hardline : ""];
}
case "css-comment": {
const isInlineComment = node.inline || node.raws.inline;
const text = options.originalText.slice(locStart(node), locEnd(node));
return isInlineComment ? text.trimEnd() : text;
}
case "css-rule": {
return [print("selector"), node.important ? " !important" : "", node.nodes ? [node.selector && node.selector.type === "selector-unknown" && lastLineHasInlineComment(node.selector.value) ? line : " ", "{", node.nodes.length > 0 ? indent([hardline, printNodeSequence(path, options, print)]) : "", hardline, "}", isDetachedRulesetDeclarationNode(node) ? ";" : ""] : ";"];
}
case "css-decl": {
const parentNode = path.getParentNode();
const {
between: rawBetween
} = node.raws;
const trimmedBetween = rawBetween.trim();
const isColon = trimmedBetween === ":";
let value = hasComposesNode(node) ? removeLines(print("value")) : print("value");
if (!isColon && lastLineHasInlineComment(trimmedBetween)) {
value = indent([hardline, dedent(value)]);
}
return [node.raws.before.replace(/[\s;]/g, ""), parentNode.type === "css-atrule" && parentNode.variable || insideICSSRuleNode(path) ? node.prop : maybeToLowerCase(node.prop), trimmedBetween.startsWith("//") ? " " : "", trimmedBetween, node.extend ? "" : " ", isLessParser(options) && node.extend && node.selector ? ["extend(", print("selector"), ")"] : "", value, node.raws.important ? node.raws.important.replace(/\s*!\s*important/i, " !important") : node.important ? " !important" : "", node.raws.scssDefault ? node.raws.scssDefault.replace(/\s*!default/i, " !default") : node.scssDefault ? " !default" : "", node.raws.scssGlobal ? node.raws.scssGlobal.replace(/\s*!global/i, " !global") : node.scssGlobal ? " !global" : "", node.nodes ? [" {", indent([softline, printNodeSequence(path, options, print)]), softline, "}"] : isTemplatePropNode(node) && !parentNode.raws.semicolon && options.originalText[locEnd(node) - 1] !== ";" ? "" : options.__isHTMLStyleAttribute && isLastNode(path, node) ? ifBreak(";") : ";"];
}
case "css-atrule": {
const parentNode = path.getParentNode();
const isTemplatePlaceholderNodeWithoutSemiColon = isTemplatePlaceholderNode(node) && !parentNode.raws.semicolon && options.originalText[locEnd(node) - 1] !== ";";
if (isLessParser(options)) {
if (node.mixin) {
return [print("selector"), node.important ? " !important" : "", isTemplatePlaceholderNodeWithoutSemiColon ? "" : ";"];
}
if (node.function) {
return [node.name, print("params"), isTemplatePlaceholderNodeWithoutSemiColon ? "" : ";"];
}
if (node.variable) {
return ["@", node.name, ": ", node.value ? print("value") : "", node.raws.between.trim() ? node.raws.between.trim() + " " : "", node.nodes ? ["{", indent([node.nodes.length > 0 ? softline : "", printNodeSequence(path, options, print)]), softline, "}"] : "", isTemplatePlaceholderNodeWithoutSemiColon ? "" : ";"];
}
}
return ["@", isDetachedRulesetCallNode(node) || node.name.endsWith(":") ? node.name : maybeToLowerCase(node.name), node.params ? [isDetachedRulesetCallNode(node) ? "" : isTemplatePlaceholderNode(node) ? node.raws.afterName === "" ? "" : node.name.endsWith(":") ? " " : /^\s*\n\s*\n/.test(node.raws.afterName) ? [hardline, hardline] : /^\s*\n/.test(node.raws.afterName) ? hardline : " " : " ", print("params")] : "", node.selector ? indent([" ", print("selector")]) : "", node.value ? group([" ", print("value"), isSCSSControlDirectiveNode(node) ? hasParensAroundNode(node) ? " " : line : ""]) : node.name === "else" ? " " : "", node.nodes ? [isSCSSControlDirectiveNode(node) ? "" : node.selector && !node.selector.nodes && typeof node.selector.value === "string" && lastLineHasInlineComment(node.selector.value) || !node.selector && typeof node.params === "string" && lastLineHasInlineComment(node.params) ? line : " ", "{", indent([node.nodes.length > 0 ? softline : "", printNodeSequence(path, options, print)]), softline, "}"] : isTemplatePlaceholderNodeWithoutSemiColon ? "" : ";"];
}
case "media-query-list": {
const parts = [];
path.each((childPath) => {
const node2 = childPath.getValue();
if (node2.type === "media-query" && node2.value === "") {
return;
}
parts.push(print());
}, "nodes");
return group(indent(join(line, parts)));
}
case "media-query": {
return [join(" ", path.map(print, "nodes")), isLastNode(path, node) ? "" : ","];
}
case "media-type": {
return adjustNumbers(adjustStrings(node.value, options));
}
case "media-feature-expression": {
if (!node.nodes) {
return node.value;
}
return ["(", ...path.map(print, "nodes"), ")"];
}
case "media-feature": {
return maybeToLowerCase(adjustStrings(node.value.replace(/ +/g, " "), options));
}
case "media-colon": {
return [node.value, " "];
}
case "media-value": {
return adjustNumbers(adjustStrings(node.value, options));
}
case "media-keyword": {
return adjustStrings(node.value, options);
}
case "media-url": {
return adjustStrings(node.value.replace(/^url\(\s+/gi, "url(").replace(/\s+\)$/g, ")"), options);
}
case "media-unknown": {
return node.value;
}
case "selector-root": {
return group([insideAtRuleNode(path, "custom-selector") ? [getAncestorNode(path, "css-atrule").customSelector, line] : "", join([",", insideAtRuleNode(path, ["extend", "custom-selector", "nest"]) ? line : hardline], path.map(print, "nodes"))]);
}
case "selector-selector": {
return group(indent(path.map(print, "nodes")));
}
case "selector-comment": {
return node.value;
}
case "selector-string": {
return adjustStrings(node.value, options);
}
case "selector-tag": {
const parentNode = path.getParentNode();
const index = parentNode && parentNode.nodes.indexOf(node);
const prevNode = index && parentNode.nodes[index - 1];
return [node.namespace ? [node.namespace === true ? "" : node.namespace.trim(), "|"] : "", prevNode.type === "selector-nesting" ? node.value : adjustNumbers(isKeyframeAtRuleKeywords(path, node.value) ? node.value.toLowerCase() : node.value)];
}
case "selector-id": {
return ["#", node.value];
}
case "selector-class": {
return [".", adjustNumbers(adjustStrings(node.value, options))];
}
case "selector-attribute": {
var _node$operator;
return ["[", node.namespace ? [node.namespace === true ? "" : node.namespace.trim(), "|"] : "", node.attribute.trim(), (_node$operator = node.operator) !== null && _node$operator !== void 0 ? _node$operator : "", node.value ? quoteAttributeValue(adjustStrings(node.value.trim(), options), options) : "", node.insensitive ? " i" : "", "]"];
}
case "selector-combinator": {
if (node.value === "+" || node.value === ">" || node.value === "~" || node.value === ">>>") {
const parentNode = path.getParentNode();
const leading2 = parentNode.type === "selector-selector" && parentNode.nodes[0] === node ? "" : line;
return [leading2, node.value, isLastNode(path, node) ? "" : " "];
}
const leading = node.value.trim().startsWith("(") ? line : "";
const value = adjustNumbers(adjustStrings(node.value.trim(), options)) || line;
return [leading, value];
}
case "selector-universal": {
return [node.namespace ? [node.namespace === true ? "" : node.namespace.trim(), "|"] : "", node.value];
}
case "selector-pseudo": {
return [maybeToLowerCase(node.value), isNonEmptyArray(node.nodes) ? group(["(", indent([softline, join([",", line], path.map(print, "nodes"))]), softline, ")"]) : ""];
}
case "selector-nesting": {
return node.value;
}
case "selector-unknown": {
const ruleAncestorNode = getAncestorNode(path, "css-rule");
if (ruleAncestorNode && ruleAncestorNode.isSCSSNesterProperty) {
return adjustNumbers(adjustStrings(maybeToLowerCase(node.value), options));
}
const parentNode = path.getParentNode();
if (parentNode.raws && parentNode.raws.selector) {
const start = locStart(parentNode);
const end = start + parentNode.raws.selector.length;
return options.originalText.slice(start, end).trim();
}
const grandParent = path.getParentNode(1);
if (parentNode.type === "value-paren_group" && grandParent && grandParent.type === "value-func" && grandParent.value === "selector") {
const start = locEnd(parentNode.open) + 1;
const end = locStart(parentNode.close);
const selector = options.originalText.slice(start, end).trim();
return lastLineHasInlineComment(selector) ? [breakParent, selector] : selector;
}
return node.value;
}
case "value-value":
case "value-root": {
return print("group");
}
case "value-comment": {
return options.originalText.slice(locStart(node), locEnd(node));
}
case "value-comma_group": {
const parentNode = path.getParentNode();
const parentParentNode = path.getParentNode(1);
const declAncestorProp = getPropOfDeclNode(path);
const isGridValue = declAncestorProp && parentNode.type === "value-value" && (declAncestorProp === "grid" || declAncestorProp.startsWith("grid-template"));
const atRuleAncestorNode = getAncestorNode(path, "css-atrule");
const isControlDirective = atRuleAncestorNode && isSCSSControlDirectiveNode(atRuleAncestorNode);
const hasInlineComment = node.groups.some((node2) => isInlineValueCommentNode(node2));
const printed = path.map(print, "groups");
const parts = [];
const insideURLFunction = insideValueFunctionNode(path, "url");
let insideSCSSInterpolationInString = false;
let didBreak = false;
for (let i = 0; i < node.groups.length; ++i) {
var _iNode$value;
parts.push(printed[i]);
const iPrevNode = node.groups[i - 1];
const iNode = node.groups[i];
const iNextNode = node.groups[i + 1];
const iNextNextNode = node.groups[i + 2];
if (insideURLFunction) {
if (iNextNode && isAdditionNode(iNextNode) || isAdditionNode(iNode)) {
parts.push(" ");
}
continue;
}
if (insideAtRuleNode(path, "forward") && iNode.type === "value-word" && iNode.value && iPrevNode !== void 0 && iPrevNode.type === "value-word" && iPrevNode.value === "as" && iNextNode.type === "value-operator" && iNextNode.value === "*") {
continue;
}
if (!iNextNode) {
continue;
}
if (iNode.type === "value-word" && iNode.value.endsWith("-") && isAtWordPlaceholderNode(iNextNode)) {
continue;
}
if (iNode.type === "value-string" && iNode.quoted) {
const positionOfOpeningInterpolation = iNode.value.lastIndexOf("#{");
const positionOfClosingInterpolation = iNode.value.lastIndexOf("}");
if (positionOfOpeningInterpolation !== -1 && positionOfClosingInterpolation !== -1) {
insideSCSSInterpolationInString = positionOfOpeningInterpolation > positionOfClosingInterpolation;
} else if (positionOfOpeningInterpolation !== -1) {
insideSCSSInterpolationInString = true;
} else if (positionOfClosingInterpolation !== -1) {
insideSCSSInterpolationInString = false;
}
}
if (insideSCSSInterpolationInString) {
continue;
}
if (isColonNode(iNode) || isColonNode(iNextNode)) {
continue;
}
if (iNode.type === "value-atword" && (iNode.value === "" || iNode.value.endsWith("["))) {
continue;
}
if (iNextNode.type === "value-word" && iNextNode.value.startsWith("]")) {
continue;
}
if (iNode.value === "~") {
continue;
}
if (iNode.value && iNode.value.includes("\\") && iNextNode && iNextNode.type !== "value-comment") {
continue;
}
if (iPrevNode && iPrevNode.value && iPrevNode.value.indexOf("\\") === iPrevNode.value.length - 1 && iNode.type === "value-operator" && iNode.value === "/") {
continue;
}
if (iNode.value === "\\") {
continue;
}
if (isPostcssSimpleVarNode(iNode, iNextNode)) {
continue;
}
if (isHashNode(iNode) || isLeftCurlyBraceNode(iNode) || isRightCurlyBraceNode(iNextNode) || isLeftCurlyBraceNode(iNextNode) && hasEmptyRawBefore(iNextNode) || isRightCurlyBraceNode(iNode) && hasEmptyRawBefore(iNextNode)) {
continue;
}
if (iNode.value === "--" && isHashNode(iNextNode)) {
continue;
}
const isMathOperator = isMathOperatorNode(iNode);
const isNextMathOperator = isMathOperatorNode(iNextNode);
if ((isMathOperator && isHashNode(iNextNode) || isNextMathOperator && isRightCurlyBraceNode(iNode)) && hasEmptyRawBefore(iNextNode)) {
continue;
}
if (!iPrevNode && isDivisionNode(iNode)) {
continue;
}
if (insideValueFunctionNode(path, "calc") && (isAdditionNode(iNode) || isAdditionNode(iNextNode) || isSubtractionNode(iNode) || isSubtractionNode(iNextNode)) && hasEmptyRawBefore(iNextNode)) {
continue;
}
const isColorAdjusterNode = (isAdditionNode(iNode) || isSubtractionNode(iNode)) && i === 0 && (iNextNode.type === "value-number" || iNextNode.isHex) && parentParentNode && isColorAdjusterFuncNode(parentParentNode) && !hasEmptyRawBefore(iNextNode);
const requireSpaceBeforeOperator = iNextNextNode && iNextNextNode.type === "value-func" || iNextNextNode && isWordNode(iNextNextNode) || iNode.type === "value-func" || isWordNode(iNode);
const requireSpaceAfterOperator = iNextNode.type === "value-func" || isWordNode(iNextNode) || iPrevNode && iPrevNode.type === "value-func" || iPrevNode && isWordNode(iPrevNode);
if (!(isMultiplicationNode(iNextNode) || isMultiplicationNode(iNode)) && !insideValueFunctionNode(path, "calc") && !isColorAdjusterNode && (isDivisionNode(iNextNode) && !requireSpaceBeforeOperator || isDivisionNode(iNode) && !requireSpaceAfterOperator || isAdditionNode(iNextNode) && !requireSpaceBeforeOperator || isAdditionNode(iNode) && !requireSpaceAfterOperator || isSubtractionNode(iNextNode) || isSubtractionNode(iNode)) && (hasEmptyRawBefore(iNextNode) || isMathOperator && (!iPrevNode || iPrevNode && isMathOperatorNode(iPrevNode)))) {
continue;
}
if ((options.parser === "scss" || options.parser === "less") && isMathOperator && iNode.value === "-" && isParenGroupNode(iNextNode) && locEnd(iNode) === locStart(iNextNode.open) && iNextNode.open.value === "(") {
continue;
}
if (isInlineValueCommentNode(iNode)) {
if (parentNode.type === "value-paren_group") {
parts.push(dedent(hardline));
continue;
}
parts.push(hardline);
continue;
}
if (isControlDirective && (isEqualityOperatorNode(iNextNode) || isRelationalOperatorNode(iNextNode) || isIfElseKeywordNode(iNextNode) || isEachKeywordNode(iNode) || isForKeywordNode(iNode))) {
parts.push(" ");
continue;
}
if (atRuleAncestorNode && atRuleAncestorNode.name.toLowerCase() === "namespace") {
parts.push(" ");
continue;
}
if (isGridValue) {
if (iNode.source && iNextNode.source && iNode.source.start.line !== iNextNode.source.start.line) {
parts.push(hardline);
didBreak = true;
} else {
parts.push(" ");
}
continue;
}
if (isNextMathOperator) {
parts.push(" ");
continue;
}
if (iNextNode && iNextNode.value === "...") {
continue;
}
if (isAtWordPlaceholderNode(iNode) && isAtWordPlaceholderNode(iNextNode) && locEnd(iNode) === locStart(iNextNode)) {
continue;
}
if (isAtWordPlaceholderNode(iNode) && isParenGroupNode(iNextNode) && locEnd(iNode) === locStart(iNextNode.open)) {
parts.push(softline);
continue;
}
if (iNode.value === "with" && isParenGroupNode(iNextNode)) {
parts.push(" ");
continue;
}
if ((_iNode$value = iNode.value) !== null && _iNode$value !== void 0 && _iNode$value.endsWith("#") && iNextNode.value === "{" && isParenGroupNode(iNextNode.group)) {
continue;
}
parts.push(line);
}
if (hasInlineComment) {
parts.push(breakParent);
}
if (didBreak) {
parts.unshift(hardline);
}
if (isControlDirective) {
return group(indent(parts));
}
if (insideURLFunctionInImportAtRuleNode(path)) {
return group(fill(parts));
}
return group(indent(fill(parts)));
}
case "value-paren_group": {
const parentNode = path.getParentNode();
if (parentNode && isURLFunctionNode(parentNode) && (node.groups.length === 1 || node.groups.length > 0 && node.groups[0].type === "value-comma_group" && node.groups[0].groups.length > 0 && node.groups[0].groups[0].type === "value-word" && node.groups[0].groups[0].value.startsWith("data:"))) {
return [node.open ? print("open") : "", join(",", path.map(print, "groups")), node.close ? print("close") : ""];
}
if (!node.open) {
const printed2 = path.map(print, "groups");
const res = [];
for (let i = 0; i < printed2.length; i++) {
if (i !== 0) {
res.push([",", line]);
}
res.push(printed2[i]);
}
return group(indent(fill(res)));
}
const isSCSSMapItem = isSCSSMapItemNode(path);
const lastItem = getLast(node.groups);
const isLastItemComment = lastItem && lastItem.type === "value-comment";
const isKey = isKeyInValuePairNode(node, parentNode);
const isConfiguration = isConfigurationNode(node, parentNode);
const shouldBreak = isConfiguration || isSCSSMapItem && !isKey;
const shouldDedent = isConfiguration || isKey;
const printed = group([node.open ? print("open") : "", indent([softline, join([line], path.map((childPath, index) => {
const child = childPath.getValue();
const isLast = index === node.groups.length - 1;
let printed2 = [print(), isLast ? "" : ","];
if (isKeyValuePairNode(child) && child.type === "value-comma_group" && child.groups && child.groups[0].type !== "value-paren_group" && child.groups[2] && child.groups[2].type === "value-paren_group") {
const parts = getDocParts(printed2[0].contents.contents);
parts[1] = group(parts[1]);
printed2 = [group(dedent(printed2))];
}
if (!isLast && child.type === "value-comma_group" && isNonEmptyArray(child.groups)) {
let last = getLast(child.groups);
if (!last.source && last.close) {
last = last.close;
}
if (last.source && isNextLineEmpty(options.originalText, last, locEnd)) {
printed2.push(hardline);
}
}
return printed2;
}, "groups"))]), ifBreak(!isLastItemComment && isSCSS(options.parser, options.originalText) && isSCSSMapItem && shouldPrintComma(options) ? "," : ""), softline, node.close ? print("close") : ""], {
shouldBreak
});
return shouldDedent ? dedent(printed) : printed;
}
case "value-func": {
return [node.value, insideAtRuleNode(path, "supports") && isMediaAndSupportsKeywords(node) ? " " : "", print("group")];
}
case "value-paren": {
return node.value;
}
case "value-number": {
return [printCssNumber(node.value), printUnit(node.unit)];
}
case "value-operator": {
return node.value;
}
case "value-word": {
if (node.isColor && node.isHex || isWideKeywords(node.value)) {
return node.value.toLowerCase();
}
return node.value;
}
case "value-colon": {
const parentNode = path.getParentNode();
const index = parentNode && parentNode.groups.indexOf(node);
const prevNode = index && parentNode.groups[index - 1];
return [node.value, prevNode && typeof prevNode.value === "string" && getLast(prevNode.value) === "\\" || insideValueFunctionNode(path, "url") ? "" : line];
}
case "value-comma": {
return [node.value, " "];
}
case "value-string": {
return printString(node.raws.quote + node.value + node.raws.quote, options);
}
case "value-atword": {
return ["@", node.value];
}
case "value-unicode-range": {
return node.value;
}
case "value-unknown": {
return node.value;
}
default:
throw new Error(`Unknown postcss type ${JSON.stringify(node.type)}`);
}
}
function printNodeSequence(path, options, print) {
const parts = [];
path.each((pathChild, i, nodes) => {
const prevNode = nodes[i - 1];
if (prevNode && prevNode.type === "css-comment" && prevNode.text.trim() === "prettier-ignore") {
const childNode = pathChild.getValue();
parts.push(options.originalText.slice(locStart(childNode), locEnd(childNode)));
} else {
parts.push(print());
}
if (i !== nodes.length - 1) {
if (nodes[i + 1].type === "css-comment" && !hasNewline(options.originalText, locStart(nodes[i + 1]), {
backwards: true
}) && !isFrontMatterNode(nodes[i]) || nodes[i + 1].type === "css-atrule" && nodes[i + 1].name === "else" && nodes[i].type !== "css-comment") {
parts.push(" ");
} else {
parts.push(options.__isHTMLStyleAttribute ? line : hardline);
if (isNextLineEmpty(options.originalText, pathChild.getValue(), locEnd) && !isFrontMatterNode(nodes[i])) {
parts.push(hardline);
}
}
}
}, "nodes");
return parts;
}
var STRING_REGEX = /(["'])(?:(?!\1)[^\\]|\\.)*\1/gs;
var NUMBER_REGEX = /(?:\d*\.\d+|\d+\.?)(?:[Ee][+-]?\d+)?/g;
var STANDARD_UNIT_REGEX = /[A-Za-z]+/g;
var WORD_PART_REGEX = /[$@]?[A-Z_a-z\u0080-\uFFFF][\w\u0080-\uFFFF-]*/g;
var ADJUST_NUMBERS_REGEX = new RegExp(STRING_REGEX.source + `|(${WORD_PART_REGEX.source})?(${NUMBER_REGEX.source})(${STANDARD_UNIT_REGEX.source})?`, "g");
function adjustStrings(value, options) {
return value.replace(STRING_REGEX, (match) => printString(match, options));
}
function quoteAttributeValue(value, options) {
const quote2 = options.singleQuote ? "'" : '"';
return value.includes('"') || value.includes("'") ? value : quote2 + value + quote2;
}
function adjustNumbers(value) {
return value.replace(ADJUST_NUMBERS_REGEX, (match, quote2, wordPart, number, unit) => !wordPart && number ? printCssNumber(number) + maybeToLowerCase(unit || "") : match);
}
function printCssNumber(rawNumber) {
return printNumber(rawNumber).replace(/\.0(?=$|e)/, "");
}
module22.exports = {
print: genericPrint,
embed,
insertPragma,
massageAstNode: clean
};
}
});
var require_options3 = __commonJS22({
"src/language-css/options.js"(exports2, module22) {
"use strict";
var commonOptions = require_common_options();
module22.exports = {
singleQuote: commonOptions.singleQuote
};
}
});
var require_parsers2 = __commonJS22({
"src/language-css/parsers.js"(exports2, module22) {
"use strict";
module22.exports = {
get css() {
return require_parser_postcss().parsers.css;
},
get less() {
return require_parser_postcss().parsers.less;
},
get scss() {
return require_parser_postcss().parsers.scss;
}
};
}
});
var require_CSS = __commonJS22({
"node_modules/linguist-languages/data/CSS.json"(exports2, module22) {
module22.exports = {
name: "CSS",
type: "markup",
tmScope: "source.css",
aceMode: "css",
codemirrorMode: "css",
codemirrorMimeType: "text/css",
color: "#563d7c",
extensions: [".css"],
languageId: 50
};
}
});
var require_PostCSS = __commonJS22({
"node_modules/linguist-languages/data/PostCSS.json"(exports2, module22) {
module22.exports = {
name: "PostCSS",
type: "markup",
color: "#dc3a0c",
tmScope: "source.postcss",
group: "CSS",
extensions: [".pcss", ".postcss"],
aceMode: "text",
languageId: 262764437
};
}
});
var require_Less = __commonJS22({
"node_modules/linguist-languages/data/Less.json"(exports2, module22) {
module22.exports = {
name: "Less",
type: "markup",
color: "#1d365d",
aliases: ["less-css"],
extensions: [".less"],
tmScope: "source.css.less",
aceMode: "less",
codemirrorMode: "css",
codemirrorMimeType: "text/css",
languageId: 198
};
}
});
var require_SCSS = __commonJS22({
"node_modules/linguist-languages/data/SCSS.json"(exports2, module22) {
module22.exports = {
name: "SCSS",
type: "markup",
color: "#c6538c",
tmScope: "source.css.scss",
aceMode: "scss",
codemirrorMode: "css",
codemirrorMimeType: "text/x-scss",
extensions: [".scss"],
languageId: 329
};
}
});
var require_language_css = __commonJS22({
"src/language-css/index.js"(exports2, module22) {
"use strict";
var createLanguage = require_create_language();
var printer = require_printer_postcss();
var options = require_options3();
var parsers = require_parsers2();
var languages = [createLanguage(require_CSS(), (data) => ({
since: "1.4.0",
parsers: ["css"],
vscodeLanguageIds: ["css"],
extensions: [...data.extensions, ".wxss"]
})), createLanguage(require_PostCSS(), () => ({
since: "1.4.0",
parsers: ["css"],
vscodeLanguageIds: ["postcss"]
})), createLanguage(require_Less(), () => ({
since: "1.4.0",
parsers: ["less"],
vscodeLanguageIds: ["less"]
})), createLanguage(require_SCSS(), () => ({
since: "1.4.0",
parsers: ["scss"],
vscodeLanguageIds: ["scss"]
}))];
var printers = {
postcss: printer
};
module22.exports = {
languages,
options,
printers,
parsers
};
}
});
var require_loc3 = __commonJS22({
"src/language-handlebars/loc.js"(exports2, module22) {
"use strict";
function locStart(node) {
return node.loc.start.offset;
}
function locEnd(node) {
return node.loc.end.offset;
}
module22.exports = {
locStart,
locEnd
};
}
});
var require_clean3 = __commonJS22({
"src/language-handlebars/clean.js"(exports2, module22) {
"use strict";
function clean(ast, newNode) {
if (ast.type === "TextNode") {
const trimmed = ast.chars.trim();
if (!trimmed) {
return null;
}
newNode.chars = trimmed.replace(/[\t\n\f\r ]+/g, " ");
}
if (ast.type === "AttrNode" && ast.name.toLowerCase() === "class") {
delete newNode.value;
}
}
clean.ignoredProperties = /* @__PURE__ */ new Set(["loc", "selfClosing"]);
module22.exports = clean;
}
});
var require_html_void_elements_evaluate = __commonJS22({
"src/language-handlebars/html-void-elements.evaluate.js"(exports2, module22) {
module22.exports = ["area", "base", "br", "col", "command", "embed", "hr", "img", "input", "keygen", "link", "meta", "param", "source", "track", "wbr"];
}
});
var require_utils9 = __commonJS22({
"src/language-handlebars/utils.js"(exports2, module22) {
"use strict";
var getLast = require_get_last();
var htmlVoidElements = require_html_void_elements_evaluate();
function isLastNodeOfSiblings(path) {
const node = path.getValue();
const parentNode = path.getParentNode(0);
if (isParentOfSomeType(path, ["ElementNode"]) && getLast(parentNode.children) === node) {
return true;
}
if (isParentOfSomeType(path, ["Block"]) && getLast(parentNode.body) === node) {
return true;
}
return false;
}
function isUppercase(string) {
return string.toUpperCase() === string;
}
function isGlimmerComponent(node) {
return isNodeOfSomeType(node, ["ElementNode"]) && typeof node.tag === "string" && !node.tag.startsWith(":") && (isUppercase(node.tag[0]) || node.tag.includes("."));
}
var voidTags = new Set(htmlVoidElements);
function isVoidTag(tag) {
return voidTags.has(tag.toLowerCase()) && !isUppercase(tag[0]);
}
function isVoid(node) {
return node.selfClosing === true || isVoidTag(node.tag) || isGlimmerComponent(node) && node.children.every((node2) => isWhitespaceNode(node2));
}
function isWhitespaceNode(node) {
return isNodeOfSomeType(node, ["TextNode"]) && !/\S/.test(node.chars);
}
function isNodeOfSomeType(node, types) {
return node && types.includes(node.type);
}
function isParentOfSomeType(path, types) {
const parentNode = path.getParentNode(0);
return isNodeOfSomeType(parentNode, types);
}
function isPreviousNodeOfSomeType(path, types) {
const previousNode = getPreviousNode(path);
return isNodeOfSomeType(previousNode, types);
}
function isNextNodeOfSomeType(path, types) {
const nextNode = getNextNode(path);
return isNodeOfSomeType(nextNode, types);
}
function getSiblingNode(path, offset) {
var _path$getParentNode2, _ref7, _ref8, _parentNode$children;
const node = path.getValue();
const parentNode = (_path$getParentNode2 = path.getParentNode(0)) !== null && _path$getParentNode2 !== void 0 ? _path$getParentNode2 : {};
const children = (_ref7 = (_ref8 = (_parentNode$children = parentNode.children) !== null && _parentNode$children !== void 0 ? _parentNode$children : parentNode.body) !== null && _ref8 !== void 0 ? _ref8 : parentNode.parts) !== null && _ref7 !== void 0 ? _ref7 : [];
const index = children.indexOf(node);
return index !== -1 && children[index + offset];
}
function getPreviousNode(path, lookBack = 1) {
return getSiblingNode(path, -lookBack);
}
function getNextNode(path) {
return getSiblingNode(path, 1);
}
function isPrettierIgnoreNode(node) {
return isNodeOfSomeType(node, ["MustacheCommentStatement"]) && typeof node.value === "string" && node.value.trim() === "prettier-ignore";
}
function hasPrettierIgnore(path) {
const node = path.getValue();
const previousPreviousNode = getPreviousNode(path, 2);
return isPrettierIgnoreNode(node) || isPrettierIgnoreNode(previousPreviousNode);
}
module22.exports = {
getNextNode,
getPreviousNode,
hasPrettierIgnore,
isLastNodeOfSiblings,
isNextNodeOfSomeType,
isNodeOfSomeType,
isParentOfSomeType,
isPreviousNodeOfSomeType,
isVoid,
isWhitespaceNode
};
}
});
var require_printer_glimmer = __commonJS22({
"src/language-handlebars/printer-glimmer.js"(exports2, module22) {
"use strict";
var {
builders: {
dedent,
fill,
group,
hardline,
ifBreak,
indent,
join,
line,
softline
},
utils: {
getDocParts,
replaceTextEndOfLine
}
} = require_doc();
var {
getPreferredQuote,
isNonEmptyArray
} = require_util();
var {
locStart,
locEnd
} = require_loc3();
var clean = require_clean3();
var {
getNextNode,
getPreviousNode,
hasPrettierIgnore,
isLastNodeOfSiblings,
isNextNodeOfSomeType,
isNodeOfSomeType,
isParentOfSomeType,
isPreviousNodeOfSomeType,
isVoid,
isWhitespaceNode
} = require_utils9();
var NEWLINES_TO_PRESERVE_MAX = 2;
function print(path, options, print2) {
const node = path.getValue();
if (!node) {
return "";
}
if (hasPrettierIgnore(path)) {
return options.originalText.slice(locStart(node), locEnd(node));
}
const favoriteQuote = options.singleQuote ? "'" : '"';
switch (node.type) {
case "Block":
case "Program":
case "Template": {
return group(path.map(print2, "body"));
}
case "ElementNode": {
const startingTag = group(printStartingTag(path, print2));
const escapeNextElementNode = options.htmlWhitespaceSensitivity === "ignore" && isNextNodeOfSomeType(path, ["ElementNode"]) ? softline : "";
if (isVoid(node)) {
return [startingTag, escapeNextElementNode];
}
const endingTag = ["", node.tag, ">"];
if (node.children.length === 0) {
return [startingTag, indent(endingTag), escapeNextElementNode];
}
if (options.htmlWhitespaceSensitivity === "ignore") {
return [startingTag, indent(printChildren(path, options, print2)), hardline, indent(endingTag), escapeNextElementNode];
}
return [startingTag, indent(group(printChildren(path, options, print2))), indent(endingTag), escapeNextElementNode];
}
case "BlockStatement": {
const pp = path.getParentNode(1);
const isElseIfLike = pp && pp.inverse && pp.inverse.body.length === 1 && pp.inverse.body[0] === node && pp.inverse.body[0].path.parts[0] === pp.path.parts[0];
if (isElseIfLike) {
return [printElseIfLikeBlock(path, print2, pp.inverse.body[0].path.parts[0]), printProgram(path, print2, options), printInverse(path, print2, options)];
}
return [printOpenBlock(path, print2), group([printProgram(path, print2, options), printInverse(path, print2, options), printCloseBlock(path, print2, options)])];
}
case "ElementModifierStatement": {
return group(["{{", printPathAndParams(path, print2), "}}"]);
}
case "MustacheStatement": {
return group([printOpeningMustache(node), printPathAndParams(path, print2), printClosingMustache(node)]);
}
case "SubExpression": {
return group(["(", printSubExpressionPathAndParams(path, print2), softline, ")"]);
}
case "AttrNode": {
const isText = node.value.type === "TextNode";
const isEmptyText = isText && node.value.chars === "";
if (isEmptyText && locStart(node.value) === locEnd(node.value)) {
return node.name;
}
const quote2 = isText ? getPreferredQuote(node.value.chars, favoriteQuote).quote : node.value.type === "ConcatStatement" ? getPreferredQuote(node.value.parts.filter((part) => part.type === "TextNode").map((part) => part.chars).join(""), favoriteQuote).quote : "";
const valueDoc = print2("value");
return [node.name, "=", quote2, node.name === "class" && quote2 ? group(indent(valueDoc)) : valueDoc, quote2];
}
case "ConcatStatement": {
return path.map(print2, "parts");
}
case "Hash": {
return join(line, path.map(print2, "pairs"));
}
case "HashPair": {
return [node.key, "=", print2("value")];
}
case "TextNode": {
let text = node.chars.replace(/{{/g, "\\{{");
const attrName = getCurrentAttributeName(path);
if (attrName) {
if (attrName === "class") {
const formattedClasses = text.trim().split(/\s+/).join(" ");
let leadingSpace2 = false;
let trailingSpace2 = false;
if (isParentOfSomeType(path, ["ConcatStatement"])) {
if (isPreviousNodeOfSomeType(path, ["MustacheStatement"]) && /^\s/.test(text)) {
leadingSpace2 = true;
}
if (isNextNodeOfSomeType(path, ["MustacheStatement"]) && /\s$/.test(text) && formattedClasses !== "") {
trailingSpace2 = true;
}
}
return [leadingSpace2 ? line : "", formattedClasses, trailingSpace2 ? line : ""];
}
return replaceTextEndOfLine(text);
}
const whitespacesOnlyRE = /^[\t\n\f\r ]*$/;
const isWhitespaceOnly = whitespacesOnlyRE.test(text);
const isFirstElement = !getPreviousNode(path);
const isLastElement = !getNextNode(path);
if (options.htmlWhitespaceSensitivity !== "ignore") {
const leadingWhitespacesRE = /^[\t\n\f\r ]*/;
const trailingWhitespacesRE = /[\t\n\f\r ]*$/;
const shouldTrimTrailingNewlines = isLastElement && isParentOfSomeType(path, ["Template"]);
const shouldTrimLeadingNewlines = isFirstElement && isParentOfSomeType(path, ["Template"]);
if (isWhitespaceOnly) {
if (shouldTrimLeadingNewlines || shouldTrimTrailingNewlines) {
return "";
}
let breaks = [line];
const newlines = countNewLines(text);
if (newlines) {
breaks = generateHardlines(newlines);
}
if (isLastNodeOfSiblings(path)) {
breaks = breaks.map((newline) => dedent(newline));
}
return breaks;
}
const [lead] = text.match(leadingWhitespacesRE);
const [tail] = text.match(trailingWhitespacesRE);
let leadBreaks = [];
if (lead) {
leadBreaks = [line];
const leadingNewlines = countNewLines(lead);
if (leadingNewlines) {
leadBreaks = generateHardlines(leadingNewlines);
}
text = text.replace(leadingWhitespacesRE, "");
}
let trailBreaks = [];
if (tail) {
if (!shouldTrimTrailingNewlines) {
trailBreaks = [line];
const trailingNewlines = countNewLines(tail);
if (trailingNewlines) {
trailBreaks = generateHardlines(trailingNewlines);
}
if (isLastNodeOfSiblings(path)) {
trailBreaks = trailBreaks.map((hardline2) => dedent(hardline2));
}
}
text = text.replace(trailingWhitespacesRE, "");
}
return [...leadBreaks, fill(getTextValueParts(text)), ...trailBreaks];
}
const lineBreaksCount = countNewLines(text);
let leadingLineBreaksCount = countLeadingNewLines(text);
let trailingLineBreaksCount = countTrailingNewLines(text);
if ((isFirstElement || isLastElement) && isWhitespaceOnly && isParentOfSomeType(path, ["Block", "ElementNode", "Template"])) {
return "";
}
if (isWhitespaceOnly && lineBreaksCount) {
leadingLineBreaksCount = Math.min(lineBreaksCount, NEWLINES_TO_PRESERVE_MAX);
trailingLineBreaksCount = 0;
} else {
if (isNextNodeOfSomeType(path, ["BlockStatement", "ElementNode"])) {
trailingLineBreaksCount = Math.max(trailingLineBreaksCount, 1);
}
if (isPreviousNodeOfSomeType(path, ["BlockStatement", "ElementNode"])) {
leadingLineBreaksCount = Math.max(leadingLineBreaksCount, 1);
}
}
let leadingSpace = "";
let trailingSpace = "";
if (trailingLineBreaksCount === 0 && isNextNodeOfSomeType(path, ["MustacheStatement"])) {
trailingSpace = " ";
}
if (leadingLineBreaksCount === 0 && isPreviousNodeOfSomeType(path, ["MustacheStatement"])) {
leadingSpace = " ";
}
if (isFirstElement) {
leadingLineBreaksCount = 0;
leadingSpace = "";
}
if (isLastElement) {
trailingLineBreaksCount = 0;
trailingSpace = "";
}
text = text.replace(/^[\t\n\f\r ]+/g, leadingSpace).replace(/[\t\n\f\r ]+$/, trailingSpace);
return [...generateHardlines(leadingLineBreaksCount), fill(getTextValueParts(text)), ...generateHardlines(trailingLineBreaksCount)];
}
case "MustacheCommentStatement": {
const start = locStart(node);
const end = locEnd(node);
const isLeftWhiteSpaceSensitive = options.originalText.charAt(start + 2) === "~";
const isRightWhitespaceSensitive = options.originalText.charAt(end - 3) === "~";
const dashes = node.value.includes("}}") ? "--" : "";
return ["{{", isLeftWhiteSpaceSensitive ? "~" : "", "!", dashes, node.value, dashes, isRightWhitespaceSensitive ? "~" : "", "}}"];
}
case "PathExpression": {
return node.original;
}
case "BooleanLiteral": {
return String(node.value);
}
case "CommentStatement": {
return [""];
}
case "StringLiteral": {
if (needsOppositeQuote(path)) {
const printFavoriteQuote = !options.singleQuote ? "'" : '"';
return printStringLiteral(node.value, printFavoriteQuote);
}
return printStringLiteral(node.value, favoriteQuote);
}
case "NumberLiteral": {
return String(node.value);
}
case "UndefinedLiteral": {
return "undefined";
}
case "NullLiteral": {
return "null";
}
default:
throw new Error("unknown glimmer type: " + JSON.stringify(node.type));
}
}
function sortByLoc(a, b) {
return locStart(a) - locStart(b);
}
function printStartingTag(path, print2) {
const node = path.getValue();
const types = ["attributes", "modifiers", "comments"].filter((property) => isNonEmptyArray(node[property]));
const attributes = types.flatMap((type) => node[type]).sort(sortByLoc);
for (const attributeType of types) {
path.each((attributePath) => {
const index = attributes.indexOf(attributePath.getValue());
attributes.splice(index, 1, [line, print2()]);
}, attributeType);
}
if (isNonEmptyArray(node.blockParams)) {
attributes.push(line, printBlockParams(node));
}
return ["<", node.tag, indent(attributes), printStartingTagEndMarker(node)];
}
function printChildren(path, options, print2) {
const node = path.getValue();
const isEmpty = node.children.every((node2) => isWhitespaceNode(node2));
if (options.htmlWhitespaceSensitivity === "ignore" && isEmpty) {
return "";
}
return path.map((childPath, childIndex) => {
const printedChild = print2();
if (childIndex === 0 && options.htmlWhitespaceSensitivity === "ignore") {
return [softline, printedChild];
}
return printedChild;
}, "children");
}
function printStartingTagEndMarker(node) {
if (isVoid(node)) {
return ifBreak([softline, "/>"], [" />", softline]);
}
return ifBreak([softline, ">"], ">");
}
function printOpeningMustache(node) {
const mustache = node.escaped === false ? "{{{" : "{{";
const strip = node.strip && node.strip.open ? "~" : "";
return [mustache, strip];
}
function printClosingMustache(node) {
const mustache = node.escaped === false ? "}}}" : "}}";
const strip = node.strip && node.strip.close ? "~" : "";
return [strip, mustache];
}
function printOpeningBlockOpeningMustache(node) {
const opening = printOpeningMustache(node);
const strip = node.openStrip.open ? "~" : "";
return [opening, strip, "#"];
}
function printOpeningBlockClosingMustache(node) {
const closing = printClosingMustache(node);
const strip = node.openStrip.close ? "~" : "";
return [strip, closing];
}
function printClosingBlockOpeningMustache(node) {
const opening = printOpeningMustache(node);
const strip = node.closeStrip.open ? "~" : "";
return [opening, strip, "/"];
}
function printClosingBlockClosingMustache(node) {
const closing = printClosingMustache(node);
const strip = node.closeStrip.close ? "~" : "";
return [strip, closing];
}
function printInverseBlockOpeningMustache(node) {
const opening = printOpeningMustache(node);
const strip = node.inverseStrip.open ? "~" : "";
return [opening, strip];
}
function printInverseBlockClosingMustache(node) {
const closing = printClosingMustache(node);
const strip = node.inverseStrip.close ? "~" : "";
return [strip, closing];
}
function printOpenBlock(path, print2) {
const node = path.getValue();
const parts = [];
const paramsDoc = printParams(path, print2);
if (paramsDoc) {
parts.push(group(paramsDoc));
}
if (isNonEmptyArray(node.program.blockParams)) {
parts.push(printBlockParams(node.program));
}
return group([printOpeningBlockOpeningMustache(node), printPath(path, print2), parts.length > 0 ? indent([line, join(line, parts)]) : "", softline, printOpeningBlockClosingMustache(node)]);
}
function printElseBlock(node, options) {
return [options.htmlWhitespaceSensitivity === "ignore" ? hardline : "", printInverseBlockOpeningMustache(node), "else", printInverseBlockClosingMustache(node)];
}
function printElseIfLikeBlock(path, print2, ifLikeKeyword) {
const node = path.getValue();
const parentNode = path.getParentNode(1);
return group([printInverseBlockOpeningMustache(parentNode), ["else", " ", ifLikeKeyword], indent([line, group(printParams(path, print2)), ...isNonEmptyArray(node.program.blockParams) ? [line, printBlockParams(node.program)] : []]), softline, printInverseBlockClosingMustache(parentNode)]);
}
function printCloseBlock(path, print2, options) {
const node = path.getValue();
if (options.htmlWhitespaceSensitivity === "ignore") {
const escape = blockStatementHasOnlyWhitespaceInProgram(node) ? softline : hardline;
return [escape, printClosingBlockOpeningMustache(node), print2("path"), printClosingBlockClosingMustache(node)];
}
return [printClosingBlockOpeningMustache(node), print2("path"), printClosingBlockClosingMustache(node)];
}
function blockStatementHasOnlyWhitespaceInProgram(node) {
return isNodeOfSomeType(node, ["BlockStatement"]) && node.program.body.every((node2) => isWhitespaceNode(node2));
}
function blockStatementHasElseIfLike(node) {
return blockStatementHasElse(node) && node.inverse.body.length === 1 && isNodeOfSomeType(node.inverse.body[0], ["BlockStatement"]) && node.inverse.body[0].path.parts[0] === node.path.parts[0];
}
function blockStatementHasElse(node) {
return isNodeOfSomeType(node, ["BlockStatement"]) && node.inverse;
}
function printProgram(path, print2, options) {
const node = path.getValue();
if (blockStatementHasOnlyWhitespaceInProgram(node)) {
return "";
}
const program = print2("program");
if (options.htmlWhitespaceSensitivity === "ignore") {
return indent([hardline, program]);
}
return indent(program);
}
function printInverse(path, print2, options) {
const node = path.getValue();
const inverse = print2("inverse");
const printed = options.htmlWhitespaceSensitivity === "ignore" ? [hardline, inverse] : inverse;
if (blockStatementHasElseIfLike(node)) {
return printed;
}
if (blockStatementHasElse(node)) {
return [printElseBlock(node, options), indent(printed)];
}
return "";
}
function getTextValueParts(value) {
return getDocParts(join(line, splitByHtmlWhitespace(value)));
}
function splitByHtmlWhitespace(string) {
return string.split(/[\t\n\f\r ]+/);
}
function getCurrentAttributeName(path) {
for (let depth = 0; depth < 2; depth++) {
const parentNode = path.getParentNode(depth);
if (parentNode && parentNode.type === "AttrNode") {
return parentNode.name.toLowerCase();
}
}
}
function countNewLines(string) {
string = typeof string === "string" ? string : "";
return string.split("\n").length - 1;
}
function countLeadingNewLines(string) {
string = typeof string === "string" ? string : "";
const newLines = (string.match(/^([^\S\n\r]*[\n\r])+/g) || [])[0] || "";
return countNewLines(newLines);
}
function countTrailingNewLines(string) {
string = typeof string === "string" ? string : "";
const newLines = (string.match(/([\n\r][^\S\n\r]*)+$/g) || [])[0] || "";
return countNewLines(newLines);
}
function generateHardlines(number = 0) {
return Array.from({
length: Math.min(number, NEWLINES_TO_PRESERVE_MAX)
}).fill(hardline);
}
function printStringLiteral(stringLiteral, favoriteQuote) {
const {
quote: quote2,
regex
} = getPreferredQuote(stringLiteral, favoriteQuote);
return [quote2, stringLiteral.replace(regex, `\\${quote2}`), quote2];
}
function needsOppositeQuote(path) {
let index = 0;
let parentNode = path.getParentNode(index);
while (parentNode && isNodeOfSomeType(parentNode, ["SubExpression"])) {
index++;
parentNode = path.getParentNode(index);
}
if (parentNode && isNodeOfSomeType(path.getParentNode(index + 1), ["ConcatStatement"]) && isNodeOfSomeType(path.getParentNode(index + 2), ["AttrNode"])) {
return true;
}
return false;
}
function printSubExpressionPathAndParams(path, print2) {
const printed = printPath(path, print2);
const params = printParams(path, print2);
if (!params) {
return printed;
}
return indent([printed, line, group(params)]);
}
function printPathAndParams(path, print2) {
const p = printPath(path, print2);
const params = printParams(path, print2);
if (!params) {
return p;
}
return [indent([p, line, params]), softline];
}
function printPath(path, print2) {
return print2("path");
}
function printParams(path, print2) {
const node = path.getValue();
const parts = [];
if (node.params.length > 0) {
const params = path.map(print2, "params");
parts.push(...params);
}
if (node.hash && node.hash.pairs.length > 0) {
const hash = print2("hash");
parts.push(hash);
}
if (parts.length === 0) {
return "";
}
return join(line, parts);
}
function printBlockParams(node) {
return ["as |", node.blockParams.join(" "), "|"];
}
module22.exports = {
print,
massageAstNode: clean
};
}
});
var require_parsers3 = __commonJS22({
"src/language-handlebars/parsers.js"(exports2, module22) {
"use strict";
module22.exports = {
get glimmer() {
return require_parser_glimmer().parsers.glimmer;
}
};
}
});
var require_Handlebars = __commonJS22({
"node_modules/linguist-languages/data/Handlebars.json"(exports2, module22) {
module22.exports = {
name: "Handlebars",
type: "markup",
color: "#f7931e",
aliases: ["hbs", "htmlbars"],
extensions: [".handlebars", ".hbs"],
tmScope: "text.html.handlebars",
aceMode: "handlebars",
languageId: 155
};
}
});
var require_language_handlebars = __commonJS22({
"src/language-handlebars/index.js"(exports2, module22) {
"use strict";
var createLanguage = require_create_language();
var printer = require_printer_glimmer();
var parsers = require_parsers3();
var languages = [createLanguage(require_Handlebars(), () => ({
since: "2.3.0",
parsers: ["glimmer"],
vscodeLanguageIds: ["handlebars"]
}))];
var printers = {
glimmer: printer
};
module22.exports = {
languages,
printers,
parsers
};
}
});
var require_pragma3 = __commonJS22({
"src/language-graphql/pragma.js"(exports2, module22) {
"use strict";
function hasPragma(text) {
return /^\s*#[^\S\n]*@(?:format|prettier)\s*(?:\n|$)/.test(text);
}
function insertPragma(text) {
return "# @format\n\n" + text;
}
module22.exports = {
hasPragma,
insertPragma
};
}
});
var require_loc4 = __commonJS22({
"src/language-graphql/loc.js"(exports2, module22) {
"use strict";
function locStart(node) {
if (typeof node.start === "number") {
return node.start;
}
return node.loc && node.loc.start;
}
function locEnd(node) {
if (typeof node.end === "number") {
return node.end;
}
return node.loc && node.loc.end;
}
module22.exports = {
locStart,
locEnd
};
}
});
var require_printer_graphql = __commonJS22({
"src/language-graphql/printer-graphql.js"(exports2, module22) {
"use strict";
var {
builders: {
join,
hardline,
line,
softline,
group,
indent,
ifBreak
}
} = require_doc();
var {
isNextLineEmpty,
isNonEmptyArray
} = require_util();
var {
insertPragma
} = require_pragma3();
var {
locStart,
locEnd
} = require_loc4();
function genericPrint(path, options, print) {
const node = path.getValue();
if (!node) {
return "";
}
if (typeof node === "string") {
return node;
}
switch (node.kind) {
case "Document": {
const parts = [];
path.each((pathChild, index, definitions) => {
parts.push(print());
if (index !== definitions.length - 1) {
parts.push(hardline);
if (isNextLineEmpty(options.originalText, pathChild.getValue(), locEnd)) {
parts.push(hardline);
}
}
}, "definitions");
return [...parts, hardline];
}
case "OperationDefinition": {
const hasOperation = options.originalText[locStart(node)] !== "{";
const hasName = Boolean(node.name);
return [hasOperation ? node.operation : "", hasOperation && hasName ? [" ", print("name")] : "", hasOperation && !hasName && isNonEmptyArray(node.variableDefinitions) ? " " : "", isNonEmptyArray(node.variableDefinitions) ? group(["(", indent([softline, join([ifBreak("", ", "), softline], path.map(print, "variableDefinitions"))]), softline, ")"]) : "", printDirectives(path, print, node), node.selectionSet ? !hasOperation && !hasName ? "" : " " : "", print("selectionSet")];
}
case "FragmentDefinition": {
return ["fragment ", print("name"), isNonEmptyArray(node.variableDefinitions) ? group(["(", indent([softline, join([ifBreak("", ", "), softline], path.map(print, "variableDefinitions"))]), softline, ")"]) : "", " on ", print("typeCondition"), printDirectives(path, print, node), " ", print("selectionSet")];
}
case "SelectionSet": {
return ["{", indent([hardline, join(hardline, printSequence(path, options, print, "selections"))]), hardline, "}"];
}
case "Field": {
return group([node.alias ? [print("alias"), ": "] : "", print("name"), node.arguments.length > 0 ? group(["(", indent([softline, join([ifBreak("", ", "), softline], printSequence(path, options, print, "arguments"))]), softline, ")"]) : "", printDirectives(path, print, node), node.selectionSet ? " " : "", print("selectionSet")]);
}
case "Name": {
return node.value;
}
case "StringValue": {
if (node.block) {
const lines = node.value.replace(/"""/g, "\\$&").split("\n");
if (lines.length === 1) {
lines[0] = lines[0].trim();
}
if (lines.every((line2) => line2 === "")) {
lines.length = 0;
}
return join(hardline, ['"""', ...lines, '"""']);
}
return ['"', node.value.replace(/["\\]/g, "\\$&").replace(/\n/g, "\\n"), '"'];
}
case "IntValue":
case "FloatValue":
case "EnumValue": {
return node.value;
}
case "BooleanValue": {
return node.value ? "true" : "false";
}
case "NullValue": {
return "null";
}
case "Variable": {
return ["$", print("name")];
}
case "ListValue": {
return group(["[", indent([softline, join([ifBreak("", ", "), softline], path.map(print, "values"))]), softline, "]"]);
}
case "ObjectValue": {
return group(["{", options.bracketSpacing && node.fields.length > 0 ? " " : "", indent([softline, join([ifBreak("", ", "), softline], path.map(print, "fields"))]), softline, ifBreak("", options.bracketSpacing && node.fields.length > 0 ? " " : ""), "}"]);
}
case "ObjectField":
case "Argument": {
return [print("name"), ": ", print("value")];
}
case "Directive": {
return ["@", print("name"), node.arguments.length > 0 ? group(["(", indent([softline, join([ifBreak("", ", "), softline], printSequence(path, options, print, "arguments"))]), softline, ")"]) : ""];
}
case "NamedType": {
return print("name");
}
case "VariableDefinition": {
return [print("variable"), ": ", print("type"), node.defaultValue ? [" = ", print("defaultValue")] : "", printDirectives(path, print, node)];
}
case "ObjectTypeExtension":
case "ObjectTypeDefinition": {
return [print("description"), node.description ? hardline : "", node.kind === "ObjectTypeExtension" ? "extend " : "", "type ", print("name"), node.interfaces.length > 0 ? [" implements ", ...printInterfaces(path, options, print)] : "", printDirectives(path, print, node), node.fields.length > 0 ? [" {", indent([hardline, join(hardline, printSequence(path, options, print, "fields"))]), hardline, "}"] : ""];
}
case "FieldDefinition": {
return [print("description"), node.description ? hardline : "", print("name"), node.arguments.length > 0 ? group(["(", indent([softline, join([ifBreak("", ", "), softline], printSequence(path, options, print, "arguments"))]), softline, ")"]) : "", ": ", print("type"), printDirectives(path, print, node)];
}
case "DirectiveDefinition": {
return [print("description"), node.description ? hardline : "", "directive ", "@", print("name"), node.arguments.length > 0 ? group(["(", indent([softline, join([ifBreak("", ", "), softline], printSequence(path, options, print, "arguments"))]), softline, ")"]) : "", node.repeatable ? " repeatable" : "", " on ", join(" | ", path.map(print, "locations"))];
}
case "EnumTypeExtension":
case "EnumTypeDefinition": {
return [print("description"), node.description ? hardline : "", node.kind === "EnumTypeExtension" ? "extend " : "", "enum ", print("name"), printDirectives(path, print, node), node.values.length > 0 ? [" {", indent([hardline, join(hardline, printSequence(path, options, print, "values"))]), hardline, "}"] : ""];
}
case "EnumValueDefinition": {
return [print("description"), node.description ? hardline : "", print("name"), printDirectives(path, print, node)];
}
case "InputValueDefinition": {
return [print("description"), node.description ? node.description.block ? hardline : line : "", print("name"), ": ", print("type"), node.defaultValue ? [" = ", print("defaultValue")] : "", printDirectives(path, print, node)];
}
case "InputObjectTypeExtension":
case "InputObjectTypeDefinition": {
return [print("description"), node.description ? hardline : "", node.kind === "InputObjectTypeExtension" ? "extend " : "", "input ", print("name"), printDirectives(path, print, node), node.fields.length > 0 ? [" {", indent([hardline, join(hardline, printSequence(path, options, print, "fields"))]), hardline, "}"] : ""];
}
case "SchemaExtension": {
return ["extend schema", printDirectives(path, print, node), ...node.operationTypes.length > 0 ? [" {", indent([hardline, join(hardline, printSequence(path, options, print, "operationTypes"))]), hardline, "}"] : []];
}
case "SchemaDefinition": {
return [print("description"), node.description ? hardline : "", "schema", printDirectives(path, print, node), " {", node.operationTypes.length > 0 ? indent([hardline, join(hardline, printSequence(path, options, print, "operationTypes"))]) : "", hardline, "}"];
}
case "OperationTypeDefinition": {
return [print("operation"), ": ", print("type")];
}
case "InterfaceTypeExtension":
case "InterfaceTypeDefinition": {
return [print("description"), node.description ? hardline : "", node.kind === "InterfaceTypeExtension" ? "extend " : "", "interface ", print("name"), node.interfaces.length > 0 ? [" implements ", ...printInterfaces(path, options, print)] : "", printDirectives(path, print, node), node.fields.length > 0 ? [" {", indent([hardline, join(hardline, printSequence(path, options, print, "fields"))]), hardline, "}"] : ""];
}
case "FragmentSpread": {
return ["...", print("name"), printDirectives(path, print, node)];
}
case "InlineFragment": {
return ["...", node.typeCondition ? [" on ", print("typeCondition")] : "", printDirectives(path, print, node), " ", print("selectionSet")];
}
case "UnionTypeExtension":
case "UnionTypeDefinition": {
return group([print("description"), node.description ? hardline : "", group([node.kind === "UnionTypeExtension" ? "extend " : "", "union ", print("name"), printDirectives(path, print, node), node.types.length > 0 ? [" =", ifBreak("", " "), indent([ifBreak([line, " "]), join([line, "| "], path.map(print, "types"))])] : ""])]);
}
case "ScalarTypeExtension":
case "ScalarTypeDefinition": {
return [print("description"), node.description ? hardline : "", node.kind === "ScalarTypeExtension" ? "extend " : "", "scalar ", print("name"), printDirectives(path, print, node)];
}
case "NonNullType": {
return [print("type"), "!"];
}
case "ListType": {
return ["[", print("type"), "]"];
}
default:
throw new Error("unknown graphql type: " + JSON.stringify(node.kind));
}
}
function printDirectives(path, print, node) {
if (node.directives.length === 0) {
return "";
}
const printed = join(line, path.map(print, "directives"));
if (node.kind === "FragmentDefinition" || node.kind === "OperationDefinition") {
return group([line, printed]);
}
return [" ", group(indent([softline, printed]))];
}
function printSequence(path, options, print, property) {
return path.map((path2, index, sequence) => {
const printed = print();
if (index < sequence.length - 1 && isNextLineEmpty(options.originalText, path2.getValue(), locEnd)) {
return [printed, hardline];
}
return printed;
}, property);
}
function canAttachComment(node) {
return node.kind && node.kind !== "Comment";
}
function printComment(commentPath) {
const comment = commentPath.getValue();
if (comment.kind === "Comment") {
return "#" + comment.value.trimEnd();
}
throw new Error("Not a comment: " + JSON.stringify(comment));
}
function printInterfaces(path, options, print) {
const node = path.getNode();
const parts = [];
const {
interfaces
} = node;
const printed = path.map((node2) => print(node2), "interfaces");
for (let index = 0; index < interfaces.length; index++) {
const interfaceNode = interfaces[index];
parts.push(printed[index]);
const nextInterfaceNode = interfaces[index + 1];
if (nextInterfaceNode) {
const textBetween = options.originalText.slice(interfaceNode.loc.end, nextInterfaceNode.loc.start);
const hasComment = textBetween.includes("#");
const separator = textBetween.replace(/#.*/g, "").trim();
parts.push(separator === "," ? "," : " &", hasComment ? line : " ");
}
}
return parts;
}
function clean(node, newNode) {
if (node.kind === "StringValue" && node.block && !node.value.includes("\n")) {
newNode.value = newNode.value.trim();
}
}
clean.ignoredProperties = /* @__PURE__ */ new Set(["loc", "comments"]);
function hasPrettierIgnore(path) {
var _node$comments;
const node = path.getValue();
return node === null || node === void 0 ? void 0 : (_node$comments = node.comments) === null || _node$comments === void 0 ? void 0 : _node$comments.some((comment) => comment.value.trim() === "prettier-ignore");
}
module22.exports = {
print: genericPrint,
massageAstNode: clean,
hasPrettierIgnore,
insertPragma,
printComment,
canAttachComment
};
}
});
var require_options4 = __commonJS22({
"src/language-graphql/options.js"(exports2, module22) {
"use strict";
var commonOptions = require_common_options();
module22.exports = {
bracketSpacing: commonOptions.bracketSpacing
};
}
});
var require_parsers4 = __commonJS22({
"src/language-graphql/parsers.js"(exports2, module22) {
"use strict";
module22.exports = {
get graphql() {
return require_parser_graphql().parsers.graphql;
}
};
}
});
var require_GraphQL = __commonJS22({
"node_modules/linguist-languages/data/GraphQL.json"(exports2, module22) {
module22.exports = {
name: "GraphQL",
type: "data",
color: "#e10098",
extensions: [".graphql", ".gql", ".graphqls"],
tmScope: "source.graphql",
aceMode: "text",
languageId: 139
};
}
});
var require_language_graphql = __commonJS22({
"src/language-graphql/index.js"(exports2, module22) {
"use strict";
var createLanguage = require_create_language();
var printer = require_printer_graphql();
var options = require_options4();
var parsers = require_parsers4();
var languages = [createLanguage(require_GraphQL(), () => ({
since: "1.5.0",
parsers: ["graphql"],
vscodeLanguageIds: ["graphql"]
}))];
var printers = {
graphql: printer
};
module22.exports = {
languages,
options,
printers,
parsers
};
}
});
var require_collapse_white_space = __commonJS22({
"node_modules/collapse-white-space/index.js"(exports2, module22) {
"use strict";
module22.exports = collapse;
function collapse(value) {
return String(value).replace(/\s+/g, " ");
}
}
});
var require_loc5 = __commonJS22({
"src/language-markdown/loc.js"(exports2, module22) {
"use strict";
function locStart(node) {
return node.position.start.offset;
}
function locEnd(node) {
return node.position.end.offset;
}
module22.exports = {
locStart,
locEnd
};
}
});
var require_constants_evaluate = __commonJS22({
"src/language-markdown/constants.evaluate.js"(exports2, module22) {
module22.exports = {
cjkPattern: "(?:[\\u02ea-\\u02eb\\u1100-\\u11ff\\u2e80-\\u2e99\\u2e9b-\\u2ef3\\u2f00-\\u2fd5\\u2ff0-\\u303f\\u3041-\\u3096\\u3099-\\u309f\\u30a1-\\u30fa\\u30fc-\\u30ff\\u3105-\\u312f\\u3131-\\u318e\\u3190-\\u3191\\u3196-\\u31ba\\u31c0-\\u31e3\\u31f0-\\u321e\\u322a-\\u3247\\u3260-\\u327e\\u328a-\\u32b0\\u32c0-\\u32cb\\u32d0-\\u3370\\u337b-\\u337f\\u33e0-\\u33fe\\u3400-\\u4db5\\u4e00-\\u9fef\\ua960-\\ua97c\\uac00-\\ud7a3\\ud7b0-\\ud7c6\\ud7cb-\\ud7fb\\uf900-\\ufa6d\\ufa70-\\ufad9\\ufe10-\\ufe1f\\ufe30-\\ufe6f\\uff00-\\uffef]|[\\ud840-\\ud868\\ud86a-\\ud86c\\ud86f-\\ud872\\ud874-\\ud879][\\udc00-\\udfff]|\\ud82c[\\udc00-\\udd1e\\udd50-\\udd52\\udd64-\\udd67]|\\ud83c[\\ude00\\ude50-\\ude51]|\\ud869[\\udc00-\\uded6\\udf00-\\udfff]|\\ud86d[\\udc00-\\udf34\\udf40-\\udfff]|\\ud86e[\\udc00-\\udc1d\\udc20-\\udfff]|\\ud873[\\udc00-\\udea1\\udeb0-\\udfff]|\\ud87a[\\udc00-\\udfe0]|\\ud87e[\\udc00-\\ude1d])(?:[\\ufe00-\\ufe0f]|\\udb40[\\udd00-\\uddef])?",
kPattern: "[\\u1100-\\u11ff\\u3001-\\u3003\\u3008-\\u3011\\u3013-\\u301f\\u302e-\\u3030\\u3037\\u30fb\\u3131-\\u318e\\u3200-\\u321e\\u3260-\\u327e\\ua960-\\ua97c\\uac00-\\ud7a3\\ud7b0-\\ud7c6\\ud7cb-\\ud7fb\\ufe45-\\ufe46\\uff61-\\uff65\\uffa0-\\uffbe\\uffc2-\\uffc7\\uffca-\\uffcf\\uffd2-\\uffd7\\uffda-\\uffdc]",
punctuationPattern: "[\\u0021-\\u002f\\u003a-\\u0040\\u005b-\\u0060\\u007b-\\u007e\\u00a1\\u00a7\\u00ab\\u00b6-\\u00b7\\u00bb\\u00bf\\u037e\\u0387\\u055a-\\u055f\\u0589-\\u058a\\u05be\\u05c0\\u05c3\\u05c6\\u05f3-\\u05f4\\u0609-\\u060a\\u060c-\\u060d\\u061b\\u061e-\\u061f\\u066a-\\u066d\\u06d4\\u0700-\\u070d\\u07f7-\\u07f9\\u0830-\\u083e\\u085e\\u0964-\\u0965\\u0970\\u09fd\\u0a76\\u0af0\\u0c77\\u0c84\\u0df4\\u0e4f\\u0e5a-\\u0e5b\\u0f04-\\u0f12\\u0f14\\u0f3a-\\u0f3d\\u0f85\\u0fd0-\\u0fd4\\u0fd9-\\u0fda\\u104a-\\u104f\\u10fb\\u1360-\\u1368\\u1400\\u166e\\u169b-\\u169c\\u16eb-\\u16ed\\u1735-\\u1736\\u17d4-\\u17d6\\u17d8-\\u17da\\u1800-\\u180a\\u1944-\\u1945\\u1a1e-\\u1a1f\\u1aa0-\\u1aa6\\u1aa8-\\u1aad\\u1b5a-\\u1b60\\u1bfc-\\u1bff\\u1c3b-\\u1c3f\\u1c7e-\\u1c7f\\u1cc0-\\u1cc7\\u1cd3\\u2010-\\u2027\\u2030-\\u2043\\u2045-\\u2051\\u2053-\\u205e\\u207d-\\u207e\\u208d-\\u208e\\u2308-\\u230b\\u2329-\\u232a\\u2768-\\u2775\\u27c5-\\u27c6\\u27e6-\\u27ef\\u2983-\\u2998\\u29d8-\\u29db\\u29fc-\\u29fd\\u2cf9-\\u2cfc\\u2cfe-\\u2cff\\u2d70\\u2e00-\\u2e2e\\u2e30-\\u2e4f\\u3001-\\u3003\\u3008-\\u3011\\u3014-\\u301f\\u3030\\u303d\\u30a0\\u30fb\\ua4fe-\\ua4ff\\ua60d-\\ua60f\\ua673\\ua67e\\ua6f2-\\ua6f7\\ua874-\\ua877\\ua8ce-\\ua8cf\\ua8f8-\\ua8fa\\ua8fc\\ua92e-\\ua92f\\ua95f\\ua9c1-\\ua9cd\\ua9de-\\ua9df\\uaa5c-\\uaa5f\\uaade-\\uaadf\\uaaf0-\\uaaf1\\uabeb\\ufd3e-\\ufd3f\\ufe10-\\ufe19\\ufe30-\\ufe52\\ufe54-\\ufe61\\ufe63\\ufe68\\ufe6a-\\ufe6b\\uff01-\\uff03\\uff05-\\uff0a\\uff0c-\\uff0f\\uff1a-\\uff1b\\uff1f-\\uff20\\uff3b-\\uff3d\\uff3f\\uff5b\\uff5d\\uff5f-\\uff65]|\\ud800[\\udd00-\\udd02\\udf9f\\udfd0]|\\ud801[\\udd6f]|\\ud802[\\udc57\\udd1f\\udd3f\\ude50-\\ude58\\ude7f\\udef0-\\udef6\\udf39-\\udf3f\\udf99-\\udf9c]|\\ud803[\\udf55-\\udf59]|\\ud804[\\udc47-\\udc4d\\udcbb-\\udcbc\\udcbe-\\udcc1\\udd40-\\udd43\\udd74-\\udd75\\uddc5-\\uddc8\\uddcd\\udddb\\udddd-\\udddf\\ude38-\\ude3d\\udea9]|\\ud805[\\udc4b-\\udc4f\\udc5b\\udc5d\\udcc6\\uddc1-\\uddd7\\ude41-\\ude43\\ude60-\\ude6c\\udf3c-\\udf3e]|\\ud806[\\udc3b\\udde2\\ude3f-\\ude46\\ude9a-\\ude9c\\ude9e-\\udea2]|\\ud807[\\udc41-\\udc45\\udc70-\\udc71\\udef7-\\udef8\\udfff]|\\ud809[\\udc70-\\udc74]|\\ud81a[\\ude6e-\\ude6f\\udef5\\udf37-\\udf3b\\udf44]|\\ud81b[\\ude97-\\ude9a\\udfe2]|\\ud82f[\\udc9f]|\\ud836[\\ude87-\\ude8b]|\\ud83a[\\udd5e-\\udd5f]"
};
}
});
var require_utils10 = __commonJS22({
"src/language-markdown/utils.js"(exports2, module22) {
"use strict";
var {
getLast
} = require_util();
var {
locStart,
locEnd
} = require_loc5();
var {
cjkPattern,
kPattern,
punctuationPattern
} = require_constants_evaluate();
var INLINE_NODE_TYPES = ["liquidNode", "inlineCode", "emphasis", "esComment", "strong", "delete", "wikiLink", "link", "linkReference", "image", "imageReference", "footnote", "footnoteReference", "sentence", "whitespace", "word", "break", "inlineMath"];
var INLINE_NODE_WRAPPER_TYPES = [...INLINE_NODE_TYPES, "tableCell", "paragraph", "heading"];
var kRegex = new RegExp(kPattern);
var punctuationRegex = new RegExp(punctuationPattern);
function splitText(text, options) {
const KIND_NON_CJK = "non-cjk";
const KIND_CJ_LETTER = "cj-letter";
const KIND_K_LETTER = "k-letter";
const KIND_CJK_PUNCTUATION = "cjk-punctuation";
const nodes = [];
const tokens = (options.proseWrap === "preserve" ? text : text.replace(new RegExp(`(${cjkPattern})
(${cjkPattern})`, "g"), "$1$2")).split(/([\t\n ]+)/);
for (const [index, token] of tokens.entries()) {
if (index % 2 === 1) {
nodes.push({
type: "whitespace",
value: /\n/.test(token) ? "\n" : " "
});
continue;
}
if ((index === 0 || index === tokens.length - 1) && token === "") {
continue;
}
const innerTokens = token.split(new RegExp(`(${cjkPattern})`));
for (const [innerIndex, innerToken] of innerTokens.entries()) {
if ((innerIndex === 0 || innerIndex === innerTokens.length - 1) && innerToken === "") {
continue;
}
if (innerIndex % 2 === 0) {
if (innerToken !== "") {
appendNode({
type: "word",
value: innerToken,
kind: KIND_NON_CJK,
hasLeadingPunctuation: punctuationRegex.test(innerToken[0]),
hasTrailingPunctuation: punctuationRegex.test(getLast(innerToken))
});
}
continue;
}
appendNode(punctuationRegex.test(innerToken) ? {
type: "word",
value: innerToken,
kind: KIND_CJK_PUNCTUATION,
hasLeadingPunctuation: true,
hasTrailingPunctuation: true
} : {
type: "word",
value: innerToken,
kind: kRegex.test(innerToken) ? KIND_K_LETTER : KIND_CJ_LETTER,
hasLeadingPunctuation: false,
hasTrailingPunctuation: false
});
}
}
return nodes;
function appendNode(node) {
const lastNode = getLast(nodes);
if (lastNode && lastNode.type === "word") {
if (lastNode.kind === KIND_NON_CJK && node.kind === KIND_CJ_LETTER && !lastNode.hasTrailingPunctuation || lastNode.kind === KIND_CJ_LETTER && node.kind === KIND_NON_CJK && !node.hasLeadingPunctuation) {
nodes.push({
type: "whitespace",
value: " "
});
} else if (!isBetween(KIND_NON_CJK, KIND_CJK_PUNCTUATION) && ![lastNode.value, node.value].some((value) => /\u3000/.test(value))) {
nodes.push({
type: "whitespace",
value: ""
});
}
}
nodes.push(node);
function isBetween(kind1, kind2) {
return lastNode.kind === kind1 && node.kind === kind2 || lastNode.kind === kind2 && node.kind === kind1;
}
}
}
function getOrderedListItemInfo(orderListItem, originalText) {
const [, numberText, marker, leadingSpaces] = originalText.slice(orderListItem.position.start.offset, orderListItem.position.end.offset).match(/^\s*(\d+)(\.|\))(\s*)/);
return {
numberText,
marker,
leadingSpaces
};
}
function hasGitDiffFriendlyOrderedList(node, options) {
if (!node.ordered) {
return false;
}
if (node.children.length < 2) {
return false;
}
const firstNumber = Number(getOrderedListItemInfo(node.children[0], options.originalText).numberText);
const secondNumber = Number(getOrderedListItemInfo(node.children[1], options.originalText).numberText);
if (firstNumber === 0 && node.children.length > 2) {
const thirdNumber = Number(getOrderedListItemInfo(node.children[2], options.originalText).numberText);
return secondNumber === 1 && thirdNumber === 1;
}
return secondNumber === 1;
}
function getFencedCodeBlockValue(node, originalText) {
const {
value
} = node;
if (node.position.end.offset === originalText.length && value.endsWith("\n") && originalText.endsWith("\n")) {
return value.slice(0, -1);
}
return value;
}
function mapAst(ast, handler) {
return function preorder(node, index, parentStack) {
const newNode = Object.assign({}, handler(node, index, parentStack));
if (newNode.children) {
newNode.children = newNode.children.map((child, index2) => preorder(child, index2, [newNode, ...parentStack]));
}
return newNode;
}(ast, null, []);
}
function isAutolink(node) {
if ((node === null || node === void 0 ? void 0 : node.type) !== "link" || node.children.length !== 1) {
return false;
}
const [child] = node.children;
return locStart(node) === locStart(child) && locEnd(node) === locEnd(child);
}
module22.exports = {
mapAst,
splitText,
punctuationPattern,
getFencedCodeBlockValue,
getOrderedListItemInfo,
hasGitDiffFriendlyOrderedList,
INLINE_NODE_TYPES,
INLINE_NODE_WRAPPER_TYPES,
isAutolink
};
}
});
var require_embed3 = __commonJS22({
"src/language-markdown/embed.js"(exports2, module22) {
"use strict";
var {
inferParserByLanguage,
getMaxContinuousCount
} = require_util();
var {
builders: {
hardline,
markAsRoot
},
utils: {
replaceEndOfLine
}
} = require_doc();
var printFrontMatter = require_print();
var {
getFencedCodeBlockValue
} = require_utils10();
function embed(path, print, textToDoc, options) {
const node = path.getValue();
if (node.type === "code" && node.lang !== null) {
const parser = inferParserByLanguage(node.lang, options);
if (parser) {
const styleUnit = options.__inJsTemplate ? "~" : "`";
const style = styleUnit.repeat(Math.max(3, getMaxContinuousCount(node.value, styleUnit) + 1));
const newOptions = {
parser
};
if (node.lang === "tsx") {
newOptions.filepath = "dummy.tsx";
}
const doc2 = textToDoc(getFencedCodeBlockValue(node, options.originalText), newOptions, {
stripTrailingHardline: true
});
return markAsRoot([style, node.lang, node.meta ? " " + node.meta : "", hardline, replaceEndOfLine(doc2), hardline, style]);
}
}
switch (node.type) {
case "front-matter":
return printFrontMatter(node, textToDoc);
case "importExport":
return [textToDoc(node.value, {
parser: "babel"
}, {
stripTrailingHardline: true
}), hardline];
case "jsx":
return textToDoc(`<$>${node.value}$>`, {
parser: "__js_expression",
rootMarker: "mdx"
}, {
stripTrailingHardline: true
});
}
return null;
}
module22.exports = embed;
}
});
var require_pragma4 = __commonJS22({
"src/language-markdown/pragma.js"(exports2, module22) {
"use strict";
var parseFrontMatter = require_parse42();
var pragmas = ["format", "prettier"];
function startWithPragma(text) {
const pragma = `@(${pragmas.join("|")})`;
const regex = new RegExp([``, `{\\s*\\/\\*\\s*${pragma}\\s*\\*\\/\\s*}`, ``].join("|"), "m");
const matched = text.match(regex);
return (matched === null || matched === void 0 ? void 0 : matched.index) === 0;
}
module22.exports = {
startWithPragma,
hasPragma: (text) => startWithPragma(parseFrontMatter(text).content.trimStart()),
insertPragma: (text) => {
const extracted = parseFrontMatter(text);
const pragma = ``;
return extracted.frontMatter ? `${extracted.frontMatter.raw}
${pragma}
${extracted.content}` : `${pragma}
${extracted.content}`;
}
};
}
});
var require_print_preprocess2 = __commonJS22({
"src/language-markdown/print-preprocess.js"(exports2, module22) {
"use strict";
var getLast = require_get_last();
var {
getOrderedListItemInfo,
mapAst,
splitText
} = require_utils10();
var isSingleCharRegex = /^.$/su;
function preprocess(ast, options) {
ast = restoreUnescapedCharacter(ast, options);
ast = mergeContinuousTexts(ast);
ast = transformInlineCode(ast, options);
ast = transformIndentedCodeblockAndMarkItsParentList(ast, options);
ast = markAlignedList(ast, options);
ast = splitTextIntoSentences(ast, options);
ast = transformImportExport(ast);
ast = mergeContinuousImportExport(ast);
return ast;
}
function transformImportExport(ast) {
return mapAst(ast, (node) => {
if (node.type !== "import" && node.type !== "export") {
return node;
}
return Object.assign(Object.assign({}, node), {}, {
type: "importExport"
});
});
}
function transformInlineCode(ast, options) {
return mapAst(ast, (node) => {
if (node.type !== "inlineCode" || options.proseWrap === "preserve") {
return node;
}
return Object.assign(Object.assign({}, node), {}, {
value: node.value.replace(/\s+/g, " ")
});
});
}
function restoreUnescapedCharacter(ast, options) {
return mapAst(ast, (node) => node.type !== "text" || node.value === "*" || node.value === "_" || !isSingleCharRegex.test(node.value) || node.position.end.offset - node.position.start.offset === node.value.length ? node : Object.assign(Object.assign({}, node), {}, {
value: options.originalText.slice(node.position.start.offset, node.position.end.offset)
}));
}
function mergeContinuousImportExport(ast) {
return mergeChildren(ast, (prevNode, node) => prevNode.type === "importExport" && node.type === "importExport", (prevNode, node) => ({
type: "importExport",
value: prevNode.value + "\n\n" + node.value,
position: {
start: prevNode.position.start,
end: node.position.end
}
}));
}
function mergeChildren(ast, shouldMerge, mergeNode) {
return mapAst(ast, (node) => {
if (!node.children) {
return node;
}
const children = node.children.reduce((current, child) => {
const lastChild = getLast(current);
if (lastChild && shouldMerge(lastChild, child)) {
current.splice(-1, 1, mergeNode(lastChild, child));
} else {
current.push(child);
}
return current;
}, []);
return Object.assign(Object.assign({}, node), {}, {
children
});
});
}
function mergeContinuousTexts(ast) {
return mergeChildren(ast, (prevNode, node) => prevNode.type === "text" && node.type === "text", (prevNode, node) => ({
type: "text",
value: prevNode.value + node.value,
position: {
start: prevNode.position.start,
end: node.position.end
}
}));
}
function splitTextIntoSentences(ast, options) {
return mapAst(ast, (node, index, [parentNode]) => {
if (node.type !== "text") {
return node;
}
let {
value
} = node;
if (parentNode.type === "paragraph") {
if (index === 0) {
value = value.trimStart();
}
if (index === parentNode.children.length - 1) {
value = value.trimEnd();
}
}
return {
type: "sentence",
position: node.position,
children: splitText(value, options)
};
});
}
function transformIndentedCodeblockAndMarkItsParentList(ast, options) {
return mapAst(ast, (node, index, parentStack) => {
if (node.type === "code") {
const isIndented = /^\n?(?: {4,}|\t)/.test(options.originalText.slice(node.position.start.offset, node.position.end.offset));
node.isIndented = isIndented;
if (isIndented) {
for (let i = 0; i < parentStack.length; i++) {
const parent = parentStack[i];
if (parent.hasIndentedCodeblock) {
break;
}
if (parent.type === "list") {
parent.hasIndentedCodeblock = true;
}
}
}
}
return node;
});
}
function markAlignedList(ast, options) {
return mapAst(ast, (node, index, parentStack) => {
if (node.type === "list" && node.children.length > 0) {
for (let i = 0; i < parentStack.length; i++) {
const parent = parentStack[i];
if (parent.type === "list" && !parent.isAligned) {
node.isAligned = false;
return node;
}
}
node.isAligned = isAligned(node);
}
return node;
});
function getListItemStart(listItem) {
return listItem.children.length === 0 ? -1 : listItem.children[0].position.start.column - 1;
}
function isAligned(list) {
if (!list.ordered) {
return true;
}
const [firstItem, secondItem] = list.children;
const firstInfo = getOrderedListItemInfo(firstItem, options.originalText);
if (firstInfo.leadingSpaces.length > 1) {
return true;
}
const firstStart = getListItemStart(firstItem);
if (firstStart === -1) {
return false;
}
if (list.children.length === 1) {
return firstStart % options.tabWidth === 0;
}
const secondStart = getListItemStart(secondItem);
if (firstStart !== secondStart) {
return false;
}
if (firstStart % options.tabWidth === 0) {
return true;
}
const secondInfo = getOrderedListItemInfo(secondItem, options.originalText);
return secondInfo.leadingSpaces.length > 1;
}
}
module22.exports = preprocess;
}
});
var require_clean4 = __commonJS22({
"src/language-markdown/clean.js"(exports2, module22) {
"use strict";
var collapseWhiteSpace = require_collapse_white_space();
var {
isFrontMatterNode
} = require_util();
var {
startWithPragma
} = require_pragma4();
var ignoredProperties = /* @__PURE__ */ new Set(["position", "raw"]);
function clean(ast, newObj, parent) {
if (ast.type === "front-matter" || ast.type === "code" || ast.type === "yaml" || ast.type === "import" || ast.type === "export" || ast.type === "jsx") {
delete newObj.value;
}
if (ast.type === "list") {
delete newObj.isAligned;
}
if (ast.type === "list" || ast.type === "listItem") {
delete newObj.spread;
delete newObj.loose;
}
if (ast.type === "text") {
return null;
}
if (ast.type === "inlineCode") {
newObj.value = ast.value.replace(/[\t\n ]+/g, " ");
}
if (ast.type === "wikiLink") {
newObj.value = ast.value.trim().replace(/[\t\n]+/g, " ");
}
if (ast.type === "definition" || ast.type === "linkReference" || ast.type === "imageReference") {
newObj.label = collapseWhiteSpace(ast.label);
}
if ((ast.type === "definition" || ast.type === "link" || ast.type === "image") && ast.title) {
newObj.title = ast.title.replace(/\\(["')])/g, "$1");
}
if (parent && parent.type === "root" && parent.children.length > 0 && (parent.children[0] === ast || isFrontMatterNode(parent.children[0]) && parent.children[1] === ast) && ast.type === "html" && startWithPragma(ast.value)) {
return null;
}
}
clean.ignoredProperties = ignoredProperties;
module22.exports = clean;
}
});
var require_printer_markdown = __commonJS22({
"src/language-markdown/printer-markdown.js"(exports2, module22) {
"use strict";
var collapseWhiteSpace = require_collapse_white_space();
var {
getLast,
getMinNotPresentContinuousCount,
getMaxContinuousCount,
getStringWidth,
isNonEmptyArray
} = require_util();
var {
builders: {
breakParent,
join,
line,
literalline,
markAsRoot,
hardline,
softline,
ifBreak,
fill,
align,
indent,
group,
hardlineWithoutBreakParent
},
utils: {
normalizeDoc,
replaceTextEndOfLine
},
printer: {
printDocToString
}
} = require_doc();
var embed = require_embed3();
var {
insertPragma
} = require_pragma4();
var {
locStart,
locEnd
} = require_loc5();
var preprocess = require_print_preprocess2();
var clean = require_clean4();
var {
getFencedCodeBlockValue,
hasGitDiffFriendlyOrderedList,
splitText,
punctuationPattern,
INLINE_NODE_TYPES,
INLINE_NODE_WRAPPER_TYPES,
isAutolink
} = require_utils10();
var TRAILING_HARDLINE_NODES = /* @__PURE__ */ new Set(["importExport"]);
var SINGLE_LINE_NODE_TYPES = ["heading", "tableCell", "link", "wikiLink"];
var SIBLING_NODE_TYPES = /* @__PURE__ */ new Set(["listItem", "definition", "footnoteDefinition"]);
function genericPrint(path, options, print) {
const node = path.getValue();
if (shouldRemainTheSameContent(path)) {
return splitText(options.originalText.slice(node.position.start.offset, node.position.end.offset), options).map((node2) => node2.type === "word" ? node2.value : node2.value === "" ? "" : printLine(path, node2.value, options));
}
switch (node.type) {
case "front-matter":
return options.originalText.slice(node.position.start.offset, node.position.end.offset);
case "root":
if (node.children.length === 0) {
return "";
}
return [normalizeDoc(printRoot(path, options, print)), !TRAILING_HARDLINE_NODES.has(getLastDescendantNode(node).type) ? hardline : ""];
case "paragraph":
return printChildren(path, options, print, {
postprocessor: fill
});
case "sentence":
return printChildren(path, options, print);
case "word": {
let escapedValue = node.value.replace(/\*/g, "\\$&").replace(new RegExp([`(^|${punctuationPattern})(_+)`, `(_+)(${punctuationPattern}|$)`].join("|"), "g"), (_, text1, underscore1, underscore2, text2) => (underscore1 ? `${text1}${underscore1}` : `${underscore2}${text2}`).replace(/_/g, "\\_"));
const isFirstSentence = (node2, name, index) => node2.type === "sentence" && index === 0;
const isLastChildAutolink = (node2, name, index) => isAutolink(node2.children[index - 1]);
if (escapedValue !== node.value && (path.match(void 0, isFirstSentence, isLastChildAutolink) || path.match(void 0, isFirstSentence, (node2, name, index) => node2.type === "emphasis" && index === 0, isLastChildAutolink))) {
escapedValue = escapedValue.replace(/^(\\?[*_])+/, (prefix) => prefix.replace(/\\/g, ""));
}
return escapedValue;
}
case "whitespace": {
const parentNode = path.getParentNode();
const index = parentNode.children.indexOf(node);
const nextNode = parentNode.children[index + 1];
const proseWrap = nextNode && /^>|^(?:[*+-]|#{1,6}|\d+[).])$/.test(nextNode.value) ? "never" : options.proseWrap;
return printLine(path, node.value, {
proseWrap
});
}
case "emphasis": {
let style;
if (isAutolink(node.children[0])) {
style = options.originalText[node.position.start.offset];
} else {
const parentNode = path.getParentNode();
const index = parentNode.children.indexOf(node);
const prevNode = parentNode.children[index - 1];
const nextNode = parentNode.children[index + 1];
const hasPrevOrNextWord = prevNode && prevNode.type === "sentence" && prevNode.children.length > 0 && getLast(prevNode.children).type === "word" && !getLast(prevNode.children).hasTrailingPunctuation || nextNode && nextNode.type === "sentence" && nextNode.children.length > 0 && nextNode.children[0].type === "word" && !nextNode.children[0].hasLeadingPunctuation;
style = hasPrevOrNextWord || getAncestorNode(path, "emphasis") ? "*" : "_";
}
return [style, printChildren(path, options, print), style];
}
case "strong":
return ["**", printChildren(path, options, print), "**"];
case "delete":
return ["~~", printChildren(path, options, print), "~~"];
case "inlineCode": {
const backtickCount = getMinNotPresentContinuousCount(node.value, "`");
const style = "`".repeat(backtickCount || 1);
const gap = backtickCount && !/^\s/.test(node.value) ? " " : "";
return [style, gap, node.value, gap, style];
}
case "wikiLink": {
let contents = "";
if (options.proseWrap === "preserve") {
contents = node.value;
} else {
contents = node.value.replace(/[\t\n]+/g, " ");
}
return ["[[", contents, "]]"];
}
case "link":
switch (options.originalText[node.position.start.offset]) {
case "<": {
const mailto = "mailto:";
const url = node.url.startsWith(mailto) && options.originalText.slice(node.position.start.offset + 1, node.position.start.offset + 1 + mailto.length) !== mailto ? node.url.slice(mailto.length) : node.url;
return ["<", url, ">"];
}
case "[":
return ["[", printChildren(path, options, print), "](", printUrl(node.url, ")"), printTitle(node.title, options), ")"];
default:
return options.originalText.slice(node.position.start.offset, node.position.end.offset);
}
case "image":
return [""), printTitle(node.title, options), ")"];
case "blockquote":
return ["> ", align("> ", printChildren(path, options, print))];
case "heading":
return ["#".repeat(node.depth) + " ", printChildren(path, options, print)];
case "code": {
if (node.isIndented) {
const alignment = " ".repeat(4);
return align(alignment, [alignment, ...replaceTextEndOfLine(node.value, hardline)]);
}
const styleUnit = options.__inJsTemplate ? "~" : "`";
const style = styleUnit.repeat(Math.max(3, getMaxContinuousCount(node.value, styleUnit) + 1));
return [style, node.lang || "", node.meta ? " " + node.meta : "", hardline, ...replaceTextEndOfLine(getFencedCodeBlockValue(node, options.originalText), hardline), hardline, style];
}
case "html": {
const parentNode = path.getParentNode();
const value = parentNode.type === "root" && getLast(parentNode.children) === node ? node.value.trimEnd() : node.value;
const isHtmlComment = /^$/s.test(value);
return replaceTextEndOfLine(value, isHtmlComment ? hardline : markAsRoot(literalline));
}
case "list": {
const nthSiblingIndex = getNthListSiblingIndex(node, path.getParentNode());
const isGitDiffFriendlyOrderedList = hasGitDiffFriendlyOrderedList(node, options);
return printChildren(path, options, print, {
processor: (childPath, index) => {
const prefix = getPrefix();
const childNode = childPath.getValue();
if (childNode.children.length === 2 && childNode.children[1].type === "html" && childNode.children[0].position.start.column !== childNode.children[1].position.start.column) {
return [prefix, printListItem(childPath, options, print, prefix)];
}
return [prefix, align(" ".repeat(prefix.length), printListItem(childPath, options, print, prefix))];
function getPrefix() {
const rawPrefix = node.ordered ? (index === 0 ? node.start : isGitDiffFriendlyOrderedList ? 1 : node.start + index) + (nthSiblingIndex % 2 === 0 ? ". " : ") ") : nthSiblingIndex % 2 === 0 ? "- " : "* ";
return node.isAligned || node.hasIndentedCodeblock ? alignListPrefix(rawPrefix, options) : rawPrefix;
}
}
});
}
case "thematicBreak": {
const counter = getAncestorCounter(path, "list");
if (counter === -1) {
return "---";
}
const nthSiblingIndex = getNthListSiblingIndex(path.getParentNode(counter), path.getParentNode(counter + 1));
return nthSiblingIndex % 2 === 0 ? "***" : "---";
}
case "linkReference":
return ["[", printChildren(path, options, print), "]", node.referenceType === "full" ? printLinkReference(node) : node.referenceType === "collapsed" ? "[]" : ""];
case "imageReference":
switch (node.referenceType) {
case "full":
return ["![", node.alt || "", "]", printLinkReference(node)];
default:
return ["![", node.alt, "]", node.referenceType === "collapsed" ? "[]" : ""];
}
case "definition": {
const lineOrSpace = options.proseWrap === "always" ? line : " ";
return group([printLinkReference(node), ":", indent([lineOrSpace, printUrl(node.url), node.title === null ? "" : [lineOrSpace, printTitle(node.title, options, false)]])]);
}
case "footnote":
return ["[^", printChildren(path, options, print), "]"];
case "footnoteReference":
return printFootnoteReference(node);
case "footnoteDefinition": {
const nextNode = path.getParentNode().children[path.getName() + 1];
const shouldInlineFootnote = node.children.length === 1 && node.children[0].type === "paragraph" && (options.proseWrap === "never" || options.proseWrap === "preserve" && node.children[0].position.start.line === node.children[0].position.end.line);
return [printFootnoteReference(node), ": ", shouldInlineFootnote ? printChildren(path, options, print) : group([align(" ".repeat(4), printChildren(path, options, print, {
processor: (childPath, index) => index === 0 ? group([softline, print()]) : print()
})), nextNode && nextNode.type === "footnoteDefinition" ? softline : ""])];
}
case "table":
return printTable(path, options, print);
case "tableCell":
return printChildren(path, options, print);
case "break":
return /\s/.test(options.originalText[node.position.start.offset]) ? [" ", markAsRoot(literalline)] : ["\\", hardline];
case "liquidNode":
return replaceTextEndOfLine(node.value, hardline);
case "importExport":
return [node.value, hardline];
case "esComment":
return ["{/* ", node.value, " */}"];
case "jsx":
return node.value;
case "math":
return ["$$", hardline, node.value ? [...replaceTextEndOfLine(node.value, hardline), hardline] : "", "$$"];
case "inlineMath": {
return options.originalText.slice(locStart(node), locEnd(node));
}
case "tableRow":
case "listItem":
default:
throw new Error(`Unknown markdown type ${JSON.stringify(node.type)}`);
}
}
function printListItem(path, options, print, listPrefix) {
const node = path.getValue();
const prefix = node.checked === null ? "" : node.checked ? "[x] " : "[ ] ";
return [prefix, printChildren(path, options, print, {
processor: (childPath, index) => {
if (index === 0 && childPath.getValue().type !== "list") {
return align(" ".repeat(prefix.length), print());
}
const alignment = " ".repeat(clamp(options.tabWidth - listPrefix.length, 0, 3));
return [alignment, align(alignment, print())];
}
})];
}
function alignListPrefix(prefix, options) {
const additionalSpaces = getAdditionalSpaces();
return prefix + " ".repeat(additionalSpaces >= 4 ? 0 : additionalSpaces);
function getAdditionalSpaces() {
const restSpaces = prefix.length % options.tabWidth;
return restSpaces === 0 ? 0 : options.tabWidth - restSpaces;
}
}
function getNthListSiblingIndex(node, parentNode) {
return getNthSiblingIndex(node, parentNode, (siblingNode) => siblingNode.ordered === node.ordered);
}
function getNthSiblingIndex(node, parentNode, condition) {
let index = -1;
for (const childNode of parentNode.children) {
if (childNode.type === node.type && condition(childNode)) {
index++;
} else {
index = -1;
}
if (childNode === node) {
return index;
}
}
}
function getAncestorCounter(path, typeOrTypes) {
const types = Array.isArray(typeOrTypes) ? typeOrTypes : [typeOrTypes];
let counter = -1;
let ancestorNode;
while (ancestorNode = path.getParentNode(++counter)) {
if (types.includes(ancestorNode.type)) {
return counter;
}
}
return -1;
}
function getAncestorNode(path, typeOrTypes) {
const counter = getAncestorCounter(path, typeOrTypes);
return counter === -1 ? null : path.getParentNode(counter);
}
function printLine(path, value, options) {
if (options.proseWrap === "preserve" && value === "\n") {
return hardline;
}
const isBreakable = options.proseWrap === "always" && !getAncestorNode(path, SINGLE_LINE_NODE_TYPES);
return value !== "" ? isBreakable ? line : " " : isBreakable ? softline : "";
}
function printTable(path, options, print) {
const node = path.getValue();
const columnMaxWidths = [];
const contents = path.map((rowPath) => rowPath.map((cellPath, columnIndex) => {
const text = printDocToString(print(), options).formatted;
const width = getStringWidth(text);
columnMaxWidths[columnIndex] = Math.max(columnMaxWidths[columnIndex] || 3, width);
return {
text,
width
};
}, "children"), "children");
const alignedTable = printTableContents(false);
if (options.proseWrap !== "never") {
return [breakParent, alignedTable];
}
const compactTable = printTableContents(true);
return [breakParent, group(ifBreak(compactTable, alignedTable))];
function printTableContents(isCompact) {
const parts = [printRow(contents[0], isCompact), printAlign(isCompact)];
if (contents.length > 1) {
parts.push(join(hardlineWithoutBreakParent, contents.slice(1).map((rowContents) => printRow(rowContents, isCompact))));
}
return join(hardlineWithoutBreakParent, parts);
}
function printAlign(isCompact) {
const align2 = columnMaxWidths.map((width, index) => {
const align3 = node.align[index];
const first = align3 === "center" || align3 === "left" ? ":" : "-";
const last = align3 === "center" || align3 === "right" ? ":" : "-";
const middle = isCompact ? "-" : "-".repeat(width - 2);
return `${first}${middle}${last}`;
});
return `| ${align2.join(" | ")} |`;
}
function printRow(rowContents, isCompact) {
const columns = rowContents.map(({
text,
width
}, columnIndex) => {
if (isCompact) {
return text;
}
const spaces = columnMaxWidths[columnIndex] - width;
const align2 = node.align[columnIndex];
let before = 0;
if (align2 === "right") {
before = spaces;
} else if (align2 === "center") {
before = Math.floor(spaces / 2);
}
const after = spaces - before;
return `${" ".repeat(before)}${text}${" ".repeat(after)}`;
});
return `| ${columns.join(" | ")} |`;
}
}
function printRoot(path, options, print) {
const ignoreRanges = [];
let ignoreStart = null;
const {
children
} = path.getValue();
for (const [index, childNode] of children.entries()) {
switch (isPrettierIgnore(childNode)) {
case "start":
if (ignoreStart === null) {
ignoreStart = {
index,
offset: childNode.position.end.offset
};
}
break;
case "end":
if (ignoreStart !== null) {
ignoreRanges.push({
start: ignoreStart,
end: {
index,
offset: childNode.position.start.offset
}
});
ignoreStart = null;
}
break;
default:
break;
}
}
return printChildren(path, options, print, {
processor: (childPath, index) => {
if (ignoreRanges.length > 0) {
const ignoreRange = ignoreRanges[0];
if (index === ignoreRange.start.index) {
return [printIgnoreComment(children[ignoreRange.start.index]), options.originalText.slice(ignoreRange.start.offset, ignoreRange.end.offset), printIgnoreComment(children[ignoreRange.end.index])];
}
if (ignoreRange.start.index < index && index < ignoreRange.end.index) {
return false;
}
if (index === ignoreRange.end.index) {
ignoreRanges.shift();
return false;
}
}
return print();
}
});
}
function printChildren(path, options, print, events = {}) {
const {
postprocessor
} = events;
const processor = events.processor || (() => print());
const node = path.getValue();
const parts = [];
let lastChildNode;
path.each((childPath, index) => {
const childNode = childPath.getValue();
const result = processor(childPath, index);
if (result !== false) {
const data = {
parts,
prevNode: lastChildNode,
parentNode: node,
options
};
if (shouldPrePrintHardline(childNode, data)) {
parts.push(hardline);
if (lastChildNode && TRAILING_HARDLINE_NODES.has(lastChildNode.type)) {
if (shouldPrePrintTripleHardline(childNode, data)) {
parts.push(hardline);
}
} else {
if (shouldPrePrintDoubleHardline(childNode, data) || shouldPrePrintTripleHardline(childNode, data)) {
parts.push(hardline);
}
if (shouldPrePrintTripleHardline(childNode, data)) {
parts.push(hardline);
}
}
}
parts.push(result);
lastChildNode = childNode;
}
}, "children");
return postprocessor ? postprocessor(parts) : parts;
}
function printIgnoreComment(node) {
if (node.type === "html") {
return node.value;
}
if (node.type === "paragraph" && Array.isArray(node.children) && node.children.length === 1 && node.children[0].type === "esComment") {
return ["{/* ", node.children[0].value, " */}"];
}
}
function getLastDescendantNode(node) {
let current = node;
while (isNonEmptyArray(current.children)) {
current = getLast(current.children);
}
return current;
}
function isPrettierIgnore(node) {
let match;
if (node.type === "html") {
match = node.value.match(/^$/);
} else {
let comment;
if (node.type === "esComment") {
comment = node;
} else if (node.type === "paragraph" && node.children.length === 1 && node.children[0].type === "esComment") {
comment = node.children[0];
}
if (comment) {
match = comment.value.match(/^prettier-ignore(?:-(start|end))?$/);
}
}
return match ? match[1] || "next" : false;
}
function shouldPrePrintHardline(node, data) {
const isFirstNode = data.parts.length === 0;
const isInlineNode = INLINE_NODE_TYPES.includes(node.type);
const isInlineHTML = node.type === "html" && INLINE_NODE_WRAPPER_TYPES.includes(data.parentNode.type);
return !isFirstNode && !isInlineNode && !isInlineHTML;
}
function shouldPrePrintDoubleHardline(node, data) {
var _data$prevNode, _data$prevNode2, _data$prevNode3;
const isSequence = (data.prevNode && data.prevNode.type) === node.type;
const isSiblingNode = isSequence && SIBLING_NODE_TYPES.has(node.type);
const isInTightListItem = data.parentNode.type === "listItem" && !data.parentNode.loose;
const isPrevNodeLooseListItem = ((_data$prevNode = data.prevNode) === null || _data$prevNode === void 0 ? void 0 : _data$prevNode.type) === "listItem" && data.prevNode.loose;
const isPrevNodePrettierIgnore = isPrettierIgnore(data.prevNode) === "next";
const isBlockHtmlWithoutBlankLineBetweenPrevHtml = node.type === "html" && ((_data$prevNode2 = data.prevNode) === null || _data$prevNode2 === void 0 ? void 0 : _data$prevNode2.type) === "html" && data.prevNode.position.end.line + 1 === node.position.start.line;
const isHtmlDirectAfterListItem = node.type === "html" && data.parentNode.type === "listItem" && ((_data$prevNode3 = data.prevNode) === null || _data$prevNode3 === void 0 ? void 0 : _data$prevNode3.type) === "paragraph" && data.prevNode.position.end.line + 1 === node.position.start.line;
return isPrevNodeLooseListItem || !(isSiblingNode || isInTightListItem || isPrevNodePrettierIgnore || isBlockHtmlWithoutBlankLineBetweenPrevHtml || isHtmlDirectAfterListItem);
}
function shouldPrePrintTripleHardline(node, data) {
const isPrevNodeList = data.prevNode && data.prevNode.type === "list";
const isIndentedCode = node.type === "code" && node.isIndented;
return isPrevNodeList && isIndentedCode;
}
function shouldRemainTheSameContent(path) {
const ancestorNode = getAncestorNode(path, ["linkReference", "imageReference"]);
return ancestorNode && (ancestorNode.type !== "linkReference" || ancestorNode.referenceType !== "full");
}
function printUrl(url, dangerousCharOrChars = []) {
const dangerousChars = [" ", ...Array.isArray(dangerousCharOrChars) ? dangerousCharOrChars : [dangerousCharOrChars]];
return new RegExp(dangerousChars.map((x) => `\\${x}`).join("|")).test(url) ? `<${url}>` : url;
}
function printTitle(title, options, printSpace = true) {
if (!title) {
return "";
}
if (printSpace) {
return " " + printTitle(title, options, false);
}
title = title.replace(/\\(["')])/g, "$1");
if (title.includes('"') && title.includes("'") && !title.includes(")")) {
return `(${title})`;
}
const singleCount = title.split("'").length - 1;
const doubleCount = title.split('"').length - 1;
const quote2 = singleCount > doubleCount ? '"' : doubleCount > singleCount ? "'" : options.singleQuote ? "'" : '"';
title = title.replace(/\\/, "\\\\");
title = title.replace(new RegExp(`(${quote2})`, "g"), "\\$1");
return `${quote2}${title}${quote2}`;
}
function clamp(value, min, max) {
return value < min ? min : value > max ? max : value;
}
function hasPrettierIgnore(path) {
const index = Number(path.getName());
if (index === 0) {
return false;
}
const prevNode = path.getParentNode().children[index - 1];
return isPrettierIgnore(prevNode) === "next";
}
function printLinkReference(node) {
return `[${collapseWhiteSpace(node.label)}]`;
}
function printFootnoteReference(node) {
return `[^${node.label}]`;
}
module22.exports = {
preprocess,
print: genericPrint,
embed,
massageAstNode: clean,
hasPrettierIgnore,
insertPragma
};
}
});
var require_options5 = __commonJS22({
"src/language-markdown/options.js"(exports2, module22) {
"use strict";
var commonOptions = require_common_options();
module22.exports = {
proseWrap: commonOptions.proseWrap,
singleQuote: commonOptions.singleQuote
};
}
});
var require_parsers5 = __commonJS22({
"src/language-markdown/parsers.js"(exports2, module22) {
"use strict";
module22.exports = {
get remark() {
return require_parser_markdown().parsers.remark;
},
get markdown() {
return require_parser_markdown().parsers.remark;
},
get mdx() {
return require_parser_markdown().parsers.mdx;
}
};
}
});
var require_Markdown = __commonJS22({
"node_modules/linguist-languages/data/Markdown.json"(exports2, module22) {
module22.exports = {
name: "Markdown",
type: "prose",
color: "#083fa1",
aliases: ["pandoc"],
aceMode: "markdown",
codemirrorMode: "gfm",
codemirrorMimeType: "text/x-gfm",
wrap: true,
extensions: [".md", ".livemd", ".markdown", ".mdown", ".mdwn", ".mdx", ".mkd", ".mkdn", ".mkdown", ".ronn", ".scd", ".workbook"],
filenames: ["contents.lr"],
tmScope: "source.gfm",
languageId: 222
};
}
});
var require_language_markdown = __commonJS22({
"src/language-markdown/index.js"(exports2, module22) {
"use strict";
var createLanguage = require_create_language();
var printer = require_printer_markdown();
var options = require_options5();
var parsers = require_parsers5();
var languages = [createLanguage(require_Markdown(), (data) => ({
since: "1.8.0",
parsers: ["markdown"],
vscodeLanguageIds: ["markdown"],
filenames: [...data.filenames, "README"],
extensions: data.extensions.filter((extension) => extension !== ".mdx")
})), createLanguage(require_Markdown(), () => ({
name: "MDX",
since: "1.15.0",
parsers: ["mdx"],
vscodeLanguageIds: ["mdx"],
filenames: [],
extensions: [".mdx"]
}))];
var printers = {
mdast: printer
};
module22.exports = {
languages,
options,
printers,
parsers
};
}
});
var require_clean5 = __commonJS22({
"src/language-html/clean.js"(exports2, module22) {
"use strict";
var {
isFrontMatterNode
} = require_util();
var ignoredProperties = /* @__PURE__ */ new Set(["sourceSpan", "startSourceSpan", "endSourceSpan", "nameSpan", "valueSpan"]);
function clean(ast, newNode) {
if (ast.type === "text" || ast.type === "comment") {
return null;
}
if (isFrontMatterNode(ast) || ast.type === "yaml" || ast.type === "toml") {
return null;
}
if (ast.type === "attribute") {
delete newNode.value;
}
if (ast.type === "docType") {
delete newNode.value;
}
}
clean.ignoredProperties = ignoredProperties;
module22.exports = clean;
}
});
var require_constants_evaluate2 = __commonJS22({
"src/language-html/constants.evaluate.js"(exports2, module22) {
module22.exports = {
CSS_DISPLAY_TAGS: {
area: "none",
base: "none",
basefont: "none",
datalist: "none",
head: "none",
link: "none",
meta: "none",
noembed: "none",
noframes: "none",
param: "block",
rp: "none",
script: "block",
source: "block",
style: "none",
template: "inline",
track: "block",
title: "none",
html: "block",
body: "block",
address: "block",
blockquote: "block",
center: "block",
div: "block",
figure: "block",
figcaption: "block",
footer: "block",
form: "block",
header: "block",
hr: "block",
legend: "block",
listing: "block",
main: "block",
p: "block",
plaintext: "block",
pre: "block",
xmp: "block",
slot: "contents",
ruby: "ruby",
rt: "ruby-text",
article: "block",
aside: "block",
h1: "block",
h2: "block",
h3: "block",
h4: "block",
h5: "block",
h6: "block",
hgroup: "block",
nav: "block",
section: "block",
dir: "block",
dd: "block",
dl: "block",
dt: "block",
ol: "block",
ul: "block",
li: "list-item",
table: "table",
caption: "table-caption",
colgroup: "table-column-group",
col: "table-column",
thead: "table-header-group",
tbody: "table-row-group",
tfoot: "table-footer-group",
tr: "table-row",
td: "table-cell",
th: "table-cell",
fieldset: "block",
button: "inline-block",
details: "block",
summary: "block",
dialog: "block",
meter: "inline-block",
progress: "inline-block",
object: "inline-block",
video: "inline-block",
audio: "inline-block",
select: "inline-block",
option: "block",
optgroup: "block"
},
CSS_DISPLAY_DEFAULT: "inline",
CSS_WHITE_SPACE_TAGS: {
listing: "pre",
plaintext: "pre",
pre: "pre",
xmp: "pre",
nobr: "nowrap",
table: "initial",
textarea: "pre-wrap"
},
CSS_WHITE_SPACE_DEFAULT: "normal"
};
}
});
var require_is_unknown_namespace = __commonJS22({
"src/language-html/utils/is-unknown-namespace.js"(exports2, module22) {
"use strict";
function isUnknownNamespace(node) {
return node.type === "element" && !node.hasExplicitNamespace && !["html", "svg"].includes(node.namespace);
}
module22.exports = isUnknownNamespace;
}
});
var require_utils11 = __commonJS22({
"src/language-html/utils/index.js"(exports2, module22) {
"use strict";
var {
inferParserByLanguage,
isFrontMatterNode
} = require_util();
var {
builders: {
line,
hardline,
join
},
utils: {
getDocParts,
replaceTextEndOfLine
}
} = require_doc();
var {
CSS_DISPLAY_TAGS,
CSS_DISPLAY_DEFAULT,
CSS_WHITE_SPACE_TAGS,
CSS_WHITE_SPACE_DEFAULT
} = require_constants_evaluate2();
var isUnknownNamespace = require_is_unknown_namespace();
var HTML_WHITESPACE = /* @__PURE__ */ new Set([" ", "\n", "\f", "\r", " "]);
var htmlTrimStart = (string) => string.replace(/^[\t\n\f\r ]+/, "");
var htmlTrimEnd = (string) => string.replace(/[\t\n\f\r ]+$/, "");
var htmlTrim = (string) => htmlTrimStart(htmlTrimEnd(string));
var htmlTrimLeadingBlankLines = (string) => string.replace(/^[\t\f\r ]*\n/g, "");
var htmlTrimPreserveIndentation = (string) => htmlTrimLeadingBlankLines(htmlTrimEnd(string));
var splitByHtmlWhitespace = (string) => string.split(/[\t\n\f\r ]+/);
var getLeadingHtmlWhitespace = (string) => string.match(/^[\t\n\f\r ]*/)[0];
var getLeadingAndTrailingHtmlWhitespace = (string) => {
const [, leadingWhitespace, text, trailingWhitespace] = string.match(/^([\t\n\f\r ]*)(.*?)([\t\n\f\r ]*)$/s);
return {
leadingWhitespace,
trailingWhitespace,
text
};
};
var hasHtmlWhitespace = (string) => /[\t\n\f\r ]/.test(string);
function shouldPreserveContent(node, options) {
if (node.type === "ieConditionalComment" && node.lastChild && !node.lastChild.isSelfClosing && !node.lastChild.endSourceSpan) {
return true;
}
if (node.type === "ieConditionalComment" && !node.complete) {
return true;
}
if (isPreLikeNode(node) && node.children.some((child) => child.type !== "text" && child.type !== "interpolation")) {
return true;
}
if (isVueNonHtmlBlock(node, options) && !isScriptLikeTag(node) && node.type !== "interpolation") {
return true;
}
return false;
}
function hasPrettierIgnore(node) {
if (node.type === "attribute") {
return false;
}
if (!node.parent) {
return false;
}
if (!node.prev) {
return false;
}
return isPrettierIgnore(node.prev);
}
function isPrettierIgnore(node) {
return node.type === "comment" && node.value.trim() === "prettier-ignore";
}
function isTextLikeNode(node) {
return node.type === "text" || node.type === "comment";
}
function isScriptLikeTag(node) {
return node.type === "element" && (node.fullName === "script" || node.fullName === "style" || node.fullName === "svg:style" || isUnknownNamespace(node) && (node.name === "script" || node.name === "style"));
}
function canHaveInterpolation(node) {
return node.children && !isScriptLikeTag(node);
}
function isWhitespaceSensitiveNode(node) {
return isScriptLikeTag(node) || node.type === "interpolation" || isIndentationSensitiveNode(node);
}
function isIndentationSensitiveNode(node) {
return getNodeCssStyleWhiteSpace(node).startsWith("pre");
}
function isLeadingSpaceSensitiveNode(node, options) {
const isLeadingSpaceSensitive = _isLeadingSpaceSensitiveNode();
if (isLeadingSpaceSensitive && !node.prev && node.parent && node.parent.tagDefinition && node.parent.tagDefinition.ignoreFirstLf) {
return node.type === "interpolation";
}
return isLeadingSpaceSensitive;
function _isLeadingSpaceSensitiveNode() {
if (isFrontMatterNode(node)) {
return false;
}
if ((node.type === "text" || node.type === "interpolation") && node.prev && (node.prev.type === "text" || node.prev.type === "interpolation")) {
return true;
}
if (!node.parent || node.parent.cssDisplay === "none") {
return false;
}
if (isPreLikeNode(node.parent)) {
return true;
}
if (!node.prev && (node.parent.type === "root" || isPreLikeNode(node) && node.parent || isScriptLikeTag(node.parent) || isVueCustomBlock(node.parent, options) || !isFirstChildLeadingSpaceSensitiveCssDisplay(node.parent.cssDisplay))) {
return false;
}
if (node.prev && !isNextLeadingSpaceSensitiveCssDisplay(node.prev.cssDisplay)) {
return false;
}
return true;
}
}
function isTrailingSpaceSensitiveNode(node, options) {
if (isFrontMatterNode(node)) {
return false;
}
if ((node.type === "text" || node.type === "interpolation") && node.next && (node.next.type === "text" || node.next.type === "interpolation")) {
return true;
}
if (!node.parent || node.parent.cssDisplay === "none") {
return false;
}
if (isPreLikeNode(node.parent)) {
return true;
}
if (!node.next && (node.parent.type === "root" || isPreLikeNode(node) && node.parent || isScriptLikeTag(node.parent) || isVueCustomBlock(node.parent, options) || !isLastChildTrailingSpaceSensitiveCssDisplay(node.parent.cssDisplay))) {
return false;
}
if (node.next && !isPrevTrailingSpaceSensitiveCssDisplay(node.next.cssDisplay)) {
return false;
}
return true;
}
function isDanglingSpaceSensitiveNode(node) {
return isDanglingSpaceSensitiveCssDisplay(node.cssDisplay) && !isScriptLikeTag(node);
}
function forceNextEmptyLine(node) {
return isFrontMatterNode(node) || node.next && node.sourceSpan.end && node.sourceSpan.end.line + 1 < node.next.sourceSpan.start.line;
}
function forceBreakContent(node) {
return forceBreakChildren(node) || node.type === "element" && node.children.length > 0 && (["body", "script", "style"].includes(node.name) || node.children.some((child) => hasNonTextChild(child))) || node.firstChild && node.firstChild === node.lastChild && node.firstChild.type !== "text" && hasLeadingLineBreak(node.firstChild) && (!node.lastChild.isTrailingSpaceSensitive || hasTrailingLineBreak(node.lastChild));
}
function forceBreakChildren(node) {
return node.type === "element" && node.children.length > 0 && (["html", "head", "ul", "ol", "select"].includes(node.name) || node.cssDisplay.startsWith("table") && node.cssDisplay !== "table-cell");
}
function preferHardlineAsLeadingSpaces(node) {
return preferHardlineAsSurroundingSpaces(node) || node.prev && preferHardlineAsTrailingSpaces(node.prev) || hasSurroundingLineBreak(node);
}
function preferHardlineAsTrailingSpaces(node) {
return preferHardlineAsSurroundingSpaces(node) || node.type === "element" && node.fullName === "br" || hasSurroundingLineBreak(node);
}
function hasSurroundingLineBreak(node) {
return hasLeadingLineBreak(node) && hasTrailingLineBreak(node);
}
function hasLeadingLineBreak(node) {
return node.hasLeadingSpaces && (node.prev ? node.prev.sourceSpan.end.line < node.sourceSpan.start.line : node.parent.type === "root" || node.parent.startSourceSpan.end.line < node.sourceSpan.start.line);
}
function hasTrailingLineBreak(node) {
return node.hasTrailingSpaces && (node.next ? node.next.sourceSpan.start.line > node.sourceSpan.end.line : node.parent.type === "root" || node.parent.endSourceSpan && node.parent.endSourceSpan.start.line > node.sourceSpan.end.line);
}
function preferHardlineAsSurroundingSpaces(node) {
switch (node.type) {
case "ieConditionalComment":
case "comment":
case "directive":
return true;
case "element":
return ["script", "select"].includes(node.name);
}
return false;
}
function getLastDescendant(node) {
return node.lastChild ? getLastDescendant(node.lastChild) : node;
}
function hasNonTextChild(node) {
return node.children && node.children.some((child) => child.type !== "text");
}
function _inferScriptParser(node) {
const {
type,
lang
} = node.attrMap;
if (type === "module" || type === "text/javascript" || type === "text/babel" || type === "application/javascript" || lang === "jsx") {
return "babel";
}
if (type === "application/x-typescript" || lang === "ts" || lang === "tsx") {
return "typescript";
}
if (type === "text/markdown") {
return "markdown";
}
if (type === "text/html") {
return "html";
}
if (type && (type.endsWith("json") || type.endsWith("importmap")) || type === "speculationrules") {
return "json";
}
if (type === "text/x-handlebars-template") {
return "glimmer";
}
}
function inferStyleParser(node, options) {
const {
lang
} = node.attrMap;
if (!lang || lang === "postcss" || lang === "css") {
return "css";
}
if (lang === "scss") {
return "scss";
}
if (lang === "less") {
return "less";
}
if (lang === "stylus") {
return inferParserByLanguage("stylus", options);
}
}
function inferScriptParser(node, options) {
if (node.name === "script" && !node.attrMap.src) {
if (!node.attrMap.lang && !node.attrMap.type) {
return "babel";
}
return _inferScriptParser(node);
}
if (node.name === "style") {
return inferStyleParser(node, options);
}
if (options && isVueNonHtmlBlock(node, options)) {
return _inferScriptParser(node) || !("src" in node.attrMap) && inferParserByLanguage(node.attrMap.lang, options);
}
}
function isBlockLikeCssDisplay(cssDisplay) {
return cssDisplay === "block" || cssDisplay === "list-item" || cssDisplay.startsWith("table");
}
function isFirstChildLeadingSpaceSensitiveCssDisplay(cssDisplay) {
return !isBlockLikeCssDisplay(cssDisplay) && cssDisplay !== "inline-block";
}
function isLastChildTrailingSpaceSensitiveCssDisplay(cssDisplay) {
return !isBlockLikeCssDisplay(cssDisplay) && cssDisplay !== "inline-block";
}
function isPrevTrailingSpaceSensitiveCssDisplay(cssDisplay) {
return !isBlockLikeCssDisplay(cssDisplay);
}
function isNextLeadingSpaceSensitiveCssDisplay(cssDisplay) {
return !isBlockLikeCssDisplay(cssDisplay);
}
function isDanglingSpaceSensitiveCssDisplay(cssDisplay) {
return !isBlockLikeCssDisplay(cssDisplay) && cssDisplay !== "inline-block";
}
function isPreLikeNode(node) {
return getNodeCssStyleWhiteSpace(node).startsWith("pre");
}
function countParents(path, predicate) {
let counter = 0;
for (let i = path.stack.length - 1; i >= 0; i--) {
const value = path.stack[i];
if (value && typeof value === "object" && !Array.isArray(value) && predicate(value)) {
counter++;
}
}
return counter;
}
function hasParent(node, fn) {
let current = node;
while (current) {
if (fn(current)) {
return true;
}
current = current.parent;
}
return false;
}
function getNodeCssStyleDisplay(node, options) {
if (node.prev && node.prev.type === "comment") {
const match = node.prev.value.match(/^\s*display:\s*([a-z]+)\s*$/);
if (match) {
return match[1];
}
}
let isInSvgForeignObject = false;
if (node.type === "element" && node.namespace === "svg") {
if (hasParent(node, (parent) => parent.fullName === "svg:foreignObject")) {
isInSvgForeignObject = true;
} else {
return node.name === "svg" ? "inline-block" : "block";
}
}
switch (options.htmlWhitespaceSensitivity) {
case "strict":
return "inline";
case "ignore":
return "block";
default: {
if (options.parser === "vue" && node.parent && node.parent.type === "root") {
return "block";
}
return node.type === "element" && (!node.namespace || isInSvgForeignObject || isUnknownNamespace(node)) && CSS_DISPLAY_TAGS[node.name] || CSS_DISPLAY_DEFAULT;
}
}
}
function getNodeCssStyleWhiteSpace(node) {
return node.type === "element" && (!node.namespace || isUnknownNamespace(node)) && CSS_WHITE_SPACE_TAGS[node.name] || CSS_WHITE_SPACE_DEFAULT;
}
function getMinIndentation(text) {
let minIndentation = Number.POSITIVE_INFINITY;
for (const lineText of text.split("\n")) {
if (lineText.length === 0) {
continue;
}
if (!HTML_WHITESPACE.has(lineText[0])) {
return 0;
}
const indentation = getLeadingHtmlWhitespace(lineText).length;
if (lineText.length === indentation) {
continue;
}
if (indentation < minIndentation) {
minIndentation = indentation;
}
}
return minIndentation === Number.POSITIVE_INFINITY ? 0 : minIndentation;
}
function dedentString(text, minIndent = getMinIndentation(text)) {
return minIndent === 0 ? text : text.split("\n").map((lineText) => lineText.slice(minIndent)).join("\n");
}
function countChars(text, char2) {
let counter = 0;
for (let i = 0; i < text.length; i++) {
if (text[i] === char2) {
counter++;
}
}
return counter;
}
function unescapeQuoteEntities(text) {
return text.replace(/'/g, "'").replace(/"/g, '"');
}
var vueRootElementsSet = /* @__PURE__ */ new Set(["template", "style", "script"]);
function isVueCustomBlock(node, options) {
return isVueSfcBlock(node, options) && !vueRootElementsSet.has(node.fullName);
}
function isVueSfcBlock(node, options) {
return options.parser === "vue" && node.type === "element" && node.parent.type === "root" && node.fullName.toLowerCase() !== "html";
}
function isVueNonHtmlBlock(node, options) {
return isVueSfcBlock(node, options) && (isVueCustomBlock(node, options) || node.attrMap.lang && node.attrMap.lang !== "html");
}
function isVueSlotAttribute(attribute) {
const attributeName = attribute.fullName;
return attributeName.charAt(0) === "#" || attributeName === "slot-scope" || attributeName === "v-slot" || attributeName.startsWith("v-slot:");
}
function isVueSfcBindingsAttribute(attribute, options) {
const element = attribute.parent;
if (!isVueSfcBlock(element, options)) {
return false;
}
const tagName = element.fullName;
const attributeName = attribute.fullName;
return tagName === "script" && attributeName === "setup" || tagName === "style" && attributeName === "vars";
}
function getTextValueParts(node, value = node.value) {
return node.parent.isWhitespaceSensitive ? node.parent.isIndentationSensitive ? replaceTextEndOfLine(value) : replaceTextEndOfLine(dedentString(htmlTrimPreserveIndentation(value)), hardline) : getDocParts(join(line, splitByHtmlWhitespace(value)));
}
function isVueScriptTag(node, options) {
return isVueSfcBlock(node, options) && node.name === "script";
}
module22.exports = {
htmlTrim,
htmlTrimPreserveIndentation,
hasHtmlWhitespace,
getLeadingAndTrailingHtmlWhitespace,
canHaveInterpolation,
countChars,
countParents,
dedentString,
forceBreakChildren,
forceBreakContent,
forceNextEmptyLine,
getLastDescendant,
getNodeCssStyleDisplay,
getNodeCssStyleWhiteSpace,
hasPrettierIgnore,
inferScriptParser,
isVueCustomBlock,
isVueNonHtmlBlock,
isVueScriptTag,
isVueSlotAttribute,
isVueSfcBindingsAttribute,
isVueSfcBlock,
isDanglingSpaceSensitiveNode,
isIndentationSensitiveNode,
isLeadingSpaceSensitiveNode,
isPreLikeNode,
isScriptLikeTag,
isTextLikeNode,
isTrailingSpaceSensitiveNode,
isWhitespaceSensitiveNode,
isUnknownNamespace,
preferHardlineAsLeadingSpaces,
preferHardlineAsTrailingSpaces,
shouldPreserveContent,
unescapeQuoteEntities,
getTextValueParts
};
}
});
var require_chars = __commonJS22({
"node_modules/angular-html-parser/lib/compiler/src/chars.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
exports2.$EOF = 0;
exports2.$BSPACE = 8;
exports2.$TAB = 9;
exports2.$LF = 10;
exports2.$VTAB = 11;
exports2.$FF = 12;
exports2.$CR = 13;
exports2.$SPACE = 32;
exports2.$BANG = 33;
exports2.$DQ = 34;
exports2.$HASH = 35;
exports2.$$ = 36;
exports2.$PERCENT = 37;
exports2.$AMPERSAND = 38;
exports2.$SQ = 39;
exports2.$LPAREN = 40;
exports2.$RPAREN = 41;
exports2.$STAR = 42;
exports2.$PLUS = 43;
exports2.$COMMA = 44;
exports2.$MINUS = 45;
exports2.$PERIOD = 46;
exports2.$SLASH = 47;
exports2.$COLON = 58;
exports2.$SEMICOLON = 59;
exports2.$LT = 60;
exports2.$EQ = 61;
exports2.$GT = 62;
exports2.$QUESTION = 63;
exports2.$0 = 48;
exports2.$7 = 55;
exports2.$9 = 57;
exports2.$A = 65;
exports2.$E = 69;
exports2.$F = 70;
exports2.$X = 88;
exports2.$Z = 90;
exports2.$LBRACKET = 91;
exports2.$BACKSLASH = 92;
exports2.$RBRACKET = 93;
exports2.$CARET = 94;
exports2.$_ = 95;
exports2.$a = 97;
exports2.$b = 98;
exports2.$e = 101;
exports2.$f = 102;
exports2.$n = 110;
exports2.$r = 114;
exports2.$t = 116;
exports2.$u = 117;
exports2.$v = 118;
exports2.$x = 120;
exports2.$z = 122;
exports2.$LBRACE = 123;
exports2.$BAR = 124;
exports2.$RBRACE = 125;
exports2.$NBSP = 160;
exports2.$PIPE = 124;
exports2.$TILDA = 126;
exports2.$AT = 64;
exports2.$BT = 96;
function isWhitespace(code) {
return code >= exports2.$TAB && code <= exports2.$SPACE || code == exports2.$NBSP;
}
exports2.isWhitespace = isWhitespace;
function isDigit(code) {
return exports2.$0 <= code && code <= exports2.$9;
}
exports2.isDigit = isDigit;
function isAsciiLetter(code) {
return code >= exports2.$a && code <= exports2.$z || code >= exports2.$A && code <= exports2.$Z;
}
exports2.isAsciiLetter = isAsciiLetter;
function isAsciiHexDigit(code) {
return code >= exports2.$a && code <= exports2.$f || code >= exports2.$A && code <= exports2.$F || isDigit(code);
}
exports2.isAsciiHexDigit = isAsciiHexDigit;
function isNewLine(code) {
return code === exports2.$LF || code === exports2.$CR;
}
exports2.isNewLine = isNewLine;
function isOctalDigit(code) {
return exports2.$0 <= code && code <= exports2.$7;
}
exports2.isOctalDigit = isOctalDigit;
}
});
var require_static_symbol = __commonJS22({
"node_modules/angular-html-parser/lib/compiler/src/aot/static_symbol.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var StaticSymbol = class {
constructor(filePath2, name, members) {
this.filePath = filePath2;
this.name = name;
this.members = members;
}
assertNoMembers() {
if (this.members.length) {
throw new Error(`Illegal state: symbol without members expected, but got ${JSON.stringify(this)}.`);
}
}
};
exports2.StaticSymbol = StaticSymbol;
var StaticSymbolCache = class {
constructor() {
this.cache = /* @__PURE__ */ new Map();
}
get(declarationFile, name, members) {
members = members || [];
const memberSuffix = members.length ? `.${members.join(".")}` : "";
const key = `"${declarationFile}".${name}${memberSuffix}`;
let result = this.cache.get(key);
if (!result) {
result = new StaticSymbol(declarationFile, name, members);
this.cache.set(key, result);
}
return result;
}
};
exports2.StaticSymbolCache = StaticSymbolCache;
}
});
var require_util3 = __commonJS22({
"node_modules/angular-html-parser/lib/compiler/src/util.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var DASH_CASE_REGEXP = /-+([a-z0-9])/g;
function dashCaseToCamelCase(input) {
return input.replace(DASH_CASE_REGEXP, (...m) => m[1].toUpperCase());
}
exports2.dashCaseToCamelCase = dashCaseToCamelCase;
function splitAtColon(input, defaultValues) {
return _splitAt(input, ":", defaultValues);
}
exports2.splitAtColon = splitAtColon;
function splitAtPeriod(input, defaultValues) {
return _splitAt(input, ".", defaultValues);
}
exports2.splitAtPeriod = splitAtPeriod;
function _splitAt(input, character, defaultValues) {
const characterIndex = input.indexOf(character);
if (characterIndex == -1)
return defaultValues;
return [input.slice(0, characterIndex).trim(), input.slice(characterIndex + 1).trim()];
}
function visitValue(value, visitor, context) {
if (Array.isArray(value)) {
return visitor.visitArray(value, context);
}
if (isStrictStringMap(value)) {
return visitor.visitStringMap(value, context);
}
if (value == null || typeof value == "string" || typeof value == "number" || typeof value == "boolean") {
return visitor.visitPrimitive(value, context);
}
return visitor.visitOther(value, context);
}
exports2.visitValue = visitValue;
function isDefined(val) {
return val !== null && val !== void 0;
}
exports2.isDefined = isDefined;
function noUndefined(val) {
return val === void 0 ? null : val;
}
exports2.noUndefined = noUndefined;
var ValueTransformer = class {
visitArray(arr, context) {
return arr.map((value) => visitValue(value, this, context));
}
visitStringMap(map, context) {
const result = {};
Object.keys(map).forEach((key) => {
result[key] = visitValue(map[key], this, context);
});
return result;
}
visitPrimitive(value, context) {
return value;
}
visitOther(value, context) {
return value;
}
};
exports2.ValueTransformer = ValueTransformer;
exports2.SyncAsync = {
assertSync: (value) => {
if (isPromise(value)) {
throw new Error(`Illegal state: value cannot be a promise`);
}
return value;
},
then: (value, cb) => {
return isPromise(value) ? value.then(cb) : cb(value);
},
all: (syncAsyncValues) => {
return syncAsyncValues.some(isPromise) ? Promise.all(syncAsyncValues) : syncAsyncValues;
}
};
function error(msg) {
throw new Error(`Internal Error: ${msg}`);
}
exports2.error = error;
function syntaxError(msg, parseErrors) {
const error2 = Error(msg);
error2[ERROR_SYNTAX_ERROR] = true;
if (parseErrors)
error2[ERROR_PARSE_ERRORS] = parseErrors;
return error2;
}
exports2.syntaxError = syntaxError;
var ERROR_SYNTAX_ERROR = "ngSyntaxError";
var ERROR_PARSE_ERRORS = "ngParseErrors";
function isSyntaxError(error2) {
return error2[ERROR_SYNTAX_ERROR];
}
exports2.isSyntaxError = isSyntaxError;
function getParseErrors(error2) {
return error2[ERROR_PARSE_ERRORS] || [];
}
exports2.getParseErrors = getParseErrors;
function escapeRegExp(s) {
return s.replace(/([.*+?^=!:${}()|[\]\/\\])/g, "\\$1");
}
exports2.escapeRegExp = escapeRegExp;
var STRING_MAP_PROTO = Object.getPrototypeOf({});
function isStrictStringMap(obj) {
return typeof obj === "object" && obj !== null && Object.getPrototypeOf(obj) === STRING_MAP_PROTO;
}
function utf8Encode(str) {
let encoded = "";
for (let index = 0; index < str.length; index++) {
let codePoint = str.charCodeAt(index);
if (codePoint >= 55296 && codePoint <= 56319 && str.length > index + 1) {
const low = str.charCodeAt(index + 1);
if (low >= 56320 && low <= 57343) {
index++;
codePoint = (codePoint - 55296 << 10) + low - 56320 + 65536;
}
}
if (codePoint <= 127) {
encoded += String.fromCharCode(codePoint);
} else if (codePoint <= 2047) {
encoded += String.fromCharCode(codePoint >> 6 & 31 | 192, codePoint & 63 | 128);
} else if (codePoint <= 65535) {
encoded += String.fromCharCode(codePoint >> 12 | 224, codePoint >> 6 & 63 | 128, codePoint & 63 | 128);
} else if (codePoint <= 2097151) {
encoded += String.fromCharCode(codePoint >> 18 & 7 | 240, codePoint >> 12 & 63 | 128, codePoint >> 6 & 63 | 128, codePoint & 63 | 128);
}
}
return encoded;
}
exports2.utf8Encode = utf8Encode;
function stringify(token) {
if (typeof token === "string") {
return token;
}
if (token instanceof Array) {
return "[" + token.map(stringify).join(", ") + "]";
}
if (token == null) {
return "" + token;
}
if (token.overriddenName) {
return `${token.overriddenName}`;
}
if (token.name) {
return `${token.name}`;
}
if (!token.toString) {
return "object";
}
const res = token.toString();
if (res == null) {
return "" + res;
}
const newLineIndex = res.indexOf("\n");
return newLineIndex === -1 ? res : res.substring(0, newLineIndex);
}
exports2.stringify = stringify;
function resolveForwardRef(type) {
if (typeof type === "function" && type.hasOwnProperty("__forward_ref__")) {
return type();
} else {
return type;
}
}
exports2.resolveForwardRef = resolveForwardRef;
function isPromise(obj) {
return !!obj && typeof obj.then === "function";
}
exports2.isPromise = isPromise;
var Version2 = class {
constructor(full) {
this.full = full;
const splits = full.split(".");
this.major = splits[0];
this.minor = splits[1];
this.patch = splits.slice(2).join(".");
}
};
exports2.Version = Version2;
var __window = typeof window !== "undefined" && window;
var __self = typeof self !== "undefined" && typeof WorkerGlobalScope !== "undefined" && self instanceof WorkerGlobalScope && self;
var __global = typeof global !== "undefined" && global;
var _global = __global || __window || __self;
exports2.global = _global;
}
});
var require_compile_metadata = __commonJS22({
"node_modules/angular-html-parser/lib/compiler/src/compile_metadata.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var static_symbol_1 = require_static_symbol();
var util_1 = require_util3();
var HOST_REG_EXP = /^(?:(?:\[([^\]]+)\])|(?:\(([^\)]+)\)))|(\@[-\w]+)$/;
function sanitizeIdentifier(name) {
return name.replace(/\W/g, "_");
}
exports2.sanitizeIdentifier = sanitizeIdentifier;
var _anonymousTypeIndex = 0;
function identifierName(compileIdentifier) {
if (!compileIdentifier || !compileIdentifier.reference) {
return null;
}
const ref = compileIdentifier.reference;
if (ref instanceof static_symbol_1.StaticSymbol) {
return ref.name;
}
if (ref["__anonymousType"]) {
return ref["__anonymousType"];
}
let identifier = util_1.stringify(ref);
if (identifier.indexOf("(") >= 0) {
identifier = `anonymous_${_anonymousTypeIndex++}`;
ref["__anonymousType"] = identifier;
} else {
identifier = sanitizeIdentifier(identifier);
}
return identifier;
}
exports2.identifierName = identifierName;
function identifierModuleUrl(compileIdentifier) {
const ref = compileIdentifier.reference;
if (ref instanceof static_symbol_1.StaticSymbol) {
return ref.filePath;
}
return `./${util_1.stringify(ref)}`;
}
exports2.identifierModuleUrl = identifierModuleUrl;
function viewClassName(compType, embeddedTemplateIndex) {
return `View_${identifierName({
reference: compType
})}_${embeddedTemplateIndex}`;
}
exports2.viewClassName = viewClassName;
function rendererTypeName(compType) {
return `RenderType_${identifierName({
reference: compType
})}`;
}
exports2.rendererTypeName = rendererTypeName;
function hostViewClassName(compType) {
return `HostView_${identifierName({
reference: compType
})}`;
}
exports2.hostViewClassName = hostViewClassName;
function componentFactoryName(compType) {
return `${identifierName({
reference: compType
})}NgFactory`;
}
exports2.componentFactoryName = componentFactoryName;
var CompileSummaryKind;
(function(CompileSummaryKind2) {
CompileSummaryKind2[CompileSummaryKind2["Pipe"] = 0] = "Pipe";
CompileSummaryKind2[CompileSummaryKind2["Directive"] = 1] = "Directive";
CompileSummaryKind2[CompileSummaryKind2["NgModule"] = 2] = "NgModule";
CompileSummaryKind2[CompileSummaryKind2["Injectable"] = 3] = "Injectable";
})(CompileSummaryKind = exports2.CompileSummaryKind || (exports2.CompileSummaryKind = {}));
function tokenName(token) {
return token.value != null ? sanitizeIdentifier(token.value) : identifierName(token.identifier);
}
exports2.tokenName = tokenName;
function tokenReference(token) {
if (token.identifier != null) {
return token.identifier.reference;
} else {
return token.value;
}
}
exports2.tokenReference = tokenReference;
var CompileStylesheetMetadata = class {
constructor({
moduleUrl,
styles,
styleUrls
} = {}) {
this.moduleUrl = moduleUrl || null;
this.styles = _normalizeArray(styles);
this.styleUrls = _normalizeArray(styleUrls);
}
};
exports2.CompileStylesheetMetadata = CompileStylesheetMetadata;
var CompileTemplateMetadata = class {
constructor({
encapsulation,
template,
templateUrl,
htmlAst,
styles,
styleUrls,
externalStylesheets,
animations,
ngContentSelectors,
interpolation,
isInline,
preserveWhitespaces
}) {
this.encapsulation = encapsulation;
this.template = template;
this.templateUrl = templateUrl;
this.htmlAst = htmlAst;
this.styles = _normalizeArray(styles);
this.styleUrls = _normalizeArray(styleUrls);
this.externalStylesheets = _normalizeArray(externalStylesheets);
this.animations = animations ? flatten(animations) : [];
this.ngContentSelectors = ngContentSelectors || [];
if (interpolation && interpolation.length != 2) {
throw new Error(`'interpolation' should have a start and an end symbol.`);
}
this.interpolation = interpolation;
this.isInline = isInline;
this.preserveWhitespaces = preserveWhitespaces;
}
toSummary() {
return {
ngContentSelectors: this.ngContentSelectors,
encapsulation: this.encapsulation,
styles: this.styles,
animations: this.animations
};
}
};
exports2.CompileTemplateMetadata = CompileTemplateMetadata;
var CompileDirectiveMetadata = class {
static create({
isHost,
type,
isComponent,
selector,
exportAs,
changeDetection,
inputs,
outputs,
host,
providers,
viewProviders,
queries,
guards,
viewQueries,
entryComponents,
template,
componentViewType,
rendererType,
componentFactory
}) {
const hostListeners = {};
const hostProperties = {};
const hostAttributes = {};
if (host != null) {
Object.keys(host).forEach((key) => {
const value = host[key];
const matches = key.match(HOST_REG_EXP);
if (matches === null) {
hostAttributes[key] = value;
} else if (matches[1] != null) {
hostProperties[matches[1]] = value;
} else if (matches[2] != null) {
hostListeners[matches[2]] = value;
}
});
}
const inputsMap = {};
if (inputs != null) {
inputs.forEach((bindConfig) => {
const parts = util_1.splitAtColon(bindConfig, [bindConfig, bindConfig]);
inputsMap[parts[0]] = parts[1];
});
}
const outputsMap = {};
if (outputs != null) {
outputs.forEach((bindConfig) => {
const parts = util_1.splitAtColon(bindConfig, [bindConfig, bindConfig]);
outputsMap[parts[0]] = parts[1];
});
}
return new CompileDirectiveMetadata({
isHost,
type,
isComponent: !!isComponent,
selector,
exportAs,
changeDetection,
inputs: inputsMap,
outputs: outputsMap,
hostListeners,
hostProperties,
hostAttributes,
providers,
viewProviders,
queries,
guards,
viewQueries,
entryComponents,
template,
componentViewType,
rendererType,
componentFactory
});
}
constructor({
isHost,
type,
isComponent,
selector,
exportAs,
changeDetection,
inputs,
outputs,
hostListeners,
hostProperties,
hostAttributes,
providers,
viewProviders,
queries,
guards,
viewQueries,
entryComponents,
template,
componentViewType,
rendererType,
componentFactory
}) {
this.isHost = !!isHost;
this.type = type;
this.isComponent = isComponent;
this.selector = selector;
this.exportAs = exportAs;
this.changeDetection = changeDetection;
this.inputs = inputs;
this.outputs = outputs;
this.hostListeners = hostListeners;
this.hostProperties = hostProperties;
this.hostAttributes = hostAttributes;
this.providers = _normalizeArray(providers);
this.viewProviders = _normalizeArray(viewProviders);
this.queries = _normalizeArray(queries);
this.guards = guards;
this.viewQueries = _normalizeArray(viewQueries);
this.entryComponents = _normalizeArray(entryComponents);
this.template = template;
this.componentViewType = componentViewType;
this.rendererType = rendererType;
this.componentFactory = componentFactory;
}
toSummary() {
return {
summaryKind: CompileSummaryKind.Directive,
type: this.type,
isComponent: this.isComponent,
selector: this.selector,
exportAs: this.exportAs,
inputs: this.inputs,
outputs: this.outputs,
hostListeners: this.hostListeners,
hostProperties: this.hostProperties,
hostAttributes: this.hostAttributes,
providers: this.providers,
viewProviders: this.viewProviders,
queries: this.queries,
guards: this.guards,
viewQueries: this.viewQueries,
entryComponents: this.entryComponents,
changeDetection: this.changeDetection,
template: this.template && this.template.toSummary(),
componentViewType: this.componentViewType,
rendererType: this.rendererType,
componentFactory: this.componentFactory
};
}
};
exports2.CompileDirectiveMetadata = CompileDirectiveMetadata;
var CompilePipeMetadata = class {
constructor({
type,
name,
pure
}) {
this.type = type;
this.name = name;
this.pure = !!pure;
}
toSummary() {
return {
summaryKind: CompileSummaryKind.Pipe,
type: this.type,
name: this.name,
pure: this.pure
};
}
};
exports2.CompilePipeMetadata = CompilePipeMetadata;
var CompileShallowModuleMetadata = class {
};
exports2.CompileShallowModuleMetadata = CompileShallowModuleMetadata;
var CompileNgModuleMetadata = class {
constructor({
type,
providers,
declaredDirectives,
exportedDirectives,
declaredPipes,
exportedPipes,
entryComponents,
bootstrapComponents,
importedModules,
exportedModules,
schemas,
transitiveModule,
id
}) {
this.type = type || null;
this.declaredDirectives = _normalizeArray(declaredDirectives);
this.exportedDirectives = _normalizeArray(exportedDirectives);
this.declaredPipes = _normalizeArray(declaredPipes);
this.exportedPipes = _normalizeArray(exportedPipes);
this.providers = _normalizeArray(providers);
this.entryComponents = _normalizeArray(entryComponents);
this.bootstrapComponents = _normalizeArray(bootstrapComponents);
this.importedModules = _normalizeArray(importedModules);
this.exportedModules = _normalizeArray(exportedModules);
this.schemas = _normalizeArray(schemas);
this.id = id || null;
this.transitiveModule = transitiveModule || null;
}
toSummary() {
const module3 = this.transitiveModule;
return {
summaryKind: CompileSummaryKind.NgModule,
type: this.type,
entryComponents: module3.entryComponents,
providers: module3.providers,
modules: module3.modules,
exportedDirectives: module3.exportedDirectives,
exportedPipes: module3.exportedPipes
};
}
};
exports2.CompileNgModuleMetadata = CompileNgModuleMetadata;
var TransitiveCompileNgModuleMetadata = class {
constructor() {
this.directivesSet = /* @__PURE__ */ new Set();
this.directives = [];
this.exportedDirectivesSet = /* @__PURE__ */ new Set();
this.exportedDirectives = [];
this.pipesSet = /* @__PURE__ */ new Set();
this.pipes = [];
this.exportedPipesSet = /* @__PURE__ */ new Set();
this.exportedPipes = [];
this.modulesSet = /* @__PURE__ */ new Set();
this.modules = [];
this.entryComponentsSet = /* @__PURE__ */ new Set();
this.entryComponents = [];
this.providers = [];
}
addProvider(provider, module3) {
this.providers.push({
provider,
module: module3
});
}
addDirective(id) {
if (!this.directivesSet.has(id.reference)) {
this.directivesSet.add(id.reference);
this.directives.push(id);
}
}
addExportedDirective(id) {
if (!this.exportedDirectivesSet.has(id.reference)) {
this.exportedDirectivesSet.add(id.reference);
this.exportedDirectives.push(id);
}
}
addPipe(id) {
if (!this.pipesSet.has(id.reference)) {
this.pipesSet.add(id.reference);
this.pipes.push(id);
}
}
addExportedPipe(id) {
if (!this.exportedPipesSet.has(id.reference)) {
this.exportedPipesSet.add(id.reference);
this.exportedPipes.push(id);
}
}
addModule(id) {
if (!this.modulesSet.has(id.reference)) {
this.modulesSet.add(id.reference);
this.modules.push(id);
}
}
addEntryComponent(ec) {
if (!this.entryComponentsSet.has(ec.componentType)) {
this.entryComponentsSet.add(ec.componentType);
this.entryComponents.push(ec);
}
}
};
exports2.TransitiveCompileNgModuleMetadata = TransitiveCompileNgModuleMetadata;
function _normalizeArray(obj) {
return obj || [];
}
var ProviderMeta = class {
constructor(token, {
useClass,
useValue,
useExisting,
useFactory,
deps,
multi
}) {
this.token = token;
this.useClass = useClass || null;
this.useValue = useValue;
this.useExisting = useExisting;
this.useFactory = useFactory || null;
this.dependencies = deps || null;
this.multi = !!multi;
}
};
exports2.ProviderMeta = ProviderMeta;
function flatten(list) {
return list.reduce((flat, item) => {
const flatItem = Array.isArray(item) ? flatten(item) : item;
return flat.concat(flatItem);
}, []);
}
exports2.flatten = flatten;
function jitSourceUrl(url) {
return url.replace(/(\w+:\/\/[\w:-]+)?(\/+)?/, "ng:///");
}
function templateSourceUrl(ngModuleType, compMeta, templateMeta) {
let url;
if (templateMeta.isInline) {
if (compMeta.type.reference instanceof static_symbol_1.StaticSymbol) {
url = `${compMeta.type.reference.filePath}.${compMeta.type.reference.name}.html`;
} else {
url = `${identifierName(ngModuleType)}/${identifierName(compMeta.type)}.html`;
}
} else {
url = templateMeta.templateUrl;
}
return compMeta.type.reference instanceof static_symbol_1.StaticSymbol ? url : jitSourceUrl(url);
}
exports2.templateSourceUrl = templateSourceUrl;
function sharedStylesheetJitUrl(meta, id) {
const pathParts = meta.moduleUrl.split(/\/\\/g);
const baseName = pathParts[pathParts.length - 1];
return jitSourceUrl(`css/${id}${baseName}.ngstyle.js`);
}
exports2.sharedStylesheetJitUrl = sharedStylesheetJitUrl;
function ngModuleJitUrl(moduleMeta) {
return jitSourceUrl(`${identifierName(moduleMeta.type)}/module.ngfactory.js`);
}
exports2.ngModuleJitUrl = ngModuleJitUrl;
function templateJitUrl(ngModuleType, compMeta) {
return jitSourceUrl(`${identifierName(ngModuleType)}/${identifierName(compMeta.type)}.ngfactory.js`);
}
exports2.templateJitUrl = templateJitUrl;
}
});
var require_parse_util = __commonJS22({
"node_modules/angular-html-parser/lib/compiler/src/parse_util.js"(exports2) {
"use strict";
Object.defineProperty(exports2, "__esModule", {
value: true
});
var chars = require_chars();
var compile_metadata_1 = require_compile_metadata();
var ParseLocation = class {
constructor(file, offset, line, col) {
this.file = file;
this.offset = offset;
this.line = line;
this.col = col;
}
toString() {
return this.offset != null ? `${this.file.url}@${this.line}:${this.col}` : this.file.url;
}
moveBy(delta) {
const source = this.file.content;
const len = source.length;
let offset = this.offset;
let line = this.line;
let col = this.col;
while (offset > 0 && delta < 0) {
offset--;
delta++;
const ch = source.charCodeAt(offset);
if (ch == chars.$LF) {
line--;
const priorLine = source.substr(0, offset - 1).lastIndexOf(String.fromCharCode(chars.$LF));
col = priorLine > 0 ? offset - priorLine : offset;
} else {
col--;
}
}
while (offset < len && delta > 0) {
const ch = source.charCodeAt(offset);
offset++;
delta--;
if (ch == chars.$LF) {
line++;
col = 0;
} else {
col++;
}
}
return new ParseLocation(this.file, offset, line, col);
}
getContext(maxChars, maxLines) {
const content = this.file.content;
let startOffset = this.offset;
if (startOffset != null) {
if (startOffset > content.length - 1) {
startOffset = content.length - 1;
}
let endOffset = startOffset;
let ctxChars = 0;
let ctxLines = 0;
while (ctxChars < maxChars && startOffset > 0) {
startOffset--;
ctxChars++;
if (content[startOffset] == "\n") {
if (++ctxLines == maxLines) {
break;
}
}
}
ctxChars = 0;
ctxLines = 0;
while (ctxChars < maxChars && endOffset < content.length - 1) {
endOffset++;
ctxChars++;
if (content[endOffset] == "\n") {
if (++ctxLines == maxLines) {
break;
}
}
}
return {
before: content.substring(startOffset, this.offset),
after: content.substring(this.offset, endOffset + 1)
};
}
return null;
}
};
exports2.ParseLocation = ParseLocation;
var ParseSourceFile = class {
constructor(content, url) {
this.content = content;
this.url = url;
}
};
exports2.ParseSourceFile = ParseSourceFile;
var ParseSourceSpan = class {
constructor(start, end, details = null) {
this.start = start;
this.end = end;
this.details = details;
}
toString() {
return this.start.file.content.substring(this.start.offset, this.end.offset);
}
};
exports2.ParseSourceSpan = ParseSourceSpan;
exports2.EMPTY_PARSE_LOCATION = new ParseLocation(new ParseSourceFile("", ""), 0, 0, 0);
exports2.EMPTY_SOURCE_SPAN = new ParseSourceSpan(exports2.EMPTY_PARSE_LOCATION, exports2.EMPTY_PARSE_LOCATION);
var ParseErrorLevel;
(function(ParseErrorLevel2) {
ParseErrorLevel2[ParseErrorLevel2["WARNING"] = 0] = "WARNING";
ParseErrorLevel2[ParseErrorLevel2["ERROR"] = 1] = "ERROR";
})(ParseErrorLevel = exports2.ParseErrorLevel || (exports2.ParseErrorLevel = {}));
var ParseError = class {
constructor(span, msg, level = ParseErrorLevel.ERROR) {
this.span = span;
this.msg = msg;
this.level = level;
}
contextualMessage() {
const ctx = this.span.start.getContext(100, 3);
return ctx ? `${this.msg} ("${ctx.before}[${ParseErrorLevel[this.level]} ->]${ctx.after}")` : this.msg;
}
toString() {
const details = this.span.details ? `, ${this.span.details}` : "";
return `${this.contextualMessage()}: ${this.span.start}${details}`;
}
};
exports2.ParseError = ParseError;
function typeSourceSpan(kind, type) {
const moduleUrl = compile_metadata_1.identifierModuleUrl(type);
const sourceFileName = moduleUrl != null ? `in ${kind} ${compile_metadata_1.identifierName(type)} in ${moduleUrl}` : `in ${kind} ${compile_metadata_1.identifierName(type)}`;
const sourceFile = new ParseSourceFile("", sourceFileName);
return new ParseSourceSpan(new ParseLocation(sourceFile, -1, -1, -1), new ParseLocation(sourceFile, -1, -1, -1));
}
exports2.typeSourceSpan = typeSourceSpan;
function r3JitTypeSourceSpan(kind, typeName, sourceUrl) {
const sourceFileName = `in ${kind} ${typeName} in ${sourceUrl}`;
const sourceFile = new ParseSourceFile("", sourceFileName);
return new ParseSourceSpan(new ParseLocation(sourceFile, -1, -1, -1), new ParseLocation(sourceFile, -1, -1, -1));
}
exports2.r3JitTypeSourceSpan = r3JitTypeSourceSpan;
}
});
var require_print_preprocess3 = __commonJS22({
"src/language-html/print-preprocess.js"(exports2, module22) {
"use strict";
var {
ParseSourceSpan
} = require_parse_util();
var {
htmlTrim,
getLeadingAndTrailingHtmlWhitespace,
hasHtmlWhitespace,
canHaveInterpolation,
getNodeCssStyleDisplay,
isDanglingSpaceSensitiveNode,
isIndentationSensitiveNode,
isLeadingSpaceSensitiveNode,
isTrailingSpaceSensitiveNode,
isWhitespaceSensitiveNode,
isVueScriptTag
} = require_utils11();
var PREPROCESS_PIPELINE = [removeIgnorableFirstLf, mergeIfConditionalStartEndCommentIntoElementOpeningTag, mergeCdataIntoText, extractInterpolation, extractWhitespaces, addCssDisplay, addIsSelfClosing, addHasHtmComponentClosingTag, addIsSpaceSensitive, mergeSimpleElementIntoText, markTsScript];
function preprocess(ast, options) {
for (const fn of PREPROCESS_PIPELINE) {
fn(ast, options);
}
return ast;
}
function removeIgnorableFirstLf(ast) {
ast.walk((node) => {
if (node.type === "element" && node.tagDefinition.ignoreFirstLf && node.children.length > 0 && node.children[0].type === "text" && node.children[0].value[0] === "\n") {
const text = node.children[0];
if (text.value.length === 1) {
node.removeChild(text);
} else {
text.value = text.value.slice(1);
}
}
});
}
function mergeIfConditionalStartEndCommentIntoElementOpeningTag(ast) {
const isTarget = (node) => node.type === "element" && node.prev && node.prev.type === "ieConditionalStartComment" && node.prev.sourceSpan.end.offset === node.startSourceSpan.start.offset && node.firstChild && node.firstChild.type === "ieConditionalEndComment" && node.firstChild.sourceSpan.start.offset === node.startSourceSpan.end.offset;
ast.walk((node) => {
if (node.children) {
for (let i = 0; i < node.children.length; i++) {
const child = node.children[i];
if (!isTarget(child)) {
continue;
}
const ieConditionalStartComment = child.prev;
const ieConditionalEndComment = child.firstChild;
node.removeChild(ieConditionalStartComment);
i--;
const startSourceSpan = new ParseSourceSpan(ieConditionalStartComment.sourceSpan.start, ieConditionalEndComment.sourceSpan.end);
const sourceSpan = new ParseSourceSpan(startSourceSpan.start, child.sourceSpan.end);
child.condition = ieConditionalStartComment.condition;
child.sourceSpan = sourceSpan;
child.startSourceSpan = startSourceSpan;
child.removeChild(ieConditionalEndComment);
}
}
});
}
function mergeNodeIntoText(ast, shouldMerge, getValue) {
ast.walk((node) => {
if (node.children) {
for (let i = 0; i < node.children.length; i++) {
const child = node.children[i];
if (child.type !== "text" && !shouldMerge(child)) {
continue;
}
if (child.type !== "text") {
child.type = "text";
child.value = getValue(child);
}
const prevChild = child.prev;
if (!prevChild || prevChild.type !== "text") {
continue;
}
prevChild.value += child.value;
prevChild.sourceSpan = new ParseSourceSpan(prevChild.sourceSpan.start, child.sourceSpan.end);
node.removeChild(child);
i--;
}
}
});
}
function mergeCdataIntoText(ast) {
return mergeNodeIntoText(ast, (node) => node.type === "cdata", (node) => ``);
}
function mergeSimpleElementIntoText(ast) {
const isSimpleElement = (node) => node.type === "element" && node.attrs.length === 0 && node.children.length === 1 && node.firstChild.type === "text" && !hasHtmlWhitespace(node.children[0].value) && !node.firstChild.hasLeadingSpaces && !node.firstChild.hasTrailingSpaces && node.isLeadingSpaceSensitive && !node.hasLeadingSpaces && node.isTrailingSpaceSensitive && !node.hasTrailingSpaces && node.prev && node.prev.type === "text" && node.next && node.next.type === "text";
ast.walk((node) => {
if (node.children) {
for (let i = 0; i < node.children.length; i++) {
const child = node.children[i];
if (!isSimpleElement(child)) {
continue;
}
const prevChild = child.prev;
const nextChild = child.next;
prevChild.value += `<${child.rawName}>` + child.firstChild.value + `${child.rawName}>` + nextChild.value;
prevChild.sourceSpan = new ParseSourceSpan(prevChild.sourceSpan.start, nextChild.sourceSpan.end);
prevChild.isTrailingSpaceSensitive = nextChild.isTrailingSpaceSensitive;
prevChild.hasTrailingSpaces = nextChild.hasTrailingSpaces;
node.removeChild(child);
i--;
node.removeChild(nextChild);
}
}
});
}
function extractInterpolation(ast, options) {
if (options.parser === "html") {
return;
}
const interpolationRegex = /{{(.+?)}}/s;
ast.walk((node) => {
if (!canHaveInterpolation(node)) {
return;
}
for (const child of node.children) {
if (child.type !== "text") {
continue;
}
let startSourceSpan = child.sourceSpan.start;
let endSourceSpan = null;
const components = child.value.split(interpolationRegex);
for (let i = 0; i < components.length; i++, startSourceSpan = endSourceSpan) {
const value = components[i];
if (i % 2 === 0) {
endSourceSpan = startSourceSpan.moveBy(value.length);
if (value.length > 0) {
node.insertChildBefore(child, {
type: "text",
value,
sourceSpan: new ParseSourceSpan(startSourceSpan, endSourceSpan)
});
}
continue;
}
endSourceSpan = startSourceSpan.moveBy(value.length + 4);
node.insertChildBefore(child, {
type: "interpolation",
sourceSpan: new ParseSourceSpan(startSourceSpan, endSourceSpan),
children: value.length === 0 ? [] : [{
type: "text",
value,
sourceSpan: new ParseSourceSpan(startSourceSpan.moveBy(2), endSourceSpan.moveBy(-2))
}]
});
}
node.removeChild(child);
}
});
}
function extractWhitespaces(ast) {
ast.walk((node) => {
if (!node.children) {
return;
}
if (node.children.length === 0 || node.children.length === 1 && node.children[0].type === "text" && htmlTrim(node.children[0].value).length === 0) {
node.hasDanglingSpaces = node.children.length > 0;
node.children = [];
return;
}
const isWhitespaceSensitive = isWhitespaceSensitiveNode(node);
const isIndentationSensitive = isIndentationSensitiveNode(node);
if (!isWhitespaceSensitive) {
for (let i = 0; i < node.children.length; i++) {
const child = node.children[i];
if (child.type !== "text") {
continue;
}
const {
leadingWhitespace,
text,
trailingWhitespace
} = getLeadingAndTrailingHtmlWhitespace(child.value);
const prevChild = child.prev;
const nextChild = child.next;
if (!text) {
node.removeChild(child);
i--;
if (leadingWhitespace || trailingWhitespace) {
if (prevChild) {
prevChild.hasTrailingSpaces = true;
}
if (nextChild) {
nextChild.hasLeadingSpaces = true;
}
}
} else {
child.value = text;
child.sourceSpan = new ParseSourceSpan(child.sourceSpan.start.moveBy(leadingWhitespace.length), child.sourceSpan.end.moveBy(-trailingWhitespace.length));
if (leadingWhitespace) {
if (prevChild) {
prevChild.hasTrailingSpaces = true;
}
child.hasLeadingSpaces = true;
}
if (trailingWhitespace) {
child.hasTrailingSpaces = true;
if (nextChild) {
nextChild.hasLeadingSpaces = true;
}
}
}
}
}
node.isWhitespaceSensitive = isWhitespaceSensitive;
node.isIndentationSensitive = isIndentationSensitive;
});
}
function addIsSelfClosing(ast) {
ast.walk((node) => {
node.isSelfClosing = !node.children || node.type === "element" && (node.tagDefinition.isVoid || node.startSourceSpan === node.endSourceSpan);
});
}
function addHasHtmComponentClosingTag(ast, options) {
ast.walk((node) => {
if (node.type !== "element") {
return;
}
node.hasHtmComponentClosingTag = node.endSourceSpan && /^<\s*\/\s*\/\s*>$/.test(options.originalText.slice(node.endSourceSpan.start.offset, node.endSourceSpan.end.offset));
});
}
function addCssDisplay(ast, options) {
ast.walk((node) => {
node.cssDisplay = getNodeCssStyleDisplay(node, options);
});
}
function addIsSpaceSensitive(ast, options) {
ast.walk((node) => {
const {
children
} = node;
if (!children) {
return;
}
if (children.length === 0) {
node.isDanglingSpaceSensitive = isDanglingSpaceSensitiveNode(node);
return;
}
for (const child of children) {
child.isLeadingSpaceSensitive = isLeadingSpaceSensitiveNode(child, options);
child.isTrailingSpaceSensitive = isTrailingSpaceSensitiveNode(child, options);
}
for (let index = 0; index < children.length; index++) {
const child = children[index];
child.isLeadingSpaceSensitive = index === 0 ? child.isLeadingSpaceSensitive : child.prev.isTrailingSpaceSensitive && child.isLeadingSpaceSensitive;
child.isTrailingSpaceSensitive = index === children.length - 1 ? child.isTrailingSpaceSensitive : child.next.isLeadingSpaceSensitive && child.isTrailingSpaceSensitive;
}
});
}
function markTsScript(ast, options) {
if (options.parser === "vue") {
const vueScriptTag = ast.children.find((child) => isVueScriptTag(child, options));
if (!vueScriptTag) {
return;
}
const {
lang
} = vueScriptTag.attrMap;
if (lang === "ts" || lang === "typescript") {
options.__should_parse_vue_template_with_ts = true;
}
}
}
module22.exports = preprocess;
}
});
var require_pragma5 = __commonJS22({
"src/language-html/pragma.js"(exports2, module22) {
"use strict";
function hasPragma(text) {
return /^\s*/.test(text);
}
function insertPragma(text) {
return "\n\n" + text.replace(/^\s*\n/, "");
}
module22.exports = {
hasPragma,
insertPragma
};
}
});
var require_loc6 = __commonJS22({
"src/language-html/loc.js"(exports2, module22) {
"use strict";
function locStart(node) {
return node.sourceSpan.start.offset;
}
function locEnd(node) {
return node.sourceSpan.end.offset;
}
module22.exports = {
locStart,
locEnd
};
}
});
var require_tag = __commonJS22({
"src/language-html/print/tag.js"(exports2, module22) {
"use strict";
var assert = require("assert");
var {
isNonEmptyArray
} = require_util();
var {
builders: {
indent,
join,
line,
softline,
hardline
},
utils: {
replaceTextEndOfLine
}
} = require_doc();
var {
locStart,
locEnd
} = require_loc6();
var {
isTextLikeNode,
getLastDescendant,
isPreLikeNode,
hasPrettierIgnore,
shouldPreserveContent,
isVueSfcBlock
} = require_utils11();
function printClosingTag(node, options) {
return [node.isSelfClosing ? "" : printClosingTagStart(node, options), printClosingTagEnd(node, options)];
}
function printClosingTagStart(node, options) {
return node.lastChild && needsToBorrowParentClosingTagStartMarker(node.lastChild) ? "" : [printClosingTagPrefix(node, options), printClosingTagStartMarker(node, options)];
}
function printClosingTagEnd(node, options) {
return (node.next ? needsToBorrowPrevClosingTagEndMarker(node.next) : needsToBorrowLastChildClosingTagEndMarker(node.parent)) ? "" : [printClosingTagEndMarker(node, options), printClosingTagSuffix(node, options)];
}
function printClosingTagPrefix(node, options) {
return needsToBorrowLastChildClosingTagEndMarker(node) ? printClosingTagEndMarker(node.lastChild, options) : "";
}
function printClosingTagSuffix(node, options) {
return needsToBorrowParentClosingTagStartMarker(node) ? printClosingTagStartMarker(node.parent, options) : needsToBorrowNextOpeningTagStartMarker(node) ? printOpeningTagStartMarker(node.next) : "";
}
function printClosingTagStartMarker(node, options) {
assert(!node.isSelfClosing);
if (shouldNotPrintClosingTag(node, options)) {
return "";
}
switch (node.type) {
case "ieConditionalComment":
return "";
case "ieConditionalStartComment":
return "]>";
case "interpolation":
return "}}";
case "element":
if (node.isSelfClosing) {
return "/>";
}
default:
return ">";
}
}
function shouldNotPrintClosingTag(node, options) {
return !node.isSelfClosing && !node.endSourceSpan && (hasPrettierIgnore(node) || shouldPreserveContent(node.parent, options));
}
function needsToBorrowPrevClosingTagEndMarker(node) {
return node.prev && node.prev.type !== "docType" && !isTextLikeNode(node.prev) && node.isLeadingSpaceSensitive && !node.hasLeadingSpaces;
}
function needsToBorrowLastChildClosingTagEndMarker(node) {
return node.lastChild && node.lastChild.isTrailingSpaceSensitive && !node.lastChild.hasTrailingSpaces && !isTextLikeNode(getLastDescendant(node.lastChild)) && !isPreLikeNode(node);
}
function needsToBorrowParentClosingTagStartMarker(node) {
return !node.next && !node.hasTrailingSpaces && node.isTrailingSpaceSensitive && isTextLikeNode(getLastDescendant(node));
}
function needsToBorrowNextOpeningTagStartMarker(node) {
return node.next && !isTextLikeNode(node.next) && isTextLikeNode(node) && node.isTrailingSpaceSensitive && !node.hasTrailingSpaces;
}
function getPrettierIgnoreAttributeCommentData(value) {
const match = value.trim().match(/^prettier-ignore-attribute(?:\s+(.+))?$/s);
if (!match) {
return false;
}
if (!match[1]) {
return true;
}
return match[1].split(/\s+/);
}
function needsToBorrowParentOpeningTagEndMarker(node) {
return !node.prev && node.isLeadingSpaceSensitive && !node.hasLeadingSpaces;
}
function printAttributes(path, options, print) {
const node = path.getValue();
if (!isNonEmptyArray(node.attrs)) {
return node.isSelfClosing ? " " : "";
}
const ignoreAttributeData = node.prev && node.prev.type === "comment" && getPrettierIgnoreAttributeCommentData(node.prev.value);
const hasPrettierIgnoreAttribute = typeof ignoreAttributeData === "boolean" ? () => ignoreAttributeData : Array.isArray(ignoreAttributeData) ? (attribute) => ignoreAttributeData.includes(attribute.rawName) : () => false;
const printedAttributes = path.map((attributePath) => {
const attribute = attributePath.getValue();
return hasPrettierIgnoreAttribute(attribute) ? replaceTextEndOfLine(options.originalText.slice(locStart(attribute), locEnd(attribute))) : print();
}, "attrs");
const forceNotToBreakAttrContent = node.type === "element" && node.fullName === "script" && node.attrs.length === 1 && node.attrs[0].fullName === "src" && node.children.length === 0;
const shouldPrintAttributePerLine = options.singleAttributePerLine && node.attrs.length > 1 && !isVueSfcBlock(node, options);
const attributeLine = shouldPrintAttributePerLine ? hardline : line;
const parts = [indent([forceNotToBreakAttrContent ? " " : line, join(attributeLine, printedAttributes)])];
if (node.firstChild && needsToBorrowParentOpeningTagEndMarker(node.firstChild) || node.isSelfClosing && needsToBorrowLastChildClosingTagEndMarker(node.parent) || forceNotToBreakAttrContent) {
parts.push(node.isSelfClosing ? " " : "");
} else {
parts.push(options.bracketSameLine ? node.isSelfClosing ? " " : "" : node.isSelfClosing ? line : softline);
}
return parts;
}
function printOpeningTagEnd(node) {
return node.firstChild && needsToBorrowParentOpeningTagEndMarker(node.firstChild) ? "" : printOpeningTagEndMarker(node);
}
function printOpeningTag(path, options, print) {
const node = path.getValue();
return [printOpeningTagStart(node, options), printAttributes(path, options, print), node.isSelfClosing ? "" : printOpeningTagEnd(node)];
}
function printOpeningTagStart(node, options) {
return node.prev && needsToBorrowNextOpeningTagStartMarker(node.prev) ? "" : [printOpeningTagPrefix(node, options), printOpeningTagStartMarker(node)];
}
function printOpeningTagPrefix(node, options) {
return needsToBorrowParentOpeningTagEndMarker(node) ? printOpeningTagEndMarker(node.parent) : needsToBorrowPrevClosingTagEndMarker(node) ? printClosingTagEndMarker(node.prev, options) : "";
}
function printOpeningTagStartMarker(node) {
switch (node.type) {
case "ieConditionalComment":
case "ieConditionalStartComment":
return `<${node.rawName}`;
}
default:
return `<${node.rawName}`;
}
}
function printOpeningTagEndMarker(node) {
assert(!node.isSelfClosing);
switch (node.type) {
case "ieConditionalComment":
return "]>";
case "element":
if (node.condition) {
return ">";
}
default:
return ">";
}
}
module22.exports = {
printClosingTag,
printClosingTagStart,
printClosingTagStartMarker,
printClosingTagEndMarker,
printClosingTagSuffix,
printClosingTagEnd,
needsToBorrowLastChildClosingTagEndMarker,
needsToBorrowParentClosingTagStartMarker,
needsToBorrowPrevClosingTagEndMarker,
printOpeningTag,
printOpeningTagStart,
printOpeningTagPrefix,
printOpeningTagStartMarker,
printOpeningTagEndMarker,
needsToBorrowNextOpeningTagStartMarker,
needsToBorrowParentOpeningTagEndMarker
};
}
});
var require_parse_srcset = __commonJS22({
"node_modules/parse-srcset/src/parse-srcset.js"(exports2, module22) {
(function(root, factory) {
if (typeof define === "function" && define.amd) {
define([], factory);
} else if (typeof module22 === "object" && module22.exports) {
module22.exports = factory();
} else {
root.parseSrcset = factory();
}
})(exports2, function() {
return function(input, options) {
var logger = options && options.logger || console;
function isSpace(c2) {
return c2 === " " || c2 === " " || c2 === "\n" || c2 === "\f" || c2 === "\r";
}
function collectCharacters(regEx) {
var chars, match = regEx.exec(input.substring(pos));
if (match) {
chars = match[0];
pos += chars.length;
return chars;
}
}
var inputLength = input.length, regexLeadingSpaces = /^[ \t\n\r\u000c]+/, regexLeadingCommasOrSpaces = /^[, \t\n\r\u000c]+/, regexLeadingNotSpaces = /^[^ \t\n\r\u000c]+/, regexTrailingCommas = /[,]+$/, regexNonNegativeInteger = /^\d+$/, regexFloatingPoint = /^-?(?:[0-9]+|[0-9]*\.[0-9]+)(?:[eE][+-]?[0-9]+)?$/, url, descriptors, currentDescriptor, state, c, pos = 0, candidates = [];
while (true) {
collectCharacters(regexLeadingCommasOrSpaces);
if (pos >= inputLength) {
return candidates;
}
url = collectCharacters(regexLeadingNotSpaces);
descriptors = [];
if (url.slice(-1) === ",") {
url = url.replace(regexTrailingCommas, "");
parseDescriptors();
} else {
tokenize();
}
}
function tokenize() {
collectCharacters(regexLeadingSpaces);
currentDescriptor = "";
state = "in descriptor";
while (true) {
c = input.charAt(pos);
if (state === "in descriptor") {
if (isSpace(c)) {
if (currentDescriptor) {
descriptors.push(currentDescriptor);
currentDescriptor = "";
state = "after descriptor";
}
} else if (c === ",") {
pos += 1;
if (currentDescriptor) {
descriptors.push(currentDescriptor);
}
parseDescriptors();
return;
} else if (c === "(") {
currentDescriptor = currentDescriptor + c;
state = "in parens";
} else if (c === "") {
if (currentDescriptor) {
descriptors.push(currentDescriptor);
}
parseDescriptors();
return;
} else {
currentDescriptor = currentDescriptor + c;
}
} else if (state === "in parens") {
if (c === ")") {
currentDescriptor = currentDescriptor + c;
state = "in descriptor";
} else if (c === "") {
descriptors.push(currentDescriptor);
parseDescriptors();
return;
} else {
currentDescriptor = currentDescriptor + c;
}
} else if (state === "after descriptor") {
if (isSpace(c)) {
} else if (c === "") {
parseDescriptors();
return;
} else {
state = "in descriptor";
pos -= 1;
}
}
pos += 1;
}
}
function parseDescriptors() {
var pError = false, w, d, h, i, candidate = {}, desc, lastChar, value, intVal, floatVal;
for (i = 0; i < descriptors.length; i++) {
desc = descriptors[i];
lastChar = desc[desc.length - 1];
value = desc.substring(0, desc.length - 1);
intVal = parseInt(value, 10);
floatVal = parseFloat(value);
if (regexNonNegativeInteger.test(value) && lastChar === "w") {
if (w || d) {
pError = true;
}
if (intVal === 0) {
pError = true;
} else {
w = intVal;
}
} else if (regexFloatingPoint.test(value) && lastChar === "x") {
if (w || d || h) {
pError = true;
}
if (floatVal < 0) {
pError = true;
} else {
d = floatVal;
}
} else if (regexNonNegativeInteger.test(value) && lastChar === "h") {
if (h || d) {
pError = true;
}
if (intVal === 0) {
pError = true;
} else {
h = intVal;
}
} else {
pError = true;
}
}
if (!pError) {
candidate.url = url;
if (w) {
candidate.w = w;
}
if (d) {
candidate.d = d;
}
if (h) {
candidate.h = h;
}
candidates.push(candidate);
} else if (logger && logger.error) {
logger.error("Invalid srcset descriptor found in '" + input + "' at '" + desc + "'.");
}
}
};
});
}
});
var require_syntax_attribute = __commonJS22({
"src/language-html/syntax-attribute.js"(exports2, module22) {
"use strict";
var parseSrcset = require_parse_srcset();
var {
builders: {
ifBreak,
join,
line
}
} = require_doc();
function printImgSrcset(value) {
const srcset = parseSrcset(value, {
logger: {
error(message) {
throw new Error(message);
}
}
});
const hasW = srcset.some(({
w
}) => w);
const hasH = srcset.some(({
h
}) => h);
const hasX = srcset.some(({
d
}) => d);
if (hasW + hasH + hasX > 1) {
throw new Error("Mixed descriptor in srcset is not supported");
}
const key = hasW ? "w" : hasH ? "h" : "d";
const unit = hasW ? "w" : hasH ? "h" : "x";
const getMax = (values) => Math.max(...values);
const urls = srcset.map((src) => src.url);
const maxUrlLength = getMax(urls.map((url) => url.length));
const descriptors = srcset.map((src) => src[key]).map((descriptor) => descriptor ? descriptor.toString() : "");
const descriptorLeftLengths = descriptors.map((descriptor) => {
const index = descriptor.indexOf(".");
return index === -1 ? descriptor.length : index;
});
const maxDescriptorLeftLength = getMax(descriptorLeftLengths);
return join([",", line], urls.map((url, index) => {
const parts = [url];
const descriptor = descriptors[index];
if (descriptor) {
const urlPadding = maxUrlLength - url.length + 1;
const descriptorPadding = maxDescriptorLeftLength - descriptorLeftLengths[index];
const alignment = " ".repeat(urlPadding + descriptorPadding);
parts.push(ifBreak(alignment, " "), descriptor + unit);
}
return parts;
}));
}
function printClassNames(value) {
return value.trim().split(/\s+/).join(" ");
}
module22.exports = {
printImgSrcset,
printClassNames
};
}
});
var require_syntax_vue = __commonJS22({
"src/language-html/syntax-vue.js"(exports2, module22) {
"use strict";
var {
builders: {
group
}
} = require_doc();
function printVueFor(value, textToDoc) {
const {
left,
operator,
right
} = parseVueFor(value);
return [group(textToDoc(`function _(${left}) {}`, {
parser: "babel",
__isVueForBindingLeft: true
})), " ", operator, " ", textToDoc(right, {
parser: "__js_expression"
}, {
stripTrailingHardline: true
})];
}
function parseVueFor(value) {
const forAliasRE = /(.*?)\s+(in|of)\s+(.*)/s;
const forIteratorRE = /,([^,\]}]*)(?:,([^,\]}]*))?$/;
const stripParensRE = /^\(|\)$/g;
const inMatch = value.match(forAliasRE);
if (!inMatch) {
return;
}
const res = {};
res.for = inMatch[3].trim();
if (!res.for) {
return;
}
const alias = inMatch[1].trim().replace(stripParensRE, "");
const iteratorMatch = alias.match(forIteratorRE);
if (iteratorMatch) {
res.alias = alias.replace(forIteratorRE, "");
res.iterator1 = iteratorMatch[1].trim();
if (iteratorMatch[2]) {
res.iterator2 = iteratorMatch[2].trim();
}
} else {
res.alias = alias;
}
const left = [res.alias, res.iterator1, res.iterator2];
if (left.some((part, index) => !part && (index === 0 || left.slice(index + 1).some(Boolean)))) {
return;
}
return {
left: left.filter(Boolean).join(","),
operator: inMatch[2],
right: res.for
};
}
function printVueBindings(value, textToDoc) {
return textToDoc(`function _(${value}) {}`, {
parser: "babel",
__isVueBindings: true
});
}
function isVueEventBindingExpression(eventBindingValue) {
const fnExpRE = /^(?:[\w$]+|\([^)]*\))\s*=>|^function\s*\(/;
const simplePathRE = /^[$A-Z_a-z][\w$]*(?:\.[$A-Z_a-z][\w$]*|\['[^']*']|\["[^"]*"]|\[\d+]|\[[$A-Z_a-z][\w$]*])*$/;
const value = eventBindingValue.trim();
return fnExpRE.test(value) || simplePathRE.test(value);
}
module22.exports = {
isVueEventBindingExpression,
printVueFor,
printVueBindings
};
}
});
var require_get_node_content = __commonJS22({
"src/language-html/get-node-content.js"(exports2, module22) {
"use strict";
var {
needsToBorrowParentClosingTagStartMarker,
printClosingTagStartMarker,
needsToBorrowLastChildClosingTagEndMarker,
printClosingTagEndMarker,
needsToBorrowParentOpeningTagEndMarker,
printOpeningTagEndMarker
} = require_tag();
function getNodeContent(node, options) {
let start = node.startSourceSpan.end.offset;
if (node.firstChild && needsToBorrowParentOpeningTagEndMarker(node.firstChild)) {
start -= printOpeningTagEndMarker(node).length;
}
let end = node.endSourceSpan.start.offset;
if (node.lastChild && needsToBorrowParentClosingTagStartMarker(node.lastChild)) {
end += printClosingTagStartMarker(node, options).length;
} else if (needsToBorrowLastChildClosingTagEndMarker(node)) {
end -= printClosingTagEndMarker(node.lastChild, options).length;
}
return options.originalText.slice(start, end);
}
module22.exports = getNodeContent;
}
});
var require_embed4 = __commonJS22({
"src/language-html/embed.js"(exports2, module22) {
"use strict";
var {
builders: {
breakParent,
group,
hardline,
indent,
line,
fill,
softline
},
utils: {
mapDoc,
replaceTextEndOfLine
}
} = require_doc();
var printFrontMatter = require_print();
var {
printClosingTag,
printClosingTagSuffix,
needsToBorrowPrevClosingTagEndMarker,
printOpeningTagPrefix,
printOpeningTag
} = require_tag();
var {
printImgSrcset,
printClassNames
} = require_syntax_attribute();
var {
printVueFor,
printVueBindings,
isVueEventBindingExpression
} = require_syntax_vue();
var {
isScriptLikeTag,
isVueNonHtmlBlock,
inferScriptParser,
htmlTrimPreserveIndentation,
dedentString,
unescapeQuoteEntities,
isVueSlotAttribute,
isVueSfcBindingsAttribute,
getTextValueParts
} = require_utils11();
var getNodeContent = require_get_node_content();
function printEmbeddedAttributeValue(node, htmlTextToDoc, options) {
const isKeyMatched = (patterns) => new RegExp(patterns.join("|")).test(node.fullName);
const getValue = () => unescapeQuoteEntities(node.value);
let shouldHug = false;
const __onHtmlBindingRoot = (root, options2) => {
const rootNode = root.type === "NGRoot" ? root.node.type === "NGMicrosyntax" && root.node.body.length === 1 && root.node.body[0].type === "NGMicrosyntaxExpression" ? root.node.body[0].expression : root.node : root.type === "JsExpressionRoot" ? root.node : root;
if (rootNode && (rootNode.type === "ObjectExpression" || rootNode.type === "ArrayExpression" || options2.parser === "__vue_expression" && (rootNode.type === "TemplateLiteral" || rootNode.type === "StringLiteral"))) {
shouldHug = true;
}
};
const printHug = (doc2) => group(doc2);
const printExpand = (doc2, canHaveTrailingWhitespace = true) => group([indent([softline, doc2]), canHaveTrailingWhitespace ? softline : ""]);
const printMaybeHug = (doc2) => shouldHug ? printHug(doc2) : printExpand(doc2);
const attributeTextToDoc = (code, opts) => htmlTextToDoc(code, Object.assign({
__onHtmlBindingRoot,
__embeddedInHtml: true
}, opts));
if (node.fullName === "srcset" && (node.parent.fullName === "img" || node.parent.fullName === "source")) {
return printExpand(printImgSrcset(getValue()));
}
if (node.fullName === "class" && !options.parentParser) {
const value = getValue();
if (!value.includes("{{")) {
return printClassNames(value);
}
}
if (node.fullName === "style" && !options.parentParser) {
const value = getValue();
if (!value.includes("{{")) {
return printExpand(attributeTextToDoc(value, {
parser: "css",
__isHTMLStyleAttribute: true
}));
}
}
if (options.parser === "vue") {
if (node.fullName === "v-for") {
return printVueFor(getValue(), attributeTextToDoc);
}
if (isVueSlotAttribute(node) || isVueSfcBindingsAttribute(node, options)) {
return printVueBindings(getValue(), attributeTextToDoc);
}
const vueEventBindingPatterns = ["^@", "^v-on:"];
const vueExpressionBindingPatterns = ["^:", "^v-bind:"];
const jsExpressionBindingPatterns = ["^v-"];
if (isKeyMatched(vueEventBindingPatterns)) {
const value = getValue();
const parser = isVueEventBindingExpression(value) ? "__js_expression" : options.__should_parse_vue_template_with_ts ? "__vue_ts_event_binding" : "__vue_event_binding";
return printMaybeHug(attributeTextToDoc(value, {
parser
}));
}
if (isKeyMatched(vueExpressionBindingPatterns)) {
return printMaybeHug(attributeTextToDoc(getValue(), {
parser: "__vue_expression"
}));
}
if (isKeyMatched(jsExpressionBindingPatterns)) {
return printMaybeHug(attributeTextToDoc(getValue(), {
parser: "__js_expression"
}));
}
}
if (options.parser === "angular") {
const ngTextToDoc = (code, opts) => attributeTextToDoc(code, Object.assign(Object.assign({}, opts), {}, {
trailingComma: "none"
}));
const ngDirectiveBindingPatterns = ["^\\*"];
const ngStatementBindingPatterns = ["^\\(.+\\)$", "^on-"];
const ngExpressionBindingPatterns = ["^\\[.+\\]$", "^bind(on)?-", "^ng-(if|show|hide|class|style)$"];
const ngI18nPatterns = ["^i18n(-.+)?$"];
if (isKeyMatched(ngStatementBindingPatterns)) {
return printMaybeHug(ngTextToDoc(getValue(), {
parser: "__ng_action"
}));
}
if (isKeyMatched(ngExpressionBindingPatterns)) {
return printMaybeHug(ngTextToDoc(getValue(), {
parser: "__ng_binding"
}));
}
if (isKeyMatched(ngI18nPatterns)) {
const value2 = getValue().trim();
return printExpand(fill(getTextValueParts(node, value2)), !value2.includes("@@"));
}
if (isKeyMatched(ngDirectiveBindingPatterns)) {
return printMaybeHug(ngTextToDoc(getValue(), {
parser: "__ng_directive"
}));
}
const interpolationRegex = /{{(.+?)}}/s;
const value = getValue();
if (interpolationRegex.test(value)) {
const parts = [];
for (const [index, part] of value.split(interpolationRegex).entries()) {
if (index % 2 === 0) {
parts.push(replaceTextEndOfLine(part));
} else {
try {
parts.push(group(["{{", indent([line, ngTextToDoc(part, {
parser: "__ng_interpolation",
__isInHtmlInterpolation: true
})]), line, "}}"]));
} catch {
parts.push("{{", replaceTextEndOfLine(part), "}}");
}
}
}
return group(parts);
}
}
return null;
}
function embed(path, print, textToDoc, options) {
const node = path.getValue();
switch (node.type) {
case "element": {
if (isScriptLikeTag(node) || node.type === "interpolation") {
return;
}
if (!node.isSelfClosing && isVueNonHtmlBlock(node, options)) {
const parser = inferScriptParser(node, options);
if (!parser) {
return;
}
const content = getNodeContent(node, options);
let isEmpty = /^\s*$/.test(content);
let doc2 = "";
if (!isEmpty) {
doc2 = textToDoc(htmlTrimPreserveIndentation(content), {
parser,
__embeddedInHtml: true
}, {
stripTrailingHardline: true
});
isEmpty = doc2 === "";
}
return [printOpeningTagPrefix(node, options), group(printOpeningTag(path, options, print)), isEmpty ? "" : hardline, doc2, isEmpty ? "" : hardline, printClosingTag(node, options), printClosingTagSuffix(node, options)];
}
break;
}
case "text": {
if (isScriptLikeTag(node.parent)) {
const parser = inferScriptParser(node.parent, options);
if (parser) {
const value = parser === "markdown" ? dedentString(node.value.replace(/^[^\S\n]*\n/, "")) : node.value;
const textToDocOptions = {
parser,
__embeddedInHtml: true
};
if (options.parser === "html" && parser === "babel") {
let sourceType = "script";
const {
attrMap
} = node.parent;
if (attrMap && (attrMap.type === "module" || attrMap.type === "text/babel" && attrMap["data-type"] === "module")) {
sourceType = "module";
}
textToDocOptions.__babelSourceType = sourceType;
}
return [breakParent, printOpeningTagPrefix(node, options), textToDoc(value, textToDocOptions, {
stripTrailingHardline: true
}), printClosingTagSuffix(node, options)];
}
} else if (node.parent.type === "interpolation") {
const textToDocOptions = {
__isInHtmlInterpolation: true,
__embeddedInHtml: true
};
if (options.parser === "angular") {
textToDocOptions.parser = "__ng_interpolation";
textToDocOptions.trailingComma = "none";
} else if (options.parser === "vue") {
textToDocOptions.parser = options.__should_parse_vue_template_with_ts ? "__vue_ts_expression" : "__vue_expression";
} else {
textToDocOptions.parser = "__js_expression";
}
return [indent([line, textToDoc(node.value, textToDocOptions, {
stripTrailingHardline: true
})]), node.parent.next && needsToBorrowPrevClosingTagEndMarker(node.parent.next) ? " " : line];
}
break;
}
case "attribute": {
if (!node.value) {
break;
}
if (/^PRETTIER_HTML_PLACEHOLDER_\d+_\d+_IN_JS$/.test(options.originalText.slice(node.valueSpan.start.offset, node.valueSpan.end.offset))) {
return [node.rawName, "=", node.value];
}
if (options.parser === "lwc") {
const interpolationRegex = /^{.*}$/s;
if (interpolationRegex.test(options.originalText.slice(node.valueSpan.start.offset, node.valueSpan.end.offset))) {
return [node.rawName, "=", node.value];
}
}
const embeddedAttributeValueDoc = printEmbeddedAttributeValue(node, (code, opts) => textToDoc(code, Object.assign({
__isInHtmlAttribute: true,
__embeddedInHtml: true
}, opts), {
stripTrailingHardline: true
}), options);
if (embeddedAttributeValueDoc) {
return [node.rawName, '="', group(mapDoc(embeddedAttributeValueDoc, (doc2) => typeof doc2 === "string" ? doc2.replace(/"/g, """) : doc2)), '"'];
}
break;
}
case "front-matter":
return printFrontMatter(node, textToDoc);
}
}
module22.exports = embed;
}
});
var require_children = __commonJS22({
"src/language-html/print/children.js"(exports2, module22) {
"use strict";
var {
builders: {
breakParent,
group,
ifBreak,
line,
softline,
hardline
},
utils: {
replaceTextEndOfLine
}
} = require_doc();
var {
locStart,
locEnd
} = require_loc6();
var {
forceBreakChildren,
forceNextEmptyLine,
isTextLikeNode,
hasPrettierIgnore,
preferHardlineAsLeadingSpaces
} = require_utils11();
var {
printOpeningTagPrefix,
needsToBorrowNextOpeningTagStartMarker,
printOpeningTagStartMarker,
needsToBorrowPrevClosingTagEndMarker,
printClosingTagEndMarker,
printClosingTagSuffix,
needsToBorrowParentClosingTagStartMarker
} = require_tag();
function printChild(childPath, options, print) {
const child = childPath.getValue();
if (hasPrettierIgnore(child)) {
return [printOpeningTagPrefix(child, options), ...replaceTextEndOfLine(options.originalText.slice(locStart(child) + (child.prev && needsToBorrowNextOpeningTagStartMarker(child.prev) ? printOpeningTagStartMarker(child).length : 0), locEnd(child) - (child.next && needsToBorrowPrevClosingTagEndMarker(child.next) ? printClosingTagEndMarker(child, options).length : 0))), printClosingTagSuffix(child, options)];
}
return print();
}
function printBetweenLine(prevNode, nextNode) {
return isTextLikeNode(prevNode) && isTextLikeNode(nextNode) ? prevNode.isTrailingSpaceSensitive ? prevNode.hasTrailingSpaces ? preferHardlineAsLeadingSpaces(nextNode) ? hardline : line : "" : preferHardlineAsLeadingSpaces(nextNode) ? hardline : softline : needsToBorrowNextOpeningTagStartMarker(prevNode) && (hasPrettierIgnore(nextNode) || nextNode.firstChild || nextNode.isSelfClosing || nextNode.type === "element" && nextNode.attrs.length > 0) || prevNode.type === "element" && prevNode.isSelfClosing && needsToBorrowPrevClosingTagEndMarker(nextNode) ? "" : !nextNode.isLeadingSpaceSensitive || preferHardlineAsLeadingSpaces(nextNode) || needsToBorrowPrevClosingTagEndMarker(nextNode) && prevNode.lastChild && needsToBorrowParentClosingTagStartMarker(prevNode.lastChild) && prevNode.lastChild.lastChild && needsToBorrowParentClosingTagStartMarker(prevNode.lastChild.lastChild) ? hardline : nextNode.hasLeadingSpaces ? line : softline;
}
function printChildren(path, options, print) {
const node = path.getValue();
if (forceBreakChildren(node)) {
return [breakParent, ...path.map((childPath) => {
const childNode = childPath.getValue();
const prevBetweenLine = !childNode.prev ? "" : printBetweenLine(childNode.prev, childNode);
return [!prevBetweenLine ? "" : [prevBetweenLine, forceNextEmptyLine(childNode.prev) ? hardline : ""], printChild(childPath, options, print)];
}, "children")];
}
const groupIds = node.children.map(() => Symbol(""));
return path.map((childPath, childIndex) => {
const childNode = childPath.getValue();
if (isTextLikeNode(childNode)) {
if (childNode.prev && isTextLikeNode(childNode.prev)) {
const prevBetweenLine2 = printBetweenLine(childNode.prev, childNode);
if (prevBetweenLine2) {
if (forceNextEmptyLine(childNode.prev)) {
return [hardline, hardline, printChild(childPath, options, print)];
}
return [prevBetweenLine2, printChild(childPath, options, print)];
}
}
return printChild(childPath, options, print);
}
const prevParts = [];
const leadingParts = [];
const trailingParts = [];
const nextParts = [];
const prevBetweenLine = childNode.prev ? printBetweenLine(childNode.prev, childNode) : "";
const nextBetweenLine = childNode.next ? printBetweenLine(childNode, childNode.next) : "";
if (prevBetweenLine) {
if (forceNextEmptyLine(childNode.prev)) {
prevParts.push(hardline, hardline);
} else if (prevBetweenLine === hardline) {
prevParts.push(hardline);
} else {
if (isTextLikeNode(childNode.prev)) {
leadingParts.push(prevBetweenLine);
} else {
leadingParts.push(ifBreak("", softline, {
groupId: groupIds[childIndex - 1]
}));
}
}
}
if (nextBetweenLine) {
if (forceNextEmptyLine(childNode)) {
if (isTextLikeNode(childNode.next)) {
nextParts.push(hardline, hardline);
}
} else if (nextBetweenLine === hardline) {
if (isTextLikeNode(childNode.next)) {
nextParts.push(hardline);
}
} else {
trailingParts.push(nextBetweenLine);
}
}
return [...prevParts, group([...leadingParts, group([printChild(childPath, options, print), ...trailingParts], {
id: groupIds[childIndex]
})]), ...nextParts];
}, "children");
}
module22.exports = {
printChildren
};
}
});
var require_element = __commonJS22({
"src/language-html/print/element.js"(exports2, module22) {
"use strict";
var {
builders: {
breakParent,
dedentToRoot,
group,
ifBreak,
indentIfBreak,
indent,
line,
softline
},
utils: {
replaceTextEndOfLine
}
} = require_doc();
var getNodeContent = require_get_node_content();
var {
shouldPreserveContent,
isScriptLikeTag,
isVueCustomBlock,
countParents,
forceBreakContent
} = require_utils11();
var {
printOpeningTagPrefix,
printOpeningTag,
printClosingTagSuffix,
printClosingTag,
needsToBorrowPrevClosingTagEndMarker,
needsToBorrowLastChildClosingTagEndMarker
} = require_tag();
var {
printChildren
} = require_children();
function printElement(path, options, print) {
const node = path.getValue();
if (shouldPreserveContent(node, options)) {
return [printOpeningTagPrefix(node, options), group(printOpeningTag(path, options, print)), ...replaceTextEndOfLine(getNodeContent(node, options)), ...printClosingTag(node, options), printClosingTagSuffix(node, options)];
}
const shouldHugContent = node.children.length === 1 && node.firstChild.type === "interpolation" && node.firstChild.isLeadingSpaceSensitive && !node.firstChild.hasLeadingSpaces && node.lastChild.isTrailingSpaceSensitive && !node.lastChild.hasTrailingSpaces;
const attrGroupId = Symbol("element-attr-group-id");
const printTag = (doc2) => group([group(printOpeningTag(path, options, print), {
id: attrGroupId
}), doc2, printClosingTag(node, options)]);
const printChildrenDoc = (childrenDoc) => {
if (shouldHugContent) {
return indentIfBreak(childrenDoc, {
groupId: attrGroupId
});
}
if ((isScriptLikeTag(node) || isVueCustomBlock(node, options)) && node.parent.type === "root" && options.parser === "vue" && !options.vueIndentScriptAndStyle) {
return childrenDoc;
}
return indent(childrenDoc);
};
const printLineBeforeChildren = () => {
if (shouldHugContent) {
return ifBreak(softline, "", {
groupId: attrGroupId
});
}
if (node.firstChild.hasLeadingSpaces && node.firstChild.isLeadingSpaceSensitive) {
return line;
}
if (node.firstChild.type === "text" && node.isWhitespaceSensitive && node.isIndentationSensitive) {
return dedentToRoot(softline);
}
return softline;
};
const printLineAfterChildren = () => {
const needsToBorrow = node.next ? needsToBorrowPrevClosingTagEndMarker(node.next) : needsToBorrowLastChildClosingTagEndMarker(node.parent);
if (needsToBorrow) {
if (node.lastChild.hasTrailingSpaces && node.lastChild.isTrailingSpaceSensitive) {
return " ";
}
return "";
}
if (shouldHugContent) {
return ifBreak(softline, "", {
groupId: attrGroupId
});
}
if (node.lastChild.hasTrailingSpaces && node.lastChild.isTrailingSpaceSensitive) {
return line;
}
if ((node.lastChild.type === "comment" || node.lastChild.type === "text" && node.isWhitespaceSensitive && node.isIndentationSensitive) && new RegExp(`\\n[\\t ]{${options.tabWidth * countParents(path, (node2) => node2.parent && node2.parent.type !== "root")}}$`).test(node.lastChild.value)) {
return "";
}
return softline;
};
if (node.children.length === 0) {
return printTag(node.hasDanglingSpaces && node.isDanglingSpaceSensitive ? line : "");
}
return printTag([forceBreakContent(node) ? breakParent : "", printChildrenDoc([printLineBeforeChildren(), printChildren(path, options, print)]), printLineAfterChildren()]);
}
module22.exports = {
printElement
};
}
});
var require_printer_html = __commonJS22({
"src/language-html/printer-html.js"(exports2, module22) {
"use strict";
var {
builders: {
fill,
group,
hardline,
literalline
},
utils: {
cleanDoc,
getDocParts,
isConcat,
replaceTextEndOfLine
}
} = require_doc();
var clean = require_clean5();
var {
countChars,
unescapeQuoteEntities,
getTextValueParts
} = require_utils11();
var preprocess = require_print_preprocess3();
var {
insertPragma
} = require_pragma5();
var {
locStart,
locEnd
} = require_loc6();
var embed = require_embed4();
var {
printClosingTagSuffix,
printClosingTagEnd,
printOpeningTagPrefix,
printOpeningTagStart
} = require_tag();
var {
printElement
} = require_element();
var {
printChildren
} = require_children();
function genericPrint(path, options, print) {
const node = path.getValue();
switch (node.type) {
case "front-matter":
return replaceTextEndOfLine(node.raw);
case "root":
if (options.__onHtmlRoot) {
options.__onHtmlRoot(node);
}
return [group(printChildren(path, options, print)), hardline];
case "element":
case "ieConditionalComment": {
return printElement(path, options, print);
}
case "ieConditionalStartComment":
case "ieConditionalEndComment":
return [printOpeningTagStart(node), printClosingTagEnd(node)];
case "interpolation":
return [printOpeningTagStart(node, options), ...path.map(print, "children"), printClosingTagEnd(node, options)];
case "text": {
if (node.parent.type === "interpolation") {
const trailingNewlineRegex = /\n[^\S\n]*$/;
const hasTrailingNewline = trailingNewlineRegex.test(node.value);
const value = hasTrailingNewline ? node.value.replace(trailingNewlineRegex, "") : node.value;
return [...replaceTextEndOfLine(value), hasTrailingNewline ? hardline : ""];
}
const printed = cleanDoc([printOpeningTagPrefix(node, options), ...getTextValueParts(node), printClosingTagSuffix(node, options)]);
if (isConcat(printed) || printed.type === "fill") {
return fill(getDocParts(printed));
}
return printed;
}
case "docType":
return [group([printOpeningTagStart(node, options), " ", node.value.replace(/^html\b/i, "html").replace(/\s+/g, " ")]), printClosingTagEnd(node, options)];
case "comment": {
return [printOpeningTagPrefix(node, options), ...replaceTextEndOfLine(options.originalText.slice(locStart(node), locEnd(node)), literalline), printClosingTagSuffix(node, options)];
}
case "attribute": {
if (node.value === null) {
return node.rawName;
}
const value = unescapeQuoteEntities(node.value);
const singleQuoteCount = countChars(value, "'");
const doubleQuoteCount = countChars(value, '"');
const quote2 = singleQuoteCount < doubleQuoteCount ? "'" : '"';
return [node.rawName, "=", quote2, ...replaceTextEndOfLine(quote2 === '"' ? value.replace(/"/g, """) : value.replace(/'/g, "'")), quote2];
}
default:
throw new Error(`Unexpected node type ${node.type}`);
}
}
module22.exports = {
preprocess,
print: genericPrint,
insertPragma,
massageAstNode: clean,
embed
};
}
});
var require_options6 = __commonJS22({
"src/language-html/options.js"(exports2, module22) {
"use strict";
var commonOptions = require_common_options();
var CATEGORY_HTML = "HTML";
module22.exports = {
bracketSameLine: commonOptions.bracketSameLine,
htmlWhitespaceSensitivity: {
since: "1.15.0",
category: CATEGORY_HTML,
type: "choice",
default: "css",
description: "How to handle whitespaces in HTML.",
choices: [{
value: "css",
description: "Respect the default value of CSS display property."
}, {
value: "strict",
description: "Whitespaces are considered sensitive."
}, {
value: "ignore",
description: "Whitespaces are considered insensitive."
}]
},
singleAttributePerLine: commonOptions.singleAttributePerLine,
vueIndentScriptAndStyle: {
since: "1.19.0",
category: CATEGORY_HTML,
type: "boolean",
default: false,
description: "Indent script and style tags in Vue files."
}
};
}
});
var require_parsers6 = __commonJS22({
"src/language-html/parsers.js"(exports2, module22) {
"use strict";
module22.exports = {
get html() {
return require_parser_html().parsers.html;
},
get vue() {
return require_parser_html().parsers.vue;
},
get angular() {
return require_parser_html().parsers.angular;
},
get lwc() {
return require_parser_html().parsers.lwc;
}
};
}
});
var require_HTML = __commonJS22({
"node_modules/linguist-languages/data/HTML.json"(exports2, module22) {
module22.exports = {
name: "HTML",
type: "markup",
tmScope: "text.html.basic",
aceMode: "html",
codemirrorMode: "htmlmixed",
codemirrorMimeType: "text/html",
color: "#e34c26",
aliases: ["xhtml"],
extensions: [".html", ".hta", ".htm", ".html.hl", ".inc", ".xht", ".xhtml"],
languageId: 146
};
}
});
var require_Vue = __commonJS22({
"node_modules/linguist-languages/data/Vue.json"(exports2, module22) {
module22.exports = {
name: "Vue",
type: "markup",
color: "#41b883",
extensions: [".vue"],
tmScope: "text.html.vue",
aceMode: "html",
languageId: 391
};
}
});
var require_language_html = __commonJS22({
"src/language-html/index.js"(exports2, module22) {
"use strict";
var createLanguage = require_create_language();
var printer = require_printer_html();
var options = require_options6();
var parsers = require_parsers6();
var languages = [createLanguage(require_HTML(), () => ({
name: "Angular",
since: "1.15.0",
parsers: ["angular"],
vscodeLanguageIds: ["html"],
extensions: [".component.html"],
filenames: []
})), createLanguage(require_HTML(), (data) => ({
since: "1.15.0",
parsers: ["html"],
vscodeLanguageIds: ["html"],
extensions: [...data.extensions, ".mjml"]
})), createLanguage(require_HTML(), () => ({
name: "Lightning Web Components",
since: "1.17.0",
parsers: ["lwc"],
vscodeLanguageIds: ["html"],
extensions: [],
filenames: []
})), createLanguage(require_Vue(), () => ({
since: "1.10.0",
parsers: ["vue"],
vscodeLanguageIds: ["vue"]
}))];
var printers = {
html: printer
};
module22.exports = {
languages,
printers,
options,
parsers
};
}
});
var require_pragma6 = __commonJS22({
"src/language-yaml/pragma.js"(exports2, module22) {
"use strict";
function isPragma(text) {
return /^\s*@(?:prettier|format)\s*$/.test(text);
}
function hasPragma(text) {
return /^\s*#[^\S\n]*@(?:prettier|format)\s*?(?:\n|$)/.test(text);
}
function insertPragma(text) {
return `# @format
${text}`;
}
module22.exports = {
isPragma,
hasPragma,
insertPragma
};
}
});
var require_loc7 = __commonJS22({
"src/language-yaml/loc.js"(exports2, module22) {
"use strict";
function locStart(node) {
return node.position.start.offset;
}
function locEnd(node) {
return node.position.end.offset;
}
module22.exports = {
locStart,
locEnd
};
}
});
var require_embed5 = __commonJS22({
"src/language-yaml/embed.js"(exports2, module22) {
"use strict";
function embed(path, print, textToDoc, options) {
const node = path.getValue();
if (node.type === "root" && options.filepath && /(?:[/\\]|^)\.(?:prettier|stylelint|lintstaged)rc$/.test(options.filepath)) {
return textToDoc(options.originalText, Object.assign(Object.assign({}, options), {}, {
parser: "json"
}));
}
}
module22.exports = embed;
}
});
var require_utils12 = __commonJS22({
"src/language-yaml/utils.js"(exports2, module22) {
"use strict";
var {
getLast,
isNonEmptyArray
} = require_util();
function getAncestorCount(path, filter) {
let counter = 0;
const pathStackLength = path.stack.length - 1;
for (let i = 0; i < pathStackLength; i++) {
const value = path.stack[i];
if (isNode(value) && filter(value)) {
counter++;
}
}
return counter;
}
function isNode(value, types) {
return value && typeof value.type === "string" && (!types || types.includes(value.type));
}
function mapNode(node, callback, parent) {
return callback("children" in node ? Object.assign(Object.assign({}, node), {}, {
children: node.children.map((childNode) => mapNode(childNode, callback, node))
}) : node, parent);
}
function defineShortcut(x, key, getter) {
Object.defineProperty(x, key, {
get: getter,
enumerable: false
});
}
function isNextLineEmpty(node, text) {
let newlineCount = 0;
const textLength = text.length;
for (let i = node.position.end.offset - 1; i < textLength; i++) {
const char2 = text[i];
if (char2 === "\n") {
newlineCount++;
}
if (newlineCount === 1 && /\S/.test(char2)) {
return false;
}
if (newlineCount === 2) {
return true;
}
}
return false;
}
function isLastDescendantNode(path) {
const node = path.getValue();
switch (node.type) {
case "tag":
case "anchor":
case "comment":
return false;
}
const pathStackLength = path.stack.length;
for (let i = 1; i < pathStackLength; i++) {
const item = path.stack[i];
const parentItem = path.stack[i - 1];
if (Array.isArray(parentItem) && typeof item === "number" && item !== parentItem.length - 1) {
return false;
}
}
return true;
}
function getLastDescendantNode(node) {
return isNonEmptyArray(node.children) ? getLastDescendantNode(getLast(node.children)) : node;
}
function isPrettierIgnore(comment) {
return comment.value.trim() === "prettier-ignore";
}
function hasPrettierIgnore(path) {
const node = path.getValue();
if (node.type === "documentBody") {
const document2 = path.getParentNode();
return hasEndComments(document2.head) && isPrettierIgnore(getLast(document2.head.endComments));
}
return hasLeadingComments(node) && isPrettierIgnore(getLast(node.leadingComments));
}
function isEmptyNode(node) {
return !isNonEmptyArray(node.children) && !hasComments(node);
}
function hasComments(node) {
return hasLeadingComments(node) || hasMiddleComments(node) || hasIndicatorComment(node) || hasTrailingComment(node) || hasEndComments(node);
}
function hasLeadingComments(node) {
return isNonEmptyArray(node === null || node === void 0 ? void 0 : node.leadingComments);
}
function hasMiddleComments(node) {
return isNonEmptyArray(node === null || node === void 0 ? void 0 : node.middleComments);
}
function hasIndicatorComment(node) {
return node === null || node === void 0 ? void 0 : node.indicatorComment;
}
function hasTrailingComment(node) {
return node === null || node === void 0 ? void 0 : node.trailingComment;
}
function hasEndComments(node) {
return isNonEmptyArray(node === null || node === void 0 ? void 0 : node.endComments);
}
function splitWithSingleSpace(text) {
const parts = [];
let lastPart;
for (const part of text.split(/( +)/)) {
if (part !== " ") {
if (lastPart === " ") {
parts.push(part);
} else {
parts.push((parts.pop() || "") + part);
}
} else if (lastPart === void 0) {
parts.unshift("");
}
lastPart = part;
}
if (lastPart === " ") {
parts.push((parts.pop() || "") + " ");
}
if (parts[0] === "") {
parts.shift();
parts.unshift(" " + (parts.shift() || ""));
}
return parts;
}
function getFlowScalarLineContents(nodeType, content, options) {
const rawLineContents = content.split("\n").map((lineContent, index, lineContents) => index === 0 && index === lineContents.length - 1 ? lineContent : index !== 0 && index !== lineContents.length - 1 ? lineContent.trim() : index === 0 ? lineContent.trimEnd() : lineContent.trimStart());
if (options.proseWrap === "preserve") {
return rawLineContents.map((lineContent) => lineContent.length === 0 ? [] : [lineContent]);
}
return rawLineContents.map((lineContent) => lineContent.length === 0 ? [] : splitWithSingleSpace(lineContent)).reduce((reduced, lineContentWords, index) => index !== 0 && rawLineContents[index - 1].length > 0 && lineContentWords.length > 0 && !(nodeType === "quoteDouble" && getLast(getLast(reduced)).endsWith("\\")) ? [...reduced.slice(0, -1), [...getLast(reduced), ...lineContentWords]] : [...reduced, lineContentWords], []).map((lineContentWords) => options.proseWrap === "never" ? [lineContentWords.join(" ")] : lineContentWords);
}
function getBlockValueLineContents(node, {
parentIndent,
isLastDescendant,
options
}) {
const content = node.position.start.line === node.position.end.line ? "" : options.originalText.slice(node.position.start.offset, node.position.end.offset).match(/^[^\n]*\n(.*)$/s)[1];
let leadingSpaceCount;
if (node.indent === null) {
const matches = content.match(/^(? *)[^\n\r ]/m);
leadingSpaceCount = matches ? matches.groups.leadingSpace.length : Number.POSITIVE_INFINITY;
} else {
leadingSpaceCount = node.indent - 1 + parentIndent;
}
const rawLineContents = content.split("\n").map((lineContent) => lineContent.slice(leadingSpaceCount));
if (options.proseWrap === "preserve" || node.type === "blockLiteral") {
return removeUnnecessaryTrailingNewlines(rawLineContents.map((lineContent) => lineContent.length === 0 ? [] : [lineContent]));
}
return removeUnnecessaryTrailingNewlines(rawLineContents.map((lineContent) => lineContent.length === 0 ? [] : splitWithSingleSpace(lineContent)).reduce((reduced, lineContentWords, index) => index !== 0 && rawLineContents[index - 1].length > 0 && lineContentWords.length > 0 && !/^\s/.test(lineContentWords[0]) && !/^\s|\s$/.test(getLast(reduced)) ? [...reduced.slice(0, -1), [...getLast(reduced), ...lineContentWords]] : [...reduced, lineContentWords], []).map((lineContentWords) => lineContentWords.reduce((reduced, word) => reduced.length > 0 && /\s$/.test(getLast(reduced)) ? [...reduced.slice(0, -1), getLast(reduced) + " " + word] : [...reduced, word], [])).map((lineContentWords) => options.proseWrap === "never" ? [lineContentWords.join(" ")] : lineContentWords));
function removeUnnecessaryTrailingNewlines(lineContents) {
if (node.chomping === "keep") {
return getLast(lineContents).length === 0 ? lineContents.slice(0, -1) : lineContents;
}
let trailingNewlineCount = 0;
for (let i = lineContents.length - 1; i >= 0; i--) {
if (lineContents[i].length === 0) {
trailingNewlineCount++;
} else {
break;
}
}
return trailingNewlineCount === 0 ? lineContents : trailingNewlineCount >= 2 && !isLastDescendant ? lineContents.slice(0, -(trailingNewlineCount - 1)) : lineContents.slice(0, -trailingNewlineCount);
}
}
function isInlineNode(node) {
if (!node) {
return true;
}
switch (node.type) {
case "plain":
case "quoteDouble":
case "quoteSingle":
case "alias":
case "flowMapping":
case "flowSequence":
return true;
default:
return false;
}
}
module22.exports = {
getLast,
getAncestorCount,
isNode,
isEmptyNode,
isInlineNode,
mapNode,
defineShortcut,
isNextLineEmpty,
isLastDescendantNode,
getBlockValueLineContents,
getFlowScalarLineContents,
getLastDescendantNode,
hasPrettierIgnore,
hasLeadingComments,
hasMiddleComments,
hasIndicatorComment,
hasTrailingComment,
hasEndComments
};
}
});
var require_print_preprocess4 = __commonJS22({
"src/language-yaml/print-preprocess.js"(exports2, module22) {
"use strict";
var {
defineShortcut,
mapNode
} = require_utils12();
function preprocess(ast) {
return mapNode(ast, defineShortcuts);
}
function defineShortcuts(node) {
switch (node.type) {
case "document":
defineShortcut(node, "head", () => node.children[0]);
defineShortcut(node, "body", () => node.children[1]);
break;
case "documentBody":
case "sequenceItem":
case "flowSequenceItem":
case "mappingKey":
case "mappingValue":
defineShortcut(node, "content", () => node.children[0]);
break;
case "mappingItem":
case "flowMappingItem":
defineShortcut(node, "key", () => node.children[0]);
defineShortcut(node, "value", () => node.children[1]);
break;
}
return node;
}
module22.exports = preprocess;
}
});
var require_misc2 = __commonJS22({
"src/language-yaml/print/misc.js"(exports2, module22) {
"use strict";
var {
builders: {
softline,
align
}
} = require_doc();
var {
hasEndComments,
isNextLineEmpty,
isNode
} = require_utils12();
var printedEmptyLineCache = /* @__PURE__ */ new WeakMap();
function printNextEmptyLine(path, originalText) {
const node = path.getValue();
const root = path.stack[0];
let isNextEmptyLinePrintedSet;
if (printedEmptyLineCache.has(root)) {
isNextEmptyLinePrintedSet = printedEmptyLineCache.get(root);
} else {
isNextEmptyLinePrintedSet = /* @__PURE__ */ new Set();
printedEmptyLineCache.set(root, isNextEmptyLinePrintedSet);
}
if (!isNextEmptyLinePrintedSet.has(node.position.end.line)) {
isNextEmptyLinePrintedSet.add(node.position.end.line);
if (isNextLineEmpty(node, originalText) && !shouldPrintEndComments(path.getParentNode())) {
return softline;
}
}
return "";
}
function shouldPrintEndComments(node) {
return hasEndComments(node) && !isNode(node, ["documentHead", "documentBody", "flowMapping", "flowSequence"]);
}
function alignWithSpaces(width, doc2) {
return align(" ".repeat(width), doc2);
}
module22.exports = {
alignWithSpaces,
shouldPrintEndComments,
printNextEmptyLine
};
}
});
var require_flow_mapping_sequence = __commonJS22({
"src/language-yaml/print/flow-mapping-sequence.js"(exports2, module22) {
"use strict";
var {
builders: {
ifBreak,
line,
softline,
hardline,
join
}
} = require_doc();
var {
isEmptyNode,
getLast,
hasEndComments
} = require_utils12();
var {
printNextEmptyLine,
alignWithSpaces
} = require_misc2();
function printFlowMapping(path, print, options) {
const node = path.getValue();
const isMapping = node.type === "flowMapping";
const openMarker = isMapping ? "{" : "[";
const closeMarker = isMapping ? "}" : "]";
let bracketSpacing = softline;
if (isMapping && node.children.length > 0 && options.bracketSpacing) {
bracketSpacing = line;
}
const lastItem = getLast(node.children);
const isLastItemEmptyMappingItem = lastItem && lastItem.type === "flowMappingItem" && isEmptyNode(lastItem.key) && isEmptyNode(lastItem.value);
return [openMarker, alignWithSpaces(options.tabWidth, [bracketSpacing, printChildren(path, print, options), options.trailingComma === "none" ? "" : ifBreak(","), hasEndComments(node) ? [hardline, join(hardline, path.map(print, "endComments"))] : ""]), isLastItemEmptyMappingItem ? "" : bracketSpacing, closeMarker];
}
function printChildren(path, print, options) {
const node = path.getValue();
const parts = path.map((childPath, index) => [print(), index === node.children.length - 1 ? "" : [",", line, node.children[index].position.start.line !== node.children[index + 1].position.start.line ? printNextEmptyLine(childPath, options.originalText) : ""]], "children");
return parts;
}
module22.exports = {
printFlowMapping,
printFlowSequence: printFlowMapping
};
}
});
var require_mapping_item = __commonJS22({
"src/language-yaml/print/mapping-item.js"(exports2, module22) {
"use strict";
var {
builders: {
conditionalGroup,
group,
hardline,
ifBreak,
join,
line
}
} = require_doc();
var {
hasLeadingComments,
hasMiddleComments,
hasTrailingComment,
hasEndComments,
isNode,
isEmptyNode,
isInlineNode
} = require_utils12();
var {
alignWithSpaces
} = require_misc2();
function printMappingItem(node, parentNode, path, print, options) {
const {
key,
value
} = node;
const isEmptyMappingKey = isEmptyNode(key);
const isEmptyMappingValue = isEmptyNode(value);
if (isEmptyMappingKey && isEmptyMappingValue) {
return ": ";
}
const printedKey = print("key");
const spaceBeforeColon = needsSpaceInFrontOfMappingValue(node) ? " " : "";
if (isEmptyMappingValue) {
if (node.type === "flowMappingItem" && parentNode.type === "flowMapping") {
return printedKey;
}
if (node.type === "mappingItem" && isAbsolutelyPrintedAsSingleLineNode(key.content, options) && !hasTrailingComment(key.content) && (!parentNode.tag || parentNode.tag.value !== "tag:yaml.org,2002:set")) {
return [printedKey, spaceBeforeColon, ":"];
}
return ["? ", alignWithSpaces(2, printedKey)];
}
const printedValue = print("value");
if (isEmptyMappingKey) {
return [": ", alignWithSpaces(2, printedValue)];
}
if (hasLeadingComments(value) || !isInlineNode(key.content)) {
return ["? ", alignWithSpaces(2, printedKey), hardline, join("", path.map(print, "value", "leadingComments").map((comment) => [comment, hardline])), ": ", alignWithSpaces(2, printedValue)];
}
if (isSingleLineNode(key.content) && !hasLeadingComments(key.content) && !hasMiddleComments(key.content) && !hasTrailingComment(key.content) && !hasEndComments(key) && !hasLeadingComments(value.content) && !hasMiddleComments(value.content) && !hasEndComments(value) && isAbsolutelyPrintedAsSingleLineNode(value.content, options)) {
return [printedKey, spaceBeforeColon, ": ", printedValue];
}
const groupId = Symbol("mappingKey");
const groupedKey = group([ifBreak("? "), group(alignWithSpaces(2, printedKey), {
id: groupId
})]);
const explicitMappingValue = [hardline, ": ", alignWithSpaces(2, printedValue)];
const implicitMappingValueParts = [spaceBeforeColon, ":"];
if (hasLeadingComments(value.content) || hasEndComments(value) && value.content && !isNode(value.content, ["mapping", "sequence"]) || parentNode.type === "mapping" && hasTrailingComment(key.content) && isInlineNode(value.content) || isNode(value.content, ["mapping", "sequence"]) && value.content.tag === null && value.content.anchor === null) {
implicitMappingValueParts.push(hardline);
} else if (value.content) {
implicitMappingValueParts.push(line);
}
implicitMappingValueParts.push(printedValue);
const implicitMappingValue = alignWithSpaces(options.tabWidth, implicitMappingValueParts);
if (isAbsolutelyPrintedAsSingleLineNode(key.content, options) && !hasLeadingComments(key.content) && !hasMiddleComments(key.content) && !hasEndComments(key)) {
return conditionalGroup([[printedKey, implicitMappingValue]]);
}
return conditionalGroup([[groupedKey, ifBreak(explicitMappingValue, implicitMappingValue, {
groupId
})]]);
}
function isAbsolutelyPrintedAsSingleLineNode(node, options) {
if (!node) {
return true;
}
switch (node.type) {
case "plain":
case "quoteSingle":
case "quoteDouble":
break;
case "alias":
return true;
default:
return false;
}
if (options.proseWrap === "preserve") {
return node.position.start.line === node.position.end.line;
}
if (/\\$/m.test(options.originalText.slice(node.position.start.offset, node.position.end.offset))) {
return false;
}
switch (options.proseWrap) {
case "never":
return !node.value.includes("\n");
case "always":
return !/[\n ]/.test(node.value);
default:
return false;
}
}
function needsSpaceInFrontOfMappingValue(node) {
return node.key.content && node.key.content.type === "alias";
}
function isSingleLineNode(node) {
if (!node) {
return true;
}
switch (node.type) {
case "plain":
case "quoteDouble":
case "quoteSingle":
return node.position.start.line === node.position.end.line;
case "alias":
return true;
default:
return false;
}
}
module22.exports = printMappingItem;
}
});
var require_block2 = __commonJS22({
"src/language-yaml/print/block.js"(exports2, module22) {
"use strict";
var {
builders: {
dedent,
dedentToRoot,
fill,
hardline,
join,
line,
literalline,
markAsRoot
},
utils: {
getDocParts
}
} = require_doc();
var {
getAncestorCount,
getBlockValueLineContents,
hasIndicatorComment,
isLastDescendantNode,
isNode
} = require_utils12();
var {
alignWithSpaces
} = require_misc2();
function printBlock(path, print, options) {
const node = path.getValue();
const parentIndent = getAncestorCount(path, (ancestorNode) => isNode(ancestorNode, ["sequence", "mapping"]));
const isLastDescendant = isLastDescendantNode(path);
const parts = [node.type === "blockFolded" ? ">" : "|"];
if (node.indent !== null) {
parts.push(node.indent.toString());
}
if (node.chomping !== "clip") {
parts.push(node.chomping === "keep" ? "+" : "-");
}
if (hasIndicatorComment(node)) {
parts.push(" ", print("indicatorComment"));
}
const lineContents = getBlockValueLineContents(node, {
parentIndent,
isLastDescendant,
options
});
const contentsParts = [];
for (const [index, lineWords] of lineContents.entries()) {
if (index === 0) {
contentsParts.push(hardline);
}
contentsParts.push(fill(getDocParts(join(line, lineWords))));
if (index !== lineContents.length - 1) {
contentsParts.push(lineWords.length === 0 ? hardline : markAsRoot(literalline));
} else if (node.chomping === "keep" && isLastDescendant) {
contentsParts.push(dedentToRoot(lineWords.length === 0 ? hardline : literalline));
}
}
if (node.indent === null) {
parts.push(dedent(alignWithSpaces(options.tabWidth, contentsParts)));
} else {
parts.push(dedentToRoot(alignWithSpaces(node.indent - 1 + parentIndent, contentsParts)));
}
return parts;
}
module22.exports = printBlock;
}
});
var require_printer_yaml = __commonJS22({
"src/language-yaml/printer-yaml.js"(exports2, module22) {
"use strict";
var {
builders: {
breakParent,
fill,
group,
hardline,
join,
line,
lineSuffix,
literalline
},
utils: {
getDocParts,
replaceTextEndOfLine
}
} = require_doc();
var {
isPreviousLineEmpty
} = require_util();
var {
insertPragma,
isPragma
} = require_pragma6();
var {
locStart
} = require_loc7();
var embed = require_embed5();
var {
getFlowScalarLineContents,
getLastDescendantNode,
hasLeadingComments,
hasMiddleComments,
hasTrailingComment,
hasEndComments,
hasPrettierIgnore,
isLastDescendantNode,
isNode,
isInlineNode
} = require_utils12();
var preprocess = require_print_preprocess4();
var {
alignWithSpaces,
printNextEmptyLine,
shouldPrintEndComments
} = require_misc2();
var {
printFlowMapping,
printFlowSequence
} = require_flow_mapping_sequence();
var printMappingItem = require_mapping_item();
var printBlock = require_block2();
function genericPrint(path, options, print) {
const node = path.getValue();
const parts = [];
if (node.type !== "mappingValue" && hasLeadingComments(node)) {
parts.push([join(hardline, path.map(print, "leadingComments")), hardline]);
}
const {
tag,
anchor
} = node;
if (tag) {
parts.push(print("tag"));
}
if (tag && anchor) {
parts.push(" ");
}
if (anchor) {
parts.push(print("anchor"));
}
let nextEmptyLine = "";
if (isNode(node, ["mapping", "sequence", "comment", "directive", "mappingItem", "sequenceItem"]) && !isLastDescendantNode(path)) {
nextEmptyLine = printNextEmptyLine(path, options.originalText);
}
if (tag || anchor) {
if (isNode(node, ["sequence", "mapping"]) && !hasMiddleComments(node)) {
parts.push(hardline);
} else {
parts.push(" ");
}
}
if (hasMiddleComments(node)) {
parts.push([node.middleComments.length === 1 ? "" : hardline, join(hardline, path.map(print, "middleComments")), hardline]);
}
const parentNode = path.getParentNode();
if (hasPrettierIgnore(path)) {
parts.push(replaceTextEndOfLine(options.originalText.slice(node.position.start.offset, node.position.end.offset).trimEnd(), literalline));
} else {
parts.push(group(printNode(node, parentNode, path, options, print)));
}
if (hasTrailingComment(node) && !isNode(node, ["document", "documentHead"])) {
parts.push(lineSuffix([node.type === "mappingValue" && !node.content ? "" : " ", parentNode.type === "mappingKey" && path.getParentNode(2).type === "mapping" && isInlineNode(node) ? "" : breakParent, print("trailingComment")]));
}
if (shouldPrintEndComments(node)) {
parts.push(alignWithSpaces(node.type === "sequenceItem" ? 2 : 0, [hardline, join(hardline, path.map((path2) => [isPreviousLineEmpty(options.originalText, path2.getValue(), locStart) ? hardline : "", print()], "endComments"))]));
}
parts.push(nextEmptyLine);
return parts;
}
function printNode(node, parentNode, path, options, print) {
switch (node.type) {
case "root": {
const {
children
} = node;
const parts = [];
path.each((childPath, index) => {
const document2 = children[index];
const nextDocument = children[index + 1];
if (index !== 0) {
parts.push(hardline);
}
parts.push(print());
if (shouldPrintDocumentEndMarker(document2, nextDocument)) {
parts.push(hardline, "...");
if (hasTrailingComment(document2)) {
parts.push(" ", print("trailingComment"));
}
} else if (nextDocument && !hasTrailingComment(nextDocument.head)) {
parts.push(hardline, "---");
}
}, "children");
const lastDescendantNode = getLastDescendantNode(node);
if (!isNode(lastDescendantNode, ["blockLiteral", "blockFolded"]) || lastDescendantNode.chomping !== "keep") {
parts.push(hardline);
}
return parts;
}
case "document": {
const nextDocument = parentNode.children[path.getName() + 1];
const parts = [];
if (shouldPrintDocumentHeadEndMarker(node, nextDocument, parentNode, options) === "head") {
if (node.head.children.length > 0 || node.head.endComments.length > 0) {
parts.push(print("head"));
}
if (hasTrailingComment(node.head)) {
parts.push(["---", " ", print(["head", "trailingComment"])]);
} else {
parts.push("---");
}
}
if (shouldPrintDocumentBody(node)) {
parts.push(print("body"));
}
return join(hardline, parts);
}
case "documentHead":
return join(hardline, [...path.map(print, "children"), ...path.map(print, "endComments")]);
case "documentBody": {
const {
children,
endComments
} = node;
let separator = "";
if (children.length > 0 && endComments.length > 0) {
const lastDescendantNode = getLastDescendantNode(node);
if (isNode(lastDescendantNode, ["blockFolded", "blockLiteral"])) {
if (lastDescendantNode.chomping !== "keep") {
separator = [hardline, hardline];
}
} else {
separator = hardline;
}
}
return [join(hardline, path.map(print, "children")), separator, join(hardline, path.map(print, "endComments"))];
}
case "directive":
return ["%", join(" ", [node.name, ...node.parameters])];
case "comment":
return ["#", node.value];
case "alias":
return ["*", node.value];
case "tag":
return options.originalText.slice(node.position.start.offset, node.position.end.offset);
case "anchor":
return ["&", node.value];
case "plain":
return printFlowScalarContent(node.type, options.originalText.slice(node.position.start.offset, node.position.end.offset), options);
case "quoteDouble":
case "quoteSingle": {
const singleQuote = "'";
const doubleQuote = '"';
const raw = options.originalText.slice(node.position.start.offset + 1, node.position.end.offset - 1);
if (node.type === "quoteSingle" && raw.includes("\\") || node.type === "quoteDouble" && /\\[^"]/.test(raw)) {
const originalQuote = node.type === "quoteDouble" ? doubleQuote : singleQuote;
return [originalQuote, printFlowScalarContent(node.type, raw, options), originalQuote];
}
if (raw.includes(doubleQuote)) {
return [singleQuote, printFlowScalarContent(node.type, node.type === "quoteDouble" ? raw.replace(/\\"/g, doubleQuote).replace(/'/g, singleQuote.repeat(2)) : raw, options), singleQuote];
}
if (raw.includes(singleQuote)) {
return [doubleQuote, printFlowScalarContent(node.type, node.type === "quoteSingle" ? raw.replace(/''/g, singleQuote) : raw, options), doubleQuote];
}
const quote2 = options.singleQuote ? singleQuote : doubleQuote;
return [quote2, printFlowScalarContent(node.type, raw, options), quote2];
}
case "blockFolded":
case "blockLiteral": {
return printBlock(path, print, options);
}
case "mapping":
case "sequence":
return join(hardline, path.map(print, "children"));
case "sequenceItem":
return ["- ", alignWithSpaces(2, node.content ? print("content") : "")];
case "mappingKey":
case "mappingValue":
return !node.content ? "" : print("content");
case "mappingItem":
case "flowMappingItem": {
return printMappingItem(node, parentNode, path, print, options);
}
case "flowMapping":
return printFlowMapping(path, print, options);
case "flowSequence":
return printFlowSequence(path, print, options);
case "flowSequenceItem":
return print("content");
default:
throw new Error(`Unexpected node type ${node.type}`);
}
}
function shouldPrintDocumentBody(document2) {
return document2.body.children.length > 0 || hasEndComments(document2.body);
}
function shouldPrintDocumentEndMarker(document2, nextDocument) {
return hasTrailingComment(document2) || nextDocument && (nextDocument.head.children.length > 0 || hasEndComments(nextDocument.head));
}
function shouldPrintDocumentHeadEndMarker(document2, nextDocument, root, options) {
if (root.children[0] === document2 && /---(?:\s|$)/.test(options.originalText.slice(locStart(document2), locStart(document2) + 4)) || document2.head.children.length > 0 || hasEndComments(document2.head) || hasTrailingComment(document2.head)) {
return "head";
}
if (shouldPrintDocumentEndMarker(document2, nextDocument)) {
return false;
}
return nextDocument ? "root" : false;
}
function printFlowScalarContent(nodeType, content, options) {
const lineContents = getFlowScalarLineContents(nodeType, content, options);
return join(hardline, lineContents.map((lineContentWords) => fill(getDocParts(join(line, lineContentWords)))));
}
function clean(node, newNode) {
if (isNode(newNode)) {
delete newNode.position;
switch (newNode.type) {
case "comment":
if (isPragma(newNode.value)) {
return null;
}
break;
case "quoteDouble":
case "quoteSingle":
newNode.type = "quote";
break;
}
}
}
module22.exports = {
preprocess,
embed,
print: genericPrint,
massageAstNode: clean,
insertPragma
};
}
});
var require_options7 = __commonJS22({
"src/language-yaml/options.js"(exports2, module22) {
"use strict";
var commonOptions = require_common_options();
module22.exports = {
bracketSpacing: commonOptions.bracketSpacing,
singleQuote: commonOptions.singleQuote,
proseWrap: commonOptions.proseWrap
};
}
});
var require_parsers7 = __commonJS22({
"src/language-yaml/parsers.js"(exports2, module22) {
"use strict";
module22.exports = {
get yaml() {
return require_parser_yaml().parsers.yaml;
}
};
}
});
var require_YAML = __commonJS22({
"node_modules/linguist-languages/data/YAML.json"(exports2, module22) {
module22.exports = {
name: "YAML",
type: "data",
color: "#cb171e",
tmScope: "source.yaml",
aliases: ["yml"],
extensions: [".yml", ".mir", ".reek", ".rviz", ".sublime-syntax", ".syntax", ".yaml", ".yaml-tmlanguage", ".yaml.sed", ".yml.mysql"],
filenames: [".clang-format", ".clang-tidy", ".gemrc", "CITATION.cff", "glide.lock", "yarn.lock"],
aceMode: "yaml",
codemirrorMode: "yaml",
codemirrorMimeType: "text/x-yaml",
languageId: 407
};
}
});
var require_language_yaml = __commonJS22({
"src/language-yaml/index.js"(exports2, module22) {
"use strict";
var createLanguage = require_create_language();
var printer = require_printer_yaml();
var options = require_options7();
var parsers = require_parsers7();
var languages = [createLanguage(require_YAML(), (data) => ({
since: "1.14.0",
parsers: ["yaml"],
vscodeLanguageIds: ["yaml", "ansible", "home-assistant"],
filenames: [...data.filenames.filter((filename) => filename !== "yarn.lock"), ".prettierrc", ".stylelintrc", ".lintstagedrc"]
}))];
module22.exports = {
languages,
printers: {
yaml: printer
},
options,
parsers
};
}
});
var require_languages = __commonJS22({
"src/languages.js"(exports2, module22) {
"use strict";
module22.exports = [require_language_js(), require_language_css(), require_language_handlebars(), require_language_graphql(), require_language_markdown(), require_language_html(), require_language_yaml()];
}
});
var require_load_plugins = __commonJS22({
"src/common/load-plugins.js"(exports2, module22) {
"use strict";
var fs2 = require("fs");
var path = require("path");
var fastGlob = require_out4();
var partition = require_partition();
var uniqByKey = require_uniq_by_key();
var internalPlugins = require_languages();
var {
default: mem2,
memClear: memClear2
} = (init_dist(), __toCommonJS(dist_exports));
var thirdParty = require_third_party();
var resolve = require_resolve2();
var memoizedLoad = mem2(load, {
cacheKey: JSON.stringify
});
var memoizedSearch = mem2(findPluginsInNodeModules);
var clearCache = () => {
memClear2(memoizedLoad);
memClear2(memoizedSearch);
};
function load(plugins2, pluginSearchDirs) {
if (!plugins2) {
plugins2 = [];
}
if (pluginSearchDirs === false) {
pluginSearchDirs = [];
} else {
pluginSearchDirs = pluginSearchDirs || [];
if (pluginSearchDirs.length === 0) {
const autoLoadDir = thirdParty.findParentDir(__dirname, "node_modules");
if (autoLoadDir) {
pluginSearchDirs = [autoLoadDir];
}
}
}
const [externalPluginNames, externalPluginInstances] = partition(plugins2, (plugin) => typeof plugin === "string");
const externalManualLoadPluginInfos = externalPluginNames.map((pluginName) => {
let requirePath;
try {
requirePath = resolve(path.resolve(process.cwd(), pluginName));
} catch {
requirePath = resolve(pluginName, {
paths: [process.cwd()]
});
}
return {
name: pluginName,
requirePath
};
});
const externalAutoLoadPluginInfos = pluginSearchDirs.flatMap((pluginSearchDir) => {
const resolvedPluginSearchDir = path.resolve(process.cwd(), pluginSearchDir);
const nodeModulesDir = path.resolve(resolvedPluginSearchDir, "node_modules");
if (!isDirectory(nodeModulesDir) && !isDirectory(resolvedPluginSearchDir)) {
throw new Error(`${pluginSearchDir} does not exist or is not a directory`);
}
return memoizedSearch(nodeModulesDir).map((pluginName) => ({
name: pluginName,
requirePath: resolve(pluginName, {
paths: [resolvedPluginSearchDir]
})
}));
});
const externalPlugins = [...uniqByKey([...externalManualLoadPluginInfos, ...externalAutoLoadPluginInfos], "requirePath").map((externalPluginInfo) => Object.assign({
name: externalPluginInfo.name
}, require(externalPluginInfo.requirePath))), ...externalPluginInstances];
return [...internalPlugins, ...externalPlugins];
}
function findPluginsInNodeModules(nodeModulesDir) {
const pluginPackageJsonPaths = fastGlob.sync(["prettier-plugin-*/package.json", "@*/prettier-plugin-*/package.json", "@prettier/plugin-*/package.json"], {
cwd: nodeModulesDir
});
return pluginPackageJsonPaths.map(path.dirname);
}
function isDirectory(dir) {
try {
return fs2.statSync(dir).isDirectory();
} catch {
return false;
}
}
module22.exports = {
loadPlugins: memoizedLoad,
clearCache
};
}
});
var {
version
} = require_package();
var core = require_core();
var {
getSupportInfo
} = require_support();
var getFileInfo = require_get_file_info();
var sharedUtil = require_util_shared();
var plugins = require_load_plugins();
var config = require_resolve_config();
var doc = require_doc();
function _withPlugins(fn, optsArgIdx = 1) {
return (...args) => {
const opts = args[optsArgIdx] || {};
args[optsArgIdx] = Object.assign(Object.assign({}, opts), {}, {
plugins: plugins.loadPlugins(opts.plugins, opts.pluginSearchDirs)
});
return fn(...args);
};
}
function withPlugins(fn, optsArgIdx) {
const resultingFn = _withPlugins(fn, optsArgIdx);
if (fn.sync) {
resultingFn.sync = _withPlugins(fn.sync, optsArgIdx);
}
return resultingFn;
}
var formatWithCursor = withPlugins(core.formatWithCursor);
module2.exports = {
formatWithCursor,
format(text, opts) {
return formatWithCursor(text, opts).formatted;
},
check(text, opts) {
const {
formatted
} = formatWithCursor(text, opts);
return formatted === text;
},
doc,
resolveConfig: config.resolveConfig,
resolveConfigFile: config.resolveConfigFile,
clearConfigCache() {
config.clearCache();
plugins.clearCache();
},
getFileInfo: withPlugins(getFileInfo),
getSupportInfo: withPlugins(getSupportInfo, 0),
version,
util: sharedUtil,
__internal: {
errors: require_errors(),
coreOptions: require_core_options(),
createIgnorer: require_create_ignorer(),
optionsModule: require_options(),
optionsNormalizer: require_options_normalizer(),
utils: {
arrayify: require_arrayify(),
getLast: require_get_last(),
partition: require_partition(),
isNonEmptyArray: require_util().isNonEmptyArray
}
},
__debug: {
parse: withPlugins(core.parse),
formatAST: withPlugins(core.formatAST),
formatDoc: withPlugins(core.formatDoc),
printToDoc: withPlugins(core.printToDoc),
printDocToString: withPlugins(core.printDocToString)
}
};
}
});
// node_modules/he/he.js
var require_he = __commonJS({
"node_modules/he/he.js"(exports, module2) {
(function(root) {
var freeExports = typeof exports == "object" && exports;
var freeModule = typeof module2 == "object" && module2 && module2.exports == freeExports && module2;
var freeGlobal = typeof global == "object" && global;
if (freeGlobal.global === freeGlobal || freeGlobal.window === freeGlobal) {
root = freeGlobal;
}
var regexAstralSymbols = /[\uD800-\uDBFF][\uDC00-\uDFFF]/g;
var regexAsciiWhitelist = /[\x01-\x7F]/g;
var regexBmpWhitelist = /[\x01-\t\x0B\f\x0E-\x1F\x7F\x81\x8D\x8F\x90\x9D\xA0-\uFFFF]/g;
var regexEncodeNonAscii = /<\u20D2|=\u20E5|>\u20D2|\u205F\u200A|\u219D\u0338|\u2202\u0338|\u2220\u20D2|\u2229\uFE00|\u222A\uFE00|\u223C\u20D2|\u223D\u0331|\u223E\u0333|\u2242\u0338|\u224B\u0338|\u224D\u20D2|\u224E\u0338|\u224F\u0338|\u2250\u0338|\u2261\u20E5|\u2264\u20D2|\u2265\u20D2|\u2266\u0338|\u2267\u0338|\u2268\uFE00|\u2269\uFE00|\u226A\u0338|\u226A\u20D2|\u226B\u0338|\u226B\u20D2|\u227F\u0338|\u2282\u20D2|\u2283\u20D2|\u228A\uFE00|\u228B\uFE00|\u228F\u0338|\u2290\u0338|\u2293\uFE00|\u2294\uFE00|\u22B4\u20D2|\u22B5\u20D2|\u22D8\u0338|\u22D9\u0338|\u22DA\uFE00|\u22DB\uFE00|\u22F5\u0338|\u22F9\u0338|\u2933\u0338|\u29CF\u0338|\u29D0\u0338|\u2A6D\u0338|\u2A70\u0338|\u2A7D\u0338|\u2A7E\u0338|\u2AA1\u0338|\u2AA2\u0338|\u2AAC\uFE00|\u2AAD\uFE00|\u2AAF\u0338|\u2AB0\u0338|\u2AC5\u0338|\u2AC6\u0338|\u2ACB\uFE00|\u2ACC\uFE00|\u2AFD\u20E5|[\xA0-\u0113\u0116-\u0122\u0124-\u012B\u012E-\u014D\u0150-\u017E\u0192\u01B5\u01F5\u0237\u02C6\u02C7\u02D8-\u02DD\u0311\u0391-\u03A1\u03A3-\u03A9\u03B1-\u03C9\u03D1\u03D2\u03D5\u03D6\u03DC\u03DD\u03F0\u03F1\u03F5\u03F6\u0401-\u040C\u040E-\u044F\u0451-\u045C\u045E\u045F\u2002-\u2005\u2007-\u2010\u2013-\u2016\u2018-\u201A\u201C-\u201E\u2020-\u2022\u2025\u2026\u2030-\u2035\u2039\u203A\u203E\u2041\u2043\u2044\u204F\u2057\u205F-\u2063\u20AC\u20DB\u20DC\u2102\u2105\u210A-\u2113\u2115-\u211E\u2122\u2124\u2127-\u2129\u212C\u212D\u212F-\u2131\u2133-\u2138\u2145-\u2148\u2153-\u215E\u2190-\u219B\u219D-\u21A7\u21A9-\u21AE\u21B0-\u21B3\u21B5-\u21B7\u21BA-\u21DB\u21DD\u21E4\u21E5\u21F5\u21FD-\u2205\u2207-\u2209\u220B\u220C\u220F-\u2214\u2216-\u2218\u221A\u221D-\u2238\u223A-\u2257\u2259\u225A\u225C\u225F-\u2262\u2264-\u228B\u228D-\u229B\u229D-\u22A5\u22A7-\u22B0\u22B2-\u22BB\u22BD-\u22DB\u22DE-\u22E3\u22E6-\u22F7\u22F9-\u22FE\u2305\u2306\u2308-\u2310\u2312\u2313\u2315\u2316\u231C-\u231F\u2322\u2323\u232D\u232E\u2336\u233D\u233F\u237C\u23B0\u23B1\u23B4-\u23B6\u23DC-\u23DF\u23E2\u23E7\u2423\u24C8\u2500\u2502\u250C\u2510\u2514\u2518\u251C\u2524\u252C\u2534\u253C\u2550-\u256C\u2580\u2584\u2588\u2591-\u2593\u25A1\u25AA\u25AB\u25AD\u25AE\u25B1\u25B3-\u25B5\u25B8\u25B9\u25BD-\u25BF\u25C2\u25C3\u25CA\u25CB\u25EC\u25EF\u25F8-\u25FC\u2605\u2606\u260E\u2640\u2642\u2660\u2663\u2665\u2666\u266A\u266D-\u266F\u2713\u2717\u2720\u2736\u2758\u2772\u2773\u27C8\u27C9\u27E6-\u27ED\u27F5-\u27FA\u27FC\u27FF\u2902-\u2905\u290C-\u2913\u2916\u2919-\u2920\u2923-\u292A\u2933\u2935-\u2939\u293C\u293D\u2945\u2948-\u294B\u294E-\u2976\u2978\u2979\u297B-\u297F\u2985\u2986\u298B-\u2996\u299A\u299C\u299D\u29A4-\u29B7\u29B9\u29BB\u29BC\u29BE-\u29C5\u29C9\u29CD-\u29D0\u29DC-\u29DE\u29E3-\u29E5\u29EB\u29F4\u29F6\u2A00-\u2A02\u2A04\u2A06\u2A0C\u2A0D\u2A10-\u2A17\u2A22-\u2A27\u2A29\u2A2A\u2A2D-\u2A31\u2A33-\u2A3C\u2A3F\u2A40\u2A42-\u2A4D\u2A50\u2A53-\u2A58\u2A5A-\u2A5D\u2A5F\u2A66\u2A6A\u2A6D-\u2A75\u2A77-\u2A9A\u2A9D-\u2AA2\u2AA4-\u2AB0\u2AB3-\u2AC8\u2ACB\u2ACC\u2ACF-\u2ADB\u2AE4\u2AE6-\u2AE9\u2AEB-\u2AF3\u2AFD\uFB00-\uFB04]|\uD835[\uDC9C\uDC9E\uDC9F\uDCA2\uDCA5\uDCA6\uDCA9-\uDCAC\uDCAE-\uDCB9\uDCBB\uDCBD-\uDCC3\uDCC5-\uDCCF\uDD04\uDD05\uDD07-\uDD0A\uDD0D-\uDD14\uDD16-\uDD1C\uDD1E-\uDD39\uDD3B-\uDD3E\uDD40-\uDD44\uDD46\uDD4A-\uDD50\uDD52-\uDD6B]/g;
var encodeMap = { "\xAD": "shy", "\u200C": "zwnj", "\u200D": "zwj", "\u200E": "lrm", "\u2063": "ic", "\u2062": "it", "\u2061": "af", "\u200F": "rlm", "\u200B": "ZeroWidthSpace", "\u2060": "NoBreak", "\u0311": "DownBreve", "\u20DB": "tdot", "\u20DC": "DotDot", " ": "Tab", "\n": "NewLine", "\u2008": "puncsp", "\u205F": "MediumSpace", "\u2009": "thinsp", "\u200A": "hairsp", "\u2004": "emsp13", "\u2002": "ensp", "\u2005": "emsp14", "\u2003": "emsp", "\u2007": "numsp", "\xA0": "nbsp", "\u205F\u200A": "ThickSpace", "\u203E": "oline", "_": "lowbar", "\u2010": "dash", "\u2013": "ndash", "\u2014": "mdash", "\u2015": "horbar", ",": "comma", ";": "semi", "\u204F": "bsemi", ":": "colon", "\u2A74": "Colone", "!": "excl", "\xA1": "iexcl", "?": "quest", "\xBF": "iquest", ".": "period", "\u2025": "nldr", "\u2026": "mldr", "\xB7": "middot", "'": "apos", "\u2018": "lsquo", "\u2019": "rsquo", "\u201A": "sbquo", "\u2039": "lsaquo", "\u203A": "rsaquo", '"': "quot", "\u201C": "ldquo", "\u201D": "rdquo", "\u201E": "bdquo", "\xAB": "laquo", "\xBB": "raquo", "(": "lpar", ")": "rpar", "[": "lsqb", "]": "rsqb", "{": "lcub", "}": "rcub", "\u2308": "lceil", "\u2309": "rceil", "\u230A": "lfloor", "\u230B": "rfloor", "\u2985": "lopar", "\u2986": "ropar", "\u298B": "lbrke", "\u298C": "rbrke", "\u298D": "lbrkslu", "\u298E": "rbrksld", "\u298F": "lbrksld", "\u2990": "rbrkslu", "\u2991": "langd", "\u2992": "rangd", "\u2993": "lparlt", "\u2994": "rpargt", "\u2995": "gtlPar", "\u2996": "ltrPar", "\u27E6": "lobrk", "\u27E7": "robrk", "\u27E8": "lang", "\u27E9": "rang", "\u27EA": "Lang", "\u27EB": "Rang", "\u27EC": "loang", "\u27ED": "roang", "\u2772": "lbbrk", "\u2773": "rbbrk", "\u2016": "Vert", "\xA7": "sect", "\xB6": "para", "@": "commat", "*": "ast", "/": "sol", "undefined": null, "&": "amp", "#": "num", "%": "percnt", "\u2030": "permil", "\u2031": "pertenk", "\u2020": "dagger", "\u2021": "Dagger", "\u2022": "bull", "\u2043": "hybull", "\u2032": "prime", "\u2033": "Prime", "\u2034": "tprime", "\u2057": "qprime", "\u2035": "bprime", "\u2041": "caret", "`": "grave", "\xB4": "acute", "\u02DC": "tilde", "^": "Hat", "\xAF": "macr", "\u02D8": "breve", "\u02D9": "dot", "\xA8": "die", "\u02DA": "ring", "\u02DD": "dblac", "\xB8": "cedil", "\u02DB": "ogon", "\u02C6": "circ", "\u02C7": "caron", "\xB0": "deg", "\xA9": "copy", "\xAE": "reg", "\u2117": "copysr", "\u2118": "wp", "\u211E": "rx", "\u2127": "mho", "\u2129": "iiota", "\u2190": "larr", "\u219A": "nlarr", "\u2192": "rarr", "\u219B": "nrarr", "\u2191": "uarr", "\u2193": "darr", "\u2194": "harr", "\u21AE": "nharr", "\u2195": "varr", "\u2196": "nwarr", "\u2197": "nearr", "\u2198": "searr", "\u2199": "swarr", "\u219D": "rarrw", "\u219D\u0338": "nrarrw", "\u219E": "Larr", "\u219F": "Uarr", "\u21A0": "Rarr", "\u21A1": "Darr", "\u21A2": "larrtl", "\u21A3": "rarrtl", "\u21A4": "mapstoleft", "\u21A5": "mapstoup", "\u21A6": "map", "\u21A7": "mapstodown", "\u21A9": "larrhk", "\u21AA": "rarrhk", "\u21AB": "larrlp", "\u21AC": "rarrlp", "\u21AD": "harrw", "\u21B0": "lsh", "\u21B1": "rsh", "\u21B2": "ldsh", "\u21B3": "rdsh", "\u21B5": "crarr", "\u21B6": "cularr", "\u21B7": "curarr", "\u21BA": "olarr", "\u21BB": "orarr", "\u21BC": "lharu", "\u21BD": "lhard", "\u21BE": "uharr", "\u21BF": "uharl", "\u21C0": "rharu", "\u21C1": "rhard", "\u21C2": "dharr", "\u21C3": "dharl", "\u21C4": "rlarr", "\u21C5": "udarr", "\u21C6": "lrarr", "\u21C7": "llarr", "\u21C8": "uuarr", "\u21C9": "rrarr", "\u21CA": "ddarr", "\u21CB": "lrhar", "\u21CC": "rlhar", "\u21D0": "lArr", "\u21CD": "nlArr", "\u21D1": "uArr", "\u21D2": "rArr", "\u21CF": "nrArr", "\u21D3": "dArr", "\u21D4": "iff", "\u21CE": "nhArr", "\u21D5": "vArr", "\u21D6": "nwArr", "\u21D7": "neArr", "\u21D8": "seArr", "\u21D9": "swArr", "\u21DA": "lAarr", "\u21DB": "rAarr", "\u21DD": "zigrarr", "\u21E4": "larrb", "\u21E5": "rarrb", "\u21F5": "duarr", "\u21FD": "loarr", "\u21FE": "roarr", "\u21FF": "hoarr", "\u2200": "forall", "\u2201": "comp", "\u2202": "part", "\u2202\u0338": "npart", "\u2203": "exist", "\u2204": "nexist", "\u2205": "empty", "\u2207": "Del", "\u2208": "in", "\u2209": "notin", "\u220B": "ni", "\u220C": "notni", "\u03F6": "bepsi", "\u220F": "prod", "\u2210": "coprod", "\u2211": "sum", "+": "plus", "\xB1": "pm", "\xF7": "div", "\xD7": "times", "<": "lt", "\u226E": "nlt", "<\u20D2": "nvlt", "=": "equals", "\u2260": "ne", "=\u20E5": "bne", "\u2A75": "Equal", ">": "gt", "\u226F": "ngt", ">\u20D2": "nvgt", "\xAC": "not", "|": "vert", "\xA6": "brvbar", "\u2212": "minus", "\u2213": "mp", "\u2214": "plusdo", "\u2044": "frasl", "\u2216": "setmn", "\u2217": "lowast", "\u2218": "compfn", "\u221A": "Sqrt", "\u221D": "prop", "\u221E": "infin", "\u221F": "angrt", "\u2220": "ang", "\u2220\u20D2": "nang", "\u2221": "angmsd", "\u2222": "angsph", "\u2223": "mid", "\u2224": "nmid", "\u2225": "par", "\u2226": "npar", "\u2227": "and", "\u2228": "or", "\u2229": "cap", "\u2229\uFE00": "caps", "\u222A": "cup", "\u222A\uFE00": "cups", "\u222B": "int", "\u222C": "Int", "\u222D": "tint", "\u2A0C": "qint", "\u222E": "oint", "\u222F": "Conint", "\u2230": "Cconint", "\u2231": "cwint", "\u2232": "cwconint", "\u2233": "awconint", "\u2234": "there4", "\u2235": "becaus", "\u2236": "ratio", "\u2237": "Colon", "\u2238": "minusd", "\u223A": "mDDot", "\u223B": "homtht", "\u223C": "sim", "\u2241": "nsim", "\u223C\u20D2": "nvsim", "\u223D": "bsim", "\u223D\u0331": "race", "\u223E": "ac", "\u223E\u0333": "acE", "\u223F": "acd", "\u2240": "wr", "\u2242": "esim", "\u2242\u0338": "nesim", "\u2243": "sime", "\u2244": "nsime", "\u2245": "cong", "\u2247": "ncong", "\u2246": "simne", "\u2248": "ap", "\u2249": "nap", "\u224A": "ape", "\u224B": "apid", "\u224B\u0338": "napid", "\u224C": "bcong", "\u224D": "CupCap", "\u226D": "NotCupCap", "\u224D\u20D2": "nvap", "\u224E": "bump", "\u224E\u0338": "nbump", "\u224F": "bumpe", "\u224F\u0338": "nbumpe", "\u2250": "doteq", "\u2250\u0338": "nedot", "\u2251": "eDot", "\u2252": "efDot", "\u2253": "erDot", "\u2254": "colone", "\u2255": "ecolon", "\u2256": "ecir", "\u2257": "cire", "\u2259": "wedgeq", "\u225A": "veeeq", "\u225C": "trie", "\u225F": "equest", "\u2261": "equiv", "\u2262": "nequiv", "\u2261\u20E5": "bnequiv", "\u2264": "le", "\u2270": "nle", "\u2264\u20D2": "nvle", "\u2265": "ge", "\u2271": "nge", "\u2265\u20D2": "nvge", "\u2266": "lE", "\u2266\u0338": "nlE", "\u2267": "gE", "\u2267\u0338": "ngE", "\u2268\uFE00": "lvnE", "\u2268": "lnE", "\u2269": "gnE", "\u2269\uFE00": "gvnE", "\u226A": "ll", "\u226A\u0338": "nLtv", "\u226A\u20D2": "nLt", "\u226B": "gg", "\u226B\u0338": "nGtv", "\u226B\u20D2": "nGt", "\u226C": "twixt", "\u2272": "lsim", "\u2274": "nlsim", "\u2273": "gsim", "\u2275": "ngsim", "\u2276": "lg", "\u2278": "ntlg", "\u2277": "gl", "\u2279": "ntgl", "\u227A": "pr", "\u2280": "npr", "\u227B": "sc", "\u2281": "nsc", "\u227C": "prcue", "\u22E0": "nprcue", "\u227D": "sccue", "\u22E1": "nsccue", "\u227E": "prsim", "\u227F": "scsim", "\u227F\u0338": "NotSucceedsTilde", "\u2282": "sub", "\u2284": "nsub", "\u2282\u20D2": "vnsub", "\u2283": "sup", "\u2285": "nsup", "\u2283\u20D2": "vnsup", "\u2286": "sube", "\u2288": "nsube", "\u2287": "supe", "\u2289": "nsupe", "\u228A\uFE00": "vsubne", "\u228A": "subne", "\u228B\uFE00": "vsupne", "\u228B": "supne", "\u228D": "cupdot", "\u228E": "uplus", "\u228F": "sqsub", "\u228F\u0338": "NotSquareSubset", "\u2290": "sqsup", "\u2290\u0338": "NotSquareSuperset", "\u2291": "sqsube", "\u22E2": "nsqsube", "\u2292": "sqsupe", "\u22E3": "nsqsupe", "\u2293": "sqcap", "\u2293\uFE00": "sqcaps", "\u2294": "sqcup", "\u2294\uFE00": "sqcups", "\u2295": "oplus", "\u2296": "ominus", "\u2297": "otimes", "\u2298": "osol", "\u2299": "odot", "\u229A": "ocir", "\u229B": "oast", "\u229D": "odash", "\u229E": "plusb", "\u229F": "minusb", "\u22A0": "timesb", "\u22A1": "sdotb", "\u22A2": "vdash", "\u22AC": "nvdash", "\u22A3": "dashv", "\u22A4": "top", "\u22A5": "bot", "\u22A7": "models", "\u22A8": "vDash", "\u22AD": "nvDash", "\u22A9": "Vdash", "\u22AE": "nVdash", "\u22AA": "Vvdash", "\u22AB": "VDash", "\u22AF": "nVDash", "\u22B0": "prurel", "\u22B2": "vltri", "\u22EA": "nltri", "\u22B3": "vrtri", "\u22EB": "nrtri", "\u22B4": "ltrie", "\u22EC": "nltrie", "\u22B4\u20D2": "nvltrie", "\u22B5": "rtrie", "\u22ED": "nrtrie", "\u22B5\u20D2": "nvrtrie", "\u22B6": "origof", "\u22B7": "imof", "\u22B8": "mumap", "\u22B9": "hercon", "\u22BA": "intcal", "\u22BB": "veebar", "\u22BD": "barvee", "\u22BE": "angrtvb", "\u22BF": "lrtri", "\u22C0": "Wedge", "\u22C1": "Vee", "\u22C2": "xcap", "\u22C3": "xcup", "\u22C4": "diam", "\u22C5": "sdot", "\u22C6": "Star", "\u22C7": "divonx", "\u22C8": "bowtie", "\u22C9": "ltimes", "\u22CA": "rtimes", "\u22CB": "lthree", "\u22CC": "rthree", "\u22CD": "bsime", "\u22CE": "cuvee", "\u22CF": "cuwed", "\u22D0": "Sub", "\u22D1": "Sup", "\u22D2": "Cap", "\u22D3": "Cup", "\u22D4": "fork", "\u22D5": "epar", "\u22D6": "ltdot", "\u22D7": "gtdot", "\u22D8": "Ll", "\u22D8\u0338": "nLl", "\u22D9": "Gg", "\u22D9\u0338": "nGg", "\u22DA\uFE00": "lesg", "\u22DA": "leg", "\u22DB": "gel", "\u22DB\uFE00": "gesl", "\u22DE": "cuepr", "\u22DF": "cuesc", "\u22E6": "lnsim", "\u22E7": "gnsim", "\u22E8": "prnsim", "\u22E9": "scnsim", "\u22EE": "vellip", "\u22EF": "ctdot", "\u22F0": "utdot", "\u22F1": "dtdot", "\u22F2": "disin", "\u22F3": "isinsv", "\u22F4": "isins", "\u22F5": "isindot", "\u22F5\u0338": "notindot", "\u22F6": "notinvc", "\u22F7": "notinvb", "\u22F9": "isinE", "\u22F9\u0338": "notinE", "\u22FA": "nisd", "\u22FB": "xnis", "\u22FC": "nis", "\u22FD": "notnivc", "\u22FE": "notnivb", "\u2305": "barwed", "\u2306": "Barwed", "\u230C": "drcrop", "\u230D": "dlcrop", "\u230E": "urcrop", "\u230F": "ulcrop", "\u2310": "bnot", "\u2312": "profline", "\u2313": "profsurf", "\u2315": "telrec", "\u2316": "target", "\u231C": "ulcorn", "\u231D": "urcorn", "\u231E": "dlcorn", "\u231F": "drcorn", "\u2322": "frown", "\u2323": "smile", "\u232D": "cylcty", "\u232E": "profalar", "\u2336": "topbot", "\u233D": "ovbar", "\u233F": "solbar", "\u237C": "angzarr", "\u23B0": "lmoust", "\u23B1": "rmoust", "\u23B4": "tbrk", "\u23B5": "bbrk", "\u23B6": "bbrktbrk", "\u23DC": "OverParenthesis", "\u23DD": "UnderParenthesis", "\u23DE": "OverBrace", "\u23DF": "UnderBrace", "\u23E2": "trpezium", "\u23E7": "elinters", "\u2423": "blank", "\u2500": "boxh", "\u2502": "boxv", "\u250C": "boxdr", "\u2510": "boxdl", "\u2514": "boxur", "\u2518": "boxul", "\u251C": "boxvr", "\u2524": "boxvl", "\u252C": "boxhd", "\u2534": "boxhu", "\u253C": "boxvh", "\u2550": "boxH", "\u2551": "boxV", "\u2552": "boxdR", "\u2553": "boxDr", "\u2554": "boxDR", "\u2555": "boxdL", "\u2556": "boxDl", "\u2557": "boxDL", "\u2558": "boxuR", "\u2559": "boxUr", "\u255A": "boxUR", "\u255B": "boxuL", "\u255C": "boxUl", "\u255D": "boxUL", "\u255E": "boxvR", "\u255F": "boxVr", "\u2560": "boxVR", "\u2561": "boxvL", "\u2562": "boxVl", "\u2563": "boxVL", "\u2564": "boxHd", "\u2565": "boxhD", "\u2566": "boxHD", "\u2567": "boxHu", "\u2568": "boxhU", "\u2569": "boxHU", "\u256A": "boxvH", "\u256B": "boxVh", "\u256C": "boxVH", "\u2580": "uhblk", "\u2584": "lhblk", "\u2588": "block", "\u2591": "blk14", "\u2592": "blk12", "\u2593": "blk34", "\u25A1": "squ", "\u25AA": "squf", "\u25AB": "EmptyVerySmallSquare", "\u25AD": "rect", "\u25AE": "marker", "\u25B1": "fltns", "\u25B3": "xutri", "\u25B4": "utrif", "\u25B5": "utri", "\u25B8": "rtrif", "\u25B9": "rtri", "\u25BD": "xdtri", "\u25BE": "dtrif", "\u25BF": "dtri", "\u25C2": "ltrif", "\u25C3": "ltri", "\u25CA": "loz", "\u25CB": "cir", "\u25EC": "tridot", "\u25EF": "xcirc", "\u25F8": "ultri", "\u25F9": "urtri", "\u25FA": "lltri", "\u25FB": "EmptySmallSquare", "\u25FC": "FilledSmallSquare", "\u2605": "starf", "\u2606": "star", "\u260E": "phone", "\u2640": "female", "\u2642": "male", "\u2660": "spades", "\u2663": "clubs", "\u2665": "hearts", "\u2666": "diams", "\u266A": "sung", "\u2713": "check", "\u2717": "cross", "\u2720": "malt", "\u2736": "sext", "\u2758": "VerticalSeparator", "\u27C8": "bsolhsub", "\u27C9": "suphsol", "\u27F5": "xlarr", "\u27F6": "xrarr", "\u27F7": "xharr", "\u27F8": "xlArr", "\u27F9": "xrArr", "\u27FA": "xhArr", "\u27FC": "xmap", "\u27FF": "dzigrarr", "\u2902": "nvlArr", "\u2903": "nvrArr", "\u2904": "nvHarr", "\u2905": "Map", "\u290C": "lbarr", "\u290D": "rbarr", "\u290E": "lBarr", "\u290F": "rBarr", "\u2910": "RBarr", "\u2911": "DDotrahd", "\u2912": "UpArrowBar", "\u2913": "DownArrowBar", "\u2916": "Rarrtl", "\u2919": "latail", "\u291A": "ratail", "\u291B": "lAtail", "\u291C": "rAtail", "\u291D": "larrfs", "\u291E": "rarrfs", "\u291F": "larrbfs", "\u2920": "rarrbfs", "\u2923": "nwarhk", "\u2924": "nearhk", "\u2925": "searhk", "\u2926": "swarhk", "\u2927": "nwnear", "\u2928": "toea", "\u2929": "tosa", "\u292A": "swnwar", "\u2933": "rarrc", "\u2933\u0338": "nrarrc", "\u2935": "cudarrr", "\u2936": "ldca", "\u2937": "rdca", "\u2938": "cudarrl", "\u2939": "larrpl", "\u293C": "curarrm", "\u293D": "cularrp", "\u2945": "rarrpl", "\u2948": "harrcir", "\u2949": "Uarrocir", "\u294A": "lurdshar", "\u294B": "ldrushar", "\u294E": "LeftRightVector", "\u294F": "RightUpDownVector", "\u2950": "DownLeftRightVector", "\u2951": "LeftUpDownVector", "\u2952": "LeftVectorBar", "\u2953": "RightVectorBar", "\u2954": "RightUpVectorBar", "\u2955": "RightDownVectorBar", "\u2956": "DownLeftVectorBar", "\u2957": "DownRightVectorBar", "\u2958": "LeftUpVectorBar", "\u2959": "LeftDownVectorBar", "\u295A": "LeftTeeVector", "\u295B": "RightTeeVector", "\u295C": "RightUpTeeVector", "\u295D": "RightDownTeeVector", "\u295E": "DownLeftTeeVector", "\u295F": "DownRightTeeVector", "\u2960": "LeftUpTeeVector", "\u2961": "LeftDownTeeVector", "\u2962": "lHar", "\u2963": "uHar", "\u2964": "rHar", "\u2965": "dHar", "\u2966": "luruhar", "\u2967": "ldrdhar", "\u2968": "ruluhar", "\u2969": "rdldhar", "\u296A": "lharul", "\u296B": "llhard", "\u296C": "rharul", "\u296D": "lrhard", "\u296E": "udhar", "\u296F": "duhar", "\u2970": "RoundImplies", "\u2971": "erarr", "\u2972": "simrarr", "\u2973": "larrsim", "\u2974": "rarrsim", "\u2975": "rarrap", "\u2976": "ltlarr", "\u2978": "gtrarr", "\u2979": "subrarr", "\u297B": "suplarr", "\u297C": "lfisht", "\u297D": "rfisht", "\u297E": "ufisht", "\u297F": "dfisht", "\u299A": "vzigzag", "\u299C": "vangrt", "\u299D": "angrtvbd", "\u29A4": "ange", "\u29A5": "range", "\u29A6": "dwangle", "\u29A7": "uwangle", "\u29A8": "angmsdaa", "\u29A9": "angmsdab", "\u29AA": "angmsdac", "\u29AB": "angmsdad", "\u29AC": "angmsdae", "\u29AD": "angmsdaf", "\u29AE": "angmsdag", "\u29AF": "angmsdah", "\u29B0": "bemptyv", "\u29B1": "demptyv", "\u29B2": "cemptyv", "\u29B3": "raemptyv", "\u29B4": "laemptyv", "\u29B5": "ohbar", "\u29B6": "omid", "\u29B7": "opar", "\u29B9": "operp", "\u29BB": "olcross", "\u29BC": "odsold", "\u29BE": "olcir", "\u29BF": "ofcir", "\u29C0": "olt", "\u29C1": "ogt", "\u29C2": "cirscir", "\u29C3": "cirE", "\u29C4": "solb", "\u29C5": "bsolb", "\u29C9": "boxbox", "\u29CD": "trisb", "\u29CE": "rtriltri", "\u29CF": "LeftTriangleBar", "\u29CF\u0338": "NotLeftTriangleBar", "\u29D0": "RightTriangleBar", "\u29D0\u0338": "NotRightTriangleBar", "\u29DC": "iinfin", "\u29DD": "infintie", "\u29DE": "nvinfin", "\u29E3": "eparsl", "\u29E4": "smeparsl", "\u29E5": "eqvparsl", "\u29EB": "lozf", "\u29F4": "RuleDelayed", "\u29F6": "dsol", "\u2A00": "xodot", "\u2A01": "xoplus", "\u2A02": "xotime", "\u2A04": "xuplus", "\u2A06": "xsqcup", "\u2A0D": "fpartint", "\u2A10": "cirfnint", "\u2A11": "awint", "\u2A12": "rppolint", "\u2A13": "scpolint", "\u2A14": "npolint", "\u2A15": "pointint", "\u2A16": "quatint", "\u2A17": "intlarhk", "\u2A22": "pluscir", "\u2A23": "plusacir", "\u2A24": "simplus", "\u2A25": "plusdu", "\u2A26": "plussim", "\u2A27": "plustwo", "\u2A29": "mcomma", "\u2A2A": "minusdu", "\u2A2D": "loplus", "\u2A2E": "roplus", "\u2A2F": "Cross", "\u2A30": "timesd", "\u2A31": "timesbar", "\u2A33": "smashp", "\u2A34": "lotimes", "\u2A35": "rotimes", "\u2A36": "otimesas", "\u2A37": "Otimes", "\u2A38": "odiv", "\u2A39": "triplus", "\u2A3A": "triminus", "\u2A3B": "tritime", "\u2A3C": "iprod", "\u2A3F": "amalg", "\u2A40": "capdot", "\u2A42": "ncup", "\u2A43": "ncap", "\u2A44": "capand", "\u2A45": "cupor", "\u2A46": "cupcap", "\u2A47": "capcup", "\u2A48": "cupbrcap", "\u2A49": "capbrcup", "\u2A4A": "cupcup", "\u2A4B": "capcap", "\u2A4C": "ccups", "\u2A4D": "ccaps", "\u2A50": "ccupssm", "\u2A53": "And", "\u2A54": "Or", "\u2A55": "andand", "\u2A56": "oror", "\u2A57": "orslope", "\u2A58": "andslope", "\u2A5A": "andv", "\u2A5B": "orv", "\u2A5C": "andd", "\u2A5D": "ord", "\u2A5F": "wedbar", "\u2A66": "sdote", "\u2A6A": "simdot", "\u2A6D": "congdot", "\u2A6D\u0338": "ncongdot", "\u2A6E": "easter", "\u2A6F": "apacir", "\u2A70": "apE", "\u2A70\u0338": "napE", "\u2A71": "eplus", "\u2A72": "pluse", "\u2A73": "Esim", "\u2A77": "eDDot", "\u2A78": "equivDD", "\u2A79": "ltcir", "\u2A7A": "gtcir", "\u2A7B": "ltquest", "\u2A7C": "gtquest", "\u2A7D": "les", "\u2A7D\u0338": "nles", "\u2A7E": "ges", "\u2A7E\u0338": "nges", "\u2A7F": "lesdot", "\u2A80": "gesdot", "\u2A81": "lesdoto", "\u2A82": "gesdoto", "\u2A83": "lesdotor", "\u2A84": "gesdotol", "\u2A85": "lap", "\u2A86": "gap", "\u2A87": "lne", "\u2A88": "gne", "\u2A89": "lnap", "\u2A8A": "gnap", "\u2A8B": "lEg", "\u2A8C": "gEl", "\u2A8D": "lsime", "\u2A8E": "gsime", "\u2A8F": "lsimg", "\u2A90": "gsiml", "\u2A91": "lgE", "\u2A92": "glE", "\u2A93": "lesges", "\u2A94": "gesles", "\u2A95": "els", "\u2A96": "egs", "\u2A97": "elsdot", "\u2A98": "egsdot", "\u2A99": "el", "\u2A9A": "eg", "\u2A9D": "siml", "\u2A9E": "simg", "\u2A9F": "simlE", "\u2AA0": "simgE", "\u2AA1": "LessLess", "\u2AA1\u0338": "NotNestedLessLess", "\u2AA2": "GreaterGreater", "\u2AA2\u0338": "NotNestedGreaterGreater", "\u2AA4": "glj", "\u2AA5": "gla", "\u2AA6": "ltcc", "\u2AA7": "gtcc", "\u2AA8": "lescc", "\u2AA9": "gescc", "\u2AAA": "smt", "\u2AAB": "lat", "\u2AAC": "smte", "\u2AAC\uFE00": "smtes", "\u2AAD": "late", "\u2AAD\uFE00": "lates", "\u2AAE": "bumpE", "\u2AAF": "pre", "\u2AAF\u0338": "npre", "\u2AB0": "sce", "\u2AB0\u0338": "nsce", "\u2AB3": "prE", "\u2AB4": "scE", "\u2AB5": "prnE", "\u2AB6": "scnE", "\u2AB7": "prap", "\u2AB8": "scap", "\u2AB9": "prnap", "\u2ABA": "scnap", "\u2ABB": "Pr", "\u2ABC": "Sc", "\u2ABD": "subdot", "\u2ABE": "supdot", "\u2ABF": "subplus", "\u2AC0": "supplus", "\u2AC1": "submult", "\u2AC2": "supmult", "\u2AC3": "subedot", "\u2AC4": "supedot", "\u2AC5": "subE", "\u2AC5\u0338": "nsubE", "\u2AC6": "supE", "\u2AC6\u0338": "nsupE", "\u2AC7": "subsim", "\u2AC8": "supsim", "\u2ACB\uFE00": "vsubnE", "\u2ACB": "subnE", "\u2ACC\uFE00": "vsupnE", "\u2ACC": "supnE", "\u2ACF": "csub", "\u2AD0": "csup", "\u2AD1": "csube", "\u2AD2": "csupe", "\u2AD3": "subsup", "\u2AD4": "supsub", "\u2AD5": "subsub", "\u2AD6": "supsup", "\u2AD7": "suphsub", "\u2AD8": "supdsub", "\u2AD9": "forkv", "\u2ADA": "topfork", "\u2ADB": "mlcp", "\u2AE4": "Dashv", "\u2AE6": "Vdashl", "\u2AE7": "Barv", "\u2AE8": "vBar", "\u2AE9": "vBarv", "\u2AEB": "Vbar", "\u2AEC": "Not", "\u2AED": "bNot", "\u2AEE": "rnmid", "\u2AEF": "cirmid", "\u2AF0": "midcir", "\u2AF1": "topcir", "\u2AF2": "nhpar", "\u2AF3": "parsim", "\u2AFD": "parsl", "\u2AFD\u20E5": "nparsl", "\u266D": "flat", "\u266E": "natur", "\u266F": "sharp", "\xA4": "curren", "\xA2": "cent", "$": "dollar", "\xA3": "pound", "\xA5": "yen", "\u20AC": "euro", "\xB9": "sup1", "\xBD": "half", "\u2153": "frac13", "\xBC": "frac14", "\u2155": "frac15", "\u2159": "frac16", "\u215B": "frac18", "\xB2": "sup2", "\u2154": "frac23", "\u2156": "frac25", "\xB3": "sup3", "\xBE": "frac34", "\u2157": "frac35", "\u215C": "frac38", "\u2158": "frac45", "\u215A": "frac56", "\u215D": "frac58", "\u215E": "frac78", "\u{1D4B6}": "ascr", "\u{1D552}": "aopf", "\u{1D51E}": "afr", "\u{1D538}": "Aopf", "\u{1D504}": "Afr", "\u{1D49C}": "Ascr", "\xAA": "ordf", "\xE1": "aacute", "\xC1": "Aacute", "\xE0": "agrave", "\xC0": "Agrave", "\u0103": "abreve", "\u0102": "Abreve", "\xE2": "acirc", "\xC2": "Acirc", "\xE5": "aring", "\xC5": "angst", "\xE4": "auml", "\xC4": "Auml", "\xE3": "atilde", "\xC3": "Atilde", "\u0105": "aogon", "\u0104": "Aogon", "\u0101": "amacr", "\u0100": "Amacr", "\xE6": "aelig", "\xC6": "AElig", "\u{1D4B7}": "bscr", "\u{1D553}": "bopf", "\u{1D51F}": "bfr", "\u{1D539}": "Bopf", "\u212C": "Bscr", "\u{1D505}": "Bfr", "\u{1D520}": "cfr", "\u{1D4B8}": "cscr", "\u{1D554}": "copf", "\u212D": "Cfr", "\u{1D49E}": "Cscr", "\u2102": "Copf", "\u0107": "cacute", "\u0106": "Cacute", "\u0109": "ccirc", "\u0108": "Ccirc", "\u010D": "ccaron", "\u010C": "Ccaron", "\u010B": "cdot", "\u010A": "Cdot", "\xE7": "ccedil", "\xC7": "Ccedil", "\u2105": "incare", "\u{1D521}": "dfr", "\u2146": "dd", "\u{1D555}": "dopf", "\u{1D4B9}": "dscr", "\u{1D49F}": "Dscr", "\u{1D507}": "Dfr", "\u2145": "DD", "\u{1D53B}": "Dopf", "\u010F": "dcaron", "\u010E": "Dcaron", "\u0111": "dstrok", "\u0110": "Dstrok", "\xF0": "eth", "\xD0": "ETH", "\u2147": "ee", "\u212F": "escr", "\u{1D522}": "efr", "\u{1D556}": "eopf", "\u2130": "Escr", "\u{1D508}": "Efr", "\u{1D53C}": "Eopf", "\xE9": "eacute", "\xC9": "Eacute", "\xE8": "egrave", "\xC8": "Egrave", "\xEA": "ecirc", "\xCA": "Ecirc", "\u011B": "ecaron", "\u011A": "Ecaron", "\xEB": "euml", "\xCB": "Euml", "\u0117": "edot", "\u0116": "Edot", "\u0119": "eogon", "\u0118": "Eogon", "\u0113": "emacr", "\u0112": "Emacr", "\u{1D523}": "ffr", "\u{1D557}": "fopf", "\u{1D4BB}": "fscr", "\u{1D509}": "Ffr", "\u{1D53D}": "Fopf", "\u2131": "Fscr", "\uFB00": "fflig", "\uFB03": "ffilig", "\uFB04": "ffllig", "\uFB01": "filig", "fj": "fjlig", "\uFB02": "fllig", "\u0192": "fnof", "\u210A": "gscr", "\u{1D558}": "gopf", "\u{1D524}": "gfr", "\u{1D4A2}": "Gscr", "\u{1D53E}": "Gopf", "\u{1D50A}": "Gfr", "\u01F5": "gacute", "\u011F": "gbreve", "\u011E": "Gbreve", "\u011D": "gcirc", "\u011C": "Gcirc", "\u0121": "gdot", "\u0120": "Gdot", "\u0122": "Gcedil", "\u{1D525}": "hfr", "\u210E": "planckh", "\u{1D4BD}": "hscr", "\u{1D559}": "hopf", "\u210B": "Hscr", "\u210C": "Hfr", "\u210D": "Hopf", "\u0125": "hcirc", "\u0124": "Hcirc", "\u210F": "hbar", "\u0127": "hstrok", "\u0126": "Hstrok", "\u{1D55A}": "iopf", "\u{1D526}": "ifr", "\u{1D4BE}": "iscr", "\u2148": "ii", "\u{1D540}": "Iopf", "\u2110": "Iscr", "\u2111": "Im", "\xED": "iacute", "\xCD": "Iacute", "\xEC": "igrave", "\xCC": "Igrave", "\xEE": "icirc", "\xCE": "Icirc", "\xEF": "iuml", "\xCF": "Iuml", "\u0129": "itilde", "\u0128": "Itilde", "\u0130": "Idot", "\u012F": "iogon", "\u012E": "Iogon", "\u012B": "imacr", "\u012A": "Imacr", "\u0133": "ijlig", "\u0132": "IJlig", "\u0131": "imath", "\u{1D4BF}": "jscr", "\u{1D55B}": "jopf", "\u{1D527}": "jfr", "\u{1D4A5}": "Jscr", "\u{1D50D}": "Jfr", "\u{1D541}": "Jopf", "\u0135": "jcirc", "\u0134": "Jcirc", "\u0237": "jmath", "\u{1D55C}": "kopf", "\u{1D4C0}": "kscr", "\u{1D528}": "kfr", "\u{1D4A6}": "Kscr", "\u{1D542}": "Kopf", "\u{1D50E}": "Kfr", "\u0137": "kcedil", "\u0136": "Kcedil", "\u{1D529}": "lfr", "\u{1D4C1}": "lscr", "\u2113": "ell", "\u{1D55D}": "lopf", "\u2112": "Lscr", "\u{1D50F}": "Lfr", "\u{1D543}": "Lopf", "\u013A": "lacute", "\u0139": "Lacute", "\u013E": "lcaron", "\u013D": "Lcaron", "\u013C": "lcedil", "\u013B": "Lcedil", "\u0142": "lstrok", "\u0141": "Lstrok", "\u0140": "lmidot", "\u013F": "Lmidot", "\u{1D52A}": "mfr", "\u{1D55E}": "mopf", "\u{1D4C2}": "mscr", "\u{1D510}": "Mfr", "\u{1D544}": "Mopf", "\u2133": "Mscr", "\u{1D52B}": "nfr", "\u{1D55F}": "nopf", "\u{1D4C3}": "nscr", "\u2115": "Nopf", "\u{1D4A9}": "Nscr", "\u{1D511}": "Nfr", "\u0144": "nacute", "\u0143": "Nacute", "\u0148": "ncaron", "\u0147": "Ncaron", "\xF1": "ntilde", "\xD1": "Ntilde", "\u0146": "ncedil", "\u0145": "Ncedil", "\u2116": "numero", "\u014B": "eng", "\u014A": "ENG", "\u{1D560}": "oopf", "\u{1D52C}": "ofr", "\u2134": "oscr", "\u{1D4AA}": "Oscr", "\u{1D512}": "Ofr", "\u{1D546}": "Oopf", "\xBA": "ordm", "\xF3": "oacute", "\xD3": "Oacute", "\xF2": "ograve", "\xD2": "Ograve", "\xF4": "ocirc", "\xD4": "Ocirc", "\xF6": "ouml", "\xD6": "Ouml", "\u0151": "odblac", "\u0150": "Odblac", "\xF5": "otilde", "\xD5": "Otilde", "\xF8": "oslash", "\xD8": "Oslash", "\u014D": "omacr", "\u014C": "Omacr", "\u0153": "oelig", "\u0152": "OElig", "\u{1D52D}": "pfr", "\u{1D4C5}": "pscr", "\u{1D561}": "popf", "\u2119": "Popf", "\u{1D513}": "Pfr", "\u{1D4AB}": "Pscr", "\u{1D562}": "qopf", "\u{1D52E}": "qfr", "\u{1D4C6}": "qscr", "\u{1D4AC}": "Qscr", "\u{1D514}": "Qfr", "\u211A": "Qopf", "\u0138": "kgreen", "\u{1D52F}": "rfr", "\u{1D563}": "ropf", "\u{1D4C7}": "rscr", "\u211B": "Rscr", "\u211C": "Re", "\u211D": "Ropf", "\u0155": "racute", "\u0154": "Racute", "\u0159": "rcaron", "\u0158": "Rcaron", "\u0157": "rcedil", "\u0156": "Rcedil", "\u{1D564}": "sopf", "\u{1D4C8}": "sscr", "\u{1D530}": "sfr", "\u{1D54A}": "Sopf", "\u{1D516}": "Sfr", "\u{1D4AE}": "Sscr", "\u24C8": "oS", "\u015B": "sacute", "\u015A": "Sacute", "\u015D": "scirc", "\u015C": "Scirc", "\u0161": "scaron", "\u0160": "Scaron", "\u015F": "scedil", "\u015E": "Scedil", "\xDF": "szlig", "\u{1D531}": "tfr", "\u{1D4C9}": "tscr", "\u{1D565}": "topf", "\u{1D4AF}": "Tscr", "\u{1D517}": "Tfr", "\u{1D54B}": "Topf", "\u0165": "tcaron", "\u0164": "Tcaron", "\u0163": "tcedil", "\u0162": "Tcedil", "\u2122": "trade", "\u0167": "tstrok", "\u0166": "Tstrok", "\u{1D4CA}": "uscr", "\u{1D566}": "uopf", "\u{1D532}": "ufr", "\u{1D54C}": "Uopf", "\u{1D518}": "Ufr", "\u{1D4B0}": "Uscr", "\xFA": "uacute", "\xDA": "Uacute", "\xF9": "ugrave", "\xD9": "Ugrave", "\u016D": "ubreve", "\u016C": "Ubreve", "\xFB": "ucirc", "\xDB": "Ucirc", "\u016F": "uring", "\u016E": "Uring", "\xFC": "uuml", "\xDC": "Uuml", "\u0171": "udblac", "\u0170": "Udblac", "\u0169": "utilde", "\u0168": "Utilde", "\u0173": "uogon", "\u0172": "Uogon", "\u016B": "umacr", "\u016A": "Umacr", "\u{1D533}": "vfr", "\u{1D567}": "vopf", "\u{1D4CB}": "vscr", "\u{1D519}": "Vfr", "\u{1D54D}": "Vopf", "\u{1D4B1}": "Vscr", "\u{1D568}": "wopf", "\u{1D4CC}": "wscr", "\u{1D534}": "wfr", "\u{1D4B2}": "Wscr", "\u{1D54E}": "Wopf", "\u{1D51A}": "Wfr", "\u0175": "wcirc", "\u0174": "Wcirc", "\u{1D535}": "xfr", "\u{1D4CD}": "xscr", "\u{1D569}": "xopf", "\u{1D54F}": "Xopf", "\u{1D51B}": "Xfr", "\u{1D4B3}": "Xscr", "\u{1D536}": "yfr", "\u{1D4CE}": "yscr", "\u{1D56A}": "yopf", "\u{1D4B4}": "Yscr", "\u{1D51C}": "Yfr", "\u{1D550}": "Yopf", "\xFD": "yacute", "\xDD": "Yacute", "\u0177": "ycirc", "\u0176": "Ycirc", "\xFF": "yuml", "\u0178": "Yuml", "\u{1D4CF}": "zscr", "\u{1D537}": "zfr", "\u{1D56B}": "zopf", "\u2128": "Zfr", "\u2124": "Zopf", "\u{1D4B5}": "Zscr", "\u017A": "zacute", "\u0179": "Zacute", "\u017E": "zcaron", "\u017D": "Zcaron", "\u017C": "zdot", "\u017B": "Zdot", "\u01B5": "imped", "\xFE": "thorn", "\xDE": "THORN", "\u0149": "napos", "\u03B1": "alpha", "\u0391": "Alpha", "\u03B2": "beta", "\u0392": "Beta", "\u03B3": "gamma", "\u0393": "Gamma", "\u03B4": "delta", "\u0394": "Delta", "\u03B5": "epsi", "\u03F5": "epsiv", "\u0395": "Epsilon", "\u03DD": "gammad", "\u03DC": "Gammad", "\u03B6": "zeta", "\u0396": "Zeta", "\u03B7": "eta", "\u0397": "Eta", "\u03B8": "theta", "\u03D1": "thetav", "\u0398": "Theta", "\u03B9": "iota", "\u0399": "Iota", "\u03BA": "kappa", "\u03F0": "kappav", "\u039A": "Kappa", "\u03BB": "lambda", "\u039B": "Lambda", "\u03BC": "mu", "\xB5": "micro", "\u039C": "Mu", "\u03BD": "nu", "\u039D": "Nu", "\u03BE": "xi", "\u039E": "Xi", "\u03BF": "omicron", "\u039F": "Omicron", "\u03C0": "pi", "\u03D6": "piv", "\u03A0": "Pi", "\u03C1": "rho", "\u03F1": "rhov", "\u03A1": "Rho", "\u03C3": "sigma", "\u03A3": "Sigma", "\u03C2": "sigmaf", "\u03C4": "tau", "\u03A4": "Tau", "\u03C5": "upsi", "\u03A5": "Upsilon", "\u03D2": "Upsi", "\u03C6": "phi", "\u03D5": "phiv", "\u03A6": "Phi", "\u03C7": "chi", "\u03A7": "Chi", "\u03C8": "psi", "\u03A8": "Psi", "\u03C9": "omega", "\u03A9": "ohm", "\u0430": "acy", "\u0410": "Acy", "\u0431": "bcy", "\u0411": "Bcy", "\u0432": "vcy", "\u0412": "Vcy", "\u0433": "gcy", "\u0413": "Gcy", "\u0453": "gjcy", "\u0403": "GJcy", "\u0434": "dcy", "\u0414": "Dcy", "\u0452": "djcy", "\u0402": "DJcy", "\u0435": "iecy", "\u0415": "IEcy", "\u0451": "iocy", "\u0401": "IOcy", "\u0454": "jukcy", "\u0404": "Jukcy", "\u0436": "zhcy", "\u0416": "ZHcy", "\u0437": "zcy", "\u0417": "Zcy", "\u0455": "dscy", "\u0405": "DScy", "\u0438": "icy", "\u0418": "Icy", "\u0456": "iukcy", "\u0406": "Iukcy", "\u0457": "yicy", "\u0407": "YIcy", "\u0439": "jcy", "\u0419": "Jcy", "\u0458": "jsercy", "\u0408": "Jsercy", "\u043A": "kcy", "\u041A": "Kcy", "\u045C": "kjcy", "\u040C": "KJcy", "\u043B": "lcy", "\u041B": "Lcy", "\u0459": "ljcy", "\u0409": "LJcy", "\u043C": "mcy", "\u041C": "Mcy", "\u043D": "ncy", "\u041D": "Ncy", "\u045A": "njcy", "\u040A": "NJcy", "\u043E": "ocy", "\u041E": "Ocy", "\u043F": "pcy", "\u041F": "Pcy", "\u0440": "rcy", "\u0420": "Rcy", "\u0441": "scy", "\u0421": "Scy", "\u0442": "tcy", "\u0422": "Tcy", "\u045B": "tshcy", "\u040B": "TSHcy", "\u0443": "ucy", "\u0423": "Ucy", "\u045E": "ubrcy", "\u040E": "Ubrcy", "\u0444": "fcy", "\u0424": "Fcy", "\u0445": "khcy", "\u0425": "KHcy", "\u0446": "tscy", "\u0426": "TScy", "\u0447": "chcy", "\u0427": "CHcy", "\u045F": "dzcy", "\u040F": "DZcy", "\u0448": "shcy", "\u0428": "SHcy", "\u0449": "shchcy", "\u0429": "SHCHcy", "\u044A": "hardcy", "\u042A": "HARDcy", "\u044B": "ycy", "\u042B": "Ycy", "\u044C": "softcy", "\u042C": "SOFTcy", "\u044D": "ecy", "\u042D": "Ecy", "\u044E": "yucy", "\u042E": "YUcy", "\u044F": "yacy", "\u042F": "YAcy", "\u2135": "aleph", "\u2136": "beth", "\u2137": "gimel", "\u2138": "daleth" };
var regexEscape = /["&'<>`]/g;
var escapeMap = {
'"': """,
"&": "&",
"'": "'",
"<": "<",
">": ">",
"`": "`"
};
var regexInvalidEntity = /(?:[xX][^a-fA-F0-9]|[^0-9xX])/;
var regexInvalidRawCodePoint = /[\0-\x08\x0B\x0E-\x1F\x7F-\x9F\uFDD0-\uFDEF\uFFFE\uFFFF]|[\uD83F\uD87F\uD8BF\uD8FF\uD93F\uD97F\uD9BF\uD9FF\uDA3F\uDA7F\uDABF\uDAFF\uDB3F\uDB7F\uDBBF\uDBFF][\uDFFE\uDFFF]|[\uD800-\uDBFF](?![\uDC00-\uDFFF])|(?:[^\uD800-\uDBFF]|^)[\uDC00-\uDFFF]/;
var regexDecode = /&(CounterClockwiseContourIntegral|DoubleLongLeftRightArrow|ClockwiseContourIntegral|NotNestedGreaterGreater|NotSquareSupersetEqual|DiacriticalDoubleAcute|NotRightTriangleEqual|NotSucceedsSlantEqual|NotPrecedesSlantEqual|CloseCurlyDoubleQuote|NegativeVeryThinSpace|DoubleContourIntegral|FilledVerySmallSquare|CapitalDifferentialD|OpenCurlyDoubleQuote|EmptyVerySmallSquare|NestedGreaterGreater|DoubleLongRightArrow|NotLeftTriangleEqual|NotGreaterSlantEqual|ReverseUpEquilibrium|DoubleLeftRightArrow|NotSquareSubsetEqual|NotDoubleVerticalBar|RightArrowLeftArrow|NotGreaterFullEqual|NotRightTriangleBar|SquareSupersetEqual|DownLeftRightVector|DoubleLongLeftArrow|leftrightsquigarrow|LeftArrowRightArrow|NegativeMediumSpace|blacktriangleright|RightDownVectorBar|PrecedesSlantEqual|RightDoubleBracket|SucceedsSlantEqual|NotLeftTriangleBar|RightTriangleEqual|SquareIntersection|RightDownTeeVector|ReverseEquilibrium|NegativeThickSpace|longleftrightarrow|Longleftrightarrow|LongLeftRightArrow|DownRightTeeVector|DownRightVectorBar|GreaterSlantEqual|SquareSubsetEqual|LeftDownVectorBar|LeftDoubleBracket|VerticalSeparator|rightleftharpoons|NotGreaterGreater|NotSquareSuperset|blacktriangleleft|blacktriangledown|NegativeThinSpace|LeftDownTeeVector|NotLessSlantEqual|leftrightharpoons|DoubleUpDownArrow|DoubleVerticalBar|LeftTriangleEqual|FilledSmallSquare|twoheadrightarrow|NotNestedLessLess|DownLeftTeeVector|DownLeftVectorBar|RightAngleBracket|NotTildeFullEqual|NotReverseElement|RightUpDownVector|DiacriticalTilde|NotSucceedsTilde|circlearrowright|NotPrecedesEqual|rightharpoondown|DoubleRightArrow|NotSucceedsEqual|NonBreakingSpace|NotRightTriangle|LessEqualGreater|RightUpTeeVector|LeftAngleBracket|GreaterFullEqual|DownArrowUpArrow|RightUpVectorBar|twoheadleftarrow|GreaterEqualLess|downharpoonright|RightTriangleBar|ntrianglerighteq|NotSupersetEqual|LeftUpDownVector|DiacriticalAcute|rightrightarrows|vartriangleright|UpArrowDownArrow|DiacriticalGrave|UnderParenthesis|EmptySmallSquare|LeftUpVectorBar|leftrightarrows|DownRightVector|downharpoonleft|trianglerighteq|ShortRightArrow|OverParenthesis|DoubleLeftArrow|DoubleDownArrow|NotSquareSubset|bigtriangledown|ntrianglelefteq|UpperRightArrow|curvearrowright|vartriangleleft|NotLeftTriangle|nleftrightarrow|LowerRightArrow|NotHumpDownHump|NotGreaterTilde|rightthreetimes|LeftUpTeeVector|NotGreaterEqual|straightepsilon|LeftTriangleBar|rightsquigarrow|ContourIntegral|rightleftarrows|CloseCurlyQuote|RightDownVector|LeftRightVector|nLeftrightarrow|leftharpoondown|circlearrowleft|SquareSuperset|OpenCurlyQuote|hookrightarrow|HorizontalLine|DiacriticalDot|NotLessGreater|ntriangleright|DoubleRightTee|InvisibleComma|InvisibleTimes|LowerLeftArrow|DownLeftVector|NotSubsetEqual|curvearrowleft|trianglelefteq|NotVerticalBar|TildeFullEqual|downdownarrows|NotGreaterLess|RightTeeVector|ZeroWidthSpace|looparrowright|LongRightArrow|doublebarwedge|ShortLeftArrow|ShortDownArrow|RightVectorBar|GreaterGreater|ReverseElement|rightharpoonup|LessSlantEqual|leftthreetimes|upharpoonright|rightarrowtail|LeftDownVector|Longrightarrow|NestedLessLess|UpperLeftArrow|nshortparallel|leftleftarrows|leftrightarrow|Leftrightarrow|LeftRightArrow|longrightarrow|upharpoonleft|RightArrowBar|ApplyFunction|LeftTeeVector|leftarrowtail|NotEqualTilde|varsubsetneqq|varsupsetneqq|RightTeeArrow|SucceedsEqual|SucceedsTilde|LeftVectorBar|SupersetEqual|hookleftarrow|DifferentialD|VerticalTilde|VeryThinSpace|blacktriangle|bigtriangleup|LessFullEqual|divideontimes|leftharpoonup|UpEquilibrium|ntriangleleft|RightTriangle|measuredangle|shortparallel|longleftarrow|Longleftarrow|LongLeftArrow|DoubleLeftTee|Poincareplane|PrecedesEqual|triangleright|DoubleUpArrow|RightUpVector|fallingdotseq|looparrowleft|PrecedesTilde|NotTildeEqual|NotTildeTilde|smallsetminus|Proportional|triangleleft|triangledown|UnderBracket|NotHumpEqual|exponentiale|ExponentialE|NotLessTilde|HilbertSpace|RightCeiling|blacklozenge|varsupsetneq|HumpDownHump|GreaterEqual|VerticalLine|LeftTeeArrow|NotLessEqual|DownTeeArrow|LeftTriangle|varsubsetneq|Intersection|NotCongruent|DownArrowBar|LeftUpVector|LeftArrowBar|risingdotseq|GreaterTilde|RoundImplies|SquareSubset|ShortUpArrow|NotSuperset|quaternions|precnapprox|backepsilon|preccurlyeq|OverBracket|blacksquare|MediumSpace|VerticalBar|circledcirc|circleddash|CircleMinus|CircleTimes|LessGreater|curlyeqprec|curlyeqsucc|diamondsuit|UpDownArrow|Updownarrow|RuleDelayed|Rrightarrow|updownarrow|RightVector|nRightarrow|nrightarrow|eqslantless|LeftCeiling|Equilibrium|SmallCircle|expectation|NotSucceeds|thickapprox|GreaterLess|SquareUnion|NotPrecedes|NotLessLess|straightphi|succnapprox|succcurlyeq|SubsetEqual|sqsupseteq|Proportion|Laplacetrf|ImaginaryI|supsetneqq|NotGreater|gtreqqless|NotElement|ThickSpace|TildeEqual|TildeTilde|Fouriertrf|rmoustache|EqualTilde|eqslantgtr|UnderBrace|LeftVector|UpArrowBar|nLeftarrow|nsubseteqq|subsetneqq|nsupseteqq|nleftarrow|succapprox|lessapprox|UpTeeArrow|upuparrows|curlywedge|lesseqqgtr|varepsilon|varnothing|RightFloor|complement|CirclePlus|sqsubseteq|Lleftarrow|circledast|RightArrow|Rightarrow|rightarrow|lmoustache|Bernoullis|precapprox|mapstoleft|mapstodown|longmapsto|dotsquare|downarrow|DoubleDot|nsubseteq|supsetneq|leftarrow|nsupseteq|subsetneq|ThinSpace|ngeqslant|subseteqq|HumpEqual|NotSubset|triangleq|NotCupCap|lesseqgtr|heartsuit|TripleDot|Leftarrow|Coproduct|Congruent|varpropto|complexes|gvertneqq|LeftArrow|LessTilde|supseteqq|MinusPlus|CircleDot|nleqslant|NotExists|gtreqless|nparallel|UnionPlus|LeftFloor|checkmark|CenterDot|centerdot|Mellintrf|gtrapprox|bigotimes|OverBrace|spadesuit|therefore|pitchfork|rationals|PlusMinus|Backslash|Therefore|DownBreve|backsimeq|backprime|DownArrow|nshortmid|Downarrow|lvertneqq|eqvparsl|imagline|imagpart|infintie|integers|Integral|intercal|LessLess|Uarrocir|intlarhk|sqsupset|angmsdaf|sqsubset|llcorner|vartheta|cupbrcap|lnapprox|Superset|SuchThat|succnsim|succneqq|angmsdag|biguplus|curlyvee|trpezium|Succeeds|NotTilde|bigwedge|angmsdah|angrtvbd|triminus|cwconint|fpartint|lrcorner|smeparsl|subseteq|urcorner|lurdshar|laemptyv|DDotrahd|approxeq|ldrushar|awconint|mapstoup|backcong|shortmid|triangle|geqslant|gesdotol|timesbar|circledR|circledS|setminus|multimap|naturals|scpolint|ncongdot|RightTee|boxminus|gnapprox|boxtimes|andslope|thicksim|angmsdaa|varsigma|cirfnint|rtriltri|angmsdab|rppolint|angmsdac|barwedge|drbkarow|clubsuit|thetasym|bsolhsub|capbrcup|dzigrarr|doteqdot|DotEqual|dotminus|UnderBar|NotEqual|realpart|otimesas|ulcorner|hksearow|hkswarow|parallel|PartialD|elinters|emptyset|plusacir|bbrktbrk|angmsdad|pointint|bigoplus|angmsdae|Precedes|bigsqcup|varkappa|notindot|supseteq|precneqq|precnsim|profalar|profline|profsurf|leqslant|lesdotor|raemptyv|subplus|notnivb|notnivc|subrarr|zigrarr|vzigzag|submult|subedot|Element|between|cirscir|larrbfs|larrsim|lotimes|lbrksld|lbrkslu|lozenge|ldrdhar|dbkarow|bigcirc|epsilon|simrarr|simplus|ltquest|Epsilon|luruhar|gtquest|maltese|npolint|eqcolon|npreceq|bigodot|ddagger|gtrless|bnequiv|harrcir|ddotseq|equivDD|backsim|demptyv|nsqsube|nsqsupe|Upsilon|nsubset|upsilon|minusdu|nsucceq|swarrow|nsupset|coloneq|searrow|boxplus|napprox|natural|asympeq|alefsym|congdot|nearrow|bigstar|diamond|supplus|tritime|LeftTee|nvinfin|triplus|NewLine|nvltrie|nvrtrie|nwarrow|nexists|Diamond|ruluhar|Implies|supmult|angzarr|suplarr|suphsub|questeq|because|digamma|Because|olcross|bemptyv|omicron|Omicron|rotimes|NoBreak|intprod|angrtvb|orderof|uwangle|suphsol|lesdoto|orslope|DownTee|realine|cudarrl|rdldhar|OverBar|supedot|lessdot|supdsub|topfork|succsim|rbrkslu|rbrksld|pertenk|cudarrr|isindot|planckh|lessgtr|pluscir|gesdoto|plussim|plustwo|lesssim|cularrp|rarrsim|Cayleys|notinva|notinvb|notinvc|UpArrow|Uparrow|uparrow|NotLess|dwangle|precsim|Product|curarrm|Cconint|dotplus|rarrbfs|ccupssm|Cedilla|cemptyv|notniva|quatint|frac35|frac38|frac45|frac56|frac58|frac78|tridot|xoplus|gacute|gammad|Gammad|lfisht|lfloor|bigcup|sqsupe|gbreve|Gbreve|lharul|sqsube|sqcups|Gcedil|apacir|llhard|lmidot|Lmidot|lmoust|andand|sqcaps|approx|Abreve|spades|circeq|tprime|divide|topcir|Assign|topbot|gesdot|divonx|xuplus|timesd|gesles|atilde|solbar|SOFTcy|loplus|timesb|lowast|lowbar|dlcorn|dlcrop|softcy|dollar|lparlt|thksim|lrhard|Atilde|lsaquo|smashp|bigvee|thinsp|wreath|bkarow|lsquor|lstrok|Lstrok|lthree|ltimes|ltlarr|DotDot|simdot|ltrPar|weierp|xsqcup|angmsd|sigmav|sigmaf|zeetrf|Zcaron|zcaron|mapsto|vsupne|thetav|cirmid|marker|mcomma|Zacute|vsubnE|there4|gtlPar|vsubne|bottom|gtrarr|SHCHcy|shchcy|midast|midcir|middot|minusb|minusd|gtrdot|bowtie|sfrown|mnplus|models|colone|seswar|Colone|mstpos|searhk|gtrsim|nacute|Nacute|boxbox|telrec|hairsp|Tcedil|nbumpe|scnsim|ncaron|Ncaron|ncedil|Ncedil|hamilt|Scedil|nearhk|hardcy|HARDcy|tcedil|Tcaron|commat|nequiv|nesear|tcaron|target|hearts|nexist|varrho|scedil|Scaron|scaron|hellip|Sacute|sacute|hercon|swnwar|compfn|rtimes|rthree|rsquor|rsaquo|zacute|wedgeq|homtht|barvee|barwed|Barwed|rpargt|horbar|conint|swarhk|roplus|nltrie|hslash|hstrok|Hstrok|rmoust|Conint|bprime|hybull|hyphen|iacute|Iacute|supsup|supsub|supsim|varphi|coprod|brvbar|agrave|Supset|supset|igrave|Igrave|notinE|Agrave|iiiint|iinfin|copysr|wedbar|Verbar|vangrt|becaus|incare|verbar|inodot|bullet|drcorn|intcal|drcrop|cularr|vellip|Utilde|bumpeq|cupcap|dstrok|Dstrok|CupCap|cupcup|cupdot|eacute|Eacute|supdot|iquest|easter|ecaron|Ecaron|ecolon|isinsv|utilde|itilde|Itilde|curarr|succeq|Bumpeq|cacute|ulcrop|nparsl|Cacute|nprcue|egrave|Egrave|nrarrc|nrarrw|subsup|subsub|nrtrie|jsercy|nsccue|Jsercy|kappav|kcedil|Kcedil|subsim|ulcorn|nsimeq|egsdot|veebar|kgreen|capand|elsdot|Subset|subset|curren|aacute|lacute|Lacute|emptyv|ntilde|Ntilde|lagran|lambda|Lambda|capcap|Ugrave|langle|subdot|emsp13|numero|emsp14|nvdash|nvDash|nVdash|nVDash|ugrave|ufisht|nvHarr|larrfs|nvlArr|larrhk|larrlp|larrpl|nvrArr|Udblac|nwarhk|larrtl|nwnear|oacute|Oacute|latail|lAtail|sstarf|lbrace|odblac|Odblac|lbrack|udblac|odsold|eparsl|lcaron|Lcaron|ograve|Ograve|lcedil|Lcedil|Aacute|ssmile|ssetmn|squarf|ldquor|capcup|ominus|cylcty|rharul|eqcirc|dagger|rfloor|rfisht|Dagger|daleth|equals|origof|capdot|equest|dcaron|Dcaron|rdquor|oslash|Oslash|otilde|Otilde|otimes|Otimes|urcrop|Ubreve|ubreve|Yacute|Uacute|uacute|Rcedil|rcedil|urcorn|parsim|Rcaron|Vdashl|rcaron|Tstrok|percnt|period|permil|Exists|yacute|rbrack|rbrace|phmmat|ccaron|Ccaron|planck|ccedil|plankv|tstrok|female|plusdo|plusdu|ffilig|plusmn|ffllig|Ccedil|rAtail|dfisht|bernou|ratail|Rarrtl|rarrtl|angsph|rarrpl|rarrlp|rarrhk|xwedge|xotime|forall|ForAll|Vvdash|vsupnE|preceq|bigcap|frac12|frac13|frac14|primes|rarrfs|prnsim|frac15|Square|frac16|square|lesdot|frac18|frac23|propto|prurel|rarrap|rangle|puncsp|frac25|Racute|qprime|racute|lesges|frac34|abreve|AElig|eqsim|utdot|setmn|urtri|Equal|Uring|seArr|uring|searr|dashv|Dashv|mumap|nabla|iogon|Iogon|sdote|sdotb|scsim|napid|napos|equiv|natur|Acirc|dblac|erarr|nbump|iprod|erDot|ucirc|awint|esdot|angrt|ncong|isinE|scnap|Scirc|scirc|ndash|isins|Ubrcy|nearr|neArr|isinv|nedot|ubrcy|acute|Ycirc|iukcy|Iukcy|xutri|nesim|caret|jcirc|Jcirc|caron|twixt|ddarr|sccue|exist|jmath|sbquo|ngeqq|angst|ccaps|lceil|ngsim|UpTee|delta|Delta|rtrif|nharr|nhArr|nhpar|rtrie|jukcy|Jukcy|kappa|rsquo|Kappa|nlarr|nlArr|TSHcy|rrarr|aogon|Aogon|fflig|xrarr|tshcy|ccirc|nleqq|filig|upsih|nless|dharl|nlsim|fjlig|ropar|nltri|dharr|robrk|roarr|fllig|fltns|roang|rnmid|subnE|subne|lAarr|trisb|Ccirc|acirc|ccups|blank|VDash|forkv|Vdash|langd|cedil|blk12|blk14|laquo|strns|diams|notin|vDash|larrb|blk34|block|disin|uplus|vdash|vBarv|aelig|starf|Wedge|check|xrArr|lates|lbarr|lBarr|notni|lbbrk|bcong|frasl|lbrke|frown|vrtri|vprop|vnsup|gamma|Gamma|wedge|xodot|bdquo|srarr|doteq|ldquo|boxdl|boxdL|gcirc|Gcirc|boxDl|boxDL|boxdr|boxdR|boxDr|TRADE|trade|rlhar|boxDR|vnsub|npart|vltri|rlarr|boxhd|boxhD|nprec|gescc|nrarr|nrArr|boxHd|boxHD|boxhu|boxhU|nrtri|boxHu|clubs|boxHU|times|colon|Colon|gimel|xlArr|Tilde|nsime|tilde|nsmid|nspar|THORN|thorn|xlarr|nsube|nsubE|thkap|xhArr|comma|nsucc|boxul|boxuL|nsupe|nsupE|gneqq|gnsim|boxUl|boxUL|grave|boxur|boxuR|boxUr|boxUR|lescc|angle|bepsi|boxvh|varpi|boxvH|numsp|Theta|gsime|gsiml|theta|boxVh|boxVH|boxvl|gtcir|gtdot|boxvL|boxVl|boxVL|crarr|cross|Cross|nvsim|boxvr|nwarr|nwArr|sqsup|dtdot|Uogon|lhard|lharu|dtrif|ocirc|Ocirc|lhblk|duarr|odash|sqsub|Hacek|sqcup|llarr|duhar|oelig|OElig|ofcir|boxvR|uogon|lltri|boxVr|csube|uuarr|ohbar|csupe|ctdot|olarr|olcir|harrw|oline|sqcap|omacr|Omacr|omega|Omega|boxVR|aleph|lneqq|lnsim|loang|loarr|rharu|lobrk|hcirc|operp|oplus|rhard|Hcirc|orarr|Union|order|ecirc|Ecirc|cuepr|szlig|cuesc|breve|reals|eDDot|Breve|hoarr|lopar|utrif|rdquo|Umacr|umacr|efDot|swArr|ultri|alpha|rceil|ovbar|swarr|Wcirc|wcirc|smtes|smile|bsemi|lrarr|aring|parsl|lrhar|bsime|uhblk|lrtri|cupor|Aring|uharr|uharl|slarr|rbrke|bsolb|lsime|rbbrk|RBarr|lsimg|phone|rBarr|rbarr|icirc|lsquo|Icirc|emacr|Emacr|ratio|simne|plusb|simlE|simgE|simeq|pluse|ltcir|ltdot|empty|xharr|xdtri|iexcl|Alpha|ltrie|rarrw|pound|ltrif|xcirc|bumpe|prcue|bumpE|asymp|amacr|cuvee|Sigma|sigma|iiint|udhar|iiota|ijlig|IJlig|supnE|imacr|Imacr|prime|Prime|image|prnap|eogon|Eogon|rarrc|mdash|mDDot|cuwed|imath|supne|imped|Amacr|udarr|prsim|micro|rarrb|cwint|raquo|infin|eplus|range|rangd|Ucirc|radic|minus|amalg|veeeq|rAarr|epsiv|ycirc|quest|sharp|quot|zwnj|Qscr|race|qscr|Qopf|qopf|qint|rang|Rang|Zscr|zscr|Zopf|zopf|rarr|rArr|Rarr|Pscr|pscr|prop|prod|prnE|prec|ZHcy|zhcy|prap|Zeta|zeta|Popf|popf|Zdot|plus|zdot|Yuml|yuml|phiv|YUcy|yucy|Yscr|yscr|perp|Yopf|yopf|part|para|YIcy|Ouml|rcub|yicy|YAcy|rdca|ouml|osol|Oscr|rdsh|yacy|real|oscr|xvee|andd|rect|andv|Xscr|oror|ordm|ordf|xscr|ange|aopf|Aopf|rHar|Xopf|opar|Oopf|xopf|xnis|rhov|oopf|omid|xmap|oint|apid|apos|ogon|ascr|Ascr|odot|odiv|xcup|xcap|ocir|oast|nvlt|nvle|nvgt|nvge|nvap|Wscr|wscr|auml|ntlg|ntgl|nsup|nsub|nsim|Nscr|nscr|nsce|Wopf|ring|npre|wopf|npar|Auml|Barv|bbrk|Nopf|nopf|nmid|nLtv|beta|ropf|Ropf|Beta|beth|nles|rpar|nleq|bnot|bNot|nldr|NJcy|rscr|Rscr|Vscr|vscr|rsqb|njcy|bopf|nisd|Bopf|rtri|Vopf|nGtv|ngtr|vopf|boxh|boxH|boxv|nges|ngeq|boxV|bscr|scap|Bscr|bsim|Vert|vert|bsol|bull|bump|caps|cdot|ncup|scnE|ncap|nbsp|napE|Cdot|cent|sdot|Vbar|nang|vBar|chcy|Mscr|mscr|sect|semi|CHcy|Mopf|mopf|sext|circ|cire|mldr|mlcp|cirE|comp|shcy|SHcy|vArr|varr|cong|copf|Copf|copy|COPY|malt|male|macr|lvnE|cscr|ltri|sime|ltcc|simg|Cscr|siml|csub|Uuml|lsqb|lsim|uuml|csup|Lscr|lscr|utri|smid|lpar|cups|smte|lozf|darr|Lopf|Uscr|solb|lopf|sopf|Sopf|lneq|uscr|spar|dArr|lnap|Darr|dash|Sqrt|LJcy|ljcy|lHar|dHar|Upsi|upsi|diam|lesg|djcy|DJcy|leqq|dopf|Dopf|dscr|Dscr|dscy|ldsh|ldca|squf|DScy|sscr|Sscr|dsol|lcub|late|star|Star|Uopf|Larr|lArr|larr|uopf|dtri|dzcy|sube|subE|Lang|lang|Kscr|kscr|Kopf|kopf|KJcy|kjcy|KHcy|khcy|DZcy|ecir|edot|eDot|Jscr|jscr|succ|Jopf|jopf|Edot|uHar|emsp|ensp|Iuml|iuml|eopf|isin|Iscr|iscr|Eopf|epar|sung|epsi|escr|sup1|sup2|sup3|Iota|iota|supe|supE|Iopf|iopf|IOcy|iocy|Escr|esim|Esim|imof|Uarr|QUOT|uArr|uarr|euml|IEcy|iecy|Idot|Euml|euro|excl|Hscr|hscr|Hopf|hopf|TScy|tscy|Tscr|hbar|tscr|flat|tbrk|fnof|hArr|harr|half|fopf|Fopf|tdot|gvnE|fork|trie|gtcc|fscr|Fscr|gdot|gsim|Gscr|gscr|Gopf|gopf|gneq|Gdot|tosa|gnap|Topf|topf|geqq|toea|GJcy|gjcy|tint|gesl|mid|Sfr|ggg|top|ges|gla|glE|glj|geq|gne|gEl|gel|gnE|Gcy|gcy|gap|Tfr|tfr|Tcy|tcy|Hat|Tau|Ffr|tau|Tab|hfr|Hfr|ffr|Fcy|fcy|icy|Icy|iff|ETH|eth|ifr|Ifr|Eta|eta|int|Int|Sup|sup|ucy|Ucy|Sum|sum|jcy|ENG|ufr|Ufr|eng|Jcy|jfr|els|ell|egs|Efr|efr|Jfr|uml|kcy|Kcy|Ecy|ecy|kfr|Kfr|lap|Sub|sub|lat|lcy|Lcy|leg|Dot|dot|lEg|leq|les|squ|div|die|lfr|Lfr|lgE|Dfr|dfr|Del|deg|Dcy|dcy|lne|lnE|sol|loz|smt|Cup|lrm|cup|lsh|Lsh|sim|shy|map|Map|mcy|Mcy|mfr|Mfr|mho|gfr|Gfr|sfr|cir|Chi|chi|nap|Cfr|vcy|Vcy|cfr|Scy|scy|ncy|Ncy|vee|Vee|Cap|cap|nfr|scE|sce|Nfr|nge|ngE|nGg|vfr|Vfr|ngt|bot|nGt|nis|niv|Rsh|rsh|nle|nlE|bne|Bfr|bfr|nLl|nlt|nLt|Bcy|bcy|not|Not|rlm|wfr|Wfr|npr|nsc|num|ocy|ast|Ocy|ofr|xfr|Xfr|Ofr|ogt|ohm|apE|olt|Rho|ape|rho|Rfr|rfr|ord|REG|ang|reg|orv|And|and|AMP|Rcy|amp|Afr|ycy|Ycy|yen|yfr|Yfr|rcy|par|pcy|Pcy|pfr|Pfr|phi|Phi|afr|Acy|acy|zcy|Zcy|piv|acE|acd|zfr|Zfr|pre|prE|psi|Psi|qfr|Qfr|zwj|Or|ge|Gg|gt|gg|el|oS|lt|Lt|LT|Re|lg|gl|eg|ne|Im|it|le|DD|wp|wr|nu|Nu|dd|lE|Sc|sc|pi|Pi|ee|af|ll|Ll|rx|gE|xi|pm|Xi|ic|pr|Pr|in|ni|mp|mu|ac|Mu|or|ap|Gt|GT|ii);|&(Aacute|Agrave|Atilde|Ccedil|Eacute|Egrave|Iacute|Igrave|Ntilde|Oacute|Ograve|Oslash|Otilde|Uacute|Ugrave|Yacute|aacute|agrave|atilde|brvbar|ccedil|curren|divide|eacute|egrave|frac12|frac14|frac34|iacute|igrave|iquest|middot|ntilde|oacute|ograve|oslash|otilde|plusmn|uacute|ugrave|yacute|AElig|Acirc|Aring|Ecirc|Icirc|Ocirc|THORN|Ucirc|acirc|acute|aelig|aring|cedil|ecirc|icirc|iexcl|laquo|micro|ocirc|pound|raquo|szlig|thorn|times|ucirc|Auml|COPY|Euml|Iuml|Ouml|QUOT|Uuml|auml|cent|copy|euml|iuml|macr|nbsp|ordf|ordm|ouml|para|quot|sect|sup1|sup2|sup3|uuml|yuml|AMP|ETH|REG|amp|deg|eth|not|reg|shy|uml|yen|GT|LT|gt|lt)(?!;)([=a-zA-Z0-9]?)|([0-9]+)(;?)|[xX]([a-fA-F0-9]+)(;?)|&([0-9a-zA-Z]+)/g;
var decodeMap = { "aacute": "\xE1", "Aacute": "\xC1", "abreve": "\u0103", "Abreve": "\u0102", "ac": "\u223E", "acd": "\u223F", "acE": "\u223E\u0333", "acirc": "\xE2", "Acirc": "\xC2", "acute": "\xB4", "acy": "\u0430", "Acy": "\u0410", "aelig": "\xE6", "AElig": "\xC6", "af": "\u2061", "afr": "\u{1D51E}", "Afr": "\u{1D504}", "agrave": "\xE0", "Agrave": "\xC0", "alefsym": "\u2135", "aleph": "\u2135", "alpha": "\u03B1", "Alpha": "\u0391", "amacr": "\u0101", "Amacr": "\u0100", "amalg": "\u2A3F", "amp": "&", "AMP": "&", "and": "\u2227", "And": "\u2A53", "andand": "\u2A55", "andd": "\u2A5C", "andslope": "\u2A58", "andv": "\u2A5A", "ang": "\u2220", "ange": "\u29A4", "angle": "\u2220", "angmsd": "\u2221", "angmsdaa": "\u29A8", "angmsdab": "\u29A9", "angmsdac": "\u29AA", "angmsdad": "\u29AB", "angmsdae": "\u29AC", "angmsdaf": "\u29AD", "angmsdag": "\u29AE", "angmsdah": "\u29AF", "angrt": "\u221F", "angrtvb": "\u22BE", "angrtvbd": "\u299D", "angsph": "\u2222", "angst": "\xC5", "angzarr": "\u237C", "aogon": "\u0105", "Aogon": "\u0104", "aopf": "\u{1D552}", "Aopf": "\u{1D538}", "ap": "\u2248", "apacir": "\u2A6F", "ape": "\u224A", "apE": "\u2A70", "apid": "\u224B", "apos": "'", "ApplyFunction": "\u2061", "approx": "\u2248", "approxeq": "\u224A", "aring": "\xE5", "Aring": "\xC5", "ascr": "\u{1D4B6}", "Ascr": "\u{1D49C}", "Assign": "\u2254", "ast": "*", "asymp": "\u2248", "asympeq": "\u224D", "atilde": "\xE3", "Atilde": "\xC3", "auml": "\xE4", "Auml": "\xC4", "awconint": "\u2233", "awint": "\u2A11", "backcong": "\u224C", "backepsilon": "\u03F6", "backprime": "\u2035", "backsim": "\u223D", "backsimeq": "\u22CD", "Backslash": "\u2216", "Barv": "\u2AE7", "barvee": "\u22BD", "barwed": "\u2305", "Barwed": "\u2306", "barwedge": "\u2305", "bbrk": "\u23B5", "bbrktbrk": "\u23B6", "bcong": "\u224C", "bcy": "\u0431", "Bcy": "\u0411", "bdquo": "\u201E", "becaus": "\u2235", "because": "\u2235", "Because": "\u2235", "bemptyv": "\u29B0", "bepsi": "\u03F6", "bernou": "\u212C", "Bernoullis": "\u212C", "beta": "\u03B2", "Beta": "\u0392", "beth": "\u2136", "between": "\u226C", "bfr": "\u{1D51F}", "Bfr": "\u{1D505}", "bigcap": "\u22C2", "bigcirc": "\u25EF", "bigcup": "\u22C3", "bigodot": "\u2A00", "bigoplus": "\u2A01", "bigotimes": "\u2A02", "bigsqcup": "\u2A06", "bigstar": "\u2605", "bigtriangledown": "\u25BD", "bigtriangleup": "\u25B3", "biguplus": "\u2A04", "bigvee": "\u22C1", "bigwedge": "\u22C0", "bkarow": "\u290D", "blacklozenge": "\u29EB", "blacksquare": "\u25AA", "blacktriangle": "\u25B4", "blacktriangledown": "\u25BE", "blacktriangleleft": "\u25C2", "blacktriangleright": "\u25B8", "blank": "\u2423", "blk12": "\u2592", "blk14": "\u2591", "blk34": "\u2593", "block": "\u2588", "bne": "=\u20E5", "bnequiv": "\u2261\u20E5", "bnot": "\u2310", "bNot": "\u2AED", "bopf": "\u{1D553}", "Bopf": "\u{1D539}", "bot": "\u22A5", "bottom": "\u22A5", "bowtie": "\u22C8", "boxbox": "\u29C9", "boxdl": "\u2510", "boxdL": "\u2555", "boxDl": "\u2556", "boxDL": "\u2557", "boxdr": "\u250C", "boxdR": "\u2552", "boxDr": "\u2553", "boxDR": "\u2554", "boxh": "\u2500", "boxH": "\u2550", "boxhd": "\u252C", "boxhD": "\u2565", "boxHd": "\u2564", "boxHD": "\u2566", "boxhu": "\u2534", "boxhU": "\u2568", "boxHu": "\u2567", "boxHU": "\u2569", "boxminus": "\u229F", "boxplus": "\u229E", "boxtimes": "\u22A0", "boxul": "\u2518", "boxuL": "\u255B", "boxUl": "\u255C", "boxUL": "\u255D", "boxur": "\u2514", "boxuR": "\u2558", "boxUr": "\u2559", "boxUR": "\u255A", "boxv": "\u2502", "boxV": "\u2551", "boxvh": "\u253C", "boxvH": "\u256A", "boxVh": "\u256B", "boxVH": "\u256C", "boxvl": "\u2524", "boxvL": "\u2561", "boxVl": "\u2562", "boxVL": "\u2563", "boxvr": "\u251C", "boxvR": "\u255E", "boxVr": "\u255F", "boxVR": "\u2560", "bprime": "\u2035", "breve": "\u02D8", "Breve": "\u02D8", "brvbar": "\xA6", "bscr": "\u{1D4B7}", "Bscr": "\u212C", "bsemi": "\u204F", "bsim": "\u223D", "bsime": "\u22CD", "bsol": "\\", "bsolb": "\u29C5", "bsolhsub": "\u27C8", "bull": "\u2022", "bullet": "\u2022", "bump": "\u224E", "bumpe": "\u224F", "bumpE": "\u2AAE", "bumpeq": "\u224F", "Bumpeq": "\u224E", "cacute": "\u0107", "Cacute": "\u0106", "cap": "\u2229", "Cap": "\u22D2", "capand": "\u2A44", "capbrcup": "\u2A49", "capcap": "\u2A4B", "capcup": "\u2A47", "capdot": "\u2A40", "CapitalDifferentialD": "\u2145", "caps": "\u2229\uFE00", "caret": "\u2041", "caron": "\u02C7", "Cayleys": "\u212D", "ccaps": "\u2A4D", "ccaron": "\u010D", "Ccaron": "\u010C", "ccedil": "\xE7", "Ccedil": "\xC7", "ccirc": "\u0109", "Ccirc": "\u0108", "Cconint": "\u2230", "ccups": "\u2A4C", "ccupssm": "\u2A50", "cdot": "\u010B", "Cdot": "\u010A", "cedil": "\xB8", "Cedilla": "\xB8", "cemptyv": "\u29B2", "cent": "\xA2", "centerdot": "\xB7", "CenterDot": "\xB7", "cfr": "\u{1D520}", "Cfr": "\u212D", "chcy": "\u0447", "CHcy": "\u0427", "check": "\u2713", "checkmark": "\u2713", "chi": "\u03C7", "Chi": "\u03A7", "cir": "\u25CB", "circ": "\u02C6", "circeq": "\u2257", "circlearrowleft": "\u21BA", "circlearrowright": "\u21BB", "circledast": "\u229B", "circledcirc": "\u229A", "circleddash": "\u229D", "CircleDot": "\u2299", "circledR": "\xAE", "circledS": "\u24C8", "CircleMinus": "\u2296", "CirclePlus": "\u2295", "CircleTimes": "\u2297", "cire": "\u2257", "cirE": "\u29C3", "cirfnint": "\u2A10", "cirmid": "\u2AEF", "cirscir": "\u29C2", "ClockwiseContourIntegral": "\u2232", "CloseCurlyDoubleQuote": "\u201D", "CloseCurlyQuote": "\u2019", "clubs": "\u2663", "clubsuit": "\u2663", "colon": ":", "Colon": "\u2237", "colone": "\u2254", "Colone": "\u2A74", "coloneq": "\u2254", "comma": ",", "commat": "@", "comp": "\u2201", "compfn": "\u2218", "complement": "\u2201", "complexes": "\u2102", "cong": "\u2245", "congdot": "\u2A6D", "Congruent": "\u2261", "conint": "\u222E", "Conint": "\u222F", "ContourIntegral": "\u222E", "copf": "\u{1D554}", "Copf": "\u2102", "coprod": "\u2210", "Coproduct": "\u2210", "copy": "\xA9", "COPY": "\xA9", "copysr": "\u2117", "CounterClockwiseContourIntegral": "\u2233", "crarr": "\u21B5", "cross": "\u2717", "Cross": "\u2A2F", "cscr": "\u{1D4B8}", "Cscr": "\u{1D49E}", "csub": "\u2ACF", "csube": "\u2AD1", "csup": "\u2AD0", "csupe": "\u2AD2", "ctdot": "\u22EF", "cudarrl": "\u2938", "cudarrr": "\u2935", "cuepr": "\u22DE", "cuesc": "\u22DF", "cularr": "\u21B6", "cularrp": "\u293D", "cup": "\u222A", "Cup": "\u22D3", "cupbrcap": "\u2A48", "cupcap": "\u2A46", "CupCap": "\u224D", "cupcup": "\u2A4A", "cupdot": "\u228D", "cupor": "\u2A45", "cups": "\u222A\uFE00", "curarr": "\u21B7", "curarrm": "\u293C", "curlyeqprec": "\u22DE", "curlyeqsucc": "\u22DF", "curlyvee": "\u22CE", "curlywedge": "\u22CF", "curren": "\xA4", "curvearrowleft": "\u21B6", "curvearrowright": "\u21B7", "cuvee": "\u22CE", "cuwed": "\u22CF", "cwconint": "\u2232", "cwint": "\u2231", "cylcty": "\u232D", "dagger": "\u2020", "Dagger": "\u2021", "daleth": "\u2138", "darr": "\u2193", "dArr": "\u21D3", "Darr": "\u21A1", "dash": "\u2010", "dashv": "\u22A3", "Dashv": "\u2AE4", "dbkarow": "\u290F", "dblac": "\u02DD", "dcaron": "\u010F", "Dcaron": "\u010E", "dcy": "\u0434", "Dcy": "\u0414", "dd": "\u2146", "DD": "\u2145", "ddagger": "\u2021", "ddarr": "\u21CA", "DDotrahd": "\u2911", "ddotseq": "\u2A77", "deg": "\xB0", "Del": "\u2207", "delta": "\u03B4", "Delta": "\u0394", "demptyv": "\u29B1", "dfisht": "\u297F", "dfr": "\u{1D521}", "Dfr": "\u{1D507}", "dHar": "\u2965", "dharl": "\u21C3", "dharr": "\u21C2", "DiacriticalAcute": "\xB4", "DiacriticalDot": "\u02D9", "DiacriticalDoubleAcute": "\u02DD", "DiacriticalGrave": "`", "DiacriticalTilde": "\u02DC", "diam": "\u22C4", "diamond": "\u22C4", "Diamond": "\u22C4", "diamondsuit": "\u2666", "diams": "\u2666", "die": "\xA8", "DifferentialD": "\u2146", "digamma": "\u03DD", "disin": "\u22F2", "div": "\xF7", "divide": "\xF7", "divideontimes": "\u22C7", "divonx": "\u22C7", "djcy": "\u0452", "DJcy": "\u0402", "dlcorn": "\u231E", "dlcrop": "\u230D", "dollar": "$", "dopf": "\u{1D555}", "Dopf": "\u{1D53B}", "dot": "\u02D9", "Dot": "\xA8", "DotDot": "\u20DC", "doteq": "\u2250", "doteqdot": "\u2251", "DotEqual": "\u2250", "dotminus": "\u2238", "dotplus": "\u2214", "dotsquare": "\u22A1", "doublebarwedge": "\u2306", "DoubleContourIntegral": "\u222F", "DoubleDot": "\xA8", "DoubleDownArrow": "\u21D3", "DoubleLeftArrow": "\u21D0", "DoubleLeftRightArrow": "\u21D4", "DoubleLeftTee": "\u2AE4", "DoubleLongLeftArrow": "\u27F8", "DoubleLongLeftRightArrow": "\u27FA", "DoubleLongRightArrow": "\u27F9", "DoubleRightArrow": "\u21D2", "DoubleRightTee": "\u22A8", "DoubleUpArrow": "\u21D1", "DoubleUpDownArrow": "\u21D5", "DoubleVerticalBar": "\u2225", "downarrow": "\u2193", "Downarrow": "\u21D3", "DownArrow": "\u2193", "DownArrowBar": "\u2913", "DownArrowUpArrow": "\u21F5", "DownBreve": "\u0311", "downdownarrows": "\u21CA", "downharpoonleft": "\u21C3", "downharpoonright": "\u21C2", "DownLeftRightVector": "\u2950", "DownLeftTeeVector": "\u295E", "DownLeftVector": "\u21BD", "DownLeftVectorBar": "\u2956", "DownRightTeeVector": "\u295F", "DownRightVector": "\u21C1", "DownRightVectorBar": "\u2957", "DownTee": "\u22A4", "DownTeeArrow": "\u21A7", "drbkarow": "\u2910", "drcorn": "\u231F", "drcrop": "\u230C", "dscr": "\u{1D4B9}", "Dscr": "\u{1D49F}", "dscy": "\u0455", "DScy": "\u0405", "dsol": "\u29F6", "dstrok": "\u0111", "Dstrok": "\u0110", "dtdot": "\u22F1", "dtri": "\u25BF", "dtrif": "\u25BE", "duarr": "\u21F5", "duhar": "\u296F", "dwangle": "\u29A6", "dzcy": "\u045F", "DZcy": "\u040F", "dzigrarr": "\u27FF", "eacute": "\xE9", "Eacute": "\xC9", "easter": "\u2A6E", "ecaron": "\u011B", "Ecaron": "\u011A", "ecir": "\u2256", "ecirc": "\xEA", "Ecirc": "\xCA", "ecolon": "\u2255", "ecy": "\u044D", "Ecy": "\u042D", "eDDot": "\u2A77", "edot": "\u0117", "eDot": "\u2251", "Edot": "\u0116", "ee": "\u2147", "efDot": "\u2252", "efr": "\u{1D522}", "Efr": "\u{1D508}", "eg": "\u2A9A", "egrave": "\xE8", "Egrave": "\xC8", "egs": "\u2A96", "egsdot": "\u2A98", "el": "\u2A99", "Element": "\u2208", "elinters": "\u23E7", "ell": "\u2113", "els": "\u2A95", "elsdot": "\u2A97", "emacr": "\u0113", "Emacr": "\u0112", "empty": "\u2205", "emptyset": "\u2205", "EmptySmallSquare": "\u25FB", "emptyv": "\u2205", "EmptyVerySmallSquare": "\u25AB", "emsp": "\u2003", "emsp13": "\u2004", "emsp14": "\u2005", "eng": "\u014B", "ENG": "\u014A", "ensp": "\u2002", "eogon": "\u0119", "Eogon": "\u0118", "eopf": "\u{1D556}", "Eopf": "\u{1D53C}", "epar": "\u22D5", "eparsl": "\u29E3", "eplus": "\u2A71", "epsi": "\u03B5", "epsilon": "\u03B5", "Epsilon": "\u0395", "epsiv": "\u03F5", "eqcirc": "\u2256", "eqcolon": "\u2255", "eqsim": "\u2242", "eqslantgtr": "\u2A96", "eqslantless": "\u2A95", "Equal": "\u2A75", "equals": "=", "EqualTilde": "\u2242", "equest": "\u225F", "Equilibrium": "\u21CC", "equiv": "\u2261", "equivDD": "\u2A78", "eqvparsl": "\u29E5", "erarr": "\u2971", "erDot": "\u2253", "escr": "\u212F", "Escr": "\u2130", "esdot": "\u2250", "esim": "\u2242", "Esim": "\u2A73", "eta": "\u03B7", "Eta": "\u0397", "eth": "\xF0", "ETH": "\xD0", "euml": "\xEB", "Euml": "\xCB", "euro": "\u20AC", "excl": "!", "exist": "\u2203", "Exists": "\u2203", "expectation": "\u2130", "exponentiale": "\u2147", "ExponentialE": "\u2147", "fallingdotseq": "\u2252", "fcy": "\u0444", "Fcy": "\u0424", "female": "\u2640", "ffilig": "\uFB03", "fflig": "\uFB00", "ffllig": "\uFB04", "ffr": "\u{1D523}", "Ffr": "\u{1D509}", "filig": "\uFB01", "FilledSmallSquare": "\u25FC", "FilledVerySmallSquare": "\u25AA", "fjlig": "fj", "flat": "\u266D", "fllig": "\uFB02", "fltns": "\u25B1", "fnof": "\u0192", "fopf": "\u{1D557}", "Fopf": "\u{1D53D}", "forall": "\u2200", "ForAll": "\u2200", "fork": "\u22D4", "forkv": "\u2AD9", "Fouriertrf": "\u2131", "fpartint": "\u2A0D", "frac12": "\xBD", "frac13": "\u2153", "frac14": "\xBC", "frac15": "\u2155", "frac16": "\u2159", "frac18": "\u215B", "frac23": "\u2154", "frac25": "\u2156", "frac34": "\xBE", "frac35": "\u2157", "frac38": "\u215C", "frac45": "\u2158", "frac56": "\u215A", "frac58": "\u215D", "frac78": "\u215E", "frasl": "\u2044", "frown": "\u2322", "fscr": "\u{1D4BB}", "Fscr": "\u2131", "gacute": "\u01F5", "gamma": "\u03B3", "Gamma": "\u0393", "gammad": "\u03DD", "Gammad": "\u03DC", "gap": "\u2A86", "gbreve": "\u011F", "Gbreve": "\u011E", "Gcedil": "\u0122", "gcirc": "\u011D", "Gcirc": "\u011C", "gcy": "\u0433", "Gcy": "\u0413", "gdot": "\u0121", "Gdot": "\u0120", "ge": "\u2265", "gE": "\u2267", "gel": "\u22DB", "gEl": "\u2A8C", "geq": "\u2265", "geqq": "\u2267", "geqslant": "\u2A7E", "ges": "\u2A7E", "gescc": "\u2AA9", "gesdot": "\u2A80", "gesdoto": "\u2A82", "gesdotol": "\u2A84", "gesl": "\u22DB\uFE00", "gesles": "\u2A94", "gfr": "\u{1D524}", "Gfr": "\u{1D50A}", "gg": "\u226B", "Gg": "\u22D9", "ggg": "\u22D9", "gimel": "\u2137", "gjcy": "\u0453", "GJcy": "\u0403", "gl": "\u2277", "gla": "\u2AA5", "glE": "\u2A92", "glj": "\u2AA4", "gnap": "\u2A8A", "gnapprox": "\u2A8A", "gne": "\u2A88", "gnE": "\u2269", "gneq": "\u2A88", "gneqq": "\u2269", "gnsim": "\u22E7", "gopf": "\u{1D558}", "Gopf": "\u{1D53E}", "grave": "`", "GreaterEqual": "\u2265", "GreaterEqualLess": "\u22DB", "GreaterFullEqual": "\u2267", "GreaterGreater": "\u2AA2", "GreaterLess": "\u2277", "GreaterSlantEqual": "\u2A7E", "GreaterTilde": "\u2273", "gscr": "\u210A", "Gscr": "\u{1D4A2}", "gsim": "\u2273", "gsime": "\u2A8E", "gsiml": "\u2A90", "gt": ">", "Gt": "\u226B", "GT": ">", "gtcc": "\u2AA7", "gtcir": "\u2A7A", "gtdot": "\u22D7", "gtlPar": "\u2995", "gtquest": "\u2A7C", "gtrapprox": "\u2A86", "gtrarr": "\u2978", "gtrdot": "\u22D7", "gtreqless": "\u22DB", "gtreqqless": "\u2A8C", "gtrless": "\u2277", "gtrsim": "\u2273", "gvertneqq": "\u2269\uFE00", "gvnE": "\u2269\uFE00", "Hacek": "\u02C7", "hairsp": "\u200A", "half": "\xBD", "hamilt": "\u210B", "hardcy": "\u044A", "HARDcy": "\u042A", "harr": "\u2194", "hArr": "\u21D4", "harrcir": "\u2948", "harrw": "\u21AD", "Hat": "^", "hbar": "\u210F", "hcirc": "\u0125", "Hcirc": "\u0124", "hearts": "\u2665", "heartsuit": "\u2665", "hellip": "\u2026", "hercon": "\u22B9", "hfr": "\u{1D525}", "Hfr": "\u210C", "HilbertSpace": "\u210B", "hksearow": "\u2925", "hkswarow": "\u2926", "hoarr": "\u21FF", "homtht": "\u223B", "hookleftarrow": "\u21A9", "hookrightarrow": "\u21AA", "hopf": "\u{1D559}", "Hopf": "\u210D", "horbar": "\u2015", "HorizontalLine": "\u2500", "hscr": "\u{1D4BD}", "Hscr": "\u210B", "hslash": "\u210F", "hstrok": "\u0127", "Hstrok": "\u0126", "HumpDownHump": "\u224E", "HumpEqual": "\u224F", "hybull": "\u2043", "hyphen": "\u2010", "iacute": "\xED", "Iacute": "\xCD", "ic": "\u2063", "icirc": "\xEE", "Icirc": "\xCE", "icy": "\u0438", "Icy": "\u0418", "Idot": "\u0130", "iecy": "\u0435", "IEcy": "\u0415", "iexcl": "\xA1", "iff": "\u21D4", "ifr": "\u{1D526}", "Ifr": "\u2111", "igrave": "\xEC", "Igrave": "\xCC", "ii": "\u2148", "iiiint": "\u2A0C", "iiint": "\u222D", "iinfin": "\u29DC", "iiota": "\u2129", "ijlig": "\u0133", "IJlig": "\u0132", "Im": "\u2111", "imacr": "\u012B", "Imacr": "\u012A", "image": "\u2111", "ImaginaryI": "\u2148", "imagline": "\u2110", "imagpart": "\u2111", "imath": "\u0131", "imof": "\u22B7", "imped": "\u01B5", "Implies": "\u21D2", "in": "\u2208", "incare": "\u2105", "infin": "\u221E", "infintie": "\u29DD", "inodot": "\u0131", "int": "\u222B", "Int": "\u222C", "intcal": "\u22BA", "integers": "\u2124", "Integral": "\u222B", "intercal": "\u22BA", "Intersection": "\u22C2", "intlarhk": "\u2A17", "intprod": "\u2A3C", "InvisibleComma": "\u2063", "InvisibleTimes": "\u2062", "iocy": "\u0451", "IOcy": "\u0401", "iogon": "\u012F", "Iogon": "\u012E", "iopf": "\u{1D55A}", "Iopf": "\u{1D540}", "iota": "\u03B9", "Iota": "\u0399", "iprod": "\u2A3C", "iquest": "\xBF", "iscr": "\u{1D4BE}", "Iscr": "\u2110", "isin": "\u2208", "isindot": "\u22F5", "isinE": "\u22F9", "isins": "\u22F4", "isinsv": "\u22F3", "isinv": "\u2208", "it": "\u2062", "itilde": "\u0129", "Itilde": "\u0128", "iukcy": "\u0456", "Iukcy": "\u0406", "iuml": "\xEF", "Iuml": "\xCF", "jcirc": "\u0135", "Jcirc": "\u0134", "jcy": "\u0439", "Jcy": "\u0419", "jfr": "\u{1D527}", "Jfr": "\u{1D50D}", "jmath": "\u0237", "jopf": "\u{1D55B}", "Jopf": "\u{1D541}", "jscr": "\u{1D4BF}", "Jscr": "\u{1D4A5}", "jsercy": "\u0458", "Jsercy": "\u0408", "jukcy": "\u0454", "Jukcy": "\u0404", "kappa": "\u03BA", "Kappa": "\u039A", "kappav": "\u03F0", "kcedil": "\u0137", "Kcedil": "\u0136", "kcy": "\u043A", "Kcy": "\u041A", "kfr": "\u{1D528}", "Kfr": "\u{1D50E}", "kgreen": "\u0138", "khcy": "\u0445", "KHcy": "\u0425", "kjcy": "\u045C", "KJcy": "\u040C", "kopf": "\u{1D55C}", "Kopf": "\u{1D542}", "kscr": "\u{1D4C0}", "Kscr": "\u{1D4A6}", "lAarr": "\u21DA", "lacute": "\u013A", "Lacute": "\u0139", "laemptyv": "\u29B4", "lagran": "\u2112", "lambda": "\u03BB", "Lambda": "\u039B", "lang": "\u27E8", "Lang": "\u27EA", "langd": "\u2991", "langle": "\u27E8", "lap": "\u2A85", "Laplacetrf": "\u2112", "laquo": "\xAB", "larr": "\u2190", "lArr": "\u21D0", "Larr": "\u219E", "larrb": "\u21E4", "larrbfs": "\u291F", "larrfs": "\u291D", "larrhk": "\u21A9", "larrlp": "\u21AB", "larrpl": "\u2939", "larrsim": "\u2973", "larrtl": "\u21A2", "lat": "\u2AAB", "latail": "\u2919", "lAtail": "\u291B", "late": "\u2AAD", "lates": "\u2AAD\uFE00", "lbarr": "\u290C", "lBarr": "\u290E", "lbbrk": "\u2772", "lbrace": "{", "lbrack": "[", "lbrke": "\u298B", "lbrksld": "\u298F", "lbrkslu": "\u298D", "lcaron": "\u013E", "Lcaron": "\u013D", "lcedil": "\u013C", "Lcedil": "\u013B", "lceil": "\u2308", "lcub": "{", "lcy": "\u043B", "Lcy": "\u041B", "ldca": "\u2936", "ldquo": "\u201C", "ldquor": "\u201E", "ldrdhar": "\u2967", "ldrushar": "\u294B", "ldsh": "\u21B2", "le": "\u2264", "lE": "\u2266", "LeftAngleBracket": "\u27E8", "leftarrow": "\u2190", "Leftarrow": "\u21D0", "LeftArrow": "\u2190", "LeftArrowBar": "\u21E4", "LeftArrowRightArrow": "\u21C6", "leftarrowtail": "\u21A2", "LeftCeiling": "\u2308", "LeftDoubleBracket": "\u27E6", "LeftDownTeeVector": "\u2961", "LeftDownVector": "\u21C3", "LeftDownVectorBar": "\u2959", "LeftFloor": "\u230A", "leftharpoondown": "\u21BD", "leftharpoonup": "\u21BC", "leftleftarrows": "\u21C7", "leftrightarrow": "\u2194", "Leftrightarrow": "\u21D4", "LeftRightArrow": "\u2194", "leftrightarrows": "\u21C6", "leftrightharpoons": "\u21CB", "leftrightsquigarrow": "\u21AD", "LeftRightVector": "\u294E", "LeftTee": "\u22A3", "LeftTeeArrow": "\u21A4", "LeftTeeVector": "\u295A", "leftthreetimes": "\u22CB", "LeftTriangle": "\u22B2", "LeftTriangleBar": "\u29CF", "LeftTriangleEqual": "\u22B4", "LeftUpDownVector": "\u2951", "LeftUpTeeVector": "\u2960", "LeftUpVector": "\u21BF", "LeftUpVectorBar": "\u2958", "LeftVector": "\u21BC", "LeftVectorBar": "\u2952", "leg": "\u22DA", "lEg": "\u2A8B", "leq": "\u2264", "leqq": "\u2266", "leqslant": "\u2A7D", "les": "\u2A7D", "lescc": "\u2AA8", "lesdot": "\u2A7F", "lesdoto": "\u2A81", "lesdotor": "\u2A83", "lesg": "\u22DA\uFE00", "lesges": "\u2A93", "lessapprox": "\u2A85", "lessdot": "\u22D6", "lesseqgtr": "\u22DA", "lesseqqgtr": "\u2A8B", "LessEqualGreater": "\u22DA", "LessFullEqual": "\u2266", "LessGreater": "\u2276", "lessgtr": "\u2276", "LessLess": "\u2AA1", "lesssim": "\u2272", "LessSlantEqual": "\u2A7D", "LessTilde": "\u2272", "lfisht": "\u297C", "lfloor": "\u230A", "lfr": "\u{1D529}", "Lfr": "\u{1D50F}", "lg": "\u2276", "lgE": "\u2A91", "lHar": "\u2962", "lhard": "\u21BD", "lharu": "\u21BC", "lharul": "\u296A", "lhblk": "\u2584", "ljcy": "\u0459", "LJcy": "\u0409", "ll": "\u226A", "Ll": "\u22D8", "llarr": "\u21C7", "llcorner": "\u231E", "Lleftarrow": "\u21DA", "llhard": "\u296B", "lltri": "\u25FA", "lmidot": "\u0140", "Lmidot": "\u013F", "lmoust": "\u23B0", "lmoustache": "\u23B0", "lnap": "\u2A89", "lnapprox": "\u2A89", "lne": "\u2A87", "lnE": "\u2268", "lneq": "\u2A87", "lneqq": "\u2268", "lnsim": "\u22E6", "loang": "\u27EC", "loarr": "\u21FD", "lobrk": "\u27E6", "longleftarrow": "\u27F5", "Longleftarrow": "\u27F8", "LongLeftArrow": "\u27F5", "longleftrightarrow": "\u27F7", "Longleftrightarrow": "\u27FA", "LongLeftRightArrow": "\u27F7", "longmapsto": "\u27FC", "longrightarrow": "\u27F6", "Longrightarrow": "\u27F9", "LongRightArrow": "\u27F6", "looparrowleft": "\u21AB", "looparrowright": "\u21AC", "lopar": "\u2985", "lopf": "\u{1D55D}", "Lopf": "\u{1D543}", "loplus": "\u2A2D", "lotimes": "\u2A34", "lowast": "\u2217", "lowbar": "_", "LowerLeftArrow": "\u2199", "LowerRightArrow": "\u2198", "loz": "\u25CA", "lozenge": "\u25CA", "lozf": "\u29EB", "lpar": "(", "lparlt": "\u2993", "lrarr": "\u21C6", "lrcorner": "\u231F", "lrhar": "\u21CB", "lrhard": "\u296D", "lrm": "\u200E", "lrtri": "\u22BF", "lsaquo": "\u2039", "lscr": "\u{1D4C1}", "Lscr": "\u2112", "lsh": "\u21B0", "Lsh": "\u21B0", "lsim": "\u2272", "lsime": "\u2A8D", "lsimg": "\u2A8F", "lsqb": "[", "lsquo": "\u2018", "lsquor": "\u201A", "lstrok": "\u0142", "Lstrok": "\u0141", "lt": "<", "Lt": "\u226A", "LT": "<", "ltcc": "\u2AA6", "ltcir": "\u2A79", "ltdot": "\u22D6", "lthree": "\u22CB", "ltimes": "\u22C9", "ltlarr": "\u2976", "ltquest": "\u2A7B", "ltri": "\u25C3", "ltrie": "\u22B4", "ltrif": "\u25C2", "ltrPar": "\u2996", "lurdshar": "\u294A", "luruhar": "\u2966", "lvertneqq": "\u2268\uFE00", "lvnE": "\u2268\uFE00", "macr": "\xAF", "male": "\u2642", "malt": "\u2720", "maltese": "\u2720", "map": "\u21A6", "Map": "\u2905", "mapsto": "\u21A6", "mapstodown": "\u21A7", "mapstoleft": "\u21A4", "mapstoup": "\u21A5", "marker": "\u25AE", "mcomma": "\u2A29", "mcy": "\u043C", "Mcy": "\u041C", "mdash": "\u2014", "mDDot": "\u223A", "measuredangle": "\u2221", "MediumSpace": "\u205F", "Mellintrf": "\u2133", "mfr": "\u{1D52A}", "Mfr": "\u{1D510}", "mho": "\u2127", "micro": "\xB5", "mid": "\u2223", "midast": "*", "midcir": "\u2AF0", "middot": "\xB7", "minus": "\u2212", "minusb": "\u229F", "minusd": "\u2238", "minusdu": "\u2A2A", "MinusPlus": "\u2213", "mlcp": "\u2ADB", "mldr": "\u2026", "mnplus": "\u2213", "models": "\u22A7", "mopf": "\u{1D55E}", "Mopf": "\u{1D544}", "mp": "\u2213", "mscr": "\u{1D4C2}", "Mscr": "\u2133", "mstpos": "\u223E", "mu": "\u03BC", "Mu": "\u039C", "multimap": "\u22B8", "mumap": "\u22B8", "nabla": "\u2207", "nacute": "\u0144", "Nacute": "\u0143", "nang": "\u2220\u20D2", "nap": "\u2249", "napE": "\u2A70\u0338", "napid": "\u224B\u0338", "napos": "\u0149", "napprox": "\u2249", "natur": "\u266E", "natural": "\u266E", "naturals": "\u2115", "nbsp": "\xA0", "nbump": "\u224E\u0338", "nbumpe": "\u224F\u0338", "ncap": "\u2A43", "ncaron": "\u0148", "Ncaron": "\u0147", "ncedil": "\u0146", "Ncedil": "\u0145", "ncong": "\u2247", "ncongdot": "\u2A6D\u0338", "ncup": "\u2A42", "ncy": "\u043D", "Ncy": "\u041D", "ndash": "\u2013", "ne": "\u2260", "nearhk": "\u2924", "nearr": "\u2197", "neArr": "\u21D7", "nearrow": "\u2197", "nedot": "\u2250\u0338", "NegativeMediumSpace": "\u200B", "NegativeThickSpace": "\u200B", "NegativeThinSpace": "\u200B", "NegativeVeryThinSpace": "\u200B", "nequiv": "\u2262", "nesear": "\u2928", "nesim": "\u2242\u0338", "NestedGreaterGreater": "\u226B", "NestedLessLess": "\u226A", "NewLine": "\n", "nexist": "\u2204", "nexists": "\u2204", "nfr": "\u{1D52B}", "Nfr": "\u{1D511}", "nge": "\u2271", "ngE": "\u2267\u0338", "ngeq": "\u2271", "ngeqq": "\u2267\u0338", "ngeqslant": "\u2A7E\u0338", "nges": "\u2A7E\u0338", "nGg": "\u22D9\u0338", "ngsim": "\u2275", "ngt": "\u226F", "nGt": "\u226B\u20D2", "ngtr": "\u226F", "nGtv": "\u226B\u0338", "nharr": "\u21AE", "nhArr": "\u21CE", "nhpar": "\u2AF2", "ni": "\u220B", "nis": "\u22FC", "nisd": "\u22FA", "niv": "\u220B", "njcy": "\u045A", "NJcy": "\u040A", "nlarr": "\u219A", "nlArr": "\u21CD", "nldr": "\u2025", "nle": "\u2270", "nlE": "\u2266\u0338", "nleftarrow": "\u219A", "nLeftarrow": "\u21CD", "nleftrightarrow": "\u21AE", "nLeftrightarrow": "\u21CE", "nleq": "\u2270", "nleqq": "\u2266\u0338", "nleqslant": "\u2A7D\u0338", "nles": "\u2A7D\u0338", "nless": "\u226E", "nLl": "\u22D8\u0338", "nlsim": "\u2274", "nlt": "\u226E", "nLt": "\u226A\u20D2", "nltri": "\u22EA", "nltrie": "\u22EC", "nLtv": "\u226A\u0338", "nmid": "\u2224", "NoBreak": "\u2060", "NonBreakingSpace": "\xA0", "nopf": "\u{1D55F}", "Nopf": "\u2115", "not": "\xAC", "Not": "\u2AEC", "NotCongruent": "\u2262", "NotCupCap": "\u226D", "NotDoubleVerticalBar": "\u2226", "NotElement": "\u2209", "NotEqual": "\u2260", "NotEqualTilde": "\u2242\u0338", "NotExists": "\u2204", "NotGreater": "\u226F", "NotGreaterEqual": "\u2271", "NotGreaterFullEqual": "\u2267\u0338", "NotGreaterGreater": "\u226B\u0338", "NotGreaterLess": "\u2279", "NotGreaterSlantEqual": "\u2A7E\u0338", "NotGreaterTilde": "\u2275", "NotHumpDownHump": "\u224E\u0338", "NotHumpEqual": "\u224F\u0338", "notin": "\u2209", "notindot": "\u22F5\u0338", "notinE": "\u22F9\u0338", "notinva": "\u2209", "notinvb": "\u22F7", "notinvc": "\u22F6", "NotLeftTriangle": "\u22EA", "NotLeftTriangleBar": "\u29CF\u0338", "NotLeftTriangleEqual": "\u22EC", "NotLess": "\u226E", "NotLessEqual": "\u2270", "NotLessGreater": "\u2278", "NotLessLess": "\u226A\u0338", "NotLessSlantEqual": "\u2A7D\u0338", "NotLessTilde": "\u2274", "NotNestedGreaterGreater": "\u2AA2\u0338", "NotNestedLessLess": "\u2AA1\u0338", "notni": "\u220C", "notniva": "\u220C", "notnivb": "\u22FE", "notnivc": "\u22FD", "NotPrecedes": "\u2280", "NotPrecedesEqual": "\u2AAF\u0338", "NotPrecedesSlantEqual": "\u22E0", "NotReverseElement": "\u220C", "NotRightTriangle": "\u22EB", "NotRightTriangleBar": "\u29D0\u0338", "NotRightTriangleEqual": "\u22ED", "NotSquareSubset": "\u228F\u0338", "NotSquareSubsetEqual": "\u22E2", "NotSquareSuperset": "\u2290\u0338", "NotSquareSupersetEqual": "\u22E3", "NotSubset": "\u2282\u20D2", "NotSubsetEqual": "\u2288", "NotSucceeds": "\u2281", "NotSucceedsEqual": "\u2AB0\u0338", "NotSucceedsSlantEqual": "\u22E1", "NotSucceedsTilde": "\u227F\u0338", "NotSuperset": "\u2283\u20D2", "NotSupersetEqual": "\u2289", "NotTilde": "\u2241", "NotTildeEqual": "\u2244", "NotTildeFullEqual": "\u2247", "NotTildeTilde": "\u2249", "NotVerticalBar": "\u2224", "npar": "\u2226", "nparallel": "\u2226", "nparsl": "\u2AFD\u20E5", "npart": "\u2202\u0338", "npolint": "\u2A14", "npr": "\u2280", "nprcue": "\u22E0", "npre": "\u2AAF\u0338", "nprec": "\u2280", "npreceq": "\u2AAF\u0338", "nrarr": "\u219B", "nrArr": "\u21CF", "nrarrc": "\u2933\u0338", "nrarrw": "\u219D\u0338", "nrightarrow": "\u219B", "nRightarrow": "\u21CF", "nrtri": "\u22EB", "nrtrie": "\u22ED", "nsc": "\u2281", "nsccue": "\u22E1", "nsce": "\u2AB0\u0338", "nscr": "\u{1D4C3}", "Nscr": "\u{1D4A9}", "nshortmid": "\u2224", "nshortparallel": "\u2226", "nsim": "\u2241", "nsime": "\u2244", "nsimeq": "\u2244", "nsmid": "\u2224", "nspar": "\u2226", "nsqsube": "\u22E2", "nsqsupe": "\u22E3", "nsub": "\u2284", "nsube": "\u2288", "nsubE": "\u2AC5\u0338", "nsubset": "\u2282\u20D2", "nsubseteq": "\u2288", "nsubseteqq": "\u2AC5\u0338", "nsucc": "\u2281", "nsucceq": "\u2AB0\u0338", "nsup": "\u2285", "nsupe": "\u2289", "nsupE": "\u2AC6\u0338", "nsupset": "\u2283\u20D2", "nsupseteq": "\u2289", "nsupseteqq": "\u2AC6\u0338", "ntgl": "\u2279", "ntilde": "\xF1", "Ntilde": "\xD1", "ntlg": "\u2278", "ntriangleleft": "\u22EA", "ntrianglelefteq": "\u22EC", "ntriangleright": "\u22EB", "ntrianglerighteq": "\u22ED", "nu": "\u03BD", "Nu": "\u039D", "num": "#", "numero": "\u2116", "numsp": "\u2007", "nvap": "\u224D\u20D2", "nvdash": "\u22AC", "nvDash": "\u22AD", "nVdash": "\u22AE", "nVDash": "\u22AF", "nvge": "\u2265\u20D2", "nvgt": ">\u20D2", "nvHarr": "\u2904", "nvinfin": "\u29DE", "nvlArr": "\u2902", "nvle": "\u2264\u20D2", "nvlt": "<\u20D2", "nvltrie": "\u22B4\u20D2", "nvrArr": "\u2903", "nvrtrie": "\u22B5\u20D2", "nvsim": "\u223C\u20D2", "nwarhk": "\u2923", "nwarr": "\u2196", "nwArr": "\u21D6", "nwarrow": "\u2196", "nwnear": "\u2927", "oacute": "\xF3", "Oacute": "\xD3", "oast": "\u229B", "ocir": "\u229A", "ocirc": "\xF4", "Ocirc": "\xD4", "ocy": "\u043E", "Ocy": "\u041E", "odash": "\u229D", "odblac": "\u0151", "Odblac": "\u0150", "odiv": "\u2A38", "odot": "\u2299", "odsold": "\u29BC", "oelig": "\u0153", "OElig": "\u0152", "ofcir": "\u29BF", "ofr": "\u{1D52C}", "Ofr": "\u{1D512}", "ogon": "\u02DB", "ograve": "\xF2", "Ograve": "\xD2", "ogt": "\u29C1", "ohbar": "\u29B5", "ohm": "\u03A9", "oint": "\u222E", "olarr": "\u21BA", "olcir": "\u29BE", "olcross": "\u29BB", "oline": "\u203E", "olt": "\u29C0", "omacr": "\u014D", "Omacr": "\u014C", "omega": "\u03C9", "Omega": "\u03A9", "omicron": "\u03BF", "Omicron": "\u039F", "omid": "\u29B6", "ominus": "\u2296", "oopf": "\u{1D560}", "Oopf": "\u{1D546}", "opar": "\u29B7", "OpenCurlyDoubleQuote": "\u201C", "OpenCurlyQuote": "\u2018", "operp": "\u29B9", "oplus": "\u2295", "or": "\u2228", "Or": "\u2A54", "orarr": "\u21BB", "ord": "\u2A5D", "order": "\u2134", "orderof": "\u2134", "ordf": "\xAA", "ordm": "\xBA", "origof": "\u22B6", "oror": "\u2A56", "orslope": "\u2A57", "orv": "\u2A5B", "oS": "\u24C8", "oscr": "\u2134", "Oscr": "\u{1D4AA}", "oslash": "\xF8", "Oslash": "\xD8", "osol": "\u2298", "otilde": "\xF5", "Otilde": "\xD5", "otimes": "\u2297", "Otimes": "\u2A37", "otimesas": "\u2A36", "ouml": "\xF6", "Ouml": "\xD6", "ovbar": "\u233D", "OverBar": "\u203E", "OverBrace": "\u23DE", "OverBracket": "\u23B4", "OverParenthesis": "\u23DC", "par": "\u2225", "para": "\xB6", "parallel": "\u2225", "parsim": "\u2AF3", "parsl": "\u2AFD", "part": "\u2202", "PartialD": "\u2202", "pcy": "\u043F", "Pcy": "\u041F", "percnt": "%", "period": ".", "permil": "\u2030", "perp": "\u22A5", "pertenk": "\u2031", "pfr": "\u{1D52D}", "Pfr": "\u{1D513}", "phi": "\u03C6", "Phi": "\u03A6", "phiv": "\u03D5", "phmmat": "\u2133", "phone": "\u260E", "pi": "\u03C0", "Pi": "\u03A0", "pitchfork": "\u22D4", "piv": "\u03D6", "planck": "\u210F", "planckh": "\u210E", "plankv": "\u210F", "plus": "+", "plusacir": "\u2A23", "plusb": "\u229E", "pluscir": "\u2A22", "plusdo": "\u2214", "plusdu": "\u2A25", "pluse": "\u2A72", "PlusMinus": "\xB1", "plusmn": "\xB1", "plussim": "\u2A26", "plustwo": "\u2A27", "pm": "\xB1", "Poincareplane": "\u210C", "pointint": "\u2A15", "popf": "\u{1D561}", "Popf": "\u2119", "pound": "\xA3", "pr": "\u227A", "Pr": "\u2ABB", "prap": "\u2AB7", "prcue": "\u227C", "pre": "\u2AAF", "prE": "\u2AB3", "prec": "\u227A", "precapprox": "\u2AB7", "preccurlyeq": "\u227C", "Precedes": "\u227A", "PrecedesEqual": "\u2AAF", "PrecedesSlantEqual": "\u227C", "PrecedesTilde": "\u227E", "preceq": "\u2AAF", "precnapprox": "\u2AB9", "precneqq": "\u2AB5", "precnsim": "\u22E8", "precsim": "\u227E", "prime": "\u2032", "Prime": "\u2033", "primes": "\u2119", "prnap": "\u2AB9", "prnE": "\u2AB5", "prnsim": "\u22E8", "prod": "\u220F", "Product": "\u220F", "profalar": "\u232E", "profline": "\u2312", "profsurf": "\u2313", "prop": "\u221D", "Proportion": "\u2237", "Proportional": "\u221D", "propto": "\u221D", "prsim": "\u227E", "prurel": "\u22B0", "pscr": "\u{1D4C5}", "Pscr": "\u{1D4AB}", "psi": "\u03C8", "Psi": "\u03A8", "puncsp": "\u2008", "qfr": "\u{1D52E}", "Qfr": "\u{1D514}", "qint": "\u2A0C", "qopf": "\u{1D562}", "Qopf": "\u211A", "qprime": "\u2057", "qscr": "\u{1D4C6}", "Qscr": "\u{1D4AC}", "quaternions": "\u210D", "quatint": "\u2A16", "quest": "?", "questeq": "\u225F", "quot": '"', "QUOT": '"', "rAarr": "\u21DB", "race": "\u223D\u0331", "racute": "\u0155", "Racute": "\u0154", "radic": "\u221A", "raemptyv": "\u29B3", "rang": "\u27E9", "Rang": "\u27EB", "rangd": "\u2992", "range": "\u29A5", "rangle": "\u27E9", "raquo": "\xBB", "rarr": "\u2192", "rArr": "\u21D2", "Rarr": "\u21A0", "rarrap": "\u2975", "rarrb": "\u21E5", "rarrbfs": "\u2920", "rarrc": "\u2933", "rarrfs": "\u291E", "rarrhk": "\u21AA", "rarrlp": "\u21AC", "rarrpl": "\u2945", "rarrsim": "\u2974", "rarrtl": "\u21A3", "Rarrtl": "\u2916", "rarrw": "\u219D", "ratail": "\u291A", "rAtail": "\u291C", "ratio": "\u2236", "rationals": "\u211A", "rbarr": "\u290D", "rBarr": "\u290F", "RBarr": "\u2910", "rbbrk": "\u2773", "rbrace": "}", "rbrack": "]", "rbrke": "\u298C", "rbrksld": "\u298E", "rbrkslu": "\u2990", "rcaron": "\u0159", "Rcaron": "\u0158", "rcedil": "\u0157", "Rcedil": "\u0156", "rceil": "\u2309", "rcub": "}", "rcy": "\u0440", "Rcy": "\u0420", "rdca": "\u2937", "rdldhar": "\u2969", "rdquo": "\u201D", "rdquor": "\u201D", "rdsh": "\u21B3", "Re": "\u211C", "real": "\u211C", "realine": "\u211B", "realpart": "\u211C", "reals": "\u211D", "rect": "\u25AD", "reg": "\xAE", "REG": "\xAE", "ReverseElement": "\u220B", "ReverseEquilibrium": "\u21CB", "ReverseUpEquilibrium": "\u296F", "rfisht": "\u297D", "rfloor": "\u230B", "rfr": "\u{1D52F}", "Rfr": "\u211C", "rHar": "\u2964", "rhard": "\u21C1", "rharu": "\u21C0", "rharul": "\u296C", "rho": "\u03C1", "Rho": "\u03A1", "rhov": "\u03F1", "RightAngleBracket": "\u27E9", "rightarrow": "\u2192", "Rightarrow": "\u21D2", "RightArrow": "\u2192", "RightArrowBar": "\u21E5", "RightArrowLeftArrow": "\u21C4", "rightarrowtail": "\u21A3", "RightCeiling": "\u2309", "RightDoubleBracket": "\u27E7", "RightDownTeeVector": "\u295D", "RightDownVector": "\u21C2", "RightDownVectorBar": "\u2955", "RightFloor": "\u230B", "rightharpoondown": "\u21C1", "rightharpoonup": "\u21C0", "rightleftarrows": "\u21C4", "rightleftharpoons": "\u21CC", "rightrightarrows": "\u21C9", "rightsquigarrow": "\u219D", "RightTee": "\u22A2", "RightTeeArrow": "\u21A6", "RightTeeVector": "\u295B", "rightthreetimes": "\u22CC", "RightTriangle": "\u22B3", "RightTriangleBar": "\u29D0", "RightTriangleEqual": "\u22B5", "RightUpDownVector": "\u294F", "RightUpTeeVector": "\u295C", "RightUpVector": "\u21BE", "RightUpVectorBar": "\u2954", "RightVector": "\u21C0", "RightVectorBar": "\u2953", "ring": "\u02DA", "risingdotseq": "\u2253", "rlarr": "\u21C4", "rlhar": "\u21CC", "rlm": "\u200F", "rmoust": "\u23B1", "rmoustache": "\u23B1", "rnmid": "\u2AEE", "roang": "\u27ED", "roarr": "\u21FE", "robrk": "\u27E7", "ropar": "\u2986", "ropf": "\u{1D563}", "Ropf": "\u211D", "roplus": "\u2A2E", "rotimes": "\u2A35", "RoundImplies": "\u2970", "rpar": ")", "rpargt": "\u2994", "rppolint": "\u2A12", "rrarr": "\u21C9", "Rrightarrow": "\u21DB", "rsaquo": "\u203A", "rscr": "\u{1D4C7}", "Rscr": "\u211B", "rsh": "\u21B1", "Rsh": "\u21B1", "rsqb": "]", "rsquo": "\u2019", "rsquor": "\u2019", "rthree": "\u22CC", "rtimes": "\u22CA", "rtri": "\u25B9", "rtrie": "\u22B5", "rtrif": "\u25B8", "rtriltri": "\u29CE", "RuleDelayed": "\u29F4", "ruluhar": "\u2968", "rx": "\u211E", "sacute": "\u015B", "Sacute": "\u015A", "sbquo": "\u201A", "sc": "\u227B", "Sc": "\u2ABC", "scap": "\u2AB8", "scaron": "\u0161", "Scaron": "\u0160", "sccue": "\u227D", "sce": "\u2AB0", "scE": "\u2AB4", "scedil": "\u015F", "Scedil": "\u015E", "scirc": "\u015D", "Scirc": "\u015C", "scnap": "\u2ABA", "scnE": "\u2AB6", "scnsim": "\u22E9", "scpolint": "\u2A13", "scsim": "\u227F", "scy": "\u0441", "Scy": "\u0421", "sdot": "\u22C5", "sdotb": "\u22A1", "sdote": "\u2A66", "searhk": "\u2925", "searr": "\u2198", "seArr": "\u21D8", "searrow": "\u2198", "sect": "\xA7", "semi": ";", "seswar": "\u2929", "setminus": "\u2216", "setmn": "\u2216", "sext": "\u2736", "sfr": "\u{1D530}", "Sfr": "\u{1D516}", "sfrown": "\u2322", "sharp": "\u266F", "shchcy": "\u0449", "SHCHcy": "\u0429", "shcy": "\u0448", "SHcy": "\u0428", "ShortDownArrow": "\u2193", "ShortLeftArrow": "\u2190", "shortmid": "\u2223", "shortparallel": "\u2225", "ShortRightArrow": "\u2192", "ShortUpArrow": "\u2191", "shy": "\xAD", "sigma": "\u03C3", "Sigma": "\u03A3", "sigmaf": "\u03C2", "sigmav": "\u03C2", "sim": "\u223C", "simdot": "\u2A6A", "sime": "\u2243", "simeq": "\u2243", "simg": "\u2A9E", "simgE": "\u2AA0", "siml": "\u2A9D", "simlE": "\u2A9F", "simne": "\u2246", "simplus": "\u2A24", "simrarr": "\u2972", "slarr": "\u2190", "SmallCircle": "\u2218", "smallsetminus": "\u2216", "smashp": "\u2A33", "smeparsl": "\u29E4", "smid": "\u2223", "smile": "\u2323", "smt": "\u2AAA", "smte": "\u2AAC", "smtes": "\u2AAC\uFE00", "softcy": "\u044C", "SOFTcy": "\u042C", "sol": "/", "solb": "\u29C4", "solbar": "\u233F", "sopf": "\u{1D564}", "Sopf": "\u{1D54A}", "spades": "\u2660", "spadesuit": "\u2660", "spar": "\u2225", "sqcap": "\u2293", "sqcaps": "\u2293\uFE00", "sqcup": "\u2294", "sqcups": "\u2294\uFE00", "Sqrt": "\u221A", "sqsub": "\u228F", "sqsube": "\u2291", "sqsubset": "\u228F", "sqsubseteq": "\u2291", "sqsup": "\u2290", "sqsupe": "\u2292", "sqsupset": "\u2290", "sqsupseteq": "\u2292", "squ": "\u25A1", "square": "\u25A1", "Square": "\u25A1", "SquareIntersection": "\u2293", "SquareSubset": "\u228F", "SquareSubsetEqual": "\u2291", "SquareSuperset": "\u2290", "SquareSupersetEqual": "\u2292", "SquareUnion": "\u2294", "squarf": "\u25AA", "squf": "\u25AA", "srarr": "\u2192", "sscr": "\u{1D4C8}", "Sscr": "\u{1D4AE}", "ssetmn": "\u2216", "ssmile": "\u2323", "sstarf": "\u22C6", "star": "\u2606", "Star": "\u22C6", "starf": "\u2605", "straightepsilon": "\u03F5", "straightphi": "\u03D5", "strns": "\xAF", "sub": "\u2282", "Sub": "\u22D0", "subdot": "\u2ABD", "sube": "\u2286", "subE": "\u2AC5", "subedot": "\u2AC3", "submult": "\u2AC1", "subne": "\u228A", "subnE": "\u2ACB", "subplus": "\u2ABF", "subrarr": "\u2979", "subset": "\u2282", "Subset": "\u22D0", "subseteq": "\u2286", "subseteqq": "\u2AC5", "SubsetEqual": "\u2286", "subsetneq": "\u228A", "subsetneqq": "\u2ACB", "subsim": "\u2AC7", "subsub": "\u2AD5", "subsup": "\u2AD3", "succ": "\u227B", "succapprox": "\u2AB8", "succcurlyeq": "\u227D", "Succeeds": "\u227B", "SucceedsEqual": "\u2AB0", "SucceedsSlantEqual": "\u227D", "SucceedsTilde": "\u227F", "succeq": "\u2AB0", "succnapprox": "\u2ABA", "succneqq": "\u2AB6", "succnsim": "\u22E9", "succsim": "\u227F", "SuchThat": "\u220B", "sum": "\u2211", "Sum": "\u2211", "sung": "\u266A", "sup": "\u2283", "Sup": "\u22D1", "sup1": "\xB9", "sup2": "\xB2", "sup3": "\xB3", "supdot": "\u2ABE", "supdsub": "\u2AD8", "supe": "\u2287", "supE": "\u2AC6", "supedot": "\u2AC4", "Superset": "\u2283", "SupersetEqual": "\u2287", "suphsol": "\u27C9", "suphsub": "\u2AD7", "suplarr": "\u297B", "supmult": "\u2AC2", "supne": "\u228B", "supnE": "\u2ACC", "supplus": "\u2AC0", "supset": "\u2283", "Supset": "\u22D1", "supseteq": "\u2287", "supseteqq": "\u2AC6", "supsetneq": "\u228B", "supsetneqq": "\u2ACC", "supsim": "\u2AC8", "supsub": "\u2AD4", "supsup": "\u2AD6", "swarhk": "\u2926", "swarr": "\u2199", "swArr": "\u21D9", "swarrow": "\u2199", "swnwar": "\u292A", "szlig": "\xDF", "Tab": " ", "target": "\u2316", "tau": "\u03C4", "Tau": "\u03A4", "tbrk": "\u23B4", "tcaron": "\u0165", "Tcaron": "\u0164", "tcedil": "\u0163", "Tcedil": "\u0162", "tcy": "\u0442", "Tcy": "\u0422", "tdot": "\u20DB", "telrec": "\u2315", "tfr": "\u{1D531}", "Tfr": "\u{1D517}", "there4": "\u2234", "therefore": "\u2234", "Therefore": "\u2234", "theta": "\u03B8", "Theta": "\u0398", "thetasym": "\u03D1", "thetav": "\u03D1", "thickapprox": "\u2248", "thicksim": "\u223C", "ThickSpace": "\u205F\u200A", "thinsp": "\u2009", "ThinSpace": "\u2009", "thkap": "\u2248", "thksim": "\u223C", "thorn": "\xFE", "THORN": "\xDE", "tilde": "\u02DC", "Tilde": "\u223C", "TildeEqual": "\u2243", "TildeFullEqual": "\u2245", "TildeTilde": "\u2248", "times": "\xD7", "timesb": "\u22A0", "timesbar": "\u2A31", "timesd": "\u2A30", "tint": "\u222D", "toea": "\u2928", "top": "\u22A4", "topbot": "\u2336", "topcir": "\u2AF1", "topf": "\u{1D565}", "Topf": "\u{1D54B}", "topfork": "\u2ADA", "tosa": "\u2929", "tprime": "\u2034", "trade": "\u2122", "TRADE": "\u2122", "triangle": "\u25B5", "triangledown": "\u25BF", "triangleleft": "\u25C3", "trianglelefteq": "\u22B4", "triangleq": "\u225C", "triangleright": "\u25B9", "trianglerighteq": "\u22B5", "tridot": "\u25EC", "trie": "\u225C", "triminus": "\u2A3A", "TripleDot": "\u20DB", "triplus": "\u2A39", "trisb": "\u29CD", "tritime": "\u2A3B", "trpezium": "\u23E2", "tscr": "\u{1D4C9}", "Tscr": "\u{1D4AF}", "tscy": "\u0446", "TScy": "\u0426", "tshcy": "\u045B", "TSHcy": "\u040B", "tstrok": "\u0167", "Tstrok": "\u0166", "twixt": "\u226C", "twoheadleftarrow": "\u219E", "twoheadrightarrow": "\u21A0", "uacute": "\xFA", "Uacute": "\xDA", "uarr": "\u2191", "uArr": "\u21D1", "Uarr": "\u219F", "Uarrocir": "\u2949", "ubrcy": "\u045E", "Ubrcy": "\u040E", "ubreve": "\u016D", "Ubreve": "\u016C", "ucirc": "\xFB", "Ucirc": "\xDB", "ucy": "\u0443", "Ucy": "\u0423", "udarr": "\u21C5", "udblac": "\u0171", "Udblac": "\u0170", "udhar": "\u296E", "ufisht": "\u297E", "ufr": "\u{1D532}", "Ufr": "\u{1D518}", "ugrave": "\xF9", "Ugrave": "\xD9", "uHar": "\u2963", "uharl": "\u21BF", "uharr": "\u21BE", "uhblk": "\u2580", "ulcorn": "\u231C", "ulcorner": "\u231C", "ulcrop": "\u230F", "ultri": "\u25F8", "umacr": "\u016B", "Umacr": "\u016A", "uml": "\xA8", "UnderBar": "_", "UnderBrace": "\u23DF", "UnderBracket": "\u23B5", "UnderParenthesis": "\u23DD", "Union": "\u22C3", "UnionPlus": "\u228E", "uogon": "\u0173", "Uogon": "\u0172", "uopf": "\u{1D566}", "Uopf": "\u{1D54C}", "uparrow": "\u2191", "Uparrow": "\u21D1", "UpArrow": "\u2191", "UpArrowBar": "\u2912", "UpArrowDownArrow": "\u21C5", "updownarrow": "\u2195", "Updownarrow": "\u21D5", "UpDownArrow": "\u2195", "UpEquilibrium": "\u296E", "upharpoonleft": "\u21BF", "upharpoonright": "\u21BE", "uplus": "\u228E", "UpperLeftArrow": "\u2196", "UpperRightArrow": "\u2197", "upsi": "\u03C5", "Upsi": "\u03D2", "upsih": "\u03D2", "upsilon": "\u03C5", "Upsilon": "\u03A5", "UpTee": "\u22A5", "UpTeeArrow": "\u21A5", "upuparrows": "\u21C8", "urcorn": "\u231D", "urcorner": "\u231D", "urcrop": "\u230E", "uring": "\u016F", "Uring": "\u016E", "urtri": "\u25F9", "uscr": "\u{1D4CA}", "Uscr": "\u{1D4B0}", "utdot": "\u22F0", "utilde": "\u0169", "Utilde": "\u0168", "utri": "\u25B5", "utrif": "\u25B4", "uuarr": "\u21C8", "uuml": "\xFC", "Uuml": "\xDC", "uwangle": "\u29A7", "vangrt": "\u299C", "varepsilon": "\u03F5", "varkappa": "\u03F0", "varnothing": "\u2205", "varphi": "\u03D5", "varpi": "\u03D6", "varpropto": "\u221D", "varr": "\u2195", "vArr": "\u21D5", "varrho": "\u03F1", "varsigma": "\u03C2", "varsubsetneq": "\u228A\uFE00", "varsubsetneqq": "\u2ACB\uFE00", "varsupsetneq": "\u228B\uFE00", "varsupsetneqq": "\u2ACC\uFE00", "vartheta": "\u03D1", "vartriangleleft": "\u22B2", "vartriangleright": "\u22B3", "vBar": "\u2AE8", "Vbar": "\u2AEB", "vBarv": "\u2AE9", "vcy": "\u0432", "Vcy": "\u0412", "vdash": "\u22A2", "vDash": "\u22A8", "Vdash": "\u22A9", "VDash": "\u22AB", "Vdashl": "\u2AE6", "vee": "\u2228", "Vee": "\u22C1", "veebar": "\u22BB", "veeeq": "\u225A", "vellip": "\u22EE", "verbar": "|", "Verbar": "\u2016", "vert": "|", "Vert": "\u2016", "VerticalBar": "\u2223", "VerticalLine": "|", "VerticalSeparator": "\u2758", "VerticalTilde": "\u2240", "VeryThinSpace": "\u200A", "vfr": "\u{1D533}", "Vfr": "\u{1D519}", "vltri": "\u22B2", "vnsub": "\u2282\u20D2", "vnsup": "\u2283\u20D2", "vopf": "\u{1D567}", "Vopf": "\u{1D54D}", "vprop": "\u221D", "vrtri": "\u22B3", "vscr": "\u{1D4CB}", "Vscr": "\u{1D4B1}", "vsubne": "\u228A\uFE00", "vsubnE": "\u2ACB\uFE00", "vsupne": "\u228B\uFE00", "vsupnE": "\u2ACC\uFE00", "Vvdash": "\u22AA", "vzigzag": "\u299A", "wcirc": "\u0175", "Wcirc": "\u0174", "wedbar": "\u2A5F", "wedge": "\u2227", "Wedge": "\u22C0", "wedgeq": "\u2259", "weierp": "\u2118", "wfr": "\u{1D534}", "Wfr": "\u{1D51A}", "wopf": "\u{1D568}", "Wopf": "\u{1D54E}", "wp": "\u2118", "wr": "\u2240", "wreath": "\u2240", "wscr": "\u{1D4CC}", "Wscr": "\u{1D4B2}", "xcap": "\u22C2", "xcirc": "\u25EF", "xcup": "\u22C3", "xdtri": "\u25BD", "xfr": "\u{1D535}", "Xfr": "\u{1D51B}", "xharr": "\u27F7", "xhArr": "\u27FA", "xi": "\u03BE", "Xi": "\u039E", "xlarr": "\u27F5", "xlArr": "\u27F8", "xmap": "\u27FC", "xnis": "\u22FB", "xodot": "\u2A00", "xopf": "\u{1D569}", "Xopf": "\u{1D54F}", "xoplus": "\u2A01", "xotime": "\u2A02", "xrarr": "\u27F6", "xrArr": "\u27F9", "xscr": "\u{1D4CD}", "Xscr": "\u{1D4B3}", "xsqcup": "\u2A06", "xuplus": "\u2A04", "xutri": "\u25B3", "xvee": "\u22C1", "xwedge": "\u22C0", "yacute": "\xFD", "Yacute": "\xDD", "yacy": "\u044F", "YAcy": "\u042F", "ycirc": "\u0177", "Ycirc": "\u0176", "ycy": "\u044B", "Ycy": "\u042B", "yen": "\xA5", "yfr": "\u{1D536}", "Yfr": "\u{1D51C}", "yicy": "\u0457", "YIcy": "\u0407", "yopf": "\u{1D56A}", "Yopf": "\u{1D550}", "yscr": "\u{1D4CE}", "Yscr": "\u{1D4B4}", "yucy": "\u044E", "YUcy": "\u042E", "yuml": "\xFF", "Yuml": "\u0178", "zacute": "\u017A", "Zacute": "\u0179", "zcaron": "\u017E", "Zcaron": "\u017D", "zcy": "\u0437", "Zcy": "\u0417", "zdot": "\u017C", "Zdot": "\u017B", "zeetrf": "\u2128", "ZeroWidthSpace": "\u200B", "zeta": "\u03B6", "Zeta": "\u0396", "zfr": "\u{1D537}", "Zfr": "\u2128", "zhcy": "\u0436", "ZHcy": "\u0416", "zigrarr": "\u21DD", "zopf": "\u{1D56B}", "Zopf": "\u2124", "zscr": "\u{1D4CF}", "Zscr": "\u{1D4B5}", "zwj": "\u200D", "zwnj": "\u200C" };
var decodeMapLegacy = { "aacute": "\xE1", "Aacute": "\xC1", "acirc": "\xE2", "Acirc": "\xC2", "acute": "\xB4", "aelig": "\xE6", "AElig": "\xC6", "agrave": "\xE0", "Agrave": "\xC0", "amp": "&", "AMP": "&", "aring": "\xE5", "Aring": "\xC5", "atilde": "\xE3", "Atilde": "\xC3", "auml": "\xE4", "Auml": "\xC4", "brvbar": "\xA6", "ccedil": "\xE7", "Ccedil": "\xC7", "cedil": "\xB8", "cent": "\xA2", "copy": "\xA9", "COPY": "\xA9", "curren": "\xA4", "deg": "\xB0", "divide": "\xF7", "eacute": "\xE9", "Eacute": "\xC9", "ecirc": "\xEA", "Ecirc": "\xCA", "egrave": "\xE8", "Egrave": "\xC8", "eth": "\xF0", "ETH": "\xD0", "euml": "\xEB", "Euml": "\xCB", "frac12": "\xBD", "frac14": "\xBC", "frac34": "\xBE", "gt": ">", "GT": ">", "iacute": "\xED", "Iacute": "\xCD", "icirc": "\xEE", "Icirc": "\xCE", "iexcl": "\xA1", "igrave": "\xEC", "Igrave": "\xCC", "iquest": "\xBF", "iuml": "\xEF", "Iuml": "\xCF", "laquo": "\xAB", "lt": "<", "LT": "<", "macr": "\xAF", "micro": "\xB5", "middot": "\xB7", "nbsp": "\xA0", "not": "\xAC", "ntilde": "\xF1", "Ntilde": "\xD1", "oacute": "\xF3", "Oacute": "\xD3", "ocirc": "\xF4", "Ocirc": "\xD4", "ograve": "\xF2", "Ograve": "\xD2", "ordf": "\xAA", "ordm": "\xBA", "oslash": "\xF8", "Oslash": "\xD8", "otilde": "\xF5", "Otilde": "\xD5", "ouml": "\xF6", "Ouml": "\xD6", "para": "\xB6", "plusmn": "\xB1", "pound": "\xA3", "quot": '"', "QUOT": '"', "raquo": "\xBB", "reg": "\xAE", "REG": "\xAE", "sect": "\xA7", "shy": "\xAD", "sup1": "\xB9", "sup2": "\xB2", "sup3": "\xB3", "szlig": "\xDF", "thorn": "\xFE", "THORN": "\xDE", "times": "\xD7", "uacute": "\xFA", "Uacute": "\xDA", "ucirc": "\xFB", "Ucirc": "\xDB", "ugrave": "\xF9", "Ugrave": "\xD9", "uml": "\xA8", "uuml": "\xFC", "Uuml": "\xDC", "yacute": "\xFD", "Yacute": "\xDD", "yen": "\xA5", "yuml": "\xFF" };
var decodeMapNumeric = { "0": "\uFFFD", "128": "\u20AC", "130": "\u201A", "131": "\u0192", "132": "\u201E", "133": "\u2026", "134": "\u2020", "135": "\u2021", "136": "\u02C6", "137": "\u2030", "138": "\u0160", "139": "\u2039", "140": "\u0152", "142": "\u017D", "145": "\u2018", "146": "\u2019", "147": "\u201C", "148": "\u201D", "149": "\u2022", "150": "\u2013", "151": "\u2014", "152": "\u02DC", "153": "\u2122", "154": "\u0161", "155": "\u203A", "156": "\u0153", "158": "\u017E", "159": "\u0178" };
var invalidReferenceCodePoints = [1, 2, 3, 4, 5, 6, 7, 8, 11, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153, 154, 155, 156, 157, 158, 159, 64976, 64977, 64978, 64979, 64980, 64981, 64982, 64983, 64984, 64985, 64986, 64987, 64988, 64989, 64990, 64991, 64992, 64993, 64994, 64995, 64996, 64997, 64998, 64999, 65e3, 65001, 65002, 65003, 65004, 65005, 65006, 65007, 65534, 65535, 131070, 131071, 196606, 196607, 262142, 262143, 327678, 327679, 393214, 393215, 458750, 458751, 524286, 524287, 589822, 589823, 655358, 655359, 720894, 720895, 786430, 786431, 851966, 851967, 917502, 917503, 983038, 983039, 1048574, 1048575, 1114110, 1114111];
var stringFromCharCode = String.fromCharCode;
var object = {};
var hasOwnProperty = object.hasOwnProperty;
var has = function(object2, propertyName) {
return hasOwnProperty.call(object2, propertyName);
};
var contains = function(array, value) {
var index = -1;
var length = array.length;
while (++index < length) {
if (array[index] == value) {
return true;
}
}
return false;
};
var merge = function(options, defaults) {
if (!options) {
return defaults;
}
var result = {};
var key2;
for (key2 in defaults) {
result[key2] = has(options, key2) ? options[key2] : defaults[key2];
}
return result;
};
var codePointToSymbol = function(codePoint, strict) {
var output = "";
if (codePoint >= 55296 && codePoint <= 57343 || codePoint > 1114111) {
if (strict) {
parseError("character reference outside the permissible Unicode range");
}
return "\uFFFD";
}
if (has(decodeMapNumeric, codePoint)) {
if (strict) {
parseError("disallowed character reference");
}
return decodeMapNumeric[codePoint];
}
if (strict && contains(invalidReferenceCodePoints, codePoint)) {
parseError("disallowed character reference");
}
if (codePoint > 65535) {
codePoint -= 65536;
output += stringFromCharCode(codePoint >>> 10 & 1023 | 55296);
codePoint = 56320 | codePoint & 1023;
}
output += stringFromCharCode(codePoint);
return output;
};
var hexEscape = function(codePoint) {
return "" + codePoint.toString(16).toUpperCase() + ";";
};
var decEscape = function(codePoint) {
return "" + codePoint + ";";
};
var parseError = function(message) {
throw Error("Parse error: " + message);
};
var encode2 = function(string, options) {
options = merge(options, encode2.options);
var strict = options.strict;
if (strict && regexInvalidRawCodePoint.test(string)) {
parseError("forbidden code point");
}
var encodeEverything = options.encodeEverything;
var useNamedReferences = options.useNamedReferences;
var allowUnsafeSymbols = options.allowUnsafeSymbols;
var escapeCodePoint = options.decimal ? decEscape : hexEscape;
var escapeBmpSymbol = function(symbol) {
return escapeCodePoint(symbol.charCodeAt(0));
};
if (encodeEverything) {
string = string.replace(regexAsciiWhitelist, function(symbol) {
if (useNamedReferences && has(encodeMap, symbol)) {
return "&" + encodeMap[symbol] + ";";
}
return escapeBmpSymbol(symbol);
});
if (useNamedReferences) {
string = string.replace(/>\u20D2/g, ">⃒").replace(/<\u20D2/g, "<⃒").replace(/fj/g, "fj");
}
if (useNamedReferences) {
string = string.replace(regexEncodeNonAscii, function(string2) {
return "&" + encodeMap[string2] + ";";
});
}
} else if (useNamedReferences) {
if (!allowUnsafeSymbols) {
string = string.replace(regexEscape, function(string2) {
return "&" + encodeMap[string2] + ";";
});
}
string = string.replace(/>\u20D2/g, ">⃒").replace(/<\u20D2/g, "<⃒");
string = string.replace(regexEncodeNonAscii, function(string2) {
return "&" + encodeMap[string2] + ";";
});
} else if (!allowUnsafeSymbols) {
string = string.replace(regexEscape, escapeBmpSymbol);
}
return string.replace(regexAstralSymbols, function($0) {
var high = $0.charCodeAt(0);
var low = $0.charCodeAt(1);
var codePoint = (high - 55296) * 1024 + low - 56320 + 65536;
return escapeCodePoint(codePoint);
}).replace(regexBmpWhitelist, escapeBmpSymbol);
};
encode2.options = {
"allowUnsafeSymbols": false,
"encodeEverything": false,
"strict": false,
"useNamedReferences": false,
"decimal": false
};
var decode = function(html, options) {
options = merge(options, decode.options);
var strict = options.strict;
if (strict && regexInvalidEntity.test(html)) {
parseError("malformed character reference");
}
return html.replace(regexDecode, function($0, $1, $2, $3, $4, $5, $6, $7, $8) {
var codePoint;
var semicolon;
var decDigits;
var hexDigits;
var reference;
var next;
if ($1) {
reference = $1;
return decodeMap[reference];
}
if ($2) {
reference = $2;
next = $3;
if (next && options.isAttributeValue) {
if (strict && next == "=") {
parseError("`&` did not start a character reference");
}
return $0;
} else {
if (strict) {
parseError(
"named character reference was not terminated by a semicolon"
);
}
return decodeMapLegacy[reference] + (next || "");
}
}
if ($4) {
decDigits = $4;
semicolon = $5;
if (strict && !semicolon) {
parseError("character reference was not terminated by a semicolon");
}
codePoint = parseInt(decDigits, 10);
return codePointToSymbol(codePoint, strict);
}
if ($6) {
hexDigits = $6;
semicolon = $7;
if (strict && !semicolon) {
parseError("character reference was not terminated by a semicolon");
}
codePoint = parseInt(hexDigits, 16);
return codePointToSymbol(codePoint, strict);
}
if (strict) {
parseError(
"named character reference was not terminated by a semicolon"
);
}
return $0;
});
};
decode.options = {
"isAttributeValue": false,
"strict": false
};
var escape = function(string) {
return string.replace(regexEscape, function($0) {
return escapeMap[$0];
});
};
var he = {
"version": "1.2.0",
"encode": encode2,
"decode": decode,
"escape": escape,
"unescape": decode
};
if (typeof define == "function" && typeof define.amd == "object" && define.amd) {
define(function() {
return he;
});
} else if (freeExports && !freeExports.nodeType) {
if (freeModule) {
freeModule.exports = he;
} else {
for (var key in he) {
has(he, key) && (freeExports[key] = he[key]);
}
}
} else {
root.he = he;
}
})(exports);
}
});
// node_modules/a-node-html-parser/dist/nodes/node.js
var require_node = __commonJS({
"node_modules/a-node-html-parser/dist/nodes/node.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
var he_1 = require_he();
var Node = function() {
function Node2(parentNode, range) {
if (parentNode === void 0) {
parentNode = null;
}
this.parentNode = parentNode;
this.childNodes = [];
Object.defineProperty(this, "range", {
enumerable: false,
writable: true,
configurable: true,
value: range !== null && range !== void 0 ? range : [-1, -1]
});
}
Node2.prototype.remove = function() {
var _this = this;
if (this.parentNode) {
var children = this.parentNode.childNodes;
this.parentNode.childNodes = children.filter(function(child) {
return _this !== child;
});
this.parentNode = null;
}
return this;
};
Object.defineProperty(Node2.prototype, "innerText", {
get: function() {
return this.rawText;
},
enumerable: false,
configurable: true
});
Object.defineProperty(Node2.prototype, "textContent", {
get: function() {
return (0, he_1.decode)(this.rawText);
},
set: function(val) {
this.rawText = (0, he_1.encode)(val);
},
enumerable: false,
configurable: true
});
return Node2;
}();
exports.default = Node;
}
});
// node_modules/a-node-html-parser/dist/nodes/type.js
var require_type = __commonJS({
"node_modules/a-node-html-parser/dist/nodes/type.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
var NodeType;
(function(NodeType2) {
NodeType2[NodeType2["ELEMENT_NODE"] = 1] = "ELEMENT_NODE";
NodeType2[NodeType2["TEXT_NODE"] = 3] = "TEXT_NODE";
NodeType2[NodeType2["COMMENT_NODE"] = 8] = "COMMENT_NODE";
})(NodeType || (NodeType = {}));
exports.default = NodeType;
}
});
// node_modules/a-node-html-parser/dist/nodes/comment.js
var require_comment = __commonJS({
"node_modules/a-node-html-parser/dist/nodes/comment.js"(exports) {
"use strict";
var __extends = exports && exports.__extends || function() {
var extendStatics = function(d, b) {
extendStatics = Object.setPrototypeOf || { __proto__: [] } instanceof Array && function(d2, b2) {
d2.__proto__ = b2;
} || function(d2, b2) {
for (var p in b2)
if (Object.prototype.hasOwnProperty.call(b2, p))
d2[p] = b2[p];
};
return extendStatics(d, b);
};
return function(d, b) {
if (typeof b !== "function" && b !== null)
throw new TypeError("Class extends value " + String(b) + " is not a constructor or null");
extendStatics(d, b);
function __() {
this.constructor = d;
}
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
};
}();
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
var node_1 = __importDefault(require_node());
var type_1 = __importDefault(require_type());
var CommentNode = function(_super) {
__extends(CommentNode2, _super);
function CommentNode2(rawText, parentNode, range) {
var _this = _super.call(this, parentNode, range) || this;
_this.rawText = rawText;
_this.nodeType = type_1.default.COMMENT_NODE;
return _this;
}
CommentNode2.prototype.clone = function() {
return new CommentNode2(this.rawText, null);
};
Object.defineProperty(CommentNode2.prototype, "text", {
get: function() {
return this.rawText;
},
enumerable: false,
configurable: true
});
CommentNode2.prototype.toString = function() {
return "");
};
return CommentNode2;
}(node_1.default);
exports.default = CommentNode;
}
});
// node_modules/domelementtype/lib/index.js
var require_lib = __commonJS({
"node_modules/domelementtype/lib/index.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.Doctype = exports.CDATA = exports.Tag = exports.Style = exports.Script = exports.Comment = exports.Directive = exports.Text = exports.Root = exports.isTag = exports.ElementType = void 0;
var ElementType;
(function(ElementType2) {
ElementType2["Root"] = "root";
ElementType2["Text"] = "text";
ElementType2["Directive"] = "directive";
ElementType2["Comment"] = "comment";
ElementType2["Script"] = "script";
ElementType2["Style"] = "style";
ElementType2["Tag"] = "tag";
ElementType2["CDATA"] = "cdata";
ElementType2["Doctype"] = "doctype";
})(ElementType = exports.ElementType || (exports.ElementType = {}));
function isTag(elem) {
return elem.type === ElementType.Tag || elem.type === ElementType.Script || elem.type === ElementType.Style;
}
exports.isTag = isTag;
exports.Root = ElementType.Root;
exports.Text = ElementType.Text;
exports.Directive = ElementType.Directive;
exports.Comment = ElementType.Comment;
exports.Script = ElementType.Script;
exports.Style = ElementType.Style;
exports.Tag = ElementType.Tag;
exports.CDATA = ElementType.CDATA;
exports.Doctype = ElementType.Doctype;
}
});
// node_modules/domhandler/lib/node.js
var require_node2 = __commonJS({
"node_modules/domhandler/lib/node.js"(exports) {
"use strict";
var __extends = exports && exports.__extends || function() {
var extendStatics = function(d, b) {
extendStatics = Object.setPrototypeOf || { __proto__: [] } instanceof Array && function(d2, b2) {
d2.__proto__ = b2;
} || function(d2, b2) {
for (var p in b2)
if (Object.prototype.hasOwnProperty.call(b2, p))
d2[p] = b2[p];
};
return extendStatics(d, b);
};
return function(d, b) {
if (typeof b !== "function" && b !== null)
throw new TypeError("Class extends value " + String(b) + " is not a constructor or null");
extendStatics(d, b);
function __() {
this.constructor = d;
}
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
};
}();
var __assign = exports && exports.__assign || function() {
__assign = Object.assign || function(t) {
for (var s, i = 1, n2 = arguments.length; i < n2; i++) {
s = arguments[i];
for (var p in s)
if (Object.prototype.hasOwnProperty.call(s, p))
t[p] = s[p];
}
return t;
};
return __assign.apply(this, arguments);
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.cloneNode = exports.hasChildren = exports.isDocument = exports.isDirective = exports.isComment = exports.isText = exports.isCDATA = exports.isTag = exports.Element = exports.Document = exports.NodeWithChildren = exports.ProcessingInstruction = exports.Comment = exports.Text = exports.DataNode = exports.Node = void 0;
var domelementtype_1 = require_lib();
var nodeTypes = /* @__PURE__ */ new Map([
[domelementtype_1.ElementType.Tag, 1],
[domelementtype_1.ElementType.Script, 1],
[domelementtype_1.ElementType.Style, 1],
[domelementtype_1.ElementType.Directive, 1],
[domelementtype_1.ElementType.Text, 3],
[domelementtype_1.ElementType.CDATA, 4],
[domelementtype_1.ElementType.Comment, 8],
[domelementtype_1.ElementType.Root, 9]
]);
var Node = function() {
function Node2(type) {
this.type = type;
this.parent = null;
this.prev = null;
this.next = null;
this.startIndex = null;
this.endIndex = null;
}
Object.defineProperty(Node2.prototype, "nodeType", {
get: function() {
var _a;
return (_a = nodeTypes.get(this.type)) !== null && _a !== void 0 ? _a : 1;
},
enumerable: false,
configurable: true
});
Object.defineProperty(Node2.prototype, "parentNode", {
get: function() {
return this.parent;
},
set: function(parent) {
this.parent = parent;
},
enumerable: false,
configurable: true
});
Object.defineProperty(Node2.prototype, "previousSibling", {
get: function() {
return this.prev;
},
set: function(prev) {
this.prev = prev;
},
enumerable: false,
configurable: true
});
Object.defineProperty(Node2.prototype, "nextSibling", {
get: function() {
return this.next;
},
set: function(next) {
this.next = next;
},
enumerable: false,
configurable: true
});
Node2.prototype.cloneNode = function(recursive) {
if (recursive === void 0) {
recursive = false;
}
return cloneNode(this, recursive);
};
return Node2;
}();
exports.Node = Node;
var DataNode = function(_super) {
__extends(DataNode2, _super);
function DataNode2(type, data) {
var _this = _super.call(this, type) || this;
_this.data = data;
return _this;
}
Object.defineProperty(DataNode2.prototype, "nodeValue", {
get: function() {
return this.data;
},
set: function(data) {
this.data = data;
},
enumerable: false,
configurable: true
});
return DataNode2;
}(Node);
exports.DataNode = DataNode;
var Text = function(_super) {
__extends(Text2, _super);
function Text2(data) {
return _super.call(this, domelementtype_1.ElementType.Text, data) || this;
}
return Text2;
}(DataNode);
exports.Text = Text;
var Comment = function(_super) {
__extends(Comment2, _super);
function Comment2(data) {
return _super.call(this, domelementtype_1.ElementType.Comment, data) || this;
}
return Comment2;
}(DataNode);
exports.Comment = Comment;
var ProcessingInstruction = function(_super) {
__extends(ProcessingInstruction2, _super);
function ProcessingInstruction2(name, data) {
var _this = _super.call(this, domelementtype_1.ElementType.Directive, data) || this;
_this.name = name;
return _this;
}
return ProcessingInstruction2;
}(DataNode);
exports.ProcessingInstruction = ProcessingInstruction;
var NodeWithChildren = function(_super) {
__extends(NodeWithChildren2, _super);
function NodeWithChildren2(type, children) {
var _this = _super.call(this, type) || this;
_this.children = children;
return _this;
}
Object.defineProperty(NodeWithChildren2.prototype, "firstChild", {
get: function() {
var _a;
return (_a = this.children[0]) !== null && _a !== void 0 ? _a : null;
},
enumerable: false,
configurable: true
});
Object.defineProperty(NodeWithChildren2.prototype, "lastChild", {
get: function() {
return this.children.length > 0 ? this.children[this.children.length - 1] : null;
},
enumerable: false,
configurable: true
});
Object.defineProperty(NodeWithChildren2.prototype, "childNodes", {
get: function() {
return this.children;
},
set: function(children) {
this.children = children;
},
enumerable: false,
configurable: true
});
return NodeWithChildren2;
}(Node);
exports.NodeWithChildren = NodeWithChildren;
var Document = function(_super) {
__extends(Document2, _super);
function Document2(children) {
return _super.call(this, domelementtype_1.ElementType.Root, children) || this;
}
return Document2;
}(NodeWithChildren);
exports.Document = Document;
var Element2 = function(_super) {
__extends(Element3, _super);
function Element3(name, attribs, children, type) {
if (children === void 0) {
children = [];
}
if (type === void 0) {
type = name === "script" ? domelementtype_1.ElementType.Script : name === "style" ? domelementtype_1.ElementType.Style : domelementtype_1.ElementType.Tag;
}
var _this = _super.call(this, type, children) || this;
_this.name = name;
_this.attribs = attribs;
return _this;
}
Object.defineProperty(Element3.prototype, "tagName", {
get: function() {
return this.name;
},
set: function(name) {
this.name = name;
},
enumerable: false,
configurable: true
});
Object.defineProperty(Element3.prototype, "attributes", {
get: function() {
var _this = this;
return Object.keys(this.attribs).map(function(name) {
var _a, _b;
return {
name,
value: _this.attribs[name],
namespace: (_a = _this["x-attribsNamespace"]) === null || _a === void 0 ? void 0 : _a[name],
prefix: (_b = _this["x-attribsPrefix"]) === null || _b === void 0 ? void 0 : _b[name]
};
});
},
enumerable: false,
configurable: true
});
return Element3;
}(NodeWithChildren);
exports.Element = Element2;
function isTag(node) {
return (0, domelementtype_1.isTag)(node);
}
exports.isTag = isTag;
function isCDATA(node) {
return node.type === domelementtype_1.ElementType.CDATA;
}
exports.isCDATA = isCDATA;
function isText(node) {
return node.type === domelementtype_1.ElementType.Text;
}
exports.isText = isText;
function isComment2(node) {
return node.type === domelementtype_1.ElementType.Comment;
}
exports.isComment = isComment2;
function isDirective(node) {
return node.type === domelementtype_1.ElementType.Directive;
}
exports.isDirective = isDirective;
function isDocument(node) {
return node.type === domelementtype_1.ElementType.Root;
}
exports.isDocument = isDocument;
function hasChildren(node) {
return Object.prototype.hasOwnProperty.call(node, "children");
}
exports.hasChildren = hasChildren;
function cloneNode(node, recursive) {
if (recursive === void 0) {
recursive = false;
}
var result;
if (isText(node)) {
result = new Text(node.data);
} else if (isComment2(node)) {
result = new Comment(node.data);
} else if (isTag(node)) {
var children = recursive ? cloneChildren(node.children) : [];
var clone_1 = new Element2(node.name, __assign({}, node.attribs), children);
children.forEach(function(child) {
return child.parent = clone_1;
});
if (node.namespace != null) {
clone_1.namespace = node.namespace;
}
if (node["x-attribsNamespace"]) {
clone_1["x-attribsNamespace"] = __assign({}, node["x-attribsNamespace"]);
}
if (node["x-attribsPrefix"]) {
clone_1["x-attribsPrefix"] = __assign({}, node["x-attribsPrefix"]);
}
result = clone_1;
} else if (isCDATA(node)) {
var children = recursive ? cloneChildren(node.children) : [];
var clone_2 = new NodeWithChildren(domelementtype_1.ElementType.CDATA, children);
children.forEach(function(child) {
return child.parent = clone_2;
});
result = clone_2;
} else if (isDocument(node)) {
var children = recursive ? cloneChildren(node.children) : [];
var clone_3 = new Document(children);
children.forEach(function(child) {
return child.parent = clone_3;
});
if (node["x-mode"]) {
clone_3["x-mode"] = node["x-mode"];
}
result = clone_3;
} else if (isDirective(node)) {
var instruction = new ProcessingInstruction(node.name, node.data);
if (node["x-name"] != null) {
instruction["x-name"] = node["x-name"];
instruction["x-publicId"] = node["x-publicId"];
instruction["x-systemId"] = node["x-systemId"];
}
result = instruction;
} else {
throw new Error("Not implemented yet: ".concat(node.type));
}
result.startIndex = node.startIndex;
result.endIndex = node.endIndex;
if (node.sourceCodeLocation != null) {
result.sourceCodeLocation = node.sourceCodeLocation;
}
return result;
}
exports.cloneNode = cloneNode;
function cloneChildren(childs) {
var children = childs.map(function(child) {
return cloneNode(child, true);
});
for (var i = 1; i < children.length; i++) {
children[i].prev = children[i - 1];
children[i - 1].next = children[i];
}
return children;
}
}
});
// node_modules/domhandler/lib/index.js
var require_lib2 = __commonJS({
"node_modules/domhandler/lib/index.js"(exports) {
"use strict";
var __createBinding = exports && exports.__createBinding || (Object.create ? function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
var desc = Object.getOwnPropertyDescriptor(m, k);
if (!desc || ("get" in desc ? !m.__esModule : desc.writable || desc.configurable)) {
desc = { enumerable: true, get: function() {
return m[k];
} };
}
Object.defineProperty(o, k2, desc);
} : function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
o[k2] = m[k];
});
var __exportStar = exports && exports.__exportStar || function(m, exports2) {
for (var p in m)
if (p !== "default" && !Object.prototype.hasOwnProperty.call(exports2, p))
__createBinding(exports2, m, p);
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.DomHandler = void 0;
var domelementtype_1 = require_lib();
var node_1 = require_node2();
__exportStar(require_node2(), exports);
var reWhitespace = /\s+/g;
var defaultOpts = {
normalizeWhitespace: false,
withStartIndices: false,
withEndIndices: false,
xmlMode: false
};
var DomHandler = function() {
function DomHandler2(callback, options, elementCB) {
this.dom = [];
this.root = new node_1.Document(this.dom);
this.done = false;
this.tagStack = [this.root];
this.lastNode = null;
this.parser = null;
if (typeof options === "function") {
elementCB = options;
options = defaultOpts;
}
if (typeof callback === "object") {
options = callback;
callback = void 0;
}
this.callback = callback !== null && callback !== void 0 ? callback : null;
this.options = options !== null && options !== void 0 ? options : defaultOpts;
this.elementCB = elementCB !== null && elementCB !== void 0 ? elementCB : null;
}
DomHandler2.prototype.onparserinit = function(parser) {
this.parser = parser;
};
DomHandler2.prototype.onreset = function() {
this.dom = [];
this.root = new node_1.Document(this.dom);
this.done = false;
this.tagStack = [this.root];
this.lastNode = null;
this.parser = null;
};
DomHandler2.prototype.onend = function() {
if (this.done)
return;
this.done = true;
this.parser = null;
this.handleCallback(null);
};
DomHandler2.prototype.onerror = function(error) {
this.handleCallback(error);
};
DomHandler2.prototype.onclosetag = function() {
this.lastNode = null;
var elem = this.tagStack.pop();
if (this.options.withEndIndices) {
elem.endIndex = this.parser.endIndex;
}
if (this.elementCB)
this.elementCB(elem);
};
DomHandler2.prototype.onopentag = function(name, attribs) {
var type = this.options.xmlMode ? domelementtype_1.ElementType.Tag : void 0;
var element = new node_1.Element(name, attribs, void 0, type);
this.addNode(element);
this.tagStack.push(element);
};
DomHandler2.prototype.ontext = function(data) {
var normalizeWhitespace = this.options.normalizeWhitespace;
var lastNode = this.lastNode;
if (lastNode && lastNode.type === domelementtype_1.ElementType.Text) {
if (normalizeWhitespace) {
lastNode.data = (lastNode.data + data).replace(reWhitespace, " ");
} else {
lastNode.data += data;
}
if (this.options.withEndIndices) {
lastNode.endIndex = this.parser.endIndex;
}
} else {
if (normalizeWhitespace) {
data = data.replace(reWhitespace, " ");
}
var node = new node_1.Text(data);
this.addNode(node);
this.lastNode = node;
}
};
DomHandler2.prototype.oncomment = function(data) {
if (this.lastNode && this.lastNode.type === domelementtype_1.ElementType.Comment) {
this.lastNode.data += data;
return;
}
var node = new node_1.Comment(data);
this.addNode(node);
this.lastNode = node;
};
DomHandler2.prototype.oncommentend = function() {
this.lastNode = null;
};
DomHandler2.prototype.oncdatastart = function() {
var text = new node_1.Text("");
var node = new node_1.NodeWithChildren(domelementtype_1.ElementType.CDATA, [text]);
this.addNode(node);
text.parent = node;
this.lastNode = text;
};
DomHandler2.prototype.oncdataend = function() {
this.lastNode = null;
};
DomHandler2.prototype.onprocessinginstruction = function(name, data) {
var node = new node_1.ProcessingInstruction(name, data);
this.addNode(node);
};
DomHandler2.prototype.handleCallback = function(error) {
if (typeof this.callback === "function") {
this.callback(error, this.dom);
} else if (error) {
throw error;
}
};
DomHandler2.prototype.addNode = function(node) {
var parent = this.tagStack[this.tagStack.length - 1];
var previousSibling = parent.children[parent.children.length - 1];
if (this.options.withStartIndices) {
node.startIndex = this.parser.startIndex;
}
if (this.options.withEndIndices) {
node.endIndex = this.parser.endIndex;
}
parent.children.push(node);
if (previousSibling) {
node.prev = previousSibling;
previousSibling.next = node;
}
node.parent = parent;
this.lastNode = null;
};
return DomHandler2;
}();
exports.DomHandler = DomHandler;
exports.default = DomHandler;
}
});
// node_modules/entities/lib/maps/entities.json
var require_entities = __commonJS({
"node_modules/entities/lib/maps/entities.json"(exports, module2) {
module2.exports = { Aacute: "\xC1", aacute: "\xE1", Abreve: "\u0102", abreve: "\u0103", ac: "\u223E", acd: "\u223F", acE: "\u223E\u0333", Acirc: "\xC2", acirc: "\xE2", acute: "\xB4", Acy: "\u0410", acy: "\u0430", AElig: "\xC6", aelig: "\xE6", af: "\u2061", Afr: "\u{1D504}", afr: "\u{1D51E}", Agrave: "\xC0", agrave: "\xE0", alefsym: "\u2135", aleph: "\u2135", Alpha: "\u0391", alpha: "\u03B1", Amacr: "\u0100", amacr: "\u0101", amalg: "\u2A3F", amp: "&", AMP: "&", andand: "\u2A55", And: "\u2A53", and: "\u2227", andd: "\u2A5C", andslope: "\u2A58", andv: "\u2A5A", ang: "\u2220", ange: "\u29A4", angle: "\u2220", angmsdaa: "\u29A8", angmsdab: "\u29A9", angmsdac: "\u29AA", angmsdad: "\u29AB", angmsdae: "\u29AC", angmsdaf: "\u29AD", angmsdag: "\u29AE", angmsdah: "\u29AF", angmsd: "\u2221", angrt: "\u221F", angrtvb: "\u22BE", angrtvbd: "\u299D", angsph: "\u2222", angst: "\xC5", angzarr: "\u237C", Aogon: "\u0104", aogon: "\u0105", Aopf: "\u{1D538}", aopf: "\u{1D552}", apacir: "\u2A6F", ap: "\u2248", apE: "\u2A70", ape: "\u224A", apid: "\u224B", apos: "'", ApplyFunction: "\u2061", approx: "\u2248", approxeq: "\u224A", Aring: "\xC5", aring: "\xE5", Ascr: "\u{1D49C}", ascr: "\u{1D4B6}", Assign: "\u2254", ast: "*", asymp: "\u2248", asympeq: "\u224D", Atilde: "\xC3", atilde: "\xE3", Auml: "\xC4", auml: "\xE4", awconint: "\u2233", awint: "\u2A11", backcong: "\u224C", backepsilon: "\u03F6", backprime: "\u2035", backsim: "\u223D", backsimeq: "\u22CD", Backslash: "\u2216", Barv: "\u2AE7", barvee: "\u22BD", barwed: "\u2305", Barwed: "\u2306", barwedge: "\u2305", bbrk: "\u23B5", bbrktbrk: "\u23B6", bcong: "\u224C", Bcy: "\u0411", bcy: "\u0431", bdquo: "\u201E", becaus: "\u2235", because: "\u2235", Because: "\u2235", bemptyv: "\u29B0", bepsi: "\u03F6", bernou: "\u212C", Bernoullis: "\u212C", Beta: "\u0392", beta: "\u03B2", beth: "\u2136", between: "\u226C", Bfr: "\u{1D505}", bfr: "\u{1D51F}", bigcap: "\u22C2", bigcirc: "\u25EF", bigcup: "\u22C3", bigodot: "\u2A00", bigoplus: "\u2A01", bigotimes: "\u2A02", bigsqcup: "\u2A06", bigstar: "\u2605", bigtriangledown: "\u25BD", bigtriangleup: "\u25B3", biguplus: "\u2A04", bigvee: "\u22C1", bigwedge: "\u22C0", bkarow: "\u290D", blacklozenge: "\u29EB", blacksquare: "\u25AA", blacktriangle: "\u25B4", blacktriangledown: "\u25BE", blacktriangleleft: "\u25C2", blacktriangleright: "\u25B8", blank: "\u2423", blk12: "\u2592", blk14: "\u2591", blk34: "\u2593", block: "\u2588", bne: "=\u20E5", bnequiv: "\u2261\u20E5", bNot: "\u2AED", bnot: "\u2310", Bopf: "\u{1D539}", bopf: "\u{1D553}", bot: "\u22A5", bottom: "\u22A5", bowtie: "\u22C8", boxbox: "\u29C9", boxdl: "\u2510", boxdL: "\u2555", boxDl: "\u2556", boxDL: "\u2557", boxdr: "\u250C", boxdR: "\u2552", boxDr: "\u2553", boxDR: "\u2554", boxh: "\u2500", boxH: "\u2550", boxhd: "\u252C", boxHd: "\u2564", boxhD: "\u2565", boxHD: "\u2566", boxhu: "\u2534", boxHu: "\u2567", boxhU: "\u2568", boxHU: "\u2569", boxminus: "\u229F", boxplus: "\u229E", boxtimes: "\u22A0", boxul: "\u2518", boxuL: "\u255B", boxUl: "\u255C", boxUL: "\u255D", boxur: "\u2514", boxuR: "\u2558", boxUr: "\u2559", boxUR: "\u255A", boxv: "\u2502", boxV: "\u2551", boxvh: "\u253C", boxvH: "\u256A", boxVh: "\u256B", boxVH: "\u256C", boxvl: "\u2524", boxvL: "\u2561", boxVl: "\u2562", boxVL: "\u2563", boxvr: "\u251C", boxvR: "\u255E", boxVr: "\u255F", boxVR: "\u2560", bprime: "\u2035", breve: "\u02D8", Breve: "\u02D8", brvbar: "\xA6", bscr: "\u{1D4B7}", Bscr: "\u212C", bsemi: "\u204F", bsim: "\u223D", bsime: "\u22CD", bsolb: "\u29C5", bsol: "\\", bsolhsub: "\u27C8", bull: "\u2022", bullet: "\u2022", bump: "\u224E", bumpE: "\u2AAE", bumpe: "\u224F", Bumpeq: "\u224E", bumpeq: "\u224F", Cacute: "\u0106", cacute: "\u0107", capand: "\u2A44", capbrcup: "\u2A49", capcap: "\u2A4B", cap: "\u2229", Cap: "\u22D2", capcup: "\u2A47", capdot: "\u2A40", CapitalDifferentialD: "\u2145", caps: "\u2229\uFE00", caret: "\u2041", caron: "\u02C7", Cayleys: "\u212D", ccaps: "\u2A4D", Ccaron: "\u010C", ccaron: "\u010D", Ccedil: "\xC7", ccedil: "\xE7", Ccirc: "\u0108", ccirc: "\u0109", Cconint: "\u2230", ccups: "\u2A4C", ccupssm: "\u2A50", Cdot: "\u010A", cdot: "\u010B", cedil: "\xB8", Cedilla: "\xB8", cemptyv: "\u29B2", cent: "\xA2", centerdot: "\xB7", CenterDot: "\xB7", cfr: "\u{1D520}", Cfr: "\u212D", CHcy: "\u0427", chcy: "\u0447", check: "\u2713", checkmark: "\u2713", Chi: "\u03A7", chi: "\u03C7", circ: "\u02C6", circeq: "\u2257", circlearrowleft: "\u21BA", circlearrowright: "\u21BB", circledast: "\u229B", circledcirc: "\u229A", circleddash: "\u229D", CircleDot: "\u2299", circledR: "\xAE", circledS: "\u24C8", CircleMinus: "\u2296", CirclePlus: "\u2295", CircleTimes: "\u2297", cir: "\u25CB", cirE: "\u29C3", cire: "\u2257", cirfnint: "\u2A10", cirmid: "\u2AEF", cirscir: "\u29C2", ClockwiseContourIntegral: "\u2232", CloseCurlyDoubleQuote: "\u201D", CloseCurlyQuote: "\u2019", clubs: "\u2663", clubsuit: "\u2663", colon: ":", Colon: "\u2237", Colone: "\u2A74", colone: "\u2254", coloneq: "\u2254", comma: ",", commat: "@", comp: "\u2201", compfn: "\u2218", complement: "\u2201", complexes: "\u2102", cong: "\u2245", congdot: "\u2A6D", Congruent: "\u2261", conint: "\u222E", Conint: "\u222F", ContourIntegral: "\u222E", copf: "\u{1D554}", Copf: "\u2102", coprod: "\u2210", Coproduct: "\u2210", copy: "\xA9", COPY: "\xA9", copysr: "\u2117", CounterClockwiseContourIntegral: "\u2233", crarr: "\u21B5", cross: "\u2717", Cross: "\u2A2F", Cscr: "\u{1D49E}", cscr: "\u{1D4B8}", csub: "\u2ACF", csube: "\u2AD1", csup: "\u2AD0", csupe: "\u2AD2", ctdot: "\u22EF", cudarrl: "\u2938", cudarrr: "\u2935", cuepr: "\u22DE", cuesc: "\u22DF", cularr: "\u21B6", cularrp: "\u293D", cupbrcap: "\u2A48", cupcap: "\u2A46", CupCap: "\u224D", cup: "\u222A", Cup: "\u22D3", cupcup: "\u2A4A", cupdot: "\u228D", cupor: "\u2A45", cups: "\u222A\uFE00", curarr: "\u21B7", curarrm: "\u293C", curlyeqprec: "\u22DE", curlyeqsucc: "\u22DF", curlyvee: "\u22CE", curlywedge: "\u22CF", curren: "\xA4", curvearrowleft: "\u21B6", curvearrowright: "\u21B7", cuvee: "\u22CE", cuwed: "\u22CF", cwconint: "\u2232", cwint: "\u2231", cylcty: "\u232D", dagger: "\u2020", Dagger: "\u2021", daleth: "\u2138", darr: "\u2193", Darr: "\u21A1", dArr: "\u21D3", dash: "\u2010", Dashv: "\u2AE4", dashv: "\u22A3", dbkarow: "\u290F", dblac: "\u02DD", Dcaron: "\u010E", dcaron: "\u010F", Dcy: "\u0414", dcy: "\u0434", ddagger: "\u2021", ddarr: "\u21CA", DD: "\u2145", dd: "\u2146", DDotrahd: "\u2911", ddotseq: "\u2A77", deg: "\xB0", Del: "\u2207", Delta: "\u0394", delta: "\u03B4", demptyv: "\u29B1", dfisht: "\u297F", Dfr: "\u{1D507}", dfr: "\u{1D521}", dHar: "\u2965", dharl: "\u21C3", dharr: "\u21C2", DiacriticalAcute: "\xB4", DiacriticalDot: "\u02D9", DiacriticalDoubleAcute: "\u02DD", DiacriticalGrave: "`", DiacriticalTilde: "\u02DC", diam: "\u22C4", diamond: "\u22C4", Diamond: "\u22C4", diamondsuit: "\u2666", diams: "\u2666", die: "\xA8", DifferentialD: "\u2146", digamma: "\u03DD", disin: "\u22F2", div: "\xF7", divide: "\xF7", divideontimes: "\u22C7", divonx: "\u22C7", DJcy: "\u0402", djcy: "\u0452", dlcorn: "\u231E", dlcrop: "\u230D", dollar: "$", Dopf: "\u{1D53B}", dopf: "\u{1D555}", Dot: "\xA8", dot: "\u02D9", DotDot: "\u20DC", doteq: "\u2250", doteqdot: "\u2251", DotEqual: "\u2250", dotminus: "\u2238", dotplus: "\u2214", dotsquare: "\u22A1", doublebarwedge: "\u2306", DoubleContourIntegral: "\u222F", DoubleDot: "\xA8", DoubleDownArrow: "\u21D3", DoubleLeftArrow: "\u21D0", DoubleLeftRightArrow: "\u21D4", DoubleLeftTee: "\u2AE4", DoubleLongLeftArrow: "\u27F8", DoubleLongLeftRightArrow: "\u27FA", DoubleLongRightArrow: "\u27F9", DoubleRightArrow: "\u21D2", DoubleRightTee: "\u22A8", DoubleUpArrow: "\u21D1", DoubleUpDownArrow: "\u21D5", DoubleVerticalBar: "\u2225", DownArrowBar: "\u2913", downarrow: "\u2193", DownArrow: "\u2193", Downarrow: "\u21D3", DownArrowUpArrow: "\u21F5", DownBreve: "\u0311", downdownarrows: "\u21CA", downharpoonleft: "\u21C3", downharpoonright: "\u21C2", DownLeftRightVector: "\u2950", DownLeftTeeVector: "\u295E", DownLeftVectorBar: "\u2956", DownLeftVector: "\u21BD", DownRightTeeVector: "\u295F", DownRightVectorBar: "\u2957", DownRightVector: "\u21C1", DownTeeArrow: "\u21A7", DownTee: "\u22A4", drbkarow: "\u2910", drcorn: "\u231F", drcrop: "\u230C", Dscr: "\u{1D49F}", dscr: "\u{1D4B9}", DScy: "\u0405", dscy: "\u0455", dsol: "\u29F6", Dstrok: "\u0110", dstrok: "\u0111", dtdot: "\u22F1", dtri: "\u25BF", dtrif: "\u25BE", duarr: "\u21F5", duhar: "\u296F", dwangle: "\u29A6", DZcy: "\u040F", dzcy: "\u045F", dzigrarr: "\u27FF", Eacute: "\xC9", eacute: "\xE9", easter: "\u2A6E", Ecaron: "\u011A", ecaron: "\u011B", Ecirc: "\xCA", ecirc: "\xEA", ecir: "\u2256", ecolon: "\u2255", Ecy: "\u042D", ecy: "\u044D", eDDot: "\u2A77", Edot: "\u0116", edot: "\u0117", eDot: "\u2251", ee: "\u2147", efDot: "\u2252", Efr: "\u{1D508}", efr: "\u{1D522}", eg: "\u2A9A", Egrave: "\xC8", egrave: "\xE8", egs: "\u2A96", egsdot: "\u2A98", el: "\u2A99", Element: "\u2208", elinters: "\u23E7", ell: "\u2113", els: "\u2A95", elsdot: "\u2A97", Emacr: "\u0112", emacr: "\u0113", empty: "\u2205", emptyset: "\u2205", EmptySmallSquare: "\u25FB", emptyv: "\u2205", EmptyVerySmallSquare: "\u25AB", emsp13: "\u2004", emsp14: "\u2005", emsp: "\u2003", ENG: "\u014A", eng: "\u014B", ensp: "\u2002", Eogon: "\u0118", eogon: "\u0119", Eopf: "\u{1D53C}", eopf: "\u{1D556}", epar: "\u22D5", eparsl: "\u29E3", eplus: "\u2A71", epsi: "\u03B5", Epsilon: "\u0395", epsilon: "\u03B5", epsiv: "\u03F5", eqcirc: "\u2256", eqcolon: "\u2255", eqsim: "\u2242", eqslantgtr: "\u2A96", eqslantless: "\u2A95", Equal: "\u2A75", equals: "=", EqualTilde: "\u2242", equest: "\u225F", Equilibrium: "\u21CC", equiv: "\u2261", equivDD: "\u2A78", eqvparsl: "\u29E5", erarr: "\u2971", erDot: "\u2253", escr: "\u212F", Escr: "\u2130", esdot: "\u2250", Esim: "\u2A73", esim: "\u2242", Eta: "\u0397", eta: "\u03B7", ETH: "\xD0", eth: "\xF0", Euml: "\xCB", euml: "\xEB", euro: "\u20AC", excl: "!", exist: "\u2203", Exists: "\u2203", expectation: "\u2130", exponentiale: "\u2147", ExponentialE: "\u2147", fallingdotseq: "\u2252", Fcy: "\u0424", fcy: "\u0444", female: "\u2640", ffilig: "\uFB03", fflig: "\uFB00", ffllig: "\uFB04", Ffr: "\u{1D509}", ffr: "\u{1D523}", filig: "\uFB01", FilledSmallSquare: "\u25FC", FilledVerySmallSquare: "\u25AA", fjlig: "fj", flat: "\u266D", fllig: "\uFB02", fltns: "\u25B1", fnof: "\u0192", Fopf: "\u{1D53D}", fopf: "\u{1D557}", forall: "\u2200", ForAll: "\u2200", fork: "\u22D4", forkv: "\u2AD9", Fouriertrf: "\u2131", fpartint: "\u2A0D", frac12: "\xBD", frac13: "\u2153", frac14: "\xBC", frac15: "\u2155", frac16: "\u2159", frac18: "\u215B", frac23: "\u2154", frac25: "\u2156", frac34: "\xBE", frac35: "\u2157", frac38: "\u215C", frac45: "\u2158", frac56: "\u215A", frac58: "\u215D", frac78: "\u215E", frasl: "\u2044", frown: "\u2322", fscr: "\u{1D4BB}", Fscr: "\u2131", gacute: "\u01F5", Gamma: "\u0393", gamma: "\u03B3", Gammad: "\u03DC", gammad: "\u03DD", gap: "\u2A86", Gbreve: "\u011E", gbreve: "\u011F", Gcedil: "\u0122", Gcirc: "\u011C", gcirc: "\u011D", Gcy: "\u0413", gcy: "\u0433", Gdot: "\u0120", gdot: "\u0121", ge: "\u2265", gE: "\u2267", gEl: "\u2A8C", gel: "\u22DB", geq: "\u2265", geqq: "\u2267", geqslant: "\u2A7E", gescc: "\u2AA9", ges: "\u2A7E", gesdot: "\u2A80", gesdoto: "\u2A82", gesdotol: "\u2A84", gesl: "\u22DB\uFE00", gesles: "\u2A94", Gfr: "\u{1D50A}", gfr: "\u{1D524}", gg: "\u226B", Gg: "\u22D9", ggg: "\u22D9", gimel: "\u2137", GJcy: "\u0403", gjcy: "\u0453", gla: "\u2AA5", gl: "\u2277", glE: "\u2A92", glj: "\u2AA4", gnap: "\u2A8A", gnapprox: "\u2A8A", gne: "\u2A88", gnE: "\u2269", gneq: "\u2A88", gneqq: "\u2269", gnsim: "\u22E7", Gopf: "\u{1D53E}", gopf: "\u{1D558}", grave: "`", GreaterEqual: "\u2265", GreaterEqualLess: "\u22DB", GreaterFullEqual: "\u2267", GreaterGreater: "\u2AA2", GreaterLess: "\u2277", GreaterSlantEqual: "\u2A7E", GreaterTilde: "\u2273", Gscr: "\u{1D4A2}", gscr: "\u210A", gsim: "\u2273", gsime: "\u2A8E", gsiml: "\u2A90", gtcc: "\u2AA7", gtcir: "\u2A7A", gt: ">", GT: ">", Gt: "\u226B", gtdot: "\u22D7", gtlPar: "\u2995", gtquest: "\u2A7C", gtrapprox: "\u2A86", gtrarr: "\u2978", gtrdot: "\u22D7", gtreqless: "\u22DB", gtreqqless: "\u2A8C", gtrless: "\u2277", gtrsim: "\u2273", gvertneqq: "\u2269\uFE00", gvnE: "\u2269\uFE00", Hacek: "\u02C7", hairsp: "\u200A", half: "\xBD", hamilt: "\u210B", HARDcy: "\u042A", hardcy: "\u044A", harrcir: "\u2948", harr: "\u2194", hArr: "\u21D4", harrw: "\u21AD", Hat: "^", hbar: "\u210F", Hcirc: "\u0124", hcirc: "\u0125", hearts: "\u2665", heartsuit: "\u2665", hellip: "\u2026", hercon: "\u22B9", hfr: "\u{1D525}", Hfr: "\u210C", HilbertSpace: "\u210B", hksearow: "\u2925", hkswarow: "\u2926", hoarr: "\u21FF", homtht: "\u223B", hookleftarrow: "\u21A9", hookrightarrow: "\u21AA", hopf: "\u{1D559}", Hopf: "\u210D", horbar: "\u2015", HorizontalLine: "\u2500", hscr: "\u{1D4BD}", Hscr: "\u210B", hslash: "\u210F", Hstrok: "\u0126", hstrok: "\u0127", HumpDownHump: "\u224E", HumpEqual: "\u224F", hybull: "\u2043", hyphen: "\u2010", Iacute: "\xCD", iacute: "\xED", ic: "\u2063", Icirc: "\xCE", icirc: "\xEE", Icy: "\u0418", icy: "\u0438", Idot: "\u0130", IEcy: "\u0415", iecy: "\u0435", iexcl: "\xA1", iff: "\u21D4", ifr: "\u{1D526}", Ifr: "\u2111", Igrave: "\xCC", igrave: "\xEC", ii: "\u2148", iiiint: "\u2A0C", iiint: "\u222D", iinfin: "\u29DC", iiota: "\u2129", IJlig: "\u0132", ijlig: "\u0133", Imacr: "\u012A", imacr: "\u012B", image: "\u2111", ImaginaryI: "\u2148", imagline: "\u2110", imagpart: "\u2111", imath: "\u0131", Im: "\u2111", imof: "\u22B7", imped: "\u01B5", Implies: "\u21D2", incare: "\u2105", in: "\u2208", infin: "\u221E", infintie: "\u29DD", inodot: "\u0131", intcal: "\u22BA", int: "\u222B", Int: "\u222C", integers: "\u2124", Integral: "\u222B", intercal: "\u22BA", Intersection: "\u22C2", intlarhk: "\u2A17", intprod: "\u2A3C", InvisibleComma: "\u2063", InvisibleTimes: "\u2062", IOcy: "\u0401", iocy: "\u0451", Iogon: "\u012E", iogon: "\u012F", Iopf: "\u{1D540}", iopf: "\u{1D55A}", Iota: "\u0399", iota: "\u03B9", iprod: "\u2A3C", iquest: "\xBF", iscr: "\u{1D4BE}", Iscr: "\u2110", isin: "\u2208", isindot: "\u22F5", isinE: "\u22F9", isins: "\u22F4", isinsv: "\u22F3", isinv: "\u2208", it: "\u2062", Itilde: "\u0128", itilde: "\u0129", Iukcy: "\u0406", iukcy: "\u0456", Iuml: "\xCF", iuml: "\xEF", Jcirc: "\u0134", jcirc: "\u0135", Jcy: "\u0419", jcy: "\u0439", Jfr: "\u{1D50D}", jfr: "\u{1D527}", jmath: "\u0237", Jopf: "\u{1D541}", jopf: "\u{1D55B}", Jscr: "\u{1D4A5}", jscr: "\u{1D4BF}", Jsercy: "\u0408", jsercy: "\u0458", Jukcy: "\u0404", jukcy: "\u0454", Kappa: "\u039A", kappa: "\u03BA", kappav: "\u03F0", Kcedil: "\u0136", kcedil: "\u0137", Kcy: "\u041A", kcy: "\u043A", Kfr: "\u{1D50E}", kfr: "\u{1D528}", kgreen: "\u0138", KHcy: "\u0425", khcy: "\u0445", KJcy: "\u040C", kjcy: "\u045C", Kopf: "\u{1D542}", kopf: "\u{1D55C}", Kscr: "\u{1D4A6}", kscr: "\u{1D4C0}", lAarr: "\u21DA", Lacute: "\u0139", lacute: "\u013A", laemptyv: "\u29B4", lagran: "\u2112", Lambda: "\u039B", lambda: "\u03BB", lang: "\u27E8", Lang: "\u27EA", langd: "\u2991", langle: "\u27E8", lap: "\u2A85", Laplacetrf: "\u2112", laquo: "\xAB", larrb: "\u21E4", larrbfs: "\u291F", larr: "\u2190", Larr: "\u219E", lArr: "\u21D0", larrfs: "\u291D", larrhk: "\u21A9", larrlp: "\u21AB", larrpl: "\u2939", larrsim: "\u2973", larrtl: "\u21A2", latail: "\u2919", lAtail: "\u291B", lat: "\u2AAB", late: "\u2AAD", lates: "\u2AAD\uFE00", lbarr: "\u290C", lBarr: "\u290E", lbbrk: "\u2772", lbrace: "{", lbrack: "[", lbrke: "\u298B", lbrksld: "\u298F", lbrkslu: "\u298D", Lcaron: "\u013D", lcaron: "\u013E", Lcedil: "\u013B", lcedil: "\u013C", lceil: "\u2308", lcub: "{", Lcy: "\u041B", lcy: "\u043B", ldca: "\u2936", ldquo: "\u201C", ldquor: "\u201E", ldrdhar: "\u2967", ldrushar: "\u294B", ldsh: "\u21B2", le: "\u2264", lE: "\u2266", LeftAngleBracket: "\u27E8", LeftArrowBar: "\u21E4", leftarrow: "\u2190", LeftArrow: "\u2190", Leftarrow: "\u21D0", LeftArrowRightArrow: "\u21C6", leftarrowtail: "\u21A2", LeftCeiling: "\u2308", LeftDoubleBracket: "\u27E6", LeftDownTeeVector: "\u2961", LeftDownVectorBar: "\u2959", LeftDownVector: "\u21C3", LeftFloor: "\u230A", leftharpoondown: "\u21BD", leftharpoonup: "\u21BC", leftleftarrows: "\u21C7", leftrightarrow: "\u2194", LeftRightArrow: "\u2194", Leftrightarrow: "\u21D4", leftrightarrows: "\u21C6", leftrightharpoons: "\u21CB", leftrightsquigarrow: "\u21AD", LeftRightVector: "\u294E", LeftTeeArrow: "\u21A4", LeftTee: "\u22A3", LeftTeeVector: "\u295A", leftthreetimes: "\u22CB", LeftTriangleBar: "\u29CF", LeftTriangle: "\u22B2", LeftTriangleEqual: "\u22B4", LeftUpDownVector: "\u2951", LeftUpTeeVector: "\u2960", LeftUpVectorBar: "\u2958", LeftUpVector: "\u21BF", LeftVectorBar: "\u2952", LeftVector: "\u21BC", lEg: "\u2A8B", leg: "\u22DA", leq: "\u2264", leqq: "\u2266", leqslant: "\u2A7D", lescc: "\u2AA8", les: "\u2A7D", lesdot: "\u2A7F", lesdoto: "\u2A81", lesdotor: "\u2A83", lesg: "\u22DA\uFE00", lesges: "\u2A93", lessapprox: "\u2A85", lessdot: "\u22D6", lesseqgtr: "\u22DA", lesseqqgtr: "\u2A8B", LessEqualGreater: "\u22DA", LessFullEqual: "\u2266", LessGreater: "\u2276", lessgtr: "\u2276", LessLess: "\u2AA1", lesssim: "\u2272", LessSlantEqual: "\u2A7D", LessTilde: "\u2272", lfisht: "\u297C", lfloor: "\u230A", Lfr: "\u{1D50F}", lfr: "\u{1D529}", lg: "\u2276", lgE: "\u2A91", lHar: "\u2962", lhard: "\u21BD", lharu: "\u21BC", lharul: "\u296A", lhblk: "\u2584", LJcy: "\u0409", ljcy: "\u0459", llarr: "\u21C7", ll: "\u226A", Ll: "\u22D8", llcorner: "\u231E", Lleftarrow: "\u21DA", llhard: "\u296B", lltri: "\u25FA", Lmidot: "\u013F", lmidot: "\u0140", lmoustache: "\u23B0", lmoust: "\u23B0", lnap: "\u2A89", lnapprox: "\u2A89", lne: "\u2A87", lnE: "\u2268", lneq: "\u2A87", lneqq: "\u2268", lnsim: "\u22E6", loang: "\u27EC", loarr: "\u21FD", lobrk: "\u27E6", longleftarrow: "\u27F5", LongLeftArrow: "\u27F5", Longleftarrow: "\u27F8", longleftrightarrow: "\u27F7", LongLeftRightArrow: "\u27F7", Longleftrightarrow: "\u27FA", longmapsto: "\u27FC", longrightarrow: "\u27F6", LongRightArrow: "\u27F6", Longrightarrow: "\u27F9", looparrowleft: "\u21AB", looparrowright: "\u21AC", lopar: "\u2985", Lopf: "\u{1D543}", lopf: "\u{1D55D}", loplus: "\u2A2D", lotimes: "\u2A34", lowast: "\u2217", lowbar: "_", LowerLeftArrow: "\u2199", LowerRightArrow: "\u2198", loz: "\u25CA", lozenge: "\u25CA", lozf: "\u29EB", lpar: "(", lparlt: "\u2993", lrarr: "\u21C6", lrcorner: "\u231F", lrhar: "\u21CB", lrhard: "\u296D", lrm: "\u200E", lrtri: "\u22BF", lsaquo: "\u2039", lscr: "\u{1D4C1}", Lscr: "\u2112", lsh: "\u21B0", Lsh: "\u21B0", lsim: "\u2272", lsime: "\u2A8D", lsimg: "\u2A8F", lsqb: "[", lsquo: "\u2018", lsquor: "\u201A", Lstrok: "\u0141", lstrok: "\u0142", ltcc: "\u2AA6", ltcir: "\u2A79", lt: "<", LT: "<", Lt: "\u226A", ltdot: "\u22D6", lthree: "\u22CB", ltimes: "\u22C9", ltlarr: "\u2976", ltquest: "\u2A7B", ltri: "\u25C3", ltrie: "\u22B4", ltrif: "\u25C2", ltrPar: "\u2996", lurdshar: "\u294A", luruhar: "\u2966", lvertneqq: "\u2268\uFE00", lvnE: "\u2268\uFE00", macr: "\xAF", male: "\u2642", malt: "\u2720", maltese: "\u2720", Map: "\u2905", map: "\u21A6", mapsto: "\u21A6", mapstodown: "\u21A7", mapstoleft: "\u21A4", mapstoup: "\u21A5", marker: "\u25AE", mcomma: "\u2A29", Mcy: "\u041C", mcy: "\u043C", mdash: "\u2014", mDDot: "\u223A", measuredangle: "\u2221", MediumSpace: "\u205F", Mellintrf: "\u2133", Mfr: "\u{1D510}", mfr: "\u{1D52A}", mho: "\u2127", micro: "\xB5", midast: "*", midcir: "\u2AF0", mid: "\u2223", middot: "\xB7", minusb: "\u229F", minus: "\u2212", minusd: "\u2238", minusdu: "\u2A2A", MinusPlus: "\u2213", mlcp: "\u2ADB", mldr: "\u2026", mnplus: "\u2213", models: "\u22A7", Mopf: "\u{1D544}", mopf: "\u{1D55E}", mp: "\u2213", mscr: "\u{1D4C2}", Mscr: "\u2133", mstpos: "\u223E", Mu: "\u039C", mu: "\u03BC", multimap: "\u22B8", mumap: "\u22B8", nabla: "\u2207", Nacute: "\u0143", nacute: "\u0144", nang: "\u2220\u20D2", nap: "\u2249", napE: "\u2A70\u0338", napid: "\u224B\u0338", napos: "\u0149", napprox: "\u2249", natural: "\u266E", naturals: "\u2115", natur: "\u266E", nbsp: "\xA0", nbump: "\u224E\u0338", nbumpe: "\u224F\u0338", ncap: "\u2A43", Ncaron: "\u0147", ncaron: "\u0148", Ncedil: "\u0145", ncedil: "\u0146", ncong: "\u2247", ncongdot: "\u2A6D\u0338", ncup: "\u2A42", Ncy: "\u041D", ncy: "\u043D", ndash: "\u2013", nearhk: "\u2924", nearr: "\u2197", neArr: "\u21D7", nearrow: "\u2197", ne: "\u2260", nedot: "\u2250\u0338", NegativeMediumSpace: "\u200B", NegativeThickSpace: "\u200B", NegativeThinSpace: "\u200B", NegativeVeryThinSpace: "\u200B", nequiv: "\u2262", nesear: "\u2928", nesim: "\u2242\u0338", NestedGreaterGreater: "\u226B", NestedLessLess: "\u226A", NewLine: "\n", nexist: "\u2204", nexists: "\u2204", Nfr: "\u{1D511}", nfr: "\u{1D52B}", ngE: "\u2267\u0338", nge: "\u2271", ngeq: "\u2271", ngeqq: "\u2267\u0338", ngeqslant: "\u2A7E\u0338", nges: "\u2A7E\u0338", nGg: "\u22D9\u0338", ngsim: "\u2275", nGt: "\u226B\u20D2", ngt: "\u226F", ngtr: "\u226F", nGtv: "\u226B\u0338", nharr: "\u21AE", nhArr: "\u21CE", nhpar: "\u2AF2", ni: "\u220B", nis: "\u22FC", nisd: "\u22FA", niv: "\u220B", NJcy: "\u040A", njcy: "\u045A", nlarr: "\u219A", nlArr: "\u21CD", nldr: "\u2025", nlE: "\u2266\u0338", nle: "\u2270", nleftarrow: "\u219A", nLeftarrow: "\u21CD", nleftrightarrow: "\u21AE", nLeftrightarrow: "\u21CE", nleq: "\u2270", nleqq: "\u2266\u0338", nleqslant: "\u2A7D\u0338", nles: "\u2A7D\u0338", nless: "\u226E", nLl: "\u22D8\u0338", nlsim: "\u2274", nLt: "\u226A\u20D2", nlt: "\u226E", nltri: "\u22EA", nltrie: "\u22EC", nLtv: "\u226A\u0338", nmid: "\u2224", NoBreak: "\u2060", NonBreakingSpace: "\xA0", nopf: "\u{1D55F}", Nopf: "\u2115", Not: "\u2AEC", not: "\xAC", NotCongruent: "\u2262", NotCupCap: "\u226D", NotDoubleVerticalBar: "\u2226", NotElement: "\u2209", NotEqual: "\u2260", NotEqualTilde: "\u2242\u0338", NotExists: "\u2204", NotGreater: "\u226F", NotGreaterEqual: "\u2271", NotGreaterFullEqual: "\u2267\u0338", NotGreaterGreater: "\u226B\u0338", NotGreaterLess: "\u2279", NotGreaterSlantEqual: "\u2A7E\u0338", NotGreaterTilde: "\u2275", NotHumpDownHump: "\u224E\u0338", NotHumpEqual: "\u224F\u0338", notin: "\u2209", notindot: "\u22F5\u0338", notinE: "\u22F9\u0338", notinva: "\u2209", notinvb: "\u22F7", notinvc: "\u22F6", NotLeftTriangleBar: "\u29CF\u0338", NotLeftTriangle: "\u22EA", NotLeftTriangleEqual: "\u22EC", NotLess: "\u226E", NotLessEqual: "\u2270", NotLessGreater: "\u2278", NotLessLess: "\u226A\u0338", NotLessSlantEqual: "\u2A7D\u0338", NotLessTilde: "\u2274", NotNestedGreaterGreater: "\u2AA2\u0338", NotNestedLessLess: "\u2AA1\u0338", notni: "\u220C", notniva: "\u220C", notnivb: "\u22FE", notnivc: "\u22FD", NotPrecedes: "\u2280", NotPrecedesEqual: "\u2AAF\u0338", NotPrecedesSlantEqual: "\u22E0", NotReverseElement: "\u220C", NotRightTriangleBar: "\u29D0\u0338", NotRightTriangle: "\u22EB", NotRightTriangleEqual: "\u22ED", NotSquareSubset: "\u228F\u0338", NotSquareSubsetEqual: "\u22E2", NotSquareSuperset: "\u2290\u0338", NotSquareSupersetEqual: "\u22E3", NotSubset: "\u2282\u20D2", NotSubsetEqual: "\u2288", NotSucceeds: "\u2281", NotSucceedsEqual: "\u2AB0\u0338", NotSucceedsSlantEqual: "\u22E1", NotSucceedsTilde: "\u227F\u0338", NotSuperset: "\u2283\u20D2", NotSupersetEqual: "\u2289", NotTilde: "\u2241", NotTildeEqual: "\u2244", NotTildeFullEqual: "\u2247", NotTildeTilde: "\u2249", NotVerticalBar: "\u2224", nparallel: "\u2226", npar: "\u2226", nparsl: "\u2AFD\u20E5", npart: "\u2202\u0338", npolint: "\u2A14", npr: "\u2280", nprcue: "\u22E0", nprec: "\u2280", npreceq: "\u2AAF\u0338", npre: "\u2AAF\u0338", nrarrc: "\u2933\u0338", nrarr: "\u219B", nrArr: "\u21CF", nrarrw: "\u219D\u0338", nrightarrow: "\u219B", nRightarrow: "\u21CF", nrtri: "\u22EB", nrtrie: "\u22ED", nsc: "\u2281", nsccue: "\u22E1", nsce: "\u2AB0\u0338", Nscr: "\u{1D4A9}", nscr: "\u{1D4C3}", nshortmid: "\u2224", nshortparallel: "\u2226", nsim: "\u2241", nsime: "\u2244", nsimeq: "\u2244", nsmid: "\u2224", nspar: "\u2226", nsqsube: "\u22E2", nsqsupe: "\u22E3", nsub: "\u2284", nsubE: "\u2AC5\u0338", nsube: "\u2288", nsubset: "\u2282\u20D2", nsubseteq: "\u2288", nsubseteqq: "\u2AC5\u0338", nsucc: "\u2281", nsucceq: "\u2AB0\u0338", nsup: "\u2285", nsupE: "\u2AC6\u0338", nsupe: "\u2289", nsupset: "\u2283\u20D2", nsupseteq: "\u2289", nsupseteqq: "\u2AC6\u0338", ntgl: "\u2279", Ntilde: "\xD1", ntilde: "\xF1", ntlg: "\u2278", ntriangleleft: "\u22EA", ntrianglelefteq: "\u22EC", ntriangleright: "\u22EB", ntrianglerighteq: "\u22ED", Nu: "\u039D", nu: "\u03BD", num: "#", numero: "\u2116", numsp: "\u2007", nvap: "\u224D\u20D2", nvdash: "\u22AC", nvDash: "\u22AD", nVdash: "\u22AE", nVDash: "\u22AF", nvge: "\u2265\u20D2", nvgt: ">\u20D2", nvHarr: "\u2904", nvinfin: "\u29DE", nvlArr: "\u2902", nvle: "\u2264\u20D2", nvlt: "<\u20D2", nvltrie: "\u22B4\u20D2", nvrArr: "\u2903", nvrtrie: "\u22B5\u20D2", nvsim: "\u223C\u20D2", nwarhk: "\u2923", nwarr: "\u2196", nwArr: "\u21D6", nwarrow: "\u2196", nwnear: "\u2927", Oacute: "\xD3", oacute: "\xF3", oast: "\u229B", Ocirc: "\xD4", ocirc: "\xF4", ocir: "\u229A", Ocy: "\u041E", ocy: "\u043E", odash: "\u229D", Odblac: "\u0150", odblac: "\u0151", odiv: "\u2A38", odot: "\u2299", odsold: "\u29BC", OElig: "\u0152", oelig: "\u0153", ofcir: "\u29BF", Ofr: "\u{1D512}", ofr: "\u{1D52C}", ogon: "\u02DB", Ograve: "\xD2", ograve: "\xF2", ogt: "\u29C1", ohbar: "\u29B5", ohm: "\u03A9", oint: "\u222E", olarr: "\u21BA", olcir: "\u29BE", olcross: "\u29BB", oline: "\u203E", olt: "\u29C0", Omacr: "\u014C", omacr: "\u014D", Omega: "\u03A9", omega: "\u03C9", Omicron: "\u039F", omicron: "\u03BF", omid: "\u29B6", ominus: "\u2296", Oopf: "\u{1D546}", oopf: "\u{1D560}", opar: "\u29B7", OpenCurlyDoubleQuote: "\u201C", OpenCurlyQuote: "\u2018", operp: "\u29B9", oplus: "\u2295", orarr: "\u21BB", Or: "\u2A54", or: "\u2228", ord: "\u2A5D", order: "\u2134", orderof: "\u2134", ordf: "\xAA", ordm: "\xBA", origof: "\u22B6", oror: "\u2A56", orslope: "\u2A57", orv: "\u2A5B", oS: "\u24C8", Oscr: "\u{1D4AA}", oscr: "\u2134", Oslash: "\xD8", oslash: "\xF8", osol: "\u2298", Otilde: "\xD5", otilde: "\xF5", otimesas: "\u2A36", Otimes: "\u2A37", otimes: "\u2297", Ouml: "\xD6", ouml: "\xF6", ovbar: "\u233D", OverBar: "\u203E", OverBrace: "\u23DE", OverBracket: "\u23B4", OverParenthesis: "\u23DC", para: "\xB6", parallel: "\u2225", par: "\u2225", parsim: "\u2AF3", parsl: "\u2AFD", part: "\u2202", PartialD: "\u2202", Pcy: "\u041F", pcy: "\u043F", percnt: "%", period: ".", permil: "\u2030", perp: "\u22A5", pertenk: "\u2031", Pfr: "\u{1D513}", pfr: "\u{1D52D}", Phi: "\u03A6", phi: "\u03C6", phiv: "\u03D5", phmmat: "\u2133", phone: "\u260E", Pi: "\u03A0", pi: "\u03C0", pitchfork: "\u22D4", piv: "\u03D6", planck: "\u210F", planckh: "\u210E", plankv: "\u210F", plusacir: "\u2A23", plusb: "\u229E", pluscir: "\u2A22", plus: "+", plusdo: "\u2214", plusdu: "\u2A25", pluse: "\u2A72", PlusMinus: "\xB1", plusmn: "\xB1", plussim: "\u2A26", plustwo: "\u2A27", pm: "\xB1", Poincareplane: "\u210C", pointint: "\u2A15", popf: "\u{1D561}", Popf: "\u2119", pound: "\xA3", prap: "\u2AB7", Pr: "\u2ABB", pr: "\u227A", prcue: "\u227C", precapprox: "\u2AB7", prec: "\u227A", preccurlyeq: "\u227C", Precedes: "\u227A", PrecedesEqual: "\u2AAF", PrecedesSlantEqual: "\u227C", PrecedesTilde: "\u227E", preceq: "\u2AAF", precnapprox: "\u2AB9", precneqq: "\u2AB5", precnsim: "\u22E8", pre: "\u2AAF", prE: "\u2AB3", precsim: "\u227E", prime: "\u2032", Prime: "\u2033", primes: "\u2119", prnap: "\u2AB9", prnE: "\u2AB5", prnsim: "\u22E8", prod: "\u220F", Product: "\u220F", profalar: "\u232E", profline: "\u2312", profsurf: "\u2313", prop: "\u221D", Proportional: "\u221D", Proportion: "\u2237", propto: "\u221D", prsim: "\u227E", prurel: "\u22B0", Pscr: "\u{1D4AB}", pscr: "\u{1D4C5}", Psi: "\u03A8", psi: "\u03C8", puncsp: "\u2008", Qfr: "\u{1D514}", qfr: "\u{1D52E}", qint: "\u2A0C", qopf: "\u{1D562}", Qopf: "\u211A", qprime: "\u2057", Qscr: "\u{1D4AC}", qscr: "\u{1D4C6}", quaternions: "\u210D", quatint: "\u2A16", quest: "?", questeq: "\u225F", quot: '"', QUOT: '"', rAarr: "\u21DB", race: "\u223D\u0331", Racute: "\u0154", racute: "\u0155", radic: "\u221A", raemptyv: "\u29B3", rang: "\u27E9", Rang: "\u27EB", rangd: "\u2992", range: "\u29A5", rangle: "\u27E9", raquo: "\xBB", rarrap: "\u2975", rarrb: "\u21E5", rarrbfs: "\u2920", rarrc: "\u2933", rarr: "\u2192", Rarr: "\u21A0", rArr: "\u21D2", rarrfs: "\u291E", rarrhk: "\u21AA", rarrlp: "\u21AC", rarrpl: "\u2945", rarrsim: "\u2974", Rarrtl: "\u2916", rarrtl: "\u21A3", rarrw: "\u219D", ratail: "\u291A", rAtail: "\u291C", ratio: "\u2236", rationals: "\u211A", rbarr: "\u290D", rBarr: "\u290F", RBarr: "\u2910", rbbrk: "\u2773", rbrace: "}", rbrack: "]", rbrke: "\u298C", rbrksld: "\u298E", rbrkslu: "\u2990", Rcaron: "\u0158", rcaron: "\u0159", Rcedil: "\u0156", rcedil: "\u0157", rceil: "\u2309", rcub: "}", Rcy: "\u0420", rcy: "\u0440", rdca: "\u2937", rdldhar: "\u2969", rdquo: "\u201D", rdquor: "\u201D", rdsh: "\u21B3", real: "\u211C", realine: "\u211B", realpart: "\u211C", reals: "\u211D", Re: "\u211C", rect: "\u25AD", reg: "\xAE", REG: "\xAE", ReverseElement: "\u220B", ReverseEquilibrium: "\u21CB", ReverseUpEquilibrium: "\u296F", rfisht: "\u297D", rfloor: "\u230B", rfr: "\u{1D52F}", Rfr: "\u211C", rHar: "\u2964", rhard: "\u21C1", rharu: "\u21C0", rharul: "\u296C", Rho: "\u03A1", rho: "\u03C1", rhov: "\u03F1", RightAngleBracket: "\u27E9", RightArrowBar: "\u21E5", rightarrow: "\u2192", RightArrow: "\u2192", Rightarrow: "\u21D2", RightArrowLeftArrow: "\u21C4", rightarrowtail: "\u21A3", RightCeiling: "\u2309", RightDoubleBracket: "\u27E7", RightDownTeeVector: "\u295D", RightDownVectorBar: "\u2955", RightDownVector: "\u21C2", RightFloor: "\u230B", rightharpoondown: "\u21C1", rightharpoonup: "\u21C0", rightleftarrows: "\u21C4", rightleftharpoons: "\u21CC", rightrightarrows: "\u21C9", rightsquigarrow: "\u219D", RightTeeArrow: "\u21A6", RightTee: "\u22A2", RightTeeVector: "\u295B", rightthreetimes: "\u22CC", RightTriangleBar: "\u29D0", RightTriangle: "\u22B3", RightTriangleEqual: "\u22B5", RightUpDownVector: "\u294F", RightUpTeeVector: "\u295C", RightUpVectorBar: "\u2954", RightUpVector: "\u21BE", RightVectorBar: "\u2953", RightVector: "\u21C0", ring: "\u02DA", risingdotseq: "\u2253", rlarr: "\u21C4", rlhar: "\u21CC", rlm: "\u200F", rmoustache: "\u23B1", rmoust: "\u23B1", rnmid: "\u2AEE", roang: "\u27ED", roarr: "\u21FE", robrk: "\u27E7", ropar: "\u2986", ropf: "\u{1D563}", Ropf: "\u211D", roplus: "\u2A2E", rotimes: "\u2A35", RoundImplies: "\u2970", rpar: ")", rpargt: "\u2994", rppolint: "\u2A12", rrarr: "\u21C9", Rrightarrow: "\u21DB", rsaquo: "\u203A", rscr: "\u{1D4C7}", Rscr: "\u211B", rsh: "\u21B1", Rsh: "\u21B1", rsqb: "]", rsquo: "\u2019", rsquor: "\u2019", rthree: "\u22CC", rtimes: "\u22CA", rtri: "\u25B9", rtrie: "\u22B5", rtrif: "\u25B8", rtriltri: "\u29CE", RuleDelayed: "\u29F4", ruluhar: "\u2968", rx: "\u211E", Sacute: "\u015A", sacute: "\u015B", sbquo: "\u201A", scap: "\u2AB8", Scaron: "\u0160", scaron: "\u0161", Sc: "\u2ABC", sc: "\u227B", sccue: "\u227D", sce: "\u2AB0", scE: "\u2AB4", Scedil: "\u015E", scedil: "\u015F", Scirc: "\u015C", scirc: "\u015D", scnap: "\u2ABA", scnE: "\u2AB6", scnsim: "\u22E9", scpolint: "\u2A13", scsim: "\u227F", Scy: "\u0421", scy: "\u0441", sdotb: "\u22A1", sdot: "\u22C5", sdote: "\u2A66", searhk: "\u2925", searr: "\u2198", seArr: "\u21D8", searrow: "\u2198", sect: "\xA7", semi: ";", seswar: "\u2929", setminus: "\u2216", setmn: "\u2216", sext: "\u2736", Sfr: "\u{1D516}", sfr: "\u{1D530}", sfrown: "\u2322", sharp: "\u266F", SHCHcy: "\u0429", shchcy: "\u0449", SHcy: "\u0428", shcy: "\u0448", ShortDownArrow: "\u2193", ShortLeftArrow: "\u2190", shortmid: "\u2223", shortparallel: "\u2225", ShortRightArrow: "\u2192", ShortUpArrow: "\u2191", shy: "\xAD", Sigma: "\u03A3", sigma: "\u03C3", sigmaf: "\u03C2", sigmav: "\u03C2", sim: "\u223C", simdot: "\u2A6A", sime: "\u2243", simeq: "\u2243", simg: "\u2A9E", simgE: "\u2AA0", siml: "\u2A9D", simlE: "\u2A9F", simne: "\u2246", simplus: "\u2A24", simrarr: "\u2972", slarr: "\u2190", SmallCircle: "\u2218", smallsetminus: "\u2216", smashp: "\u2A33", smeparsl: "\u29E4", smid: "\u2223", smile: "\u2323", smt: "\u2AAA", smte: "\u2AAC", smtes: "\u2AAC\uFE00", SOFTcy: "\u042C", softcy: "\u044C", solbar: "\u233F", solb: "\u29C4", sol: "/", Sopf: "\u{1D54A}", sopf: "\u{1D564}", spades: "\u2660", spadesuit: "\u2660", spar: "\u2225", sqcap: "\u2293", sqcaps: "\u2293\uFE00", sqcup: "\u2294", sqcups: "\u2294\uFE00", Sqrt: "\u221A", sqsub: "\u228F", sqsube: "\u2291", sqsubset: "\u228F", sqsubseteq: "\u2291", sqsup: "\u2290", sqsupe: "\u2292", sqsupset: "\u2290", sqsupseteq: "\u2292", square: "\u25A1", Square: "\u25A1", SquareIntersection: "\u2293", SquareSubset: "\u228F", SquareSubsetEqual: "\u2291", SquareSuperset: "\u2290", SquareSupersetEqual: "\u2292", SquareUnion: "\u2294", squarf: "\u25AA", squ: "\u25A1", squf: "\u25AA", srarr: "\u2192", Sscr: "\u{1D4AE}", sscr: "\u{1D4C8}", ssetmn: "\u2216", ssmile: "\u2323", sstarf: "\u22C6", Star: "\u22C6", star: "\u2606", starf: "\u2605", straightepsilon: "\u03F5", straightphi: "\u03D5", strns: "\xAF", sub: "\u2282", Sub: "\u22D0", subdot: "\u2ABD", subE: "\u2AC5", sube: "\u2286", subedot: "\u2AC3", submult: "\u2AC1", subnE: "\u2ACB", subne: "\u228A", subplus: "\u2ABF", subrarr: "\u2979", subset: "\u2282", Subset: "\u22D0", subseteq: "\u2286", subseteqq: "\u2AC5", SubsetEqual: "\u2286", subsetneq: "\u228A", subsetneqq: "\u2ACB", subsim: "\u2AC7", subsub: "\u2AD5", subsup: "\u2AD3", succapprox: "\u2AB8", succ: "\u227B", succcurlyeq: "\u227D", Succeeds: "\u227B", SucceedsEqual: "\u2AB0", SucceedsSlantEqual: "\u227D", SucceedsTilde: "\u227F", succeq: "\u2AB0", succnapprox: "\u2ABA", succneqq: "\u2AB6", succnsim: "\u22E9", succsim: "\u227F", SuchThat: "\u220B", sum: "\u2211", Sum: "\u2211", sung: "\u266A", sup1: "\xB9", sup2: "\xB2", sup3: "\xB3", sup: "\u2283", Sup: "\u22D1", supdot: "\u2ABE", supdsub: "\u2AD8", supE: "\u2AC6", supe: "\u2287", supedot: "\u2AC4", Superset: "\u2283", SupersetEqual: "\u2287", suphsol: "\u27C9", suphsub: "\u2AD7", suplarr: "\u297B", supmult: "\u2AC2", supnE: "\u2ACC", supne: "\u228B", supplus: "\u2AC0", supset: "\u2283", Supset: "\u22D1", supseteq: "\u2287", supseteqq: "\u2AC6", supsetneq: "\u228B", supsetneqq: "\u2ACC", supsim: "\u2AC8", supsub: "\u2AD4", supsup: "\u2AD6", swarhk: "\u2926", swarr: "\u2199", swArr: "\u21D9", swarrow: "\u2199", swnwar: "\u292A", szlig: "\xDF", Tab: " ", target: "\u2316", Tau: "\u03A4", tau: "\u03C4", tbrk: "\u23B4", Tcaron: "\u0164", tcaron: "\u0165", Tcedil: "\u0162", tcedil: "\u0163", Tcy: "\u0422", tcy: "\u0442", tdot: "\u20DB", telrec: "\u2315", Tfr: "\u{1D517}", tfr: "\u{1D531}", there4: "\u2234", therefore: "\u2234", Therefore: "\u2234", Theta: "\u0398", theta: "\u03B8", thetasym: "\u03D1", thetav: "\u03D1", thickapprox: "\u2248", thicksim: "\u223C", ThickSpace: "\u205F\u200A", ThinSpace: "\u2009", thinsp: "\u2009", thkap: "\u2248", thksim: "\u223C", THORN: "\xDE", thorn: "\xFE", tilde: "\u02DC", Tilde: "\u223C", TildeEqual: "\u2243", TildeFullEqual: "\u2245", TildeTilde: "\u2248", timesbar: "\u2A31", timesb: "\u22A0", times: "\xD7", timesd: "\u2A30", tint: "\u222D", toea: "\u2928", topbot: "\u2336", topcir: "\u2AF1", top: "\u22A4", Topf: "\u{1D54B}", topf: "\u{1D565}", topfork: "\u2ADA", tosa: "\u2929", tprime: "\u2034", trade: "\u2122", TRADE: "\u2122", triangle: "\u25B5", triangledown: "\u25BF", triangleleft: "\u25C3", trianglelefteq: "\u22B4", triangleq: "\u225C", triangleright: "\u25B9", trianglerighteq: "\u22B5", tridot: "\u25EC", trie: "\u225C", triminus: "\u2A3A", TripleDot: "\u20DB", triplus: "\u2A39", trisb: "\u29CD", tritime: "\u2A3B", trpezium: "\u23E2", Tscr: "\u{1D4AF}", tscr: "\u{1D4C9}", TScy: "\u0426", tscy: "\u0446", TSHcy: "\u040B", tshcy: "\u045B", Tstrok: "\u0166", tstrok: "\u0167", twixt: "\u226C", twoheadleftarrow: "\u219E", twoheadrightarrow: "\u21A0", Uacute: "\xDA", uacute: "\xFA", uarr: "\u2191", Uarr: "\u219F", uArr: "\u21D1", Uarrocir: "\u2949", Ubrcy: "\u040E", ubrcy: "\u045E", Ubreve: "\u016C", ubreve: "\u016D", Ucirc: "\xDB", ucirc: "\xFB", Ucy: "\u0423", ucy: "\u0443", udarr: "\u21C5", Udblac: "\u0170", udblac: "\u0171", udhar: "\u296E", ufisht: "\u297E", Ufr: "\u{1D518}", ufr: "\u{1D532}", Ugrave: "\xD9", ugrave: "\xF9", uHar: "\u2963", uharl: "\u21BF", uharr: "\u21BE", uhblk: "\u2580", ulcorn: "\u231C", ulcorner: "\u231C", ulcrop: "\u230F", ultri: "\u25F8", Umacr: "\u016A", umacr: "\u016B", uml: "\xA8", UnderBar: "_", UnderBrace: "\u23DF", UnderBracket: "\u23B5", UnderParenthesis: "\u23DD", Union: "\u22C3", UnionPlus: "\u228E", Uogon: "\u0172", uogon: "\u0173", Uopf: "\u{1D54C}", uopf: "\u{1D566}", UpArrowBar: "\u2912", uparrow: "\u2191", UpArrow: "\u2191", Uparrow: "\u21D1", UpArrowDownArrow: "\u21C5", updownarrow: "\u2195", UpDownArrow: "\u2195", Updownarrow: "\u21D5", UpEquilibrium: "\u296E", upharpoonleft: "\u21BF", upharpoonright: "\u21BE", uplus: "\u228E", UpperLeftArrow: "\u2196", UpperRightArrow: "\u2197", upsi: "\u03C5", Upsi: "\u03D2", upsih: "\u03D2", Upsilon: "\u03A5", upsilon: "\u03C5", UpTeeArrow: "\u21A5", UpTee: "\u22A5", upuparrows: "\u21C8", urcorn: "\u231D", urcorner: "\u231D", urcrop: "\u230E", Uring: "\u016E", uring: "\u016F", urtri: "\u25F9", Uscr: "\u{1D4B0}", uscr: "\u{1D4CA}", utdot: "\u22F0", Utilde: "\u0168", utilde: "\u0169", utri: "\u25B5", utrif: "\u25B4", uuarr: "\u21C8", Uuml: "\xDC", uuml: "\xFC", uwangle: "\u29A7", vangrt: "\u299C", varepsilon: "\u03F5", varkappa: "\u03F0", varnothing: "\u2205", varphi: "\u03D5", varpi: "\u03D6", varpropto: "\u221D", varr: "\u2195", vArr: "\u21D5", varrho: "\u03F1", varsigma: "\u03C2", varsubsetneq: "\u228A\uFE00", varsubsetneqq: "\u2ACB\uFE00", varsupsetneq: "\u228B\uFE00", varsupsetneqq: "\u2ACC\uFE00", vartheta: "\u03D1", vartriangleleft: "\u22B2", vartriangleright: "\u22B3", vBar: "\u2AE8", Vbar: "\u2AEB", vBarv: "\u2AE9", Vcy: "\u0412", vcy: "\u0432", vdash: "\u22A2", vDash: "\u22A8", Vdash: "\u22A9", VDash: "\u22AB", Vdashl: "\u2AE6", veebar: "\u22BB", vee: "\u2228", Vee: "\u22C1", veeeq: "\u225A", vellip: "\u22EE", verbar: "|", Verbar: "\u2016", vert: "|", Vert: "\u2016", VerticalBar: "\u2223", VerticalLine: "|", VerticalSeparator: "\u2758", VerticalTilde: "\u2240", VeryThinSpace: "\u200A", Vfr: "\u{1D519}", vfr: "\u{1D533}", vltri: "\u22B2", vnsub: "\u2282\u20D2", vnsup: "\u2283\u20D2", Vopf: "\u{1D54D}", vopf: "\u{1D567}", vprop: "\u221D", vrtri: "\u22B3", Vscr: "\u{1D4B1}", vscr: "\u{1D4CB}", vsubnE: "\u2ACB\uFE00", vsubne: "\u228A\uFE00", vsupnE: "\u2ACC\uFE00", vsupne: "\u228B\uFE00", Vvdash: "\u22AA", vzigzag: "\u299A", Wcirc: "\u0174", wcirc: "\u0175", wedbar: "\u2A5F", wedge: "\u2227", Wedge: "\u22C0", wedgeq: "\u2259", weierp: "\u2118", Wfr: "\u{1D51A}", wfr: "\u{1D534}", Wopf: "\u{1D54E}", wopf: "\u{1D568}", wp: "\u2118", wr: "\u2240", wreath: "\u2240", Wscr: "\u{1D4B2}", wscr: "\u{1D4CC}", xcap: "\u22C2", xcirc: "\u25EF", xcup: "\u22C3", xdtri: "\u25BD", Xfr: "\u{1D51B}", xfr: "\u{1D535}", xharr: "\u27F7", xhArr: "\u27FA", Xi: "\u039E", xi: "\u03BE", xlarr: "\u27F5", xlArr: "\u27F8", xmap: "\u27FC", xnis: "\u22FB", xodot: "\u2A00", Xopf: "\u{1D54F}", xopf: "\u{1D569}", xoplus: "\u2A01", xotime: "\u2A02", xrarr: "\u27F6", xrArr: "\u27F9", Xscr: "\u{1D4B3}", xscr: "\u{1D4CD}", xsqcup: "\u2A06", xuplus: "\u2A04", xutri: "\u25B3", xvee: "\u22C1", xwedge: "\u22C0", Yacute: "\xDD", yacute: "\xFD", YAcy: "\u042F", yacy: "\u044F", Ycirc: "\u0176", ycirc: "\u0177", Ycy: "\u042B", ycy: "\u044B", yen: "\xA5", Yfr: "\u{1D51C}", yfr: "\u{1D536}", YIcy: "\u0407", yicy: "\u0457", Yopf: "\u{1D550}", yopf: "\u{1D56A}", Yscr: "\u{1D4B4}", yscr: "\u{1D4CE}", YUcy: "\u042E", yucy: "\u044E", yuml: "\xFF", Yuml: "\u0178", Zacute: "\u0179", zacute: "\u017A", Zcaron: "\u017D", zcaron: "\u017E", Zcy: "\u0417", zcy: "\u0437", Zdot: "\u017B", zdot: "\u017C", zeetrf: "\u2128", ZeroWidthSpace: "\u200B", Zeta: "\u0396", zeta: "\u03B6", zfr: "\u{1D537}", Zfr: "\u2128", ZHcy: "\u0416", zhcy: "\u0436", zigrarr: "\u21DD", zopf: "\u{1D56B}", Zopf: "\u2124", Zscr: "\u{1D4B5}", zscr: "\u{1D4CF}", zwj: "\u200D", zwnj: "\u200C" };
}
});
// node_modules/entities/lib/maps/legacy.json
var require_legacy = __commonJS({
"node_modules/entities/lib/maps/legacy.json"(exports, module2) {
module2.exports = { Aacute: "\xC1", aacute: "\xE1", Acirc: "\xC2", acirc: "\xE2", acute: "\xB4", AElig: "\xC6", aelig: "\xE6", Agrave: "\xC0", agrave: "\xE0", amp: "&", AMP: "&", Aring: "\xC5", aring: "\xE5", Atilde: "\xC3", atilde: "\xE3", Auml: "\xC4", auml: "\xE4", brvbar: "\xA6", Ccedil: "\xC7", ccedil: "\xE7", cedil: "\xB8", cent: "\xA2", copy: "\xA9", COPY: "\xA9", curren: "\xA4", deg: "\xB0", divide: "\xF7", Eacute: "\xC9", eacute: "\xE9", Ecirc: "\xCA", ecirc: "\xEA", Egrave: "\xC8", egrave: "\xE8", ETH: "\xD0", eth: "\xF0", Euml: "\xCB", euml: "\xEB", frac12: "\xBD", frac14: "\xBC", frac34: "\xBE", gt: ">", GT: ">", Iacute: "\xCD", iacute: "\xED", Icirc: "\xCE", icirc: "\xEE", iexcl: "\xA1", Igrave: "\xCC", igrave: "\xEC", iquest: "\xBF", Iuml: "\xCF", iuml: "\xEF", laquo: "\xAB", lt: "<", LT: "<", macr: "\xAF", micro: "\xB5", middot: "\xB7", nbsp: "\xA0", not: "\xAC", Ntilde: "\xD1", ntilde: "\xF1", Oacute: "\xD3", oacute: "\xF3", Ocirc: "\xD4", ocirc: "\xF4", Ograve: "\xD2", ograve: "\xF2", ordf: "\xAA", ordm: "\xBA", Oslash: "\xD8", oslash: "\xF8", Otilde: "\xD5", otilde: "\xF5", Ouml: "\xD6", ouml: "\xF6", para: "\xB6", plusmn: "\xB1", pound: "\xA3", quot: '"', QUOT: '"', raquo: "\xBB", reg: "\xAE", REG: "\xAE", sect: "\xA7", shy: "\xAD", sup1: "\xB9", sup2: "\xB2", sup3: "\xB3", szlig: "\xDF", THORN: "\xDE", thorn: "\xFE", times: "\xD7", Uacute: "\xDA", uacute: "\xFA", Ucirc: "\xDB", ucirc: "\xFB", Ugrave: "\xD9", ugrave: "\xF9", uml: "\xA8", Uuml: "\xDC", uuml: "\xFC", Yacute: "\xDD", yacute: "\xFD", yen: "\xA5", yuml: "\xFF" };
}
});
// node_modules/entities/lib/maps/xml.json
var require_xml = __commonJS({
"node_modules/entities/lib/maps/xml.json"(exports, module2) {
module2.exports = { amp: "&", apos: "'", gt: ">", lt: "<", quot: '"' };
}
});
// node_modules/entities/lib/maps/decode.json
var require_decode = __commonJS({
"node_modules/entities/lib/maps/decode.json"(exports, module2) {
module2.exports = { "0": 65533, "128": 8364, "130": 8218, "131": 402, "132": 8222, "133": 8230, "134": 8224, "135": 8225, "136": 710, "137": 8240, "138": 352, "139": 8249, "140": 338, "142": 381, "145": 8216, "146": 8217, "147": 8220, "148": 8221, "149": 8226, "150": 8211, "151": 8212, "152": 732, "153": 8482, "154": 353, "155": 8250, "156": 339, "158": 382, "159": 376 };
}
});
// node_modules/entities/lib/decode_codepoint.js
var require_decode_codepoint = __commonJS({
"node_modules/entities/lib/decode_codepoint.js"(exports) {
"use strict";
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
var decode_json_1 = __importDefault(require_decode());
var fromCodePoint = String.fromCodePoint || function(codePoint) {
var output = "";
if (codePoint > 65535) {
codePoint -= 65536;
output += String.fromCharCode(codePoint >>> 10 & 1023 | 55296);
codePoint = 56320 | codePoint & 1023;
}
output += String.fromCharCode(codePoint);
return output;
};
function decodeCodePoint(codePoint) {
if (codePoint >= 55296 && codePoint <= 57343 || codePoint > 1114111) {
return "\uFFFD";
}
if (codePoint in decode_json_1.default) {
codePoint = decode_json_1.default[codePoint];
}
return fromCodePoint(codePoint);
}
exports.default = decodeCodePoint;
}
});
// node_modules/entities/lib/decode.js
var require_decode2 = __commonJS({
"node_modules/entities/lib/decode.js"(exports) {
"use strict";
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.decodeHTML = exports.decodeHTMLStrict = exports.decodeXML = void 0;
var entities_json_1 = __importDefault(require_entities());
var legacy_json_1 = __importDefault(require_legacy());
var xml_json_1 = __importDefault(require_xml());
var decode_codepoint_1 = __importDefault(require_decode_codepoint());
var strictEntityRe = /&(?:[a-zA-Z0-9]+|#[xX][\da-fA-F]+|#\d+);/g;
exports.decodeXML = getStrictDecoder(xml_json_1.default);
exports.decodeHTMLStrict = getStrictDecoder(entities_json_1.default);
function getStrictDecoder(map) {
var replace = getReplacer(map);
return function(str) {
return String(str).replace(strictEntityRe, replace);
};
}
var sorter = function(a, b) {
return a < b ? 1 : -1;
};
exports.decodeHTML = function() {
var legacy = Object.keys(legacy_json_1.default).sort(sorter);
var keys = Object.keys(entities_json_1.default).sort(sorter);
for (var i = 0, j = 0; i < keys.length; i++) {
if (legacy[j] === keys[i]) {
keys[i] += ";?";
j++;
} else {
keys[i] += ";";
}
}
var re = new RegExp("&(?:" + keys.join("|") + "|#[xX][\\da-fA-F]+;?|#\\d+;?)", "g");
var replace = getReplacer(entities_json_1.default);
function replacer(str) {
if (str.substr(-1) !== ";")
str += ";";
return replace(str);
}
return function(str) {
return String(str).replace(re, replacer);
};
}();
function getReplacer(map) {
return function replace(str) {
if (str.charAt(1) === "#") {
var secondChar = str.charAt(2);
if (secondChar === "X" || secondChar === "x") {
return decode_codepoint_1.default(parseInt(str.substr(3), 16));
}
return decode_codepoint_1.default(parseInt(str.substr(2), 10));
}
return map[str.slice(1, -1)] || str;
};
}
}
});
// node_modules/entities/lib/encode.js
var require_encode = __commonJS({
"node_modules/entities/lib/encode.js"(exports) {
"use strict";
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.escapeUTF8 = exports.escape = exports.encodeNonAsciiHTML = exports.encodeHTML = exports.encodeXML = void 0;
var xml_json_1 = __importDefault(require_xml());
var inverseXML = getInverseObj(xml_json_1.default);
var xmlReplacer = getInverseReplacer(inverseXML);
exports.encodeXML = getASCIIEncoder(inverseXML);
var entities_json_1 = __importDefault(require_entities());
var inverseHTML = getInverseObj(entities_json_1.default);
var htmlReplacer = getInverseReplacer(inverseHTML);
exports.encodeHTML = getInverse(inverseHTML, htmlReplacer);
exports.encodeNonAsciiHTML = getASCIIEncoder(inverseHTML);
function getInverseObj(obj) {
return Object.keys(obj).sort().reduce(function(inverse, name) {
inverse[obj[name]] = "&" + name + ";";
return inverse;
}, {});
}
function getInverseReplacer(inverse) {
var single = [];
var multiple = [];
for (var _i = 0, _a = Object.keys(inverse); _i < _a.length; _i++) {
var k = _a[_i];
if (k.length === 1) {
single.push("\\" + k);
} else {
multiple.push(k);
}
}
single.sort();
for (var start = 0; start < single.length - 1; start++) {
var end = start;
while (end < single.length - 1 && single[end].charCodeAt(1) + 1 === single[end + 1].charCodeAt(1)) {
end += 1;
}
var count = 1 + end - start;
if (count < 3)
continue;
single.splice(start, count, single[start] + "-" + single[end]);
}
multiple.unshift("[" + single.join("") + "]");
return new RegExp(multiple.join("|"), "g");
}
var reNonASCII = /(?:[\x80-\uD7FF\uE000-\uFFFF]|[\uD800-\uDBFF][\uDC00-\uDFFF]|[\uD800-\uDBFF](?![\uDC00-\uDFFF])|(?:[^\uD800-\uDBFF]|^)[\uDC00-\uDFFF])/g;
var getCodePoint = String.prototype.codePointAt != null ? function(str) {
return str.codePointAt(0);
} : function(c) {
return (c.charCodeAt(0) - 55296) * 1024 + c.charCodeAt(1) - 56320 + 65536;
};
function singleCharReplacer(c) {
return "" + (c.length > 1 ? getCodePoint(c) : c.charCodeAt(0)).toString(16).toUpperCase() + ";";
}
function getInverse(inverse, re) {
return function(data) {
return data.replace(re, function(name) {
return inverse[name];
}).replace(reNonASCII, singleCharReplacer);
};
}
var reEscapeChars = new RegExp(xmlReplacer.source + "|" + reNonASCII.source, "g");
function escape(data) {
return data.replace(reEscapeChars, singleCharReplacer);
}
exports.escape = escape;
function escapeUTF8(data) {
return data.replace(xmlReplacer, singleCharReplacer);
}
exports.escapeUTF8 = escapeUTF8;
function getASCIIEncoder(obj) {
return function(data) {
return data.replace(reEscapeChars, function(c) {
return obj[c] || singleCharReplacer(c);
});
};
}
}
});
// node_modules/entities/lib/index.js
var require_lib3 = __commonJS({
"node_modules/entities/lib/index.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.decodeXMLStrict = exports.decodeHTML5Strict = exports.decodeHTML4Strict = exports.decodeHTML5 = exports.decodeHTML4 = exports.decodeHTMLStrict = exports.decodeHTML = exports.decodeXML = exports.encodeHTML5 = exports.encodeHTML4 = exports.escapeUTF8 = exports.escape = exports.encodeNonAsciiHTML = exports.encodeHTML = exports.encodeXML = exports.encode = exports.decodeStrict = exports.decode = void 0;
var decode_1 = require_decode2();
var encode_1 = require_encode();
function decode(data, level) {
return (!level || level <= 0 ? decode_1.decodeXML : decode_1.decodeHTML)(data);
}
exports.decode = decode;
function decodeStrict(data, level) {
return (!level || level <= 0 ? decode_1.decodeXML : decode_1.decodeHTMLStrict)(data);
}
exports.decodeStrict = decodeStrict;
function encode2(data, level) {
return (!level || level <= 0 ? encode_1.encodeXML : encode_1.encodeHTML)(data);
}
exports.encode = encode2;
var encode_2 = require_encode();
Object.defineProperty(exports, "encodeXML", { enumerable: true, get: function() {
return encode_2.encodeXML;
} });
Object.defineProperty(exports, "encodeHTML", { enumerable: true, get: function() {
return encode_2.encodeHTML;
} });
Object.defineProperty(exports, "encodeNonAsciiHTML", { enumerable: true, get: function() {
return encode_2.encodeNonAsciiHTML;
} });
Object.defineProperty(exports, "escape", { enumerable: true, get: function() {
return encode_2.escape;
} });
Object.defineProperty(exports, "escapeUTF8", { enumerable: true, get: function() {
return encode_2.escapeUTF8;
} });
Object.defineProperty(exports, "encodeHTML4", { enumerable: true, get: function() {
return encode_2.encodeHTML;
} });
Object.defineProperty(exports, "encodeHTML5", { enumerable: true, get: function() {
return encode_2.encodeHTML;
} });
var decode_2 = require_decode2();
Object.defineProperty(exports, "decodeXML", { enumerable: true, get: function() {
return decode_2.decodeXML;
} });
Object.defineProperty(exports, "decodeHTML", { enumerable: true, get: function() {
return decode_2.decodeHTML;
} });
Object.defineProperty(exports, "decodeHTMLStrict", { enumerable: true, get: function() {
return decode_2.decodeHTMLStrict;
} });
Object.defineProperty(exports, "decodeHTML4", { enumerable: true, get: function() {
return decode_2.decodeHTML;
} });
Object.defineProperty(exports, "decodeHTML5", { enumerable: true, get: function() {
return decode_2.decodeHTML;
} });
Object.defineProperty(exports, "decodeHTML4Strict", { enumerable: true, get: function() {
return decode_2.decodeHTMLStrict;
} });
Object.defineProperty(exports, "decodeHTML5Strict", { enumerable: true, get: function() {
return decode_2.decodeHTMLStrict;
} });
Object.defineProperty(exports, "decodeXMLStrict", { enumerable: true, get: function() {
return decode_2.decodeXML;
} });
}
});
// node_modules/dom-serializer/lib/foreignNames.js
var require_foreignNames = __commonJS({
"node_modules/dom-serializer/lib/foreignNames.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.attributeNames = exports.elementNames = void 0;
exports.elementNames = /* @__PURE__ */ new Map([
["altglyph", "altGlyph"],
["altglyphdef", "altGlyphDef"],
["altglyphitem", "altGlyphItem"],
["animatecolor", "animateColor"],
["animatemotion", "animateMotion"],
["animatetransform", "animateTransform"],
["clippath", "clipPath"],
["feblend", "feBlend"],
["fecolormatrix", "feColorMatrix"],
["fecomponenttransfer", "feComponentTransfer"],
["fecomposite", "feComposite"],
["feconvolvematrix", "feConvolveMatrix"],
["fediffuselighting", "feDiffuseLighting"],
["fedisplacementmap", "feDisplacementMap"],
["fedistantlight", "feDistantLight"],
["fedropshadow", "feDropShadow"],
["feflood", "feFlood"],
["fefunca", "feFuncA"],
["fefuncb", "feFuncB"],
["fefuncg", "feFuncG"],
["fefuncr", "feFuncR"],
["fegaussianblur", "feGaussianBlur"],
["feimage", "feImage"],
["femerge", "feMerge"],
["femergenode", "feMergeNode"],
["femorphology", "feMorphology"],
["feoffset", "feOffset"],
["fepointlight", "fePointLight"],
["fespecularlighting", "feSpecularLighting"],
["fespotlight", "feSpotLight"],
["fetile", "feTile"],
["feturbulence", "feTurbulence"],
["foreignobject", "foreignObject"],
["glyphref", "glyphRef"],
["lineargradient", "linearGradient"],
["radialgradient", "radialGradient"],
["textpath", "textPath"]
]);
exports.attributeNames = /* @__PURE__ */ new Map([
["definitionurl", "definitionURL"],
["attributename", "attributeName"],
["attributetype", "attributeType"],
["basefrequency", "baseFrequency"],
["baseprofile", "baseProfile"],
["calcmode", "calcMode"],
["clippathunits", "clipPathUnits"],
["diffuseconstant", "diffuseConstant"],
["edgemode", "edgeMode"],
["filterunits", "filterUnits"],
["glyphref", "glyphRef"],
["gradienttransform", "gradientTransform"],
["gradientunits", "gradientUnits"],
["kernelmatrix", "kernelMatrix"],
["kernelunitlength", "kernelUnitLength"],
["keypoints", "keyPoints"],
["keysplines", "keySplines"],
["keytimes", "keyTimes"],
["lengthadjust", "lengthAdjust"],
["limitingconeangle", "limitingConeAngle"],
["markerheight", "markerHeight"],
["markerunits", "markerUnits"],
["markerwidth", "markerWidth"],
["maskcontentunits", "maskContentUnits"],
["maskunits", "maskUnits"],
["numoctaves", "numOctaves"],
["pathlength", "pathLength"],
["patterncontentunits", "patternContentUnits"],
["patterntransform", "patternTransform"],
["patternunits", "patternUnits"],
["pointsatx", "pointsAtX"],
["pointsaty", "pointsAtY"],
["pointsatz", "pointsAtZ"],
["preservealpha", "preserveAlpha"],
["preserveaspectratio", "preserveAspectRatio"],
["primitiveunits", "primitiveUnits"],
["refx", "refX"],
["refy", "refY"],
["repeatcount", "repeatCount"],
["repeatdur", "repeatDur"],
["requiredextensions", "requiredExtensions"],
["requiredfeatures", "requiredFeatures"],
["specularconstant", "specularConstant"],
["specularexponent", "specularExponent"],
["spreadmethod", "spreadMethod"],
["startoffset", "startOffset"],
["stddeviation", "stdDeviation"],
["stitchtiles", "stitchTiles"],
["surfacescale", "surfaceScale"],
["systemlanguage", "systemLanguage"],
["tablevalues", "tableValues"],
["targetx", "targetX"],
["targety", "targetY"],
["textlength", "textLength"],
["viewbox", "viewBox"],
["viewtarget", "viewTarget"],
["xchannelselector", "xChannelSelector"],
["ychannelselector", "yChannelSelector"],
["zoomandpan", "zoomAndPan"]
]);
}
});
// node_modules/dom-serializer/lib/index.js
var require_lib4 = __commonJS({
"node_modules/dom-serializer/lib/index.js"(exports) {
"use strict";
var __assign = exports && exports.__assign || function() {
__assign = Object.assign || function(t) {
for (var s, i = 1, n2 = arguments.length; i < n2; i++) {
s = arguments[i];
for (var p in s)
if (Object.prototype.hasOwnProperty.call(s, p))
t[p] = s[p];
}
return t;
};
return __assign.apply(this, arguments);
};
var __createBinding = exports && exports.__createBinding || (Object.create ? function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
Object.defineProperty(o, k2, { enumerable: true, get: function() {
return m[k];
} });
} : function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
o[k2] = m[k];
});
var __setModuleDefault = exports && exports.__setModuleDefault || (Object.create ? function(o, v) {
Object.defineProperty(o, "default", { enumerable: true, value: v });
} : function(o, v) {
o["default"] = v;
});
var __importStar = exports && exports.__importStar || function(mod) {
if (mod && mod.__esModule)
return mod;
var result = {};
if (mod != null) {
for (var k in mod)
if (k !== "default" && Object.prototype.hasOwnProperty.call(mod, k))
__createBinding(result, mod, k);
}
__setModuleDefault(result, mod);
return result;
};
Object.defineProperty(exports, "__esModule", { value: true });
var ElementType = __importStar(require_lib());
var entities_1 = require_lib3();
var foreignNames_1 = require_foreignNames();
var unencodedElements = /* @__PURE__ */ new Set([
"style",
"script",
"xmp",
"iframe",
"noembed",
"noframes",
"plaintext",
"noscript"
]);
function formatAttributes(attributes, opts) {
if (!attributes)
return;
return Object.keys(attributes).map(function(key) {
var _a, _b;
var value = (_a = attributes[key]) !== null && _a !== void 0 ? _a : "";
if (opts.xmlMode === "foreign") {
key = (_b = foreignNames_1.attributeNames.get(key)) !== null && _b !== void 0 ? _b : key;
}
if (!opts.emptyAttrs && !opts.xmlMode && value === "") {
return key;
}
return key + '="' + (opts.decodeEntities !== false ? entities_1.encodeXML(value) : value.replace(/"/g, """)) + '"';
}).join(" ");
}
var singleTag = /* @__PURE__ */ new Set([
"area",
"base",
"basefont",
"br",
"col",
"command",
"embed",
"frame",
"hr",
"img",
"input",
"isindex",
"keygen",
"link",
"meta",
"param",
"source",
"track",
"wbr"
]);
function render(node, options) {
if (options === void 0) {
options = {};
}
var nodes = "length" in node ? node : [node];
var output = "";
for (var i = 0; i < nodes.length; i++) {
output += renderNode(nodes[i], options);
}
return output;
}
exports.default = render;
function renderNode(node, options) {
switch (node.type) {
case ElementType.Root:
return render(node.children, options);
case ElementType.Directive:
case ElementType.Doctype:
return renderDirective(node);
case ElementType.Comment:
return renderComment(node);
case ElementType.CDATA:
return renderCdata(node);
case ElementType.Script:
case ElementType.Style:
case ElementType.Tag:
return renderTag(node, options);
case ElementType.Text:
return renderText(node, options);
}
}
var foreignModeIntegrationPoints = /* @__PURE__ */ new Set([
"mi",
"mo",
"mn",
"ms",
"mtext",
"annotation-xml",
"foreignObject",
"desc",
"title"
]);
var foreignElements = /* @__PURE__ */ new Set(["svg", "math"]);
function renderTag(elem, opts) {
var _a;
if (opts.xmlMode === "foreign") {
elem.name = (_a = foreignNames_1.elementNames.get(elem.name)) !== null && _a !== void 0 ? _a : elem.name;
if (elem.parent && foreignModeIntegrationPoints.has(elem.parent.name)) {
opts = __assign(__assign({}, opts), { xmlMode: false });
}
}
if (!opts.xmlMode && foreignElements.has(elem.name)) {
opts = __assign(__assign({}, opts), { xmlMode: "foreign" });
}
var tag = "<" + elem.name;
var attribs = formatAttributes(elem.attribs, opts);
if (attribs) {
tag += " " + attribs;
}
if (elem.children.length === 0 && (opts.xmlMode ? opts.selfClosingTags !== false : opts.selfClosingTags && singleTag.has(elem.name))) {
if (!opts.xmlMode)
tag += " ";
tag += "/>";
} else {
tag += ">";
if (elem.children.length > 0) {
tag += render(elem.children, opts);
}
if (opts.xmlMode || !singleTag.has(elem.name)) {
tag += "" + elem.name + ">";
}
}
return tag;
}
function renderDirective(elem) {
return "<" + elem.data + ">";
}
function renderText(elem, opts) {
var data = elem.data || "";
if (opts.decodeEntities !== false && !(!opts.xmlMode && elem.parent && unencodedElements.has(elem.parent.name))) {
data = entities_1.encodeXML(data);
}
return data;
}
function renderCdata(elem) {
return "";
}
function renderComment(elem) {
return "";
}
}
});
// node_modules/domutils/lib/stringify.js
var require_stringify = __commonJS({
"node_modules/domutils/lib/stringify.js"(exports) {
"use strict";
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.innerText = exports.textContent = exports.getText = exports.getInnerHTML = exports.getOuterHTML = void 0;
var domhandler_1 = require_lib2();
var dom_serializer_1 = __importDefault(require_lib4());
var domelementtype_1 = require_lib();
function getOuterHTML(node, options) {
return (0, dom_serializer_1.default)(node, options);
}
exports.getOuterHTML = getOuterHTML;
function getInnerHTML(node, options) {
return (0, domhandler_1.hasChildren)(node) ? node.children.map(function(node2) {
return getOuterHTML(node2, options);
}).join("") : "";
}
exports.getInnerHTML = getInnerHTML;
function getText(node) {
if (Array.isArray(node))
return node.map(getText).join("");
if ((0, domhandler_1.isTag)(node))
return node.name === "br" ? "\n" : getText(node.children);
if ((0, domhandler_1.isCDATA)(node))
return getText(node.children);
if ((0, domhandler_1.isText)(node))
return node.data;
return "";
}
exports.getText = getText;
function textContent(node) {
if (Array.isArray(node))
return node.map(textContent).join("");
if ((0, domhandler_1.hasChildren)(node) && !(0, domhandler_1.isComment)(node)) {
return textContent(node.children);
}
if ((0, domhandler_1.isText)(node))
return node.data;
return "";
}
exports.textContent = textContent;
function innerText(node) {
if (Array.isArray(node))
return node.map(innerText).join("");
if ((0, domhandler_1.hasChildren)(node) && (node.type === domelementtype_1.ElementType.Tag || (0, domhandler_1.isCDATA)(node))) {
return innerText(node.children);
}
if ((0, domhandler_1.isText)(node))
return node.data;
return "";
}
exports.innerText = innerText;
}
});
// node_modules/domutils/lib/traversal.js
var require_traversal = __commonJS({
"node_modules/domutils/lib/traversal.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.prevElementSibling = exports.nextElementSibling = exports.getName = exports.hasAttrib = exports.getAttributeValue = exports.getSiblings = exports.getParent = exports.getChildren = void 0;
var domhandler_1 = require_lib2();
var emptyArray = [];
function getChildren(elem) {
var _a;
return (_a = elem.children) !== null && _a !== void 0 ? _a : emptyArray;
}
exports.getChildren = getChildren;
function getParent(elem) {
return elem.parent || null;
}
exports.getParent = getParent;
function getSiblings(elem) {
var _a, _b;
var parent = getParent(elem);
if (parent != null)
return getChildren(parent);
var siblings = [elem];
var prev = elem.prev, next = elem.next;
while (prev != null) {
siblings.unshift(prev);
_a = prev, prev = _a.prev;
}
while (next != null) {
siblings.push(next);
_b = next, next = _b.next;
}
return siblings;
}
exports.getSiblings = getSiblings;
function getAttributeValue(elem, name) {
var _a;
return (_a = elem.attribs) === null || _a === void 0 ? void 0 : _a[name];
}
exports.getAttributeValue = getAttributeValue;
function hasAttrib(elem, name) {
return elem.attribs != null && Object.prototype.hasOwnProperty.call(elem.attribs, name) && elem.attribs[name] != null;
}
exports.hasAttrib = hasAttrib;
function getName(elem) {
return elem.name;
}
exports.getName = getName;
function nextElementSibling(elem) {
var _a;
var next = elem.next;
while (next !== null && !(0, domhandler_1.isTag)(next))
_a = next, next = _a.next;
return next;
}
exports.nextElementSibling = nextElementSibling;
function prevElementSibling(elem) {
var _a;
var prev = elem.prev;
while (prev !== null && !(0, domhandler_1.isTag)(prev))
_a = prev, prev = _a.prev;
return prev;
}
exports.prevElementSibling = prevElementSibling;
}
});
// node_modules/domutils/lib/manipulation.js
var require_manipulation = __commonJS({
"node_modules/domutils/lib/manipulation.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.prepend = exports.prependChild = exports.append = exports.appendChild = exports.replaceElement = exports.removeElement = void 0;
function removeElement(elem) {
if (elem.prev)
elem.prev.next = elem.next;
if (elem.next)
elem.next.prev = elem.prev;
if (elem.parent) {
var childs = elem.parent.children;
childs.splice(childs.lastIndexOf(elem), 1);
}
}
exports.removeElement = removeElement;
function replaceElement(elem, replacement) {
var prev = replacement.prev = elem.prev;
if (prev) {
prev.next = replacement;
}
var next = replacement.next = elem.next;
if (next) {
next.prev = replacement;
}
var parent = replacement.parent = elem.parent;
if (parent) {
var childs = parent.children;
childs[childs.lastIndexOf(elem)] = replacement;
}
}
exports.replaceElement = replaceElement;
function appendChild(elem, child) {
removeElement(child);
child.next = null;
child.parent = elem;
if (elem.children.push(child) > 1) {
var sibling = elem.children[elem.children.length - 2];
sibling.next = child;
child.prev = sibling;
} else {
child.prev = null;
}
}
exports.appendChild = appendChild;
function append(elem, next) {
removeElement(next);
var parent = elem.parent;
var currNext = elem.next;
next.next = currNext;
next.prev = elem;
elem.next = next;
next.parent = parent;
if (currNext) {
currNext.prev = next;
if (parent) {
var childs = parent.children;
childs.splice(childs.lastIndexOf(currNext), 0, next);
}
} else if (parent) {
parent.children.push(next);
}
}
exports.append = append;
function prependChild(elem, child) {
removeElement(child);
child.parent = elem;
child.prev = null;
if (elem.children.unshift(child) !== 1) {
var sibling = elem.children[1];
sibling.prev = child;
child.next = sibling;
} else {
child.next = null;
}
}
exports.prependChild = prependChild;
function prepend(elem, prev) {
removeElement(prev);
var parent = elem.parent;
if (parent) {
var childs = parent.children;
childs.splice(childs.indexOf(elem), 0, prev);
}
if (elem.prev) {
elem.prev.next = prev;
}
prev.parent = parent;
prev.prev = elem.prev;
prev.next = elem;
elem.prev = prev;
}
exports.prepend = prepend;
}
});
// node_modules/domutils/lib/querying.js
var require_querying = __commonJS({
"node_modules/domutils/lib/querying.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.findAll = exports.existsOne = exports.findOne = exports.findOneChild = exports.find = exports.filter = void 0;
var domhandler_1 = require_lib2();
function filter(test, node, recurse, limit) {
if (recurse === void 0) {
recurse = true;
}
if (limit === void 0) {
limit = Infinity;
}
if (!Array.isArray(node))
node = [node];
return find(test, node, recurse, limit);
}
exports.filter = filter;
function find(test, nodes, recurse, limit) {
var result = [];
for (var _i = 0, nodes_1 = nodes; _i < nodes_1.length; _i++) {
var elem = nodes_1[_i];
if (test(elem)) {
result.push(elem);
if (--limit <= 0)
break;
}
if (recurse && (0, domhandler_1.hasChildren)(elem) && elem.children.length > 0) {
var children = find(test, elem.children, recurse, limit);
result.push.apply(result, children);
limit -= children.length;
if (limit <= 0)
break;
}
}
return result;
}
exports.find = find;
function findOneChild(test, nodes) {
return nodes.find(test);
}
exports.findOneChild = findOneChild;
function findOne(test, nodes, recurse) {
if (recurse === void 0) {
recurse = true;
}
var elem = null;
for (var i = 0; i < nodes.length && !elem; i++) {
var checked = nodes[i];
if (!(0, domhandler_1.isTag)(checked)) {
continue;
} else if (test(checked)) {
elem = checked;
} else if (recurse && checked.children.length > 0) {
elem = findOne(test, checked.children);
}
}
return elem;
}
exports.findOne = findOne;
function existsOne(test, nodes) {
return nodes.some(function(checked) {
return (0, domhandler_1.isTag)(checked) && (test(checked) || checked.children.length > 0 && existsOne(test, checked.children));
});
}
exports.existsOne = existsOne;
function findAll(test, nodes) {
var _a;
var result = [];
var stack = nodes.filter(domhandler_1.isTag);
var elem;
while (elem = stack.shift()) {
var children = (_a = elem.children) === null || _a === void 0 ? void 0 : _a.filter(domhandler_1.isTag);
if (children && children.length > 0) {
stack.unshift.apply(stack, children);
}
if (test(elem))
result.push(elem);
}
return result;
}
exports.findAll = findAll;
}
});
// node_modules/domutils/lib/legacy.js
var require_legacy2 = __commonJS({
"node_modules/domutils/lib/legacy.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.getElementsByTagType = exports.getElementsByTagName = exports.getElementById = exports.getElements = exports.testElement = void 0;
var domhandler_1 = require_lib2();
var querying_1 = require_querying();
var Checks = {
tag_name: function(name) {
if (typeof name === "function") {
return function(elem) {
return (0, domhandler_1.isTag)(elem) && name(elem.name);
};
} else if (name === "*") {
return domhandler_1.isTag;
}
return function(elem) {
return (0, domhandler_1.isTag)(elem) && elem.name === name;
};
},
tag_type: function(type) {
if (typeof type === "function") {
return function(elem) {
return type(elem.type);
};
}
return function(elem) {
return elem.type === type;
};
},
tag_contains: function(data) {
if (typeof data === "function") {
return function(elem) {
return (0, domhandler_1.isText)(elem) && data(elem.data);
};
}
return function(elem) {
return (0, domhandler_1.isText)(elem) && elem.data === data;
};
}
};
function getAttribCheck(attrib, value) {
if (typeof value === "function") {
return function(elem) {
return (0, domhandler_1.isTag)(elem) && value(elem.attribs[attrib]);
};
}
return function(elem) {
return (0, domhandler_1.isTag)(elem) && elem.attribs[attrib] === value;
};
}
function combineFuncs(a, b) {
return function(elem) {
return a(elem) || b(elem);
};
}
function compileTest(options) {
var funcs = Object.keys(options).map(function(key) {
var value = options[key];
return Object.prototype.hasOwnProperty.call(Checks, key) ? Checks[key](value) : getAttribCheck(key, value);
});
return funcs.length === 0 ? null : funcs.reduce(combineFuncs);
}
function testElement(options, node) {
var test = compileTest(options);
return test ? test(node) : true;
}
exports.testElement = testElement;
function getElements(options, nodes, recurse, limit) {
if (limit === void 0) {
limit = Infinity;
}
var test = compileTest(options);
return test ? (0, querying_1.filter)(test, nodes, recurse, limit) : [];
}
exports.getElements = getElements;
function getElementById(id, nodes, recurse) {
if (recurse === void 0) {
recurse = true;
}
if (!Array.isArray(nodes))
nodes = [nodes];
return (0, querying_1.findOne)(getAttribCheck("id", id), nodes, recurse);
}
exports.getElementById = getElementById;
function getElementsByTagName(tagName, nodes, recurse, limit) {
if (recurse === void 0) {
recurse = true;
}
if (limit === void 0) {
limit = Infinity;
}
return (0, querying_1.filter)(Checks.tag_name(tagName), nodes, recurse, limit);
}
exports.getElementsByTagName = getElementsByTagName;
function getElementsByTagType(type, nodes, recurse, limit) {
if (recurse === void 0) {
recurse = true;
}
if (limit === void 0) {
limit = Infinity;
}
return (0, querying_1.filter)(Checks.tag_type(type), nodes, recurse, limit);
}
exports.getElementsByTagType = getElementsByTagType;
}
});
// node_modules/domutils/lib/helpers.js
var require_helpers = __commonJS({
"node_modules/domutils/lib/helpers.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.uniqueSort = exports.compareDocumentPosition = exports.removeSubsets = void 0;
var domhandler_1 = require_lib2();
function removeSubsets(nodes) {
var idx = nodes.length;
while (--idx >= 0) {
var node = nodes[idx];
if (idx > 0 && nodes.lastIndexOf(node, idx - 1) >= 0) {
nodes.splice(idx, 1);
continue;
}
for (var ancestor = node.parent; ancestor; ancestor = ancestor.parent) {
if (nodes.includes(ancestor)) {
nodes.splice(idx, 1);
break;
}
}
}
return nodes;
}
exports.removeSubsets = removeSubsets;
function compareDocumentPosition(nodeA, nodeB) {
var aParents = [];
var bParents = [];
if (nodeA === nodeB) {
return 0;
}
var current = (0, domhandler_1.hasChildren)(nodeA) ? nodeA : nodeA.parent;
while (current) {
aParents.unshift(current);
current = current.parent;
}
current = (0, domhandler_1.hasChildren)(nodeB) ? nodeB : nodeB.parent;
while (current) {
bParents.unshift(current);
current = current.parent;
}
var maxIdx = Math.min(aParents.length, bParents.length);
var idx = 0;
while (idx < maxIdx && aParents[idx] === bParents[idx]) {
idx++;
}
if (idx === 0) {
return 1;
}
var sharedParent = aParents[idx - 1];
var siblings = sharedParent.children;
var aSibling = aParents[idx];
var bSibling = bParents[idx];
if (siblings.indexOf(aSibling) > siblings.indexOf(bSibling)) {
if (sharedParent === nodeB) {
return 4 | 16;
}
return 4;
}
if (sharedParent === nodeA) {
return 2 | 8;
}
return 2;
}
exports.compareDocumentPosition = compareDocumentPosition;
function uniqueSort(nodes) {
nodes = nodes.filter(function(node, i, arr) {
return !arr.includes(node, i + 1);
});
nodes.sort(function(a, b) {
var relative = compareDocumentPosition(a, b);
if (relative & 2) {
return -1;
} else if (relative & 4) {
return 1;
}
return 0;
});
return nodes;
}
exports.uniqueSort = uniqueSort;
}
});
// node_modules/domutils/lib/feeds.js
var require_feeds = __commonJS({
"node_modules/domutils/lib/feeds.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.getFeed = void 0;
var stringify_1 = require_stringify();
var legacy_1 = require_legacy2();
function getFeed(doc) {
var feedRoot = getOneElement(isValidFeed, doc);
return !feedRoot ? null : feedRoot.name === "feed" ? getAtomFeed(feedRoot) : getRssFeed(feedRoot);
}
exports.getFeed = getFeed;
function getAtomFeed(feedRoot) {
var _a;
var childs = feedRoot.children;
var feed = {
type: "atom",
items: (0, legacy_1.getElementsByTagName)("entry", childs).map(function(item) {
var _a2;
var children = item.children;
var entry = { media: getMediaElements(children) };
addConditionally(entry, "id", "id", children);
addConditionally(entry, "title", "title", children);
var href2 = (_a2 = getOneElement("link", children)) === null || _a2 === void 0 ? void 0 : _a2.attribs.href;
if (href2) {
entry.link = href2;
}
var description = fetch("summary", children) || fetch("content", children);
if (description) {
entry.description = description;
}
var pubDate = fetch("updated", children);
if (pubDate) {
entry.pubDate = new Date(pubDate);
}
return entry;
})
};
addConditionally(feed, "id", "id", childs);
addConditionally(feed, "title", "title", childs);
var href = (_a = getOneElement("link", childs)) === null || _a === void 0 ? void 0 : _a.attribs.href;
if (href) {
feed.link = href;
}
addConditionally(feed, "description", "subtitle", childs);
var updated = fetch("updated", childs);
if (updated) {
feed.updated = new Date(updated);
}
addConditionally(feed, "author", "email", childs, true);
return feed;
}
function getRssFeed(feedRoot) {
var _a, _b;
var childs = (_b = (_a = getOneElement("channel", feedRoot.children)) === null || _a === void 0 ? void 0 : _a.children) !== null && _b !== void 0 ? _b : [];
var feed = {
type: feedRoot.name.substr(0, 3),
id: "",
items: (0, legacy_1.getElementsByTagName)("item", feedRoot.children).map(function(item) {
var children = item.children;
var entry = { media: getMediaElements(children) };
addConditionally(entry, "id", "guid", children);
addConditionally(entry, "title", "title", children);
addConditionally(entry, "link", "link", children);
addConditionally(entry, "description", "description", children);
var pubDate = fetch("pubDate", children);
if (pubDate)
entry.pubDate = new Date(pubDate);
return entry;
})
};
addConditionally(feed, "title", "title", childs);
addConditionally(feed, "link", "link", childs);
addConditionally(feed, "description", "description", childs);
var updated = fetch("lastBuildDate", childs);
if (updated) {
feed.updated = new Date(updated);
}
addConditionally(feed, "author", "managingEditor", childs, true);
return feed;
}
var MEDIA_KEYS_STRING = ["url", "type", "lang"];
var MEDIA_KEYS_INT = [
"fileSize",
"bitrate",
"framerate",
"samplingrate",
"channels",
"duration",
"height",
"width"
];
function getMediaElements(where) {
return (0, legacy_1.getElementsByTagName)("media:content", where).map(function(elem) {
var attribs = elem.attribs;
var media = {
medium: attribs.medium,
isDefault: !!attribs.isDefault
};
for (var _i = 0, MEDIA_KEYS_STRING_1 = MEDIA_KEYS_STRING; _i < MEDIA_KEYS_STRING_1.length; _i++) {
var attrib = MEDIA_KEYS_STRING_1[_i];
if (attribs[attrib]) {
media[attrib] = attribs[attrib];
}
}
for (var _a = 0, MEDIA_KEYS_INT_1 = MEDIA_KEYS_INT; _a < MEDIA_KEYS_INT_1.length; _a++) {
var attrib = MEDIA_KEYS_INT_1[_a];
if (attribs[attrib]) {
media[attrib] = parseInt(attribs[attrib], 10);
}
}
if (attribs.expression) {
media.expression = attribs.expression;
}
return media;
});
}
function getOneElement(tagName, node) {
return (0, legacy_1.getElementsByTagName)(tagName, node, true, 1)[0];
}
function fetch(tagName, where, recurse) {
if (recurse === void 0) {
recurse = false;
}
return (0, stringify_1.textContent)((0, legacy_1.getElementsByTagName)(tagName, where, recurse, 1)).trim();
}
function addConditionally(obj, prop, tagName, where, recurse) {
if (recurse === void 0) {
recurse = false;
}
var val = fetch(tagName, where, recurse);
if (val)
obj[prop] = val;
}
function isValidFeed(value) {
return value === "rss" || value === "feed" || value === "rdf:RDF";
}
}
});
// node_modules/domutils/lib/index.js
var require_lib5 = __commonJS({
"node_modules/domutils/lib/index.js"(exports) {
"use strict";
var __createBinding = exports && exports.__createBinding || (Object.create ? function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
Object.defineProperty(o, k2, { enumerable: true, get: function() {
return m[k];
} });
} : function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
o[k2] = m[k];
});
var __exportStar = exports && exports.__exportStar || function(m, exports2) {
for (var p in m)
if (p !== "default" && !Object.prototype.hasOwnProperty.call(exports2, p))
__createBinding(exports2, m, p);
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.hasChildren = exports.isDocument = exports.isComment = exports.isText = exports.isCDATA = exports.isTag = void 0;
__exportStar(require_stringify(), exports);
__exportStar(require_traversal(), exports);
__exportStar(require_manipulation(), exports);
__exportStar(require_querying(), exports);
__exportStar(require_legacy2(), exports);
__exportStar(require_helpers(), exports);
__exportStar(require_feeds(), exports);
var domhandler_1 = require_lib2();
Object.defineProperty(exports, "isTag", { enumerable: true, get: function() {
return domhandler_1.isTag;
} });
Object.defineProperty(exports, "isCDATA", { enumerable: true, get: function() {
return domhandler_1.isCDATA;
} });
Object.defineProperty(exports, "isText", { enumerable: true, get: function() {
return domhandler_1.isText;
} });
Object.defineProperty(exports, "isComment", { enumerable: true, get: function() {
return domhandler_1.isComment;
} });
Object.defineProperty(exports, "isDocument", { enumerable: true, get: function() {
return domhandler_1.isDocument;
} });
Object.defineProperty(exports, "hasChildren", { enumerable: true, get: function() {
return domhandler_1.hasChildren;
} });
}
});
// node_modules/boolbase/index.js
var require_boolbase = __commonJS({
"node_modules/boolbase/index.js"(exports, module2) {
module2.exports = {
trueFunc: function trueFunc() {
return true;
},
falseFunc: function falseFunc() {
return false;
}
};
}
});
// node_modules/css-what/lib/commonjs/types.js
var require_types = __commonJS({
"node_modules/css-what/lib/commonjs/types.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.AttributeAction = exports.IgnoreCaseMode = exports.SelectorType = void 0;
var SelectorType;
(function(SelectorType2) {
SelectorType2["Attribute"] = "attribute";
SelectorType2["Pseudo"] = "pseudo";
SelectorType2["PseudoElement"] = "pseudo-element";
SelectorType2["Tag"] = "tag";
SelectorType2["Universal"] = "universal";
SelectorType2["Adjacent"] = "adjacent";
SelectorType2["Child"] = "child";
SelectorType2["Descendant"] = "descendant";
SelectorType2["Parent"] = "parent";
SelectorType2["Sibling"] = "sibling";
SelectorType2["ColumnCombinator"] = "column-combinator";
})(SelectorType = exports.SelectorType || (exports.SelectorType = {}));
exports.IgnoreCaseMode = {
Unknown: null,
QuirksMode: "quirks",
IgnoreCase: true,
CaseSensitive: false
};
var AttributeAction;
(function(AttributeAction2) {
AttributeAction2["Any"] = "any";
AttributeAction2["Element"] = "element";
AttributeAction2["End"] = "end";
AttributeAction2["Equals"] = "equals";
AttributeAction2["Exists"] = "exists";
AttributeAction2["Hyphen"] = "hyphen";
AttributeAction2["Not"] = "not";
AttributeAction2["Start"] = "start";
})(AttributeAction = exports.AttributeAction || (exports.AttributeAction = {}));
}
});
// node_modules/css-what/lib/commonjs/parse.js
var require_parse = __commonJS({
"node_modules/css-what/lib/commonjs/parse.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.parse = exports.isTraversal = void 0;
var types_1 = require_types();
var reName = /^[^\\#]?(?:\\(?:[\da-f]{1,6}\s?|.)|[\w\-\u00b0-\uFFFF])+/;
var reEscape = /\\([\da-f]{1,6}\s?|(\s)|.)/gi;
var actionTypes = /* @__PURE__ */ new Map([
[126, types_1.AttributeAction.Element],
[94, types_1.AttributeAction.Start],
[36, types_1.AttributeAction.End],
[42, types_1.AttributeAction.Any],
[33, types_1.AttributeAction.Not],
[124, types_1.AttributeAction.Hyphen]
]);
var unpackPseudos = /* @__PURE__ */ new Set([
"has",
"not",
"matches",
"is",
"where",
"host",
"host-context"
]);
function isTraversal(selector) {
switch (selector.type) {
case types_1.SelectorType.Adjacent:
case types_1.SelectorType.Child:
case types_1.SelectorType.Descendant:
case types_1.SelectorType.Parent:
case types_1.SelectorType.Sibling:
case types_1.SelectorType.ColumnCombinator:
return true;
default:
return false;
}
}
exports.isTraversal = isTraversal;
var stripQuotesFromPseudos = /* @__PURE__ */ new Set(["contains", "icontains"]);
function funescape(_, escaped, escapedWhitespace) {
var high = parseInt(escaped, 16) - 65536;
return high !== high || escapedWhitespace ? escaped : high < 0 ? String.fromCharCode(high + 65536) : String.fromCharCode(high >> 10 | 55296, high & 1023 | 56320);
}
function unescapeCSS(str) {
return str.replace(reEscape, funescape);
}
function isQuote(c) {
return c === 39 || c === 34;
}
function isWhitespace(c) {
return c === 32 || c === 9 || c === 10 || c === 12 || c === 13;
}
function parse2(selector) {
var subselects = [];
var endIndex = parseSelector(subselects, "".concat(selector), 0);
if (endIndex < selector.length) {
throw new Error("Unmatched selector: ".concat(selector.slice(endIndex)));
}
return subselects;
}
exports.parse = parse2;
function parseSelector(subselects, selector, selectorIndex) {
var tokens = [];
function getName(offset) {
var match = selector.slice(selectorIndex + offset).match(reName);
if (!match) {
throw new Error("Expected name, found ".concat(selector.slice(selectorIndex)));
}
var name = match[0];
selectorIndex += offset + name.length;
return unescapeCSS(name);
}
function stripWhitespace(offset) {
selectorIndex += offset;
while (selectorIndex < selector.length && isWhitespace(selector.charCodeAt(selectorIndex))) {
selectorIndex++;
}
}
function readValueWithParenthesis() {
selectorIndex += 1;
var start = selectorIndex;
var counter = 1;
for (; counter > 0 && selectorIndex < selector.length; selectorIndex++) {
if (selector.charCodeAt(selectorIndex) === 40 && !isEscaped(selectorIndex)) {
counter++;
} else if (selector.charCodeAt(selectorIndex) === 41 && !isEscaped(selectorIndex)) {
counter--;
}
}
if (counter) {
throw new Error("Parenthesis not matched");
}
return unescapeCSS(selector.slice(start, selectorIndex - 1));
}
function isEscaped(pos) {
var slashCount = 0;
while (selector.charCodeAt(--pos) === 92)
slashCount++;
return (slashCount & 1) === 1;
}
function ensureNotTraversal() {
if (tokens.length > 0 && isTraversal(tokens[tokens.length - 1])) {
throw new Error("Did not expect successive traversals.");
}
}
function addTraversal(type) {
if (tokens.length > 0 && tokens[tokens.length - 1].type === types_1.SelectorType.Descendant) {
tokens[tokens.length - 1].type = type;
return;
}
ensureNotTraversal();
tokens.push({ type });
}
function addSpecialAttribute(name, action2) {
tokens.push({
type: types_1.SelectorType.Attribute,
name,
action: action2,
value: getName(1),
namespace: null,
ignoreCase: "quirks"
});
}
function finalizeSubselector() {
if (tokens.length && tokens[tokens.length - 1].type === types_1.SelectorType.Descendant) {
tokens.pop();
}
if (tokens.length === 0) {
throw new Error("Empty sub-selector");
}
subselects.push(tokens);
}
stripWhitespace(0);
if (selector.length === selectorIndex) {
return selectorIndex;
}
loop:
while (selectorIndex < selector.length) {
var firstChar = selector.charCodeAt(selectorIndex);
switch (firstChar) {
case 32:
case 9:
case 10:
case 12:
case 13: {
if (tokens.length === 0 || tokens[0].type !== types_1.SelectorType.Descendant) {
ensureNotTraversal();
tokens.push({ type: types_1.SelectorType.Descendant });
}
stripWhitespace(1);
break;
}
case 62: {
addTraversal(types_1.SelectorType.Child);
stripWhitespace(1);
break;
}
case 60: {
addTraversal(types_1.SelectorType.Parent);
stripWhitespace(1);
break;
}
case 126: {
addTraversal(types_1.SelectorType.Sibling);
stripWhitespace(1);
break;
}
case 43: {
addTraversal(types_1.SelectorType.Adjacent);
stripWhitespace(1);
break;
}
case 46: {
addSpecialAttribute("class", types_1.AttributeAction.Element);
break;
}
case 35: {
addSpecialAttribute("id", types_1.AttributeAction.Equals);
break;
}
case 91: {
stripWhitespace(1);
var name_1 = void 0;
var namespace = null;
if (selector.charCodeAt(selectorIndex) === 124) {
name_1 = getName(1);
} else if (selector.startsWith("*|", selectorIndex)) {
namespace = "*";
name_1 = getName(2);
} else {
name_1 = getName(0);
if (selector.charCodeAt(selectorIndex) === 124 && selector.charCodeAt(selectorIndex + 1) !== 61) {
namespace = name_1;
name_1 = getName(1);
}
}
stripWhitespace(0);
var action = types_1.AttributeAction.Exists;
var possibleAction = actionTypes.get(selector.charCodeAt(selectorIndex));
if (possibleAction) {
action = possibleAction;
if (selector.charCodeAt(selectorIndex + 1) !== 61) {
throw new Error("Expected `=`");
}
stripWhitespace(2);
} else if (selector.charCodeAt(selectorIndex) === 61) {
action = types_1.AttributeAction.Equals;
stripWhitespace(1);
}
var value = "";
var ignoreCase = null;
if (action !== "exists") {
if (isQuote(selector.charCodeAt(selectorIndex))) {
var quote2 = selector.charCodeAt(selectorIndex);
var sectionEnd = selectorIndex + 1;
while (sectionEnd < selector.length && (selector.charCodeAt(sectionEnd) !== quote2 || isEscaped(sectionEnd))) {
sectionEnd += 1;
}
if (selector.charCodeAt(sectionEnd) !== quote2) {
throw new Error("Attribute value didn't end");
}
value = unescapeCSS(selector.slice(selectorIndex + 1, sectionEnd));
selectorIndex = sectionEnd + 1;
} else {
var valueStart = selectorIndex;
while (selectorIndex < selector.length && (!isWhitespace(selector.charCodeAt(selectorIndex)) && selector.charCodeAt(selectorIndex) !== 93 || isEscaped(selectorIndex))) {
selectorIndex += 1;
}
value = unescapeCSS(selector.slice(valueStart, selectorIndex));
}
stripWhitespace(0);
var forceIgnore = selector.charCodeAt(selectorIndex) | 32;
if (forceIgnore === 115) {
ignoreCase = false;
stripWhitespace(1);
} else if (forceIgnore === 105) {
ignoreCase = true;
stripWhitespace(1);
}
}
if (selector.charCodeAt(selectorIndex) !== 93) {
throw new Error("Attribute selector didn't terminate");
}
selectorIndex += 1;
var attributeSelector = {
type: types_1.SelectorType.Attribute,
name: name_1,
action,
value,
namespace,
ignoreCase
};
tokens.push(attributeSelector);
break;
}
case 58: {
if (selector.charCodeAt(selectorIndex + 1) === 58) {
tokens.push({
type: types_1.SelectorType.PseudoElement,
name: getName(2).toLowerCase(),
data: selector.charCodeAt(selectorIndex) === 40 ? readValueWithParenthesis() : null
});
continue;
}
var name_2 = getName(1).toLowerCase();
var data = null;
if (selector.charCodeAt(selectorIndex) === 40) {
if (unpackPseudos.has(name_2)) {
if (isQuote(selector.charCodeAt(selectorIndex + 1))) {
throw new Error("Pseudo-selector ".concat(name_2, " cannot be quoted"));
}
data = [];
selectorIndex = parseSelector(data, selector, selectorIndex + 1);
if (selector.charCodeAt(selectorIndex) !== 41) {
throw new Error("Missing closing parenthesis in :".concat(name_2, " (").concat(selector, ")"));
}
selectorIndex += 1;
} else {
data = readValueWithParenthesis();
if (stripQuotesFromPseudos.has(name_2)) {
var quot = data.charCodeAt(0);
if (quot === data.charCodeAt(data.length - 1) && isQuote(quot)) {
data = data.slice(1, -1);
}
}
data = unescapeCSS(data);
}
}
tokens.push({ type: types_1.SelectorType.Pseudo, name: name_2, data });
break;
}
case 44: {
finalizeSubselector();
tokens = [];
stripWhitespace(1);
break;
}
default: {
if (selector.startsWith("/*", selectorIndex)) {
var endIndex = selector.indexOf("*/", selectorIndex + 2);
if (endIndex < 0) {
throw new Error("Comment was not terminated");
}
selectorIndex = endIndex + 2;
if (tokens.length === 0) {
stripWhitespace(0);
}
break;
}
var namespace = null;
var name_3 = void 0;
if (firstChar === 42) {
selectorIndex += 1;
name_3 = "*";
} else if (firstChar === 124) {
name_3 = "";
if (selector.charCodeAt(selectorIndex + 1) === 124) {
addTraversal(types_1.SelectorType.ColumnCombinator);
stripWhitespace(2);
break;
}
} else if (reName.test(selector.slice(selectorIndex))) {
name_3 = getName(0);
} else {
break loop;
}
if (selector.charCodeAt(selectorIndex) === 124 && selector.charCodeAt(selectorIndex + 1) !== 124) {
namespace = name_3;
if (selector.charCodeAt(selectorIndex + 1) === 42) {
name_3 = "*";
selectorIndex += 2;
} else {
name_3 = getName(1);
}
}
tokens.push(name_3 === "*" ? { type: types_1.SelectorType.Universal, namespace } : { type: types_1.SelectorType.Tag, name: name_3, namespace });
}
}
}
finalizeSubselector();
return selectorIndex;
}
}
});
// node_modules/css-what/lib/commonjs/stringify.js
var require_stringify2 = __commonJS({
"node_modules/css-what/lib/commonjs/stringify.js"(exports) {
"use strict";
var __spreadArray = exports && exports.__spreadArray || function(to, from, pack) {
if (pack || arguments.length === 2)
for (var i = 0, l = from.length, ar; i < l; i++) {
if (ar || !(i in from)) {
if (!ar)
ar = Array.prototype.slice.call(from, 0, i);
ar[i] = from[i];
}
}
return to.concat(ar || Array.prototype.slice.call(from));
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.stringify = void 0;
var types_1 = require_types();
var attribValChars = ["\\", '"'];
var pseudoValChars = __spreadArray(__spreadArray([], attribValChars, true), ["(", ")"], false);
var charsToEscapeInAttributeValue = new Set(attribValChars.map(function(c) {
return c.charCodeAt(0);
}));
var charsToEscapeInPseudoValue = new Set(pseudoValChars.map(function(c) {
return c.charCodeAt(0);
}));
var charsToEscapeInName = new Set(__spreadArray(__spreadArray([], pseudoValChars, true), [
"~",
"^",
"$",
"*",
"+",
"!",
"|",
":",
"[",
"]",
" ",
"."
], false).map(function(c) {
return c.charCodeAt(0);
}));
function stringify(selector) {
return selector.map(function(token) {
return token.map(stringifyToken).join("");
}).join(", ");
}
exports.stringify = stringify;
function stringifyToken(token, index, arr) {
switch (token.type) {
case types_1.SelectorType.Child:
return index === 0 ? "> " : " > ";
case types_1.SelectorType.Parent:
return index === 0 ? "< " : " < ";
case types_1.SelectorType.Sibling:
return index === 0 ? "~ " : " ~ ";
case types_1.SelectorType.Adjacent:
return index === 0 ? "+ " : " + ";
case types_1.SelectorType.Descendant:
return " ";
case types_1.SelectorType.ColumnCombinator:
return index === 0 ? "|| " : " || ";
case types_1.SelectorType.Universal:
return token.namespace === "*" && index + 1 < arr.length && "name" in arr[index + 1] ? "" : "".concat(getNamespace(token.namespace), "*");
case types_1.SelectorType.Tag:
return getNamespacedName(token);
case types_1.SelectorType.PseudoElement:
return "::".concat(escapeName(token.name, charsToEscapeInName)).concat(token.data === null ? "" : "(".concat(escapeName(token.data, charsToEscapeInPseudoValue), ")"));
case types_1.SelectorType.Pseudo:
return ":".concat(escapeName(token.name, charsToEscapeInName)).concat(token.data === null ? "" : "(".concat(typeof token.data === "string" ? escapeName(token.data, charsToEscapeInPseudoValue) : stringify(token.data), ")"));
case types_1.SelectorType.Attribute: {
if (token.name === "id" && token.action === types_1.AttributeAction.Equals && token.ignoreCase === "quirks" && !token.namespace) {
return "#".concat(escapeName(token.value, charsToEscapeInName));
}
if (token.name === "class" && token.action === types_1.AttributeAction.Element && token.ignoreCase === "quirks" && !token.namespace) {
return ".".concat(escapeName(token.value, charsToEscapeInName));
}
var name_1 = getNamespacedName(token);
if (token.action === types_1.AttributeAction.Exists) {
return "[".concat(name_1, "]");
}
return "[".concat(name_1).concat(getActionValue(token.action), '="').concat(escapeName(token.value, charsToEscapeInAttributeValue), '"').concat(token.ignoreCase === null ? "" : token.ignoreCase ? " i" : " s", "]");
}
}
}
function getActionValue(action) {
switch (action) {
case types_1.AttributeAction.Equals:
return "";
case types_1.AttributeAction.Element:
return "~";
case types_1.AttributeAction.Start:
return "^";
case types_1.AttributeAction.End:
return "$";
case types_1.AttributeAction.Any:
return "*";
case types_1.AttributeAction.Not:
return "!";
case types_1.AttributeAction.Hyphen:
return "|";
case types_1.AttributeAction.Exists:
throw new Error("Shouldn't be here");
}
}
function getNamespacedName(token) {
return "".concat(getNamespace(token.namespace)).concat(escapeName(token.name, charsToEscapeInName));
}
function getNamespace(namespace) {
return namespace !== null ? "".concat(namespace === "*" ? "*" : escapeName(namespace, charsToEscapeInName), "|") : "";
}
function escapeName(str, charsToEscape) {
var lastIdx = 0;
var ret = "";
for (var i = 0; i < str.length; i++) {
if (charsToEscape.has(str.charCodeAt(i))) {
ret += "".concat(str.slice(lastIdx, i), "\\").concat(str.charAt(i));
lastIdx = i + 1;
}
}
return ret.length > 0 ? ret + str.slice(lastIdx) : str;
}
}
});
// node_modules/css-what/lib/commonjs/index.js
var require_commonjs = __commonJS({
"node_modules/css-what/lib/commonjs/index.js"(exports) {
"use strict";
var __createBinding = exports && exports.__createBinding || (Object.create ? function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
var desc = Object.getOwnPropertyDescriptor(m, k);
if (!desc || ("get" in desc ? !m.__esModule : desc.writable || desc.configurable)) {
desc = { enumerable: true, get: function() {
return m[k];
} };
}
Object.defineProperty(o, k2, desc);
} : function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
o[k2] = m[k];
});
var __exportStar = exports && exports.__exportStar || function(m, exports2) {
for (var p in m)
if (p !== "default" && !Object.prototype.hasOwnProperty.call(exports2, p))
__createBinding(exports2, m, p);
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.stringify = exports.parse = exports.isTraversal = void 0;
__exportStar(require_types(), exports);
var parse_1 = require_parse();
Object.defineProperty(exports, "isTraversal", { enumerable: true, get: function() {
return parse_1.isTraversal;
} });
Object.defineProperty(exports, "parse", { enumerable: true, get: function() {
return parse_1.parse;
} });
var stringify_1 = require_stringify2();
Object.defineProperty(exports, "stringify", { enumerable: true, get: function() {
return stringify_1.stringify;
} });
}
});
// node_modules/css-select/lib/procedure.js
var require_procedure = __commonJS({
"node_modules/css-select/lib/procedure.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.isTraversal = exports.procedure = void 0;
exports.procedure = {
universal: 50,
tag: 30,
attribute: 1,
pseudo: 0,
"pseudo-element": 0,
"column-combinator": -1,
descendant: -1,
child: -1,
parent: -1,
sibling: -1,
adjacent: -1,
_flexibleDescendant: -1
};
function isTraversal(t) {
return exports.procedure[t.type] < 0;
}
exports.isTraversal = isTraversal;
}
});
// node_modules/css-select/lib/sort.js
var require_sort = __commonJS({
"node_modules/css-select/lib/sort.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
var css_what_1 = require_commonjs();
var procedure_1 = require_procedure();
var attributes = {
exists: 10,
equals: 8,
not: 7,
start: 6,
end: 6,
any: 5,
hyphen: 4,
element: 4
};
function sortByProcedure(arr) {
var procs = arr.map(getProcedure);
for (var i = 1; i < arr.length; i++) {
var procNew = procs[i];
if (procNew < 0)
continue;
for (var j = i - 1; j >= 0 && procNew < procs[j]; j--) {
var token = arr[j + 1];
arr[j + 1] = arr[j];
arr[j] = token;
procs[j + 1] = procs[j];
procs[j] = procNew;
}
}
}
exports.default = sortByProcedure;
function getProcedure(token) {
var proc = procedure_1.procedure[token.type];
if (token.type === css_what_1.SelectorType.Attribute) {
proc = attributes[token.action];
if (proc === attributes.equals && token.name === "id") {
proc = 9;
}
if (token.ignoreCase) {
proc >>= 1;
}
} else if (token.type === css_what_1.SelectorType.Pseudo) {
if (!token.data) {
proc = 3;
} else if (token.name === "has" || token.name === "contains") {
proc = 0;
} else if (Array.isArray(token.data)) {
proc = 0;
for (var i = 0; i < token.data.length; i++) {
if (token.data[i].length !== 1)
continue;
var cur = getProcedure(token.data[i][0]);
if (cur === 0) {
proc = 0;
break;
}
if (cur > proc)
proc = cur;
}
if (token.data.length > 1 && proc > 0)
proc -= 1;
} else {
proc = 1;
}
}
return proc;
}
}
});
// node_modules/css-select/lib/attributes.js
var require_attributes = __commonJS({
"node_modules/css-select/lib/attributes.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.attributeRules = void 0;
var boolbase_1 = require_boolbase();
var reChars = /[-[\]{}()*+?.,\\^$|#\s]/g;
function escapeRegex(value) {
return value.replace(reChars, "\\$&");
}
var caseInsensitiveAttributes = /* @__PURE__ */ new Set([
"accept",
"accept-charset",
"align",
"alink",
"axis",
"bgcolor",
"charset",
"checked",
"clear",
"codetype",
"color",
"compact",
"declare",
"defer",
"dir",
"direction",
"disabled",
"enctype",
"face",
"frame",
"hreflang",
"http-equiv",
"lang",
"language",
"link",
"media",
"method",
"multiple",
"nohref",
"noresize",
"noshade",
"nowrap",
"readonly",
"rel",
"rev",
"rules",
"scope",
"scrolling",
"selected",
"shape",
"target",
"text",
"type",
"valign",
"valuetype",
"vlink"
]);
function shouldIgnoreCase(selector, options) {
return typeof selector.ignoreCase === "boolean" ? selector.ignoreCase : selector.ignoreCase === "quirks" ? !!options.quirksMode : !options.xmlMode && caseInsensitiveAttributes.has(selector.name);
}
exports.attributeRules = {
equals: function(next, data, options) {
var adapter = options.adapter;
var name = data.name;
var value = data.value;
if (shouldIgnoreCase(data, options)) {
value = value.toLowerCase();
return function(elem) {
var attr = adapter.getAttributeValue(elem, name);
return attr != null && attr.length === value.length && attr.toLowerCase() === value && next(elem);
};
}
return function(elem) {
return adapter.getAttributeValue(elem, name) === value && next(elem);
};
},
hyphen: function(next, data, options) {
var adapter = options.adapter;
var name = data.name;
var value = data.value;
var len = value.length;
if (shouldIgnoreCase(data, options)) {
value = value.toLowerCase();
return function hyphenIC(elem) {
var attr = adapter.getAttributeValue(elem, name);
return attr != null && (attr.length === len || attr.charAt(len) === "-") && attr.substr(0, len).toLowerCase() === value && next(elem);
};
}
return function hyphen(elem) {
var attr = adapter.getAttributeValue(elem, name);
return attr != null && (attr.length === len || attr.charAt(len) === "-") && attr.substr(0, len) === value && next(elem);
};
},
element: function(next, data, options) {
var adapter = options.adapter;
var name = data.name, value = data.value;
if (/\s/.test(value)) {
return boolbase_1.falseFunc;
}
var regex = new RegExp("(?:^|\\s)".concat(escapeRegex(value), "(?:$|\\s)"), shouldIgnoreCase(data, options) ? "i" : "");
return function element(elem) {
var attr = adapter.getAttributeValue(elem, name);
return attr != null && attr.length >= value.length && regex.test(attr) && next(elem);
};
},
exists: function(next, _a, _b) {
var name = _a.name;
var adapter = _b.adapter;
return function(elem) {
return adapter.hasAttrib(elem, name) && next(elem);
};
},
start: function(next, data, options) {
var adapter = options.adapter;
var name = data.name;
var value = data.value;
var len = value.length;
if (len === 0) {
return boolbase_1.falseFunc;
}
if (shouldIgnoreCase(data, options)) {
value = value.toLowerCase();
return function(elem) {
var attr = adapter.getAttributeValue(elem, name);
return attr != null && attr.length >= len && attr.substr(0, len).toLowerCase() === value && next(elem);
};
}
return function(elem) {
var _a;
return !!((_a = adapter.getAttributeValue(elem, name)) === null || _a === void 0 ? void 0 : _a.startsWith(value)) && next(elem);
};
},
end: function(next, data, options) {
var adapter = options.adapter;
var name = data.name;
var value = data.value;
var len = -value.length;
if (len === 0) {
return boolbase_1.falseFunc;
}
if (shouldIgnoreCase(data, options)) {
value = value.toLowerCase();
return function(elem) {
var _a;
return ((_a = adapter.getAttributeValue(elem, name)) === null || _a === void 0 ? void 0 : _a.substr(len).toLowerCase()) === value && next(elem);
};
}
return function(elem) {
var _a;
return !!((_a = adapter.getAttributeValue(elem, name)) === null || _a === void 0 ? void 0 : _a.endsWith(value)) && next(elem);
};
},
any: function(next, data, options) {
var adapter = options.adapter;
var name = data.name, value = data.value;
if (value === "") {
return boolbase_1.falseFunc;
}
if (shouldIgnoreCase(data, options)) {
var regex_1 = new RegExp(escapeRegex(value), "i");
return function anyIC(elem) {
var attr = adapter.getAttributeValue(elem, name);
return attr != null && attr.length >= value.length && regex_1.test(attr) && next(elem);
};
}
return function(elem) {
var _a;
return !!((_a = adapter.getAttributeValue(elem, name)) === null || _a === void 0 ? void 0 : _a.includes(value)) && next(elem);
};
},
not: function(next, data, options) {
var adapter = options.adapter;
var name = data.name;
var value = data.value;
if (value === "") {
return function(elem) {
return !!adapter.getAttributeValue(elem, name) && next(elem);
};
} else if (shouldIgnoreCase(data, options)) {
value = value.toLowerCase();
return function(elem) {
var attr = adapter.getAttributeValue(elem, name);
return (attr == null || attr.length !== value.length || attr.toLowerCase() !== value) && next(elem);
};
}
return function(elem) {
return adapter.getAttributeValue(elem, name) !== value && next(elem);
};
}
};
}
});
// node_modules/nth-check/lib/parse.js
var require_parse2 = __commonJS({
"node_modules/nth-check/lib/parse.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.parse = void 0;
var whitespace = /* @__PURE__ */ new Set([9, 10, 12, 13, 32]);
var ZERO = "0".charCodeAt(0);
var NINE = "9".charCodeAt(0);
function parse2(formula) {
formula = formula.trim().toLowerCase();
if (formula === "even") {
return [2, 0];
} else if (formula === "odd") {
return [2, 1];
}
var idx = 0;
var a = 0;
var sign = readSign();
var number = readNumber();
if (idx < formula.length && formula.charAt(idx) === "n") {
idx++;
a = sign * (number !== null && number !== void 0 ? number : 1);
skipWhitespace();
if (idx < formula.length) {
sign = readSign();
skipWhitespace();
number = readNumber();
} else {
sign = number = 0;
}
}
if (number === null || idx < formula.length) {
throw new Error("n-th rule couldn't be parsed ('".concat(formula, "')"));
}
return [a, sign * number];
function readSign() {
if (formula.charAt(idx) === "-") {
idx++;
return -1;
}
if (formula.charAt(idx) === "+") {
idx++;
}
return 1;
}
function readNumber() {
var start = idx;
var value = 0;
while (idx < formula.length && formula.charCodeAt(idx) >= ZERO && formula.charCodeAt(idx) <= NINE) {
value = value * 10 + (formula.charCodeAt(idx) - ZERO);
idx++;
}
return idx === start ? null : value;
}
function skipWhitespace() {
while (idx < formula.length && whitespace.has(formula.charCodeAt(idx))) {
idx++;
}
}
}
exports.parse = parse2;
}
});
// node_modules/nth-check/lib/compile.js
var require_compile = __commonJS({
"node_modules/nth-check/lib/compile.js"(exports) {
"use strict";
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.generate = exports.compile = void 0;
var boolbase_1 = __importDefault(require_boolbase());
function compile(parsed) {
var a = parsed[0];
var b = parsed[1] - 1;
if (b < 0 && a <= 0)
return boolbase_1.default.falseFunc;
if (a === -1)
return function(index) {
return index <= b;
};
if (a === 0)
return function(index) {
return index === b;
};
if (a === 1)
return b < 0 ? boolbase_1.default.trueFunc : function(index) {
return index >= b;
};
var absA = Math.abs(a);
var bMod = (b % absA + absA) % absA;
return a > 1 ? function(index) {
return index >= b && index % absA === bMod;
} : function(index) {
return index <= b && index % absA === bMod;
};
}
exports.compile = compile;
function generate(parsed) {
var a = parsed[0];
var b = parsed[1] - 1;
var n2 = 0;
if (a < 0) {
var aPos_1 = -a;
var minValue_1 = (b % aPos_1 + aPos_1) % aPos_1;
return function() {
var val = minValue_1 + aPos_1 * n2++;
return val > b ? null : val;
};
}
if (a === 0)
return b < 0 ? function() {
return null;
} : function() {
return n2++ === 0 ? b : null;
};
if (b < 0) {
b += a * Math.ceil(-b / a);
}
return function() {
return a * n2++ + b;
};
}
exports.generate = generate;
}
});
// node_modules/nth-check/lib/index.js
var require_lib6 = __commonJS({
"node_modules/nth-check/lib/index.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.sequence = exports.generate = exports.compile = exports.parse = void 0;
var parse_js_1 = require_parse2();
Object.defineProperty(exports, "parse", { enumerable: true, get: function() {
return parse_js_1.parse;
} });
var compile_js_1 = require_compile();
Object.defineProperty(exports, "compile", { enumerable: true, get: function() {
return compile_js_1.compile;
} });
Object.defineProperty(exports, "generate", { enumerable: true, get: function() {
return compile_js_1.generate;
} });
function nthCheck(formula) {
return (0, compile_js_1.compile)((0, parse_js_1.parse)(formula));
}
exports.default = nthCheck;
function sequence(formula) {
return (0, compile_js_1.generate)((0, parse_js_1.parse)(formula));
}
exports.sequence = sequence;
}
});
// node_modules/css-select/lib/pseudo-selectors/filters.js
var require_filters = __commonJS({
"node_modules/css-select/lib/pseudo-selectors/filters.js"(exports) {
"use strict";
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.filters = void 0;
var nth_check_1 = __importDefault(require_lib6());
var boolbase_1 = require_boolbase();
function getChildFunc(next, adapter) {
return function(elem) {
var parent = adapter.getParent(elem);
return parent != null && adapter.isTag(parent) && next(elem);
};
}
exports.filters = {
contains: function(next, text, _a) {
var adapter = _a.adapter;
return function contains(elem) {
return next(elem) && adapter.getText(elem).includes(text);
};
},
icontains: function(next, text, _a) {
var adapter = _a.adapter;
var itext = text.toLowerCase();
return function icontains(elem) {
return next(elem) && adapter.getText(elem).toLowerCase().includes(itext);
};
},
"nth-child": function(next, rule, _a) {
var adapter = _a.adapter, equals = _a.equals;
var func = (0, nth_check_1.default)(rule);
if (func === boolbase_1.falseFunc)
return boolbase_1.falseFunc;
if (func === boolbase_1.trueFunc)
return getChildFunc(next, adapter);
return function nthChild(elem) {
var siblings = adapter.getSiblings(elem);
var pos = 0;
for (var i = 0; i < siblings.length; i++) {
if (equals(elem, siblings[i]))
break;
if (adapter.isTag(siblings[i])) {
pos++;
}
}
return func(pos) && next(elem);
};
},
"nth-last-child": function(next, rule, _a) {
var adapter = _a.adapter, equals = _a.equals;
var func = (0, nth_check_1.default)(rule);
if (func === boolbase_1.falseFunc)
return boolbase_1.falseFunc;
if (func === boolbase_1.trueFunc)
return getChildFunc(next, adapter);
return function nthLastChild(elem) {
var siblings = adapter.getSiblings(elem);
var pos = 0;
for (var i = siblings.length - 1; i >= 0; i--) {
if (equals(elem, siblings[i]))
break;
if (adapter.isTag(siblings[i])) {
pos++;
}
}
return func(pos) && next(elem);
};
},
"nth-of-type": function(next, rule, _a) {
var adapter = _a.adapter, equals = _a.equals;
var func = (0, nth_check_1.default)(rule);
if (func === boolbase_1.falseFunc)
return boolbase_1.falseFunc;
if (func === boolbase_1.trueFunc)
return getChildFunc(next, adapter);
return function nthOfType(elem) {
var siblings = adapter.getSiblings(elem);
var pos = 0;
for (var i = 0; i < siblings.length; i++) {
var currentSibling = siblings[i];
if (equals(elem, currentSibling))
break;
if (adapter.isTag(currentSibling) && adapter.getName(currentSibling) === adapter.getName(elem)) {
pos++;
}
}
return func(pos) && next(elem);
};
},
"nth-last-of-type": function(next, rule, _a) {
var adapter = _a.adapter, equals = _a.equals;
var func = (0, nth_check_1.default)(rule);
if (func === boolbase_1.falseFunc)
return boolbase_1.falseFunc;
if (func === boolbase_1.trueFunc)
return getChildFunc(next, adapter);
return function nthLastOfType(elem) {
var siblings = adapter.getSiblings(elem);
var pos = 0;
for (var i = siblings.length - 1; i >= 0; i--) {
var currentSibling = siblings[i];
if (equals(elem, currentSibling))
break;
if (adapter.isTag(currentSibling) && adapter.getName(currentSibling) === adapter.getName(elem)) {
pos++;
}
}
return func(pos) && next(elem);
};
},
root: function(next, _rule, _a) {
var adapter = _a.adapter;
return function(elem) {
var parent = adapter.getParent(elem);
return (parent == null || !adapter.isTag(parent)) && next(elem);
};
},
scope: function(next, rule, options, context) {
var equals = options.equals;
if (!context || context.length === 0) {
return exports.filters.root(next, rule, options);
}
if (context.length === 1) {
return function(elem) {
return equals(context[0], elem) && next(elem);
};
}
return function(elem) {
return context.includes(elem) && next(elem);
};
},
hover: dynamicStatePseudo("isHovered"),
visited: dynamicStatePseudo("isVisited"),
active: dynamicStatePseudo("isActive")
};
function dynamicStatePseudo(name) {
return function dynamicPseudo(next, _rule, _a) {
var adapter = _a.adapter;
var func = adapter[name];
if (typeof func !== "function") {
return boolbase_1.falseFunc;
}
return function active(elem) {
return func(elem) && next(elem);
};
};
}
}
});
// node_modules/css-select/lib/pseudo-selectors/pseudos.js
var require_pseudos = __commonJS({
"node_modules/css-select/lib/pseudo-selectors/pseudos.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.verifyPseudoArgs = exports.pseudos = void 0;
exports.pseudos = {
empty: function(elem, _a) {
var adapter = _a.adapter;
return !adapter.getChildren(elem).some(function(elem2) {
return adapter.isTag(elem2) || adapter.getText(elem2) !== "";
});
},
"first-child": function(elem, _a) {
var adapter = _a.adapter, equals = _a.equals;
var firstChild = adapter.getSiblings(elem).find(function(elem2) {
return adapter.isTag(elem2);
});
return firstChild != null && equals(elem, firstChild);
},
"last-child": function(elem, _a) {
var adapter = _a.adapter, equals = _a.equals;
var siblings = adapter.getSiblings(elem);
for (var i = siblings.length - 1; i >= 0; i--) {
if (equals(elem, siblings[i]))
return true;
if (adapter.isTag(siblings[i]))
break;
}
return false;
},
"first-of-type": function(elem, _a) {
var adapter = _a.adapter, equals = _a.equals;
var siblings = adapter.getSiblings(elem);
var elemName = adapter.getName(elem);
for (var i = 0; i < siblings.length; i++) {
var currentSibling = siblings[i];
if (equals(elem, currentSibling))
return true;
if (adapter.isTag(currentSibling) && adapter.getName(currentSibling) === elemName) {
break;
}
}
return false;
},
"last-of-type": function(elem, _a) {
var adapter = _a.adapter, equals = _a.equals;
var siblings = adapter.getSiblings(elem);
var elemName = adapter.getName(elem);
for (var i = siblings.length - 1; i >= 0; i--) {
var currentSibling = siblings[i];
if (equals(elem, currentSibling))
return true;
if (adapter.isTag(currentSibling) && adapter.getName(currentSibling) === elemName) {
break;
}
}
return false;
},
"only-of-type": function(elem, _a) {
var adapter = _a.adapter, equals = _a.equals;
var elemName = adapter.getName(elem);
return adapter.getSiblings(elem).every(function(sibling) {
return equals(elem, sibling) || !adapter.isTag(sibling) || adapter.getName(sibling) !== elemName;
});
},
"only-child": function(elem, _a) {
var adapter = _a.adapter, equals = _a.equals;
return adapter.getSiblings(elem).every(function(sibling) {
return equals(elem, sibling) || !adapter.isTag(sibling);
});
}
};
function verifyPseudoArgs(func, name, subselect) {
if (subselect === null) {
if (func.length > 2) {
throw new Error("pseudo-selector :".concat(name, " requires an argument"));
}
} else if (func.length === 2) {
throw new Error("pseudo-selector :".concat(name, " doesn't have any arguments"));
}
}
exports.verifyPseudoArgs = verifyPseudoArgs;
}
});
// node_modules/css-select/lib/pseudo-selectors/aliases.js
var require_aliases = __commonJS({
"node_modules/css-select/lib/pseudo-selectors/aliases.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.aliases = void 0;
exports.aliases = {
"any-link": ":is(a, area, link)[href]",
link: ":any-link:not(:visited)",
disabled: ":is(\n :is(button, input, select, textarea, optgroup, option)[disabled],\n optgroup[disabled] > option,\n fieldset[disabled]:not(fieldset[disabled] legend:first-of-type *)\n )",
enabled: ":not(:disabled)",
checked: ":is(:is(input[type=radio], input[type=checkbox])[checked], option:selected)",
required: ":is(input, select, textarea)[required]",
optional: ":is(input, select, textarea):not([required])",
selected: "option:is([selected], select:not([multiple]):not(:has(> option[selected])) > :first-of-type)",
checkbox: "[type=checkbox]",
file: "[type=file]",
password: "[type=password]",
radio: "[type=radio]",
reset: "[type=reset]",
image: "[type=image]",
submit: "[type=submit]",
parent: ":not(:empty)",
header: ":is(h1, h2, h3, h4, h5, h6)",
button: ":is(button, input[type=button])",
input: ":is(input, textarea, select, button)",
text: "input:is(:not([type!='']), [type=text])"
};
}
});
// node_modules/css-select/lib/pseudo-selectors/subselects.js
var require_subselects = __commonJS({
"node_modules/css-select/lib/pseudo-selectors/subselects.js"(exports) {
"use strict";
var __spreadArray = exports && exports.__spreadArray || function(to, from, pack) {
if (pack || arguments.length === 2)
for (var i = 0, l = from.length, ar; i < l; i++) {
if (ar || !(i in from)) {
if (!ar)
ar = Array.prototype.slice.call(from, 0, i);
ar[i] = from[i];
}
}
return to.concat(ar || Array.prototype.slice.call(from));
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.subselects = exports.getNextSiblings = exports.ensureIsTag = exports.PLACEHOLDER_ELEMENT = void 0;
var boolbase_1 = require_boolbase();
var procedure_1 = require_procedure();
exports.PLACEHOLDER_ELEMENT = {};
function ensureIsTag(next, adapter) {
if (next === boolbase_1.falseFunc)
return boolbase_1.falseFunc;
return function(elem) {
return adapter.isTag(elem) && next(elem);
};
}
exports.ensureIsTag = ensureIsTag;
function getNextSiblings(elem, adapter) {
var siblings = adapter.getSiblings(elem);
if (siblings.length <= 1)
return [];
var elemIndex = siblings.indexOf(elem);
if (elemIndex < 0 || elemIndex === siblings.length - 1)
return [];
return siblings.slice(elemIndex + 1).filter(adapter.isTag);
}
exports.getNextSiblings = getNextSiblings;
var is = function(next, token, options, context, compileToken) {
var opts = {
xmlMode: !!options.xmlMode,
adapter: options.adapter,
equals: options.equals
};
var func = compileToken(token, opts, context);
return function(elem) {
return func(elem) && next(elem);
};
};
exports.subselects = {
is,
matches: is,
where: is,
not: function(next, token, options, context, compileToken) {
var opts = {
xmlMode: !!options.xmlMode,
adapter: options.adapter,
equals: options.equals
};
var func = compileToken(token, opts, context);
if (func === boolbase_1.falseFunc)
return next;
if (func === boolbase_1.trueFunc)
return boolbase_1.falseFunc;
return function not(elem) {
return !func(elem) && next(elem);
};
},
has: function(next, subselect, options, _context, compileToken) {
var adapter = options.adapter;
var opts = {
xmlMode: !!options.xmlMode,
adapter,
equals: options.equals
};
var context = subselect.some(function(s) {
return s.some(procedure_1.isTraversal);
}) ? [exports.PLACEHOLDER_ELEMENT] : void 0;
var compiled = compileToken(subselect, opts, context);
if (compiled === boolbase_1.falseFunc)
return boolbase_1.falseFunc;
if (compiled === boolbase_1.trueFunc) {
return function(elem) {
return adapter.getChildren(elem).some(adapter.isTag) && next(elem);
};
}
var hasElement = ensureIsTag(compiled, adapter);
var _a = compiled.shouldTestNextSiblings, shouldTestNextSiblings = _a === void 0 ? false : _a;
if (context) {
return function(elem) {
context[0] = elem;
var childs = adapter.getChildren(elem);
var nextElements = shouldTestNextSiblings ? __spreadArray(__spreadArray([], childs, true), getNextSiblings(elem, adapter), true) : childs;
return next(elem) && adapter.existsOne(hasElement, nextElements);
};
}
return function(elem) {
return next(elem) && adapter.existsOne(hasElement, adapter.getChildren(elem));
};
}
};
}
});
// node_modules/css-select/lib/pseudo-selectors/index.js
var require_pseudo_selectors = __commonJS({
"node_modules/css-select/lib/pseudo-selectors/index.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.compilePseudoSelector = exports.aliases = exports.pseudos = exports.filters = void 0;
var boolbase_1 = require_boolbase();
var css_what_1 = require_commonjs();
var filters_1 = require_filters();
Object.defineProperty(exports, "filters", { enumerable: true, get: function() {
return filters_1.filters;
} });
var pseudos_1 = require_pseudos();
Object.defineProperty(exports, "pseudos", { enumerable: true, get: function() {
return pseudos_1.pseudos;
} });
var aliases_1 = require_aliases();
Object.defineProperty(exports, "aliases", { enumerable: true, get: function() {
return aliases_1.aliases;
} });
var subselects_1 = require_subselects();
function compilePseudoSelector(next, selector, options, context, compileToken) {
var name = selector.name, data = selector.data;
if (Array.isArray(data)) {
return subselects_1.subselects[name](next, data, options, context, compileToken);
}
if (name in aliases_1.aliases) {
if (data != null) {
throw new Error("Pseudo ".concat(name, " doesn't have any arguments"));
}
var alias = (0, css_what_1.parse)(aliases_1.aliases[name]);
return subselects_1.subselects.is(next, alias, options, context, compileToken);
}
if (name in filters_1.filters) {
return filters_1.filters[name](next, data, options, context);
}
if (name in pseudos_1.pseudos) {
var pseudo_1 = pseudos_1.pseudos[name];
(0, pseudos_1.verifyPseudoArgs)(pseudo_1, name, data);
return pseudo_1 === boolbase_1.falseFunc ? boolbase_1.falseFunc : next === boolbase_1.trueFunc ? function(elem) {
return pseudo_1(elem, options, data);
} : function(elem) {
return pseudo_1(elem, options, data) && next(elem);
};
}
throw new Error("unmatched pseudo-class :".concat(name));
}
exports.compilePseudoSelector = compilePseudoSelector;
}
});
// node_modules/css-select/lib/general.js
var require_general = __commonJS({
"node_modules/css-select/lib/general.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.compileGeneralSelector = void 0;
var attributes_1 = require_attributes();
var pseudo_selectors_1 = require_pseudo_selectors();
var css_what_1 = require_commonjs();
function compileGeneralSelector(next, selector, options, context, compileToken) {
var adapter = options.adapter, equals = options.equals;
switch (selector.type) {
case css_what_1.SelectorType.PseudoElement: {
throw new Error("Pseudo-elements are not supported by css-select");
}
case css_what_1.SelectorType.ColumnCombinator: {
throw new Error("Column combinators are not yet supported by css-select");
}
case css_what_1.SelectorType.Attribute: {
if (selector.namespace != null) {
throw new Error("Namespaced attributes are not yet supported by css-select");
}
if (!options.xmlMode || options.lowerCaseAttributeNames) {
selector.name = selector.name.toLowerCase();
}
return attributes_1.attributeRules[selector.action](next, selector, options);
}
case css_what_1.SelectorType.Pseudo: {
return (0, pseudo_selectors_1.compilePseudoSelector)(next, selector, options, context, compileToken);
}
case css_what_1.SelectorType.Tag: {
if (selector.namespace != null) {
throw new Error("Namespaced tag names are not yet supported by css-select");
}
var name_1 = selector.name;
if (!options.xmlMode || options.lowerCaseTags) {
name_1 = name_1.toLowerCase();
}
return function tag(elem) {
return adapter.getName(elem) === name_1 && next(elem);
};
}
case css_what_1.SelectorType.Descendant: {
if (options.cacheResults === false || typeof WeakSet === "undefined") {
return function descendant(elem) {
var current = elem;
while (current = adapter.getParent(current)) {
if (adapter.isTag(current) && next(current)) {
return true;
}
}
return false;
};
}
var isFalseCache_1 = /* @__PURE__ */ new WeakSet();
return function cachedDescendant(elem) {
var current = elem;
while (current = adapter.getParent(current)) {
if (!isFalseCache_1.has(current)) {
if (adapter.isTag(current) && next(current)) {
return true;
}
isFalseCache_1.add(current);
}
}
return false;
};
}
case "_flexibleDescendant": {
return function flexibleDescendant(elem) {
var current = elem;
do {
if (adapter.isTag(current) && next(current))
return true;
} while (current = adapter.getParent(current));
return false;
};
}
case css_what_1.SelectorType.Parent: {
return function parent(elem) {
return adapter.getChildren(elem).some(function(elem2) {
return adapter.isTag(elem2) && next(elem2);
});
};
}
case css_what_1.SelectorType.Child: {
return function child(elem) {
var parent = adapter.getParent(elem);
return parent != null && adapter.isTag(parent) && next(parent);
};
}
case css_what_1.SelectorType.Sibling: {
return function sibling(elem) {
var siblings = adapter.getSiblings(elem);
for (var i = 0; i < siblings.length; i++) {
var currentSibling = siblings[i];
if (equals(elem, currentSibling))
break;
if (adapter.isTag(currentSibling) && next(currentSibling)) {
return true;
}
}
return false;
};
}
case css_what_1.SelectorType.Adjacent: {
if (adapter.prevElementSibling) {
return function adjacent(elem) {
var previous = adapter.prevElementSibling(elem);
return previous != null && next(previous);
};
}
return function adjacent(elem) {
var siblings = adapter.getSiblings(elem);
var lastElement;
for (var i = 0; i < siblings.length; i++) {
var currentSibling = siblings[i];
if (equals(elem, currentSibling))
break;
if (adapter.isTag(currentSibling)) {
lastElement = currentSibling;
}
}
return !!lastElement && next(lastElement);
};
}
case css_what_1.SelectorType.Universal: {
if (selector.namespace != null && selector.namespace !== "*") {
throw new Error("Namespaced universal selectors are not yet supported by css-select");
}
return next;
}
}
}
exports.compileGeneralSelector = compileGeneralSelector;
}
});
// node_modules/css-select/lib/compile.js
var require_compile2 = __commonJS({
"node_modules/css-select/lib/compile.js"(exports) {
"use strict";
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.compileToken = exports.compileUnsafe = exports.compile = void 0;
var css_what_1 = require_commonjs();
var boolbase_1 = require_boolbase();
var sort_1 = __importDefault(require_sort());
var procedure_1 = require_procedure();
var general_1 = require_general();
var subselects_1 = require_subselects();
function compile(selector, options, context) {
var next = compileUnsafe(selector, options, context);
return (0, subselects_1.ensureIsTag)(next, options.adapter);
}
exports.compile = compile;
function compileUnsafe(selector, options, context) {
var token = typeof selector === "string" ? (0, css_what_1.parse)(selector) : selector;
return compileToken(token, options, context);
}
exports.compileUnsafe = compileUnsafe;
function includesScopePseudo(t) {
return t.type === "pseudo" && (t.name === "scope" || Array.isArray(t.data) && t.data.some(function(data) {
return data.some(includesScopePseudo);
}));
}
var DESCENDANT_TOKEN = { type: css_what_1.SelectorType.Descendant };
var FLEXIBLE_DESCENDANT_TOKEN = {
type: "_flexibleDescendant"
};
var SCOPE_TOKEN = {
type: css_what_1.SelectorType.Pseudo,
name: "scope",
data: null
};
function absolutize(token, _a, context) {
var adapter = _a.adapter;
var hasContext = !!(context === null || context === void 0 ? void 0 : context.every(function(e) {
var parent = adapter.isTag(e) && adapter.getParent(e);
return e === subselects_1.PLACEHOLDER_ELEMENT || parent && adapter.isTag(parent);
}));
for (var _i = 0, token_1 = token; _i < token_1.length; _i++) {
var t = token_1[_i];
if (t.length > 0 && (0, procedure_1.isTraversal)(t[0]) && t[0].type !== "descendant") {
} else if (hasContext && !t.some(includesScopePseudo)) {
t.unshift(DESCENDANT_TOKEN);
} else {
continue;
}
t.unshift(SCOPE_TOKEN);
}
}
function compileToken(token, options, context) {
var _a;
token = token.filter(function(t) {
return t.length > 0;
});
token.forEach(sort_1.default);
context = (_a = options.context) !== null && _a !== void 0 ? _a : context;
var isArrayContext = Array.isArray(context);
var finalContext = context && (Array.isArray(context) ? context : [context]);
absolutize(token, options, finalContext);
var shouldTestNextSiblings = false;
var query = token.map(function(rules) {
if (rules.length >= 2) {
var first = rules[0], second = rules[1];
if (first.type !== "pseudo" || first.name !== "scope") {
} else if (isArrayContext && second.type === "descendant") {
rules[1] = FLEXIBLE_DESCENDANT_TOKEN;
} else if (second.type === "adjacent" || second.type === "sibling") {
shouldTestNextSiblings = true;
}
}
return compileRules(rules, options, finalContext);
}).reduce(reduceRules, boolbase_1.falseFunc);
query.shouldTestNextSiblings = shouldTestNextSiblings;
return query;
}
exports.compileToken = compileToken;
function compileRules(rules, options, context) {
var _a;
return rules.reduce(function(previous, rule) {
return previous === boolbase_1.falseFunc ? boolbase_1.falseFunc : (0, general_1.compileGeneralSelector)(previous, rule, options, context, compileToken);
}, (_a = options.rootFunc) !== null && _a !== void 0 ? _a : boolbase_1.trueFunc);
}
function reduceRules(a, b) {
if (b === boolbase_1.falseFunc || a === boolbase_1.trueFunc) {
return a;
}
if (a === boolbase_1.falseFunc || b === boolbase_1.trueFunc) {
return b;
}
return function combine(elem) {
return a(elem) || b(elem);
};
}
}
});
// node_modules/css-select/lib/index.js
var require_lib7 = __commonJS({
"node_modules/css-select/lib/index.js"(exports) {
"use strict";
var __createBinding = exports && exports.__createBinding || (Object.create ? function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
var desc = Object.getOwnPropertyDescriptor(m, k);
if (!desc || ("get" in desc ? !m.__esModule : desc.writable || desc.configurable)) {
desc = { enumerable: true, get: function() {
return m[k];
} };
}
Object.defineProperty(o, k2, desc);
} : function(o, m, k, k2) {
if (k2 === void 0)
k2 = k;
o[k2] = m[k];
});
var __setModuleDefault = exports && exports.__setModuleDefault || (Object.create ? function(o, v) {
Object.defineProperty(o, "default", { enumerable: true, value: v });
} : function(o, v) {
o["default"] = v;
});
var __importStar = exports && exports.__importStar || function(mod) {
if (mod && mod.__esModule)
return mod;
var result = {};
if (mod != null) {
for (var k in mod)
if (k !== "default" && Object.prototype.hasOwnProperty.call(mod, k))
__createBinding(result, mod, k);
}
__setModuleDefault(result, mod);
return result;
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.aliases = exports.pseudos = exports.filters = exports.is = exports.selectOne = exports.selectAll = exports.prepareContext = exports._compileToken = exports._compileUnsafe = exports.compile = void 0;
var DomUtils = __importStar(require_lib5());
var boolbase_1 = require_boolbase();
var compile_1 = require_compile2();
var subselects_1 = require_subselects();
var defaultEquals = function(a, b) {
return a === b;
};
var defaultOptions = {
adapter: DomUtils,
equals: defaultEquals
};
function convertOptionFormats(options) {
var _a, _b, _c, _d;
var opts = options !== null && options !== void 0 ? options : defaultOptions;
(_a = opts.adapter) !== null && _a !== void 0 ? _a : opts.adapter = DomUtils;
(_b = opts.equals) !== null && _b !== void 0 ? _b : opts.equals = (_d = (_c = opts.adapter) === null || _c === void 0 ? void 0 : _c.equals) !== null && _d !== void 0 ? _d : defaultEquals;
return opts;
}
function wrapCompile(func) {
return function addAdapter(selector, options, context) {
var opts = convertOptionFormats(options);
return func(selector, opts, context);
};
}
exports.compile = wrapCompile(compile_1.compile);
exports._compileUnsafe = wrapCompile(compile_1.compileUnsafe);
exports._compileToken = wrapCompile(compile_1.compileToken);
function getSelectorFunc(searchFunc) {
return function select(query, elements, options) {
var opts = convertOptionFormats(options);
if (typeof query !== "function") {
query = (0, compile_1.compileUnsafe)(query, opts, elements);
}
var filteredElements = prepareContext(elements, opts.adapter, query.shouldTestNextSiblings);
return searchFunc(query, filteredElements, opts);
};
}
function prepareContext(elems, adapter, shouldTestNextSiblings) {
if (shouldTestNextSiblings === void 0) {
shouldTestNextSiblings = false;
}
if (shouldTestNextSiblings) {
elems = appendNextSiblings(elems, adapter);
}
return Array.isArray(elems) ? adapter.removeSubsets(elems) : adapter.getChildren(elems);
}
exports.prepareContext = prepareContext;
function appendNextSiblings(elem, adapter) {
var elems = Array.isArray(elem) ? elem.slice(0) : [elem];
var elemsLength = elems.length;
for (var i = 0; i < elemsLength; i++) {
var nextSiblings = (0, subselects_1.getNextSiblings)(elems[i], adapter);
elems.push.apply(elems, nextSiblings);
}
return elems;
}
exports.selectAll = getSelectorFunc(function(query, elems, options) {
return query === boolbase_1.falseFunc || !elems || elems.length === 0 ? [] : options.adapter.findAll(query, elems);
});
exports.selectOne = getSelectorFunc(function(query, elems, options) {
return query === boolbase_1.falseFunc || !elems || elems.length === 0 ? null : options.adapter.findOne(query, elems);
});
function is(elem, query, options) {
var opts = convertOptionFormats(options);
return (typeof query === "function" ? query : (0, compile_1.compile)(query, opts))(elem);
}
exports.is = is;
exports.default = exports.selectAll;
var pseudo_selectors_1 = require_pseudo_selectors();
Object.defineProperty(exports, "filters", { enumerable: true, get: function() {
return pseudo_selectors_1.filters;
} });
Object.defineProperty(exports, "pseudos", { enumerable: true, get: function() {
return pseudo_selectors_1.pseudos;
} });
Object.defineProperty(exports, "aliases", { enumerable: true, get: function() {
return pseudo_selectors_1.aliases;
} });
}
});
// node_modules/a-node-html-parser/dist/back.js
var require_back = __commonJS({
"node_modules/a-node-html-parser/dist/back.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
function arr_back(arr) {
return arr[arr.length - 1];
}
exports.default = arr_back;
}
});
// node_modules/a-node-html-parser/dist/matcher.js
var require_matcher = __commonJS({
"node_modules/a-node-html-parser/dist/matcher.js"(exports) {
"use strict";
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
var type_1 = __importDefault(require_type());
function isTag(node) {
return node && node.nodeType === type_1.default.ELEMENT_NODE;
}
function getAttributeValue(elem, name) {
return isTag(elem) ? elem.getAttribute(name) : void 0;
}
function getName(elem) {
return (elem && elem.rawTagName || "").toLowerCase();
}
function getChildren(node) {
return node && node.childNodes;
}
function getParent(node) {
return node ? node.parentNode : null;
}
function getText(node) {
return node.text;
}
function removeSubsets(nodes) {
var idx = nodes.length;
var node;
var ancestor;
var replace;
while (--idx > -1) {
node = ancestor = nodes[idx];
nodes[idx] = null;
replace = true;
while (ancestor) {
if (nodes.indexOf(ancestor) > -1) {
replace = false;
nodes.splice(idx, 1);
break;
}
ancestor = getParent(ancestor);
}
if (replace) {
nodes[idx] = node;
}
}
return nodes;
}
function existsOne(test, elems) {
return elems.some(function(elem) {
return isTag(elem) ? test(elem) || existsOne(test, getChildren(elem)) : false;
});
}
function getSiblings(node) {
var parent = getParent(node);
return parent && getChildren(parent);
}
function hasAttrib(elem, name) {
return getAttributeValue(elem, name) !== void 0;
}
function findOne(test, elems) {
var elem = null;
for (var i = 0, l = elems.length; i < l && !elem; i++) {
var el = elems[i];
if (test(el)) {
elem = el;
} else {
var childs = getChildren(el);
if (childs && childs.length > 0) {
elem = findOne(test, childs);
}
}
}
return elem;
}
function findAll(test, nodes) {
var result = [];
for (var i = 0, j = nodes.length; i < j; i++) {
if (!isTag(nodes[i]))
continue;
if (test(nodes[i]))
result.push(nodes[i]);
var childs = getChildren(nodes[i]);
if (childs)
result = result.concat(findAll(test, childs));
}
return result;
}
exports.default = {
isTag,
getAttributeValue,
getName,
getChildren,
getParent,
getText,
removeSubsets,
existsOne,
getSiblings,
hasAttrib,
findOne,
findAll
};
}
});
// node_modules/a-node-html-parser/dist/void-tag.js
var require_void_tag = __commonJS({
"node_modules/a-node-html-parser/dist/void-tag.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
var VoidTag = function() {
function VoidTag2(addClosingSlash, tags) {
if (addClosingSlash === void 0) {
addClosingSlash = false;
}
this.addClosingSlash = addClosingSlash;
if (Array.isArray(tags)) {
this.voidTags = tags.reduce(function(set, tag) {
return set.add(tag.toLowerCase());
}, /* @__PURE__ */ new Set());
} else {
this.voidTags = ["area", "base", "br", "col", "embed", "hr", "img", "input", "link", "meta", "param", "source", "track", "wbr"].reduce(function(set, tag) {
return set.add(tag);
}, /* @__PURE__ */ new Set());
}
}
VoidTag2.prototype.formatNode = function(tag, attrs, innerHTML) {
var addClosingSlash = this.addClosingSlash;
var closingSpace = addClosingSlash && attrs && !attrs.endsWith(" ") ? " " : "";
var closingSlash = addClosingSlash ? "".concat(closingSpace, "/") : "";
return this.isVoidElement(tag.toLowerCase()) ? "<".concat(tag).concat(attrs).concat(closingSlash, ">") : "<".concat(tag).concat(attrs, ">").concat(innerHTML, "").concat(tag, ">");
};
VoidTag2.prototype.isVoidElement = function(tag) {
return this.voidTags.has(tag);
};
return VoidTag2;
}();
exports.default = VoidTag;
}
});
// node_modules/a-node-html-parser/dist/nodes/text.js
var require_text = __commonJS({
"node_modules/a-node-html-parser/dist/nodes/text.js"(exports) {
"use strict";
var __extends = exports && exports.__extends || function() {
var extendStatics = function(d, b) {
extendStatics = Object.setPrototypeOf || { __proto__: [] } instanceof Array && function(d2, b2) {
d2.__proto__ = b2;
} || function(d2, b2) {
for (var p in b2)
if (Object.prototype.hasOwnProperty.call(b2, p))
d2[p] = b2[p];
};
return extendStatics(d, b);
};
return function(d, b) {
if (typeof b !== "function" && b !== null)
throw new TypeError("Class extends value " + String(b) + " is not a constructor or null");
extendStatics(d, b);
function __() {
this.constructor = d;
}
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
};
}();
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
var he_1 = require_he();
var node_1 = __importDefault(require_node());
var type_1 = __importDefault(require_type());
var TextNode = function(_super) {
__extends(TextNode2, _super);
function TextNode2(rawText, parentNode, range) {
var _this = _super.call(this, parentNode, range) || this;
_this.nodeType = type_1.default.TEXT_NODE;
_this._rawText = rawText;
return _this;
}
TextNode2.prototype.clone = function() {
return new TextNode2(this._rawText, null);
};
Object.defineProperty(TextNode2.prototype, "rawText", {
get: function() {
return this._rawText;
},
set: function(text) {
this._rawText = text;
this._trimmedRawText = void 0;
this._trimmedText = void 0;
},
enumerable: false,
configurable: true
});
Object.defineProperty(TextNode2.prototype, "trimmedRawText", {
get: function() {
if (this._trimmedRawText !== void 0)
return this._trimmedRawText;
this._trimmedRawText = trimText(this.rawText);
return this._trimmedRawText;
},
enumerable: false,
configurable: true
});
Object.defineProperty(TextNode2.prototype, "trimmedText", {
get: function() {
if (this._trimmedText !== void 0)
return this._trimmedText;
this._trimmedText = trimText(this.text);
return this._trimmedText;
},
enumerable: false,
configurable: true
});
Object.defineProperty(TextNode2.prototype, "text", {
get: function() {
return (0, he_1.decode)(this.rawText);
},
enumerable: false,
configurable: true
});
Object.defineProperty(TextNode2.prototype, "isWhitespace", {
get: function() {
return /^(\s| )*$/.test(this.rawText);
},
enumerable: false,
configurable: true
});
TextNode2.prototype.toString = function() {
return this.rawText;
};
return TextNode2;
}(node_1.default);
exports.default = TextNode;
function trimText(text) {
var i = 0;
var startPos;
var endPos;
while (i >= 0 && i < text.length) {
if (/\S/.test(text[i])) {
if (startPos === void 0) {
startPos = i;
i = text.length;
} else {
endPos = i;
i = void 0;
}
}
if (startPos === void 0)
i++;
else
i--;
}
if (startPos === void 0)
startPos = 0;
if (endPos === void 0)
endPos = text.length - 1;
var hasLeadingSpace = startPos > 0 && /[^\S\r\n]/.test(text[startPos - 1]);
var hasTrailingSpace = endPos < text.length - 1 && /[^\S\r\n]/.test(text[endPos + 1]);
return (hasLeadingSpace ? " " : "") + text.slice(startPos, endPos + 1) + (hasTrailingSpace ? " " : "");
}
}
});
// node_modules/a-node-html-parser/dist/nodes/html.js
var require_html = __commonJS({
"node_modules/a-node-html-parser/dist/nodes/html.js"(exports) {
"use strict";
var __extends = exports && exports.__extends || function() {
var extendStatics = function(d, b) {
extendStatics = Object.setPrototypeOf || { __proto__: [] } instanceof Array && function(d2, b2) {
d2.__proto__ = b2;
} || function(d2, b2) {
for (var p in b2)
if (Object.prototype.hasOwnProperty.call(b2, p))
d2[p] = b2[p];
};
return extendStatics(d, b);
};
return function(d, b) {
if (typeof b !== "function" && b !== null)
throw new TypeError("Class extends value " + String(b) + " is not a constructor or null");
extendStatics(d, b);
function __() {
this.constructor = d;
}
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
};
}();
var __assign = exports && exports.__assign || function() {
__assign = Object.assign || function(t) {
for (var s, i = 1, n2 = arguments.length; i < n2; i++) {
s = arguments[i];
for (var p in s)
if (Object.prototype.hasOwnProperty.call(s, p))
t[p] = s[p];
}
return t;
};
return __assign.apply(this, arguments);
};
var __spreadArray = exports && exports.__spreadArray || function(to, from, pack) {
if (pack || arguments.length === 2)
for (var i = 0, l = from.length, ar; i < l; i++) {
if (ar || !(i in from)) {
if (!ar)
ar = Array.prototype.slice.call(from, 0, i);
ar[i] = from[i];
}
}
return to.concat(ar || Array.prototype.slice.call(from));
};
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.parse = exports.base_parse = void 0;
var css_select_1 = require_lib7();
var he_1 = __importDefault(require_he());
var back_1 = __importDefault(require_back());
var matcher_1 = __importDefault(require_matcher());
var void_tag_1 = __importDefault(require_void_tag());
var comment_1 = __importDefault(require_comment());
var node_1 = __importDefault(require_node());
var text_1 = __importDefault(require_text());
var type_1 = __importDefault(require_type());
function decode(val) {
return JSON.parse(JSON.stringify(he_1.default.decode(val)));
}
var Htags = ["h1", "h2", "h3", "h4", "h5", "h6", "header", "hgroup"];
var Dtags = ["details", "dialog", "dd", "div", "dt"];
var Ftags = ["fieldset", "figcaption", "figure", "footer", "form"];
var tableTags = ["table", "td", "tr"];
var htmlTags = ["address", "article", "aside", "blockquote", "br", "hr", "li", "main", "nav", "ol", "p", "pre", "section", "ul"];
var kBlockElements = /* @__PURE__ */ new Set();
function addToKBlockElement() {
var args = [];
for (var _i = 0; _i < arguments.length; _i++) {
args[_i] = arguments[_i];
}
var addToSet = function(array) {
for (var index = 0; index < array.length; index++) {
var element = array[index];
kBlockElements.add(element);
kBlockElements.add(element.toUpperCase());
}
};
for (var _a = 0, args_1 = args; _a < args_1.length; _a++) {
var arg = args_1[_a];
addToSet(arg);
}
}
addToKBlockElement(Htags, Dtags, Ftags, tableTags, htmlTags);
var DOMTokenList = function() {
function DOMTokenList2(valuesInit, afterUpdate) {
if (valuesInit === void 0) {
valuesInit = [];
}
if (afterUpdate === void 0) {
afterUpdate = function() {
return null;
};
}
this._set = new Set(valuesInit);
this._afterUpdate = afterUpdate;
}
DOMTokenList2.prototype._validate = function(c) {
if (/\s/.test(c)) {
throw new Error("DOMException in DOMTokenList.add: The token '".concat(c, "' contains HTML space characters, which are not valid in tokens."));
}
};
DOMTokenList2.prototype.add = function(c) {
this._validate(c);
this._set.add(c);
this._afterUpdate(this);
};
DOMTokenList2.prototype.replace = function(c1, c2) {
this._validate(c2);
this._set.delete(c1);
this._set.add(c2);
this._afterUpdate(this);
};
DOMTokenList2.prototype.remove = function(c) {
this._set.delete(c) && this._afterUpdate(this);
};
DOMTokenList2.prototype.toggle = function(c) {
this._validate(c);
if (this._set.has(c))
this._set.delete(c);
else
this._set.add(c);
this._afterUpdate(this);
};
DOMTokenList2.prototype.contains = function(c) {
return this._set.has(c);
};
Object.defineProperty(DOMTokenList2.prototype, "length", {
get: function() {
return this._set.size;
},
enumerable: false,
configurable: true
});
DOMTokenList2.prototype.values = function() {
return this._set.values();
};
Object.defineProperty(DOMTokenList2.prototype, "value", {
get: function() {
return Array.from(this._set.values());
},
enumerable: false,
configurable: true
});
DOMTokenList2.prototype.toString = function() {
return Array.from(this._set.values()).join(" ");
};
return DOMTokenList2;
}();
var HTMLElement2 = function(_super) {
__extends(HTMLElement3, _super);
function HTMLElement3(tagName, keyAttrs, rawAttrs, parentNode, range, voidTag) {
if (rawAttrs === void 0) {
rawAttrs = "";
}
if (voidTag === void 0) {
voidTag = new void_tag_1.default();
}
var _this = _super.call(this, parentNode, range) || this;
_this.rawAttrs = rawAttrs;
_this.voidTag = voidTag;
_this.nodeType = type_1.default.ELEMENT_NODE;
_this.rawTagName = tagName;
_this.rawAttrs = rawAttrs || "";
_this.id = keyAttrs.id || "";
_this.childNodes = [];
_this.classList = new DOMTokenList(
keyAttrs.class ? keyAttrs.class.split(/\s+/) : [],
function(classList) {
return _this.setAttribute("class", classList.toString());
}
);
if (keyAttrs.id) {
if (!rawAttrs) {
_this.rawAttrs = 'id="'.concat(keyAttrs.id, '"');
}
}
if (keyAttrs.class) {
if (!rawAttrs) {
var cls = 'class="'.concat(_this.classList.toString(), '"');
if (_this.rawAttrs) {
_this.rawAttrs += " ".concat(cls);
} else {
_this.rawAttrs = cls;
}
}
}
return _this;
}
HTMLElement3.prototype.quoteAttribute = function(attr) {
if (attr == null) {
return "null";
}
return JSON.stringify(attr.replace(/"/g, """));
};
HTMLElement3.prototype.removeChild = function(node) {
this.childNodes = this.childNodes.filter(function(child) {
return child !== node;
});
return this;
};
HTMLElement3.prototype.exchangeChild = function(oldNode, newNode) {
var children = this.childNodes;
this.childNodes = children.map(function(child) {
if (child === oldNode) {
return newNode;
}
return child;
});
return this;
};
Object.defineProperty(HTMLElement3.prototype, "tagName", {
get: function() {
return this.rawTagName ? this.rawTagName.toUpperCase() : this.rawTagName;
},
set: function(newname) {
this.rawTagName = newname.toLowerCase();
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "localName", {
get: function() {
return this.rawTagName.toLowerCase();
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "isVoidElement", {
get: function() {
return this.voidTag.isVoidElement(this.localName);
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "rawText", {
get: function() {
return this.childNodes.reduce(function(pre, cur) {
return pre += cur.rawText;
}, "");
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "textContent", {
get: function() {
return decode(this.rawText);
},
set: function(val) {
var content = [new text_1.default(val, this)];
this.childNodes = content;
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "text", {
get: function() {
return decode(this.rawText);
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "structuredText", {
get: function() {
var currentBlock = [];
var blocks = [currentBlock];
function dfs(node) {
if (node.nodeType === type_1.default.ELEMENT_NODE) {
if (kBlockElements.has(node.rawTagName)) {
if (currentBlock.length > 0) {
blocks.push(currentBlock = []);
}
node.childNodes.forEach(dfs);
if (currentBlock.length > 0) {
blocks.push(currentBlock = []);
}
} else {
node.childNodes.forEach(dfs);
}
} else if (node.nodeType === type_1.default.TEXT_NODE) {
if (node.isWhitespace) {
currentBlock.prependWhitespace = true;
} else {
var text = node.trimmedText;
if (currentBlock.prependWhitespace) {
text = " ".concat(text);
currentBlock.prependWhitespace = false;
}
currentBlock.push(text);
}
}
}
dfs(this);
return blocks.map(function(block) {
return block.join("").replace(/\s{2,}/g, " ");
}).join("\n").replace(/\s+$/, "");
},
enumerable: false,
configurable: true
});
HTMLElement3.prototype.toString = function() {
var tag = this.rawTagName;
if (tag) {
var attrs = this.rawAttrs ? " ".concat(this.rawAttrs) : "";
return this.voidTag.formatNode(tag, attrs, this.innerHTML);
}
return this.innerHTML;
};
Object.defineProperty(HTMLElement3.prototype, "innerHTML", {
get: function() {
return this.childNodes.map(function(child) {
return child.toString();
}).join("");
},
set: function(content) {
var r = parse2(content);
var nodes = r.childNodes.length ? r.childNodes : [new text_1.default(content, this)];
resetParent(nodes, this);
resetParent(this.childNodes, null);
this.childNodes = nodes;
},
enumerable: false,
configurable: true
});
HTMLElement3.prototype.set_content = function(content, options) {
if (options === void 0) {
options = {};
}
if (content instanceof node_1.default) {
content = [content];
} else if (typeof content == "string") {
var r = parse2(content, options);
content = r.childNodes.length ? r.childNodes : [new text_1.default(content, this)];
}
resetParent(this.childNodes, null);
resetParent(content, this);
this.childNodes = content;
return this;
};
HTMLElement3.prototype.replaceWith = function() {
var _this = this;
var nodes = [];
for (var _i = 0; _i < arguments.length; _i++) {
nodes[_i] = arguments[_i];
}
var parent = this.parentNode;
var content = nodes.map(function(node) {
if (node instanceof node_1.default) {
return [node];
} else if (typeof node == "string") {
var r = parse2(node);
return r.childNodes.length ? r.childNodes : [new text_1.default(node, _this)];
}
return [];
}).flat();
var idx = parent.childNodes.findIndex(function(child) {
return child === _this;
});
resetParent([this], null);
parent.childNodes = __spreadArray(__spreadArray(__spreadArray([], parent.childNodes.slice(0, idx), true), resetParent(content, parent), true), parent.childNodes.slice(idx + 1), true);
};
Object.defineProperty(HTMLElement3.prototype, "outerHTML", {
get: function() {
return this.toString();
},
enumerable: false,
configurable: true
});
HTMLElement3.prototype.trimRight = function(pattern) {
for (var i = 0; i < this.childNodes.length; i++) {
var childNode = this.childNodes[i];
if (childNode.nodeType === type_1.default.ELEMENT_NODE) {
childNode.trimRight(pattern);
} else {
var index = childNode.rawText.search(pattern);
if (index > -1) {
childNode.rawText = childNode.rawText.substr(0, index);
this.childNodes.length = i + 1;
}
}
}
return this;
};
Object.defineProperty(HTMLElement3.prototype, "structure", {
get: function() {
var res = [];
var indention = 0;
function write(str) {
res.push(" ".repeat(indention) + str);
}
function dfs(node) {
var idStr = node.id ? "#".concat(node.id) : "";
var classStr = node.classList.length ? ".".concat(node.classList.value.join(".")) : "";
write("".concat(node.rawTagName).concat(idStr).concat(classStr));
indention++;
node.childNodes.forEach(function(childNode) {
if (childNode.nodeType === type_1.default.ELEMENT_NODE) {
dfs(childNode);
} else if (childNode.nodeType === type_1.default.TEXT_NODE) {
if (!childNode.isWhitespace) {
write("#text");
}
}
});
indention--;
}
dfs(this);
return res.join("\n");
},
enumerable: false,
configurable: true
});
HTMLElement3.prototype.removeWhitespace = function() {
var _this = this;
var o = 0;
this.childNodes.forEach(function(node) {
if (node.nodeType === type_1.default.TEXT_NODE) {
if (node.isWhitespace) {
return;
}
node.rawText = node.trimmedRawText;
} else if (node.nodeType === type_1.default.ELEMENT_NODE) {
node.removeWhitespace();
}
_this.childNodes[o++] = node;
});
this.childNodes.length = o;
return this;
};
HTMLElement3.prototype.querySelectorAll = function(selector) {
return (0, css_select_1.selectAll)(selector, this, {
xmlMode: true,
adapter: matcher_1.default
});
};
HTMLElement3.prototype.querySelector = function(selector) {
return (0, css_select_1.selectOne)(selector, this, {
xmlMode: true,
adapter: matcher_1.default
});
};
HTMLElement3.prototype.getElementsByTagName = function(tagName) {
var upperCasedTagName = tagName.toUpperCase();
var re = [];
var stack = [];
var currentNodeReference = this;
var index = 0;
while (index !== void 0) {
var child = void 0;
do {
child = currentNodeReference.childNodes[index++];
} while (index < currentNodeReference.childNodes.length && child === void 0);
if (child === void 0) {
currentNodeReference = currentNodeReference.parentNode;
index = stack.pop();
continue;
}
if (child.nodeType === type_1.default.ELEMENT_NODE) {
if (tagName === "*" || child.tagName === upperCasedTagName)
re.push(child);
if (child.childNodes.length > 0) {
stack.push(index);
currentNodeReference = child;
index = 0;
}
}
}
return re;
};
HTMLElement3.prototype.getElementById = function(id) {
var stack = [];
var currentNodeReference = this;
var index = 0;
while (index !== void 0) {
var child = void 0;
do {
child = currentNodeReference.childNodes[index++];
} while (index < currentNodeReference.childNodes.length && child === void 0);
if (child === void 0) {
currentNodeReference = currentNodeReference.parentNode;
index = stack.pop();
continue;
}
if (child.nodeType === type_1.default.ELEMENT_NODE) {
if (child.id === id) {
return child;
}
;
if (child.childNodes.length > 0) {
stack.push(index);
currentNodeReference = child;
index = 0;
}
}
}
return null;
};
HTMLElement3.prototype.closest = function(selector) {
var mapChild = /* @__PURE__ */ new Map();
var el = this;
var old = null;
function findOne(test, elems) {
var elem = null;
for (var i = 0, l = elems.length; i < l && !elem; i++) {
var el_1 = elems[i];
if (test(el_1)) {
elem = el_1;
} else {
var child = mapChild.get(el_1);
if (child) {
elem = findOne(test, [child]);
}
}
}
return elem;
}
while (el) {
mapChild.set(el, old);
old = el;
el = el.parentNode;
}
el = this;
while (el) {
var e = (0, css_select_1.selectOne)(selector, el, {
xmlMode: true,
adapter: __assign(__assign({}, matcher_1.default), { getChildren: function(node) {
var child = mapChild.get(node);
return child && [child];
}, getSiblings: function(node) {
return [node];
}, findOne, findAll: function() {
return [];
} })
});
if (e) {
return e;
}
el = el.parentNode;
}
return null;
};
HTMLElement3.prototype.appendChild = function(node) {
node.remove();
this.childNodes.push(node);
node.parentNode = this;
return node;
};
Object.defineProperty(HTMLElement3.prototype, "firstChild", {
get: function() {
return this.childNodes[0];
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "lastChild", {
get: function() {
return (0, back_1.default)(this.childNodes);
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "attrs", {
get: function() {
if (this._attrs) {
return this._attrs;
}
this._attrs = {};
var attrs = this.rawAttributes;
for (var key in attrs) {
var val = attrs[key] || "";
this._attrs[key.toLowerCase()] = decode(val);
}
return this._attrs;
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "attributes", {
get: function() {
var ret_attrs = {};
var attrs = this.rawAttributes;
for (var key in attrs) {
var val = attrs[key] || "";
ret_attrs[key] = decode(val);
}
return ret_attrs;
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "rawAttributes", {
get: function() {
if (this._rawAttrs) {
return this._rawAttrs;
}
var attrs = {};
if (this.rawAttrs) {
var re = /([a-zA-Z()[\]#@][a-zA-Z0-9-_:()[\]#\\$]*)(?:\s*=\s*((?:'[^']*')|(?:"[^"]*")|\S+))?/g;
var match = void 0;
while (match = re.exec(this.rawAttrs)) {
var key = match[1];
var val = match[2] || null;
if (val && (val[0] === "'" || val[0] === '"'))
val = val.slice(1, val.length - 1);
attrs[key] = val;
}
}
this._rawAttrs = attrs;
return attrs;
},
enumerable: false,
configurable: true
});
HTMLElement3.prototype.removeAttribute = function(key) {
var attrs = this.rawAttributes;
delete attrs[key];
if (this._attrs) {
delete this._attrs[key];
}
this.rawAttrs = Object.keys(attrs).map(function(name) {
var val = JSON.stringify(attrs[name]);
if (val === void 0 || val === "null") {
return name;
}
return "".concat(name, "=").concat(val);
}).join(" ");
if (key === "id") {
this.id = "";
}
return this;
};
HTMLElement3.prototype.hasAttribute = function(key) {
return key.toLowerCase() in this.attrs;
};
HTMLElement3.prototype.getAttribute = function(key) {
return this.attrs[key.toLowerCase()];
};
HTMLElement3.prototype.setAttribute = function(key, value) {
var _this = this;
if (arguments.length < 2) {
throw new Error("Failed to execute 'setAttribute' on 'Element'");
}
var k2 = key.toLowerCase();
var attrs = this.rawAttributes;
for (var k in attrs) {
if (k.toLowerCase() === k2) {
key = k;
break;
}
}
attrs[key] = String(value);
if (this._attrs) {
this._attrs[k2] = decode(attrs[key]);
}
this.rawAttrs = Object.keys(attrs).map(function(name) {
var val = _this.quoteAttribute(attrs[name]);
if (val === "null" || val === '""')
return name;
return "".concat(name, "=").concat(val);
}).join(" ");
if (key === "id") {
this.id = value;
}
};
HTMLElement3.prototype.setAttributes = function(attributes) {
var _this = this;
if (this._attrs) {
delete this._attrs;
}
if (this._rawAttrs) {
delete this._rawAttrs;
}
this.rawAttrs = Object.keys(attributes).map(function(name) {
var val = attributes[name];
if (val === "null" || val === '""')
return name;
return "".concat(name, "=").concat(_this.quoteAttribute(String(val)));
}).join(" ");
return this;
};
HTMLElement3.prototype.insertAdjacentHTML = function(where, html) {
var _a, _b, _c;
var _this = this;
if (arguments.length < 2) {
throw new Error("2 arguments required");
}
var p = parse2(html);
if (where === "afterend") {
var idx = this.parentNode.childNodes.findIndex(function(child) {
return child === _this;
});
resetParent(p.childNodes, this.parentNode);
(_a = this.parentNode.childNodes).splice.apply(_a, __spreadArray([idx + 1, 0], p.childNodes, false));
} else if (where === "afterbegin") {
resetParent(p.childNodes, this);
(_b = this.childNodes).unshift.apply(_b, p.childNodes);
} else if (where === "beforeend") {
p.childNodes.forEach(function(n2) {
_this.appendChild(n2);
});
} else if (where === "beforebegin") {
var idx = this.parentNode.childNodes.findIndex(function(child) {
return child === _this;
});
resetParent(p.childNodes, this.parentNode);
(_c = this.parentNode.childNodes).splice.apply(_c, __spreadArray([idx, 0], p.childNodes, false));
} else {
throw new Error("The value provided ('".concat(where, "') is not one of 'beforebegin', 'afterbegin', 'beforeend', or 'afterend'"));
}
return this;
};
Object.defineProperty(HTMLElement3.prototype, "nextSibling", {
get: function() {
if (this.parentNode) {
var children = this.parentNode.childNodes;
var i = 0;
while (i < children.length) {
var child = children[i++];
if (this === child)
return children[i] || null;
}
return null;
}
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "nextElementSibling", {
get: function() {
if (this.parentNode) {
var children = this.parentNode.childNodes;
var i = 0;
var find = false;
while (i < children.length) {
var child = children[i++];
if (find) {
if (child instanceof HTMLElement3) {
return child || null;
}
} else if (this === child) {
find = true;
}
}
return null;
}
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "previousSibling", {
get: function() {
if (this.parentNode) {
var children = this.parentNode.childNodes;
var i = children.length;
while (i > 0) {
var child = children[--i];
if (this === child)
return children[i - 1] || null;
}
return null;
}
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "previousElementSibling", {
get: function() {
if (this.parentNode) {
var children = this.parentNode.childNodes;
var i = children.length;
var find = false;
while (i > 0) {
var child = children[--i];
if (find) {
if (child instanceof HTMLElement3) {
return child || null;
}
} else if (this === child) {
find = true;
}
}
return null;
}
},
enumerable: false,
configurable: true
});
Object.defineProperty(HTMLElement3.prototype, "classNames", {
get: function() {
return this.classList.toString();
},
enumerable: false,
configurable: true
});
HTMLElement3.prototype.clone = function() {
return parse2(this.toString()).firstChild;
};
return HTMLElement3;
}(node_1.default);
exports.default = HTMLElement2;
var kMarkupPattern = /|<(\/?)([a-zA-Z][-.:0-9_a-zA-Z]*)((?:\s+[^>]*?(?:(?:'[^']*')|(?:"[^"]*"))?)*)\s*(\/?)>/g;
var kAttributePattern = /(?:^|\s)(id|class)\s*=\s*((?:'[^']*')|(?:"[^"]*")|\S+)/gi;
var kSelfClosingElements = {
area: true,
AREA: true,
base: true,
BASE: true,
br: true,
BR: true,
col: true,
COL: true,
hr: true,
HR: true,
img: true,
IMG: true,
input: true,
INPUT: true,
link: true,
LINK: true,
meta: true,
META: true,
source: true,
SOURCE: true,
embed: true,
EMBED: true,
param: true,
PARAM: true,
track: true,
TRACK: true,
wbr: true,
WBR: true
};
var kElementsClosedByOpening = {
li: { li: true, LI: true },
LI: { li: true, LI: true },
p: { p: true, div: true, P: true, DIV: true },
P: { p: true, div: true, P: true, DIV: true },
b: { div: true, DIV: true },
B: { div: true, DIV: true },
td: { td: true, th: true, TD: true, TH: true },
TD: { td: true, th: true, TD: true, TH: true },
th: { td: true, th: true, TD: true, TH: true },
TH: { td: true, th: true, TD: true, TH: true },
h1: { h1: true, H1: true },
H1: { h1: true, H1: true },
h2: { h2: true, H2: true },
H2: { h2: true, H2: true },
h3: { h3: true, H3: true },
H3: { h3: true, H3: true },
h4: { h4: true, H4: true },
H4: { h4: true, H4: true },
h5: { h5: true, H5: true },
H5: { h5: true, H5: true },
h6: { h6: true, H6: true },
H6: { h6: true, H6: true }
};
var kElementsClosedByClosing = {
li: { ul: true, ol: true, UL: true, OL: true },
LI: { ul: true, ol: true, UL: true, OL: true },
a: { div: true, DIV: true },
A: { div: true, DIV: true },
b: { div: true, DIV: true },
B: { div: true, DIV: true },
i: { div: true, DIV: true },
I: { div: true, DIV: true },
p: { div: true, DIV: true },
P: { div: true, DIV: true },
td: { tr: true, table: true, TR: true, TABLE: true },
TD: { tr: true, table: true, TR: true, TABLE: true },
th: { tr: true, table: true, TR: true, TABLE: true },
TH: { tr: true, table: true, TR: true, TABLE: true }
};
var frameflag = "documentfragmentcontainer";
function base_parse(data, options) {
var _a, _b;
if (options === void 0) {
options = { lowerCaseTagName: false, comment: false };
}
var voidTag = new void_tag_1.default((_a = options === null || options === void 0 ? void 0 : options.voidTag) === null || _a === void 0 ? void 0 : _a.closingSlash, (_b = options === null || options === void 0 ? void 0 : options.voidTag) === null || _b === void 0 ? void 0 : _b.tags);
var elements = options.blockTextElements || {
script: true,
noscript: true,
style: true,
pre: true
};
var element_names = Object.keys(elements);
var kBlockTextElements = element_names.map(function(it) {
return new RegExp("^".concat(it, "$"), "i");
});
var kIgnoreElements = element_names.filter(function(it) {
return elements[it];
}).map(function(it) {
return new RegExp("^".concat(it, "$"), "i");
});
function element_should_be_ignore(tag) {
return kIgnoreElements.some(function(it) {
return it.test(tag);
});
}
function is_block_text_element(tag) {
return kBlockTextElements.some(function(it) {
return it.test(tag);
});
}
var createRange = function(startPos, endPos) {
return [startPos - frameFlagOffset, endPos - frameFlagOffset];
};
var root = new HTMLElement2(null, {}, "", null, [0, data.length], voidTag);
var currentParent = root;
var stack = [root];
var lastTextPos = -1;
var noNestedTagIndex = void 0;
var match;
data = "<".concat(frameflag, ">").concat(data, "").concat(frameflag, ">");
var lowerCaseTagName = options.lowerCaseTagName, fixNestedATags = options.fixNestedATags;
var dataEndPos = data.length - (frameflag.length + 2);
var frameFlagOffset = frameflag.length + 2;
while (match = kMarkupPattern.exec(data)) {
var matchText = match[0], leadingSlash = match[1], tagName = match[2], attributes = match[3], closingSlash = match[4];
var matchLength = matchText.length;
var tagStartPos = kMarkupPattern.lastIndex - matchLength;
var tagEndPos = kMarkupPattern.lastIndex;
if (lastTextPos > -1) {
if (lastTextPos + matchLength < tagEndPos) {
var text = data.substring(lastTextPos, tagStartPos);
currentParent.appendChild(new text_1.default(text, currentParent, createRange(lastTextPos, tagStartPos)));
}
}
lastTextPos = kMarkupPattern.lastIndex;
if (tagName === frameflag)
continue;
if (matchText[1] === "!") {
if (options.comment) {
var text = data.substring(tagStartPos + 4, tagEndPos - 3);
currentParent.appendChild(new comment_1.default(text, currentParent, createRange(tagStartPos, tagEndPos)));
}
continue;
}
if (lowerCaseTagName)
tagName = tagName.toLowerCase();
if (!leadingSlash) {
var attrs = {};
for (var attMatch = void 0; attMatch = kAttributePattern.exec(attributes); ) {
var key = attMatch[1], val = attMatch[2];
var isQuoted = val[0] === "'" || val[0] === '"';
attrs[key.toLowerCase()] = isQuoted ? val.slice(1, val.length - 1) : val;
}
var parentTagName = currentParent.rawTagName;
if (!closingSlash && kElementsClosedByOpening[parentTagName]) {
if (kElementsClosedByOpening[parentTagName][tagName]) {
stack.pop();
currentParent = (0, back_1.default)(stack);
}
}
if (fixNestedATags && (tagName === "a" || tagName === "A")) {
if (noNestedTagIndex !== void 0) {
stack.splice(noNestedTagIndex);
currentParent = (0, back_1.default)(stack);
}
noNestedTagIndex = stack.length;
}
var tagEndPos_1 = kMarkupPattern.lastIndex;
var tagStartPos_1 = tagEndPos_1 - matchLength;
currentParent = currentParent.appendChild(
new HTMLElement2(tagName, attrs, attributes.slice(1), null, createRange(tagStartPos_1, tagEndPos_1), voidTag)
);
stack.push(currentParent);
if (is_block_text_element(tagName)) {
var closeMarkup = "".concat(tagName, ">");
var closeIndex = lowerCaseTagName ? data.toLocaleLowerCase().indexOf(closeMarkup, kMarkupPattern.lastIndex) : data.indexOf(closeMarkup, kMarkupPattern.lastIndex);
var textEndPos = closeIndex === -1 ? dataEndPos : closeIndex;
if (element_should_be_ignore(tagName)) {
var text = data.substring(tagEndPos_1, textEndPos);
if (text.length > 0 && /\S/.test(text)) {
currentParent.appendChild(new text_1.default(text, currentParent, createRange(tagEndPos_1, textEndPos)));
}
}
if (closeIndex === -1) {
lastTextPos = kMarkupPattern.lastIndex = data.length + 1;
} else {
lastTextPos = kMarkupPattern.lastIndex = closeIndex + closeMarkup.length;
leadingSlash = "/";
}
}
}
if (leadingSlash || closingSlash || kSelfClosingElements[tagName]) {
while (true) {
if (noNestedTagIndex != null && (tagName === "a" || tagName === "A"))
noNestedTagIndex = void 0;
if (currentParent.rawTagName === tagName) {
currentParent.range[1] = createRange(-1, Math.max(lastTextPos, tagEndPos))[1];
stack.pop();
currentParent = (0, back_1.default)(stack);
break;
} else {
var parentTagName = currentParent.tagName;
if (kElementsClosedByClosing[parentTagName]) {
if (kElementsClosedByClosing[parentTagName][tagName]) {
stack.pop();
currentParent = (0, back_1.default)(stack);
continue;
}
}
break;
}
}
}
}
return stack;
}
exports.base_parse = base_parse;
function parse2(data, options) {
if (options === void 0) {
options = { lowerCaseTagName: false, comment: false };
}
var stack = base_parse(data, options);
var root = stack[0];
var _loop_1 = function() {
var last = stack.pop();
var oneBefore = (0, back_1.default)(stack);
if (last.parentNode && last.parentNode.parentNode) {
if (last.parentNode === oneBefore && last.tagName === oneBefore.tagName) {
if (options.parseNoneClosedTags !== true) {
oneBefore.removeChild(last);
last.childNodes.forEach(function(child) {
oneBefore.parentNode.appendChild(child);
});
stack.pop();
}
} else {
if (options.parseNoneClosedTags !== true) {
oneBefore.removeChild(last);
last.childNodes.forEach(function(child) {
oneBefore.appendChild(child);
});
}
}
} else {
}
};
while (stack.length > 1) {
_loop_1();
}
return root;
}
exports.parse = parse2;
function resetParent(nodes, parent) {
return nodes.map(function(node) {
node.parentNode = parent;
return node;
});
}
}
});
// node_modules/a-node-html-parser/dist/parse.js
var require_parse3 = __commonJS({
"node_modules/a-node-html-parser/dist/parse.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
exports.default = void 0;
var html_1 = require_html();
Object.defineProperty(exports, "default", { enumerable: true, get: function() {
return html_1.parse;
} });
}
});
// node_modules/a-node-html-parser/dist/valid.js
var require_valid = __commonJS({
"node_modules/a-node-html-parser/dist/valid.js"(exports) {
"use strict";
Object.defineProperty(exports, "__esModule", { value: true });
var html_1 = require_html();
function valid(data, options) {
if (options === void 0) {
options = { lowerCaseTagName: false, comment: false };
}
var stack = (0, html_1.base_parse)(data, options);
return Boolean(stack.length === 1);
}
exports.default = valid;
}
});
// node_modules/a-node-html-parser/dist/index.js
var require_dist = __commonJS({
"node_modules/a-node-html-parser/dist/index.js"(exports) {
"use strict";
var __importDefault = exports && exports.__importDefault || function(mod) {
return mod && mod.__esModule ? mod : { "default": mod };
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.NodeType = exports.TextNode = exports.Node = exports.valid = exports.CommentNode = exports.HTMLElement = exports.parse = void 0;
var comment_1 = __importDefault(require_comment());
exports.CommentNode = comment_1.default;
var html_1 = __importDefault(require_html());
exports.HTMLElement = html_1.default;
var node_1 = __importDefault(require_node());
exports.Node = node_1.default;
var text_1 = __importDefault(require_text());
exports.TextNode = text_1.default;
var type_1 = __importDefault(require_type());
exports.NodeType = type_1.default;
var parse_1 = __importDefault(require_parse3());
var valid_1 = __importDefault(require_valid());
exports.valid = valid_1.default;
function parse2(data, options) {
if (options === void 0) {
options = {
lowerCaseTagName: false,
comment: false
};
}
return (0, parse_1.default)(data, options);
}
exports.default = parse2;
exports.parse = parse2;
parse2.parse = parse_1.default;
parse2.HTMLElement = html_1.default;
parse2.CommentNode = comment_1.default;
parse2.valid = valid_1.default;
parse2.Node = node_1.default;
parse2.TextNode = text_1.default;
parse2.NodeType = type_1.default;
}
});
// convert.ts
var acorn = __toESM(require_acorn());
var fs = __toESM(require("fs"));
// node_modules/magic-string/dist/magic-string.es.mjs
var import_sourcemap_codec = __toESM(require_sourcemap_codec_umd(), 1);
var BitSet = class {
constructor(arg) {
this.bits = arg instanceof BitSet ? arg.bits.slice() : [];
}
add(n2) {
this.bits[n2 >> 5] |= 1 << (n2 & 31);
}
has(n2) {
return !!(this.bits[n2 >> 5] & 1 << (n2 & 31));
}
};
var Chunk = class {
constructor(start, end, content) {
this.start = start;
this.end = end;
this.original = content;
this.intro = "";
this.outro = "";
this.content = content;
this.storeName = false;
this.edited = false;
{
this.previous = null;
this.next = null;
}
}
appendLeft(content) {
this.outro += content;
}
appendRight(content) {
this.intro = this.intro + content;
}
clone() {
const chunk = new Chunk(this.start, this.end, this.original);
chunk.intro = this.intro;
chunk.outro = this.outro;
chunk.content = this.content;
chunk.storeName = this.storeName;
chunk.edited = this.edited;
return chunk;
}
contains(index) {
return this.start < index && index < this.end;
}
eachNext(fn) {
let chunk = this;
while (chunk) {
fn(chunk);
chunk = chunk.next;
}
}
eachPrevious(fn) {
let chunk = this;
while (chunk) {
fn(chunk);
chunk = chunk.previous;
}
}
edit(content, storeName, contentOnly) {
this.content = content;
if (!contentOnly) {
this.intro = "";
this.outro = "";
}
this.storeName = storeName;
this.edited = true;
return this;
}
prependLeft(content) {
this.outro = content + this.outro;
}
prependRight(content) {
this.intro = content + this.intro;
}
split(index) {
const sliceIndex = index - this.start;
const originalBefore = this.original.slice(0, sliceIndex);
const originalAfter = this.original.slice(sliceIndex);
this.original = originalBefore;
const newChunk = new Chunk(index, this.end, originalAfter);
newChunk.outro = this.outro;
this.outro = "";
this.end = index;
if (this.edited) {
newChunk.edit("", false);
this.content = "";
} else {
this.content = originalBefore;
}
newChunk.next = this.next;
if (newChunk.next)
newChunk.next.previous = newChunk;
newChunk.previous = this;
this.next = newChunk;
return newChunk;
}
toString() {
return this.intro + this.content + this.outro;
}
trimEnd(rx) {
this.outro = this.outro.replace(rx, "");
if (this.outro.length)
return true;
const trimmed = this.content.replace(rx, "");
if (trimmed.length) {
if (trimmed !== this.content) {
this.split(this.start + trimmed.length).edit("", void 0, true);
}
return true;
} else {
this.edit("", void 0, true);
this.intro = this.intro.replace(rx, "");
if (this.intro.length)
return true;
}
}
trimStart(rx) {
this.intro = this.intro.replace(rx, "");
if (this.intro.length)
return true;
const trimmed = this.content.replace(rx, "");
if (trimmed.length) {
if (trimmed !== this.content) {
this.split(this.end - trimmed.length);
this.edit("", void 0, true);
}
return true;
} else {
this.edit("", void 0, true);
this.outro = this.outro.replace(rx, "");
if (this.outro.length)
return true;
}
}
};
function getBtoa() {
if (typeof window !== "undefined" && typeof window.btoa === "function") {
return (str) => window.btoa(unescape(encodeURIComponent(str)));
} else if (typeof Buffer === "function") {
return (str) => Buffer.from(str, "utf-8").toString("base64");
} else {
return () => {
throw new Error("Unsupported environment: `window.btoa` or `Buffer` should be supported.");
};
}
}
var btoa2 = /* @__PURE__ */ getBtoa();
var SourceMap = class {
constructor(properties) {
this.version = 3;
this.file = properties.file;
this.sources = properties.sources;
this.sourcesContent = properties.sourcesContent;
this.names = properties.names;
this.mappings = (0, import_sourcemap_codec.encode)(properties.mappings);
}
toString() {
return JSON.stringify(this);
}
toUrl() {
return "data:application/json;charset=utf-8;base64," + btoa2(this.toString());
}
};
function guessIndent(code) {
const lines = code.split("\n");
const tabbed = lines.filter((line) => /^\t+/.test(line));
const spaced = lines.filter((line) => /^ {2,}/.test(line));
if (tabbed.length === 0 && spaced.length === 0) {
return null;
}
if (tabbed.length >= spaced.length) {
return " ";
}
const min = spaced.reduce((previous, current) => {
const numSpaces = /^ +/.exec(current)[0].length;
return Math.min(numSpaces, previous);
}, Infinity);
return new Array(min + 1).join(" ");
}
function getRelativePath(from, to) {
const fromParts = from.split(/[/\\]/);
const toParts = to.split(/[/\\]/);
fromParts.pop();
while (fromParts[0] === toParts[0]) {
fromParts.shift();
toParts.shift();
}
if (fromParts.length) {
let i = fromParts.length;
while (i--)
fromParts[i] = "..";
}
return fromParts.concat(toParts).join("/");
}
var toString = Object.prototype.toString;
function isObject(thing) {
return toString.call(thing) === "[object Object]";
}
function getLocator(source) {
const originalLines = source.split("\n");
const lineOffsets = [];
for (let i = 0, pos = 0; i < originalLines.length; i++) {
lineOffsets.push(pos);
pos += originalLines[i].length + 1;
}
return function locate(index) {
let i = 0;
let j = lineOffsets.length;
while (i < j) {
const m = i + j >> 1;
if (index < lineOffsets[m]) {
j = m;
} else {
i = m + 1;
}
}
const line = i - 1;
const column = index - lineOffsets[line];
return { line, column };
};
}
var Mappings = class {
constructor(hires) {
this.hires = hires;
this.generatedCodeLine = 0;
this.generatedCodeColumn = 0;
this.raw = [];
this.rawSegments = this.raw[this.generatedCodeLine] = [];
this.pending = null;
}
addEdit(sourceIndex, content, loc, nameIndex) {
if (content.length) {
const segment = [this.generatedCodeColumn, sourceIndex, loc.line, loc.column];
if (nameIndex >= 0) {
segment.push(nameIndex);
}
this.rawSegments.push(segment);
} else if (this.pending) {
this.rawSegments.push(this.pending);
}
this.advance(content);
this.pending = null;
}
addUneditedChunk(sourceIndex, chunk, original, loc, sourcemapLocations) {
let originalCharIndex = chunk.start;
let first = true;
while (originalCharIndex < chunk.end) {
if (this.hires || first || sourcemapLocations.has(originalCharIndex)) {
this.rawSegments.push([this.generatedCodeColumn, sourceIndex, loc.line, loc.column]);
}
if (original[originalCharIndex] === "\n") {
loc.line += 1;
loc.column = 0;
this.generatedCodeLine += 1;
this.raw[this.generatedCodeLine] = this.rawSegments = [];
this.generatedCodeColumn = 0;
first = true;
} else {
loc.column += 1;
this.generatedCodeColumn += 1;
first = false;
}
originalCharIndex += 1;
}
this.pending = null;
}
advance(str) {
if (!str)
return;
const lines = str.split("\n");
if (lines.length > 1) {
for (let i = 0; i < lines.length - 1; i++) {
this.generatedCodeLine++;
this.raw[this.generatedCodeLine] = this.rawSegments = [];
}
this.generatedCodeColumn = 0;
}
this.generatedCodeColumn += lines[lines.length - 1].length;
}
};
var n = "\n";
var warned = {
insertLeft: false,
insertRight: false,
storeName: false
};
var MagicString = class {
constructor(string, options = {}) {
const chunk = new Chunk(0, string.length, string);
Object.defineProperties(this, {
original: { writable: true, value: string },
outro: { writable: true, value: "" },
intro: { writable: true, value: "" },
firstChunk: { writable: true, value: chunk },
lastChunk: { writable: true, value: chunk },
lastSearchedChunk: { writable: true, value: chunk },
byStart: { writable: true, value: {} },
byEnd: { writable: true, value: {} },
filename: { writable: true, value: options.filename },
indentExclusionRanges: { writable: true, value: options.indentExclusionRanges },
sourcemapLocations: { writable: true, value: new BitSet() },
storedNames: { writable: true, value: {} },
indentStr: { writable: true, value: void 0 }
});
this.byStart[0] = chunk;
this.byEnd[string.length] = chunk;
}
addSourcemapLocation(char2) {
this.sourcemapLocations.add(char2);
}
append(content) {
if (typeof content !== "string")
throw new TypeError("outro content must be a string");
this.outro += content;
return this;
}
appendLeft(index, content) {
if (typeof content !== "string")
throw new TypeError("inserted content must be a string");
this._split(index);
const chunk = this.byEnd[index];
if (chunk) {
chunk.appendLeft(content);
} else {
this.intro += content;
}
return this;
}
appendRight(index, content) {
if (typeof content !== "string")
throw new TypeError("inserted content must be a string");
this._split(index);
const chunk = this.byStart[index];
if (chunk) {
chunk.appendRight(content);
} else {
this.outro += content;
}
return this;
}
clone() {
const cloned = new MagicString(this.original, { filename: this.filename });
let originalChunk = this.firstChunk;
let clonedChunk = cloned.firstChunk = cloned.lastSearchedChunk = originalChunk.clone();
while (originalChunk) {
cloned.byStart[clonedChunk.start] = clonedChunk;
cloned.byEnd[clonedChunk.end] = clonedChunk;
const nextOriginalChunk = originalChunk.next;
const nextClonedChunk = nextOriginalChunk && nextOriginalChunk.clone();
if (nextClonedChunk) {
clonedChunk.next = nextClonedChunk;
nextClonedChunk.previous = clonedChunk;
clonedChunk = nextClonedChunk;
}
originalChunk = nextOriginalChunk;
}
cloned.lastChunk = clonedChunk;
if (this.indentExclusionRanges) {
cloned.indentExclusionRanges = this.indentExclusionRanges.slice();
}
cloned.sourcemapLocations = new BitSet(this.sourcemapLocations);
cloned.intro = this.intro;
cloned.outro = this.outro;
return cloned;
}
generateDecodedMap(options) {
options = options || {};
const sourceIndex = 0;
const names = Object.keys(this.storedNames);
const mappings = new Mappings(options.hires);
const locate = getLocator(this.original);
if (this.intro) {
mappings.advance(this.intro);
}
this.firstChunk.eachNext((chunk) => {
const loc = locate(chunk.start);
if (chunk.intro.length)
mappings.advance(chunk.intro);
if (chunk.edited) {
mappings.addEdit(
sourceIndex,
chunk.content,
loc,
chunk.storeName ? names.indexOf(chunk.original) : -1
);
} else {
mappings.addUneditedChunk(sourceIndex, chunk, this.original, loc, this.sourcemapLocations);
}
if (chunk.outro.length)
mappings.advance(chunk.outro);
});
return {
file: options.file ? options.file.split(/[/\\]/).pop() : null,
sources: [options.source ? getRelativePath(options.file || "", options.source) : null],
sourcesContent: options.includeContent ? [this.original] : [null],
names,
mappings: mappings.raw
};
}
generateMap(options) {
return new SourceMap(this.generateDecodedMap(options));
}
_ensureindentStr() {
if (this.indentStr === void 0) {
this.indentStr = guessIndent(this.original);
}
}
_getRawIndentString() {
this._ensureindentStr();
return this.indentStr;
}
getIndentString() {
this._ensureindentStr();
return this.indentStr === null ? " " : this.indentStr;
}
indent(indentStr, options) {
const pattern = /^[^\r\n]/gm;
if (isObject(indentStr)) {
options = indentStr;
indentStr = void 0;
}
if (indentStr === void 0) {
this._ensureindentStr();
indentStr = this.indentStr || " ";
}
if (indentStr === "")
return this;
options = options || {};
const isExcluded = {};
if (options.exclude) {
const exclusions = typeof options.exclude[0] === "number" ? [options.exclude] : options.exclude;
exclusions.forEach((exclusion) => {
for (let i = exclusion[0]; i < exclusion[1]; i += 1) {
isExcluded[i] = true;
}
});
}
let shouldIndentNextCharacter = options.indentStart !== false;
const replacer = (match) => {
if (shouldIndentNextCharacter)
return `${indentStr}${match}`;
shouldIndentNextCharacter = true;
return match;
};
this.intro = this.intro.replace(pattern, replacer);
let charIndex = 0;
let chunk = this.firstChunk;
while (chunk) {
const end = chunk.end;
if (chunk.edited) {
if (!isExcluded[charIndex]) {
chunk.content = chunk.content.replace(pattern, replacer);
if (chunk.content.length) {
shouldIndentNextCharacter = chunk.content[chunk.content.length - 1] === "\n";
}
}
} else {
charIndex = chunk.start;
while (charIndex < end) {
if (!isExcluded[charIndex]) {
const char2 = this.original[charIndex];
if (char2 === "\n") {
shouldIndentNextCharacter = true;
} else if (char2 !== "\r" && shouldIndentNextCharacter) {
shouldIndentNextCharacter = false;
if (charIndex === chunk.start) {
chunk.prependRight(indentStr);
} else {
this._splitChunk(chunk, charIndex);
chunk = chunk.next;
chunk.prependRight(indentStr);
}
}
}
charIndex += 1;
}
}
charIndex = chunk.end;
chunk = chunk.next;
}
this.outro = this.outro.replace(pattern, replacer);
return this;
}
insert() {
throw new Error(
"magicString.insert(...) is deprecated. Use prependRight(...) or appendLeft(...)"
);
}
insertLeft(index, content) {
if (!warned.insertLeft) {
console.warn(
"magicString.insertLeft(...) is deprecated. Use magicString.appendLeft(...) instead"
);
warned.insertLeft = true;
}
return this.appendLeft(index, content);
}
insertRight(index, content) {
if (!warned.insertRight) {
console.warn(
"magicString.insertRight(...) is deprecated. Use magicString.prependRight(...) instead"
);
warned.insertRight = true;
}
return this.prependRight(index, content);
}
move(start, end, index) {
if (index >= start && index <= end)
throw new Error("Cannot move a selection inside itself");
this._split(start);
this._split(end);
this._split(index);
const first = this.byStart[start];
const last = this.byEnd[end];
const oldLeft = first.previous;
const oldRight = last.next;
const newRight = this.byStart[index];
if (!newRight && last === this.lastChunk)
return this;
const newLeft = newRight ? newRight.previous : this.lastChunk;
if (oldLeft)
oldLeft.next = oldRight;
if (oldRight)
oldRight.previous = oldLeft;
if (newLeft)
newLeft.next = first;
if (newRight)
newRight.previous = last;
if (!first.previous)
this.firstChunk = last.next;
if (!last.next) {
this.lastChunk = first.previous;
this.lastChunk.next = null;
}
first.previous = newLeft;
last.next = newRight || null;
if (!newLeft)
this.firstChunk = first;
if (!newRight)
this.lastChunk = last;
return this;
}
overwrite(start, end, content, options) {
options = options || {};
return this.update(start, end, content, { ...options, overwrite: !options.contentOnly });
}
update(start, end, content, options) {
if (typeof content !== "string")
throw new TypeError("replacement content must be a string");
while (start < 0)
start += this.original.length;
while (end < 0)
end += this.original.length;
if (end > this.original.length)
throw new Error("end is out of bounds");
if (start === end)
throw new Error(
"Cannot overwrite a zero-length range \u2013 use appendLeft or prependRight instead"
);
this._split(start);
this._split(end);
if (options === true) {
if (!warned.storeName) {
console.warn(
"The final argument to magicString.overwrite(...) should be an options object. See https://github.com/rich-harris/magic-string"
);
warned.storeName = true;
}
options = { storeName: true };
}
const storeName = options !== void 0 ? options.storeName : false;
const overwrite = options !== void 0 ? options.overwrite : false;
if (storeName) {
const original = this.original.slice(start, end);
Object.defineProperty(this.storedNames, original, {
writable: true,
value: true,
enumerable: true
});
}
const first = this.byStart[start];
const last = this.byEnd[end];
if (first) {
let chunk = first;
while (chunk !== last) {
if (chunk.next !== this.byStart[chunk.end]) {
throw new Error("Cannot overwrite across a split point");
}
chunk = chunk.next;
chunk.edit("", false);
}
first.edit(content, storeName, !overwrite);
} else {
const newChunk = new Chunk(start, end, "").edit(content, storeName);
last.next = newChunk;
newChunk.previous = last;
}
return this;
}
prepend(content) {
if (typeof content !== "string")
throw new TypeError("outro content must be a string");
this.intro = content + this.intro;
return this;
}
prependLeft(index, content) {
if (typeof content !== "string")
throw new TypeError("inserted content must be a string");
this._split(index);
const chunk = this.byEnd[index];
if (chunk) {
chunk.prependLeft(content);
} else {
this.intro = content + this.intro;
}
return this;
}
prependRight(index, content) {
if (typeof content !== "string")
throw new TypeError("inserted content must be a string");
this._split(index);
const chunk = this.byStart[index];
if (chunk) {
chunk.prependRight(content);
} else {
this.outro = content + this.outro;
}
return this;
}
remove(start, end) {
while (start < 0)
start += this.original.length;
while (end < 0)
end += this.original.length;
if (start === end)
return this;
if (start < 0 || end > this.original.length)
throw new Error("Character is out of bounds");
if (start > end)
throw new Error("end must be greater than start");
this._split(start);
this._split(end);
let chunk = this.byStart[start];
while (chunk) {
chunk.intro = "";
chunk.outro = "";
chunk.edit("");
chunk = end > chunk.end ? this.byStart[chunk.end] : null;
}
return this;
}
lastChar() {
if (this.outro.length)
return this.outro[this.outro.length - 1];
let chunk = this.lastChunk;
do {
if (chunk.outro.length)
return chunk.outro[chunk.outro.length - 1];
if (chunk.content.length)
return chunk.content[chunk.content.length - 1];
if (chunk.intro.length)
return chunk.intro[chunk.intro.length - 1];
} while (chunk = chunk.previous);
if (this.intro.length)
return this.intro[this.intro.length - 1];
return "";
}
lastLine() {
let lineIndex = this.outro.lastIndexOf(n);
if (lineIndex !== -1)
return this.outro.substr(lineIndex + 1);
let lineStr = this.outro;
let chunk = this.lastChunk;
do {
if (chunk.outro.length > 0) {
lineIndex = chunk.outro.lastIndexOf(n);
if (lineIndex !== -1)
return chunk.outro.substr(lineIndex + 1) + lineStr;
lineStr = chunk.outro + lineStr;
}
if (chunk.content.length > 0) {
lineIndex = chunk.content.lastIndexOf(n);
if (lineIndex !== -1)
return chunk.content.substr(lineIndex + 1) + lineStr;
lineStr = chunk.content + lineStr;
}
if (chunk.intro.length > 0) {
lineIndex = chunk.intro.lastIndexOf(n);
if (lineIndex !== -1)
return chunk.intro.substr(lineIndex + 1) + lineStr;
lineStr = chunk.intro + lineStr;
}
} while (chunk = chunk.previous);
lineIndex = this.intro.lastIndexOf(n);
if (lineIndex !== -1)
return this.intro.substr(lineIndex + 1) + lineStr;
return this.intro + lineStr;
}
slice(start = 0, end = this.original.length) {
while (start < 0)
start += this.original.length;
while (end < 0)
end += this.original.length;
let result = "";
let chunk = this.firstChunk;
while (chunk && (chunk.start > start || chunk.end <= start)) {
if (chunk.start < end && chunk.end >= end) {
return result;
}
chunk = chunk.next;
}
if (chunk && chunk.edited && chunk.start !== start)
throw new Error(`Cannot use replaced character ${start} as slice start anchor.`);
const startChunk = chunk;
while (chunk) {
if (chunk.intro && (startChunk !== chunk || chunk.start === start)) {
result += chunk.intro;
}
const containsEnd = chunk.start < end && chunk.end >= end;
if (containsEnd && chunk.edited && chunk.end !== end)
throw new Error(`Cannot use replaced character ${end} as slice end anchor.`);
const sliceStart = startChunk === chunk ? start - chunk.start : 0;
const sliceEnd = containsEnd ? chunk.content.length + end - chunk.end : chunk.content.length;
result += chunk.content.slice(sliceStart, sliceEnd);
if (chunk.outro && (!containsEnd || chunk.end === end)) {
result += chunk.outro;
}
if (containsEnd) {
break;
}
chunk = chunk.next;
}
return result;
}
snip(start, end) {
const clone = this.clone();
clone.remove(0, start);
clone.remove(end, clone.original.length);
return clone;
}
_split(index) {
if (this.byStart[index] || this.byEnd[index])
return;
let chunk = this.lastSearchedChunk;
const searchForward = index > chunk.end;
while (chunk) {
if (chunk.contains(index))
return this._splitChunk(chunk, index);
chunk = searchForward ? this.byStart[chunk.end] : this.byEnd[chunk.start];
}
}
_splitChunk(chunk, index) {
if (chunk.edited && chunk.content.length) {
const loc = getLocator(this.original)(index);
throw new Error(
`Cannot split a chunk that has already been edited (${loc.line}:${loc.column} \u2013 "${chunk.original}")`
);
}
const newChunk = chunk.split(index);
this.byEnd[index] = chunk;
this.byStart[index] = newChunk;
this.byEnd[newChunk.end] = newChunk;
if (chunk === this.lastChunk)
this.lastChunk = newChunk;
this.lastSearchedChunk = chunk;
return true;
}
toString() {
let str = this.intro;
let chunk = this.firstChunk;
while (chunk) {
str += chunk.toString();
chunk = chunk.next;
}
return str + this.outro;
}
isEmpty() {
let chunk = this.firstChunk;
do {
if (chunk.intro.length && chunk.intro.trim() || chunk.content.length && chunk.content.trim() || chunk.outro.length && chunk.outro.trim())
return false;
} while (chunk = chunk.next);
return true;
}
length() {
let chunk = this.firstChunk;
let length = 0;
do {
length += chunk.intro.length + chunk.content.length + chunk.outro.length;
} while (chunk = chunk.next);
return length;
}
trimLines() {
return this.trim("[\\r\\n]");
}
trim(charType) {
return this.trimStart(charType).trimEnd(charType);
}
trimEndAborted(charType) {
const rx = new RegExp((charType || "\\s") + "+$");
this.outro = this.outro.replace(rx, "");
if (this.outro.length)
return true;
let chunk = this.lastChunk;
do {
const end = chunk.end;
const aborted = chunk.trimEnd(rx);
if (chunk.end !== end) {
if (this.lastChunk === chunk) {
this.lastChunk = chunk.next;
}
this.byEnd[chunk.end] = chunk;
this.byStart[chunk.next.start] = chunk.next;
this.byEnd[chunk.next.end] = chunk.next;
}
if (aborted)
return true;
chunk = chunk.previous;
} while (chunk);
return false;
}
trimEnd(charType) {
this.trimEndAborted(charType);
return this;
}
trimStartAborted(charType) {
const rx = new RegExp("^" + (charType || "\\s") + "+");
this.intro = this.intro.replace(rx, "");
if (this.intro.length)
return true;
let chunk = this.firstChunk;
do {
const end = chunk.end;
const aborted = chunk.trimStart(rx);
if (chunk.end !== end) {
if (chunk === this.lastChunk)
this.lastChunk = chunk.next;
this.byEnd[chunk.end] = chunk;
this.byStart[chunk.next.start] = chunk.next;
this.byEnd[chunk.next.end] = chunk.next;
}
if (aborted)
return true;
chunk = chunk.next;
} while (chunk);
return false;
}
trimStart(charType) {
this.trimStartAborted(charType);
return this;
}
hasChanged() {
return this.original !== this.toString();
}
_replaceRegexp(searchValue, replacement) {
function getReplacement(match, str) {
if (typeof replacement === "string") {
return replacement.replace(/\$(\$|&|\d+)/g, (_, i) => {
if (i === "$")
return "$";
if (i === "&")
return match[0];
const num = +i;
if (num < match.length)
return match[+i];
return `$${i}`;
});
} else {
return replacement(...match, match.index, str, match.groups);
}
}
function matchAll(re, str) {
let match;
const matches = [];
while (match = re.exec(str)) {
matches.push(match);
}
return matches;
}
if (searchValue.global) {
const matches = matchAll(searchValue, this.original);
matches.forEach((match) => {
if (match.index != null)
this.overwrite(
match.index,
match.index + match[0].length,
getReplacement(match, this.original)
);
});
} else {
const match = this.original.match(searchValue);
if (match && match.index != null)
this.overwrite(
match.index,
match.index + match[0].length,
getReplacement(match, this.original)
);
}
return this;
}
_replaceString(string, replacement) {
const { original } = this;
const index = original.indexOf(string);
if (index !== -1) {
this.overwrite(index, index + string.length, replacement);
}
return this;
}
replace(searchValue, replacement) {
if (typeof searchValue === "string") {
return this._replaceString(searchValue, replacement);
}
return this._replaceRegexp(searchValue, replacement);
}
_replaceAllString(string, replacement) {
const { original } = this;
const stringLength = string.length;
for (let index = original.indexOf(string); index !== -1; index = original.indexOf(string, index + stringLength)) {
this.overwrite(index, index + stringLength, replacement);
}
return this;
}
replaceAll(searchValue, replacement) {
if (typeof searchValue === "string") {
return this._replaceAllString(searchValue, replacement);
}
if (!searchValue.global) {
throw new TypeError(
"MagicString.prototype.replaceAll called with a non-global RegExp argument"
);
}
return this._replaceRegexp(searchValue, replacement);
}
};
// convert.ts
var prettier = __toESM(require_prettier());
var htmlParse = require_dist().parse;
var assumeBooleanAttributes = ["hidden", "checked"];
var filePath = process.argv[2];
var stat = fs.lstatSync(filePath);
if (!stat.isFile()) {
console.error("The input file does not exist.");
process.exit();
}
var useLit1 = false;
if (process.argv.includes("-1")) {
useLit1 = true;
}
var useOptionalChaining = true;
if (process.argv.includes("-disable-optional-chaining")) {
useOptionalChaining = false;
}
var outputSuffix = "";
if (process.argv.includes("-out")) {
outputSuffix = ".out.js";
}
convertFile(filePath, useLit1, useOptionalChaining, outputSuffix);
function char(str, i) {
if (i >= str.length) {
return void 0;
}
return str.charAt(i);
}
function splitExpression(expression) {
let current;
const result = [];
for (var i = 0; i < expression.length; i++) {
if (!current || current.type === "text") {
if (char(expression, i) === "[" && char(expression, i + 1) === "[") {
if (current) {
result.push(current);
}
current = { type: "oneway", value: "" };
i++;
} else if (char(expression, i) === "{" && char(expression, i + 1) === "{") {
if (current) {
result.push(current);
}
current = { type: "twoway", value: "" };
i++;
} else {
if (!current) {
current = { type: "text", value: "" };
}
current.value += char(expression, i);
}
} else {
if (current.type === "oneway" && char(expression, i) === "]" && char(expression, i + 1) === "]") {
result.push(current);
i++;
current = void 0;
} else if (current.type === "twoway" && char(expression, i) === "}" && char(expression, i + 1) === "}") {
result.push(current);
i++;
current = void 0;
} else {
current.value += char(expression, i);
}
}
}
return result;
}
function convertFile(filename, useLit12, useOptionalChaining2, outputSuffix2) {
const jsInputFile = filename;
let jsOutputFile = jsInputFile + outputSuffix2;
const jsContents = fs.readFileSync(jsInputFile, { encoding: "UTF-8" });
const tsOutput = new MagicString(jsContents);
const polymerJs = acorn.parse(jsContents, { sourceType: "module" });
const initValues = [];
const computedProperties = [];
const observedProperties = [];
let valueInitPosition = -1;
let newMethodInjectPosition = -1;
let usesRepeat = false;
let usesHeaderRenderer = false;
let usesBodyRenderer = false;
let usesFooterRenderer = false;
let usesUnsafeCss = false;
function error(message, ...optionalParams) {
console.error("ERROR", message, optionalParams);
}
function warn(message, ...optionalParams) {
console.warn("WARNING", message, optionalParams);
}
const body = polymerJs.body;
const modifyClass = (node, resolve) => {
const className = node.id.name;
const parentClass = node.superClass;
let tag = "";
let template = "";
newMethodInjectPosition = node.body.end - 1;
const newSuper = getSuperClass(node.superClass);
if (!newSuper || !newSuper?.includes("LitElement")) {
return;
}
if (newSuper) {
tsOutput.overwrite(node.superClass.start, node.superClass.end, newSuper);
}
for (const classContent of node.body.body) {
if (classContent.type == "MethodDefinition" && classContent.key.name == "template") {
const taggedTemplate = classContent.value.body.body[0].argument;
template = taggedTemplate.quasi.quasis[0].value.raw;
const modifiedTemplate = modifyTemplate(template);
const html = modifiedTemplate.htmls.join("\n");
const hasStyleIncludes = modifiedTemplate.styleIncludes.length > 0;
const hasStyles = modifiedTemplate.styles.length > 0;
if (hasStyleIncludes) {
usesUnsafeCss = true;
}
const stylesGetter = ` static get styles() {
${hasStyleIncludes ? `const includedStyles = {};` : ""}
${modifiedTemplate.styleIncludes.map(
(include) => `includedStyles["${include}"] = ${nullSafe(
`document.querySelector(
"dom-module[id='${include}']"
).firstElementChild.content.firstElementChild.innerText`,
["document"],
'""'
)};`
).join("\n")}
return [
${modifiedTemplate.styleIncludes.map((include) => `unsafeCSS(includedStyles["${include}"])`).join(", ")}${modifiedTemplate.styleIncludes.length > 0 ? "," : ""}
${modifiedTemplate.styles.map((css) => `css\`${css}\``).join(",")}
];
}`;
const renderMethod = `render() {
return html\`${html}\`;
}`;
tsOutput.overwrite(classContent.start, classContent.end, (hasStyles ? stylesGetter : "") + renderMethod);
} else if (classContent.type == "MethodDefinition" && classContent.key.name == "is") {
tag = classContent.value.body.body[0].argument.value;
} else if (classContent.type == "MethodDefinition" && classContent.key.name == "_attachDom") {
tsOutput.overwrite(
classContent.start,
classContent.end,
`createRenderRoot() {
// Do not use a shadow root
return this;
}
`
);
} else if (classContent.type == "MethodDefinition" && classContent.key.name == "ready") {
const src = getSource(classContent);
tsOutput.overwrite(
classContent.start,
classContent.end,
src.replace("ready()", "firstUpdated(_changedProperties)").replace("super.ready()", "super.firstUpdated(_changedProperties)")
);
} else if (classContent.type === "MethodDefinition" && classContent.kind === "get" && classContent.key.name === "properties") {
if (classContent.value.type === "FunctionExpression" && classContent.value.body.type == "BlockStatement") {
const returnStatment = classContent.value.body.body;
returnStatment[0].argument.properties.forEach((prop) => {
const propName = prop.key.name;
if (prop.value.type === "Identifier") {
} else {
prop.value.properties.forEach((typeValue) => {
const keyNode = typeValue.key;
const valueNode = typeValue.value;
if (keyNode.type === "Identifier") {
if (keyNode.name === "value") {
if (valueNode.type === "Literal") {
const initValue = valueNode.raw;
initValues.push({
name: propName,
value: initValue
});
} else if (valueNode.type === "FunctionExpression") {
if (valueNode?.body?.body[0]?.type === "ReturnStatement") {
const arrayExpression = getSource(valueNode.body.body[0].argument);
initValues.push({
name: propName,
value: arrayExpression
});
}
} else if (valueNode.type === "ArrayExpression") {
initValues.push({
name: propName,
value: getSource(valueNode)
});
} else {
warn("UNKNOWN type of 'value' for property", propName, ":", valueNode.type);
}
removeIncludingTrailingComma(typeValue);
} else if (keyNode.name === "computed") {
computedProperties.push({
name: propName,
value: resolveExpression(valueNode.value, true, resolve)
});
removeIncludingTrailingComma(typeValue);
} else if (keyNode.name === "observer") {
const observer = valueNode.value;
if (observer.includes("(")) {
tsOutput.prependLeft(valueNode.start, "/* TODO: Convert this complex observer manually */\n");
} else {
observedProperties.push({
name: propName,
value: observer
});
removeIncludingTrailingComma(typeValue);
}
} else if (keyNode.name === "reflectToAttribute") {
removeIncludingTrailingComma(typeValue);
if (valueNode.type === "Literal" && valueNode.raw === "true") {
tsOutput.prependLeft(typeValue.start, "reflect: true,");
}
}
}
});
}
});
}
} else if (classContent.type === "MethodDefinition" && classContent.kind === "constructor") {
const constructorNode = classContent;
valueInitPosition = constructorNode.value.body.end - 1;
} else {
}
}
return { className, parentClass, template, tag };
};
const removeIncludingTrailingComma = (node) => {
if (jsContents.substring(node.end, node.end + 1) === ",") {
tsOutput.remove(node.start, node.end + 1);
} else {
tsOutput.remove(node.start, node.end);
}
};
const rewriteTextNode = (node, resolver) => {
const bindingRe = /\[\[(.+?)\]\]/g;
const bindingRe2 = /\{\{(.+?)\}\}/g;
var result = node.rawText;
result = result.replace(bindingRe, (_fullMatch, variableName) => {
const resolved = resolveExpression(variableName, true, resolver);
return `\${${resolved}}`;
});
result = result.replace(bindingRe2, (_fullMatch, variableName) => {
const resolved = resolveExpression(variableName, true, resolver);
return `\${${resolved}}`;
});
node.rawText = result;
};
const rewriteElement = (element, resolver) => {
if (element.tagName === "TEMPLATE" && element.getAttribute("is") === "dom-if") {
const polymerExpression = element.getAttribute("if");
replaceWithLitIf(element, polymerExpression, resolver, element);
return;
} else if (element.tagName === "DOM-IF") {
const template = element.childNodes.filter((el) => el.tagName === "TEMPLATE")[0];
const polymerExpression = element.getAttribute("if");
replaceWithLitIf(element, polymerExpression, resolver, template);
return;
} else if (element.tagName === "DOM-REPEAT") {
const template = element.childNodes.filter((el) => el.tagName === "TEMPLATE")[0];
const polymerItemsExpression = element.getAttribute("items");
replaceWithLitRepeat(element, polymerItemsExpression, resolver, template);
return;
} else if (element.tagName === "TEMPLATE" && element.getAttribute("is") === "dom-repeat") {
const polymerItemsExpression = element.getAttribute("items");
replaceWithLitRepeat(element, polymerItemsExpression, resolver, element);
return;
} else if (element.tagName === "VAADIN-GRID-COLUMN") {
const templates = element.childNodes.filter((child) => child.tagName === "TEMPLATE");
const headerTemplate = templates.find((template) => template.getAttribute("class") === "header");
const bodyTemplate = templates.find((template) => !template.hasAttribute("class"));
const footerTemplate = templates.find((template) => template.getAttribute("class") === "footer");
if (headerTemplate) {
usesHeaderRenderer = true;
headerTemplate.childNodes.forEach((child) => rewriteHtmlNode(child, resolver));
element.setAttribute(
`\${columnHeaderRenderer(
(column) =>
html\`${headerTemplate.innerHTML}\`
)}
`,
""
);
headerTemplate.remove();
}
if (bodyTemplate) {
usesBodyRenderer = true;
const itemResolver = (originalExpression, expr, makeNullSafe, resolver2, undefinedValue) => {
if (originalExpression.startsWith("item.")) {
return makeNullSafe ? nullSafe(originalExpression, ["item", "this"], undefinedValue) : originalExpression;
}
return resolver2(originalExpression, expr, true, itemResolver, undefinedValue, ["this"]);
};
bodyTemplate.childNodes.forEach((child) => rewriteHtmlNode(child, itemResolver));
element.setAttribute(
`\${columnBodyRenderer(
(item) =>
html\`${bodyTemplate.innerHTML}\`
)}
`,
""
);
bodyTemplate.remove();
}
if (footerTemplate) {
usesFooterRenderer = true;
footerTemplate.childNodes.forEach((child) => rewriteHtmlNode(child, resolver));
element.setAttribute(
`\${columnFooterRenderer(
(column) =>
html\`${footerTemplate.innerHTML}\`
)}
`,
""
);
footerTemplate.remove();
}
} else if (element.attributes) {
for (const key of Object.keys(element.attributes)) {
const value = element.attributes[key];
if (key.startsWith("on-")) {
const eventName = key.substring(3);
const eventHandler = value;
element.removeAttribute(key);
element.setAttribute("@" + eventName, `\${this.${eventHandler}}`);
} else if (value.includes("[[") && value.includes("]]") || value.includes("{{") && value.includes("}}")) {
const expressions = splitExpression(value);
if (key.endsWith("$")) {
let attributeKey = key.substring(0, key.length - 1);
if (attributeKey.endsWith("\\")) {
attributeKey = attributeKey.substring(0, attributeKey.length - 1);
}
if (assumeBooleanAttributes.includes(attributeKey)) {
attributeKey = "?" + attributeKey;
}
element.setAttribute(attributeKey, "${" + resolveExpressions(expressions, true, resolver) + "}");
element.removeAttribute(key);
} else {
element.setAttribute("." + key, "${" + resolveExpressions(expressions, true, resolver) + "}");
element.removeAttribute(key);
}
if (expressions.length === 1 && expressions[0].type === "twoway") {
const eventName = key + "-changed";
const attributeKey = "@" + eventName;
const attributeValue = `\${(e) => (${resolveExpressions(expressions, false, resolver)} = e.target.value)}`;
element.setAttribute(attributeKey, attributeValue);
}
}
}
}
for (const child of element.childNodes) {
rewriteHtmlNode(child, resolver);
}
};
const modifyTemplate = (inputHtml) => {
const root = htmlParse(inputHtml, {
lowerCaseTagName: true,
script: true,
style: true,
pre: true,
comment: true
});
const htmls = [];
const styles = [];
const styleIncludes = [];
for (const child of root.childNodes) {
if (child.tagName == "CUSTOM-STYLE" || child.tagName == "STYLE") {
let style;
if (child.tagName == "CUSTOM-STYLE") {
style = child.childNodes[1];
} else {
style = child;
}
const includes = style.getAttribute("include");
if (includes) {
styleIncludes.push(...includes.split(" "));
}
const css = style.innerHTML;
styles.push(css);
child.remove();
} else {
rewriteHtmlNode(child, baseResolver);
}
}
htmls.push(root.innerHTML);
const ret = { htmls, styles, styleIncludes };
return ret;
};
const getSource = (node) => {
return jsContents.substring(node.start, node.end);
};
const skipImports = ["@polymer/polymer/polymer-element.js", "@polymer/polymer/lib/utils/html-tag.js"];
for (const node of body) {
if (node.type === "ClassDeclaration") {
modifyClass(node, baseResolver);
} else if (node.type === "ImportDeclaration") {
removeImport(node, "html", "PolymerElement");
removeImport(node, "@polymer/polymer/lib/elements/dom-if.js");
removeImport(node, "@polymer/polymer/lib/elements/dom-repeat.js");
} else if (node.type === "ExportNamedDeclaration") {
if (node.declaration.type === "ClassDeclaration") {
modifyClass(node.declaration, baseResolver);
} else {
warn("Unhandled root node", node.type, getSource(node));
}
} else if (!getSource(node).includes("customElements.define") && !getSource(node).includes("window.Vaadin.")) {
warn("Unhandled root node", node.type, getSource(node));
}
}
const litImport = useLit12 ? "lit-element" : "lit";
tsOutput.prepend(`import { html, LitElement, css } from "${litImport}";
`);
if (usesRepeat) {
tsOutput.prepend(`import { repeat } from "lit/directives/repeat.js";
`);
}
if (usesUnsafeCss) {
tsOutput.prepend(`import { unsafeCSS } from "${litImport}";
`);
}
const usedRenderers = [];
if (usesBodyRenderer)
usedRenderers.push("columnBodyRenderer");
if (usesFooterRenderer)
usedRenderers.push("columnFooterRenderer");
if (usesHeaderRenderer)
usedRenderers.push("columnHeaderRenderer");
if (usedRenderers.length !== 0) {
tsOutput.prepend(`import { ${usedRenderers.join(", ")} } from "@vaadin/grid/lit.js";
`);
}
const valueInitCode = initValues.map((initValue) => {
return `this.${initValue.name} = ${initValue.value};`;
}).join("\n");
const computedPropertiesCode = computedProperties.map((computedProperty) => {
return `get ${computedProperty.name}() {
return ${computedProperty.value};
}`;
}).join("\n");
const observedPropertiesCode = observedProperties.map((observedProperty) => {
const variable = observedProperty.name;
const observer = observedProperty.value;
return `set ${variable}(newValue) {
const oldValue = this.${variable};
this._${variable} = newValue;
if (oldValue !== newValue) {
this.${observer}(newValue, oldValue);
this.requestUpdateInternal("${variable}", oldValue, this.constructor.properties.${variable});
}
}
get ${variable}() {
return this._${variable};
}
`;
}).join("\n");
if (valueInitCode.length != 0) {
if (valueInitPosition !== -1) {
tsOutput.prependRight(valueInitPosition, valueInitCode);
} else {
const constructorCode = `constructor() {
super();
${valueInitCode}
}`;
tsOutput.prependRight(newMethodInjectPosition, constructorCode);
}
}
if (observedPropertiesCode.length != 0) {
tsOutput.prependRight(newMethodInjectPosition, observedPropertiesCode);
}
if (computedPropertiesCode.length != 0) {
tsOutput.prependRight(newMethodInjectPosition, computedPropertiesCode);
}
function resolveExpressions(expressions, makeNullSafe, resolver, undefinedValue = "undefined", qualifiedPrefixes = ["this"]) {
if (expressions.length === 1) {
return resolveExpression(expressions[0].value, makeNullSafe, resolver, undefinedValue, qualifiedPrefixes);
}
const resolved = expressions.map((bindingOrText) => {
if (bindingOrText.type === "text") {
return `'${bindingOrText.value}'`;
} else {
return "(" + resolveExpression(
bindingOrText.value,
makeNullSafe,
resolver,
"''",
qualifiedPrefixes
) + ")";
}
});
const result = resolved.join("+");
return result;
}
function resolveExpression(expression, makeNullSafe, resolver, undefinedValue = "undefined", qualifiedPrefixes = ["this"]) {
try {
const expr = acorn.parse(expression).body[0];
return resolver(expression, expr, makeNullSafe, resolver, undefinedValue, qualifiedPrefixes);
} catch (e) {
throw new Error("Unable to parse expression: " + expression);
}
}
function baseResolver(originalExpression, expr, makeNullSafe, resolve, undefinedValue, qualifiedPrefixes) {
if (expr.type === "ExpressionStatement") {
return resolve(originalExpression, expr.expression, makeNullSafe, resolve, undefinedValue, qualifiedPrefixes);
} else if (expr.type === "MemberExpression") {
const result = prependThisIfNeeded(qualifiedPrefixes, originalExpression.substring(expr.start, expr.end));
return makeNullSafe ? nullSafe(result, qualifiedPrefixes, undefinedValue) : result;
} else if (expr.type === "Literal") {
return expr.raw;
} else if (expr.type === "Identifier") {
const result = prependThisIfNeeded(qualifiedPrefixes, expr.name);
return makeNullSafe ? nullSafe(result, qualifiedPrefixes, undefinedValue) : result;
} else if (expr.type === "UnaryExpression") {
return expr.operator + "(" + resolve(originalExpression, expr.argument, makeNullSafe, resolve, undefinedValue, qualifiedPrefixes) + ")";
} else if (expr.type === "CallExpression") {
const args = expr.arguments.map(
(argument) => resolve(originalExpression, argument, makeNullSafe, resolve, undefinedValue, qualifiedPrefixes)
).join(", ");
const caller = resolve(originalExpression, expr.callee, makeNullSafe, resolve, undefinedValue, qualifiedPrefixes);
const retval2 = "(" + caller + ")(" + args + ")";
return retval2;
}
const retval = originalExpression.substring(expr.start, expr.end);
warn("Unresolved expression", expr, "returning", retval);
return retval;
}
let output = tsOutput.toString();
output = output.replace(/this.\$\[['"]([^;., ()]*['"]\])/g, `this.renderRoot.querySelector("#$1")`);
output = output.replace(/this\.\$\.([^;., ()]*)/g, `this.renderRoot.querySelector("#$1")`);
const prettified = prettier.format(output, {
parser: "typescript"
});
fs.writeFileSync(jsOutputFile, prettified);
function removeImport(node, ...identifiersOrFrom) {
const remove = [];
if (identifiersOrFrom.includes(node.source.value)) {
tsOutput.remove(node.start, node.end);
return;
}
node.specifiers.forEach((specifier) => {
if (identifiersOrFrom.includes(specifier?.imported?.name)) {
remove.push(specifier);
}
});
if (remove.length === 0) {
return;
}
if (remove.length === node.specifiers.length) {
tsOutput.remove(node.start, node.end);
} else {
error("Unable to remove only part of an import");
remove.forEach((specifier) => removeIncludingTrailingComma(specifier));
}
}
function rewriteHtmlNode(child, resolver) {
if (child.nodeType === 1) {
rewriteElement(child, resolver);
} else if (child.nodeType === 3) {
rewriteTextNode(child, resolver);
} else {
warn("unhandled child", child);
}
}
function replaceWithLitIf(element, polymerExpression, resolver, template) {
const expression = polymerExpression.substring(2, polymerExpression.length - 2);
const litExpression = resolveExpression(expression, true, resolver);
template.childNodes.forEach((child) => rewriteElement(child, resolver));
const litIf = `\${${litExpression} ? html\`${template.innerHTML}\` : html\`\`}`;
element.replaceWith(litIf);
}
function replaceWithLitRepeat(element, polymerItemsExpression, outerResolver, template) {
const expression = polymerItemsExpression.substring(2, polymerItemsExpression.length - 2);
const litExpression = resolveExpression(expression, true, outerResolver, "[]");
const itemResolver = (originalExpression, node, makeNullSafe, resolver, undefinedValue, qualifiedPrefixes) => {
return resolver(originalExpression, node, makeNullSafe, outerResolver, undefinedValue, [
...qualifiedPrefixes,
"item",
"index"
]);
};
template.childNodes.forEach((child) => rewriteElement(child, itemResolver));
const litRepeat = `\${(${litExpression}).map((item, index) => html\`${template.innerHTML}\`)}`;
element.replaceWith(litRepeat);
}
function nullSafe(name, assumedNonNull, undefinedValue) {
if (useOptionalChaining2) {
const parts = name.split(".");
let result;
if (assumedNonNull.includes(parts[0])) {
if (parts.length === 1) {
result = parts[0];
} else {
result = `${parts[0]}.${parts.slice(1).join("?.")}`;
}
} else {
result = parts.join("?.");
}
if (undefinedValue !== "undefined") {
return `(${result} ?? ${undefinedValue})`;
} else {
return result;
}
} else {
const parts = name.split(".");
let condition = "";
let lastPart = parts.length - 1;
if (undefinedValue !== "undefined") {
lastPart++;
}
for (var i = 1; i <= lastPart; i++) {
let accessor = parts[0];
for (var j = 1; j < i; j++) {
accessor += "." + parts[j];
}
if (condition !== "") {
condition += " && ";
}
if (!assumedNonNull.includes(accessor)) {
condition += accessor;
}
}
if (condition) {
return `(${condition}) ? ${name} : ${undefinedValue}`;
} else {
return name;
}
}
}
function prependThisIfNeeded(qualifiedPrefixes, variable) {
const parts = variable.split(/\./);
if (qualifiedPrefixes.includes(parts[0])) {
return variable;
}
return "this." + variable;
}
function getSuperClass(superClass) {
if (superClass.type === "CallExpression") {
return getSource(superClass).replace("PolymerElement", "LitElement");
} else if (superClass.type === "Identifier") {
return "LitElement";
} else {
warn("Unknown super class type", superClass);
}
return void 0;
}
}
/*! https://mths.be/he v1.2.0 by @mathias | MIT license */
© 2015 - 2025 Weber Informatics LLC | Privacy Policy