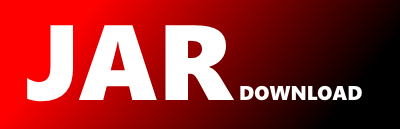
ltl-dsl.0.1.3.source-code.LtlBaseListener Maven / Gradle / Ivy
Show all versions of ltl-dsl Show documentation
// Generated from /builds/stups/prob/ltl-pattern-parser/src/main/antlr/Ltl.g4 by ANTLR 4.7.2
package de.prob.ltl.parser;
import de.prob.ltl.parser.prolog.LtlPrologTermGenerator;
import de.prob.ltl.parser.pattern.PatternManager;
import de.prob.ltl.parser.semantic.SemanticCheck;
import de.prob.ltl.parser.symboltable.SymbolTableManager;
import de.prob.parserbase.ProBParserBase;
import de.prob.prolog.output.StructuredPrologOutput;
import de.prob.prolog.term.PrologTerm;
import org.antlr.v4.runtime.ParserRuleContext;
import org.antlr.v4.runtime.tree.ErrorNode;
import org.antlr.v4.runtime.tree.TerminalNode;
/**
* This class provides an empty implementation of {@link LtlListener},
* which can be extended to create a listener which only needs to handle a subset
* of the available methods.
*/
public class LtlBaseListener implements LtlListener {
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterStart(LtlParser.StartContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitStart(LtlParser.StartContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterStart_pattern_def(LtlParser.Start_pattern_defContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitStart_pattern_def(LtlParser.Start_pattern_defContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterBody(LtlParser.BodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitBody(LtlParser.BodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterVarArgument(LtlParser.VarArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitVarArgument(LtlParser.VarArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterNumArgument(LtlParser.NumArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitNumArgument(LtlParser.NumArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSeqArgument(LtlParser.SeqArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSeqArgument(LtlParser.SeqArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterParArgument(LtlParser.ParArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitParArgument(LtlParser.ParArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterExprArgument(LtlParser.ExprArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitExprArgument(LtlParser.ExprArgumentContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPattern_def(LtlParser.Pattern_defContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPattern_def(LtlParser.Pattern_defContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterVarParam(LtlParser.VarParamContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitVarParam(LtlParser.VarParamContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterNumVarParam(LtlParser.NumVarParamContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitNumVarParam(LtlParser.NumVarParamContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSeqVarParam(LtlParser.SeqVarParamContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSeqVarParam(LtlParser.SeqVarParamContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPattern_call(LtlParser.Pattern_callContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPattern_call(LtlParser.Pattern_callContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterScope_call(LtlParser.Scope_callContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitScope_call(LtlParser.Scope_callContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterVar_def(LtlParser.Var_defContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitVar_def(LtlParser.Var_defContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterVar_assign(LtlParser.Var_assignContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitVar_assign(LtlParser.Var_assignContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSeqDefinition(LtlParser.SeqDefinitionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSeqDefinition(LtlParser.SeqDefinitionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSeqVarExtension(LtlParser.SeqVarExtensionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSeqVarExtension(LtlParser.SeqVarExtensionContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSeq_call(LtlParser.Seq_callContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSeq_call(LtlParser.Seq_callContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLoop(LtlParser.LoopContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLoop(LtlParser.LoopContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterLoop_body(LtlParser.Loop_bodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitLoop_body(LtlParser.Loop_bodyContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterUnaryCombinedExpr(LtlParser.UnaryCombinedExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitUnaryCombinedExpr(LtlParser.UnaryCombinedExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterUnaryLtlExpr(LtlParser.UnaryLtlExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitUnaryLtlExpr(LtlParser.UnaryLtlExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterOnceExpr(LtlParser.OnceExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitOnceExpr(LtlParser.OnceExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterNextExpr(LtlParser.NextExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitNextExpr(LtlParser.NextExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAtomExpr(LtlParser.AtomExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAtomExpr(LtlParser.AtomExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterOrExpr(LtlParser.OrExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitOrExpr(LtlParser.OrExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterGloballyExpr(LtlParser.GloballyExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitGloballyExpr(LtlParser.GloballyExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterHistoricallyExpr(LtlParser.HistoricallyExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitHistoricallyExpr(LtlParser.HistoricallyExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterNotExpr(LtlParser.NotExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitNotExpr(LtlParser.NotExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterImpliesExpr(LtlParser.ImpliesExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitImpliesExpr(LtlParser.ImpliesExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterFinallyExpr(LtlParser.FinallyExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitFinallyExpr(LtlParser.FinallyExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterYesterdayExpr(LtlParser.YesterdayExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitYesterdayExpr(LtlParser.YesterdayExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterAndExpr(LtlParser.AndExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitAndExpr(LtlParser.AndExprContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterVariableCallAtom(LtlParser.VariableCallAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitVariableCallAtom(LtlParser.VariableCallAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPatternCallAtom(LtlParser.PatternCallAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPatternCallAtom(LtlParser.PatternCallAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterScopeCallAtom(LtlParser.ScopeCallAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitScopeCallAtom(LtlParser.ScopeCallAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterSeqCallAtom(LtlParser.SeqCallAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitSeqCallAtom(LtlParser.SeqCallAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterPredicateAtom(LtlParser.PredicateAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitPredicateAtom(LtlParser.PredicateAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterActionAtom(LtlParser.ActionAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitActionAtom(LtlParser.ActionAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterEnabledAtom(LtlParser.EnabledAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitEnabledAtom(LtlParser.EnabledAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterParAtom(LtlParser.ParAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitParAtom(LtlParser.ParAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterBooleanAtom(LtlParser.BooleanAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitBooleanAtom(LtlParser.BooleanAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterStateAtom(LtlParser.StateAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitStateAtom(LtlParser.StateAtomContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void enterEveryRule(ParserRuleContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void exitEveryRule(ParserRuleContext ctx) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void visitTerminal(TerminalNode node) { }
/**
* {@inheritDoc}
*
* The default implementation does nothing.
*/
@Override public void visitErrorNode(ErrorNode node) { }
}