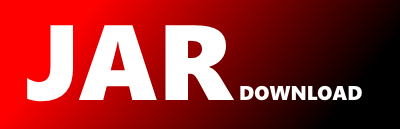
ltl-dsl.0.1.3.source-code.LtlListener Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ltl-dsl Show documentation
Show all versions of ltl-dsl Show documentation
Parser for a LTL Pattern DSL
// Generated from /builds/stups/prob/ltl-pattern-parser/src/main/antlr/Ltl.g4 by ANTLR 4.7.2
package de.prob.ltl.parser;
import de.prob.ltl.parser.prolog.LtlPrologTermGenerator;
import de.prob.ltl.parser.pattern.PatternManager;
import de.prob.ltl.parser.semantic.SemanticCheck;
import de.prob.ltl.parser.symboltable.SymbolTableManager;
import de.prob.parserbase.ProBParserBase;
import de.prob.prolog.output.StructuredPrologOutput;
import de.prob.prolog.term.PrologTerm;
import org.antlr.v4.runtime.tree.ParseTreeListener;
/**
* This interface defines a complete listener for a parse tree produced by
* {@link LtlParser}.
*/
public interface LtlListener extends ParseTreeListener {
/**
* Enter a parse tree produced by {@link LtlParser#start}.
* @param ctx the parse tree
*/
void enterStart(LtlParser.StartContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#start}.
* @param ctx the parse tree
*/
void exitStart(LtlParser.StartContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#start_pattern_def}.
* @param ctx the parse tree
*/
void enterStart_pattern_def(LtlParser.Start_pattern_defContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#start_pattern_def}.
* @param ctx the parse tree
*/
void exitStart_pattern_def(LtlParser.Start_pattern_defContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#body}.
* @param ctx the parse tree
*/
void enterBody(LtlParser.BodyContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#body}.
* @param ctx the parse tree
*/
void exitBody(LtlParser.BodyContext ctx);
/**
* Enter a parse tree produced by the {@code varArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void enterVarArgument(LtlParser.VarArgumentContext ctx);
/**
* Exit a parse tree produced by the {@code varArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void exitVarArgument(LtlParser.VarArgumentContext ctx);
/**
* Enter a parse tree produced by the {@code numArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void enterNumArgument(LtlParser.NumArgumentContext ctx);
/**
* Exit a parse tree produced by the {@code numArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void exitNumArgument(LtlParser.NumArgumentContext ctx);
/**
* Enter a parse tree produced by the {@code seqArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void enterSeqArgument(LtlParser.SeqArgumentContext ctx);
/**
* Exit a parse tree produced by the {@code seqArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void exitSeqArgument(LtlParser.SeqArgumentContext ctx);
/**
* Enter a parse tree produced by the {@code parArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void enterParArgument(LtlParser.ParArgumentContext ctx);
/**
* Exit a parse tree produced by the {@code parArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void exitParArgument(LtlParser.ParArgumentContext ctx);
/**
* Enter a parse tree produced by the {@code exprArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void enterExprArgument(LtlParser.ExprArgumentContext ctx);
/**
* Exit a parse tree produced by the {@code exprArgument}
* labeled alternative in {@link LtlParser#argument}.
* @param ctx the parse tree
*/
void exitExprArgument(LtlParser.ExprArgumentContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#pattern_def}.
* @param ctx the parse tree
*/
void enterPattern_def(LtlParser.Pattern_defContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#pattern_def}.
* @param ctx the parse tree
*/
void exitPattern_def(LtlParser.Pattern_defContext ctx);
/**
* Enter a parse tree produced by the {@code varParam}
* labeled alternative in {@link LtlParser#pattern_def_param}.
* @param ctx the parse tree
*/
void enterVarParam(LtlParser.VarParamContext ctx);
/**
* Exit a parse tree produced by the {@code varParam}
* labeled alternative in {@link LtlParser#pattern_def_param}.
* @param ctx the parse tree
*/
void exitVarParam(LtlParser.VarParamContext ctx);
/**
* Enter a parse tree produced by the {@code numVarParam}
* labeled alternative in {@link LtlParser#pattern_def_param}.
* @param ctx the parse tree
*/
void enterNumVarParam(LtlParser.NumVarParamContext ctx);
/**
* Exit a parse tree produced by the {@code numVarParam}
* labeled alternative in {@link LtlParser#pattern_def_param}.
* @param ctx the parse tree
*/
void exitNumVarParam(LtlParser.NumVarParamContext ctx);
/**
* Enter a parse tree produced by the {@code seqVarParam}
* labeled alternative in {@link LtlParser#pattern_def_param}.
* @param ctx the parse tree
*/
void enterSeqVarParam(LtlParser.SeqVarParamContext ctx);
/**
* Exit a parse tree produced by the {@code seqVarParam}
* labeled alternative in {@link LtlParser#pattern_def_param}.
* @param ctx the parse tree
*/
void exitSeqVarParam(LtlParser.SeqVarParamContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#pattern_call}.
* @param ctx the parse tree
*/
void enterPattern_call(LtlParser.Pattern_callContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#pattern_call}.
* @param ctx the parse tree
*/
void exitPattern_call(LtlParser.Pattern_callContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#scope_call}.
* @param ctx the parse tree
*/
void enterScope_call(LtlParser.Scope_callContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#scope_call}.
* @param ctx the parse tree
*/
void exitScope_call(LtlParser.Scope_callContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#var_def}.
* @param ctx the parse tree
*/
void enterVar_def(LtlParser.Var_defContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#var_def}.
* @param ctx the parse tree
*/
void exitVar_def(LtlParser.Var_defContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#var_assign}.
* @param ctx the parse tree
*/
void enterVar_assign(LtlParser.Var_assignContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#var_assign}.
* @param ctx the parse tree
*/
void exitVar_assign(LtlParser.Var_assignContext ctx);
/**
* Enter a parse tree produced by the {@code seqDefinition}
* labeled alternative in {@link LtlParser#seq_def}.
* @param ctx the parse tree
*/
void enterSeqDefinition(LtlParser.SeqDefinitionContext ctx);
/**
* Exit a parse tree produced by the {@code seqDefinition}
* labeled alternative in {@link LtlParser#seq_def}.
* @param ctx the parse tree
*/
void exitSeqDefinition(LtlParser.SeqDefinitionContext ctx);
/**
* Enter a parse tree produced by the {@code seqVarExtension}
* labeled alternative in {@link LtlParser#seq_def}.
* @param ctx the parse tree
*/
void enterSeqVarExtension(LtlParser.SeqVarExtensionContext ctx);
/**
* Exit a parse tree produced by the {@code seqVarExtension}
* labeled alternative in {@link LtlParser#seq_def}.
* @param ctx the parse tree
*/
void exitSeqVarExtension(LtlParser.SeqVarExtensionContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#seq_call}.
* @param ctx the parse tree
*/
void enterSeq_call(LtlParser.Seq_callContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#seq_call}.
* @param ctx the parse tree
*/
void exitSeq_call(LtlParser.Seq_callContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#loop}.
* @param ctx the parse tree
*/
void enterLoop(LtlParser.LoopContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#loop}.
* @param ctx the parse tree
*/
void exitLoop(LtlParser.LoopContext ctx);
/**
* Enter a parse tree produced by {@link LtlParser#loop_body}.
* @param ctx the parse tree
*/
void enterLoop_body(LtlParser.Loop_bodyContext ctx);
/**
* Exit a parse tree produced by {@link LtlParser#loop_body}.
* @param ctx the parse tree
*/
void exitLoop_body(LtlParser.Loop_bodyContext ctx);
/**
* Enter a parse tree produced by the {@code unaryCombinedExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterUnaryCombinedExpr(LtlParser.UnaryCombinedExprContext ctx);
/**
* Exit a parse tree produced by the {@code unaryCombinedExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitUnaryCombinedExpr(LtlParser.UnaryCombinedExprContext ctx);
/**
* Enter a parse tree produced by the {@code unaryLtlExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterUnaryLtlExpr(LtlParser.UnaryLtlExprContext ctx);
/**
* Exit a parse tree produced by the {@code unaryLtlExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitUnaryLtlExpr(LtlParser.UnaryLtlExprContext ctx);
/**
* Enter a parse tree produced by the {@code onceExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterOnceExpr(LtlParser.OnceExprContext ctx);
/**
* Exit a parse tree produced by the {@code onceExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitOnceExpr(LtlParser.OnceExprContext ctx);
/**
* Enter a parse tree produced by the {@code nextExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterNextExpr(LtlParser.NextExprContext ctx);
/**
* Exit a parse tree produced by the {@code nextExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitNextExpr(LtlParser.NextExprContext ctx);
/**
* Enter a parse tree produced by the {@code atomExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterAtomExpr(LtlParser.AtomExprContext ctx);
/**
* Exit a parse tree produced by the {@code atomExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitAtomExpr(LtlParser.AtomExprContext ctx);
/**
* Enter a parse tree produced by the {@code orExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterOrExpr(LtlParser.OrExprContext ctx);
/**
* Exit a parse tree produced by the {@code orExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitOrExpr(LtlParser.OrExprContext ctx);
/**
* Enter a parse tree produced by the {@code globallyExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterGloballyExpr(LtlParser.GloballyExprContext ctx);
/**
* Exit a parse tree produced by the {@code globallyExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitGloballyExpr(LtlParser.GloballyExprContext ctx);
/**
* Enter a parse tree produced by the {@code historicallyExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterHistoricallyExpr(LtlParser.HistoricallyExprContext ctx);
/**
* Exit a parse tree produced by the {@code historicallyExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitHistoricallyExpr(LtlParser.HistoricallyExprContext ctx);
/**
* Enter a parse tree produced by the {@code notExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterNotExpr(LtlParser.NotExprContext ctx);
/**
* Exit a parse tree produced by the {@code notExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitNotExpr(LtlParser.NotExprContext ctx);
/**
* Enter a parse tree produced by the {@code impliesExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterImpliesExpr(LtlParser.ImpliesExprContext ctx);
/**
* Exit a parse tree produced by the {@code impliesExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitImpliesExpr(LtlParser.ImpliesExprContext ctx);
/**
* Enter a parse tree produced by the {@code finallyExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterFinallyExpr(LtlParser.FinallyExprContext ctx);
/**
* Exit a parse tree produced by the {@code finallyExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitFinallyExpr(LtlParser.FinallyExprContext ctx);
/**
* Enter a parse tree produced by the {@code yesterdayExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterYesterdayExpr(LtlParser.YesterdayExprContext ctx);
/**
* Exit a parse tree produced by the {@code yesterdayExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitYesterdayExpr(LtlParser.YesterdayExprContext ctx);
/**
* Enter a parse tree produced by the {@code andExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void enterAndExpr(LtlParser.AndExprContext ctx);
/**
* Exit a parse tree produced by the {@code andExpr}
* labeled alternative in {@link LtlParser#expr}.
* @param ctx the parse tree
*/
void exitAndExpr(LtlParser.AndExprContext ctx);
/**
* Enter a parse tree produced by the {@code variableCallAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterVariableCallAtom(LtlParser.VariableCallAtomContext ctx);
/**
* Exit a parse tree produced by the {@code variableCallAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitVariableCallAtom(LtlParser.VariableCallAtomContext ctx);
/**
* Enter a parse tree produced by the {@code patternCallAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterPatternCallAtom(LtlParser.PatternCallAtomContext ctx);
/**
* Exit a parse tree produced by the {@code patternCallAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitPatternCallAtom(LtlParser.PatternCallAtomContext ctx);
/**
* Enter a parse tree produced by the {@code scopeCallAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterScopeCallAtom(LtlParser.ScopeCallAtomContext ctx);
/**
* Exit a parse tree produced by the {@code scopeCallAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitScopeCallAtom(LtlParser.ScopeCallAtomContext ctx);
/**
* Enter a parse tree produced by the {@code seqCallAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterSeqCallAtom(LtlParser.SeqCallAtomContext ctx);
/**
* Exit a parse tree produced by the {@code seqCallAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitSeqCallAtom(LtlParser.SeqCallAtomContext ctx);
/**
* Enter a parse tree produced by the {@code predicateAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterPredicateAtom(LtlParser.PredicateAtomContext ctx);
/**
* Exit a parse tree produced by the {@code predicateAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitPredicateAtom(LtlParser.PredicateAtomContext ctx);
/**
* Enter a parse tree produced by the {@code actionAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterActionAtom(LtlParser.ActionAtomContext ctx);
/**
* Exit a parse tree produced by the {@code actionAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitActionAtom(LtlParser.ActionAtomContext ctx);
/**
* Enter a parse tree produced by the {@code enabledAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterEnabledAtom(LtlParser.EnabledAtomContext ctx);
/**
* Exit a parse tree produced by the {@code enabledAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitEnabledAtom(LtlParser.EnabledAtomContext ctx);
/**
* Enter a parse tree produced by the {@code parAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterParAtom(LtlParser.ParAtomContext ctx);
/**
* Exit a parse tree produced by the {@code parAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitParAtom(LtlParser.ParAtomContext ctx);
/**
* Enter a parse tree produced by the {@code booleanAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterBooleanAtom(LtlParser.BooleanAtomContext ctx);
/**
* Exit a parse tree produced by the {@code booleanAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitBooleanAtom(LtlParser.BooleanAtomContext ctx);
/**
* Enter a parse tree produced by the {@code stateAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void enterStateAtom(LtlParser.StateAtomContext ctx);
/**
* Exit a parse tree produced by the {@code stateAtom}
* labeled alternative in {@link LtlParser#atom}.
* @param ctx the parse tree
*/
void exitStateAtom(LtlParser.StateAtomContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy