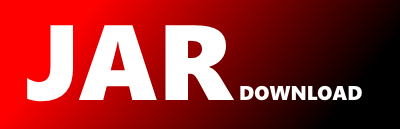
webclient.js-i2b2.cells.CRC.i2b2_msgs.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of i2b2-shrine Show documentation
Show all versions of i2b2-shrine Show documentation
Standalone service to to run the AKTIN broker and aggregator
together with an i2b2 query frontend.
The newest version!
/**
* @projectDescription Messages used by the CRC cell communicator object
* @inherits i2b2.CRC.cfg
* @namespace i2b2.CRC.cfg.msgs
* @author Nick Benik, Griffin Weber MD PhD
* @version 1.3
* ----------------------------------------------------------------------------------------
* updated 9-15-08: RC4 launch [Nick Benik]
*/
// create the communicator Object
i2b2.CRC.ajax = i2b2.hive.communicatorFactory("CRC");
// create namespaces to hold all the communicator messages and parsing routines
i2b2.CRC.cfg.msgs = {};
i2b2.CRC.cfg.parsers = {};
// ================================================================================================== //
i2b2.CRC.cfg.msgs.getQueryMasterList_fromUserId = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' {{{sec_project}}} \n'+
' US \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' {{{crc_user_type}}} \n'+
' \n'+
' \n'+
' {{{crc_user_by}}} \n'+
' {{{sec_project}}} \n'+
' {{{crc_max_records}}} \n'+
' \n'+
' \n'+
' \n';
i2b2.CRC.cfg.parsers.getQueryMasterList_fromUserId = function() {
if (!this.error) {
this.model = [];
var qm = this.refXML.getElementsByTagName('query_master');
for(var i=0; i<1*qm.length; i++) {
var o = new Object;
o.xmlOrig = qm[i];
o.id = i2b2.h.getXNodeVal(qm[i],'query_master_id');
o.name = i2b2.h.getXNodeVal(qm[i],'name');
o.userid = i2b2.h.getXNodeVal(qm[i],'user_id');
o.group = i2b2.h.getXNodeVal(qm[i],'group_id');
o.created = i2b2.h.getXNodeVal(qm[i],'create_date');
var dStr = '';
var d = o.created.match(/^[0-9\-]*/).toString();
if (d) {
d = d.replace(/-/g,'/');
d = new Date(Date.parse(d));
if (d) {
dStr = ' [' + (d.getMonth()+1) + '-' + d.getDate() + '-' + d.getFullYear().toString() + ']';
}
}
o.name += dStr + ' ['+o.userid+']';
// encapsulate into an SDX package
var sdxDataPack = i2b2.sdx.Master.EncapsulateData('QM',o);
this.model.push(sdxDataPack);
}
} else {
this.model = false;
console.error("[getQueryMasterList_fromUserId] Could not parse() data!");
}
return this;
}
i2b2.CRC.ajax._addFunctionCall( "getQueryMasterList_fromUserId",
"{{{URL}}}request",
i2b2.CRC.cfg.msgs.getQueryMasterList_fromUserId,
null,
i2b2.CRC.cfg.parsers.getQueryMasterList_fromUserId);
// ================================================================================================== //
i2b2.CRC.cfg.msgs.getNameInfo = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' {{{sec_project}}} \n'+
' US \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' {{{crc_user_type}}} \n'+
' \n'+
' \n'+
' {{{crc_find_string}}} \n'+
' {{{crc_create_date}}} \n'+
' {{{crc_sort_order}}} \n'+
' \n'+
' \n'+
' \n';
i2b2.CRC.cfg.parsers.getNameInfo = function() {
if (!this.error) {
this.model = [];
var qm = this.refXML.getElementsByTagName('query_master');
for(var i=0; i<1*qm.length; i++) {
var o = new Object;
o.xmlOrig = qm[i];
o.id = i2b2.h.getXNodeVal(qm[i],'query_master_id');
o.name = i2b2.h.getXNodeVal(qm[i],'name');
o.userid = i2b2.h.getXNodeVal(qm[i],'user_id');
o.group = i2b2.h.getXNodeVal(qm[i],'group_id');
o.created = i2b2.h.getXNodeVal(qm[i],'create_date');
var dStr = '';
var d = o.created.match(/^[0-9\-]*/).toString();
if (d) {
d = d.replace(/-/g,'/');
d = new Date(Date.parse(d));
if (d) {
dStr = ' [' + (d.getMonth()+1) + '-' + d.getDate() + '-' + d.getFullYear().toString() + ']';
}
}
o.name += dStr + ' ['+o.userid+']';
// encapsulate into an SDX package
var sdxDataPack = i2b2.sdx.Master.EncapsulateData('QM',o);
this.model.push(sdxDataPack);
}
} else {
this.model = false;
console.error("[getNameInfo] Could not parse() data!");
}
return this;
}
i2b2.CRC.ajax._addFunctionCall( "getNameInfo",
"{{{URL}}}getNameInfo",
i2b2.CRC.cfg.msgs.getNameInfo,
null,
i2b2.CRC.cfg.parsers.getNameInfo);
// ================================================================================================== //
i2b2.CRC.cfg.msgs.getQueryInstanceList_fromQueryMasterId = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' CRC_QRY_getQueryInstanceList_fromQueryMasterId \n'+
' \n'+
' \n'+
' {{{qm_key_value}}} \n'+
' \n'+
' \n'+
' ';
i2b2.CRC.cfg.parsers.getQueryInstanceList_fromQueryMasterId = function(){
if (!this.error) {
this.model = [];
var qi = this.refXML.getElementsByTagName('query_instance');
for(var i1=0; i1<1*qi.length; i1++) {
var o = new Object;
o.xmlOrig = qi[i1];
o.query_master_id = i2b2.h.getXNodeVal(qi[i1],'query_master_id');
o.query_instance_id = i2b2.h.getXNodeVal(qi[i1],'query_instance_id');
o.id = o.query_instance_id;
o.batch_mode = i2b2.h.getXNodeVal(qi[i1],'batch_mode');
o.start_date = i2b2.h.getXNodeVal(qi[i1],'start_date');
o.end_date = i2b2.h.getXNodeVal(qi[i1],'end_date');
o.query_status_type = i2b2.h.getXNodeVal(qi[i1],'query_status_type');
var sdxDataPack = i2b2.sdx.Master.EncapsulateData('QI',o);
this.model.push(sdxDataPack);
}
} else {
this.model = false;
console.error("[getQueryInstanceList_fromQueryMasterId] Could not parse() data!");
}
return this;
}
i2b2.CRC.ajax._addFunctionCall( "getQueryInstanceList_fromQueryMasterId",
"{{{URL}}}request",
i2b2.CRC.cfg.msgs.getQueryInstanceList_fromQueryMasterId,
null,
i2b2.CRC.cfg.parsers.getQueryInstanceList_fromQueryMasterId);
// ================================================================================================== //
i2b2.CRC.cfg.msgs.getQueryResultInstanceList_fromQueryResultInstanceId = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' CRC_QRY_getResultDocument_fromResultInstanceId \n'+
' \n'+
' \n'+
' {{{qr_key_value}}} \n'+
' \n'+
' \n'+
' ';
i2b2.CRC.cfg.parsers.getQueryResultInstanceList_fromQueryResultInstanceId = function(){
if (!this.error) {
this.model = [];
var qi = this.refXML.getElementsByTagName('query_result_instance');
for(var i1=0; i1<1*qi.length; i1++) {
var o = new Object;
o.xmlOrig = qi[i1];
o.result_instance_id = i2b2.h.getXNodeVal(qi[i1],'result_instance_id');
o.query_instance_id = i2b2.h.getXNodeVal(qi[i1],'query_instance_id');
o.id = o.query_instance_id;
o.batch_mode = i2b2.h.getXNodeVal(qi[i1],'batch_mode');
o.start_date = i2b2.h.getXNodeVal(qi[i1],'start_date');
o.end_date = i2b2.h.getXNodeVal(qi[i1],'end_date');
o.query_status_type = i2b2.h.getXNodeVal(qi[i1],'query_status_type');
var sdxDataPack = i2b2.sdx.Master.EncapsulateData('QI',o);
this.model.push(sdxDataPack);
}
} else {
this.model = false;
console.error("[getQueryResultInstanceList_fromQueryResultInstanceId] Could not parse() data!");
}
return this;
}
i2b2.CRC.ajax._addFunctionCall( "getQueryResultInstanceList_fromQueryResultInstanceId",
"{{{URL}}}request",
i2b2.CRC.cfg.msgs.getQueryResultInstanceList_fromQueryResultInstanceId,
null,
i2b2.CRC.cfg.parsers.getQueryResultInstanceList_fromQueryResultInstanceId);
// ================================================================================================== //
i2b2.CRC.cfg.msgs.getQueryResultInstanceList_fromQueryInstanceId = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' CRC_QRY_getQueryResultInstanceList_fromQueryInstanceId \n'+
' \n'+
' \n'+
' {{{qi_key_value}}} \n'+
' \n'+
' \n'+
' ';
i2b2.CRC.cfg.parsers.getQueryResultInstanceList_fromQueryInstanceId = function(){
if (!this.error) {
this.model = [];
// extract records from XML msg
var ps = this.refXML.getElementsByTagName('query_result_instance');
for(var i1=0; i1 10) {
o.title = "Encounter Set - "+o.size+" encounters";
} else {
if (i2b2.h.isSHRINE()) {
o.title = "Encounter Set - 10 encounters or less";
} else {
o.title = "Encounter Set - "+o.size+" encounters";
}
}
}
o.PRS_id = i2b2.h.getXNodeVal(ps[i1],'result_instance_id');
o.titleCRC = o.title;
// o.title = pn.parent.sdxInfo.sdxDisplayName + ' [PATIENTSET_'+o.PRS_id+']';
o.result_instance_id = o.PRS_id;
var sdxDataNode = i2b2.sdx.Master.EncapsulateData('ENS',o);
break;
case "PATIENTSET":
// create the title using shrine setting
try {
var t = i2b2.h.XPath(temp,'self::description')[0].firstChild.nodeValue;
} catch(e) { var t = null; }
if (t) {
o.title = t;
} else {
if (o.size > 10) {
o.title = "Patient Set - "+o.size+" patients";
} else {
if (i2b2.h.isSHRINE()) {
o.title = "Patient Set - 10 patients or less";
} else {
o.title = "Patient Set - "+o.size+" patients";
}
}
}
o.PRS_id = i2b2.h.getXNodeVal(ps[i1],'result_instance_id');
o.titleCRC = o.title;
// o.title = pn.parent.sdxInfo.sdxDisplayName + ' [PATIENTSET_'+o.PRS_id+']';
o.result_instance_id = o.PRS_id;
var sdxDataNode = i2b2.sdx.Master.EncapsulateData('PRS',o);
break;
case "PATIENT_COUNT_XML":
// create the title using shrine setting
try {
var t = i2b2.h.XPath(temp,'self::description')[0].firstChild.nodeValue;
} catch(e) { var t = null; }
if (t) {
o.title = t;
} else {
if (o.size > 10) {
o.title = "Patient Count - "+o.size+" patients";
} else {
if (i2b2.h.isSHRINE()) {
o.title = "Patient Count - 10 patients or less";
} else {
o.title = "Patient Count - "+o.size+" patients";
}
}
}
o.PRC_id = i2b2.h.getXNodeVal(ps[i1],'result_instance_id');
o.titleCRC = o.title;
// o.title = pn.parent.sdxInfo.sdxDisplayName + ' [PATIENT_COUNT_XML_'+o.PRC_id+']';
o.result_instance_id = o.PRC_id;
var sdxDataNode = i2b2.sdx.Master.EncapsulateData('PRC',o);
break;
}
this.model.push(sdxDataNode);
}
} else {
this.model = false;
console.error("[getQueryResultInstanceList_fromQueryInstanceId] Could not parse() data!");
}
return this;
}
i2b2.CRC.ajax._addFunctionCall( "getQueryResultInstanceList_fromQueryInstanceId",
"{{{URL}}}request",
i2b2.CRC.cfg.msgs.getQueryResultInstanceList_fromQueryInstanceId,
null,
i2b2.CRC.cfg.parsers.getQueryResultInstanceList_fromQueryInstanceId);
// ================================================================================================== //
i2b2.CRC.cfg.msgs.getRequestXml_fromQueryMasterId = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' CRC_QRY_getRequestXml_fromQueryMasterId \n'+
' \n'+
' \n'+
' {{{qm_key_value}}} \n'+
' \n'+
' \n'+
' ';
i2b2.CRC.ajax._addFunctionCall("getRequestXml_fromQueryMasterId","{{{URL}}}request", i2b2.CRC.cfg.msgs.getRequestXml_fromQueryMasterId);
// ================================================================================================== //
i2b2.CRC.cfg.msgs.runQueryInstance_fromQueryDefinition = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' optimize_without_temp_table \n'+
' CRC_QRY_runQueryInstance_fromQueryDefinition \n'+
' \n'+
' \n'+
' {{{psm_query_definition}}}\n'+
' {{{psm_result_output}}}\n'+
' \n'+
' {{{shrine_topic}}}\n'+
' \n'+
' \n';
i2b2.CRC.ajax._addFunctionCall( "runQueryInstance_fromQueryDefinition",
"{{{URL}}}request",
i2b2.CRC.cfg.msgs.runQueryInstance_fromQueryDefinition,
["psm_result_output","psm_query_definition","shrine_topic"]);
// ================================================================================================== //1
i2b2.CRC.cfg.msgs.deleteQueryMaster = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' CRC_QRY_deleteQueryMaster \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' {{{qm_key_value}}} \n'+
' \n'+
' \n'+
' \n';
i2b2.CRC.ajax._addFunctionCall("deleteQueryMaster","{{{URL}}}request", i2b2.CRC.cfg.msgs.deleteQueryMaster);
// ================================================================================================== //
i2b2.CRC.cfg.msgs.renameQueryMaster = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' CRC_QRY_renameQueryMaster \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' {{{qm_key_value}}} \n'+
' {{{qm_name}}} \n'+
' \n'+
' \n'+
' ';
i2b2.CRC.ajax._addFunctionCall("renameQueryMaster","{{{URL}}}request", i2b2.CRC.cfg.msgs.renameQueryMaster);
// ================================================================================================== //
i2b2.CRC.cfg.msgs.getPDO_fromInputList = '\r'+
'\r'+
' \n'+
' {{{proxy_info}}}'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{patient_limit}}} \n'+
' {{{result_wait_time}}}000 \n'+
' getPDO_fromInputList \n'+
' \n'+
' \n'+
' {{{PDO_Request}}}'+
' \n'+
' \n'+
' ';
i2b2.CRC.cfg.parsers.getPDO_fromInputList = function(){
if (!this.error) {
this.model = {
patients: [],
events: [],
observations: []
};
// extract event records
var ps = this.refXML.getElementsByTagName('event');
for(var i1=0; i1\r'+
' \n'+
' {{{proxy_info}}}'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' get_observationfact_by_primary_key \n'+
' \n'+
' \n'+
' {{{PDO_Request}}}'+
' \n'+
' \n'+
'';
i2b2.CRC.cfg.parsers.getIbservationfact_byPrimaryKey = function(){
if (!this.error) {
this.model = {
observations: []
};
// extract observation records
var ps = this.refXML.getElementsByTagName('observation');
for(var i1=0; i1\r'+
' \n'+
' {{{proxy_info}}}'+
' \n'+
' i2b2_QueryTool \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' i2b2_DataRepositoryCell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' PHS \n'+
' \n'+
' \n'+
' Q04 \n'+
' EQQ \n'+
' \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' messageId \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{sec_user}}} \n'+
' 0 \n'+
' 0 \n'+
' CRC_QRY_getResultType \n'+
' \n'+
' \n'+
'';
i2b2.CRC.cfg.parsers.getQRY_getResultType = function(){
if (!this.error) {
this.model = {
result: []
};
// extract event records
var ps = this.refXML.getElementsByTagName('query_result_type');
for(var i1=0; i1
© 2015 - 2025 Weber Informatics LLC | Privacy Policy