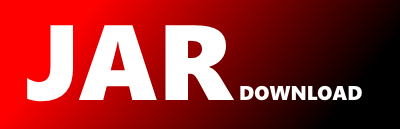
webclient.js-i2b2.cells.ONT.i2b2_msgs.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of i2b2-shrine Show documentation
Show all versions of i2b2-shrine Show documentation
Standalone service to to run the AKTIN broker and aggregator
together with an i2b2 query frontend.
The newest version!
/**
* @projectDescription Messages used by the ONT cell communicator object.
* @inherits i2b2.ONT.cfg
* @namespace i2b2.ONT.cfg.msgs
* @author Nick Benik, Griffin Weber MD PhD
* @version 1.3
* ----------------------------------------------------------------------------------------
* updated 9-15-08: RC4 launch [Nick Benik]
*/
// create the communicator Object
i2b2.ONT.ajax = i2b2.hive.communicatorFactory("ONT");
i2b2.ONT.cfg.msgs = {};
i2b2.ONT.cfg.parsers = {};
i2b2.ONT.cfg.parsers.ExtractConcepts = function(){
if (!this.error) {
this.model = [];
// extract records from XML msg
var c = this.refXML.getElementsByTagName('concept');
for(var i=0; i<1*c.length; i++) {
var o = new Object;
o.xmlOrig = c[i];
o.name = i2b2.h.getXNodeVal(c[i],'name');
o.hasChildren = i2b2.h.getXNodeVal(c[i],'visualattributes');
if(typeof o.hasChildren !== "undefined")
o.hasChildren = i2b2.h.getXNodeVal(c[i],'visualattributes').substring(0,2);
o.level = i2b2.h.getXNodeVal(c[i],'level');
o.key = i2b2.h.getXNodeVal(c[i],'key');
o.tooltip = i2b2.h.getXNodeVal(c[i],'tooltip');
o.synonym = i2b2.h.getXNodeVal(c[i],'synonym_cd');
o.visual_attributes = i2b2.h.getXNodeVal(c[i],'visualattributes');
o.totalnum = i2b2.h.getXNodeVal(c[i],'totalnum');
o.basecode = i2b2.h.getXNodeVal(c[i],'basecode');;
o.fact_table_column = i2b2.h.getXNodeVal(c[i],'facttablecolumn');
o.table_name = i2b2.h.getXNodeVal(c[i],'tablename');
o.column_name = i2b2.h.getXNodeVal(c[i],'columnname');
o.column_datatype = i2b2.h.getXNodeVal(c[i],'columndatatype');
o.operator = i2b2.h.getXNodeVal(c[i],'operator');
o.dim_code = i2b2.h.getXNodeVal(c[i],'dimcode');
o.protected_access = i2b2.h.getXNodeVal(c[i],'protected_access');
// encapsulate the data node into SDX package
var sdxDataPack = i2b2.sdx.Master.EncapsulateData('CONCPT',o);
// save extracted info
this.model.push(sdxDataPack);
}
} else {
this.model = false;
console.error("[ExtractConcepts] Could not parse() data!");
}
return this;
};
// ================================================================================================== //
i2b2.ONT.cfg.msgs.GetChildConcepts = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' {{{version}}} \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' {{{version}}} \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{concept_key_value}}} \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.ajax._addFunctionCall( "GetChildConcepts",
"{{{URL}}}getChildren",
i2b2.ONT.cfg.msgs.GetChildConcepts,
null,
i2b2.ONT.cfg.parsers.ExtractConcepts);
// ================================================================================================== //
i2b2.ONT.cfg.msgs.GetChildModifiers = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{modifier_key_value}}} \n'+
' {{{modifier_applied_path}}} \n'+
' {{{modifier_applied_concept}}} \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.ajax._addFunctionCall( "GetChildModifiers",
"{{{URL}}}getModifierChildren",
i2b2.ONT.cfg.msgs.GetChildModifiers,
null,
i2b2.ONT.cfg.parsers.GetModifiers);
// ================================================================================================== //
i2b2.ONT.cfg.msgs.GetCategories = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' \n'+
' ';
/*
i2b2.ONT.cfg.parsers.GetCategories = function(){
if (!this.error) {
this.model = [];
// extract records from XML msg
var c = this.refXML.getElementsByTagName('concept');
for(var i=0; i<1*c.length; i++) {
var o = new Object;
o.xmlOrig = c[i];
o.level = i2b2.h.getXNodeVal(c[i],'level');
o.key = i2b2.h.getXNodeVal(c[i],'key');
o.name = i2b2.h.getXNodeVal(c[i],'name');
o.total_num = i2b2.h.getXNodeVal(c[i],'totalnum');
// save extracted info
this.model.push(0);
}
} else {
this.model = false;
console.error("[GetCategories] Could not parse() data!");
}
return this;
};
*/
i2b2.ONT.ajax._addFunctionCall( "GetCategories",
"{{{URL}}}getCategories",
i2b2.ONT.cfg.msgs.GetCategories,
null,
i2b2.ONT.cfg.parsers.ExtractConcepts);
// ================================================================================================== //
i2b2.ONT.cfg.msgs.GetModifiers = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{concept_key_value}}} \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.cfg.parsers.GetModifiers = function(){
if (!this.error) {
this.model = [];
// extract records from XML msg
var c = this.refXML.getElementsByTagName('modifier');
for(var i=0; i<1*c.length; i++) {
var o = new Object;
o.xmlOrig = c[i];
o.level = i2b2.h.getXNodeVal(c[i],'level');
o.basecode = i2b2.h.getXNodeVal(c[i],'basecode');
o.name = i2b2.h.getXNodeVal(c[i],'name');
o.total_num = i2b2.h.getXNodeVal(c[i],'totalnum');
// save extracted info
this.model.push(o);
}
} else {
this.model = false;
console.error("[GetModifiers] Could not parse() data!");
return null;
}
return this;
};
i2b2.ONT.ajax._addFunctionCall( "GetModifiers",
"{{{URL}}}getModifiers",
i2b2.ONT.cfg.msgs.GetModifiers,
null,
i2b2.ONT.cfg.parsers.GetModifiers);
// ================================================================================================== //
i2b2.ONT.cfg.msgs.GetSchemes = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.cfg.parsers.GetSchemes = function(){
if (!this.error) {
this.model = [];
// extract records from XML msg
var c = this.refXML.getElementsByTagName('concept');
for(var i=0; i<1*c.length; i++) {
var o = new Object;
o.xmlOrig = c[i];
o.level = i2b2.h.getXNodeVal(c[i],'level');
o.key = i2b2.h.getXNodeVal(c[i],'key');
o.name = i2b2.h.getXNodeVal(c[i],'name');
o.total_num = i2b2.h.getXNodeVal(c[i],'totalnum');
// save extracted info
this.model.push(o);
}
} else {
this.model = false;
console.error("[GetSchemes] Could not parse() data!");
}
return this;
};
i2b2.ONT.ajax._addFunctionCall( "GetSchemes",
"{{{URL}}}getSchemes",
i2b2.ONT.cfg.msgs.GetSchemes,
null,
i2b2.ONT.cfg.parsers.GetSchemes);
// ================================================================================================== //
i2b2.ONT.cfg.msgs.GetNameInfo = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{ont_search_string}}} \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.ajax._addFunctionCall( "GetNameInfo",
"{{{URL}}}getNameInfo",
i2b2.ONT.cfg.msgs.GetNameInfo,
null,
i2b2.ONT.cfg.parsers.ExtractConcepts);
// ================================================================================================== //
i2b2.ONT.cfg.msgs.GetModifierCodeInfo = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{modifier_key_value}}} \n'+
' {{{ont_search_string}}} \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.ajax._addFunctionCall( "GetModifierCodeInfo",
"{{{URL}}}getModifierCodeInfo",
i2b2.ONT.cfg.msgs.GetModifierCodeInfo,
null,
i2b2.ONT.cfg.parsers.GetModifiers);
// ================================================================================================== //
i2b2.ONT.cfg.msgs.GetModifierNameInfo = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{modifier_key_value}}} \n'+
' {{{ont_search_string}}} \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.ajax._addFunctionCall( "GetModifierNameInfo",
"{{{URL}}}getModifierNameInfo",
i2b2.ONT.cfg.msgs.GetModifierNameInfo,
null,
i2b2.ONT.cfg.parsers.GetModifiers);
// ================================================================================================== //
i2b2.ONT.cfg.msgs.GetCodeInfo = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{ont_search_coding}}}{{{ont_search_string}}} \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.ajax._addFunctionCall( "GetCodeInfo",
"{{{URL}}}getCodeInfo",
i2b2.ONT.cfg.msgs.GetCodeInfo,
null,
i2b2.ONT.cfg.parsers.ExtractConcepts);
// ================================================================================================== //=
i2b2.ONT.cfg.msgs.GetTermInfo = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{concept_key_value}}} \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.ajax._addFunctionCall( "GetTermInfo",
"{{{URL}}}getTermInfo",
i2b2.ONT.cfg.msgs.GetTermInfo,
null,
i2b2.ONT.cfg.parsers.ExtractConcepts);
// ================================================================================================== //=
i2b2.ONT.cfg.msgs.GetModifierInfo = '\n'+
'\n'+
' \n'+
' {{{proxy_info}}}\n'+
' 1.1 \n'+
' 2.4 \n'+
' \n'+
' i2b2 Ontology \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' \n'+
' Ontology Cell \n'+
' ' + i2b2.ClientVersion + ' \n'+
' \n'+
' \n'+
' i2b2 Hive \n'+
' \n'+
' {{{header_msg_datetime}}} \n'+
' \n'+
' {{{sec_domain}}} \n'+
' {{{sec_user}}} \n'+
' {{{sec_pass_node}}}\n'+
' \n'+
' \n'+
' {{{header_msg_id}}} \n'+
' 0 \n'+
' \n'+
' \n'+
' P \n'+
' I \n'+
' \n'+
' AL \n'+
' AL \n'+
' US \n'+
' {{{sec_project}}} \n'+
' \n'+
' \n'+
' {{{result_wait_time}}}000 \n'+
' \n'+
' \n'+
' \n'+
' {{{modifier_key_value}}} \n'+
' {{{modifier_applied_path}}} \n'+
' \n'+
' \n'+
' ';
i2b2.ONT.ajax._addFunctionCall( "GetModifierInfo",
"{{{URL}}}getModifierInfo",
i2b2.ONT.cfg.msgs.GetModifierInfo,
null,
i2b2.ONT.cfg.parsers.GetModifiers);
© 2015 - 2025 Weber Informatics LLC | Privacy Policy