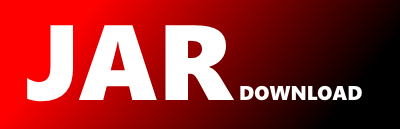
webclient.js-i2b2.cells.plugins.standard.Timeline.Timeline_ctrlr.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of i2b2-shrine Show documentation
Show all versions of i2b2-shrine Show documentation
Standalone service to to run the AKTIN broker and aggregator
together with an i2b2 query frontend.
The newest version!
/**
* @projectDescription Visual display of PDO results in a timeline format.
* @inherits i2b2
* @namespace i2b2.Timeline
* @author Nick Benik, Griffin Weber MD PhD
* @version 1.3
* ----------------------------------------------------------------------------------------
* updated 11-06-08: Initial Launch [Griffin Weber]
* updated 01-13-09: Performance tuning, added details dialogs [Nick Benik]
* updated 11-09-15: Hid "
" in any of the concept names
*/
i2b2.Timeline.Init = function(loadedDiv) {
// register DIV as valid DragDrop target for Patient Record Sets (PRS) objects
var op_trgt = {dropTarget:true};
i2b2.sdx.Master.AttachType("Timeline-CONCPTDROP", "CONCPT", op_trgt);
i2b2.sdx.Master.AttachType("Timeline-PRSDROP", "PRS", op_trgt);
// drop event handlers used by this plugin
i2b2.sdx.Master.setHandlerCustom("Timeline-CONCPTDROP", "CONCPT", "DropHandler", i2b2.Timeline.conceptDropped);
i2b2.sdx.Master.setHandlerCustom("Timeline-PRSDROP", "PRS", "DropHandler", i2b2.Timeline.prsDropped);
// array to store concepts
i2b2.Timeline.model.concepts = [];
// set initial pagination values
i2b2.Timeline.model.pgstart = 1;
i2b2.Timeline.model.pgsize = 10;
// set initial zoom values
i2b2.Timeline.model.zoomScale = 1.0;
i2b2.Timeline.model.zoomPan = 1.0;
i2b2.Timeline.model.showMetadataDialog = true;
// manage YUI tabs
this.yuiTabs = new YAHOO.widget.TabView("Timeline-TABS", {activeIndex:0});
this.yuiTabs.on('activeTabChange', function(ev) {
//Tabs have changed
if (ev.newValue.get('id')=="Timeline-TAB1") {
// user switched to Results tab
if ((i2b2.Timeline.model.concepts.length>0) && i2b2.Timeline.model.prsRecord) {
// contact PDO only if we have data
if (i2b2.Timeline.model.dirtyResultsData) {
// recalculate the results only if the input data has changed
$('Timeline-pgstart').value = '1';
$('Timeline-pgsize').value = '10';
i2b2.Timeline.pgGo(0);
}
}
}
});
};
i2b2.Timeline.setShowMetadataDialog = function(sdxData) {
i2b2.Timeline.model.showMetadataDialog = sdxData;
}
i2b2.Timeline.Unload = function() {
// purge old data
i2b2.Timeline.model = {};
i2b2.Timeline.model.prsRecord = false;
i2b2.Timeline.model.conceptRecord = false;
i2b2.Timeline.model.dirtyResultsData = true;
try { i2b2.Timeline.yuiPanel.destroy(); } catch(e) {}
return true;
};
i2b2.Timeline.prsDropped = function(sdxData) {
sdxData = sdxData[0]; // only interested in first record
// save the info to our local data model
i2b2.Timeline.model.prsRecord = sdxData;
// let the user know that the drop was successful by displaying the name of the patient set
$("Timeline-PRSDROP").innerHTML = i2b2.h.Escape(sdxData.sdxInfo.sdxDisplayName);
// temporarly change background color to give GUI feedback of a successful drop occuring
$("Timeline-PRSDROP").style.background = "#CFB";
setTimeout("$('Timeline-PRSDROP').style.background='#DEEBEF'", 250);
// optimization to prevent requerying the hive for new results if the input dataset has not changed
i2b2.Timeline.model.dirtyResultsData = true;
};
i2b2.Timeline.conceptDropped = function(sdxData, showDialog) {
showDialog = typeof showDialog !== 'undefined' ? showDialog : true;
sdxData = sdxData[0]; // only interested in first record
if(sdxData.sdxInfo.sdxType == "QM"){ // item is a previous query
if(showDialog)
alert("Previous query item being dropped is not supported.");
return false;
}
if (sdxData.origData.isModifier) {
if(showDialog)
alert("Modifier item being dropped is not supported.");
return false;
}
if (typeof sdxData.origData.table_name == 'undefined'){ // BUG FIX: WEBCLIENT-138
var results = i2b2.ONT.ajax.GetTermInfo("ONT", {ont_max_records:'max="1"', ont_synonym_records:'false', ont_hidden_records: 'false', concept_key_value: sdxData.origData.key}).parse();
if(results.model.length > 0){
sdxData = results.model[0];
}
}
if (sdxData.origData.table_name.toUpperCase() == "PATIENT_DIMENSION"){
if(showDialog)
alert("Patient dimension item being dropped is not supported.");
return false;
}
// save the info to our local data model
i2b2.Timeline.model.concepts.push(sdxData);
var cdetails = i2b2.ONT.ajax.GetTermInfo("CRC:QueryTool", {concept_key_value:sdxData.origData.key, ont_synonym_records: true, ont_hidden_records: true} );
try { new ActiveXObject ("MSXML2.DOMDocument.6.0"); isActiveXSupported = true; } catch (e) { isActiveXSupported = false; }
if (isActiveXSupported) {
//Internet Explorer
xmlDocRet = new ActiveXObject("Microsoft.XMLDOM");
xmlDocRet.async = "false";
xmlDocRet.loadXML(cdetails.msgResponse);
xmlDocRet.setProperty("SelectionLanguage", "XPath");
var c = i2b2.h.XPath(xmlDocRet, 'descendant::concept');
} else {
var c = i2b2.h.XPath(cdetails.refXML, 'descendant::concept');
}
if (c.length > 0) {
sdxData.origData.xmlOrig = c[0];
}
var sdxDataNode = i2b2.sdx.Master.EncapsulateData('CONCPT',sdxData.origData);
//var sdxRenderData = i2b2.sdx.Master.RenderHTML(tvTree.id, sdxDataNode, renderOptions);
var lvMetaDatas1 = i2b2.h.XPath(sdxData.origData.xmlOrig, 'metadataxml/ValueMetadata[string-length(Version)>0]');
if ( (lvMetaDatas1.length > 0) && (i2b2.Timeline.model.showMetadataDialog)) {
//bring up popup
i2b2.Timeline.view.modalLabValues.show(this, sdxData.origData.key, sdxData, false);
// this.showModValues(sdxConcept.origData.key, sdxRenderData);
}
// sort and display the concept list
i2b2.Timeline.conceptsRender();
// optimization to prevent requerying the hive for new results if the input dataset has not changed
i2b2.Timeline.model.dirtyResultsData = true;
};
i2b2.Timeline.conceptDelete = function(concptIndex) {
// remove the selected concept
i2b2.Timeline.model.concepts.splice(concptIndex,1);
// sort and display the concept list
i2b2.Timeline.conceptsRender();
// optimization to prevent requerying the hive for new results if the input dataset has not changed
i2b2.Timeline.model.dirtyResultsData = true;
};
i2b2.Timeline.Resize = function() {
var h = parseInt( $('anaPluginViewFrame').style.height ) - 61 - 17;
$$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.results-timelineBox")[0].style.height = h + 'px';
try { i2b2.Timeline.yuiPanel.destroy(); } catch(e) {}
};
i2b2.Timeline.wasHidden = function() {
try { i2b2.Timeline.yuiPanel.destroy(); } catch(e) {}
}
i2b2.Timeline.conceptsRender = function() {
var s = '';
// are there any concepts in the list
if (i2b2.Timeline.model.concepts.length) {
// sort the concepts in alphabetical order
i2b2.Timeline.model.concepts.sort(function() {return arguments[0].sdxInfo.sdxDisplayName > arguments[1].sdxInfo.sdxDisplayName});
// draw the list of concepts
for (var i1 = 0; i1 < i2b2.Timeline.model.concepts.length; i1++) {
if (i1 > 0) { s += ''; }
var values = i2b2.Timeline.model.concepts[i1].LabValues;
var tt = "";
if (undefined != values) {
switch(values.MatchBy) {
case "FLAG":
tt = ' = '+i2b2.h.Escape(values.ValueFlag);
break;
case "VALUE":
if (values.GeneralValueType=="ENUM") {
var sEnum = [];
for (var i2=0;i2' + i2b2.h.Escape(i2b2.h.HideBreak(i2b2.Timeline.model.concepts[i1].sdxInfo.sdxDisplayName)) + tt + '';
}
// show the delete message
$("Timeline-DeleteMsg").style.display = 'block';
} else {
// no concepts selected yet
s = 'Drop one or more Concepts here';
$("Timeline-DeleteMsg").style.display = 'none';
}
// update html
$("Timeline-CONCPTDROP").innerHTML = s;
};
i2b2.Timeline.showObservation = function(localkey) {
try { i2b2.Timeline.yuiPanel.destroy(); } catch(e) {}
var t = i2b2.Timeline.model.observation_PKs[localkey];
// Get the blob
var msg_filter = '\n' +
' '+t.event_id+' \n'+
' '+t.patient_id+' \n'+
' '+t.concept_id+' \n'+
' '+t.observer_id+' \n'+
' \n \n';
i2b2.Timeline.model.pData = "";
// callback processor
var scopedCallback = new i2b2_scopedCallback();
scopedCallback.scope = this;
scopedCallback.callback = function(results) {
// THIS function is used to process the AJAX results of the getChild call
// results data object contains the following attributes:
// refXML: xmlDomObject <--- for data processing
// msgRequest: xml (string)
// msgResponse: xml (string)
// error: boolean
// errorStatus: string [only with error=true]
// errorMsg: string [only with error=true]
// check for errors
if (results.error) {
alert('The results from the server could not be understood. Press F12 for more information.');
console.error("Bad Results from Cell Communicator: ",results);
return false;
}
var s = '';
var patients = {};
// get all the patient records
i2b2.Timeline.model.pData = i2b2.h.getXNodeVal(results.refXML, 'observation_blob');
//end get blob
var disp = "\nevent_id: " + t.event_id + "
" +
"\npatient_id: " + t.patient_id + "
" +
"\nconcept_id: " + t.concept_id + "
" +
"\nobserver_id: " + t.observer_id + "
" +
"\nstart_date: " + t.start_date_key;
if ( i2b2.h.getXNodeVal(results.refXML, 'end_date') != undefined)
disp += "
\nend_date:" + i2b2.h.getXNodeVal(results.refXML, 'end_date') ;
if ( i2b2.h.getXNodeVal(results.refXML, 'tval_char') != undefined)
disp += "
\ntval_char:" + i2b2.h.getXNodeVal(results.refXML, 'tval_char') ;
if ( i2b2.h.getXNodeVal(results.refXML, 'nval_num') != undefined)
disp += "
\nnval_num:" + i2b2.h.getXNodeVal(results.refXML, 'nval_num') ;
if ( i2b2.h.getXNodeVal(results.refXML, 'valueflag_cd') != undefined)
disp += "
\nvalueflag_cd:" + i2b2.h.getXNodeVal(results.refXML, 'valueflag_cd') ;
if ( i2b2.h.getXNodeVal(results.refXML, 'units_cd') != undefined)
disp += "
\nunits_cd:" + i2b2.h.getXNodeVal(results.refXML, 'units_cd') ;
if ( i2b2.h.getXNodeVal(results.refXML, 'modifier_cd') != undefined)
disp += "
\nmodifier_cd:" + i2b2.h.getXNodeVal(results.refXML, 'modifier_cd') ;
if (i2b2.Timeline.model.pData != undefined)
{
disp += "
" + i2b2.Timeline.model.pData + "
";
}
i2b2.Timeline.yuiPanel = new YAHOO.widget.Panel("Timeline-InfoPanel", { width:"330px",height:"560px",
zindex: 10000,
constraintoviewport: true,
autofillheight: "body",
draggable: true,
visible: true,
x: 17,
y: 45,
context: ["TIMELINEOBS-"+localkey,"tr","bl", ["beforeShow", "windowResize", "windowScroll"]] } );
i2b2.Timeline.yuiPanel.setHeader("Observation Details");
i2b2.Timeline.yuiPanel.setBody(disp);
i2b2.Timeline.yuiPanel.render(document.body);
var resize = new YAHOO.util.Resize('Timeline-InfoPanel', {
handles: ['br'],
autoRatio: false,
minWidth: 300,
minHeight: 100,
status: false
});
/*
resize.on('resize', function(args) {
var panelHeight = args.height;
this.cfg.setProperty("height", panelHeight + "px");
}, panel, true);
resize.on('startResize', function(args) {
if (this.cfg.getProperty("constraintoviewport")) {
var D = YAHOO.util.Dom;
var clientRegion = D.getClientRegion();
var elRegion = D.getRegion(this.element);
resize.set("maxWidth", clientRegion.right - elRegion.left - YAHOO.widget.Overlay.VIEWPORT_OFFSET);
resize.set("maxHeight", clientRegion.bottom - elRegion.top - YAHOO.widget.Overlay.VIEWPORT_OFFSET);
} else {
resize.set("maxWidth", null);
resize.set("maxHeight", null);
}
}, panel, true);
*/
}
// AJAX CALL USING THE EXISTING CRC CELL COMMUNICATOR
i2b2.CRC.ajax.getIbservationfact_byPrimaryKey("Plugin:Timeline", {PDO_Request:msg_filter}, scopedCallback);
}
i2b2.Timeline.pgGo = function(dir) {
var formStart = parseInt($('Timeline-pgstart').value);
var formSize = parseInt($('Timeline-pgsize').value);
if (!formStart) {formStart = 1;}
if (!formSize) {formSize = 10;}
if (formSize<1) {formSize = 1;}
formStart = formStart + formSize * dir;
if (formStart<1) {formStart = 1;}
i2b2.Timeline.model.pgstart = formStart;
i2b2.Timeline.model.pgsize = formSize;
$('Timeline-pgstart').value = formStart;
$('Timeline-pgsize').value = formSize;
i2b2.Timeline.model.dirtyResultsData = true;
//remove old results
$$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.results-directions")[0].hide();
$('Timeline-results-scaleLbl1').innerHTML = '';
$('Timeline-results-scaleLbl2').innerHTML = '';
$('Timeline-results-scaleLbl3').innerHTML = '';
$$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.results-timeline")[0].innerHTML = 'Please wait while the timeline is being drawn...';
$$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.results-finished")[0].show();
//reset zoom key
$$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.zoomKeyRange")[0].style.width = '90px';
$$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.zoomKeyRange")[0].style.left = '0px';
// give a brief pause for the GUI to catch up
setTimeout('i2b2.Timeline.getResults();', 50);
};
i2b2.Timeline.updateZoomScaleLabels = function() {
var z = i2b2.Timeline.model.zoomScale*1.0;
var p = i2b2.Timeline.model.zoomPan*1.0;
// update zoom key
$$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.zoomKeyRange")[0].style.width = (90/z) + 'px';
$$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.zoomKeyRange")[0].style.left = ((p*90)-(90/z)) + 'px';
// calculate date labels
var first_time = i2b2.Timeline.model.first_time;
var last_time = i2b2.Timeline.model.last_time;
var lf = last_time - first_time;
var t3 = first_time + lf*p;
var t1 = t3 - lf/z;
var t2 = (t1+t3)/2;
var d1 = new Date(t1);
var d2 = new Date(t2);
var d3 = new Date(t3);
// update labels
$('Timeline-results-scaleLbl1').innerHTML = (d1.getMonth()+1) + '/' + d1.getDate() + '/' + d1.getFullYear();
$('Timeline-results-scaleLbl2').innerHTML = (d2.getMonth()+1) + '/' + d2.getDate() + '/' + d2.getFullYear();
$('Timeline-results-scaleLbl3').innerHTML = (d3.getMonth()+1) + '/' + d3.getDate() + '/' + d3.getFullYear();
}
i2b2.Timeline.zoom = function(op) {
if (op == '+') {
i2b2.Timeline.model.zoomScale *= 2.0;
}
if (op == '-') {
i2b2.Timeline.model.zoomScale *= 0.5;
}
if (op == '<') {
i2b2.Timeline.model.zoomPan -= 0.25/(i2b2.Timeline.model.zoomScale*1.0);
}
if (op == '>') {
i2b2.Timeline.model.zoomPan += 0.25/(i2b2.Timeline.model.zoomScale*1.0);
}
if (i2b2.Timeline.model.zoomScale < 1) {
i2b2.Timeline.model.zoomScale = 1.0;
}
if (i2b2.Timeline.model.zoomPan > 1) {
i2b2.Timeline.model.zoomPan = 1.0;
}
if (i2b2.Timeline.model.zoomPan < 1/(i2b2.Timeline.model.zoomScale*1.0)) {
i2b2.Timeline.model.zoomPan = 1/(i2b2.Timeline.model.zoomScale*1.0);
}
i2b2.Timeline.updateZoomScaleLabels();
var z = i2b2.Timeline.model.zoomScale*1.0;
var p = i2b2.Timeline.model.zoomPan*1.0;
p = 100.0 * (1 - z*p);
z = 100.0 * z;
var o = $$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.results-finished DIV.ptObsZoom");
for (var i=0; iFLAG\n';
s += '\t\t\t\tEQ \n';
s += '\t\t\t\t'+i2b2.h.Escape(lvd.ValueFlag)+' \n';
break;
case "VALUE":
if (lvd.GeneralValueType=="ENUM") {
var sEnum = [];
for (var i2=0;i2TEXT\n';
s += '\t\t\t\t'+sEnum+' \n';
s += '\t\t\t\tIN \n';
} else if (lvd.GeneralValueType=="STRING") {
s += '\t\t\t\tTEXT \n';
s += '\t\t\t\t'+lvd.StringOp+' \n';
s += '\t\t\t\t \n';
} else if (lvd.GeneralValueType=="LARGESTRING") {
if (lvd.DbOp) {
s += '\t\t\t\tCONTAINS[database] \n';
} else {
s += '\t\t\t\tCONTAINS \n';
}
s += '\t\t\t\tLARGETEXT \n';
s += '\t\t\t\t \n';
} else {
s += '\t\t\t\t'+lvd.GeneralValueType+' \n';
s += '\t\t\t\t'+lvd.UnitsCtrl+' \n';
s += '\t\t\t\t'+lvd.NumericOp+' \n';
if (lvd.NumericOp == 'BETWEEN') {
s += '\t\t\t\t'+i2b2.h.Escape(lvd.ValueLow)+' and '+i2b2.h.Escape(lvd.ValueHigh)+' \n';
} else {
s += '\t\t\t\t'+i2b2.h.Escape(lvd.Value)+' \n';
}
}
break;
case "":
break;
}
s += '\t\t\t\n';
return s;
}
i2b2.Timeline.getResults = function() {
if (i2b2.Timeline.model.dirtyResultsData) {
// translate the concept XML for injection as PDO item XML
var filterList = '';
for (var i1=0; i1\n'+
' 0 \n'+
' 0 \n'+
' 0 \n'+
' - \n'+
'
'+cdata.level+' \n'+
' '+cdata.key+' \n'+
' '+cdata.tablename+' \n'+
' '+cdata.dimcode+' \n'+
' '+cdata.synonym+' \n';
if (i2b2.Timeline.model.concepts[i1].LabValues) {
//s += '\t\t\t\n';
filterList += i2b2.Timeline.getValues( i2b2.Timeline.model.concepts[i1].LabValues);
}
filterList +=' \n'+
' \n';
}
var pgstart = i2b2.Timeline.model.pgstart;
var pgend = pgstart + i2b2.Timeline.model.pgsize - 1;
var msg_filter = '\n' +
' \n'+
' '+i2b2.Timeline.model.prsRecord.sdxInfo.sdxKeyValue+' \n'+
' \n'+
' \n'+
'\n'+
filterList+
' \n'+
'\n'+
' \n'+
' \n'+
' \n';
// callback processor
var scopedCallback = new i2b2_scopedCallback();
scopedCallback.scope = this;
scopedCallback.callback = function(results) {
// THIS function is used to process the AJAX results of the getChild call
// results data object contains the following attributes:
// refXML: xmlDomObject <--- for data processing
// msgRequest: xml (string)
// msgResponse: xml (string)
// error: boolean
// errorStatus: string [only with error=true]
// errorMsg: string [only with error=true]
// check for errors
if (results.error) {
alert('The results from the server could not be understood. Press F12 for more information.');
console.error("Bad Results from Cell Communicator: ",results);
return false;
}
var s = '';
var patients = {};
// get all the patient records
var pData = i2b2.h.XPath(results.refXML, '//patient');
for (var i1=0; i1 last_date) {last_date = o.end_date;}
}
}
}
i2b2.Timeline.model.patients = patients;
var first_time = first_date.getTime()*1.0;
var last_time = last_date.getTime()*1.0;
var lf = last_time - first_time + 1;
i2b2.Timeline.model.first_time = first_time;
i2b2.Timeline.model.last_time = last_time;
var observation_keys = new Array();
for (var patientID in patients) {
s += '';
s += '' + patients[patientID].name + '';
s += '';
for (i1=0; i1';
s += '' + i2b2.h.Escape(i2b2.h.HideBreak(i2b2.Timeline.model.concepts[i1].sdxInfo.sdxDisplayName)) + ' ';
s += ' ';
s += '';
s += ' ';
s += '';
}
}
// save the DB key lookup table
i2b2.Timeline.model.observation_PKs = observation_keys;
s += '
';
s += '';
}
i2b2.Timeline.model.zoomScale = 1.0;
i2b2.Timeline.model.zoomPan = 1.0;
i2b2.Timeline.updateZoomScaleLabels();
$$("DIV#Timeline-mainDiv DIV#Timeline-TABS DIV.results-timeline")[0].innerHTML = s;
// optimization - only requery when the input data is changed
i2b2.Timeline.model.dirtyResultsData = false;
}
// AJAX CALL USING THE EXISTING CRC CELL COMMUNICATOR
i2b2.CRC.ajax.getPDO_fromInputList("Plugin:Timeline", {PDO_Request:msg_filter}, scopedCallback);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy