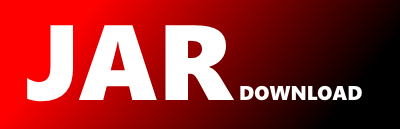
deeProcessApplicationValidator.3.0.0.source-code.bpmn-navigated-viewer.js Maven / Gradle / Ivy
Show all versions of viadeeProcessApplicationValidator Show documentation
/*!
* bpmn-js - bpmn-navigated-viewer v0.20.6
* Copyright 2014, 2015 camunda Services GmbH and other contributors
*
* Released under the bpmn.io license
* http://bpmn.io/license
*
* Source Code: https://github.com/bpmn-io/bpmn-js
*
* Date: 2017-05-04
*/
(function(f){if(typeof exports==="object"&&typeof module!=="undefined"){module.exports=f()}else if(typeof define==="function"&&define.amd){define([],f)}else{var g;if(typeof window!=="undefined"){g=window}else if(typeof global!=="undefined"){g=global}else if(typeof self!=="undefined"){g=self}else{g=this}g.BpmnJS = f()}})(function(){var define,module,exports;return (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o area
* must not be changed.
*
* @see http://bpmn.io/license for more information.
*/
'use strict';
var assign = _dereq_(115),
omit = _dereq_(118),
isNumber = _dereq_(111);
var domify = _dereq_(124),
domQuery = _dereq_(126),
domRemove = _dereq_(127);
var innerSVG = _dereq_(151);
var Diagram = _dereq_(157),
BpmnModdle = _dereq_(16);
var inherits = _dereq_(30);
var Importer = _dereq_(9);
function checkValidationError(err) {
// check if we can help the user by indicating wrong BPMN 2.0 xml
// (in case he or the exporting tool did not get that right)
var pattern = /unparsable content <([^>]+)> detected([\s\S]*)$/;
var match = pattern.exec(err.message);
if (match) {
err.message =
'unparsable content <' + match[1] + '> detected; ' +
'this may indicate an invalid BPMN 2.0 diagram file' + match[2];
}
return err;
}
var DEFAULT_OPTIONS = {
width: '100%',
height: '100%',
position: 'relative'
};
/**
* Ensure the passed argument is a proper unit (defaulting to px)
*/
function ensureUnit(val) {
return val + (isNumber(val) ? 'px' : '');
}
/**
* A viewer for BPMN 2.0 diagrams.
*
* Have a look at {@link NavigatedViewer} or {@link Modeler} for bundles that include
* additional features.
*
*
* ## Extending the Viewer
*
* In order to extend the viewer pass extension modules to bootstrap via the
* `additionalModules` option. An extension module is an object that exposes
* named services.
*
* The following example depicts the integration of a simple
* logging component that integrates with interaction events:
*
*
* ```javascript
*
* // logging component
* function InteractionLogger(eventBus) {
* eventBus.on('element.hover', function(event) {
* console.log()
* })
* }
*
* InteractionLogger.$inject = [ 'eventBus' ]; // minification save
*
* // extension module
* var extensionModule = {
* __init__: [ 'interactionLogger' ],
* interactionLogger: [ 'type', InteractionLogger ]
* };
*
* // extend the viewer
* var bpmnViewer = new Viewer({ additionalModules: [ extensionModule ] });
* bpmnViewer.importXML(...);
* ```
*
* @param {Object} [options] configuration options to pass to the viewer
* @param {DOMElement} [options.container] the container to render the viewer in, defaults to body.
* @param {String|Number} [options.width] the width of the viewer
* @param {String|Number} [options.height] the height of the viewer
* @param {Object} [options.moddleExtensions] extension packages to provide
* @param {Array} [options.modules] a list of modules to override the default modules
* @param {Array} [options.additionalModules] a list of modules to use with the default modules
*/
function Viewer(options) {
options = assign({}, DEFAULT_OPTIONS, options);
this.moddle = this._createModdle(options);
this.container = this._createContainer(options);
/* */
addProjectLogo(this.container);
/* */
this._init(this.container, this.moddle, options);
}
inherits(Viewer, Diagram);
module.exports = Viewer;
/**
* Parse and render a BPMN 2.0 diagram.
*
* Once finished the viewer reports back the result to the
* provided callback function with (err, warnings).
*
* ## Life-Cycle Events
*
* During import the viewer will fire life-cycle events:
*
* * import.parse.start (about to read model from xml)
* * import.parse.complete (model read; may have worked or not)
* * import.render.start (graphical import start)
* * import.render.complete (graphical import finished)
* * import.done (everything done)
*
* You can use these events to hook into the life-cycle.
*
* @param {String} xml the BPMN 2.0 xml
* @param {Function} [done] invoked with (err, warnings=[])
*/
Viewer.prototype.importXML = function(xml, done) {
// done is optional
done = done || function() {};
var self = this;
// hook in pre-parse listeners +
// allow xml manipulation
xml = this._emit('import.parse.start', { xml: xml }) || xml;
this.moddle.fromXML(xml, 'bpmn:Definitions', function(err, definitions, context) {
// hook in post parse listeners +
// allow definitions manipulation
definitions = self._emit('import.parse.complete', {
error: err,
definitions: definitions,
context: context
}) || definitions;
if (err) {
err = checkValidationError(err);
self._emit('import.done', { error: err });
return done(err);
}
var parseWarnings = context.warnings;
self.importDefinitions(definitions, function(err, importWarnings) {
var allWarnings = [].concat(parseWarnings, importWarnings || []);
self._emit('import.done', { error: err, warnings: allWarnings });
done(err, allWarnings);
});
});
};
/**
* Export the currently displayed BPMN 2.0 diagram as
* a BPMN 2.0 XML document.
*
* @param {Object} [options] export options
* @param {Boolean} [options.format=false] output formated XML
* @param {Boolean} [options.preamble=true] output preamble
*
* @param {Function} done invoked with (err, xml)
*/
Viewer.prototype.saveXML = function(options, done) {
if (!done) {
done = options;
options = {};
}
var definitions = this.definitions;
if (!definitions) {
return done(new Error('no definitions loaded'));
}
this.moddle.toXML(definitions, options, done);
};
/**
* Export the currently displayed BPMN 2.0 diagram as
* an SVG image.
*
* @param {Object} [options]
* @param {Function} done invoked with (err, svgStr)
*/
Viewer.prototype.saveSVG = function(options, done) {
if (!done) {
done = options;
options = {};
}
var canvas = this.get('canvas');
var contentNode = canvas.getDefaultLayer(),
defsNode = domQuery('defs', canvas._svg);
var contents = innerSVG(contentNode),
defs = (defsNode && defsNode.outerHTML) || '';
var bbox = contentNode.getBBox();
var svg =
'\n' +
'\n' +
'\n' +
'';
done(null, svg);
};
/**
* Get a named diagram service.
*
* @example
*
* var elementRegistry = viewer.get('elementRegistry');
* var startEventShape = elementRegistry.get('StartEvent_1');
*
* @param {String} name
*
* @return {Object} diagram service instance
*
* @method Viewer#get
*/
/**
* Invoke a function in the context of this viewer.
*
* @example
*
* viewer.invoke(function(elementRegistry) {
* var startEventShape = elementRegistry.get('StartEvent_1');
* });
*
* @param {Function} fn to be invoked
*
* @return {Object} the functions return value
*
* @method Viewer#invoke
*/
/**
* Remove all drawn elements from the viewer.
*
* After calling this method the viewer can still
* be reused for opening another diagram.
*
* @method Viewer#clear
*/
Viewer.prototype.importDefinitions = function(definitions, done) {
// use try/catch to not swallow synchronous exceptions
// that may be raised during model parsing
try {
if (this.definitions) {
// clear existing rendered diagram
this.clear();
}
// update definitions
this.definitions = definitions;
// perform graphical import
Importer.importBpmnDiagram(this, definitions, done);
} catch (e) {
// handle synchronous errors
done(e);
}
};
Viewer.prototype.getModules = function() {
return this._modules;
};
/**
* Destroy the viewer instance and remove all its
* remainders from the document tree.
*/
Viewer.prototype.destroy = function() {
// diagram destroy
Diagram.prototype.destroy.call(this);
// dom detach
domRemove(this.container);
};
/**
* Register an event listener
*
* Remove a previously added listener via {@link #off(event, callback)}.
*
* @param {String} event
* @param {Number} [priority]
* @param {Function} callback
* @param {Object} [that]
*/
Viewer.prototype.on = function(event, priority, callback, target) {
return this.get('eventBus').on(event, priority, callback, target);
};
/**
* De-register an event listener
*
* @param {String} event
* @param {Function} callback
*/
Viewer.prototype.off = function(event, callback) {
this.get('eventBus').off(event, callback);
};
Viewer.prototype.attachTo = function(parentNode) {
if (!parentNode) {
throw new Error('parentNode required');
}
// ensure we detach from the
// previous, old parent
this.detach();
// unwrap jQuery if provided
if (parentNode.get && parentNode.constructor.prototype.jquery) {
parentNode = parentNode.get(0);
}
if (typeof parentNode === 'string') {
parentNode = domQuery(parentNode);
}
var container = this._container;
parentNode.appendChild(container);
this._emit('attach', {});
};
Viewer.prototype.detach = function() {
var container = this._container,
parentNode = container.parentNode;
if (!parentNode) {
return;
}
this._emit('detach', {});
parentNode.removeChild(container);
};
Viewer.prototype._init = function(container, moddle, options) {
this._container = container;
var baseModules = options.modules || this.getModules(),
additionalModules = options.additionalModules || [],
staticModules = [
{
bpmnjs: [ 'value', this ],
moddle: [ 'value', moddle ]
}
];
var diagramModules = [].concat(staticModules, baseModules, additionalModules);
var diagramOptions = assign(omit(options, 'additionalModules'), {
canvas: assign({}, options.canvas, { container: container }),
modules: diagramModules
});
// invoke diagram constructor
Diagram.call(this, diagramOptions);
if (options && options.container) {
this.attachTo(options.container);
}
};
/**
* Emit an event on the underlying {@link EventBus}
*
* @param {String} type
* @param {Object} event
*
* @return {Object} event processing result (if any)
*/
Viewer.prototype._emit = function(type, event) {
return this.get('eventBus').fire(type, event);
};
Viewer.prototype._createContainer = function(options) {
var container = domify('');
assign(container.style, {
width: ensureUnit(options.width),
height: ensureUnit(options.height),
position: options.position
});
return container;
};
Viewer.prototype._createModdle = function(options) {
var moddleOptions = assign({}, this._moddleExtensions, options.moddleExtensions);
return new BpmnModdle(moddleOptions);
};
// modules the viewer is composed of
Viewer.prototype._modules = [
_dereq_(3),
_dereq_(179),
_dereq_(178),
_dereq_(174)
];
// default moddle extensions the viewer is composed of
Viewer.prototype._moddleExtensions = {};
/* */
var PoweredBy = _dereq_(15),
domEvent = _dereq_(125);
/**
* Adds the project logo to the diagram container as
* required by the bpmn.io license.
*
* @see http://bpmn.io/license
*
* @param {Element} container
*/
function addProjectLogo(container) {
var logoData = PoweredBy.BPMNIO_LOGO;
var linkMarkup =
'' +
'
' +
'';
var linkElement = domify(linkMarkup);
container.appendChild(linkElement);
domEvent.bind(linkElement, 'click', function(event) {
PoweredBy.open();
event.preventDefault();
});
}
/* */
},{"111":111,"115":115,"118":118,"124":124,"125":125,"126":126,"127":127,"15":15,"151":151,"157":157,"16":16,"174":174,"178":178,"179":179,"3":3,"30":30,"9":9}],3:[function(_dereq_,module,exports){
module.exports = {
__depends__: [
_dereq_(6),
_dereq_(11)
]
};
},{"11":11,"6":6}],4:[function(_dereq_,module,exports){
'use strict';
var inherits = _dereq_(30),
isObject = _dereq_(112),
assign = _dereq_(115),
forEach = _dereq_(35),
every = _dereq_(32),
some = _dereq_(38);
var BaseRenderer = _dereq_(165),
TextUtil = _dereq_(199),
DiUtil = _dereq_(12);
var getBusinessObject = _dereq_(14).getBusinessObject,
is = _dereq_(14).is;
var RenderUtil = _dereq_(197);
var componentsToPath = RenderUtil.componentsToPath,
createLine = RenderUtil.createLine;
var domQuery = _dereq_(126);
var svgAppend = _dereq_(145),
svgAttr = _dereq_(147),
svgCreate = _dereq_(150),
svgClasses = _dereq_(148);
var rotate = _dereq_(198).rotate,
transform = _dereq_(198).transform,
translate = _dereq_(198).translate;
var TASK_BORDER_RADIUS = 10;
var INNER_OUTER_DIST = 3;
var LABEL_STYLE = {
fontFamily: 'Arial, sans-serif',
fontSize: 12
};
function BpmnRenderer(eventBus, styles, pathMap, canvas, priority) {
BaseRenderer.call(this, eventBus, priority);
var textUtil = new TextUtil({
style: LABEL_STYLE,
size: { width: 100 }
});
var markers = {};
var computeStyle = styles.computeStyle;
function addMarker(id, options) {
var attrs = assign({
fill: 'black',
strokeWidth: 1,
strokeLinecap: 'round',
strokeDasharray: 'none'
}, options.attrs);
var ref = options.ref || { x: 0, y: 0 };
var scale = options.scale || 1;
// fix for safari / chrome / firefox bug not correctly
// resetting stroke dash array
if (attrs.strokeDasharray === 'none') {
attrs.strokeDasharray = [10000, 1];
}
var marker = svgCreate('marker');
svgAttr(options.element, attrs);
svgAppend(marker, options.element);
svgAttr(marker, {
id: id,
viewBox: '0 0 20 20',
refX: ref.x,
refY: ref.y,
markerWidth: 20 * scale,
markerHeight: 20 * scale,
orient: 'auto'
});
var defs = domQuery('defs', canvas._svg);
if (!defs) {
defs = svgCreate('defs');
svgAppend(canvas._svg, defs);
}
svgAppend(defs, marker);
markers[id] = marker;
}
function marker(type, fill, stroke) {
var id = type + '-' + fill + '-' + stroke;
if (!markers[id]) {
createMarker(type, fill, stroke);
}
return 'url(#' + id + ')';
}
function createMarker(type, fill, stroke) {
var id = type + '-' + fill + '-' + stroke;
if (type === 'sequenceflow-end') {
var sequenceflowEnd = svgCreate('path');
svgAttr(sequenceflowEnd, { d: 'M 1 5 L 11 10 L 1 15 Z' });
addMarker(id, {
element: sequenceflowEnd,
ref: { x: 11, y: 10 },
scale: 0.5,
attrs: {
fill: stroke,
stroke: stroke
}
});
}
if (type === 'messageflow-start') {
var messageflowStart = svgCreate('circle');
svgAttr(messageflowStart, { cx: 6, cy: 6, r: 3.5 });
addMarker(id, {
element: messageflowStart,
attrs: {
fill: fill,
stroke: stroke
},
ref: { x: 6, y: 6 }
});
}
if (type === 'messageflow-end') {
var messageflowEnd = svgCreate('path');
svgAttr(messageflowEnd, { d: 'm 1 5 l 0 -3 l 7 3 l -7 3 z' });
addMarker(id, {
element: messageflowEnd,
attrs: {
fill: fill,
stroke: stroke,
strokeLinecap: 'butt'
},
ref: { x: 8.5, y: 5 }
});
}
if (type === 'association-start') {
var associationStart = svgCreate('path');
svgAttr(associationStart, { d: 'M 11 5 L 1 10 L 11 15' });
addMarker(id, {
element: associationStart,
attrs: {
fill: 'none',
stroke: stroke,
strokeWidth: 1.5
},
ref: { x: 1, y: 10 },
scale: 0.5
});
}
if (type === 'association-end') {
var associationEnd = svgCreate('path');
svgAttr(associationEnd, { d: 'M 1 5 L 11 10 L 1 15' });
addMarker(id, {
element: associationEnd,
attrs: {
fill: 'none',
stroke: stroke,
strokeWidth: 1.5
},
ref: { x: 12, y: 10 },
scale: 0.5
});
}
if (type === 'conditional-flow-marker') {
var conditionalflowMarker = svgCreate('path');
svgAttr(conditionalflowMarker, { d: 'M 0 10 L 8 6 L 16 10 L 8 14 Z' });
addMarker(id, {
element: conditionalflowMarker,
attrs: {
fill: fill,
stroke: stroke
},
ref: { x: -1, y: 10 },
scale: 0.5
});
}
if (type === 'conditional-default-flow-marker') {
var conditionaldefaultflowMarker = svgCreate('path');
svgAttr(conditionaldefaultflowMarker, { d: 'M 6 4 L 10 16' });
addMarker(id, {
element: conditionaldefaultflowMarker,
attrs: {
stroke: stroke
},
ref: { x: 0, y: 10 },
scale: 0.5
});
}
}
function drawCircle(parentGfx, width, height, offset, attrs) {
if (isObject(offset)) {
attrs = offset;
offset = 0;
}
offset = offset || 0;
attrs = computeStyle(attrs, {
stroke: 'black',
strokeWidth: 2,
fill: 'white'
});
var cx = width / 2,
cy = height / 2;
var circle = svgCreate('circle');
svgAttr(circle, {
cx: cx,
cy: cy,
r: Math.round((width + height) / 4 - offset)
});
svgAttr(circle, attrs);
svgAppend(parentGfx, circle);
return circle;
}
function drawRect(parentGfx, width, height, r, offset, attrs) {
if (isObject(offset)) {
attrs = offset;
offset = 0;
}
offset = offset || 0;
attrs = computeStyle(attrs, {
stroke: 'black',
strokeWidth: 2,
fill: 'white'
});
var rect = svgCreate('rect');
svgAttr(rect, {
x: offset,
y: offset,
width: width - offset * 2,
height: height - offset * 2,
rx: r,
ry: r
});
svgAttr(rect, attrs);
svgAppend(parentGfx, rect);
return rect;
}
function drawDiamond(parentGfx, width, height, attrs) {
var x_2 = width / 2;
var y_2 = height / 2;
var points = [{ x: x_2, y: 0 }, { x: width, y: y_2 }, { x: x_2, y: height }, { x: 0, y: y_2 }];
var pointsString = points.map(function(point) {
return point.x + ',' + point.y;
}).join(' ');
attrs = computeStyle(attrs, {
stroke: 'black',
strokeWidth: 2,
fill: 'white'
});
var polygon = svgCreate('polygon');
svgAttr(polygon, {
points: pointsString
});
svgAttr(polygon, attrs);
svgAppend(parentGfx, polygon);
return polygon;
}
function drawLine(parentGfx, waypoints, attrs) {
attrs = computeStyle(attrs, [ 'no-fill' ], {
stroke: 'black',
strokeWidth: 2,
fill: 'none'
});
var line = createLine(waypoints, attrs);
svgAppend(parentGfx, line);
return line;
}
function drawPath(parentGfx, d, attrs) {
attrs = computeStyle(attrs, [ 'no-fill' ], {
strokeWidth: 2,
stroke: 'black'
});
var path = svgCreate('path');
svgAttr(path, { d: d });
svgAttr(path, attrs);
svgAppend(parentGfx, path);
return path;
}
function drawMarker(type, parentGfx, path, attrs) {
return drawPath(parentGfx, path, assign({ 'data-marker': type }, attrs));
}
function as(type) {
return function(parentGfx, element) {
return handlers[type](parentGfx, element);
};
}
function renderer(type) {
return handlers[type];
}
function renderEventContent(element, parentGfx) {
var event = getSemantic(element);
var isThrowing = isThrowEvent(event);
if (isTypedEvent(event, 'bpmn:MessageEventDefinition')) {
return renderer('bpmn:MessageEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:TimerEventDefinition')) {
return renderer('bpmn:TimerEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:ConditionalEventDefinition')) {
return renderer('bpmn:ConditionalEventDefinition')(parentGfx, element);
}
if (isTypedEvent(event, 'bpmn:SignalEventDefinition')) {
return renderer('bpmn:SignalEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:CancelEventDefinition') &&
isTypedEvent(event, 'bpmn:TerminateEventDefinition', { parallelMultiple: false })) {
return renderer('bpmn:MultipleEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:CancelEventDefinition') &&
isTypedEvent(event, 'bpmn:TerminateEventDefinition', { parallelMultiple: true })) {
return renderer('bpmn:ParallelMultipleEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:EscalationEventDefinition')) {
return renderer('bpmn:EscalationEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:LinkEventDefinition')) {
return renderer('bpmn:LinkEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:ErrorEventDefinition')) {
return renderer('bpmn:ErrorEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:CancelEventDefinition')) {
return renderer('bpmn:CancelEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:CompensateEventDefinition')) {
return renderer('bpmn:CompensateEventDefinition')(parentGfx, element, isThrowing);
}
if (isTypedEvent(event, 'bpmn:TerminateEventDefinition')) {
return renderer('bpmn:TerminateEventDefinition')(parentGfx, element, isThrowing);
}
return null;
}
function renderLabel(parentGfx, label, options) {
var text = textUtil.createText(label || '', options);
svgClasses(text).add('djs-label');
svgAppend(parentGfx, text);
return text;
}
function renderEmbeddedLabel(parentGfx, element, align) {
var semantic = getSemantic(element);
return renderLabel(parentGfx, semantic.name, {
box: element,
align: align,
padding: 5,
style: {
fill: getStrokeColor(element)
}
});
}
function renderExternalLabel(parentGfx, element) {
var semantic = getSemantic(element);
var box = {
width: 90,
height: 30,
x: element.width / 2 + element.x,
y: element.height / 2 + element.y
};
return renderLabel(parentGfx, semantic.name, {
box: box,
fitBox: true,
style: { fontSize: '11px' }
});
}
function renderLaneLabel(parentGfx, text, element) {
var textBox = renderLabel(parentGfx, text, {
box: { height: 30, width: element.height },
align: 'center-middle',
style: {
fill: getStrokeColor(element)
}
});
var top = -1 * element.height;
transform(textBox, 0, -top, 270);
}
function createPathFromConnection(connection) {
var waypoints = connection.waypoints;
var pathData = 'm ' + waypoints[0].x + ',' + waypoints[0].y;
for (var i = 1; i < waypoints.length; i++) {
pathData += 'L' + waypoints[i].x + ',' + waypoints[i].y + ' ';
}
return pathData;
}
var handlers = this.handlers = {
'bpmn:Event': function(parentGfx, element, attrs) {
return drawCircle(parentGfx, element.width, element.height, attrs);
},
'bpmn:StartEvent': function(parentGfx, element) {
var attrs = {
fill: getFillColor(element),
stroke: getStrokeColor(element)
};
var semantic = getSemantic(element);
if (!semantic.isInterrupting) {
attrs = {
strokeDasharray: '6',
strokeLinecap: 'round'
};
}
var circle = renderer('bpmn:Event')(parentGfx, element, attrs);
renderEventContent(element, parentGfx);
return circle;
},
'bpmn:MessageEventDefinition': function(parentGfx, element, isThrowing) {
var pathData = pathMap.getScaledPath('EVENT_MESSAGE', {
xScaleFactor: 0.9,
yScaleFactor: 0.9,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.235,
my: 0.315
}
});
var fill = isThrowing ? getStrokeColor(element) : getFillColor(element);
var stroke = isThrowing ? getFillColor(element) : getStrokeColor(element);
var messagePath = drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: fill,
stroke: stroke
});
return messagePath;
},
'bpmn:TimerEventDefinition': function(parentGfx, element) {
var circle = drawCircle(parentGfx, element.width, element.height, 0.2 * element.height, {
strokeWidth: 2,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
var pathData = pathMap.getScaledPath('EVENT_TIMER_WH', {
xScaleFactor: 0.75,
yScaleFactor: 0.75,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.5,
my: 0.5
}
});
drawPath(parentGfx, pathData, {
strokeWidth: 2,
strokeLinecap: 'square',
stroke: getStrokeColor(element)
});
for (var i = 0;i < 12;i++) {
var linePathData = pathMap.getScaledPath('EVENT_TIMER_LINE', {
xScaleFactor: 0.75,
yScaleFactor: 0.75,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.5,
my: 0.5
}
});
var width = element.width / 2;
var height = element.height / 2;
drawPath(parentGfx, linePathData, {
strokeWidth: 1,
strokeLinecap: 'square',
transform: 'rotate(' + (i * 30) + ',' + height + ',' + width + ')',
stroke: getStrokeColor(element)
});
}
return circle;
},
'bpmn:EscalationEventDefinition': function(parentGfx, event, isThrowing) {
var pathData = pathMap.getScaledPath('EVENT_ESCALATION', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: event.width,
containerHeight: event.height,
position: {
mx: 0.5,
my: 0.2
}
});
var fill = isThrowing ? getStrokeColor(event) : 'none';
return drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: fill,
stroke: getStrokeColor(event)
});
},
'bpmn:ConditionalEventDefinition': function(parentGfx, event) {
var pathData = pathMap.getScaledPath('EVENT_CONDITIONAL', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: event.width,
containerHeight: event.height,
position: {
mx: 0.5,
my: 0.222
}
});
return drawPath(parentGfx, pathData, {
strokeWidth: 1,
stroke: getStrokeColor(event)
});
},
'bpmn:LinkEventDefinition': function(parentGfx, event, isThrowing) {
var pathData = pathMap.getScaledPath('EVENT_LINK', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: event.width,
containerHeight: event.height,
position: {
mx: 0.57,
my: 0.263
}
});
var fill = isThrowing ? getStrokeColor(event) : 'none';
return drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: fill,
stroke: getStrokeColor(event)
});
},
'bpmn:ErrorEventDefinition': function(parentGfx, event, isThrowing) {
var pathData = pathMap.getScaledPath('EVENT_ERROR', {
xScaleFactor: 1.1,
yScaleFactor: 1.1,
containerWidth: event.width,
containerHeight: event.height,
position: {
mx: 0.2,
my: 0.722
}
});
var fill = isThrowing ? getStrokeColor(event) : 'none';
return drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: fill,
stroke: getStrokeColor(event)
});
},
'bpmn:CancelEventDefinition': function(parentGfx, event, isThrowing) {
var pathData = pathMap.getScaledPath('EVENT_CANCEL_45', {
xScaleFactor: 1.0,
yScaleFactor: 1.0,
containerWidth: event.width,
containerHeight: event.height,
position: {
mx: 0.638,
my: -0.055
}
});
var fill = isThrowing ? 'black' : 'none';
var path = drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: fill
});
rotate(path, 45);
return path;
},
'bpmn:CompensateEventDefinition': function(parentGfx, event, isThrowing) {
var pathData = pathMap.getScaledPath('EVENT_COMPENSATION', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: event.width,
containerHeight: event.height,
position: {
mx: 0.22,
my: 0.5
}
});
var fill = isThrowing ? getStrokeColor(event) : 'none';
return drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: fill,
stroke: getStrokeColor(event)
});
},
'bpmn:SignalEventDefinition': function(parentGfx, event, isThrowing) {
var pathData = pathMap.getScaledPath('EVENT_SIGNAL', {
xScaleFactor: 0.9,
yScaleFactor: 0.9,
containerWidth: event.width,
containerHeight: event.height,
position: {
mx: 0.5,
my: 0.2
}
});
var fill = isThrowing ? getStrokeColor(event) : 'none';
return drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: fill,
stroke: getStrokeColor(event)
});
},
'bpmn:MultipleEventDefinition': function(parentGfx, event, isThrowing) {
var pathData = pathMap.getScaledPath('EVENT_MULTIPLE', {
xScaleFactor: 1.1,
yScaleFactor: 1.1,
containerWidth: event.width,
containerHeight: event.height,
position: {
mx: 0.222,
my: 0.36
}
});
var fill = isThrowing ? 'black' : 'none';
return drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: fill
});
},
'bpmn:ParallelMultipleEventDefinition': function(parentGfx, event) {
var pathData = pathMap.getScaledPath('EVENT_PARALLEL_MULTIPLE', {
xScaleFactor: 1.2,
yScaleFactor: 1.2,
containerWidth: event.width,
containerHeight: event.height,
position: {
mx: 0.458,
my: 0.194
}
});
return drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: getStrokeColor(event),
stroke: getStrokeColor(event)
});
},
'bpmn:EndEvent': function(parentGfx, element) {
var circle = renderer('bpmn:Event')(parentGfx, element, {
strokeWidth: 4,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
renderEventContent(element, parentGfx, true);
return circle;
},
'bpmn:TerminateEventDefinition': function(parentGfx, element) {
var circle = drawCircle(parentGfx, element.width, element.height, 8, {
strokeWidth: 4,
fill: getStrokeColor(element),
stroke: getStrokeColor(element)
});
return circle;
},
'bpmn:IntermediateEvent': function(parentGfx, element) {
var outer = renderer('bpmn:Event')(parentGfx, element, {
strokeWidth: 1,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
/* inner */ drawCircle(parentGfx, element.width, element.height, INNER_OUTER_DIST, {
strokeWidth: 1,
fill: getFillColor(element, 'none'),
stroke: getStrokeColor(element)
});
renderEventContent(element, parentGfx);
return outer;
},
'bpmn:IntermediateCatchEvent': as('bpmn:IntermediateEvent'),
'bpmn:IntermediateThrowEvent': as('bpmn:IntermediateEvent'),
'bpmn:Activity': function(parentGfx, element, attrs) {
return drawRect(parentGfx, element.width, element.height, TASK_BORDER_RADIUS, attrs);
},
'bpmn:Task': function(parentGfx, element) {
var attrs = {
fill: getFillColor(element),
stroke: getStrokeColor(element)
};
var rect = renderer('bpmn:Activity')(parentGfx, element, attrs);
renderEmbeddedLabel(parentGfx, element, 'center-middle');
attachTaskMarkers(parentGfx, element);
return rect;
},
'bpmn:ServiceTask': function(parentGfx, element) {
var task = renderer('bpmn:Task')(parentGfx, element);
var pathDataBG = pathMap.getScaledPath('TASK_TYPE_SERVICE', {
abspos: {
x: 12,
y: 18
}
});
/* service bg */ drawPath(parentGfx, pathDataBG, {
strokeWidth: 1,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
var fillPathData = pathMap.getScaledPath('TASK_TYPE_SERVICE_FILL', {
abspos: {
x: 17.2,
y: 18
}
});
/* service fill */ drawPath(parentGfx, fillPathData, {
strokeWidth: 0,
fill: getFillColor(element)
});
var pathData = pathMap.getScaledPath('TASK_TYPE_SERVICE', {
abspos: {
x: 17,
y: 22
}
});
/* service */ drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
return task;
},
'bpmn:UserTask': function(parentGfx, element) {
var task = renderer('bpmn:Task')(parentGfx, element);
var x = 15;
var y = 12;
var pathData = pathMap.getScaledPath('TASK_TYPE_USER_1', {
abspos: {
x: x,
y: y
}
});
/* user path */ drawPath(parentGfx, pathData, {
strokeWidth: 0.5,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
var pathData2 = pathMap.getScaledPath('TASK_TYPE_USER_2', {
abspos: {
x: x,
y: y
}
});
/* user2 path */ drawPath(parentGfx, pathData2, {
strokeWidth: 0.5,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
var pathData3 = pathMap.getScaledPath('TASK_TYPE_USER_3', {
abspos: {
x: x,
y: y
}
});
/* user3 path */ drawPath(parentGfx, pathData3, {
strokeWidth: 0.5,
fill: getStrokeColor(element),
stroke: getStrokeColor(element)
});
return task;
},
'bpmn:ManualTask': function(parentGfx, element) {
var task = renderer('bpmn:Task')(parentGfx, element);
var pathData = pathMap.getScaledPath('TASK_TYPE_MANUAL', {
abspos: {
x: 17,
y: 15
}
});
/* manual path */ drawPath(parentGfx, pathData, {
strokeWidth: 0.5, // 0.25,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
return task;
},
'bpmn:SendTask': function(parentGfx, element) {
var task = renderer('bpmn:Task')(parentGfx, element);
var pathData = pathMap.getScaledPath('TASK_TYPE_SEND', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: 21,
containerHeight: 14,
position: {
mx: 0.285,
my: 0.357
}
});
/* send path */ drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: getStrokeColor(element),
stroke: getFillColor(element)
});
return task;
},
'bpmn:ReceiveTask' : function(parentGfx, element) {
var semantic = getSemantic(element);
var task = renderer('bpmn:Task')(parentGfx, element);
var pathData;
if (semantic.instantiate) {
drawCircle(parentGfx, 28, 28, 20 * 0.22, { strokeWidth: 1 });
pathData = pathMap.getScaledPath('TASK_TYPE_INSTANTIATING_SEND', {
abspos: {
x: 7.77,
y: 9.52
}
});
} else {
pathData = pathMap.getScaledPath('TASK_TYPE_SEND', {
xScaleFactor: 0.9,
yScaleFactor: 0.9,
containerWidth: 21,
containerHeight: 14,
position: {
mx: 0.3,
my: 0.4
}
});
}
/* receive path */ drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
return task;
},
'bpmn:ScriptTask': function(parentGfx, element) {
var task = renderer('bpmn:Task')(parentGfx, element);
var pathData = pathMap.getScaledPath('TASK_TYPE_SCRIPT', {
abspos: {
x: 15,
y: 20
}
});
/* script path */ drawPath(parentGfx, pathData, {
strokeWidth: 1,
stroke: getStrokeColor(element)
});
return task;
},
'bpmn:BusinessRuleTask': function(parentGfx, element) {
var task = renderer('bpmn:Task')(parentGfx, element);
var headerPathData = pathMap.getScaledPath('TASK_TYPE_BUSINESS_RULE_HEADER', {
abspos: {
x: 8,
y: 8
}
});
var businessHeaderPath = drawPath(parentGfx, headerPathData);
svgAttr(businessHeaderPath, {
strokeWidth: 1,
fill: getFillColor(element, '#aaaaaa'),
stroke: getStrokeColor(element)
});
var headerData = pathMap.getScaledPath('TASK_TYPE_BUSINESS_RULE_MAIN', {
abspos: {
x: 8,
y: 8
}
});
var businessPath = drawPath(parentGfx, headerData);
svgAttr(businessPath, {
strokeWidth: 1,
stroke: getStrokeColor(element)
});
return task;
},
'bpmn:SubProcess': function(parentGfx, element, attrs) {
attrs = assign({
fillOpacity: 0.95,
fill: getFillColor(element),
stroke: getStrokeColor(element)
}, attrs);
var rect = renderer('bpmn:Activity')(parentGfx, element, attrs);
var expanded = DiUtil.isExpanded(element);
var isEventSubProcess = DiUtil.isEventSubProcess(element);
if (isEventSubProcess) {
svgAttr(rect, {
strokeDasharray: '1,2'
});
}
renderEmbeddedLabel(parentGfx, element, expanded ? 'center-top' : 'center-middle');
if (expanded) {
attachTaskMarkers(parentGfx, element);
} else {
attachTaskMarkers(parentGfx, element, ['SubProcessMarker']);
}
return rect;
},
'bpmn:AdHocSubProcess': function(parentGfx, element) {
return renderer('bpmn:SubProcess')(parentGfx, element);
},
'bpmn:Transaction': function(parentGfx, element) {
var outer = renderer('bpmn:SubProcess')(parentGfx, element);
var innerAttrs = styles.style([ 'no-fill', 'no-events' ], {
stroke: getStrokeColor(element)
});
/* inner path */ drawRect(parentGfx, element.width, element.height, TASK_BORDER_RADIUS - 2, INNER_OUTER_DIST, innerAttrs);
return outer;
},
'bpmn:CallActivity': function(parentGfx, element) {
return renderer('bpmn:SubProcess')(parentGfx, element, {
strokeWidth: 5
});
},
'bpmn:Participant': function(parentGfx, element) {
var attrs = {
fillOpacity: 0.95,
fill: getFillColor(element),
stroke: getStrokeColor(element)
};
var lane = renderer('bpmn:Lane')(parentGfx, element, attrs);
var expandedPool = DiUtil.isExpanded(element);
if (expandedPool) {
drawLine(parentGfx, [
{ x: 30, y: 0 },
{ x: 30, y: element.height }
], {
stroke: getStrokeColor(element)
});
var text = getSemantic(element).name;
renderLaneLabel(parentGfx, text, element);
} else {
// Collapsed pool draw text inline
var text2 = getSemantic(element).name;
renderLabel(parentGfx, text2, {
box: element, align: 'center-middle',
style: {
fill: getStrokeColor(element)
}
});
}
var participantMultiplicity = !!(getSemantic(element).participantMultiplicity);
if (participantMultiplicity) {
renderer('ParticipantMultiplicityMarker')(parentGfx, element);
}
return lane;
},
'bpmn:Lane': function(parentGfx, element, attrs) {
var rect = drawRect(parentGfx, element.width, element.height, 0, assign({
fill: getFillColor(element),
stroke: getStrokeColor(element)
}, attrs));
var semantic = getSemantic(element);
if (semantic.$type === 'bpmn:Lane') {
var text = semantic.name;
renderLaneLabel(parentGfx, text, element);
}
return rect;
},
'bpmn:InclusiveGateway': function(parentGfx, element) {
var attrs = {
fill: getFillColor(element),
stroke: getStrokeColor(element)
};
var diamond = drawDiamond(parentGfx, element.width, element.height, attrs);
/* circle path */
drawCircle(parentGfx, element.width, element.height, element.height * 0.24, {
strokeWidth: 2.5,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
return diamond;
},
'bpmn:ExclusiveGateway': function(parentGfx, element) {
var attrs = {
fill: getFillColor(element),
stroke: getStrokeColor(element)
};
var diamond = drawDiamond(parentGfx, element.width, element.height, attrs);
var pathData = pathMap.getScaledPath('GATEWAY_EXCLUSIVE', {
xScaleFactor: 0.4,
yScaleFactor: 0.4,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.32,
my: 0.3
}
});
if ((getDi(element).isMarkerVisible)) {
drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: getStrokeColor(element),
stroke: getStrokeColor(element)
});
}
return diamond;
},
'bpmn:ComplexGateway': function(parentGfx, element) {
var attrs = {
fill: getFillColor(element),
stroke: getStrokeColor(element)
};
var diamond = drawDiamond(parentGfx, element.width, element.height, attrs);
var pathData = pathMap.getScaledPath('GATEWAY_COMPLEX', {
xScaleFactor: 0.5,
yScaleFactor:0.5,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.46,
my: 0.26
}
});
/* complex path */ drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: getStrokeColor(element),
stroke: getStrokeColor(element)
});
return diamond;
},
'bpmn:ParallelGateway': function(parentGfx, element) {
var attrs = {
fill: getFillColor(element),
stroke: getStrokeColor(element)
};
var diamond = drawDiamond(parentGfx, element.width, element.height, attrs);
var pathData = pathMap.getScaledPath('GATEWAY_PARALLEL', {
xScaleFactor: 0.6,
yScaleFactor:0.6,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.46,
my: 0.2
}
});
/* parallel path */ drawPath(parentGfx, pathData, {
strokeWidth: 1,
fill: getStrokeColor(element),
stroke: getStrokeColor(element)
});
return diamond;
},
'bpmn:EventBasedGateway': function(parentGfx, element) {
var semantic = getSemantic(element);
var attrs = {
fill: getFillColor(element),
stroke: getStrokeColor(element)
};
var diamond = drawDiamond(parentGfx, element.width, element.height, attrs);
/* outer circle path */ drawCircle(parentGfx, element.width, element.height, element.height * 0.20, {
strokeWidth: 1,
fill: 'none',
stroke: getStrokeColor(element)
});
var type = semantic.eventGatewayType;
var instantiate = !!semantic.instantiate;
function drawEvent() {
var pathData = pathMap.getScaledPath('GATEWAY_EVENT_BASED', {
xScaleFactor: 0.18,
yScaleFactor: 0.18,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.36,
my: 0.44
}
});
var attrs = {
strokeWidth: 2,
fill: getFillColor(element, 'none'),
stroke: getStrokeColor(element)
};
/* event path */ drawPath(parentGfx, pathData, attrs);
}
if (type === 'Parallel') {
var pathData = pathMap.getScaledPath('GATEWAY_PARALLEL', {
xScaleFactor: 0.4,
yScaleFactor:0.4,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.474,
my: 0.296
}
});
var parallelPath = drawPath(parentGfx, pathData);
svgAttr(parallelPath, {
strokeWidth: 1,
fill: 'none'
});
} else if (type === 'Exclusive') {
if (!instantiate) {
var innerCircle = drawCircle(parentGfx, element.width, element.height, element.height * 0.26);
svgAttr(innerCircle, {
strokeWidth: 1,
fill: 'none',
stroke: getStrokeColor(element)
});
}
drawEvent();
}
return diamond;
},
'bpmn:Gateway': function(parentGfx, element) {
return drawDiamond(parentGfx, element.width, element.height);
},
'bpmn:SequenceFlow': function(parentGfx, element) {
var pathData = createPathFromConnection(element);
var fill = getFillColor(element),
stroke = getStrokeColor(element);
var attrs = {
strokeLinejoin: 'round',
markerEnd: marker('sequenceflow-end', fill, stroke),
stroke: getStrokeColor(element)
};
var path = drawPath(parentGfx, pathData, attrs);
var sequenceFlow = getSemantic(element);
var source = element.source.businessObject;
// conditional flow marker
if (sequenceFlow.conditionExpression && source.$instanceOf('bpmn:Activity')) {
svgAttr(path, {
markerStart: marker('conditional-flow-marker', fill, stroke)
});
}
// default marker
if (source.default && (source.$instanceOf('bpmn:Gateway') || source.$instanceOf('bpmn:Activity')) &&
source.default === sequenceFlow) {
svgAttr(path, {
markerStart: marker('conditional-default-flow-marker', fill, stroke)
});
}
return path;
},
'bpmn:Association': function(parentGfx, element, attrs) {
var semantic = getSemantic(element);
var fill = getFillColor(element),
stroke = getStrokeColor(element);
attrs = assign({
strokeDasharray: '0.5, 5',
strokeLinecap: 'round',
strokeLinejoin: 'round',
stroke: getStrokeColor(element)
}, attrs || {});
if (semantic.associationDirection === 'One' ||
semantic.associationDirection === 'Both') {
attrs.markerEnd = marker('association-end', fill, stroke);
}
if (semantic.associationDirection === 'Both') {
attrs.markerStart = marker('association-start', fill, stroke);
}
return drawLine(parentGfx, element.waypoints, attrs);
},
'bpmn:DataInputAssociation': function(parentGfx, element) {
var fill = getFillColor(element),
stroke = getStrokeColor(element);
return renderer('bpmn:Association')(parentGfx, element, {
markerEnd: marker('association-end', fill, stroke)
});
},
'bpmn:DataOutputAssociation': function(parentGfx, element) {
var fill = getFillColor(element),
stroke = getStrokeColor(element);
return renderer('bpmn:Association')(parentGfx, element, {
markerEnd: marker('association-end', fill, stroke)
});
},
'bpmn:MessageFlow': function(parentGfx, element) {
var semantic = getSemantic(element),
di = getDi(element);
var fill = getFillColor(element),
stroke = getStrokeColor(element);
var pathData = createPathFromConnection(element);
var attrs = {
markerEnd: marker('messageflow-end', fill, stroke),
markerStart: marker('messageflow-start', fill, stroke),
strokeDasharray: '10, 12',
strokeLinecap: 'round',
strokeLinejoin: 'round',
strokeWidth: '1.5px',
stroke: getStrokeColor(element)
};
var path = drawPath(parentGfx, pathData, attrs);
if (semantic.messageRef) {
var midPoint = path.getPointAtLength(path.getTotalLength() / 2);
var markerPathData = pathMap.getScaledPath('MESSAGE_FLOW_MARKER', {
abspos: {
x: midPoint.x,
y: midPoint.y
}
});
var messageAttrs = { strokeWidth: 1 };
if (di.messageVisibleKind === 'initiating') {
messageAttrs.fill = 'white';
messageAttrs.stroke = 'black';
} else {
messageAttrs.fill = '#888';
messageAttrs.stroke = 'white';
}
drawPath(parentGfx, markerPathData, messageAttrs);
}
return path;
},
'bpmn:DataObject': function(parentGfx, element) {
var pathData = pathMap.getScaledPath('DATA_OBJECT_PATH', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.474,
my: 0.296
}
});
var elementObject = drawPath(parentGfx, pathData, {
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
var semantic = getSemantic(element);
if (isCollection(semantic)) {
renderDataItemCollection(parentGfx, element);
}
return elementObject;
},
'bpmn:DataObjectReference': as('bpmn:DataObject'),
'bpmn:DataInput': function(parentGfx, element) {
var arrowPathData = pathMap.getRawPath('DATA_ARROW');
// page
var elementObject = renderer('bpmn:DataObject')(parentGfx, element);
/* input arrow path */ drawPath(parentGfx, arrowPathData, { strokeWidth: 1 });
return elementObject;
},
'bpmn:DataOutput': function(parentGfx, element) {
var arrowPathData = pathMap.getRawPath('DATA_ARROW');
// page
var elementObject = renderer('bpmn:DataObject')(parentGfx, element);
/* output arrow path */ drawPath(parentGfx, arrowPathData, {
strokeWidth: 1,
fill: 'black'
});
return elementObject;
},
'bpmn:DataStoreReference': function(parentGfx, element) {
var DATA_STORE_PATH = pathMap.getScaledPath('DATA_STORE', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0,
my: 0.133
}
});
var elementStore = drawPath(parentGfx, DATA_STORE_PATH, {
strokeWidth: 2,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
return elementStore;
},
'bpmn:BoundaryEvent': function(parentGfx, element) {
var semantic = getSemantic(element),
cancel = semantic.cancelActivity;
var attrs = {
strokeWidth: 1,
fill: getFillColor(element),
stroke: getStrokeColor(element)
};
if (!cancel) {
attrs.strokeDasharray = '6';
attrs.strokeLinecap = 'round';
}
var outer = renderer('bpmn:Event')(parentGfx, element, attrs);
/* inner path */ drawCircle(parentGfx, element.width, element.height, INNER_OUTER_DIST, assign(attrs, { fill: 'none' }));
renderEventContent(element, parentGfx);
return outer;
},
'bpmn:Group': function(parentGfx, element) {
return drawRect(parentGfx, element.width, element.height, TASK_BORDER_RADIUS, {
strokeWidth: 1,
strokeDasharray: '8,3,1,3',
fill: 'none',
pointerEvents: 'none'
});
},
'label': function(parentGfx, element) {
return renderExternalLabel(parentGfx, element);
},
'bpmn:TextAnnotation': function(parentGfx, element) {
var style = {
'fill': 'none',
'stroke': 'none'
};
var textElement = drawRect(parentGfx, element.width, element.height, 0, 0, style);
var textPathData = pathMap.getScaledPath('TEXT_ANNOTATION', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.0,
my: 0.0
}
});
drawPath(parentGfx, textPathData, {
stroke: getStrokeColor(element)
});
var text = getSemantic(element).text || '';
renderLabel(parentGfx, text, { box: element, align: 'left-middle', padding: 5 });
return textElement;
},
'ParticipantMultiplicityMarker': function(parentGfx, element) {
var markerPath = pathMap.getScaledPath('MARKER_PARALLEL', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: ((element.width / 2) / element.width),
my: (element.height - 15) / element.height
}
});
drawMarker('participant-multiplicity', parentGfx, markerPath);
},
'SubProcessMarker': function(parentGfx, element) {
var markerRect = drawRect(parentGfx, 14, 14, 0, {
strokeWidth: 1,
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
// Process marker is placed in the middle of the box
// therefore fixed values can be used here
translate(markerRect, element.width / 2 - 7.5, element.height - 20);
var markerPath = pathMap.getScaledPath('MARKER_SUB_PROCESS', {
xScaleFactor: 1.5,
yScaleFactor: 1.5,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: (element.width / 2 - 7.5) / element.width,
my: (element.height - 20) / element.height
}
});
drawMarker('sub-process', parentGfx, markerPath, {
fill: getFillColor(element),
stroke: getStrokeColor(element)
});
},
'ParallelMarker': function(parentGfx, element, position) {
var markerPath = pathMap.getScaledPath('MARKER_PARALLEL', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: ((element.width / 2 + position.parallel) / element.width),
my: (element.height - 20) / element.height
}
});
drawMarker('parallel', parentGfx, markerPath);
},
'SequentialMarker': function(parentGfx, element, position) {
var markerPath = pathMap.getScaledPath('MARKER_SEQUENTIAL', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: ((element.width / 2 + position.seq) / element.width),
my: (element.height - 19) / element.height
}
});
drawMarker('sequential', parentGfx, markerPath);
},
'CompensationMarker': function(parentGfx, element, position) {
var markerMath = pathMap.getScaledPath('MARKER_COMPENSATION', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: ((element.width / 2 + position.compensation) / element.width),
my: (element.height - 13) / element.height
}
});
drawMarker('compensation', parentGfx, markerMath, { strokeWidth: 1 });
},
'LoopMarker': function(parentGfx, element, position) {
var markerPath = pathMap.getScaledPath('MARKER_LOOP', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: ((element.width / 2 + position.loop) / element.width),
my: (element.height - 7) / element.height
}
});
drawMarker('loop', parentGfx, markerPath, {
strokeWidth: 1,
fill: 'none',
strokeLinecap: 'round',
strokeMiterlimit: 0.5
});
},
'AdhocMarker': function(parentGfx, element, position) {
var markerPath = pathMap.getScaledPath('MARKER_ADHOC', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: ((element.width / 2 + position.adhoc) / element.width),
my: (element.height - 15) / element.height
}
});
drawMarker('adhoc', parentGfx, markerPath, {
strokeWidth: 1,
fill: 'black'
});
}
};
function attachTaskMarkers(parentGfx, element, taskMarkers) {
var obj = getSemantic(element);
var subprocess = taskMarkers && taskMarkers.indexOf('SubProcessMarker') !== -1;
var position;
if (subprocess) {
position = {
seq: -21,
parallel: -22,
compensation: -42,
loop: -18,
adhoc: 10
};
} else {
position = {
seq: -3,
parallel: -6,
compensation: -27,
loop: 0,
adhoc: 10
};
}
forEach(taskMarkers, function(marker) {
renderer(marker)(parentGfx, element, position);
});
if (obj.isForCompensation) {
renderer('CompensationMarker')(parentGfx, element, position);
}
if (obj.$type === 'bpmn:AdHocSubProcess') {
renderer('AdhocMarker')(parentGfx, element, position);
}
var loopCharacteristics = obj.loopCharacteristics,
isSequential = loopCharacteristics && loopCharacteristics.isSequential;
if (loopCharacteristics) {
if (isSequential === undefined) {
renderer('LoopMarker')(parentGfx, element, position);
}
if (isSequential === false) {
renderer('ParallelMarker')(parentGfx, element, position);
}
if (isSequential === true) {
renderer('SequentialMarker')(parentGfx, element, position);
}
}
}
function renderDataItemCollection(parentGfx, element) {
var yPosition = (element.height - 16) / element.height;
var pathData = pathMap.getScaledPath('DATA_OBJECT_COLLECTION_PATH', {
xScaleFactor: 1,
yScaleFactor: 1,
containerWidth: element.width,
containerHeight: element.height,
position: {
mx: 0.451,
my: yPosition
}
});
/* collection path */ drawPath(parentGfx, pathData, {
strokeWidth: 2
});
}
}
inherits(BpmnRenderer, BaseRenderer);
BpmnRenderer.$inject = [ 'eventBus', 'styles', 'pathMap', 'canvas' ];
module.exports = BpmnRenderer;
BpmnRenderer.prototype.canRender = function(element) {
return is(element, 'bpmn:BaseElement');
};
BpmnRenderer.prototype.drawShape = function(parentGfx, element) {
var type = element.type;
var h = this.handlers[type];
/* jshint -W040 */
return h(parentGfx, element);
};
BpmnRenderer.prototype.drawConnection = function(parentGfx, element) {
var type = element.type;
var h = this.handlers[type];
/* jshint -W040 */
return h(parentGfx, element);
};
BpmnRenderer.prototype.getShapePath = function(element) {
if (is(element, 'bpmn:Event')) {
return getCirclePath(element);
}
if (is(element, 'bpmn:Activity')) {
return getRoundRectPath(element, TASK_BORDER_RADIUS);
}
if (is(element, 'bpmn:Gateway')) {
return getDiamondPath(element);
}
return getRectPath(element);
};
///////// helper functions /////////////////////////////
/**
* Checks if eventDefinition of the given element matches with semantic type.
*
* @return {boolean} true if element is of the given semantic type
*/
function isTypedEvent(event, eventDefinitionType, filter) {
function matches(definition, filter) {
return every(filter, function(val, key) {
// we want a == conversion here, to be able to catch
// undefined == false and friends
/* jshint -W116 */
return definition[key] == val;
});
}
return some(event.eventDefinitions, function(definition) {
return definition.$type === eventDefinitionType && matches(event, filter);
});
}
function isThrowEvent(event) {
return (event.$type === 'bpmn:IntermediateThrowEvent') || (event.$type === 'bpmn:EndEvent');
}
function isCollection(element) {
var dataObject = element.dataObjectRef;
return element.isCollection || (dataObject && dataObject.isCollection);
}
function getDi(element) {
return element.businessObject.di;
}
function getSemantic(element) {
return element.businessObject;
}
/////// cropping path customizations /////////////////////////
function getCirclePath(shape) {
var cx = shape.x + shape.width / 2,
cy = shape.y + shape.height / 2,
radius = shape.width / 2;
var circlePath = [
['M', cx, cy],
['m', 0, -radius],
['a', radius, radius, 0, 1, 1, 0, 2 * radius],
['a', radius, radius, 0, 1, 1, 0, -2 * radius],
['z']
];
return componentsToPath(circlePath);
}
function getRoundRectPath(shape, borderRadius) {
var x = shape.x,
y = shape.y,
width = shape.width,
height = shape.height;
var roundRectPath = [
['M', x + borderRadius, y],
['l', width - borderRadius * 2, 0],
['a', borderRadius, borderRadius, 0, 0, 1, borderRadius, borderRadius],
['l', 0, height - borderRadius * 2],
['a', borderRadius, borderRadius, 0, 0, 1, -borderRadius, borderRadius],
['l', borderRadius * 2 - width, 0],
['a', borderRadius, borderRadius, 0, 0, 1, -borderRadius, -borderRadius],
['l', 0, borderRadius * 2 - height],
['a', borderRadius, borderRadius, 0, 0, 1, borderRadius, -borderRadius],
['z']
];
return componentsToPath(roundRectPath);
}
function getDiamondPath(shape) {
var width = shape.width,
height = shape.height,
x = shape.x,
y = shape.y,
halfWidth = width / 2,
halfHeight = height / 2;
var diamondPath = [
['M', x + halfWidth, y],
['l', halfWidth, halfHeight],
['l', -halfWidth, halfHeight],
['l', -halfWidth, -halfHeight],
['z']
];
return componentsToPath(diamondPath);
}
function getRectPath(shape) {
var x = shape.x,
y = shape.y,
width = shape.width,
height = shape.height;
var rectPath = [
['M', x, y],
['l', width, 0],
['l', 0, height],
['l', -width, 0],
['z']
];
return componentsToPath(rectPath);
}
function getFillColor(element, defaultColor) {
var bo = getBusinessObject(element);
return bo.di.get('fill') || defaultColor || 'white';
}
function getStrokeColor(element, defaultColor) {
var bo = getBusinessObject(element);
return bo.di.get('stroke') || defaultColor || 'black';
}
},{"112":112,"115":115,"12":12,"126":126,"14":14,"145":145,"147":147,"148":148,"150":150,"165":165,"197":197,"198":198,"199":199,"30":30,"32":32,"35":35,"38":38}],5:[function(_dereq_,module,exports){
'use strict';
/**
* Map containing SVG paths needed by BpmnRenderer.
*/
function PathMap() {
/**
* Contains a map of path elements
*
* Path definition
* A parameterized path is defined like this:
*
* 'GATEWAY_PARALLEL': {
* d: 'm {mx},{my} {e.x0},0 0,{e.x1} {e.x1},0 0,{e.y0} -{e.x1},0 0,{e.y1} ' +
'-{e.x0},0 0,-{e.y1} -{e.x1},0 0,-{e.y0} {e.x1},0 z',
* height: 17.5,
* width: 17.5,
* heightElements: [2.5, 7.5],
* widthElements: [2.5, 7.5]
* }
*
* It's important to specify a correct height and width for the path as the scaling
* is based on the ratio between the specified height and width in this object and the
* height and width that is set as scale target (Note x,y coordinates will be scaled with
* individual ratios).
* The 'heightElements' and 'widthElements' array must contain the values that will be scaled.
* The scaling is based on the computed ratios.
* Coordinates on the y axis should be in the heightElement's array, they will be scaled using
* the computed ratio coefficient.
* In the parameterized path the scaled values can be accessed through the 'e' object in {} brackets.
*
* - The values for the y axis can be accessed in the path string using {e.y0}, {e.y1}, ....
* - The values for the x axis can be accessed in the path string using {e.x0}, {e.x1}, ....
*
* The numbers x0, x1 respectively y0, y1, ... map to the corresponding array index.
*
*/
this.pathMap = {
'EVENT_MESSAGE': {
d: 'm {mx},{my} l 0,{e.y1} l {e.x1},0 l 0,-{e.y1} z l {e.x0},{e.y0} l {e.x0},-{e.y0}',
height: 36,
width: 36,
heightElements: [6, 14],
widthElements: [10.5, 21]
},
'EVENT_SIGNAL': {
d: 'M {mx},{my} l {e.x0},{e.y0} l -{e.x1},0 Z',
height: 36,
width: 36,
heightElements: [18],
widthElements: [10, 20]
},
'EVENT_ESCALATION': {
d: 'M {mx},{my} l {e.x0},{e.y0} l -{e.x0},-{e.y1} l -{e.x0},{e.y1} Z',
height: 36,
width: 36,
heightElements: [20, 7],
widthElements: [8]
},
'EVENT_CONDITIONAL': {
d: 'M {e.x0},{e.y0} l {e.x1},0 l 0,{e.y2} l -{e.x1},0 Z ' +
'M {e.x2},{e.y3} l {e.x0},0 ' +
'M {e.x2},{e.y4} l {e.x0},0 ' +
'M {e.x2},{e.y5} l {e.x0},0 ' +
'M {e.x2},{e.y6} l {e.x0},0 ' +
'M {e.x2},{e.y7} l {e.x0},0 ' +
'M {e.x2},{e.y8} l {e.x0},0 ',
height: 36,
width: 36,
heightElements: [8.5, 14.5, 18, 11.5, 14.5, 17.5, 20.5, 23.5, 26.5],
widthElements: [10.5, 14.5, 12.5]
},
'EVENT_LINK': {
d: 'm {mx},{my} 0,{e.y0} -{e.x1},0 0,{e.y1} {e.x1},0 0,{e.y0} {e.x0},-{e.y2} -{e.x0},-{e.y2} z',
height: 36,
width: 36,
heightElements: [4.4375, 6.75, 7.8125],
widthElements: [9.84375, 13.5]
},
'EVENT_ERROR': {
d: 'm {mx},{my} {e.x0},-{e.y0} {e.x1},-{e.y1} {e.x2},{e.y2} {e.x3},-{e.y3} -{e.x4},{e.y4} -{e.x5},-{e.y5} z',
height: 36,
width: 36,
heightElements: [0.023, 8.737, 8.151, 16.564, 10.591, 8.714],
widthElements: [0.085, 6.672, 6.97, 4.273, 5.337, 6.636]
},
'EVENT_CANCEL_45': {
d: 'm {mx},{my} -{e.x1},0 0,{e.x0} {e.x1},0 0,{e.y1} {e.x0},0 ' +
'0,-{e.y1} {e.x1},0 0,-{e.y0} -{e.x1},0 0,-{e.y1} -{e.x0},0 z',
height: 36,
width: 36,
heightElements: [4.75, 8.5],
widthElements: [4.75, 8.5]
},
'EVENT_COMPENSATION': {
d: 'm {mx},{my} {e.x0},-{e.y0} 0,{e.y1} z m {e.x1},-{e.y2} {e.x2},-{e.y3} 0,{e.y1} -{e.x2},-{e.y3} z',
height: 36,
width: 36,
heightElements: [6.5, 13, 0.4, 6.1],
widthElements: [9, 9.3, 8.7]
},
'EVENT_TIMER_WH': {
d: 'M {mx},{my} l {e.x0},-{e.y0} m -{e.x0},{e.y0} l {e.x1},{e.y1} ',
height: 36,
width: 36,
heightElements: [10, 2],
widthElements: [3, 7]
},
'EVENT_TIMER_LINE': {
d: 'M {mx},{my} ' +
'm {e.x0},{e.y0} l -{e.x1},{e.y1} ',
height: 36,
width: 36,
heightElements: [10, 3],
widthElements: [0, 0]
},
'EVENT_MULTIPLE': {
d:'m {mx},{my} {e.x1},-{e.y0} {e.x1},{e.y0} -{e.x0},{e.y1} -{e.x2},0 z',
height: 36,
width: 36,
heightElements: [6.28099, 12.56199],
widthElements: [3.1405, 9.42149, 12.56198]
},
'EVENT_PARALLEL_MULTIPLE': {
d:'m {mx},{my} {e.x0},0 0,{e.y1} {e.x1},0 0,{e.y0} -{e.x1},0 0,{e.y1} ' +
'-{e.x0},0 0,-{e.y1} -{e.x1},0 0,-{e.y0} {e.x1},0 z',
height: 36,
width: 36,
heightElements: [2.56228, 7.68683],
widthElements: [2.56228, 7.68683]
},
'GATEWAY_EXCLUSIVE': {
d:'m {mx},{my} {e.x0},{e.y0} {e.x1},{e.y0} {e.x2},0 {e.x4},{e.y2} ' +
'{e.x4},{e.y1} {e.x2},0 {e.x1},{e.y3} {e.x0},{e.y3} ' +
'{e.x3},0 {e.x5},{e.y1} {e.x5},{e.y2} {e.x3},0 z',
height: 17.5,
width: 17.5,
heightElements: [8.5, 6.5312, -6.5312, -8.5],
widthElements: [6.5, -6.5, 3, -3, 5, -5]
},
'GATEWAY_PARALLEL': {
d:'m {mx},{my} 0,{e.y1} -{e.x1},0 0,{e.y0} {e.x1},0 0,{e.y1} {e.x0},0 ' +
'0,-{e.y1} {e.x1},0 0,-{e.y0} -{e.x1},0 0,-{e.y1} -{e.x0},0 z',
height: 30,
width: 30,
heightElements: [5, 12.5],
widthElements: [5, 12.5]
},
'GATEWAY_EVENT_BASED': {
d:'m {mx},{my} {e.x0},{e.y0} {e.x0},{e.y1} {e.x1},{e.y2} {e.x2},0 z',
height: 11,
width: 11,
heightElements: [-6, 6, 12, -12],
widthElements: [9, -3, -12]
},
'GATEWAY_COMPLEX': {
d:'m {mx},{my} 0,{e.y0} -{e.x0},-{e.y1} -{e.x1},{e.y2} {e.x0},{e.y1} -{e.x2},0 0,{e.y3} ' +
'{e.x2},0 -{e.x0},{e.y1} l {e.x1},{e.y2} {e.x0},-{e.y1} 0,{e.y0} {e.x3},0 0,-{e.y0} {e.x0},{e.y1} ' +
'{e.x1},-{e.y2} -{e.x0},-{e.y1} {e.x2},0 0,-{e.y3} -{e.x2},0 {e.x0},-{e.y1} -{e.x1},-{e.y2} ' +
'-{e.x0},{e.y1} 0,-{e.y0} -{e.x3},0 z',
height: 17.125,
width: 17.125,
heightElements: [4.875, 3.4375, 2.125, 3],
widthElements: [3.4375, 2.125, 4.875, 3]
},
'DATA_OBJECT_PATH': {
d:'m 0,0 {e.x1},0 {e.x0},{e.y0} 0,{e.y1} -{e.x2},0 0,-{e.y2} {e.x1},0 0,{e.y0} {e.x0},0',
height: 61,
width: 51,
heightElements: [10, 50, 60],
widthElements: [10, 40, 50, 60]
},
'DATA_OBJECT_COLLECTION_PATH': {
d:'m {mx}, {my} ' +
'm 0 15 l 0 -15 ' +
'm 4 15 l 0 -15 ' +
'm 4 15 l 0 -15 ',
height: 61,
width: 51,
heightElements: [12],
widthElements: [1, 6, 12, 15]
},
'DATA_ARROW': {
d:'m 5,9 9,0 0,-3 5,5 -5,5 0,-3 -9,0 z',
height: 61,
width: 51,
heightElements: [],
widthElements: []
},
'DATA_STORE': {
d:'m {mx},{my} ' +
'l 0,{e.y2} ' +
'c {e.x0},{e.y1} {e.x1},{e.y1} {e.x2},0 ' +
'l 0,-{e.y2} ' +
'c -{e.x0},-{e.y1} -{e.x1},-{e.y1} -{e.x2},0' +
'c {e.x0},{e.y1} {e.x1},{e.y1} {e.x2},0 ' +
'm -{e.x2},{e.y0}' +
'c {e.x0},{e.y1} {e.x1},{e.y1} {e.x2},0' +
'm -{e.x2},{e.y0}' +
'c {e.x0},{e.y1} {e.x1},{e.y1} {e.x2},0',
height: 61,
width: 61,
heightElements: [7, 10, 45],
widthElements: [2, 58, 60]
},
'TEXT_ANNOTATION': {
d: 'm {mx}, {my} m 10,0 l -10,0 l 0,{e.y0} l 10,0',
height: 30,
width: 10,
heightElements: [30],
widthElements: [10]
},
'MARKER_SUB_PROCESS': {
d: 'm{mx},{my} m 7,2 l 0,10 m -5,-5 l 10,0',
height: 10,
width: 10,
heightElements: [],
widthElements: []
},
'MARKER_PARALLEL': {
d: 'm{mx},{my} m 3,2 l 0,10 m 3,-10 l 0,10 m 3,-10 l 0,10',
height: 10,
width: 10,
heightElements: [],
widthElements: []
},
'MARKER_SEQUENTIAL': {
d: 'm{mx},{my} m 0,3 l 10,0 m -10,3 l 10,0 m -10,3 l 10,0',
height: 10,
width: 10,
heightElements: [],
widthElements: []
},
'MARKER_COMPENSATION': {
d: 'm {mx},{my} 7,-5 0,10 z m 7.1,-0.3 6.9,-4.7 0,10 -6.9,-4.7 z',
height: 10,
width: 21,
heightElements: [],
widthElements: []
},
'MARKER_LOOP': {
d: 'm {mx},{my} c 3.526979,0 6.386161,-2.829858 6.386161,-6.320661 0,-3.490806 -2.859182,-6.320661 ' +
'-6.386161,-6.320661 -3.526978,0 -6.38616,2.829855 -6.38616,6.320661 0,1.745402 ' +
'0.714797,3.325567 1.870463,4.469381 0.577834,0.571908 1.265885,1.034728 2.029916,1.35457 ' +
'l -0.718163,-3.909793 m 0.718163,3.909793 -3.885211,0.802902',
height: 13.9,
width: 13.7,
heightElements: [],
widthElements: []
},
'MARKER_ADHOC': {
d: 'm {mx},{my} m 0.84461,2.64411 c 1.05533,-1.23780996 2.64337,-2.07882 4.29653,-1.97997996 2.05163,0.0805 ' +
'3.85579,1.15803 5.76082,1.79107 1.06385,0.34139996 2.24454,0.1438 3.18759,-0.43767 0.61743,-0.33642 ' +
'1.2775,-0.64078 1.7542,-1.17511 0,0.56023 0,1.12046 0,1.6807 -0.98706,0.96237996 -2.29792,1.62393996 ' +
'-3.6918,1.66181996 -1.24459,0.0927 -2.46671,-0.2491 -3.59505,-0.74812 -1.35789,-0.55965 ' +
'-2.75133,-1.33436996 -4.27027,-1.18121996 -1.37741,0.14601 -2.41842,1.13685996 -3.44288,1.96782996 z',
height: 4,
width: 15,
heightElements: [],
widthElements: []
},
'TASK_TYPE_SEND': {
d: 'm {mx},{my} l 0,{e.y1} l {e.x1},0 l 0,-{e.y1} z l {e.x0},{e.y0} l {e.x0},-{e.y0}',
height: 14,
width: 21,
heightElements: [6, 14],
widthElements: [10.5, 21]
},
'TASK_TYPE_SCRIPT': {
d: 'm {mx},{my} c 9.966553,-6.27276 -8.000926,-7.91932 2.968968,-14.938 l -8.802728,0 ' +
'c -10.969894,7.01868 6.997585,8.66524 -2.968967,14.938 z ' +
'm -7,-12 l 5,0 ' +
'm -4.5,3 l 4.5,0 ' +
'm -3,3 l 5,0' +
'm -4,3 l 5,0',
height: 15,
width: 12.6,
heightElements: [6, 14],
widthElements: [10.5, 21]
},
'TASK_TYPE_USER_1': {
d: 'm {mx},{my} c 0.909,-0.845 1.594,-2.049 1.594,-3.385 0,-2.554 -1.805,-4.62199999 ' +
'-4.357,-4.62199999 -2.55199998,0 -4.28799998,2.06799999 -4.28799998,4.62199999 0,1.348 ' +
'0.974,2.562 1.89599998,3.405 -0.52899998,0.187 -5.669,2.097 -5.794,4.7560005 v 6.718 ' +
'h 17 v -6.718 c 0,-2.2980005 -5.5279996,-4.5950005 -6.0509996,-4.7760005 z' +
'm -8,6 l 0,5.5 m 11,0 l 0,-5'
},
'TASK_TYPE_USER_2': {
d: 'm {mx},{my} m 2.162,1.009 c 0,2.4470005 -2.158,4.4310005 -4.821,4.4310005 ' +
'-2.66499998,0 -4.822,-1.981 -4.822,-4.4310005 '
},
'TASK_TYPE_USER_3': {
d: 'm {mx},{my} m -6.9,-3.80 c 0,0 2.25099998,-2.358 4.27399998,-1.177 2.024,1.181 4.221,1.537 ' +
'4.124,0.965 -0.098,-0.57 -0.117,-3.79099999 -4.191,-4.13599999 -3.57499998,0.001 ' +
'-4.20799998,3.36699999 -4.20699998,4.34799999 z'
},
'TASK_TYPE_MANUAL': {
d: 'm {mx},{my} c 0.234,-0.01 5.604,0.008 8.029,0.004 0.808,0 1.271,-0.172 1.417,-0.752 0.227,-0.898 ' +
'-0.334,-1.314 -1.338,-1.316 -2.467,-0.01 -7.886,-0.004 -8.108,-0.004 -0.014,-0.079 0.016,-0.533 0,-0.61 ' +
'0.195,-0.042 8.507,0.006 9.616,0.002 0.877,-0.007 1.35,-0.438 1.353,-1.208 0.003,-0.768 -0.479,-1.09 ' +
'-1.35,-1.091 -2.968,-0.002 -9.619,-0.013 -9.619,-0.013 v -0.591 c 0,0 5.052,-0.016 7.225,-0.016 ' +
'0.888,-0.002 1.354,-0.416 1.351,-1.193 -0.006,-0.761 -0.492,-1.196 -1.361,-1.196 -3.473,-0.005 ' +
'-10.86,-0.003 -11.0829995,-0.003 -0.022,-0.047 -0.045,-0.094 -0.069,-0.139 0.3939995,-0.319 ' +
'2.0409995,-1.626 2.4149995,-2.017 0.469,-0.4870005 0.519,-1.1650005 0.162,-1.6040005 -0.414,-0.511 ' +
'-0.973,-0.5 -1.48,-0.236 -1.4609995,0.764 -6.5999995,3.6430005 -7.7329995,4.2710005 -0.9,0.499 ' +
'-1.516,1.253 -1.882,2.19 -0.37000002,0.95 -0.17,2.01 -0.166,2.979 0.004,0.718 -0.27300002,1.345 ' +
'-0.055,2.063 0.629,2.087 2.425,3.312 4.859,3.318 4.6179995,0.014 9.2379995,-0.139 13.8569995,-0.158 ' +
'0.755,-0.004 1.171,-0.301 1.182,-1.033 0.012,-0.754 -0.423,-0.969 -1.183,-0.973 -1.778,-0.01 ' +
'-5.824,-0.004 -6.04,-0.004 10e-4,-0.084 0.003,-0.586 10e-4,-0.67 z'
},
'TASK_TYPE_INSTANTIATING_SEND': {
d: 'm {mx},{my} l 0,8.4 l 12.6,0 l 0,-8.4 z l 6.3,3.6 l 6.3,-3.6'
},
'TASK_TYPE_SERVICE': {
d: 'm {mx},{my} v -1.71335 c 0.352326,-0.0705 0.703932,-0.17838 1.047628,-0.32133 ' +
'0.344416,-0.14465 0.665822,-0.32133 0.966377,-0.52145 l 1.19431,1.18005 1.567487,-1.57688 ' +
'-1.195028,-1.18014 c 0.403376,-0.61394 0.683079,-1.29908 0.825447,-2.01824 l 1.622133,-0.01 ' +
'v -2.2196 l -1.636514,0.01 c -0.07333,-0.35153 -0.178319,-0.70024 -0.323564,-1.04372 ' +
'-0.145244,-0.34406 -0.321407,-0.6644 -0.522735,-0.96217 l 1.131035,-1.13631 -1.583305,-1.56293 ' +
'-1.129598,1.13589 c -0.614052,-0.40108 -1.302883,-0.68093 -2.022633,-0.82247 l 0.0093,-1.61852 ' +
'h -2.241173 l 0.0042,1.63124 c -0.353763,0.0736 -0.705369,0.17977 -1.049785,0.32371 -0.344415,0.14437 ' +
'-0.665102,0.32092 -0.9635006,0.52046 l -1.1698628,-1.15823 -1.5667691,1.5792 1.1684265,1.15669 ' +
'c -0.4026573,0.61283 -0.68308,1.29797 -0.8247287,2.01713 l -1.6588041,0.003 v 2.22174 ' +
'l 1.6724648,-0.006 c 0.073327,0.35077 0.1797598,0.70243 0.3242851,1.04472 0.1452428,0.34448 ' +
'0.3214064,0.6644 0.5227339,0.96066 l -1.1993431,1.19723 1.5840256,1.56011 1.1964668,-1.19348 ' +
'c 0.6140517,0.40346 1.3028827,0.68232 2.0233517,0.82331 l 7.19e-4,1.69892 h 2.226848 z ' +
'm 0.221462,-3.9957 c -1.788948,0.7502 -3.8576,-0.0928 -4.6097055,-1.87438 -0.7521065,-1.78321 ' +
'0.090598,-3.84627 1.8802645,-4.59604 1.78823,-0.74936 3.856881,0.0929 4.608987,1.87437 ' +
'0.752106,1.78165 -0.0906,3.84612 -1.879546,4.59605 z'
},
'TASK_TYPE_SERVICE_FILL': {
d: 'm {mx},{my} c -1.788948,0.7502 -3.8576,-0.0928 -4.6097055,-1.87438 -0.7521065,-1.78321 ' +
'0.090598,-3.84627 1.8802645,-4.59604 1.78823,-0.74936 3.856881,0.0929 4.608987,1.87437 ' +
'0.752106,1.78165 -0.0906,3.84612 -1.879546,4.59605 z'
},
'TASK_TYPE_BUSINESS_RULE_HEADER': {
d: 'm {mx},{my} 0,4 20,0 0,-4 z'
},
'TASK_TYPE_BUSINESS_RULE_MAIN': {
d: 'm {mx},{my} 0,12 20,0 0,-12 z' +
'm 0,8 l 20,0 ' +
'm -13,-4 l 0,8'
},
'MESSAGE_FLOW_MARKER': {
d: 'm {mx},{my} m -10.5 ,-7 l 0,14 l 21,0 l 0,-14 z l 10.5,6 l 10.5,-6'
}
};
this.getRawPath = function getRawPath(pathId) {
return this.pathMap[pathId].d;
};
/**
* Scales the path to the given height and width.
* Use case
* Use case is to scale the content of elements (event, gateways) based
* on the element bounding box's size.
*
* Why not transform
* Scaling a path with transform() will also scale the stroke and IE does not support
* the option 'non-scaling-stroke' to prevent this.
* Also there are use cases where only some parts of a path should be
* scaled.
*
* @param {String} pathId The ID of the path.
* @param {Object} param
* Example param object scales the path to 60% size of the container (data.width, data.height).
*
* {
* xScaleFactor: 0.6,
* yScaleFactor:0.6,
* containerWidth: data.width,
* containerHeight: data.height,
* position: {
* mx: 0.46,
* my: 0.2,
* }
* }
*
*
* - targetpathwidth = xScaleFactor * containerWidth
* - targetpathheight = yScaleFactor * containerHeight
* - Position is used to set the starting coordinate of the path. M is computed:
*
* - position.x * containerWidth
* - position.y * containerHeight
*
* Center of the container position: {
* mx: 0.5,
* my: 0.5,
* }
* Upper left corner of the container
* position: {
* mx: 0.0,
* my: 0.0,
* }
*
*
*
*
*/
this.getScaledPath = function getScaledPath(pathId, param) {
var rawPath = this.pathMap[pathId];
// positioning
// compute the start point of the path
var mx, my;
if (param.abspos) {
mx = param.abspos.x;
my = param.abspos.y;
} else {
mx = param.containerWidth * param.position.mx;
my = param.containerHeight * param.position.my;
}
var coordinates = {}; //map for the scaled coordinates
if (param.position) {
// path
var heightRatio = (param.containerHeight / rawPath.height) * param.yScaleFactor;
var widthRatio = (param.containerWidth / rawPath.width) * param.xScaleFactor;
//Apply height ratio
for (var heightIndex = 0; heightIndex < rawPath.heightElements.length; heightIndex++) {
coordinates['y' + heightIndex] = rawPath.heightElements[heightIndex] * heightRatio;
}
//Apply width ratio
for (var widthIndex = 0; widthIndex < rawPath.widthElements.length; widthIndex++) {
coordinates['x' + widthIndex] = rawPath.widthElements[widthIndex] * widthRatio;
}
}
//Apply value to raw path
var path = format(
rawPath.d, {
mx: mx,
my: my,
e: coordinates
}
);
return path;
};
}
module.exports = PathMap;
////////// helpers //////////
// copied from https://github.com/adobe-webplatform/Snap.svg/blob/master/src/svg.js
var tokenRegex = /\{([^\}]+)\}/g,
objNotationRegex = /(?:(?:^|\.)(.+?)(?=\[|\.|$|\()|\[('|")(.+?)\2\])(\(\))?/g; // matches .xxxxx or ["xxxxx"] to run over object properties
function replacer(all, key, obj) {
var res = obj;
key.replace(objNotationRegex, function(all, name, quote, quotedName, isFunc) {
name = name || quotedName;
if (res) {
if (name in res) {
res = res[name];
}
typeof res == 'function' && isFunc && (res = res());
}
});
res = (res == null || res == obj ? all : res) + '';
return res;
}
function format(str, obj) {
return String(str).replace(tokenRegex, function(all, key) {
return replacer(all, key, obj);
});
}
},{}],6:[function(_dereq_,module,exports){
module.exports = {
__init__: [ 'bpmnRenderer' ],
bpmnRenderer: [ 'type', _dereq_(4) ],
pathMap: [ 'type', _dereq_(5) ]
};
},{"4":4,"5":5}],7:[function(_dereq_,module,exports){
'use strict';
var assign = _dereq_(115),
map = _dereq_(36);
var LabelUtil = _dereq_(13);
var TextUtil = _dereq_(199);
var is = _dereq_(14).is;
var hasExternalLabel = LabelUtil.hasExternalLabel,
getExternalLabelBounds = LabelUtil.getExternalLabelBounds,
isExpanded = _dereq_(12).isExpanded,
elementToString = _dereq_(10).elementToString;
function elementData(semantic, attrs) {
return assign({
id: semantic.id,
type: semantic.$type,
businessObject: semantic
}, attrs);
}
function collectWaypoints(waypoints) {
return map(waypoints, function(p) {
return { x: p.x, y: p.y };
});
}
function notYetDrawn(translate, semantic, refSemantic, property) {
return new Error(translate('element {element} referenced by {referenced}#{property} not yet drawn', {
element: elementToString(refSemantic),
referenced: elementToString(semantic),
property: property
}));
}
/**
* An importer that adds bpmn elements to the canvas
*
* @param {EventBus} eventBus
* @param {Canvas} canvas
* @param {ElementFactory} elementFactory
* @param {ElementRegistry} elementRegistry
*/
function BpmnImporter(eventBus, canvas, elementFactory, elementRegistry, translate) {
this._eventBus = eventBus;
this._canvas = canvas;
this._elementFactory = elementFactory;
this._elementRegistry = elementRegistry;
this._translate = translate;
this._textUtil = new TextUtil();
}
BpmnImporter.$inject = [ 'eventBus', 'canvas', 'elementFactory', 'elementRegistry', 'translate' ];
module.exports = BpmnImporter;
/**
* Add bpmn element (semantic) to the canvas onto the
* specified parent shape.
*/
BpmnImporter.prototype.add = function(semantic, parentElement) {
var di = semantic.di,
element,
translate = this._translate,
hidden;
// ROOT ELEMENT
// handle the special case that we deal with a
// invisible root element (process or collaboration)
if (is(di, 'bpmndi:BPMNPlane')) {
// add a virtual element (not being drawn)
element = this._elementFactory.createRoot(elementData(semantic));
this._canvas.setRootElement(element);
}
// SHAPE
else if (is(di, 'bpmndi:BPMNShape')) {
var collapsed = !isExpanded(semantic);
hidden = parentElement && (parentElement.hidden || parentElement.collapsed);
var bounds = semantic.di.bounds;
element = this._elementFactory.createShape(elementData(semantic, {
collapsed: collapsed,
hidden: hidden,
x: Math.round(bounds.x),
y: Math.round(bounds.y),
width: Math.round(bounds.width),
height: Math.round(bounds.height)
}));
if (is(semantic, 'bpmn:BoundaryEvent')) {
this._attachBoundary(semantic, element);
}
this._canvas.addShape(element, parentElement);
}
// CONNECTION
else if (is(di, 'bpmndi:BPMNEdge')) {
var source = this._getSource(semantic),
target = this._getTarget(semantic);
hidden = parentElement && (parentElement.hidden || parentElement.collapsed);
element = this._elementFactory.createConnection(elementData(semantic, {
hidden: hidden,
source: source,
target: target,
waypoints: collectWaypoints(semantic.di.waypoint)
}));
if (is(semantic, 'bpmn:DataAssociation')) {
// render always on top; this ensures DataAssociations
// are rendered correctly across different "hacks" people
// love to model such as cross participant / sub process
// associations
parentElement = null;
}
this._canvas.addConnection(element, parentElement);
} else {
throw new Error(translate('unknown di {di} for element {semantic}', {
di: elementToString(di),
semantic: elementToString(semantic)
}));
}
// (optional) LABEL
if (hasExternalLabel(semantic)) {
this.addLabel(semantic, element);
}
this._eventBus.fire('bpmnElement.added', { element: element });
return element;
};
/**
* Attach the boundary element to the given host
*
* @param {ModdleElement} boundarySemantic
* @param {djs.model.Base} boundaryElement
*/
BpmnImporter.prototype._attachBoundary = function(boundarySemantic, boundaryElement) {
var translate = this._translate;
var hostSemantic = boundarySemantic.attachedToRef;
if (!hostSemantic) {
throw new Error(translate('missing {semantic}#attachedToRef', {
semantic: elementToString(boundarySemantic)
}));
}
var host = this._elementRegistry.get(hostSemantic.id),
attachers = host && host.attachers;
if (!host) {
throw notYetDrawn(translate, boundarySemantic, hostSemantic, 'attachedToRef');
}
// wire element.host <> host.attachers
boundaryElement.host = host;
if (!attachers) {
host.attachers = attachers = [];
}
if (attachers.indexOf(boundaryElement) === -1) {
attachers.push(boundaryElement);
}
};
/**
* add label for an element
*/
BpmnImporter.prototype.addLabel = function(semantic, element) {
var bounds,
text,
label;
bounds = getExternalLabelBounds(semantic, element);
text = semantic.name;
if (text) {
// get corrected bounds from actual layouted text
bounds = getLayoutedBounds(bounds, text, this._textUtil);
}
label = this._elementFactory.createLabel(elementData(semantic, {
id: semantic.id + '_label',
labelTarget: element,
type: 'label',
hidden: element.hidden || !semantic.name,
x: Math.round(bounds.x),
y: Math.round(bounds.y),
width: Math.round(bounds.width),
height: Math.round(bounds.height)
}));
return this._canvas.addShape(label, element.parent);
};
/**
* Return the drawn connection end based on the given side.
*
* @throws {Error} if the end is not yet drawn
*/
BpmnImporter.prototype._getEnd = function(semantic, side) {
var element,
refSemantic,
type = semantic.$type,
translate = this._translate;
refSemantic = semantic[side + 'Ref'];
// handle mysterious isMany DataAssociation#sourceRef
if (side === 'source' && type === 'bpmn:DataInputAssociation') {
refSemantic = refSemantic && refSemantic[0];
}
// fix source / target for DataInputAssociation / DataOutputAssociation
if (side === 'source' && type === 'bpmn:DataOutputAssociation' ||
side === 'target' && type === 'bpmn:DataInputAssociation') {
refSemantic = semantic.$parent;
}
element = refSemantic && this._getElement(refSemantic);
if (element) {
return element;
}
if (refSemantic) {
throw notYetDrawn(translate, semantic, refSemantic, side + 'Ref');
} else {
throw new Error(translate('{semantic}#{side} Ref not specified', {
semantic: elementToString(semantic),
side: side
}));
}
};
BpmnImporter.prototype._getSource = function(semantic) {
return this._getEnd(semantic, 'source');
};
BpmnImporter.prototype._getTarget = function(semantic) {
return this._getEnd(semantic, 'target');
};
BpmnImporter.prototype._getElement = function(semantic) {
return this._elementRegistry.get(semantic.id);
};
// TODO(nikku): repeating code (search for )
var EXTERNAL_LABEL_STYLE = {
fontFamily: 'Arial, sans-serif',
fontSize: '11px'
};
function getLayoutedBounds(bounds, text, textUtil) {
var layoutedLabelDimensions = textUtil.getDimensions(text, {
box: {
width: 90,
height: 30,
x: bounds.width / 2 + bounds.x,
y: bounds.height / 2 + bounds.y
},
style: EXTERNAL_LABEL_STYLE
});
// resize label shape to fit label text
return {
x: Math.round(bounds.x + bounds.width / 2 - layoutedLabelDimensions.width / 2),
y: Math.round(bounds.y),
width: Math.ceil(layoutedLabelDimensions.width),
height: Math.ceil(layoutedLabelDimensions.height)
};
}
},{"10":10,"115":115,"12":12,"13":13,"14":14,"199":199,"36":36}],8:[function(_dereq_,module,exports){
'use strict';
var filter = _dereq_(33),
find = _dereq_(34),
forEach = _dereq_(35);
var Refs = _dereq_(140);
var elementToString = _dereq_(10).elementToString;
var diRefs = new Refs({ name: 'bpmnElement', enumerable: true }, { name: 'di' });
/**
* Returns true if an element has the given meta-model type
*
* @param {ModdleElement} element
* @param {String} type
*
* @return {Boolean}
*/
function is(element, type) {
return element.$instanceOf(type);
}
/**
* Find a suitable display candidate for definitions where the DI does not
* correctly specify one.
*/
function findDisplayCandidate(definitions) {
return find(definitions.rootElements, function(e) {
return is(e, 'bpmn:Process') || is(e, 'bpmn:Collaboration');
});
}
function BpmnTreeWalker(handler, translate) {
// list of containers already walked
var handledElements = {};
// list of elements to handle deferred to ensure
// prerequisites are drawn
var deferred = [];
///// Helpers /////////////////////////////////
function contextual(fn, ctx) {
return function(e) {
fn(e, ctx);
};
}
function handled(element) {
handledElements[element.id] = element;
}
function isHandled(element) {
return handledElements[element.id];
}
function visit(element, ctx) {
var gfx = element.gfx;
// avoid multiple rendering of elements
if (gfx) {
throw new Error(
translate('already rendered {element}', { element: elementToString(element) })
);
}
// call handler
return handler.element(element, ctx);
}
function visitRoot(element, diagram) {
return handler.root(element, diagram);
}
function visitIfDi(element, ctx) {
try {
var gfx = element.di && visit(element, ctx);
handled(element);
return gfx;
} catch (e) {
logError(e.message, { element: element, error: e });
console.error(translate('failed to import {element}', { element: elementToString(element) }));
console.error(e);
}
}
function logError(message, context) {
handler.error(message, context);
}
////// DI handling ////////////////////////////
function registerDi(di) {
var bpmnElement = di.bpmnElement;
if (bpmnElement) {
if (bpmnElement.di) {
logError(
translate('multiple DI elements defined for {element}', {
element: elementToString(bpmnElement)
}),
{ element: bpmnElement }
);
} else {
diRefs.bind(bpmnElement, 'di');
bpmnElement.di = di;
}
} else {
logError(
translate('no bpmnElement referenced in {element}', {
element: elementToString(di)
}),
{ element: di }
);
}
}
function handleDiagram(diagram) {
handlePlane(diagram.plane);
}
function handlePlane(plane) {
registerDi(plane);
forEach(plane.planeElement, handlePlaneElement);
}
function handlePlaneElement(planeElement) {
registerDi(planeElement);
}
////// Semantic handling //////////////////////
/**
* Handle definitions and return the rendered diagram (if any)
*
* @param {ModdleElement} definitions to walk and import
* @param {ModdleElement} [diagram] specific diagram to import and display
*
* @throws {Error} if no diagram to display could be found
*/
function handleDefinitions(definitions, diagram) {
// make sure we walk the correct bpmnElement
var diagrams = definitions.diagrams;
if (diagram && diagrams.indexOf(diagram) === -1) {
throw new Error(translate('diagram not part of bpmn:Definitions'));
}
if (!diagram && diagrams && diagrams.length) {
diagram = diagrams[0];
}
// no diagram -> nothing to import
if (!diagram) {
throw new Error(translate('no diagram to display'));
}
// load DI from selected diagram only
handleDiagram(diagram);
var plane = diagram.plane;
if (!plane) {
throw new Error(translate(
'no plane for {element}',
{ element: elementToString(diagram) }
));
}
var rootElement = plane.bpmnElement;
// ensure we default to a suitable display candidate (process or collaboration),
// even if non is specified in DI
if (!rootElement) {
rootElement = findDisplayCandidate(definitions);
if (!rootElement) {
throw new Error(translate('no process or collaboration to display'));
} else {
logError(
translate('correcting missing bpmnElement on {plane} to {rootElement}', {
plane: elementToString(plane),
rootElement: elementToString(rootElement)
})
);
// correct DI on the fly
plane.bpmnElement = rootElement;
registerDi(plane);
}
}
var ctx = visitRoot(rootElement, plane);
if (is(rootElement, 'bpmn:Process')) {
handleProcess(rootElement, ctx);
} else if (is(rootElement, 'bpmn:Collaboration')) {
handleCollaboration(rootElement, ctx);
// force drawing of everything not yet drawn that is part of the target DI
handleUnhandledProcesses(definitions.rootElements, ctx);
} else {
throw new Error(
translate('unsupported bpmnElement for {plane}: {rootElement}', {
plane: elementToString(plane),
rootElement: elementToString(rootElement)
})
);
}
// handle all deferred elements
handleDeferred(deferred);
}
function handleDeferred(deferred) {
forEach(deferred, function(d) { d(); });
}
function handleProcess(process, context) {
handleFlowElementsContainer(process, context);
handleIoSpecification(process.ioSpecification, context);
handleArtifacts(process.artifacts, context);
// log process handled
handled(process);
}
function handleUnhandledProcesses(rootElements) {
// walk through all processes that have not yet been drawn and draw them
// if they contain lanes with DI information.
// we do this to pass the free-floating lane test cases in the MIWG test suite
var processes = filter(rootElements, function(e) {
return !isHandled(e) && is(e, 'bpmn:Process') && e.laneSets;
});
processes.forEach(contextual(handleProcess));
}
function handleMessageFlow(messageFlow, context) {
visitIfDi(messageFlow, context);
}
function handleMessageFlows(messageFlows, context) {
forEach(messageFlows, contextual(handleMessageFlow, context));
}
function handleDataAssociation(association, context) {
visitIfDi(association, context);
}
function handleDataInput(dataInput, context) {
visitIfDi(dataInput, context);
}
function handleDataOutput(dataOutput, context) {
visitIfDi(dataOutput, context);
}
function handleArtifact(artifact, context) {
// bpmn:TextAnnotation
// bpmn:Group
// bpmn:Association
visitIfDi(artifact, context);
}
function handleArtifacts(artifacts, context) {
forEach(artifacts, function(e) {
if (is(e, 'bpmn:Association')) {
deferred.push(function() {
handleArtifact(e, context);
});
} else {
handleArtifact(e, context);
}
});
}
function handleIoSpecification(ioSpecification, context) {
if (!ioSpecification) {
return;
}
forEach(ioSpecification.dataInputs, contextual(handleDataInput, context));
forEach(ioSpecification.dataOutputs, contextual(handleDataOutput, context));
}
function handleSubProcess(subProcess, context) {
handleFlowElementsContainer(subProcess, context);
handleArtifacts(subProcess.artifacts, context);
}
function handleFlowNode(flowNode, context) {
var childCtx = visitIfDi(flowNode, context);
if (is(flowNode, 'bpmn:SubProcess')) {
handleSubProcess(flowNode, childCtx || context);
}
if (is(flowNode, 'bpmn:Activity')) {
handleIoSpecification(flowNode.ioSpecification, context);
}
// defer handling of associations
// affected types:
//
// * bpmn:Activity
// * bpmn:ThrowEvent
// * bpmn:CatchEvent
//
deferred.push(function() {
forEach(flowNode.dataInputAssociations, contextual(handleDataAssociation, context));
forEach(flowNode.dataOutputAssociations, contextual(handleDataAssociation, context));
});
}
function handleSequenceFlow(sequenceFlow, context) {
visitIfDi(sequenceFlow, context);
}
function handleDataElement(dataObject, context) {
visitIfDi(dataObject, context);
}
function handleBoundaryEvent(dataObject, context) {
visitIfDi(dataObject, context);
}
function handleLane(lane, context) {
var newContext = visitIfDi(lane, context);
if (lane.childLaneSet) {
handleLaneSet(lane.childLaneSet, newContext || context);
}
wireFlowNodeRefs(lane);
}
function handleLaneSet(laneSet, context) {
forEach(laneSet.lanes, contextual(handleLane, context));
}
function handleLaneSets(laneSets, context) {
forEach(laneSets, contextual(handleLaneSet, context));
}
function handleFlowElementsContainer(container, context) {
if (container.laneSets) {
handleLaneSets(container.laneSets, context);
}
handleFlowElements(container.flowElements, context);
}
function handleFlowElements(flowElements, context) {
forEach(flowElements, function(e) {
if (is(e, 'bpmn:SequenceFlow')) {
deferred.push(function() {
handleSequenceFlow(e, context);
});
} else if (is(e, 'bpmn:BoundaryEvent')) {
deferred.unshift(function() {
handleBoundaryEvent(e, context);
});
} else if (is(e, 'bpmn:FlowNode')) {
handleFlowNode(e, context);
} else if (is(e, 'bpmn:DataObject')) {
// SKIP (assume correct referencing via DataObjectReference)
} else if (is(e, 'bpmn:DataStoreReference')) {
handleDataElement(e, context);
} else if (is(e, 'bpmn:DataObjectReference')) {
handleDataElement(e, context);
} else {
logError(
translate('unrecognized flowElement {element} in context {context}', {
element: elementToString(e),
context: (context ? elementToString(context.businessObject) : 'null')
}),
{ element: e, context: context }
);
}
});
}
function handleParticipant(participant, context) {
var newCtx = visitIfDi(participant, context);
var process = participant.processRef;
if (process) {
handleProcess(process, newCtx || context);
}
}
function handleCollaboration(collaboration) {
forEach(collaboration.participants, contextual(handleParticipant));
handleArtifacts(collaboration.artifacts);
// handle message flows latest in the process
deferred.push(function() {
handleMessageFlows(collaboration.messageFlows);
});
}
function wireFlowNodeRefs(lane) {
// wire the virtual flowNodeRefs <-> relationship
forEach(lane.flowNodeRef, function(flowNode) {
var lanes = flowNode.get('lanes');
if (lanes) {
lanes.push(lane);
}
});
}
///// API ////////////////////////////////
return {
handleDefinitions: handleDefinitions
};
}
module.exports = BpmnTreeWalker;
},{"10":10,"140":140,"33":33,"34":34,"35":35}],9:[function(_dereq_,module,exports){
'use strict';
var BpmnTreeWalker = _dereq_(8);
/**
* Import the definitions into a diagram.
*
* Errors and warnings are reported through the specified callback.
*
* @param {Diagram} diagram
* @param {ModdleElement} definitions
* @param {Function} done the callback, invoked with (err, [ warning ]) once the import is done
*/
function importBpmnDiagram(diagram, definitions, done) {
var importer = diagram.get('bpmnImporter'),
eventBus = diagram.get('eventBus'),
translate = diagram.get('translate');
var error,
warnings = [];
/**
* Walk the diagram semantically, importing (=drawing)
* all elements you encounter.
*
* @param {ModdleElement} definitions
*/
function render(definitions) {
var visitor = {
root: function(element) {
return importer.add(element);
},
element: function(element, parentShape) {
return importer.add(element, parentShape);
},
error: function(message, context) {
warnings.push({ message: message, context: context });
}
};
var walker = new BpmnTreeWalker(visitor, translate);
// traverse BPMN 2.0 document model,
// starting at definitions
walker.handleDefinitions(definitions);
}
eventBus.fire('import.render.start', { definitions: definitions });
try {
render(definitions);
} catch (e) {
error = e;
}
eventBus.fire('import.render.complete', {
error: error,
warnings: warnings
});
done(error, warnings);
}
module.exports.importBpmnDiagram = importBpmnDiagram;
},{"8":8}],10:[function(_dereq_,module,exports){
'use strict';
module.exports.elementToString = function(e) {
if (!e) {
return '';
}
return '<' + e.$type + (e.id ? ' id="' + e.id : '') + '" />';
};
},{}],11:[function(_dereq_,module,exports){
module.exports = {
__depends__: [
_dereq_(179)
],
bpmnImporter: [ 'type', _dereq_(7) ]
};
},{"179":179,"7":7}],12:[function(_dereq_,module,exports){
'use strict';
var is = _dereq_(14).is,
getBusinessObject = _dereq_(14).getBusinessObject;
var forEach = _dereq_(35);
module.exports.isExpanded = function(element) {
if (is(element, 'bpmn:CallActivity')) {
return false;
}
if (is(element, 'bpmn:SubProcess')) {
return !!getBusinessObject(element).di.isExpanded;
}
if (is(element, 'bpmn:Participant')) {
return !!getBusinessObject(element).processRef;
}
return true;
};
module.exports.isInterrupting = function(element) {
return element && getBusinessObject(element).isInterrupting !== false;
};
module.exports.isEventSubProcess = function(element) {
return element && !!getBusinessObject(element).triggeredByEvent;
};
function hasEventDefinition(element, eventType) {
var bo = getBusinessObject(element),
hasEventDefinition = false;
if (bo.eventDefinitions) {
forEach(bo.eventDefinitions, function(event) {
if (is(event, eventType)) {
hasEventDefinition = true;
}
});
}
return hasEventDefinition;
}
module.exports.hasEventDefinition = hasEventDefinition;
module.exports.hasErrorEventDefinition = function(element) {
return hasEventDefinition(element, 'bpmn:ErrorEventDefinition');
};
module.exports.hasEscalationEventDefinition = function(element) {
return hasEventDefinition(element, 'bpmn:EscalationEventDefinition');
};
module.exports.hasCompensateEventDefinition = function(element) {
return hasEventDefinition(element, 'bpmn:CompensateEventDefinition');
};
},{"14":14,"35":35}],13:[function(_dereq_,module,exports){
'use strict';
var assign = _dereq_(115);
var is = _dereq_(14).is;
var DEFAULT_LABEL_SIZE = module.exports.DEFAULT_LABEL_SIZE = {
width: 90,
height: 20
};
var FLOW_LABEL_INDENT = module.exports.FLOW_LABEL_INDENT = 15;
/**
* Returns true if the given semantic has an external label
*
* @param {BpmnElement} semantic
* @return {Boolean} true if has label
*/
module.exports.hasExternalLabel = function(semantic) {
return is(semantic, 'bpmn:Event') ||
is(semantic, 'bpmn:Gateway') ||
is(semantic, 'bpmn:DataStoreReference') ||
is(semantic, 'bpmn:DataObjectReference') ||
is(semantic, 'bpmn:SequenceFlow') ||
is(semantic, 'bpmn:MessageFlow');
};
/**
* Get the position for sequence flow labels
*
* @param {Array} waypoints
* @return {Point} the label position
*/
function getFlowLabelPosition(waypoints) {
// get the waypoints mid
var mid = waypoints.length / 2 - 1;
var first = waypoints[Math.floor(mid)];
var second = waypoints[Math.ceil(mid + 0.01)];
// get position
var position = getWaypointsMid(waypoints);
// calculate angle
var angle = Math.atan( (second.y - first.y) / (second.x - first.x) );
var x = position.x,
y = position.y;
if ( Math.abs(angle) < Math.PI / 2 ) {
y -= FLOW_LABEL_INDENT;
} else {
x += FLOW_LABEL_INDENT;
}
return { x: x, y: y };
}
module.exports.getFlowLabelPosition = getFlowLabelPosition;
/**
* Get the middle of a number of waypoints
*
* @param {Array} waypoints
* @return {Point} the mid point
*/
function getWaypointsMid(waypoints) {
var mid = waypoints.length / 2 - 1;
var first = waypoints[Math.floor(mid)];
var second = waypoints[Math.ceil(mid + 0.01)];
return {
x: first.x + (second.x - first.x) / 2,
y: first.y + (second.y - first.y) / 2
};
}
module.exports.getWaypointsMid = getWaypointsMid;
function getExternalLabelMid(element) {
if (element.waypoints) {
return getFlowLabelPosition(element.waypoints);
} else {
return {
x: element.x + element.width / 2,
y: element.y + element.height + DEFAULT_LABEL_SIZE.height / 2
};
}
}
module.exports.getExternalLabelMid = getExternalLabelMid;
/**
* Returns the bounds of an elements label, parsed from the elements DI or
* generated from its bounds.
*
* @param {BpmnElement} semantic
* @param {djs.model.Base} element
*/
module.exports.getExternalLabelBounds = function(semantic, element) {
var mid,
size,
bounds,
di = semantic.di,
label = di.label;
if (label && label.bounds) {
bounds = label.bounds;
size = {
width: Math.max(DEFAULT_LABEL_SIZE.width, bounds.width),
height: bounds.height
};
mid = {
x: bounds.x + bounds.width / 2,
y: bounds.y + bounds.height / 2
};
} else {
mid = getExternalLabelMid(element);
size = DEFAULT_LABEL_SIZE;
}
return assign({
x: mid.x - size.width / 2,
y: mid.y - size.height / 2
}, size);
};
},{"115":115,"14":14}],14:[function(_dereq_,module,exports){
'use strict';
/**
* Is an element of the given BPMN type?
*
* @param {djs.model.Base|ModdleElement} element
* @param {String} type
*
* @return {Boolean}
*/
function is(element, type) {
var bo = getBusinessObject(element);
return bo && (typeof bo.$instanceOf === 'function') && bo.$instanceOf(type);
}
module.exports.is = is;
/**
* Return the business object for a given element.
*
* @param {djs.model.Base|ModdleElement} element
*
* @return {ModdleElement}
*/
function getBusinessObject(element) {
return (element && element.businessObject) || element;
}
module.exports.getBusinessObject = getBusinessObject;
},{}],15:[function(_dereq_,module,exports){
/**
* This file must not be changed or exchanged.
*
* @see http://bpmn.io/license for more information.
*/
'use strict';
var domify = _dereq_(124);
var domDelegate = _dereq_(123);
/* jshint -W101 */
// inlined ../resources/bpmnjs.png
var logoData = module.exports.BPMNIO_LOGO = 'iVBORw0KGgoAAAANSUhEUgAAADQAAAA0CAMAAADypuvZAAAAGXRFWHRTb2Z0d2FyZQBBZG9iZSBJbWFnZVJlYWR5ccllPAAAADBQTFRFiMte9PrwldFwfcZPqtqN0+zEyOe1XLgjvuKncsJAZ70y6fXh3vDT////UrQV////G2zN+AAAABB0Uk5T////////////////////AOAjXRkAAAHDSURBVHjavJZJkoUgDEBJmAX8979tM8u3E6x20VlYJfFFMoL4vBDxATxZcakIOJTWSmxvKWVIkJ8jHvlRv1F2LFrVISCZI+tCtQx+XfewgVTfyY3plPiQEAzI3zWy+kR6NBhFBYeBuscJLOUuA2WVLpCjVIaFzrNQZArxAZKUQm6gsj37L9Cb7dnIBUKxENaaMJQqMpDXvSL+ktxdGRm2IsKgJGGPg7atwUG5CcFUEuSv+CwQqizTrvDTNXdMU2bMiDWZd8d7QIySWVRsb2vBBioxOFt4OinPBapL+neAb5KL5IJ8szOza2/DYoipUCx+CjO0Bpsv0V6mktNZ+k8rlABlWG0FrOpKYVo8DT3dBeLEjUBAj7moDogVii7nSS9QzZnFcOVBp1g2PyBQ3Vr5aIapN91VJy33HTJLC1iX2FY6F8gRdaAeIEfVONgtFCzZTmoLEdOjBDfsIOA6128gw3eu1shAajdZNAORxuQDJN5A5PbEG6gNIu24QJD5iNyRMZIr6bsHbCtCU/OaOaSvgkUyDMdDa1BXGf5HJ1To+/Ym6mCKT02Y+/Sa126ZKyd3jxhzpc1r8zVL6YM1Qy/kR4ABAFJ6iQUnivhAAAAAAElFTkSuQmCC';
/* jshint +W101 */
function css(attrs) {
return attrs.join(';');
}
var LIGHTBOX_STYLES = css([
'z-index: 1001',
'position: fixed',
'top: 0',
'left: 0',
'right: 0',
'bottom: 0'
]);
var BACKDROP_STYLES = css([
'width: 100%',
'height: 100%',
'background: rgba(0,0,0,0.2)'
]);
var NOTICE_STYLES = css([
'position: absolute',
'left: 50%',
'top: 40%',
'margin: 0 -130px',
'width: 260px',
'padding: 10px',
'background: white',
'border: solid 1px #AAA',
'border-radius: 3px',
'font-family: Helvetica, Arial, sans-serif',
'font-size: 14px',
'line-height: 1.2em'
]);
var LIGHTBOX_MARKUP =
'' +
'' +
'' +
'' +
'
' +
'' +
'Web-based tooling for BPMN, DMN and CMMN diagrams ' +
'powered by bpmn.io.' +
'' +
'';
var lightbox;
function open() {
if (!lightbox) {
lightbox = domify(LIGHTBOX_MARKUP);
domDelegate.bind(lightbox, '.backdrop', 'click', function(event) {
document.body.removeChild(lightbox);
});
}
document.body.appendChild(lightbox);
}
module.exports.open = open;
},{"123":123,"124":124}],16:[function(_dereq_,module,exports){
module.exports = _dereq_(18);
},{"18":18}],17:[function(_dereq_,module,exports){
'use strict';
var isString = _dereq_(113),
isFunction = _dereq_(109),
assign = _dereq_(115);
var Moddle = _dereq_(131),
XmlReader = _dereq_(129),
XmlWriter = _dereq_(130);
/**
* A sub class of {@link Moddle} with support for import and export of BPMN 2.0 xml files.
*
* @class BpmnModdle
* @extends Moddle
*
* @param {Object|Array} packages to use for instantiating the model
* @param {Object} [options] additional options to pass over
*/
function BpmnModdle(packages, options) {
Moddle.call(this, packages, options);
}
BpmnModdle.prototype = Object.create(Moddle.prototype);
module.exports = BpmnModdle;
/**
* Instantiates a BPMN model tree from a given xml string.
*
* @param {String} xmlStr
* @param {String} [typeName='bpmn:Definitions'] name of the root element
* @param {Object} [options] options to pass to the underlying reader
* @param {Function} done callback that is invoked with (err, result, parseContext)
* once the import completes
*/
BpmnModdle.prototype.fromXML = function(xmlStr, typeName, options, done) {
if (!isString(typeName)) {
done = options;
options = typeName;
typeName = 'bpmn:Definitions';
}
if (isFunction(options)) {
done = options;
options = {};
}
var reader = new XmlReader(assign({ model: this, lax: true }, options));
var rootHandler = reader.handler(typeName);
reader.fromXML(xmlStr, rootHandler, done);
};
/**
* Serializes a BPMN 2.0 object tree to XML.
*
* @param {String} element the root element, typically an instance of `bpmn:Definitions`
* @param {Object} [options] to pass to the underlying writer
* @param {Function} done callback invoked with (err, xmlStr) once the import completes
*/
BpmnModdle.prototype.toXML = function(element, options, done) {
if (isFunction(options)) {
done = options;
options = {};
}
var writer = new XmlWriter(options);
try {
var result = writer.toXML(element);
done(null, result);
} catch (e) {
done(e);
}
};
},{"109":109,"113":113,"115":115,"129":129,"130":130,"131":131}],18:[function(_dereq_,module,exports){
'use strict';
var assign = _dereq_(115);
var BpmnModdle = _dereq_(17);
var packages = {
bpmn: _dereq_(20),
bpmndi: _dereq_(21),
dc: _dereq_(22),
di: _dereq_(23),
bioc: _dereq_(19)
};
module.exports = function(additionalPackages, options) {
return new BpmnModdle(assign({}, packages, additionalPackages), options);
};
},{"115":115,"17":17,"19":19,"20":20,"21":21,"22":22,"23":23}],19:[function(_dereq_,module,exports){
module.exports={
"name": "bpmn.io colors for BPMN",
"uri": "http://bpmn.io/schema/bpmn/biocolor/1.0",
"prefix": "bioc",
"types": [
{
"name": "ColoredShape",
"extends": [ "bpmndi:BPMNShape" ],
"properties": [
{
"name": "stroke",
"isAttr": true,
"type": "String"
},
{
"name": "fill",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "ColoredEdge",
"extends": [ "bpmndi:BPMNEdge" ],
"properties": [
{
"name": "stroke",
"isAttr": true,
"type": "String"
},
{
"name": "fill",
"isAttr": true,
"type": "String"
}
]
}
],
"emumerations": [],
"associations": []
}
},{}],20:[function(_dereq_,module,exports){
module.exports={
"name": "BPMN20",
"uri": "http://www.omg.org/spec/BPMN/20100524/MODEL",
"associations": [],
"types": [
{
"name": "Interface",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "operations",
"type": "Operation",
"isMany": true
},
{
"name": "implementationRef",
"type": "String",
"isAttr": true
}
]
},
{
"name": "Operation",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "inMessageRef",
"type": "Message",
"isReference": true
},
{
"name": "outMessageRef",
"type": "Message",
"isReference": true
},
{
"name": "errorRef",
"type": "Error",
"isMany": true,
"isReference": true
},
{
"name": "implementationRef",
"type": "String",
"isAttr": true
}
]
},
{
"name": "EndPoint",
"superClass": [
"RootElement"
]
},
{
"name": "Auditing",
"superClass": [
"BaseElement"
]
},
{
"name": "GlobalTask",
"superClass": [
"CallableElement"
],
"properties": [
{
"name": "resources",
"type": "ResourceRole",
"isMany": true
}
]
},
{
"name": "Monitoring",
"superClass": [
"BaseElement"
]
},
{
"name": "Performer",
"superClass": [
"ResourceRole"
]
},
{
"name": "Process",
"superClass": [
"FlowElementsContainer",
"CallableElement"
],
"properties": [
{
"name": "processType",
"type": "ProcessType",
"isAttr": true
},
{
"name": "isClosed",
"isAttr": true,
"type": "Boolean"
},
{
"name": "auditing",
"type": "Auditing"
},
{
"name": "monitoring",
"type": "Monitoring"
},
{
"name": "properties",
"type": "Property",
"isMany": true
},
{
"name": "laneSets",
"type": "LaneSet",
"isMany": true,
"replaces": "FlowElementsContainer#laneSets"
},
{
"name": "flowElements",
"type": "FlowElement",
"isMany": true,
"replaces": "FlowElementsContainer#flowElements"
},
{
"name": "artifacts",
"type": "Artifact",
"isMany": true
},
{
"name": "resources",
"type": "ResourceRole",
"isMany": true
},
{
"name": "correlationSubscriptions",
"type": "CorrelationSubscription",
"isMany": true
},
{
"name": "supports",
"type": "Process",
"isMany": true,
"isReference": true
},
{
"name": "definitionalCollaborationRef",
"type": "Collaboration",
"isAttr": true,
"isReference": true
},
{
"name": "isExecutable",
"isAttr": true,
"type": "Boolean"
}
]
},
{
"name": "LaneSet",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "lanes",
"type": "Lane",
"isMany": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "Lane",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "partitionElementRef",
"type": "BaseElement",
"isAttr": true,
"isReference": true
},
{
"name": "partitionElement",
"type": "BaseElement"
},
{
"name": "flowNodeRef",
"type": "FlowNode",
"isMany": true,
"isReference": true
},
{
"name": "childLaneSet",
"type": "LaneSet",
"xml": {
"serialize": "xsi:type"
}
}
]
},
{
"name": "GlobalManualTask",
"superClass": [
"GlobalTask"
]
},
{
"name": "ManualTask",
"superClass": [
"Task"
]
},
{
"name": "UserTask",
"superClass": [
"Task"
],
"properties": [
{
"name": "renderings",
"type": "Rendering",
"isMany": true
},
{
"name": "implementation",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "Rendering",
"superClass": [
"BaseElement"
]
},
{
"name": "HumanPerformer",
"superClass": [
"Performer"
]
},
{
"name": "PotentialOwner",
"superClass": [
"HumanPerformer"
]
},
{
"name": "GlobalUserTask",
"superClass": [
"GlobalTask"
],
"properties": [
{
"name": "implementation",
"isAttr": true,
"type": "String"
},
{
"name": "renderings",
"type": "Rendering",
"isMany": true
}
]
},
{
"name": "Gateway",
"isAbstract": true,
"superClass": [
"FlowNode"
],
"properties": [
{
"name": "gatewayDirection",
"type": "GatewayDirection",
"default": "Unspecified",
"isAttr": true
}
]
},
{
"name": "EventBasedGateway",
"superClass": [
"Gateway"
],
"properties": [
{
"name": "instantiate",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "eventGatewayType",
"type": "EventBasedGatewayType",
"isAttr": true,
"default": "Exclusive"
}
]
},
{
"name": "ComplexGateway",
"superClass": [
"Gateway"
],
"properties": [
{
"name": "activationCondition",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "default",
"type": "SequenceFlow",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ExclusiveGateway",
"superClass": [
"Gateway"
],
"properties": [
{
"name": "default",
"type": "SequenceFlow",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "InclusiveGateway",
"superClass": [
"Gateway"
],
"properties": [
{
"name": "default",
"type": "SequenceFlow",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ParallelGateway",
"superClass": [
"Gateway"
]
},
{
"name": "RootElement",
"isAbstract": true,
"superClass": [
"BaseElement"
]
},
{
"name": "Relationship",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "type",
"isAttr": true,
"type": "String"
},
{
"name": "direction",
"type": "RelationshipDirection",
"isAttr": true
},
{
"name": "source",
"isMany": true,
"isReference": true,
"type": "Element"
},
{
"name": "target",
"isMany": true,
"isReference": true,
"type": "Element"
}
]
},
{
"name": "BaseElement",
"isAbstract": true,
"properties": [
{
"name": "id",
"isAttr": true,
"type": "String",
"isId": true
},
{
"name": "documentation",
"type": "Documentation",
"isMany": true
},
{
"name": "extensionDefinitions",
"type": "ExtensionDefinition",
"isMany": true,
"isReference": true
},
{
"name": "extensionElements",
"type": "ExtensionElements"
}
]
},
{
"name": "Extension",
"properties": [
{
"name": "mustUnderstand",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "definition",
"type": "ExtensionDefinition",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ExtensionDefinition",
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "extensionAttributeDefinitions",
"type": "ExtensionAttributeDefinition",
"isMany": true
}
]
},
{
"name": "ExtensionAttributeDefinition",
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "type",
"isAttr": true,
"type": "String"
},
{
"name": "isReference",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "extensionDefinition",
"type": "ExtensionDefinition",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ExtensionElements",
"properties": [
{
"name": "valueRef",
"isAttr": true,
"isReference": true,
"type": "Element"
},
{
"name": "values",
"type": "Element",
"isMany": true
},
{
"name": "extensionAttributeDefinition",
"type": "ExtensionAttributeDefinition",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "Documentation",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "text",
"type": "String",
"isBody": true
},
{
"name": "textFormat",
"default": "text/plain",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "Event",
"isAbstract": true,
"superClass": [
"FlowNode",
"InteractionNode"
],
"properties": [
{
"name": "properties",
"type": "Property",
"isMany": true
}
]
},
{
"name": "IntermediateCatchEvent",
"superClass": [
"CatchEvent"
]
},
{
"name": "IntermediateThrowEvent",
"superClass": [
"ThrowEvent"
]
},
{
"name": "EndEvent",
"superClass": [
"ThrowEvent"
]
},
{
"name": "StartEvent",
"superClass": [
"CatchEvent"
],
"properties": [
{
"name": "isInterrupting",
"default": true,
"isAttr": true,
"type": "Boolean"
}
]
},
{
"name": "ThrowEvent",
"isAbstract": true,
"superClass": [
"Event"
],
"properties": [
{
"name": "dataInputs",
"type": "DataInput",
"isMany": true
},
{
"name": "dataInputAssociations",
"type": "DataInputAssociation",
"isMany": true
},
{
"name": "inputSet",
"type": "InputSet"
},
{
"name": "eventDefinitions",
"type": "EventDefinition",
"isMany": true
},
{
"name": "eventDefinitionRef",
"type": "EventDefinition",
"isMany": true,
"isReference": true
}
]
},
{
"name": "CatchEvent",
"isAbstract": true,
"superClass": [
"Event"
],
"properties": [
{
"name": "parallelMultiple",
"isAttr": true,
"type": "Boolean",
"default": false
},
{
"name": "dataOutputs",
"type": "DataOutput",
"isMany": true
},
{
"name": "dataOutputAssociations",
"type": "DataOutputAssociation",
"isMany": true
},
{
"name": "outputSet",
"type": "OutputSet"
},
{
"name": "eventDefinitions",
"type": "EventDefinition",
"isMany": true
},
{
"name": "eventDefinitionRef",
"type": "EventDefinition",
"isMany": true,
"isReference": true
}
]
},
{
"name": "BoundaryEvent",
"superClass": [
"CatchEvent"
],
"properties": [
{
"name": "cancelActivity",
"default": true,
"isAttr": true,
"type": "Boolean"
},
{
"name": "attachedToRef",
"type": "Activity",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "EventDefinition",
"isAbstract": true,
"superClass": [
"RootElement"
]
},
{
"name": "CancelEventDefinition",
"superClass": [
"EventDefinition"
]
},
{
"name": "ErrorEventDefinition",
"superClass": [
"EventDefinition"
],
"properties": [
{
"name": "errorRef",
"type": "Error",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "TerminateEventDefinition",
"superClass": [
"EventDefinition"
]
},
{
"name": "EscalationEventDefinition",
"superClass": [
"EventDefinition"
],
"properties": [
{
"name": "escalationRef",
"type": "Escalation",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "Escalation",
"properties": [
{
"name": "structureRef",
"type": "ItemDefinition",
"isAttr": true,
"isReference": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "escalationCode",
"isAttr": true,
"type": "String"
}
],
"superClass": [
"RootElement"
]
},
{
"name": "CompensateEventDefinition",
"superClass": [
"EventDefinition"
],
"properties": [
{
"name": "waitForCompletion",
"isAttr": true,
"type": "Boolean"
},
{
"name": "activityRef",
"type": "Activity",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "TimerEventDefinition",
"superClass": [
"EventDefinition"
],
"properties": [
{
"name": "timeDate",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "timeCycle",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "timeDuration",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
}
]
},
{
"name": "LinkEventDefinition",
"superClass": [
"EventDefinition"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "target",
"type": "LinkEventDefinition",
"isAttr": true,
"isReference": true
},
{
"name": "source",
"type": "LinkEventDefinition",
"isMany": true,
"isReference": true
}
]
},
{
"name": "MessageEventDefinition",
"superClass": [
"EventDefinition"
],
"properties": [
{
"name": "messageRef",
"type": "Message",
"isAttr": true,
"isReference": true
},
{
"name": "operationRef",
"type": "Operation",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ConditionalEventDefinition",
"superClass": [
"EventDefinition"
],
"properties": [
{
"name": "condition",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
}
]
},
{
"name": "SignalEventDefinition",
"superClass": [
"EventDefinition"
],
"properties": [
{
"name": "signalRef",
"type": "Signal",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "Signal",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "structureRef",
"type": "ItemDefinition",
"isAttr": true,
"isReference": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "ImplicitThrowEvent",
"superClass": [
"ThrowEvent"
]
},
{
"name": "DataState",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "ItemAwareElement",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "itemSubjectRef",
"type": "ItemDefinition",
"isAttr": true,
"isReference": true
},
{
"name": "dataState",
"type": "DataState"
}
]
},
{
"name": "DataAssociation",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "assignment",
"type": "Assignment",
"isMany": true
},
{
"name": "sourceRef",
"type": "ItemAwareElement",
"isMany": true,
"isReference": true
},
{
"name": "targetRef",
"type": "ItemAwareElement",
"isReference": true
},
{
"name": "transformation",
"type": "FormalExpression",
"xml": {
"serialize": "property"
}
}
]
},
{
"name": "DataInput",
"superClass": [
"ItemAwareElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "isCollection",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "inputSetRef",
"type": "InputSet",
"isVirtual": true,
"isMany": true,
"isReference": true
},
{
"name": "inputSetWithOptional",
"type": "InputSet",
"isVirtual": true,
"isMany": true,
"isReference": true
},
{
"name": "inputSetWithWhileExecuting",
"type": "InputSet",
"isVirtual": true,
"isMany": true,
"isReference": true
}
]
},
{
"name": "DataOutput",
"superClass": [
"ItemAwareElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "isCollection",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "outputSetRef",
"type": "OutputSet",
"isVirtual": true,
"isMany": true,
"isReference": true
},
{
"name": "outputSetWithOptional",
"type": "OutputSet",
"isVirtual": true,
"isMany": true,
"isReference": true
},
{
"name": "outputSetWithWhileExecuting",
"type": "OutputSet",
"isVirtual": true,
"isMany": true,
"isReference": true
}
]
},
{
"name": "InputSet",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "dataInputRefs",
"type": "DataInput",
"isMany": true,
"isReference": true
},
{
"name": "optionalInputRefs",
"type": "DataInput",
"isMany": true,
"isReference": true
},
{
"name": "whileExecutingInputRefs",
"type": "DataInput",
"isMany": true,
"isReference": true
},
{
"name": "outputSetRefs",
"type": "OutputSet",
"isMany": true,
"isReference": true
}
]
},
{
"name": "OutputSet",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "dataOutputRefs",
"type": "DataOutput",
"isMany": true,
"isReference": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "inputSetRefs",
"type": "InputSet",
"isMany": true,
"isReference": true
},
{
"name": "optionalOutputRefs",
"type": "DataOutput",
"isMany": true,
"isReference": true
},
{
"name": "whileExecutingOutputRefs",
"type": "DataOutput",
"isMany": true,
"isReference": true
}
]
},
{
"name": "Property",
"superClass": [
"ItemAwareElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "DataInputAssociation",
"superClass": [
"DataAssociation"
]
},
{
"name": "DataOutputAssociation",
"superClass": [
"DataAssociation"
]
},
{
"name": "InputOutputSpecification",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "dataInputs",
"type": "DataInput",
"isMany": true
},
{
"name": "dataOutputs",
"type": "DataOutput",
"isMany": true
},
{
"name": "inputSets",
"type": "InputSet",
"isMany": true
},
{
"name": "outputSets",
"type": "OutputSet",
"isMany": true
}
]
},
{
"name": "DataObject",
"superClass": [
"FlowElement",
"ItemAwareElement"
],
"properties": [
{
"name": "isCollection",
"default": false,
"isAttr": true,
"type": "Boolean"
}
]
},
{
"name": "InputOutputBinding",
"properties": [
{
"name": "inputDataRef",
"type": "InputSet",
"isAttr": true,
"isReference": true
},
{
"name": "outputDataRef",
"type": "OutputSet",
"isAttr": true,
"isReference": true
},
{
"name": "operationRef",
"type": "Operation",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "Assignment",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "from",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "to",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
}
]
},
{
"name": "DataStore",
"superClass": [
"RootElement",
"ItemAwareElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "capacity",
"isAttr": true,
"type": "Integer"
},
{
"name": "isUnlimited",
"default": true,
"isAttr": true,
"type": "Boolean"
}
]
},
{
"name": "DataStoreReference",
"superClass": [
"ItemAwareElement",
"FlowElement"
],
"properties": [
{
"name": "dataStoreRef",
"type": "DataStore",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "DataObjectReference",
"superClass": [
"ItemAwareElement",
"FlowElement"
],
"properties": [
{
"name": "dataObjectRef",
"type": "DataObject",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ConversationLink",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "sourceRef",
"type": "InteractionNode",
"isAttr": true,
"isReference": true
},
{
"name": "targetRef",
"type": "InteractionNode",
"isAttr": true,
"isReference": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "ConversationAssociation",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "innerConversationNodeRef",
"type": "ConversationNode",
"isAttr": true,
"isReference": true
},
{
"name": "outerConversationNodeRef",
"type": "ConversationNode",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "CallConversation",
"superClass": [
"ConversationNode"
],
"properties": [
{
"name": "calledCollaborationRef",
"type": "Collaboration",
"isAttr": true,
"isReference": true
},
{
"name": "participantAssociations",
"type": "ParticipantAssociation",
"isMany": true
}
]
},
{
"name": "Conversation",
"superClass": [
"ConversationNode"
]
},
{
"name": "SubConversation",
"superClass": [
"ConversationNode"
],
"properties": [
{
"name": "conversationNodes",
"type": "ConversationNode",
"isMany": true
}
]
},
{
"name": "ConversationNode",
"isAbstract": true,
"superClass": [
"InteractionNode",
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "participantRefs",
"type": "Participant",
"isMany": true,
"isReference": true
},
{
"name": "messageFlowRefs",
"type": "MessageFlow",
"isMany": true,
"isReference": true
},
{
"name": "correlationKeys",
"type": "CorrelationKey",
"isMany": true
}
]
},
{
"name": "GlobalConversation",
"superClass": [
"Collaboration"
]
},
{
"name": "PartnerEntity",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "participantRef",
"type": "Participant",
"isMany": true,
"isReference": true
}
]
},
{
"name": "PartnerRole",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "participantRef",
"type": "Participant",
"isMany": true,
"isReference": true
}
]
},
{
"name": "CorrelationProperty",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "correlationPropertyRetrievalExpression",
"type": "CorrelationPropertyRetrievalExpression",
"isMany": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "type",
"type": "ItemDefinition",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "Error",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "structureRef",
"type": "ItemDefinition",
"isAttr": true,
"isReference": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "errorCode",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "CorrelationKey",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "correlationPropertyRef",
"type": "CorrelationProperty",
"isMany": true,
"isReference": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "Expression",
"superClass": [
"BaseElement"
],
"isAbstract": false,
"properties": [
{
"name": "body",
"type": "String",
"isBody": true
}
]
},
{
"name": "FormalExpression",
"superClass": [
"Expression"
],
"properties": [
{
"name": "language",
"isAttr": true,
"type": "String"
},
{
"name": "evaluatesToTypeRef",
"type": "ItemDefinition",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "Message",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "itemRef",
"type": "ItemDefinition",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ItemDefinition",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "itemKind",
"type": "ItemKind",
"isAttr": true
},
{
"name": "structureRef",
"type": "String",
"isAttr": true
},
{
"name": "isCollection",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "import",
"type": "Import",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "FlowElement",
"isAbstract": true,
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "auditing",
"type": "Auditing"
},
{
"name": "monitoring",
"type": "Monitoring"
},
{
"name": "categoryValueRef",
"type": "CategoryValue",
"isMany": true,
"isReference": true
}
]
},
{
"name": "SequenceFlow",
"superClass": [
"FlowElement"
],
"properties": [
{
"name": "isImmediate",
"isAttr": true,
"type": "Boolean"
},
{
"name": "conditionExpression",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "sourceRef",
"type": "FlowNode",
"isAttr": true,
"isReference": true
},
{
"name": "targetRef",
"type": "FlowNode",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "FlowElementsContainer",
"isAbstract": true,
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "laneSets",
"type": "LaneSet",
"isMany": true
},
{
"name": "flowElements",
"type": "FlowElement",
"isMany": true
}
]
},
{
"name": "CallableElement",
"isAbstract": true,
"superClass": [
"RootElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "ioSpecification",
"type": "InputOutputSpecification",
"xml": {
"serialize": "property"
}
},
{
"name": "supportedInterfaceRef",
"type": "Interface",
"isMany": true,
"isReference": true
},
{
"name": "ioBinding",
"type": "InputOutputBinding",
"isMany": true,
"xml": {
"serialize": "property"
}
}
]
},
{
"name": "FlowNode",
"isAbstract": true,
"superClass": [
"FlowElement"
],
"properties": [
{
"name": "incoming",
"type": "SequenceFlow",
"isMany": true,
"isReference": true
},
{
"name": "outgoing",
"type": "SequenceFlow",
"isMany": true,
"isReference": true
},
{
"name": "lanes",
"type": "Lane",
"isVirtual": true,
"isMany": true,
"isReference": true
}
]
},
{
"name": "CorrelationPropertyRetrievalExpression",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "messagePath",
"type": "FormalExpression"
},
{
"name": "messageRef",
"type": "Message",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "CorrelationPropertyBinding",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "dataPath",
"type": "FormalExpression"
},
{
"name": "correlationPropertyRef",
"type": "CorrelationProperty",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "Resource",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "resourceParameters",
"type": "ResourceParameter",
"isMany": true
}
]
},
{
"name": "ResourceParameter",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "isRequired",
"isAttr": true,
"type": "Boolean"
},
{
"name": "type",
"type": "ItemDefinition",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "CorrelationSubscription",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "correlationKeyRef",
"type": "CorrelationKey",
"isAttr": true,
"isReference": true
},
{
"name": "correlationPropertyBinding",
"type": "CorrelationPropertyBinding",
"isMany": true
}
]
},
{
"name": "MessageFlow",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "sourceRef",
"type": "InteractionNode",
"isAttr": true,
"isReference": true
},
{
"name": "targetRef",
"type": "InteractionNode",
"isAttr": true,
"isReference": true
},
{
"name": "messageRef",
"type": "Message",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "MessageFlowAssociation",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "innerMessageFlowRef",
"type": "MessageFlow",
"isAttr": true,
"isReference": true
},
{
"name": "outerMessageFlowRef",
"type": "MessageFlow",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "InteractionNode",
"isAbstract": true,
"properties": [
{
"name": "incomingConversationLinks",
"type": "ConversationLink",
"isVirtual": true,
"isMany": true,
"isReference": true
},
{
"name": "outgoingConversationLinks",
"type": "ConversationLink",
"isVirtual": true,
"isMany": true,
"isReference": true
}
]
},
{
"name": "Participant",
"superClass": [
"InteractionNode",
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "interfaceRef",
"type": "Interface",
"isMany": true,
"isReference": true
},
{
"name": "participantMultiplicity",
"type": "ParticipantMultiplicity"
},
{
"name": "endPointRefs",
"type": "EndPoint",
"isMany": true,
"isReference": true
},
{
"name": "processRef",
"type": "Process",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ParticipantAssociation",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "innerParticipantRef",
"type": "Participant",
"isAttr": true,
"isReference": true
},
{
"name": "outerParticipantRef",
"type": "Participant",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ParticipantMultiplicity",
"properties": [
{
"name": "minimum",
"default": 0,
"isAttr": true,
"type": "Integer"
},
{
"name": "maximum",
"default": 1,
"isAttr": true,
"type": "Integer"
}
]
},
{
"name": "Collaboration",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "isClosed",
"isAttr": true,
"type": "Boolean"
},
{
"name": "participants",
"type": "Participant",
"isMany": true
},
{
"name": "messageFlows",
"type": "MessageFlow",
"isMany": true
},
{
"name": "artifacts",
"type": "Artifact",
"isMany": true
},
{
"name": "conversations",
"type": "ConversationNode",
"isMany": true
},
{
"name": "conversationAssociations",
"type": "ConversationAssociation"
},
{
"name": "participantAssociations",
"type": "ParticipantAssociation",
"isMany": true
},
{
"name": "messageFlowAssociations",
"type": "MessageFlowAssociation",
"isMany": true
},
{
"name": "correlationKeys",
"type": "CorrelationKey",
"isMany": true
},
{
"name": "choreographyRef",
"type": "Choreography",
"isMany": true,
"isReference": true
},
{
"name": "conversationLinks",
"type": "ConversationLink",
"isMany": true
}
]
},
{
"name": "ChoreographyActivity",
"isAbstract": true,
"superClass": [
"FlowNode"
],
"properties": [
{
"name": "participantRefs",
"type": "Participant",
"isMany": true,
"isReference": true
},
{
"name": "initiatingParticipantRef",
"type": "Participant",
"isAttr": true,
"isReference": true
},
{
"name": "correlationKeys",
"type": "CorrelationKey",
"isMany": true
},
{
"name": "loopType",
"type": "ChoreographyLoopType",
"default": "None",
"isAttr": true
}
]
},
{
"name": "CallChoreography",
"superClass": [
"ChoreographyActivity"
],
"properties": [
{
"name": "calledChoreographyRef",
"type": "Choreography",
"isAttr": true,
"isReference": true
},
{
"name": "participantAssociations",
"type": "ParticipantAssociation",
"isMany": true
}
]
},
{
"name": "SubChoreography",
"superClass": [
"ChoreographyActivity",
"FlowElementsContainer"
],
"properties": [
{
"name": "artifacts",
"type": "Artifact",
"isMany": true
}
]
},
{
"name": "ChoreographyTask",
"superClass": [
"ChoreographyActivity"
],
"properties": [
{
"name": "messageFlowRef",
"type": "MessageFlow",
"isMany": true,
"isReference": true
}
]
},
{
"name": "Choreography",
"superClass": [
"FlowElementsContainer",
"Collaboration"
]
},
{
"name": "GlobalChoreographyTask",
"superClass": [
"Choreography"
],
"properties": [
{
"name": "initiatingParticipantRef",
"type": "Participant",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "TextAnnotation",
"superClass": [
"Artifact"
],
"properties": [
{
"name": "text",
"type": "String"
},
{
"name": "textFormat",
"default": "text/plain",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "Group",
"superClass": [
"Artifact"
],
"properties": [
{
"name": "categoryValueRef",
"type": "CategoryValue",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "Association",
"superClass": [
"Artifact"
],
"properties": [
{
"name": "associationDirection",
"type": "AssociationDirection",
"isAttr": true
},
{
"name": "sourceRef",
"type": "BaseElement",
"isAttr": true,
"isReference": true
},
{
"name": "targetRef",
"type": "BaseElement",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "Category",
"superClass": [
"RootElement"
],
"properties": [
{
"name": "categoryValue",
"type": "CategoryValue",
"isMany": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "Artifact",
"isAbstract": true,
"superClass": [
"BaseElement"
]
},
{
"name": "CategoryValue",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "categorizedFlowElements",
"type": "FlowElement",
"isVirtual": true,
"isMany": true,
"isReference": true
},
{
"name": "value",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "Activity",
"isAbstract": true,
"superClass": [
"FlowNode"
],
"properties": [
{
"name": "isForCompensation",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "default",
"type": "SequenceFlow",
"isAttr": true,
"isReference": true
},
{
"name": "ioSpecification",
"type": "InputOutputSpecification",
"xml": {
"serialize": "property"
}
},
{
"name": "boundaryEventRefs",
"type": "BoundaryEvent",
"isMany": true,
"isReference": true
},
{
"name": "properties",
"type": "Property",
"isMany": true
},
{
"name": "dataInputAssociations",
"type": "DataInputAssociation",
"isMany": true
},
{
"name": "dataOutputAssociations",
"type": "DataOutputAssociation",
"isMany": true
},
{
"name": "startQuantity",
"default": 1,
"isAttr": true,
"type": "Integer"
},
{
"name": "resources",
"type": "ResourceRole",
"isMany": true
},
{
"name": "completionQuantity",
"default": 1,
"isAttr": true,
"type": "Integer"
},
{
"name": "loopCharacteristics",
"type": "LoopCharacteristics"
}
]
},
{
"name": "ServiceTask",
"superClass": [
"Task"
],
"properties": [
{
"name": "implementation",
"isAttr": true,
"type": "String"
},
{
"name": "operationRef",
"type": "Operation",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "SubProcess",
"superClass": [
"Activity",
"FlowElementsContainer",
"InteractionNode"
],
"properties": [
{
"name": "triggeredByEvent",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "artifacts",
"type": "Artifact",
"isMany": true
}
]
},
{
"name": "LoopCharacteristics",
"isAbstract": true,
"superClass": [
"BaseElement"
]
},
{
"name": "MultiInstanceLoopCharacteristics",
"superClass": [
"LoopCharacteristics"
],
"properties": [
{
"name": "isSequential",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "behavior",
"type": "MultiInstanceBehavior",
"default": "All",
"isAttr": true
},
{
"name": "loopCardinality",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "loopDataInputRef",
"type": "ItemAwareElement",
"isReference": true
},
{
"name": "loopDataOutputRef",
"type": "ItemAwareElement",
"isReference": true
},
{
"name": "inputDataItem",
"type": "DataInput",
"xml": {
"serialize": "property"
}
},
{
"name": "outputDataItem",
"type": "DataOutput",
"xml": {
"serialize": "property"
}
},
{
"name": "complexBehaviorDefinition",
"type": "ComplexBehaviorDefinition",
"isMany": true
},
{
"name": "completionCondition",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "oneBehaviorEventRef",
"type": "EventDefinition",
"isAttr": true,
"isReference": true
},
{
"name": "noneBehaviorEventRef",
"type": "EventDefinition",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "StandardLoopCharacteristics",
"superClass": [
"LoopCharacteristics"
],
"properties": [
{
"name": "testBefore",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "loopCondition",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "loopMaximum",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
}
]
},
{
"name": "CallActivity",
"superClass": [
"Activity"
],
"properties": [
{
"name": "calledElement",
"type": "String",
"isAttr": true
}
]
},
{
"name": "Task",
"superClass": [
"Activity",
"InteractionNode"
]
},
{
"name": "SendTask",
"superClass": [
"Task"
],
"properties": [
{
"name": "implementation",
"isAttr": true,
"type": "String"
},
{
"name": "operationRef",
"type": "Operation",
"isAttr": true,
"isReference": true
},
{
"name": "messageRef",
"type": "Message",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ReceiveTask",
"superClass": [
"Task"
],
"properties": [
{
"name": "implementation",
"isAttr": true,
"type": "String"
},
{
"name": "instantiate",
"default": false,
"isAttr": true,
"type": "Boolean"
},
{
"name": "operationRef",
"type": "Operation",
"isAttr": true,
"isReference": true
},
{
"name": "messageRef",
"type": "Message",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ScriptTask",
"superClass": [
"Task"
],
"properties": [
{
"name": "scriptFormat",
"isAttr": true,
"type": "String"
},
{
"name": "script",
"type": "String"
}
]
},
{
"name": "BusinessRuleTask",
"superClass": [
"Task"
],
"properties": [
{
"name": "implementation",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "AdHocSubProcess",
"superClass": [
"SubProcess"
],
"properties": [
{
"name": "completionCondition",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "ordering",
"type": "AdHocOrdering",
"isAttr": true
},
{
"name": "cancelRemainingInstances",
"default": true,
"isAttr": true,
"type": "Boolean"
}
]
},
{
"name": "Transaction",
"superClass": [
"SubProcess"
],
"properties": [
{
"name": "protocol",
"isAttr": true,
"type": "String"
},
{
"name": "method",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "GlobalScriptTask",
"superClass": [
"GlobalTask"
],
"properties": [
{
"name": "scriptLanguage",
"isAttr": true,
"type": "String"
},
{
"name": "script",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "GlobalBusinessRuleTask",
"superClass": [
"GlobalTask"
],
"properties": [
{
"name": "implementation",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "ComplexBehaviorDefinition",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "condition",
"type": "FormalExpression"
},
{
"name": "event",
"type": "ImplicitThrowEvent"
}
]
},
{
"name": "ResourceRole",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "resourceRef",
"type": "Resource",
"isReference": true
},
{
"name": "resourceParameterBindings",
"type": "ResourceParameterBinding",
"isMany": true
},
{
"name": "resourceAssignmentExpression",
"type": "ResourceAssignmentExpression"
},
{
"name": "name",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "ResourceParameterBinding",
"properties": [
{
"name": "expression",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
},
{
"name": "parameterRef",
"type": "ResourceParameter",
"isAttr": true,
"isReference": true
}
]
},
{
"name": "ResourceAssignmentExpression",
"properties": [
{
"name": "expression",
"type": "Expression",
"xml": {
"serialize": "xsi:type"
}
}
],
"superClass": [
"BaseElement"
]
},
{
"name": "Import",
"properties": [
{
"name": "importType",
"isAttr": true,
"type": "String"
},
{
"name": "location",
"isAttr": true,
"type": "String"
},
{
"name": "namespace",
"isAttr": true,
"type": "String"
}
]
},
{
"name": "Definitions",
"superClass": [
"BaseElement"
],
"properties": [
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "targetNamespace",
"isAttr": true,
"type": "String"
},
{
"name": "expressionLanguage",
"default": "http://www.w3.org/1999/XPath",
"isAttr": true,
"type": "String"
},
{
"name": "typeLanguage",
"default": "http://www.w3.org/2001/XMLSchema",
"isAttr": true,
"type": "String"
},
{
"name": "imports",
"type": "Import",
"isMany": true
},
{
"name": "extensions",
"type": "Extension",
"isMany": true
},
{
"name": "rootElements",
"type": "RootElement",
"isMany": true
},
{
"name": "diagrams",
"isMany": true,
"type": "bpmndi:BPMNDiagram"
},
{
"name": "exporter",
"isAttr": true,
"type": "String"
},
{
"name": "relationships",
"type": "Relationship",
"isMany": true
},
{
"name": "exporterVersion",
"isAttr": true,
"type": "String"
}
]
}
],
"emumerations": [
{
"name": "ProcessType",
"literalValues": [
{
"name": "None"
},
{
"name": "Public"
},
{
"name": "Private"
}
]
},
{
"name": "GatewayDirection",
"literalValues": [
{
"name": "Unspecified"
},
{
"name": "Converging"
},
{
"name": "Diverging"
},
{
"name": "Mixed"
}
]
},
{
"name": "EventBasedGatewayType",
"literalValues": [
{
"name": "Parallel"
},
{
"name": "Exclusive"
}
]
},
{
"name": "RelationshipDirection",
"literalValues": [
{
"name": "None"
},
{
"name": "Forward"
},
{
"name": "Backward"
},
{
"name": "Both"
}
]
},
{
"name": "ItemKind",
"literalValues": [
{
"name": "Physical"
},
{
"name": "Information"
}
]
},
{
"name": "ChoreographyLoopType",
"literalValues": [
{
"name": "None"
},
{
"name": "Standard"
},
{
"name": "MultiInstanceSequential"
},
{
"name": "MultiInstanceParallel"
}
]
},
{
"name": "AssociationDirection",
"literalValues": [
{
"name": "None"
},
{
"name": "One"
},
{
"name": "Both"
}
]
},
{
"name": "MultiInstanceBehavior",
"literalValues": [
{
"name": "None"
},
{
"name": "One"
},
{
"name": "All"
},
{
"name": "Complex"
}
]
},
{
"name": "AdHocOrdering",
"literalValues": [
{
"name": "Parallel"
},
{
"name": "Sequential"
}
]
}
],
"prefix": "bpmn",
"xml": {
"tagAlias": "lowerCase",
"typePrefix": "t"
}
}
},{}],21:[function(_dereq_,module,exports){
module.exports={
"name": "BPMNDI",
"uri": "http://www.omg.org/spec/BPMN/20100524/DI",
"types": [
{
"name": "BPMNDiagram",
"properties": [
{
"name": "plane",
"type": "BPMNPlane",
"redefines": "di:Diagram#rootElement"
},
{
"name": "labelStyle",
"type": "BPMNLabelStyle",
"isMany": true
}
],
"superClass": [
"di:Diagram"
]
},
{
"name": "BPMNPlane",
"properties": [
{
"name": "bpmnElement",
"isAttr": true,
"isReference": true,
"type": "bpmn:BaseElement",
"redefines": "di:DiagramElement#modelElement"
}
],
"superClass": [
"di:Plane"
]
},
{
"name": "BPMNShape",
"properties": [
{
"name": "bpmnElement",
"isAttr": true,
"isReference": true,
"type": "bpmn:BaseElement",
"redefines": "di:DiagramElement#modelElement"
},
{
"name": "isHorizontal",
"isAttr": true,
"type": "Boolean"
},
{
"name": "isExpanded",
"isAttr": true,
"type": "Boolean"
},
{
"name": "isMarkerVisible",
"isAttr": true,
"type": "Boolean"
},
{
"name": "label",
"type": "BPMNLabel"
},
{
"name": "isMessageVisible",
"isAttr": true,
"type": "Boolean"
},
{
"name": "participantBandKind",
"type": "ParticipantBandKind",
"isAttr": true
},
{
"name": "choreographyActivityShape",
"type": "BPMNShape",
"isAttr": true,
"isReference": true
}
],
"superClass": [
"di:LabeledShape"
]
},
{
"name": "BPMNEdge",
"properties": [
{
"name": "label",
"type": "BPMNLabel"
},
{
"name": "bpmnElement",
"isAttr": true,
"isReference": true,
"type": "bpmn:BaseElement",
"redefines": "di:DiagramElement#modelElement"
},
{
"name": "sourceElement",
"isAttr": true,
"isReference": true,
"type": "di:DiagramElement",
"redefines": "di:Edge#source"
},
{
"name": "targetElement",
"isAttr": true,
"isReference": true,
"type": "di:DiagramElement",
"redefines": "di:Edge#target"
},
{
"name": "messageVisibleKind",
"type": "MessageVisibleKind",
"isAttr": true,
"default": "initiating"
}
],
"superClass": [
"di:LabeledEdge"
]
},
{
"name": "BPMNLabel",
"properties": [
{
"name": "labelStyle",
"type": "BPMNLabelStyle",
"isAttr": true,
"isReference": true,
"redefines": "di:DiagramElement#style"
}
],
"superClass": [
"di:Label"
]
},
{
"name": "BPMNLabelStyle",
"properties": [
{
"name": "font",
"type": "dc:Font"
}
],
"superClass": [
"di:Style"
]
}
],
"emumerations": [
{
"name": "ParticipantBandKind",
"literalValues": [
{
"name": "top_initiating"
},
{
"name": "middle_initiating"
},
{
"name": "bottom_initiating"
},
{
"name": "top_non_initiating"
},
{
"name": "middle_non_initiating"
},
{
"name": "bottom_non_initiating"
}
]
},
{
"name": "MessageVisibleKind",
"literalValues": [
{
"name": "initiating"
},
{
"name": "non_initiating"
}
]
}
],
"associations": [],
"prefix": "bpmndi"
}
},{}],22:[function(_dereq_,module,exports){
module.exports={
"name": "DC",
"uri": "http://www.omg.org/spec/DD/20100524/DC",
"types": [
{
"name": "Boolean"
},
{
"name": "Integer"
},
{
"name": "Real"
},
{
"name": "String"
},
{
"name": "Font",
"properties": [
{
"name": "name",
"type": "String",
"isAttr": true
},
{
"name": "size",
"type": "Real",
"isAttr": true
},
{
"name": "isBold",
"type": "Boolean",
"isAttr": true
},
{
"name": "isItalic",
"type": "Boolean",
"isAttr": true
},
{
"name": "isUnderline",
"type": "Boolean",
"isAttr": true
},
{
"name": "isStrikeThrough",
"type": "Boolean",
"isAttr": true
}
]
},
{
"name": "Point",
"properties": [
{
"name": "x",
"type": "Real",
"default": "0",
"isAttr": true
},
{
"name": "y",
"type": "Real",
"default": "0",
"isAttr": true
}
]
},
{
"name": "Bounds",
"properties": [
{
"name": "x",
"type": "Real",
"default": "0",
"isAttr": true
},
{
"name": "y",
"type": "Real",
"default": "0",
"isAttr": true
},
{
"name": "width",
"type": "Real",
"isAttr": true
},
{
"name": "height",
"type": "Real",
"isAttr": true
}
]
}
],
"prefix": "dc",
"associations": []
}
},{}],23:[function(_dereq_,module,exports){
module.exports={
"name": "DI",
"uri": "http://www.omg.org/spec/DD/20100524/DI",
"types": [
{
"name": "DiagramElement",
"isAbstract": true,
"properties": [
{
"name": "id",
"type": "String",
"isAttr": true,
"isId": true
},
{
"name": "extension",
"type": "Extension"
},
{
"name": "owningDiagram",
"type": "Diagram",
"isReadOnly": true,
"isVirtual": true,
"isReference": true
},
{
"name": "owningElement",
"type": "DiagramElement",
"isReadOnly": true,
"isVirtual": true,
"isReference": true
},
{
"name": "modelElement",
"isReadOnly": true,
"isVirtual": true,
"isReference": true,
"type": "Element"
},
{
"name": "style",
"type": "Style",
"isReadOnly": true,
"isVirtual": true,
"isReference": true
},
{
"name": "ownedElement",
"type": "DiagramElement",
"isReadOnly": true,
"isVirtual": true,
"isMany": true
}
]
},
{
"name": "Node",
"isAbstract": true,
"superClass": [
"DiagramElement"
]
},
{
"name": "Edge",
"isAbstract": true,
"superClass": [
"DiagramElement"
],
"properties": [
{
"name": "source",
"type": "DiagramElement",
"isReadOnly": true,
"isVirtual": true,
"isReference": true
},
{
"name": "target",
"type": "DiagramElement",
"isReadOnly": true,
"isVirtual": true,
"isReference": true
},
{
"name": "waypoint",
"isUnique": false,
"isMany": true,
"type": "dc:Point",
"xml": {
"serialize": "xsi:type"
}
}
]
},
{
"name": "Diagram",
"isAbstract": true,
"properties": [
{
"name": "id",
"type": "String",
"isAttr": true,
"isId": true
},
{
"name": "rootElement",
"type": "DiagramElement",
"isReadOnly": true,
"isVirtual": true
},
{
"name": "name",
"isAttr": true,
"type": "String"
},
{
"name": "documentation",
"isAttr": true,
"type": "String"
},
{
"name": "resolution",
"isAttr": true,
"type": "Real"
},
{
"name": "ownedStyle",
"type": "Style",
"isReadOnly": true,
"isVirtual": true,
"isMany": true
}
]
},
{
"name": "Shape",
"isAbstract": true,
"superClass": [
"Node"
],
"properties": [
{
"name": "bounds",
"type": "dc:Bounds"
}
]
},
{
"name": "Plane",
"isAbstract": true,
"superClass": [
"Node"
],
"properties": [
{
"name": "planeElement",
"type": "DiagramElement",
"subsettedProperty": "DiagramElement-ownedElement",
"isMany": true
}
]
},
{
"name": "LabeledEdge",
"isAbstract": true,
"superClass": [
"Edge"
],
"properties": [
{
"name": "ownedLabel",
"type": "Label",
"isReadOnly": true,
"subsettedProperty": "DiagramElement-ownedElement",
"isVirtual": true,
"isMany": true
}
]
},
{
"name": "LabeledShape",
"isAbstract": true,
"superClass": [
"Shape"
],
"properties": [
{
"name": "ownedLabel",
"type": "Label",
"isReadOnly": true,
"subsettedProperty": "DiagramElement-ownedElement",
"isVirtual": true,
"isMany": true
}
]
},
{
"name": "Label",
"isAbstract": true,
"superClass": [
"Node"
],
"properties": [
{
"name": "bounds",
"type": "dc:Bounds"
}
]
},
{
"name": "Style",
"isAbstract": true,
"properties": [
{
"name": "id",
"type": "String",
"isAttr": true,
"isId": true
}
]
},
{
"name": "Extension",
"properties": [
{
"name": "values",
"type": "Element",
"isMany": true
}
]
}
],
"associations": [],
"prefix": "di",
"xml": {
"tagAlias": "lowerCase"
}
}
},{}],24:[function(_dereq_,module,exports){
var matches = _dereq_(27)
module.exports = function (element, selector, checkYoSelf, root) {
element = checkYoSelf ? {parentNode: element} : element
root = root || document
// Make sure `element !== document` and `element != null`
// otherwise we get an illegal invocation
while ((element = element.parentNode) && element !== document) {
if (matches(element, selector))
return element
// After `matches` on the edge case that
// the selector matches the root
// (when the root is not the document)
if (element === root)
return
}
}
},{"27":27}],25:[function(_dereq_,module,exports){
/**
* Module dependencies.
*/
try {
var closest = _dereq_(24);
} catch(err) {
var closest = _dereq_(24);
}
try {
var event = _dereq_(26);
} catch(err) {
var event = _dereq_(26);
}
/**
* Delegate event `type` to `selector`
* and invoke `fn(e)`. A callback function
* is returned which may be passed to `.unbind()`.
*
* @param {Element} el
* @param {String} selector
* @param {String} type
* @param {Function} fn
* @param {Boolean} capture
* @return {Function}
* @api public
*/
exports.bind = function(el, selector, type, fn, capture){
return event.bind(el, type, function(e){
var target = e.target || e.srcElement;
e.delegateTarget = closest(target, selector, true, el);
if (e.delegateTarget) fn.call(el, e);
}, capture);
};
/**
* Unbind event `type`'s callback `fn`.
*
* @param {Element} el
* @param {String} type
* @param {Function} fn
* @param {Boolean} capture
* @api public
*/
exports.unbind = function(el, type, fn, capture){
event.unbind(el, type, fn, capture);
};
},{"24":24,"26":26}],26:[function(_dereq_,module,exports){
var bind = window.addEventListener ? 'addEventListener' : 'attachEvent',
unbind = window.removeEventListener ? 'removeEventListener' : 'detachEvent',
prefix = bind !== 'addEventListener' ? 'on' : '';
/**
* Bind `el` event `type` to `fn`.
*
* @param {Element} el
* @param {String} type
* @param {Function} fn
* @param {Boolean} capture
* @return {Function}
* @api public
*/
exports.bind = function(el, type, fn, capture){
el[bind](prefix + type, fn, capture || false);
return fn;
};
/**
* Unbind `el` event `type`'s callback `fn`.
*
* @param {Element} el
* @param {String} type
* @param {Function} fn
* @param {Boolean} capture
* @return {Function}
* @api public
*/
exports.unbind = function(el, type, fn, capture){
el[unbind](prefix + type, fn, capture || false);
return fn;
};
},{}],27:[function(_dereq_,module,exports){
/**
* Module dependencies.
*/
try {
var query = _dereq_(28);
} catch (err) {
var query = _dereq_(28);
}
/**
* Element prototype.
*/
var proto = Element.prototype;
/**
* Vendor function.
*/
var vendor = proto.matches
|| proto.webkitMatchesSelector
|| proto.mozMatchesSelector
|| proto.msMatchesSelector
|| proto.oMatchesSelector;
/**
* Expose `match()`.
*/
module.exports = match;
/**
* Match `el` to `selector`.
*
* @param {Element} el
* @param {String} selector
* @return {Boolean}
* @api public
*/
function match(el, selector) {
if (!el || el.nodeType !== 1) return false;
if (vendor) return vendor.call(el, selector);
var nodes = query.all(selector, el.parentNode);
for (var i = 0; i < nodes.length; ++i) {
if (nodes[i] == el) return true;
}
return false;
}
},{"28":28}],28:[function(_dereq_,module,exports){
function one(selector, el) {
return el.querySelector(selector);
}
exports = module.exports = function(selector, el){
el = el || document;
return one(selector, el);
};
exports.all = function(selector, el){
el = el || document;
return el.querySelectorAll(selector);
};
exports.engine = function(obj){
if (!obj.one) throw new Error('.one callback required');
if (!obj.all) throw new Error('.all callback required');
one = obj.one;
exports.all = obj.all;
return exports;
};
},{}],29:[function(_dereq_,module,exports){
/**
* Expose `parse`.
*/
module.exports = parse;
/**
* Tests for browser support.
*/
var innerHTMLBug = false;
var bugTestDiv;
if (typeof document !== 'undefined') {
bugTestDiv = document.createElement('div');
// Setup
bugTestDiv.innerHTML = '
a';
// Make sure that link elements get serialized correctly by innerHTML
// This requires a wrapper element in IE
innerHTMLBug = !bugTestDiv.getElementsByTagName('link').length;
bugTestDiv = undefined;
}
/**
* Wrap map from jquery.
*/
var map = {
legend: [1, ''],
tr: [2, '', '
'],
col: [2, '', '
'],
// for script/link/style tags to work in IE6-8, you have to wrap
// in a div with a non-whitespace character in front, ha!
_default: innerHTMLBug ? [1, 'X', ''] : [0, '', '']
};
map.td =
map.th = [3, '', '
'];
map.option =
map.optgroup = [1, ''];
map.thead =
map.tbody =
map.colgroup =
map.caption =
map.tfoot = [1, '', '
'];
map.polyline =
map.ellipse =
map.polygon =
map.circle =
map.text =
map.line =
map.path =
map.rect =
map.g = [1, ''];
/**
* Parse `html` and return a DOM Node instance, which could be a TextNode,
* HTML DOM Node of some kind ( for example), or a DocumentFragment
* instance, depending on the contents of the `html` string.
*
* @param {String} html - HTML string to "domify"
* @param {Document} doc - The `document` instance to create the Node for
* @return {DOMNode} the TextNode, DOM Node, or DocumentFragment instance
* @api private
*/
function parse(html, doc) {
if ('string' != typeof html) throw new TypeError('String expected');
// default to the global `document` object
if (!doc) doc = document;
// tag name
var m = /<([\w:]+)/.exec(html);
if (!m) return doc.createTextNode(html);
html = html.replace(/^\s+|\s+$/g, ''); // Remove leading/trailing whitespace
var tag = m[1];
// body support
if (tag == 'body') {
var el = doc.createElement('html');
el.innerHTML = html;
return el.removeChild(el.lastChild);
}
// wrap map
var wrap = map[tag] || map._default;
var depth = wrap[0];
var prefix = wrap[1];
var suffix = wrap[2];
var el = doc.createElement('div');
el.innerHTML = prefix + html + suffix;
while (depth--) el = el.lastChild;
// one element
if (el.firstChild == el.lastChild) {
return el.removeChild(el.firstChild);
}
// several elements
var fragment = doc.createDocumentFragment();
while (el.firstChild) {
fragment.appendChild(el.removeChild(el.firstChild));
}
return fragment;
}
},{}],30:[function(_dereq_,module,exports){
if (typeof Object.create === 'function') {
// implementation from standard node.js 'util' module
module.exports = function inherits(ctor, superCtor) {
ctor.super_ = superCtor
ctor.prototype = Object.create(superCtor.prototype, {
constructor: {
value: ctor,
enumerable: false,
writable: true,
configurable: true
}
});
};
} else {
// old school shim for old browsers
module.exports = function inherits(ctor, superCtor) {
ctor.super_ = superCtor
var TempCtor = function () {}
TempCtor.prototype = superCtor.prototype
ctor.prototype = new TempCtor()
ctor.prototype.constructor = ctor
}
}
},{}],31:[function(_dereq_,module,exports){
/**
* Gets the last element of `array`.
*
* @static
* @memberOf _
* @category Array
* @param {Array} array The array to query.
* @returns {*} Returns the last element of `array`.
* @example
*
* _.last([1, 2, 3]);
* // => 3
*/
function last(array) {
var length = array ? array.length : 0;
return length ? array[length - 1] : undefined;
}
module.exports = last;
},{}],32:[function(_dereq_,module,exports){
var arrayEvery = _dereq_(43),
baseCallback = _dereq_(51),
baseEvery = _dereq_(56),
isArray = _dereq_(108),
isIterateeCall = _dereq_(97);
/**
* Checks if `predicate` returns truthy for **all** elements of `collection`.
* The predicate is bound to `thisArg` and invoked with three arguments:
* (value, index|key, collection).
*
* If a property name is provided for `predicate` the created `_.property`
* style callback returns the property value of the given element.
*
* If a value is also provided for `thisArg` the created `_.matchesProperty`
* style callback returns `true` for elements that have a matching property
* value, else `false`.
*
* If an object is provided for `predicate` the created `_.matches` style
* callback returns `true` for elements that have the properties of the given
* object, else `false`.
*
* @static
* @memberOf _
* @alias all
* @category Collection
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function|Object|string} [predicate=_.identity] The function invoked
* per iteration.
* @param {*} [thisArg] The `this` binding of `predicate`.
* @returns {boolean} Returns `true` if all elements pass the predicate check,
* else `false`.
* @example
*
* _.every([true, 1, null, 'yes'], Boolean);
* // => false
*
* var users = [
* { 'user': 'barney', 'active': false },
* { 'user': 'fred', 'active': false }
* ];
*
* // using the `_.matches` callback shorthand
* _.every(users, { 'user': 'barney', 'active': false });
* // => false
*
* // using the `_.matchesProperty` callback shorthand
* _.every(users, 'active', false);
* // => true
*
* // using the `_.property` callback shorthand
* _.every(users, 'active');
* // => false
*/
function every(collection, predicate, thisArg) {
var func = isArray(collection) ? arrayEvery : baseEvery;
if (thisArg && isIterateeCall(collection, predicate, thisArg)) {
predicate = undefined;
}
if (typeof predicate != 'function' || thisArg !== undefined) {
predicate = baseCallback(predicate, thisArg, 3);
}
return func(collection, predicate);
}
module.exports = every;
},{"108":108,"43":43,"51":51,"56":56,"97":97}],33:[function(_dereq_,module,exports){
var arrayFilter = _dereq_(44),
baseCallback = _dereq_(51),
baseFilter = _dereq_(57),
isArray = _dereq_(108);
/**
* Iterates over elements of `collection`, returning an array of all elements
* `predicate` returns truthy for. The predicate is bound to `thisArg` and
* invoked with three arguments: (value, index|key, collection).
*
* If a property name is provided for `predicate` the created `_.property`
* style callback returns the property value of the given element.
*
* If a value is also provided for `thisArg` the created `_.matchesProperty`
* style callback returns `true` for elements that have a matching property
* value, else `false`.
*
* If an object is provided for `predicate` the created `_.matches` style
* callback returns `true` for elements that have the properties of the given
* object, else `false`.
*
* @static
* @memberOf _
* @alias select
* @category Collection
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function|Object|string} [predicate=_.identity] The function invoked
* per iteration.
* @param {*} [thisArg] The `this` binding of `predicate`.
* @returns {Array} Returns the new filtered array.
* @example
*
* _.filter([4, 5, 6], function(n) {
* return n % 2 == 0;
* });
* // => [4, 6]
*
* var users = [
* { 'user': 'barney', 'age': 36, 'active': true },
* { 'user': 'fred', 'age': 40, 'active': false }
* ];
*
* // using the `_.matches` callback shorthand
* _.pluck(_.filter(users, { 'age': 36, 'active': true }), 'user');
* // => ['barney']
*
* // using the `_.matchesProperty` callback shorthand
* _.pluck(_.filter(users, 'active', false), 'user');
* // => ['fred']
*
* // using the `_.property` callback shorthand
* _.pluck(_.filter(users, 'active'), 'user');
* // => ['barney']
*/
function filter(collection, predicate, thisArg) {
var func = isArray(collection) ? arrayFilter : baseFilter;
predicate = baseCallback(predicate, thisArg, 3);
return func(collection, predicate);
}
module.exports = filter;
},{"108":108,"44":44,"51":51,"57":57}],34:[function(_dereq_,module,exports){
var baseEach = _dereq_(55),
createFind = _dereq_(85);
/**
* Iterates over elements of `collection`, returning the first element
* `predicate` returns truthy for. The predicate is bound to `thisArg` and
* invoked with three arguments: (value, index|key, collection).
*
* If a property name is provided for `predicate` the created `_.property`
* style callback returns the property value of the given element.
*
* If a value is also provided for `thisArg` the created `_.matchesProperty`
* style callback returns `true` for elements that have a matching property
* value, else `false`.
*
* If an object is provided for `predicate` the created `_.matches` style
* callback returns `true` for elements that have the properties of the given
* object, else `false`.
*
* @static
* @memberOf _
* @alias detect
* @category Collection
* @param {Array|Object|string} collection The collection to search.
* @param {Function|Object|string} [predicate=_.identity] The function invoked
* per iteration.
* @param {*} [thisArg] The `this` binding of `predicate`.
* @returns {*} Returns the matched element, else `undefined`.
* @example
*
* var users = [
* { 'user': 'barney', 'age': 36, 'active': true },
* { 'user': 'fred', 'age': 40, 'active': false },
* { 'user': 'pebbles', 'age': 1, 'active': true }
* ];
*
* _.result(_.find(users, function(chr) {
* return chr.age < 40;
* }), 'user');
* // => 'barney'
*
* // using the `_.matches` callback shorthand
* _.result(_.find(users, { 'age': 1, 'active': true }), 'user');
* // => 'pebbles'
*
* // using the `_.matchesProperty` callback shorthand
* _.result(_.find(users, 'active', false), 'user');
* // => 'fred'
*
* // using the `_.property` callback shorthand
* _.result(_.find(users, 'active'), 'user');
* // => 'barney'
*/
var find = createFind(baseEach);
module.exports = find;
},{"55":55,"85":85}],35:[function(_dereq_,module,exports){
var arrayEach = _dereq_(42),
baseEach = _dereq_(55),
createForEach = _dereq_(86);
/**
* Iterates over elements of `collection` invoking `iteratee` for each element.
* The `iteratee` is bound to `thisArg` and invoked with three arguments:
* (value, index|key, collection). Iteratee functions may exit iteration early
* by explicitly returning `false`.
*
* **Note:** As with other "Collections" methods, objects with a "length" property
* are iterated like arrays. To avoid this behavior `_.forIn` or `_.forOwn`
* may be used for object iteration.
*
* @static
* @memberOf _
* @alias each
* @category Collection
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function} [iteratee=_.identity] The function invoked per iteration.
* @param {*} [thisArg] The `this` binding of `iteratee`.
* @returns {Array|Object|string} Returns `collection`.
* @example
*
* _([1, 2]).forEach(function(n) {
* console.log(n);
* }).value();
* // => logs each value from left to right and returns the array
*
* _.forEach({ 'a': 1, 'b': 2 }, function(n, key) {
* console.log(n, key);
* });
* // => logs each value-key pair and returns the object (iteration order is not guaranteed)
*/
var forEach = createForEach(arrayEach, baseEach);
module.exports = forEach;
},{"42":42,"55":55,"86":86}],36:[function(_dereq_,module,exports){
var arrayMap = _dereq_(45),
baseCallback = _dereq_(51),
baseMap = _dereq_(69),
isArray = _dereq_(108);
/**
* Creates an array of values by running each element in `collection` through
* `iteratee`. The `iteratee` is bound to `thisArg` and invoked with three
* arguments: (value, index|key, collection).
*
* If a property name is provided for `iteratee` the created `_.property`
* style callback returns the property value of the given element.
*
* If a value is also provided for `thisArg` the created `_.matchesProperty`
* style callback returns `true` for elements that have a matching property
* value, else `false`.
*
* If an object is provided for `iteratee` the created `_.matches` style
* callback returns `true` for elements that have the properties of the given
* object, else `false`.
*
* Many lodash methods are guarded to work as iteratees for methods like
* `_.every`, `_.filter`, `_.map`, `_.mapValues`, `_.reject`, and `_.some`.
*
* The guarded methods are:
* `ary`, `callback`, `chunk`, `clone`, `create`, `curry`, `curryRight`,
* `drop`, `dropRight`, `every`, `fill`, `flatten`, `invert`, `max`, `min`,
* `parseInt`, `slice`, `sortBy`, `take`, `takeRight`, `template`, `trim`,
* `trimLeft`, `trimRight`, `trunc`, `random`, `range`, `sample`, `some`,
* `sum`, `uniq`, and `words`
*
* @static
* @memberOf _
* @alias collect
* @category Collection
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function|Object|string} [iteratee=_.identity] The function invoked
* per iteration.
* @param {*} [thisArg] The `this` binding of `iteratee`.
* @returns {Array} Returns the new mapped array.
* @example
*
* function timesThree(n) {
* return n * 3;
* }
*
* _.map([1, 2], timesThree);
* // => [3, 6]
*
* _.map({ 'a': 1, 'b': 2 }, timesThree);
* // => [3, 6] (iteration order is not guaranteed)
*
* var users = [
* { 'user': 'barney' },
* { 'user': 'fred' }
* ];
*
* // using the `_.property` callback shorthand
* _.map(users, 'user');
* // => ['barney', 'fred']
*/
function map(collection, iteratee, thisArg) {
var func = isArray(collection) ? arrayMap : baseMap;
iteratee = baseCallback(iteratee, thisArg, 3);
return func(collection, iteratee);
}
module.exports = map;
},{"108":108,"45":45,"51":51,"69":69}],37:[function(_dereq_,module,exports){
var arrayReduce = _dereq_(47),
baseEach = _dereq_(55),
createReduce = _dereq_(87);
/**
* Reduces `collection` to a value which is the accumulated result of running
* each element in `collection` through `iteratee`, where each successive
* invocation is supplied the return value of the previous. If `accumulator`
* is not provided the first element of `collection` is used as the initial
* value. The `iteratee` is bound to `thisArg` and invoked with four arguments:
* (accumulator, value, index|key, collection).
*
* Many lodash methods are guarded to work as iteratees for methods like
* `_.reduce`, `_.reduceRight`, and `_.transform`.
*
* The guarded methods are:
* `assign`, `defaults`, `defaultsDeep`, `includes`, `merge`, `sortByAll`,
* and `sortByOrder`
*
* @static
* @memberOf _
* @alias foldl, inject
* @category Collection
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function} [iteratee=_.identity] The function invoked per iteration.
* @param {*} [accumulator] The initial value.
* @param {*} [thisArg] The `this` binding of `iteratee`.
* @returns {*} Returns the accumulated value.
* @example
*
* _.reduce([1, 2], function(total, n) {
* return total + n;
* });
* // => 3
*
* _.reduce({ 'a': 1, 'b': 2 }, function(result, n, key) {
* result[key] = n * 3;
* return result;
* }, {});
* // => { 'a': 3, 'b': 6 } (iteration order is not guaranteed)
*/
var reduce = createReduce(arrayReduce, baseEach);
module.exports = reduce;
},{"47":47,"55":55,"87":87}],38:[function(_dereq_,module,exports){
var arraySome = _dereq_(48),
baseCallback = _dereq_(51),
baseSome = _dereq_(76),
isArray = _dereq_(108),
isIterateeCall = _dereq_(97);
/**
* Checks if `predicate` returns truthy for **any** element of `collection`.
* The function returns as soon as it finds a passing value and does not iterate
* over the entire collection. The predicate is bound to `thisArg` and invoked
* with three arguments: (value, index|key, collection).
*
* If a property name is provided for `predicate` the created `_.property`
* style callback returns the property value of the given element.
*
* If a value is also provided for `thisArg` the created `_.matchesProperty`
* style callback returns `true` for elements that have a matching property
* value, else `false`.
*
* If an object is provided for `predicate` the created `_.matches` style
* callback returns `true` for elements that have the properties of the given
* object, else `false`.
*
* @static
* @memberOf _
* @alias any
* @category Collection
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function|Object|string} [predicate=_.identity] The function invoked
* per iteration.
* @param {*} [thisArg] The `this` binding of `predicate`.
* @returns {boolean} Returns `true` if any element passes the predicate check,
* else `false`.
* @example
*
* _.some([null, 0, 'yes', false], Boolean);
* // => true
*
* var users = [
* { 'user': 'barney', 'active': true },
* { 'user': 'fred', 'active': false }
* ];
*
* // using the `_.matches` callback shorthand
* _.some(users, { 'user': 'barney', 'active': false });
* // => false
*
* // using the `_.matchesProperty` callback shorthand
* _.some(users, 'active', false);
* // => true
*
* // using the `_.property` callback shorthand
* _.some(users, 'active');
* // => true
*/
function some(collection, predicate, thisArg) {
var func = isArray(collection) ? arraySome : baseSome;
if (thisArg && isIterateeCall(collection, predicate, thisArg)) {
predicate = undefined;
}
if (typeof predicate != 'function' || thisArg !== undefined) {
predicate = baseCallback(predicate, thisArg, 3);
}
return func(collection, predicate);
}
module.exports = some;
},{"108":108,"48":48,"51":51,"76":76,"97":97}],39:[function(_dereq_,module,exports){
var baseDelay = _dereq_(53),
restParam = _dereq_(40);
/**
* Defers invoking the `func` until the current call stack has cleared. Any
* additional arguments are provided to `func` when it's invoked.
*
* @static
* @memberOf _
* @category Function
* @param {Function} func The function to defer.
* @param {...*} [args] The arguments to invoke the function with.
* @returns {number} Returns the timer id.
* @example
*
* _.defer(function(text) {
* console.log(text);
* }, 'deferred');
* // logs 'deferred' after one or more milliseconds
*/
var defer = restParam(function(func, args) {
return baseDelay(func, 1, args);
});
module.exports = defer;
},{"40":40,"53":53}],40:[function(_dereq_,module,exports){
/** Used as the `TypeError` message for "Functions" methods. */
var FUNC_ERROR_TEXT = 'Expected a function';
/* Native method references for those with the same name as other `lodash` methods. */
var nativeMax = Math.max;
/**
* Creates a function that invokes `func` with the `this` binding of the
* created function and arguments from `start` and beyond provided as an array.
*
* **Note:** This method is based on the [rest parameter](https://developer.mozilla.org/Web/JavaScript/Reference/Functions/rest_parameters).
*
* @static
* @memberOf _
* @category Function
* @param {Function} func The function to apply a rest parameter to.
* @param {number} [start=func.length-1] The start position of the rest parameter.
* @returns {Function} Returns the new function.
* @example
*
* var say = _.restParam(function(what, names) {
* return what + ' ' + _.initial(names).join(', ') +
* (_.size(names) > 1 ? ', & ' : '') + _.last(names);
* });
*
* say('hello', 'fred', 'barney', 'pebbles');
* // => 'hello fred, barney, & pebbles'
*/
function restParam(func, start) {
if (typeof func != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
start = nativeMax(start === undefined ? (func.length - 1) : (+start || 0), 0);
return function() {
var args = arguments,
index = -1,
length = nativeMax(args.length - start, 0),
rest = Array(length);
while (++index < length) {
rest[index] = args[start + index];
}
switch (start) {
case 0: return func.call(this, rest);
case 1: return func.call(this, args[0], rest);
case 2: return func.call(this, args[0], args[1], rest);
}
var otherArgs = Array(start + 1);
index = -1;
while (++index < start) {
otherArgs[index] = args[index];
}
otherArgs[start] = rest;
return func.apply(this, otherArgs);
};
}
module.exports = restParam;
},{}],41:[function(_dereq_,module,exports){
(function (global){
var cachePush = _dereq_(80),
getNative = _dereq_(93);
/** Native method references. */
var Set = getNative(global, 'Set');
/* Native method references for those with the same name as other `lodash` methods. */
var nativeCreate = getNative(Object, 'create');
/**
*
* Creates a cache object to store unique values.
*
* @private
* @param {Array} [values] The values to cache.
*/
function SetCache(values) {
var length = values ? values.length : 0;
this.data = { 'hash': nativeCreate(null), 'set': new Set };
while (length--) {
this.push(values[length]);
}
}
// Add functions to the `Set` cache.
SetCache.prototype.push = cachePush;
module.exports = SetCache;
}).call(this,typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {})
},{"80":80,"93":93}],42:[function(_dereq_,module,exports){
/**
* A specialized version of `_.forEach` for arrays without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Array} array The array to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array} Returns `array`.
*/
function arrayEach(array, iteratee) {
var index = -1,
length = array.length;
while (++index < length) {
if (iteratee(array[index], index, array) === false) {
break;
}
}
return array;
}
module.exports = arrayEach;
},{}],43:[function(_dereq_,module,exports){
/**
* A specialized version of `_.every` for arrays without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Array} array The array to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {boolean} Returns `true` if all elements pass the predicate check,
* else `false`.
*/
function arrayEvery(array, predicate) {
var index = -1,
length = array.length;
while (++index < length) {
if (!predicate(array[index], index, array)) {
return false;
}
}
return true;
}
module.exports = arrayEvery;
},{}],44:[function(_dereq_,module,exports){
/**
* A specialized version of `_.filter` for arrays without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Array} array The array to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {Array} Returns the new filtered array.
*/
function arrayFilter(array, predicate) {
var index = -1,
length = array.length,
resIndex = -1,
result = [];
while (++index < length) {
var value = array[index];
if (predicate(value, index, array)) {
result[++resIndex] = value;
}
}
return result;
}
module.exports = arrayFilter;
},{}],45:[function(_dereq_,module,exports){
/**
* A specialized version of `_.map` for arrays without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Array} array The array to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array} Returns the new mapped array.
*/
function arrayMap(array, iteratee) {
var index = -1,
length = array.length,
result = Array(length);
while (++index < length) {
result[index] = iteratee(array[index], index, array);
}
return result;
}
module.exports = arrayMap;
},{}],46:[function(_dereq_,module,exports){
/**
* Appends the elements of `values` to `array`.
*
* @private
* @param {Array} array The array to modify.
* @param {Array} values The values to append.
* @returns {Array} Returns `array`.
*/
function arrayPush(array, values) {
var index = -1,
length = values.length,
offset = array.length;
while (++index < length) {
array[offset + index] = values[index];
}
return array;
}
module.exports = arrayPush;
},{}],47:[function(_dereq_,module,exports){
/**
* A specialized version of `_.reduce` for arrays without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Array} array The array to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @param {*} [accumulator] The initial value.
* @param {boolean} [initFromArray] Specify using the first element of `array`
* as the initial value.
* @returns {*} Returns the accumulated value.
*/
function arrayReduce(array, iteratee, accumulator, initFromArray) {
var index = -1,
length = array.length;
if (initFromArray && length) {
accumulator = array[++index];
}
while (++index < length) {
accumulator = iteratee(accumulator, array[index], index, array);
}
return accumulator;
}
module.exports = arrayReduce;
},{}],48:[function(_dereq_,module,exports){
/**
* A specialized version of `_.some` for arrays without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Array} array The array to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {boolean} Returns `true` if any element passes the predicate check,
* else `false`.
*/
function arraySome(array, predicate) {
var index = -1,
length = array.length;
while (++index < length) {
if (predicate(array[index], index, array)) {
return true;
}
}
return false;
}
module.exports = arraySome;
},{}],49:[function(_dereq_,module,exports){
var keys = _dereq_(116);
/**
* A specialized version of `_.assign` for customizing assigned values without
* support for argument juggling, multiple sources, and `this` binding `customizer`
* functions.
*
* @private
* @param {Object} object The destination object.
* @param {Object} source The source object.
* @param {Function} customizer The function to customize assigned values.
* @returns {Object} Returns `object`.
*/
function assignWith(object, source, customizer) {
var index = -1,
props = keys(source),
length = props.length;
while (++index < length) {
var key = props[index],
value = object[key],
result = customizer(value, source[key], key, object, source);
if ((result === result ? (result !== value) : (value === value)) ||
(value === undefined && !(key in object))) {
object[key] = result;
}
}
return object;
}
module.exports = assignWith;
},{"116":116}],50:[function(_dereq_,module,exports){
var baseCopy = _dereq_(52),
keys = _dereq_(116);
/**
* The base implementation of `_.assign` without support for argument juggling,
* multiple sources, and `customizer` functions.
*
* @private
* @param {Object} object The destination object.
* @param {Object} source The source object.
* @returns {Object} Returns `object`.
*/
function baseAssign(object, source) {
return source == null
? object
: baseCopy(source, keys(source), object);
}
module.exports = baseAssign;
},{"116":116,"52":52}],51:[function(_dereq_,module,exports){
var baseMatches = _dereq_(70),
baseMatchesProperty = _dereq_(71),
bindCallback = _dereq_(78),
identity = _dereq_(121),
property = _dereq_(122);
/**
* The base implementation of `_.callback` which supports specifying the
* number of arguments to provide to `func`.
*
* @private
* @param {*} [func=_.identity] The value to convert to a callback.
* @param {*} [thisArg] The `this` binding of `func`.
* @param {number} [argCount] The number of arguments to provide to `func`.
* @returns {Function} Returns the callback.
*/
function baseCallback(func, thisArg, argCount) {
var type = typeof func;
if (type == 'function') {
return thisArg === undefined
? func
: bindCallback(func, thisArg, argCount);
}
if (func == null) {
return identity;
}
if (type == 'object') {
return baseMatches(func);
}
return thisArg === undefined
? property(func)
: baseMatchesProperty(func, thisArg);
}
module.exports = baseCallback;
},{"121":121,"122":122,"70":70,"71":71,"78":78}],52:[function(_dereq_,module,exports){
/**
* Copies properties of `source` to `object`.
*
* @private
* @param {Object} source The object to copy properties from.
* @param {Array} props The property names to copy.
* @param {Object} [object={}] The object to copy properties to.
* @returns {Object} Returns `object`.
*/
function baseCopy(source, props, object) {
object || (object = {});
var index = -1,
length = props.length;
while (++index < length) {
var key = props[index];
object[key] = source[key];
}
return object;
}
module.exports = baseCopy;
},{}],53:[function(_dereq_,module,exports){
/** Used as the `TypeError` message for "Functions" methods. */
var FUNC_ERROR_TEXT = 'Expected a function';
/**
* The base implementation of `_.delay` and `_.defer` which accepts an index
* of where to slice the arguments to provide to `func`.
*
* @private
* @param {Function} func The function to delay.
* @param {number} wait The number of milliseconds to delay invocation.
* @param {Object} args The arguments provide to `func`.
* @returns {number} Returns the timer id.
*/
function baseDelay(func, wait, args) {
if (typeof func != 'function') {
throw new TypeError(FUNC_ERROR_TEXT);
}
return setTimeout(function() { func.apply(undefined, args); }, wait);
}
module.exports = baseDelay;
},{}],54:[function(_dereq_,module,exports){
var baseIndexOf = _dereq_(65),
cacheIndexOf = _dereq_(79),
createCache = _dereq_(84);
/** Used as the size to enable large array optimizations. */
var LARGE_ARRAY_SIZE = 200;
/**
* The base implementation of `_.difference` which accepts a single array
* of values to exclude.
*
* @private
* @param {Array} array The array to inspect.
* @param {Array} values The values to exclude.
* @returns {Array} Returns the new array of filtered values.
*/
function baseDifference(array, values) {
var length = array ? array.length : 0,
result = [];
if (!length) {
return result;
}
var index = -1,
indexOf = baseIndexOf,
isCommon = true,
cache = (isCommon && values.length >= LARGE_ARRAY_SIZE) ? createCache(values) : null,
valuesLength = values.length;
if (cache) {
indexOf = cacheIndexOf;
isCommon = false;
values = cache;
}
outer:
while (++index < length) {
var value = array[index];
if (isCommon && value === value) {
var valuesIndex = valuesLength;
while (valuesIndex--) {
if (values[valuesIndex] === value) {
continue outer;
}
}
result.push(value);
}
else if (indexOf(values, value, 0) < 0) {
result.push(value);
}
}
return result;
}
module.exports = baseDifference;
},{"65":65,"79":79,"84":84}],55:[function(_dereq_,module,exports){
var baseForOwn = _dereq_(63),
createBaseEach = _dereq_(82);
/**
* The base implementation of `_.forEach` without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array|Object|string} Returns `collection`.
*/
var baseEach = createBaseEach(baseForOwn);
module.exports = baseEach;
},{"63":63,"82":82}],56:[function(_dereq_,module,exports){
var baseEach = _dereq_(55);
/**
* The base implementation of `_.every` without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {boolean} Returns `true` if all elements pass the predicate check,
* else `false`
*/
function baseEvery(collection, predicate) {
var result = true;
baseEach(collection, function(value, index, collection) {
result = !!predicate(value, index, collection);
return result;
});
return result;
}
module.exports = baseEvery;
},{"55":55}],57:[function(_dereq_,module,exports){
var baseEach = _dereq_(55);
/**
* The base implementation of `_.filter` without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {Array} Returns the new filtered array.
*/
function baseFilter(collection, predicate) {
var result = [];
baseEach(collection, function(value, index, collection) {
if (predicate(value, index, collection)) {
result.push(value);
}
});
return result;
}
module.exports = baseFilter;
},{"55":55}],58:[function(_dereq_,module,exports){
/**
* The base implementation of `_.find`, `_.findLast`, `_.findKey`, and `_.findLastKey`,
* without support for callback shorthands and `this` binding, which iterates
* over `collection` using the provided `eachFunc`.
*
* @private
* @param {Array|Object|string} collection The collection to search.
* @param {Function} predicate The function invoked per iteration.
* @param {Function} eachFunc The function to iterate over `collection`.
* @param {boolean} [retKey] Specify returning the key of the found element
* instead of the element itself.
* @returns {*} Returns the found element or its key, else `undefined`.
*/
function baseFind(collection, predicate, eachFunc, retKey) {
var result;
eachFunc(collection, function(value, key, collection) {
if (predicate(value, key, collection)) {
result = retKey ? key : value;
return false;
}
});
return result;
}
module.exports = baseFind;
},{}],59:[function(_dereq_,module,exports){
/**
* The base implementation of `_.findIndex` and `_.findLastIndex` without
* support for callback shorthands and `this` binding.
*
* @private
* @param {Array} array The array to search.
* @param {Function} predicate The function invoked per iteration.
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {number} Returns the index of the matched value, else `-1`.
*/
function baseFindIndex(array, predicate, fromRight) {
var length = array.length,
index = fromRight ? length : -1;
while ((fromRight ? index-- : ++index < length)) {
if (predicate(array[index], index, array)) {
return index;
}
}
return -1;
}
module.exports = baseFindIndex;
},{}],60:[function(_dereq_,module,exports){
var arrayPush = _dereq_(46),
isArguments = _dereq_(107),
isArray = _dereq_(108),
isArrayLike = _dereq_(95),
isObjectLike = _dereq_(100);
/**
* The base implementation of `_.flatten` with added support for restricting
* flattening and specifying the start index.
*
* @private
* @param {Array} array The array to flatten.
* @param {boolean} [isDeep] Specify a deep flatten.
* @param {boolean} [isStrict] Restrict flattening to arrays-like objects.
* @param {Array} [result=[]] The initial result value.
* @returns {Array} Returns the new flattened array.
*/
function baseFlatten(array, isDeep, isStrict, result) {
result || (result = []);
var index = -1,
length = array.length;
while (++index < length) {
var value = array[index];
if (isObjectLike(value) && isArrayLike(value) &&
(isStrict || isArray(value) || isArguments(value))) {
if (isDeep) {
// Recursively flatten arrays (susceptible to call stack limits).
baseFlatten(value, isDeep, isStrict, result);
} else {
arrayPush(result, value);
}
} else if (!isStrict) {
result[result.length] = value;
}
}
return result;
}
module.exports = baseFlatten;
},{"100":100,"107":107,"108":108,"46":46,"95":95}],61:[function(_dereq_,module,exports){
var createBaseFor = _dereq_(83);
/**
* The base implementation of `baseForIn` and `baseForOwn` which iterates
* over `object` properties returned by `keysFunc` invoking `iteratee` for
* each property. Iteratee functions may exit iteration early by explicitly
* returning `false`.
*
* @private
* @param {Object} object The object to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @param {Function} keysFunc The function to get the keys of `object`.
* @returns {Object} Returns `object`.
*/
var baseFor = createBaseFor();
module.exports = baseFor;
},{"83":83}],62:[function(_dereq_,module,exports){
var baseFor = _dereq_(61),
keysIn = _dereq_(117);
/**
* The base implementation of `_.forIn` without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Object} object The object to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Object} Returns `object`.
*/
function baseForIn(object, iteratee) {
return baseFor(object, iteratee, keysIn);
}
module.exports = baseForIn;
},{"117":117,"61":61}],63:[function(_dereq_,module,exports){
var baseFor = _dereq_(61),
keys = _dereq_(116);
/**
* The base implementation of `_.forOwn` without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Object} object The object to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Object} Returns `object`.
*/
function baseForOwn(object, iteratee) {
return baseFor(object, iteratee, keys);
}
module.exports = baseForOwn;
},{"116":116,"61":61}],64:[function(_dereq_,module,exports){
var toObject = _dereq_(105);
/**
* The base implementation of `get` without support for string paths
* and default values.
*
* @private
* @param {Object} object The object to query.
* @param {Array} path The path of the property to get.
* @param {string} [pathKey] The key representation of path.
* @returns {*} Returns the resolved value.
*/
function baseGet(object, path, pathKey) {
if (object == null) {
return;
}
if (pathKey !== undefined && pathKey in toObject(object)) {
path = [pathKey];
}
var index = 0,
length = path.length;
while (object != null && index < length) {
object = object[path[index++]];
}
return (index && index == length) ? object : undefined;
}
module.exports = baseGet;
},{"105":105}],65:[function(_dereq_,module,exports){
var indexOfNaN = _dereq_(94);
/**
* The base implementation of `_.indexOf` without support for binary searches.
*
* @private
* @param {Array} array The array to search.
* @param {*} value The value to search for.
* @param {number} fromIndex The index to search from.
* @returns {number} Returns the index of the matched value, else `-1`.
*/
function baseIndexOf(array, value, fromIndex) {
if (value !== value) {
return indexOfNaN(array, fromIndex);
}
var index = fromIndex - 1,
length = array.length;
while (++index < length) {
if (array[index] === value) {
return index;
}
}
return -1;
}
module.exports = baseIndexOf;
},{"94":94}],66:[function(_dereq_,module,exports){
var baseIsEqualDeep = _dereq_(67),
isObject = _dereq_(112),
isObjectLike = _dereq_(100);
/**
* The base implementation of `_.isEqual` without support for `this` binding
* `customizer` functions.
*
* @private
* @param {*} value The value to compare.
* @param {*} other The other value to compare.
* @param {Function} [customizer] The function to customize comparing values.
* @param {boolean} [isLoose] Specify performing partial comparisons.
* @param {Array} [stackA] Tracks traversed `value` objects.
* @param {Array} [stackB] Tracks traversed `other` objects.
* @returns {boolean} Returns `true` if the values are equivalent, else `false`.
*/
function baseIsEqual(value, other, customizer, isLoose, stackA, stackB) {
if (value === other) {
return true;
}
if (value == null || other == null || (!isObject(value) && !isObjectLike(other))) {
return value !== value && other !== other;
}
return baseIsEqualDeep(value, other, baseIsEqual, customizer, isLoose, stackA, stackB);
}
module.exports = baseIsEqual;
},{"100":100,"112":112,"67":67}],67:[function(_dereq_,module,exports){
var equalArrays = _dereq_(88),
equalByTag = _dereq_(89),
equalObjects = _dereq_(90),
isArray = _dereq_(108),
isTypedArray = _dereq_(114);
/** `Object#toString` result references. */
var argsTag = '[object Arguments]',
arrayTag = '[object Array]',
objectTag = '[object Object]';
/** Used for native method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
* of values.
*/
var objToString = objectProto.toString;
/**
* A specialized version of `baseIsEqual` for arrays and objects which performs
* deep comparisons and tracks traversed objects enabling objects with circular
* references to be compared.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Function} [customizer] The function to customize comparing objects.
* @param {boolean} [isLoose] Specify performing partial comparisons.
* @param {Array} [stackA=[]] Tracks traversed `value` objects.
* @param {Array} [stackB=[]] Tracks traversed `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function baseIsEqualDeep(object, other, equalFunc, customizer, isLoose, stackA, stackB) {
var objIsArr = isArray(object),
othIsArr = isArray(other),
objTag = arrayTag,
othTag = arrayTag;
if (!objIsArr) {
objTag = objToString.call(object);
if (objTag == argsTag) {
objTag = objectTag;
} else if (objTag != objectTag) {
objIsArr = isTypedArray(object);
}
}
if (!othIsArr) {
othTag = objToString.call(other);
if (othTag == argsTag) {
othTag = objectTag;
} else if (othTag != objectTag) {
othIsArr = isTypedArray(other);
}
}
var objIsObj = objTag == objectTag,
othIsObj = othTag == objectTag,
isSameTag = objTag == othTag;
if (isSameTag && !(objIsArr || objIsObj)) {
return equalByTag(object, other, objTag);
}
if (!isLoose) {
var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
if (objIsWrapped || othIsWrapped) {
return equalFunc(objIsWrapped ? object.value() : object, othIsWrapped ? other.value() : other, customizer, isLoose, stackA, stackB);
}
}
if (!isSameTag) {
return false;
}
// Assume cyclic values are equal.
// For more information on detecting circular references see https://es5.github.io/#JO.
stackA || (stackA = []);
stackB || (stackB = []);
var length = stackA.length;
while (length--) {
if (stackA[length] == object) {
return stackB[length] == other;
}
}
// Add `object` and `other` to the stack of traversed objects.
stackA.push(object);
stackB.push(other);
var result = (objIsArr ? equalArrays : equalObjects)(object, other, equalFunc, customizer, isLoose, stackA, stackB);
stackA.pop();
stackB.pop();
return result;
}
module.exports = baseIsEqualDeep;
},{"108":108,"114":114,"88":88,"89":89,"90":90}],68:[function(_dereq_,module,exports){
var baseIsEqual = _dereq_(66),
toObject = _dereq_(105);
/**
* The base implementation of `_.isMatch` without support for callback
* shorthands and `this` binding.
*
* @private
* @param {Object} object The object to inspect.
* @param {Array} matchData The propery names, values, and compare flags to match.
* @param {Function} [customizer] The function to customize comparing objects.
* @returns {boolean} Returns `true` if `object` is a match, else `false`.
*/
function baseIsMatch(object, matchData, customizer) {
var index = matchData.length,
length = index,
noCustomizer = !customizer;
if (object == null) {
return !length;
}
object = toObject(object);
while (index--) {
var data = matchData[index];
if ((noCustomizer && data[2])
? data[1] !== object[data[0]]
: !(data[0] in object)
) {
return false;
}
}
while (++index < length) {
data = matchData[index];
var key = data[0],
objValue = object[key],
srcValue = data[1];
if (noCustomizer && data[2]) {
if (objValue === undefined && !(key in object)) {
return false;
}
} else {
var result = customizer ? customizer(objValue, srcValue, key) : undefined;
if (!(result === undefined ? baseIsEqual(srcValue, objValue, customizer, true) : result)) {
return false;
}
}
}
return true;
}
module.exports = baseIsMatch;
},{"105":105,"66":66}],69:[function(_dereq_,module,exports){
var baseEach = _dereq_(55),
isArrayLike = _dereq_(95);
/**
* The base implementation of `_.map` without support for callback shorthands
* and `this` binding.
*
* @private
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @returns {Array} Returns the new mapped array.
*/
function baseMap(collection, iteratee) {
var index = -1,
result = isArrayLike(collection) ? Array(collection.length) : [];
baseEach(collection, function(value, key, collection) {
result[++index] = iteratee(value, key, collection);
});
return result;
}
module.exports = baseMap;
},{"55":55,"95":95}],70:[function(_dereq_,module,exports){
var baseIsMatch = _dereq_(68),
getMatchData = _dereq_(92),
toObject = _dereq_(105);
/**
* The base implementation of `_.matches` which does not clone `source`.
*
* @private
* @param {Object} source The object of property values to match.
* @returns {Function} Returns the new function.
*/
function baseMatches(source) {
var matchData = getMatchData(source);
if (matchData.length == 1 && matchData[0][2]) {
var key = matchData[0][0],
value = matchData[0][1];
return function(object) {
if (object == null) {
return false;
}
return object[key] === value && (value !== undefined || (key in toObject(object)));
};
}
return function(object) {
return baseIsMatch(object, matchData);
};
}
module.exports = baseMatches;
},{"105":105,"68":68,"92":92}],71:[function(_dereq_,module,exports){
var baseGet = _dereq_(64),
baseIsEqual = _dereq_(66),
baseSlice = _dereq_(75),
isArray = _dereq_(108),
isKey = _dereq_(98),
isStrictComparable = _dereq_(101),
last = _dereq_(31),
toObject = _dereq_(105),
toPath = _dereq_(106);
/**
* The base implementation of `_.matchesProperty` which does not clone `srcValue`.
*
* @private
* @param {string} path The path of the property to get.
* @param {*} srcValue The value to compare.
* @returns {Function} Returns the new function.
*/
function baseMatchesProperty(path, srcValue) {
var isArr = isArray(path),
isCommon = isKey(path) && isStrictComparable(srcValue),
pathKey = (path + '');
path = toPath(path);
return function(object) {
if (object == null) {
return false;
}
var key = pathKey;
object = toObject(object);
if ((isArr || !isCommon) && !(key in object)) {
object = path.length == 1 ? object : baseGet(object, baseSlice(path, 0, -1));
if (object == null) {
return false;
}
key = last(path);
object = toObject(object);
}
return object[key] === srcValue
? (srcValue !== undefined || (key in object))
: baseIsEqual(srcValue, object[key], undefined, true);
};
}
module.exports = baseMatchesProperty;
},{"101":101,"105":105,"106":106,"108":108,"31":31,"64":64,"66":66,"75":75,"98":98}],72:[function(_dereq_,module,exports){
/**
* The base implementation of `_.property` without support for deep paths.
*
* @private
* @param {string} key The key of the property to get.
* @returns {Function} Returns the new function.
*/
function baseProperty(key) {
return function(object) {
return object == null ? undefined : object[key];
};
}
module.exports = baseProperty;
},{}],73:[function(_dereq_,module,exports){
var baseGet = _dereq_(64),
toPath = _dereq_(106);
/**
* A specialized version of `baseProperty` which supports deep paths.
*
* @private
* @param {Array|string} path The path of the property to get.
* @returns {Function} Returns the new function.
*/
function basePropertyDeep(path) {
var pathKey = (path + '');
path = toPath(path);
return function(object) {
return baseGet(object, path, pathKey);
};
}
module.exports = basePropertyDeep;
},{"106":106,"64":64}],74:[function(_dereq_,module,exports){
/**
* The base implementation of `_.reduce` and `_.reduceRight` without support
* for callback shorthands and `this` binding, which iterates over `collection`
* using the provided `eachFunc`.
*
* @private
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function} iteratee The function invoked per iteration.
* @param {*} accumulator The initial value.
* @param {boolean} initFromCollection Specify using the first or last element
* of `collection` as the initial value.
* @param {Function} eachFunc The function to iterate over `collection`.
* @returns {*} Returns the accumulated value.
*/
function baseReduce(collection, iteratee, accumulator, initFromCollection, eachFunc) {
eachFunc(collection, function(value, index, collection) {
accumulator = initFromCollection
? (initFromCollection = false, value)
: iteratee(accumulator, value, index, collection);
});
return accumulator;
}
module.exports = baseReduce;
},{}],75:[function(_dereq_,module,exports){
/**
* The base implementation of `_.slice` without an iteratee call guard.
*
* @private
* @param {Array} array The array to slice.
* @param {number} [start=0] The start position.
* @param {number} [end=array.length] The end position.
* @returns {Array} Returns the slice of `array`.
*/
function baseSlice(array, start, end) {
var index = -1,
length = array.length;
start = start == null ? 0 : (+start || 0);
if (start < 0) {
start = -start > length ? 0 : (length + start);
}
end = (end === undefined || end > length) ? length : (+end || 0);
if (end < 0) {
end += length;
}
length = start > end ? 0 : ((end - start) >>> 0);
start >>>= 0;
var result = Array(length);
while (++index < length) {
result[index] = array[index + start];
}
return result;
}
module.exports = baseSlice;
},{}],76:[function(_dereq_,module,exports){
var baseEach = _dereq_(55);
/**
* The base implementation of `_.some` without support for callback shorthands
* and `this` binding.
*
* @private
* @param {Array|Object|string} collection The collection to iterate over.
* @param {Function} predicate The function invoked per iteration.
* @returns {boolean} Returns `true` if any element passes the predicate check,
* else `false`.
*/
function baseSome(collection, predicate) {
var result;
baseEach(collection, function(value, index, collection) {
result = predicate(value, index, collection);
return !result;
});
return !!result;
}
module.exports = baseSome;
},{"55":55}],77:[function(_dereq_,module,exports){
/**
* Converts `value` to a string if it's not one. An empty string is returned
* for `null` or `undefined` values.
*
* @private
* @param {*} value The value to process.
* @returns {string} Returns the string.
*/
function baseToString(value) {
return value == null ? '' : (value + '');
}
module.exports = baseToString;
},{}],78:[function(_dereq_,module,exports){
var identity = _dereq_(121);
/**
* A specialized version of `baseCallback` which only supports `this` binding
* and specifying the number of arguments to provide to `func`.
*
* @private
* @param {Function} func The function to bind.
* @param {*} thisArg The `this` binding of `func`.
* @param {number} [argCount] The number of arguments to provide to `func`.
* @returns {Function} Returns the callback.
*/
function bindCallback(func, thisArg, argCount) {
if (typeof func != 'function') {
return identity;
}
if (thisArg === undefined) {
return func;
}
switch (argCount) {
case 1: return function(value) {
return func.call(thisArg, value);
};
case 3: return function(value, index, collection) {
return func.call(thisArg, value, index, collection);
};
case 4: return function(accumulator, value, index, collection) {
return func.call(thisArg, accumulator, value, index, collection);
};
case 5: return function(value, other, key, object, source) {
return func.call(thisArg, value, other, key, object, source);
};
}
return function() {
return func.apply(thisArg, arguments);
};
}
module.exports = bindCallback;
},{"121":121}],79:[function(_dereq_,module,exports){
var isObject = _dereq_(112);
/**
* Checks if `value` is in `cache` mimicking the return signature of
* `_.indexOf` by returning `0` if the value is found, else `-1`.
*
* @private
* @param {Object} cache The cache to search.
* @param {*} value The value to search for.
* @returns {number} Returns `0` if `value` is found, else `-1`.
*/
function cacheIndexOf(cache, value) {
var data = cache.data,
result = (typeof value == 'string' || isObject(value)) ? data.set.has(value) : data.hash[value];
return result ? 0 : -1;
}
module.exports = cacheIndexOf;
},{"112":112}],80:[function(_dereq_,module,exports){
var isObject = _dereq_(112);
/**
* Adds `value` to the cache.
*
* @private
* @name push
* @memberOf SetCache
* @param {*} value The value to cache.
*/
function cachePush(value) {
var data = this.data;
if (typeof value == 'string' || isObject(value)) {
data.set.add(value);
} else {
data.hash[value] = true;
}
}
module.exports = cachePush;
},{"112":112}],81:[function(_dereq_,module,exports){
var bindCallback = _dereq_(78),
isIterateeCall = _dereq_(97),
restParam = _dereq_(40);
/**
* Creates a `_.assign`, `_.defaults`, or `_.merge` function.
*
* @private
* @param {Function} assigner The function to assign values.
* @returns {Function} Returns the new assigner function.
*/
function createAssigner(assigner) {
return restParam(function(object, sources) {
var index = -1,
length = object == null ? 0 : sources.length,
customizer = length > 2 ? sources[length - 2] : undefined,
guard = length > 2 ? sources[2] : undefined,
thisArg = length > 1 ? sources[length - 1] : undefined;
if (typeof customizer == 'function') {
customizer = bindCallback(customizer, thisArg, 5);
length -= 2;
} else {
customizer = typeof thisArg == 'function' ? thisArg : undefined;
length -= (customizer ? 1 : 0);
}
if (guard && isIterateeCall(sources[0], sources[1], guard)) {
customizer = length < 3 ? undefined : customizer;
length = 1;
}
while (++index < length) {
var source = sources[index];
if (source) {
assigner(object, source, customizer);
}
}
return object;
});
}
module.exports = createAssigner;
},{"40":40,"78":78,"97":97}],82:[function(_dereq_,module,exports){
var getLength = _dereq_(91),
isLength = _dereq_(99),
toObject = _dereq_(105);
/**
* Creates a `baseEach` or `baseEachRight` function.
*
* @private
* @param {Function} eachFunc The function to iterate over a collection.
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {Function} Returns the new base function.
*/
function createBaseEach(eachFunc, fromRight) {
return function(collection, iteratee) {
var length = collection ? getLength(collection) : 0;
if (!isLength(length)) {
return eachFunc(collection, iteratee);
}
var index = fromRight ? length : -1,
iterable = toObject(collection);
while ((fromRight ? index-- : ++index < length)) {
if (iteratee(iterable[index], index, iterable) === false) {
break;
}
}
return collection;
};
}
module.exports = createBaseEach;
},{"105":105,"91":91,"99":99}],83:[function(_dereq_,module,exports){
var toObject = _dereq_(105);
/**
* Creates a base function for `_.forIn` or `_.forInRight`.
*
* @private
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {Function} Returns the new base function.
*/
function createBaseFor(fromRight) {
return function(object, iteratee, keysFunc) {
var iterable = toObject(object),
props = keysFunc(object),
length = props.length,
index = fromRight ? length : -1;
while ((fromRight ? index-- : ++index < length)) {
var key = props[index];
if (iteratee(iterable[key], key, iterable) === false) {
break;
}
}
return object;
};
}
module.exports = createBaseFor;
},{"105":105}],84:[function(_dereq_,module,exports){
(function (global){
var SetCache = _dereq_(41),
getNative = _dereq_(93);
/** Native method references. */
var Set = getNative(global, 'Set');
/* Native method references for those with the same name as other `lodash` methods. */
var nativeCreate = getNative(Object, 'create');
/**
* Creates a `Set` cache object to optimize linear searches of large arrays.
*
* @private
* @param {Array} [values] The values to cache.
* @returns {null|Object} Returns the new cache object if `Set` is supported, else `null`.
*/
function createCache(values) {
return (nativeCreate && Set) ? new SetCache(values) : null;
}
module.exports = createCache;
}).call(this,typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {})
},{"41":41,"93":93}],85:[function(_dereq_,module,exports){
var baseCallback = _dereq_(51),
baseFind = _dereq_(58),
baseFindIndex = _dereq_(59),
isArray = _dereq_(108);
/**
* Creates a `_.find` or `_.findLast` function.
*
* @private
* @param {Function} eachFunc The function to iterate over a collection.
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {Function} Returns the new find function.
*/
function createFind(eachFunc, fromRight) {
return function(collection, predicate, thisArg) {
predicate = baseCallback(predicate, thisArg, 3);
if (isArray(collection)) {
var index = baseFindIndex(collection, predicate, fromRight);
return index > -1 ? collection[index] : undefined;
}
return baseFind(collection, predicate, eachFunc);
};
}
module.exports = createFind;
},{"108":108,"51":51,"58":58,"59":59}],86:[function(_dereq_,module,exports){
var bindCallback = _dereq_(78),
isArray = _dereq_(108);
/**
* Creates a function for `_.forEach` or `_.forEachRight`.
*
* @private
* @param {Function} arrayFunc The function to iterate over an array.
* @param {Function} eachFunc The function to iterate over a collection.
* @returns {Function} Returns the new each function.
*/
function createForEach(arrayFunc, eachFunc) {
return function(collection, iteratee, thisArg) {
return (typeof iteratee == 'function' && thisArg === undefined && isArray(collection))
? arrayFunc(collection, iteratee)
: eachFunc(collection, bindCallback(iteratee, thisArg, 3));
};
}
module.exports = createForEach;
},{"108":108,"78":78}],87:[function(_dereq_,module,exports){
var baseCallback = _dereq_(51),
baseReduce = _dereq_(74),
isArray = _dereq_(108);
/**
* Creates a function for `_.reduce` or `_.reduceRight`.
*
* @private
* @param {Function} arrayFunc The function to iterate over an array.
* @param {Function} eachFunc The function to iterate over a collection.
* @returns {Function} Returns the new each function.
*/
function createReduce(arrayFunc, eachFunc) {
return function(collection, iteratee, accumulator, thisArg) {
var initFromArray = arguments.length < 3;
return (typeof iteratee == 'function' && thisArg === undefined && isArray(collection))
? arrayFunc(collection, iteratee, accumulator, initFromArray)
: baseReduce(collection, baseCallback(iteratee, thisArg, 4), accumulator, initFromArray, eachFunc);
};
}
module.exports = createReduce;
},{"108":108,"51":51,"74":74}],88:[function(_dereq_,module,exports){
var arraySome = _dereq_(48);
/**
* A specialized version of `baseIsEqualDeep` for arrays with support for
* partial deep comparisons.
*
* @private
* @param {Array} array The array to compare.
* @param {Array} other The other array to compare.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Function} [customizer] The function to customize comparing arrays.
* @param {boolean} [isLoose] Specify performing partial comparisons.
* @param {Array} [stackA] Tracks traversed `value` objects.
* @param {Array} [stackB] Tracks traversed `other` objects.
* @returns {boolean} Returns `true` if the arrays are equivalent, else `false`.
*/
function equalArrays(array, other, equalFunc, customizer, isLoose, stackA, stackB) {
var index = -1,
arrLength = array.length,
othLength = other.length;
if (arrLength != othLength && !(isLoose && othLength > arrLength)) {
return false;
}
// Ignore non-index properties.
while (++index < arrLength) {
var arrValue = array[index],
othValue = other[index],
result = customizer ? customizer(isLoose ? othValue : arrValue, isLoose ? arrValue : othValue, index) : undefined;
if (result !== undefined) {
if (result) {
continue;
}
return false;
}
// Recursively compare arrays (susceptible to call stack limits).
if (isLoose) {
if (!arraySome(other, function(othValue) {
return arrValue === othValue || equalFunc(arrValue, othValue, customizer, isLoose, stackA, stackB);
})) {
return false;
}
} else if (!(arrValue === othValue || equalFunc(arrValue, othValue, customizer, isLoose, stackA, stackB))) {
return false;
}
}
return true;
}
module.exports = equalArrays;
},{"48":48}],89:[function(_dereq_,module,exports){
/** `Object#toString` result references. */
var boolTag = '[object Boolean]',
dateTag = '[object Date]',
errorTag = '[object Error]',
numberTag = '[object Number]',
regexpTag = '[object RegExp]',
stringTag = '[object String]';
/**
* A specialized version of `baseIsEqualDeep` for comparing objects of
* the same `toStringTag`.
*
* **Note:** This function only supports comparing values with tags of
* `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {string} tag The `toStringTag` of the objects to compare.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function equalByTag(object, other, tag) {
switch (tag) {
case boolTag:
case dateTag:
// Coerce dates and booleans to numbers, dates to milliseconds and booleans
// to `1` or `0` treating invalid dates coerced to `NaN` as not equal.
return +object == +other;
case errorTag:
return object.name == other.name && object.message == other.message;
case numberTag:
// Treat `NaN` vs. `NaN` as equal.
return (object != +object)
? other != +other
: object == +other;
case regexpTag:
case stringTag:
// Coerce regexes to strings and treat strings primitives and string
// objects as equal. See https://es5.github.io/#x15.10.6.4 for more details.
return object == (other + '');
}
return false;
}
module.exports = equalByTag;
},{}],90:[function(_dereq_,module,exports){
var keys = _dereq_(116);
/** Used for native method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* A specialized version of `baseIsEqualDeep` for objects with support for
* partial deep comparisons.
*
* @private
* @param {Object} object The object to compare.
* @param {Object} other The other object to compare.
* @param {Function} equalFunc The function to determine equivalents of values.
* @param {Function} [customizer] The function to customize comparing values.
* @param {boolean} [isLoose] Specify performing partial comparisons.
* @param {Array} [stackA] Tracks traversed `value` objects.
* @param {Array} [stackB] Tracks traversed `other` objects.
* @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
*/
function equalObjects(object, other, equalFunc, customizer, isLoose, stackA, stackB) {
var objProps = keys(object),
objLength = objProps.length,
othProps = keys(other),
othLength = othProps.length;
if (objLength != othLength && !isLoose) {
return false;
}
var index = objLength;
while (index--) {
var key = objProps[index];
if (!(isLoose ? key in other : hasOwnProperty.call(other, key))) {
return false;
}
}
var skipCtor = isLoose;
while (++index < objLength) {
key = objProps[index];
var objValue = object[key],
othValue = other[key],
result = customizer ? customizer(isLoose ? othValue : objValue, isLoose? objValue : othValue, key) : undefined;
// Recursively compare objects (susceptible to call stack limits).
if (!(result === undefined ? equalFunc(objValue, othValue, customizer, isLoose, stackA, stackB) : result)) {
return false;
}
skipCtor || (skipCtor = key == 'constructor');
}
if (!skipCtor) {
var objCtor = object.constructor,
othCtor = other.constructor;
// Non `Object` object instances with different constructors are not equal.
if (objCtor != othCtor &&
('constructor' in object && 'constructor' in other) &&
!(typeof objCtor == 'function' && objCtor instanceof objCtor &&
typeof othCtor == 'function' && othCtor instanceof othCtor)) {
return false;
}
}
return true;
}
module.exports = equalObjects;
},{"116":116}],91:[function(_dereq_,module,exports){
var baseProperty = _dereq_(72);
/**
* Gets the "length" property value of `object`.
*
* **Note:** This function is used to avoid a [JIT bug](https://bugs.webkit.org/show_bug.cgi?id=142792)
* that affects Safari on at least iOS 8.1-8.3 ARM64.
*
* @private
* @param {Object} object The object to query.
* @returns {*} Returns the "length" value.
*/
var getLength = baseProperty('length');
module.exports = getLength;
},{"72":72}],92:[function(_dereq_,module,exports){
var isStrictComparable = _dereq_(101),
pairs = _dereq_(119);
/**
* Gets the propery names, values, and compare flags of `object`.
*
* @private
* @param {Object} object The object to query.
* @returns {Array} Returns the match data of `object`.
*/
function getMatchData(object) {
var result = pairs(object),
length = result.length;
while (length--) {
result[length][2] = isStrictComparable(result[length][1]);
}
return result;
}
module.exports = getMatchData;
},{"101":101,"119":119}],93:[function(_dereq_,module,exports){
var isNative = _dereq_(110);
/**
* Gets the native function at `key` of `object`.
*
* @private
* @param {Object} object The object to query.
* @param {string} key The key of the method to get.
* @returns {*} Returns the function if it's native, else `undefined`.
*/
function getNative(object, key) {
var value = object == null ? undefined : object[key];
return isNative(value) ? value : undefined;
}
module.exports = getNative;
},{"110":110}],94:[function(_dereq_,module,exports){
/**
* Gets the index at which the first occurrence of `NaN` is found in `array`.
*
* @private
* @param {Array} array The array to search.
* @param {number} fromIndex The index to search from.
* @param {boolean} [fromRight] Specify iterating from right to left.
* @returns {number} Returns the index of the matched `NaN`, else `-1`.
*/
function indexOfNaN(array, fromIndex, fromRight) {
var length = array.length,
index = fromIndex + (fromRight ? 0 : -1);
while ((fromRight ? index-- : ++index < length)) {
var other = array[index];
if (other !== other) {
return index;
}
}
return -1;
}
module.exports = indexOfNaN;
},{}],95:[function(_dereq_,module,exports){
var getLength = _dereq_(91),
isLength = _dereq_(99);
/**
* Checks if `value` is array-like.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is array-like, else `false`.
*/
function isArrayLike(value) {
return value != null && isLength(getLength(value));
}
module.exports = isArrayLike;
},{"91":91,"99":99}],96:[function(_dereq_,module,exports){
/** Used to detect unsigned integer values. */
var reIsUint = /^\d+$/;
/**
* Used as the [maximum length](http://ecma-international.org/ecma-262/6.0/#sec-number.max_safe_integer)
* of an array-like value.
*/
var MAX_SAFE_INTEGER = 9007199254740991;
/**
* Checks if `value` is a valid array-like index.
*
* @private
* @param {*} value The value to check.
* @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
* @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
*/
function isIndex(value, length) {
value = (typeof value == 'number' || reIsUint.test(value)) ? +value : -1;
length = length == null ? MAX_SAFE_INTEGER : length;
return value > -1 && value % 1 == 0 && value < length;
}
module.exports = isIndex;
},{}],97:[function(_dereq_,module,exports){
var isArrayLike = _dereq_(95),
isIndex = _dereq_(96),
isObject = _dereq_(112);
/**
* Checks if the provided arguments are from an iteratee call.
*
* @private
* @param {*} value The potential iteratee value argument.
* @param {*} index The potential iteratee index or key argument.
* @param {*} object The potential iteratee object argument.
* @returns {boolean} Returns `true` if the arguments are from an iteratee call, else `false`.
*/
function isIterateeCall(value, index, object) {
if (!isObject(object)) {
return false;
}
var type = typeof index;
if (type == 'number'
? (isArrayLike(object) && isIndex(index, object.length))
: (type == 'string' && index in object)) {
var other = object[index];
return value === value ? (value === other) : (other !== other);
}
return false;
}
module.exports = isIterateeCall;
},{"112":112,"95":95,"96":96}],98:[function(_dereq_,module,exports){
var isArray = _dereq_(108),
toObject = _dereq_(105);
/** Used to match property names within property paths. */
var reIsDeepProp = /\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\n\\]|\\.)*?\1)\]/,
reIsPlainProp = /^\w*$/;
/**
* Checks if `value` is a property name and not a property path.
*
* @private
* @param {*} value The value to check.
* @param {Object} [object] The object to query keys on.
* @returns {boolean} Returns `true` if `value` is a property name, else `false`.
*/
function isKey(value, object) {
var type = typeof value;
if ((type == 'string' && reIsPlainProp.test(value)) || type == 'number') {
return true;
}
if (isArray(value)) {
return false;
}
var result = !reIsDeepProp.test(value);
return result || (object != null && value in toObject(object));
}
module.exports = isKey;
},{"105":105,"108":108}],99:[function(_dereq_,module,exports){
/**
* Used as the [maximum length](http://ecma-international.org/ecma-262/6.0/#sec-number.max_safe_integer)
* of an array-like value.
*/
var MAX_SAFE_INTEGER = 9007199254740991;
/**
* Checks if `value` is a valid array-like length.
*
* **Note:** This function is based on [`ToLength`](http://ecma-international.org/ecma-262/6.0/#sec-tolength).
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
*/
function isLength(value) {
return typeof value == 'number' && value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
}
module.exports = isLength;
},{}],100:[function(_dereq_,module,exports){
/**
* Checks if `value` is object-like.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is object-like, else `false`.
*/
function isObjectLike(value) {
return !!value && typeof value == 'object';
}
module.exports = isObjectLike;
},{}],101:[function(_dereq_,module,exports){
var isObject = _dereq_(112);
/**
* Checks if `value` is suitable for strict equality comparisons, i.e. `===`.
*
* @private
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` if suitable for strict
* equality comparisons, else `false`.
*/
function isStrictComparable(value) {
return value === value && !isObject(value);
}
module.exports = isStrictComparable;
},{"112":112}],102:[function(_dereq_,module,exports){
var toObject = _dereq_(105);
/**
* A specialized version of `_.pick` which picks `object` properties specified
* by `props`.
*
* @private
* @param {Object} object The source object.
* @param {string[]} props The property names to pick.
* @returns {Object} Returns the new object.
*/
function pickByArray(object, props) {
object = toObject(object);
var index = -1,
length = props.length,
result = {};
while (++index < length) {
var key = props[index];
if (key in object) {
result[key] = object[key];
}
}
return result;
}
module.exports = pickByArray;
},{"105":105}],103:[function(_dereq_,module,exports){
var baseForIn = _dereq_(62);
/**
* A specialized version of `_.pick` which picks `object` properties `predicate`
* returns truthy for.
*
* @private
* @param {Object} object The source object.
* @param {Function} predicate The function invoked per iteration.
* @returns {Object} Returns the new object.
*/
function pickByCallback(object, predicate) {
var result = {};
baseForIn(object, function(value, key, object) {
if (predicate(value, key, object)) {
result[key] = value;
}
});
return result;
}
module.exports = pickByCallback;
},{"62":62}],104:[function(_dereq_,module,exports){
var isArguments = _dereq_(107),
isArray = _dereq_(108),
isIndex = _dereq_(96),
isLength = _dereq_(99),
keysIn = _dereq_(117);
/** Used for native method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* A fallback implementation of `Object.keys` which creates an array of the
* own enumerable property names of `object`.
*
* @private
* @param {Object} object The object to query.
* @returns {Array} Returns the array of property names.
*/
function shimKeys(object) {
var props = keysIn(object),
propsLength = props.length,
length = propsLength && object.length;
var allowIndexes = !!length && isLength(length) &&
(isArray(object) || isArguments(object));
var index = -1,
result = [];
while (++index < propsLength) {
var key = props[index];
if ((allowIndexes && isIndex(key, length)) || hasOwnProperty.call(object, key)) {
result.push(key);
}
}
return result;
}
module.exports = shimKeys;
},{"107":107,"108":108,"117":117,"96":96,"99":99}],105:[function(_dereq_,module,exports){
var isObject = _dereq_(112);
/**
* Converts `value` to an object if it's not one.
*
* @private
* @param {*} value The value to process.
* @returns {Object} Returns the object.
*/
function toObject(value) {
return isObject(value) ? value : Object(value);
}
module.exports = toObject;
},{"112":112}],106:[function(_dereq_,module,exports){
var baseToString = _dereq_(77),
isArray = _dereq_(108);
/** Used to match property names within property paths. */
var rePropName = /[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\n\\]|\\.)*?)\2)\]/g;
/** Used to match backslashes in property paths. */
var reEscapeChar = /\\(\\)?/g;
/**
* Converts `value` to property path array if it's not one.
*
* @private
* @param {*} value The value to process.
* @returns {Array} Returns the property path array.
*/
function toPath(value) {
if (isArray(value)) {
return value;
}
var result = [];
baseToString(value).replace(rePropName, function(match, number, quote, string) {
result.push(quote ? string.replace(reEscapeChar, '$1') : (number || match));
});
return result;
}
module.exports = toPath;
},{"108":108,"77":77}],107:[function(_dereq_,module,exports){
var isArrayLike = _dereq_(95),
isObjectLike = _dereq_(100);
/** Used for native method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/** Native method references. */
var propertyIsEnumerable = objectProto.propertyIsEnumerable;
/**
* Checks if `value` is classified as an `arguments` object.
*
* @static
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
* @example
*
* _.isArguments(function() { return arguments; }());
* // => true
*
* _.isArguments([1, 2, 3]);
* // => false
*/
function isArguments(value) {
return isObjectLike(value) && isArrayLike(value) &&
hasOwnProperty.call(value, 'callee') && !propertyIsEnumerable.call(value, 'callee');
}
module.exports = isArguments;
},{"100":100,"95":95}],108:[function(_dereq_,module,exports){
var getNative = _dereq_(93),
isLength = _dereq_(99),
isObjectLike = _dereq_(100);
/** `Object#toString` result references. */
var arrayTag = '[object Array]';
/** Used for native method references. */
var objectProto = Object.prototype;
/**
* Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
* of values.
*/
var objToString = objectProto.toString;
/* Native method references for those with the same name as other `lodash` methods. */
var nativeIsArray = getNative(Array, 'isArray');
/**
* Checks if `value` is classified as an `Array` object.
*
* @static
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
* @example
*
* _.isArray([1, 2, 3]);
* // => true
*
* _.isArray(function() { return arguments; }());
* // => false
*/
var isArray = nativeIsArray || function(value) {
return isObjectLike(value) && isLength(value.length) && objToString.call(value) == arrayTag;
};
module.exports = isArray;
},{"100":100,"93":93,"99":99}],109:[function(_dereq_,module,exports){
var isObject = _dereq_(112);
/** `Object#toString` result references. */
var funcTag = '[object Function]';
/** Used for native method references. */
var objectProto = Object.prototype;
/**
* Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
* of values.
*/
var objToString = objectProto.toString;
/**
* Checks if `value` is classified as a `Function` object.
*
* @static
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
* @example
*
* _.isFunction(_);
* // => true
*
* _.isFunction(/abc/);
* // => false
*/
function isFunction(value) {
// The use of `Object#toString` avoids issues with the `typeof` operator
// in older versions of Chrome and Safari which return 'function' for regexes
// and Safari 8 which returns 'object' for typed array constructors.
return isObject(value) && objToString.call(value) == funcTag;
}
module.exports = isFunction;
},{"112":112}],110:[function(_dereq_,module,exports){
var isFunction = _dereq_(109),
isObjectLike = _dereq_(100);
/** Used to detect host constructors (Safari > 5). */
var reIsHostCtor = /^\[object .+?Constructor\]$/;
/** Used for native method references. */
var objectProto = Object.prototype;
/** Used to resolve the decompiled source of functions. */
var fnToString = Function.prototype.toString;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/** Used to detect if a method is native. */
var reIsNative = RegExp('^' +
fnToString.call(hasOwnProperty).replace(/[\\^$.*+?()[\]{}|]/g, '\\$&')
.replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
);
/**
* Checks if `value` is a native function.
*
* @static
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is a native function, else `false`.
* @example
*
* _.isNative(Array.prototype.push);
* // => true
*
* _.isNative(_);
* // => false
*/
function isNative(value) {
if (value == null) {
return false;
}
if (isFunction(value)) {
return reIsNative.test(fnToString.call(value));
}
return isObjectLike(value) && reIsHostCtor.test(value);
}
module.exports = isNative;
},{"100":100,"109":109}],111:[function(_dereq_,module,exports){
var isObjectLike = _dereq_(100);
/** `Object#toString` result references. */
var numberTag = '[object Number]';
/** Used for native method references. */
var objectProto = Object.prototype;
/**
* Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
* of values.
*/
var objToString = objectProto.toString;
/**
* Checks if `value` is classified as a `Number` primitive or object.
*
* **Note:** To exclude `Infinity`, `-Infinity`, and `NaN`, which are classified
* as numbers, use the `_.isFinite` method.
*
* @static
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
* @example
*
* _.isNumber(8.4);
* // => true
*
* _.isNumber(NaN);
* // => true
*
* _.isNumber('8.4');
* // => false
*/
function isNumber(value) {
return typeof value == 'number' || (isObjectLike(value) && objToString.call(value) == numberTag);
}
module.exports = isNumber;
},{"100":100}],112:[function(_dereq_,module,exports){
/**
* Checks if `value` is the [language type](https://es5.github.io/#x8) of `Object`.
* (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
*
* @static
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is an object, else `false`.
* @example
*
* _.isObject({});
* // => true
*
* _.isObject([1, 2, 3]);
* // => true
*
* _.isObject(1);
* // => false
*/
function isObject(value) {
// Avoid a V8 JIT bug in Chrome 19-20.
// See https://code.google.com/p/v8/issues/detail?id=2291 for more details.
var type = typeof value;
return !!value && (type == 'object' || type == 'function');
}
module.exports = isObject;
},{}],113:[function(_dereq_,module,exports){
var isObjectLike = _dereq_(100);
/** `Object#toString` result references. */
var stringTag = '[object String]';
/** Used for native method references. */
var objectProto = Object.prototype;
/**
* Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
* of values.
*/
var objToString = objectProto.toString;
/**
* Checks if `value` is classified as a `String` primitive or object.
*
* @static
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
* @example
*
* _.isString('abc');
* // => true
*
* _.isString(1);
* // => false
*/
function isString(value) {
return typeof value == 'string' || (isObjectLike(value) && objToString.call(value) == stringTag);
}
module.exports = isString;
},{"100":100}],114:[function(_dereq_,module,exports){
var isLength = _dereq_(99),
isObjectLike = _dereq_(100);
/** `Object#toString` result references. */
var argsTag = '[object Arguments]',
arrayTag = '[object Array]',
boolTag = '[object Boolean]',
dateTag = '[object Date]',
errorTag = '[object Error]',
funcTag = '[object Function]',
mapTag = '[object Map]',
numberTag = '[object Number]',
objectTag = '[object Object]',
regexpTag = '[object RegExp]',
setTag = '[object Set]',
stringTag = '[object String]',
weakMapTag = '[object WeakMap]';
var arrayBufferTag = '[object ArrayBuffer]',
float32Tag = '[object Float32Array]',
float64Tag = '[object Float64Array]',
int8Tag = '[object Int8Array]',
int16Tag = '[object Int16Array]',
int32Tag = '[object Int32Array]',
uint8Tag = '[object Uint8Array]',
uint8ClampedTag = '[object Uint8ClampedArray]',
uint16Tag = '[object Uint16Array]',
uint32Tag = '[object Uint32Array]';
/** Used to identify `toStringTag` values of typed arrays. */
var typedArrayTags = {};
typedArrayTags[float32Tag] = typedArrayTags[float64Tag] =
typedArrayTags[int8Tag] = typedArrayTags[int16Tag] =
typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] =
typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] =
typedArrayTags[uint32Tag] = true;
typedArrayTags[argsTag] = typedArrayTags[arrayTag] =
typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] =
typedArrayTags[dateTag] = typedArrayTags[errorTag] =
typedArrayTags[funcTag] = typedArrayTags[mapTag] =
typedArrayTags[numberTag] = typedArrayTags[objectTag] =
typedArrayTags[regexpTag] = typedArrayTags[setTag] =
typedArrayTags[stringTag] = typedArrayTags[weakMapTag] = false;
/** Used for native method references. */
var objectProto = Object.prototype;
/**
* Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
* of values.
*/
var objToString = objectProto.toString;
/**
* Checks if `value` is classified as a typed array.
*
* @static
* @memberOf _
* @category Lang
* @param {*} value The value to check.
* @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
* @example
*
* _.isTypedArray(new Uint8Array);
* // => true
*
* _.isTypedArray([]);
* // => false
*/
function isTypedArray(value) {
return isObjectLike(value) && isLength(value.length) && !!typedArrayTags[objToString.call(value)];
}
module.exports = isTypedArray;
},{"100":100,"99":99}],115:[function(_dereq_,module,exports){
var assignWith = _dereq_(49),
baseAssign = _dereq_(50),
createAssigner = _dereq_(81);
/**
* Assigns own enumerable properties of source object(s) to the destination
* object. Subsequent sources overwrite property assignments of previous sources.
* If `customizer` is provided it's invoked to produce the assigned values.
* The `customizer` is bound to `thisArg` and invoked with five arguments:
* (objectValue, sourceValue, key, object, source).
*
* **Note:** This method mutates `object` and is based on
* [`Object.assign`](http://ecma-international.org/ecma-262/6.0/#sec-object.assign).
*
* @static
* @memberOf _
* @alias extend
* @category Object
* @param {Object} object The destination object.
* @param {...Object} [sources] The source objects.
* @param {Function} [customizer] The function to customize assigned values.
* @param {*} [thisArg] The `this` binding of `customizer`.
* @returns {Object} Returns `object`.
* @example
*
* _.assign({ 'user': 'barney' }, { 'age': 40 }, { 'user': 'fred' });
* // => { 'user': 'fred', 'age': 40 }
*
* // using a customizer callback
* var defaults = _.partialRight(_.assign, function(value, other) {
* return _.isUndefined(value) ? other : value;
* });
*
* defaults({ 'user': 'barney' }, { 'age': 36 }, { 'user': 'fred' });
* // => { 'user': 'barney', 'age': 36 }
*/
var assign = createAssigner(function(object, source, customizer) {
return customizer
? assignWith(object, source, customizer)
: baseAssign(object, source);
});
module.exports = assign;
},{"49":49,"50":50,"81":81}],116:[function(_dereq_,module,exports){
var getNative = _dereq_(93),
isArrayLike = _dereq_(95),
isObject = _dereq_(112),
shimKeys = _dereq_(104);
/* Native method references for those with the same name as other `lodash` methods. */
var nativeKeys = getNative(Object, 'keys');
/**
* Creates an array of the own enumerable property names of `object`.
*
* **Note:** Non-object values are coerced to objects. See the
* [ES spec](http://ecma-international.org/ecma-262/6.0/#sec-object.keys)
* for more details.
*
* @static
* @memberOf _
* @category Object
* @param {Object} object The object to query.
* @returns {Array} Returns the array of property names.
* @example
*
* function Foo() {
* this.a = 1;
* this.b = 2;
* }
*
* Foo.prototype.c = 3;
*
* _.keys(new Foo);
* // => ['a', 'b'] (iteration order is not guaranteed)
*
* _.keys('hi');
* // => ['0', '1']
*/
var keys = !nativeKeys ? shimKeys : function(object) {
var Ctor = object == null ? undefined : object.constructor;
if ((typeof Ctor == 'function' && Ctor.prototype === object) ||
(typeof object != 'function' && isArrayLike(object))) {
return shimKeys(object);
}
return isObject(object) ? nativeKeys(object) : [];
};
module.exports = keys;
},{"104":104,"112":112,"93":93,"95":95}],117:[function(_dereq_,module,exports){
var isArguments = _dereq_(107),
isArray = _dereq_(108),
isIndex = _dereq_(96),
isLength = _dereq_(99),
isObject = _dereq_(112);
/** Used for native method references. */
var objectProto = Object.prototype;
/** Used to check objects for own properties. */
var hasOwnProperty = objectProto.hasOwnProperty;
/**
* Creates an array of the own and inherited enumerable property names of `object`.
*
* **Note:** Non-object values are coerced to objects.
*
* @static
* @memberOf _
* @category Object
* @param {Object} object The object to query.
* @returns {Array} Returns the array of property names.
* @example
*
* function Foo() {
* this.a = 1;
* this.b = 2;
* }
*
* Foo.prototype.c = 3;
*
* _.keysIn(new Foo);
* // => ['a', 'b', 'c'] (iteration order is not guaranteed)
*/
function keysIn(object) {
if (object == null) {
return [];
}
if (!isObject(object)) {
object = Object(object);
}
var length = object.length;
length = (length && isLength(length) &&
(isArray(object) || isArguments(object)) && length) || 0;
var Ctor = object.constructor,
index = -1,
isProto = typeof Ctor == 'function' && Ctor.prototype === object,
result = Array(length),
skipIndexes = length > 0;
while (++index < length) {
result[index] = (index + '');
}
for (var key in object) {
if (!(skipIndexes && isIndex(key, length)) &&
!(key == 'constructor' && (isProto || !hasOwnProperty.call(object, key)))) {
result.push(key);
}
}
return result;
}
module.exports = keysIn;
},{"107":107,"108":108,"112":112,"96":96,"99":99}],118:[function(_dereq_,module,exports){
var arrayMap = _dereq_(45),
baseDifference = _dereq_(54),
baseFlatten = _dereq_(60),
bindCallback = _dereq_(78),
keysIn = _dereq_(117),
pickByArray = _dereq_(102),
pickByCallback = _dereq_(103),
restParam = _dereq_(40);
/**
* The opposite of `_.pick`; this method creates an object composed of the
* own and inherited enumerable properties of `object` that are not omitted.
*
* @static
* @memberOf _
* @category Object
* @param {Object} object The source object.
* @param {Function|...(string|string[])} [predicate] The function invoked per
* iteration or property names to omit, specified as individual property
* names or arrays of property names.
* @param {*} [thisArg] The `this` binding of `predicate`.
* @returns {Object} Returns the new object.
* @example
*
* var object = { 'user': 'fred', 'age': 40 };
*
* _.omit(object, 'age');
* // => { 'user': 'fred' }
*
* _.omit(object, _.isNumber);
* // => { 'user': 'fred' }
*/
var omit = restParam(function(object, props) {
if (object == null) {
return {};
}
if (typeof props[0] != 'function') {
var props = arrayMap(baseFlatten(props), String);
return pickByArray(object, baseDifference(keysIn(object), props));
}
var predicate = bindCallback(props[0], props[1], 3);
return pickByCallback(object, function(value, key, object) {
return !predicate(value, key, object);
});
});
module.exports = omit;
},{"102":102,"103":103,"117":117,"40":40,"45":45,"54":54,"60":60,"78":78}],119:[function(_dereq_,module,exports){
var keys = _dereq_(116),
toObject = _dereq_(105);
/**
* Creates a two dimensional array of the key-value pairs for `object`,
* e.g. `[[key1, value1], [key2, value2]]`.
*
* @static
* @memberOf _
* @category Object
* @param {Object} object The object to query.
* @returns {Array} Returns the new array of key-value pairs.
* @example
*
* _.pairs({ 'barney': 36, 'fred': 40 });
* // => [['barney', 36], ['fred', 40]] (iteration order is not guaranteed)
*/
function pairs(object) {
object = toObject(object);
var index = -1,
props = keys(object),
length = props.length,
result = Array(length);
while (++index < length) {
var key = props[index];
result[index] = [key, object[key]];
}
return result;
}
module.exports = pairs;
},{"105":105,"116":116}],120:[function(_dereq_,module,exports){
var baseFlatten = _dereq_(60),
bindCallback = _dereq_(78),
pickByArray = _dereq_(102),
pickByCallback = _dereq_(103),
restParam = _dereq_(40);
/**
* Creates an object composed of the picked `object` properties. Property
* names may be specified as individual arguments or as arrays of property
* names. If `predicate` is provided it's invoked for each property of `object`
* picking the properties `predicate` returns truthy for. The predicate is
* bound to `thisArg` and invoked with three arguments: (value, key, object).
*
* @static
* @memberOf _
* @category Object
* @param {Object} object The source object.
* @param {Function|...(string|string[])} [predicate] The function invoked per
* iteration or property names to pick, specified as individual property
* names or arrays of property names.
* @param {*} [thisArg] The `this` binding of `predicate`.
* @returns {Object} Returns the new object.
* @example
*
* var object = { 'user': 'fred', 'age': 40 };
*
* _.pick(object, 'user');
* // => { 'user': 'fred' }
*
* _.pick(object, _.isString);
* // => { 'user': 'fred' }
*/
var pick = restParam(function(object, props) {
if (object == null) {
return {};
}
return typeof props[0] == 'function'
? pickByCallback(object, bindCallback(props[0], props[1], 3))
: pickByArray(object, baseFlatten(props));
});
module.exports = pick;
},{"102":102,"103":103,"40":40,"60":60,"78":78}],121:[function(_dereq_,module,exports){
/**
* This method returns the first argument provided to it.
*
* @static
* @memberOf _
* @category Utility
* @param {*} value Any value.
* @returns {*} Returns `value`.
* @example
*
* var object = { 'user': 'fred' };
*
* _.identity(object) === object;
* // => true
*/
function identity(value) {
return value;
}
module.exports = identity;
},{}],122:[function(_dereq_,module,exports){
var baseProperty = _dereq_(72),
basePropertyDeep = _dereq_(73),
isKey = _dereq_(98);
/**
* Creates a function that returns the property value at `path` on a
* given object.
*
* @static
* @memberOf _
* @category Utility
* @param {Array|string} path The path of the property to get.
* @returns {Function} Returns the new function.
* @example
*
* var objects = [
* { 'a': { 'b': { 'c': 2 } } },
* { 'a': { 'b': { 'c': 1 } } }
* ];
*
* _.map(objects, _.property('a.b.c'));
* // => [2, 1]
*
* _.pluck(_.sortBy(objects, _.property(['a', 'b', 'c'])), 'a.b.c');
* // => [1, 2]
*/
function property(path) {
return isKey(path) ? baseProperty(path) : basePropertyDeep(path);
}
module.exports = property;
},{"72":72,"73":73,"98":98}],123:[function(_dereq_,module,exports){
module.exports = _dereq_(25);
},{"25":25}],124:[function(_dereq_,module,exports){
module.exports = _dereq_(29);
},{"29":29}],125:[function(_dereq_,module,exports){
module.exports = _dereq_(26);
},{"26":26}],126:[function(_dereq_,module,exports){
module.exports = _dereq_(28);
},{"28":28}],127:[function(_dereq_,module,exports){
module.exports = function(el) {
el.parentNode && el.parentNode.removeChild(el);
};
},{}],128:[function(_dereq_,module,exports){
'use strict';
function capitalize(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
function lower(string) {
return string.charAt(0).toLowerCase() + string.slice(1);
}
function hasLowerCaseAlias(pkg) {
return pkg.xml && pkg.xml.tagAlias === 'lowerCase';
}
module.exports.aliasToName = function(alias, pkg) {
if (hasLowerCaseAlias(pkg)) {
return capitalize(alias);
} else {
return alias;
}
};
module.exports.nameToAlias = function(name, pkg) {
if (hasLowerCaseAlias(pkg)) {
return lower(name);
} else {
return name;
}
};
module.exports.DEFAULT_NS_MAP = {
'xsi': 'http://www.w3.org/2001/XMLSchema-instance'
};
var XSI_TYPE = module.exports.XSI_TYPE = 'xsi:type';
function serializeFormat(element) {
return element.xml && element.xml.serialize;
}
module.exports.serializeAsType = function(element) {
return serializeFormat(element) === XSI_TYPE;
};
module.exports.serializeAsProperty = function(element) {
return serializeFormat(element) === 'property';
};
},{}],129:[function(_dereq_,module,exports){
'use strict';
var reduce = _dereq_(37),
forEach = _dereq_(35),
find = _dereq_(34),
assign = _dereq_(115),
defer = _dereq_(39);
var Stack = _dereq_(144),
SaxParser = _dereq_(143).parser,
Moddle = _dereq_(131),
parseNameNs = _dereq_(136).parseName,
Types = _dereq_(139),
coerceType = Types.coerceType,
isSimpleType = Types.isSimple,
common = _dereq_(128),
XSI_TYPE = common.XSI_TYPE,
XSI_URI = common.DEFAULT_NS_MAP.xsi,
serializeAsType = common.serializeAsType,
aliasToName = common.aliasToName;
function parseNodeAttributes(node) {
var nodeAttrs = node.attributes;
return reduce(nodeAttrs, function(result, v, k) {
var name, ns;
if (!v.local) {
name = v.prefix;
} else {
ns = parseNameNs(v.name, v.prefix);
name = ns.name;
}
result[name] = v.value;
return result;
}, {});
}
function normalizeType(node, attr, model) {
var nameNs = parseNameNs(attr.value);
var uri = node.ns[nameNs.prefix || ''],
localName = nameNs.localName,
pkg = uri && model.getPackage(uri),
typePrefix;
if (pkg) {
typePrefix = pkg.xml && pkg.xml.typePrefix;
if (typePrefix && localName.indexOf(typePrefix) === 0) {
localName = localName.slice(typePrefix.length);
}
attr.value = pkg.prefix + ':' + localName;
}
}
/**
* Normalizes namespaces for a node given an optional default namespace and a
* number of mappings from uris to default prefixes.
*
* @param {XmlNode} node
* @param {Model} model the model containing all registered namespaces
* @param {Uri} defaultNsUri
*/
function normalizeNamespaces(node, model, defaultNsUri) {
var uri, prefix;
uri = node.uri || defaultNsUri;
if (uri) {
var pkg = model.getPackage(uri);
if (pkg) {
prefix = pkg.prefix;
} else {
prefix = node.prefix;
}
node.prefix = prefix;
node.uri = uri;
}
forEach(node.attributes, function(attr) {
// normalize xsi:type attributes because the
// assigned type may or may not be namespace prefixed
if (attr.uri === XSI_URI && attr.local === 'type') {
normalizeType(node, attr, model);
}
normalizeNamespaces(attr, model, null);
});
}
function error(message) {
return new Error(message);
}
/**
* Get the moddle descriptor for a given instance or type.
*
* @param {ModdleElement|Function} element
*
* @return {Object} the moddle descriptor
*/
function getModdleDescriptor(element) {
return element.$descriptor;
}
/**
* A parse context.
*
* @class
*
* @param {Object} options
* @param {ElementHandler} options.rootHandler the root handler for parsing a document
* @param {boolean} [options.lax=false] whether or not to ignore invalid elements
*/
function Context(options) {
/**
* @property {ElementHandler} rootHandler
*/
/**
* @property {Boolean} lax
*/
assign(this, options);
this.elementsById = {};
this.references = [];
this.warnings = [];
/**
* Add an unresolved reference.
*
* @param {Object} reference
*/
this.addReference = function(reference) {
this.references.push(reference);
};
/**
* Add a processed element.
*
* @param {ModdleElement} element
*/
this.addElement = function(element) {
if (!element) {
throw error('expected element');
}
var elementsById = this.elementsById;
var descriptor = getModdleDescriptor(element);
var idProperty = descriptor.idProperty,
id;
if (idProperty) {
id = element.get(idProperty.name);
if (id) {
if (elementsById[id]) {
throw error('duplicate ID <' + id + '>');
}
elementsById[id] = element;
}
}
};
/**
* Add an import warning.
*
* @param {Object} warning
* @param {String} warning.message
* @param {Error} [warning.error]
*/
this.addWarning = function(warning) {
this.warnings.push(warning);
};
}
function BaseHandler() {}
BaseHandler.prototype.handleEnd = function() {};
BaseHandler.prototype.handleText = function() {};
BaseHandler.prototype.handleNode = function() {};
/**
* A simple pass through handler that does nothing except for
* ignoring all input it receives.
*
* This is used to ignore unknown elements and
* attributes.
*/
function NoopHandler() { }
NoopHandler.prototype = Object.create(BaseHandler.prototype);
NoopHandler.prototype.handleNode = function() {
return this;
};
function BodyHandler() {}
BodyHandler.prototype = Object.create(BaseHandler.prototype);
BodyHandler.prototype.handleText = function(text) {
this.body = (this.body || '') + text;
};
function ReferenceHandler(property, context) {
this.property = property;
this.context = context;
}
ReferenceHandler.prototype = Object.create(BodyHandler.prototype);
ReferenceHandler.prototype.handleNode = function(node) {
if (this.element) {
throw error('expected no sub nodes');
} else {
this.element = this.createReference(node);
}
return this;
};
ReferenceHandler.prototype.handleEnd = function() {
this.element.id = this.body;
};
ReferenceHandler.prototype.createReference = function(node) {
return {
property: this.property.ns.name,
id: ''
};
};
function ValueHandler(propertyDesc, element) {
this.element = element;
this.propertyDesc = propertyDesc;
}
ValueHandler.prototype = Object.create(BodyHandler.prototype);
ValueHandler.prototype.handleEnd = function() {
var value = this.body || '',
element = this.element,
propertyDesc = this.propertyDesc;
value = coerceType(propertyDesc.type, value);
if (propertyDesc.isMany) {
element.get(propertyDesc.name).push(value);
} else {
element.set(propertyDesc.name, value);
}
};
function BaseElementHandler() {}
BaseElementHandler.prototype = Object.create(BodyHandler.prototype);
BaseElementHandler.prototype.handleNode = function(node) {
var parser = this,
element = this.element;
if (!element) {
element = this.element = this.createElement(node);
this.context.addElement(element);
} else {
parser = this.handleChild(node);
}
return parser;
};
/**
* @class XMLReader.ElementHandler
*
*/
function ElementHandler(model, type, context) {
this.model = model;
this.type = model.getType(type);
this.context = context;
}
ElementHandler.prototype = Object.create(BaseElementHandler.prototype);
ElementHandler.prototype.addReference = function(reference) {
this.context.addReference(reference);
};
ElementHandler.prototype.handleEnd = function() {
var value = this.body,
element = this.element,
descriptor = getModdleDescriptor(element),
bodyProperty = descriptor.bodyProperty;
if (bodyProperty && value !== undefined) {
value = coerceType(bodyProperty.type, value);
element.set(bodyProperty.name, value);
}
};
/**
* Create an instance of the model from the given node.
*
* @param {Element} node the xml node
*/
ElementHandler.prototype.createElement = function(node) {
var attributes = parseNodeAttributes(node),
Type = this.type,
descriptor = getModdleDescriptor(Type),
context = this.context,
instance = new Type({});
forEach(attributes, function(value, name) {
var prop = descriptor.propertiesByName[name],
values;
if (prop && prop.isReference) {
if (!prop.isMany) {
context.addReference({
element: instance,
property: prop.ns.name,
id: value
});
} else {
// IDREFS: parse references as whitespace-separated list
values = value.split(' ');
forEach(values, function(v) {
context.addReference({
element: instance,
property: prop.ns.name,
id: v
});
});
}
} else {
if (prop) {
value = coerceType(prop.type, value);
}
instance.set(name, value);
}
});
return instance;
};
ElementHandler.prototype.getPropertyForNode = function(node) {
var nameNs = parseNameNs(node.local, node.prefix);
var type = this.type,
model = this.model,
descriptor = getModdleDescriptor(type);
var propertyName = nameNs.name,
property = descriptor.propertiesByName[propertyName],
elementTypeName,
elementType,
typeAnnotation;
// search for properties by name first
if (property) {
if (serializeAsType(property)) {
typeAnnotation = node.attributes[XSI_TYPE];
// xsi type is optional, if it does not exists the
// default type is assumed
if (typeAnnotation) {
elementTypeName = typeAnnotation.value;
// TODO: extract real name from attribute
elementType = model.getType(elementTypeName);
return assign({}, property, { effectiveType: getModdleDescriptor(elementType).name });
}
}
// search for properties by name first
return property;
}
var pkg = model.getPackage(nameNs.prefix);
if (pkg) {
elementTypeName = nameNs.prefix + ':' + aliasToName(nameNs.localName, descriptor.$pkg);
elementType = model.getType(elementTypeName);
// search for collection members later
property = find(descriptor.properties, function(p) {
return !p.isVirtual && !p.isReference && !p.isAttribute && elementType.hasType(p.type);
});
if (property) {
return assign({}, property, { effectiveType: getModdleDescriptor(elementType).name });
}
} else {
// parse unknown element (maybe extension)
property = find(descriptor.properties, function(p) {
return !p.isReference && !p.isAttribute && p.type === 'Element';
});
if (property) {
return property;
}
}
throw error('unrecognized element <' + nameNs.name + '>');
};
ElementHandler.prototype.toString = function() {
return 'ElementDescriptor[' + getModdleDescriptor(this.type).name + ']';
};
ElementHandler.prototype.valueHandler = function(propertyDesc, element) {
return new ValueHandler(propertyDesc, element);
};
ElementHandler.prototype.referenceHandler = function(propertyDesc) {
return new ReferenceHandler(propertyDesc, this.context);
};
ElementHandler.prototype.handler = function(type) {
if (type === 'Element') {
return new GenericElementHandler(this.model, type, this.context);
} else {
return new ElementHandler(this.model, type, this.context);
}
};
/**
* Handle the child element parsing
*
* @param {Element} node the xml node
*/
ElementHandler.prototype.handleChild = function(node) {
var propertyDesc, type, element, childHandler;
propertyDesc = this.getPropertyForNode(node);
element = this.element;
type = propertyDesc.effectiveType || propertyDesc.type;
if (isSimpleType(type)) {
return this.valueHandler(propertyDesc, element);
}
if (propertyDesc.isReference) {
childHandler = this.referenceHandler(propertyDesc).handleNode(node);
} else {
childHandler = this.handler(type).handleNode(node);
}
var newElement = childHandler.element;
// child handles may decide to skip elements
// by not returning anything
if (newElement !== undefined) {
if (propertyDesc.isMany) {
element.get(propertyDesc.name).push(newElement);
} else {
element.set(propertyDesc.name, newElement);
}
if (propertyDesc.isReference) {
assign(newElement, {
element: element
});
this.context.addReference(newElement);
} else {
// establish child -> parent relationship
newElement.$parent = element;
}
}
return childHandler;
};
function GenericElementHandler(model, type, context) {
this.model = model;
this.context = context;
}
GenericElementHandler.prototype = Object.create(BaseElementHandler.prototype);
GenericElementHandler.prototype.createElement = function(node) {
var name = node.name,
prefix = node.prefix,
uri = node.ns[prefix],
attributes = node.attributes;
return this.model.createAny(name, uri, attributes);
};
GenericElementHandler.prototype.handleChild = function(node) {
var handler = new GenericElementHandler(this.model, 'Element', this.context).handleNode(node),
element = this.element;
var newElement = handler.element,
children;
if (newElement !== undefined) {
children = element.$children = element.$children || [];
children.push(newElement);
// establish child -> parent relationship
newElement.$parent = element;
}
return handler;
};
GenericElementHandler.prototype.handleText = function(text) {
this.body = this.body || '' + text;
};
GenericElementHandler.prototype.handleEnd = function() {
if (this.body) {
this.element.$body = this.body;
}
};
/**
* A reader for a meta-model
*
* @param {Object} options
* @param {Model} options.model used to read xml files
* @param {Boolean} options.lax whether to make parse errors warnings
*/
function XMLReader(options) {
if (options instanceof Moddle) {
options = {
model: options
};
}
assign(this, { lax: false }, options);
}
/**
* Parse the given XML into a moddle document tree.
*
* @param {String} xml
* @param {ElementHandler|Object} options or rootHandler
* @param {Function} done
*/
XMLReader.prototype.fromXML = function(xml, options, done) {
var rootHandler = options.rootHandler;
if (options instanceof ElementHandler) {
// root handler passed via (xml, { rootHandler: ElementHandler }, ...)
rootHandler = options;
options = {};
} else {
if (typeof options === 'string') {
// rootHandler passed via (xml, 'someString', ...)
rootHandler = this.handler(options);
options = {};
} else if (typeof rootHandler === 'string') {
// rootHandler passed via (xml, { rootHandler: 'someString' }, ...)
rootHandler = this.handler(rootHandler);
}
}
var model = this.model,
lax = this.lax;
var context = new Context(assign({}, options, { rootHandler: rootHandler })),
parser = new SaxParser(true, { xmlns: true, trim: true }),
stack = new Stack();
rootHandler.context = context;
// push root handler
stack.push(rootHandler);
function resolveReferences() {
var elementsById = context.elementsById;
var references = context.references;
var i, r;
for (i = 0; !!(r = references[i]); i++) {
var element = r.element;
var reference = elementsById[r.id];
var property = getModdleDescriptor(element).propertiesByName[r.property];
if (!reference) {
context.addWarning({
message: 'unresolved reference <' + r.id + '>',
element: r.element,
property: r.property,
value: r.id
});
}
if (property.isMany) {
var collection = element.get(property.name),
idx = collection.indexOf(r);
// we replace an existing place holder (idx != -1) or
// append to the collection instead
if (idx === -1) {
idx = collection.length;
}
if (!reference) {
// remove unresolvable reference
collection.splice(idx, 1);
} else {
// add or update reference in collection
collection[idx] = reference;
}
} else {
element.set(property.name, reference);
}
}
}
function handleClose(tagName) {
stack.pop().handleEnd();
}
function handleOpen(node) {
var handler = stack.peek();
normalizeNamespaces(node, model);
try {
stack.push(handler.handleNode(node));
} catch (e) {
var line = this.line,
column = this.column;
var message =
'unparsable content <' + node.name + '> detected\n\t' +
'line: ' + line + '\n\t' +
'column: ' + column + '\n\t' +
'nested error: ' + e.message;
if (lax) {
context.addWarning({
message: message,
error: e
});
console.warn('could not parse node');
console.warn(e);
stack.push(new NoopHandler());
} else {
console.error('could not parse document');
console.error(e);
throw error(message);
}
}
}
function handleText(text) {
stack.peek().handleText(text);
}
parser.onopentag = handleOpen;
parser.oncdata = parser.ontext = handleText;
parser.onclosetag = handleClose;
parser.onend = resolveReferences;
// deferred parse XML to make loading really ascnchronous
// this ensures the execution environment (node or browser)
// is kept responsive and that certain optimization strategies
// can kick in
defer(function() {
var error;
try {
parser.write(xml).close();
} catch (e) {
error = e;
}
done(error, error ? undefined : rootHandler.element, context);
});
};
XMLReader.prototype.handler = function(name) {
return new ElementHandler(this.model, name);
};
module.exports = XMLReader;
module.exports.ElementHandler = ElementHandler;
},{"115":115,"128":128,"131":131,"136":136,"139":139,"143":143,"144":144,"34":34,"35":35,"37":37,"39":39}],130:[function(_dereq_,module,exports){
'use strict';
var map = _dereq_(36),
forEach = _dereq_(35),
isString = _dereq_(113),
filter = _dereq_(33),
assign = _dereq_(115);
var Types = _dereq_(139),
parseNameNs = _dereq_(136).parseName,
common = _dereq_(128),
nameToAlias = common.nameToAlias,
serializeAsType = common.serializeAsType,
serializeAsProperty = common.serializeAsProperty;
var XML_PREAMBLE = '\n',
ESCAPE_CHARS = /(<|>|'|"|&|\n\r|\n)/g,
DEFAULT_NS_MAP = common.DEFAULT_NS_MAP,
XSI_TYPE = common.XSI_TYPE;
function nsName(ns) {
if (isString(ns)) {
return ns;
} else {
return (ns.prefix ? ns.prefix + ':' : '') + ns.localName;
}
}
function getNsAttrs(namespaces) {
function isUsed(ns) {
return namespaces.used[ns.uri];
}
function toAttr(ns) {
var name = 'xmlns' + (ns.prefix ? ':' + ns.prefix : '');
return { name: name, value: ns.uri };
}
var allNs = [].concat(namespaces.wellknown, namespaces.custom);
return map(filter(allNs, isUsed), toAttr);
}
function getElementNs(ns, descriptor) {
if (descriptor.isGeneric) {
return descriptor.name;
} else {
return assign({ localName: nameToAlias(descriptor.ns.localName, descriptor.$pkg) }, ns);
}
}
function getPropertyNs(ns, descriptor) {
return assign({ localName: descriptor.ns.localName }, ns);
}
function getSerializableProperties(element) {
var descriptor = element.$descriptor;
return filter(descriptor.properties, function(p) {
var name = p.name;
if (p.isVirtual) {
return false;
}
// do not serialize defaults
if (!element.hasOwnProperty(name)) {
return false;
}
var value = element[name];
// do not serialize default equals
if (value === p.default) {
return false;
}
// do not serialize null properties
if (value === null) {
return false;
}
return p.isMany ? value.length : true;
});
}
var ESCAPE_MAP = {
'\n': '10',
'\n\r': '10',
'"': '34',
'\'': '39',
'<': '60',
'>': '62',
'&': '38'
};
/**
* Escape a string attribute to not contain any bad values (line breaks, '"', ...)
*
* @param {String} str the string to escape
* @return {String} the escaped string
*/
function escapeAttr(str) {
// ensure we are handling strings here
str = isString(str) ? str : '' + str;
return str.replace(ESCAPE_CHARS, function(str) {
return '' + ESCAPE_MAP[str] + ';';
});
}
function filterAttributes(props) {
return filter(props, function(p) { return p.isAttr; });
}
function filterContained(props) {
return filter(props, function(p) { return !p.isAttr; });
}
function ReferenceSerializer(parent, ns) {
this.ns = ns;
}
ReferenceSerializer.prototype.build = function(element) {
this.element = element;
return this;
};
ReferenceSerializer.prototype.serializeTo = function(writer) {
writer
.appendIndent()
.append('<' + nsName(this.ns) + '>' + this.element.id + '' + nsName(this.ns) + '>')
.appendNewLine();
};
function BodySerializer() {}
BodySerializer.prototype.serializeValue = BodySerializer.prototype.serializeTo = function(writer) {
var escape = this.escape;
if (escape) {
writer.append('');
}
};
BodySerializer.prototype.build = function(prop, value) {
this.value = value;
if (prop.type === 'String' && value.search(ESCAPE_CHARS) !== -1) {
this.escape = true;
}
return this;
};
function ValueSerializer(ns) {
this.ns = ns;
}
ValueSerializer.prototype = new BodySerializer();
ValueSerializer.prototype.serializeTo = function(writer) {
writer
.appendIndent()
.append('<' + nsName(this.ns) + '>');
this.serializeValue(writer);
writer
.append( '' + nsName(this.ns) + '>')
.appendNewLine();
};
function ElementSerializer(parent, ns) {
this.body = [];
this.attrs = [];
this.parent = parent;
this.ns = ns;
}
ElementSerializer.prototype.build = function(element) {
this.element = element;
var otherAttrs = this.parseNsAttributes(element);
if (!this.ns) {
this.ns = this.nsTagName(element.$descriptor);
}
if (element.$descriptor.isGeneric) {
this.parseGeneric(element);
} else {
var properties = getSerializableProperties(element);
this.parseAttributes(filterAttributes(properties));
this.parseContainments(filterContained(properties));
this.parseGenericAttributes(element, otherAttrs);
}
return this;
};
ElementSerializer.prototype.nsTagName = function(descriptor) {
var effectiveNs = this.logNamespaceUsed(descriptor.ns);
return getElementNs(effectiveNs, descriptor);
};
ElementSerializer.prototype.nsPropertyTagName = function(descriptor) {
var effectiveNs = this.logNamespaceUsed(descriptor.ns);
return getPropertyNs(effectiveNs, descriptor);
};
ElementSerializer.prototype.isLocalNs = function(ns) {
return ns.uri === this.ns.uri;
};
/**
* Get the actual ns attribute name for the given element.
*
* @param {Object} element
* @param {Boolean} [inherited=false]
*
* @return {Object} nsName
*/
ElementSerializer.prototype.nsAttributeName = function(element) {
var ns;
if (isString(element)) {
ns = parseNameNs(element);
} else {
ns = element.ns;
}
// return just local name for inherited attributes
if (element.inherited) {
return { localName: ns.localName };
}
// parse + log effective ns
var effectiveNs = this.logNamespaceUsed(ns);
// strip prefix if same namespace like parent
if (this.isLocalNs(effectiveNs)) {
return { localName: ns.localName };
} else {
return assign({ localName: ns.localName }, effectiveNs);
}
};
ElementSerializer.prototype.parseGeneric = function(element) {
var self = this,
body = this.body,
attrs = this.attrs;
forEach(element, function(val, key) {
if (key === '$body') {
body.push(new BodySerializer().build({ type: 'String' }, val));
} else
if (key === '$children') {
forEach(val, function(child) {
body.push(new ElementSerializer(self).build(child));
});
} else
if (key.indexOf('$') !== 0) {
attrs.push({ name: key, value: escapeAttr(val) });
}
});
};
/**
* Parse namespaces and return a list of left over generic attributes
*
* @param {Object} element
* @return {Array