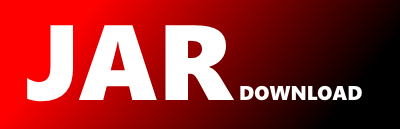
protege.code-generation.2.0.0.source-code.factory.header Maven / Gradle / Ivy
Show all versions of code-generation Show documentation
package ${factoryPackage};
import ${package}.impl.*;
${factoryExtraImport}
import java.util.Collection;
import org.protege.owl.codegeneration.CodeGenerationFactory;
import org.protege.owl.codegeneration.WrappedIndividual;
import org.protege.owl.codegeneration.impl.FactoryHelper;
import org.protege.owl.codegeneration.impl.ProtegeJavaMapping;
import org.protege.owl.codegeneration.inference.CodeGenerationInference;
import org.protege.owl.codegeneration.inference.SimpleInference;
import org.semanticweb.owlapi.model.OWLClass;
import org.semanticweb.owlapi.model.OWLOntology;
import org.semanticweb.owlapi.model.OWLOntologyStorageException;
/**
* A class that serves as the entry point to the generated code providing access
* to existing individuals in the ontology and the ability to create new individuals in the ontology.
*
* Generated by Protege (http://protege.stanford.edu).
* Source Class: ${factoryClass}
* @version generated on ${date} by ${user}
*/
public class ${factoryClass} implements CodeGenerationFactory {
private OWLOntology ontology;
private ProtegeJavaMapping javaMapping = new ProtegeJavaMapping();
private FactoryHelper delegate;
private CodeGenerationInference inference;
public ${factoryClass}(OWLOntology ontology) {
this(ontology, new SimpleInference(ontology));
}
public ${factoryClass}(OWLOntology ontology, CodeGenerationInference inference) {
this.ontology = ontology;
this.inference = inference;
javaMapping.initialize(ontology, inference);
delegate = new FactoryHelper(ontology, inference);
}
public OWLOntology getOwlOntology() {
return ontology;
}
public void saveOwlOntology() throws OWLOntologyStorageException {
ontology.getOWLOntologyManager().saveOntology(ontology);
}
public void flushOwlReasoner() {
delegate.flushOwlReasoner();
}
public boolean canAs(WrappedIndividual resource, Class extends WrappedIndividual> javaInterface) {
return javaMapping.canAs(resource, javaInterface);
}
public X as(WrappedIndividual resource, Class extends X> javaInterface) {
return javaMapping.as(resource, javaInterface);
}
public Class> getJavaInterfaceFromOwlClass(OWLClass cls) {
return javaMapping.getJavaInterfaceFromOwlClass(cls);
}
public OWLClass getOwlClassFromJavaInterface(Class> javaInterface) {
return javaMapping.getOwlClassFromJavaInterface(javaInterface);
}
public CodeGenerationInference getInference() {
return inference;
}