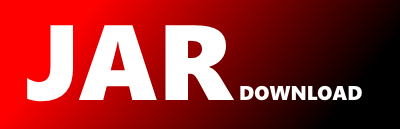
chrome.content.js.lib.RDFa.1.2.0.js Maven / Gradle / Ivy
/** @preserve green-turtle version 1.2.0 Copyright (c) 2011-2013, R. Alexander Milowski All rights reserved. */
/**
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met:
Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
if (typeof GreenTurtle == "undefined") {
var GreenTurtle = (function() {
var env = {};
env.version = "1.2.0";
function URIResolver() {
}
URIResolver.SCHEME = /^[A-Za-z][A-Za-z0-9\+\-\.]*\:/;
URIResolver.prototype.parseURI = function(uri) {
var match = URIResolver.SCHEME.exec(uri);
if (!match) {
throw "Bad URI value, no scheme: "+uri;
}
var parsed = { spec: uri };
parsed.scheme = match[0].substring(0,match[0].length-1);
parsed.schemeSpecificPart = parsed.spec.substring(match[0].length);
if (parsed.schemeSpecificPart.charAt(0)=='/' && parsed.schemeSpecificPart.charAt(1)=='/') {
this.parseGeneric(parsed);
} else {
parsed.isGeneric = false;
}
parsed.normalize = function() {
if (!this.isGeneric) {
return;
}
if (this.segments.length==0) {
return;
}
// edge case of ending in "/."
if (this.path.length>1 && this.path.substring(this.path.length-2)=="/.") {
this.path = this.path.substring(0,this.path.length-1);
this.segments.splice(this.segments.length-1,1);
this.schemeSpecificPart = "//"+this.authority+this.path;
if (typeof this.query != "undefined") {
this.schemeSpecificPart += "?" + this.query;
}
if (typeof this.fragment != "undefined") {
this.schemeSpecificPart += "#" + this.fragment;
}
this.spec = this.scheme+":"+this.schemeSpecificPart;
return;
}
var end = this.path.charAt(this.path.length-1);
if (end!="/") {
end = "";
}
for (var i=0; i0 && this.segments[i]=="..") {
this.segments.splice(i-1,2);
i -= 2;
}
if (this.segments[i]==".") {
this.segments.splice(i,1);
i--;
}
}
this.path = this.segments.length==0 ? "/" : "/"+this.segments.join("/")+end;
this.schemeSpecificPart = "//"+this.authority+this.path;
if (typeof this.query != "undefined") {
this.schemeSpecificPart += "?" + this.query;
}
if (typeof this.fragment != "undefined") {
this.schemeSpecificPart += "#" + this.fragment;
}
this.spec = this.scheme+":"+this.schemeSpecificPart;
}
parsed.resolve = function(href) {
if (!href) {
return this.spec;
}
if (href.charAt(0)=='#') {
var lastHash = this.spec.lastIndexOf('#');
return lastHash<0 ? this.spec+href : this.spec.substring(0,lastHash)+href;
}
if (!this.isGeneric) {
throw "Cannot resolve uri against non-generic URI: "+this.spec;
}
var colon = href.indexOf(':');
if (href.charAt(0)=='/') {
return this.scheme+"://"+this.authority+href;
} else if (href.charAt(0)=='.' && href.charAt(1)=='/') {
if (this.path.charAt(this.path.length-1)=='/') {
return this.scheme+"://"+this.authority+this.path+href.substring(2);
} else {
var last = this.path.lastIndexOf('/');
return this.scheme+"://"+this.authority+this.path.substring(0,last)+href.substring(1);
}
} else if (URIResolver.SCHEME.test(href)) {
return href;
} else if (href.charAt(0)=="?") {
return this.scheme+"://"+this.authority+this.path+href;
} else {
if (this.path.charAt(this.path.length-1)=='/') {
return this.scheme+"://"+this.authority+this.path+href;
} else {
var last = this.path.lastIndexOf('/');
return this.scheme+"://"+this.authority+this.path.substring(0,last+1)+href;
}
}
};
parsed.relativeTo = function(otherURI) {
if (otherURI.scheme!=this.scheme) {
return this.spec;
}
if (!this.isGeneric) {
throw "A non generic URI cannot be made relative: "+this.spec;
}
if (!otherURI.isGeneric) {
throw "Cannot make a relative URI against a non-generic URI: "+otherURI.spec;
}
if (otherURI.authority!=this.authority) {
return this.spec;
}
var i=0;
for (; i=0) {
parsed.fragment = parsed.path.substring(hash+1);
parsed.path = parsed.path.substring(0,hash);
}
var questionMark = parsed.path.indexOf('?');
if (questionMark>=0) {
parsed.query = parsed.path.substring(questionMark+1);
parsed.path = parsed.path.substring(0,questionMark);
}
if (parsed.path=="/" || parsed.path=="") {
parsed.segments = [];
} else {
parsed.segments = parsed.path.split(/\//);
if (parsed.segments.length>0 && parsed.segments[0]=='' && parsed.path.length>1 && parsed.path.charAt(1)!='/') {
// empty segment at the start, remove it
parsed.segments.shift();
}
if (parsed.segments.length>0 && parsed.path.length>0 && parsed.path.charAt(parsed.path.length-1)=='/' && parsed.segments[parsed.segments.length-1]=='') {
// we may have an empty the end
// check to see if it is legimate
if (parsed.path.length>1 && parsed.path.charAt(parsed.path.length-2)!='/') {
parsed.segments.pop();
}
}
// check for non-escaped characters
for (var i=0; i0) {
throw "Unecaped character "+check[j].charAt(0)+" ("+check[j].charCodeAt(0)+") in URI "+parsed.spec;
}
}
}
}
parsed.isGeneric = true;
}
RDFaProcessor.prototype = new URIResolver();
RDFaProcessor.prototype.constructor=RDFaProcessor;
function RDFaProcessor(targetObject) {
if (targetObject) {
this.target = targetObject;
} else {
this.target = {
graph: {
subjects: {},
prefixes: {},
terms: {}
}
};
}
this.theOne = "_:"+(new Date()).getTime();
this.language = null;
this.vocabulary = null;
this.blankCounter = 0;
this.langAttributes = [ { namespaceURI: "http://www.w3.org/XML/1998/namespace", localName: "lang" } ];
this.inXHTMLMode = false;
this.absURIRE = /[\w\_\-]+:\S+/;
this.finishedHandlers = [];
this.init();
}
RDFaProcessor.prototype.newBlankNode = function() {
this.blankCounter++;
return "_:"+this.blankCounter;
}
RDFaProcessor.XMLLiteralURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#XMLLiteral";
RDFaProcessor.HTMLLiteralURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#HTML";
RDFaProcessor.PlainLiteralURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#PlainLiteral";
RDFaProcessor.objectURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#object";
RDFaProcessor.typeURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#type";
RDFaProcessor.nameChar = '[-A-Z_a-z\u00C0-\u00D6\u00D8-\u00F6\u00F8-\u02FF\u0370-\u037D\u037F-\u1FFF\u200C-\u200D\u2070-\u218F\u2C00-\u2FEF\u3001-\uD7FF\uF900-\uFDCF\uFDF0-\uFFFD\u10000-\uEFFFF\.0-9\u00B7\u0300-\u036F\u203F-\u2040]';
RDFaProcessor.nameStartChar = '[\u0041-\u005A\u0061-\u007A\u00C0-\u00D6\u00D8-\u00F6\u00F8-\u00FF\u0100-\u0131\u0134-\u013E\u0141-\u0148\u014A-\u017E\u0180-\u01C3\u01CD-\u01F0\u01F4-\u01F5\u01FA-\u0217\u0250-\u02A8\u02BB-\u02C1\u0386\u0388-\u038A\u038C\u038E-\u03A1\u03A3-\u03CE\u03D0-\u03D6\u03DA\u03DC\u03DE\u03E0\u03E2-\u03F3\u0401-\u040C\u040E-\u044F\u0451-\u045C\u045E-\u0481\u0490-\u04C4\u04C7-\u04C8\u04CB-\u04CC\u04D0-\u04EB\u04EE-\u04F5\u04F8-\u04F9\u0531-\u0556\u0559\u0561-\u0586\u05D0-\u05EA\u05F0-\u05F2\u0621-\u063A\u0641-\u064A\u0671-\u06B7\u06BA-\u06BE\u06C0-\u06CE\u06D0-\u06D3\u06D5\u06E5-\u06E6\u0905-\u0939\u093D\u0958-\u0961\u0985-\u098C\u098F-\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09DC-\u09DD\u09DF-\u09E1\u09F0-\u09F1\u0A05-\u0A0A\u0A0F-\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32-\u0A33\u0A35-\u0A36\u0A38-\u0A39\u0A59-\u0A5C\u0A5E\u0A72-\u0A74\u0A85-\u0A8B\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0\u0AB2-\u0AB3\u0AB5-\u0AB9\u0ABD\u0AE0\u0B05-\u0B0C\u0B0F-\u0B10\u0B13-\u0B28\u0B2A-\u0B30\u0B32-\u0B33\u0B36-\u0B39\u0B3D\u0B5C-\u0B5D\u0B5F-\u0B61\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95\u0B99-\u0B9A\u0B9C\u0B9E-\u0B9F\u0BA3-\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB5\u0BB7-\u0BB9\u0C05-\u0C0C\u0C0E-\u0C10\u0C12-\u0C28\u0C2A-\u0C33\u0C35-\u0C39\u0C60-\u0C61\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CDE\u0CE0-\u0CE1\u0D05-\u0D0C\u0D0E-\u0D10\u0D12-\u0D28\u0D2A-\u0D39\u0D60-\u0D61\u0E01-\u0E2E\u0E30\u0E32-\u0E33\u0E40-\u0E45\u0E81-\u0E82\u0E84\u0E87-\u0E88\u0E8A\u0E8D\u0E94-\u0E97\u0E99-\u0E9F\u0EA1-\u0EA3\u0EA5\u0EA7\u0EAA-\u0EAB\u0EAD-\u0EAE\u0EB0\u0EB2-\u0EB3\u0EBD\u0EC0-\u0EC4\u0F40-\u0F47\u0F49-\u0F69\u10A0-\u10C5\u10D0-\u10F6\u1100\u1102-\u1103\u1105-\u1107\u1109\u110B-\u110C\u110E-\u1112\u113C\u113E\u1140\u114C\u114E\u1150\u1154-\u1155\u1159\u115F-\u1161\u1163\u1165\u1167\u1169\u116D-\u116E\u1172-\u1173\u1175\u119E\u11A8\u11AB\u11AE-\u11AF\u11B7-\u11B8\u11BA\u11BC-\u11C2\u11EB\u11F0\u11F9\u1E00-\u1E9B\u1EA0-\u1EF9\u1F00-\u1F15\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u2126\u212A-\u212B\u212E\u2180-\u2182\u3041-\u3094\u30A1-\u30FA\u3105-\u312C\uAC00-\uD7A3\u4E00-\u9FA5\u3007\u3021-\u3029_]';
RDFaProcessor.NCNAME = new RegExp('^' + RDFaProcessor.nameStartChar + RDFaProcessor.nameChar + '*$');
RDFaProcessor.trim = function(str) {
return str.replace(/^\s\s*/, '').replace(/\s\s*$/, '');
}
RDFaProcessor.prototype.tokenize = function(str) {
return RDFaProcessor.trim(str).split(/\s+/);
}
RDFaProcessor.prototype.parseSafeCURIEOrCURIEOrURI = function(value,prefixes,base) {
value = RDFaProcessor.trim(value);
if (value.charAt(0)=='[' && value.charAt(value.length-1)==']') {
value = value.substring(1,value.length-1);
value = value.trim(value);
if (value.length==0) {
return null;
}
if (value=="_:") {
// the one node
return this.theOne;
}
return this.parseCURIE(value,prefixes,base);
} else {
return this.parseCURIEOrURI(value,prefixes,base);
}
}
RDFaProcessor.prototype.parseCURIE = function(value,prefixes,base) {
var colon = value.indexOf(":");
if (colon>=0) {
var prefix = value.substring(0,colon);
if (prefix=="") {
// default prefix
var uri = prefixes[""];
return uri ? uri+value.substring(colon+1) : null;
} else if (prefix=="_") {
// blank node
return "_:"+value.substring(colon+1);
} else if (RDFaProcessor.NCNAME.test(prefix)) {
var uri = prefixes[prefix];
if (uri) {
return uri+value.substring(colon+1);
}
}
}
return null;
}
RDFaProcessor.prototype.parseCURIEOrURI = function(value,prefixes,base) {
var curie = this.parseCURIE(value,prefixes,base);
if (curie) {
return curie;
}
return this.resolveAndNormalize(base,value);
}
RDFaProcessor.prototype.parsePredicate = function(value,defaultVocabulary,terms,prefixes,base,ignoreTerms) {
if (value=="") {
return null;
}
var predicate = this.parseTermOrCURIEOrAbsURI(value,defaultVocabulary,ignoreTerms ? null : terms,prefixes,base);
if (predicate && predicate.indexOf("_:")==0) {
return null;
}
return predicate;
}
RDFaProcessor.prototype.parseTermOrCURIEOrURI = function(value,defaultVocabulary,terms,prefixes,base) {
//alert("Parsing "+value+" with default vocab "+defaultVocabulary);
value = RDFaProcessor.trim(value);
var curie = this.parseCURIE(value,prefixes,base);
if (curie) {
return curie;
} else {
var term = terms[value];
if (term) {
return term;
}
var lcvalue = value.toLowerCase();
term = terms[lcvalue];
if (term) {
return term;
}
if (defaultVocabulary && !this.absURIRE.exec(value)) {
return defaultVocabulary+value
}
}
return this.resolveAndNormalize(base,value);
}
RDFaProcessor.prototype.parseTermOrCURIEOrAbsURI = function(value,defaultVocabulary,terms,prefixes,base) {
//alert("Parsing "+value+" with default vocab "+defaultVocabulary);
value = RDFaProcessor.trim(value);
var curie = this.parseCURIE(value,prefixes,base);
if (curie) {
return curie;
} else if (terms) {
if (defaultVocabulary && !this.absURIRE.exec(value)) {
return defaultVocabulary+value
}
var term = terms[value];
if (term) {
return term;
}
var lcvalue = value.toLowerCase();
term = terms[lcvalue];
if (term) {
return term;
}
}
if (this.absURIRE.exec(value)) {
return this.resolveAndNormalize(base,value);
}
return null;
}
RDFaProcessor.prototype.resolveAndNormalize = function(base,href) {
var u = base.resolve(href);
var parsed = this.parseURI(u);
parsed.normalize();
return parsed.spec;
}
RDFaProcessor.prototype.parsePrefixMappings = function(str,target) {
var values = this.tokenize(str);
var prefix = null;
var uri = null;
for (var i=0; i=0) {
baseURI = baseURI.substring(0,hash);
}
if (options && options.baseURIMap) {
baseURI = options.baseURIMap(baseURI);
}
return baseURI;
}
queue.push({ current: node, context: this.push(null,removeHash(node.baseURI))});
while (queue.length>0) {
var item = queue.shift();
if (item.parent) {
// Sequence Step 14: list triple generation
if (item.context.parent && item.context.parent.listMapping==item.listMapping) {
// Skip a child context with exactly the same mapping
continue;
}
//console.log("Generating lists for "+item.subject+", tag "+item.parent.localName);
for (var predicate in item.listMapping) {
var list = item.listMapping[predicate];
if (list.length==0) {
this.addTriple(item.parent,item.subject,predicate,{ type: RDFaProcessor.objectURI, value: "http://www.w3.org/1999/02/22-rdf-syntax-ns#nil" });
continue;
}
var bnodes = [];
for (var i=0; i0) {
vocabulary = value;
var baseSubject = base.spec;
//this.newSubject(current,baseSubject);
this.addTriple(current,baseSubject,"http://www.w3.org/ns/rdfa#usesVocabulary",{ type: RDFaProcessor.objectURI , value: vocabulary});
} else {
vocabulary = this.vocabulary;
}
}
// Sequence Step 3: IRI mappings
// handle xmlns attributes
for (var i=0; i0) {
language = value;
} else {
language = null;
}
}
var relAtt = current.getAttributeNode("rel");
var revAtt = current.getAttributeNode("rev");
var typeofAtt = current.getAttributeNode("typeof");
var propertyAtt = current.getAttributeNode("property");
var datatypeAtt = current.getAttributeNode("datatype");
var datetimeAtt = this.inHTMLMode ? current.getAttributeNode("datetime") : null;
var contentAtt = current.getAttributeNode("content");
var aboutAtt = current.getAttributeNode("about");
var srcAtt = current.getAttributeNode("src");
var resourceAtt = current.getAttributeNode("resource");
var hrefAtt = current.getAttributeNode("href");
var inlistAtt = current.getAttributeNode("inlist");
var relAttPredicates = [];
if (relAtt) {
var values = this.tokenize(relAtt.value);
for (var i=0; i=0) {
var prefix = value.substring(0,colon);
if (prefix=="") {
// default prefix
var uri = dataContext.prefixes[""];
return uri ? uri+value.substring(colon+1) : null;
} else if (prefix=="_") {
// blank node
return "_:"+value.substring(colon+1);
} else if (DocumentData.NCNAME.test(prefix)) {
var uri = dataContext.prefixes[prefix];
if (uri) {
return uri+value.substring(colon+1);
}
}
}
return resolve ? dataContext.baseURI.resolve(value) : value;
}
};
this.base = null;
this.toString = function(requestOptions, replace) {
var options = requestOptions && requestOptions.shorten ? { graph: this, shorten: true, prefixesUsed: {} } : null;
s = "";
for (var subject in this.subjects) {
var snode = this.subjects[subject];
s += snode.toString(options, replace);
s += "\n";
}
var prolog = requestOptions && requestOptions.baseURI ? "@base <"+baseURI+"> .\n" : "";
if (options && options.shorten) {
for (var prefix in options.prefixesUsed) {
prolog += "@prefix "+prefix+" <"+this.prefixes[prefix]+"> .\n";
}
}
return prolog.length==0 ? s : prolog+"\n"+s;
};
this.clear = function() {
this.subjects = {};
this.prefixes = {};
this.terms = {};
}
this.clear();
Object.defineProperty(this,"tripleCount",{
enumerable: true,
configurable: false,
get: function() {
var count = 0;
for (var s in this.subjects) {
var snode = this.subjects[s];
for (var p in snode.predicates) {
count += snode.predicates[p].objects.length;
}
}
return count;
}
});
}
RDFaGraph.prototype.expand = function(curie) {
return this.curieParser.parse(curie,true);
}
RDFaGraph.prototype.shorten = function(uri,prefixesUsed) {
for (prefix in this.prefixes) {
var mapped = this.prefixes[prefix];
if (uri.indexOf(mapped)==0) {
if (prefixesUsed) {
prefixesUsed[prefix] = mapped;
}
return prefix+":"+uri.substring(mapped.length);
}
}
return null;
}
function RDFaSubject(graph,subject) {
this.graph = graph;
// TODO: subject or id?
this.subject = subject
this.id = subject;
this.predicates = {};
this.origins = [];
this.types = [];
}
RDFaSubject.prototype.toString = function(options, replace) {
var s = null;
if (this.subject.substring(0,2)=="_:") {
s = this.subject;
} else if (options && options.shorten) {
s = this.graph.shorten(this.subject,options.prefixesUsed);
if (!s) {
s = "<" + this.subject + ">";
}
} else {
s = "<" + this.subject + ">";
//replace the base uri of subject to server url
//Fuqi Song, April 2014
if(replace){
var pref = "http://";
var begin = s.indexOf(pref);
var domain = s.substring(begin, s.indexOf("/", pref.length+begin));
s= s.replace(domain, "http://localhost:8080/kgram/ldp");
}
//end
}
var first = true;
for (var predicate in this.predicates) {
if (!first) {
s += ";\n";
} else {
first = false;
}
s += " " + this.predicates[predicate].toString(options);
}
s += " .";
return s;
}
RDFaSubject.prototype.toObject = function() {
var o = { subject: this.subject, predicates: {} };
for (var predicate in this.predicates) {
var pnode = this.predicates[predicate];
var p = { predicate: predicate, objects: [] };
o.predicates[predicate] = p;
for (var i=0; i"
}
} else {
s = "<" + this.predicate + ">"
}
s += " ";
for (var i=0; i0) {
s += ", ";
}
// TODO: handle HTML literal
if (this.objects[i].type=="http://www.w3.org/1999/02/22-rdf-syntax-ns#object") {
if (this.objects[i].value.substring(0,2)=="_:") {
s += this.objects[i].value;
} else if (options && options.shorten && options.graph) {
s = options.graph.shorten(this.objects[i].value,options.prefixesUsed);
if (!s) {
s = "<" + this.objects[i].value + ">"
}
} else {
s += "<" + this.objects[i].value + ">";
}
} else if (this.objects[i].type=="http://www.w3.org/2001/XMLSchema#integer" ||
this.objects[i].type=="http://www.w3.org/2001/XMLSchema#decimal" ||
this.objects[i].type=="http://www.w3.org/2001/XMLSchema#double" ||
this.objects[i].type=="http://www.w3.org/2001/XMLSchema#boolean") {
s += this.objects[i].value;
} else if (this.objects[i].type=="http://www.w3.org/1999/02/22-rdf-syntax-ns#XMLLiteral") {
var serializer = new XMLSerializer();
var value = "";
for (var x=0; x';
} else {
var l = this.objects[i].value;
if (l.indexOf("\n")>=0 || l.indexOf("\r")>=0) {
//** Modified by Fuqi Song, to replace all occurances, not only the first one**
//s += '"""' + l.replace(/"""/,"\\\"\\\"\\\"") + '"""';
s += '"""' + l.replace(/"""/g,"\\\"\\\"\\\"") + '"""';
} else {
//s += '"' + l.replace(/"/,"\\\"") + '"';
//** Modified by Fuqi Song, to replace all occurances, not only the first one**
//27 mars 2014
s += '"' + l.replace(/"/g,"\\\"") + '"';
}
if (this.objects[i].type!="http://www.w3.org/1999/02/22-rdf-syntax-ns#PlainLiteral") {
s += "^^<"+this.objects[i].type+">";
} else if (this.objects[i].language) {
s += "@"+this.objects[i].language;
}
}
}
return s;
}
GraphRDFaProcessor.prototype = new RDFaProcessor();
GraphRDFaProcessor.prototype.constructor=RDFaProcessor;
function GraphRDFaProcessor(target) {
RDFaProcessor.call(this,target);
}
GraphRDFaProcessor.prototype.getObjectSize = function(obj) {
var size = 0;
for (var key in obj) {
if (obj.hasOwnProperty(key)) {
size++;
}
}
return size;
};
GraphRDFaProcessor.prototype.init = function() {
var thisObj = this;
this.finishedHandlers.push(function(node) {
for (var subject in thisObj.target.graph.subjects) {
var snode = thisObj.target.graph.subjects[subject];
if (thisObj.getObjectSize(snode.predicates)==0) {
delete thisObj.target.graph.subjects[subject];
}
}
});
}
GraphRDFaProcessor.prototype.newSubjectOrigin = function(origin,subject) {
var snode = this.newSubject(null,subject);
for (var i=0; imax) {
max = n;
}
}
}
}
if (graph) {
var blankMap = {};
var subjectMap = mergeBlank ?
function(u) { return u; } :
function(u) {
var mapSubject = blankMap[u];
if (!mapSubject && /_:([0-9]+)/.test(u)) {
max++;
mapSubject = "_:"+max;
blankMap[u] = mapSubject;
}
return mapSubject ? mapSubject : u;
};
for (var subject in graph) {
var mapSubject = subjectMap(subject);
var snode = graph[subject];
subject = mapSubject ? mapSubject : subject;
var target = this._data_.graph.subjects[subject];
if (target) {
for (var predicate in snode.predicates) {
var pnode = snode.predicates[predicate];
var targetPredicate = target.predicates[predicate];
if (targetPredicate) {
for (var i=0; i=0) {
return NodeFilter.FILTER_ACCEPT;
}
}
return NodeFilter.FILTER_SKIP;
}
},
false);
var results = [];
results.item = function(index) {
return this[index];
}
while (walker.nextNode()) {
results.push(walker.currentNode);
}
return results;
}
Element.prototype.getFirstElementByType = function() {
var typeList = arguments;
var walker = this.ownerDocument.createTreeWalker(this,NodeFilter.SHOW_ELEMENT,
{ acceptNode: function(e) {
if (!e.data) { return NodeFilter.FILTER_SKIP; }
for (var i=0; i=0) {
return NodeFilter.FILTER_ACCEPT;
}
}
return NodeFilter.FILTER_SKIP;
}
},
false);
return walker.nextNode() ? walker.currentNode : null;
}
DocumentData.attach = function(target,options) {
Object.defineProperty(target,"data", {
value: new DocumentData(options && options.baseURI ? options.baseURI : target.nodeType==Node.DOCUMENT_NODE ? target.documentElement.baseURI : target.graph.baseURI),
writable: false,
configurable: false,
enumerable: true
});
target.getElementsByType = function(type) {
return this.getElementsByProperty("http://www.w3.org/1999/02/22-rdf-syntax-ns#type",type);
};
target.getElementsBySubject = function(subject) {
var nodes = [];
nodes.item = function(index) {
return this[index];
};
subject = this.data._data_.graph.curieParser.parse(subject,true);
var snode = this.data._data_.graph.subjects[subject];
if (snode) {
for (var i=0; i=0) {
return subject;
}
}
return null;
};
}
TurtleParser.prototype = new URIResolver();
TurtleParser.prototype.constructor=TurtleParser;
function TurtleParser() {
this.reset();
this.onError = function(lineNumber,msg) {
console.log(lineNumber+": "+msg);
};
}
TurtleParser.commentRE = /^#.*/;
TurtleParser.wsRE = /^\s+/;
TurtleParser.iriRE = /^\<((?:(?:[^\x00-\x20<>"{}|^`\\]|\\u[0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f]|\\U[0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f])*))\>/;
TurtleParser.singleQuoteLiteralRE = /^'((?:[^'\n\r\\]*|\\'|\\)*)'/;
TurtleParser.doubleQuoteLiteralRE = /^\"((?:[^"\n\r\\]*|\\"|\\)*)\"/;
TurtleParser.longDoubleQuoteLiteralRE = /^\"\"\"((?:[^"\\]*|\\"|\\|\"(?!\")|\"\"(?!\"))*)\"\"\"/;
TurtleParser.longSingleQuoteLiteralRE = /^'''((?:[^'\\]*|\\'|\\|'(?!')|''(?!'))*)'''/;
TurtleParser.typeRE = /^\^\^/;
TurtleParser.dotRE = /^\./;
TurtleParser.openSquareBracketRE = /^\[/;
TurtleParser.closeSquareBracketRE = /^\]/;
// PN_CHARS_BASE
// [A-Z]|[a-z]|[\u00C0-\u00D6]|[\u00D8-\u00F6]|[\u00F8-\u02FF]|[\u0370-\u037D]|[\u037F-\u1FFF]|[\u200C-\u200D]|[\u2070-\u218F]|[\u2C00-\u2FEF]|[\u3001-\uD7FF]|[\uF900-\uFDCF]|[\uFDF0-\uFFFD]
// [\ud800-\udfff][\ud800-\udfff] - surrogate pairs
// PN_CHARS_U
// [A-Z]|[a-z]|[\u00C0-\u00D6]|[\u00D8-\u00F6]|[\u00F8-\u02FF]|[\u0370-\u037D]|[\u037F-\u1FFF]|[\u200C-\u200D]|[\u2070-\u218F]|[\u2C00-\u2FEF]|[\u3001-\uD7FF]|[\uF900-\uFDCF]|[\uFDF0-\uFFFD]
// [\ud800-\udfff][\ud800-\udfff] - surrogate pairs
// [_]
// PN_CHARS
// [A-Z]|[a-z]|[\u00C0-\u00D6]|[\u00D8-\u00F6]|[\u00F8-\u02FF]|[\u0370-\u037D]|[\u037F-\u1FFF]|[\u200C-\u200D]|[\u2070-\u218F]|[\u2C00-\u2FEF]|[\u3001-\uD7FF]|[\uF900-\uFDCF]|[\uFDF0-\uFFFD]
// [_]
// [\-\u00B7]|[0-9]|[\u0300-\u036F]|[\u203F-\u2040]
TurtleParser.prefixRE = /^((?:(?:[A-Z]|[a-z]|[\u00C0-\u00D6]|[\u00D8-\u00F6]|[\u00F8-\u02FF]|[\u0370-\u037D]|[\u037F-\u1FFF]|[\u200C-\u200D]|[\u2070-\u218F]|[\u2C00-\u2FEF]|[\u3001-\uD7FF]|[\uF900-\uFDCF]|[\uFDF0-\uFFFD]|[\ud800-\udfff][\ud800-\udfff])(?:(?:[A-Z]|[a-z]|[\u00C0-\u00D6]|[\u00D8-\u00F6]|[\u00F8-\u02FF]|[\u0370-\u037D]|[\u037F-\u1FFF]|[\u200C-\u200D]|[\u2070-\u218F]|[\u2C00-\u2FEF]|[\u3001-\uD7FF]|[\uF900-\uFDCF]|[\uFDF0-\uFFFD]|[\ud800-\udfff][\ud800-\udfff]|[_\.\-\u00B7]|[0-9]|[\u0300-\u036F]|[\u203F-\u2040])*(?:[A-Z]|[a-z]|[\u00C0-\u00D6]|[\u00D8-\u00F6]|[\u00F8-\u02FF]|[\u0370-\u037D]|[\u037F-\u1FFF]|[\u200C-\u200D]|[\u2070-\u218F]|[\u2C00-\u2FEF]|[\u3001-\uD7FF]|[\uF900-\uFDCF]|[\uFDF0-\uFFFD]|[\ud800-\udfff][\ud800-\udfff]|[_\-\u00B7]|[0-9]|[\u0300-\u036F]|[\u203F-\u2040]))?)?):/;
TurtleParser.blankNodeRE = /^(_:)/;
TurtleParser.localNameRE = /^((?:[A-Z]|[a-z]|[\u00C0-\u00D6]|[\u00D8-\u00F6]|[\u00F8-\u02FF]|[\u0370-\u037D]|[\u037F-\u1FFF]|[\u200C-\u200D]|[\u2070-\u218F]|[\u2C00-\u2FEF]|[\u3001-\uD7FF]|[\uF900-\uFDCF]|[\uFDF0-\uFFFD]|[\ud800-\udfff][\ud800-\udfff]|[_:]|[0-9]|%[0-9A-Fa-f][0-9A-Fa-f]|\\[_~\.\-!$&'()*+,;=\/?#@%])(?:(?:[A-Z]|[a-z]|[\u00C0-\u00D6]|[\u00D8-\u00F6]|[\u00F8-\u02FF]|[\u0370-\u037D]|[\u037F-\u1FFF]|[\u200C-\u200D]|[\u2070-\u218F]|[\u2C00-\u2FEF]|[\u3001-\uD7FF]|[\uF900-\uFDCF]|[\uFDF0-\uFFFD]|[\ud800-\udfff][\ud800-\udfff]|[_\-\.:\u00b7]|[\u0300-\u036F]|[\u203F-\u2040]|[0-9]|%[0-9A-Fa-f][0-9A-Fa-f]|\\[_~\.\-!$&'()*+,;=/?#@%])*(?:[A-Z]|[a-z]|[\u00C0-\u00D6]|[\u00D8-\u00F6]|[\u00F8-\u02FF]|[\u0370-\u037D]|[\u037F-\u1FFF]|[\u200C-\u200D]|[\u2070-\u218F]|[\u2C00-\u2FEF]|[\u3001-\uD7FF]|[\uF900-\uFDCF]|[\uFDF0-\uFFFD]|[\ud800-\udfff][\ud800-\udfff]|[_\-:\u00b7]|[\u0300-\u036F]|[\u203F-\u2040]|[0-9]|%[0-9A-Fa-f][0-9A-Fa-f]|\\[_~\.\-!$&'()*+,;=/?#@%]))?)/;
TurtleParser.langRE = /^@([a-zA-Z]+(?:-[a-zA-Z0-9]+)*)/;
TurtleParser.prefixIDRE = /^@prefix/;
TurtleParser.baseRE = /^@base/;
TurtleParser.sparqlPrefixRE = /^[Pp][Rr][Ee][Ff][Ii][Xx]/;
TurtleParser.sparqlBaseRE = /^[Bb][Aa][Ss][Ee]/;
TurtleParser.semicolonRE = /^;/;
TurtleParser.commaRE = /^,/;
TurtleParser.aRE = /^a/;
TurtleParser.openParenRE = /^\(/;
TurtleParser.closeParenRE = /^\)/;
TurtleParser.integerRE = /^([+-]?[0-9]+)/;
TurtleParser.decimalRE = /^([+-]?[0-9]*\.[0-9]+)/;
TurtleParser.doubleRE = /^([+-]?(?:[0-9]+\.[0-9]*[eE][+-]?[0-9]+|\.[0-9]+[eE][+-]?[0-9]+|[0-9]+[eE][+-]?[0-9]+))/;
TurtleParser.booleanRE = /^(true|false)/;
TurtleParser.typeURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#type";
TurtleParser.objectURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#object";
TurtleParser.plainLiteralURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#PlainLiteral";
TurtleParser.nilURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#nil";
TurtleParser.firstURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#first";
TurtleParser.restURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#rest";
TurtleParser.xsdStringURI = "http://www.w3.org/2001/XMLSchema#string";
TurtleParser.xsdIntegerURI = "http://www.w3.org/2001/XMLSchema#integer";
TurtleParser.xsdDecimalURI = "http://www.w3.org/2001/XMLSchema#decimal";
TurtleParser.xsdDoubleURI = "http://www.w3.org/2001/XMLSchema#double";
TurtleParser.xsdBooleanURI = "http://www.w3.org/2001/XMLSchema#boolean";
TurtleParser.prototype.reset = function() {
this.context = new RDFaGraph();
this.blankNodeCounter = 0;
this.errorCount = 0;
this.lineNumber = 1;
}
TurtleParser.dumpGraph = function(graph) {
for (var subject in graph) {
var snode = graph[subject];
console.log(snode.toString());
}
}
TurtleParser.prototype.newBlankNode = function() {
this.blankNodeCounter++;
return "_:"+this.blankNodeCounter;
}
TurtleParser.prototype._match = function(re,text) {
var match = re.exec(text);
return match ? { text: match[0], remaining: text.substring(match[0].length), values: match.length>1 ? match.splice(1) : null} : null;
}
TurtleParser.prototype._trim = function(text) {
var match = null;
do {
match = TurtleParser.wsRE.exec(text);
if (match) {
this.lineNumber += (match[0].split(/\n/).length-1);
text = text.substring(match[0].length);
}
match = TurtleParser.commentRE.exec(text);
if (match) {
text = text.substring(match[0].length);
}
} while (match);
return text;
}
TurtleParser.prototype.reportError = function(msg) {
this.onError(this.lineNumber,msg);
}
TurtleParser.prototype.parse = function(text,baseURI) {
if (baseURI) {
this.context.baseURI = this.parseURI(baseURI);
}
while (text.length>0) {
text = this._trim(text);
if (text.length>0) {
text = this.parseStatement(text);
}
}
}
TurtleParser.prototype.parseStatement = function(text) {
var match = this._match(TurtleParser.prefixIDRE,text);
if (match) {
// prefix
var remaining = this._trim(match.remaining);
match = this.parsePrefixName(remaining);
if (!match) {
this.reportError("Cannot parse prefix after @prefix: "+text.substring(20)+"...");
this.errorCount++;
return remaining;
}
var prefix = match.prefix;
remaining = this._trim(match.remaining);
match = this.parseIRIReference(remaining);
if (!match) {
this.reportError("Cannot parse IRI after @prefix: "+remaining.substring(20)+"...");
this.errorCount++;
return remaining;
}
this.context.prefixes[prefix] = this.context.baseURI ? this.context.baseURI.resolve(match.iri) : match.iri;
try {
this.parseURI(this.context.prefixes[prefix]);
} catch (ex) {
this.reportError(ex.toString());
this.errorCount++;
}
remaining = this._trim(match.remaining);
match = this._match(TurtleParser.dotRE,remaining);
if (match) {
return match.remaining;
} else {
this.reportError("Missing end . for @prefix statement. Found: "+remaining.substring(0,20)+"...");
this.errorCount++;
return remaining;
}
}
match = this._match(TurtleParser.baseRE,text);
if (match) {
// base
var remaining = this._trim(match.remaining);
match = this.parseIRIReference(remaining);
if (!match) {
this.reportError("Cannot parse IRI after @base: "+remaining.substring(0,20)+"...");
this.errorCount++;
return remaining;
}
try {
this.context.baseURI = this.context.baseURI ? this.parseURI(this.context.baseURI.resolve(match.iri)) : this.parseURI(match.iri);
} catch (ex) {
this.reportError(ex+"; IRI: "+match.iri);
this.errorCount++;
}
remaining = this._trim(match.remaining);
match = this._match(TurtleParser.dotRE,remaining);
if (match) {
return match.remaining;
} else {
this.reportError("Missing end . for @base statement. Found: "+remaining.substring(0,20)+"...");
this.errorCount++;
return remaining;
}
}
match = this._match(TurtleParser.sparqlPrefixRE,text);
if (match) {
// sparql prefix
var remaining = this._trim(match.remaining);
match = this.parsePrefixName(remaining);
if (!match) {
this.reportError("Cannot parse prefix after PREFIX: "+text.substring(0,20)+"...");
this.errorCount++;
return remaining;
}
var prefix = match.prefix;
remaining = this._trim(match.remaining);
match = this.parseIRIReference(remaining);
if (!match) {
this.reportError("Cannot parse IRI after PREFIX: "+remaining.substring(0,20)+"...");
this.errorCount++;
return remaining;
}
this.context.prefixes[prefix] = this.context.baseURI ? this.context.baseURI.resolve(match.iri) : match.iri;
try {
this.parseURI(this.context.prefixes[prefix]);
} catch (ex) {
this.reportError(ex.toString());
this.errorCount++;
}
return match.remaining;
}
match = this._match(TurtleParser.sparqlBaseRE,text);
if (match) {
// sparql base
var remaining = this._trim(match.remaining);
match = this.parseIRIReference(remaining);
if (!match) {
this.reportError("Cannot parse IRI after BASE: "+remaining.substring(0,20)+"...");
this.errorCount++;
return remaining;
}
try {
this.context.baseURI = this.context.baseURI ? this.parseURI(this.context.baseURI.resolve(match.iri)) : this.parseURI(match.iri);
} catch (ex) {
this.reportError(ex+"; IRI: "+match.iri);
this.errorCount++;
}
return match.remaining;
}
// triples
text = this.parseTriples(text);
text = this._trim(text);
match = this._match(TurtleParser.dotRE,text);
if (match) {
return match.remaining;
} else {
this.reportError("Missing end . triples. Found: "+text.substring(0,20)+"...");
this.errorCount++;
return text;
}
}
TurtleParser.prototype.parseTriples = function(text) {
var match = this.parseIRI(text);
if (match) {
return this.parsePredicateObjectList(match.iri,this._trim(match.remaining));
}
match = this.parseBlankNode(text);
if (match) {
return this.parsePredicateObjectList(match.iri,this._trim(match.remaining));
}
// collection as subject
match = this._match(TurtleParser.openParenRE,text);
if (match) {
// collection
var subject = null;
var remaining = match.remaining;
var currentSubject = null;
do {
remaining = this._trim(remaining);
// try closing the collection
match = this._match(TurtleParser.closeParenRE,remaining);
if (match) {
remaining = match.remaining;
if (currentSubject) {
this.addTriple(currentSubject,TurtleParser.restURI,{ type: TurtleParser.objectURI, value: TurtleParser.nilURI});
} else {
subject = this.newBlankNode();
}
break;
}
var nextSubject = this.newBlankNode();
if (!currentSubject) {
subject = nextSubject;
} else {
this.addTriple(currentSubject,TurtleParser.restURI,{ type: TurtleParser.objectURI, value: nextSubject});
}
currentSubject = nextSubject;
remaining = this.parseObject(currentSubject,TurtleParser.firstURI,remaining);
} while (remaining.length>0);
return this.parsePredicateObjectList(subject,this._trim(remaining));
}
// blank node property list as subject
match = this._match(TurtleParser.openSquareBracketRE,text);
if (match) {
var subject = this.newBlankNode();
var remaining = this._trim(match.remaining);
// test for empty node
match = this._match(TurtleParser.closeSquareBracketRE,remaining);
if (match) {
remaining = match.remaining;
} else {
remaining = this.parsePredicateObjectList(subject,remaining);
match = this._match(TurtleParser.closeSquareBracketRE,this._trim(remaining));
if (match) {
remaining = match.remaining;
} else {
this.reportError("Missing end square bracket ']'.");
}
}
return this.parsePredicateObjectList(subject,this._trim(remaining),true);
}
if (!match) {
// end the parse
this.reportError("Terminating: Cannot parse at "+text.substring(0,20)+" ...");
this.errorCount++;
return "";
}
return this.parsePredicateObjectList(match.iri,this._trim(match.remaining));
}
TurtleParser.prototype.parsePredicateObjectList = function(subject,text,allowEmpty) {
var more = true;
var remaining = null;
do {
var match = this.parseIRI(text);
if (!match) {
match = this._match(TurtleParser.aRE,text);
if (match) {
match.iri = TurtleParser.typeURI;
}
}
if (!match) {
if (allowEmpty) {
return text;
}
this.reportError("Terminating: Cannot parse predicate IRI.");
this.errorCount++;
return "";
}
remaining = this.parseObjectList(subject,match.iri,this._trim(match.remaining));
match = this._match(TurtleParser.semicolonRE,this._trim(remaining));
if (match) {
do {
text = this._trim(match.remaining);
match = this._match(TurtleParser.semicolonRE,text);
} while (match);
allowEmpty = true;
} else {
more = false;
}
} while (more);
return remaining;
}
TurtleParser.prototype.parseObjectList = function(subject,predicate,text) {
var more = true;
var remaining = null;
do {
remaining = this.parseObject(subject,predicate,text);
var match = this._match(TurtleParser.commaRE,this._trim(remaining));
if (match) {
text = this._trim(match.remaining);
} else {
more = false;
}
} while (more);
return remaining;
}
TurtleParser.prototype.parseObject = function(subject,predicate,text) {
var match = this.parseIRI(text);
if (match) {
// object reference, generate triple
this.addTriple(subject,predicate,{ type: TurtleParser.objectURI, value: match.iri});
return match.remaining;
}
var match = this.parseBlankNode(text);
if (match) {
// object reference, generate triple
this.addTriple(subject,predicate,{ type: TurtleParser.objectURI, value: match.iri});
return match.remaining;
}
match = this._match(TurtleParser.openParenRE,text);
if (match) {
// collection
var collectionSubject = subject;
var collectionPredicate = predicate;
var remaining = match.remaining;
do {
remaining = this._trim(remaining);
// try closing the collection
match = this._match(TurtleParser.closeParenRE,remaining);
if (match) {
this.addTriple(collectionSubject,collectionSubject==subject ? predicate : TurtleParser.restURI,{ type: TurtleParser.objectURI, value: TurtleParser.nilURI});
return match.remaining;
}
var nextSubject = this.newBlankNode();
// there must be an object
if (collectionSubject==subject) {
this.addTriple(subject,predicate,{ type: TurtleParser.objectURI, value: nextSubject});
} else {
this.addTriple(collectionSubject,TurtleParser.restURI,{ type: TurtleParser.objectURI, value: nextSubject});
}
collectionSubject = nextSubject;
collectionPredicate = TurtleParser.firstURI;
remaining = this.parseObject(collectionSubject,collectionPredicate,remaining);
} while (remaining.length>0);
}
match = this._match(TurtleParser.openSquareBracketRE,text);
if (match) {
var newSubject = this.newBlankNode();
this.addTriple(subject,predicate,{ type: TurtleParser.objectURI, value: newSubject});
var remaining = this.parsePredicateObjectList(newSubject,this._trim(match.remaining),true);
remaining = this._trim(remaining);
match = this._match(TurtleParser.closeSquareBracketRE,remaining);
if (match) {
return match.remaining;
} else {
this.reportError("Missing close square bracket ']' for blank node "+newSubject+" predicate object list");
this.errorCount++;
return remaining;
}
}
var match = this.parseLiteral(text);
if (match) {
this.addTriple(subject,predicate,{ type: match.type ? match.type : TurtleParser.plainLiteralURI, value: match.literal, language: match.language});
return match.remaining;
}
this.reportError("Terminating: Cannot parse literal at "+text.substring(0,20));
this.errorCount++;
return "";
}
TurtleParser.prototype.parsePrefixName = function(text) {
var match = this._match(TurtleParser.prefixRE,text);
if (match) {
match.prefix = match.values[0];
}
return match;
}
TurtleParser.prototype.parseIRIReference = function(text) {
var match = this._match(TurtleParser.iriRE,text);
if (match) {
match.iri = TurtleParser.expandURI(match.values[0]);
}
return match;
}
TurtleParser.prototype.parseIRI = function(text) {
var match = this._match(TurtleParser.iriRE,text);
if (match) {
var expanded = TurtleParser.expandURI(match.values[0]);
match.iri = this.context.baseURI ? this.context.baseURI.resolve(expanded) : expanded;
try {
this.parseURI(match.iri);
} catch (ex) {
this.reportError(ex.toString());
this.errorCount++;
}
return match;
}
match = this._match(TurtleParser.prefixRE,text);
if (match) {
var prefix = match.values[0];
var ns = this.context.prefixes[prefix];
if (!ns) {
this.reportError("No prefix mapping for "+prefix);
this.errorCount++;
return null;
}
var remaining = match.remaining;
match = this._match(TurtleParser.localNameRE,remaining);
if (match) {
match.iri = ns+TurtleParser.expandName(match.values[0]);
try {
this.parseURI(match.iri);
} catch (ex) {
this.reportError(ex.toString());
this.errorCount++;
}
return match;
} else {
return { iri: ns, remaining: remaining };
}
}
return null;
}
TurtleParser.prototype.parseBlankNode = function(text) {
var match = this._match(TurtleParser.blankNodeRE,text);
if (match) {
var remaining = match.remaining;
match = this._match(TurtleParser.localNameRE,remaining);
if (match) {
match.iri = "_:"+TurtleParser.expandName(match.values[0]);
return match;
}
return null;
}
return null;
}
TurtleParser.prototype.parseLiteral = function(text) {
var match = this._match(TurtleParser.longDoubleQuoteLiteralRE,text);
if (!match) {
match = this._match(TurtleParser.longSingleQuoteLiteralRE,text);
}
if (!match) {
match = this._match(TurtleParser.singleQuoteLiteralRE,text);
}
if (!match) {
match = this._match(TurtleParser.doubleQuoteLiteralRE,text);
}
if (match) {
var literal = null;
try {
literal = TurtleParser.expandLiteral(match.values[0]);
} catch (ex) {
this.reportError(ex.toString());
this.errorCount++;
}
var remaining = match.remaining;
match = this._match(TurtleParser.langRE,remaining);
if (match) {
match.literal = literal;
match.language = match.values[0];
return match;
}
match = this._match(TurtleParser.typeRE,remaining);
if (match) {
var remaining = match.remaining;
match = this.parseIRI(remaining);
if (match) {
match.literal = literal;
match.type = match.iri;
return match;
} else {
this.reportError("Missing type URI after ^^");
this.errorCount++;
return { literal: literal, remaining: remaining}
}
}
return { literal: literal, remaining: remaining};
}
match = this._match(TurtleParser.doubleRE,text);
if (match) {
match.literal = match.values[0];
match.type = TurtleParser.xsdDoubleURI;
return match;
}
match = this._match(TurtleParser.decimalRE,text);
if (match) {
match.literal = match.values[0];
match.type = TurtleParser.xsdDecimalURI;
return match;
}
match = this._match(TurtleParser.integerRE,text);
if (match) {
match.literal = match.values[0];
match.type = TurtleParser.xsdIntegerURI;
return match;
}
match = this._match(TurtleParser.booleanRE,text);
if (match) {
match.literal = match.values[0];
match.type = TurtleParser.xsdBooleanURI;
return match;
}
return null;
}
TurtleParser.prototype.newSubject = function(subject) {
var snode = this.context.subjects[subject];
if (!snode) {
snode = new RDFaSubject(this.context,subject);
this.context.subjects[subject] = snode;
}
return snode;
}
TurtleParser.expandHex = function(hex) {
var check = hex.split(/[0-9A-Fa-f]+/);
for (var j=0; j0) {
throw "Bad hex in escape: "+hex;
}
}
var code = parseInt(hex,16);
if (isNaN(code)) {
throw "Bad hex in escape: "+hex;
}
if (code<0x10000) {
return String.fromCharCode(code);
} else {
// Evil: generate surrogate pairs
var n = code - 0x10000;
var h = n >> 10;
var l = n & 0x3ff;
return String.fromCharCode(h + 0xd800) + String.fromCharCode(l + 0xdc00);
}
}
TurtleParser.escapedSequenceRE = /(\\t|\\b|\\n|\\r|\\f|\\"|\\'|\\\\|(?:\\U[0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f])|(?:\\u[0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f]))/;
TurtleParser.expandLiteral = function(literal) {
var parts = literal.split(TurtleParser.escapedSequenceRE);
var s = "";
for (var i=0; i=0) {
throw "Bad escape "+parts[i].substring(pos,pos+2);
}
s += parts[i];
}
}
return s;
}
TurtleParser.escapedURIRE = /((?:\\U[0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f])|(?:\\u[0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f][0-9A-Fa-f]))/;
TurtleParser.expandURI = function(uri) {
var parts = uri.split(TurtleParser.escapedURIRE);
var s = "";
for (var i=0; i=0) {
throw "Bad escape "+parts[i].substring(pos,pos+2);
}
s += parts[i];
}
}
return s;
}
TurtleParser.escapedNameCharRE = /(\\[_~\.\-!$&'()*+,;=/?#@%])/;
TurtleParser.expandName = function(name) {
var parts = name.split(TurtleParser.escapedNameCharRE);
var s = "";
for (var i=0; i=0) {
baseURI = baseURI.substring(0,hash);
}
return this.parseURI(baseURI);
}
MicrodataProcessor.trim = function(str) {
return str.replace(/^\s\s*/, '').replace(/\s\s*$/, '');
}
MicrodataProcessor.tokenize = function(str) {
return MicrodataProcessor.trim(str).split(/\s+/);
}
MicrodataProcessor.prototype.getVocabulary = function(uri) {
for (var i=0; i=0) {
return makeVocab(uri.substring(0,hash+1));
}
var lastSlash = uri.lastIndexOf("/");
if (lastSlash>=0) {
return makeVocab(uri.substring(0,lastSlash+1));
}
return makeVocab(uri);
}
MicrodataProcessor.prototype.getProperty = function(value,vocabulary) {
if (MicrodataProcessor.absoluteURIRE.exec(value)) {
return value;
}
return vocabulary ? vocabulary.getProperty(value) : null;
}
MicrodataProcessor.valueMappings = {
"meta" : function(node,base) { return node.getAttribute("content"); },
"audio" : function(node,base) { return base.resolve(node.getAttribute("src")); },
"a" : function(node,base) { return base.resolve(node.getAttribute("href")); },
"object" : function(node,base) { return base.resolve(node.getAttribute("data")); },
"time" : function(node,base) { var datetime = node.getAttribute("datetime"); return datetime ? datetime : node.textContent; }
};
MicrodataProcessor.valueMappings["embed"] = MicrodataProcessor.valueMappings["audio"];
MicrodataProcessor.valueMappings["iframe"] = MicrodataProcessor.valueMappings["audio"];
MicrodataProcessor.valueMappings["img"] = MicrodataProcessor.valueMappings["audio"];
MicrodataProcessor.valueMappings["source"] = MicrodataProcessor.valueMappings["audio"];
MicrodataProcessor.valueMappings["track"] = MicrodataProcessor.valueMappings["audio"];
MicrodataProcessor.valueMappings["video"] = MicrodataProcessor.valueMappings["audio"];
MicrodataProcessor.valueMappings["area"] = MicrodataProcessor.valueMappings["a"];
MicrodataProcessor.valueMappings["link"] = MicrodataProcessor.valueMappings["a"];
MicrodataProcessor.prototype.getValue = function(node,base) {
var converter = MicrodataProcessor.valueMappings[node.localName];
return converter ? converter(node,base) : node.textContent;
}
MicrodataProcessor.objectURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#object";
MicrodataProcessor.PlainLiteralURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#PlainLiteral";
MicrodataProcessor.typeURI = "http://www.w3.org/1999/02/22-rdf-syntax-ns#type";
MicrodataProcessor.prototype.process = function(node) {
if (node.nodeType==Node.DOCUMENT_NODE) {
node = node.documentElement;
}
var queue = [];
queue.push({ current: node, context: this.push(null,null) });
while (queue.length>0) {
var item = queue.shift();
var current = item.current;
var context = item.context;
var base = this.createBaseURI(current.baseURI);
var subject = null;
var vocabulary = context.vocabulary;
var itemScope = current.hasAttribute("itemscope");
if (itemScope) {
//console.log("Item at "+current.tagName+", parent.subject="+context.subject);
var itemType = current.getAttribute("itemtype");
var itemId = current.getAttribute("itemid");
if (itemType) {
vocabulary = this.getVocabulary(itemType);
subject = itemId ? itemId : this.newBlankNode();
this.addTriple(current,subject,MicrodataProcessor.typeURI, {type: MicrodataProcessor.objectURI, value: itemType});
}
}
var itemProp = current.getAttribute("itemprop");
if (itemProp) {
//console.log("Property "+itemProp+" at "+current.tagName+", parent.subject="+context.subject);
var tokens = MicrodataProcessor.tokenize(itemProp);
if (itemScope) {
// Only make a triple if there is both a parent subject and new subject
if (context.parent.subject && subject) {
// make a triple with the new subject as the object
this.addTriple(current, context.subject, prop, { type: MicrodataProcessor.objectURI, value: subject});
}
} else if (vocabulary) {
if (!subject) {
subject = context.subject;
}
if (subject) {
var value = this.getValue(current,base);
// make a triple with the new subject and predicate
for (var i=0; i0 ? this.getProperty(tokens[i],vocabulary) : null;
if (!prop) {
continue;
}
this.addTriple(current, subject, prop, { type: MicrodataProcessor.PlainLiteralURI, value: value});
}
}
}
}
for (var child = current.lastChild; child; child = child.previousSibling) {
if (child.nodeType==Node.ELEMENT_NODE) {
queue.unshift({ current: child, context: this.push(context,subject,vocabulary)});
}
}
}
}
MicrodataProcessor.prototype.push = function(parent,subject,vocabulary) {
return {
parent: parent,
subject: subject ? subject : (parent ? parent.subject : null),
vocabulary: vocabulary ? vocabulary : (parent ? parent.vocabulary : null)
};
}
MicrodataProcessor.prototype.addTriple = function(origin,subject,predicate,object) {
}
GraphMicrodataProcessor.prototype = new MicrodataProcessor();
GraphMicrodataProcessor.prototype.constructor=MicrodataProcessor;
function GraphMicrodataProcessor(targetGraph) {
MicrodataProcessor.call(this);
this.graph = targetGraph;
}
GraphMicrodataProcessor.prototype.addTriple = function(origin,subject,predicate,object) {
var snode = this.graph.subjects[subject];
if (!snode) {
snode = new RDFaSubject(this.graph,subject);
this.graph.subjects[subject] = snode;
}
var pnode = snode.predicates[predicate];
if (!pnode) {
pnode = new RDFaPredicate(predicate);
snode.predicates[predicate] = pnode;
}
for (var i=0; i0) {
throw base ? "Errors during parsing "+base+" of type "+mediaType : "Errors during parsing of type "+mediaType;
}
return parser.context;
},
createDocumentData: function(baseURI) {
return new DocumentData(baseURI ? baseURI : window ? window.location.href : "about:blank");
}
};
Object.defineProperty(implementation,"processors", {
value: {},
writable: false,
configurable: false,
enumerable: true
});
implementation.processors["text/turtle"] = {
createParser: function() {
return new TurtleParser();
},
process: function(node,options) {
if (!this.enabled) {
return;
}
var owner = node.nodeType==Node.DOCUMENT_NODE ? node : node.ownerDocument;
if (!owner.data) {
return;
}
var success = true;
var scripts = owner.getElementsByTagNameNS("http://www.w3.org/1999/xhtml","script");
for (var i=0; i0) {
success = false;
} else {
owner.data.merge(parser.context.subjects,{ prefixes: parser.context.prefixes});
}
}
return success;
},
enabled: true
};
implementation.processors["microdata"] = {
process: function(node,options) {
if (!this.enabled) {
return;
}
var owner = node.nodeType==Node.DOCUMENT_NODE ? node : node.ownerDocument;
if (!owner.data) {
return false;
}
var processor = new GraphMicrodataProcessor(owner.data.graph);
processor.process(node);
return true;
},
enabled: false
};
Object.defineProperty(env,"implementation", {
value: implementation,
writable: false,
configurable: false,
enumerable: true
});
env.attach = function(document,options) {
implementation.attach(document,options);
};
return env;
})();
}
GreenTurtle.attach(document);
© 2015 - 2024 Weber Informatics LLC | Privacy Policy