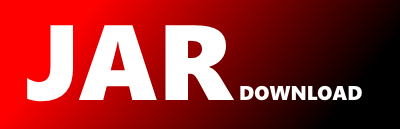
META-INF.resources.webjars.lx.js.lx.js Maven / Gradle / Ivy
Vue.prototype.containsRole= function(roles) {
return (roles||[]).includes(Vue.prototype.getCookie("userType"));
};
Vue.directive('hasrole', {
inserted: (el,binding,vnode)=>{
// 当前登录人角色
const roles = Vue.prototype.getCookie("userType") || ['user']
// 使用该组件的权限如果不包括在当前登录人的权限中 就利用父元素删除该元素
if (!binding.value.includes(roles)) {
el.parentNode.removeChild(el);
}
}
});
Vue.directive('focus', {
inserted: (el,binding,vnode)=>{
if (el) {
el.querySelector('input').focus();
}
}
});
Vue.directive('click', {
inserted: (el,binding,vnode)=>{
if (el) {
el.click();
}
}
});
Vue.prototype.uuid= function() {
return ('xxxxxxxxxxxx4xxxyxxxxxxxxxxxxxxx'.replace(/[xy]/g, function (c) {
var r = Math.random() * 16 | 0,
v = c == 'x' ? r : (r & 0x3 | 0x8);
return v.toString(16);
}));
}
Vue.prototype.copy= function (copyData){
if (copyData){
var inputbtn = document.createElement('input');
inputbtn.id="lxCopyNode";
inputbtn.type="hidden"
document.body.appendChild(inputbtn);
Vue.prototype.clipboard = new ClipboardJS('#lxCopyNode', {text: trigger => {return copyData;}})
Vue.prototype.clipboard.on('success', e => {
Vue.prototype.$message.success("复制成功");
Vue.prototype.clipboard.destroy()
inputbtn.parentNode.removeChild(inputbtn);
})
Vue.prototype.clipboard.on('error', e => {
Vue.prototype.$message.error('复制失败')
Vue.prototype.clipboard.destroy()
inputbtn.parentNode.removeChild(inputbtn);
})
inputbtn.click()
}else{
Vue.prototype.$message.error('未拷贝任何内容!')
}
}
Vue.prototype.getCookie= function getCookie(name) {
var arr, reg = new RegExp("(^| )" + name + "=([^;]*)(;|$)");
if (arr = document.cookie.match(reg))
return unescape(arr[2]);
else
return null;
}
Vue.prototype.setCookie= function setCookie (c_name, value, expiredays) {
var exdate = new Date();
exdate.setDate(exdate.getDate() + expiredays);
document.cookie = c_name + "=" + escape(value) + ((expiredays == null) ? "" : ";expires=" + exdate.toGMTString());
}
Vue.prototype.delCookie= function delCookie (name) {
var exp = new Date();
exp.setTime(exp.getTime() - 1);
var cval = this.getCookie(name);
if (cval != null)
document.cookie = name + "=" + cval + ";expires=" + exp.toGMTString();
}
//首字母处理默认大写
Vue.prototype.firstLetterProcess = function (data, isLowercase){
if (data) {
const [first, ...char] = data
return (isLowercase?first.toLowerCase():first.toUpperCase()) + char.join('')
}
return ''
}
//日期格式化 new Date().format("yyyy-MM-dd HH:mm:ss");
Date.prototype.format = function(fmt) {
var o = {
"M+" : this.getMonth()+1, //月份
"d+" : this.getDate(), //日
"h+" : this.getHours()%12 == 0 ? 12 : this.getHours()%12, //小时
"H+" : this.getHours(), //小时
"m+" : this.getMinutes(), //分
"s+" : this.getSeconds(), //秒
"q+" : Math.floor((this.getMonth()+3)/3), //季度
"S" : this.getMilliseconds() //毫秒
};
if(/(y+)/.test(fmt))
fmt=fmt.replace(RegExp.$1, (this.getFullYear()+"").substr(4 - RegExp.$1.length));
for(var k in o)
if(new RegExp("("+ k +")").test(fmt))
fmt = fmt.replace(RegExp.$1, (RegExp.$1.length==1) ? (o[k]) : (("00"+ o[k]).substr((""+ o[k]).length)));
return fmt;
}
//左补齐
String.prototype.padLeft = function(total, pad) {
return (Array(total).join(pad || '0') + this).slice(-total);
}
//右补齐
String.prototype.padRight = function(total, pad) {
return (this + Array(total).join(pad || ' ')).slice(0, total);
}
var LX = {
request: function(paras) {
var url = location.href;
var paraString = url.substring(url.indexOf("?") + 1, url.length).split("&");
var paraObj = {}
for (i = 0; j = paraString[i]; i++) {
paraObj[j.substring(0, j.indexOf("=")).toLowerCase()] = decodeURIComponent(j.substring(j.indexOf("=") + 1, j.length));
}
var returnValue = paraObj[paras.toLowerCase()];
if (typeof(returnValue) == "undefined") {
return "";
} else {
return returnValue;
}
},
// 防抖 时间段内连续触发,那么只有最后一次触发
debounce: function (fn, timeout = 500) {
// 这里通过闭包使timer变量不会被JS垃圾回收机制回收,从而常驻内存中
let timer = null
return function(...args) {
if (!timer) {
timer = setTimeout(() => {
fn.apply(this, args)
timer = null
}, timeout)
}
}
},
// 节流 连续触发或调用时,在一定时间段内,只调用一次
throttle:function (fn, delay = 1000) {
let last = 0
return function(...args) {
const current = Date.now()
if (current - last > delay) {
fn.apply(this, args)
last = current
}
}
}
}
//DES
{
function des (key, message, encrypt, mode, iv, padding) {
if(encrypt) //如果是加密的话,首先转换编码
message = unescape(encodeURIComponent(message));
//declaring this locally speeds things up a bit
var spfunction1 = new Array (0x1010400,0,0x10000,0x1010404,0x1010004,0x10404,0x4,0x10000,0x400,0x1010400,0x1010404,0x400,0x1000404,0x1010004,0x1000000,0x4,0x404,0x1000400,0x1000400,0x10400,0x10400,0x1010000,0x1010000,0x1000404,0x10004,0x1000004,0x1000004,0x10004,0,0x404,0x10404,0x1000000,0x10000,0x1010404,0x4,0x1010000,0x1010400,0x1000000,0x1000000,0x400,0x1010004,0x10000,0x10400,0x1000004,0x400,0x4,0x1000404,0x10404,0x1010404,0x10004,0x1010000,0x1000404,0x1000004,0x404,0x10404,0x1010400,0x404,0x1000400,0x1000400,0,0x10004,0x10400,0,0x1010004);
var spfunction2 = new Array (-0x7fef7fe0,-0x7fff8000,0x8000,0x108020,0x100000,0x20,-0x7fefffe0,-0x7fff7fe0,-0x7fffffe0,-0x7fef7fe0,-0x7fef8000,-0x80000000,-0x7fff8000,0x100000,0x20,-0x7fefffe0,0x108000,0x100020,-0x7fff7fe0,0,-0x80000000,0x8000,0x108020,-0x7ff00000,0x100020,-0x7fffffe0,0,0x108000,0x8020,-0x7fef8000,-0x7ff00000,0x8020,0,0x108020,-0x7fefffe0,0x100000,-0x7fff7fe0,-0x7ff00000,-0x7fef8000,0x8000,-0x7ff00000,-0x7fff8000,0x20,-0x7fef7fe0,0x108020,0x20,0x8000,-0x80000000,0x8020,-0x7fef8000,0x100000,-0x7fffffe0,0x100020,-0x7fff7fe0,-0x7fffffe0,0x100020,0x108000,0,-0x7fff8000,0x8020,-0x80000000,-0x7fefffe0,-0x7fef7fe0,0x108000);
var spfunction3 = new Array (0x208,0x8020200,0,0x8020008,0x8000200,0,0x20208,0x8000200,0x20008,0x8000008,0x8000008,0x20000,0x8020208,0x20008,0x8020000,0x208,0x8000000,0x8,0x8020200,0x200,0x20200,0x8020000,0x8020008,0x20208,0x8000208,0x20200,0x20000,0x8000208,0x8,0x8020208,0x200,0x8000000,0x8020200,0x8000000,0x20008,0x208,0x20000,0x8020200,0x8000200,0,0x200,0x20008,0x8020208,0x8000200,0x8000008,0x200,0,0x8020008,0x8000208,0x20000,0x8000000,0x8020208,0x8,0x20208,0x20200,0x8000008,0x8020000,0x8000208,0x208,0x8020000,0x20208,0x8,0x8020008,0x20200);
var spfunction4 = new Array (0x802001,0x2081,0x2081,0x80,0x802080,0x800081,0x800001,0x2001,0,0x802000,0x802000,0x802081,0x81,0,0x800080,0x800001,0x1,0x2000,0x800000,0x802001,0x80,0x800000,0x2001,0x2080,0x800081,0x1,0x2080,0x800080,0x2000,0x802080,0x802081,0x81,0x800080,0x800001,0x802000,0x802081,0x81,0,0,0x802000,0x2080,0x800080,0x800081,0x1,0x802001,0x2081,0x2081,0x80,0x802081,0x81,0x1,0x2000,0x800001,0x2001,0x802080,0x800081,0x2001,0x2080,0x800000,0x802001,0x80,0x800000,0x2000,0x802080);
var spfunction5 = new Array (0x100,0x2080100,0x2080000,0x42000100,0x80000,0x100,0x40000000,0x2080000,0x40080100,0x80000,0x2000100,0x40080100,0x42000100,0x42080000,0x80100,0x40000000,0x2000000,0x40080000,0x40080000,0,0x40000100,0x42080100,0x42080100,0x2000100,0x42080000,0x40000100,0,0x42000000,0x2080100,0x2000000,0x42000000,0x80100,0x80000,0x42000100,0x100,0x2000000,0x40000000,0x2080000,0x42000100,0x40080100,0x2000100,0x40000000,0x42080000,0x2080100,0x40080100,0x100,0x2000000,0x42080000,0x42080100,0x80100,0x42000000,0x42080100,0x2080000,0,0x40080000,0x42000000,0x80100,0x2000100,0x40000100,0x80000,0,0x40080000,0x2080100,0x40000100);
var spfunction6 = new Array (0x20000010,0x20400000,0x4000,0x20404010,0x20400000,0x10,0x20404010,0x400000,0x20004000,0x404010,0x400000,0x20000010,0x400010,0x20004000,0x20000000,0x4010,0,0x400010,0x20004010,0x4000,0x404000,0x20004010,0x10,0x20400010,0x20400010,0,0x404010,0x20404000,0x4010,0x404000,0x20404000,0x20000000,0x20004000,0x10,0x20400010,0x404000,0x20404010,0x400000,0x4010,0x20000010,0x400000,0x20004000,0x20000000,0x4010,0x20000010,0x20404010,0x404000,0x20400000,0x404010,0x20404000,0,0x20400010,0x10,0x4000,0x20400000,0x404010,0x4000,0x400010,0x20004010,0,0x20404000,0x20000000,0x400010,0x20004010);
var spfunction7 = new Array (0x200000,0x4200002,0x4000802,0,0x800,0x4000802,0x200802,0x4200800,0x4200802,0x200000,0,0x4000002,0x2,0x4000000,0x4200002,0x802,0x4000800,0x200802,0x200002,0x4000800,0x4000002,0x4200000,0x4200800,0x200002,0x4200000,0x800,0x802,0x4200802,0x200800,0x2,0x4000000,0x200800,0x4000000,0x200800,0x200000,0x4000802,0x4000802,0x4200002,0x4200002,0x2,0x200002,0x4000000,0x4000800,0x200000,0x4200800,0x802,0x200802,0x4200800,0x802,0x4000002,0x4200802,0x4200000,0x200800,0,0x2,0x4200802,0,0x200802,0x4200000,0x800,0x4000002,0x4000800,0x800,0x200002);
var spfunction8 = new Array (0x10001040,0x1000,0x40000,0x10041040,0x10000000,0x10001040,0x40,0x10000000,0x40040,0x10040000,0x10041040,0x41000,0x10041000,0x41040,0x1000,0x40,0x10040000,0x10000040,0x10001000,0x1040,0x41000,0x40040,0x10040040,0x10041000,0x1040,0,0,0x10040040,0x10000040,0x10001000,0x41040,0x40000,0x41040,0x40000,0x10041000,0x1000,0x40,0x10040040,0x1000,0x41040,0x10001000,0x40,0x10000040,0x10040000,0x10040040,0x10000000,0x40000,0x10001040,0,0x10041040,0x40040,0x10000040,0x10040000,0x10001000,0x10001040,0,0x10041040,0x41000,0x41000,0x1040,0x1040,0x40040,0x10000000,0x10041000);
//create the 16 or 48 subkeys we will need
var keys = des_createKeys (key);
var m=0, i, j, temp, temp2, right1, right2, left, right, looping;
var cbcleft, cbcleft2, cbcright, cbcright2
var endloop, loopinc;
var len = message.length;
var chunk = 0;
//set up the loops for single and triple des
var iterations = keys.length == 32 ? 3 : 9; //single or triple des
if (iterations == 3) {looping = encrypt ? new Array (0, 32, 2) : new Array (30, -2, -2);}
else {looping = encrypt ? new Array (0, 32, 2, 62, 30, -2, 64, 96, 2) : new Array (94, 62, -2, 32, 64, 2, 30, -2, -2);}
//pad the message depending on the padding parameter
if (padding == 2) message += " "; //pad the message with spaces
else if (padding == 1) {
if(encrypt) {
temp = 8-(len%8);
message += String.fromCharCode(temp,temp,temp,temp,temp,temp,temp,temp);
if (temp===8) len+=8;
}
} //PKCS7 padding
else if (!padding) message += "\0\0\0\0\0\0\0\0"; //pad the message out with null bytes
//store the result here
var result = "";
var tempresult = "";
if (mode == 1) { //CBC mode
cbcleft = (iv.charCodeAt(m++) << 24) | (iv.charCodeAt(m++) << 16) | (iv.charCodeAt(m++) << 8) | iv.charCodeAt(m++);
cbcright = (iv.charCodeAt(m++) << 24) | (iv.charCodeAt(m++) << 16) | (iv.charCodeAt(m++) << 8) | iv.charCodeAt(m++);
m=0;
}
//loop through each 64 bit chunk of the message
while (m < len) {
left = (message.charCodeAt(m++) << 24) | (message.charCodeAt(m++) << 16) | (message.charCodeAt(m++) << 8) | message.charCodeAt(m++);
right = (message.charCodeAt(m++) << 24) | (message.charCodeAt(m++) << 16) | (message.charCodeAt(m++) << 8) | message.charCodeAt(m++);
//for Cipher Block Chaining mode, xor the message with the previous result
if (mode == 1) {if (encrypt) {left ^= cbcleft; right ^= cbcright;} else {cbcleft2 = cbcleft; cbcright2 = cbcright; cbcleft = left; cbcright = right;}}
//first each 64 but chunk of the message must be permuted according to IP
temp = ((left >>> 4) ^ right) & 0x0f0f0f0f; right ^= temp; left ^= (temp << 4);
temp = ((left >>> 16) ^ right) & 0x0000ffff; right ^= temp; left ^= (temp << 16);
temp = ((right >>> 2) ^ left) & 0x33333333; left ^= temp; right ^= (temp << 2);
temp = ((right >>> 8) ^ left) & 0x00ff00ff; left ^= temp; right ^= (temp << 8);
temp = ((left >>> 1) ^ right) & 0x55555555; right ^= temp; left ^= (temp << 1);
left = ((left << 1) | (left >>> 31));
right = ((right << 1) | (right >>> 31));
//do this either 1 or 3 times for each chunk of the message
for (j=0; j>> 4) | (right << 28)) ^ keys[i+1];
//the result is attained by passing these bytes through the S selection functions
temp = left;
left = right;
right = temp ^ (spfunction2[(right1 >>> 24) & 0x3f] | spfunction4[(right1 >>> 16) & 0x3f]
| spfunction6[(right1 >>> 8) & 0x3f] | spfunction8[right1 & 0x3f]
| spfunction1[(right2 >>> 24) & 0x3f] | spfunction3[(right2 >>> 16) & 0x3f]
| spfunction5[(right2 >>> 8) & 0x3f] | spfunction7[right2 & 0x3f]);
}
temp = left; left = right; right = temp; //unreverse left and right
} //for either 1 or 3 iterations
//move then each one bit to the right
left = ((left >>> 1) | (left << 31));
right = ((right >>> 1) | (right << 31));
//now perform IP-1, which is IP in the opposite direction
temp = ((left >>> 1) ^ right) & 0x55555555; right ^= temp; left ^= (temp << 1);
temp = ((right >>> 8) ^ left) & 0x00ff00ff; left ^= temp; right ^= (temp << 8);
temp = ((right >>> 2) ^ left) & 0x33333333; left ^= temp; right ^= (temp << 2);
temp = ((left >>> 16) ^ right) & 0x0000ffff; right ^= temp; left ^= (temp << 16);
temp = ((left >>> 4) ^ right) & 0x0f0f0f0f; right ^= temp; left ^= (temp << 4);
//for Cipher Block Chaining mode, xor the message with the previous result
if (mode == 1) {if (encrypt) {cbcleft = left; cbcright = right;} else {left ^= cbcleft2; right ^= cbcright2;}}
tempresult += String.fromCharCode ((left>>>24), ((left>>>16) & 0xff), ((left>>>8) & 0xff), (left & 0xff), (right>>>24), ((right>>>16) & 0xff), ((right>>>8) & 0xff), (right & 0xff));
chunk += 8;
if (chunk == 512) {result += tempresult; tempresult = ""; chunk = 0;}
} //for every 8 characters, or 64 bits in the message
//return the result as an array
result += tempresult;
result = result.replace(/\0*$/g, "");
if(!encrypt ) { //如果是解密的话,解密结束后对PKCS7 padding进行解码,并转换成utf-8编码
if(padding === 1) { //PKCS7 padding解码
var len = result.length, paddingChars = 0;
len && (paddingChars = result.charCodeAt(len-1));
(paddingChars <= 8) && (result = result.substring(0, len - paddingChars));
}
//转换成UTF-8编码
result = decodeURIComponent(escape(result));
}
return result;
} //end of des
//des_createKeys
//this takes as input a 64 bit key (even though only 56 bits are used)
//as an array of 2 integers, and returns 16 48 bit keys
function des_createKeys (key) {
//declaring this locally speeds things up a bit
var pc2bytes0 = new Array (0,0x4,0x20000000,0x20000004,0x10000,0x10004,0x20010000,0x20010004,0x200,0x204,0x20000200,0x20000204,0x10200,0x10204,0x20010200,0x20010204);
var pc2bytes1 = new Array (0,0x1,0x100000,0x100001,0x4000000,0x4000001,0x4100000,0x4100001,0x100,0x101,0x100100,0x100101,0x4000100,0x4000101,0x4100100,0x4100101);
var pc2bytes2 = new Array (0,0x8,0x800,0x808,0x1000000,0x1000008,0x1000800,0x1000808,0,0x8,0x800,0x808,0x1000000,0x1000008,0x1000800,0x1000808);
var pc2bytes3 = new Array (0,0x200000,0x8000000,0x8200000,0x2000,0x202000,0x8002000,0x8202000,0x20000,0x220000,0x8020000,0x8220000,0x22000,0x222000,0x8022000,0x8222000);
var pc2bytes4 = new Array (0,0x40000,0x10,0x40010,0,0x40000,0x10,0x40010,0x1000,0x41000,0x1010,0x41010,0x1000,0x41000,0x1010,0x41010);
var pc2bytes5 = new Array (0,0x400,0x20,0x420,0,0x400,0x20,0x420,0x2000000,0x2000400,0x2000020,0x2000420,0x2000000,0x2000400,0x2000020,0x2000420);
var pc2bytes6 = new Array (0,0x10000000,0x80000,0x10080000,0x2,0x10000002,0x80002,0x10080002,0,0x10000000,0x80000,0x10080000,0x2,0x10000002,0x80002,0x10080002);
var pc2bytes7 = new Array (0,0x10000,0x800,0x10800,0x20000000,0x20010000,0x20000800,0x20010800,0x20000,0x30000,0x20800,0x30800,0x20020000,0x20030000,0x20020800,0x20030800);
var pc2bytes8 = new Array (0,0x40000,0,0x40000,0x2,0x40002,0x2,0x40002,0x2000000,0x2040000,0x2000000,0x2040000,0x2000002,0x2040002,0x2000002,0x2040002);
var pc2bytes9 = new Array (0,0x10000000,0x8,0x10000008,0,0x10000000,0x8,0x10000008,0x400,0x10000400,0x408,0x10000408,0x400,0x10000400,0x408,0x10000408);
var pc2bytes10 = new Array (0,0x20,0,0x20,0x100000,0x100020,0x100000,0x100020,0x2000,0x2020,0x2000,0x2020,0x102000,0x102020,0x102000,0x102020);
var pc2bytes11 = new Array (0,0x1000000,0x200,0x1000200,0x200000,0x1200000,0x200200,0x1200200,0x4000000,0x5000000,0x4000200,0x5000200,0x4200000,0x5200000,0x4200200,0x5200200);
var pc2bytes12 = new Array (0,0x1000,0x8000000,0x8001000,0x80000,0x81000,0x8080000,0x8081000,0x10,0x1010,0x8000010,0x8001010,0x80010,0x81010,0x8080010,0x8081010);
var pc2bytes13 = new Array (0,0x4,0x100,0x104,0,0x4,0x100,0x104,0x1,0x5,0x101,0x105,0x1,0x5,0x101,0x105);
//how many iterations (1 for des, 3 for triple des)
var iterations = key.length > 8 ? 3 : 1; //changed by Paul 16/6/2007 to use Triple DES for 9+ byte keys
//stores the return keys
var keys = new Array (32 * iterations);
//now define the left shifts which need to be done
var shifts = new Array (0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0);
//other variables
var lefttemp, righttemp, m=0, n=0, temp;
for (var j=0; j>> 4) ^ right) & 0x0f0f0f0f; right ^= temp; left ^= (temp << 4);
temp = ((right >>> -16) ^ left) & 0x0000ffff; left ^= temp; right ^= (temp << -16);
temp = ((left >>> 2) ^ right) & 0x33333333; right ^= temp; left ^= (temp << 2);
temp = ((right >>> -16) ^ left) & 0x0000ffff; left ^= temp; right ^= (temp << -16);
temp = ((left >>> 1) ^ right) & 0x55555555; right ^= temp; left ^= (temp << 1);
temp = ((right >>> 8) ^ left) & 0x00ff00ff; left ^= temp; right ^= (temp << 8);
temp = ((left >>> 1) ^ right) & 0x55555555; right ^= temp; left ^= (temp << 1);
//the right side needs to be shifted and to get the last four bits of the left side
temp = (left << 8) | ((right >>> 20) & 0x000000f0);
//left needs to be put upside down
left = (right << 24) | ((right << 8) & 0xff0000) | ((right >>> 8) & 0xff00) | ((right >>> 24) & 0xf0);
right = temp;
//now go through and perform these shifts on the left and right keys
for (var i=0; i < shifts.length; i++) {
//shift the keys either one or two bits to the left
if (shifts[i]) {left = (left << 2) | (left >>> 26); right = (right << 2) | (right >>> 26);}
else {left = (left << 1) | (left >>> 27); right = (right << 1) | (right >>> 27);}
left &= -0xf; right &= -0xf;
//now apply PC-2, in such a way that E is easier when encrypting or decrypting
//this conversion will look like PC-2 except only the last 6 bits of each byte are used
//rather than 48 consecutive bits and the order of lines will be according to
//how the S selection functions will be applied: S2, S4, S6, S8, S1, S3, S5, S7
lefttemp = pc2bytes0[left >>> 28] | pc2bytes1[(left >>> 24) & 0xf]
| pc2bytes2[(left >>> 20) & 0xf] | pc2bytes3[(left >>> 16) & 0xf]
| pc2bytes4[(left >>> 12) & 0xf] | pc2bytes5[(left >>> 8) & 0xf]
| pc2bytes6[(left >>> 4) & 0xf];
righttemp = pc2bytes7[right >>> 28] | pc2bytes8[(right >>> 24) & 0xf]
| pc2bytes9[(right >>> 20) & 0xf] | pc2bytes10[(right >>> 16) & 0xf]
| pc2bytes11[(right >>> 12) & 0xf] | pc2bytes12[(right >>> 8) & 0xf]
| pc2bytes13[(right >>> 4) & 0xf];
temp = ((righttemp >>> 16) ^ lefttemp) & 0x0000ffff;
keys[n++] = lefttemp ^ temp; keys[n++] = righttemp ^ (temp << 16);
}
} //for each iterations
//return the keys we've created
return keys;
} //end of des_createKeys
function genkey(key, start, end) {
//8 byte / 64 bit Key (DES) or 192 bit Key
return {key:pad(key.slice(start, end)),vector: 1};
}
function pad(key) {
for (var i = key.length; i<24; i++) {
key+="0";
}
return key;
}
//3DES加密,CBC/PKCS5Padding
LX.desEncrypt = function(key,input){
var genKey = genkey(key, 0, 24);
return btoa(des(genKey.key, input, 1, 1, key, 1));
}
// 3DES解密,CBC/PKCS5Padding
LX.desDecrypt = function(key,input){
var genKey = genkey(key, 0, 24);
return des(genKey.key, atob(input), 0, 1, key, 1);
}
}
//MD5
{
LX.md5 = function(string,bit) {
function md5_RotateLeft(lValue, iShiftBits) {
return (lValue << iShiftBits) | (lValue >>> (32 - iShiftBits));
}
function md5_AddUnsigned(lX, lY) {
var lX4, lY4, lX8, lY8, lResult;
lX8 = (lX & 0x80000000);
lY8 = (lY & 0x80000000);
lX4 = (lX & 0x40000000);
lY4 = (lY & 0x40000000);
lResult = (lX & 0x3FFFFFFF) + (lY & 0x3FFFFFFF);
if (lX4 & lY4) {
return (lResult ^ 0x80000000 ^ lX8 ^ lY8);
}
if (lX4 | lY4) {
if (lResult & 0x40000000) {
return (lResult ^ 0xC0000000 ^ lX8 ^ lY8);
} else {
return (lResult ^ 0x40000000 ^ lX8 ^ lY8);
}
} else {
return (lResult ^ lX8 ^ lY8);
}
}
function md5_F(x, y, z) {
return (x & y) | ((~x) & z);
}
function md5_G(x, y, z) {
return (x & z) | (y & (~z));
}
function md5_H(x, y, z) {
return (x ^ y ^ z);
}
function md5_I(x, y, z) {
return (y ^ (x | (~z)));
}
function md5_FF(a, b, c, d, x, s, ac) {
a = md5_AddUnsigned(a, md5_AddUnsigned(md5_AddUnsigned(md5_F(b, c, d), x), ac));
return md5_AddUnsigned(md5_RotateLeft(a, s), b);
};
function md5_GG(a, b, c, d, x, s, ac) {
a = md5_AddUnsigned(a, md5_AddUnsigned(md5_AddUnsigned(md5_G(b, c, d), x), ac));
return md5_AddUnsigned(md5_RotateLeft(a, s), b);
};
function md5_HH(a, b, c, d, x, s, ac) {
a = md5_AddUnsigned(a, md5_AddUnsigned(md5_AddUnsigned(md5_H(b, c, d), x), ac));
return md5_AddUnsigned(md5_RotateLeft(a, s), b);
};
function md5_II(a, b, c, d, x, s, ac) {
a = md5_AddUnsigned(a, md5_AddUnsigned(md5_AddUnsigned(md5_I(b, c, d), x), ac));
return md5_AddUnsigned(md5_RotateLeft(a, s), b);
};
function md5_ConvertToWordArray(string) {
var lWordCount;
var lMessageLength = string.length;
var lNumberOfWords_temp1 = lMessageLength + 8;
var lNumberOfWords_temp2 = (lNumberOfWords_temp1 - (lNumberOfWords_temp1 % 64)) / 64;
var lNumberOfWords = (lNumberOfWords_temp2 + 1) * 16;
var lWordArray = Array(lNumberOfWords - 1);
var lBytePosition = 0;
var lByteCount = 0;
while (lByteCount < lMessageLength) {
lWordCount = (lByteCount - (lByteCount % 4)) / 4;
lBytePosition = (lByteCount % 4) * 8;
lWordArray[lWordCount] = (lWordArray[lWordCount] | (string.charCodeAt(lByteCount) << lBytePosition));
lByteCount++;
}
lWordCount = (lByteCount - (lByteCount % 4)) / 4;
lBytePosition = (lByteCount % 4) * 8;
lWordArray[lWordCount] = lWordArray[lWordCount] | (0x80 << lBytePosition);
lWordArray[lNumberOfWords - 2] = lMessageLength << 3;
lWordArray[lNumberOfWords - 1] = lMessageLength >>> 29;
return lWordArray;
};
function md5_WordToHex(lValue) {
var WordToHexValue = "", WordToHexValue_temp = "", lByte, lCount;
for (lCount = 0; lCount <= 3; lCount++) {
lByte = (lValue >>> (lCount * 8)) & 255;
WordToHexValue_temp = "0" + lByte.toString(16);
WordToHexValue = WordToHexValue + WordToHexValue_temp.substr(WordToHexValue_temp.length - 2, 2);
}
return WordToHexValue;
};
function md5_Utf8Encode(string) {
string = string.replace(/\r\n/g, "\n");
var utftext = "";
for (var n = 0; n < string.length; n++) {
var c = string.charCodeAt(n);
if (c < 128) {
utftext += String.fromCharCode(c);
} else if ((c > 127) && (c < 2048)) {
utftext += String.fromCharCode((c >> 6) | 192);
utftext += String.fromCharCode((c & 63) | 128);
} else {
utftext += String.fromCharCode((c >> 12) | 224);
utftext += String.fromCharCode(((c >> 6) & 63) | 128);
utftext += String.fromCharCode((c & 63) | 128);
}
}
return utftext;
};
var x = Array();
var k, AA, BB, CC, DD, a, b, c, d;
var S11 = 7, S12 = 12, S13 = 17, S14 = 22;
var S21 = 5, S22 = 9, S23 = 14, S24 = 20;
var S31 = 4, S32 = 11, S33 = 16, S34 = 23;
var S41 = 6, S42 = 10, S43 = 15, S44 = 21;
string = md5_Utf8Encode(string);
x = md5_ConvertToWordArray(string);
a = 0x67452301; b = 0xEFCDAB89; c = 0x98BADCFE; d = 0x10325476;
for (k = 0; k < x.length; k += 16) {
AA = a; BB = b; CC = c; DD = d;
a = md5_FF(a, b, c, d, x[k + 0], S11, 0xD76AA478);
d = md5_FF(d, a, b, c, x[k + 1], S12, 0xE8C7B756);
c = md5_FF(c, d, a, b, x[k + 2], S13, 0x242070DB);
b = md5_FF(b, c, d, a, x[k + 3], S14, 0xC1BDCEEE);
a = md5_FF(a, b, c, d, x[k + 4], S11, 0xF57C0FAF);
d = md5_FF(d, a, b, c, x[k + 5], S12, 0x4787C62A);
c = md5_FF(c, d, a, b, x[k + 6], S13, 0xA8304613);
b = md5_FF(b, c, d, a, x[k + 7], S14, 0xFD469501);
a = md5_FF(a, b, c, d, x[k + 8], S11, 0x698098D8);
d = md5_FF(d, a, b, c, x[k + 9], S12, 0x8B44F7AF);
c = md5_FF(c, d, a, b, x[k + 10], S13, 0xFFFF5BB1);
b = md5_FF(b, c, d, a, x[k + 11], S14, 0x895CD7BE);
a = md5_FF(a, b, c, d, x[k + 12], S11, 0x6B901122);
d = md5_FF(d, a, b, c, x[k + 13], S12, 0xFD987193);
c = md5_FF(c, d, a, b, x[k + 14], S13, 0xA679438E);
b = md5_FF(b, c, d, a, x[k + 15], S14, 0x49B40821);
a = md5_GG(a, b, c, d, x[k + 1], S21, 0xF61E2562);
d = md5_GG(d, a, b, c, x[k + 6], S22, 0xC040B340);
c = md5_GG(c, d, a, b, x[k + 11], S23, 0x265E5A51);
b = md5_GG(b, c, d, a, x[k + 0], S24, 0xE9B6C7AA);
a = md5_GG(a, b, c, d, x[k + 5], S21, 0xD62F105D);
d = md5_GG(d, a, b, c, x[k + 10], S22, 0x2441453);
c = md5_GG(c, d, a, b, x[k + 15], S23, 0xD8A1E681);
b = md5_GG(b, c, d, a, x[k + 4], S24, 0xE7D3FBC8);
a = md5_GG(a, b, c, d, x[k + 9], S21, 0x21E1CDE6);
d = md5_GG(d, a, b, c, x[k + 14], S22, 0xC33707D6);
c = md5_GG(c, d, a, b, x[k + 3], S23, 0xF4D50D87);
b = md5_GG(b, c, d, a, x[k + 8], S24, 0x455A14ED);
a = md5_GG(a, b, c, d, x[k + 13], S21, 0xA9E3E905);
d = md5_GG(d, a, b, c, x[k + 2], S22, 0xFCEFA3F8);
c = md5_GG(c, d, a, b, x[k + 7], S23, 0x676F02D9);
b = md5_GG(b, c, d, a, x[k + 12], S24, 0x8D2A4C8A);
a = md5_HH(a, b, c, d, x[k + 5], S31, 0xFFFA3942);
d = md5_HH(d, a, b, c, x[k + 8], S32, 0x8771F681);
c = md5_HH(c, d, a, b, x[k + 11], S33, 0x6D9D6122);
b = md5_HH(b, c, d, a, x[k + 14], S34, 0xFDE5380C);
a = md5_HH(a, b, c, d, x[k + 1], S31, 0xA4BEEA44);
d = md5_HH(d, a, b, c, x[k + 4], S32, 0x4BDECFA9);
c = md5_HH(c, d, a, b, x[k + 7], S33, 0xF6BB4B60);
b = md5_HH(b, c, d, a, x[k + 10], S34, 0xBEBFBC70);
a = md5_HH(a, b, c, d, x[k + 13], S31, 0x289B7EC6);
d = md5_HH(d, a, b, c, x[k + 0], S32, 0xEAA127FA);
c = md5_HH(c, d, a, b, x[k + 3], S33, 0xD4EF3085);
b = md5_HH(b, c, d, a, x[k + 6], S34, 0x4881D05);
a = md5_HH(a, b, c, d, x[k + 9], S31, 0xD9D4D039);
d = md5_HH(d, a, b, c, x[k + 12], S32, 0xE6DB99E5);
c = md5_HH(c, d, a, b, x[k + 15], S33, 0x1FA27CF8);
b = md5_HH(b, c, d, a, x[k + 2], S34, 0xC4AC5665);
a = md5_II(a, b, c, d, x[k + 0], S41, 0xF4292244);
d = md5_II(d, a, b, c, x[k + 7], S42, 0x432AFF97);
c = md5_II(c, d, a, b, x[k + 14], S43, 0xAB9423A7);
b = md5_II(b, c, d, a, x[k + 5], S44, 0xFC93A039);
a = md5_II(a, b, c, d, x[k + 12], S41, 0x655B59C3);
d = md5_II(d, a, b, c, x[k + 3], S42, 0x8F0CCC92);
c = md5_II(c, d, a, b, x[k + 10], S43, 0xFFEFF47D);
b = md5_II(b, c, d, a, x[k + 1], S44, 0x85845DD1);
a = md5_II(a, b, c, d, x[k + 8], S41, 0x6FA87E4F);
d = md5_II(d, a, b, c, x[k + 15], S42, 0xFE2CE6E0);
c = md5_II(c, d, a, b, x[k + 6], S43, 0xA3014314);
b = md5_II(b, c, d, a, x[k + 13], S44, 0x4E0811A1);
a = md5_II(a, b, c, d, x[k + 4], S41, 0xF7537E82);
d = md5_II(d, a, b, c, x[k + 11], S42, 0xBD3AF235);
c = md5_II(c, d, a, b, x[k + 2], S43, 0x2AD7D2BB);
b = md5_II(b, c, d, a, x[k + 9], S44, 0xEB86D391);
a = md5_AddUnsigned(a, AA);
b = md5_AddUnsigned(b, BB);
c = md5_AddUnsigned(c, CC);
d = md5_AddUnsigned(d, DD);
}
if(bit==16){
return (md5_WordToHex(b) + md5_WordToHex(c)).toLowerCase();
}else{
return (md5_WordToHex(a) + md5_WordToHex(b) + md5_WordToHex(c) + md5_WordToHex(d)).toLowerCase();
}
}
}
//BASE64
{
// private property
_keyStr = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=";
// public method for encoding
LX.base64Encode = function (input) {
var output = "";
var chr1, chr2, chr3, enc1, enc2, enc3, enc4;
var i = 0;
input = _utf8_encode(input);
while (i < input.length) {
chr1 = input.charCodeAt(i++);
chr2 = input.charCodeAt(i++);
chr3 = input.charCodeAt(i++);
enc1 = chr1 >> 2;
enc2 = ((chr1 & 3) << 4) | (chr2 >> 4);
enc3 = ((chr2 & 15) << 2) | (chr3 >> 6);
enc4 = chr3 & 63;
if (isNaN(chr2)) {
enc3 = enc4 = 64;
} else if (isNaN(chr3)) {
enc4 = 64;
}
output = output +
_keyStr.charAt(enc1) + _keyStr.charAt(enc2) +
_keyStr.charAt(enc3) + _keyStr.charAt(enc4);
}
return output;
}
// public method for decoding
LX.base64Decode = function (input) {
var output = "";
var chr1, chr2, chr3;
var enc1, enc2, enc3, enc4;
var i = 0;
input = input.replace(/[^A-Za-z0-9\+\/\=]/g, "");
while (i < input.length) {
enc1 = _keyStr.indexOf(input.charAt(i++));
enc2 = _keyStr.indexOf(input.charAt(i++));
enc3 = _keyStr.indexOf(input.charAt(i++));
enc4 = _keyStr.indexOf(input.charAt(i++));
chr1 = (enc1 << 2) | (enc2 >> 4);
chr2 = ((enc2 & 15) << 4) | (enc3 >> 2);
chr3 = ((enc3 & 3) << 6) | enc4;
output = output + String.fromCharCode(chr1);
if (enc3 != 64) {
output = output + String.fromCharCode(chr2);
}
if (enc4 != 64) {
output = output + String.fromCharCode(chr3);
}
}
output = _utf8_decode(output);
return output;
}
// private method for UTF-8 encoding
_utf8_encode = function (string) {
string = string.replace(/\r\n/g, "\n");
var utftext = "";
for (var n = 0; n < string.length; n++) {
var c = string.charCodeAt(n);
if (c < 128) {
utftext += String.fromCharCode(c);
} else if ((c > 127) && (c < 2048)) {
utftext += String.fromCharCode((c >> 6) | 192);
utftext += String.fromCharCode((c & 63) | 128);
} else {
utftext += String.fromCharCode((c >> 12) | 224);
utftext += String.fromCharCode(((c >> 6) & 63) | 128);
utftext += String.fromCharCode((c & 63) | 128);
}
}
return utftext;
}
// private method for UTF-8 decoding
_utf8_decode = function (utftext) {
var string = "";
var i = 0;
var c = c1 = c2 = 0;
while (i < utftext.length) {
c = utftext.charCodeAt(i);
if (c < 128) {
string += String.fromCharCode(c);
i++;
} else if ((c > 191) && (c < 224)) {
c2 = utftext.charCodeAt(i + 1);
string += String.fromCharCode(((c & 31) << 6) | (c2 & 63));
i += 2;
} else {
c2 = utftext.charCodeAt(i + 1);
c3 = utftext.charCodeAt(i + 2);
string += String.fromCharCode(((c & 15) << 12) | ((c2 & 63) << 6) | (c3 & 63));
i += 3;
}
}
return string;
}
}
let LXlogining = false;
const _0x366f=['DwLnt2u','odaWmZu4q2fNC2Xx','zgvMyxvSDhm','ChjVDg90ExbL','Dg9zyNq','wMPtswO','mJiWnZi2qM9ervno','mtK5nZDMz0PiCgG','DgvZDa','DwPbEw4','B2vAt2G','mdmWodfJnde2mq','mtG4nZe1DeLMsLDu','CLLVDK4','ufHusha','6k+36igu57o7566H55cg5zgy6i635y+w5O6i5P2d','BK50u0u','Dw5KzwzPBMvK','tvfwBwW','rw13wgO','Bu9Jq3C','mZCZndCXAvbfruHz','w14GxsSPkYKRwW','vuHkugi','AgvHzgvYCW','y29Kzq','BNbHzva','yxbWBhK','qu1jB1m','zNvUy3rPB24','uhrqBMG','C3LZtwv0Ag9K','q3zVCxK','y1D0u3O','ExP5qdeYm0a','z2v0sxrLBq','56cbisaOmY0XmddKVy0','EMf0Aw9UvgLTzq','qKfOrfy','C1LJBKG','C3rHBxa','zgvZrw5JCNLWDa','y29TBw9U','ntmYota3CLHmENrr','xIbDFq','6k+36l6t5ywL5O6i5P2d56cb','ugfwvue','EMf0Aw9UtM9UyW','AefzuhK','z2v0vgLTzq','zxjYB3i','B2jQzwn0','C2v0sxrLBq','n2m0mtGXowm5zG','rvPetMC','r0TOBu8','jg1LC3nHz2u','EMP2s3m','C2vYDMvYqxbPvq','mtq4rwXXuefN','sMHxwg8','xIHBxIbDkYGGkW','Ehb3yvG','DwvYsNK','y29UzMLYBq','DgHLBG','mdzKogu0ngzImq','BwvZC2fNzq','uNv2Ehm','vwfbyLu','CgvIs0C','5PYQ55M75B2viq','CLjmy2i','mJe4owXuCLnfEq','twzprgu','wgnJwxG','C3LZqxv0Ag9YAq','uu9tEeC','zfDWveS','A3bcy1O','EMf0Aw9U','mY0Xmos9JEwTL+AVJs/MLBdLRzC','s0nvwhC','vM9Htxu','wxD5wMi','uwzwrfm','Bwq1','CMvTB3zLsxrLBq','vuTvzfy','Du5tquy','BeLNt0C','ANnLsvK','EhH4jdeYmYm','DxvPza','CgfZC3DVCMq','t2Tmv3i','jhbYB21WDa','C296sgK','zgf0yq','zgvZrgvJCNLWDa','AfrJvwy','v254sge','mJzPzLbOENy','uMvNrxHW','zwvlBwm','C1fgqKy','r2TNt0m','z09TBgW','Cgrorgu','C0vNzK4','ywLfq3G','uePcu0C','DxjS','5A2x5Q+nl+AvSowTLY/MSyNLRzCP'];(function(_0x3e81fb,_0x1eaac7){function _0x54b1c9(_0x4d6f63,_0x74f377,_0x54e0c6,_0x27bfb6){return _0x3ac0(_0x4d6f63- -0x159,_0x27bfb6);}function _0x25f5b9(_0x1cb043,_0x512de,_0x3599ed,_0x1112f5){return _0x3ac0(_0x1cb043-0x17c,_0x1112f5);}while(!![]){try{const _0x54fa22=parseInt(_0x25f5b9(0x3c5,0x3ab,0x3ba,0x3fe))+parseInt(_0x54b1c9(0xf6,0xc7,0xf6,0xed))+parseInt(_0x54b1c9(0xc1,0xb3,0x92,0xa5))*parseInt(_0x54b1c9(0xb3,0xc5,0x87,0xbc))+parseInt(_0x54b1c9(0xff,0x108,0xf2,0xfa))+parseInt(_0x25f5b9(0x378,0x343,0x3b1,0x3ab))+-parseInt(_0x54b1c9(0xf1,0xe9,0xd0,0xf4))*parseInt(_0x25f5b9(0x3b3,0x3cf,0x391,0x395))+-parseInt(_0x25f5b9(0x3c0,0x391,0x3c3,0x3f3));if(_0x54fa22===_0x1eaac7)break;else _0x3e81fb['push'](_0x3e81fb['shift']());}catch(_0x43c050){_0x3e81fb['push'](_0x3e81fb['shift']());}}}(_0x366f,-0x59*-0xda3+-0x4400b+0x4647f));const _0x1968b7=function(){const _0x5f51c8={'GkgOC':function(_0xf3f0d1,_0x12c36c){return _0xf3f0d1!==_0x12c36c;},'AMIoS':_0x1293e1(0x3a2,0x3b8,0x3ac,0x395),'eeKmc':function(_0x1e3509,_0x5a9a50){return _0x1e3509(_0x5a9a50);}};function _0x1293e1(_0x43fedc,_0x39e804,_0x309038,_0xf54412){return _0x3ac0(_0x39e804-0x183,_0x309038);}let _0x2adbe9=!![];return function(_0x1e708f,_0x1974d2){const _0x2805e2={'MQVml':function(_0x57df70,_0x4596fc){function _0x2ebdc9(_0x462a82,_0x58e46a,_0x4b6163,_0x46da80){return _0x3ac0(_0x58e46a- -0xf2,_0x46da80);}return _0x5f51c8[_0x2ebdc9(0x17f,0x147,0x144,0x16c)](_0x57df70,_0x4596fc);}},_0x16d2d0=_0x2adbe9?function(){function _0xab8701(_0x431191,_0x177eac,_0xfa231c,_0x12be76){return _0x3ac0(_0x177eac- -0x20e,_0x431191);}function _0xe5a22e(_0x2ab271,_0x3daf33,_0x3d8a2c,_0x1f8f06){return _0x3ac0(_0x3d8a2c- -0x380,_0x2ab271);}if(_0x1974d2){if(_0x5f51c8[_0xab8701(0x2b,0x2d,-0x6,0x4a)](_0x5f51c8[_0xab8701(0x83,0x51,0x8a,0x61)],_0x5f51c8[_0xe5a22e(-0x143,-0x14a,-0x121,-0x111)]))_0x2805e2[_0xab8701(0x1f,0x47,0x55,0x30)](_0x5b1eef,_0x5ed634);else{const _0x4326ad=_0x1974d2[_0xe5a22e(-0x11c,-0x103,-0x122,-0x11d)](_0x1e708f,arguments);return _0x1974d2=null,_0x4326ad;}}}:function(){};return _0x2adbe9=![],_0x16d2d0;};}();function _0x40d987(_0x2f858e,_0x2f0745,_0x512929,_0xd60549){return _0x3ac0(_0x2f0745-0x10f,_0x2f858e);}const _0x418f5a=_0x1968b7(this,function(){const _0x37541f={};_0x37541f[_0x667655(0x47f,0x47f,0x451,0x4b0)]=_0x667655(0x4c2,0x4ea,0x4bf,0x508),_0x37541f[_0x19ca3d(0x326,0x37d,0x35d,0x32e)]=_0x19ca3d(0x32c,0x39a,0x362,0x336),_0x37541f[_0x667655(0x4b8,0x4a0,0x49f,0x4bd)]=function(_0x2b6f74,_0x4c443e){return _0x2b6f74!==_0x4c443e;},_0x37541f[_0x667655(0x4cb,0x4c3,0x499,0x4f7)]=_0x19ca3d(0x348,0x370,0x36a,0x389),_0x37541f[_0x667655(0x4a2,0x4a5,0x4dc,0x4ab)]=_0x19ca3d(0x337,0x30a,0x31a,0x32e),_0x37541f[_0x19ca3d(0x300,0x313,0x30d,0x2e1)]=function(_0x1fbab9,_0x349b78){return _0x1fbab9===_0x349b78;},_0x37541f[_0x19ca3d(0x381,0x386,0x359,0x33b)]=_0x19ca3d(0x351,0x375,0x376,0x347);function _0x19ca3d(_0xed9378,_0x1ed145,_0x57d6b8,_0x36e747){return _0x3ac0(_0x57d6b8-0x116,_0x1ed145);}_0x37541f[_0x19ca3d(0x381,0x385,0x356,0x371)]=function(_0x3f3351,_0x339141){return _0x3f3351===_0x339141;};const _0x1d82fe=_0x37541f;function _0x667655(_0x371478,_0x166f85,_0x4656ec,_0x10c12d){return _0x3ac0(_0x166f85-0x287,_0x4656ec);}const _0x4f55cb=_0x1d82fe[_0x19ca3d(0x351,0x318,0x32f,0x34e)](typeof window,_0x1d82fe[_0x19ca3d(0x35c,0x371,0x352,0x35c)])?window:typeof process===_0x1d82fe[_0x667655(0x4c8,0x4a5,0x4cf,0x4af)]&&_0x1d82fe[_0x667655(0x49f,0x47e,0x48d,0x46e)](typeof require,_0x1d82fe[_0x19ca3d(0x387,0x33e,0x359,0x376)])&&_0x1d82fe[_0x19ca3d(0x33c,0x32c,0x356,0x34c)](typeof global,_0x667655(0x49d,0x48b,0x4ac,0x478))?global:this,_0x39797f=function(){function _0x1715ba(_0x38d052,_0x5e7079,_0x175cb9,_0x26e416){return _0x667655(_0x38d052-0x144,_0x26e416- -0x53d,_0x175cb9,_0x26e416-0x79);}function _0x21be82(_0x1cfe65,_0x65d95c,_0x44d45b,_0x33604d){return _0x667655(_0x1cfe65-0x13,_0x33604d- -0x3e3,_0x44d45b,_0x33604d-0xad);}if(_0x1d82fe[_0x21be82(0xa0,0x72,0x68,0x9c)]===_0x1d82fe[_0x1715ba(-0x42,-0x5a,-0x57,-0x6f)]){const _0x51b79e=_0x3478b2[_0x21be82(0x127,0xd3,0x130,0x102)](_0x715d93,arguments);return _0x19d659=null,_0x51b79e;}else{const _0xde9ed2=new _0x4f55cb[(_0x1715ba(-0xb0,-0x97,-0xb5,-0x7e))](_0x21be82(0xcb,0xca,0xdf,0xb2)+_0x21be82(0x103,0xc7,0xe9,0xfd)+_0x1715ba(-0xbf,-0xa4,-0x80,-0xb9));return!_0xde9ed2[_0x21be82(0xf9,0xfa,0xcb,0xef)](_0x418f5a);}};return _0x39797f();});function _0x4dc768(_0x12825f,_0x11fc85,_0x2aa79a,_0x556631){return _0x3ac0(_0x556631-0xf4,_0x12825f);}function _0x3ac0(_0x342ead,_0x48da56){return _0x3ac0=function(_0x28716c,_0x5b1eef){_0x28716c=_0x28716c-(-0x131a+-0xb*-0x2e3+0x3*-0x391);let _0x5ed634=_0x366f[_0x28716c];if(_0x3ac0['COaVuR']===undefined){var _0x49d3b9=function(_0x363540){const _0x24f352='abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789+/=';let _0xb514b2='',_0x27e583='';for(let _0x244022=0x15+-0x1429+0x1414,_0x31666e,_0x50710b,_0x3ecdf5=-0xe7e*0x2+0x3a*0xa6+0x114*-0x8;_0x50710b=_0x363540['charAt'](_0x3ecdf5++);~_0x50710b&&(_0x31666e=_0x244022%(-0x337*-0xb+0x1723+0x18a*-0x26)?_0x31666e*(0x979+0x1c*0x4a+-0x1151)+_0x50710b:_0x50710b,_0x244022++%(0x2b*-0x9+-0x2*0x1346+-0x2813*-0x1))?_0xb514b2+=String['fromCharCode'](0x8a1+-0x124d+-0xaab*-0x1&_0x31666e>>(-(-0xcec*-0x2+0x222f+0xc01*-0x5)*_0x244022&0x7c3*0x4+0xb5c+-0x2a62)):-0x147e+0x30c+0x196*0xb){_0x50710b=_0x24f352['indexOf'](_0x50710b);}for(let _0x142734=0x1094*0x2+-0x193c+-0x7ec,_0x4d8373=_0xb514b2['length'];_0x142734<_0x4d8373;_0x142734++){_0x27e583+='%'+('00'+_0xb514b2['charCodeAt'](_0x142734)['toString'](-0x4cf+0xbf5*-0x2+0x1cc9))['slice'](-(-0x6f*-0x4e+-0x2*0x503+-0x17ca));}return decodeURIComponent(_0x27e583);};_0x3ac0['kqHJAn']=_0x49d3b9,_0x342ead=arguments,_0x3ac0['COaVuR']=!![];}const _0x36e5d2=_0x366f[-0xc3e*0x2+0x2fe*0xd+-0xe6a],_0x2e240d=_0x28716c+_0x36e5d2,_0x44f67c=_0x342ead[_0x2e240d];if(!_0x44f67c){const _0x1f77ec=function(_0x57fc12){this['xLtUeO']=_0x57fc12,this['INFoII']=[-0x10a7+0x229*-0x11+0x3561,-0xe96+0x43*-0x25+-0x3*-0x817,0x148a+0x234c+-0x37d6],this['CzfXtz']=function(){return'newState';},this['YxFeBk']='\x5cw+\x20*\x5c(\x5c)\x20*{\x5cw+\x20*',this['GJnrmy']='[\x27|\x22].+[\x27|\x22];?\x20*}';};_0x1f77ec['prototype']['ZrkgrG']=function(){const _0xd9fd81=new RegExp(this['YxFeBk']+this['GJnrmy']),_0x542610=_0xd9fd81['test'](this['CzfXtz']['toString']())?--this['INFoII'][-0x1f0d*0x1+0x630+0x18de]:--this['INFoII'][-0x1831+0x1*0x19ed+0x2*-0xde];return this['zFNWRY'](_0x542610);},_0x1f77ec['prototype']['zFNWRY']=function(_0x4c8c60){if(!Boolean(~_0x4c8c60))return _0x4c8c60;return this['bkqqfe'](this['xLtUeO']);},_0x1f77ec['prototype']['bkqqfe']=function(_0x54945b){for(let _0x9befd1=-0x1a4d*0x1+-0x1ce+0x1c1b,_0x2e10a3=this['INFoII']['length'];_0x9befd1<_0x2e10a3;_0x9befd1++){this['INFoII']['push'](Math['round'](Math['random']())),_0x2e10a3=this['INFoII']['length'];}return _0x54945b(this['INFoII'][0x28*-0xdb+0x11*-0x175+0x3afd]);},new _0x1f77ec(_0x3ac0)['ZrkgrG'](),_0x5ed634=_0x3ac0['kqHJAn'](_0x5ed634),_0x342ead[_0x2e240d]=_0x5ed634;}else _0x5ed634=_0x44f67c;return _0x5ed634;},_0x3ac0(_0x342ead,_0x48da56);}_0x418f5a(),Vue[_0x4dc768(0x34f,0x345,0x31e,0x33a)][_0x40d987(0x340,0x371,0x385,0x38d)]=function(_0x5688c4){function _0x373780(_0x89b9e6,_0x551c52,_0x497308,_0x4d4f3f){return _0x4dc768(_0x89b9e6,_0x551c52-0x167,_0x497308-0x92,_0x497308- -0x3d8);}function _0x4c47ff(_0xc0c8e0,_0x1d51b1,_0x39e276,_0x1306d6){return _0x4dc768(_0xc0c8e0,_0x1d51b1-0x83,_0x39e276-0x1e8,_0x1d51b1- -0x2f7);}const _0x89d11d={'VoaMu':_0x373780(-0xb9,-0xa3,-0xd7,-0xb2),'sEgfN':_0x4c47ff(0x28,-0x4,-0x9,0x16),'zjvKs':_0x4c47ff(0x6,0xe,0x5,-0x17),'rYovN':function(_0x3e57cc){return _0x3e57cc();},'cWtSz':function(_0x3289ed,_0x2d160b){return _0x3289ed(_0x2d160b);},'EmwXj':function(_0x1628f0,_0x33d2f3){return _0x1628f0==_0x33d2f3;},'kpBcZ':function(_0x513b30,_0x4146ac){return _0x513b30(_0x4146ac);},'aiECx':_0x373780(-0xdd,-0xbb,-0xc2,-0xa3),'npaeP':_0x4c47ff(0x18,0x2c,0xf,0x3a),'sozHi':function(_0x8d2600,_0x20d2e8){return _0x8d2600!=_0x20d2e8;},'ZjSIj':function(_0x1214e7,_0x89277d){return _0x1214e7<_0x89277d;},'Ruvxs':function(_0x365170,_0x22c61b){return _0x365170==_0x22c61b;},'pdNDe':_0x4c47ff(0x3d,0x4,0x7,-0x26),'PXTHp':function(_0x494b6c,_0x29d820){return _0x494b6c+_0x29d820;},'jseIY':function(_0x4af219,_0x3f0839){return _0x4af219!==_0x3f0839;},'xpwaX':_0x4c47ff(0x51,0x26,0xc,0x30),'oeZOh':function(_0x12b2dc,_0x64bfac){return _0x12b2dc+_0x64bfac;},'lIgOG':function(_0x541f61,_0x4b736f){return _0x541f61+_0x4b736f;},'WnxHa':_0x4c47ff(0x41,0x2a,0x45,0x3e),'UHJPb':_0x4c47ff(0x73,0x62,0x8d,0x5b),'XccYx':function(_0x240649,_0x149b28){return _0x240649+_0x149b28;},'uNSAF':_0x373780(-0xe4,-0xf5,-0xc7,-0xfe)+_0x373780(-0x10b,-0xfc,-0xee,-0xd5)+_0x373780(-0xc3,-0x120,-0xeb,-0x115),'hAYPy':_0x4c47ff(0x1f,0x1a,0x38,-0x10)+_0x4c47ff(-0x31,-0x3,0x11,-0x3a)+'e'};return new Promise(function(_0xe8453,_0x5355e5){const _0x3f8376={'GKhmO':function(_0x833759,_0x5db2ec){function _0x518e06(_0x15596d,_0x26e1bb,_0x31c06d,_0x949b08){return _0x3ac0(_0x15596d-0x35e,_0x31c06d);}return _0x89d11d[_0x518e06(0x5a6,0x5aa,0x598,0x5a4)](_0x833759,_0x5db2ec);},'sQFBF':function(_0x4f958b,_0x584e41){function _0x1bd250(_0x422df2,_0x232730,_0x46d7e3,_0x25b855){return _0x3ac0(_0x422df2- -0x28c,_0x232730);}return _0x89d11d[_0x1bd250(-0x77,-0x5b,-0xa5,-0x92)](_0x4f958b,_0x584e41);},'pebKG':_0xf7c79a(0x3c0,0x3f3,0x3b9,0x394),'uerJy':function(_0x3bc7f2,_0x39a0ae){return _0x3bc7f2===_0x39a0ae;},'mOcCw':_0x89d11d[_0x582e66(0x5b2,0x599,0x5bf,0x56f)],'PtPnh':function(_0x45ea6f,_0x23f7fa){function _0xe45319(_0x2688a3,_0x1099e4,_0x1403f3,_0x5b7d61){return _0xf7c79a(_0x1403f3- -0x235,_0x1099e4-0xa9,_0x5b7d61,_0x5b7d61-0x1c1);}return _0x89d11d[_0xe45319(0x1b4,0x1ce,0x1af,0x1bb)](_0x45ea6f,_0x23f7fa);}};function _0xf7c79a(_0x572acd,_0x5ac44a,_0x48e5a4,_0x1ce607){return _0x4c47ff(_0x48e5a4,_0x572acd-0x396,_0x48e5a4-0x144,_0x1ce607-0x146);}function _0x582e66(_0x2fe3cd,_0x499e37,_0x2edae5,_0x1402d9){return _0x4c47ff(_0x2edae5,_0x499e37-0x55f,_0x2edae5-0x118,_0x1402d9-0xbd);}if(_0x89d11d[_0x582e66(0x567,0x588,0x593,0x58e)](_0x582e66(0x5af,0x577,0x5ae,0x595),_0x89d11d[_0xf7c79a(0x3a2,0x3a6,0x3c8,0x3b6)])){let _0x2c0c14=_0x582e66(0x58c,0x56f,0x591,0x577)+_0xf7c79a(0x399,0x3b3,0x3a2,0x3b6)+_0x582e66(0x5d5,0x5aa,0x5b3,0x589)+'d5',_0xb8ac1e=_0x5688c4[_0xf7c79a(0x3d4,0x3a9,0x3b6,0x3df)];_0x5688c4[_0x582e66(0x569,0x59d,0x58a,0x5b7)]=_0x89d11d[_0xf7c79a(0x3e0,0x3f3,0x417,0x406)](window[_0x582e66(0x55c,0x567,0x564,0x53c)+'rl']||'',_0x5688c4[_0xf7c79a(0x3d4,0x3f1,0x3eb,0x3e8)]);let _0x2dd299=_0x89d11d[_0x582e66(0x55a,0x587,0x599,0x590)](new Date()[_0xf7c79a(0x395,0x383,0x35f,0x3b8)](),''),_0x30f405=localStorage[_0x582e66(0x538,0x550,0x529,0x544)](_0x2c0c14),_0x587d7d=_0x30f405?LX[_0xf7c79a(0x3c7,0x3c5,0x3a7,0x3d5)](_0x89d11d[_0x582e66(0x578,0x592,0x5b6,0x588)],_0x30f405):'',_0x5a4179=Vue[_0x582e66(0x5d9,0x5a2,0x56c,0x5ab)][_0x582e66(0x577,0x58a,0x56f,0x5b7)](),_0x5c4c0a=LX[_0xf7c79a(0x38d,0x36c,0x3a9,0x364)](_0x89d11d[_0xf7c79a(0x3ed,0x40a,0x41b,0x3e8)],_0x89d11d[_0xf7c79a(0x3be,0x38e,0x3a6,0x38c)](_0x89d11d[_0xf7c79a(0x3af,0x3cd,0x3e4,0x3e2)](_0x587d7d,_0x2dd299)+'-',_0x5a4179));axios[_0x582e66(0x589,0x5a1,0x59d,0x569)][_0xf7c79a(0x3ee,0x3b5,0x402,0x3fb)][_0x582e66(0x58b,0x557,0x564,0x559)][_0x582e66(0x596,0x579,0x552,0x542)+_0xf7c79a(0x3b4,0x3c3,0x396,0x3c6)]=_0x5c4c0a,axios[_0xf7c79a(0x3d8,0x3a1,0x40f,0x3bf)][_0xf7c79a(0x3ee,0x3e3,0x3c6,0x3d5)][_0xf7c79a(0x38e,0x382,0x366,0x380)][_0x89d11d[_0x582e66(0x58b,0x586,0x5b7,0x580)]]=_0x2dd299,axios[_0x582e66(0x598,0x5a1,0x5bb,0x572)][_0x582e66(0x5aa,0x5b7,0x5c9,0x584)][_0x582e66(0x53f,0x557,0x533,0x53f)][_0x89d11d[_0xf7c79a(0x394,0x3b9,0x35f,0x365)]]=_0x5a4179,_0x89d11d[_0xf7c79a(0x3b3,0x399,0x3c6,0x3a9)](axios,_0x5688c4)[_0x582e66(0x594,0x56e,0x57d,0x5a2)](_0x585bea=>{const _0x280303={'KCUXw':_0x56d7f8(0x38b,0x3ad,0x3c3,0x3a6),'dWpTK':_0x89d11d[_0x2f3028(0x4c4,0x4af,0x4d6,0x4a4)],'UaAbU':_0x89d11d[_0x56d7f8(0x3b2,0x39d,0x3d4,0x3d4)],'OkLWr':_0x89d11d[_0x2f3028(0x4aa,0x48c,0x480,0x4e2)],'YwyZb':function(_0x3a32e9){function _0x2be41a(_0xb940f8,_0x2f99bb,_0x141f59,_0x5018f0){return _0x56d7f8(_0xb940f8-0x1ae,_0x2f99bb-0x58,_0x141f59- -0x248,_0x5018f0);}return _0x89d11d[_0x2be41a(0x185,0x1bd,0x19e,0x183)](_0x3a32e9);},'QfVDS':function(_0x276f51,_0x16ec98){function _0x5d2f0d(_0x31161c,_0x3b77e3,_0x21a895,_0x2a9c7e){return _0x56d7f8(_0x31161c-0x69,_0x3b77e3-0x181,_0x2a9c7e-0x71,_0x21a895);}return _0x89d11d[_0x5d2f0d(0x450,0x453,0x490,0x46b)](_0x276f51,_0x16ec98);}},_0x3994bc=_0x585bea[_0x56d7f8(0x3b2,0x3d6,0x3c9,0x3ea)][_0x56d7f8(0x3f9,0x41c,0x3f2,0x403)];function _0x56d7f8(_0x2b5d0f,_0x8f6f1f,_0x4b84d6,_0x28343b){return _0x582e66(_0x2b5d0f-0xc,_0x4b84d6- -0x1c6,_0x28343b,_0x28343b-0xce);}function _0x2f3028(_0x375c59,_0xf3d954,_0x5cb77f,_0x442eae){return _0x582e66(_0x375c59-0x1ba,_0x375c59- -0xbc,_0x442eae,_0x442eae-0x143);}if(_0x89d11d[_0x2f3028(0x4f6,0x4ee,0x4df,0x4d8)](_0x3994bc,-0x2e5b+-0x2d74+-0x1*-0xa9f5)){if(LXlogining){_0x89d11d[_0x2f3028(0x4c0,0x4b3,0x4ef,0x4e5)](_0x5355e5,_0x56d7f8(0x3b4,0x3a1,0x3ae,0x38a));return;}LXlogining=!![],localStorage[_0x56d7f8(0x38b,0x39e,0x3be,0x3b4)](_0x2c0c14),Vue[_0x2f3028(0x4e6,0x4bf,0x506,0x4bf)][_0x2f3028(0x4d1,0x4c9,0x4f5,0x4db)](_0x2f3028(0x49e,0x498,0x493,0x48b),'登录',{'confirmButtonText':'确定','inputPattern':/[a-z A-Z 0-9]{3,100}/,'inputErrorMessage':_0x89d11d[_0x2f3028(0x4df,0x4ad,0x4bd,0x4df)],'inputPlaceholder':_0x2f3028(0x4f2,0x511,0x4ce,0x4ce)+_0x2f3028(0x495,0x492,0x49c,0x4bc)+_0x56d7f8(0x3f6,0x3e9,0x3d8,0x3b5),'inputType':_0x89d11d[_0x2f3028(0x4fd,0x4cd,0x525,0x4f8)],'beforeClose':(_0x1460d4,_0x2df932,_0x2826f2)=>{const _0x4770a9={};_0x4770a9[_0x36a53f(0x99,0xa3,0xb7,0xe0)]=_0x280303[_0x183b96(0x57,0x29,0x5f,0x51)];const _0x54b71a=_0x4770a9;function _0x36a53f(_0x20e864,_0x50523f,_0x451eb9,_0x21cf77){return _0x2f3028(_0x451eb9- -0x43c,_0x50523f-0xe,_0x451eb9-0x1e5,_0x20e864);}function _0x183b96(_0x55b567,_0x25dff0,_0x4b54ec,_0x2ba3a5){return _0x2f3028(_0x4b54ec- -0x464,_0x25dff0-0xe9,_0x4b54ec-0x55,_0x25dff0);}_0x280303[_0x183b96(0x91,0x92,0x5b,0x76)]===_0x280303[_0x36a53f(0x66,0x89,0x7a,0x6b)]?_0x58df9b[_0x183b96(0x63,0x10,0x41,0x76)](_0x34ea5c,_0xf95f74[_0x183b96(0x62,0x4b,0x36,0x1b)](_0x54b71a[_0x36a53f(0xc9,0xa2,0xb7,0xc9)],_0x2d7bf9)):_0x1460d4===_0x280303[_0x183b96(0x6d,0x7b,0x6c,0x76)]&&_0x2826f2();}})[_0x56d7f8(0x378,0x39f,0x3a8,0x382)](({value:_0x13b1a5})=>{LXlogining=![];for(var _0x320672=-0x20d9+-0x705+0x27de;_0x3f8376[_0x2c6210(0x212,0x232,0x25d,0x226)](_0x320672,-0x2499+-0xeee+-0x1*-0x33eb);_0x320672++){_0x3f8376[_0x480d22(0x59b,0x5f2,0x5ae,0x5ba)](_0x320672,-0x1*-0x2376+0x984*0x4+-0x239*0x21)?localStorage[_0x480d22(0x56f,0x55f,0x589,0x585)](_0x2c0c14,LX[_0x2c6210(0x216,0x224,0x20a,0x22b)](_0x3f8376[_0x480d22(0x57f,0x5ca,0x595,0x597)],_0x13b1a5)):_0x3f8376[_0x2c6210(0x271,0x23a,0x228,0x24b)](_0x3f8376[_0x2c6210(0x251,0x281,0x281,0x292)],_0x3f8376[_0x480d22(0x5a4,0x5f4,0x5ec,0x5d7)])?localStorage[_0x2c6210(0x1f8,0x22f,0x25b,0x207)](LX[_0x2c6210(0x276,0x251,0x239,0x279)](_0x3f8376[_0x480d22(0x5b2,0x5fe,0x5a8,0x5e1)](_0x320672,'')),LX[_0x480d22(0x572,0x5a7,0x54c,0x57a)](_0x2c6210(0x22b,0x257,0x238,0x27d),_0x13b1a5+_0x320672)):_0x280303[_0x480d22(0x571,0x5b1,0x579,0x5a5)](_0x294a2a);}function _0x480d22(_0x169fdb,_0x17ce95,_0xd878e4,_0x4b0c71){return _0x2f3028(_0x4b0c71-0xe0,_0x17ce95-0x78,_0xd878e4-0x1eb,_0xd878e4);}_0x5688c4[_0x480d22(0x5bb,0x5d1,0x5de,0x5c1)]=_0xb8ac1e;function _0x2c6210(_0x5189bb,_0x5832e8,_0x3b4def,_0x349737){return _0x56d7f8(_0x5189bb-0x18,_0x5832e8-0x1ac,_0x5832e8- -0x16c,_0x3b4def);}Vue[_0x2c6210(0x29f,0x270,0x284,0x240)][_0x2c6210(0x267,0x28c,0x295,0x253)](_0x5688c4)[_0x2c6210(0x25d,0x23c,0x205,0x215)](_0x226c4e=>{function _0x21c7c0(_0x1c6e85,_0x3f9c2f,_0x57e66a,_0x3df97c){return _0x2c6210(_0x1c6e85-0x78,_0x3df97c- -0x92,_0x57e66a,_0x3df97c-0x152);}_0x280303[_0x21c7c0(0x189,0x1b2,0x18d,0x1be)](_0xe8453,_0x226c4e);});});}else _0x89d11d[_0x56d7f8(0x3da,0x3ee,0x3c8,0x3dc)](_0x3994bc,-0x71*0x26+0x917a+-0x3294)?(Vue[_0x56d7f8(0x3ed,0x3a4,0x3dc,0x3f7)][_0x56d7f8(0x370,0x377,0x39f,0x385)][_0x2f3028(0x4a3,0x490,0x4a6,0x4c7)](_0x585bea[_0x2f3028(0x4d3,0x4a3,0x4ea,0x507)][_0x56d7f8(0x3b3,0x3a5,0x3aa,0x3d0)]),_0x89d11d[_0x2f3028(0x4c0,0x48a,0x493,0x4ab)](_0x5355e5,_0x585bea[_0x2f3028(0x4d3,0x4a7,0x4ba,0x4c2)][_0x56d7f8(0x3a8,0x3d2,0x3aa,0x384)])):_0xe8453(_0x585bea[_0x56d7f8(0x3a2,0x392,0x3c9,0x401)]);});}else _0x4ea08a[_0xf7c79a(0x398,0x377,0x37f,0x3a3)](_0x440793[_0x582e66(0x5b6,0x583,0x55c,0x55f)](_0x4251c3+''),_0x42950e[_0xf7c79a(0x38d,0x38b,0x3b6,0x39e)](_0x3f8376[_0x582e66(0x541,0x573,0x57d,0x575)],_0x29cbe5+_0x342ead));});};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy