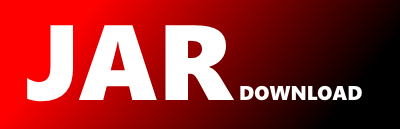
acanvasio.expretau.expretau-parser.1.1.0.source-code.ExpretauParserVisitor Maven / Gradle / Ivy
// Generated from ExpretauParser.g4 by ANTLR 4.8
package io.github.datacanvasio.expretau.antlr4;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link ExpretauParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface ExpretauParserVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by the {@code Or}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOr(ExpretauParser.OrContext ctx);
/**
* Visit a parse tree produced by the {@code MulDiv}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMulDiv(ExpretauParser.MulDivContext ctx);
/**
* Visit a parse tree produced by the {@code AddSub}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAddSub(ExpretauParser.AddSubContext ctx);
/**
* Visit a parse tree produced by the {@code Var}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVar(ExpretauParser.VarContext ctx);
/**
* Visit a parse tree produced by the {@code PosNeg}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPosNeg(ExpretauParser.PosNegContext ctx);
/**
* Visit a parse tree produced by the {@code StringOp}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringOp(ExpretauParser.StringOpContext ctx);
/**
* Visit a parse tree produced by the {@code Index}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIndex(ExpretauParser.IndexContext ctx);
/**
* Visit a parse tree produced by the {@code Int}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInt(ExpretauParser.IntContext ctx);
/**
* Visit a parse tree produced by the {@code Str}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStr(ExpretauParser.StrContext ctx);
/**
* Visit a parse tree produced by the {@code Not}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNot(ExpretauParser.NotContext ctx);
/**
* Visit a parse tree produced by the {@code Relation}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRelation(ExpretauParser.RelationContext ctx);
/**
* Visit a parse tree produced by the {@code StrIndex}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStrIndex(ExpretauParser.StrIndexContext ctx);
/**
* Visit a parse tree produced by the {@code Bool}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBool(ExpretauParser.BoolContext ctx);
/**
* Visit a parse tree produced by the {@code And}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAnd(ExpretauParser.AndContext ctx);
/**
* Visit a parse tree produced by the {@code Pars}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPars(ExpretauParser.ParsContext ctx);
/**
* Visit a parse tree produced by the {@code Real}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReal(ExpretauParser.RealContext ctx);
/**
* Visit a parse tree produced by the {@code Fun}
* labeled alternative in {@link ExpretauParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFun(ExpretauParser.FunContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy