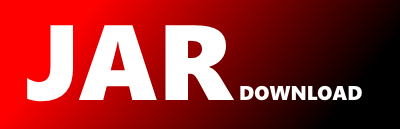
-m.mmm-code-java-parser.0.9.1.source-code.JavaSourceCodeParserListener Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mmm-code-java-parser Show documentation
Show all versions of mmm-code-java-parser Show documentation
Parser for Java Source-Code.
// Generated from JavaSourceCodeParser.g4 by ANTLR 4.7
package io.github.mmm.code.java.parser.base;
import org.antlr.v4.runtime.tree.ParseTreeListener;
/**
* This interface defines a complete listener for a parse tree produced by
* {@link JavaSourceCodeParser}.
*/
public interface JavaSourceCodeParserListener extends ParseTreeListener {
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#compilationUnit}.
* @param ctx the parse tree
*/
void enterCompilationUnit(JavaSourceCodeParser.CompilationUnitContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#compilationUnit}.
* @param ctx the parse tree
*/
void exitCompilationUnit(JavaSourceCodeParser.CompilationUnitContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#packageDeclaration}.
* @param ctx the parse tree
*/
void enterPackageDeclaration(JavaSourceCodeParser.PackageDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#packageDeclaration}.
* @param ctx the parse tree
*/
void exitPackageDeclaration(JavaSourceCodeParser.PackageDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#importDeclaration}.
* @param ctx the parse tree
*/
void enterImportDeclaration(JavaSourceCodeParser.ImportDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#importDeclaration}.
* @param ctx the parse tree
*/
void exitImportDeclaration(JavaSourceCodeParser.ImportDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeDeclaration}.
* @param ctx the parse tree
*/
void enterTypeDeclaration(JavaSourceCodeParser.TypeDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeDeclaration}.
* @param ctx the parse tree
*/
void exitTypeDeclaration(JavaSourceCodeParser.TypeDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#modifier}.
* @param ctx the parse tree
*/
void enterModifier(JavaSourceCodeParser.ModifierContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#modifier}.
* @param ctx the parse tree
*/
void exitModifier(JavaSourceCodeParser.ModifierContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#classOrInterfaceModifier}.
* @param ctx the parse tree
*/
void enterClassOrInterfaceModifier(JavaSourceCodeParser.ClassOrInterfaceModifierContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#classOrInterfaceModifier}.
* @param ctx the parse tree
*/
void exitClassOrInterfaceModifier(JavaSourceCodeParser.ClassOrInterfaceModifierContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#variableModifier}.
* @param ctx the parse tree
*/
void enterVariableModifier(JavaSourceCodeParser.VariableModifierContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#variableModifier}.
* @param ctx the parse tree
*/
void exitVariableModifier(JavaSourceCodeParser.VariableModifierContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#classDeclaration}.
* @param ctx the parse tree
*/
void enterClassDeclaration(JavaSourceCodeParser.ClassDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#classDeclaration}.
* @param ctx the parse tree
*/
void exitClassDeclaration(JavaSourceCodeParser.ClassDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeParameters}.
* @param ctx the parse tree
*/
void enterTypeParameters(JavaSourceCodeParser.TypeParametersContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeParameters}.
* @param ctx the parse tree
*/
void exitTypeParameters(JavaSourceCodeParser.TypeParametersContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeParameter}.
* @param ctx the parse tree
*/
void enterTypeParameter(JavaSourceCodeParser.TypeParameterContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeParameter}.
* @param ctx the parse tree
*/
void exitTypeParameter(JavaSourceCodeParser.TypeParameterContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeBound}.
* @param ctx the parse tree
*/
void enterTypeBound(JavaSourceCodeParser.TypeBoundContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeBound}.
* @param ctx the parse tree
*/
void exitTypeBound(JavaSourceCodeParser.TypeBoundContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#enumDeclaration}.
* @param ctx the parse tree
*/
void enterEnumDeclaration(JavaSourceCodeParser.EnumDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#enumDeclaration}.
* @param ctx the parse tree
*/
void exitEnumDeclaration(JavaSourceCodeParser.EnumDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#enumConstants}.
* @param ctx the parse tree
*/
void enterEnumConstants(JavaSourceCodeParser.EnumConstantsContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#enumConstants}.
* @param ctx the parse tree
*/
void exitEnumConstants(JavaSourceCodeParser.EnumConstantsContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#enumConstant}.
* @param ctx the parse tree
*/
void enterEnumConstant(JavaSourceCodeParser.EnumConstantContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#enumConstant}.
* @param ctx the parse tree
*/
void exitEnumConstant(JavaSourceCodeParser.EnumConstantContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#enumBodyDeclarations}.
* @param ctx the parse tree
*/
void enterEnumBodyDeclarations(JavaSourceCodeParser.EnumBodyDeclarationsContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#enumBodyDeclarations}.
* @param ctx the parse tree
*/
void exitEnumBodyDeclarations(JavaSourceCodeParser.EnumBodyDeclarationsContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#interfaceDeclaration}.
* @param ctx the parse tree
*/
void enterInterfaceDeclaration(JavaSourceCodeParser.InterfaceDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#interfaceDeclaration}.
* @param ctx the parse tree
*/
void exitInterfaceDeclaration(JavaSourceCodeParser.InterfaceDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#classBody}.
* @param ctx the parse tree
*/
void enterClassBody(JavaSourceCodeParser.ClassBodyContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#classBody}.
* @param ctx the parse tree
*/
void exitClassBody(JavaSourceCodeParser.ClassBodyContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#interfaceBody}.
* @param ctx the parse tree
*/
void enterInterfaceBody(JavaSourceCodeParser.InterfaceBodyContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#interfaceBody}.
* @param ctx the parse tree
*/
void exitInterfaceBody(JavaSourceCodeParser.InterfaceBodyContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#classBodyDeclaration}.
* @param ctx the parse tree
*/
void enterClassBodyDeclaration(JavaSourceCodeParser.ClassBodyDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#classBodyDeclaration}.
* @param ctx the parse tree
*/
void exitClassBodyDeclaration(JavaSourceCodeParser.ClassBodyDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#memberDeclaration}.
* @param ctx the parse tree
*/
void enterMemberDeclaration(JavaSourceCodeParser.MemberDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#memberDeclaration}.
* @param ctx the parse tree
*/
void exitMemberDeclaration(JavaSourceCodeParser.MemberDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#methodDeclaration}.
* @param ctx the parse tree
*/
void enterMethodDeclaration(JavaSourceCodeParser.MethodDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#methodDeclaration}.
* @param ctx the parse tree
*/
void exitMethodDeclaration(JavaSourceCodeParser.MethodDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#methodBody}.
* @param ctx the parse tree
*/
void enterMethodBody(JavaSourceCodeParser.MethodBodyContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#methodBody}.
* @param ctx the parse tree
*/
void exitMethodBody(JavaSourceCodeParser.MethodBodyContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeTypeOrVoid}.
* @param ctx the parse tree
*/
void enterTypeTypeOrVoid(JavaSourceCodeParser.TypeTypeOrVoidContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeTypeOrVoid}.
* @param ctx the parse tree
*/
void exitTypeTypeOrVoid(JavaSourceCodeParser.TypeTypeOrVoidContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#genericMethodDeclaration}.
* @param ctx the parse tree
*/
void enterGenericMethodDeclaration(JavaSourceCodeParser.GenericMethodDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#genericMethodDeclaration}.
* @param ctx the parse tree
*/
void exitGenericMethodDeclaration(JavaSourceCodeParser.GenericMethodDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#genericConstructorDeclaration}.
* @param ctx the parse tree
*/
void enterGenericConstructorDeclaration(JavaSourceCodeParser.GenericConstructorDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#genericConstructorDeclaration}.
* @param ctx the parse tree
*/
void exitGenericConstructorDeclaration(JavaSourceCodeParser.GenericConstructorDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#constructorDeclaration}.
* @param ctx the parse tree
*/
void enterConstructorDeclaration(JavaSourceCodeParser.ConstructorDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#constructorDeclaration}.
* @param ctx the parse tree
*/
void exitConstructorDeclaration(JavaSourceCodeParser.ConstructorDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#fieldDeclaration}.
* @param ctx the parse tree
*/
void enterFieldDeclaration(JavaSourceCodeParser.FieldDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#fieldDeclaration}.
* @param ctx the parse tree
*/
void exitFieldDeclaration(JavaSourceCodeParser.FieldDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#interfaceBodyDeclaration}.
* @param ctx the parse tree
*/
void enterInterfaceBodyDeclaration(JavaSourceCodeParser.InterfaceBodyDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#interfaceBodyDeclaration}.
* @param ctx the parse tree
*/
void exitInterfaceBodyDeclaration(JavaSourceCodeParser.InterfaceBodyDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#interfaceMemberDeclaration}.
* @param ctx the parse tree
*/
void enterInterfaceMemberDeclaration(JavaSourceCodeParser.InterfaceMemberDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#interfaceMemberDeclaration}.
* @param ctx the parse tree
*/
void exitInterfaceMemberDeclaration(JavaSourceCodeParser.InterfaceMemberDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#constDeclaration}.
* @param ctx the parse tree
*/
void enterConstDeclaration(JavaSourceCodeParser.ConstDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#constDeclaration}.
* @param ctx the parse tree
*/
void exitConstDeclaration(JavaSourceCodeParser.ConstDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#constantDeclarator}.
* @param ctx the parse tree
*/
void enterConstantDeclarator(JavaSourceCodeParser.ConstantDeclaratorContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#constantDeclarator}.
* @param ctx the parse tree
*/
void exitConstantDeclarator(JavaSourceCodeParser.ConstantDeclaratorContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#interfaceMethodDeclaration}.
* @param ctx the parse tree
*/
void enterInterfaceMethodDeclaration(JavaSourceCodeParser.InterfaceMethodDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#interfaceMethodDeclaration}.
* @param ctx the parse tree
*/
void exitInterfaceMethodDeclaration(JavaSourceCodeParser.InterfaceMethodDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#interfaceMethodModifier}.
* @param ctx the parse tree
*/
void enterInterfaceMethodModifier(JavaSourceCodeParser.InterfaceMethodModifierContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#interfaceMethodModifier}.
* @param ctx the parse tree
*/
void exitInterfaceMethodModifier(JavaSourceCodeParser.InterfaceMethodModifierContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#genericInterfaceMethodDeclaration}.
* @param ctx the parse tree
*/
void enterGenericInterfaceMethodDeclaration(JavaSourceCodeParser.GenericInterfaceMethodDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#genericInterfaceMethodDeclaration}.
* @param ctx the parse tree
*/
void exitGenericInterfaceMethodDeclaration(JavaSourceCodeParser.GenericInterfaceMethodDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#variableDeclarators}.
* @param ctx the parse tree
*/
void enterVariableDeclarators(JavaSourceCodeParser.VariableDeclaratorsContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#variableDeclarators}.
* @param ctx the parse tree
*/
void exitVariableDeclarators(JavaSourceCodeParser.VariableDeclaratorsContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#variableDeclarator}.
* @param ctx the parse tree
*/
void enterVariableDeclarator(JavaSourceCodeParser.VariableDeclaratorContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#variableDeclarator}.
* @param ctx the parse tree
*/
void exitVariableDeclarator(JavaSourceCodeParser.VariableDeclaratorContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#variableDeclaratorId}.
* @param ctx the parse tree
*/
void enterVariableDeclaratorId(JavaSourceCodeParser.VariableDeclaratorIdContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#variableDeclaratorId}.
* @param ctx the parse tree
*/
void exitVariableDeclaratorId(JavaSourceCodeParser.VariableDeclaratorIdContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#variableInitializer}.
* @param ctx the parse tree
*/
void enterVariableInitializer(JavaSourceCodeParser.VariableInitializerContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#variableInitializer}.
* @param ctx the parse tree
*/
void exitVariableInitializer(JavaSourceCodeParser.VariableInitializerContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#arrayInitializer}.
* @param ctx the parse tree
*/
void enterArrayInitializer(JavaSourceCodeParser.ArrayInitializerContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#arrayInitializer}.
* @param ctx the parse tree
*/
void exitArrayInitializer(JavaSourceCodeParser.ArrayInitializerContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#classOrInterfaceType}.
* @param ctx the parse tree
*/
void enterClassOrInterfaceType(JavaSourceCodeParser.ClassOrInterfaceTypeContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#classOrInterfaceType}.
* @param ctx the parse tree
*/
void exitClassOrInterfaceType(JavaSourceCodeParser.ClassOrInterfaceTypeContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeArgument}.
* @param ctx the parse tree
*/
void enterTypeArgument(JavaSourceCodeParser.TypeArgumentContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeArgument}.
* @param ctx the parse tree
*/
void exitTypeArgument(JavaSourceCodeParser.TypeArgumentContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#qualifiedNameList}.
* @param ctx the parse tree
*/
void enterQualifiedNameList(JavaSourceCodeParser.QualifiedNameListContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#qualifiedNameList}.
* @param ctx the parse tree
*/
void exitQualifiedNameList(JavaSourceCodeParser.QualifiedNameListContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#formalParameters}.
* @param ctx the parse tree
*/
void enterFormalParameters(JavaSourceCodeParser.FormalParametersContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#formalParameters}.
* @param ctx the parse tree
*/
void exitFormalParameters(JavaSourceCodeParser.FormalParametersContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#formalParameterList}.
* @param ctx the parse tree
*/
void enterFormalParameterList(JavaSourceCodeParser.FormalParameterListContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#formalParameterList}.
* @param ctx the parse tree
*/
void exitFormalParameterList(JavaSourceCodeParser.FormalParameterListContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#formalParameter}.
* @param ctx the parse tree
*/
void enterFormalParameter(JavaSourceCodeParser.FormalParameterContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#formalParameter}.
* @param ctx the parse tree
*/
void exitFormalParameter(JavaSourceCodeParser.FormalParameterContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#lastFormalParameter}.
* @param ctx the parse tree
*/
void enterLastFormalParameter(JavaSourceCodeParser.LastFormalParameterContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#lastFormalParameter}.
* @param ctx the parse tree
*/
void exitLastFormalParameter(JavaSourceCodeParser.LastFormalParameterContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#qualifiedName}.
* @param ctx the parse tree
*/
void enterQualifiedName(JavaSourceCodeParser.QualifiedNameContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#qualifiedName}.
* @param ctx the parse tree
*/
void exitQualifiedName(JavaSourceCodeParser.QualifiedNameContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#literal}.
* @param ctx the parse tree
*/
void enterLiteral(JavaSourceCodeParser.LiteralContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#literal}.
* @param ctx the parse tree
*/
void exitLiteral(JavaSourceCodeParser.LiteralContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#integerLiteral}.
* @param ctx the parse tree
*/
void enterIntegerLiteral(JavaSourceCodeParser.IntegerLiteralContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#integerLiteral}.
* @param ctx the parse tree
*/
void exitIntegerLiteral(JavaSourceCodeParser.IntegerLiteralContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#annotation}.
* @param ctx the parse tree
*/
void enterAnnotation(JavaSourceCodeParser.AnnotationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#annotation}.
* @param ctx the parse tree
*/
void exitAnnotation(JavaSourceCodeParser.AnnotationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#elementValuePairs}.
* @param ctx the parse tree
*/
void enterElementValuePairs(JavaSourceCodeParser.ElementValuePairsContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#elementValuePairs}.
* @param ctx the parse tree
*/
void exitElementValuePairs(JavaSourceCodeParser.ElementValuePairsContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#elementValuePair}.
* @param ctx the parse tree
*/
void enterElementValuePair(JavaSourceCodeParser.ElementValuePairContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#elementValuePair}.
* @param ctx the parse tree
*/
void exitElementValuePair(JavaSourceCodeParser.ElementValuePairContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#elementValue}.
* @param ctx the parse tree
*/
void enterElementValue(JavaSourceCodeParser.ElementValueContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#elementValue}.
* @param ctx the parse tree
*/
void exitElementValue(JavaSourceCodeParser.ElementValueContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#elementValueArrayInitializer}.
* @param ctx the parse tree
*/
void enterElementValueArrayInitializer(JavaSourceCodeParser.ElementValueArrayInitializerContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#elementValueArrayInitializer}.
* @param ctx the parse tree
*/
void exitElementValueArrayInitializer(JavaSourceCodeParser.ElementValueArrayInitializerContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#annotationTypeDeclaration}.
* @param ctx the parse tree
*/
void enterAnnotationTypeDeclaration(JavaSourceCodeParser.AnnotationTypeDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#annotationTypeDeclaration}.
* @param ctx the parse tree
*/
void exitAnnotationTypeDeclaration(JavaSourceCodeParser.AnnotationTypeDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#annotationTypeBody}.
* @param ctx the parse tree
*/
void enterAnnotationTypeBody(JavaSourceCodeParser.AnnotationTypeBodyContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#annotationTypeBody}.
* @param ctx the parse tree
*/
void exitAnnotationTypeBody(JavaSourceCodeParser.AnnotationTypeBodyContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#annotationTypeElementDeclaration}.
* @param ctx the parse tree
*/
void enterAnnotationTypeElementDeclaration(JavaSourceCodeParser.AnnotationTypeElementDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#annotationTypeElementDeclaration}.
* @param ctx the parse tree
*/
void exitAnnotationTypeElementDeclaration(JavaSourceCodeParser.AnnotationTypeElementDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#annotationTypeElementRest}.
* @param ctx the parse tree
*/
void enterAnnotationTypeElementRest(JavaSourceCodeParser.AnnotationTypeElementRestContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#annotationTypeElementRest}.
* @param ctx the parse tree
*/
void exitAnnotationTypeElementRest(JavaSourceCodeParser.AnnotationTypeElementRestContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#annotationMethodOrConstantRest}.
* @param ctx the parse tree
*/
void enterAnnotationMethodOrConstantRest(JavaSourceCodeParser.AnnotationMethodOrConstantRestContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#annotationMethodOrConstantRest}.
* @param ctx the parse tree
*/
void exitAnnotationMethodOrConstantRest(JavaSourceCodeParser.AnnotationMethodOrConstantRestContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#annotationMethodRest}.
* @param ctx the parse tree
*/
void enterAnnotationMethodRest(JavaSourceCodeParser.AnnotationMethodRestContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#annotationMethodRest}.
* @param ctx the parse tree
*/
void exitAnnotationMethodRest(JavaSourceCodeParser.AnnotationMethodRestContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#annotationConstantRest}.
* @param ctx the parse tree
*/
void enterAnnotationConstantRest(JavaSourceCodeParser.AnnotationConstantRestContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#annotationConstantRest}.
* @param ctx the parse tree
*/
void exitAnnotationConstantRest(JavaSourceCodeParser.AnnotationConstantRestContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#defaultValue}.
* @param ctx the parse tree
*/
void enterDefaultValue(JavaSourceCodeParser.DefaultValueContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#defaultValue}.
* @param ctx the parse tree
*/
void exitDefaultValue(JavaSourceCodeParser.DefaultValueContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#block}.
* @param ctx the parse tree
*/
void enterBlock(JavaSourceCodeParser.BlockContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#block}.
* @param ctx the parse tree
*/
void exitBlock(JavaSourceCodeParser.BlockContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#blockStatement}.
* @param ctx the parse tree
*/
void enterBlockStatement(JavaSourceCodeParser.BlockStatementContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#blockStatement}.
* @param ctx the parse tree
*/
void exitBlockStatement(JavaSourceCodeParser.BlockStatementContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#localVariableDeclaration}.
* @param ctx the parse tree
*/
void enterLocalVariableDeclaration(JavaSourceCodeParser.LocalVariableDeclarationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#localVariableDeclaration}.
* @param ctx the parse tree
*/
void exitLocalVariableDeclaration(JavaSourceCodeParser.LocalVariableDeclarationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#statement}.
* @param ctx the parse tree
*/
void enterStatement(JavaSourceCodeParser.StatementContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#statement}.
* @param ctx the parse tree
*/
void exitStatement(JavaSourceCodeParser.StatementContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#catchClause}.
* @param ctx the parse tree
*/
void enterCatchClause(JavaSourceCodeParser.CatchClauseContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#catchClause}.
* @param ctx the parse tree
*/
void exitCatchClause(JavaSourceCodeParser.CatchClauseContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#catchType}.
* @param ctx the parse tree
*/
void enterCatchType(JavaSourceCodeParser.CatchTypeContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#catchType}.
* @param ctx the parse tree
*/
void exitCatchType(JavaSourceCodeParser.CatchTypeContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#finallyBlock}.
* @param ctx the parse tree
*/
void enterFinallyBlock(JavaSourceCodeParser.FinallyBlockContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#finallyBlock}.
* @param ctx the parse tree
*/
void exitFinallyBlock(JavaSourceCodeParser.FinallyBlockContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#resourceSpecification}.
* @param ctx the parse tree
*/
void enterResourceSpecification(JavaSourceCodeParser.ResourceSpecificationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#resourceSpecification}.
* @param ctx the parse tree
*/
void exitResourceSpecification(JavaSourceCodeParser.ResourceSpecificationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#resources}.
* @param ctx the parse tree
*/
void enterResources(JavaSourceCodeParser.ResourcesContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#resources}.
* @param ctx the parse tree
*/
void exitResources(JavaSourceCodeParser.ResourcesContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#resource}.
* @param ctx the parse tree
*/
void enterResource(JavaSourceCodeParser.ResourceContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#resource}.
* @param ctx the parse tree
*/
void exitResource(JavaSourceCodeParser.ResourceContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#switchBlockStatementGroup}.
* @param ctx the parse tree
*/
void enterSwitchBlockStatementGroup(JavaSourceCodeParser.SwitchBlockStatementGroupContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#switchBlockStatementGroup}.
* @param ctx the parse tree
*/
void exitSwitchBlockStatementGroup(JavaSourceCodeParser.SwitchBlockStatementGroupContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#switchLabel}.
* @param ctx the parse tree
*/
void enterSwitchLabel(JavaSourceCodeParser.SwitchLabelContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#switchLabel}.
* @param ctx the parse tree
*/
void exitSwitchLabel(JavaSourceCodeParser.SwitchLabelContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#forControl}.
* @param ctx the parse tree
*/
void enterForControl(JavaSourceCodeParser.ForControlContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#forControl}.
* @param ctx the parse tree
*/
void exitForControl(JavaSourceCodeParser.ForControlContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#forInit}.
* @param ctx the parse tree
*/
void enterForInit(JavaSourceCodeParser.ForInitContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#forInit}.
* @param ctx the parse tree
*/
void exitForInit(JavaSourceCodeParser.ForInitContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#enhancedForControl}.
* @param ctx the parse tree
*/
void enterEnhancedForControl(JavaSourceCodeParser.EnhancedForControlContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#enhancedForControl}.
* @param ctx the parse tree
*/
void exitEnhancedForControl(JavaSourceCodeParser.EnhancedForControlContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#parExpression}.
* @param ctx the parse tree
*/
void enterParExpression(JavaSourceCodeParser.ParExpressionContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#parExpression}.
* @param ctx the parse tree
*/
void exitParExpression(JavaSourceCodeParser.ParExpressionContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#expressionList}.
* @param ctx the parse tree
*/
void enterExpressionList(JavaSourceCodeParser.ExpressionListContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#expressionList}.
* @param ctx the parse tree
*/
void exitExpressionList(JavaSourceCodeParser.ExpressionListContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#expression}.
* @param ctx the parse tree
*/
void enterExpression(JavaSourceCodeParser.ExpressionContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#expression}.
* @param ctx the parse tree
*/
void exitExpression(JavaSourceCodeParser.ExpressionContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#lambdaExpression}.
* @param ctx the parse tree
*/
void enterLambdaExpression(JavaSourceCodeParser.LambdaExpressionContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#lambdaExpression}.
* @param ctx the parse tree
*/
void exitLambdaExpression(JavaSourceCodeParser.LambdaExpressionContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#lambdaParameters}.
* @param ctx the parse tree
*/
void enterLambdaParameters(JavaSourceCodeParser.LambdaParametersContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#lambdaParameters}.
* @param ctx the parse tree
*/
void exitLambdaParameters(JavaSourceCodeParser.LambdaParametersContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#lambdaBody}.
* @param ctx the parse tree
*/
void enterLambdaBody(JavaSourceCodeParser.LambdaBodyContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#lambdaBody}.
* @param ctx the parse tree
*/
void exitLambdaBody(JavaSourceCodeParser.LambdaBodyContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#primary}.
* @param ctx the parse tree
*/
void enterPrimary(JavaSourceCodeParser.PrimaryContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#primary}.
* @param ctx the parse tree
*/
void exitPrimary(JavaSourceCodeParser.PrimaryContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#methodReference}.
* @param ctx the parse tree
*/
void enterMethodReference(JavaSourceCodeParser.MethodReferenceContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#methodReference}.
* @param ctx the parse tree
*/
void exitMethodReference(JavaSourceCodeParser.MethodReferenceContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#classType}.
* @param ctx the parse tree
*/
void enterClassType(JavaSourceCodeParser.ClassTypeContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#classType}.
* @param ctx the parse tree
*/
void exitClassType(JavaSourceCodeParser.ClassTypeContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#creator}.
* @param ctx the parse tree
*/
void enterCreator(JavaSourceCodeParser.CreatorContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#creator}.
* @param ctx the parse tree
*/
void exitCreator(JavaSourceCodeParser.CreatorContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#createdName}.
* @param ctx the parse tree
*/
void enterCreatedName(JavaSourceCodeParser.CreatedNameContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#createdName}.
* @param ctx the parse tree
*/
void exitCreatedName(JavaSourceCodeParser.CreatedNameContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#innerCreator}.
* @param ctx the parse tree
*/
void enterInnerCreator(JavaSourceCodeParser.InnerCreatorContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#innerCreator}.
* @param ctx the parse tree
*/
void exitInnerCreator(JavaSourceCodeParser.InnerCreatorContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#arrayCreatorRest}.
* @param ctx the parse tree
*/
void enterArrayCreatorRest(JavaSourceCodeParser.ArrayCreatorRestContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#arrayCreatorRest}.
* @param ctx the parse tree
*/
void exitArrayCreatorRest(JavaSourceCodeParser.ArrayCreatorRestContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#classCreatorRest}.
* @param ctx the parse tree
*/
void enterClassCreatorRest(JavaSourceCodeParser.ClassCreatorRestContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#classCreatorRest}.
* @param ctx the parse tree
*/
void exitClassCreatorRest(JavaSourceCodeParser.ClassCreatorRestContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#explicitGenericInvocation}.
* @param ctx the parse tree
*/
void enterExplicitGenericInvocation(JavaSourceCodeParser.ExplicitGenericInvocationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#explicitGenericInvocation}.
* @param ctx the parse tree
*/
void exitExplicitGenericInvocation(JavaSourceCodeParser.ExplicitGenericInvocationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeArgumentsOrDiamond}.
* @param ctx the parse tree
*/
void enterTypeArgumentsOrDiamond(JavaSourceCodeParser.TypeArgumentsOrDiamondContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeArgumentsOrDiamond}.
* @param ctx the parse tree
*/
void exitTypeArgumentsOrDiamond(JavaSourceCodeParser.TypeArgumentsOrDiamondContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#nonWildcardTypeArgumentsOrDiamond}.
* @param ctx the parse tree
*/
void enterNonWildcardTypeArgumentsOrDiamond(JavaSourceCodeParser.NonWildcardTypeArgumentsOrDiamondContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#nonWildcardTypeArgumentsOrDiamond}.
* @param ctx the parse tree
*/
void exitNonWildcardTypeArgumentsOrDiamond(JavaSourceCodeParser.NonWildcardTypeArgumentsOrDiamondContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#nonWildcardTypeArguments}.
* @param ctx the parse tree
*/
void enterNonWildcardTypeArguments(JavaSourceCodeParser.NonWildcardTypeArgumentsContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#nonWildcardTypeArguments}.
* @param ctx the parse tree
*/
void exitNonWildcardTypeArguments(JavaSourceCodeParser.NonWildcardTypeArgumentsContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeList}.
* @param ctx the parse tree
*/
void enterTypeList(JavaSourceCodeParser.TypeListContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeList}.
* @param ctx the parse tree
*/
void exitTypeList(JavaSourceCodeParser.TypeListContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeType}.
* @param ctx the parse tree
*/
void enterTypeType(JavaSourceCodeParser.TypeTypeContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeType}.
* @param ctx the parse tree
*/
void exitTypeType(JavaSourceCodeParser.TypeTypeContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#primitiveType}.
* @param ctx the parse tree
*/
void enterPrimitiveType(JavaSourceCodeParser.PrimitiveTypeContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#primitiveType}.
* @param ctx the parse tree
*/
void exitPrimitiveType(JavaSourceCodeParser.PrimitiveTypeContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#typeArguments}.
* @param ctx the parse tree
*/
void enterTypeArguments(JavaSourceCodeParser.TypeArgumentsContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#typeArguments}.
* @param ctx the parse tree
*/
void exitTypeArguments(JavaSourceCodeParser.TypeArgumentsContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#superSuffix}.
* @param ctx the parse tree
*/
void enterSuperSuffix(JavaSourceCodeParser.SuperSuffixContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#superSuffix}.
* @param ctx the parse tree
*/
void exitSuperSuffix(JavaSourceCodeParser.SuperSuffixContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#explicitGenericInvocationSuffix}.
* @param ctx the parse tree
*/
void enterExplicitGenericInvocationSuffix(JavaSourceCodeParser.ExplicitGenericInvocationSuffixContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#explicitGenericInvocationSuffix}.
* @param ctx the parse tree
*/
void exitExplicitGenericInvocationSuffix(JavaSourceCodeParser.ExplicitGenericInvocationSuffixContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#arguments}.
* @param ctx the parse tree
*/
void enterArguments(JavaSourceCodeParser.ArgumentsContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#arguments}.
* @param ctx the parse tree
*/
void exitArguments(JavaSourceCodeParser.ArgumentsContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#documentation}.
* @param ctx the parse tree
*/
void enterDocumentation(JavaSourceCodeParser.DocumentationContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#documentation}.
* @param ctx the parse tree
*/
void exitDocumentation(JavaSourceCodeParser.DocumentationContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#documentationContent}.
* @param ctx the parse tree
*/
void enterDocumentationContent(JavaSourceCodeParser.DocumentationContentContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#documentationContent}.
* @param ctx the parse tree
*/
void exitDocumentationContent(JavaSourceCodeParser.DocumentationContentContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#skipWhitespace}.
* @param ctx the parse tree
*/
void enterSkipWhitespace(JavaSourceCodeParser.SkipWhitespaceContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#skipWhitespace}.
* @param ctx the parse tree
*/
void exitSkipWhitespace(JavaSourceCodeParser.SkipWhitespaceContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#description}.
* @param ctx the parse tree
*/
void enterDescription(JavaSourceCodeParser.DescriptionContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#description}.
* @param ctx the parse tree
*/
void exitDescription(JavaSourceCodeParser.DescriptionContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#descriptionLine}.
* @param ctx the parse tree
*/
void enterDescriptionLine(JavaSourceCodeParser.DescriptionLineContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#descriptionLine}.
* @param ctx the parse tree
*/
void exitDescriptionLine(JavaSourceCodeParser.DescriptionLineContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#descriptionLineStart}.
* @param ctx the parse tree
*/
void enterDescriptionLineStart(JavaSourceCodeParser.DescriptionLineStartContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#descriptionLineStart}.
* @param ctx the parse tree
*/
void exitDescriptionLineStart(JavaSourceCodeParser.DescriptionLineStartContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#descriptionLineNoSpaceNoAt}.
* @param ctx the parse tree
*/
void enterDescriptionLineNoSpaceNoAt(JavaSourceCodeParser.DescriptionLineNoSpaceNoAtContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#descriptionLineNoSpaceNoAt}.
* @param ctx the parse tree
*/
void exitDescriptionLineNoSpaceNoAt(JavaSourceCodeParser.DescriptionLineNoSpaceNoAtContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#descriptionLineElement}.
* @param ctx the parse tree
*/
void enterDescriptionLineElement(JavaSourceCodeParser.DescriptionLineElementContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#descriptionLineElement}.
* @param ctx the parse tree
*/
void exitDescriptionLineElement(JavaSourceCodeParser.DescriptionLineElementContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#descriptionLineText}.
* @param ctx the parse tree
*/
void enterDescriptionLineText(JavaSourceCodeParser.DescriptionLineTextContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#descriptionLineText}.
* @param ctx the parse tree
*/
void exitDescriptionLineText(JavaSourceCodeParser.DescriptionLineTextContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#descriptionNewline}.
* @param ctx the parse tree
*/
void enterDescriptionNewline(JavaSourceCodeParser.DescriptionNewlineContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#descriptionNewline}.
* @param ctx the parse tree
*/
void exitDescriptionNewline(JavaSourceCodeParser.DescriptionNewlineContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#tagSection}.
* @param ctx the parse tree
*/
void enterTagSection(JavaSourceCodeParser.TagSectionContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#tagSection}.
* @param ctx the parse tree
*/
void exitTagSection(JavaSourceCodeParser.TagSectionContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#blockTag}.
* @param ctx the parse tree
*/
void enterBlockTag(JavaSourceCodeParser.BlockTagContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#blockTag}.
* @param ctx the parse tree
*/
void exitBlockTag(JavaSourceCodeParser.BlockTagContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#blockTagName}.
* @param ctx the parse tree
*/
void enterBlockTagName(JavaSourceCodeParser.BlockTagNameContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#blockTagName}.
* @param ctx the parse tree
*/
void exitBlockTagName(JavaSourceCodeParser.BlockTagNameContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#blockTagContent}.
* @param ctx the parse tree
*/
void enterBlockTagContent(JavaSourceCodeParser.BlockTagContentContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#blockTagContent}.
* @param ctx the parse tree
*/
void exitBlockTagContent(JavaSourceCodeParser.BlockTagContentContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#blockTagText}.
* @param ctx the parse tree
*/
void enterBlockTagText(JavaSourceCodeParser.BlockTagTextContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#blockTagText}.
* @param ctx the parse tree
*/
void exitBlockTagText(JavaSourceCodeParser.BlockTagTextContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#blockTagTextElement}.
* @param ctx the parse tree
*/
void enterBlockTagTextElement(JavaSourceCodeParser.BlockTagTextElementContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#blockTagTextElement}.
* @param ctx the parse tree
*/
void exitBlockTagTextElement(JavaSourceCodeParser.BlockTagTextElementContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#inlineTag}.
* @param ctx the parse tree
*/
void enterInlineTag(JavaSourceCodeParser.InlineTagContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#inlineTag}.
* @param ctx the parse tree
*/
void exitInlineTag(JavaSourceCodeParser.InlineTagContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#inlineTagName}.
* @param ctx the parse tree
*/
void enterInlineTagName(JavaSourceCodeParser.InlineTagNameContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#inlineTagName}.
* @param ctx the parse tree
*/
void exitInlineTagName(JavaSourceCodeParser.InlineTagNameContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#inlineTagContent}.
* @param ctx the parse tree
*/
void enterInlineTagContent(JavaSourceCodeParser.InlineTagContentContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#inlineTagContent}.
* @param ctx the parse tree
*/
void exitInlineTagContent(JavaSourceCodeParser.InlineTagContentContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#braceExpression}.
* @param ctx the parse tree
*/
void enterBraceExpression(JavaSourceCodeParser.BraceExpressionContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#braceExpression}.
* @param ctx the parse tree
*/
void exitBraceExpression(JavaSourceCodeParser.BraceExpressionContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#braceContent}.
* @param ctx the parse tree
*/
void enterBraceContent(JavaSourceCodeParser.BraceContentContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#braceContent}.
* @param ctx the parse tree
*/
void exitBraceContent(JavaSourceCodeParser.BraceContentContext ctx);
/**
* Enter a parse tree produced by {@link JavaSourceCodeParser#braceText}.
* @param ctx the parse tree
*/
void enterBraceText(JavaSourceCodeParser.BraceTextContext ctx);
/**
* Exit a parse tree produced by {@link JavaSourceCodeParser#braceText}.
* @param ctx the parse tree
*/
void exitBraceText(JavaSourceCodeParser.BraceTextContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy