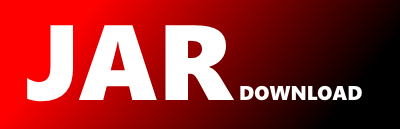
joynr.infrastructure.GlobalCapabilitiesDirectoryProvider.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libjoynr-js Show documentation
Show all versions of libjoynr-js Show documentation
JOYnr JavaScript libjoynr-js
/*
* PLEASE NOTE. THIS IS A GENERATED FILE!
* Generation date: Wed Jan 27 16:29:10 CET 2016
*/
(function(undefined){
var checkImpl = function() {
var missingInImplementation = [];
if (! this.add.checkOperation()) missingInImplementation.push("Operation:add");
if (! this.lookup.checkOperation()) missingInImplementation.push("Operation:lookup");
if (! this.remove.checkOperation()) missingInImplementation.push("Operation:remove");
return missingInImplementation;
};
/**
* @name GlobalCapabilitiesDirectoryProvider
* @constructor
*
* @classdesc
* PLEASE NOTE. THIS IS A GENERATED FILE!
*
Generation date: Wed Jan 27 16:29:10 CET 2016
*
The GlobalCapabilitiesDirectory
is a joynr
* internal interface. When a provider that is globally available is
* registered with joynr, the framework creates an entry for that provider
* in the global capabilities directory. These
* CapabilityInformation
entries contain access
* information as well as supported QoS. The information is later used in
* the arbitration process to pick a provider for a proxy.
* @see CapabilityInformation
*
* @summary Constructor of GlobalCapabilitiesDirectoryProvider object
*
* @param {Object} [implementation] the implementation of the provider
* @param {Object} [implementation.ATTRIBUTENAME] the definition of attribute implementation
* @param {Function} [implementation.ATTRIBUTENAME.set] the getter function with the
* signature "function(value){}" that stores the given attribute value
* @param {Function} [implementation.ATTRIBUTENAME.get] the getter function with the
* signature "function(){}" that returns the current attribute value
* @param {Function} [implementation.OPERATIONNAME] the operation function
* @param {Object} [implementation.EVENTNAME] the definition of the event implementation
*
* @returns {GlobalCapabilitiesDirectoryProvider} a GlobalCapabilitiesDirectoryProvider object to communicate with the joynr infrastructure
*/
var GlobalCapabilitiesDirectoryProvider = null;
GlobalCapabilitiesDirectoryProvider = function GlobalCapabilitiesDirectoryProvider(
passedImplementation,
dependencies
) {
if (!(this instanceof GlobalCapabilitiesDirectoryProvider)) {
// in case someone calls constructor without new keyword (e.g. var c = Constructor({..}))
return new GlobalCapabilitiesDirectoryProvider(
passedImplementation,
dependencies);
}
var implementation = passedImplementation || {};
// defining provider members
/**
* @function GlobalCapabilitiesDirectoryProvider#add
* @summary The add operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Registers a list of capability information with the
* directory.
*
* @param {Array.} capabilities -
* the list of capabilities to register
*/
/**
* @function GlobalCapabilitiesDirectoryProvider#add
* @summary The add operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Registers capability information with the directory.
*
* @param {CapabilityInformation} capability -
* the capability to register
*/
this.add = new dependencies.ProviderOperation(this, implementation.add, "add", [
{
inputParameter: [
{
name : "capabilities",
type : "joynr.types.CapabilityInformation[]"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
},
{
inputParameter: [
{
name : "capability",
type : "joynr.types.CapabilityInformation"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
}
]);
/**
* @function GlobalCapabilitiesDirectoryProvider#lookup
* @summary The lookup operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Looks up a list of capabilities for a given domain,
* interface name and provider QoS.
*
* @param {String} domain -
* the name of the domain
* @param {String} interfaceName -
* the interface name of the capability
* @returns {Array.} result -
* a list of matching capabilities
*/
/**
* @function GlobalCapabilitiesDirectoryProvider#lookup
* @summary The lookup operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Looks up the capability information for a given
* participant ID.
*
* @param {String} participantId -
* the participant ID identifying the provider
* @returns {CapabilityInformation} result -
* the matching capability
*/
this.lookup = new dependencies.ProviderOperation(this, implementation.lookup, "lookup", [
{
inputParameter: [
{
name : "domain",
type : "String"
},
{
name : "interfaceName",
type : "String"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
{
name : "result",
type : "joynr.types.CapabilityInformation[]"
}
]
},
{
inputParameter: [
{
name : "participantId",
type : "String"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
{
name : "result",
type : "joynr.types.CapabilityInformation"
}
]
}
]);
/**
* @function GlobalCapabilitiesDirectoryProvider#remove
* @summary The remove operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Unregisters a list of capabilities from the directory.
*
* @param {Array.} participantIds -
* the list of participant IDs, for which the
* capability has to be removed
*/
/**
* @function GlobalCapabilitiesDirectoryProvider#remove
* @summary The remove operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Unregisters a capability from the directory.
*
* @param {String} participantId -
* the participant ID for which the capability has
* to be removed
*/
this.remove = new dependencies.ProviderOperation(this, implementation.remove, "remove", [
{
inputParameter: [
{
name : "participantIds",
type : "String[]"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
},
{
inputParameter: [
{
name : "participantId",
type : "String"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
}
]);
Object.defineProperty(this, 'checkImplementation', {
enumerable: false,
configurable: false,
writable: false,
value: checkImpl
});
this.interfaceName = "infrastructure/GlobalCapabilitiesDirectory";
this.id = dependencies.uuid();
return Object.freeze(this);
};
// AMD support
if (typeof define === 'function' && define.amd) {
define("joynr/infrastructure/GlobalCapabilitiesDirectoryProvider", [
"joynr/types/CapabilityInformation"
], function () {
return GlobalCapabilitiesDirectoryProvider;
}
);
} else if (typeof exports !== 'undefined' ) {
if ((module !== undefined) && module.exports) {
require("../../joynr/types/CapabilityInformation");
exports = module.exports = GlobalCapabilitiesDirectoryProvider;
}
else {
// support CommonJS module 1.1.1 spec (`exports` cannot be a function)
exports.GlobalCapabilitiesDirectoryProvider = GlobalCapabilitiesDirectoryProvider;
}
} else {
window.GlobalCapabilitiesDirectoryProvider = GlobalCapabilitiesDirectoryProvider;
}
})();
© 2015 - 2025 Weber Informatics LLC | Privacy Policy