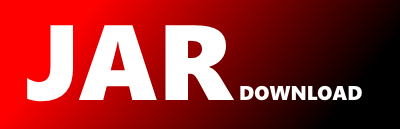
joynr.system.RoutingProvider.js Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libjoynr-js Show documentation
Show all versions of libjoynr-js Show documentation
JOYnr JavaScript libjoynr-js
/*
* PLEASE NOTE. THIS IS A GENERATED FILE!
* Generation date: Wed Jan 27 16:29:10 CET 2016
*/
(function(undefined){
var checkImpl = function() {
var missingInImplementation = [];
if (! this.addNextHop.checkOperation()) missingInImplementation.push("Operation:addNextHop");
if (! this.resolveNextHop.checkOperation()) missingInImplementation.push("Operation:resolveNextHop");
if (! this.removeNextHop.checkOperation()) missingInImplementation.push("Operation:removeNextHop");
return missingInImplementation;
};
/**
* @name RoutingProvider
* @constructor
*
* @classdesc
* PLEASE NOTE. THIS IS A GENERATED FILE!
*
Generation date: Wed Jan 27 16:29:10 CET 2016
*
The Routing
interface is a joynr internal
* interface. joynr uses a hierarchy of MessageRouter
s to
* route messages from source to destination. The Routing
* interface is used to update routing information between parent and
* child MessageRouter
s.
*
* @summary Constructor of RoutingProvider object
*
* @param {Object} [implementation] the implementation of the provider
* @param {Object} [implementation.ATTRIBUTENAME] the definition of attribute implementation
* @param {Function} [implementation.ATTRIBUTENAME.set] the getter function with the
* signature "function(value){}" that stores the given attribute value
* @param {Function} [implementation.ATTRIBUTENAME.get] the getter function with the
* signature "function(){}" that returns the current attribute value
* @param {Function} [implementation.OPERATIONNAME] the operation function
* @param {Object} [implementation.EVENTNAME] the definition of the event implementation
*
* @returns {RoutingProvider} a RoutingProvider object to communicate with the joynr infrastructure
*/
var RoutingProvider = null;
RoutingProvider = function RoutingProvider(
passedImplementation,
dependencies
) {
if (!(this instanceof RoutingProvider)) {
// in case someone calls constructor without new keyword (e.g. var c = Constructor({..}))
return new RoutingProvider(
passedImplementation,
dependencies);
}
var implementation = passedImplementation || {};
// defining provider members
/**
* @function RoutingProvider#addNextHop
* @summary The addNextHop operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Adds a hop to the parent routing table.
*
* The overloaded methods (one for each concrete Address type) is
* needed since polymorphism is currently not supported by joynr.
*
* @param {String} participantId -
* the ID of the target participant
* @param {ChannelAddress} channelAddress -
* the messaging address of the next hop towards
* the corresponding participant ID
*/
/**
* @function RoutingProvider#addNextHop
* @summary The addNextHop operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Adds a hop to the parent routing table.
*
* The overloaded methods (one for each concrete Address type) is
* needed since polymorphism is currently not supported by joynr.
*
* @param {String} participantId -
* the ID of the target participant
* @param {CommonApiDbusAddress} commonApiDbusAddress -
* the messaging address of the next hop towards
* the corresponding participant ID
*/
/**
* @function RoutingProvider#addNextHop
* @summary The addNextHop operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Adds a hop to the parent routing table.
*
* The overloaded methods (one for each concrete Address type) is
* needed since polymorphism is currently not supported by joynr.
*
* @param {String} participantId -
* the ID of the target participant
* @param {BrowserAddress} browserAddress -
* the messaging address of the next hop towards
* the corresponding participant ID
*/
/**
* @function RoutingProvider#addNextHop
* @summary The addNextHop operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Adds a hop to the parent routing table.
*
* The overloaded methods (one for each concrete Address type) is
* needed since polymorphism is currently not supported by joynr.
*
* @param {String} participantId -
* the ID of the target participant
* @param {WebSocketAddress} webSocketAddress -
* the messaging address of the next hop towards
* the corresponding participant ID
*/
/**
* @function RoutingProvider#addNextHop
* @summary The addNextHop operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Adds a hop to the parent routing table.
*
* The overloaded methods (one for each concrete Address type) is
* needed since polymorphism is currently not supported by joynr.
*
* @param {String} participantId -
* the ID of the target participant
* @param {WebSocketClientAddress} webSocketClientAddress -
* the messaging address of the next hop towards
* the corresponding participant ID
*/
this.addNextHop = new dependencies.ProviderOperation(this, implementation.addNextHop, "addNextHop", [
{
inputParameter: [
{
name : "participantId",
type : "String"
},
{
name : "channelAddress",
type : "joynr.system.RoutingTypes.ChannelAddress"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
},
{
inputParameter: [
{
name : "participantId",
type : "String"
},
{
name : "commonApiDbusAddress",
type : "joynr.system.RoutingTypes.CommonApiDbusAddress"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
},
{
inputParameter: [
{
name : "participantId",
type : "String"
},
{
name : "browserAddress",
type : "joynr.system.RoutingTypes.BrowserAddress"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
},
{
inputParameter: [
{
name : "participantId",
type : "String"
},
{
name : "webSocketAddress",
type : "joynr.system.RoutingTypes.WebSocketAddress"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
},
{
inputParameter: [
{
name : "participantId",
type : "String"
},
{
name : "webSocketClientAddress",
type : "joynr.system.RoutingTypes.WebSocketClientAddress"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
}
]);
/**
* @function RoutingProvider#resolveNextHop
* @summary The resolveNextHop operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
Asks the parent routing table whether it is able to
* resolve the destination participant ID.
*
* @param {String} participantId -
* the ID of the target participant to resolve
* @returns {Boolean} resolved -
* true, if the participant ID could be resolved
*/
this.resolveNextHop = new dependencies.ProviderOperation(this, implementation.resolveNextHop, "resolveNextHop", [
{
inputParameter: [
{
name : "participantId",
type : "String"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
{
name : "resolved",
type : "Boolean"
}
]
}
]);
/**
* @function RoutingProvider#removeNextHop
* @summary The removeNextHop operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
Removes a hop from the parent routing table.
*
* @param {String} participantId -
* the ID of the target participant
*/
this.removeNextHop = new dependencies.ProviderOperation(this, implementation.removeNextHop, "removeNextHop", [
{
inputParameter: [
{
name : "participantId",
type : "String"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
}
]);
Object.defineProperty(this, 'checkImplementation', {
enumerable: false,
configurable: false,
writable: false,
value: checkImpl
});
this.interfaceName = "system/Routing";
this.id = dependencies.uuid();
return Object.freeze(this);
};
// AMD support
if (typeof define === 'function' && define.amd) {
define("joynr/system/RoutingProvider", [
"joynr/system/RoutingTypes/WebSocketClientAddress",
"joynr/system/RoutingTypes/ChannelAddress",
"joynr/system/RoutingTypes/WebSocketAddress",
"joynr/system/RoutingTypes/CommonApiDbusAddress",
"joynr/system/RoutingTypes/BrowserAddress"
], function () {
return RoutingProvider;
}
);
} else if (typeof exports !== 'undefined' ) {
if ((module !== undefined) && module.exports) {
require("../../joynr/system/RoutingTypes/WebSocketClientAddress");
require("../../joynr/system/RoutingTypes/ChannelAddress");
require("../../joynr/system/RoutingTypes/WebSocketAddress");
require("../../joynr/system/RoutingTypes/CommonApiDbusAddress");
require("../../joynr/system/RoutingTypes/BrowserAddress");
exports = module.exports = RoutingProvider;
}
else {
// support CommonJS module 1.1.1 spec (`exports` cannot be a function)
exports.RoutingProvider = RoutingProvider;
}
} else {
window.RoutingProvider = RoutingProvider;
}
})();
© 2015 - 2025 Weber Informatics LLC | Privacy Policy