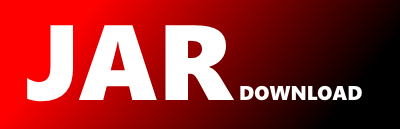
joynr.infrastructure.GlobalCapabilitiesDirectoryProvider.js Maven / Gradle / Ivy
/*
* PLEASE NOTE. THIS IS A GENERATED FILE!
* Generation date: Thu Aug 18 09:35:51 CEST 2016
*/
(function(undefined){
var checkImpl = function() {
var missingInImplementation = [];
if (! this.add.checkOperation()) missingInImplementation.push("Operation:add");
if (! this.lookup.checkOperation()) missingInImplementation.push("Operation:lookup");
if (! this.touch.checkOperation()) missingInImplementation.push("Operation:touch");
if (! this.remove.checkOperation()) missingInImplementation.push("Operation:remove");
return missingInImplementation;
};
/**
* @name GlobalCapabilitiesDirectoryProvider
* @constructor
*
* @classdesc
* PLEASE NOTE. THIS IS A GENERATED FILE!
*
Generation date: Thu Aug 18 09:35:51 CEST 2016
*
The GlobalCapabilitiesDirectory is a joynr
* internal interface. The joynr framework uses it to register
* providers, which are available at a global scope, with the backend.
* A provider is registered along with parameters which will be used
* during the arbitration process to select a suitable provider for
* a proxy. These information are stored in GlobalDiscoveryEntry objects.
* @see GlobalDiscoveryEntry
*
* @summary Constructor of GlobalCapabilitiesDirectoryProvider object
*
* @param {Object} [implementation] the implementation of the provider
* @param {Object} [implementation.ATTRIBUTENAME] the definition of attribute implementation
* @param {Function} [implementation.ATTRIBUTENAME.set] the getter function with the
* signature "function(value){}" that stores the given attribute value
* @param {Function} [implementation.ATTRIBUTENAME.get] the getter function with the
* signature "function(){}" that returns the current attribute value
* @param {Function} [implementation.OPERATIONNAME] the operation function
* @param {Object} [implementation.EVENTNAME] the definition of the event implementation
*
* @returns {GlobalCapabilitiesDirectoryProvider} a GlobalCapabilitiesDirectoryProvider object to communicate with the joynr infrastructure
*/
var GlobalCapabilitiesDirectoryProvider = null;
GlobalCapabilitiesDirectoryProvider = function GlobalCapabilitiesDirectoryProvider(
passedImplementation,
dependencies
) {
if (!(this instanceof GlobalCapabilitiesDirectoryProvider)) {
// in case someone calls constructor without new keyword (e.g. var c = Constructor({..}))
return new GlobalCapabilitiesDirectoryProvider(
passedImplementation,
dependencies);
}
var implementation = passedImplementation || {};
// defining provider members
/**
* @function GlobalCapabilitiesDirectoryProvider#add
* @summary The add operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Registers several providers with the backend.
*
* @param {Object} settings the arguments object for this function call
* @param {Array.} settings.globalDiscoveryEntries -
* A list which stores information about each provider instance
* that shall be registered with the backend.
GlobalDiscoveryEntry
*/
/**
* @function GlobalCapabilitiesDirectoryProvider#add
* @summary The add operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Registers a single provider with the backend.
*
* @param {Object} settings the arguments object for this function call
* @param {GlobalDiscoveryEntry} settings.globalDiscoveryEntry -
* Information about the provider which shall be registered with
* the backend.
GlobalDiscoveryEntry
*/
this.add = new dependencies.ProviderOperation(this, implementation.add, "add", [
{
inputParameter: [
{
name : "globalDiscoveryEntries",
type : "joynr.types.GlobalDiscoveryEntry[]"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
},
{
inputParameter: [
{
name : "globalDiscoveryEntry",
type : "joynr.types.GlobalDiscoveryEntry"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
}
]);
/**
* @function GlobalCapabilitiesDirectoryProvider#lookup
* @summary The lookup operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Looks up a list of providers for a domain and an interface name. The domain
* and interface name correspond to the attributes stored in GlobalDiscoveryEntry.
* @see GlobalDiscoveryEntry
*
* @param {Object} settings the arguments object for this function call
* @param {Array.} settings.domains -
* Domain names for which providers shall be returned.
* @param {String} settings.interfaceName -
* The name of the requested provider interface.
* @returns {GlobalCapabilitiesDirectoryProvider#LookupReturned}
* {Array.} result
*/
/**
* @typedef {Object} GlobalCapabilitiesDirectoryProvider#LookupReturned
* @property {Array.} result
* Information about all providers which were found for the given
* domain and interface name.
*/
/**
* @function GlobalCapabilitiesDirectoryProvider#lookup
* @summary The lookup operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Looks up a provider for a given participant Id.
*
* @param {Object} settings the arguments object for this function call
* @param {String} settings.participantId -
* The participant Id which identifies the requested provider.
* @returns {GlobalCapabilitiesDirectoryProvider#LookupReturned}
* {GlobalDiscoveryEntry} result
*/
/**
* @typedef {Object} GlobalCapabilitiesDirectoryProvider#LookupReturned
* @property {GlobalDiscoveryEntry} result
* Information about the found provider.
*/
this.lookup = new dependencies.ProviderOperation(this, implementation.lookup, "lookup", [
{
inputParameter: [
{
name : "domains",
type : "String[]"
},
{
name : "interfaceName",
type : "String"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
{
name : "result",
type : "joynr.types.GlobalDiscoveryEntry[]"
}
]
},
{
inputParameter: [
{
name : "participantId",
type : "String"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
{
name : "result",
type : "joynr.types.GlobalDiscoveryEntry"
}
]
}
]);
/**
* @function GlobalCapabilitiesDirectoryProvider#touch
* @summary The touch operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
Updates the last seen date for a specific cluster controller.
*
* @param {Object} settings the arguments object for this function call
* @param {String} settings.clusterControllerId -
* Identifies the cluster controller for which the last
* seen date shall be updated.
*/
this.touch = new dependencies.ProviderOperation(this, implementation.touch, "touch", [
{
inputParameter: [
{
name : "clusterControllerId",
type : "String"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
}
]);
/**
* @function GlobalCapabilitiesDirectoryProvider#remove
* @summary The remove operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Unregisters providers from the backend.
*
* @param {Object} settings the arguments object for this function call
* @param {Array.} settings.participantIds -
* The participand Ids which identify the providers that shall be removed
* from the backend.
*/
/**
* @function GlobalCapabilitiesDirectoryProvider#remove
* @summary The remove operation is GENERATED FROM THE INTERFACE DESCRIPTION
*
method overloading: different call semantics possible
*
Unregisters a provider from the backend.
*
* @param {Object} settings the arguments object for this function call
* @param {String} settings.participantId -
* The participant Id which identifies the provider that shall be removed
* from the backend.
*/
this.remove = new dependencies.ProviderOperation(this, implementation.remove, "remove", [
{
inputParameter: [
{
name : "participantIds",
type : "String[]"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
},
{
inputParameter: [
{
name : "participantId",
type : "String"
}
],
error: {
type: "no error enumeration given"
},
outputParameter: [
]
}
]);
Object.defineProperty(this, 'checkImplementation', {
enumerable: false,
configurable: false,
writable: false,
value: checkImpl
});
this.interfaceName = "infrastructure/GlobalCapabilitiesDirectory";
this.id = dependencies.uuid();
return Object.freeze(this);
};
/**
* @name GlobalCapabilitiesDirectoryProvider#MAJOR_VERSION
* @constant {Number}
* @default 0
* @summary The MAJOR_VERSION of the provider is GENERATED FROM THE INTERFACE DESCRIPTION
*/
Object.defineProperty(GlobalCapabilitiesDirectoryProvider, 'MAJOR_VERSION', {
enumerable: false,
configurable: false,
writable: false,
readable: true,
value: 0
});
/**
* @name GlobalCapabilitiesDirectoryProvider#MINOR_VERSION
* @constant {Number}
* @default 1
* @summary The MINOR_VERSION of the provider is GENERATED FROM THE INTERFACE DESCRIPTION
*/
Object.defineProperty(GlobalCapabilitiesDirectoryProvider, 'MINOR_VERSION', {
enumerable: false,
configurable: false,
writable: false,
readable: true,
value: 1
});
// AMD support
if (typeof define === 'function' && define.amd) {
define("joynr/infrastructure/GlobalCapabilitiesDirectoryProvider", [
"joynr/types/GlobalDiscoveryEntry"
], function () {
return GlobalCapabilitiesDirectoryProvider;
}
);
} else if (typeof exports !== 'undefined' ) {
if ((module !== undefined) && module.exports) {
require("../../joynr/types/GlobalDiscoveryEntry");
exports = module.exports = GlobalCapabilitiesDirectoryProvider;
}
else {
// support CommonJS module 1.1.1 spec (`exports` cannot be a function)
exports.GlobalCapabilitiesDirectoryProvider = GlobalCapabilitiesDirectoryProvider;
}
} else {
window.GlobalCapabilitiesDirectoryProvider = GlobalCapabilitiesDirectoryProvider;
}
})();
© 2015 - 2025 Weber Informatics LLC | Privacy Policy