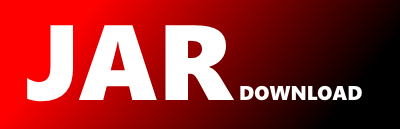
joynr.types.GlobalDiscoveryEntry.js Maven / Gradle / Ivy
/**
* This is the generated struct type GlobalDiscoveryEntry: DOCS GENERATED FROM INTERFACE DESCRIPTION
* Generation date: Mon Dec 05 09:32:59 CET 2016
*/
(function(undefined) {
/**
* @name GlobalDiscoveryEntry
* @constructor
*
* @classdesc
* This is the generated struct type GlobalDiscoveryEntry: DOCS GENERATED FROM INTERFACE DESCRIPTION
*
Generation date: Mon Dec 05 09:32:59 CET 2016
*
GlobalDiscoveryEntry stores information about a provider instance and extends
* DiscoveryEntry by adding transport middleware specific address information.
* GlobalDiscoveryEntry objects are used to register a new provider instance with the backend.
* In addition, objects of GlobalDiscoveryEntry will be returned by the backend during the
* arbitration process in order to select a suitable provider for a proxy.
* @see DiscoveryEntry
*
* @param {Object} members - an object containing the individual member elements
* @param {Version} members.providerVersion - semantic version information
* @param {String} members.domain - the domain to register the provider with
* @param {String} members.interfaceName - the name of the provider interface
* @param {String} members.participantId - the participant ID of the provider
* @param {ProviderQos} members.qos - the qos of the provider
* @param {Number} members.lastSeenDateMs - the date in millis since epoch when the source for this provider last
* contacted the directory
* @param {Number} members.expiryDateMs - the date in millis since epoch when this entry can be purged from the
* directory and caches
* @param {String} members.publicKeyId - the ID of the public key to be used to encrypt messages to
* this provider
* @param {String} members.address - the serialized transport-middleware-specific address where the provider can
* be reached. The address is a subclass of Address
and is stored as a JSON string.
* @returns {GlobalDiscoveryEntry} a new instance of a GlobalDiscoveryEntry
*/
var GlobalDiscoveryEntry = function GlobalDiscoveryEntry(members) {
if (!(this instanceof GlobalDiscoveryEntry)) {
// in case someone calls constructor without new keyword (e.g. var c = Constructor({..}))
return new GlobalDiscoveryEntry(members);
}
/**
* Used for serialization.
* @name GlobalDiscoveryEntry#_typeName
* @type String
* @readonly
*/
Object.defineProperty(this, "_typeName", {
configurable : false,
writable : false,
enumerable : true,
value : GlobalDiscoveryEntry._typeName
});
/**
* Parent class.
* @name GlobalDiscoveryEntry#_extends
* @type String
* @readonly
*/
Object.defineProperty(this, "_extends", {
configurable : false,
writable : false,
enumerable : false,
value : "joynr.types.DiscoveryEntry"
});
/**
* semantic version information
* @name GlobalDiscoveryEntry#providerVersion
* @type Version
*/
/**
* the domain to register the provider with
* @name GlobalDiscoveryEntry#domain
* @type String
*/
/**
* the name of the provider interface
* @name GlobalDiscoveryEntry#interfaceName
* @type String
*/
/**
* the participant ID of the provider
* @name GlobalDiscoveryEntry#participantId
* @type String
*/
/**
* the qos of the provider
* @name GlobalDiscoveryEntry#qos
* @type ProviderQos
*/
/**
* the date in millis since epoch when the source for this provider last
* contacted the directory
* @name GlobalDiscoveryEntry#lastSeenDateMs
* @type Number
*/
/**
* the date in millis since epoch when this entry can be purged from the
* directory and caches
* @name GlobalDiscoveryEntry#expiryDateMs
* @type Number
*/
/**
* the ID of the public key to be used to encrypt messages to
* this provider
* @name GlobalDiscoveryEntry#publicKeyId
* @type String
*/
/**
* the serialized transport-middleware-specific address where the provider can
* be reached. The address is a subclass of Address
and is stored as a JSON string.
* @name GlobalDiscoveryEntry#address
* @type String
*/
if (members !== undefined) {
this.providerVersion = members.providerVersion;
this.domain = members.domain;
this.interfaceName = members.interfaceName;
this.participantId = members.participantId;
this.qos = members.qos;
this.lastSeenDateMs = members.lastSeenDateMs;
this.expiryDateMs = members.expiryDateMs;
this.publicKeyId = members.publicKeyId;
this.address = members.address;
}
};
Object.defineProperty(GlobalDiscoveryEntry, "_typeName", {
configurable : false,
writable : false,
enumerable : false,
value : "joynr.types.GlobalDiscoveryEntry"
});
Object.defineProperty(GlobalDiscoveryEntry, 'checkMembers', {
enumerable: false,
configurable: false,
writable: false,
readable: true,
value: function checkMembers(instance, check) {
check(instance.providerVersion, ["Object", "Version"], "members.providerVersion");
check(instance.domain, "String", "members.domain");
check(instance.interfaceName, "String", "members.interfaceName");
check(instance.participantId, "String", "members.participantId");
check(instance.qos, ["Object", "ProviderQos"], "members.qos");
check(instance.lastSeenDateMs, "Number", "members.lastSeenDateMs");
check(instance.expiryDateMs, "Number", "members.expiryDateMs");
check(instance.publicKeyId, "String", "members.publicKeyId");
check(instance.address, "String", "members.address");
}
});
/**
* @name GlobalDiscoveryEntry#MAJOR_VERSION
* @constant {Number}
* @default 0
* @summary The MAJOR_VERSION of the struct type GlobalDiscoveryEntry is GENERATED FROM THE INTERFACE DESCRIPTION
*/
Object.defineProperty(GlobalDiscoveryEntry, 'MAJOR_VERSION', {
enumerable: false,
configurable: false,
writable: false,
readable: true,
value: 0
});
/**
* @name GlobalDiscoveryEntry#MINOR_VERSION
* @constant {Number}
* @default 0
* @summary The MINOR_VERSION of the struct type GlobalDiscoveryEntry is GENERATED FROM THE INTERFACE DESCRIPTION
*/
Object.defineProperty(GlobalDiscoveryEntry, 'MINOR_VERSION', {
enumerable: false,
configurable: false,
writable: false,
readable: true,
value: 0
});
var preparePrototype = function(joynr) {
GlobalDiscoveryEntry.prototype = new joynr.JoynrObject();
GlobalDiscoveryEntry.prototype.constructor = GlobalDiscoveryEntry;
Object.defineProperty(GlobalDiscoveryEntry.prototype, 'equals', {
enumerable: false,
configurable: false,
writable: false,
readable: true,
value: function equals(other) {
var i;
if (this === other) {
return true;
}
if (other === undefined || other === null) {
return false;
}
if (other._typeName === undefined || this._typeName !== other._typeName) {
return false;
}
if (this.providerVersion === undefined || this.providerVersion === null) {
if (other.providerVersion !== null && other.providerVersion !== undefined) {
return false;
}
} else if (!this.providerVersion.equals(other.providerVersion)){
return false;
}
if (this.domain === undefined || this.domain === null) {
if (other.domain !== null && other.domain !== undefined) {
return false;
}
} else if (this.domain !== other.domain){
return false;
}
if (this.interfaceName === undefined || this.interfaceName === null) {
if (other.interfaceName !== null && other.interfaceName !== undefined) {
return false;
}
} else if (this.interfaceName !== other.interfaceName){
return false;
}
if (this.participantId === undefined || this.participantId === null) {
if (other.participantId !== null && other.participantId !== undefined) {
return false;
}
} else if (this.participantId !== other.participantId){
return false;
}
if (this.qos === undefined || this.qos === null) {
if (other.qos !== null && other.qos !== undefined) {
return false;
}
} else if (!this.qos.equals(other.qos)){
return false;
}
if (this.lastSeenDateMs === undefined || this.lastSeenDateMs === null) {
if (other.lastSeenDateMs !== null && other.lastSeenDateMs !== undefined) {
return false;
}
} else if (this.lastSeenDateMs !== other.lastSeenDateMs){
return false;
}
if (this.expiryDateMs === undefined || this.expiryDateMs === null) {
if (other.expiryDateMs !== null && other.expiryDateMs !== undefined) {
return false;
}
} else if (this.expiryDateMs !== other.expiryDateMs){
return false;
}
if (this.publicKeyId === undefined || this.publicKeyId === null) {
if (other.publicKeyId !== null && other.publicKeyId !== undefined) {
return false;
}
} else if (this.publicKeyId !== other.publicKeyId){
return false;
}
if (this.address === undefined || this.address === null) {
if (other.address !== null && other.address !== undefined) {
return false;
}
} else if (this.address !== other.address){
return false;
}
return true;
}
});
};
var memberTypes = {
providerVersion: function() { return "joynr.types.Version"; },
domain: function() { return "String"; },
interfaceName: function() { return "String"; },
participantId: function() { return "String"; },
qos: function() { return "joynr.types.ProviderQos"; },
lastSeenDateMs: function() { return "Long"; },
expiryDateMs: function() { return "Long"; },
publicKeyId: function() { return "String"; },
address: function() { return "String"; }
};
Object.defineProperty(GlobalDiscoveryEntry, 'getMemberType', {
enumerable: false,
value: function getMemberType(memberName) {
if (memberTypes[memberName] !== undefined) {
return memberTypes[memberName]();
}
return undefined;
}
});
// AMD support
if (typeof define === 'function' && define.amd) {
define("joynr/types/GlobalDiscoveryEntry", ["joynr"], function (joynr) {
preparePrototype(joynr);
joynr.addType("joynr.types.GlobalDiscoveryEntry", GlobalDiscoveryEntry);
return GlobalDiscoveryEntry;
});
} else if (typeof exports !== 'undefined' ) {
if ((module !== undefined) && module.exports) {
exports = module.exports = GlobalDiscoveryEntry;
} else {
// support CommonJS module 1.1.1 spec (`exports` cannot be a function)
exports.GlobalDiscoveryEntry = GlobalDiscoveryEntry;
}
var joynr = requirejs("joynr");
preparePrototype(joynr);
joynr.addType("joynr.types.GlobalDiscoveryEntry", GlobalDiscoveryEntry);
} else {
preparePrototype(window.joynr);
window.joynr.addType("joynr.types.GlobalDiscoveryEntry", GlobalDiscoveryEntry);
window.GlobalDiscoveryEntry = GlobalDiscoveryEntry;
}
})();
© 2015 - 2025 Weber Informatics LLC | Privacy Policy