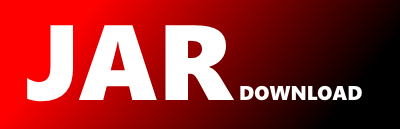
e.ultra.kontainer.0.76.4.source-code.KontainerBuilder.kt Maven / Gradle / Ivy
Show all versions of kontainer Show documentation
package de.peekandpoke.ultra.kontainer
import kotlin.reflect.KClass
@Suppress("Detekt:TooManyFunctions")
class KontainerBuilder internal constructor(builder: KontainerBuilder.() -> Unit) {
private val configValues = mutableMapOf()
private val definitions = mutableMapOf, ServiceDefinition>()
private val injectionTypeUpgrade = InjectionTypeUpgrade()
class InjectionTypeUpgrade {
fun adjust(def: ServiceDefinition): ServiceDefinition {
return adjust(newDef = def, existingDef = def.overwrites)
}
fun adjust(newDef: ServiceDefinition, existingDef: ServiceDefinition?): ServiceDefinition {
return when (existingDef) {
// When there is no existing definition, we can use the new definition as is
null -> newDef
else -> when (existingDef.injectionType) {
// When the existing definition is a prototype,
InjectionType.Prototype ->
// Then we need to upgrade the new definition to a prototype as well
newDef.withType(InjectionType.Prototype)
// When the existing definition is a dynamic
InjectionType.Dynamic ->
// Then the type will stay dynamic
newDef.withType(InjectionType.Dynamic)
// When the existing definition is a singleton
InjectionType.Singleton ->
// Then we can use it as is
newDef
}
}
}
}
init {
builder(this)
//
// The kontainer is always present (dynamic as it changes with every new Kontainer instance)
//
addDynamic(Kontainer::class, ServiceProducer.forKontainer())
//
// The InjectionContext is always present and dynamic
//
// NOTICE: We provide a dummy ServiceProducer below.
// The InjectionContext is injected via [ParameterProvider.ForInjectionContext]
//
addDynamic(InjectionContext::class, ServiceProducer.forInstance(InjectionContext.kontainerRoot))
//
// The blueprint is always present (singleton as it always stays the same)
//
addSingleton(KontainerBlueprint::class, ServiceProducer.forKontainerBlueprint())
}
/**
* Builds a [KontainerBlueprint] from the current configuration
*/
internal fun build(
config: KontainerBlueprint.Config = KontainerBlueprint.Config.default
): KontainerBlueprint {
return KontainerBlueprint(
config = config,
configValues = configValues.toMap(),
definitions = definitions.toMap(),
)
}
/**
* Performs injection type upgrade.
*
* See [injectionTypeUpgrade] and [InjectionTypeUpgrade].
*/
private fun ServiceDefinition.withTypeUpgrade() = injectionTypeUpgrade.adjust(this)
/**
* Add a service with the given parameters
*/
private fun add(
serviceCls: KClass,
type: InjectionType,
producer: ServiceProducer,
): KontainerBuilder = apply {
val service = ServiceDefinition(
serviceCls = serviceCls,
injectionType = type,
producer = producer,
overwrites = definitions[serviceCls]
).withTypeUpgrade()
definitions[service.serviceCls] = service
}
/**
* Adds a singleton service definition
*/
private fun addSingleton(
serviceCls: KClass,
producer: ServiceProducer,
): KontainerBuilder {
return add(serviceCls, InjectionType.Singleton, producer)
}
/**
* Adds a prototype service definition
*/
private fun addPrototype(
srv: KClass,
producer: ServiceProducer,
): KontainerBuilder {
return add(srv, InjectionType.Prototype, producer)
}
/**
* Adds a dynamic service definition
*/
private fun addDynamic(
srv: KClass,
producer: ServiceProducer,
): KontainerBuilder {
return add(srv, InjectionType.Dynamic, producer)
}
// /////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// Config values
// //
/**
* Sets an injectable config value
*/
fun config(id: String, value: Int): KontainerBuilder = apply {
configValues[id] = value
}
/**
* Sets an injectable config value
*/
fun config(id: String, value: Long): KontainerBuilder = apply {
configValues[id] = value
}
/**
* Sets an injectable config value
*/
fun config(id: String, value: Float): KontainerBuilder = apply {
configValues[id] = value
}
/**
* Sets an injectable config value
*/
fun config(id: String, value: Double): KontainerBuilder = apply {
configValues[id] = value
}
/**
* Sets an injectable config value
*/
fun config(id: String, value: String): KontainerBuilder = apply {
configValues[id] = value
}
/**
* Sets an injectable config value
*/
fun config(id: String, value: Boolean): KontainerBuilder = apply {
configValues[id] = value
}
// /////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// Modules
// //
/**
* Imports a module
*/
fun module(
module: KontainerModule
): KontainerBuilder = apply {
module.apply(this)
}
/**
* Imports a parameterized module
*/
fun module(
module: ParameterizedKontainerModule
,
param: P,
): KontainerBuilder = apply {
module.apply(this, param)
}
/**
* Imports a parameterized module
*/
fun module(
module: ParameterizedKontainerModule2,
p1: P1,
p2: P2,
): KontainerBuilder = apply {
module.apply(this, p1, p2)
}
/**
* Imports a parameterized module
*/
fun module(
module: ParameterizedKontainerModule3,
p1: P1,
p2: P2,
p3: P3,
): KontainerBuilder = apply {
module.apply(this, p1, p2, p3)
}
// /////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// Singleton Instances
// //
/**
* Register an already existing [instance] as a service
*
* The service can by injected by the type [SRV] and its base types
*/
fun instance(
instance: SRV,
): KontainerBuilder {
@Suppress("UNCHECKED_CAST")
return addSingleton(
serviceCls = instance::class as KClass,
ServiceProducer.forInstance(instance)
)
}
/**
* Register an already existing [instance] as a service
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun instance(
srv: KClass,
instance: IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forInstance(instance))
}
// /////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// Singleton Services
// //
/**
* Registers a singleton service
*
* The service can by injected by the type [SRV] and its base types
*/
fun singleton(
srv: KClass,
): KontainerBuilder {
return singleton(srv, srv)
}
/**
* Registers a singleton service
*
* The service can by injected by the type [SRV] and its base types
*/
fun singleton(
srv: KClass,
impl: KClass,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forClass(impl))
}
/**
* Registers a singleton service with variable number of parameters
*
* The service can by injected by the type [SRV] and its base types
*/
fun > singleton(
srv: KClass,
factory: FAC,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory method with 0 injected parameters
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton0(
srv: KClass,
factory: () -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13, P14) -> IMPL
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13, P14, P15) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a singleton via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun singleton(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13, P14, P15, P16) -> IMPL,
): KontainerBuilder {
return addSingleton(srv, ServiceProducer.forFactory(factory))
}
// /////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// Prototype Services
// //
/**
* Registers a prototype service
*
* The service can be injected by the type [SRV] or its base types
*/
fun prototype(
srv: KClass,
): KontainerBuilder {
return prototype(srv, srv)
}
/**
* Registers a prototype service
*
* The service can be injected by the type [SRV] or its base types.
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
impl: KClass,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forClass(impl))
}
/**
* Registers a prototype service with variable number of parameters
*
* The service can by injected by the type [SRV] and its base types
*/
fun > prototype(
srv: KClass,
factory: FAC,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a Prototype via a factory method with 0 injected parameters
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype0(
srv: KClass,
factory: () -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13, P14) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13, P14, P15) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a prototype via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun prototype(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13, P14, P15, P16) -> IMPL,
): KontainerBuilder {
return addPrototype(srv, ServiceProducer.forFactory(factory))
}
// /////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// Dynamic Services
// //
/**
* Registers a dynamic service
*
* The service can be injected by the type [SRV] and its base types.
*/
fun dynamic(
srv: KClass,
): KontainerBuilder {
return dynamic(srv, srv)
}
/**
* Registers a dynamic service [SRV] with a default implementation [IMPL]
*
* The service can be injected by the type [SRV] and its base types.
* The actual default implementation is registered with type [IMPL].
*/
fun dynamic(
srv: KClass,
impl: KClass,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forClass(impl))
}
/**
* Registers a dynamic service with variable number of parameters
*
* The service can by injected by the type [SRV] and its base types
*/
fun > dynamic(
srv: KClass,
factory: FAC,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic singleton via a factory method with 0 injected parameters
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic0(
srv: KClass,
factory: () -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13, P14) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13, P14, P15) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
/**
* Create a dynamic service via a factory
*
* The service can by injected by the type [SRV] and its base types
* The actual implementation will have the type [IMPL]
*/
fun dynamic(
srv: KClass,
factory: (P1, P2, P3, P4, P5, P6, P7, P8, P9, P10, P11, P12, P13, P14, P15, P16) -> IMPL,
): KontainerBuilder {
return addDynamic(srv, ServiceProducer.forFactory(factory))
}
}