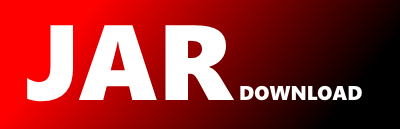
e.ultra.mutator.0.77.0.source-code.MapMutator.kt Maven / Gradle / Ivy
package de.peekandpoke.ultra.mutator
fun Map.mutator(
onModify: OnModify
© 2015 - 2025 Weber Informatics LLC | Privacy Policy
package de.peekandpoke.ultra.mutator
fun Map.mutator(
onModify: OnModify