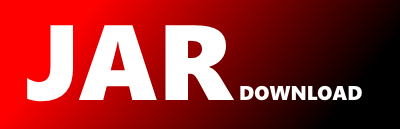
dev-ui.qwc-open-webui.js Maven / Gradle / Ivy
import { LitElement, html, css } from 'lit';
import { JsonRpc } from 'jsonrpc';
import '@vaadin/checkbox';
import '@vaadin/progress-bar';
import { envVarMappings } from 'build-time-data';
class OpenWebUI extends LitElement {
jsonRpc = new JsonRpc(this);
static styles = css`
:host {
margin: 20px;
display: flex;
flex-direction: column;
align-items: left;
justify-content: center;
font-family: Arial, sans-serif;
}
div {
background-color: #f9f9f9;
padding: 20px;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0,0,0,0.1);
}
label {
font-weight: bold;
}
.large-input {
width: 500px;
}
button {
padding: 10px 20px;
border: none;
border-radius: 5px;
background-color: #007BFF;
color: white;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
button:disabled {
background-color: #cccccc;
cursor: not-allowed;
}
button:disabled:hover {
background-color: #cccccc;
}
h2 {
color: #333;
}
a {
color: #007BFF;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
.hide {
visibility: hidden;
}
.show {
visibility: visible;
}
`;
static properties = {
container: {state: true},
image: {state: true},
requestGpu: {state: true},
portBindings: {state: true},
envVars: {state: true},
envVarMappings: {state: true},
volumes: {state: true},
url: {state: true},
signedUp: {state: true},
autoSingup: {state: true},
username: {state: true},
email: {state: true},
password: {state: true},
_progressBarClass: {state: true},
}
constructor() {
super();
this.image = "ghcr.io/open-webui/open-webui:main";
this.requestGpu = false;
this.portBindings = {3000 : 8080};
this.envVars = {};
this.envVarMappings = envVarMappings;
this.volumes = {"open-webui": "/app/backend/data"};
this.signedUp = false;
this.autoSignup = true;
this.username = "quarkus";
this.email = "[email protected]";
this.password = "quarkus";
Object.entries(this.envVarMappings).forEach(([envVarKey, quarkusConfigPropertyKey]) => {
this.jsonRpc.getConfigValue({key: quarkusConfigPropertyKey}).then((resp) => {
const envVarValue = resp.result;
if (envVarValue) {
this.envVars[envVarKey] = envVarValue;
}
});
});
this._hideProgressBar();
this._refresh();
}
render() {
return html`
${this._renderContainerInfo()}
${this._renderCreateOptions()}
`;
}
_renderCreateOptions() {
return html`
this.image = e.target.value}">
{
this.requestGpu = event.target.checked;
this.requestUpdate();
}}">
Auto Signup
{
this.autoSignup = event.target.checked;
this.requestUpdate();
}}">
this.username = e.target.value}">
this.email = e.target.value}">
this.password = e.target.value}">
Port Bindings
${Object.entries(this.portBindings).map(([key, value], index) => html`
this._changePortBindingKey(index, e.target.value)}">
this._changePortBindingValue(index, e.target.value)}">
`)}
Volumes
${Object.entries(this.volumes).map(([key, value], index) => html`
this._changeVolumeKey(index, e.target.value)}">
this._changeVolumeValue(index, e.target.value)}">
`)}
Environment Variables
${Object.entries(this.envVars).map(([key, value], index) => html`
this._changeEnvVarKey(index, e.target.value)}">
this._changeEnvVarValue(index, e.target.value)}">
`)}
Open WebUI container
${this.container.id}
${this.container.name}
${this.container.config.image}
${this.container.config.cmd.join(" ")}